text
stringlengths 55
456k
| metadata
dict |
---|---|
# content-disposition
[![NPM Version][npm-image]][npm-url]
[![NPM Downloads][downloads-image]][downloads-url]
[![Node.js Version][node-version-image]][node-version-url]
[![Build Status][github-actions-ci-image]][github-actions-ci-url]
[![Test Coverage][coveralls-image]][coveralls-url]
Create and parse HTTP `Content-Disposition` header
## Installation
```sh
$ npm install content-disposition
```
## API
```js
var contentDisposition = require('content-disposition')
```
### contentDisposition(filename, options)
Create an attachment `Content-Disposition` header value using the given file name,
if supplied. The `filename` is optional and if no file name is desired, but you
want to specify `options`, set `filename` to `undefined`.
```js
res.setHeader('Content-Disposition', contentDisposition('∫ maths.pdf'))
```
**note** HTTP headers are of the ISO-8859-1 character set. If you are writing this
header through a means different from `setHeader` in Node.js, you'll want to specify
the `'binary'` encoding in Node.js.
#### Options
`contentDisposition` accepts these properties in the options object.
##### fallback
If the `filename` option is outside ISO-8859-1, then the file name is actually
stored in a supplemental field for clients that support Unicode file names and
a ISO-8859-1 version of the file name is automatically generated.
This specifies the ISO-8859-1 file name to override the automatic generation or
disables the generation all together, defaults to `true`.
- A string will specify the ISO-8859-1 file name to use in place of automatic
generation.
- `false` will disable including a ISO-8859-1 file name and only include the
Unicode version (unless the file name is already ISO-8859-1).
- `true` will enable automatic generation if the file name is outside ISO-8859-1.
If the `filename` option is ISO-8859-1 and this option is specified and has a
different value, then the `filename` option is encoded in the extended field
and this set as the fallback field, even though they are both ISO-8859-1.
##### type
Specifies the disposition type, defaults to `"attachment"`. This can also be
`"inline"`, or any other value (all values except inline are treated like
`attachment`, but can convey additional information if both parties agree to
it). The type is normalized to lower-case.
### contentDisposition.parse(string)
```js
var disposition = contentDisposition.parse('attachment; filename="EURO rates.txt"; filename*=UTF-8\'\'%e2%82%ac%20rates.txt')
```
Parse a `Content-Disposition` header string. This automatically handles extended
("Unicode") parameters by decoding them and providing them under the standard
parameter name. This will return an object with the following properties (examples
are shown for the string `'attachment; filename="EURO rates.txt"; filename*=UTF-8\'\'%e2%82%ac%20rates.txt'`):
- `type`: The disposition type (always lower case). Example: `'attachment'`
- `parameters`: An object of the parameters in the disposition (name of parameter
always lower case and extended versions replace non-extended versions). Example:
`{filename: "€ rates.txt"}`
## Examples
### Send a file for download
```js
var contentDisposition = require('content-disposition')
var destroy = require('destroy')
var fs = require('fs')
var http = require('http')
var onFinished = require('on-finished')
var filePath = '/path/to/public/plans.pdf'
http.createServer(function onRequest (req, res) {
// set headers
res.setHeader('Content-Type', 'application/pdf')
res.setHeader('Content-Disposition', contentDisposition(filePath))
// send file
var stream = fs.createReadStream(filePath)
stream.pipe(res)
onFinished(res, function () {
destroy(stream)
})
})
```
## Testing
```sh
$ npm test
```
## References
- [RFC 2616: Hypertext Transfer Protocol -- HTTP/1.1][rfc-2616]
- [RFC 5987: Character Set and Language Encoding for Hypertext Transfer Protocol (HTTP) Header Field Parameters][rfc-5987]
- [RFC 6266: Use of the Content-Disposition Header Field in the Hypertext Transfer Protocol (HTTP)][rfc-6266]
- [Test Cases for HTTP Content-Disposition header field (RFC 6266) and the Encodings defined in RFCs 2047, 2231 and 5987][tc-2231]
[rfc-2616]: https://tools.ietf.org/html/rfc2616
[rfc-5987]: https://tools.ietf.org/html/rfc5987
[rfc-6266]: https://tools.ietf.org/html/rfc6266
[tc-2231]: http://greenbytes.de/tech/tc2231/
## License
[MIT](LICENSE)
[npm-image]: https://img.shields.io/npm/v/content-disposition.svg
[npm-url]: https://npmjs.org/package/content-disposition
[node-version-image]: https://img.shields.io/node/v/content-disposition.svg
[node-version-url]: https://nodejs.org/en/download
[coveralls-image]: https://img.shields.io/coveralls/jshttp/content-disposition.svg
[coveralls-url]: https://coveralls.io/r/jshttp/content-disposition?branch=master
[downloads-image]: https://img.shields.io/npm/dm/content-disposition.svg
[downloads-url]: https://npmjs.org/package/content-disposition
[github-actions-ci-image]: https://img.shields.io/github/workflow/status/jshttp/content-disposition/ci/master?label=ci
[github-actions-ci-url]: https://github.com/jshttp/content-disposition?query=workflow%3Aci | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/content-disposition/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/content-disposition/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 5200
} |
1.0.5 / 2023-01-29
==================
* perf: skip value escaping when unnecessary
1.0.4 / 2017-09-11
==================
* perf: skip parameter parsing when no parameters
1.0.3 / 2017-09-10
==================
* perf: remove argument reassignment
1.0.2 / 2016-05-09
==================
* perf: enable strict mode
1.0.1 / 2015-02-13
==================
* Improve missing `Content-Type` header error message
1.0.0 / 2015-02-01
==================
* Initial implementation, derived from `[email protected]` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/content-type/HISTORY.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/content-type/HISTORY.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 522
} |
# content-type
[![NPM Version][npm-version-image]][npm-url]
[![NPM Downloads][npm-downloads-image]][npm-url]
[![Node.js Version][node-image]][node-url]
[![Build Status][ci-image]][ci-url]
[![Coverage Status][coveralls-image]][coveralls-url]
Create and parse HTTP Content-Type header according to RFC 7231
## Installation
```sh
$ npm install content-type
```
## API
```js
var contentType = require('content-type')
```
### contentType.parse(string)
```js
var obj = contentType.parse('image/svg+xml; charset=utf-8')
```
Parse a `Content-Type` header. This will return an object with the following
properties (examples are shown for the string `'image/svg+xml; charset=utf-8'`):
- `type`: The media type (the type and subtype, always lower case).
Example: `'image/svg+xml'`
- `parameters`: An object of the parameters in the media type (name of parameter
always lower case). Example: `{charset: 'utf-8'}`
Throws a `TypeError` if the string is missing or invalid.
### contentType.parse(req)
```js
var obj = contentType.parse(req)
```
Parse the `Content-Type` header from the given `req`. Short-cut for
`contentType.parse(req.headers['content-type'])`.
Throws a `TypeError` if the `Content-Type` header is missing or invalid.
### contentType.parse(res)
```js
var obj = contentType.parse(res)
```
Parse the `Content-Type` header set on the given `res`. Short-cut for
`contentType.parse(res.getHeader('content-type'))`.
Throws a `TypeError` if the `Content-Type` header is missing or invalid.
### contentType.format(obj)
```js
var str = contentType.format({
type: 'image/svg+xml',
parameters: { charset: 'utf-8' }
})
```
Format an object into a `Content-Type` header. This will return a string of the
content type for the given object with the following properties (examples are
shown that produce the string `'image/svg+xml; charset=utf-8'`):
- `type`: The media type (will be lower-cased). Example: `'image/svg+xml'`
- `parameters`: An object of the parameters in the media type (name of the
parameter will be lower-cased). Example: `{charset: 'utf-8'}`
Throws a `TypeError` if the object contains an invalid type or parameter names.
## License
[MIT](LICENSE)
[ci-image]: https://badgen.net/github/checks/jshttp/content-type/master?label=ci
[ci-url]: https://github.com/jshttp/content-type/actions/workflows/ci.yml
[coveralls-image]: https://badgen.net/coveralls/c/github/jshttp/content-type/master
[coveralls-url]: https://coveralls.io/r/jshttp/content-type?branch=master
[node-image]: https://badgen.net/npm/node/content-type
[node-url]: https://nodejs.org/en/download
[npm-downloads-image]: https://badgen.net/npm/dm/content-type
[npm-url]: https://npmjs.org/package/content-type
[npm-version-image]: https://badgen.net/npm/v/content-type | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/content-type/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/content-type/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2781
} |
# convert-source-map [![Build Status][ci-image]][ci-url]
Converts a source-map from/to different formats and allows adding/changing properties.
```js
var convert = require('convert-source-map');
var json = convert
.fromComment('//# sourceMappingURL=data:application/json;base64,eyJ2ZXJzaW9uIjozLCJmaWxlIjoiYnVpbGQvZm9vLm1pbi5qcyIsInNvdXJjZXMiOlsic3JjL2Zvby5qcyJdLCJuYW1lcyI6W10sIm1hcHBpbmdzIjoiQUFBQSIsInNvdXJjZVJvb3QiOiIvIn0=')
.toJSON();
var modified = convert
.fromComment('//# sourceMappingURL=data:application/json;base64,eyJ2ZXJzaW9uIjozLCJmaWxlIjoiYnVpbGQvZm9vLm1pbi5qcyIsInNvdXJjZXMiOlsic3JjL2Zvby5qcyJdLCJuYW1lcyI6W10sIm1hcHBpbmdzIjoiQUFBQSIsInNvdXJjZVJvb3QiOiIvIn0=')
.setProperty('sources', [ 'SRC/FOO.JS' ])
.toJSON();
console.log(json);
console.log(modified);
```
```json
{"version":3,"file":"build/foo.min.js","sources":["src/foo.js"],"names":[],"mappings":"AAAA","sourceRoot":"/"}
{"version":3,"file":"build/foo.min.js","sources":["SRC/FOO.JS"],"names":[],"mappings":"AAAA","sourceRoot":"/"}
```
## Upgrading
Prior to v2.0.0, the `fromMapFileComment` and `fromMapFileSource` functions took a String directory path and used that to resolve & read the source map file from the filesystem. However, this made the library limited to nodejs environments and broke on sources with querystrings.
In v2.0.0, you now need to pass a function that does the file reading. It will receive the source filename as a String that you can resolve to a filesystem path, URL, or anything else.
If you are using `convert-source-map` in nodejs and want the previous behavior, you'll use a function like such:
```diff
+ var fs = require('fs'); // Import the fs module to read a file
+ var path = require('path'); // Import the path module to resolve a path against your directory
- var conv = convert.fromMapFileSource(css, '../my-dir');
+ var conv = convert.fromMapFileSource(css, function (filename) {
+ return fs.readFileSync(path.resolve('../my-dir', filename), 'utf-8');
+ });
```
## API
### fromObject(obj)
Returns source map converter from given object.
### fromJSON(json)
Returns source map converter from given json string.
### fromURI(uri)
Returns source map converter from given uri encoded json string.
### fromBase64(base64)
Returns source map converter from given base64 encoded json string.
### fromComment(comment)
Returns source map converter from given base64 or uri encoded json string prefixed with `//# sourceMappingURL=...`.
### fromMapFileComment(comment, readMap)
Returns source map converter from given `filename` by parsing `//# sourceMappingURL=filename`.
`readMap` must be a function which receives the source map filename and returns either a String or Buffer of the source map (if read synchronously), or a `Promise` containing a String or Buffer of the source map (if read asynchronously).
If `readMap` doesn't return a `Promise`, `fromMapFileComment` will return a source map converter synchronously.
If `readMap` returns a `Promise`, `fromMapFileComment` will also return `Promise`. The `Promise` will be either resolved with the source map converter or rejected with an error.
#### Examples
**Synchronous read in Node.js:**
```js
var convert = require('convert-source-map');
var fs = require('fs');
function readMap(filename) {
return fs.readFileSync(filename, 'utf8');
}
var json = convert
.fromMapFileComment('//# sourceMappingURL=map-file-comment.css.map', readMap)
.toJSON();
console.log(json);
```
**Asynchronous read in Node.js:**
```js
var convert = require('convert-source-map');
var { promises: fs } = require('fs'); // Notice the `promises` import
function readMap(filename) {
return fs.readFile(filename, 'utf8');
}
var converter = await convert.fromMapFileComment('//# sourceMappingURL=map-file-comment.css.map', readMap)
var json = converter.toJSON();
console.log(json);
```
**Asynchronous read in the browser:**
```js
var convert = require('convert-source-map');
async function readMap(url) {
const res = await fetch(url);
return res.text();
}
const converter = await convert.fromMapFileComment('//# sourceMappingURL=map-file-comment.css.map', readMap)
var json = converter.toJSON();
console.log(json);
```
### fromSource(source)
Finds last sourcemap comment in file and returns source map converter or returns `null` if no source map comment was found.
### fromMapFileSource(source, readMap)
Finds last sourcemap comment in file and returns source map converter or returns `null` if no source map comment was found.
`readMap` must be a function which receives the source map filename and returns either a String or Buffer of the source map (if read synchronously), or a `Promise` containing a String or Buffer of the source map (if read asynchronously).
If `readMap` doesn't return a `Promise`, `fromMapFileSource` will return a source map converter synchronously.
If `readMap` returns a `Promise`, `fromMapFileSource` will also return `Promise`. The `Promise` will be either resolved with the source map converter or rejected with an error.
### toObject()
Returns a copy of the underlying source map.
### toJSON([space])
Converts source map to json string. If `space` is given (optional), this will be passed to
[JSON.stringify](https://developer.mozilla.org/en-US/docs/JavaScript/Reference/Global_Objects/JSON/stringify) when the
JSON string is generated.
### toURI()
Converts source map to uri encoded json string.
### toBase64()
Converts source map to base64 encoded json string.
### toComment([options])
Converts source map to an inline comment that can be appended to the source-file.
By default, the comment is formatted like: `//# sourceMappingURL=...`, which you would
normally see in a JS source file.
When `options.encoding == 'uri'`, the data will be uri encoded, otherwise they will be base64 encoded.
When `options.multiline == true`, the comment is formatted like: `/*# sourceMappingURL=... */`, which you would find in a CSS source file.
### addProperty(key, value)
Adds given property to the source map. Throws an error if property already exists.
### setProperty(key, value)
Sets given property to the source map. If property doesn't exist it is added, otherwise its value is updated.
### getProperty(key)
Gets given property of the source map.
### removeComments(src)
Returns `src` with all source map comments removed
### removeMapFileComments(src)
Returns `src` with all source map comments pointing to map files removed.
### commentRegex
Provides __a fresh__ RegExp each time it is accessed. Can be used to find source map comments.
Breaks down a source map comment into groups: Groups: 1: media type, 2: MIME type, 3: charset, 4: encoding, 5: data.
### mapFileCommentRegex
Provides __a fresh__ RegExp each time it is accessed. Can be used to find source map comments pointing to map files.
### generateMapFileComment(file, [options])
Returns a comment that links to an external source map via `file`.
By default, the comment is formatted like: `//# sourceMappingURL=...`, which you would normally see in a JS source file.
When `options.multiline == true`, the comment is formatted like: `/*# sourceMappingURL=... */`, which you would find in a CSS source file.
[ci-url]: https://github.com/thlorenz/convert-source-map/actions?query=workflow:ci
[ci-image]: https://img.shields.io/github/workflow/status/thlorenz/convert-source-map/CI?style=flat-square | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/convert-source-map/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/convert-source-map/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 7420
} |
1.0.6 / 2015-02-03
==================
* use `npm test` instead of `make test` to run tests
* clearer assertion messages when checking input
1.0.5 / 2014-09-05
==================
* add license to package.json
1.0.4 / 2014-06-25
==================
* corrected avoidance of timing attacks (thanks @tenbits!)
1.0.3 / 2014-01-28
==================
* [incorrect] fix for timing attacks
1.0.2 / 2014-01-28
==================
* fix missing repository warning
* fix typo in test
1.0.1 / 2013-04-15
==================
* Revert "Changed underlying HMAC algo. to sha512."
* Revert "Fix for timing attacks on MAC verification."
0.0.1 / 2010-01-03
==================
* Initial release | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/cookie-signature/History.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/cookie-signature/History.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 694
} |
# cookie-signature
Sign and unsign cookies.
## Example
```js
var cookie = require('cookie-signature');
var val = cookie.sign('hello', 'tobiiscool');
val.should.equal('hello.DGDUkGlIkCzPz+C0B064FNgHdEjox7ch8tOBGslZ5QI');
var val = cookie.sign('hello', 'tobiiscool');
cookie.unsign(val, 'tobiiscool').should.equal('hello');
cookie.unsign(val, 'luna').should.be.false;
```
## License
(The MIT License)
Copyright (c) 2012 LearnBoost <[email protected]>
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/cookie-signature/Readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/cookie-signature/Readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1489
} |
# cookie
[![NPM Version][npm-version-image]][npm-url]
[![NPM Downloads][npm-downloads-image]][npm-url]
[![Node.js Version][node-image]][node-url]
[![Build Status][ci-image]][ci-url]
[![Coverage Status][coveralls-image]][coveralls-url]
Basic HTTP cookie parser and serializer for HTTP servers.
## Installation
This is a [Node.js](https://nodejs.org/en/) module available through the
[npm registry](https://www.npmjs.com/). Installation is done using the
[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
```sh
$ npm install cookie
```
## API
```js
var cookie = require('cookie');
```
### cookie.parse(str, options)
Parse an HTTP `Cookie` header string and returning an object of all cookie name-value pairs.
The `str` argument is the string representing a `Cookie` header value and `options` is an
optional object containing additional parsing options.
```js
var cookies = cookie.parse('foo=bar; equation=E%3Dmc%5E2');
// { foo: 'bar', equation: 'E=mc^2' }
```
#### Options
`cookie.parse` accepts these properties in the options object.
##### decode
Specifies a function that will be used to decode a cookie's value. Since the value of a cookie
has a limited character set (and must be a simple string), this function can be used to decode
a previously-encoded cookie value into a JavaScript string or other object.
The default function is the global `decodeURIComponent`, which will decode any URL-encoded
sequences into their byte representations.
**note** if an error is thrown from this function, the original, non-decoded cookie value will
be returned as the cookie's value.
### cookie.serialize(name, value, options)
Serialize a cookie name-value pair into a `Set-Cookie` header string. The `name` argument is the
name for the cookie, the `value` argument is the value to set the cookie to, and the `options`
argument is an optional object containing additional serialization options.
```js
var setCookie = cookie.serialize('foo', 'bar');
// foo=bar
```
#### Options
`cookie.serialize` accepts these properties in the options object.
##### domain
Specifies the value for the [`Domain` `Set-Cookie` attribute][rfc-6265-5.2.3]. By default, no
domain is set, and most clients will consider the cookie to apply to only the current domain.
##### encode
Specifies a function that will be used to encode a cookie's value. Since value of a cookie
has a limited character set (and must be a simple string), this function can be used to encode
a value into a string suited for a cookie's value.
The default function is the global `encodeURIComponent`, which will encode a JavaScript string
into UTF-8 byte sequences and then URL-encode any that fall outside of the cookie range.
##### expires
Specifies the `Date` object to be the value for the [`Expires` `Set-Cookie` attribute][rfc-6265-5.2.1].
By default, no expiration is set, and most clients will consider this a "non-persistent cookie" and
will delete it on a condition like exiting a web browser application.
**note** the [cookie storage model specification][rfc-6265-5.3] states that if both `expires` and
`maxAge` are set, then `maxAge` takes precedence, but it is possible not all clients by obey this,
so if both are set, they should point to the same date and time.
##### httpOnly
Specifies the `boolean` value for the [`HttpOnly` `Set-Cookie` attribute][rfc-6265-5.2.6]. When truthy,
the `HttpOnly` attribute is set, otherwise it is not. By default, the `HttpOnly` attribute is not set.
**note** be careful when setting this to `true`, as compliant clients will not allow client-side
JavaScript to see the cookie in `document.cookie`.
##### maxAge
Specifies the `number` (in seconds) to be the value for the [`Max-Age` `Set-Cookie` attribute][rfc-6265-5.2.2].
The given number will be converted to an integer by rounding down. By default, no maximum age is set.
**note** the [cookie storage model specification][rfc-6265-5.3] states that if both `expires` and
`maxAge` are set, then `maxAge` takes precedence, but it is possible not all clients by obey this,
so if both are set, they should point to the same date and time.
##### partitioned
Specifies the `boolean` value for the [`Partitioned` `Set-Cookie`](rfc-cutler-httpbis-partitioned-cookies)
attribute. When truthy, the `Partitioned` attribute is set, otherwise it is not. By default, the
`Partitioned` attribute is not set.
**note** This is an attribute that has not yet been fully standardized, and may change in the future.
This also means many clients may ignore this attribute until they understand it.
More information about can be found in [the proposal](https://github.com/privacycg/CHIPS).
##### path
Specifies the value for the [`Path` `Set-Cookie` attribute][rfc-6265-5.2.4]. By default, the path
is considered the ["default path"][rfc-6265-5.1.4].
##### priority
Specifies the `string` to be the value for the [`Priority` `Set-Cookie` attribute][rfc-west-cookie-priority-00-4.1].
- `'low'` will set the `Priority` attribute to `Low`.
- `'medium'` will set the `Priority` attribute to `Medium`, the default priority when not set.
- `'high'` will set the `Priority` attribute to `High`.
More information about the different priority levels can be found in
[the specification][rfc-west-cookie-priority-00-4.1].
**note** This is an attribute that has not yet been fully standardized, and may change in the future.
This also means many clients may ignore this attribute until they understand it.
##### sameSite
Specifies the `boolean` or `string` to be the value for the [`SameSite` `Set-Cookie` attribute][rfc-6265bis-09-5.4.7].
- `true` will set the `SameSite` attribute to `Strict` for strict same site enforcement.
- `false` will not set the `SameSite` attribute.
- `'lax'` will set the `SameSite` attribute to `Lax` for lax same site enforcement.
- `'none'` will set the `SameSite` attribute to `None` for an explicit cross-site cookie.
- `'strict'` will set the `SameSite` attribute to `Strict` for strict same site enforcement.
More information about the different enforcement levels can be found in
[the specification][rfc-6265bis-09-5.4.7].
**note** This is an attribute that has not yet been fully standardized, and may change in the future.
This also means many clients may ignore this attribute until they understand it.
##### secure
Specifies the `boolean` value for the [`Secure` `Set-Cookie` attribute][rfc-6265-5.2.5]. When truthy,
the `Secure` attribute is set, otherwise it is not. By default, the `Secure` attribute is not set.
**note** be careful when setting this to `true`, as compliant clients will not send the cookie back to
the server in the future if the browser does not have an HTTPS connection.
## Example
The following example uses this module in conjunction with the Node.js core HTTP server
to prompt a user for their name and display it back on future visits.
```js
var cookie = require('cookie');
var escapeHtml = require('escape-html');
var http = require('http');
var url = require('url');
function onRequest(req, res) {
// Parse the query string
var query = url.parse(req.url, true, true).query;
if (query && query.name) {
// Set a new cookie with the name
res.setHeader('Set-Cookie', cookie.serialize('name', String(query.name), {
httpOnly: true,
maxAge: 60 * 60 * 24 * 7 // 1 week
}));
// Redirect back after setting cookie
res.statusCode = 302;
res.setHeader('Location', req.headers.referer || '/');
res.end();
return;
}
// Parse the cookies on the request
var cookies = cookie.parse(req.headers.cookie || '');
// Get the visitor name set in the cookie
var name = cookies.name;
res.setHeader('Content-Type', 'text/html; charset=UTF-8');
if (name) {
res.write('<p>Welcome back, <b>' + escapeHtml(name) + '</b>!</p>');
} else {
res.write('<p>Hello, new visitor!</p>');
}
res.write('<form method="GET">');
res.write('<input placeholder="enter your name" name="name"> <input type="submit" value="Set Name">');
res.end('</form>');
}
http.createServer(onRequest).listen(3000);
```
## Testing
```sh
$ npm test
```
## Benchmark
```
$ npm run bench
> [email protected] bench
> node benchmark/index.js
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
modules@108
napi@9
[email protected]
[email protected]
[email protected]
[email protected]+quic
[email protected]
tz@2023c
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
> node benchmark/parse-top.js
cookie.parse - top sites
14 tests completed.
parse accounts.google.com x 2,588,913 ops/sec ±0.74% (186 runs sampled)
parse apple.com x 2,370,002 ops/sec ±0.69% (186 runs sampled)
parse cloudflare.com x 2,213,102 ops/sec ±0.88% (188 runs sampled)
parse docs.google.com x 2,194,157 ops/sec ±1.03% (184 runs sampled)
parse drive.google.com x 2,265,084 ops/sec ±0.79% (187 runs sampled)
parse en.wikipedia.org x 457,099 ops/sec ±0.81% (186 runs sampled)
parse linkedin.com x 504,407 ops/sec ±0.89% (186 runs sampled)
parse maps.google.com x 1,230,959 ops/sec ±0.98% (186 runs sampled)
parse microsoft.com x 926,294 ops/sec ±0.88% (184 runs sampled)
parse play.google.com x 2,311,338 ops/sec ±0.83% (185 runs sampled)
parse support.google.com x 1,508,850 ops/sec ±0.86% (186 runs sampled)
parse www.google.com x 1,022,582 ops/sec ±1.32% (182 runs sampled)
parse youtu.be x 332,136 ops/sec ±1.02% (185 runs sampled)
parse youtube.com x 323,833 ops/sec ±0.77% (183 runs sampled)
> node benchmark/parse.js
cookie.parse - generic
6 tests completed.
simple x 3,214,032 ops/sec ±1.61% (183 runs sampled)
decode x 587,237 ops/sec ±1.16% (187 runs sampled)
unquote x 2,954,618 ops/sec ±1.35% (183 runs sampled)
duplicates x 857,008 ops/sec ±0.89% (187 runs sampled)
10 cookies x 292,133 ops/sec ±0.89% (187 runs sampled)
100 cookies x 22,610 ops/sec ±0.68% (187 runs sampled)
```
## References
- [RFC 6265: HTTP State Management Mechanism][rfc-6265]
- [Same-site Cookies][rfc-6265bis-09-5.4.7]
[rfc-cutler-httpbis-partitioned-cookies]: https://tools.ietf.org/html/draft-cutler-httpbis-partitioned-cookies/
[rfc-west-cookie-priority-00-4.1]: https://tools.ietf.org/html/draft-west-cookie-priority-00#section-4.1
[rfc-6265bis-09-5.4.7]: https://tools.ietf.org/html/draft-ietf-httpbis-rfc6265bis-09#section-5.4.7
[rfc-6265]: https://tools.ietf.org/html/rfc6265
[rfc-6265-5.1.4]: https://tools.ietf.org/html/rfc6265#section-5.1.4
[rfc-6265-5.2.1]: https://tools.ietf.org/html/rfc6265#section-5.2.1
[rfc-6265-5.2.2]: https://tools.ietf.org/html/rfc6265#section-5.2.2
[rfc-6265-5.2.3]: https://tools.ietf.org/html/rfc6265#section-5.2.3
[rfc-6265-5.2.4]: https://tools.ietf.org/html/rfc6265#section-5.2.4
[rfc-6265-5.2.5]: https://tools.ietf.org/html/rfc6265#section-5.2.5
[rfc-6265-5.2.6]: https://tools.ietf.org/html/rfc6265#section-5.2.6
[rfc-6265-5.3]: https://tools.ietf.org/html/rfc6265#section-5.3
## License
[MIT](LICENSE)
[ci-image]: https://badgen.net/github/checks/jshttp/cookie/master?label=ci
[ci-url]: https://github.com/jshttp/cookie/actions/workflows/ci.yml
[coveralls-image]: https://badgen.net/coveralls/c/github/jshttp/cookie/master
[coveralls-url]: https://coveralls.io/r/jshttp/cookie?branch=master
[node-image]: https://badgen.net/npm/node/cookie
[node-url]: https://nodejs.org/en/download
[npm-downloads-image]: https://badgen.net/npm/dm/cookie
[npm-url]: https://npmjs.org/package/cookie
[npm-version-image]: https://badgen.net/npm/v/cookie | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/cookie/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/cookie/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 11748
} |
# Security Policies and Procedures
## Reporting a Bug
The `cookie` team and community take all security bugs seriously. Thank
you for improving the security of the project. We appreciate your efforts and
responsible disclosure and will make every effort to acknowledge your
contributions.
Report security bugs by emailing the current owner(s) of `cookie`. This
information can be found in the npm registry using the command
`npm owner ls cookie`.
If unsure or unable to get the information from the above, open an issue
in the [project issue tracker](https://github.com/jshttp/cookie/issues)
asking for the current contact information.
To ensure the timely response to your report, please ensure that the entirety
of the report is contained within the email body and not solely behind a web
link or an attachment.
At least one owner will acknowledge your email within 48 hours, and will send a
more detailed response within 48 hours indicating the next steps in handling
your report. After the initial reply to your report, the owners will
endeavor to keep you informed of the progress towards a fix and full
announcement, and may ask for additional information or guidance. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/cookie/SECURITY.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/cookie/SECURITY.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1179
} |
# cross-spawn
[![NPM version][npm-image]][npm-url] [![Downloads][downloads-image]][npm-url] [![Build Status][ci-image]][ci-url] [![Build status][appveyor-image]][appveyor-url]
[npm-url]:https://npmjs.org/package/cross-spawn
[downloads-image]:https://img.shields.io/npm/dm/cross-spawn.svg
[npm-image]:https://img.shields.io/npm/v/cross-spawn.svg
[ci-url]:https://github.com/moxystudio/node-cross-spawn/actions/workflows/ci.yaml
[ci-image]:https://github.com/moxystudio/node-cross-spawn/actions/workflows/ci.yaml/badge.svg
[appveyor-url]:https://ci.appveyor.com/project/satazor/node-cross-spawn
[appveyor-image]:https://img.shields.io/appveyor/ci/satazor/node-cross-spawn/master.svg
A cross platform solution to node's spawn and spawnSync.
## Installation
Node.js version 8 and up:
`$ npm install cross-spawn`
Node.js version 7 and under:
`$ npm install cross-spawn@6`
## Why
Node has issues when using spawn on Windows:
- It ignores [PATHEXT](https://github.com/joyent/node/issues/2318)
- It does not support [shebangs](https://en.wikipedia.org/wiki/Shebang_(Unix))
- Has problems running commands with [spaces](https://github.com/nodejs/node/issues/7367)
- Has problems running commands with posix relative paths (e.g.: `./my-folder/my-executable`)
- Has an [issue](https://github.com/moxystudio/node-cross-spawn/issues/82) with command shims (files in `node_modules/.bin/`), where arguments with quotes and parenthesis would result in [invalid syntax error](https://github.com/moxystudio/node-cross-spawn/blob/e77b8f22a416db46b6196767bcd35601d7e11d54/test/index.test.js#L149)
- No `options.shell` support on node `<v4.8`
All these issues are handled correctly by `cross-spawn`.
There are some known modules, such as [win-spawn](https://github.com/ForbesLindesay/win-spawn), that try to solve this but they are either broken or provide faulty escaping of shell arguments.
## Usage
Exactly the same way as node's [`spawn`](https://nodejs.org/api/child_process.html#child_process_child_process_spawn_command_args_options) or [`spawnSync`](https://nodejs.org/api/child_process.html#child_process_child_process_spawnsync_command_args_options), so it's a drop in replacement.
```js
const spawn = require('cross-spawn');
// Spawn NPM asynchronously
const child = spawn('npm', ['list', '-g', '-depth', '0'], { stdio: 'inherit' });
// Spawn NPM synchronously
const result = spawn.sync('npm', ['list', '-g', '-depth', '0'], { stdio: 'inherit' });
```
## Caveats
### Using `options.shell` as an alternative to `cross-spawn`
Starting from node `v4.8`, `spawn` has a `shell` option that allows you run commands from within a shell. This new option solves
the [PATHEXT](https://github.com/joyent/node/issues/2318) issue but:
- It's not supported in node `<v4.8`
- You must manually escape the command and arguments which is very error prone, specially when passing user input
- There are a lot of other unresolved issues from the [Why](#why) section that you must take into account
If you are using the `shell` option to spawn a command in a cross platform way, consider using `cross-spawn` instead. You have been warned.
### `options.shell` support
While `cross-spawn` adds support for `options.shell` in node `<v4.8`, all of its enhancements are disabled.
This mimics the Node.js behavior. More specifically, the command and its arguments will not be automatically escaped nor shebang support will be offered. This is by design because if you are using `options.shell` you are probably targeting a specific platform anyway and you don't want things to get into your way.
### Shebangs support
While `cross-spawn` handles shebangs on Windows, its support is limited. More specifically, it just supports `#!/usr/bin/env <program>` where `<program>` must not contain any arguments.
If you would like to have the shebang support improved, feel free to contribute via a pull-request.
Remember to always test your code on Windows!
## Tests
`$ npm test`
`$ npm test -- --watch` during development
## License
Released under the [MIT License](https://www.opensource.org/licenses/mit-license.php). | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/cross-spawn/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/cross-spawn/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 4116
} |
# cssesc [](https://travis-ci.org/mathiasbynens/cssesc) [](https://codecov.io/gh/mathiasbynens/cssesc)
A JavaScript library for escaping CSS strings and identifiers while generating the shortest possible ASCII-only output.
This is a JavaScript library for [escaping text for use in CSS strings or identifiers](https://mathiasbynens.be/notes/css-escapes) while generating the shortest possible valid ASCII-only output. [Here’s an online demo.](https://mothereff.in/css-escapes)
[A polyfill for the CSSOM `CSS.escape()` method is available in a separate repository.](https://mths.be/cssescape) (In comparison, _cssesc_ is much more powerful.)
Feel free to fork if you see possible improvements!
## Installation
Via [npm](https://www.npmjs.com/):
```bash
npm install cssesc
```
In a browser:
```html
<script src="cssesc.js"></script>
```
In [Node.js](https://nodejs.org/):
```js
const cssesc = require('cssesc');
```
In Ruby using [the `ruby-cssesc` wrapper gem](https://github.com/borodean/ruby-cssesc):
```bash
gem install ruby-cssesc
```
```ruby
require 'ruby-cssesc'
CSSEsc.escape('I ♥ Ruby', is_identifier: true)
```
In Sass using [`sassy-escape`](https://github.com/borodean/sassy-escape):
```bash
gem install sassy-escape
```
```scss
body {
content: escape('I ♥ Sass', $is-identifier: true);
}
```
## API
### `cssesc(value, options)`
This function takes a value and returns an escaped version of the value where any characters that are not printable ASCII symbols are escaped using the shortest possible (but valid) [escape sequences for use in CSS strings or identifiers](https://mathiasbynens.be/notes/css-escapes).
```js
cssesc('Ich ♥ Bücher');
// → 'Ich \\2665 B\\FC cher'
cssesc('foo 𝌆 bar');
// → 'foo \\1D306 bar'
```
By default, `cssesc` returns a string that can be used as part of a CSS string. If the target is a CSS identifier rather than a CSS string, use the `isIdentifier: true` setting (see below).
The optional `options` argument accepts an object with the following options:
#### `isIdentifier`
The default value for the `isIdentifier` option is `false`. This means that the input text will be escaped for use in a CSS string literal. If you want to use the result as a CSS identifier instead (in a selector, for example), set this option to `true`.
```js
cssesc('123a2b');
// → '123a2b'
cssesc('123a2b', {
'isIdentifier': true
});
// → '\\31 23a2b'
```
#### `quotes`
The default value for the `quotes` option is `'single'`. This means that any occurences of `'` in the input text will be escaped as `\'`, so that the output can be used in a CSS string literal wrapped in single quotes.
```js
cssesc('Lorem ipsum "dolor" sit \'amet\' etc.');
// → 'Lorem ipsum "dolor" sit \\\'amet\\\' etc.'
// → "Lorem ipsum \"dolor\" sit \\'amet\\' etc."
cssesc('Lorem ipsum "dolor" sit \'amet\' etc.', {
'quotes': 'single'
});
// → 'Lorem ipsum "dolor" sit \\\'amet\\\' etc.'
// → "Lorem ipsum \"dolor\" sit \\'amet\\' etc."
```
If you want to use the output as part of a CSS string literal wrapped in double quotes, set the `quotes` option to `'double'`.
```js
cssesc('Lorem ipsum "dolor" sit \'amet\' etc.', {
'quotes': 'double'
});
// → 'Lorem ipsum \\"dolor\\" sit \'amet\' etc.'
// → "Lorem ipsum \\\"dolor\\\" sit 'amet' etc."
```
#### `wrap`
The `wrap` option takes a boolean value (`true` or `false`), and defaults to `false` (disabled). When enabled, the output will be a valid CSS string literal wrapped in quotes. The type of quotes can be specified through the `quotes` setting.
```js
cssesc('Lorem ipsum "dolor" sit \'amet\' etc.', {
'quotes': 'single',
'wrap': true
});
// → '\'Lorem ipsum "dolor" sit \\\'amet\\\' etc.\''
// → "\'Lorem ipsum \"dolor\" sit \\\'amet\\\' etc.\'"
cssesc('Lorem ipsum "dolor" sit \'amet\' etc.', {
'quotes': 'double',
'wrap': true
});
// → '"Lorem ipsum \\"dolor\\" sit \'amet\' etc."'
// → "\"Lorem ipsum \\\"dolor\\\" sit \'amet\' etc.\""
```
#### `escapeEverything`
The `escapeEverything` option takes a boolean value (`true` or `false`), and defaults to `false` (disabled). When enabled, all the symbols in the output will be escaped, even printable ASCII symbols.
```js
cssesc('lolwat"foo\'bar', {
'escapeEverything': true
});
// → '\\6C\\6F\\6C\\77\\61\\74\\"\\66\\6F\\6F\\\'\\62\\61\\72'
// → "\\6C\\6F\\6C\\77\\61\\74\\\"\\66\\6F\\6F\\'\\62\\61\\72"
```
#### Overriding the default options globally
The global default settings can be overridden by modifying the `css.options` object. This saves you from passing in an `options` object for every call to `encode` if you want to use the non-default setting.
```js
// Read the global default setting for `escapeEverything`:
cssesc.options.escapeEverything;
// → `false` by default
// Override the global default setting for `escapeEverything`:
cssesc.options.escapeEverything = true;
// Using the global default setting for `escapeEverything`, which is now `true`:
cssesc('foo © bar ≠ baz 𝌆 qux');
// → '\\66\\6F\\6F\\ \\A9\\ \\62\\61\\72\\ \\2260\\ \\62\\61\\7A\\ \\1D306\\ \\71\\75\\78'
```
### `cssesc.version`
A string representing the semantic version number.
### Using the `cssesc` binary
To use the `cssesc` binary in your shell, simply install cssesc globally using npm:
```bash
npm install -g cssesc
```
After that you will be able to escape text for use in CSS strings or identifiers from the command line:
```bash
$ cssesc 'föo ♥ bår 𝌆 baz'
f\F6o \2665 b\E5r \1D306 baz
```
If the output needs to be a CSS identifier rather than part of a string literal, use the `-i`/`--identifier` option:
```bash
$ cssesc --identifier 'föo ♥ bår 𝌆 baz'
f\F6o\ \2665\ b\E5r\ \1D306\ baz
```
See `cssesc --help` for the full list of options.
## Support
This library supports the Node.js and browser versions mentioned in [`.babelrc`](https://github.com/mathiasbynens/cssesc/blob/master/.babelrc). For a version that supports a wider variety of legacy browsers and environments out-of-the-box, [see v0.1.0](https://github.com/mathiasbynens/cssesc/releases/tag/v0.1.0).
## Author
| [](https://twitter.com/mathias "Follow @mathias on Twitter") |
|---|
| [Mathias Bynens](https://mathiasbynens.be/) |
## License
This library is available under the [MIT](https://mths.be/mit) license. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/cssesc/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/cssesc/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 6516
} |
# CSSType
[](https://www.npmjs.com/package/csstype)
TypeScript and Flow definitions for CSS, generated by [data from MDN](https://github.com/mdn/data). It provides autocompletion and type checking for CSS properties and values.
**TypeScript**
```ts
import type * as CSS from 'csstype';
const style: CSS.Properties = {
colour: 'white', // Type error on property
textAlign: 'middle', // Type error on value
};
```
**Flow**
```js
// @flow strict
import * as CSS from 'csstype';
const style: CSS.Properties<> = {
colour: 'white', // Type error on property
textAlign: 'middle', // Type error on value
};
```
_Further examples below will be in TypeScript!_
## Getting started
```sh
$ npm install csstype
```
## Table of content
- [Style types](#style-types)
- [At-rule types](#at-rule-types)
- [Pseudo types](#pseudo-types)
- [Generics](#generics)
- [Usage](#usage)
- [What should I do when I get type errors?](#what-should-i-do-when-i-get-type-errors)
- [Version 3.0](#version-30)
- [Contributing](#contributing)
## Style types
Properties are categorized in different uses and in several technical variations to provide typings that suits as many as possible.
| | Default | `Hyphen` | `Fallback` | `HyphenFallback` |
| -------------- | -------------------- | -------------------------- | ---------------------------- | ---------------------------------- |
| **All** | `Properties` | `PropertiesHyphen` | `PropertiesFallback` | `PropertiesHyphenFallback` |
| **`Standard`** | `StandardProperties` | `StandardPropertiesHyphen` | `StandardPropertiesFallback` | `StandardPropertiesHyphenFallback` |
| **`Vendor`** | `VendorProperties` | `VendorPropertiesHyphen` | `VendorPropertiesFallback` | `VendorPropertiesHyphenFallback` |
| **`Obsolete`** | `ObsoleteProperties` | `ObsoletePropertiesHyphen` | `ObsoletePropertiesFallback` | `ObsoletePropertiesHyphenFallback` |
| **`Svg`** | `SvgProperties` | `SvgPropertiesHyphen` | `SvgPropertiesFallback` | `SvgPropertiesHyphenFallback` |
Categories:
- **All** - Includes `Standard`, `Vendor`, `Obsolete` and `Svg`
- **`Standard`** - Current properties and extends subcategories `StandardLonghand` and `StandardShorthand` _(e.g. `StandardShorthandProperties`)_
- **`Vendor`** - Vendor prefixed properties and extends subcategories `VendorLonghand` and `VendorShorthand` _(e.g. `VendorShorthandProperties`)_
- **`Obsolete`** - Removed or deprecated properties
- **`Svg`** - SVG-specific properties
Variations:
- **Default** - JavaScript (camel) cased property names
- **`Hyphen`** - CSS (kebab) cased property names
- **`Fallback`** - Also accepts array of values e.g. `string | string[]`
## At-rule types
At-rule interfaces with descriptors.
**TypeScript**: These will be found in the `AtRule` namespace, e.g. `AtRule.Viewport`.
**Flow**: These will be prefixed with `AtRule$`, e.g. `AtRule$Viewport`.
| | Default | `Hyphen` | `Fallback` | `HyphenFallback` |
| -------------------- | -------------- | -------------------- | ---------------------- | ---------------------------- |
| **`@counter-style`** | `CounterStyle` | `CounterStyleHyphen` | `CounterStyleFallback` | `CounterStyleHyphenFallback` |
| **`@font-face`** | `FontFace` | `FontFaceHyphen` | `FontFaceFallback` | `FontFaceHyphenFallback` |
| **`@viewport`** | `Viewport` | `ViewportHyphen` | `ViewportFallback` | `ViewportHyphenFallback` |
## Pseudo types
String literals of pseudo classes and pseudo elements
- `Pseudos`
Extends:
- `AdvancedPseudos`
Function-like pseudos e.g. `:not(:first-child)`. The string literal contains the value excluding the parenthesis: `:not`. These are separated because they require an argument that results in infinite number of variations.
- `SimplePseudos`
Plain pseudos e.g. `:hover` that can only be **one** variation.
## Generics
All interfaces has two optional generic argument to define length and time: `CSS.Properties<TLength = string | 0, TTime = string>`
- **Length** is the first generic parameter and defaults to `string | 0` because `0` is the only [length where the unit identifier is optional](https://drafts.csswg.org/css-values-3/#lengths). You can specify this, e.g. `string | number`, for platforms and libraries that accepts any numeric value as length with a specific unit.
```tsx
const style: CSS.Properties<string | number> = {
width: 100,
};
```
- **Time** is the second generic argument and defaults to `string`. You can specify this, e.g. `string | number`, for platforms and libraries that accepts any numeric value as length with a specific unit.
```tsx
const style: CSS.Properties<string | number, number> = {
transitionDuration: 1000,
};
```
## Usage
```ts
import type * as CSS from 'csstype';
const style: CSS.Properties = {
width: '10px',
margin: '1em',
};
```
In some cases, like for CSS-in-JS libraries, an array of values is a way to provide fallback values in CSS. Using `CSS.PropertiesFallback` instead of `CSS.Properties` will add the possibility to use any property value as an array of values.
```ts
import type * as CSS from 'csstype';
const style: CSS.PropertiesFallback = {
display: ['-webkit-flex', 'flex'],
color: 'white',
};
```
There's even string literals for pseudo selectors and elements.
```ts
import type * as CSS from 'csstype';
const pseudos: { [P in CSS.SimplePseudos]?: CSS.Properties } = {
':hover': {
display: 'flex',
},
};
```
Hyphen cased (kebab cased) properties are provided in `CSS.PropertiesHyphen` and `CSS.PropertiesHyphenFallback`. It's not **not** added by default in `CSS.Properties`. To allow both of them, you can simply extend with `CSS.PropertiesHyphen` or/and `CSS.PropertiesHyphenFallback`.
```ts
import type * as CSS from 'csstype';
interface Style extends CSS.Properties, CSS.PropertiesHyphen {}
const style: Style = {
'flex-grow': 1,
'flex-shrink': 0,
'font-weight': 'normal',
backgroundColor: 'white',
};
```
Adding type checked CSS properties to a `HTMLElement`.
```ts
import type * as CSS from 'csstype';
const style: CSS.Properties = {
color: 'red',
margin: '1em',
};
let button = document.createElement('button');
Object.assign(button.style, style);
```
## What should I do when I get type errors?
The goal is to have as perfect types as possible and we're trying to do our best. But with CSS Custom Properties, the CSS specification changing frequently and vendors implementing their own specifications with new releases sometimes causes type errors even if it should work. Here's some steps you could take to get it fixed:
_If you're using CSS Custom Properties you can step directly to step 3._
1. **First of all, make sure you're doing it right.** A type error could also indicate that you're not :wink:
- Some CSS specs that some vendors has implemented could have been officially rejected or haven't yet received any official acceptance and are therefor not included
- If you're using TypeScript, [type widening](https://blog.mariusschulz.com/2017/02/04/TypeScript-2-1-literal-type-widening) could be the reason you get `Type 'string' is not assignable to...` errors
2. **Have a look in [issues](https://github.com/frenic/csstype/issues) to see if an issue already has been filed. If not, create a new one.** To help us out, please refer to any information you have found.
3. Fix the issue locally with **TypeScript** (Flow further down):
- The recommended way is to use **module augmentation**. Here's a few examples:
```ts
// My css.d.ts file
import type * as CSS from 'csstype';
declare module 'csstype' {
interface Properties {
// Add a missing property
WebkitRocketLauncher?: string;
// Add a CSS Custom Property
'--theme-color'?: 'black' | 'white';
// Allow namespaced CSS Custom Properties
[index: `--theme-${string}`]: any;
// Allow any CSS Custom Properties
[index: `--${string}`]: any;
// ...or allow any other property
[index: string]: any;
}
}
```
- The alternative way is to use **type assertion**. Here's a few examples:
```ts
const style: CSS.Properties = {
// Add a missing property
['WebkitRocketLauncher' as any]: 'launching',
// Add a CSS Custom Property
['--theme-color' as any]: 'black',
};
```
Fix the issue locally with **Flow**:
- Use **type assertion**. Here's a few examples:
```js
const style: $Exact<CSS.Properties<*>> = {
// Add a missing property
[('WebkitRocketLauncher': any)]: 'launching',
// Add a CSS Custom Property
[('--theme-color': any)]: 'black',
};
```
## Version 3.0
- **All property types are exposed with namespace**
TypeScript: `Property.AlignContent` (was `AlignContentProperty` before)
Flow: `Property$AlignContent`
- **All at-rules are exposed with namespace**
TypeScript: `AtRule.FontFace` (was `FontFace` before)
Flow: `AtRule$FontFace`
- **Data types are NOT exposed**
E.g. `Color` and `Box`. Because the generation of data types may suddenly be removed or renamed.
- **TypeScript hack for autocompletion**
Uses `(string & {})` for literal string unions and `(number & {})` for literal number unions ([related issue](https://github.com/microsoft/TypeScript/issues/29729)). Utilize `PropertyValue<T>` to unpack types from e.g. `(string & {})` to `string`.
- **New generic for time**
Read more on the ["Generics"](#generics) section.
- **Flow types improvements**
Flow Strict enabled and exact types are used.
## Contributing
**Never modify `index.d.ts` and `index.js.flow` directly. They are generated automatically and committed so that we can easily follow any change it results in.** Therefor it's important that you run `$ git config merge.ours.driver true` after you've forked and cloned. That setting prevents merge conflicts when doing rebase.
### Commands
- `npm run build` Generates typings and type checks them
- `npm run watch` Runs build on each save
- `npm run test` Runs the tests
- `npm run lazy` Type checks, lints and formats everything | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/csstype/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/csstype/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 10517
} |
# d3-array
Data in JavaScript is often represented by an iterable (such as an [array](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array), [set](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Set) or [generator](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Generator)), and so iterable manipulation is a common task when analyzing or visualizing data. For example, you might take a contiguous slice (subset) of an array, filter an array using a predicate function, or map an array to a parallel set of values using a transform function. Before looking at the methods that d3-array provides, familiarize yourself with the powerful [array methods built-in to JavaScript](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array).
JavaScript includes **mutation methods** that modify the array:
* [*array*.pop](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/pop) - Remove the last element from the array.
* [*array*.push](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/push) - Add one or more elements to the end of the array.
* [*array*.reverse](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/reverse) - Reverse the order of the elements of the array.
* [*array*.shift](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/shift) - Remove the first element from the array.
* [*array*.sort](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/sort) - Sort the elements of the array.
* [*array*.splice](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/splice) - Add or remove elements from the array.
* [*array*.unshift](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/unshift) - Add one or more elements to the front of the array.
There are also **access methods** that return some representation of the array:
* [*array*.concat](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/concat) - Join the array with other array(s) or value(s).
* [*array*.join](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/join) - Join all elements of the array into a string.
* [*array*.slice](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/slice) - Extract a section of the array.
* [*array*.indexOf](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/indexOf) - Find the first occurrence of a value within the array.
* [*array*.lastIndexOf](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/lastIndexOf) - Find the last occurrence of a value within the array.
And finally **iteration methods** that apply functions to elements in the array:
* [*array*.filter](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/filter) - Create a new array with only the elements for which a predicate is true.
* [*array*.forEach](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach) - Call a function for each element in the array.
* [*array*.every](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/every) - See if every element in the array satisfies a predicate.
* [*array*.map](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/map) - Create a new array with the result of calling a function on every element in the array.
* [*array*.some](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/some) - See if at least one element in the array satisfies a predicate.
* [*array*.reduce](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/reduce) - Apply a function to reduce the array to a single value (from left-to-right).
* [*array*.reduceRight](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/reduceRight) - Apply a function to reduce the array to a single value (from right-to-left).
## Installing
If you use npm, `npm install d3-array`. You can also download the [latest release on GitHub](https://github.com/d3/d3-array/releases/latest). For vanilla HTML in modern browsers, import d3-array from jsDelivr:
```html
<script type="module">
import {min} from "https://cdn.jsdelivr.net/npm/d3-array@3/+esm";
const m = min(array);
</script>
```
For legacy environments, you can load d3-array’s UMD bundle; a `d3` global is exported:
```html
<script src="https://cdn.jsdelivr.net/npm/d3-array@3"></script>
<script>
const m = d3.min(array);
</script>
```
## API Reference
* [Statistics](#statistics)
* [Search](#search)
* [Transformations](#transformations)
* [Iterables](#iterables)
* [Sets](#sets)
* [Bins](#bins)
* [Interning](#interning)
### Statistics
Methods for computing basic summary statistics.
<a name="min" href="#min">#</a> d3.<b>min</b>(<i>iterable</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/min.js), [Examples](https://observablehq.com/@d3/d3-extent)
Returns the minimum value in the given *iterable* using natural order. If the iterable contains no comparable values, returns undefined. An optional *accessor* function may be specified, which is equivalent to calling Array.from before computing the minimum value.
Unlike the built-in [Math.min](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Math/min), this method ignores undefined, null and NaN values; this is useful for ignoring missing data. In addition, elements are compared using natural order rather than numeric order. For example, the minimum of the strings [“20”, “3”] is “20”, while the minimum of the numbers [20, 3] is 3.
See also [extent](#extent).
<a name="minIndex" href="#minIndex">#</a> d3.<b>minIndex</b>(<i>iterable</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/minIndex.js), [Examples](https://observablehq.com/@d3/d3-extent)
Returns the index of the minimum value in the given *iterable* using natural order. If the iterable contains no comparable values, returns -1. An optional *accessor* function may be specified, which is equivalent to calling Array.from before computing the minimum value.
Unlike the built-in [Math.min](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Math/min), this method ignores undefined, null and NaN values; this is useful for ignoring missing data. In addition, elements are compared using natural order rather than numeric order. For example, the minimum of the strings [“20”, “3”] is “20”, while the minimum of the numbers [20, 3] is 3.
<a name="max" href="#max">#</a> d3.<b>max</b>(<i>iterable</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/max.js), [Examples](https://observablehq.com/@d3/d3-extent)
Returns the maximum value in the given *iterable* using natural order. If the iterable contains no comparable values, returns undefined. An optional *accessor* function may be specified, which is equivalent to calling Array.from before computing the maximum value.
Unlike the built-in [Math.max](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Math/max), this method ignores undefined values; this is useful for ignoring missing data. In addition, elements are compared using natural order rather than numeric order. For example, the maximum of the strings [“20”, “3”] is “3”, while the maximum of the numbers [20, 3] is 20.
See also [extent](#extent).
<a name="maxIndex" href="#maxIndex">#</a> d3.<b>maxIndex</b>(<i>iterable</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/maxIndex.js), [Examples](https://observablehq.com/@d3/d3-extent)
Returns the index of the maximum value in the given *iterable* using natural order. If the iterable contains no comparable values, returns -1. An optional *accessor* function may be specified, which is equivalent to calling Array.from before computing the maximum value.
Unlike the built-in [Math.max](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Math/max), this method ignores undefined values; this is useful for ignoring missing data. In addition, elements are compared using natural order rather than numeric order. For example, the maximum of the strings [“20”, “3”] is “3”, while the maximum of the numbers [20, 3] is 20.
<a name="extent" href="#extent">#</a> d3.<b>extent</b>(<i>iterable</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/extent.js), [Examples](https://observablehq.com/@d3/d3-extent)
Returns the [minimum](#min) and [maximum](#max) value in the given *iterable* using natural order. If the iterable contains no comparable values, returns [undefined, undefined]. An optional *accessor* function may be specified, which is equivalent to calling Array.from before computing the extent.
<a name="mode" href="#mode">#</a> d3.<b>mode</b>(<i>iterable</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/mode.js), [Examples](https://observablehq.com/@d3/d3-mode)
Returns the mode of the given *iterable*, *i.e.* the value which appears the most often. In case of equality, returns the first of the relevant values. If the iterable contains no comparable values, returns undefined. An optional *accessor* function may be specified, which is equivalent to calling Array.from before computing the mode. This method ignores undefined, null and NaN values; this is useful for ignoring missing data.
<a name="sum" href="#sum">#</a> d3.<b>sum</b>(<i>iterable</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/sum.js), [Examples](https://observablehq.com/@d3/d3-sum)
Returns the sum of the given *iterable* of numbers. If the iterable contains no numbers, returns 0. An optional *accessor* function may be specified, which is equivalent to calling Array.from before computing the sum. This method ignores undefined and NaN values; this is useful for ignoring missing data.
<a name="mean" href="#mean">#</a> d3.<b>mean</b>(<i>iterable</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/mean.js), [Examples](https://observablehq.com/@d3/d3-mean-d3-median-and-friends)
Returns the mean of the given *iterable* of numbers. If the iterable contains no numbers, returns undefined. An optional *accessor* function may be specified, which is equivalent to calling Array.from before computing the mean. This method ignores undefined and NaN values; this is useful for ignoring missing data.
<a name="median" href="#median">#</a> d3.<b>median</b>(<i>iterable</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/median.js), [Examples](https://observablehq.com/@d3/d3-mean-d3-median-and-friends)
Returns the median of the given *iterable* of numbers using the [R-7 method](https://en.wikipedia.org/wiki/Quantile#Estimating_quantiles_from_a_sample). If the iterable contains no numbers, returns undefined. An optional *accessor* function may be specified, which is equivalent to calling Array.from before computing the median. This method ignores undefined and NaN values; this is useful for ignoring missing data.
<a name="medianIndex" href="#medianIndex">#</a> d3.<b>medianIndex</b>(<i>array</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/median.js)
Similar to *median*, but returns the index of the element to the left of the median.
<a name="cumsum" href="#cumsum">#</a> d3.<b>cumsum</b>(<i>iterable</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/cumsum.js), [Examples](https://observablehq.com/@d3/d3-cumsum)
Returns the cumulative sum of the given *iterable* of numbers, as a Float64Array of the same length. If the iterable contains no numbers, returns zeros. An optional *accessor* function may be specified, which is equivalent to calling Array.from before computing the cumulative sum. This method ignores undefined and NaN values; this is useful for ignoring missing data.
<a name="quantile" href="#quantile">#</a> d3.<b>quantile</b>(<i>iterable</i>, <i>p</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/quantile.js), [Examples](https://observablehq.com/@d3/d3-mean-d3-median-and-friends)
Returns the *p*-quantile of the given *iterable* of numbers, where *p* is a number in the range [0, 1]. For example, the median can be computed using *p* = 0.5, the first quartile at *p* = 0.25, and the third quartile at *p* = 0.75. This particular implementation uses the [R-7 method](http://en.wikipedia.org/wiki/Quantile#Quantiles_of_a_population), which is the default for the R programming language and Excel. For example:
```js
var a = [0, 10, 30];
d3.quantile(a, 0); // 0
d3.quantile(a, 0.5); // 10
d3.quantile(a, 1); // 30
d3.quantile(a, 0.25); // 5
d3.quantile(a, 0.75); // 20
d3.quantile(a, 0.1); // 2
```
An optional *accessor* function may be specified, which is equivalent to calling *array*.map(*accessor*) before computing the quantile.
<a name="quantileIndex" href="#quantileIndex">#</a> d3.<b>quantileIndex</b>(<i>array</i>, <i>p</i>[, <i>accessor</i>]) [Source](https://github.com/d3/d3-array/blob/main/src/quantile.js "Source")
Similar to *quantile*, but returns the index to the left of *p*.
<a name="quantileSorted" href="#quantileSorted">#</a> d3.<b>quantileSorted</b>(<i>array</i>, <i>p</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/quantile.js), [Examples](https://observablehq.com/@d3/d3-mean-d3-median-and-friends)
Similar to *quantile*, but expects the input to be a **sorted** *array* of values. In contrast with *quantile*, the accessor is only called on the elements needed to compute the quantile.
<a name="rank" href="#rank">#</a> d3.<b>rank</b>(<i>iterable</i>[, <i>comparator</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/rank.js), [Examples](https://observablehq.com/@d3/rank)
<br><a name="rank" href="#rank">#</a> d3.<b>rank</b>(<i>iterable</i>[, <i>accessor</i>])
Returns an array with the rank of each value in the *iterable*, *i.e.* the zero-based index of the value when the iterable is sorted. Nullish values are sorted to the end and ranked NaN. An optional *comparator* or *accessor* function may be specified; the latter is equivalent to calling *array*.map(*accessor*) before computing the ranks. If *comparator* is not specified, it defaults to [ascending](#ascending). Ties (equivalent values) all get the same rank, defined as the first time the value is found.
```js
d3.rank([{x: 1}, {}, {x: 2}, {x: 0}], d => d.x); // [1, NaN, 2, 0]
d3.rank(["b", "c", "b", "a"]); // [1, 3, 1, 0]
d3.rank([1, 2, 3], d3.descending); // [2, 1, 0]
```
<a name="variance" href="#variance">#</a> d3.<b>variance</b>(<i>iterable</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/variance.js), [Examples](https://observablehq.com/@d3/d3-mean-d3-median-and-friends)
Returns an [unbiased estimator of the population variance](http://mathworld.wolfram.com/SampleVariance.html) of the given *iterable* of numbers using [Welford’s algorithm](https://en.wikipedia.org/wiki/Algorithms_for_calculating_variance#Welford's_online_algorithm). If the iterable has fewer than two numbers, returns undefined. An optional *accessor* function may be specified, which is equivalent to calling Array.from before computing the variance. This method ignores undefined and NaN values; this is useful for ignoring missing data.
<a name="deviation" href="#deviation">#</a> d3.<b>deviation</b>(<i>iterable</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/deviation.js), [Examples](https://observablehq.com/@d3/d3-mean-d3-median-and-friends)
Returns the standard deviation, defined as the square root of the [bias-corrected variance](#variance), of the given *iterable* of numbers. If the iterable has fewer than two numbers, returns undefined. An optional *accessor* function may be specified, which is equivalent to calling Array.from before computing the standard deviation. This method ignores undefined and NaN values; this is useful for ignoring missing data.
<a name="fsum" href="#fsum">#</a> d3.<b>fsum</b>([<i>values</i>][, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/fsum.js), [Examples](https://observablehq.com/@d3/d3-fsum)
Returns a full precision summation of the given *values*.
```js
d3.fsum([.1, .1, .1, .1, .1, .1, .1, .1, .1, .1]); // 1
d3.sum([.1, .1, .1, .1, .1, .1, .1, .1, .1, .1]); // 0.9999999999999999
```
Although slower, d3.fsum can replace d3.sum wherever greater precision is needed. Uses <a href="#adder">d3.Adder</a>.
<a name="fcumsum" href="#fcumsum">#</a> d3.<b>fcumsum</b>([<i>values</i>][, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/fsum.js), [Examples](https://observablehq.com/@d3/d3-fcumsum)
Returns a full precision cumulative sum of the given *values*.
```js
d3.fcumsum([1, 1e-14, -1]); // [1, 1.00000000000001, 1e-14]
d3.cumsum([1, 1e-14, -1]); // [1, 1.00000000000001, 9.992e-15]
```
Although slower, d3.fcumsum can replace d3.cumsum when greater precision is needed. Uses <a href="#adder">d3.Adder</a>.
<a name="adder" href="#adder">#</a> new d3.<b>Adder</b>()
Creates a full precision adder for [IEEE 754](https://en.wikipedia.org/wiki/IEEE_754) floating point numbers, setting its initial value to 0.
<a name="adder_add" href="#adder_add">#</a> *adder*.<b>add</b>(<i>number</i>)
Adds the specified *number* to the adder’s current value and returns the adder.
<a name="adder_valueOf" href="#adder_valueOf">#</a> *adder*.<b>valueOf</b>()
Returns the IEEE 754 double precision representation of the adder’s current value. Most useful as the short-hand notation `+adder`.
### Search
Methods for searching arrays for a specific element.
<a name="least" href="#least">#</a> d3.<b>least</b>(<i>iterable</i>[, <i>comparator</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/least.js), [Examples](https://observablehq.com/@d3/d3-least)
<br><a name="least" href="#least">#</a> d3.<b>least</b>(<i>iterable</i>[, <i>accessor</i>])
Returns the least element of the specified *iterable* according to the specified *comparator* or *accessor*. If the given *iterable* contains no comparable elements (*i.e.*, the comparator returns NaN when comparing each element to itself), returns undefined. If *comparator* is not specified, it defaults to [ascending](#ascending). For example:
```js
const array = [{foo: 42}, {foo: 91}];
d3.least(array, (a, b) => a.foo - b.foo); // {foo: 42}
d3.least(array, (a, b) => b.foo - a.foo); // {foo: 91}
d3.least(array, a => a.foo); // {foo: 42}
```
This function is similar to [min](#min), except it allows the use of a comparator rather than an accessor.
<a name="leastIndex" href="#leastIndex">#</a> d3.<b>leastIndex</b>(<i>iterable</i>[, <i>comparator</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/leastIndex.js), [Examples](https://observablehq.com/@d3/d3-least)
<br><a name="leastIndex" href="#leastIndex">#</a> d3.<b>leastIndex</b>(<i>iterable</i>[, <i>accessor</i>])
Returns the index of the least element of the specified *iterable* according to the specified *comparator* or *accessor*. If the given *iterable* contains no comparable elements (*i.e.*, the comparator returns NaN when comparing each element to itself), returns -1. If *comparator* is not specified, it defaults to [ascending](#ascending). For example:
```js
const array = [{foo: 42}, {foo: 91}];
d3.leastIndex(array, (a, b) => a.foo - b.foo); // 0
d3.leastIndex(array, (a, b) => b.foo - a.foo); // 1
d3.leastIndex(array, a => a.foo); // 0
```
This function is similar to [minIndex](#minIndex), except it allows the use of a comparator rather than an accessor.
<a name="greatest" href="#greatest">#</a> d3.<b>greatest</b>(<i>iterable</i>[, <i>comparator</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/greatest.js), [Examples](https://observablehq.com/@d3/d3-least)
<br><a name="greatest" href="#greatest">#</a> d3.<b>greatest</b>(<i>iterable</i>[, <i>accessor</i>])
Returns the greatest element of the specified *iterable* according to the specified *comparator* or *accessor*. If the given *iterable* contains no comparable elements (*i.e.*, the comparator returns NaN when comparing each element to itself), returns undefined. If *comparator* is not specified, it defaults to [ascending](#ascending). For example:
```js
const array = [{foo: 42}, {foo: 91}];
d3.greatest(array, (a, b) => a.foo - b.foo); // {foo: 91}
d3.greatest(array, (a, b) => b.foo - a.foo); // {foo: 42}
d3.greatest(array, a => a.foo); // {foo: 91}
```
This function is similar to [max](#max), except it allows the use of a comparator rather than an accessor.
<a name="greatestIndex" href="#greatestIndex">#</a> d3.<b>greatestIndex</b>(<i>iterable</i>[, <i>comparator</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/greatestIndex.js), [Examples](https://observablehq.com/@d3/d3-least)
<br><a name="greatestIndex" href="#greatestIndex">#</a> d3.<b>greatestIndex</b>(<i>iterable</i>[, <i>accessor</i>])
Returns the index of the greatest element of the specified *iterable* according to the specified *comparator* or *accessor*. If the given *iterable* contains no comparable elements (*i.e.*, the comparator returns NaN when comparing each element to itself), returns -1. If *comparator* is not specified, it defaults to [ascending](#ascending). For example:
```js
const array = [{foo: 42}, {foo: 91}];
d3.greatestIndex(array, (a, b) => a.foo - b.foo); // 1
d3.greatestIndex(array, (a, b) => b.foo - a.foo); // 0
d3.greatestIndex(array, a => a.foo); // 1
```
This function is similar to [maxIndex](#maxIndex), except it allows the use of a comparator rather than an accessor.
<a name="bisectLeft" href="#bisectLeft">#</a> d3.<b>bisectLeft</b>(<i>array</i>, <i>x</i>[, <i>lo</i>[, <i>hi</i>]]) · [Source](https://github.com/d3/d3-array/blob/main/src/bisect.js)
Returns the insertion point for *x* in *array* to maintain sorted order. The arguments *lo* and *hi* may be used to specify a subset of the array which should be considered; by default the entire array is used. If *x* is already present in *array*, the insertion point will be before (to the left of) any existing entries. The return value is suitable for use as the first argument to [splice](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/splice) assuming that *array* is already sorted. The returned insertion point *i* partitions the *array* into two halves so that all *v* < *x* for *v* in *array*.slice(*lo*, *i*) for the left side and all *v* >= *x* for *v* in *array*.slice(*i*, *hi*) for the right side.
<a name="bisect" href="#bisect">#</a> d3.<b>bisect</b>(<i>array</i>, <i>x</i>[, <i>lo</i>[, <i>hi</i>]]) · [Source](https://github.com/d3/d3-array/blob/main/src/bisect.js), [Examples](https://observablehq.com/@d3/d3-bisect)
<br><a name="bisectRight" href="#bisectRight">#</a> d3.<b>bisectRight</b>(<i>array</i>, <i>x</i>[, <i>lo</i>[, <i>hi</i>]])
Similar to [bisectLeft](#bisectLeft), but returns an insertion point which comes after (to the right of) any existing entries of *x* in *array*. The returned insertion point *i* partitions the *array* into two halves so that all *v* <= *x* for *v* in *array*.slice(*lo*, *i*) for the left side and all *v* > *x* for *v* in *array*.slice(*i*, *hi*) for the right side.
<a name="bisectCenter" href="#bisectCenter">#</a> d3.<b>bisectCenter</b>(<i>array</i>, <i>x</i>[, <i>lo</i>[, <i>hi</i>]]) · [Source](https://github.com/d3/d3-array/blob/main/src/bisect.js), [Examples](https://observablehq.com/@d3/multi-line-chart)
Returns the index of the value closest to *x* in the given *array* of numbers. The arguments *lo* (inclusive) and *hi* (exclusive) may be used to specify a subset of the array which should be considered; by default the entire array is used.
See [*bisector*.center](#bisector_center).
<a name="bisector" href="#bisector">#</a> d3.<b>bisector</b>(<i>accessor</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/bisector.js)
<br><a name="bisector" href="#bisector">#</a> d3.<b>bisector</b>(<i>comparator</i>)
Returns a new bisector using the specified *accessor* or *comparator* function. This method can be used to bisect arrays of objects instead of being limited to simple arrays of primitives. For example, given the following array of objects:
```js
var data = [
{date: new Date(2011, 1, 1), value: 0.5},
{date: new Date(2011, 2, 1), value: 0.6},
{date: new Date(2011, 3, 1), value: 0.7},
{date: new Date(2011, 4, 1), value: 0.8}
];
```
A suitable bisect function could be constructed as:
```js
var bisectDate = d3.bisector(function(d) { return d.date; }).right;
```
This is equivalent to specifying a comparator:
```js
var bisectDate = d3.bisector(function(d, x) { return d.date - x; }).right;
```
And then applied as *bisectDate*(*array*, *date*), returning an index. Note that the comparator is always passed the search value *x* as the second argument. Use a comparator rather than an accessor if you want values to be sorted in an order different than natural order, such as in descending rather than ascending order.
<a name="bisector_left" href="#bisector_left">#</a> <i>bisector</i>.<b>left</b>(<i>array</i>, <i>x</i>[, <i>lo</i>[, <i>hi</i>]]) · [Source](https://github.com/d3/d3-array/blob/main/src/bisector.js)
Equivalent to [bisectLeft](#bisectLeft), but uses this bisector’s associated comparator.
<a name="bisector_right" href="#bisector_right">#</a> <i>bisector</i>.<b>right</b>(<i>array</i>, <i>x</i>[, <i>lo</i>[, <i>hi</i>]]) · [Source](https://github.com/d3/d3-array/blob/main/src/bisector.js)
Equivalent to [bisectRight](#bisectRight), but uses this bisector’s associated comparator.
<a name="bisector_center" href="#bisector_center">#</a> <i>bisector</i>.<b>center</b>(<i>array</i>, <i>x</i>[, <i>lo</i>[, <i>hi</i>]]) · [Source](https://github.com/d3/d3-array/blob/main/src/bisector.js)
Returns the index of the closest value to *x* in the given sorted *array*. This expects that the bisector’s associated accessor returns a quantitative value, or that the bisector’s associated comparator returns a signed distance; otherwise, this method is equivalent to *bisector*.left.
<a name="quickselect" href="#quickselect">#</a> d3.<b>quickselect</b>(<i>array</i>, <i>k</i>, <i>left</i> = 0, <i>right</i> = <i>array</i>.length - 1, <i>compare</i> = ascending) · [Source](https://github.com/d3/d3-array/blob/main/src/quickselect.js), [Examples](https://observablehq.com/@d3/d3-quickselect)
See [mourner/quickselect](https://github.com/mourner/quickselect/blob/master/README.md).
<a name="ascending" href="#ascending">#</a> d3.<b>ascending</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/ascending.js), [Examples](https://observablehq.com/@d3/d3-ascending)
Returns -1 if *a* is less than *b*, or 1 if *a* is greater than *b*, or 0. This is the comparator function for natural order, and can be used in conjunction with the built-in [*array*.sort](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/sort) method to arrange elements in ascending order. It is implemented as:
```js
function ascending(a, b) {
return a == null || b == null ? NaN : a < b ? -1 : a > b ? 1 : a >= b ? 0 : NaN;
}
```
Note that if no comparator function is specified to the built-in sort method, the default order is lexicographic (alphabetical), not natural! This can lead to surprising behavior when sorting an array of numbers.
<a name="descending" href="#descending">#</a> d3.<b>descending</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/descending.js), [Examples](https://observablehq.com/@d3/d3-ascending)
Returns -1 if *a* is greater than *b*, or 1 if *a* is less than *b*, or 0. This is the comparator function for reverse natural order, and can be used in conjunction with the built-in array sort method to arrange elements in descending order. It is implemented as:
```js
function descending(a, b) {
return a == null || b == null ? NaN : b < a ? -1 : b > a ? 1 : b >= a ? 0 : NaN;
}
```
Note that if no comparator function is specified to the built-in sort method, the default order is lexicographic (alphabetical), not natural! This can lead to surprising behavior when sorting an array of numbers.
### Transformations
Methods for transforming arrays and for generating new arrays.
<a name="group" href="#group">#</a> d3.<b>group</b>(<i>iterable</i>, <i>...keys</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/group.js), [Examples](https://observablehq.com/@d3/d3-group-d3-rollup)
Groups the specified *iterable* of values into an [InternMap](#InternMap) from *key* to array of value. For example, given some data:
```js
data = [
{name: "jim", amount: "34.0", date: "11/12/2015"},
{name: "carl", amount: "120.11", date: "11/12/2015"},
{name: "stacy", amount: "12.01", date: "01/04/2016"},
{name: "stacy", amount: "34.05", date: "01/04/2016"}
]
```
To group the data by name:
```js
d3.group(data, d => d.name)
```
This produces:
```js
Map(3) {
"jim" => Array(1)
"carl" => Array(1)
"stacy" => Array(2)
}
```
If more than one *key* is specified, a nested InternMap is returned. For example:
```js
d3.group(data, d => d.name, d => d.date)
```
This produces:
```js
Map(3) {
"jim" => Map(1) {
"11/12/2015" => Array(1)
}
"carl" => Map(1) {
"11/12/2015" => Array(1)
}
"stacy" => Map(1) {
"01/04/2016" => Array(2)
}
}
```
To convert a Map to an Array, use [Array.from](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/from). For example:
```js
Array.from(d3.group(data, d => d.name))
```
This produces:
```js
[
["jim", Array(1)],
["carl", Array(1)],
["stacy", Array(2)]
]
```
You can also simultaneously convert the [*key*, *value*] to some other representation by passing a map function to Array.from:
```js
Array.from(d3.group(data, d => d.name), ([key, value]) => ({key, value}))
```
This produces:
```js
[
{key: "jim", value: Array(1)},
{key: "carl", value: Array(1)},
{key: "stacy", value: Array(2)}
]
```
[*selection*.data](https://github.com/d3/d3-selection/blob/main/README.md#selection_data) accepts iterables directly, meaning that you can use a Map (or Set or other iterable) to perform a data join without first needing to convert to an array.
<a name="groups" href="#groups">#</a> d3.<b>groups</b>(<i>iterable</i>, <i>...keys</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/group.js), [Examples](https://observablehq.com/@d3/d3-group-d3-rollup)
Equivalent to [group](#group), but returns nested arrays instead of nested maps.
<a name="flatGroup" href="#flatGroup">#</a> d3.<b>flatGroup</b>(<i>iterable</i>, <i>...keys</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/group.js), [Examples](https://observablehq.com/@d3/d3-flatgroup)
Equivalent to [group](#group), but returns a flat array of [*key0*, *key1*, …, *values*] instead of nested maps.
<a name="index" href="#index">#</a> d3.<b>index</b>(<i>iterable</i>, <i>...keys</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/group.js), [Examples](https://observablehq.com/@d3/d3-group)
Equivalent to [group](#group) but returns a unique value per compound key instead of an array, throwing if the key is not unique.
For example, given the data defined above,
```js
d3.index(data, d => d.amount)
```
returns
```js
Map(4) {
"34.0" => Object {name: "jim", amount: "34.0", date: "11/12/2015"}
"120.11" => Object {name: "carl", amount: "120.11", date: "11/12/2015"}
"12.01" => Object {name: "stacy", amount: "12.01", date: "01/04/2016"}
"34.05" => Object {name: "stacy", amount: "34.05", date: "01/04/2016"}
}
```
On the other hand,
```js
d3.index(data, d => d.name)
```
throws an error because two objects share the same name.
<a name="indexes" href="#indexes">#</a> d3.<b>indexes</b>(<i>iterable</i>, <i>...keys</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/group.js), [Examples](https://observablehq.com/@d3/d3-group)
Equivalent to [index](#index), but returns nested arrays instead of nested maps.
<a name="rollup" href="#rollup">#</a> d3.<b>rollup</b>(<i>iterable</i>, <i>reduce</i>, <i>...keys</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/group.js), [Examples](https://observablehq.com/@d3/d3-group-d3-rollup)
[Groups](#group) and reduces the specified *iterable* of values into an InternMap from *key* to value. For example, given some data:
```js
data = [
{name: "jim", amount: "34.0", date: "11/12/2015"},
{name: "carl", amount: "120.11", date: "11/12/2015"},
{name: "stacy", amount: "12.01", date: "01/04/2016"},
{name: "stacy", amount: "34.05", date: "01/04/2016"}
]
```
To count the number of elements by name:
```js
d3.rollup(data, v => v.length, d => d.name)
```
This produces:
```js
Map(3) {
"jim" => 1
"carl" => 1
"stacy" => 2
}
```
If more than one *key* is specified, a nested Map is returned. For example:
```js
d3.rollup(data, v => v.length, d => d.name, d => d.date)
```
This produces:
```js
Map(3) {
"jim" => Map(1) {
"11/12/2015" => 1
}
"carl" => Map(1) {
"11/12/2015" => 1
}
"stacy" => Map(1) {
"01/04/2016" => 2
}
}
```
To convert a Map to an Array, use [Array.from](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/from). See [d3.group](#group) for examples.
<a name="rollups" href="#rollups">#</a> d3.<b>rollups</b>(<i>iterable</i>, <i>reduce</i>, <i>...keys</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/group.js), [Examples](https://observablehq.com/@d3/d3-group-d3-rollup)
Equivalent to [rollup](#rollup), but returns nested arrays instead of nested maps.
<a name="flatRollup" href="#flatRollup">#</a> d3.<b>flatRollup</b>(<i>iterable</i>, <i>reduce</i>, <i>...keys</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/group.js), [Examples](https://observablehq.com/@d3/d3-flatgroup)
Equivalent to [rollup](#rollup), but returns a flat array of [*key0*, *key1*, …, *value*] instead of nested maps.
<a name="groupSort" href="#groupSort">#</a> d3.<b>groupSort</b>(<i>iterable</i>, <i>comparator</i>, <i>key</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/groupSort.js), [Examples](https://observablehq.com/@d3/d3-groupsort)
<br><a name="groupSort" href="#groupSort">#</a> d3.<b>groupSort</b>(<i>iterable</i>, <i>accessor</i>, <i>key</i>)
Groups the specified *iterable* of elements according to the specified *key* function, sorts the groups according to the specified *comparator*, and then returns an array of keys in sorted order. For example, if you had a table of barley yields for different varieties, sites, and years, to sort the barley varieties by ascending median yield:
```js
d3.groupSort(barley, g => d3.median(g, d => d.yield), d => d.variety)
```
For descending order, negate the group value:
```js
d3.groupSort(barley, g => -d3.median(g, d => d.yield), d => d.variety)
```
If a *comparator* is passed instead of an *accessor* (i.e., if the second argument is a function that takes exactly two arguments), it will be asked to compare two groups *a* and *b* and should return a negative value if *a* should be before *b*, a positive value if *a* should be after *b*, or zero for a partial ordering.
<a name="count" href="#count">#</a> d3.<b>count</b>(<i>iterable</i>[, <i>accessor</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/count.js), [Examples](https://observablehq.com/@d3/d3-count)
Returns the number of valid number values (*i.e.*, not null, NaN, or undefined) in the specified *iterable*; accepts an accessor.
For example:
```js
d3.count([{n: "Alice", age: NaN}, {n: "Bob", age: 18}, {n: "Other"}], d => d.age) // 1
```
<a name="cross" href="#cross">#</a> d3.<b>cross</b>(<i>...iterables</i>[, <i>reducer</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/cross.js), [Examples](https://observablehq.com/@d3/d3-cross)
Returns the [Cartesian product](https://en.wikipedia.org/wiki/Cartesian_product) of the specified *iterables*. For example, if two iterables *a* and *b* are specified, for each element *i* in the iterable *a* and each element *j* in the iterable *b*, in order, invokes the specified *reducer* function passing the element *i* and element *j*. If a *reducer* is not specified, it defaults to a function which creates a two-element array for each pair:
```js
function pair(a, b) {
return [a, b];
}
```
For example:
```js
d3.cross([1, 2], ["x", "y"]); // returns [[1, "x"], [1, "y"], [2, "x"], [2, "y"]]
d3.cross([1, 2], ["x", "y"], (a, b) => a + b); // returns ["1x", "1y", "2x", "2y"]
```
<a name="merge" href="#merge">#</a> d3.<b>merge</b>(<i>iterables</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/merge.js), [Examples](https://observablehq.com/@d3/d3-merge)
Merges the specified iterable of *iterables* into a single array. This method is similar to the built-in array concat method; the only difference is that it is more convenient when you have an array of arrays.
```js
d3.merge([[1], [2, 3]]); // returns [1, 2, 3]
```
<a name="pairs" href="#pairs">#</a> d3.<b>pairs</b>(<i>iterable</i>[, <i>reducer</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/pairs.js), [Examples](https://observablehq.com/@d3/d3-pairs)
For each adjacent pair of elements in the specified *iterable*, in order, invokes the specified *reducer* function passing the element *i* and element *i* - 1. If a *reducer* is not specified, it defaults to a function which creates a two-element array for each pair:
```js
function pair(a, b) {
return [a, b];
}
```
For example:
```js
d3.pairs([1, 2, 3, 4]); // returns [[1, 2], [2, 3], [3, 4]]
d3.pairs([1, 2, 3, 4], (a, b) => b - a); // returns [1, 1, 1];
```
If the specified iterable has fewer than two elements, returns the empty array.
<a name="permute" href="#permute">#</a> d3.<b>permute</b>(<i>source</i>, <i>keys</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/permute.js), [Examples](https://observablehq.com/@d3/d3-permute)
Returns a permutation of the specified *source* object (or array) using the specified iterable of *keys*. The returned array contains the corresponding property of the source object for each key in *keys*, in order. For example:
```js
permute(["a", "b", "c"], [1, 2, 0]); // returns ["b", "c", "a"]
```
It is acceptable to have more keys than source elements, and for keys to be duplicated or omitted.
This method can also be used to extract the values from an object into an array with a stable order. Extracting keyed values in order can be useful for generating data arrays in nested selections. For example:
```js
let object = {yield: 27, variety: "Manchuria", year: 1931, site: "University Farm"};
let fields = ["site", "variety", "yield"];
d3.permute(object, fields); // returns ["University Farm", "Manchuria", 27]
```
<a name="shuffle" href="#shuffle">#</a> d3.<b>shuffle</b>(<i>array</i>[, <i>start</i>[, <i>stop</i>]]) · [Source](https://github.com/d3/d3-array/blob/main/src/shuffle.js), [Examples](https://observablehq.com/@d3/d3-shuffle)
Randomizes the order of the specified *array* in-place using the [Fisher–Yates shuffle](https://bost.ocks.org/mike/shuffle/) and returns the *array*. If *start* is specified, it is the starting index (inclusive) of the *array* to shuffle; if *start* is not specified, it defaults to zero. If *stop* is specified, it is the ending index (exclusive) of the *array* to shuffle; if *stop* is not specified, it defaults to *array*.length. For example, to shuffle the first ten elements of the *array*: shuffle(*array*, 0, 10).
<a name="shuffler" href="#shuffler">#</a> d3.<b>shuffler</b>(<i>random</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/shuffle.js)
Returns a [shuffle function](#shuffle) given the specified random source. For example, using [d3.randomLcg](https://github.com/d3/d3-random/blob/main/README.md#randomLcg):
```js
const random = d3.randomLcg(0.9051667019185816);
const shuffle = d3.shuffler(random);
shuffle([0, 1, 2, 3, 4, 5, 6, 7, 8, 9]); // returns [7, 4, 5, 3, 9, 0, 6, 1, 2, 8]
```
<a name="ticks" href="#ticks">#</a> d3.<b>ticks</b>(<i>start</i>, <i>stop</i>, <i>count</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/ticks.js), [Examples](https://observablehq.com/@d3/d3-ticks)
Returns an array of approximately *count* + 1 uniformly-spaced, nicely-rounded values between *start* and *stop* (inclusive). Each value is a power of ten multiplied by 1, 2 or 5. See also [d3.tickIncrement](#tickIncrement), [d3.tickStep](#tickStep) and [*linear*.ticks](https://github.com/d3/d3-scale/blob/main/README.md#linear_ticks).
Ticks are inclusive in the sense that they may include the specified *start* and *stop* values if (and only if) they are exact, nicely-rounded values consistent with the inferred [step](#tickStep). More formally, each returned tick *t* satisfies *start* ≤ *t* and *t* ≤ *stop*.
<a name="tickIncrement" href="#tickIncrement">#</a> d3.<b>tickIncrement</b>(<i>start</i>, <i>stop</i>, <i>count</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/ticks.js), [Examples](https://observablehq.com/@d3/d3-ticks)
Like [d3.tickStep](#tickStep), except requires that *start* is always less than or equal to *stop*, and if the tick step for the given *start*, *stop* and *count* would be less than one, returns the negative inverse tick step instead. This method is always guaranteed to return an integer, and is used by [d3.ticks](#ticks) to guarantee that the returned tick values are represented as precisely as possible in IEEE 754 floating point.
<a name="tickStep" href="#tickStep">#</a> d3.<b>tickStep</b>(<i>start</i>, <i>stop</i>, <i>count</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/ticks.js), [Examples](https://observablehq.com/@d3/d3-ticks)
Returns the difference between adjacent tick values if the same arguments were passed to [d3.ticks](#ticks): a nicely-rounded value that is a power of ten multiplied by 1, 2 or 5. Note that due to the limited precision of IEEE 754 floating point, the returned value may not be exact decimals; use [d3-format](https://github.com/d3/d3-format) to format numbers for human consumption.
<a name="nice" href="#nice">#</a> d3.<b>nice</b>(<i>start</i>, <i>stop</i>, <i>count</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/nice.js)
Returns a new interval [*niceStart*, *niceStop*] covering the given interval [*start*, *stop*] and where *niceStart* and *niceStop* are guaranteed to align with the corresponding [tick step](#tickStep). Like [d3.tickIncrement](#tickIncrement), this requires that *start* is less than or equal to *stop*.
<a name="range" href="#range">#</a> d3.<b>range</b>([<i>start</i>, ]<i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/range.js), [Examples](https://observablehq.com/@d3/d3-range)
Returns an array containing an arithmetic progression, similar to the Python built-in [range](http://docs.python.org/library/functions.html#range). This method is often used to iterate over a sequence of uniformly-spaced numeric values, such as the indexes of an array or the ticks of a linear scale. (See also [d3.ticks](#ticks) for nicely-rounded values.)
If *step* is omitted, it defaults to 1. If *start* is omitted, it defaults to 0. The *stop* value is exclusive; it is not included in the result. If *step* is positive, the last element is the largest *start* + *i* \* *step* less than *stop*; if *step* is negative, the last element is the smallest *start* + *i* \* *step* greater than *stop*. If the returned array would contain an infinite number of values, an empty range is returned.
The arguments are not required to be integers; however, the results are more predictable if they are. The values in the returned array are defined as *start* + *i* \* *step*, where *i* is an integer from zero to one minus the total number of elements in the returned array. For example:
```js
d3.range(0, 1, 0.2) // [0, 0.2, 0.4, 0.6000000000000001, 0.8]
```
This unexpected behavior is due to IEEE 754 double-precision floating point, which defines 0.2 * 3 = 0.6000000000000001. Use [d3-format](https://github.com/d3/d3-format) to format numbers for human consumption with appropriate rounding; see also [linear.tickFormat](https://github.com/d3/d3-scale/blob/main/README.md#linear_tickFormat) in [d3-scale](https://github.com/d3/d3-scale).
Likewise, if the returned array should have a specific length, consider using [array.map](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Array/map) on an integer range. For example:
```js
d3.range(0, 1, 1 / 49); // BAD: returns 50 elements!
d3.range(49).map(function(d) { return d / 49; }); // GOOD: returns 49 elements.
```
<a name="transpose" href="#transpose">#</a> d3.<b>transpose</b>(<i>matrix</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/transpose.js), [Examples](https://observablehq.com/@d3/d3-transpose)
Uses the [zip](#zip) operator as a two-dimensional [matrix transpose](http://en.wikipedia.org/wiki/Transpose).
<a name="zip" href="#zip">#</a> d3.<b>zip</b>(<i>arrays…</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/zip.js), [Examples](https://observablehq.com/@d3/d3-transpose)
Returns an array of arrays, where the *i*th array contains the *i*th element from each of the argument *arrays*. The returned array is truncated in length to the shortest array in *arrays*. If *arrays* contains only a single array, the returned array contains one-element arrays. With no arguments, the returned array is empty.
```js
d3.zip([1, 2], [3, 4]); // returns [[1, 3], [2, 4]]
```
#### Blur
<a name="blur" href="#blur">#</a> d3.<b>blur</b>(*data*, *radius*) · [Source](https://github.com/d3/d3-array/blob/main/src/blur.js), [Examples](https://observablehq.com/@d3/d3-blur)
Blurs an array of *data* in-place by applying three iterations of a moving average transform, for a fast approximation of a gaussian kernel of the given *radius*, a non-negative number, and returns the array.
```js
const randomWalk = d3.cumsum({length: 1000}, () => Math.random() - 0.5);
blur(randomWalk, 5);
```
Copy the data if you don’t want to smooth it in-place:
```js
const smoothed = blur(randomWalk.slice(), 5);
```
<a name="blur2" href="#blur2">#</a> d3.<b>blur2</b>({*data*, *width*[, *height*]}, *rx*[, *ry*]) · [Source](https://github.com/d3/d3-array/blob/main/src/blur.js), [Examples](https://observablehq.com/@d3/d3-blur)
Blurs a matrix of the given *width* and *height* in-place, by applying an horizontal blur of radius *rx* and a vertical blur or radius *ry* (which defaults to *rx*). The matrix *data* is stored in a flat array, used to determine the *height* if it is not specified. Returns the blurred {data, width, height}.
```js
data = [
1, 0, 0,
0, 0, 0,
0, 0, 1
];
blur2({data, width: 3}, 1);
```
<a name="blurImage" href="#blurImage">#</a> d3.<b>blurImage</b>(*imageData*, *rx*[, *ry*]) · [Source](https://github.com/d3/d3-array/blob/main/src/blur.js), [Examples](https://observablehq.com/@d3/d3-blurimage)
Blurs an [ImageData](https://developer.mozilla.org/en-US/docs/Web/API/ImageData) structure in-place, blurring each of the RGBA layers independently by applying an horizontal blur of radius *rx* and a vertical blur or radius *ry* (which defaults to *rx*). Returns the blurred ImageData.
```js
const imData = context.getImageData(0, 0, width, height);
blurImage(imData, 5);
```
### Iterables
These are equivalent to built-in array methods, but work with any iterable including Map, Set, and Generator.
<a name="every" href="#every">#</a> d3.<b>every</b>(<i>iterable</i>, <i>test</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/every.js)
Returns true if the given *test* function returns true for every value in the given *iterable*. This method returns as soon as *test* returns a non-truthy value or all values are iterated over. Equivalent to [*array*.every](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/every):
```js
d3.every(new Set([1, 3, 5, 7]), x => x & 1) // true
```
<a name="some" href="#some">#</a> d3.<b>some</b>(<i>iterable</i>, <i>test</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/some.js)
Returns true if the given *test* function returns true for any value in the given *iterable*. This method returns as soon as *test* returns a truthy value or all values are iterated over. Equivalent to [*array*.some](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/some):
```js
d3.some(new Set([0, 2, 3, 4]), x => x & 1) // true
```
<a name="filter" href="#filter">#</a> d3.<b>filter</b>(<i>iterable</i>, <i>test</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/filter.js)
Returns a new array containing the values from *iterable*, in order, for which the given *test* function returns true. Equivalent to [*array*.filter](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/filter):
```js
d3.filter(new Set([0, 2, 3, 4]), x => x & 1) // [3]
```
<a name="map" href="#map">#</a> d3.<b>map</b>(<i>iterable</i>, <i>mapper</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/map.js)
Returns a new array containing the mapped values from *iterable*, in order, as defined by given *mapper* function. Equivalent to [*array*.map](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map) and [Array.from](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/from):
```js
d3.map(new Set([0, 2, 3, 4]), x => x & 1) // [0, 0, 1, 0]
```
<a name="reduce" href="#reduce">#</a> d3.<b>reduce</b>(<i>iterable</i>, <i>reducer</i>[, <i>initialValue</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/reduce.js)
Returns the reduced value defined by given *reducer* function, which is repeatedly invoked for each value in *iterable*, being passed the current reduced value and the next value. Equivalent to [*array*.reduce](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/reduce):
```js
d3.reduce(new Set([0, 2, 3, 4]), (p, v) => p + v, 0) // 9
```
<a name="reverse" href="#reverse">#</a> d3.<b>reverse</b>(<i>iterable</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/reverse.js)
Returns an array containing the values in the given *iterable* in reverse order. Equivalent to [*array*.reverse](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/reverse), except that it does not mutate the given *iterable*:
```js
d3.reverse(new Set([0, 2, 3, 1])) // [1, 3, 2, 0]
```
<a name="sort" href="#sort">#</a> d3.<b>sort</b>(<i>iterable</i>, <i>comparator</i> = d3.ascending) · [Source](https://github.com/d3/d3-array/blob/main/src/sort.js)
<br><a name="sort" href="#sort">#</a> d3.<b>sort</b>(<i>iterable</i>, ...<i>accessors</i>)
Returns an array containing the values in the given *iterable* in the sorted order defined by the given *comparator* or *accessor* function. If *comparator* is not specified, it defaults to [d3.ascending](#ascending). Equivalent to [*array*.sort](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/sort), except that it does not mutate the given *iterable*, and the comparator defaults to natural order instead of lexicographic order:
```js
d3.sort(new Set([0, 2, 3, 1])) // [0, 1, 2, 3]
```
If an *accessor* (a function that does not take exactly two arguments) is specified,
```js
d3.sort(data, d => d.value)
```
it is equivalent to a *comparator* using [natural order](#ascending):
```js
d3.sort(data, (a, b) => d3.ascending(a.value, b.value))
```
The *accessor* is only invoked once per element, and thus the returned sorted order is consistent even if the accessor is nondeterministic.
Multiple accessors may be specified to break ties:
```js
d3.sort(points, ({x}) => x, ({y}) => y)
```
This is equivalent to:
```js
d3.sort(data, (a, b) => d3.ascending(a.x, b.x) || d3.ascending(a.y, b.y))
```
### Sets
This methods implement basic set operations for any iterable.
<a name="difference" href="#difference">#</a> d3.<b>difference</b>(<i>iterable</i>, ...<i>others</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/difference.js)
Returns a new InternSet containing every value in *iterable* that is not in any of the *others* iterables.
```js
d3.difference([0, 1, 2, 0], [1]) // Set {0, 2}
```
<a name="union" href="#union">#</a> d3.<b>union</b>(...<i>iterables</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/union.js)
Returns a new InternSet containing every (distinct) value that appears in any of the given *iterables*. The order of values in the returned set is based on their first occurrence in the given *iterables*.
```js
d3.union([0, 2, 1, 0], [1, 3]) // Set {0, 2, 1, 3}
```
<a name="intersection" href="#intersection">#</a> d3.<b>intersection</b>(...<i>iterables</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/intersection.js)
Returns a new InternSet containing every (distinct) value that appears in all of the given *iterables*. The order of values in the returned set is based on their first occurrence in the given *iterables*.
```js
d3.intersection([0, 2, 1, 0], [1, 3]) // Set {1}
```
<a name="superset" href="#superset">#</a> d3.<b>superset</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/superset.js)
Returns true if *a* is a superset of *b*: if every value in the given iterable *b* is also in the given iterable *a*.
```js
d3.superset([0, 2, 1, 3, 0], [1, 3]) // true
```
<a name="subset" href="#subset">#</a> d3.<b>subset</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/subset.js)
Returns true if *a* is a subset of *b*: if every value in the given iterable *a* is also in the given iterable *b*.
```js
d3.subset([1, 3], [0, 2, 1, 3, 0]) // true
```
<a name="disjoint" href="#disjoint">#</a> d3.<b>disjoint</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/disjoint.js)
Returns true if *a* and *b* are disjoint: if *a* and *b* contain no shared value.
```js
d3.disjoint([1, 3], [2, 4]) // true
```
### Bins
[<img src="https://raw.githubusercontent.com/d3/d3-array/main/img/histogram.png" width="480" height="250" alt="Histogram">](http://bl.ocks.org/mbostock/3048450)
Binning groups discrete samples into a smaller number of consecutive, non-overlapping intervals. They are often used to visualize the distribution of numerical data as histograms.
<a name="bin" href="#bin">#</a> d3.<b>bin</b>() · [Source](https://github.com/d3/d3-array/blob/main/src/bin.js), [Examples](https://observablehq.com/@d3/d3-bin)
Constructs a new bin generator with the default settings.
<a name="_bin" href="#_bin">#</a> <i>bin</i>(<i>data</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/bin.js), [Examples](https://observablehq.com/@d3/d3-bin)
Bins the given iterable of *data* samples. Returns an array of bins, where each bin is an array containing the associated elements from the input *data*. Thus, the `length` of the bin is the number of elements in that bin. Each bin has two additional attributes:
* `x0` - the lower bound of the bin (inclusive).
* `x1` - the upper bound of the bin (exclusive, except for the last bin).
Any null or non-comparable values in the given *data*, or those outside the [domain](#bin_domain), are ignored.
<a name="bin_value" href="#bin_value">#</a> <i>bin</i>.<b>value</b>([<i>value</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/bin.js), [Examples](https://observablehq.com/@d3/d3-bin)
If *value* is specified, sets the value accessor to the specified function or constant and returns this bin generator. If *value* is not specified, returns the current value accessor, which defaults to the identity function.
When bins are [generated](#_bin), the value accessor will be invoked for each element in the input data array, being passed the element `d`, the index `i`, and the array `data` as three arguments. The default value accessor assumes that the input data are orderable (comparable), such as numbers or dates. If your data are not, then you should specify an accessor that returns the corresponding orderable value for a given datum.
This is similar to mapping your data to values before invoking the bin generator, but has the benefit that the input data remains associated with the returned bins, thereby making it easier to access other fields of the data.
<a name="bin_domain" href="#bin_domain">#</a> <i>bin</i>.<b>domain</b>([<i>domain</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/bin.js), [Examples](https://observablehq.com/@d3/d3-bin)
If *domain* is specified, sets the domain accessor to the specified function or array and returns this bin generator. If *domain* is not specified, returns the current domain accessor, which defaults to [extent](#extent). The bin domain is defined as an array [*min*, *max*], where *min* is the minimum observable value and *max* is the maximum observable value; both values are inclusive. Any value outside of this domain will be ignored when the bins are [generated](#_bin).
For example, if you are using the bin generator in conjunction with a [linear scale](https://github.com/d3/d3-scale/blob/main/README.md#linear-scales) `x`, you might say:
```js
var bin = d3.bin()
.domain(x.domain())
.thresholds(x.ticks(20));
```
You can then compute the bins from an array of numbers like so:
```js
var bins = bin(numbers);
```
If the default [extent](#extent) domain is used and the [thresholds](#bin_thresholds) are specified as a count (rather than explicit values), then the computed domain will be [niced](#nice) such that all bins are uniform width.
Note that the domain accessor is invoked on the materialized array of [values](#bin_value), not on the input data array.
<a name="bin_thresholds" href="#bin_thresholds">#</a> <i>bin</i>.<b>thresholds</b>([<i>count</i>]) · [Source](https://github.com/d3/d3-array/blob/main/src/bin.js), [Examples](https://observablehq.com/@d3/d3-bin)
<br><a name="bin_thresholds" href="#bin_thresholds">#</a> <i>bin</i>.<b>thresholds</b>([<i>thresholds</i>])
If *thresholds* is specified, sets the [threshold generator](#bin-thresholds) to the specified function or array and returns this bin generator. If *thresholds* is not specified, returns the current threshold generator, which by default implements [Sturges’ formula](#thresholdSturges). (Thus by default, the values to be binned must be numbers!) Thresholds are defined as an array of values [*x0*, *x1*, …]. Any value less than *x0* will be placed in the first bin; any value greater than or equal to *x0* but less than *x1* will be placed in the second bin; and so on. Thus, the [generated bins](#_bin) will have *thresholds*.length + 1 bins. See [bin thresholds](#bin-thresholds) for more information.
Any threshold values outside the [domain](#bin_domain) are ignored. The first *bin*.x0 is always equal to the minimum domain value, and the last *bin*.x1 is always equal to the maximum domain value.
If a *count* is specified instead of an array of *thresholds*, then the [domain](#bin_domain) will be uniformly divided into approximately *count* bins; see [ticks](#ticks).
#### Bin Thresholds
These functions are typically not used directly; instead, pass them to [*bin*.thresholds](#bin_thresholds).
<a name="thresholdFreedmanDiaconis" href="#thresholdFreedmanDiaconis">#</a> d3.<b>thresholdFreedmanDiaconis</b>(<i>values</i>, <i>min</i>, <i>max</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/threshold/freedmanDiaconis.js), [Examples](https://observablehq.com/@d3/d3-bin)
Returns the number of bins according to the [Freedman–Diaconis rule](https://en.wikipedia.org/wiki/Histogram#Mathematical_definition); the input *values* must be numbers.
<a name="thresholdScott" href="#thresholdScott">#</a> d3.<b>thresholdScott</b>(<i>values</i>, <i>min</i>, <i>max</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/threshold/scott.js), [Examples](https://observablehq.com/@d3/d3-bin)
Returns the number of bins according to [Scott’s normal reference rule](https://en.wikipedia.org/wiki/Histogram#Mathematical_definition); the input *values* must be numbers.
<a name="thresholdSturges" href="#thresholdSturges">#</a> d3.<b>thresholdSturges</b>(<i>values</i>) · [Source](https://github.com/d3/d3-array/blob/main/src/threshold/sturges.js), [Examples](https://observablehq.com/@d3/d3-bin)
Returns the number of bins according to [Sturges’ formula](https://en.wikipedia.org/wiki/Histogram#Mathematical_definition); the input *values* must be numbers.
You may also implement your own threshold generator taking three arguments: the array of input [*values*](#bin_value) derived from the data, and the [observable domain](#bin_domain) represented as *min* and *max*. The generator may then return either the array of numeric thresholds or the *count* of bins; in the latter case the domain is divided uniformly into approximately *count* bins; see [ticks](#ticks).
For instance, when binning date values, you might want to use the ticks from a time scale ([Example](https://observablehq.com/@d3/d3-bin-time-thresholds)).
### Interning
<a name="InternMap" href="#InternMap">#</a> new d3.<b>InternMap</b>([<i>iterable</i>][, <i>key</i>]) · [Source](https://github.com/mbostock/internmap/blob/main/src/index.js), [Examples](https://observablehq.com/d/d4c5f6ad343866b9)
<br><a name="InternSet" href="#InternSet">#</a> new d3.<b>InternSet</b>([<i>iterable</i>][, <i>key</i>]) · [Source](https://github.com/mbostock/internmap/blob/main/src/index.js), [Examples](https://observablehq.com/d/d4c5f6ad343866b9)
The [InternMap and InternSet](https://github.com/mbostock/internmap) classes extend the native JavaScript Map and Set classes, respectively, allowing Dates and other non-primitive keys by bypassing the [SameValueZero algorithm](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Equality_comparisons_and_sameness) when determining key equality. d3.group, d3.rollup and d3.index use an InternMap rather than a native Map. These two classes are exported for convenience. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/d3-array/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/d3-array/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 62813
} |
# d3-color
Even though your browser understands a lot about colors, it doesn’t offer much help in manipulating colors through JavaScript. The d3-color module therefore provides representations for various color spaces, allowing specification, conversion and manipulation. (Also see [d3-interpolate](https://github.com/d3/d3-interpolate) for color interpolation.)
For example, take the color named “steelblue”:
```js
const c = d3.color("steelblue"); // {r: 70, g: 130, b: 180, opacity: 1}
```
Let’s try converting it to HSL:
```js
const c = d3.hsl("steelblue"); // {h: 207.27…, s: 0.44, l: 0.4902…, opacity: 1}
```
Now rotate the hue by 90°, bump up the saturation, and format as a string for CSS:
```js
c.h += 90;
c.s += 0.2;
c + ""; // rgb(198, 45, 205)
```
To fade the color slightly:
```js
c.opacity = 0.8;
c + ""; // rgba(198, 45, 205, 0.8)
```
In addition to the ubiquitous and machine-friendly [RGB](#rgb) and [HSL](#hsl) color space, d3-color supports color spaces that are designed for humans:
* [CIELAB](#lab) (*a.k.a.* “Lab”)
* [CIELCh<sub>ab</sub>](#lch) (*a.k.a.* “LCh” or “HCL”)
* Dave Green’s [Cubehelix](#cubehelix)
Cubehelix features monotonic lightness, while CIELAB and its polar form CIELCh<sub>ab</sub> are perceptually uniform.
## Extensions
For additional color spaces, see:
* [d3-cam16](https://github.com/d3/d3-cam16)
* [d3-cam02](https://github.com/connorgr/d3-cam02)
* [d3-hsv](https://github.com/d3/d3-hsv)
* [d3-hcg](https://github.com/d3/d3-hcg)
* [d3-hsluv](https://github.com/petulla/d3-hsluv)
To measure color differences, see:
* [d3-color-difference](https://github.com/Evercoder/d3-color-difference)
## Installing
If you use npm, `npm install d3-color`. You can also download the [latest release on GitHub](https://github.com/d3/d3-color/releases/latest). For vanilla HTML in modern browsers, import d3-color from Skypack:
```html
<script type="module">
import {rgb} from "https://cdn.skypack.dev/d3-color@3";
const steelblue = d3.rgb("steelblue");
</script>
```
For legacy environments, you can load d3-color’s UMD bundle from an npm-based CDN such as jsDelivr; a `d3` global is exported:
```html
<script src="https://cdn.jsdelivr.net/npm/d3-color@3"></script>
<script>
const steelblue = d3.rgb("steelblue");
</script>
```
[Try d3-color in your browser.](https://observablehq.com/collection/@d3/d3-color)
## API Reference
<a name="color" href="#color">#</a> d3.<b>color</b>(<i>specifier</i>) [<>](https://github.com/d3/d3-color/blob/master/src/color.js "Source")
Parses the specified [CSS Color Module Level 3](http://www.w3.org/TR/css3-color/#colorunits) *specifier* string, returning an [RGB](#rgb) or [HSL](#hsl) color, along with [CSS Color Module Level 4 hex](https://www.w3.org/TR/css-color-4/#hex-notation) *specifier* strings. If the specifier was not valid, null is returned. Some examples:
* `rgb(255, 255, 255)`
* `rgb(10%, 20%, 30%)`
* `rgba(255, 255, 255, 0.4)`
* `rgba(10%, 20%, 30%, 0.4)`
* `hsl(120, 50%, 20%)`
* `hsla(120, 50%, 20%, 0.4)`
* `#ffeeaa`
* `#fea`
* `#ffeeaa22`
* `#fea2`
* `steelblue`
The list of supported [named colors](http://www.w3.org/TR/SVG/types.html#ColorKeywords) is specified by CSS.
Note: this function may also be used with `instanceof` to test if an object is a color instance. The same is true of color subclasses, allowing you to test whether a color is in a particular color space.
<a name="color_opacity" href="#color_opacity">#</a> *color*.<b>opacity</b>
This color’s opacity, typically in the range [0, 1].
<a name="color_rgb" href="#color_rgb">#</a> *color*.<b>rgb</b>() [<>](https://github.com/d3/d3-color/blob/master/src/color.js "Source")
Returns the [RGB equivalent](#rgb) of this color. For RGB colors, that’s `this`.
<a name="color_copy" href="#color_copy">#</a> <i>color</i>.<b>copy</b>([<i>values</i>]) [<>](https://github.com/d3/d3-color/blob/master/src/color.js "Source")
Returns a copy of this color. If *values* is specified, any enumerable own properties of *values* are assigned to the new returned color. For example, to derive a copy of a *color* with opacity 0.5, say
```js
color.copy({opacity: 0.5})
```
<a name="color_brighter" href="#color_brighter">#</a> *color*.<b>brighter</b>([<i>k</i>]) [<>](https://github.com/d3/d3-color/blob/master/src/color.js "Source")
Returns a brighter copy of this color. If *k* is specified, it controls how much brighter the returned color should be. If *k* is not specified, it defaults to 1. The behavior of this method is dependent on the implementing color space.
<a name="color_darker" href="#color_darker">#</a> *color*.<b>darker</b>([<i>k</i>]) [<>](https://github.com/d3/d3-color/blob/master/src/color.js "Source")
Returns a darker copy of this color. If *k* is specified, it controls how much darker the returned color should be. If *k* is not specified, it defaults to 1. The behavior of this method is dependent on the implementing color space.
<a name="color_displayable" href="#color_displayable">#</a> *color*.<b>displayable</b>() [<>](https://github.com/d3/d3-color/blob/master/src/color.js "Source")
Returns true if and only if the color is displayable on standard hardware. For example, this returns false for an RGB color if any channel value is less than zero or greater than 255 when rounded, or if the opacity is not in the range [0, 1].
<a name="color_formatHex" href="#color_formatHex">#</a> *color*.<b>formatHex</b>() [<>](https://github.com/d3/d3-color/blob/master/src/color.js "Source")
Returns a hexadecimal string representing this color in RGB space, such as `#f7eaba`. If this color is not displayable, a suitable displayable color is returned instead. For example, RGB channel values greater than 255 are clamped to 255.
<a name="color_formatHsl" href="#color_formatHsl">#</a> *color*.<b>formatHsl</b>() [<>](https://github.com/d3/d3-color/blob/master/src/color.js "Source")
Returns a string representing this color according to the [CSS Color Module Level 3 specification](https://www.w3.org/TR/css-color-3/#hsl-color), such as `hsl(257, 50%, 80%)` or `hsla(257, 50%, 80%, 0.2)`. If this color is not displayable, a suitable displayable color is returned instead by clamping S and L channel values to the interval [0, 100].
<a name="color_formatRgb" href="#color_formatRgb">#</a> *color*.<b>formatRgb</b>() [<>](https://github.com/d3/d3-color/blob/master/src/color.js "Source")
Returns a string representing this color according to the [CSS Object Model specification](https://drafts.csswg.org/cssom/#serialize-a-css-component-value), such as `rgb(247, 234, 186)` or `rgba(247, 234, 186, 0.2)`. If this color is not displayable, a suitable displayable color is returned instead by clamping RGB channel values to the interval [0, 255].
<a name="color_toString" href="#color_toString">#</a> *color*.<b>toString</b>() [<>](https://github.com/d3/d3-color/blob/master/src/color.js "Source")
An alias for [*color*.formatRgb](#color_formatRgb).
<a name="rgb" href="#rgb">#</a> d3.<b>rgb</b>(<i>r</i>, <i>g</i>, <i>b</i>[, <i>opacity</i>]) [<>](https://github.com/d3/d3-color/blob/master/src/color.js "Source")<br>
<a href="#rgb">#</a> d3.<b>rgb</b>(<i>specifier</i>)<br>
<a href="#rgb">#</a> d3.<b>rgb</b>(<i>color</i>)<br>
Constructs a new [RGB](https://en.wikipedia.org/wiki/RGB_color_model) color. The channel values are exposed as `r`, `g` and `b` properties on the returned instance. Use the [RGB color picker](http://bl.ocks.org/mbostock/78d64ca7ef013b4dcf8f) to explore this color space.
If *r*, *g* and *b* are specified, these represent the channel values of the returned color; an *opacity* may also be specified. If a CSS Color Module Level 3 *specifier* string is specified, it is parsed and then converted to the RGB color space. See [color](#color) for examples. If a [*color*](#color) instance is specified, it is converted to the RGB color space using [*color*.rgb](#color_rgb). Note that unlike [*color*.rgb](#color_rgb) this method *always* returns a new instance, even if *color* is already an RGB color.
<a name="rgb_clamp" href="#rgb_clamp">#</a> *rgb*.<b>clamp</b>() [<>](https://github.com/d3/d3-color/blob/master/src/color.js "Source")
Returns a new RGB color where the `r`, `g`, and `b` channels are clamped to the range [0, 255] and rounded to the nearest integer value, and the `opacity` is clamped to the range [0, 1].
<a name="hsl" href="#hsl">#</a> d3.<b>hsl</b>(<i>h</i>, <i>s</i>, <i>l</i>[, <i>opacity</i>]) [<>](https://github.com/d3/d3-color/blob/master/src/color.js "Source")<br>
<a href="#hsl">#</a> d3.<b>hsl</b>(<i>specifier</i>)<br>
<a href="#hsl">#</a> d3.<b>hsl</b>(<i>color</i>)<br>
Constructs a new [HSL](https://en.wikipedia.org/wiki/HSL_and_HSV) color. The channel values are exposed as `h`, `s` and `l` properties on the returned instance. Use the [HSL color picker](http://bl.ocks.org/mbostock/debaad4fcce9bcee14cf) to explore this color space.
If *h*, *s* and *l* are specified, these represent the channel values of the returned color; an *opacity* may also be specified. If a CSS Color Module Level 3 *specifier* string is specified, it is parsed and then converted to the HSL color space. See [color](#color) for examples. If a [*color*](#color) instance is specified, it is converted to the RGB color space using [*color*.rgb](#color_rgb) and then converted to HSL. (Colors already in the HSL color space skip the conversion to RGB.)
<a name="hsl_clamp" href="#hsl_clamp">#</a> *hsl*.<b>clamp</b>() [<>](https://github.com/d3/d3-color/blob/master/src/color.js "Source")
Returns a new HSL color where the `h` channel is clamped to the range [0, 360), and the `s`, `l`, and `opacity` channels are clamped to the range [0, 1].
<a name="lab" href="#lab">#</a> d3.<b>lab</b>(<i>l</i>, <i>a</i>, <i>b</i>[, <i>opacity</i>]) [<>](https://github.com/d3/d3-color/blob/master/src/lab.js "Source")<br>
<a href="#lab">#</a> d3.<b>lab</b>(<i>specifier</i>)<br>
<a href="#lab">#</a> d3.<b>lab</b>(<i>color</i>)<br>
Constructs a new [CIELAB](https://en.wikipedia.org/wiki/Lab_color_space#CIELAB) color. The channel values are exposed as `l`, `a` and `b` properties on the returned instance. Use the [CIELAB color picker](http://bl.ocks.org/mbostock/9f37cc207c0cb166921b) to explore this color space. The value of *l* is typically in the range [0, 100], while *a* and *b* are typically in [-160, +160].
If *l*, *a* and *b* are specified, these represent the channel values of the returned color; an *opacity* may also be specified. If a CSS Color Module Level 3 *specifier* string is specified, it is parsed and then converted to the CIELAB color space. See [color](#color) for examples. If a [*color*](#color) instance is specified, it is converted to the RGB color space using [*color*.rgb](#color_rgb) and then converted to CIELAB. (Colors already in the CIELAB color space skip the conversion to RGB, and colors in the HCL color space are converted directly to CIELAB.)
<a name="gray" href="#gray">#</a> d3.<b>gray</b>(<i>l</i>[, <i>opacity</i>]) [<>](https://github.com/d3/d3-color/blob/master/src/lab.js "Source")<br>
Constructs a new [CIELAB](#lab) color with the specified *l* value and *a* = *b* = 0.
<a name="hcl" href="#hcl">#</a> d3.<b>hcl</b>(<i>h</i>, <i>c</i>, <i>l</i>[, <i>opacity</i>]) [<>](https://github.com/d3/d3-color/blob/master/src/lab.js "Source")<br>
<a href="#hcl">#</a> d3.<b>hcl</b>(<i>specifier</i>)<br>
<a href="#hcl">#</a> d3.<b>hcl</b>(<i>color</i>)<br>
Equivalent to [d3.lch](#lch), but with reversed argument order.
<a name="lch" href="#lch">#</a> d3.<b>lch</b>(<i>l</i>, <i>c</i>, <i>h</i>[, <i>opacity</i>]) [<>](https://github.com/d3/d3-color/blob/master/src/lab.js "Source")<br>
<a href="#lch">#</a> d3.<b>lch</b>(<i>specifier</i>)<br>
<a href="#lch">#</a> d3.<b>lch</b>(<i>color</i>)<br>
Constructs a new [CIELCh<sub>ab</sub>](https://en.wikipedia.org/wiki/CIELAB_color_space#Cylindrical_representation:_CIELCh_or_CIEHLC) color. The channel values are exposed as `l`, `c` and `h` properties on the returned instance. Use the [CIELCh<sub>ab</sub> color picker](http://bl.ocks.org/mbostock/3e115519a1b495e0bd95) to explore this color space. The value of *l* is typically in the range [0, 100], *c* is typically in [0, 230], and *h* is typically in [0, 360).
If *l*, *c*, and *h* are specified, these represent the channel values of the returned color; an *opacity* may also be specified. If a CSS Color Module Level 3 *specifier* string is specified, it is parsed and then converted to CIELCh<sub>ab</sub> color space. See [color](#color) for examples. If a [*color*](#color) instance is specified, it is converted to the RGB color space using [*color*.rgb](#color_rgb) and then converted to CIELCh<sub>ab</sub>. (Colors already in CIELCh<sub>ab</sub> color space skip the conversion to RGB, and colors in CIELAB color space are converted directly to CIELCh<sub>ab</sub>.)
<a name="cubehelix" href="#cubehelix">#</a> d3.<b>cubehelix</b>(<i>h</i>, <i>s</i>, <i>l</i>[, <i>opacity</i>]) [<>](https://github.com/d3/d3-color/blob/master/src/cubehelix.js "Source")<br>
<a href="#cubehelix">#</a> d3.<b>cubehelix</b>(<i>specifier</i>)<br>
<a href="#cubehelix">#</a> d3.<b>cubehelix</b>(<i>color</i>)<br>
Constructs a new [Cubehelix](http://www.mrao.cam.ac.uk/~dag/CUBEHELIX/) color. The channel values are exposed as `h`, `s` and `l` properties on the returned instance. Use the [Cubehelix color picker](http://bl.ocks.org/mbostock/ba8d75e45794c27168b5) to explore this color space.
If *h*, *s* and *l* are specified, these represent the channel values of the returned color; an *opacity* may also be specified. If a CSS Color Module Level 3 *specifier* string is specified, it is parsed and then converted to the Cubehelix color space. See [color](#color) for examples. If a [*color*](#color) instance is specified, it is converted to the RGB color space using [*color*.rgb](#color_rgb) and then converted to Cubehelix. (Colors already in the Cubehelix color space skip the conversion to RGB.) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/d3-color/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/d3-color/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 14003
} |
# d3-ease
*Easing* is a method of distorting time to control apparent motion in animation. It is most commonly used for [slow-in, slow-out](https://en.wikipedia.org/wiki/12_basic_principles_of_animation#Slow_In_and_Slow_Out). By easing time, [animated transitions](https://github.com/d3/d3-transition) are smoother and exhibit more plausible motion.
The easing types in this module implement the [ease method](#ease_ease), which takes a normalized time *t* and returns the corresponding “eased” time *tʹ*. Both the normalized time and the eased time are typically in the range [0,1], where 0 represents the start of the animation and 1 represents the end; some easing types, such as [elastic](#easeElastic), may return eased times slightly outside this range. A good easing type should return 0 if *t* = 0 and 1 if *t* = 1. See the [easing explorer](https://observablehq.com/@d3/easing) for a visual demonstration.
These easing types are largely based on work by [Robert Penner](http://robertpenner.com/easing/).
## Installing
If you use npm, `npm install d3-ease`. You can also download the [latest release on GitHub](https://github.com/d3/d3-ease/releases/latest). For vanilla HTML in modern browsers, import d3-ease from Skypack:
```html
<script type="module">
import {easeCubic} from "https://cdn.skypack.dev/d3-ease@3";
const e = easeCubic(0.25);
</script>
```
For legacy environments, you can load d3-ease’s UMD bundle from an npm-based CDN such as jsDelivr; a `d3` global is exported:
```html
<script src="https://cdn.jsdelivr.net/npm/d3-ease@3"></script>
<script>
const e = d3.easeCubic(0.25);
</script>
```
[Try d3-ease in your browser.](https://observablehq.com/@d3/easing-animations)
## API Reference
<a name="_ease" href="#_ease">#</a> <i>ease</i>(<i>t</i>)
Given the specified normalized time *t*, typically in the range [0,1], returns the “eased” time *tʹ*, also typically in [0,1]. 0 represents the start of the animation and 1 represents the end. A good implementation returns 0 if *t* = 0 and 1 if *t* = 1. See the [easing explorer](https://observablehq.com/@d3/easing) for a visual demonstration. For example, to apply [cubic](#easeCubic) easing:
```js
const te = d3.easeCubic(t);
```
Similarly, to apply custom [elastic](#easeElastic) easing:
```js
// Before the animation starts, create your easing function.
const customElastic = d3.easeElastic.period(0.4);
// During the animation, apply the easing function.
const te = customElastic(t);
```
<a name="easeLinear" href="#easeLinear">#</a> d3.<b>easeLinear</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/linear.js "Source")
Linear easing; the identity function; *linear*(*t*) returns *t*.
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/linear.png" alt="linear">](https://observablehq.com/@d3/easing#linear)
<a name="easePolyIn" href="#easePolyIn">#</a> d3.<b>easePolyIn</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/poly.js#L3 "Source")
Polynomial easing; raises *t* to the specified [exponent](#poly_exponent). If the exponent is not specified, it defaults to 3, equivalent to [cubicIn](#easeCubicIn).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/polyIn.png" alt="polyIn">](https://observablehq.com/@d3/easing#polyIn)
<a name="easePolyOut" href="#easePolyOut">#</a> d3.<b>easePolyOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/poly.js#L15 "Source")
Reverse polynomial easing; equivalent to 1 - [polyIn](#easePolyIn)(1 - *t*). If the [exponent](#poly_exponent) is not specified, it defaults to 3, equivalent to [cubicOut](#easeCubicOut).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/polyOut.png" alt="polyOut">](https://observablehq.com/@d3/easing#polyOut)
<a name="easePoly" href="#easePoly">#</a> d3.<b>easePoly</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/poly.js "Source")
<br><a name="easePolyInOut" href="#easePolyInOut">#</a> d3.<b>easePolyInOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/poly.js#L27 "Source")
Symmetric polynomial easing; scales [polyIn](#easePolyIn) for *t* in [0, 0.5] and [polyOut](#easePolyOut) for *t* in [0.5, 1]. If the [exponent](#poly_exponent) is not specified, it defaults to 3, equivalent to [cubic](#easeCubic).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/polyInOut.png" alt="polyInOut">](https://observablehq.com/@d3/easing#polyInOut)
<a name="poly_exponent" href="#poly_exponent">#</a> <i>poly</i>.<b>exponent</b>(<i>e</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/poly.js#L1 "Source")
Returns a new polynomial easing with the specified exponent *e*. For example, to create equivalents of [linear](#easeLinear), [quad](#easeQuad), and [cubic](#easeCubic):
```js
const linear = d3.easePoly.exponent(1);
const quad = d3.easePoly.exponent(2);
const cubic = d3.easePoly.exponent(3);
```
<a name="easeQuadIn" href="#easeQuadIn">#</a> d3.<b>easeQuadIn</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/quad.js#L1 "Source")
Quadratic easing; equivalent to [polyIn](#easePolyIn).[exponent](#poly_exponent)(2).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/quadIn.png" alt="quadIn">](https://observablehq.com/@d3/easing#quadIn)
<a name="easeQuadOut" href="#easeQuadOut">#</a> d3.<b>easeQuadOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/quad.js#L5 "Source")
Reverse quadratic easing; equivalent to 1 - [quadIn](#easeQuadIn)(1 - *t*). Also equivalent to [polyOut](#easePolyOut).[exponent](#poly_exponent)(2).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/quadOut.png" alt="quadOut">](https://observablehq.com/@d3/easing#quadOut)
<a name="easeQuad" href="#easeQuad">#</a> d3.<b>easeQuad</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/quad.js "Source")
<br><a name="easeQuadInOut" href="#easeQuadInOut">#</a> d3.<b>easeQuadInOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/quad.js#L9 "Source")
Symmetric quadratic easing; scales [quadIn](#easeQuadIn) for *t* in [0, 0.5] and [quadOut](#easeQuadOut) for *t* in [0.5, 1]. Also equivalent to [poly](#easePoly).[exponent](#poly_exponent)(2).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/quadInOut.png" alt="quadInOut">](https://observablehq.com/@d3/easing#quadInOut)
<a name="easeCubicIn" href="#easeCubicIn">#</a> d3.<b>easeCubicIn</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/cubic.js#L1 "Source")
Cubic easing; equivalent to [polyIn](#easePolyIn).[exponent](#poly_exponent)(3).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/cubicIn.png" alt="cubicIn">](https://observablehq.com/@d3/easing#cubicIn)
<a name="easeCubicOut" href="#easeCubicOut">#</a> d3.<b>easeCubicOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/cubic.js#L5 "Source")
Reverse cubic easing; equivalent to 1 - [cubicIn](#easeCubicIn)(1 - *t*). Also equivalent to [polyOut](#easePolyOut).[exponent](#poly_exponent)(3).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/cubicOut.png" alt="cubicOut">](https://observablehq.com/@d3/easing#cubicOut)
<a name="easeCubic" href="#easeCubic">#</a> d3.<b>easeCubic</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/cubic.js "Source")
<br><a name="easeCubicInOut" href="#easeCubicInOut">#</a> d3.<b>easeCubicInOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/cubic.js#L9 "Source")
Symmetric cubic easing; scales [cubicIn](#easeCubicIn) for *t* in [0, 0.5] and [cubicOut](#easeCubicOut) for *t* in [0.5, 1]. Also equivalent to [poly](#easePoly).[exponent](#poly_exponent)(3).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/cubicInOut.png" alt="cubicInOut">](https://observablehq.com/@d3/easing#cubicInOut)
<a name="easeSinIn" href="#easeSinIn">#</a> d3.<b>easeSinIn</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/sin.js#L4 "Source")
Sinusoidal easing; returns sin(*t*).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/sinIn.png" alt="sinIn">](https://observablehq.com/@d3/easing#sinIn)
<a name="easeSinOut" href="#easeSinOut">#</a> d3.<b>easeSinOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/sin.js#L8 "Source")
Reverse sinusoidal easing; equivalent to 1 - [sinIn](#easeSinIn)(1 - *t*).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/sinOut.png" alt="sinOut">](https://observablehq.com/@d3/easing#sinOut)
<a name="easeSin" href="#easeSin">#</a> d3.<b>easeSin</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/sin.js "Source")
<br><a name="easeSinInOut" href="#easeSinInOut">#</a> d3.<b>easeSinInOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/sin.js#L12 "Source")
Symmetric sinusoidal easing; scales [sinIn](#easeSinIn) for *t* in [0, 0.5] and [sinOut](#easeSinOut) for *t* in [0.5, 1].
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/sinInOut.png" alt="sinInOut">](https://observablehq.com/@d3/easing#sinInOut)
<a name="easeExpIn" href="#easeExpIn">#</a> d3.<b>easeExpIn</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/exp.js#L1 "Source")
Exponential easing; raises 2 to the exponent 10 \* (*t* - 1).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/expIn.png" alt="expIn">](https://observablehq.com/@d3/easing#expIn)
<a name="easeExpOut" href="#easeExpOut">#</a> d3.<b>easeExpOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/exp.js#L5 "Source")
Reverse exponential easing; equivalent to 1 - [expIn](#easeExpIn)(1 - *t*).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/expOut.png" alt="expOut">](https://observablehq.com/@d3/easing#expOut)
<a name="easeExp" href="#easeExp">#</a> d3.<b>easeExp</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/exp.js "Source")
<br><a name="easeExpInOut" href="#easeExpInOut">#</a> d3.<b>easeExpInOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/exp.js#L9 "Source")
Symmetric exponential easing; scales [expIn](#easeExpIn) for *t* in [0, 0.5] and [expOut](#easeExpOut) for *t* in [0.5, 1].
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/expInOut.png" alt="expInOut">](https://observablehq.com/@d3/easing#expInOut)
<a name="easeCircleIn" href="#easeCircleIn">#</a> d3.<b>easeCircleIn</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/circle.js#L1 "Source")
Circular easing.
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/circleIn.png" alt="circleIn">](https://observablehq.com/@d3/easing#circleIn)
<a name="easeCircleOut" href="#easeCircleOut">#</a> d3.<b>easeCircleOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/circle.js#L5 "Source")
Reverse circular easing; equivalent to 1 - [circleIn](#easeCircleIn)(1 - *t*).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/circleOut.png" alt="circleOut">](https://observablehq.com/@d3/easing#circleOut)
<a name="easeCircle" href="#easeCircle">#</a> d3.<b>easeCircle</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/circle.js "Source")
<br><a name="easeCircleInOut" href="#easeCircleInOut">#</a> d3.<b>easeCircleInOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/circle.js#L9 "Source")
Symmetric circular easing; scales [circleIn](#easeCircleIn) for *t* in [0, 0.5] and [circleOut](#easeCircleOut) for *t* in [0.5, 1].
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/circleInOut.png" alt="circleInOut">](https://observablehq.com/@d3/easing#circleInOut)
<a name="easeElasticIn" href="#easeElasticIn">#</a> d3.<b>easeElasticIn</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/elastic.js#L5 "Source")
Elastic easing, like a rubber band. The [amplitude](#elastic_amplitude) and [period](#elastic_period) of the oscillation are configurable; if not specified, they default to 1 and 0.3, respectively.
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/elasticIn.png" alt="elasticIn">](https://observablehq.com/@d3/easing#elasticIn)
<a name="easeElastic" href="#easeElastic">#</a> d3.<b>easeElastic</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/elastic.js "Source")
<br><a name="easeElasticOut" href="#easeElasticOut">#</a> d3.<b>easeElasticOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/elastic.js#L18 "Source")
Reverse elastic easing; equivalent to 1 - [elasticIn](#easeElasticIn)(1 - *t*).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/elasticOut.png" alt="elasticOut">](https://observablehq.com/@d3/easing#elasticOut)
<a name="easeElasticInOut" href="#easeElasticInOut">#</a> d3.<b>easeElasticInOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/elastic.js#L31 "Source")
Symmetric elastic easing; scales [elasticIn](#easeElasticIn) for *t* in [0, 0.5] and [elasticOut](#easeElasticOut) for *t* in [0.5, 1].
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/elasticInOut.png" alt="elasticInOut">](https://observablehq.com/@d3/easing#elasticInOut)
<a name="elastic_amplitude" href="#elastic_amplitude">#</a> <i>elastic</i>.<b>amplitude</b>(<i>a</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/elastic.js#L40 "Source")
Returns a new elastic easing with the specified amplitude *a*.
<a name="elastic_period" href="#elastic_period">#</a> <i>elastic</i>.<b>period</b>(<i>p</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/elastic.js#L41 "Source")
Returns a new elastic easing with the specified period *p*.
<a name="easeBackIn" href="#easeBackIn">#</a> d3.<b>easeBackIn</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/back.js#L3 "Source")
[Anticipatory](https://en.wikipedia.org/wiki/12_basic_principles_of_animation#Anticipation) easing, like a dancer bending his knees before jumping off the floor. The degree of [overshoot](#back_overshoot) is configurable; if not specified, it defaults to 1.70158.
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/backIn.png" alt="backIn">](https://observablehq.com/@d3/easing#backIn)
<a name="easeBackOut" href="#easeBackOut">#</a> d3.<b>easeBackOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/back.js#L15 "Source")
Reverse anticipatory easing; equivalent to 1 - [backIn](#easeBackIn)(1 - *t*).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/backOut.png" alt="backOut">](https://observablehq.com/@d3/easing#backOut)
<a name="easeBack" href="#easeBack">#</a> d3.<b>easeBack</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/back.js "Source")
<br><a name="easeBackInOut" href="#easeBackInOut">#</a> d3.<b>easeBackInOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/back.js#L27 "Source")
Symmetric anticipatory easing; scales [backIn](#easeBackIn) for *t* in [0, 0.5] and [backOut](#easeBackOut) for *t* in [0.5, 1].
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/backInOut.png" alt="backInOut">](https://observablehq.com/@d3/easing#backInOut)
<a name="back_overshoot" href="#back_overshoot">#</a> <i>back</i>.<b>overshoot</b>(<i>s</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/back.js#L1 "Source")
Returns a new back easing with the specified overshoot *s*.
<a name="easeBounceIn" href="#easeBounceIn">#</a> d3.<b>easeBounceIn</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/bounce.js#L12 "Source")
Bounce easing, like a rubber ball.
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/bounceIn.png" alt="bounceIn">](https://observablehq.com/@d3/easing#bounceIn)
<a name="easeBounce" href="#easeBounce">#</a> d3.<b>easeBounce</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/bounce.js "Source")
<br><a name="easeBounceOut" href="#easeBounceOut">#</a> d3.<b>easeBounceOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/bounce.js#L16 "Source")
Reverse bounce easing; equivalent to 1 - [bounceIn](#easeBounceIn)(1 - *t*).
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/bounceOut.png" alt="bounceOut">](https://observablehq.com/@d3/easing#bounceOut)
<a name="easeBounceInOut" href="#easeBounceInOut">#</a> d3.<b>easeBounceInOut</b>(<i>t</i>) [<>](https://github.com/d3/d3-ease/blob/master/src/bounce.js#L20 "Source")
Symmetric bounce easing; scales [bounceIn](#easeBounceIn) for *t* in [0, 0.5] and [bounceOut](#easeBounceOut) for *t* in [0.5, 1].
[<img src="https://raw.githubusercontent.com/d3/d3-ease/master/img/bounceInOut.png" alt="bounceInOut">](https://observablehq.com/@d3/easing#bounceInOut) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/d3-ease/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/d3-ease/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 16796
} |
# d3-format
Ever noticed how sometimes JavaScript doesn’t display numbers the way you expect? Like, you tried to print tenths with a simple loop:
```js
for (let i = 0; i < 10; ++i) {
console.log(0.1 * i);
}
```
And you got this:
```js
0
0.1
0.2
0.30000000000000004
0.4
0.5
0.6000000000000001
0.7000000000000001
0.8
0.9
```
Welcome to [binary floating point](https://en.wikipedia.org/wiki/Double-precision_floating-point_format)! ಠ_ಠ
Yet rounding error is not the only reason to customize number formatting. A table of numbers should be formatted consistently for comparison; above, 0.0 would be better than 0. Large numbers should have grouped digits (e.g., 42,000) or be in scientific or metric notation (4.2e+4, 42k). Currencies should have fixed precision ($3.50). Reported numerical results should be rounded to significant digits (4021 becomes 4000). Number formats should appropriate to the reader’s locale (42.000,00 or 42,000.00). The list goes on.
Formatting numbers for human consumption is the purpose of d3-format, which is modeled after Python 3’s [format specification mini-language](https://docs.python.org/3/library/string.html#format-specification-mini-language) ([PEP 3101](https://www.python.org/dev/peps/pep-3101/)). Revisiting the example above:
```js
const f = d3.format(".1f");
for (let i = 0; i < 10; ++i) {
console.log(f(0.1 * i));
}
```
Now you get this:
```js
0.0
0.1
0.2
0.3
0.4
0.5
0.6
0.7
0.8
0.9
```
But d3-format is much more than an alias for [number.toFixed](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Number/toFixed)! A few more examples:
```js
d3.format(".0%")(0.123); // rounded percentage, "12%"
d3.format("($.2f")(-3.5); // localized fixed-point currency, "(£3.50)"
d3.format("+20")(42); // space-filled and signed, " +42"
d3.format(".^20")(42); // dot-filled and centered, ".........42........."
d3.format(".2s")(42e6); // SI-prefix with two significant digits, "42M"
d3.format("#x")(48879); // prefixed lowercase hexadecimal, "0xbeef"
d3.format(",.2r")(4223); // grouped thousands with two significant digits, "4,200"
```
See [*locale*.format](#locale_format) for a detailed specification, and try running [d3.formatSpecifier](#formatSpecifier) on the above formats to decode their meaning.
## Installing
If you use npm, `npm install d3-format`. You can also download the [latest release on GitHub](https://github.com/d3/d3-format/releases/latest). In modern browsers, you can import d3-format from Skypack:
```html
<script type="module">
import {format} from "https://cdn.skypack.dev/d3-format@3";
const f = format(".2s");
</script>
```
For legacy environments, you can load d3-format’s UMD bundle from an npm-based CDN such as jsDelivr; a `d3` global is exported:
```html
<script src="https://cdn.jsdelivr.net/npm/d3-format@3"></script>
<script>
var f = d3.format(".2s");
</script>
```
Locale files are published to npm and can be loaded using [d3.json](https://github.com/d3/d3-fetch/blob/master/README.md#json). For example, to set Russian as the default locale:
```js
const locale = await d3.json("https://cdn.jsdelivr.net/npm/d3-format@3/locale/ru-RU.json");
d3.formatDefaultLocale(locale);
const f = d3.format("$,");
console.log(f(1234.56)); // 1 234,56 руб.
```
[Try d3-format in your browser.](https://observablehq.com/@d3/d3-format)
## API Reference
<a name="format" href="#format">#</a> d3.<b>format</b>(<i>specifier</i>) [<>](https://github.com/d3/d3-format/blob/main/src/defaultLocale.js#L4 "Source")
An alias for [*locale*.format](#locale_format) on the [default locale](#formatDefaultLocale).
<a name="formatPrefix" href="#formatPrefix">#</a> d3.<b>formatPrefix</b>(<i>specifier</i>, <i>value</i>) [<>](https://github.com/d3/d3-format/blob/main/src/defaultLocale.js#L5 "Source")
An alias for [*locale*.formatPrefix](#locale_formatPrefix) on the [default locale](#formatDefaultLocale).
<a name="locale_format" href="#locale_format">#</a> <i>locale</i>.<b>format</b>(<i>specifier</i>) [<>](https://github.com/d3/d3-format/blob/main/src/locale.js#L18 "Source")
Returns a new format function for the given string *specifier*. The returned function takes a number as the only argument, and returns a string representing the formatted number. The general form of a specifier is:
```
[[fill]align][sign][symbol][0][width][,][.precision][~][type]
```
The *fill* can be any character. The presence of a fill character is signaled by the *align* character following it, which must be one of the following:
* `>` - Forces the field to be right-aligned within the available space. (Default behavior).
* `<` - Forces the field to be left-aligned within the available space.
* `^` - Forces the field to be centered within the available space.
* `=` - like `>`, but with any sign and symbol to the left of any padding.
The *sign* can be:
* `-` - nothing for zero or positive and a minus sign for negative. (Default behavior.)
* `+` - a plus sign for zero or positive and a minus sign for negative.
* `(` - nothing for zero or positive and parentheses for negative.
* ` ` (space) - a space for zero or positive and a minus sign for negative.
The *symbol* can be:
* `$` - apply currency symbols per the locale definition.
* `#` - for binary, octal, or hexadecimal notation, prefix by `0b`, `0o`, or `0x`, respectively.
The *zero* (`0`) option enables zero-padding; this implicitly sets *fill* to `0` and *align* to `=`. The *width* defines the minimum field width; if not specified, then the width will be determined by the content. The *comma* (`,`) option enables the use of a group separator, such as a comma for thousands.
Depending on the *type*, the *precision* either indicates the number of digits that follow the decimal point (types `f` and `%`), or the number of significant digits (types ``, `e`, `g`, `r`, `s` and `p`). If the precision is not specified, it defaults to 6 for all types except `` (none), which defaults to 12. Precision is ignored for integer formats (types `b`, `o`, `d`, `x`, and `X`) and character data (type `c`). See [precisionFixed](#precisionFixed) and [precisionRound](#precisionRound) for help picking an appropriate precision.
The `~` option trims insignificant trailing zeros across all format types. This is most commonly used in conjunction with types `r`, `e`, `s` and `%`. For example:
```js
d3.format("s")(1500); // "1.50000k"
d3.format("~s")(1500); // "1.5k"
```
The available *type* values are:
* `e` - exponent notation.
* `f` - fixed point notation.
* `g` - either decimal or exponent notation, rounded to significant digits.
* `r` - decimal notation, rounded to significant digits.
* `s` - decimal notation with an [SI prefix](#locale_formatPrefix), rounded to significant digits.
* `%` - multiply by 100, and then decimal notation with a percent sign.
* `p` - multiply by 100, round to significant digits, and then decimal notation with a percent sign.
* `b` - binary notation, rounded to integer.
* `o` - octal notation, rounded to integer.
* `d` - decimal notation, rounded to integer.
* `x` - hexadecimal notation, using lower-case letters, rounded to integer.
* `X` - hexadecimal notation, using upper-case letters, rounded to integer.
* `c` - character data, for a string of text.
The type `` (none) is also supported as shorthand for `~g` (with a default precision of 12 instead of 6), and the type `n` is shorthand for `,g`. For the `g`, `n` and `` (none) types, decimal notation is used if the resulting string would have *precision* or fewer digits; otherwise, exponent notation is used. For example:
```js
d3.format(".2")(42); // "42"
d3.format(".2")(4.2); // "4.2"
d3.format(".1")(42); // "4e+1"
d3.format(".1")(4.2); // "4"
```
<a name="locale_formatPrefix" href="#locale_formatPrefix">#</a> <i>locale</i>.<b>formatPrefix</b>(<i>specifier</i>, <i>value</i>) [<>](https://github.com/d3/d3-format/blob/main/src/locale.js#L127 "Source")
Equivalent to [*locale*.format](#locale_format), except the returned function will convert values to the units of the appropriate [SI prefix](https://en.wikipedia.org/wiki/Metric_prefix#List_of_SI_prefixes) for the specified numeric reference *value* before formatting in fixed point notation. The following prefixes are supported:
* `y` - yocto, 10⁻²⁴
* `z` - zepto, 10⁻²¹
* `a` - atto, 10⁻¹⁸
* `f` - femto, 10⁻¹⁵
* `p` - pico, 10⁻¹²
* `n` - nano, 10⁻⁹
* `µ` - micro, 10⁻⁶
* `m` - milli, 10⁻³
* `` (none) - 10⁰
* `k` - kilo, 10³
* `M` - mega, 10⁶
* `G` - giga, 10⁹
* `T` - tera, 10¹²
* `P` - peta, 10¹⁵
* `E` - exa, 10¹⁸
* `Z` - zetta, 10²¹
* `Y` - yotta, 10²⁴
Unlike [*locale*.format](#locale_format) with the `s` format type, this method returns a formatter with a consistent SI prefix, rather than computing the prefix dynamically for each number. In addition, the *precision* for the given *specifier* represents the number of digits past the decimal point (as with `f` fixed point notation), not the number of significant digits. For example:
```js
const f = d3.formatPrefix(",.0", 1e-6);
f(0.00042); // "420µ"
f(0.0042); // "4,200µ"
```
This method is useful when formatting multiple numbers in the same units for easy comparison. See [precisionPrefix](#precisionPrefix) for help picking an appropriate precision, and [bl.ocks.org/9764126](http://bl.ocks.org/mbostock/9764126) for an example.
<a name="formatSpecifier" href="#formatSpecifier">#</a> d3.<b>formatSpecifier</b>(<i>specifier</i>) [<>](https://github.com/d3/d3-format/blob/main/src/formatSpecifier.js "Source")
Parses the specified *specifier*, returning an object with exposed fields that correspond to the [format specification mini-language](#locale_format) and a toString method that reconstructs the specifier. For example, `formatSpecifier("s")` returns:
```js
FormatSpecifier {
"fill": " ",
"align": ">",
"sign": "-",
"symbol": "",
"zero": false,
"width": undefined,
"comma": false,
"precision": undefined,
"trim": false,
"type": "s"
}
```
This method is useful for understanding how format specifiers are parsed and for deriving new specifiers. For example, you might compute an appropriate precision based on the numbers you want to format using [precisionFixed](#precisionFixed) and then create a new format:
```js
const s = d3.formatSpecifier("f");
s.precision = d3.precisionFixed(0.01);
const f = d3.format(s);
f(42); // "42.00";
```
<a name="FormatSpecifier" href="#FormatSpecifier">#</a> new d3.<b>FormatSpecifier</b>(<i>specifier</i>) [<>](https://github.com/d3/d3-format/blob/main/src/formatSpecifier.js "Source")
Given the specified *specifier* object, returning an object with exposed fields that correspond to the [format specification mini-language](#locale_format) and a toString method that reconstructs the specifier. For example, `new FormatSpecifier({type: "s"})` returns:
```js
FormatSpecifier {
"fill": " ",
"align": ">",
"sign": "-",
"symbol": "",
"zero": false,
"width": undefined,
"comma": false,
"precision": undefined,
"trim": false,
"type": "s"
}
```
<a name="precisionFixed" href="#precisionFixed">#</a> d3.<b>precisionFixed</b>(<i>step</i>) [<>](https://github.com/d3/d3-format/blob/main/src/precisionFixed.js "Source")
Returns a suggested decimal precision for fixed point notation given the specified numeric *step* value. The *step* represents the minimum absolute difference between values that will be formatted. (This assumes that the values to be formatted are also multiples of *step*.) For example, given the numbers 1, 1.5, and 2, the *step* should be 0.5 and the suggested precision is 1:
```js
const p = d3.precisionFixed(0.5);
const f = d3.format("." + p + "f");
f(1); // "1.0"
f(1.5); // "1.5"
f(2); // "2.0"
```
Whereas for the numbers 1, 2 and 3, the *step* should be 1 and the suggested precision is 0:
```js
const p = d3.precisionFixed(1);
const f = d3.format("." + p + "f");
f(1); // "1"
f(2); // "2"
f(3); // "3"
```
Note: for the `%` format type, subtract two:
```js
const p = Math.max(0, d3.precisionFixed(0.05) - 2);
const f = d3.format("." + p + "%");
f(0.45); // "45%"
f(0.50); // "50%"
f(0.55); // "55%"
```
<a name="precisionPrefix" href="#precisionPrefix">#</a> d3.<b>precisionPrefix</b>(<i>step</i>, <i>value</i>) [<>](https://github.com/d3/d3-format/blob/main/src/precisionPrefix.js "Source")
Returns a suggested decimal precision for use with [*locale*.formatPrefix](#locale_formatPrefix) given the specified numeric *step* and reference *value*. The *step* represents the minimum absolute difference between values that will be formatted, and *value* determines which SI prefix will be used. (This assumes that the values to be formatted are also multiples of *step*.) For example, given the numbers 1.1e6, 1.2e6, and 1.3e6, the *step* should be 1e5, the *value* could be 1.3e6, and the suggested precision is 1:
```js
const p = d3.precisionPrefix(1e5, 1.3e6);
const f = d3.formatPrefix("." + p, 1.3e6);
f(1.1e6); // "1.1M"
f(1.2e6); // "1.2M"
f(1.3e6); // "1.3M"
```
<a name="precisionRound" href="#precisionRound">#</a> d3.<b>precisionRound</b>(<i>step</i>, <i>max</i>) [<>](https://github.com/d3/d3-format/blob/main/src/precisionRound.js "Source")
Returns a suggested decimal precision for format types that round to significant digits given the specified numeric *step* and *max* values. The *step* represents the minimum absolute difference between values that will be formatted, and the *max* represents the largest absolute value that will be formatted. (This assumes that the values to be formatted are also multiples of *step*.) For example, given the numbers 0.99, 1.0, and 1.01, the *step* should be 0.01, the *max* should be 1.01, and the suggested precision is 3:
```js
const p = d3.precisionRound(0.01, 1.01);
const f = d3.format("." + p + "r");
f(0.99); // "0.990"
f(1.0); // "1.00"
f(1.01); // "1.01"
```
Whereas for the numbers 0.9, 1.0, and 1.1, the *step* should be 0.1, the *max* should be 1.1, and the suggested precision is 2:
```js
const p = d3.precisionRound(0.1, 1.1);
const f = d3.format("." + p + "r");
f(0.9); // "0.90"
f(1.0); // "1.0"
f(1.1); // "1.1"
```
Note: for the `e` format type, subtract one:
```js
const p = Math.max(0, d3.precisionRound(0.01, 1.01) - 1);
const f = d3.format("." + p + "e");
f(0.01); // "1.00e-2"
f(1.01); // "1.01e+0"
```
### Locales
<a name="formatLocale" href="#formatLocale">#</a> d3.<b>formatLocale</b>(<i>definition</i>) [<>](https://github.com/d3/d3-format/blob/main/src/locale.js "Source")
Returns a *locale* object for the specified *definition* with [*locale*.format](#locale_format) and [*locale*.formatPrefix](#locale_formatPrefix) methods. The *definition* must include the following properties:
* `decimal` - the decimal point (e.g., `"."`).
* `thousands` - the group separator (e.g., `","`).
* `grouping` - the array of group sizes (e.g., `[3]`), cycled as needed.
* `currency` - the currency prefix and suffix (e.g., `["$", ""]`).
* `numerals` - optional; an array of ten strings to replace the numerals 0-9.
* `percent` - optional; the percent sign (defaults to `"%"`).
* `minus` - optional; the minus sign (defaults to `"−"`).
* `nan` - optional; the not-a-number value (defaults `"NaN"`).
Note that the *thousands* property is a misnomer, as the grouping definition allows groups other than thousands.
<a name="formatDefaultLocale" href="#formatDefaultLocale">#</a> d3.<b>formatDefaultLocale</b>(<i>definition</i>) [<>](https://github.com/d3/d3-format/blob/main/src/defaultLocale.js "Source")
Equivalent to [d3.formatLocale](#formatLocale), except it also redefines [d3.format](#format) and [d3.formatPrefix](#formatPrefix) to the new locale’s [*locale*.format](#locale_format) and [*locale*.formatPrefix](#locale_formatPrefix). If you do not set a default locale, it defaults to [U.S. English](https://github.com/d3/d3-format/blob/main/locale/en-US.json). | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/d3-format/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/d3-format/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 15914
} |
# d3-interpolate
This module provides a variety of interpolation methods for blending between two values. Values may be numbers, colors, strings, arrays, or even deeply-nested objects. For example:
```js
const i = d3.interpolateNumber(10, 20);
i(0.0); // 10
i(0.2); // 12
i(0.5); // 15
i(1.0); // 20
```
The returned function `i` is called an *interpolator*. Given a starting value *a* and an ending value *b*, it takes a parameter *t* in the domain [0, 1] and returns the corresponding interpolated value between *a* and *b*. An interpolator typically returns a value equivalent to *a* at *t* = 0 and a value equivalent to *b* at *t* = 1.
You can interpolate more than just numbers. To find the perceptual midpoint between steelblue and brown:
```js
d3.interpolateLab("steelblue", "brown")(0.5); // "rgb(142, 92, 109)"
```
Here’s a more elaborate example demonstrating type inference used by [interpolate](#interpolate):
```js
const i = d3.interpolate({colors: ["red", "blue"]}, {colors: ["white", "black"]});
i(0.0); // {colors: ["rgb(255, 0, 0)", "rgb(0, 0, 255)"]}
i(0.5); // {colors: ["rgb(255, 128, 128)", "rgb(0, 0, 128)"]}
i(1.0); // {colors: ["rgb(255, 255, 255)", "rgb(0, 0, 0)"]}
```
Note that the generic value interpolator detects not only nested objects and arrays, but also color strings and numbers embedded in strings!
## Installing
If you use npm, `npm install d3-interpolate`. You can also download the [latest release on GitHub](https://github.com/d3/d3-interpolate/releases/latest). For vanilla HTML in modern browsers, import d3-interpolate from Skypack:
```html
<script type="module">
import {interpolateRgb} from "https://cdn.skypack.dev/d3-interpolate@3";
const interpolate = interpolateRgb("steelblue", "brown");
</script>
```
For legacy environments, you can load d3-interpolate’s UMD bundle from an npm-based CDN such as jsDelivr; a `d3` global is exported. (If using [color interpolation](#color-spaces), also load [d3-color](https://github.com/d3/d3-color).)
```html
<script src="https://cdn.jsdelivr.net/npm/d3-color@3"></script>
<script src="https://cdn.jsdelivr.net/npm/d3-interpolate@3"></script>
<script>
const interpolate = d3.interpolateRgb("steelblue", "brown");
</script>
```
## API Reference
<a name="interpolate" href="#interpolate">#</a> d3.<b>interpolate</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/value.js), [Examples](https://observablehq.com/@d3/d3-interpolate)
Returns an interpolator between the two arbitrary values *a* and *b*. The interpolator implementation is based on the type of the end value *b*, using the following algorithm:
1. If *b* is null, undefined or a boolean, use the constant *b*.
2. If *b* is a number, use [interpolateNumber](#interpolateNumber).
3. If *b* is a [color](https://github.com/d3/d3-color/blob/master/README.md#color) or a string coercible to a color, use [interpolateRgb](#interpolateRgb).
4. If *b* is a [date](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date), use [interpolateDate](#interpolateDate).
5. If *b* is a string, use [interpolateString](#interpolateString).
6. If *b* is a [typed array](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/TypedArray) of numbers, use [interpolateNumberArray](#interpolateNumberArray).
7. If *b* is a generic [array](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/isArray), use [interpolateArray](#interpolateArray).
8. If *b* is coercible to a number, use [interpolateNumber](#interpolateNumber).
9. Use [interpolateObject](#interpolateObject).
Based on the chosen interpolator, *a* is coerced to the suitable corresponding type.
<a name="interpolateNumber" href="#interpolateNumber">#</a> d3.<b>interpolateNumber</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/number.js), [Examples](https://observablehq.com/@d3/d3-interpolatenumber)
Returns an interpolator between the two numbers *a* and *b*. The returned interpolator is equivalent to:
```js
function interpolator(t) {
return a * (1 - t) + b * t;
}
```
Caution: avoid interpolating to or from the number zero when the interpolator is used to generate a string. When very small values are stringified, they may be converted to scientific notation, which is an invalid attribute or style property value in older browsers. For example, the number `0.0000001` is converted to the string `"1e-7"`. This is particularly noticeable with interpolating opacity. To avoid scientific notation, start or end the transition at 1e-6: the smallest value that is not stringified in scientific notation.
<a name="interpolateRound" href="#interpolateRound">#</a> d3.<b>interpolateRound</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/round.js), [Examples](https://observablehq.com/@d3/d3-interpolatenumber)
Returns an interpolator between the two numbers *a* and *b*; the interpolator is similar to [interpolateNumber](#interpolateNumber), except it will round the resulting value to the nearest integer.
<a name="interpolateString" href="#interpolateString">#</a> d3.<b>interpolateString</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/string.js), [Examples](https://observablehq.com/@d3/d3-interpolatestring)
Returns an interpolator between the two strings *a* and *b*. The string interpolator finds numbers embedded in *a* and *b*, where each number is of the form understood by JavaScript. A few examples of numbers that will be detected within a string: `-1`, `42`, `3.14159`, and `6.0221413e+23`.
For each number embedded in *b*, the interpolator will attempt to find a corresponding number in *a*. If a corresponding number is found, a numeric interpolator is created using [interpolateNumber](#interpolateNumber). The remaining parts of the string *b* are used as a template: the static parts of the string *b* remain constant for the interpolation, with the interpolated numeric values embedded in the template.
For example, if *a* is `"300 12px sans-serif"`, and *b* is `"500 36px Comic-Sans"`, two embedded numbers are found. The remaining static parts (of string *b*) are a space between the two numbers (`" "`), and the suffix (`"px Comic-Sans"`). The result of the interpolator at *t* = 0.5 is `"400 24px Comic-Sans"`.
<a name="interpolateDate" href="#interpolateDate">#</a> d3.<b>interpolateDate</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/date.js), [Examples](https://observablehq.com/@d3/d3-interpolatedate)
Returns an interpolator between the two [dates](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date) *a* and *b*.
Note: **no defensive copy** of the returned date is created; the same Date instance is returned for every evaluation of the interpolator. No copy is made for performance reasons; interpolators are often part of the inner loop of [animated transitions](https://github.com/d3/d3-transition).
<a name="interpolateArray" href="#interpolateArray">#</a> d3.<b>interpolateArray</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/array.js), [Examples](https://observablehq.com/@d3/d3-interpolateobject)
Returns an interpolator between the two arrays *a* and *b*. If *b* is a typed array (e.g., Float64Array), [interpolateNumberArray](#interpolateNumberArray) is called instead.
Internally, an array template is created that is the same length as *b*. For each element in *b*, if there exists a corresponding element in *a*, a generic interpolator is created for the two elements using [interpolate](#interpolate). If there is no such element, the static value from *b* is used in the template. Then, for the given parameter *t*, the template’s embedded interpolators are evaluated. The updated array template is then returned.
For example, if *a* is the array `[0, 1]` and *b* is the array `[1, 10, 100]`, then the result of the interpolator for *t* = 0.5 is the array `[0.5, 5.5, 100]`.
Note: **no defensive copy** of the template array is created; modifications of the returned array may adversely affect subsequent evaluation of the interpolator. No copy is made for performance reasons; interpolators are often part of the inner loop of [animated transitions](https://github.com/d3/d3-transition).
<a name="interpolateNumberArray" href="#interpolateNumberArray">#</a> d3.<b>interpolateNumberArray</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/numberArray.js), [Examples](https://observablehq.com/@d3/d3-interpolatenumberarray)
Returns an interpolator between the two arrays of numbers *a* and *b*. Internally, an array template is created that is the same type and length as *b*. For each element in *b*, if there exists a corresponding element in *a*, the values are directly interpolated in the array template. If there is no such element, the static value from *b* is copied. The updated array template is then returned.
Note: For performance reasons, **no defensive copy** is made of the template array and the arguments *a* and *b*; modifications of these arrays may affect subsequent evaluation of the interpolator.
<a name="interpolateObject" href="#interpolateObject">#</a> d3.<b>interpolateObject</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/object.js), [Examples](https://observablehq.com/@d3/d3-interpolateobject)
Returns an interpolator between the two objects *a* and *b*. Internally, an object template is created that has the same properties as *b*. For each property in *b*, if there exists a corresponding property in *a*, a generic interpolator is created for the two elements using [interpolate](#interpolate). If there is no such property, the static value from *b* is used in the template. Then, for the given parameter *t*, the template's embedded interpolators are evaluated and the updated object template is then returned.
For example, if *a* is the object `{x: 0, y: 1}` and *b* is the object `{x: 1, y: 10, z: 100}`, the result of the interpolator for *t* = 0.5 is the object `{x: 0.5, y: 5.5, z: 100}`.
Object interpolation is particularly useful for *dataspace interpolation*, where data is interpolated rather than attribute values. For example, you can interpolate an object which describes an arc in a pie chart, and then use [d3.arc](https://github.com/d3/d3-shape/blob/master/README.md#arc) to compute the new SVG path data.
Note: **no defensive copy** of the template object is created; modifications of the returned object may adversely affect subsequent evaluation of the interpolator. No copy is made for performance reasons; interpolators are often part of the inner loop of [animated transitions](https://github.com/d3/d3-transition).
<a name="interpolateTransformCss" href="#interpolateTransformCss">#</a> d3.<b>interpolateTransformCss</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/transform/index.js#L62), [Examples](https://observablehq.com/@d3/d3-interpolatetransformcss)
Returns an interpolator between the two 2D CSS transforms represented by *a* and *b*. Each transform is decomposed to a standard representation of translate, rotate, *x*-skew and scale; these component transformations are then interpolated. This behavior is standardized by CSS: see [matrix decomposition for animation](http://www.w3.org/TR/css3-2d-transforms/#matrix-decomposition).
<a name="interpolateTransformSvg" href="#interpolateTransformSvg">#</a> d3.<b>interpolateTransformSvg</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/transform/index.js#L63), [Examples](https://observablehq.com/@d3/d3-interpolatetransformcss)
Returns an interpolator between the two 2D SVG transforms represented by *a* and *b*. Each transform is decomposed to a standard representation of translate, rotate, *x*-skew and scale; these component transformations are then interpolated. This behavior is standardized by CSS: see [matrix decomposition for animation](http://www.w3.org/TR/css3-2d-transforms/#matrix-decomposition).
<a name="interpolateZoom" href="#interpolateZoom">#</a> d3.<b>interpolateZoom</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/zoom.js), [Examples](https://observablehq.com/@d3/d3-interpolatezoom)
Returns an interpolator between the two views *a* and *b* of a two-dimensional plane, based on [“Smooth and efficient zooming and panning”](http://www.win.tue.nl/~vanwijk/zoompan.pdf) by Jarke J. van Wijk and Wim A.A. Nuij. Each view is defined as an array of three numbers: *cx*, *cy* and *width*. The first two coordinates *cx*, *cy* represent the center of the viewport; the last coordinate *width* represents the size of the viewport.
The returned interpolator exposes a *duration* property which encodes the recommended transition duration in milliseconds. This duration is based on the path length of the curved trajectory through *x,y* space. If you want a slower or faster transition, multiply this by an arbitrary scale factor (<i>V</i> as described in the original paper).
<a name="interpolateZoom_rho" href="#interpolateZoom_rho">#</a> *interpolateZoom*.<b>rho</b>(<i>rho</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/zoom.js)<!-- , [Examples](https://observablehq.com/@d3/interpolatezoom-rho) -->
Given a [zoom interpolator](#interpolateZoom), returns a new zoom interpolator using the specified curvature *rho*. When *rho* is close to 0, the interpolator is almost linear. The default curvature is sqrt(2).
<a name="interpolateDiscrete" href="#interpolateDiscrete">#</a> d3.<b>interpolateDiscrete</b>(<i>values</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/discrete.js), [Examples](https://observablehq.com/@d3/d3-interpolatediscrete)
Returns a discrete interpolator for the given array of *values*. The returned interpolator maps *t* in [0, 1 / *n*) to *values*[0], *t* in [1 / *n*, 2 / *n*) to *values*[1], and so on, where *n* = *values*.length. In effect, this is a lightweight [quantize scale](https://github.com/d3/d3-scale/blob/master/README.md#quantize-scales) with a fixed domain of [0, 1].
### Sampling
<a name="quantize" href="#quantize">#</a> d3.<b>quantize</b>(<i>interpolator</i>, <i>n</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/quantize.js), [Examples](https://observablehq.com/@d3/d3-quantize)
Returns *n* uniformly-spaced samples from the specified *interpolator*, where *n* is an integer greater than one. The first sample is always at *t* = 0, and the last sample is always at *t* = 1. This can be useful in generating a fixed number of samples from a given interpolator, such as to derive the range of a [quantize scale](https://github.com/d3/d3-scale/blob/master/README.md#quantize-scales) from a [continuous interpolator](https://github.com/d3/d3-scale-chromatic/blob/master/README.md#interpolateWarm).
Caution: this method will not work with interpolators that do not return defensive copies of their output, such as [d3.interpolateArray](#interpolateArray), [d3.interpolateDate](#interpolateDate) and [d3.interpolateObject](#interpolateObject). For those interpolators, you must wrap the interpolator and create a copy for each returned value.
### Color Spaces
<a name="interpolateRgb" href="#interpolateRgb">#</a> d3.<b>interpolateRgb</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/rgb.js), [Examples](https://observablehq.com/@d3/working-with-color)
<img src="https://raw.githubusercontent.com/d3/d3-interpolate/master/img/rgb.png" width="100%" height="40" alt="rgb">
Or, with a corrected [gamma](#interpolate_gamma) of 2.2:
<img src="https://raw.githubusercontent.com/d3/d3-interpolate/master/img/rgbGamma.png" width="100%" height="40" alt="rgbGamma">
Returns an RGB color space interpolator between the two colors *a* and *b* with a configurable [gamma](#interpolate_gamma). If the gamma is not specified, it defaults to 1.0. The colors *a* and *b* need not be in RGB; they will be converted to RGB using [d3.rgb](https://github.com/d3/d3-color/blob/master/README.md#rgb). The return value of the interpolator is an RGB string.
<a href="#interpolateRgbBasis" name="interpolateRgbBasis">#</a> d3.<b>interpolateRgbBasis</b>(<i>colors</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/rgb.js#L54), [Examples](https://observablehq.com/@d3/working-with-color)
Returns a uniform nonrational B-spline interpolator through the specified array of *colors*, which are converted to [RGB color space](https://github.com/d3/d3-color/blob/master/README.md#rgb). Implicit control points are generated such that the interpolator returns *colors*[0] at *t* = 0 and *colors*[*colors*.length - 1] at *t* = 1. Opacity interpolation is not currently supported. See also [d3.interpolateBasis](#interpolateBasis), and see [d3-scale-chromatic](https://github.com/d3/d3-scale-chromatic) for examples.
<a href="#interpolateRgbBasisClosed" name="interpolateRgbBasisClosed">#</a> d3.<b>interpolateRgbBasisClosed</b>(<i>colors</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/rgb.js#L55), [Examples](https://observablehq.com/@d3/working-with-color)
Returns a uniform nonrational B-spline interpolator through the specified array of *colors*, which are converted to [RGB color space](https://github.com/d3/d3-color/blob/master/README.md#rgb). The control points are implicitly repeated such that the resulting spline has cyclical C² continuity when repeated around *t* in [0,1]; this is useful, for example, to create cyclical color scales. Opacity interpolation is not currently supported. See also [d3.interpolateBasisClosed](#interpolateBasisClosed), and see [d3-scale-chromatic](https://github.com/d3/d3-scale-chromatic) for examples.
<a name="interpolateHsl" href="#interpolateHsl">#</a> d3.<b>interpolateHsl</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/hsl.js), [Examples](https://observablehq.com/@d3/working-with-color)
<img src="https://raw.githubusercontent.com/d3/d3-interpolate/master/img/hsl.png" width="100%" height="40" alt="hsl">
Returns an HSL color space interpolator between the two colors *a* and *b*. The colors *a* and *b* need not be in HSL; they will be converted to HSL using [d3.hsl](https://github.com/d3/d3-color/blob/master/README.md#hsl). If either color’s hue or saturation is NaN, the opposing color’s channel value is used. The shortest path between hues is used. The return value of the interpolator is an RGB string.
<a name="interpolateHslLong" href="#interpolateHslLong">#</a> d3.<b>interpolateHslLong</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/hsl.js#L21), [Examples](https://observablehq.com/@d3/working-with-color)
<img src="https://raw.githubusercontent.com/d3/d3-interpolate/master/img/hslLong.png" width="100%" height="40" alt="hslLong">
Like [interpolateHsl](#interpolateHsl), but does not use the shortest path between hues.
<a name="interpolateLab" href="#interpolateLab">#</a> d3.<b>interpolateLab</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/lab.js), [Examples](https://observablehq.com/@d3/working-with-color)
<img src="https://raw.githubusercontent.com/d3/d3-interpolate/master/img/lab.png" width="100%" height="40" alt="lab">
Returns a [CIELAB color space](https://en.wikipedia.org/wiki/Lab_color_space#CIELAB) interpolator between the two colors *a* and *b*. The colors *a* and *b* need not be in CIELAB; they will be converted to CIELAB using [d3.lab](https://github.com/d3/d3-color/blob/master/README.md#lab). The return value of the interpolator is an RGB string.
<a name="interpolateHcl" href="#interpolateHcl">#</a> d3.<b>interpolateHcl</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/hcl.js), [Examples](https://observablehq.com/@d3/working-with-color)
<img src="https://raw.githubusercontent.com/d3/d3-interpolate/master/img/hcl.png" width="100%" height="40" alt="hcl">
Returns a [CIELCh<sub>ab</sub> color space](https://en.wikipedia.org/wiki/CIELAB_color_space#Cylindrical_representation:_CIELCh_or_CIEHLC) interpolator between the two colors *a* and *b*. The colors *a* and *b* need not be in CIELCh<sub>ab</sub>; they will be converted to CIELCh<sub>ab</sub> using [d3.hcl](https://github.com/d3/d3-color/blob/master/README.md#hcl). If either color’s hue or chroma is NaN, the opposing color’s channel value is used. The shortest path between hues is used. The return value of the interpolator is an RGB string.
<a name="interpolateHclLong" href="#interpolateHclLong">#</a> d3.<b>interpolateHclLong</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/hcl.js#L21), [Examples](https://observablehq.com/@d3/working-with-color)
<img src="https://raw.githubusercontent.com/d3/d3-interpolate/master/img/hclLong.png" width="100%" height="40" alt="hclLong">
Like [interpolateHcl](#interpolateHcl), but does not use the shortest path between hues.
<a name="interpolateCubehelix" href="#interpolateCubehelix">#</a> d3.<b>interpolateCubehelix</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/cubehelix.js), [Examples](https://observablehq.com/@d3/working-with-color)
<img src="https://raw.githubusercontent.com/d3/d3-interpolate/master/img/cubehelix.png" width="100%" height="40" alt="cubehelix">
Or, with a [gamma](#interpolate_gamma) of 3.0 to emphasize high-intensity values:
<img src="https://raw.githubusercontent.com/d3/d3-interpolate/master/img/cubehelixGamma.png" width="100%" height="40" alt="cubehelixGamma">
Returns a Cubehelix color space interpolator between the two colors *a* and *b* using a configurable [gamma](#interpolate_gamma). If the gamma is not specified, it defaults to 1.0. The colors *a* and *b* need not be in Cubehelix; they will be converted to Cubehelix using [d3.cubehelix](https://github.com/d3/d3-color/blob/master/README.md#cubehelix). If either color’s hue or saturation is NaN, the opposing color’s channel value is used. The shortest path between hues is used. The return value of the interpolator is an RGB string.
<a name="interpolateCubehelixLong" href="#interpolateCubehelixLong">#</a> d3.<b>interpolateCubehelixLong</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/cubehelix.js#L29), [Examples](https://observablehq.com/@d3/working-with-color)
<img src="https://raw.githubusercontent.com/d3/d3-interpolate/master/img/cubehelixLong.png" width="100%" height="40" alt="cubehelixLong">
Or, with a [gamma](#interpolate_gamma) of 3.0 to emphasize high-intensity values:
<img src="https://raw.githubusercontent.com/d3/d3-interpolate/master/img/cubehelixGammaLong.png" width="100%" height="40" alt="cubehelixGammaLong">
Like [interpolateCubehelix](#interpolateCubehelix), but does not use the shortest path between hues.
<a name="interpolate_gamma" href="#interpolate_gamma">#</a> <i>interpolate</i>.<b>gamma</b>(<i>gamma</i>)
Given that *interpolate* is one of [interpolateRgb](#interpolateRgb), [interpolateCubehelix](#interpolateCubehelix) or [interpolateCubehelixLong](#interpolateCubehelixLong), returns a new interpolator factory of the same type using the specified *gamma*. For example, to interpolate from purple to orange with a gamma of 2.2 in RGB space:
```js
const interpolator = d3.interpolateRgb.gamma(2.2)("purple", "orange");
```
See Eric Brasseur’s article, [Gamma error in picture scaling](http://www.ericbrasseur.org/gamma.html), for more on gamma correction.
<a name="interpolateHue" href="#interpolateHue">#</a> d3.<b>interpolateHue</b>(<i>a</i>, <i>b</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/hue.js), [Examples](https://observablehq.com/@d3/working-with-color)
Returns an interpolator between the two hue angles *a* and *b*. If either hue is NaN, the opposing value is used. The shortest path between hues is used. The return value of the interpolator is a number in [0, 360).
### Splines
Whereas standard interpolators blend from a starting value *a* at *t* = 0 to an ending value *b* at *t* = 1, spline interpolators smoothly blend multiple input values for *t* in [0,1] using piecewise polynomial functions. Only cubic uniform nonrational [B-splines](https://en.wikipedia.org/wiki/B-spline) are currently supported, also known as basis splines.
<a href="#interpolateBasis" name="interpolateBasis">#</a> d3.<b>interpolateBasis</b>(<i>values</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/basis.js), [Examples](https://observablehq.com/@d3/d3-interpolatebasis)
Returns a uniform nonrational B-spline interpolator through the specified array of *values*, which must be numbers. Implicit control points are generated such that the interpolator returns *values*[0] at *t* = 0 and *values*[*values*.length - 1] at *t* = 1. See also [d3.curveBasis](https://github.com/d3/d3-shape/blob/master/README.md#curveBasis).
<a href="#interpolateBasisClosed" name="interpolateBasisClosed">#</a> d3.<b>interpolateBasisClosed</b>(<i>values</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/basisClosed.js), [Examples](https://observablehq.com/@d3/d3-interpolatebasis)
Returns a uniform nonrational B-spline interpolator through the specified array of *values*, which must be numbers. The control points are implicitly repeated such that the resulting one-dimensional spline has cyclical C² continuity when repeated around *t* in [0,1]. See also [d3.curveBasisClosed](https://github.com/d3/d3-shape/blob/master/README.md#curveBasisClosed).
### Piecewise
<a name="piecewise" href="#piecewise">#</a> d3.<b>piecewise</b>([<i>interpolate</i>, ]<i>values</i>) · [Source](https://github.com/d3/d3-interpolate/blob/master/src/piecewise.js), [Examples](https://observablehq.com/@d3/d3-piecewise)
Returns a piecewise interpolator, composing interpolators for each adjacent pair of *values*. The returned interpolator maps *t* in [0, 1 / (*n* - 1)] to *interpolate*(*values*[0], *values*[1]), *t* in [1 / (*n* - 1), 2 / (*n* - 1)] to *interpolate*(*values*[1], *values*[2]), and so on, where *n* = *values*.length. In effect, this is a lightweight [linear scale](https://github.com/d3/d3-scale/blob/master/README.md#linear-scales). For example, to blend through red, green and blue:
```js
const interpolate = d3.piecewise(d3.interpolateRgb.gamma(2.2), ["red", "green", "blue"]);
```
If *interpolate* is not specified, defaults to [d3.interpolate](#interpolate). | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/d3-interpolate/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/d3-interpolate/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 26798
} |
# d3-path
Say you have some code that draws to a 2D canvas:
```js
function drawCircle(context, radius) {
context.moveTo(radius, 0);
context.arc(0, 0, radius, 0, 2 * Math.PI);
}
```
The d3-path module lets you take this exact code and additionally render to [SVG](http://www.w3.org/TR/SVG/paths.html). It works by [serializing](#path_toString) [CanvasPathMethods](http://www.w3.org/TR/2dcontext/#canvaspathmethods) calls to [SVG path data](http://www.w3.org/TR/SVG/paths.html#PathData). For example:
```js
const context = d3.path();
drawCircle(context, 40);
pathElement.setAttribute("d", context.toString());
```
Now code you write once can be used with both Canvas (for performance) and SVG (for convenience). For a practical example, see [d3-shape](https://github.com/d3/d3-shape).
## Installing
If you use npm, `npm install d3-path`. You can also download the [latest release on GitHub](https://github.com/d3/d3-path/releases/latest). In modern browsers, you can import d3-path from jsDelivr:
```html
<script type="module">
import {path} from "https://cdn.jsdelivr.net/npm/d3-path@3/+esm";
const p = path();
p.moveTo(1, 2);
p.lineTo(3, 4);
p.closePath();
</script>
```
For legacy environments, you can load d3-path’s UMD bundle from an npm-based CDN such as jsDelivr; a `d3` global is exported:
```html
<script src="https://cdn.jsdelivr.net/npm/d3-path@3"></script>
<script>
const path = d3.path();
</script>
```
## API Reference
<a name="path" href="#path">#</a> d3.<b>path</b>() · [Source](https://github.com/d3/d3-path/blob/master/src/path.js), [Examples](https://observablehq.com/@d3/d3-path)
Constructs a new path serializer that implements [CanvasPathMethods](http://www.w3.org/TR/2dcontext/#canvaspathmethods).
<a name="path_moveTo" href="#path_moveTo">#</a> <i>path</i>.<b>moveTo</b>(<i>x</i>, <i>y</i>)
Move to the specified point ⟨*x*, *y*⟩. Equivalent to [*context*.moveTo](http://www.w3.org/TR/2dcontext/#dom-context-2d-moveto) and SVG’s [“moveto” command](http://www.w3.org/TR/SVG/paths.html#PathDataMovetoCommands).
<a name="path_closePath" href="#path_closePath">#</a> <i>path</i>.<b>closePath</b>()
Ends the current subpath and causes an automatic straight line to be drawn from the current point to the initial point of the current subpath. Equivalent to [*context*.closePath](http://www.w3.org/TR/2dcontext/#dom-context-2d-closepath) and SVG’s [“closepath” command](http://www.w3.org/TR/SVG/paths.html#PathDataClosePathCommand).
<a name="path_lineTo" href="#path_lineTo">#</a> <i>path</i>.<b>lineTo</b>(<i>x</i>, <i>y</i>)
Draws a straight line from the current point to the specified point ⟨*x*, *y*⟩. Equivalent to [*context*.lineTo](http://www.w3.org/TR/2dcontext/#dom-context-2d-lineto) and SVG’s [“lineto” command](http://www.w3.org/TR/SVG/paths.html#PathDataLinetoCommands).
<a name="path_quadraticCurveTo" href="#path_quadraticCurveTo">#</a> <i>path</i>.<b>quadraticCurveTo</b>(<i>cpx</i>, <i>cpy</i>, <i>x</i>, <i>y</i>)
Draws a quadratic Bézier segment from the current point to the specified point ⟨*x*, *y*⟩, with the specified control point ⟨*cpx*, *cpy*⟩. Equivalent to [*context*.quadraticCurveTo](http://www.w3.org/TR/2dcontext/#dom-context-2d-quadraticcurveto) and SVG’s [quadratic Bézier curve commands](http://www.w3.org/TR/SVG/paths.html#PathDataQuadraticBezierCommands).
<a name="path_bezierCurveTo" href="#path_bezierCurveTo">#</a> <i>path</i>.<b>bezierCurveTo</b>(<i>cpx1</i>, <i>cpy1</i>, <i>cpx2</i>, <i>cpy2</i>, <i>x</i>, <i>y</i>)
Draws a cubic Bézier segment from the current point to the specified point ⟨*x*, *y*⟩, with the specified control points ⟨*cpx1*, *cpy1*⟩ and ⟨*cpx2*, *cpy2*⟩. Equivalent to [*context*.bezierCurveTo](http://www.w3.org/TR/2dcontext/#dom-context-2d-beziercurveto) and SVG’s [cubic Bézier curve commands](http://www.w3.org/TR/SVG/paths.html#PathDataCubicBezierCommands).
<a name="path_arcTo" href="#path_arcTo">#</a> <i>path</i>.<b>arcTo</b>(<i>x1</i>, <i>y1</i>, <i>x2</i>, <i>y2</i>, <i>radius</i>)
Draws a circular arc segment with the specified *radius* that starts tangent to the line between the current point and the specified point ⟨*x1*, *y1*⟩ and ends tangent to the line between the specified points ⟨*x1*, *y1*⟩ and ⟨*x2*, *y2*⟩. If the first tangent point is not equal to the current point, a straight line is drawn between the current point and the first tangent point. Equivalent to [*context*.arcTo](http://www.w3.org/TR/2dcontext/#dom-context-2d-arcto) and uses SVG’s [elliptical arc curve commands](http://www.w3.org/TR/SVG/paths.html#PathDataEllipticalArcCommands).
<a name="path_arc" href="#path_arc">#</a> <i>path</i>.<b>arc</b>(<i>x</i>, <i>y</i>, <i>radius</i>, <i>startAngle</i>, <i>endAngle</i>[, <i>anticlockwise</i>])
Draws a circular arc segment with the specified center ⟨*x*, *y*⟩, *radius*, *startAngle* and *endAngle*. If *anticlockwise* is true, the arc is drawn in the anticlockwise direction; otherwise, it is drawn in the clockwise direction. If the current point is not equal to the starting point of the arc, a straight line is drawn from the current point to the start of the arc. Equivalent to [*context*.arc](http://www.w3.org/TR/2dcontext/#dom-context-2d-arc) and uses SVG’s [elliptical arc curve commands](http://www.w3.org/TR/SVG/paths.html#PathDataEllipticalArcCommands).
<a name="path_rect" href="#path_rect">#</a> <i>path</i>.<b>rect</b>(<i>x</i>, <i>y</i>, <i>w</i>, <i>h</i>)
Creates a new subpath containing just the four points ⟨*x*, *y*⟩, ⟨*x* + *w*, *y*⟩, ⟨*x* + *w*, *y* + *h*⟩, ⟨*x*, *y* + *h*⟩, with those four points connected by straight lines, and then marks the subpath as closed. Equivalent to [*context*.rect](http://www.w3.org/TR/2dcontext/#dom-context-2d-rect) and uses SVG’s [“lineto” commands](http://www.w3.org/TR/SVG/paths.html#PathDataLinetoCommands).
<a name="path_toString" href="#path_toString">#</a> <i>path</i>.<b>toString</b>()
Returns the string representation of this *path* according to SVG’s [path data specification](http://www.w3.org/TR/SVG/paths.html#PathData).
<a name="pathRound" href="#pathRound">#</a> d3.<b>pathRound</b>(*digits* = 3) · [Source](https://github.com/d3/d3-path/blob/master/src/path.js), [Examples](https://observablehq.com/@d3/d3-path)
Like [d3.path](#path), except limits the digits after the decimal to the specified number of *digits*. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/d3-path/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/d3-path/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 6357
} |
# d3-scale
Scales are a convenient abstraction for a fundamental task in visualization: mapping a dimension of abstract data to a visual representation. Although most often used for position-encoding quantitative data, such as mapping a measurement in meters to a position in pixels for dots in a scatterplot, scales can represent virtually any visual encoding, such as diverging colors, stroke widths, or symbol size. Scales can also be used with virtually any type of data, such as named categorical data or discrete data that requires sensible breaks.
For [continuous](#continuous-scales) quantitative data, you typically want a [linear scale](#linear-scales). (For time series data, a [time scale](#time-scales).) If the distribution calls for it, consider transforming data using a [power](#power-scales) or [log](#log-scales) scale. A [quantize scale](#quantize-scales) may aid differentiation by rounding continuous data to a fixed set of discrete values; similarly, a [quantile scale](#quantile-scales) computes quantiles from a sample population, and a [threshold scale](#threshold-scales) allows you to specify arbitrary breaks in continuous data.
For discrete ordinal (ordered) or categorical (unordered) data, an [ordinal scale](#ordinal-scales) specifies an explicit mapping from a set of data values to a corresponding set of visual attributes (such as colors). The related [band](#band-scales) and [point](#point-scales) scales are useful for position-encoding ordinal data, such as bars in a bar chart or dots in an categorical scatterplot.
This repository does not provide color schemes; see [d3-scale-chromatic](https://github.com/d3/d3-scale-chromatic) for color schemes designed to work with d3-scale.
Scales have no intrinsic visual representation. However, most scales can [generate](#continuous_ticks) and [format](#continuous_tickFormat) ticks for reference marks to aid in the construction of axes.
For a longer introduction, see these recommended tutorials:
* [Introducing d3-scale](https://medium.com/@mbostock/introducing-d3-scale-61980c51545f) by Mike Bostock
* Chapter 7. Scales of [*Interactive Data Visualization for the Web*](http://alignedleft.com/work/d3-book) by Scott Murray
* [d3: scales, and color.](https://jckr.github.io/blog/2011/08/11/d3-scales-and-color/) by Jérôme Cukier
## Installing
If you use npm, `npm install d3-scale`. You can also download the [latest release on GitHub](https://github.com/d3/d3-scale/releases/latest). For vanilla HTML in modern browsers, import d3-scale from Skypack:
```html
<script type="module">
import {scaleLinear} from "https://cdn.skypack.dev/d3-scale@4";
const x = scaleLinear();
</script>
```
For legacy environments, you can load d3-scale’s UMD bundle from an npm-based CDN such as jsDelivr; a `d3` global is exported:
```html
<script src="https://cdn.jsdelivr.net/npm/d3-array@3"></script>
<script src="https://cdn.jsdelivr.net/npm/d3-color@3"></script>
<script src="https://cdn.jsdelivr.net/npm/d3-format@3"></script>
<script src="https://cdn.jsdelivr.net/npm/d3-interpolate@3"></script>
<script src="https://cdn.jsdelivr.net/npm/d3-time@3"></script>
<script src="https://cdn.jsdelivr.net/npm/d3-time-format@4"></script>
<script src="https://cdn.jsdelivr.net/npm/d3-scale@4"></script>
<script>
const x = d3.scaleLinear();
</script>
```
(You can omit d3-time and d3-time-format if you’re not using [d3.scaleTime](#scaleTime) or [d3.scaleUtc](#scaleUtc).)
## API Reference
* [Continuous](#continuous-scales) ([Linear](#linear-scales), [Power](#power-scales), [Log](#log-scales), [Identity](#identity-scales), [Time](#time-scales), [Radial](#radial-scales))
* [Sequential](#sequential-scales)
* [Diverging](#diverging-scales)
* [Quantize](#quantize-scales)
* [Quantile](#quantile-scales)
* [Threshold](#threshold-scales)
* [Ordinal](#ordinal-scales) ([Band](#band-scales), [Point](#point-scales))
### Continuous Scales
Continuous scales map a continuous, quantitative input [domain](#continuous_domain) to a continuous output [range](#continuous_range). If the range is also numeric, the mapping may be [inverted](#continuous_invert). A continuous scale is not constructed directly; instead, try a [linear](#linear-scales), [power](#power-scales), [log](#log-scales), [identity](#identity-scales), [radial](#radial-scales), [time](#time-scales) or [sequential color](#sequential-scales) scale.
<a name="_continuous" href="#_continuous">#</a> <i>continuous</i>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/continuous.js), [Examples](https://observablehq.com/@d3/continuous-scales)
Given a *value* from the [domain](#continuous_domain), returns the corresponding value from the [range](#continuous_range). If the given *value* is outside the domain, and [clamping](#continuous_clamp) is not enabled, the mapping may be extrapolated such that the returned value is outside the range. For example, to apply a position encoding:
```js
var x = d3.scaleLinear()
.domain([10, 130])
.range([0, 960]);
x(20); // 80
x(50); // 320
```
Or to apply a color encoding:
```js
var color = d3.scaleLinear()
.domain([10, 100])
.range(["brown", "steelblue"]);
color(20); // "#9a3439"
color(50); // "#7b5167"
```
Or, in shorthand:
```js
var x = d3.scaleLinear([10, 130], [0, 960]);
var color = d3.scaleLinear([10, 100], ["brown", "steelblue"]);
```
<a name="continuous_invert" href="#continuous_invert">#</a> <i>continuous</i>.<b>invert</b>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/continuous.js), [Examples](https://observablehq.com/@d3/continuous-scales)
Given a *value* from the [range](#continuous_range), returns the corresponding value from the [domain](#continuous_domain). Inversion is useful for interaction, say to determine the data value corresponding to the position of the mouse. For example, to invert a position encoding:
```js
var x = d3.scaleLinear()
.domain([10, 130])
.range([0, 960]);
x.invert(80); // 20
x.invert(320); // 50
```
If the given *value* is outside the range, and [clamping](#continuous_clamp) is not enabled, the mapping may be extrapolated such that the returned value is outside the domain. This method is only supported if the range is numeric. If the range is not numeric, returns NaN.
For a valid value *y* in the range, <i>continuous</i>(<i>continuous</i>.invert(<i>y</i>)) approximately equals *y*; similarly, for a valid value *x* in the domain, <i>continuous</i>.invert(<i>continuous</i>(<i>x</i>)) approximately equals *x*. The scale and its inverse may not be exact due to the limitations of floating point precision.
<a name="continuous_domain" href="#continuous_domain">#</a> <i>continuous</i>.<b>domain</b>([<i>domain</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/continuous.js), [Examples](https://observablehq.com/@d3/continuous-scales)
If *domain* is specified, sets the scale’s domain to the specified array of numbers. The array must contain two or more elements. If the elements in the given array are not numbers, they will be coerced to numbers. If *domain* is not specified, returns a copy of the scale’s current domain.
Although continuous scales typically have two values each in their domain and range, specifying more than two values produces a piecewise scale. For example, to create a [diverging color scale](#diverging-scales) that interpolates between white and red for negative values, and white and green for positive values, say:
```js
var color = d3.scaleLinear()
.domain([-1, 0, 1])
.range(["red", "white", "green"]);
color(-0.5); // "rgb(255, 128, 128)"
color(+0.5); // "rgb(128, 192, 128)"
```
Internally, a piecewise scale performs a [binary search](https://github.com/d3/d3-array/blob/master/README.md#bisect) for the range interpolator corresponding to the given domain value. Thus, the domain must be in ascending or descending order. If the domain and range have different lengths *N* and *M*, only the first *min(N,M)* elements in each are observed.
<a name="continuous_range" href="#continuous_range">#</a> <i>continuous</i>.<b>range</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/continuous.js), [Examples](https://observablehq.com/@d3/continuous-scales)
If *range* is specified, sets the scale’s range to the specified array of values. The array must contain two or more elements. Unlike the [domain](#continuous_domain), elements in the given array need not be numbers; any value that is supported by the underlying [interpolator](#continuous_interpolate) will work, though note that numeric ranges are required for [invert](#continuous_invert). If *range* is not specified, returns a copy of the scale’s current range. See [*continuous*.interpolate](#continuous_interpolate) for more examples.
<a name="continuous_rangeRound" href="#continuous_rangeRound">#</a> <i>continuous</i>.<b>rangeRound</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/continuous.js), [Examples](https://observablehq.com/@d3/continuous-scales)
Sets the scale’s [*range*](#continuous_range) to the specified array of values while also setting the scale’s [interpolator](#continuous_interpolate) to [interpolateRound](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolateRound). This is a convenience method equivalent to:
```js
continuous
.range(range)
.interpolate(d3.interpolateRound);
```
The rounding interpolator is sometimes useful for avoiding antialiasing artifacts, though also consider the [shape-rendering](https://developer.mozilla.org/en-US/docs/Web/SVG/Attribute/shape-rendering) “crispEdges” styles. Note that this interpolator can only be used with numeric ranges.
<a name="continuous_clamp" href="#continuous_clamp">#</a> <i>continuous</i>.<b>clamp</b>(<i>clamp</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/continuous.js), [Examples](https://observablehq.com/@d3/continuous-scales)
If *clamp* is specified, enables or disables clamping accordingly. If clamping is disabled and the scale is passed a value outside the [domain](#continuous_domain), the scale may return a value outside the [range](#continuous_range) through extrapolation. If clamping is enabled, the return value of the scale is always within the scale’s range. Clamping similarly applies to [*continuous*.invert](#continuous_invert). For example:
```js
var x = d3.scaleLinear()
.domain([10, 130])
.range([0, 960]);
x(-10); // -160, outside range
x.invert(-160); // -10, outside domain
x.clamp(true);
x(-10); // 0, clamped to range
x.invert(-160); // 10, clamped to domain
```
If *clamp* is not specified, returns whether or not the scale currently clamps values to within the range.
<a name="continuous_unknown" href="#continuous_unknown">#</a> <i>continuous</i>.<b>unknown</b>([<i>value</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/continuous.js), [Examples](https://observablehq.com/@d3/continuous-scales)
If *value* is specified, sets the output value of the scale for undefined (or NaN) input values and returns this scale. If *value* is not specified, returns the current unknown value, which defaults to undefined.
<a name="continuous_interpolate" href="#continuous_interpolate">#</a> <i>continuous</i>.<b>interpolate</b>(<i>interpolate</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/continuous.js), [Examples](https://observablehq.com/@d3/continuous-scales)
If *interpolate* is specified, sets the scale’s [range](#continuous_range) interpolator factory. This interpolator factory is used to create interpolators for each adjacent pair of values from the range; these interpolators then map a normalized domain parameter *t* in [0, 1] to the corresponding value in the range. If *factory* is not specified, returns the scale’s current interpolator factory, which defaults to [d3.interpolate](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolate). See [d3-interpolate](https://github.com/d3/d3-interpolate) for more interpolators.
For example, consider a diverging color scale with three colors in the range:
```js
var color = d3.scaleLinear()
.domain([-100, 0, +100])
.range(["red", "white", "green"]);
```
Two interpolators are created internally by the scale, equivalent to:
```js
var i0 = d3.interpolate("red", "white"),
i1 = d3.interpolate("white", "green");
```
A common reason to specify a custom interpolator is to change the color space of interpolation. For example, to use [HCL](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolateHcl):
```js
var color = d3.scaleLinear()
.domain([10, 100])
.range(["brown", "steelblue"])
.interpolate(d3.interpolateHcl);
```
Or for [Cubehelix](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolateCubehelix) with a custom gamma:
```js
var color = d3.scaleLinear()
.domain([10, 100])
.range(["brown", "steelblue"])
.interpolate(d3.interpolateCubehelix.gamma(3));
```
Note: the [default interpolator](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolate) **may reuse return values**. For example, if the range values are objects, then the value interpolator always returns the same object, modifying it in-place. If the scale is used to set an attribute or style, this is typically acceptable (and desirable for performance); however, if you need to store the scale’s return value, you must specify your own interpolator or make a copy as appropriate.
<a name="continuous_ticks" href="#continuous_ticks">#</a> <i>continuous</i>.<b>ticks</b>([<i>count</i>])
Returns approximately *count* representative values from the scale’s [domain](#continuous_domain). If *count* is not specified, it defaults to 10. The returned tick values are uniformly spaced, have human-readable values (such as multiples of powers of 10), and are guaranteed to be within the extent of the domain. Ticks are often used to display reference lines, or tick marks, in conjunction with the visualized data. The specified *count* is only a hint; the scale may return more or fewer values depending on the domain. See also d3-array’s [ticks](https://github.com/d3/d3-array/blob/master/README.md#ticks).
<a name="continuous_tickFormat" href="#continuous_tickFormat">#</a> <i>continuous</i>.<b>tickFormat</b>([<i>count</i>[, <i>specifier</i>]]) · [Source](https://github.com/d3/d3-scale/blob/master/src/tickFormat.js), [Examples](https://observablehq.com/@d3/scale-ticks)
Returns a [number format](https://github.com/d3/d3-format) function suitable for displaying a tick value, automatically computing the appropriate precision based on the fixed interval between tick values. The specified *count* should have the same value as the count that is used to generate the [tick values](#continuous_ticks).
An optional *specifier* allows a [custom format](https://github.com/d3/d3-format/blob/master/README.md#locale_format) where the precision of the format is automatically set by the scale as appropriate for the tick interval. For example, to format percentage change, you might say:
```js
var x = d3.scaleLinear()
.domain([-1, 1])
.range([0, 960]);
var ticks = x.ticks(5),
tickFormat = x.tickFormat(5, "+%");
ticks.map(tickFormat); // ["-100%", "-50%", "+0%", "+50%", "+100%"]
```
If *specifier* uses the format type `s`, the scale will return a [SI-prefix format](https://github.com/d3/d3-format/blob/master/README.md#locale_formatPrefix) based on the largest value in the domain. If the *specifier* already specifies a precision, this method is equivalent to [*locale*.format](https://github.com/d3/d3-format/blob/master/README.md#locale_format).
See also [d3.tickFormat](#tickFormat).
<a name="continuous_nice" href="#continuous_nice">#</a> <i>continuous</i>.<b>nice</b>([<i>count</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/nice.js), [Examples](https://observablehq.com/@d3/d3-scalelinear)
Extends the [domain](#continuous_domain) so that it starts and ends on nice round values. This method typically modifies the scale’s domain, and may only extend the bounds to the nearest round value. An optional tick *count* argument allows greater control over the step size used to extend the bounds, guaranteeing that the returned [ticks](#continuous_ticks) will exactly cover the domain. Nicing is useful if the domain is computed from data, say using [extent](https://github.com/d3/d3-array/blob/master/README.md#extent), and may be irregular. For example, for a domain of [0.201479…, 0.996679…], a nice domain might be [0.2, 1.0]. If the domain has more than two values, nicing the domain only affects the first and last value. See also d3-array’s [tickStep](https://github.com/d3/d3-array/blob/master/README.md#tickStep).
Nicing a scale only modifies the current domain; it does not automatically nice domains that are subsequently set using [*continuous*.domain](#continuous_domain). You must re-nice the scale after setting the new domain, if desired.
<a name="continuous_copy" href="#continuous_copy">#</a> <i>continuous</i>.<b>copy</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/continuous.js), [Examples](https://observablehq.com/@d3/continuous-scales)
Returns an exact copy of this scale. Changes to this scale will not affect the returned scale, and vice versa.
<a name="tickFormat" href="#tickFormat">#</a> d3.<b>tickFormat</b>(<i>start</i>, <i>stop</i>, <i>count</i>[, <i>specifier</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/tickFormat.js), [Examples](https://observablehq.com/@d3/scale-ticks)
Returns a [number format](https://github.com/d3/d3-format) function suitable for displaying a tick value, automatically computing the appropriate precision based on the fixed interval between tick values, as determined by [d3.tickStep](https://github.com/d3/d3-array/blob/master/README.md#tickStep).
An optional *specifier* allows a [custom format](https://github.com/d3/d3-format/blob/master/README.md#locale_format) where the precision of the format is automatically set by the scale as appropriate for the tick interval. For example, to format percentage change, you might say:
```js
var tickFormat = d3.tickFormat(-1, 1, 5, "+%");
tickFormat(-0.5); // "-50%"
```
If *specifier* uses the format type `s`, the scale will return a [SI-prefix format](https://github.com/d3/d3-format/blob/master/README.md#locale_formatPrefix) based on the larger absolute value of *start* and *stop*. If the *specifier* already specifies a precision, this method is equivalent to [*locale*.format](https://github.com/d3/d3-format/blob/master/README.md#locale_format).
#### Linear Scales
<a name="scaleLinear" href="#scaleLinear">#</a> d3.<b>scaleLinear</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/linear.js), [Examples](https://observablehq.com/@d3/d3-scalelinear)
Constructs a new [continuous scale](#continuous-scales) with the specified [domain](#continuous_domain) and [range](#continuous_range), the [default](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolate) [interpolator](#continuous_interpolate) and [clamping](#continuous_clamp) disabled. If either *domain* or *range* are not specified, each defaults to [0, 1]. Linear scales are a good default choice for continuous quantitative data because they preserve proportional differences. Each range value *y* can be expressed as a function of the domain value *x*: *y* = *mx* + *b*.
#### Power Scales
Power scales are similar to [linear scales](#linear-scales), except an exponential transform is applied to the input domain value before the output range value is computed. Each range value *y* can be expressed as a function of the domain value *x*: *y* = *mx^k* + *b*, where *k* is the [exponent](#pow_exponent) value. Power scales also support negative domain values, in which case the input value and the resulting output value are multiplied by -1.
<a name="scalePow" href="#scalePow">#</a> d3.<b>scalePow</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/pow.js), [Examples](https://observablehq.com/@d3/continuous-scales)
Constructs a new [continuous scale](#continuous-scales) with the specified [domain](#continuous_domain) and [range](#continuous_range), the [exponent](#pow_exponent) 1, the [default](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolate) [interpolator](#continuous_interpolate) and [clamping](#continuous_clamp) disabled. If either *domain* or *range* are not specified, each defaults to [0, 1]. (Note that this is effectively a [linear](#linear-scales) scale until you set a different exponent.)
<a name="_pow" href="#_pow">#</a> <i>pow</i>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/pow.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*](#_continuous).
<a name="pow_invert" href="#pow_invert">#</a> <i>pow</i>.<b>invert</b>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/pow.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.invert](#continuous_invert).
<a name="pow_exponent" href="#pow_exponent">#</a> <i>pow</i>.<b>exponent</b>([<i>exponent</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/pow.js), [Examples](https://observablehq.com/@d3/continuous-scales)
If *exponent* is specified, sets the current exponent to the given numeric value. If *exponent* is not specified, returns the current exponent, which defaults to 1. (Note that this is effectively a [linear](#linear-scales) scale until you set a different exponent.)
<a name="pow_domain" href="#pow_domain">#</a> <i>pow</i>.<b>domain</b>([<i>domain</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/pow.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.domain](#continuous_domain).
<a name="pow_range" href="#pow_range">#</a> <i>pow</i>.<b>range</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/pow.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.range](#continuous_range).
<a name="pow_rangeRound" href="#pow_rangeRound">#</a> <i>pow</i>.<b>rangeRound</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/pow.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.rangeRound](#continuous_rangeRound).
<a name="pow_clamp" href="#pow_clamp">#</a> <i>pow</i>.<b>clamp</b>(<i>clamp</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/pow.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.clamp](#continuous_clamp).
<a name="pow_interpolate" href="#pow_interpolate">#</a> <i>pow</i>.<b>interpolate</b>(<i>interpolate</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/pow.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.interpolate](#continuous_interpolate).
<a name="pow_ticks" href="#pow_ticks">#</a> <i>pow</i>.<b>ticks</b>([<i>count</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/pow.js), [Examples](https://observablehq.com/@d3/scale-ticks)
See [*continuous*.ticks](#continuous_ticks).
<a name="pow_tickFormat" href="#pow_tickFormat">#</a> <i>pow</i>.<b>tickFormat</b>([<i>count</i>[, <i>specifier</i>]]) · [Source](https://github.com/d3/d3-scale/blob/master/src/pow.js), [Examples](https://observablehq.com/@d3/scale-ticks)
See [*continuous*.tickFormat](#continuous_tickFormat).
<a name="pow_nice" href="#pow_nice">#</a> <i>pow</i>.<b>nice</b>([<i>count</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/pow.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.nice](#continuous_nice).
<a name="pow_copy" href="#pow_copy">#</a> <i>pow</i>.<b>copy</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/pow.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.copy](#continuous_copy).
<a name="scaleSqrt" href="#scaleSqrt">#</a> d3.<b>scaleSqrt</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/pow.js), [Examples](https://observablehq.com/@d3/continuous-scales)
Constructs a new [continuous](#continuous-scales) [power scale](#power-scales) with the specified [domain](#continuous_domain) and [range](#continuous_range), the [exponent](#pow_exponent) 0.5, the [default](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolate) [interpolator](#continuous_interpolate) and [clamping](#continuous_clamp) disabled. If either *domain* or *range* are not specified, each defaults to [0, 1]. This is a convenience method equivalent to `d3.scalePow(…).exponent(0.5)`.
#### Log Scales
Log scales are similar to [linear scales](#linear-scales), except a logarithmic transform is applied to the input domain value before the output range value is computed. The mapping to the range value *y* can be expressed as a function of the domain value *x*: *y* = *m* log(<i>x</i>) + *b*.
As log(0) = -∞, a log scale domain must be **strictly-positive or strictly-negative**; the domain must not include or cross zero. A log scale with a positive domain has a well-defined behavior for positive values, and a log scale with a negative domain has a well-defined behavior for negative values. (For a negative domain, input and output values are implicitly multiplied by -1.) The behavior of the scale is undefined if you pass a negative value to a log scale with a positive domain or vice versa.
<a name="scaleLog" href="#scaleLog">#</a> d3.<b>scaleLog</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/log.js), [Examples](https://observablehq.com/@d3/continuous-scales)
Constructs a new [continuous scale](#continuous-scales) with the specified [domain](#log_domain) and [range](#log_range), the [base](#log_base) 10, the [default](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolate) [interpolator](#log_interpolate) and [clamping](#log_clamp) disabled. If *domain* is not specified, it defaults to [1, 10]. If *range* is not specified, it defaults to [0, 1].
<a name="_log" href="#_log">#</a> <i>log</i>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/log.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*](#_continuous).
<a name="log_invert" href="#log_invert">#</a> <i>log</i>.<b>invert</b>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/log.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.invert](#continuous_invert).
<a name="log_base" href="#log_base">#</a> <i>log</i>.<b>base</b>([<i>base</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/log.js), [Examples](https://observablehq.com/@d3/continuous-scales)
If *base* is specified, sets the base for this logarithmic scale to the specified value. If *base* is not specified, returns the current base, which defaults to 10.
<a name="log_domain" href="#log_domain">#</a> <i>log</i>.<b>domain</b>([<i>domain</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/log.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.domain](#continuous_domain).
<a name="log_range" href="#log_range">#</a> <i>log</i>.<b>range</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/continuous.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.range](#continuous_range).
<a name="log_rangeRound" href="#log_rangeRound">#</a> <i>log</i>.<b>rangeRound</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/log.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.rangeRound](#continuous_rangeRound).
<a name="log_clamp" href="#log_clamp">#</a> <i>log</i>.<b>clamp</b>(<i>clamp</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/log.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.clamp](#continuous_clamp).
<a name="log_interpolate" href="#log_interpolate">#</a> <i>log</i>.<b>interpolate</b>(<i>interpolate</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/log.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.interpolate](#continuous_interpolate).
<a name="log_ticks" href="#log_ticks">#</a> <i>log</i>.<b>ticks</b>([<i>count</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/log.js), [Examples](https://observablehq.com/@d3/scale-ticks)
Like [*continuous*.ticks](#continuous_ticks), but customized for a log scale. If the [base](#log_base) is an integer, the returned ticks are uniformly spaced within each integer power of base; otherwise, one tick per power of base is returned. The returned ticks are guaranteed to be within the extent of the domain. If the orders of magnitude in the [domain](#log_domain) is greater than *count*, then at most one tick per power is returned. Otherwise, the tick values are unfiltered, but note that you can use [*log*.tickFormat](#log_tickFormat) to filter the display of tick labels. If *count* is not specified, it defaults to 10.
<a name="log_tickFormat" href="#log_tickFormat">#</a> <i>log</i>.<b>tickFormat</b>([<i>count</i>[, <i>specifier</i>]]) · [Source](https://github.com/d3/d3-scale/blob/master/src/log.js), [Examples](https://observablehq.com/@d3/scale-ticks)
Like [*continuous*.tickFormat](#continuous_tickFormat), but customized for a log scale. The specified *count* typically has the same value as the count that is used to generate the [tick values](#continuous_ticks). If there are too many ticks, the formatter may return the empty string for some of the tick labels; however, note that the ticks are still shown. To disable filtering, specify a *count* of Infinity. When specifying a count, you may also provide a format *specifier* or format function. For example, to get a tick formatter that will display 20 ticks of a currency, say `log.tickFormat(20, "$,f")`. If the specifier does not have a defined precision, the precision will be set automatically by the scale, returning the appropriate format. This provides a convenient way of specifying a format whose precision will be automatically set by the scale.
<a name="log_nice" href="#log_nice">#</a> <i>log</i>.<b>nice</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/log.js), [Examples](https://observablehq.com/@d3/d3-scalelinear)
Like [*continuous*.nice](#continuous_nice), except extends the domain to integer powers of [base](#log_base). For example, for a domain of [0.201479…, 0.996679…], and base 10, the nice domain is [0.1, 1]. If the domain has more than two values, nicing the domain only affects the first and last value.
<a name="log_copy" href="#log_copy">#</a> <i>log</i>.<b>copy</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/log.js), [Examples](https://observablehq.com/@d3/continuous-scales)
See [*continuous*.copy](#continuous_copy).
#### Symlog Scales
See [A bi-symmetric log transformation for wide-range data](https://www.researchgate.net/profile/John_Webber4/publication/233967063_A_bi-symmetric_log_transformation_for_wide-range_data/links/0fcfd50d791c85082e000000.pdf) by Webber for more.
<a name="scaleSymlog" href="#scaleSymlog">#</a> d3.<b>scaleSymlog</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/symlog.js), [Examples](https://observablehq.com/@d3/continuous-scales)
Constructs a new [continuous scale](#continuous-scales) with the specified [domain](#continuous_domain) and [range](#continuous_range), the [constant](#symlog_constant) 1, the [default](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolate) [interpolator](#continuous_interpolate) and [clamping](#continuous_clamp) disabled. If *domain* is not specified, it defaults to [0, 1]. If *range* is not specified, it defaults to [0, 1].
<a name="symlog_constant" href="#symlog_constant">#</a> <i>symlog</i>.<b>constant</b>([<i>constant</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/symlog.js), [Examples](https://observablehq.com/@d3/continuous-scales)
If *constant* is specified, sets the symlog constant to the specified number and returns this scale; otherwise returns the current value of the symlog constant, which defaults to 1. See “A bi-symmetric log transformation for wide-range data” by Webber for more.
#### Identity Scales
Identity scales are a special case of [linear scales](#linear-scales) where the domain and range are identical; the scale and its invert method are thus the identity function. These scales are occasionally useful when working with pixel coordinates, say in conjunction with an axis. Identity scales do not support [rangeRound](#continuous_rangeRound), [clamp](#continuous_clamp) or [interpolate](#continuous_interpolate).
<a name="scaleIdentity" href="#scaleIdentity">#</a> d3.<b>scaleIdentity</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/identity.js), [Examples](https://observablehq.com/@d3/d3-scalelinear)
Constructs a new identity scale with the specified [domain](#continuous_domain) and [range](#continuous_range). If *range* is not specified, it defaults to [0, 1].
#### Radial Scales
Radial scales are a variant of [linear scales](#linear-scales) where the range is internally squared so that an input value corresponds linearly to the squared output value. These scales are useful when you want the input value to correspond to the area of a graphical mark and the mark is specified by radius, as in a radial bar chart. Radial scales do not support [interpolate](#continuous_interpolate).
<a name="scaleRadial" href="#scaleRadial">#</a> d3.<b>scaleRadial</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/radial.js), [Examples](https://observablehq.com/@d3/radial-stacked-bar-chart)
Constructs a new radial scale with the specified [domain](#continuous_domain) and [range](#continuous_range). If *domain* or *range* is not specified, each defaults to [0, 1].
#### Time Scales
Time scales are a variant of [linear scales](#linear-scales) that have a temporal domain: domain values are coerced to [dates](https://developer.mozilla.org/en/JavaScript/Reference/Global_Objects/Date) rather than numbers, and [invert](#continuous_invert) likewise returns a date. Time scales implement [ticks](#time_ticks) based on [calendar intervals](https://github.com/d3/d3-time), taking the pain out of generating axes for temporal domains.
For example, to create a position encoding:
```js
var x = d3.scaleTime()
.domain([new Date(2000, 0, 1), new Date(2000, 0, 2)])
.range([0, 960]);
x(new Date(2000, 0, 1, 5)); // 200
x(new Date(2000, 0, 1, 16)); // 640
x.invert(200); // Sat Jan 01 2000 05:00:00 GMT-0800 (PST)
x.invert(640); // Sat Jan 01 2000 16:00:00 GMT-0800 (PST)
```
For a valid value *y* in the range, <i>time</i>(<i>time</i>.invert(<i>y</i>)) equals *y*; similarly, for a valid value *x* in the domain, <i>time</i>.invert(<i>time</i>(<i>x</i>)) equals *x*. The invert method is useful for interaction, say to determine the value in the domain that corresponds to the pixel location under the mouse.
<a name="scaleTime" href="#scaleTime">#</a> d3.<b>scaleTime</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/time.js), [Examples](https://observablehq.com/@d3/d3-scaletime)
Constructs a new time scale with the specified [domain](#time_domain) and [range](#time_range), the [default](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolate) [interpolator](#time_interpolate) and [clamping](#time_clamp) disabled. If *domain* is not specified, it defaults to [2000-01-01, 2000-01-02]. If *range* is not specified, it defaults to [0, 1].
<a name="_time" href="#_time">#</a> <i>time</i>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/time.js), [Examples](https://observablehq.com/@d3/d3-scaletime)
See [*continuous*](#_continuous).
<a name="time_invert" href="#time_invert">#</a> <i>time</i>.<b>invert</b>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/time.js), [Examples](https://observablehq.com/@d3/d3-scaletime)
See [*continuous*.invert](#continuous_invert).
<a name="time_domain" href="#time_domain">#</a> <i>time</i>.<b>domain</b>([<i>domain</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/time.js), [Examples](https://observablehq.com/@d3/d3-scaletime)
See [*continuous*.domain](#continuous_domain).
<a name="time_range" href="#time_range">#</a> <i>time</i>.<b>range</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/time.js), [Examples](https://observablehq.com/@d3/d3-scaletime)
See [*continuous*.range](#continuous_range).
<a name="time_rangeRound" href="#time_rangeRound">#</a> <i>time</i>.<b>rangeRound</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/time.js), [Examples](https://observablehq.com/@d3/d3-scaletime)
See [*continuous*.rangeRound](#continuous_rangeRound).
<a name="time_clamp" href="#time_clamp">#</a> <i>time</i>.<b>clamp</b>(<i>clamp</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/time.js), [Examples](https://observablehq.com/@d3/d3-scaletime)
See [*continuous*.clamp](#continuous_clamp).
<a name="time_interpolate" href="#time_interpolate">#</a> <i>time</i>.<b>interpolate</b>(<i>interpolate</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/time.js), [Examples](https://observablehq.com/@d3/d3-scaletime)
See [*continuous*.interpolate](#continuous_interpolate).
<a name="time_ticks" href="#time_ticks">#</a> <i>time</i>.<b>ticks</b>([<i>count</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/time.js), [Examples](https://observablehq.com/@d3/d3-scaletime)
<br><a name="time_ticks" href="#time_ticks">#</a> <i>time</i>.<b>ticks</b>([<i>interval</i>])
Returns representative dates from the scale’s [domain](#time_domain). The returned tick values are uniformly-spaced (mostly), have sensible values (such as every day at midnight), and are guaranteed to be within the extent of the domain. Ticks are often used to display reference lines, or tick marks, in conjunction with the visualized data.
An optional *count* may be specified to affect how many ticks are generated. If *count* is not specified, it defaults to 10. The specified *count* is only a hint; the scale may return more or fewer values depending on the domain. For example, to create ten default ticks, say:
```js
var x = d3.scaleTime();
x.ticks(10);
// [Sat Jan 01 2000 00:00:00 GMT-0800 (PST),
// Sat Jan 01 2000 03:00:00 GMT-0800 (PST),
// Sat Jan 01 2000 06:00:00 GMT-0800 (PST),
// Sat Jan 01 2000 09:00:00 GMT-0800 (PST),
// Sat Jan 01 2000 12:00:00 GMT-0800 (PST),
// Sat Jan 01 2000 15:00:00 GMT-0800 (PST),
// Sat Jan 01 2000 18:00:00 GMT-0800 (PST),
// Sat Jan 01 2000 21:00:00 GMT-0800 (PST),
// Sun Jan 02 2000 00:00:00 GMT-0800 (PST)]
```
The following time intervals are considered for automatic ticks:
* 1-, 5-, 15- and 30-second.
* 1-, 5-, 15- and 30-minute.
* 1-, 3-, 6- and 12-hour.
* 1- and 2-day.
* 1-week.
* 1- and 3-month.
* 1-year.
In lieu of a *count*, a [time *interval*](https://github.com/d3/d3-time/blob/master/README.md#intervals) may be explicitly specified. To prune the generated ticks for a given time *interval*, use [*interval*.every](https://github.com/d3/d3-time/blob/master/README.md#interval_every). For example, to generate ticks at 15-[minute](https://github.com/d3/d3-time/blob/master/README.md#minute) intervals:
```js
var x = d3.scaleTime()
.domain([new Date(2000, 0, 1, 0), new Date(2000, 0, 1, 2)]);
x.ticks(d3.timeMinute.every(15));
// [Sat Jan 01 2000 00:00:00 GMT-0800 (PST),
// Sat Jan 01 2000 00:15:00 GMT-0800 (PST),
// Sat Jan 01 2000 00:30:00 GMT-0800 (PST),
// Sat Jan 01 2000 00:45:00 GMT-0800 (PST),
// Sat Jan 01 2000 01:00:00 GMT-0800 (PST),
// Sat Jan 01 2000 01:15:00 GMT-0800 (PST),
// Sat Jan 01 2000 01:30:00 GMT-0800 (PST),
// Sat Jan 01 2000 01:45:00 GMT-0800 (PST),
// Sat Jan 01 2000 02:00:00 GMT-0800 (PST)]
```
Alternatively, pass a test function to [*interval*.filter](https://github.com/d3/d3-time/blob/master/README.md#interval_filter):
```js
x.ticks(d3.timeMinute.filter(function(d) {
return d.getMinutes() % 15 === 0;
}));
```
Note: in some cases, such as with day ticks, specifying a *step* can result in irregular spacing of ticks because time intervals have varying length.
<a name="time_tickFormat" href="#time_tickFormat">#</a> <i>time</i>.<b>tickFormat</b>([<i>count</i>[, <i>specifier</i>]]) · [Source](https://github.com/d3/d3-scale/blob/master/src/time.js), [Examples](https://observablehq.com/@d3/scale-ticks)
<br><a href="#time_tickFormat">#</a> <i>time</i>.<b>tickFormat</b>([<i>interval</i>[, <i>specifier</i>]])
Returns a time format function suitable for displaying [tick](#time_ticks) values. The specified *count* or *interval* is currently ignored, but is accepted for consistency with other scales such as [*continuous*.tickFormat](#continuous_tickFormat). If a format *specifier* is specified, this method is equivalent to [format](https://github.com/d3/d3-time-format/blob/master/README.md#format). If *specifier* is not specified, the default time format is returned. The default multi-scale time format chooses a human-readable representation based on the specified date as follows:
* `%Y` - for year boundaries, such as `2011`.
* `%B` - for month boundaries, such as `February`.
* `%b %d` - for week boundaries, such as `Feb 06`.
* `%a %d` - for day boundaries, such as `Mon 07`.
* `%I %p` - for hour boundaries, such as `01 AM`.
* `%I:%M` - for minute boundaries, such as `01:23`.
* `:%S` - for second boundaries, such as `:45`.
* `.%L` - milliseconds for all other times, such as `.012`.
Although somewhat unusual, this default behavior has the benefit of providing both local and global context: for example, formatting a sequence of ticks as [11 PM, Mon 07, 01 AM] reveals information about hours, dates, and day simultaneously, rather than just the hours [11 PM, 12 AM, 01 AM]. See [d3-time-format](https://github.com/d3/d3-time-format) if you’d like to roll your own conditional time format.
<a name="time_nice" href="#time_nice">#</a> <i>time</i>.<b>nice</b>([<i>count</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/time.js), [Examples](https://observablehq.com/@d3/d3-scaletime)
<br><a name="time_nice" href="#time_nice">#</a> <i>time</i>.<b>nice</b>([<i>interval</i>])
Extends the [domain](#time_domain) so that it starts and ends on nice round values. This method typically modifies the scale’s domain, and may only extend the bounds to the nearest round value. See [*continuous*.nice](#continuous_nice) for more.
An optional tick *count* argument allows greater control over the step size used to extend the bounds, guaranteeing that the returned [ticks](#time_ticks) will exactly cover the domain. Alternatively, a [time *interval*](https://github.com/d3/d3-time/blob/master/README.md#intervals) may be specified to explicitly set the ticks. If an *interval* is specified, an optional *step* may also be specified to skip some ticks. For example, `time.nice(d3.timeSecond.every(10))` will extend the domain to an even ten seconds (0, 10, 20, <i>etc.</i>). See [*time*.ticks](#time_ticks) and [*interval*.every](https://github.com/d3/d3-time/blob/master/README.md#interval_every) for further detail.
Nicing is useful if the domain is computed from data, say using [extent](https://github.com/d3/d3-array/blob/master/README.md#extent), and may be irregular. For example, for a domain of [2009-07-13T00:02, 2009-07-13T23:48], the nice domain is [2009-07-13, 2009-07-14]. If the domain has more than two values, nicing the domain only affects the first and last value.
<a name="time_copy" href="#time_copy">#</a> <i>time</i>.<b>copy</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/time.js), [Examples](https://observablehq.com/@d3/d3-scaletime)
See [*continuous*.copy](#continuous_copy).
<a name="scaleUtc" href="#scaleUtc">#</a> d3.<b>scaleUtc</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/utcTime.js), [Examples](https://observablehq.com/@d3/d3-scaletime)
Equivalent to [scaleTime](#scaleTime), but the returned time scale operates in [Coordinated Universal Time](https://en.wikipedia.org/wiki/Coordinated_Universal_Time) rather than local time.
### Sequential Scales
Sequential scales, like [diverging scales](#diverging-scales), are similar to [continuous scales](#continuous-scales) in that they map a continuous, numeric input domain to a continuous output range. However, unlike continuous scales, the input domain and output range of a sequential scale always has exactly two elements, and the output range is typically specified as an interpolator rather than an array of values. These scales do not expose [invert](#continuous_invert) and [interpolate](#continuous_interpolate) methods.
<a name="scaleSequential" href="#scaleSequential">#</a> d3.<b>scaleSequential</b>([[<i>domain</i>, ]<i>interpolator</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/sequential.js), [Examples](https://observablehq.com/@d3/sequential-scales)
Constructs a new sequential scale with the specified [*domain*](#sequential_domain) and [*interpolator*](#sequential_interpolator) function or array. If *domain* is not specified, it defaults to [0, 1]. If *interpolator* is not specified, it defaults to the identity function. When the scale is [applied](#_sequential), the interpolator will be invoked with a value typically in the range [0, 1], where 0 represents the minimum value and 1 represents the maximum value. For example, to implement the ill-advised [HSL](https://github.com/d3/d3-color/blob/master/README.md#hsl) rainbow scale:
```js
var rainbow = d3.scaleSequential(function(t) {
return d3.hsl(t * 360, 1, 0.5) + "";
});
```
A more aesthetically-pleasing and perceptually-effective cyclical hue encoding is to use [d3.interpolateRainbow](https://github.com/d3/d3-scale-chromatic/blob/master/README.md#interpolateRainbow):
```js
var rainbow = d3.scaleSequential(d3.interpolateRainbow);
```
If *interpolator* is an array, it represents the scale’s two-element output range and is converted to an interpolator function using [d3.interpolate](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolate).
<a name="_sequential" href="#_sequential">#</a> <i>sequential</i>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/sequential.js), [Examples](https://observablehq.com/@d3/sequential-scales)
See [*continuous*](#_continuous).
<a name="sequential_domain" href="#sequential_domain">#</a> <i>sequential</i>.<b>domain</b>([<i>domain</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/sequential.js), [Examples](https://observablehq.com/@d3/sequential-scales)
See [*continuous*.domain](#continuous_domain). Note that a sequential scale’s domain must be numeric and must contain exactly two values.
<a name="sequential_clamp" href="#sequential_clamp">#</a> <i>sequential</i>.<b>clamp</b>([<i>clamp</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/sequential.js), [Examples](https://observablehq.com/@d3/sequential-scales)
See [*continuous*.clamp](#continuous_clamp).
<a name="sequential_interpolator" href="#sequential_interpolator">#</a> <i>sequential</i>.<b>interpolator</b>([<i>interpolator</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/sequential.js), [Examples](https://observablehq.com/@d3/sequential-scales)
If *interpolator* is specified, sets the scale’s interpolator to the specified function. If *interpolator* is not specified, returns the scale’s current interpolator.
<a name="sequential_range" href="#sequential_range">#</a> <i>sequential</i>.<b>range</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/sequential.js), [Examples](https://observablehq.com/@d3/sequential-scales)
See [*continuous*.range](#continuous_range). If *range* is specified, the given two-element array is converted to an interpolator function using [d3.interpolate](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolate).
<a name="sequential_rangeRound" href="#sequential_rangeRound">#</a> <i>sequential</i>.<b>rangeRound</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/sequential.js), [Examples](https://observablehq.com/@d3/sequential-scales)
See [*continuous*.rangeRound](#continuous_rangeRound). If *range* is specified, implicitly uses [d3.interpolateRound](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolateRound) as the interpolator.
<a name="sequential_copy" href="#sequential_copy">#</a> <i>sequential</i>.<b>copy</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/sequential.js), [Examples](https://observablehq.com/@d3/sequential-scales)
See [*continuous*.copy](#continuous_copy).
<a name="scaleSequentialLog" href="#scaleSequentialLog">#</a> d3.<b>scaleSequentialLog</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/sequential.js), [Examples](https://observablehq.com/@d3/sequential-scales)
A [sequential scale](#sequential-scales) with a logarithmic transform, analogous to a [log scale](#log-scales).
<a name="scaleSequentialPow" href="#scaleSequentialPow">#</a> d3.<b>scaleSequentialPow</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/sequential.js), [Examples](https://observablehq.com/@d3/sequential-scales)
A [sequential scale](#sequential-scales) with an exponential transform, analogous to a [power scale](#pow-scales).
<a name="scaleSequentialSqrt" href="#scaleSequentialSqrt">#</a> d3.<b>scaleSequentialSqrt</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/sequential.js), [Examples](https://observablehq.com/@d3/sequential-scales)
A [sequential scale](#sequential-scales) with a square-root transform, analogous to a [d3.scaleSqrt](#scaleSqrt).
<a name="scaleSequentialSymlog" href="#scaleSequentialSymlog">#</a> d3.<b>scaleSequentialSymlog</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/sequential.js), [Examples](https://observablehq.com/@d3/sequential-scales)
A [sequential scale](#sequential-scales) with a symmetric logarithmic transform, analogous to a [symlog scale](#symlog-scales).
<a name="scaleSequentialQuantile" href="#scaleSequentialQuantile">#</a> d3.<b>scaleSequentialQuantile</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/sequentialQuantile.js), [Examples](https://observablehq.com/@d3/sequential-scales)
A [sequential scale](#sequential-scales) using a *p*-quantile transform, analogous to a [quantile scale](#quantile-scales).
<a name="sequentialQuantile_quantiles" href="#sequentialQuantile_quantiles">#</a> <i>sequentialQuantile</i>.<b>quantiles</b>(<i>n</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/sequentialQuantile.js), [Examples](https://observablehq.com/@d3/sequential-scales)
Returns an array of *n* + 1 quantiles. For example, if *n* = 4, returns an array of five numbers: the minimum value, the first quartile, the median, the third quartile, and the maximum.
### Diverging Scales
Diverging scales, like [sequential scales](#sequential-scales), are similar to [continuous scales](#continuous-scales) in that they map a continuous, numeric input domain to a continuous output range. However, unlike continuous scales, the input domain and output range of a diverging scale always has exactly three elements, and the output range is typically specified as an interpolator rather than an array of values. These scales do not expose [invert](#continuous_invert) and [interpolate](#continuous_interpolate) methods.
<a name="scaleDiverging" href="#scaleDiverging">#</a> d3.<b>scaleDiverging</b>([[<i>domain</i>, ]<i>interpolator</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/diverging.js), [Examples](https://observablehq.com/@d3/diverging-scales)
Constructs a new diverging scale with the specified [*domain*](#diverging_domain) and [*interpolator*](#diverging_interpolator) function or array. If *domain* is not specified, it defaults to [0, 0.5, 1]. If *interpolator* is not specified, it defaults to the identity function. When the scale is [applied](#_diverging), the interpolator will be invoked with a value typically in the range [0, 1], where 0 represents the extreme negative value, 0.5 represents the neutral value, and 1 represents the extreme positive value. For example, using [d3.interpolateSpectral](https://github.com/d3/d3-scale-chromatic/blob/master/README.md#interpolateSpectral):
```js
var spectral = d3.scaleDiverging(d3.interpolateSpectral);
```
If *interpolator* is an array, it represents the scale’s three-element output range and is converted to an interpolator function using [d3.interpolate](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolate) and [d3.piecewise](https://github.com/d3/d3-interpolate/blob/master/README.md#piecewise).
<a name="_diverging" href="#_diverging">#</a> <i>diverging</i>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/diverging.js), [Examples](https://observablehq.com/@d3/diverging-scales)
See [*continuous*](#_continuous).
<a name="diverging_domain" href="#diverging_domain">#</a> <i>diverging</i>.<b>domain</b>([<i>domain</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/diverging.js), [Examples](https://observablehq.com/@d3/diverging-scales)
See [*continuous*.domain](#continuous_domain). Note that a diverging scale’s domain must be numeric and must contain exactly three values. The default domain is [0, 0.5, 1].
<a name="diverging_clamp" href="#diverging_clamp">#</a> <i>diverging</i>.<b>clamp</b>([<i>clamp</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/diverging.js), [Examples](https://observablehq.com/@d3/diverging-scales)
See [*continuous*.clamp](#continuous_clamp).
<a name="diverging_interpolator" href="#diverging_interpolator">#</a> <i>diverging</i>.<b>interpolator</b>([<i>interpolator</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/diverging.js), [Examples](https://observablehq.com/@d3/diverging-scales)
If *interpolator* is specified, sets the scale’s interpolator to the specified function. If *interpolator* is not specified, returns the scale’s current interpolator.
<a name="diverging_range" href="#diverging_range">#</a> <i>diverging</i>.<b>range</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/diverging.js), [Examples](https://observablehq.com/@d3/diverging-scales)
See [*continuous*.range](#continuous_range). If *range* is specified, the given three-element array is converted to an interpolator function using [d3.interpolate](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolate) and [d3.piecewise](https://github.com/d3/d3-interpolate/blob/master/README.md#piecewise).
<a name="diverging_rangeRound" href="#diverging_rangeRound">#</a> <i>diverging</i>.<b>rangeRound</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/diverging.js), [Examples](https://observablehq.com/@d3/diverging-scales)
See [*continuous*.range](#continuous_rangeRound). If *range* is specified, implicitly uses [d3.interpolateRound](https://github.com/d3/d3-interpolate/blob/master/README.md#interpolateRound) as the interpolator.
<a name="diverging_copy" href="#diverging_copy">#</a> <i>diverging</i>.<b>copy</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/diverging.js), [Examples](https://observablehq.com/@d3/diverging-scales)
See [*continuous*.copy](#continuous_copy).
<a name="diverging_unknown" href="#diverging_unknown">#</a> <i>diverging</i>.<b>unknown</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/diverging.js), [Examples](https://observablehq.com/@d3/diverging-scales)
See [*continuous*.unknown](#continuous_unknown).
<a name="scaleDivergingLog" href="#scaleDivergingLog">#</a> d3.<b>scaleDivergingLog</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/diverging.js), [Examples](https://observablehq.com/@d3/diverging-scales)
A [diverging scale](#diverging-scales) with a logarithmic transform, analogous to a [log scale](#log-scales).
<a name="scaleDivergingPow" href="#scaleDivergingPow">#</a> d3.<b>scaleDivergingPow</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/diverging.js), [Examples](https://observablehq.com/@d3/diverging-scales)
A [diverging scale](#diverging-scales) with an exponential transform, analogous to a [power scale](#pow-scales).
<a name="scaleDivergingSqrt" href="#scaleDivergingSqrt">#</a> d3.<b>scaleDivergingSqrt</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/diverging.js), [Examples](https://observablehq.com/@d3/diverging-scales)
A [diverging scale](#diverging-scales) with a square-root transform, analogous to a [d3.scaleSqrt](#scaleSqrt).
<a name="scaleDivergingSymlog" href="#scaleDivergingSymlog">#</a> d3.<b>scaleDivergingSymlog</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/diverging.js), [Examples](https://observablehq.com/@d3/diverging-scales)
A [diverging scale](#diverging-scales) with a symmetric logarithmic transform, analogous to a [symlog scale](#symlog-scales).
### Quantize Scales
Quantize scales are similar to [linear scales](#linear-scales), except they use a discrete rather than continuous range. The continuous input domain is divided into uniform segments based on the number of values in (*i.e.*, the cardinality of) the output range. Each range value *y* can be expressed as a quantized linear function of the domain value *x*: *y* = *m round(x)* + *b*. See [this choropleth](https://observablehq.com/@d3/choropleth) for an example.
<a name="scaleQuantize" href="#scaleQuantize">#</a> d3.<b>scaleQuantize</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/quantize.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
Constructs a new quantize scale with the specified [*domain*](#quantize_domain) and [*range*](#quantize_range). If either *domain* or *range* is not specified, each defaults to [0, 1]. Thus, the default quantize scale is equivalent to the [Math.round](https://developer.mozilla.org/en/JavaScript/Reference/Global_Objects/Math/round) function.
<a name="_quantize" href="#_quantize">#</a> <i>quantize</i>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/quantize.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
Given a *value* in the input [domain](#quantize_domain), returns the corresponding value in the output [range](#quantize_range). For example, to apply a color encoding:
```js
var color = d3.scaleQuantize()
.domain([0, 1])
.range(["brown", "steelblue"]);
color(0.49); // "brown"
color(0.51); // "steelblue"
```
Or dividing the domain into three equally-sized parts with different range values to compute an appropriate stroke width:
```js
var width = d3.scaleQuantize()
.domain([10, 100])
.range([1, 2, 4]);
width(20); // 1
width(50); // 2
width(80); // 4
```
<a name="quantize_invertExtent" href="#quantize_invertExtent">#</a> <i>quantize</i>.<b>invertExtent</b>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/quantize.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
Returns the extent of values in the [domain](#quantize_domain) [<i>x0</i>, <i>x1</i>] for the corresponding *value* in the [range](#quantize_range): the inverse of [*quantize*](#_quantize). This method is useful for interaction, say to determine the value in the domain that corresponds to the pixel location under the mouse.
```js
var width = d3.scaleQuantize()
.domain([10, 100])
.range([1, 2, 4]);
width.invertExtent(2); // [40, 70]
```
<a name="quantize_domain" href="#quantize_domain">#</a> <i>quantize</i>.<b>domain</b>([<i>domain</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/quantize.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
If *domain* is specified, sets the scale’s domain to the specified two-element array of numbers. If the elements in the given array are not numbers, they will be coerced to numbers. The numbers must be in ascending order or the behavior of the scale is undefined. If *domain* is not specified, returns the scale’s current domain.
<a name="quantize_range" href="#quantize_range">#</a> <i>quantize</i>.<b>range</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/quantize.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
If *range* is specified, sets the scale’s range to the specified array of values. The array may contain any number of discrete values. The elements in the given array need not be numbers; any value or type will work. If *range* is not specified, returns the scale’s current range.
<a name="quantize_ticks" href="#quantize_ticks">#</a> <i>quantize</i>.<b>ticks</b>([<i>count</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/quantize.js), [Examples](https://observablehq.com/@d3/scale-ticks)
Equivalent to [*continuous*.ticks](#continuous_ticks).
<a name="quantize_tickFormat" href="#quantize_tickFormat">#</a> <i>quantize</i>.<b>tickFormat</b>([<i>count</i>[, <i>specifier</i>]]) · [Source](https://github.com/d3/d3-scale/blob/master/src/linear.js), [Examples](https://observablehq.com/@d3/scale-ticks)
Equivalent to [*continuous*.tickFormat](#continuous_tickFormat).
<a name="quantize_nice" href="#quantize_nice">#</a> <i>quantize</i>.<b>nice</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/quantize.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
Equivalent to [*continuous*.nice](#continuous_nice).
<a name="quantize_thresholds" href="#quantize_thresholds">#</a> <i>quantize</i>.<b>thresholds</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/quantize.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
Returns the array of computed thresholds within the [domain](#quantize_domain).
<a name="quantize_copy" href="#quantize_copy">#</a> <i>quantize</i>.<b>copy</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/quantize.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
Returns an exact copy of this scale. Changes to this scale will not affect the returned scale, and vice versa.
### Quantile Scales
Quantile scales map a sampled input domain to a discrete range. The domain is considered continuous and thus the scale will accept any reasonable input value; however, the domain is specified as a discrete set of sample values. The number of values in (the cardinality of) the output range determines the number of quantiles that will be computed from the domain. To compute the quantiles, the domain is sorted, and treated as a [population of discrete values](https://en.wikipedia.org/wiki/Quantile#Quantiles_of_a_population); see d3-array’s [quantile](https://github.com/d3/d3-array/blob/master/README.md#quantile). See [this quantile choropleth](https://observablehq.com/@d3/quantile-choropleth) for an example.
<a name="scaleQuantile" href="#scaleQuantile">#</a> d3.<b>scaleQuantile</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/quantile.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
Constructs a new quantile scale with the specified [*domain*](#quantile_domain) and [*range*](#quantile_range). If either *domain* or *range* is not specified, each defaults to the empty array. The quantile scale is invalid until both a domain and range are specified.
<a name="_quantile" href="#_quantile">#</a> <i>quantile</i>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/quantile.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
Given a *value* in the input [domain](#quantile_domain), returns the corresponding value in the output [range](#quantile_range).
<a name="quantile_invertExtent" href="#quantile_invertExtent">#</a> <i>quantile</i>.<b>invertExtent</b>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/quantile.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
Returns the extent of values in the [domain](#quantile_domain) [<i>x0</i>, <i>x1</i>] for the corresponding *value* in the [range](#quantile_range): the inverse of [*quantile*](#_quantile). This method is useful for interaction, say to determine the value in the domain that corresponds to the pixel location under the mouse.
<a name="quantile_domain" href="#quantile_domain">#</a> <i>quantile</i>.<b>domain</b>([<i>domain</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/quantile.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
If *domain* is specified, sets the domain of the quantile scale to the specified set of discrete numeric values. The array must not be empty, and must contain at least one numeric value; NaN, null and undefined values are ignored and not considered part of the sample population. If the elements in the given array are not numbers, they will be coerced to numbers. A copy of the input array is sorted and stored internally. If *domain* is not specified, returns the scale’s current domain.
<a name="quantile_range" href="#quantile_range">#</a> <i>quantile</i>.<b>range</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/quantile.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
If *range* is specified, sets the discrete values in the range. The array must not be empty, and may contain any type of value. The number of values in (the cardinality, or length, of) the *range* array determines the number of quantiles that are computed. For example, to compute quartiles, *range* must be an array of four elements such as [0, 1, 2, 3]. If *range* is not specified, returns the current range.
<a name="quantile_quantiles" href="#quantile_quantiles">#</a> <i>quantile</i>.<b>quantiles</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/quantile.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
Returns the quantile thresholds. If the [range](#quantile_range) contains *n* discrete values, the returned array will contain *n* - 1 thresholds. Values less than the first threshold are considered in the first quantile; values greater than or equal to the first threshold but less than the second threshold are in the second quantile, and so on. Internally, the thresholds array is used with [bisect](https://github.com/d3/d3-array/blob/master/README.md#bisect) to find the output quantile associated with the given input value.
<a name="quantile_copy" href="#quantile_copy">#</a> <i>quantile</i>.<b>copy</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/quantile.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
Returns an exact copy of this scale. Changes to this scale will not affect the returned scale, and vice versa.
### Threshold Scales
Threshold scales are similar to [quantize scales](#quantize-scales), except they allow you to map arbitrary subsets of the domain to discrete values in the range. The input domain is still continuous, and divided into slices based on a set of threshold values. See [this choropleth](https://observablehq.com/@d3/threshold-choropleth) for an example.
<a name="scaleThreshold" href="#scaleThreshold">#</a> d3.<b>scaleThreshold</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/threshold.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
Constructs a new threshold scale with the specified [*domain*](#threshold_domain) and [*range*](#threshold_range). If *domain* is not specified, it defaults to [0.5]. If *range* is not specified, it defaults to [0, 1]. Thus, the default threshold scale is equivalent to the [Math.round](https://developer.mozilla.org/en/JavaScript/Reference/Global_Objects/Math/round) function for numbers; for example threshold(0.49) returns 0, and threshold(0.51) returns 1.
<a name="_threshold" href="#_threshold">#</a> <i>threshold</i>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/threshold.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
Given a *value* in the input [domain](#threshold_domain), returns the corresponding value in the output [range](#threshold_range). For example:
```js
var color = d3.scaleThreshold()
.domain([0, 1])
.range(["red", "white", "green"]);
color(-1); // "red"
color(0); // "white"
color(0.5); // "white"
color(1); // "green"
color(1000); // "green"
```
<a name="threshold_invertExtent" href="#threshold_invertExtent">#</a> <i>threshold</i>.<b>invertExtent</b>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/threshold.js), [Examples](https://observablehq.com/@d3/choropleth)
Returns the extent of values in the [domain](#threshold_domain) [<i>x0</i>, <i>x1</i>] for the corresponding *value* in the [range](#threshold_range), representing the inverse mapping from range to domain. This method is useful for interaction, say to determine the value in the domain that corresponds to the pixel location under the mouse. For example:
```js
var color = d3.scaleThreshold()
.domain([0, 1])
.range(["red", "white", "green"]);
color.invertExtent("red"); // [undefined, 0]
color.invertExtent("white"); // [0, 1]
color.invertExtent("green"); // [1, undefined]
```
<a name="threshold_domain" href="#threshold_domain">#</a> <i>threshold</i>.<b>domain</b>([<i>domain</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/threshold.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
If *domain* is specified, sets the scale’s domain to the specified array of values. The values must be in ascending order or the behavior of the scale is undefined. The values are typically numbers, but any naturally ordered values (such as strings) will work; a threshold scale can be used to encode any type that is ordered. If the number of values in the scale’s range is N+1, the number of values in the scale’s domain must be N. If there are fewer than N elements in the domain, the additional values in the range are ignored. If there are more than N elements in the domain, the scale may return undefined for some inputs. If *domain* is not specified, returns the scale’s current domain.
<a name="threshold_range" href="#threshold_range">#</a> <i>threshold</i>.<b>range</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/threshold.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
If *range* is specified, sets the scale’s range to the specified array of values. If the number of values in the scale’s domain is N, the number of values in the scale’s range must be N+1. If there are fewer than N+1 elements in the range, the scale may return undefined for some inputs. If there are more than N+1 elements in the range, the additional values are ignored. The elements in the given array need not be numbers; any value or type will work. If *range* is not specified, returns the scale’s current range.
<a name="threshold_copy" href="#threshold_copy">#</a> <i>threshold</i>.<b>copy</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/threshold.js), [Examples](https://observablehq.com/@d3/quantile-quantize-and-threshold-scales)
Returns an exact copy of this scale. Changes to this scale will not affect the returned scale, and vice versa.
### Ordinal Scales
Unlike [continuous scales](#continuous-scales), ordinal scales have a discrete domain and range. For example, an ordinal scale might map a set of named categories to a set of colors, or determine the horizontal positions of columns in a column chart.
<a name="scaleOrdinal" href="#scaleOrdinal">#</a> d3.<b>scaleOrdinal</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/ordinal.js), [Examples](https://observablehq.com/@d3/d3-scaleordinal)
Constructs a new ordinal scale with the specified [*domain*](#ordinal_domain) and [*range*](#ordinal_range). If *domain* is not specified, it defaults to the empty array. If *range* is not specified, it defaults to the empty array; an ordinal scale always returns undefined until a non-empty range is defined.
<a name="_ordinal" href="#_ordinal">#</a> <i>ordinal</i>(<i>value</i>) · [Source](https://github.com/d3/d3-scale/blob/master/src/ordinal.js), [Examples](https://observablehq.com/@d3/d3-scaleordinal)
Given a *value* in the input [domain](#ordinal_domain), returns the corresponding value in the output [range](#ordinal_range). If the given *value* is not in the scale’s [domain](#ordinal_domain), returns the [unknown](#ordinal_unknown); or, if the unknown value is [implicit](#scaleImplicit) (the default), then the *value* is implicitly added to the domain and the next-available value in the range is assigned to *value*, such that this and subsequent invocations of the scale given the same input *value* return the same output value.
<a name="ordinal_domain" href="#ordinal_domain">#</a> <i>ordinal</i>.<b>domain</b>([<i>domain</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/ordinal.js), [Examples](https://observablehq.com/@d3/d3-scaleordinal)
If *domain* is specified, sets the domain to the specified array of values. The first element in *domain* will be mapped to the first element in the range, the second domain value to the second range value, and so on. Domain values are stored internally in an [InternMap](https://github.com/mbostock/internmap) from primitive value to index; the resulting index is then used to retrieve a value from the range. Thus, an ordinal scale’s values must be coercible to a primitive value, and the primitive domain value uniquely identifies the corresponding range value. If *domain* is not specified, this method returns the current domain.
Setting the domain on an ordinal scale is optional if the [unknown value](#ordinal_unknown) is [implicit](#scaleImplicit) (the default). In this case, the domain will be inferred implicitly from usage by assigning each unique value passed to the scale a new value from the range. Note that an explicit domain is recommended to ensure deterministic behavior, as inferring the domain from usage will be dependent on ordering.
<a name="ordinal_range" href="#ordinal_range">#</a> <i>ordinal</i>.<b>range</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/ordinal.js), [Examples](https://observablehq.com/@d3/d3-scaleordinal)
If *range* is specified, sets the range of the ordinal scale to the specified array of values. The first element in the domain will be mapped to the first element in *range*, the second domain value to the second range value, and so on. If there are fewer elements in the range than in the domain, the scale will reuse values from the start of the range. If *range* is not specified, this method returns the current range.
<a name="ordinal_unknown" href="#ordinal_unknown">#</a> <i>ordinal</i>.<b>unknown</b>([<i>value</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/ordinal.js), [Examples](https://observablehq.com/@d3/d3-scaleordinal)
If *value* is specified, sets the output value of the scale for unknown input values and returns this scale. If *value* is not specified, returns the current unknown value, which defaults to [implicit](#scaleImplicit). The implicit value enables implicit domain construction; see [*ordinal*.domain](#ordinal_domain).
<a name="ordinal_copy" href="#ordinal_copy">#</a> <i>ordinal</i>.<b>copy</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/ordinal.js), [Examples](https://observablehq.com/@d3/d3-scaleordinal)
Returns an exact copy of this ordinal scale. Changes to this scale will not affect the returned scale, and vice versa.
<a name="scaleImplicit" href="#scaleImplicit">#</a> d3.<b>scaleImplicit</b> · [Source](https://github.com/d3/d3-scale/blob/master/src/ordinal.js), [Examples](https://observablehq.com/@d3/d3-scaleordinal)
A special value for [*ordinal*.unknown](#ordinal_unknown) that enables implicit domain construction: unknown values are implicitly added to the domain.
#### Band Scales
Band scales are like [ordinal scales](#ordinal-scales) except the output range is continuous and numeric. Discrete output values are automatically computed by the scale by dividing the continuous range into uniform bands. Band scales are typically used for bar charts with an ordinal or categorical dimension. The [unknown value](#ordinal_unknown) of a band scale is effectively undefined: they do not allow implicit domain construction.
<img src="https://raw.githubusercontent.com/d3/d3-scale/master/img/band.png" width="751" height="238" alt="band">
<a name="scaleBand" href="#scaleBand">#</a> d3.<b>scaleBand</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scaleband)
Constructs a new band scale with the specified [*domain*](#band_domain) and [*range*](#band_range), no [padding](#band_padding), no [rounding](#band_round) and center [alignment](#band_align). If *domain* is not specified, it defaults to the empty domain. If *range* is not specified, it defaults to the unit range [0, 1].
<a name="_band" href="#_band">#</a> <i>band</i>(*value*) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scaleband)
Given a *value* in the input [domain](#band_domain), returns the start of the corresponding band derived from the output [range](#band_range). If the given *value* is not in the scale’s domain, returns undefined.
<a name="band_domain" href="#band_domain">#</a> <i>band</i>.<b>domain</b>([<i>domain</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scaleband)
If *domain* is specified, sets the domain to the specified array of values. The first element in *domain* will be mapped to the first band, the second domain value to the second band, and so on. Domain values are stored internally in an [InternMap](https://github.com/mbostock/internmap) from primitive value to index; the resulting index is then used to determine the band. Thus, a band scale’s values must be coercible to a primitive value, and the primitive domain value uniquely identifies the corresponding band. If *domain* is not specified, this method returns the current domain.
<a name="band_range" href="#band_range">#</a> <i>band</i>.<b>range</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scaleband)
If *range* is specified, sets the scale’s range to the specified two-element array of numbers. If the elements in the given array are not numbers, they will be coerced to numbers. If *range* is not specified, returns the scale’s current range, which defaults to [0, 1].
<a name="band_rangeRound" href="#band_rangeRound">#</a> <i>band</i>.<b>rangeRound</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scaleband)
Sets the scale’s [*range*](#band_range) to the specified two-element array of numbers while also enabling [rounding](#band_round). This is a convenience method equivalent to:
```js
band
.range(range)
.round(true);
```
Rounding is sometimes useful for avoiding antialiasing artifacts, though also consider the [shape-rendering](https://developer.mozilla.org/en-US/docs/Web/SVG/Attribute/shape-rendering) “crispEdges” styles.
<a name="band_round" href="#band_round">#</a> <i>band</i>.<b>round</b>([<i>round</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scaleband)
If *round* is specified, enables or disables rounding accordingly. If rounding is enabled, the start and stop of each band will be integers. Rounding is sometimes useful for avoiding antialiasing artifacts, though also consider the [shape-rendering](https://developer.mozilla.org/en-US/docs/Web/SVG/Attribute/shape-rendering) “crispEdges” styles. Note that if the width of the domain is not a multiple of the cardinality of the range, there may be leftover unused space, even without padding! Use [*band*.align](#band_align) to specify how the leftover space is distributed.
<a name="band_paddingInner" href="#band_paddingInner">#</a> <i>band</i>.<b>paddingInner</b>([<i>padding</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scaleband)
If *padding* is specified, sets the inner padding to the specified number which must be less than or equal to 1. If *padding* is not specified, returns the current inner padding which defaults to 0. The inner padding specifies the proportion of the range that is reserved for blank space between bands; a value of 0 means no blank space between bands, and a value of 1 means a [bandwidth](#band_bandwidth) of zero.
<a name="band_paddingOuter" href="#band_paddingOuter">#</a> <i>band</i>.<b>paddingOuter</b>([<i>padding</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scaleband)
If *padding* is specified, sets the outer padding to the specified number which is typically in the range [0, 1]. If *padding* is not specified, returns the current outer padding which defaults to 0. The outer padding specifies the amount of blank space, in terms of multiples of the [step](#band_step), to reserve before the first band and after the last band.
<a name="band_padding" href="#band_padding">#</a> <i>band</i>.<b>padding</b>([<i>padding</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scaleband)
A convenience method for setting the [inner](#band_paddingInner) and [outer](#band_paddingOuter) padding to the same *padding* value. If *padding* is not specified, returns the inner padding.
<a name="band_align" href="#band_align">#</a> <i>band</i>.<b>align</b>([<i>align</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scaleband)
If *align* is specified, sets the alignment to the specified value which must be in the range [0, 1]. If *align* is not specified, returns the current alignment which defaults to 0.5. The alignment specifies how outer padding is distributed in the range. A value of 0.5 indicates that the outer padding should be equally distributed before the first band and after the last band; *i.e.*, the bands should be centered within the range. A value of 0 or 1 may be used to shift the bands to one side, say to position them adjacent to an axis. For more, [see this explainer](https://observablehq.com/@d3/band-align).
<a name="band_bandwidth" href="#band_bandwidth">#</a> <i>band</i>.<b>bandwidth</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scaleband)
Returns the width of each band.
<a name="band_step" href="#band_step">#</a> <i>band</i>.<b>step</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scaleband)
Returns the distance between the starts of adjacent bands.
<a name="band_copy" href="#band_copy">#</a> <i>band</i>.<b>copy</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scaleband)
Returns an exact copy of this scale. Changes to this scale will not affect the returned scale, and vice versa.
#### Point Scales
Point scales are a variant of [band scales](#band-scales) with the bandwidth fixed to zero. Point scales are typically used for scatterplots with an ordinal or categorical dimension. The [unknown value](#ordinal_unknown) of a point scale is always undefined: they do not allow implicit domain construction.
<img src="https://raw.githubusercontent.com/d3/d3-scale/master/img/point.png" width="648" height="155" alt="point">
<a name="scalePoint" href="#scalePoint">#</a> d3.<b>scalePoint</b>([[<i>domain</i>, ]<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scalepoint)
Constructs a new point scale with the specified [*domain*](#point_domain) and [*range*](#point_range), no [padding](#point_padding), no [rounding](#point_round) and center [alignment](#point_align). If *domain* is not specified, it defaults to the empty domain. If *range* is not specified, it defaults to the unit range [0, 1].
<a name="_point" href="#_point">#</a> <i>point</i>(*value*) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scalepoint)
Given a *value* in the input [domain](#point_domain), returns the corresponding point derived from the output [range](#point_range). If the given *value* is not in the scale’s domain, returns undefined.
<a name="point_domain" href="#point_domain">#</a> <i>point</i>.<b>domain</b>([<i>domain</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scalepoint)
If *domain* is specified, sets the domain to the specified array of values. The first element in *domain* will be mapped to the first point, the second domain value to the second point, and so on. Domain values are stored internally in an [InternMap](https://github.com/mbostock/internmap) from primitive value to index; the resulting index is then used to determine the point. Thus, a point scale’s values must be coercible to a primitive value, and the primitive domain value uniquely identifies the corresponding point. If *domain* is not specified, this method returns the current domain.
<a name="point_range" href="#point_range">#</a> <i>point</i>.<b>range</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scalepoint)
If *range* is specified, sets the scale’s range to the specified two-element array of numbers. If the elements in the given array are not numbers, they will be coerced to numbers. If *range* is not specified, returns the scale’s current range, which defaults to [0, 1].
<a name="point_rangeRound" href="#point_rangeRound">#</a> <i>point</i>.<b>rangeRound</b>([<i>range</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scalepoint)
Sets the scale’s [*range*](#point_range) to the specified two-element array of numbers while also enabling [rounding](#point_round). This is a convenience method equivalent to:
```js
point
.range(range)
.round(true);
```
Rounding is sometimes useful for avoiding antialiasing artifacts, though also consider the [shape-rendering](https://developer.mozilla.org/en-US/docs/Web/SVG/Attribute/shape-rendering) “crispEdges” styles.
<a name="point_round" href="#point_round">#</a> <i>point</i>.<b>round</b>([<i>round</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scalepoint)
If *round* is specified, enables or disables rounding accordingly. If rounding is enabled, the position of each point will be integers. Rounding is sometimes useful for avoiding antialiasing artifacts, though also consider the [shape-rendering](https://developer.mozilla.org/en-US/docs/Web/SVG/Attribute/shape-rendering) “crispEdges” styles. Note that if the width of the domain is not a multiple of the cardinality of the range, there may be leftover unused space, even without padding! Use [*point*.align](#point_align) to specify how the leftover space is distributed.
<a name="point_padding" href="#point_padding">#</a> <i>point</i>.<b>padding</b>([<i>padding</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scalepoint)
If *padding* is specified, sets the outer padding to the specified number which is typically in the range [0, 1]. If *padding* is not specified, returns the current outer padding which defaults to 0. The outer padding specifies the amount of blank space, in terms of multiples of the [step](#band_step), to reserve before the first point and after the last point. Equivalent to [*band*.paddingOuter](#band_paddingOuter).
<a name="point_align" href="#point_align">#</a> <i>point</i>.<b>align</b>([<i>align</i>]) · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scalepoint)
If *align* is specified, sets the alignment to the specified value which must be in the range [0, 1]. If *align* is not specified, returns the current alignment which defaults to 0.5. The alignment specifies how any leftover unused space in the range is distributed. A value of 0.5 indicates that the leftover space should be equally distributed before the first point and after the last point; *i.e.*, the points should be centered within the range. A value of 0 or 1 may be used to shift the points to one side, say to position them adjacent to an axis.
<a name="point_bandwidth" href="#point_bandwidth">#</a> <i>point</i>.<b>bandwidth</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scalepoint)
Returns zero.
<a name="point_step" href="#point_step">#</a> <i>point</i>.<b>step</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scalepoint)
Returns the distance between the starts of adjacent points.
<a name="point_copy" href="#point_copy">#</a> <i>point</i>.<b>copy</b>() · [Source](https://github.com/d3/d3-scale/blob/master/src/band.js), [Examples](https://observablehq.com/@d3/d3-scalepoint)
Returns an exact copy of this scale. Changes to this scale will not affect the returned scale, and vice versa. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/d3-scale/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/d3-scale/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 91249
} |
# d3-shape
Visualizations typically consist of discrete graphical marks, such as [symbols](#symbols), [arcs](#arcs), [lines](#lines) and [areas](#areas). While the rectangles of a bar chart may be easy enough to generate directly using [SVG](http://www.w3.org/TR/SVG/paths.html#PathData) or [Canvas](http://www.w3.org/TR/2dcontext/#canvaspathmethods), other shapes are complex, such as rounded annular sectors and centripetal Catmull–Rom splines. This module provides a variety of shape generators for your convenience.
As with other aspects of D3, these shapes are driven by data: each shape generator exposes accessors that control how the input data are mapped to a visual representation. For example, you might define a line generator for a time series by [scaling](https://github.com/d3/d3-scale) fields of your data to fit the chart:
```js
const line = d3.line()
.x(d => x(d.date))
.y(d => y(d.value));
```
This line generator can then be used to compute the `d` attribute of an SVG path element:
```js
path.datum(data).attr("d", line);
```
Or you can use it to render to a Canvas 2D context:
```js
line.context(context)(data);
```
For more, read [Introducing d3-shape](https://medium.com/@mbostock/introducing-d3-shape-73f8367e6d12).
## Installing
If you use npm, `npm install d3-shape`. You can also download the [latest release on GitHub](https://github.com/d3/d3-shape/releases/latest). For vanilla HTML in modern browsers, import d3-shape from jsDelivr:
```html
<script type="module">
import {line} from "https://cdn.jsdelivr.net/npm/d3-shape@3/+esm";
const l = line();
</script>
```
For legacy environments, you can load d3-shape’s UMD bundle; a `d3` global is exported:
```html
<script src="https://cdn.jsdelivr.net/npm/d3-path@3"></script>
<script src="https://cdn.jsdelivr.net/npm/d3-shape@3"></script>
<script>
const l = d3.line();
</script>
```
## API Reference
* [Arcs](#arcs)
* [Pies](#pies)
* [Lines](#lines)
* [Areas](#areas)
* [Curves](#curves)
* [Custom Curves](#custom-curves)
* [Links](#links)
* [Symbols](#symbols)
* [Custom Symbol Types](#custom-symbol-types)
* [Stacks](#stacks)
Note: all the methods that accept arrays also accept iterables and convert them to arrays internally.
### Arcs
[<img alt="Pie Chart" src="./img/pie.png" width="295" height="295">](https://observablehq.com/@d3/pie-chart)[<img alt="Donut Chart" src="./img/donut.png" width="295" height="295">](https://observablehq.com/@d3/donut-chart)
The arc generator produces a [circular](https://en.wikipedia.org/wiki/Circular_sector) or [annular](https://en.wikipedia.org/wiki/Annulus_\(mathematics\)) sector, as in a pie or donut chart. If the absolute difference between the [start](#arc_startAngle) and [end](#arc_endAngle) angles (the *angular span*) is greater than [τ](https://en.wikipedia.org/wiki/Turn_\(geometry\)#Tau_proposal), the arc generator will produce a complete circle or annulus. If it is less than τ, the arc’s angular length will be equal to the absolute difference between the two angles (going clockwise if the signed difference is positive and anticlockwise if it is negative). If the absolute difference is less than τ, the arc may have [rounded corners](#arc_cornerRadius) and [angular padding](#arc_padAngle). Arcs are always centered at ⟨0,0⟩; use a transform (see: [SVG](http://www.w3.org/TR/SVG/coords.html#TransformAttribute), [Canvas](http://www.w3.org/TR/2dcontext/#transformations)) to move the arc to a different position.
See also the [pie generator](#pies), which computes the necessary angles to represent an array of data as a pie or donut chart; these angles can then be passed to an arc generator.
<a name="arc" href="#arc">#</a> d3.<b>arc</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/arc.js)
Constructs a new arc generator with the default settings.
<a name="_arc" href="#_arc">#</a> <i>arc</i>(<i>arguments…</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/arc.js)
Generates an arc for the given *arguments*. The *arguments* are arbitrary; they are simply propagated to the arc generator’s accessor functions along with the `this` object. For example, with the default settings, an object with radii and angles is expected:
```js
const arc = d3.arc();
arc({
innerRadius: 0,
outerRadius: 100,
startAngle: 0,
endAngle: Math.PI / 2
}); // "M0,-100A100,100,0,0,1,100,0L0,0Z"
```
If the radii and angles are instead defined as constants, you can generate an arc without any arguments:
```js
const arc = d3.arc()
.innerRadius(0)
.outerRadius(100)
.startAngle(0)
.endAngle(Math.PI / 2);
arc(); // "M0,-100A100,100,0,0,1,100,0L0,0Z"
```
If the arc generator has a [context](#arc_context), then the arc is rendered to this context as a sequence of [path method](http://www.w3.org/TR/2dcontext/#canvaspathmethods) calls and this function returns void. Otherwise, a [path data](http://www.w3.org/TR/SVG/paths.html#PathData) string is returned.
<a name="arc_centroid" href="#arc_centroid">#</a> <i>arc</i>.<b>centroid</b>(<i>arguments…</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/arc.js)
Computes the midpoint [*x*, *y*] of the center line of the arc that would be [generated](#_arc) by the given *arguments*. The *arguments* are arbitrary; they are simply propagated to the arc generator’s accessor functions along with the `this` object. To be consistent with the generated arc, the accessors must be deterministic, *i.e.*, return the same value given the same arguments. The midpoint is defined as ([startAngle](#arc_startAngle) + [endAngle](#arc_endAngle)) / 2 and ([innerRadius](#arc_innerRadius) + [outerRadius](#arc_outerRadius)) / 2. For example:
[<img alt="Circular Sector Centroids" src="./img/centroid-circular-sector.png" width="250" height="250">](https://observablehq.com/@d3/pie-settings)[<img alt="Annular Sector Centroids" src="./img/centroid-annular-sector.png" width="250" height="250">](https://observablehq.com/@d3/pie-settings)
Note that this is **not the geometric center** of the arc, which may be outside the arc; this method is merely a convenience for positioning labels.
<a name="arc_innerRadius" href="#arc_innerRadius">#</a> <i>arc</i>.<b>innerRadius</b>([<i>radius</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/arc.js)
If *radius* is specified, sets the inner radius to the specified function or number and returns this arc generator. If *radius* is not specified, returns the current inner radius accessor, which defaults to:
```js
function innerRadius(d) {
return d.innerRadius;
}
```
Specifying the inner radius as a function is useful for constructing a stacked polar bar chart, often in conjunction with a [sqrt scale](https://github.com/d3/d3-scale#sqrt). More commonly, a constant inner radius is used for a donut or pie chart. If the outer radius is smaller than the inner radius, the inner and outer radii are swapped. A negative value is treated as zero.
<a name="arc_outerRadius" href="#arc_outerRadius">#</a> <i>arc</i>.<b>outerRadius</b>([<i>radius</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/arc.js)
If *radius* is specified, sets the outer radius to the specified function or number and returns this arc generator. If *radius* is not specified, returns the current outer radius accessor, which defaults to:
```js
function outerRadius(d) {
return d.outerRadius;
}
```
Specifying the outer radius as a function is useful for constructing a coxcomb or polar bar chart, often in conjunction with a [sqrt scale](https://github.com/d3/d3-scale#sqrt). More commonly, a constant outer radius is used for a pie or donut chart. If the outer radius is smaller than the inner radius, the inner and outer radii are swapped. A negative value is treated as zero.
<a name="arc_cornerRadius" href="#arc_cornerRadius">#</a> <i>arc</i>.<b>cornerRadius</b>([<i>radius</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/arc.js)
If *radius* is specified, sets the corner radius to the specified function or number and returns this arc generator. If *radius* is not specified, returns the current corner radius accessor, which defaults to:
```js
function cornerRadius() {
return 0;
}
```
If the corner radius is greater than zero, the corners of the arc are rounded using circles of the given radius. For a circular sector, the two outer corners are rounded; for an annular sector, all four corners are rounded. The corner circles are shown in this diagram:
[<img alt="Rounded Circular Sectors" src="./img/rounded-circular-sector.png" width="250" height="250">](https://observablehq.com/@d3/pie-settings)[<img alt="Rounded Annular Sectors" src="./img/rounded-annular-sector.png" width="250" height="250">](https://observablehq.com/@d3/pie-settings)
The corner radius may not be larger than ([outerRadius](#arc_outerRadius) - [innerRadius](#arc_innerRadius)) / 2. In addition, for arcs whose angular span is less than π, the corner radius may be reduced as two adjacent rounded corners intersect. This is occurs more often with the inner corners. See the [arc corners animation](https://observablehq.com/@d3/arc-corners) for illustration.
<a name="arc_startAngle" href="#arc_startAngle">#</a> <i>arc</i>.<b>startAngle</b>([<i>angle</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/arc.js)
If *angle* is specified, sets the start angle to the specified function or number and returns this arc generator. If *angle* is not specified, returns the current start angle accessor, which defaults to:
```js
function startAngle(d) {
return d.startAngle;
}
```
The *angle* is specified in radians, with 0 at -*y* (12 o’clock) and positive angles proceeding clockwise. If |endAngle - startAngle| ≥ τ, a complete circle or annulus is generated rather than a sector.
<a name="arc_endAngle" href="#arc_endAngle">#</a> <i>arc</i>.<b>endAngle</b>([<i>angle</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/arc.js)
If *angle* is specified, sets the end angle to the specified function or number and returns this arc generator. If *angle* is not specified, returns the current end angle accessor, which defaults to:
```js
function endAngle(d) {
return d.endAngle;
}
```
The *angle* is specified in radians, with 0 at -*y* (12 o’clock) and positive angles proceeding clockwise. If |endAngle - startAngle| ≥ τ, a complete circle or annulus is generated rather than a sector.
<a name="arc_padAngle" href="#arc_padAngle">#</a> <i>arc</i>.<b>padAngle</b>([<i>angle</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/arc.js)
If *angle* is specified, sets the pad angle to the specified function or number and returns this arc generator. If *angle* is not specified, returns the current pad angle accessor, which defaults to:
```js
function padAngle() {
return d && d.padAngle;
}
```
The pad angle is converted to a fixed linear distance separating adjacent arcs, defined as [padRadius](#arc_padRadius) * padAngle. This distance is subtracted equally from the [start](#arc_startAngle) and [end](#arc_endAngle) of the arc. If the arc forms a complete circle or annulus, as when |endAngle - startAngle| ≥ τ, the pad angle is ignored.
If the [inner radius](#arc_innerRadius) or angular span is small relative to the pad angle, it may not be possible to maintain parallel edges between adjacent arcs. In this case, the inner edge of the arc may collapse to a point, similar to a circular sector. For this reason, padding is typically only applied to annular sectors (*i.e.*, when innerRadius is positive), as shown in this diagram:
[<img alt="Padded Circular Sectors" src="./img/padded-circular-sector.png" width="250" height="250">](https://observablehq.com/@d3/pie-settings)[<img alt="Padded Annular Sectors" src="./img/padded-annular-sector.png" width="250" height="250">](https://observablehq.com/@d3/pie-settings)
The recommended minimum inner radius when using padding is outerRadius \* padAngle / sin(θ), where θ is the angular span of the smallest arc before padding. For example, if the outer radius is 200 pixels and the pad angle is 0.02 radians, a reasonable θ is 0.04 radians, and a reasonable inner radius is 100 pixels. See the [arc padding animation](https://observablehq.com/@d3/arc-pad-angle) for illustration.
Often, the pad angle is not set directly on the arc generator, but is instead computed by the [pie generator](#pies) so as to ensure that the area of padded arcs is proportional to their value; see [*pie*.padAngle](#pie_padAngle). See the [pie padding animation](https://observablehq.com/@d3/arc-pad-angle) for illustration. If you apply a constant pad angle to the arc generator directly, it tends to subtract disproportionately from smaller arcs, introducing distortion.
<a name="arc_padRadius" href="#arc_padRadius">#</a> <i>arc</i>.<b>padRadius</b>([<i>radius</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/arc.js)
If *radius* is specified, sets the pad radius to the specified function or number and returns this arc generator. If *radius* is not specified, returns the current pad radius accessor, which defaults to null, indicating that the pad radius should be automatically computed as sqrt([innerRadius](#arc_innerRadius) * innerRadius + [outerRadius](#arc_outerRadius) * outerRadius). The pad radius determines the fixed linear distance separating adjacent arcs, defined as padRadius * [padAngle](#arc_padAngle).
<a name="arc_context" href="#arc_context">#</a> <i>arc</i>.<b>context</b>([<i>context</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/arc.js)
If *context* is specified, sets the context and returns this arc generator. If *context* is not specified, returns the current context, which defaults to null. If the context is not null, then the [generated arc](#_arc) is rendered to this context as a sequence of [path method](http://www.w3.org/TR/2dcontext/#canvaspathmethods) calls. Otherwise, a [path data](http://www.w3.org/TR/SVG/paths.html#PathData) string representing the generated arc is returned.
<a name="arc_digits" href="#arc_digits">#</a> <i>arc</i>.<b>digits</b>([<i>digits</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/arc.js)
If *digits* is specified, sets the maximum number of digits after the decimal separator and returns this arc generator. If *digits* is not specified, returns the current maximum fraction digits, which defaults to 3. This option only applies when the associated [*context*](#arc_context) is null, as when this arc generator is used to produce [path data](http://www.w3.org/TR/SVG/paths.html#PathData).
### Pies
The pie generator does not produce a shape directly, but instead computes the necessary angles to represent a tabular dataset as a pie or donut chart; these angles can then be passed to an [arc generator](#arcs).
<a name="pie" href="#pie">#</a> d3.<b>pie</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/pie.js)
Constructs a new pie generator with the default settings.
<a name="_pie" href="#_pie">#</a> <i>pie</i>(<i>data</i>[, <i>arguments…</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/pie.js)
Generates a pie for the given array of *data*, returning an array of objects representing each datum’s arc angles. Any additional *arguments* are arbitrary; they are simply propagated to the pie generator’s accessor functions along with the `this` object. The length of the returned array is the same as *data*, and each element *i* in the returned array corresponds to the element *i* in the input data. Each object in the returned array has the following properties:
* `data` - the input datum; the corresponding element in the input data array.
* `value` - the numeric [value](#pie_value) of the arc.
* `index` - the zero-based [sorted index](#pie_sort) of the arc.
* `startAngle` - the [start angle](#pie_startAngle) of the arc.
* `endAngle` - the [end angle](#pie_endAngle) of the arc.
* `padAngle` - the [pad angle](#pie_padAngle) of the arc.
This representation is designed to work with the arc generator’s default [startAngle](#arc_startAngle), [endAngle](#arc_endAngle) and [padAngle](#arc_padAngle) accessors. The angular units are arbitrary, but if you plan to use the pie generator in conjunction with an [arc generator](#arcs), you should specify angles in radians, with 0 at -*y* (12 o’clock) and positive angles proceeding clockwise.
Given a small dataset of numbers, here is how to compute the arc angles to render this data as a pie chart:
```js
const data = [1, 1, 2, 3, 5, 8, 13, 21];
const arcs = d3.pie()(data);
```
The first pair of parens, `pie()`, [constructs](#pie) a default pie generator. The second, `pie()(data)`, [invokes](#_pie) this generator on the dataset, returning an array of objects:
```json
[
{"data": 1, "value": 1, "index": 6, "startAngle": 6.050474740247008, "endAngle": 6.166830023713296, "padAngle": 0},
{"data": 1, "value": 1, "index": 7, "startAngle": 6.166830023713296, "endAngle": 6.283185307179584, "padAngle": 0},
{"data": 2, "value": 2, "index": 5, "startAngle": 5.817764173314431, "endAngle": 6.050474740247008, "padAngle": 0},
{"data": 3, "value": 3, "index": 4, "startAngle": 5.468698322915565, "endAngle": 5.817764173314431, "padAngle": 0},
{"data": 5, "value": 5, "index": 3, "startAngle": 4.886921905584122, "endAngle": 5.468698322915565, "padAngle": 0},
{"data": 8, "value": 8, "index": 2, "startAngle": 3.956079637853813, "endAngle": 4.886921905584122, "padAngle": 0},
{"data": 13, "value": 13, "index": 1, "startAngle": 2.443460952792061, "endAngle": 3.956079637853813, "padAngle": 0},
{"data": 21, "value": 21, "index": 0, "startAngle": 0.000000000000000, "endAngle": 2.443460952792061, "padAngle": 0}
]
```
Note that the returned array is in the same order as the data, even though this pie chart is [sorted](#pie_sortValues) by descending value, starting with the arc for the last datum (value 21) at 12 o’clock.
<a name="pie_value" href="#pie_value">#</a> <i>pie</i>.<b>value</b>([<i>value</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/pie.js)
If *value* is specified, sets the value accessor to the specified function or number and returns this pie generator. If *value* is not specified, returns the current value accessor, which defaults to:
```js
function value(d) {
return d;
}
```
When a pie is [generated](#_pie), the value accessor will be invoked for each element in the input data array, being passed the element `d`, the index `i`, and the array `data` as three arguments. The default value accessor assumes that the input data are numbers, or that they are coercible to numbers using [valueOf](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/valueOf). If your data are not simply numbers, then you should specify an accessor that returns the corresponding numeric value for a given datum. For example:
```js
const data = [
{"number": 4, "name": "Locke"},
{"number": 8, "name": "Reyes"},
{"number": 15, "name": "Ford"},
{"number": 16, "name": "Jarrah"},
{"number": 23, "name": "Shephard"},
{"number": 42, "name": "Kwon"}
];
const arcs = d3.pie()
.value(d => d.number)
(data);
```
This is similar to [mapping](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map) your data to values before invoking the pie generator:
```js
const arcs = d3.pie()(data.map(d => d.number));
```
The benefit of an accessor is that the input data remains associated with the returned objects, thereby making it easier to access other fields of the data, for example to set the color or to add text labels.
<a name="pie_sort" href="#pie_sort">#</a> <i>pie</i>.<b>sort</b>([<i>compare</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/pie.js)
If *compare* is specified, sets the data comparator to the specified function and returns this pie generator. If *compare* is not specified, returns the current data comparator, which defaults to null. If both the data comparator and the value comparator are null, then arcs are positioned in the original input order. Otherwise, the data is sorted according to the data comparator, and the resulting order is used. Setting the data comparator implicitly sets the [value comparator](#pie_sortValues) to null.
The *compare* function takes two arguments *a* and *b*, each elements from the input data array. If the arc for *a* should be before the arc for *b*, then the comparator must return a number less than zero; if the arc for *a* should be after the arc for *b*, then the comparator must return a number greater than zero; returning zero means that the relative order of *a* and *b* is unspecified. For example, to sort arcs by their associated name:
```js
pie.sort((a, b) => a.name.localeCompare(b.name));
```
Sorting does not affect the order of the [generated arc array](#_pie) which is always in the same order as the input data array; it merely affects the computed angles of each arc. The first arc starts at the [start angle](#pie_startAngle) and the last arc ends at the [end angle](#pie_endAngle).
<a name="pie_sortValues" href="#pie_sortValues">#</a> <i>pie</i>.<b>sortValues</b>([<i>compare</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/pie.js)
If *compare* is specified, sets the value comparator to the specified function and returns this pie generator. If *compare* is not specified, returns the current value comparator, which defaults to descending value. The default value comparator is implemented as:
```js
function compare(a, b) {
return b - a;
}
```
If both the data comparator and the value comparator are null, then arcs are positioned in the original input order. Otherwise, the data is sorted according to the data comparator, and the resulting order is used. Setting the value comparator implicitly sets the [data comparator](#pie_sort) to null.
The value comparator is similar to the [data comparator](#pie_sort), except the two arguments *a* and *b* are values derived from the input data array using the [value accessor](#pie_value), not the data elements. If the arc for *a* should be before the arc for *b*, then the comparator must return a number less than zero; if the arc for *a* should be after the arc for *b*, then the comparator must return a number greater than zero; returning zero means that the relative order of *a* and *b* is unspecified. For example, to sort arcs by ascending value:
```js
pie.sortValues((a, b) => a - b);
```
Sorting does not affect the order of the [generated arc array](#_pie) which is always in the same order as the input data array; it merely affects the computed angles of each arc. The first arc starts at the [start angle](#pie_startAngle) and the last arc ends at the [end angle](#pie_endAngle).
<a name="pie_startAngle" href="#pie_startAngle">#</a> <i>pie</i>.<b>startAngle</b>([<i>angle</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/pie.js)
If *angle* is specified, sets the overall start angle of the pie to the specified function or number and returns this pie generator. If *angle* is not specified, returns the current start angle accessor, which defaults to:
```js
function startAngle() {
return 0;
}
```
The start angle here means the *overall* start angle of the pie, *i.e.*, the start angle of the first arc. The start angle accessor is invoked once, being passed the same arguments and `this` context as the [pie generator](#_pie). The units of *angle* are arbitrary, but if you plan to use the pie generator in conjunction with an [arc generator](#arcs), you should specify an angle in radians, with 0 at -*y* (12 o’clock) and positive angles proceeding clockwise.
<a name="pie_endAngle" href="#pie_endAngle">#</a> <i>pie</i>.<b>endAngle</b>([<i>angle</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/pie.js)
If *angle* is specified, sets the overall end angle of the pie to the specified function or number and returns this pie generator. If *angle* is not specified, returns the current end angle accessor, which defaults to:
```js
function endAngle() {
return 2 * Math.PI;
}
```
The end angle here means the *overall* end angle of the pie, *i.e.*, the end angle of the last arc. The end angle accessor is invoked once, being passed the same arguments and `this` context as the [pie generator](#_pie). The units of *angle* are arbitrary, but if you plan to use the pie generator in conjunction with an [arc generator](#arcs), you should specify an angle in radians, with 0 at -*y* (12 o’clock) and positive angles proceeding clockwise.
The value of the end angle is constrained to [startAngle](#pie_startAngle) ± τ, such that |endAngle - startAngle| ≤ τ.
<a name="pie_padAngle" href="#pie_padAngle">#</a> <i>pie</i>.<b>padAngle</b>([<i>angle</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/pie.js)
If *angle* is specified, sets the pad angle to the specified function or number and returns this pie generator. If *angle* is not specified, returns the current pad angle accessor, which defaults to:
```js
function padAngle() {
return 0;
}
```
The pad angle here means the angular separation between each adjacent arc. The total amount of padding reserved is the specified *angle* times the number of elements in the input data array, and at most |endAngle - startAngle|; the remaining space is then divided proportionally by [value](#pie_value) such that the relative area of each arc is preserved. See the [pie padding animation](https://observablehq.com/@d3/arc-pad-angle) for illustration. The pad angle accessor is invoked once, being passed the same arguments and `this` context as the [pie generator](#_pie). The units of *angle* are arbitrary, but if you plan to use the pie generator in conjunction with an [arc generator](#arcs), you should specify an angle in radians.
### Lines
[<img width="295" height="154" alt="Line Chart" src="./img/line.png">](https://observablehq.com/@d3/line-chart)
The line generator produces a [spline](https://en.wikipedia.org/wiki/Spline_\(mathematics\)) or [polyline](https://en.wikipedia.org/wiki/Polygonal_chain), as in a line chart. Lines also appear in many other visualization types, such as the links in [hierarchical edge bundling](https://observablehq.com/@d3/hierarchical-edge-bundling).
<a name="line" href="#line">#</a> d3.<b>line</b>([<i>x</i>][, <i>y</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/line.js), [Examples](https://observablehq.com/@d3/d3-line)
Constructs a new line generator with the default settings. If *x* or *y* are specified, sets the corresponding accessors to the specified function or number and returns this line generator.
<a name="_line" href="#_line">#</a> <i>line</i>(<i>data</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/line.js), [Examples](https://observablehq.com/@d3/d3-line)
Generates a line for the given array of *data*. Depending on this line generator’s associated [curve](#line_curve), the given input *data* may need to be sorted by *x*-value before being passed to the line generator. If the line generator has a [context](#line_context), then the line is rendered to this context as a sequence of [path method](http://www.w3.org/TR/2dcontext/#canvaspathmethods) calls and this function returns void. Otherwise, a [path data](http://www.w3.org/TR/SVG/paths.html#PathData) string is returned.
<a name="line_x" href="#line_x">#</a> <i>line</i>.<b>x</b>([<i>x</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/line.js), [Examples](https://observablehq.com/@d3/d3-line)
If *x* is specified, sets the x accessor to the specified function or number and returns this line generator. If *x* is not specified, returns the current x accessor, which defaults to:
```js
function x(d) {
return d[0];
}
```
When a line is [generated](#_line), the x accessor will be invoked for each [defined](#line_defined) element in the input data array, being passed the element `d`, the index `i`, and the array `data` as three arguments. The default x accessor assumes that the input data are two-element arrays of numbers. If your data are in a different format, or if you wish to transform the data before rendering, then you should specify a custom accessor. For example, if `x` is a [time scale](https://github.com/d3/d3-scale#time-scales) and `y` is a [linear scale](https://github.com/d3/d3-scale#linear-scales):
```js
const data = [
{date: new Date(2007, 3, 24), value: 93.24},
{date: new Date(2007, 3, 25), value: 95.35},
{date: new Date(2007, 3, 26), value: 98.84},
{date: new Date(2007, 3, 27), value: 99.92},
{date: new Date(2007, 3, 30), value: 99.80},
{date: new Date(2007, 4, 1), value: 99.47},
…
];
const line = d3.line()
.x(d => x(d.date))
.y(d => y(d.value));
```
<a name="line_y" href="#line_y">#</a> <i>line</i>.<b>y</b>([<i>y</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/line.js), [Examples](https://observablehq.com/@d3/d3-line)
If *y* is specified, sets the y accessor to the specified function or number and returns this line generator. If *y* is not specified, returns the current y accessor, which defaults to:
```js
function y(d) {
return d[1];
}
```
When a line is [generated](#_line), the y accessor will be invoked for each [defined](#line_defined) element in the input data array, being passed the element `d`, the index `i`, and the array `data` as three arguments. The default y accessor assumes that the input data are two-element arrays of numbers. See [*line*.x](#line_x) for more information.
<a name="line_defined" href="#line_defined">#</a> <i>line</i>.<b>defined</b>([<i>defined</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/line.js), [Examples](https://observablehq.com/@d3/d3-line)
If *defined* is specified, sets the defined accessor to the specified function or boolean and returns this line generator. If *defined* is not specified, returns the current defined accessor, which defaults to:
```js
function defined() {
return true;
}
```
The default accessor thus assumes that the input data is always defined. When a line is [generated](#_line), the defined accessor will be invoked for each element in the input data array, being passed the element `d`, the index `i`, and the array `data` as three arguments. If the given element is defined (*i.e.*, if the defined accessor returns a truthy value for this element), the [x](#line_x) and [y](#line_y) accessors will subsequently be evaluated and the point will be added to the current line segment. Otherwise, the element will be skipped, the current line segment will be ended, and a new line segment will be generated for the next defined point. As a result, the generated line may have several discrete segments. For example:
[<img src="./img/line-defined.png" width="480" height="250" alt="Line with Missing Data">](https://observablehq.com/@d3/line-with-missing-data)
Note that if a line segment consists of only a single point, it may appear invisible unless rendered with rounded or square [line caps](https://developer.mozilla.org/en-US/docs/Web/SVG/Attribute/stroke-linecap). In addition, some curves such as [curveCardinalOpen](#curveCardinalOpen) only render a visible segment if it contains multiple points.
<a name="line_curve" href="#line_curve">#</a> <i>line</i>.<b>curve</b>([<i>curve</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/line.js), [Examples](https://observablehq.com/@d3/d3-line)
If *curve* is specified, sets the [curve factory](#curves) and returns this line generator. If *curve* is not specified, returns the current curve factory, which defaults to [curveLinear](#curveLinear).
<a name="line_context" href="#line_context">#</a> <i>line</i>.<b>context</b>([<i>context</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/line.js), [Examples](https://observablehq.com/@d3/d3-line)
If *context* is specified, sets the context and returns this line generator. If *context* is not specified, returns the current context, which defaults to null. If the context is not null, then the [generated line](#_line) is rendered to this context as a sequence of [path method](http://www.w3.org/TR/2dcontext/#canvaspathmethods) calls. Otherwise, a [path data](http://www.w3.org/TR/SVG/paths.html#PathData) string representing the generated line is returned.
<a name="line_digits" href="#line_digits">#</a> <i>line</i>.<b>digits</b>([<i>digits</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/line.js)
If *digits* is specified, sets the maximum number of digits after the decimal separator and returns this line generator. If *digits* is not specified, returns the current maximum fraction digits, which defaults to 3. This option only applies when the associated [*context*](#line_context) is null, as when this line generator is used to produce [path data](http://www.w3.org/TR/SVG/paths.html#PathData).
<a name="lineRadial" href="#lineRadial">#</a> d3.<b>lineRadial</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/lineRadial.js), [Examples](https://observablehq.com/@d3/d3-lineradial)
<img alt="Radial Line" width="250" height="250" src="./img/line-radial.png">
Constructs a new radial line generator with the default settings. A radial line generator is equivalent to the standard Cartesian [line generator](#line), except the [x](#line_x) and [y](#line_y) accessors are replaced with [angle](#lineRadial_angle) and [radius](#lineRadial_radius) accessors. Radial lines are always positioned relative to ⟨0,0⟩; use a transform (see: [SVG](http://www.w3.org/TR/SVG/coords.html#TransformAttribute), [Canvas](http://www.w3.org/TR/2dcontext/#transformations)) to change the origin.
<a name="_lineRadial" href="#_lineRadial">#</a> <i>lineRadial</i>(<i>data</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/lineRadial.js#L4), [Examples](https://observablehq.com/@d3/d3-lineradial)
Equivalent to [*line*](#_line).
<a name="lineRadial_angle" href="#lineRadial_angle">#</a> <i>lineRadial</i>.<b>angle</b>([<i>angle</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/lineRadial.js#L7), [Examples](https://observablehq.com/@d3/d3-lineradial)
Equivalent to [*line*.x](#line_x), except the accessor returns the angle in radians, with 0 at -*y* (12 o’clock).
<a name="lineRadial_radius" href="#lineRadial_radius">#</a> <i>lineRadial</i>.<b>radius</b>([<i>radius</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/lineRadial.js#L8), [Examples](https://observablehq.com/@d3/d3-lineradial)
Equivalent to [*line*.y](#line_y), except the accessor returns the radius: the distance from the origin ⟨0,0⟩.
<a name="lineRadial_defined" href="#lineRadial_defined">#</a> <i>lineRadial</i>.<b>defined</b>([<i>defined</i>])
Equivalent to [*line*.defined](#line_defined).
<a name="lineRadial_curve" href="#lineRadial_curve">#</a> <i>lineRadial</i>.<b>curve</b>([<i>curve</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/lineRadial.js), [Examples](https://observablehq.com/@d3/d3-lineradial)
Equivalent to [*line*.curve](#line_curve). Note that [curveMonotoneX](#curveMonotoneX) or [curveMonotoneY](#curveMonotoneY) are not recommended for radial lines because they assume that the data is monotonic in *x* or *y*, which is typically untrue of radial lines.
<a name="lineRadial_context" href="#lineRadial_context">#</a> <i>lineRadial</i>.<b>context</b>([<i>context</i>])
Equivalent to [*line*.context](#line_context).
### Areas
[<img alt="Area Chart" width="295" height="154" src="./img/area.png">](https://observablehq.com/@d3/area-chart)[<img alt="Stacked Area Chart" width="295" height="154" src="./img/area-stacked.png">](https://observablehq.com/@d3/stacked-area-chart)[<img alt="Difference Chart" width="295" height="154" src="./img/area-difference.png">](https://observablehq.com/@d3/difference-chart)
The area generator produces an area, as in an area chart. An area is defined by two bounding [lines](#lines), either splines or polylines. Typically, the two lines share the same [*x*-values](#area_x) ([x0](#area_x0) = [x1](#area_x1)), differing only in *y*-value ([y0](#area_y0) and [y1](#area_y1)); most commonly, y0 is defined as a constant representing [zero](http://www.vox.com/2015/11/19/9758062/y-axis-zero-chart). The first line (the <i>topline</i>) is defined by x1 and y1 and is rendered first; the second line (the <i>baseline</i>) is defined by x0 and y0 and is rendered second, with the points in reverse order. With a [curveLinear](#curveLinear) [curve](#area_curve), this produces a clockwise polygon.
<a name="area" href="#area">#</a> d3.<b>area</b>([<i>x</i>][, <i>y0</i>][, <i>y1</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
Constructs a new area generator with the default settings. If *x*, *y0* or *y1* are specified, sets the corresponding accessors to the specified function or number and returns this area generator.
<a name="_area" href="#_area">#</a> <i>area</i>(<i>data</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
Generates an area for the given array of *data*. Depending on this area generator’s associated [curve](#area_curve), the given input *data* may need to be sorted by *x*-value before being passed to the area generator. If the area generator has a [context](#line_context), then the area is rendered to this context as a sequence of [path method](http://www.w3.org/TR/2dcontext/#canvaspathmethods) calls and this function returns void. Otherwise, a [path data](http://www.w3.org/TR/SVG/paths.html#PathData) string is returned.
<a name="area_x" href="#area_x">#</a> <i>area</i>.<b>x</b>([<i>x</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
If *x* is specified, sets [x0](#area_x0) to *x* and [x1](#area_x1) to null and returns this area generator. If *x* is not specified, returns the current x0 accessor.
<a name="area_x0" href="#area_x0">#</a> <i>area</i>.<b>x0</b>([<i>x</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
If *x* is specified, sets the x0 accessor to the specified function or number and returns this area generator. If *x* is not specified, returns the current x0 accessor, which defaults to:
```js
function x(d) {
return d[0];
}
```
When an area is [generated](#_area), the x0 accessor will be invoked for each [defined](#area_defined) element in the input data array, being passed the element `d`, the index `i`, and the array `data` as three arguments. The default x0 accessor assumes that the input data are two-element arrays of numbers. If your data are in a different format, or if you wish to transform the data before rendering, then you should specify a custom accessor. For example, if `x` is a [time scale](https://github.com/d3/d3-scale#time-scales) and `y` is a [linear scale](https://github.com/d3/d3-scale#linear-scales):
```js
const data = [
{date: new Date(2007, 3, 24), value: 93.24},
{date: new Date(2007, 3, 25), value: 95.35},
{date: new Date(2007, 3, 26), value: 98.84},
{date: new Date(2007, 3, 27), value: 99.92},
{date: new Date(2007, 3, 30), value: 99.80},
{date: new Date(2007, 4, 1), value: 99.47},
…
];
const area = d3.area()
.x(d => x(d.date))
.y1(d => y(d.value))
.y0(y(0));
```
<a name="area_x1" href="#area_x1">#</a> <i>area</i>.<b>x1</b>([<i>x</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
If *x* is specified, sets the x1 accessor to the specified function or number and returns this area generator. If *x* is not specified, returns the current x1 accessor, which defaults to null, indicating that the previously-computed [x0](#area_x0) value should be reused for the x1 value.
When an area is [generated](#_area), the x1 accessor will be invoked for each [defined](#area_defined) element in the input data array, being passed the element `d`, the index `i`, and the array `data` as three arguments. See [*area*.x0](#area_x0) for more information.
<a name="area_y" href="#area_y">#</a> <i>area</i>.<b>y</b>([<i>y</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
If *y* is specified, sets [y0](#area_y0) to *y* and [y1](#area_y1) to null and returns this area generator. If *y* is not specified, returns the current y0 accessor.
<a name="area_y0" href="#area_y0">#</a> <i>area</i>.<b>y0</b>([<i>y</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
If *y* is specified, sets the y0 accessor to the specified function or number and returns this area generator. If *y* is not specified, returns the current y0 accessor, which defaults to:
```js
function y() {
return 0;
}
```
When an area is [generated](#_area), the y0 accessor will be invoked for each [defined](#area_defined) element in the input data array, being passed the element `d`, the index `i`, and the array `data` as three arguments. See [*area*.x0](#area_x0) for more information.
<a name="area_y1" href="#area_y1">#</a> <i>area</i>.<b>y1</b>([<i>y</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
If *y* is specified, sets the y1 accessor to the specified function or number and returns this area generator. If *y* is not specified, returns the current y1 accessor, which defaults to:
```js
function y(d) {
return d[1];
}
```
A null accessor is also allowed, indicating that the previously-computed [y0](#area_y0) value should be reused for the y1 value. When an area is [generated](#_area), the y1 accessor will be invoked for each [defined](#area_defined) element in the input data array, being passed the element `d`, the index `i`, and the array `data` as three arguments. See [*area*.x0](#area_x0) for more information.
<a name="area_defined" href="#area_defined">#</a> <i>area</i>.<b>defined</b>([<i>defined</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
If *defined* is specified, sets the defined accessor to the specified function or boolean and returns this area generator. If *defined* is not specified, returns the current defined accessor, which defaults to:
```js
function defined() {
return true;
}
```
The default accessor thus assumes that the input data is always defined. When an area is [generated](#_area), the defined accessor will be invoked for each element in the input data array, being passed the element `d`, the index `i`, and the array `data` as three arguments. If the given element is defined (*i.e.*, if the defined accessor returns a truthy value for this element), the [x0](#area_x0), [x1](#area_x1), [y0](#area_y0) and [y1](#area_y1) accessors will subsequently be evaluated and the point will be added to the current area segment. Otherwise, the element will be skipped, the current area segment will be ended, and a new area segment will be generated for the next defined point. As a result, the generated area may have several discrete segments. For example:
[<img src="./img/area-defined.png" width="480" height="250" alt="Area with Missing Data">](https://observablehq.com/@d3/area-with-missing-data)
Note that if an area segment consists of only a single point, it may appear invisible unless rendered with rounded or square [line caps](https://developer.mozilla.org/en-US/docs/Web/SVG/Attribute/stroke-linecap). In addition, some curves such as [curveCardinalOpen](#curveCardinalOpen) only render a visible segment if it contains multiple points.
<a name="area_curve" href="#area_curve">#</a> <i>area</i>.<b>curve</b>([<i>curve</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
If *curve* is specified, sets the [curve factory](#curves) and returns this area generator. If *curve* is not specified, returns the current curve factory, which defaults to [curveLinear](#curveLinear).
<a name="area_context" href="#area_context">#</a> <i>area</i>.<b>context</b>([<i>context</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
If *context* is specified, sets the context and returns this area generator. If *context* is not specified, returns the current context, which defaults to null. If the context is not null, then the [generated area](#_area) is rendered to this context as a sequence of [path method](http://www.w3.org/TR/2dcontext/#canvaspathmethods) calls. Otherwise, a [path data](http://www.w3.org/TR/SVG/paths.html#PathData) string representing the generated area is returned.
<a name="area_digits" href="#area_digits">#</a> <i>area</i>.<b>digits</b>([<i>digits</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
If *digits* is specified, sets the maximum number of digits after the decimal separator and returns this area generator. If *digits* is not specified, returns the current maximum fraction digits, which defaults to 3. This option only applies when the associated [*context*](#area_context) is null, as when this area generator is used to produce [path data](http://www.w3.org/TR/SVG/paths.html#PathData).
<a name="area_lineX0" href="#area_lineX0">#</a> <i>area</i>.<b>lineX0</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
<br><a name="area_lineY0" href="#area_lineY0">#</a> <i>area</i>.<b>lineY0</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
Returns a new [line generator](#lines) that has this area generator’s current [defined accessor](#area_defined), [curve](#area_curve) and [context](#area_context). The line’s [*x*-accessor](#line_x) is this area’s [*x0*-accessor](#area_x0), and the line’s [*y*-accessor](#line_y) is this area’s [*y0*-accessor](#area_y0).
<a name="area_lineX1" href="#area_lineX1">#</a> <i>area</i>.<b>lineX1</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
Returns a new [line generator](#lines) that has this area generator’s current [defined accessor](#area_defined), [curve](#area_curve) and [context](#area_context). The line’s [*x*-accessor](#line_x) is this area’s [*x1*-accessor](#area_x1), and the line’s [*y*-accessor](#line_y) is this area’s [*y0*-accessor](#area_y0).
<a name="area_lineY1" href="#area_lineY1">#</a> <i>area</i>.<b>lineY1</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/area.js)
Returns a new [line generator](#lines) that has this area generator’s current [defined accessor](#area_defined), [curve](#area_curve) and [context](#area_context). The line’s [*x*-accessor](#line_x) is this area’s [*x0*-accessor](#area_x0), and the line’s [*y*-accessor](#line_y) is this area’s [*y1*-accessor](#area_y1).
<a name="areaRadial" href="#areaRadial">#</a> d3.<b>areaRadial</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/areaRadial.js)
<img alt="Radial Area" width="250" height="250" src="./img/area-radial.png">
Constructs a new radial area generator with the default settings. A radial area generator is equivalent to the standard Cartesian [area generator](#area), except the [x](#area_x) and [y](#area_y) accessors are replaced with [angle](#areaRadial_angle) and [radius](#areaRadial_radius) accessors. Radial areas are always positioned relative to ⟨0,0⟩; use a transform (see: [SVG](http://www.w3.org/TR/SVG/coords.html#TransformAttribute), [Canvas](http://www.w3.org/TR/2dcontext/#transformations)) to change the origin.
<a name="_areaRadial" href="#_areaRadial">#</a> <i>areaRadial</i>(<i>data</i>)
Equivalent to [*area*](#_area).
<a name="areaRadial_angle" href="#areaRadial_angle">#</a> <i>areaRadial</i>.<b>angle</b>([<i>angle</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/areaRadial.js)
Equivalent to [*area*.x](#area_x), except the accessor returns the angle in radians, with 0 at -*y* (12 o’clock).
<a name="areaRadial_startAngle" href="#areaRadial_startAngle">#</a> <i>areaRadial</i>.<b>startAngle</b>([<i>angle</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/areaRadial.js)
Equivalent to [*area*.x0](#area_x0), except the accessor returns the angle in radians, with 0 at -*y* (12 o’clock). Note: typically [angle](#areaRadial_angle) is used instead of setting separate start and end angles.
<a name="areaRadial_endAngle" href="#areaRadial_endAngle">#</a> <i>areaRadial</i>.<b>endAngle</b>([<i>angle</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/areaRadial.js)
Equivalent to [*area*.x1](#area_x1), except the accessor returns the angle in radians, with 0 at -*y* (12 o’clock). Note: typically [angle](#areaRadial_angle) is used instead of setting separate start and end angles.
<a name="areaRadial_radius" href="#areaRadial_radius">#</a> <i>areaRadial</i>.<b>radius</b>([<i>radius</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/areaRadial.js)
Equivalent to [*area*.y](#area_y), except the accessor returns the radius: the distance from the origin ⟨0,0⟩.
<a name="areaRadial_innerRadius" href="#areaRadial_innerRadius">#</a> <i>areaRadial</i>.<b>innerRadius</b>([<i>radius</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/areaRadial.js)
Equivalent to [*area*.y0](#area_y0), except the accessor returns the radius: the distance from the origin ⟨0,0⟩.
<a name="areaRadial_outerRadius" href="#areaRadial_outerRadius">#</a> <i>areaRadial</i>.<b>outerRadius</b>([<i>radius</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/areaRadial.js)
Equivalent to [*area*.y1](#area_y1), except the accessor returns the radius: the distance from the origin ⟨0,0⟩.
<a name="areaRadial_defined" href="#areaRadial_defined">#</a> <i>areaRadial</i>.<b>defined</b>([<i>defined</i>])
Equivalent to [*area*.defined](#area_defined).
<a name="areaRadial_curve" href="#areaRadial_curve">#</a> <i>areaRadial</i>.<b>curve</b>([<i>curve</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/areaRadial.js)
Equivalent to [*area*.curve](#area_curve). Note that [curveMonotoneX](#curveMonotoneX) or [curveMonotoneY](#curveMonotoneY) are not recommended for radial areas because they assume that the data is monotonic in *x* or *y*, which is typically untrue of radial areas.
<a name="areaRadial_context" href="#areaRadial_context">#</a> <i>areaRadial</i>.<b>context</b>([<i>context</i>])
Equivalent to [*line*.context](#line_context).
<a name="areaRadial_lineStartAngle" href="#areaRadial_lineStartAngle">#</a> <i>areaRadial</i>.<b>lineStartAngle</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/areaRadial.js)
<br><a name="areaRadial_lineInnerRadius" href="#areaRadial_lineInnerRadius">#</a> <i>areaRadial</i>.<b>lineInnerRadius</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/areaRadial.js)
Returns a new [radial line generator](#lineRadial) that has this radial area generator’s current [defined accessor](#areaRadial_defined), [curve](#areaRadial_curve) and [context](#areaRadial_context). The line’s [angle accessor](#lineRadial_angle) is this area’s [start angle accessor](#areaRadial_startAngle), and the line’s [radius accessor](#lineRadial_radius) is this area’s [inner radius accessor](#areaRadial_innerRadius).
<a name="areaRadial_lineEndAngle" href="#areaRadial_lineEndAngle">#</a> <i>areaRadial</i>.<b>lineEndAngle</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/areaRadial.js)
Returns a new [radial line generator](#lineRadial) that has this radial area generator’s current [defined accessor](#areaRadial_defined), [curve](#areaRadial_curve) and [context](#areaRadial_context). The line’s [angle accessor](#lineRadial_angle) is this area’s [end angle accessor](#areaRadial_endAngle), and the line’s [radius accessor](#lineRadial_radius) is this area’s [inner radius accessor](#areaRadial_innerRadius).
<a name="areaRadial_lineOuterRadius" href="#areaRadial_lineOuterRadius">#</a> <i>areaRadial</i>.<b>lineOuterRadius</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/areaRadial.js)
Returns a new [radial line generator](#lineRadial) that has this radial area generator’s current [defined accessor](#areaRadial_defined), [curve](#areaRadial_curve) and [context](#areaRadial_context). The line’s [angle accessor](#lineRadial_angle) is this area’s [start angle accessor](#areaRadial_startAngle), and the line’s [radius accessor](#lineRadial_radius) is this area’s [outer radius accessor](#areaRadial_outerRadius).
### Curves
While [lines](#lines) are defined as a sequence of two-dimensional [*x*, *y*] points, and [areas](#areas) are similarly defined by a topline and a baseline, there remains the task of transforming this discrete representation into a continuous shape: *i.e.*, how to interpolate between the points. A variety of curves are provided for this purpose.
Curves are typically not constructed or used directly, instead being passed to [*line*.curve](#line_curve) and [*area*.curve](#area_curve). For example:
```js
const line = d3.line(d => d.date, d => d.value)
.curve(d3.curveCatmullRom.alpha(0.5));
```
<a name="curveBasis" href="#curveBasis">#</a> d3.<b>curveBasis</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/basis.js)
<img src="./img/basis.png" width="888" height="240" alt="basis">
Produces a cubic [basis spline](https://en.wikipedia.org/wiki/B-spline) using the specified control points. The first and last points are triplicated such that the spline starts at the first point and ends at the last point, and is tangent to the line between the first and second points, and to the line between the penultimate and last points.
<a name="curveBasisClosed" href="#curveBasisClosed">#</a> d3.<b>curveBasisClosed</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/basisClosed.js)
<img src="./img/basisClosed.png" width="888" height="240" alt="basisClosed">
Produces a closed cubic [basis spline](https://en.wikipedia.org/wiki/B-spline) using the specified control points. When a line segment ends, the first three control points are repeated, producing a closed loop with C2 continuity.
<a name="curveBasisOpen" href="#curveBasisOpen">#</a> d3.<b>curveBasisOpen</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/basisOpen.js)
<img src="./img/basisOpen.png" width="888" height="240" alt="basisOpen">
Produces a cubic [basis spline](https://en.wikipedia.org/wiki/B-spline) using the specified control points. Unlike [basis](#basis), the first and last points are not repeated, and thus the curve typically does not intersect these points.
<a name="curveBumpX" href="#curveBumpX">#</a> d3.<b>curveBumpX</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/bump.js)
<img src="./img/bumpX.png" width="888" height="240" alt="bumpX">
Produces a Bézier curve between each pair of points, with horizontal tangents at each point.
<a name="curveBumpY" href="#curveBumpY">#</a> d3.<b>curveBumpY</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/bump.js)
<img src="./img/bumpY.png" width="888" height="240" alt="bumpY">
Produces a Bézier curve between each pair of points, with vertical tangents at each point.
<a name="curveBundle" href="#curveBundle">#</a> d3.<b>curveBundle</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/bundle.js)
<img src="./img/bundle.png" width="888" height="240" alt="bundle">
Produces a straightened cubic [basis spline](https://en.wikipedia.org/wiki/B-spline) using the specified control points, with the spline straightened according to the curve’s [*beta*](#curveBundle_beta), which defaults to 0.85. This curve is typically used in [hierarchical edge bundling](https://observablehq.com/@d3/hierarchical-edge-bundling) to disambiguate connections, as proposed by [Danny Holten](https://www.win.tue.nl/vis1/home/dholten/) in [Hierarchical Edge Bundles: Visualization of Adjacency Relations in Hierarchical Data](https://www.win.tue.nl/vis1/home/dholten/papers/bundles_infovis.pdf). This curve does not implement [*curve*.areaStart](#curve_areaStart) and [*curve*.areaEnd](#curve_areaEnd); it is intended to work with [d3.line](#lines), not [d3.area](#areas).
<a name="curveBundle_beta" href="#curveBundle_beta">#</a> <i>bundle</i>.<b>beta</b>(<i>beta</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/bundle.js)
Returns a bundle curve with the specified *beta* in the range [0, 1], representing the bundle strength. If *beta* equals zero, a straight line between the first and last point is produced; if *beta* equals one, a standard [basis](#basis) spline is produced. For example:
```js
const line = d3.line().curve(d3.curveBundle.beta(0.5));
```
<a name="curveCardinal" href="#curveCardinal">#</a> d3.<b>curveCardinal</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/cardinal.js)
<img src="./img/cardinal.png" width="888" height="240" alt="cardinal">
Produces a cubic [cardinal spline](https://en.wikipedia.org/wiki/Cubic_Hermite_spline#Cardinal_spline) using the specified control points, with one-sided differences used for the first and last piece. The default [tension](#curveCardinal_tension) is 0.
<a name="curveCardinalClosed" href="#curveCardinalClosed">#</a> d3.<b>curveCardinalClosed</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/cardinalClosed.js)
<img src="./img/cardinalClosed.png" width="888" height="240" alt="cardinalClosed">
Produces a closed cubic [cardinal spline](https://en.wikipedia.org/wiki/Cubic_Hermite_spline#Cardinal_spline) using the specified control points. When a line segment ends, the first three control points are repeated, producing a closed loop. The default [tension](#curveCardinal_tension) is 0.
<a name="curveCardinalOpen" href="#curveCardinalOpen">#</a> d3.<b>curveCardinalOpen</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/cardinalOpen.js)
<img src="./img/cardinalOpen.png" width="888" height="240" alt="cardinalOpen">
Produces a cubic [cardinal spline](https://en.wikipedia.org/wiki/Cubic_Hermite_spline#Cardinal_spline) using the specified control points. Unlike [curveCardinal](#curveCardinal), one-sided differences are not used for the first and last piece, and thus the curve starts at the second point and ends at the penultimate point. The default [tension](#curveCardinal_tension) is 0.
<a name="curveCardinal_tension" href="#curveCardinal_tension">#</a> <i>cardinal</i>.<b>tension</b>(<i>tension</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/cardinalOpen.js)
Returns a cardinal curve with the specified *tension* in the range [0, 1]. The *tension* determines the length of the tangents: a *tension* of one yields all zero tangents, equivalent to [curveLinear](#curveLinear); a *tension* of zero produces a uniform [Catmull–Rom](#curveCatmullRom) spline. For example:
```js
const line = d3.line().curve(d3.curveCardinal.tension(0.5));
```
<a name="curveCatmullRom" href="#curveCatmullRom">#</a> d3.<b>curveCatmullRom</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/catmullRom.js)
<img src="./img/catmullRom.png" width="888" height="240" alt="catmullRom">
Produces a cubic Catmull–Rom spline using the specified control points and the parameter [*alpha*](#curveCatmullRom_alpha), which defaults to 0.5, as proposed by Yuksel et al. in [On the Parameterization of Catmull–Rom Curves](http://www.cemyuksel.com/research/catmullrom_param/), with one-sided differences used for the first and last piece.
<a name="curveCatmullRomClosed" href="#curveCatmullRomClosed">#</a> d3.<b>curveCatmullRomClosed</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/catmullRomClosed.js)
<img src="./img/catmullRomClosed.png" width="888" height="330" alt="catmullRomClosed">
Produces a closed cubic Catmull–Rom spline using the specified control points and the parameter [*alpha*](#curveCatmullRom_alpha), which defaults to 0.5, as proposed by Yuksel et al. When a line segment ends, the first three control points are repeated, producing a closed loop.
<a name="curveCatmullRomOpen" href="#curveCatmullRomOpen">#</a> d3.<b>curveCatmullRomOpen</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/catmullRomOpen.js)
<img src="./img/catmullRomOpen.png" width="888" height="240" alt="catmullRomOpen">
Produces a cubic Catmull–Rom spline using the specified control points and the parameter [*alpha*](#curveCatmullRom_alpha), which defaults to 0.5, as proposed by Yuksel et al. Unlike [curveCatmullRom](#curveCatmullRom), one-sided differences are not used for the first and last piece, and thus the curve starts at the second point and ends at the penultimate point.
<a name="curveCatmullRom_alpha" href="#curveCatmullRom_alpha">#</a> <i>catmullRom</i>.<b>alpha</b>(<i>alpha</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/catmullRom.js)
Returns a cubic Catmull–Rom curve with the specified *alpha* in the range [0, 1]. If *alpha* is zero, produces a uniform spline, equivalent to [curveCardinal](#curveCardinal) with a tension of zero; if *alpha* is one, produces a chordal spline; if *alpha* is 0.5, produces a [centripetal spline](https://en.wikipedia.org/wiki/Centripetal_Catmull–Rom_spline). Centripetal splines are recommended to avoid self-intersections and overshoot. For example:
```js
const line = d3.line().curve(d3.curveCatmullRom.alpha(0.5));
```
<a name="curveLinear" href="#curveLinear">#</a> d3.<b>curveLinear</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/linear.js)
<img src="./img/linear.png" width="888" height="240" alt="linear">
Produces a polyline through the specified points.
<a name="curveLinearClosed" href="#curveLinearClosed">#</a> d3.<b>curveLinearClosed</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/linearClosed.js)
<img src="./img/linearClosed.png" width="888" height="240" alt="linearClosed">
Produces a closed polyline through the specified points by repeating the first point when the line segment ends.
<a name="curveMonotoneX" href="#curveMonotoneX">#</a> d3.<b>curveMonotoneX</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/monotone.js)
<img src="./img/monotoneX.png" width="888" height="240" alt="monotoneX">
Produces a cubic spline that [preserves monotonicity](https://en.wikipedia.org/wiki/Monotone_cubic_interpolation) in *y*, assuming monotonicity in *x*, as proposed by Steffen in [A simple method for monotonic interpolation in one dimension](http://adsabs.harvard.edu/full/1990A%26A...239..443S): “a smooth curve with continuous first-order derivatives that passes through any given set of data points without spurious oscillations. Local extrema can occur only at grid points where they are given by the data, but not in between two adjacent grid points.”
<a name="curveMonotoneY" href="#curveMonotoneY">#</a> d3.<b>curveMonotoneY</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/monotone.js)
<img src="./img/monotoneY.png" width="888" height="240" alt="monotoneY">
Produces a cubic spline that [preserves monotonicity](https://en.wikipedia.org/wiki/Monotone_cubic_interpolation) in *x*, assuming monotonicity in *y*, as proposed by Steffen in [A simple method for monotonic interpolation in one dimension](http://adsabs.harvard.edu/full/1990A%26A...239..443S): “a smooth curve with continuous first-order derivatives that passes through any given set of data points without spurious oscillations. Local extrema can occur only at grid points where they are given by the data, but not in between two adjacent grid points.”
<a name="curveNatural" href="#curveNatural">#</a> d3.<b>curveNatural</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/natural.js)
<img src="./img/natural.png" width="888" height="240" alt="natural">
Produces a [natural](https://en.wikipedia.org/wiki/Spline_interpolation) [cubic spline](http://mathworld.wolfram.com/CubicSpline.html) with the second derivative of the spline set to zero at the endpoints.
<a name="curveStep" href="#curveStep">#</a> d3.<b>curveStep</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/step.js)
<img src="./img/step.png" width="888" height="240" alt="step">
Produces a piecewise constant function (a [step function](https://en.wikipedia.org/wiki/Step_function)) consisting of alternating horizontal and vertical lines. The *y*-value changes at the midpoint of each pair of adjacent *x*-values.
<a name="curveStepAfter" href="#curveStepAfter">#</a> d3.<b>curveStepAfter</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/step.js)
<img src="./img/stepAfter.png" width="888" height="240" alt="stepAfter">
Produces a piecewise constant function (a [step function](https://en.wikipedia.org/wiki/Step_function)) consisting of alternating horizontal and vertical lines. The *y*-value changes after the *x*-value.
<a name="curveStepBefore" href="#curveStepBefore">#</a> d3.<b>curveStepBefore</b>(<i>context</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/step.js)
<img src="./img/stepBefore.png" width="888" height="240" alt="stepBefore">
Produces a piecewise constant function (a [step function](https://en.wikipedia.org/wiki/Step_function)) consisting of alternating horizontal and vertical lines. The *y*-value changes before the *x*-value.
### Custom Curves
Curves are typically not used directly, instead being passed to [*line*.curve](#line_curve) and [*area*.curve](#area_curve). However, you can define your own curve implementation should none of the built-in curves satisfy your needs using the following interface. You can also use this low-level interface with a built-in curve type as an alternative to the line and area generators.
<a name="curve_areaStart" href="#curve_areaStart">#</a> <i>curve</i>.<b>areaStart</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/step.js#L7)
Indicates the start of a new area segment. Each area segment consists of exactly two [line segments](#curve_lineStart): the topline, followed by the baseline, with the baseline points in reverse order.
<a name="curve_areaEnd" href="#curve_areaEnd">#</a> <i>curve</i>.<b>areaEnd</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/step.js)
Indicates the end of the current area segment.
<a name="curve_lineStart" href="#curve_lineStart">#</a> <i>curve</i>.<b>lineStart</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/step.js)
Indicates the start of a new line segment. Zero or more [points](#curve_point) will follow.
<a name="curve_lineEnd" href="#curve_lineEnd">#</a> <i>curve</i>.<b>lineEnd</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/step.js)
Indicates the end of the current line segment.
<a name="curve_point" href="#curve_point">#</a> <i>curve</i>.<b>point</b>(<i>x</i>, <i>y</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/curve/step.js)
Indicates a new point in the current line segment with the given *x*- and *y*-values.
### Links
[<img alt="Tidy Tree" src="https://raw.githubusercontent.com/d3/d3-hierarchy/master/img/tree.png">](https://observablehq.com/@d3/tidy-tree)
The **link** shape generates a smooth cubic Bézier curve from a source point to a target point. The tangents of the curve at the start and end are either [vertical](#linkVertical), [horizontal](#linkHorizontal) or [radial](#linkRadial).
<a name="link" href="#link">#</a> d3.<b>link</b>(<i>curve</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/link.js)
Returns a new [link generator](#_link) using the specified <i>curve</i>. For example, to visualize [links](https://github.com/d3/d3-hierarchy/blob/master/README.md#node_links) in a [tree diagram](https://github.com/d3/d3-hierarchy/blob/master/README.md#tree) rooted on the top edge of the display, you might say:
```js
const link = d3.link(d3.curveBumpY)
.x(d => d.x)
.y(d => d.y);
```
<a name="linkVertical" href="#linkVertical">#</a> d3.<b>linkVertical</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/link.js)
Shorthand for [d3.link](#link) with [d3.curveBumpY](#curveBumpY); suitable for visualizing [links](https://github.com/d3/d3-hierarchy/blob/master/README.md#node_links) in a [tree diagram](https://github.com/d3/d3-hierarchy/blob/master/README.md#tree) rooted on the top edge of the display. Equivalent to:
```js
const link = d3.link(d3.curveBumpY);
```
<a name="linkHorizontal" href="#linkHorizontal">#</a> d3.<b>linkHorizontal</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/link.js)
Shorthand for [d3.link](#link) with [d3.curveBumpX](#curveBumpX); suitable for visualizing [links](https://github.com/d3/d3-hierarchy/blob/master/README.md#node_links) in a [tree diagram](https://github.com/d3/d3-hierarchy/blob/master/README.md#tree) rooted on the left edge of the display. Equivalent to:
```js
const link = d3.link(d3.curveBumpX);
```
<a href="#_link" name="_link">#</a> <i>link</i>(<i>arguments…</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/link.js)
Generates a link for the given *arguments*. The *arguments* are arbitrary; they are simply propagated to the link generator’s accessor functions along with the `this` object. For example, with the default settings, an object expected:
```js
link({
source: [100, 100],
target: [300, 300]
});
```
<a name="link_source" href="#link_source">#</a> <i>link</i>.<b>source</b>([<i>source</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/link.js)
If *source* is specified, sets the source accessor to the specified function and returns this link generator. If *source* is not specified, returns the current source accessor, which defaults to:
```js
function source(d) {
return d.source;
}
```
<a name="link_target" href="#link_target">#</a> <i>link</i>.<b>target</b>([<i>target</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/link.js)
If *target* is specified, sets the target accessor to the specified function and returns this link generator. If *target* is not specified, returns the current target accessor, which defaults to:
```js
function target(d) {
return d.target;
}
```
<a name="link_x" href="#link_x">#</a> <i>link</i>.<b>x</b>([<i>x</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/link.js)
If *x* is specified, sets the *x*-accessor to the specified function or number and returns this link generator. If *x* is not specified, returns the current *x*-accessor, which defaults to:
```js
function x(d) {
return d[0];
}
```
<a name="link_y" href="#link_y">#</a> <i>link</i>.<b>y</b>([<i>y</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/link.js)
If *y* is specified, sets the *y*-accessor to the specified function or number and returns this link generator. If *y* is not specified, returns the current *y*-accessor, which defaults to:
```js
function y(d) {
return d[1];
}
```
<a name="link_context" href="#link_context">#</a> <i>link</i>.<b>context</b>([<i>context</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/link.js)
If *context* is specified, sets the context and returns this link generator. If *context* is not specified, returns the current context, which defaults to null. If the context is not null, then the [generated link](#_link) is rendered to this context as a sequence of [path method](http://www.w3.org/TR/2dcontext/#canvaspathmethods) calls. Otherwise, a [path data](http://www.w3.org/TR/SVG/paths.html#PathData) string representing the generated link is returned. See also [d3-path](https://github.com/d3/d3-path).
<a name="link_digits" href="#link_digits">#</a> <i>link</i>.<b>digits</b>([<i>digits</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/link.js)
If *digits* is specified, sets the maximum number of digits after the decimal separator and returns this link generator. If *digits* is not specified, returns the current maximum fraction digits, which defaults to 3. This option only applies when the associated [*context*](#link_context) is null, as when this link generator is used to produce [path data](http://www.w3.org/TR/SVG/paths.html#PathData).
<a name="linkRadial" href="#linkRadial">#</a> d3.<b>linkRadial</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/link.js)
Returns a new [link generator](#_link) with radial tangents. For example, to visualize [links](https://github.com/d3/d3-hierarchy/blob/master/README.md#node_links) in a [tree diagram](https://github.com/d3/d3-hierarchy/blob/master/README.md#tree) rooted in the center of the display, you might say:
```js
const link = d3.linkRadial()
.angle(d => d.x)
.radius(d => d.y);
```
<a name="linkRadial_angle" href="#linkRadial_angle">#</a> <i>linkRadial</i>.<b>angle</b>([<i>angle</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/link.js)
Equivalent to [*link*.x](#link_x), except the accessor returns the angle in radians, with 0 at -*y* (12 o’clock).
<a name="linkRadial_radius" href="#linkRadial_radius">#</a> <i>linkRadial</i>.<b>radius</b>([<i>radius</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/link.js)
Equivalent to [*link*.y](#link_y), except the accessor returns the radius: the distance from the origin ⟨0,0⟩.
### Symbols
Symbols provide a categorical shape encoding as is commonly used in scatterplots. Symbols are always centered at ⟨0,0⟩; use a transform (see: [SVG](http://www.w3.org/TR/SVG/coords.html#TransformAttribute), [Canvas](http://www.w3.org/TR/2dcontext/#transformations)) to move the symbol to a different position.
<a name="symbol" href="#symbol">#</a> d3.<b>symbol</b>([<i>type</i>][, <i>size</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol.js), [Examples](https://observablehq.com/@d3/fitted-symbols)
Constructs a new symbol generator of the specified [type](#symbol_type) and [size](#symbol_size). If not specified, *type* defaults to a circle, and *size* defaults to 64.
<a name="_symbol" href="#_symbol">#</a> <i>symbol</i>(<i>arguments</i>…) · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol.js)
Generates a symbol for the given *arguments*. The *arguments* are arbitrary; they are simply propagated to the symbol generator’s accessor functions along with the `this` object. For example, with the default settings, no arguments are needed to produce a circle with area 64 square pixels. If the symbol generator has a [context](#symbol_context), then the symbol is rendered to this context as a sequence of [path method](http://www.w3.org/TR/2dcontext/#canvaspathmethods) calls and this function returns void. Otherwise, a [path data](http://www.w3.org/TR/SVG/paths.html#PathData) string is returned.
<a name="symbol_type" href="#symbol_type">#</a> <i>symbol</i>.<b>type</b>([<i>type</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol.js)
If *type* is specified, sets the symbol type to the specified function or symbol type and returns this symbol generator. If *type* is a function, the symbol generator’s arguments and *this* are passed through. (See [*selection*.attr](https://github.com/d3/d3-selection/blob/master/README.md#selection_attr) if you are using d3-selection.) If *type* is not specified, returns the current symbol type accessor, which defaults to:
```js
function type() {
return circle;
}
```
See [symbolsFill](#symbolsFill) and [symbolsStroke](#symbolsStroke) for built-in symbol types. To implement a custom symbol type, pass an object that implements [*symbolType*.draw](#symbolType_draw).
<a name="symbol_size" href="#symbol_size">#</a> <i>symbol</i>.<b>size</b>([<i>size</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol.js)
If *size* is specified, sets the size to the specified function or number and returns this symbol generator. If *size* is a function, the symbol generator’s arguments and *this* are passed through. (See [*selection*.attr](https://github.com/d3/d3-selection/blob/master/README.md#selection_attr) if you are using d3-selection.) If *size* is not specified, returns the current size accessor, which defaults to:
```js
function size() {
return 64;
}
```
Specifying the size as a function is useful for constructing a scatterplot with a size encoding. If you wish to scale the symbol to fit a given bounding box, rather than by area, try [SVG’s getBBox](https://observablehq.com/d/1fac2626b9e1b65f).
<a name="symbol_context" href="#symbol_context">#</a> <i>symbol</i>.<b>context</b>([<i>context</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol.js)
If *context* is specified, sets the context and returns this symbol generator. If *context* is not specified, returns the current context, which defaults to null. If the context is not null, then the [generated symbol](#_symbol) is rendered to this context as a sequence of [path method](http://www.w3.org/TR/2dcontext/#canvaspathmethods) calls. Otherwise, a [path data](http://www.w3.org/TR/SVG/paths.html#PathData) string representing the generated symbol is returned.
<a name="symbol_digits" href="#symbol_digits">#</a> <i>symbol</i>.<b>digits</b>([<i>digits</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol.js)
If *digits* is specified, sets the maximum number of digits after the decimal separator and returns this symbol generator. If *digits* is not specified, returns the current maximum fraction digits, which defaults to 3. This option only applies when the associated [*context*](#symbol_context) is null, as when this symbol generator is used to produce [path data](http://www.w3.org/TR/SVG/paths.html#PathData).
<a name="symbolsFill" href="#symbolsFill">#</a> d3.<b>symbolsFill</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol.js)
<a href="#symbolCircle"><img src="./img/circle.png" width="100" height="100"></a><a href="#symbolCross"><img src="./img/cross.png" width="100" height="100"></a><a href="#symbolDiamond"><img src="./img/diamond.png" width="100" height="100"></a><a href="#symbolSquare"><img src="./img/square.png" width="100" height="100"></a><a href="#symbolStar"><img src="./img/star.png" width="100" height="100"></a><a href="#symbolTriangle"><img src="./img/triangle.png" width="100" height="100"><a href="#symbolWye"><img src="./img/wye.png" width="100" height="100"></a>
An array containing a set of symbol types designed for filling: [circle](#symbolCircle), [cross](#symbolCross), [diamond](#symbolDiamond), [square](#symbolSquare), [star](#symbolStar), [triangle](#symbolTriangle), and [wye](#symbolWye). Useful for constructing the range of an [ordinal scale](https://github.com/d3/d3-scale#ordinal-scales) should you wish to use a shape encoding for categorical data.
<a name="symbolsStroke" href="#symbolsStroke">#</a> d3.<b>symbolsStroke</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol.js)
An array containing a set of symbol types designed for stroking: [circle](#symbolCircle), [plus](#symbolPlus), [times](#symbolTimes), [triangle2](#symbolTriangle2), [asterisk](#symbolAsterisk), [square2](#symbolSquare2), and [diamond2](#symbolDiamond2). Useful for constructing the range of an [ordinal scale](https://github.com/d3/d3-scale#ordinal-scales) should you wish to use a shape encoding for categorical data.
<a name="symbolAsterisk" href="#symbolAsterisk">#</a> d3.<b>symbolAsterisk</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol/asterisk.js)
The asterisk symbol type; intended for stroking.
<a name="symbolCircle" href="#symbolCircle">#</a> d3.<b>symbolCircle</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol/circle.js)
The circle symbol type; intended for either filling or stroking.
<a name="symbolCross" href="#symbolCross">#</a> d3.<b>symbolCross</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol/cross.js)
The Greek cross symbol type, with arms of equal length; intended for filling.
<a name="symbolDiamond" href="#symbolDiamond">#</a> d3.<b>symbolDiamond</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol/diamond.js)
The rhombus symbol type; intended for filling.
<a name="symbolDiamond2" href="#symbolDiamond2">#</a> d3.<b>symbolDiamond2</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol/diamond.js)
The rotated square symbol type; intended for stroking.
<a name="symbolPlus" href="#symbolPlus">#</a> d3.<b>symbolPlus</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol/plus.js)
The plus symbol type; intended for stroking.
<a name="symbolSquare" href="#symbolSquare">#</a> d3.<b>symbolSquare</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol/square.js)
The square symbol type; intended for filling.
<a name="symbolSquare2" href="#symbolSquare2">#</a> d3.<b>symbolSquare2</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol/square2.js)
The square2 symbol type; intended for stroking.
<a name="symbolStar" href="#symbolStar">#</a> d3.<b>symbolStar</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol/star.js)
The pentagonal star (pentagram) symbol type; intended for filling.
<a name="symbolTriangle" href="#symbolTriangle">#</a> d3.<b>symbolTriangle</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol/triangle.js)
The up-pointing triangle symbol type; intended for filling.
<a name="symbolTriangle2" href="#symbolTriangle2">#</a> d3.<b>symbolTriangle2</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol/triangle2.js)
The up-pointing triangle symbol type; intended for stroking.
<a name="symbolWye" href="#symbolWye">#</a> d3.<b>symbolWye</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol/wye.js)
The Y-shape symbol type; intended for filling.
<a name="symbolTimes" href="#symbolTimes">#</a> d3.<b>symbolTimes</b> · [Source](https://github.com/d3/d3-shape/blob/main/src/symbol/times.js)
The X-shape symbol type; intended for stroking.
<a name="pointRadial" href="#pointRadial">#</a> d3.<b>pointRadial</b>(<i>angle</i>, <i>radius</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/pointRadial.js), [Examples](https://observablehq.com/@d3/radial-area-chart)
Returns the point [<i>x</i>, <i>y</i>] for the given *angle* in radians, with 0 at -*y* (12 o’clock) and positive angles proceeding clockwise, and the given *radius*.
### Custom Symbol Types
Symbol types are typically not used directly, instead being passed to [*symbol*.type](#symbol_type). However, you can define your own symbol type implementation should none of the built-in types satisfy your needs using the following interface. You can also use this low-level interface with a built-in symbol type as an alternative to the symbol generator.
<a name="symbolType_draw" href="#symbolType_draw">#</a> <i>symbolType</i>.<b>draw</b>(<i>context</i>, <i>size</i>)
Renders this symbol type to the specified *context* with the specified *size* in square pixels. The *context* implements the [CanvasPathMethods](http://www.w3.org/TR/2dcontext/#canvaspathmethods) interface. (Note that this is a subset of the CanvasRenderingContext2D interface!)
### Stacks
[<img alt="Stacked Bar Chart" src="./img/stacked-bar.png" width="295" height="154">](https://observablehq.com/@d3/stacked-bar-chart)[<img alt="Streamgraph" src="./img/stacked-stream.png" width="295" height="154">](https://observablehq.com/@mbostock/streamgraph-transitions)
Some shape types can be stacked, placing one shape adjacent to another. For example, a bar chart of monthly sales might be broken down into a multi-series bar chart by product category, stacking bars vertically. This is equivalent to subdividing a bar chart by an ordinal dimension (such as product category) and applying a color encoding.
Stacked charts can show overall value and per-category value simultaneously; however, it is typically harder to compare across categories, as only the bottom layer of the stack is aligned. So, chose the [stack order](#stack_order) carefully, and consider a [streamgraph](#stackOffsetWiggle). (See also [grouped charts](https://observablehq.com/@d3/grouped-bar-chart).)
Like the [pie generator](#pies), the stack generator does not produce a shape directly. Instead it computes positions which you can then pass to an [area generator](#areas) or use directly, say to position bars.
<a name="stack" href="#stack">#</a> d3.<b>stack</b>() · [Source](https://github.com/d3/d3-shape/blob/main/src/stack.js)
Constructs a new stack generator with the default settings.
<a name="_stack" href="#_stack">#</a> <i>stack</i>(<i>data</i>[, <i>arguments…</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/stack.js)
Generates a stack for the given array of *data*, returning an array representing each series. Any additional *arguments* are arbitrary; they are simply propagated to accessors along with the `this` object.
The series are determined by the [keys accessor](#stack_keys); each series *i* in the returned array corresponds to the *i*th key. Each series is an array of points, where each point *j* corresponds to the *j*th element in the input *data*. Lastly, each point is represented as an array [*y0*, *y1*] where *y0* is the lower value (baseline) and *y1* is the upper value (topline); the difference between *y0* and *y1* corresponds to the computed [value](#stack_value) for this point. The key for each series is available as *series*.key, and the [index](#stack_order) as *series*.index. The input data element for each point is available as *point*.data.
For example, consider the following table representing monthly sales of fruits:
Month | Apples | Bananas | Cherries | Durians
--------|--------|---------|----------|---------
1/2015 | 3840 | 1920 | 960 | 400
2/2015 | 1600 | 1440 | 960 | 400
3/2015 | 640 | 960 | 640 | 400
4/2015 | 320 | 480 | 640 | 400
This might be represented in JavaScript as an array of objects:
```js
const data = [
{month: new Date(2015, 0, 1), apples: 3840, bananas: 1920, cherries: 960, durians: 400},
{month: new Date(2015, 1, 1), apples: 1600, bananas: 1440, cherries: 960, durians: 400},
{month: new Date(2015, 2, 1), apples: 640, bananas: 960, cherries: 640, durians: 400},
{month: new Date(2015, 3, 1), apples: 320, bananas: 480, cherries: 640, durians: 400}
];
```
To produce a stack for this data:
```js
const stack = d3.stack()
.keys(["apples", "bananas", "cherries", "durians"])
.order(d3.stackOrderNone)
.offset(d3.stackOffsetNone);
const series = stack(data);
```
The resulting array has one element per *series*. Each series has one point per month, and each point has a lower and upper value defining the baseline and topline:
```js
[
[[ 0, 3840], [ 0, 1600], [ 0, 640], [ 0, 320]], // apples
[[3840, 5760], [1600, 3040], [ 640, 1600], [ 320, 800]], // bananas
[[5760, 6720], [3040, 4000], [1600, 2240], [ 800, 1440]], // cherries
[[6720, 7120], [4000, 4400], [2240, 2640], [1440, 1840]], // durians
]
```
Each series in then typically passed to an [area generator](#areas) to render an area chart, or used to construct rectangles for a bar chart.
<a name="stack_keys" href="#stack_keys">#</a> <i>stack</i>.<b>keys</b>([<i>keys</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/stack.js)
If *keys* is specified, sets the keys accessor to the specified function or array and returns this stack generator. If *keys* is not specified, returns the current keys accessor, which defaults to the empty array. A series (layer) is [generated](#_stack) for each key. Keys are typically strings, but they may be arbitrary values. The series’ key is passed to the [value accessor](#stack_value), along with each data point, to compute the point’s value.
<a name="stack_value" href="#stack_value">#</a> <i>stack</i>.<b>value</b>([<i>value</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/stack.js)
If *value* is specified, sets the value accessor to the specified function or number and returns this stack generator. If *value* is not specified, returns the current value accessor, which defaults to:
```js
function value(d, key) {
return d[key];
}
```
Thus, by default the stack generator assumes that the input data is an array of objects, with each object exposing named properties with numeric values; see [*stack*](#_stack) for an example.
<a name="stack_order" href="#stack_order">#</a> <i>stack</i>.<b>order</b>([<i>order</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/stack.js)
If *order* is specified, sets the order accessor to the specified function or array and returns this stack generator. If *order* is not specified, returns the current order accessor, which defaults to [stackOrderNone](#stackOrderNone); this uses the order given by the [key accessor](#stack_key). See [stack orders](#stack-orders) for the built-in orders.
If *order* is a function, it is passed the generated series array and must return an array of numeric indexes representing the stack order. For example, the default order is defined as:
```js
function orderNone(series) {
let n = series.length;
const o = new Array(n);
while (--n >= 0) o[n] = n;
return o;
}
```
The stack order is computed prior to the [offset](#stack_offset); thus, the lower value for all points is zero at the time the order is computed. The index attribute for each series is also not set until after the order is computed.
<a name="stack_offset" href="#stack_offset">#</a> <i>stack</i>.<b>offset</b>([<i>offset</i>]) · [Source](https://github.com/d3/d3-shape/blob/main/src/stack.js)
If *offset* is specified, sets the offset accessor to the specified function and returns this stack generator. If *offset* is not specified, returns the current offset acccesor, which defaults to [stackOffsetNone](#stackOffsetNone); this uses a zero baseline. See [stack offsets](#stack-offsets) for the built-in offsets.
The offset function is passed the generated series array and the order index array; it is then responsible for updating the lower and upper values in the series array. For example, the default offset is defined as:
```js
function offsetNone(series, order) {
if (!((n = series.length) > 1)) return;
for (let i = 1, s0, s1 = series[order[0]], n, m = s1.length; i < n; ++i) {
s0 = s1, s1 = series[order[i]];
for (let j = 0; j < m; ++j) {
s1[j][1] += s1[j][0] = s0[j][1];
}
}
}
```
### Stack Orders
Stack orders are typically not used directly, but are instead passed to [*stack*.order](#stack_order).
<a name="stackOrderAppearance" href="#stackOrderAppearance">#</a> d3.<b>stackOrderAppearance</b>(<i>series</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/order/appearance.js)
Returns a series order such that the earliest series (according to the maximum value) is at the bottom.
<a name="stackOrderAscending" href="#stackOrderAscending">#</a> d3.<b>stackOrderAscending</b>(<i>series</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/order/ascending.js)
Returns a series order such that the smallest series (according to the sum of values) is at the bottom.
<a name="stackOrderDescending" href="#stackOrderDescending">#</a> d3.<b>stackOrderDescending</b>(<i>series</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/order/descending.js)
Returns a series order such that the largest series (according to the sum of values) is at the bottom.
<a name="stackOrderInsideOut" href="#stackOrderInsideOut">#</a> d3.<b>stackOrderInsideOut</b>(<i>series</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/order/insideOut.js)
Returns a series order such that the earliest series (according to the maximum value) are on the inside and the later series are on the outside. This order is recommended for streamgraphs in conjunction with the [wiggle offset](#stackOffsetWiggle). See [Stacked Graphs—Geometry & Aesthetics](http://leebyron.com/streamgraph/) by Byron & Wattenberg for more information.
<a name="stackOrderNone" href="#stackOrderNone">#</a> d3.<b>stackOrderNone</b>(<i>series</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/order/none.js)
Returns the given series order [0, 1, … *n* - 1] where *n* is the number of elements in *series*. Thus, the stack order is given by the [key accessor](#stack_keys).
<a name="stackOrderReverse" href="#stackOrderReverse">#</a> d3.<b>stackOrderReverse</b>(<i>series</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/order/reverse.js)
Returns the reverse of the given series order [*n* - 1, *n* - 2, … 0] where *n* is the number of elements in *series*. Thus, the stack order is given by the reverse of the [key accessor](#stack_keys).
### Stack Offsets
Stack offsets are typically not used directly, but are instead passed to [*stack*.offset](#stack_offset).
<a name="stackOffsetExpand" href="#stackOffsetExpand">#</a> d3.<b>stackOffsetExpand</b>(<i>series</i>, <i>order</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/offset/expand.js)
Applies a zero baseline and normalizes the values for each point such that the topline is always one.
<a name="stackOffsetDiverging" href="#stackOffsetDiverging">#</a> d3.<b>stackOffsetDiverging</b>(<i>series</i>, <i>order</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/offset/diverging.js)
Positive values are stacked above zero, negative values are [stacked below zero](https://observablehq.com/@d3/diverging-stacked-bar-chart), and zero values are stacked at zero.
<a name="stackOffsetNone" href="#stackOffsetNone">#</a> d3.<b>stackOffsetNone</b>(<i>series</i>, <i>order</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/offset/none.js)
Applies a zero baseline.
<a name="stackOffsetSilhouette" href="#stackOffsetSilhouette">#</a> d3.<b>stackOffsetSilhouette</b>(<i>series</i>, <i>order</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/offset/silhouette.js)
Shifts the baseline down such that the center of the streamgraph is always at zero.
<a name="stackOffsetWiggle" href="#stackOffsetWiggle">#</a> d3.<b>stackOffsetWiggle</b>(<i>series</i>, <i>order</i>) · [Source](https://github.com/d3/d3-shape/blob/main/src/offset/wiggle.js)
Shifts the baseline so as to minimize the weighted wiggle of layers. This offset is recommended for streamgraphs in conjunction with the [inside-out order](#stackOrderInsideOut). See [Stacked Graphs—Geometry & Aesthetics](http://leebyron.com/streamgraph/) by Bryon & Wattenberg for more information. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/d3-shape/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/d3-shape/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 94546
} |
# d3-time-format
This module provides a JavaScript implementation of the venerable [strptime](http://pubs.opengroup.org/onlinepubs/009695399/functions/strptime.html) and [strftime](http://pubs.opengroup.org/onlinepubs/007908799/xsh/strftime.html) functions from the C standard library, and can be used to parse or format [dates](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date) in a variety of locale-specific representations. To format a date, create a [formatter](#locale_format) from a specifier (a string with the desired format *directives*, indicated by `%`); then pass a date to the formatter, which returns a string. For example, to convert the current date to a human-readable string:
```js
const formatTime = d3.timeFormat("%B %d, %Y");
formatTime(new Date); // "June 30, 2015"
```
Likewise, to convert a string back to a date, create a [parser](#locale_parse):
```js
const parseTime = d3.timeParse("%B %d, %Y");
parseTime("June 30, 2015"); // Tue Jun 30 2015 00:00:00 GMT-0700 (PDT)
```
You can implement more elaborate conditional time formats, too. For example, here’s a [multi-scale time format](https://bl.ocks.org/mbostock/4149176) using [time intervals](https://github.com/d3/d3-time):
```js
const formatMillisecond = d3.timeFormat(".%L"),
formatSecond = d3.timeFormat(":%S"),
formatMinute = d3.timeFormat("%I:%M"),
formatHour = d3.timeFormat("%I %p"),
formatDay = d3.timeFormat("%a %d"),
formatWeek = d3.timeFormat("%b %d"),
formatMonth = d3.timeFormat("%B"),
formatYear = d3.timeFormat("%Y");
function multiFormat(date) {
return (d3.timeSecond(date) < date ? formatMillisecond
: d3.timeMinute(date) < date ? formatSecond
: d3.timeHour(date) < date ? formatMinute
: d3.timeDay(date) < date ? formatHour
: d3.timeMonth(date) < date ? (d3.timeWeek(date) < date ? formatDay : formatWeek)
: d3.timeYear(date) < date ? formatMonth
: formatYear)(date);
}
```
This module is used by D3 [time scales](https://github.com/d3/d3-scale/blob/main/README.md#time-scales) to generate human-readable ticks.
## Installing
If you use npm, `npm install d3-time-format`. You can also download the [latest release on GitHub](https://github.com/d3/d3-time-format/releases/latest). For vanilla HTML in modern browsers, import d3-time-format from Skypack:
```html
<script type="module">
import {timeFormat} from "https://cdn.skypack.dev/d3-time-format@4";
const format = timeFormat("%x");
</script>
```
For legacy environments, you can load d3-time-format’s UMD bundle from an npm-based CDN such as jsDelivr; a `d3` global is exported:
```html
<script src="https://cdn.jsdelivr.net/npm/d3-array@3"></script>
<script src="https://cdn.jsdelivr.net/npm/d3-time@3"></script>
<script src="https://cdn.jsdelivr.net/npm/d3-time-format@4"></script>
<script>
const format = d3.timeFormat("%x");
</script>
Locale files are published to npm and can be loaded using [d3.json](https://github.com/d3/d3-fetch/blob/main/README.md#json). For example, to set Russian as the default locale:
```js
d3.json("https://cdn.jsdelivr.net/npm/d3-time-format@3/locale/ru-RU.json").then(locale => {
d3.timeFormatDefaultLocale(locale);
const format = d3.timeFormat("%c");
console.log(format(new Date)); // понедельник, 5 декабря 2016 г. 10:31:59
});
```
## API Reference
<a name="timeFormat" href="#timeFormat">#</a> d3.<b>timeFormat</b>(<i>specifier</i>) · [Source](https://github.com/d3/d3-time-format/blob/main/src/defaultLocale.js)
An alias for [*locale*.format](#locale_format) on the [default locale](#timeFormatDefaultLocale).
<a name="timeParse" href="#timeParse">#</a> d3.<b>timeParse</b>(<i>specifier</i>) · [Source](https://github.com/d3/d3-time-format/blob/main/src/defaultLocale.js)
An alias for [*locale*.parse](#locale_parse) on the [default locale](#timeFormatDefaultLocale).
<a name="utcFormat" href="#utcFormat">#</a> d3.<b>utcFormat</b>(<i>specifier</i>) · [Source](https://github.com/d3/d3-time-format/blob/main/src/defaultLocale.js)
An alias for [*locale*.utcFormat](#locale_utcFormat) on the [default locale](#timeFormatDefaultLocale).
<a name="utcParse" href="#utcParse">#</a> d3.<b>utcParse</b>(<i>specifier</i>) · [Source](https://github.com/d3/d3-time-format/blob/main/src/defaultLocale.js)
An alias for [*locale*.utcParse](#locale_utcParse) on the [default locale](#timeFormatDefaultLocale).
<a name="isoFormat" href="#isoFormat">#</a> d3.<b>isoFormat</b> · [Source](https://github.com/d3/d3-time-format/blob/main/src/isoFormat.js)
The full [ISO 8601](https://en.wikipedia.org/wiki/ISO_8601) UTC time formatter. Where available, this method will use [Date.toISOString](https://developer.mozilla.org/en-US/docs/JavaScript/Reference/Global_Objects/Date/toISOString) to format.
<a name="isoParse" href="#isoParse">#</a> d3.<b>isoParse</b> · [Source](https://github.com/d3/d3-time-format/blob/main/src/isoParse.js)
The full [ISO 8601](https://en.wikipedia.org/wiki/ISO_8601) UTC time parser. Where available, this method will use the [Date constructor](https://developer.mozilla.org/en-US/docs/JavaScript/Reference/Global_Objects/Date) to parse strings. If you depend on strict validation of the input format according to ISO 8601, you should construct a [UTC parser function](#utcParse):
```js
const strictIsoParse = d3.utcParse("%Y-%m-%dT%H:%M:%S.%LZ");
```
<a name="locale_format" href="#locale_format">#</a> <i>locale</i>.<b>format</b>(<i>specifier</i>) · [Source](https://github.com/d3/d3-time-format/blob/main/src/locale.js)
Returns a new formatter for the given string *specifier*. The specifier string may contain the following directives:
* `%a` - abbreviated weekday name.*
* `%A` - full weekday name.*
* `%b` - abbreviated month name.*
* `%B` - full month name.*
* `%c` - the locale’s date and time, such as `%x, %X`.*
* `%d` - zero-padded day of the month as a decimal number [01,31].
* `%e` - space-padded day of the month as a decimal number [ 1,31]; equivalent to `%_d`.
* `%f` - microseconds as a decimal number [000000, 999999].
* `%g` - ISO 8601 week-based year without century as a decimal number [00,99].
* `%G` - ISO 8601 week-based year with century as a decimal number.
* `%H` - hour (24-hour clock) as a decimal number [00,23].
* `%I` - hour (12-hour clock) as a decimal number [01,12].
* `%j` - day of the year as a decimal number [001,366].
* `%m` - month as a decimal number [01,12].
* `%M` - minute as a decimal number [00,59].
* `%L` - milliseconds as a decimal number [000, 999].
* `%p` - either AM or PM.*
* `%q` - quarter of the year as a decimal number [1,4].
* `%Q` - milliseconds since UNIX epoch.
* `%s` - seconds since UNIX epoch.
* `%S` - second as a decimal number [00,61].
* `%u` - Monday-based (ISO 8601) weekday as a decimal number [1,7].
* `%U` - Sunday-based week of the year as a decimal number [00,53].
* `%V` - ISO 8601 week of the year as a decimal number [01, 53].
* `%w` - Sunday-based weekday as a decimal number [0,6].
* `%W` - Monday-based week of the year as a decimal number [00,53].
* `%x` - the locale’s date, such as `%-m/%-d/%Y`.*
* `%X` - the locale’s time, such as `%-I:%M:%S %p`.*
* `%y` - year without century as a decimal number [00,99].
* `%Y` - year with century as a decimal number, such as `1999`.
* `%Z` - time zone offset, such as `-0700`, `-07:00`, `-07`, or `Z`.
* `%%` - a literal percent sign (`%`).
Directives marked with an asterisk (\*) may be affected by the [locale definition](#locales).
For `%U`, all days in a new year preceding the first Sunday are considered to be in week 0. For `%W`, all days in a new year preceding the first Monday are considered to be in week 0. Week numbers are computed using [*interval*.count](https://github.com/d3/d3-time/blob/main/README.md#interval_count). For example, 2015-52 and 2016-00 represent Monday, December 28, 2015, while 2015-53 and 2016-01 represent Monday, January 4, 2016. This differs from the [ISO week date](https://en.wikipedia.org/wiki/ISO_week_date) specification (`%V`), which uses a more complicated definition!
For `%V`,`%g` and `%G`, per the [strftime man page](http://man7.org/linux/man-pages/man3/strftime.3.html):
> In this system, weeks start on a Monday, and are numbered from 01, for the first week, up to 52 or 53, for the last week. Week 1 is the first week where four or more days fall within the new year (or, synonymously, week 01 is: the first week of the year that contains a Thursday; or, the week that has 4 January in it). If the ISO week number belongs to the previous or next year, that year is used instead.
The `%` sign indicating a directive may be immediately followed by a padding modifier:
* `0` - zero-padding
* `_` - space-padding
* `-` - disable padding
If no padding modifier is specified, the default is `0` for all directives except `%e`, which defaults to `_`. (In some implementations of strftime and strptime, a directive may include an optional field width or precision; this feature is not yet implemented.)
The returned function formats a specified *[date](https://developer.mozilla.org/en/JavaScript/Reference/Global_Objects/Date)*, returning the corresponding string.
```js
const formatMonth = d3.timeFormat("%B"),
formatDay = d3.timeFormat("%A"),
date = new Date(2014, 4, 1); // Thu May 01 2014 00:00:00 GMT-0700 (PDT)
formatMonth(date); // "May"
formatDay(date); // "Thursday"
```
<a name="locale_parse" href="#locale_parse">#</a> <i>locale</i>.<b>parse</b>(<i>specifier</i>) · [Source](https://github.com/d3/d3-time-format/blob/main/src/locale.js)
Returns a new parser for the given string *specifier*. The specifier string may contain the same directives as [*locale*.format](#locale_format). The `%d` and `%e` directives are considered equivalent for parsing.
The returned function parses a specified *string*, returning the corresponding [date](https://developer.mozilla.org/en/JavaScript/Reference/Global_Objects/Date) or null if the string could not be parsed according to this format’s specifier. Parsing is strict: if the specified <i>string</i> does not exactly match the associated specifier, this method returns null. For example, if the associated specifier is `%Y-%m-%dT%H:%M:%SZ`, then the string `"2011-07-01T19:15:28Z"` will be parsed as expected, but `"2011-07-01T19:15:28"`, `"2011-07-01 19:15:28"` and `"2011-07-01"` will return null. (Note that the literal `Z` here is different from the time zone offset directive `%Z`.) If a more flexible parser is desired, try multiple formats sequentially until one returns non-null.
<a name="locale_utcFormat" href="#locale_utcFormat">#</a> <i>locale</i>.<b>utcFormat</b>(<i>specifier</i>) · [Source](https://github.com/d3/d3-time-format/blob/main/src/locale.js)
Equivalent to [*locale*.format](#locale_format), except all directives are interpreted as [Coordinated Universal Time (UTC)](https://en.wikipedia.org/wiki/Coordinated_Universal_Time) rather than local time.
<a name="locale_utcParse" href="#locale_utcParse">#</a> <i>locale</i>.<b>utcParse</b>(<i>specifier</i>) · [Source](https://github.com/d3/d3-time-format/blob/main/src/locale.js)
Equivalent to [*locale*.parse](#locale_parse), except all directives are interpreted as [Coordinated Universal Time (UTC)](https://en.wikipedia.org/wiki/Coordinated_Universal_Time) rather than local time.
### Locales
<a name="timeFormatLocale" href="#timeFormatLocale">#</a> d3.<b>timeFormatLocale</b>(<i>definition</i>) · [Source](https://github.com/d3/d3-time-format/blob/main/src/locale.js)
Returns a *locale* object for the specified *definition* with [*locale*.format](#locale_format), [*locale*.parse](#locale_parse), [*locale*.utcFormat](#locale_utcFormat), [*locale*.utcParse](#locale_utcParse) methods. The *definition* must include the following properties:
* `dateTime` - the date and time (`%c`) format specifier (<i>e.g.</i>, `"%a %b %e %X %Y"`).
* `date` - the date (`%x`) format specifier (<i>e.g.</i>, `"%m/%d/%Y"`).
* `time` - the time (`%X`) format specifier (<i>e.g.</i>, `"%H:%M:%S"`).
* `periods` - the A.M. and P.M. equivalents (<i>e.g.</i>, `["AM", "PM"]`).
* `days` - the full names of the weekdays, starting with Sunday.
* `shortDays` - the abbreviated names of the weekdays, starting with Sunday.
* `months` - the full names of the months (starting with January).
* `shortMonths` - the abbreviated names of the months (starting with January).
For an example, see [Localized Time Axis II](https://bl.ocks.org/mbostock/805115ebaa574e771db1875a6d828949).
<a name="timeFormatDefaultLocale" href="#timeFormatDefaultLocale">#</a> d3.<b>timeFormatDefaultLocale</b>(<i>definition</i>) · [Source](https://github.com/d3/d3-time-format/blob/main/src/defaultLocale.js)
Equivalent to [d3.timeFormatLocale](#timeFormatLocale), except it also redefines [d3.timeFormat](#timeFormat), [d3.timeParse](#timeParse), [d3.utcFormat](#utcFormat) and [d3.utcParse](#utcParse) to the new locale’s [*locale*.format](#locale_format), [*locale*.parse](#locale_parse), [*locale*.utcFormat](#locale_utcFormat) and [*locale*.utcParse](#locale_utcParse). If you do not set a default locale, it defaults to [U.S. English](https://github.com/d3/d3-time-format/blob/main/locale/en-US.json).
For an example, see [Localized Time Axis](https://bl.ocks.org/mbostock/6f1cc065d4d172bcaf322e399aa8d62f). | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/d3-time-format/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/d3-time-format/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 13343
} |
# d3-time
When visualizing time series data, analyzing temporal patterns, or working with time in general, the irregularities of conventional time units quickly become apparent. In the [Gregorian calendar](https://en.wikipedia.org/wiki/Gregorian_calendar), for example, most months have 31 days but some have 28, 29 or 30; most years have 365 days but [leap years](https://en.wikipedia.org/wiki/Leap_year) have 366; and with [daylight saving](https://en.wikipedia.org/wiki/Daylight_saving_time), most days have 24 hours but some have 23 or 25. Adding to complexity, daylight saving conventions vary around the world.
As a result of these temporal peculiarities, it can be difficult to perform seemingly-trivial tasks. For example, if you want to compute the number of days that have passed between two dates, you can’t simply subtract and divide by 24 hours (86,400,000 ms):
```js
start = new Date(2015, 02, 01) // 2015-03-01T00:00
end = new Date(2015, 03, 01) // 2015-04-01T00:00
(end - start) / 864e5 // 30.958333333333332, oops! 🤯
```
You can, however, use [d3.timeDay](#timeDay).[count](#interval_count):
```js
d3.timeDay.count(start, end) // 31 😌
```
The [day](#day) [interval](#api-reference) is one of several provided by d3-time. Each interval represents a conventional unit of time—[hours](#timeHour), [weeks](#timeWeek), [months](#timeMonth), *etc.*—and has methods to calculate boundary dates. For example, [d3.timeDay](#timeDay) computes midnight (typically 12:00 AM local time) of the corresponding day. In addition to [rounding](#interval_round) and [counting](#interval_count), intervals can also be used to generate arrays of boundary dates. For example, to compute each Sunday in the current month:
```js
start = d3.timeMonth.floor() // 2015-01-01T00:00
stop = d3.timeMonth.ceil() // 2015-02-01T00:00
d3.timeWeek.range(start, stop) // [2015-01-07T00:00, 2015-01-14T00:00, 2015-01-21T00:00, 2015-01-28T00:00]
```
The d3-time module does not implement its own calendaring system; it merely implements a convenient API for calendar math on top of ECMAScript [Date](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date). Thus, it ignores leap seconds and can only work with the local time zone and [Coordinated Universal Time](https://en.wikipedia.org/wiki/Coordinated_Universal_Time) (UTC).
This module is used by D3’s time scales to generate sensible ticks, by D3’s time format, and can also be used directly to do things like [calendar layouts](http://bl.ocks.org/mbostock/4063318).
## Installing
If you use npm, `npm install d3-time`. You can also download the [latest release on GitHub](https://github.com/d3/d3-time/releases/latest). For vanilla HTML in modern browsers, import d3-time from Skypack:
```html
<script type="module">
import {timeDay} from "https://cdn.skypack.dev/d3-time@3";
const day = timeDay();
</script>
```
For legacy environments, you can load d3-time’s UMD bundle from an npm-based CDN such as jsDelivr; a `d3` global is exported:
```html
<script src="https://cdn.jsdelivr.net/npm/d3-array@3"></script>
<script src="https://cdn.jsdelivr.net/npm/d3-time@3"></script>
<script>
const day = d3.timeDay();
</script>
```
[Try d3-time in your browser.](https://observablehq.com/collection/@d3/d3-time)
## API Reference
<a name="_interval" href="#_interval">#</a> <i>interval</i>([<i>date</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/interval.js)
Equivalent to [*interval*.floor](#interval_floor), except if *date* is not specified, it defaults to the current time. For example, [d3.timeYear](#timeYear)(*date*) and d3.timeYear.floor(*date*) are equivalent.
```js
monday = d3.timeMonday() // the latest preceeding Monday, local time
```
<a name="interval_floor" href="#interval_floor">#</a> <i>interval</i>.<b>floor</b>(<i>date</i>) · [Source](https://github.com/d3/d3-time/blob/main/src/interval.js)
Returns a new date representing the latest interval boundary date before or equal to *date*. For example, [d3.timeDay](#timeDay).floor(*date*) typically returns 12:00 AM local time on the given *date*.
This method is idempotent: if the specified *date* is already floored to the current interval, a new date with an identical time is returned. Furthermore, the returned date is the minimum expressible value of the associated interval, such that *interval*.floor(*interval*.floor(*date*) - 1) returns the preceeding interval boundary date.
Note that the `==` and `===` operators do not compare by value with [Date](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date) objects, and thus you cannot use them to tell whether the specified *date* has already been floored. Instead, coerce to a number and then compare:
```js
// Returns true if the specified date is a day boundary.
function isDay(date) {
return +d3.timeDay.floor(date) === +date;
}
```
This is more reliable than testing whether the time is 12:00 AM, as in some time zones midnight may not exist due to daylight saving.
<a name="interval_round" href="#interval_round">#</a> <i>interval</i>.<b>round</b>(<i>date</i>) · [Source](https://github.com/d3/d3-time/blob/main/src/interval.js)
Returns a new date representing the closest interval boundary date to *date*. For example, [d3.timeDay](#timeDay).round(*date*) typically returns 12:00 AM local time on the given *date* if it is on or before noon, and 12:00 AM of the following day if it is after noon.
This method is idempotent: if the specified *date* is already rounded to the current interval, a new date with an identical time is returned.
<a name="interval_ceil" href="#interval_ceil">#</a> <i>interval</i>.<b>ceil</b>(<i>date</i>) · [Source](https://github.com/d3/d3-time/blob/main/src/interval.js)
Returns a new date representing the earliest interval boundary date after or equal to *date*. For example, [d3.timeDay](#timeDay).ceil(*date*) typically returns 12:00 AM local time on the date following the given *date*.
This method is idempotent: if the specified *date* is already ceilinged to the current interval, a new date with an identical time is returned. Furthermore, the returned date is the maximum expressible value of the associated interval, such that *interval*.ceil(*interval*.ceil(*date*) + 1) returns the following interval boundary date.
<a name="interval_offset" href="#interval_offset">#</a> <i>interval</i>.<b>offset</b>(<i>date</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/interval.js)
Returns a new date equal to *date* plus *step* intervals. If *step* is not specified it defaults to 1. If *step* is negative, then the returned date will be before the specified *date*; if *step* is zero, then a copy of the specified *date* is returned; if *step* is not an integer, it is [floored](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/floor). This method does not round the specified *date* to the interval. For example, if *date* is today at 5:34 PM, then [d3.timeDay](#timeDay).offset(*date*, 1) returns 5:34 PM tomorrow (even if daylight saving changes!).
<a name="interval_range" href="#interval_range">#</a> <i>interval</i>.<b>range</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/interval.js)
Returns an array of dates representing every interval boundary after or equal to *start* (inclusive) and before *stop* (exclusive). If *step* is specified, then every *step*th boundary will be returned; for example, for the [d3.timeDay](#timeDay) interval a *step* of 2 will return every other day. If *step* is not an integer, it is [floored](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/floor).
The first date in the returned array is the earliest boundary after or equal to *start*; subsequent dates are [offset](#interval_offset) by *step* intervals and [floored](#interval_floor). Thus, two overlapping ranges may be consistent. For example, this range contains odd days:
```js
d3.timeDay.range(new Date(2015, 0, 1), new Date(2015, 0, 7), 2) // [2015-01-01T00:00, 2015-01-03T00:00, 2015-01-05T00:00]
```
While this contains even days:
```js
d3.timeDay.range(new Date(2015, 0, 2), new Date(2015, 0, 8), 2) // [2015-01-02T00:00, 2015-01-04T00:00, 2015-01-06T00:00]
```
To make ranges consistent when a *step* is specified, use [*interval*.every](#interval_every) instead.
<a name="interval_filter" href="#interval_filter">#</a> <i>interval</i>.<b>filter</b>(<i>test</i>) · [Source](https://github.com/d3/d3-time/blob/main/src/interval.js)
Returns a new interval that is a filtered subset of this interval using the specified *test* function. The *test* function is passed a date and should return true if and only if the specified date should be considered part of the interval. For example, to create an interval that returns the 1st, 11th, 21th and 31th (if it exists) of each month:
```js
d3.timeDay.filter(d => (d.getDate() - 1) % 10 === 0)
```
The returned filtered interval does not support [*interval*.count](#interval_count). See also [*interval*.every](#interval_every).
<a name="interval_every" href="#interval_every">#</a> <i>interval</i>.<b>every</b>(<i>step</i>) · [Source](https://github.com/d3/d3-time/blob/main/src/interval.js)
Returns a [filtered](#interval_filter) view of this interval representing every *step*th date. The meaning of *step* is dependent on this interval’s parent interval as defined by the field function. For example, [d3.timeMinute](#timeMinute).every(15) returns an interval representing every fifteen minutes, starting on the hour: :00, :15, :30, :45, <i>etc.</i> Note that for some intervals, the resulting dates may not be uniformly-spaced; [d3.timeDay](#timeDay)’s parent interval is [d3.timeMonth](#timeMonth), and thus the interval number resets at the start of each month. If *step* is not valid, returns null. If *step* is one, returns this interval.
This method can be used in conjunction with [*interval*.range](#interval_range) to ensure that two overlapping ranges are consistent. For example, this range contains odd days:
```js
d3.timeDay.every(2).range(new Date(2015, 0, 1), new Date(2015, 0, 7)) // [2015-01-01T00:00, 2015-01-03T00:00, 2015-01-05T00:00]
```
As does this one:
```js
d3.timeDay.every(2).range(new Date(2015, 0, 2), new Date(2015, 0, 8)) // [2015-01-03T00:00, 2015-01-05T00:00, 2015-01-07T00:00]
```
The returned filtered interval does not support [*interval*.count](#interval_count). See also [*interval*.filter](#interval_filter).
<a name="interval_count" href="#interval_count">#</a> <i>interval</i>.<b>count</b>(<i>start</i>, <i>end</i>) · [Source](https://github.com/d3/d3-time/blob/main/src/interval.js)
Returns the number of interval boundaries after *start* (exclusive) and before or equal to *end* (inclusive). Note that this behavior is slightly different than [*interval*.range](#interval_range) because its purpose is to return the zero-based number of the specified *end* date relative to the specified *start* date. For example, to compute the current zero-based day-of-year number:
```js
d3.timeDay.count(d3.timeYear(now), now) // 177
```
Likewise, to compute the current zero-based week-of-year number for weeks that start on Sunday:
```js
d3.timeSunday.count(d3.timeYear(now), now) // 25
```
<a name="timeInterval" href="#timeInterval">#</a> d3.<b>timeInterval</b>(<i>floor</i>, <i>offset</i>[, <i>count</i>[, <i>field</i>]]) · [Source](https://github.com/d3/d3-time/blob/main/src/interval.js)
Constructs a new custom interval given the specified *floor* and *offset* functions and an optional *count* function.
The *floor* function takes a single date as an argument and rounds it down to the nearest interval boundary.
The *offset* function takes a date and an integer step as arguments and advances the specified date by the specified number of boundaries; the step may be positive, negative or zero.
The optional *count* function takes a start date and an end date, already floored to the current interval, and returns the number of boundaries between the start (exclusive) and end (inclusive). If a *count* function is not specified, the returned interval does not expose [*interval*.count](#interval_count) or [*interval*.every](#interval_every) methods. Note: due to an internal optimization, the specified *count* function must not invoke *interval*.count on other time intervals.
The optional *field* function takes a date, already floored to the current interval, and returns the field value of the specified date, corresponding to the number of boundaries between this date (exclusive) and the latest previous parent boundary. For example, for the [d3.timeDay](#timeDay) interval, this returns the number of days since the start of the month. If a *field* function is not specified, it defaults to counting the number of interval boundaries since the UNIX epoch of January 1, 1970 UTC. The *field* function defines the behavior of [*interval*.every](#interval_every).
### Intervals
The following intervals are provided:
<a name="timeMillisecond" href="#timeMillisecond">#</a> d3.<b>timeMillisecond</b> · [Source](https://github.com/d3/d3-time/blob/main/src/millisecond.js "Source")
<br><a href="#timeMillisecond">#</a> d3.<b>utcMillisecond</b>
Milliseconds; the shortest available time unit.
<a name="timeSecond" href="#timeSecond">#</a> d3.<b>timeSecond</b> · [Source](https://github.com/d3/d3-time/blob/main/src/second.js "Source")
<br><a href="#timeSecond">#</a> d3.<b>utcSecond</b>
Seconds (e.g., 01:23:45.0000 AM); 1,000 milliseconds.
<a name="timeMinute" href="#timeMinute">#</a> d3.<b>timeMinute</b> · [Source](https://github.com/d3/d3-time/blob/main/src/minute.js "Source")
<br><a href="#timeMinute">#</a> d3.<b>utcMinute</b> · [Source](https://github.com/d3/d3-time/blob/main/src/utcMinute.js "Source")
Minutes (e.g., 01:02:00 AM); 60 seconds. Note that ECMAScript [ignores leap seconds](http://www.ecma-international.org/ecma-262/5.1/#sec-15.9.1.1).
<a name="timeHour" href="#timeHour">#</a> d3.<b>timeHour</b> · [Source](https://github.com/d3/d3-time/blob/main/src/hour.js "Source")
<br><a href="#timeHour">#</a> d3.<b>utcHour</b> · [Source](https://github.com/d3/d3-time/blob/main/src/utcHour.js "Source")
Hours (e.g., 01:00 AM); 60 minutes. Note that advancing time by one hour in local time can return the same hour or skip an hour due to daylight saving.
<a name="timeDay" href="#timeDay">#</a> d3.<b>timeDay</b> · [Source](https://github.com/d3/d3-time/blob/main/src/day.js "Source")
<br><a href="#timeDay">#</a> d3.<b>utcDay</b> · [Source](https://github.com/d3/d3-time/blob/main/src/utcDay.js "Source")
<br><a href="#timeDay">#</a> d3.<b>unixDay</b> · [Source](https://github.com/d3/d3-time/blob/main/src/unixDay.js "Source")
Days (e.g., February 7, 2012 at 12:00 AM); typically 24 hours. Days in local time may range from 23 to 25 hours due to daylight saving. d3.unixDay is like [d3.utcDay](#timeDay), except it counts days since the UNIX epoch (January 1, 1970) such that *interval*.every returns uniformly-spaced dates rather than varying based on day-of-month.
<a name="timeWeek" href="#timeWeek">#</a> d3.<b>timeWeek</b> · [Source](https://github.com/d3/d3-time/blob/main/src/week.js "Source")
<br><a href="#timeWeek">#</a> d3.<b>utcWeek</b> · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js "Source")
Alias for [d3.timeSunday](#timeSunday); 7 days and typically 168 hours. Weeks in local time may range from 167 to 169 hours due to daylight saving.
<a name="timeSunday" href="#timeSunday">#</a> d3.<b>timeSunday</b> · [Source](https://github.com/d3/d3-time/blob/main/src/week.js)
<br><a href="#timeSunday">#</a> d3.<b>utcSunday</b> · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js)
Sunday-based weeks (e.g., February 5, 2012 at 12:00 AM).
<a name="timeMonday" href="#timeMonday">#</a> d3.<b>timeMonday</b> · [Source](https://github.com/d3/d3-time/blob/main/src/week.js)
<br><a href="#timeMonday">#</a> d3.<b>utcMonday</b> · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js)
Monday-based weeks (e.g., February 6, 2012 at 12:00 AM).
<a name="timeTuesday" href="#timeTuesday">#</a> d3.<b>timeTuesday</b> · [Source](https://github.com/d3/d3-time/blob/main/src/week.js)
<br><a href="#timeTuesday">#</a> d3.<b>utcTuesday</b> · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js)
Tuesday-based weeks (e.g., February 7, 2012 at 12:00 AM).
<a name="timeWednesday" href="#timeWednesday">#</a> d3.<b>timeWednesday</b> · [Source](https://github.com/d3/d3-time/blob/main/src/week.js)
<br><a href="#timeWednesday">#</a> d3.<b>utcWednesday</b> · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js)
Wednesday-based weeks (e.g., February 8, 2012 at 12:00 AM).
<a name="timeThursday" href="#timeThursday">#</a> d3.<b>timeThursday</b> · [Source](https://github.com/d3/d3-time/blob/main/src/week.js)
<br><a href="#timeThursday">#</a> d3.<b>utcThursday</b> · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js)
Thursday-based weeks (e.g., February 9, 2012 at 12:00 AM).
<a name="timeFriday" href="#timeFriday">#</a> d3.<b>timeFriday</b> · [Source](https://github.com/d3/d3-time/blob/main/src/week.js)
<br><a href="#timeFriday">#</a> d3.<b>utcFriday</b> · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js)
Friday-based weeks (e.g., February 10, 2012 at 12:00 AM).
<a name="timeSaturday" href="#timeSaturday">#</a> d3.<b>timeSaturday</b> · [Source](https://github.com/d3/d3-time/blob/main/src/week.js)
<br><a href="#timeSaturday">#</a> d3.<b>utcSaturday</b> · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js)
Saturday-based weeks (e.g., February 11, 2012 at 12:00 AM).
<a name="timeMonth" href="#timeMonth">#</a> d3.<b>timeMonth</b> · [Source](https://github.com/d3/d3-time/blob/main/src/month.js "Source")
<br><a href="#timeMonth">#</a> d3.<b>utcMonth</b> · [Source](https://github.com/d3/d3-time/blob/main/src/utcMonth.js "Source")
Months (e.g., February 1, 2012 at 12:00 AM); ranges from 28 to 31 days.
<a name="timeYear" href="#timeYear">#</a> d3.<b>timeYear</b> · [Source](https://github.com/d3/d3-time/blob/main/src/year.js "Source")
<br><a href="#timeYear">#</a> d3.<b>utcYear</b> · [Source](https://github.com/d3/d3-time/blob/main/src/utcYear.js "Source")
Years (e.g., January 1, 2012 at 12:00 AM); ranges from 365 to 366 days.
### Ranges
For convenience, aliases for [*interval*.range](#interval_range) are also provided as plural forms of the corresponding interval.
<a name="timeMilliseconds" href="#timeMilliseconds">#</a> d3.<b>timeMilliseconds</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/millisecond.js)
<br><a href="#timeMilliseconds">#</a> d3.<b>utcMilliseconds</b>(<i>start</i>, <i>stop</i>[, <i>step</i>])
Aliases for [d3.timeMillisecond](#timeMillisecond).[range](#interval_range) and [d3.utcMillisecond](#timeMillisecond).[range](#interval_range).
<a name="timeSeconds" href="#timeSeconds">#</a> d3.<b>timeSeconds</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/second.js)
<br><a href="#timeSeconds">#</a> d3.<b>utcSeconds</b>(<i>start</i>, <i>stop</i>[, <i>step</i>])
Aliases for [d3.timeSecond](#timeSecond).[range](#interval_range) and [d3.utcSecond](#timeSecond).[range](#interval_range).
<a name="timeMinutes" href="#timeMinutes">#</a> d3.<b>timeMinutes</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/minute.js)
<br><a href="#timeMinutes">#</a> d3.<b>utcMinutes</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/utcMinute.js)
Aliases for [d3.timeMinute](#timeMinute).[range](#interval_range) and [d3.utcMinute](#timeMinute).[range](#interval_range).
<a name="timeHours" href="#timeHours">#</a> d3.<b>timeHours</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/hour.js)
<br><a href="#timeHours">#</a> d3.<b>utcHours</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/utcHour.js)
Aliases for [d3.timeHour](#timeHour).[range](#interval_range) and [d3.utcHour](#timeHour).[range](#interval_range).
<a name="timeDays" href="#timeDays">#</a> d3.<b>timeDays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/day.js)
<br><a href="#timeDays">#</a> d3.<b>utcDays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/utcDay.js)
<br><a href="#timeDays">#</a> d3.<b>unixDays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/unixDay.js)
Aliases for [d3.timeDay](#timeDay).[range](#interval_range), [d3.utcDay](#timeDay).[range](#interval_range), and [d3.unixDay](#timeDay).[range](#interval_range).
<a name="timeWeeks" href="#timeWeeks">#</a> d3.<b>timeWeeks</b>(<i>start</i>, <i>stop</i>[, <i>step</i>])
<br><a href="#timeWeeks">#</a> d3.<b>utcWeeks</b>(<i>start</i>, <i>stop</i>[, <i>step</i>])
Aliases for [d3.timeWeek](#timeWeek).[range](#interval_range) and [d3.utcWeek](#timeWeek).[range](#interval_range).
<a name="timeSundays" href="#timeSundays">#</a> d3.<b>timeSundays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/week.js)
<br><a href="#timeSundays">#</a> d3.<b>utcSundays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js)
Aliases for [d3.timeSunday](#timeSunday).[range](#interval_range) and [d3.utcSunday](#timeSunday).[range](#interval_range).
<a name="timeMondays" href="#timeMondays">#</a> d3.<b>timeMondays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/week.js)
<br><a href="#timeMondays">#</a> d3.<b>utcMondays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js)
Aliases for [d3.timeMonday](#timeMonday).[range](#interval_range) and [d3.utcMonday](#timeMonday).[range](#interval_range).
<a name="timeTuesdays" href="#timeTuesdays">#</a> d3.<b>timeTuesdays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/week.js)
<br><a href="#timeTuesdays">#</a> d3.<b>utcTuesdays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js)
Aliases for [d3.timeTuesday](#timeTuesday).[range](#interval_range) and [d3.utcTuesday](#timeTuesday).[range](#interval_range).
<a name="timeWednesdays" href="#timeWednesdays">#</a> d3.<b>timeWednesdays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/week.js)
<br><a href="#timeWednesdays">#</a> d3.<b>utcWednesdays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js)
Aliases for [d3.timeWednesday](#timeWednesday).[range](#interval_range) and [d3.utcWednesday](#timeWednesday).[range](#interval_range).
<a name="timeThursdays" href="#timeThursdays">#</a> d3.<b>timeThursdays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/week.js)
<br><a href="#timeThursdays">#</a> d3.<b>utcThursdays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js)
Aliases for [d3.timeThursday](#timeThursday).[range](#interval_range) and [d3.utcThursday](#timeThursday).[range](#interval_range).
<a name="timeFridays" href="#timeFridays">#</a> d3.<b>timeFridays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/week.js)
<br><a href="#timeFridays">#</a> d3.<b>utcFridays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js)
Aliases for [d3.timeFriday](#timeFriday).[range](#interval_range) and [d3.utcFriday](#timeFriday).[range](#interval_range).
<a name="timeSaturdays" href="#timeSaturdays">#</a> d3.<b>timeSaturdays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/week.js)
<br><a href="#timeSaturdays">#</a> d3.<b>utcSaturdays</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/utcWeek.js)
Aliases for [d3.timeSaturday](#timeSaturday).[range](#interval_range) and [d3.utcSaturday](#timeSaturday).[range](#interval_range).
<a name="timeMonths" href="#timeMonths">#</a> d3.<b>timeMonths</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/month.js)
<br><a href="#timeMonths">#</a> d3.<b>utcMonths</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/utcMonth.js)
Aliases for [d3.timeMonth](#timeMonth).[range](#interval_range) and [d3.utcMonth](#timeMonth).[range](#interval_range).
<a name="timeYears" href="#timeYears">#</a> d3.<b>timeYears</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/year.js)
<br><a href="#timeYears">#</a> d3.<b>utcYears</b>(<i>start</i>, <i>stop</i>[, <i>step</i>]) · [Source](https://github.com/d3/d3-time/blob/main/src/utcYear.js)
Aliases for [d3.timeYear](#timeYear).[range](#interval_range) and [d3.utcYear](#timeYear).[range](#interval_range).
### Ticks
<a name="timeTicks" href="#timeTicks">#</a> d3.<b>timeTicks</b>(<i>start</i>, <i>stop</i>, <i>count</i>) · [Source](https://github.com/d3/d3-time/blob/main/src/ticks.js)
Equivalent to [d3.utcTicks](#utcTicks), but in local time.
<a name="timeTickInterval" href="#timeTickInterval">#</a> d3.<b>timeTickInterval</b>(<i>start</i>, <i>stop</i>, <i>count</i>) · [Source](https://github.com/d3/d3-time/blob/main/src/ticks.js)
Returns the time interval that would be used by [d3.timeTicks](#timeTicks) given the same arguments.
<a name="utcTicks" href="#utcTicks">#</a> d3.<b>utcTicks</b>(<i>start</i>, <i>stop</i>, <i>count</i>) · [Source](https://github.com/d3/d3-time/blob/main/src/ticks.js)
Returns an array of approximately *count* dates at regular intervals between *start* and *stop* (inclusive). If *stop* is before *start*, dates are returned in reverse chronological order; otherwise dates are returned in chronological order. The following UTC time intervals are considered:
* 1 second
* 5 seconds
* 15 seconds
* 30 seconds
* 1 minute
* 5 minutes
* 15 minutes
* 30 minutes
* 1 hour
* 3 hours
* 6 hours
* 12 hours
* 1 day
* 2 days
* 1 week
* 1 month
* 3 months
* 1 year
Multiples of milliseconds (for small ranges) and years (for large ranges) are also considered, following the rules of [d3.ticks](https://github.com/d3/d3-array/blob/main/README.md#ticks). The interval producing the number of dates that is closest to *count* is used. For example:
```js
start = new Date(Date.UTC(1970, 2, 1))
stop = new Date(Date.UTC(1996, 2, 19))
count = 4
d3.utcTicks(start, stop, count) // [1975-01-01, 1980-01-01, 1985-01-01, 1990-01-01, 1995-01-01]
```
If *count* is a time interval, this function behaves similarly to [*interval*.range](#interval_range) except that both *start* and *stop* are inclusive and it may return dates in reverse chronological order if *stop* is before *start*.
<a name="utcTickInterval" href="#utcTickInterval">#</a> d3.<b>utcTickInterval</b>(<i>start</i>, <i>stop</i>, <i>count</i>) · [Source](https://github.com/d3/d3-time/blob/main/src/ticks.js)
Returns the time interval that would be used by [d3.utcTicks](#utcTicks) given the same arguments. If there is no associated interval, such as when *start* or *stop* is invalid, returns null. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/d3-time/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/d3-time/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 27863
} |
# d3-timer
This module provides an efficient queue capable of managing thousands of concurrent animations, while guaranteeing consistent, synchronized timing with concurrent or staged animations. Internally, it uses [requestAnimationFrame](https://developer.mozilla.org/en-US/docs/Web/API/window/requestAnimationFrame) for fluid animation (if available), switching to [setTimeout](https://developer.mozilla.org/en-US/docs/Web/API/WindowTimers/setTimeout) for delays longer than 24ms.
## Installing
If you use npm, `npm install d3-timer`. You can also download the [latest release on GitHub](https://github.com/d3/d3-timer/releases/latest). For vanilla HTML in modern browsers, import d3-timer from Skypack:
```html
<script type="module">
import {timer} from "https://cdn.skypack.dev/d3-timer@3";
const t = timer(callback);
</script>
```
For legacy environments, you can load d3-timer’s UMD bundle from an npm-based CDN such as jsDelivr; a `d3` global is exported:
```html
<script src="https://cdn.jsdelivr.net/npm/d3-timer@3"></script>
<script>
const timer = d3.timer(callback);
</script>
```
## API Reference
<a name="now" href="#now">#</a> d3.<b>now</b>() [<>](https://github.com/d3/d3-timer/blob/master/src/timer.js "Source")
Returns the current time as defined by [performance.now](https://developer.mozilla.org/en-US/docs/Web/API/Performance/now) if available, and [Date.now](https://developer.mozilla.org/en-US/docs/JavaScript/Reference/Global_Objects/Date/now) if not. The current time is updated at the start of a frame; it is thus consistent during the frame, and any timers scheduled during the same frame will be synchronized. If this method is called outside of a frame, such as in response to a user event, the current time is calculated and then fixed until the next frame, again ensuring consistent timing during event handling.
<a name="timer" href="#timer">#</a> d3.<b>timer</b>(<i>callback</i>[, <i>delay</i>[, <i>time</i>]]) [<>](https://github.com/d3/d3-timer/blob/master/src/timer.js "Source")
Schedules a new timer, invoking the specified *callback* repeatedly until the timer is [stopped](#timer_stop). An optional numeric *delay* in milliseconds may be specified to invoke the given *callback* after a delay; if *delay* is not specified, it defaults to zero. The delay is relative to the specified *time* in milliseconds; if *time* is not specified, it defaults to [now](#now).
The *callback* is passed the (apparent) *elapsed* time since the timer became active. For example:
```js
const t = d3.timer((elapsed) => {
console.log(elapsed);
if (elapsed > 200) t.stop();
}, 150);
```
This produces roughly the following console output:
```
3
25
48
65
85
106
125
146
167
189
209
```
(The exact values may vary depending on your JavaScript runtime and what else your computer is doing.) Note that the first *elapsed* time is 3ms: this is the elapsed time since the timer started, not since the timer was scheduled. Here the timer started 150ms after it was scheduled due to the specified delay. The apparent *elapsed* time may be less than the true *elapsed* time if the page is backgrounded and [requestAnimationFrame](https://developer.mozilla.org/en-US/docs/Web/API/window/requestAnimationFrame) is paused; in the background, apparent time is frozen.
If [timer](#timer) is called within the callback of another timer, the new timer callback (if eligible as determined by the specified *delay* and *time*) will be invoked immediately at the end of the current frame, rather than waiting until the next frame. Within a frame, timer callbacks are guaranteed to be invoked in the order they were scheduled, regardless of their start time.
<a name="timer_restart" href="#timer_restart">#</a> <i>timer</i>.<b>restart</b>(<i>callback</i>[, <i>delay</i>[, <i>time</i>]]) [<>](https://github.com/d3/d3-timer/blob/master/src/timer.js "Source")
Restart a timer with the specified *callback* and optional *delay* and *time*. This is equivalent to stopping this timer and creating a new timer with the specified arguments, although this timer retains the original invocation priority.
<a name="timer_stop" href="#timer_stop">#</a> <i>timer</i>.<b>stop</b>() [<>](https://github.com/d3/d3-timer/blob/master/src/timer.js "Source")
Stops this timer, preventing subsequent callbacks. This method has no effect if the timer has already stopped.
<a name="timerFlush" href="#timerFlush">#</a> d3.<b>timerFlush</b>() [<>](https://github.com/d3/d3-timer/blob/master/src/timer.js "Source")
Immediately invoke any eligible timer callbacks. Note that zero-delay timers are normally first executed after one frame (~17ms). This can cause a brief flicker because the browser renders the page twice: once at the end of the first event loop, then again immediately on the first timer callback. By flushing the timer queue at the end of the first event loop, you can run any zero-delay timers immediately and avoid the flicker.
<a name="timeout" href="#timeout">#</a> d3.<b>timeout</b>(<i>callback</i>[, <i>delay</i>[, <i>time</i>]]) [<>](https://github.com/d3/d3-timer/blob/master/src/timeout.js "Source")
Like [timer](#timer), except the timer automatically [stops](#timer_stop) on its first callback. A suitable replacement for [setTimeout](https://developer.mozilla.org/en-US/docs/Web/API/WindowTimers/setTimeout) that is guaranteed to not run in the background. The *callback* is passed the elapsed time.
<a name="interval" href="#interval">#</a> d3.<b>interval</b>(<i>callback</i>[, <i>delay</i>[, <i>time</i>]]) [<>](https://github.com/d3/d3-timer/blob/master/src/interval.js "Source")
Like [timer](#timer), except the *callback* is invoked only every *delay* milliseconds; if *delay* is not specified, this is equivalent to [timer](#timer). A suitable replacement for [setInterval](https://developer.mozilla.org/en-US/docs/Web/API/WindowTimers/setInterval) that is guaranteed to not run in the background. The *callback* is passed the elapsed time. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/d3-timer/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/d3-timer/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 5984
} |
# Change Log
All notable changes to this project will be documented in this file.
This project adheres to [Semantic Versioning].
This change log follows the format documented in [Keep a CHANGELOG].
[semantic versioning]: http://semver.org/
[keep a changelog]: http://keepachangelog.com/
## v3.6.0 - 2024-03-18
On this release worked @kossnocorp and @world1dan. Also, thanks to [@seated](https://github.com/seated) [for sponsoring me](https://github.com/sponsors/kossnocorp).
### Fixed
- [Fixed weeks in the Belarisuan locale's `formatDistance`.](https://github.com/date-fns/date-fns/pull/3720)
### Added
- [Added CDN versions of modules compatible with older browsers.](https://github.com/date-fns/date-fns/pull/3737) [See the CDN guide.](https://date-fns.org/docs/CDN)
## v3.5.0 - 2024-03-15
Kudos to @fturmel, @kossnocorp, @makstyle119, @tan75, @marcreichel, @tareknatsheh and @audunru for working on the release. Also, thanks to [@seated](https://github.com/seated) [for sponsoring me](https://github.com/sponsors/kossnocorp).
### Fixed
- [Fixed functions that use current date internally and made them work with date extensions like `UTCDate`.](https://github.com/date-fns/date-fns/issues/3730)
- [Fixed `daysToWeeks` returning negative 0.](https://github.com/date-fns/date-fns/commit/882ced61c692c7c4a79eaaec6eb07cb9c8c9195b)
- [Fixed German grammar for the "half a minute" string.](https://github.com/date-fns/date-fns/pull/3715)
### Added
- [Added the Northern Sámi (`se`) locale.](https://github.com/date-fns/date-fns/pull/3724)
- Added the `constructNow` function that creates the current date using the passed reference date's constructor.
## v3.4.0 - 2024-03-11
Kudos to @kossnocorp, @sakamossan and @Revan99 for working on the release. Also, thanks to [@seated](https://github.com/seated) [for sponsoring me](https://github.com/sponsors/kossnocorp).
### Added
- [Added `roundToNearestHours` function.](https://github.com/date-fns/date-fns/pull/2752)
- [Added Central Kurdish (`ckb`) locale.](https://github.com/date-fns/date-fns/pull/3421)
## v3.3.1 - 2024-01-22
Kudos to @kossnocorp and @fturmel for working on the release.
### Fixed
- Fixed DST issue in `getOverlappingDaysInIntervals`, resulting in an inconsistent number of days returned for intervals starting and ending in different DST periods.
- Fixed functions incorrectly using `trunc` instead of `round`. The bug was introduced in v3.3.0. The affected functions: `differenceInCalendarDays`, `differenceInCalendarISOWeeks`, `differenceInCalendarWeeks`, `getISOWeek`, `getWeek`, and `getISOWeeksInYear`.
## v3.3.0 - 2024-01-20
On this release worked @kossnocorp, @TheKvikk, @fturmel and @ckcherry23.
### Fixed
- Fixed the bug in `getOverlappingDaysInIntervals` caused by incorrect sorting of interval components that led to 0 for timestamps of different lengths.
- Fixed bugs when working with negative numbers caused by using `Math.floor` (`-1.1` → `-2`) instead of `Math.trunc` (`-1.1` → `-1`). Most of the conversion functions (i.e., `hoursToMinutes`) were affected when passing some negative fractional input. Also, some other functions that could be possibly affected by unfortunate timezone/date combinations were fixed.
The functions that were affected: `format`, `parse`, `getUnixTime`, `daysToWeeks`, `hoursToMilliseconds`, `hoursToMinutes`, `hoursToSeconds`, `milliseconds`, `minutesToMilliseconds`, `millisecondsToMinutes`, `monthsToYears`, `millisecondsToHours`, `millisecondsToSeconds`, `minutesToHours`, `minutesToSeconds`, `yearsToQuarters`, `yearsToMonths`, `yearsToDays`, `weeksToDays`, `secondsToMinutes`, `secondsToHours`, `quartersToYears`, `quartersToMonths` and `monthsToQuarters`.
- [Fixed the Czech locale's `formatDistance` to include `1` in `formatDistance`.](https://github.com/date-fns/date-fns/pull/3269)
- Fixed `differenceInSeconds` and other functions relying on rounding options that can produce a negative 0.
- [Added a preprocessor to the locales API, enabling fixing a long-standing bug in the French locale.](https://github.com/date-fns/date-fns/pull/3662) ([#1391](https://github.com/date-fns/date-fns/issues/1391))
- Added missing `yearsToDays` to the FP submodule.
- Made functions using rounding methods always return `0` instead of `-0`.
### Added
- [Added `format` alias `formatDate` with corresponding `FormatDateOptions` interface](https://github.com/date-fns/date-fns/pull/3653).
## v3.2.0 - 2024-01-09
This release is brought to you by @kossnocorp, @fturmel, @grossbart, @MelvinVermeer, and @jcarstairs-scottlogic.
### Fixed
- Fixed types compatability with Lodash's `flow` and fp-ts's `pipe`. ([#3641](https://github.com/date-fns/date-fns/issues/3641))
- [Fixed inconsistent behavior of `roundToNearestMinutes`.](https://github.com/date-fns/date-fns/pull/3132)
### Added
- Added exports of `format`, `lightFormat`, and `parse` internals that enable 3rd-parties to consume those.
## v3.1.0 - 2024-01-05
This release is brought to you by @kossnocorp, @makstyle119 and @dmgawel.
### Fixed
- [Fixed the plural form of weeks in Swedish](https://github.com/date-fns/date-fns/pull/3448).
### Added
- [Added `yearsToDays` function](https://github.com/date-fns/date-fns/pull/3540).
- Added warning about using protected tokens like `Y` or `D` without passing a corresponding option. [See #2950](https://github.com/date-fns/date-fns/issues/2950).
## v3.0.6 - 2023-12-22
On this release worked @imwh0im, @jamcry and @tyrw.
### Fixed
- [Fixed bug in `areIntervalsOverlapping` caused by incorrect sorting](https://github.com/date-fns/date-fns/pull/3628) ([#3614](https://github.com/date-fns/date-fns/issues/3614))
## v3.0.5 - 2023-12-21
This release is brought to you by @goku4199.
### Fixed
- [Fixed internal `toDate` not processing string arguments properly](https://github.com/date-fns/date-fns/pull/3626)
## v3.0.4 - 2023-12-21
This release is brought to you by @kossnocorp.
### Fixed
- Fixed isWithinInterval bug caused by incorrectly sorting dates ([#3623](https://github.com/date-fns/date-fns/issues/3623)).
## v3.0.3 - 2023-12-21
### Fixed
- Rolled back pointing ESM types to the same `d.ts` files. Instead now it copies the content to avoid [the Masquerading as CJS problem](https://github.com/arethetypeswrong/arethetypeswrong.github.io/blob/main/docs/problems/FalseCJS.md) reported by "Are the types wrong?".
## v3.0.2 - 2023-12-21
### Fixed
- Fixed [yet another issue caused by ESM types](https://github.com/date-fns/date-fns/issues/3620) by pointing to the same `d.ts` files.
- [Added `package.json` to exports](https://github.com/date-fns/date-fns/pull/3601) to provide access to tooling.
- [Fixed TypeScript 5.4 build break](https://github.com/date-fns/date-fns/pull/3598) by using the latest type names.
## v3.0.1 - 2023-12-20
### Fixed
- [Fixed an error](https://github.com/date-fns/date-fns/pull/3618) in certain environments caused by `d.mts` files exporting only types.
## v3.0.0 - 2023-12-18
### Changed
- **BREAKING**: date-fns is now a dual-package with the support of both ESM and CommonJS. The files exports are now explicitly in the `package.json`. The ESM files now have `.mjs` extension.
- **BREAKING**: The package now has a flat structure, meaning functions are now named `node_modules/date-fns/add.mjs`, locales are `node_modules/date-fns/locale/enUS.mjs`, etc.
- **BREAKING**: Now all file content’s exported via named exports instead of `export default`, which will require change direct imports i.e. `const addDays = require(‘date-fns/addDays’)` to `const { addDays } = require(‘date-fns/addDays’)`.
- **BREAKING**: TypeScript types are now completely rewritten, check out the `d.ts` files for more information.
- **BREAKING**: `constants` now is not exported via the index, so to import one use `import { daysInYear } from "date-fns/constants";`. It improves compatibility with setups that modularize imports [like Next.js](https://twitter.com/kossnocorp/status/1731181274579325260).
- **BREAKING**: Functions now don’t check the number of passed arguments, delegating this task to type checkers. The functions are now slimmer because of this.
- **BREAKING** The arguments are not explicitly converted to the target types. Instead, they are passed as is, delegating this task to type checkers.
- **BREAKING**: Functions that accept `Interval` arguments now do not throw an error if the start is before the end and handle it as a negative interval. If one of the properties in an `Invalid Date`, these functions also do not throw and handle them as invalid intervals.
- `areIntervalsOverlapping` normalize intervals before comparison, so `{ start: a, end: b }` is practically equivalent to `{ start: b, end: a }`. When comparing intervals with one of the properties being `Invalid Date`, the function will return false unless the others are valid and equal, given the `inclusive` option is passed. Otherwise, and when even one of the intervals has both properties invalid, the function will always return `false`.
- `getOverlappingDaysInIntervals` now normalizes intervals before comparison, so `{ start: a, end: b }` is practically equivalent to `{ start: b, end: a }`. If any of the intervals’ properties is an `Invalid Date`, the function will always return 0.
- `isWithinInterval` now normalizes intervals before comparison, so `{ start: a, end: b }` is practically equivalent to `{ start: b, end: a }`. If any of the intervals’ properties is an `Invalid Date`, the function will always return false.
- `intervalToDuration` now returns negative durations for negative intervals. If one or both of the interval properties are invalid, the function will return an empty object.
- The eachXOfInterval functions (`eachDayOfInterval`, `eachHourOfInterval`, `eachMinuteOfInterval`, `eachMonthOfInterval`, `eachWeekendOfInterval`, `eachWeekendOfMonth`, `eachWeekendOfYear`, `eachWeekOfInterval`, `eachYearOfInterval`) now return a reversed array if the passed interval’s start is after the end. Invalid properties will result in an empty array. Functions that accept the `step` option now also allow negative, 0, and NaN values and return reversed results if the step is negative and an empty array otherwise.
- **BREAKING**: `intervalToDuration` now skips 0 values in the resulting duration, resulting in more compact objects with only relevant properties.
- **BREAKING**: `roundToNearestMinutes` now returns `Invalid Date` instead of throwing an error when `nearestTo` option is less than 1 or more than 30.
- **BREAKING**: IE is no longer supported.
- **BREAKING**: Now all functions use `Math.trunc` rounding method where rounding is required. The behavior is configurable on a per-function basis.
- **BREAKING**: Undocumented `onlyNumeric` option was removed from `nn` and `sv` locales. If you relied on it, [please contact me](mailto:[email protected]).
- **BREAKING**: Flow is not supported anymore. If you relied on it, [please contact me](mailto:[email protected]).
- **BREAKING**: The locales now use regular functions instead of the UTC version, which should not break any code unless you used locales directly.
### Added
- All functions that accept date arguments now also accept strings.
- All functions now export options interfaces.
- Now functions allow passing custom Date extensions like [UTCDate](https://github.com/date-fns/utc). They will detect and use the arguments constructor to generate the result of the same class.
- `eachMonthOfInterval`, `eachQuarterOfInterval`, `eachWeekOfInterval`, and `eachYearOfInterval` now accept the `step` option like most of the eachXOfInterval functions.
- A new `interval` function that validates interval, emulating the v2 interval functions behavior.
- `differenceInX` functions now accept options and allow setting up `roundingMethod` that configures how the result is rounded. `Math.trunc` is the default method.
## v2.30.0
Kudos to @kossnocorp and @Andarist for working on the release.
### Changes
- Fixed increased build size after enabling compatibility with older browsers in the previous release. This was done by adding @babel/runtime as a dependency. [See more details](https://github.com/date-fns/date-fns/issues/3208#issuecomment-1528592465).
## v2.29.3 - 2022-09-13
This release is prepared by our own @leshakoss.
### Fixed
- [Fixed Ukrainian (`uk`) locale grammar for `formatDistance`.](https://github.com/date-fns/date-fns/pull/3175)
- [Improved browser compatibility by transforming the code with `@babel/preset-env`.](https://github.com/date-fns/date-fns/pull/3167)
## v2.29.2 - 2022-08-18
This release is brought to you by @nopears, @vadimpopa and @leshakoss.
### Fixed
- [Fixed `sv` locale abbreviated months matcher.](https://github.com/date-fns/date-fns/pull/3160)
- [Fixed `uk` locale abbreviated months matcher.](https://github.com/date-fns/date-fns/pull/3139)
- [Fixed a breaking change in `intervalToDuration` by removing a recently introduced RangeError.](https://github.com/date-fns/date-fns/pull/3153)
## v2.29.1 - 2022-08-18
Thanks to @fturmel for working on the release.
### Fixed
- [Fixed TypeScript and flow types for daysInYear constant.](https://github.com/date-fns/date-fns/pull/3125)
## v2.29.0 - 2022-07-22
On this release worked @tan75, @kossnocorp, @nopears, @Balastrong, @cpapazoglou, @dovca, @aliasgar55, @tomchentw, @JuanM04, @alexandresaura, @fturmel, @aezell, @andersravn, @TiagoPortfolio, @SukkaW, @Zebreus, @aviskarkc10, @maic66, @a-korzun, @Mejans, @davidspiess, @alexgul1, @matroskin062, @undecaf, @mprovenc, @jooola and @leshakoss.
### Added
- [Added `intlFormatDistance` function`.](https://github.com/date-fns/date-fns/pull/2173)
- [Added `setDefaultOptions` and `getDefaultOptions` functions that allow you to set default default locale, `weekStartsOn` and `firstWeekContainsDate`.](https://github.com/date-fns/date-fns/pull/3069)
- [Added `roundingMethod` option to `roundToNearestMinutes`.](https://github.com/date-fns/date-fns/pull/3091)
- [Added Swiss Italian locale (`it-CH`).](https://github.com/date-fns/date-fns/pull/2886)
- [Added Occitan (`oc`) locale.](https://github.com/date-fns/date-fns/pull/2106) ([#2061](https://github.com/date-fns/date-fns/issues/2061))
- [Added Belarusian Classic (`be-tarask`) locale.](https://github.com/date-fns/date-fns/pull/3115)
### Fixed
- [Fixed Azerbaijani (`az`) locale for `formatDistance`.](https://github.com/date-fns/date-fns/pull/2924)
- [Fixed Czech (`cs`) locale for `parse`.](https://github.com/date-fns/date-fns/pull/3059)
- [Fixed TypeScript types for constants.](https://github.com/date-fns/date-fns/pull/2941)
- [Fixed long formatters in the South African English locale (`en-ZA`).](https://github.com/date-fns/date-fns/pull/3014)
- [Fixed a typo in the Icelandic locale (`is`) for `format`.](https://github.com/date-fns/date-fns/pull/2974)
- [Fixed weekday format for `formatRelative` in the Portuguese locale (`pt`).](https://github.com/date-fns/date-fns/pull/2992)
- [Fixed `intervalToDuration` being off by 1 day sometimes.](https://github.com/date-fns/date-fns/pull/2616)
- [Fixed ordinal number formatting in Italian locale (`it`).](https://github.com/date-fns/date-fns/pull/1617)
- [Fixed issue parsing months in Croatian (`hr`), Georgian (`ka`) and Serbian (`sr` and `sr-Latn`) locales.](https://github.com/date-fns/date-fns/pull/2898)
### Changed
- [Replaced `git.io` links with full URLs in error messages.](https://github.com/date-fns/date-fns/pull/3021)
- [_Internal_: removed "v2.0.0 breaking changes" section from individual function docs](https://github.com/date-fns/date-fns/pull/2905)
## v2.28.0 - 2021-12-28
Kudos to @tan75, @fturmel, @arcanar7, @jeffjose, @helmut-lang, @zrev2220, @jooola, @minitesh, @cowboy-bebug, @mesqueeb, @JuanM04, @zhirzh, @damon02 and @leshakoss for working on the release.
### Added
- [Added West Frisian (`fy`) locale.](https://github.com/date-fns/date-fns/pull/2183)
- [Added Uzbek Cyrillic locale (`uz-Cyrl`).](https://github.com/date-fns/date-fns/pull/2811)
### Fixed
- [add the missing accent mark for Saturday in Spanish locale (`es`) for `format`.](https://github.com/date-fns/date-fns/pull/2869)
- [allowed `K` token to be used with `a` or `b` in `parse`.](https://github.com/date-fns/date-fns/pull/2814)
## v2.27.0 - 2021-11-30
Kudos to @tan75, @hg-pyun, @07akioni, @razvanmitre, @Haqverdi, @pgcalixto, @janziemba, @fturmel, @JuanM04, @zhirzh, @seanghay, @bulutfatih, @nodeadtree, @cHaLkdusT, @a-korzun, @fishmandev, @wingclover, @Zacharias3690, @kossnocorp and @leshakoss for working on the release.
### Fixed
- [Fixed translation for quarters in `format` in Chinese Simplified locale (`zh-CN`).](https://github.com/date-fns/date-fns/pull/2771)
- [Fixed `P` token in `format` for Romanian locale (`ro`).](https://github.com/date-fns/date-fns/pull/2213)
- [Fixed era and month formatters in Azerbaijani locale (`az`).](https://github.com/date-fns/date-fns/pull/1632)
- [Fixed `formatRelative` patterns in Georgian locale (`ka`).](https://github.com/date-fns/date-fns/pull/2797)
- [Fixed regular expressions for `parse` in Estonian locale (`er`).](https://github.com/date-fns/date-fns/pull/2038)
- [Fixed the format of zeros in `formatDuration` in Czech locale (`cs`).](https://github.com/date-fns/date-fns/pull/2579)
- [Fixed ordinal formatting for years, weeks, hours, minutes and seconds in `fr`, `fr-CA` and `fr-CH` locales.](https://github.com/date-fns/date-fns/pull/2626)
- [Fixed constants not having proper TypeScript and Flow types.](https://github.com/date-fns/date-fns/pull/2791)
- [Fixed translation for Monday in Turkish locale (`tr`).](https://github.com/date-fns/date-fns/pull/2720)
- [Fixed `eachMinuteOfInterval` not handling intervals less than a minute correctly.](https://github.com/date-fns/date-fns/pull/2603)
- [Fixed flow types for `closestTo` and `closestIndexTo`.](https://github.com/date-fns/date-fns/pull/2781)
### Added
- [Added Khmer locale (`km`).](https://github.com/date-fns/date-fns/pull/2713)
## v2.26.0 - 2021-11-19
Thanks to @kossnocorp, @leshakoss, @tan75, @gaplo, @AbdAllahAbdElFattah13, @fturmel, @kentaro84207, @V-Gutierrez, @atefBB, @jhonatanmacazana, @zhirzh, @Haqverdi, @mandaputtra, @micnic and @rikkalo for working on the release.
### Fixed
- [Fixed `formatRelative` format for `lastWeek` in Spanish locale.](https://github.com/date-fns/date-fns/pull/2753)
- [Fixed translation for October in Hindi locale.](https://github.com/date-fns/date-fns/pull/2729)
- [Fixed Azerbaijani locale to use correct era matchers for `parse`.](https://github.com/date-fns/date-fns/pull/1633)
- [Added the functions that use `weekStartsOn` and `firstWeekContainsDate` that were missing from the `Locale` documentation page.](https://github.com/date-fns/date-fns/pull/2652)
### Changed
- [Changed abbreviation for August from "Ags" to "Agt" in Indonesian locale.](https://github.com/date-fns/date-fns/pull/2658)
### Added
- [Added Irish English locale (`en-IE`).](https://github.com/date-fns/date-fns/pull/2772)
- [Added Arabic locale (`ar`).](https://github.com/date-fns/date-fns/pull/2721) ([#1670](https://github.com/date-fns/date-fns/issues/1670))
- [Added Hong Kong Traditional Chinese locale (zh-HK).](https://github.com/date-fns/date-fns/pull/2686) ([#2684](https://github.com/date-fns/date-fns/issues/2684))
- [Added Egyptian Arabic locale (ar-EG).](https://github.com/date-fns/date-fns/pull/2699)
## v2.25.0 - 2021-10-05
This release is brought to you by @kossnocorp, @gierschv, @fturmel, @redbmk, @mprovenc, @artyom-ivanov and @tan75.
### Added
- [Added Japanese Hiragana locale (`ja-Hira`).](https://github.com/date-fns/date-fns/pull/2663)
- [Added standalone months support to `de` and `de-AT` locales.](https://github.com/date-fns/date-fns/pull/2602)
## v2.24.0 - 2021-09-17
Kudos to [Sasha Koss](http://github.com/kossnocorp), [Lucas Silva](http://github.com/LucasHFS), [Jan Ziemba](http://github.com/janziemba), [Anastasia Kobzar](http://github.com/rikkalo), [Deepak Gupta](http://github.com/Mr-DG-Wick), [Jonas L](http://github.com/jooola), [Kentaro Suzuki](http://github.com/kentaro84207), [Koussay Haj Kacem](http://github.com/essana3), [fturmel](http://github.com/fturmel), [Tan75](http://github.com/tan75) and [Adriaan Callaerts](http://github.com/call-a3) for working on the release.
### Fixed
- [Fixed an edge case in the Slovak locale caused by unescaped character.](https://github.com/date-fns/date-fns/pull/2540) ([#2083](https://github.com/date-fns/date-fns/issues/2083))
### Changed
- [Used `1` instead of `ein` for German `formatDuration` to make it consistent with other locales and formats.](https://github.com/date-fns/date-fns/pull/2576) ([#2505](https://github.com/date-fns/date-fns/issues/2505))
- [Made Norwegian `formatDuration` consistent with other locales by using numeric representation instead of written.](https://github.com/date-fns/date-fns/pull/2631) ([#2469](https://github.com/date-fns/date-fns/issues/2469))
- [Use the word "sekunda" instead of "vteřina" for second in the Czech locale.](https://github.com/date-fns/date-fns/pull/2577)
- [Made Flemish short date format corresponds to the Flemish government.](https://github.com/date-fns/date-fns/pull/2535)
### Added
- [Added `roundingMethod` option to `differenceInHours`, `differenceInMinutes`, `differenceInQuarters`, `differenceInSeconds` and `differenceInWeeks` with `trunc` as the default method.](https://github.com/date-fns/date-fns/pull/2571) ([#2555](https://github.com/date-fns/date-fns/issues/2555))
- [Added new functions: `previousDay`, `previousMonday`, `previousTuesday`, `previousWednesday`, `previousThursday`, `previousFriday`, `previousSaturday` and `previousSunday`.](https://github.com/date-fns/date-fns/pull/2522)
## v2.23.0 - 2021-07-23
Thanks to [Liam Tait](http://github.com/Liam-Tait), [fturmel](http://github.com/fturmel), [Takuya Uehara](http://github.com/indigolain), [Branislav Lazic](http://github.com/BranislavLazic), [Seyyed Morteza Moosavi](http://github.com/smmoosavi), [Felipe Armoni](http://github.com/komyg), [Sasha Koss](http://github.com/kossnocorp), [Michael Mok](http://github.com/pmmmwh), [Tan75](http://github.com/tan75) and [Maxim Topciu](http://github.com/maximtop) for working on the release.
### Changed
- [Improved `nextDay` performance by roughly 50%.](https://github.com/date-fns/date-fns/pull/2524)
- [Added more ordinal formatting to the Japanese locale.](https://github.com/date-fns/date-fns/pull/2471)
### Added
- [Added a new `clamp` function that allows to bound a date to an interval.](https://github.com/date-fns/date-fns/pull/2498)
- [Added Bosnian locale (bs).](https://github.com/date-fns/date-fns/pull/2495)
- [Allowed passing `undefined` in the duration to add and sub functions.](https://github.com/date-fns/date-fns/pull/2515)
## v2.22.1 - 2021-05-28
Thanks to [Sasha Koss](http://github.com/kossnocorp) for working on the release.
### Fixed
- Fixed constant typings. ([#2491](https://github.com/date-fns/date-fns/issues/2491))
## v2.22.0 - 2021-05-28
[Sasha Koss](http://github.com/kossnocorp), [Lucas Silva](http://github.com/LucasHFS), [Lay](http://github.com/brownsugar), [jwbth](http://github.com/jwbth), [fturmel](http://github.com/fturmel), [Tan75](http://github.com/tan75) and [Anastasia Kobzar](http://github.com/rikkalo) worked on this release.
### Fixed
- [Fixed Taiwanese locale to use traditional Chinese and removed unnecessary spaces.](https://github.com/date-fns/date-fns/pull/2436)
- [Fixed Russian locale to use correct long formats.](https://github.com/date-fns/date-fns/pull/2478)
### Added
- [Added 18 new conversion functions](https://github.com/date-fns/date-fns/pull/2433):
- `daysToWeeks`
- `hoursToMilliseconds`
- `hoursToMinutes`
- `hoursToSeconds`
- `millisecondsToHours`
- `millisecondsToMinutes`
- `millisecondsToSeconds`
- `minutesToHours`
- `minutesToMilliseconds`
- `minutesToSeconds`
- `monthsToQuarters`
- `monthsToYears`
- `quartersToMonths`
- `quartersToYears`
- `secondsToHours`
- `secondsToMilliseconds`
- `secondsToMinutes`
- `weeksToDays`
- `yearsToMonths`
- `yearsToQuarters`
## v2.21.3 - 2021-05-08
This release is brought to you by [Maxim Topciu](http://github.com/maximtop).
### Fixed
- [Fixed IE11 support by babelifing the shorthand properties.](https://github.com/date-fns/date-fns/pull/2467)
## v2.21.2 - 2021-05-05
Kudos to [Aleksei Korzun](http://github.com/a-korzun), [Maxim Topciu](http://github.com/maximtop), [Jonas L](http://github.com/jooola), [Mohammad ali Ali panah](http://github.com/always-maap) and [Tan75](http://github.com/tan75) for working on the release.
### Fixed
- [`differenceInBusinessDays` now returns `NaN` instead of `Invalid Date` when an invalid argument is passed to the function.](https://github.com/date-fns/date-fns/pull/2414)
- [Fixed `weekStartsOn` in Persian locale.](https://github.com/date-fns/date-fns/pull/2430)
## v2.21.1 - 2021-04-15
Thanks to [Sasha Koss](http://github.com/kossnocorp) for working on the release.
### Fixed
- [Fixed a breaking change introduced by using modern default argument value syntax (see https://github.com/Hacker0x01/react-datepicker/issues/2870).](https://github.com/date-fns/date-fns/pull/2423)
## v2.21.0 - 2021-04-14
This release is brought to you by [Aleksei Korzun](http://github.com/a-korzun), [Tan75](http://github.com/tan75), [Rubens Mariuzzo](http://github.com/rmariuzzo), [Christoph Stenglein](http://github.com/cstenglein) and [Clément Tamisier](http://github.com/ctamisier).
### Fixed
- [Made `formatDistanceStrict` return `12 months` instead of `1 year` when `unit: 'month'`.](https://github.com/date-fns/date-fns/pull/2411)
### Added
- [Added Haitian Creole (`ht`) locale.](https://github.com/date-fns/date-fns/pull/2396)
- [Added Austrian German (`de-AT`) locale.](https://github.com/date-fns/date-fns/pull/2362)
## v2.20.3 - 2021-04-13
Kudos to [fturmel](http://github.com/fturmel) for working on the release.
### Fixed
- [Fixed broken tree-shaking caused by missing links to corresponding ESM.](https://github.com/date-fns/date-fns/pull/2339) ([#2207](https://github.com/date-fns/date-fns/issues/2207))
## v2.20.2 - 2021-04-12
Kudos to [Maxim Topciu](http://github.com/maximtop) for working on the release.
### Fixed
- [Fixed IE11 incompatibility caused by the usage of spread syntax.](https://github.com/date-fns/date-fns/pull/2407) ([#2408](https://github.com/date-fns/date-fns/issues/2408))
## v2.20.1 - 2021-04-09
This release is brought to you by [Sasha Koss](http://github.com/kossnocorp) and [Tan75](http://github.com/tan75).
### Fixed
- Fixed `isDate` Flow typings that we broke in `v2.20.0`.
## v2.20.0 - 2021-04-08
This release is brought to you by [Sasha Koss](http://github.com/kossnocorp), [Maxim Topciu](http://github.com/maximtop), [tu4mo](http://github.com/tu4mo), [Tan75](http://github.com/tan75), [Ardit Dine](http://github.com/arditdine), [Carl Rosell](http://github.com/CarlRosell), [Roman Mahotskyi](http://github.com/enheit), [Mateusz Krzak](http://github.com/mateuszkrzak), [fgottschalk](http://github.com/fgottschalk), [Anastasia Kobzar](http://github.com/rikkalo), [Bilguun Ochirbat](http://github.com/bilguun0203), [Lesha Koss](http://github.com/leshakoss), [YuLe](http://github.com/yuler) and [guyroberts21](http://github.com/guyroberts21).
### Fixed
- [Made `formatDistanceStrict` and `formatDistanceToNowStrict` always return `1 year` instead of `12 months`.](https://github.com/date-fns/date-fns/pull/2391) ([#2388](https://github.com/date-fns/date-fns/issues/2388))
- Fixed `nextDay`, `nextMonday` and `nextTuesday` missing in exports and type definitions. ([#2325](https://github.com/date-fns/date-fns/issues/2325))
- [Fixed a DST bug in `formatDistanceStrict`.](https://github.com/date-fns/date-fns/pull/2329) ([#2307](https://github.com/date-fns/date-fns/issues/2307))
### Added
- [Added new `eachMinuteOfInterval` function.](https://github.com/date-fns/date-fns/pull/2382)
- [Added Albanian (`sq`) locale](https://github.com/date-fns/date-fns/pull/2290)
- [Added Mongolian (`mn`) locale](https://github.com/date-fns/date-fns/pull/1961)
- [Added `nextWednesday`, `nextThursday`, `nextFriday`, `nextSaturday` and `nextSunday`.](https://github.com/date-fns/date-fns/pull/2291)
## v2.19.0 - 2021-03-05
[Tan75](http://github.com/tan75) worked on this release.
### Fixed
- [Assigned the correct `firstWeekContainsDate` value (`4`) for the French locale.](https://github.com/date-fns/date-fns/pull/2273) ([#2148](https://github.com/date-fns/date-fns/issues/2148))
- [Fixed torsdag abbreviation in the Swedish locale.](https://github.com/date-fns/date-fns/pull/2220)
- [Fixed a bug in `differenceInMonths` and `intervalToDuration` that occurs when dealing with the 28th of February.](https://github.com/date-fns/date-fns/pull/2256) ([#2255](https://github.com/date-fns/date-fns/issues/2255))
### Added
- [Added new functions: `nextDay`, `nextMonday` and `nextTuesday` that allows getting the next day of the week, Monday or Tuesday respectively.](https://github.com/date-fns/date-fns/pull/2214)
## v2.18.0 - 2021-03-01
Thanks to [Tan75](http://github.com/tan75) and [Lesha Koss](http://github.com/leshakoss).
### Fixed
- [Fixed documentation missing for `intlFormat`.](https://github.com/date-fns/date-fns/pull/2259) ([#2258](https://github.com/date-fns/date-fns/issues/2258))
- [Fixed date formats in the Latvian locale.](https://github.com/date-fns/date-fns/pull/2205) ([#2202](https://github.com/date-fns/date-fns/issues/2202))
### Added
- [Added support of positive and negative offsets in `parseJSON`.](https://github.com/date-fns/date-fns/pull/2200) ([#2149](https://github.com/date-fns/date-fns/issues/2149))
## [2.17.0] - 2021-02-05
Kudos to [@shaykav](https://github.com/date-fns/date-fns/pull/1952), [@davidgape89](https://github.com/davidgape89), [@rikkalo](https://github.com/rikkalo), [@tan75](https://github.com/tan75), [@talgautb](https://github.com/talgautb), [@owenl131](https://github.com/owenl131), [@kylesezhi](https://github.com/kylesezhi), [@inigoiparragirre](https://github.com/inigoiparragirre), [@gius](https://github.com/gius), [@Endeauvirr](https://github.com/Endeauvirr) and [@frankyston](https://github.com/frankyston).
### Fixed
- [Fixed Russian locale parsing issue](https://github.com/date-fns/date-fns/pull/1950).
- [Fixed `differenceInMonths` for edge cases, such as the end of February dates](https://github.com/date-fns/date-fns/pull/2185).
- [Fixed suffixes for the Kazakh locale](https://github.com/date-fns/date-fns/pull/2010).
- [Fixed `formatDuration` week translation in `pt` and `pt-BR` locales](https://github.com/date-fns/date-fns/pull/2125).
- [Made Japanese locale to use the correct value for the start of the week](https://github.com/date-fns/date-fns/pull/2099).
- [Adjusted date formats in the Basque locale](https://github.com/date-fns/date-fns/pull/2080).
- [Fixed the short and medium date formats in the Czech locale](https://github.com/date-fns/date-fns/pull/2111).
- [Adjusted the Polish translations of `formatDistance`](https://github.com/date-fns/date-fns/pull/2187).
- [Fixed the week's abbreviations in the Brazilian Portuguese](https://github.com/date-fns/date-fns/pull/2170).
### Added
- [Added `intlFormat`](https://github.com/date-fns/date-fns/pull/2172) a lightweight formatting function that uses [Intl API](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Intl). Eventually, it will become the default formatting function, so it's highly recommended for new code.
- [Added `en-ZA` locale](https://github.com/date-fns/date-fns/pull/1952).
- [Added an ability to format lowercase am/pm with `aaa` and `bbb` tokens](https://github.com/date-fns/date-fns/pull/2016).
- [Added ordinal formatting for Japanese year values](https://github.com/date-fns/date-fns/pull/2177/files).
## [2.16.1] - 2020-07-31
Kudos to [@aleksaps](https://github.com/aleksaps), [@leedriscoll](https://github.com/leedriscoll) and [@BanForFun](https://github.com/BanForFun) for pull-requests!
### Fixed
- [Fixed a typo in Scottish Gaelic (gd) locale](https://github.com/date-fns/date-fns/pull/1925).
- [Fixed typos in Serbian Latin locale](https://github.com/date-fns/date-fns/pull/1928).
- [Fixed greek grammar for Saturday on `formatRelative`](https://github.com/date-fns/date-fns/pull/1930).
- Removed locale snapshots from the npm package making it lighter.
## [2.16.0] - 2020-08-27
Kudos to [@jvpelt](https://github.com/jvpelt), [@piotrl](https://github.com/piotrl), [@yotamofek](https://github.com/yotamofek), [@dwaxweiler](https://github.com/dwaxweiler), [@leedriscoll](https://github.com/leedriscoll) and [@bradevans](https://github.com/bradevans) for working on the release. Also thanks to [@PascalHonegger](https://github.com/PascalHonegger), [@pickfire](https://github.com/pickfire), [@TheJaredWilcurt](https://github.com/TheJaredWilcurt), [@SidKH](https://github.com/SidKH) and [@nfantone](https://github.com/nfantone) for improving the documentation.
### Fixed
- [Added correct translations for Welsh `1 minute` and `2 days`](https://github.com/date-fns/date-fns/pull/1903).
- [Fixed `formatRFC3339` formatting timezone offset with minutes](https://github.com/date-fns/date-fns/pull/1890).
- [Added missing locale type definition for `formatDuration`](https://github.com/date-fns/date-fns/pull/1881)
- [Fixed Scottish Gaelic locale issues](https://github.com/date-fns/date-fns/pull/1914).
### Changed
- [Used shorter Hebrew alternative for "about"](https://github.com/date-fns/date-fns/pull/1893).
- [Improved string arguments warning after upgrading to v2](https://github.com/date-fns/date-fns/pull/1910).
### Added
- [Added Luxembourgish (lb) locale](https://github.com/date-fns/date-fns/pull/1900).
## [2.15.0] - 2020-07-17
Thanks to [@belgamo](https://github.com/belgamo), [@Matsuuu](https://github.com/Matsuuu), [@Imballinst](https://github.com/Imballinst), [@arsnyder16](https://github.com/arsnyder16), [@pankajupadhyay29](https://github.com/pankajupadhyay29), [@DCBN](https://github.com/DCBN), [@leedriscoll](https://github.com/leedriscoll), [@gottsohn](https://github.com/gottsohn), [@mukuljainx](https://github.com/mukuljainx) and [@dtriana](https://github.com/dtriana) for working on the release. Also kudos to [@KidkArolis](https://github.com/KidkArolis), [@imgx64](https://github.com/imgx64), [@fjc0k](https://github.com/fjc0k), [@wmonk](https://github.com/wmonk), [@djD-REK](https://github.com/djD-REK), [@dandv](https://github.com/dandv), [@psimk](https://github.com/psimk) and [@brimworks](https://github.com/brimworks) for improving the documentation.
### Fixed
- [Fixed behavior of `addBusinessDays` when input date is a weekend day](https://github.com/date-fns/date-fns/pull/1790).
- [Fixed `parseISO` not returning `Invalid Date` on incorrect string when there are spaces in it](https://github.com/date-fns/date-fns/pull/1791).
- [Fixed `es` round-tripping dates with Wednesday](https://github.com/date-fns/date-fns/pull/1792).
- [Fixed round-trip bug with `d`/`EEEE` ordering in tokens like `PPPPP`](https://github.com/date-fns/date-fns/pull/1795).
- [Fixed issues with parsing values in Japanese](https://github.com/date-fns/date-fns/pull/1807).
- [Fixed Hungarian breaking IE11](https://github.com/date-fns/date-fns/pull/1842).
- [Fixed Spanish accents in Saturday and Wednesday](https://github.com/date-fns/date-fns/pull/1872).
### Changed
- [Improved the message of protected tokens error](https://github.com/date-fns/date-fns/pull/1641).
### Added
- [Added Swiss-French `fr-CH` locale](https://github.com/date-fns/date-fns/pull/1809).
- [Added Flemish `nl-BE` locale](https://github.com/date-fns/date-fns/pull/1812).
- [Added Scottish Gaelic `gd` locale](https://github.com/date-fns/date-fns/pull/1832).
- [Added New Zealand English `en-NZ` locale](https://github.com/date-fns/date-fns/pull/1835).
- [Added `isMatch` function](https://github.com/date-fns/date-fns/pull/1868).
## [2.14.0] - 2020-05-18
Kudos to [@julamb](https://github.com/julamb), [@JacobSoderblom](https://github.com/JacobSoderblom), [@justingrant](http://github.com/justingrant), [@dragunoff](https://github.com/dragunoff), [@jmate0321](https://github.com/jmate0321), [@gbhasha](https://github.com/gbhasha), [@rasck](https://github.com/rasck), [@AlbertoPdRF](https://github.com/AlbertoPdRF), [@sebastianhaberey](https://github.com/sebastianhaberey) and [@giogonzo](https://github.com/giogonzo) for working on the release!
### Fixed
- [Fixed DST issues with `add`, `addDays` and `addMonths`](https://github.com/date-fns/date-fns/pull/1760).
- [Fixed "quarter" translation in the Bulgarian locale](https://github.com/date-fns/date-fns/pull/1763).
- [Fixed `formatDistance` strings in the Hungarian locale](https://github.com/date-fns/date-fns/pull/1765).
- [Fixed Danish month abbreviations](https://github.com/date-fns/date-fns/pull/1774).
- [Fixed parsing of mei in the Dutch locale](https://github.com/date-fns/date-fns/pull/1774).
- [Fixed missing preposition in `formatLong` in the Spanish locale](https://github.com/date-fns/date-fns/pull/1775).
- [Fixed `formatRelative` in the Italian locale](https://github.com/date-fns/date-fns/pull/1777).
### Added
- [Added `eachQuarterOfInterval`](https://github.com/date-fns/date-fns/pull/1715).
- [Added Basque (`eu`) locale](https://github.com/date-fns/date-fns/pull/1759).
- [Added Indian English (`en-IN`) locale](https://github.com/date-fns/date-fns/pull/1767).
- [Added `eachHourOfInterval`](https://github.com/date-fns/date-fns/pull/1776).
## [2.13.0] - 2020-05-06
Thanks to [@JorenVos](https://github.com/JorenVos), [@developergouli](https://github.com/developergouli), [@rhlowe](https://github.com/rhlowe) and [@justingrant](http://github.com/justingrant) for working on the release!
### Fixed
- [Fixed mei abbreviation in the Dutch locale](https://github.com/date-fns/date-fns/pull/1752).
- [Fixed `differenceInDays` DST behavior broken in 2.12.0](https://github.com/date-fns/date-fns/pull/1754).
### Added
- [Added Kannada locale support](https://github.com/date-fns/date-fns/pull/1747).
- [Added `formatISODuration` function](https://github.com/date-fns/date-fns/pull/1713).
- [Added `intervalToDuration` function](https://github.com/date-fns/date-fns/pull/1713).
## [2.12.0] - 2020-04-09
Kudos to [@leshakoss](http://github.com/leshakoss), [@skyuplam](https://github.com/skyuplam), [@so99ynoodles](https://github.com/so99ynoodles), [@dkozickis](https://github.com/dkozickis), [@belgamo](https://github.com/belgamo), [@akgondber](https://github.com/akgondber), [@dcousens](https://github.com/dcousens) and [@BoomDev](https://github.com/BoomDev) for working on the release!
### Fixed
- [Fixed minulý štvrtok in Slovak locale](https://github.com/date-fns/date-fns/pull/1701).
- Fixed date ordinalNumber for [ja/zh-CN/zh-TW](https://github.com/date-fns/date-fns/pull/1690) and [ko](https://github.com/date-fns/date-fns/pull/1696).
- [Fixed quarters parsing](https://github.com/date-fns/date-fns/pull/1694).
- [Fixed `setDay` with `weekStartsOn` != 0](https://github.com/date-fns/date-fns/pull/1639).
- [Fixed differenceInDays across DST](https://github.com/date-fns/date-fns/pull/1630).
- [Fixed required arguments exception message](https://github.com/date-fns/date-fns/pull/1674).
### Added
- [Added new function `formatDistanceToNowStrict`](https://github.com/date-fns/date-fns/pull/1679).
## [2.11.1] - 2020-03-26
### Fixed
- Rebuilt TypeScript and flow types.
## [2.11.0] - 2020-03-13
Kudos to [@oakhan3](https://github.com/oakhan3), [@Mukhammadali](https://github.com/Mukhammadali), [@altrim](https://github.com/altrim), [@leepowellcouk](https://github.com/leepowellcouk), [@amatzon](@https://github.com/amatzon), [@bryanMt](https://github.com/bryanMt), [@kalekseev](https://github.com/kalekseev), [@eugene-platov](https://github.com/eugene-platov) and [@tjrobinson](https://github.com/tjrobinson) for working on the release.
### Fixed
- [Fixed a bug in `differenceInYears` causing incorrect results when the left date is a leap day](https://github.com/date-fns/date-fns/pull/1654).
- [Fixed `parseISO` to work correctly around time shift dates](https://github.com/date-fns/date-fns/pull/1667).
- [Fixed `format` to work correctly with GMT-0752/GMT-0456 and similar timezones](https://github.com/date-fns/date-fns/pull/1666).
### Changed
- [Changed `getDay` typings to return `0|1|2|3|4|5|6` instead of `number`](https://github.com/date-fns/date-fns/pull/1668).
- [Improved Chinese locale](https://github.com/date-fns/date-fns/pull/1664):
- Change date format to meet the national standard (GB/T 7408-2005).
- Improve `ordinalNumber` function behavior.
- Add prefix in `formatRelative` depending on if it's a current week or not.
### Added
- [Added Uzbek `uz` locale](https://github.com/date-fns/date-fns/pull/1648).
- [Updated Macedonian locale for v2](https://github.com/date-fns/date-fns/pull/1649).
- [Added Maltese `mt` locale](https://github.com/date-fns/date-fns/pull/1658).
## [2.10.0] - 2020-02-25
### Fixed
- [Fixed `formatISO` when formatting time with timezones with minute offsets > 0](https://github.com/date-fns/date-fns/pull/1599). Kudos to [@dcRUSTy](https://github.com/dcRUSTy).
### Fixed
- Fixed a bug in setDay when using weekStartsOn that is not 0
### Added
- [Added `weeks` to `Duration`](https://github.com/date-fns/date-fns/pull/1592).
- [Added `weeks` support to `add` and `sub`](https://github.com/date-fns/date-fns/pull/1592).
- [Added details message in `throwProtectedError`](https://github.com/date-fns/date-fns/pull/1592).
## [2.9.0] - 2020-01-08
Thanks to [@mborgbrant](https://github.com/mborgbrant), [@saintplay](https://github.com/saintplay), [@mrenty](https://github.com/mrenty), [@kibertoad](https://github.com/kibertoad), [@levibuzolic](https://github.com/levibuzolic), [@Anshuman71](https://github.com/Anshuman71), [@talgautb](https://github.com/talgautb), [@filipjuza](https://github.com/filipjuza), [@tobyzerner](https://github.com/tobyzerner), [@emil9453](https://github.com/emil9453), [@fintara](https://github.com/fintara), [@pascaliske](https://github.com/pascaliske), [@rramiachraf](https://github.com/rramiachraf), [@marnusw](https://github.com/marnusw) and [@Imballinst](https://github.com/Imballinst) for working on the release.
### Fixed
- [Fixed a bug with addBusinessDays returning the Tuesday when adding 1 day on weekends. Now it returns the Monday](https://github.com/date-fns/date-fns/pull/1588).
- [Added missing timezone to `formatISO`](https://github.com/date-fns/date-fns/pull/1576).
- [Removed dots from short day period names in the Kazakh locale](https://github.com/date-fns/date-fns/pull/1512).
- [Fixed typo in formatDistance in the Czech locale](https://github.com/date-fns/date-fns/pull/1540).
- [Fixed shortenings in the Bulgarian locale](https://github.com/date-fns/date-fns/pull/1560).
- [Fixed regex for the May in the Portuguese locale](https://github.com/date-fns/date-fns/pull/1565).
### Added
- [Added `eachMonthOfInterval` and `eachYearOfInterval`](https://github.com/date-fns/date-fns/pull/618).
- [Added `inclusive` option to `areIntervalsOverlapping](https://github.com/date-fns/date-fns/pull/643).
- [Added `isExists` function that checks if the given date is exists](https://github.com/date-fns/date-fns/pull/682).
- [Added `add` function to add seconds, minutes, hours, weeks, years in single call](https://github.com/date-fns/date-fns/pull/1581).
- [Added `sub` function, the opposite of `add`](https://github.com/date-fns/date-fns/pull/1583).
- [Added `Duration` type used in `add` and `sub`](https://github.com/date-fns/date-fns/pull/1583).
- [Added Azerbaijani (az) locale](https://github.com/date-fns/date-fns/pull/1547).
- [Added Moroccan Arabic (ar-MA) locale](https://github.com/date-fns/date-fns/pull/1578).
### Changed
- [Reduced the total minified build size by 1Kb/4%](https://github.com/date-fns/date-fns/pull/1563).
- [Made all properties in `Locale` type optional](https://github.com/date-fns/date-fns/pull/1542).
- [Added missing properties to `Locale` type](https://github.com/date-fns/date-fns/pull/1542).
- [Add the locale code to `Locale` type](https://github.com/date-fns/date-fns/pull/1580).
- [Added support of space time separator to `parseJSON`](https://github.com/date-fns/date-fns/pull/1579).
- [Allowed up to 7 digits in milliseconds in `parseJSON`](https://github.com/date-fns/date-fns/pull/1579).
## [2.8.1] - 2019-11-22
Thanks to [@Imballinst](https://github.com/Imballinst) for the bug fix!
### Fixed
- [Add colon between the hour and minutes for `formatRFC3339`](https://github.com/date-fns/date-fns/pull/1549). [See #1548](https://github.com/date-fns/date-fns/issues/1548).
## [2.8.0] - 2019-11-19
Kudos to [@NaridaL](https://github.com/NaridaL), [@Zyten](https://github.com/Zyten), [@Imballinst](https://github.com/Imballinst), [@leshakoss](https://github.com/leshakoss) and [@Neorth](https://github.com/Neorth) for working on the release.
### Fixed
- [Remove the next week preposition in the Swedish locale](https://github.com/date-fns/date-fns/pull/1538).
### Added
- [Added Malay (ms) locale](https://github.com/date-fns/date-fns/pull/1537).
- [Added `formatISO`, `formatISO9075`, `formatRFC3339`, and `formatRFC7231` functions](https://github.com/date-fns/date-fns/pull/1536).
## [2.7.0] - 2019-11-07
Thanks to [@mzgajner](https://github.com/mzgajner), [@NaridaL](https://github.com/NaridaL), [@Zyten](https://github.com/Zyten), [@leshakoss](https://github.com/leshakoss), [@fintara](https://github.com/fintara), [@kpr-hellofresh](https://github.com/kpr-hellofresh) for contributing to the release.
### Fixed
- [Fixed a mistake in the Slovenian locale](https://github.com/date-fns/date-fns/pull/1529).
- [Fixed incorrect behavior of `parseISO` in Firefox caused by differences in `getTimezoneOffset`](https://github.com/date-fns/date-fns/pull/1495).
### Changed
- [Make object arguments types more elaborate in Flow type definitions](https://github.com/date-fns/date-fns/pull/1519).
- [Get rid of deprecated Function in Flow type definitions](https://github.com/date-fns/date-fns/pull/1520).
- [Allow `parseJSON` to accept strings without trailing 'Z' symbol and with up to 6 digits in the milliseconds' field](https://github.com/date-fns/date-fns/pull/1499).
### Added
- [Added Bulgarian (bg) locale](https://github.com/date-fns/date-fns/pull/1522).
## [2.6.0] - 2019-10-22
Kudos to [@marnusw](https://github.com/marnusw), [@cdrikd](https://github.com/cdrikd) and [@rogyvoje](https://github.com/rogyvoje) for working on the release!
### Added
- [Added `parseJSON` - lightweight function (just 411 B) that parses dates formatted with `toJSON`](https://github.com/date-fns/date-fns/pull/1463).
- [Added the language code to each locale](https://github.com/date-fns/date-fns/pull/1489).
- [Added `subBusinessDays` function](https://github.com/date-fns/date-fns/pull/1491).
- [Added both Serbian - cyrillic (sr) and latin (sr-Latn) locales](https://github.com/date-fns/date-fns/pull/1494).
## [2.5.1] - 2019-10-18
Thanks to [@mitchellbutler](https://github.com/mitchellbutler) for the bug fix!
### Fixed
- [Fixed infinite loop in `addBusinessDays`](https://github.com/date-fns/date-fns/pull/1486).
## [2.5.0] - 2019-10-16
Kudos to [@dkozickis](https://github.com/dkozickis), [@drugoi](https://github.com/drugoi), [@kranthilakum](https://github.com/kranthilakum), [@102](https://github.com/102), [@gpetrioli](https://github.com/gpetrioli) and [@JulienMalige](https://github.com/JulienMalige) for making the release happen.
### Fixed
- [Fixed compatibility with IE11 by removing `findIndex` from the code](https://github.com/date-fns/date-fns/pull/1457).
- [Fixed Greek locale patterns](https://github.com/date-fns/date-fns/pull/1480).
### Added
- [Added Kazakh (kk) locale](https://github.com/date-fns/date-fns/pull/1460).
- [Added Telugu (te) locale](https://github.com/date-fns/date-fns/pull/1464).
- [Added Canadian French (fr-CA) locale](https://github.com/date-fns/date-fns/issues/1465).
- [Added Australian English (en-AU) locale](https://github.com/date-fns/date-fns/pull/1470).
- [Exported `Interval` and `Locale` types from Flow typings](https://github.com/date-fns/date-fns/pull/1475).
## [2.4.1] - 2019-09-28
Thanks to [@mrclayman](https://github.com/mrclayman) for reporting the issue and [@leshakoss](https://github.com/leshakoss) for fixing it.
### Fixed
- [Fixed am/pm mixup in the Czech locale](https://github.com/date-fns/date-fns/pull/1453).
## [2.4.0] - 2019-09-27
This release is brought to you by these amazing people: [@lovelovedokidoki](https://github.com/lovelovedokidoki), [@alexigityan](https://github.com/alexigityan), [@kalekseev](https://github.com/kalekseev) and [@andybangs](https://github.com/andybangs). You rock!
### Fixed
- [Fixed Vietnamese parsing patterns](https://github.com/date-fns/date-fns/pull/1445).
- [Fixed Czech parsing regexes](https://github.com/date-fns/date-fns/pull/1446).
- [Fixed offset for Eastern Hemisphere in `parseISO`](https://github.com/date-fns/date-fns/pull/1450).
### Added
- [Added Armenian locale support](https://github.com/date-fns/date-fns/pull/1448).
## [2.3.0] - 2019-09-24
Huge thanks to [@lovelovedokidoki](https://github.com/lovelovedokidoki) who improved 8 (!) locales in an unstoppable open-source rampage and [@VesterDe](https://github.com/VesterDe) for fixing Slovenian locale 👏
### Fixed
- [Fixed the translation of "yesterday" in the Slovenian locale](https://github.com/date-fns/date-fns/pull/1420).
- [Fixed French parsing issues with June and August](https://github.com/date-fns/date-fns/pull/1430).
- [Improved Turkish parsing](https://github.com/date-fns/date-fns/pull/1432).
- [Fixed "March" in Dutch parsing patterns](https://github.com/date-fns/date-fns/pull/1433).
- [Fixed Hindi parsing patterns](https://github.com/date-fns/date-fns/pull/1434).
### Added
- [Added Finnish matching patterns](https://github.com/date-fns/date-fns/pull/1425).
- [Accept abbreviated March, June, July in Norwegian locales](https://github.com/date-fns/date-fns/pull/1431).
- [Added parsing for Greek months with long formatting](https://github.com/date-fns/date-fns/pull/1435).
## [2.2.1] - 2019-09-12
Kudos to date-fns contributors: [@mzgajner](https://github.com/mzgajner), [@sibiraj-s](https://github.com/sibiraj-s), [@mukeshmandiwal](https://github.com/mukeshmandiwal), [@SneakyFish5](https://github.com/SneakyFish5) and [@CarterLi](https://github.com/CarterLi).
### Added
- [Added new `set` function](https://github.com/date-fns/date-fns/pull/1398).
- [Updated Slovenian (sl) locale for v2](https://github.com/date-fns/date-fns/pull/1418).
- [Added Tamil (ta) locale](https://github.com/date-fns/date-fns/pull/1411).
- [Added Hindi (hi) locale](https://github.com/date-fns/date-fns/pull/1409).
- [Added support of `\n` in `format`, `lightFormat` and `parse`](https://github.com/date-fns/date-fns/pull/1417).
## [2.1.0] - 2019-09-06
Thanks to date-fns contributors: [@ManadayM](https://github.com/ManadayM), [@illuminist](https://github.com/illuminist), [@visualfanatic](https://github.com/visualfanatic), [@vsaarinen](https://github.com/vsaarinen) and at last but not the least [@leshakoss](https://github.com/leshakoss)!
### Fixed
- [Set start of the week to Sunday for Thai locale](https://github.com/date-fns/date-fns/pull/1402).
- [Fixed month matching in Polish locale](https://github.com/date-fns/date-fns/pull/1404).
- [Fixed `eachWeekendOfInterval` skipping the first date in the supplied interval](https://github.com/date-fns/date-fns/pull/1407).
### Added
- [Added Gujarati locale](https://github.com/date-fns/date-fns/pull/1400).
## [2.0.1] - 2019-08-23
### Fixed
- [Fix](https://github.com/date-fns/date-fns/pull/1046) `getWeekOfMonth` with `options.weekStartsOn` set to 1 [not working for Sundays](https://github.com/date-fns/date-fns/issues/1040). Kudos to [@waseemahmad31](https://github.com/waseemahmad31)!
## [2.0.0] - 2019-08-20
If you're upgrading from v2 alpha or beta, [see the pre-release changelog](https://gist.github.com/kossnocorp/a307a464760b405bb78ef5020a4ab136).
### Fixed
- Fixed the `toDate` bug occurring when parsing ISO-8601 style dates (but not valid ISO format)
with a trailing Z (e.g `2012-01Z`), it returned Invalid Date for FireFox/IE11 [#510](https://github.com/date-fns/date-fns/issue/510)
- Fixed `differenceIn...` functions returning negative zero in some cases:
[#692](https://github.com/date-fns/date-fns/issues/692)
- `isDate` now works properly with dates passed across iframes [#754](https://github.com/date-fns/date-fns/pull/754).
- Fixed a few bugs that appeared in timezones with offsets that include seconds (e.g. GMT+00:57:44).
See PR [#789](https://github.com/date-fns/date-fns/pull/789).
- [Fixed DST issue](https://github.com/date-fns/date-fns/pull/1003). See [#972](https://github.com/date-fns/date-fns/issues/972) and [#992](https://github.com/date-fns/date-fns/issues/992) for more details.
- Fixed DST issue in `eachDayOfInterval` that caused time in the days
after DST change to have the shift as well.
- Fixed bug in Galician locale caused by incorrect usage of `getHours`
instead of `getUTCHours`.
### Changed
- **BREAKING**: now functions don't accept string arguments, but only
numbers or dates. When a string is passed, it will result in
an unexpected result (`Invalid Date`, `NaN`, etc).
From now on a string should be parsed using `parseISO` (ISO 8601)
or `parse`.
In v1 we've used `new Date()` to parse strings, but it resulted in many
hard-to-track bugs caused by inconsistencies in different browsers.
To address that we've implemented our ISO 8601 parser but that made
library to significantly grow in size. To prevent inevitable bugs
and keep the library tiny, we made this trade-off.
See [this post](https://blog.date-fns.org/post/we-cut-date-fns-v2-minimal-build-size-down-to-300-bytes-and-now-its-the-smallest-date-library-18f2nvh2z0yal) for more details.
```javascript
// Before v2.0.0
addDays("2016-01-01", 1);
// v2.0.0 onward
addDays(parseISO("2016-01-01"), 1);
```
- **BREAKING**: new format string API for `format` function
which is based on [Unicode Technical Standard #35](https://www.unicode.org/reports/tr35/tr35-dates.html#Date_Field_Symbol_Table).
See [this post](https://blog.date-fns.org/post/unicode-tokens-in-date-fns-v2-sreatyki91jg) for more details.
| Unit | v2 Pattern | v1 Pattern | Result examples |
| ------------------------------- | ---------- | ---------- | --------------------------------- |
| Era | G..GGG | | AD, BC |
| | GGGG | | Anno Domini, Before Christ |
| | GGGGG | | A, B |
| Calendar year | y | | 44, 1, 1900, 2017 |
| | yo | | 44th, 1st, 0th, 17th |
| | yy | YY | 44, 01, 00, 17 |
| | yyy | | 044, 001, 1900, 2017 |
| | yyyy | YYYY | 0044, 0001, 1900, 2017 |
| | yyyyy | | ... |
| Local week-numbering year | Y | | 44, 1, 1900, 2017 |
| | Yo | | 44th, 1st, 1900th, 2017th |
| | YY | | 44, 01, 00, 17 |
| | YYY | | 044, 001, 1900, 2017 |
| | YYYY | | 0044, 0001, 1900, 2017 |
| | YYYYY | | ... |
| ISO week-numbering year | R | | -43, 0, 1, 1900, 2017 |
| | RR | GG | -43, 00, 01, 1900, 2017 |
| | RRR | | -043, 000, 001, 1900, 2017 |
| | RRRR | GGGG | -0043, 0000, 0001, 1900, 2017 |
| | RRRRR | | ... |
| Extended year | u | | -43, 0, 1, 1900, 2017 |
| | uu | | -43, 01, 1900, 2017 |
| | uuu | | -043, 001, 1900, 2017 |
| | uuuu | | -0043, 0001, 1900, 2017 |
| | uuuuu | | ... |
| Quarter (formatting) | Q | | 1, 2, 3, 4 |
| | Qo | | 1st, 2nd, 3rd, 4th |
| | QQ | | 01, 02, 03, 04 |
| | QQQ | | Q1, Q2, Q3, Q4 |
| | QQQQ | | 1st quarter, 2nd quarter, ... |
| | QQQQQ | | 1, 2, 3, 4 |
| Quarter (stand-alone) | q | Q | 1, 2, 3, 4 |
| | qo | Qo | 1st, 2nd, 3rd, 4th |
| | qq | | 01, 02, 03, 04 |
| | qqq | | Q1, Q2, Q3, Q4 |
| | qqqq | | 1st quarter, 2nd quarter, ... |
| | qqqqq | | 1, 2, 3, 4 |
| Month (formatting) | M | | 1, 2, ..., 12 |
| | Mo | | 1st, 2nd, ..., 12th |
| | MM | | 01, 02, ..., 12 |
| | MMM | | Jan, Feb, ..., Dec |
| | MMMM | | January, February, ..., December |
| | MMMMM | | J, F, ..., D |
| Month (stand-alone) | L | M | 1, 2, ..., 12 |
| | Lo | | 1st, 2nd, ..., 12th |
| | LL | MM | 01, 02, ..., 12 |
| | LLL | MMM | Jan, Feb, ..., Dec |
| | LLLL | MMMM | January, February, ..., December |
| | LLLLL | | J, F, ..., D |
| Local week of year | w | | 1, 2, ..., 53 |
| | wo | | 1st, 2nd, ..., 53th |
| | ww | | 01, 02, ..., 53 |
| ISO week of year | I | W | 1, 2, ..., 53 |
| | Io | Wo | 1st, 2nd, ..., 53th |
| | II | WW | 01, 02, ..., 53 |
| Day of month | d | D | 1, 2, ..., 31 |
| | do | Do | 1st, 2nd, ..., 31st |
| | dd | DD | 01, 02, ..., 31 |
| Day of year | D | DDD | 1, 2, ..., 365, 366 |
| | Do | DDDo | 1st, 2nd, ..., 365th, 366th |
| | DD | | 01, 02, ..., 365, 366 |
| | DDD | DDDD | 001, 002, ..., 365, 366 |
| | DDDD | | ... |
| Day of week (formatting) | E..EEE | | Mon, Tue, Wed, ..., Su |
| | EEEE | | Monday, Tuesday, ..., Sunday |
| | EEEEE | | M, T, W, T, F, S, S |
| | EEEEEE | | Mo, Tu, We, Th, Fr, Sa, Su |
| ISO day of week (formatting) | i | E | 1, 2, 3, ..., 7 |
| | io | do | 1st, 2nd, ..., 7th |
| | ii | | 01, 02, ..., 07 |
| | iii | ddd | Mon, Tue, Wed, ..., Su |
| | iiii | dddd | Monday, Tuesday, ..., Sunday |
| | iiiii | | M, T, W, T, F, S, S |
| | iiiiii | dd | Mo, Tu, We, Th, Fr, Sa, Su |
| Local day of week (formatting) | e | | 2, 3, 4, ..., 1 |
| | eo | | 2nd, 3rd, ..., 1st |
| | ee | | 02, 03, ..., 01 |
| | eee | | Mon, Tue, Wed, ..., Su |
| | eeee | | Monday, Tuesday, ..., Sunday |
| | eeeee | | M, T, W, T, F, S, S |
| | eeeeee | | Mo, Tu, We, Th, Fr, Sa, Su |
| Local day of week (stand-alone) | c | | 2, 3, 4, ..., 1 |
| | co | | 2nd, 3rd, ..., 1st |
| | cc | | 02, 03, ..., 01 |
| | ccc | | Mon, Tue, Wed, ..., Su |
| | cccc | | Monday, Tuesday, ..., Sunday |
| | ccccc | | M, T, W, T, F, S, S |
| | cccccc | | Mo, Tu, We, Th, Fr, Sa, Su |
| AM, PM | a..aaa | A | AM, PM |
| | aaaa | aa | a.m., p.m. |
| | aaaaa | | a, p |
| AM, PM, noon, midnight | b..bbb | | AM, PM, noon, midnight |
| | bbbb | | a.m., p.m., noon, midnight |
| | bbbbb | | a, p, n, mi |
| Flexible day period | B..BBB | | at night, in the morning, ... |
| | BBBB | | at night, in the morning, ... |
| | BBBBB | | at night, in the morning, ... |
| Hour [1-12] | h | | 1, 2, ..., 11, 12 |
| | ho | | 1st, 2nd, ..., 11th, 12th |
| | hh | | 01, 02, ..., 11, 12 |
| Hour [0-23] | H | | 0, 1, 2, ..., 23 |
| | Ho | | 0th, 1st, 2nd, ..., 23rd |
| | HH | | 00, 01, 02, ..., 23 |
| Hour [0-11] | K | | 1, 2, ..., 11, 0 |
| | Ko | | 1st, 2nd, ..., 11th, 0th |
| | KK | | 1, 2, ..., 11, 0 |
| Hour [1-24] | k | | 24, 1, 2, ..., 23 |
| | ko | | 24th, 1st, 2nd, ..., 23rd |
| | kk | | 24, 01, 02, ..., 23 |
| Minute | m | | 0, 1, ..., 59 |
| | mo | | 0th, 1st, ..., 59th |
| | mm | | 00, 01, ..., 59 |
| Second | s | | 0, 1, ..., 59 |
| | so | | 0th, 1st, ..., 59th |
| | ss | | 00, 01, ..., 59 |
| Fraction of second | S | | 0, 1, ..., 9 |
| | SS | | 00, 01, ..., 99 |
| | SSS | | 000, 0001, ..., 999 |
| | SSSS | | ... |
| Timezone (ISO-8601 w/ Z) | X | | -08, +0530, Z |
| | XX | | -0800, +0530, Z |
| | XXX | | -08:00, +05:30, Z |
| | XXXX | | -0800, +0530, Z, +123456 |
| | XXXXX | | -08:00, +05:30, Z, +12:34:56 |
| Timezone (ISO-8601 w/o Z) | x | | -08, +0530, +00 |
| | xx | ZZ | -0800, +0530, +0000 |
| | xxx | Z | -08:00, +05:30, +00:00 |
| | xxxx | | -0800, +0530, +0000, +123456 |
| | xxxxx | | -08:00, +05:30, +00:00, +12:34:56 |
| Timezone (GMT) | O...OOO | | GMT-8, GMT+5:30, GMT+0 |
| | OOOO | | GMT-08:00, GMT+05:30, GMT+00:00 |
| Timezone (specific non-locat.) | z...zzz | | GMT-8, GMT+5:30, GMT+0 |
| | zzzz | | GMT-08:00, GMT+05:30, GMT+00:00 |
| Seconds timestamp | t | X | 512969520 |
| | tt | | ... |
| Milliseconds timestamp | T | x | 512969520900 |
| | TT | | ... |
| Long localized date | P | | 5/29/53 |
| | PP | | May 29, 1453 |
| | PPP | | May 29th, 1453 |
| | PPPP | | Sunday, May 29th, 1453 |
| Long localized time | p | | 12:00 AM |
| | pp | | 12:00:00 AM |
| | ppp | | 12:00:00 AM GMT+2 |
| | pppp | | 12:00:00 AM GMT+02:00 |
| Combination of date and time | Pp | | 5/29/53, 12:00 AM |
| | PPpp | | May 29, 1453, 12:00 AM |
| | PPPppp | | May 29th, 1453 at ... |
| | PPPPpppp | | Sunday, May 29th, 1453 at ... |
Characters are now escaped using single quote symbols (`'`) instead of square brackets.
`format` now throws RangeError if it encounters an unescaped latin character
that isn't a valid formatting token.
To use `YY` and `YYYY` tokens that represent week-numbering years,
you should set `useAdditionalWeekYearTokens` option:
```javascript
format(Date.now(), "YY", { useAdditionalWeekYearTokens: true });
//=> '86'
```
To use `D` and `DD` tokens which represent days of the year,
set `useAdditionalDayOfYearTokens` option:
```javascript
format(Date.now(), "D", { useAdditionalDayOfYearTokens: true });
//=> '364'
```
- **BREAKING**: function submodules now use camelCase naming schema:
```javascript
// Before v2.0.0
import differenceInCalendarISOYears from "date-fns/difference_in_calendar_iso_years";
// v2.0.0 onward
import differenceInCalendarISOYears from "date-fns/differenceInCalendarISOYears";
```
- **BREAKING**: min and max functions now accept an array of dates
rather than spread arguments.
```javascript
// Before v2.0.0
var date1 = new Date(1989, 6 /* Jul */, 10);
var date2 = new Date(1987, 1 /* Feb */, 11);
var minDate = min(date1, date2);
var maxDate = max(date1, date2);
// v2.0.0 onward:
var dates = [
new Date(1989, 6 /* Jul */, 10),
new Date(1987, 1 /* Feb */, 11),
];
var minDate = min(dates);
var maxDate = max(dates);
```
- **BREAKING**: make the second argument of `format` required for the sake of explicitness.
```javascript
// Before v2.0.0
format(new Date(2016, 0, 1));
// v2.0.0 onward
format(new Date(2016, 0, 1), "yyyy-MM-dd'T'HH:mm:ss.SSSxxx");
```
- **BREAKING** renamed ISO week-numbering year helpers:
- `addISOYears` → `addISOWeekYears`
- `differenceInCalendarISOYears` → `differenceInCalendarISOWeekYears`
- `differenceInISOYears` → `differenceInISOWeekYears`
- `endOfISOYear` → `endOfISOWeekYear`
- `getISOYear` → `getISOWeekYear`
- `isSameISOYear` → `isSameISOWeekYear`
- `lastDayOfISOYear` → `lastDayOfISOWeekYear`
- `setISOYear` → `setISOWeekYear`
- `subISOYears` → `subISOWeekYears`
i.e. "ISO year" renamed to "ISO week year", which is short for
[ISO week-numbering year](https://en.wikipedia.org/wiki/ISO_week_date).
It makes them consistent with locale-dependent week-numbering year helpers,
e.g., `startOfWeekYear`.
- **BREAKING**: functions renamed:
- `areRangesOverlapping` → `areIntervalsOverlapping`
- `eachDay` → `eachDayOfInterval`
- `getOverlappingDaysInRanges` → `getOverlappingDaysInIntervals`
- `isWithinRange` → `isWithinInterval`
This change was made to mirror the use of the word "interval" in standard ISO 8601:2004 terminology:
```
2.1.3
time interval
part of the time axis limited by two instants
```
Also these functions now accept an object with `start` and `end` properties
instead of two arguments as an interval. All these functions
throw `RangeError` if the start of the interval is after its end
or if any date in the interval is `Invalid Date`.
```javascript
// Before v2.0.0
areRangesOverlapping(
new Date(2014, 0, 10),
new Date(2014, 0, 20),
new Date(2014, 0, 17),
new Date(2014, 0, 21),
);
eachDay(new Date(2014, 0, 10), new Date(2014, 0, 20));
getOverlappingDaysInRanges(
new Date(2014, 0, 10),
new Date(2014, 0, 20),
new Date(2014, 0, 17),
new Date(2014, 0, 21),
);
isWithinRange(
new Date(2014, 0, 3),
new Date(2014, 0, 1),
new Date(2014, 0, 7),
);
// v2.0.0 onward
areIntervalsOverlapping(
{ start: new Date(2014, 0, 10), end: new Date(2014, 0, 20) },
{ start: new Date(2014, 0, 17), end: new Date(2014, 0, 21) },
);
eachDayOfInterval({
start: new Date(2014, 0, 10),
end: new Date(2014, 0, 20),
});
getOverlappingDaysInIntervals(
{ start: new Date(2014, 0, 10), end: new Date(2014, 0, 20) },
{ start: new Date(2014, 0, 17), end: new Date(2014, 0, 21) },
);
isWithinInterval(new Date(2014, 0, 3), {
start: new Date(2014, 0, 1),
end: new Date(2014, 0, 7),
});
```
- **BREAKING**: functions renamed:
- `distanceInWords` → `formatDistance`
- `distanceInWordsStrict` → `formatDistanceStrict`
- `distanceInWordsToNow` → `formatDistanceToNow`
to make them consistent with `format` and `formatRelative`.
- **BREAKING**: The order of arguments of `distanceInWords` and `distanceInWordsStrict`
is swapped to make them consistent with `differenceIn...` functions.
```javascript
// Before v2.0.0
distanceInWords(
new Date(1986, 3, 4, 10, 32, 0),
new Date(1986, 3, 4, 11, 32, 0),
{ addSuffix: true },
); //=> 'in about 1 hour'
// v2.0.0 onward
formatDistance(
new Date(1986, 3, 4, 11, 32, 0),
new Date(1986, 3, 4, 10, 32, 0),
{ addSuffix: true },
); //=> 'in about 1 hour'
```
- **BREAKING**: `partialMethod` option in `formatDistanceStrict` is renamed to `roundingMethod`.
```javascript
// Before v2.0.0
distanceInWordsStrict(
new Date(1986, 3, 4, 10, 32, 0),
new Date(1986, 3, 4, 10, 33, 1),
{ partialMethod: "ceil" },
); //=> '2 minutes'
// v2.0.0 onward
formatDistanceStrict(
new Date(1986, 3, 4, 10, 33, 1),
new Date(1986, 3, 4, 10, 32, 0),
{ roundingMethod: "ceil" },
); //=> '2 minutes'
```
- **BREAKING**: in `formatDistanceStrict`, if `roundingMethod` is not specified,
it now defaults to `round` instead of `floor`.
- **BREAKING**: `unit` option in `formatDistanceStrict` now accepts one of the strings:
'second', 'minute', 'hour', 'day', 'month' or 'year' instead of 's', 'm', 'h', 'd', 'M' or 'Y'
```javascript
// Before v2.0.0
distanceInWordsStrict(
new Date(1986, 3, 4, 10, 32, 0),
new Date(1986, 3, 4, 10, 33, 1),
{ unit: "m" },
);
// v2.0.0 onward
formatDistanceStrict(
new Date(1986, 3, 4, 10, 33, 1),
new Date(1986, 3, 4, 10, 32, 0),
{ unit: "minute" },
);
```
- **BREAKING**: `parse` that previously used to convert strings and
numbers to dates now parses only strings in an arbitrary format
specified as an argument. Use `toDate` to coerce numbers and `parseISO`
to parse ISO 8601 strings.
```javascript
// Before v2.0.0
parse("2016-01-01");
parse(1547005581366);
parse(new Date()); // Clone the date
// v2.0.0 onward
parse("2016-01-01", "yyyy-MM-dd", new Date());
parseISO("2016-01-01");
toDate(1547005581366);
toDate(new Date()); // Clone the date
```
- **BREAKING**: `toDate` (previously `parse`) now doesn't accept string
arguments but only numbers and dates. `toDate` called with an invalid
argument will return `Invalid Date`.
- **BREAKING**: new locale format.
See [docs/Locale](https://date-fns.org/docs/Locale).
Locales renamed:
- `en` → `en-US`
- `zh_cn` → `zh-CN`
- `zh_tw` → `zh-TW`
```javascript
// Before v2.0.0
import locale from "date-fns/locale/zh_cn";
// v2.0.0 onward
import locale from "date-fns/locale/zh-CN";
```
- **BREAKING**: now `closestTo` and `closestIndexTo` don't throw an exception
when the second argument is not an array, and return Invalid Date instead.
- **BREAKING**: now `isValid` doesn't throw an exception
if the first argument is not an instance of Date.
Instead, argument is converted beforehand using `toDate`.
Examples:
| `isValid` argument | Before v2.0.0 | v2.0.0 onward |
| ------------------------- | ------------- | ------------- |
| `new Date()` | `true` | `true` |
| `new Date('2016-01-01')` | `true` | `true` |
| `new Date('')` | `false` | `false` |
| `new Date(1488370835081)` | `true` | `true` |
| `new Date(NaN)` | `false` | `false` |
| `'2016-01-01'` | `TypeError` | `false` |
| `''` | `TypeError` | `false` |
| `1488370835081` | `TypeError` | `true` |
| `NaN` | `TypeError` | `false` |
We introduce this change to make _date-fns_ consistent with ECMAScript behavior
that try to coerce arguments to the expected type
(which is also the case with other _date-fns_ functions).
- **BREAKING**: functions now throw `RangeError` if optional values passed to `options`
are not `undefined` or have expected values.
This change is introduced for consistency with ECMAScript standard library which does the same.
- **BREAKING**: `format`, `formatDistance` (previously `distanceInWords`) and
`formatDistanceStrict` (previously `distanceInWordsStrict`) now throw
`RangeError` if one of the passed arguments is invalid. It reflects behavior of
`toISOString` and Intl API. See [#1032](https://github.com/date-fns/date-fns/pull/1032).
- **BREAKING**: all functions now implicitly convert arguments by following rules:
| | date | number | string | boolean |
| --------- | ------------ | ------ | ----------- | ------- |
| 0 | new Date(0) | 0 | '0' | false |
| '0' | Invalid Date | 0 | '0' | false |
| 1 | new Date(1) | 1 | '1' | true |
| '1' | Invalid Date | 1 | '1' | true |
| true | Invalid Date | NaN | 'true' | true |
| false | Invalid Date | NaN | 'false' | false |
| null | Invalid Date | NaN | 'null' | false |
| undefined | Invalid Date | NaN | 'undefined' | false |
| NaN | Invalid Date | NaN | 'NaN' | false |
Notes:
- as before, arguments expected to be `Date` are converted to `Date` using _date-fns'_ `toDate` function;
- arguments expected to be numbers are converted to integer numbers using our custom `toInteger` implementation
(see [#765](https://github.com/date-fns/date-fns/pull/765));
- arguments expected to be strings are converted to strings using JavaScript's `String` function;
- arguments expected to be booleans are converted to boolean using JavaScript's `Boolean` function.
`null` and `undefined` passed to optional arguments (i.e. properties of `options` argument)
are ignored as if no argument was passed.
If any resulting argument is invalid (i.e. `NaN` for numbers and `Invalid Date` for dates),
an invalid value will be returned:
- `false` for functions that return booleans (expect `isValid`);
- `Invalid Date` for functions that return dates;
- and `NaN` for functions that return numbers.
See tests and PRs [#460](https://github.com/date-fns/date-fns/pull/460) and
[#765](https://github.com/date-fns/date-fns/pull/765) for exact behavior.
- **BREAKING**: all functions now check if the passed number of arguments is less
than the number of required arguments and will throw `TypeError` exception if so.
- **BREAKING**: all functions that accept numbers as arguments, now coerce
values using `Number()` and also round off decimals. Positive decimals are
rounded using `Math.floor`, decimals less than zero are rounded using
`Math.ceil`.
- **BREAKING**: The Bower & UMD/CDN package versions are no longer supported.
- **BREAKING**: `null` now is not a valid date. `isValid(null)` returns `false`;
`toDate(null)` returns an invalid date. Since `toDate` is used internally
by all the functions, operations over `null` will also return an invalid date.
[See #537](https://github.com/date-fns/date-fns/issues/537) for the reasoning.
- `toDate` (previously `parse`) and `isValid` functions now accept `any` type
as the first argument.
- [Exclude `docs.json` from the npm package](https://github.com/date-fns/date-fns/pull/837). Kudos to [@hawkrives](https://github.com/hawkrives).
### Added
- FP functions like those in [lodash](https://github.com/lodash/lodash/wiki/FP-Guide),
that support [currying](https://en.wikipedia.org/wiki/Currying), and, as a consequence,
functional-style [function composing](https://medium.com/making-internets/why-using-chain-is-a-mistake-9bc1f80d51ba).
Functions with options (`format`, `parse`, etc.) have two FP counterparts:
one that has the options object as its first argument and one that hasn't.
The name of the former has `WithOptions` added to the end of its name.
In FP functions, the order of arguments is reversed.
See [FP Guide](https://date-fns.org/docs/FP-Guide) for more information.
```javascript
import addYears from "date-fns/fp/addYears";
import formatWithOptions from "date-fns/fp/formatWithOptions";
import eo from "date-fns/locale/eo";
// If FP function has not received enough arguments, it returns another function
const addFiveYears = addYears(5);
// Several arguments can be curried at once
const dateToString = formatWithOptions({ locale: eo }, "d MMMM yyyy");
const dates = [
new Date(2017, 0 /* Jan */, 1),
new Date(2017, 1 /* Feb */, 11),
new Date(2017, 6 /* Jul */, 2),
];
const formattedDates = dates.map((date) => dateToString(addFiveYears(date)));
//=> ['1 januaro 2022', '11 februaro 2022', '2 julio 2022']
```
- Added support for [ECMAScript Modules](http://www.ecma-international.org/ecma-262/6.0/#sec-modules).
It allows usage with bundlers that support tree-shaking,
like [rollup.js](http://rollupjs.org) and [webpack](https://webpack.js.org):
```javascript
// Without tree-shaking:
import format from "date-fns/format";
import parse from "date-fns/parse";
// With tree-shaking:
import { format, parse } from "date-fns";
```
Also, ESM functions provide default export, they can be used with TypeScript
to import functions in more idiomatic way:
```typescript
// Before
import * as format from "date-fns/format";
// Now
import format from "date-fns/format";
```
- `formatRelative` function. See [formatRelative](https://date-fns.org/docs/formatRelative)
- Flow typings for `index.js`, `fp/index.js`, `locale/index.js`, and their ESM equivalents.
See PR [#558](https://github.com/date-fns/date-fns/pull/558)
- New locale-dependent week-numbering year helpers:
- `getWeek`
- `getWeekYear`
- `setWeek`
- `setWeekYear`
- `startOfWeekYear`
- Added `eachWeekOfInterval`, the weekly equivalent of `eachDayOfInterval`
- [Added `getUnixTime` function](https://github.com/date-fns/date-fns/pull/870). Kudos to [@Kingwl](https://github.com/Kingwl).
- [New decade helpers](https://github.com/date-fns/date-fns/pull/839). Thanks to [@y-nk](https://github.com/y-nk)!
- `getDecade`
- `startOfDecade`
- `endOfDecade`
- `lastDayOfDecade`
- [New `roundToNearestMinutes` function](https://github.com/date-fns/date-fns/pull/928). Kudos to [@xkizer](https://github.com/xkizer).
- Added new function `fromUnixTime`. Thansk to [@xkizer](https://github.com/xkizer).
- New interval, month, and year helpers to fetch a list of all Saturdays and Sundays (weekends) for a given date interval. `eachWeekendOfInterval` is the handler function while the other two are wrapper functions. Kudos to [@laekettavong](https://github.com/laekettavong)!
- `eachWeekendOfInterval`
- `eachWeekendOfMonth`
- `eachWeekendOfYear`
- Build-efficient `lightFormat` that only supports the popular subset of tokens. See [#1050](https://github.com/date-fns/date-fns/pull/1015).
- `parseISO` function that parses ISO 8601 strings. See [#1023](https://github.com/date-fns/date-fns/pull/1023).
- Add constants that can be imported directly from `date-fns` or the submodule `date-fns/constants`:
- `maxTime`
- `minTime`
- New locales:
- [Norwegian Nynorsk locale (nn)](https://github.com/date-fns/date-fns/pull/1172)
by [@draperunner](https://github.com/draperunner).
- [Ukrainian locale (ua)](https://github.com/date-fns/date-fns/pull/532)
by [@korzhyk](https://github.com/korzhyk).
- [Vietnamese locale (vi)](https://github.com/date-fns/date-fns/pull/546)
by [@trongthanh](https://github.com/trongthanh).
- [Persian locale (fa-IR)](https://github.com/date-fns/date-fns/pull/1113)
by [@mort3za](https://github.com/mort3za).
- [Latvian locale (lv)](https://github.com/date-fns/date-fns/pull/1175)
by [@prudolfs](https://github.com/prudolfs).
- [Bengali locale (bb)](https://github.com/date-fns/date-fns/pull/845)
by [@nutboltu](https://github.com/nutboltu) and [@touhidrahman](https://github.com/touhidrahman).
- [Hungarian (hu) and Lithuanian (lt) locales](https://github.com/date-fns/date-fns/pull/864)
by [@izifortune](https://github.com/izifortune) and [pardoeryanair](https://github.com/pardoeryanair).
- [Canadian English locale (en-CA)](https://github.com/date-fns/date-fns/pull/688)
by [@markowsiak](https://github.com/markowsiak).
- [Great Britain English locale (en-GB)](https://github.com/date-fns/date-fns/pull/563)
by [@glintik](https://github.com/glintik).
- [Uighur locale (ug)](https://github.com/date-fns/date-fns/pull/1080)
by [@abduwaly](https://github.com/abduwaly).
- [Added new function `differenceInBusinessDays`](https://github.com/date-fns/date-fns/pull/1194)
which calculates the difference in business days. Kudos to [@ThorrStevens](https://github.com/ThorrStevens)!
- [Added new function `addBusinessDays`](https://github.com/date-fns/date-fns/pull/1154),
similar to `addDays` but ignoring weekends. Thanks to [@ThorrStevens](https://github.com/ThorrStevens)!
## [1.30.1] - 2018-12-10
### Fixed
- [Fixed DST issue](https://github.com/date-fns/date-fns/pull/1005). See [#972](https://github.com/date-fns/date-fns/issues/972) and [#992](https://github.com/date-fns/date-fns/issues/992) for more details. This fix was backported from v2.
- Fix a few bugs that appear in timezones with offsets that include seconds (e.g. GMT+00:57:44). See PR [#789](https://github.com/date-fns/date-fns/issues/789). This fix was backported from v2.
- [Fixed misspelled January in the Thai locale](https://github.com/date-fns/date-fns/pull/913). Thanks to [@ratchapol-an](https://github.com/ratchapol-an)!
### Added
- [Added Serbian locale](https://github.com/date-fns/date-fns/pull/717). Kudos to [@mawi12345](https://github.com/mawi12345)!
- [Added Belarusian locale](https://github.com/date-fns/date-fns/pull/716). Kudos to [@mawi12345](https://github.com/mawi12345) again!
### Changed
- [Improved ja translation of distanceInWords](https://github.com/date-fns/date-fns/pull/880). Thanks to [@kudohamu](https://github.com/kudohamu)!
## [1.30.0] - 2018-12-10
⚠️ The release got failed.
## [1.29.0] - 2017-10-11
### Fixed
- Fixed Italian translations for `formatDistance`. ([see the issue: #550](https://github.com/date-fns/date-fns/issues/550); [see the PR: #552](https://github.com/date-fns/date-fns/pull/552))
Thanks to [@giofilo](https://github.com/giofilo)!
### Added
- [Hungarian locale (hu)](https://github.com/date-fns/date-fns/pull/503)
(thanks to László Horváth [@horvathlg](https://github.com/horvathlg))
- [Slovenian locale (sl)](https://github.com/date-fns/date-fns/pull/505)
(thanks to Adam Stradovnik [@Neoglyph](https://github.com/Neoglyph))
- Added `step` to `eachDay` function. Thanks to [@BDav24](https://github.com/BDav24).
See PR [#487](https://github.com/date-fns/date-fns/pull/487).
## [1.28.5] - 2017-05-19
### Fixed
- Fixed a.m./p.m. formatters in Chinese Simplified locale.
Thanks to [@fnlctrl](https://github.com/fnlctrl).
See PR [#486](https://github.com/date-fns/date-fns/pull/486)
## [1.28.4] - 2017-04-26
### Fixed
- Fixed accents on weekdays in the Italian locale.
See PR [#481](https://github.com/date-fns/date-fns/pull/481).
Thanks to [@albertorestifo](https://github.com/albertorestifo)
- Fixed typo in `ddd` format token in Spanish language locale.
Kudos to [@fjaguero](https://github.com/fjaguero).
See PR [#482](https://github.com/date-fns/date-fns/pull/482)
## [1.28.3] - 2017-04-14
### Fixed
- Fixed ordinal numbers for Danish language locale. Thanks to [@kgram](https://github.com/kgram).
See PR [#474](https://github.com/date-fns/date-fns/pull/474)
## [1.28.2] - 2017-03-27
### Fixed
- Fixed `dd` and `ddd` formatters in Polish language locale. Kudos to [@justrag](https://github.com/justrag).
See PR: [#467](https://github.com/date-fns/date-fns/pull/467)
## [1.28.1] - 2017-03-19
### Fixed
- Fixed DST border bug in `addMilliseconds`, `addSeconds`, `addMinutes`, `addHours`,
`subMilliseconds`, `subSeconds`, `subMinutes` and `subHours`.
See issue [#465](https://github.com/date-fns/date-fns/issues/465)
- Minor fix for Indonesian locale. Thanks to [@bentinata](https://github.com/bentinata).
See PR: [#458](https://github.com/date-fns/date-fns/pull/458)
## [1.28.0] - 2017-02-27
### Added
- [Romanian locale (ro)](https://github.com/date-fns/date-fns/pull/446)
(thanks to Sergiu Munteanu [@jsergiu](https://github.com/jsergiu))
### Fixed
- All functions now convert all their arguments to the respective types.
See PR: [#443](https://github.com/date-fns/date-fns/pull/443)
- Fixes for ordinals (1er, 2, 3, …) in French locale.
Thanks to [@fbonzon](https://github.com/fbonzon).
See PR: [#449](https://github.com/date-fns/date-fns/pull/449)
## [1.27.2] - 2017-02-01
### Fixed
- Various fixes for Dutch locale. See PR: [#416](https://github.com/date-fns/date-fns/pull/416).
Thanks to Ruben Stolk [@rubenstolk](https://github.com/rubenstolk)
## [1.27.1] - 2017-01-20
### Fixed
- Added generation of TypeScript locale sub-modules, allowing import of locales in TypeScript.
## [1.27.0] - 2017-01-19
### Added
- [Macedonian locale (mk)](https://github.com/date-fns/date-fns/pull/398)
(thanks to Petar Vlahu [@vlahupetar](https://github.com/vlahupetar))
## [1.26.0] - 2017-01-15
### Added
- `getTime`
### Fixed
- Various fixes for Japanese locale. See PR: [395](https://github.com/date-fns/date-fns/pull/395).
Thanks to Yamagishi Kazutoshi [@ykzts](https://github.com/ykzts)
## [1.25.0] - 2017-01-11
### Added
- [Bulgarian locale (bg)](https://github.com/date-fns/date-fns/pull/357)
(thanks to Nikolay Stoynov [@arvigeus](https://github.com/arvigeus))
- [Czech locale (cs)](https://github.com/date-fns/date-fns/pull/386)
(thanks to David Rus [@davidrus](https://github.com/davidrus))
## [1.24.0] - 2017-01-06
### Added
- [Modern Standard Arabic locale (ar)](https://github.com/date-fns/date-fns/pull/367)
(thanks to Abdallah Hassan [@AbdallahAHO](https://github.com/AbdallahAHO))
## [1.23.0] - 2017-01-05
### Added
- Auto generate TypeScript and flow typings from documentation on release.
Thanks to [@mattlewis92](https://github.com/mattlewis92).
See related PRs: [#355](https://github.com/date-fns/date-fns/pull/355),
[#370](https://github.com/date-fns/date-fns/pull/370)
- [Croatian locale (hr)](https://github.com/date-fns/date-fns/pull/365)
(thanks to Matija Marohnić [@silvenon](https://github.com/silvenon))
- [Thai locale (th)](https://github.com/date-fns/date-fns/pull/362)
(thanks to Athiwat Hirunworawongkun [@athivvat](https://github.com/athivvat))
- [Finnish locale (fi)](https://github.com/date-fns/date-fns/pull/361)
(thanks to Pyry-Samuli Lahti [@Pyppe](https://github.com/Pyppe))
## [1.22.0] - 2016-12-28
### Added
- [Icelandic locale (is)](https://github.com/date-fns/date-fns/pull/356)
(thanks to Derek Blank [@derekblank](https://github.com/derekblank))
## [1.21.1] - 2016-12-18
### Fixed
- Fixed `isBefore` and `isAfter` documentation mistakes.
## [1.21.0] - 2016-12-16
### Added
- [Filipino locale (fil)](https://github.com/date-fns/date-fns/pull/339)
(thanks to Ian De La Cruz [@RIanDeLaCruz](https://github.com/RIanDeLaCruz))
- [Danish locale (da)](https://github.com/date-fns/date-fns/pull/343)
(kudos to Anders B. Hansen [@Andersbiha](https://github.com/Andersbiha))
## [1.20.1] - 2016-12-14
### Fixed
- Fixed documentation for `getOverlappingDaysInRanges`.
## [1.20.0] - 2016-12-13
### Added
- `areRangesOverlapping` and `getOverlappingDaysInRanges`
Thanks to Joanna T [@asia-t](https://github.com/asia-t).
See PR: [#331](https://github.com/date-fns/date-fns/pull/331)
## [1.19.0] - 2016-12-13
### Added
- [Greek locale (el)](https://github.com/date-fns/date-fns/pull/334)
(kudos to Theodoros Orfanidis [@teoulas](https://github.com/teoulas))
- [Slovak locale (sk)](https://github.com/date-fns/date-fns/pull/336)
(kudos to Marek Suscak [@mareksuscak](https://github.com/mareksuscak))
- Added yarn support.
Thanks to Uladzimir Havenchyk [@havenchyk](https://github.com/havenchyk).
See PR: [#288](https://github.com/date-fns/date-fns/pull/288)
## [1.18.0] - 2016-12-12
### Added
- [Turkish locale (tr)](https://github.com/date-fns/date-fns/pull/329)
(kudos to Alpcan Aydın [@alpcanaydin](https://github.com/alpcanaydin))
- [Korean locale (ko)](https://github.com/date-fns/date-fns/pull/327)
(thanks to Hong Chulju [@angdev](https://github.com/angdev))
### Fixed
- `SS` and `SSS` formats in `format` are now correctly displayed with leading zeros.
Thanks to Paul Dijou [@pauldijou](https://github.com/pauldijou).
See PR: [#330](https://github.com/date-fns/date-fns/pull/330)
## [1.17.0] - 2016-12-10
### Added
- [Polish locale (pl)](https://github.com/date-fns/date-fns/pull/294)
(thanks to Mateusz Derks [@ertrzyiks](https://github.com/ertrzyiks))
- [Portuguese locale (pt)](https://github.com/date-fns/date-fns/pull/316)
(thanks to Dário Freire [@dfreire](https://github.com/dfreire))
- [Swedish locale (sv)](https://github.com/date-fns/date-fns/pull/311)
(thanks to Johannes Ulén [@ejulen](https://github.com/ejulen))
- [French locale (fr)](https://github.com/date-fns/date-fns/pull/281)
(thanks to Jean Dupouy [@izeau](https://github.com/izeau))
- Performance tests. See PR: [#289](https://github.com/date-fns/date-fns/pull/289)
### Fixed
- Fixed TypeScript and flow typings for `isValid`.
See PR: [#310](https://github.com/date-fns/date-fns/pull/310)
- Fixed incorrect locale tests that could potentially lead to `format` bugs.
Kudos to Mateusz Derks [@ertrzyiks](https://github.com/ertrzyiks).
See related PRs: [#312](https://github.com/date-fns/date-fns/pull/312),
[#320](https://github.com/date-fns/date-fns/pull/320)
- Minor language fixes in the documentation.
Thanks to Vedad Šoše [@vedadsose](https://github.com/vedadsose) ([#314](https://github.com/date-fns/date-fns/pull/314))
and Asia [@asia-t](https://github.com/asia-t) ([#318](https://github.com/date-fns/date-fns/pull/318))
### Changed
- `format` now returns `String('Invalid Date')` if the passed date is invalid.
See PR: [#323](https://github.com/date-fns/date-fns/pull/323)
- `distanceInWords`, `distanceInWordsToNow`, `distanceInWordsStrict` and `format` functions now
check if the passed locale is valid, and fallback to English locale otherwise.
See PR: [#321](https://github.com/date-fns/date-fns/pull/321)
- _Internal_: use a loop instead of `Object.keys` in `buildFormattingTokensRegExp`
to improve compatibility with older browsers.
See PR: [#322](https://github.com/date-fns/date-fns/pull/322)
## [1.16.0] - 2016-12-08
### Added
- [Italian locale (it)](https://github.com/date-fns/date-fns/pull/298)
(thanks to Alberto Restifo [@albertorestifo](https://github.com/albertorestifo))
- For German `buildDistanceInWordsLocale`, add nominative case translations (for distances without a suffix).
Kudos to Asia [@asia-t](https://github.com/asia-t).
See related PR: [#295](https://github.com/date-fns/date-fns/pull/295)
## [1.15.1] - 2016-12-07
### Fixed
- Fixed TypeScript imports from individual modules.
Thanks to [@mattlewis92](https://github.com/mattlewis92).
See related PR: [#287](https://github.com/date-fns/date-fns/pull/287)
## [1.15.0] - 2016-12-07
### Added
- [Indonesian locale (id)](https://github.com/date-fns/date-fns/pull/299)
(thanks to Rahmat Budiharso [@rbudiharso](https://github.com/rbudiharso))
- [Catalan locale (ca)](https://github.com/date-fns/date-fns/pull/300)
(thanks to Guillermo Grau [@guigrpa](https://github.com/guigrpa))
### Fixed
- Fixed some inaccuracies in Spanish locale.
Kudos to [@guigrpa](https://github.com/guigrpa).
See related PR: [#302](https://github.com/date-fns/date-fns/pull/302)
## [1.14.1] - 2016-12-06
### Fixed
- Fixed broken test for Norwegian Bokmål locale.
## [1.14.0] - 2016-12-06
### Added
- [Norwegian Bokmål locale (nb)](https://github.com/date-fns/date-fns/pull/291)
(thanks to Hans-Kristian Koren [@Hanse](https://github.com/Hanse))
## [1.13.0] - 2016-12-06
### Added
- [Chinese Traditional locale (zh_tw)](https://github.com/date-fns/date-fns/pull/283)
(thanks to tonypai [@tpai](https://github.com/tpai)).
- [Dutch language locale (nl)](https://github.com/date-fns/date-fns/pull/278)
(kudos to Jorik Tangelder [@jtangelder](https://github.com/jtangelder))
## [1.12.1] - 2016-12-05
### Fixed
- Added `distanceInWordsStrict` to the list of supported functions in I18n doc.
## [1.12.0] - 2016-12-05
### Added
- [Spanish language locale (es)](https://github.com/date-fns/date-fns/pull/269)
(thanks to Juan Angosto [@juanangosto](https://github.com/juanangosto)).
### Fixed
- Fixed flow typings for some of the functions.
See PR: [#273](https://github.com/date-fns/date-fns/pull/273)
## [1.11.2] - 2016-11-28
### Fixed
- Bug in `parse` when it sometimes parses ISO week-numbering dates incorrectly.
See PR: [#262](https://github.com/date-fns/date-fns/pull/262)
- Bug in some functions which caused them to handle dates earlier than 100 AD incorrectly.
See PR: [#263](https://github.com/date-fns/date-fns/pull/263)
## [1.11.1] - 2016-11-24
### Fixed
- Include TypeScript typings with npm package.
## [1.11.0] - 2016-11-23
### Added
- `distanceInWordsStrict`.
Kudos to [@STRML](https://github.com/STRML).
See related PR: [#254](https://github.com/date-fns/date-fns/pull/254)
- [TypeScript](https://www.typescriptlang.org/) typings for all functions.
Kudos to [@mattlewis92](https://github.com/mattlewis92).
See related PR: [#255](https://github.com/date-fns/date-fns/pull/255)
## [1.10.0] - 2016-11-01
### Added
- `parse` now can parse dates that are ISO 8601 centuries (e.g., `19` and `+0019`).
```javascript
var result = parse("19");
//=> Mon Jan 01 1900 00:00:00
```
- In `parse`, added ability to specify the number of additional digits
for extended year or century format (possible values are 0, 1 or 2; default is 2).
```javascript
parse("+002016-11-01");
parse("+02016-11-01", { additionalDigits: 1 });
parse("+2016-11-01", { additionalDigits: 0 });
```
## [1.9.0] - 2016-10-25
### Added
- Got index.js imports to work with SystemJS.
## [1.8.1] - 2016-10-24
### Fixed
- Added Japanese and German language locales to the list in I18n doc.
## [1.8.0] - 2016-10-23
### Added
- [Japanese language locale (ja)](https://github.com/date-fns/date-fns/pull/241)
(thanks to Thomas Eilmsteiner [@DeMuu](https://github.com/DeMuu) again!)
- `getISODay`
- `setISODay`
## [1.7.0] - 2016-10-20
### Added
- [German language locale (de)](https://github.com/date-fns/date-fns/pull/237)
(thanks to Thomas Eilmsteiner [@DeMuu](https://github.com/DeMuu)).
## [1.6.0] - 2016-10-16
### Added
- [Chinese Simplified locale (zh_cn)](https://github.com/date-fns/date-fns/pull/235)
(kudos to Changyu [@KingMario](https://github.com/KingMario) Geng).
## [1.5.2] - 2016-10-13
### Fixed
- Incorrectly generated docs for `format`.
- Fixed typo in I18n doc.
## [1.5.1] - 2016-10-12
### Fixed
- A change log entry for [1.5.0] is added.
## [1.5.0] - 2016-10-12
### Added
- [The initial I18n support](https://date-fns.org/docs/I18n)
## [1.4.0] - 2016-10-09
### Added
- Basic [SystemJS](https://github.com/systemjs/systemjs) support.
### Fixed
- Fixed incorrect behaviour of `YYYY` and `YY` for years prior to 1000:
now `format(new Date('0001-01-01'), 'YYYY-MM-DD')` returns `0001-01-01`
instead of `1-01-01`.
## [1.3.0] - 2016-05-26
### Added
- `closestIndexTo`
## [1.2.0] - 2016-05-23
### Added
- Added an ability to pass negative numbers to `setDay`.
## [1.1.1] - 2016-05-19
### Fixed
- Fixed [Flow](http://flowtype.org/) declarations for some of the functions.
## [1.1.0] - 2016-05-19
### Added
- [Flow](http://flowtype.org/) declarations for each function
in [the ".js.flow" style](http://flowtype.org/docs/declarations.html#declaration-files).
Kudos to [@JohnyDays](https://github.com/JohnyDays). See related PRs:
- [#205](https://github.com/date-fns/date-fns/pull/205)
- [#207](https://github.com/date-fns/date-fns/pull/207)
## [1.0.0] - 2016-05-18
### Fixed
- `format` now returns the correct result for key `E`.
- Prevent `startOf...`, `endOf...` and `lastDayOf...` functions
to return dates with an incorrect time when the date is modifying
into another time zone.
- `parse` now parses years from 1 AD to 99 AD correctly.
- Fix a bug in `getISOWeek` appearing because of a changing time zone
(e.g., when the given date is in DST and the start of the ISO year is not).
### Changed
- **BREAKING**: all functions are moved to the root of the library, so they
are now accessible with `require('date-fns/name_of_function')` or
`import nameOfFunction from 'date-fns/name_of_function'`.
```javascript
// Before v1.0.0
var addMonths = require("date-fns/src/add_months");
// v1.0.0 onward
var addMonths = require("date-fns/add_months");
```
- **BREAKING**: functions that had the last optional argument `weekStartsAt`
(i.e. `endOfWeek`, `isSameWeek`, `lastDayOfWeek`, `setDay`, `startOfWeek`)
now instead receive the object `options` with the property `options.weekStartsOn`
as the last argument.
```javascript
// Before v1.0.0
var result = endOfWeek(new Date(2014, 8, 2), 1);
// v1.0.0 onward
var result = endOfWeek(new Date(2014, 8, 2), { weekStartsOn: 1 });
```
- **BREAKING**: remove the function `getTimeSinceMidnight` that was used inside
the other functions.
- **BREAKING**: `differenceInDays` now returns the number of full days instead
of calendar days.
- **BREAKING**: `eachDay` and `isWithinRange` now throw an exception
when the given range boundaries are invalid.
- Faster `isLeapYear`.
- _Internal_: make the documentation more verbose.
- _Internal_: convert the tests from Chai to power-assert allowing them
to run against IE8.
### Added
- `addISOYears`
- `closestTo`
- `differenceInCalendarDays`
- `differenceInCalendarISOWeeks`
- `differenceInCalendarISOYears`
- `differenceInCalendarMonths`
- `differenceInCalendarQuarters`
- `differenceInCalendarWeeks`
- `differenceInCalendarYears`
- `differenceInHours`
- `differenceInISOYears`
- `differenceInMilliseconds`
- `differenceInMinutes`
- `differenceInMonths`
- `differenceInQuarters`
- `differenceInSeconds`
- `differenceInWeeks`
- `differenceInYears`
- `distanceInWords`
- `distanceInWordsToNow`
- `endOfISOWeek`
- `endOfISOYear`
- `endOfToday`
- `endOfTomorrow`
- `endOfYesterday`
- `getDaysInYear`
- `isDate`
- `isFriday`
- `isMonday`
- `isSameISOWeek`
- `isSameISOYear`
- `isSaturday`
- `isSunday`
- `isThisHour`
- `isThisISOWeek`
- `isThisISOYear`
- `isThisMinute`
- `isThisMonth`
- `isThisQuarter`
- `isThisSecond`
- `isThisWeek`
- `isThisYear`
- `isThursday`
- `isTomorrow`
- `isTuesday`
- `isValid`
- `isWednesday`
- `isYesterday`
- `lastDayOfISOWeek`
- `lastDayOfISOYear`
- `startOfISOWeek`
- `startOfToday`
- `startOfTomorrow`
- `startOfYesterday`
- `subISOYears`
- Add `Qo`, `W`, `Wo`, `WW`, `GG`, `GGGG`, `Z`, `ZZ`, `X`, `x` keys to `format`.
## [0.17.0] - 2015-09-29
### Fixed
- Fixed a lot of bugs appearing when date is modifying into other time zone
(e.g., when adding months and original date is in DST but new date is not).
- Prevent instances of Date to lose milliseconds value when passed to.
`parse` in IE10.
### Changed
- `setISOWeek` now keeps time from original date.
- _Internal_: reuse `getDaysInMonth` inside of `addMonths`.
### Added
- `differenceInDays`
- `getTimeSinceMidnight`
- `format` now has new format key `aa`, which returns `a.m.`/`p.m.`
as opposed to `a` that returns `am`/`pm`.
- Complete UMD package (for Bower and CDN).
## [0.16.0] - 2015-09-01
### Changed
- Use `parse` to clean date arguments in all functions.
- `parse` now fallbacks to `new Date` when the argument
is not an ISO formatted date.
- _Internal_: reuse `getDaysInMonth` inside of `setMonth`.
### Added
- `addQuarters`
- `addWeeks`
- `endOfQuarter`
- `getDate`
- `getDay`
- `getDaysInMonth`
- `getHours`
- `getISOWeeksInYear`
- `getMilliseconds`
- `getMinutes`
- `getMonth`
- `getSeconds`
- `getYear`
- `isLeapYear`
- `isSameHour`
- `isSameMinute`
- `isSameQuarter`
- `isSameSecond`
- `lastDayOfQuarter`
- `lastDayOfWeek`
- `max`
- `min`
- `setDate`
- `setDay`
- `setHours`
- `setMilliseconds`
- `setMinutes`
- `setSeconds`
- `startOfQuarter`
- `subQuarters`
- `subWeeks`
## [0.15.0] - 2015-08-26
### Changed
- `format` now returns `a.m.`/`p.m.` instead of `am`/`pm`.
- `setMonth` now sets last day of month if original date was last day
of longer month.
- _Internal_: Fix code style according to ESLint.
- _Internal_: Make tests run through all time zones.
### Added
- `getQuarter`
- `setQuarter`
- `getDayOfYear`
- `setDayOfYear`
- `isPast`
- `addSeconds`
- `subSeconds`
- `startOfSecond`
- `endOfSecond`
- `startOfMinute`
- `endOfMinute`
- `addMilliseconds`
- `subMilliseconds`
- `endOfYear`
- `addYears`
- `subYears`
- `lastDayOfYear`
- `lastDayOfMonth`
## [0.14.11] - 2015-08-21
### Fixed
- `format` now uses `parse` to avoid time zone bugs.
### Changed
- `setIsoWeek` now sets time to the start of the day.
## [0.14.10] - 2015-07-29
### Fixed
- `format` now behaves correctly with 12:00 am.
- `format` now behaves correctly with ordinal numbers.
### Added
- `compareAsc`
- `compareDesc`
- `addHours`
- `subHours`
- `isSameDay`
- `parse`
- `getISOYear`
- `setISOYear`
- `startOfISOYear`
- `getISOWeek`
- `setISOWeek`
## [0.14.9] - 2015-01-14
### Fixed
- `addMonths` now correctly behaves with February
(see [#18](https://github.com/js-fns/date-fns/pull/18)).
## [0.14.8] - 2014-12-25
### Fixed
- `format` function now behaves correctly with `pm`/`am`.
## [0.14.6] - 2014-12-04
### Fixed
- Fix broken Bower support.
## [0.14.0] - 2014-11-05
### Added
- Bower package.
## [0.13.0] - 2014-10-22
### Added
- `addMinutes`
- `subMinutes`
- `isEqual`
- `isBefore`
- `isAfter`
## [0.12.1] - 2014-10-19
### Fixed
- Incorrect rounding in `DDD` formatter.
## [0.12.0] - 2014-10-15
### Added
- `isSameYear`
## [0.11.0] - 2014-10-15
### Added
- `isWithinRange`
## [0.10.0] - 2014-10-13
### Added
- `format`
- `startOfYear`
## [0.9.0] - 2014-10-10
### Changed
- _Internal_: simplify `isWeekend`
### Added
- `isFuture`
## [0.8.0] - 2014-10-09
### Changed
- _Internal_: reuse `addDays` inside of `subDays`.
### Added
- `addMonths`
- `subMonths`
- `setMonth`
- `setYear`
## [0.7.0] - 2014-10-08
### Added
- `isSameWeek`
## [0.6.0] - 2014-10-07
### Fixed
- Inconsistent behavior of `endOfMonth`.
### Added
- `isFirstDayOfMonth`
- `isLastDayOfMonth`
- `isSameMonth`
## [0.5.0] - 2014-10-07
### Added
- `addDays`
- `subDays`
## [0.4.0] - 2014-10-07
### Added
- `startOfWeek`
- `endOfWeek`
- `eachDay`
## [0.3.0] - 2014-10-06
### Changed
- `startOfDay` now sets milliseconds as well.
### Added
- `endOfDay`
- `startOfMonth`
- `endOfMonth`
## [0.2.0] - 2014-10-06
### Added
- `isToday`
- `isWeekend`
## 0.1.0 - 2014-10-06
### Added
- `startOfDay`
[unreleased]: https://github.com/date-fns/date-fns/compare/v2.16.1...HEAD
[2.16.1]: https://github.com/date-fns/date-fns/compare/v2.16.0...v2.16.1
[2.16.0]: https://github.com/date-fns/date-fns/compare/v2.15.0...v2.16.0
[2.15.0]: https://github.com/date-fns/date-fns/compare/v2.14.0...v2.15.0
[2.14.0]: https://github.com/date-fns/date-fns/compare/v2.13.0...v2.14.0
[2.13.0]: https://github.com/date-fns/date-fns/compare/v2.12.0...v2.13.0
[2.12.0]: https://github.com/date-fns/date-fns/compare/v2.11.1...v2.12.0
[2.11.1]: https://github.com/date-fns/date-fns/compare/v2.11.0...v2.11.1
[2.11.0]: https://github.com/date-fns/date-fns/compare/v2.10.0...v2.11.0
[2.10.0]: https://github.com/date-fns/date-fns/compare/v2.9.0...v2.10.0
[2.9.0]: https://github.com/date-fns/date-fns/compare/v2.8.1...v2.9.0
[2.8.1]: https://github.com/date-fns/date-fns/compare/v2.8.0...v2.8.1
[2.8.0]: https://github.com/date-fns/date-fns/compare/v2.7.0...v2.8.0
[2.7.0]: https://github.com/date-fns/date-fns/compare/v2.6.0...v2.7.0
[2.6.0]: https://github.com/date-fns/date-fns/compare/v2.5.1...v2.6.0
[2.5.1]: https://github.com/date-fns/date-fns/compare/v2.5.0...v2.5.1
[2.5.0]: https://github.com/date-fns/date-fns/compare/v2.4.1...v2.5.0
[2.4.1]: https://github.com/date-fns/date-fns/compare/v2.4.0...v2.4.1
[2.4.0]: https://github.com/date-fns/date-fns/compare/v2.3.0...v2.4.0
[2.3.0]: https://github.com/date-fns/date-fns/compare/v2.2.1...v2.3.0
[2.2.1]: https://github.com/date-fns/date-fns/compare/v2.1.0...v2.2.1
[2.1.0]: https://github.com/date-fns/date-fns/compare/v2.0.1...v2.1.0
[2.0.1]: https://github.com/date-fns/date-fns/compare/v2.0.0...v2.0.1
[2.0.0]: https://github.com/date-fns/date-fns/compare/v1.28.5...v2.0.0
[1.28.5]: https://github.com/date-fns/date-fns/compare/v1.28.4...v1.28.5
[1.28.4]: https://github.com/date-fns/date-fns/compare/v1.28.3...v1.28.4
[1.28.3]: https://github.com/date-fns/date-fns/compare/v1.28.2...v1.28.3
[1.28.2]: https://github.com/date-fns/date-fns/compare/v1.28.1...v1.28.2
[1.28.1]: https://github.com/date-fns/date-fns/compare/v1.28.0...v1.28.1
[1.28.0]: https://github.com/date-fns/date-fns/compare/v1.27.2...v1.28.0
[1.27.2]: https://github.com/date-fns/date-fns/compare/v1.27.1...v1.27.2
[1.27.1]: https://github.com/date-fns/date-fns/compare/v1.27.0...v1.27.1
[1.27.0]: https://github.com/date-fns/date-fns/compare/v1.26.0...v1.27.0
[1.26.0]: https://github.com/date-fns/date-fns/compare/v1.25.0...v1.26.0
[1.25.0]: https://github.com/date-fns/date-fns/compare/v1.24.0...v1.25.0
[1.24.0]: https://github.com/date-fns/date-fns/compare/v1.23.0...v1.24.0
[1.23.0]: https://github.com/date-fns/date-fns/compare/v1.22.0...v1.23.0
[1.22.0]: https://github.com/date-fns/date-fns/compare/v1.21.1...v1.22.0
[1.21.1]: https://github.com/date-fns/date-fns/compare/v1.21.0...v1.21.1
[1.21.0]: https://github.com/date-fns/date-fns/compare/v1.20.1...v1.21.0
[1.20.1]: https://github.com/date-fns/date-fns/compare/v1.20.0...v1.20.1
[1.20.0]: https://github.com/date-fns/date-fns/compare/v1.19.0...v1.20.0
[1.19.0]: https://github.com/date-fns/date-fns/compare/v1.18.0...v1.19.0
[1.18.0]: https://github.com/date-fns/date-fns/compare/v1.17.0...v1.18.0
[1.17.0]: https://github.com/date-fns/date-fns/compare/v1.16.0...v1.17.0
[1.16.0]: https://github.com/date-fns/date-fns/compare/v1.15.1...v1.16.0
[1.15.1]: https://github.com/date-fns/date-fns/compare/v1.15.0...v1.15.1
[1.15.0]: https://github.com/date-fns/date-fns/compare/v1.14.1...v1.15.0
[1.14.1]: https://github.com/date-fns/date-fns/compare/v1.14.0...v1.14.1
[1.14.0]: https://github.com/date-fns/date-fns/compare/v1.13.0...v1.14.0
[1.13.0]: https://github.com/date-fns/date-fns/compare/v1.12.1...v1.13.0
[1.12.1]: https://github.com/date-fns/date-fns/compare/v1.12.0...v1.12.1
[1.12.0]: https://github.com/date-fns/date-fns/compare/v1.11.2...v1.12.0
[1.11.2]: https://github.com/date-fns/date-fns/compare/v1.11.1...v1.11.2
[1.11.1]: https://github.com/date-fns/date-fns/compare/v1.11.0...v1.11.1
[1.11.0]: https://github.com/date-fns/date-fns/compare/v1.10.0...v1.11.0
[1.10.0]: https://github.com/date-fns/date-fns/compare/v1.9.0...v1.10.0
[1.9.0]: https://github.com/date-fns/date-fns/compare/v1.8.1...v1.9.0
[1.8.1]: https://github.com/date-fns/date-fns/compare/v1.8.0...v1.8.1
[1.8.0]: https://github.com/date-fns/date-fns/compare/v1.7.0...v1.8.0
[1.7.0]: https://github.com/date-fns/date-fns/compare/v1.6.0...v1.7.0
[1.6.0]: https://github.com/date-fns/date-fns/compare/v1.5.2...v1.6.0
[1.5.2]: https://github.com/date-fns/date-fns/compare/v1.5.1...v1.5.2
[1.5.1]: https://github.com/date-fns/date-fns/compare/v1.5.0...v1.5.1
[1.5.0]: https://github.com/date-fns/date-fns/compare/v1.4.0...v1.5.0
[1.4.0]: https://github.com/date-fns/date-fns/compare/v1.3.0...v1.4.0
[1.3.0]: https://github.com/date-fns/date-fns/compare/v1.2.0...v1.3.0
[1.2.0]: https://github.com/date-fns/date-fns/compare/v1.1.1...v1.2.0
[1.1.1]: https://github.com/date-fns/date-fns/compare/v1.1.0...v1.1.1
[1.1.0]: https://github.com/date-fns/date-fns/compare/v1.0.0...v1.1.0
[1.0.0]: https://github.com/date-fns/date-fns/compare/v0.17.0...v1.0.0
[0.17.0]: https://github.com/date-fns/date-fns/compare/v0.16.0...v0.17.0
[0.16.0]: https://github.com/date-fns/date-fns/compare/v0.15.0...v0.16.0
[0.15.0]: https://github.com/date-fns/date-fns/compare/v0.14.11...v0.15.0
[0.14.11]: https://github.com/date-fns/date-fns/compare/v0.14.10...v0.14.11
[0.14.10]: https://github.com/date-fns/date-fns/compare/v0.14.9...v0.14.10
[0.14.9]: https://github.com/date-fns/date-fns/compare/v0.14.8...v0.14.9
[0.14.8]: https://github.com/date-fns/date-fns/compare/v0.14.6...v0.14.8
[0.14.6]: https://github.com/date-fns/date-fns/compare/v0.14.0...v0.14.6
[0.14.0]: https://github.com/date-fns/date-fns/compare/v0.13.0...v0.14.0
[0.13.0]: https://github.com/date-fns/date-fns/compare/v0.12.1...v0.13.0
[0.12.1]: https://github.com/date-fns/date-fns/compare/v0.12.0...v0.12.1
[0.12.0]: https://github.com/date-fns/date-fns/compare/v0.11.0...v0.12.0
[0.11.0]: https://github.com/date-fns/date-fns/compare/v0.10.0...v0.11.0
[0.10.0]: https://github.com/date-fns/date-fns/compare/v0.9.0...v0.10.0
[0.9.0]: https://github.com/date-fns/date-fns/compare/v0.8.0...v0.9.0
[0.8.0]: https://github.com/date-fns/date-fns/compare/v0.7.0...v0.8.0
[0.7.0]: https://github.com/date-fns/date-fns/compare/v0.6.0...v0.7.0
[0.6.0]: https://github.com/date-fns/date-fns/compare/v0.5.0...v0.6.0
[0.5.0]: https://github.com/date-fns/date-fns/compare/v0.4.0...v0.5.0
[0.4.0]: https://github.com/date-fns/date-fns/compare/v0.3.0...v0.4.0
[0.3.0]: https://github.com/date-fns/date-fns/compare/v0.2.0...v0.3.0
[0.2.0]: https://github.com/date-fns/date-fns/compare/v0.1.0...v0.2.0 | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/date-fns/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/date-fns/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 116219
} |
MIT License
Copyright (c) 2021 Sasha Koss and Lesha Koss https://kossnocorp.mit-license.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/date-fns/LICENSE.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/date-fns/LICENSE.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1116
} |
🎉️ **NEW**: [date-fns v3 is out!](https://blog.date-fns.org/v3-is-out/)
<img alt="date-fns" title="date-fns" src="https://raw.githubusercontent.com/date-fns/date-fns/master/docs/logotype.svg" width="150" />
date-fns provides the most comprehensive, yet simple and consistent toolset for manipulating JavaScript dates in a browser & Node.js
👉 [Documentation](https://date-fns.org/)
👉 [Blog](https://blog.date-fns.org/)
<hr>
It's like [Lodash](https://lodash.com) for dates
- It has [**200+ functions** for all occasions](https://date-fns.org/docs/Getting-Started/).
- **Modular**: Pick what you need. Works with webpack, Browserify, or Rollup and also supports tree-shaking.
- **Native dates**: Uses existing native type. It doesn't extend core objects for safety's sake.
- **Immutable & Pure**: Built using pure functions and always returns a new date instance.
- **TypeScript**: The library is 100% TypeScript with brand-new handcrafted types.
- **I18n**: Dozens of locales. Include only what you need.
- [and many more benefits](https://date-fns.org/)
```js
import { compareAsc, format } from "date-fns";
format(new Date(2014, 1, 11), "yyyy-MM-dd");
//=> '2014-02-11'
const dates = [
new Date(1995, 6, 2),
new Date(1987, 1, 11),
new Date(1989, 6, 10),
];
dates.sort(compareAsc);
//=> [
// Wed Feb 11 1987 00:00:00,
// Mon Jul 10 1989 00:00:00,
// Sun Jul 02 1995 00:00:00
// ]
```
The library is available as an [npm package](https://www.npmjs.com/package/date-fns).
To install the package run:
```bash
npm install date-fns --save
```
## Docs
[See date-fns.org](https://date-fns.org/) for more details, API,
and other docs.
<br />
<!-- END OF README-JOB SECTION -->
## License
[MIT © Sasha Koss](https://kossnocorp.mit-license.org/) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/date-fns/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/date-fns/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1763
} |
# Security Policy
## Supported Versions
Security updates are applied only to the latest release.
## Reporting a Vulnerability
If you have discovered a security vulnerability in this project, please report it privately. **Do not disclose it as a public issue.**
This gives us time to work with you to fix the issue before public exposure, reducing the chance that the exploit will be used before a patch is released.
Please disclose it to [Sasha Koss](mailto:[email protected]). This project is maintained by a team of volunteers
on a reasonable-effort basis. As such, please give us at least 90 days to work on a fix before public exposure. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/date-fns/SECURITY.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/date-fns/SECURITY.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 641
} |
# debug
[](#backers)
[](#sponsors)
<img width="647" src="https://user-images.githubusercontent.com/71256/29091486-fa38524c-7c37-11e7-895f-e7ec8e1039b6.png">
A tiny JavaScript debugging utility modelled after Node.js core's debugging
technique. Works in Node.js and web browsers.
## Installation
```bash
$ npm install debug
```
## Usage
`debug` exposes a function; simply pass this function the name of your module, and it will return a decorated version of `console.error` for you to pass debug statements to. This will allow you to toggle the debug output for different parts of your module as well as the module as a whole.
Example [_app.js_](./examples/node/app.js):
```js
var debug = require('debug')('http')
, http = require('http')
, name = 'My App';
// fake app
debug('booting %o', name);
http.createServer(function(req, res){
debug(req.method + ' ' + req.url);
res.end('hello\n');
}).listen(3000, function(){
debug('listening');
});
// fake worker of some kind
require('./worker');
```
Example [_worker.js_](./examples/node/worker.js):
```js
var a = require('debug')('worker:a')
, b = require('debug')('worker:b');
function work() {
a('doing lots of uninteresting work');
setTimeout(work, Math.random() * 1000);
}
work();
function workb() {
b('doing some work');
setTimeout(workb, Math.random() * 2000);
}
workb();
```
The `DEBUG` environment variable is then used to enable these based on space or
comma-delimited names.
Here are some examples:
<img width="647" alt="screen shot 2017-08-08 at 12 53 04 pm" src="https://user-images.githubusercontent.com/71256/29091703-a6302cdc-7c38-11e7-8304-7c0b3bc600cd.png">
<img width="647" alt="screen shot 2017-08-08 at 12 53 38 pm" src="https://user-images.githubusercontent.com/71256/29091700-a62a6888-7c38-11e7-800b-db911291ca2b.png">
<img width="647" alt="screen shot 2017-08-08 at 12 53 25 pm" src="https://user-images.githubusercontent.com/71256/29091701-a62ea114-7c38-11e7-826a-2692bedca740.png">
#### Windows command prompt notes
##### CMD
On Windows the environment variable is set using the `set` command.
```cmd
set DEBUG=*,-not_this
```
Example:
```cmd
set DEBUG=* & node app.js
```
##### PowerShell (VS Code default)
PowerShell uses different syntax to set environment variables.
```cmd
$env:DEBUG = "*,-not_this"
```
Example:
```cmd
$env:DEBUG='app';node app.js
```
Then, run the program to be debugged as usual.
npm script example:
```js
"windowsDebug": "@powershell -Command $env:DEBUG='*';node app.js",
```
## Namespace Colors
Every debug instance has a color generated for it based on its namespace name.
This helps when visually parsing the debug output to identify which debug instance
a debug line belongs to.
#### Node.js
In Node.js, colors are enabled when stderr is a TTY. You also _should_ install
the [`supports-color`](https://npmjs.org/supports-color) module alongside debug,
otherwise debug will only use a small handful of basic colors.
<img width="521" src="https://user-images.githubusercontent.com/71256/29092181-47f6a9e6-7c3a-11e7-9a14-1928d8a711cd.png">
#### Web Browser
Colors are also enabled on "Web Inspectors" that understand the `%c` formatting
option. These are WebKit web inspectors, Firefox ([since version
31](https://hacks.mozilla.org/2014/05/editable-box-model-multiple-selection-sublime-text-keys-much-more-firefox-developer-tools-episode-31/))
and the Firebug plugin for Firefox (any version).
<img width="524" src="https://user-images.githubusercontent.com/71256/29092033-b65f9f2e-7c39-11e7-8e32-f6f0d8e865c1.png">
## Millisecond diff
When actively developing an application it can be useful to see when the time spent between one `debug()` call and the next. Suppose for example you invoke `debug()` before requesting a resource, and after as well, the "+NNNms" will show you how much time was spent between calls.
<img width="647" src="https://user-images.githubusercontent.com/71256/29091486-fa38524c-7c37-11e7-895f-e7ec8e1039b6.png">
When stdout is not a TTY, `Date#toISOString()` is used, making it more useful for logging the debug information as shown below:
<img width="647" src="https://user-images.githubusercontent.com/71256/29091956-6bd78372-7c39-11e7-8c55-c948396d6edd.png">
## Conventions
If you're using this in one or more of your libraries, you _should_ use the name of your library so that developers may toggle debugging as desired without guessing names. If you have more than one debuggers you _should_ prefix them with your library name and use ":" to separate features. For example "bodyParser" from Connect would then be "connect:bodyParser". If you append a "*" to the end of your name, it will always be enabled regardless of the setting of the DEBUG environment variable. You can then use it for normal output as well as debug output.
## Wildcards
The `*` character may be used as a wildcard. Suppose for example your library has
debuggers named "connect:bodyParser", "connect:compress", "connect:session",
instead of listing all three with
`DEBUG=connect:bodyParser,connect:compress,connect:session`, you may simply do
`DEBUG=connect:*`, or to run everything using this module simply use `DEBUG=*`.
You can also exclude specific debuggers by prefixing them with a "-" character.
For example, `DEBUG=*,-connect:*` would include all debuggers except those
starting with "connect:".
## Environment Variables
When running through Node.js, you can set a few environment variables that will
change the behavior of the debug logging:
| Name | Purpose |
|-----------|-------------------------------------------------|
| `DEBUG` | Enables/disables specific debugging namespaces. |
| `DEBUG_HIDE_DATE` | Hide date from debug output (non-TTY). |
| `DEBUG_COLORS`| Whether or not to use colors in the debug output. |
| `DEBUG_DEPTH` | Object inspection depth. |
| `DEBUG_SHOW_HIDDEN` | Shows hidden properties on inspected objects. |
__Note:__ The environment variables beginning with `DEBUG_` end up being
converted into an Options object that gets used with `%o`/`%O` formatters.
See the Node.js documentation for
[`util.inspect()`](https://nodejs.org/api/util.html#util_util_inspect_object_options)
for the complete list.
## Formatters
Debug uses [printf-style](https://wikipedia.org/wiki/Printf_format_string) formatting.
Below are the officially supported formatters:
| Formatter | Representation |
|-----------|----------------|
| `%O` | Pretty-print an Object on multiple lines. |
| `%o` | Pretty-print an Object all on a single line. |
| `%s` | String. |
| `%d` | Number (both integer and float). |
| `%j` | JSON. Replaced with the string '[Circular]' if the argument contains circular references. |
| `%%` | Single percent sign ('%'). This does not consume an argument. |
### Custom formatters
You can add custom formatters by extending the `debug.formatters` object.
For example, if you wanted to add support for rendering a Buffer as hex with
`%h`, you could do something like:
```js
const createDebug = require('debug')
createDebug.formatters.h = (v) => {
return v.toString('hex')
}
// …elsewhere
const debug = createDebug('foo')
debug('this is hex: %h', new Buffer('hello world'))
// foo this is hex: 68656c6c6f20776f726c6421 +0ms
```
## Browser Support
You can build a browser-ready script using [browserify](https://github.com/substack/node-browserify),
or just use the [browserify-as-a-service](https://wzrd.in/) [build](https://wzrd.in/standalone/debug@latest),
if you don't want to build it yourself.
Debug's enable state is currently persisted by `localStorage`.
Consider the situation shown below where you have `worker:a` and `worker:b`,
and wish to debug both. You can enable this using `localStorage.debug`:
```js
localStorage.debug = 'worker:*'
```
And then refresh the page.
```js
a = debug('worker:a');
b = debug('worker:b');
setInterval(function(){
a('doing some work');
}, 1000);
setInterval(function(){
b('doing some work');
}, 1200);
```
In Chromium-based web browsers (e.g. Brave, Chrome, and Electron), the JavaScript console will—by default—only show messages logged by `debug` if the "Verbose" log level is _enabled_.
<img width="647" src="https://user-images.githubusercontent.com/7143133/152083257-29034707-c42c-4959-8add-3cee850e6fcf.png">
## Output streams
By default `debug` will log to stderr, however this can be configured per-namespace by overriding the `log` method:
Example [_stdout.js_](./examples/node/stdout.js):
```js
var debug = require('debug');
var error = debug('app:error');
// by default stderr is used
error('goes to stderr!');
var log = debug('app:log');
// set this namespace to log via console.log
log.log = console.log.bind(console); // don't forget to bind to console!
log('goes to stdout');
error('still goes to stderr!');
// set all output to go via console.info
// overrides all per-namespace log settings
debug.log = console.info.bind(console);
error('now goes to stdout via console.info');
log('still goes to stdout, but via console.info now');
```
## Extend
You can simply extend debugger
```js
const log = require('debug')('auth');
//creates new debug instance with extended namespace
const logSign = log.extend('sign');
const logLogin = log.extend('login');
log('hello'); // auth hello
logSign('hello'); //auth:sign hello
logLogin('hello'); //auth:login hello
```
## Set dynamically
You can also enable debug dynamically by calling the `enable()` method :
```js
let debug = require('debug');
console.log(1, debug.enabled('test'));
debug.enable('test');
console.log(2, debug.enabled('test'));
debug.disable();
console.log(3, debug.enabled('test'));
```
print :
```
1 false
2 true
3 false
```
Usage :
`enable(namespaces)`
`namespaces` can include modes separated by a colon and wildcards.
Note that calling `enable()` completely overrides previously set DEBUG variable :
```
$ DEBUG=foo node -e 'var dbg = require("debug"); dbg.enable("bar"); console.log(dbg.enabled("foo"))'
=> false
```
`disable()`
Will disable all namespaces. The functions returns the namespaces currently
enabled (and skipped). This can be useful if you want to disable debugging
temporarily without knowing what was enabled to begin with.
For example:
```js
let debug = require('debug');
debug.enable('foo:*,-foo:bar');
let namespaces = debug.disable();
debug.enable(namespaces);
```
Note: There is no guarantee that the string will be identical to the initial
enable string, but semantically they will be identical.
## Checking whether a debug target is enabled
After you've created a debug instance, you can determine whether or not it is
enabled by checking the `enabled` property:
```javascript
const debug = require('debug')('http');
if (debug.enabled) {
// do stuff...
}
```
You can also manually toggle this property to force the debug instance to be
enabled or disabled.
## Usage in child processes
Due to the way `debug` detects if the output is a TTY or not, colors are not shown in child processes when `stderr` is piped. A solution is to pass the `DEBUG_COLORS=1` environment variable to the child process.
For example:
```javascript
worker = fork(WORKER_WRAP_PATH, [workerPath], {
stdio: [
/* stdin: */ 0,
/* stdout: */ 'pipe',
/* stderr: */ 'pipe',
'ipc',
],
env: Object.assign({}, process.env, {
DEBUG_COLORS: 1 // without this settings, colors won't be shown
}),
});
worker.stderr.pipe(process.stderr, { end: false });
```
## Authors
- TJ Holowaychuk
- Nathan Rajlich
- Andrew Rhyne
- Josh Junon
## Backers
Support us with a monthly donation and help us continue our activities. [[Become a backer](https://opencollective.com/debug#backer)]
<a href="https://opencollective.com/debug/backer/0/website" target="_blank"><img src="https://opencollective.com/debug/backer/0/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/1/website" target="_blank"><img src="https://opencollective.com/debug/backer/1/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/2/website" target="_blank"><img src="https://opencollective.com/debug/backer/2/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/3/website" target="_blank"><img src="https://opencollective.com/debug/backer/3/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/4/website" target="_blank"><img src="https://opencollective.com/debug/backer/4/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/5/website" target="_blank"><img src="https://opencollective.com/debug/backer/5/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/6/website" target="_blank"><img src="https://opencollective.com/debug/backer/6/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/7/website" target="_blank"><img src="https://opencollective.com/debug/backer/7/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/8/website" target="_blank"><img src="https://opencollective.com/debug/backer/8/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/9/website" target="_blank"><img src="https://opencollective.com/debug/backer/9/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/10/website" target="_blank"><img src="https://opencollective.com/debug/backer/10/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/11/website" target="_blank"><img src="https://opencollective.com/debug/backer/11/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/12/website" target="_blank"><img src="https://opencollective.com/debug/backer/12/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/13/website" target="_blank"><img src="https://opencollective.com/debug/backer/13/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/14/website" target="_blank"><img src="https://opencollective.com/debug/backer/14/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/15/website" target="_blank"><img src="https://opencollective.com/debug/backer/15/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/16/website" target="_blank"><img src="https://opencollective.com/debug/backer/16/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/17/website" target="_blank"><img src="https://opencollective.com/debug/backer/17/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/18/website" target="_blank"><img src="https://opencollective.com/debug/backer/18/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/19/website" target="_blank"><img src="https://opencollective.com/debug/backer/19/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/20/website" target="_blank"><img src="https://opencollective.com/debug/backer/20/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/21/website" target="_blank"><img src="https://opencollective.com/debug/backer/21/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/22/website" target="_blank"><img src="https://opencollective.com/debug/backer/22/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/23/website" target="_blank"><img src="https://opencollective.com/debug/backer/23/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/24/website" target="_blank"><img src="https://opencollective.com/debug/backer/24/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/25/website" target="_blank"><img src="https://opencollective.com/debug/backer/25/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/26/website" target="_blank"><img src="https://opencollective.com/debug/backer/26/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/27/website" target="_blank"><img src="https://opencollective.com/debug/backer/27/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/28/website" target="_blank"><img src="https://opencollective.com/debug/backer/28/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/29/website" target="_blank"><img src="https://opencollective.com/debug/backer/29/avatar.svg"></a>
## Sponsors
Become a sponsor and get your logo on our README on Github with a link to your site. [[Become a sponsor](https://opencollective.com/debug#sponsor)]
<a href="https://opencollective.com/debug/sponsor/0/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/0/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/1/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/1/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/2/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/2/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/3/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/3/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/4/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/4/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/5/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/5/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/6/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/6/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/7/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/7/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/8/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/8/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/9/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/9/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/10/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/10/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/11/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/11/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/12/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/12/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/13/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/13/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/14/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/14/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/15/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/15/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/16/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/16/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/17/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/17/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/18/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/18/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/19/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/19/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/20/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/20/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/21/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/21/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/22/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/22/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/23/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/23/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/24/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/24/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/25/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/25/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/26/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/26/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/27/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/27/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/28/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/28/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/29/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/29/avatar.svg"></a>
## License
(The MIT License)
Copyright (c) 2014-2017 TJ Holowaychuk <[email protected]>
Copyright (c) 2018-2021 Josh Junon
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/debug/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/debug/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 22108
} |
#### 2.5.1
* 30/09/2020
* Correct initial `sqrt` estimate.
#### 2.5.0
* 16/10/2018
* Add default export to *decimal.d.ts*.
* Add `Symbol.for('nodejs.util.inspect.custom')` to *decimal.mjs*.
#### 2.4.1
* 24/05/2018
* Add `browser` field to *package.json*.
#### 2.4.0
* 22/05/2018
* Amend *.mjs* exports.
* Remove extension from `main` field in *package.json*.
#### 2.3.1
* 13/11/2017
* Add constructor properties to typings.
* Amend `LN10` section of *doc/API.html*.
#### 2.3.0
* 26/09/2017
* Add *bignumber.mjs*.
#### 2.2.5
* 08/09/2017
* #5 Fix import.
#### 2.2.4
* 15/08/2017
* Add TypeScript type declaration file, *decimal.d.ts*
* Correct `toPositive` and `toNegative` examples
#### 2.2.3
* 04/05/2017
* Fix *README* badge
#### 2.2.2
05/04/2017
* `Decimal.default` to `Decimal['default']` IE8 issue
#### 2.2.1
10/03/2017
* Remove `tonum` from documentation
#### 2.2.0
10/01/2017
* Add `exponent` method
#### 2.0.2
12/12/2016
* npm publish
#### 2.0.1
12/12/2016
* Filename-casing issue
#### 2.0.0
11/12/2016
* Make `LN10` configurable at runtime
* Reduce `LN10` default precision
* Remove `ceil`, `floor`, `min`, `max` and `truncated`
* Rename `divToInt` to `idiv`, `toSD` to `tosd`, `toDP` to `todp`, `isInt` to `isint`, `isNeg` to `isneg`, `isPos` to `ispos` and `round` to `toInteger`
* Rename some test files
* Add `set` as alias to `config`
* Support ES6 import shims
* Add to README
#### 1.0.4
28/02/2016
* Add to README
#### 1.0.3
25/02/2016
* Add to README
#### 1.0.2
25/02/2016
* Correct url
* Amend .travis.yml as Node.js v0.6 doesn't include `process.hrtime` which is used in testing.
#### 1.0.0
24/02/2016
* Initial release | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/decimal.js-light/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/decimal.js-light/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1656
} |
The MIT Expat Licence.
Copyright (c) 2020 Michael Mclaughlin
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/decimal.js-light/LICENCE.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/decimal.js-light/LICENCE.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1085
} |

The light version of [decimal.js](https://github.com/MikeMcl/decimal.js/), an arbitrary-precision Decimal type for JavaScript.
[](https://travis-ci.org/MikeMcl/decimal.js-light)
<br />
This library is the newest of the family of libraries: [bignumber.js](https://github.com/MikeMcl/bignumber.js/), [big.js](https://github.com/MikeMcl/big.js/), [decimal.js](https://github.com/MikeMcl/decimal.js/) and *decimal.js-light*.<br>
The API is more or less a subset of the API of *decimal.js*.
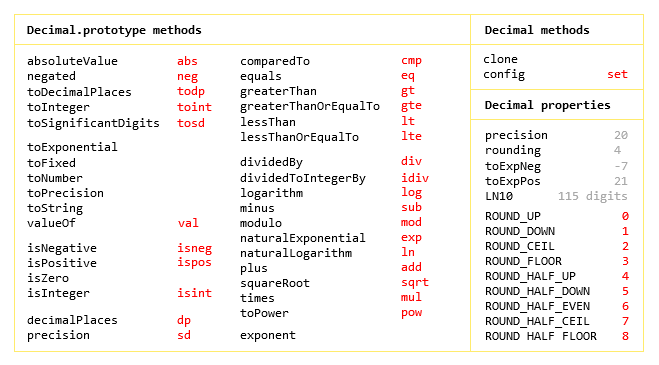
__Differences between this library and *decimal.js*__
Size of *decimal.js* minified: 32.1 KB.<br>
Size of *decimal.js-light* minified: 12.7 KB.
This library does not include `NaN`, `Infinity` or `-0` as legitimate values, or work with values in other bases.
Here, the `Decimal.round` property is just the default rounding mode for `toDecimalPlaces`, `toExponential`, `toFixed`, `toPrecision` and `toSignificantDigits`. It does not apply to arithmetic operations, which are simply truncated at the required precision.
If rounding is required just apply it explicitly, for example
```js
x = new Decimal(2);
y = new Decimal(3);
// decimal.js
x.dividedBy(y).toString(); // '0.66666666666666666667'
// decimal.js-light
x.dividedBy(y).toString(); // '0.66666666666666666666'
x.dividedBy(y).toDecimalPlaces(19).toString(); // '0.6666666666666666667'
```
The `naturalExponential`, `naturalLogarithm`, `logarithm`, and `toPower` methods in this library have by default a limited precision of around 100 digits. This limit can be increased at runtime using the `LN10` (the natural logarithm of ten) configuration object property.
For example, if a maximum precision of 400 digits is required for these operations use
```js
// 415 digits
Decimal.set({
LN10: '2.302585092994045684017991454684364207601101488628772976033327900967572609677352480235997205089598298341967784042286248633409525465082806756666287369098781689482907208325554680843799894826233198528393505308965377732628846163366222287698219886746543667474404243274365155048934314939391479619404400222105101714174800368808401264708068556774321622835522011480466371565912137345074785694768346361679210180644507064800027'
});
```
Also, in this library the `e` property of a Decimal is the base 10000000 exponent, not the base 10 exponent as in *decimal.js*.<br>
Use the `exponent` method to get the base 10 exponent.
## Quickstart
Browser:
```html
<script src='path/to/decimal.js-light'></script>
```
Node package manager:
```shell
$ npm install --save decimal.js-light
```
```js
// Node.js
var Decimal = require('decimal.js-light');
// Adjust the global configuration if required (these are the defaults)
Decimal.set({
precision: 20,
rounding: Decimal.ROUND_HALF_UP,
toExpNeg: -7,
toExpPos: 21
});
phi = new Decimal('1.61803398874989484820458683436563811772030917980576');
phi.toFixed(10); // '1.6180339887'
phi.times(2).minus(1).toPower(2).plus('1e-19').equals(5); // true
```
See the [documentation](http://mikemcl.github.io/decimal.js-light) for further information.
[TypeScript](https://github.com/Microsoft/TypeScript) type declaration file contributed by [TANAKA Koichi](https://github.com/MugeSo). | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/decimal.js-light/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/decimal.js-light/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 3486
} |
# MIT License
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/dedent/LICENSE.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/dedent/LICENSE.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1037
} |
<h1 align="center">dedent</h1>
<p align="center">A string tag that strips indentation from multi-line strings. ⬅️</p>
<p align="center">
<a href="#contributors" target="_blank">
<!-- prettier-ignore-start -->
<!-- ALL-CONTRIBUTORS-BADGE:START - Do not remove or modify this section -->
<img alt="All Contributors: 18 👪" src="https://img.shields.io/badge/all_contributors-18_👪-21bb42.svg" />
<!-- ALL-CONTRIBUTORS-BADGE:END -->
<!-- prettier-ignore-end -->
</a>
<a href="https://codecov.io/gh/dmnd/dedent" target="_blank">
<img alt="Codecov Test Coverage" src="https://codecov.io/gh/dmnd/dedent/branch/main/graph/badge.svg"/>
</a>
<a href="https://github.com/dmnd/dedent/blob/main/.github/CODE_OF_CONDUCT.md" target="_blank">
<img alt="Contributor Covenant" src="https://img.shields.io/badge/code_of_conduct-enforced-21bb42" />
</a>
<a href="https://github.com/dmnd/dedent/blob/main/LICENSE.md" target="_blank">
<img alt="License: MIT" src="https://img.shields.io/github/license/dmnd/dedent?color=21bb42">
</a>
<img alt="Style: Prettier" src="https://img.shields.io/badge/style-prettier-21bb42.svg" />
<img alt="TypeScript: Strict" src="https://img.shields.io/badge/typescript-strict-21bb42.svg" />
<img alt="npm package version" src="https://img.shields.io/npm/v/dedent?color=21bb42" />
<img alt="Contributor Covenant" src="https://img.shields.io/badge/code_of_conduct-enforced-21bb42" />
</p>
## Usage
```shell
npm i dedent
```
```js
import dedent from "dedent";
function usageExample() {
const first = dedent`A string that gets so long you need to break it over
multiple lines. Luckily dedent is here to keep it
readable without lots of spaces ending up in the string
itself.`;
const second = dedent`
Leading and trailing lines will be trimmed, so you can write something like
this and have it work as you expect:
* how convenient it is
* that I can use an indented list
- and still have it do the right thing
That's all.
`;
const third = dedent(`
Wait! I lied. Dedent can also be used as a function.
`);
return first + "\n\n" + second + "\n\n" + third;
}
console.log(usageExample());
```
```plaintext
A string that gets so long you need to break it over
multiple lines. Luckily dedent is here to keep it
readable without lots of spaces ending up in the string
itself.
Leading and trailing lines will be trimmed, so you can write something like
this and have it work as you expect:
* how convenient it is
* that I can use an indented list
- and still have it do the right thing
That's all.
Wait! I lied. Dedent can also be used as a function.
```
## Options
You can customize the options `dedent` runs with by calling its `withOptions` method with an object:
<!-- prettier-ignore -->
```js
import dedent from 'dedent';
dedent.withOptions({ /* ... */ })`input`;
dedent.withOptions({ /* ... */ })(`input`);
```
`options` returns a new `dedent` function, so if you'd like to reuse the same options, you can create a dedicated `dedent` function:
<!-- prettier-ignore -->
```js
import dedent from 'dedent';
const dedenter = dedent.withOptions({ /* ... */ });
dedenter`input`;
dedenter(`input`);
```
### `escapeSpecialCharacters`
JavaScript string tags by default add an extra `\` escape in front of some special characters such as `$` dollar signs.
`dedent` will escape those special characters when called as a string tag.
If you'd like to change the behavior, an `escapeSpecialCharacters` option is available.
It defaults to:
- `false`: when `dedent` is called as a function
- `true`: when `dedent` is called as a string tag
```js
import dedent from "dedent";
// "$hello!"
dedent`
$hello!
`;
// "\$hello!"
dedent.withOptions({ escapeSpecialCharacters: false })`
$hello!
`;
// "$hello!"
dedent.withOptions({ escapeSpecialCharacters: true })`
$hello!
`;
```
For more context, see [🚀 Feature: Add an option to disable special character escaping](https://github.com/dmnd/dedent/issues/63).
## License
MIT
## Contributors
<!-- spellchecker: disable -->
<!-- ALL-CONTRIBUTORS-LIST:START - Do not remove or modify this section -->
<!-- prettier-ignore-start -->
<!-- markdownlint-disable -->
<table>
<tbody>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://adrianjost.dev/"><img src="https://avatars.githubusercontent.com/u/22987140?v=4?s=100" width="100px;" alt="Adrian Jost"/><br /><sub><b>Adrian Jost</b></sub></a><br /><a href="https://github.com/dmnd/dedent/commits?author=adrianjost" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://m811.com/"><img src="https://avatars.githubusercontent.com/u/156837?v=4?s=100" width="100px;" alt="Andri Möll"/><br /><sub><b>Andri Möll</b></sub></a><br /><a href="https://github.com/dmnd/dedent/issues?q=author%3Amoll" title="Bug reports">🐛</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://bennypowers.dev/"><img src="https://avatars.githubusercontent.com/u/1466420?v=4?s=100" width="100px;" alt="Benny Powers - עם ישראל חי!"/><br /><sub><b>Benny Powers - עם ישראל חי!</b></sub></a><br /><a href="#tool-bennypowers" title="Tools">🔧</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/phenomnomnominal"><img src="https://avatars.githubusercontent.com/u/1086286?v=4?s=100" width="100px;" alt="Craig Spence"/><br /><sub><b>Craig Spence</b></sub></a><br /><a href="https://github.com/dmnd/dedent/commits?author=phenomnomnominal" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://synthesis.com/"><img src="https://avatars.githubusercontent.com/u/4427?v=4?s=100" width="100px;" alt="Desmond Brand"/><br /><sub><b>Desmond Brand</b></sub></a><br /><a href="https://github.com/dmnd/dedent/issues?q=author%3Admnd" title="Bug reports">🐛</a> <a href="https://github.com/dmnd/dedent/commits?author=dmnd" title="Code">💻</a> <a href="https://github.com/dmnd/dedent/commits?author=dmnd" title="Documentation">📖</a> <a href="#ideas-dmnd" title="Ideas, Planning, & Feedback">🤔</a> <a href="#infra-dmnd" title="Infrastructure (Hosting, Build-Tools, etc)">🚇</a> <a href="#maintenance-dmnd" title="Maintenance">🚧</a> <a href="#projectManagement-dmnd" title="Project Management">📆</a> <a href="#tool-dmnd" title="Tools">🔧</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/G-Rath"><img src="https://avatars.githubusercontent.com/u/3151613?v=4?s=100" width="100px;" alt="Gareth Jones"/><br /><sub><b>Gareth Jones</b></sub></a><br /><a href="https://github.com/dmnd/dedent/commits?author=G-Rath" title="Code">💻</a> <a href="https://github.com/dmnd/dedent/issues?q=author%3AG-Rath" title="Bug reports">🐛</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/otakustay"><img src="https://avatars.githubusercontent.com/u/639549?v=4?s=100" width="100px;" alt="Gray Zhang"/><br /><sub><b>Gray Zhang</b></sub></a><br /><a href="https://github.com/dmnd/dedent/issues?q=author%3Aotakustay" title="Bug reports">🐛</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://haroen.me/"><img src="https://avatars.githubusercontent.com/u/6270048?v=4?s=100" width="100px;" alt="Haroen Viaene"/><br /><sub><b>Haroen Viaene</b></sub></a><br /><a href="https://github.com/dmnd/dedent/commits?author=Haroenv" title="Code">💻</a> <a href="#maintenance-Haroenv" title="Maintenance">🚧</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://blog.cometkim.kr/"><img src="https://avatars.githubusercontent.com/u/9696352?v=4?s=100" width="100px;" alt="Hyeseong Kim"/><br /><sub><b>Hyeseong Kim</b></sub></a><br /><a href="#tool-cometkim" title="Tools">🔧</a> <a href="#infra-cometkim" title="Infrastructure (Hosting, Build-Tools, etc)">🚇</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/jlarmstrongiv"><img src="https://avatars.githubusercontent.com/u/20903247?v=4?s=100" width="100px;" alt="John L. Armstrong IV"/><br /><sub><b>John L. Armstrong IV</b></sub></a><br /><a href="https://github.com/dmnd/dedent/issues?q=author%3Ajlarmstrongiv" title="Bug reports">🐛</a></td>
<td align="center" valign="top" width="14.28%"><a href="http://www.joshuakgoldberg.com/"><img src="https://avatars.githubusercontent.com/u/3335181?v=4?s=100" width="100px;" alt="Josh Goldberg ✨"/><br /><sub><b>Josh Goldberg ✨</b></sub></a><br /><a href="https://github.com/dmnd/dedent/issues?q=author%3AJoshuaKGoldberg" title="Bug reports">🐛</a> <a href="https://github.com/dmnd/dedent/commits?author=JoshuaKGoldberg" title="Code">💻</a> <a href="https://github.com/dmnd/dedent/commits?author=JoshuaKGoldberg" title="Documentation">📖</a> <a href="#ideas-JoshuaKGoldberg" title="Ideas, Planning, & Feedback">🤔</a> <a href="#infra-JoshuaKGoldberg" title="Infrastructure (Hosting, Build-Tools, etc)">🚇</a> <a href="#maintenance-JoshuaKGoldberg" title="Maintenance">🚧</a> <a href="#projectManagement-JoshuaKGoldberg" title="Project Management">📆</a> <a href="#tool-JoshuaKGoldberg" title="Tools">🔧</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://pratapvardhan.com/"><img src="https://avatars.githubusercontent.com/u/3757165?v=4?s=100" width="100px;" alt="Pratap Vardhan"/><br /><sub><b>Pratap Vardhan</b></sub></a><br /><a href="https://github.com/dmnd/dedent/commits?author=pratapvardhan" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/lydell"><img src="https://avatars.githubusercontent.com/u/2142817?v=4?s=100" width="100px;" alt="Simon Lydell"/><br /><sub><b>Simon Lydell</b></sub></a><br /><a href="https://github.com/dmnd/dedent/issues?q=author%3Alydell" title="Bug reports">🐛</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/yinm"><img src="https://avatars.githubusercontent.com/u/13295106?v=4?s=100" width="100px;" alt="Yusuke Iinuma"/><br /><sub><b>Yusuke Iinuma</b></sub></a><br /><a href="https://github.com/dmnd/dedent/commits?author=yinm" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/yvele"><img src="https://avatars.githubusercontent.com/u/4225430?v=4?s=100" width="100px;" alt="Yves M."/><br /><sub><b>Yves M.</b></sub></a><br /><a href="#tool-yvele" title="Tools">🔧</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/d07RiV"><img src="https://avatars.githubusercontent.com/u/3448203?v=4?s=100" width="100px;" alt="d07riv"/><br /><sub><b>d07riv</b></sub></a><br /><a href="https://github.com/dmnd/dedent/issues?q=author%3Ad07RiV" title="Bug reports">🐛</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://mizdra.net/"><img src="https://avatars.githubusercontent.com/u/9639995?v=4?s=100" width="100px;" alt="mizdra"/><br /><sub><b>mizdra</b></sub></a><br /><a href="https://github.com/dmnd/dedent/commits?author=mizdra" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/sirian"><img src="https://avatars.githubusercontent.com/u/897643?v=4?s=100" width="100px;" alt="sirian"/><br /><sub><b>sirian</b></sub></a><br /><a href="https://github.com/dmnd/dedent/issues?q=author%3Asirian" title="Bug reports">🐛</a></td>
</tr>
</tbody>
</table>
<!-- markdownlint-restore -->
<!-- prettier-ignore-end -->
<!-- ALL-CONTRIBUTORS-LIST:END -->
<!-- spellchecker: enable -->
> 💙 This package was templated with [create-typescript-app](https://github.com/JoshuaKGoldberg/create-typescript-app). | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/dedent/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/dedent/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 11736
} |
# Changelog
All notable changes to this project will be documented in this file.
The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/)
and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html).
## [v1.1.4](https://github.com/ljharb/define-data-property/compare/v1.1.3...v1.1.4) - 2024-02-13
### Commits
- [Refactor] use `es-define-property` [`90f2f4c`](https://github.com/ljharb/define-data-property/commit/90f2f4cc20298401e71c28e1e08888db12021453)
- [Dev Deps] update `@types/object.getownpropertydescriptors` [`cd929d9`](https://github.com/ljharb/define-data-property/commit/cd929d9a04f5f2fdcfa9d5be140940b91a083153)
## [v1.1.3](https://github.com/ljharb/define-data-property/compare/v1.1.2...v1.1.3) - 2024-02-12
### Commits
- [types] hand-write d.ts instead of emitting it [`0cbc988`](https://github.com/ljharb/define-data-property/commit/0cbc988203c105f2d97948327c7167ebd33bd318)
- [meta] simplify `exports` [`690781e`](https://github.com/ljharb/define-data-property/commit/690781eed28bbf2d6766237efda0ba6dd591609e)
- [Dev Deps] update `hasown`; clean up DT packages [`6cdfd1c`](https://github.com/ljharb/define-data-property/commit/6cdfd1cb2d91d791bfd18cda5d5cab232fd5d8fc)
- [actions] cleanup [`3142bc6`](https://github.com/ljharb/define-data-property/commit/3142bc6a4bc406a51f5b04f31e98562a27f35ffd)
- [meta] add `funding` [`8474423`](https://github.com/ljharb/define-data-property/commit/847442391a79779af3e0f1bf0b5bb923552b7804)
- [Deps] update `get-intrinsic` [`3e9be00`](https://github.com/ljharb/define-data-property/commit/3e9be00e07784ba34e7c77d8bc0fdbc832ad61de)
## [v1.1.2](https://github.com/ljharb/define-data-property/compare/v1.1.1...v1.1.2) - 2024-02-05
### Commits
- [Dev Deps] update @types packages, `object-inspect`, `tape`, `typescript` [`df41bf8`](https://github.com/ljharb/define-data-property/commit/df41bf84ca3456be6226055caab44e38e3a7fd2f)
- [Dev Deps] update DT packages, `aud`, `npmignore`, `tape`, typescript` [`fab0e4e`](https://github.com/ljharb/define-data-property/commit/fab0e4ec709ee02b79f42d6db3ee5f26e0a34b8a)
- [Dev Deps] use `hasown` instead of `has` [`aa51ef9`](https://github.com/ljharb/define-data-property/commit/aa51ef93f6403d49d9bb72a807bcdb6e418978c0)
- [Refactor] use `es-errors`, so things that only need those do not need `get-intrinsic` [`d89be50`](https://github.com/ljharb/define-data-property/commit/d89be50571175888d391238605122679f7e65ffc)
- [Deps] update `has-property-descriptors` [`7af887c`](https://github.com/ljharb/define-data-property/commit/7af887c9083b59b195b0079e04815cfed9fcee2b)
- [Deps] update `get-intrinsic` [`bb8728e`](https://github.com/ljharb/define-data-property/commit/bb8728ec42cd998505a7157ae24853a560c20646)
## [v1.1.1](https://github.com/ljharb/define-data-property/compare/v1.1.0...v1.1.1) - 2023-10-12
### Commits
- [Tests] fix tests in ES3 engines [`5c6920e`](https://github.com/ljharb/define-data-property/commit/5c6920edd1f52f675b02f417e539c28135b43f94)
- [Dev Deps] update `@types/es-value-fixtures`, `@types/for-each`, `@types/gopd`, `@types/has-property-descriptors`, `tape`, `typescript` [`7d82dfc`](https://github.com/ljharb/define-data-property/commit/7d82dfc20f778b4465bba06335dd53f6f431aea3)
- [Fix] IE 8 has a broken `Object.defineProperty` [`0672e1a`](https://github.com/ljharb/define-data-property/commit/0672e1af2a9fcc787e7c23b96dea60d290df5548)
- [meta] emit types on prepack [`73acb1f`](https://github.com/ljharb/define-data-property/commit/73acb1f903c21b314ec7156bf10f73c7910530c0)
- [Dev Deps] update `tape`, `typescript` [`9489a77`](https://github.com/ljharb/define-data-property/commit/9489a7738bf2ecf0ac71d5b78ec4ca6ad7ba0142)
## [v1.1.0](https://github.com/ljharb/define-data-property/compare/v1.0.1...v1.1.0) - 2023-09-13
### Commits
- [New] add `loose` arg [`155235a`](https://github.com/ljharb/define-data-property/commit/155235a4c4d7741f6de01cd87c99599a56654b72)
- [New] allow `null` to be passed for the non* args [`7d2fa5f`](https://github.com/ljharb/define-data-property/commit/7d2fa5f06be0392736c13b126f7cd38979f34792)
## [v1.0.1](https://github.com/ljharb/define-data-property/compare/v1.0.0...v1.0.1) - 2023-09-12
### Commits
- [meta] add TS types [`43d763c`](https://github.com/ljharb/define-data-property/commit/43d763c6c883f652de1c9c02ef6216ee507ffa69)
- [Dev Deps] update `@types/tape`, `typescript` [`f444985`](https://github.com/ljharb/define-data-property/commit/f444985811c36f3e6448a03ad2f9b7898917f4c7)
- [meta] add `safe-publish-latest`, [`172bb10`](https://github.com/ljharb/define-data-property/commit/172bb10890896ebb160e64398f6ee55760107bee)
## v1.0.0 - 2023-09-12
### Commits
- Initial implementation, tests, readme [`5b43d6b`](https://github.com/ljharb/define-data-property/commit/5b43d6b44e675a904810467a7d4e0adb7efc3196)
- Initial commit [`35e577a`](https://github.com/ljharb/define-data-property/commit/35e577a6ba59a98befa97776d70d90f3bea9009d)
- npm init [`82a0a04`](https://github.com/ljharb/define-data-property/commit/82a0a04a321ca7de220af02d41e2745e8a9962ed)
- Only apps should have lockfiles [`96df244`](https://github.com/ljharb/define-data-property/commit/96df244a3c6f426f9a2437be825d1c6f5dd7158e)
- [meta] use `npmignore` to autogenerate an npmignore file [`a87ff18`](https://github.com/ljharb/define-data-property/commit/a87ff18cb79e14c2eb5720486c4759fd9a189375) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/define-data-property/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/define-data-property/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 5389
} |
# define-data-property <sup>[![Version Badge][npm-version-svg]][package-url]</sup>
[![github actions][actions-image]][actions-url]
[![coverage][codecov-image]][codecov-url]
[![License][license-image]][license-url]
[![Downloads][downloads-image]][downloads-url]
[![npm badge][npm-badge-png]][package-url]
Define a data property on an object. Will fall back to assignment in an engine without descriptors.
The three `non*` argument can also be passed `null`, which will use the existing state if available.
The `loose` argument will mean that if you attempt to set a non-normal data property, in an environment without descriptor support, it will fall back to normal assignment.
## Usage
```javascript
var defineDataProperty = require('define-data-property');
var assert = require('assert');
var obj = {};
defineDataProperty(obj, 'key', 'value');
defineDataProperty(
obj,
'key2',
'value',
true, // nonEnumerable, optional
false, // nonWritable, optional
true, // nonConfigurable, optional
false // loose, optional
);
assert.deepEqual(
Object.getOwnPropertyDescriptors(obj),
{
key: {
configurable: true,
enumerable: true,
value: 'value',
writable: true,
},
key2: {
configurable: false,
enumerable: false,
value: 'value',
writable: true,
},
}
);
```
[package-url]: https://npmjs.org/package/define-data-property
[npm-version-svg]: https://versionbadg.es/ljharb/define-data-property.svg
[deps-svg]: https://david-dm.org/ljharb/define-data-property.svg
[deps-url]: https://david-dm.org/ljharb/define-data-property
[dev-deps-svg]: https://david-dm.org/ljharb/define-data-property/dev-status.svg
[dev-deps-url]: https://david-dm.org/ljharb/define-data-property#info=devDependencies
[npm-badge-png]: https://nodei.co/npm/define-data-property.png?downloads=true&stars=true
[license-image]: https://img.shields.io/npm/l/define-data-property.svg
[license-url]: LICENSE
[downloads-image]: https://img.shields.io/npm/dm/define-data-property.svg
[downloads-url]: https://npm-stat.com/charts.html?package=define-data-property
[codecov-image]: https://codecov.io/gh/ljharb/define-data-property/branch/main/graphs/badge.svg
[codecov-url]: https://app.codecov.io/gh/ljharb/define-data-property/
[actions-image]: https://img.shields.io/endpoint?url=https://github-actions-badge-u3jn4tfpocch.runkit.sh/ljharb/define-data-property
[actions-url]: https://github.com/ljharb/define-data-property/actions | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/define-data-property/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/define-data-property/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2430
} |
2.0.0 / 2018-10-26
==================
* Drop support for Node.js 0.6
* Replace internal `eval` usage with `Function` constructor
* Use instance methods on `process` to check for listeners
1.1.2 / 2018-01-11
==================
* perf: remove argument reassignment
* Support Node.js 0.6 to 9.x
1.1.1 / 2017-07-27
==================
* Remove unnecessary `Buffer` loading
* Support Node.js 0.6 to 8.x
1.1.0 / 2015-09-14
==================
* Enable strict mode in more places
* Support io.js 3.x
* Support io.js 2.x
* Support web browser loading
- Requires bundler like Browserify or webpack
1.0.1 / 2015-04-07
==================
* Fix `TypeError`s when under `'use strict'` code
* Fix useless type name on auto-generated messages
* Support io.js 1.x
* Support Node.js 0.12
1.0.0 / 2014-09-17
==================
* No changes
0.4.5 / 2014-09-09
==================
* Improve call speed to functions using the function wrapper
* Support Node.js 0.6
0.4.4 / 2014-07-27
==================
* Work-around v8 generating empty stack traces
0.4.3 / 2014-07-26
==================
* Fix exception when global `Error.stackTraceLimit` is too low
0.4.2 / 2014-07-19
==================
* Correct call site for wrapped functions and properties
0.4.1 / 2014-07-19
==================
* Improve automatic message generation for function properties
0.4.0 / 2014-07-19
==================
* Add `TRACE_DEPRECATION` environment variable
* Remove non-standard grey color from color output
* Support `--no-deprecation` argument
* Support `--trace-deprecation` argument
* Support `deprecate.property(fn, prop, message)`
0.3.0 / 2014-06-16
==================
* Add `NO_DEPRECATION` environment variable
0.2.0 / 2014-06-15
==================
* Add `deprecate.property(obj, prop, message)`
* Remove `supports-color` dependency for node.js 0.8
0.1.0 / 2014-06-15
==================
* Add `deprecate.function(fn, message)`
* Add `process.on('deprecation', fn)` emitter
* Automatically generate message when omitted from `deprecate()`
0.0.1 / 2014-06-15
==================
* Fix warning for dynamic calls at singe call site
0.0.0 / 2014-06-15
==================
* Initial implementation | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/depd/History.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/depd/History.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2255
} |
# depd
[![NPM Version][npm-version-image]][npm-url]
[![NPM Downloads][npm-downloads-image]][npm-url]
[![Node.js Version][node-image]][node-url]
[![Linux Build][travis-image]][travis-url]
[![Windows Build][appveyor-image]][appveyor-url]
[![Coverage Status][coveralls-image]][coveralls-url]
Deprecate all the things
> With great modules comes great responsibility; mark things deprecated!
## Install
This module is installed directly using `npm`:
```sh
$ npm install depd
```
This module can also be bundled with systems like
[Browserify](http://browserify.org/) or [webpack](https://webpack.github.io/),
though by default this module will alter it's API to no longer display or
track deprecations.
## API
<!-- eslint-disable no-unused-vars -->
```js
var deprecate = require('depd')('my-module')
```
This library allows you to display deprecation messages to your users.
This library goes above and beyond with deprecation warnings by
introspection of the call stack (but only the bits that it is interested
in).
Instead of just warning on the first invocation of a deprecated
function and never again, this module will warn on the first invocation
of a deprecated function per unique call site, making it ideal to alert
users of all deprecated uses across the code base, rather than just
whatever happens to execute first.
The deprecation warnings from this module also include the file and line
information for the call into the module that the deprecated function was
in.
**NOTE** this library has a similar interface to the `debug` module, and
this module uses the calling file to get the boundary for the call stacks,
so you should always create a new `deprecate` object in each file and not
within some central file.
### depd(namespace)
Create a new deprecate function that uses the given namespace name in the
messages and will display the call site prior to the stack entering the
file this function was called from. It is highly suggested you use the
name of your module as the namespace.
### deprecate(message)
Call this function from deprecated code to display a deprecation message.
This message will appear once per unique caller site. Caller site is the
first call site in the stack in a different file from the caller of this
function.
If the message is omitted, a message is generated for you based on the site
of the `deprecate()` call and will display the name of the function called,
similar to the name displayed in a stack trace.
### deprecate.function(fn, message)
Call this function to wrap a given function in a deprecation message on any
call to the function. An optional message can be supplied to provide a custom
message.
### deprecate.property(obj, prop, message)
Call this function to wrap a given property on object in a deprecation message
on any accessing or setting of the property. An optional message can be supplied
to provide a custom message.
The method must be called on the object where the property belongs (not
inherited from the prototype).
If the property is a data descriptor, it will be converted to an accessor
descriptor in order to display the deprecation message.
### process.on('deprecation', fn)
This module will allow easy capturing of deprecation errors by emitting the
errors as the type "deprecation" on the global `process`. If there are no
listeners for this type, the errors are written to STDERR as normal, but if
there are any listeners, nothing will be written to STDERR and instead only
emitted. From there, you can write the errors in a different format or to a
logging source.
The error represents the deprecation and is emitted only once with the same
rules as writing to STDERR. The error has the following properties:
- `message` - This is the message given by the library
- `name` - This is always `'DeprecationError'`
- `namespace` - This is the namespace the deprecation came from
- `stack` - This is the stack of the call to the deprecated thing
Example `error.stack` output:
```
DeprecationError: my-cool-module deprecated oldfunction
at Object.<anonymous> ([eval]-wrapper:6:22)
at Module._compile (module.js:456:26)
at evalScript (node.js:532:25)
at startup (node.js:80:7)
at node.js:902:3
```
### process.env.NO_DEPRECATION
As a user of modules that are deprecated, the environment variable `NO_DEPRECATION`
is provided as a quick solution to silencing deprecation warnings from being
output. The format of this is similar to that of `DEBUG`:
```sh
$ NO_DEPRECATION=my-module,othermod node app.js
```
This will suppress deprecations from being output for "my-module" and "othermod".
The value is a list of comma-separated namespaces. To suppress every warning
across all namespaces, use the value `*` for a namespace.
Providing the argument `--no-deprecation` to the `node` executable will suppress
all deprecations (only available in Node.js 0.8 or higher).
**NOTE** This will not suppress the deperecations given to any "deprecation"
event listeners, just the output to STDERR.
### process.env.TRACE_DEPRECATION
As a user of modules that are deprecated, the environment variable `TRACE_DEPRECATION`
is provided as a solution to getting more detailed location information in deprecation
warnings by including the entire stack trace. The format of this is the same as
`NO_DEPRECATION`:
```sh
$ TRACE_DEPRECATION=my-module,othermod node app.js
```
This will include stack traces for deprecations being output for "my-module" and
"othermod". The value is a list of comma-separated namespaces. To trace every
warning across all namespaces, use the value `*` for a namespace.
Providing the argument `--trace-deprecation` to the `node` executable will trace
all deprecations (only available in Node.js 0.8 or higher).
**NOTE** This will not trace the deperecations silenced by `NO_DEPRECATION`.
## Display

When a user calls a function in your library that you mark deprecated, they
will see the following written to STDERR (in the given colors, similar colors
and layout to the `debug` module):
```
bright cyan bright yellow
| | reset cyan
| | | |
▼ ▼ ▼ ▼
my-cool-module deprecated oldfunction [eval]-wrapper:6:22
▲ ▲ ▲ ▲
| | | |
namespace | | location of mycoolmod.oldfunction() call
| deprecation message
the word "deprecated"
```
If the user redirects their STDERR to a file or somewhere that does not support
colors, they see (similar layout to the `debug` module):
```
Sun, 15 Jun 2014 05:21:37 GMT my-cool-module deprecated oldfunction at [eval]-wrapper:6:22
▲ ▲ ▲ ▲ ▲
| | | | |
timestamp of message namespace | | location of mycoolmod.oldfunction() call
| deprecation message
the word "deprecated"
```
## Examples
### Deprecating all calls to a function
This will display a deprecated message about "oldfunction" being deprecated
from "my-module" on STDERR.
```js
var deprecate = require('depd')('my-cool-module')
// message automatically derived from function name
// Object.oldfunction
exports.oldfunction = deprecate.function(function oldfunction () {
// all calls to function are deprecated
})
// specific message
exports.oldfunction = deprecate.function(function () {
// all calls to function are deprecated
}, 'oldfunction')
```
### Conditionally deprecating a function call
This will display a deprecated message about "weirdfunction" being deprecated
from "my-module" on STDERR when called with less than 2 arguments.
```js
var deprecate = require('depd')('my-cool-module')
exports.weirdfunction = function () {
if (arguments.length < 2) {
// calls with 0 or 1 args are deprecated
deprecate('weirdfunction args < 2')
}
}
```
When calling `deprecate` as a function, the warning is counted per call site
within your own module, so you can display different deprecations depending
on different situations and the users will still get all the warnings:
```js
var deprecate = require('depd')('my-cool-module')
exports.weirdfunction = function () {
if (arguments.length < 2) {
// calls with 0 or 1 args are deprecated
deprecate('weirdfunction args < 2')
} else if (typeof arguments[0] !== 'string') {
// calls with non-string first argument are deprecated
deprecate('weirdfunction non-string first arg')
}
}
```
### Deprecating property access
This will display a deprecated message about "oldprop" being deprecated
from "my-module" on STDERR when accessed. A deprecation will be displayed
when setting the value and when getting the value.
```js
var deprecate = require('depd')('my-cool-module')
exports.oldprop = 'something'
// message automatically derives from property name
deprecate.property(exports, 'oldprop')
// explicit message
deprecate.property(exports, 'oldprop', 'oldprop >= 0.10')
```
## License
[MIT](LICENSE)
[appveyor-image]: https://badgen.net/appveyor/ci/dougwilson/nodejs-depd/master?label=windows
[appveyor-url]: https://ci.appveyor.com/project/dougwilson/nodejs-depd
[coveralls-image]: https://badgen.net/coveralls/c/github/dougwilson/nodejs-depd/master
[coveralls-url]: https://coveralls.io/r/dougwilson/nodejs-depd?branch=master
[node-image]: https://badgen.net/npm/node/depd
[node-url]: https://nodejs.org/en/download/
[npm-downloads-image]: https://badgen.net/npm/dm/depd
[npm-url]: https://npmjs.org/package/depd
[npm-version-image]: https://badgen.net/npm/v/depd
[travis-image]: https://badgen.net/travis/dougwilson/nodejs-depd/master?label=linux
[travis-url]: https://travis-ci.org/dougwilson/nodejs-depd | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/depd/Readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/depd/Readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 9961
} |
# destroy
[![NPM version][npm-image]][npm-url]
[![Build Status][github-actions-ci-image]][github-actions-ci-url]
[![Test coverage][coveralls-image]][coveralls-url]
[![License][license-image]][license-url]
[![Downloads][downloads-image]][downloads-url]
Destroy a stream.
This module is meant to ensure a stream gets destroyed, handling different APIs
and Node.js bugs.
## API
```js
var destroy = require('destroy')
```
### destroy(stream [, suppress])
Destroy the given stream, and optionally suppress any future `error` events.
In most cases, this is identical to a simple `stream.destroy()` call. The rules
are as follows for a given stream:
1. If the `stream` is an instance of `ReadStream`, then call `stream.destroy()`
and add a listener to the `open` event to call `stream.close()` if it is
fired. This is for a Node.js bug that will leak a file descriptor if
`.destroy()` is called before `open`.
2. If the `stream` is an instance of a zlib stream, then call `stream.destroy()`
and close the underlying zlib handle if open, otherwise call `stream.close()`.
This is for consistency across Node.js versions and a Node.js bug that will
leak a native zlib handle.
3. If the `stream` is not an instance of `Stream`, then nothing happens.
4. If the `stream` has a `.destroy()` method, then call it.
The function returns the `stream` passed in as the argument.
## Example
```js
var destroy = require('destroy')
var fs = require('fs')
var stream = fs.createReadStream('package.json')
// ... and later
destroy(stream)
```
[npm-image]: https://img.shields.io/npm/v/destroy.svg?style=flat-square
[npm-url]: https://npmjs.org/package/destroy
[github-tag]: http://img.shields.io/github/tag/stream-utils/destroy.svg?style=flat-square
[github-url]: https://github.com/stream-utils/destroy/tags
[coveralls-image]: https://img.shields.io/coveralls/stream-utils/destroy.svg?style=flat-square
[coveralls-url]: https://coveralls.io/r/stream-utils/destroy?branch=master
[license-image]: http://img.shields.io/npm/l/destroy.svg?style=flat-square
[license-url]: LICENSE.md
[downloads-image]: http://img.shields.io/npm/dm/destroy.svg?style=flat-square
[downloads-url]: https://npmjs.org/package/destroy
[github-actions-ci-image]: https://img.shields.io/github/workflow/status/stream-utils/destroy/ci/master?label=ci&style=flat-square
[github-actions-ci-url]: https://github.com/stream-utils/destroy/actions/workflows/ci.yml | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/destroy/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/destroy/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2458
} |
## detect-node
> This is a fork of `detect-node`.
Differences:
- uses named export {isNode}
- has d.ts integrated
- supports ESM
### Install
```shell
npm install --save detect-node-es
```
### Usage:
```diff
-var isNode = require('detect-node');
+var {isNode} = require('detect-node-es');
if (isNode) {
console.log("Running under Node.JS");
} else {
alert("Hello from browser (or whatever not-a-node env)");
}
```
The check is performed as:
```js
module.exports = false;
// Only Node.JS has a process variable that is of [[Class]] process
try {
module.exports = Object.prototype.toString.call(global.process) === '[object process]'
} catch(e) {}
```
Thanks to Ingvar Stepanyan for the initial idea. This check is both **the most reliable I could find** and it does not use `process` env directly, which would cause browserify to include it into the build. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/detect-node-es/Readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/detect-node-es/Readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 870
} |
didYouMean.js - A simple JavaScript matching engine
===================================================
[Available on GitHub](https://github.com/dcporter/didyoumean.js).
A super-simple, highly optimized JS library for matching human-quality input to a list of potential
matches. You can use it to suggest a misspelled command-line utility option to a user, or to offer
links to nearby valid URLs on your 404 page. (The examples below are taken from a personal project,
my [HTML5 business card](http://dcporter.aws.af.cm/me), which uses didYouMean.js to suggest correct
URLs from misspelled ones, such as [dcporter.aws.af.cm/me/instagarm](http://dcporter.aws.af.cm/me/instagarm).)
Uses the [Levenshtein distance algorithm](https://en.wikipedia.org/wiki/Levenshtein_distance).
didYouMean.js works in the browser as well as in node.js. To install it for use in node:
```
npm install didyoumean
```
Examples
--------
Matching against a list of strings:
```
var input = 'insargrm'
var list = ['facebook', 'twitter', 'instagram', 'linkedin'];
console.log(didYouMean(input, list));
> 'instagram'
// The method matches 'insargrm' to 'instagram'.
input = 'google plus';
console.log(didYouMean(input, list));
> null
// The method was unable to find 'google plus' in the list of options.
```
Matching against a list of objects:
```
var input = 'insargrm';
var list = [ { id: 'facebook' }, { id: 'twitter' }, { id: 'instagram' }, { id: 'linkedin' } ];
var key = 'id';
console.log(didYouMean(input, list, key));
> 'instagram'
// The method returns the matching value.
didYouMean.returnWinningObject = true;
console.log(didYouMean(input, list, key));
> { id: 'instagram' }
// The method returns the matching object.
```
didYouMean(str, list, [key])
----------------------------
- str: The string input to match.
- list: An array of strings or objects to match against.
- key (OPTIONAL): If your list array contains objects, you must specify the key which contains the string
to match against.
Returns: the closest matching string, or null if no strings exceed the threshold.
Options
-------
Options are set on the didYouMean function object. You may change them at any time.
### threshold
By default, the method will only return strings whose edit distance is less than 40% (0.4x) of their length.
For example, if a ten-letter string is five edits away from its nearest match, the method will return null.
You can control this by setting the "threshold" value on the didYouMean function. For example, to set the
edit distance threshold to 50% of the input string's length:
```
didYouMean.threshold = 0.5;
```
To return the nearest match no matter the threshold, set this value to null.
### thresholdAbsolute
This option behaves the same as threshold, but instead takes an integer number of edit steps. For example,
if thresholdAbsolute is set to 20 (the default), then the method will only return strings whose edit distance
is less than 20. Both options apply.
### caseSensitive
By default, the method will perform case-insensitive comparisons. If you wish to force case sensitivity, set
the "caseSensitive" value to true:
```
didYouMean.caseSensitive = true;
```
### nullResultValue
By default, the method will return null if there is no sufficiently close match. You can change this value here.
### returnWinningObject
By default, the method will return the winning string value (if any). If your list contains objects rather
than strings, you may set returnWinningObject to true.
```
didYouMean.returnWinningObject = true;
```
This option has no effect on lists of strings.
### returnFirstMatch
By default, the method will search all values and return the closest match. If you're simply looking for a "good-
enough" match, you can set your thresholds appropriately and set returnFirstMatch to true to substantially speed
things up.
License
-------
didYouMean copyright (c) 2013-2014 Dave Porter.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License
[here](http://www.apache.org/licenses/LICENSE-2.0).
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/didyoumean/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/didyoumean/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 4510
} |
# `dlv(obj, keypath)` [](https://npmjs.com/package/dlv) [](https://travis-ci.org/developit/dlv)
> Safely get a dot-notated path within a nested object, with ability to return a default if the full key path does not exist or the value is undefined
### Why?
Smallest possible implementation: only **130 bytes.**
You could write this yourself, but then you'd have to write [tests].
Supports ES Modules, CommonJS and globals.
### Installation
`npm install --save dlv`
### Usage
`delve(object, keypath, [default])`
```js
import delve from 'dlv';
let obj = {
a: {
b: {
c: 1,
d: undefined,
e: null
}
}
};
//use string dot notation for keys
delve(obj, 'a.b.c') === 1;
//or use an array key
delve(obj, ['a', 'b', 'c']) === 1;
delve(obj, 'a.b') === obj.a.b;
//returns undefined if the full key path does not exist and no default is specified
delve(obj, 'a.b.f') === undefined;
//optional third parameter for default if the full key in path is missing
delve(obj, 'a.b.f', 'foo') === 'foo';
//or if the key exists but the value is undefined
delve(obj, 'a.b.d', 'foo') === 'foo';
//Non-truthy defined values are still returned if they exist at the full keypath
delve(obj, 'a.b.e', 'foo') === null;
//undefined obj or key returns undefined, unless a default is supplied
delve(undefined, 'a.b.c') === undefined;
delve(undefined, 'a.b.c', 'foo') === 'foo';
delve(obj, undefined, 'foo') === 'foo';
```
### Setter Counterparts
- [dset](https://github.com/lukeed/dset) by [@lukeed](https://github.com/lukeed) is the spiritual "set" counterpart of `dlv` and very fast.
- [bury](https://github.com/kalmbach/bury) by [@kalmbach](https://github.com/kalmbach) does the opposite of `dlv` and is implemented in a very similar manner.
### License
[MIT](https://oss.ninja/mit/developit/)
[preact]: https://github.com/developit/preact
[tests]: https://github.com/developit/dlv/blob/master/test.js | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/dlv/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/dlv/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2019
} |
# dom-helpers
tiny modular DOM lib for ie9+
## Install
```sh
npm i -S dom-helpers
```
Mostly just naive wrappers around common DOM API inconsistencies, Cross browser work is minimal and mostly taken from jQuery. This library doesn't do a lot to normalize behavior across browsers, it mostly seeks to provide a common interface, and eliminate the need to write the same damn `if (ie9)` statements in every project.
For example `on()` works in all browsers ie9+ but it uses the native event system so actual event oddities will continue to exist. If you need **robust** cross-browser support, use jQuery. If you are just tired of rewriting:
```js
if (document.addEventListener)
return (node, eventName, handler, capture) =>
node.addEventListener(eventName, handler, capture || false)
else if (document.attachEvent)
return (node, eventName, handler) =>
node.attachEvent('on' + eventName, handler)
```
over and over again, or you need a ok `getComputedStyle` polyfill but don't want to include all of jQuery, use this.
dom-helpers does expect certain, polyfillable, es5 features to be present for which you can use `es5-shim` where needed
The real advantage to this collection is that any method can be required individually, meaning bundlers like webpack will only include the exact methods you use. This is great for environments where jQuery doesn't make sense, such as `React` where you only occasionally need to do direct DOM manipulation.
All methods are exported as a flat namesapce
```js
var helpers = require('dom-helpers')
var offset = require('dom-helpers/offset')
// style is a function
require('dom-helpers/css')(node, { width: '40px' })
```
- dom-helpers
- `ownerDocument(element)`: returns the element's document owner
- `ownerWindow(element)`: returns the element's document window
- `activeElement`: return focused element safely
- `querySelectorAll(element, selector)`: optimized qsa, uses `getElementBy{Id|TagName|ClassName}` if it can.
- `contains(container, element)`
- `height(element, useClientHeight)`
- `width(element, useClientWidth)`
- `matches(element, selector)`
- `offset(element)` -> `{ top: Number, left: Number, height: Number, width: Number}`
- `offsetParent(element)`: return the parent node that the element is offset from
- `position(element, [offsetParent]`: return "offset" of the node to its offsetParent, optionally you can specify the offset parent if different than the "real" one
- `scrollTop(element, [value])`
- `scrollLeft(element, [value])`
- `scrollParent(element)`
- `addClass(element, className)`
- `removeClass(element, className)`
- `hasClass(element, className)`
- `toggleClass(element, className)`
- `style(element, propName)` or `style(element, objectOfPropValues)`
- `getComputedStyle(element)` -> `getPropertyValue(name)`
- `animate(node, properties, duration, easing, callback)` programmatically start css transitions
- `transitionEnd(node, handler, [duration], [padding])` listens for transition end, and ensures that the handler if called even if the transition fails to fire its end event. Will attempt to read duration from the element, otherwise one can be provided
- `addEventListener(node, eventName, handler, [options])`:
- `removeEventListener(node, eventName, handler, [options])`:
- `listen(node, eventName, handler, [options])`: wraps `addEventlistener` and returns a function that calls `removeEventListener` for you
- `filter(selector, fn)`: returns a function handler that only fires when the target matches or is contained in the selector ex: `on(list, 'click', filter('li > a', handler))`
- `requestAnimationFrame(cb)` returns an ID for canceling
- `cancelAnimationFrame(id)`
- `scrollbarSize([recalc])` returns the scrollbar's width size in pixels
- `scrollTo(element, [scrollParent])` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/dom-helpers/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/dom-helpers/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 3843
} |
# Changelog
All notable changes to this project will be documented in this file. See [standard-version](https://github.com/conventional-changelog/standard-version) for commit guidelines.
## [Unreleased](https://github.com/motdotla/dotenv/compare/v16.4.7...master)
## [16.4.7](https://github.com/motdotla/dotenv/compare/v16.4.6...v16.4.7 (2024-12-03)
### Changed
- Ignore `.tap` folder when publishing. (oops, sorry about that everyone. - @motdotla) [#848](https://github.com/motdotla/dotenv/pull/848)
## [16.4.6](https://github.com/motdotla/dotenv/compare/v16.4.5...v16.4.6) (2024-12-02)
### Changed
- Clean up stale dev dependencies [#847](https://github.com/motdotla/dotenv/pull/847)
- Various README updates clarifying usage and alternative solutions using [dotenvx](https://github.com/dotenvx/dotenvx)
## [16.4.5](https://github.com/motdotla/dotenv/compare/v16.4.4...v16.4.5) (2024-02-19)
### Changed
- 🐞 Fix recent regression when using `path` option. return to historical behavior: do not attempt to auto find `.env` if `path` set. (regression was introduced in `16.4.3`) [#814](https://github.com/motdotla/dotenv/pull/814)
## [16.4.4](https://github.com/motdotla/dotenv/compare/v16.4.3...v16.4.4) (2024-02-13)
### Changed
- 🐞 Replaced chaining operator `?.` with old school `&&` (fixing node 12 failures) [#812](https://github.com/motdotla/dotenv/pull/812)
## [16.4.3](https://github.com/motdotla/dotenv/compare/v16.4.2...v16.4.3) (2024-02-12)
### Changed
- Fixed processing of multiple files in `options.path` [#805](https://github.com/motdotla/dotenv/pull/805)
## [16.4.2](https://github.com/motdotla/dotenv/compare/v16.4.1...v16.4.2) (2024-02-10)
### Changed
- Changed funding link in package.json to [`dotenvx.com`](https://dotenvx.com)
## [16.4.1](https://github.com/motdotla/dotenv/compare/v16.4.0...v16.4.1) (2024-01-24)
- Patch support for array as `path` option [#797](https://github.com/motdotla/dotenv/pull/797)
## [16.4.0](https://github.com/motdotla/dotenv/compare/v16.3.2...v16.4.0) (2024-01-23)
- Add `error.code` to error messages around `.env.vault` decryption handling [#795](https://github.com/motdotla/dotenv/pull/795)
- Add ability to find `.env.vault` file when filename(s) passed as an array [#784](https://github.com/motdotla/dotenv/pull/784)
## [16.3.2](https://github.com/motdotla/dotenv/compare/v16.3.1...v16.3.2) (2024-01-18)
### Added
- Add debug message when no encoding set [#735](https://github.com/motdotla/dotenv/pull/735)
### Changed
- Fix output typing for `populate` [#792](https://github.com/motdotla/dotenv/pull/792)
- Use subarray instead of slice [#793](https://github.com/motdotla/dotenv/pull/793)
## [16.3.1](https://github.com/motdotla/dotenv/compare/v16.3.0...v16.3.1) (2023-06-17)
### Added
- Add missing type definitions for `processEnv` and `DOTENV_KEY` options. [#756](https://github.com/motdotla/dotenv/pull/756)
## [16.3.0](https://github.com/motdotla/dotenv/compare/v16.2.0...v16.3.0) (2023-06-16)
### Added
- Optionally pass `DOTENV_KEY` to options rather than relying on `process.env.DOTENV_KEY`. Defaults to `process.env.DOTENV_KEY` [#754](https://github.com/motdotla/dotenv/pull/754)
## [16.2.0](https://github.com/motdotla/dotenv/compare/v16.1.4...v16.2.0) (2023-06-15)
### Added
- Optionally write to your own target object rather than `process.env`. Defaults to `process.env`. [#753](https://github.com/motdotla/dotenv/pull/753)
- Add import type URL to types file [#751](https://github.com/motdotla/dotenv/pull/751)
## [16.1.4](https://github.com/motdotla/dotenv/compare/v16.1.3...v16.1.4) (2023-06-04)
### Added
- Added `.github/` to `.npmignore` [#747](https://github.com/motdotla/dotenv/pull/747)
## [16.1.3](https://github.com/motdotla/dotenv/compare/v16.1.2...v16.1.3) (2023-05-31)
### Removed
- Removed `browser` keys for `path`, `os`, and `crypto` in package.json. These were set to false incorrectly as of 16.1. Instead, if using dotenv on the front-end make sure to include polyfills for `path`, `os`, and `crypto`. [node-polyfill-webpack-plugin](https://github.com/Richienb/node-polyfill-webpack-plugin) provides these.
## [16.1.2](https://github.com/motdotla/dotenv/compare/v16.1.1...v16.1.2) (2023-05-31)
### Changed
- Exposed private function `_configDotenv` as `configDotenv`. [#744](https://github.com/motdotla/dotenv/pull/744)
## [16.1.1](https://github.com/motdotla/dotenv/compare/v16.1.0...v16.1.1) (2023-05-30)
### Added
- Added type definition for `decrypt` function
### Changed
- Fixed `{crypto: false}` in `packageJson.browser`
## [16.1.0](https://github.com/motdotla/dotenv/compare/v16.0.3...v16.1.0) (2023-05-30)
### Added
- Add `populate` convenience method [#733](https://github.com/motdotla/dotenv/pull/733)
- Accept URL as path option [#720](https://github.com/motdotla/dotenv/pull/720)
- Add dotenv to `npm fund` command
- Spanish language README [#698](https://github.com/motdotla/dotenv/pull/698)
- Add `.env.vault` support. 🎉 ([#730](https://github.com/motdotla/dotenv/pull/730))
ℹ️ `.env.vault` extends the `.env` file format standard with a localized encrypted vault file. Package it securely with your production code deploys. It's cloud agnostic so that you can deploy your secrets anywhere – without [risky third-party integrations](https://techcrunch.com/2023/01/05/circleci-breach/). [read more](https://github.com/motdotla/dotenv#-deploying)
### Changed
- Fixed "cannot resolve 'fs'" error on tools like Replit [#693](https://github.com/motdotla/dotenv/pull/693)
## [16.0.3](https://github.com/motdotla/dotenv/compare/v16.0.2...v16.0.3) (2022-09-29)
### Changed
- Added library version to debug logs ([#682](https://github.com/motdotla/dotenv/pull/682))
## [16.0.2](https://github.com/motdotla/dotenv/compare/v16.0.1...v16.0.2) (2022-08-30)
### Added
- Export `env-options.js` and `cli-options.js` in package.json for use with downstream [dotenv-expand](https://github.com/motdotla/dotenv-expand) module
## [16.0.1](https://github.com/motdotla/dotenv/compare/v16.0.0...v16.0.1) (2022-05-10)
### Changed
- Minor README clarifications
- Development ONLY: updated devDependencies as recommended for development only security risks ([#658](https://github.com/motdotla/dotenv/pull/658))
## [16.0.0](https://github.com/motdotla/dotenv/compare/v15.0.1...v16.0.0) (2022-02-02)
### Added
- _Breaking:_ Backtick support 🎉 ([#615](https://github.com/motdotla/dotenv/pull/615))
If you had values containing the backtick character, please quote those values with either single or double quotes.
## [15.0.1](https://github.com/motdotla/dotenv/compare/v15.0.0...v15.0.1) (2022-02-02)
### Changed
- Properly parse empty single or double quoted values 🐞 ([#614](https://github.com/motdotla/dotenv/pull/614))
## [15.0.0](https://github.com/motdotla/dotenv/compare/v14.3.2...v15.0.0) (2022-01-31)
`v15.0.0` is a major new release with some important breaking changes.
### Added
- _Breaking:_ Multiline parsing support (just works. no need for the flag.)
### Changed
- _Breaking:_ `#` marks the beginning of a comment (UNLESS the value is wrapped in quotes. Please update your `.env` files to wrap in quotes any values containing `#`. For example: `SECRET_HASH="something-with-a-#-hash"`).
..Understandably, (as some teams have noted) this is tedious to do across the entire team. To make it less tedious, we recommend using [dotenv cli](https://github.com/dotenv-org/cli) going forward. It's an optional plugin that will keep your `.env` files in sync between machines, environments, or team members.
### Removed
- _Breaking:_ Remove multiline option (just works out of the box now. no need for the flag.)
## [14.3.2](https://github.com/motdotla/dotenv/compare/v14.3.1...v14.3.2) (2022-01-25)
### Changed
- Preserve backwards compatibility on values containing `#` 🐞 ([#603](https://github.com/motdotla/dotenv/pull/603))
## [14.3.1](https://github.com/motdotla/dotenv/compare/v14.3.0...v14.3.1) (2022-01-25)
### Changed
- Preserve backwards compatibility on exports by re-introducing the prior in-place exports 🐞 ([#606](https://github.com/motdotla/dotenv/pull/606))
## [14.3.0](https://github.com/motdotla/dotenv/compare/v14.2.0...v14.3.0) (2022-01-24)
### Added
- Add `multiline` option 🎉 ([#486](https://github.com/motdotla/dotenv/pull/486))
## [14.2.0](https://github.com/motdotla/dotenv/compare/v14.1.1...v14.2.0) (2022-01-17)
### Added
- Add `dotenv_config_override` cli option
- Add `DOTENV_CONFIG_OVERRIDE` command line env option
## [14.1.1](https://github.com/motdotla/dotenv/compare/v14.1.0...v14.1.1) (2022-01-17)
### Added
- Add React gotcha to FAQ on README
## [14.1.0](https://github.com/motdotla/dotenv/compare/v14.0.1...v14.1.0) (2022-01-17)
### Added
- Add `override` option 🎉 ([#595](https://github.com/motdotla/dotenv/pull/595))
## [14.0.1](https://github.com/motdotla/dotenv/compare/v14.0.0...v14.0.1) (2022-01-16)
### Added
- Log error on failure to load `.env` file ([#594](https://github.com/motdotla/dotenv/pull/594))
## [14.0.0](https://github.com/motdotla/dotenv/compare/v13.0.1...v14.0.0) (2022-01-16)
### Added
- _Breaking:_ Support inline comments for the parser 🎉 ([#568](https://github.com/motdotla/dotenv/pull/568))
## [13.0.1](https://github.com/motdotla/dotenv/compare/v13.0.0...v13.0.1) (2022-01-16)
### Changed
* Hide comments and newlines from debug output ([#404](https://github.com/motdotla/dotenv/pull/404))
## [13.0.0](https://github.com/motdotla/dotenv/compare/v12.0.4...v13.0.0) (2022-01-16)
### Added
* _Breaking:_ Add type file for `config.js` ([#539](https://github.com/motdotla/dotenv/pull/539))
## [12.0.4](https://github.com/motdotla/dotenv/compare/v12.0.3...v12.0.4) (2022-01-16)
### Changed
* README updates
* Minor order adjustment to package json format
## [12.0.3](https://github.com/motdotla/dotenv/compare/v12.0.2...v12.0.3) (2022-01-15)
### Changed
* Simplified jsdoc for consistency across editors
## [12.0.2](https://github.com/motdotla/dotenv/compare/v12.0.1...v12.0.2) (2022-01-15)
### Changed
* Improve embedded jsdoc type documentation
## [12.0.1](https://github.com/motdotla/dotenv/compare/v12.0.0...v12.0.1) (2022-01-15)
### Changed
* README updates and clarifications
## [12.0.0](https://github.com/motdotla/dotenv/compare/v11.0.0...v12.0.0) (2022-01-15)
### Removed
- _Breaking:_ drop support for Flow static type checker ([#584](https://github.com/motdotla/dotenv/pull/584))
### Changed
- Move types/index.d.ts to lib/main.d.ts ([#585](https://github.com/motdotla/dotenv/pull/585))
- Typescript cleanup ([#587](https://github.com/motdotla/dotenv/pull/587))
- Explicit typescript inclusion in package.json ([#566](https://github.com/motdotla/dotenv/pull/566))
## [11.0.0](https://github.com/motdotla/dotenv/compare/v10.0.0...v11.0.0) (2022-01-11)
### Changed
- _Breaking:_ drop support for Node v10 ([#558](https://github.com/motdotla/dotenv/pull/558))
- Patch debug option ([#550](https://github.com/motdotla/dotenv/pull/550))
## [10.0.0](https://github.com/motdotla/dotenv/compare/v9.0.2...v10.0.0) (2021-05-20)
### Added
- Add generic support to parse function
- Allow for import "dotenv/config.js"
- Add support to resolve home directory in path via ~
## [9.0.2](https://github.com/motdotla/dotenv/compare/v9.0.1...v9.0.2) (2021-05-10)
### Changed
- Support windows newlines with debug mode
## [9.0.1](https://github.com/motdotla/dotenv/compare/v9.0.0...v9.0.1) (2021-05-08)
### Changed
- Updates to README
## [9.0.0](https://github.com/motdotla/dotenv/compare/v8.6.0...v9.0.0) (2021-05-05)
### Changed
- _Breaking:_ drop support for Node v8
## [8.6.0](https://github.com/motdotla/dotenv/compare/v8.5.1...v8.6.0) (2021-05-05)
### Added
- define package.json in exports
## [8.5.1](https://github.com/motdotla/dotenv/compare/v8.5.0...v8.5.1) (2021-05-05)
### Changed
- updated dev dependencies via npm audit
## [8.5.0](https://github.com/motdotla/dotenv/compare/v8.4.0...v8.5.0) (2021-05-05)
### Added
- allow for `import "dotenv/config"`
## [8.4.0](https://github.com/motdotla/dotenv/compare/v8.3.0...v8.4.0) (2021-05-05)
### Changed
- point to exact types file to work with VS Code
## [8.3.0](https://github.com/motdotla/dotenv/compare/v8.2.0...v8.3.0) (2021-05-05)
### Changed
- _Breaking:_ drop support for Node v8 (mistake to be released as minor bump. later bumped to 9.0.0. see above.)
## [8.2.0](https://github.com/motdotla/dotenv/compare/v8.1.0...v8.2.0) (2019-10-16)
### Added
- TypeScript types
## [8.1.0](https://github.com/motdotla/dotenv/compare/v8.0.0...v8.1.0) (2019-08-18)
### Changed
- _Breaking:_ drop support for Node v6 ([#392](https://github.com/motdotla/dotenv/issues/392))
# [8.0.0](https://github.com/motdotla/dotenv/compare/v7.0.0...v8.0.0) (2019-05-02)
### Changed
- _Breaking:_ drop support for Node v6 ([#302](https://github.com/motdotla/dotenv/issues/392))
## [7.0.0] - 2019-03-12
### Fixed
- Fix removing unbalanced quotes ([#376](https://github.com/motdotla/dotenv/pull/376))
### Removed
- Removed `load` alias for `config` for consistency throughout code and documentation.
## [6.2.0] - 2018-12-03
### Added
- Support preload configuration via environment variables ([#351](https://github.com/motdotla/dotenv/issues/351))
## [6.1.0] - 2018-10-08
### Added
- `debug` option for `config` and `parse` methods will turn on logging
## [6.0.0] - 2018-06-02
### Changed
- _Breaking:_ drop support for Node v4 ([#304](https://github.com/motdotla/dotenv/pull/304))
## [5.0.0] - 2018-01-29
### Added
- Testing against Node v8 and v9
- Documentation on trim behavior of values
- Documentation on how to use with `import`
### Changed
- _Breaking_: default `path` is now `path.resolve(process.cwd(), '.env')`
- _Breaking_: does not write over keys already in `process.env` if the key has a falsy value
- using `const` and `let` instead of `var`
### Removed
- Testing against Node v7
## [4.0.0] - 2016-12-23
### Changed
- Return Object with parsed content or error instead of false ([#165](https://github.com/motdotla/dotenv/pull/165)).
### Removed
- `verbose` option removed in favor of returning result.
## [3.0.0] - 2016-12-20
### Added
- `verbose` option will log any error messages. Off by default.
- parses email addresses correctly
- allow importing config method directly in ES6
### Changed
- Suppress error messages by default ([#154](https://github.com/motdotla/dotenv/pull/154))
- Ignoring more files for NPM to make package download smaller
### Fixed
- False positive test due to case-sensitive variable ([#124](https://github.com/motdotla/dotenv/pull/124))
### Removed
- `silent` option removed in favor of `verbose`
## [2.0.0] - 2016-01-20
### Added
- CHANGELOG to ["make it easier for users and contributors to see precisely what notable changes have been made between each release"](http://keepachangelog.com/). Linked to from README
- LICENSE to be more explicit about what was defined in `package.json`. Linked to from README
- Testing nodejs v4 on travis-ci
- added examples of how to use dotenv in different ways
- return parsed object on success rather than boolean true
### Changed
- README has shorter description not referencing ruby gem since we don't have or want feature parity
### Removed
- Variable expansion and escaping so environment variables are encouraged to be fully orthogonal
## [1.2.0] - 2015-06-20
### Added
- Preload hook to require dotenv without including it in your code
### Changed
- clarified license to be "BSD-2-Clause" in `package.json`
### Fixed
- retain spaces in string vars
## [1.1.0] - 2015-03-31
### Added
- Silent option to silence `console.log` when `.env` missing
## [1.0.0] - 2015-03-13
### Removed
- support for multiple `.env` files. should always use one `.env` file for the current environment
[7.0.0]: https://github.com/motdotla/dotenv/compare/v6.2.0...v7.0.0
[6.2.0]: https://github.com/motdotla/dotenv/compare/v6.1.0...v6.2.0
[6.1.0]: https://github.com/motdotla/dotenv/compare/v6.0.0...v6.1.0
[6.0.0]: https://github.com/motdotla/dotenv/compare/v5.0.0...v6.0.0
[5.0.0]: https://github.com/motdotla/dotenv/compare/v4.0.0...v5.0.0
[4.0.0]: https://github.com/motdotla/dotenv/compare/v3.0.0...v4.0.0
[3.0.0]: https://github.com/motdotla/dotenv/compare/v2.0.0...v3.0.0
[2.0.0]: https://github.com/motdotla/dotenv/compare/v1.2.0...v2.0.0
[1.2.0]: https://github.com/motdotla/dotenv/compare/v1.1.0...v1.2.0
[1.1.0]: https://github.com/motdotla/dotenv/compare/v1.0.0...v1.1.0
[1.0.0]: https://github.com/motdotla/dotenv/compare/v0.4.0...v1.0.0 | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/dotenv/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/dotenv/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 16606
} |
<div align="center">
🎉 announcing <a href="https://github.com/dotenvx/dotenvx">dotenvx</a>. <em>run anywhere, multi-environment, encrypted envs</em>.
</div>
<div align="center">
<p>
<sup>
<a href="https://github.com/sponsors/motdotla">Dotenv es apoyado por la comunidad.</a>
</sup>
</p>
<sup>Gracias espaciales a:</sup>
<br>
<br>
<a href="https://www.warp.dev/?utm_source=github&utm_medium=referral&utm_campaign=dotenv_p_20220831">
<div>
<img src="https://res.cloudinary.com/dotenv-org/image/upload/v1661980709/warp_hi8oqj.png" width="230" alt="Warp">
</div>
<b>Warp es una rápida e impresionante terminal basada en Rust, reinventado para funcionar como una aplicación moderna.</b>
<div>
<sup>Haga más en la CLI con edición de texto real, resultado básado en bloques, y busqueda de comandos de IA.</sup>
</div>
</a>
<br>
<a href="https://retool.com/?utm_source=sponsor&utm_campaign=dotenv">
<div>
<img src="https://res.cloudinary.com/dotenv-org/image/upload/c_scale,w_300/v1664466968/logo-full-black_vidfqf.png" width="270" alt="Retool">
</div>
<b>Retool ayuda a los desarrolladores a crear software interno personalizado, como aplicaciones CRUD y paneles de administración, realmente rápido.</b>
<div>
<sup>Construya Interfaces de Usuario de forma visual con componentes flexibles, conéctese a cualquier fuente de datos, y escriba lógica de negocio en JavaScript.</sup>
</div>
</a>
<br>
<a href="https://workos.com/?utm_campaign=github_repo&utm_medium=referral&utm_content=dotenv&utm_source=github">
<div>
<img src="https://res.cloudinary.com/dotenv-org/image/upload/c_scale,w_400/v1665605496/68747470733a2f2f73696e647265736f726875732e636f6d2f6173736574732f7468616e6b732f776f726b6f732d6c6f676f2d77686974652d62672e737667_zdmsbu.svg" width="270" alt="WorkOS">
</div>
<b>Su Apliación, Lista para la Empresa.</b>
<div>
<sup>Agrega Inicio de Sesión Único, Autenticación Multi-Factor, y mucho más, en minutos en lugar de meses.</sup>
</div>
</a>
<hr>
<br>
<br>
<br>
<br>
</div>
# dotenv [](https://www.npmjs.com/package/dotenv)
<img src="https://raw.githubusercontent.com/motdotla/dotenv/master/dotenv.svg" alt="dotenv" align="right" width="200" />
Dotenv es un módulo de dependencia cero que carga las variables de entorno desde un archivo `.env` en [`process.env`](https://nodejs.org/docs/latest/api/process.html#process_process_env). El almacenamiento de la configuración del entorno separado del código está basado en la metodología [The Twelve-Factor App](http://12factor.net/config).
[](https://github.com/feross/standard)
[](LICENSE)
## Instalación
```bash
# instalación local (recomendado)
npm install dotenv --save
```
O installación con yarn? `yarn add dotenv`
## Uso
Cree un archivo `.env` en la raíz de su proyecto:
```dosini
S3_BUCKET="YOURS3BUCKET"
SECRET_KEY="YOURSECRETKEYGOESHERE"
```
Tan prónto como sea posible en su aplicación, importe y configure dotenv:
```javascript
require('dotenv').config()
console.log(process.env) // elimine esto después que haya confirmado que esta funcionando
```
.. o usa ES6?
```javascript
import * as dotenv from 'dotenv' // vea en https://github.com/motdotla/dotenv#como-uso-dotenv-con-import
// REVISAR LINK DE REFERENCIA DE IMPORTACIÓN
dotenv.config()
import express from 'express'
```
Eso es todo. `process.env` ahora tiene las claves y los valores que definiste en tu archivo `.env`:
```javascript
require('dotenv').config()
...
s3.getBucketCors({Bucket: process.env.S3_BUCKET}, function(err, data) {})
```
### Valores multilínea
Si necesita variables de varias líneas, por ejemplo, claves privadas, ahora se admiten en la versión (`>= v15.0.0`) con saltos de línea:
```dosini
PRIVATE_KEY="-----BEGIN RSA PRIVATE KEY-----
...
Kh9NV...
...
-----END RSA PRIVATE KEY-----"
```
Alternativamente, puede usar comillas dobles y usar el carácter `\n`:
```dosini
PRIVATE_KEY="-----BEGIN RSA PRIVATE KEY-----\nKh9NV...\n-----END RSA PRIVATE KEY-----\n"
```
### Comentarios
Los comentarios pueden ser agregados en tu archivo o en la misma línea:
```dosini
# This is a comment
SECRET_KEY=YOURSECRETKEYGOESHERE # comment
SECRET_HASH="something-with-a-#-hash"
```
Los comentarios comienzan donde existe un `#`, entonces, si su valor contiene un `#`, enciérrelo entre comillas. Este es un cambio importante desde la versión `>= v15.0.0` en adelante.
### Análisis
El motor que analiza el contenido de su archivo que contiene variables de entorno está disponible para su uso. Este Acepta una Cadena o un Búfer y devolverá un Objeto con las claves y los valores analizados.
```javascript
const dotenv = require('dotenv')
const buf = Buffer.from('BASICO=basico')
const config = dotenv.parse(buf) // devolverá un objeto
console.log(typeof config, config) // objeto { BASICO : 'basico' }
```
### Precarga
Puede usar el `--require` (`-r`) [opción de línea de comando](https://nodejs.org/api/cli.html#-r---require-module) para precargar dotenv. Al hacer esto, no necesita requerir ni cargar dotnev en el código de su aplicación.
```bash
$ node -r dotenv/config tu_script.js
```
Las opciones de configuración a continuación se admiten como argumentos de línea de comandos en el formato `dotenv_config_<option>=value`
```bash
$ node -r dotenv/config tu_script.js dotenv_config_path=/custom/path/to/.env dotenv_config_debug=true
```
Además, puede usar variables de entorno para establecer opciones de configuración. Los argumentos de línea de comandos precederán a estos.
```bash
$ DOTENV_CONFIG_<OPTION>=value node -r dotenv/config tu_script.js
```
```bash
$ DOTENV_CONFIG_ENCODING=latin1 DOTENV_CONFIG_DEBUG=true node -r dotenv/config tu_script.js dotenv_config_path=/custom/path/to/.env
```
### Expansión Variable
Necesitaras agregar el valor de otro variable en una de sus variables? Usa [dotenv-expand](https://github.com/motdotla/dotenv-expand).
### Sincronizando
Necesitas mentener sincronizados los archivos `.env` entre maquinas, entornos, o miembros del equipo? Usa
[dotenv-vault](https://github.com/dotenv-org/dotenv-vault).
## Ejemplos
Vea [ejemplos](https://github.com/dotenv-org/examples) sobre el uso de dotenv con varios frameworks, lenguajes y configuraciones.
* [nodejs](https://github.com/dotenv-org/examples/tree/master/dotenv-nodejs)
* [nodejs (depurar en)](https://github.com/dotenv-org/examples/tree/master/dotenv-nodejs-debug)
* [nodejs (anular en)](https://github.com/dotenv-org/examples/tree/master/dotenv-nodejs-override)
* [esm](https://github.com/dotenv-org/examples/tree/master/dotenv-esm)
* [esm (precarga)](https://github.com/dotenv-org/examples/tree/master/dotenv-esm-preload)
* [typescript](https://github.com/dotenv-org/examples/tree/master/dotenv-typescript)
* [typescript parse](https://github.com/dotenv-org/examples/tree/master/dotenv-typescript-parse)
* [typescript config](https://github.com/dotenv-org/examples/tree/master/dotenv-typescript-config)
* [webpack](https://github.com/dotenv-org/examples/tree/master/dotenv-webpack)
* [webpack (plugin)](https://github.com/dotenv-org/examples/tree/master/dotenv-webpack2)
* [react](https://github.com/dotenv-org/examples/tree/master/dotenv-react)
* [react (typescript)](https://github.com/dotenv-org/examples/tree/master/dotenv-react-typescript)
* [express](https://github.com/dotenv-org/examples/tree/master/dotenv-express)
* [nestjs](https://github.com/dotenv-org/examples/tree/master/dotenv-nestjs)
## Documentación
Dotenv expone dos funciones:
* `configuración`
* `analizar`
### Configuración
`Configuración` leerá su archivo `.env`, analizará el contenido, lo asignará a [`process.env`](https://nodejs.org/docs/latest/api/process.html#process_process_env),
y devolverá un Objeto con una clave `parsed` que contiene el contenido cargado o una clave `error` si falla.
```js
const result = dotenv.config()
if (result.error) {
throw result.error
}
console.log(result.parsed)
```
Adicionalmente, puede pasar opciones a `configuracion`.
#### Opciones
##### Ruta
Por defecto: `path.resolve(process.cwd(), '.env')`
Especifique una ruta personalizada si el archivo que contiene las variables de entorno se encuentra localizado en otro lugar.
```js
require('dotenv').config({ path: '/personalizado/ruta/a/.env' })
```
##### Codificación
Por defecto: `utf8`
Especifique la codificación del archivo que contiene las variables de entorno.
```js
require('dotenv').config({ encoding: 'latin1' })
```
##### Depurar
Por defecto: `false`
Active el registro de ayuda para depurar por qué ciertas claves o valores no se inician como lo esperabas.
```js
require('dotenv').config({ debug: process.env.DEBUG })
```
##### Anular
Por defecto: `false`
Anule cualquier variable de entorno que ya se haya configurada en su maquina con los valores de su archivo .env.
```js
require('dotenv').config({ override: true })
```
### Analizar
El motor que analiza el contenido del archivo que contiene las variables de entorno está disponible para su uso. Acepta una Cadena o un Búfer y retornará un objecto con los valores analizados.
```js
const dotenv = require('dotenv')
const buf = Buffer.from('BASICO=basico')
const config = dotenv.parse(buf) // devolverá un objeto
console.log(typeof config, config) // objeto { BASICO : 'basico' }
```
#### Opciones
##### Depurar
Por defecto: `false`
Active el registro de ayuda para depurar por qué ciertas claves o valores no se inician como lo esperabas.
```js
const dotenv = require('dotenv')
const buf = Buffer.from('hola mundo')
const opt = { debug: true }
const config = dotenv.parse(buf, opt)
// espere por un mensaje de depuración porque el búfer no esta listo KEY=VAL
```
## FAQ
### ¿Por qué el archivo `.env` no carga mis variables de entorno correctamente?
Lo más probable es que su archivo `.env` no esté en el lugar correcto. [Vea este stack overflow](https://stackoverflow.com/questions/42335016/dotenv-file-is-not-loading-environment-variables).
Active el modo de depuración y vuelva a intentarlo...
```js
require('dotenv').config({ debug: true })
```
Recibirá un error apropiado en su consola.
### ¿Debo confirmar mi archivo `.env`?
No. Recomendamos **enfáticamente** no enviar su archivo `.env` a la versión de control. Solo debe incluir los valores especificos del entorno, como la base de datos, contraseñas o claves API.
### ¿Debería tener multiples archivos `.env`?
No. Recomendamos **enfáticamente** no tener un archivo `.env` "principal" y un archivo `.env` de "entorno" como `.env.test`. Su configuración debe variar entre implementaciones y no debe compartir valores entre entornos.
> En una Aplicación de Doce Factores, las variables de entorno son controles diferenciados, cada uno totalmente independiente a otras variables de entorno. Nunca se agrupan como "entornos", sino que se gestionan de manera independiente para cada despliegue. Este es un modelo que se escala sin problemas a medida que la aplicación se expande de forma natural en más despliegues a lo largo de su vida.
>
> – [La Apliación de los Doce Factores](https://12factor.net/es/)
### ¿Qué reglas sigue el motor de análisis?
El motor de análisis actualmente admite las siguientes reglas:
- `BASICO=basico` se convierte en `{BASICO: 'basico'}`
- las líneas vacías se saltan
- las líneas que comienzan con `#` se tratan como comentarios
- `#` marca el comienzo de un comentario (a menos que el valor esté entre comillas)
- valores vacíos se convierten en cadenas vacías (`VACIO=` se convierte en `{VACIO: ''}`)
- las comillas internas se mantienen (piensa en JSON) (`JSON={"foo": "bar"}` se convierte en `{JSON:"{\"foo\": \"bar\"}"`)
- los espacios en blanco se eliminan de ambos extremos de los valores no citanos (aprende más en [`trim`](https://developer.mozilla.org/es/docs/Web/JavaScript/Reference/Global_Objects/String/Trim)) (`FOO= algo ` se convierte en `{FOO: 'algo'}`)
- los valores entre comillas simples y dobles se escapan (`CITA_SIMPLE='citado'` se convierte en `{CITA_SIMPLE: "citado"}`)
- los valores entre comillas simples y dobles mantienen los espacios en blanco en ambos extremos (`FOO=" algo "` se convierte en `{FOO: ' algo '}`)
- los valores entre comillas dobles expanden nuevas líneas (`MULTILINEA="nueva\nlínea"` se convierte en
```
{MULTILINEA: 'nueva
línea'}
```
- se admite la comilla simple invertida (`` SIGNO_ACENTO=`Esto tiene comillas 'simples' y "dobles" en su interior.` ``)
### ¿Qué sucede con las variables de entorno que ya estaban configuradas?
Por defecto, nunca modificaremos ninguna variable de entorno que ya haya sido establecida. En particular, si hay una variable en su archivo `.env` que colisiona con una que ya existe en su entorno, entonces esa variable se omitirá.
Si por el contrario, quieres anular `process.env` utiliza la opción `override`.
```javascript
require('dotenv').config({ override: true })
```
### ¿Por qué mis variables de entorno no aparecen para React?
Su código React se ejecuta en Webpack, donde el módulo `fs` o incluso el propio `process` global no son accesibles fuera-de-la-caja. El módulo `process.env` sólo puede ser inyectado a través de la configuración de Webpack.
Si estás usando [`react-scripts`](https://www.npmjs.com/package/react-scripts), el cual se distribuye a través de [`create-react-app`](https://create-react-app.dev/), tiene dotenv incorporado pero con una singularidad. Escriba sus variables de entorno con `REACT_APP_`. Vea [este stack overflow](https://stackoverflow.com/questions/42182577/is-it-possible-to-use-dotenv-in-a-react-project) para más detalles.
Si estás utilizando otros frameworks (por ejemplo, Next.js, Gatsby...), debes consultar su documentación para saber cómo injectar variables de entorno en el cliente.
### ¿Puedo personalizar/escribir plugins para dotenv?
Sí! `dotenv.config()` devuelve un objeto que representa el archivo `.env` analizado. Esto te da todo lo que necesitas para poder establecer valores en `process.env`. Por ejemplo:
```js
const dotenv = require('dotenv')
const variableExpansion = require('dotenv-expand')
const miEnv = dotenv.config()
variableExpansion(miEnv)
```
### Cómo uso dotnev con `import`?
Simplemente..
```javascript
// index.mjs (ESM)
import * as dotenv from 'dotenv' // vea https://github.com/motdotla/dotenv#como-uso-dotenv-con-import
dotenv.config()
import express from 'express'
```
Un poco de historia...
> Cuando se ejecuta un módulo que contiene una sentencia `import`, los módulos que importa serán cargados primero, y luego se ejecuta cada bloque del módulo en un recorrido en profundidad del gráfico de dependencias, evitando los ciclos al saltarse todo lo que ya se ha ejecutado.
>
> – [ES6 en Profundidad: Módulos](https://hacks.mozilla.org/2015/08/es6-in-depth-modules/)
¿Qué significa esto en lenguaje sencillo? Significa que se podrías pensar que lo siguiente funcionaría pero no lo hará.
```js
// notificarError.mjs
import { Cliente } from 'mejor-servicio-para-notificar-error'
export default new Client(process.env.CLAVE_API)
// index.mjs
import dotenv from 'dotenv'
dotenv.config()
import notificarError from './notificarError.mjs'
notificarError.report(new Error('ejemplo documentado'))
```
`process.env.CLAVE_API` será vacio.
En su lugar, el código anterior debe ser escrito como...
```js
// notificarError.mjs
import { Cliente } from 'mejor-servicio-para-notificar-errores'
export default new Client(process.env.CLAVE_API)
// index.mjs
import * as dotenv from 'dotenv'
dotenv.config()
import notificarError from './notificarError.mjs'
notificarError.report(new Error('ejemplo documentado'))
```
¿Esto tiene algo de sentido? Esto es poco poco intuitivo, pero es como funciona la importación de módulos en ES6. Aquí hay un ejemplo [ejemplo práctico de esta trampa](https://github.com/dotenv-org/examples/tree/master/dotenv-es6-import-pitfall).
Existen dos arternativas a este planteamiento:
1. Precarga dotenv: `node --require dotenv/config index.js` (_Nota: no es necesario usar `import` dotenv con este método_)
2. Cree un archivo separado que ejecutará `config` primero como se describe en [este comentario #133](https://github.com/motdotla/dotenv/issues/133#issuecomment-255298822)
### ¿Qué pasa con la expansión de variable?
Prueba [dotenv-expand](https://github.com/motdotla/dotenv-expand)
### ¿Qué pasa con la sincronización y la seguridad de los archivos .env?
Vea [dotenv-vault](https://github.com/dotenv-org/dotenv-vault)
## Guía de contribución
Vea [CONTRIBUTING.md](CONTRIBUTING.md)
## REGISTRO DE CAMBIOS
Vea [CHANGELOG.md](CHANGELOG.md)
## ¿Quiénes utilizan dotenv?
[Estos módulos npm dependen de él.](https://www.npmjs.com/browse/depended/dotenv)
Los proyectos que lo amplían suelen utilizar la [palabra clave "dotenv" en npm](https://www.npmjs.com/search?q=keywords:dotenv). | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/dotenv/README-es.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/dotenv/README-es.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 17039
} |
<div align="center">
🎉 announcing <a href="https://github.com/dotenvx/dotenvx">dotenvx</a>. <em>run anywhere, multi-environment, encrypted envs</em>.
</div>
<div align="center">
<p>
<sup>
<a href="https://github.com/sponsors/motdotla">Dotenv is supported by the community.</a>
</sup>
</p>
<sup>Special thanks to:</sup>
<br>
<br>
<a href="https://www.warp.dev/?utm_source=github&utm_medium=referral&utm_campaign=dotenv_p_20220831">
<div>
<img src="https://res.cloudinary.com/dotenv-org/image/upload/v1661980709/warp_hi8oqj.png" width="230" alt="Warp">
</div>
<b>Warp is a blazingly fast, Rust-based terminal reimagined to work like a modern app.</b>
<div>
<sup>Get more done in the CLI with real text editing, block-based output, and AI command search.</sup>
</div>
</a>
<br>
<a href="https://workos.com/?utm_campaign=github_repo&utm_medium=referral&utm_content=dotenv&utm_source=github">
<div>
<img src="https://res.cloudinary.com/dotenv-org/image/upload/c_scale,w_400/v1665605496/68747470733a2f2f73696e647265736f726875732e636f6d2f6173736574732f7468616e6b732f776f726b6f732d6c6f676f2d77686974652d62672e737667_zdmsbu.svg" width="270" alt="WorkOS">
</div>
<b>Your App, Enterprise Ready.</b>
<div>
<sup>Add Single Sign-On, Multi-Factor Auth, and more, in minutes instead of months.</sup>
</div>
</a>
<hr>
</div>
# dotenv [](https://www.npmjs.com/package/dotenv)
<img src="https://raw.githubusercontent.com/motdotla/dotenv/master/dotenv.svg" alt="dotenv" align="right" width="200" />
Dotenv is a zero-dependency module that loads environment variables from a `.env` file into [`process.env`](https://nodejs.org/docs/latest/api/process.html#process_process_env). Storing configuration in the environment separate from code is based on [The Twelve-Factor App](https://12factor.net/config) methodology.
[](https://github.com/feross/standard)
[](LICENSE)
[](https://codecov.io/gh/motdotla/dotenv-expand)
* [🌱 Install](#-install)
* [🏗️ Usage (.env)](#%EF%B8%8F-usage)
* [🌴 Multiple Environments 🆕](#-manage-multiple-environments)
* [🚀 Deploying (encryption) 🆕](#-deploying)
* [📚 Examples](#-examples)
* [📖 Docs](#-documentation)
* [❓ FAQ](#-faq)
* [⏱️ Changelog](./CHANGELOG.md)
## 🌱 Install
```bash
# install locally (recommended)
npm install dotenv --save
```
Or installing with yarn? `yarn add dotenv`
## 🏗️ Usage
<a href="https://www.youtube.com/watch?v=YtkZR0NFd1g">
<div align="right">
<img src="https://img.youtube.com/vi/YtkZR0NFd1g/hqdefault.jpg" alt="how to use dotenv video tutorial" align="right" width="330" />
<img src="https://simpleicons.vercel.app/youtube/ff0000" alt="youtube/@dotenvorg" align="right" width="24" />
</div>
</a>
Create a `.env` file in the root of your project (if using a monorepo structure like `apps/backend/app.js`, put it in the root of the folder where your `app.js` process runs):
```dosini
S3_BUCKET="YOURS3BUCKET"
SECRET_KEY="YOURSECRETKEYGOESHERE"
```
As early as possible in your application, import and configure dotenv:
```javascript
require('dotenv').config()
console.log(process.env) // remove this after you've confirmed it is working
```
.. [or using ES6?](#how-do-i-use-dotenv-with-import)
```javascript
import 'dotenv/config'
```
That's it. `process.env` now has the keys and values you defined in your `.env` file:
```javascript
require('dotenv').config()
// or import 'dotenv/config' if you're using ES6
...
s3.getBucketCors({Bucket: process.env.S3_BUCKET}, function(err, data) {})
```
### Multiline values
If you need multiline variables, for example private keys, those are now supported (`>= v15.0.0`) with line breaks:
```dosini
PRIVATE_KEY="-----BEGIN RSA PRIVATE KEY-----
...
Kh9NV...
...
-----END RSA PRIVATE KEY-----"
```
Alternatively, you can double quote strings and use the `\n` character:
```dosini
PRIVATE_KEY="-----BEGIN RSA PRIVATE KEY-----\nKh9NV...\n-----END RSA PRIVATE KEY-----\n"
```
### Comments
Comments may be added to your file on their own line or inline:
```dosini
# This is a comment
SECRET_KEY=YOURSECRETKEYGOESHERE # comment
SECRET_HASH="something-with-a-#-hash"
```
Comments begin where a `#` exists, so if your value contains a `#` please wrap it in quotes. This is a breaking change from `>= v15.0.0` and on.
### Parsing
The engine which parses the contents of your file containing environment variables is available to use. It accepts a String or Buffer and will return an Object with the parsed keys and values.
```javascript
const dotenv = require('dotenv')
const buf = Buffer.from('BASIC=basic')
const config = dotenv.parse(buf) // will return an object
console.log(typeof config, config) // object { BASIC : 'basic' }
```
### Preload
> Note: Consider using [`dotenvx`](https://github.com/dotenvx/dotenvx) instead of preloading. I am now doing (and recommending) so.
>
> It serves the same purpose (you do not need to require and load dotenv), adds better debugging, and works with ANY language, framework, or platform. – [motdotla](https://github.com/motdotla)
You can use the `--require` (`-r`) [command line option](https://nodejs.org/api/cli.html#-r---require-module) to preload dotenv. By doing this, you do not need to require and load dotenv in your application code.
```bash
$ node -r dotenv/config your_script.js
```
The configuration options below are supported as command line arguments in the format `dotenv_config_<option>=value`
```bash
$ node -r dotenv/config your_script.js dotenv_config_path=/custom/path/to/.env dotenv_config_debug=true
```
Additionally, you can use environment variables to set configuration options. Command line arguments will precede these.
```bash
$ DOTENV_CONFIG_<OPTION>=value node -r dotenv/config your_script.js
```
```bash
$ DOTENV_CONFIG_ENCODING=latin1 DOTENV_CONFIG_DEBUG=true node -r dotenv/config your_script.js dotenv_config_path=/custom/path/to/.env
```
### Variable Expansion
You need to add the value of another variable in one of your variables? Use [dotenv-expand](https://github.com/motdotla/dotenv-expand).
### Command Substitution
Use [dotenvx](https://github.com/dotenvx/dotenvx) to use command substitution.
Add the output of a command to one of your variables in your .env file.
```ini
# .env
DATABASE_URL="postgres://$(whoami)@localhost/my_database"
```
```js
// index.js
console.log('DATABASE_URL', process.env.DATABASE_URL)
```
```sh
$ dotenvx run --debug -- node index.js
[[email protected]] injecting env (1) from .env
DATABASE_URL postgres://yourusername@localhost/my_database
```
### Syncing
You need to keep `.env` files in sync between machines, environments, or team members? Use [dotenvx](https://github.com/dotenvx/dotenvx) to encrypt your `.env` files and safely include them in source control. This still subscribes to the twelve-factor app rules by generating a decryption key separate from code.
### Multiple Environments
Use [dotenvx](https://github.com/dotenvx/dotenvx) to generate `.env.ci`, `.env.production` files, and more.
### Deploying
You need to deploy your secrets in a cloud-agnostic manner? Use [dotenvx](https://github.com/dotenvx/dotenvx) to generate a private decryption key that is set on your production server.
## 🌴 Manage Multiple Environments
Use [dotenvx](https://github.com/dotenvx/dotenvx)
Run any environment locally. Create a `.env.ENVIRONMENT` file and use `--env-file` to load it. It's straightforward, yet flexible.
```bash
$ echo "HELLO=production" > .env.production
$ echo "console.log('Hello ' + process.env.HELLO)" > index.js
$ dotenvx run --env-file=.env.production -- node index.js
Hello production
> ^^
```
or with multiple .env files
```bash
$ echo "HELLO=local" > .env.local
$ echo "HELLO=World" > .env
$ echo "console.log('Hello ' + process.env.HELLO)" > index.js
$ dotenvx run --env-file=.env.local --env-file=.env -- node index.js
Hello local
```
[more environment examples](https://dotenvx.com/docs/quickstart/environments)
## 🚀 Deploying
Use [dotenvx](https://github.com/dotenvx/dotenvx).
Add encryption to your `.env` files with a single command. Pass the `--encrypt` flag.
```
$ dotenvx set HELLO Production --encrypt -f .env.production
$ echo "console.log('Hello ' + process.env.HELLO)" > index.js
$ DOTENV_PRIVATE_KEY_PRODUCTION="<.env.production private key>" dotenvx run -- node index.js
[dotenvx] injecting env (2) from .env.production
Hello Production
```
[learn more](https://github.com/dotenvx/dotenvx?tab=readme-ov-file#encryption)
## 📚 Examples
See [examples](https://github.com/dotenv-org/examples) of using dotenv with various frameworks, languages, and configurations.
* [nodejs](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-nodejs)
* [nodejs (debug on)](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-nodejs-debug)
* [nodejs (override on)](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-nodejs-override)
* [nodejs (processEnv override)](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-custom-target)
* [esm](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-esm)
* [esm (preload)](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-esm-preload)
* [typescript](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-typescript)
* [typescript parse](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-typescript-parse)
* [typescript config](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-typescript-config)
* [webpack](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-webpack)
* [webpack (plugin)](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-webpack2)
* [react](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-react)
* [react (typescript)](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-react-typescript)
* [express](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-express)
* [nestjs](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-nestjs)
* [fastify](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-fastify)
## 📖 Documentation
Dotenv exposes four functions:
* `config`
* `parse`
* `populate`
* `decrypt`
### Config
`config` will read your `.env` file, parse the contents, assign it to
[`process.env`](https://nodejs.org/docs/latest/api/process.html#process_process_env),
and return an Object with a `parsed` key containing the loaded content or an `error` key if it failed.
```js
const result = dotenv.config()
if (result.error) {
throw result.error
}
console.log(result.parsed)
```
You can additionally, pass options to `config`.
#### Options
##### path
Default: `path.resolve(process.cwd(), '.env')`
Specify a custom path if your file containing environment variables is located elsewhere.
```js
require('dotenv').config({ path: '/custom/path/to/.env' })
```
By default, `config` will look for a file called .env in the current working directory.
Pass in multiple files as an array, and they will be parsed in order and combined with `process.env` (or `option.processEnv`, if set). The first value set for a variable will win, unless the `options.override` flag is set, in which case the last value set will win. If a value already exists in `process.env` and the `options.override` flag is NOT set, no changes will be made to that value.
```js
require('dotenv').config({ path: ['.env.local', '.env'] })
```
##### encoding
Default: `utf8`
Specify the encoding of your file containing environment variables.
```js
require('dotenv').config({ encoding: 'latin1' })
```
##### debug
Default: `false`
Turn on logging to help debug why certain keys or values are not being set as you expect.
```js
require('dotenv').config({ debug: process.env.DEBUG })
```
##### override
Default: `false`
Override any environment variables that have already been set on your machine with values from your .env file(s). If multiple files have been provided in `option.path` the override will also be used as each file is combined with the next. Without `override` being set, the first value wins. With `override` set the last value wins.
```js
require('dotenv').config({ override: true })
```
##### processEnv
Default: `process.env`
Specify an object to write your secrets to. Defaults to `process.env` environment variables.
```js
const myObject = {}
require('dotenv').config({ processEnv: myObject })
console.log(myObject) // values from .env
console.log(process.env) // this was not changed or written to
```
### Parse
The engine which parses the contents of your file containing environment
variables is available to use. It accepts a String or Buffer and will return
an Object with the parsed keys and values.
```js
const dotenv = require('dotenv')
const buf = Buffer.from('BASIC=basic')
const config = dotenv.parse(buf) // will return an object
console.log(typeof config, config) // object { BASIC : 'basic' }
```
#### Options
##### debug
Default: `false`
Turn on logging to help debug why certain keys or values are not being set as you expect.
```js
const dotenv = require('dotenv')
const buf = Buffer.from('hello world')
const opt = { debug: true }
const config = dotenv.parse(buf, opt)
// expect a debug message because the buffer is not in KEY=VAL form
```
### Populate
The engine which populates the contents of your .env file to `process.env` is available for use. It accepts a target, a source, and options. This is useful for power users who want to supply their own objects.
For example, customizing the source:
```js
const dotenv = require('dotenv')
const parsed = { HELLO: 'world' }
dotenv.populate(process.env, parsed)
console.log(process.env.HELLO) // world
```
For example, customizing the source AND target:
```js
const dotenv = require('dotenv')
const parsed = { HELLO: 'universe' }
const target = { HELLO: 'world' } // empty object
dotenv.populate(target, parsed, { override: true, debug: true })
console.log(target) // { HELLO: 'universe' }
```
#### options
##### Debug
Default: `false`
Turn on logging to help debug why certain keys or values are not being populated as you expect.
##### override
Default: `false`
Override any environment variables that have already been set.
## ❓ FAQ
### Why is the `.env` file not loading my environment variables successfully?
Most likely your `.env` file is not in the correct place. [See this stack overflow](https://stackoverflow.com/questions/42335016/dotenv-file-is-not-loading-environment-variables).
Turn on debug mode and try again..
```js
require('dotenv').config({ debug: true })
```
You will receive a helpful error outputted to your console.
### Should I commit my `.env` file?
No. We **strongly** recommend against committing your `.env` file to version
control. It should only include environment-specific values such as database
passwords or API keys. Your production database should have a different
password than your development database.
### Should I have multiple `.env` files?
We recommend creating one `.env` file per environment. Use `.env` for local/development, `.env.production` for production and so on. This still follows the twelve factor principles as each is attributed individually to its own environment. Avoid custom set ups that work in inheritance somehow (`.env.production` inherits values form `.env` for example). It is better to duplicate values if necessary across each `.env.environment` file.
> In a twelve-factor app, env vars are granular controls, each fully orthogonal to other env vars. They are never grouped together as “environments”, but instead are independently managed for each deploy. This is a model that scales up smoothly as the app naturally expands into more deploys over its lifetime.
>
> – [The Twelve-Factor App](http://12factor.net/config)
### What rules does the parsing engine follow?
The parsing engine currently supports the following rules:
- `BASIC=basic` becomes `{BASIC: 'basic'}`
- empty lines are skipped
- lines beginning with `#` are treated as comments
- `#` marks the beginning of a comment (unless when the value is wrapped in quotes)
- empty values become empty strings (`EMPTY=` becomes `{EMPTY: ''}`)
- inner quotes are maintained (think JSON) (`JSON={"foo": "bar"}` becomes `{JSON:"{\"foo\": \"bar\"}"`)
- whitespace is removed from both ends of unquoted values (see more on [`trim`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/Trim)) (`FOO= some value ` becomes `{FOO: 'some value'}`)
- single and double quoted values are escaped (`SINGLE_QUOTE='quoted'` becomes `{SINGLE_QUOTE: "quoted"}`)
- single and double quoted values maintain whitespace from both ends (`FOO=" some value "` becomes `{FOO: ' some value '}`)
- double quoted values expand new lines (`MULTILINE="new\nline"` becomes
```
{MULTILINE: 'new
line'}
```
- backticks are supported (`` BACKTICK_KEY=`This has 'single' and "double" quotes inside of it.` ``)
### What happens to environment variables that were already set?
By default, we will never modify any environment variables that have already been set. In particular, if there is a variable in your `.env` file which collides with one that already exists in your environment, then that variable will be skipped.
If instead, you want to override `process.env` use the `override` option.
```javascript
require('dotenv').config({ override: true })
```
### How come my environment variables are not showing up for React?
Your React code is run in Webpack, where the `fs` module or even the `process` global itself are not accessible out-of-the-box. `process.env` can only be injected through Webpack configuration.
If you are using [`react-scripts`](https://www.npmjs.com/package/react-scripts), which is distributed through [`create-react-app`](https://create-react-app.dev/), it has dotenv built in but with a quirk. Preface your environment variables with `REACT_APP_`. See [this stack overflow](https://stackoverflow.com/questions/42182577/is-it-possible-to-use-dotenv-in-a-react-project) for more details.
If you are using other frameworks (e.g. Next.js, Gatsby...), you need to consult their documentation for how to inject environment variables into the client.
### Can I customize/write plugins for dotenv?
Yes! `dotenv.config()` returns an object representing the parsed `.env` file. This gives you everything you need to continue setting values on `process.env`. For example:
```js
const dotenv = require('dotenv')
const variableExpansion = require('dotenv-expand')
const myEnv = dotenv.config()
variableExpansion(myEnv)
```
### How do I use dotenv with `import`?
Simply..
```javascript
// index.mjs (ESM)
import 'dotenv/config' // see https://github.com/motdotla/dotenv#how-do-i-use-dotenv-with-import
import express from 'express'
```
A little background..
> When you run a module containing an `import` declaration, the modules it imports are loaded first, then each module body is executed in a depth-first traversal of the dependency graph, avoiding cycles by skipping anything already executed.
>
> – [ES6 In Depth: Modules](https://hacks.mozilla.org/2015/08/es6-in-depth-modules/)
What does this mean in plain language? It means you would think the following would work but it won't.
`errorReporter.mjs`:
```js
import { Client } from 'best-error-reporting-service'
export default new Client(process.env.API_KEY)
```
`index.mjs`:
```js
// Note: this is INCORRECT and will not work
import * as dotenv from 'dotenv'
dotenv.config()
import errorReporter from './errorReporter.mjs'
errorReporter.report(new Error('documented example'))
```
`process.env.API_KEY` will be blank.
Instead, `index.mjs` should be written as..
```js
import 'dotenv/config'
import errorReporter from './errorReporter.mjs'
errorReporter.report(new Error('documented example'))
```
Does that make sense? It's a bit unintuitive, but it is how importing of ES6 modules work. Here is a [working example of this pitfall](https://github.com/dotenv-org/examples/tree/master/usage/dotenv-es6-import-pitfall).
There are two alternatives to this approach:
1. Preload dotenv: `node --require dotenv/config index.js` (_Note: you do not need to `import` dotenv with this approach_)
2. Create a separate file that will execute `config` first as outlined in [this comment on #133](https://github.com/motdotla/dotenv/issues/133#issuecomment-255298822)
### Why am I getting the error `Module not found: Error: Can't resolve 'crypto|os|path'`?
You are using dotenv on the front-end and have not included a polyfill. Webpack < 5 used to include these for you. Do the following:
```bash
npm install node-polyfill-webpack-plugin
```
Configure your `webpack.config.js` to something like the following.
```js
require('dotenv').config()
const path = require('path');
const webpack = require('webpack')
const NodePolyfillPlugin = require('node-polyfill-webpack-plugin')
module.exports = {
mode: 'development',
entry: './src/index.ts',
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist'),
},
plugins: [
new NodePolyfillPlugin(),
new webpack.DefinePlugin({
'process.env': {
HELLO: JSON.stringify(process.env.HELLO)
}
}),
]
};
```
Alternatively, just use [dotenv-webpack](https://github.com/mrsteele/dotenv-webpack) which does this and more behind the scenes for you.
### What about variable expansion?
Try [dotenv-expand](https://github.com/motdotla/dotenv-expand)
### What about syncing and securing .env files?
Use [dotenvx](https://github.com/dotenvx/dotenvx)
### What if I accidentally commit my `.env` file to code?
Remove it, [remove git history](https://docs.github.com/en/authentication/keeping-your-account-and-data-secure/removing-sensitive-data-from-a-repository) and then install the [git pre-commit hook](https://github.com/dotenvx/dotenvx#pre-commit) to prevent this from ever happening again.
```
brew install dotenvx/brew/dotenvx
dotenvx precommit --install
```
### How can I prevent committing my `.env` file to a Docker build?
Use the [docker prebuild hook](https://dotenvx.com/docs/features/prebuild).
```bash
# Dockerfile
...
RUN curl -fsS https://dotenvx.sh/ | sh
...
RUN dotenvx prebuild
CMD ["dotenvx", "run", "--", "node", "index.js"]
```
## Contributing Guide
See [CONTRIBUTING.md](CONTRIBUTING.md)
## CHANGELOG
See [CHANGELOG.md](CHANGELOG.md)
## Who's using dotenv?
[These npm modules depend on it.](https://www.npmjs.com/browse/depended/dotenv)
Projects that expand it often use the [keyword "dotenv" on npm](https://www.npmjs.com/search?q=keywords:dotenv). | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/dotenv/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/dotenv/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 22974
} |
## Drizzle Kit
DrizzleKit - is a CLI migrator tool for DrizzleORM. It is probably one and only tool that lets you completely automatically generate SQL migrations and covers ~95% of the common cases like deletions and renames by prompting user input.
<https://github.com/drizzle-team/drizzle-kit-mirror> - is a mirror repository for issues.
## Documentation
Check the full documenation on [the website](https://orm.drizzle.team/kit-docs/overview)
### How it works
`drizzle-kit` will traverse `schema folder` or `schema file`, generate schema snapshot and compare it to the previous version, if there's one.
Based on the difference it will generate all needed SQL migrations and if there are any `automatically unresolvable` cases like `renames` it will prompt user for input.
For schema file:
```typescript
// ./src/db/schema.ts
import { integer, pgTable, serial, text, varchar } from "drizzle-orm/pg-core";
const users = pgTable("users", {
id: serial("id").primaryKey(),
fullName: varchar("full_name", { length: 256 }),
}, (table) => ({
nameIdx: index("name_idx", table.fullName),
})
);
export const authOtp = pgTable("auth_otp", {
id: serial("id").primaryKey(),
phone: varchar("phone", { length: 256 }),
userId: integer("user_id").references(() => users.id),
});
```
It will generate:
```SQL
CREATE TABLE IF NOT EXISTS auth_otp (
"id" SERIAL PRIMARY KEY,
"phone" character varying(256),
"user_id" INT
);
CREATE TABLE IF NOT EXISTS users (
"id" SERIAL PRIMARY KEY,
"full_name" character varying(256)
);
DO $$ BEGIN
ALTER TABLE auth_otp ADD CONSTRAINT auth_otp_user_id_fkey FOREIGN KEY ("user_id") REFERENCES users(id);
EXCEPTION
WHEN duplicate_object THEN null;
END $$;
CREATE INDEX IF NOT EXISTS users_full_name_index ON users (full_name);
```
### Installation & configuration
```shell
npm install -D drizzle-kit
```
Running with CLI options
```jsonc
// package.json
{
"scripts": {
"generate": "drizzle-kit generate --out migrations-folder --schema src/db/schema.ts"
}
}
```
```shell
npm run generate
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/drizzle-kit/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/drizzle-kit/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2060
} |
<div align="center">
<img src="./misc/readme/logo-github-sq-dark.svg#gh-dark-mode-only" />
<img src="./misc/readme/logo-github-sq-light.svg#gh-light-mode-only" />
</div>
<br/>
<div align="center">
<h3>Headless ORM for NodeJS, TypeScript and JavaScript 🚀</h3>
<a href="https://orm.drizzle.team">Website</a> •
<a href="https://orm.drizzle.team/docs/overview">Documentation</a> •
<a href="https://x.com/drizzleorm">Twitter</a> •
<a href="https://driz.link/discord">Discord</a>
</div>
<br/>
<br/>
### What's Drizzle?
Drizzle is a modern TypeScript ORM developers [wanna use in their next project](https://stateofdb.com/tools/drizzle).
It is [lightweight](https://bundlephobia.com/package/drizzle-orm) at only ~7.4kb minified+gzipped, and it's tree shakeable with exactly 0 dependencies.
**Drizzle supports every PostgreSQL, MySQL and SQLite database**, including serverless ones like [Turso](https://orm.drizzle.team/docs/get-started-sqlite#turso), [Neon](https://orm.drizzle.team/docs/get-started-postgresql#neon), [Xata](xata.io), [PlanetScale](https://orm.drizzle.team/docs/get-started-mysql#planetscale), [Cloudflare D1](https://orm.drizzle.team/docs/get-started-sqlite#cloudflare-d1), [FlyIO LiteFS](https://fly.io/docs/litefs/), [Vercel Postgres](https://orm.drizzle.team/docs/get-started-postgresql#vercel-postgres), [Supabase](https://orm.drizzle.team/docs/get-started-postgresql#supabase) and [AWS Data API](https://orm.drizzle.team/docs/get-started-postgresql#aws-data-api). No bells and whistles, no Rust binaries, no serverless adapters, everything just works out of the box.
**Drizzle is serverless-ready by design**. It works in every major JavaScript runtime like NodeJS, Bun, Deno, Cloudflare Workers, Supabase functions, any Edge runtime, and even in browsers.
With Drizzle you can be [**fast out of the box**](https://orm.drizzle.team/benchmarks) and save time and costs while never introducing any data proxies into your infrastructure.
While you can use Drizzle as a JavaScript library, it shines with TypeScript. It lets you [**declare SQL schemas**](https://orm.drizzle.team/docs/sql-schema-declaration) and build both [**relational**](https://orm.drizzle.team/docs/rqb) and [**SQL-like queries**](https://orm.drizzle.team/docs/select), while keeping the balance between type-safety and extensibility for toolmakers to build on top.
### Ecosystem
While Drizzle ORM remains a thin typed layer on top of SQL, we made a set of tools for people to have best possible developer experience.
Drizzle comes with a powerful [**Drizzle Kit**](https://orm.drizzle.team/kit-docs/overview) CLI companion for you to have hassle-free migrations. It can generate SQL migration files for you or apply schema changes directly to the database.
We also have [**Drizzle Studio**](https://orm.drizzle.team/drizzle-studio/overview) for you to effortlessly browse and manipulate data in your database of choice.
### Documentation
Check out the full documentation on [the website](https://orm.drizzle.team/docs/overview).
### Our sponsors ❤️
<p align="center">
<a href="https://drizzle.team" target="_blank">
<img src='https://api.drizzle.team/v2/sponsors/svg'/>
</a>
</p> | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/drizzle-orm/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/drizzle-orm/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 3205
} |
<div align='center'>
<h1>drizzle-zod <a href=''><img alt='npm' src='https://img.shields.io/npm/v/drizzle-zod?label='></a></h1>
<img alt='npm' src='https://img.shields.io/npm/dm/drizzle-zod'>
<img alt='npm bundle size' src='https://img.shields.io/bundlephobia/min/drizzle-zod'>
<a href='https://discord.gg/yfjTbVXMW4'><img alt='Discord' src='https://img.shields.io/discord/1043890932593987624'></a>
<img alt='License' src='https://img.shields.io/npm/l/drizzle-zod'>
<h6><i>If you know SQL, you know Drizzle ORM</i></h6>
<hr />
</div>
`drizzle-zod` is a plugin for [Drizzle ORM](https://github.com/drizzle-team/drizzle-orm) that allows you to generate [Zod](https://zod.dev/) schemas from Drizzle ORM schemas.
**Features**
- Create a select schema for tables, views and enums.
- Create insert and update schemas for tables.
- Supports all dialects: PostgreSQL, MySQL and SQLite.
# Usage
```ts
import { pgEnum, pgTable, serial, text, timestamp } from 'drizzle-orm/pg-core';
import { createInsertSchema, createSelectSchema } from 'drizzle-zod';
import { z } from 'zod';
const users = pgTable('users', {
id: serial('id').primaryKey(),
name: text('name').notNull(),
email: text('email').notNull(),
role: text('role', { enum: ['admin', 'user'] }).notNull(),
createdAt: timestamp('created_at').notNull().defaultNow(),
});
// Schema for inserting a user - can be used to validate API requests
const insertUserSchema = createInsertSchema(users);
// Schema for updating a user - can be used to validate API requests
const updateUserSchema = createUpdateSchema(users);
// Schema for selecting a user - can be used to validate API responses
const selectUserSchema = createSelectSchema(users);
// Overriding the fields
const insertUserSchema = createInsertSchema(users, {
role: z.string(),
});
// Refining the fields - useful if you want to change the fields before they become nullable/optional in the final schema
const insertUserSchema = createInsertSchema(users, {
id: (schema) => schema.positive(),
email: (schema) => schema.email(),
role: z.string(),
});
// Usage
const user = insertUserSchema.parse({
name: 'John Doe',
email: '[email protected]',
role: 'admin',
});
// Zod schema type is also inferred from the table schema, so you have full type safety
const requestSchema = insertUserSchema.pick({ name: true, email: true });
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/drizzle-zod/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/drizzle-zod/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2361
} |
# East Asian Width
Get [East Asian Width](http://www.unicode.org/reports/tr11/) from a character.
'F'(Fullwidth), 'H'(Halfwidth), 'W'(Wide), 'Na'(Narrow), 'A'(Ambiguous) or 'N'(Natural).
Original Code is [東アジアの文字幅 (East Asian Width) の判定 - 中途](http://d.hatena.ne.jp/takenspc/20111126#1322252878).
## Install
$ npm install eastasianwidth
## Usage
var eaw = require('eastasianwidth');
console.log(eaw.eastAsianWidth('₩')) // 'F'
console.log(eaw.eastAsianWidth('。')) // 'H'
console.log(eaw.eastAsianWidth('뀀')) // 'W'
console.log(eaw.eastAsianWidth('a')) // 'Na'
console.log(eaw.eastAsianWidth('①')) // 'A'
console.log(eaw.eastAsianWidth('ف')) // 'N'
console.log(eaw.characterLength('₩')) // 2
console.log(eaw.characterLength('。')) // 1
console.log(eaw.characterLength('뀀')) // 2
console.log(eaw.characterLength('a')) // 1
console.log(eaw.characterLength('①')) // 2
console.log(eaw.characterLength('ف')) // 1
console.log(eaw.length('あいうえお')) // 10
console.log(eaw.length('abcdefg')) // 7
console.log(eaw.length('¢₩。ᅵㄅ뀀¢⟭a⊙①بف')) // 19 | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/eastasianwidth/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/eastasianwidth/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1107
} |
# EE First
[![NPM version][npm-image]][npm-url]
[![Build status][travis-image]][travis-url]
[![Test coverage][coveralls-image]][coveralls-url]
[![License][license-image]][license-url]
[![Downloads][downloads-image]][downloads-url]
[![Gittip][gittip-image]][gittip-url]
Get the first event in a set of event emitters and event pairs,
then clean up after itself.
## Install
```sh
$ npm install ee-first
```
## API
```js
var first = require('ee-first')
```
### first(arr, listener)
Invoke `listener` on the first event from the list specified in `arr`. `arr` is
an array of arrays, with each array in the format `[ee, ...event]`. `listener`
will be called only once, the first time any of the given events are emitted. If
`error` is one of the listened events, then if that fires first, the `listener`
will be given the `err` argument.
The `listener` is invoked as `listener(err, ee, event, args)`, where `err` is the
first argument emitted from an `error` event, if applicable; `ee` is the event
emitter that fired; `event` is the string event name that fired; and `args` is an
array of the arguments that were emitted on the event.
```js
var ee1 = new EventEmitter()
var ee2 = new EventEmitter()
first([
[ee1, 'close', 'end', 'error'],
[ee2, 'error']
], function (err, ee, event, args) {
// listener invoked
})
```
#### .cancel()
The group of listeners can be cancelled before being invoked and have all the event
listeners removed from the underlying event emitters.
```js
var thunk = first([
[ee1, 'close', 'end', 'error'],
[ee2, 'error']
], function (err, ee, event, args) {
// listener invoked
})
// cancel and clean up
thunk.cancel()
```
[npm-image]: https://img.shields.io/npm/v/ee-first.svg?style=flat-square
[npm-url]: https://npmjs.org/package/ee-first
[github-tag]: http://img.shields.io/github/tag/jonathanong/ee-first.svg?style=flat-square
[github-url]: https://github.com/jonathanong/ee-first/tags
[travis-image]: https://img.shields.io/travis/jonathanong/ee-first.svg?style=flat-square
[travis-url]: https://travis-ci.org/jonathanong/ee-first
[coveralls-image]: https://img.shields.io/coveralls/jonathanong/ee-first.svg?style=flat-square
[coveralls-url]: https://coveralls.io/r/jonathanong/ee-first?branch=master
[license-image]: http://img.shields.io/npm/l/ee-first.svg?style=flat-square
[license-url]: LICENSE.md
[downloads-image]: http://img.shields.io/npm/dm/ee-first.svg?style=flat-square
[downloads-url]: https://npmjs.org/package/ee-first
[gittip-image]: https://img.shields.io/gittip/jonathanong.svg?style=flat-square
[gittip-url]: https://www.gittip.com/jonathanong/ | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/ee-first/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/ee-first/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2616
} |
### Made by [@kilianvalkhof](https://twitter.com/kilianvalkhof)
#### Other projects:
- 💻 [Polypane](https://polypane.app) - Develop responsive websites and apps twice as fast on multiple screens at once
- 🖌️ [Superposition](https://superposition.design) - Kickstart your design system by extracting design tokens from your website
- 🗒️ [FromScratch](https://fromscratch.rocks) - A smart but simple autosaving scratchpad
---
# Electron-to-Chromium [](https://www.npmjs.com/package/electron-to-chromium) [](https://travis-ci.org/Kilian/electron-to-chromium) [](https://www.npmjs.com/package/electron-to-chromium) [](https://codecov.io/gh/Kilian/electron-to-chromium)[](https://app.fossa.io/projects/git%2Bgithub.com%2FKilian%2Felectron-to-chromium?ref=badge_shield)
This repository provides a mapping of Electron versions to the Chromium version that it uses.
This package is used in [Browserslist](https://github.com/ai/browserslist), so you can use e.g. `electron >= 1.4` in [Autoprefixer](https://github.com/postcss/autoprefixer), [Stylelint](https://github.com/stylelint/stylelint), [babel-preset-env](https://github.com/babel/babel-preset-env) and [eslint-plugin-compat](https://github.com/amilajack/eslint-plugin-compat).
**Supported by:**
<a href="https://m.do.co/c/bb22ea58e765">
<img src="https://opensource.nyc3.cdn.digitaloceanspaces.com/attribution/assets/SVG/DO_Logo_horizontal_blue.svg" width="201px">
</a>
## Install
Install using `npm install electron-to-chromium`.
## Usage
To include Electron-to-Chromium, require it:
```js
var e2c = require('electron-to-chromium');
```
### Properties
The Electron-to-Chromium object has 4 properties to use:
#### `versions`
An object of key-value pairs with a _major_ Electron version as the key, and the corresponding major Chromium version as the value.
```js
var versions = e2c.versions;
console.log(versions['1.4']);
// returns "53"
```
#### `fullVersions`
An object of key-value pairs with a Electron version as the key, and the corresponding full Chromium version as the value.
```js
var versions = e2c.fullVersions;
console.log(versions['1.4.11']);
// returns "53.0.2785.143"
```
#### `chromiumVersions`
An object of key-value pairs with a _major_ Chromium version as the key, and the corresponding major Electron version as the value.
```js
var versions = e2c.chromiumVersions;
console.log(versions['54']);
// returns "1.4"
```
#### `fullChromiumVersions`
An object of key-value pairs with a Chromium version as the key, and an array of the corresponding major Electron versions as the value.
```js
var versions = e2c.fullChromiumVersions;
console.log(versions['54.0.2840.101']);
// returns ["1.5.1", "1.5.0"]
```
### Functions
#### `electronToChromium(query)`
Arguments:
* Query: string or number, required. A major or full Electron version.
A function that returns the corresponding Chromium version for a given Electron function. Returns a string.
If you provide it with a major Electron version, it will return a major Chromium version:
```js
var chromeVersion = e2c.electronToChromium('1.4');
// chromeVersion is "53"
```
If you provide it with a full Electron version, it will return the full Chromium version.
```js
var chromeVersion = e2c.electronToChromium('1.4.11');
// chromeVersion is "53.0.2785.143"
```
If a query does not match a Chromium version, it will return `undefined`.
```js
var chromeVersion = e2c.electronToChromium('9000');
// chromeVersion is undefined
```
#### `chromiumToElectron(query)`
Arguments:
* Query: string or number, required. A major or full Chromium version.
Returns a string with the corresponding Electron version for a given Chromium query.
If you provide it with a major Chromium version, it will return a major Electron version:
```js
var electronVersion = e2c.chromiumToElectron('54');
// electronVersion is "1.4"
```
If you provide it with a full Chrome version, it will return an array of full Electron versions.
```js
var electronVersions = e2c.chromiumToElectron('56.0.2924.87');
// electronVersions is ["1.6.3", "1.6.2", "1.6.1", "1.6.0"]
```
If a query does not match an Electron version, it will return `undefined`.
```js
var electronVersion = e2c.chromiumToElectron('10');
// electronVersion is undefined
```
#### `electronToBrowserList(query)` **DEPRECATED**
Arguments:
* Query: string or number, required. A major Electron version.
_**Deprecated**: Browserlist already includes electron-to-chromium._
A function that returns a [Browserslist](https://github.com/ai/browserslist) query that matches the given major Electron version. Returns a string.
If you provide it with a major Electron version, it will return a Browserlist query string that matches the Chromium capabilities:
```js
var query = e2c.electronToBrowserList('1.4');
// query is "Chrome >= 53"
```
If a query does not match a Chromium version, it will return `undefined`.
```js
var query = e2c.electronToBrowserList('9000');
// query is undefined
```
### Importing just versions, fullVersions, chromiumVersions and fullChromiumVersions
All lists can be imported on their own, if file size is a concern.
#### `versions`
```js
var versions = require('electron-to-chromium/versions');
```
#### `fullVersions`
```js
var fullVersions = require('electron-to-chromium/full-versions');
```
#### `chromiumVersions`
```js
var chromiumVersions = require('electron-to-chromium/chromium-versions');
```
#### `fullChromiumVersions`
```js
var fullChromiumVersions = require('electron-to-chromium/full-chromium-versions');
```
## Updating
This package will be updated with each new Electron release.
To update the list, run `npm run build.js`. Requires internet access as it downloads from the canonical list of Electron versions.
To verify correct behaviour, run `npm test`.
## License
[](https://app.fossa.io/projects/git%2Bgithub.com%2FKilian%2Felectron-to-chromium?ref=badge_large) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/electron-to-chromium/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/electron-to-chromium/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 6446
} |
<br />
<div align="center">
<p align="center">
<a href="https://www.embla-carousel.com/"><img width="100" height="100" src="https://www.embla-carousel.com/embla-logo.svg" alt="Embla Carousel">
</a>
</p>
<p align="center">
<a href="https://opensource.org/licenses/MIT"><img src="https://img.shields.io/npm/l/embla-carousel?color=%238ab4f8"></a>
<a href="https://www.npmjs.com/package/embla-carousel-react"><img src="https://img.shields.io/npm/v/embla-carousel-react.svg?color=%23c1a8e2"></a>
<a href="https://github.com/davidjerleke/embla-carousel/actions?query=workflow%3A%22Continuous+Integration%22"><img src="https://img.shields.io/github/actions/workflow/status/davidjerleke/embla-carousel/cd.yml?color=%238ab4f8"></a>
<a href="https://prettier.io"><img src="https://img.shields.io/badge/code_style-prettier-ff69b4.svg?color=%23c1a8e2"></a>
<a href="https://bundlephobia.com/result?p=embla-carousel-react@latest"><img src="https://img.shields.io/bundlephobia/minzip/embla-carousel-react?color=%238ab4f8&label=gzip%20size">
</a>
</p>
<strong>
<h2 align="center">Embla Carousel React</h2>
</strong>
<p align="center">
<strong>Embla Carousel</strong> is a bare bones carousel library with great fluid motion and awesome swipe precision. It's library agnostic, dependency free and 100% open source.
</p>
<br>
<p align="center">
<strong>
<code> <a href="https://www.embla-carousel.com/examples/predefined/">Examples</a> </code>
</strong>
</p>
<p align="center">
<strong>
<code> <a href="https://www.embla-carousel.com/examples/generator/">Generator</a> </code>
</strong>
</p>
<p align="center">
<strong>
<code> <a href="https://www.embla-carousel.com/get-started/#choose-installation-type">Installation</a> </code>
</strong>
</p>
</div>
<br>
<div align="center">
<strong>
<h2 align="center">Ready for</h2>
</strong>
<p align="center">
<a href="https://www.embla-carousel.com/get-started/module/">
<img src="https://www.embla-carousel.com/javascript-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/module/">
<img src="https://www.embla-carousel.com/typescript-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/react/">
<img src="https://www.embla-carousel.com/react-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/vue/">
<img src="https://www.embla-carousel.com/vue-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/svelte/">
<img src="https://www.embla-carousel.com/svelte-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/solid/">
<img src="https://www.embla-carousel.com/solid-logo.svg" width="40" height="40" />
</a>
<a href="https://github.com/donaldxdonald/embla-carousel-angular">
<img src="https://www.embla-carousel.com/angular-logo.svg" width="40" height="40" />
</a>
</p>
</div>
<br>
<div align="center">
<strong>
<h2 align="center">Contributors</h2>
</strong>
<p align="center">
Thank you to all contributors for making <a href="https://www.embla-carousel.com/">Embla Carousel</a> awesome! <a href="https://github.com/davidjerleke/embla-carousel/blob/master/CONTRIBUTING.md">Contributions</a> are welcome.
</p>
<p align="center">
<a href="https://github.com/davidjerleke">
<img src="https://avatars2.githubusercontent.com/u/11529148?s=120&v=4" title="davidjerleke" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/Ronit-gurjar">
<img src="https://avatars2.githubusercontent.com/u/92150685?s=120&v=4" title="Ronit-gurjar" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/zaaakher">
<img src="https://avatars2.githubusercontent.com/u/46135573?s=120&v=4" title="zaaakher" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/xiel">
<img src="https://avatars2.githubusercontent.com/u/615522?s=120&v=4" title="xiel" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/javiergonzalezGenially">
<img src="https://avatars2.githubusercontent.com/u/78730098?s=120&v=4" title="javiergonzalezGenially" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/meirroth">
<img src="https://avatars2.githubusercontent.com/u/12494197?s=120&v=4" title="meirroth" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/hamidrezahanafi">
<img src="https://avatars2.githubusercontent.com/u/91487491?s=120&v=4" title="hamidrezahanafi" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/negezor">
<img src="https://avatars2.githubusercontent.com/u/9392723?s=120&v=4" title="negezor" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/th-km">
<img src="https://avatars2.githubusercontent.com/u/35410212?s=120&v=4" title="th-km" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/openscript">
<img src="https://avatars2.githubusercontent.com/u/1105080?s=120&v=4" title="openscript" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/nwidynski">
<img src="https://avatars2.githubusercontent.com/u/25958801?s=120&v=4" title="nwidynski" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/wopian">
<img src="https://avatars2.githubusercontent.com/u/3440094?s=120&v=4" title="wopian" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/horseeyephil">
<img src="https://avatars2.githubusercontent.com/u/32337092?s=120&v=4" title="horseeyephil" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/pipisasa">
<img src="https://avatars2.githubusercontent.com/u/54534600?s=120&v=4" title="pipisasa" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/ranno-lauri">
<img src="https://avatars2.githubusercontent.com/u/87007115?s=120&v=4" title="ranno-lauri" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/ruijdacd">
<img src="https://avatars2.githubusercontent.com/u/9107610?s=120&v=4" title="ruijdacd" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/rojadesign">
<img src="https://avatars2.githubusercontent.com/u/35687281?s=120&v=4" title="rojadesign" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/sadeghbarati">
<img src="https://avatars2.githubusercontent.com/u/17789047?s=120&v=4" title="sadeghbarati" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/S-Shingler">
<img src="https://avatars2.githubusercontent.com/u/48463809?s=120&v=4" title="S-Shingler" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/Yonom">
<img src="https://avatars2.githubusercontent.com/u/1394504?s=120&v=4" title="Yonom" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/smultar">
<img src="https://avatars2.githubusercontent.com/u/6223536?s=120&v=4" title="smultar" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/dev-suraj-kumar">
<img src="https://avatars2.githubusercontent.com/u/184739775?s=120&v=4" title="dev-suraj-kumar" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/tlo-johnson">
<img src="https://avatars2.githubusercontent.com/u/8763144?s=120&v=4" title="tlo-johnson" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/anzbert">
<img src="https://avatars2.githubusercontent.com/u/38823700?s=120&v=4" title="anzbert" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/sarussss">
<img src="https://avatars2.githubusercontent.com/u/15656996?s=120&v=4" title="sarussss" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/silllli">
<img src="https://avatars2.githubusercontent.com/u/9334305?s=120&v=4" title="silllli" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/nikrowell">
<img src="https://avatars2.githubusercontent.com/u/260039?s=120&v=4" title="nikrowell" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/mujahidfa">
<img src="https://avatars2.githubusercontent.com/u/17759705?s=120&v=4" title="mujahidfa" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/romellem">
<img src="https://avatars2.githubusercontent.com/u/8504000?s=120&v=4" title="romellem" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/junlarsen">
<img src="https://avatars2.githubusercontent.com/u/42585241?s=120&v=4" title="junlarsen" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/LucasMariniFalbo">
<img src="https://avatars2.githubusercontent.com/u/9245477?s=120&v=4" title="LucasMariniFalbo" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/LiamMartens">
<img src="https://avatars2.githubusercontent.com/u/5265324?s=120&v=4" title="LiamMartens" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/S1r-Lanzelot">
<img src="https://avatars2.githubusercontent.com/u/4487160?s=120&v=4" title="S1r-Lanzelot" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/ishaqibrahimbot">
<img src="https://avatars2.githubusercontent.com/u/74908398?s=120&v=4" title="ishaqibrahimbot" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/fcasibu">
<img src="https://avatars2.githubusercontent.com/u/75290989?s=120&v=4" title="fcasibu" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/cundd">
<img src="https://avatars2.githubusercontent.com/u/743122?s=120&v=4" title="cundd" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/sedlukha">
<img src="https://avatars2.githubusercontent.com/u/14075940?s=120&v=4" title="sedlukha" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/allen-garvey">
<img src="https://avatars2.githubusercontent.com/u/9314727?s=120&v=4" title="allen-garvey" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/lesha1201">
<img src="https://avatars2.githubusercontent.com/u/10157660?s=120&v=4" title="lesha1201" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/SaizFerri">
<img src="https://avatars2.githubusercontent.com/u/19834971?s=120&v=4" title="SaizFerri" width="50" height="50" style="max-width: 100%" />
</a>
</p>
</div>
<br>
<div align="center">
<strong>
<h2 align="center">Special Thanks</h2>
</strong>
<p align="center">
<sup>
<a href="https://github.com/gunnarx2">gunnarx2</a> - React wrapper <a href="https://www.embla-carousel.com/get-started/react/">useEmblaCarousel</a>.
</sup>
<br>
<sup>
<a href="https://github.com/LiamMartens">LiamMartens</a> - Solid wrapper <a href="https://www.embla-carousel.com/get-started/solid/">createEmblaCarousel</a>.
</sup>
<br>
<sup>
<a href="https://github.com/donaldxdonald">donaldxdonald</a>, <a href="https://github.com/zip-fa">zip-fa</a>, <a href="https://github.com/JeanMeche">JeanMeche</a> - Angular wrapper <a href="https://github.com/donaldxdonald/embla-carousel-angular?tab=readme-ov-file#installation">EmblaCarouselDirective</a>.
</sup>
<br>
<sup>
<a href="https://github.com/xiel">xiel</a> - Plugin <a href="https://github.com/xiel/embla-carousel-wheel-gestures">Embla Carousel Wheel Gestures</a>.
</sup>
<br>
<sup>
<a href="https://github.com/zaaakher">zaaakher</a> - Contributing <a href="https://github.com/davidjerleke/embla-carousel/blob/master/CONTRIBUTING.md">guidelines</a>.
</sup>
<br>
<sup>
<a href="https://github.com/sarussss">sarussss</a> - Answering questions.
</sup>
</p>
</div>
<br>
<h2 align="center">Open Source</h2>
<p align="center">
Embla is <a href="https://github.com/davidjerleke/embla-carousel/blob/master/LICENSE">MIT licensed</a> 💖.<br><br>
<sup>Embla Carousel - Copyright © 2019-present.</sup><br />
<sup>Package created by David Jerleke.</sup>
</p>
<p align="center">
<strong>· · ·</strong>
</p>
<p align="center">
Thanks <a href="https://www.browserstack.com">BrowserStack</a>.
</p>
<p align="center">
<a href="https://www.browserstack.com">
<img src="https://www.embla-carousel.com/browserstack-logo.svg" width="45" height="45" />
</a>
</p> | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/embla-carousel-react/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/embla-carousel-react/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 13265
} |
<br />
<div align="center">
<p align="center">
<a href="https://www.embla-carousel.com/"><img width="100" height="100" src="https://www.embla-carousel.com/embla-logo.svg" alt="Embla Carousel">
</a>
</p>
<p align="center">
<a href="https://opensource.org/licenses/MIT"><img src="https://img.shields.io/npm/l/embla-carousel?color=%238ab4f8"></a>
<a href="https://www.npmjs.com/package/embla-carousel-reactive-utils"><img src="https://img.shields.io/npm/v/embla-carousel-reactive-utils.svg?color=%23c1a8e2"></a>
<a href="https://github.com/davidjerleke/embla-carousel/actions?query=workflow%3A%22Continuous+Integration%22"><img src="https://img.shields.io/github/actions/workflow/status/davidjerleke/embla-carousel/cd.yml?color=%238ab4f8"></a>
<a href="https://prettier.io"><img src="https://img.shields.io/badge/code_style-prettier-ff69b4.svg?color=%23c1a8e2"></a>
<a href="https://bundlephobia.com/result?p=embla-carousel-reactive-utils@latest"><img src="https://img.shields.io/bundlephobia/minzip/embla-carousel-reactive-utils?color=%238ab4f8&label=gzip%20size">
</a>
</p>
<strong>
<h2 align="center">Embla Carousel Reactive Utils</h2>
</strong>
<p align="center">
<strong>Embla Carousel</strong> is a bare bones carousel library with great fluid motion and awesome swipe precision. It's library agnostic, dependency free and 100% open source.
</p>
<br>
<p align="center">
<strong>
<code> <a href="https://www.embla-carousel.com/examples/predefined/">Examples</a> </code>
</strong>
</p>
<p align="center">
<strong>
<code> <a href="https://www.embla-carousel.com/examples/generator/">Generator</a> </code>
</strong>
</p>
<p align="center">
<strong>
<code> <a href="https://www.embla-carousel.com/get-started/#choose-installation-type">Installation</a> </code>
</strong>
</p>
</div>
<br>
<div align="center">
<strong>
<h2 align="center">Ready for</h2>
</strong>
<p align="center">
<a href="https://www.embla-carousel.com/get-started/module/">
<img src="https://www.embla-carousel.com/javascript-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/module/">
<img src="https://www.embla-carousel.com/typescript-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/react/">
<img src="https://www.embla-carousel.com/react-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/vue/">
<img src="https://www.embla-carousel.com/vue-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/svelte/">
<img src="https://www.embla-carousel.com/svelte-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/solid/">
<img src="https://www.embla-carousel.com/solid-logo.svg" width="40" height="40" />
</a>
<a href="https://github.com/donaldxdonald/embla-carousel-angular">
<img src="https://www.embla-carousel.com/angular-logo.svg" width="40" height="40" />
</a>
</p>
</div>
<br>
<div align="center">
<strong>
<h2 align="center">Contributors</h2>
</strong>
<p align="center">
Thank you to all contributors for making <a href="https://www.embla-carousel.com/">Embla Carousel</a> awesome! <a href="https://github.com/davidjerleke/embla-carousel/blob/master/CONTRIBUTING.md">Contributions</a> are welcome.
</p>
<p align="center">
<a href="https://github.com/davidjerleke">
<img src="https://avatars2.githubusercontent.com/u/11529148?s=120&v=4" title="davidjerleke" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/Ronit-gurjar">
<img src="https://avatars2.githubusercontent.com/u/92150685?s=120&v=4" title="Ronit-gurjar" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/zaaakher">
<img src="https://avatars2.githubusercontent.com/u/46135573?s=120&v=4" title="zaaakher" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/xiel">
<img src="https://avatars2.githubusercontent.com/u/615522?s=120&v=4" title="xiel" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/javiergonzalezGenially">
<img src="https://avatars2.githubusercontent.com/u/78730098?s=120&v=4" title="javiergonzalezGenially" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/meirroth">
<img src="https://avatars2.githubusercontent.com/u/12494197?s=120&v=4" title="meirroth" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/hamidrezahanafi">
<img src="https://avatars2.githubusercontent.com/u/91487491?s=120&v=4" title="hamidrezahanafi" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/negezor">
<img src="https://avatars2.githubusercontent.com/u/9392723?s=120&v=4" title="negezor" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/th-km">
<img src="https://avatars2.githubusercontent.com/u/35410212?s=120&v=4" title="th-km" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/openscript">
<img src="https://avatars2.githubusercontent.com/u/1105080?s=120&v=4" title="openscript" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/nwidynski">
<img src="https://avatars2.githubusercontent.com/u/25958801?s=120&v=4" title="nwidynski" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/wopian">
<img src="https://avatars2.githubusercontent.com/u/3440094?s=120&v=4" title="wopian" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/horseeyephil">
<img src="https://avatars2.githubusercontent.com/u/32337092?s=120&v=4" title="horseeyephil" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/pipisasa">
<img src="https://avatars2.githubusercontent.com/u/54534600?s=120&v=4" title="pipisasa" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/ranno-lauri">
<img src="https://avatars2.githubusercontent.com/u/87007115?s=120&v=4" title="ranno-lauri" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/ruijdacd">
<img src="https://avatars2.githubusercontent.com/u/9107610?s=120&v=4" title="ruijdacd" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/rojadesign">
<img src="https://avatars2.githubusercontent.com/u/35687281?s=120&v=4" title="rojadesign" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/sadeghbarati">
<img src="https://avatars2.githubusercontent.com/u/17789047?s=120&v=4" title="sadeghbarati" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/S-Shingler">
<img src="https://avatars2.githubusercontent.com/u/48463809?s=120&v=4" title="S-Shingler" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/Yonom">
<img src="https://avatars2.githubusercontent.com/u/1394504?s=120&v=4" title="Yonom" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/smultar">
<img src="https://avatars2.githubusercontent.com/u/6223536?s=120&v=4" title="smultar" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/dev-suraj-kumar">
<img src="https://avatars2.githubusercontent.com/u/184739775?s=120&v=4" title="dev-suraj-kumar" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/tlo-johnson">
<img src="https://avatars2.githubusercontent.com/u/8763144?s=120&v=4" title="tlo-johnson" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/anzbert">
<img src="https://avatars2.githubusercontent.com/u/38823700?s=120&v=4" title="anzbert" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/sarussss">
<img src="https://avatars2.githubusercontent.com/u/15656996?s=120&v=4" title="sarussss" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/silllli">
<img src="https://avatars2.githubusercontent.com/u/9334305?s=120&v=4" title="silllli" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/nikrowell">
<img src="https://avatars2.githubusercontent.com/u/260039?s=120&v=4" title="nikrowell" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/mujahidfa">
<img src="https://avatars2.githubusercontent.com/u/17759705?s=120&v=4" title="mujahidfa" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/romellem">
<img src="https://avatars2.githubusercontent.com/u/8504000?s=120&v=4" title="romellem" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/junlarsen">
<img src="https://avatars2.githubusercontent.com/u/42585241?s=120&v=4" title="junlarsen" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/LucasMariniFalbo">
<img src="https://avatars2.githubusercontent.com/u/9245477?s=120&v=4" title="LucasMariniFalbo" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/LiamMartens">
<img src="https://avatars2.githubusercontent.com/u/5265324?s=120&v=4" title="LiamMartens" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/S1r-Lanzelot">
<img src="https://avatars2.githubusercontent.com/u/4487160?s=120&v=4" title="S1r-Lanzelot" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/ishaqibrahimbot">
<img src="https://avatars2.githubusercontent.com/u/74908398?s=120&v=4" title="ishaqibrahimbot" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/fcasibu">
<img src="https://avatars2.githubusercontent.com/u/75290989?s=120&v=4" title="fcasibu" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/cundd">
<img src="https://avatars2.githubusercontent.com/u/743122?s=120&v=4" title="cundd" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/sedlukha">
<img src="https://avatars2.githubusercontent.com/u/14075940?s=120&v=4" title="sedlukha" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/allen-garvey">
<img src="https://avatars2.githubusercontent.com/u/9314727?s=120&v=4" title="allen-garvey" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/lesha1201">
<img src="https://avatars2.githubusercontent.com/u/10157660?s=120&v=4" title="lesha1201" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/SaizFerri">
<img src="https://avatars2.githubusercontent.com/u/19834971?s=120&v=4" title="SaizFerri" width="50" height="50" style="max-width: 100%" />
</a>
</p>
</div>
<br>
<div align="center">
<strong>
<h2 align="center">Special Thanks</h2>
</strong>
<p align="center">
<sup>
<a href="https://github.com/gunnarx2">gunnarx2</a> - React wrapper <a href="https://www.embla-carousel.com/get-started/react/">useEmblaCarousel</a>.
</sup>
<br>
<sup>
<a href="https://github.com/LiamMartens">LiamMartens</a> - Solid wrapper <a href="https://www.embla-carousel.com/get-started/solid/">createEmblaCarousel</a>.
</sup>
<br>
<sup>
<a href="https://github.com/donaldxdonald">donaldxdonald</a>, <a href="https://github.com/zip-fa">zip-fa</a>, <a href="https://github.com/JeanMeche">JeanMeche</a> - Angular wrapper <a href="https://github.com/donaldxdonald/embla-carousel-angular?tab=readme-ov-file#installation">EmblaCarouselDirective</a>.
</sup>
<br>
<sup>
<a href="https://github.com/xiel">xiel</a> - Plugin <a href="https://github.com/xiel/embla-carousel-wheel-gestures">Embla Carousel Wheel Gestures</a>.
</sup>
<br>
<sup>
<a href="https://github.com/zaaakher">zaaakher</a> - Contributing <a href="https://github.com/davidjerleke/embla-carousel/blob/master/CONTRIBUTING.md">guidelines</a>.
</sup>
<br>
<sup>
<a href="https://github.com/sarussss">sarussss</a> - Answering questions.
</sup>
</p>
</div>
<br>
<h2 align="center">Open Source</h2>
<p align="center">
Embla is <a href="https://github.com/davidjerleke/embla-carousel/blob/master/LICENSE">MIT licensed</a> 💖.<br><br>
<sup>Embla Carousel - Copyright © 2019-present.</sup><br />
<sup>Package created by David Jerleke.</sup>
</p>
<p align="center">
<strong>· · ·</strong>
</p>
<p align="center">
Thanks <a href="https://www.browserstack.com">BrowserStack</a>.
</p>
<p align="center">
<a href="https://www.browserstack.com">
<img src="https://www.embla-carousel.com/browserstack-logo.svg" width="45" height="45" />
</a>
</p> | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/embla-carousel-reactive-utils/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/embla-carousel-reactive-utils/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 13310
} |
<br />
<div align="center">
<p align="center">
<a href="https://www.embla-carousel.com/"><img width="100" height="100" src="https://www.embla-carousel.com/embla-logo.svg" alt="Embla Carousel">
</a>
</p>
<p align="center">
<a href="https://opensource.org/licenses/MIT"><img src="https://img.shields.io/npm/l/embla-carousel?color=%238ab4f8"></a>
<a href="https://www.npmjs.com/package/embla-carousel"><img src="https://img.shields.io/npm/v/embla-carousel.svg?color=%23c1a8e2"></a>
<a href="https://github.com/davidjerleke/embla-carousel/actions?query=workflow%3A%22Continuous+Integration%22"><img src="https://img.shields.io/github/actions/workflow/status/davidjerleke/embla-carousel/cd.yml?color=%238ab4f8"></a>
<a href="https://prettier.io"><img src="https://img.shields.io/badge/code_style-prettier-ff69b4.svg?color=%23c1a8e2"></a>
<a href="https://bundlephobia.com/result?p=embla-carousel@latest"><img src="https://img.shields.io/bundlephobia/minzip/embla-carousel?color=%238ab4f8&label=gzip%20size">
</a>
</p>
<strong>
<h2 align="center">Embla Carousel</h2>
</strong>
<p align="center">
<strong>Embla Carousel</strong> is a bare bones carousel library with great fluid motion and awesome swipe precision. It's library agnostic, dependency free and 100% open source.
</p>
<br>
<p align="center">
<strong>
<code> <a href="https://www.embla-carousel.com/examples/predefined/">Examples</a> </code>
</strong>
</p>
<p align="center">
<strong>
<code> <a href="https://www.embla-carousel.com/examples/generator/">Generator</a> </code>
</strong>
</p>
<p align="center">
<strong>
<code> <a href="https://www.embla-carousel.com/get-started/#choose-installation-type">Installation</a> </code>
</strong>
</p>
</div>
<br>
<div align="center">
<strong>
<h2 align="center">Ready for</h2>
</strong>
<p align="center">
<a href="https://www.embla-carousel.com/get-started/module/">
<img src="https://www.embla-carousel.com/javascript-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/module/">
<img src="https://www.embla-carousel.com/typescript-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/react/">
<img src="https://www.embla-carousel.com/react-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/vue/">
<img src="https://www.embla-carousel.com/vue-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/svelte/">
<img src="https://www.embla-carousel.com/svelte-logo.svg" width="40" height="40" />
</a>
<a href="https://www.embla-carousel.com/get-started/solid/">
<img src="https://www.embla-carousel.com/solid-logo.svg" width="40" height="40" />
</a>
<a href="https://github.com/donaldxdonald/embla-carousel-angular">
<img src="https://www.embla-carousel.com/angular-logo.svg" width="40" height="40" />
</a>
</p>
</div>
<br>
<div align="center">
<strong>
<h2 align="center">Contributors</h2>
</strong>
<p align="center">
Thank you to all contributors for making <a href="https://www.embla-carousel.com/">Embla Carousel</a> awesome! <a href="https://github.com/davidjerleke/embla-carousel/blob/master/CONTRIBUTING.md">Contributions</a> are welcome.
</p>
<p align="center">
<a href="https://github.com/davidjerleke">
<img src="https://avatars2.githubusercontent.com/u/11529148?s=120&v=4" title="davidjerleke" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/Ronit-gurjar">
<img src="https://avatars2.githubusercontent.com/u/92150685?s=120&v=4" title="Ronit-gurjar" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/zaaakher">
<img src="https://avatars2.githubusercontent.com/u/46135573?s=120&v=4" title="zaaakher" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/xiel">
<img src="https://avatars2.githubusercontent.com/u/615522?s=120&v=4" title="xiel" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/javiergonzalezGenially">
<img src="https://avatars2.githubusercontent.com/u/78730098?s=120&v=4" title="javiergonzalezGenially" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/meirroth">
<img src="https://avatars2.githubusercontent.com/u/12494197?s=120&v=4" title="meirroth" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/hamidrezahanafi">
<img src="https://avatars2.githubusercontent.com/u/91487491?s=120&v=4" title="hamidrezahanafi" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/negezor">
<img src="https://avatars2.githubusercontent.com/u/9392723?s=120&v=4" title="negezor" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/th-km">
<img src="https://avatars2.githubusercontent.com/u/35410212?s=120&v=4" title="th-km" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/openscript">
<img src="https://avatars2.githubusercontent.com/u/1105080?s=120&v=4" title="openscript" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/nwidynski">
<img src="https://avatars2.githubusercontent.com/u/25958801?s=120&v=4" title="nwidynski" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/wopian">
<img src="https://avatars2.githubusercontent.com/u/3440094?s=120&v=4" title="wopian" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/horseeyephil">
<img src="https://avatars2.githubusercontent.com/u/32337092?s=120&v=4" title="horseeyephil" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/pipisasa">
<img src="https://avatars2.githubusercontent.com/u/54534600?s=120&v=4" title="pipisasa" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/ranno-lauri">
<img src="https://avatars2.githubusercontent.com/u/87007115?s=120&v=4" title="ranno-lauri" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/ruijdacd">
<img src="https://avatars2.githubusercontent.com/u/9107610?s=120&v=4" title="ruijdacd" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/rojadesign">
<img src="https://avatars2.githubusercontent.com/u/35687281?s=120&v=4" title="rojadesign" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/sadeghbarati">
<img src="https://avatars2.githubusercontent.com/u/17789047?s=120&v=4" title="sadeghbarati" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/S-Shingler">
<img src="https://avatars2.githubusercontent.com/u/48463809?s=120&v=4" title="S-Shingler" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/Yonom">
<img src="https://avatars2.githubusercontent.com/u/1394504?s=120&v=4" title="Yonom" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/smultar">
<img src="https://avatars2.githubusercontent.com/u/6223536?s=120&v=4" title="smultar" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/dev-suraj-kumar">
<img src="https://avatars2.githubusercontent.com/u/184739775?s=120&v=4" title="dev-suraj-kumar" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/tlo-johnson">
<img src="https://avatars2.githubusercontent.com/u/8763144?s=120&v=4" title="tlo-johnson" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/anzbert">
<img src="https://avatars2.githubusercontent.com/u/38823700?s=120&v=4" title="anzbert" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/sarussss">
<img src="https://avatars2.githubusercontent.com/u/15656996?s=120&v=4" title="sarussss" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/silllli">
<img src="https://avatars2.githubusercontent.com/u/9334305?s=120&v=4" title="silllli" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/nikrowell">
<img src="https://avatars2.githubusercontent.com/u/260039?s=120&v=4" title="nikrowell" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/mujahidfa">
<img src="https://avatars2.githubusercontent.com/u/17759705?s=120&v=4" title="mujahidfa" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/romellem">
<img src="https://avatars2.githubusercontent.com/u/8504000?s=120&v=4" title="romellem" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/junlarsen">
<img src="https://avatars2.githubusercontent.com/u/42585241?s=120&v=4" title="junlarsen" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/LucasMariniFalbo">
<img src="https://avatars2.githubusercontent.com/u/9245477?s=120&v=4" title="LucasMariniFalbo" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/LiamMartens">
<img src="https://avatars2.githubusercontent.com/u/5265324?s=120&v=4" title="LiamMartens" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/S1r-Lanzelot">
<img src="https://avatars2.githubusercontent.com/u/4487160?s=120&v=4" title="S1r-Lanzelot" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/ishaqibrahimbot">
<img src="https://avatars2.githubusercontent.com/u/74908398?s=120&v=4" title="ishaqibrahimbot" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/fcasibu">
<img src="https://avatars2.githubusercontent.com/u/75290989?s=120&v=4" title="fcasibu" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/cundd">
<img src="https://avatars2.githubusercontent.com/u/743122?s=120&v=4" title="cundd" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/sedlukha">
<img src="https://avatars2.githubusercontent.com/u/14075940?s=120&v=4" title="sedlukha" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/allen-garvey">
<img src="https://avatars2.githubusercontent.com/u/9314727?s=120&v=4" title="allen-garvey" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/lesha1201">
<img src="https://avatars2.githubusercontent.com/u/10157660?s=120&v=4" title="lesha1201" width="50" height="50" style="max-width: 100%" />
</a><a href="https://github.com/SaizFerri">
<img src="https://avatars2.githubusercontent.com/u/19834971?s=120&v=4" title="SaizFerri" width="50" height="50" style="max-width: 100%" />
</a>
</p>
</div>
<br>
<div align="center">
<strong>
<h2 align="center">Special Thanks</h2>
</strong>
<p align="center">
<sup>
<a href="https://github.com/gunnarx2">gunnarx2</a> - React wrapper <a href="https://www.embla-carousel.com/get-started/react/">useEmblaCarousel</a>.
</sup>
<br>
<sup>
<a href="https://github.com/LiamMartens">LiamMartens</a> - Solid wrapper <a href="https://www.embla-carousel.com/get-started/solid/">createEmblaCarousel</a>.
</sup>
<br>
<sup>
<a href="https://github.com/donaldxdonald">donaldxdonald</a>, <a href="https://github.com/zip-fa">zip-fa</a>, <a href="https://github.com/JeanMeche">JeanMeche</a> - Angular wrapper <a href="https://github.com/donaldxdonald/embla-carousel-angular?tab=readme-ov-file#installation">EmblaCarouselDirective</a>.
</sup>
<br>
<sup>
<a href="https://github.com/xiel">xiel</a> - Plugin <a href="https://github.com/xiel/embla-carousel-wheel-gestures">Embla Carousel Wheel Gestures</a>.
</sup>
<br>
<sup>
<a href="https://github.com/zaaakher">zaaakher</a> - Contributing <a href="https://github.com/davidjerleke/embla-carousel/blob/master/CONTRIBUTING.md">guidelines</a>.
</sup>
<br>
<sup>
<a href="https://github.com/sarussss">sarussss</a> - Answering questions.
</sup>
</p>
</div>
<br>
<h2 align="center">Open Source</h2>
<p align="center">
Embla is <a href="https://github.com/davidjerleke/embla-carousel/blob/master/LICENSE">MIT licensed</a> 💖.<br><br>
<sup>Embla Carousel - Copyright © 2019-present.</sup><br />
<sup>Package created by David Jerleke.</sup>
</p>
<p align="center">
<strong>· · ·</strong>
</p>
<p align="center">
Thanks <a href="https://www.browserstack.com">BrowserStack</a>.
</p>
<p align="center">
<a href="https://www.browserstack.com">
<img src="https://www.embla-carousel.com/browserstack-logo.svg" width="45" height="45" />
</a>
</p> | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/embla-carousel/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/embla-carousel/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 13235
} |
# emoji-regex [](https://travis-ci.org/mathiasbynens/emoji-regex)
_emoji-regex_ offers a regular expression to match all emoji symbols and sequences (including textual representations of emoji) as per the Unicode Standard.
This repository contains a script that generates this regular expression based on [Unicode data](https://github.com/node-unicode/node-unicode-data). Because of this, the regular expression can easily be updated whenever new emoji are added to the Unicode standard.
## Installation
Via [npm](https://www.npmjs.com/):
```bash
npm install emoji-regex
```
In [Node.js](https://nodejs.org/):
```js
const emojiRegex = require('emoji-regex/RGI_Emoji.js');
// Note: because the regular expression has the global flag set, this module
// exports a function that returns the regex rather than exporting the regular
// expression itself, to make it impossible to (accidentally) mutate the
// original regular expression.
const text = `
\u{231A}: ⌚ default emoji presentation character (Emoji_Presentation)
\u{2194}\u{FE0F}: ↔️ default text presentation character rendered as emoji
\u{1F469}: 👩 emoji modifier base (Emoji_Modifier_Base)
\u{1F469}\u{1F3FF}: 👩🏿 emoji modifier base followed by a modifier
`;
const regex = emojiRegex();
let match;
while (match = regex.exec(text)) {
const emoji = match[0];
console.log(`Matched sequence ${ emoji } — code points: ${ [...emoji].length }`);
}
```
Console output:
```
Matched sequence ⌚ — code points: 1
Matched sequence ⌚ — code points: 1
Matched sequence ↔️ — code points: 2
Matched sequence ↔️ — code points: 2
Matched sequence 👩 — code points: 1
Matched sequence 👩 — code points: 1
Matched sequence 👩🏿 — code points: 2
Matched sequence 👩🏿 — code points: 2
```
## Regular expression flavors
The package comes with three distinct regular expressions:
```js
// This is the recommended regular expression to use. It matches all
// emoji recommended for general interchange, as defined via the
// `RGI_Emoji` property in the Unicode Standard.
// https://unicode.org/reports/tr51/#def_rgi_set
// When in doubt, use this!
const emojiRegexRGI = require('emoji-regex/RGI_Emoji.js');
// This is the old regular expression, prior to `RGI_Emoji` being
// standardized. In addition to all `RGI_Emoji` sequences, it matches
// some emoji you probably don’t want to match (such as emoji component
// symbols that are not meant to be used separately).
const emojiRegex = require('emoji-regex/index.js');
// This regular expression matches even more emoji than the previous
// one, including emoji that render as text instead of icons (i.e.
// emoji that are not `Emoji_Presentation` symbols and that aren’t
// forced to render as emoji by a variation selector).
const emojiRegexText = require('emoji-regex/text.js');
```
Additionally, in environments which support ES2015 Unicode escapes, you may `require` ES2015-style versions of the regexes:
```js
const emojiRegexRGI = require('emoji-regex/es2015/RGI_Emoji.js');
const emojiRegex = require('emoji-regex/es2015/index.js');
const emojiRegexText = require('emoji-regex/es2015/text.js');
```
## For maintainers
### How to update emoji-regex after new Unicode Standard releases
1. Update the Unicode data dependency in `package.json` by running the following commands:
```sh
# Example: updating from Unicode v12 to Unicode v13.
npm uninstall @unicode/unicode-12.0.0
npm install @unicode/unicode-13.0.0 --save-dev
````
1. Generate the new output:
```sh
npm run build
```
1. Verify that tests still pass:
```sh
npm test
```
1. Send a pull request with the changes, and get it reviewed & merged.
1. On the `main` branch, bump the emoji-regex version number in `package.json`:
```sh
npm version patch -m 'Release v%s'
```
Instead of `patch`, use `minor` or `major` [as needed](https://semver.org/).
Note that this produces a Git commit + tag.
1. Push the release commit and tag:
```sh
git push
```
Our CI then automatically publishes the new release to npm.
## Author
| [](https://twitter.com/mathias "Follow @mathias on Twitter") |
|---|
| [Mathias Bynens](https://mathiasbynens.be/) |
## License
_emoji-regex_ is available under the [MIT](https://mths.be/mit) license. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/emoji-regex/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/emoji-regex/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 4445
} |
# Encode URL
Encode a URL to a percent-encoded form, excluding already-encoded sequences.
## Installation
```sh
npm install encodeurl
```
## API
```js
var encodeUrl = require('encodeurl')
```
### encodeUrl(url)
Encode a URL to a percent-encoded form, excluding already-encoded sequences.
This function accepts a URL and encodes all the non-URL code points (as UTF-8 byte sequences). It will not encode the "%" character unless it is not part of a valid sequence (`%20` will be left as-is, but `%foo` will be encoded as `%25foo`).
This encode is meant to be "safe" and does not throw errors. It will try as hard as it can to properly encode the given URL, including replacing any raw, unpaired surrogate pairs with the Unicode replacement character prior to encoding.
## Examples
### Encode a URL containing user-controlled data
```js
var encodeUrl = require('encodeurl')
var escapeHtml = require('escape-html')
http.createServer(function onRequest (req, res) {
// get encoded form of inbound url
var url = encodeUrl(req.url)
// create html message
var body = '<p>Location ' + escapeHtml(url) + ' not found</p>'
// send a 404
res.statusCode = 404
res.setHeader('Content-Type', 'text/html; charset=UTF-8')
res.setHeader('Content-Length', String(Buffer.byteLength(body, 'utf-8')))
res.end(body, 'utf-8')
})
```
### Encode a URL for use in a header field
```js
var encodeUrl = require('encodeurl')
var escapeHtml = require('escape-html')
var url = require('url')
http.createServer(function onRequest (req, res) {
// parse inbound url
var href = url.parse(req)
// set new host for redirect
href.host = 'localhost'
href.protocol = 'https:'
href.slashes = true
// create location header
var location = encodeUrl(url.format(href))
// create html message
var body = '<p>Redirecting to new site: ' + escapeHtml(location) + '</p>'
// send a 301
res.statusCode = 301
res.setHeader('Content-Type', 'text/html; charset=UTF-8')
res.setHeader('Content-Length', String(Buffer.byteLength(body, 'utf-8')))
res.setHeader('Location', location)
res.end(body, 'utf-8')
})
```
## Similarities
This function is _similar_ to the intrinsic function `encodeURI`. However, it will not encode:
* The `\`, `^`, or `|` characters
* The `%` character when it's part of a valid sequence
* `[` and `]` (for IPv6 hostnames)
* Replaces raw, unpaired surrogate pairs with the Unicode replacement character
As a result, the encoding aligns closely with the behavior in the [WHATWG URL specification][whatwg-url]. However, this package only encodes strings and does not do any URL parsing or formatting.
It is expected that any output from `new URL(url)` will not change when used with this package, as the output has already been encoded. Additionally, if we were to encode before `new URL(url)`, we do not expect the before and after encoded formats to be parsed any differently.
## Testing
```sh
$ npm test
$ npm run lint
```
## References
- [RFC 3986: Uniform Resource Identifier (URI): Generic Syntax][rfc-3986]
- [WHATWG URL Living Standard][whatwg-url]
[rfc-3986]: https://tools.ietf.org/html/rfc3986
[whatwg-url]: https://url.spec.whatwg.org/
## License
[MIT](LICENSE) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/encodeurl/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/encodeurl/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 3220
} |
# Changelog
All notable changes to this project will be documented in this file.
The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/)
and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html).
## v1.0.0 - 2024-02-12
### Commits
- Initial implementation, tests, readme, types [`3e154e1`](https://github.com/ljharb/es-define-property/commit/3e154e11a2fee09127220f5e503bf2c0a31dd480)
- Initial commit [`07d98de`](https://github.com/ljharb/es-define-property/commit/07d98de34a4dc31ff5e83a37c0c3f49e0d85cd50)
- npm init [`c4eb634`](https://github.com/ljharb/es-define-property/commit/c4eb6348b0d3886aac36cef34ad2ee0665ea6f3e)
- Only apps should have lockfiles [`7af86ec`](https://github.com/ljharb/es-define-property/commit/7af86ec1d311ec0b17fdfe616a25f64276903856) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/es-define-property/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/es-define-property/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 821
} |
# es-define-property <sup>[![Version Badge][npm-version-svg]][package-url]</sup>
[![github actions][actions-image]][actions-url]
[![coverage][codecov-image]][codecov-url]
[![License][license-image]][license-url]
[![Downloads][downloads-image]][downloads-url]
[![npm badge][npm-badge-png]][package-url]
`Object.defineProperty`, but not IE 8's broken one.
## Example
```js
const assert = require('assert');
const $defineProperty = require('es-define-property');
if ($defineProperty) {
assert.equal($defineProperty, Object.defineProperty);
} else if (Object.defineProperty) {
assert.equal($defineProperty, false, 'this is IE 8');
} else {
assert.equal($defineProperty, false, 'this is an ES3 engine');
}
```
## Tests
Simply clone the repo, `npm install`, and run `npm test`
## Security
Please email [@ljharb](https://github.com/ljharb) or see https://tidelift.com/security if you have a potential security vulnerability to report.
[package-url]: https://npmjs.org/package/es-define-property
[npm-version-svg]: https://versionbadg.es/ljharb/es-define-property.svg
[deps-svg]: https://david-dm.org/ljharb/es-define-property.svg
[deps-url]: https://david-dm.org/ljharb/es-define-property
[dev-deps-svg]: https://david-dm.org/ljharb/es-define-property/dev-status.svg
[dev-deps-url]: https://david-dm.org/ljharb/es-define-property#info=devDependencies
[npm-badge-png]: https://nodei.co/npm/es-define-property.png?downloads=true&stars=true
[license-image]: https://img.shields.io/npm/l/es-define-property.svg
[license-url]: LICENSE
[downloads-image]: https://img.shields.io/npm/dm/es-define-property.svg
[downloads-url]: https://npm-stat.com/charts.html?package=es-define-property
[codecov-image]: https://codecov.io/gh/ljharb/es-define-property/branch/main/graphs/badge.svg
[codecov-url]: https://app.codecov.io/gh/ljharb/es-define-property/
[actions-image]: https://img.shields.io/endpoint?url=https://github-actions-badge-u3jn4tfpocch.runkit.sh/ljharb/es-define-property
[actions-url]: https://github.com/ljharb/es-define-property/actions | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/es-define-property/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/es-define-property/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2055
} |
# Changelog
All notable changes to this project will be documented in this file.
The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/)
and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html).
## [v1.3.0](https://github.com/ljharb/es-errors/compare/v1.2.1...v1.3.0) - 2024-02-05
### Commits
- [New] add `EvalError` and `URIError` [`1927627`](https://github.com/ljharb/es-errors/commit/1927627ba68cb6c829d307231376c967db53acdf)
## [v1.2.1](https://github.com/ljharb/es-errors/compare/v1.2.0...v1.2.1) - 2024-02-04
### Commits
- [Fix] add missing `exports` entry [`5bb5f28`](https://github.com/ljharb/es-errors/commit/5bb5f280f98922701109d6ebb82eea2257cecc7e)
## [v1.2.0](https://github.com/ljharb/es-errors/compare/v1.1.0...v1.2.0) - 2024-02-04
### Commits
- [New] add `ReferenceError` [`6d8cf5b`](https://github.com/ljharb/es-errors/commit/6d8cf5bbb6f3f598d02cf6f30e468ba2caa8e143)
## [v1.1.0](https://github.com/ljharb/es-errors/compare/v1.0.0...v1.1.0) - 2024-02-04
### Commits
- [New] add base Error [`2983ab6`](https://github.com/ljharb/es-errors/commit/2983ab65f7bc5441276cb021dc3aa03c78881698)
## v1.0.0 - 2024-02-03
### Commits
- Initial implementation, tests, readme, type [`8f47631`](https://github.com/ljharb/es-errors/commit/8f476317e9ad76f40ad648081829b1a1a3a1288b)
- Initial commit [`ea5d099`](https://github.com/ljharb/es-errors/commit/ea5d099ef18e550509ab9e2be000526afd81c385)
- npm init [`6f5ebf9`](https://github.com/ljharb/es-errors/commit/6f5ebf9cead474dadd72b9e63dad315820a089ae)
- Only apps should have lockfiles [`e1a0aeb`](https://github.com/ljharb/es-errors/commit/e1a0aeb7b80f5cfc56be54d6b2100e915d47def8)
- [meta] add `sideEffects` flag [`a9c7d46`](https://github.com/ljharb/es-errors/commit/a9c7d460a492f1d8a241c836bc25a322a19cc043) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/es-errors/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/es-errors/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1832
} |
# es-errors <sup>[![Version Badge][npm-version-svg]][package-url]</sup>
[![github actions][actions-image]][actions-url]
[![coverage][codecov-image]][codecov-url]
[![License][license-image]][license-url]
[![Downloads][downloads-image]][downloads-url]
[![npm badge][npm-badge-png]][package-url]
A simple cache for a few of the JS Error constructors.
## Example
```js
const assert = require('assert');
const Base = require('es-errors');
const Eval = require('es-errors/eval');
const Range = require('es-errors/range');
const Ref = require('es-errors/ref');
const Syntax = require('es-errors/syntax');
const Type = require('es-errors/type');
const URI = require('es-errors/uri');
assert.equal(Base, Error);
assert.equal(Eval, EvalError);
assert.equal(Range, RangeError);
assert.equal(Ref, ReferenceError);
assert.equal(Syntax, SyntaxError);
assert.equal(Type, TypeError);
assert.equal(URI, URIError);
```
## Tests
Simply clone the repo, `npm install`, and run `npm test`
## Security
Please email [@ljharb](https://github.com/ljharb) or see https://tidelift.com/security if you have a potential security vulnerability to report.
[package-url]: https://npmjs.org/package/es-errors
[npm-version-svg]: https://versionbadg.es/ljharb/es-errors.svg
[deps-svg]: https://david-dm.org/ljharb/es-errors.svg
[deps-url]: https://david-dm.org/ljharb/es-errors
[dev-deps-svg]: https://david-dm.org/ljharb/es-errors/dev-status.svg
[dev-deps-url]: https://david-dm.org/ljharb/es-errors#info=devDependencies
[npm-badge-png]: https://nodei.co/npm/es-errors.png?downloads=true&stars=true
[license-image]: https://img.shields.io/npm/l/es-errors.svg
[license-url]: LICENSE
[downloads-image]: https://img.shields.io/npm/dm/es-errors.svg
[downloads-url]: https://npm-stat.com/charts.html?package=es-errors
[codecov-image]: https://codecov.io/gh/ljharb/es-errors/branch/main/graphs/badge.svg
[codecov-url]: https://app.codecov.io/gh/ljharb/es-errors/
[actions-image]: https://img.shields.io/endpoint?url=https://github-actions-badge-u3jn4tfpocch.runkit.sh/ljharb/es-errors
[actions-url]: https://github.com/ljharb/es-errors/actions | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/es-errors/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/es-errors/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2113
} |
# esbuild-register
[](https://npm.im/esbuild-register) [](https://npm.im/esbuild-register)
## Install
```bash
npm i esbuild esbuild-register -D
# Or Yarn
yarn add esbuild esbuild-register --dev
# Or pnpm
pnpm add esbuild esbuild-register -D
```
## Usage
```bash
node -r esbuild-register file.ts
```
It will use `jsxFactory`, `jsxFragmentFactory` and `target` options from your `tsconfig.json`
### Experimental loader support
When using in a project with `type: "module"` in `package.json`, you need the `--loader` flag to load TypeScript files:
```bash
node --loader esbuild-register/loader -r esbuild-register ./file.ts
```
## Programmatic Usage
```ts
const { register } = require('esbuild-register/dist/node')
const { unregister } = register({
// ...options
})
// Unregister the require hook if you don't need it anymore
unregister()
```
## Sponsors
[](https://github.com/sponsors/egoist)
## License
MIT © [EGOIST](https://egoist.dev) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/esbuild-register/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/esbuild-register/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1137
} |
MIT License
Copyright (c) 2020 Evan Wallace
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/esbuild/LICENSE.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/esbuild/LICENSE.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1068
} |
# esbuild
This is a JavaScript bundler and minifier. See https://github.com/evanw/esbuild and the [JavaScript API documentation](https://esbuild.github.io/api/) for details. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/esbuild/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/esbuild/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 174
} |
# escalade [](https://github.com/lukeed/escalade/actions) [](https://licenses.dev/npm/escalade) [](https://codecov.io/gh/lukeed/escalade)
> A tiny (183B to 210B) and [fast](#benchmarks) utility to ascend parent directories
With [escalade](https://en.wikipedia.org/wiki/Escalade), you can scale parent directories until you've found what you're looking for.<br>Given an input file or directory, `escalade` will continue executing your callback function until either:
1) the callback returns a truthy value
2) `escalade` has reached the system root directory (eg, `/`)
> **Important:**<br>Please note that `escalade` only deals with direct ancestry – it will not dive into parents' sibling directories.
---
**Notice:** As of v3.1.0, `escalade` now includes [Deno support](http://deno.land/x/escalade)! Please see [Deno Usage](#deno) below.
---
## Install
```
$ npm install --save escalade
```
## Modes
There are two "versions" of `escalade` available:
#### "async"
> **Node.js:** >= 8.x<br>
> **Size (gzip):** 210 bytes<br>
> **Availability:** [CommonJS](https://unpkg.com/escalade/dist/index.js), [ES Module](https://unpkg.com/escalade/dist/index.mjs)
This is the primary/default mode. It makes use of `async`/`await` and [`util.promisify`](https://nodejs.org/api/util.html#util_util_promisify_original).
#### "sync"
> **Node.js:** >= 6.x<br>
> **Size (gzip):** 183 bytes<br>
> **Availability:** [CommonJS](https://unpkg.com/escalade/sync/index.js), [ES Module](https://unpkg.com/escalade/sync/index.mjs)
This is the opt-in mode, ideal for scenarios where `async` usage cannot be supported.
## Usage
***Example Structure***
```
/Users/lukeed
└── oss
├── license
└── escalade
├── package.json
└── test
└── fixtures
├── index.js
└── foobar
└── demo.js
```
***Example Usage***
```js
//~> demo.js
import { join } from 'path';
import escalade from 'escalade';
const input = join(__dirname, 'demo.js');
// or: const input = __dirname;
const pkg = await escalade(input, (dir, names) => {
console.log('~> dir:', dir);
console.log('~> names:', names);
console.log('---');
if (names.includes('package.json')) {
// will be resolved into absolute
return 'package.json';
}
});
//~> dir: /Users/lukeed/oss/escalade/test/fixtures/foobar
//~> names: ['demo.js']
//---
//~> dir: /Users/lukeed/oss/escalade/test/fixtures
//~> names: ['index.js', 'foobar']
//---
//~> dir: /Users/lukeed/oss/escalade/test
//~> names: ['fixtures']
//---
//~> dir: /Users/lukeed/oss/escalade
//~> names: ['package.json', 'test']
//---
console.log(pkg);
//=> /Users/lukeed/oss/escalade/package.json
// Now search for "missing123.txt"
// (Assume it doesn't exist anywhere!)
const missing = await escalade(input, (dir, names) => {
console.log('~> dir:', dir);
return names.includes('missing123.txt') && 'missing123.txt';
});
//~> dir: /Users/lukeed/oss/escalade/test/fixtures/foobar
//~> dir: /Users/lukeed/oss/escalade/test/fixtures
//~> dir: /Users/lukeed/oss/escalade/test
//~> dir: /Users/lukeed/oss/escalade
//~> dir: /Users/lukeed/oss
//~> dir: /Users/lukeed
//~> dir: /Users
//~> dir: /
console.log(missing);
//=> undefined
```
> **Note:** To run the above example with "sync" mode, import from `escalade/sync` and remove the `await` keyword.
## API
### escalade(input, callback)
Returns: `string|void` or `Promise<string|void>`
When your `callback` locates a file, `escalade` will resolve/return with an absolute path.<br>
If your `callback` was never satisfied, then `escalade` will resolve/return with nothing (undefined).
> **Important:**<br>The `sync` and `async` versions share the same API.<br>The **only** difference is that `sync` is not Promise-based.
#### input
Type: `string`
The path from which to start ascending.
This may be a file or a directory path.<br>However, when `input` is a file, `escalade` will begin with its parent directory.
> **Important:** Unless given an absolute path, `input` will be resolved from `process.cwd()` location.
#### callback
Type: `Function`
The callback to execute for each ancestry level. It always is given two arguments:
1) `dir` - an absolute path of the current parent directory
2) `names` - a list (`string[]`) of contents _relative to_ the `dir` parent
> **Note:** The `names` list can contain names of files _and_ directories.
When your callback returns a _falsey_ value, then `escalade` will continue with `dir`'s parent directory, re-invoking your callback with new argument values.
When your callback returns a string, then `escalade` stops iteration immediately.<br>
If the string is an absolute path, then it's left as is. Otherwise, the string is resolved into an absolute path _from_ the `dir` that housed the satisfying condition.
> **Important:** Your `callback` can be a `Promise/AsyncFunction` when using the "async" version of `escalade`.
## Benchmarks
> Running on Node.js v10.13.0
```
# Load Time
find-up 3.891ms
escalade 0.485ms
escalade/sync 0.309ms
# Levels: 6 (target = "foo.txt"):
find-up x 24,856 ops/sec ±6.46% (55 runs sampled)
escalade x 73,084 ops/sec ±4.23% (73 runs sampled)
find-up.sync x 3,663 ops/sec ±1.12% (83 runs sampled)
escalade/sync x 9,360 ops/sec ±0.62% (88 runs sampled)
# Levels: 12 (target = "package.json"):
find-up x 29,300 ops/sec ±10.68% (70 runs sampled)
escalade x 73,685 ops/sec ± 5.66% (66 runs sampled)
find-up.sync x 1,707 ops/sec ± 0.58% (91 runs sampled)
escalade/sync x 4,667 ops/sec ± 0.68% (94 runs sampled)
# Levels: 18 (target = "missing123.txt"):
find-up x 21,818 ops/sec ±17.37% (14 runs sampled)
escalade x 67,101 ops/sec ±21.60% (20 runs sampled)
find-up.sync x 1,037 ops/sec ± 2.86% (88 runs sampled)
escalade/sync x 1,248 ops/sec ± 0.50% (93 runs sampled)
```
## Deno
As of v3.1.0, `escalade` is available on the Deno registry.
Please note that the [API](#api) is identical and that there are still [two modes](#modes) from which to choose:
```ts
// Choose "async" mode
import escalade from 'https://deno.land/escalade/async.ts';
// Choose "sync" mode
import escalade from 'https://deno.land/escalade/sync.ts';
```
> **Important:** The `allow-read` permission is required!
## Related
- [premove](https://github.com/lukeed/premove) - A tiny (247B) utility to remove items recursively
- [totalist](https://github.com/lukeed/totalist) - A tiny (195B to 224B) utility to recursively list all (total) files in a directory
- [mk-dirs](https://github.com/lukeed/mk-dirs) - A tiny (420B) utility to make a directory and its parents, recursively
## License
MIT © [Luke Edwards](https://lukeed.com) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/escalade/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/escalade/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 6923
} |
# escape-html
Escape string for use in HTML
## Example
```js
var escape = require('escape-html');
var html = escape('foo & bar');
// -> foo & bar
```
## Benchmark
```
$ npm run-script bench
> [email protected] bench nodejs-escape-html
> node benchmark/index.js
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
modules@11
[email protected]
1 test completed.
2 tests completed.
3 tests completed.
no special characters x 19,435,271 ops/sec ±0.85% (187 runs sampled)
single special character x 6,132,421 ops/sec ±0.67% (194 runs sampled)
many special characters x 3,175,826 ops/sec ±0.65% (193 runs sampled)
```
## License
MIT | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/escape-html/Readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/escape-html/Readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 703
} |
1.8.1 / 2017-09-12
==================
* perf: replace regular expression with substring
1.8.0 / 2017-02-18
==================
* Use SHA1 instead of MD5 for ETag hashing
- Improves performance for larger entities
- Works with FIPS 140-2 OpenSSL configuration
1.7.0 / 2015-06-08
==================
* Always include entity length in ETags for hash length extensions
* Generate non-Stats ETags using MD5 only (no longer CRC32)
* Improve stat performance by removing hashing
* Remove base64 padding in ETags to shorten
* Use MD5 instead of MD4 in weak ETags over 1KB
1.6.0 / 2015-05-10
==================
* Improve support for JXcore
* Remove requirement of `atime` in the stats object
* Support "fake" stats objects in environments without `fs`
1.5.1 / 2014-11-19
==================
* deps: [email protected]
- Minor fixes
1.5.0 / 2014-10-14
==================
* Improve string performance
* Slightly improve speed for weak ETags over 1KB
1.4.0 / 2014-09-21
==================
* Support "fake" stats objects
* Support Node.js 0.6
1.3.1 / 2014-09-14
==================
* Use the (new and improved) `crc` for crc32
1.3.0 / 2014-08-29
==================
* Default strings to strong ETags
* Improve speed for weak ETags over 1KB
1.2.1 / 2014-08-29
==================
* Use the (much faster) `buffer-crc32` for crc32
1.2.0 / 2014-08-24
==================
* Add support for file stat objects
1.1.0 / 2014-08-24
==================
* Add fast-path for empty entity
* Add weak ETag generation
* Shrink size of generated ETags
1.0.1 / 2014-08-24
==================
* Fix behavior of string containing Unicode
1.0.0 / 2014-05-18
==================
* Initial release | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/etag/HISTORY.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/etag/HISTORY.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1731
} |
# etag
[![NPM Version][npm-image]][npm-url]
[![NPM Downloads][downloads-image]][downloads-url]
[![Node.js Version][node-version-image]][node-version-url]
[![Build Status][travis-image]][travis-url]
[![Test Coverage][coveralls-image]][coveralls-url]
Create simple HTTP ETags
This module generates HTTP ETags (as defined in RFC 7232) for use in
HTTP responses.
## Installation
This is a [Node.js](https://nodejs.org/en/) module available through the
[npm registry](https://www.npmjs.com/). Installation is done using the
[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
```sh
$ npm install etag
```
## API
<!-- eslint-disable no-unused-vars -->
```js
var etag = require('etag')
```
### etag(entity, [options])
Generate a strong ETag for the given entity. This should be the complete
body of the entity. Strings, `Buffer`s, and `fs.Stats` are accepted. By
default, a strong ETag is generated except for `fs.Stats`, which will
generate a weak ETag (this can be overwritten by `options.weak`).
<!-- eslint-disable no-undef -->
```js
res.setHeader('ETag', etag(body))
```
#### Options
`etag` accepts these properties in the options object.
##### weak
Specifies if the generated ETag will include the weak validator mark (that
is, the leading `W/`). The actual entity tag is the same. The default value
is `false`, unless the `entity` is `fs.Stats`, in which case it is `true`.
## Testing
```sh
$ npm test
```
## Benchmark
```bash
$ npm run-script bench
> [email protected] bench nodejs-etag
> node benchmark/index.js
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
modules@48
[email protected]
> node benchmark/body0-100b.js
100B body
4 tests completed.
buffer - strong x 258,647 ops/sec ±1.07% (180 runs sampled)
buffer - weak x 263,812 ops/sec ±0.61% (184 runs sampled)
string - strong x 259,955 ops/sec ±1.19% (185 runs sampled)
string - weak x 264,356 ops/sec ±1.09% (184 runs sampled)
> node benchmark/body1-1kb.js
1KB body
4 tests completed.
buffer - strong x 189,018 ops/sec ±1.12% (182 runs sampled)
buffer - weak x 190,586 ops/sec ±0.81% (186 runs sampled)
string - strong x 144,272 ops/sec ±0.96% (188 runs sampled)
string - weak x 145,380 ops/sec ±1.43% (187 runs sampled)
> node benchmark/body2-5kb.js
5KB body
4 tests completed.
buffer - strong x 92,435 ops/sec ±0.42% (188 runs sampled)
buffer - weak x 92,373 ops/sec ±0.58% (189 runs sampled)
string - strong x 48,850 ops/sec ±0.56% (186 runs sampled)
string - weak x 49,380 ops/sec ±0.56% (190 runs sampled)
> node benchmark/body3-10kb.js
10KB body
4 tests completed.
buffer - strong x 55,989 ops/sec ±0.93% (188 runs sampled)
buffer - weak x 56,148 ops/sec ±0.55% (190 runs sampled)
string - strong x 27,345 ops/sec ±0.43% (188 runs sampled)
string - weak x 27,496 ops/sec ±0.45% (190 runs sampled)
> node benchmark/body4-100kb.js
100KB body
4 tests completed.
buffer - strong x 7,083 ops/sec ±0.22% (190 runs sampled)
buffer - weak x 7,115 ops/sec ±0.26% (191 runs sampled)
string - strong x 3,068 ops/sec ±0.34% (190 runs sampled)
string - weak x 3,096 ops/sec ±0.35% (190 runs sampled)
> node benchmark/stats.js
stat
4 tests completed.
real - strong x 871,642 ops/sec ±0.34% (189 runs sampled)
real - weak x 867,613 ops/sec ±0.39% (190 runs sampled)
fake - strong x 401,051 ops/sec ±0.40% (189 runs sampled)
fake - weak x 400,100 ops/sec ±0.47% (188 runs sampled)
```
## License
[MIT](LICENSE)
[npm-image]: https://img.shields.io/npm/v/etag.svg
[npm-url]: https://npmjs.org/package/etag
[node-version-image]: https://img.shields.io/node/v/etag.svg
[node-version-url]: https://nodejs.org/en/download/
[travis-image]: https://img.shields.io/travis/jshttp/etag/master.svg
[travis-url]: https://travis-ci.org/jshttp/etag
[coveralls-image]: https://img.shields.io/coveralls/jshttp/etag/master.svg
[coveralls-url]: https://coveralls.io/r/jshttp/etag?branch=master
[downloads-image]: https://img.shields.io/npm/dm/etag.svg
[downloads-url]: https://npmjs.org/package/etag | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/etag/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/etag/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 4173
} |
# EventEmitter3
[](https://www.npmjs.com/package/eventemitter3)[](https://travis-ci.org/primus/eventemitter3)[](https://david-dm.org/primus/eventemitter3)[](https://coveralls.io/r/primus/eventemitter3?branch=master)[](https://webchat.freenode.net/?channels=primus)
[](https://saucelabs.com/u/eventemitter3)
EventEmitter3 is a high performance EventEmitter. It has been micro-optimized
for various of code paths making this, one of, if not the fastest EventEmitter
available for Node.js and browsers. The module is API compatible with the
EventEmitter that ships by default with Node.js but there are some slight
differences:
- Domain support has been removed.
- We do not `throw` an error when you emit an `error` event and nobody is
listening.
- The `newListener` and `removeListener` events have been removed as they
are useful only in some uncommon use-cases.
- The `setMaxListeners`, `getMaxListeners`, `prependListener` and
`prependOnceListener` methods are not available.
- Support for custom context for events so there is no need to use `fn.bind`.
- The `removeListener` method removes all matching listeners, not only the
first.
It's a drop in replacement for existing EventEmitters, but just faster. Free
performance, who wouldn't want that? The EventEmitter is written in EcmaScript 3
so it will work in the oldest browsers and node versions that you need to
support.
## Installation
```bash
$ npm install --save eventemitter3
```
## CDN
Recommended CDN:
```text
https://unpkg.com/eventemitter3@latest/umd/eventemitter3.min.js
```
## Usage
After installation the only thing you need to do is require the module:
```js
var EventEmitter = require('eventemitter3');
```
And you're ready to create your own EventEmitter instances. For the API
documentation, please follow the official Node.js documentation:
http://nodejs.org/api/events.html
### Contextual emits
We've upgraded the API of the `EventEmitter.on`, `EventEmitter.once` and
`EventEmitter.removeListener` to accept an extra argument which is the `context`
or `this` value that should be set for the emitted events. This means you no
longer have the overhead of an event that required `fn.bind` in order to get a
custom `this` value.
```js
var EE = new EventEmitter()
, context = { foo: 'bar' };
function emitted() {
console.log(this === context); // true
}
EE.once('event-name', emitted, context);
EE.on('another-event', emitted, context);
EE.removeListener('another-event', emitted, context);
```
### Tests and benchmarks
This module is well tested. You can run:
- `npm test` to run the tests under Node.js.
- `npm run test-browser` to run the tests in real browsers via Sauce Labs.
We also have a set of benchmarks to compare EventEmitter3 with some available
alternatives. To run the benchmarks run `npm run benchmark`.
Tests and benchmarks are not included in the npm package. If you want to play
with them you have to clone the GitHub repository.
Note that you will have to run an additional `npm i` in the benchmarks folder
before `npm run benchmark`.
## License
[MIT](LICENSE) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/eventemitter3/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/eventemitter3/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 3635
} |
1.18.1 / 2024-10-08
==========
* deps: [email protected]
- Fix object assignment of `hasOwnProperty`
* deps: [email protected]
- Allow leading dot for domain
- Although not permitted in the spec, some users expect this to work and user agents ignore the leading dot according to spec
- Add fast path for `serialize` without options, use `obj.hasOwnProperty` when parsing
* deps: [email protected]
- perf: parse cookies ~10% faster
- fix: narrow the validation of cookies to match RFC6265
- fix: add `main` to `package.json` for rspack
1.18.0 / 2024-01-28
===================
* Add debug log for pathname mismatch
* Add `partitioned` to `cookie` options
* Add `priority` to `cookie` options
* Fix handling errors from setting cookie
* Support any type in `secret` that `crypto.createHmac` supports
* deps: [email protected]
- Fix `expires` option to reject invalid dates
- perf: improve default decode speed
- perf: remove slow string split in parse
* deps: [email protected]
1.17.3 / 2022-05-11
===================
* Fix resaving already-saved new session at end of request
* deps: [email protected]
1.17.2 / 2021-05-19
===================
* Fix `res.end` patch to always commit headers
* deps: [email protected]
* deps: [email protected]
1.17.1 / 2020-04-16
===================
* Fix internal method wrapping error on failed reloads
1.17.0 / 2019-10-10
===================
* deps: [email protected]
- Add `SameSite=None` support
* deps: [email protected]
1.16.2 / 2019-06-12
===================
* Fix restoring `cookie.originalMaxAge` when store returns `Date`
* deps: parseurl@~1.3.3
1.16.1 / 2019-04-11
===================
* Fix error passing `data` option to `Cookie` constructor
* Fix uncaught error from bad session data
1.16.0 / 2019-04-10
===================
* Catch invalid `cookie.maxAge` value earlier
* Deprecate setting `cookie.maxAge` to a `Date` object
* Fix issue where `resave: false` may not save altered sessions
* Remove `utils-merge` dependency
* Use `safe-buffer` for improved Buffer API
* Use `Set-Cookie` as cookie header name for compatibility
* deps: depd@~2.0.0
- Replace internal `eval` usage with `Function` constructor
- Use instance methods on `process` to check for listeners
- perf: remove argument reassignment
* deps: on-headers@~1.0.2
- Fix `res.writeHead` patch missing return value
1.15.6 / 2017-09-26
===================
* deps: [email protected]
* deps: parseurl@~1.3.2
- perf: reduce overhead for full URLs
- perf: unroll the "fast-path" `RegExp`
* deps: uid-safe@~2.1.5
- perf: remove only trailing `=`
* deps: [email protected]
1.15.5 / 2017-08-02
===================
* Fix `TypeError` when `req.url` is an empty string
* deps: depd@~1.1.1
- Remove unnecessary `Buffer` loading
1.15.4 / 2017-07-18
===================
* deps: [email protected]
1.15.3 / 2017-05-17
===================
* deps: [email protected]
- deps: [email protected]
1.15.2 / 2017-03-26
===================
* deps: [email protected]
- Fix `DEBUG_MAX_ARRAY_LENGTH`
* deps: uid-safe@~2.1.4
- Remove `base64-url` dependency
1.15.1 / 2017-02-10
===================
* deps: [email protected]
- Fix deprecation messages in WebStorm and other editors
- Undeprecate `DEBUG_FD` set to `1` or `2`
1.15.0 / 2017-01-22
===================
* Fix detecting modified session when session contains "cookie" property
* Fix resaving already-saved reloaded session at end of request
* deps: [email protected]
- perf: use `Buffer.from` when available
* deps: [email protected]
- Allow colors in workers
- Deprecated `DEBUG_FD` environment variable
- Use same color for same namespace
- Fix error when running under React Native
- deps: [email protected]
* perf: remove unreachable branch in set-cookie method
1.14.2 / 2016-10-30
===================
* deps: [email protected]
- Fix deprecation warning in Node.js 7.x
* deps: uid-safe@~2.1.3
- deps: [email protected]
1.14.1 / 2016-08-24
===================
* Fix not always resetting session max age before session save
* Fix the cookie `sameSite` option to actually alter the `Set-Cookie`
* deps: uid-safe@~2.1.2
- deps: [email protected]
1.14.0 / 2016-07-01
===================
* Correctly inherit from `EventEmitter` class in `Store` base class
* Fix issue where `Set-Cookie` `Expires` was not always updated
* Methods are no longer enumerable on `req.session` object
* deps: [email protected]
- Add `sameSite` option
- Improve error message when `encode` is not a function
- Improve error message when `expires` is not a `Date`
- perf: enable strict mode
- perf: use for loop in parse
- perf: use string concatination for serialization
* deps: parseurl@~1.3.1
- perf: enable strict mode
* deps: uid-safe@~2.1.1
- Use `random-bytes` for byte source
- deps: [email protected]
* perf: enable strict mode
* perf: remove argument reassignment
1.13.0 / 2016-01-10
===================
* Fix `rolling: true` to not set cookie when no session exists
- Better `saveUninitialized: false` + `rolling: true` behavior
* deps: [email protected]
1.12.1 / 2015-10-29
===================
* deps: [email protected]
- Fix cookie `Max-Age` to never be a floating point number
1.12.0 / 2015-10-25
===================
* Support the value `'auto'` in the `cookie.secure` option
* deps: [email protected]
- Throw on invalid values provided to `serialize`
* deps: depd@~1.1.0
- Enable strict mode in more places
- Support web browser loading
* deps: on-headers@~1.0.1
- perf: enable strict mode
1.11.3 / 2015-05-22
===================
* deps: [email protected]
- Slight optimizations
* deps: [email protected]
1.11.2 / 2015-05-10
===================
* deps: debug@~2.2.0
- deps: [email protected]
* deps: uid-safe@~2.0.0
1.11.1 / 2015-04-08
===================
* Fix mutating `options.secret` value
1.11.0 / 2015-04-07
===================
* Support an array in `secret` option for key rotation
* deps: depd@~1.0.1
1.10.4 / 2015-03-15
===================
* deps: debug@~2.1.3
- Fix high intensity foreground color for bold
- deps: [email protected]
1.10.3 / 2015-02-16
===================
* deps: [email protected]
* deps: [email protected]
- Use `crypto.randomBytes`, if available
- deps: [email protected]
1.10.2 / 2015-01-31
===================
* deps: [email protected]
- Fix error branch that would throw
- deps: [email protected]
1.10.1 / 2015-01-08
===================
* deps: [email protected]
- Remove dependency on `mz`
1.10.0 / 2015-01-05
===================
* Add `store.touch` interface for session stores
* Fix `MemoryStore` expiration with `resave: false`
* deps: debug@~2.1.1
1.9.3 / 2014-12-02
==================
* Fix error when `req.sessionID` contains a non-string value
1.9.2 / 2014-11-22
==================
* deps: [email protected]
- Minor fixes
1.9.1 / 2014-10-22
==================
* Remove unnecessary empty write call
- Fixes Node.js 0.11.14 behavior change
- Helps work-around Node.js 0.10.1 zlib bug
1.9.0 / 2014-09-16
==================
* deps: debug@~2.1.0
- Implement `DEBUG_FD` env variable support
* deps: depd@~1.0.0
1.8.2 / 2014-09-15
==================
* Use `crc` instead of `buffer-crc32` for speed
* deps: [email protected]
1.8.1 / 2014-09-08
==================
* Keep `req.session.save` non-enumerable
* Prevent session prototype methods from being overwritten
1.8.0 / 2014-09-07
==================
* Do not resave already-saved session at end of request
* deps: [email protected]
* deps: debug@~2.0.0
1.7.6 / 2014-08-18
==================
* Fix exception on `res.end(null)` calls
1.7.5 / 2014-08-10
==================
* Fix parsing original URL
* deps: on-headers@~1.0.0
* deps: parseurl@~1.3.0
1.7.4 / 2014-08-05
==================
* Fix response end delay for non-chunked responses
1.7.3 / 2014-08-05
==================
* Fix `res.end` patch to call correct upstream `res.write`
1.7.2 / 2014-07-27
==================
* deps: [email protected]
- Work-around v8 generating empty stack traces
1.7.1 / 2014-07-26
==================
* deps: [email protected]
- Fix exception when global `Error.stackTraceLimit` is too low
1.7.0 / 2014-07-22
==================
* Improve session-ending error handling
- Errors are passed to `next(err)` instead of `console.error`
* deps: [email protected]
* deps: [email protected]
- Add `TRACE_DEPRECATION` environment variable
- Remove non-standard grey color from color output
- Support `--no-deprecation` argument
- Support `--trace-deprecation` argument
1.6.5 / 2014-07-11
==================
* Do not require `req.originalUrl`
* deps: [email protected]
- Add support for multiple wildcards in namespaces
1.6.4 / 2014-07-07
==================
* Fix blank responses for stores with synchronous operations
1.6.3 / 2014-07-04
==================
* Fix resave deprecation message
1.6.2 / 2014-07-04
==================
* Fix confusing option deprecation messages
1.6.1 / 2014-06-28
==================
* Fix saveUninitialized deprecation message
1.6.0 / 2014-06-28
==================
* Add deprecation message to undefined `resave` option
* Add deprecation message to undefined `saveUninitialized` option
* Fix `res.end` patch to return correct value
* Fix `res.end` patch to handle multiple `res.end` calls
* Reject cookies with missing signatures
1.5.2 / 2014-06-26
==================
* deps: [email protected]
- fix for timing attacks
1.5.1 / 2014-06-21
==================
* Move hard-to-track-down `req.secret` deprecation message
1.5.0 / 2014-06-19
==================
* Debug name is now "express-session"
* Deprecate integration with `cookie-parser` middleware
* Deprecate looking for secret in `req.secret`
* Directly read cookies; `cookie-parser` no longer required
* Directly set cookies; `res.cookie` no longer required
* Generate session IDs with `uid-safe`, faster and even less collisions
1.4.0 / 2014-06-17
==================
* Add `genid` option to generate custom session IDs
* Add `saveUninitialized` option to control saving uninitialized sessions
* Add `unset` option to control unsetting `req.session`
* Generate session IDs with `rand-token` by default; reduce collisions
* deps: [email protected]
1.3.1 / 2014-06-14
==================
* Add description in package for npmjs.org listing
1.3.0 / 2014-06-14
==================
* Integrate with express "trust proxy" by default
* deps: [email protected]
1.2.1 / 2014-05-27
==================
* Fix `resave` such that `resave: true` works
1.2.0 / 2014-05-19
==================
* Add `resave` option to control saving unmodified sessions
1.1.0 / 2014-05-12
==================
* Add `name` option; replacement for `key` option
* Use `setImmediate` in MemoryStore for node.js >= 0.10
1.0.4 / 2014-04-27
==================
* deps: [email protected]
1.0.3 / 2014-04-19
==================
* Use `res.cookie()` instead of `res.setHeader()`
* deps: [email protected]
1.0.2 / 2014-02-23
==================
* Add missing dependency to `package.json`
1.0.1 / 2014-02-15
==================
* Add missing dependencies to `package.json`
1.0.0 / 2014-02-15
==================
* Genesis from `connect` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express-session/HISTORY.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express-session/HISTORY.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 11415
} |
# express-session
[![NPM Version][npm-version-image]][npm-url]
[![NPM Downloads][npm-downloads-image]][node-url]
[![Build Status][ci-image]][ci-url]
[![Test Coverage][coveralls-image]][coveralls-url]
## Installation
This is a [Node.js](https://nodejs.org/en/) module available through the
[npm registry](https://www.npmjs.com/). Installation is done using the
[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
```sh
$ npm install express-session
```
## API
```js
var session = require('express-session')
```
### session(options)
Create a session middleware with the given `options`.
**Note** Session data is _not_ saved in the cookie itself, just the session ID.
Session data is stored server-side.
**Note** Since version 1.5.0, the [`cookie-parser` middleware](https://www.npmjs.com/package/cookie-parser)
no longer needs to be used for this module to work. This module now directly reads
and writes cookies on `req`/`res`. Using `cookie-parser` may result in issues
if the `secret` is not the same between this module and `cookie-parser`.
**Warning** The default server-side session storage, `MemoryStore`, is _purposely_
not designed for a production environment. It will leak memory under most
conditions, does not scale past a single process, and is meant for debugging and
developing.
For a list of stores, see [compatible session stores](#compatible-session-stores).
#### Options
`express-session` accepts these properties in the options object.
##### cookie
Settings object for the session ID cookie. The default value is
`{ path: '/', httpOnly: true, secure: false, maxAge: null }`.
The following are options that can be set in this object.
##### cookie.domain
Specifies the value for the `Domain` `Set-Cookie` attribute. By default, no domain
is set, and most clients will consider the cookie to apply to only the current
domain.
##### cookie.expires
Specifies the `Date` object to be the value for the `Expires` `Set-Cookie` attribute.
By default, no expiration is set, and most clients will consider this a
"non-persistent cookie" and will delete it on a condition like exiting a web browser
application.
**Note** If both `expires` and `maxAge` are set in the options, then the last one
defined in the object is what is used.
**Note** The `expires` option should not be set directly; instead only use the `maxAge`
option.
##### cookie.httpOnly
Specifies the `boolean` value for the `HttpOnly` `Set-Cookie` attribute. When truthy,
the `HttpOnly` attribute is set, otherwise it is not. By default, the `HttpOnly`
attribute is set.
**Note** be careful when setting this to `true`, as compliant clients will not allow
client-side JavaScript to see the cookie in `document.cookie`.
##### cookie.maxAge
Specifies the `number` (in milliseconds) to use when calculating the `Expires`
`Set-Cookie` attribute. This is done by taking the current server time and adding
`maxAge` milliseconds to the value to calculate an `Expires` datetime. By default,
no maximum age is set.
**Note** If both `expires` and `maxAge` are set in the options, then the last one
defined in the object is what is used.
##### cookie.partitioned
Specifies the `boolean` value for the [`Partitioned` `Set-Cookie`](rfc-cutler-httpbis-partitioned-cookies)
attribute. When truthy, the `Partitioned` attribute is set, otherwise it is not.
By default, the `Partitioned` attribute is not set.
**Note** This is an attribute that has not yet been fully standardized, and may
change in the future. This also means many clients may ignore this attribute until
they understand it.
More information about can be found in [the proposal](https://github.com/privacycg/CHIPS).
##### cookie.path
Specifies the value for the `Path` `Set-Cookie`. By default, this is set to `'/'`, which
is the root path of the domain.
##### cookie.priority
Specifies the `string` to be the value for the [`Priority` `Set-Cookie` attribute][rfc-west-cookie-priority-00-4.1].
- `'low'` will set the `Priority` attribute to `Low`.
- `'medium'` will set the `Priority` attribute to `Medium`, the default priority when not set.
- `'high'` will set the `Priority` attribute to `High`.
More information about the different priority levels can be found in
[the specification][rfc-west-cookie-priority-00-4.1].
**Note** This is an attribute that has not yet been fully standardized, and may change in the future.
This also means many clients may ignore this attribute until they understand it.
##### cookie.sameSite
Specifies the `boolean` or `string` to be the value for the `SameSite` `Set-Cookie` attribute.
By default, this is `false`.
- `true` will set the `SameSite` attribute to `Strict` for strict same site enforcement.
- `false` will not set the `SameSite` attribute.
- `'lax'` will set the `SameSite` attribute to `Lax` for lax same site enforcement.
- `'none'` will set the `SameSite` attribute to `None` for an explicit cross-site cookie.
- `'strict'` will set the `SameSite` attribute to `Strict` for strict same site enforcement.
More information about the different enforcement levels can be found in
[the specification][rfc-6265bis-03-4.1.2.7].
**Note** This is an attribute that has not yet been fully standardized, and may change in
the future. This also means many clients may ignore this attribute until they understand it.
**Note** There is a [draft spec](https://tools.ietf.org/html/draft-west-cookie-incrementalism-01)
that requires that the `Secure` attribute be set to `true` when the `SameSite` attribute has been
set to `'none'`. Some web browsers or other clients may be adopting this specification.
##### cookie.secure
Specifies the `boolean` value for the `Secure` `Set-Cookie` attribute. When truthy,
the `Secure` attribute is set, otherwise it is not. By default, the `Secure`
attribute is not set.
**Note** be careful when setting this to `true`, as compliant clients will not send
the cookie back to the server in the future if the browser does not have an HTTPS
connection.
Please note that `secure: true` is a **recommended** option. However, it requires
an https-enabled website, i.e., HTTPS is necessary for secure cookies. If `secure`
is set, and you access your site over HTTP, the cookie will not be set. If you
have your node.js behind a proxy and are using `secure: true`, you need to set
"trust proxy" in express:
```js
var app = express()
app.set('trust proxy', 1) // trust first proxy
app.use(session({
secret: 'keyboard cat',
resave: false,
saveUninitialized: true,
cookie: { secure: true }
}))
```
For using secure cookies in production, but allowing for testing in development,
the following is an example of enabling this setup based on `NODE_ENV` in express:
```js
var app = express()
var sess = {
secret: 'keyboard cat',
cookie: {}
}
if (app.get('env') === 'production') {
app.set('trust proxy', 1) // trust first proxy
sess.cookie.secure = true // serve secure cookies
}
app.use(session(sess))
```
The `cookie.secure` option can also be set to the special value `'auto'` to have
this setting automatically match the determined security of the connection. Be
careful when using this setting if the site is available both as HTTP and HTTPS,
as once the cookie is set on HTTPS, it will no longer be visible over HTTP. This
is useful when the Express `"trust proxy"` setting is properly setup to simplify
development vs production configuration.
##### genid
Function to call to generate a new session ID. Provide a function that returns
a string that will be used as a session ID. The function is given `req` as the
first argument if you want to use some value attached to `req` when generating
the ID.
The default value is a function which uses the `uid-safe` library to generate IDs.
**NOTE** be careful to generate unique IDs so your sessions do not conflict.
```js
app.use(session({
genid: function(req) {
return genuuid() // use UUIDs for session IDs
},
secret: 'keyboard cat'
}))
```
##### name
The name of the session ID cookie to set in the response (and read from in the
request).
The default value is `'connect.sid'`.
**Note** if you have multiple apps running on the same hostname (this is just
the name, i.e. `localhost` or `127.0.0.1`; different schemes and ports do not
name a different hostname), then you need to separate the session cookies from
each other. The simplest method is to simply set different `name`s per app.
##### proxy
Trust the reverse proxy when setting secure cookies (via the "X-Forwarded-Proto"
header).
The default value is `undefined`.
- `true` The "X-Forwarded-Proto" header will be used.
- `false` All headers are ignored and the connection is considered secure only
if there is a direct TLS/SSL connection.
- `undefined` Uses the "trust proxy" setting from express
##### resave
Forces the session to be saved back to the session store, even if the session
was never modified during the request. Depending on your store this may be
necessary, but it can also create race conditions where a client makes two
parallel requests to your server and changes made to the session in one
request may get overwritten when the other request ends, even if it made no
changes (this behavior also depends on what store you're using).
The default value is `true`, but using the default has been deprecated,
as the default will change in the future. Please research into this setting
and choose what is appropriate to your use-case. Typically, you'll want
`false`.
How do I know if this is necessary for my store? The best way to know is to
check with your store if it implements the `touch` method. If it does, then
you can safely set `resave: false`. If it does not implement the `touch`
method and your store sets an expiration date on stored sessions, then you
likely need `resave: true`.
##### rolling
Force the session identifier cookie to be set on every response. The expiration
is reset to the original [`maxAge`](#cookiemaxage), resetting the expiration
countdown.
The default value is `false`.
With this enabled, the session identifier cookie will expire in
[`maxAge`](#cookiemaxage) since the last response was sent instead of in
[`maxAge`](#cookiemaxage) since the session was last modified by the server.
This is typically used in conjuction with short, non-session-length
[`maxAge`](#cookiemaxage) values to provide a quick timeout of the session data
with reduced potential of it occurring during on going server interactions.
**Note** When this option is set to `true` but the `saveUninitialized` option is
set to `false`, the cookie will not be set on a response with an uninitialized
session. This option only modifies the behavior when an existing session was
loaded for the request.
##### saveUninitialized
Forces a session that is "uninitialized" to be saved to the store. A session is
uninitialized when it is new but not modified. Choosing `false` is useful for
implementing login sessions, reducing server storage usage, or complying with
laws that require permission before setting a cookie. Choosing `false` will also
help with race conditions where a client makes multiple parallel requests
without a session.
The default value is `true`, but using the default has been deprecated, as the
default will change in the future. Please research into this setting and
choose what is appropriate to your use-case.
**Note** if you are using Session in conjunction with PassportJS, Passport
will add an empty Passport object to the session for use after a user is
authenticated, which will be treated as a modification to the session, causing
it to be saved. *This has been fixed in PassportJS 0.3.0*
##### secret
**Required option**
This is the secret used to sign the session ID cookie. The secret can be any type
of value that is supported by Node.js `crypto.createHmac` (like a string or a
`Buffer`). This can be either a single secret, or an array of multiple secrets. If
an array of secrets is provided, only the first element will be used to sign the
session ID cookie, while all the elements will be considered when verifying the
signature in requests. The secret itself should be not easily parsed by a human and
would best be a random set of characters. A best practice may include:
- The use of environment variables to store the secret, ensuring the secret itself
does not exist in your repository.
- Periodic updates of the secret, while ensuring the previous secret is in the
array.
Using a secret that cannot be guessed will reduce the ability to hijack a session to
only guessing the session ID (as determined by the `genid` option).
Changing the secret value will invalidate all existing sessions. In order to rotate
the secret without invalidating sessions, provide an array of secrets, with the new
secret as first element of the array, and including previous secrets as the later
elements.
**Note** HMAC-256 is used to sign the session ID. For this reason, the secret should
contain at least 32 bytes of entropy.
##### store
The session store instance, defaults to a new `MemoryStore` instance.
##### unset
Control the result of unsetting `req.session` (through `delete`, setting to `null`,
etc.).
The default value is `'keep'`.
- `'destroy'` The session will be destroyed (deleted) when the response ends.
- `'keep'` The session in the store will be kept, but modifications made during
the request are ignored and not saved.
### req.session
To store or access session data, simply use the request property `req.session`,
which is (generally) serialized as JSON by the store, so nested objects
are typically fine. For example below is a user-specific view counter:
```js
// Use the session middleware
app.use(session({ secret: 'keyboard cat', cookie: { maxAge: 60000 }}))
// Access the session as req.session
app.get('/', function(req, res, next) {
if (req.session.views) {
req.session.views++
res.setHeader('Content-Type', 'text/html')
res.write('<p>views: ' + req.session.views + '</p>')
res.write('<p>expires in: ' + (req.session.cookie.maxAge / 1000) + 's</p>')
res.end()
} else {
req.session.views = 1
res.end('welcome to the session demo. refresh!')
}
})
```
#### Session.regenerate(callback)
To regenerate the session simply invoke the method. Once complete,
a new SID and `Session` instance will be initialized at `req.session`
and the `callback` will be invoked.
```js
req.session.regenerate(function(err) {
// will have a new session here
})
```
#### Session.destroy(callback)
Destroys the session and will unset the `req.session` property.
Once complete, the `callback` will be invoked.
```js
req.session.destroy(function(err) {
// cannot access session here
})
```
#### Session.reload(callback)
Reloads the session data from the store and re-populates the
`req.session` object. Once complete, the `callback` will be invoked.
```js
req.session.reload(function(err) {
// session updated
})
```
#### Session.save(callback)
Save the session back to the store, replacing the contents on the store with the
contents in memory (though a store may do something else--consult the store's
documentation for exact behavior).
This method is automatically called at the end of the HTTP response if the
session data has been altered (though this behavior can be altered with various
options in the middleware constructor). Because of this, typically this method
does not need to be called.
There are some cases where it is useful to call this method, for example,
redirects, long-lived requests or in WebSockets.
```js
req.session.save(function(err) {
// session saved
})
```
#### Session.touch()
Updates the `.maxAge` property. Typically this is
not necessary to call, as the session middleware does this for you.
### req.session.id
Each session has a unique ID associated with it. This property is an
alias of [`req.sessionID`](#reqsessionid-1) and cannot be modified.
It has been added to make the session ID accessible from the `session`
object.
### req.session.cookie
Each session has a unique cookie object accompany it. This allows
you to alter the session cookie per visitor. For example we can
set `req.session.cookie.expires` to `false` to enable the cookie
to remain for only the duration of the user-agent.
#### Cookie.maxAge
Alternatively `req.session.cookie.maxAge` will return the time
remaining in milliseconds, which we may also re-assign a new value
to adjust the `.expires` property appropriately. The following
are essentially equivalent
```js
var hour = 3600000
req.session.cookie.expires = new Date(Date.now() + hour)
req.session.cookie.maxAge = hour
```
For example when `maxAge` is set to `60000` (one minute), and 30 seconds
has elapsed it will return `30000` until the current request has completed,
at which time `req.session.touch()` is called to reset
`req.session.cookie.maxAge` to its original value.
```js
req.session.cookie.maxAge // => 30000
```
#### Cookie.originalMaxAge
The `req.session.cookie.originalMaxAge` property returns the original
`maxAge` (time-to-live), in milliseconds, of the session cookie.
### req.sessionID
To get the ID of the loaded session, access the request property
`req.sessionID`. This is simply a read-only value set when a session
is loaded/created.
## Session Store Implementation
Every session store _must_ be an `EventEmitter` and implement specific
methods. The following methods are the list of **required**, **recommended**,
and **optional**.
* Required methods are ones that this module will always call on the store.
* Recommended methods are ones that this module will call on the store if
available.
* Optional methods are ones this module does not call at all, but helps
present uniform stores to users.
For an example implementation view the [connect-redis](http://github.com/visionmedia/connect-redis) repo.
### store.all(callback)
**Optional**
This optional method is used to get all sessions in the store as an array. The
`callback` should be called as `callback(error, sessions)`.
### store.destroy(sid, callback)
**Required**
This required method is used to destroy/delete a session from the store given
a session ID (`sid`). The `callback` should be called as `callback(error)` once
the session is destroyed.
### store.clear(callback)
**Optional**
This optional method is used to delete all sessions from the store. The
`callback` should be called as `callback(error)` once the store is cleared.
### store.length(callback)
**Optional**
This optional method is used to get the count of all sessions in the store.
The `callback` should be called as `callback(error, len)`.
### store.get(sid, callback)
**Required**
This required method is used to get a session from the store given a session
ID (`sid`). The `callback` should be called as `callback(error, session)`.
The `session` argument should be a session if found, otherwise `null` or
`undefined` if the session was not found (and there was no error). A special
case is made when `error.code === 'ENOENT'` to act like `callback(null, null)`.
### store.set(sid, session, callback)
**Required**
This required method is used to upsert a session into the store given a
session ID (`sid`) and session (`session`) object. The callback should be
called as `callback(error)` once the session has been set in the store.
### store.touch(sid, session, callback)
**Recommended**
This recommended method is used to "touch" a given session given a
session ID (`sid`) and session (`session`) object. The `callback` should be
called as `callback(error)` once the session has been touched.
This is primarily used when the store will automatically delete idle sessions
and this method is used to signal to the store the given session is active,
potentially resetting the idle timer.
## Compatible Session Stores
The following modules implement a session store that is compatible with this
module. Please make a PR to add additional modules :)
[![★][aerospike-session-store-image] aerospike-session-store][aerospike-session-store-url] A session store using [Aerospike](http://www.aerospike.com/).
[aerospike-session-store-url]: https://www.npmjs.com/package/aerospike-session-store
[aerospike-session-store-image]: https://badgen.net/github/stars/aerospike/aerospike-session-store-expressjs?label=%E2%98%85
[![★][better-sqlite3-session-store-image] better-sqlite3-session-store][better-sqlite3-session-store-url] A session store based on [better-sqlite3](https://github.com/JoshuaWise/better-sqlite3).
[better-sqlite3-session-store-url]: https://www.npmjs.com/package/better-sqlite3-session-store
[better-sqlite3-session-store-image]: https://badgen.net/github/stars/timdaub/better-sqlite3-session-store?label=%E2%98%85
[![★][cassandra-store-image] cassandra-store][cassandra-store-url] An Apache Cassandra-based session store.
[cassandra-store-url]: https://www.npmjs.com/package/cassandra-store
[cassandra-store-image]: https://badgen.net/github/stars/webcc/cassandra-store?label=%E2%98%85
[![★][cluster-store-image] cluster-store][cluster-store-url] A wrapper for using in-process / embedded
stores - such as SQLite (via knex), leveldb, files, or memory - with node cluster (desirable for Raspberry Pi 2
and other multi-core embedded devices).
[cluster-store-url]: https://www.npmjs.com/package/cluster-store
[cluster-store-image]: https://badgen.net/github/stars/coolaj86/cluster-store?label=%E2%98%85
[![★][connect-arango-image] connect-arango][connect-arango-url] An ArangoDB-based session store.
[connect-arango-url]: https://www.npmjs.com/package/connect-arango
[connect-arango-image]: https://badgen.net/github/stars/AlexanderArvidsson/connect-arango?label=%E2%98%85
[![★][connect-azuretables-image] connect-azuretables][connect-azuretables-url] An [Azure Table Storage](https://azure.microsoft.com/en-gb/services/storage/tables/)-based session store.
[connect-azuretables-url]: https://www.npmjs.com/package/connect-azuretables
[connect-azuretables-image]: https://badgen.net/github/stars/mike-goodwin/connect-azuretables?label=%E2%98%85
[![★][connect-cloudant-store-image] connect-cloudant-store][connect-cloudant-store-url] An [IBM Cloudant](https://cloudant.com/)-based session store.
[connect-cloudant-store-url]: https://www.npmjs.com/package/connect-cloudant-store
[connect-cloudant-store-image]: https://badgen.net/github/stars/adriantanasa/connect-cloudant-store?label=%E2%98%85
[![★][connect-cosmosdb-image] connect-cosmosdb][connect-cosmosdb-url] An Azure [Cosmos DB](https://azure.microsoft.com/en-us/products/cosmos-db/)-based session store.
[connect-cosmosdb-url]: https://www.npmjs.com/package/connect-cosmosdb
[connect-cosmosdb-image]: https://badgen.net/github/stars/thekillingspree/connect-cosmosdb?label=%E2%98%85
[![★][connect-couchbase-image] connect-couchbase][connect-couchbase-url] A [couchbase](http://www.couchbase.com/)-based session store.
[connect-couchbase-url]: https://www.npmjs.com/package/connect-couchbase
[connect-couchbase-image]: https://badgen.net/github/stars/christophermina/connect-couchbase?label=%E2%98%85
[![★][connect-datacache-image] connect-datacache][connect-datacache-url] An [IBM Bluemix Data Cache](http://www.ibm.com/cloud-computing/bluemix/)-based session store.
[connect-datacache-url]: https://www.npmjs.com/package/connect-datacache
[connect-datacache-image]: https://badgen.net/github/stars/adriantanasa/connect-datacache?label=%E2%98%85
[![★][@google-cloud/connect-datastore-image] @google-cloud/connect-datastore][@google-cloud/connect-datastore-url] A [Google Cloud Datastore](https://cloud.google.com/datastore/docs/concepts/overview)-based session store.
[@google-cloud/connect-datastore-url]: https://www.npmjs.com/package/@google-cloud/connect-datastore
[@google-cloud/connect-datastore-image]: https://badgen.net/github/stars/GoogleCloudPlatform/cloud-datastore-session-node?label=%E2%98%85
[![★][connect-db2-image] connect-db2][connect-db2-url] An IBM DB2-based session store built using [ibm_db](https://www.npmjs.com/package/ibm_db) module.
[connect-db2-url]: https://www.npmjs.com/package/connect-db2
[connect-db2-image]: https://badgen.net/github/stars/wallali/connect-db2?label=%E2%98%85
[![★][connect-dynamodb-image] connect-dynamodb][connect-dynamodb-url] A DynamoDB-based session store.
[connect-dynamodb-url]: https://www.npmjs.com/package/connect-dynamodb
[connect-dynamodb-image]: https://badgen.net/github/stars/ca98am79/connect-dynamodb?label=%E2%98%85
[![★][@google-cloud/connect-firestore-image] @google-cloud/connect-firestore][@google-cloud/connect-firestore-url] A [Google Cloud Firestore](https://cloud.google.com/firestore/docs/overview)-based session store.
[@google-cloud/connect-firestore-url]: https://www.npmjs.com/package/@google-cloud/connect-firestore
[@google-cloud/connect-firestore-image]: https://badgen.net/github/stars/googleapis/nodejs-firestore-session?label=%E2%98%85
[![★][connect-hazelcast-image] connect-hazelcast][connect-hazelcast-url] Hazelcast session store for Connect and Express.
[connect-hazelcast-url]: https://www.npmjs.com/package/connect-hazelcast
[connect-hazelcast-image]: https://badgen.net/github/stars/huseyinbabal/connect-hazelcast?label=%E2%98%85
[![★][connect-loki-image] connect-loki][connect-loki-url] A Loki.js-based session store.
[connect-loki-url]: https://www.npmjs.com/package/connect-loki
[connect-loki-image]: https://badgen.net/github/stars/Requarks/connect-loki?label=%E2%98%85
[![★][connect-lowdb-image] connect-lowdb][connect-lowdb-url] A lowdb-based session store.
[connect-lowdb-url]: https://www.npmjs.com/package/connect-lowdb
[connect-lowdb-image]: https://badgen.net/github/stars/travishorn/connect-lowdb?label=%E2%98%85
[![★][connect-memcached-image] connect-memcached][connect-memcached-url] A memcached-based session store.
[connect-memcached-url]: https://www.npmjs.com/package/connect-memcached
[connect-memcached-image]: https://badgen.net/github/stars/balor/connect-memcached?label=%E2%98%85
[![★][connect-memjs-image] connect-memjs][connect-memjs-url] A memcached-based session store using
[memjs](https://www.npmjs.com/package/memjs) as the memcached client.
[connect-memjs-url]: https://www.npmjs.com/package/connect-memjs
[connect-memjs-image]: https://badgen.net/github/stars/liamdon/connect-memjs?label=%E2%98%85
[![★][connect-ml-image] connect-ml][connect-ml-url] A MarkLogic Server-based session store.
[connect-ml-url]: https://www.npmjs.com/package/connect-ml
[connect-ml-image]: https://badgen.net/github/stars/bluetorch/connect-ml?label=%E2%98%85
[![★][connect-monetdb-image] connect-monetdb][connect-monetdb-url] A MonetDB-based session store.
[connect-monetdb-url]: https://www.npmjs.com/package/connect-monetdb
[connect-monetdb-image]: https://badgen.net/github/stars/MonetDB/npm-connect-monetdb?label=%E2%98%85
[![★][connect-mongo-image] connect-mongo][connect-mongo-url] A MongoDB-based session store.
[connect-mongo-url]: https://www.npmjs.com/package/connect-mongo
[connect-mongo-image]: https://badgen.net/github/stars/kcbanner/connect-mongo?label=%E2%98%85
[![★][connect-mongodb-session-image] connect-mongodb-session][connect-mongodb-session-url] Lightweight MongoDB-based session store built and maintained by MongoDB.
[connect-mongodb-session-url]: https://www.npmjs.com/package/connect-mongodb-session
[connect-mongodb-session-image]: https://badgen.net/github/stars/mongodb-js/connect-mongodb-session?label=%E2%98%85
[![★][connect-mssql-v2-image] connect-mssql-v2][connect-mssql-v2-url] A Microsoft SQL Server-based session store based on [connect-mssql](https://www.npmjs.com/package/connect-mssql).
[connect-mssql-v2-url]: https://www.npmjs.com/package/connect-mssql-v2
[connect-mssql-v2-image]: https://badgen.net/github/stars/jluboff/connect-mssql-v2?label=%E2%98%85
[![★][connect-neo4j-image] connect-neo4j][connect-neo4j-url] A [Neo4j](https://neo4j.com)-based session store.
[connect-neo4j-url]: https://www.npmjs.com/package/connect-neo4j
[connect-neo4j-image]: https://badgen.net/github/stars/MaxAndersson/connect-neo4j?label=%E2%98%85
[![★][connect-ottoman-image] connect-ottoman][connect-ottoman-url] A [couchbase ottoman](http://www.couchbase.com/)-based session store.
[connect-ottoman-url]: https://www.npmjs.com/package/connect-ottoman
[connect-ottoman-image]: https://badgen.net/github/stars/noiissyboy/connect-ottoman?label=%E2%98%85
[![★][connect-pg-simple-image] connect-pg-simple][connect-pg-simple-url] A PostgreSQL-based session store.
[connect-pg-simple-url]: https://www.npmjs.com/package/connect-pg-simple
[connect-pg-simple-image]: https://badgen.net/github/stars/voxpelli/node-connect-pg-simple?label=%E2%98%85
[![★][connect-redis-image] connect-redis][connect-redis-url] A Redis-based session store.
[connect-redis-url]: https://www.npmjs.com/package/connect-redis
[connect-redis-image]: https://badgen.net/github/stars/tj/connect-redis?label=%E2%98%85
[![★][connect-session-firebase-image] connect-session-firebase][connect-session-firebase-url] A session store based on the [Firebase Realtime Database](https://firebase.google.com/docs/database/)
[connect-session-firebase-url]: https://www.npmjs.com/package/connect-session-firebase
[connect-session-firebase-image]: https://badgen.net/github/stars/benweier/connect-session-firebase?label=%E2%98%85
[![★][connect-session-knex-image] connect-session-knex][connect-session-knex-url] A session store using
[Knex.js](http://knexjs.org/), which is a SQL query builder for PostgreSQL, MySQL, MariaDB, SQLite3, and Oracle.
[connect-session-knex-url]: https://www.npmjs.com/package/connect-session-knex
[connect-session-knex-image]: https://badgen.net/github/stars/llambda/connect-session-knex?label=%E2%98%85
[![★][connect-session-sequelize-image] connect-session-sequelize][connect-session-sequelize-url] A session store using
[Sequelize.js](http://sequelizejs.com/), which is a Node.js / io.js ORM for PostgreSQL, MySQL, SQLite and MSSQL.
[connect-session-sequelize-url]: https://www.npmjs.com/package/connect-session-sequelize
[connect-session-sequelize-image]: https://badgen.net/github/stars/mweibel/connect-session-sequelize?label=%E2%98%85
[![★][connect-sqlite3-image] connect-sqlite3][connect-sqlite3-url] A [SQLite3](https://github.com/mapbox/node-sqlite3) session store modeled after the TJ's `connect-redis` store.
[connect-sqlite3-url]: https://www.npmjs.com/package/connect-sqlite3
[connect-sqlite3-image]: https://badgen.net/github/stars/rawberg/connect-sqlite3?label=%E2%98%85
[![★][connect-typeorm-image] connect-typeorm][connect-typeorm-url] A [TypeORM](https://github.com/typeorm/typeorm)-based session store.
[connect-typeorm-url]: https://www.npmjs.com/package/connect-typeorm
[connect-typeorm-image]: https://badgen.net/github/stars/makepost/connect-typeorm?label=%E2%98%85
[![★][couchdb-expression-image] couchdb-expression][couchdb-expression-url] A [CouchDB](https://couchdb.apache.org/)-based session store.
[couchdb-expression-url]: https://www.npmjs.com/package/couchdb-expression
[couchdb-expression-image]: https://badgen.net/github/stars/tkshnwesper/couchdb-expression?label=%E2%98%85
[![★][dynamodb-store-image] dynamodb-store][dynamodb-store-url] A DynamoDB-based session store.
[dynamodb-store-url]: https://www.npmjs.com/package/dynamodb-store
[dynamodb-store-image]: https://badgen.net/github/stars/rafaelrpinto/dynamodb-store?label=%E2%98%85
[![★][dynamodb-store-v3-image] dynamodb-store-v3][dynamodb-store-v3-url] Implementation of a session store using DynamoDB backed by the [AWS SDK for JavaScript v3](https://github.com/aws/aws-sdk-js-v3).
[dynamodb-store-v3-url]: https://www.npmjs.com/package/dynamodb-store-v3
[dynamodb-store-v3-image]: https://badgen.net/github/stars/FryDay/dynamodb-store-v3?label=%E2%98%85
[![★][express-etcd-image] express-etcd][express-etcd-url] An [etcd](https://github.com/stianeikeland/node-etcd) based session store.
[express-etcd-url]: https://www.npmjs.com/package/express-etcd
[express-etcd-image]: https://badgen.net/github/stars/gildean/express-etcd?label=%E2%98%85
[![★][express-mysql-session-image] express-mysql-session][express-mysql-session-url] A session store using native
[MySQL](https://www.mysql.com/) via the [node-mysql](https://github.com/felixge/node-mysql) module.
[express-mysql-session-url]: https://www.npmjs.com/package/express-mysql-session
[express-mysql-session-image]: https://badgen.net/github/stars/chill117/express-mysql-session?label=%E2%98%85
[![★][express-nedb-session-image] express-nedb-session][express-nedb-session-url] A NeDB-based session store.
[express-nedb-session-url]: https://www.npmjs.com/package/express-nedb-session
[express-nedb-session-image]: https://badgen.net/github/stars/louischatriot/express-nedb-session?label=%E2%98%85
[![★][express-oracle-session-image] express-oracle-session][express-oracle-session-url] A session store using native
[oracle](https://www.oracle.com/) via the [node-oracledb](https://www.npmjs.com/package/oracledb) module.
[express-oracle-session-url]: https://www.npmjs.com/package/express-oracle-session
[express-oracle-session-image]: https://badgen.net/github/stars/slumber86/express-oracle-session?label=%E2%98%85
[![★][express-session-cache-manager-image] express-session-cache-manager][express-session-cache-manager-url]
A store that implements [cache-manager](https://www.npmjs.com/package/cache-manager), which supports
a [variety of storage types](https://www.npmjs.com/package/cache-manager#store-engines).
[express-session-cache-manager-url]: https://www.npmjs.com/package/express-session-cache-manager
[express-session-cache-manager-image]: https://badgen.net/github/stars/theogravity/express-session-cache-manager?label=%E2%98%85
[![★][express-session-etcd3-image] express-session-etcd3][express-session-etcd3-url] An [etcd3](https://github.com/mixer/etcd3) based session store.
[express-session-etcd3-url]: https://www.npmjs.com/package/express-session-etcd3
[express-session-etcd3-image]: https://badgen.net/github/stars/willgm/express-session-etcd3?label=%E2%98%85
[![★][express-session-level-image] express-session-level][express-session-level-url] A [LevelDB](https://github.com/Level/levelup) based session store.
[express-session-level-url]: https://www.npmjs.com/package/express-session-level
[express-session-level-image]: https://badgen.net/github/stars/tgohn/express-session-level?label=%E2%98%85
[![★][express-session-rsdb-image] express-session-rsdb][express-session-rsdb-url] Session store based on Rocket-Store: A very simple, super fast and yet powerfull, flat file database.
[express-session-rsdb-url]: https://www.npmjs.com/package/express-session-rsdb
[express-session-rsdb-image]: https://badgen.net/github/stars/paragi/express-session-rsdb?label=%E2%98%85
[![★][express-sessions-image] express-sessions][express-sessions-url] A session store supporting both MongoDB and Redis.
[express-sessions-url]: https://www.npmjs.com/package/express-sessions
[express-sessions-image]: https://badgen.net/github/stars/konteck/express-sessions?label=%E2%98%85
[![★][firestore-store-image] firestore-store][firestore-store-url] A [Firestore](https://github.com/hendrysadrak/firestore-store)-based session store.
[firestore-store-url]: https://www.npmjs.com/package/firestore-store
[firestore-store-image]: https://badgen.net/github/stars/hendrysadrak/firestore-store?label=%E2%98%85
[![★][fortune-session-image] fortune-session][fortune-session-url] A [Fortune.js](https://github.com/fortunejs/fortune)
based session store. Supports all backends supported by Fortune (MongoDB, Redis, Postgres, NeDB).
[fortune-session-url]: https://www.npmjs.com/package/fortune-session
[fortune-session-image]: https://badgen.net/github/stars/aliceklipper/fortune-session?label=%E2%98%85
[![★][hazelcast-store-image] hazelcast-store][hazelcast-store-url] A Hazelcast-based session store built on the [Hazelcast Node Client](https://www.npmjs.com/package/hazelcast-client).
[hazelcast-store-url]: https://www.npmjs.com/package/hazelcast-store
[hazelcast-store-image]: https://badgen.net/github/stars/jackspaniel/hazelcast-store?label=%E2%98%85
[![★][level-session-store-image] level-session-store][level-session-store-url] A LevelDB-based session store.
[level-session-store-url]: https://www.npmjs.com/package/level-session-store
[level-session-store-image]: https://badgen.net/github/stars/toddself/level-session-store?label=%E2%98%85
[![★][lowdb-session-store-image] lowdb-session-store][lowdb-session-store-url] A [lowdb](https://www.npmjs.com/package/lowdb)-based session store.
[lowdb-session-store-url]: https://www.npmjs.com/package/lowdb-session-store
[lowdb-session-store-image]: https://badgen.net/github/stars/fhellwig/lowdb-session-store?label=%E2%98%85
[![★][medea-session-store-image] medea-session-store][medea-session-store-url] A Medea-based session store.
[medea-session-store-url]: https://www.npmjs.com/package/medea-session-store
[medea-session-store-image]: https://badgen.net/github/stars/BenjaminVadant/medea-session-store?label=%E2%98%85
[![★][memorystore-image] memorystore][memorystore-url] A memory session store made for production.
[memorystore-url]: https://www.npmjs.com/package/memorystore
[memorystore-image]: https://badgen.net/github/stars/roccomuso/memorystore?label=%E2%98%85
[![★][mssql-session-store-image] mssql-session-store][mssql-session-store-url] A SQL Server-based session store.
[mssql-session-store-url]: https://www.npmjs.com/package/mssql-session-store
[mssql-session-store-image]: https://badgen.net/github/stars/jwathen/mssql-session-store?label=%E2%98%85
[![★][nedb-session-store-image] nedb-session-store][nedb-session-store-url] An alternate NeDB-based (either in-memory or file-persisted) session store.
[nedb-session-store-url]: https://www.npmjs.com/package/nedb-session-store
[nedb-session-store-image]: https://badgen.net/github/stars/JamesMGreene/nedb-session-store?label=%E2%98%85
[![★][@quixo3/prisma-session-store-image] @quixo3/prisma-session-store][@quixo3/prisma-session-store-url] A session store for the [Prisma Framework](https://www.prisma.io).
[@quixo3/prisma-session-store-url]: https://www.npmjs.com/package/@quixo3/prisma-session-store
[@quixo3/prisma-session-store-image]: https://badgen.net/github/stars/kleydon/prisma-session-store?label=%E2%98%85
[![★][restsession-image] restsession][restsession-url] Store sessions utilizing a RESTful API
[restsession-url]: https://www.npmjs.com/package/restsession
[restsession-image]: https://badgen.net/github/stars/jankal/restsession?label=%E2%98%85
[![★][sequelstore-connect-image] sequelstore-connect][sequelstore-connect-url] A session store using [Sequelize.js](http://sequelizejs.com/).
[sequelstore-connect-url]: https://www.npmjs.com/package/sequelstore-connect
[sequelstore-connect-image]: https://badgen.net/github/stars/MattMcFarland/sequelstore-connect?label=%E2%98%85
[![★][session-file-store-image] session-file-store][session-file-store-url] A file system-based session store.
[session-file-store-url]: https://www.npmjs.com/package/session-file-store
[session-file-store-image]: https://badgen.net/github/stars/valery-barysok/session-file-store?label=%E2%98%85
[![★][session-pouchdb-store-image] session-pouchdb-store][session-pouchdb-store-url] Session store for PouchDB / CouchDB. Accepts embedded, custom, or remote PouchDB instance and realtime synchronization.
[session-pouchdb-store-url]: https://www.npmjs.com/package/session-pouchdb-store
[session-pouchdb-store-image]: https://badgen.net/github/stars/solzimer/session-pouchdb-store?label=%E2%98%85
[![★][@cyclic.sh/session-store-image] @cyclic.sh/session-store][@cyclic.sh/session-store-url] A DynamoDB-based session store for [Cyclic.sh](https://www.cyclic.sh/) apps.
[@cyclic.sh/session-store-url]: https://www.npmjs.com/package/@cyclic.sh/session-store
[@cyclic.sh/session-store-image]: https://badgen.net/github/stars/cyclic-software/session-store?label=%E2%98%85
[![★][@databunker/session-store-image] @databunker/session-store][@databunker/session-store-url] A [Databunker](https://databunker.org/)-based encrypted session store.
[@databunker/session-store-url]: https://www.npmjs.com/package/@databunker/session-store
[@databunker/session-store-image]: https://badgen.net/github/stars/securitybunker/databunker-session-store?label=%E2%98%85
[![★][sessionstore-image] sessionstore][sessionstore-url] A session store that works with various databases.
[sessionstore-url]: https://www.npmjs.com/package/sessionstore
[sessionstore-image]: https://badgen.net/github/stars/adrai/sessionstore?label=%E2%98%85
[![★][tch-nedb-session-image] tch-nedb-session][tch-nedb-session-url] A file system session store based on NeDB.
[tch-nedb-session-url]: https://www.npmjs.com/package/tch-nedb-session
[tch-nedb-session-image]: https://badgen.net/github/stars/tomaschyly/NeDBSession?label=%E2%98%85
## Examples
### View counter
A simple example using `express-session` to store page views for a user.
```js
var express = require('express')
var parseurl = require('parseurl')
var session = require('express-session')
var app = express()
app.use(session({
secret: 'keyboard cat',
resave: false,
saveUninitialized: true
}))
app.use(function (req, res, next) {
if (!req.session.views) {
req.session.views = {}
}
// get the url pathname
var pathname = parseurl(req).pathname
// count the views
req.session.views[pathname] = (req.session.views[pathname] || 0) + 1
next()
})
app.get('/foo', function (req, res, next) {
res.send('you viewed this page ' + req.session.views['/foo'] + ' times')
})
app.get('/bar', function (req, res, next) {
res.send('you viewed this page ' + req.session.views['/bar'] + ' times')
})
app.listen(3000)
```
### User login
A simple example using `express-session` to keep a user log in session.
```js
var escapeHtml = require('escape-html')
var express = require('express')
var session = require('express-session')
var app = express()
app.use(session({
secret: 'keyboard cat',
resave: false,
saveUninitialized: true
}))
// middleware to test if authenticated
function isAuthenticated (req, res, next) {
if (req.session.user) next()
else next('route')
}
app.get('/', isAuthenticated, function (req, res) {
// this is only called when there is an authentication user due to isAuthenticated
res.send('hello, ' + escapeHtml(req.session.user) + '!' +
' <a href="/logout">Logout</a>')
})
app.get('/', function (req, res) {
res.send('<form action="/login" method="post">' +
'Username: <input name="user"><br>' +
'Password: <input name="pass" type="password"><br>' +
'<input type="submit" text="Login"></form>')
})
app.post('/login', express.urlencoded({ extended: false }), function (req, res) {
// login logic to validate req.body.user and req.body.pass
// would be implemented here. for this example any combo works
// regenerate the session, which is good practice to help
// guard against forms of session fixation
req.session.regenerate(function (err) {
if (err) next(err)
// store user information in session, typically a user id
req.session.user = req.body.user
// save the session before redirection to ensure page
// load does not happen before session is saved
req.session.save(function (err) {
if (err) return next(err)
res.redirect('/')
})
})
})
app.get('/logout', function (req, res, next) {
// logout logic
// clear the user from the session object and save.
// this will ensure that re-using the old session id
// does not have a logged in user
req.session.user = null
req.session.save(function (err) {
if (err) next(err)
// regenerate the session, which is good practice to help
// guard against forms of session fixation
req.session.regenerate(function (err) {
if (err) next(err)
res.redirect('/')
})
})
})
app.listen(3000)
```
## Debugging
This module uses the [debug](https://www.npmjs.com/package/debug) module
internally to log information about session operations.
To see all the internal logs, set the `DEBUG` environment variable to
`express-session` when launching your app (`npm start`, in this example):
```sh
$ DEBUG=express-session npm start
```
On Windows, use the corresponding command;
```sh
> set DEBUG=express-session & npm start
```
## License
[MIT](LICENSE)
[rfc-6265bis-03-4.1.2.7]: https://tools.ietf.org/html/draft-ietf-httpbis-rfc6265bis-03#section-4.1.2.7
[rfc-cutler-httpbis-partitioned-cookies]: https://tools.ietf.org/html/draft-cutler-httpbis-partitioned-cookies/
[rfc-west-cookie-priority-00-4.1]: https://tools.ietf.org/html/draft-west-cookie-priority-00#section-4.1
[ci-image]: https://badgen.net/github/checks/expressjs/session/master?label=ci
[ci-url]: https://github.com/expressjs/session/actions?query=workflow%3Aci
[coveralls-image]: https://badgen.net/coveralls/c/github/expressjs/session/master
[coveralls-url]: https://coveralls.io/r/expressjs/session?branch=master
[node-url]: https://nodejs.org/en/download
[npm-downloads-image]: https://badgen.net/npm/dm/express-session
[npm-url]: https://npmjs.org/package/express-session
[npm-version-image]: https://badgen.net/npm/v/express-session | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express-session/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express-session/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 45372
} |
4.21.2 / 2024-11-06
==========
* deps: [email protected]
- Fix backtracking protection
* deps: [email protected]
- Throws an error on invalid path values
4.21.1 / 2024-10-08
==========
* Backported a fix for [CVE-2024-47764](https://nvd.nist.gov/vuln/detail/CVE-2024-47764)
4.21.0 / 2024-09-11
==========
* Deprecate `res.location("back")` and `res.redirect("back")` magic string
* deps: [email protected]
* includes [email protected]
* deps: [email protected]
* deps: [email protected]
4.20.0 / 2024-09-10
==========
* deps: [email protected]
* Remove link renderization in html while redirecting
* deps: [email protected]
* Remove link renderization in html while redirecting
* deps: [email protected]
* add `depth` option to customize the depth level in the parser
* IMPORTANT: The default `depth` level for parsing URL-encoded data is now `32` (previously was `Infinity`)
* Remove link renderization in html while using `res.redirect`
* deps: [email protected]
- Adds support for named matching groups in the routes using a regex
- Adds backtracking protection to parameters without regexes defined
* deps: encodeurl@~2.0.0
- Removes encoding of `\`, `|`, and `^` to align better with URL spec
* Deprecate passing `options.maxAge` and `options.expires` to `res.clearCookie`
- Will be ignored in v5, clearCookie will set a cookie with an expires in the past to instruct clients to delete the cookie
4.19.2 / 2024-03-25
==========
* Improved fix for open redirect allow list bypass
4.19.1 / 2024-03-20
==========
* Allow passing non-strings to res.location with new encoding handling checks
4.19.0 / 2024-03-20
==========
* Prevent open redirect allow list bypass due to encodeurl
* deps: [email protected]
4.18.3 / 2024-02-29
==========
* Fix routing requests without method
* deps: [email protected]
- Fix strict json error message on Node.js 19+
- deps: content-type@~1.0.5
- deps: [email protected]
* deps: [email protected]
- Add `partitioned` option
4.18.2 / 2022-10-08
===================
* Fix regression routing a large stack in a single route
* deps: [email protected]
- deps: [email protected]
- perf: remove unnecessary object clone
* deps: [email protected]
4.18.1 / 2022-04-29
===================
* Fix hanging on large stack of sync routes
4.18.0 / 2022-04-25
===================
* Add "root" option to `res.download`
* Allow `options` without `filename` in `res.download`
* Deprecate string and non-integer arguments to `res.status`
* Fix behavior of `null`/`undefined` as `maxAge` in `res.cookie`
* Fix handling very large stacks of sync middleware
* Ignore `Object.prototype` values in settings through `app.set`/`app.get`
* Invoke `default` with same arguments as types in `res.format`
* Support proper 205 responses using `res.send`
* Use `http-errors` for `res.format` error
* deps: [email protected]
- Fix error message for json parse whitespace in `strict`
- Fix internal error when inflated body exceeds limit
- Prevent loss of async hooks context
- Prevent hanging when request already read
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
* deps: [email protected]
- Add `priority` option
- Fix `expires` option to reject invalid dates
* deps: [email protected]
- Replace internal `eval` usage with `Function` constructor
- Use instance methods on `process` to check for listeners
* deps: [email protected]
- Remove set content headers that break response
- deps: [email protected]
- deps: [email protected]
* deps: [email protected]
- Prevent loss of async hooks context
* deps: [email protected]
* deps: [email protected]
- Fix emitted 416 error missing headers property
- Limit the headers removed for 304 response
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
* deps: [email protected]
- deps: [email protected]
* deps: [email protected]
- Remove code 306
- Rename `425 Unordered Collection` to standard `425 Too Early`
4.17.3 / 2022-02-16
===================
* deps: accepts@~1.3.8
- deps: mime-types@~2.1.34
- deps: [email protected]
* deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
* deps: [email protected]
* deps: [email protected]
* Fix handling of `__proto__` keys
* pref: remove unnecessary regexp for trust proxy
4.17.2 / 2021-12-16
===================
* Fix handling of `undefined` in `res.jsonp`
* Fix handling of `undefined` when `"json escape"` is enabled
* Fix incorrect middleware execution with unanchored `RegExp`s
* Fix `res.jsonp(obj, status)` deprecation message
* Fix typo in `res.is` JSDoc
* deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: type-is@~1.6.18
* deps: [email protected]
- deps: [email protected]
* deps: [email protected]
- Fix `maxAge` option to reject invalid values
* deps: proxy-addr@~2.0.7
- Use `req.socket` over deprecated `req.connection`
- deps: [email protected]
- deps: [email protected]
* deps: [email protected]
* deps: [email protected]
* deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- pref: ignore empty http tokens
* deps: [email protected]
- deps: [email protected]
* deps: [email protected]
4.17.1 / 2019-05-25
===================
* Revert "Improve error message for `null`/`undefined` to `res.status`"
4.17.0 / 2019-05-16
===================
* Add `express.raw` to parse bodies into `Buffer`
* Add `express.text` to parse bodies into string
* Improve error message for non-strings to `res.sendFile`
* Improve error message for `null`/`undefined` to `res.status`
* Support multiple hosts in `X-Forwarded-Host`
* deps: accepts@~1.3.7
* deps: [email protected]
- Add encoding MIK
- Add petabyte (`pb`) support
- Fix parsing array brackets after index
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: type-is@~1.6.17
* deps: [email protected]
* deps: [email protected]
- Add `SameSite=None` support
* deps: finalhandler@~1.1.2
- Set stricter `Content-Security-Policy` header
- deps: parseurl@~1.3.3
- deps: statuses@~1.5.0
* deps: parseurl@~1.3.3
* deps: proxy-addr@~2.0.5
- deps: [email protected]
* deps: [email protected]
- Fix parsing array brackets after index
* deps: range-parser@~1.2.1
* deps: [email protected]
- Set stricter CSP header in redirect & error responses
- deps: http-errors@~1.7.2
- deps: [email protected]
- deps: [email protected]
- deps: range-parser@~1.2.1
- deps: statuses@~1.5.0
- perf: remove redundant `path.normalize` call
* deps: [email protected]
- Set stricter CSP header in redirect response
- deps: parseurl@~1.3.3
- deps: [email protected]
* deps: [email protected]
* deps: statuses@~1.5.0
- Add `103 Early Hints`
* deps: type-is@~1.6.18
- deps: mime-types@~2.1.24
- perf: prevent internal `throw` on invalid type
4.16.4 / 2018-10-10
===================
* Fix issue where `"Request aborted"` may be logged in `res.sendfile`
* Fix JSDoc for `Router` constructor
* deps: [email protected]
- Fix deprecation warnings on Node.js 10+
- Fix stack trace for strict json parse error
- deps: depd@~1.1.2
- deps: http-errors@~1.6.3
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: type-is@~1.6.16
* deps: proxy-addr@~2.0.4
- deps: [email protected]
* deps: [email protected]
* deps: [email protected]
4.16.3 / 2018-03-12
===================
* deps: accepts@~1.3.5
- deps: mime-types@~2.1.18
* deps: depd@~1.1.2
- perf: remove argument reassignment
* deps: encodeurl@~1.0.2
- Fix encoding `%` as last character
* deps: [email protected]
- Fix 404 output for bad / missing pathnames
- deps: encodeurl@~1.0.2
- deps: statuses@~1.4.0
* deps: proxy-addr@~2.0.3
- deps: [email protected]
* deps: [email protected]
- Fix incorrect end tag in default error & redirects
- deps: depd@~1.1.2
- deps: encodeurl@~1.0.2
- deps: statuses@~1.4.0
* deps: [email protected]
- Fix incorrect end tag in redirects
- deps: encodeurl@~1.0.2
- deps: [email protected]
* deps: statuses@~1.4.0
* deps: type-is@~1.6.16
- deps: mime-types@~2.1.18
4.16.2 / 2017-10-09
===================
* Fix `TypeError` in `res.send` when given `Buffer` and `ETag` header set
* perf: skip parsing of entire `X-Forwarded-Proto` header
4.16.1 / 2017-09-29
===================
* deps: [email protected]
* deps: [email protected]
- Fix regression when `root` is incorrectly set to a file
- deps: [email protected]
4.16.0 / 2017-09-28
===================
* Add `"json escape"` setting for `res.json` and `res.jsonp`
* Add `express.json` and `express.urlencoded` to parse bodies
* Add `options` argument to `res.download`
* Improve error message when autoloading invalid view engine
* Improve error messages when non-function provided as middleware
* Skip `Buffer` encoding when not generating ETag for small response
* Use `safe-buffer` for improved Buffer API
* deps: accepts@~1.3.4
- deps: mime-types@~2.1.16
* deps: content-type@~1.0.4
- perf: remove argument reassignment
- perf: skip parameter parsing when no parameters
* deps: etag@~1.8.1
- perf: replace regular expression with substring
* deps: [email protected]
- Use `res.headersSent` when available
* deps: parseurl@~1.3.2
- perf: reduce overhead for full URLs
- perf: unroll the "fast-path" `RegExp`
* deps: proxy-addr@~2.0.2
- Fix trimming leading / trailing OWS in `X-Forwarded-For`
- deps: forwarded@~0.1.2
- deps: [email protected]
- perf: reduce overhead when no `X-Forwarded-For` header
* deps: [email protected]
- Fix parsing & compacting very deep objects
* deps: [email protected]
- Add 70 new types for file extensions
- Add `immutable` option
- Fix missing `</html>` in default error & redirects
- Set charset as "UTF-8" for .js and .json
- Use instance methods on steam to check for listeners
- deps: [email protected]
- perf: improve path validation speed
* deps: [email protected]
- Add 70 new types for file extensions
- Add `immutable` option
- Set charset as "UTF-8" for .js and .json
- deps: [email protected]
* deps: [email protected]
* deps: [email protected]
* deps: vary@~1.1.2
- perf: improve header token parsing speed
* perf: re-use options object when generating ETags
* perf: remove dead `.charset` set in `res.jsonp`
4.15.5 / 2017-09-24
===================
* deps: [email protected]
* deps: finalhandler@~1.0.6
- deps: [email protected]
- deps: parseurl@~1.3.2
* deps: [email protected]
- Fix handling of modified headers with invalid dates
- perf: improve ETag match loop
- perf: improve `If-None-Match` token parsing
* deps: [email protected]
- Fix handling of modified headers with invalid dates
- deps: [email protected]
- deps: etag@~1.8.1
- deps: [email protected]
- perf: improve `If-Match` token parsing
* deps: [email protected]
- deps: parseurl@~1.3.2
- deps: [email protected]
- perf: improve slash collapsing
4.15.4 / 2017-08-06
===================
* deps: [email protected]
* deps: depd@~1.1.1
- Remove unnecessary `Buffer` loading
* deps: finalhandler@~1.0.4
- deps: [email protected]
* deps: proxy-addr@~1.1.5
- Fix array argument being altered
- deps: [email protected]
* deps: [email protected]
* deps: [email protected]
- deps: [email protected]
- deps: depd@~1.1.1
- deps: http-errors@~1.6.2
* deps: [email protected]
- deps: [email protected]
4.15.3 / 2017-05-16
===================
* Fix error when `res.set` cannot add charset to `Content-Type`
* deps: [email protected]
- Fix `DEBUG_MAX_ARRAY_LENGTH`
- deps: [email protected]
* deps: finalhandler@~1.0.3
- Fix missing `</html>` in HTML document
- deps: [email protected]
* deps: proxy-addr@~1.1.4
- deps: [email protected]
* deps: [email protected]
- deps: [email protected]
- deps: [email protected]
* deps: [email protected]
- deps: [email protected]
* deps: type-is@~1.6.15
- deps: mime-types@~2.1.15
* deps: vary@~1.1.1
- perf: hoist regular expression
4.15.2 / 2017-03-06
===================
* deps: [email protected]
- Fix regression parsing keys starting with `[`
4.15.1 / 2017-03-05
===================
* deps: [email protected]
- Fix issue when `Date.parse` does not return `NaN` on invalid date
- Fix strict violation in broken environments
* deps: [email protected]
- Fix issue when `Date.parse` does not return `NaN` on invalid date
- deps: [email protected]
4.15.0 / 2017-03-01
===================
* Add debug message when loading view engine
* Add `next("router")` to exit from router
* Fix case where `router.use` skipped requests routes did not
* Remove usage of `res._headers` private field
- Improves compatibility with Node.js 8 nightly
* Skip routing when `req.url` is not set
* Use `%o` in path debug to tell types apart
* Use `Object.create` to setup request & response prototypes
* Use `setprototypeof` module to replace `__proto__` setting
* Use `statuses` instead of `http` module for status messages
* deps: [email protected]
- Allow colors in workers
- Deprecated `DEBUG_FD` environment variable set to `3` or higher
- Fix error when running under React Native
- Use same color for same namespace
- deps: [email protected]
* deps: etag@~1.8.0
- Use SHA1 instead of MD5 for ETag hashing
- Works with FIPS 140-2 OpenSSL configuration
* deps: finalhandler@~1.0.0
- Fix exception when `err` cannot be converted to a string
- Fully URL-encode the pathname in the 404
- Only include the pathname in the 404 message
- Send complete HTML document
- Set `Content-Security-Policy: default-src 'self'` header
- deps: [email protected]
* deps: [email protected]
- Fix false detection of `no-cache` request directive
- Fix incorrect result when `If-None-Match` has both `*` and ETags
- Fix weak `ETag` matching to match spec
- perf: delay reading header values until needed
- perf: enable strict mode
- perf: hoist regular expressions
- perf: remove duplicate conditional
- perf: remove unnecessary boolean coercions
- perf: skip checking modified time if ETag check failed
- perf: skip parsing `If-None-Match` when no `ETag` header
- perf: use `Date.parse` instead of `new Date`
* deps: [email protected]
- Fix array parsing from skipping empty values
- Fix compacting nested arrays
* deps: [email protected]
- Fix false detection of `no-cache` request directive
- Fix incorrect result when `If-None-Match` has both `*` and ETags
- Fix weak `ETag` matching to match spec
- Remove usage of `res._headers` private field
- Support `If-Match` and `If-Unmodified-Since` headers
- Use `res.getHeaderNames()` when available
- Use `res.headersSent` when available
- deps: [email protected]
- deps: etag@~1.8.0
- deps: [email protected]
- deps: http-errors@~1.6.1
* deps: [email protected]
- Fix false detection of `no-cache` request directive
- Fix incorrect result when `If-None-Match` has both `*` and ETags
- Fix weak `ETag` matching to match spec
- Remove usage of `res._headers` private field
- Send complete HTML document in redirect response
- Set default CSP header in redirect response
- Support `If-Match` and `If-Unmodified-Since` headers
- Use `res.getHeaderNames()` when available
- Use `res.headersSent` when available
- deps: [email protected]
* perf: add fast match path for `*` route
* perf: improve `req.ips` performance
4.14.1 / 2017-01-28
===================
* deps: [email protected]
* deps: [email protected]
- Fix exception when `err.headers` is not an object
- deps: statuses@~1.3.1
- perf: hoist regular expressions
- perf: remove duplicate validation path
* deps: proxy-addr@~1.1.3
- deps: [email protected]
* deps: [email protected]
- deps: http-errors@~1.5.1
- deps: [email protected]
- deps: statuses@~1.3.1
* deps: serve-static@~1.11.2
- deps: [email protected]
* deps: type-is@~1.6.14
- deps: mime-types@~2.1.13
4.14.0 / 2016-06-16
===================
* Add `acceptRanges` option to `res.sendFile`/`res.sendfile`
* Add `cacheControl` option to `res.sendFile`/`res.sendfile`
* Add `options` argument to `req.range`
- Includes the `combine` option
* Encode URL in `res.location`/`res.redirect` if not already encoded
* Fix some redirect handling in `res.sendFile`/`res.sendfile`
* Fix Windows absolute path check using forward slashes
* Improve error with invalid arguments to `req.get()`
* Improve performance for `res.json`/`res.jsonp` in most cases
* Improve `Range` header handling in `res.sendFile`/`res.sendfile`
* deps: accepts@~1.3.3
- Fix including type extensions in parameters in `Accept` parsing
- Fix parsing `Accept` parameters with quoted equals
- Fix parsing `Accept` parameters with quoted semicolons
- Many performance improvements
- deps: mime-types@~2.1.11
- deps: [email protected]
* deps: content-type@~1.0.2
- perf: enable strict mode
* deps: [email protected]
- Add `sameSite` option
- Fix cookie `Max-Age` to never be a floating point number
- Improve error message when `encode` is not a function
- Improve error message when `expires` is not a `Date`
- Throw better error for invalid argument to parse
- Throw on invalid values provided to `serialize`
- perf: enable strict mode
- perf: hoist regular expression
- perf: use for loop in parse
- perf: use string concatenation for serialization
* deps: [email protected]
- Change invalid or non-numeric status code to 500
- Overwrite status message to match set status code
- Prefer `err.statusCode` if `err.status` is invalid
- Set response headers from `err.headers` object
- Use `statuses` instead of `http` module for status messages
* deps: proxy-addr@~1.1.2
- Fix accepting various invalid netmasks
- Fix IPv6-mapped IPv4 validation edge cases
- IPv4 netmasks must be contiguous
- IPv6 addresses cannot be used as a netmask
- deps: [email protected]
* deps: [email protected]
- Add `decoder` option in `parse` function
* deps: range-parser@~1.2.0
- Add `combine` option to combine overlapping ranges
- Fix incorrectly returning -1 when there is at least one valid range
- perf: remove internal function
* deps: [email protected]
- Add `acceptRanges` option
- Add `cacheControl` option
- Attempt to combine multiple ranges into single range
- Correctly inherit from `Stream` class
- Fix `Content-Range` header in 416 responses when using `start`/`end` options
- Fix `Content-Range` header missing from default 416 responses
- Fix redirect error when `path` contains raw non-URL characters
- Fix redirect when `path` starts with multiple forward slashes
- Ignore non-byte `Range` headers
- deps: http-errors@~1.5.0
- deps: range-parser@~1.2.0
- deps: statuses@~1.3.0
- perf: remove argument reassignment
* deps: serve-static@~1.11.1
- Add `acceptRanges` option
- Add `cacheControl` option
- Attempt to combine multiple ranges into single range
- Fix redirect error when `req.url` contains raw non-URL characters
- Ignore non-byte `Range` headers
- Use status code 301 for redirects
- deps: [email protected]
* deps: type-is@~1.6.13
- Fix type error when given invalid type to match against
- deps: mime-types@~2.1.11
* deps: vary@~1.1.0
- Only accept valid field names in the `field` argument
* perf: use strict equality when possible
4.13.4 / 2016-01-21
===================
* deps: [email protected]
- perf: enable strict mode
* deps: [email protected]
- Throw on invalid values provided to `serialize`
* deps: depd@~1.1.0
- Support web browser loading
- perf: enable strict mode
* deps: escape-html@~1.0.3
- perf: enable strict mode
- perf: optimize string replacement
- perf: use faster string coercion
* deps: [email protected]
- deps: escape-html@~1.0.3
* deps: [email protected]
- perf: enable strict mode
* deps: methods@~1.1.2
- perf: enable strict mode
* deps: parseurl@~1.3.1
- perf: enable strict mode
* deps: proxy-addr@~1.0.10
- deps: [email protected]
- perf: enable strict mode
* deps: range-parser@~1.0.3
- perf: enable strict mode
* deps: [email protected]
- deps: depd@~1.1.0
- deps: destroy@~1.0.4
- deps: escape-html@~1.0.3
- deps: range-parser@~1.0.3
* deps: serve-static@~1.10.2
- deps: escape-html@~1.0.3
- deps: parseurl@~1.3.0
- deps: [email protected]
4.13.3 / 2015-08-02
===================
* Fix infinite loop condition using `mergeParams: true`
* Fix inner numeric indices incorrectly altering parent `req.params`
4.13.2 / 2015-07-31
===================
* deps: accepts@~1.2.12
- deps: mime-types@~2.1.4
* deps: [email protected]
- perf: enable strict mode
* deps: [email protected]
- Fix regression with escaped round brackets and matching groups
* deps: type-is@~1.6.6
- deps: mime-types@~2.1.4
4.13.1 / 2015-07-05
===================
* deps: accepts@~1.2.10
- deps: mime-types@~2.1.2
* deps: [email protected]
- Fix dropping parameters like `hasOwnProperty`
- Fix various parsing edge cases
* deps: type-is@~1.6.4
- deps: mime-types@~2.1.2
- perf: enable strict mode
- perf: remove argument reassignment
4.13.0 / 2015-06-20
===================
* Add settings to debug output
* Fix `res.format` error when only `default` provided
* Fix issue where `next('route')` in `app.param` would incorrectly skip values
* Fix hiding platform issues with `decodeURIComponent`
- Only `URIError`s are a 400
* Fix using `*` before params in routes
* Fix using capture groups before params in routes
* Simplify `res.cookie` to call `res.append`
* Use `array-flatten` module for flattening arrays
* deps: accepts@~1.2.9
- deps: mime-types@~2.1.1
- perf: avoid argument reassignment & argument slice
- perf: avoid negotiator recursive construction
- perf: enable strict mode
- perf: remove unnecessary bitwise operator
* deps: [email protected]
- perf: deduce the scope of try-catch deopt
- perf: remove argument reassignments
* deps: [email protected]
* deps: etag@~1.7.0
- Always include entity length in ETags for hash length extensions
- Generate non-Stats ETags using MD5 only (no longer CRC32)
- Improve stat performance by removing hashing
- Improve support for JXcore
- Remove base64 padding in ETags to shorten
- Support "fake" stats objects in environments without fs
- Use MD5 instead of MD4 in weak ETags over 1KB
* deps: [email protected]
- Fix a false-positive when unpiping in Node.js 0.8
- Support `statusCode` property on `Error` objects
- Use `unpipe` module for unpiping requests
- deps: [email protected]
- deps: on-finished@~2.3.0
- perf: enable strict mode
- perf: remove argument reassignment
* deps: [email protected]
- Add weak `ETag` matching support
* deps: on-finished@~2.3.0
- Add defined behavior for HTTP `CONNECT` requests
- Add defined behavior for HTTP `Upgrade` requests
- deps: [email protected]
* deps: [email protected]
* deps: [email protected]
- Allow Node.js HTTP server to set `Date` response header
- Fix incorrectly removing `Content-Location` on 304 response
- Improve the default redirect response headers
- Send appropriate headers on default error response
- Use `http-errors` for standard emitted errors
- Use `statuses` instead of `http` module for status messages
- deps: [email protected]
- deps: etag@~1.7.0
- deps: [email protected]
- deps: on-finished@~2.3.0
- perf: enable strict mode
- perf: remove unnecessary array allocations
* deps: serve-static@~1.10.0
- Add `fallthrough` option
- Fix reading options from options prototype
- Improve the default redirect response headers
- Malformed URLs now `next()` instead of 400
- deps: [email protected]
- deps: [email protected]
- perf: enable strict mode
- perf: remove argument reassignment
* deps: type-is@~1.6.3
- deps: mime-types@~2.1.1
- perf: reduce try block size
- perf: remove bitwise operations
* perf: enable strict mode
* perf: isolate `app.render` try block
* perf: remove argument reassignments in application
* perf: remove argument reassignments in request prototype
* perf: remove argument reassignments in response prototype
* perf: remove argument reassignments in routing
* perf: remove argument reassignments in `View`
* perf: skip attempting to decode zero length string
* perf: use saved reference to `http.STATUS_CODES`
4.12.4 / 2015-05-17
===================
* deps: accepts@~1.2.7
- deps: mime-types@~2.0.11
- deps: [email protected]
* deps: debug@~2.2.0
- deps: [email protected]
* deps: depd@~1.0.1
* deps: etag@~1.6.0
- Improve support for JXcore
- Support "fake" stats objects in environments without `fs`
* deps: [email protected]
- deps: debug@~2.2.0
- deps: on-finished@~2.2.1
* deps: on-finished@~2.2.1
- Fix `isFinished(req)` when data buffered
* deps: proxy-addr@~1.0.8
- deps: [email protected]
* deps: [email protected]
- Fix allowing parameters like `constructor`
* deps: [email protected]
- deps: debug@~2.2.0
- deps: depd@~1.0.1
- deps: etag@~1.6.0
- deps: [email protected]
- deps: on-finished@~2.2.1
* deps: serve-static@~1.9.3
- deps: [email protected]
* deps: type-is@~1.6.2
- deps: mime-types@~2.0.11
4.12.3 / 2015-03-17
===================
* deps: accepts@~1.2.5
- deps: mime-types@~2.0.10
* deps: debug@~2.1.3
- Fix high intensity foreground color for bold
- deps: [email protected]
* deps: [email protected]
- deps: debug@~2.1.3
* deps: proxy-addr@~1.0.7
- deps: [email protected]
* deps: [email protected]
- Fix error when parameter `hasOwnProperty` is present
* deps: [email protected]
- Throw errors early for invalid `extensions` or `index` options
- deps: debug@~2.1.3
* deps: serve-static@~1.9.2
- deps: [email protected]
* deps: type-is@~1.6.1
- deps: mime-types@~2.0.10
4.12.2 / 2015-03-02
===================
* Fix regression where `"Request aborted"` is logged using `res.sendFile`
4.12.1 / 2015-03-01
===================
* Fix constructing application with non-configurable prototype properties
* Fix `ECONNRESET` errors from `res.sendFile` usage
* Fix `req.host` when using "trust proxy" hops count
* Fix `req.protocol`/`req.secure` when using "trust proxy" hops count
* Fix wrong `code` on aborted connections from `res.sendFile`
* deps: [email protected]
4.12.0 / 2015-02-23
===================
* Fix `"trust proxy"` setting to inherit when app is mounted
* Generate `ETag`s for all request responses
- No longer restricted to only responses for `GET` and `HEAD` requests
* Use `content-type` to parse `Content-Type` headers
* deps: accepts@~1.2.4
- Fix preference sorting to be stable for long acceptable lists
- deps: mime-types@~2.0.9
- deps: [email protected]
* deps: [email protected]
* deps: [email protected]
- Always read the stat size from the file
- Fix mutating passed-in `options`
- deps: [email protected]
* deps: serve-static@~1.9.1
- deps: [email protected]
* deps: type-is@~1.6.0
- fix argument reassignment
- fix false-positives in `hasBody` `Transfer-Encoding` check
- support wildcard for both type and subtype (`*/*`)
- deps: mime-types@~2.0.9
4.11.2 / 2015-02-01
===================
* Fix `res.redirect` double-calling `res.end` for `HEAD` requests
* deps: accepts@~1.2.3
- deps: mime-types@~2.0.8
* deps: proxy-addr@~1.0.6
- deps: [email protected]
* deps: type-is@~1.5.6
- deps: mime-types@~2.0.8
4.11.1 / 2015-01-20
===================
* deps: [email protected]
- Fix root path disclosure
* deps: serve-static@~1.8.1
- Fix redirect loop in Node.js 0.11.14
- Fix root path disclosure
- deps: [email protected]
4.11.0 / 2015-01-13
===================
* Add `res.append(field, val)` to append headers
* Deprecate leading `:` in `name` for `app.param(name, fn)`
* Deprecate `req.param()` -- use `req.params`, `req.body`, or `req.query` instead
* Deprecate `app.param(fn)`
* Fix `OPTIONS` responses to include the `HEAD` method properly
* Fix `res.sendFile` not always detecting aborted connection
* Match routes iteratively to prevent stack overflows
* deps: accepts@~1.2.2
- deps: mime-types@~2.0.7
- deps: [email protected]
* deps: [email protected]
- deps: debug@~2.1.1
- deps: etag@~1.5.1
- deps: [email protected]
- deps: on-finished@~2.2.0
* deps: serve-static@~1.8.0
- deps: [email protected]
4.10.8 / 2015-01-13
===================
* Fix crash from error within `OPTIONS` response handler
* deps: proxy-addr@~1.0.5
- deps: [email protected]
4.10.7 / 2015-01-04
===================
* Fix `Allow` header for `OPTIONS` to not contain duplicate methods
* Fix incorrect "Request aborted" for `res.sendFile` when `HEAD` or 304
* deps: debug@~2.1.1
* deps: [email protected]
- deps: debug@~2.1.1
- deps: on-finished@~2.2.0
* deps: methods@~1.1.1
* deps: on-finished@~2.2.0
* deps: serve-static@~1.7.2
- Fix potential open redirect when mounted at root
* deps: type-is@~1.5.5
- deps: mime-types@~2.0.7
4.10.6 / 2014-12-12
===================
* Fix exception in `req.fresh`/`req.stale` without response headers
4.10.5 / 2014-12-10
===================
* Fix `res.send` double-calling `res.end` for `HEAD` requests
* deps: accepts@~1.1.4
- deps: mime-types@~2.0.4
* deps: type-is@~1.5.4
- deps: mime-types@~2.0.4
4.10.4 / 2014-11-24
===================
* Fix `res.sendfile` logging standard write errors
4.10.3 / 2014-11-23
===================
* Fix `res.sendFile` logging standard write errors
* deps: etag@~1.5.1
* deps: proxy-addr@~1.0.4
- deps: [email protected]
* deps: [email protected]
- Fix `arrayLimit` behavior
4.10.2 / 2014-11-09
===================
* Correctly invoke async router callback asynchronously
* deps: accepts@~1.1.3
- deps: mime-types@~2.0.3
* deps: type-is@~1.5.3
- deps: mime-types@~2.0.3
4.10.1 / 2014-10-28
===================
* Fix handling of URLs containing `://` in the path
* deps: [email protected]
- Fix parsing of mixed objects and values
4.10.0 / 2014-10-23
===================
* Add support for `app.set('views', array)`
- Views are looked up in sequence in array of directories
* Fix `res.send(status)` to mention `res.sendStatus(status)`
* Fix handling of invalid empty URLs
* Use `content-disposition` module for `res.attachment`/`res.download`
- Sends standards-compliant `Content-Disposition` header
- Full Unicode support
* Use `path.resolve` in view lookup
* deps: debug@~2.1.0
- Implement `DEBUG_FD` env variable support
* deps: depd@~1.0.0
* deps: etag@~1.5.0
- Improve string performance
- Slightly improve speed for weak ETags over 1KB
* deps: [email protected]
- Terminate in progress response only on error
- Use `on-finished` to determine request status
- deps: debug@~2.1.0
- deps: on-finished@~2.1.1
* deps: on-finished@~2.1.1
- Fix handling of pipelined requests
* deps: [email protected]
- Fix parsing of mixed implicit and explicit arrays
* deps: [email protected]
- deps: debug@~2.1.0
- deps: depd@~1.0.0
- deps: etag@~1.5.0
- deps: on-finished@~2.1.1
* deps: serve-static@~1.7.1
- deps: [email protected]
4.9.8 / 2014-10-17
==================
* Fix `res.redirect` body when redirect status specified
* deps: accepts@~1.1.2
- Fix error when media type has invalid parameter
- deps: [email protected]
4.9.7 / 2014-10-10
==================
* Fix using same param name in array of paths
4.9.6 / 2014-10-08
==================
* deps: accepts@~1.1.1
- deps: mime-types@~2.0.2
- deps: [email protected]
* deps: serve-static@~1.6.4
- Fix redirect loop when index file serving disabled
* deps: type-is@~1.5.2
- deps: mime-types@~2.0.2
4.9.5 / 2014-09-24
==================
* deps: etag@~1.4.0
* deps: proxy-addr@~1.0.3
- Use `forwarded` npm module
* deps: [email protected]
- deps: etag@~1.4.0
* deps: serve-static@~1.6.3
- deps: [email protected]
4.9.4 / 2014-09-19
==================
* deps: [email protected]
- Fix issue with object keys starting with numbers truncated
4.9.3 / 2014-09-18
==================
* deps: proxy-addr@~1.0.2
- Fix a global leak when multiple subnets are trusted
- deps: [email protected]
4.9.2 / 2014-09-17
==================
* Fix regression for empty string `path` in `app.use`
* Fix `router.use` to accept array of middleware without path
* Improve error message for bad `app.use` arguments
4.9.1 / 2014-09-16
==================
* Fix `app.use` to accept array of middleware without path
* deps: [email protected]
* deps: etag@~1.3.1
* deps: [email protected]
- deps: [email protected]
- deps: etag@~1.3.1
- deps: range-parser@~1.0.2
* deps: serve-static@~1.6.2
- deps: [email protected]
4.9.0 / 2014-09-08
==================
* Add `res.sendStatus`
* Invoke callback for sendfile when client aborts
- Applies to `res.sendFile`, `res.sendfile`, and `res.download`
- `err` will be populated with request aborted error
* Support IP address host in `req.subdomains`
* Use `etag` to generate `ETag` headers
* deps: accepts@~1.1.0
- update `mime-types`
* deps: [email protected]
* deps: debug@~2.0.0
* deps: [email protected]
- Set `X-Content-Type-Options: nosniff` header
- deps: debug@~2.0.0
* deps: [email protected]
* deps: [email protected]
- Throw error when parameter format invalid on parse
* deps: [email protected]
- Fix issue where first empty value in array is discarded
* deps: range-parser@~1.0.2
* deps: [email protected]
- Add `lastModified` option
- Use `etag` to generate `ETag` header
- deps: debug@~2.0.0
- deps: [email protected]
* deps: serve-static@~1.6.1
- Add `lastModified` option
- deps: [email protected]
* deps: type-is@~1.5.1
- fix `hasbody` to be true for `content-length: 0`
- deps: [email protected]
- deps: mime-types@~2.0.1
* deps: vary@~1.0.0
- Accept valid `Vary` header string as `field`
4.8.8 / 2014-09-04
==================
* deps: [email protected]
- Fix a path traversal issue when using `root`
- Fix malicious path detection for empty string path
* deps: serve-static@~1.5.4
- deps: [email protected]
4.8.7 / 2014-08-29
==================
* deps: [email protected]
- Remove unnecessary cloning
4.8.6 / 2014-08-27
==================
* deps: [email protected]
- Array parsing fix
- Performance improvements
4.8.5 / 2014-08-18
==================
* deps: [email protected]
- deps: [email protected]
- deps: [email protected]
* deps: serve-static@~1.5.3
- deps: [email protected]
4.8.4 / 2014-08-14
==================
* deps: [email protected]
* deps: [email protected]
- Work around `fd` leak in Node.js 0.10 for `fs.ReadStream`
* deps: serve-static@~1.5.2
- deps: [email protected]
4.8.3 / 2014-08-10
==================
* deps: parseurl@~1.3.0
* deps: [email protected]
* deps: serve-static@~1.5.1
- Fix parsing of weird `req.originalUrl` values
- deps: parseurl@~1.3.0
- deps: [email protected]
4.8.2 / 2014-08-07
==================
* deps: [email protected]
- Fix parsing array of objects
4.8.1 / 2014-08-06
==================
* fix incorrect deprecation warnings on `res.download`
* deps: [email protected]
- Accept urlencoded square brackets
- Accept empty values in implicit array notation
4.8.0 / 2014-08-05
==================
* add `res.sendFile`
- accepts a file system path instead of a URL
- requires an absolute path or `root` option specified
* deprecate `res.sendfile` -- use `res.sendFile` instead
* support mounted app as any argument to `app.use()`
* deps: [email protected]
- Complete rewrite
- Limits array length to 20
- Limits object depth to 5
- Limits parameters to 1,000
* deps: [email protected]
- Add `extensions` option
* deps: serve-static@~1.5.0
- Add `extensions` option
- deps: [email protected]
4.7.4 / 2014-08-04
==================
* fix `res.sendfile` regression for serving directory index files
* deps: [email protected]
- Fix incorrect 403 on Windows and Node.js 0.11
- Fix serving index files without root dir
* deps: serve-static@~1.4.4
- deps: [email protected]
4.7.3 / 2014-08-04
==================
* deps: [email protected]
- Fix incorrect 403 on Windows and Node.js 0.11
* deps: serve-static@~1.4.3
- Fix incorrect 403 on Windows and Node.js 0.11
- deps: [email protected]
4.7.2 / 2014-07-27
==================
* deps: [email protected]
- Work-around v8 generating empty stack traces
* deps: [email protected]
- deps: [email protected]
* deps: serve-static@~1.4.2
4.7.1 / 2014-07-26
==================
* deps: [email protected]
- Fix exception when global `Error.stackTraceLimit` is too low
* deps: [email protected]
- deps: [email protected]
* deps: serve-static@~1.4.1
4.7.0 / 2014-07-25
==================
* fix `req.protocol` for proxy-direct connections
* configurable query parser with `app.set('query parser', parser)`
- `app.set('query parser', 'extended')` parse with "qs" module
- `app.set('query parser', 'simple')` parse with "querystring" core module
- `app.set('query parser', false)` disable query string parsing
- `app.set('query parser', true)` enable simple parsing
* deprecate `res.json(status, obj)` -- use `res.status(status).json(obj)` instead
* deprecate `res.jsonp(status, obj)` -- use `res.status(status).jsonp(obj)` instead
* deprecate `res.send(status, body)` -- use `res.status(status).send(body)` instead
* deps: [email protected]
* deps: [email protected]
- Add `TRACE_DEPRECATION` environment variable
- Remove non-standard grey color from color output
- Support `--no-deprecation` argument
- Support `--trace-deprecation` argument
* deps: [email protected]
- Respond after request fully read
- deps: [email protected]
* deps: parseurl@~1.2.0
- Cache URLs based on original value
- Remove no-longer-needed URL mis-parse work-around
- Simplify the "fast-path" `RegExp`
* deps: [email protected]
- Add `dotfiles` option
- Cap `maxAge` value to 1 year
- deps: [email protected]
- deps: [email protected]
* deps: serve-static@~1.4.0
- deps: parseurl@~1.2.0
- deps: [email protected]
* perf: prevent multiple `Buffer` creation in `res.send`
4.6.1 / 2014-07-12
==================
* fix `subapp.mountpath` regression for `app.use(subapp)`
4.6.0 / 2014-07-11
==================
* accept multiple callbacks to `app.use()`
* add explicit "Rosetta Flash JSONP abuse" protection
- previous versions are not vulnerable; this is just explicit protection
* catch errors in multiple `req.param(name, fn)` handlers
* deprecate `res.redirect(url, status)` -- use `res.redirect(status, url)` instead
* fix `res.send(status, num)` to send `num` as json (not error)
* remove unnecessary escaping when `res.jsonp` returns JSON response
* support non-string `path` in `app.use(path, fn)`
- supports array of paths
- supports `RegExp`
* router: fix optimization on router exit
* router: refactor location of `try` blocks
* router: speed up standard `app.use(fn)`
* deps: [email protected]
- Add support for multiple wildcards in namespaces
* deps: [email protected]
- deps: [email protected]
* deps: [email protected]
- add `CONNECT`
* deps: parseurl@~1.1.3
- faster parsing of href-only URLs
* deps: [email protected]
* deps: [email protected]
- deps: [email protected]
* deps: serve-static@~1.3.2
- deps: parseurl@~1.1.3
- deps: [email protected]
* perf: fix arguments reassign deopt in some `res` methods
4.5.1 / 2014-07-06
==================
* fix routing regression when altering `req.method`
4.5.0 / 2014-07-04
==================
* add deprecation message to non-plural `req.accepts*`
* add deprecation message to `res.send(body, status)`
* add deprecation message to `res.vary()`
* add `headers` option to `res.sendfile`
- use to set headers on successful file transfer
* add `mergeParams` option to `Router`
- merges `req.params` from parent routes
* add `req.hostname` -- correct name for what `req.host` returns
* deprecate things with `depd` module
* deprecate `req.host` -- use `req.hostname` instead
* fix behavior when handling request without routes
* fix handling when `route.all` is only route
* invoke `router.param()` only when route matches
* restore `req.params` after invoking router
* use `finalhandler` for final response handling
* use `media-typer` to alter content-type charset
* deps: accepts@~1.0.7
* deps: [email protected]
- Accept string for `maxage` (converted by `ms`)
- Include link in default redirect response
* deps: serve-static@~1.3.0
- Accept string for `maxAge` (converted by `ms`)
- Add `setHeaders` option
- Include HTML link in redirect response
- deps: [email protected]
* deps: type-is@~1.3.2
4.4.5 / 2014-06-26
==================
* deps: [email protected]
- fix for timing attacks
4.4.4 / 2014-06-20
==================
* fix `res.attachment` Unicode filenames in Safari
* fix "trim prefix" debug message in `express:router`
* deps: accepts@~1.0.5
* deps: [email protected]
4.4.3 / 2014-06-11
==================
* fix persistence of modified `req.params[name]` from `app.param()`
* deps: [email protected]
- deps: [email protected]
* deps: [email protected]
* deps: [email protected]
- Do not throw uncatchable error on file open race condition
- Use `escape-html` for HTML escaping
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
* deps: [email protected]
- Do not throw uncatchable error on file open race condition
- deps: [email protected]
4.4.2 / 2014-06-09
==================
* fix catching errors from top-level handlers
* use `vary` module for `res.vary`
* deps: [email protected]
* deps: [email protected]
* deps: [email protected]
- fix "event emitter leak" warnings
- deps: [email protected]
- deps: [email protected]
* deps: [email protected]
- fix "event emitter leak" warnings
- deps: [email protected]
* deps: [email protected]
4.4.1 / 2014-06-02
==================
* deps: [email protected]
* deps: [email protected]
- Send `max-age` in `Cache-Control` in correct format
* deps: [email protected]
- use `escape-html` for escaping
- deps: [email protected]
4.4.0 / 2014-05-30
==================
* custom etag control with `app.set('etag', val)`
- `app.set('etag', function(body, encoding){ return '"etag"' })` custom etag generation
- `app.set('etag', 'weak')` weak tag
- `app.set('etag', 'strong')` strong etag
- `app.set('etag', false)` turn off
- `app.set('etag', true)` standard etag
* mark `res.send` ETag as weak and reduce collisions
* update accepts to 1.0.2
- Fix interpretation when header not in request
* update send to 0.4.0
- Calculate ETag with md5 for reduced collisions
- Ignore stream errors after request ends
- deps: [email protected]
* update serve-static to 1.2.0
- Calculate ETag with md5 for reduced collisions
- Ignore stream errors after request ends
- deps: [email protected]
4.3.2 / 2014-05-28
==================
* fix handling of errors from `router.param()` callbacks
4.3.1 / 2014-05-23
==================
* revert "fix behavior of multiple `app.VERB` for the same path"
- this caused a regression in the order of route execution
4.3.0 / 2014-05-21
==================
* add `req.baseUrl` to access the path stripped from `req.url` in routes
* fix behavior of multiple `app.VERB` for the same path
* fix issue routing requests among sub routers
* invoke `router.param()` only when necessary instead of every match
* proper proxy trust with `app.set('trust proxy', trust)`
- `app.set('trust proxy', 1)` trust first hop
- `app.set('trust proxy', 'loopback')` trust loopback addresses
- `app.set('trust proxy', '10.0.0.1')` trust single IP
- `app.set('trust proxy', '10.0.0.1/16')` trust subnet
- `app.set('trust proxy', '10.0.0.1, 10.0.0.2')` trust list
- `app.set('trust proxy', false)` turn off
- `app.set('trust proxy', true)` trust everything
* set proper `charset` in `Content-Type` for `res.send`
* update type-is to 1.2.0
- support suffix matching
4.2.0 / 2014-05-11
==================
* deprecate `app.del()` -- use `app.delete()` instead
* deprecate `res.json(obj, status)` -- use `res.json(status, obj)` instead
- the edge-case `res.json(status, num)` requires `res.status(status).json(num)`
* deprecate `res.jsonp(obj, status)` -- use `res.jsonp(status, obj)` instead
- the edge-case `res.jsonp(status, num)` requires `res.status(status).jsonp(num)`
* fix `req.next` when inside router instance
* include `ETag` header in `HEAD` requests
* keep previous `Content-Type` for `res.jsonp`
* support PURGE method
- add `app.purge`
- add `router.purge`
- include PURGE in `app.all`
* update debug to 0.8.0
- add `enable()` method
- change from stderr to stdout
* update methods to 1.0.0
- add PURGE
4.1.2 / 2014-05-08
==================
* fix `req.host` for IPv6 literals
* fix `res.jsonp` error if callback param is object
4.1.1 / 2014-04-27
==================
* fix package.json to reflect supported node version
4.1.0 / 2014-04-24
==================
* pass options from `res.sendfile` to `send`
* preserve casing of headers in `res.header` and `res.set`
* support unicode file names in `res.attachment` and `res.download`
* update accepts to 1.0.1
- deps: [email protected]
* update cookie to 0.1.2
- Fix for maxAge == 0
- made compat with expires field
* update send to 0.3.0
- Accept API options in options object
- Coerce option types
- Control whether to generate etags
- Default directory access to 403 when index disabled
- Fix sending files with dots without root set
- Include file path in etag
- Make "Can't set headers after they are sent." catchable
- Send full entity-body for multi range requests
- Set etags to "weak"
- Support "If-Range" header
- Support multiple index paths
- deps: [email protected]
* update serve-static to 1.1.0
- Accept options directly to `send` module
- Resolve relative paths at middleware setup
- Use parseurl to parse the URL from request
- deps: [email protected]
* update type-is to 1.1.0
- add non-array values support
- add `multipart` as a shorthand
4.0.0 / 2014-04-09
==================
* remove:
- node 0.8 support
- connect and connect's patches except for charset handling
- express(1) - moved to [express-generator](https://github.com/expressjs/generator)
- `express.createServer()` - it has been deprecated for a long time. Use `express()`
- `app.configure` - use logic in your own app code
- `app.router` - is removed
- `req.auth` - use `basic-auth` instead
- `req.accepted*` - use `req.accepts*()` instead
- `res.location` - relative URL resolution is removed
- `res.charset` - include the charset in the content type when using `res.set()`
- all bundled middleware except `static`
* change:
- `app.route` -> `app.mountpath` when mounting an express app in another express app
- `json spaces` no longer enabled by default in development
- `req.accepts*` -> `req.accepts*s` - i.e. `req.acceptsEncoding` -> `req.acceptsEncodings`
- `req.params` is now an object instead of an array
- `res.locals` is no longer a function. It is a plain js object. Treat it as such.
- `res.headerSent` -> `res.headersSent` to match node.js ServerResponse object
* refactor:
- `req.accepts*` with [accepts](https://github.com/expressjs/accepts)
- `req.is` with [type-is](https://github.com/expressjs/type-is)
- [path-to-regexp](https://github.com/component/path-to-regexp)
* add:
- `app.router()` - returns the app Router instance
- `app.route()` - Proxy to the app's `Router#route()` method to create a new route
- Router & Route - public API
3.21.2 / 2015-07-31
===================
* deps: [email protected]
- deps: body-parser@~1.13.3
- deps: compression@~1.5.2
- deps: errorhandler@~1.4.2
- deps: method-override@~2.3.5
- deps: serve-index@~1.7.2
- deps: type-is@~1.6.6
- deps: vhost@~3.0.1
* deps: vary@~1.0.1
- Fix setting empty header from empty `field`
- perf: enable strict mode
- perf: remove argument reassignments
3.21.1 / 2015-07-05
===================
* deps: basic-auth@~1.0.3
* deps: [email protected]
- deps: body-parser@~1.13.2
- deps: compression@~1.5.1
- deps: errorhandler@~1.4.1
- deps: morgan@~1.6.1
- deps: [email protected]
- deps: [email protected]
- deps: serve-index@~1.7.1
- deps: type-is@~1.6.4
3.21.0 / 2015-06-18
===================
* deps: [email protected]
- perf: enable strict mode
- perf: hoist regular expression
- perf: parse with regular expressions
- perf: remove argument reassignment
* deps: [email protected]
- deps: body-parser@~1.13.1
- deps: [email protected]
- deps: compression@~1.5.0
- deps: [email protected]
- deps: cookie-parser@~1.3.5
- deps: csurf@~1.8.3
- deps: errorhandler@~1.4.0
- deps: express-session@~1.11.3
- deps: [email protected]
- deps: [email protected]
- deps: morgan@~1.6.0
- deps: serve-favicon@~2.3.0
- deps: serve-index@~1.7.0
- deps: serve-static@~1.10.0
- deps: type-is@~1.6.3
* deps: [email protected]
- perf: deduce the scope of try-catch deopt
- perf: remove argument reassignments
* deps: [email protected]
* deps: etag@~1.7.0
- Always include entity length in ETags for hash length extensions
- Generate non-Stats ETags using MD5 only (no longer CRC32)
- Improve stat performance by removing hashing
- Improve support for JXcore
- Remove base64 padding in ETags to shorten
- Support "fake" stats objects in environments without fs
- Use MD5 instead of MD4 in weak ETags over 1KB
* deps: [email protected]
- Add weak `ETag` matching support
* deps: [email protected]
- Work in global strict mode
* deps: [email protected]
- Allow Node.js HTTP server to set `Date` response header
- Fix incorrectly removing `Content-Location` on 304 response
- Improve the default redirect response headers
- Send appropriate headers on default error response
- Use `http-errors` for standard emitted errors
- Use `statuses` instead of `http` module for status messages
- deps: [email protected]
- deps: etag@~1.7.0
- deps: [email protected]
- deps: on-finished@~2.3.0
- perf: enable strict mode
- perf: remove unnecessary array allocations
3.20.3 / 2015-05-17
===================
* deps: [email protected]
- deps: body-parser@~1.12.4
- deps: compression@~1.4.4
- deps: connect-timeout@~1.6.2
- deps: debug@~2.2.0
- deps: depd@~1.0.1
- deps: errorhandler@~1.3.6
- deps: [email protected]
- deps: method-override@~2.3.3
- deps: morgan@~1.5.3
- deps: [email protected]
- deps: response-time@~2.3.1
- deps: serve-favicon@~2.2.1
- deps: serve-index@~1.6.4
- deps: serve-static@~1.9.3
- deps: type-is@~1.6.2
* deps: debug@~2.2.0
- deps: [email protected]
* deps: depd@~1.0.1
* deps: proxy-addr@~1.0.8
- deps: [email protected]
* deps: [email protected]
- deps: debug@~2.2.0
- deps: depd@~1.0.1
- deps: etag@~1.6.0
- deps: [email protected]
- deps: on-finished@~2.2.1
3.20.2 / 2015-03-16
===================
* deps: [email protected]
- deps: body-parser@~1.12.2
- deps: compression@~1.4.3
- deps: connect-timeout@~1.6.1
- deps: debug@~2.1.3
- deps: errorhandler@~1.3.5
- deps: express-session@~1.10.4
- deps: [email protected]
- deps: method-override@~2.3.2
- deps: morgan@~1.5.2
- deps: [email protected]
- deps: serve-index@~1.6.3
- deps: serve-static@~1.9.2
- deps: type-is@~1.6.1
* deps: debug@~2.1.3
- Fix high intensity foreground color for bold
- deps: [email protected]
* deps: [email protected]
* deps: proxy-addr@~1.0.7
- deps: [email protected]
* deps: [email protected]
- Throw errors early for invalid `extensions` or `index` options
- deps: debug@~2.1.3
3.20.1 / 2015-02-28
===================
* Fix `req.host` when using "trust proxy" hops count
* Fix `req.protocol`/`req.secure` when using "trust proxy" hops count
3.20.0 / 2015-02-18
===================
* Fix `"trust proxy"` setting to inherit when app is mounted
* Generate `ETag`s for all request responses
- No longer restricted to only responses for `GET` and `HEAD` requests
* Use `content-type` to parse `Content-Type` headers
* deps: [email protected]
- Use `content-type` to parse `Content-Type` headers
- deps: body-parser@~1.12.0
- deps: compression@~1.4.1
- deps: connect-timeout@~1.6.0
- deps: cookie-parser@~1.3.4
- deps: [email protected]
- deps: csurf@~1.7.0
- deps: errorhandler@~1.3.4
- deps: express-session@~1.10.3
- deps: http-errors@~1.3.1
- deps: response-time@~2.3.0
- deps: serve-index@~1.6.2
- deps: serve-static@~1.9.1
- deps: type-is@~1.6.0
* deps: [email protected]
* deps: [email protected]
- Always read the stat size from the file
- Fix mutating passed-in `options`
- deps: [email protected]
3.19.2 / 2015-02-01
===================
* deps: [email protected]
- deps: compression@~1.3.1
- deps: csurf@~1.6.6
- deps: errorhandler@~1.3.3
- deps: express-session@~1.10.2
- deps: serve-index@~1.6.1
- deps: type-is@~1.5.6
* deps: proxy-addr@~1.0.6
- deps: [email protected]
3.19.1 / 2015-01-20
===================
* deps: [email protected]
- deps: body-parser@~1.10.2
- deps: serve-static@~1.8.1
* deps: [email protected]
- Fix root path disclosure
3.19.0 / 2015-01-09
===================
* Fix `OPTIONS` responses to include the `HEAD` method property
* Use `readline` for prompt in `express(1)`
* deps: [email protected]
* deps: [email protected]
- deps: body-parser@~1.10.1
- deps: compression@~1.3.0
- deps: connect-timeout@~1.5.0
- deps: csurf@~1.6.4
- deps: debug@~2.1.1
- deps: errorhandler@~1.3.2
- deps: express-session@~1.10.1
- deps: [email protected]
- deps: method-override@~2.3.1
- deps: morgan@~1.5.1
- deps: serve-favicon@~2.2.0
- deps: serve-index@~1.6.0
- deps: serve-static@~1.8.0
- deps: type-is@~1.5.5
* deps: debug@~2.1.1
* deps: methods@~1.1.1
* deps: proxy-addr@~1.0.5
- deps: [email protected]
* deps: [email protected]
- deps: debug@~2.1.1
- deps: etag@~1.5.1
- deps: [email protected]
- deps: on-finished@~2.2.0
3.18.6 / 2014-12-12
===================
* Fix exception in `req.fresh`/`req.stale` without response headers
3.18.5 / 2014-12-11
===================
* deps: [email protected]
- deps: compression@~1.2.2
- deps: express-session@~1.9.3
- deps: http-errors@~1.2.8
- deps: serve-index@~1.5.3
- deps: type-is@~1.5.4
3.18.4 / 2014-11-23
===================
* deps: [email protected]
- deps: body-parser@~1.9.3
- deps: compression@~1.2.1
- deps: errorhandler@~1.2.3
- deps: express-session@~1.9.2
- deps: [email protected]
- deps: serve-favicon@~2.1.7
- deps: serve-static@~1.5.1
- deps: type-is@~1.5.3
* deps: etag@~1.5.1
* deps: proxy-addr@~1.0.4
- deps: [email protected]
3.18.3 / 2014-11-09
===================
* deps: [email protected]
- Correctly invoke async callback asynchronously
- deps: csurf@~1.6.3
3.18.2 / 2014-10-28
===================
* deps: [email protected]
- Fix handling of URLs containing `://` in the path
- deps: body-parser@~1.9.2
- deps: [email protected]
3.18.1 / 2014-10-22
===================
* Fix internal `utils.merge` deprecation warnings
* deps: [email protected]
- deps: body-parser@~1.9.1
- deps: express-session@~1.9.1
- deps: [email protected]
- deps: morgan@~1.4.1
- deps: [email protected]
- deps: serve-static@~1.7.1
* deps: [email protected]
- deps: on-finished@~2.1.1
3.18.0 / 2014-10-17
===================
* Use `content-disposition` module for `res.attachment`/`res.download`
- Sends standards-compliant `Content-Disposition` header
- Full Unicode support
* Use `etag` module to generate `ETag` headers
* deps: [email protected]
- Use `http-errors` module for creating errors
- Use `utils-merge` module for merging objects
- deps: body-parser@~1.9.0
- deps: compression@~1.2.0
- deps: connect-timeout@~1.4.0
- deps: debug@~2.1.0
- deps: depd@~1.0.0
- deps: express-session@~1.9.0
- deps: [email protected]
- deps: method-override@~2.3.0
- deps: morgan@~1.4.0
- deps: response-time@~2.2.0
- deps: serve-favicon@~2.1.6
- deps: serve-index@~1.5.0
- deps: serve-static@~1.7.0
* deps: debug@~2.1.0
- Implement `DEBUG_FD` env variable support
* deps: depd@~1.0.0
* deps: [email protected]
- deps: debug@~2.1.0
- deps: depd@~1.0.0
- deps: etag@~1.5.0
3.17.8 / 2014-10-15
===================
* deps: [email protected]
- deps: compression@~1.1.2
- deps: csurf@~1.6.2
- deps: errorhandler@~1.2.2
3.17.7 / 2014-10-08
===================
* deps: [email protected]
- Fix accepting non-object arguments to `logger`
- deps: serve-static@~1.6.4
3.17.6 / 2014-10-02
===================
* deps: [email protected]
- deps: morgan@~1.3.2
- deps: type-is@~1.5.2
3.17.5 / 2014-09-24
===================
* deps: [email protected]
- deps: body-parser@~1.8.4
- deps: serve-favicon@~2.1.5
- deps: serve-static@~1.6.3
* deps: proxy-addr@~1.0.3
- Use `forwarded` npm module
* deps: [email protected]
- deps: etag@~1.4.0
3.17.4 / 2014-09-19
===================
* deps: [email protected]
- deps: body-parser@~1.8.3
- deps: [email protected]
3.17.3 / 2014-09-18
===================
* deps: proxy-addr@~1.0.2
- Fix a global leak when multiple subnets are trusted
- deps: [email protected]
3.17.2 / 2014-09-15
===================
* Use `crc` instead of `buffer-crc32` for speed
* deps: [email protected]
- deps: body-parser@~1.8.2
- deps: [email protected]
- deps: express-session@~1.8.2
- deps: morgan@~1.3.1
- deps: serve-favicon@~2.1.3
- deps: serve-static@~1.6.2
* deps: [email protected]
* deps: [email protected]
- deps: [email protected]
- deps: etag@~1.3.1
- deps: range-parser@~1.0.2
3.17.1 / 2014-09-08
===================
* Fix error in `req.subdomains` on empty host
3.17.0 / 2014-09-08
===================
* Support `X-Forwarded-Host` in `req.subdomains`
* Support IP address host in `req.subdomains`
* deps: [email protected]
- deps: body-parser@~1.8.1
- deps: compression@~1.1.0
- deps: connect-timeout@~1.3.0
- deps: cookie-parser@~1.3.3
- deps: [email protected]
- deps: csurf@~1.6.1
- deps: debug@~2.0.0
- deps: errorhandler@~1.2.0
- deps: express-session@~1.8.1
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: method-override@~2.2.0
- deps: morgan@~1.3.0
- deps: [email protected]
- deps: serve-favicon@~2.1.3
- deps: serve-index@~1.2.1
- deps: serve-static@~1.6.1
- deps: type-is@~1.5.1
- deps: vhost@~3.0.0
* deps: [email protected]
* deps: debug@~2.0.0
* deps: [email protected]
* deps: [email protected]
- Throw error when parameter format invalid on parse
* deps: range-parser@~1.0.2
* deps: [email protected]
- Add `lastModified` option
- Use `etag` to generate `ETag` header
- deps: debug@~2.0.0
- deps: [email protected]
* deps: vary@~1.0.0
- Accept valid `Vary` header string as `field`
3.16.10 / 2014-09-04
====================
* deps: [email protected]
- deps: serve-static@~1.5.4
* deps: [email protected]
- Fix a path traversal issue when using `root`
- Fix malicious path detection for empty string path
3.16.9 / 2014-08-29
===================
* deps: [email protected]
- deps: body-parser@~1.6.7
- deps: [email protected]
3.16.8 / 2014-08-27
===================
* deps: [email protected]
- deps: body-parser@~1.6.6
- deps: csurf@~1.4.1
- deps: [email protected]
3.16.7 / 2014-08-18
===================
* deps: [email protected]
- deps: body-parser@~1.6.5
- deps: express-session@~1.7.6
- deps: morgan@~1.2.3
- deps: serve-static@~1.5.3
* deps: [email protected]
- deps: [email protected]
- deps: [email protected]
3.16.6 / 2014-08-14
===================
* deps: [email protected]
- deps: body-parser@~1.6.4
- deps: [email protected]
- deps: serve-static@~1.5.2
* deps: [email protected]
- Work around `fd` leak in Node.js 0.10 for `fs.ReadStream`
3.16.5 / 2014-08-11
===================
* deps: [email protected]
- Fix backwards compatibility in `logger`
3.16.4 / 2014-08-10
===================
* Fix original URL parsing in `res.location`
* deps: [email protected]
- Fix `query` middleware breaking with argument
- deps: body-parser@~1.6.3
- deps: compression@~1.0.11
- deps: connect-timeout@~1.2.2
- deps: express-session@~1.7.5
- deps: method-override@~2.1.3
- deps: on-headers@~1.0.0
- deps: parseurl@~1.3.0
- deps: [email protected]
- deps: response-time@~2.0.1
- deps: serve-index@~1.1.6
- deps: serve-static@~1.5.1
* deps: parseurl@~1.3.0
3.16.3 / 2014-08-07
===================
* deps: [email protected]
- deps: [email protected]
3.16.2 / 2014-08-07
===================
* deps: [email protected]
- deps: body-parser@~1.6.2
- deps: [email protected]
3.16.1 / 2014-08-06
===================
* deps: [email protected]
- deps: body-parser@~1.6.1
- deps: [email protected]
3.16.0 / 2014-08-05
===================
* deps: [email protected]
- deps: body-parser@~1.6.0
- deps: compression@~1.0.10
- deps: csurf@~1.4.0
- deps: express-session@~1.7.4
- deps: [email protected]
- deps: serve-static@~1.5.0
* deps: [email protected]
- Add `extensions` option
3.15.3 / 2014-08-04
===================
* fix `res.sendfile` regression for serving directory index files
* deps: [email protected]
- deps: serve-index@~1.1.5
- deps: serve-static@~1.4.4
* deps: [email protected]
- Fix incorrect 403 on Windows and Node.js 0.11
- Fix serving index files without root dir
3.15.2 / 2014-07-27
===================
* deps: [email protected]
- deps: body-parser@~1.5.2
- deps: [email protected]
- deps: express-session@~1.7.2
- deps: morgan@~1.2.2
- deps: serve-static@~1.4.2
* deps: [email protected]
- Work-around v8 generating empty stack traces
* deps: [email protected]
- deps: [email protected]
3.15.1 / 2014-07-26
===================
* deps: [email protected]
- deps: body-parser@~1.5.1
- deps: [email protected]
- deps: express-session@~1.7.1
- deps: morgan@~1.2.1
- deps: serve-index@~1.1.4
- deps: serve-static@~1.4.1
* deps: [email protected]
- Fix exception when global `Error.stackTraceLimit` is too low
* deps: [email protected]
- deps: [email protected]
3.15.0 / 2014-07-22
===================
* Fix `req.protocol` for proxy-direct connections
* Pass options from `res.sendfile` to `send`
* deps: [email protected]
- deps: body-parser@~1.5.0
- deps: compression@~1.0.9
- deps: connect-timeout@~1.2.1
- deps: [email protected]
- deps: [email protected]
- deps: express-session@~1.7.0
- deps: [email protected]
- deps: method-override@~2.1.2
- deps: morgan@~1.2.0
- deps: [email protected]
- deps: parseurl@~1.2.0
- deps: serve-static@~1.4.0
* deps: [email protected]
* deps: [email protected]
- Add `TRACE_DEPRECATION` environment variable
- Remove non-standard grey color from color output
- Support `--no-deprecation` argument
- Support `--trace-deprecation` argument
* deps: parseurl@~1.2.0
- Cache URLs based on original value
- Remove no-longer-needed URL mis-parse work-around
- Simplify the "fast-path" `RegExp`
* deps: [email protected]
- Add `dotfiles` option
- Cap `maxAge` value to 1 year
- deps: [email protected]
- deps: [email protected]
3.14.0 / 2014-07-11
===================
* add explicit "Rosetta Flash JSONP abuse" protection
- previous versions are not vulnerable; this is just explicit protection
* deprecate `res.redirect(url, status)` -- use `res.redirect(status, url)` instead
* fix `res.send(status, num)` to send `num` as json (not error)
* remove unnecessary escaping when `res.jsonp` returns JSON response
* deps: [email protected]
- support empty password
- support empty username
* deps: [email protected]
- deps: [email protected]
- deps: express-session@~1.6.4
- deps: method-override@~2.1.0
- deps: parseurl@~1.1.3
- deps: serve-static@~1.3.1
* deps: [email protected]
- Add support for multiple wildcards in namespaces
* deps: [email protected]
- add `CONNECT`
* deps: parseurl@~1.1.3
- faster parsing of href-only URLs
3.13.0 / 2014-07-03
===================
* add deprecation message to `app.configure`
* add deprecation message to `req.auth`
* use `basic-auth` to parse `Authorization` header
* deps: [email protected]
- deps: csurf@~1.3.0
- deps: express-session@~1.6.1
- deps: [email protected]
- deps: serve-static@~1.3.0
* deps: [email protected]
- Accept string for `maxage` (converted by `ms`)
- Include link in default redirect response
3.12.1 / 2014-06-26
===================
* deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: express-session@~1.5.2
- deps: type-is@~1.3.2
* deps: [email protected]
- fix for timing attacks
3.12.0 / 2014-06-21
===================
* use `media-typer` to alter content-type charset
* deps: [email protected]
- deprecate `connect(middleware)` -- use `app.use(middleware)` instead
- deprecate `connect.createServer()` -- use `connect()` instead
- fix `res.setHeader()` patch to work with get -> append -> set pattern
- deps: compression@~1.0.8
- deps: errorhandler@~1.1.1
- deps: express-session@~1.5.0
- deps: serve-index@~1.1.3
3.11.0 / 2014-06-19
===================
* deprecate things with `depd` module
* deps: [email protected]
* deps: [email protected]
- deprecate `verify` option to `json` -- use `body-parser` npm module instead
- deprecate `verify` option to `urlencoded` -- use `body-parser` npm module instead
- deprecate things with `depd` module
- use `finalhandler` for final response handling
- use `media-typer` to parse `content-type` for charset
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
3.10.5 / 2014-06-11
===================
* deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
* deps: [email protected]
* deps: [email protected]
- Do not throw uncatchable error on file open race condition
- Use `escape-html` for HTML escaping
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
3.10.4 / 2014-06-09
===================
* deps: [email protected]
- fix "event emitter leak" warnings
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
* deps: [email protected]
* deps: [email protected]
- fix "event emitter leak" warnings
- deps: [email protected]
- deps: [email protected]
3.10.3 / 2014-06-05
===================
* use `vary` module for `res.vary`
* deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
* deps: [email protected]
3.10.2 / 2014-06-03
===================
* deps: [email protected]
- deps: [email protected]
3.10.1 / 2014-06-03
===================
* deps: [email protected]
- deps: [email protected]
* deps: [email protected]
3.10.0 / 2014-06-02
===================
* deps: [email protected]
- deprecate `methodOverride()` -- use `method-override` npm module instead
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
* deps: [email protected]
* deps: [email protected]
- Send `max-age` in `Cache-Control` in correct format
3.9.0 / 2014-05-30
==================
* custom etag control with `app.set('etag', val)`
- `app.set('etag', function(body, encoding){ return '"etag"' })` custom etag generation
- `app.set('etag', 'weak')` weak tag
- `app.set('etag', 'strong')` strong etag
- `app.set('etag', false)` turn off
- `app.set('etag', true)` standard etag
* Include ETag in HEAD requests
* mark `res.send` ETag as weak and reduce collisions
* update connect to 2.18.0
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
* update send to 0.4.0
- Calculate ETag with md5 for reduced collisions
- Ignore stream errors after request ends
- deps: [email protected]
3.8.1 / 2014-05-27
==================
* update connect to 2.17.3
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
3.8.0 / 2014-05-21
==================
* keep previous `Content-Type` for `res.jsonp`
* set proper `charset` in `Content-Type` for `res.send`
* update connect to 2.17.1
- fix `res.charset` appending charset when `content-type` has one
- deps: [email protected]
- deps: [email protected]
- deps: [email protected]
3.7.0 / 2014-05-18
==================
* proper proxy trust with `app.set('trust proxy', trust)`
- `app.set('trust proxy', 1)` trust first hop
- `app.set('trust proxy', 'loopback')` trust loopback addresses
- `app.set('trust proxy', '10.0.0.1')` trust single IP
- `app.set('trust proxy', '10.0.0.1/16')` trust subnet
- `app.set('trust proxy', '10.0.0.1, 10.0.0.2')` trust list
- `app.set('trust proxy', false)` turn off
- `app.set('trust proxy', true)` trust everything
* update connect to 2.16.2
- deprecate `res.headerSent` -- use `res.headersSent`
- deprecate `res.on("header")` -- use on-headers module instead
- fix edge-case in `res.appendHeader` that would append in wrong order
- json: use body-parser
- urlencoded: use body-parser
- dep: [email protected]
- dep: [email protected]
- dep: [email protected]
- dep: [email protected]
- dep: [email protected]
3.6.0 / 2014-05-09
==================
* deprecate `app.del()` -- use `app.delete()` instead
* deprecate `res.json(obj, status)` -- use `res.json(status, obj)` instead
- the edge-case `res.json(status, num)` requires `res.status(status).json(num)`
* deprecate `res.jsonp(obj, status)` -- use `res.jsonp(status, obj)` instead
- the edge-case `res.jsonp(status, num)` requires `res.status(status).jsonp(num)`
* support PURGE method
- add `app.purge`
- add `router.purge`
- include PURGE in `app.all`
* update connect to 2.15.0
* Add `res.appendHeader`
* Call error stack even when response has been sent
* Patch `res.headerSent` to return Boolean
* Patch `res.headersSent` for node.js 0.8
* Prevent default 404 handler after response sent
* dep: [email protected]
* dep: [email protected]
* dep: debug@^0.8.0
* dep: [email protected]
* dep: [email protected]
* dep: [email protected]
* dep: [email protected]
* dep: [email protected]
* update debug to 0.8.0
* add `enable()` method
* change from stderr to stdout
* update methods to 1.0.0
- add PURGE
* update mkdirp to 0.5.0
3.5.3 / 2014-05-08
==================
* fix `req.host` for IPv6 literals
* fix `res.jsonp` error if callback param is object
3.5.2 / 2014-04-24
==================
* update connect to 2.14.5
* update cookie to 0.1.2
* update mkdirp to 0.4.0
* update send to 0.3.0
3.5.1 / 2014-03-25
==================
* pin less-middleware in generated app
3.5.0 / 2014-03-06
==================
* bump deps
3.4.8 / 2014-01-13
==================
* prevent incorrect automatic OPTIONS responses #1868 @dpatti
* update binary and examples for jade 1.0 #1876 @yossi, #1877 @reqshark, #1892 @matheusazzi
* throw 400 in case of malformed paths @rlidwka
3.4.7 / 2013-12-10
==================
* update connect
3.4.6 / 2013-12-01
==================
* update connect (raw-body)
3.4.5 / 2013-11-27
==================
* update connect
* res.location: remove leading ./ #1802 @kapouer
* res.redirect: fix `res.redirect('toString') #1829 @michaelficarra
* res.send: always send ETag when content-length > 0
* router: add Router.all() method
3.4.4 / 2013-10-29
==================
* update connect
* update supertest
* update methods
* express(1): replace bodyParser() with urlencoded() and json() #1795 @chirag04
3.4.3 / 2013-10-23
==================
* update connect
3.4.2 / 2013-10-18
==================
* update connect
* downgrade commander
3.4.1 / 2013-10-15
==================
* update connect
* update commander
* jsonp: check if callback is a function
* router: wrap encodeURIComponent in a try/catch #1735 (@lxe)
* res.format: now includes charset @1747 (@sorribas)
* res.links: allow multiple calls @1746 (@sorribas)
3.4.0 / 2013-09-07
==================
* add res.vary(). Closes #1682
* update connect
3.3.8 / 2013-09-02
==================
* update connect
3.3.7 / 2013-08-28
==================
* update connect
3.3.6 / 2013-08-27
==================
* Revert "remove charset from json responses. Closes #1631" (causes issues in some clients)
* add: req.accepts take an argument list
3.3.4 / 2013-07-08
==================
* update send and connect
3.3.3 / 2013-07-04
==================
* update connect
3.3.2 / 2013-07-03
==================
* update connect
* update send
* remove .version export
3.3.1 / 2013-06-27
==================
* update connect
3.3.0 / 2013-06-26
==================
* update connect
* add support for multiple X-Forwarded-Proto values. Closes #1646
* change: remove charset from json responses. Closes #1631
* change: return actual booleans from req.accept* functions
* fix jsonp callback array throw
3.2.6 / 2013-06-02
==================
* update connect
3.2.5 / 2013-05-21
==================
* update connect
* update node-cookie
* add: throw a meaningful error when there is no default engine
* change generation of ETags with res.send() to GET requests only. Closes #1619
3.2.4 / 2013-05-09
==================
* fix `req.subdomains` when no Host is present
* fix `req.host` when no Host is present, return undefined
3.2.3 / 2013-05-07
==================
* update connect / qs
3.2.2 / 2013-05-03
==================
* update qs
3.2.1 / 2013-04-29
==================
* add app.VERB() paths array deprecation warning
* update connect
* update qs and remove all ~ semver crap
* fix: accept number as value of Signed Cookie
3.2.0 / 2013-04-15
==================
* add "view" constructor setting to override view behaviour
* add req.acceptsEncoding(name)
* add req.acceptedEncodings
* revert cookie signature change causing session race conditions
* fix sorting of Accept values of the same quality
3.1.2 / 2013-04-12
==================
* add support for custom Accept parameters
* update cookie-signature
3.1.1 / 2013-04-01
==================
* add X-Forwarded-Host support to `req.host`
* fix relative redirects
* update mkdirp
* update buffer-crc32
* remove legacy app.configure() method from app template.
3.1.0 / 2013-01-25
==================
* add support for leading "." in "view engine" setting
* add array support to `res.set()`
* add node 0.8.x to travis.yml
* add "subdomain offset" setting for tweaking `req.subdomains`
* add `res.location(url)` implementing `res.redirect()`-like setting of Location
* use app.get() for x-powered-by setting for inheritance
* fix colons in passwords for `req.auth`
3.0.6 / 2013-01-04
==================
* add http verb methods to Router
* update connect
* fix mangling of the `res.cookie()` options object
* fix jsonp whitespace escape. Closes #1132
3.0.5 / 2012-12-19
==================
* add throwing when a non-function is passed to a route
* fix: explicitly remove Transfer-Encoding header from 204 and 304 responses
* revert "add 'etag' option"
3.0.4 / 2012-12-05
==================
* add 'etag' option to disable `res.send()` Etags
* add escaping of urls in text/plain in `res.redirect()`
for old browsers interpreting as html
* change crc32 module for a more liberal license
* update connect
3.0.3 / 2012-11-13
==================
* update connect
* update cookie module
* fix cookie max-age
3.0.2 / 2012-11-08
==================
* add OPTIONS to cors example. Closes #1398
* fix route chaining regression. Closes #1397
3.0.1 / 2012-11-01
==================
* update connect
3.0.0 / 2012-10-23
==================
* add `make clean`
* add "Basic" check to req.auth
* add `req.auth` test coverage
* add cb && cb(payload) to `res.jsonp()`. Closes #1374
* add backwards compat for `res.redirect()` status. Closes #1336
* add support for `res.json()` to retain previously defined Content-Types. Closes #1349
* update connect
* change `res.redirect()` to utilize a pathname-relative Location again. Closes #1382
* remove non-primitive string support for `res.send()`
* fix view-locals example. Closes #1370
* fix route-separation example
3.0.0rc5 / 2012-09-18
==================
* update connect
* add redis search example
* add static-files example
* add "x-powered-by" setting (`app.disable('x-powered-by')`)
* add "application/octet-stream" redirect Accept test case. Closes #1317
3.0.0rc4 / 2012-08-30
==================
* add `res.jsonp()`. Closes #1307
* add "verbose errors" option to error-pages example
* add another route example to express(1) so people are not so confused
* add redis online user activity tracking example
* update connect dep
* fix etag quoting. Closes #1310
* fix error-pages 404 status
* fix jsonp callback char restrictions
* remove old OPTIONS default response
3.0.0rc3 / 2012-08-13
==================
* update connect dep
* fix signed cookies to work with `connect.cookieParser()` ("s:" prefix was missing) [tnydwrds]
* fix `res.render()` clobbering of "locals"
3.0.0rc2 / 2012-08-03
==================
* add CORS example
* update connect dep
* deprecate `.createServer()` & remove old stale examples
* fix: escape `res.redirect()` link
* fix vhost example
3.0.0rc1 / 2012-07-24
==================
* add more examples to view-locals
* add scheme-relative redirects (`res.redirect("//foo.com")`) support
* update cookie dep
* update connect dep
* update send dep
* fix `express(1)` -h flag, use -H for hogan. Closes #1245
* fix `res.sendfile()` socket error handling regression
3.0.0beta7 / 2012-07-16
==================
* update connect dep for `send()` root normalization regression
3.0.0beta6 / 2012-07-13
==================
* add `err.view` property for view errors. Closes #1226
* add "jsonp callback name" setting
* add support for "/foo/:bar*" non-greedy matches
* change `res.sendfile()` to use `send()` module
* change `res.send` to use "response-send" module
* remove `app.locals.use` and `res.locals.use`, use regular middleware
3.0.0beta5 / 2012-07-03
==================
* add "make check" support
* add route-map example
* add `res.json(obj, status)` support back for BC
* add "methods" dep, remove internal methods module
* update connect dep
* update auth example to utilize cores pbkdf2
* updated tests to use "supertest"
3.0.0beta4 / 2012-06-25
==================
* Added `req.auth`
* Added `req.range(size)`
* Added `res.links(obj)`
* Added `res.send(body, status)` support back for backwards compat
* Added `.default()` support to `res.format()`
* Added 2xx / 304 check to `req.fresh`
* Revert "Added + support to the router"
* Fixed `res.send()` freshness check, respect res.statusCode
3.0.0beta3 / 2012-06-15
==================
* Added hogan `--hjs` to express(1) [nullfirm]
* Added another example to content-negotiation
* Added `fresh` dep
* Changed: `res.send()` always checks freshness
* Fixed: expose connects mime module. Closes #1165
3.0.0beta2 / 2012-06-06
==================
* Added `+` support to the router
* Added `req.host`
* Changed `req.param()` to check route first
* Update connect dep
3.0.0beta1 / 2012-06-01
==================
* Added `res.format()` callback to override default 406 behaviour
* Fixed `res.redirect()` 406. Closes #1154
3.0.0alpha5 / 2012-05-30
==================
* Added `req.ip`
* Added `{ signed: true }` option to `res.cookie()`
* Removed `res.signedCookie()`
* Changed: dont reverse `req.ips`
* Fixed "trust proxy" setting check for `req.ips`
3.0.0alpha4 / 2012-05-09
==================
* Added: allow `[]` in jsonp callback. Closes #1128
* Added `PORT` env var support in generated template. Closes #1118 [benatkin]
* Updated: connect 2.2.2
3.0.0alpha3 / 2012-05-04
==================
* Added public `app.routes`. Closes #887
* Added _view-locals_ example
* Added _mvc_ example
* Added `res.locals.use()`. Closes #1120
* Added conditional-GET support to `res.send()`
* Added: coerce `res.set()` values to strings
* Changed: moved `static()` in generated apps below router
* Changed: `res.send()` only set ETag when not previously set
* Changed connect 2.2.1 dep
* Changed: `make test` now runs unit / acceptance tests
* Fixed req/res proto inheritance
3.0.0alpha2 / 2012-04-26
==================
* Added `make benchmark` back
* Added `res.send()` support for `String` objects
* Added client-side data exposing example
* Added `res.header()` and `req.header()` aliases for BC
* Added `express.createServer()` for BC
* Perf: memoize parsed urls
* Perf: connect 2.2.0 dep
* Changed: make `expressInit()` middleware self-aware
* Fixed: use app.get() for all core settings
* Fixed redis session example
* Fixed session example. Closes #1105
* Fixed generated express dep. Closes #1078
3.0.0alpha1 / 2012-04-15
==================
* Added `app.locals.use(callback)`
* Added `app.locals` object
* Added `app.locals(obj)`
* Added `res.locals` object
* Added `res.locals(obj)`
* Added `res.format()` for content-negotiation
* Added `app.engine()`
* Added `res.cookie()` JSON cookie support
* Added "trust proxy" setting
* Added `req.subdomains`
* Added `req.protocol`
* Added `req.secure`
* Added `req.path`
* Added `req.ips`
* Added `req.fresh`
* Added `req.stale`
* Added comma-delimited / array support for `req.accepts()`
* Added debug instrumentation
* Added `res.set(obj)`
* Added `res.set(field, value)`
* Added `res.get(field)`
* Added `app.get(setting)`. Closes #842
* Added `req.acceptsLanguage()`
* Added `req.acceptsCharset()`
* Added `req.accepted`
* Added `req.acceptedLanguages`
* Added `req.acceptedCharsets`
* Added "json replacer" setting
* Added "json spaces" setting
* Added X-Forwarded-Proto support to `res.redirect()`. Closes #92
* Added `--less` support to express(1)
* Added `express.response` prototype
* Added `express.request` prototype
* Added `express.application` prototype
* Added `app.path()`
* Added `app.render()`
* Added `res.type()` to replace `res.contentType()`
* Changed: `res.redirect()` to add relative support
* Changed: enable "jsonp callback" by default
* Changed: renamed "case sensitive routes" to "case sensitive routing"
* Rewrite of all tests with mocha
* Removed "root" setting
* Removed `res.redirect('home')` support
* Removed `req.notify()`
* Removed `app.register()`
* Removed `app.redirect()`
* Removed `app.is()`
* Removed `app.helpers()`
* Removed `app.dynamicHelpers()`
* Fixed `res.sendfile()` with non-GET. Closes #723
* Fixed express(1) public dir for windows. Closes #866
2.5.9/ 2012-04-02
==================
* Added support for PURGE request method [pbuyle]
* Fixed `express(1)` generated app `app.address()` before `listening` [mmalecki]
2.5.8 / 2012-02-08
==================
* Update mkdirp dep. Closes #991
2.5.7 / 2012-02-06
==================
* Fixed `app.all` duplicate DELETE requests [mscdex]
2.5.6 / 2012-01-13
==================
* Updated hamljs dev dep. Closes #953
2.5.5 / 2012-01-08
==================
* Fixed: set `filename` on cached templates [matthewleon]
2.5.4 / 2012-01-02
==================
* Fixed `express(1)` eol on 0.4.x. Closes #947
2.5.3 / 2011-12-30
==================
* Fixed `req.is()` when a charset is present
2.5.2 / 2011-12-10
==================
* Fixed: express(1) LF -> CRLF for windows
2.5.1 / 2011-11-17
==================
* Changed: updated connect to 1.8.x
* Removed sass.js support from express(1)
2.5.0 / 2011-10-24
==================
* Added ./routes dir for generated app by default
* Added npm install reminder to express(1) app gen
* Added 0.5.x support
* Removed `make test-cov` since it wont work with node 0.5.x
* Fixed express(1) public dir for windows. Closes #866
2.4.7 / 2011-10-05
==================
* Added mkdirp to express(1). Closes #795
* Added simple _json-config_ example
* Added shorthand for the parsed request's pathname via `req.path`
* Changed connect dep to 1.7.x to fix npm issue...
* Fixed `res.redirect()` __HEAD__ support. [reported by xerox]
* Fixed `req.flash()`, only escape args
* Fixed absolute path checking on windows. Closes #829 [reported by andrewpmckenzie]
2.4.6 / 2011-08-22
==================
* Fixed multiple param callback regression. Closes #824 [reported by TroyGoode]
2.4.5 / 2011-08-19
==================
* Added support for routes to handle errors. Closes #809
* Added `app.routes.all()`. Closes #803
* Added "basepath" setting to work in conjunction with reverse proxies etc.
* Refactored `Route` to use a single array of callbacks
* Added support for multiple callbacks for `app.param()`. Closes #801
Closes #805
* Changed: removed .call(self) for route callbacks
* Dependency: `qs >= 0.3.1`
* Fixed `res.redirect()` on windows due to `join()` usage. Closes #808
2.4.4 / 2011-08-05
==================
* Fixed `res.header()` intention of a set, even when `undefined`
* Fixed `*`, value no longer required
* Fixed `res.send(204)` support. Closes #771
2.4.3 / 2011-07-14
==================
* Added docs for `status` option special-case. Closes #739
* Fixed `options.filename`, exposing the view path to template engines
2.4.2. / 2011-07-06
==================
* Revert "removed jsonp stripping" for XSS
2.4.1 / 2011-07-06
==================
* Added `res.json()` JSONP support. Closes #737
* Added _extending-templates_ example. Closes #730
* Added "strict routing" setting for trailing slashes
* Added support for multiple envs in `app.configure()` calls. Closes #735
* Changed: `res.send()` using `res.json()`
* Changed: when cookie `path === null` don't default it
* Changed; default cookie path to "home" setting. Closes #731
* Removed _pids/logs_ creation from express(1)
2.4.0 / 2011-06-28
==================
* Added chainable `res.status(code)`
* Added `res.json()`, an explicit version of `res.send(obj)`
* Added simple web-service example
2.3.12 / 2011-06-22
==================
* \#express is now on freenode! come join!
* Added `req.get(field, param)`
* Added links to Japanese documentation, thanks @hideyukisaito!
* Added; the `express(1)` generated app outputs the env
* Added `content-negotiation` example
* Dependency: connect >= 1.5.1 < 2.0.0
* Fixed view layout bug. Closes #720
* Fixed; ignore body on 304. Closes #701
2.3.11 / 2011-06-04
==================
* Added `npm test`
* Removed generation of dummy test file from `express(1)`
* Fixed; `express(1)` adds express as a dep
* Fixed; prune on `prepublish`
2.3.10 / 2011-05-27
==================
* Added `req.route`, exposing the current route
* Added _package.json_ generation support to `express(1)`
* Fixed call to `app.param()` function for optional params. Closes #682
2.3.9 / 2011-05-25
==================
* Fixed bug-ish with `../' in `res.partial()` calls
2.3.8 / 2011-05-24
==================
* Fixed `app.options()`
2.3.7 / 2011-05-23
==================
* Added route `Collection`, ex: `app.get('/user/:id').remove();`
* Added support for `app.param(fn)` to define param logic
* Removed `app.param()` support for callback with return value
* Removed module.parent check from express(1) generated app. Closes #670
* Refactored router. Closes #639
2.3.6 / 2011-05-20
==================
* Changed; using devDependencies instead of git submodules
* Fixed redis session example
* Fixed markdown example
* Fixed view caching, should not be enabled in development
2.3.5 / 2011-05-20
==================
* Added export `.view` as alias for `.View`
2.3.4 / 2011-05-08
==================
* Added `./examples/say`
* Fixed `res.sendfile()` bug preventing the transfer of files with spaces
2.3.3 / 2011-05-03
==================
* Added "case sensitive routes" option.
* Changed; split methods supported per rfc [slaskis]
* Fixed route-specific middleware when using the same callback function several times
2.3.2 / 2011-04-27
==================
* Fixed view hints
2.3.1 / 2011-04-26
==================
* Added `app.match()` as `app.match.all()`
* Added `app.lookup()` as `app.lookup.all()`
* Added `app.remove()` for `app.remove.all()`
* Added `app.remove.VERB()`
* Fixed template caching collision issue. Closes #644
* Moved router over from connect and started refactor
2.3.0 / 2011-04-25
==================
* Added options support to `res.clearCookie()`
* Added `res.helpers()` as alias of `res.locals()`
* Added; json defaults to UTF-8 with `res.send()`. Closes #632. [Daniel * Dependency `connect >= 1.4.0`
* Changed; auto set Content-Type in res.attachement [Aaron Heckmann]
* Renamed "cache views" to "view cache". Closes #628
* Fixed caching of views when using several apps. Closes #637
* Fixed gotcha invoking `app.param()` callbacks once per route middleware.
Closes #638
* Fixed partial lookup precedence. Closes #631
Shaw]
2.2.2 / 2011-04-12
==================
* Added second callback support for `res.download()` connection errors
* Fixed `filename` option passing to template engine
2.2.1 / 2011-04-04
==================
* Added `layout(path)` helper to change the layout within a view. Closes #610
* Fixed `partial()` collection object support.
Previously only anything with `.length` would work.
When `.length` is present one must still be aware of holes,
however now `{ collection: {foo: 'bar'}}` is valid, exposes
`keyInCollection` and `keysInCollection`.
* Performance improved with better view caching
* Removed `request` and `response` locals
* Changed; errorHandler page title is now `Express` instead of `Connect`
2.2.0 / 2011-03-30
==================
* Added `app.lookup.VERB()`, ex `app.lookup.put('/user/:id')`. Closes #606
* Added `app.match.VERB()`, ex `app.match.put('/user/12')`. Closes #606
* Added `app.VERB(path)` as alias of `app.lookup.VERB()`.
* Dependency `connect >= 1.2.0`
2.1.1 / 2011-03-29
==================
* Added; expose `err.view` object when failing to locate a view
* Fixed `res.partial()` call `next(err)` when no callback is given [reported by aheckmann]
* Fixed; `res.send(undefined)` responds with 204 [aheckmann]
2.1.0 / 2011-03-24
==================
* Added `<root>/_?<name>` partial lookup support. Closes #447
* Added `request`, `response`, and `app` local variables
* Added `settings` local variable, containing the app's settings
* Added `req.flash()` exception if `req.session` is not available
* Added `res.send(bool)` support (json response)
* Fixed stylus example for latest version
* Fixed; wrap try/catch around `res.render()`
2.0.0 / 2011-03-17
==================
* Fixed up index view path alternative.
* Changed; `res.locals()` without object returns the locals
2.0.0rc3 / 2011-03-17
==================
* Added `res.locals(obj)` to compliment `res.local(key, val)`
* Added `res.partial()` callback support
* Fixed recursive error reporting issue in `res.render()`
2.0.0rc2 / 2011-03-17
==================
* Changed; `partial()` "locals" are now optional
* Fixed `SlowBuffer` support. Closes #584 [reported by tyrda01]
* Fixed .filename view engine option [reported by drudge]
* Fixed blog example
* Fixed `{req,res}.app` reference when mounting [Ben Weaver]
2.0.0rc / 2011-03-14
==================
* Fixed; expose `HTTPSServer` constructor
* Fixed express(1) default test charset. Closes #579 [reported by secoif]
* Fixed; default charset to utf-8 instead of utf8 for lame IE [reported by NickP]
2.0.0beta3 / 2011-03-09
==================
* Added support for `res.contentType()` literal
The original `res.contentType('.json')`,
`res.contentType('application/json')`, and `res.contentType('json')`
will work now.
* Added `res.render()` status option support back
* Added charset option for `res.render()`
* Added `.charset` support (via connect 1.0.4)
* Added view resolution hints when in development and a lookup fails
* Added layout lookup support relative to the page view.
For example while rendering `./views/user/index.jade` if you create
`./views/user/layout.jade` it will be used in favour of the root layout.
* Fixed `res.redirect()`. RFC states absolute url [reported by unlink]
* Fixed; default `res.send()` string charset to utf8
* Removed `Partial` constructor (not currently used)
2.0.0beta2 / 2011-03-07
==================
* Added res.render() `.locals` support back to aid in migration process
* Fixed flash example
2.0.0beta / 2011-03-03
==================
* Added HTTPS support
* Added `res.cookie()` maxAge support
* Added `req.header()` _Referrer_ / _Referer_ special-case, either works
* Added mount support for `res.redirect()`, now respects the mount-point
* Added `union()` util, taking place of `merge(clone())` combo
* Added stylus support to express(1) generated app
* Added secret to session middleware used in examples and generated app
* Added `res.local(name, val)` for progressive view locals
* Added default param support to `req.param(name, default)`
* Added `app.disabled()` and `app.enabled()`
* Added `app.register()` support for omitting leading ".", either works
* Added `res.partial()`, using the same interface as `partial()` within a view. Closes #539
* Added `app.param()` to map route params to async/sync logic
* Added; aliased `app.helpers()` as `app.locals()`. Closes #481
* Added extname with no leading "." support to `res.contentType()`
* Added `cache views` setting, defaulting to enabled in "production" env
* Added index file partial resolution, eg: partial('user') may try _views/user/index.jade_.
* Added `req.accepts()` support for extensions
* Changed; `res.download()` and `res.sendfile()` now utilize Connect's
static file server `connect.static.send()`.
* Changed; replaced `connect.utils.mime()` with npm _mime_ module
* Changed; allow `req.query` to be pre-defined (via middleware or other parent
* Changed view partial resolution, now relative to parent view
* Changed view engine signature. no longer `engine.render(str, options, callback)`, now `engine.compile(str, options) -> Function`, the returned function accepts `fn(locals)`.
* Fixed `req.param()` bug returning Array.prototype methods. Closes #552
* Fixed; using `Stream#pipe()` instead of `sys.pump()` in `res.sendfile()`
* Fixed; using _qs_ module instead of _querystring_
* Fixed; strip unsafe chars from jsonp callbacks
* Removed "stream threshold" setting
1.0.8 / 2011-03-01
==================
* Allow `req.query` to be pre-defined (via middleware or other parent app)
* "connect": ">= 0.5.0 < 1.0.0". Closes #547
* Removed the long deprecated __EXPRESS_ENV__ support
1.0.7 / 2011-02-07
==================
* Fixed `render()` setting inheritance.
Mounted apps would not inherit "view engine"
1.0.6 / 2011-02-07
==================
* Fixed `view engine` setting bug when period is in dirname
1.0.5 / 2011-02-05
==================
* Added secret to generated app `session()` call
1.0.4 / 2011-02-05
==================
* Added `qs` dependency to _package.json_
* Fixed namespaced `require()`s for latest connect support
1.0.3 / 2011-01-13
==================
* Remove unsafe characters from JSONP callback names [Ryan Grove]
1.0.2 / 2011-01-10
==================
* Removed nested require, using `connect.router`
1.0.1 / 2010-12-29
==================
* Fixed for middleware stacked via `createServer()`
previously the `foo` middleware passed to `createServer(foo)`
would not have access to Express methods such as `res.send()`
or props like `req.query` etc.
1.0.0 / 2010-11-16
==================
* Added; deduce partial object names from the last segment.
For example by default `partial('forum/post', postObject)` will
give you the _post_ object, providing a meaningful default.
* Added http status code string representation to `res.redirect()` body
* Added; `res.redirect()` supporting _text/plain_ and _text/html_ via __Accept__.
* Added `req.is()` to aid in content negotiation
* Added partial local inheritance [suggested by masylum]. Closes #102
providing access to parent template locals.
* Added _-s, --session[s]_ flag to express(1) to add session related middleware
* Added _--template_ flag to express(1) to specify the
template engine to use.
* Added _--css_ flag to express(1) to specify the
stylesheet engine to use (or just plain css by default).
* Added `app.all()` support [thanks aheckmann]
* Added partial direct object support.
You may now `partial('user', user)` providing the "user" local,
vs previously `partial('user', { object: user })`.
* Added _route-separation_ example since many people question ways
to do this with CommonJS modules. Also view the _blog_ example for
an alternative.
* Performance; caching view path derived partial object names
* Fixed partial local inheritance precedence. [reported by Nick Poulden] Closes #454
* Fixed jsonp support; _text/javascript_ as per mailinglist discussion
1.0.0rc4 / 2010-10-14
==================
* Added _NODE_ENV_ support, _EXPRESS_ENV_ is deprecated and will be removed in 1.0.0
* Added route-middleware support (very helpful, see the [docs](http://expressjs.com/guide.html#Route-Middleware))
* Added _jsonp callback_ setting to enable/disable jsonp autowrapping [Dav Glass]
* Added callback query check on response.send to autowrap JSON objects for simple webservice implementations [Dav Glass]
* Added `partial()` support for array-like collections. Closes #434
* Added support for swappable querystring parsers
* Added session usage docs. Closes #443
* Added dynamic helper caching. Closes #439 [suggested by maritz]
* Added authentication example
* Added basic Range support to `res.sendfile()` (and `res.download()` etc)
* Changed; `express(1)` generated app using 2 spaces instead of 4
* Default env to "development" again [aheckmann]
* Removed _context_ option is no more, use "scope"
* Fixed; exposing _./support_ libs to examples so they can run without installs
* Fixed mvc example
1.0.0rc3 / 2010-09-20
==================
* Added confirmation for `express(1)` app generation. Closes #391
* Added extending of flash formatters via `app.flashFormatters`
* Added flash formatter support. Closes #411
* Added streaming support to `res.sendfile()` using `sys.pump()` when >= "stream threshold"
* Added _stream threshold_ setting for `res.sendfile()`
* Added `res.send()` __HEAD__ support
* Added `res.clearCookie()`
* Added `res.cookie()`
* Added `res.render()` headers option
* Added `res.redirect()` response bodies
* Added `res.render()` status option support. Closes #425 [thanks aheckmann]
* Fixed `res.sendfile()` responding with 403 on malicious path
* Fixed `res.download()` bug; when an error occurs remove _Content-Disposition_
* Fixed; mounted apps settings now inherit from parent app [aheckmann]
* Fixed; stripping Content-Length / Content-Type when 204
* Fixed `res.send()` 204. Closes #419
* Fixed multiple _Set-Cookie_ headers via `res.header()`. Closes #402
* Fixed bug messing with error handlers when `listenFD()` is called instead of `listen()`. [thanks guillermo]
1.0.0rc2 / 2010-08-17
==================
* Added `app.register()` for template engine mapping. Closes #390
* Added `res.render()` callback support as second argument (no options)
* Added callback support to `res.download()`
* Added callback support for `res.sendfile()`
* Added support for middleware access via `express.middlewareName()` vs `connect.middlewareName()`
* Added "partials" setting to docs
* Added default expresso tests to `express(1)` generated app. Closes #384
* Fixed `res.sendfile()` error handling, defer via `next()`
* Fixed `res.render()` callback when a layout is used [thanks guillermo]
* Fixed; `make install` creating ~/.node_libraries when not present
* Fixed issue preventing error handlers from being defined anywhere. Closes #387
1.0.0rc / 2010-07-28
==================
* Added mounted hook. Closes #369
* Added connect dependency to _package.json_
* Removed "reload views" setting and support code
development env never caches, production always caches.
* Removed _param_ in route callbacks, signature is now
simply (req, res, next), previously (req, res, params, next).
Use _req.params_ for path captures, _req.query_ for GET params.
* Fixed "home" setting
* Fixed middleware/router precedence issue. Closes #366
* Fixed; _configure()_ callbacks called immediately. Closes #368
1.0.0beta2 / 2010-07-23
==================
* Added more examples
* Added; exporting `Server` constructor
* Added `Server#helpers()` for view locals
* Added `Server#dynamicHelpers()` for dynamic view locals. Closes #349
* Added support for absolute view paths
* Added; _home_ setting defaults to `Server#route` for mounted apps. Closes #363
* Added Guillermo Rauch to the contributor list
* Added support for "as" for non-collection partials. Closes #341
* Fixed _install.sh_, ensuring _~/.node_libraries_ exists. Closes #362 [thanks jf]
* Fixed `res.render()` exceptions, now passed to `next()` when no callback is given [thanks guillermo]
* Fixed instanceof `Array` checks, now `Array.isArray()`
* Fixed express(1) expansion of public dirs. Closes #348
* Fixed middleware precedence. Closes #345
* Fixed view watcher, now async [thanks aheckmann]
1.0.0beta / 2010-07-15
==================
* Re-write
- much faster
- much lighter
- Check [ExpressJS.com](http://expressjs.com) for migration guide and updated docs
0.14.0 / 2010-06-15
==================
* Utilize relative requires
* Added Static bufferSize option [aheckmann]
* Fixed caching of view and partial subdirectories [aheckmann]
* Fixed mime.type() comments now that ".ext" is not supported
* Updated haml submodule
* Updated class submodule
* Removed bin/express
0.13.0 / 2010-06-01
==================
* Added node v0.1.97 compatibility
* Added support for deleting cookies via Request#cookie('key', null)
* Updated haml submodule
* Fixed not-found page, now using charset utf-8
* Fixed show-exceptions page, now using charset utf-8
* Fixed view support due to fs.readFile Buffers
* Changed; mime.type() no longer accepts ".type" due to node extname() changes
0.12.0 / 2010-05-22
==================
* Added node v0.1.96 compatibility
* Added view `helpers` export which act as additional local variables
* Updated haml submodule
* Changed ETag; removed inode, modified time only
* Fixed LF to CRLF for setting multiple cookies
* Fixed cookie compilation; values are now urlencoded
* Fixed cookies parsing; accepts quoted values and url escaped cookies
0.11.0 / 2010-05-06
==================
* Added support for layouts using different engines
- this.render('page.html.haml', { layout: 'super-cool-layout.html.ejs' })
- this.render('page.html.haml', { layout: 'foo' }) // assumes 'foo.html.haml'
- this.render('page.html.haml', { layout: false }) // no layout
* Updated ext submodule
* Updated haml submodule
* Fixed EJS partial support by passing along the context. Issue #307
0.10.1 / 2010-05-03
==================
* Fixed binary uploads.
0.10.0 / 2010-04-30
==================
* Added charset support via Request#charset (automatically assigned to 'UTF-8' when respond()'s
encoding is set to 'utf8' or 'utf-8').
* Added "encoding" option to Request#render(). Closes #299
* Added "dump exceptions" setting, which is enabled by default.
* Added simple ejs template engine support
* Added error response support for text/plain, application/json. Closes #297
* Added callback function param to Request#error()
* Added Request#sendHead()
* Added Request#stream()
* Added support for Request#respond(304, null) for empty response bodies
* Added ETag support to Request#sendfile()
* Added options to Request#sendfile(), passed to fs.createReadStream()
* Added filename arg to Request#download()
* Performance enhanced due to pre-reversing plugins so that plugins.reverse() is not called on each request
* Performance enhanced by preventing several calls to toLowerCase() in Router#match()
* Changed; Request#sendfile() now streams
* Changed; Renamed Request#halt() to Request#respond(). Closes #289
* Changed; Using sys.inspect() instead of JSON.encode() for error output
* Changed; run() returns the http.Server instance. Closes #298
* Changed; Defaulting Server#host to null (INADDR_ANY)
* Changed; Logger "common" format scale of 0.4f
* Removed Logger "request" format
* Fixed; Catching ENOENT in view caching, preventing error when "views/partials" is not found
* Fixed several issues with http client
* Fixed Logger Content-Length output
* Fixed bug preventing Opera from retaining the generated session id. Closes #292
0.9.0 / 2010-04-14
==================
* Added DSL level error() route support
* Added DSL level notFound() route support
* Added Request#error()
* Added Request#notFound()
* Added Request#render() callback function. Closes #258
* Added "max upload size" setting
* Added "magic" variables to collection partials (\_\_index\_\_, \_\_length\_\_, \_\_isFirst\_\_, \_\_isLast\_\_). Closes #254
* Added [haml.js](http://github.com/visionmedia/haml.js) submodule; removed haml-js
* Added callback function support to Request#halt() as 3rd/4th arg
* Added preprocessing of route param wildcards using param(). Closes #251
* Added view partial support (with collections etc.)
* Fixed bug preventing falsey params (such as ?page=0). Closes #286
* Fixed setting of multiple cookies. Closes #199
* Changed; view naming convention is now NAME.TYPE.ENGINE (for example page.html.haml)
* Changed; session cookie is now httpOnly
* Changed; Request is no longer global
* Changed; Event is no longer global
* Changed; "sys" module is no longer global
* Changed; moved Request#download to Static plugin where it belongs
* Changed; Request instance created before body parsing. Closes #262
* Changed; Pre-caching views in memory when "cache view contents" is enabled. Closes #253
* Changed; Pre-caching view partials in memory when "cache view partials" is enabled
* Updated support to node --version 0.1.90
* Updated dependencies
* Removed set("session cookie") in favour of use(Session, { cookie: { ... }})
* Removed utils.mixin(); use Object#mergeDeep()
0.8.0 / 2010-03-19
==================
* Added coffeescript example app. Closes #242
* Changed; cache api now async friendly. Closes #240
* Removed deprecated 'express/static' support. Use 'express/plugins/static'
0.7.6 / 2010-03-19
==================
* Added Request#isXHR. Closes #229
* Added `make install` (for the executable)
* Added `express` executable for setting up simple app templates
* Added "GET /public/*" to Static plugin, defaulting to <root>/public
* Added Static plugin
* Fixed; Request#render() only calls cache.get() once
* Fixed; Namespacing View caches with "view:"
* Fixed; Namespacing Static caches with "static:"
* Fixed; Both example apps now use the Static plugin
* Fixed set("views"). Closes #239
* Fixed missing space for combined log format
* Deprecated Request#sendfile() and 'express/static'
* Removed Server#running
0.7.5 / 2010-03-16
==================
* Added Request#flash() support without args, now returns all flashes
* Updated ext submodule
0.7.4 / 2010-03-16
==================
* Fixed session reaper
* Changed; class.js replacing js-oo Class implementation (quite a bit faster, no browser cruft)
0.7.3 / 2010-03-16
==================
* Added package.json
* Fixed requiring of haml / sass due to kiwi removal
0.7.2 / 2010-03-16
==================
* Fixed GIT submodules (HAH!)
0.7.1 / 2010-03-16
==================
* Changed; Express now using submodules again until a PM is adopted
* Changed; chat example using millisecond conversions from ext
0.7.0 / 2010-03-15
==================
* Added Request#pass() support (finds the next matching route, or the given path)
* Added Logger plugin (default "common" format replaces CommonLogger)
* Removed Profiler plugin
* Removed CommonLogger plugin
0.6.0 / 2010-03-11
==================
* Added seed.yml for kiwi package management support
* Added HTTP client query string support when method is GET. Closes #205
* Added support for arbitrary view engines.
For example "foo.engine.html" will now require('engine'),
the exports from this module are cached after the first require().
* Added async plugin support
* Removed usage of RESTful route funcs as http client
get() etc, use http.get() and friends
* Removed custom exceptions
0.5.0 / 2010-03-10
==================
* Added ext dependency (library of js extensions)
* Removed extname() / basename() utils. Use path module
* Removed toArray() util. Use arguments.values
* Removed escapeRegexp() util. Use RegExp.escape()
* Removed process.mixin() dependency. Use utils.mixin()
* Removed Collection
* Removed ElementCollection
* Shameless self promotion of ebook "Advanced JavaScript" (http://dev-mag.com) ;)
0.4.0 / 2010-02-11
==================
* Added flash() example to sample upload app
* Added high level restful http client module (express/http)
* Changed; RESTful route functions double as HTTP clients. Closes #69
* Changed; throwing error when routes are added at runtime
* Changed; defaulting render() context to the current Request. Closes #197
* Updated haml submodule
0.3.0 / 2010-02-11
==================
* Updated haml / sass submodules. Closes #200
* Added flash message support. Closes #64
* Added accepts() now allows multiple args. fixes #117
* Added support for plugins to halt. Closes #189
* Added alternate layout support. Closes #119
* Removed Route#run(). Closes #188
* Fixed broken specs due to use(Cookie) missing
0.2.1 / 2010-02-05
==================
* Added "plot" format option for Profiler (for gnuplot processing)
* Added request number to Profiler plugin
* Fixed binary encoding for multipart file uploads, was previously defaulting to UTF8
* Fixed issue with routes not firing when not files are present. Closes #184
* Fixed process.Promise -> events.Promise
0.2.0 / 2010-02-03
==================
* Added parseParam() support for name[] etc. (allows for file inputs with "multiple" attr) Closes #180
* Added Both Cache and Session option "reapInterval" may be "reapEvery". Closes #174
* Added expiration support to cache api with reaper. Closes #133
* Added cache Store.Memory#reap()
* Added Cache; cache api now uses first class Cache instances
* Added abstract session Store. Closes #172
* Changed; cache Memory.Store#get() utilizing Collection
* Renamed MemoryStore -> Store.Memory
* Fixed use() of the same plugin several time will always use latest options. Closes #176
0.1.0 / 2010-02-03
==================
* Changed; Hooks (before / after) pass request as arg as well as evaluated in their context
* Updated node support to 0.1.27 Closes #169
* Updated dirname(__filename) -> __dirname
* Updated libxmljs support to v0.2.0
* Added session support with memory store / reaping
* Added quick uid() helper
* Added multi-part upload support
* Added Sass.js support / submodule
* Added production env caching view contents and static files
* Added static file caching. Closes #136
* Added cache plugin with memory stores
* Added support to StaticFile so that it works with non-textual files.
* Removed dirname() helper
* Removed several globals (now their modules must be required)
0.0.2 / 2010-01-10
==================
* Added view benchmarks; currently haml vs ejs
* Added Request#attachment() specs. Closes #116
* Added use of node's parseQuery() util. Closes #123
* Added `make init` for submodules
* Updated Haml
* Updated sample chat app to show messages on load
* Updated libxmljs parseString -> parseHtmlString
* Fixed `make init` to work with older versions of git
* Fixed specs can now run independent specs for those who can't build deps. Closes #127
* Fixed issues introduced by the node url module changes. Closes 126.
* Fixed two assertions failing due to Collection#keys() returning strings
* Fixed faulty Collection#toArray() spec due to keys() returning strings
* Fixed `make test` now builds libxmljs.node before testing
0.0.1 / 2010-01-03
==================
* Initial release | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express/History.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express/History.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 115152
} |
[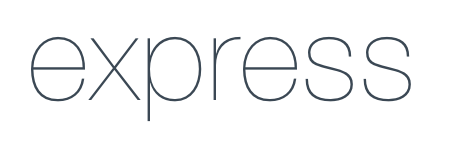](http://expressjs.com/)
**Fast, unopinionated, minimalist web framework for [Node.js](http://nodejs.org).**
**This project has a [Code of Conduct][].**
## Table of contents
* [Installation](#Installation)
* [Features](#Features)
* [Docs & Community](#docs--community)
* [Quick Start](#Quick-Start)
* [Running Tests](#Running-Tests)
* [Philosophy](#Philosophy)
* [Examples](#Examples)
* [Contributing to Express](#Contributing)
* [TC (Technical Committee)](#tc-technical-committee)
* [Triagers](#triagers)
* [License](#license)
[![NPM Version][npm-version-image]][npm-url]
[![NPM Install Size][npm-install-size-image]][npm-install-size-url]
[![NPM Downloads][npm-downloads-image]][npm-downloads-url]
[![OpenSSF Scorecard Badge][ossf-scorecard-badge]][ossf-scorecard-visualizer]
```js
const express = require('express')
const app = express()
app.get('/', function (req, res) {
res.send('Hello World')
})
app.listen(3000)
```
## Installation
This is a [Node.js](https://nodejs.org/en/) module available through the
[npm registry](https://www.npmjs.com/).
Before installing, [download and install Node.js](https://nodejs.org/en/download/).
Node.js 0.10 or higher is required.
If this is a brand new project, make sure to create a `package.json` first with
the [`npm init` command](https://docs.npmjs.com/creating-a-package-json-file).
Installation is done using the
[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
```console
$ npm install express
```
Follow [our installing guide](http://expressjs.com/en/starter/installing.html)
for more information.
## Features
* Robust routing
* Focus on high performance
* Super-high test coverage
* HTTP helpers (redirection, caching, etc)
* View system supporting 14+ template engines
* Content negotiation
* Executable for generating applications quickly
## Docs & Community
* [Website and Documentation](http://expressjs.com/) - [[website repo](https://github.com/expressjs/expressjs.com)]
* [#express](https://web.libera.chat/#express) on [Libera Chat](https://libera.chat) IRC
* [GitHub Organization](https://github.com/expressjs) for Official Middleware & Modules
* Visit the [Wiki](https://github.com/expressjs/express/wiki)
* [Google Group](https://groups.google.com/group/express-js) for discussion
* [Gitter](https://gitter.im/expressjs/express) for support and discussion
**PROTIP** Be sure to read [Migrating from 3.x to 4.x](https://github.com/expressjs/express/wiki/Migrating-from-3.x-to-4.x) as well as [New features in 4.x](https://github.com/expressjs/express/wiki/New-features-in-4.x).
## Quick Start
The quickest way to get started with express is to utilize the executable [`express(1)`](https://github.com/expressjs/generator) to generate an application as shown below:
Install the executable. The executable's major version will match Express's:
```console
$ npm install -g express-generator@4
```
Create the app:
```console
$ express /tmp/foo && cd /tmp/foo
```
Install dependencies:
```console
$ npm install
```
Start the server:
```console
$ npm start
```
View the website at: http://localhost:3000
## Philosophy
The Express philosophy is to provide small, robust tooling for HTTP servers, making
it a great solution for single page applications, websites, hybrids, or public
HTTP APIs.
Express does not force you to use any specific ORM or template engine. With support for over
14 template engines via [Consolidate.js](https://github.com/tj/consolidate.js),
you can quickly craft your perfect framework.
## Examples
To view the examples, clone the Express repo and install the dependencies:
```console
$ git clone https://github.com/expressjs/express.git --depth 1
$ cd express
$ npm install
```
Then run whichever example you want:
```console
$ node examples/content-negotiation
```
## Contributing
[![Linux Build][github-actions-ci-image]][github-actions-ci-url]
[![Windows Build][appveyor-image]][appveyor-url]
[![Test Coverage][coveralls-image]][coveralls-url]
The Express.js project welcomes all constructive contributions. Contributions take many forms,
from code for bug fixes and enhancements, to additions and fixes to documentation, additional
tests, triaging incoming pull requests and issues, and more!
See the [Contributing Guide](Contributing.md) for more technical details on contributing.
### Security Issues
If you discover a security vulnerability in Express, please see [Security Policies and Procedures](Security.md).
### Running Tests
To run the test suite, first install the dependencies, then run `npm test`:
```console
$ npm install
$ npm test
```
## People
The original author of Express is [TJ Holowaychuk](https://github.com/tj)
[List of all contributors](https://github.com/expressjs/express/graphs/contributors)
### TC (Technical Committee)
* [UlisesGascon](https://github.com/UlisesGascon) - **Ulises Gascón** (he/him)
* [jonchurch](https://github.com/jonchurch) - **Jon Church**
* [wesleytodd](https://github.com/wesleytodd) - **Wes Todd**
* [LinusU](https://github.com/LinusU) - **Linus Unnebäck**
* [blakeembrey](https://github.com/blakeembrey) - **Blake Embrey**
* [sheplu](https://github.com/sheplu) - **Jean Burellier**
* [crandmck](https://github.com/crandmck) - **Rand McKinney**
* [ctcpip](https://github.com/ctcpip) - **Chris de Almeida**
<details>
<summary>TC emeriti members</summary>
#### TC emeriti members
* [dougwilson](https://github.com/dougwilson) - **Douglas Wilson**
* [hacksparrow](https://github.com/hacksparrow) - **Hage Yaapa**
* [jonathanong](https://github.com/jonathanong) - **jongleberry**
* [niftylettuce](https://github.com/niftylettuce) - **niftylettuce**
* [troygoode](https://github.com/troygoode) - **Troy Goode**
</details>
### Triagers
* [aravindvnair99](https://github.com/aravindvnair99) - **Aravind Nair**
* [carpasse](https://github.com/carpasse) - **Carlos Serrano**
* [CBID2](https://github.com/CBID2) - **Christine Belzie**
* [enyoghasim](https://github.com/enyoghasim) - **David Enyoghasim**
* [UlisesGascon](https://github.com/UlisesGascon) - **Ulises Gascón** (he/him)
* [mertcanaltin](https://github.com/mertcanaltin) - **Mert Can Altin**
* [0ss](https://github.com/0ss) - **Salah**
* [import-brain](https://github.com/import-brain) - **Eric Cheng** (he/him)
* [3imed-jaberi](https://github.com/3imed-jaberi) - **Imed Jaberi**
* [dakshkhetan](https://github.com/dakshkhetan) - **Daksh Khetan** (he/him)
* [lucasraziel](https://github.com/lucasraziel) - **Lucas Soares Do Rego**
* [IamLizu](https://github.com/IamLizu) - **S M Mahmudul Hasan** (he/him)
* [Sushmeet](https://github.com/Sushmeet) - **Sushmeet Sunger**
<details>
<summary>Triagers emeriti members</summary>
#### Emeritus Triagers
* [AuggieH](https://github.com/AuggieH) - **Auggie Hudak**
* [G-Rath](https://github.com/G-Rath) - **Gareth Jones**
* [MohammadXroid](https://github.com/MohammadXroid) - **Mohammad Ayashi**
* [NawafSwe](https://github.com/NawafSwe) - **Nawaf Alsharqi**
* [NotMoni](https://github.com/NotMoni) - **Moni**
* [VigneshMurugan](https://github.com/VigneshMurugan) - **Vignesh Murugan**
* [davidmashe](https://github.com/davidmashe) - **David Ashe**
* [digitaIfabric](https://github.com/digitaIfabric) - **David**
* [e-l-i-s-e](https://github.com/e-l-i-s-e) - **Elise Bonner**
* [fed135](https://github.com/fed135) - **Frederic Charette**
* [firmanJS](https://github.com/firmanJS) - **Firman Abdul Hakim**
* [getspooky](https://github.com/getspooky) - **Yasser Ameur**
* [ghinks](https://github.com/ghinks) - **Glenn**
* [ghousemohamed](https://github.com/ghousemohamed) - **Ghouse Mohamed**
* [gireeshpunathil](https://github.com/gireeshpunathil) - **Gireesh Punathil**
* [jake32321](https://github.com/jake32321) - **Jake Reed**
* [jonchurch](https://github.com/jonchurch) - **Jon Church**
* [lekanikotun](https://github.com/lekanikotun) - **Troy Goode**
* [marsonya](https://github.com/marsonya) - **Lekan Ikotun**
* [mastermatt](https://github.com/mastermatt) - **Matt R. Wilson**
* [maxakuru](https://github.com/maxakuru) - **Max Edell**
* [mlrawlings](https://github.com/mlrawlings) - **Michael Rawlings**
* [rodion-arr](https://github.com/rodion-arr) - **Rodion Abdurakhimov**
* [sheplu](https://github.com/sheplu) - **Jean Burellier**
* [tarunyadav1](https://github.com/tarunyadav1) - **Tarun yadav**
* [tunniclm](https://github.com/tunniclm) - **Mike Tunnicliffe**
</details>
## License
[MIT](LICENSE)
[appveyor-image]: https://badgen.net/appveyor/ci/dougwilson/express/master?label=windows
[appveyor-url]: https://ci.appveyor.com/project/dougwilson/express
[coveralls-image]: https://badgen.net/coveralls/c/github/expressjs/express/master
[coveralls-url]: https://coveralls.io/r/expressjs/express?branch=master
[github-actions-ci-image]: https://badgen.net/github/checks/expressjs/express/master?label=linux
[github-actions-ci-url]: https://github.com/expressjs/express/actions/workflows/ci.yml
[npm-downloads-image]: https://badgen.net/npm/dm/express
[npm-downloads-url]: https://npmcharts.com/compare/express?minimal=true
[npm-install-size-image]: https://badgen.net/packagephobia/install/express
[npm-install-size-url]: https://packagephobia.com/result?p=express
[npm-url]: https://npmjs.org/package/express
[npm-version-image]: https://badgen.net/npm/v/express
[ossf-scorecard-badge]: https://api.scorecard.dev/projects/github.com/expressjs/express/badge
[ossf-scorecard-visualizer]: https://ossf.github.io/scorecard-visualizer/#/projects/github.com/expressjs/express
[Code of Conduct]: https://github.com/expressjs/express/blob/master/Code-Of-Conduct.md | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express/Readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express/Readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 9802
} |
# Reproducing a build
## Clone version
```
git clone https://github.com/planttheidea/fast-equals.git
cd fast-equals
git checkout {version}
```
Replace `{version}` above with the appropriate package version. If you want to compare a version older than `1.6.2`, you'll need to use a commit hash directly.
## Install
```
yarn install
```
We use `yarn` for our package management, so to ensure that exact dependencies you should also use it.
## Build artifacts
```
yarn run build
```
**NOTE**: To get an exact checksum match with the versions released on npm, it may be necessary to change line endings. For example, on Linux you might run:
```
unix2dos dist/fast-equals.min.js
```
## Get checksum
```
sha256sum dist/fast-equals.min.js
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fast-equals/BUILD.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fast-equals/BUILD.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 747
} |
# fast-equals CHANGELOG
## 5.0.1
### Bugfixes
- Fix reference to `metaOverride` in typings and documentation (holdover from temporary API in v5 beta)
## 5.0.0
### Breaking changes
#### `constructor` equality now required
To align with other implementations common in the community, but also to be more functionally correct, the two objects being compared now must have equal `constructor`s.
#### `Map` / `Set` comparisons no longer support IE11
In previous verisons, `.forEach()` was used to ensure that support for `Symbol` was not required, as IE11 did not have `Symbol` and therefore both `Map` and `Set` did not have iterator-based methods such as `.values()` or `.entries()`. Since IE11 is no longer a supported browser, and support for those methods is present in all browsers and Node for quite a while, the comparison has moved to use these methods. This results in a ~20% performance increase.
#### `createCustomEqual` contract has changed
To better facilitate strict comparisons, but also to allow for `meta` use separate from caching, the contract for `createCustomEqual` has changed. See the [README documentation](./README.md#createcustomequal) for more details, but froma high-level:
- `meta` is no longer passed through to equality comparators, but rather a general `state` object which contains `meta`
- `cache` now also lives on the `state` object, which allows for use of the `meta` property separate from but in parallel with the circular cache
- `equals` is now on `state`, which prevents the need to pass through the separate `isEqual` method for the equality comparator
#### `createCustomCircularEqual` has been removed
You can create a custom circular equality comparator through `createCustomEqual` now by providing `circular: true` to the options.
#### Custom `meta` values are no longer passed at callsite
To use `meta` properties for comparisons, they must be returned in a `createState` method.
#### Deep links have changed
If you were deep-linking into a specific asset type (ESM / CJS / UMD), they have changed location.
**NOTE**: You may no longer need to deep-link, as [the build resolution has improved](#better-build-system-resolution).
### Enhancements
#### New "strict" comparators available
The following new comparators are available:
- `strictDeepEqual`
- `strictShallowEqual`
- `strictCircularDeepEqual`
- `strictCircularShallowEqual`
This will perform the same comparisons as their non-strict counterparts, but will verify additional properties (non-enumerable properties on objects, keyed objects on `Array` / `Map` / `Set`) and that the descriptors for the properties align.
#### `TypedArray` support
Support for comparing all typed array values is now supported, and you can provide a custom comparator via the new `areTypedArraysEqual` option in the `createCustomEqual` configuration.
#### Better build system resolution
The library now leverages the `exports` property in the `package.json` to provide builds specific to your method of consumption (ESM / CommonJS / UMD). There is still a minified UMD version available if you want to use it instead.
#### `arePrimitiveWrappersEqual` option added to `createCustomEqual` configuration
If you want a custom comparator for primitive wrappers (`new Boolean()` / `new Number()` / `new String()`) it is now available.
## 4.0.3
- Remove unnecessary second strict equality check for objects in edge-case scenarios
## 4.0.2
- [#85](https://github.com/planttheidea/fast-equals/issues/85) - `createCustomCircularEqual` typing is incorrect
## 4.0.1
- [#81](https://github.com/planttheidea/fast-equals/issues/81) - Fix typing issues related to importing in `index.d.ts` file
## 4.0.0
### Breaking Changes
#### Certain ES2015 features are now required
In previous versions, there were automatic fallbacks for certain ES2015 features if they did not exist:
- [`RegExp.prototype.flags`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/RegExp/flags)
- [`WeakMap`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/WeakMap)
Due to the omnipresence of support in both browser and NodeJS, these have been deprecated. There is still an option if you require support for these legacy environments, however; see [`createCustomEqual`](./README.md#createcustomequal) and [`createCustomCircularEqual`](./README.md#createcustomcircularequal) for more details.
#### `createCustomEqual` contract has changed
To allow more flexibility and customizability for a variety of edge cases, `createCustomEqual` now allows override of specific type value comparisons in addition to the general comparator it did prior. See [the documentation](./README.md#createcustomequal) for more details.
### Enhancements
#### `createCustomCircularEqual` added
Like `createCustomEqual`, it will create a custom equality comparator, with the exception that it will handle circular references. See [the documentation](./README.md#createcustomcircularequal) for more details.
#### Cross-realm comparisons are now supported
Prior to `4.x.x.`, `instanceof` was used internally for checking of object classes, which only worked when comparing objects from the same [Realm](https://262.ecma-international.org/6.0/#sec-code-realms). This has changed to instead use an object's [StringTag](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Symbol/toStringTag), which is not realm-specific.
#### TypeScript typings improved
For better typing in edge-case scenarios like custom comparators with `meta` values, typings have been refactored for accuracy and better narrow flow-through.
## 3.0.3
- Fix [#77](https://github.com/planttheidea/fast-equals/issues/73) - better circular object validation
## 3.0.2
- Fix [#73](https://github.com/planttheidea/fast-equals/issues/73) - support comparison of primitive wrappers
- [#76](https://github.com/planttheidea/fast-equals/pull/76) - improve speed and accuracy of `RegExp` comparison in modern environments
## 3.0.1
- Fix [#71](https://github.com/planttheidea/fast-equals/pull/71) - use generic types for better type flow-through
## 3.0.0
### Breaking changes
When creating a custom equality comparator via `createCustomEqual`, the equality method has an expanded contract:
```ts
// Before
type EqualityComparator = (objectA: any, objectB: any, meta: any) => boolean;
// After
type InternalEqualityComparator = (
objectA: any,
objectB: any,
indexOrKeyA: any,
indexOrKeyB: any,
parentA: any,
parentB: any,
meta: any,
) => boolean;
```
If you have a custom equality comparator, you can ignore the differences by just passing additional `undefined` parameters, or you can use the parameters to further improve / clarify the logic.
- Add [#57](https://github.com/planttheidea/fast-equals/pull/57) - support additional metadata for custom equality comparators
## 2.0.4
- Fix [#58](https://github.com/planttheidea/fast-equals/issues/58) - duplicate entries in `Map` / `Set` can create false equality success
- [#60](https://github.com/planttheidea/fast-equals/issues/60) - Add documentation for key equality of `Map` being a part of `deepEqual`
## 2.0.3
- Fix [#50](https://github.com/planttheidea/fast-equals/pull/50) - copy-pasta in cacheable check
## 2.0.2
- Optimize iterables comparisons to not double-iterate
- Optimize loop-based comparisons for speed
- Improve cache handling in circular handlers
- Improve stability of memory by reducing variable instantiation
## 2.0.1
- Fix [#41](https://github.com/planttheidea/fast-equals/pull/41) - prevent `.rpt2_cache` directory from being published for better CI environment support (thanks [@herberttn](https://github.com/herberttn))
## 2.0.0
### Breaking changes
- There are longer `fast-equals/es`, `fast-equals/lib`, `fast-equals/mjs` locations
- Instead, there are 3 builds in `dist` for different consumption types:
- `fast-equals.js` (UMD / `browser`)
- `fast-equals.esm.js` (ESM / `module`)
- `fast-equals.cjs.js` (CommonJS / `main`)
- There is no default export anymore, only the previously-existing named exports
- To get all into a namespace, use `import * as fe from 'fast-equals`
### Updates
- Rewritten completely in TypeScript
- Improve speed of `Map` / `Set` comparisons
- Improve speed of React element comparisons
### Fixes
- Consider pure objects (`Object.create(null)`) to be plain objects
- Fix typings for `createCustomEqual`
## 1.6.3
- Check the size of the iterable before converting to arrays
## 1.6.2
- Fix [#23](https://github.com/planttheidea/fast-equals/issues/23) - false positives for map
- Replace `uglify` with `terser`
- Use `rollup` to build all the distributables (`main`, `module`, and `browser`)
- Maintain `lib` and `es` transpilations in case consumers were deep-linking
## 1.6.1
- Upgrade to `babel@7`
- Add `"sideEffects": false` to `package.json` for better tree-shaking in `webpack`
## 1.6.0
- Add ESM support for NodeJS with separate [`.mjs` extension](https://nodejs.org/api/esm.html) exports
## 1.5.3
- Fix `Map` / `Set` comparison to not require order to match to be equal
## 1.5.2
- Improve speed of object comparison through custom `hasKey` method
## 1.5.1
- Fix lack of support for `unicode` and `sticky` RegExp flag checks
## 1.5.0
- Add [`circularDeepEqual`](README.md#circulardeepequal) and [`circularShallowEqual`](README.md#circularshallowequal) methods
- Add `meta` third parameter to `comparator` calls, for use with `createCustomEqual` method
## 1.4.1
- Fix issue where `lastIndex` was not being tested on `RegExp` objects
## 1.4.0
- Add support for comparing promise-like objects (strict equality only)
## 1.3.1
- Make `react` comparison more accurate, and a touch faster
## 1.3.0
- Add support for deep-equal comparisons between `react` elements
- Add comparison with `react-fast-compare`
- Use `rollup` for `dist` file builds
## 1.2.1
- Fix errors from TypeScript typings in strict mode (thanks [@HitoriSensei](https://github.com/HitoriSensei))
## 1.2.0
- Surface `isSameValueZero` as [`sameValueZeroEqual`](#samevaluezeroequal) option
## 1.1.0
- Add TypeScript typings (thanks [@josh-sachs](https://github.com/josh-sachs))
## 1.0.6
- Support invalid date equality via `isSameValueZero`
## 1.0.5
- Replace `isStrictlyEqual` with `isSameValueZero` to ensure that `shallowEqual` accounts for `NaN` equality
## 1.0.4
- Only check values when comparing `Set` objects (improves performance of `Set` check by ~12%)
## 1.0.3
- Make `Map` and `Set` comparisons more explicit
## 1.0.2
- Fix symmetrical comparison of iterables
- Reduce footprint
## 1.0.1
- Prevent babel transpilation of `typeof` into helper for faster runtime
## 1.0.0
- Initial release
```
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fast-equals/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fast-equals/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 10789
} |
# fast-equals
<img src="https://img.shields.io/badge/build-passing-brightgreen.svg"/>
<img src="https://img.shields.io/badge/coverage-100%25-brightgreen.svg"/>
<img src="https://img.shields.io/badge/license-MIT-blue.svg"/>
Perform [blazing fast](#benchmarks) equality comparisons (either deep or shallow) on two objects passed, while also maintaining a high degree of flexibility for various implementation use-cases. It has no dependencies, and is ~1.8kB when minified and gzipped.
The following types are handled out-of-the-box:
- Plain objects (including `react` elements and `Arguments`)
- Arrays
- Typed Arrays
- `Date` objects
- `RegExp` objects
- `Map` / `Set` iterables
- `Promise` objects
- Primitive wrappers (`new Boolean()` / `new Number()` / `new String()`)
- Custom class instances, including subclasses of native classes
Methods are available for deep, shallow, or referential equality comparison. In addition, you can opt into support for circular objects, or performing a "strict" comparison with unconventional property definition, or both. You can also customize any specific type comparison based on your application's use-cases.
## Table of contents
- [fast-equals](#fast-equals)
- [Table of contents](#table-of-contents)
- [Usage](#usage)
- [Specific builds](#specific-builds)
- [Available methods](#available-methods)
- [deepEqual](#deepequal)
- [Comparing `Map`s](#comparing-maps)
- [shallowEqual](#shallowequal)
- [sameValueZeroEqual](#samevaluezeroequal)
- [circularDeepEqual](#circulardeepequal)
- [circularShallowEqual](#circularshallowequal)
- [strictDeepEqual](#strictdeepequal)
- [strictShallowEqual](#strictshallowequal)
- [strictCircularDeepEqual](#strictcirculardeepequal)
- [strictCircularShallowEqual](#strictcircularshallowequal)
- [createCustomEqual](#createcustomequal)
- [Recipes](#recipes)
- [Benchmarks](#benchmarks)
- [Development](#development)
## Usage
```ts
import { deepEqual } from 'fast-equals';
console.log(deepEqual({ foo: 'bar' }, { foo: 'bar' })); // true
```
### Specific builds
By default, npm should resolve the correct build of the package based on your consumption (ESM vs CommonJS). However, if you want to force use of a specific build, they can be located here:
- ESM => `fast-equals/dist/esm/index.mjs`
- CommonJS => `fast-equals/dist/cjs/index.cjs`
- UMD => `fast-equals/dist/umd/index.js`
- Minified UMD => `fast-equals/dist/min/index.js`
If you are having issues loading a specific build type, [please file an issue](https://github.com/planttheidea/fast-equals/issues).
## Available methods
### deepEqual
Performs a deep equality comparison on the two objects passed and returns a boolean representing the value equivalency of the objects.
```ts
import { deepEqual } from 'fast-equals';
const objectA = { foo: { bar: 'baz' } };
const objectB = { foo: { bar: 'baz' } };
console.log(objectA === objectB); // false
console.log(deepEqual(objectA, objectB)); // true
```
#### Comparing `Map`s
`Map` objects support complex keys (objects, Arrays, etc.), however [the spec for key lookups in `Map` are based on `SameZeroValue`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Map#key_equality). If the spec were followed for comparison, the following would always be `false`:
```ts
const mapA = new Map([[{ foo: 'bar' }, { baz: 'quz' }]]);
const mapB = new Map([[{ foo: 'bar' }, { baz: 'quz' }]]);
deepEqual(mapA, mapB);
```
To support true deep equality of all contents, `fast-equals` will perform a deep equality comparison for key and value parirs. Therefore, the above would be `true`.
### shallowEqual
Performs a shallow equality comparison on the two objects passed and returns a boolean representing the value equivalency of the objects.
```ts
import { shallowEqual } from 'fast-equals';
const nestedObject = { bar: 'baz' };
const objectA = { foo: nestedObject };
const objectB = { foo: nestedObject };
const objectC = { foo: { bar: 'baz' } };
console.log(objectA === objectB); // false
console.log(shallowEqual(objectA, objectB)); // true
console.log(shallowEqual(objectA, objectC)); // false
```
### sameValueZeroEqual
Performs a [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero) comparison on the two objects passed and returns a boolean representing the value equivalency of the objects. In simple terms, this means either strictly equal or both `NaN`.
```ts
import { sameValueZeroEqual } from 'fast-equals';
const mainObject = { foo: NaN, bar: 'baz' };
const objectA = 'baz';
const objectB = NaN;
const objectC = { foo: NaN, bar: 'baz' };
console.log(sameValueZeroEqual(mainObject.bar, objectA)); // true
console.log(sameValueZeroEqual(mainObject.foo, objectB)); // true
console.log(sameValueZeroEqual(mainObject, objectC)); // false
```
### circularDeepEqual
Performs the same comparison as `deepEqual` but supports circular objects. It is slower than `deepEqual`, so only use if you know circular objects are present.
```ts
function Circular(value) {
this.me = {
deeply: {
nested: {
reference: this,
},
},
value,
};
}
console.log(circularDeepEqual(new Circular('foo'), new Circular('foo'))); // true
console.log(circularDeepEqual(new Circular('foo'), new Circular('bar'))); // false
```
Just as with `deepEqual`, [both keys and values are compared for deep equality](#comparing-maps).
### circularShallowEqual
Performs the same comparison as `shallowequal` but supports circular objects. It is slower than `shallowEqual`, so only use if you know circular objects are present.
```ts
const array = ['foo'];
array.push(array);
console.log(circularShallowEqual(array, ['foo', array])); // true
console.log(circularShallowEqual(array, [array])); // false
```
### strictDeepEqual
Performs the same comparison as `deepEqual` but performs a strict comparison of the objects. In this includes:
- Checking symbol properties
- Checking non-enumerable properties in object comparisons
- Checking full descriptor of properties on the object to match
- Checking non-index properties on arrays
- Checking non-key properties on `Map` / `Set` objects
```ts
const array = [{ foo: 'bar' }];
const otherArray = [{ foo: 'bar' }];
array.bar = 'baz';
otherArray.bar = 'baz';
console.log(strictDeepEqual(array, otherArray)); // true;
console.log(strictDeepEqual(array, [{ foo: 'bar' }])); // false;
```
### strictShallowEqual
Performs the same comparison as `shallowEqual` but performs a strict comparison of the objects. In this includes:
- Checking non-enumerable properties in object comparisons
- Checking full descriptor of properties on the object to match
- Checking non-index properties on arrays
- Checking non-key properties on `Map` / `Set` objects
```ts
const array = ['foo'];
const otherArray = ['foo'];
array.bar = 'baz';
otherArray.bar = 'baz';
console.log(strictDeepEqual(array, otherArray)); // true;
console.log(strictDeepEqual(array, ['foo'])); // false;
```
### strictCircularDeepEqual
Performs the same comparison as `circularDeepEqual` but performs a strict comparison of the objects. In this includes:
- Checking `Symbol` properties on the object
- Checking non-enumerable properties in object comparisons
- Checking full descriptor of properties on the object to match
- Checking non-index properties on arrays
- Checking non-key properties on `Map` / `Set` objects
```ts
function Circular(value) {
this.me = {
deeply: {
nested: {
reference: this,
},
},
value,
};
}
const first = new Circular('foo');
Object.defineProperty(first, 'bar', {
enumerable: false,
value: 'baz',
});
const second = new Circular('foo');
Object.defineProperty(second, 'bar', {
enumerable: false,
value: 'baz',
});
console.log(circularDeepEqual(first, second)); // true
console.log(circularDeepEqual(first, new Circular('foo'))); // false
```
### strictCircularShallowEqual
Performs the same comparison as `circularShallowEqual` but performs a strict comparison of the objects. In this includes:
- Checking non-enumerable properties in object comparisons
- Checking full descriptor of properties on the object to match
- Checking non-index properties on arrays
- Checking non-key properties on `Map` / `Set` objects
```ts
const array = ['foo'];
const otherArray = ['foo'];
array.push(array);
otherArray.push(otherArray);
array.bar = 'baz';
otherArray.bar = 'baz';
console.log(circularShallowEqual(array, otherArray)); // true
console.log(circularShallowEqual(array, ['foo', array])); // false
```
### createCustomEqual
Creates a custom equality comparator that will be used on nested values in the object. Unlike `deepEqual` and `shallowEqual`, this is a factory method that receives the default options used internally, and allows you to override the defaults as needed. This is generally for extreme edge-cases, or supporting legacy environments.
The signature is as follows:
```ts
interface Cache<Key extends object, Value> {
delete(key: Key): boolean;
get(key: Key): Value | undefined;
set(key: Key, value: any): any;
}
interface ComparatorConfig<Meta> {
areArraysEqual: TypeEqualityComparator<any[], Meta>;
areDatesEqual: TypeEqualityComparator<Date, Meta>;
areMapsEqual: TypeEqualityComparator<Map<any, any>, Meta>;
areObjectsEqual: TypeEqualityComparator<Record<string, any>, Meta>;
arePrimitiveWrappersEqual: TypeEqualityComparator<
boolean | string | number,
Meta
>;
areRegExpsEqual: TypeEqualityComparator<RegExp, Meta>;
areSetsEqual: TypeEqualityComparator<Set<any>, Meta>;
areTypedArraysEqual: TypeEqualityComparatory<TypedArray, Meta>;
}
function createCustomEqual<Meta>(options: {
circular?: boolean;
createCustomConfig?: (
defaultConfig: ComparatorConfig<Meta>,
) => Partial<ComparatorConfig<Meta>>;
createInternalComparator?: (
compare: <A, B>(a: A, b: B, state: State<Meta>) => boolean,
) => (
a: any,
b: any,
indexOrKeyA: any,
indexOrKeyB: any,
parentA: any,
parentB: any,
state: State<Meta>,
) => boolean;
createState?: () => { cache?: Cache; meta?: Meta };
strict?: boolean;
}): <A, B>(a: A, b: B) => boolean;
```
Create a custom equality comparator. This allows complete control over building a bespoke equality method, in case your use-case requires a higher degree of performance, legacy environment support, or any other non-standard usage. The [recipes](#recipes) provide examples of use in different use-cases, but if you have a specific goal in mind and would like assistance feel free to [file an issue](https://github.com/planttheidea/fast-equals/issues).
_**NOTE**: `Map` implementations compare equality for both keys and value. When using a custom comparator and comparing equality of the keys, the iteration index is provided as both `indexOrKeyA` and `indexOrKeyB` to help use-cases where ordering of keys matters to equality._
#### Recipes
Some recipes have been created to provide examples of use-cases for `createCustomEqual`. Even if not directly applicable to the problem you are solving, they can offer guidance of how to structure your solution.
- [Legacy environment support for `RegExp` comparators](./recipes/legacy-regexp-support.md)
- [Explicit property check](./recipes/explicit-property-check.md)
- [Using `meta` in comparison](./recipes//using-meta-in-comparison.md)
- [Comparing non-standard properties](./recipes/non-standard-properties.md)
- [Strict property descriptor comparison](./recipes/strict-property-descriptor-check.md)
- [Legacy environment support for circualr equal comparators](./recipes/legacy-circular-equal-support.md)
## Benchmarks
All benchmarks were performed on an i9-11900H Ubuntu Linux 22.04 laptop with 64GB of memory using NodeJS version `16.14.2`, and are based on averages of running comparisons based deep equality on the following object types:
- Primitives (`String`, `Number`, `null`, `undefined`)
- `Function`
- `Object`
- `Array`
- `Date`
- `RegExp`
- `react` elements
- A mixed object with a combination of all the above types
```bash
Testing mixed objects equal...
┌─────────┬─────────────────────────────────┬────────────────┐
│ (index) │ Package │ Ops/sec │
├─────────┼─────────────────────────────────┼────────────────┤
│ 0 │ 'fast-equals' │ 1249567.730326 │
│ 1 │ 'fast-deep-equal' │ 1182463.587514 │
│ 2 │ 'react-fast-compare' │ 1152487.319161 │
│ 3 │ 'shallow-equal-fuzzy' │ 1092360.712389 │
│ 4 │ 'fast-equals (circular)' │ 676669.92003 │
│ 5 │ 'underscore.isEqual' │ 429430.837497 │
│ 6 │ 'lodash.isEqual' │ 237915.684734 │
│ 7 │ 'fast-equals (strict)' │ 181386.38032 │
│ 8 │ 'fast-equals (strict circular)' │ 156779.745875 │
│ 9 │ 'deep-eql' │ 139155.099209 │
│ 10 │ 'deep-equal' │ 1026.527229 │
└─────────┴─────────────────────────────────┴────────────────┘
Testing mixed objects not equal...
┌─────────┬─────────────────────────────────┬────────────────┐
│ (index) │ Package │ Ops/sec │
├─────────┼─────────────────────────────────┼────────────────┤
│ 0 │ 'fast-equals' │ 3255824.097237 │
│ 1 │ 'react-fast-compare' │ 2654721.726058 │
│ 2 │ 'fast-deep-equal' │ 2582218.974752 │
│ 3 │ 'fast-equals (circular)' │ 2474303.26566 │
│ 4 │ 'fast-equals (strict)' │ 1088066.604881 │
│ 5 │ 'fast-equals (strict circular)' │ 949253.614181 │
│ 6 │ 'nano-equal' │ 939170.554148 │
│ 7 │ 'underscore.isEqual' │ 738852.197879 │
│ 8 │ 'lodash.isEqual' │ 307306.622212 │
│ 9 │ 'deep-eql' │ 156250.110401 │
│ 10 │ 'assert.deepStrictEqual' │ 22839.454561 │
│ 11 │ 'deep-equal' │ 4034.45114 │
└─────────┴─────────────────────────────────┴────────────────┘
```
Caveats that impact the benchmark (and accuracy of comparison):
- `Map`s, `Promise`s, and `Set`s were excluded from the benchmark entirely because no library other than `deep-eql` fully supported their comparison
- `fast-deep-equal`, `react-fast-compare` and `nano-equal` throw on objects with `null` as prototype (`Object.create(null)`)
- `assert.deepStrictEqual` does not support `NaN` or `SameValueZero` equality for dates
- `deep-eql` does not support `SameValueZero` equality for zero equality (positive and negative zero are not equal)
- `deep-equal` does not support `NaN` and does not strictly compare object type, or date / regexp values, nor uses `SameValueZero` equality for dates
- `fast-deep-equal` does not support `NaN` or `SameValueZero` equality for dates
- `nano-equal` does not strictly compare object property structure, array length, or object type, nor `SameValueZero` equality for dates
- `react-fast-compare` does not support `NaN` or `SameValueZero` equality for dates, and does not compare `function` equality
- `shallow-equal-fuzzy` does not strictly compare object type or regexp values, nor `SameValueZero` equality for dates
- `underscore.isEqual` does not support `SameValueZero` equality for primitives or dates
All of these have the potential of inflating the respective library's numbers in comparison to `fast-equals`, but it was the closest apples-to-apples comparison I could create of a reasonable sample size. It should be noted that `react` elements can be circular objects, however simple elements are not; I kept the `react` comparison very basic to allow it to be included.
## Development
Standard practice, clone the repo and `npm i` to get the dependencies. The following npm scripts are available:
- benchmark => run benchmark tests against other equality libraries
- build => build `main`, `module`, and `browser` distributables with `rollup`
- clean => run `rimraf` on the `dist` folder
- dev => start webpack playground App
- dist => run `build`
- lint => run ESLint on all files in `src` folder (also runs on `dev` script)
- lint:fix => run `lint` script, but with auto-fixer
- prepublish:compile => run `lint`, `test:coverage`, `transpile:lib`, `transpile:es`, and `dist` scripts
- start => run `dev`
- test => run AVA with NODE_ENV=test on all files in `test` folder
- test:coverage => run same script as `test` with code coverage calculation via `nyc`
- test:watch => run same script as `test` but keep persistent watcher | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fast-equals/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fast-equals/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 16653
} |
# fast-glob
> It's a very fast and efficient [glob][glob_definition] library for [Node.js][node_js].
This package provides methods for traversing the file system and returning pathnames that matched a defined set of a specified pattern according to the rules used by the Unix Bash shell with some simplifications, meanwhile results are returned in **arbitrary order**. Quick, simple, effective.
## Table of Contents
<details>
<summary><strong>Details</strong></summary>
* [Highlights](#highlights)
* [Old and modern mode](#old-and-modern-mode)
* [Pattern syntax](#pattern-syntax)
* [Basic syntax](#basic-syntax)
* [Advanced syntax](#advanced-syntax)
* [Installation](#installation)
* [API](#api)
* [Asynchronous](#asynchronous)
* [Synchronous](#synchronous)
* [Stream](#stream)
* [patterns](#patterns)
* [[options]](#options)
* [Helpers](#helpers)
* [generateTasks](#generatetaskspatterns-options)
* [isDynamicPattern](#isdynamicpatternpattern-options)
* [escapePath](#escapepathpath)
* [convertPathToPattern](#convertpathtopatternpath)
* [Options](#options-3)
* [Common](#common)
* [concurrency](#concurrency)
* [cwd](#cwd)
* [deep](#deep)
* [followSymbolicLinks](#followsymboliclinks)
* [fs](#fs)
* [ignore](#ignore)
* [suppressErrors](#suppresserrors)
* [throwErrorOnBrokenSymbolicLink](#throwerroronbrokensymboliclink)
* [Output control](#output-control)
* [absolute](#absolute)
* [markDirectories](#markdirectories)
* [objectMode](#objectmode)
* [onlyDirectories](#onlydirectories)
* [onlyFiles](#onlyfiles)
* [stats](#stats)
* [unique](#unique)
* [Matching control](#matching-control)
* [braceExpansion](#braceexpansion)
* [caseSensitiveMatch](#casesensitivematch)
* [dot](#dot)
* [extglob](#extglob)
* [globstar](#globstar)
* [baseNameMatch](#basenamematch)
* [FAQ](#faq)
* [What is a static or dynamic pattern?](#what-is-a-static-or-dynamic-pattern)
* [How to write patterns on Windows?](#how-to-write-patterns-on-windows)
* [Why are parentheses match wrong?](#why-are-parentheses-match-wrong)
* [How to exclude directory from reading?](#how-to-exclude-directory-from-reading)
* [How to use UNC path?](#how-to-use-unc-path)
* [Compatible with `node-glob`?](#compatible-with-node-glob)
* [Benchmarks](#benchmarks)
* [Server](#server)
* [Nettop](#nettop)
* [Changelog](#changelog)
* [License](#license)
</details>
## Highlights
* Fast. Probably the fastest.
* Supports multiple and negative patterns.
* Synchronous, Promise and Stream API.
* Object mode. Can return more than just strings.
* Error-tolerant.
## Old and modern mode
This package works in two modes, depending on the environment in which it is used.
* **Old mode**. Node.js below 10.10 or when the [`stats`](#stats) option is *enabled*.
* **Modern mode**. Node.js 10.10+ and the [`stats`](#stats) option is *disabled*.
The modern mode is faster. Learn more about the [internal mechanism][nodelib_fs_scandir_old_and_modern_modern].
## Pattern syntax
> :warning: Always use forward-slashes in glob expressions (patterns and [`ignore`](#ignore) option). Use backslashes for escaping characters.
There is more than one form of syntax: basic and advanced. Below is a brief overview of the supported features. Also pay attention to our [FAQ](#faq).
> :book: This package uses [`micromatch`][micromatch] as a library for pattern matching.
### Basic syntax
* An asterisk (`*`) — matches everything except slashes (path separators), hidden files (names starting with `.`).
* A double star or globstar (`**`) — matches zero or more directories.
* Question mark (`?`) – matches any single character except slashes (path separators).
* Sequence (`[seq]`) — matches any character in sequence.
> :book: A few additional words about the [basic matching behavior][picomatch_matching_behavior].
Some examples:
* `src/**/*.js` — matches all files in the `src` directory (any level of nesting) that have the `.js` extension.
* `src/*.??` — matches all files in the `src` directory (only first level of nesting) that have a two-character extension.
* `file-[01].js` — matches files: `file-0.js`, `file-1.js`.
### Advanced syntax
* [Escapes characters][micromatch_backslashes] (`\\`) — matching special characters (`$^*+?()[]`) as literals.
* [POSIX character classes][picomatch_posix_brackets] (`[[:digit:]]`).
* [Extended globs][micromatch_extglobs] (`?(pattern-list)`).
* [Bash style brace expansions][micromatch_braces] (`{}`).
* [Regexp character classes][micromatch_regex_character_classes] (`[1-5]`).
* [Regex groups][regular_expressions_brackets] (`(a|b)`).
> :book: A few additional words about the [advanced matching behavior][micromatch_extended_globbing].
Some examples:
* `src/**/*.{css,scss}` — matches all files in the `src` directory (any level of nesting) that have the `.css` or `.scss` extension.
* `file-[[:digit:]].js` — matches files: `file-0.js`, `file-1.js`, …, `file-9.js`.
* `file-{1..3}.js` — matches files: `file-1.js`, `file-2.js`, `file-3.js`.
* `file-(1|2)` — matches files: `file-1.js`, `file-2.js`.
## Installation
```console
npm install fast-glob
```
## API
### Asynchronous
```js
fg(patterns, [options])
fg.async(patterns, [options])
fg.glob(patterns, [options])
```
Returns a `Promise` with an array of matching entries.
```js
const fg = require('fast-glob');
const entries = await fg(['.editorconfig', '**/index.js'], { dot: true });
// ['.editorconfig', 'services/index.js']
```
### Synchronous
```js
fg.sync(patterns, [options])
fg.globSync(patterns, [options])
```
Returns an array of matching entries.
```js
const fg = require('fast-glob');
const entries = fg.sync(['.editorconfig', '**/index.js'], { dot: true });
// ['.editorconfig', 'services/index.js']
```
### Stream
```js
fg.stream(patterns, [options])
fg.globStream(patterns, [options])
```
Returns a [`ReadableStream`][node_js_stream_readable_streams] when the `data` event will be emitted with matching entry.
```js
const fg = require('fast-glob');
const stream = fg.stream(['.editorconfig', '**/index.js'], { dot: true });
for await (const entry of stream) {
// .editorconfig
// services/index.js
}
```
#### patterns
* Required: `true`
* Type: `string | string[]`
Any correct pattern(s).
> :1234: [Pattern syntax](#pattern-syntax)
>
> :warning: This package does not respect the order of patterns. First, all the negative patterns are applied, and only then the positive patterns. If you want to get a certain order of records, use sorting or split calls.
#### [options]
* Required: `false`
* Type: [`Options`](#options-3)
See [Options](#options-3) section.
### Helpers
#### `generateTasks(patterns, [options])`
Returns the internal representation of patterns ([`Task`](./src/managers/tasks.ts) is a combining patterns by base directory).
```js
fg.generateTasks('*');
[{
base: '.', // Parent directory for all patterns inside this task
dynamic: true, // Dynamic or static patterns are in this task
patterns: ['*'],
positive: ['*'],
negative: []
}]
```
##### patterns
* Required: `true`
* Type: `string | string[]`
Any correct pattern(s).
##### [options]
* Required: `false`
* Type: [`Options`](#options-3)
See [Options](#options-3) section.
#### `isDynamicPattern(pattern, [options])`
Returns `true` if the passed pattern is a dynamic pattern.
> :1234: [What is a static or dynamic pattern?](#what-is-a-static-or-dynamic-pattern)
```js
fg.isDynamicPattern('*'); // true
fg.isDynamicPattern('abc'); // false
```
##### pattern
* Required: `true`
* Type: `string`
Any correct pattern.
##### [options]
* Required: `false`
* Type: [`Options`](#options-3)
See [Options](#options-3) section.
#### `escapePath(path)`
Returns the path with escaped special characters depending on the platform.
* Posix:
* `*?|(){}[]`;
* `!` at the beginning of line;
* `@+!` before the opening parenthesis;
* `\\` before non-special characters;
* Windows:
* `(){}[]`
* `!` at the beginning of line;
* `@+!` before the opening parenthesis;
* Characters like `*?|` cannot be used in the path ([windows_naming_conventions][windows_naming_conventions]), so they will not be escaped;
```js
fg.escapePath('!abc');
// \\!abc
fg.escapePath('[OpenSource] mrmlnc – fast-glob (Deluxe Edition) 2014') + '/*.flac'
// \\[OpenSource\\] mrmlnc – fast-glob \\(Deluxe Edition\\) 2014/*.flac
fg.posix.escapePath('C:\\Program Files (x86)\\**\\*');
// C:\\\\Program Files \\(x86\\)\\*\\*\\*
fg.win32.escapePath('C:\\Program Files (x86)\\**\\*');
// Windows: C:\\Program Files \\(x86\\)\\**\\*
```
#### `convertPathToPattern(path)`
Converts a path to a pattern depending on the platform, including special character escaping.
* Posix. Works similarly to the `fg.posix.escapePath` method.
* Windows. Works similarly to the `fg.win32.escapePath` method, additionally converting backslashes to forward slashes in cases where they are not escape characters (`!()+@{}[]`).
```js
fg.convertPathToPattern('[OpenSource] mrmlnc – fast-glob (Deluxe Edition) 2014') + '/*.flac';
// \\[OpenSource\\] mrmlnc – fast-glob \\(Deluxe Edition\\) 2014/*.flac
fg.convertPathToPattern('C:/Program Files (x86)/**/*');
// Posix: C:/Program Files \\(x86\\)/\\*\\*/\\*
// Windows: C:/Program Files \\(x86\\)/**/*
fg.convertPathToPattern('C:\\Program Files (x86)\\**\\*');
// Posix: C:\\\\Program Files \\(x86\\)\\*\\*\\*
// Windows: C:/Program Files \\(x86\\)/**/*
fg.posix.convertPathToPattern('\\\\?\\c:\\Program Files (x86)') + '/**/*';
// Posix: \\\\\\?\\\\c:\\\\Program Files \\(x86\\)/**/* (broken pattern)
fg.win32.convertPathToPattern('\\\\?\\c:\\Program Files (x86)') + '/**/*';
// Windows: //?/c:/Program Files \\(x86\\)/**/*
```
## Options
### Common options
#### concurrency
* Type: `number`
* Default: `os.cpus().length`
Specifies the maximum number of concurrent requests from a reader to read directories.
> :book: The higher the number, the higher the performance and load on the file system. If you want to read in quiet mode, set the value to a comfortable number or `1`.
<details>
<summary>More details</summary>
In Node, there are [two types of threads][nodejs_thread_pool]: Event Loop (code) and a Thread Pool (fs, dns, …). The thread pool size controlled by the `UV_THREADPOOL_SIZE` environment variable. Its default size is 4 ([documentation][libuv_thread_pool]). The pool is one for all tasks within a single Node process.
Any code can make 4 real concurrent accesses to the file system. The rest of the FS requests will wait in the queue.
> :book: Each new instance of FG in the same Node process will use the same Thread pool.
But this package also has the `concurrency` option. This option allows you to control the number of concurrent accesses to the FS at the package level. By default, this package has a value equal to the number of cores available for the current Node process. This allows you to set a value smaller than the pool size (`concurrency: 1`) or, conversely, to prepare tasks for the pool queue more quickly (`concurrency: Number.POSITIVE_INFINITY`).
So, in fact, this package can **only make 4 concurrent requests to the FS**. You can increase this value by using an environment variable (`UV_THREADPOOL_SIZE`), but in practice this does not give a multiple advantage.
</details>
#### cwd
* Type: `string`
* Default: `process.cwd()`
The current working directory in which to search.
#### deep
* Type: `number`
* Default: `Infinity`
Specifies the maximum depth of a read directory relative to the start directory.
For example, you have the following tree:
```js
dir/
└── one/ // 1
└── two/ // 2
└── file.js // 3
```
```js
// With base directory
fg.sync('dir/**', { onlyFiles: false, deep: 1 }); // ['dir/one']
fg.sync('dir/**', { onlyFiles: false, deep: 2 }); // ['dir/one', 'dir/one/two']
// With cwd option
fg.sync('**', { onlyFiles: false, cwd: 'dir', deep: 1 }); // ['one']
fg.sync('**', { onlyFiles: false, cwd: 'dir', deep: 2 }); // ['one', 'one/two']
```
> :book: If you specify a pattern with some base directory, this directory will not participate in the calculation of the depth of the found directories. Think of it as a [`cwd`](#cwd) option.
#### followSymbolicLinks
* Type: `boolean`
* Default: `true`
Indicates whether to traverse descendants of symbolic link directories when expanding `**` patterns.
> :book: Note that this option does not affect the base directory of the pattern. For example, if `./a` is a symlink to directory `./b` and you specified `['./a**', './b/**']` patterns, then directory `./a` will still be read.
> :book: If the [`stats`](#stats) option is specified, the information about the symbolic link (`fs.lstat`) will be replaced with information about the entry (`fs.stat`) behind it.
#### fs
* Type: `FileSystemAdapter`
* Default: `fs.*`
Custom implementation of methods for working with the file system.
```ts
export interface FileSystemAdapter {
lstat?: typeof fs.lstat;
stat?: typeof fs.stat;
lstatSync?: typeof fs.lstatSync;
statSync?: typeof fs.statSync;
readdir?: typeof fs.readdir;
readdirSync?: typeof fs.readdirSync;
}
```
#### ignore
* Type: `string[]`
* Default: `[]`
An array of glob patterns to exclude matches. This is an alternative way to use negative patterns.
```js
dir/
├── package-lock.json
└── package.json
```
```js
fg.sync(['*.json', '!package-lock.json']); // ['package.json']
fg.sync('*.json', { ignore: ['package-lock.json'] }); // ['package.json']
```
#### suppressErrors
* Type: `boolean`
* Default: `false`
By default this package suppress only `ENOENT` errors. Set to `true` to suppress any error.
> :book: Can be useful when the directory has entries with a special level of access.
#### throwErrorOnBrokenSymbolicLink
* Type: `boolean`
* Default: `false`
Throw an error when symbolic link is broken if `true` or safely return `lstat` call if `false`.
> :book: This option has no effect on errors when reading the symbolic link directory.
### Output control
#### absolute
* Type: `boolean`
* Default: `false`
Return the absolute path for entries.
```js
fg.sync('*.js', { absolute: false }); // ['index.js']
fg.sync('*.js', { absolute: true }); // ['/home/user/index.js']
```
> :book: This option is required if you want to use negative patterns with absolute path, for example, `!${__dirname}/*.js`.
#### markDirectories
* Type: `boolean`
* Default: `false`
Mark the directory path with the final slash.
```js
fg.sync('*', { onlyFiles: false, markDirectories: false }); // ['index.js', 'controllers']
fg.sync('*', { onlyFiles: false, markDirectories: true }); // ['index.js', 'controllers/']
```
#### objectMode
* Type: `boolean`
* Default: `false`
Returns objects (instead of strings) describing entries.
```js
fg.sync('*', { objectMode: false }); // ['src/index.js']
fg.sync('*', { objectMode: true }); // [{ name: 'index.js', path: 'src/index.js', dirent: <fs.Dirent> }]
```
The object has the following fields:
* name (`string`) — the last part of the path (basename)
* path (`string`) — full path relative to the pattern base directory
* dirent ([`fs.Dirent`][node_js_fs_class_fs_dirent]) — instance of `fs.Dirent`
> :book: An object is an internal representation of entry, so getting it does not affect performance.
#### onlyDirectories
* Type: `boolean`
* Default: `false`
Return only directories.
```js
fg.sync('*', { onlyDirectories: false }); // ['index.js', 'src']
fg.sync('*', { onlyDirectories: true }); // ['src']
```
> :book: If `true`, the [`onlyFiles`](#onlyfiles) option is automatically `false`.
#### onlyFiles
* Type: `boolean`
* Default: `true`
Return only files.
```js
fg.sync('*', { onlyFiles: false }); // ['index.js', 'src']
fg.sync('*', { onlyFiles: true }); // ['index.js']
```
#### stats
* Type: `boolean`
* Default: `false`
Enables an [object mode](#objectmode) with an additional field:
* stats ([`fs.Stats`][node_js_fs_class_fs_stats]) — instance of `fs.Stats`
```js
fg.sync('*', { stats: false }); // ['src/index.js']
fg.sync('*', { stats: true }); // [{ name: 'index.js', path: 'src/index.js', dirent: <fs.Dirent>, stats: <fs.Stats> }]
```
> :book: Returns `fs.stat` instead of `fs.lstat` for symbolic links when the [`followSymbolicLinks`](#followsymboliclinks) option is specified.
>
> :warning: Unlike [object mode](#objectmode) this mode requires additional calls to the file system. On average, this mode is slower at least twice. See [old and modern mode](#old-and-modern-mode) for more details.
#### unique
* Type: `boolean`
* Default: `true`
Ensures that the returned entries are unique.
```js
fg.sync(['*.json', 'package.json'], { unique: false }); // ['package.json', 'package.json']
fg.sync(['*.json', 'package.json'], { unique: true }); // ['package.json']
```
If `true` and similar entries are found, the result is the first found.
### Matching control
#### braceExpansion
* Type: `boolean`
* Default: `true`
Enables Bash-like brace expansion.
> :1234: [Syntax description][bash_hackers_syntax_expansion_brace] or more [detailed description][micromatch_braces].
```js
dir/
├── abd
├── acd
└── a{b,c}d
```
```js
fg.sync('a{b,c}d', { braceExpansion: false }); // ['a{b,c}d']
fg.sync('a{b,c}d', { braceExpansion: true }); // ['abd', 'acd']
```
#### caseSensitiveMatch
* Type: `boolean`
* Default: `true`
Enables a [case-sensitive][wikipedia_case_sensitivity] mode for matching files.
```js
dir/
├── file.txt
└── File.txt
```
```js
fg.sync('file.txt', { caseSensitiveMatch: false }); // ['file.txt', 'File.txt']
fg.sync('file.txt', { caseSensitiveMatch: true }); // ['file.txt']
```
#### dot
* Type: `boolean`
* Default: `false`
Allow patterns to match entries that begin with a period (`.`).
> :book: Note that an explicit dot in a portion of the pattern will always match dot files.
```js
dir/
├── .editorconfig
└── package.json
```
```js
fg.sync('*', { dot: false }); // ['package.json']
fg.sync('*', { dot: true }); // ['.editorconfig', 'package.json']
```
#### extglob
* Type: `boolean`
* Default: `true`
Enables Bash-like `extglob` functionality.
> :1234: [Syntax description][micromatch_extglobs].
```js
dir/
├── README.md
└── package.json
```
```js
fg.sync('*.+(json|md)', { extglob: false }); // []
fg.sync('*.+(json|md)', { extglob: true }); // ['README.md', 'package.json']
```
#### globstar
* Type: `boolean`
* Default: `true`
Enables recursively repeats a pattern containing `**`. If `false`, `**` behaves exactly like `*`.
```js
dir/
└── a
└── b
```
```js
fg.sync('**', { onlyFiles: false, globstar: false }); // ['a']
fg.sync('**', { onlyFiles: false, globstar: true }); // ['a', 'a/b']
```
#### baseNameMatch
* Type: `boolean`
* Default: `false`
If set to `true`, then patterns without slashes will be matched against the basename of the path if it contains slashes.
```js
dir/
└── one/
└── file.md
```
```js
fg.sync('*.md', { baseNameMatch: false }); // []
fg.sync('*.md', { baseNameMatch: true }); // ['one/file.md']
```
## FAQ
## What is a static or dynamic pattern?
All patterns can be divided into two types:
* **static**. A pattern is considered static if it can be used to get an entry on the file system without using matching mechanisms. For example, the `file.js` pattern is a static pattern because we can just verify that it exists on the file system.
* **dynamic**. A pattern is considered dynamic if it cannot be used directly to find occurrences without using a matching mechanisms. For example, the `*` pattern is a dynamic pattern because we cannot use this pattern directly.
A pattern is considered dynamic if it contains the following characters (`…` — any characters or their absence) or options:
* The [`caseSensitiveMatch`](#casesensitivematch) option is disabled
* `\\` (the escape character)
* `*`, `?`, `!` (at the beginning of line)
* `[…]`
* `(…|…)`
* `@(…)`, `!(…)`, `*(…)`, `?(…)`, `+(…)` (respects the [`extglob`](#extglob) option)
* `{…,…}`, `{…..…}` (respects the [`braceExpansion`](#braceexpansion) option)
## How to write patterns on Windows?
Always use forward-slashes in glob expressions (patterns and [`ignore`](#ignore) option). Use backslashes for escaping characters. With the [`cwd`](#cwd) option use a convenient format.
**Bad**
```ts
[
'directory\\*',
path.join(process.cwd(), '**')
]
```
**Good**
```ts
[
'directory/*',
fg.convertPathToPattern(process.cwd()) + '/**'
]
```
> :book: Use the [`.convertPathToPattern`](#convertpathtopatternpath) package to convert Windows-style path to a Unix-style path.
Read more about [matching with backslashes][micromatch_backslashes].
## Why are parentheses match wrong?
```js
dir/
└── (special-*file).txt
```
```js
fg.sync(['(special-*file).txt']) // []
```
Refers to Bash. You need to escape special characters:
```js
fg.sync(['\\(special-*file\\).txt']) // ['(special-*file).txt']
```
Read more about [matching special characters as literals][picomatch_matching_special_characters_as_literals]. Or use the [`.escapePath`](#escapepathpath).
## How to exclude directory from reading?
You can use a negative pattern like this: `!**/node_modules` or `!**/node_modules/**`. Also you can use [`ignore`](#ignore) option. Just look at the example below.
```js
first/
├── file.md
└── second/
└── file.txt
```
If you don't want to read the `second` directory, you must write the following pattern: `!**/second` or `!**/second/**`.
```js
fg.sync(['**/*.md', '!**/second']); // ['first/file.md']
fg.sync(['**/*.md'], { ignore: ['**/second/**'] }); // ['first/file.md']
```
> :warning: When you write `!**/second/**/*` it means that the directory will be **read**, but all the entries will not be included in the results.
You have to understand that if you write the pattern to exclude directories, then the directory will not be read under any circumstances.
## How to use UNC path?
You cannot use [Uniform Naming Convention (UNC)][unc_path] paths as patterns (due to syntax) directly, but you can use them as [`cwd`](#cwd) directory or use the `fg.convertPathToPattern` method.
```ts
// cwd
fg.sync('*', { cwd: '\\\\?\\C:\\Python27' /* or //?/C:/Python27 */ });
fg.sync('Python27/*', { cwd: '\\\\?\\C:\\' /* or //?/C:/ */ });
// .convertPathToPattern
fg.sync(fg.convertPathToPattern('\\\\?\\c:\\Python27') + '/*');
```
## Compatible with `node-glob`?
| node-glob | fast-glob |
| :----------: | :-------: |
| `cwd` | [`cwd`](#cwd) |
| `root` | – |
| `dot` | [`dot`](#dot) |
| `nomount` | – |
| `mark` | [`markDirectories`](#markdirectories) |
| `nosort` | – |
| `nounique` | [`unique`](#unique) |
| `nobrace` | [`braceExpansion`](#braceexpansion) |
| `noglobstar` | [`globstar`](#globstar) |
| `noext` | [`extglob`](#extglob) |
| `nocase` | [`caseSensitiveMatch`](#casesensitivematch) |
| `matchBase` | [`baseNameMatch`](#basenamematch) |
| `nodir` | [`onlyFiles`](#onlyfiles) |
| `ignore` | [`ignore`](#ignore) |
| `follow` | [`followSymbolicLinks`](#followsymboliclinks) |
| `realpath` | – |
| `absolute` | [`absolute`](#absolute) |
## Benchmarks
You can see results [here](https://github.com/mrmlnc/fast-glob/actions/workflows/benchmark.yml?query=branch%3Amaster) for every commit into the `main` branch.
* **Product benchmark** – comparison with the main competitors.
* **Regress benchmark** – regression between the current version and the version from the npm registry.
## Changelog
See the [Releases section of our GitHub project][github_releases] for changelog for each release version.
## License
This software is released under the terms of the MIT license.
[bash_hackers_syntax_expansion_brace]: https://wiki.bash-hackers.org/syntax/expansion/brace
[github_releases]: https://github.com/mrmlnc/fast-glob/releases
[glob_definition]: https://en.wikipedia.org/wiki/Glob_(programming)
[glob_linux_man]: http://man7.org/linux/man-pages/man3/glob.3.html
[micromatch_backslashes]: https://github.com/micromatch/micromatch#backslashes
[micromatch_braces]: https://github.com/micromatch/braces
[micromatch_extended_globbing]: https://github.com/micromatch/micromatch#extended-globbing
[micromatch_extglobs]: https://github.com/micromatch/micromatch#extglobs
[micromatch_regex_character_classes]: https://github.com/micromatch/micromatch#regex-character-classes
[micromatch]: https://github.com/micromatch/micromatch
[node_js_fs_class_fs_dirent]: https://nodejs.org/api/fs.html#fs_class_fs_dirent
[node_js_fs_class_fs_stats]: https://nodejs.org/api/fs.html#fs_class_fs_stats
[node_js_stream_readable_streams]: https://nodejs.org/api/stream.html#stream_readable_streams
[node_js]: https://nodejs.org/en
[nodelib_fs_scandir_old_and_modern_modern]: https://github.com/nodelib/nodelib/blob/master/packages/fs/fs.scandir/README.md#old-and-modern-mode
[npm_normalize_path]: https://www.npmjs.com/package/normalize-path
[npm_unixify]: https://www.npmjs.com/package/unixify
[picomatch_matching_behavior]: https://github.com/micromatch/picomatch#matching-behavior-vs-bash
[picomatch_matching_special_characters_as_literals]: https://github.com/micromatch/picomatch#matching-special-characters-as-literals
[picomatch_posix_brackets]: https://github.com/micromatch/picomatch#posix-brackets
[regular_expressions_brackets]: https://www.regular-expressions.info/brackets.html
[unc_path]: https://learn.microsoft.com/openspecs/windows_protocols/ms-dtyp/62e862f4-2a51-452e-8eeb-dc4ff5ee33cc
[wikipedia_case_sensitivity]: https://en.wikipedia.org/wiki/Case_sensitivity
[nodejs_thread_pool]: https://nodejs.org/en/docs/guides/dont-block-the-event-loop
[libuv_thread_pool]: http://docs.libuv.org/en/v1.x/threadpool.html
[windows_naming_conventions]: https://learn.microsoft.com/en-us/windows/win32/fileio/naming-a-file#naming-conventions | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fast-glob/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fast-glob/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 25944
} |
# fastq
![ci][ci-url]
[![npm version][npm-badge]][npm-url]
Fast, in memory work queue.
Benchmarks (1 million tasks):
* setImmediate: 812ms
* fastq: 854ms
* async.queue: 1298ms
* neoAsync.queue: 1249ms
Obtained on node 12.16.1, on a dedicated server.
If you need zero-overhead series function call, check out
[fastseries](http://npm.im/fastseries). For zero-overhead parallel
function call, check out [fastparallel](http://npm.im/fastparallel).
[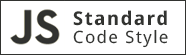](https://github.com/feross/standard)
* <a href="#install">Installation</a>
* <a href="#usage">Usage</a>
* <a href="#api">API</a>
* <a href="#license">Licence & copyright</a>
## Install
`npm i fastq --save`
## Usage (callback API)
```js
'use strict'
const queue = require('fastq')(worker, 1)
queue.push(42, function (err, result) {
if (err) { throw err }
console.log('the result is', result)
})
function worker (arg, cb) {
cb(null, arg * 2)
}
```
## Usage (promise API)
```js
const queue = require('fastq').promise(worker, 1)
async function worker (arg) {
return arg * 2
}
async function run () {
const result = await queue.push(42)
console.log('the result is', result)
}
run()
```
### Setting "this"
```js
'use strict'
const that = { hello: 'world' }
const queue = require('fastq')(that, worker, 1)
queue.push(42, function (err, result) {
if (err) { throw err }
console.log(this)
console.log('the result is', result)
})
function worker (arg, cb) {
console.log(this)
cb(null, arg * 2)
}
```
### Using with TypeScript (callback API)
```ts
'use strict'
import * as fastq from "fastq";
import type { queue, done } from "fastq";
type Task = {
id: number
}
const q: queue<Task> = fastq(worker, 1)
q.push({ id: 42})
function worker (arg: Task, cb: done) {
console.log(arg.id)
cb(null)
}
```
### Using with TypeScript (promise API)
```ts
'use strict'
import * as fastq from "fastq";
import type { queueAsPromised } from "fastq";
type Task = {
id: number
}
const q: queueAsPromised<Task> = fastq.promise(asyncWorker, 1)
q.push({ id: 42}).catch((err) => console.error(err))
async function asyncWorker (arg: Task): Promise<void> {
// No need for a try-catch block, fastq handles errors automatically
console.log(arg.id)
}
```
## API
* <a href="#fastqueue"><code>fastqueue()</code></a>
* <a href="#push"><code>queue#<b>push()</b></code></a>
* <a href="#unshift"><code>queue#<b>unshift()</b></code></a>
* <a href="#pause"><code>queue#<b>pause()</b></code></a>
* <a href="#resume"><code>queue#<b>resume()</b></code></a>
* <a href="#idle"><code>queue#<b>idle()</b></code></a>
* <a href="#length"><code>queue#<b>length()</b></code></a>
* <a href="#getQueue"><code>queue#<b>getQueue()</b></code></a>
* <a href="#kill"><code>queue#<b>kill()</b></code></a>
* <a href="#killAndDrain"><code>queue#<b>killAndDrain()</b></code></a>
* <a href="#error"><code>queue#<b>error()</b></code></a>
* <a href="#concurrency"><code>queue#<b>concurrency</b></code></a>
* <a href="#drain"><code>queue#<b>drain</b></code></a>
* <a href="#empty"><code>queue#<b>empty</b></code></a>
* <a href="#saturated"><code>queue#<b>saturated</b></code></a>
* <a href="#promise"><code>fastqueue.promise()</code></a>
-------------------------------------------------------
<a name="fastqueue"></a>
### fastqueue([that], worker, concurrency)
Creates a new queue.
Arguments:
* `that`, optional context of the `worker` function.
* `worker`, worker function, it would be called with `that` as `this`,
if that is specified.
* `concurrency`, number of concurrent tasks that could be executed in
parallel.
-------------------------------------------------------
<a name="push"></a>
### queue.push(task, done)
Add a task at the end of the queue. `done(err, result)` will be called
when the task was processed.
-------------------------------------------------------
<a name="unshift"></a>
### queue.unshift(task, done)
Add a task at the beginning of the queue. `done(err, result)` will be called
when the task was processed.
-------------------------------------------------------
<a name="pause"></a>
### queue.pause()
Pause the processing of tasks. Currently worked tasks are not
stopped.
-------------------------------------------------------
<a name="resume"></a>
### queue.resume()
Resume the processing of tasks.
-------------------------------------------------------
<a name="idle"></a>
### queue.idle()
Returns `false` if there are tasks being processed or waiting to be processed.
`true` otherwise.
-------------------------------------------------------
<a name="length"></a>
### queue.length()
Returns the number of tasks waiting to be processed (in the queue).
-------------------------------------------------------
<a name="getQueue"></a>
### queue.getQueue()
Returns all the tasks be processed (in the queue). Returns empty array when there are no tasks
-------------------------------------------------------
<a name="kill"></a>
### queue.kill()
Removes all tasks waiting to be processed, and reset `drain` to an empty
function.
-------------------------------------------------------
<a name="killAndDrain"></a>
### queue.killAndDrain()
Same than `kill` but the `drain` function will be called before reset to empty.
-------------------------------------------------------
<a name="error"></a>
### queue.error(handler)
Set a global error handler. `handler(err, task)` will be called
each time a task is completed, `err` will be not null if the task has thrown an error.
-------------------------------------------------------
<a name="concurrency"></a>
### queue.concurrency
Property that returns the number of concurrent tasks that could be executed in
parallel. It can be altered at runtime.
-------------------------------------------------------
<a name="drain"></a>
### queue.drain
Function that will be called when the last
item from the queue has been processed by a worker.
It can be altered at runtime.
-------------------------------------------------------
<a name="empty"></a>
### queue.empty
Function that will be called when the last
item from the queue has been assigned to a worker.
It can be altered at runtime.
-------------------------------------------------------
<a name="saturated"></a>
### queue.saturated
Function that will be called when the queue hits the concurrency
limit.
It can be altered at runtime.
-------------------------------------------------------
<a name="promise"></a>
### fastqueue.promise([that], worker(arg), concurrency)
Creates a new queue with `Promise` apis. It also offers all the methods
and properties of the object returned by [`fastqueue`](#fastqueue) with the modified
[`push`](#pushPromise) and [`unshift`](#unshiftPromise) methods.
Node v10+ is required to use the promisified version.
Arguments:
* `that`, optional context of the `worker` function.
* `worker`, worker function, it would be called with `that` as `this`,
if that is specified. It MUST return a `Promise`.
* `concurrency`, number of concurrent tasks that could be executed in
parallel.
<a name="pushPromise"></a>
#### queue.push(task) => Promise
Add a task at the end of the queue. The returned `Promise` will be fulfilled (rejected)
when the task is completed successfully (unsuccessfully).
This promise could be ignored as it will not lead to a `'unhandledRejection'`.
<a name="unshiftPromise"></a>
#### queue.unshift(task) => Promise
Add a task at the beginning of the queue. The returned `Promise` will be fulfilled (rejected)
when the task is completed successfully (unsuccessfully).
This promise could be ignored as it will not lead to a `'unhandledRejection'`.
<a name="drained"></a>
#### queue.drained() => Promise
Wait for the queue to be drained. The returned `Promise` will be resolved when all tasks in the queue have been processed by a worker.
This promise could be ignored as it will not lead to a `'unhandledRejection'`.
## License
ISC
[ci-url]: https://github.com/mcollina/fastq/workflows/ci/badge.svg
[npm-badge]: https://badge.fury.io/js/fastq.svg
[npm-url]: https://badge.fury.io/js/fastq | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fastq/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fastq/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 8167
} |
# fill-range [](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=W8YFZ425KND68) [](https://www.npmjs.com/package/fill-range) [](https://npmjs.org/package/fill-range) [](https://npmjs.org/package/fill-range) [](https://travis-ci.org/jonschlinkert/fill-range)
> Fill in a range of numbers or letters, optionally passing an increment or `step` to use, or create a regex-compatible range with `options.toRegex`
Please consider following this project's author, [Jon Schlinkert](https://github.com/jonschlinkert), and consider starring the project to show your :heart: and support.
## Install
Install with [npm](https://www.npmjs.com/):
```sh
$ npm install --save fill-range
```
## Usage
Expands numbers and letters, optionally using a `step` as the last argument. _(Numbers may be defined as JavaScript numbers or strings)_.
```js
const fill = require('fill-range');
// fill(from, to[, step, options]);
console.log(fill('1', '10')); //=> ['1', '2', '3', '4', '5', '6', '7', '8', '9', '10']
console.log(fill('1', '10', { toRegex: true })); //=> [1-9]|10
```
**Params**
* `from`: **{String|Number}** the number or letter to start with
* `to`: **{String|Number}** the number or letter to end with
* `step`: **{String|Number|Object|Function}** Optionally pass a [step](#optionsstep) to use.
* `options`: **{Object|Function}**: See all available [options](#options)
## Examples
By default, an array of values is returned.
**Alphabetical ranges**
```js
console.log(fill('a', 'e')); //=> ['a', 'b', 'c', 'd', 'e']
console.log(fill('A', 'E')); //=> [ 'A', 'B', 'C', 'D', 'E' ]
```
**Numerical ranges**
Numbers can be defined as actual numbers or strings.
```js
console.log(fill(1, 5)); //=> [ 1, 2, 3, 4, 5 ]
console.log(fill('1', '5')); //=> [ 1, 2, 3, 4, 5 ]
```
**Negative ranges**
Numbers can be defined as actual numbers or strings.
```js
console.log(fill('-5', '-1')); //=> [ '-5', '-4', '-3', '-2', '-1' ]
console.log(fill('-5', '5')); //=> [ '-5', '-4', '-3', '-2', '-1', '0', '1', '2', '3', '4', '5' ]
```
**Steps (increments)**
```js
// numerical ranges with increments
console.log(fill('0', '25', 4)); //=> [ '0', '4', '8', '12', '16', '20', '24' ]
console.log(fill('0', '25', 5)); //=> [ '0', '5', '10', '15', '20', '25' ]
console.log(fill('0', '25', 6)); //=> [ '0', '6', '12', '18', '24' ]
// alphabetical ranges with increments
console.log(fill('a', 'z', 4)); //=> [ 'a', 'e', 'i', 'm', 'q', 'u', 'y' ]
console.log(fill('a', 'z', 5)); //=> [ 'a', 'f', 'k', 'p', 'u', 'z' ]
console.log(fill('a', 'z', 6)); //=> [ 'a', 'g', 'm', 's', 'y' ]
```
## Options
### options.step
**Type**: `number` (formatted as a string or number)
**Default**: `undefined`
**Description**: The increment to use for the range. Can be used with letters or numbers.
**Example(s)**
```js
// numbers
console.log(fill('1', '10', 2)); //=> [ '1', '3', '5', '7', '9' ]
console.log(fill('1', '10', 3)); //=> [ '1', '4', '7', '10' ]
console.log(fill('1', '10', 4)); //=> [ '1', '5', '9' ]
// letters
console.log(fill('a', 'z', 5)); //=> [ 'a', 'f', 'k', 'p', 'u', 'z' ]
console.log(fill('a', 'z', 7)); //=> [ 'a', 'h', 'o', 'v' ]
console.log(fill('a', 'z', 9)); //=> [ 'a', 'j', 's' ]
```
### options.strictRanges
**Type**: `boolean`
**Default**: `false`
**Description**: By default, `null` is returned when an invalid range is passed. Enable this option to throw a `RangeError` on invalid ranges.
**Example(s)**
The following are all invalid:
```js
fill('1.1', '2'); // decimals not supported in ranges
fill('a', '2'); // incompatible range values
fill(1, 10, 'foo'); // invalid "step" argument
```
### options.stringify
**Type**: `boolean`
**Default**: `undefined`
**Description**: Cast all returned values to strings. By default, integers are returned as numbers.
**Example(s)**
```js
console.log(fill(1, 5)); //=> [ 1, 2, 3, 4, 5 ]
console.log(fill(1, 5, { stringify: true })); //=> [ '1', '2', '3', '4', '5' ]
```
### options.toRegex
**Type**: `boolean`
**Default**: `undefined`
**Description**: Create a regex-compatible source string, instead of expanding values to an array.
**Example(s)**
```js
// alphabetical range
console.log(fill('a', 'e', { toRegex: true })); //=> '[a-e]'
// alphabetical with step
console.log(fill('a', 'z', 3, { toRegex: true })); //=> 'a|d|g|j|m|p|s|v|y'
// numerical range
console.log(fill('1', '100', { toRegex: true })); //=> '[1-9]|[1-9][0-9]|100'
// numerical range with zero padding
console.log(fill('000001', '100000', { toRegex: true }));
//=> '0{5}[1-9]|0{4}[1-9][0-9]|0{3}[1-9][0-9]{2}|0{2}[1-9][0-9]{3}|0[1-9][0-9]{4}|100000'
```
### options.transform
**Type**: `function`
**Default**: `undefined`
**Description**: Customize each value in the returned array (or [string](#optionstoRegex)). _(you can also pass this function as the last argument to `fill()`)_.
**Example(s)**
```js
// add zero padding
console.log(fill(1, 5, value => String(value).padStart(4, '0')));
//=> ['0001', '0002', '0003', '0004', '0005']
```
## About
<details>
<summary><strong>Contributing</strong></summary>
Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new).
</details>
<details>
<summary><strong>Running Tests</strong></summary>
Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
```sh
$ npm install && npm test
```
</details>
<details>
<summary><strong>Building docs</strong></summary>
_(This project's readme.md is generated by [verb](https://github.com/verbose/verb-generate-readme), please don't edit the readme directly. Any changes to the readme must be made in the [.verb.md](.verb.md) readme template.)_
To generate the readme, run the following command:
```sh
$ npm install -g verbose/verb#dev verb-generate-readme && verb
```
</details>
### Contributors
| **Commits** | **Contributor** |
| --- | --- |
| 116 | [jonschlinkert](https://github.com/jonschlinkert) |
| 4 | [paulmillr](https://github.com/paulmillr) |
| 2 | [realityking](https://github.com/realityking) |
| 2 | [bluelovers](https://github.com/bluelovers) |
| 1 | [edorivai](https://github.com/edorivai) |
| 1 | [wtgtybhertgeghgtwtg](https://github.com/wtgtybhertgeghgtwtg) |
### Author
**Jon Schlinkert**
* [GitHub Profile](https://github.com/jonschlinkert)
* [Twitter Profile](https://twitter.com/jonschlinkert)
* [LinkedIn Profile](https://linkedin.com/in/jonschlinkert)
Please consider supporting me on Patreon, or [start your own Patreon page](https://patreon.com/invite/bxpbvm)!
<a href="https://www.patreon.com/jonschlinkert">
<img src="https://c5.patreon.com/external/logo/[email protected]" height="50">
</a>
### License
Copyright © 2019, [Jon Schlinkert](https://github.com/jonschlinkert).
Released under the [MIT License](LICENSE).
***
_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.8.0, on April 08, 2019._ | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fill-range/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fill-range/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 7485
} |
v1.3.1 / 2024-09-11
==================
* deps: encodeurl@~2.0.0
v1.3.0 / 2024-09-03
==================
* ignore status message for HTTP/2 (#53)
v1.2.1 / 2024-09-02
==================
* Gracefully handle when handling an error and socket is null
1.2.0 / 2022-03-22
==================
* Remove set content headers that break response
* deps: [email protected]
* deps: [email protected]
- Rename `425 Unordered Collection` to standard `425 Too Early`
1.1.2 / 2019-05-09
==================
* Set stricter `Content-Security-Policy` header
* deps: parseurl@~1.3.3
* deps: statuses@~1.5.0
1.1.1 / 2018-03-06
==================
* Fix 404 output for bad / missing pathnames
* deps: encodeurl@~1.0.2
- Fix encoding `%` as last character
* deps: statuses@~1.4.0
1.1.0 / 2017-09-24
==================
* Use `res.headersSent` when available
1.0.6 / 2017-09-22
==================
* deps: [email protected]
1.0.5 / 2017-09-15
==================
* deps: parseurl@~1.3.2
- perf: reduce overhead for full URLs
- perf: unroll the "fast-path" `RegExp`
1.0.4 / 2017-08-03
==================
* deps: [email protected]
1.0.3 / 2017-05-16
==================
* deps: [email protected]
- deps: [email protected]
1.0.2 / 2017-04-22
==================
* deps: [email protected]
- deps: [email protected]
1.0.1 / 2017-03-21
==================
* Fix missing `</html>` in HTML document
* deps: [email protected]
- Fix: `DEBUG_MAX_ARRAY_LENGTH`
1.0.0 / 2017-02-15
==================
* Fix exception when `err` cannot be converted to a string
* Fully URL-encode the pathname in the 404 message
* Only include the pathname in the 404 message
* Send complete HTML document
* Set `Content-Security-Policy: default-src 'self'` header
* deps: [email protected]
- Allow colors in workers
- Deprecated `DEBUG_FD` environment variable set to `3` or higher
- Fix error when running under React Native
- Use same color for same namespace
- deps: [email protected]
0.5.1 / 2016-11-12
==================
* Fix exception when `err.headers` is not an object
* deps: statuses@~1.3.1
* perf: hoist regular expressions
* perf: remove duplicate validation path
0.5.0 / 2016-06-15
==================
* Change invalid or non-numeric status code to 500
* Overwrite status message to match set status code
* Prefer `err.statusCode` if `err.status` is invalid
* Set response headers from `err.headers` object
* Use `statuses` instead of `http` module for status messages
- Includes all defined status messages
0.4.1 / 2015-12-02
==================
* deps: escape-html@~1.0.3
- perf: enable strict mode
- perf: optimize string replacement
- perf: use faster string coercion
0.4.0 / 2015-06-14
==================
* Fix a false-positive when unpiping in Node.js 0.8
* Support `statusCode` property on `Error` objects
* Use `unpipe` module for unpiping requests
* deps: [email protected]
* deps: on-finished@~2.3.0
- Add defined behavior for HTTP `CONNECT` requests
- Add defined behavior for HTTP `Upgrade` requests
- deps: [email protected]
* perf: enable strict mode
* perf: remove argument reassignment
0.3.6 / 2015-05-11
==================
* deps: debug@~2.2.0
- deps: [email protected]
0.3.5 / 2015-04-22
==================
* deps: on-finished@~2.2.1
- Fix `isFinished(req)` when data buffered
0.3.4 / 2015-03-15
==================
* deps: debug@~2.1.3
- Fix high intensity foreground color for bold
- deps: [email protected]
0.3.3 / 2015-01-01
==================
* deps: debug@~2.1.1
* deps: on-finished@~2.2.0
0.3.2 / 2014-10-22
==================
* deps: on-finished@~2.1.1
- Fix handling of pipelined requests
0.3.1 / 2014-10-16
==================
* deps: debug@~2.1.0
- Implement `DEBUG_FD` env variable support
0.3.0 / 2014-09-17
==================
* Terminate in progress response only on error
* Use `on-finished` to determine request status
0.2.0 / 2014-09-03
==================
* Set `X-Content-Type-Options: nosniff` header
* deps: debug@~2.0.0
0.1.0 / 2014-07-16
==================
* Respond after request fully read
- prevents hung responses and socket hang ups
* deps: [email protected]
0.0.3 / 2014-07-11
==================
* deps: [email protected]
- Add support for multiple wildcards in namespaces
0.0.2 / 2014-06-19
==================
* Handle invalid status codes
0.0.1 / 2014-06-05
==================
* deps: [email protected]
0.0.0 / 2014-06-05
==================
* Extracted from connect/express | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/finalhandler/HISTORY.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/finalhandler/HISTORY.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 4548
} |
# finalhandler
[![NPM Version][npm-image]][npm-url]
[![NPM Downloads][downloads-image]][downloads-url]
[![Node.js Version][node-image]][node-url]
[![Build Status][github-actions-ci-image]][github-actions-ci-url]
[![Test Coverage][coveralls-image]][coveralls-url]
Node.js function to invoke as the final step to respond to HTTP request.
## Installation
This is a [Node.js](https://nodejs.org/en/) module available through the
[npm registry](https://www.npmjs.com/). Installation is done using the
[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
```sh
$ npm install finalhandler
```
## API
```js
var finalhandler = require('finalhandler')
```
### finalhandler(req, res, [options])
Returns function to be invoked as the final step for the given `req` and `res`.
This function is to be invoked as `fn(err)`. If `err` is falsy, the handler will
write out a 404 response to the `res`. If it is truthy, an error response will
be written out to the `res` or `res` will be terminated if a response has already
started.
When an error is written, the following information is added to the response:
* The `res.statusCode` is set from `err.status` (or `err.statusCode`). If
this value is outside the 4xx or 5xx range, it will be set to 500.
* The `res.statusMessage` is set according to the status code.
* The body will be the HTML of the status code message if `env` is
`'production'`, otherwise will be `err.stack`.
* Any headers specified in an `err.headers` object.
The final handler will also unpipe anything from `req` when it is invoked.
#### options.env
By default, the environment is determined by `NODE_ENV` variable, but it can be
overridden by this option.
#### options.onerror
Provide a function to be called with the `err` when it exists. Can be used for
writing errors to a central location without excessive function generation. Called
as `onerror(err, req, res)`.
## Examples
### always 404
```js
var finalhandler = require('finalhandler')
var http = require('http')
var server = http.createServer(function (req, res) {
var done = finalhandler(req, res)
done()
})
server.listen(3000)
```
### perform simple action
```js
var finalhandler = require('finalhandler')
var fs = require('fs')
var http = require('http')
var server = http.createServer(function (req, res) {
var done = finalhandler(req, res)
fs.readFile('index.html', function (err, buf) {
if (err) return done(err)
res.setHeader('Content-Type', 'text/html')
res.end(buf)
})
})
server.listen(3000)
```
### use with middleware-style functions
```js
var finalhandler = require('finalhandler')
var http = require('http')
var serveStatic = require('serve-static')
var serve = serveStatic('public')
var server = http.createServer(function (req, res) {
var done = finalhandler(req, res)
serve(req, res, done)
})
server.listen(3000)
```
### keep log of all errors
```js
var finalhandler = require('finalhandler')
var fs = require('fs')
var http = require('http')
var server = http.createServer(function (req, res) {
var done = finalhandler(req, res, { onerror: logerror })
fs.readFile('index.html', function (err, buf) {
if (err) return done(err)
res.setHeader('Content-Type', 'text/html')
res.end(buf)
})
})
server.listen(3000)
function logerror (err) {
console.error(err.stack || err.toString())
}
```
## License
[MIT](LICENSE)
[npm-image]: https://img.shields.io/npm/v/finalhandler.svg
[npm-url]: https://npmjs.org/package/finalhandler
[node-image]: https://img.shields.io/node/v/finalhandler.svg
[node-url]: https://nodejs.org/en/download
[coveralls-image]: https://img.shields.io/coveralls/pillarjs/finalhandler.svg
[coveralls-url]: https://coveralls.io/r/pillarjs/finalhandler?branch=master
[downloads-image]: https://img.shields.io/npm/dm/finalhandler.svg
[downloads-url]: https://npmjs.org/package/finalhandler
[github-actions-ci-image]: https://github.com/pillarjs/finalhandler/actions/workflows/ci.yml/badge.svg
[github-actions-ci-url]: https://github.com/pillarjs/finalhandler/actions/workflows/ci.yml | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/finalhandler/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/finalhandler/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 4119
} |
# Security Policies and Procedures
## Reporting a Bug
The `finalhandler` team and community take all security bugs seriously. Thank
you for improving the security of Express. We appreciate your efforts and
responsible disclosure and will make every effort to acknowledge your
contributions.
Report security bugs by emailing the current owner(s) of `finalhandler`. This
information can be found in the npm registry using the command
`npm owner ls finalhandler`.
If unsure or unable to get the information from the above, open an issue
in the [project issue tracker](https://github.com/pillarjs/finalhandler/issues)
asking for the current contact information.
To ensure the timely response to your report, please ensure that the entirety
of the report is contained within the email body and not solely behind a web
link or an attachment.
At least one owner will acknowledge your email within 48 hours, and will send a
more detailed response within 48 hours indicating the next steps in handling
your report. After the initial reply to your report, the owners will
endeavor to keep you informed of the progress towards a fix and full
announcement, and may ask for additional information or guidance. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/finalhandler/SECURITY.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/finalhandler/SECURITY.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1201
} |
# foreground-child
Run a child as if it's the foreground process. Give it stdio. Exit
when it exits.
Mostly this module is here to support some use cases around
wrapping child processes for test coverage and such. But it's
also generally useful any time you want one program to execute
another as if it's the "main" process, for example, if a program
takes a `--cmd` argument to execute in some way.
## USAGE
```js
import { foregroundChild } from 'foreground-child'
// hybrid module, this also works:
// const { foregroundChild } = require('foreground-child')
// cats out this file
const child = foregroundChild('cat', [__filename])
// At this point, it's best to just do nothing else.
// return or whatever.
// If the child gets a signal, or just exits, then this
// parent process will exit in the same way.
```
You can provide custom spawn options by passing an object after
the program and arguments:
```js
const child = foregroundChild(`cat ${__filename}`, { shell: true })
```
A callback can optionally be provided, if you want to perform an
action before your foreground-child exits:
```js
const child = foregroundChild('cat', [__filename], spawnOptions, () => {
doSomeActions()
})
```
The callback can return a Promise in order to perform
asynchronous actions. If the callback does not return a promise,
then it must complete its actions within a single JavaScript
tick.
```js
const child = foregroundChild('cat', [__filename], async () => {
await doSomeAsyncActions()
})
```
If the callback throws or rejects, then it will be unhandled, and
node will exit in error.
If the callback returns a string value, then that will be used as
the signal to exit the parent process. If it returns a number,
then that number will be used as the parent exit status code. If
it returns boolean `false`, then the parent process will not be
terminated. If it returns `undefined`, then it will exit with the
same signal/code as the child process.
## Caveats
The "normal" standard IO file descriptors (0, 1, and 2 for stdin,
stdout, and stderr respectively) are shared with the child process.
Additionally, if there is an IPC channel set up in the parent, then
messages are proxied to the child on file descriptor 3.
In Node, it's possible to also map arbitrary file descriptors
into a child process. In these cases, foreground-child will not
map the file descriptors into the child. If file descriptors 0,
1, or 2 are used for the IPC channel, then strange behavior may
happen (like printing IPC messages to stderr, for example).
Note that a SIGKILL will always kill the parent process, but
will not proxy the signal to the child process, because SIGKILL
cannot be caught. In order to address this, a special "watchdog"
child process is spawned which will send a SIGKILL to the child
process if it does not terminate within half a second after the
watchdog receives a SIGHUP due to its parent terminating.
On Windows, issuing a `process.kill(process.pid, signal)` with a
fatal termination signal may cause the process to exit with a `1`
status code rather than reporting the signal properly. This
module tries to do the right thing, but on Windows systems, you
may see that incorrect result. There is as far as I'm aware no
workaround for this.
## util: `foreground-child/proxy-signals`
If you just want to proxy the signals to a child process that the
main process receives, you can use the `proxy-signals` export
from this package.
```js
import { proxySignals } from 'foreground-child/proxy-signals'
const childProcess = spawn('command', ['some', 'args'])
proxySignals(childProcess)
```
Now, any fatal signal received by the current process will be
proxied to the child process.
It doesn't go in the other direction; ie, signals sent to the
child process will not affect the parent. For that, listen to the
child `exit` or `close` events, and handle them appropriately.
## util: `foreground-child/watchdog`
If you are spawning a child process, and want to ensure that it
isn't left dangling if the parent process exits, you can use the
watchdog utility exported by this module.
```js
import { watchdog } from 'foreground-child/watchdog'
const childProcess = spawn('command', ['some', 'args'])
const watchdogProcess = watchdog(childProcess)
// watchdogProcess is a reference to the process monitoring the
// parent and child. There's usually no reason to do anything
// with it, as it's silent and will terminate
// automatically when it's no longer needed.
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/foreground-child/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/foreground-child/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 4487
} |
0.2.0 / 2021-05-31
==================
* Use `req.socket` over deprecated `req.connection`
0.1.2 / 2017-09-14
==================
* perf: improve header parsing
* perf: reduce overhead when no `X-Forwarded-For` header
0.1.1 / 2017-09-10
==================
* Fix trimming leading / trailing OWS
* perf: hoist regular expression
0.1.0 / 2014-09-21
==================
* Initial release | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/forwarded/HISTORY.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/forwarded/HISTORY.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 399
} |
# forwarded
[![NPM Version][npm-image]][npm-url]
[![NPM Downloads][downloads-image]][downloads-url]
[![Node.js Version][node-version-image]][node-version-url]
[![Build Status][ci-image]][ci-url]
[![Test Coverage][coveralls-image]][coveralls-url]
Parse HTTP X-Forwarded-For header
## Installation
This is a [Node.js](https://nodejs.org/en/) module available through the
[npm registry](https://www.npmjs.com/). Installation is done using the
[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
```sh
$ npm install forwarded
```
## API
```js
var forwarded = require('forwarded')
```
### forwarded(req)
```js
var addresses = forwarded(req)
```
Parse the `X-Forwarded-For` header from the request. Returns an array
of the addresses, including the socket address for the `req`, in reverse
order (i.e. index `0` is the socket address and the last index is the
furthest address, typically the end-user).
## Testing
```sh
$ npm test
```
## License
[MIT](LICENSE)
[ci-image]: https://badgen.net/github/checks/jshttp/forwarded/master?label=ci
[ci-url]: https://github.com/jshttp/forwarded/actions?query=workflow%3Aci
[npm-image]: https://img.shields.io/npm/v/forwarded.svg
[npm-url]: https://npmjs.org/package/forwarded
[node-version-image]: https://img.shields.io/node/v/forwarded.svg
[node-version-url]: https://nodejs.org/en/download/
[coveralls-image]: https://img.shields.io/coveralls/jshttp/forwarded/master.svg
[coveralls-url]: https://coveralls.io/r/jshttp/forwarded?branch=master
[downloads-image]: https://img.shields.io/npm/dm/forwarded.svg
[downloads-url]: https://npmjs.org/package/forwarded | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/forwarded/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/forwarded/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1653
} |
# Fraction.js - ℚ in JavaScript
[](https://npmjs.org/package/fraction.js "View this project on npm")
[](http://opensource.org/licenses/MIT)
Tired of inprecise numbers represented by doubles, which have to store rational and irrational numbers like PI or sqrt(2) the same way? Obviously the following problem is preventable:
```javascript
1 / 98 * 98 // = 0.9999999999999999
```
If you need more precision or just want a fraction as a result, just include *Fraction.js*:
```javascript
var Fraction = require('fraction.js');
// or
import Fraction from 'fraction.js';
```
and give it a trial:
```javascript
Fraction(1).div(98).mul(98) // = 1
```
Internally, numbers are represented as *numerator / denominator*, which adds just a little overhead. However, the library is written with performance and accuracy in mind, which makes it the perfect basis for [Polynomial.js](https://github.com/infusion/Polynomial.js) and [Math.js](https://github.com/josdejong/mathjs).
Convert decimal to fraction
===
The simplest job for fraction.js is to get a fraction out of a decimal:
```javascript
var x = new Fraction(1.88);
var res = x.toFraction(true); // String "1 22/25"
```
Examples / Motivation
===
A simple example might be
```javascript
var f = new Fraction("9.4'31'"); // 9.4313131313131...
f.mul([-4, 3]).mod("4.'8'"); // 4.88888888888888...
```
The result is
```javascript
console.log(f.toFraction()); // -4154 / 1485
```
You could of course also access the sign (s), numerator (n) and denominator (d) on your own:
```javascript
f.s * f.n / f.d = -1 * 4154 / 1485 = -2.797306...
```
If you would try to calculate it yourself, you would come up with something like:
```javascript
(9.4313131 * (-4 / 3)) % 4.888888 = -2.797308133...
```
Quite okay, but yea - not as accurate as it could be.
Laplace Probability
===
Simple example. What's the probability of throwing a 3, and 1 or 4, and 2 or 4 or 6 with a fair dice?
P({3}):
```javascript
var p = new Fraction([3].length, 6).toString(); // 0.1(6)
```
P({1, 4}):
```javascript
var p = new Fraction([1, 4].length, 6).toString(); // 0.(3)
```
P({2, 4, 6}):
```javascript
var p = new Fraction([2, 4, 6].length, 6).toString(); // 0.5
```
Convert degrees/minutes/seconds to precise rational representation:
===
57+45/60+17/3600
```javascript
var deg = 57; // 57°
var min = 45; // 45 Minutes
var sec = 17; // 17 Seconds
new Fraction(deg).add(min, 60).add(sec, 3600).toString() // -> 57.7547(2)
```
Rational approximation of irrational numbers
===
Now it's getting messy ;d To approximate a number like *sqrt(5) - 2* with a numerator and denominator, you can reformat the equation as follows: *pow(n / d + 2, 2) = 5*.
Then the following algorithm will generate the rational number besides the binary representation.
```javascript
var x = "/", s = "";
var a = new Fraction(0),
b = new Fraction(1);
for (var n = 0; n <= 10; n++) {
var c = a.add(b).div(2);
console.log(n + "\t" + a + "\t" + b + "\t" + c + "\t" + x);
if (c.add(2).pow(2) < 5) {
a = c;
x = "1";
} else {
b = c;
x = "0";
}
s+= x;
}
console.log(s)
```
The result is
```
n a[n] b[n] c[n] x[n]
0 0/1 1/1 1/2 /
1 0/1 1/2 1/4 0
2 0/1 1/4 1/8 0
3 1/8 1/4 3/16 1
4 3/16 1/4 7/32 1
5 7/32 1/4 15/64 1
6 15/64 1/4 31/128 1
7 15/64 31/128 61/256 0
8 15/64 61/256 121/512 0
9 15/64 121/512 241/1024 0
10 241/1024 121/512 483/2048 1
```
Thus the approximation after 11 iterations of the bisection method is *483 / 2048* and the binary representation is 0.00111100011 (see [WolframAlpha](http://www.wolframalpha.com/input/?i=sqrt%285%29-2+binary))
I published another example on how to approximate PI with fraction.js on my [blog](http://www.xarg.org/2014/03/precise-calculations-in-javascript/) (Still not the best idea to approximate irrational numbers, but it illustrates the capabilities of Fraction.js perfectly).
Get the exact fractional part of a number
---
```javascript
var f = new Fraction("-6.(3416)");
console.log("" + f.mod(1).abs()); // 0.(3416)
```
Mathematical correct modulo
---
The behaviour on negative congruences is different to most modulo implementations in computer science. Even the *mod()* function of Fraction.js behaves in the typical way. To solve the problem of having the mathematical correct modulo with Fraction.js you could come up with this:
```javascript
var a = -1;
var b = 10.99;
console.log(new Fraction(a)
.mod(b)); // Not correct, usual Modulo
console.log(new Fraction(a)
.mod(b).add(b).mod(b)); // Correct! Mathematical Modulo
```
fmod() impreciseness circumvented
---
It turns out that Fraction.js outperforms almost any fmod() implementation, including JavaScript itself, [php.js](http://phpjs.org/functions/fmod/), C++, Python, Java and even Wolframalpha due to the fact that numbers like 0.05, 0.1, ... are infinite decimal in base 2.
The equation *fmod(4.55, 0.05)* gives *0.04999999999999957*, wolframalpha says *1/20*. The correct answer should be **zero**, as 0.05 divides 4.55 without any remainder.
Parser
===
Any function (see below) as well as the constructor of the *Fraction* class parses its input and reduce it to the smallest term.
You can pass either Arrays, Objects, Integers, Doubles or Strings.
Arrays / Objects
---
```javascript
new Fraction(numerator, denominator);
new Fraction([numerator, denominator]);
new Fraction({n: numerator, d: denominator});
```
Integers
---
```javascript
new Fraction(123);
```
Doubles
---
```javascript
new Fraction(55.4);
```
**Note:** If you pass a double as it is, Fraction.js will perform a number analysis based on Farey Sequences. If you concern performance, cache Fraction.js objects and pass arrays/objects.
The method is really precise, but too large exact numbers, like 1234567.9991829 will result in a wrong approximation. If you want to keep the number as it is, convert it to a string, as the string parser will not perform any further observations. If you have problems with the approximation, in the file `examples/approx.js` is a different approximation algorithm, which might work better in some more specific use-cases.
Strings
---
```javascript
new Fraction("123.45");
new Fraction("123/45"); // A rational number represented as two decimals, separated by a slash
new Fraction("123:45"); // A rational number represented as two decimals, separated by a colon
new Fraction("4 123/45"); // A rational number represented as a whole number and a fraction
new Fraction("123.'456'"); // Note the quotes, see below!
new Fraction("123.(456)"); // Note the brackets, see below!
new Fraction("123.45'6'"); // Note the quotes, see below!
new Fraction("123.45(6)"); // Note the brackets, see below!
```
Two arguments
---
```javascript
new Fraction(3, 2); // 3/2 = 1.5
```
Repeating decimal places
---
*Fraction.js* can easily handle repeating decimal places. For example *1/3* is *0.3333...*. There is only one repeating digit. As you can see in the examples above, you can pass a number like *1/3* as "0.'3'" or "0.(3)", which are synonym. There are no tests to parse something like 0.166666666 to 1/6! If you really want to handle this number, wrap around brackets on your own with the function below for example: 0.1(66666666)
Assume you want to divide 123.32 / 33.6(567). [WolframAlpha](http://www.wolframalpha.com/input/?i=123.32+%2F+%2812453%2F370%29) states that you'll get a period of 1776 digits. *Fraction.js* comes to the same result. Give it a try:
```javascript
var f = new Fraction("123.32");
console.log("Bam: " + f.div("33.6(567)"));
```
To automatically make a number like "0.123123123" to something more Fraction.js friendly like "0.(123)", I hacked this little brute force algorithm in a 10 minutes. Improvements are welcome...
```javascript
function formatDecimal(str) {
var comma, pre, offset, pad, times, repeat;
if (-1 === (comma = str.indexOf(".")))
return str;
pre = str.substr(0, comma + 1);
str = str.substr(comma + 1);
for (var i = 0; i < str.length; i++) {
offset = str.substr(0, i);
for (var j = 0; j < 5; j++) {
pad = str.substr(i, j + 1);
times = Math.ceil((str.length - offset.length) / pad.length);
repeat = new Array(times + 1).join(pad); // Silly String.repeat hack
if (0 === (offset + repeat).indexOf(str)) {
return pre + offset + "(" + pad + ")";
}
}
}
return null;
}
var f, x = formatDecimal("13.0123123123"); // = 13.0(123)
if (x !== null) {
f = new Fraction(x);
}
```
Attributes
===
The Fraction object allows direct access to the numerator, denominator and sign attributes. It is ensured that only the sign-attribute holds sign information so that a sign comparison is only necessary against this attribute.
```javascript
var f = new Fraction('-1/2');
console.log(f.n); // Numerator: 1
console.log(f.d); // Denominator: 2
console.log(f.s); // Sign: -1
```
Functions
===
Fraction abs()
---
Returns the actual number without any sign information
Fraction neg()
---
Returns the actual number with flipped sign in order to get the additive inverse
Fraction add(n)
---
Returns the sum of the actual number and the parameter n
Fraction sub(n)
---
Returns the difference of the actual number and the parameter n
Fraction mul(n)
---
Returns the product of the actual number and the parameter n
Fraction div(n)
---
Returns the quotient of the actual number and the parameter n
Fraction pow(exp)
---
Returns the power of the actual number, raised to an possible rational exponent. If the result becomes non-rational the function returns `null`.
Fraction mod(n)
---
Returns the modulus (rest of the division) of the actual object and n (this % n). It's a much more precise [fmod()](#fmod-impreciseness-circumvented) if you like. Please note that *mod()* is just like the modulo operator of most programming languages. If you want a mathematical correct modulo, see [here](#mathematical-correct-modulo).
Fraction mod()
---
Returns the modulus (rest of the division) of the actual object (numerator mod denominator)
Fraction gcd(n)
---
Returns the fractional greatest common divisor
Fraction lcm(n)
---
Returns the fractional least common multiple
Fraction ceil([places=0-16])
---
Returns the ceiling of a rational number with Math.ceil
Fraction floor([places=0-16])
---
Returns the floor of a rational number with Math.floor
Fraction round([places=0-16])
---
Returns the rational number rounded with Math.round
Fraction roundTo(multiple)
---
Rounds a fraction to the closest multiple of another fraction.
Fraction inverse()
---
Returns the multiplicative inverse of the actual number (n / d becomes d / n) in order to get the reciprocal
Fraction simplify([eps=0.001])
---
Simplifies the rational number under a certain error threshold. Ex. `0.333` will be `1/3` with `eps=0.001`
boolean equals(n)
---
Check if two numbers are equal
int compare(n)
---
Compare two numbers.
```
result < 0: n is greater than actual number
result > 0: n is smaller than actual number
result = 0: n is equal to the actual number
```
boolean divisible(n)
---
Check if two numbers are divisible (n divides this)
double valueOf()
---
Returns a decimal representation of the fraction
String toString([decimalPlaces=15])
---
Generates an exact string representation of the actual object. For repeated decimal places all digits are collected within brackets, like `1/3 = "0.(3)"`. For all other numbers, up to `decimalPlaces` significant digits are collected - which includes trailing zeros if the number is getting truncated. However, `1/2 = "0.5"` without trailing zeros of course.
**Note:** As `valueOf()` and `toString()` are provided, `toString()` is only called implicitly in a real string context. Using the plus-operator like `"123" + new Fraction` will call valueOf(), because JavaScript tries to combine two primitives first and concatenates them later, as string will be the more dominant type. `alert(new Fraction)` or `String(new Fraction)` on the other hand will do what you expect. If you really want to have control, you should call `toString()` or `valueOf()` explicitly!
String toLatex(excludeWhole=false)
---
Generates an exact LaTeX representation of the actual object. You can see a [live demo](http://www.xarg.org/2014/03/precise-calculations-in-javascript/) on my blog.
The optional boolean parameter indicates if you want to exclude the whole part. "1 1/3" instead of "4/3"
String toFraction(excludeWhole=false)
---
Gets a string representation of the fraction
The optional boolean parameter indicates if you want to exclude the whole part. "1 1/3" instead of "4/3"
Array toContinued()
---
Gets an array of the fraction represented as a continued fraction. The first element always contains the whole part.
```javascript
var f = new Fraction('88/33');
var c = f.toContinued(); // [2, 1, 2]
```
Fraction clone()
---
Creates a copy of the actual Fraction object
Exceptions
===
If a really hard error occurs (parsing error, division by zero), *fraction.js* throws exceptions! Please make sure you handle them correctly.
Installation
===
Installing fraction.js is as easy as cloning this repo or use the following command:
```
npm install fraction.js
```
Using Fraction.js with the browser
===
```html
<script src="fraction.js"></script>
<script>
console.log(Fraction("123/456"));
</script>
```
Using Fraction.js with TypeScript
===
```js
import Fraction from "fraction.js";
console.log(Fraction("123/456"));
```
Coding Style
===
As every library I publish, fraction.js is also built to be as small as possible after compressing it with Google Closure Compiler in advanced mode. Thus the coding style orientates a little on maxing-out the compression rate. Please make sure you keep this style if you plan to extend the library.
Precision
===
Fraction.js tries to circumvent floating point errors, by having an internal representation of numerator and denominator. As it relies on JavaScript, there is also a limit. The biggest number representable is `Number.MAX_SAFE_INTEGER / 1` and the smallest is `-1 / Number.MAX_SAFE_INTEGER`, with `Number.MAX_SAFE_INTEGER=9007199254740991`. If this is not enough, there is `bigfraction.js` shipped experimentally, which relies on `BigInt` and should become the new Fraction.js eventually.
Testing
===
If you plan to enhance the library, make sure you add test cases and all the previous tests are passing. You can test the library with
```
npm test
```
Copyright and licensing
===
Copyright (c) 2023, [Robert Eisele](https://raw.org/)
Licensed under the MIT license. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fraction.js/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fraction.js/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 14995
} |
The MIT License (MIT)
Copyright (c) 2018 Framer B.V.
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/framer-motion/LICENSE.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/framer-motion/LICENSE.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1077
} |
<p align="center">
<img width="100" height="100" alt="Motion logo" src="https://user-images.githubusercontent.com/7850794/164965523-3eced4c4-6020-467e-acde-f11b7900ad62.png" />
</p>
<h1 align="center">Motion for React</h1>
<br>
<p align="center">
<a href="https://www.npmjs.com/package/framer-motion" target="_blank">
<img src="https://img.shields.io/npm/v/framer-motion.svg?style=flat-square" />
</a>
<a href="https://www.npmjs.com/package/framer-motion" target="_blank">
<img src="https://img.shields.io/npm/dm/framer-motion.svg?style=flat-square" />
</a>
<a href="https://twitter.com/motiondotdev" target="_blank">
<img src="https://img.shields.io/twitter/follow/framer.svg?style=social&label=Follow" />
</a>
<a href="https://discord.gg/DfkSpYe" target="_blank">
<img src="https://img.shields.io/discord/308323056592486420.svg?logo=discord&logoColor=white" alt="Chat on Discord">
</a>
</p>
<br>
<hr>
<br>
Motion for React is an open source, production-ready library that’s designed for all creative developers.
It's the only animation library with a hybrid engine, combining the power of JavaScript animations with the performance of native browser APIs.
It looks like this:
```jsx
<motion.div animate={{ x: 0 }} />
```
It does all this:
- [Springs](https://motion.dev/docs/react-transitions#spring)
- [Keyframes](https://motion.dev/docs/react-animation#keyframes)
- [Layout animations](https://motion.dev/docs/react-layout-animations)
- [Shared layout animations](https://motion.dev/docs/react-layout-animations#shared-layout-animations)
- [Gestures (drag/tap/hover)](https://motion.dev/docs/react-gestures)
- [Scroll animations](https://motion.dev/docs/react-scroll-animations)
- [SVG paths](https://motion.dev/docs/react-animation#svg-line-drawing)
- [Exit animations](https://motion.dev/docs/react-animation#exit-animations)
- [Server-side rendering](https://motion.dev/docs/react-motion-component#server-side-rendering)
- [Independent transforms](https://motion.dev/docs/react-motion-component#style)
- [Orchestrate animations across components](https://motion.dev/docs/react-animation#orchestration)
- [CSS variables](https://motion.dev/docs/react-animation#css-variables)
...and a whole lot more.
## Get started
### 🐇 Quick start
Install `motion` via your package manager:
```
npm install motion
```
Then import the `motion` component:
```jsx
import { motion } from "motion/react"
export function Component({ isVisible }) {
return <motion.div animate={{ opacity: isVisible ? 1 : 0 }} />
}
```
### 💎 Contribute
- Want to contribute to Motion? Our [contributing guide](https://github.com/framer/motion/blob/master/CONTRIBUTING.md) has you covered.
### 👩🏻⚖️ License
- Motion for React is MIT licensed.
## ✨ Sponsors
Motion is sustainable thanks to the kind support of its sponsors.
### Partners
#### Framer
Motion powers Framer animations, the web builder for creative pros. Design and ship your dream site. Zero code, maximum speed.
<a href="https://www.framer.com?utm_source=motion-readme">
<img alt="Framer" src="https://github.com/user-attachments/assets/0404c7a1-c29d-4785-89ae-aae315f3c759" width="300px" height="200px">
</a>
### Platinum
<a href="https://tailwindcss.com"><img alt="Tailwind" src="https://github.com/user-attachments/assets/c0496f09-b8ee-4bc4-85ab-83a071bbbdec" width="300px" height="200px"></a>
<a href="https://emilkowal.ski"><img alt="Emil Kowalski" src="https://github.com/user-attachments/assets/29f56b1a-37fb-4695-a6a6-151f6c24864f" width="300px" height="200px"></a>
<a href="https://linear.app"><img alt="Linear" src="https://github.com/user-attachments/assets/a93710bb-d8ed-40e3-b0fb-1c5b3e2b16bb" width="300px" height="200px"></a>
### Gold
<a href="https://liveblocks.io"><img alt="Liveblocks" src="https://github.com/user-attachments/assets/31436a47-951e-4eab-9a68-bdd54ccf9444" width="225px" height="150px"></a>
### Silver
<a href="https://www.frontend.fyi/?utm_source=motion"><img alt="Frontend.fyi" src="https://github.com/user-attachments/assets/07d23aa5-69db-44a0-849d-90177e6fc817" width="150px" height="100px"></a>
<a href="https://statamic.com"><img alt="Statamic" src="https://github.com/user-attachments/assets/5d28f090-bdd9-4b31-b134-fb2b94ca636f" width="150px" height="100px"></a>
<a href="https://firecrawl.dev"><img alt="Firecrawl" src="https://github.com/user-attachments/assets/cba90e54-1329-4353-8fba-85beef4d2ee9" width="150px" height="100px"></a>
<a href="https://puzzmo.com"><img alt="Puzzmo" src="https://github.com/user-attachments/assets/aa2d5586-e5e2-43b9-8446-db456e4b0758" width="150px" height="100px"></a>
<a href="https://buildui.com"><img alt="Build UI" src="https://github.com/user-attachments/assets/024bfcd5-50e8-4b3d-a115-d5c6d6030d1c" width="150px" height="100px"></a>
<a href="https://hover.dev"><img alt="Hover" src="https://github.com/user-attachments/assets/4715b555-d2ac-4cb7-9f35-d36d708827b3" width="150px" height="100px"></a>
### Personal
- [Nusu](https://x.com/nusualabuga)
- [OlegWock](https://sinja.io)
- [Lambert Weller](https://github.com/l-mbert)
- [Jake LeBoeuf](https://jklb.wf)
- [Han Lee](https://github.com/hahnlee) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/framer-motion/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/framer-motion/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 5224
} |
0.5.2 / 2017-09-13
==================
* Fix regression matching multiple ETags in `If-None-Match`
* perf: improve `If-None-Match` token parsing
0.5.1 / 2017-09-11
==================
* Fix handling of modified headers with invalid dates
* perf: improve ETag match loop
0.5.0 / 2017-02-21
==================
* Fix incorrect result when `If-None-Match` has both `*` and ETags
* Fix weak `ETag` matching to match spec
* perf: delay reading header values until needed
* perf: skip checking modified time if ETag check failed
* perf: skip parsing `If-None-Match` when no `ETag` header
* perf: use `Date.parse` instead of `new Date`
0.4.0 / 2017-02-05
==================
* Fix false detection of `no-cache` request directive
* perf: enable strict mode
* perf: hoist regular expressions
* perf: remove duplicate conditional
* perf: remove unnecessary boolean coercions
0.3.0 / 2015-05-12
==================
* Add weak `ETag` matching support
0.2.4 / 2014-09-07
==================
* Support Node.js 0.6
0.2.3 / 2014-09-07
==================
* Move repository to jshttp
0.2.2 / 2014-02-19
==================
* Revert "Fix for blank page on Safari reload"
0.2.1 / 2014-01-29
==================
* Fix for blank page on Safari reload
0.2.0 / 2013-08-11
==================
* Return stale for `Cache-Control: no-cache`
0.1.0 / 2012-06-15
==================
* Add `If-None-Match: *` support
0.0.1 / 2012-06-10
==================
* Initial release | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fresh/HISTORY.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fresh/HISTORY.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1499
} |
# fresh
[![NPM Version][npm-image]][npm-url]
[![NPM Downloads][downloads-image]][downloads-url]
[![Node.js Version][node-version-image]][node-version-url]
[![Build Status][travis-image]][travis-url]
[![Test Coverage][coveralls-image]][coveralls-url]
HTTP response freshness testing
## Installation
This is a [Node.js](https://nodejs.org/en/) module available through the
[npm registry](https://www.npmjs.com/). Installation is done using the
[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
```
$ npm install fresh
```
## API
<!-- eslint-disable no-unused-vars -->
```js
var fresh = require('fresh')
```
### fresh(reqHeaders, resHeaders)
Check freshness of the response using request and response headers.
When the response is still "fresh" in the client's cache `true` is
returned, otherwise `false` is returned to indicate that the client
cache is now stale and the full response should be sent.
When a client sends the `Cache-Control: no-cache` request header to
indicate an end-to-end reload request, this module will return `false`
to make handling these requests transparent.
## Known Issues
This module is designed to only follow the HTTP specifications, not
to work-around all kinda of client bugs (especially since this module
typically does not recieve enough information to understand what the
client actually is).
There is a known issue that in certain versions of Safari, Safari
will incorrectly make a request that allows this module to validate
freshness of the resource even when Safari does not have a
representation of the resource in the cache. The module
[jumanji](https://www.npmjs.com/package/jumanji) can be used in
an Express application to work-around this issue and also provides
links to further reading on this Safari bug.
## Example
### API usage
<!-- eslint-disable no-redeclare, no-undef -->
```js
var reqHeaders = { 'if-none-match': '"foo"' }
var resHeaders = { 'etag': '"bar"' }
fresh(reqHeaders, resHeaders)
// => false
var reqHeaders = { 'if-none-match': '"foo"' }
var resHeaders = { 'etag': '"foo"' }
fresh(reqHeaders, resHeaders)
// => true
```
### Using with Node.js http server
```js
var fresh = require('fresh')
var http = require('http')
var server = http.createServer(function (req, res) {
// perform server logic
// ... including adding ETag / Last-Modified response headers
if (isFresh(req, res)) {
// client has a fresh copy of resource
res.statusCode = 304
res.end()
return
}
// send the resource
res.statusCode = 200
res.end('hello, world!')
})
function isFresh (req, res) {
return fresh(req.headers, {
'etag': res.getHeader('ETag'),
'last-modified': res.getHeader('Last-Modified')
})
}
server.listen(3000)
```
## License
[MIT](LICENSE)
[npm-image]: https://img.shields.io/npm/v/fresh.svg
[npm-url]: https://npmjs.org/package/fresh
[node-version-image]: https://img.shields.io/node/v/fresh.svg
[node-version-url]: https://nodejs.org/en/
[travis-image]: https://img.shields.io/travis/jshttp/fresh/master.svg
[travis-url]: https://travis-ci.org/jshttp/fresh
[coveralls-image]: https://img.shields.io/coveralls/jshttp/fresh/master.svg
[coveralls-url]: https://coveralls.io/r/jshttp/fresh?branch=master
[downloads-image]: https://img.shields.io/npm/dm/fresh.svg
[downloads-url]: https://npmjs.org/package/fresh | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fresh/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fresh/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 3373
} |
# fsevents
Native access to MacOS FSEvents in [Node.js](https://nodejs.org/)
The FSEvents API in MacOS allows applications to register for notifications of
changes to a given directory tree. It is a very fast and lightweight alternative
to kqueue.
This is a low-level library. For a cross-platform file watching module that
uses fsevents, check out [Chokidar](https://github.com/paulmillr/chokidar).
## Usage
```sh
npm install fsevents
```
Supports only **Node.js v8.16 and higher**.
```js
const fsevents = require('fsevents');
// To start observation
const stop = fsevents.watch(__dirname, (path, flags, id) => {
const info = fsevents.getInfo(path, flags);
});
// To end observation
stop();
```
> **Important note:** The API behaviour is slightly different from typical JS APIs. The `stop` function **must** be
> retrieved and stored somewhere, even if you don't plan to stop the watcher. If you forget it, the garbage collector
> will eventually kick in, the watcher will be unregistered, and your callbacks won't be called anymore.
The callback passed as the second parameter to `.watch` get's called whenever the operating system detects a
a change in the file system. It takes three arguments:
###### `fsevents.watch(dirname: string, (path: string, flags: number, id: string) => void): () => Promise<undefined>`
* `path: string` - the item in the filesystem that have been changed
* `flags: number` - a numeric value describing what the change was
* `id: string` - an unique-id identifying this specific event
Returns closer callback which when called returns a Promise resolving when the watcher process has been shut down.
###### `fsevents.getInfo(path: string, flags: number, id: string): FsEventInfo`
The `getInfo` function takes the `path`, `flags` and `id` arguments and converts those parameters into a structure
that is easier to digest to determine what the change was.
The `FsEventsInfo` has the following shape:
```js
/**
* @typedef {'created'|'modified'|'deleted'|'moved'|'root-changed'|'cloned'|'unknown'} FsEventsEvent
* @typedef {'file'|'directory'|'symlink'} FsEventsType
*/
{
"event": "created", // {FsEventsEvent}
"path": "file.txt",
"type": "file", // {FsEventsType}
"changes": {
"inode": true, // Had iNode Meta-Information changed
"finder": false, // Had Finder Meta-Data changed
"access": false, // Had access permissions changed
"xattrs": false // Had xAttributes changed
},
"flags": 0x100000000
}
```
## Changelog
- v2.3 supports Apple Silicon ARM CPUs
- v2 supports node 8.16+ and reduces package size massively
- v1.2.8 supports node 6+
- v1.2.7 supports node 4+
## Troubleshooting
- I'm getting `EBADPLATFORM` `Unsupported platform for fsevents` error.
- It's fine, nothing is broken. fsevents is macos-only. Other platforms are skipped. If you want to hide this warning, report a bug to NPM bugtracker asking them to hide ebadplatform warnings by default.
## License
The MIT License Copyright (C) 2010-2020 by Philipp Dunkel, Ben Noordhuis, Elan Shankar, Paul Miller — see LICENSE file.
Visit our [GitHub page](https://github.com/fsevents/fsevents) and [NPM Page](https://npmjs.org/package/fsevents) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fsevents/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fsevents/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 3200
} |
# Changelog
All notable changes to this project will be documented in this file.
The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/)
and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html).
## [v1.1.2](https://github.com/ljharb/function-bind/compare/v1.1.1...v1.1.2) - 2023-10-12
### Merged
- Point to the correct file [`#16`](https://github.com/ljharb/function-bind/pull/16)
### Commits
- [Tests] migrate tests to Github Actions [`4f8b57c`](https://github.com/ljharb/function-bind/commit/4f8b57c02f2011fe9ae353d5e74e8745f0988af8)
- [Tests] remove `jscs` [`90eb2ed`](https://github.com/ljharb/function-bind/commit/90eb2edbeefd5b76cd6c3a482ea3454db169b31f)
- [meta] update `.gitignore` [`53fcdc3`](https://github.com/ljharb/function-bind/commit/53fcdc371cd66634d6e9b71c836a50f437e89fed)
- [Tests] up to `node` `v11.10`, `v10.15`, `v9.11`, `v8.15`, `v6.16`, `v4.9`; use `nvm install-latest-npm`; run audit script in tests [`1fe8f6e`](https://github.com/ljharb/function-bind/commit/1fe8f6e9aed0dfa8d8b3cdbd00c7f5ea0cd2b36e)
- [meta] add `auto-changelog` [`1921fcb`](https://github.com/ljharb/function-bind/commit/1921fcb5b416b63ffc4acad051b6aad5722f777d)
- [Robustness] remove runtime dependency on all builtins except `.apply` [`f743e61`](https://github.com/ljharb/function-bind/commit/f743e61aa6bb2360358c04d4884c9db853d118b7)
- Docs: enable badges; update wording [`503cb12`](https://github.com/ljharb/function-bind/commit/503cb12d998b5f91822776c73332c7adcd6355dd)
- [readme] update badges [`290c5db`](https://github.com/ljharb/function-bind/commit/290c5dbbbda7264efaeb886552a374b869a4bb48)
- [Tests] switch to nyc for coverage [`ea360ba`](https://github.com/ljharb/function-bind/commit/ea360ba907fc2601ed18d01a3827fa2d3533cdf8)
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `tape` [`cae5e9e`](https://github.com/ljharb/function-bind/commit/cae5e9e07a5578dc6df26c03ee22851ce05b943c)
- [meta] add `funding` field; create FUNDING.yml [`c9f4274`](https://github.com/ljharb/function-bind/commit/c9f4274aa80ea3aae9657a3938fdba41a3b04ca6)
- [Tests] fix eslint errors from #15 [`f69aaa2`](https://github.com/ljharb/function-bind/commit/f69aaa2beb2fdab4415bfb885760a699d0b9c964)
- [actions] fix permissions [`99a0cd9`](https://github.com/ljharb/function-bind/commit/99a0cd9f3b5bac223a0d572f081834cd73314be7)
- [meta] use `npmignore` to autogenerate an npmignore file [`f03b524`](https://github.com/ljharb/function-bind/commit/f03b524ca91f75a109a5d062f029122c86ecd1ae)
- [Dev Deps] update `@ljharb/eslint‑config`, `eslint`, `tape` [`7af9300`](https://github.com/ljharb/function-bind/commit/7af930023ae2ce7645489532821e4fbbcd7a2280)
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `covert`, `tape` [`64a9127`](https://github.com/ljharb/function-bind/commit/64a9127ab0bd331b93d6572eaf6e9971967fc08c)
- [Tests] use `aud` instead of `npm audit` [`e75069c`](https://github.com/ljharb/function-bind/commit/e75069c50010a8fcce2a9ce2324934c35fdb4386)
- [Dev Deps] update `@ljharb/eslint-config`, `aud`, `tape` [`d03555c`](https://github.com/ljharb/function-bind/commit/d03555ca59dea3b71ce710045e4303b9e2619e28)
- [meta] add `safe-publish-latest` [`9c8f809`](https://github.com/ljharb/function-bind/commit/9c8f8092aed027d7e80c94f517aa892385b64f09)
- [Dev Deps] update `@ljharb/eslint-config`, `tape` [`baf6893`](https://github.com/ljharb/function-bind/commit/baf6893e27f5b59abe88bc1995e6f6ed1e527397)
- [meta] create SECURITY.md [`4db1779`](https://github.com/ljharb/function-bind/commit/4db17799f1f28ae294cb95e0081ca2b591c3911b)
- [Tests] add `npm run audit` [`c8b38ec`](https://github.com/ljharb/function-bind/commit/c8b38ec40ed3f85dabdee40ed4148f1748375bc2)
- Revert "Point to the correct file" [`05cdf0f`](https://github.com/ljharb/function-bind/commit/05cdf0fa205c6a3c5ba40bbedd1dfa9874f915c9)
## [v1.1.1](https://github.com/ljharb/function-bind/compare/v1.1.0...v1.1.1) - 2017-08-28
### Commits
- [Tests] up to `node` `v8`; newer npm breaks on older node; fix scripts [`817f7d2`](https://github.com/ljharb/function-bind/commit/817f7d28470fdbff8ef608d4d565dd4d1430bc5e)
- [Dev Deps] update `eslint`, `jscs`, `tape`, `@ljharb/eslint-config` [`854288b`](https://github.com/ljharb/function-bind/commit/854288b1b6f5c555f89aceb9eff1152510262084)
- [Dev Deps] update `tape`, `jscs`, `eslint`, `@ljharb/eslint-config` [`83e639f`](https://github.com/ljharb/function-bind/commit/83e639ff74e6cd6921285bccec22c1bcf72311bd)
- Only apps should have lockfiles [`5ed97f5`](https://github.com/ljharb/function-bind/commit/5ed97f51235c17774e0832e122abda0f3229c908)
- Use a SPDX-compliant “license” field. [`5feefea`](https://github.com/ljharb/function-bind/commit/5feefea0dc0193993e83e5df01ded424403a5381)
## [v1.1.0](https://github.com/ljharb/function-bind/compare/v1.0.2...v1.1.0) - 2016-02-14
### Commits
- Update `eslint`, `tape`; use my personal shared `eslint` config [`9c9062a`](https://github.com/ljharb/function-bind/commit/9c9062abbe9dd70b59ea2c3a3c3a81f29b457097)
- Add `npm run eslint` [`dd96c56`](https://github.com/ljharb/function-bind/commit/dd96c56720034a3c1ffee10b8a59a6f7c53e24ad)
- [New] return the native `bind` when available. [`82186e0`](https://github.com/ljharb/function-bind/commit/82186e03d73e580f95ff167e03f3582bed90ed72)
- [Dev Deps] update `tape`, `jscs`, `eslint`, `@ljharb/eslint-config` [`a3dd767`](https://github.com/ljharb/function-bind/commit/a3dd76720c795cb7f4586b0544efabf8aa107b8b)
- Update `eslint` [`3dae2f7`](https://github.com/ljharb/function-bind/commit/3dae2f7423de30a2d20313ddb1edc19660142fe9)
- Update `tape`, `covert`, `jscs` [`a181eee`](https://github.com/ljharb/function-bind/commit/a181eee0cfa24eb229c6e843a971f36e060a2f6a)
- [Tests] up to `node` `v5.6`, `v4.3` [`964929a`](https://github.com/ljharb/function-bind/commit/964929a6a4ddb36fb128de2bcc20af5e4f22e1ed)
- Test up to `io.js` `v2.1` [`2be7310`](https://github.com/ljharb/function-bind/commit/2be7310f2f74886a7124ca925be411117d41d5ea)
- Update `tape`, `jscs`, `eslint`, `@ljharb/eslint-config` [`45f3d68`](https://github.com/ljharb/function-bind/commit/45f3d6865c6ca93726abcef54febe009087af101)
- [Dev Deps] update `tape`, `jscs` [`6e1340d`](https://github.com/ljharb/function-bind/commit/6e1340d94642deaecad3e717825db641af4f8b1f)
- [Tests] up to `io.js` `v3.3`, `node` `v4.1` [`d9bad2b`](https://github.com/ljharb/function-bind/commit/d9bad2b778b1b3a6dd2876087b88b3acf319f8cc)
- Update `eslint` [`935590c`](https://github.com/ljharb/function-bind/commit/935590caa024ab356102e4858e8fc315b2ccc446)
- [Dev Deps] update `jscs`, `eslint`, `@ljharb/eslint-config` [`8c9a1ef`](https://github.com/ljharb/function-bind/commit/8c9a1efd848e5167887aa8501857a0940a480c57)
- Test on `io.js` `v2.2` [`9a3a38c`](https://github.com/ljharb/function-bind/commit/9a3a38c92013aed6e108666e7bd40969b84ac86e)
- Run `travis-ci` tests on `iojs` and `node` v0.12; speed up builds; allow 0.8 failures. [`69afc26`](https://github.com/ljharb/function-bind/commit/69afc2617405b147dd2a8d8ae73ca9e9283f18b4)
- [Dev Deps] Update `tape`, `eslint` [`36c1be0`](https://github.com/ljharb/function-bind/commit/36c1be0ab12b45fe5df6b0fdb01a5d5137fd0115)
- Update `tape`, `jscs` [`98d8303`](https://github.com/ljharb/function-bind/commit/98d8303cd5ca1c6b8f985469f86b0d44d7d45f6e)
- Update `jscs` [`9633a4e`](https://github.com/ljharb/function-bind/commit/9633a4e9fbf82051c240855166e468ba8ba0846f)
- Update `tape`, `jscs` [`c80ef0f`](https://github.com/ljharb/function-bind/commit/c80ef0f46efc9791e76fa50de4414092ac147831)
- Test up to `io.js` `v3.0` [`7e2c853`](https://github.com/ljharb/function-bind/commit/7e2c8537d52ab9cf5a655755561d8917684c0df4)
- Test on `io.js` `v2.4` [`5a199a2`](https://github.com/ljharb/function-bind/commit/5a199a27ba46795ba5eaf0845d07d4b8232895c9)
- Test on `io.js` `v2.3` [`a511b88`](https://github.com/ljharb/function-bind/commit/a511b8896de0bddf3b56862daa416c701f4d0453)
- Fixing a typo from 822b4e1938db02dc9584aa434fd3a45cb20caf43 [`732d6b6`](https://github.com/ljharb/function-bind/commit/732d6b63a9b33b45230e630dbcac7a10855d3266)
- Update `jscs` [`da52a48`](https://github.com/ljharb/function-bind/commit/da52a4886c06d6490f46ae30b15e4163ba08905d)
- Lock covert to v1.0.0. [`d6150fd`](https://github.com/ljharb/function-bind/commit/d6150fda1e6f486718ebdeff823333d9e48e7430)
## [v1.0.2](https://github.com/ljharb/function-bind/compare/v1.0.1...v1.0.2) - 2014-10-04
## [v1.0.1](https://github.com/ljharb/function-bind/compare/v1.0.0...v1.0.1) - 2014-10-03
### Merged
- make CI build faster [`#3`](https://github.com/ljharb/function-bind/pull/3)
### Commits
- Using my standard jscs.json [`d8ee94c`](https://github.com/ljharb/function-bind/commit/d8ee94c993eff0a84cf5744fe6a29627f5cffa1a)
- Adding `npm run lint` [`7571ab7`](https://github.com/ljharb/function-bind/commit/7571ab7dfdbd99b25a1dbb2d232622bd6f4f9c10)
- Using consistent indentation [`e91a1b1`](https://github.com/ljharb/function-bind/commit/e91a1b13a61e99ec1e530e299b55508f74218a95)
- Updating jscs [`7e17892`](https://github.com/ljharb/function-bind/commit/7e1789284bc629bc9c1547a61c9b227bbd8c7a65)
- Using consistent quotes [`c50b57f`](https://github.com/ljharb/function-bind/commit/c50b57fcd1c5ec38320979c837006069ebe02b77)
- Adding keywords [`cb94631`](https://github.com/ljharb/function-bind/commit/cb946314eed35f21186a25fb42fc118772f9ee00)
- Directly export a function expression instead of using a declaration, and relying on hoisting. [`5a33c5f`](https://github.com/ljharb/function-bind/commit/5a33c5f45642de180e0d207110bf7d1843ceb87c)
- Naming npm URL and badge in README; use SVG [`2aef8fc`](https://github.com/ljharb/function-bind/commit/2aef8fcb79d54e63a58ae557c4e60949e05d5e16)
- Naming deps URLs in README [`04228d7`](https://github.com/ljharb/function-bind/commit/04228d766670ee45ca24e98345c1f6a7621065b5)
- Naming travis-ci URLs in README; using SVG [`62c810c`](https://github.com/ljharb/function-bind/commit/62c810c2f54ced956cd4d4ab7b793055addfe36e)
- Make sure functions are invoked correctly (also passing coverage tests) [`2b289b4`](https://github.com/ljharb/function-bind/commit/2b289b4dfbf037ffcfa4dc95eb540f6165e9e43a)
- Removing the strict mode pragmas; they make tests fail. [`1aa701d`](https://github.com/ljharb/function-bind/commit/1aa701d199ddc3782476e8f7eef82679be97b845)
- Adding myself as a contributor [`85fd57b`](https://github.com/ljharb/function-bind/commit/85fd57b0860e5a7af42de9a287f3f265fc6d72fc)
- Adding strict mode pragmas [`915b08e`](https://github.com/ljharb/function-bind/commit/915b08e084c86a722eafe7245e21db74aa21ca4c)
- Adding devDeps URLs to README [`4ccc731`](https://github.com/ljharb/function-bind/commit/4ccc73112c1769859e4ca3076caf4086b3cba2cd)
- Fixing the description. [`a7a472c`](https://github.com/ljharb/function-bind/commit/a7a472cf649af515c635cf560fc478fbe48999c8)
- Using a function expression instead of a function declaration. [`b5d3e4e`](https://github.com/ljharb/function-bind/commit/b5d3e4ea6aaffc63888953eeb1fbc7ff45f1fa14)
- Updating tape [`f086be6`](https://github.com/ljharb/function-bind/commit/f086be6029fb56dde61a258c1340600fa174d1e0)
- Updating jscs [`5f9bdb3`](https://github.com/ljharb/function-bind/commit/5f9bdb375ab13ba48f30852aab94029520c54d71)
- Updating jscs [`9b409ba`](https://github.com/ljharb/function-bind/commit/9b409ba6118e23395a4e5d83ef39152aab9d3bfc)
- Run coverage as part of tests. [`8e1b6d4`](https://github.com/ljharb/function-bind/commit/8e1b6d459f047d1bd4fee814e01247c984c80bd0)
- Run linter as part of tests [`c1ca83f`](https://github.com/ljharb/function-bind/commit/c1ca83f832df94587d09e621beba682fabfaa987)
- Updating covert [`701e837`](https://github.com/ljharb/function-bind/commit/701e83774b57b4d3ef631e1948143f43a72f4bb9)
## [v1.0.0](https://github.com/ljharb/function-bind/compare/v0.2.0...v1.0.0) - 2014-08-09
### Commits
- Make sure old and unstable nodes don't fail Travis [`27adca3`](https://github.com/ljharb/function-bind/commit/27adca34a4ab6ad67b6dfde43942a1b103ce4d75)
- Fixing an issue when the bound function is called as a constructor in ES3. [`e20122d`](https://github.com/ljharb/function-bind/commit/e20122d267d92ce553859b280cbbea5d27c07731)
- Adding `npm run coverage` [`a2e29c4`](https://github.com/ljharb/function-bind/commit/a2e29c4ecaef9e2f6cd1603e868c139073375502)
- Updating tape [`b741168`](https://github.com/ljharb/function-bind/commit/b741168b12b235b1717ff696087645526b69213c)
- Upgrading tape [`63631a0`](https://github.com/ljharb/function-bind/commit/63631a04c7fbe97cc2fa61829cc27246d6986f74)
- Updating tape [`363cb46`](https://github.com/ljharb/function-bind/commit/363cb46dafb23cb3e347729a22f9448051d78464)
## v0.2.0 - 2014-03-23
### Commits
- Updating test coverage to match es5-shim. [`aa94d44`](https://github.com/ljharb/function-bind/commit/aa94d44b8f9d7f69f10e060db7709aa7a694e5d4)
- initial [`942ee07`](https://github.com/ljharb/function-bind/commit/942ee07e94e542d91798137bc4b80b926137e066)
- Setting the bound function's length properly. [`079f46a`](https://github.com/ljharb/function-bind/commit/079f46a2d3515b7c0b308c2c13fceb641f97ca25)
- Ensuring that some older browsers will throw when given a regex. [`36ac55b`](https://github.com/ljharb/function-bind/commit/36ac55b87f460d4330253c92870aa26fbfe8227f)
- Removing npm scripts that don't have dependencies [`9d2be60`](https://github.com/ljharb/function-bind/commit/9d2be600002cb8bc8606f8f3585ad3e05868c750)
- Updating tape [`297a4ac`](https://github.com/ljharb/function-bind/commit/297a4acc5464db381940aafb194d1c88f4e678f3)
- Skipping length tests for now. [`d9891ea`](https://github.com/ljharb/function-bind/commit/d9891ea4d2aaffa69f408339cdd61ff740f70565)
- don't take my tea [`dccd930`](https://github.com/ljharb/function-bind/commit/dccd930bfd60ea10cb178d28c97550c3bc8c1e07) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/function-bind/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/function-bind/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 13802
} |
# function-bind <sup>[![Version Badge][npm-version-svg]][package-url]</sup>
[![github actions][actions-image]][actions-url]
<!--[![coverage][codecov-image]][codecov-url]-->
[![dependency status][deps-svg]][deps-url]
[![dev dependency status][dev-deps-svg]][dev-deps-url]
[![License][license-image]][license-url]
[![Downloads][downloads-image]][downloads-url]
[![npm badge][npm-badge-png]][package-url]
Implementation of function.prototype.bind
Old versions of phantomjs, Internet Explorer < 9, and node < 0.6 don't support `Function.prototype.bind`.
## Example
```js
Function.prototype.bind = require("function-bind")
```
## Installation
`npm install function-bind`
## Contributors
- Raynos
## MIT Licenced
[package-url]: https://npmjs.org/package/function-bind
[npm-version-svg]: https://versionbadg.es/Raynos/function-bind.svg
[deps-svg]: https://david-dm.org/Raynos/function-bind.svg
[deps-url]: https://david-dm.org/Raynos/function-bind
[dev-deps-svg]: https://david-dm.org/Raynos/function-bind/dev-status.svg
[dev-deps-url]: https://david-dm.org/Raynos/function-bind#info=devDependencies
[npm-badge-png]: https://nodei.co/npm/function-bind.png?downloads=true&stars=true
[license-image]: https://img.shields.io/npm/l/function-bind.svg
[license-url]: LICENSE
[downloads-image]: https://img.shields.io/npm/dm/function-bind.svg
[downloads-url]: https://npm-stat.com/charts.html?package=function-bind
[codecov-image]: https://codecov.io/gh/Raynos/function-bind/branch/main/graphs/badge.svg
[codecov-url]: https://app.codecov.io/gh/Raynos/function-bind/
[actions-image]: https://img.shields.io/endpoint?url=https://github-actions-badge-u3jn4tfpocch.runkit.sh/Raynos/function-bind
[actions-url]: https://github.com/Raynos/function-bind/actions | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/function-bind/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/function-bind/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1754
} |
# gensync
This module allows for developers to write common code that can share
implementation details, hiding whether an underlying request happens
synchronously or asynchronously. This is in contrast with many current Node
APIs which explicitly implement the same API twice, once with calls to
synchronous functions, and once with asynchronous functions.
Take for example `fs.readFile` and `fs.readFileSync`, if you're writing an API
that loads a file and then performs a synchronous operation on the data, it
can be frustrating to maintain two parallel functions.
## Example
```js
const fs = require("fs");
const gensync = require("gensync");
const readFile = gensync({
sync: fs.readFileSync,
errback: fs.readFile,
});
const myOperation = gensync(function* (filename) {
const code = yield* readFile(filename, "utf8");
return "// some custom prefix\n" + code;
});
// Load and add the prefix synchronously:
const result = myOperation.sync("./some-file.js");
// Load and add the prefix asynchronously with promises:
myOperation.async("./some-file.js").then(result => {
});
// Load and add the prefix asynchronously with promises:
myOperation.errback("./some-file.js", (err, result) => {
});
```
This could even be exposed as your official API by doing
```js
// Using the common 'Sync' suffix for sync functions, and 'Async' suffix for
// promise-returning versions.
exports.myOperationSync = myOperation.sync;
exports.myOperationAsync = myOperation.async;
exports.myOperation = myOperation.errback;
```
or potentially expose one of the async versions as the default, with a
`.sync` property on the function to expose the synchronous version.
```js
module.exports = myOperation.errback;
module.exports.sync = myOperation.sync;
````
## API
### gensync(generatorFnOrOptions)
Returns a function that can be "await"-ed in another `gensync` generator
function, or executed via
* `.sync(...args)` - Returns the computed value, or throws.
* `.async(...args)` - Returns a promise for the computed value.
* `.errback(...args, (err, result) => {})` - Calls the callback with the computed value, or error.
#### Passed a generator
Wraps the generator to populate the `.sync`/`.async`/`.errback` helpers above to
allow for evaluation of the generator for the final value.
##### Example
```js
const readFile = function* () {
return 42;
};
const readFileAndMore = gensync(function* (){
const val = yield* readFile();
return 42 + val;
});
// In general cases
const code = readFileAndMore.sync("./file.js", "utf8");
readFileAndMore.async("./file.js", "utf8").then(code => {})
readFileAndMore.errback("./file.js", "utf8", (err, code) => {});
// In a generator being called indirectly with .sync/.async/.errback
const code = yield* readFileAndMore("./file.js", "utf8");
```
#### Passed an options object
* `opts.sync`
Example: `(...args) => 4`
A function that will be called when `.sync()` is called on the `gensync()`
result, or when the result is passed to `yield*` in another generator that
is being run synchronously.
Also called for `.async()` calls if no async handlers are provided.
* `opts.async`
Example: `async (...args) => 4`
A function that will be called when `.async()` or `.errback()` is called on
the `gensync()` result, or when the result is passed to `yield*` in another
generator that is being run asynchronously.
* `opts.errback`
Example: `(...args, cb) => cb(null, 4)`
A function that will be called when `.async()` or `.errback()` is called on
the `gensync()` result, or when the result is passed to `yield*` in another
generator that is being run asynchronously.
This option allows for simpler compatibility with many existing Node APIs,
and also avoids introducing the extra even loop turns that promises introduce
to access the result value.
* `opts.name`
Example: `"readFile"`
A string name to apply to the returned function. If no value is provided,
the name of `errback`/`async`/`sync` functions will be used, with any
`Sync` or `Async` suffix stripped off. If the callback is simply named
with ES6 inference (same name as the options property), the name is ignored.
* `opts.arity`
Example: `4`
A number for the length to set on the returned function. If no value
is provided, the length will be carried over from the `sync` function's
`length` value.
##### Example
```js
const readFile = gensync({
sync: fs.readFileSync,
errback: fs.readFile,
});
const code = readFile.sync("./file.js", "utf8");
readFile.async("./file.js", "utf8").then(code => {})
readFile.errback("./file.js", "utf8", (err, code) => {});
```
### gensync.all(iterable)
`Promise.all`-like combinator that works with an iterable of generator objects
that could be passed to `yield*` within a gensync generator.
#### Example
```js
const loadFiles = gensync(function* () {
return yield* gensync.all([
readFile("./one.js"),
readFile("./two.js"),
readFile("./three.js"),
]);
});
```
### gensync.race(iterable)
`Promise.race`-like combinator that works with an iterable of generator objects
that could be passed to `yield*` within a gensync generator.
#### Example
```js
const loadFiles = gensync(function* () {
return yield* gensync.race([
readFile("./one.js"),
readFile("./two.js"),
readFile("./three.js"),
]);
});
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/gensync/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/gensync/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 5349
} |
# Changelog
All notable changes to this project will be documented in this file.
The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/)
and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html).
## [v1.2.4](https://github.com/ljharb/get-intrinsic/compare/v1.2.3...v1.2.4) - 2024-02-05
### Commits
- [Refactor] use all 7 <+ ES6 Errors from `es-errors` [`bcac811`](https://github.com/ljharb/get-intrinsic/commit/bcac811abdc1c982e12abf848a410d6aae148d14)
## [v1.2.3](https://github.com/ljharb/get-intrinsic/compare/v1.2.2...v1.2.3) - 2024-02-03
### Commits
- [Refactor] use `es-errors`, so things that only need those do not need `get-intrinsic` [`f11db9c`](https://github.com/ljharb/get-intrinsic/commit/f11db9c4fb97d87bbd53d3c73ac6b3db3613ad3b)
- [Dev Deps] update `aud`, `es-abstract`, `mock-property`, `npmignore` [`b7ac7d1`](https://github.com/ljharb/get-intrinsic/commit/b7ac7d1616fefb03877b1aed0c8f8d61aad32b6c)
- [meta] simplify `exports` [`faa0cc6`](https://github.com/ljharb/get-intrinsic/commit/faa0cc618e2830ffb51a8202490b0c215d965cbc)
- [meta] add missing `engines.node` [`774dd0b`](https://github.com/ljharb/get-intrinsic/commit/774dd0b3e8f741c3f05a6322d124d6087f146af1)
- [Dev Deps] update `tape` [`5828e8e`](https://github.com/ljharb/get-intrinsic/commit/5828e8e4a04e69312e87a36c0ea39428a7a4c3d8)
- [Robustness] use null objects for lookups [`eb9a11f`](https://github.com/ljharb/get-intrinsic/commit/eb9a11fa9eb3e13b193fcc05a7fb814341b1a7b7)
- [meta] add `sideEffects` flag [`89bcc7a`](https://github.com/ljharb/get-intrinsic/commit/89bcc7a42e19bf07b7c21e3094d5ab177109e6d2)
## [v1.2.2](https://github.com/ljharb/get-intrinsic/compare/v1.2.1...v1.2.2) - 2023-10-20
### Commits
- [Dev Deps] update `@ljharb/eslint-config`, `aud`, `call-bind`, `es-abstract`, `mock-property`, `object-inspect`, `tape` [`f51bcf2`](https://github.com/ljharb/get-intrinsic/commit/f51bcf26412d58d17ce17c91c9afd0ad271f0762)
- [Refactor] use `hasown` instead of `has` [`18d14b7`](https://github.com/ljharb/get-intrinsic/commit/18d14b799bea6b5765e1cec91890830cbcdb0587)
- [Deps] update `function-bind` [`6e109c8`](https://github.com/ljharb/get-intrinsic/commit/6e109c81e03804cc5e7824fb64353cdc3d8ee2c7)
## [v1.2.1](https://github.com/ljharb/get-intrinsic/compare/v1.2.0...v1.2.1) - 2023-05-13
### Commits
- [Fix] avoid a crash in envs without `__proto__` [`7bad8d0`](https://github.com/ljharb/get-intrinsic/commit/7bad8d061bf8721733b58b73a2565af2b6756b64)
- [Dev Deps] update `es-abstract` [`c60e6b7`](https://github.com/ljharb/get-intrinsic/commit/c60e6b7b4cf9660c7f27ed970970fd55fac48dc5)
## [v1.2.0](https://github.com/ljharb/get-intrinsic/compare/v1.1.3...v1.2.0) - 2023-01-19
### Commits
- [actions] update checkout action [`ca6b12f`](https://github.com/ljharb/get-intrinsic/commit/ca6b12f31eaacea4ea3b055e744cd61623385ffb)
- [Dev Deps] update `@ljharb/eslint-config`, `es-abstract`, `object-inspect`, `tape` [`41a3727`](https://github.com/ljharb/get-intrinsic/commit/41a3727d0026fa04273ae216a5f8e12eefd72da8)
- [Fix] ensure `Error.prototype` is undeniable [`c511e97`](https://github.com/ljharb/get-intrinsic/commit/c511e97ae99c764c4524b540dee7a70757af8da3)
- [Dev Deps] update `aud`, `es-abstract`, `tape` [`1bef8a8`](https://github.com/ljharb/get-intrinsic/commit/1bef8a8fd439ebb80863199b6189199e0851ac67)
- [Dev Deps] update `aud`, `es-abstract` [`0d41f16`](https://github.com/ljharb/get-intrinsic/commit/0d41f16bcd500bc28b7bfc98043ebf61ea081c26)
- [New] add `BigInt64Array` and `BigUint64Array` [`a6cca25`](https://github.com/ljharb/get-intrinsic/commit/a6cca25f29635889b7e9bd669baf9e04be90e48c)
- [Tests] use `gopd` [`ecf7722`](https://github.com/ljharb/get-intrinsic/commit/ecf7722240d15cfd16edda06acf63359c10fb9bd)
## [v1.1.3](https://github.com/ljharb/get-intrinsic/compare/v1.1.2...v1.1.3) - 2022-09-12
### Commits
- [Dev Deps] update `es-abstract`, `es-value-fixtures`, `tape` [`07ff291`](https://github.com/ljharb/get-intrinsic/commit/07ff291816406ebe5a12d7f16965bde0942dd688)
- [Fix] properly check for % signs [`50ac176`](https://github.com/ljharb/get-intrinsic/commit/50ac1760fe99c227e64eabde76e9c0e44cd881b5)
## [v1.1.2](https://github.com/ljharb/get-intrinsic/compare/v1.1.1...v1.1.2) - 2022-06-08
### Fixed
- [Fix] properly validate against extra % signs [`#16`](https://github.com/ljharb/get-intrinsic/issues/16)
### Commits
- [actions] reuse common workflows [`0972547`](https://github.com/ljharb/get-intrinsic/commit/0972547efd0abc863fe4c445a6ca7eb4f8c6901d)
- [meta] use `npmignore` to autogenerate an npmignore file [`5ba0b51`](https://github.com/ljharb/get-intrinsic/commit/5ba0b51d8d8d4f1c31d426d74abc0770fd106bad)
- [actions] use `node/install` instead of `node/run`; use `codecov` action [`c364492`](https://github.com/ljharb/get-intrinsic/commit/c364492af4af51333e6f81c0bf21fd3d602c3661)
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `auto-changelog`, `es-abstract`, `object-inspect`, `tape` [`dc04dad`](https://github.com/ljharb/get-intrinsic/commit/dc04dad86f6e5608775a2640cb0db5927ae29ed9)
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `es-abstract`, `object-inspect`, `safe-publish-latest`, `tape` [`1c14059`](https://github.com/ljharb/get-intrinsic/commit/1c1405984e86dd2dc9366c15d8a0294a96a146a5)
- [Tests] use `mock-property` [`b396ef0`](https://github.com/ljharb/get-intrinsic/commit/b396ef05bb73b1d699811abd64b0d9b97997fdda)
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `auto-changelog`, `object-inspect`, `tape` [`c2c758d`](https://github.com/ljharb/get-intrinsic/commit/c2c758d3b90af4fef0a76910d8d3c292ec8d1d3e)
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `es-abstract`, `es-value-fixtures`, `object-inspect`, `tape` [`29e3c09`](https://github.com/ljharb/get-intrinsic/commit/29e3c091c2bf3e17099969847e8729d0e46896de)
- [actions] update codecov uploader [`8cbc141`](https://github.com/ljharb/get-intrinsic/commit/8cbc1418940d7a8941f3a7985cbc4ac095c5e13d)
- [Dev Deps] update `@ljharb/eslint-config`, `es-abstract`, `es-value-fixtures`, `object-inspect`, `tape` [`10b6f5c`](https://github.com/ljharb/get-intrinsic/commit/10b6f5c02593fb3680c581d696ac124e30652932)
- [readme] add github actions/codecov badges [`4e25400`](https://github.com/ljharb/get-intrinsic/commit/4e25400d9f51ae9eb059cbe22d9144e70ea214e8)
- [Tests] use `for-each` instead of `foreach` [`c05b957`](https://github.com/ljharb/get-intrinsic/commit/c05b957ad9a7bc7721af7cc9e9be1edbfe057496)
- [Dev Deps] update `es-abstract` [`29b05ae`](https://github.com/ljharb/get-intrinsic/commit/29b05aec3e7330e9ad0b8e0f685a9112c20cdd97)
- [meta] use `prepublishOnly` script for npm 7+ [`95c285d`](https://github.com/ljharb/get-intrinsic/commit/95c285da810516057d3bbfa871176031af38f05d)
- [Deps] update `has-symbols` [`593cb4f`](https://github.com/ljharb/get-intrinsic/commit/593cb4fb38e7922e40e42c183f45274b636424cd)
- [readme] fix repo URLs [`1c8305b`](https://github.com/ljharb/get-intrinsic/commit/1c8305b5365827c9b6fc785434aac0e1328ff2f5)
- [Deps] update `has-symbols` [`c7138b6`](https://github.com/ljharb/get-intrinsic/commit/c7138b6c6d73132d859471fb8c13304e1e7c8b20)
- [Dev Deps] remove unused `has-bigints` [`bd63aff`](https://github.com/ljharb/get-intrinsic/commit/bd63aff6ad8f3a986c557fcda2914187bdaab359)
## [v1.1.1](https://github.com/ljharb/get-intrinsic/compare/v1.1.0...v1.1.1) - 2021-02-03
### Fixed
- [meta] export `./package.json` [`#9`](https://github.com/ljharb/get-intrinsic/issues/9)
### Commits
- [readme] flesh out the readme; use `evalmd` [`d12f12c`](https://github.com/ljharb/get-intrinsic/commit/d12f12c15345a0a0772cc65a7c64369529abd614)
- [eslint] set up proper globals config [`5a8c098`](https://github.com/ljharb/get-intrinsic/commit/5a8c0984e3319d1ac0e64b102f8ec18b64e79f36)
- [Dev Deps] update `eslint` [`7b9a5c0`](https://github.com/ljharb/get-intrinsic/commit/7b9a5c0d31a90ca1a1234181c74988fb046701cd)
## [v1.1.0](https://github.com/ljharb/get-intrinsic/compare/v1.0.2...v1.1.0) - 2021-01-25
### Fixed
- [Refactor] delay `Function` eval until syntax-derived values are requested [`#3`](https://github.com/ljharb/get-intrinsic/issues/3)
### Commits
- [Tests] migrate tests to Github Actions [`2ab762b`](https://github.com/ljharb/get-intrinsic/commit/2ab762b48164aea8af37a40ba105bbc8246ab8c4)
- [meta] do not publish github action workflow files [`5e7108e`](https://github.com/ljharb/get-intrinsic/commit/5e7108e4768b244d48d9567ba4f8a6cab9c65b8e)
- [Tests] add some coverage [`01ac7a8`](https://github.com/ljharb/get-intrinsic/commit/01ac7a87ac29738567e8524cd8c9e026b1fa8cb3)
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `call-bind`, `es-abstract`, `tape`; add `call-bind` [`911b672`](https://github.com/ljharb/get-intrinsic/commit/911b672fbffae433a96924c6ce013585e425f4b7)
- [Refactor] rearrange evalled constructors a bit [`7e7e4bf`](https://github.com/ljharb/get-intrinsic/commit/7e7e4bf583f3799c8ac1c6c5e10d2cb553957347)
- [meta] add Automatic Rebase and Require Allow Edits workflows [`0199968`](https://github.com/ljharb/get-intrinsic/commit/01999687a263ffce0a3cb011dfbcb761754aedbc)
## [v1.0.2](https://github.com/ljharb/get-intrinsic/compare/v1.0.1...v1.0.2) - 2020-12-17
### Commits
- [Fix] Throw for non‑existent intrinsics [`68f873b`](https://github.com/ljharb/get-intrinsic/commit/68f873b013c732a05ad6f5fc54f697e55515461b)
- [Fix] Throw for non‑existent segments in the intrinsic path [`8325dee`](https://github.com/ljharb/get-intrinsic/commit/8325deee43128f3654d3399aa9591741ebe17b21)
- [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `has-bigints`, `object-inspect` [`0c227a7`](https://github.com/ljharb/get-intrinsic/commit/0c227a7d8b629166f25715fd242553892e458525)
- [meta] do not lint coverage output [`70d2419`](https://github.com/ljharb/get-intrinsic/commit/70d24199b620043cd9110fc5f426d214ebe21dc9)
## [v1.0.1](https://github.com/ljharb/get-intrinsic/compare/v1.0.0...v1.0.1) - 2020-10-30
### Commits
- [Tests] gather coverage data on every job [`d1d280d`](https://github.com/ljharb/get-intrinsic/commit/d1d280dec714e3f0519cc877dbcb193057d9cac6)
- [Fix] add missing dependencies [`5031771`](https://github.com/ljharb/get-intrinsic/commit/5031771bb1095b38be88ce7c41d5de88718e432e)
- [Tests] use `es-value-fixtures` [`af48765`](https://github.com/ljharb/get-intrinsic/commit/af48765a23c5323fb0b6b38dbf00eb5099c7bebc)
## v1.0.0 - 2020-10-29
### Commits
- Implementation [`bbce57c`](https://github.com/ljharb/get-intrinsic/commit/bbce57c6f33d05b2d8d3efa273ceeb3ee01127bb)
- Tests [`17b4f0d`](https://github.com/ljharb/get-intrinsic/commit/17b4f0d56dea6b4059b56fc30ef3ee4d9500ebc2)
- Initial commit [`3153294`](https://github.com/ljharb/get-intrinsic/commit/31532948de363b0a27dd9fd4649e7b7028ec4b44)
- npm init [`fb326c4`](https://github.com/ljharb/get-intrinsic/commit/fb326c4d2817c8419ec31de1295f06bb268a7902)
- [meta] add Automatic Rebase and Require Allow Edits workflows [`48862fb`](https://github.com/ljharb/get-intrinsic/commit/48862fb2508c8f6a57968e6d08b7c883afc9d550)
- [meta] add `auto-changelog` [`5f28ad0`](https://github.com/ljharb/get-intrinsic/commit/5f28ad019e060a353d8028f9f2591a9cc93074a1)
- [meta] add "funding"; create `FUNDING.yml` [`c2bbdde`](https://github.com/ljharb/get-intrinsic/commit/c2bbddeba73a875be61484ee4680b129a6d4e0a1)
- [Tests] add `npm run lint` [`0a84b98`](https://github.com/ljharb/get-intrinsic/commit/0a84b98b22b7cf7a748666f705b0003a493c35fd)
- Only apps should have lockfiles [`9586c75`](https://github.com/ljharb/get-intrinsic/commit/9586c75866c1ee678e4d5d4dbbdef6997e511b05) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/get-intrinsic/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/get-intrinsic/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 11631
} |
# get-intrinsic <sup>[![Version Badge][npm-version-svg]][package-url]</sup>
[![github actions][actions-image]][actions-url]
[![coverage][codecov-image]][codecov-url]
[![dependency status][deps-svg]][deps-url]
[![dev dependency status][dev-deps-svg]][dev-deps-url]
[![License][license-image]][license-url]
[![Downloads][downloads-image]][downloads-url]
[![npm badge][npm-badge-png]][package-url]
Get and robustly cache all JS language-level intrinsics at first require time.
See the syntax described [in the JS spec](https://tc39.es/ecma262/#sec-well-known-intrinsic-objects) for reference.
## Example
```js
var GetIntrinsic = require('get-intrinsic');
var assert = require('assert');
// static methods
assert.equal(GetIntrinsic('%Math.pow%'), Math.pow);
assert.equal(Math.pow(2, 3), 8);
assert.equal(GetIntrinsic('%Math.pow%')(2, 3), 8);
delete Math.pow;
assert.equal(GetIntrinsic('%Math.pow%')(2, 3), 8);
// instance methods
var arr = [1];
assert.equal(GetIntrinsic('%Array.prototype.push%'), Array.prototype.push);
assert.deepEqual(arr, [1]);
arr.push(2);
assert.deepEqual(arr, [1, 2]);
GetIntrinsic('%Array.prototype.push%').call(arr, 3);
assert.deepEqual(arr, [1, 2, 3]);
delete Array.prototype.push;
GetIntrinsic('%Array.prototype.push%').call(arr, 4);
assert.deepEqual(arr, [1, 2, 3, 4]);
// missing features
delete JSON.parse; // to simulate a real intrinsic that is missing in the environment
assert.throws(() => GetIntrinsic('%JSON.parse%'));
assert.equal(undefined, GetIntrinsic('%JSON.parse%', true));
```
## Tests
Simply clone the repo, `npm install`, and run `npm test`
## Security
Please email [@ljharb](https://github.com/ljharb) or see https://tidelift.com/security if you have a potential security vulnerability to report.
[package-url]: https://npmjs.org/package/get-intrinsic
[npm-version-svg]: https://versionbadg.es/ljharb/get-intrinsic.svg
[deps-svg]: https://david-dm.org/ljharb/get-intrinsic.svg
[deps-url]: https://david-dm.org/ljharb/get-intrinsic
[dev-deps-svg]: https://david-dm.org/ljharb/get-intrinsic/dev-status.svg
[dev-deps-url]: https://david-dm.org/ljharb/get-intrinsic#info=devDependencies
[npm-badge-png]: https://nodei.co/npm/get-intrinsic.png?downloads=true&stars=true
[license-image]: https://img.shields.io/npm/l/get-intrinsic.svg
[license-url]: LICENSE
[downloads-image]: https://img.shields.io/npm/dm/get-intrinsic.svg
[downloads-url]: https://npm-stat.com/charts.html?package=get-intrinsic
[codecov-image]: https://codecov.io/gh/ljharb/get-intrinsic/branch/main/graphs/badge.svg
[codecov-url]: https://app.codecov.io/gh/ljharb/get-intrinsic/
[actions-image]: https://img.shields.io/endpoint?url=https://github-actions-badge-u3jn4tfpocch.runkit.sh/ljharb/get-intrinsic
[actions-url]: https://github.com/ljharb/get-intrinsic/actions | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/get-intrinsic/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/get-intrinsic/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2790
} |
# get-nonce
just returns a **nonce** (number used once). No batteries included in those 46 bytes of this library.
---
- ✅ build in `webpack` support via `__webpack_nonce__`
# API
- `getNonce(): string|undefined` - returns the current `nonce`
- `setNonce(newValue)` - set's nonce value
## Why?
Why we need a library to access `__webpack_nonce__`? Abstractions!
"I", as a library author, don't want to "predict" the platform "you" going to use.
"I", as well, want an easier way to test and control `nonce` value.
Like - `nonce` is supported out of the box only by webpack, what you are going to do?
This is why this "man-in-the-middle" was created.
Yep, think about `left-pad` :)
## Webpack
> https://webpack.js.org/guides/csp/
To activate the feature set a **webpack_nonce** variable needs to be included in your entry script.
```
__webpack_nonce__ = uuid(); // for example
```
Without `webpack` `__webpack_nonce__` is actually just a global variable,
which makes it actually bundler independent,
however "other bundlers" are able to replicate it only setting it as a global variable
(as here in tests) which violates a "secure" nature of `nonce`.
`get-nonce` is not global.
## Used in
- `react-style-singleton` <- `react-remove-scroll` <- `react-focus-on`
## Inspiration
- [this issue](https://github.com/theKashey/react-remove-scroll/issues/21)
- [styled-components](https://github.com/styled-components/styled-components/blob/147b0e9a1f10786551b13fd27452fcd5c678d5e0/packages/styled-components/src/utils/nonce.js)
# Licence
MIT | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/get-nonce/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/get-nonce/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1551
} |
<p align="center">
<img width="160" src=".github/logo.webp">
</p>
<h1 align="center">
<sup>get-tsconfig</sup>
<br>
<a href="https://npm.im/get-tsconfig"><img src="https://badgen.net/npm/v/get-tsconfig"></a> <a href="https://npm.im/get-tsconfig"><img src="https://badgen.net/npm/dm/get-tsconfig"></a>
</h1>
Find and parse `tsconfig.json` files.
### Features
- Zero dependency (not even TypeScript)
- Tested against TypeScript for correctness
- Supports comments & dangling commas in `tsconfig.json`
- Resolves [`extends`](https://www.typescriptlang.org/tsconfig/#extends)
- Fully typed `tsconfig.json`
- Validates and throws parsing errors
- Tiny! `7 kB` Minified + Gzipped
<br>
<p align="center">
<a href="https://github.com/sponsors/privatenumber/sponsorships?tier_id=398771"><img width="412" src="https://raw.githubusercontent.com/privatenumber/sponsors/master/banners/assets/donate.webp"></a>
<a href="https://github.com/sponsors/privatenumber/sponsorships?tier_id=397608"><img width="412" src="https://raw.githubusercontent.com/privatenumber/sponsors/master/banners/assets/sponsor.webp"></a>
</p>
<p align="center"><sup><i>Already a sponsor?</i> Join the discussion in the <a href="https://github.com/pvtnbr/get-tsconfig">Development repo</a>!</sup></p>
## Install
```bash
npm install get-tsconfig
```
## Why?
For TypeScript related tooling to correctly parse `tsconfig.json` file without depending on TypeScript.
## API
### getTsconfig(searchPath?, configName?, cache?)
Searches for a `tsconfig.json` file and parses it. Returns `null` if a config file cannot be found, or an object containing the path and parsed TSConfig object if found.
Returns:
```ts
type TsconfigResult = {
/**
* The path to the tsconfig.json file
*/
path: string
/**
* The resolved tsconfig.json file
*/
config: TsConfigJsonResolved
}
```
#### searchPath
Type: `string`
Default: `process.cwd()`
Accepts a path to a file or directory to search up for a `tsconfig.json` file.
#### configName
Type: `string`
Default: `tsconfig.json`
The file name of the TypeScript config file.
#### cache
Type: `Map<string, any>`
Default: `new Map()`
Optional cache for fs operations.
#### Example
```ts
import { getTsconfig } from 'get-tsconfig'
// Searches for tsconfig.json starting in the current directory
console.log(getTsconfig())
// Find tsconfig.json from a TypeScript file path
console.log(getTsconfig('./path/to/index.ts'))
// Find tsconfig.json from a directory file path
console.log(getTsconfig('./path/to/directory'))
// Explicitly pass in tsconfig.json path
console.log(getTsconfig('./path/to/tsconfig.json'))
// Search for jsconfig.json - https://code.visualstudio.com/docs/languages/jsconfig
console.log(getTsconfig('.', 'jsconfig.json'))
```
---
### parseTsconfig(tsconfigPath, cache?)
The `tsconfig.json` parser used internally by `getTsconfig`. Returns the parsed tsconfig as `TsConfigJsonResolved`.
#### tsconfigPath
Type: `string`
Required path to the tsconfig file.
#### cache
Type: `Map<string, any>`
Default: `new Map()`
Optional cache for fs operations.
#### Example
```ts
import { parseTsconfig } from 'get-tsconfig'
// Must pass in a path to an existing tsconfig.json file
console.log(parseTsconfig('./path/to/tsconfig.custom.json'))
```
### createFileMatcher(tsconfig: TsconfigResult, caseSensitivePaths?: boolean)
Given a `tsconfig.json` file, it returns a file-matcher function that determines whether it should apply to a file path.
```ts
type FileMatcher = (filePath: string) => TsconfigResult['config'] | undefined
```
#### tsconfig
Type: `TsconfigResult`
Pass in the return value from `getTsconfig`, or a `TsconfigResult` object.
#### caseSensitivePaths
Type: `boolean`
By default, it uses [`is-fs-case-sensitive`](https://github.com/privatenumber/is-fs-case-sensitive) to detect whether the file-system is case-sensitive.
Pass in `true` to make it case-sensitive.
#### Example
For example, if it's called with a `tsconfig.json` file that has `include`/`exclude`/`files` defined, the file-matcher will return the config for files that match `include`/`files`, and return `undefined` for files that don't match or match `exclude`.
```ts
const tsconfig = getTsconfig()
const fileMatcher = tsconfig && createFileMatcher(tsconfig)
/*
* Returns tsconfig.json if it matches the file,
* undefined if not
*/
const configForFile = fileMatcher?.('/path/to/file.ts')
const distCode = compileTypescript({
code: sourceCode,
tsconfig: configForFile
})
```
---
### createPathsMatcher(tsconfig: TsconfigResult)
Given a tsconfig with [`compilerOptions.paths`](https://www.typescriptlang.org/tsconfig#paths) defined, it returns a matcher function.
The matcher function accepts an [import specifier (the path to resolve)](https://nodejs.org/api/esm.html#terminology), checks it against `compilerOptions.paths`, and returns an array of possible paths to check:
```ts
function pathsMatcher(specifier: string): string[]
```
This function only returns possible paths and doesn't actually do any resolution. This helps increase compatibility wtih file/build systems which usually have their own resolvers.
#### Example
```ts
import { getTsconfig, createPathsMatcher } from 'get-tsconfig'
const tsconfig = getTsconfig()
const pathsMatcher = createPathsMatcher(tsconfig)
const exampleResolver = (request: string) => {
if (pathsMatcher) {
const tryPaths = pathsMatcher(request)
// Check if paths in `tryPaths` exist
}
}
```
## FAQ
### How can I use TypeScript to parse `tsconfig.json`?
This package is a re-implementation of TypeScript's `tsconfig.json` parser.
However, if you already have TypeScript as a dependency, you can simply use it's API:
```ts
import {
sys as tsSys,
findConfigFile,
readConfigFile,
parseJsonConfigFileContent
} from 'typescript'
// Find tsconfig.json file
const tsconfigPath = findConfigFile(process.cwd(), tsSys.fileExists, 'tsconfig.json')
// Read tsconfig.json file
const tsconfigFile = readConfigFile(tsconfigPath, tsSys.readFile)
// Resolve extends
const parsedTsconfig = parseJsonConfigFileContent(
tsconfigFile.config,
tsSys,
path.dirname(tsconfigPath)
)
```
## Sponsors
<p align="center">
<a href="https://github.com/sponsors/privatenumber">
<img src="https://cdn.jsdelivr.net/gh/privatenumber/sponsors/sponsorkit/sponsors.svg">
</a>
</p> | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/get-tsconfig/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/get-tsconfig/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 6418
} |
<p align="center">
<a href="https://gulpjs.com">
<img height="257" width="114" src="https://raw.githubusercontent.com/gulpjs/artwork/master/gulp-2x.png">
</a>
</p>
# glob-parent
[![NPM version][npm-image]][npm-url] [![Downloads][downloads-image]][npm-url] [![Build Status][ci-image]][ci-url] [![Coveralls Status][coveralls-image]][coveralls-url]
Extract the non-magic parent path from a glob string.
## Usage
```js
var globParent = require('glob-parent');
globParent('path/to/*.js'); // 'path/to'
globParent('/root/path/to/*.js'); // '/root/path/to'
globParent('/*.js'); // '/'
globParent('*.js'); // '.'
globParent('**/*.js'); // '.'
globParent('path/{to,from}'); // 'path'
globParent('path/!(to|from)'); // 'path'
globParent('path/?(to|from)'); // 'path'
globParent('path/+(to|from)'); // 'path'
globParent('path/*(to|from)'); // 'path'
globParent('path/@(to|from)'); // 'path'
globParent('path/**/*'); // 'path'
// if provided a non-glob path, returns the nearest dir
globParent('path/foo/bar.js'); // 'path/foo'
globParent('path/foo/'); // 'path/foo'
globParent('path/foo'); // 'path' (see issue #3 for details)
```
## API
### `globParent(maybeGlobString, [options])`
Takes a string and returns the part of the path before the glob begins. Be aware of Escaping rules and Limitations below.
#### options
```js
{
// Disables the automatic conversion of slashes for Windows
flipBackslashes: true;
}
```
## Escaping
The following characters have special significance in glob patterns and must be escaped if you want them to be treated as regular path characters:
- `?` (question mark) unless used as a path segment alone
- `*` (asterisk)
- `|` (pipe)
- `(` (opening parenthesis)
- `)` (closing parenthesis)
- `{` (opening curly brace)
- `}` (closing curly brace)
- `[` (opening bracket)
- `]` (closing bracket)
**Example**
```js
globParent('foo/[bar]/'); // 'foo'
globParent('foo/\\[bar]/'); // 'foo/[bar]'
```
## Limitations
### Braces & Brackets
This library attempts a quick and imperfect method of determining which path
parts have glob magic without fully parsing/lexing the pattern. There are some
advanced use cases that can trip it up, such as nested braces where the outer
pair is escaped and the inner one contains a path separator. If you find
yourself in the unlikely circumstance of being affected by this or need to
ensure higher-fidelity glob handling in your library, it is recommended that you
pre-process your input with [expand-braces] and/or [expand-brackets].
### Windows
Backslashes are not valid path separators for globs. If a path with backslashes
is provided anyway, for simple cases, glob-parent will replace the path
separator for you and return the non-glob parent path (now with
forward-slashes, which are still valid as Windows path separators).
This cannot be used in conjunction with escape characters.
```js
// BAD
globParent('C:\\Program Files \\(x86\\)\\*.ext'); // 'C:/Program Files /(x86/)'
// GOOD
globParent('C:/Program Files\\(x86\\)/*.ext'); // 'C:/Program Files (x86)'
```
If you are using escape characters for a pattern without path parts (i.e.
relative to `cwd`), prefix with `./` to avoid confusing glob-parent.
```js
// BAD
globParent('foo \\[bar]'); // 'foo '
globParent('foo \\[bar]*'); // 'foo '
// GOOD
globParent('./foo \\[bar]'); // 'foo [bar]'
globParent('./foo \\[bar]*'); // '.'
```
## License
ISC
<!-- prettier-ignore-start -->
[downloads-image]: https://img.shields.io/npm/dm/glob-parent.svg?style=flat-square
[npm-url]: https://www.npmjs.com/package/glob-parent
[npm-image]: https://img.shields.io/npm/v/glob-parent.svg?style=flat-square
[ci-url]: https://github.com/gulpjs/glob-parent/actions?query=workflow:dev
[ci-image]: https://img.shields.io/github/workflow/status/gulpjs/glob-parent/dev?style=flat-square
[coveralls-url]: https://coveralls.io/r/gulpjs/glob-parent
[coveralls-image]: https://img.shields.io/coveralls/gulpjs/glob-parent/master.svg?style=flat-square
<!-- prettier-ignore-end -->
<!-- prettier-ignore-start -->
[expand-braces]: https://github.com/jonschlinkert/expand-braces
[expand-brackets]: https://github.com/jonschlinkert/expand-brackets
<!-- prettier-ignore-end --> | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/glob-parent/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/glob-parent/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 4197
} |
# Glob
Match files using the patterns the shell uses.
The most correct and second fastest glob implementation in
JavaScript. (See **Comparison to Other JavaScript Glob
Implementations** at the bottom of this readme.)

## Usage
Install with npm
```
npm i glob
```
**Note** the npm package name is _not_ `node-glob` that's a
different thing that was abandoned years ago. Just `glob`.
```js
// load using import
import { glob, globSync, globStream, globStreamSync, Glob } from 'glob'
// or using commonjs, that's fine, too
const {
glob,
globSync,
globStream,
globStreamSync,
Glob,
} = require('glob')
// the main glob() and globSync() resolve/return array of filenames
// all js files, but don't look in node_modules
const jsfiles = await glob('**/*.js', { ignore: 'node_modules/**' })
// pass in a signal to cancel the glob walk
const stopAfter100ms = await glob('**/*.css', {
signal: AbortSignal.timeout(100),
})
// multiple patterns supported as well
const images = await glob(['css/*.{png,jpeg}', 'public/*.{png,jpeg}'])
// but of course you can do that with the glob pattern also
// the sync function is the same, just returns a string[] instead
// of Promise<string[]>
const imagesAlt = globSync('{css,public}/*.{png,jpeg}')
// you can also stream them, this is a Minipass stream
const filesStream = globStream(['**/*.dat', 'logs/**/*.log'])
// construct a Glob object if you wanna do it that way, which
// allows for much faster walks if you have to look in the same
// folder multiple times.
const g = new Glob('**/foo', {})
// glob objects are async iterators, can also do globIterate() or
// g.iterate(), same deal
for await (const file of g) {
console.log('found a foo file:', file)
}
// pass a glob as the glob options to reuse its settings and caches
const g2 = new Glob('**/bar', g)
// sync iteration works as well
for (const file of g2) {
console.log('found a bar file:', file)
}
// you can also pass withFileTypes: true to get Path objects
// these are like a Dirent, but with some more added powers
// check out http://npm.im/path-scurry for more info on their API
const g3 = new Glob('**/baz/**', { withFileTypes: true })
g3.stream().on('data', path => {
console.log(
'got a path object',
path.fullpath(),
path.isDirectory(),
path.readdirSync().map(e => e.name),
)
})
// if you use stat:true and withFileTypes, you can sort results
// by things like modified time, filter by permission mode, etc.
// All Stats fields will be available in that case. Slightly
// slower, though.
// For example:
const results = await glob('**', { stat: true, withFileTypes: true })
const timeSortedFiles = results
.sort((a, b) => a.mtimeMs - b.mtimeMs)
.map(path => path.fullpath())
const groupReadableFiles = results
.filter(path => path.mode & 0o040)
.map(path => path.fullpath())
// custom ignores can be done like this, for example by saying
// you'll ignore all markdown files, and all folders named 'docs'
const customIgnoreResults = await glob('**', {
ignore: {
ignored: p => /\.md$/.test(p.name),
childrenIgnored: p => p.isNamed('docs'),
},
})
// another fun use case, only return files with the same name as
// their parent folder, plus either `.ts` or `.js`
const folderNamedModules = await glob('**/*.{ts,js}', {
ignore: {
ignored: p => {
const pp = p.parent
return !(p.isNamed(pp.name + '.ts') || p.isNamed(pp.name + '.js'))
},
},
})
// find all files edited in the last hour, to do this, we ignore
// all of them that are more than an hour old
const newFiles = await glob('**', {
// need stat so we have mtime
stat: true,
// only want the files, not the dirs
nodir: true,
ignore: {
ignored: p => {
return new Date() - p.mtime > 60 * 60 * 1000
},
// could add similar childrenIgnored here as well, but
// directory mtime is inconsistent across platforms, so
// probably better not to, unless you know the system
// tracks this reliably.
},
})
```
**Note** Glob patterns should always use `/` as a path separator,
even on Windows systems, as `\` is used to escape glob
characters. If you wish to use `\` as a path separator _instead
of_ using it as an escape character on Windows platforms, you may
set `windowsPathsNoEscape:true` in the options. In this mode,
special glob characters cannot be escaped, making it impossible
to match a literal `*` `?` and so on in filenames.
## Command Line Interface
```
$ glob -h
Usage:
glob [options] [<pattern> [<pattern> ...]]
Expand the positional glob expression arguments into any matching file system
paths found.
-c<command> --cmd=<command>
Run the command provided, passing the glob expression
matches as arguments.
-A --all By default, the glob cli command will not expand any
arguments that are an exact match to a file on disk.
This prevents double-expanding, in case the shell
expands an argument whose filename is a glob
expression.
For example, if 'app/*.ts' would match 'app/[id].ts',
then on Windows powershell or cmd.exe, 'glob app/*.ts'
will expand to 'app/[id].ts', as expected. However, in
posix shells such as bash or zsh, the shell will first
expand 'app/*.ts' to a list of filenames. Then glob
will look for a file matching 'app/[id].ts' (ie,
'app/i.ts' or 'app/d.ts'), which is unexpected.
Setting '--all' prevents this behavior, causing glob to
treat ALL patterns as glob expressions to be expanded,
even if they are an exact match to a file on disk.
When setting this option, be sure to enquote arguments
so that the shell will not expand them prior to passing
them to the glob command process.
-a --absolute Expand to absolute paths
-d --dot-relative Prepend './' on relative matches
-m --mark Append a / on any directories matched
-x --posix Always resolve to posix style paths, using '/' as the
directory separator, even on Windows. Drive letter
absolute matches on Windows will be expanded to their
full resolved UNC maths, eg instead of 'C:\foo\bar', it
will expand to '//?/C:/foo/bar'.
-f --follow Follow symlinked directories when expanding '**'
-R --realpath Call 'fs.realpath' on all of the results. In the case
of an entry that cannot be resolved, the entry is
omitted. This incurs a slight performance penalty, of
course, because of the added system calls.
-s --stat Call 'fs.lstat' on all entries, whether required or not
to determine if it's a valid match.
-b --match-base Perform a basename-only match if the pattern does not
contain any slash characters. That is, '*.js' would be
treated as equivalent to '**/*.js', matching js files
in all directories.
--dot Allow patterns to match files/directories that start
with '.', even if the pattern does not start with '.'
--nobrace Do not expand {...} patterns
--nocase Perform a case-insensitive match. This defaults to
'true' on macOS and Windows platforms, and false on all
others.
Note: 'nocase' should only be explicitly set when it is
known that the filesystem's case sensitivity differs
from the platform default. If set 'true' on
case-insensitive file systems, then the walk may return
more or less results than expected.
--nodir Do not match directories, only files.
Note: to *only* match directories, append a '/' at the
end of the pattern.
--noext Do not expand extglob patterns, such as '+(a|b)'
--noglobstar Do not expand '**' against multiple path portions. Ie,
treat it as a normal '*' instead.
--windows-path-no-escape
Use '\' as a path separator *only*, and *never* as an
escape character. If set, all '\' characters are
replaced with '/' in the pattern.
-D<n> --max-depth=<n> Maximum depth to traverse from the current working
directory
-C<cwd> --cwd=<cwd> Current working directory to execute/match in
-r<root> --root=<root> A string path resolved against the 'cwd', which is used
as the starting point for absolute patterns that start
with '/' (but not drive letters or UNC paths on
Windows).
Note that this *doesn't* necessarily limit the walk to
the 'root' directory, and doesn't affect the cwd
starting point for non-absolute patterns. A pattern
containing '..' will still be able to traverse out of
the root directory, if it is not an actual root
directory on the filesystem, and any non-absolute
patterns will still be matched in the 'cwd'.
To start absolute and non-absolute patterns in the same
path, you can use '--root=' to set it to the empty
string. However, be aware that on Windows systems, a
pattern like 'x:/*' or '//host/share/*' will *always*
start in the 'x:/' or '//host/share/' directory,
regardless of the --root setting.
--platform=<platform> Defaults to the value of 'process.platform' if
available, or 'linux' if not. Setting --platform=win32
on non-Windows systems may cause strange behavior!
-i<ignore> --ignore=<ignore>
Glob patterns to ignore Can be set multiple times
-v --debug Output a huge amount of noisy debug information about
patterns as they are parsed and used to match files.
-h --help Show this usage information
```
## `glob(pattern: string | string[], options?: GlobOptions) => Promise<string[] | Path[]>`
Perform an asynchronous glob search for the pattern(s) specified.
Returns
[Path](https://isaacs.github.io/path-scurry/classes/PathBase)
objects if the `withFileTypes` option is set to `true`. See below
for full options field desciptions.
## `globSync(pattern: string | string[], options?: GlobOptions) => string[] | Path[]`
Synchronous form of `glob()`.
Alias: `glob.sync()`
## `globIterate(pattern: string | string[], options?: GlobOptions) => AsyncGenerator<string>`
Return an async iterator for walking glob pattern matches.
Alias: `glob.iterate()`
## `globIterateSync(pattern: string | string[], options?: GlobOptions) => Generator<string>`
Return a sync iterator for walking glob pattern matches.
Alias: `glob.iterate.sync()`, `glob.sync.iterate()`
## `globStream(pattern: string | string[], options?: GlobOptions) => Minipass<string | Path>`
Return a stream that emits all the strings or `Path` objects and
then emits `end` when completed.
Alias: `glob.stream()`
## `globStreamSync(pattern: string | string[], options?: GlobOptions) => Minipass<string | Path>`
Syncronous form of `globStream()`. Will read all the matches as
fast as you consume them, even all in a single tick if you
consume them immediately, but will still respond to backpressure
if they're not consumed immediately.
Alias: `glob.stream.sync()`, `glob.sync.stream()`
## `hasMagic(pattern: string | string[], options?: GlobOptions) => boolean`
Returns `true` if the provided pattern contains any "magic" glob
characters, given the options provided.
Brace expansion is not considered "magic" unless the
`magicalBraces` option is set, as brace expansion just turns one
string into an array of strings. So a pattern like `'x{a,b}y'`
would return `false`, because `'xay'` and `'xby'` both do not
contain any magic glob characters, and it's treated the same as
if you had called it on `['xay', 'xby']`. When
`magicalBraces:true` is in the options, brace expansion _is_
treated as a pattern having magic.
## `escape(pattern: string, options?: GlobOptions) => string`
Escape all magic characters in a glob pattern, so that it will
only ever match literal strings
If the `windowsPathsNoEscape` option is used, then characters are
escaped by wrapping in `[]`, because a magic character wrapped in
a character class can only be satisfied by that exact character.
Slashes (and backslashes in `windowsPathsNoEscape` mode) cannot
be escaped or unescaped.
## `unescape(pattern: string, options?: GlobOptions) => string`
Un-escape a glob string that may contain some escaped characters.
If the `windowsPathsNoEscape` option is used, then square-brace
escapes are removed, but not backslash escapes. For example, it
will turn the string `'[*]'` into `*`, but it will not turn
`'\\*'` into `'*'`, because `\` is a path separator in
`windowsPathsNoEscape` mode.
When `windowsPathsNoEscape` is not set, then both brace escapes
and backslash escapes are removed.
Slashes (and backslashes in `windowsPathsNoEscape` mode) cannot
be escaped or unescaped.
## Class `Glob`
An object that can perform glob pattern traversals.
### `const g = new Glob(pattern: string | string[], options: GlobOptions)`
Options object is required.
See full options descriptions below.
Note that a previous `Glob` object can be passed as the
`GlobOptions` to another `Glob` instantiation to re-use settings
and caches with a new pattern.
Traversal functions can be called multiple times to run the walk
again.
### `g.stream()`
Stream results asynchronously,
### `g.streamSync()`
Stream results synchronously.
### `g.iterate()`
Default async iteration function. Returns an AsyncGenerator that
iterates over the results.
### `g.iterateSync()`
Default sync iteration function. Returns a Generator that
iterates over the results.
### `g.walk()`
Returns a Promise that resolves to the results array.
### `g.walkSync()`
Returns a results array.
### Properties
All options are stored as properties on the `Glob` object.
- `opts` The options provided to the constructor.
- `patterns` An array of parsed immutable `Pattern` objects.
## Options
Exported as `GlobOptions` TypeScript interface. A `GlobOptions`
object may be provided to any of the exported methods, and must
be provided to the `Glob` constructor.
All options are optional, boolean, and false by default, unless
otherwise noted.
All resolved options are added to the Glob object as properties.
If you are running many `glob` operations, you can pass a Glob
object as the `options` argument to a subsequent operation to
share the previously loaded cache.
- `cwd` String path or `file://` string or URL object. The
current working directory in which to search. Defaults to
`process.cwd()`. See also: "Windows, CWDs, Drive Letters, and
UNC Paths", below.
This option may be either a string path or a `file://` URL
object or string.
- `root` A string path resolved against the `cwd` option, which
is used as the starting point for absolute patterns that start
with `/`, (but not drive letters or UNC paths on Windows).
Note that this _doesn't_ necessarily limit the walk to the
`root` directory, and doesn't affect the cwd starting point for
non-absolute patterns. A pattern containing `..` will still be
able to traverse out of the root directory, if it is not an
actual root directory on the filesystem, and any non-absolute
patterns will be matched in the `cwd`. For example, the
pattern `/../*` with `{root:'/some/path'}` will return all
files in `/some`, not all files in `/some/path`. The pattern
`*` with `{root:'/some/path'}` will return all the entries in
the cwd, not the entries in `/some/path`.
To start absolute and non-absolute patterns in the same
path, you can use `{root:''}`. However, be aware that on
Windows systems, a pattern like `x:/*` or `//host/share/*` will
_always_ start in the `x:/` or `//host/share` directory,
regardless of the `root` setting.
- `windowsPathsNoEscape` Use `\\` as a path separator _only_, and
_never_ as an escape character. If set, all `\\` characters are
replaced with `/` in the pattern.
Note that this makes it **impossible** to match against paths
containing literal glob pattern characters, but allows matching
with patterns constructed using `path.join()` and
`path.resolve()` on Windows platforms, mimicking the (buggy!)
behavior of Glob v7 and before on Windows. Please use with
caution, and be mindful of [the caveat below about Windows
paths](#windows). (For legacy reasons, this is also set if
`allowWindowsEscape` is set to the exact value `false`.)
- `dot` Include `.dot` files in normal matches and `globstar`
matches. Note that an explicit dot in a portion of the pattern
will always match dot files.
- `magicalBraces` Treat brace expansion like `{a,b}` as a "magic"
pattern. Has no effect if {@link nobrace} is set.
Only has effect on the {@link hasMagic} function, no effect on
glob pattern matching itself.
- `dotRelative` Prepend all relative path strings with `./` (or
`.\` on Windows).
Without this option, returned relative paths are "bare", so
instead of returning `'./foo/bar'`, they are returned as
`'foo/bar'`.
Relative patterns starting with `'../'` are not prepended with
`./`, even if this option is set.
- `mark` Add a `/` character to directory matches. Note that this
requires additional stat calls.
- `nobrace` Do not expand `{a,b}` and `{1..3}` brace sets.
- `noglobstar` Do not match `**` against multiple filenames. (Ie,
treat it as a normal `*` instead.)
- `noext` Do not match "extglob" patterns such as `+(a|b)`.
- `nocase` Perform a case-insensitive match. This defaults to
`true` on macOS and Windows systems, and `false` on all others.
**Note** `nocase` should only be explicitly set when it is
known that the filesystem's case sensitivity differs from the
platform default. If set `true` on case-sensitive file
systems, or `false` on case-insensitive file systems, then the
walk may return more or less results than expected.
- `maxDepth` Specify a number to limit the depth of the directory
traversal to this many levels below the `cwd`.
- `matchBase` Perform a basename-only match if the pattern does
not contain any slash characters. That is, `*.js` would be
treated as equivalent to `**/*.js`, matching all js files in
all directories.
- `nodir` Do not match directories, only files. (Note: to match
_only_ directories, put a `/` at the end of the pattern.)
Note: when `follow` and `nodir` are both set, then symbolic
links to directories are also omitted.
- `stat` Call `lstat()` on all entries, whether required or not
to determine whether it's a valid match. When used with
`withFileTypes`, this means that matches will include data such
as modified time, permissions, and so on. Note that this will
incur a performance cost due to the added system calls.
- `ignore` string or string[], or an object with `ignore` and
`ignoreChildren` methods.
If a string or string[] is provided, then this is treated as a
glob pattern or array of glob patterns to exclude from matches.
To ignore all children within a directory, as well as the entry
itself, append `'/**'` to the ignore pattern.
**Note** `ignore` patterns are _always_ in `dot:true` mode,
regardless of any other settings.
If an object is provided that has `ignored(path)` and/or
`childrenIgnored(path)` methods, then these methods will be
called to determine whether any Path is a match or if its
children should be traversed, respectively.
- `follow` Follow symlinked directories when expanding `**`
patterns. This can result in a lot of duplicate references in
the presence of cyclic links, and make performance quite bad.
By default, a `**` in a pattern will follow 1 symbolic link if
it is not the first item in the pattern, or none if it is the
first item in the pattern, following the same behavior as Bash.
Note: when `follow` and `nodir` are both set, then symbolic
links to directories are also omitted.
- `realpath` Set to true to call `fs.realpath` on all of the
results. In the case of an entry that cannot be resolved, the
entry is omitted. This incurs a slight performance penalty, of
course, because of the added system calls.
- `absolute` Set to true to always receive absolute paths for
matched files. Set to `false` to always receive relative paths
for matched files.
By default, when this option is not set, absolute paths are
returned for patterns that are absolute, and otherwise paths
are returned that are relative to the `cwd` setting.
This does _not_ make an extra system call to get the realpath,
it only does string path resolution.
`absolute` may not be used along with `withFileTypes`.
- `posix` Set to true to use `/` as the path separator in
returned results. On posix systems, this has no effect. On
Windows systems, this will return `/` delimited path results,
and absolute paths will be returned in their full resolved UNC
path form, eg insted of `'C:\\foo\\bar'`, it will return
`//?/C:/foo/bar`.
- `platform` Defaults to value of `process.platform` if
available, or `'linux'` if not. Setting `platform:'win32'` on
non-Windows systems may cause strange behavior.
- `withFileTypes` Return [PathScurry](http://npm.im/path-scurry)
`Path` objects instead of strings. These are similar to a
NodeJS `Dirent` object, but with additional methods and
properties.
`withFileTypes` may not be used along with `absolute`.
- `signal` An AbortSignal which will cancel the Glob walk when
triggered.
- `fs` An override object to pass in custom filesystem methods.
See [PathScurry docs](http://npm.im/path-scurry) for what can
be overridden.
- `scurry` A [PathScurry](http://npm.im/path-scurry) object used
to traverse the file system. If the `nocase` option is set
explicitly, then any provided `scurry` object must match this
setting.
- `includeChildMatches` boolean, default `true`. Do not match any
children of any matches. For example, the pattern `**\/foo`
would match `a/foo`, but not `a/foo/b/foo` in this mode.
This is especially useful for cases like "find all
`node_modules` folders, but not the ones in `node_modules`".
In order to support this, the `Ignore` implementation must
support an `add(pattern: string)` method. If using the default
`Ignore` class, then this is fine, but if this is set to
`false`, and a custom `Ignore` is provided that does not have
an `add()` method, then it will throw an error.
**Caveat** It _only_ ignores matches that would be a descendant
of a previous match, and only if that descendant is matched
_after_ the ancestor is encountered. Since the file system walk
happens in indeterminate order, it's possible that a match will
already be added before its ancestor, if multiple or braced
patterns are used.
For example:
```js
const results = await glob(
[
// likely to match first, since it's just a stat
'a/b/c/d/e/f',
// this pattern is more complicated! It must to various readdir()
// calls and test the results against a regular expression, and that
// is certainly going to take a little bit longer.
//
// So, later on, it encounters a match at 'a/b/c/d/e', but it's too
// late to ignore a/b/c/d/e/f, because it's already been emitted.
'a/[bdf]/?/[a-z]/*',
],
{ includeChildMatches: false },
)
```
It's best to only set this to `false` if you can be reasonably
sure that no components of the pattern will potentially match
one another's file system descendants, or if the occasional
included child entry will not cause problems.
## Glob Primer
Much more information about glob pattern expansion can be found
by running `man bash` and searching for `Pattern Matching`.
"Globs" are the patterns you type when you do stuff like `ls
*.js` on the command line, or put `build/*` in a `.gitignore`
file.
Before parsing the path part patterns, braced sections are
expanded into a set. Braced sections start with `{` and end with
`}`, with 2 or more comma-delimited sections within. Braced
sections may contain slash characters, so `a{/b/c,bcd}` would
expand into `a/b/c` and `abcd`.
The following characters have special magic meaning when used in
a path portion. With the exception of `**`, none of these match
path separators (ie, `/` on all platforms, and `\` on Windows).
- `*` Matches 0 or more characters in a single path portion.
When alone in a path portion, it must match at least 1
character. If `dot:true` is not specified, then `*` will not
match against a `.` character at the start of a path portion.
- `?` Matches 1 character. If `dot:true` is not specified, then
`?` will not match against a `.` character at the start of a
path portion.
- `[...]` Matches a range of characters, similar to a RegExp
range. If the first character of the range is `!` or `^` then
it matches any character not in the range. If the first
character is `]`, then it will be considered the same as `\]`,
rather than the end of the character class.
- `!(pattern|pattern|pattern)` Matches anything that does not
match any of the patterns provided. May _not_ contain `/`
characters. Similar to `*`, if alone in a path portion, then
the path portion must have at least one character.
- `?(pattern|pattern|pattern)` Matches zero or one occurrence of
the patterns provided. May _not_ contain `/` characters.
- `+(pattern|pattern|pattern)` Matches one or more occurrences of
the patterns provided. May _not_ contain `/` characters.
- `*(a|b|c)` Matches zero or more occurrences of the patterns
provided. May _not_ contain `/` characters.
- `@(pattern|pat*|pat?erN)` Matches exactly one of the patterns
provided. May _not_ contain `/` characters.
- `**` If a "globstar" is alone in a path portion, then it
matches zero or more directories and subdirectories searching
for matches. It does not crawl symlinked directories, unless
`{follow:true}` is passed in the options object. A pattern
like `a/b/**` will only match `a/b` if it is a directory.
Follows 1 symbolic link if not the first item in the pattern,
or 0 if it is the first item, unless `follow:true` is set, in
which case it follows all symbolic links.
`[:class:]` patterns are supported by this implementation, but
`[=c=]` and `[.symbol.]` style class patterns are not.
### Dots
If a file or directory path portion has a `.` as the first
character, then it will not match any glob pattern unless that
pattern's corresponding path part also has a `.` as its first
character.
For example, the pattern `a/.*/c` would match the file at
`a/.b/c`. However the pattern `a/*/c` would not, because `*` does
not start with a dot character.
You can make glob treat dots as normal characters by setting
`dot:true` in the options.
### Basename Matching
If you set `matchBase:true` in the options, and the pattern has
no slashes in it, then it will seek for any file anywhere in the
tree with a matching basename. For example, `*.js` would match
`test/simple/basic.js`.
### Empty Sets
If no matching files are found, then an empty array is returned.
This differs from the shell, where the pattern itself is
returned. For example:
```sh
$ echo a*s*d*f
a*s*d*f
```
## Comparisons to other fnmatch/glob implementations
While strict compliance with the existing standards is a
worthwhile goal, some discrepancies exist between node-glob and
other implementations, and are intentional.
The double-star character `**` is supported by default, unless
the `noglobstar` flag is set. This is supported in the manner of
bsdglob and bash 5, where `**` only has special significance if
it is the only thing in a path part. That is, `a/**/b` will match
`a/x/y/b`, but `a/**b` will not.
Note that symlinked directories are not traversed as part of a
`**`, though their contents may match against subsequent portions
of the pattern. This prevents infinite loops and duplicates and
the like. You can force glob to traverse symlinks with `**` by
setting `{follow:true}` in the options.
There is no equivalent of the `nonull` option. A pattern that
does not find any matches simply resolves to nothing. (An empty
array, immediately ended stream, etc.)
If brace expansion is not disabled, then it is performed before
any other interpretation of the glob pattern. Thus, a pattern
like `+(a|{b),c)}`, which would not be valid in bash or zsh, is
expanded **first** into the set of `+(a|b)` and `+(a|c)`, and
those patterns are checked for validity. Since those two are
valid, matching proceeds.
The character class patterns `[:class:]` (posix standard named
classes) style class patterns are supported and unicode-aware,
but `[=c=]` (locale-specific character collation weight), and
`[.symbol.]` (collating symbol), are not.
### Repeated Slashes
Unlike Bash and zsh, repeated `/` are always coalesced into a
single path separator.
### Comments and Negation
Previously, this module let you mark a pattern as a "comment" if
it started with a `#` character, or a "negated" pattern if it
started with a `!` character.
These options were deprecated in version 5, and removed in
version 6.
To specify things that should not match, use the `ignore` option.
## Windows
**Please only use forward-slashes in glob expressions.**
Though windows uses either `/` or `\` as its path separator, only
`/` characters are used by this glob implementation. You must use
forward-slashes **only** in glob expressions. Back-slashes will
always be interpreted as escape characters, not path separators.
Results from absolute patterns such as `/foo/*` are mounted onto
the root setting using `path.join`. On windows, this will by
default result in `/foo/*` matching `C:\foo\bar.txt`.
To automatically coerce all `\` characters to `/` in pattern
strings, **thus making it impossible to escape literal glob
characters**, you may set the `windowsPathsNoEscape` option to
`true`.
### Windows, CWDs, Drive Letters, and UNC Paths
On posix systems, when a pattern starts with `/`, any `cwd`
option is ignored, and the traversal starts at `/`, plus any
non-magic path portions specified in the pattern.
On Windows systems, the behavior is similar, but the concept of
an "absolute path" is somewhat more involved.
#### UNC Paths
A UNC path may be used as the start of a pattern on Windows
platforms. For example, a pattern like: `//?/x:/*` will return
all file entries in the root of the `x:` drive. A pattern like
`//ComputerName/Share/*` will return all files in the associated
share.
UNC path roots are always compared case insensitively.
#### Drive Letters
A pattern starting with a drive letter, like `c:/*`, will search
in that drive, regardless of any `cwd` option provided.
If the pattern starts with `/`, and is not a UNC path, and there
is an explicit `cwd` option set with a drive letter, then the
drive letter in the `cwd` is used as the root of the directory
traversal.
For example, `glob('/tmp', { cwd: 'c:/any/thing' })` will return
`['c:/tmp']` as the result.
If an explicit `cwd` option is not provided, and the pattern
starts with `/`, then the traversal will run on the root of the
drive provided as the `cwd` option. (That is, it is the result of
`path.resolve('/')`.)
## Race Conditions
Glob searching, by its very nature, is susceptible to race
conditions, since it relies on directory walking.
As a result, it is possible that a file that exists when glob
looks for it may have been deleted or modified by the time it
returns the result.
By design, this implementation caches all readdir calls that it
makes, in order to cut down on system overhead. However, this
also makes it even more susceptible to races, especially if the
cache object is reused between glob calls.
Users are thus advised not to use a glob result as a guarantee of
filesystem state in the face of rapid changes. For the vast
majority of operations, this is never a problem.
### See Also:
- `man sh`
- `man bash` [Pattern
Matching](https://www.gnu.org/software/bash/manual/html_node/Pattern-Matching.html)
- `man 3 fnmatch`
- `man 5 gitignore`
- [minimatch documentation](https://github.com/isaacs/minimatch)
## Glob Logo
Glob's logo was created by [Tanya
Brassie](http://tanyabrassie.com/). Logo files can be found
[here](https://github.com/isaacs/node-glob/tree/master/logo).
The logo is licensed under a [Creative Commons
Attribution-ShareAlike 4.0 International
License](https://creativecommons.org/licenses/by-sa/4.0/).
## Contributing
Any change to behavior (including bugfixes) must come with a
test.
Patches that fail tests or reduce performance will be rejected.
```sh
# to run tests
npm test
# to re-generate test fixtures
npm run test-regen
# run the benchmarks
npm run bench
# to profile javascript
npm run prof
```
## Comparison to Other JavaScript Glob Implementations
**tl;dr**
- If you want glob matching that is as faithful as possible to
Bash pattern expansion semantics, and as fast as possible
within that constraint, _use this module_.
- If you are reasonably sure that the patterns you will encounter
are relatively simple, and want the absolutely fastest glob
matcher out there, _use [fast-glob](http://npm.im/fast-glob)_.
- If you are reasonably sure that the patterns you will encounter
are relatively simple, and want the convenience of
automatically respecting `.gitignore` files, _use
[globby](http://npm.im/globby)_.
There are some other glob matcher libraries on npm, but these
three are (in my opinion, as of 2023) the best.
---
**full explanation**
Every library reflects a set of opinions and priorities in the
trade-offs it makes. Other than this library, I can personally
recommend both [globby](http://npm.im/globby) and
[fast-glob](http://npm.im/fast-glob), though they differ in their
benefits and drawbacks.
Both have very nice APIs and are reasonably fast.
`fast-glob` is, as far as I am aware, the fastest glob
implementation in JavaScript today. However, there are many
cases where the choices that `fast-glob` makes in pursuit of
speed mean that its results differ from the results returned by
Bash and other sh-like shells, which may be surprising.
In my testing, `fast-glob` is around 10-20% faster than this
module when walking over 200k files nested 4 directories
deep[1](#fn-webscale). However, there are some inconsistencies
with Bash matching behavior that this module does not suffer
from:
- `**` only matches files, not directories
- `..` path portions are not handled unless they appear at the
start of the pattern
- `./!(<pattern>)` will not match any files that _start_ with
`<pattern>`, even if they do not match `<pattern>`. For
example, `!(9).txt` will not match `9999.txt`.
- Some brace patterns in the middle of a pattern will result in
failing to find certain matches.
- Extglob patterns are allowed to contain `/` characters.
Globby exhibits all of the same pattern semantics as fast-glob,
(as it is a wrapper around fast-glob) and is slightly slower than
node-glob (by about 10-20% in the benchmark test set, or in other
words, anywhere from 20-50% slower than fast-glob). However, it
adds some API conveniences that may be worth the costs.
- Support for `.gitignore` and other ignore files.
- Support for negated globs (ie, patterns starting with `!`
rather than using a separate `ignore` option).
The priority of this module is "correctness" in the sense of
performing a glob pattern expansion as faithfully as possible to
the behavior of Bash and other sh-like shells, with as much speed
as possible.
Note that prior versions of `node-glob` are _not_ on this list.
Former versions of this module are far too slow for any cases
where performance matters at all, and were designed with APIs
that are extremely dated by current JavaScript standards.
---
<small id="fn-webscale">[1]: In the cases where this module
returns results and `fast-glob` doesn't, it's even faster, of
course.</small>

### Benchmark Results
First number is time, smaller is better.
Second number is the count of results returned.
```
--- pattern: '**' ---
~~ sync ~~
node fast-glob sync 0m0.598s 200364
node globby sync 0m0.765s 200364
node current globSync mjs 0m0.683s 222656
node current glob syncStream 0m0.649s 222656
~~ async ~~
node fast-glob async 0m0.350s 200364
node globby async 0m0.509s 200364
node current glob async mjs 0m0.463s 222656
node current glob stream 0m0.411s 222656
--- pattern: '**/..' ---
~~ sync ~~
node fast-glob sync 0m0.486s 0
node globby sync 0m0.769s 200364
node current globSync mjs 0m0.564s 2242
node current glob syncStream 0m0.583s 2242
~~ async ~~
node fast-glob async 0m0.283s 0
node globby async 0m0.512s 200364
node current glob async mjs 0m0.299s 2242
node current glob stream 0m0.312s 2242
--- pattern: './**/0/**/0/**/0/**/0/**/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.490s 10
node globby sync 0m0.517s 10
node current globSync mjs 0m0.540s 10
node current glob syncStream 0m0.550s 10
~~ async ~~
node fast-glob async 0m0.290s 10
node globby async 0m0.296s 10
node current glob async mjs 0m0.278s 10
node current glob stream 0m0.302s 10
--- pattern: './**/[01]/**/[12]/**/[23]/**/[45]/**/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.500s 160
node globby sync 0m0.528s 160
node current globSync mjs 0m0.556s 160
node current glob syncStream 0m0.573s 160
~~ async ~~
node fast-glob async 0m0.283s 160
node globby async 0m0.301s 160
node current glob async mjs 0m0.306s 160
node current glob stream 0m0.322s 160
--- pattern: './**/0/**/0/**/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.502s 5230
node globby sync 0m0.527s 5230
node current globSync mjs 0m0.544s 5230
node current glob syncStream 0m0.557s 5230
~~ async ~~
node fast-glob async 0m0.285s 5230
node globby async 0m0.305s 5230
node current glob async mjs 0m0.304s 5230
node current glob stream 0m0.310s 5230
--- pattern: '**/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.580s 200023
node globby sync 0m0.771s 200023
node current globSync mjs 0m0.685s 200023
node current glob syncStream 0m0.649s 200023
~~ async ~~
node fast-glob async 0m0.349s 200023
node globby async 0m0.509s 200023
node current glob async mjs 0m0.427s 200023
node current glob stream 0m0.388s 200023
--- pattern: '{**/*.txt,**/?/**/*.txt,**/?/**/?/**/*.txt,**/?/**/?/**/?/**/*.txt,**/?/**/?/**/?/**/?/**/*.txt}' ---
~~ sync ~~
node fast-glob sync 0m0.589s 200023
node globby sync 0m0.771s 200023
node current globSync mjs 0m0.716s 200023
node current glob syncStream 0m0.684s 200023
~~ async ~~
node fast-glob async 0m0.351s 200023
node globby async 0m0.518s 200023
node current glob async mjs 0m0.462s 200023
node current glob stream 0m0.468s 200023
--- pattern: '**/5555/0000/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.496s 1000
node globby sync 0m0.519s 1000
node current globSync mjs 0m0.539s 1000
node current glob syncStream 0m0.567s 1000
~~ async ~~
node fast-glob async 0m0.285s 1000
node globby async 0m0.299s 1000
node current glob async mjs 0m0.305s 1000
node current glob stream 0m0.301s 1000
--- pattern: './**/0/**/../[01]/**/0/../**/0/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.484s 0
node globby sync 0m0.507s 0
node current globSync mjs 0m0.577s 4880
node current glob syncStream 0m0.586s 4880
~~ async ~~
node fast-glob async 0m0.280s 0
node globby async 0m0.298s 0
node current glob async mjs 0m0.327s 4880
node current glob stream 0m0.324s 4880
--- pattern: '**/????/????/????/????/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.547s 100000
node globby sync 0m0.673s 100000
node current globSync mjs 0m0.626s 100000
node current glob syncStream 0m0.618s 100000
~~ async ~~
node fast-glob async 0m0.315s 100000
node globby async 0m0.414s 100000
node current glob async mjs 0m0.366s 100000
node current glob stream 0m0.345s 100000
--- pattern: './{**/?{/**/?{/**/?{/**/?,,,,},,,,},,,,},,,}/**/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.588s 100000
node globby sync 0m0.670s 100000
node current globSync mjs 0m0.717s 200023
node current glob syncStream 0m0.687s 200023
~~ async ~~
node fast-glob async 0m0.343s 100000
node globby async 0m0.418s 100000
node current glob async mjs 0m0.519s 200023
node current glob stream 0m0.451s 200023
--- pattern: '**/!(0|9).txt' ---
~~ sync ~~
node fast-glob sync 0m0.573s 160023
node globby sync 0m0.731s 160023
node current globSync mjs 0m0.680s 180023
node current glob syncStream 0m0.659s 180023
~~ async ~~
node fast-glob async 0m0.345s 160023
node globby async 0m0.476s 160023
node current glob async mjs 0m0.427s 180023
node current glob stream 0m0.388s 180023
--- pattern: './{*/**/../{*/**/../{*/**/../{*/**/../{*/**,,,,},,,,},,,,},,,,},,,,}/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.483s 0
node globby sync 0m0.512s 0
node current globSync mjs 0m0.811s 200023
node current glob syncStream 0m0.773s 200023
~~ async ~~
node fast-glob async 0m0.280s 0
node globby async 0m0.299s 0
node current glob async mjs 0m0.617s 200023
node current glob stream 0m0.568s 200023
--- pattern: './*/**/../*/**/../*/**/../*/**/../*/**/../*/**/../*/**/../*/**/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.485s 0
node globby sync 0m0.507s 0
node current globSync mjs 0m0.759s 200023
node current glob syncStream 0m0.740s 200023
~~ async ~~
node fast-glob async 0m0.281s 0
node globby async 0m0.297s 0
node current glob async mjs 0m0.544s 200023
node current glob stream 0m0.464s 200023
--- pattern: './*/**/../*/**/../*/**/../*/**/../*/**/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.486s 0
node globby sync 0m0.513s 0
node current globSync mjs 0m0.734s 200023
node current glob syncStream 0m0.696s 200023
~~ async ~~
node fast-glob async 0m0.286s 0
node globby async 0m0.296s 0
node current glob async mjs 0m0.506s 200023
node current glob stream 0m0.483s 200023
--- pattern: './0/**/../1/**/../2/**/../3/**/../4/**/../5/**/../6/**/../7/**/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.060s 0
node globby sync 0m0.074s 0
node current globSync mjs 0m0.067s 0
node current glob syncStream 0m0.066s 0
~~ async ~~
node fast-glob async 0m0.060s 0
node globby async 0m0.075s 0
node current glob async mjs 0m0.066s 0
node current glob stream 0m0.067s 0
--- pattern: './**/?/**/?/**/?/**/?/**/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.568s 100000
node globby sync 0m0.651s 100000
node current globSync mjs 0m0.619s 100000
node current glob syncStream 0m0.617s 100000
~~ async ~~
node fast-glob async 0m0.332s 100000
node globby async 0m0.409s 100000
node current glob async mjs 0m0.372s 100000
node current glob stream 0m0.351s 100000
--- pattern: '**/*/**/*/**/*/**/*/**' ---
~~ sync ~~
node fast-glob sync 0m0.603s 200113
node globby sync 0m0.798s 200113
node current globSync mjs 0m0.730s 222137
node current glob syncStream 0m0.693s 222137
~~ async ~~
node fast-glob async 0m0.356s 200113
node globby async 0m0.525s 200113
node current glob async mjs 0m0.508s 222137
node current glob stream 0m0.455s 222137
--- pattern: './**/*/**/*/**/*/**/*/**/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.622s 200000
node globby sync 0m0.792s 200000
node current globSync mjs 0m0.722s 200000
node current glob syncStream 0m0.695s 200000
~~ async ~~
node fast-glob async 0m0.369s 200000
node globby async 0m0.527s 200000
node current glob async mjs 0m0.502s 200000
node current glob stream 0m0.481s 200000
--- pattern: '**/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.588s 200023
node globby sync 0m0.771s 200023
node current globSync mjs 0m0.684s 200023
node current glob syncStream 0m0.658s 200023
~~ async ~~
node fast-glob async 0m0.352s 200023
node globby async 0m0.516s 200023
node current glob async mjs 0m0.432s 200023
node current glob stream 0m0.384s 200023
--- pattern: './**/**/**/**/**/**/**/**/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.589s 200023
node globby sync 0m0.766s 200023
node current globSync mjs 0m0.682s 200023
node current glob syncStream 0m0.652s 200023
~~ async ~~
node fast-glob async 0m0.352s 200023
node globby async 0m0.523s 200023
node current glob async mjs 0m0.436s 200023
node current glob stream 0m0.380s 200023
--- pattern: '**/*/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.592s 200023
node globby sync 0m0.776s 200023
node current globSync mjs 0m0.691s 200023
node current glob syncStream 0m0.659s 200023
~~ async ~~
node fast-glob async 0m0.357s 200023
node globby async 0m0.513s 200023
node current glob async mjs 0m0.471s 200023
node current glob stream 0m0.424s 200023
--- pattern: '**/*/**/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.585s 200023
node globby sync 0m0.766s 200023
node current globSync mjs 0m0.694s 200023
node current glob syncStream 0m0.664s 200023
~~ async ~~
node fast-glob async 0m0.350s 200023
node globby async 0m0.514s 200023
node current glob async mjs 0m0.472s 200023
node current glob stream 0m0.424s 200023
--- pattern: '**/[0-9]/**/*.txt' ---
~~ sync ~~
node fast-glob sync 0m0.544s 100000
node globby sync 0m0.636s 100000
node current globSync mjs 0m0.626s 100000
node current glob syncStream 0m0.621s 100000
~~ async ~~
node fast-glob async 0m0.322s 100000
node globby async 0m0.404s 100000
node current glob async mjs 0m0.360s 100000
node current glob stream 0m0.352s 100000
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/glob/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/glob/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 48382
} |
# globals [](https://travis-ci.org/sindresorhus/globals)
> Global identifiers from different JavaScript environments
Extracted from [JSHint](https://github.com/jshint/jshint/blob/3a8efa979dbb157bfb5c10b5826603a55a33b9ad/src/vars.js) and [ESLint](https://github.com/eslint/eslint/blob/b648406218f8a2d7302b98f5565e23199f44eb31/conf/environments.json) and merged.
It's just a [JSON file](globals.json), so use it in whatever environment you like.
**This module [no longer accepts](https://github.com/sindresorhus/globals/issues/82) new environments. If you need it for ESLint, just [create a plugin](http://eslint.org/docs/developer-guide/working-with-plugins#environments-in-plugins).**
## Install
```
$ npm install globals
```
## Usage
```js
const globals = require('globals');
console.log(globals.browser);
/*
{
addEventListener: false,
applicationCache: false,
ArrayBuffer: false,
atob: false,
...
}
*/
```
Each global is given a value of `true` or `false`. A value of `true` indicates that the variable may be overwritten. A value of `false` indicates that the variable should be considered read-only. This information is used by static analysis tools to flag incorrect behavior. We assume all variables should be `false` unless we hear otherwise.
## License
MIT © [Sindre Sorhus](https://sindresorhus.com) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/globals/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/globals/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1405
} |
# Changelog
All notable changes to this project will be documented in this file.
The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/)
and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html).
## [v1.0.1](https://github.com/ljharb/gopd/compare/v1.0.0...v1.0.1) - 2022-11-01
### Commits
- [Fix] actually export gOPD instead of dP [`4b624bf`](https://github.com/ljharb/gopd/commit/4b624bfbeff788c5e3ff16d9443a83627847234f)
## v1.0.0 - 2022-11-01
### Commits
- Initial implementation, tests, readme [`0911e01`](https://github.com/ljharb/gopd/commit/0911e012cd642092bd88b732c161c58bf4f20bea)
- Initial commit [`b84e33f`](https://github.com/ljharb/gopd/commit/b84e33f5808a805ac57ff88d4247ad935569acbe)
- [actions] add reusable workflows [`12ae28a`](https://github.com/ljharb/gopd/commit/12ae28ae5f50f86e750215b6e2188901646d0119)
- npm init [`280118b`](https://github.com/ljharb/gopd/commit/280118badb45c80b4483836b5cb5315bddf6e582)
- [meta] add `auto-changelog` [`bb78de5`](https://github.com/ljharb/gopd/commit/bb78de5639a180747fb290c28912beaaf1615709)
- [meta] create FUNDING.yml; add `funding` in package.json [`11c22e6`](https://github.com/ljharb/gopd/commit/11c22e6355bb01f24e7fac4c9bb3055eb5b25002)
- [meta] use `npmignore` to autogenerate an npmignore file [`4f4537a`](https://github.com/ljharb/gopd/commit/4f4537a843b39f698c52f072845092e6fca345bb)
- Only apps should have lockfiles [`c567022`](https://github.com/ljharb/gopd/commit/c567022a18573aa7951cf5399445d9840e23e98b) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/gopd/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/gopd/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1540
} |
Subsets and Splits