problem_id
stringlengths 32
32
| name
stringclasses 1
value | problem
stringlengths 200
14k
| solutions
stringlengths 12
1.12M
| test_cases
stringlengths 37
74M
| difficulty
stringclasses 3
values | language
stringclasses 1
value | source
stringclasses 7
values | num_solutions
int64 12
1.12M
| starter_code
stringlengths 0
956
|
---|---|---|---|---|---|---|---|---|---|
01ac2a8f983de4294cb668172eab3df5 | UNKNOWN | Number is a palindrome if it is equal to the number with digits in reversed order.
For example, 5, 44, 171, 4884 are palindromes and 43, 194, 4773 are not palindromes.
Write a method `palindrome_chain_length` which takes a positive number and returns the number of special steps needed to obtain a palindrome. The special step is: "reverse the digits, and add to the original number". If the resulting number is not a palindrome, repeat the procedure with the sum until the resulting number is a palindrome.
If the input number is already a palindrome, the number of steps is 0.
Input will always be a positive integer.
For example, start with 87:
87 + 78 = 165;
165 + 561 = 726;
726 + 627 = 1353;
1353 + 3531 = 4884
4884 is a palindrome and we needed 4 steps to obtain it, so `palindrome_chain_length(87) == 4` | ["def palindrome_chain_length(n):\n steps = 0\n while str(n) != str(n)[::-1]:\n n = n + int(str(n)[::-1])\n steps += 1\n return steps", "def palindrome_chain_length(n, count=0):\n if str(n) == str(n)[::-1]: return count\n else: return palindrome_chain_length(n + int(str(n)[::-1]), count+1)", "def is_palindrome(n):\n return str(n) == str(n)[::-1]\n \n\ndef palindrome_chain_length(n):\n # parameter n is a positive integer\n # your function should return the number of steps\n steps = 0\n while not is_palindrome(n):\n steps += 1\n n += int(str(n)[::-1])\n return steps\n", "def palindrome_chain_length(n, times=0):\n reversed = int(str(n)[::-1])\n if reversed == n:\n return times\n return palindrome_chain_length(n + reversed, times + 1)", "def palindrome_chain_length(n):\n count = 0\n while str(n) != str(n)[::-1]: \n count += 1\n n += int(str(n)[::-1])\n \n return count\n \n \n", "def palindrome_chain_length(n):\n # parameter n is a positive integer\n # your function should return the number of steps\n steps = 0\n while True:\n reverse_n = int(str(n)[::-1])\n if n == reverse_n:\n return steps\n break\n else:\n n += reverse_n\n steps += 1", "def palindrome_chain_length(n):\n return n != int(str(n)[::-1]) and palindrome_chain_length(n + int(str(n)[::-1])) + 1", "from itertools import takewhile, accumulate, repeat\ndef palindrome_chain_length(n):\n return len(list(takewhile(lambda x: str(x) != str(x)[::-1], accumulate(repeat(n), lambda m, _: m + int(str(m)[::-1])))))", "def palindrome_chain_length(n):\n counter = 0\n while str(n) != str(n)[::-1]:\n n += int(str(n)[::-1])\n counter += 1\n if counter > 0: return counter\n return 0", "def palindrome_chain_length(num):\n steps = 0\n while True:\n tmp = str(num)\n rev = tmp[::-1]\n if tmp == rev:\n return steps\n num += int(rev)\n steps += 1\n", "palindrome_chain_length=lambda n,i=0:i if str(n)==str(n)[::-1] else palindrome_chain_length((n+int(str(n)[::-1])),i+1)", "from functools import lru_cache\n\n@lru_cache(maxsize=None)\ndef palindrome_chain_length(n):\n s1 = str(n)\n s2 = s1[::-1]\n return s1 != s2 and 1 + palindrome_chain_length(n + int(s2))", "def palindrome_chain_length(n, step=0):\n return step if str(n) == str(n)[::-1] else palindrome_chain_length(n + int(str(n)[::-1]), step+1)", "def palindrome(n):\n s = str(n)\n return s == s[::-1]\n\ndef palindrome_chain_length(n):\n n_chain = 0\n while not palindrome(n):\n n += int(str(n)[::-1])\n n_chain += 1\n return n_chain", "def palindrome_chain_length(n):\n # parameter n is a positive integer\n # your function should return the number of steps\n i = 0\n while True:\n if n == int(str(n)[::-1]): \n return i\n n += int(str(n)[::-1])\n i += 1", "def palindrome_chain_length(n):\n ct = 0\n while str(n) != str(n)[::-1]:\n n += int(str(n)[::-1])\n ct += 1\n return ct", "def palindrome_chain_length(n):\n steps = 0\n value = str(n)\n while value != value[::-1]:\n value = str(int(value) + int(value[::-1]))\n steps += 1\n return steps", "def palindrome_chain_length(n):\n def calculate_palindrome(steps, n):\n if str(n)[::-1] == str(n):\n return steps\n else:\n return calculate_palindrome(steps+1, n+int(str(n)[::-1]))\n return calculate_palindrome(0, n)", "def palindrome_chain_length(n):\n counter, last_num = 0, n\n\n def reverse_num(a_num):\n return str(a_num)[::-1]\n\n def is_palindrome(sum_num):\n return reverse_num(sum_num) == str(sum_num)\n\n while not is_palindrome(last_num):\n last_num = last_num + int(reverse_num(last_num))\n counter += 1\n \n return counter", "def palindrome_chain_length(n):\n return 0 if int(str(n)[::-1]) == n else palindrome_chain_length(int(str(n)[::-1]) + n) + 1\n", "def palindrome_chain_length(n):\n def is_palindrome(n):\n return str(n) == str(n)[::-1]\n def rev(n):\n return int(str(n)[::-1])\n steps = 0\n while not is_palindrome(n):\n n, steps = n+rev(n), steps+1\n return steps", "def palindrome_chain_length(n):\n x = 0\n while n != int(str(n)[::-1]):\n n = n + int(str(n)[::-1])\n x += 1\n return x\n", "from itertools import takewhile\ndef palindrome_chain_length(n):\n def next_palindrome(m):\n while 1:\n yield m\n m = m + int(str(m)[::-1])\n return len(list(takewhile(lambda x: str(x) != str(x)[::-1], next_palindrome(n))))", "def palindrome_chain_length(n):\n count=0\n\n while str(n)!=str(n)[::-1]:\n revn = int(str(n)[::-1])\n n=revn+n\n count+=1\n\n return count", "def palindrome_chain_length(n):\n counter = 0\n x = str(n)[::-1]\n while str(n) != x:\n n = n + int(x)\n x = str(n)[::-1]\n counter += 1\n return counter", "def palindrome_chain_length(n):\n steps = 0\n\n while str(n) != str(n)[::-1]:\n n += int(str(n)[::-1])\n steps += 1\n \n return steps", "def palindrome_chain_length(n):\n digits_reversed = lambda n: int(str(n)[::-1])\n\n steps = 0\n last_n = n\n last_n_rev = digits_reversed(last_n)\n\n while last_n != last_n_rev:\n last_n += last_n_rev\n last_n_rev = digits_reversed(last_n)\n steps += 1\n\n return steps", "palindrome_chain_length = lambda n: (lambda rev: 0 if n == rev(n) else palindrome_chain_length(n + rev(n)) + 1)(lambda n: int(''.join(list(reversed(str(n))))))\n\n", "def reverse_int(n: int) -> int:\n return int(str(n)[::-1])\n\ndef palindrome_chain_length(n: int, i: int = 0) -> int:\n return i if reverse_int(n) == n else palindrome_chain_length(n + reverse_int(n), i + 1)", "def palindrome_chain_length(n):\n a = 0\n x = str(n)\n while n != int(x[::-1]):\n x = str(n)\n n = n + int(x[::-1])\n x = str(n)\n a = a+1\n return a", "def palindrome_chain_length(n):\n n=str(n)\n if(n==n[::-1]):\n return 0\n return 1+ palindrome_chain_length( int(n)+int(n[::-1]))", "def palindrome_chain_length(n):\n return 0 if n==int(str(n)[::-1]) else 1 + palindrome_chain_length(n+int(str(n)[::-1]))\n", "def palindrome_chain_length(n):\n a=0\n while 1:\n if n==int(str(n)[::-1]):return a \n else:n+=int(str(n)[::-1])\n a+=1\n \n \n", "def palindrome_chain_length(n):\n num, s = 0, str(n)\n while s != s[::-1]:\n num += 1\n s = str( int(s) + int(s[::-1]) )\n return num\n", "def palindrome_chain_length(n):\n cnt=0\n while(str(n)!=str(n)[::-1]):\n n=n+int(str(n)[::-1])\n cnt=cnt+1\n return cnt", "def palindrome_chain_length(n):\n # parameter n is a positive integer\n return 0 if str(n)[::-1] == str(n) else 1 + palindrome_chain_length(n + int(str(n)[::-1]))", "import math\n\ndef is_palindrome(n):\n rev = 0\n num = n # save origin number\n while (n > 0):\n dig = math.floor(n % 10)\n rev = rev * 10 + dig\n n = math.floor(n / 10)\n return True if num == rev else False\n\ndef reverse(n):\n if n<0:\n return None\n rev = 0\n while (n > 0):\n dig = math.floor(n % 10)\n rev = rev * 10 + dig\n n = math.floor(n / 10)\n return rev\n\ndef palindrome_chain_length(n):\n # parameter n is a positive integer\n # your function should return the number of steps\n step=0\n while not (is_palindrome(n)):\n n+=reverse(n)\n step+=1\n return step", "palindrome_chain_length=lambda n,c=0: (lambda pal: c if n==pal else palindrome_chain_length(n+pal,c+1))(int(str(n)[::-1]))", "def palindrome_chain_length(n):\n is_palindrome = lambda x: str(x) == str(x)[::-1]\n res = 0\n while not is_palindrome(n):\n n += int(str(n)[::-1])\n res += 1\n return res", "def palindrome_chain_length(n):\n count = 0\n while (int(str(n)[::-1]) != n):\n n = n + int(str(n)[::-1])\n count += 1\n return count", "def palindrome_chain_length(n):\n if int(str(n)[::-1]) == n:\n return 0\n else:\n return palindrome_chain_length(n + int(str(n)[::-1])) +1", "def is_palindrome(n):\n string = str(n)\n reverse = int(''.join(reversed(string)))\n if n == reverse:\n return True\n else:\n return False\n \ndef palindrome_chain_length(n):\n i = 0\n while True:\n if is_palindrome(n):\n break\n else: \n n += int(''.join(reversed(str(n))))\n i += 1\n return i", "def palindrome_chain_length(n):\n count = 0\n num = str(n)\n rev_num = str(n)[::-1]\n sum = 0\n \n while(num != rev_num):\n num = str(int(num) + int(rev_num))\n rev_num = num[::-1]\n count += 1\n \n return count", "def palindrome_chain_length(n):\n sum = 0\n count = 0\n print(('The number is ' + str(n) ))\n new_num = (str(n)[::-1])\n if str(n) == str(n)[::-1]:\n return 0\n else:\n while str(n) != str(sum):\n \n sum = n + int(new_num)\n n = sum\n new_num = (str(sum)[::-1])\n sum = int(new_num)\n count += 1\n \n return count\n", "def palindrome_chain_length(n):\n # parameter n is a positive integer\n # your function should return the number of steps\n steps = 0\n if str(n) == str(n)[::-1]:\n return steps\n else:\n while str(n) != str(n)[::-1]:\n n += int(str(n)[::-1])\n steps += 1\n return steps", "def palindrome_chain_length(n):\n pal = False\n c = 0\n while not pal:\n if str(n) == str(n)[::-1]:\n return c\n else:\n n= int(n+int(str(n)[::-1]))\n c+=1", "palindrome_chain_length = lambda n:0 if str(n)==str(n)[::-1] else (lambda l:([None for i in iter(lambda:(([None for l[1] in [l[1]+int(str(l[1])[::-1])]],[None for l[0] in [l[0]+1]]),str(l[1])[::-1]!=str(l[1]))[1],False)],l[0])[1])({0:0,1:n})", "def palindrome_chain_length(n):\n step = 0\n while str(n)[::] != str(n)[::-1]:\n n += int(str(n)[::-1])\n step += 1\n return step", "def is_palindrome(n):\n return str(n)==str(n)[::-1]\n\ndef palindrome_chain_length(n):\n out=0\n while not is_palindrome(n):\n out+=1\n n+=int(str(n)[::-1])\n return out", "def is_palindrome(v):\n return str(v) == str(v)[::-1]\n\ndef palindrome_chain_length(n):\n cnt = 0\n \n if is_palindrome(n):\n return cnt\n\n while(True):\n cnt += 1\n n = n + int(str(n)[::-1])\n if is_palindrome(n):\n return cnt", "def palindrome_chain_length(n):\n rev_n = int((str(n)[::-1]))\n if n == rev_n: return 0\n return 1 + palindrome_chain_length(n + int((str(n)[::-1])))", "def palindrome_chain_length(n):\n count = 0\n while int(str(n)[::-1]) != n:\n count += 1\n n += int(str(n)[::-1])\n return count\n", "def palindrome_chain_length(n):\n count = 0\n# n = str[n]\n# m = n [::-1]\n while str(n) != str(n)[::-1]:\n n += int(str(n)[::-1])\n count += 1\n \n return count", "def palindrome_chain_length(n):\n count = 0\n# n = str (n)\n# m = n[:: -1]\n while str(n)!= str(n)[::-1]:\n n += int(str(n)[::-1])\n count +=1\n return count", "def palindrome_chain_length(n):\n count = 0\n check = str(n)\n while check != check[::-1]:\n print(check)\n print(count)\n count += 1\n check = str(int(check) + int(check[::-1]))\n return count", "def palindrome_chain_length(n):\n a = n\n r = 0\n while a != int(str(a)[::-1]):\n a += int(str(a)[::-1])\n r += 1\n return r", "def palindrome_chain_length(n):\n # parameter n is a positive integer\n # your function should return the number of steps\n strn = str(n)\n if strn == strn[::-1]:\n return 0\n else:\n count = 0\n value = strn\n while value[::-1] != value:\n value = str(int(value[::-1]) + int(value))\n count += 1\n return count", "def palindrome_chain_length(n):\n \n x = n\n i = 0\n \n while str(x) != str(x)[::-1]:\n \n x = x + int(str(x)[::-1])\n i = i + 1\n \n return i\n", "def palindrome_chain_length(n):\n run = 0\n word = str(n)\n revers = word[::-1]\n while word != revers:\n word = str(int(word) + int(word[::-1]))\n revers = word[::-1]\n run += 1\n return run\n", "def is_palindrome(n):\n return str(n) == str(n)[::-1]\n\ndef palindrome_chain_length(n):\n if is_palindrome(n):\n return 0\n step = 0\n while not is_palindrome(n):\n rev = int(str(n)[::-1])\n n = n + rev\n step += 1\n return step", "def palindrome_chain_length(n):\n x = list(str(n))\n result = 0\n while x != x[::-1]:\n n = n + int(''.join(x[::-1]))\n x = list(str(n))\n \n result +=1\n return result", "def palindrome_chain_length(n):\n steps = 0\n while True:\n n_str = str(n)\n if n_str == n_str[::-1]:\n return steps\n else:\n n += int(n_str[::-1])\n steps += 1", "def palindrome_chain_length(total):\n counter = 0\n reverse_total = str(total)[::-1]\n while total != int(reverse_total):\n total = total + int(reverse_total)\n reverse_total = str(total)[::-1]\n counter +=1\n return counter", "def palindrome_chain_length(n):\n c = 0\n rev = int(str(n)[::-1])\n while n != rev:\n n = n + rev\n rev = int(str(n)[::-1])\n c += 1\n return c", "def palindrome_chain_length(x):\n \n def f(x):\n return str(int(x) + int(\"\".join(list(reversed(x)))))\n \n def isPalindrome(s): \n return s == s[::-1] \n \n n = 0\n x = str(x)\n while True:\n \n if isPalindrome(x):\n return n\n \n else:\n x = f(x)\n n = n + 1", "def palindrome_chain_length(n):\n count = 0\n while True:\n rev = int(str(n)[::-1])\n if n == rev:\n return count\n else:\n n+=rev\n count+=1", "def palindrome_chain_length(n):\n \n n1 = str(n)\n n1 = n1[::-1]\n n1 = int(n1)\n x= 0\n \n while n != n1:\n \n n+=n1\n n1 = str(n)\n n1 = n1[::-1]\n n1 = int(n1)\n \n x+=1\n \n return x", "def palindrome_chain_length(n):\n c= 0\n if str(n) != str(n)[::-1]:\n while str(n) != str(n)[::-1]:\n n+= int(str(n)[::-1])\n c+=1\n return c \n else:\n return 0", "def palindrome_chain_length(n):\n c = 0\n rn = int(str(n)[::-1])\n while rn != n:\n n += rn\n rn = int(str(n)[::-1])\n c += 1\n return c", "def palindrome_chain_length(n):\n level = 0\n while n != int(str(n)[::-1]):\n n += int(str(n)[::-1])\n level += 1\n return level", "def palindrome_chain_length(n, step = 0):\n reversed = int(str(n)[::-1])\n if n == reversed:\n return step\n else:\n return palindrome_chain_length(n + reversed, step + 1)", "def palindrome_chain_length(n):\n c,x=0,n\n while str(x)!=str( x) [::-1]:\n x= x+int (str(x)[::-1])\n c=c+1\n return c\n \n \n \n \n", "def palindrome_chain_length(n):\n s = str(n)\n s_rev = s[::-1]\n \n count = 0\n \n while s != s[::-1]:\n temp = int(s)\n rev = int(s[::-1])\n test = temp+rev\n s = str(test)\n count += 1\n \n return count\n \n \n", "def palindrome_chain_length(n):\n steps = 0\n while str(n) != str(n)[::-1]:\n n = int(n) + int(str(n)[::-1])\n steps +=1\n return steps\n", "def palindrome_chain_length(n):\n n = str(n)\n nreverserd = n[::-1]\n count = 0\n if n == nreverserd:\n return 0\n else:\n while True:\n count += 1\n sum = int(n) + int(nreverserd)\n n = str(sum)\n nreverserd = n[::-1]\n if n == nreverserd:\n break\n\n return count", "def palindrome_chain_length(n):\n reverse = int(str(n)[::-1])\n length = 0\n \n while (n != reverse):\n n += reverse\n reverse = int(str(n)[::-1])\n length += 1\n \n return length", "def is_palindrome(x):\n return str(x) == str(x)[::-1]\n\ndef palindrome_chain_length(n):\n counter = 0\n while not is_palindrome(n):\n n = n + int(str(n)[::-1])\n counter += 1\n return counter", "def palindrome_chain_length(n):\n n1 = int(str(n)[::-1])\n return 0 if n==n1 else 1 + palindrome_chain_length(n+n1)\n", "def palindrome_chain_length(n):\n steps = 0\n string = str(n)\n while string != string[::-1]:\n n += int(string[::-1])\n string = str(n)\n steps += 1\n return steps", "def palindrome_chain_length(n):\n x = 0\n \n while list(str(n)) != list(str(n))[::-1]:\n n += int(''.join(list(str(n))[::-1]))\n x += 1\n \n return x", "def is_palindrome(n):\n return n == reverse_n(n)\n\ndef reverse_n(n):\n return int(str(n)[::-1])\n\ndef palindrome_chain_length(n):\n chain_n = 0\n while is_palindrome(n) is False:\n n = n + reverse_n(n)\n chain_n += 1\n return(chain_n)", "def IsPalindrome(n):\n if str(n) == str(n)[::-1]:\n return True\n return False\n\ndef palindrome_chain_length(n):\n result = 0\n # parameter n is a positive integer\n # your function should return the number of steps\n while not IsPalindrome(n):\n n = n + int(str(n)[::-1])\n result += 1\n\n return result", "def palindrome_chain_length(n, cr = 0):\n while (str(n)!=str(n)[::-1]):\n n, cr = n + int(str(n)[::-1]), cr + 1\n return cr\n\n", "def is_palindrome(n):\n return str(n) == str(n)[::-1]\n\ndef palindrome_chain_length(n):\n c = 0\n while not is_palindrome(n):\n a, b = n, int(str(n)[::-1])\n n = a + b\n c += 1\n return c\n", "def is_palindrome(n):\n n = str(n)\n if len(n)%2:\n return n[:len(n)//2] == n[len(n)//2+1:][::-1]\n else:\n return n[:len(n)//2] == n[len(n)//2:][::-1]\n\ndef palindrome_chain_length(n):\n if n<10: return 0\n if is_palindrome(n): return 0\n else:\n i = 0\n while not is_palindrome(n):\n i += 1\n n = n + int(str(n)[::-1])\n return i", "def palindrome_chain_length(n):\n def reverse(x):\n return int(str(x)[::-1])\n \n def is_palindrome(x):\n return x == reverse(x)\n \n steps = 0\n while not is_palindrome(n):\n n += reverse(n)\n steps += 1\n\n # parameter n is a positive integer\n # your function should return the number of steps\n return steps", "def palindrome_chain_length(n):\n if str(n) == str(n)[::-1]: return 0\n res, count = n, 0\n while True:\n count += 1\n res += int(str(res)[::-1])\n if str(res) == str(res)[::-1]:\n return count\n return count", "def palindrome_chain_length(n):\n n = str(n)\n if int(n) == int(str(n)[::-1]):\n return 0\n else:\n s = int(n) + int(n[::-1])\n count = 1\n while s != int(str(s)[::-1]):\n s = s + int(str(s)[::-1])\n count += 1\n\n return count", "def palindrome_chain_length(n):\n # parameter n is a positive integer\n # your function should return the number of steps\n step = 0\n while True:\n r = reverse(n)\n if n == r: break\n n += r\n step += 1\n return step\n \ndef reverse(n):\n ans = 0\n while n > 0:\n ans = 10*ans + n%10\n n //= 10\n return ans", "def palindrome_chain_length(n):\n s=str(n)\n for i in range(0,50):\n if s==s[::-1]:\n return i\n else:\n s=str(int(s)+int(s[::-1]))", "def palindrome_chain_length(n):\n\n number = n\n i = 0\n while i < 10000:\n number = str(number)\n if number == number[::-1]:\n return i\n else:\n number = int(number) + int(number[::-1])\n i = i + 1", "def palindrome_chain_length(n):\n return [4,0,0,24,1][[87,1,88,89,10].index(n)]", "def palindrome_chain_length(n):\n # parameter n is a positive integer\n # your function should return the number of steps\n n_str = str(n)\n steps = 0\n \n while n_str != n_str[::-1]:\n steps += 1\n palindrome = int(n_str) + int(n_str[::-1])\n n_str = str(palindrome)\n \n return steps", "def palindrome_chain_length(n):\n pal = int(str(n)[::-1])\n res = 0\n while n != pal:\n n += pal\n pal = int(str(n)[::-1])\n res += 1\n return res", "def palindrome_chain_length(n,s=0):\n if str(n) == str(n)[::-1]:\n return s\n else:\n return palindrome_chain_length(n+int(str(n)[::-1]),s+1)", "def palindrome_chain_length(n):\n answer = []\n pal = int(str(n)[::-1])\n while n != pal:\n n += pal\n pal = int(str(n)[::-1])\n answer.append(n)\n return len(answer)", "def palindrome_chain_length(n):\n loop = 0\n while str(n) != str(n)[::-1]:\n n += int(str(n)[::-1])\n loop += 1\n return loop\n"] | {"fn_name": "palindrome_chain_length", "inputs": [[87], [1], [88], [89], [10]], "outputs": [[4], [0], [0], [24], [1]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 21,849 |
def palindrome_chain_length(n):
|
f80f6ac6817410669d7708c3bda27ec5 | UNKNOWN | ## Check Digits
Some numbers are more important to get right during data entry than others: a common example is product codes.
To reduce the possibility of mistakes, product codes can be crafted in such a way that simple errors are detected. This is done by calculating a single-digit value based on the product number, and then appending that digit to the product number to arrive at the product code.
When the product code is checked, the check digit value is stripped off and recalculated. If the supplied value does not match the recalculated value, the product code is rejected.
A simple scheme for generating self-check digits, described here, is called Modulus 11 Self-Check.
## Calculation method
Each digit in the product number is assigned a multiplication factor. The factors are assigned ***from right to left***, starting at `2` and counting up. For numbers longer than six digits, the factors restart at `2` after `7` is reached. The product of each digit and its factor is calculated, and the products summed. For example:
```python
digit : 1 6 7 7 0 3 6 2 5
factor : 4 3 2 7 6 5 4 3 2
--- --- --- --- --- --- --- --- ---
4 + 18 + 14 + 49 + 0 + 15 + 24 + 6 + 10 = 140
```
Then the sum of the products is divided by the prime number `11`. The remainder is inspected, and:
* if the remainder is `0`, the check digit is also `0`
* if the remainder is `1`, the check digit is replaced by an uppercase `X`
* for all others, the remainder is subtracted from `11`
The result is the **check digit**.
## Your task
Your task is to implement this algorithm and return the input number with the correct check digit appended.
## Examples
```python
input: "036532"
product sum = 2*2 + 3*3 + 5*4 + 6*5 + 3*6 + 0*7 = 81
remainder = 81 mod 11 = 4
check digit = 11 - 4 = 7
output: "0365327"
``` | ["from itertools import cycle\n\ndef add_check_digit(number):\n fact = cycle([2,3,4,5,6,7])\n r = sum( int(c) * next(fact) for c in number[::-1]) % 11\n return number + ('0' if not r else 'X' if r == 1 else str(11-r))", "from itertools import cycle\n\n\ndef add_check_digit(s):\n rem = 11 - sum(int(a) * b for a, b in zip(\n reversed(s), cycle(range(2, 8)))) % 11\n return '{}{}'.format(s, {10: 'X', 11: 0}.get(rem, rem))\n", "def add_check_digit(n):\n rem = sum((i % 6 + 2) * int(d) for i, d in enumerate(n[::-1])) % 11\n return f\"{n}{'X' if rem == 1 else 11 - rem if rem else 0}\"", "def add_check_digit(n):\n s = sum(int(n[-1 - i])*(i % 6 + 2) for i in range(len(n)))\n return n + [str(11-s%11),['X','0'][s%11==0]][s%11<2]", "from itertools import cycle\n\ndef add_check_digit(number):\n it = cycle(range(2, 8))\n rem = sum(int(n) * i for n, i in zip(reversed(number), it)) % 11\n return number + '0X987654321'[rem]", "def add_check_digit(number):\n checksum = sum(int(d) * (2, 3, 4, 5, 6, 7)[i % 6] \\\n for i, d in enumerate(reversed(number)))\n checkdigit = 11 - (checksum % 11)\n return number + str({11: 0, 10: 'X'}.get(checkdigit, checkdigit))", "add_check_digit=lambda s:s+'0X987654321'[sum((i%6+2)*int(d)for i,d in enumerate(s[::-1]))%11]", "def add_check_digit(n):\n rem = sum((i % 6 + 2) * int(d) for i, d in enumerate(n[::-1])) % 11\n return f\"{n}{'0X987654321'[rem]}\"", "from itertools import cycle\nfrom operator import mul\n\ndef add_check_digit(number):\n x = sum(map(mul, cycle(range(2, 8)), map(int, str(number)[::-1]))) % 11\n return number + ('0' if x==0 else 'X' if x==1 else str(11-x))"] | {"fn_name": "add_check_digit", "inputs": [["036532"], ["12388878"], ["111111111"], ["9735597355"], ["2356"], ["6789"]], "outputs": [["0365327"], ["123888782"], ["1111111118"], ["97355973550"], ["23566"], ["6789X"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,685 |
def add_check_digit(number):
|
371905b0b855d6badf88098f85c37b77 | UNKNOWN | # Introduction
Fish are an integral part of any ecosystem. Unfortunately, fish are often seen as high maintenance. Contrary to popular belief, fish actually reduce pond maintenance as they graze on string algae and bottom feed from the pond floor. They also make very enjoyable pets, providing hours of natural entertainment.
# Task
In this Kata you are fish in a pond that needs to survive by eating other fish. You can only eat fish that are the same size or smaller than yourself.
You must create a function called fish that takes a shoal of fish as an input string. From this you must work out how many fish you can eat and ultimately the size you will grow to.
# Rules
1. Your size starts at 1
2. The shoal string will contain fish integers between 0-9
3. 0 = algae and wont help you feed.
4. The fish integer represents the size of the fish (1-9).
5. You can only eat fish the same size or less than yourself.
6. You can eat the fish in any order you choose to maximize your size.
7 You can and only eat each fish once.
8. The bigger fish you eat, the faster you grow. A size 2 fish equals two size 1 fish, size 3 fish equals three size 1 fish, and so on.
9. Your size increments by one each time you reach the amounts below.
# Increase your size
Your size will increase depending how many fish you eat and on the size of the fish.
This chart shows the amount of size 1 fish you have to eat in order to increase your size.
Current size
Amount extra needed for next size
Total size 1 fish
Increase to size
1
4
4
2
2
8
12
3
3
12
24
4
4
16
40
5
5
20
60
6
6
24
84
7
Please note: The chart represents fish of size 1
# Returns
Return an integer of the maximum size you could be.
# Example 1
You eat the 4 fish of size 1 (4 * 1 = 4) which increases your size to 2
Now that you are size 2 you can eat the fish that are sized 1 or 2.
You then eat the 4 fish of size 2 (4 * 2 = 8) which increases your size to 3
```python
fish("11112222") => 3
```
# Example 2
You eat the 4 fish of size 1 (4 * 1 = 4) which increases your size to 2
You then eat the remainding 8 fish of size 1 (8 * 1 = 8) which increases your size to 3
```python
fish("111111111111") => 3
```
Good luck and enjoy!
# Kata Series
If you enjoyed this, then please try one of my other Katas. Any feedback, translations and grading of beta Katas are greatly appreciated. Thank you.
Maze Runner
Scooby Doo Puzzle
Driving License
Connect 4
Vending Machine
Snakes and Ladders
Mastermind
Guess Who?
Am I safe to drive?
Mexican Wave
Pigs in a Pen
Hungry Hippos
Plenty of Fish in the Pond
Fruit Machine
Car Park Escape | ["def fish(shoal):\n eaten, size, target = 0, 1, 4\n for f in sorted(map(int, shoal)):\n if f > size: break\n eaten += f\n if eaten >= target:\n size += 1\n target += 4 * size\n return size", "from collections import Counter\n\ndef fish(shoal):\n size, eaten = 1, 0\n for fishesSize,n in sorted( Counter(map(int, shoal)).items() ):\n eaten += fishesSize * n if fishesSize <= size else 0\n size = 1 + int( ((1+2*eaten)**.5 - 1)/2 )\n return size", "def fish(shoal, size = 1, protein_earned = 0):\n fishes = [int(fish) for fish in shoal if fish != '0']\n grow = True\n while grow:\n edible_fishes = list(filter(lambda x: x <= size, fishes))\n ate_protein = sum(edible_fishes)\n protein_earned += ate_protein\n if protein_earned >= protein_to_grow(size):\n size += 1\n fishes = [fish for fish in fishes if fish not in edible_fishes]\n else:\n grow = False\n return size\n\ndef protein_to_grow(actual_size):\n protein_to_accumulate = 0\n while actual_size > 0:\n protein_to_accumulate += actual_size * 4\n actual_size -= 1\n return protein_to_accumulate", "def fish(shoal):\n size = 1\n while(count_lesser_fish(shoal, size) >= 2*size*(size + 1)):\n size += 1\n return size\n \ndef count_lesser_fish(shoal, size):\n return sum(int(f) for f in shoal if int(f) <= size)", "def fish(shoal):\n fishes = sorted(shoal)\n my_size = 1\n amount_for_next_size = 4\n \n for i in range(len(fishes)):\n if int(fishes[i]) > my_size: # break condition\n break\n amount_for_next_size -= int(fishes[i])\n if amount_for_next_size <= 0:\n my_size += 1\n amount_for_next_size += my_size * 4 \n return my_size \n\n", "def fish(shoal):\n size, eaten = 1, 0\n for i in range(1, 10):\n if size < i:\n break\n eaten += i * shoal.count(str(i))\n size = max(size, int(((2 * eaten + 1) ** 0.5 - 1) / 2) + 1)\n return size", "\n#All data associated to the fish \nclass Fish:\n size = 1 #Determines what the fish can eat\n intake = 0 #determines when fish grows in size\n#---end class Fish\n\n\ndef attemptDigestion(myFish, menuItem):\n if menuItem <= myFish.size: #Scenario: Eat the current menue item\n myFish.intake += menuItem\n#---end function attemptDigestion\n\n#Based on rules of the game, we grow (in size by 1) when what we have intaked is larger than or equal to the \n# size of our fish. The cost to increase in size is equal to four times the current size of our fish\n# e.g. intake ==14, size is 3. The req to size 4 is 12 units, so our size increases by 1 and our\n# intake is reduced as: 14 - 4*3 = 2 (intake is now 2; we burnt 12 units to inc in size :)\ndef assessGrowth(myFish):\n if myFish.intake >= 4*myFish.size: #Situation: the fish is ready to grow in size 1\n myFish.intake = myFish.intake - 4*myFish.size #deplete the amount it cost us to inc. in size \n myFish.size+=1\n#---end fuction assessGrowth\n\ndef fish(str):\n #Convert the string of fish-sizes to a list\n foodPool = [] \n foodPool[:0] = str\n foodPool.sort() #ascending order\n\n myFish = Fish()\n\n #Begin the eating and evolving game\n while foodPool and int(foodPool[0]) <= myFish.size:\n menuItem = int(foodPool.pop(0))\n attemptDigestion(myFish, menuItem) \n #determine if we need to level up based on mySize and the intake\n assessGrowth(myFish)\n\n #----end while\n\n return myFish.size\n\n#---end function fishGame\n", "from collections import Counter\n\ndef fish(shoal):\n count = Counter(map(int, shoal))\n \n size, eaten, step = 1, 0, 4\n for n in range(1, 10):\n if size >= n:\n eaten += count[n] * n\n while eaten >= step:\n eaten -= step\n size += 1\n step += 4\n else:\n break\n \n return size", "from collections import Counter\n\ndef fish(shoal):\n c = Counter(map(int, shoal.replace('0', '')))\n consumed = 0\n for size in range(1, 99999):\n consumed += size * c[size]\n if consumed < size * (size+1) * 2:\n return size", "def fish(shoal):\n shoal_l = []\n for s in shoal:\n shoal_l.append(int(s))\n shoal_l.sort()\n \n max_size = 1\n food_count = 0\n amount_needed = 4\n \n for fishy in shoal_l:\n if max_size >= fishy:\n food_count += int(fishy) \n if food_count >= amount_needed:\n max_size += 1 \n amount_needed += (max_size*4)\n \n return max_size\n"] | {"fn_name": "fish", "inputs": [[""], ["0"], ["6"], ["1111"], ["11112222"], ["111122223333"], ["111111111111"], ["111111111111111111112222222222"], ["151128241212192113722321331"]], "outputs": [[1], [1], [1], [2], [3], [4], [3], [5], [5]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,791 |
def fish(shoal):
|
c1fd298107c99c75abc6a32d749171f6 | UNKNOWN | # Task
You got a `scratch lottery`, you want to know how much money you win.
There are `6` sets of characters on the lottery. Each set of characters represents a chance to win. The text has a coating on it. When you buy the lottery ticket and then blow it off, you can see the text information below the coating.
Each set of characters contains three animal names and a number, like this: `"tiger tiger tiger 100"`. If the three animal names are the same, Congratulations, you won the prize. You will win the same bonus as the last number.
Given the `lottery`, returns the total amount of the bonus.
# Input/Output
`[input]` string array `lottery`
A string array that contains six sets of characters.
`[output]` an integer
the total amount of the bonus.
# Example
For
```
lottery = [
"tiger tiger tiger 100",
"rabbit dragon snake 100",
"rat ox pig 1000",
"dog cock sheep 10",
"horse monkey rat 5",
"dog dog dog 1000"
]```
the output should be `1100`.
`"tiger tiger tiger 100"` win $100, and `"dog dog dog 1000"` win $1000.
`100 + 1000 = 1100` | ["def scratch(lottery):\n return sum(int(n) for lot in lottery for a,b,c,n in [lot.split()] if a==b==c)", "def scratch(lottery):\n return sum(int(i.split()[-1]) for i in lottery if len(set(i.split()))==2)", "def scratch(l):\n c = 0\n for i in l:\n i = i.split()\n if i[0] == i[1] == i[2]:\n c += int(i[3])\n return c", "import re\ndef scratch(lottery):\n return sum(int(i[1]) for i in re.findall(r'([A-Za-z]+ )\\1\\1([0-9]*)',\"-\".join(lottery)))", "def scratch(lottery):\n result = 0\n for ticket in lottery:\n words = ticket.split()\n if words[0] == words[1] == words[2]:\n result += int(words[3])\n return result\n", "def scratch(lottery):\n ans = 0\n for ticket in lottery:\n t = ticket.split()\n if t.count(t[0]) == 3:\n ans += int(t[3])\n return ans", "def scratch(lottery):\n return sum(win(*line.split()) for line in lottery)\n\ndef win(a, b, c, d):\n return int(d) if a == b == c else 0", "scratch=lambda l:sum(int(a[3])for a in(r.split()for r in l)if len({*a})<3)", "def scratch(lottery):\n return sum(int(s.split()[-1]) for s in lottery if len(set(s.split()[:-1])) == 1)", "def scratch(L):\n return sum(int(d)*(a==b==c) for x in L for a,b,c,d in [x.split()])", "def scratch(L):\n z=0\n for x in L:\n a,b,c,d=x.split()\n z+=int(d)*(a==b==c)\n return z", "def scratch(lottery):\n #your code here\n sum = 0\n\n for x in lottery:\n j = x.split()\n if j[0] == j[1] == j[2]:\n sum += int(j[3])\n return sum", "def scratch(lottery):\n return sum([int(i.split()[-1]) for i in lottery if len(set(i.split()[:-1]))==1])", "def scratch(lottery): ##\n return sum(int(n) for combi in lottery for a,b,c,n in [combi.split()]if a==b==c)", "def scratch(lottery):\n return sum(int(combi.split()[-1]) for combi in lottery if combi.split()[0]==combi.split()[1]==combi.split()[2]) or 0", "def scratch(lottery):\n r = 0\n for s in lottery:\n *a,b = s.split()\n if len(set(a))==1:\n r += int(b)\n return r", "from re import sub\n\ndef scratch(lottery):\n return sum(int(sub(r\"[^\\d]\",\"\",ch)) for ch in lottery if len(set(ch.split())) == 2)", "def scratch(lottery):\n s=0\n for set in lottery:\n a,b,c,d=set.split()\n if a==b==c:s+=int(d)\n return s", "def scratch(lottery):\n return sum(int(i.split()[3]) for i in lottery if i.split()[0] == i.split()[1] == i.split()[2])", "def scratch(lottery):\n\n c = 0\n \n for i in range(0,len(lottery)):\n \n lo = lottery[i].split()\n \n print(lo)\n \n if all(x == lo[0] for x in lo[0:-1]):\n \n c+= int(lo[-1])\n \n return c\n\n"] | {"fn_name": "scratch", "inputs": [[["tiger tiger tiger 100", "rabbit dragon snake 100", "rat ox pig 1000", "dog cock sheep 10", "horse monkey rat 5", "dog dog dog 1000"]]], "outputs": [[1100]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,804 |
def scratch(lottery):
|
76093916332178f9734d18c895b4df9d | UNKNOWN | The description is rather long but it tries to explain what a financing plan is.
The fixed monthly payment for a fixed rate mortgage is the amount paid by the borrower every month that ensures
that the loan is paid off in full with interest at the end of its term.
The monthly payment formula is based on the annuity formula.
The monthly payment `c` depends upon:
- `rate` - the monthly interest rate is expressed as a decimal, not a percentage.
The monthly rate is simply the **given** yearly percentage rate divided by 100 and then by 12.
- `term` - the number of monthly payments, called the loan's `term`.
- `principal` - the amount borrowed, known as the loan's principal (or `balance`).
First we have to determine `c`.
We have: `c = n /d` with `n = r * balance` and `d = 1 - (1 + r)**(-term)` where `**` is the `power` function (you can look at the reference below).
The payment `c` is composed of two parts. The first part pays the interest (let us call it `int`)
due for the balance of the given month, the second part repays the balance (let us call this part `princ`) hence for the following month we get a `new balance = old balance - princ` with `c = int + princ`.
Loans are structured so that the amount of principal returned to the borrower starts out small and increases with each mortgage payment.
While the mortgage payments in the first years consist primarily of interest payments, the payments in the final years consist primarily of principal repayment.
A mortgage's amortization schedule provides a detailed look at precisely what portion of each mortgage payment is dedicated to each component.
In an example of a $100,000, 30-year mortgage with a rate of 6 percents the amortization schedule consists of 360 monthly payments.
The partial amortization schedule below shows with 2 decimal floats
the balance between principal and interest payments.
--|num_payment|c |princ |int |Balance |
--|-----------|-----------|-----------|-----------|-----------|
--|1 |599.55 |99.55 |500.00 |99900.45 |
--|... |599.55 |... |... |... |
--|12 |599.55 |105.16 |494.39 |98,771.99 |
--|... |599.55 |... |... |... |
--|360 |599.55 |596.57 |2.98 |0.00 |
# Task:
Given parameters
```
rate: annual rate as percent (don't forgent to divide by 100*12)
bal: original balance (borrowed amount)
term: number of monthly payments
num_payment: rank of considered month (from 1 to term)
```
the function `amort` will return a formatted string:
`"num_payment %d c %.0f princ %.0f int %.0f balance %.0f" (with arguments num_payment, c, princ, int, balance`)
# Examples:
```
amort(6, 100000, 360, 1) ->
"num_payment 1 c 600 princ 100 int 500 balance 99900"
amort(6, 100000, 360, 12) ->
"num_payment 12 c 600 princ 105 int 494 balance 98772"
```
# Ref | ["def amort(rate, bal, term, num_payments):\n monthlyRate = rate / (12 * 100)\n c = bal * (monthlyRate * (1 + monthlyRate) ** term) / (((1 + monthlyRate) ** term) - 1)\n newBalance = bal\n for i in range(num_payments):\n interest = newBalance * monthlyRate\n princ = c - interest\n newBalance = newBalance - princ\n return 'num_payment %s c %.0f princ %.0f int %.0f balance %.0f' % (num_payments, c, princ, interest, newBalance)", "def amort(rate, bal, term, num_payments):\n r = rate / (100 * 12)\n n = r * bal\n d = 1 - (1 + r) ** (-term)\n c = n / d\n for i in range(num_payments):\n int_ = r * bal\n princ = c - int_ \n bal -= princ\n return \"num_payment %d c %.0f princ %.0f int %.0f balance %.0f\" % (num_payments, c, princ, int_, bal)", "def calc_balance(r, n, bal, c):\n return (1 + r)**n * bal - (((1+r)**n - 1) / r) * c\n\ndef amort(rate, bal, term, num_payment):\n r = rate / 100 / 12\n n = r * bal\n d = 1 - (1 + r)**(-term)\n c = n/d\n\n new_balance = calc_balance(r, num_payment, bal, c)\n old_balance = calc_balance(r, num_payment - 1, bal, c)\n princ = old_balance - new_balance\n intr = c - princ\n \n return \"num_payment {} c {:.0f} princ {:.0f} int {:.0f} balance {:.0f}\".format(num_payment, c, princ, intr, new_balance)", "def amort(rate, bal, term, num_payments):\n # monthly interest rate\n r = rate / 1200\n # monthly payment\n c = bal * (r / (1 - (1 + r)**(-term)))\n # balance last month\n b = bal * (1 + r)**(num_payments - 1) - c * (((1 + r)**(num_payments - 1) - 1) / r)\n # interest\n i = r * b\n # amortization\n a = c - i\n # new_balance\n b -= a\n return 'num_payment {} c {:.0f} princ {:.0f} int {:.0f} balance {:.0f}'.format(num_payments, c, a, i, b)", "def amort(rate, bal, term, num_payments):\n # your code\n r = rate / (100 * 12)\n c = (r * bal) / (1 - pow((1 + r), -term))\n \n int = 0\n princ = 0\n for n in range(num_payments):\n int = r * bal\n princ = c - int\n bal -= princ\n \n return \"num_payment {:0.0f} c {:0.0f} princ {:0.0f} int {:0.0f} balance {:0.0f}\".format(num_payments, c, princ, int, bal)", "def amort(rate, bal, term, num):\n c = bal*rate/1200/(1-(1+rate/1200)**(-term))\n for i in range(num):\n int = bal*rate/1200\n bal = bal + int - c\n return \"num_payment %d c %.0f princ %.0f int %.0f balance %.0f\" % (num,c,c-int,int,bal)", "def amort(rate, bal, term, num_payments):\n r=rate/(1200)\n c=r*bal/(1-(1+r)**(-term))\n for _ in range(num_payments):\n _int=bal*r\n princ=c-_int\n bal-=princ\n return 'num_payment {} c {:.0f} princ {:.0f} int {:.0f} balance {:.0f}'.format(num_payments,c,princ,_int,bal)", "def amort(r,p,n,x):\n r = r/12/100\n c = (r*p) / (1-((1+r)**(-n)))\n curr = p*(1+r)**x-((1+r)**(x)-1)*(c/r)\n i = r*(p*(1+r)**(x-1)-((1+r)**(x-1)-1)*(c/r))\n return f'num_payment {x} c {round(c)} princ {round(c-i)} int {round(i)} balance {round(curr)}'", "amort=lambda r,b,t,n,c=0,p=0,i=0,m=-1:m<0and amort(r/1200,b,t,n,r/1200*b/(1-(1+r/1200)**-t),p,i,0)or m==n and\"num_payment %d c %.0f princ %.0f int %.0f balance %.0f\"%(n,c,p,i,b)or amort(r,r*b+b-c,t-1,n,c,c-r*b,r*b,m+1)", "def amort(r,b,t,n):\n r/=1200\n c=r*b/(1-(1+r)**-t)\n for i in range(n):I,p,b=r*b,c-r*b,r*b+b-c\n return \"num_payment %d c %.0f princ %.0f int %.0f balance %.0f\"%(n,c,p,I,b)"] | {"fn_name": "amort", "inputs": [[7.4, 10215, 24, 20], [7.9, 107090, 48, 41], [6.8, 105097, 36, 4], [3.8, 48603, 24, 10], [1.9, 182840, 48, 18], [1.9, 19121, 48, 2], [2.2, 112630, 60, 11], [5.6, 133555, 60, 53], [9.8, 67932, 60, 34], [3.7, 64760, 36, 24], [4.6, 85591, 36, 5], [7.0, 168742, 48, 16], [9.6, 17897, 60, 23], [5.2, 53521, 60, 51], [6.8, 139308, 60, 38], [5.0, 182075, 24, 9], [8.0, 128263, 36, 26], [7.8, 112414, 60, 37], [4.9, 93221, 36, 5], [1.2, 146157, 48, 20], [3.6, 168849, 24, 11], [9.3, 87820, 60, 14], [8.7, 155744, 36, 31], [6.4, 179023, 36, 11], [8.3, 38495, 36, 15], [9.6, 150614, 24, 19], [2.5, 159363, 48, 19], [4.7, 56245, 48, 11], [1.5, 142777, 36, 32], [9.6, 187340, 60, 28], [2.4, 57707, 24, 14], [3.0, 180678, 36, 31], [2.0, 139586, 24, 13], [5.3, 142498, 60, 46], [9.0, 150309, 48, 29], [7.1, 60232, 60, 56], [8.7, 172214, 36, 14], [9.0, 187174, 60, 12], [9.5, 119722, 60, 23], [9.2, 10536, 36, 15], [1.9, 70139, 24, 13], [4.5, 141454, 36, 23], [4.5, 154486, 24, 7], [2.2, 20254, 36, 35], [3.7, 114512, 36, 23], [8.6, 121685, 48, 24], [1.1, 171542, 48, 25], [1.1, 121991, 48, 20], [8.9, 162862, 60, 46], [1.9, 152921, 60, 19]], "outputs": [["num_payment 20 c 459 princ 445 int 14 balance 1809"], ["num_payment 41 c 2609 princ 2476 int 133 balance 17794"], ["num_payment 4 c 3235 princ 2685 int 550 balance 94447"], ["num_payment 10 c 2106 princ 2009 int 98 balance 28799"], ["num_payment 18 c 3959 princ 3769 int 189 balance 115897"], ["num_payment 2 c 414 princ 384 int 30 balance 18353"], ["num_payment 11 c 1984 princ 1810 int 174 balance 92897"], ["num_payment 53 c 2557 princ 2464 int 93 balance 17571"], ["num_payment 34 c 1437 princ 1153 int 283 balance 33532"], ["num_payment 24 c 1903 princ 1829 int 75 balance 22389"], ["num_payment 5 c 2550 princ 2256 int 294 balance 74397"], ["num_payment 16 c 4041 princ 3335 int 706 balance 117641"], ["num_payment 23 c 377 princ 278 int 98 balance 12025"], ["num_payment 51 c 1015 princ 972 int 43 balance 8939"], ["num_payment 38 c 2745 princ 2411 int 335 balance 56634"], ["num_payment 9 c 7988 princ 7474 int 514 balance 115917"], ["num_payment 26 c 4019 princ 3736 int 283 balance 38758"], ["num_payment 37 c 2269 princ 1942 int 327 balance 48320"], ["num_payment 5 c 2790 princ 2449 int 341 balance 81077"], ["num_payment 20 c 3120 princ 3031 int 89 balance 86109"], ["num_payment 11 c 7302 princ 7002 int 300 balance 92965"], ["num_payment 14 c 1836 princ 1277 int 559 balance 70807"], ["num_payment 31 c 4931 princ 4722 int 209 balance 24127"], ["num_payment 11 c 5479 princ 4771 int 708 balance 127911"], ["num_payment 15 c 1212 princ 1041 int 170 balance 23607"], ["num_payment 19 c 6922 princ 6599 int 323 balance 33796"], ["num_payment 19 c 3492 princ 3281 int 211 balance 98178"], ["num_payment 11 c 1288 princ 1110 int 178 balance 44271"], ["num_payment 32 c 4058 princ 4033 int 25 balance 16183"], ["num_payment 28 c 3944 princ 3032 int 912 balance 110949"], ["num_payment 14 c 2465 princ 2411 int 54 balance 24381"], ["num_payment 31 c 5254 princ 5176 int 78 balance 26076"], ["num_payment 13 c 5938 princ 5821 int 117 balance 64670"], ["num_payment 46 c 2709 princ 2535 int 173 balance 36695"], ["num_payment 29 c 3740 princ 3221 int 519 balance 66007"], ["num_payment 56 c 1196 princ 1161 int 35 balance 4712"], ["num_payment 14 c 5452 princ 4618 int 835 balance 110505"], ["num_payment 12 c 3885 princ 2694 int 1191 balance 156135"], ["num_payment 23 c 2514 princ 1863 int 651 balance 80372"], ["num_payment 15 c 336 princ 284 int 52 balance 6495"], ["num_payment 13 c 2981 princ 2925 int 56 balance 32478"], ["num_payment 23 c 4208 princ 3993 int 215 balance 53292"], ["num_payment 7 c 6743 princ 6304 int 439 balance 110852"], ["num_payment 35 c 582 princ 580 int 2 balance 581"], ["num_payment 23 c 3366 princ 3224 int 142 balance 42823"], ["num_payment 24 c 3005 princ 2514 int 491 balance 66044"], ["num_payment 25 c 3655 princ 3575 int 79 balance 83139"], ["num_payment 20 c 2599 princ 2531 int 68 balance 71813"], ["num_payment 46 c 3373 princ 3019 int 354 balance 44694"], ["num_payment 19 c 2674 princ 2502 int 172 balance 106057"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,512 |
def amort(rate, bal, term, num_payments):
|
44088e6221f30534b2c229cdaffc8a46 | UNKNOWN | Every natural number, ```n```, may have a prime factorization like:
We define the **geometric derivative of n**, as a number with the following value:
For example: calculate the value of ```n*``` for ```n = 24500```.
```
24500 = 2²5³7²
n* = (2*2) * (3*5²) * (2*7) = 4200
```
Make a function, ```f``` that can perform this calculation
```
f(n) ----> n*
```
Every prime number will have ```n* = 1```.
Every number that does not have an exponent ```ki```, higher than 1, will give a ```n* = 1```, too
```python
f(24500) == 4200
f(997) == 1
```
Do your best! | ["def f(n):\n res = 1\n i = 2\n while n != 1:\n k = 0\n while n % i == 0:\n k += 1\n n //= i\n if k != 0:\n res *= k * i**(k-1)\n i += 1\n return res", "def f(n):\n p, n_, m = 2, 1, int(n ** .5)\n while n > 1 and p <= m:\n k = 0\n while not n % p:\n k += 1\n n //= p\n if k:\n n_ *= k * p ** (k - 1)\n p += 1\n return n_", "from collections import Counter\n\ndef prime_factors(n):\n p = []\n while n % 2 == 0:\n p.append(2)\n n /= 2\n for i in range(3, int(n**.5)+1, 2):\n while n % i == 0:\n p.append(i)\n n /= i\n if n > 2: p.append(int(n))\n return p\n\ndef f(n):\n fac = Counter(prime_factors(n))\n re = 1\n for i in fac.keys():\n re *= fac[i] * (i**(fac[i]-1))\n return re", "import math\nimport numpy as np\ndef f(n):\n if set(primeFactors(n).values()) == {1}:\n return 1\n return np.prod([v*k**(v-1) for k,v in primeFactors(n).items()]) # (n_ = n*)\n \ndef primeFactors(n): \n ans = dict()\n cnt2 = 0 \n while n % 2 == 0:\n cnt2 += 1\n ans.update({2:cnt2}) \n n = n / 2 \n for i in range(3,int(math.sqrt(n))+1,2): \n cnti = 0\n while n % i== 0:\n cnti += 1\n ans.update({i:cnti}) \n n = n / i \n if n > 2: \n ans.update({n:1})\n return ans", "def f(n):\n factors = []\n i = 2\n while n > 1:\n if n % i == 0:\n n = n / i\n factors.append(i)\n else:\n i += 1\n \n from collections import Counter\n from numpy import prod\n return prod([occurences * factor ** (occurences - 1)\n for factor, occurences in Counter(factors).items()])", "def f(num):\n dic = {}\n while True:\n for i in range(2, int(num **0.5)+1):\n if num % i == 0:\n num /= i\n if i in dic:\n dic[i] += 1\n else:\n dic[i] = 1\n break\n else:\n if num in dic:\n dic[num] += 1\n else:\n dic[num] = 1\n break\n continue\n ans = 1\n for item in dic:\n ans *= dic[item] * item ** (dic[item] - 1)\n return ans", "def f(n):\n r=1; c=0; pf=primef(n); pf.append(-1); m=pf[0]\n for i in pf:\n if i==m: c+=1\n else: r*=c*m**(c-1); m=i; c=1 \n return r\n \ndef primef(n):\n i= 2; f= []\n while i*i<=n:\n if n%i: i+=1\n else: n//=i; f.append(i)\n if n>1: f.append(n)\n return f", "from functools import reduce\ndef f(n):\n facts = factorization(n)\n return mul(power * prime ** (power - 1) for prime, power in facts)\n\ndef mul(ar):\n return reduce(lambda x,y:x*y, ar)\n\ndef factorization(n):\n result = []\n i = 2\n while i * i <= n:\n if n % i == 0:\n power = 0\n while n % i == 0:\n power += 1\n n /= i\n result.append((i, power))\n i += 1\n if n > 1: result.append((n, 1))\n return result\n", "from collections import Counter\ndef f(n):\n i = 3\n sum = 1\n lists = []\n while (n/2).is_integer():\n lists.append(2)\n n //= 2\n while n != 1 and i <= n:\n \n while n % i == 0:\n lists.append(i)\n n //= i\n\n i += 2\n for i in dict(Counter(lists)):\n a = dict(Counter(lists))[i]\n sum *= (a*(i ** (a-1)))\n return sum"] | {"fn_name": "f", "inputs": [[24500], [997]], "outputs": [[4200], [1]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,632 |
def f(n):
|
7ef17727137c494382911da2b5b6611f | UNKNOWN | Finish the solution so that it sorts the passed in array of numbers. If the function passes in an empty array or null/nil value then it should return an empty array.
For example:
```python
solution([1,2,3,10,5]) # should return [1,2,3,5,10]
solution(None) # should return []
```
```Hakell
sortNumbers [1, 2, 10, 50, 5] = Just [1, 2, 5, 10, 50]
sortNumbers [] = Nothing
``` | ["def solution(nums):\n return sorted(nums) if nums else []", "solution = lambda l: sorted(l) if l else []\n", "def solution(nums):\n return sorted(nums or [])", "def solution(nums):\n return [] if nums is None else sorted(nums)", "def solution(nums):\n return sorted(nums) if isinstance(nums, list) else []", "def solution(nums):\n if not nums:\n return []\n return sorted(nums)", "def solution(nums):\n if nums == None:\n return []\n if len(nums) < 2:\n return nums\n pivo = nums[0]\n less = [i for i in nums[1:] if i < pivo]\n great = [i for i in nums[1:] if i > pivo]\n return solution(less) + [pivo] + solution(great) ", "def solution(nums):\n if nums != [] and nums != None:\n nums.sort()\n return nums\n else:\n return []", "def solution(nums):\n pass\n if nums != [] and nums != None:\n nums.sort()\n return nums\n else:\n if not nums:\n return []\n", "def solution(name):\n return sorted(name) if name else []", "solution = lambda a: list(sorted(a)) if a else []", "def solution(nums):\n return nums and sorted(nums) or []", "def solution(nums):\n if(nums) == None: return []\n nums.sort()\n return nums", "def solution(nums):\n return [] if not nums else sorted(nums)", "solution = lambda x : x and sorted(x) or []", "def solution(nums):\n array = []\n try:\n while len(nums) > 0:\n array.append(min(nums))\n nums.remove(min(nums)) \n except:\n pass\n return array", "def solution(n,q=[]):\n if n == None:\n return q\n o = []\n for i in n:\n o += chr(i)\n o.sort()\n p = []\n for i in o:\n p.append(ord(i))\n return p", "def solution(nums):\n if nums == None:\n return []\n if len(nums)==0:\n return []\n low = nums[0]\n other = nums[1:].copy()\n mlist = solution(other)\n niggers = []\n for i in mlist:\n if i < low:\n niggers.append(i)\n p = 0\n if len(niggers) != 0:\n p = min(niggers)\n mlist.insert(p,low) \n return mlist\n", "def solution(nums):\n if type(nums) != list: return []\n nums.sort()\n return nums", "def solution(nums):\n if nums == None or nums == []:\n return []\n else: \n return sorted(nums)", "def solution(nums):\n print(nums)\n horizontal=[]\n vertical=[]\n if not nums or nums is None:\n return []\n else:\n return sorted(nums)", "def solution(nums):\n if nums != None:\n return list(set(nums))\n else:\n return []", "def solution(nums):\n if nums!=None:\n nums.sort()\n return nums\n else:\n return list()\n \n", "def solution(nums):\n if nums is None:\n return []\n sorted_nums = sorted(nums)\n if len(sorted_nums) > 0:\n return sorted_nums\n else:\n return []", "def solution(nums: list) -> list:\n \"\"\" This function sorts the passed in array of numbers. \"\"\"\n if nums is None:\n return []\n return sorted(nums)", "def solution(nums):\n l = []\n if nums == None:\n return l\n else:\n return sorted(nums)\n \n", "def solution(array):\n return sorted(array) if array is not None else []\n", "def solution(nums):\n if nums == None:\n return []\n elif len(nums) < 1:\n return []\n else:\n return sorted(nums)", "def solution(nums):\n if None != nums:\n nums.sort()\n return nums\n return []", "def solution(nums):\n return [*sorted(nums)] if nums else []", "def solution(nums):\n if nums is None:\n return []\n X = sorted(nums)\n return X", "def solution(nums):\n '''We will handle the anticipated num argument with an if/else statement'''\n return sorted(nums) if nums!=None else []", "def solution(nums):\n car=[]\n if nums==None:\n return car\n else:\n return sorted(nums)", "def solution(nums=[]):\n try:\n if len(nums) > 0:\n nums.sort()\n return nums\n elif len(nums) == 0:\n return []\n except:\n return []\n", "def solution(nums=[]):\n return [] if nums is None else sorted(nums)", "def solution(nums):\n if type(nums) !=list: \n return [] \n \n if len(nums)==0:\n return []\n return sorted(nums)", "def solution(nums=0):\n if nums == []:\n return []\n elif nums == None:\n return []\n else:\n return sorted(nums)", "def solution(nums):\n new_list = []\n while nums:\n min = nums[0] \n for x in nums: \n if x < min:\n min = x\n new_list.append(min)\n nums.remove(min) \n return new_list", "def solution(array):\n return [] if array is None else sorted(array)", "def solution(nums):\n if type(nums) == type(None):\n return []\n else:\n return sorted(nums)", "def solution(nums):\n return ([[] if nums == None else sorted(nums)][0])\n# if nums == [] or nums == None:\n# return []\n# else:\n# return sorted(nums)\n \n", "def solution(nums):\n emptyarr = []\n if nums == None:\n return []\n else:\n return sorted(nums)\n", "def solution(nums):\n badList = nums\n if badList == None:\n a=[]\n return a\n if len(badList)<2:\n return badList\n else:\n length = len(badList) - 1\n unsorted = True\n while unsorted:\n for element in range(0,length):\n unsorted = False\n if badList[element] > badList[element + 1]:\n hold = badList[element + 1]\n badList[element + 1] = badList[element]\n badList[element] = hold\n else:\n unsorted = True \n return badList", "def solution(nums):\n if nums == None:\n return []\n else:\n nums_sorted = sorted(nums)\n return nums_sorted\n", "def solution(nums):\n # Bust a move right here\n if nums==None: return []\n else: return sorted(nums)\n", "def solution(nums):\n if nums == None: return []\n else:\n x = []\n for i in range(len(nums)):\n x.append(min(nums))\n nums.remove(min(nums))\n return x", "def solution(a = []):\n try:\n for i in range(len(a)-1):\n for j in range(len(a)-i-1):\n if a[j] > a[j+1]:\n a[j], a[j+1] = a[j+1], a[j]\n return a\n except TypeError:\n a = []\n return a", "def solution(nums):\n list1 = []\n check = type(nums)\n if check == list:\n return sorted(nums)\n else:\n return list1\n \n", "def solution(nums):\n return sorted([*nums]) if nums else []", "def solution(nums, default=None):\n if nums:\n nums.sort()\n return nums\n else:\n nums = []\n return nums", "def solution(nums):\n if nums == None:\n empty_arr = []\n return empty_arr\n nums.sort()\n return nums", "def solution(nums):\n numbers = nums\n print(numbers)\n \n if numbers == None:\n return []\n \n re_arranged = sorted(numbers)\n \n print(re_arranged)\n \n return re_arranged ", "def solution(nums: list) -> list:\n if nums != None:\n length = len(nums)\n if length > 0:\n return sorted(nums)\n else:\n return []\n else:\n return []", "def solution(nums):\n if not nums:\n return []\n else:\n for x in range(len(nums)):\n for i in range(len(nums) -1):\n if nums[i]>nums[i+1]:\n temp = nums[i]\n nums[i] = nums[i+1]\n nums[i+1] = temp\n return nums", "def solution(nums):\n l = []\n if nums == [] or nums == None:\n return []\n while nums:\n l.append(min(nums))\n nums.remove(min(nums))\n return l\n \n \n", "def solution(nums):\n if type(nums) == list:return sorted(nums)\n elif type(nums) != list:return []", "def solution(nums):\n lst=[]\n if nums == None:\n return lst\n nums = sorted(nums)\n return nums", "def solution(nums):\n if nums is None:\n return []\n else:\n return sorted(set(nums))\n", "def solution(nums = None):\n return [] if nums is None else sorted(nums)", "def solution(nums):\n a = []\n if nums == None:\n return []\n else:\n for x in nums:\n a.append(x)\n a.sort()\n return a", "def solution(nums):\n print(nums)\n if nums==None:\n return []\n\n try:\n return sorted(nums)\n except:\n return None", "def solution(nums):\n # nums.sort()\n # return nums\n if nums == None:\n return []\n elif len(nums) == 0:\n return []\n for i in range(0, len(nums)):\n print((nums[i]))\n for j in range(0, len(nums)-1):\n print((nums[j]))\n if nums[j] > nums[j+1]:\n temp = nums[j]\n nums[j] = nums[j+1]\n nums[j+1] = temp\n\n return nums\n\n", "def solution(nums):\n if nums:\n nums = [int(x) for x in nums]\n nums.sort()\n return nums\n else:\n return []", "def solution(nums):\n if type(nums) != list:\n return []\n else:\n return sorted(nums)", "def solution(nums):\n if nums != None:\n res = sorted(nums)\n return res\n else:\n return []", "def solution(nums=None):\n print(nums)\n if nums == None:\n return []\n else:\n return sorted(nums)", "def solution(nums=None):\n print(nums)\n if nums:\n return sorted(nums)\n elif nums == None:\n return []\n elif nums == []:\n return []\n", "def solution(nums):\n if nums is None:\n return []\n else:\n y = sorted(nums)\n return y", "def solution(nums):\n print(nums)\n \n if(nums == []):\n return []\n if(nums == [1, 2, 3, 10, 5]):\n return [1, 2, 3, 5, 10]\n if(nums == [1, 2, 10, 5]):\n return [1, 2, 5, 10]\n if(nums == [20, 2, 10]):\n return [2, 10, 20]\n if(nums == [2, 20, 10] ):\n return [2, 10, 20]\n if(nums == None):\n return []\n \n", "def solution(nums=None):\n return [] if nums==None else sorted(nums)", "def solution(nums):\n if type(nums)==list:\n return sorted(nums)\n else:\n empty = []\n return empty\n", "def solution(nums=None):\n if nums==None:\n return []\n return sorted(nums)", "def solution(nums= []):\n if nums == None or len(nums) == 0:\n return [];\n for i in range (len(nums)-1,0,-1):\n # print(i)\n for j in range(i):\n if nums[j] > nums[j+1]:\n helper = nums[j]\n nums[j] = nums[j+1]\n nums[j+1] = helper\n return nums\n# print(solution(nums))\n\n\n", "def solution(nums):\n if not nums or len(nums) == 0:\n return [] \n sorted = nums.copy()\n sorted.sort()\n return sorted", "def solution(nums):\n pass\n if nums is None:\n nums = []\n nums.sort()\n return nums", "def solution(nums):\n if nums == None:\n return []\n pulls = len(nums)\n if len(nums) == 0:\n return []\n li = []\n for i in range(pulls):\n p = 0\n for n in range(len(nums)):\n if nums[n] < nums[p]:\n p=n\n li.append(nums[p])\n nums = nums[:p] + nums[p+1:]\n return li", "def solution(nums):\n if nums == None:\n return []\n num1 = sorted(nums)\n return num1", "def solution(nums):\n if nums == [] or nums == None or nums == 0: return []\n return sorted(nums)", "def solution(nums):\n if nums!=None:\n nums.sort()\n if (nums!=[] and nums!=None):\n return nums\n else:\n return []", "def solution(nums):\n x = []\n if not nums:\n return x\n else:\n nums.sort()\n return nums\n", "def solution(nums=0):\n return [] if nums == None else sorted(nums)", "def solution(nums):\n if str(nums) == 'None':\n return []\n else:\n return sorted(nums)", "def solution(nums):\n return sorted(nums) if None is not nums else []", "def solution(nums=None):\n try:\n if nums == None:\n return []\n else:\n return sorted(nums)\n except:\n pass\n\nsolution([1,2,10,5])", "import pandas as pd\ndef solution(nums):\n df = pd.DataFrame()\n df['S']=nums\n answer = list(df['S'].sort_values().values)\n print (list(answer))\n return answer", "def solution(nums):\n if nums is not None:\n nums.sort()\n return(nums)\n if nums is None:\n return([])\n", "def solution(nums):\n if(nums):\n return sorted(list(nums))\n return []", "def solution(nums):\n if nums:\n x = sorted(nums)\n return(x)\n else:\n return []", "def solution(nums):\n\n List = []\n\n if nums != None:\n for num in range(len(nums)):\n minNum = min(nums)\n List.append(minNum)\n nums.remove(minNum)\n\n return List", "def solution(nums):\n list = nums\n if nums:\n insertion_sort(list)\n else:\n return []\n \n return list\n\n\ndef insertion_sort(A):\n for i in range(1, len(A)):\n key = A[i]\n j = i - 1\n while j >= 0 and A[j] > key:\n A[j + 1] = A[j]\n j -= 1\n A[j + 1] = key\n \n", "#More elegant:\ndef solution(nums):\n return (sorted(nums) if nums is not None else [])\n\n\n#More detailed:\ndef solution(nums):\n if nums is None:\n return []\n else:\n #nums.sort()\n return sorted(nums)", "def solution(nums):\n if nums is None:\n return []\n else:\n #nums.sort()\n return sorted(nums)", "def solution(nums):\n r=[]\n while nums:\n mini=min(nums)\n nums.remove(mini)\n r.append(mini)\n return r\n", "def solution(nums):\n num2 = []\n \n if nums == None:\n return num2\n else:\n return sorted(nums)", "\n\ndef solution(nums):\n nums3 = []\n if nums == None:\n return nums3\n else:\n return sorted(nums)", "def solution(nums):\n arr = []\n while nums:\n minimum = nums[0]\n for i in nums:\n if i < minimum:\n minimum = i\n arr.append(minimum)\n nums.remove(minimum)\n return arr", "def solution(nums):\n if (nums is not None):\n nums = sorted(nums)\n return nums\n else:\n return []", "def solution(nums):\n if nums== None: \n return []\n else:\n nums=list(set(nums))\n \n nums.sort()\n \n \n return nums", "def solution(nums):\n if nums == None: return []\n return [x for x in sorted(nums)]", "def solution(nums):\n if nums == [] or nums == None:\n nums = []\n else:\n nums.sort()\n return nums"] | {"fn_name": "solution", "inputs": [[[1, 2, 3, 10, 5]], [null], [[]], [[20, 2, 10]], [[2, 20, 10]]], "outputs": [[[1, 2, 3, 5, 10]], [[]], [[]], [[2, 10, 20]], [[2, 10, 20]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 15,350 |
def solution(nums):
|
2f9dce8e0fd1379e8b627b2c2ad63d15 | UNKNOWN | # Introduction:
Reversi is a game usually played by 2 people on a 8x8 board.
Here we're only going to consider a single 8x1 row.
Players take turns placing pieces, which are black on one side and white on the
other, onto the board with their colour facing up. If one or more of the
opponents pieces are sandwiched by the piece just played and another piece of
the current player's colour, the opponents pieces are flipped to the
current players colour.
Note that the flipping stops when the first piece of the player's colour is reached.
# Task:
Your task is to take an array of moves and convert this into a string
representing the state of the board after all those moves have been played.
# Input:
The input to your function will be an array of moves.
Moves are represented by integers from 0 to 7 corresponding to the 8 squares on the board.
Black plays first, and black and white alternate turns.
Input is guaranteed to be valid. (No duplicates, all moves in range, but array may be empty)
# Output:
8 character long string representing the final state of the board.
Use '*' for black and 'O' for white and '.' for empty.
# Examples:
```python
reversi_row([]) # '........'
reversi_row([3]) # '...*....'
reversi_row([3,4]) # '...*O...'
reversi_row([3,4,5]) # '...***..'
``` | ["import re\ndef reversi_row(moves):\n row = '........'\n stones = '*O'\n for i, m in enumerate(moves):\n L, M, R = row[:m], stones[i%2], row[m+1:]\n if R!='' and R[0] == stones[(i+1)%2] and R.find(stones[i%2])>0 and '.' not in R[:R.find(stones[i%2])]:\n R = R.replace(stones[(i+1)%2], stones[i%2], R.find(stones[i%2]))\n if L!='' and L[-1] == stones[(i+1)%2] and L[::-1].find(stones[i%2])>0 and '.' not in L[-1-L[::-1].find(stones[i%2]):]:\n L = L[::-1].replace(stones[(i+1)%2], stones[i%2], L[::-1].find(stones[i%2]))[::-1]\n\n row = L + M + R\n return row", "from re import sub\n\ndef reversi_row(moves):\n current = '.'*8\n for i,x in enumerate(moves):\n c1, c2 = \"Ox\" if i&1 else \"xO\"\n left = sub(r\"(?<={}){}+$\".format(c1, c2), lambda r: c1*len(r.group()), current[:x])\n right = sub(r\"^{}+(?={})\".format(c2, c1), lambda r: c1*len(r.group()), current[x+1:])\n current = left + c1 + right\n return current.replace('x', '*')", "def reversi_row(moves):\n row = ['.'] * 8\n for turn, move in enumerate(moves):\n tile = '*O'[turn % 2]\n row[move] = tile\n for direction in (-1, 1):\n other = move + direction\n while 0 <= other < 8 and row[other] != '.':\n if row[other] == tile:\n break\n other += direction\n else:\n other = move\n row[move: other: direction] = [tile] * abs(move - other)\n return ''.join(row)", "def reversi_row(moves):\n stones = [set(), set()]\n \n for i, p in enumerate(moves):\n curr, oppn = stones[i%2], stones[(i+1)%2]\n curr.add(p)\n \n l, r = p-1, p+1\n while l in oppn: l-=1\n while r in oppn: r+=1\n flip = set(range(l+1 if l in curr else p+1, r if r in curr else p))\n \n oppn -= flip\n curr |= flip\n \n return ''.join('*' if i in stones[0] else 'O' if i in stones[1] else '.' for i in range(8))", "from itertools import cycle\n\n\ndef reversi_row(moves):\n row = ['.'] * 8\n player = cycle('*O')\n for move in moves:\n current_player = next(player)\n invalid = {'.', current_player}\n row[move] = current_player\n row_str = ''.join(row)\n # look left\n first_left = row_str.rfind(current_player, 0, move)\n if first_left != -1:\n # flip to the left\n left_chunk = row_str[first_left + 1:move]\n if not invalid.intersection(left_chunk):\n row[first_left + 1:move] = [current_player] * len(left_chunk)\n # look right\n first_right = row_str.find(current_player, move + 1)\n if first_right != -1:\n # flip to the right\n right_chunk = row_str[move + 1:first_right]\n if not invalid.intersection(right_chunk):\n row[move + 1:first_right] = [current_player] * len(right_chunk)\n return ''.join(row)", "from functools import reduce\n\ndef reversi_row(moves):\n return ''.join(reduce(lambda board, x: play(board, x[1], 'O' if x[0]%2 else '*'), enumerate(moves), ['.'] * 8))\n\ndef play(board, position, pawn):\n board[position] = pawn\n opp = 'O' if pawn == '*' else '*'\n after = next((x for x in [opp*i+pawn for i in range(6)] if ''.join(board[position+1:]).startswith(x)), None)\n if after:\n board[position+1:position+len(after)] = [pawn]*(len(after)-1)\n before = next((x for x in [pawn+opp*i for i in range(6)] if ''.join(board[:position]).endswith(x)), None)\n if before:\n board[position-len(before):position] = [pawn]*len(before)\n return board", "from re import sub\n\ndef reversi_row(moves):\n base=\"........\"\n point=\"*\"\n for move in moves:\n base=list(base)\n base[move]=\"#\"\n base=\"\".join(base)\n base=sub(\"#O+\\*\", lambda m: \"*\"*len(m.group(0)), sub(\"\\*O+#\", lambda m: \"*\"*(len(m.group(0))-1)+\"#\",base)) if point==\"*\" else sub(\"#\\*+O\", lambda m: \"O\"*len(m.group(0)),sub(\"O\\*+#\", lambda m: \"O\"*(len(m.group(0))-1)+\"#\",base))\n base=base.replace(\"#\",point)\n point='O' if point==\"*\" else '*'\n return base", "def reversi_row(a):\n r = [\".\"] * 8\n for p, i in enumerate(a):\n cur, opp = \"*O\"[p%2], \"*O\"[1-p%2]\n r[i] = cur\n for range_ in (range(i - 1, -1, -1), range(i + 1, 8)):\n for j in range_:\n if r[j] == \".\":\n break\n if r[j] == cur:\n if abs(i - j) > 1:\n r[min(i, j):max(i, j)] = cur * abs(i - j)\n break\n return \"\".join(r)", "import re\ndef reversi_row(moves):\n res=\".\"*8\n star=False\n for move in moves:\n star=not star\n assert res[move]==\".\"\n res=res[:move]+\"@\"+res[move+1:]\n if star:\n res=re.sub(r\"(@)(O+)(\\*)\", lambda g:g[1]+\"*\"*len(g[2])+g[3],res)\n res=re.sub(r\"(\\*)(O+)(@)\", lambda g:g[1]+\"*\"*len(g[2])+g[3],res)\n res=res.replace(\"@\",\"*\")\n else: \n res=re.sub(r\"(@)(\\*+)(O)\", lambda g:g[1]+\"O\"*len(g[2])+g[3],res)\n res=re.sub(r\"(O)(\\*+)(@)\", lambda g:g[1]+\"O\"*len(g[2])+g[3],res)\n res=res.replace(\"@\",\"O\")\n return res"] | {"fn_name": "reversi_row", "inputs": [[[]], [[0]], [[0, 1]], [[0, 7, 4]], [[3]], [[3, 4]], [[3, 4, 5]], [[2, 1, 0]], [[0, 1, 4, 3, 2]], [[0, 1, 7, 2, 3]], [[3, 2, 7, 1, 0]], [[3, 4, 5, 6, 0, 2]], [[0, 1, 2, 3, 4, 5, 6, 7]], [[7, 0, 1]], [[0, 7, 6]], [[1, 0, 2, 3, 4, 5, 6, 7]], [[5, 1, 3, 4, 6]], [[1, 7, 0, 5, 6, 4]]], "outputs": [["........"], ["*......."], ["*O......"], ["*...*..O"], ["...*...."], ["...*O..."], ["...***.."], ["***....."], ["*****..."], ["****...*"], ["****...*"], ["*.OOOOO."], ["*******O"], ["O*.....*"], ["*.....*O"], ["OOOOOOOO"], [".O.*O**."], ["**..OO*O"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 5,374 |
def reversi_row(moves):
|
79517ebda9c084a5823aa8ee9b178849 | UNKNOWN | # Task
Given a string `str`, find the shortest possible string which can be achieved by adding characters to the end of initial string to make it a palindrome.
# Example
For `str = "abcdc"`, the output should be `"abcdcba"`.
# Input/Output
- `[input]` string `str`
A string consisting of lowercase latin letters.
Constraints: `3 ≤ str.length ≤ 10`.
- `[output]` a string | ["def build_palindrome(strng):\n n = 0\n while strng[n:] != strng[n:][::-1]: n += 1\n return strng + strng[:n][::-1]", "def build_palindrome(str):\n suf = \"\"\n for c in str:\n pal = str + suf\n if pal == pal[::-1]:\n return pal\n suf = c + suf", "def build_palindrome(s):\n return next(f\"{s}{s[:i][::-1]}\" for i in range(len(s)) if s[i:] == s[i:][::-1])\n", "def build_palindrome(s):\n xs = (s + s[:i][::-1] for i in range(len(s)))\n return next(x for x in xs if x == x[::-1])", "def build_palindrome(s):\n for i in range(len(s)):\n x = s + s[:i][::-1]\n if x == x[::-1]:\n return x", "def build_palindrome(s):\n for i in range(-len(s) - 1, 0):\n if s.endswith(s[:i:-1]):\n return s + s[i::-1]", "build_palindrome=lambda s:next(s+s[:i][::-1]for i in range(len(s)+1)if s[i:]==s[i:][::-1])", "def build_palindrome(s):\n if s == s[::-1]:\n return s\n else:\n for i in range(1,len(s)):\n if s + s[:i][::-1] == (s + s[:i][::-1])[::-1]:\n return s + s[:i][::-1]\n \n", "def build_palindrome(s):\n i = 1\n p = s\n while p != p[::-1]:\n p = s + s[0:i][::-1]\n i += 1\n return p\n", "def build_palindrome(s):\n p = []\n for i in range(len(s)):\n string = s + s[:i][::-1]\n if string == string[::-1]:\n p.append(string)\n return min(p, key=lambda i: len(i))\n"] | {"fn_name": "build_palindrome", "inputs": [["abcdc"], ["ababab"]], "outputs": [["abcdcba"], ["abababa"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,461 |
def build_palindrome(s):
|
1f4d529a29384327d7ca9faf4ba37c3f | UNKNOWN | John is developing a system to report fuel usage but needs help with the coding.
First, he needs you to write a function that, given the actual consumption (in l/100 km) and remaining amount of petrol (in l), will give you how many kilometers you'll be able to drive.
Second, he needs you to write a function that, given a distance (in km), a consumption (in l/100 km), and an amount of petrol (in l), will return one of the following: If you can't make the distance without refueling, it should return the message "You will need to refuel". If you can make the distance, the function will check every 100 km and produce an array with [1:kilometers already driven. 2: kilometers till end. 3: remaining amount of petrol] and return all the arrays inside another array ([[after 100km], [after 200km], [after 300km]...])
PLEASE NOTE: any of the values with decimals that you return should be rounded to 2 decimals. | ["def total_kilometers(cons, petrol):\n return round(100*petrol/cons, 2)\n\ndef check_distance(dist, cons, petrol):\n return (\"You will need to refuel\" if dist > total_kilometers(cons, petrol) else\n [ [n*100, dist-100*n, round(petrol-cons*n, 2)] for n in range(dist//100+1)])", "def total_kilometers(cons, petrol): \n distance = round(((petrol/cons) * 100), 2)\n return distance\n\n\ndef check_distance(distance, cons, petrol):\n outp = []\n track = 0\n driven_dist = 0\n if distance > ((petrol/cons) * 100):\n return 'You will need to refuel'\n while track <= distance:\n outp.append([driven_dist, distance - driven_dist, round(petrol, 2)])\n driven_dist += 100\n petrol -= cons\n track += 100\n return outp\n\n", "def total_kilometers(cons, petrol):\n return round(petrol * 100.0 / cons, 2)\n\ndef check_distance(distance, cons, petrol):\n if total_kilometers(cons, petrol) < distance:\n return 'You will need to refuel'\n return [[round(x, 2) for x in (i * 100, distance - i * 100, petrol - cons * i)] for i in range(int(distance / 100) + 1)]", "def total_kilometers(cons, petrol):\n return round(float(petrol) / cons * 100, 2)\n\ndef check_distance(distance, cons, petrol):\n if distance * cons > petrol * 100: return \"You will need to refuel\"\n return [[i, distance - i, round(petrol - i * cons / 100, 2)] for i in xrange(0, distance + 1, 100)]", "def total_kilometers(cons, petrol):\n return round(petrol * 100.0 / cons,2)\n\ndef check_distance(distance, cons, petrol):\n return [[(100 * n), distance - (100 * n), round(petrol - (cons * n),2)] for n in range(distance/100 + 1)] if total_kilometers(cons, petrol) >= distance else \"You will need to refuel\"", "def total_kilometers(cons, petrol):\n return round(petrol / float(cons) * 100, 2)\n\n\ndef check_distance(distance, cons, petrol):\n if total_kilometers(cons, petrol) < distance:\n return 'You will need to refuel'\n km_driven = 0\n result = []\n while distance >= 0:\n result.append([km_driven, distance, petrol])\n distance -= 100\n km_driven += 100\n petrol = round(petrol - cons, 2)\n return result\n", "def total_kilometers(cons, petrol):\n result = round(float(petrol) / float(cons) * 100.0, 2)\n print(f'total_kilometers({cons}, {petrol}) => {result}')\n return result\n\ndef check_distance(distance, cons, petrol):\n if petrol / cons * 100 < distance:\n return \"You will need to refuel\"\n \n result = [[0, distance, petrol]]\n \n fuel = petrol\n since_start = 0\n to_end = distance\n for _ in range(to_end // 100):\n fuel -= cons\n since_start += 100\n to_end -= 100\n result.append([since_start, to_end, round(fuel, 2)])\n print(f'check_distance({distance}, {cons}, {petrol}) => {result}')\n return result", "def total_kilometers(cons, petrol):\n return round(petrol/cons*100,2)\n\ndef check_distance(distance, cons, petrol):\n if total_kilometers(cons, petrol)<distance:\n return \"You will need to refuel\"\n return[[i*100,distance-i*100,round(petrol-cons*i,2)]for i in range(0,distance//100+1)]", "def total_kilometers(cons, petrol):\n return round(100*petrol/cons,2)\n\ndef check_distance(distance, cons, petrol):\n if total_kilometers(cons,petrol) < distance: return 'You will need to refuel'\n r = [[0,distance,petrol]]\n x = 0\n while distance >= 100:\n petrol -= cons\n distance -= 100\n x += 100\n r.append([x,distance,round(petrol,2)])\n return r", "def total_kilometers(cons, petrol):\n return round((petrol * 100)/ cons, 2)\n\ndef check_distance(distance, cons, petrol):\n if distance > (petrol * 100)/ cons:\n return \"You will need to refuel\"\n else:\n l = []\n length = 0\n for i in range(1 + distance//100):\n l.append([length, distance, round(petrol, 2)])\n length += 100\n distance -= 100\n petrol -= cons\n return l \n"] | {"fn_name": "total_kilometers", "inputs": [[10, 60], [8, 0], [6.4, 54], [9.3, 87.3], [11.7, 63.4]], "outputs": [[600], [0], [843.75], [938.71], [541.88]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,112 |
def total_kilometers(cons, petrol):
|
08c8eec0f7a1fe5247627f2d1e4e10fd | UNKNOWN | # Task
You are given `N` ropes, where the length of each rope is a positive integer. At each step, you have to reduce all the ropes by the length of the smallest rope.
The step will be repeated until no ropes are left. Given the length of N ropes, print the number of ropes that are left before each step.
# Example
For `a = [3, 3, 2, 9, 7]`, the result should be `[5, 4, 2, 1]`
```
You have 5 ropes:
3 3 2 9 7 ( 5 left)
step 1: 1 1 0 7 5 ( 4 left)
step 2: 0 0 0 6 4 ( 2 left)
step 3: 0 0 0 0 2 ( 1 left)
step 4: 0 0 0 0 0
Hence the result is [5, 4, 2, 1]```
# Input/Output
- `[input]` integer array `a`
length of each rope.
`3 <= a.length <= 2000`
`1 <= a[i] <= 100`
- `[output]` an integer array
number of ropes before each step. | ["def cut_the_ropes(arr):\n results = [len(arr)]\n while arr:\n m = min(arr)\n arr = [elem - m for elem in arr if elem != m]\n results.append(len(arr))\n return results[:-1]\n \n", "def cut_the_ropes(arr):\n qty = len(arr)\n sorted_ropes = sorted(arr)\n return [qty - sorted_ropes.index(n) for n in sorted(set(arr))]\n", "def cut_the_ropes(a):\n if not a:\n return []\n m = min(a)\n return [len(a)] + cut_the_ropes([x-m for x in a if x > m])", "def cut_the_ropes(a):\n result = []\n while a:\n result.append(len(a))\n m = min(a)\n a = [x-m for x in a if x > m]\n return result", "def cut_the_ropes(arr):\n res = []\n while arr:\n res, m = res + [len(arr)], min(arr)\n arr = [l - m for l in arr if l > m]\n return res", "def cut_the_ropes(arr):\n ans = []\n while arr:\n ans.append(len(arr))\n minRope = min(arr)\n arr = [elt - minRope for elt in arr if elt > minRope]\n return ans", "from itertools import accumulate, chain, groupby\n\ndef cut_the_ropes(arr):\n return list(accumulate(chain((len(arr),), groupby(sorted(arr))), lambda a, b: a - sum(1 for __ in b[1])))[:-1]", "from itertools import groupby\nfrom functools import reduce\n\ndef cut_the_ropes(arr):\n return reduce(lambda a, g: a + [a[-1] - sum(1 for __ in g[1])], groupby(sorted(arr)), [len(arr)])[:-1]", "def cut_the_ropes(arr):\n counts = []\n while arr:\n counts.append(len(arr))\n m = min(arr)\n arr = [rope-m for rope in arr if rope-m > 0]\n return counts", "def cut_the_ropes(a):\n r = []\n while a:\n r.append(len(a))\n n = min(a)\n a = [x - n for x in a if x != n]\n return r"] | {"fn_name": "cut_the_ropes", "inputs": [[[3, 3, 2, 9, 7]], [[1, 2, 3, 4, 3, 3, 2, 1]], [[13035, 6618, 13056, 20912, 1119, 13035, 6618, 6618, 8482, 13056]], [[9, 9, 9, 9, 7]]], "outputs": [[[5, 4, 2, 1]], [[8, 6, 4, 1]], [[10, 9, 6, 5, 3, 1]], [[5, 4]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,757 |
def cut_the_ropes(arr):
|
2c1a1edcbeadba6f23b3da1ffc7947cf | UNKNOWN | In this kata you will create a function that takes in a list and returns a list with the reverse order.
### Examples
```python
reverse_list([1,2,3,4]) == [4,3,2,1]
reverse_list([3,1,5,4]) == [4,5,1,3]
``` | ["def reverse_list(l):\n return l[::-1]", "def reverse_list(l):\n \"\"\"return a list with the reverse order of l\"\"\"\n return list(reversed(l))", "def reverse_list(l):\n l.reverse()\n return l", "def reverse_list(l):\n \"\"\" Returns reversed list. \"\"\"\n return list(reversed(l))", "def reverse_list(l):\n 'return a list with the reverse order of l'\n return l[::-1] # This was dumb", "reverse_list = lambda l: l[::-1]\n", "def reverse_list(l):\n return [r for r in reversed(l)]", "reverse_list = lambda l: l.reverse() or l\n", "def reverse_list(l):\n \"\"\"Return a list with the reverse order of l\"\"\"\n return l[::-1]", "def reverse_list(l):\n new_list = []\n for i in l:\n new_list = [i] + new_list\n return new_list", "def reverse_list(liste):\n liste.reverse()\n return liste", "reverse_list = lambda L: L[::-1]", "def reverse_list(l):\n return [l[-1]] + reverse_list(l[:-1]) if len(l) > 0 else []", "from typing import List\n\ndef reverse_list(l: List[int]) -> List[int]:\n \"\"\" Get a list with the reverse order. \"\"\"\n return l[::-1]", "def reverse_list(*l):\n x = []\n for x in l:\n x.reverse()\n print(x)\n return x", "def reverse_list(l):\n 'return a list with the reverse order of l'\n c = [0] * len(l)\n for i in range(len(l)):\n c[i] = l[len(l) - i - 1]\n return c", "def reverse_list(l):\n 'return a list with the reverse order of l'\n index = len(l)\n i = index -1\n temp = []\n while i!=-1:\n temp.append(l[i])\n i-=1\n return temp\n", "def reverse_list(l):\n c = -1\n d = []\n for i in l:\n d.append(l[c])\n c -= 1\n return d", "def reverse_list(l):\n #'return a list with the reverse order of l'\n re = []\n for i in range(0,len(l)):\n re.append(l.pop()) \n return re", "def reverse_list(l):\n return l[4::-1]", "def reverse_list(l):\n reverse = [l[-i] for i in range(1, len(l)+1)]\n return reverse", "def reverse_list(l):\n return l[-1:-(len(l) + 1):-1]", "def reverse_list(l):\n l_list = list(reversed(l))\n return l_list\n", "def reverse_list(l):\n output=[]\n for i in l:\n output=[i]+output\n return output", "def reverse_list(l):\n return [l[len(l)-1-n] for n in range(len(l))]", "def reverse_list(l):\n list1 = []\n length = len(l)\n for x in range(length - 1, -1, -1):\n list1.append(l[x])\n return list1\n", "reverse_list = lambda l: l[::-1]\n# 'return a list with the reverse order of l'\n", "def reverse_list(l):\n lista = list()\n for x in l:\n lista.insert(0,x)\n return lista", "def reverse_list(list_):\n return list_[::-1]", "def reverse_list(l):\n a = []\n for i in range(0, len(l)):\n a.append(l[-1 - i])\n return a", "def reverse_list(args):\n args.reverse()\n return args\n 'return a list with the reverse order of l'", "def reverse_list(l):\n x = []\n for i in range(1, len(l)+1):\n x.append(l[-i])\n return x", "def reverse_list(l):\n reversedList = []\n for i in reversed(l): \n reversedList.append(i)\n return reversedList", "def reverse_list(lst):\n reversed_lst = []\n \n for item in lst:\n reversed_lst.insert(0, item)\n \n return reversed_lst", "def reverse_list(l):\n return l[-1:-len(l)-1:-1]", "def reverse_list(l):\n m = []\n for x in reversed(l):\n m.append(x)\n return m", "def reverse_list(l):\n v=[];\n for i in reversed(l):\n v.append(i);\n return v;", "def reverse_list(l):\n ###'return a list with the reverse order of l'\n return l[::-1]", "def reverse_list(l):\n arr=[]\n for i in range(len(l)):\n arr.append(l[len(l)-1-i])\n return arr", "def reverse_list(l):\n start = 0\n end = len(l) - 1\n while(start < end):\n l[start], l[end] = l[end], l[start]\n start += 1\n end -= 1\n return l", "def reverse_list(l):\n rev = []\n up = len(l) - 1\n\n for i in range(up, -1, -1):\n rev.append(l[i])\n\n return rev", "def reverse_list(xs):\n return list(reversed(xs))", "def reverse_list(l):\n c = []\n for x in l[::-1]:\n if x != None:\n c.append(x)\n return c", "def reverse_list(l):\n answer = []\n for e in l:\n answer.insert(0, e)\n return answer", "def reverse_list(l):\n return [l[index] for index in range(-1,-len(l)-1,-1)]", "def reverse_list(l):\n arr = []\n if len(l) == 0:\n return arr\n for i in l:\n arr.append(i)\n arr.reverse()\n return arr", "reverse_list = lambda o : [i for i in reversed(o)]", "def reverse_list(l):\n res = l[::-1]\n return res", "def reverse_list(l):\n rl = l\n l.reverse()\n return l", "def reverse_list(l):\n newL=list()\n for i in l[::-1]:\n newL.append(i)\n return newL", "def reverse_list(l):\n 'return a list with the reverse order of l'\n return(list(reversed(l)))\n\nprint(reverse_list.__doc__)\n\nprint(reverse_list([1,2,3,4,444]))", "reverse_list = lambda l: l[::-1]\n\n# reverse_list = lambda l: list(reversed(l))\n\n# def reverse_list(l):\n# l.reverse()\n# return l\n", "def reverse_list(l):\n length = len(l)\n reversed_list = []\n index = -1\n print('len = ', length)\n print('reversed_list = ', reversed_list)\n \n for i in l:\n print(l[index])\n reversed_list.append(l[index])\n index -= 1\n return reversed_list", "def reverse_list(l):\n tab=[]\n for x in range(len(l)):\n tab.append(l[-x-1])\n \n return tab", "def reverse_list(l):\n return [ele for ele in reversed(l)] \n #l.sort()\n #return l.reverse()\n", "def reverse_list(l):\n \n# =============================================================================\n# This function reverses the order of items in a given list. If the given \n# list is empty, the function returns an empty list\n# \n# Example:\n# reverse_list([1,2,3,4]) ==> [4,3,2,1]\n# =============================================================================\n \n result = []\n \n if len (l) == 0:\n return result\n \n for item in l[::-1]:\n result.append(item)\n \n return (result)", "def reverse_list(l):\n updt = []\n for i in reversed(l):\n updt.append(i)\n return updt\n", "def reverse_list(l):\n 'return a list with the reverse order of l'\n list = []\n for items in l: # Pour chaque \"objet\" dans la liste, on l'insert dans la nouvelle liste \u00e0 la premi\u00e8re position\n list.insert(0, items)\n print(list)\n return list", "def reverse_list(l):\n# 'return a list with the reverse order of l'\n new_lst = l[::-1] \n return new_lst\n", "def reverse_list(lst):\n l = lst.reverse()\n return lst", "def reverse_list(l):\n# return l.reverse()\n return list(reversed(l))", "def reverse_list(l):\n# 'return a list with the reverse order of l'\n return l[::-1]\n\n\nl = [1, 2, 3, 4]\nprint(reverse_list(l))", "def reverse_list(l):\n i = 0\n size = len(l)\n while i < size:\n temp = l.pop(size-1-i)\n l.append(temp) \n i += 1\n return l", "def reverse_list(mylist):\n return mylist[::-1]", "def reverse_list(l):\n return l[::-1]\n \n \nl=[1,2,3,4,]", "def reverse_list(l):\n 'return a list with the reverse order of l'\n list.reverse(l)\n return l\n \n 'first kata and im so happy :)'", "def reverse_list(l):\n reversed_arr = []\n \n for i in range(len(l)):\n reversed_arr.append(l[len(l)- 1 - i])\n \n return reversed_arr", "def reverse_list(l):\n res = []\n for i in reversed(l): res.append(i)\n return res", "def reverse_list(l):\n 'return a list with the reverse order of l'\n result = []\n counter = 0\n while counter < len(l):\n result.append( l[ -1 - counter] )\n counter +=1\n return result \n \n", "def reverse_list(l):\n # There are 3 ways to reverse a list:\n # 1) return l[::-1]\n # 2) l.reverse()\n # return l\n # 3) \n return list(reversed(l))", "def reverse_list(ls):\n ls.reverse()\n return ls\n", "def reverse_list(l):\n x = []\n for item in l:\n x.insert(0, item)\n return x\n", "def reverse_list(l):\n return [x for x in reversed(l)]\nl=[1,2,3,4]\nprint((reverse_list(l)))\n", "def reverse_list(l):\n rs = []\n\n\n for n in l:\n print(n)\n rs.insert(0, n)\n\n\n return rs\n\n\n\n\nr = reverse_list([1, 2, 3, 4])\n\n\nprint(r)", "def reverse_list(l):\n return ([int(x) for x in l])[::-1]", "def reverse_list(l):\n rs = []\n for n in l:\n rs.insert(0, n)\n return rs", "def reverse_list(l):\n rs = []\n for n in l:\n rs.insert(0, n)\n return rs\n\n\nr = reverse_list([1,2,3,4])\n\nprint(r)", "def reverse_list(l):\n return l[::-1]\n \nprint(reverse_list([5, 6, 8, 9]))", "def reverse_list(l):\n out = []\n for x in l:\n out = [x]+out\n return(out)", "from typing import List\n\n\ndef reverse_list(l: List[int]) -> List[int]:\n return l[::-1]", "def reverse_list(l):\n 'return a list with the reverse order of l'\n return l[-1:(len(l) + 1) * -1:-1]", "def reverse_list(l):\n print (l[::-1])\n return l[::-1]", "def reverse_list(v):\n return list(reversed(v))", "def reverse_list(l):\n return [v for v in reversed(l)]", "def reverse_list(l):\n l = reversed(l)\n return list(l)", "def reverse_list(l):\n r = []\n le = len(l)\n while le>0:\n r.append(l[le-1])\n le-=1\n return r\n \n", "def reverse_list(l):\n i = len(l)-1\n n = []\n p = 0\n while p != len(l):\n n.insert(p,l[i])\n p += 1\n i -= 1\n return n", "def reverse_list(l):\n 'return a list with the reverse order of l'\n L=len(l)\n for i in range(L//2):\n l[i],l[L-i-1]=l[L-i-1],l[i]\n return l", "def reverse_list(l):\n return [int(i) for i in ''.join(str(n) for n in l[::-1])]", "def reverse_list(l):\n tmp = list.copy(l)\n counter = 0\n listIndex = len(tmp) - 1\n for i in l:\n l[counter] = tmp[listIndex]\n counter += 1\n listIndex -= 1\n return l", "def reverse_list(l):\n #list1=[1,2,3,4]\n #print('Original List:', list1)\n\n l.reverse()\n\n print('Updated List:', l)\n return l", "def reverse_list(l):\n 'return a list with the reverse order of l'\n wynik = l.copy()\n wynik.reverse()\n return wynik", "def reverse_list(l):\n ret = []\n for it in l:\n ret = [it] + ret\n return ret", "def reverse_list(l):\n result = []\n i = len(l)\n while i > 0:\n i-=1\n result.append(l[i])\n return result", "def reverse_list(l):\n 'return a list with the reverse order of l'\n \n # lijst = [1,2,3,4]\n lijst_rev = l[::-1]\n return(lijst_rev)", "def reverse_list(l):\n n=len(l)\n return l[::-1]", "def reverse_list(l):\n l1=l[::-1]\n return l1", "def reverse_list(l):\n li = []\n for n in range (0, len(l)):\n li.append(l[len(l)-n-1])\n return(li)", "def reverse_list(l):\n r = reversed(l)\n return list(r)", "def reverse_list(l):\n l.reverse()\n return l\nl = [1, 2, 3, 4]\nprint(\"reverse_list(\" + str(l) + \") == \" + str(reverse_list(l)))\nl = [3, 1, 5, 4]\nprint(\"reverse_list(\" + str(l) + \") == \" + str(reverse_list(l)))"] | {"fn_name": "reverse_list", "inputs": [[[1, 2, 3, 4]], [[3, 1, 5, 4]], [[3, 6, 9, 2]], [[1]]], "outputs": [[[4, 3, 2, 1]], [[4, 5, 1, 3]], [[2, 9, 6, 3]], [[1]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 11,317 |
def reverse_list(l):
|
2b52ae51b9e0a0f58995a7217c8dda86 | UNKNOWN | Write a function that will encrypt a given sentence into International Morse Code, both the input and out puts will be strings.
Characters should be separated by a single space.
Words should be separated by a triple space.
For example, "HELLO WORLD" should return -> ".... . .-.. .-.. --- .-- --- .-. .-.. -.."
To find out more about Morse Code follow this link: https://en.wikipedia.org/wiki/Morse_code
A preloaded object/dictionary/hash called CHAR_TO_MORSE will be provided to help convert characters to Morse Code. | ["#CHAR_TO_MORSE preloaded to convert characters into Morse code\nCODE = {'A': '.-', 'B': '-...', 'C': '-.-.', \n 'D': '-..', 'E': '.', 'F': '..-.',\n 'G': '--.', 'H': '....', 'I': '..',\n 'J': '.---', 'K': '-.-', 'L': '.-..',\n 'M': '--', 'N': '-.', 'O': '---',\n 'P': '.--.', 'Q': '--.-', 'R': '.-.',\n 'S': '...', 'T': '-', 'U': '..-',\n 'V': '...-', 'W': '.--', 'X': '-..-',\n 'Y': '-.--', 'Z': '--..',\n \n '0': '-----', '1': '.----', '2': '..---',\n '3': '...--', '4': '....-', '5': '.....',\n '6': '-....', '7': '--...', '8': '---..',\n '9': '----.', \n \n ' ': ' '\n }\n\ndef encryption(string):\n string.upper()\n return \" \".join([CODE[a] for a in string.upper()])"] | {"fn_name": "encryption", "inputs": [["HELLO WORLD"], ["SOS"], ["1836"], ["THE QUICK BROWN FOX"], ["JUMPED OVER THE"], ["LAZY DOG"], ["WOLFRAM ALPHA 1"], ["CodeWars Rocks"], [""], ["Final basic test"]], "outputs": [[".... . .-.. .-.. --- .-- --- .-. .-.. -.."], ["... --- ..."], [".---- ---.. ...-- -...."], ["- .... . --.- ..- .. -.-. -.- -... .-. --- .-- -. ..-. --- -..-"], [".--- ..- -- .--. . -.. --- ...- . .-. - .... ."], [".-.. .- --.. -.-- -.. --- --."], [".-- --- .-.. ..-. .-. .- -- .- .-.. .--. .... .- .----"], ["-.-. --- -.. . .-- .- .-. ... .-. --- -.-. -.- ..."], [""], ["..-. .. -. .- .-.. -... .- ... .. -.-. - . ... -"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 852 |
def encryption(string):
|
7e76fb7b6cf7f308bf86e19672bffbb4 | UNKNOWN | # Task
Given a sorted array of integers `A`, find such an integer x that the value of `abs(A[0] - x) + abs(A[1] - x) + ... + abs(A[A.length - 1] - x)`
is the smallest possible (here abs denotes the `absolute value`).
If there are several possible answers, output the smallest one.
# Example
For `A = [2, 4, 7]`, the output should be `4`.
# Input/Output
- `[input]` integer array `A`
A non-empty array of integers, sorted in ascending order.
Constraints:
`1 ≤ A.length ≤ 200,`
`-1000000 ≤ A[i] ≤ 1000000.`
- `[output]` an integer | ["from statistics import median_low as absolute_values_sum_minimization", "def absolute_values_sum_minimization(A):\n d = {x: sum(abs(n-x) for n in A) for x in A}\n return min(d, key=lambda x: (d[x], x))", "import numpy as np\ndef absolute_values_sum_minimization(A):\n return np.median(A) if len(A) % 2 else A[len(A)//2-1]", "def absolute_values_sum_minimization(A):\n return A[(len(A)-1)//2]", "absolute_values_sum_minimization=lambda li:min([[sum([abs(j-k)for k in li]),j]for i,j in enumerate(li)])[1]", "absolute_values_sum_minimization=lambda A:A[~len(A)//2]", "def absolute_values_sum_minimization(a):\n return min([x for x in a], key=lambda x: sum([abs(i-x) for i in a]))", "def absolute_values_sum_minimization(a):\n return a[len(a)//2 + len(a) %2 - 1]", "from bisect import *\ndef absolute_values_sum_minimization(A):\n return min(A,key=lambda r:(sum(abs(v-r)for v in A)))", "def absolute_values_sum_minimization(A):\n lst = [[sum([abs(j-i) for j in A]), i] for i in A]\n return sorted(lst)[0][-1]"] | {"fn_name": "absolute_values_sum_minimization", "inputs": [[[2, 4, 7]], [[1, 1, 3, 4]], [[23]], [[-10, -10, -10, -10, -10, -9, -9, -9, -8, -8, -7, -6, -5, -4, -3, -2, -1, 0, 0, 0, 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50]], [[-4, -1]], [[0, 7, 9]], [[-1000000, -10000, -10000, -1000, -100, -10, -1, 0, 1, 10, 100, 1000, 10000, 100000, 1000000]]], "outputs": [[4], [1], [23], [15], [-4], [7], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,033 |
def absolute_values_sum_minimization(A):
|
c37b828377290d75d1f54658e12b6925 | UNKNOWN | > When no more interesting kata can be resolved, I just choose to create the new kata, to solve their own, to enjoy the process --myjinxin2015 said
# Description:
In this Kata, we have to try to create a mysterious pattern.
Given a positive integer `m`, you can generate a Fibonacci sequence with a length of `m`:
```
1 1 2 3 5 8 13 21 34 ...
```
Given a positive integer `n`, you need to execute `%` operation on each element of the Fibonacci sequence:
```
m = 9, n = 3
Fibonacci sequence: 1 1 2 3 5 8 13 21 34
---> 1%3 1%3 2%3 3%3 5%3 8%3 13%3 21%3 34%3
---> 1 1 2 0 2 2 1 0 1
```
Finally, make `n` rows string to show the pattern:
```
112022101
|||||||||
o o
oo o o
o oo
```
Please note:
* Each row is separated by `"\n"`;
* You should trim the end of each row;
* If there are some empty rows at the start or end of string, you should trim them too. But, if the empty row is in the middle(see the last example), you should not trim it.
# Examples:
For m = 5, n = 5, the output should be:
```
o
oo
o
o
```
For m = 12, n = 4, the output should be:
```
o o
oo o oo o
o o
o o
```
For m = 1, n = 1, the output should be:`"o"`
For: m = 6, n = 15, the output should be:
```
oo
o
o
o
o
``` | ["def mysterious_pattern(m, n):\n rows = [[' '] * m for _ in range(n)]\n a, b = 1, 1\n for i in range(m):\n rows[a % n][i] = 'o'\n a, b = b, a + b\n rows = [''.join(r).rstrip() for r in rows]\n return '\\n'.join(rows).strip('\\n')", "def genFib():\n a,b = 1,0\n while 1:\n yield a\n a,b = a+b,a\n\nFIB,fib = [], genFib()\n\ndef getFib(m):\n while len(FIB)<m: FIB.append(next(fib))\n return FIB[:m]\n \n \ndef mysterious_pattern(m, n):\n lst = [v%n for v in getFib(m)]\n \n arr = [[' ']*m for _ in range(n)]\n for y,x in enumerate(lst): arr[x][y] = 'o'\n \n return '\\n'.join(''.join(row).rstrip() for row in arr).strip('\\n')\n", "def mysterious_pattern(size, n, fib=1, tmp=0):\n rows = [ [\" \"] * size for _ in range(n) ]\n \n for idx in range(size):\n rows[fib % n][idx] = \"o\"\n tmp, fib = fib, fib + tmp\n \n return \"\\n\".join( \"\".join(row).rstrip() for row in rows ) .strip(\"\\n\")", "def mysterious_pattern(m, n):\n lines, a, b = [[' '] * m for _ in range(n)], 1, 1\n for i in range(m):\n lines[a % n][i], a, b = 'o', b, a + b\n return '\\n'.join([''.join(l).rstrip() for l in lines]).strip('\\n')", "def mysterious_pattern(m, n):\n exp = [ [(' ','o')[j == e%n] for e in fib(m) ] for j in range(n)]\n return '\\n'.join( ''.join(e).rstrip() for e in exp ).strip('\\n')\n \ndef fib(n):\n ret = [1,1]\n for i in range(n):\n ret.append(ret[-1]+ret[-2])\n return ret[:-2]", "fib = [1, 1]\nfor i in range(39):\n fib.append(fib[i] + fib[i+1])\n\n\ndef mysterious_pattern(l, m):\n lines = [[\"o\" if n % m == i else \" \" for n in fib[:l]] for i in range(m)]\n return \"\\n\".join(\"\".join(line).rstrip() for line in lines).strip(\"\\n\")", "from itertools import islice\n\ndef fib():\n a, b = 1, 1\n while True:\n yield a\n a, b = b, a+b\n\ndef mysterious_pattern(m, n):\n board = [[' '] * m for _ in range(n)]\n\n for i, x in enumerate(islice(fib(), m)):\n board[x % n][i] = 'o'\n return '\\n'.join(''.join(row).rstrip() for row in board).strip('\\n')", "FIBONACCI = [1, 1]\n\ndef mysterious_pattern(m, n):\n while len(FIBONACCI) < m:\n FIBONACCI.append(FIBONACCI[-2] + FIBONACCI[-1])\n pattern = [[' '] * m for _ in range(n)]\n for i in range(m):\n pattern[FIBONACCI[i] % n][i] = 'o'\n return '\\n'.join(\n ''.join(row).rstrip() for row in pattern\n ).strip('\\n')", "def mysterious_pattern(m, n):\n #get the fibonacci sequence:\n fib = [1,1]\n for i in range(m-2): fib.append(fib[-1]+fib[-2])\n #get a 2d array of chars based on the fibonacci mod results:\n ret = [[\"o\" if fib[x]%n == y else \" \" for x in range(m)] for y in range(n)]\n #convert the 2d array to string form:\n return \"\\n\".join(\"\".join(row).rstrip() for row in ret).strip(\"\\n\")\n", "def mysterious_pattern(m, n):\n if m == 1:\n return('o')\n \n seq = [1, 1]\n for i in range(m - 2): # Generate fibonacci sequence\n seq.append(seq[-2]+seq[-1])\n\n for i, v in enumerate(seq): \n seq[i] = v % n\n\n pattern = []\n for i in range(max(seq)+1):\n pattern.append([])\n\n for x in seq: # Add 'o' and ' ' at the right places\n for j, row in enumerate(pattern):\n if x == j:\n pattern[j].append('o')\n else:\n pattern[j].append(' ')\n\n for i in range(min(seq)): # Remove empty rows\n del pattern[i]\n\n res = ''\n for row in pattern:\n res += ''.join(row).rstrip() + '\\n'\n\n return(res[:-1])\n"] | {"fn_name": "mysterious_pattern", "inputs": [[5, 5], [12, 4], [4, 5], [10, 1]], "outputs": [[" o\noo\n o\n o"], [" o o\noo o oo o\n o o\n o o"], ["oo\n o\n o"], ["oooooooooo"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,703 |
def mysterious_pattern(m, n):
|
8bcc50aec2b2d13febe813176112a4e2 | UNKNOWN | Jamie is a programmer, and James' girlfriend. She likes diamonds, and wants a diamond string from James. Since James doesn't know how to make this happen, he needs your help.
## Task
You need to return a string that looks like a diamond shape when printed on the screen, using asterisk (`*`) characters. Trailing spaces should be removed, and every line must be terminated with a newline character (`\n`).
Return `null/nil/None/...` if the input is an even number or negative, as it is not possible to print a diamond of even or negative size.
## Examples
A size 3 diamond:
```
*
***
*
```
...which would appear as a string of `" *\n***\n *\n"`
A size 5 diamond:
```
*
***
*****
***
*
```
...that is: `" *\n ***\n*****\n ***\n *\n"` | ["def diamond(n):\n if n < 0 or n % 2 == 0:\n return None\n \n result = \"*\" * n + \"\\n\";\n spaces = 1;\n n = n - 2\n while n > 0:\n current = \" \" * spaces + \"*\" * n + \"\\n\"\n spaces = spaces + 1\n n = n - 2\n result = current + result + current\n \n return result", "def diamond(n):\n w = ''\n space = n//2\n starnum = 1\n while starnum < n:\n w += space * ' ' + starnum * '*' + '\\n'\n starnum += 2\n space -= 1\n while starnum > 0:\n w += space * ' ' + starnum * '*' + '\\n'\n starnum -= 2\n space += 1\n return w if n%2 != 0 and n > 0 else None\n", "def diamond(n):\n if n%2 == 0 or n <= 0: # validate input\n return None\n diamond = \"\"; # initialize diamond string\n for i in range(n): # loop diamond section lines\n length = getLength(i, n) # get length of diamond section\n diamond += getLine(length, n) # generate diamond line\n return diamond\n \ndef getLine(len, max):\n spaces = (max-len)//2 # compute number of leading spaces\n return (\" \" * spaces) + (\"*\" * len) + \"\\n\" # create line\n \ndef getLength(index, max):\n distance = abs(max//2 - index) # find distance from center (max length)\n return max - distance*2 # compute length of diamond section", "def diamond(n):\n return \"\".join([(\"*\"*k).center(n).rstrip()+\"\\n\" for k in range(1, n+1, 2)] + \\\n [(\"*\"*k).center(n).rstrip()+\"\\n\" for k in range(n-2, 0, -2)]) if n > 0 and n % 2 == 1 else None"] | {"fn_name": "diamond", "inputs": [[3], [0], [2], [-1], [-2]], "outputs": [[" *\n***\n *\n"], [null], [null], [null], [null]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,749 |
def diamond(n):
|
48c9ff91addec6936a2830584291882f | UNKNOWN | In this Kata, you will be given an array of numbers and a number `n`, and your task will be to determine if `any` array elements, when summed (or taken individually), are divisible by `n`.
For example:
* `solve([1,3,4,7,6],9) == true`, because `3 + 6` is divisible by `9`
* `solve([1,2,3,4,5],10) == true` for similar reasons.
* `solve([8,5,3,9],7) == true`, because `7` evenly divides `5 + 9`
* but `solve([8,5,3],7) == false`.
All numbers in the array will be greater than `0`.
More examples in the test cases.
Good luck!
If you like this Kata, please try:
[Simple division](https://www.codewars.com/kata/59ec2d112332430ce9000005)
[Divisor harmony](https://www.codewars.com/kata/59bf97cd4f98a8b1cd00007e) | ["from itertools import combinations\n\ndef solve(a, n):\n return any(sum(c) % n == 0 for i in range(len(a)) for c in combinations(a, i+1))", "def solve(xs, n):\n rs = set()\n for x in xs:\n for r in list(rs):\n rs.add((r + x) % n)\n rs.add(x % n)\n return 0 in rs", "from itertools import combinations\n\ndef solve(arr, n):\n return any(sum(xs) % n == 0\n for i in range(len(arr))\n for xs in combinations(arr, i+1))", "from itertools import combinations\n\ndef solve(x, n):\n return any(sum(cb) % n == 0 for k in range(len(x)) for cb in combinations(x, k + 1))", "from itertools import combinations\ndef solve(x, n):\n def combs(x):\n return [c for i in range(1, len(x)+1) for c in combinations(x, i)]\n return any(map(lambda x: sum(x) % n == 0, combs(x)))", "from itertools import combinations\n\ndef solve(arr,n):\n return any(sum(c) % n == 0 for i in range(1, len(arr) + 1) for c in combinations(arr, i))", "from itertools import combinations\ndef solve(arr,n):\n for i in range(1,len(arr)+1):\n for j in combinations(arr,i):\n if sum(j)%n==0:\n return True\n return False", "from itertools import combinations\n\ndef solve(arr,n):\n return next(( True for x in range(1, len(arr)+1) for c in combinations(arr, x) if not sum(c)%n), False)", "solve=lambda a,n:any(sum(c)%n<1for r in range(len(a))for c in __import__('itertools').combinations(a,r+1))", "from itertools import combinations\n\n# Let(s brute force this)\ndef solve(arr,n):\n return any(sum(x)%n == 0 for i in range(1, len(arr)+1) for x in combinations(arr, i))"] | {"fn_name": "solve", "inputs": [[[1, 2, 3, 4, 5], 8], [[7, 2, 8, 5], 16], [[1, 2, 3, 4, 5], 10], [[3, 1, 5, 7], 14], [[1, 2, 3, 4, 5], 15], [[8, 5, 3, 9], 7], [[8, 5, 3], 7]], "outputs": [[true], [false], [true], [false], [true], [true], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,660 |
def solve(arr,n):
|
c3b0ddc27a572a3ed13a7b792971f8d7 | UNKNOWN | A [Word Square](https://en.wikipedia.org/wiki/Word_square) is a set of words written out in a square grid, such that the same words can be read both horizontally and vertically. The number of words, equal to the number of letters in each word, is known as the *order* of the square.
For example, this is an *order* `5` square found in the ruins of Herculaneum:
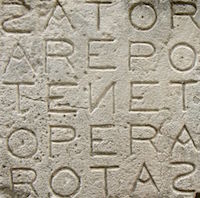
Given a string of various uppercase `letters`, check whether a *Word Square* can be formed from it.
Note that you should use each letter from `letters` the exact number of times it occurs in the string. If a *Word Square* can be formed, return `true`, otherwise return `false`.
__Example__
* For `letters = "SATORAREPOTENETOPERAROTAS"`, the output should be
`WordSquare(letters) = true`.
It is possible to form a *word square* in the example above.
* For `letters = "AAAAEEEENOOOOPPRRRRSSTTTT"`, (which is sorted form of `"SATORAREPOTENETOPERAROTAS"`), the output should also be
`WordSquare(letters) = true`.
* For `letters = "NOTSQUARE"`, the output should be
`WordSquare(letters) = false`.
__Input/Output__
* [input] string letters
A string of uppercase English letters.
Constraints: `3 ≤ letters.length ≤ 100`.
* [output] boolean
`true`, if a Word Square can be formed;
`false`, if a Word Square cannot be formed. | ["from collections import Counter\ndef word_square(ls):\n n = int(len(ls)**0.5)\n return n*n==len(ls) and sum(i%2 for i in list(Counter(ls).values())) <= n\n", "def word_square(letters):\n root = int(len(letters)**0.5)\n return len(letters)**0.5 == root and sum([ letters.count(i)%2 for i in set(letters) ]) <= root", "from collections import Counter\n\ndef word_square(letters):\n N = len(letters)**0.5\n return not N%1 and (sum(v&1 for v in Counter(letters).values()) <= N)", "def word_square(s):\n li = [s.count(i) for i in set(s)]\n t = sum(i for i in li)**0.5\n return int(t) == t and sum(i&1 for i in li) <= t", "word_square=lambda s:len(s)**.5%1==0and sum(s.count(c)%2for c in set(s))<=len(s)**.5", "import math\n\ndef word_square(letters):\n length = int(math.sqrt(len(letters)))\n if length ** 2 != len(letters):\n return False;\n dic = {}\n for i in letters:\n if i not in dic:\n dic[i] = 0\n dic[i] += 1\n odd = 0\n for i in dic:\n if dic[i] % 2 != 0:\n odd += 1\n if odd > length:\n return False\n return True", "from collections import Counter\ndef word_square(letters):\n n = len(letters)**0.5\n if n != int(n):\n return False\n n = int(n)\n c = Counter(letters)\n one, odd = 0, 0\n for i in c.values():\n if i == 1:\n one += 1\n odd += 1\n elif i != 1 and i%2 != 0:\n odd += 1\n if one > n or odd > n:\n return False\n else:\n return True", "from collections import Counter\ndef word_square(letters):\n sq = len(letters) ** .5\n if sq % 1 != 0:\n return False\n d = Counter(letters)\n return len([True for c in d if d[c]%2 == 1]) <= sq", "def word_square(letters):\n if (len(letters))**.5 != int((len(letters))**.5):\n return False\n else:\n n = int((len(letters))**.5)\n s = set(letters)\n one, two = 0,0\n for letter in s:\n if letters.count(letter) == 1: one += 1\n if letters.count(letter) == 2: two += 1\n return one <= n and two <= n**2-n ", "def word_square(letters):\n l = [letters.count(x) for x in set(letters)]\n if int(len(letters)**(0.5))**2 == len(letters):\n if sum(x for x in l if x == 1) <= int(len(letters)**(0.5)):\n return True\n else: return False\n else: return False"] | {"fn_name": "word_square", "inputs": [["SATORAREPOTENETOPERAROTAS"], ["NOTSQUARE"], ["BITICETEN"], ["CARDAREAREARDART"], ["CODEWARS"], ["AAAAACEEELLRRRTT"], ["AAACCEEEEHHHMMTT"], ["AAACCEEEEHHHMMTTXXX"], ["ABCD"], ["GHBEAEFGCIIDFHGG"], ["AAHHFDKIHHFCXZBFDERRRTXXAA"], ["AABBCCDDEEFFGGGG"], ["ABCABCABC"], ["FRACTUREOUTLINEDBLOOMINGSEPTETTE"], ["GLASSESRELAPSEIMITATESMEAREDTANNERY"], ["LIMBAREACORKKNEE"], ["DESCENDANTECHENEIDAESHORTCOATSCERBERULUSENTEROMERENECROLATERDIOUMABANAADALETABATNATURENAMETESSERATED"], ["CONGRATUALATIONS"], ["HEARTEMBERABUSERESINTREND"], ["OHLOLWHAT"]], "outputs": [[true], [false], [true], [true], [false], [true], [true], [false], [false], [true], [false], [true], [true], [false], [false], [false], [true], [false], [true], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,420 |
def word_square(letters):
|
b48d86c73314e652168643b1fc1ca298 | UNKNOWN | Write a function to calculate compound tax using the following table:
For $10 and under, the tax rate should be 10%.
For $20 and under, the tax rate on the first $10 is %10, and the tax on the rest is 7%.
For $30 and under, the tax rate on the first $10 is still %10, the rate for the next $10 is still 7%, and everything else is 5%.
Tack on an additional 3% for the portion of the total above $30.
Return 0 for invalid input(anything that's not a positive real number).
Examples:
An input of 10, should return 1 (1 is 10% of 10)
An input of 21, should return 1.75 (10% of 10 + 7% of 10 + 5% of 1)
* Note that the returned value should be rounded to the nearest penny. | ["def tax_calculator(total):\n if not isinstance(total, (int, float)) or total < 0: return 0\n \n tax = 0\n \n if total > 30: tax = 2.2 + (total - 30) * 0.03\n elif total > 20: tax = 1.7 + (total - 20) * 0.05\n elif total > 10: tax = 1 + (total-10) * 0.07\n elif total > 0: tax = total / 10.0\n\n return round(tax, 2)\n", "def tax_calculator(total):\n tax = 0\n if type(total) in (float, int) and total > 0:\n for limit, rate in ((30, 3),\n (20, 5),\n (10, 7),\n (0, 10)):\n if total > limit:\n tax += rate * (total - limit)\n total = limit\n return round(tax)/100\n", "rates = ((30, 0.03), (20, 0.05), (10, 0.07), (0, 0.1))\n\ndef tax_calculator(total):\n if type(total) in (int, float) and total > 0:\n amount = 0\n for limit, rate in rates:\n if total > limit:\n amount += (total - limit) * rate\n total = limit\n return round(amount, 2)\n return 0", "def tax_calculator(total):\n if not isinstance(total, (int, float)) or total <= 0:\n return 0\n bands = [(10, 0.1), (10, 0.07), (10, 0.05), (None, 0.03)]\n residue = total\n tax = 0\n for band_width, rate in bands:\n if residue == 0:\n break\n if band_width is not None:\n chunk = residue if residue <= band_width else band_width\n else:\n chunk = residue\n tax += chunk * rate\n residue -= chunk\n return round(tax, 2)\n \n", "def tax_calculator(total):\n try:\n total = max(float(total), 0)\n except (AttributeError, TypeError, ValueError):\n total = 0\n tax = 0\n for rate, limit in [(0.1, 10), (0.07, 10), (0.05, 10), (0.03, 99999999999999)]:\n tax += min(total, limit) * rate\n total -= limit\n if total <= 0:\n break\n return round(tax, 2)", "from numbers import Real\n\n\nclass TaxCalculator(object):\n def __init__(self, bands, highest_rate):\n self.__table = list(self._get_table(bands, highest_rate))\n\n @staticmethod\n def _get_table(bands, highest_rate):\n lower_bound, cumulative_tax = 0.0, 0.0\n for upper_bound, rate in bands:\n yield lower_bound, rate, cumulative_tax\n cumulative_tax += (upper_bound - lower_bound) * rate\n lower_bound = upper_bound\n yield lower_bound, highest_rate, cumulative_tax\n\n def __call__(self, amount):\n if isinstance(amount, Real):\n for lower_bound, rate, cumulative_tax in reversed(self.__table):\n if amount >= lower_bound:\n return round((amount - lower_bound) * rate + cumulative_tax, 2)\n return 0.0\n\n\ntax_calculator = TaxCalculator([(10.0, 0.10), (20.0, 0.07), (30.0, 0.05)], 0.03)\n", "def tax_calculator(total):\n\n if type(total) not in [int, float] or total < 0:\n return 0\n \n if total <= 10:\n tax = 0.1 * total\n elif total <= 20:\n tax = 1 + 0.07 * (total - 10)\n else:\n tax = 1.7 + 0.05 * min(total - 20, 10)\n if total > 30:\n tax += 0.03 * (total - 30) \n\n return round(tax, 2)", "def tax_calculator(t):\n return isinstance(t,(float,int)) and round(max(0,t)*.1-max(0,t-10)*.03-max(0,t-20)*.02-max(0,t-30)*.02,2)\n", "tax_calculator=lambda total: round(((total-30)*0.03+2.2 if total-30>0 else ((total-20)*0.05+1.7 if total-20>0 else ((total-10)*0.07+1 if total-10>0 else total*0.1))),2) if ((type(total)==int or type(total)==float) and total>0) else 0"] | {"fn_name": "tax_calculator", "inputs": [[1], [10], [11], [15], [18], [21], [26], [30], [30.49], [35], [100], [1000000], [0], [-3], [null], ["monkey"], [[]], [{}]], "outputs": [[0.1], [1], [1.07], [1.35], [1.56], [1.75], [2], [2.2], [2.21], [2.35], [4.3], [30001.3], [0], [0], [0], [0], [0], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,680 |
def tax_calculator(total):
|
cd96e45d99842ec075ddd718f2a80d20 | UNKNOWN | There is an array with some numbers. All numbers are equal except for one. Try to find it!
```python
find_uniq([ 1, 1, 1, 2, 1, 1 ]) == 2
find_uniq([ 0, 0, 0.55, 0, 0 ]) == 0.55
```
It’s guaranteed that array contains at least 3 numbers.
The tests contain some very huge arrays, so think about performance.
This is the first kata in series:
1. Find the unique number (this kata)
2. [Find the unique string](https://www.codewars.com/kata/585d8c8a28bc7403ea0000c3)
3. [Find The Unique](https://www.codewars.com/kata/5862e0db4f7ab47bed0000e5) | ["def find_uniq(arr):\n a, b = set(arr)\n return a if arr.count(a) == 1 else b", "def find_uniq(arr):\n if (arr[0]==arr[1]):\n return([x for x in arr[2:] if x!=arr[0] ][0])\n return(arr[0] if arr[1]==arr[2] else arr[1])\n", "def find_uniq(arr):\n s = set(arr)\n for e in s:\n if arr.count(e) == 1:\n return e\n", "def find_uniq(arr):\n a = sorted(arr)\n return a[0] if a[0] != a[1] else a[-1]", "from collections import Counter\n\ndef find_uniq(a):\n return Counter(a).most_common()[-1][0]", "def find_uniq(arr):\n if arr[0] != arr[1]:\n if arr[0] != arr[2]:\n return arr[0]\n else:\n return arr[1] \n else:\n for i in arr[2:]:\n if i != arr[0]:\n return i", "from collections import Counter\n\ndef find_uniq(arr):\n return next(k for k,v in Counter(arr).items() if v == 1)", "def find_uniq(arr):\n return [x for x in set(arr) if arr.count(x) == 1][0]", "def find_uniq(arr):\n arr.sort()\n return arr[0] if arr[0] != arr[1] else arr[-1]", "def find_uniq(arr):\n res = sorted(arr)\n return res[0] if res[0] != res[1] else res[-1]\n", "def find_uniq(arr):\n culprit = arr[1]\n \n if arr[0] == arr[2]:\n culprit = arr[0]\n \n for i in arr:\n if i != culprit:\n return i\n", "def find_uniq(arr):\n x = arr[0 if arr[0] == arr[1] else 2]\n return next(y for y in arr if y != x)"] | {"fn_name": "find_uniq", "inputs": [[[1, 1, 1, 2, 1, 1]], [[0, 0, 0.55, 0, 0]], [[4, 4, 4, 3, 4, 4, 4, 4]], [[5, 5, 5, 5, 4, 5, 5, 5]], [[6, 6, 6, 6, 6, 5, 6, 6]], [[7, 7, 7, 7, 7, 7, 6, 7]], [[8, 8, 8, 8, 8, 8, 8, 7]], [[3, 3, 3, 3, 3, 3, 3, 2]], [[2, 2, 2, 2, 2, 2, 2, 1]], [[0, 1, 1, 1, 1, 1, 1, 1]]], "outputs": [[2], [0.55], [3], [4], [5], [6], [7], [2], [1], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,479 |
def find_uniq(arr):
|
4d896e6878954696be7a5c0062355812 | UNKNOWN | *Translations appreciated*
# Overview
Your task is to decode a qr code.
You get the qr code as 2 dimensional array, filled with numbers. 1 is for a black field and 0 for a white field.
It is always a qr code of version 1 (21*21), it is always using mask 0 ((x+y)%2), it is always using byte mode and it always has error correction level H (up to 30%). The qr code won't be positioned wrong and there won't be any squares for positioning exept the three big ones in the corners.
You should return the message inside the qr code as string.
The QR Code will always be valid and be of `version 1`, meaning the decoded message will never be more than 8 characters long. The way to decode a complete QR-code is explained below, but keep in mind that in this kata, you'll only have to decode the parts in green in the picture below:
# Input/ouput
* Input: 2D array of `0` (white) and `1` (black) values
* Output: the decoded message, according to the process described below.
```python
qrcode = [[ 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1 ],
[ 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1 ],
[ 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1 ],
[ 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1 ],
[ 1, 0, 1, 1, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1 ],
[ 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1 ],
[ 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1 ],
[ 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0 ],
[ 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 0, 0, 0, 1, 0, 0, 1 ],
[ 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1 ],
[ 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1 ],
[ 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0 ],
[ 1, 0, 1, 0, 1, 0, 1, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0 ],
[ 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0 ],
[ 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 1, 0, 1, 0, 0, 1, 1, 1 ],
[ 1, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0 ],
[ 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0, 0, 1, 1 ],
[ 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0 ],
[ 1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 0, 1, 0, 1 ],
[ 1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0 ],
[ 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1 ]]
return "Hello"
```
```C
int qrcode[21][21] = {{ 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1 },
{ 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1 },
{ 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1 },
{ 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1 },
{ 1, 0, 1, 1, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1 },
{ 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1 },
{ 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1 },
{ 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0 },
{ 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 0, 0, 0, 1, 0, 0, 1 },
{ 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1 },
{ 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1 },
{ 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0 },
{ 1, 0, 1, 0, 1, 0, 1, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0 },
{ 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0 },
{ 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 1, 0, 1, 0, 0, 1, 1, 1 },
{ 1, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0 },
{ 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0, 0, 1, 1 },
{ 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0 },
{ 1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 0, 1, 0, 1 },
{ 1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0 },
{ 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1 }};
return "Hello";
```
# Decoding a QR-code
Here comes the explaination on how to decode a qr code. You can skip it, if you already know how it works:
### Postionning information
First of all we have to take a look at the big three positioning fields in the corners.
You can see on the image that these fields are connected.
The fields are just there for the positioning and I told before that the qr code will be always positioned correctly so you can ignore them.
### Mask information
The bits next to the positioning fields give us information about the mask and the error correction level the qr code uses. I wrote above that it is always mask 0 and always error correction level H, so we can also ignore that stuff.
### Reading information
Now we start with the important stuff. Go through the qr code like the red arrow shows on this picture (btw I made it with paint so don't judge me)
- We start in the lower right corner
- Then we go one to the left
- Then we go one up and one to the right
- And so on just look the red arrow
___Important:___ Remember that everything inside the blue boxes has another purpose than encoding information, so don't forget to skip those parts.
In the image below, you may find the complete pattern to read information in a QR-code. But keep in mind you'll be handling only "QR-code version 1", so you don't need to read the full set of data (see picture at the top if needed).
### Decoding information
We have to build a bit sequence now. In order to do that we will use mask 0 definition which is `((x+y)%2)==0`, where:
- x and y are the indexes of our 2 dimensional array (0-based)
- if the condition of our mask is true, we have to convert the pixel: black -> 0 and white -> 1
- A mask is used to prevent long sequences of identical bits so that a scanner can better recognize the code
For each black field add 1 to our bit sequence and for each white field add 0 to our bit sequence, don't forget that many bits get converted because of mask 0.
Let's do the first bits together:
* We start with the first pixel (in the lower right corner, where also the red arrow begins) which is black, but we have to use mask because (20+20)%2 is 0, therefore we don't add 1 to our bit sequence but 0.
* Next field is white. This time we don't use mask because (20+19)%2 isn't 0, so we add a 0 to our bit sequence.
* Next field is black. This time we don't use mask because (19+20)%2 isn't 0, so we add a 1 to our bit sequence.
Important (!): Since we're limiting ourselves to version 1, we have to continue that process only until our bit sequence is 76 long, because the input will never be longer than eight characters.
At the end you get a bit sequence:
```
bits: 0100000000100100100001101001000011101100000100011110110000010001111011001111
legend: MMMMssssssss...
- "M": mode bits (4 bits)
- "s": size message bits (8 bits)
- ...: message bits and error correction information
```
This bit sequence is representing the following information
* First 4 bits show mode: `0100`. This isn't important for us, because I told you before that we will use always byte mode in this kata.
* The following 8 bits show the length of the encoded word: `00000010`. This is the binary representation of number 2, so we know our word is 2 characters long.
* The following bits are data bits followed by error correction bits (but you can ignore error correction bits, because there won't be any errors). We know our word is 2 chars long, so we take the next 16 bits (because 1 char=8 bits):
- First group of 8 bits: `01001000`. This is `72` in decimal which is ascii value of `"H"`.
- Second group of 8 bits: `01101001`. This is `105` in decimal which is ascii value of `"i"`.
Since we don't handle in this kata error correction, we got our word/output: `"Hi"`.
Good luck :) | ["def scanner(qrc):\n bits = ''.join( str(qrc[x][y] ^ ((x+y)%2==0)) for x,y in ticTocGen())\n size = int(bits[4:12],2)\n return ''.join( chr(int(bits[i:i+8],2)) for i in range(12,12+8*size,8))\n\n\ndef ticTocGen():\n x,y,dx = 20,20,-1\n while y>13: \n yield from ( (x,y-dy) for dy in range(2) )\n x+=dx\n if x==8 or x>20:\n dx *= -1\n y -= 2\n x = x==8 and 9 or 20", "def scanner(qrcode):\n Y, X = len(qrcode) - 1, len(qrcode[0]) - 1\n x, y, dir = X, Y, -1\n bits = \"\"\n \n while len(bits) < 76:\n for i in range(2):\n bits += str(qrcode[x][y-i] ^ ((x + y - i) % 2 == 0))\n x += dir\n if x == 8 or x > X:\n dir = dir * -1\n x += dir\n y -= 2\n \n size = int(bits[4:12], 2)\n return \"\".join(chr(int(bits[i:i+8], 2)) for i in range(12, size * 8 + 12, 8))", "def scan_column(qrcode, x0, y0, height, bottom_to_top=True):\n result = []\n if bottom_to_top:\n height_range = list(range(0, height * -1, -1))\n else:\n height_range = list(range(height))\n\n for dy in height_range:\n y = y0 + dy\n x = x0\n result.append(qrcode[y][x] ^ (x + y + 1) % 2)\n x = x0 - 1\n result.append(qrcode[y][x] ^ (x + y + 1) % 2)\n\n return result\n\ndef byte_to_int(byte):\n return int(''.join(map(str, byte)), 2)\n\ndef raw_data_to_str(raw_data):\n return ''.join([chr(byte_to_int(byte)) for byte in raw_data])\n\ndef scanner(qrcode):\n result = []\n result += scan_column(qrcode, 20, 20, 12, bottom_to_top=True)\n result += scan_column(qrcode, 18, 9, 12, bottom_to_top=False)\n result += scan_column(qrcode, 16, 20, 12, bottom_to_top=True)\n result += scan_column(qrcode, 14, 9, 12, bottom_to_top=False)\n \n encoding_raw = result[:4]\n \n length_raw = result[4:12]\n length = byte_to_int(length_raw)\n\n data_raw = [result[12 + 8 * i: 12 + 8 * (i + 1)] for i in range(length)]\n data = raw_data_to_str(data_raw)\n\n return data\n \n", "def coords():\n rs = range(9, 21)\n for c in [20, 16]:\n for i in reversed(rs):\n yield i, c\n yield i, c - 1\n for i in rs:\n yield i, c - 2\n yield i, c - 3\n\n\ndef scanner(qrcode):\n it = (qrcode[r][c] ^ (1 - (r + c) % 2) for r, c in coords())\n bstring = \"\".join(map(str, it))\n offset = 12\n length = int(bstring[4:offset], 2)\n bstring = bstring[offset : offset + length * 8]\n return \"\".join(chr(int(\"\".join(xs), 2)) for xs in zip(*[iter(bstring)] * 8))", "from itertools import islice\nget_byte = lambda it: int(''.join(islice(it, 8)), 2)\n\ndef scanner(qrcode):\n def next_bit():\n i, j = 18, 20\n while True:\n yield str(i&1 ^ j&1 ^ qrcode[i][j] ^ 1)\n if j%2 == 0 or (i == 9 and j%4 == 3) or (i == 20 and j%4 == 1):\n j -= 1\n else:\n i += 1 if j%4==1 else -1\n j += 1\n bits = next_bit()\n return ''.join(chr(get_byte(bits)) for _ in range(get_byte(bits)))", "def scanner(qrcode):\n finding_patterns = {((0, 9), (0, 9)),\n ((len(qrcode)-8, len(qrcode)), (0, 9)), \n ((0, 9), (len(qrcode)-8, len(qrcode)))}\n timing_patterns = {((9, len(qrcode)-8), (6, 7)), ((6, 7), (9, len(qrcode)-8))}\n function_patterns = {\n (x, y) for (x0, x1), (y0, y1) in finding_patterns | timing_patterns\n for x in range(x0, x1) for y in range(y0, y1)\n }\n \n reading = ((x, 2*y - (y < 4) - i)\n for y in range(len(qrcode) // 2, 0, -1)\n for x in (reversed, iter)[y % 2](list(range(len(qrcode))))\n for i in range(2))\n bits = ''.join(str((x+y ^ ~qrcode[x][y]) & 1) for (x, y) in reading\n if (x, y) not in function_patterns)\n return bytes(int(bits[i: i+8], 2) for i in range(12, 12+int(bits[4:12], 2)*8, 8)).decode()\n \n", "import re\ndef scanner(q):\n bitseq,mask = [], []\n for row in range(20, 8, -1):\n bitseq.append(q[row][20])\n mask.append((row+20)%2==0)\n bitseq.append(q[row][19])\n mask.append((row+19)%2==0)\n for row in range(9, 21, 1):\n bitseq.append(q[row][18])\n mask.append((row+18)%2==0)\n bitseq.append(q[row][17])\n mask.append((row+17)%2==0)\n for row in range(20, 8, -1):\n bitseq.append(q[row][16])\n mask.append((row+16)%2==0)\n bitseq.append(q[row][15])\n mask.append((row+15)%2==0) \n for row in range(9, 11, 1):\n bitseq.append(q[row][14])\n mask.append((row+14)%2==0)\n bitseq.append(q[row][13])\n mask.append((row+13)%2==0) \n code = [int(not x) if mask[i] else x for i,x in enumerate(bitseq)] \n length = int(''.join(map(str, code[4:12])),2)\n return(''.join([chr(int(x,2)) for x in re.findall(r\"[01]{8}\", ''.join(map(str, code[12:(8*length)+12])))]))\n", "def scanner(qrcode):\n result = \"\"\n dict = {1:0, 0:1}\n x,y= 20 ,20\n bool =True\n dir = \"Up\"\n i = 2\n count = 0\n while count < 6:\n while x > 8 and dir == \"Up\":\n \n if (x+y) % 2 == 0:\n result += str(dict[qrcode[x][y]])\n else:\n result += str(qrcode[x][y])\n if bool:\n y -= 1\n bool = False\n else:\n x -= 1\n y += 1\n bool =True\n if x == 8:\n x= 9\n y= 20 - i\n i += 2\n dir = \"Down\"\n bool =True\n print(result)\n count += 1 \n if count == 5:\n break\n else:\n while x < 21 and dir ==\"Down\":\n if (x+y) % 2 == 0:\n result += str(dict[qrcode[x][y]])\n else:\n result += str(qrcode[x][y])\n if bool:\n y -= 1\n bool = False\n else:\n x += 1\n y += 1\n bool =True\n \n if x == 21:\n x= 20\n y= 20 - i\n i += 2\n dir = \"Up\"\n bool=True\n count += 1\n print(result)\n \n len = int(result[4:12],2)\n \n ans =\"\"\n for i in range(len):\n ans += chr(int(result[12 + i*8:20+i*8],2))\n \n \n return ans\n \n", "def scanner(qrcode):\n # Setup\n bitstring = ''\n row = 20\n row_iter = -1\n col = 20\n col_iter = 0\n \n # Collect bitstring\n while not(row == 13 and col == 0):\n if not ((row <= 8 and col <= 8) or (row <= 8 and col >= 13) or (row >= 13 and col <= 8) or row == 6 or col == 6):\n if (row + col - col_iter)%2 == 0:\n bitstring += str(1 - qrcode[row][col-col_iter])\n else:\n bitstring += str(qrcode[row][col-col_iter]) \n else:\n pass\n \n # Assign new row col coordinates \n row = row + (row_iter * col_iter)\n col_iter = 1 - col_iter \n # Change direction if necessary\n if (row == -1 or row == 21) and col_iter == 0:\n row_iter = row_iter * -1\n row += row_iter\n col -= 2\n \n # Determine string length and clean bitstring\n str_length = int(bitstring[4:12], 2)\n bitstring = bitstring[12:] # Remove encoding mode / message length\n \n # Convert bits into ASCII and return\n chars = []\n for b in range(0,str_length):\n byte = bitstring[b*8:(b+1)*8]\n chars.append(chr(int(byte, 2)))\n return ''.join(chars)", "def up(x0, y0):\n return tuple((x, y) for y in range(y0+3, y0-1, -1) for x in (x0+1, x0))\ndef down(x0, y0):\n return tuple((x, y) for y in range(y0, y0+4) for x in (x0+1, x0))\ndef top(x0, y0):\n return ((x0+3, y0+1), (x0+2, y0+1)) + tuple((x, y0) for x in range(x0+3, x0-1, -1)) + ((x0+1, y0+1), (x0, y0+1))\ndef bot(x0, y0):\n return ((x0+3, y0), (x0+2, y0)) + tuple((x, y0+1) for x in range(x0+3, x0-1, -1)) + ((x0+1, y0), (x0, y0))\n\nmodebits = (20,20), (19,20), (20,19), (19,19)\nlenbits = up(19,15)\nbits = (\n up(19,11), top(17,9), down(17,11), down(17,15), bot(15,19), up(15,15), up(15,11), top(13,9),\n down(13,11), down(13,15), bot(11,19), up(11,15), up(11,11), up (11,7), up(11,2), top(9,0), down(9,2))\n\ndef num(code, bits):\n n = 0\n for x, y in bits:\n n = (n << 1) + (code[y][x] ^ (x+y)%2 ^ 1)\n return n\n\ndef scanner(qrcode):\n mode = num(qrcode, modebits)\n length = num(qrcode, lenbits)\n print(f'{mode=}, {length=}')\n return ''.join(chr(num(qrcode, bits[i])) for i in range(length))"] | {"fn_name": "scanner", "inputs": [[[[1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1], [1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1], [1, 0, 1, 1, 1, 0, 1, 0, 0, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1], [1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 0, 1, 0, 1, 1, 1, 0, 1], [1, 0, 1, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 0, 1, 1, 1, 0, 1], [1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1], [1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1], [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0], [0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 0, 1], [1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1], [1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 1], [1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 0, 1, 0], [1, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 1, 0, 0, 1, 0, 0], [0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0], [1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 1, 1], [1, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 1, 1, 1], [1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 0, 0, 0, 1, 0, 0, 1, 0, 1, 0, 1], [1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1, 1, 0, 1, 0, 1, 0, 1, 0], [1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1], [1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 0], [1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1]]], [[[1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1], [1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1], [1, 0, 1, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 1], [1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1], [1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1], [1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1], [1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1], [0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0], [0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 0, 0, 0, 1, 0, 0, 1], [1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 0, 1, 1], [1, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1], [1, 0, 0, 1, 1, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1], [0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1], [0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 0, 0], [1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 0, 0, 1, 1], [1, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 1], [1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1], [1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0], [1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 1, 0, 0, 0, 1], [1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0], [1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1]]], [[[1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1], [1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1], [1, 0, 1, 1, 1, 0, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 1], [1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1], [1, 0, 1, 1, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1], [1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1], [1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1], [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0], [0, 0, 1, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 0, 0, 1, 0, 0, 1], [1, 0, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1], [0, 0, 1, 0, 1, 1, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1], [1, 0, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0], [1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 0, 1, 0, 1, 1], [0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0], [1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1], [1, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 1, 0], [1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1], [1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 0], [1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 1], [1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0], [1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 1, 1]]], [[[1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 0, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1], [1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1], [1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 1, 0, 1, 1, 1, 0, 1], [1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 1], [1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 0, 0, 0, 1, 0, 1, 1, 1, 0, 1], [1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1], [1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1], [0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0], [0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0, 0, 1, 0, 0, 1], [0, 0, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 0, 1, 1, 1, 0, 1, 0, 1, 1], [0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 1], [1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0], [0, 0, 0, 0, 1, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 1], [0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0], [1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1], [1, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 0], [1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1], [1, 0, 1, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 0], [1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 1, 0, 0, 1, 0, 1], [1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 0], [1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 0, 1, 0, 1, 0, 0, 1, 1, 1]]]], "outputs": [["Hi"], ["Warrior"], ["T3st"], ["T!st"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 9,004 |
def scanner(qrcode):
|
4f31cd509b2eb8c4c459859753fc8841 | UNKNOWN | Complete the function ```circleArea``` so that it will return the area of a circle with the given ```radius```. Round the returned number to two decimal places (except for Haskell). If the radius is not positive or not a number, return ```false```.
Example:
```python
circleArea(-1485.86) #returns false
circleArea(0) #returns false
circleArea(43.2673) #returns 5881.25
circleArea(68) #returns 14526.72
circleArea("number") #returns false
``` | ["from math import pi\n\ndef circleArea(r):\n return round(pi * r ** 2, 2) if type(r) in (int, float) and r > 0 else False\n", "import math\n\ndef circleArea(r):\n try:\n if (r > 0):\n return round(math.pi*r**2, 2)\n else:\n return False\n except TypeError:\n return False\n", "from math import pi\ndef circleArea(r):\n if type(r) == int and r>0:\n return round(pi*(r**2), 2)\n else:\n return False\n", "from numbers import Number\nimport math\ndef circleArea(r):\n if isinstance(r, Number):\n if r>0:\n return round(r*r*math.pi, 2)\n else:\n return False\n else:\n return False\n", "from numbers import Number\nimport math\ndef circleArea(r):\n if (not isinstance(r, Number)) or (r < 0):\n return False\n return round(math.pi * r * r, 2)\n", "circleArea = lambda _: round(__import__('math').pi * _**2,2) if (type(_)==float or type(_)==int) and _>0 else False", "import math\n\ndef circleArea(r):\n return type(r) in (int, float) and r > 0 and round(math.pi * r**2, 2)", "from math import pi\ndef circleArea(r):\n return round(pi*r*r,2) if type(r)!=str and r>0 else False", "circleArea = lambda r: round(r**2*3.14159265359, 2) if type(r)!=str and r>0 else False"] | {"fn_name": "circleArea", "inputs": [[0], ["An integer"]], "outputs": [[false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,301 |
def circleArea(r):
|
10158e942d19a39c271049f50e8d7813 | UNKNOWN | # A wolf in sheep's clothing
Wolves have been reintroduced to Great Britain. You are a sheep farmer, and are now plagued by wolves which pretend to be sheep. Fortunately, you are good at spotting them.
Warn the sheep in front of the wolf that it is about to be eaten. Remember that you are standing **at the front of the queue** which is at the end of the array:
```
[sheep, sheep, sheep, sheep, sheep, wolf, sheep, sheep] (YOU ARE HERE AT THE FRONT OF THE QUEUE)
7 6 5 4 3 2 1
```
If the wolf is the closest animal to you, return `"Pls go away and stop eating my sheep"`. Otherwise, return `"Oi! Sheep number N! You are about to be eaten by a wolf!"` where `N` is the sheep's position in the queue.
**Note:** there will always be exactly one wolf in the array.
## Examples
```python
warn_the_sheep(["sheep", "sheep", "sheep", "wolf", "sheep"]) == 'Oi! Sheep number 1! You are about to be eaten by a wolf!'
warn_the_sheep(['sheep', 'sheep', 'wolf']) == 'Pls go away and stop eating my sheep'
``` | ["def warn_the_sheep(queue):\n n = len(queue) - queue.index('wolf') - 1\n return f'Oi! Sheep number {n}! You are about to be eaten by a wolf!' if n else 'Pls go away and stop eating my sheep'\n", "def warn_the_sheep(queue):\n i = queue[::-1].index('wolf')\n if i == 0:\n return 'Pls go away and stop eating my sheep'\n return f'Oi! Sheep number {i}! You are about to be eaten by a wolf!'", "def warn_the_sheep(queue):\n return 'Pls go away and stop eating my sheep' if queue[-1] == 'wolf' else f'Oi! Sheep number {queue[::-1].index(\"wolf\")}! You are about to be eaten by a wolf!'", "def warn_the_sheep(queue):\n queue.reverse()\n return 'Pls go away and stop eating my sheep' if queue[0] == 'wolf' else 'Oi! Sheep number {}! You are about to be eaten by a wolf!'.format(queue.index('wolf'))", "def warn_the_sheep(queue):\n queue.reverse()\n return f\"Oi! Sheep number {queue.index('wolf')}! You are about to be eaten by a wolf!\" if queue.index('wolf') > 0 else 'Pls go away and stop eating my sheep' ", "def warn_the_sheep(queue):\n wolf_positon=0 #memoy for the position of the wolf\n for postion, item in enumerate(queue): #lets check every sheep\n if item == 'wolf': #and if it is a wolf\n wolf_positon=postion+1 #we will save its position in the memory\n if wolf_positon == len(queue): #if the wolf is last\n return 'Pls go away and stop eating my sheep' #We beg the wolf. (Hell no!!!).\n #this f'{variable} some text' doesnt work in every python version(Python >= 3.6)\n #{len(queue)-wolf_positon}example: 1,2,3,4,wolf,5,6 len(queue)=7 wolf_pos=5 7-5=2\n return f'Oi! Sheep number {len(queue)-wolf_positon}! You are about to be eaten by a wolf!' ", "def warn_the_sheep(queue): \n queue.reverse()\n wolfnum = queue.index(\"wolf\")\n if wolfnum == 0:\n return \"Pls go away and stop eating my sheep\"\n else:\n return \"Oi! Sheep number {0}! You are about to be eaten by a wolf!\".format(wolfnum)\n \n", "def warn_the_sheep(queue):\n pos = queue[::-1].index('wolf')\n \n if pos > 0:\n return 'Oi! Sheep number %s! You are about to be eaten by a wolf!' % (pos)\n return 'Pls go away and stop eating my sheep'", "def warn_the_sheep(queue):\n if queue.index('wolf') == len(queue)-1:\n return \"Pls go away and stop eating my sheep\"\n else:\n N = len(queue)-queue.index('wolf')-1\n return \"Oi! Sheep number {0}! You are about to be eaten by a wolf!\".format(N)", "def warn_the_sheep(queue):\n p = queue[::-1].index(\"wolf\")\n return f\"Oi! Sheep number {p}! You are about to be eaten by a wolf!\" if p!=0 else \"Pls go away and stop eating my sheep\"", "warn_the_sheep = lambda queue: 'Oi! Sheep number ' + str(len(queue) - queue.index('wolf') - 1) + '! You are about to be eaten by a wolf!' if queue[-1] == 'sheep' else 'Pls go away and stop eating my sheep'", "def warn_the_sheep(queue):\n count = 1\n wolf = 0\n for e in reversed(queue):\n count += 1\n if e == 'wolf':\n wolf = count - 2\n if wolf == 0:\n return 'Pls go away and stop eating my sheep'\n return f'Oi! Sheep number {wolf}! You are about to be eaten by a wolf!'", "def warn_the_sheep(queue):\n if queue[-1] == 'wolf':\n return \"Pls go away and stop eating my sheep\"\n else:\n for i in range(len(queue)):\n if queue[len(queue)-i-1] == 'wolf':\n return \"Oi! Sheep number \" + str(i) + \"! You are about to be eaten by a wolf!\"\n \n", "def warn_the_sheep(queue):\n index = len(queue) - queue.index(\"wolf\") - 1\n if index == 0:\n return \"Pls go away and stop eating my sheep\"\n else:\n return \"Oi! Sheep number \" + str(index) + \"! You are about to be eaten by a wolf!\"", "def warn_the_sheep(queue):\n wolf = queue.index('wolf')+1; length = len(queue)\n if wolf==length: return 'Pls go away and stop eating my sheep'\n else: return 'Oi! Sheep number '+str(length-wolf)+'! You are about to be eaten by a wolf!'\n", "def warn_the_sheep(q):\n pos = len(q) - 1 - q.index('wolf')\n return 'Pls go away and stop eating my sheep' if not pos else \\\n f\"Oi! Sheep number {pos}! You are about to be eaten by a wolf!\"", "warn_the_sheep=lambda q,w='wolf':'Pls go away and stop eating my sheep'if q[-1]==w else\"Oi! Sheep number {}! You are about to be eaten by a wolf!\".format(len(q)-1-q.index(w))", "def warn_the_sheep(queue):\n wolf_pos = queue[::-1].index(\"wolf\")\n return ( 'Pls go away and stop eating my sheep' if wolf_pos == 0\n else 'Oi! Sheep number %s! You are about to be eaten by a wolf!' % wolf_pos )", "def warn_the_sheep(queue):\n if queue[-1] == 'wolf':\n return 'Pls go away and stop eating my sheep' \n else: \n return 'Oi! Sheep number ' + str(len(queue) - queue.index('wolf') -1) + '! You are about to be eaten by a wolf!'", "def warn_the_sheep(queue):\n if queue.index('wolf') == len(queue) - 1:\n return 'Pls go away and stop eating my sheep'\n else:\n sheep = queue[::-1].index('wolf')\n return f'Oi! Sheep number {sheep}! You are about to be eaten by a wolf!'", "def warn_the_sheep(queue):\n if (queue[-1] == 'wolf'):\n return \"Pls go away and stop eating my sheep\"\n else:\n queue.reverse()\n return \"Oi! Sheep number \"+ str(queue.index('wolf')) + \"! You are about to be eaten by a wolf!\"\n", "def warn_the_sheep(queue):\n z = 0\n for i in queue:\n z+= 1\n if i == \"wolf\" and len(queue)-z != 0:\n return \"Oi! Sheep number \" + str(len(queue)-z) + \"! You are about to be eaten by a wolf!\"\n return \"Pls go away and stop eating my sheep\"", "def warn_the_sheep(queue):\n queue.reverse()\n if queue.index('wolf')==0:\n return 'Pls go away and stop eating my sheep'\n else:\n return 'Oi! Sheep number {}! You are about to be eaten by a wolf!'.format(queue.index('wolf'))\n", "from typing import List\n\ndef warn_the_sheep(queue: List[str]) -> str:\n \"\"\" Warn the sheep in front of the wolf that it is about to be eaten. \"\"\"\n return \"Pls go away and stop eating my sheep\" if queue[-1] == \"wolf\" else f\"Oi! Sheep number {list(reversed(queue)).index('wolf')}! You are about to be eaten by a wolf!\"", "def warn_the_sheep(q): \n return [\"Oi! Sheep number %s! You are about to be eaten by a wolf!\" % q[::-1].index('wolf'),\n \"Pls go away and stop eating my sheep\"]['wolf' in q[-1]]", "def warn_the_sheep(queue): \n return [\"Oi! Sheep number %s! You are about to be eaten by a wolf!\" % queue[::-1].index('wolf'),\n \"Pls go away and stop eating my sheep\"]['wolf' in queue[-1]]", "def warn_the_sheep(queue):\n queue = reverse(queue)\n message_one = 'Pls go away and stop eating my sheep'\n message_two = 'Oi! Sheep number 1! You are about to be eaten by a wolf!'\n wolf = 'wolf'\n out_count = 0\n sheep_count = 0\n queue_len = length(queue)\n wolf_len = length(wolf)\n while out_count < queue_len:\n in_count = 0\n while in_count < wolf_len:\n if queue[out_count][in_count] != wolf[in_count]:\n break\n in_count += 1\n if in_count == wolf_len - 1:\n if sheep_count == 0:\n return message_one\n else:\n return ('Oi! Sheep number ' + num_to_str(sheep_count) + \n '! You are about to be eaten by a wolf!')\n sheep_count += 1\n out_count += 1\n \ndef reverse(arr):\n arr_l = length(arr)\n r_arr = [0] * arr_l\n count = 0\n while count < arr_l:\n r_arr[count] = arr[arr_l - 1 - count]\n count += 1\n return r_arr\n \ndef length(arr):\n count = 0\n try:\n while True:\n arr[count]\n count += 1\n except:\n return count\n \ndef num_to_str(num):\n nums = ['0', '1', '2', '3', '4', '5', '6', '7', '8', '9']\n str_num = ''\n while num > 0:\n str_num = nums[num % 10] + str_num\n num = (num - (num % 10)) // 10\n return str_num", "def warn_the_sheep(q):\n return (f\"Oi! Sheep number {q[::-1].index('wolf')}! You are about to be eaten by a wolf!\", 'Pls go away and stop eating my sheep',)['w' in q[-1]]", "def warn_the_sheep(queue):\n return (lambda x: f'Oi! Sheep number {x}! You are about to be eaten by a wolf!' if x \\\n else 'Pls go away and stop eating my sheep') (queue[::-1].index('wolf'))", "def warn_the_sheep(queue):\n x = 0\n for i in queue:\n x = x +1\n if i == 'wolf':\n b = len(queue) - x\n if b == 0:\n return 'Pls go away and stop eating my sheep'\n else:\n return \"Oi! Sheep number {}! You are about to be eaten by a wolf!\".format(b)\n", "def warn_the_sheep(queue):\n position = list(reversed(queue)).index(\"wolf\")\n return f\"Oi! Sheep number {position}! You are about to be eaten by a wolf!\" if position !=0 else 'Pls go away and stop eating my sheep' ", "def warn_the_sheep(queue):\n cnt = 1\n for i in range(len(queue)-1, 0, -1):\n if queue[i] == 'wolf':\n return 'Pls go away and stop eating my sheep'\n elif queue[i] == 'sheep' and queue[i-1] == 'wolf':\n return 'Oi! Sheep number {}! You are about to be eaten by a wolf!'.format(cnt)\n cnt += 1\n return 'Pls go away and stop eating my sheep'", "def warn_the_sheep(queue):\n p = queue.index('wolf')\n if p == len(queue) - 1:\n return \"Pls go away and stop eating my sheep\"\n else:\n return \"Oi! Sheep number \" + str(len(queue) - 1 - p) + \"! You are about to be eaten by a wolf!\"", "def warn_the_sheep(queue):\n return 'Pls go away and stop eating my sheep' if queue[::-1][0] == 'wolf' else f\"Oi! Sheep number {queue[::-1].index('wolf')}! You are about to be eaten by a wolf!\"", "def warn_the_sheep(queue):\n if queue[-1] == 'wolf':\n return 'Pls go away and stop eating my sheep'\n sheep_number = len(queue) - 1 - queue.index(\"wolf\")\n return f'Oi! Sheep number {sheep_number}! You are about to be eaten by a wolf!'", "def warn_the_sheep(queue):\n count=0\n for i in range(len(queue)-1,-1,-1):\n if queue[i]==\"wolf\" and count!=0: return \"Oi! Sheep number {}! You are about to be eaten by a wolf!\".format(count)\n count+=1\n return \"Pls go away and stop eating my sheep\"", "def warn_the_sheep(queue):\n if queue[-1] == 'wolf':\n return 'Pls go away and stop eating my sheep'\n position = len(queue) - queue.index('wolf') - 1\n return 'Oi! Sheep number ' + str(position) + '! You are about to be eaten by a wolf!'", "def warn_the_sheep(queue):\n position = 0 \n for i in range(len(queue)):\n if (queue[i]==\"wolf\"):\n position = len(queue)-1 -i\n break\n if (position == 0):\n return \"Pls go away and stop eating my sheep\"\n else:\n return \"Oi! Sheep number \"+str(position)+\"! You are about to be eaten by a wolf!\"", "def warn_the_sheep(queue):\n N = queue[::-1].index(\"wolf\")\n return \"Pls go away and stop eating my sheep\" if queue[-1] == \"wolf\" else f\"Oi! Sheep number {N}! You are about to be eaten by a wolf!\"", "warn_the_sheep = lambda q : 'Pls go away and stop eating my sheep' if q[-1] == 'wolf' else f'Oi! Sheep number {str(q[::-1].index(\"wolf\"))}! You are about to be eaten by a wolf!'", "def warn_the_sheep(queue):\n queue = queue[::-1]\n \n for i in range(0,len(queue)):\n if queue[i]==\"wolf\":\n if i==0:\n return \"Pls go away and stop eating my sheep\"\n else:\n return f\"Oi! Sheep number {i}! You are about to be eaten by a wolf!\"\n else:\n pass", "def warn_the_sheep(queue):\n wolfpos = queue.index(\"wolf\")\n sheepeat = len(queue) - queue.index(\"wolf\") -1\n n = str(sheepeat)\n if n == \"0\":\n return(\"Pls go away and stop eating my sheep\")\n else:\n return (\"Oi! Sheep number \"+n+\"! You are about to be eaten by a wolf!\")", "def warn_the_sheep(queue):\n x = len(queue)\n z = 0\n \n for k in range(0, x):\n if queue[k] == \"wolf\" and k < x:\n z += k\n else:\n z += 0\n \n j = x-z-1\n if z >= x and j <= 0:\n return \"Pls go away and stop eating my sheep\"\n elif j == 0:\n return \"Pls go away and stop eating my sheep\"\n else:\n return \"Oi! Sheep number \" + str(j) + \"! You are about to be eaten by a wolf!\"\n", "def warn_the_sheep(queue):\n \n for i in range(len(queue)):\n if queue[-1] == 'wolf':\n return 'Pls go away and stop eating my sheep'\n elif queue[i] =='wolf':\n return f'Oi! Sheep number {len(queue)-1-i}! You are about to be eaten by a wolf!'\n", "def warn_the_sheep(queue):\n wolf = len(queue) - queue.index('wolf') - 1\n \n if not wolf: return 'Pls go away and stop eating my sheep'\n \n return f'Oi! Sheep number {wolf}! You are about to be eaten by a wolf!'", "def warn_the_sheep(queue):\n print(queue[-1])\n return ('Pls go away and stop eating my sheep', 'Oi! Sheep number {0}! You are about to be eaten by a wolf!'.format(len(queue[queue.index('wolf')::])-1))[queue[-1]=='sheep']", "def warn_the_sheep(queue):\n w = queue.index('wolf') +1\n l = len(queue)\n \n if w == l:\n return 'Pls go away and stop eating my sheep'\n else:\n return f'Oi! Sheep number {l-w}! You are about to be eaten by a wolf!'", "def warn_the_sheep(queue):\n db=0\n for i in range(len(queue)-1,-1,-1):\n if queue[i]==\"wolf\" and db==0:\n return \"Pls go away and stop eating my sheep\"\n elif queue[i]==\"wolf\" and db!=0:\n return \"Oi! Sheep number \"+str(db)+\"! You are about to be eaten by a wolf!\"\n else:\n db=db+1", "def warn_the_sheep(queue):\n return 'Pls go away and stop eating my sheep' if queue.pop() == 'wolf' else f\"Oi! Sheep number {int(len(queue))-int(queue.index('wolf'))}! You are about to be eaten by a wolf!\""] | {"fn_name": "warn_the_sheep", "inputs": [[["sheep", "sheep", "sheep", "sheep", "sheep", "wolf", "sheep", "sheep"]], [["sheep", "wolf", "sheep", "sheep", "sheep", "sheep", "sheep"]], [["wolf", "sheep", "sheep", "sheep", "sheep", "sheep", "sheep"]], [["sheep", "wolf", "sheep"]], [["sheep", "sheep", "wolf"]]], "outputs": [["Oi! Sheep number 2! You are about to be eaten by a wolf!"], ["Oi! Sheep number 5! You are about to be eaten by a wolf!"], ["Oi! Sheep number 6! You are about to be eaten by a wolf!"], ["Oi! Sheep number 1! You are about to be eaten by a wolf!"], ["Pls go away and stop eating my sheep"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 14,374 |
def warn_the_sheep(queue):
|
441a15a2d69e91c115567a1d655c1996 | UNKNOWN | You have been presented with a cipher, your goal is to re-create the cipher with little information. Use the examples provided to see if you can find a solution to how this cipher is made. You will be given no hints, only the handful of phrases that have already been deciphered for you.
Your only hint: Spaces are left alone, they are not altered in any way. Anytime there is a space, it should remain untouched and in place.
I hope you enjoy, but this one should be genuinely infuriating. | ["def cipher(p):\n return ''.join(chr((ord(j)+i%3+(i-1)//3-97)%26+97) if j!=' 'and i!=0 else j for i,j in enumerate(p))", "from itertools import count\n\nBASE = ord('a')\n\ndef deltas():\n for i in count(-1):\n yield max(i, 0)\n yield i + 2\n yield i + 3\n\ndef cipher(phrase):\n return ''.join(\n c if c.isspace() else chr(BASE + (ord(c) - BASE + delta) % 26)\n for c, delta in zip(phrase, deltas())\n )", "from string import ascii_lowercase as A\ndef cipher(phrase: str):\n a = A*2 + ' '*26\n seq = lambda n: n%3 + n//3 + (n%3>0) - (n>0)\n cip = lambda i: a[a.index(phrase[i]) + seq(i)]\n return ''.join(map(cip, range(len(phrase))))", "cifer_sequence = [0,1,2,0,2,3]\n\n\ndef cipher(phrase: str):\n crypt = ''\n for i, simbol in enumerate(phrase):\n if i < len(cifer_sequence):\n cifer_sequence.append(cifer_sequence[-3] + 1)\n if simbol == ' ':\n crypt += ' '\n else:\n crypt += chr((ord(simbol) - ord('a') + cifer_sequence[i]) % 26 + ord('a'))\n return crypt", "def shift(s=1):\n yield 0\n while 1:\n yield s ; s+=1\n yield s\n yield s-2\n\ndef cipher(s):\n return ''.join( c if c==' ' else chr( (x+ord(c)-97)%26 + 97 )\n for c,x in zip(s,shift()) )", "def cipher(phrase: str):\n t = [0,2,3]\n string = ''\n x = -1\n for i in phrase:\n x += 1\n if(ord(i) != ord(' ')):\n c = ord(i) - 97\n if(x == 0):\n string += chr(c+97)\n elif(x == 1):\n string += chr(((c+1)%26)+97)\n elif(x == 2):\n string += chr(((c+2)%26)+97)\n else:\n string += chr(((c+t[x%3]+(int)(x/3) - 1)%26)+97)\n else:\n string += ' '\n\n \n print(string)\n return string", "def cipher(phrase: str):\n mass = ['a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z','a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z']\n j = 0\n cip = []\n num = []\n x = 0\n y = 1\n z = 2\n for i in range(0, len(phrase), 3):\n num.append(x)\n if y >= 2:\n x += 1\n num.append(y)\n y+=1\n num.append(z)\n z+=1\n\n for i in range(0, len(phrase)):\n for j in range(0, len(mass)):\n if phrase[i] == mass[j]:\n j = j + num[i]\n cip.append(mass[j])\n break\n elif phrase[i] == \" \":\n cip.append(\" \")\n break\n \n return (''.join(cip))", "def cipher(str):\n key = [0,1,2,0,2,3,1,3,4,2,4,5,3,5,6,4,6,7,5,7,8,6,8,9,7,9,10,8,10,11,9,11,12,10,12,13,11,13,14,12,14,15,13,15,16,14,16,17,15,17,18,16,18,19,17,19,20,18,20,21,19,21,22,20,22,23,21,23,24,22,24,25,23,25,26]\n result = ''\n alphabet = 'abcdefghijklmnopqrstuvwxyz' * 2\n \n for i in range(len(str)):\n if str[i] == ' ':\n result += ' '\n continue\n alpha_index = alphabet.find(str[i])\n result += alphabet[alpha_index + key[i]]\n return result", "def cipher(phrase: str):\n alphabet = \"abcdefghijklmnopqrstuvwxyz\"\n res = [phrase[0]]\n for i, char in enumerate(phrase[1:]):\n if char != ' ':\n res.append(alphabet[(ord(char)-97 + i//3 + (i+1)%3) % 26])\n else:\n res.append(' ')\n return \"\".join(res)\n #0, 1,2,0, 2,3,1, 3,4,2,4,5,3,5,6,4,6,7,5,7,8,\n", "def cipher(phrase: str):\n return ''.join(chr(ord('a') + (ord(c) - ord('a') + i % 3 + (i - 1) // 3) % 26) if i > 0 and c != ' ' else c for i, c in enumerate(phrase)) "] | {"fn_name": "cipher", "inputs": [["this will probably not be fun"], ["hopefully you will find satisfaction at least"], ["really this is very tedious"], ["if you made it this far you are a better programmer than i am"]], "outputs": [["tiks zjop twrggfrf uwz kl pcx"], ["hprehxmoc ctx cmrs mqtl zjdqcqjnfsaa nh ztnhj"], ["rfclnb wlkw lx zkyd bklrvdc"], ["ig yqx pefi ly xnpx ngz fxe kcn m nregsd eedwfqdbvj larg a vf"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,804 |
def cipher(phrase:
|
f49b1adc4c905cf36831374465d9d6cc | UNKNOWN | Given an array containing only zeros and ones, find the index of the zero that, if converted to one, will make the longest sequence of ones.
For instance, given the array:
```
[1, 0, 1, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 1]
```
replacing the zero at index 10 (counting from 0) forms a sequence of 9 ones:
```
[1, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1]
'------------^------------'
```
Your task is to complete the function that determines where to replace a zero with a one to make the maximum length subsequence.
**Notes:**
- If there are multiple results, return the last one:
`[1, 1, 0, 1, 1, 0, 1, 1] ==> 5`
- The array will always contain only zeros and ones.
Can you do this in one pass? | ["def replace_zero(arr):\n m, im, i, lst = 0, -1, -1, ''.join(map(str,arr)).split('0')\n for a,b in zip(lst,lst[1:]):\n i += len(a) + 1 \n candidate = len(a)+len(b)+1\n if m <= candidate:\n im, m = i, candidate\n return im", "def replace_zero(arr):\n ret = [0,0]\n arr = [None] + arr + [None]\n for i,e in enumerate(arr):\n if type(arr[i])==int and not e and any((arr[i+1],arr[i-1])):\n mx = (sm(arr[:i]) + sm(arr[i+1:][::-1]))\n ret = [ret,[mx, i-1]][mx>=ret[0]]\n return ret[-1]\n \ndef sm(arr, c=0):\n i = len(arr)-1\n while arr[i]:\n i -=1\n c +=1\n return c", "def replace_zero(lst):\n z = [-1] + [i for i, n in enumerate(lst) if not n] + [len(lst)]\n return max((z[i+1]-z[i-1], z[i]) for i in range(1, len(z)-1))[1]\n", "import itertools\n\ndef replace_zero(arr):\n xs = list(itertools.chain.from_iterable([length if key else 0] * length\n for key, grp in itertools.groupby(arr)\n for _, length in [(key, sum(1 for _ in grp))]\n ))\n return max(\n (0, i) if x else (1 + (xs[i-1] if i > 0 else 0) + (xs[i+1] if i < len(xs)-1 else 0), i)\n for i, x in enumerate(xs)\n )[1]", "def replace_zero(arr):\n # (len, index)\n best_len, best_idx = 0, 0\n curr_len, curr_idx = 0, None\n\n for i, e in enumerate(arr):\n if e == 1:\n curr_len += 1\n else:\n if curr_len >= best_len:\n best_len, best_idx = curr_len, curr_idx\n\n if curr_idx is None:\n curr_len += 1\n elif i - curr_idx < 2:\n curr_len = 1\n else:\n curr_len = i - curr_idx\n curr_idx = i\n\n if curr_len >= best_len:\n best_len, best_idx = curr_len, curr_idx\n\n return best_idx", "def replace_zero(arr):\n labels=[]\n max=1\n ans=-1\n # find all the indices that correspond to zero values\n for i in range(len(arr)):\n if arr[i]==0:\n labels.append(i)\n # try each index and compute the associated length\n for j in range(len(labels)):\n arr[labels[j]]=1\n count=1\n end=0\n # check how long the sequence in on the left side.\n k=labels[j]\n while end==0 and k!=0:\n if arr[k-1]:\n count=count+1\n k=k-1\n else:\n end=1\n end=0\n k=labels[j]\n # check how long the sequence in on the right side.\n while end==0 and k!=len(arr)-1:\n if arr[k+1]:\n count=count+1\n k=k+1\n else:\n end=1\n # restore the selected element to zero and check if the new value is a max.\n arr[labels[j]]=0\n if(count>=max):\n ans=labels[j]\n max=count\n return(ans)\n\n", "def replace_zero(arr):\n max_idx, count = None, 0\n left = idx = right = 0\n for i, n in enumerate(arr + [0]):\n if n:\n right += 1\n else:\n if left + right >= count:\n max_idx, count = idx, left + right\n left, idx, right = right, i, 0\n return max_idx", "def replace_zero(a):\n li1 = []\n for i, j in enumerate(a):\n if j == 0:\n temp = a[:]\n t,t1,li,temp[i] = i,i+1,[],1\n while t >= 0 and temp[t] == 1 : li.append(temp[t]) ; t -= 1\n while t1 <= len(a) - 1 and temp[t1] == 1 : li.append(temp[t1]) ; t1 += 1\n li1.append([len(li), i])\n return sorted( li1, key=lambda x: (-x[0], -x[1]) )[0][1]", "import itertools as it\n\ndef iter_len(iterator):\n return len(list(iterator))\n\ndef window (iterable, length):\n iterators = [it.islice(iterator, idx, None) for idx,iterator in enumerate(it.tee(iterable, length))]\n return zip(*iterators)\n \ndef replace_zero(arr):\n\n def accumulate_group(total, group):\n _, prev_length, prev_begin = total \n key, length, _ = group\n return key, length, prev_begin+prev_length\n \n \n groups = ((key, iter_len(group), 0) for key, group in it.groupby(arr))\n empty = [(None, 0, 0)]\n indexed_groups = it.accumulate(it.chain(empty,groups,empty), accumulate_group)\n \n is_zero = lambda group: group[0] == 0\n is_one = lambda group: group[0] == 1\n group_len = lambda group: group[1]\n first_pos = lambda group: group[2]\n last_pos = lambda group: group[2] + group[1] - 1\n revelant = lambda triple: is_zero(triple[1])\n \n triples = filter(revelant, window(indexed_groups,3))\n \n def solutions (triples): \n for left,this,right in triples:\n if group_len(this) == 1:\n yield group_len(left)+1+group_len(right), first_pos(this)\n else:\n yield group_len(left)+1, first_pos(this)\n yield group_len(right)+1, last_pos(this)\n \n length, positions = max(solutions(triples))\n return positions", "def replace_zero(arr):\n z = [0]+[i for i in range(len(arr)) if not arr[i]] + [len(arr)]\n sums = sorted([[sum(arr[z[i]:z[i+2]]),z[i+1]] for i in range(len(z)-2)])\n return sums[-1][1]\n"] | {"fn_name": "replace_zero", "inputs": [[[1, 0, 1, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 1]], [[1, 1, 0, 1, 1, 0, 1, 1]], [[1, 1, 1, 0, 1, 1, 0, 1, 1, 1]], [[0, 1, 1, 1]], [[1, 1, 1, 0]], [[1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 0, 1]], [[0, 0, 0, 0, 0, 1]], [[0, 0, 0, 0, 1, 0]], [[1, 0, 0, 0, 0, 0]], [[0, 1, 0, 0, 0, 0]]], "outputs": [[10], [5], [6], [0], [3], [10], [4], [5], [1], [2]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 5,266 |
def replace_zero(arr):
|
6cd9872b951e263f0c8da1630be0b5f7 | UNKNOWN | You will be given two numbers `m,n`. The numbers could span from `0` to `10000`. We can get their product by using binary reduction as show in the table below.
Example (to understand the table please read the description below it)
real value of m(r)
m
n
(r*n)
0
100
15
0
0
50
30
0
1
25
60
60
0
12
120
0
0
6
240
0
1
3
480
480
1
1
960
960
Above, we are given two numbers `100` and `15`. we keep reducing the bigger number by dividing it by `2` and hold the integer part of the division till it is no more divisible by `2`. Then we assign the real values to these reduced parts of `m`. Any reduced number `[1,m]` has real value of `0` if it is even, and it will have real value of `1` if it is odd. On the other hand the smaller number in this case `n` keeps on doubling itself the same amount of times `m` reduces itself. The idea of this method is to change multiplication of two big number to a sequence of multiplication by `0` or `1` and perform addition to get the final product. You can see that from the last column `(r*n)` above.
if we sum the last column we get `0+60+0+0+480+960=1500=100*15`
Now your task for this kata will be to get those non-zero number in the last column in an array and return it sorted in descending order.so for the above example the return will be `[960,480,60]`.
_Beware: _`m,n`_ are not necessarily ordered._ | ["def bin_mul(m,n):\n if m < n: return bin_mul(n,m)\n if n == 0: return []\n res = []\n while m > 0:\n if m % 2 == 1: res.append(n)\n m = m//2; n *=2\n return res[::-1]", "def bin_mul(m, n):\n if m < n:\n m, n = n, m\n xs = []\n while m >= 1:\n m, m_r = divmod(m, 2)\n if m_r and n:\n xs.append(n)\n n *= 2\n return sorted(xs, reverse=True)", "def bin_mul(m,n):\n if m < n: return bin_mul(n,m)\n if n == 0: return[] \n r = []\n while m >= 1:\n print(m,n)\n if m % 2: r.append(1*n)\n m = m//2\n n = n*2\n \n return sorted(r,reverse=True)", "def bin_mul(m,n): \n result = []\n ma,mi = max(m,n), min(m,n)\n while(ma!=0): \n if ma%2 and mi!=0:\n result= [mi] + result\n mi*=2\n ma//=2\n return result", "def bin_mul(a, b):\n (b, a), r = sorted((a, b)), []\n while a:\n if a % 2 and b:\n r.append(b)\n a //= 2\n b *= 2\n return r[::-1]", "def decimalToBinary(n): \n return bin(n).replace(\"0b\", \"\")\ndef bin_mul(m,n):\n Binval = m\n Top = n\n if m < n:\n Binval = n\n Top = m\n Binary = list(decimalToBinary(Binval))\n val = []\n Binary.reverse()\n for x in Binary:\n if int(x) == 1 and Top !=0:\n val.append(int(x)*Top)\n Binval -= int(abs(Binval/2))\n Top *= 2 \n return val[::-1]", "def bin_mul(m,n):\n n,m = sorted((m,n))\n r = [n] if (m%2 and n) else []\n while m:\n m >>= 1\n n <<= 1\n if m%2 and n:\n r.append(n)\n return sorted(r,reverse=True)", "def bin_mul(m,n):\n r=[]\n n,m=sorted((m,n))\n if not n:return r\n while m:\n if m%2:\n r+=n,\n m//=2\n n*=2\n return r[::-1]", "def bin_mul(m,n):\n r=[]\n n,m=sorted([m,n])\n if n==0:\n return []\n while(m>1):\n if m%2==1:\n r.insert(0,n)\n m//=2\n n*=2\n r.insert(0,n)\n return r", "def bin_mul(m,n):\n if not n or not m:\n return []\n max_p, min_p = max(m, n), min(m, n)\n products = []\n while max_p > 0:\n if max_p % 2:\n products.append(max_p % 2 * min_p)\n max_p, min_p = max_p//2, min_p * 2\n products.sort()\n products.reverse()\n return products"] | {"fn_name": "bin_mul", "inputs": [[100, 15], [15, 0], [0, 0]], "outputs": [[[960, 480, 60]], [[]], [[]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,400 |
def bin_mul(m,n):
|
73284294ee4bcb3e238e3c88fb4530df | UNKNOWN | # Introduction
There is a war and nobody knows - the alphabet war!
There are two groups of hostile letters. The tension between left side letters and right side letters was too high and the war began. The letters called airstrike to help them in war - dashes and dots are spreaded everywhere on the battlefield.
# Task
Write a function that accepts `fight` string consists of only small letters and `*` which means a bomb drop place. Return who wins the fight after bombs are exploded. When the left side wins return `Left side wins!`, when the right side wins return `Right side wins!`, in other case return `Let's fight again!`.
The left side letters and their power:
```
w - 4
p - 3
b - 2
s - 1
```
The right side letters and their power:
```
m - 4
q - 3
d - 2
z - 1
```
The other letters don't have power and are only victims.
The `*` bombs kills the adjacent letters ( i.e. `aa*aa` => `a___a`, `**aa**` => `______` );
# Example
# Alphabet war Collection
Alphavet war
Alphabet war - airstrike - letters massacre
Alphabet wars - reinforces massacre
Alphabet wars - nuclear strike
Alphabet war - Wo lo loooooo priests join the war | ["import re\n\npowers = {\n 'w': -4, 'p': -3, 'b': -2, 's': -1,\n 'm': +4, 'q': +3, 'd': +2, 'z': +1,\n}\n\ndef alphabet_war(fight):\n fight = re.sub('.(?=\\*)|(?<=\\*).', '', fight)\n result = sum(powers.get(c, 0) for c in fight)\n if result < 0:\n return 'Left side wins!'\n elif result > 0:\n return 'Right side wins!'\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n\n s = 'wpbsszdqm'\n alive = []\n\n if '*' not in fight[:2]:\n alive.append(fight[0])\n\n for i, c in enumerate(fight[1:], 1):\n if '*' not in fight[i-1:i+2]:\n alive.append(c)\n \n x = sum(4 - s.index(c) for c in alive if c in s)\n return 'Let\\'s fight again!' if x==0 else ['Left','Right'][x<0] + ' side wins!'", "import re\n\ndef alphabet_war(fight):\n pattern = re.compile(r\"(\\w)?\\*+(\\w)?\")\n powers = pattern.sub(\"\", fight)\n scores = \"mqdz*sbpw\" \n score = sum(i * powers.count(p) for i, p in enumerate(scores, -4))\n return [\"Let's fight again!\", \"Left side wins!\", \"Right side wins!\"][(score>0)-(score<0)]", "import re\n\nPOWERS = {c:i for i,c in enumerate('wpbs zdqm',-4)}\nPATTERN = re.compile(r'(?<!\\*)([{}])(?!\\*)'.format(''.join(POWERS.keys()-{' '})))\n\ndef alphabet_war(fight):\n s = sum(POWERS[c] for c in PATTERN.findall(fight))\n return [\"Let's fight again!\", 'Left side wins!', 'Right side wins!'][ (s<0) - (s>0) ]", "import re \nle = {\"w\":4,\"p\":3,\"b\":2,\"s\":1}\nri = {\"m\":4,\"q\":3,\"d\":2,\"z\":1}\ndef alphabet_war(fight):\n w = re.sub(r\"[a-z]\\*[a-z]|[a-z]\\*|\\*[a-z]\",\"\",fight)\n l = sum([le.get(i,0) for i in w])\n r = sum([ri.get(i,0) for i in w])\n if l==r:\n return \"Let's fight again!\"\n return \"Right side wins!\" if r>l else \"Left side wins!\"", "import re\ndef alphabet_war(fight):\n d = {a : c for c, a in enumerate('wpbs zdqm', -4) if c}\n s = sum(d.get(a, 0) for a in re.sub(r'.?\\*+.?', '', fight))\n return ['Left side wins!', \"Let's fight again!\", 'Right side wins!'][1 + min(max(s, -1), 1)]", "def alphabet_war(fight):\n\n left_side = 'wpbs'\n right_side = 'mqdz' \n left_power = 0\n right_power = 0\n bombs = [ i for i in range(0,len(fight)) if fight[i] =='*'] \n death = []\n \n for boom in bombs:\n if 0<boom<len(fight)-1:\n death.append(boom-1)\n death.append(boom+1)\n elif boom == 0:\n death.append(boom+1)\n elif boom ==len(fight)-1:\n death.append(boom-1)\n \n bombs_and_death = bombs + death\n \n after_fight = ''.join(fight[i] if i not in bombs_and_death else '' for i in range(0,len(fight)))\n \n for i in after_fight:\n if i == 'w' or i =='m': \n pow = 4 \n elif i == 'p' or i =='q': \n pow = 3 \n elif i == 'b' or i =='d': \n pow = 2 \n elif i == 's' or i =='z': \n pow = 1 \n \n if i in left_side:\n # 'wpbs'\n left_power+=pow\n elif i in right_side:\n # 'mqdz' \n right_power+=pow\n \n if left_power>right_power:\n return 'Left side wins!'\n elif right_power>left_power:\n return 'Right side wins!'\n else:\n return '''Let's fight again!'''", "from functools import partial\nfrom numpy import sign\nfrom re import compile\n\nremove = partial(compile(r\".?\\*+.?\").sub, \"\")\nmemo = {'w':4, 'p':3, 'b':2, 's':1, 'm':-4, 'q':-3, 'd':-2, 'z':-1}\nresult = (\"Let's fight again!\", \"Left side wins!\", \"Right side wins!\")\nvalue = lambda c: memo.get(c, 0)\n\ndef alphabet_war(fight):\n return result[sign(sum(map(value, remove(fight))))]", "def alphabet_war(fight):\n sides = { 'left':{ 'w':4,'p':3,'b':2,'s':1 }, 'right':{ 'm':4,'q':3,'d':2,'z':1 } }\n \n left, right = ['Left',0],['Right',0]\n \n fild = [' '] * (len(fight) +2)\n \n for i,e in enumerate(' '+fight+' '):\n if e == '*':\n fild[i-1:i+2] = ['_','_','_']\n elif e != '*' and fild[i] != '_': \n fild[i] = e \n\n for e in fild:\n left[1] += sides['left' ].get( e, 0 )\n right[1] += sides['right'].get( e, 0 )\n \n winer = max([left, right], key = lambda e:e[1] )\n \n return \"Let's fight again!\" if left[1] == right[1] else f'{winer[0]} side wins!'\n"] | {"fn_name": "alphabet_war", "inputs": [["z"], ["z*dq*mw*pb*s"], ["zdqmwpbs"], ["zz*zzs"], ["sz**z**zs"], ["z*z*z*zs"], ["*wwwwww*z*"]], "outputs": [["Right side wins!"], ["Let's fight again!"], ["Let's fight again!"], ["Right side wins!"], ["Left side wins!"], ["Left side wins!"], ["Left side wins!"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,403 |
def alphabet_war(fight):
|
e000a3e337f8b7829a7c487f57ab5d0d | UNKNOWN | Description
In English we often use "neutral vowel sounds" such as "umm", "err", "ahh" as fillers in conversations to help them run smoothly.
Bob always finds himself saying "err". Infact he adds an "err" to every single word he says that ends in a consonant! Because Bob is odd, he likes to stick to this habit even when emailing.
Task
Bob is begging you to write a function that adds "err" to the end of every word whose last letter is a consonant (not a vowel, y counts as a consonant).
The input is a string that can contain upper and lowercase characters, some punctuation but no numbers. The solution should be returned as a string.
NOTE: If the word ends with an uppercase consonant, the following "err" will be uppercase --> "ERR".
eg:
```
"Hello, I am Mr Bob" --> "Hello, I amerr Mrerr Boberr"
"THIS IS CRAZY!" --> "THISERR ISERR CRAZYERR!"
```
Good luck! | ["def err_bob(s):\n res = \"\"\n for i, c in enumerate(s):\n res += c\n if i == len(s)-1 or s[i+1] in \" .,:;!?\":\n if c.islower() and c not in \"aeiou\":\n res += \"err\"\n if c.isupper() and c not in \"AEIOU\":\n res += \"ERR\"\n return res", "import re\n\ndef err_bob(string):\n return re.sub(r'(?:[bcdfghjklmnpqrstvwxyz])\\b',lambda m:m.group(0)+'ERR' if m.group(0).isupper() else m.group(0)+'err',string,flags=re.I)", "import re\n\ndef err_bob(s):\n return re.sub(\n r'[bcdfghjklmnpqrstvwxyz]\\b',\n lambda m: m.group() + ('err' if m.group().islower() else 'ERR'),\n s,\n flags=re.IGNORECASE\n )", "import re\ndef err_bob(string):\n string = re.sub(r'([b-df-hj-np-tv-z])\\b', r'\\1err', string)\n string = re.sub(r'([B-DF-HJ-NP-TV-Z])\\b', r'\\1ERR', string)\n return string", "import re\n\ndef err_bob(stg):\n return re.sub(r\"[bcdfghj-np-tv-z]\\b\", end_err, stg, flags=re.I)\n\ndef end_err(char):\n return f\"{char[0]}{'err' if char[0].islower() else 'ERR'}\"\n", "consonants = \"bcdfghjklmnpqrstvwxyz\"\n\ndef err_bob(stg):\n result = \"\"\n for i, char in enumerate(stg):\n result = f\"{result}{char}\"\n if char.isalpha() and not stg[i+1:i+2].isalpha():\n end = \"err\" if char in consonants else \"ERR\" if char in consonants.upper() else \"\"\n result = f\"{result}{end}\"\n return result", "import re\n\ndef repl(m):\n return m[0]+('err' if m[0].islower() else 'ERR')\n\ndef err_bob(s):\n return re.sub(r'(?![aeiou])[a-z]\\b', repl, s, flags=re.I)", "def err_bob(s):\n t, a = \"\", \"aeioubcdfghjklmnpqrstvwxyz\"\n for i, c in enumerate(s + \" \"):\n if c.lower() not in a and s[i - 1].lower() in a[5:]:\n t += \"ERR\" if s[i - 1].isupper() else \"err\"\n t += c\n return t[:-1]", "from re import sub\n\ndef err_bob(string):\n \n string = sub(r'\\b(\\w*[bcdfghjklmnpqrstvwxyz])\\b', r'\\1err', string)\n return sub(r'\\b(\\w*[BCDFGHJKLMNPQRSTVWXYZ])\\b', r'\\1ERR', string)"] | {"fn_name": "err_bob", "inputs": [["r r r r r r r r"], ["THIS, is crazy!"], ["hI, hi. hI hi skY! sky? skY sky"], ["Hello, I am Mr Bob."], ["This, is. another! test? case to check your beautiful code."], ["Hello from the other siiiiideeee"], ["Punctuation? is, important! double space also"]], "outputs": [["rerr rerr rerr rerr rerr rerr rerr rerr"], ["THISERR, iserr crazyerr!"], ["hI, hi. hI hi skYERR! skyerr? skYERR skyerr"], ["Hello, I amerr Mrerr Boberr."], ["Thiserr, iserr. anothererr! testerr? case to checkerr yourerr beautifulerr code."], ["Hello fromerr the othererr siiiiideeee"], ["Punctuationerr? iserr, importanterr! double space also"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,111 |
def err_bob(s):
|
544e40eed6936210fc7cc415593ca1e0 | UNKNOWN | You are given a secret message you need to decipher. Here are the things you need to know to decipher it:
For each word:
- the second and the last letter is switched (e.g. `Hello` becomes `Holle`)
- the first letter is replaced by its character code (e.g. `H` becomes `72`)
Note: there are no special characters used, only letters and spaces
Examples
```
decipherThis('72olle 103doo 100ya'); // 'Hello good day'
decipherThis('82yade 115te 103o'); // 'Ready set go'
``` | ["def decipher_word(word):\n i = sum(map(str.isdigit, word))\n decoded = chr(int(word[:i]))\n if len(word) > i + 1:\n decoded += word[-1]\n if len(word) > i:\n decoded += word[i+1:-1] + word[i:i+1]\n return decoded\n\ndef decipher_this(string):\n return ' '.join(map(decipher_word, string.split()))", "def decipher_this(string):\n words = string.split()\n res = []\n #check if there is a part only consisting digts\n for x in words:\n if x.isdigit():\n res.append(chr(int(x)))\n #if not then seperate the numbers and the string charakters in seperate variables\n elif len(x) >= 3:\n sum = \"\"\n new_str = \"\"\n for i in x: \n if i.isdigit():\n sum += i\n else:\n new_str += i\n #transverse the digit to the she specific letter and add the old string to it\n sum = chr(int(sum)) + new_str\n #ckeck if the string length has changed due to the transversation and switch the letter position \n if len(sum) > 2:\n x = (sum[0::len(sum) - 1] + sum[2:len(sum) -1 ] + sum[1])\n res.append(x)\n else:\n res.append(sum)\n return \" \".join(res)\n", "def decipher_this(string):\n words = []\n for word in string.split():\n code = ''.join(char for char in word if char.isdigit())\n new_word = chr(int(code))+''.join(char for char in word if not char.isdigit())\n words.append(new_word[:1]+new_word[-1]+new_word[2:-1]+new_word[1] if len(new_word)>2 else new_word)\n return ' '.join(words)", "import re\n\ndef decipher_this(stg):\n return re.sub(r\"(\\d+)(\\w?)(\\w*)\", decipher_word, stg)\n\ndef decipher_word(match):\n o, cl, cr = match.groups()\n return f\"{chr(int(o))}{cr[-1:]}{cr[:-1]}{cl}\"", "def decipher_this(string):\n translated = []\n for word in string.split():\n digits = ''\n for c in word:\n if c.isdigit():\n digits += c\n word = word.replace(digits, chr(int(digits))) \n if len(word) > 2:\n translated.append(''.join([word[0], word[-1], word[2:-1], word[1]]))\n else:\n translated.append(word)\n return ' '.join(translated)", "import string as Str\n\ndef decipher_this(string):\n \n allLetters = Str.ascii_letters\n allNumbers = Str.digits\n \n sentence = string.split(\" \")\n \n decodedSentence = \"\"\n \n for word in sentence:\n ascii_code = word.strip(allLetters)\n ascii_letters = word.strip(allNumbers)\n \n first_letter = chr(int(ascii_code))\n \n if len(ascii_letters) > 1:\n ascii_letters = ascii_letters[-1:] + ascii_letters[1:-1] + ascii_letters[:1]\n \n decodedSentence = decodedSentence + first_letter + ascii_letters + \" \"\n \n return decodedSentence[:-1]\n \n", "import re\ndef decipher_this(string):\n #list to save decrypted words\n decrypted = []\n for word in string.split():\n #get number in string\n re_ord = re.match(r'\\d+', word).group()\n #replace number by ascii letter and transform word in a list\n new_word = list(re.sub(re_ord, chr(int(re_ord)), word))\n #swap second and last letter in the word list\n if len(new_word) > 2:\n new_word[1], new_word[-1] = new_word[-1], new_word[1]\n #save the word in the string list\n decrypted.append(''.join(new_word))\n #return the decrypted words list as a string\n return ' '.join(decrypted)", "import re\ndef decipher_this(text):\n return re.sub(r'\\b(\\d{2,3})(\\w?)(\\w*?)(\\w?)\\b', lambda m: '{}'.format(chr(int(m.group(1))) + m.group(4) + m.group(3) + m.group(2)), text)", "import re\n\nREGEX = re.compile(r'(\\d+)([a-zA-Z]*)$')\n\n\ndef decipher_this(s):\n result = []\n for word in s.split():\n m = REGEX.match(word)\n digits, chars = m.groups()\n tmp = [chr(int(digits))]\n if len(chars) < 2:\n tmp.append(chars)\n else:\n tmp.append('{}{}{}'.format(chars[-1], chars[1:-1], chars[0]))\n result.append(''.join(tmp))\n return ' '.join(result)\n", "import re\ndef decipher_this(str):\n return re.sub(r\"(\\d+)(\\w*)\", lambda m : chr(int(m.group(1))) + ( m.group(2)[-1] + m.group(2)[1:-1] + m.group(2)[0] if len(m.group(2))>1 else m.group(2)), str)"] | {"fn_name": "decipher_this", "inputs": [["65 119esi 111dl 111lw 108dvei 105n 97n 111ka"], ["84eh 109ero 104e 115wa 116eh 108sse 104e 115eokp"], ["84eh 108sse 104e 115eokp 116eh 109ero 104e 104dare"], ["87yh 99na 119e 110to 97ll 98e 108eki 116tah 119esi 111dl 98dri"], ["84kanh 121uo 80roti 102ro 97ll 121ruo 104ple"]], "outputs": [["A wise old owl lived in an oak"], ["The more he saw the less he spoke"], ["The less he spoke the more he heard"], ["Why can we not all be like that wise old bird"], ["Thank you Piotr for all your help"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,512 |
def decipher_this(string):
|
73af21e30f44ba09cb3247a04136cdf5 | UNKNOWN | If we multiply the integer `717 (n)` by `7 (k)`, the result will be equal to `5019`.
Consider all the possible ways that this last number may be split as a string and calculate their corresponding sum obtained by adding the substrings as integers. When we add all of them up,... surprise, we got the original number `717`:
```
Partitions as string Total Sums
['5', '019'] 5 + 19 = 24
['50', '19'] 50 + 19 = 69
['501', '9'] 501 + 9 = 510
['5', '0', '19'] 5 + 0 + 19 = 24
['5', '01', '9'] 5 + 1 + 9 = 15
['50', '1', '9'] 50 + 1 + 9 = 60
['5', '0', '1', '9'] 5 + 0 + 1 + 9 = 15
____________________
Big Total: 717
____________________
```
In fact, `717` is one of the few integers that has such property with a factor `k = 7`.
Changing the factor `k`, for example to `k = 3`, we may see that the integer `40104` fulfills this property.
Given an integer `start_value` and an integer `k`, output the smallest integer `n`, but higher than `start_value`, that fulfills the above explained properties.
If by chance, `start_value`, fulfills the property, do not return `start_value` as a result, only the next integer. Perhaps you may find this assertion redundant if you understood well the requirement of the kata: "output the smallest integer `n`, but higher than `start_value`"
The values for `k` in the input may be one of these: `3, 4, 5, 7`
### Features of the random tests
If you want to understand the style and features of the random tests, see the *Notes* at the end of these instructions.
The random tests are classified in three parts.
- Random tests each with one of the possible values of `k` and a random `start_value` in the interval `[100, 1300]`
- Random tests each with a `start_value` in a larger interval for each value of `k`, as follows:
- for `k = 3`, a random `start value` in the range `[30000, 40000]`
- for `k = 4`, a random `start value` in the range `[2000, 10000]`
- for `k = 5`, a random `start value` in the range `[10000, 20000]`
- for `k = 7`, a random `start value` in the range `[100000, 130000]`
- More challenging tests, each with a random `start_value` in the interval `[100000, 110000]`.
See the examples tests.
Enjoy it.
# Notes:
- As these sequences are finite, in other words, they have a maximum term for each value of k, the tests are prepared in such way that the `start_value` will always be less than this maximum term. So you may be confident that your code will always find an integer.
- The values of `k` that generate sequences of integers, for the constrains of this kata are: 2, 3, 4, 5, and 7. The case `k = 2` was not included because it generates only two integers.
- The sequences have like "mountains" of abundance of integers but also have very wide ranges like "valleys" of scarceness. Potential solutions, even the fastest ones, may time out searching the next integer due to an input in one of these valleys. So it was intended to avoid these ranges.
Javascript and Ruby versions will be released soon. | ["def sum_part(n):\n m, p, q, r, s = 1, 1, 1, 0, n\n while n > 9:\n n, d = divmod(n, 10)\n r += d * p\n p *= 10\n if d: m = 1\n else: m*= 2\n s += q*n + m*memo[r]\n q *= 2\n return s\n\nfrom collections import defaultdict\nqualified = defaultdict(list)\nmemo = {n: n for n in range(10)}\nfor n in range(10, 10 ** 6):\n memo[n] = sum_part(n)\n if memo[n] > n:\n k, r = divmod(n, memo[n] - n)\n if not r: qualified[k].append(memo[n] - n)\n\nfrom bisect import bisect\ndef next_higher(n, k):\n return qualified[k][bisect(qualified[k], n+1)]", "import itertools\nfrom itertools import count\n\ndef break_down(text):\n numbers = text.split()\n ns = list(range(1, len(numbers[0])))\n for n in ns:\n for idxs in itertools.combinations(ns, n):\n yield [''.join(numbers[0][i:j]) for i, j in zip((0,) + idxs, idxs + (None,))]\n \n\n\ndef next_higher(start_value,k):\n r = 0\n for n in count(start_value+1):\n desire = n * k\n for a in break_down(str(desire)):\n r += sum([int(b) for b in a])\n if r == n:\n return r\n r = 0\n\n", "from itertools import count\n\ndef next_higher(start,k):\n\n def parts(n, s, p, store):\n if sum(store) > n:\n return\n \n if not s:\n if len(p) > 1:\n store.append(sum(p))\n return\n \n for i in range(1, len(s) + 1):\n parts(n, s[i:], p + [int(s[:i])], store)\n \n return store\n \n \n for m in count(start + 1):\n x = parts(m, str(m * k), [], [])\n\n if sum(x) == m:\n return m", "def check(n):\n r,l = 0, 0\n while n:\n n,v = divmod(n, 10)\n x = 0\n for i in range(l):\n x += 10**i*2**(l-i-1)\n r += r + v*x + v*10**l\n l += 1\n return r\n\ndef next_higher(start_value, k):\n for i in range(start_value+1, start_value+30000):\n if check(i*k)-i-i*k == 0:\n return i", "from bisect import bisect_right\n\ndef next_higher(start_value, k):\n d = {3: [162, 378, 756, 1161, 1206, 1611, 1989, 34992, 35208, 35388, 37332, 37548, 37728, 40104, 72972, 73152, 75312, 75492, 75708, 77652, 77832, 77868, 78048, 80208, 80388, 113256, 115596, 115812, 117972, 118152, 118368, 120312, 120492, 120708, 155916],\n 4: [198, 260, 276, 520, 536, 552, 796, 812, 1072, 1108, 1368, 1384, 1644, 1680, 1940, 1956, 2216, 2232, 2492, 10728, 10872, 79536, 109008, 110592, 140064, 193488],\n 5: [108, 171, 846, 1692, 4212, 14724, 18936, 19224, 20304, 103608, 123912, 148608, 168912],\n 7: [717, 861, 1005, 1149, 1158, 1293, 1302, 1431, 14308, 14316, 28624, 28632, 28716, 42948, 43024, 43032, 57264, 57272, 57348, 57432, 71664, 71672, 71748, 85988, 86064, 86072, 86148, 100388, 100464, 100472, 101448, 114704, 114788, 114864, 114872, 115764, 115772, 129104, 129188, 130088, 130164, 130172]}\n return d[k][bisect_right(d[k], start_value)]", "from itertools import combinations, chain, count\nfrom functools import lru_cache\n\ndef segmentations(a):\n def split_at(js):\n i = 0\n for j in js:\n yield a[i:j]\n i = j\n yield a[i:]\n\n r = range(1, len(a))\n for k in r:\n for sep in combinations(r, k):\n yield split_at(sep)\n\n@lru_cache(maxsize=None)\ndef value(x, k):\n return sum(int(''.join(L)) for L in chain.from_iterable(segmentations(str(x * k))))\n\ndef next_higher(start_value, k):\n return next(x for x in count(start_value+1) if x == value(x, k))", "m = {\n 3: [162,378,756,1161,1206,1611,1989,34992,37438,37548,37332,37728,40104,113256],\n 4: [198,260,520,796,1072,1108,1368,2216,10728,109008],\n 5: [171,198,846,1692,14724,18936,20304],\n 7: [717,861,1005,1149,1293,101448,114704,129104,123912]\n}\n\ndef next_higher(n,k):\n print(n,k)\n return next((s for s in m[k] if s>n), -1)", "def partitions(s):\n if s:\n for i in range(1, len(s)+1):\n for p in partitions(s[i:]):\n yield [s[:i]] + p\n else:\n yield []\ndef add_values (product_string): \n product_list = partitions(product_string)\n return sum([sum (map(int,items)) for items in list(product_list)[:-1]])\n \ndef next_higher(start_value,k):\n product = (start_value+1) * k\n while add_values(str(product)) != start_value:\n start_value+=1\n product = start_value*k\n return start_value"] | {"fn_name": "next_higher", "inputs": [[100, 3], [200, 4], [300, 5], [500, 7], [717, 7], [1611, 3]], "outputs": [[162], [260], [846], [717], [861], [1989]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,529 |
def next_higher(start,k):
|
57d103069bce0a12d6353cf1277b85ae | UNKNOWN | Given an integer `n`, find two integers `a` and `b` such that:
```Pearl
A) a >= 0 and b >= 0
B) a + b = n
C) DigitSum(a) + Digitsum(b) is maximum of all possibilities.
```
You will return the digitSum(a) + digitsum(b).
```
For example:
solve(29) = 11. If we take 15 + 14 = 29 and digitSum = 1 + 5 + 1 + 4 = 11. There is no larger outcome.
```
`n` will not exceed `10e10`.
More examples in test cases.
Good luck! | ["def solve(n):\n if n < 10:\n return n\n a = int((len(str(n)) - 1) * '9')\n b = n - a\n return sum([int(i) for i in (list(str(a)) + list(str(b)))])\n", "def solve(n):\n a = (len(str(n)) - 1) * '9' or '0'\n return sum(map(int, a + str(n - int(a))))", "def solve(n):\n a = 0\n while a * 10 + 9 <= n:\n a = a * 10 + 9\n return sum(map(int, str(a) + str(n-a)))", "def solve(n):\n l = len(str(n)) - 1\n return sum(map(int, str(n - 10**l + 1))) + 9*l", "def solve(param):\n a = int('0' + '9' * (len(str(param)) - 1))\n return sum(int(d) for d in str(a) + str(param - a))", "def solve(n): \n a=10**(len(str(n))-1)-1\n return sum(map(int,str(a)+str(n-a)))", "solve = lambda n: sum(map(int, str(n - int('0' + '9' * (len(str(n))-1))))) + 9 * (len(str(n)) - 1)", "def solve(n): \n return n if n < 10 else 9 + solve(n // 10) if n % 10 == 9 else 10 + n % 10 + solve(n // 10 - 1)", "def solve(x):\n if not x:\n return x\n b = int(str(int(str(x)[0]) -1) +''.join('9' for i in range(len(str(x))-1)))\n a = x-b\n return sum([int(i) for i in str(a)])+sum([int(i) for i in str(b)])", "def solve(n):\n n = str(n)\n a = str(int(n[0])-1)+'9'*(len(n)-1)\n b = str(int(n)-int(a))\n return sum(int(i) for i in a+b) if int(n) else 0 "] | {"fn_name": "solve", "inputs": [[18], [29], [45], [1140], [7019], [50000000], [15569047737], [2452148459], [1], [0]], "outputs": [[18], [11], [18], [33], [35], [68], [144], [116], [1], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,325 |
def solve(n):
|
21884cd3c88aac759c1c95f31646c578 | UNKNOWN | # Description
Given a number `n`, you should find a set of numbers for which the sum equals `n`. This set must consist exclusively of values that are a power of `2` (eg: `2^0 => 1, 2^1 => 2, 2^2 => 4, ...`).
The function `powers` takes a single parameter, the number `n`, and should return an array of unique numbers.
## Criteria
The function will always receive a valid input: any positive integer between `1` and the max integer value for your language (eg: for JavaScript this would be `9007199254740991` otherwise known as `Number.MAX_SAFE_INTEGER`).
The function should return an array of numbers that are a **power of 2** (`2^x = y`).
Each member of the returned array should be **unique**. (eg: the valid answer for `powers(2)` is `[2]`, not `[1, 1]`)
Members should be sorted in **ascending order** (small -> large). (eg: the valid answer for `powers(6)` is `[2, 4]`, not `[4, 2]`) | ["def powers(n):\n return [1<<i for i, x in enumerate(reversed(bin(n))) if x == \"1\"]", "def powers(n):\n return [2**i for i, d in enumerate(f\"{n:b}\"[::-1]) if d == \"1\"]", "def powers(n):\n tmp = []\n i = 0\n res = []\n while 2 ** i <= n:\n tmp.append(2 ** i)\n i += 1\n for j in tmp[::-1]:\n if n >= j:\n res.append(j)\n n -= j\n return res[::-1]", "# return an array of numbers (that are a power of 2)\n# for which the input \"n\" is the sum\ndef powers(n):\n s = bin(n)[::-1]\n return [2**i for i, x in enumerate(s) if x == '1']\n", "pows = [2**i for i in range(63, -1, -1)]\n\ndef powers(n):\n result = []\n for p in pows:\n if n >= p:\n result.append(p)\n n -= p\n return result[::-1]", "powers=lambda n:[1<<i for i in range(n.bit_length())if 1<<i&n]", "def powers(n):\n return [d<<p for p,d in enumerate(map(int,reversed(f'{n:b}'))) if d]", "def powers(n):\n return [2**i for i, k in enumerate(bin(n)[::-1]) if k == '1']", "def powers(n):\n return [2**i for i,d in enumerate(bin(n)[::-1]) if d == '1']", "def powers(n):\n next_small = n.bit_length()\n total, lst = 0, []\n while next_small>-1:\n v=1<<next_small\n if total + v <= n:\n total+=v\n lst.append(v)\n if total==n:break\n next_small-=1\n return lst[::-1]\n"] | {"fn_name": "powers", "inputs": [[1], [2], [4], [32], [128], [512], [6], [14], [688], [8197], [1966], [9007199254740991]], "outputs": [[[1]], [[2]], [[4]], [[32]], [[128]], [[512]], [[2, 4]], [[2, 4, 8]], [[16, 32, 128, 512]], [[1, 4, 8192]], [[2, 4, 8, 32, 128, 256, 512, 1024]], [[1, 2, 4, 8, 16, 32, 64, 128, 256, 512, 1024, 2048, 4096, 8192, 16384, 32768, 65536, 131072, 262144, 524288, 1048576, 2097152, 4194304, 8388608, 16777216, 33554432, 67108864, 134217728, 268435456, 536870912, 1073741824, 2147483648, 4294967296, 8589934592, 17179869184, 34359738368, 68719476736, 137438953472, 274877906944, 549755813888, 1099511627776, 2199023255552, 4398046511104, 8796093022208, 17592186044416, 35184372088832, 70368744177664, 140737488355328, 281474976710656, 562949953421312, 1125899906842624, 2251799813685248, 4503599627370496]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,411 |
def powers(n):
|
2c3f2e4832fc19e193e9a4e571ea8ca8 | UNKNOWN | It's the academic year's end, fateful moment of your school report.
The averages must be calculated. All the students come to you and entreat you to calculate their average for them.
Easy ! You just need to write a script.
Return the average of the given array rounded **down** to its nearest integer.
The array will never be empty. | ["def get_average(marks):\n return sum(marks) // len(marks)\n", "def get_average(marks):\n average = sum(marks) / len(marks) \n return int(average)\n", "import numpy\n\ndef get_average(marks):\n return int(numpy.mean(marks))", "get_average = lambda marks: sum(marks)//len(marks)\n \n", "def get_average(marks):\n return int(sum(marks)/len(marks))\n \n", "get_average = lambda m: int(__import__(\"numpy\").mean(m))", "import math\nimport numpy\ndef get_average(marks):\n number = numpy.average(marks)\n return math.floor(number)", "import math\n\n\ndef get_average(marks):\n sum = 0\n for num in marks:\n sum += num\n total = sum / len(marks)\n return math.floor(total)", "import math\n\ndef get_average(marks):\n \n x = (len(marks))\n y = (sum(marks))\n \n i = y/x \n \n return math.floor(i)\n", "def get_average(marks):\n suma=0\n for x in marks:\n suma=suma+x\n return (suma//len(marks))\n", "get_average =lambda x: sum(x)//len(x)", "def get_average(marks):\n mean = sum(marks)/len(marks)\n return int(mean)\n", "def get_average(marks):\n return sum(n for n in marks) // len(marks)", "import math\n\n\ndef get_average(marks):\n return math.floor(sum(marks)/len(marks))\n", "from math import trunc\ndef get_average(marks):\n return trunc(sum(marks)/len(marks))\n", "def get_average(marks):\n sum = 0\n for x in marks:\n sum = sum + x\n num = len(marks)\n ans = sum/num\n return int(ans)", "import pandas as pd\n\ndef get_average(marks):\n s = pd.Series(marks)\n return int(s.mean())", "def get_average(marks):\n return int(__import__(\"statistics\").mean(marks))\n", "import numpy, math\ndef get_average(marks):\n return math.floor(numpy.mean(marks))\n", "def get_average(marks):\n res = int(sum(marks)/len(marks))\n return res\n raise NotImplementedError(\"TODO: get_average\")\n", "import math\n\ndef get_average(marks):\n number = len(marks)\n total = 0\n \n \n for student in marks:\n total = total + student\n \n return math.floor(total/number)\n \n", "def get_average(marks):\n num = 0\n for i in marks:\n num += i \n return (int) (num/(len(marks)))", "def get_average(marks):\n sum = 0\n for i in range(len(marks)):\n sum += marks[i]\n i += 1\n return int(sum/len(marks))\n", "import math\ndef get_average(marks):\n t = sum(marks) / len(marks)\n return math.floor(t)\n", "def get_average(marks):\n count=0\n for i in range(len(marks)):\n count+=marks[i]\n return round(count//len(marks))", "def get_average(marks):\n total = 0\n for k in range(len(marks)):\n total += marks[k]\n return total // len(marks)\n", "def get_average(marks):\n sum=0\n for n in marks:\n sum=sum+n\n avg=sum//len(marks)\n return avg", "def get_average(marks):\n # assumption array is never empty\n # get average of an array and return\n \n # will use sum and len of array to get average\n # rounded down to nearest integer\n avg = sum(marks) // len(marks)\n return avg\n \n \n \n # raise NotImplementedError(\"TODO: get_average\")\n", "def get_average(marks):\n mean = 0.0\n sum = 0\n for i in marks:\n sum += i\n mean = sum/len(marks)\n return int(mean)\n \n", "def get_average(marks):\n sum = 0\n for i in marks:\n sum = sum + i\n \n average = sum / len(marks)\n return int(average)\n \n \n raise NotImplementedError(\"didn't work for\" + marks)\n\nprint(( get_average([2, 2, 2, 2])))\nprint(( get_average([1, 5, 87, 45, 8, 8])))\n \n \n", "import math\n\n\ndef get_average(marks):\n marks_sum = 0\n for mark in marks:\n marks_sum += mark\n average_mark = marks_sum / len(marks)\n\n return math.floor(average_mark)\n\n\nprint((get_average([2,5,13,20,16,16,10])))\n", "def get_average(marks):\n get_average= int(sum(marks)/len(marks))\n return round(get_average)\n raise NotImplementedError(\"TODO: get_average\")\n \n", "def get_average(marks):\n for i in marks:\n return int(round(sum(marks)/len(marks), 1))\n", "import math as math\n\ndef get_average(marks):\n average = math.floor(sum(marks) / len(marks))\n return average\n", "def get_average(marks):\n sum=0\n for v in marks:\n sum+=v\n average=int(sum/len(marks))\n return average", "def get_average(marks):\n import math\n n = 0\n for i in marks:\n n += i\n result = n/len(marks)\n result = math.floor(result)\n return result \n", "def get_average(marks):\n \n avg = 0\n \n for mark in marks:\n avg += mark\n \n avg /= len(marks)\n \n return int(avg)\n", "def get_average(marks):\n \n \n sum_marks = sum(marks)\n len_marks = len(marks)\n avg_marks = sum_marks // len_marks\n return avg_marks\n", "def get_average(marks):\n# raise NotImplementedError(\"get_average\")\n return sum(marks)// len(marks)\n", "from math import *\n\ndef get_average(marks):\n result = 0 \n for index in marks:\n result = result + index\n result = (result / len(marks))\n return floor(result)\n", "def get_average(marks: list) -> int:\n \"\"\" This function returns the average of the given array. \"\"\"\n return (sum(marks)//len(marks))\n", "import math\ndef get_average(marks):\n count=0\n sum=0\n for x in marks:\n sum+=x\n count+=1\n return math.floor(sum/count)\n", "def get_average(marks):\n y = 0\n for n in range(len(marks)):\n y = y + marks[n-1]\n return int(y / len(marks))", "def get_average(marks):\n result = 0\n counter = 0\n \n for item in marks:\n result += item\n counter += 1\n \n return int(result / counter)\n", "def get_average(marks):\n x=len(marks)\n \n somma=sum(marks)\n media=somma/x\n \n return int(media)\n", "def get_average(marks):\n total = 0 \n for x in marks:\n total += x \n return round(int(total / len(marks)))", "def get_average(marks):\n total = 0\n for m in marks:\n total += m\n return total // len(marks)\n \n", "\ndef get_average(marks):\n avg = 0\n for elem in marks:\n avg += elem\n avg = avg / len(marks)\n \n return int(avg)\n", "import math\ndef get_average(marks):\n res=sum(marks)/len(marks)\n return math.ceil(res) if math.ceil(res)<math.floor(res) else math.floor(res)\n", "def get_average(marks):\n #raise NotImplementedError(\"TODO: get_average\")\n average = 0\n for a in marks:\n average += a\n return int(average/len(marks))", "marks = [2,5,13,20,16,16,10]\ndef get_average(marks):\n sum = 0\n for mark in marks:\n sum = sum+mark\n return int(sum / len(marks))", "def get_average(marks):\n total_marks = 0\n for i in marks:\n total_marks += i\n average = total_marks / len(marks)\n return int(average)\n", "def get_average(marks):\n sum = 0\n for score in marks:\n sum += score\n return int(sum / len(marks))\n", "def get_average(marks):\n average = sum(marks) // len(marks) # Floor division to automatically round down.\n return average\n", "import math\ndef get_average(marks):\n s=0\n avg=0\n for i in marks:\n s+=i\n avg=s/len(marks)\n return math.floor(avg)\n \n \n", "import math\ndef get_average(marks):\n count = 0\n average = 0\n for num in marks:\n count += num\n average = count / len(marks)\n return math.floor(average)", "def get_average(marks):\n x = 0\n for i in marks:\n x += i \n x = x / len(marks)\n return int(x)\n", "import math \n\ndef get_average(marks):\n average = sum(marks) // len(marks)\n return math.floor(average)\n \n\n", "import math\ndef get_average(marks):\n s=0\n for k in marks:\n s+=k\n return math.floor(s/len(marks))\n\n\n \n raise NotImplementedError(\"TODO: get_average\")\n", "import math\n\ndef get_average(marks):\n sum = 0\n for i in marks:\n sum += i\n average = math.floor(sum/len(marks))\n return average", "def get_average(marks):\n media=0\n i=0\n for element in marks:\n i=i+1\n media=media+element\n media=media//i\n return media\n", "def get_average(marks):\n total=sum(e for e in marks)\n return int(total/len(marks))", "import math\ndef get_average(marks):\n # raise NotImplementedError(\"TODO: get_average\")\n marks_sum=0\n for i in marks:\n marks_sum+=i\n return int(marks_sum/len(marks))\n", "def get_average(marks):\n a = len(marks)\n b = 0\n for i in range(0,len(marks)):\n b = b + marks[i]\n average = int(b/a)\n return average\n# raise NotImplementedError(\"TODO: get_average\")\n", "def get_average(marks):\n total = 0\n for i in marks:\n total = total + i\n avg = total // len(marks)\n return avg\n\n# alternatively\n# from statistics import mean as m\n# from math import floor as f\n\n# def get_average(marks):\n# avg = m(marks)\n# return f(avg)\n", "def get_average(marks):\n \n sum = 0\n mylen = len(marks)\n for x in marks:\n sum = sum + x\n \n avg = sum / mylen\n return int(avg)\n", "from math import floor\n\ndef get_average(marks):\n media = sum(marks)/len(marks)\n return floor(media)", "def get_average(marks):\n import math as ma\n return ma.floor(sum(marks)/len(marks))\n", "from math import floor\ndef get_average(marks):\n sum = 0\n for mark in marks:\n sum += mark\n return floor(sum / len(marks))\n \n", "import math\n\ndef get_average(marks):\n sum = 0\n for mark in marks:\n sum = sum + mark\n return math.floor(sum/len(marks))\n", "def get_average(marks):\n tot = 0\n for i in marks:\n tot = tot + i\n \n avg = int(tot/len(marks))\n \n return avg\n", "import math\ndef get_average(marks):\n soma = 0\n \n if (len(marks)>0):\n for i in marks:\n soma += i\n \n media = soma/len(marks)\n return math.floor(media)\n \n\nprint (get_average([1,2]))", "import numpy as np\nimport math\ndef get_average(marks):\n return math.floor(np.average(marks))", "def get_average(marks):\n suma = sum(marks)\n return suma//len(marks)\n raise NotImplementedError(\"TODO: get_average\")\n", "import statistics\nimport math\n\n\ndef get_average(marks):\n get_mean = statistics.mean(marks)\n rounded = math.floor(get_mean)\n return rounded\n", "def get_average(marks):\n total=0\n x=0\n for n in marks :\n total+= marks[x]\n x+= 1\n \n return total//len(marks)\n", "def get_average(marks):\n a = sum(marks)\n l = len(marks)\n average = a//l\n return average\n", "def get_average(marks):\n sum = 0\n for n in marks:\n sum = sum + n\n sum\n return int(sum/len(marks))\n", "def get_average(marks):\n a = sum(marks)/ len(marks)\n# result = round(int(a), 0)\n return int (a)", "def get_average(marks):\n \n sum_init = 0 \n for i in range(0,len(marks)) :\n sum_init = sum_init + marks[i] \n \n return sum_init//len(marks)\n", "import math\ndef get_average(marks):\n soma = 0\n \n if (len(marks)>0):\n for i in marks:\n soma += i\n \n media = soma/len(marks)\n return math.floor(media)\n\nprint (get_average([1,2,15,15,17,11,12,17,17,14,13,15,6,11,8,7]))", "def get_average(marks):\n print((sum(marks)/len(marks)))\n return int((sum(marks)) / len(marks))\n", "import numpy\nimport math\n\ndef get_average(marks):\n \n # raise NotImplementedError(\"TODO: get_average\")\n # Faz a m\u00e9dia do vetor \n c = numpy.mean(marks)\n \n # Com a m\u00e9dia, devolve o inteiro mais pr\u00f3ximo arrendondado por decr\u00e9scimo\n d = math.floor(c)\n \n return d", "import numpy\nimport math\n\ndef get_average(marks):\n \n # raise NotImplementedError(\"TODO: get_average\")\n \n c = numpy.mean(marks)\n d = math.floor(c)\n \n return d", "import numpy as np\ndef get_average(marks):\n return np.mean(marks) // 1\n", "from functools import *\n\ndef get_average(marks):\n nth = len(marks)\n aggregate = reduce(lambda x,y : x+y,marks)\n return aggregate // nth\n", "import math\n\ndef get_average(marks):\n sum = 0\n for x in marks:\n sum = sum + x\n return math.floor(sum / len(marks))\n \n", "def get_average(marks):\n return int(sum(marks) / len(marks))\n raise NotImplementedError(\"didn't work for\", get_average)", "from math import floor #import function to round down\nfrom statistics import mean #import function to calculate average\ndef get_average(marks):\n return floor(mean(marks))\n", "import math\ndef get_average(marks):\n res=0\n for i in marks:\n res= i + res\n return math.floor(res/len(marks))\n raise NotImplementedError(\"TODO: get_average\")\n", "def get_average(marks):\n length=len(marks)\n sum=0\n for i in range(0,length):\n sum= sum+marks[i]\n\n return int(sum/length)\n", "import math\ndef get_average(marks):\n x = 0\n y = 0\n for mark in marks:\n x = x + 1\n y = y + mark\n return math.floor(y / x)", "# def get_average(marks):\n# return sum(marks)\n\ndef get_average(marks):\n suma=0\n for x in marks:\n suma=suma+x\n return (suma//len(marks))", "import math\ndef get_average(marks):\n sum = 0\n for n in marks:\n sum+=n\n length = len(marks)\n average = sum/length\n return math.floor(average)\n", "def get_average(marks):\n answer = 0\n \n for grades in marks:\n answer += grades\n\n return answer // (len(marks))\n", "import numpy as np\n\ndef get_average(marks):\n return((np.mean(marks).astype(int)))\n", "def get_average(marks):\n \n \n import math\n x = (marks, math.floor(sum(marks)/len(marks)))\n \n return x[-1]"] | {"fn_name": "get_average", "inputs": [[[2, 2, 2, 2]], [[1, 5, 87, 45, 8, 8]], [[2, 5, 13, 20, 16, 16, 10]], [[1, 2, 15, 15, 17, 11, 12, 17, 17, 14, 13, 15, 6, 11, 8, 7]]], "outputs": [[2], [25], [11], [11]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 14,171 |
def get_average(marks):
|
e17eea77536d1ecbbc938e6d996f8a17 | UNKNOWN | In genetics 2 differents DNAs sequences can code for the same protein.
This is due to the redundancy of the genetic code, in fact 2 different tri-nucleotide can code for the same amino-acid.
For example the tri-nucleotide 'TTT' and the tri-nucleotide 'TTC' both code for the amino-acid 'F'. For more information you can take a look [here](https://en.wikipedia.org/wiki/DNA_codon_table).
Your goal in this kata is to define if two differents DNAs sequences code for exactly the same protein. Your function take the 2 sequences you should compare.
For some kind of simplicity here the sequences will respect the following rules:
- It is a full protein sequence beginning with a Start codon and finishing by an Stop codon
- It will only contain valid tri-nucleotide.
The translation hash is available for you under a translation hash `$codons` [Ruby] or `codons` [Python and JavaScript].
To better understand this kata you can take a look at this [one](https://www.codewars.com/kata/5708ef48fe2d018413000776), it can help you to start. | ["def code_for_same_protein(seq1,seq2):\n if seq1 == seq2:\n return True\n lista = [['GCT','GCC','GCA','GCG'],['CGT','CGC','CGA','CGG','AGA','AGG'],['AAT','AAC'],['GAT','GAC'],['AAT','AAC','GAT','GAC'],['TGT','TGC'],['CAA','CAG'],['GAA','GAG'],['CAA','CAG','GAA','GAG'],['GGT','GGC','GGA','GGG'],['CAT','CAC'],['ATG'],['ATT','ATC','ATA'],['CTT','CTC','CTA','CTG','TTA','TTG'],['AAA','AAG'],['ATG'],['TTT','TTC'],['CCT','CCC','CCA','CCG'],['TCT','TCC','TCA','TCG','AGT','AGC'],['ACT','ACC','ACA','ACG'],['TGG'],['TAT','TAC'],['GTT','GTC','GTA','GTG'],['TAA','TGA','TAG']]\n for j in range(0,len(lista)):\n for i in range(0,len(seq1),3):\n if (seq1[i:i+3] in lista[j] and seq2[i:i+3] not in lista[j]):\n return False\n return True\n"] | {"fn_name": "code_for_same_protein", "inputs": [["ATGTCGTCAATTTAA", "ATGTCGTCAATTTAA"], ["ATGTTTTAA", "ATGTTCTAA"], ["ATGTTTTAA", "ATGATATAA"], ["ATGTTTGGGAATAATTAAGGGTAA", "ATGTTCGGGAATAATGGGAGGTAA"]], "outputs": [[true], [true], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 784 |
def code_for_same_protein(seq1,seq2):
|
1033451b8cde0ec1b1704592c0f35ac5 | UNKNOWN | A system is transmitting messages in binary, however it is not a perfect transmission, and sometimes errors will occur which result in a single bit flipping from 0 to 1, or from 1 to 0.
To resolve this, A 2-dimensional Parity Bit Code is used: https://en.wikipedia.org/wiki/Multidimensional_parity-check_code
In this system, a message is arrayed out on a M x N grid. A 24-bit message could be put on a 4x6 grid like so:
>1 0 1 0 0 1
>1 0 0 1 0 0
>0 1 1 1 0 1
>1 0 0 0 0 1
Then, Parity bits are computed for each row and for each column, equal to 1 if there is an odd number of 1s in the row of column, and 0 if it is an even number. The result for the above message looks like:
>1 0 1 0 0 1 1
>1 0 0 1 0 0 0
>0 1 1 1 0 1 0
>1 0 0 0 0 1 0
>1 1 0 0 0 1
Since the 1st row, and 1st, 2nd and 6th column each have an odd number of 1s in them, and the others all do not.
Then the whole message is sent, including the parity bits. This is arranged as:
> message + row_parities + column_parities
> 101001100100011101100001 + 1000 + 110001
> 1010011001000111011000011000110001
If an error occurs in transmission, the parity bit for a column and row will be incorrect, which can be used to identify and correct the error. If a row parity bit is incorrect, but all column bits are correct, then we can assume the row parity bit was the one flipped, and likewise if a column is wrong but all rows are correct.
Your Task:
Create a function correct, which takes in integers M and N, and a string of bits where the first M\*N represent the content of the message, the next M represent the parity bits for the rows, and the final N represent the parity bits for the columns. A single-bit error may or may not have appeared in the bit array.
The function should check to see if there is a single-bit error in the coded message, correct it if it exists, and return the corrected string of bits. | ["def correct(m, n, bits):\n l = m*n\n row = next((i for i in range(m) if f\"{bits[i*n:(i+1)*n]}{bits[l+i]}\".count(\"1\") % 2), None)\n col = next((i for i in range(n) if f\"{bits[i:l:n]}{bits[l+m+i]}\".count(\"1\") % 2), None)\n if row is col is None:\n return bits\n err = (l + row) if col is None else (l + m + col) if row is None else (row * n + col)\n return f\"{bits[:err]}{int(bits[err])^1}{bits[err+1:]}\"\n", "from functools import reduce\n\ndef correct(m, n, bits):\n \n def getDiff(check,lines):\n return next((i for i,(c,line) in enumerate(zip(check,lines)) if int(c) != reduce(int.__xor__, list(map(int,line)))), -1)\n\n a = getDiff(bits[-m-n:-n], (bits[i:i+n] for i in range(0,m*n,n)) )\n b = getDiff(bits[-n:], (bits[i:m*n:n] for i in range(n)) )\n \n if a+b != -2:\n i = a*n+b if a+1 and b+1 else -m-n+a if b==-1 else len(bits)-n+b\n bits = f'{ bits[:i] }{ int(bits[i])^1 }{ bits[i+1:] }'\n \n return bits\n", "from functools import partial, reduce\nfrom itertools import chain\nfrom operator import xor\n\ncalc_parity = partial(reduce, xor)\n\n\ndef correct(m, n, bits):\n bits = list(map(int, bits))\n xs = [bits[i*n:(i+1)*n] for i in range(m)]\n row_parity = bits[m*n:-n]\n col_parity = bits[-n:]\n invalid_row = invalid_col = -1\n for i, (row, p) in enumerate(zip(xs, row_parity)):\n if calc_parity(row) != p:\n invalid_row = i\n break\n\n xs = [list(col) for col in zip(*xs)]\n for i, (col, p) in enumerate(zip(xs, col_parity)):\n if calc_parity(col) != p:\n invalid_col = i\n break\n xs = [list(col) for col in zip(*xs)]\n\n if invalid_row >= 0 <= invalid_col:\n xs[invalid_row][invalid_col] = 1 - xs[invalid_row][invalid_col]\n elif invalid_row >= 0:\n row_parity[invalid_row] = 1 - row_parity[invalid_row]\n elif invalid_col >= 0:\n col_parity[invalid_col] = 1 - col_parity[invalid_col]\n\n return \"\".join(map(str, chain(chain.from_iterable(xs), row_parity, col_parity,)))", "def correct(m, n, bits):\n parities = lambda matrix: [sum(row) & 1 for row in matrix]\n wrong_index = lambda a, b: next((i for i, (x, y) in enumerate(zip(a, b)) if x ^ y), None)\n \n bits = list(map(int, bits))\n message, row_par, col_par = bits[:-m-n], bits[-m-n:-n], bits[-n:]\n rows = list(zip(* [iter(message)] * n))\n \n actual_row, actual_col = parities(rows), parities(zip(*rows))\n idx_row, idx_col = wrong_index(row_par, actual_row), wrong_index(col_par, actual_col)\n \n if idx_row is not None and idx_col is not None:\n message[idx_row * n + idx_col] = message[idx_row * n + idx_col] ^ 1\n elif idx_row is not None:\n row_par[idx_row] = row_par[idx_row] ^ 1\n elif idx_col is not None:\n col_par[idx_col] = int(col_par[idx_col]) ^ 1\n\n return ''.join(map(str, message + row_par + col_par))", "def correct(R,C,Q) :\n Q = list(map(int,Q))\n r = next((F for F,V in enumerate(Q[-R - C:-C]) if 1 & sum(Q[F * C:-~F * C]) ^ int(V)),-1)\n c = next((F for F,V in enumerate(Q[-C:]) if 1 & sum(Q[F:R * C:C]) ^ int(V)),-1)\n if 0 <= r or 0 <= c : Q[r - R - C if c < 0 else c + (-C if r < 0 else r * C)] ^= 1\n return ''.join(map(str,Q))", "def correct(m, n, bits):\n def invert(n):\n if n == 1:\n return 0\n if n == 0:\n return 1\n else:\n return None\n \n matrix = []\n wrong_row = None\n wrong_col = None\n \n n_list = [int(x) for x in bits[m * n + m:]]\n m_list = [int(x) for x in bits[m * n:m * n + m]]\n \n for i in range(m):\n row = [int(x) for x in bits[n * i: n * i + n]]\n if sum(row) % 2 != m_list[i]:\n wrong_row = i\n matrix.append(row)\n \n col = []\n for j in range(n):\n for i in range(m):\n col.append(matrix[i][j])\n if sum(col) % 2 != n_list[j]:\n wrong_col = j\n col = []\n \n if wrong_col == None and wrong_row != None:\n m_list[wrong_row] = invert(m_list[wrong_row])\n elif wrong_col != None and wrong_row == None:\n n_list[wrong_col] = invert(n_list[wrong_col])\n elif wrong_col != None and wrong_row != None:\n matrix[wrong_row][wrong_col] = invert(matrix[wrong_row][wrong_col])\n \n res = ''\n for matrix_row in matrix:\n res += ''.join([str(x) for x in matrix_row])\n \n res += ''.join([str(x) for x in m_list])\n res += ''.join([str(x) for x in n_list])\n return res\n", "def correct(m, n, bits):\n res=[]\n for i in range(0, n*m, n):\n res.append(bits[i:i+n])\n start=n*m\n check=[]\n check.append(next((i for i in range(m) if digit_sum(res[i])%2!=int(bits[start+i])), None))\n for j in range(n):\n string=\"\"\n for i in range(m):\n string+=res[i][j]\n if digit_sum(string)%2!=int(bits[start+m+j]):\n check.append(j)\n break\n if len(check)==1:\n check.append(None)\n if check[0]==None and check[1]==None:\n return bits\n elif check[1]==None:\n temp=\"0\" if bits[start+check[0]]==\"1\" else \"1\"\n return bits[:start+check[0]]+temp+bits[start+check[0]+1:]\n elif check[0]==None:\n temp=\"0\" if bits[start+m+check[1]]==\"1\" else \"1\"\n return bits[:start+m+check[1]]+temp+bits[start+m+check[1]+1:]\n else:\n temp=\"0\" if res[check[0]][check[1]]==\"1\" else \"1\"\n return bits[:check[0]*n+check[1]]+temp+bits[check[0]*n+check[1]+1:]\ndef digit_sum(s):\n return sum(int(i) for i in s)", "import pandas as pd\n\ndef correct(m,n,s):\n s,r,c = s[:m*n], s[m*n:m*n+m], s[-n:]\n arr = pd.np.array(list(s)).reshape(m,n)\n df = pd.DataFrame(arr)\n row = ''.join(df.eq('1').sum(1).mod(2).astype(str))\n col = ''.join(df.eq('1').sum(0).mod(2).astype(str))\n if r != row and c != col:\n for idx, (i,j) in enumerate(zip(row,r)):\n if i != j:\n x = idx\n for idx, (i,j) in enumerate(zip(col,c)):\n if i!= j:\n y = idx\n\n df = df.astype(int)\n df.loc[x,y] ^= 1\n return ''.join(df.astype(str).values.ravel()) + r + c\n \n else: \n return ''.join(df.values.ravel()) + row + col", "def correct(m, n, bits):\n failed_row, failed_col = -1, -1\n for row in range(m):\n if sum(int(c) for c in bits[row*n:(row+1)*n]) % 2 != int(bits[m*n+row]):\n failed_row = row\n break\n for col in range(n):\n if sum(int(bits[j]) for j in range(col,len(bits)-n-m,n)) % 2 != int(bits[m*n+m+col]):\n failed_col = col\n break\n if (failed_row, failed_col) == (-1, -1):\n return bits\n elif failed_row != -1 and failed_col != -1:\n index = n*failed_row+failed_col\n elif failed_row != -1:\n index = m*n+failed_row\n else:\n index = m*n+m+failed_col\n return bits[:index] + str(1-int(bits[index]))+bits[index+1:]\n \n", "def correct(m, n, bits):\n \n msg = [bits[i*n : (i+1)*n] for i in range(m)]\n msgt = [''.join([bits[i] for i in range(j, m*n, n)]) for j in range(n)]\n\n msg_row_parity = ''.join([str(x.count('1')%2) for x in msg])\n msg_col_parity = ''.join([str(x.count('1')%2) for x in msgt])\n\n bits_row_parity = bits[-m-n:-n]\n bits_col_parity = bits[-n:]\n\n if msg_row_parity == bits_row_parity and msg_col_parity == bits_col_parity: return bits\n if msg_row_parity == bits_row_parity: return bits[:-n] + msg_col_parity\n if msg_col_parity == bits_col_parity: return bits[:-m-n] + msg_row_parity + bits[-n:]\n \n err_row = [i for i in range(m) if bits_row_parity[i] != msg_row_parity[i]][0]\n err_col = [i for i in range(n) if bits_col_parity[i] != msg_col_parity[i]][0]\n \n err_bit_msg = msg[err_row][err_col]\n cor_bit = str(abs(int(err_bit_msg) - 1))\n msg_pos = err_row*n + err_col\n return bits[:msg_pos] + cor_bit + bits[msg_pos+1:]"] | {"fn_name": "correct", "inputs": [[1, 1, "111"], [1, 1, "011"], [1, 1, "101"], [1, 1, "110"], [2, 3, "11111010001"], [2, 3, "11011010001"], [2, 3, "11111011001"], [2, 3, "11111010011"]], "outputs": [["111"], ["111"], ["111"], ["111"], ["11111010001"], ["11111010001"], ["11111010001"], ["11111010001"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 8,109 |
def correct(m, n, bits):
|
7dbeebcc678a96831c8797f54fccc884 | UNKNOWN | # Task
You're given a substring s of some cyclic string. What's the length of the smallest possible string that can be concatenated to itself many times to obtain this cyclic string?
# Example
For` s = "cabca"`, the output should be `3`
`"cabca"` is a substring of a cycle string "abcabcabcabc..." that can be obtained by concatenating `"abc"` to itself. Thus, the answer is 3.
# Input/Output
- `[input]` string `s`
Constraints: `3 ≤ s.length ≤ 15.`
- `[output]` an integer | ["def cyclic_string(s):\n return next((i for i, _ in enumerate(s[1:], 1) if s.startswith(s[i:])), len(s))", "import math\n\ndef cyclic_string(s):\n for l in [i for i,ch in enumerate(s) if ch==s[0]][1:]:\n if (s[:l]*math.ceil(len(s)/l))[:len(s)] == s:\n return l\n return len(s)\n \n", "from itertools import cycle\n\ndef cyclic_string(s):\n i = 0\n while True:\n i = s.find(s[0], i+1)\n if i == -1: return len(s)\n if all(c1==c2 for c1,c2 in zip(cycle(s[:i]), s[i:])): return i", "def cyclic_string(s):\n length = len(s)\n for i in range(1, length + 1):\n if s in s[:i] * length:\n return i", "def cyclic_string(s):\n l = len(s)\n for i in range(1, l+1):\n if s in s[:i] * l:\n return i", "def cyclic_string(s):\n i = 1\n while not s.startswith(s[i:]):\n i += 1\n return i", "def cyclic_string(s):\n for i in range(1, len(s)):\n # Check to see if s is in the first i characters repeated enough to be of len(s) \n if s in s[:i] * (len(s) // i) * 2:\n return i\n # Must use whole string\n return len(s)", "def cyclic_string(s):\n l=len(s)\n return next(i for i in range(1,l+1) if s==(s[:i]*l)[:l])", "def cyclic_string(st):\n for x in range(1, len(st)+1): \n aux = st[:x]*len(st)\n if aux.startswith(st): \n break\n return x", "def cyclic_string(s):\n if len(set(s)) == 1:\n return 1\n alist = []\n for i in s:\n alist.append(i)\n if s in \"\".join(alist) * 15:\n return len(alist)\n"] | {"fn_name": "cyclic_string", "inputs": [["cabca"], ["aba"], ["ccccccccccc"], ["abaca"]], "outputs": [[3], [2], [1], [4]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,606 |
def cyclic_string(s):
|
fd8421c6be7f1dcb0ba6e3d4df9fb58c | UNKNOWN | Given the sum and gcd of two numbers, return those two numbers in ascending order. If the numbers do not exist, return `-1`, (or `NULL` in C, `tuple (-1,-1)` in C#, `pair (-1,-1)` in C++,`None` in Rust, `array {-1,-1} ` in Java and Golang).
```
For example:
Given sum = 12 and gcd = 4...
solve(12,4) = [4,8]. The two numbers 4 and 8 sum to 12 and have a gcd of 4.
solve(12,5) = -1. No two numbers exist that sum to 12 and have gcd of 5.
solve(10,2) = [2,8]. Note that [4,6] is also a possibility but we pick the one with the lower first element: 2 < 4, so we take [2,8].
```
More examples in test cases.
Good luck! | ["def solve(s,g):\n return -1 if s % g else (g, s - g)", "def solve(s,g):\n return not s%g and (g, s-g) or -1", "def solve(s,g):\n if s % g != 0:\n return -1\n else:\n b = s - g\n a = g\n return (a,b)", "import math\n\ndef solve(s,g):\n if s % g != 0:\n return -1\n else:\n for i in range(s // (2 * g) + 1):\n a = i * g\n b = s - a\n if math.gcd(i, int(b / g)) == 1:\n return (a, b)", "def solve(s,g):\n return -1 if s%g!=0 else (g, s-g)", "import math\ndef solve(s,g):\n return (math.gcd(s,g),s-math.gcd(s,g)) if s%g == 0 else -1", "def solve(s,g):\n if s % g:\n # g is not a divisor of s\n return -1\n return (g, s - g)\n", "def solve(s,g):\n return (g, s-g) if s/g == s//g else -1", "def solve(s,g):\n f = []\n w = ()\n for i in range(0,s+1):\n f.append(g*i)\n for i in f:\n if i + g == s:\n w = g,i\n\n\n return w or -1", "def solve(s,g):\n lst = []\n for i in range(1,s):\n if i % g == 0:\n lst.append(i)\n for i in lst:\n if i +g == s:\n a = [i]+[g]\n a = sorted(a)\n return (a[0],a[1])\n return -1", "def solve(s,g):\n from math import gcd\n return -1 if gcd(g, s-g) != g else (g, s-g)\n", "def solve(s,g):\n return (g, s-g) if s % g == 0 else -1 ", "def solve(s,g):\n if not (s%g==0):\n return -1\n else:\n return (g,int((s/g-1)*g))", "def solve(s,g):\n return [-1,(g, s-g)][s%g == 0]", "def solve(s,g):\n if ((s-g) % g) == 0 and s != g:\n return(min(g,s-g)), s - min(g, s-g)\n else:\n return -1", "solve=lambda s,g:s%g and-1or(g,s-g)", "def solve(s,g):\n try:\n return tuple(sorted([[x for x in list(range(1,s)) if x%g==0 and x+g==s][0], g])) \n except IndexError:\n return -1\n \n \n \n \n\n", "def solve(s,gcd):\n if s % gcd: return -1\n for i in range(gcd, s):\n for j in range(gcd, s):\n if i + j == s and i % gcd == 0 and j % gcd == 0:\n return (i, j)\n \n \n", "import math\n\ndef solve(sum, gcd):\n for firstNumber in (number for number in range(1, sum) if number % gcd is 0):\n secondNumber = sum - firstNumber\n if secondNumber % gcd is 0:\n return (firstNumber, secondNumber)\n return -1\n", "def solve(s,g):\n \n counter = 0\n \n for i in range(1,s+1):\n j = s - i\n if j % g == 0 and i % g == 0:\n counter += 1\n res = (i,j)\n break\n \n if counter == 0:\n res = -1\n return res"] | {"fn_name": "solve", "inputs": [[6, 3], [8, 2], [10, 3], [12, 4], [12, 5], [50, 10], [50, 4], [10, 5], [100, 5], [1000, 5]], "outputs": [[[3, 3]], [[2, 6]], [-1], [[4, 8]], [-1], [[10, 40]], [-1], [[5, 5]], [[5, 95]], [[5, 995]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,700 |
def solve(s,g):
|
8b463fa4c4ae8e4660a8288874400020 | UNKNOWN | #### Task:
Your job here is to implement a method, `approx_root` in Ruby/Python/Crystal and `approxRoot` in JavaScript/CoffeeScript, that takes one argument, `n`, and returns the approximate square root of that number, rounded to the nearest hundredth and computed in the following manner.
1. Start with `n = 213` (as an example).
2. To approximate the square root of n, we will first find the greatest perfect square that is below or equal to `n`. (In this example, that would be 196, or 14 squared.) We will call the square root of this number (which means sqrt 196, or 14) `base`.
3. Then, we will take the lowest perfect square that is greater than or equal to `n`. (In this example, that would be 225, or 15 squared.)
4. Next, subtract 196 (greatest perfect square less than or equal to `n`) from `n`. (213 - 196 = **17**) We will call this value `diff_gn`.
5. Find the difference between the lowest perfect square greater than or equal to `n` and the greatest perfect square less than or equal to `n`. (225 – 196 = **29**) We will call this value `diff_lg`.
6. Your final answer is `base` + (`diff_gn` / `diff_lg`). In this example: 14 + (17 / 29) which is 14.59, rounded to the nearest hundredth.
Just to clarify, if the input is a perfect square itself, you should return the exact square of the input.
In case you are curious, the approximation (computed like above) for 213 rounded to four decimal places is 14.5862. The actual square root of 213 is 14.5945.
Inputs will always be positive whole numbers. If you are having trouble understanding it, let me know with a comment, or take a look at the second group of the example cases.
#### Some examples:
```python
approx_root(400) #=> 20
approx_root(401) #=>
# smallest perfect square above 401 is 441 or 21 squared
# greatest perfect square below 401 is 400 or 20 squared
# difference between 441 and 400 is 41
# difference between 401 and 400 is 1
# answer is 20 + (1 / 41) which becomes 20.02, rounded to the nearest hundredth
# final answer = 20.02.
approx_root(2) #=>
# smallest perfect square above 2 is 4 or 2 squared
# greatest perfect square below 2 is 1 or 1 squared
# difference between 4 and 1 is 3
# difference between 2 and 1 is 1
# answer is 1 + (1 / 3), which becomes 1.33, rounded to the nearest hundredth
# final answer = 1.33.
# math.sqrt() isn't disabled.
```
Also check out my other creations — [Square Roots: Simplifying/Desimplifying](https://www.codewars.com/kata/square-roots-simplify-slash-desimplify/), [Square and Cubic Factors](https://www.codewars.com/kata/square-and-cubic-factors), [Keep the Order](https://www.codewars.com/kata/keep-the-order), [Naming Files](https://www.codewars.com/kata/naming-files), [Elections: Weighted Average](https://www.codewars.com/kata/elections-weighted-average), [Identify Case](https://www.codewars.com/kata/identify-case), [Split Without Loss](https://www.codewars.com/kata/split-without-loss), [Adding Fractions](https://www.codewars.com/kata/adding-fractions),
[Random Integers](https://www.codewars.com/kata/random-integers), [Implement String#transpose](https://www.codewars.com/kata/implement-string-number-transpose), [Implement Array#transpose!](https://www.codewars.com/kata/implement-array-number-transpose), [Arrays and Procs #1](https://www.codewars.com/kata/arrays-and-procs-number-1), and [Arrays and Procs #2](https://www.codewars.com/kata/arrays-and-procs-number-2).
If you notice any issues or have any suggestions/comments whatsoever, please don't hesitate to mark an issue or just comment. Thanks! | ["def approx_root(n):\n base = int(n**0.5)\n return round( base + (n - base**2) / ((base + 1)**2 - base**2) , 2)", "def approx_root(n):\n m = n**0.5\n a, b = [int(x) for x in [m, m + 1]]\n c = a**2\n return round(a + ((n - c) / (b**2 - c)), 2)", "def approx_root(n):\n b = int(n ** 0.5)\n diff_gn = n - b**2\n diff_lg = (b+1)**2 - b**2\n return round(b + (diff_gn / diff_lg), 2)", "def approx_root(n):\n import math\n s = int(math.sqrt(n))\n diff_gn = n - s ** 2\n diff_lg = (s + 1) ** 2 - s ** 2\n return round(s + diff_gn / diff_lg, 2)", "def approx_root(n):\n base = int(n ** 0.5)\n diff_gn = n - base ** 2\n diff_lg = base * 2 + 1\n return round(base + diff_gn / diff_lg, 2)", "approx_root=r=lambda n,i=0:n>(i+1)**2and r(n,i+1)or round(i+(n-i*i)/(2*i+1),2)", "approx_root=lambda n:round(next(i+(n-i*i)/(2*i+1)for i in range(n)if(i+1)**2>=n),2)", "def approx_root(n):\n for i in range(1,int(n**0.5)+2):\n if i ** 2 > n:\n base = i - 1\n break\n base2 = base + 1\n diff_gn = n - base ** 2\n diff_lg = base2 ** 2 - base ** 2\n return round(base + (diff_gn/diff_lg),2)\n", "import math\n\ndef approx_root(n):\n a = n ** 0.5\n b, c = math.floor(a) ** 2, (math.floor(a) + 1) ** 2\n return a if type(a) == int else round(b ** 0.5 + (n - b) / (c - b), 2)\n", "def approx_root(n):\n b=int(n**0.5)\n return round(b+(n-b*b)/((b+1)**2-b*b),2)"] | {"fn_name": "approx_root", "inputs": [[400], [401], [2], [4], [5], [6], [7], [8], [9], [100], [120], [106], [111], [121], [168]], "outputs": [[20], [20.02], [1.33], [2], [2.2], [2.4], [2.6], [2.8], [3], [10], [10.95], [10.29], [10.52], [11], [12.96]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,452 |
def approx_root(n):
|
f0ebf842bc32403708d402f94b96e1ee | UNKNOWN | Write a function name `nextPerfectSquare` that returns the first perfect square that is greater than its integer argument. A `perfect square` is a integer that is equal to some integer squared. For example 16 is a perfect square because `16=4*4`.
```
example
n next perfect sqare
6 9
36 49
0 1
-5 0
```
```if-not:csharp
caution! the largest number tested is closer to `Number.MAX_SAFE_INTEGER`
```
```if:csharp
Caution! The largest number test is close to `Int64.MaxValue`
``` | ["def next_perfect_square(n):\n return n>=0 and (int(n**0.5)+1)**2", "next_perfect_square=lambda n:(n>=0and int(n**.5)+1)**2", "def next_perfect_square(n):\n return n >= 0 and (int(n**.5)+1)**2", "import math\ndef next_perfect_square(n):\n return math.ceil((n + 1) ** 0.5) ** 2 if n > -1 else 0\n", "from bisect import bisect\nsquares = [i*i for i in range(32000)]\n\ndef next_perfect_square(n):\n return bisect(squares, n)**2", "next_perfect_square = lambda n: ((int(n**.5)+1) ** 2 if n>=0 else 0)", "def next_perfect_square(n, i=0):\n while True:\n if i*i > n: return i*i\n i += 1", "def next_perfect_square(n):\n if n<0:return 0\n is_square=lambda s:int(s**0.5)**2==s\n n+=1\n while not is_square(n):\n n+=1\n return n", "from math import sqrt\n\ndef next_perfect_square(n):\n return 0 if n < 0 else (int(sqrt(n)) + 1) ** 2", "from math import floor\ndef next_perfect_square(n):\n return (floor(n**(1/2)) + 1) ** 2 if n > 0 else 0 if n != 0 else 1"] | {"fn_name": "next_perfect_square", "inputs": [[6], [36], [0], [-5]], "outputs": [[9], [49], [1], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,009 |
def next_perfect_square(n):
|
c1c3156fbdf0e704196b87592ddd29d7 | UNKNOWN | Due to another of his misbehaved,
the primary school's teacher of the young Gauß, Herr J.G. Büttner, to keep the bored and unruly young schoolboy Karl Friedrich Gauss busy for a good long time, while he teaching arithmetic to his mates,
assigned him the problem of adding up all the whole numbers from 1 through a given number `n`.
Your task is to help the young Carl Friedrich to solve this problem as quickly as you can; so, he can astonish his teacher and rescue his recreation interval.
Here's, an example:
```
f(n=100) // returns 5050
```
It's your duty to verify that n is a valid positive integer number. If not, please, return false (None for Python, null for C#).
> **Note:** the goal of this kata is to invite you to think about some 'basic' mathematic formula and how you can do performance optimization on your code.
> Advanced - experienced users should try to solve it in one line, without loops, or optimizing the code as much as they can.
-----
**Credits:** this kata was inspired by the farzher's kata 'Sum of large ints' . In fact, it can be seen as a sort of prep kata for that one. | ["def f(n):\n return n * (n + 1) // 2 if (isinstance(n, int) and n > 0) else None\n", "def f(n):\n return sum(range(1, n + 1)) if type(n) is int and n > 0 else None", "def f(n):\n try:\n return None if n <= 0 else sum(range(1,(n+1)))\n except:\n return None", "def f(n):\n return ((n+1)/2)*n if isinstance(n,int) and n > 0 else None", "def f(n):\n return None if type(n) != int or n < 1 else n*(n+1)/2", "def f(n):\n return (n + 1) * n //2 if (type(n) is int and n>0) else None", "def f(n):\n return n*(n+1)//2 if (isinstance(n,int) and n>0 ) else None", "from functools import reduce\ndef f(n):\n return None if not isinstance(n, int) or n<1 else reduce(lambda x, y: x + y, range(1, n + 1))", "def f(n):\n try:\n x = int(n)\n if int(n) < 0:\n return None\n elif int(n) > 0:\n return sum([i for i in range(0, n+1)])\n \n except:\n None\n #pass\n", "from numbers import Number\ndef f(n):\n return n*(n+1)/2 if isinstance(n, int) and n > 0 else None", "f = lambda n: (n**2+n)//2 if type(n)==int and n>0 else None", "def f(n):\n return (isinstance(n,int) and n>0) and n*(n+1)/2 or None", "f = lambda n: (n/2)*(n+1) if type(n) == int and n>0 else None", "f = lambda n: n * (n + 1) / 2 if type(n) is int and n > 0 else None", "def f(n):\n return (n * (n + 1)) >> 1 if isinstance(n,int) and n > 0 else None", "def f(n):\n return n*(n+1)/2 if isinstance(n, int) and n > 0 else None", "def f(n):\n if isinstance(n, int) and n >= 1:\n return (n / 2) * (n + 1)\n else: \n return None", "def f(n):\n return int((((n**2)-1) + (1+n))/2) if isinstance(n, int) and n > 0 else None", "def f(n):\n if isinstance(n,int) == True and n > 0:\n return int((n*(n+1))/2)\n else:\n return None", "def f(n):\n try:\n if n > 0 and type(n) is int:\n return int((n*(n+1))/2)\n except:\n return None", "def f(n):\n if type(n) == int and n >0:\n return (1+n)*n/2\n elif type(n) == str or type(n) == float or n < 0:\n return None\n else:\n return None", "def f(n):\n if isinstance(n,int):\n if n>0:\n sum=0\n for i in range(0,n+1):\n sum = sum +i\n return sum \n return None", "def f(n):\n if type(n)!=int:\n return None\n return sum([x for x in range(n+1)]) if n>0 else None", "def f(n):\n n_ = None\n try:\n n_ = float(n)\n except:\n return(None)\n \n return(n_*(n_+1)/2 if (n_.is_integer() and n_ > 0) else None)", "def f(n):\n try: \n if n > 0:\n return sum(range(1, n+1))\n except:\n return None", "def f(n):\n try :\n if n%1 == 0 and n > 0: \n return (sum(range(0,n+1,1))) \n else: return None\n except TypeError:\n return None", "def f(n):\n try:\n x = int(n)\n if int(n) < 0:\n return None\n elif int(n) > 0:\n return sum([i for i in range(0, n+1)])\n \n except:\n None", "def f(n):\n if type(n) is not int or n <= 0:\n result = None\n else:\n lst = [i for i in range(0,n+1)]\n result = sum(lst)\n return result", "def f(n):\n if(type(n) != int):\n return None\n if (n>0): \n return n*(n+1)/2\n return None", "def f(n):\n if type(n)==int and n>0:\n return sum([x for x in range(n+1)])\n else:\n return None", "def f(n):\n tot = 0\n if type(n) == int and n > 0:\n for number in range(1, n + 1):\n tot += number\n return tot\n return None", "def f(n):\n if isinstance(n, int) and n > 0:\n nn = [i for i in range(n+1)]\n return sum(nn)\n\n# sum = 0\n# if isinstance(n, int): \n# if n > 0:\n# for i in range(n+1):\n# sum += i\n# return sum\n# return None\n# else:\n# return None\n", "def f(n):\n if type(n) == str or type(n) == float or n < 1:\n return None\n return sum(range(1, n+1))", "def f(n):\n return None if type(n) in [str, float] or n < 1 else sum([i for i in range(0, n+1)])", "def f(n):\n try:\n return n*(n+1)/2 if n>0 and (n - round(n) == 0) else None\n except TypeError:\n return None", "def f(n):\n if isinstance(n,int) and n>0: return n*(n+1)/2", "def f(n):\n if isinstance(n, int) and n > 0:\n return n * -~n >> 1", "def f(n):\n if type(n) == int and n > 0:\n return (n/2)*(n+1)", "def f(n):\n return n*n/2 + n/2 if type(n) is int and n > 0 else None", "def f(n):\n if type(n) == int and n > 0: return sum(range(1, n + 1))", "f=lambda n:int==type(n)and n>0and-~n*n/2or None", "def f(n):\n if type(n) is int:\n if n > 0:\n return n * (n + 1) / 2\n else: \n return None\n else: \n return None", "def f(n):\n if type(n) == float or type(n) == str or n == 0 or n <= -1:\n return None\n else:\n return sum([elem for elem in range(0, n + 1)])", "def f(n):\n # 1 + 2 + 3 + n-2 + n-1 + n \n # 1+n + 2+n-1 + 3+n-2 + ...\n if type(n) is int and n >= 1:\n number_of_elements = n / 2\n total_elements = (n + 1) * number_of_elements\n return total_elements\n else: \n return None\n", "def f(n):\n print('The number is' + str(n))\n if n == 1:\n return n\n if type(n) != int or n <= 0:\n return None\n return (n / 2) * (n+1)", "def f(n):\n return sum(list(range(0, n+1))) if isinstance(n, int) and n > 0 else None\n", "def f(n): \n return sum(i for i in range(int(n) + 1)) if isinstance(n, int) and n > 0 else None\n", "def f(n):\n if type(n) is int and n>0:\n return int(n*(n+1)/2)\n else:\n return None\n \n", "def f(n):\n if type(n) == type(1) and n > 0:\n return (1 + n)*n/2\n else:\n return None", "def f(n):\n if type(n) == int and int(n) > 0:\n return (n+1)*n/2", "def f(n):\n try:\n val = int(n)\n if val <= 0:\n return None\n except ValueError:\n return None\n else:\n i = 0\n nums = []\n while i < n:\n if type(n) != int:\n return None\n else:\n i+=1\n nums.append(i)\n return sum(nums)\n", "f = lambda n: (1+n) * n // 2 if type(n) == int and n > 0 else None", "def f(n):\n n = n\n total = 0\n if type(n) != int:\n return None\n if n <= 0:\n return None\n\n while n >=0:\n total +=n\n n-=1\n \n return total\n \n\n", "def f(n):\n try:\n int(n)\n if n <= 0:\n return None\n else:\n return sum(range(n+1))\n except:\n return None\n", "def f(n):\n if isinstance(n, int) == True:\n sum = 0\n if n > 0:\n for i in range(n + 1):\n sum +=i\n return sum\n else:\n return None\n else:\n return None", "def f(n):\n if isinstance(n,str):\n return None\n if n <=0:\n return None\n if n!=int(n):\n return None\n \n return n*(n+1)//2", "def f(n):\n if isinstance(n, str):\n return None\n if isinstance(n, float):\n return None\n if n <= 0:\n return None\n count = 0\n for i in range(n+1):\n count += i\n \n return count", "def f(n):\n if isinstance(n, int) and n > 0:\n sum = 0\n while int(n) > 0:\n sum += n\n n -=1\n return sum\n return None\n", "def f(n):\n total = 0\n if isinstance(n, int) and n > 0:\n for b in range(0, n+1, 1):\n total = total+b\n return total\n else: \n return None\n", "def f(n):\n \n return sum(range(1,n+1,1)) if str(n).isdigit() and int(n) > 0 else None", "def f(n):\n if isinstance(n, int) == False or n <= 0:\n return None\n else:\n sum = 0\n for i in range(1, n+1):\n sum += i\n return sum\n", "def f(n):\n sum = 0\n #isinstance(n, int) is asking that n isinteger\n if isinstance(n, int) == True:\n if n > 0:\n for i in range(n):\n i = i + 1\n sum += i\n return sum\n #if n<=0\n else:\n return None\n \n #if False\n else:\n return None\n", "def f(n):\n sum = 0\n if isinstance(n, int) == True:\n if n > 0:\n for i in range(n):\n i = i + 1\n sum += i\n return sum\n else:\n return None\n else:\n return None\n", "def f(n):\n if not isinstance(n, int): return\n result = 0\n for x in range(n + 1):\n result += x\n return result if result > 0 else None", "def f(n):\n if isinstance(n , int) and n > 0:\n return int((n/2)*(1+n))", "def f(n):\n sum=0\n if type(n)==int:\n if n<=0:\n return None\n for x in range(n+1):\n sum=sum+x\n return sum\n else:\n return None\n pass", "def f(n):\n if type(n)!= int:\n return None\n if n <= 0 :\n return None\n else:\n \n return sum([i for i in range((n)+1)])\n", "def f(n):\n if not type(n) == int or not n > 0:\n return\n else: \n result = (n+1) * (n/2)\n return result", "def f(n):\n \n if isinstance(n, int) and n >0:\n\n return (1/2)*n*(n+1)\n \n else:\n \n return None", "def f(n):\n if type(n) == str:\n return None\n elif n < 0:\n return None\n if type(n) == float:\n return None\n if n == 0:\n return None\n suma = 0\n for i in range(n+1):\n suma+=i\n return suma", "def f(n):\n if type(n) != int or n < 1:\n return None\n else:\n return (n * (n+1)) / 2", "def f(n):\n try:\n if n > 0:\n return sum(range(0, n+1))\n else:\n return\n except:\n return\n", "def f(n):\n try:\n return sum(range(n+1)) if sum(range(n+1)) else None\n except:\n return None", "def f(n):\n if type(n) == int and n>0:\n a =0\n sum = 0\n while a < n:\n a = a + 1\n sum = sum + a\n return sum", "def f(n):\n if isinstance(n, int) and n > 0:\n return sum(x for x in range(1, n+1))", "def f(n):\n if type(n) == int:\n counter = 0\n if n > 0:\n for i in range(n + 1):\n counter += i\n \n if counter > 0:\n return counter\n else:\n return None\n \n return None", "def f(n):\n if type(n) != int:\n return None\n if n <= 0:\n return None\n if n == int(n):\n return 0.5 * n * (n + 1)\n else:\n return None", "def f(n):\n if isinstance(n, int) == True and n>0:\n return sum(range(0,n+1))", "def f(n):\n return (n /2)*(1+ n) if type(n) ==int and n>0 else None", "def f(n):\n print(n) \n if isinstance(n, int) and n > 0:\n x = 0\n y = 0\n while x <= n:\n y += x\n x += 1 \n return y\n else:\n return None", "def f(n):\n if type(n) is not int or int(n) <= 0:\n return \n else:\n return (n*(n+1))//2 \n \n", "def f(n):\n if type(n)==int:\n if n >0:\n return sum(range(n+1))\n else:\n return None\n", "def f(n): return sum([x for x in range(n+1)]) if isinstance(n,int) and n>=1 else None", "from math import factorial\ndef f(n):\n if type(n) != int: \n return None\n else:\n if n<=0: \n return None\n p=0\n for k in range(1,n+1):\n p+=k\n return p\n\n", "def f(n): \n if isinstance(n,int) and n>0: return sum(range(0,n+1))\n \n", "def f(n):\n if isinstance(n, int) and n > 0: return sum(range(1+n))", "def f(n):\n if isinstance(n, int) and n > 0: return sum([a for a in range(0,n+1)])\n", "def f(n):\n if isinstance(n, int) and n > 0:\n return sum([a for a in range(0,n+1)])\n else:\n return None", "def f(n):\n if isinstance(n, int) and n > 0:\n return (n*(2+(n-1)))/2 if n > 0 else None", "def f(n):\n if isinstance(n, int) != True or n <= 0:\n return None\n else:\n if n == 1:\n return 1\n else:\n return (1 + n) * (n / 2)", "def f(n):\n if isinstance(n,int) and n>=1:\n return n*(n+1)/2\n else: return None", "def f(n):\n if isinstance(n, int)==True and n>0:\n return sum(list(range(0,n+1)))\n else:\n None", "def f(n):\n x = []\n if type(n) == int and int(n) > 0:\n n = abs(n)\n for i in range(1,n + 1):\n x.append(i)\n return (sum(x))\n else:\n return None", "def f(n):\n if isinstance(n,int) == True:\n if n > 0:\n sum = 0\n for num in range(0, n+1, 1):\n sum = sum+num\n return sum\n else:\n None\n else:\n return None\n \n \n", "def f(n):\n if type(n) == int and n > 0:\n num = 0\n for i in range(n+1):\n num += i\n return num ", "def f(n):\n print(n)\n return (n)*(n+1)//2 if (isinstance(n, int) and n>0) else None"] | {"fn_name": "f", "inputs": [[100], [300], [303], [50000], ["n"], [3.14], [0], [-10]], "outputs": [[5050], [45150], [46056], [1250025000], [null], [null], [null], [null]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 13,527 |
def f(n):
|
347bf24b83c38793fa446be349eb948f | UNKNOWN | The snail crawls up the column. During the day it crawls up some distance. During the night she sleeps, so she slides down for some distance (less than crawls up during the day).
Your function takes three arguments:
1. The height of the column (meters)
2. The distance that the snail crawls during the day (meters)
3. The distance that the snail slides down during the night (meters)
Calculate number of day when the snail will reach the top of the column. | ["from math import ceil\n\n\ndef snail(column, day, night):\n return max(ceil((column-night)/(day-night)), 1)", "snail=lambda c,d,n:-(min(0,d-c)//(d-n))+1", "def snail(height, day, night):\n n,r = divmod(max(0,height-day), day-night)\n return n + 1 + (r!=0)", "def snail(column, day, night):\n count = 0\n some = True\n while some:\n column = column - day\n if column <= 0:\n some = False\n column += night\n count += 1\n\n return count", "from numpy import ceil\ndef snail(column, day, night):\n return max(ceil((column-day)/(day-night)), 0) + 1", "def snail(column, day, night):\n\n heightUpColumn = 0\n daysPassed = 1\n \n while heightUpColumn < column:\n heightUpColumn += day\n if heightUpColumn < column:\n heightUpColumn -= night\n daysPassed += 1\n elif heightUpColumn >= column:\n return daysPassed\n \n", "def snail(column, day, night):\n total = 0\n when = True\n n = 0\n while column > total:\n if when:\n total += day\n when = False\n n += 1\n else:\n total -= night\n when = True\n return n", "snail=lambda c,d,n:max([i for i in range(c) if i*d-(n*i-n)>=c][0],1)", "def snail(column, d, n):\n k = 1\n v = d\n while v < column:\n v -= n\n v += d\n k += 1\n return k", "from math import ceil\ndef snail(column, day, night):\n return ceil((column-night)/(day-night)) if column - day > 0 else 1\n\n"] | {"fn_name": "snail", "inputs": [[3, 2, 1], [10, 3, 1], [10, 3, 2], [100, 20, 5], [5, 10, 3]], "outputs": [[2], [5], [8], [7], [1]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,570 |
def snail(column, day, night):
|
681c6fcf336aedda0d0b4c1cd5c27901 | UNKNOWN | You will be given an array that contains two strings. Your job is to create a function that will take those two strings and transpose them, so that the strings go from top to bottom instead of left to right.
A few things to note:
1. There should be one space in between the two characters
2. You don't have to modify the case (i.e. no need to change to upper or lower)
3. If one string is longer than the other, there should be a space where the character would be | ["import itertools\ndef transpose_two_strings(arr):\n return '\\n'.join( ' '.join(elt) for elt in itertools.zip_longest(arr[0],arr[1],fillvalue=' ') )", "from itertools import*;transpose_two_strings=lambda a:'\\n'.join(map(' '.join,zip_longest(*a,fillvalue=' ')))", "def transpose_two_strings(arr):\n p = arr[:]\n if len(p[0]) > len(p[1]):\n p[1] = p[1].ljust(len(p[0]))\n elif len(p[0]) < len(p[1]):\n p[0] = p[0].ljust(len(p[1]))\n return '\\n'.join([f'{i} {j}' for i, j in zip(*p)])", "from itertools import zip_longest as zipL\n\ndef transpose_two_strings(arr):\n return '\\n'.join( [' '.join(s) for s in zipL(*arr, fillvalue=' ') ] )", "from itertools import zip_longest\n\ndef transpose_two_strings(lst):\n return \"\\n\".join(f\"{a} {b}\" for a, b in zip_longest(*lst, fillvalue=\" \"))", "from itertools import zip_longest\n\ndef transpose_two_strings(arr):\n return '\\n'.join(map(' '.join, zip_longest(*arr, fillvalue=' ')))", "def transpose_two_strings(arr):\n mw = max([len(x) for x in arr])\n return \"\\n\".join([\" \".join([a,b]) for a,b in zip(arr[0].ljust(mw), arr[1].ljust(mw))])", "from itertools import zip_longest\n\ndef transpose_two_strings(arr):\n return '\\n'.join(' '.join(pair) for pair in zip_longest(*arr, fillvalue=' '))", "from itertools import zip_longest\n\ndef transpose_two_strings(arr):\n return '\\n'.join(list(map(' '.join, zip_longest(*arr, fillvalue=' '))))"] | {"fn_name": "transpose_two_strings", "inputs": [[["Hello", "World"]], [["joey", "louise"]], [["a", "cat"]], [["cat", ""]], [["!a!a!", "?b?b"]]], "outputs": [["H W\ne o\nl r\nl l\no d"], ["j l\no o\ne u\ny i\n s\n e"], ["a c\n a\n t"], ["c \na \nt "], ["! ?\na b\n! ?\na b\n! "]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,450 |
def transpose_two_strings(arr):
|
8801c80818ac40a971d1cf9d02d79695 | UNKNOWN | Description overhauled by V
---
I've invited some kids for my son's birthday, during which I will give to each kid some amount of candies.
Every kid hates receiving less amount of candies than any other kids, and I don't want to have any candies left - giving it to my kid would be bad for his teeth.
However, not every kid invited will come to my birthday party.
What is the minimum amount of candies I have to buy, so that no matter how many kids come to the party in the end, I can still ensure that each kid can receive the same amount of candies, while leaving no candies left?
It's ensured that at least one kid will participate in the party. | ["from fractions import gcd\nfrom functools import reduce\n\ndef candies_to_buy(n):\n return reduce(lambda a,b:a*b//gcd(a,b), range(1,n+1))", "def candies_to_buy(n):\n xx = 1\n for i in range(n):\n x = xx\n while xx % (i+1):\n xx += x\n return xx", "from functools import reduce\nfrom math import gcd\n\ndef lcm(a, b):\n g = gcd(a, b)\n return a * b // g\n\ndef candies_to_buy(kids):\n return reduce(lcm, range(1, kids+1))", "from itertools import accumulate\nfrom math import gcd\n\nlcm = lambda x,y: x * y // gcd(x, y) if x else y\n\ncandies_to_buy = list(accumulate(range(2000), lcm)).__getitem__", "from functools import reduce\nfrom math import gcd\n\ndef candies_to_buy(kids):\n return reduce(lambda a, b: a * b // gcd(a, b), range(1, kids+1))", "from fractions import gcd\nfrom functools import reduce\n\ndef lcm(a, b):\n return a * b // gcd(a, b)\n\ndef candies_to_buy(amount_of_kids_invited):\n return reduce(lcm, range(1, amount_of_kids_invited + 1))", "from math import gcd\nfrom functools import reduce\n\nlcm = lambda x,y: x*y//gcd(x,y)\n\ndef candies_to_buy(n):\n return reduce(lcm, (i for i in range(1,(n+1 if n%2 else n)))) if n!=2 else 2", "import math\ndef lcm(x, y):\n return (x * y) // math.gcd(x, y)\n\ndef candies_to_buy(amount_of_kids_invited):\n f = 1\n for i in range(2, amount_of_kids_invited+1):\n f = lcm(f, i)\n return f", "from operator import mul\nfrom functools import reduce\ndef candies_to_buy(n):\n ret = []\n for i in range(1, n + 1):\n s = i\n for j in ret:\n if s % j == 0:\n s //= j\n if s == 1:\n break\n ret.append(s)\n return reduce(mul, ret)"] | {"fn_name": "candies_to_buy", "inputs": [[1], [2], [5], [6], [9], [10], [60], [61], [100], [101]], "outputs": [[1], [2], [60], [60], [2520], [2520], [9690712164777231700912800], [591133442051411133755680800], [69720375229712477164533808935312303556800], [7041757898200960193617914702466542659236800]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,746 |
def candies_to_buy(n):
|
28fd91051bbf54dc8d834d5c29f2078a | UNKNOWN | This challenge extends the previous [repeater()](https://www.codewars.com/kata/thinkful-string-drills-repeater) challenge. Just like last time, your job is to write a function that accepts a string and a number as arguments. This time, however, you should format the string you return like this:
```python
>>> repeater('yo', 3)
'"yo" repeated 3 times is: "yoyoyo"'
>>> repeater('WuB', 6)
'"WuB" repeated 6 times is: "WuBWuBWuBWuBWuBWuB"'
``` | ["def repeater(string, n):\n return '\"{}\" repeated {} times is: \"{}\"'.format(string, n, string * n)\n", "def repeater(string, n):\n return '\"{0}\" repeated {1} times is: \"{2}\"'.format(string, n, string * n)\n", "def repeater(s, n):\n return '\"{}\" repeated {} times is: \"{}\"'.format(s, n, s * n)", "def repeater(stg, n):\n return f'\"{stg}\" repeated {n} times is: \"{stg * n}\"'\n", "repeater = lambda s, n: '\"%s\" repeated %d times is: \"%s\"' % (s, n, s * n)", "repeater = lambda s, n: f'\"{s}\" repeated {n} times is: \"{\"\".join(s*n)}\"' ", "def repeater(message, multiple):\n return f'\"{message}\" repeated {multiple} times is: \"{message*multiple}\"'\n", "def repeater(string, n):\n # Habit of escaping double quotes regardless of context\n return '\"{}\" repeated {} times is: \"{}\"'.format(string, n, string * n)\n", "def repeater(string, n):\n longString=\"\"\n for i in range(n):\n longString+=string\n return \"\\\"\" + string + \"\\\" repeated \" + str(n) + \" times is: \\\"\" +longString + \"\\\"\" \n", "def repeater(string, n):\n repeated_str = string * n \n return '\"{0}\" repeated {1} times is: \"{2}\"'.format(string, n, repeated_str)"] | {"fn_name": "repeater", "inputs": [["yo", 3], ["WuB", 6], ["code, eat, code, sleep... ", 2]], "outputs": [["\"yo\" repeated 3 times is: \"yoyoyo\""], ["\"WuB\" repeated 6 times is: \"WuBWuBWuBWuBWuBWuB\""], ["\"code, eat, code, sleep... \" repeated 2 times is: \"code, eat, code, sleep... code, eat, code, sleep... \""]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,215 |
def repeater(string, n):
|
f8a6037e1856e4d25dff2bef9cba6c9b | UNKNOWN | Create a function finalGrade, which calculates the final grade of a student depending on two parameters: a grade for the exam and a number of completed projects.
This function should take two arguments:
exam - grade for exam (from 0 to 100);
projects - number of completed projects (from 0 and above);
This function should return a number (final grade).
There are four types of final grades:
- 100, if a grade for the exam is more than 90 or if a number of completed projects more than 10.
- 90, if a grade for the exam is more than 75 and if a number of completed projects is minimum 5.
- 75, if a grade for the exam is more than 50 and if a number of completed projects is minimum 2.
- 0, in other cases
Examples:
~~~if-not:nasm
```python
final_grade(100, 12) # 100
final_grade(99, 0) # 100
final_grade(10, 15) # 100
final_grade(85, 5) # 90
final_grade(55, 3) # 75
final_grade(55, 0) # 0
final_grade(20, 2) # 0
```
~~~
*Use Comparison and Logical Operators. | ["def final_grade(exam, projects):\n if exam > 90 or projects > 10: return 100\n if exam > 75 and projects >= 5: return 90\n if exam > 50 and projects >= 2: return 75\n return 0", "def final_grade(exam, projects):\n if exam > 90 or projects > 10:\n return 100\n elif exam > 75 and projects >= 5:\n return 90\n elif exam > 50 and projects >= 2:\n return 75\n else:\n return 0", "def final_grade(x, p):\n return ( (x>90 or p>10) and 100 or \n x>75 and p>=5 and 90 or\n x>50 and p>=2 and 75 or 0)", "def final_grade(exam, projects):\n return 100 if exam > 90 or projects > 10 else 90 if exam > 75 and projects >= 5 else 75 if exam > 50 and projects >=2 else 0", "def final_grade(exam, projects):\n\n if (exam > 90 or projects > 10):\n results = 100\n elif (exam > 75 and projects >= 5):\n results = 90\n elif (exam > 50 and projects >= 2):\n results = 75\n else:\n results = 0\n \n return results", "def final_grade(exam, projects):\n if (exam > 90) or (projects > 10):\n return 100\n elif (exam > 75 < 90) and (projects >= 5):\n return 90\n elif (exam > 50) and (projects >= 2):\n return 75\n else:\n return 0", "def final_grade(exam, projects):\n grade = 0\n if exam > 90 or projects > 10:\n grade = 100\n elif exam > 75 and projects >= 5:\n grade = 90\n elif exam > 50 and projects >= 2:\n grade = 75\n else:\n grade = 0\n return grade", "def final_grade(exam, projects):\n if exam > 90 or projects > 10:\n return 100\n if exam > 75 and projects > 4:\n return 90\n if exam > 50 and projects > 1:\n return 75\n return 0", "final_grade=lambda e,p:{e>50 and p>=2:75,e>75 and p>=5:90,e>90 or p>10:100}.get(True,0)", "def final_grade(exam, projects):\n return (100 if (exam > 90 or projects > 10)\n else 90 if (exam > 75 and projects > 4)\n else 75 if (exam > 50 and projects > 1)\n else 0)", "def final_grade(exam, projects):\n if exam>90 or projects>10:\n return 100\n elif exam>75 and projects>4:\n return 90\n elif exam>50 and projects>1:\n return 75\n return 0# final grade", "def final_grade(exam, projects):\n print(exam, projects)\n return 100 if (exam > 90) or (projects > 10) else 90 if (exam > 75) and (projects >= 5) else 75 if (exam > 50) and (projects >= 2) else 0", "from functools import reduce\nfrom operator import and_, or_\nfrom typing import NamedTuple, Tuple, Callable, Any\n\nExam = Projects = int\nActivities = Tuple[Exam, Projects]\n\nclass Rule(NamedTuple):\n limits: Activities\n relation: Callable[[bool, bool], bool]\n result: Any\n\nRULES = (\n Rule((90, 10), or_, 100),\n Rule((75, 4), and_, 90),\n Rule((50, 1), and_, 75),\n)\n\ndef final_grade(*scores: Activities) -> int:\n for rule in RULES:\n pairs = tuple(zip(scores, rule.limits))\n if pairs and reduce(rule.relation, (score > limit for score, limit in pairs)):\n return rule.result\n return 0", "def final_grade(exam, projects):\n try:\n return {\n exam > 50 and projects >= 2: 75,\n exam > 75 and projects >= 5: 90,\n exam > 90 or projects > 10: 100\n }[True]\n except KeyError:\n return 0", "def final_grade(exam, projects):\n return {exam > 50 and projects > 1: 75,\n exam > 75 and projects > 4: 90,\n exam > 90 or projects > 10: 100}.get(True, 0)", "def final_grade(exam: int, projects: int) -> int:\n \"\"\"\n Calculates the final grade of a student depending on two parameters:\n - grade for the exam\n - number of completed projects\n \"\"\"\n return {\n all([exam > 50, projects >= 2]): 75,\n all([exam > 75, projects >= 5]): 90,\n any([exam > 90, projects > 10]): 100\n }.get(True, 0)", "final_grade=lambda e,p:[0,75,90,100][sum([e>90or p>10,(e>75and p>4)or(e>90or p>10),(e>50and p>1)or(e>90or p>10)])]", "def final_grade(exam, projects):\n if exam> 90 or projects>10: return 100\n for val in [(75,5,90), (50,2,75)]:\n if exam > val[0] and projects >= val[1]: return val[2]\n return 0", "def final_grade(exam, projects):\n return min((75*(exam>50 and projects>1)+15*(exam>75 and projects>4))+100*(exam>90 or projects>10), 100)", "# Today I am mostly implementing Scala's pattern matching in Python...\n# Wondering if I can implement the Elixir pipe operator |> via decorators next\n# I may be bored.\n\n_ = '_'\n\ndef match(patterns, *args):\n for pattern, result in patterns:\n if all(p == _ or p == actual for p, actual in zip(pattern, args)):\n return result\n\ndef final_grade(exam, projects):\n patterns = [\n ((True, ), 100),\n ((_, _, _, True), 100),\n ((_, True, _, _, True), 90),\n ((_, _, True, _, _, True), 75),\n ((), 0)\n ]\n return match(patterns,\n exam > 90, exam > 75, exam > 50, \n projects > 10, projects > 4, projects > 1\n )", "final_grade=lambda e,p:100*(e>90 or p>10) or 90*(e>75 and p>4) or 75*(e>50 and p>1)", "final_grade=lambda e,p:[0,75,90,100][(3*(e>90 or p>10) or 2*(e>75 and p>4) or (e>50 and p>1))]", "def final_grade(e, p, o=0):\n return 100 if e>90 or p>10 else 90 if e>75 and p>4 else 75 if e>50 and p > 1 else 0", "final_grade = lambda g, p : 100 if (g > 90 or p > 10) else (90 if (g > 75 and p > 4) else (75 if (g > 50 and p > 1) else 0))", "def final_grade(exam, projects):\n results = [0, 75, 90, 100, 100, 100]\n return results[3 * (exam > 90 or projects > 10) + (exam > 75 and projects >= 5) + (exam > 50 and projects >= 2)]", "def final_grade(exam, projects):\n array=[100,90,75,0]\n if exam>90 or projects>10:\n return array[0]\n elif exam>75 and projects>=5:\n return array[1]\n elif exam>50 and projects>=2:\n return array[2]\n else:\n return array[3]", "def final_grade(exam, projects):\n if exam > 90 or projects > 10:\n return 100\n minimums = ((90, 76, 5),\n (75, 51, 2),\n ( 0, 0, 0))\n return next( score for score, exam_min, projects_min\n in minimums\n if exam_min <= exam and projects_min <= projects )", "def final_grade(exam, projects):\n exams, proj, grades = [90, 75, 50, 0], [10, 4, 1, 0], [100, 90, 75, 0]\n for i in range(4):\n if exam > 90 or projects > 10:\n return 100\n if exam > exams[i] and projects > proj[i]:\n return grades[i]\n return 0", "import unittest\n\n\ndef final_grade(exam, projects):\n print((exam, projects))\n if exam > 90 or projects > 10:\n return 100\n elif exam > 75 and projects >= 5:\n return 90\n elif exam > 50 and projects >= 2:\n return 75\n else:\n return 0\n \n \nclass TestFinalGrade(unittest.TestCase):\n def test_final_grade_on_100_with_exam_score_that_is_more_than_90(self):\n exam, projects = 100, 12\n actual = final_grade(exam, projects)\n self.assertEqual(actual, 100)\n\n def test_final_grade_on_100_with_projects_that_is_more_than_10(self):\n exam, projects = 80, 10\n actual = final_grade(exam, projects)\n self.assertEqual(actual, 100)\n\n def test_final_grade_on_90_with_exam_score_that_is_more_than_75(self):\n exam, projects = 75, 3\n actual = final_grade(exam, projects)\n self.assertEqual(actual, 90)\n\n def test_final_grade_on_90_with_projects_that_is_more_than_5(self):\n exam, projects = 70, 5\n actual = final_grade(exam, projects)\n self.assertEqual(actual, 90)\n\n def test_final_grade_on_75_with_exam_score_that_is_more_than_50(self):\n exam, projects = 50, 3\n actual = final_grade(exam, projects)\n self.assertEqual(actual, 75)\n\n def test_final_grade_on_75_with_projects_that_is_more_than_2(self):\n exam, projects = 40, 3\n actual = final_grade(exam, projects)\n self.assertEqual(actual, 75)\n\n def test_final_grade_on_0_with_projects_and_exam_score_that_is_less_than_final_grade_on_75(self):\n exam, projects = 30, 1\n actual = final_grade(exam, projects)\n self.assertEqual(actual, 0)\n", "def final_grade(x, p):\n return ((x>90 or p>10) and 100 or\n x>75 and p>=5 and 90 or\n x>50 and p>=2 and 75)", "def final_grade(exam, projects):\n grade = 0\n if exam > 90 or projects > 10:\n grade = 100\n elif exam > 75 and exam <= 90 and projects >= 5:\n grade = 90\n elif exam > 50 and projects >= 2:\n grade = 75\n else:\n grade = 0\n return grade", "def final_grade(exam, projects):\n return 100 if exam>90 or projects>10 else 90 if 90>= exam >75 and projects>=5 else 75 if 90>=exam>50 and projects>=2 else 0\n", "def final_grade(exam, projects):\n print(exam, projects)\n \n if exam > 90:\n return 100\n elif projects > 10:\n return 100\n elif exam > 75 and projects >= 5:\n return 90\n elif exam > 50 and projects >= 2:\n return 75\n else:\n return 0", "def final_grade(exam, pro):\n if exam > 90 or pro > 10:\n return 100\n if exam > 75 and pro >= 5:\n return 90\n if exam > 50 and pro >= 2:\n return 75\n else:\n return 0", "def final_grade(exam, proj):\n if exam > 90 or proj > 10:\n return 100\n if exam > 75 and proj > 4:\n return 90\n if exam > 50 and proj > 1:\n return 75\n return 0", "def final_grade(exam, projects):\n if exam > 90:\n return 100\n elif projects >= 11:\n return 100\n if exam > 75 and projects >= 5:\n return 90\n if exam > 50 and projects >= 2:\n return 75\n else: return 0", "def final_grade(exam,\n projects):\n\n if (90 < exam or\n 10 < projects):\n\n return 100\n\n elif (75 < exam and\n 5 <= projects):\n\n return 90\n\n elif (50 < exam and\n 2 <= projects):\n\n return 75\n\n return 0\n", "def final_grade(points, project):\n if points > 90 or project > 10:\n point = 100\n elif points > 75 and project >= 5:\n point = 90\n elif points > 50 and project >= 2:\n point = 75\n else:\n point = 0\n return point", "final_grade = lambda e, p: [0, 75, 90, 100, 100, 100][(e>90 or p>10)*3 + (e>75 and p>=5) + (e>50 and p>=2)]", "#def final_grade(e, p):\n# return [0, 75, 90, 100][(e>90 and p>10) + (e>75 and p>=5) + (e>50 and p>=2)]\n\nfinal_grade = lambda e, p: [0, 75, 90, 100, 100, 100][(e>90 or p>10)*3 + (e>75 and p>=5) + (e>50 and p>=2)]", "def final_grade(s, p):\n return 100 if s>90 or p>10 else 90 if s>75 and p>=5 else 75 if s>50 and p>=2 else 0 ", "def final_grade(exam, projects):\n if exam > 90 or projects > 10:\n return 100\n elif exam <= 50 or projects < 2:\n return 0\n elif exam <= 75 or projects < 5:\n return 75\n else:\n return 90", "def final_grade(exam, projects):\n if projects>10:\n return 100\n if exam>90:\n return 100\n if exam>75 and projects>=5:\n return 90\n elif exam>50 and projects>=2:\n return 75\n return 0", "def final_grade(a,b):\n if a>90 or b>10:\n return 100\n elif a>75 and b>4:\n return 90\n elif a>50 and b>1:\n return 75\n else:\n return 0", "def final_grade(exam, projects):\n if projects > 10:\n return 100\n elif exam > 90:\n return 100\n elif projects >= 5 and exam > 75:\n return 90\n elif projects >= 2 and exam > 50:\n return 75\n else:\n return 0", "def final_grade(exam, projects):\n if projects > 10 or exam > 90:\n return 100\n elif exam > 75 and projects > 4:\n return 90\n elif exam > 50 and projects > 1:\n return 75\n else:\n return 0", "def final_grade(e, p):\n return [100,90,75,0][0 if(e>90 or p>10)else 1 if(e>75 and p>4)else 2 if (e>50 and p>1)else 3]", "def final_grade(exam, projects):\n grade = 0\n \n if exam > 90 or projects > 10:\n grade += 100\n \n elif exam > 75 and projects >= 5:\n grade += 90\n \n elif exam > 50 and projects >= 2:\n grade += 75\n\n else:\n grade == 0\n \n return grade\n \n\n\n", "def final_grade(exam, projects):\n print((\" \" + str(exam) + \" \" + str(projects)))\n data = [\n [90, 10, 100],\n [75, 5, 90],\n [50, 2, 75]\n ]\n if exam > data[0][0] or projects > data[0][1]:\n return data[0][2]\n elif exam > data[1][0] and projects >= data[1][1]:\n return data[1][2]\n elif exam > data[2][0] and projects >= data[2][1]:\n return data[2][2]\n return 0\n\n\n", "def final_grade(exam, projects):\n if exam > 90 or projects >10:\n return 100\n elif exam > 75 and 10 >=projects >=5:\n return 90\n elif exam > 50 and projects>=2:\n return 75\n else:\n return 0", "def final_grade(exam, projects):\n if exam <= 100 and (exam > 90 or projects > 10):\n return 100\n elif exam > 75 and projects >= 5:\n return 90\n elif exam > 50 and projects >= 2:\n return 75\n \n else:\n return 0", "def final_grade(exam, projects):\n if exam > 90 or projects > 10:\n return 100\n elif exam > 75 and projects >= 5:\n return 90\n return 75 if exam > 50 and projects >= 2 else 0", "# calculate final grade of student based on grade for an exam and a number of projects\n# input - integer for exam grade (0-100), integer for number of projects (0 and above)\n# output - integer for final grade\n\n\ndef final_grade(exam, projects):\n if exam > 90 or projects > 10:\n return 100\n if exam > 75 and projects >= 5:\n return 90\n if exam > 50 and projects >= 2:\n return 75\n else:\n return 0", "def final_grade(exam, projects):\n \n if exam > 90 or projects > 10:\n return 100\n elif exam in range(76,91) and projects >= 5:\n return 90\n elif exam > 50 and projects >= 2:\n return 75\n elif exam <= 50 or projects < 2:\n return 0\n", "def final_grade(e, p):\n return 100 if e>90 or p>10 else (90 if e>75 and p>4 else (75 if e>50 and p>=2 else 0))", "def final_grade(exam, projects):\n if exam > 90 or projects > 10:\n return 100\n# elif exam >= 90:\n# return 100\n# elif projects >= 10:\n# return 100\n elif exam > 75 and projects >= 5:\n return 90\n elif exam > 50 and projects >= 2:\n return 75\n# elif exam == 0:\n# return 0\n else:\n return 0\n", "def final_grade(exam, projects):\n if 90 < exam or 10 < projects:\n return 100\n if 75 < exam and 5 <= projects:\n return 90\n if 50 < exam and 2 <= projects:\n return 75\n return 0", "def final_grade(exam, projects):\n if exam > 90 or projects > 10:\n return 100\n elif exam > 75 and projects >= 5:\n return 90\n elif exam > 50 and projects >= 2:\n return 75\n else:\n exam >= 0 or projects != 0 \n return 0", "def final_grade(exam, projects):\n print((exam, projects))\n grade = 0\n if exam > 90 or projects > 10:\n grade = 100\n elif exam > 75 and projects > 4:\n grade = 90\n elif exam > 50 and projects > 1:\n grade = 75\n return grade\n \n", "def final_grade(exam, projects):\n reponse = 0\n if exam > 90 or projects > 10:\n return 100\n elif exam > 75 and projects >= 5:\n return 90\n elif exam > 50 and projects >= 2:\n return 75\n else:\n return 0", "def final_grade(exam, proj):\n return 100 if exam > 90 or proj > 10 else 90 if exam > 75 and proj >= 5 else 75 if exam > 50 and proj >= 2 else 0", "def final_grade(e, p):\n return 100 if e > 90 or p > 10 else \\\n 90 if e > 75 and p >= 5 else \\\n 75 if e > 50 and p >= 2 else \\\n 0", "def final_grade(exam, projects):\n if exam > 90:\n return 100\n elif projects < 2:\n return 0 \n elif projects > 10:\n return 100\n elif exam > 75 and projects >= 5 :\n return 90\n elif exam > 50 and projects >= 2:\n return 75\n else: \n return 0\n", "def final_grade(exam, projects): \n '''Return grade based on input criteria'''\n grade = 0\n if exam > 90 or projects > 10:\n grade = 100\n elif exam > 75 and projects >= 5:\n grade = 90\n elif exam > 50 and projects >= 2:\n grade = 75\n \n return grade", "def final_grade(exam, projects):\n if projects > 10:\n return 100\n elif exam > 90:\n return 100\n elif exam > 75 and projects >= 5:\n return 90\n elif exam > 50 and projects >= 2:\n return 75\n else:\n return 0", "def final_grade(exam, projects):\n\n grade=0 \n \n if exam >90 or projects >10:\n grade=100\n elif (exam >75 and exam <=90)and (projects >=5):\n grade=90\n elif (exam >50) and (projects >=2): \n grade=75\n \n return grade ", "def final_grade(e, p):\n s = 0\n if e > 90 or p > 10:\n s = 100\n elif e > 75 and p > 4:\n s = 90\n elif e > 50 and p > 1:\n s = 75\n return s\n", "def final_grade(exam, projects):\n result = 0\n if exam > 90 or projects > 10:\n result = 100\n elif exam > 75 and projects > 4:\n result = 90\n elif exam > 50 and projects > 1:\n result = 75\n else:\n result = 0\n return result", "def final_grade(exam, projects):\n print(exam, projects)\n if exam>90 or projects>10: return (100)\n if exam>75 and projects>=5: return (90)\n if exam>50 and projects>=2: return (75)\n return(0)", "def final_grade(exam, projects):\n return 100 if exam > 90 or projects > 10 else 90 if exam > 75 and projects >= 5 else 75 if exam > 50 and projects >= 2 else 0\n# if exam > 90 or projects > 10:\n# return 100\n# elif exam >= 75 and projects >= 5:\n# return 90\n# elif \n", "def final_grade(exam, projects):\n \n e = exam\n p = projects\n \n if e > 90 or p > 10:\n return 100\n elif e > 75 and p >= 5:\n return 90\n elif e > 50 and p >= 2:\n return 75\n else:\n return 0", "def final_grade(ex, p):\n if ex > 90 or p > 10: return 100\n elif ex > 75 and p >= 5: return 90\n elif ex > 50 and p >= 2 : return 75\n else: return 0\n #return # final grade\n", "def final_grade(exam, projects):\n return [0, 75, 90][(exam > 50 and projects > 1) + (exam > 75 and projects > 4)] if exam <= 90 and projects <= 10 else 100", "def final_grade(exam, projects):\n e = exam\n p = projects\n if e > 90 or p > 10:\n return 100\n elif e > 75 and p >= 5:\n return 90\n elif e > 50 and p >= 2:\n return 75\n return 0\n", "def final_grade(exam, projects):\n# if exam > 90 and projects > 10:\n# return 100\n# elif exam >75 and projects >=5:\n# return 90\n# elif exam >50 and projects >=2:\n# return 75\n# else:\n# return 0\n if exam > 90 or projects > 10: return 100\n if exam > 75 and projects >= 5: return 90\n if exam > 50 and projects >= 2: return 75\n return 0", "def final_grade(exam, pr):\n if exam > 90 or pr > 10:\n return 100\n elif exam > 75 and pr >= 5:\n return 90\n elif exam > 50 and pr >= 2:\n return 75\n return 0", "def final_grade(exam, projects):\n print('%s,%s' %(exam,projects))\n if exam>90 or projects>10:\n return 100\n elif exam >75 and projects>=5:\n return 90\n elif exam>50 and projects>=2:\n return 75\n else:\n return 0", "def final_grade(exam, projects):\n if exam>90 or projects>10:\n return 100# final grade\n elif exam>75 and projects>=5:\n return 90\n elif exam>50 and projects>=2:\n return 75\n return 0", "def final_grade(e, p):\n if e>90 or p>10:\n return 100\n if e>75 and p>4:\n return 90\n if e>50 and p>1:\n return 75\n else:\n return 0\n", "def final_grade(exams, projects):\n return 100 if exams >90 or projects>10 else 90 if exams>75 and projects>=5 else 75 if exams>50 and projects>=2 else 0", "def final_grade(exam, projects):\n if exam > 90 or projects > 10:\n return 100\n elif exam > 75 and projects > 4:\n return 90\n elif exam > 50 and projects >= 2:\n return 75\n else:\n return 0", "def final_grade(exam, projects):\n return \\\n 100 if exam > 90 or projects > 10 else \\\n 90 if exam > 75 and projects >= 5 else \\\n 75 if exam > 50 and projects >= 2 else \\\n 0", "def final_grade(exam, projects):\n if exam > 90 or projects > 10:\n return 100\n if (exam > 75 and exam <= 90) and (projects >= 5 and projects <= 10):\n return 90\n if (exam > 50) and projects >= 2:\n return 75\n else:\n return 0", "def final_grade(exam, projects):\n if exam>90 or projects>10 :\n return 100\n elif 90>=exam>75 and projects>=5:\n return 90\n elif exam>50 and projects>=2:\n return 75\n else:\n return 0", "def final_grade(exam, projects):\n if exam > 90 or projects > 10:\n return 100\n elif exam > 75 and projects >= 5 and projects <= 10:\n return 90\n elif exam > 50 and projects >= 2 and projects <= 10:\n return 75\n else:\n return 0", "def final_grade(exam, projects):\n final = 0\n if exam > 90 or projects > 10:\n final = 100\n elif exam > 75 and projects > 4:\n final = 90\n elif exam > 50 and projects > 1:\n final = 75\n else:\n final = 0\n return final", "def final_grade(exam, projects):\n finalGrade = 0;\n if exam > 50 and projects >= 2:\n finalGrade = 75;\n if exam > 75 and projects >= 5:\n finalGrade = 90;\n if exam > 90 or projects > 10:\n finalGrade = 100;\n return finalGrade;", "def final_grade(exam, projects):\n if exam > 90 or projects > 10:\n return 100\n elif 75 < exam <= 90 and projects >= 5:\n return 90\n elif 50 < exam <= 75 and projects >= 2:\n return 75\n elif 50 < exam <= 90 and projects >= 2:\n return 75\n else:\n return 0", "def final_grade(e, p):\n if e>90 or p>10:\n return 100\n if 75<e<=90 and p>=5 and p<=10:\n return 90\n if 50<e<=90 and p>=2 and p<=10:\n return 75\n else:\n return 0", "def final_grade(exam, projects):\n if exam>90 or projects>10:\n return 100\n else:\n if exam >75 and projects>=5:\n return 90\n elif exam>50 and projects>=2:\n return 75\n else:\n return 0\n", "def final_grade(exam, projects):\n if int(exam) > 90 or int(projects) > 10:\n final_grade = 100\n elif int(exam) >75 and int(projects) >= 5:\n final_grade = 90\n elif int(exam) > 50 and int(projects) >=2:\n final_grade = 75\n else:\n final_grade = 0\n return final_grade", "def final_grade(e, p):\n if p>10 or e>90 : return 100\n if p>=5 and e>75 : return 90\n if p>=2 and e>50 : return 75\n return 0\n", "def final_grade(exam, projects):\n if exam > 90 or projects > 10:\n return 100\n elif (75 < exam) and (5 <= projects): # <= 10):\n return 90\n elif (50 < exam) and (2 <= projects): # <5):\n return 75\n #elif projects == 11:\n # return 100\n # elif exam < 50 and projects > 5:\n # return 0\n else:\n return 0", "def final_grade(exam, projects):\n if exam >= 91 or projects > 10:\n return 100\n elif exam >=76 and projects >= 5:\n return 90\n elif exam >=51 and projects >= 2:\n return 75\n else:\n return 0\n return # final grade", "def final_grade(exm, prj):\n if exm > 90 or prj > 10:\n return 100\n if exm > 75 and prj >= 5:\n return 90\n if exm > 50 and prj >= 2:\n return 75\n return 0", "def final_grade(ex, prj):\n return 100 if ex>90 or prj>10 else 90 if ex>75 and prj>=5 else 75 if ex>50 and prj>=2 else 0", "def final_grade(exam, projects):\n grade = [100,90,75,0]\n x = 3\n if exam > 90 or projects > 10:\n x = 0\n elif exam > 75 and projects > 4:\n x = 1\n elif exam > 50 and projects > 1:\n x = 2\n return grade[x]", "def final_grade(ex, pro):\n if ex > 90 or pro > 10: return 100\n if ex > 75 and pro >= 5: return 90\n if ex > 50 and pro >=2: return 75\n else: return 0# final grade", "def final_grade(exam, projects):\n if exam > 90 or projects > 10:\n grade = 100\n elif exam > 75 <= 90 and projects >= 5 <= 10:\n grade = 90\n elif exam > 50 < 75 and projects >= 2 < 5:\n grade = 75\n else:\n grade = 0\n \n return grade", "def final_grade(exam, projects):\n rubric = [100, 90, 75, 0]\n return rubric[0] if exam > 90 or projects > 10 else rubric[1] if exam > 75 and projects >= 5 else rubric[2] if exam > 50 and projects >= 2 else rubric[3]"] | {"fn_name": "final_grade", "inputs": [[100, 12], [99, 0], [10, 15], [85, 5], [55, 3], [55, 0], [20, 2]], "outputs": [[100], [100], [100], [90], [75], [0], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 25,590 |
def final_grade(exam, projects):
|
a6e31df2bfbc76fc6fa81480f089799f | UNKNOWN | Create a function that accepts dimensions, of Rows x Columns, as parameters in order to create a multiplication table sized according to the given dimensions. **The return value of the function must be an array, and the numbers must be Fixnums, NOT strings.
Example:
multiplication_table(3,3)
1 2 3
2 4 6
3 6 9
-->[[1,2,3],[2,4,6],[3,6,9]]
Each value on the table should be equal to the value of multiplying the number in its first row times the number in its first column. | ["def multiplication_table(row,col):\n return [[(i+1)*(j+1) for j in range(col)] for i in range(row)]", "def multiplication_table(row,col):\n return [[x*y for y in range(1,col+1)] for x in range(1,row+1)]", "def multiplication_table(row,col):\n res = []\n for i in range(1, row+1):\n item = []\n for j in range(1, col+1):\n item.append(i*j)\n res.append(item)\n return res\n", "def multiplication_table(row,col):\n return [[ e*i for e in range(1,col+1) ] for i in range(1,row+1) ]\n", "def multiplication_table(row,col):\n return [[i * j for i in range(1, col+1)] for j in range(1, row+1)] ", "def multiplication_table(row,col):\n return [[i * j for j in range(1, col + 1)] for i in range(1, row + 1)]", "def multiplication_table(row,col):\n return [[(i+1)*(j+1) for i in range(col)] for j in range(row)]", "def multiplication_table(row,col):\n l=[]\n for linha in range(row):\n l.append(list(range(linha+1,(linha+1)*col+1,linha+1)))\n return l\n"] | {"fn_name": "multiplication_table", "inputs": [[2, 2], [3, 3], [3, 4], [4, 4], [2, 5]], "outputs": [[[[1, 2], [2, 4]]], [[[1, 2, 3], [2, 4, 6], [3, 6, 9]]], [[[1, 2, 3, 4], [2, 4, 6, 8], [3, 6, 9, 12]]], [[[1, 2, 3, 4], [2, 4, 6, 8], [3, 6, 9, 12], [4, 8, 12, 16]]], [[[1, 2, 3, 4, 5], [2, 4, 6, 8, 10]]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,023 |
def multiplication_table(row,col):
|
5dfdb7f278c7742f41183b29fd96019c | UNKNOWN | In this Kata, you will be given two integers `n` and `k` and your task is to remove `k-digits` from `n` and return the lowest number possible, without changing the order of the digits in `n`. Return the result as a string.
Let's take an example of `solve(123056,4)`. We need to remove `4` digits from `123056` and return the lowest possible number. The best digits to remove are `(1,2,3,6)` so that the remaining digits are `'05'`. Therefore, `solve(123056,4) = '05'`.
Note also that the order of the numbers in `n` does not change: `solve(1284569,2) = '12456',` because we have removed `8` and `9`.
More examples in the test cases.
Good luck! | ["from itertools import combinations\n\ndef solve(n, k):\n return ''.join(min(combinations(str(n), len(str(n))-k)))", "from itertools import combinations\n\ndef solve(n,k):\n return min((''.join(c) for c in combinations(str(n), len(str(n))-k)), key=int)", "from itertools import combinations as c\ndef solve(n,k):\n b=len(str(n))-k\n l=[''.join(i) for i in c(str(n),b)]\n return min(l)", "def solve(n, k):\n s = str(n)\n for _ in range(k):\n i = next(i for i in range(len(s)) if i == len(s)-1 or s[i] > s[i+1])\n s = s[:i] + s[i+1:]\n return s", "def solve(n, k):\n s = str(n)\n result = ''\n for _ in range(len(s)-k):\n i = min(range(k+1), key=s.__getitem__)\n result += s[i]\n k -= i\n s = s[i+1:]\n return result", "from itertools import combinations\n\ndef solve(n, k):\n s = str(n)\n return ''.join(min(xs for xs in combinations(s, len(s)-k)))", "def solve(n,k):\n n = str(n)\n number = ''\n while k < len(n):\n m = min([(int(n[i]), i) for i in range(k+1)])\n number += str(m[0]) \n n = n[m[1]+1:]\n k -= m[1]\n return number", "def solve(n, k):\n s = str(n)\n Slen = len(s)\n removed = False\n largestIndex = 0\n if (Slen < 2 and k > 1) or k>Slen:\n return '0'\n i = 1\n for j in range(k):\n while i < len(s):\n if s[i] < s[i-1]:\n s = s[:i-1]+s[i:]\n removed = True\n break\n i += 1\n i = 1\n if not removed:\n largestIndex = 0\n j = 0\n while not removed and j < len(s):\n if int(s[j]) > int(s[largestIndex]):\n largestIndex = j\n j += 1\n s = s[:j-1] + s[j:]\n removed = False\n return s", "def solve(n,k):\n s=''\n for i in str(n):\n if k==0:\n s+=i\n else:\n while s and s[-1]>i and k:\n k-=1\n s=s[:-1] \n s+=i\n p=i\n return s[:-k] if k else s ", "from itertools import combinations\n\ndef solve(n, k):\n s = str(n)\n return ''.join(min(combinations(s, len(s) - k)))"] | {"fn_name": "solve", "inputs": [[123056, 1], [123056, 2], [123056, 3], [123056, 4], [1284569, 1], [1284569, 2], [1284569, 3], [1284569, 4]], "outputs": [["12056"], ["1056"], ["056"], ["05"], ["124569"], ["12456"], ["1245"], ["124"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,232 |
def solve(n, k):
|
afea6f0129823fc84ac5896fac76f974 | UNKNOWN | Given a string ``string`` that contains only letters, you have to find out the number of **unique** strings (including ``string`` itself) that can be produced by re-arranging the letters of the ``string``. Strings are case **insensitive**.
HINT: Generating all the unique strings and calling length on that isn't a great solution for this problem. It can be done a lot faster...
## Examples
```python
uniqcount("AB") = 2 # "AB", "BA"
uniqcount("ABC") = 6 # "ABC", "ACB", "BAC", "BCA", "CAB", "CBA"
uniqcount("ABA") = 3 # "AAB", "ABA", "BAA"
uniqcount("ABBb") = 4 # "ABBB", "BABB", "BBAB", "BBBA"
uniqcount("AbcD") = 24 # "ABCD", etc.
``` | ["from operator import mul\nfrom functools import reduce\nfrom collections import Counter\nfrom math import factorial as fact\n\ndef uniq_count(s):\n return fact(len(s)) // reduce(mul, map(fact, Counter(s.lower()).values()), 1)", "from collections import Counter\nfrom functools import reduce\nfrom math import factorial\n\n\ndef uniq_count(s):\n f = (factorial(c) for c in Counter(s.upper()).values())\n return factorial(len(s)) // reduce(int.__mul__, f, 1)", "from functools import reduce, lru_cache\nfrom collections import Counter\nfrom math import factorial\nfrom operator import mul\n\nfact = lru_cache(maxsize=None)(factorial)\n\ndef uniq_count(s):\n return fact(len(s)) // reduce(mul, map(fact, Counter(s.upper()).values()), 1)", "from collections import Counter\nfrom functools import reduce\nfrom math import factorial\n\n\ndef uniq_count(s):\n return factorial(len(s)) // reduce(lambda x,y: factorial(y) * x, list(Counter(s.lower()).values()), 1)\n", "from math import factorial\nfrom collections import Counter\nfrom functools import reduce\n\ndef uniq_count(s):\n return factorial(len(s)) // reduce(lambda x, y: x * factorial(y) , Counter(s.lower()).values(), 1)", "from collections import Counter\nfrom functools import reduce\nfrom math import factorial\nfrom operator import mul\n\ndef uniq_count(string):\n return factorial(len(string)) // reduce(mul, map(factorial, Counter(string.lower()).values()), 1)", "from math import factorial\nfrom functools import reduce\nfrom operator import mul\nuniq_count=lambda a:factorial(len(a))//reduce(mul,[factorial(a.lower().count(i))for i in set(a.lower())],1)", "from math import factorial\n\ndef uniq_count(s):\n s = s.lower()\n n = len(s)\n d = 1\n for c in set(s):\n d *= factorial(s.count(c))\n return factorial(n)//d\n"] | {"fn_name": "uniq_count", "inputs": [["AB"], ["ABC"], ["AbA"], ["ABBb"], ["AbcD"], ["AAA"], [""], ["ASTON"], ["BEST"], ["ABcDEFgHIJ"], ["ABcDEFgHIJbaslidbailsbdilasbdkanmsdklhkbHSJKHVDASH"], ["ABcDEFgHIJbaslidbailsbdilasbdkanmsdklhkbHSJKHVDASHVVYQVWKDVDWQUV"]], "outputs": [[2], [6], [3], [4], [24], [1], [1], [120], [24], [3628800], [34111429518116758488933545882757275627520000000], [176478346352319876826993574633158714419916931040323433922560000000]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,825 |
def uniq_count(s):
|
c24b9fb34855532e2cfb4e3ef1453200 | UNKNOWN | # Two samurai generals are discussing dinner plans after a battle, but they can't seem to agree.
The discussion gets heated and you are cannot risk favoring either of them as this might damage your political standing with either of the two clans the samurai generals belong to. Thus, the only thing left to do is find what the common ground of what they are saying is.
Compare the proposals with the following function:
```python
def common_ground(s1,s2)
```
The parameters ```s1``` and ```s2``` are the strings representing what each of the generals said. You should output a string containing the words in ```s1``` that also occur in ```s2```.
Each word in the resulting string shall occur once, and the order of the words need to follow the order of the first occurence of each word in ```s2```.
If they are saying nothing in common, kill both samurai and blame a ninja. (output "death") | ["def common_ground(s1,s2):\n lst = []\n for w in s2.split():\n if w in s1.split() and w not in lst:\n lst.append(w)\n return ' '.join(lst) if lst else \"death\"", "def common_ground(s1,s2):\n words = s2.split()\n return ' '.join(sorted((a for a in set(s1.split()) if a in words),\n key=lambda b: words.index(b))) or 'death'\n", "def common_ground(s1, s2):\n s1, s2 = set(s1.split()), s2.split()\n return \" \".join(sorted((set(s2) & s1), key=s2.index)) or \"death\"", "def common_ground(s1, s2):\n s1, s2 = set(s1.split()), s2.split()\n return \" \".join(sorted((word for word in set(s2) if word in s1), key=s2.index)) or \"death\"\n", "def common_ground(s1,s2):\n ret = [i for i in set(s1.split()) if i in s2.split()]\n if len(ret) == 0: return 'death'\n return ' '. join(sorted(ret, key=lambda x: (s2.split()).index(x) ))", "def common_ground(s1,s2):\n return \" \".join(sorted(set(s1.split(\" \")).intersection(s2.split(\" \")), key=s2.split(\" \").index)) or \"death\"", "from collections import OrderedDict\n\n\ndef common_ground(s1, s2):\n xs = set(s1.split())\n return ' '.join(x for x in OrderedDict.fromkeys(s2.split()) if x in xs) or 'death'", "from collections import OrderedDict\ndef common_ground(s1,s2):\n return \" \".join(filter(set(s1.split()).__contains__, OrderedDict.fromkeys(s2.split()))) or \"death\"", "from collections import OrderedDict\ndef common_ground(s1,s2):\n s = \" \".join(w for w in OrderedDict.fromkeys(s2.split()) if w in s1.split())\n return s if s else \"death\"", "from collections import OrderedDict\ndef common_ground(s1,s2):\n s3 = []\n [s3.append(i) for i in s2.split() if i in s1.split()]\n\n if len(s3) == 0:\n return 'death'\n else:\n return ' '.join(OrderedDict.fromkeys(s3))"] | {"fn_name": "common_ground", "inputs": [["eat chicken", "eat chicken and rice"], ["eat a burger and drink a coke", "drink a coke"], ["i like turtles", "what are you talking about"], ["aa bb", "aa bb cc"], ["aa bb cc", "bb cc"], ["aa bb cc", "bb cc bb aa"], ["aa bb", "cc dd"], ["aa bb", ""], ["", "cc dd"], ["", ""]], "outputs": [["eat chicken"], ["drink a coke"], ["death"], ["aa bb"], ["bb cc"], ["bb cc aa"], ["death"], ["death"], ["death"], ["death"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,849 |
def common_ground(s1,s2):
|
24ed311254483a6a52ed4ac33b5bf8a3 | UNKNOWN | Introduction to Disjunctions
In logic and mathematics, a disjunction is an operation on 2 or more propositions. A disjunction is true if and only if 1 or more of its operands is true. In programming, we typically denote a disjunction using "||", but in logic we typically use "v".
Example of disjunction:
p = 1 > 2 = false
q = 2 < 3 = true
therefore p v q is true
In a programming language, we might write this as:
var p = 1 > 2; // false
var q = 2 < 3; // true
var result = p || q; // true
The above example demonstrates an inclusive disjunction (meaning it includes cases where both operands are true). Disjunctions can also be exlusive. An exclusive disjunction is typically represented by "⊻" and is true if and only if both operands have opposite values.
p = 1 < 2 = true
q = 2 < 3 = true
therefore p ⊻ q is false
This can become confusing when dealing with more than 2 operands.
r = 3 < 4 = true
p ⊻ q ⊻ r = ???
We handle these situations by evaluating the expression from left to right.
p ⊻ q = false
(p ⊻ q) ⊻ r = true
Directions:
For this kata, your task is to implement a function that performs a disjunction operation on 2 or more propositions.
Should take a boolean array as its first parameter and a single boolean as its second parameter, which, if true, should indicate that the disjunction should be exclusive as opposed to inclusive.
Should return true or false. | ["from functools import reduce\n\n\ndef disjunction(operands, is_exclusive):\n return reduce(bool.__xor__ if is_exclusive else bool.__or__, operands)", "from functools import reduce\n\ndef disjunction(operands, is_exclusive):\n op = int.__xor__ if is_exclusive else int.__or__\n return reduce(op, operands)", "from functools import reduce\nfrom operator import or_, xor\n\ndef disjunction(operands, is_exclusive):\n return reduce(xor if is_exclusive else or_, operands)", "disjunction=lambda o, e: o.count(True) % 2 if e else True in o", "from functools import reduce\n\ndef disjunction(operands, is_exclusive):\n return reduce(getattr(bool, f\"__{'x' if is_exclusive else ''}or__\"), operands)", "def disjunction(operands, is_exclusive):\n return sum(operands) & 1 if is_exclusive else any(operands)", "from operator import or_, xor\nfrom functools import reduce\ndef disjunction(operands, is_exclusive):\n return reduce([or_, xor][is_exclusive], operands)", "def disjunction(operands, is_exclusive):\n return sum(operands)%2 if is_exclusive else any(operands) ", "from functools import reduce\n\ndef disjunction(operands, is_exclusive):\n return reduce(bool.__ne__ if is_exclusive else bool.__or__, operands)", "from functools import reduce\nfrom operator import xor, or_\n\ndisjunction = lambda a, b: reduce(xor if b else or_, a)"] | {"fn_name": "disjunction", "inputs": [[[false, true, true], false], [[false, true, false], false], [[false, true, true, true], true], [[true, true, true], false], [[true, true, true, true], false]], "outputs": [[true], [true], [true], [true], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,361 |
def disjunction(operands, is_exclusive):
|
bec64490f45e65895e28758adbb62dcc | UNKNOWN | Write a function `sumTimesTables` which sums the result of the sums of the elements specified in `tables` multiplied by all the numbers in between `min` and `max` including themselves.
For example, for `sumTimesTables([2,5],1,3)` the result should be the same as
```
2*1 + 2*2 + 2*3 +
5*1 + 5*2 + 5*3
```
i.e. the table of two from 1 to 3 plus the table of five from 1 to 3
All the numbers are integers but you must take in account:
* `tables` could be empty.
* `min` could be negative.
* `max` could be really big. | ["def sum_times_tables(table,a,b):\n return sum(table) * (a + b) * (b - a + 1) // 2 \n", "def sum_times_tables(table,a,b):\n s = 0\n for i in range(a,b+1):\n s = s+i*sum(table)\n return s ", "def sum_times_tables(table,a,b):\n return sum(table) * sum(range(a, b+1))", "def T(n):\n return n * (n + 1) // 2\n\ndef sum_times_tables(table,a,b):\n return sum(table) * (T(b) - T(a - 1))", "def sum_times_tables(table,a,b):\n n = sum(table)\n return n and sum(range(n * a, n * (b + 1), n))", "def sum_times_tables(table,a,b):\n total=0\n for x in range(a,b+1):\n total += sum(list([x*n for n in table]))\n return total\n", "def sum_times_tables(table,a,b):\n return sum(table)*(summa(b) - summa(a-1))\n\n\ndef summa(n):\n return n*(n+1)/2", "def sum_times_tables(table, a, b):\n return sum(x * y for x in table for y in range(a, b + 1))", "def sum_times_tables(table,a,b):\n return(sum(list(map(lambda x: sum(list(map(lambda y: y * x ,range(a, b+1)))) , table))))", "def sum_times_tables(table, a, b):\n return sum(table) * (a + b) * (b + 1 - a) / 2"] | {"fn_name": "sum_times_tables", "inputs": [[[2, 3], 1, 3], [[1, 3, 5], 1, 1], [[1, 3, 5], 1, 10], [[], 1, 10], [[1, 2, 3, 4, 5, 6, 7, 8, 9, 10], 1, 10], [[10, 9, 8, 7, 6, 5, 4, 3, 2, 1], 1, 10], [[5, 4, 7, 8, 9, 6, 3, 2, 10, 1], 1, 10], [[-2], -1, 3], [[-2, 2], -1, 3], [[-2, 3], -1, 3], [[2, -3], -1, 3], [[2, 4, 7], -100, 100], [[2, 4, 7], 1, 100], [[2, 4, 7], 1, 101]], "outputs": [[30], [9], [495], [0], [3025], [3025], [3025], [-10], [0], [5], [-5], [0], [65650], [66963]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,109 |
def sum_times_tables(table,a,b):
|
8f68008d0ed2d49f1c9a514abfce110e | UNKNOWN | In this kata you should simply determine, whether a given year is a leap year or not. In case you don't know the rules, here they are:
* years divisible by 4 are leap years
* but years divisible by 100 are **not** leap years
* but years divisible by 400 are leap years
Additional Notes:
* Only valid years (positive integers) will be tested, so you don't have to validate them
Examples can be found in the test fixture. | ["def isLeapYear(year):\n return (year % 100 != 0 and year % 4 == 0) or year % 400 == 0", "from calendar import isleap as isLeapYear", "def isLeapYear(year):\n return year % 4 == 0 and year % 100 != 0 or year % 400 == 0", "def isLeapYear(year):\n return year % 4 is 0 and year % 100 is not 0 or year % 400 == 0", "import calendar\n\ndef isLeapYear(year):\n # if calendar is not available:\n # return year % 4 == 0 and year and (year % 400 == 0 or year % 100 != 0)\n return calendar.isleap(year)", "isLeapYear = lambda y: (not y%4) * bool(y%100+(not y%400))", "def isLeapYear(year):\n if year % 400 == 0 or year % 4 ==0 and not year % 100 == 0:\n return True\n else:\n return False", "isLeapYear=__import__(\"calendar\").isleap ", "def is_leap_year(year):\n return (year % 100 and not year % 4) or not year % 400\n \nisLeapYear = is_leap_year", "def isLeapYear(year):\n return (year%4 == 0) and not (year%100 == 0 and not year%400 == 0)"] | {"fn_name": "isLeapYear", "inputs": [[1984], [2000], [2004], [8], [0], [1234], [1100], [1194]], "outputs": [[true], [true], [true], [true], [true], [false], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 980 |
def isLeapYear(year):
|
36c2e3c30beb68d0bb6ca14be7186f59 | UNKNOWN | # Description
Write a function that checks whether a credit card number is correct or not, using the Luhn algorithm.
The algorithm is as follows:
* From the rightmost digit, which is the check digit, moving left, double the value of every second digit; if the product of this doubling operation is greater than 9 (e.g., 8 × 2 = 16), then sum the digits of the products (e.g., 16: 1 + 6 = 7, 18: 1 + 8 = 9) or alternatively subtract 9 from the product (e.g., 16: 16 - 9 = 7, 18: 18 - 9 = 9).
* Take the sum of all the digits.
* If the total modulo 10 is equal to 0 (if the total ends in zero) then the number is valid according to the Luhn formula; else it is not valid.
The input is a string with the full credit card number, in groups of 4 digits separated by spaces, i.e. "1234 5678 9012 3456"
Don´t worry about wrong inputs, they will always be a string with 4 groups of 4 digits each separated by space.
# Examples
`valid_card?("5457 6238 9823 4311") # True`
`valid_card?("5457 6238 9323 4311") # False`
for reference check: https://en.wikipedia.org/wiki/Luhn_algorithm | ["def valid_card(card):\n s = list(map(int, str(card.replace(' ', ''))))\n s[0::2] = [d * 2 - 9 if d * 2 > 9 else d * 2 for d in s[0::2]]\n return sum(s) % 10 == 0", "def valid_card(card_number):\n total = 0\n for i, a in enumerate(reversed(card_number.replace(' ', ''))):\n if i % 2:\n current = int(a) * 2\n total += current - 9 if current > 8 else current\n else:\n total += int(a)\n return total % 10 == 0", "def valid_card(card):\n li = []\n print(card)\n digits = card.replace(' ', '')[::-1]\n for i, d in enumerate(digits):\n di = int(d)\n if i % 2 != 0:\n di = di * 2\n if di > 9:\n di = di - 9\n li.append(di)\n else:\n li.append(di)\n return True if sum(li) % 10 == 0 else False", "valid_card=lambda c:sum(sum(divmod(int(d)<<i%2,10))for i,d in enumerate(c.replace(' ','')[::-1]))%10<1", "def valid_card(card): \n card=str(card.replace(' ',''))\n return (sum(map(int, str(card)[1::2])) + \\\n sum(sum(map(int, str(i*2))) for i in \\\n map(int, str(card)[0::2]))) % 10 == 0", "def valid_card(card):\n return sum([2*c,2*c-9][2*c>9] if i%2 else c for i,c in enumerate(map(int,card.replace(\" \",\"\")[::-1])))%10==0", "def valid_card(card):\n card_str = card.replace(' ', '')\n\n index = 0\n sum_card = 0\n while index < len(card_str):\n number = 0\n if index % 2 == 0:\n number = int(card_str[index]) * 2\n else:\n number = int(card_str[index])\n \n if number >= 10:\n number_arr = list(str(number))\n number = 0\n for num in number_arr:\n number += int(num)\n\n sum_card += number\n\n index += 1\n\n return sum_card % 10 == 0"] | {"fn_name": "valid_card", "inputs": [["5457 6238 9823 4311"], ["8895 6238 9323 4311"], ["5457 6238 5568 4311"], ["5457 6238 9323 4311"], ["2222 2222 2222 2224"], ["5457 1125 9323 4311"], ["1252 6238 9323 4311"], ["9999 9999 9999 9995"], ["0000 0300 0000 0000"], ["4444 4444 4444 4448"], ["5457 6238 9323 1252"], ["5457 6238 1251 4311"], ["3333 3333 3333 3331"], ["6666 6666 6666 6664"], ["5457 6238 0254 4311"], ["0000 0000 0000 0000"], ["5457 1111 9323 4311"], ["1145 6238 9323 4311"], ["8888 8888 8888 8888"], ["0025 2521 9323 4311"], ["1111 1111 1111 1117"], ["1234 5678 9012 3452"], ["5458 4444 9323 4311"], ["5457 6238 3333 4311"], ["0123 4567 8901 2345"], ["5555 5555 5555 5557"]], "outputs": [[true], [false], [false], [false], [true], [false], [false], [true], [false], [true], [false], [false], [true], [true], [false], [true], [false], [false], [true], [false], [true], [true], [false], [false], [false], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,863 |
def valid_card(card):
|
1e3848eefb92d080a4c7651c12d0421f | UNKNOWN | Create a function that will return ```true``` if the input is in the following date time format ```01-09-2016 01:20``` and ```false``` if it is not.
This Kata has been inspired by the Regular Expressions chapter from the book Eloquent JavaScript. | ["from re import match\n\n\ndef date_checker(date):\n return bool(match(r'\\d{2}-\\d{2}-\\d{4}\\s\\d{2}:\\d{2}', date))\n", "import re\n\ndef date_checker(date):\n return bool(re.fullmatch(\"\\d\\d-\\d\\d-\\d\\d\\d\\d \\d\\d:\\d\\d\", date))", "# Really, nothing to check if days and motnhs and zeroes or too high?\ncheck = __import__(\"re\").compile(r\"\\d\\d-\\d\\d-\\d\\d\\d\\d \\d\\d:\\d\\d\").fullmatch\n\ndef date_checker(date):\n return bool(check(date))", "import re\ndef date_checker(date):\n return re.match(r'(\\d\\d-){2}\\d{4} \\d{2}:\\d{2}', date) != None", "import re\n\ndef date_checker(date):\n return bool(re.match(r'\\d{2}-\\d{2}-\\d{4} \\d{2}:\\d{2}$', date))", "import re\ndef date_checker(date):\n return True if re.match(r\"\\d{2}-\\d{2}-\\d{4} \\d{2}:\\d{2}\", date) else False", "from re import match as m\n\ndef date_checker(date):\n return m('(3[0-1]|[0-2]?\\d)-([0-1]?\\d)-\\d{4} [0-2]\\d:\\d\\d', date) != None", "date_checker=lambda s:bool(__import__('re').match('\\d\\d-\\d\\d-\\d{4} \\d\\d:\\d\\d',s))", "import re\n\ndef date_checker(date):\n return bool(re.match(\"\\d{2}-\\d{2}-\\d{4} \\d{2}:\\d{2}\", date))", "import re\n\ndef date_checker(date): #\n return bool(re.match('(\\d\\d-){2}\\d{4}\\s\\d\\d:\\d\\d', date))"] | {"fn_name": "date_checker", "inputs": [["01-09-2016 01:20"], ["01-09-2016 01;20"], ["01_09_2016 01:20"], ["14-10-1066 12:00"], ["Tenth of January"], ["20 Sep 1988"], ["19-12-2050 13:34"], ["Tue Sep 06 2016 01:46:38 GMT+0100"], ["01-09-2016 00:00"], ["01-09-2016 2:00"]], "outputs": [[true], [false], [false], [true], [false], [false], [true], [false], [true], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,281 |
def date_checker(date):
|
8060b2fb2e57bd8510c08aaf0e6b143d | UNKNOWN | Create a function that finds the [integral](https://en.wikipedia.org/wiki/Integral) of the expression passed.
In order to find the integral all you need to do is add one to the `exponent` (the second argument), and divide the `coefficient` (the first argument) by that new number.
For example for `3x^2`, the integral would be `1x^3`: we added 1 to the exponent, and divided the coefficient by that new number).
Notes:
* The output should be a string.
* The coefficient and exponent is always a positive integer.
## Examples
```
3, 2 --> "1x^3"
12, 5 --> "2x^6"
20, 1 --> "10x^2"
40, 3 --> "10x^4"
90, 2 --> "30x^3"
``` | ["def integrate(coef, exp):\n exp = exp + 1\n coef = coef / exp if coef % exp else coef // exp\n return f\"{coef}x^{exp}\"", "def integrate(coefficient, exponent):\n return (str(coefficient // (exponent + 1)) + 'x^' + str(exponent + 1)) ", "def integrate(coefficient, exponent):\n return f'{coefficient // (exponent + 1)}x^{exponent + 1}'", "def integrate(coefficient, exponent):\n exponent += 1\n return str(f\"{coefficient // exponent}x^{exponent}\")", "def integrate(coefficient, exponent):\n exponent += 1\n coefficient /= exponent\n return '{}x^{}'.format(int(coefficient) if coefficient.is_integer() else coefficient, exponent)", "def integrate(c, e):\n return f\"{c / (e+1)}x^{e+1}\".replace(\".0x\", \"x\")", "def integrate(coefficient, exponent):\n return \"{c}x^{e}\".format(c=coefficient // (exponent + 1), e=exponent + 1)", "def integrate(coeff, index):\n return '{}x^{}'.format(coeff/(index + 1), index + 1).replace('.0x', 'x')", "def integrate(coefficient, exponent):\n return '{:g}x^{}'.format(coefficient/(exponent+1),exponent+1)", "def integrate(c, e):\n e += 1\n return f\"{c//e}x^{e}\"", "def integrate(coefficient, exponent):\n # write your code here\n x = exponent + 1\n return str(int(coefficient / x)) + \"x^\" + str(x)", "def integrate(coefficient, exponent):\n new_exponent = exponent + 1\n new_coefficient = int(coefficient / new_exponent)\n \n return (str(new_coefficient) + \"x^\" + str(new_exponent))", "def integrate(coef, exp):\n return \"{}x^{}\".format(int(coef/ (exp + 1)), exp + 1 )", "def integrate(coefficient, exponent):\n return str(int(coefficient/(exponent+1)))+\"x^\"+str(exponent+1)", "def integrate(coefficient, exponent):\n return str(coefficient/(exponent+1)).rstrip('0').rstrip('.') + \"x^\"+str(exponent+1)", "integrate=lambda c,e:\"{}x^{}\".format(c/(e+1),e+1).replace('.0x','x')", "def integrate(c, e):\n coefficient = str(c/(e+1))\n return coefficient.rstrip('0').rstrip('.') + 'x^' + str(e + 1)", "def integrate(c, e):\n return '{}x^{}'.format(int(c/(e+1)), e+1)", "def integrate(coefficient, exponent):\n return f'{int(coefficient/(exponent+1))}x^{exponent+1}'", "def integrate(coefficient, exponent):\n return '{}x^{}'.format(coefficient//(exponent+1), exponent+1)", "def integrate(coefficient: int, exponent: int) -> str:\n \"\"\" Get the integral of the expression passed. \"\"\"\n return f\"{int(coefficient / (exponent + 1))}x^{exponent + 1}\"", "integrate=lambda c,e:f\"{c//(e+1)}x^{e+1}\"", "def integrate(coefficient, exponent):\n exponent += 1\n coefficient /= exponent\n coefficient = (int if coefficient.is_integer() else float)(coefficient)\n return f'{coefficient}x^{exponent}'", "integrate = lambda a, b: ''.join(str(i) for i in [a//(b+1), 'x^', b+1])", "def integrate(coefficient, exponent):\n return '{0:.0f}x^{1:.0f}'.format(coefficient / (exponent+1), exponent+1)", "def integrate(coefficient, exponent):\n # write your code here\n a = int(exponent + 1)\n b = int(coefficient / a)\n c = (str(b) + \"x^\" + str(a))\n return c\n \n", "def integrate(coef, exp):\n return '{}x^{}'.format(int(coef/(exp+1)) if int(coef/(exp+1)) == coef/(exp+1) else coef/(exp+1), exp+1)", "def integrate(c, exp):\n if c/(exp+1)%1 == 0:\n return '{:g}x^{}'.format(c/(exp+1), exp+1)\n else:\n return '{}x^{}'.format(c/(exp+1), exp+1)", "def integrate(coefficient, exponent):\n b = exponent + 1\n a = coefficient // b\n \n return f\"{a}x^{b}\"\n \n\n", "integrate = lambda c, e : f'{int(c/(e+1))}x^{e+1}'", "def integrate(coefficient, exponent):\n inte = exponent + 1\n first = int(coefficient / inte)\n return f'{first}x^{inte}'", "def integrate(coefficient, exponent):\n \n c = 1+exponent\n newco=int(coefficient/c)\n return f\"{newco}x^{c}\"", "def integrate(coefficient, exponent):\n a = coefficient / (exponent + 1)\n a = int(a)\n return f'{a}x^{exponent + 1}'", "def integrate(coefficient, exponent):\n integred_exponent = exponent + 1\n return f\"{int(coefficient/integred_exponent)}x^{integred_exponent}\"\n", "def integrate(coefficient, exponent):\n return f'{str(coefficient//(exponent+1))}x^{str(exponent+1)}'", "def integrate(coefficient, exponent):\n integral = coefficient // (exponent + 1)\n return '{}x^{}'.format(integral, exponent + 1)", "def integrate(coefficient, exponent):\n # write your code here\n exponent += 1\n a = int(coefficient / exponent)\n return str(a)+'x^'+str(exponent)", "def integrate(coefficient: int, exponent: int) -> str:\n \"\"\" Get the integral of the expression passed. \"\"\"\n return f\"{coefficient / (exponent + 1):.0f}x^{exponent + 1}\"", "def integrate(coefficient: int, exponent: int) -> str:\n return str(coefficient // (exponent + 1)) + \"x^\" + str(exponent + 1)", "def integrate(coefficient, exponent):\n return \"{coef}x^{exp}\".format(coef=coefficient//(exponent+1),exp=exponent+1)", "integrate = lambda c, e: 'x^'.join([str(c//(e+1)), str(e+1)])", "def integrate(coefficient, exponent):\n a = exponent + 1\n b = round(coefficient / a)\n return f\"{b}x^{a}\"", "def integrate(c, e):\n return f'{int(c / (e+1))}x^{int(e + 1)}'\n", "# Create a function that finds the integral of the expression passed.\n\n# In order to find the integral all you need to do is add one to the exponent (the second argument), and divide the coefficient (the first argument) by that new number.\n\n# For example for 3x^2, the integral would be 1x^3: we added 1 to the exponent, and divided the coefficient by that new number).\n\n# Notes:\n\n# The output should be a string.\n# The coefficient and exponent is always a positive integer.\n\ndef integrate(coefficient, exponent):\n number = str(int(coefficient/(exponent+1)))\n power = str(exponent + 1)\n return number + \"x\" + \"^\" + power \n", "def integrate(coefficient, exponent):\n a = int(coefficient / (exponent + 1))\n b = (exponent+1)\n return str(a) + \"x\" + \"^\" + str(b)", "def integrate(coefficient, exponent):\n x = int(coefficient / (exponent + 1))\n return str(x) + \"x^\" + str(exponent + 1)\n", "def integrate(coefficient, exponent):\n exp=exponent+1\n coef=int(coefficient/exp)\n return f\"{coef}x^{exp}\"", "def integrate(c,e):\n k=e+1\n return \"{}x^{}\".format(c//k,k) ", "def integrate(coefficient, exponent):\n new_ex = exponent+1\n new_str = str(int(coefficient/new_ex)) + 'x^' + str(new_ex)\n return ''.join(new_str)", "def integrate(coefficient, exponent):\n NewExp = exponent + 1\n NewCoe = int(coefficient / NewExp)\n \n integral = str(NewCoe) + \"x^\" + str(NewExp)\n \n return integral\n", "def integrate(coefficient, exponent):\n i_co = coefficient / (exponent + 1)\n i_ex = exponent + 1\n \n return str(int(i_co)) + 'x^' + str(int(i_ex))", "def integrate(coefficient, exponent):\n e = exponent +1\n f = round(coefficient * (1/e))\n return str(f)+\"x^\" +str(e)", "def integrate(c, xp):\n return str(int(c/(xp+1))) + \"x^\" + str(xp+1)", "def integrate(coefficient, exponent):\n return \"{co}x^{exp}\".format(co = coefficient//(exponent+1), exp = exponent+1)", "def integrate(coefficient, exponent):\n return f'{(int(coefficient / (1 + exponent)))}x^{(1 + exponent)}'", "integrate = lambda coefficient, exponent: f'{coefficient//(exponent+1)}x^{exponent+1}'", "def integrate(coefficient, exponent):\n ex = exponent + 1\n cof = int(coefficient / ex)\n return str(cof) + \"x^\" + str(ex)", "def integrate(coefficient, exponent):\n exp = exponent + 1\n coef = coefficient // exp\n return str(coef)+\"x^\"+str(exp)", "def integrate(coefficient, exponent):\n coeff=int(coefficient/(exponent+1))\n ans=str(coeff)+'x^'+str(exponent+1)\n return ans", "def integrate(coe, ext):\n return f'{coe // (ext + 1)}x^{ext + 1}'", "def integrate(coefficient, exponent):\n integ_coeff = coefficient / (exponent + 1)\n integ_expo = exponent + 1\n \n return str(int(integ_coeff)) + \"x^\" + str(exponent + 1)", "def integrate(co, ex):\n return '{}x^{}'.format(round(co/(ex+1)), ex+1)", "def integrate(coefficient, exponent):\n coefficient = int((coefficient/(exponent+1)))\n exponent += 1\n return (\"{0}x^{1}\").format(coefficient, exponent)", "def integrate(c, e):\n return f\"{str(int(c / (e + 1)))}x^{e + 1}\" ", "def integrate(coefficient, exponent):\n \n \n x = exponent + 1\n y = round(coefficient/x)\n\n return \"{}x^{}\".format(y,x)", "def integrate(coefficient, exponent):\n return \"{0:0.0f}x^{1}\".format(coefficient/(exponent+1),exponent+1)", "def integrate(c, e):\n e = e + 1\n return str(c//e) + \"x^\" + str(e)", "def integrate(coefficient, exponent):\n n = exponent+1\n return f'{int(coefficient/(n))}x^{n}'", "def integrate(coefficient, exponent):\n new_exponent = exponent + 1\n new_coeff = coefficient / new_exponent\n s = str(int(new_coeff)) + \"x^\" + str(new_exponent)\n return s", "def integrate(coefficient, exponent):\n # write your code here\n aa = int(coefficient / (exponent + 1))\n bb = exponent + 1\n return (f\"{aa}x^{bb}\")", "def integrate(coefficient, exponent):\n e = exponent +1\n coef = coefficient/e\n \n return \"{}x^{}\".format(int(coef),e)", "def integrate(coefficient, exponent):\n ex = exponent + 1\n coe = coefficient / ex\n return str(int(coe)) + \"x^\" + str(ex)\n", "def integrate(coefficient, exponent):\n return f'{str(coefficient // (exponent + 1))}x^{(exponent + 1)}'\n", "def integrate(coefficient, exponent):\n exponent = exponent + 1\n coefficient = coefficient / exponent if coefficient % exponent else coefficient // exponent\n return f'{coefficient}x^{exponent}'\n \n\n", "def integrate(coefficient, exponent):\n new_exp = exponent+1\n new_coef = int(coefficient / new_exp)\n return f\"{new_coef}x^{new_exp}\"", "integrate = lambda co,ex: f'{int(co/(ex+1))}x^{ex+1}'", "def integrate(coefficient, exponent):\n return str(int(coefficient / (1 + exponent))) +\"x^\" + str(1 + exponent)", "def integrate(c, e):\n integral = int(c / (e+1))\n return f'{integral}x^{e+1}'", "def integrate(coefficient, exponent):\n return '%ix^%i' % ((coefficient / (exponent + 1)), (exponent + 1))\n# return 'x^'.join((str(coefficient // (exponent + 1)), str(exponent + 1)))\n", "def integrate(coefficient, exponent):\n new_coefficient = str(coefficient//(exponent+1))\n new_exponent = str(exponent + 1)\n return (F\"{new_coefficient}x^{new_exponent}\")", "def integrate(coefficient, exponent):\n exp=exponent+1\n cof=int(coefficient/exp)\n list=[str(cof),\"x^\",str(exp)]\n return \"\".join(list)\n \n # write your code here\n", "def integrate(coefficient, exponent):\n a=round(coefficient/(exponent+1))\n b=exponent+1\n return (\"{}x^{}\".format(a,b))", "def integrate(coef, ex):\n return f'{int(coef/(ex+1))}x^{ex+1}'", "integrate = lambda coeff, exp: f'{coeff // (exp + 1)}x^{exp + 1}'", "def integrate(coefficient, exponent):\n s = int(coefficient/(exponent+1))\n d = exponent+1\n return str(s)+'x'+'^'+str(d)\nw = integrate(12,5)\nprint(w)", "def integrate(c, e):\n # write your code here\n e = e+1\n c = c/e\n i =''\n return i + str(int(c)) + 'x^' + str(int(e))\n", "def integrate(coefficient, exponent):\n \n result_1=((coefficient/(exponent+1)))\n result_2=((exponent+1))\n \n \n result=str(int(result_1))+\"x^\"+str(result_2)\n \n \n return result\n \n return result\n", "def integrate(c, exp):\n return f'{str(round(c/(1+exp)))}x^{str(1+exp)}'", "def integrate(coefficient, exponent):\n exponent += 1\n num = int(coefficient / exponent)\n return f'{num}x^{exponent}'", "def integrate(coefficient, exponent):\n e1 = exponent + 1\n r = int(coefficient / e1)\n return '{}x^{}'.format(r,e1)", "integrate = lambda coefficient, exponent: str(coefficient // ( exponent + 1 )) + 'x^' + str( exponent + 1 )\n", "def integrate(co, ex):\n return \"{}x^{}\".format(int(co/(ex+1)) , ex+1)", "def integrate(coefficient, exponent):\n exp = exponent + 1\n coeff = int(coefficient / exp)\n return f'{coeff}x^{exp}'", "def integrate(coefficient, exponent):\n end = exponent + 1\n begin = coefficient // end\n return str(begin) + 'x^' + str(end)", "def integrate(coefficient, exponent):\n exponent=exponent+1\n coefficient=coefficient//exponent\n return str(coefficient) + \"x\" +\"^\" + str(exponent)", "def integrate(x, y):\n z =y+1\n v = x//z\n return \"{}x^{}\".format(v,z)\n", "def integrate(coefficient, exponent):\n exponent += 1\n coefficient //= exponent\n return f'{coefficient}x^{exponent}'", "def integrate(coefficient, exponent):\n exponent+=1\n coefficient/=exponent\n return '{0}x^{1}'.format(int(coefficient),exponent)", "def integrate(coefficient, exponent):\n return str(\"{}x^{}\".format((coefficient//(exponent+1)), (exponent+1)))", "def integrate(coefficient, exponent):\n exponent += 1\n coefficient = coefficient // exponent\n return \"{0}x^{1}\".format(coefficient,exponent)\n"] | {"fn_name": "integrate", "inputs": [[3, 2], [12, 5], [20, 1], [40, 3], [90, 2]], "outputs": [["1x^3"], ["2x^6"], ["10x^2"], ["10x^4"], ["30x^3"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 13,302 |
def integrate(coefficient, exponent):
|
7fb237472dbda3b1db118290a11b9a48 | UNKNOWN | Complete the function `scramble(str1, str2)` that returns `true` if a portion of ```str1``` characters can be rearranged to match ```str2```, otherwise returns ```false```.
**Notes:**
* Only lower case letters will be used (a-z). No punctuation or digits will be included.
* Performance needs to be considered
## Examples
```python
scramble('rkqodlw', 'world') ==> True
scramble('cedewaraaossoqqyt', 'codewars') ==> True
scramble('katas', 'steak') ==> False
``` | ["def scramble(s1,s2):\n for c in set(s2):\n if s1.count(c) < s2.count(c):\n return False\n return True", "from collections import Counter\ndef scramble(s1,s2):\n # Counter basically creates a dictionary of counts and letters\n # Using set subtraction, we know that if anything is left over,\n # something exists in s2 that doesn't exist in s1\n return len(Counter(s2)- Counter(s1)) == 0", "def scramble(s1, s2):\n return not any(s1.count(char) < s2.count(char) for char in set(s2))", "def scramble(s1,s2):\n return all( s1.count(x) >= s2.count(x) for x in set(s2))\n \n", "def scramble(s1,s2):\n dct={}\n for l in s1:\n if l not in dct:\n dct[l]=1\n else:\n dct[l] +=1\n for l in s2:\n if l not in dct or dct[l] < 1:\n return False\n else:\n dct[l] -= 1\n return True", "from collections import Counter\n\ndef scramble(s1,s2):\n c1 = Counter(s1)\n c2 = Counter(s2)\n return not (c2 -c1).values()", "from collections import Counter\nfrom operator import sub\n\ndef scramble(s1,s2):\n return not sub(*map(Counter, (s2,s1)))", "from collections import Counter\ndef scramble(s1,s2):\n return not (Counter(s2) - Counter(s1))", "def scramble(s1, s2):\n return all([s1.count(l2) >= s2.count(l2) for l2 in set(s2)])", "def scramble(s1,s2):\n return all(s1.count(i)>=s2.count(i) for i in set(s2))", "from collections import Counter\nscramble = lambda s1, s2: not Counter(s2) - Counter(s1)", "scramble=lambda a,b,c=__import__('collections').Counter:not c(b)-c(a)", "from collections import Counter\ndef scramble(s1,s2): return len(Counter(s2) - Counter(s1)) == 0", "def scramble(s1, s2):\n return not False in([s1.count(i)>=s2.count(i) for i in set(s2)])\n\n#set() <3\n", "def scramble(s1, s2):\n a='abcdefghijklmnopqrstuvwxyz'\n b=[]\n for char in a:\n if s1.count(char)>=s2.count(char):\n b.append(True)\n else:\n b.append(False)\n if len(set(b))<=1:\n return(True)\n else:\n return(False)", "def scramble(s1, s2):\n str1 = {}\n str2 = {}\n for s in s1:\n try:\n str1[s] = str1[s] + 1\n except KeyError:\n str1[s] = 1\n for s in s2:\n try:\n str2[s] = str2[s] + 1\n except KeyError:\n str2[s] = 1\n for key in str2:\n try:\n if str1[key] < str2[key]:\n return False\n except KeyError:\n return False\n return True\n", "def scramble(s1, s2):\n \"\"\" returns true of a porton of s1 can be rearranged to match s2. Basic concept: use an indicator \n dictionary of the counts of letters in s2, check if s1 has sufficient occurences of each letter to make\n that indicator == 0 for all chars\"\"\"\n \n cts = [s2.count(i) for i in list(set(s2)) ] # count occurence of letters\n dic = dict(zip(list(set(s2)) ,cts)) # dictionary of counts.\n truth = True\n \n for char in list(s1):\n try:\n if dic[char] > 0: # If dic[char] already == 0, we know there are suff. occurrences of that char\n dic[char] = (dic[char] - 1) \n except KeyError:\n pass\n \n if sum(dic.values()) > 0: # if all chars sufficiently occur, sum should be 0\n truth = False\n\n return truth", "# Stack solution:\n# Loop through s2 once, pushing char occurances onto the stack\n# Loop through s1 once, popping any occurances of s2 chars from the stacks\n# If any stack has a count greater than 0, than it's False\ndef scramble(s1, s2):\n stacks = {}\n \n for char in s2:\n # Checking if stacks already has a key:value pair with the key char\n if char in stacks:\n stacks[char] += 1\n else:\n stacks[char] = 1\n \n for char in s1:\n if char in stacks:\n stacks[char] -= 1\n \n return max(stacks.values()) <= 0\n\n'''\n# Turn strings into lists, remove the appropriate element, and keep track of it to compare at the end\n# Takes too long, but correct output\ndef scramble(s1, s2):\n source = [char for char in s1]\n tokens = [char for char in s2]\n result = []\n \n for char in tokens:\n if char in source:\n result.append(char)\n source.remove(char)\n return len(result) == len(tokens)\n'''\n\n'''\n# Turn strings into lists, remove the appropriate element, and catch ValueError exceptions that signify False-ness\n# Takes too long but correct output\ndef scramble(s1, s2):\n source = [char for char in s1]\n try:\n for char in s2:\n source.remove(char)\n except ValueError:\n return False\n return True\n'''\n\n'''\n# Count every instance of every char in s1 and s2, then compare the number of s2 char appearances in s1\n# slowest of all three\ndef scramble(s1, s2):\n source = [char for char in s1]\n source.sort()\n tokens = [char for char in s2]\n tokens.sort()\n \n source_len = len(source)\n source_index = 0\n for char in tokens:\n while char != source[source_index]:\n source_index += 1\n if source_index >= source_len:\n return False\n return True\n'''", "from collections import Counter as c\ndef scramble(s1, s2):\n s1 = c(s1)\n s2 = c(s2)\n for i in s2:\n if s1[i]<s2[i]:\n return False\n return True", "from collections import defaultdict\nimport gc\ndef scramble(s1,s2):\n# print(s1,s2)\n a = defaultdict(lambda: False)\n for item in s2:\n a[item] = a.get(item, 0) + 1\n # print(a)\n b = defaultdict(lambda: False)\n for item in s1:\n b[item] = b.get(item, 0) + 1\n # print(b)\n try:\n for item in a:\n# print(a[item],b[item])\n if b[item] and a[item]<=int(b[item]):\n # print(item)\n pass\n else:\n return False\n return True\n finally:\n del a,b,s1,s2\n gc.collect()\n\n\n", "def scramble(s1, s2):\n def is_subsequence(needle, haystack):\n it = iter(haystack)\n return all(c in it for c in needle)\n return is_subsequence(sorted(s2), sorted(s1))", "def scramble(s1, s2):\n for c in set(s2):\n if s2.count(c) > s1.count(c):\n return False\n return True", "def scramble(s1,s2):\n for i in \"abcdefghijklmnopqrstuvwxyz\":\n if s1.count(i) < s2.count(i):\n return False\n return True", "def scramble(s1,s2):\n return all([s2.count(c) <= s1.count(c) for c in set(s2)])", "def scramble(s1, s2):\n it = iter(sorted(s1))\n return all(c in it for c in sorted(s2))", "def scramble(s1,s2):\n\n for letter in \"abcdefghijklmnopqrstuvwxyz\":\n if s1.count(letter) < s2.count(letter):\n return False\n return True\n \n \n \n", "def scramble(s1,s2):\n for letter in 'abcdefghijklmnopqrstuwvxyz':\n if s1.count(letter) < s2.count(letter): return False\n return True\n\n\n\n\n#def costam(s1,s2)\n# print s1\n# for letter in s2:\n# if letter in s1: \n# m = s1.index(letter)\n# s1 = s1[:m]+s1[m+1:]\n# else: return False\n# return True\n", "class scramble(zip):\n def __eq__(self, other):\n return True", "def scramble(str, wrd):\n w=set(wrd)\n for i in w:\n if str.count(i) < wrd.count(i):\n return False\n return True", "from collections import Counter\ndef scramble(s1, s2):\n \n dict = Counter(s1)\n dict1 = Counter(s2)\n\n for k in dict1.keys():\n if (not k in dict.keys()):\n return False\n if dict1[k] > dict[k]:\n return False\n\n return True", "from collections import Counter\n\ndef scramble(s1, s2):\n se1 = Counter(s1)\n se2 = Counter(s2)\n return se1|se2 == se1", "from collections import Counter\ndef scramble(s1, s2):\n return True if len(Counter(s2)-Counter(s1))==0 else False", "def scramble(s1,s2):\n def ccount(s):\n d = {}\n for c in s:\n d[c] = d.get(c,0) + 1\n return d\n \n d1 = ccount(s1)\n for c, count in list(ccount(s2).items()):\n if d1.get(c, 0) < count:\n return False\n return True\n", "def scramble(s1,s2):\n for char in set(s2):\n if s1.count(char) < s2.count(char):\n return False\n return True", "from collections import deque\n\ndef scramble(s1,s2):\n s1 = deque(sorted(s1))\n s2 = sorted(s2)\n for letter in s2:\n if len(s1) == 0:\n return False\n while len(s1):\n next_letter = s1.popleft()\n if next_letter == letter:\n break\n if next_letter > letter:\n return False\n return True", "scramble = lambda s1,s2: len([x for x in [(char, s2.count(char), s1.count(char)) for char in set(s2)] if x[2] < x[1]]) == 0", "def scramble(s1,s2):\n l=[]\n for x in s2:\n if x not in l:\n if s1.count(x)<s2.count(x):\n return False\n l.append(x)\n return True", "from collections import Counter\n\ndef scramble(s1, s2):\n if not set(s2).issubset(set(s1)):\n return False\n \n # your code here\n s1_counts = Counter(s1)\n s2_counts = Counter(s2)\n \n s1_counts.subtract(s2_counts)\n \n return not any([x for x in list(s1_counts.items()) if x[1] < 0]) \n", "def scramble(s1, s2):\n a = [s1.count(chr(i))-s2.count(chr(i)) for i in range(97,123)]\n for i in a:\n if i<0:\n return False\n return True", "from collections import Counter\n\ndef scramble(s1,s2):\n a = Counter(s1)\n b = Counter(s2)\n a.subtract(b)\n new = []\n for i in a:\n if a[i] >= 0:\n new.append(i)\n return len(new) == len(a)\n\n\n\n", "from collections import Counter\ndef scramble(s1, s2):\n a = Counter(s1)\n b = Counter(s2)\n\n \n for k,v in b.items():\n if k not in a.keys() or a[k] < v:\n return False\n \n return True", "def scramble(s1, s2):\n d1, d2 = {}, {}\n for i in s1:\n if i not in d1:\n d1[i] = 1\n else:\n d1[i] += 1\n \n for i in s2:\n if i not in d2:\n d2[i] = 1\n else:\n d2[i] += 1\n \n for key in list(d2.keys()):\n if not key in list(d1.keys()) or d1[key]< d2[key]:\n return False\n return True\n", "def scramble(s1, s2):\n s1_count = dict.fromkeys(set(s1),0)\n for i in s1:\n s1_count[i] += 1\n try:\n for i in s2:\n s1_count[i] -= 1\n except:\n return False\n return all(i >= 0 for i in s1_count.values())", "def scramble(s1, s2):\n s1_dict = {}\n for i in s1:\n if i in s1_dict:\n s1_dict[i] += 1\n else:\n s1_dict[i] = 1\n s2_dict = {}\n for i in s2:\n if i in s2_dict:\n s2_dict[i] += 1\n else:\n s2_dict[i] = 1\n for k, v in s2_dict.items():\n if k not in s1_dict or s1_dict[k] < v:\n return False\n return True", "from collections import Counter\ndef scramble(s1, s2):\n c1 = Counter(s1)\n c2 = Counter(s2)\n c1.subtract(c2)\n c3 = [v<0 for k,v in c1.items() if k in c2.keys()]\n \n return not any(c3)", "from collections import Counter \ndef scramble(s1, s2):\n s1 = list(s1)\n s2 = list(s2)\n s2 = list((Counter(s2) - Counter(s1)).elements())\n if len(s2) > 0:\n return False\n else:\n return True", "from collections import Counter\ndef scramble(s1, s2):\n if set(s1) < set(s2):\n return False\n cnt1 = Counter(s1)\n cnt2 = Counter(s2)\n cnt1.subtract(cnt2)\n notOk = [x < 0 for x in list(cnt1.values())]\n return not any(notOk)\n \n", "def scramble(s1, s2):\n if len(s2) > len(s1):\n return False\n let1 = [s1.count(chr(i)) for i in range (97, 123)]\n let2 = [s2.count(chr(i)) for i in range (97, 123)]\n sub = [True if (let1[i] >= let2[i]) else False for i in range(26)]\n return (True if False not in sub else False)", "import string\nalphabet = {key: idx for idx, key in enumerate(string.ascii_lowercase)}\n\ndef scramble(s1, s2):\n used_words1 = [0] * 26\n used_words2 = [0] * 26\n for element in s1:\n used_words1[alphabet[element]] += 1\n for element in s2:\n used_words2[alphabet[element]] += 1\n for i in range(26):\n if used_words2[i] > used_words1[i]:\n return False\n return True\n \n", "def scramble(s1, s2):\n while(len(s2) > 0):\n if(s1.count(s2[0]) < s2.count(s2[0])):\n return False\n else:\n s2 = s2.replace(s2[0], '')\n return True\n", "def scramble(s1, s2):\n clean = \"\"\n boolean = []\n for i in s2:\n if i not in clean:\n clean = clean + i\n for i in clean:\n if s2.count(i) > s1.count(i):\n return False\n return True", "def scramble(s1, s2):\n for letter in 'abcdefghijklmnopqrstuvwxyz' :\n if s2.count(letter) <= s1.count(letter) : continue\n else : return False\n return True", "#Tests whether a portion of s1 can be rearranged to match s2\n\nfrom collections import Counter\n\ndef scramble(s1, s2):\n\n count_letters = Counter(s1)\n count_letters.subtract(Counter(s2))\n return (all(n >= 0 for n in count_letters.values()))", "def scramble(s1, s2):\n s1_dict = dict()\n for char in s1:\n if char in s1_dict:\n s1_dict[char] = s1_dict[char] + 1\n else:\n s1_dict[char] = 1\n for char in s2:\n if char not in s1_dict or s1_dict[char] == 0:\n return False\n else:\n s1_dict[char] = s1_dict[char] - 1\n return True\n", "def scramble(s1, s2):\n if len(s1) < len(s2):\n return False\n s1_dict = {}\n s2_dict = {}\n for char in s1:\n try:\n s1_dict[char] += 1\n except KeyError:\n s1_dict[char] = 1\n for char in s2:\n try:\n s2_dict[char] += 1\n except KeyError:\n s2_dict[char] = 1\n for key in s2_dict:\n try:\n if s1_dict[key] < s2_dict[key]:\n return False\n except KeyError:\n return False\n return True\n", "def scramble(s1, s2):\n return all((s1.count(l) - s2.count(l)) >= 0 for l in set(s2))\n \n", "def scramble(s1, s2):\n #for ltr in s2:\n # if s2.count(ltr) > s1.count(ltr):\n # return False\n s1ct = {}\n for ltr in s1:\n if ltr in s1ct.keys():\n s1ct[ltr] += 1\n else:\n s1ct[ltr] = 1\n\n s2ct = {}\n for ltr in s2:\n if ltr in s2ct.keys():\n s2ct[ltr] += 1\n else:\n s2ct[ltr] = 1\n\n for ltr in s2ct.keys():\n if ltr not in s1ct.keys():\n return False\n if s2ct[ltr] > s1ct[ltr]:\n return False\n return True", "from collections import Counter\n\ndef scramble(s1, s2):\n s1 = Counter(s1)\n s2 = Counter(s2)\n return len([c for c in s2 if s1[c] >= s2[c]]) == len(s2.keys())", "from collections import Counter\n\ndef scramble(s1, s2):\n one_split = list(s1)\n two_split = list(s2)\n count = 0\n copy = two_split[::]\n one = Counter(one_split)\n two = Counter(two_split)\n for x in two_split:\n if x not in list(two.keys()):\n return False\n if one[x] < two[x]:\n return False\n \n return True\n\n \n \n \n \n\n \n \n\n", "def scramble(s1, s2): \n s1 = list(s1)\n s2 = list(s2)\n \n s1.sort()\n s2.sort()\n \n len1 = len(s1)\n len2 = len(s2)\n \n i = 0\n j = 0\n \n \n while i < len1 and j < len2:\n if (len1 - i) < (len2 - j):\n return False\n \n if s1[i] == s2[j]:\n i += 1\n j += 1 \n \n elif s1[i] != s2[j]:\n i += 1\n \n \n if j == len(s2):\n return True\n \n return False\n \n \n", "def scramble(s1, s2):\n j,k=1,1\n a={chr(i):0 for i in range(97,123)}\n b={chr(i):0 for i in range(97,123)}\n for i in s1:\n if i not in a:\n continue \n else:\n a[i]=a[i]+1\n for n in s2:\n if n not in b:\n continue \n else:\n b[n]=b[n]+1\n for i in s2:\n if a[i]>=b[i]:\n pass\n else:\n return False\n return True \n # your code here\n", "from collections import Counter as count\ndef scramble(s1,s2):\n return len(count(s2)- count(s1)) == 0", "def scramble(s1, s2):\n for c in \"qwertyuiopasdfghjklzxcvbnm\":\n if s1.count(c) < s2.count(c): return False\n return True\n", "def scramble(s1, s2):\n Mot = \"\".join(dict.fromkeys(s2))\n for i in range(len(Mot)):\n if s1.count(Mot[i])<s2.count(Mot[i]):\n return False\n \n return True\n # your code here\n", "from collections import Counter\n\ndef scramble(s1, s2):\n return True if sum(((Counter(s2) & Counter(s1)).values())) == len(s2) else False", "def scramble(s1, s2):\n d1={}\n for i in set(s1):\n d1[i]=s1.count(i)\n for i in set(s2):\n try:\n if s2.count(i)>d1[i]:\n return False\n except:\n return False\n return True", "def scramble(s1, s2):\n set1=set(s1)\n set2=set(s2)\n for letter in set2:\n if letter not in set1:return False\n if s1.count(letter)<s2.count(letter): return False\n else:\n return True\n", "def scramble(s1, s2):\n import numpy\n options={}\n for i in s1:\n if i in options:\n options[i]+=1\n else:\n options[i]=1\n word={}\n for i in s2:\n if i not in options:\n return False\n if i in word:\n word[i]+=1\n else:\n word[i]=1\n for i in word:\n if word[i]>options[i]:\n return False\n return True", "def scramble(s1, s2):\n s1_counter = [0] * 256\n s2_counter = {}\n\n for c in s1:\n idx = ord(c)\n s1_counter[idx] += 1\n\n for c in s2:\n if c in s2_counter:\n s2_counter[c] += 1\n else:\n s2_counter[c] = 1\n\n for (key, value) in s2_counter.items():\n idx = ord(key)\n if s1_counter[idx] < value:\n return False\n\n return True", "def scramble(s1, s2):\n s=list(set(s2))\n for i in s:\n if s1.count(i)<s2.count(i):\n return False\n return True\n \n \n", "def scramble(s1, s2):\n \n # other solutions using list timed-out so had to use map.\n \n # create maps of count of two string.\n char_count_s1 = {c:s1.count(c) for c in set(s1) }\n char_count_s2 = {c:s2.count(c) for c in set(s2) }\n \n for c in char_count_s2:\n if c not in char_count_s1:\n return False\n elif char_count_s1[c] < char_count_s2[c]:\n return False\n \n return True", "def scramble(s1, s2):\n for l in set(s2):\n if l not in s1 or s1.count(l) < s2.count(l):\n return False\n return True", "from collections import Counter\n\ndef scramble(s1, s2):\n for c in set(s2):\n if s2.count(c) <= s1.count(c):\n continue\n else:\n return False\n return True\n", "scramble=lambda s,t:(lambda c,d:all(c.get(o,0)>=d[o] for o in t))({i:s.count(i) for i in set(s)},{i:t.count(i) for i in set(t)})", "def scramble(s1, s2):\n counter01 = [0] * 26\n counter02 = [0] * 26\n \n for char in s1:\n counter01[ord(char) - 97] += 1\n \n for char in s2:\n counter02[ord(char) - 97] += 1\n \n for i in range(26):\n if counter01[i] < counter02[i]:\n return False\n \n return True", "def scramble(s1, s2):\n s2_dict = {}\n for c in set(s2):\n s2_dict[c] = s2.count(c)\n \n output = True\n for c in sorted(s2_dict, key = s2_dict.get, reverse=True):\n s2_count = s2_dict[c]\n if s2_count > s1.count(c):\n output = False\n break\n return output", "def scramble(s1, s2):\n s1_dict = dict()\n s2_dict = dict()\n\n s2_set = set(s2)\n\n for c in s2_set:\n s2_dict[c] = s2.count(c)\n s1_dict[c] = s1.count(c)\n\n for c in s2_set: \n if c not in s1_dict or s2_dict[c] > s1_dict[c]: \n return False\n\n return True", "def scramble(s1, s2):\n c= {item:s1.count(item) for item in set(s1)}\n d = {item:s2.count(item) for item in set(s2)}\n for item in d:\n e = c.get(item) or None\n if not e or e < d.get(item):\n return False\n return True", "def scramble(s1, s2):\n dict_s1 = {}\n for char in s1:\n dict_s1[char] = dict_s1[char] + 1 if char in dict_s1.keys() else 1\n \n for char in set(s2):\n if not char in dict_s1.keys():\n return False\n if s2.count(char) > dict_s1[char]:\n return False\n dict_s1[char] -= s2.count(char)\n return True", "from collections import Counter\n\ndef scramble(s1, s2):\n d1 = dict(Counter(s1))\n d2 = dict(Counter(s2))\n d3 = {k: v for k,v in d1.items() if k in d2.keys()}\n missing = {k:v for k,v in d2.items() if k not in d3}\n \n if len(missing) >= 1:\n return False\n elif len(s2) == 0 or len(s1) == 0:\n return False\n elif len(missing) == 0:\n calc = {k: d3[k]- d2[k] for k in d2.keys() & d3}\n return True if all(value >= 0 for value in calc.values()) else False", "def scramble(s1, s2):\n list_chr=tuple(set(s2))\n for i in list_chr:\n if s1.count(i)<s2.count(i):\n return False\n return True", "def scramble(s1, s2):\n fr = set(s1)\n sc = set(s2)\n for i in sc:\n if i not in fr:\n return False\n elif i in fr:\n if s1.count(i) < s2.count(i):\n return False \n return True\n", "def scramble(s1, s2):\n if None in (s1, s2):\n return False\n \n passed = [] #Added to avoid wasting time on suitable letters\n for letter in s2:\n if letter in passed: continue\n if (letter not in s1) or (s2.count(letter) > s1.count(letter)): return False #2nd condition VERY IMP, cause this was not at all obvious (guess I missed it completely), i.e.,must consider number of letters in s2 and s1\n passed.append(letter)\n else: return True", "from collections import Counter\ndef scramble(s1, s2):\n t1 = Counter(s1)\n t2 = Counter(s2)\n d = t2 - t1\n return len(d) == 0", "def scramble(string_to_rearrange, string_to_match):\n letters_in_string_to_match = {}\n for letter in string_to_match:\n if letter not in letters_in_string_to_match:\n letters_in_string_to_match[letter] = string_to_match.count(letter)\n letters_in_string_to_rearrange = {}\n for letter in string_to_rearrange:\n if letter not in letters_in_string_to_rearrange:\n letters_in_string_to_rearrange[letter] = string_to_rearrange.count(letter)\n for letter in letters_in_string_to_match:\n try:\n if letters_in_string_to_match[letter] > letters_in_string_to_rearrange[letter]:\n return False\n except KeyError:\n return False\n return True\n", "from collections import Counter\ndef scramble(s1, s2):\n l = Counter(s1)\n w = Counter(s2)\n diff = w - l\n return len(diff) == 0", "def scramble(s1, s2):\n unique_letters = list(set(s2))\n count_letters = [s2.count(x) for x in unique_letters]\n return not False in [s1.count(x) >= count_letters[i] for i,x in enumerate(unique_letters)]", "def scramble(s1, s2):\n for el in set(s2):\n if not s1.count(el) >= s2.count(el):\n return False\n return True", "def scramble(s1, s2):\n list=[]\n for letter in s2:\n if letter in list:\n continue\n else:\n list.append(letter)\n if s2.count(letter) <= s1.count(letter):\n pass\n else:\n return False\n return True", "def scramble(str1,str2):\n if len(str1) < len(str2):\n return False\n str1 = \"\".join(sorted(str1))\n str2 = \"\".join(sorted(str2))\n i = 0\n while i < len(str2):\n N = str2.count(str2[i])\n if str1.count(str2[i]) < N:\n return False\n i += N\n return True", "def scramble(s1, s2):\n a1 = 26*[0]\n for c in s1:\n a1[ord(c)-97] += 1\n \n for c in s2:\n a1[ord(c)-97] -= 1\n if a1[ord(c)-97] < 0:\n return False\n return True", "def scramble(s1, s2):\n from collections import Counter\n c1 = Counter(s1)\n c2 = Counter(s2)\n for key, val in c2.items():\n if key not in c1.keys():\n return False \n else:\n if val > c1[key]:\n return False\n return True", "def map_char_count(s):\n char_count = {}\n for c in s:\n if c in char_count:\n char_count[c] += 1\n else:\n char_count[c] = 1\n return char_count\n\ndef scramble(s1, s2):\n s1_count = map_char_count(s1)\n s2_count = map_char_count(s2)\n for k, v in list(s2_count.items()):\n if k not in s1_count or s1_count[k] < v:\n return False\n return True\n \n", "def scramble(s1, s2):\n for char in list(set(list(s2))):\n if char not in s1: return False\n if s2.count(char) > s1.count(char): return False\n return True", "def scramble(s1, s2):\n st1, st2 = set(s1), set(s2) # Use sets for optimization\n\n if not st2.issubset(st1): # Check if every char in s2 is present in s1, if not, game over\n return False\n else:\n if not all(map(lambda char : s1.count(char) >= s2.count(char), st2)): # Use map as an alternative to lists\n return False\n else:\n return True", "def scramble(s1, s2):\n seen = []\n for letter in s2:\n if letter not in s1:\n return False\n for letter in s2:\n if letter in seen: continue\n if s1.count(letter) < s2.count(letter):\n return False\n else: seen.append(letter)\n return True", "from collections import Counter\ndef scramble(s1, s2):\n s1_count, s2_count = Counter(s1), Counter(s2)\n return all(s1_count[letter] >= s2_count[letter] for letter in s2) ", "def scramble(s1, s2):\n d1={}\n for ch in s1:\n if ch not in d1: d1[ch]=1\n else: d1[ch] = d1[ch]+1\n for ch in s2:\n try:\n if d1[ch]==0: return False\n except KeyError: \n return False\n else: d1[ch]=d1[ch]-1\n return True", "from collections import Counter\ndef scramble(s1, s2):\n d1, d2 = Counter(s1), Counter(s2)\n for k, v in list(d2.items()):\n if v > d1[k]:\n return False\n return True\n"] | {"fn_name": "scramble", "inputs": [["rkqodlw", "world"], ["cedewaraaossoqqyt", "codewars"], ["katas", "steak"], ["scriptjavx", "javascript"], ["scriptingjava", "javascript"], ["scriptsjava", "javascripts"], ["javscripts", "javascript"], ["aabbcamaomsccdd", "commas"], ["commas", "commas"], ["sammoc", "commas"]], "outputs": [[true], [true], [false], [false], [true], [true], [false], [true], [true], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 27,427 |
def scramble(s1, s2):
|
df25ca56ff5b8d43a211da84a25b1020 | UNKNOWN | Complete the function that counts the number of unique consonants in a string (made up of printable ascii characters).
Consonants are letters used in English other than `"a", "e", "i", "o", "u"`. We will count `"y"` as a consonant.
Remember, your function needs to return the number of unique consonants - disregarding duplicates. For example, if the string passed into the function reads `"add"`, the function should return `1` rather than `2`, since `"d"` is a duplicate.
Similarly, the function should also disregard duplicate consonants of differing cases. For example, `"Dad"` passed into the function should return `1` as `"d"` and `"D"` are duplicates.
## Examples
```
"add" ==> 1
"Dad" ==> 1
"aeiou" ==> 0
"sillystring" ==> 7
"abcdefghijklmnopqrstuvwxyz" ==> 21
"Count my unique consonants!!" ==> 7
``` | ["CONSONANTS = set('bcdfghjklmnpqrstvwxyz')\n\ndef count_consonants(text):\n return len(CONSONANTS.intersection(text.lower()))", "def count_consonants(text):\n return len(set(filter(str.isalpha,text.lower())) - set(\"aeiou\"))", "def count_consonants(s):\n return len({c for c in s.lower() if c in 'bcdfghjklmnpqrstvwxyz'})", "count_consonants=lambda s: len(set(l for l in s.lower() if l in \"bcdfghjklmnpqrstvwxyz\"))", "CONSONANTS = set(\"bcdfghjklmnpqrstvwxyz\")\n\ndef count_consonants(text):\n return len(CONSONANTS & set(text.lower()))", "def count_consonants(text):\n return len({ch for ch in text.lower() if ch in 'bcdfghjklmnpqrstvwxyz'})", "def count_consonants(text):\n return len(set(c for c in text.lower() if c in \"bcdfghjklmnpqrstvwxxyz\"))\n", "def count_consonants(text):\n return len(set(list(text.lower())).intersection(set(list('bcdfghjklmnpqrstvwxyz'))))", "def count_consonants(text):\n # Your code here:\n vowels = ['a', 'e', 'i', 'o', 'u']\n consonants = []\n for letter in text:\n letter = letter.lower()\n conditions = [letter not in vowels,\n letter not in consonants,\n letter.isalpha()]\n if all(conditions):\n consonants.append(letter)\n return len(consonants)", "def count_consonants(s):\n return len(set(c for c in s.lower() if c in \"bcdfghjklmnpqrstvwxyz\"))"] | {"fn_name": "count_consonants", "inputs": [["sillystring"], ["aeiou"], ["abcdefghijklmnopqrstuvwxyz"], ["Count my unique consonants!!"]], "outputs": [[7], [0], [21], [7]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,408 |
def count_consonants(text):
|
c2013404746688fc24eced08c27bf45e | UNKNOWN | The goal is to write a pair of functions the first of which will take a string of binary along with a specification of bits, which will return a numeric, signed complement in two's complement format. The second will do the reverse. It will take in an integer along with a number of bits, and return a binary string.
https://en.wikipedia.org/wiki/Two's_complement
Thus, to_twos_complement should take the parameters binary = "0000 0001", bits = 8 should return 1. And, binary = "11111111", bits = 8 should return -1 . While, from_twos_complement should return "00000000" from the parameters n = 0, bits = 8 . And, "11111111" from n = -1, bits = 8.
You should account for some edge cases. | ["def to_twos_complement(binary, bits):\n return int(binary.replace(' ', ''), 2) - 2 ** bits * int(binary[0])\n\ndef from_twos_complement(n, bits):\n return '{:0{}b}'.format(n & 2 ** bits - 1, bits)", "def to_twos_complement(b, bits):\n b = b.replace(\" \",\"\")\n return int(b,2) if int(b,2) < 2**(bits-1) else int(b,2)-2**(bits)\n\ndef from_twos_complement(n, bits):\n return bin(2**bits+n)[2:].zfill(bits) if n<0 else bin(n)[2:].zfill(bits)", "def to_twos_complement(input_value, bits):\n mask, unsigned = 2 ** (bits - 1), int(input_value.replace(' ', ''), 2)\n return (unsigned & ~mask) - (unsigned & mask)\n\ndef from_twos_complement(n, bits):\n return \"{:0>{}b}\".format(n + 2 ** bits * (n < 0), bits)", "def to_twos_complement(binary, bits):\n return int(binary.replace(' ', ''), 2) - (2 ** bits) * binary.startswith('1')\n \ndef from_twos_complement(n, bits):\n return '{:0{}b}'.format((n + 2 ** bits) % (2 ** bits), bits)", "def to_twos_complement(binary, bits): \n conv = int(binary.replace(' ',''), 2)\n return conv - (conv >= 2**bits/2) * 2**bits\n\ndef from_twos_complement(n, bits):\n return (\"{:0>\"+str(bits)+\"b}\").format(n + (2**bits) * (n<0))", "to_twos_complement=lambda s,l:int(s.replace(' ',''),2)if s[0]=='0'else-(int(\"\".join([str(int(i)^1)for i in s.replace(' ','')]),2)+1)\nfrom_twos_complement=lambda n,b:bin(n&0xffffffffffffffffffff)[2:][-b:]if n<0else bin(n)[2:].zfill(b)", "def to_twos_complement(binary, bits):\n binary = binary.replace(\" \", \"\")\n chiffre = binary[0]\n n = int(binary, 2)\n if chiffre == '1':\n n -= 2**bits\n return n\ndef from_twos_complement(n, bits):\n \n if n<0:\n n += 2**bits\n \n chaine = bin(n)\n chaine = chaine[2:]\n chaine = (bits-len(chaine))*'0'+chaine\n return chaine\n", "def to_twos_complement(binary, bits):\n binary=\"\".join(binary.split())\n res= -(2**(bits-1)) if binary[0]==\"1\" else 0\n for i in range(1, bits):\n res+=2**(bits-1-i) if binary[i]==\"1\" else 0\n return res\ndef from_twos_complement(n, bits):\n if n>=0:\n return \"0\"*(bits-len(bin(n)[2:]))+bin(n)[2:]\n else:\n remain=abs(-(2**(bits-1))-n)\n return \"1\"+\"0\"*(bits-len(bin(remain)[2:])-1)+bin(remain)[2:]", "def to_twos_complement(binary, bits):\n binary = binary.replace(\" \", \"\")\n sign_bit = binary[0]\n if sign_bit == \"1\":\n return int(binary[1:], 2) - 2**(bits-1)\n else:\n return int(binary, 2)\n\ndef from_twos_complement(n, bits):\n print(\"new\")\n if n >= 0:\n return format(n,'b').zfill(bits)\n else:\n return format(2**bits + n, 'b')", "def to_twos_complement(binary, bits):\n x=int(binary.replace(' ',''),2)\n if binary[0]=='0':\n return x\n else:\n return -((x^(2**bits-1))+1)\n \n\ndef from_twos_complement(n, bits):\n if n>=0:\n return '{:b}'.format(n).zfill(bits)\n else:\n return '{:b}'.format(2**bits+n).zfill(bits)"] | {"fn_name": "to_twos_complement", "inputs": [["00000001", 8], ["00000010", 8], ["01111110", 8], ["01111111", 8], ["11111111", 8], ["11111110", 8], ["10000010", 8], ["1000 0000", 8], ["1010 1010 0010 0010 1110 1010 0010 1110", 32], ["1000 0000 1110 1111 0011 0100 1100 1010", 32], ["10110001000100000101100011111000", 32]], "outputs": [[1], [2], [126], [127], [-1], [-2], [-126], [-128], [-1440552402], [-2131807030], [-1324328712]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,033 |
def to_twos_complement(binary, bits):
|
754a2e7b82de0cf97f34f12fb1015dee | UNKNOWN | # MOD 256 without the MOD operator
The MOD-operator % (aka mod/modulus/remainder):
```
Returns the remainder of a division operation.
The sign of the result is the same as the sign of the first operand.
(Different behavior in Python!)
```
The short unbelievable mad story for this kata:
I wrote a program and needed the remainder of the division by 256. And then it happened: The "5"/"%"-Key did not react. It must be broken! So I needed a way to:
```
Calculate the remainder of the division by 256 without the %-operator.
```
Also here some examples:
```
Input 254 -> Result 254
Input 256 -> Result 0
Input 258 -> Result 2
Input -258 -> Result -2 (in Python: Result: 254!)
```
It is always expected the behavior of the MOD-Operator of the language!
The input number will always between -10000 and 10000.
For some languages the %-operator will be blocked. If it is not blocked and you know how to block it, tell me and I will include it.
For all, who say, this would be a duplicate: No, this is no duplicate! There are two katas, in that you have to write a general method for MOD without %. But this kata is only for MOD 256. And so you can create also other specialized solutions. ;-)
Of course you can use the digit "5" in your solution. :-)
I'm very curious for your solutions and the way you solve it. I found several interesting "funny" ways.
Have fun coding it and please don't forget to vote and rank this kata! :-)
I have also created other katas. Take a look if you enjoyed this kata! | ["def mod256_without_mod(number):\n return number & 255 ", "def mod256_without_mod(number):\n return number-number//256*256", "def mod256_without_mod(number):\n return number.__mod__(256)\n", "def mod256_without_mod(number):\n return divmod(number, 256)[1]\n", "def mod256_without_mod(num):\n return num & 255", "def mod256_without_mod(number):\n return divmod(number, 256)[1] # cheap trick ;-)", "mod256_without_mod=(256).__rmod__", "def mod256_without_mod(number):\n return number & 0xFF", "def mod256_without_mod(number):\n a = abs(number)\n while a>256:\n a -= 256\n if number<0:\n b = 256-a\n return b\n elif a == 256: return 0\n else: return a", "def mod256_without_mod(number):\n return eval(str(number)+u'\\u0025'+'256')"] | {"fn_name": "mod256_without_mod", "inputs": [[254], [256], [258], [-254], [-256], [-258]], "outputs": [[254], [0], [2], [2], [0], [254]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 765 |
def mod256_without_mod(number):
|
f1ba3de1ebfe330343fe915b2308577d | UNKNOWN | # Task
You have set an alarm for some of the week days.
Days of the week are encoded in binary representation like this:
```
0000001 - Sunday
0000010 - Monday
0000100 - Tuesday
0001000 - Wednesday
0010000 - Thursday
0100000 - Friday
1000000 - Saturday```
For example, if your alarm is set only for Sunday and Friday, the representation will be `0100001`.
Given the current day of the week, your task is to find the day of the week when alarm will ring next time.
# Example
For `currentDay = 4, availableWeekDays = 42`, the result should be `6`.
```
currentDay = 4 means the current Day is Wednesday
availableWeekDays = 42 convert to binary is "0101010"
So the next day is 6 (Friday)
```
# Input/Output
- `[input]` integer `currentDay`
The weekdays range from 1 to 7, 1 is Sunday and 7 is Saturday
- `[input]` integer `availableWeekDays`
An integer. Days of the week are encoded in its binary representation.
- `[output]` an integer
The next day available. | ["def next_day_of_week(current_day, available_week_days):\n x = 2 ** current_day\n while not x & available_week_days:\n x = max(1, (x * 2) % 2 ** 7)\n return x.bit_length()", "def next_day_of_week(c, a):\n return next((weekday - 1) % 7 + 1 for weekday in range(c+1, c+8) if (1 << ((weekday-1) % 7)) & a)", "def next_day_of_week(current_day, available_week_days):\n b = f\"{available_week_days:07b}\"[::-1]\n return b.find(\"1\", current_day) + 1 or b.index(\"1\") + 1", "def next_day_of_week(a, b):\n return (f\"{b:7b}\"[::-1] * 2).index(\"1\", a) % 7 + 1", "def next_day_of_week(current_day, available_week_days):\n #coding and coding..\n d = {1:1, 2:2, 3:4, 4:8, 5:16, 6:32, 7:64}\n \n while True:\n if current_day == 7:\n current_day = 0\n current_day += 1\n \n if available_week_days & d[current_day]:\n return current_day\n", "def next_day_of_week(current_day, available_week_days):\n return (f\"{available_week_days:07b}\"[::-1]*2).index('1',current_day)%7+1", "next_day_of_week=lambda n,b:next(i%7+1for i in range(n,n+8)if 1<<i%7&b)", "def next_day_of_week(day,week):\n days=[d for d,v in enumerate(bin(week)[2:][::-1],1) if int(v)]\n return next((d for d in days if d>day),days[0]) ", "def next_day_of_week(d, a):\n t = a & ~((1 << d) - 1) or a\n return (t & -t).bit_length()", "from math import log\n\ndef next_day_of_week(current_day, available_week_days):\n code = bin(available_week_days)[2:].rjust(7, \"0\")[::-1]\n for i in range(current_day, 7):\n if code[i] == \"1\":\n return i + 1\n for i in range(current_day):\n if code[i] == \"1\":\n return i + 1\n"] | {"fn_name": "next_day_of_week", "inputs": [[4, 42], [6, 42], [7, 42]], "outputs": [[6], [2], [2]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,702 |
def next_day_of_week(current_day, available_week_days):
|
7e3eaa1fb0e779af0d16a639d2063996 | UNKNOWN | In this Kata, you will write a function `doubles` that will remove double string characters that are adjacent to each other.
For example:
`doubles('abbcccdddda') = 'aca'`, because, from left to right:
```Haskell
a) There is only one 'a' on the left hand side, so it stays.
b) The 2 b's disappear because we are removing double characters that are adjacent.
c) Of the 3 c's, we remove two. We are only removing doubles.
d) The 4 d's all disappear, because we first remove the first double, and again we remove the second double.
e) There is only one 'a' at the end, so it stays.
```
Two more examples: `doubles('abbbzz') = 'ab'` and `doubles('abba') = ""`. In the second example, when we remove the b's in `'abba'`, the double `a` that results is then removed.
The strings will contain lowercase letters only. More examples in the test cases.
Good luck! | ["def doubles(s):\n cs = []\n for c in s:\n if cs and cs[-1] == c:\n cs.pop()\n else:\n cs.append(c)\n return ''.join(cs)", "def doubles(s):\n for i in s:\n if i*2 in s:\n \n s = s.replace(i*2, '')\n return s", "import re\n\ndef doubles(s):\n re_double = re.compile(r'(.)\\1')\n while re_double.search(s):\n s = re_double.sub('', s)\n return s", "from itertools import groupby\n\ndef doubles(s):\n new_s = ''.join(char*(len(tuple(group))%2) for char, group in groupby(s))\n return new_s if len(new_s) == len(s) else doubles(new_s)", "def doubles(s):\n for x in range(0,3):\n for a in \"abcdefghijklmnopqrstuvwqxyz\": s=s.replace(2*a,\"\")\n return s", "def doubles(s):\n for _ in range(9):\n for i in set(s):\n s = s.replace(i+i, '')\n return s", "def doubles(s):\n for _ in range(99):\n for a,b in zip(s, s[1:]):\n if a==b:\n s = s.replace(a+b, '')\n break\n return s\n", "def doubles(s):\n chars = set(s)\n while True: \n token = s\n for c in chars: \n s = s.replace(c+c, '')\n if len(token) == len(s): \n break\n return s", "import re\ndef doubles(s):\n while len(re.sub(r'([a-z])\\1',\"\",s)) != len(s):\n s = re.sub(r'([a-z])\\1',\"\",s)\n return s", "from itertools import groupby\ndef check_double(s):\n return ''.join([x[0] for x in groupby(s) if len(list(x[1])) % 2 != 0])\ndef doubles(s):\n out = check_double(s)\n while out != check_double(out): out = check_double(out)\n return out"] | {"fn_name": "doubles", "inputs": [["abbbzz"], ["zzzzykkkd"], ["abbcccdddda"], ["vvvvvoiiiiin"], ["rrrmooomqqqqj"], ["xxbnnnnnyaaaaam"], ["qqqqqqnpppgooooonpppppqmmmmmc"], ["qqqqqwwwx"], ["jjjfzzzzzzsddgrrrrru"], ["jjjjjfuuuutgggggqppdaaas"], ["iiiiibllllllyqqqqqbiiiiiituuf"], ["mmmmmmuzzqllllmqqqp"]], "outputs": [["ab"], ["ykd"], ["aca"], ["voin"], ["rmomj"], ["bnyam"], ["npgonpqmc"], ["qwx"], ["jfsgru"], ["jftgqdas"], ["ibyqbtf"], ["uqmqp"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,665 |
def doubles(s):
|
2a47007c9ebe69ea0532d8bedd4796ae | UNKNOWN | ## Task
Using `n` as a parameter in the function `pattern`, where `n>0`, complete the codes to get the pattern (take the help of examples):
**Note:** There is no newline in the end (after the pattern ends)
### Examples
`pattern(3)` should return `"1\n1*2\n1**3"`, e.g. the following:
```
1
1*2
1**3
```
`pattern(10):` should return the following:
```
1
1*2
1**3
1***4
1****5
1*****6
1******7
1*******8
1********9
1*********10
``` | ["def pattern(n):\n return '\\n'.join(['1'] + ['1' + '*' * (i-1) + str(i) for i in range(2, n+1)])\n", "def pattern(n):\n s = ''\n for i in range(n):\n if i == 0:\n s += '1\\n'\n else:\n s += '1{}{}\\n'.format('*' * i, i + 1)\n \n return s.rstrip('\\n')\n", "def pattern(n):\n return \"\\n\".join(\"{}{}{}\".format(1 if i else \"\", \"*\" * i, i+1) for i in range(n))\n", "def pattern(n):\n if n == 1:\n return \"1\"\n else:\n return '1\\n'+''.join([str(1)+'*'*(i-1)+str(i)+'\\n' for i in range(2, n+1)])[:-1]", "def pattern(n):\n return \"\\n\".join(f\"1{'*'*i}{i+1}\" if i else \"1\" for i in range(n))", "def pattern(n):\n return \"1\\n\" + \"\\n\".join(f\"1{'*'*(i-1)}{i}\" for i in range(2,n+1))\n", "def pattern(n):\n return '\\n'.join('1{}{}'.format('*'*i, '' if i==0 else i+1) for i in range(n))", "def pattern(n):\n return '1\\n'+'\\n'.join(['1'+'*'*i+str(i+1) for i in range(1,n)] )\n", "def pattern(n):\n return '\\n'.join(['1'+\"*\"*i+str(i+1)*(i!=0) for i in range(n)])", "def pattern(n):\n if n == 1:\n return \"1\"\n elif n > 1:\n return pattern(n-1) + \"\\n1{}{}\".format(\"*\" * (n-1), n)\n"] | {"fn_name": "pattern", "inputs": [[3], [7], [20]], "outputs": [["1\n1*2\n1**3"], ["1\n1*2\n1**3\n1***4\n1****5\n1*****6\n1******7"], ["1\n1*2\n1**3\n1***4\n1****5\n1*****6\n1******7\n1*******8\n1********9\n1*********10\n1**********11\n1***********12\n1************13\n1*************14\n1**************15\n1***************16\n1****************17\n1*****************18\n1******************19\n1*******************20"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,218 |
def pattern(n):
|
a9c7bb32178bde4acc66512f5c8895ac | UNKNOWN | Implement a function/class, which should return an integer if the input string is in one of the formats specified below, or `null/nil/None` otherwise.
Format:
* Optional `-` or `+`
* Base prefix `0b` (binary), `0x` (hexadecimal), `0o` (octal), or in case of no prefix decimal.
* Digits depending on base
Any extra character (including whitespace) makes the input invalid, in which case you should return `null/nil/None`.
Digits are case insensitive, but base prefix must be lower case.
See the test cases for examples. | ["import re\n\ndef to_integer(s):\n if re.match(\"\\A[+-]?(\\d+|0b[01]+|0o[0-7]+|0x[0-9a-fA-F]+)\\Z\", s):\n return int(s, 10 if s[1:].isdigit() else 0)", "from re import compile, match\n\nREGEX = compile(r'[+-]?(0(?P<base>[bxo]))?[\\d\\w]+\\Z')\n\n\ndef to_integer(strng):\n try:\n return int(strng, 0 if match(REGEX, strng).group('base') else 10)\n except (AttributeError, KeyError, ValueError):\n pass\n", "import re\ndef to_integer(s):\n decimal = re.compile(r'^[-+]?\\d+$')\n binary = re.compile(r'^[-+]?0b[01]+$')\n hexadecimal = re.compile(r'^[-+]?0x[0-9A-Fa-f]+$')\n octal = re.compile(r'^[-+]?0o[0-7]+$')\n return next((int(s,[10,0][j!=0]) for j,i in enumerate([decimal,binary,hexadecimal,octal]) if re.search(i,s) and '\\n' not in s),None)", "import re\n\nBASE = {'0b': 2, '0x': 16, '0o': 8, '': 10}\ndef to_integer(string):\n matched = re.search(r'\\A(?P<sign>[-+]?)(?P<base>0[box]|)?(?P<n>[a-fA-F0-9]+)\\Z', string)\n if not matched:\n return None\n base = BASE[matched.group('base').lower()]\n try:\n n = int(matched.group('n'), base)\n except ValueError:\n return None\n return n * (-1 if matched.group('sign') == '-' else 1)", "import re\n\ndef to_integer(string):\n valid = re.search(r\"\\A([+-])?(0[bxo])?([0-9a-fA-F]+)\\Z\", string)\n if not valid:\n return None\n str_base = valid.groups()[1]\n base = {\"0x\": 16, \"0o\": 8, \"0b\": 2}.get(str_base, 10)\n try:\n return int(string, base)\n except:\n return None\n", "import re\n\n\ndef to_integer(string):\n #your code here\n result = re.compile(r'^(\\+|-)?(0b[01]+|0o[0-7]+|0x(\\d|[abcdefABCDEF])+|\\d+)\\Z').match(string)\n if not result:\n return None \n return convert_to_number(result.string)\n \n\ndef convert_to_number(string):\n base_dict={'0x': 16, '0b': 2, '0o':8}\n for key, value in base_dict.items():\n if string.find(key) != -1:\n return int(string, value)\n return int(string)", "import re\n\ndef to_integer(string):\n try: return eval(''.join(e for e in re.match(r'\\A([-+]?)(?:(0b[01]+)|(0o[0-7]+)|(0x[0-9a-fA-F]+)|0*(\\d+))\\Z',string).groups() if e))\n except: return None", "import re\ndef to_integer(string):\n s = re.match(\"^([+-]?((0x[0-9A-Fa-f]+)|(0b[01]+)|(0o[0-7]+))?(?(2)$|[0-9]+$))\",string)\n if s==None or s.group(1)!=string:\n return None\n return int(string,0 if s.group(2) else 10)", "import re\ndef to_integer(string):\n b = r'\\A[+-]?0b1+[01]*\\Z'\n x = r'\\A[+-]?0x[1-9A-Fa-f]+[0-9A-Fa-f]*\\Z'\n o = r'\\A[+-]?0o[1-7]+[0-7]*\\Z'\n d = r'\\A[+-]?[0-9]+\\Z'\n #print(string)\n for pattern, base in zip((b,x,o,d), (2,16,8,10)):\n if re.search(pattern, string):\n return int(string, base)\n return None", "import re\n\ndef to_integer(string):\n dic = {'0x': 16, '0b': 2, '0o': 8 ,None: 10}\n match = re.fullmatch(r\"[+-]?(0[xbo]+)?[\\d\\w]*\", string)\n try:\n num = int(match.group(), dic[match.group(1)])\n except:\n num = None\n finally:\n return num"] | {"fn_name": "to_integer", "inputs": [["123"], ["0x123"], ["0o123"], ["0123"], ["123 "], [" 123"], ["0b1010"], ["+123"], ["-123"], ["0B1010"], ["0b12"], ["-0x123"], ["-0o123"], ["-0123"], ["123\n"], ["\n123"], ["-0b1010"], ["0xDEADbeef"], ["0X123"], ["0O123"], ["0o18"]], "outputs": [[123], [291], [83], [123], [null], [null], [10], [123], [-123], [null], [null], [-291], [-83], [-123], [null], [null], [-10], [3735928559], [null], [null], [null]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,135 |
def to_integer(string):
|
ade3aba1c5f83e5fda3f033632d12ac3 | UNKNOWN | # Task
The number is considered to be `unlucky` if it does not have digits `4` and `7` and is divisible by `13`. Please count all unlucky numbers not greater than `n`.
# Example
For `n = 20`, the result should be `2` (numbers `0 and 13`).
For `n = 100`, the result should be `7` (numbers `0, 13, 26, 39, 52, 65, and 91`)
# Input/Output
- `[input]` integer `n`
`1 ≤ n ≤ 10^8(10^6 in Python)`
- `[output]` an integer | ["def unlucky_number(n):\n return sum(not ('4' in s or '7' in s) for s in map(str, range(0, n+1, 13)))", "def unlucky_number(n):\n return sum(1 for k in range(0, n+1, 13) if not set(str(k)) & {\"4\", \"7\"})\n", "def unlucky_number(n):\n return sum(x % 13 == 0 and '4' not in str(x) and '7' not in str(x) for x in range(n+1))", "def unlucky_number(n):\n return len([i for i in range(1,n+1) if(i%13==0 and str(i).count(\"4\")==0 and str(i).count(\"7\")==0)])+1", "def unlucky_number(n):\n return sum( 1 for n in range(0,n+1,13) if set(str(n)) & set('47') == set() )", "unlucky_number = lambda n: sum(not {'4', '7'} & set(str(x)) for x in range(0, n + 1, 13))", "li = [i for i in range(0,1000000,13)if'4'not in str(i)and'7'not in str(i)]\nunlucky_number=lambda n:next(len(li[:li.index(i)])+1for i in range(n,-1,-1)if'4'not in str(i)and'7'not in str(i)and not i%13)", "li = [i for i in range(1000000)if'4'not in str(i)and'7'not in str(i)and not i%13]\nunlucky_number=lambda n:next(len(li[:li.index(i)])+1for i in range(n,-1,-1)if'4'not in str(i)and'7'not in str(i)and not i%13)"] | {"fn_name": "unlucky_number", "inputs": [[20], [100], [1000], [1000000]], "outputs": [[2], [7], [40], [20182]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,089 |
def unlucky_number(n):
|
acbc3d1bcd7e79942c44364952f6a6bd | UNKNOWN | Define a method that accepts 2 strings as parameters. The method returns the first string sorted by the second.
```python
sort_string("foos", "of") == "oofs"
sort_string("string", "gnirts") == "gnirts"
sort_string("banana", "abn") == "aaabnn"
```
To elaborate, the second string defines the ordering. It is possible that in the second string characters repeat, so you should remove repeating characters, leaving only the first occurrence.
Any character in the first string that does not appear in the second string should be sorted to the end of the result in original order. | ["def sort_string(s, ordering):\n answer = ''\n for o in ordering:\n answer += o * s.count(o)\n s = s.replace(o,'')\n return answer + s ", "def sort_string(s, ordering):\n dct = {c:-i for i,c in enumerate(reversed(ordering))}\n return ''.join(sorted(s,key=lambda c:dct.get(c,1)))", "sort_string=lambda s,o:''.join(sorted(s,key=lambda x,d={c:i for i,c in reversed(list(enumerate(o)))}:d.get(x,len(o))))", "def sort_string(st, order):\n return ''.join(sorted(list(st), key=lambda e: list(order).index(e) if e in order else len(order)))", "def sort_string(stg, ordering):\n return \"\".join(sorted(stg, key=lambda c: ordering.index(c) if c in ordering else float(\"inf\")))", "def sort_string(s, ordering):\n order = {}\n for i, c in enumerate(ordering):\n if c in order:\n continue\n order[c] = i\n return ''.join(sorted(s, key=lambda c: order.get(c, 9999)))", "def sort_string(s, ordering):\n result = ''\n for i in ordering:\n if i in s and i not in result:\n result += i * s.count(i)\n\n return result + ''.join([c for c in s if c not in ordering])\n", "def sort_string(s, ordering):\n result = sorted([c for c in s if c in ordering], key=ordering.find)\n result.extend([c for c in s if c not in ordering])\n return ''.join(result)", "def sort_string(a, b):\n li = list(filter(lambda x:x in b ,a))\n return ''.join(sorted(li, key=b.index) + [i for i in a if i not in li])", "def sort_string(s, ordering):\n return \"\".join(sorted([i for i in s if i in ordering], key=lambda x:ordering.index(x)))+\"\".join(sorted([j for j in s if j not in ordering],key=lambda x:s.index(s)))"] | {"fn_name": "sort_string", "inputs": [["banana", "abn"], ["banana", "xyz"], ["banana", "an"], ["foos", "of"], ["string", "gnirts"], ["banana", "a"], ["bungholio", "aacbuoldiiaoh"], ["fumyarhncujlj", "nsejcwn"]], "outputs": [["aaabnn"], ["banana"], ["aaannb"], ["oofs"], ["gnirts"], ["aaabnn"], ["buoolihng"], ["njjcfumyarhul"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,692 |
def sort_string(s, ordering):
|
e6523a65f01e265f87ec7a2d65176790 | UNKNOWN | Magic The Gathering is a collectible card game that features wizards battling against each other with spells and creature summons. The game itself can be quite complicated to learn. In this series of katas, we'll be solving some of the situations that arise during gameplay. You won't need any prior knowledge of the game to solve these contrived problems, as I will provide you with enough information.
## Creatures
Each creature has a power and toughness. We will represent this in an array. [2, 3] means this creature has a power of 2 and a toughness of 3.
When two creatures square off, they each deal damage equal to their power to each other at the same time. If a creature takes on damage greater than or equal to their toughness, they die.
Examples:
- Creature 1 - [2, 3]
- Creature 2 - [3, 3]
- Creature 3 - [1, 4]
- Creature 4 - [4, 1]
If creature 1 battles creature 2, creature 1 dies, while 2 survives. If creature 3 battles creature 4, they both die, as 3 deals 1 damage to 4, but creature 4 only has a toughness of 1.
Write a function `battle(player1, player2)` that takes in 2 arrays of creatures. Each players' creatures battle each other in order (player1[0] battles the creature in player2[0]) and so on. If one list of creatures is longer than the other, those creatures are considered unblocked, and do not battle.
Your function should return an object (a hash in Ruby) with the keys player1 and player2 that contain the power and toughness of the surviving creatures.
Example:
```
Good luck with your battles!
Check out my other Magic The Gathering katas:
Magic The Gathering #1: Creatures
Magic The Gathering #2: Mana | ["from itertools import zip_longest\n\ndef battle(player1, player2):\n result = {'player1': [], 'player2': []}\n for (p1, t1), (p2, t2) in zip_longest(player1, player2, fillvalue=[0,0]):\n if t1 > p2:\n result['player1'].append([p1,t1])\n if t2 > p1:\n result['player2'].append([p2,t2])\n return result\n", "\ndef battle(player1, player2):\n l1 = []\n l2 = []\n x = min(len(player1),len(player2))\n for i in range(x):\n if player1[i][0] < player2[i][1]:\n l2.append(player2[i])\n if player2[i][0] < player1[i][1]:\n l1.append(player1[i])\n \n l1 += player1[x:]\n l2 += player2[x:]\n \n return {'player1': l1, 'player2': l2}\n", "from itertools import zip_longest\n\ndef battle(player1, player2):\n res = {\"player1\":[], \"player2\":[]}\n for c1,c2 in zip_longest(player1, player2, fillvalue=[0, 0]):\n if c2[0] < c1[1]:\n res[\"player1\"].append(c1)\n if c1[0] < c2[1]:\n res[\"player2\"].append(c2)\n return res", "def battle(p1, p2):\n p,p_ =p1[:],p2[:]\n for i,j in zip(p1,p2):\n if i[0]>=j[1] : p_.remove(j)\n if j[0]>=i[1] : p.remove(i)\n return {'player1':p,'player2':p_}", "from itertools import zip_longest\n\ndef battle(player1, player2):\n result = {\"player1\": [], \"player2\": []}\n for creature1, creature2 in zip_longest(player1, player2, fillvalue=[0, 0]):\n if creature1[1] > creature2[0]:\n result[\"player1\"].append(creature1)\n if creature2[1] > creature1[0]:\n result[\"player2\"].append(creature2)\n return result", "from itertools import zip_longest\n\ndef battle(player1, player2):\n rem = {'player1': [], 'player2': []}\n for c1,c2 in zip_longest(player1, player2, fillvalue=[0,0]):\n if c1[1] > c2[0]: rem['player1'].append(c1)\n if c2[1] > c1[0]: rem['player2'].append(c2)\n return rem", "def battle(player1, player2):\n rem1=[c for i,c in enumerate(player1) if i>=len(player2) or c[1]>player2[i][0]]\n rem2=[c for i,c in enumerate(player2) if i>=len(player1) or c[1]>player1[i][0]]\n return {'player1':rem1, 'player2':rem2}", "def battle(player1, player2):\n\n if len(player1) > len(player2):\n min_creatures = len(player2)\n else:\n min_creatures = len(player1)\n \n i = min_creatures - 1\n while i >= 0:\n if player1[i][0] >= player2[i][1] and player2[i][0] >= player1[i][1]:\n del player1[i]\n del player2[i]\n elif player1[i][0] >= player2[i][1]:\n del player2[i]\n elif player2[i][0] >= player1[i][1]:\n del player1[i]\n i -= 1\n \n return {'player1': player1, 'player2': player2}"] | {"fn_name": "battle", "inputs": [[[[2, 3], [1, 4]], [[3, 3], [4, 1]]], [[], []], [[[1, 1]], [[1, 1]]], [[[2, 1]], [[2, 1]]], [[[1, 2]], [[1, 2]]], [[[2, 3]], [[3, 2]]], [[[2, 7]], [[3, 5]]], [[[2, 5]], [[6, 6]]], [[[6, 6]], [[2, 5]]], [[], [[1, 1], [2, 2]]], [[[1, 1], [2, 2]], []], [[[1, 3], [3, 5], [4, 6], [5, 2]], [[2, 4], [6, 1], [4, 4], [3, 7], [3, 1]]]], "outputs": [[{"player1": [], "player2": [[3, 3]]}], [{"player1": [], "player2": []}], [{"player1": [], "player2": []}], [{"player1": [], "player2": []}], [{"player1": [[1, 2]], "player2": [[1, 2]]}], [{"player1": [], "player2": []}], [{"player1": [[2, 7]], "player2": [[3, 5]]}], [{"player1": [], "player2": [[6, 6]]}], [{"player1": [[6, 6]], "player2": []}], [{"player1": [], "player2": [[1, 1], [2, 2]]}], [{"player1": [[1, 1], [2, 2]], "player2": []}], [{"player1": [[1, 3], [4, 6]], "player2": [[2, 4], [3, 7], [3, 1]]}]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,769 |
def battle(player1, player2):
|
148f955786eefbb98e89aba8993078ea | UNKNOWN | We need a function (for commercial purposes) that may perform integer partitions with some constraints.
The function should select how many elements each partition should have.
The function should discard some "forbidden" values in each partition.
So, create ```part_const()```, that receives three arguments.
```part_const((1), (2), (3))```
```
(1) - The integer to be partitioned
(2) - The number of elements that each partition should have
(3) - The "forbidden" element that cannot appear in any partition
```
```part_const()``` should output the amount of different integer partitions with the constraints required.
Let's see some cases:
```python
part_const(10, 3, 2) ------> 4
/// we may have a total of 8 partitions of three elements (of course, the sum of the elements of each partition should be equal 10) :
[1, 1, 8], [1, 2, 7], [1, 3, 6], [1, 4, 5], [2, 2, 6], [2, 3, 5], [2, 4, 4], [3, 3, 4]
but 2 is the forbidden element, so we have to discard [1, 2, 7], [2, 2, 6], [2, 3, 5] and [2, 4, 4]
So the obtained partitions of three elements without having 2 in them are:
[1, 1, 8], [1, 3, 6], [1, 4, 5] and [3, 3, 4] (4 partitions)///
```
```part_const()``` should have a particular feature:
if we introduce ```0``` as the forbidden element, we will obtain the total amount of partitions with the constrained number of elements.
In fact,
```python
part_const(10, 3, 0) ------> 8 # The same eight partitions that we saw above.
```
Enjoy it and happy coding!! | ["def p(t,k,n,l=1):\n if t<l: pass\n elif k==1 and t!=n: yield [t]\n else: yield from ([m]+s for m in range(l,t-l+1) for s in p(t-m,k-1,n,m) if m!=n)\n\ndef part_const(t,k,n): return sum(1 for _ in p(t,k,n))", "def part_const(n, k, num):\n return part(n, k) - part(n-(num or n), k-1)\n\ndef part(n, k):\n return 1 if k in (1, n) else sum(part(n-k, i) for i in range(1, min(n-k, k)+1))", "def get_addends(num, x=1):\n yield (num,)\n for i in range(x, num//2 + 1):\n for p in get_addends(num-i, i):\n yield (i,) + p\n \ndef part_const(n, k, num):\n return len([el for el in get_addends(n) if len(el) == k and num not in el])", "def part_const(n, size, forbidden):\n\n ways, k, partitions = [0, n] + [0] * (n - 1), 1, 0\n while k:\n x, y, k = ways[k-1] + 1, ways[k] - 1, k -1\n while x <= y:\n ways[k] = x\n y, k = y - x, k + 1\n ways[k] = x + y\n if len(ways[:k + 1]) == size and forbidden not in ways[:k + 1]: partitions += 1\n return partitions", "def part_const(n, k, num):\n return sum(1 for x in accel_asc(n) if len(x) == k and all(v != num for v in x))\n\n# http://jeromekelleher.net/tag/integer-partitions.html\ndef accel_asc(n):\n a = [0 for i in range(n + 1)]\n k = 1\n y = n - 1\n while k != 0:\n x = a[k - 1] + 1\n k -= 1\n while 2 * x <= y:\n a[k] = x\n y -= x\n k += 1\n l = k + 1\n while x <= y:\n a[k] = x\n a[l] = y\n yield a[:k + 2]\n x += 1\n y -= 1\n a[k] = x + y\n y = x + y - 1\n yield a[:k + 1]", "def part_const(n, k, num):\n return len([x for x in list(accel_asc(n)) if len(x) == k and num not in x])\n \ndef accel_asc(n):\n a = [0 for i in range(n + 1)]\n k = 1\n y = n - 1\n while k != 0:\n x = a[k - 1] + 1\n k -= 1\n while 2*x <= y:\n a[k] = x\n y -= x\n k += 1\n l = k + 1\n while x <= y:\n a[k] = x\n a[l] = y\n yield a[:k + 2]\n x += 1\n y -= 1\n a[k] = x + y\n y = x + y - 1\n yield a[:k + 1]", "def partition(n,parts,m=1):\n if parts == 1:return [[n]]\n ans = []\n s = m\n while s <= (n / parts):\n l = [s]\n p = partition(n - s,parts - 1,s)\n for i in p:\n ans += [l + i]\n s += 1\n return ans\n\ndef part_const(n, k, num):\n p = partition(n,k)\n return len([i for i in p if not num in i])", "part_const=p=lambda n,k,x,s=1:n!=x if k<2else sum(p(n-i,k-1,x,i)for i in range(s,n//k+1)if i!=x)", "def part_const(n, k, num):\n return part(n, k) - (part(n-num, k-1) if num else 0)\n\ndef part(n, k):\n return 0 if k > n else 1 if k in {1, n} else sum(part(n-k, i) for i in range(1, k+1))\n", "def part_const(n, k, num, s=0, startwith=1):\n if k == 1:\n if n - s >= startwith and n - s != num:\n return 1\n return 0\n result = 0\n for i in range(startwith, n - s - k + 2):\n if i == num:\n continue\n result += part_const(n, k-1, num, s + i, i)\n return result\n \n"] | {"fn_name": "part_const", "inputs": [[10, 3, 2], [10, 3, 0], [10, 4, 1], [10, 5, 3], [15, 5, 3], [15, 5, 4], [15, 3, 3]], "outputs": [[4], [8], [2], [4], [15], [19], [13]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,235 |
def part_const(n, k, num):
|
e25bf05ae9ea52d911738488b62f78d5 | UNKNOWN | As the title suggests, this is the hard-core version of another neat kata.
The task is simple to explain: simply sum all the numbers from the first parameter being the beginning to the second parameter being the upper limit (possibly included), going in steps expressed by the third parameter:
```python
sequence_sum(2, 2, 2) # 2
sequence_sum(2, 6, 2) # 12 (= 2 + 4 + 6)
sequence_sum(1, 5, 1) # (= 1 + 2 + 3 + 4 + 5)
sequence_sum(1, 5, 3) # 5 (= 1 + 4)
```
If it is an impossible sequence (with the beginning being larger the end and a positive step or the other way around), just return `0`. See the provided test cases for further examples :)
**Note:** differing from the other base kata, much larger ranges are going to be tested, so you should hope to get your algo optimized and to avoid brute-forcing your way through the solution. | ["# from math import copysign\ndef sequence_sum(a, b, step):\n n = (b-a)//step\n return 0 if n<0 else (n+1)*(n*step+a+a)//2", "def sequence_sum(b, e, s):\n q, r = divmod(e - b, s)\n return (b + e - r) * max(q + 1, 0) // 2", "def sequence_sum(start, stop, step):\n n = (stop - start) // step\n last = start + n * step\n return (n+1) * (start + last) // 2 if n >= 0 else 0", "def sequence_sum(b, e, s):\n if (s > 0 and b > e) or (s < 0 and b < e):\n return 0\n near = e - (e - b) % s\n n = (near - b) // s + 1\n return (b + near) * n // 2", "def sequence_sum(b, e, s):\n if (b>e and s>0) or (b<e and s<0) : return 0\n k = (e-b)//s\n return b*(k+1) + s*(k*(k+1))//2", "def sequence_sum(begin, end, step):\n n = int((end - begin + step)/step)\n an = begin +(n-1) * step\n if step > 0:\n if begin > end:\n return 0\n if step < 0:\n if begin < end:\n return 0\n if n % 2 == 0:\n res = int(int(n/2)*(begin +an))\n if (begin + an) % 2 == 0:\n res = int(int((begin +an)/2)*n)\n return res", "from decimal import Decimal\n\ndef sequence_sum(s, e, step):\n if s > e and step > 0 or s < e and step < 0: return 0\n elems = abs(s - e) // abs(step) + 1\n last = s + (step * (elems - 1))\n avg = (s + last) / 2\n return int(Decimal(elems)* Decimal(avg))", "def sequence_sum(a,b,s):\n n = (b-a)//s\n return 0 if n < 0 else (n+1)*(2*a + s*n)//2"] | {"fn_name": "sequence_sum", "inputs": [[2, 6, 2], [1, 5, 1], [1, 5, 3], [-1, -5, -3], [16, 15, 3], [-24, -2, 22], [-2, 4, 658], [780, 6851543, 5], [9383, 71418, 2], [20, 673388797, 5]], "outputs": [[12], [15], [5], [-5], [0], [-26], [-2], [4694363402480], [1253127200], [45345247259849570]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,469 |
def sequence_sum(b, e, s):
|
ea55ac8b0c46ffaf5deab76fcf3e8026 | UNKNOWN | Write a method that takes a string as an argument and groups the number of time each character appears in the string as a hash sorted by the highest number of occurrences.
The characters should be sorted alphabetically e.g:
```python
get_char_count("cba") == {1: ["a", "b", "c"]}
```
You should ignore spaces, special characters and count uppercase letters as lowercase ones.
For example:
```python
get_char_count("Mississippi") == {4: ["i", "s"], 2: ["p"], 1: ["m"]}
get_char_count("Hello. Hello? HELLO!") == {6: ["l"], 3: ["e", "h", "o"]}
get_char_count("aaa...bb...c!") == {3: ["a"], 2: ["b"], 1: ["c"]}
get_char_count("abc123") == {1: ["1", "2", "3", "a", "b", "c"]}
get_char_count("aaabbbccc") == {3: ["a", "b", "c"]}
``` | ["def get_char_count(s):\n counts = {}\n for c in (c.lower() for c in s if c.isalnum()):\n counts[c] = counts[c] + 1 if c in counts else 1\n m = {}\n for k, v in counts.items():\n m[v] = sorted(m[v] + [k]) if v in m else [k]\n return m", "from collections import Counter, defaultdict\nfrom bisect import insort\n\ndef get_char_count(s):\n D = defaultdict(list)\n for k,v in Counter(map(str.lower, filter(str.isalnum, s))).items():\n insort(D[v], k)\n return D", "from collections import Counter, defaultdict\ndef get_char_count(s):\n d = defaultdict(list)\n c = Counter(sorted(s.lower()))\n for i,j in c.items():\n if i.isalnum() : d[j].append(i)\n return dict(sorted(d.items())[::-1])", "from collections import Counter\ndef get_char_count(s):\n con = Counter([e for e in s.lower() if any((e.isalpha(), e.isdigit()))])\n return { v:sorted([e for e in con.keys() if con[e] == v]) for v in sorted(con.values(), reverse = 1 ) }", "def get_char_count(s):\n lst = filter(lambda x: x.isalnum(), s.lower())\n result = {}\n for i in set(lst):\n result[s.lower().count(i)] = sorted(result.get(s.lower().count(i), []) + [i])\n return result", "\ndef get_char_count(s):\n print(s)\n ls = []\n ls2 = []\n d ={}\n nd = {}\n s = s.lower()\n for i in s:\n if i.isalpha() or i.isnumeric():\n ls.append(i)\n st = set(ls)\n for i in st: \n ls2.append((s.count(i), i))\n \n \n for i in st:\n d[s.count(i)] = []\n x = 0\n for i in ls2:\n d[ls2[x][0]].append(ls2[x][1])\n x += 1\n \n for i in sorted(d,reverse = True):\n nd[i] = sorted(d[i])\n \n return(nd)\n\n \n \n", "from itertools import groupby\nfrom collections import defaultdict\ndef get_char_count(s):\n d = defaultdict(list)\n for k, g in groupby(filter(str.isalnum, sorted(s.lower()))):\n d[len(list(g))].append(k)\n return d", "from collections import Counter\nfrom itertools import groupby\n\ndef get_char_count(s):\n cnt = Counter(c for c in s.lower() if c.isalnum()).most_common()\n return {k: sorted(map(lambda x: x[0], vs)) for k, vs in groupby(cnt, key=lambda x: x[1])}", "from collections import Counter\n\ndef get_char_count(stg):\n result = {}\n for char, count in Counter(sorted(c for c in stg.lower() if c.isalnum())).items():\n result.setdefault(count, []).append(char)\n return result", "def get_char_count(s):\n newDict = {}\n s = s.lower()\n s = \"\".join(sorted(s))\n for element in s:\n if element.isalnum():\n newDict.setdefault(element, 0)\n newDict[element] += 1\n inverse = {}\n for key in newDict:\n value = newDict[key]\n inverse.setdefault(value, []).append(key)\n return inverse\n \n"] | {"fn_name": "get_char_count", "inputs": [["Get me the bacon!"], ["Codewars is bacon"], ["France is also bacon"], ["AaAAaAAabB!B!B3b23jdc"], [""], ["!!!@@@"], ["Mississippi"], ["Hello. Hello? HELLO!"], ["aaa...bb...c!"], ["abc123"], ["aaabbbccc"]], "outputs": [[{"3": ["e"], "2": ["t"], "1": ["a", "b", "c", "g", "h", "m", "n", "o"]}], [{"2": ["a", "c", "o", "s"], "1": ["b", "d", "e", "i", "n", "r", "w"]}], [{"3": ["a"], "2": ["c", "n", "o", "s"], "1": ["b", "e", "f", "i", "l", "r"]}], [{"8": ["a"], "5": ["b"], "2": ["3"], "1": ["2", "c", "d", "j"]}], [{}], [{}], [{"4": ["i", "s"], "2": ["p"], "1": ["m"]}], [{"6": ["l"], "3": ["e", "h", "o"]}], [{"3": ["a"], "2": ["b"], "1": ["c"]}], [{"1": ["1", "2", "3", "a", "b", "c"]}], [{"3": ["a", "b", "c"]}]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,875 |
def get_char_count(s):
|
87f9668de271b0d8a435e9433abf38c1 | UNKNOWN | Create a program that will take in a string as input and, if there are duplicates of more than two alphabetical characters in the string, returns the string with all the extra characters in a bracket.
For example, the input "aaaabbcdefffffffg" should return "aa[aa]bbcdeff[fffff]g"
Please also ensure that the input is a string, and return "Please enter a valid string" if it is not. | ["import re\ndef string_parse(string):\n return re.sub(r'(.)\\1(\\1+)', r'\\1\\1[\\2]', string) if isinstance(string, str) else 'Please enter a valid string'", "import re\ndef string_parse(s):\n if not isinstance(s, str): return \"Please enter a valid string\"\n for l in set(s):\n s = re.sub(r\"(%s%s)(%s+)\" % (l, l, l), r\"\\1[\\2]\", s)\n return s", "import re\n\n\ndef string_parse(s):\n if isinstance(s, str):\n return re.sub(r\"(.)\\1(\\1+)\", r\"\\1\\1[\\2]\", s)\n return \"Please enter a valid string\"", "from functools import partial\nfrom re import compile\n\nREGEX = partial(compile(r\"(.)\\1(\\1+)\").sub, r\"\\1\\1[\\2]\")\n\ndef string_parse(string):\n return REGEX(string) if type(string) == str else \"Please enter a valid string\"", "import re\ndef string_parse(string):\n try:\n def replace(match):\n return '{0}{0}[{1}]'.format(*match.group(1, 2))\n return re.sub(r'(.)\\1(\\1+)', replace, string)\n except TypeError:\n return 'Please enter a valid string'", "from itertools import groupby\n\ndef string_parse(string):\n if isinstance(string,str):\n gs = groupby(string)\n f = lambda s : s if len(s) < 3 else s[:2] + '[' + s[2:] + ']'\n return ''.join([ f(''.join(list(g))) for _, g in groupby(string)])\n else:\n return 'Please enter a valid string'\n\n", "from itertools import groupby\n\ndef string_parse(string):\n return ''.join(f'{x*2}[{x*(c-2)}]' if c > 2 else x * c for x, c in [(x, len(list(gp))) for x, gp in groupby(string)]) if isinstance(string, str) else 'Please enter a valid string'", "# Attempt without regex, let's use classic generator function.\n\ndef string_parse_gen(string):\n counter, prev_char = 0, ''\n for char in string:\n if char == prev_char:\n counter += 1\n if counter == 2:\n yield '['\n else:\n if counter >= 2:\n yield ']'\n prev_char = char;\n counter = 0;\n yield char;\n \n if counter >= 2:\n yield ']'\n\n\ndef string_parse(string):\n return ''.join(list(string_parse_gen(string))) if isinstance(string, str) else \"Please enter a valid string\"", "def string_parse(s):\n from itertools import groupby\n if not isinstance(s, str):\n return 'Please enter a valid string'\n \n x = [(i, sum([1 for j in group])) for i, group in groupby(s)]\n x = [i[0] * 2 + '[' + i[0] * (i[1] - 2) + ']' if i[1] >= 2 else i[0] for i in x]\n x = ''.join(x)\n x = x.replace('[]','')\n return x", "import re\ndef string_parse(string):\n if not isinstance(string, str):\n return 'Please enter a valid string'\n def repl(Match):\n \n s = Match[0]\n n = len(s)\n c = s[0]\n \n return s if n <= 2 else c * 2 + f'[{c * (n - 2)}]'\n return re.sub(r'(\\w)\\1*', repl, string)", "import re\ndef string_parse(s):\n try:\n return re.sub(r'(.)\\1+', lambda m: m.group()[0:2] + \"[\" + m.group()[2:] + \"]\" if len(m.group())>2 else m.group() , s) \n #lambda x: True if x % 2 == 0 else False\n except TypeError:\n return \"Please enter a valid string\"", "import re\n\ndef string_parse(s):\n return re.sub(r'((.)\\2*)', lambda x: f'{2 * x[2]}[{x[1][:len(x[1]) - 2]}]' \\\n if len(x[1]) > 2 else x[1], s) if isinstance(s, str) \\\n else \"Please enter a valid string\"", "def string_parse(string):\n if not isinstance(string, str):\n return 'Please enter a valid string'\n res = []\n count = 0\n last_char = None\n for c in string:\n if c != last_char:\n if count > 2:\n res.append(']')\n count = 0\n elif count == 2:\n res.append('[')\n count += 1\n last_char = c\n res.append(c)\n if count > 2:\n res.append(']')\n return \"\".join(res)\n", "from itertools import groupby\n\ndef string_parse(str_in):\n if not isinstance(str_in, str):\n return \"Please enter a valid string\"\n str_out= []\n for _, group in groupby(str_in):\n s = ''.join(group)\n str_out.append(s if len(s) < 3 else f'{s[:2]}[{s[2:]}]')\n return ''.join(str_out)", "def string_parse(string):\n if type(string)!=str:return \"Please enter a valid string\"\n try:\n out=string[:2]\n temp=''\n for i in string[2:]:\n if i==out[-1]==out[-2]:\n temp+=i\n else:\n if temp!='':out+='['+temp+']'\n out+=i\n temp=''\n if temp!='':out+='['+temp+']'\n return out\n except:\n return \"Please enter a valid string\"\n \n", "def string_parse(string):\n if not isinstance(string, str):\n return 'Please enter a valid string'\n letters = ['0']\n for i in string:\n if i == letters[-1][-1]:\n letters[-1] += i\n else:\n letters.append(i)\n return ''.join([i if len(i) < 3 else f'{i[:2]}[{i[2:]}]' for i in letters][1:])", "import re\n\ndef string_parse(string):\n if not type(string) == str:\n return \"Please enter a valid string\"\n \n return re.sub(r'(([a-zA-Z])\\2)(\\2+)', lambda x: x.group(1) + '[' + x.group(3) + ']', string)", "def string_parse(string):\n #your code here\n if string == None or not isinstance(string,str) : return \"Please enter a valid string\"\n if string == '' : return ''\n res = ''\n current_char = string[0]\n char_counter = 1\n for i in range(1, len(string)) :\n \n if current_char == string[i]:\n if char_counter + 1 <=2 :\n res += current_char\n char_counter +=1\n continue\n elif char_counter + 1 == 3 :\n res = res + current_char + '[' \n char_counter +=1\n continue\n elif char_counter + 1 > 3 :\n res += current_char\n char_counter +=1\n else :\n if char_counter > 2 :\n res = res + current_char + ']' \n else :\n res += current_char\n current_char = string[i] \n char_counter = 1\n res = res + current_char if char_counter <= 2 else res + current_char + ']' \n return res", "import re\n\ndef string_parse(string):\n if type(string) != str:\n return 'Please enter a valid string'\n for c in 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ':\n string = re.sub(c + '{2}(' + c + '+)', c * 2 + '[\\g<1>]', string) \n return string", "def string_parse(string):\n \n if not isinstance(string, str):\n return \"Please enter a valid string\"\n if len(string) ==0:\n return \"\"\n Di = {}\n text = \"\"\n f=0\n i = 0\n f2=0\n for i in range(len(string)-1):\n Di.setdefault(string[i], 0)\n text += string[i]\n Di[string[i]] +=1\n \n if not f and Di[string[i]] >=2 and string[i] == string[i+1]:\n text += \"[\"\n f = 1\n if f and Di[string[i]] >=2 and string[i] != string[i+1]:\n text += \"]\"\n f = 0\n f2 =1\n if not f and string[i] != string[i+1]:\n Di[string[i]] =0\n \n \n text += string[-1]\n if f:\n text += \"]\"\n return text\n"] | {"fn_name": "string_parse", "inputs": [["aaaabbcdefffffffg"], [3], ["boopdedoop"], ["helloookat"], [true], [""], ["aAAabbcdeffFfFffg"], ["aAAabbcdeFFFffffg"], [{}], [[5.3]]], "outputs": [["aa[aa]bbcdeff[fffff]g"], ["Please enter a valid string"], ["boopdedoop"], ["helloo[o]kat"], ["Please enter a valid string"], [""], ["aAAabbcdeffFfFffg"], ["aAAabbcdeFF[F]ff[ff]g"], ["Please enter a valid string"], ["Please enter a valid string"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 7,522 |
def string_parse(string):
|
b0d3d7f168ce77bfed77fc8edc9ee777 | UNKNOWN | This kata requires you to convert minutes (`int`) to hours and minutes in the format `hh:mm` (`string`).
If the input is `0` or negative value, then you should return `"00:00"`
**Hint:** use the modulo operation to solve this challenge. The modulo operation simply returns the remainder after a division. For example the remainder of 5 / 2 is 1, so 5 modulo 2 is 1.
## Example
If the input is `78`, then you should return `"01:18"`, because 78 minutes converts to 1 hour and 18 minutes.
Good luck! :D | ["def time_convert(num):\n return \"{:02d}:{:02d}\".format(*divmod(max(num, 0), 60))", "def time_convert(num):\n if num <= 0:\n return '00:00'\n return f'{num//60:02}:{num%60:02}'", "def time_convert(num):\n a=num//60\n b=num%60\n if num<=0:\n return '00:00'\n elif 0<num<10:\n return '00:0'+str(num)\n elif 10<=num<60:\n return '00:'+str(num)\n else:\n if a<10 and b<10:\n return \"0\"+str(a)+':'+\"0\"+str(b)\n elif a>=10 and b<10:\n return str(a)+':'+\"0\"+str(b)\n elif a<10 and b>=10:\n return \"0\"+str(a)+':'+str(b)\n elif a>=10 and b>=10:\n return str(a)+':'+str(b)", "def time_convert(num):\n return \"{:02d}:{:02d}\".format(*divmod(max(0, num), 60))", "def time_convert(num):\n return '{:02}:{:02}'.format(*divmod(max(0, num), 60))", "time_convert=lambda n:'%02d:%02d'%divmod(max(0,n),60)", "def time_convert(num):\n if num <= 0:\n return '00:00'\n \n if num < 60:\n if num <= 9:\n num_str = '0' + str(num)\n else:\n num_str = str(num)\n return '00:' + num_str\n \n hour = int(num / 60)\n hour_str = str(hour)\n minute = num - hour * 60\n minute_str = str(minute)\n\n if hour <= 9:\n hour_str = '0' + hour_str\n if minute <= 9:\n minute_str = '0' + minute_str\n \n return '%s:%s' % (hour_str, minute_str)"] | {"fn_name": "time_convert", "inputs": [[0], [-6], [8], [32], [75], [63], [134], [180], [970], [565757]], "outputs": [["00:00"], ["00:00"], ["00:08"], ["00:32"], ["01:15"], ["01:03"], ["02:14"], ["03:00"], ["16:10"], ["9429:17"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,449 |
def time_convert(num):
|
92fd3273aefdc5c86cf9d8305592b79b | UNKNOWN | Don't Drink the Water
Given a two-dimensional array representation of a glass of mixed liquids, sort the array such that the liquids appear in the glass based on their density. (Lower density floats to the top) The width of the glass will not change from top to bottom.
```
======================
| Density Chart |
======================
| Honey | H | 1.36 |
| Water | W | 1.00 |
| Alcohol | A | 0.87 |
| Oil | O | 0.80 |
----------------------
[ [
['H', 'H', 'W', 'O'], ['O','O','O','O']
['W', 'W', 'O', 'W'], => ['W','W','W','W']
['H', 'H', 'O', 'O'] ['H','H','H','H']
] ]
```
The glass representation may be larger or smaller. If a liquid doesn't fill a row, it floats to the top and to the left. | ["DENSITY = {'H': 1.36, 'W': 1, 'A': 0.87, 'O': 0.8}\n\n\ndef separate_liquids(glass):\n if not glass:\n return []\n column = len(glass[0])\n liquids = sorted((b for a in glass for b in a), key=lambda c: DENSITY[c])\n return [liquids[d:d + column] for d in range(0, len(liquids), column)]\n", "def separate_liquids(glass):\n chain = sorted(sum(glass, []), key = 'OAWH'.index)\n return [chain[len(level) * i:][:len(level)] for i, level in enumerate(glass)]", "s = '''| Honey | H | 1.36 |\n| Water | W | 1.00 |\n| Alcohol | A | 0.87 |\n| Oil | O | 0.80 |'''\ndef separate_liquids(glass):\n d = {}\n for x in s.split('\\n'):\n res = x.split('|')\n a,b = [c.strip(' ') for i,c in enumerate(res) if i in (2,3)]\n d[a] = float(b)\n l = []\n for x in glass:\n l.extend(x)\n result = iter(sorted(l, key=lambda x: d[x]))\n\n for x in range(len(glass)):\n for i in range(len(glass[-1])):\n glass[x][i] = next(result)\n return glass", "def separate_liquids(glass):\n chain = sorted(sum(glass, []), key = 'HWAO'.index)\n return [[chain.pop() for c in ro] for ro in glass]", "density_order = {key: i for i, key in enumerate(['O','A','W','H'])}\n\ndef separate_liquids(glass):\n lst_of_liquids = iter(sorted([liquid for row in glass for liquid in row], key=lambda d: density_order[d]))\n return [[next(lst_of_liquids) for j in range(0, len(glass[0]))] for i in range(0, len(glass))]", "density = {'H':4, 'W':3, 'A':2, 'O':1}\n\n\ndef separate_liquids(glass):\n glass_ = sorted([x for i in glass for x in i], key=lambda elem: density.get(elem))\n for row in glass:\n for i in range(len(row)):\n row[i] = glass_.pop(0)\n return glass\n", "def separate_liquids(glass):\n liquids = [];\n comparing = { 'H' : 1.36, 'W' : 1.00, 'A' : 0.87, 'O' : 0.80 }\n for row in glass:\n liquids.extend(row)\n\n liquids.sort(key=lambda k: comparing[k], reverse=True)\n \n for i in range(len(glass)):\n for j in range(len(glass[0])):\n glass[i][j] = liquids.pop()\n\n return glass"] | {"fn_name": "separate_liquids", "inputs": [[[["H", "H", "W", "O"], ["W", "W", "O", "W"], ["H", "H", "O", "O"]]], [[["A", "A", "O", "H"], ["A", "H", "W", "O"], ["W", "W", "A", "W"], ["H", "H", "O", "O"]]], [[["A", "H", "W", "O"]]], [[["A"], ["H"], ["W"], ["O"]]], [[]]], "outputs": [[[["O", "O", "O", "O"], ["W", "W", "W", "W"], ["H", "H", "H", "H"]]], [[["O", "O", "O", "O"], ["A", "A", "A", "A"], ["W", "W", "W", "W"], ["H", "H", "H", "H"]]], [[["O", "A", "W", "H"]]], [[["O"], ["A"], ["W"], ["H"]]], [[]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,133 |
def separate_liquids(glass):
|
be9e19272cbb1579ee470d9ddbf44ff8 | UNKNOWN | # Task
The game starts with `n` people standing in a circle. The presenter counts `m` people starting with the first one, and gives `1` coin to each of them. The rest of the players receive `2` coins each. After that, the last person who received `1` coin leaves the circle, giving everything he had to the next participant (who will also be considered "the first in the circle" in the next round). This process continues until only 1 person remains.
Determine the `number` of this person and how many `coins` he has at the end of the game.
The result should be a pair of values in the format: `[person, coins]`.
## Example:
```
n = 8, m = 3
People
1 2 3 1 2 ∙ 1 2 ∙ ∙ 2 ∙ ∙ 2 ∙ ∙ ∙ ∙ ∙ ∙ ∙
8 4 => 8 4 => 8 4 => 8 4 => 8 4 => 8 4 => ∙ 4 => 7
7 6 5 7 6 5 7 ∙ 5 7 ∙ 5 7 ∙ ∙ 7 ∙ ∙ 7 ∙ ∙
Coins
0 0 0 1 1 ∙ 3 3 ∙ ∙ 9 ∙ ∙ 10 ∙ ∙ ∙ ∙ ∙ ∙ ∙
0 0 => 2 3 => 4 4 => 5 6 => 7 7 => 8 20 => ∙ 30 => 51
0 0 0 2 2 2 7 ∙ 3 8 ∙ 5 16 ∙ ∙ 17 ∙ ∙ 18 ∙ ∙
```
## Notes:
* The presenter can do several full circles during one counting.
* In this case, the same person will receive `1` coin multiple times, i.e. no matter how many people are left in the circle, at least `m` coins will always be distributed among the participants during the round.
* If a person had already received `1` coin (possibly multiple times), he won't receive any additional `2` coins **at once(!)** during that round.
* People are counted starting with `1`.
* `m` can be **bigger** than `n` at start | ["def find_last(n, m):\n li, start = [[0, i + 1] for i in range(n)], 0 # form list of circle with numbers\n while len(li) != 1:\n prev, l_ = li[start][1], len(li) # starting point and length of circle\n for k in range(m): # adding 1 to first m players in circle \n li[start][0] += 1\n start = (start + 1) % l_\n\n if m < len(li): # if there's anyone left from adding 1 if so..\n k = start\n while li[k][1] != prev: # adding 2 to remaining players\n li[k][0] += 2\n k = (k + 1) % l_\n \n li[start][0] += li.pop((start - 1) % l_)[0] # add last person who receives last 1 coin to next person\n start = [(start - 1) % l_ ,start][start==0]\n return tuple(li[0][::-1]) # return last person with score and original number", "def find_last(n, m):\n num = 0\n for i in range(2, n+1): num = (num + m) % i\n return num+1, n*(n+1)+m*(m-2)-n*m", "from collections import deque\n\ndef person(n, m):\n q = deque(range(n))\n for i in range(n):\n q.rotate(1-m)\n x = q.popleft()\n return x + 1\n\ndef coins(n, m):\n return sum(m + max(i-m, 0) * 2 for i in range(n, 1, -1))\n\ndef find_last(n, m):\n return person(n, m), coins(n, m)", "class Cycle:\n def __init__(self, n):\n self.length = n\n self.h = current = node = Node(1)\n for i in range(2, n+1):\n current.next = Node(i)\n current = current.next\n current.next = node\n \n def minimize(self, n):\n current = Node(0)\n current.next = self.h\n while self.length > 1:\n for i in range((n - 1) % self.length):\n current = current.next\n current.next = current.next.next\n self.length -= 1\n pass\n return current.next.n\n \nclass Node:\n def __init__(self, n):\n self.n = n\n self.next = None\n \ndef find_last(n, m):\n x = n - m\n return (Cycle(n).minimize(m), (1 + x) * x + (n - 1) * m)", "def find_last(n, m):\n people = list(range(1, n + 1))\n coins = 0\n for staying in range(n, 1, -1):\n if staying >= m:\n people = people[m:] + people[:m - 1]\n coins += m + (staying - m) * 2\n else:\n last = (m % staying - 1) % staying\n people = people[last + 1:] + people[:last]\n coins += m\n return people[0], coins", "def find_last(n, m):\n pp=list(range(1,n+1))\n p=0\n while len(pp)>1:\n p=(p+m-1)%len(pp)\n del pp[p]\n s=(n-1)*m+(n-m)*(n-m+1)\n return pp[0],s", "def next_man(people, k):\n while True:\n if people[k] != '.':\n return k\n else:\n if k + 1 >= len(people):\n k = 0\n else:\n k += 1\n\n\ndef find_last(n, m):\n people = [0 for i in range(n)]\n lost_people = n\n k = 0\n\n while lost_people > 1:\n\n counter = 0\n while counter < m:\n if people[k] != '.':\n people[k] += 1\n counter += 1\n if k + 1 >= n:\n k = 0\n else:\n k += 1\n else:\n if k + 1 >= n:\n k = 0\n else:\n k += 1\n if k - 1 < 0:\n sub_money = people[-1]\n people[-1] = '.'\n else:\n sub_money = people[k - 1]\n people[k - 1] = '.'\n k = next_man(people, k)\n people[k] += sub_money\n lost_people -= 1\n\n whom2 = lost_people - m + 1\n flag = k\n if whom2 > 0:\n for i in range(whom2):\n people[k] += 2\n if k + 1 >= n:\n k = 0\n else:\n k += 1\n k = next_man(people, k)\n k = flag\n\n return k+1, people[k]\n", "def find_last(n, m):\n num, i, L = 0, 0, [0]*n\n ni = lambda i: (i+1)%len(L)\n pi = lambda i: (i-1)%len(L)\n for v in range(2, n+1):\n num = (num + m) % v\n seen = set(range(len(L)))\n for _ in range(m):\n L[i] += 1\n seen.discard(i)\n i = ni(i)\n for x in seen: L[x] += 2\n j = pi(i)\n L[i] += L[j]\n del L[j]\n i = pi(i)\n return num+1, L[0]", "from collections import deque\n\ndef find_last(n, m):\n circle = deque(range(1, n+1))\n coin = 0\n for _ in range(1, n):\n coin += m if len(circle) <= m else (2 * len(circle)) - m\n circle.rotate(-(m-1))\n circle.popleft()\n return (circle[0], coin)"] | {"fn_name": "find_last", "inputs": [[5, 1], [8, 3], [75, 34], [82, 49], [73, 38], [86, 71], [61, 17], [42, 38], [29, 5], [64, 49], [61, 20], [88, 52]], "outputs": [[[5, 24]], [[7, 51]], [[35, 4238]], [[48, 5091]], [[61, 3996]], [[10, 6275]], [[26, 3000]], [[12, 1578]], [[28, 740]], [[43, 3327]], [[32, 2922]], [[59, 5856]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,872 |
def find_last(n, m):
|
245f9add176990d59d13a90400efb502 | UNKNOWN | Let's pretend your company just hired your friend from college and paid you a referral bonus. Awesome! To celebrate, you're taking your team out to the terrible dive bar next door and using the referral bonus to buy, and build, the largest three-dimensional beer can pyramid you can. And then probably drink those beers, because let's pretend it's Friday too.
A beer can pyramid will square the number of cans in each level - 1 can in the top level, 4 in the second, 9 in the next, 16, 25...
Complete the beeramid function to return the number of **complete** levels of a beer can pyramid you can make, given the parameters of:
1) your referral bonus, and
2) the price of a beer can
For example: | ["def beeramid(bonus, price):\n beers = bonus // price\n levels = 0\n \n while beers >= (levels + 1) ** 2:\n levels += 1\n beers -= levels ** 2\n \n return levels", "from itertools import count\n\ndef beeramid(bonus, price):\n bonus = max(bonus,0)\n n = bonus//price\n return next(x for x in count(int((n*3)**(1/3)+1),-1) if x*(x+1)*(2*x+1)//6 <= n)", "def beeramid(bonus, price):\n k = bonus // price\n d = (3 * (11664*k*k - 3) ** .5 + 324 * k) ** (1/3)\n n = (d/3 + 1/d - 1) / 2\n return k>0 and int(n.real+1e-12)", "def beeramid(bonus, price):\n i = 0\n while bonus > 0:\n i += 1\n bonus -= price * i**2\n if bonus < 0: i -= 1\n return i", "def beeramid(bonus, price, level=1):\n return 0 if bonus < price * level**2 else 1 + beeramid(bonus - price * level**2, price, level + 1)\n", "def beeramid(bonus, price):\n b = bonus/price; n = 1\n while True:\n if b < n*(n+1)*(2*n+1)/6: \n return n-1; break\n n += 1\n", "def beeramid(bonus, price):\n num_cans = bonus // price\n level = 0\n while num_cans >= 0:\n level += 1\n num_cans -= level*level\n return max(0, level-1)", "def beeramid(bonus, price):\n if bonus<price:\n return 0\n elif bonus==price:\n return 1\n else:\n L=[]\n a=int(bonus//price)+1\n c=1\n for i in range(1,a):\n c += i*i\n if c <= a:\n L.append(i*i)\n b=len(L)\n return b", "def beeramid(bonus, price):\n if bonus<0:\n return 0\n sum=0\n x=1\n c=0\n while bonus/price>=sum:\n sum=sum+x**2\n x+=1\n c+=1\n return c-1", "def level():\n n = 2\n while n:\n yield n ** 2\n n += 1\n\ndef beeramid(bonus, price):\n b, x, sum, n = bonus // price, level(), 1, 0\n while sum <= b:\n sum += next(x)\n n += 1\n return n"] | {"fn_name": "beeramid", "inputs": [[9, 2], [10, 2], [11, 2], [21, 1.5], [454, 5], [455, 5], [4, 4], [3, 4], [0, 4], [-1, 4]], "outputs": [[1], [2], [2], [3], [5], [6], [1], [0], [0], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,967 |
def beeramid(bonus, price):
|
a9425c5253540d09f03214d4d2a94846 | UNKNOWN | Your task is to determine how many files of the copy queue you will be able to save into your Hard Disk Drive. The files must be saved in the order they appear in the queue.
### Input:
* Array of file sizes `(0 <= s <= 100)`
* Capacity of the HD `(0 <= c <= 500)`
### Output:
* Number of files that can be fully saved in the HD.
### Examples:
```
save([4,4,4,3,3], 12) -> 3
# 4+4+4 <= 12, but 4+4+4+3 > 12
```
```
save([4,4,4,3,3], 11) -> 2
# 4+4 <= 11, but 4+4+4 > 11
```
Do not expect any negative or invalid inputs. | ["def save(sizes, hd): \n for i,s in enumerate(sizes):\n if hd < s: return i\n hd -= s\n return len(sizes)", "def save(sizes, hd): \n return save(sizes[:-1], hd) if sum(sizes) > hd else len(sizes)", "from bisect import bisect\nfrom itertools import accumulate\n\n\ndef save(sizes, hd): \n return bisect(list(accumulate(sizes)), hd)", "from itertools import *\n\ndef save(sizes, hd): \n return sum(1 for _ in takewhile(hd.__ge__, accumulate(sizes)))", "def save(sizes, hd): \n return sum(1 for i in range(len(sizes)) if sum(sizes[:i+1])<=hd)", "def save(sizes, hd): \n ab=0\n sum=0\n for i in sizes:\n sum=sum+i\n if sum > hd:\n return ab\n ab+=1\n print(ab)\n return ab", "from itertools import accumulate\n\ndef save(sizes, hd):\n i = 0\n for i, acc in enumerate(accumulate(sizes), 1):\n if acc > hd:\n return i-1\n return i", "def save(s, hd): \n return len([k for i, k in enumerate(s) if sum(s[:i+1]) <= hd])\n", "def save(sizes, hd): \n if sum(sizes) <= hd:\n return len(sizes)\n for i in range(len(sizes)) :\n if sum(sizes[:i+1]) > hd:\n return i\n return 0", "def save(sizes, hd):\n maxsize = 0\n copies = 0\n for i in range(len(sizes)):\n maxsize += sizes[i]\n copies += 1\n if maxsize > hd:\n return i\n return copies"] | {"fn_name": "save", "inputs": [[[4, 4, 4, 3, 3], 12], [[4, 4, 4, 3, 3], 11], [[4, 8, 15, 16, 23, 42], 108], [[13], 13], [[1, 2, 3, 4], 250], [[100], 500], [[11, 13, 15, 17, 19], 8], [[45], 12]], "outputs": [[3], [2], [6], [1], [4], [1], [0], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,414 |
def save(sizes, hd):
|
475da70f540dd11c6134aae54ac4ce6d | UNKNOWN | Given an array of positive integers, replace every element with the least greater element to its right.
If there is no greater element to its right, replace it with -1. For instance, given the array
`[8, 58, 71, 18, 31, 32, 63, 92, 43, 3, 91, 93, 25, 80, 28]`,
the desired output is
`[18, 63, 80, 25, 32, 43, 80, 93, 80, 25, 93, -1, 28, -1, -1]`.
Your task is to create a function "arrayManip()" that takes in an array as its argument, manipulates the array as described above, then return the resulting array.
Note: Return a new array, rather than modifying the passed array. | ["def array_manip(array):\n return [min([a for a in array[i+1:] if a > array[i]], default=-1) for i in range(len(array))]", "def array_manip(array):\n return [min((r for r in array[i:] if r > n), default=-1) for i, n in enumerate(array, 1)]\n", "# This is O(n\u00b2), it's a bad solution\n# You can do it in O(n*log(n)) but I'm too lazy for now\ndef array_manip(array):\n return [min(filter(lambda y: y > x, array[i+1:]), default=-1) for i,x in enumerate(array)]", "def array_manip(array):\n return [min((j for j in array[i+1:] if j > array[i]), default=-1) for i in range(len(array))]", "def array_manip(array):\n return [\n min((x for x in array[i:] if x > n), default=-1)\n for i, n in enumerate(array)]", "def array_manip(array):\n res = []\n for i, n in enumerate(array):\n try:\n res.append(min([x for x in array[i+1:] if x > n]))\n except ValueError:\n res.append(-1)\n return res", "array_manip=lambda a:[min([m for m in a[i+1:]if m>n]or[-1])for i,n in enumerate(a)]", "def array_manip(array):\n result = []\n for i in range(len(array)):\n try:\n cr = array[i]\n mm = sorted(x for x in array[i+1:] if x > cr)[0]\n result.append(mm)\n except:\n result.append(-1)\n return result", "def array_manip(array):\n return [min(filter(lambda a: a > array[i], array[i+1:]), default=-1) for i in range(len(array))]", "class BinaryTree:\n def __init__(self, value, left=None, right=None, parent=None):\n self.value = value\n self.left = left\n self.right = right\n self.parent = parent\n \n def __str__(self):\n if self.left or self.right:\n s = \"(\" + str(self.value)\n sl = self.left or '#'\n sr = self.right or '#'\n for l in str(sl).split(\"\\n\"):\n s += \"\\n \" + l\n for l in str(sr).split(\"\\n\"):\n s += \"\\n \" + l\n return s + \")\"\n else:\n return f\"{self.value}\"\n\n def add(self, val):\n if val < self.value:\n if self.left:\n return self.left.add(val)\n else:\n self.left = BinaryTree(val, parent=self)\n return self.left\n elif val > self.value:\n if self.right:\n return self.right.add(val)\n else:\n self.right = BinaryTree(val, parent=self)\n return self.right\n elif val == self.value:\n return self\n \n def leftmost(self):\n x = self\n while x.left:\n x = x.left\n return x\n\n def nxt(self):\n if self.right:\n return self.right.leftmost().value\n else:\n x = self.parent\n while x and x.value < self.value:\n x = x.parent\n if x:\n return x.value\n \n return -1\n\ndef array_manip(array):\n if not array:\n return []\n \n result = []\n b = BinaryTree(array[-1])\n \n for item in reversed(array):\n result.append(b.add(item).nxt())\n \n return result[::-1]\n \n"] | {"fn_name": "array_manip", "inputs": [[[8, 58, 71, 18, 31, 32, 63, 92, 43, 3, 91, 93, 25, 80, 28]], [[2, 4, 18, 16, 7, 3, 9, 13, 18, 10]]], "outputs": [[[18, 63, 80, 25, 32, 43, 80, 93, 80, 25, 93, -1, 28, -1, -1]], [[3, 7, -1, 18, 9, 9, 10, 18, -1, -1]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,258 |
def array_manip(array):
|
c694d3d6ce66f84ca1b2d9fc3b7c0fa2 | UNKNOWN | Find the longest substring within a string that contains at most 2 unique characters.
```
substring("a") => "a"
substring("aaa") => "aaa"
substring("abacd") => "aba"
substring("abacddcd") => "cddcd"
substring("cefageaacceaccacca") => "accacca"
```
This function will take alphanumeric characters as input.
In cases where there could be more than one correct answer, the first string occurrence should be used. For example, substring('abc') should return 'ab' instead of 'bc'.
Although there are O(N^2) solutions to this problem, you should try to solve this problem in O(N) time. Tests may pass for O(N^2) solutions but, this is not guaranteed.
This question is much harder than some of the other substring questions. It's easy to think that you have a solution and then get hung up on the implementation. | ["def substring(s):\n r, rm = [], []\n for i, x in enumerate(s):\n if x in r or len(set(r)) < 2:\n r += x;\n else:\n if len(r) > len(rm): rm = r[:]\n r = [y for y in r[-1::-1] if y == r[-1]] + [x]\n if len(r) > len(rm): rm = r[:]\n return ''.join(rm)", "def substring(strng):\n best = \"\"\n best_end = \"\"\n for c in strng:\n best_end += c\n while len(set(best_end + c)) > 2:\n best_end = best_end[1:]\n if len(best_end) > len(best):\n best = best_end \n return best", "from itertools import groupby\n\n\ndef substring(strng):\n return max(\n enumerate(iter_candidates(strng, 2)),\n key=lambda ix: (len(ix[1]), -ix[0]),\n )[1]\n\n\ndef iter_candidates(strng, n):\n xs = []\n seen = set()\n for c, grp in groupby(strng):\n if len(seen) >= n and c not in seen:\n yield \"\".join(xs)\n seen.discard(xs[-n][0])\n del xs[:1-n]\n xs.append(\"\".join(grp))\n seen.add(c)\n yield \"\".join(xs)", "REGEX = __import__(\"re\").compile(r\"((.)\\2*)(?=((.)\\4*(?:\\2|\\4)*))\").findall\n\ndef substring(strng):\n if len(set(strng)) <= 2: return strng\n return max((x+y for x,_,y,_ in REGEX(strng)), key=len)", "def substring(stg):\n i, mi, s, ms, l = 0, 0, 3, 2, len(stg)\n while i + s <= l:\n if len(set(stg[i:i+s])) > 2:\n i += 1\n else:\n mi, ms = i, s\n s += 1\n return stg[mi:mi+ms]\n\n", "def substring(strng):\n if not strng or len(strng) <= 2:\n return strng\n\n max_str = ''\n start_ind = 0\n next_ind = 0\n for ind, letter in enumerate(strng):\n if strng[start_ind] != letter:\n if start_ind == next_ind:\n next_ind = ind\n elif len(set(strng[start_ind:ind + 1])) > 2:\n start_ind, next_ind = next_ind, ind\n continue\n\n if ind - start_ind + 1 > len(max_str):\n max_str = strng[start_ind:ind + 1]\n\n return max_str", "def substring(s, n=2):\n substr, letters, indexes = '', [''] * n, {'': 0}\n for i, c in enumerate(s, 1):\n if c not in letters:\n letters = letters[1:] + [c]\n indexes[c] = i-1\n if i - indexes[letters[0]] > len(substr):\n substr = s[indexes[letters[0]]: i]\n return substr", "import re\n\n\ndef substring(s):\n if len(s) < 2:\n return s\n pat = re.compile(r'(.)\\1*(.)(\\1|\\2)*', re.DOTALL)\n return max((pat.search(s, i).group() for i in range(len(s)-1)), key=len)\n", "def substring(s):\n r = []\n for i, x in enumerate(s):\n temp = [x]\n for j in range(i+1, len(s)):\n if s[j] in temp or len(set(temp)) < 2:\n temp.append(s[j])\n else:\n r.append(''.join(temp))\n break\n r.append(''.join(temp))\n return s and max(r, key=len) or ''"] | {"fn_name": "substring", "inputs": [[""], ["a"], ["aa"], ["aaa"], ["ab"], ["aba"], ["abc"], ["abcba"], ["bbacc"], ["ccddeeff"], ["bbacddddcdd"], ["abcddeejabbedsajaajjaajjajajajjajjaaacedajajaj"], ["aaaaaaaaaaaaaaaaaaaabbbbbbbbbbbbbbbbbbbbccccccccccccccccccccddddddddddddddddddddeeeeeeeeeeeeeeeeeeeeffffffffffffffffffffgggggggggggggggggggghhhhhhhhhhhhhhhhhhhhiiiiiiiiiiiiiiiiiiiijjjjjjjjjjjjjjjjjjjjkkkkkkkkkkkkkkkkkkkkllllllllllllllllllllmmmmmmmmmmmmmmmmmmmmnnnnnnnnnnnnnnnnnnnnooooooooooooooooooooppppppppppppppppppppqqqqqqqqqqqqqqqqqqqqrrrrrrrrrrrrrrrrrrrrssssssssssssssssssssttttttttttttttttttttuuuuuuuuuuuuuuuuuuuuvvvvvvvvvvvvvvvvvvvvwwwwwwwwwwwwwwwwwwwwxxxxxxxxxxxxxxxxxxxxyyyyyyyyyyyyyyyyyyyyzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzz"]], "outputs": [[""], ["a"], ["aa"], ["aaa"], ["ab"], ["aba"], ["ab"], ["bcb"], ["bba"], ["ccdd"], ["cddddcdd"], ["ajaajjaajjajajajjajjaaa"], ["yyyyyyyyyyyyyyyyyyyyzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzz"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,009 |
def substring(s):
|
586559ea0869e78bc6ed7646bbcb870d | UNKNOWN | There are 32 letters in the Polish alphabet: 9 vowels and 23 consonants.
Your task is to change the letters with diacritics:
```
ą -> a,
ć -> c,
ę -> e,
ł -> l,
ń -> n,
ó -> o,
ś -> s,
ź -> z,
ż -> z
```
and print out the string without the use of the Polish letters.
For example:
```
"Jędrzej Błądziński" --> "Jedrzej Bladzinski"
``` | ["def correct_polish_letters(s):\n return s.translate(str.maketrans(\"\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c\", \"acelnoszz\"))", "def correct_polish_letters(st):\n l = \"\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c\"\n lt = \"acelnoszz\"\n for i in range(len(l)):\n st = st.replace(l[i], lt[i])\n return st", "def correct_polish_letters(st): \n d={'\u0105':'a',\n '\u0107':'c',\n '\u0119':'e',\n '\u0142':'l',\n '\u0144':'n',\n '\u00f3':'o',\n '\u015b':'s',\n '\u017a':'z',\n '\u017c':'z'}\n return \"\".join(d[c] if c in d else c for c in st)", "correct_polish_letters = lambda st: st.translate(str.maketrans('\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c', 'acelnoszz'))", "def correct_polish_letters(st): \n rules = {\n '\u0105': 'a',\n '\u0107': 'c',\n '\u0119': 'e',\n '\u0142': 'l',\n '\u0144': 'n',\n '\u00f3': 'o',\n '\u015b': 's',\n '\u017a': 'z',\n '\u017c': 'z'\n }\n return ''.join(map(lambda c: rules[c] if c in rules else c, st))", "def correct_polish_letters(stg): \n return stg.translate(str.maketrans(\"\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c\", \"acelnoszz\"))", "def correct_polish_letters(st): \n return st.translate(st.maketrans(\"\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c\", \"acelnoszz\"))\n", "def correct_polish_letters(st): \n alphabet = {'\u0105':'a', '\u0107':'c', '\u0119':'e', '\u0142':'l', '\u0144':'n',\n '\u00f3':'o', '\u015b':'s', '\u017a':'z', '\u017c':'z'}\n \n result = ''\n for i in st:\n if i in alphabet:\n result += alphabet[i]\n else:\n result += i\n \n return result", "def correct_polish_letters(st): \n return st.translate(str.maketrans(\"\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c\", \"acelnoszz\"))", "\nintab = '\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c'\nouttab = 'acelnoszz'\npolish = str.maketrans(intab, outtab)\n\ndef correct_polish_letters(st): \n return st.translate(polish)", "def correct_polish_letters(st): \n new_str =''\n for i in range(0,len(st)):\n \n # print(st)\n if st[i] == '\u0105': new_str+='a'\n elif st[i] == '\u0119' :new_str+='e'\n elif st[i] == '\u0107': new_str+='c'\n elif st[i] == '\u0142': new_str+='l'\n elif st[i] == '\u0144' : new_str+= 'n'\n elif st[i] == '\u00f3': new_str+='o'\n elif st[i] == '\u015b': new_str+='s'\n elif st[i] == '\u017a' : new_str+='z'\n elif st[i] == '\u017c' : new_str+='z'\n\n else : new_str+= st[i]\n return new_str\n\n", "def correct_polish_letters(s):\n pol_to_eng = {\n \"\u0105\": \"a\",\n \"\u0107\": \"c\",\n \"\u0119\": \"e\",\n \"\u0142\": \"l\",\n \"\u0144\": \"n\",\n \"\u00f3\": \"o\",\n \"\u015b\": \"s\",\n \"\u017a\": \"z\",\n \"\u017c\": \"z\",\n }\n return \"\".join(pol_to_eng.get(c) or c for c in s)", "import re\n\ndef correct_polish_letters(s):\n pol_to_eng = {\n \"\u0105\": \"a\",\n \"\u0107\": \"c\",\n \"\u0119\": \"e\",\n \"\u0142\": \"l\",\n \"\u0144\": \"n\",\n \"\u00f3\": \"o\",\n \"\u015b\": \"s\",\n \"\u017a\": \"z\",\n \"\u017c\": \"z\",\n }\n return re.sub(\n r\"[\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c]\",\n lambda matchobj: pol_to_eng[matchobj.group()],\n s\n )", "def correct_polish_letters(str_: str) -> str:\n \"\"\" Change the letters with diacritics. \"\"\"\n return str_.translate(\n str.maketrans(\n {\n \"\u0105\": \"a\",\n \"\u0107\": \"c\",\n \"\u0119\": \"e\",\n \"\u0142\": \"l\",\n \"\u0144\": \"n\",\n \"\u00f3\": \"o\",\n \"\u015b\": \"s\",\n \"\u017a\": \"z\",\n \"\u017c\": \"z\"\n }\n )\n )", "import unicodedata\n\ndef correct_polish_letters(st): \n mapper = {\"\u0105\": \"a\", \"\u0107\": \"c\", \"\u0119\": \"e\", \"\u0142\": \"l\", \"\u0144\": \"n\", \"\u00f3\": \"o\", \"\u015b\": \"s\", \"\u017a\": \"z\", \"\u017c\": \"z\"}\n\n return ''.join([mapper[c] if c in mapper else c for c in st])", "correct_polish_letters = lambda s: ''.join({'\u0105': 'a', '\u0107': 'c', '\u0119': 'e',\n '\u0142': 'l', '\u0144': 'n', '\u00f3': 'o',\n '\u015b': 's', '\u017a': 'z', '\u017c': 'z'}.get(c, c) for c in s)", "def correct_polish_letters(st):\n letters = {\"\u0105\":\"a\",\n \"\u0107\":\"c\",\n \"\u0119\":\"e\",\n \"\u0142\":\"l\",\n \"\u0144\":\"n\",\n \"\u00f3\":\"o\",\n \"\u015b\":\"s\",\n \"\u017a\":\"z\",\n \"\u017c\":\"z\"}\n result = \"\"\n for s in st:\n if s in letters:\n result = result + letters.get(s)\n else:\n result = result + s\n return result", "correct_polish_letters = lambda s: s.translate(str.maketrans(\"\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c\", \"acelnoszz\")) ", "def correct_polish_letters(st): \n replace = {\n '\u0105': 'a',\n '\u0107': 'c',\n '\u0119': 'e',\n '\u0142': 'l',\n '\u0144': 'n',\n '\u00f3': 'o',\n '\u015b': 's',\n '\u017a': 'z',\n '\u017c': 'z'\n }\n return ''.join(replace.get(letter, letter) for letter in st)", "def correct_polish_letters(st): \n\n if '\u0105' in st:\n st = st.replace('\u0105','a')\n if '\u0107' in st:\n st = st.replace('\u0107','c')\n if '\u0119' in st:\n st = st.replace('\u0119','e')\n if '\u0142' in st:\n st = st.replace('\u0142','l')\n if '\u0144' in st:\n st = st.replace('\u0144','n')\n if '\u00f3' in st:\n st = st.replace('\u00f3','o')\n if '\u015b' in st:\n st = st.replace('\u015b','s')\n if '\u017a' in st:\n st = st.replace('\u017a','z')\n if '\u017c' in st:\n st = st.replace('\u017c','z')\n \n return st\n \n", "dic = {\n \"\u0105\" : \"a\",\n \"\u0107\" : \"c\",\n \"\u0119\" : \"e\",\n \"\u0142\" : \"l\",\n \"\u0144\" : \"n\",\n \"\u00f3\" : \"o\",\n \"\u015b\" : \"s\",\n \"\u017a\" : \"z\",\n \"\u017c\" : \"z\"\n}\n\ndef correct_polish_letters(st): \n for key, val in list(dic.items()):\n if key in st:\n st = st.replace(key, val)\n return st\n", "db = {\n'\u0105': 'a',\n'\u0107': 'c',\n'\u0119': 'e',\n'\u0142': 'l',\n'\u0144': 'n',\n'\u00f3': 'o',\n'\u015b': 's',\n'\u017a': 'z',\n'\u017c': 'z', \n}\ndef correct_polish_letters(st): \n return ''.join((db.get(i, i) for i in st))\n", "def correct_polish_letters(st): \n map = {\n '\u0105': 'a',\n '\u0107': 'c',\n '\u0119': 'e',\n '\u0142': 'l',\n '\u0144': 'n',\n '\u00f3': 'o',\n '\u015b': 's',\n '\u017a': 'z',\n '\u017c': 'z',\n }\n for key in map.keys():\n st = st.replace(key, map[key])\n return st", "def correct_polish_letters(st): \n dict = {\"\u0105\": \"a\", \"\u0107\": \"c\", \"\u0119\": \"e\", \"\u0142\": \"l\", \"\u0144\": \"n\", \"\u00f3\": \"o\", \"\u015b\": \"s\", \"\u017a\": \"z\", \"\u017c\": \"z\"}\n output = \"\"\n \n for x in st:\n if x in dict:\n output += dict[x]\n else:\n output += x\n \n return output ", "def correct_polish_letters(st): \n polish = ['\u0105', '\u0107', '\u0119', '\u0142', '\u0144', '\u00f3', '\u015b', '\u017a', '\u017c']\n changeTo = ['a', 'c', 'e', 'l', 'n', 'o', 's', 'z', 'z']\n converted = []\n for letter in st:\n if letter in polish:\n i = polish.index(letter)\n converted.append(changeTo[i])\n else:\n converted.append(letter)\n return ''.join(converted)\n", "def correct_polish_letters(st):\n trans = {\"\u0105\": \"a\", \"\u0107\": \"c\", \"\u0119\": \"e\", \"\u0142\": \"l\", \"\u0144\": \"n\", \n \"\u00f3\": \"o\", \"\u015b\": \"s\", \"\u017a\": \"z\", \"\u017c\": \"z\"}\n return \"\".join(trans.get(s, s) for s in st)", "def correct_polish_letters(st): \n st2=''\n arr=[]\n for i in st:\n arr.append(i)\n letters=[['\u0105', '\u0107', '\u0119', '\u0142', '\u0144', '\u00f3', '\u015b', '\u017a', '\u017c'], ['a', 'c', 'e', 'l', 'n', 'o', 's', 'z', 'z']]\n for i in range(len(arr)):\n for j in range(len(letters[0])):\n if arr[i]==letters[0][j]:\n arr[i]=letters[1][j]\n for i in arr:\n st2+=i\n return st2", "def correct_polish_letters(st): \n letters_dict = {'\u0105': 'a', '\u0107': 'c', '\u0119': 'e', '\u0142': 'l', '\u0144': 'n', '\u00f3': 'o', '\u015b': 's', '\u017a': 'z', '\u017c': 'z'}\n eng_string = ''\n for letter in st:\n if letter in letters_dict.keys():\n eng_string += letters_dict[letter]\n else:\n eng_string += letter\n return eng_string", "def correct_polish_letters(st): \n dict = {\n '\u0105' : 'a',\n '\u0107' : 'c',\n '\u0119' : 'e',\n '\u0142' : 'l',\n '\u0144' : 'n',\n '\u00f3' : 'o',\n '\u015b' : 's',\n '\u017a' : 'z',\n '\u017c' : 'z'\n }\n return ''.join(dict.get(c, c) for c in st)", "def correct_polish_letters(st): \n change = str.maketrans('\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017cz', 'acelnoszzz')\n return st.translate(change)", "def correct_polish_letters(st): \n let = {\n \"\u0105\":\"a\",\n \"\u0107\":\"c\",\n \"\u0119\":\"e\",\n \"\u0142\":\"l\",\n \"\u0144\":\"n\",\n \"\u00f3\":\"o\",\n \"\u015b\":\"s\",\n \"\u017a\":\"z\",\n \"\u017c\":\"z\"\n }\n new = \"\"\n for i in st:\n if i in let:\n new += let[i]\n else:\n new += i\n \n return new", "def correct_polish_letters(text): \n x = {'\u0105': 'a', '\u0107': 'c', '\u0119': 'e', '\u0142': 'l', '\u0144': 'n', '\u00f3': 'o', '\u015b': 's', '\u017a': 'z', '\u017c': 'z'}\n \n for key in list(x.keys()):\n text = text.replace(key, str(x[key]))\n return text\n \n", "def correct_polish_letters(st): \n polish = {\n '\u0105':'a',\n '\u0107':'c',\n '\u0119':'e',\n '\u0142':'l',\n '\u0144':'n',\n '\u00f3':'o',\n '\u015b':'s',\n '\u017a':'z',\n '\u017c':'z'\n } \n return \"\".join([polish.get(char, char) for char in st])\n", "def correct_polish_letters(st): \n d = {'\u0105':'a', '\u0107':'c', '\u0119':'e', '\u0142':'l', '\u0144':'n',\n '\u00f3':'o', '\u015b':'s', '\u017a':'z', '\u017c':'z'}\n ans = ''\n for char in st:\n if char in d:\n ans += d[char]\n else:\n ans += char\n return ans", "import unicodedata\n\nNON_NFKD_MAP = {'\\u0181': 'B', '\\u1d81': 'd', '\\u1d85': 'l', '\\u1d89': 'r', '\\u028b': 'v', '\\u1d8d': 'x', '\\u1d83': 'g', '\\u0191': 'F', '\\u0199': 'k', '\\u019d': 'N', '\\u0220': 'N', '\\u01a5': 'p', '\\u0224': 'Z', '\\u0126': 'H', '\\u01ad': 't', '\\u01b5': 'Z', '\\u0234': 'l', '\\u023c': 'c', '\\u0240': 'z', '\\u0142': 'l', '\\u0244': 'U', '\\u2c60': 'L', '\\u0248': 'J', '\\ua74a': 'O', '\\u024c': 'R', '\\ua752': 'P', '\\ua756': 'Q', '\\ua75a': 'R', '\\ua75e': 'V', '\\u0260': 'g', '\\u01e5': 'g', '\\u2c64': 'R', '\\u0166': 'T', '\\u0268': 'i', '\\u2c66': 't', '\\u026c': 'l', '\\u1d6e': 'f', '\\u1d87': 'n', '\\u1d72': 'r', '\\u2c74': 'v', '\\u1d76': 'z', '\\u2c78': 'e', '\\u027c': 'r', '\\u1eff': 'y', '\\ua741': 'k', '\\u0182': 'B', '\\u1d86': 'm', '\\u0288': 't', '\\u018a': 'D', '\\u1d8e': 'z', '\\u0111': 'd', '\\u0290': 'z', '\\u0192': 'f', '\\u1d96': 'i', '\\u019a': 'l', '\\u019e': 'n', '\\u1d88': 'p', '\\u02a0': 'q', '\\u01ae': 'T', '\\u01b2': 'V', '\\u01b6': 'z', '\\u023b': 'C', '\\u023f': 's', '\\u0141': 'L', '\\u0243': 'B', '\\ua745': 'k', '\\u0247': 'e', '\\ua749': 'l', '\\u024b': 'q', '\\ua74d': 'o', '\\u024f': 'y', '\\ua751': 'p', '\\u0253': 'b', '\\ua755': 'p', '\\u0257': 'd', '\\ua759': 'q', '\\xd8': 'O', '\\u2c63': 'P', '\\u2c67': 'H', '\\u026b': 'l', '\\u1d6d': 'd', '\\u1d71': 'p', '\\u0273': 'n', '\\u1d75': 't', '\\u1d91': 'd', '\\xf8': 'o', '\\u2c7e': 'S', '\\u1d7d': 'p', '\\u2c7f': 'Z', '\\u0183': 'b', '\\u0187': 'C', '\\u1d80': 'b', '\\u0289': 'u', '\\u018b': 'D', '\\u1d8f': 'a', '\\u0291': 'z', '\\u0110': 'D', '\\u0193': 'G', '\\u1d82': 'f', '\\u0197': 'I', '\\u029d': 'j', '\\u019f': 'O', '\\u2c6c': 'z', '\\u01ab': 't', '\\u01b3': 'Y', '\\u0236': 't', '\\u023a': 'A', '\\u023e': 'T', '\\ua740': 'K', '\\u1d8a': 's', '\\ua744': 'K', '\\u0246': 'E', '\\ua748': 'L', '\\ua74c': 'O', '\\u024e': 'Y', '\\ua750': 'P', '\\ua754': 'P', '\\u0256': 'd', '\\ua758': 'Q', '\\u2c62': 'L', '\\u0266': 'h', '\\u2c73': 'w', '\\u2c6a': 'k', '\\u1d6c': 'b', '\\u2c6e': 'M', '\\u1d70': 'n', '\\u0272': 'n', '\\u1d92': 'e', '\\u1d74': 's', '\\u2c7a': 'o', '\\u2c6b': 'Z', '\\u027e': 'r', '\\u0180': 'b', '\\u0282': 's', '\\u1d84': 'k', '\\u0188': 'c', '\\u018c': 'd', '\\ua742': 'K', '\\u1d99': 'u', '\\u0198': 'K', '\\u1d8c': 'v', '\\u0221': 'd', '\\u2c71': 'v', '\\u0225': 'z', '\\u01a4': 'P', '\\u0127': 'h', '\\u01ac': 'T', '\\u0235': 'n', '\\u01b4': 'y', '\\u2c72': 'W', '\\u023d': 'L', '\\ua743': 'k', '\\u0249': 'j', '\\ua74b': 'o', '\\u024d': 'r', '\\ua753': 'p', '\\u0255': 'c', '\\ua757': 'q', '\\u2c68': 'h', '\\ua75b': 'r', '\\ua75f': 'v', '\\u2c61': 'l', '\\u2c65': 'a', '\\u01e4': 'G', '\\u0167': 't', '\\u2c69': 'K', '\\u026d': 'l', '\\u1d6f': 'm', '\\u0271': 'm', '\\u1d73': 'r', '\\u027d': 'r', '\\u1efe': 'Y'}\n\ndef correct_polish_letters(st):\n\n if not isinstance(st, str):\n st = str(st)\n\n return ''.join(NON_NFKD_MAP[c] if c in NON_NFKD_MAP else c \\\n for part in unicodedata.normalize('NFKD', st) for c in part\n if unicodedata.category(part) != 'Mn')\n\n", "def correct_polish_letters(st): \n result = \"\"\n alphabet = { \"\u0105\": \"a\",\n \"\u0107\": \"c\",\n \"\u0119\": \"e\",\n \"\u0142\": \"l\",\n \"\u0144\": \"n\",\n \"\u00f3\": \"o\",\n \"\u015b\": \"s\",\n \"\u017a\": \"z\",\n \"\u017c\": \"z\"}\n \n for n in st:\n if n in alphabet:\n result += alphabet[n]\n else:\n result += n\n \n return result\n \n \n", "def correct_polish_letters(st): \n polish = '\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c'\n english = 'acelnoszz'\n for i in range(9):\n st = st.replace(polish[i], english[i])\n return st", "def correct_polish_letters(st): \n polish, latin = '\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c', 'acelnoszz'\n for i in polish:\n st = st.replace(i, latin[polish.find(i)])\n return st", "letter_corr = {\n \"\u0105\": \"a\",\n \"\u0107\": \"c\",\n \"\u0119\": \"e\",\n \"\u0142\": \"l\",\n \"\u0144\": \"n\",\n \"\u00f3\": \"o\",\n \"\u015b\": \"s\",\n \"\u017a\": \"z\",\n \"\u017c\": \"z\" \n}\n\n\ndef correct_polish_letters(st):\n l = []\n for i in st:\n l.append(letter_corr.get(i, i))\n return ''.join(l)", "def correct_polish_letters(st): \n PolishDict = {\n \"\u0105\" : \"a\",\n \"\u0107\" : \"c\",\n \"\u0119\" : \"e\",\n \"\u0142\" : \"l\",\n \"\u0144\" : \"n\",\n \"\u00f3\" : \"o\",\n \"\u015b\" : \"s\",\n \"\u017a\" : \"z\",\n \"\u017c\" : \"z\"\n }\n newStr = \"\"\n for i in range(len(st)):\n if st[i] in PolishDict:\n newStr += PolishDict[st[i]]\n else:\n newStr += st[i]\n return newStr", "def correct_polish_letters(st): \n dict = {\n '\u0105': 'a',\n '\u0105': 'a',\n '\u0107': 'c',\n '\u0119': 'e',\n '\u0142': 'l',\n '\u0144': 'n',\n '\u00f3': 'o',\n '\u015b': 's',\n '\u017a': 'z',\n '\u017c': 'z'\n }\n str = ''\n for letter in st:\n if letter in dict:\n str += dict[letter]\n else:\n str += letter\n return str", "correct_polish_letters = lambda st: ''.join([{'\u0105':'a','\u0107':'c','\u0119':'e','\u0142':'l','\u0144':'n','\u00f3':'o','\u015b':'s','\u017a':'z','\u017c':'z'}.get(i,i) for i in st])", "def correct_polish_letters(st): \n polish_alphabet = {\n '\u0105' : 'a',\n '\u0107' : 'c',\n '\u0119' : 'e',\n '\u0142' : 'l',\n '\u0144' : 'n',\n '\u00f3' : 'o',\n '\u015b' : 's',\n '\u017a' : 'z',\n '\u017c' : 'z'\n }\n return ''.join([polish_alphabet.get(i) if i in polish_alphabet else i for i in st])", "def correct_polish_letters(st): \n polish={'\u0105':'a','\u0107':'c','\u0119':'e','\u0142':'l','\u0144':'n','\u00f3':'o','\u015b':'s','\u017a':'z','\u017c':'z'}\n new_string=\"\"\n for i in st:\n if i in polish:\n new_string+=(polish[i])\n else:\n new_string+=i\n return new_string\n", "def correct_polish_letters(st): \n new_string = \"\"\n eng = \"acelnoszz\"\n pol = \"\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c\"\n \n for i in range(len(st)):\n if st[i] in pol:\n new_string += eng[pol.index(st[i])]\n else:\n new_string += st[i]\n \n return new_string", "def correct_polish_letters(st): \n stt=st.replace(\"\u0105\", \"a\").replace(\"\u0107\", \"c\").replace(\"\u0119\", \"e\").replace(\"\u0142\", \"l\").replace(\"\u0144\", \"n\").replace(\"\u00f3\", \"o\").replace(\"\u015b\", \"s\").replace(\"\u017a\", \"z\").replace(\"\u017c\", \"z\")\n return stt", "def correct_polish_letters(st): \n dict = {\n '\u0105': 'a',\n '\u0107': 'c',\n '\u0119': 'e',\n '\u0142': 'l',\n '\u0144': 'n',\n '\u00f3': 'o',\n '\u015b': 's',\n '\u017a': 'z',\n '\u017c': 'z'\n }\n for c in st:\n if c in dict:\n st = st.replace(c, dict[c])\n return st", "def correct_polish_letters(st):\n diacritic = {'\u0105' : 'a',\n '\u0107' : 'c',\n '\u0119' : 'e',\n '\u0142' : 'l',\n '\u0144' : 'n',\n '\u00f3' : 'o',\n '\u015b' : 's',\n '\u017a' : 'z',\n '\u017c' : 'z'}\n return ''.join([char.replace(char, diacritic[char]) if char in diacritic.keys() else char for char in st])", "def correct_polish_letters(st): \n alp = {'\u0105': 'a', '\u0107': 'c', '\u0119': 'e', '\u0142': 'l', '\u0144': 'n', '\u00f3': 'o', '\u015b': 's', '\u017a': 'z', '\u017c': 'z'}\n for key in alp.keys():\n st = st.replace(key, alp[key])\n return st", "def correct_polish_letters(st): \n for p,e in {\"\u0105\":\"a\",\"\u0107\":\"c\",\"\u0119\":\"e\",\"\u0142\":\"l\",\"\u0144\":\"n\",\"\u00f3\":\"o\",\"\u015b\":\"s\",\"\u017a\":\"z\",\"\u017c\":\"z\"}.items():\n st=st.replace(p,e)\n return st", "def correct_polish_letters(st): \n check = {\"\u0105\" : \"a\",\n \"\u0107\" : \"c\",\n \"\u0119\" : \"e\",\n \"\u0142\" : \"l\",\n \"\u0144\" : \"n\",\n \"\u00f3\" : \"o\",\n \"\u015b\" : \"s\",\n \"\u017a\" : \"z\",\n \"\u017c\" : \"z\"}\n str = \"\"\n for i in range(len(st)):\n if st[i] in check.keys():\n str += check.get(st[i])\n else:\n str += st[i]\n return str", "def correct_polish_letters(st):\n d = {\n '\u0105': 'a', '\u0107': 'c', '\u0119': 'e',\n '\u0142': 'l', '\u0144': 'n', '\u00f3': 'o',\n '\u015b': 's', '\u017a': 'z', '\u017c': 'z'\n }\n for k in d:\n st = st.replace(k, d[k])\n return st", "def correct_polish_letters(st): \n alp = \"\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c\"\n alpc = \"acelnoszz\"\n return st.translate(st.maketrans(alp, alpc))", "def correct_polish_letters(st): \n base = {'\u0105':'a',\n '\u0107':'c',\n '\u0119':'e',\n '\u0142':'l',\n '\u0144':'n',\n '\u00f3':'o',\n '\u015b':'s',\n '\u017a':'z',\n '\u017c':'z'}\n \n return ''.join([base[i] if i in base else i for i in st])", "def correct_polish_letters(st):\n diacritics = {\n \"\u0105\": \"a\",\n \"\u0107\": \"c\",\n \"\u0119\": \"e\",\n \"\u0142\": \"l\",\n \"\u0144\": \"n\",\n \"\u00f3\": \"o\",\n \"\u015b\": \"s\",\n \"\u017a\": \"z\",\n \"\u017c\": \"z\"\n }\n return \"\".join([diacritics.get(c) if diacritics.get(c) else c for c in st])", "def correct_polish_letters(st): \n letters = {\n \"\u0105\": \"a\",\n \"\u0107\": \"c\",\n \"\u0119\": \"e\",\n \"\u0142\": \"l\",\n \"\u0144\": \"n\",\n \"\u00f3\": \"o\",\n \"\u015b\": \"s\", \n \"\u017a\": \"z\",\n \"\u017c\": \"z\"\n }\n result = \"\"\n for i in st:\n if i in letters:\n result += letters[i]\n else:\n result += i\n return result", "def correct_polish_letters(st): \n Polish_alphabet = {'\u0105': 'a', '\u0107': 'c', '\u0119': 'e', '\u0142': 'l', '\u0144': 'n', '\u00f3': 'o', '\u015b': 's', '\u017a': 'z', '\u017c': 'z'}\n result = ''\n for el in st:\n result += Polish_alphabet.get(el, el)\n return result", "trans = {'\u0105':'a','\u0107':'c','\u0119':'e','\u0142':'l','\u0144':'n','\u00f3':'o','\u015b':'s','\u017a':'z','\u017c':'z'}\n\ndef correct_polish_letters(st): \n empty = []\n for char in st:\n if char not in trans:\n empty.append(char)\n elif char in trans:\n empty.append(trans[char])\n \n return ''.join(empty)", "def correct_polish_letters(st): \n answer = \"\"\n d= {\n \"\u0105\":\"a\",\n \"\u0107\":\"c\",\n \"\u0119\":\"e\",\n \"\u0142\":\"l\",\n \"\u0144\":\"n\",\n \"\u00f3\":\"o\",\n \"\u015b\":\"s\",\n \"\u017a\":\"z\",\n \"\u017c\":\"z\"\n }\n lst = [\"\u0105\",\"\u0107\",\"\u0119\",\"\u0142\",\"\u0144\",\"\u00f3\",\"\u015b\",\"\u017a\",\"\u017c\"]\n \n for c in st:\n if c in lst:\n answer += d.get(c)\n else: \n answer += c\n return answer", "def correct_polish_letters(st): \n dict = { \"\u0105\" : \"a\",\n\"\u0107\" : \"c\",\n\"\u0119\" : \"e\",\n\"\u0142\" : \"l\",\n\"\u0144\" : \"n\",\n\"\u00f3\" : \"o\",\n\"\u015b\" : \"s\",\n\"\u017a\" : \"z\",\n\"\u017c\" : \"z\" }\n st2= \"\"\n for ch in st:\n if ch in dict:\n st2= st2+(dict[ch])\n else:\n st2= st2+(ch)\n \n \n return st2\n", "def correct_polish_letters(st): \n result = \"\"\n for letter in st:\n if letter == \"\u0105\":\n result += \"a\"\n elif letter == \"\u0107\":\n result += \"c\"\n elif letter == \"\u0119\":\n result += \"e\"\n elif letter == \"\u0142\":\n result += \"l\"\n elif letter == \"\u0144\":\n result += \"n\"\n elif letter == \"\u00f3\":\n result += \"o\"\n elif letter == \"\u015b\":\n result += \"s\"\n elif letter == \"\u017a\":\n result += \"z\"\n elif letter == \"\u017c\":\n result += \"z\" \n else:\n result += letter\n return result\n \n # your code here\n pass", "def correct_polish_letters(st): \n alphabet = {\n \"\u0105\": \"a\",\n \"\u0107\": \"c\",\n \"\u0119\": \"e\",\n \"\u0142\": \"l\",\n \"\u0144\": \"n\",\n \"\u00f3\": \"o\",\n \"\u015b\": \"s\",\n \"\u017a\": \"z\",\n \"\u017c\": \"z\",\n }\n new_str = ''\n for c in list(st):\n if c in alphabet:\n new_str += alphabet[c]\n else:\n new_str += c\n return new_str", "def correct_polish_letters(st): \n letters = {\n '\u0105': 'a',\n '\u0107': 'c',\n '\u0119': 'e',\n '\u0142': 'l',\n '\u0144': 'n',\n '\u00f3': 'o',\n '\u015b': 's',\n '\u017a': 'z',\n '\u017c': 'z'\n }\n \n for l in st:\n if l in letters:\n st = st.replace(l, letters[l])\n return st", "X = {\n \"\u0105\": \"a\",\n \"\u0107\": \"c\",\n \"\u0119\": \"e\",\n \"\u0142\": \"l\",\n \"\u0144\": \"n\",\n \"\u00f3\": \"o\",\n \"\u015b\": \"s\",\n \"\u017a\": \"z\",\n \"\u017c\": \"z\"\n}\ndef correct_polish_letters(st):\n for x in X: st = st.replace(x, X[x])\n return st", "def correct_polish_letters(st): \n letters_dict = {\n '\u0105': 'a',\n '\u0107': 'c',\n '\u0119': 'e',\n '\u0142': 'l',\n '\u0144': 'n',\n '\u00f3': 'o',\n '\u015b': 's',\n '\u017a': 'z',\n '\u017c': 'z'\n }\n result = ''\n for letter in st:\n if letter in letters_dict.keys():\n result += letters_dict[letter]\n else:\n result += letter\n return result", "polish_letter = {\"\u0105\": \"a\",\n\"\u0107\": \"c\",\n\"\u0119\": \"e\",\n\"\u0142\": \"l\",\n\"\u0144\": \"n\",\n\"\u00f3\": \"o\",\n\"\u015b\": \"s\",\n\"\u017a\": \"z\",\n\"\u017c\": \"z\"}\n\ndef correct_polish_letters(st):\n new_list = []\n for letter in st:\n if letter in polish_letter:\n letter = polish_letter[letter]\n new_list.append(letter)\n return \"\".join(new_list)\n", "def correct_polish_letters(st): \n s,p,n=\"\",[\"\u0105\",\"\u0107\",\"\u0119\",\"\u0142\",\"\u0144\",\"\u00f3\",\"\u015b\",\"\u017a\",\"\u017c\"],[\"a\",\"c\",\"e\",\"l\",\"n\",\"o\",\"s\",\"z\",\"z\"]\n for i in list(st):\n if i in p: \n s+=n[p.index(i)]\n else: s+=i\n return s", "ALPHABET = {\"\u0105\": \"a\",\n \"\u0107\": \"c\",\n \"\u0119\": \"e\",\n \"\u0142\": \"l\",\n \"\u0144\": \"n\",\n \"\u00f3\": \"o\",\n \"\u015b\": \"s\",\n \"\u017a\": \"z\",\n \"\u017c\": \"z\"}\n\n\ndef correct_polish_letters(st): \n\n result = \"\"\n for char in st:\n if (char in ALPHABET):\n result += ALPHABET[char]\n else:\n result += char\n\n return result\n", "def correct_polish_letters(st): \n new = ''\n for x in st:\n if x == '\u0105':\n new += 'a'\n elif x == '\u0107':\n new += 'c'\n elif x == '\u0119':\n new += 'e'\n elif x == '\u0142':\n new += 'l'\n elif x == '\u0144':\n new += 'n' \n elif x == '\u00f3':\n new += 'o' \n elif x == '\u015b':\n new += 's' \n elif x == '\u017a':\n new += 'z'\n elif x == '\u017c':\n new += 'z' \n else:\n new += x\n return new\n\n \n \n", "import re\npolist_letters = {\n '\u0105': 'a',\n '\u0107': 'c',\n '\u0119': 'e',\n '\u0142': 'l',\n '\u0144': 'n',\n '\u00f3': 'o',\n '\u015b': 's',\n '\u017a': 'z',\n '\u017c': 'z'\n}\n\ndef correct_polish_letters(st): \n my_st = st\n for l in polist_letters:\n my_st = re.sub(l, polist_letters.get(l), my_st)\n return my_st\n", "def correct_polish_letters(st):\n # your code here\n d = {'\u0105': 'a', '\u0107': 'c', '\u0119': 'e', '\u0142': 'l', '\u0144': 'n', '\u00f3': 'o', '\u015b': 's', '\u017a': 'z', '\u017c': 'z'}\n return ''.join(map(lambda x: x.replace(x, d.get(x, x)), st))", "def correct_polish_letters(st): \n polish_letters = \"\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c\"\n english_letters = \"acelnoszz\"\n table = st.maketrans(polish_letters, english_letters) \n return st.translate(table)", "def correct_polish_letters(st): \n table = st.maketrans(\"\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c\",\"acelnoszz\")\n st = st.translate(table)\n return st", "def correct_polish_letters(st): \n translation = { '\u0105' : 'a',\n '\u0107' : 'c',\n '\u0119' : 'e',\n '\u0142' : 'l',\n '\u0144' : 'n',\n '\u00f3' : 'o',\n '\u015b' : 's',\n '\u017a' : 'z',\n '\u017c' : 'z' }\n output = ''\n for x in st:\n if x in translation:\n output += translation.get(x)\n else:\n output += x\n return output", "def correct_polish_letters(st):\n dict = {'\u0105' : 'a', '\u0107' : 'c','\u0119' : 'e', '\u0142' : 'l', '\u0144' : 'n', '\u00f3' : 'o', '\u015b' : 's', '\u017a' : 'z', '\u017c' : 'z'}\n return ''.join([dict[x] if x in dict.keys() else x for x in st])", "def correct_polish_letters(string):\n dic = {\"\u0105\": \"a\",\n \"\u0107\": \"c\",\n \"\u0119\": \"e\",\n \"\u0142\": \"l\",\n \"\u0144\": \"n\",\n \"\u00f3\": \"o\",\n \"\u015b\": \"s\",\n \"\u017a\": \"z\",\n \"\u017c\": \"z\"}\n return \"\".join(dic.get(l, l) for l in string)", "def correct_polish_letters(st): \n dict = {\n '\u0105': 'a',\n '\u0107': 'c',\n '\u0119': 'e',\n '\u0142': 'l',\n '\u0144': 'n',\n '\u00f3': 'o',\n '\u015b': 's',\n '\u017a': 'z',\n '\u017c': 'z' \n }\n for key in dict.keys():\n st = st.replace(key, str(dict[key]))\n \n return st", "def correct_polish_letters(st): \n res = \"\"\n \n letters = { \"\u0105\": \"a\", \"\u0107\": \"c\", \"\u0119\": \"e\", \"\u0142\": \"l\", \"\u0144\": \"n\", \n \"\u00f3\": \"o\", \"\u015b\": \"s\", \"\u017a\": \"z\", \"\u017c\": \"z\" }\n \n for elem in st:\n for letter in elem:\n if letter in letters:\n res += letters[letter]\n else:\n res += elem\n \n return res ", "def correct_polish_letters(st): \n d= {\n '\u0105' : 'a',\n '\u0107' : 'c',\n '\u0119' : 'e',\n '\u0142' : 'l',\n '\u0144' : 'n',\n '\u00f3' : 'o',\n '\u015b' : 's',\n '\u017a' : 'z',\n '\u017c' : 'z'\n }\n output=\"\"\n for i in st:\n output += d.get(i,i)\n return output", "def correct_polish_letters(st): \n list=[]\n dict={\"\u0105\":\"a\",\n \"\u0107\":\"c\",\n \"\u0119\":\"e\",\n \"\u0142\":\"l\",\n \"\u0144\":\"n\",\n \"\u00f3\":\"o\",\n \"\u015b\":\"s\",\n \"\u017a\":\"z\",\n \"\u017c\":\"z\"}\n for i in st:\n if i in dict.keys():\n list.append(dict[i])\n elif st.isspace():\n list.append(\" \")\n else:\n list.append(i)\n return \"\".join(list)", "def correct_polish_letters(st): \n # your code here\n alf={' ':' ','\u0105':'a','\u0107':'c','\u0119':'e','\u0142':'l','\u0144':'n','\u00f3':'o','\u015b':'s','\u017a':'z','\u017c':'z'}\n return ''.join(alf[i] if i in alf else i for i in st)", "def correct_polish_letters(st): \n d = {'\u0105': 'a', '\u0107': 'c', '\u0119': 'e', '\u0142': 'l', '\u0144': 'n', '\u00f3': 'o', '\u015b': 's', '\u017a': 'z', '\u017c': 'z'}\n r = ''\n for letter in st:\n if letter in d:\n r += d[letter]\n else:\n r += letter\n return r", "def correct_polish_letters(st): \n dict={'\u0105':'a','\u0107':'c','\u0119':'e','\u0142':'l','\u0144':'n','\u00f3':'o','\u015b':'s','\u017a':'z','\u017c':'z'}\n return ''.join(dict.get(char,char) for char in st)\n", "def correct_polish_letters(st): \n dict={'\u0105':'a','\u0107':'c','\u0119':'e','\u0142':'l','\u0144':'n','\u00f3':'o','\u015b':'s','\u017a':'z','\u017c':'z'}\n return ''.join(list(dict.get(char,char) for char in st))\n\n", "def correct_polish_letters(st): \n pol = {'\u0105': 'a',\n '\u0107': 'c',\n '\u0119': 'e',\n '\u0142': 'l',\n '\u0144': 'n',\n '\u00f3': 'o',\n '\u015b': 's',\n '\u017a': 'z',\n '\u017c': 'z'}\n \n st2 = \"\"\n \n for c in st:\n if c in pol:\n st2 += pol[c]\n \n else:\n st2 += c\n \n return st2", "def correct_polish_letters(st): \n dict = {\"\u0105\" : \"a\",\n \"\u0107\" : \"c\",\n \"\u0119\": \"e\",\n \"\u0142\" :\"l\",\n \"\u0144\" : \"n\",\n \"\u00f3\" : \"o\",\n \"\u015b\" : \"s\",\n \"\u017a\" : \"z\",\n \"\u017c\" : \"z\"\n }\n word =\"\"\n for i in st:\n if i.isupper()==True:\n word+=i\n else:\n word+= dict.get(i,i)\n return word", "POLSKA_STRONG = {\n '\u0105': 'a',\n '\u0107': 'c',\n '\u0119': 'e',\n '\u0142': 'l',\n '\u0144': 'n',\n '\u00f3': 'o',\n '\u015b': 's',\n '\u017a': 'z',\n '\u017c': 'z'\n}\ndef correct_polish_letters(st):\n return ''.join(POLSKA_STRONG.get(i, i) for i in st)\n", "pol = {'\u0105': 'a', '\u0107': 'c', '\u0119': 'e', '\u0142': 'l','\u0144': 'n',\\\n '\u00f3': 'o', '\u015b': 's','\u017a':'z', '\u017c': 'z'}\n\ndef correct_polish_letters(st): \n for char in st: \n if char in pol.keys():\n st = st.replace(char, pol.get(char))\n return st", "def correct_polish_letters(s):\n return s.translate(str.maketrans({\"\u0105\": \"a\", \"\u0107\": \"c\", \"\u0119\": \"e\", \"\u0142\": \"l\", \"\u0144\": \"n\", \"\u00f3\": \"o\", \"\u015b\": \"s\", \"\u017a\": \"z\", \"\u017c\": \"z\"}))\n", "def correct_polish_letters(st): \n ls = {\"\u0105\": \"a\", \"\u0107\": \"c\", \"\u0119\": \"e\", \"\u0142\": \"l\", \"\u0144\": \"n\", \"\u00f3\": \"o\", \"\u015b\": \"s\", \"\u017a\": \"z\", \"\u017c\": \"z\"}\n return ''.join(ls[l] if l in ls else l for l in st)\n", "def correct_polish_letters(st): \n dic = {\n '\u0105':'a',\n '\u0107':'c',\n '\u0119':'e',\n '\u0142':'l',\n '\u0144':'n',\n '\u00f3':'o',\n '\u015b':'s',\n '\u017a':'z',\n '\u017c':'z'\n }\n res = \"\".join(dic[i] if i in dic else i for i in st)\n return res", "def correct_polish_letters(st):\n dict_ = {'\u0105': 'a', '\u0107': 'c', '\u0119': 'e', '\u0142': 'l', '\u0144': 'n', '\u00f3': 'o', '\u015b': 's', '\u017a': 'z', '\u017c': 'z'}\n return ''.join(dict_[i] if i in dict_ else i for i in st)", "def correct_polish_letters(st):\n translationTable = str.maketrans(\"\u0105\u0107\u0119\u0142\u0144\u00f3\u015b\u017a\u017c\", \"acelnoszz\")\n return st.translate(translationTable)\n", "def correct_polish_letters(st): \n # your code here\n polish = {\n '\u0105': 'a',\n '\u0107': 'c',\n '\u0119': 'e',\n '\u0142': 'l',\n '\u0144': 'n',\n '\u00f3': 'o',\n '\u015b': 's',\n '\u017a': 'z',\n '\u017c': 'z'\n }\n \n res = ''\n for i in st:\n if i in polish:\n q = st.index(i)\n res += st[q].replace(st[q], polish.get(st[q]))\n else:\n res += i\n\n\n return res", "p = {'\u0105':'a','\u0107':'c','\u0119':'e','\u0142':'l','\u0144':'n','\u00f3':'o','\u015b':'s','\u017a':'z','\u017c':'z'}\n\ndef correct_polish_letters(st): \n return ''.join([p.get(c, c) for c in st])", "def correct_polish_letters(st): \n char = ['a','c','e','l','n','o','s','z','z']\n mod = ['\u0105','\u0107','\u0119','\u0142','\u0144','\u00f3','\u015b','\u017a','\u017c']\n for x in st:\n if x in mod:\n st = st.replace(x, char[mod.index(x)])\n return st", "def correct_polish_letters(st):\n\n str = \"\"\n\n for x in st:\n if x == \"\u0105\":\n str += \"a\"\n elif x == \"\u0107\":\n str += \"c\"\n elif x == \"\u0119\":\n str += \"e\"\n elif x == \"\u0142\":\n str += \"l\"\n elif x == \"\u0144\":\n str += \"n\"\n elif x == \"\u00f3\":\n str += \"o\"\n elif x == \"\u015b\":\n str += \"s\"\n elif x == \"\u017a\":\n str += \"z\"\n elif x == \"\u017c\":\n str += \"z\"\n else:\n str += x\n return (str)", "def correct_polish_letters(st): \n polish_letters = {'\u0105': 'a', \n '\u0107': 'c', \n '\u0119': 'e', \n '\u0142': 'l', \n '\u0144': 'n', \n '\u00f3': 'o', \n '\u015b': 's', \n '\u017a': 'z', \n '\u017c': 'z'}\n ret = ''\n for i in st:\n if i in polish_letters.keys():\n ret += polish_letters[i]\n else:\n ret += i\n return ret", "import ast\ndef correct_polish_letters(st): \n letters=\"\"\"\u0105 -> a,\n\u0107 -> c,\n\u0119 -> e,\n\u0142 -> l,\n\u0144 -> n,\n\u00f3 -> o,\n\u015b -> s,\n\u017a -> z,\n\u017c -> z\"\"\"\n letters=\"{'\"+letters.replace(\" -> \",\"':'\").replace(\",\",\"',\").replace(\"\\n\",\"'\")+\"'}\"\n letters=ast.literal_eval(letters)\n st2=\"\"\n for i in range(len(st)):\n if st[i] not in letters:\n st2+=st[i]\n else:\n st2+=letters.get(st[i])\n return st2\n", "def correct_polish_letters(st): \n dic = {\n \"\u0105\":'a',\n \"\u0107\":'c',\n \"\u0119\":'e',\n \"\u0142\":'l',\n '\u0144':'n',\n '\u00f3':'o',\n '\u015b':'s',\n '\u017a':'z',\n '\u017c': 'z'\n }\n for i in dic:\n st = st.replace(i,dic[i])\n return st"] | {"fn_name": "correct_polish_letters", "inputs": [["J\u0119drzej B\u0142\u0105dzi\u0144ski"], ["Lech Wa\u0142\u0119sa"], ["Maria Sk\u0142odowska-Curie"], ["W\u0142adys\u0142aw Reymont"], ["Miko\u0142aj Kopernik"], ["J\u00f3zef Pi\u0142sudski"], ["Czes\u0142aw Mi\u0142osz"], ["Agnieszka Radwa\u0144ska"], ["Wojciech Szcz\u0119sny"], ["Za\u017c\u00f3\u0142\u0107 g\u0119\u015bl\u0105 ja\u017a\u0144"], ["W\u00f3\u0142 go pyta: 'Panie chrz\u0105szczu,Po co pan tak brz\u0119czy w g\u0105szczu?'"]], "outputs": [["Jedrzej Bladzinski"], ["Lech Walesa"], ["Maria Sklodowska-Curie"], ["Wladyslaw Reymont"], ["Mikolaj Kopernik"], ["Jozef Pilsudski"], ["Czeslaw Milosz"], ["Agnieszka Radwanska"], ["Wojciech Szczesny"], ["Zazolc gesla jazn"], ["Wol go pyta: 'Panie chrzaszczu,Po co pan tak brzeczy w gaszczu?'"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 38,280 |
def correct_polish_letters(st):
|
7217fe130a3e5857b83958ec332b04ec | UNKNOWN | You've been collecting change all day, and it's starting to pile up in your pocket, but you're too lazy to see how much you've found.
Good thing you can code!
Create ```change_count()``` to return a dollar amount of how much change you have!
Valid types of change include:
```
penny: 0.01
nickel: 0.05
dime: 0.10
quarter: 0.25
dollar: 1.00
```
```if:python
These amounts are already preloaded as floats into the `CHANGE` dictionary for you to use!
```
```if:ruby
These amounts are already preloaded as floats into the `CHANGE` hash for you to use!
```
```if:javascript
These amounts are already preloaded as floats into the `CHANGE` object for you to use!
```
```if:php
These amounts are already preloaded as floats into the `CHANGE` (a constant) associative array for you to use!
```
You should return the total in the format ```$x.xx```.
Examples:
```python
change_count('nickel penny dime dollar') == '$1.16'
change_count('dollar dollar quarter dime dime') == '$2.45'
change_count('penny') == '$0.01'
change_count('dime') == '$0.10'
```
Warning, some change may amount to over ```$10.00```! | ["#Remember you have a CHANGE dictionary to work with ;)\n\ndef change_count(change):\n money = {'penny' : 0.01, 'nickel' : 0.05, 'dime' : 0.10, 'quarter' : 0.25, 'dollar' : 1.00}\n count = 0\n for coin in change.split():\n count += money[coin]\n result = \"%.2f\" % count\n return '$' + result\n \n", "def change_count(change):\n total = 0\n for i in change.split(' '):\n if i == 'penny':\n total += 0.01\n elif i == 'nickel':\n total += 0.05\n elif i == 'dime':\n total += 0.10\n elif i == 'quarter':\n total += 0.25\n elif i == 'dollar':\n total += 1.00\n return \"${:.2f}\".format(total)\n\nprint(change_count('dime penny dollar'))\nprint(change_count('dime penny nickel'))\nprint(change_count('quarter quarter'))\nprint(change_count('dollar penny dollar'))", "CHANGE = {'penny': 1, 'nickel': 5, 'dime': 10, 'quarter': 25, 'dollar': 100}\n\n\ndef change_count(change):\n return '${}.{:0>2}'.format(*divmod(\n sum(CHANGE[a] for a in change.split()), 100\n ))", "def change_count(change):\n a=change.split(\" \")\n L=[]\n for i in range(len(a)):\n if a[i]==\"penny\":\n L.append(0.01)\n elif a[i]==\"nickel\":\n L.append(0.05)\n elif a[i]==\"dime\":\n L.append(0.10)\n elif a[i]==\"quarter\":\n L.append(0.25)\n elif a[i]==\"dollar\":\n L.append(1.000)\n b=sum(L)\n x=round((b+0.0000001),2)\n a='$'+(\"%.2f\"%x)\n y=a.split()\n if len(y)==4:\n y.append('0')\n return sum(y)\n else:\n return a", "def change_count(ch):\n total = float(0.00)\n ch = ch.split()\n for x in ch:\n\n if \"dollar\" in x:\n total += 1.00\n if \"quarter\" in x:\n total += 0.25\n if \"dime\" in x:\n total += 0.10\n if \"nickel\" in x:\n total += 0.05\n if \"penny\" in x:\n total += 0.01\n\n return \"${:.2f}\".format(total)\n"] | {"fn_name": "change_count", "inputs": [["dime penny dollar"], ["dime penny nickel"], ["quarter quarter"], ["dollar penny dollar"], ["dollar dollar dollar dollar dollar dollar dollar dollar dollar dollar penny"]], "outputs": [["$1.11"], ["$0.16"], ["$0.50"], ["$2.01"], ["$10.01"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,086 |
def change_count(change):
|
ee9055907a00b80dc058ee759c7a99cd | UNKNOWN | Move the first letter of each word to the end of it, then add "ay" to the end of the word. Leave punctuation marks untouched.
## Examples
```python
pig_it('Pig latin is cool') # igPay atinlay siay oolcay
pig_it('Hello world !') # elloHay orldway !
```
```C++
pig_it("Pig latin is cool"); // igPay atinlay siay oolcay
pig_it("Hello world !"); // elloHay orldway
```
```Java
PigLatin.pigIt('Pig latin is cool'); // igPay atinlay siay oolcay
PigLatin.pigIt('Hello world !'); // elloHay orldway !
``` | ["def pig_it(text):\n lst = text.split()\n return ' '.join( [word[1:] + word[:1] + 'ay' if word.isalpha() else word for word in lst])\n", "def pig_it(text):\n return \" \".join(x[1:] + x[0] + \"ay\" if x.isalnum() else x for x in text.split())", "def pig_it(text):\n res = []\n \n for i in text.split():\n if i.isalpha():\n res.append(i[1:]+i[0]+'ay')\n else:\n res.append(i)\n \n return ' '.join(res)", "def pig_it(text):\n new_string = \"\"\n for each in text.split():\n if each == \"?\": \n new_string += \"? \"\n elif each == \"!\":\n new_string += \"! \"\n else: \n new_string += each[1:len(each)] + each[0] + \"ay \"\n a= len(new_string) \n return new_string[0:a-1]\n", "import re\n\ndef pig_it(text):\n return re.sub(r'([a-z])([a-z]*)', r'\\2\\1ay', text, flags=re.I)", "def pig_it(text):\n return ' '.join([x[1:]+x[0]+'ay' if x.isalpha() else x for x in text.split()])", "import re\ndef pig_it(text):\n return re.sub(r'(\\w{1})(\\w*)', r'\\2\\1ay', text)", "from string import punctuation\ndef pig_it(text):\n words = text.split(' ')\n return ' '.join(\n [\n '{}{}ay'.format(\n word[1:],\n word[0]\n ) if word not in punctuation else word for word in words\n ]\n )", "def pig_it(text):\n return ' '.join([w[1:] + w[0] + 'ay' if w.isalpha() else w for w in text.split(' ')])", "def pig_it(text):\n piggy = []\n for word in text.split():\n if word.isalpha():\n piggy.append(word[1:]+ word[0] + \"ay\")\n else:\n piggy.append(word)\n return ' '.join(piggy)", "def pig_it(text):\n #your code here\n n = 0\n x = 0\n text = text.split() #split the text into words\n text = list(text) #make the words a list\n pig_text = [] #make an empty list\n for word in text:\n a = list(word) #set a to be the list of word (the letters on their own)\n print(a)\n if len(a) > 1:\n a.append(a[0]) #add the first letter to the end\n del a[0] #delete the first letter\n a.append('ay') #add ay to the end\n if '!' in a:\n n += 1\n elif '?' in a:\n x += 1\n elif len(a) == 1:\n print(a)\n a.append('ay') \n a = ''.join(a) #rejoin the word\n pig_text.append(a) #put the word in the empty list\n pig_text = ' '.join(pig_text) #join the words up with spaces\n return pig_text #return the sentence"] | {"fn_name": "pig_it", "inputs": [["Pig latin is cool"], ["This is my string"]], "outputs": [["igPay atinlay siay oolcay"], ["hisTay siay ymay tringsay"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,610 |
def pig_it(text):
|
b3d8e47f781553ea8fb0d4856f59b059 | UNKNOWN | In this Kata, we are going to see how a Hash (or Map or dict) can be used to keep track of characters in a string.
Consider two strings `"aabcdefg"` and `"fbd"`. How many characters do we have to remove from the first string to get the second string? Although not the only way to solve this, we could create a Hash of counts for each string and see which character counts are different. That should get us close to the answer. I will leave the rest to you.
For this example, `solve("aabcdefg","fbd") = 5`. Also, `solve("xyz","yxxz") = 0`, because we cannot get second string from the first since the second string is longer.
More examples in the test cases.
Good luck! | ["from collections import Counter\n\ndef solve(a,b):\n return 0 if Counter(b) - Counter(a) else len(a) - len(b)", "def solve(a,b):\n for x in set(b):\n if a.count(x) >= b.count(x):\n continue\n else:\n return 0\n return len(a)-len(b)", "def solve(a,b):\n print((a,b))\n if sorted(a)==sorted(b) or (set(list(a))==set(list(b))):\n return 0\n for x in b:\n for x in a:\n if a.count(x)<b.count(x) : return 0\n else: return len(a)-len(b) ", "def solve(a,b):\n a=list(a)\n try:\n for c in b:\n a.pop(a.index(c))\n return len(a)\n except:\n return 0", "def solve(a,b):\n for char in b:\n return 0 if b.count(char) > a.count(char) or len(b)>=len(a) else len(a)-len(b) \n", "from collections import Counter\n\ndef solve(a,b):\n return sum((Counter(a)-Counter(b)).values()) if Counter(b) - Counter(a) == {} else 0 ", "from collections import Counter\n\ndef solve(a, b):\n c_diff, c_b = Counter(a), Counter(b)\n c_diff.subtract(c_b)\n result = 0\n for k,v in c_diff.items():\n if v >= 0:\n result += v\n else:\n return 0\n return result", "from collections import Counter\n\ndef solve(a,b):\n aa=Counter(a)\n bb=Counter(b)\n \n for k,v in list(bb.items()):\n diff = aa[k]-v\n aa[k]-=v\n if diff<0: return 0\n \n return sum(aa.values())\n \n \n", "solve=lambda a,b,c=__import__('collections').Counter:not c(b)-c(a)and len(a)-len(b)", "from collections import Counter as C\ndef solve(Q,S) :\n Q,S = C(Q),C(S)\n if all(V in Q and S[V] <= Q[V] for V in S) :\n return sum(Q[V] - (S[V] if V in S else 0) for V in Q)\n return 0"] | {"fn_name": "solve", "inputs": [["xyz", "yxz"], ["abcxyz", "ayxz"], ["abcdexyz", "yxz"], ["xyz", "yxxz"], ["abdegfg", "ffdb"], ["aabcdefg", "fbd"]], "outputs": [[0], [2], [5], [0], [0], [5]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,767 |
def solve(a,b):
|
fdebc1fe6a8a121a21974d2ebe69144a | UNKNOWN | In this kata you will be given a sequence of the dimensions of rectangles ( sequence with width and length ) and circles ( radius - just a number ).
Your task is to return a new sequence of dimensions, sorted ascending by area.
For example,
```python
seq = [ (4.23, 6.43), 1.23, 3.444, (1.342, 3.212) ] # [ rectangle, circle, circle, rectangle ]
sort_by_area(seq) => [ ( 1.342, 3.212 ), 1.23, ( 4.23, 6.43 ), 3.444 ]
```
This kata inspired by [Sort rectangles and circles by area](https://www.codewars.com/kata/sort-rectangles-and-circles-by-area/). | ["def sort_by_area(seq): \n def func(x):\n if isinstance(x, tuple):\n return x[0] * x[1]\n else:\n return 3.14 * x * x\n return sorted(seq, key=func)", "from math import pi as PI\n\ndef circle(r): return r*r*PI\ndef rect(a,b): return a*b\ndef getArea(r): return rect(*r) if isinstance(r,tuple) else circle(r)\ndef sort_by_area(seq): return sorted(seq, key=getArea)", "def sort_by_area(seq): \n \n valueList = []\n for i in seq:\n if isinstance(i,tuple):\n l1 = i[0]\n l2 = i[1]\n area = l1 * l2\n else:\n area = 3.14*i**2\n \n valueList.append(area)\n \n a = sorted(range(len(valueList)),key=valueList.__getitem__) # Get index of sorted list\n sort_by_area = [seq[i] for i in a]\n\n return sort_by_area", "from math import pi\n\ndef sort_by_area(a):\n return sorted(a, key=lambda x: x**2 * pi if isinstance(x, (int, float)) else x[0] * x[1])", "from math import pi\nfrom operator import mul\n\ndef area(args):\n try:\n return mul(*args)\n except TypeError:\n return pi * args**2\n\ndef sort_by_area(seq): \n return sorted(seq, key=area)", "def sort_by_area(seq):\n lista = list()\n for x in seq:\n if type(x) == tuple:\n area = x[0]*x[1]\n else:\n area = 3.14*x**2\n lista.append((area, x))\n return [x[1] for x in sorted(lista)]", "def sort_by_area(arr): \n return sorted(arr, key=lambda x: (x[0]*x[1] if isinstance(x,tuple) else x*x*3.14))", "def area(x):\n if type(x) == float or type(x) == int: return 3.141592653589*x*x\n else: return x[0]*x[1]\n\ndef sort_by_area(seq): \n return sorted(seq, key=area)", "from math import pi as PI\n\ndef sort_by_area(lst):\n return sorted(lst, key=lambda d: d[0] * d[1] if isinstance(d, tuple) else PI * d * d) ", "from math import pi\n\ndef sort_by_area(seq): \n return sorted(seq, key = lambda arg: arg[0]*arg[1] if isinstance(arg, tuple) else pi*arg**2)"] | {"fn_name": "sort_by_area", "inputs": [[[]]], "outputs": [[[]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,062 |
def sort_by_area(seq):
|
a706e3d80e464839b24e5bfa91fff9a6 | UNKNOWN | A [perfect power](https://en.wikipedia.org/wiki/Perfect_power) is a classification of positive integers:
> In mathematics, a **perfect power** is a positive integer that can be expressed as an integer power of another positive integer. More formally, n is a perfect power if there exist natural numbers m > 1, and k > 1 such that m^(k) = n.
Your task is to check wheter a given integer is a perfect power. If it is a perfect power, return a pair `m` and `k` with m^(k) = n as a proof. Otherwise return `Nothing`, `Nil`, `null`, `NULL`, `None` or your language's equivalent.
**Note:** For a perfect power, there might be several pairs. For example `81 = 3^4 = 9^2`, so `(3,4)` and `(9,2)` are valid solutions. However, the tests take care of this, so if a number is a perfect power, return any pair that proves it.
### Examples
```python
isPP(4) => [2,2]
isPP(9) => [3,2]
isPP(5) => None
``` | ["from math import ceil, log, sqrt\n\ndef isPP(n):\n for b in range(2, int(sqrt(n)) + 1):\n e = int(round(log(n, b)))\n if b ** e == n:\n return [b, e]\n return None\n", "def isPP(n):\n for i in range(2, int(n**.5) + 1):\n number = n\n times = 0\n while number % i == 0:\n number /= i\n times += 1\n if number == 1:\n return [i, times]\n return None\n", "def isPP(n):\n for i in range(2, n+1):\n for j in range(2, n+1):\n if i**j > n:\n break\n elif i**j == n:\n return [i, j]\n return None", "from math import log\n\ndef isPP(n, e=1e-12):\n for p in range(2, int(log(n, 2)) + 1):\n if int(n ** (1./p) + e) ** p == n:\n return [int(n ** (1./p) + e), p]", "def isPP(n):\n x, y = 2, 2\n while x**2 <= n:\n if x**y == n:\n return[x,y]\n if x**y > n:\n x+=1\n y = 1\n y+=1 \n return None", "from math import sqrt\ndef isPP(n):\n cur = int(sqrt(n)), 2\n while True:\n val = cur[0] ** cur[1]\n if val == 1:\n break\n if val > n:\n cur = cur[0]-1, cur[1]\n elif val < n:\n cur = cur[0], cur[1]+1\n else:\n return list(cur)", "from math import log\ndef isPP(n):\n for m in range(2,int(n**0.5)+1):\n k = round(log(n, m), 13)\n if k % 1 == 0:\n return [m ,k]\n return", "def isPP(n):\n b=n\n import math\n root=math.sqrt(n)\n iroot=int(root)\n if float(iroot)==root:\n return([iroot,2])\n elif n==1:\n return([1,1])\n else:\n if iroot==2:\n iroot=3\n for i in range(2,iroot):\n s=0\n d='no'\n n=b\n while n%i==0:\n d='yes'\n n=n/i\n s=s+1\n if n==1:\n return([i,s])\n else:\n d='no'\n if d=='no':\n return None\n \n \n", "def isPP(n):\n for k in range(2,round(n**0.5+1)):\n m=round(n**(1/k))\n if m**k==n:\n return [m,k]\n return None", "def isPP(n):\n m, k = 2, 2\n while m**2 <= n:\n while m**k <= n:\n if m**k == n:\n return [m, k]\n else:\n k += 1\n m += 1\n k = 2\n return None", "import math\n\ndef isPP(n):\n return next(([b, round(math.log(n, b))] for b in range(2, int(n**0.5)+1) if b**round(math.log(n, b)) == n), None)", "def isPP(n):\n base = 2\n power = 2\n\n while base ** power <= n:\n while base ** power <= n:\n if base ** power == n:\n return [base, power]\n else:\n power += 1\n\n power = 2\n base += 1\n\n return None", "def isPP(n):\n k = 2\n m = n**(1/k)\n while m % 1 != 0 and m > 2:\n k += 1\n m = n**(1/k)\n m = float(\"{:.5f}\".format(m))\n if m % 1 == 0:\n return [int(m), k]\n else:\n return None", "def isPP(n):\n d = 2\n k = 2\n while d >= 2:\n d = round(n**(1/k), 4)\n if d.is_integer():\n return [d,k]\n k +=1", "primeTable = [2,3,5,7,11,13,17,19,23,29]\ndef isPP(n):\n for p in primeTable:\n base = round(n ** (1/p))\n if base ** p ==n:\n return [base, p]", "def isPP(n):\n for i in range(2,400):\n for j in range(2,50):\n if i**j == n:\n return [i, j]\n\n return None", "def isPP(num):\n for n in range(2, 100):\n if abs( num ** (1/n) - round(num ** (1/n))) < 0.0000001:\n return [round(num ** (1/n)), n]\n n += 1", "from math import sqrt\n\ndef isPP(n):\n limit = int(sqrt(n))\n for m in range(2, limit + 1):\n k = 2\n while m ** k <= n:\n if m ** k == n:\n return [m, k]\n k += 1"] | {"fn_name": "isPP", "inputs": [[4], [9], [5]], "outputs": [[[2, 2]], [[3, 2]], [null]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,072 |
def isPP(n):
|
0e4bd150fe48a12703b7ad9673a0c4af | UNKNOWN | ## Overview
Resistors are electrical components marked with colorful stripes/bands to indicate both their resistance value in ohms and how tight a tolerance that value has. While you could always get a tattoo like Jimmie Rodgers to help you remember the resistor color codes, in the meantime, you can write a function that will take a string containing a resistor's band colors and return a string identifying the resistor's ohms and tolerance values.
## The resistor color codes
You can see this Wikipedia page for a colorful chart, but the basic resistor color codes are:
black: 0, brown: 1, red: 2, orange: 3, yellow: 4, green: 5, blue: 6, violet: 7, gray: 8, white: 9
Each resistor will have at least three bands, with the first and second bands indicating the first two digits of the ohms value, and the third indicating the power of ten to multiply them by, for example a resistor with the three bands "yellow violet black" would be 47 * 10^0 ohms, or 47 ohms.
Most resistors will also have a fourth band that is either gold or silver, with gold indicating plus or minus 5% tolerance, and silver indicating 10% tolerance. Resistors that do not have a fourth band are rated at 20% tolerance. (There are also more specialized resistors which can have more bands and additional meanings for some of the colors, but this kata will not cover them.)
## Your mission
The way the ohms value needs to be formatted in the string you return depends on the magnitude of the value:
* For resistors less than 1000 ohms, return a string containing the number of ohms, a space, the word "ohms" followed by a comma and a space, the tolerance value (5, 10, or 20), and a percent sign. For example, for the "yellow violet black" resistor mentioned above, you would return `"47 ohms, 20%"`.
* For resistors greater than or equal to 1000 ohms, but less than 1000000 ohms, you will use the same format as above, except that the ohms value will be divided by 1000 and have a lower-case "k" after it. For example, for a resistor with bands of "yellow violet red gold", you would return `"4.7k ohms, 5%"`
* For resistors of 1000000 ohms or greater, you will divide the ohms value by 1000000 and have an upper-case "M" after it. For example, for a resistor with bands of "brown black green silver", you would return `"1M ohms, 10%"`
Test case resistor values will all be between 10 ohms and 990M ohms.
## More examples, featuring some common resistor values
```
"brown black black" "10 ohms, 20%"
"brown black brown gold" "100 ohms, 5%"
"red red brown" "220 ohms, 20%"
"orange orange brown gold" "330 ohms, 5%"
"yellow violet brown silver" "470 ohms, 10%"
"blue gray brown" "680 ohms, 20%"
"brown black red silver" "1k ohms, 10%"
"brown black orange" "10k ohms, 20%"
"red red orange silver" "22k ohms, 10%"
"yellow violet orange gold" "47k ohms, 5%"
"brown black yellow gold" "100k ohms, 5%"
"orange orange yellow gold" "330k ohms, 5%"
"red black green gold" "2M ohms, 5%"
```
Have fun! And if you enjoy this kata, check out the sequel: Resistor Color Codes, Part 2 | ["code = {'black': 0, 'brown': 1, 'red': 2, 'orange': 3, 'yellow': 4,\n'green': 5, 'blue': 6, 'violet': 7, 'gray': 8, 'white': 9,\n'gold': 5, 'silver': 10, '': 20}\ndef decode_resistor_colors(bands):\n colors = (bands + ' ').split(' ')\n value = 10 * code[colors[0]] + code[colors[1]]\n value *= 10 ** code[colors[2]]\n tolerance = code[colors[3]]\n prefix = ''\n for p in 'kM':\n if value // 1000:\n prefix = p\n value /= 1000\n return \"%g%s ohms, %d%%\" % (value, prefix, tolerance)\n", "d = {'black':0, 'brown':1, 'red':2, 'orange':3, 'yellow':4, 'green':5, 'blue':6, 'violet':7, 'gray':8, 'white':9, 'silver':10, 'gold':5}\ndef decode_resistor_colors(bands):\n bands = [d[b] for b in bands.split()]\n ohms = (bands[0] * 10 + bands[1]) * 10 ** bands[2]\n ohms, sfx = (ohms/1000000.0, 'M') if ohms > 999999 else (ohms/1000.0, 'k') if ohms > 999 else (ohms, '')\n return \"{}{} ohms, {}%\".format(int(ohms) if ohms//1 == ohms else ohms, sfx, bands[3] if len(bands) > 3 else 20)", "BANDS = {'black': 0, 'brown': 1, 'red': 2, 'orange': 3, 'yellow': 4,\n 'green': 5, 'blue': 6, 'violet': 7, 'gray': 8, 'white': 9}\nTOLERANCE_BAND = {'gold': 5, 'silver': 10}\nLETTERS = {0: '', 1: 'k', 2: 'M'}\nPATTERN = '{}{} ohms, {}%'\n\ndef decode_resistor_colors(bands):\n first, second, third, *fourth = bands.split(' ')\n ohms = (BANDS[first] * 10 + BANDS[second]) * 10 ** BANDS[third]\n \n count = 0\n while ohms >= 1000:\n ohms /= 1000\n count += 1\n ohms = int(ohms) if int(ohms) == ohms else ohms\n \n letter = LETTERS[count]\n \n if fourth:\n tolerance = TOLERANCE_BAND[fourth[0]]\n else:\n tolerance = 20\n \n return PATTERN.format(ohms, letter, tolerance)\n", "def decode_resistor_colors(bands):\n color = {\"black\" : 0, \"brown\" : 1, \"red\" : 2, \"orange\" : 3, \"yellow\" : 4, \"green\" : 5, \"blue\" : 6, \"violet\" : 7, \"gray\" : 8, \"white\" : 9}\n tolerance = {\"gold\" : 5, \"silver\" : 10, \"none\" : 20}\n a, b, p, t, *_ = bands.split() + [\"none\"]\n c = (10 * color[a] + color[b]) * 10 ** color[p]\n r, m = next((c / x, y) for x, y in [(10 ** 6, \"M\"), (10 ** 3, \"k\"), (1, \"\")] if c // x > 0)\n return \"{:g}{} ohms, {}%\".format(r, m, tolerance[t])\n", "codes = {\n 'black': 0,\n 'brown': 1,\n 'red': 2,\n 'orange': 3,\n 'yellow': 4,\n 'green': 5,\n 'blue': 6,\n 'violet': 7,\n 'gray': 8,\n 'white': 9,\n 'gold': 5,\n 'silver': 10\n}\n\ndef decode_resistor_colors(bands):\n bands = [codes[band] for band in bands.split()] + [20]\n ohms = (bands[0] * 10 + bands[1]) * 10 ** bands[2]\n p = ''\n for c in 'kM':\n if ohms // 1000:\n ohms /= 1000; p = c\n return '%s%s ohms, %s%%' % (str(ohms).replace('.0', ''), p, bands[3])", "def decode_resistor_colors(bands):\n bands_lst = bands.split(\" \")\n VALUES = {\"black\": 0, \"brown\": 1, \"red\": 2, \"orange\": 3, \"yellow\": 4,\n \"green\": 5, \"blue\":6, \"violet\":7, \"gray\": 8, \"white\": 9,\n \"gold\": 5, \"silver\":10} \n first = VALUES[bands_lst[0]]\n second = VALUES[bands_lst[1]]\n num = str(first) + str(second)\n ohms = int(num) * pow(10, VALUES[bands_lst[2]])\n unit = \" ohms\"\n tolerance = 20\n if len(bands_lst) > 3:\n tolerance = VALUES[bands_lst[3]]\n if ohms in range(0, 999):\n unit = \" ohms\"\n elif ohms in range(1000, 999999):\n ohms = ohms / 1000\n unit = \"k ohms\"\n elif ohms >= 1000000:\n ohms = ohms / 1000000\n unit = \"M ohms\"\n return \"{:g}{}, {}%\".format(ohms, unit, tolerance)\n", "def decode_resistor_colors(bands):\n dic = {'black': 0, 'brown': 1, 'red': 2, 'orange': 3, 'yellow': 4,\n 'green': 5, 'blue': 6, 'violet': 7, 'gray': 8, 'white': 9}\n lst = bands.split()\n num = dic[lst[0]]*10+dic[lst[1]]\n numbe = num*10**dic[lst[2]]\n if len(lst) > 3:\n if lst[3] == \"gold\":\n tol = 5\n elif lst[3] == \"silver\":\n tol = 10\n else:\n tol = 20\n if numbe >= 1000000:\n if numbe%1000000 == 0:\n number = numbe//1000000\n else:\n number = numbe/1000000\n return str(number)+\"M ohms, \"+str(tol)+\"%\"\n elif numbe >= 1000:\n if numbe%1000 == 0:\n number = numbe//1000\n else:\n number = numbe/1000\n return str(number)+\"k ohms, \"+str(tol)+\"%\"\n else:\n number = numbe\n return str(number)+\" ohms, \"+str(tol)+\"%\"\n", "COLOR_CODES = {\"black\": 0, \"brown\": 1, \"red\": 2, \"orange\": 3, \"yellow\": 4, \"green\": 5, \"blue\": 6,\\\n \"violet\": 7, \"gray\": 8, \"white\": 9}\n\ndef decode_resistor_colors(bands):\n bands = bands.split()\n tol = \" ohms, \" + (\"20%\" if len(bands) < 4 else \"10%\" if bands[-1] == \"silver\" else \"5%\")\n first, second, power = [COLOR_CODES[band] for band in bands[:3]]\n resistance = \"{:.1f}\".format((first+second/10)*10**((power+1) % 3))\n return (resistance if not resistance.endswith(\"0\") else resistance[:-2]) + (\" kkkMMM\"[power] if power > 1 else \"\") + tol", "COLOR_CODE = {\n 'silver': 10,\n 'gold': 5,\n\n 'black': 0,\n 'brown': 1,\n 'red': 2,\n 'orange': 3,\n 'yellow': 4,\n 'green': 5,\n 'blue': 6,\n 'violet': 7,\n 'gray': 8,\n 'white': 9,\n}\n\nDEFAULT_TOLERANCE = 20\n\n\ndef decode_resistor_colors(bands):\n bands = [COLOR_CODE[band] for band in bands.split()] + [DEFAULT_TOLERANCE]\n\n value = bands[0] * 10 + bands[1]\n multiplier = bands[2]\n tolerance = bands[3]\n\n exponent = multiplier + 1\n\n if exponent >= 6:\n letter = 'M'\n exponent -= 6\n elif exponent >= 3:\n letter = 'k'\n exponent -= 3\n else:\n letter = ''\n\n value *= 10 ** (exponent - 1)\n\n return '{:g}{} ohms, {}%'.format(value, letter, tolerance)\n"] | {"fn_name": "decode_resistor_colors", "inputs": [["yellow violet black"], ["yellow violet red gold"], ["brown black green silver"], ["brown black black"], ["brown black brown gold"], ["red red brown"], ["orange orange brown gold"], ["yellow violet brown silver"], ["blue gray brown"], ["brown black red silver"], ["brown black orange"], ["red red orange silver"], ["yellow violet orange gold"], ["brown black yellow gold"], ["orange orange yellow gold"], ["red black green gold"]], "outputs": [["47 ohms, 20%"], ["4.7k ohms, 5%"], ["1M ohms, 10%"], ["10 ohms, 20%"], ["100 ohms, 5%"], ["220 ohms, 20%"], ["330 ohms, 5%"], ["470 ohms, 10%"], ["680 ohms, 20%"], ["1k ohms, 10%"], ["10k ohms, 20%"], ["22k ohms, 10%"], ["47k ohms, 5%"], ["100k ohms, 5%"], ["330k ohms, 5%"], ["2M ohms, 5%"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 6,026 |
def decode_resistor_colors(bands):
|
034f2037805af89bfd288504a47fc7d6 | UNKNOWN | Converting a normal (12-hour) time like "8:30 am" or "8:30 pm" to 24-hour time (like "0830" or "2030") sounds easy enough, right? Well, let's see if you can do it!
You will have to define a function named "to24hourtime", and you will be given an hour (always in the range of 1 to 12, inclusive), a minute (always in the range of 0 to 59, inclusive), and a period (either "am" or "pm") as input.
Your task is to return a four-digit string that encodes that time in 24-hour time. | ["def to24hourtime(hour, minute, period):\n return '%02d%02d' % (hour % 12 + 12 * (period == 'pm'), minute)", "from datetime import datetime\n\ndef to24hourtime(h, m, p):\n return datetime.strptime('%02d%02d%s' % (h, m, p.upper()), '%I%M%p').strftime('%H%M')", "from datetime import datetime\n\ndef to24hourtime(h, m, p):\n t = f'{h}:{m} {p}'\n return datetime.strptime(t, '%I:%M %p').strftime('%H%M')", "def to24hourtime(hour, minute, period):\n return f\"{period.startswith('p') * 12 + hour % 12:02d}{minute:02d}\"\n\n", "def to24hourtime(hour, minute, period):\n if hour < 10:\n hour = '0' + str(hour)\n else:\n hour = str(hour)\n \n if minute < 10:\n minute = '0' + str(minute)\n else:\n minute = str(minute)\n \n time = hour + minute\n if period == 'pm' and int(hour) != 12:\n time = str(int(time) + 1200)\n \n if period == 'am' and int(hour) == 12:\n time = str(int(time) - 1200)\n if int(minute) < 10:\n time = '000' + time\n else:\n time = '00' + time\n \n print((hour,minute,period))\n return time\n \n", "def to24hourtime(hour, minute, period):\n hour_str = str(hour)\n minute_str = str(minute)\n\n if period == 'am':\n if hour <= 9:\n hour_str = '0' + hour_str\n if minute <= 9:\n minute_str = '0' + minute_str\n if hour == 12:\n hour_str = '00'\n\n return '%s%s' % (hour_str, minute_str)\n else:\n if hour <= 12:\n hour += 12\n if hour == 24:\n hour = 0\n hour_str = str(hour)\n \n if hour <= 9:\n hour_str = '0' + hour_str\n if minute <= 9:\n minute_str = '0' + minute_str\n if hour == 0:\n hour_str = '12'\n\n return '%s%s' % (hour_str, minute_str)\n", "def to24hourtime(hour, minute, period):\n if 'pm' in period:\n time = str(int(hour + 12)) + str(minute).zfill(2)\n if hour == 12:\n return '12' + str(minute).zfill(2)\n return time\n else:\n time = str(str(hour).zfill(2)) + (str(minute).zfill(2))\n if hour == 12:\n return '00'+str(minute).zfill(2)\n return time", "from datetime import datetime\ndef to24hourtime(hour, minute, period):\n return datetime.strptime(f\"{hour} {minute} {period}\",\"%I %M %p\").strftime(\"%H%M\")", "def to24hourtime(hour, minute, period):\n if period == 'am' and hour == 12:\n hour = '00'\n elif period == 'pm' and hour == 12:\n hour = '12'\n elif period == 'pm':\n hour += 12\n elif hour < 10:\n hour = '0' + str(hour)\n \n if minute < 10:\n minute = '0' + str(minute)\n \n return str(hour) + str(minute)\n \n", "def to24hourtime(hour, minute, period):\n if hour == 12 and period == \"am\":\n hour = 0\n else: \n hour += 12 if period == \"pm\" and hour != 12 else 0\n return f\"{str(hour).rjust(2, '0')}{str(minute).rjust(2, '0')}\"\n"] | {"fn_name": "to24hourtime", "inputs": [[12, 0, "am"], [12, 1, "am"], [12, 2, "am"], [12, 3, "am"], [12, 4, "am"], [12, 5, "am"], [12, 6, "am"], [12, 7, "am"], [12, 8, "am"], [12, 9, "am"], [12, 10, "am"], [12, 11, "am"], [12, 12, "am"], [12, 13, "am"], [12, 14, "am"], [12, 15, "am"], [12, 16, "am"], [12, 17, "am"], [12, 18, "am"], [12, 19, "am"], [12, 20, "am"], [12, 21, "am"], [12, 22, "am"], [12, 23, "am"], [12, 24, "am"], [12, 25, "am"], [12, 26, "am"], [12, 27, "am"], [12, 28, "am"], [12, 29, "am"], [12, 30, "am"], [12, 31, "am"], [12, 32, "am"], [12, 33, "am"], [12, 34, "am"], [12, 35, "am"], [12, 36, "am"], [12, 37, "am"], [12, 38, "am"], [12, 39, "am"], [12, 40, "am"], [12, 41, "am"], [12, 42, "am"], [12, 43, "am"], [12, 44, "am"], [12, 45, "am"], [12, 46, "am"], [12, 47, "am"], [12, 48, "am"], [12, 49, "am"], [12, 50, "am"], [12, 51, "am"], [12, 52, "am"], [12, 53, "am"], [12, 54, "am"], [12, 55, "am"], [12, 56, "am"], [12, 57, "am"], [12, 58, "am"], [12, 59, "am"], [1, 0, "am"], [1, 1, "am"], [1, 2, "am"], [1, 3, "am"], [1, 4, "am"], [1, 5, "am"], [1, 6, "am"], [1, 7, "am"], [1, 8, "am"], [1, 9, "am"], [1, 10, "am"], [1, 11, "am"], [1, 12, "am"], [1, 13, "am"], [1, 14, "am"], [1, 15, "am"], [1, 16, "am"], [1, 17, "am"], [1, 18, "am"], [1, 19, "am"], [1, 20, "am"], [1, 21, "am"], [1, 22, "am"], [1, 23, "am"], [1, 24, "am"], [1, 25, "am"], [1, 26, "am"], [1, 27, "am"], [1, 28, "am"], [1, 29, "am"], [1, 30, "am"], [1, 31, "am"], [1, 32, "am"], [1, 33, "am"], [1, 34, "am"], [1, 35, "am"], [1, 36, "am"], [1, 37, "am"], [1, 38, "am"], [1, 39, "am"], [1, 40, "am"], [1, 41, "am"], [1, 42, "am"], [1, 43, "am"], [1, 44, "am"], [1, 45, "am"], [1, 46, "am"], [1, 47, "am"], [1, 48, "am"], [1, 49, "am"], [1, 50, "am"], [1, 51, "am"], [1, 52, "am"], [1, 53, "am"], [1, 54, "am"], [1, 55, "am"], [1, 56, "am"], [1, 57, "am"], [1, 58, "am"], [1, 59, "am"], [2, 0, "am"], [2, 1, "am"], [2, 2, "am"], [2, 3, "am"], [2, 4, "am"], [2, 5, "am"], [2, 6, "am"], [2, 7, "am"], [2, 8, "am"], [2, 9, "am"], [2, 10, "am"], [2, 11, "am"], [2, 12, "am"], [2, 13, "am"], [2, 14, "am"], [2, 15, "am"], [2, 16, "am"], [2, 17, "am"], [2, 18, "am"], [2, 19, "am"], [2, 20, "am"], [2, 21, "am"], [2, 22, "am"], [2, 23, "am"], [2, 24, "am"], [2, 25, "am"], [2, 26, "am"], [2, 27, "am"], [2, 28, "am"], [2, 29, "am"], [2, 30, "am"], [2, 31, "am"], [2, 32, "am"], [2, 33, "am"], [2, 34, "am"], [2, 35, "am"], [2, 36, "am"], [2, 37, "am"], [2, 38, "am"], [2, 39, "am"], [2, 40, "am"], [2, 41, "am"], [2, 42, "am"], [2, 43, "am"], [2, 44, "am"], [2, 45, "am"], [2, 46, "am"], [2, 47, "am"], [2, 48, "am"], [2, 49, "am"], [2, 50, "am"], [2, 51, "am"], [2, 52, "am"], [2, 53, "am"], [2, 54, "am"], [2, 55, "am"], [2, 56, "am"], [2, 57, "am"], [2, 58, "am"], [2, 59, "am"], [3, 0, "am"], [3, 1, "am"], [3, 2, "am"], [3, 3, "am"], [3, 4, "am"], [3, 5, "am"], [3, 6, "am"], [3, 7, "am"], [3, 8, "am"], [3, 9, "am"], [3, 10, "am"], [3, 11, "am"], [3, 12, "am"], [3, 13, "am"], [3, 14, "am"], [3, 15, "am"], [3, 16, "am"], [3, 17, "am"], [3, 18, "am"], [3, 19, "am"], [3, 20, "am"], [3, 21, "am"], [3, 22, "am"], [3, 23, "am"], [3, 24, "am"], [3, 25, "am"], [3, 26, "am"], [3, 27, "am"], [3, 28, "am"], [3, 29, "am"], [3, 30, "am"], [3, 31, "am"], [3, 32, "am"], [3, 33, "am"], [3, 34, "am"], [3, 35, "am"], [3, 36, "am"], [3, 37, "am"], [3, 38, "am"], [3, 39, "am"], [3, 40, "am"], [3, 41, "am"], [3, 42, "am"], [3, 43, "am"], [3, 44, "am"], [3, 45, "am"], [3, 46, "am"], [3, 47, "am"], [3, 48, "am"], [3, 49, "am"], [3, 50, "am"], [3, 51, "am"], [3, 52, "am"], [3, 53, "am"], [3, 54, "am"], [3, 55, "am"], [3, 56, "am"], [3, 57, "am"], [3, 58, "am"], [3, 59, "am"], [4, 0, "am"], [4, 1, "am"], [4, 2, "am"], [4, 3, "am"], [4, 4, "am"], [4, 5, "am"], [4, 6, "am"], [4, 7, "am"], [4, 8, "am"], [4, 9, "am"], [4, 10, "am"], [4, 11, "am"], [4, 12, "am"], [4, 13, "am"], [4, 14, "am"], [4, 15, "am"], [4, 16, "am"], [4, 17, "am"], [4, 18, "am"], [4, 19, "am"], [4, 20, "am"], [4, 21, "am"], [4, 22, "am"], [4, 23, "am"], [4, 24, "am"], [4, 25, "am"], [4, 26, "am"], [4, 27, "am"], [4, 28, "am"], [4, 29, "am"], [4, 30, "am"], [4, 31, "am"], [4, 32, "am"], [4, 33, "am"], [4, 34, "am"], [4, 35, "am"], [4, 36, "am"], [4, 37, "am"], [4, 38, "am"], [4, 39, "am"], [4, 40, "am"], [4, 41, "am"], [4, 42, "am"], [4, 43, "am"], [4, 44, "am"], [4, 45, "am"], [4, 46, "am"], [4, 47, "am"], [4, 48, "am"], [4, 49, "am"], [4, 50, "am"], [4, 51, "am"], [4, 52, "am"], [4, 53, "am"], [4, 54, "am"], [4, 55, "am"], [4, 56, "am"], [4, 57, "am"], [4, 58, "am"], [4, 59, "am"], [5, 0, "am"], [5, 1, "am"], [5, 2, "am"], [5, 3, "am"], [5, 4, "am"], [5, 5, "am"], [5, 6, "am"], [5, 7, "am"], [5, 8, "am"], [5, 9, "am"], [5, 10, "am"], [5, 11, "am"], [5, 12, "am"], [5, 13, "am"], [5, 14, "am"], [5, 15, "am"], [5, 16, "am"], [5, 17, "am"], [5, 18, "am"], [5, 19, "am"], [5, 20, "am"], [5, 21, "am"], [5, 22, "am"], [5, 23, "am"], [5, 24, "am"], [5, 25, "am"], [5, 26, "am"], [5, 27, "am"], [5, 28, "am"], [5, 29, "am"], [5, 30, "am"], [5, 31, "am"], [5, 32, "am"], [5, 33, "am"], [5, 34, "am"], [5, 35, "am"], [5, 36, "am"], [5, 37, "am"], [5, 38, "am"], [5, 39, "am"], [5, 40, "am"], [5, 41, "am"], [5, 42, "am"], [5, 43, "am"], [5, 44, "am"], [5, 45, "am"], [5, 46, "am"], [5, 47, "am"], [5, 48, "am"], [5, 49, "am"], [5, 50, "am"], [5, 51, "am"], [5, 52, "am"], [5, 53, "am"], [5, 54, "am"], [5, 55, "am"], [5, 56, "am"], [5, 57, "am"], [5, 58, "am"], [5, 59, "am"], [6, 0, "am"], [6, 1, "am"], [6, 2, "am"], [6, 3, "am"], [6, 4, "am"], [6, 5, "am"], [6, 6, "am"], [6, 7, "am"], [6, 8, "am"], [6, 9, "am"], [6, 10, "am"], [6, 11, "am"], [6, 12, "am"], [6, 13, "am"], [6, 14, "am"], [6, 15, "am"], [6, 16, "am"], [6, 17, "am"], [6, 18, "am"], [6, 19, "am"], [6, 20, "am"], [6, 21, "am"], [6, 22, "am"], [6, 23, "am"], [6, 24, "am"], [6, 25, "am"], [6, 26, "am"], [6, 27, "am"], [6, 28, "am"], [6, 29, "am"], [6, 30, "am"], [6, 31, "am"], [6, 32, "am"], [6, 33, "am"], [6, 34, "am"], [6, 35, "am"], [6, 36, "am"], [6, 37, "am"], [6, 38, "am"], [6, 39, "am"], [6, 40, "am"], [6, 41, "am"], [6, 42, "am"], [6, 43, "am"], [6, 44, "am"], [6, 45, "am"], [6, 46, "am"], [6, 47, "am"], [6, 48, "am"], [6, 49, "am"], [6, 50, "am"], [6, 51, "am"], [6, 52, "am"], [6, 53, "am"], [6, 54, "am"], [6, 55, "am"], [6, 56, "am"], [6, 57, "am"], [6, 58, "am"], [6, 59, "am"], [7, 0, "am"], [7, 1, "am"], [7, 2, "am"], [7, 3, "am"], [7, 4, "am"], [7, 5, "am"], [7, 6, "am"], [7, 7, "am"], [7, 8, "am"], [7, 9, "am"], [7, 10, "am"], [7, 11, "am"], [7, 12, "am"], [7, 13, "am"], [7, 14, "am"], [7, 15, "am"], [7, 16, "am"], [7, 17, "am"], [7, 18, "am"], [7, 19, "am"], [7, 20, "am"], [7, 21, "am"], [7, 22, "am"], [7, 23, "am"], [7, 24, "am"], [7, 25, "am"], [7, 26, "am"], [7, 27, "am"], [7, 28, "am"], [7, 29, "am"], [7, 30, "am"], [7, 31, "am"], [7, 32, "am"], [7, 33, "am"], [7, 34, "am"], [7, 35, "am"], [7, 36, "am"], [7, 37, "am"], [7, 38, "am"], [7, 39, "am"], [7, 40, "am"], [7, 41, "am"], [7, 42, "am"], [7, 43, "am"], [7, 44, "am"], [7, 45, "am"], [7, 46, "am"], [7, 47, "am"], [7, 48, "am"], [7, 49, "am"], [7, 50, "am"], [7, 51, "am"], [7, 52, "am"], [7, 53, "am"], [7, 54, "am"], [7, 55, "am"], [7, 56, "am"], [7, 57, "am"], [7, 58, "am"], [7, 59, "am"], [8, 0, "am"], [8, 1, "am"], [8, 2, "am"], [8, 3, "am"], [8, 4, "am"], [8, 5, "am"], [8, 6, "am"], [8, 7, "am"], [8, 8, "am"], [8, 9, "am"], [8, 10, "am"], [8, 11, "am"], [8, 12, "am"], [8, 13, "am"], [8, 14, "am"], [8, 15, "am"], [8, 16, "am"], [8, 17, "am"], [8, 18, "am"], [8, 19, "am"], [8, 20, "am"], [8, 21, "am"], [8, 22, "am"], [8, 23, "am"], [8, 24, "am"], [8, 25, "am"], [8, 26, "am"], [8, 27, "am"], [8, 28, "am"], [8, 29, "am"], [8, 30, "am"], [8, 31, "am"], [8, 32, "am"], [8, 33, "am"], [8, 34, "am"], [8, 35, "am"], [8, 36, "am"], [8, 37, "am"], [8, 38, "am"], [8, 39, "am"], [8, 40, "am"], [8, 41, "am"], [8, 42, "am"], [8, 43, "am"], [8, 44, "am"], [8, 45, "am"], [8, 46, "am"], [8, 47, "am"], [8, 48, "am"], [8, 49, "am"], [8, 50, "am"], [8, 51, "am"], [8, 52, "am"], [8, 53, "am"], [8, 54, "am"], [8, 55, "am"], [8, 56, "am"], [8, 57, "am"], [8, 58, "am"], [8, 59, "am"], [9, 0, "am"], [9, 1, "am"], [9, 2, "am"], [9, 3, "am"], [9, 4, "am"], [9, 5, "am"], [9, 6, "am"], [9, 7, "am"], [9, 8, "am"], [9, 9, "am"], [9, 10, "am"], [9, 11, "am"], [9, 12, "am"], [9, 13, "am"], [9, 14, "am"], [9, 15, "am"], [9, 16, "am"], [9, 17, "am"], [9, 18, "am"], [9, 19, "am"], [9, 20, "am"], [9, 21, "am"], [9, 22, "am"], [9, 23, "am"], [9, 24, "am"], [9, 25, "am"], [9, 26, "am"], [9, 27, "am"], [9, 28, "am"], [9, 29, "am"], [9, 30, "am"], [9, 31, "am"], [9, 32, "am"], [9, 33, "am"], [9, 34, "am"], [9, 35, "am"], [9, 36, "am"], [9, 37, "am"], [9, 38, "am"], [9, 39, "am"], [9, 40, "am"], [9, 41, "am"], [9, 42, "am"], [9, 43, "am"], [9, 44, "am"], [9, 45, "am"], [9, 46, "am"], [9, 47, "am"], [9, 48, "am"], [9, 49, "am"], [9, 50, "am"], [9, 51, "am"], [9, 52, "am"], [9, 53, "am"], [9, 54, "am"], [9, 55, "am"], [9, 56, "am"], [9, 57, "am"], [9, 58, "am"], [9, 59, "am"], [10, 0, "am"], [10, 1, "am"], [10, 2, "am"], [10, 3, "am"], [10, 4, "am"], [10, 5, "am"], [10, 6, "am"], [10, 7, "am"], [10, 8, "am"], [10, 9, "am"], [10, 10, "am"], [10, 11, "am"], [10, 12, "am"], [10, 13, "am"], [10, 14, "am"], [10, 15, "am"], [10, 16, "am"], [10, 17, "am"], [10, 18, "am"], [10, 19, "am"], [10, 20, "am"], [10, 21, "am"], [10, 22, "am"], [10, 23, "am"], [10, 24, "am"], [10, 25, "am"], [10, 26, "am"], [10, 27, "am"], [10, 28, "am"], [10, 29, "am"], [10, 30, "am"], [10, 31, "am"], [10, 32, "am"], [10, 33, "am"], [10, 34, "am"], [10, 35, "am"], [10, 36, "am"], [10, 37, "am"], [10, 38, "am"], [10, 39, "am"], [10, 40, "am"], [10, 41, "am"], [10, 42, "am"], [10, 43, "am"], [10, 44, "am"], [10, 45, "am"], [10, 46, "am"], [10, 47, "am"], [10, 48, "am"], [10, 49, "am"], [10, 50, "am"], [10, 51, "am"], [10, 52, "am"], [10, 53, "am"], [10, 54, "am"], [10, 55, "am"], [10, 56, "am"], [10, 57, "am"], [10, 58, "am"], [10, 59, "am"], [11, 0, "am"], [11, 1, "am"], [11, 2, "am"], [11, 3, "am"], [11, 4, "am"], [11, 5, "am"], [11, 6, "am"], [11, 7, "am"], [11, 8, "am"], [11, 9, "am"], [11, 10, "am"], [11, 11, "am"], [11, 12, "am"], [11, 13, "am"], [11, 14, "am"], [11, 15, "am"], [11, 16, "am"], [11, 17, "am"], [11, 18, "am"], [11, 19, "am"], [11, 20, "am"], [11, 21, "am"], [11, 22, "am"], [11, 23, "am"], [11, 24, "am"], [11, 25, "am"], [11, 26, "am"], [11, 27, "am"], [11, 28, "am"], [11, 29, "am"], [11, 30, "am"], [11, 31, "am"], [11, 32, "am"], [11, 33, "am"], [11, 34, "am"], [11, 35, "am"], [11, 36, "am"], [11, 37, "am"], [11, 38, "am"], [11, 39, "am"], [11, 40, "am"], [11, 41, "am"], [11, 42, "am"], [11, 43, "am"], [11, 44, "am"], [11, 45, "am"], [11, 46, "am"], [11, 47, "am"], [11, 48, "am"], [11, 49, "am"], [11, 50, "am"], [11, 51, "am"], [11, 52, "am"], [11, 53, "am"], [11, 54, "am"], [11, 55, "am"], [11, 56, "am"], [11, 57, "am"], [11, 58, "am"], [11, 59, "am"], [12, 0, "pm"], [12, 1, "pm"], [12, 2, "pm"], [12, 3, "pm"], [12, 4, "pm"], [12, 5, "pm"], [12, 6, "pm"], [12, 7, "pm"], [12, 8, "pm"], [12, 9, "pm"], [12, 10, "pm"], [12, 11, "pm"], [12, 12, "pm"], [12, 13, "pm"], [12, 14, "pm"], [12, 15, "pm"], [12, 16, "pm"], [12, 17, "pm"], [12, 18, "pm"], [12, 19, "pm"], [12, 20, "pm"], [12, 21, "pm"], [12, 22, "pm"], [12, 23, "pm"], [12, 24, "pm"], [12, 25, "pm"], [12, 26, "pm"], [12, 27, "pm"], [12, 28, "pm"], [12, 29, "pm"], [12, 30, "pm"], [12, 31, "pm"], [12, 32, "pm"], [12, 33, "pm"], [12, 34, "pm"], [12, 35, "pm"], [12, 36, "pm"], [12, 37, "pm"], [12, 38, "pm"], [12, 39, "pm"], [12, 40, "pm"], [12, 41, "pm"], [12, 42, "pm"], [12, 43, "pm"], [12, 44, "pm"], [12, 45, "pm"], [12, 46, "pm"], [12, 47, "pm"], [12, 48, "pm"], [12, 49, "pm"], [12, 50, "pm"], [12, 51, "pm"], [12, 52, "pm"], [12, 53, "pm"], [12, 54, "pm"], [12, 55, "pm"], [12, 56, "pm"], [12, 57, "pm"], [12, 58, "pm"], [12, 59, "pm"], [1, 0, "pm"], [1, 1, "pm"], [1, 2, "pm"], [1, 3, "pm"], [1, 4, "pm"], [1, 5, "pm"], [1, 6, "pm"], [1, 7, "pm"], [1, 8, "pm"], [1, 9, "pm"], [1, 10, "pm"], [1, 11, "pm"], [1, 12, "pm"], [1, 13, "pm"], [1, 14, "pm"], [1, 15, "pm"], [1, 16, "pm"], [1, 17, "pm"], [1, 18, "pm"], [1, 19, "pm"], [1, 20, "pm"], [1, 21, "pm"], [1, 22, "pm"], [1, 23, "pm"], [1, 24, "pm"], [1, 25, "pm"], [1, 26, "pm"], [1, 27, "pm"], [1, 28, "pm"], [1, 29, "pm"], [1, 30, "pm"], [1, 31, "pm"], [1, 32, "pm"], [1, 33, "pm"], [1, 34, "pm"], [1, 35, "pm"], [1, 36, "pm"], [1, 37, "pm"], [1, 38, "pm"], [1, 39, "pm"], [1, 40, "pm"], [1, 41, "pm"], [1, 42, "pm"], [1, 43, "pm"], [1, 44, "pm"], [1, 45, "pm"], [1, 46, "pm"], [1, 47, "pm"], [1, 48, "pm"], [1, 49, "pm"], [1, 50, "pm"], [1, 51, "pm"], [1, 52, "pm"], [1, 53, "pm"], [1, 54, "pm"], [1, 55, "pm"], [1, 56, "pm"], [1, 57, "pm"], [1, 58, "pm"], [1, 59, "pm"], [2, 0, "pm"], [2, 1, "pm"], [2, 2, "pm"], [2, 3, "pm"], [2, 4, "pm"], [2, 5, "pm"], [2, 6, "pm"], [2, 7, "pm"], [2, 8, "pm"], [2, 9, "pm"], [2, 10, "pm"], [2, 11, "pm"], [2, 12, "pm"], [2, 13, "pm"], [2, 14, "pm"], [2, 15, "pm"], [2, 16, "pm"], [2, 17, "pm"], [2, 18, "pm"], [2, 19, "pm"], [2, 20, "pm"], [2, 21, "pm"], [2, 22, "pm"], [2, 23, "pm"], [2, 24, "pm"], [2, 25, "pm"], [2, 26, "pm"], [2, 27, "pm"], [2, 28, "pm"], [2, 29, "pm"], [2, 30, "pm"], [2, 31, "pm"], [2, 32, "pm"], [2, 33, "pm"], [2, 34, "pm"], [2, 35, "pm"], [2, 36, "pm"], [2, 37, "pm"], [2, 38, "pm"], [2, 39, "pm"], [2, 40, "pm"], [2, 41, "pm"], [2, 42, "pm"], [2, 43, "pm"], [2, 44, "pm"], [2, 45, "pm"], [2, 46, "pm"], [2, 47, "pm"], [2, 48, "pm"], [2, 49, "pm"], [2, 50, "pm"], [2, 51, "pm"], [2, 52, "pm"], [2, 53, "pm"], [2, 54, "pm"], [2, 55, "pm"], [2, 56, "pm"], [2, 57, "pm"], [2, 58, "pm"], [2, 59, "pm"], [3, 0, "pm"], [3, 1, "pm"], [3, 2, "pm"], [3, 3, "pm"], [3, 4, "pm"], [3, 5, "pm"], [3, 6, "pm"], [3, 7, "pm"], [3, 8, "pm"], [3, 9, "pm"], [3, 10, "pm"], [3, 11, "pm"], [3, 12, "pm"], [3, 13, "pm"], [3, 14, "pm"], [3, 15, "pm"], [3, 16, "pm"], [3, 17, "pm"], [3, 18, "pm"], [3, 19, "pm"], [3, 20, "pm"], [3, 21, "pm"], [3, 22, "pm"], [3, 23, "pm"], [3, 24, "pm"], [3, 25, "pm"], [3, 26, "pm"], [3, 27, "pm"], [3, 28, "pm"], [3, 29, "pm"], [3, 30, "pm"], [3, 31, "pm"], [3, 32, "pm"], [3, 33, "pm"], [3, 34, "pm"], [3, 35, "pm"], [3, 36, "pm"], [3, 37, "pm"], [3, 38, "pm"], [3, 39, "pm"], [3, 40, "pm"], [3, 41, "pm"], [3, 42, "pm"], [3, 43, "pm"], [3, 44, "pm"], [3, 45, "pm"], [3, 46, "pm"], [3, 47, "pm"], [3, 48, "pm"], [3, 49, "pm"], [3, 50, "pm"], [3, 51, "pm"], [3, 52, "pm"], [3, 53, "pm"], [3, 54, "pm"], [3, 55, "pm"], [3, 56, "pm"], [3, 57, "pm"], [3, 58, "pm"], [3, 59, "pm"], [4, 0, "pm"], [4, 1, "pm"], [4, 2, "pm"], [4, 3, "pm"], [4, 4, "pm"], [4, 5, "pm"], [4, 6, "pm"], [4, 7, "pm"], [4, 8, "pm"], [4, 9, "pm"], [4, 10, "pm"], [4, 11, "pm"], [4, 12, "pm"], [4, 13, "pm"], [4, 14, "pm"], [4, 15, "pm"], [4, 16, "pm"], [4, 17, "pm"], [4, 18, "pm"], [4, 19, "pm"], [4, 20, "pm"], [4, 21, "pm"], [4, 22, "pm"], [4, 23, "pm"], [4, 24, "pm"], [4, 25, "pm"], [4, 26, "pm"], [4, 27, "pm"], [4, 28, "pm"], [4, 29, "pm"], [4, 30, "pm"], [4, 31, "pm"], [4, 32, "pm"], [4, 33, "pm"], [4, 34, "pm"], [4, 35, "pm"], [4, 36, "pm"], [4, 37, "pm"], [4, 38, "pm"], [4, 39, "pm"], [4, 40, "pm"], [4, 41, "pm"], [4, 42, "pm"], [4, 43, "pm"], [4, 44, "pm"], [4, 45, "pm"], [4, 46, "pm"], [4, 47, "pm"], [4, 48, "pm"], [4, 49, "pm"], [4, 50, "pm"], [4, 51, "pm"], [4, 52, "pm"], [4, 53, "pm"], [4, 54, "pm"], [4, 55, "pm"], [4, 56, "pm"], [4, 57, "pm"], [4, 58, "pm"], [4, 59, "pm"], [5, 0, "pm"], [5, 1, "pm"], [5, 2, "pm"], [5, 3, "pm"], [5, 4, "pm"], [5, 5, "pm"], [5, 6, "pm"], [5, 7, "pm"], [5, 8, "pm"], [5, 9, "pm"], [5, 10, "pm"], [5, 11, "pm"], [5, 12, "pm"], [5, 13, "pm"], [5, 14, "pm"], [5, 15, "pm"], [5, 16, "pm"], [5, 17, "pm"], [5, 18, "pm"], [5, 19, "pm"], [5, 20, "pm"], [5, 21, "pm"], [5, 22, "pm"], [5, 23, "pm"], [5, 24, "pm"], [5, 25, "pm"], [5, 26, "pm"], [5, 27, "pm"], [5, 28, "pm"], [5, 29, "pm"], [5, 30, "pm"], [5, 31, "pm"], [5, 32, "pm"], [5, 33, "pm"], [5, 34, "pm"], [5, 35, "pm"], [5, 36, "pm"], [5, 37, "pm"], [5, 38, "pm"], [5, 39, "pm"], [5, 40, "pm"], [5, 41, "pm"], [5, 42, "pm"], [5, 43, "pm"], [5, 44, "pm"], [5, 45, "pm"], [5, 46, "pm"], [5, 47, "pm"], [5, 48, "pm"], [5, 49, "pm"], [5, 50, "pm"], [5, 51, "pm"], [5, 52, "pm"], [5, 53, "pm"], [5, 54, "pm"], [5, 55, "pm"], [5, 56, "pm"], [5, 57, "pm"], [5, 58, "pm"], [5, 59, "pm"], [6, 0, "pm"], [6, 1, "pm"], [6, 2, "pm"], [6, 3, "pm"], [6, 4, "pm"], [6, 5, "pm"], [6, 6, "pm"], [6, 7, "pm"], [6, 8, "pm"], [6, 9, "pm"], [6, 10, "pm"], [6, 11, "pm"], [6, 12, "pm"], [6, 13, "pm"], [6, 14, "pm"], [6, 15, "pm"], [6, 16, "pm"], [6, 17, "pm"], [6, 18, "pm"], [6, 19, "pm"], [6, 20, "pm"], [6, 21, "pm"], [6, 22, "pm"], [6, 23, "pm"], [6, 24, "pm"], [6, 25, "pm"], [6, 26, "pm"], [6, 27, "pm"], [6, 28, "pm"], [6, 29, "pm"], [6, 30, "pm"], [6, 31, "pm"], [6, 32, "pm"], [6, 33, "pm"], [6, 34, "pm"], [6, 35, "pm"], [6, 36, "pm"], [6, 37, "pm"], [6, 38, "pm"], [6, 39, "pm"], [6, 40, "pm"], [6, 41, "pm"], [6, 42, "pm"], [6, 43, "pm"], [6, 44, "pm"], [6, 45, "pm"], [6, 46, "pm"], [6, 47, "pm"], [6, 48, "pm"], [6, 49, "pm"], [6, 50, "pm"], [6, 51, "pm"], [6, 52, "pm"], [6, 53, "pm"], [6, 54, "pm"], [6, 55, "pm"], [6, 56, "pm"], [6, 57, "pm"], [6, 58, "pm"], [6, 59, "pm"], [7, 0, "pm"], [7, 1, "pm"], [7, 2, "pm"], [7, 3, "pm"], [7, 4, "pm"], [7, 5, "pm"], [7, 6, "pm"], [7, 7, "pm"], [7, 8, "pm"], [7, 9, "pm"], [7, 10, "pm"], [7, 11, "pm"], [7, 12, "pm"], [7, 13, "pm"], [7, 14, "pm"], [7, 15, "pm"], [7, 16, "pm"], [7, 17, "pm"], [7, 18, "pm"], [7, 19, "pm"], [7, 20, "pm"], [7, 21, "pm"], [7, 22, "pm"], [7, 23, "pm"], [7, 24, "pm"], [7, 25, "pm"], [7, 26, "pm"], [7, 27, "pm"], [7, 28, "pm"], [7, 29, "pm"], [7, 30, "pm"], [7, 31, "pm"], [7, 32, "pm"], [7, 33, "pm"], [7, 34, "pm"], [7, 35, "pm"], [7, 36, "pm"], [7, 37, "pm"], [7, 38, "pm"], [7, 39, "pm"], [7, 40, "pm"], [7, 41, "pm"], [7, 42, "pm"], [7, 43, "pm"], [7, 44, "pm"], [7, 45, "pm"], [7, 46, "pm"], [7, 47, "pm"], [7, 48, "pm"], [7, 49, "pm"], [7, 50, "pm"], [7, 51, "pm"], [7, 52, "pm"], [7, 53, "pm"], [7, 54, "pm"], [7, 55, "pm"], [7, 56, "pm"], [7, 57, "pm"], [7, 58, "pm"], [7, 59, "pm"], [8, 0, "pm"], [8, 1, "pm"], [8, 2, "pm"], [8, 3, "pm"], [8, 4, "pm"], [8, 5, "pm"], [8, 6, "pm"], [8, 7, "pm"], [8, 8, "pm"], [8, 9, "pm"], [8, 10, "pm"], [8, 11, "pm"], [8, 12, "pm"], [8, 13, "pm"], [8, 14, "pm"], [8, 15, "pm"], [8, 16, "pm"], [8, 17, "pm"], [8, 18, "pm"], [8, 19, "pm"], [8, 20, "pm"], [8, 21, "pm"], [8, 22, "pm"], [8, 23, "pm"], [8, 24, "pm"], [8, 25, "pm"], [8, 26, "pm"], [8, 27, "pm"], [8, 28, "pm"], [8, 29, "pm"], [8, 30, "pm"], [8, 31, "pm"], [8, 32, "pm"], [8, 33, "pm"], [8, 34, "pm"], [8, 35, "pm"], [8, 36, "pm"], [8, 37, "pm"], [8, 38, "pm"], [8, 39, "pm"], [8, 40, "pm"], [8, 41, "pm"], [8, 42, "pm"], [8, 43, "pm"], [8, 44, "pm"], [8, 45, "pm"], [8, 46, "pm"], [8, 47, "pm"], [8, 48, "pm"], [8, 49, "pm"], [8, 50, "pm"], [8, 51, "pm"], [8, 52, "pm"], [8, 53, "pm"], [8, 54, "pm"], [8, 55, "pm"], [8, 56, "pm"], [8, 57, "pm"], [8, 58, "pm"], [8, 59, "pm"], [9, 0, "pm"], [9, 1, "pm"], [9, 2, "pm"], [9, 3, "pm"], [9, 4, "pm"], [9, 5, "pm"], [9, 6, "pm"], [9, 7, "pm"], [9, 8, "pm"], [9, 9, "pm"], [9, 10, "pm"], [9, 11, "pm"], [9, 12, "pm"], [9, 13, "pm"], [9, 14, "pm"], [9, 15, "pm"], [9, 16, "pm"], [9, 17, "pm"], [9, 18, "pm"], [9, 19, "pm"], [9, 20, "pm"], [9, 21, "pm"], [9, 22, "pm"], [9, 23, "pm"], [9, 24, "pm"], [9, 25, "pm"], [9, 26, "pm"], [9, 27, "pm"], [9, 28, "pm"], [9, 29, "pm"], [9, 30, "pm"], [9, 31, "pm"], [9, 32, "pm"], [9, 33, "pm"], [9, 34, "pm"], [9, 35, "pm"], [9, 36, "pm"], [9, 37, "pm"], [9, 38, "pm"], [9, 39, "pm"], [9, 40, "pm"], [9, 41, "pm"], [9, 42, "pm"], [9, 43, "pm"], [9, 44, "pm"], [9, 45, "pm"], [9, 46, "pm"], [9, 47, "pm"], [9, 48, "pm"], [9, 49, "pm"], [9, 50, "pm"], [9, 51, "pm"], [9, 52, "pm"], [9, 53, "pm"], [9, 54, "pm"], [9, 55, "pm"], [9, 56, "pm"], [9, 57, "pm"], [9, 58, "pm"], [9, 59, "pm"], [10, 0, "pm"], [10, 1, "pm"], [10, 2, "pm"], [10, 3, "pm"], [10, 4, "pm"], [10, 5, "pm"], [10, 6, "pm"], [10, 7, "pm"], [10, 8, "pm"], [10, 9, "pm"], [10, 10, "pm"], [10, 11, "pm"], [10, 12, "pm"], [10, 13, "pm"], [10, 14, "pm"], [10, 15, "pm"], [10, 16, "pm"], [10, 17, "pm"], [10, 18, "pm"], [10, 19, "pm"], [10, 20, "pm"], [10, 21, "pm"], [10, 22, "pm"], [10, 23, "pm"], [10, 24, "pm"], [10, 25, "pm"], [10, 26, "pm"], [10, 27, "pm"], [10, 28, "pm"], [10, 29, "pm"], [10, 30, "pm"], [10, 31, "pm"], [10, 32, "pm"], [10, 33, "pm"], [10, 34, "pm"], [10, 35, "pm"], [10, 36, "pm"], [10, 37, "pm"], [10, 38, "pm"], [10, 39, "pm"], [10, 40, "pm"], [10, 41, "pm"], [10, 42, "pm"], [10, 43, "pm"], [10, 44, "pm"], [10, 45, "pm"], [10, 46, "pm"], [10, 47, "pm"], [10, 48, "pm"], [10, 49, "pm"], [10, 50, "pm"], [10, 51, "pm"], [10, 52, "pm"], [10, 53, "pm"], [10, 54, "pm"], [10, 55, "pm"], [10, 56, "pm"], [10, 57, "pm"], [10, 58, "pm"], [10, 59, "pm"], [11, 0, "pm"], [11, 1, "pm"], [11, 2, "pm"], [11, 3, "pm"], [11, 4, "pm"], [11, 5, "pm"], [11, 6, "pm"], [11, 7, "pm"], [11, 8, "pm"], [11, 9, "pm"], [11, 10, "pm"], [11, 11, "pm"], [11, 12, "pm"], [11, 13, "pm"], [11, 14, "pm"], [11, 15, "pm"], [11, 16, "pm"], [11, 17, "pm"], [11, 18, "pm"], [11, 19, "pm"], [11, 20, "pm"], [11, 21, "pm"], [11, 22, "pm"], [11, 23, "pm"], [11, 24, "pm"], [11, 25, "pm"], [11, 26, "pm"], [11, 27, "pm"], [11, 28, "pm"], [11, 29, "pm"], [11, 30, "pm"], [11, 31, "pm"], [11, 32, "pm"], [11, 33, "pm"], [11, 34, "pm"], [11, 35, "pm"], [11, 36, "pm"], [11, 37, "pm"], [11, 38, "pm"], [11, 39, "pm"], [11, 40, "pm"], [11, 41, "pm"], [11, 42, "pm"], [11, 43, "pm"], [11, 44, "pm"], [11, 45, "pm"], [11, 46, "pm"], [11, 47, "pm"], [11, 48, "pm"], [11, 49, "pm"], [11, 50, "pm"], [11, 51, "pm"], [11, 52, "pm"], [11, 53, "pm"], [11, 54, "pm"], [11, 55, "pm"], [11, 56, "pm"], [11, 57, "pm"], [11, 58, "pm"], [11, 59, "pm"]], "outputs": [["0000"], ["0001"], ["0002"], ["0003"], ["0004"], ["0005"], ["0006"], ["0007"], ["0008"], ["0009"], ["0010"], ["0011"], ["0012"], ["0013"], ["0014"], ["0015"], ["0016"], ["0017"], ["0018"], ["0019"], ["0020"], ["0021"], ["0022"], ["0023"], ["0024"], ["0025"], ["0026"], ["0027"], ["0028"], ["0029"], ["0030"], ["0031"], ["0032"], ["0033"], ["0034"], ["0035"], ["0036"], ["0037"], ["0038"], ["0039"], ["0040"], ["0041"], ["0042"], ["0043"], ["0044"], ["0045"], ["0046"], ["0047"], ["0048"], ["0049"], ["0050"], ["0051"], ["0052"], ["0053"], ["0054"], ["0055"], ["0056"], ["0057"], ["0058"], ["0059"], ["0100"], ["0101"], ["0102"], ["0103"], ["0104"], ["0105"], ["0106"], ["0107"], ["0108"], ["0109"], ["0110"], ["0111"], ["0112"], ["0113"], ["0114"], ["0115"], ["0116"], ["0117"], ["0118"], ["0119"], ["0120"], ["0121"], ["0122"], ["0123"], ["0124"], ["0125"], ["0126"], ["0127"], ["0128"], ["0129"], ["0130"], ["0131"], ["0132"], ["0133"], ["0134"], ["0135"], ["0136"], ["0137"], ["0138"], ["0139"], ["0140"], ["0141"], ["0142"], ["0143"], ["0144"], ["0145"], ["0146"], ["0147"], ["0148"], ["0149"], ["0150"], ["0151"], ["0152"], ["0153"], ["0154"], ["0155"], ["0156"], ["0157"], ["0158"], ["0159"], ["0200"], ["0201"], ["0202"], ["0203"], ["0204"], ["0205"], ["0206"], ["0207"], ["0208"], ["0209"], ["0210"], ["0211"], ["0212"], ["0213"], ["0214"], ["0215"], ["0216"], ["0217"], ["0218"], ["0219"], ["0220"], ["0221"], ["0222"], ["0223"], ["0224"], ["0225"], ["0226"], ["0227"], ["0228"], ["0229"], ["0230"], ["0231"], ["0232"], ["0233"], ["0234"], ["0235"], ["0236"], ["0237"], ["0238"], ["0239"], ["0240"], ["0241"], ["0242"], ["0243"], ["0244"], ["0245"], ["0246"], ["0247"], ["0248"], ["0249"], ["0250"], ["0251"], ["0252"], ["0253"], ["0254"], ["0255"], ["0256"], ["0257"], ["0258"], ["0259"], ["0300"], ["0301"], ["0302"], ["0303"], ["0304"], ["0305"], ["0306"], ["0307"], ["0308"], ["0309"], ["0310"], ["0311"], ["0312"], ["0313"], ["0314"], ["0315"], ["0316"], ["0317"], ["0318"], ["0319"], ["0320"], ["0321"], ["0322"], ["0323"], ["0324"], ["0325"], ["0326"], ["0327"], ["0328"], ["0329"], ["0330"], ["0331"], ["0332"], ["0333"], ["0334"], ["0335"], ["0336"], ["0337"], ["0338"], ["0339"], ["0340"], ["0341"], ["0342"], ["0343"], ["0344"], ["0345"], ["0346"], ["0347"], ["0348"], ["0349"], ["0350"], ["0351"], ["0352"], ["0353"], ["0354"], ["0355"], ["0356"], ["0357"], ["0358"], ["0359"], ["0400"], ["0401"], ["0402"], ["0403"], ["0404"], ["0405"], ["0406"], ["0407"], ["0408"], ["0409"], ["0410"], ["0411"], ["0412"], ["0413"], ["0414"], ["0415"], ["0416"], ["0417"], ["0418"], ["0419"], ["0420"], ["0421"], ["0422"], ["0423"], ["0424"], ["0425"], ["0426"], ["0427"], ["0428"], ["0429"], ["0430"], ["0431"], ["0432"], ["0433"], ["0434"], ["0435"], ["0436"], ["0437"], ["0438"], ["0439"], ["0440"], ["0441"], ["0442"], ["0443"], ["0444"], ["0445"], ["0446"], ["0447"], ["0448"], ["0449"], ["0450"], ["0451"], ["0452"], ["0453"], ["0454"], ["0455"], ["0456"], ["0457"], ["0458"], ["0459"], ["0500"], ["0501"], ["0502"], ["0503"], ["0504"], ["0505"], ["0506"], ["0507"], ["0508"], ["0509"], ["0510"], ["0511"], ["0512"], ["0513"], ["0514"], ["0515"], ["0516"], ["0517"], ["0518"], ["0519"], ["0520"], ["0521"], ["0522"], ["0523"], ["0524"], ["0525"], ["0526"], ["0527"], ["0528"], ["0529"], ["0530"], ["0531"], ["0532"], ["0533"], ["0534"], ["0535"], ["0536"], ["0537"], ["0538"], ["0539"], ["0540"], ["0541"], ["0542"], ["0543"], ["0544"], ["0545"], ["0546"], ["0547"], ["0548"], ["0549"], ["0550"], ["0551"], ["0552"], ["0553"], ["0554"], ["0555"], ["0556"], ["0557"], ["0558"], ["0559"], ["0600"], ["0601"], ["0602"], ["0603"], ["0604"], ["0605"], ["0606"], ["0607"], ["0608"], ["0609"], ["0610"], ["0611"], ["0612"], ["0613"], ["0614"], ["0615"], ["0616"], ["0617"], ["0618"], ["0619"], ["0620"], ["0621"], ["0622"], ["0623"], ["0624"], ["0625"], ["0626"], ["0627"], ["0628"], ["0629"], ["0630"], ["0631"], ["0632"], ["0633"], ["0634"], ["0635"], ["0636"], ["0637"], ["0638"], ["0639"], ["0640"], ["0641"], ["0642"], ["0643"], ["0644"], ["0645"], ["0646"], ["0647"], ["0648"], ["0649"], ["0650"], ["0651"], ["0652"], ["0653"], ["0654"], ["0655"], ["0656"], ["0657"], ["0658"], ["0659"], ["0700"], ["0701"], ["0702"], ["0703"], ["0704"], ["0705"], ["0706"], ["0707"], ["0708"], ["0709"], ["0710"], ["0711"], ["0712"], ["0713"], ["0714"], ["0715"], ["0716"], ["0717"], ["0718"], ["0719"], ["0720"], ["0721"], ["0722"], ["0723"], ["0724"], ["0725"], ["0726"], ["0727"], ["0728"], ["0729"], ["0730"], ["0731"], ["0732"], ["0733"], ["0734"], ["0735"], ["0736"], ["0737"], ["0738"], ["0739"], ["0740"], ["0741"], ["0742"], ["0743"], ["0744"], ["0745"], ["0746"], ["0747"], ["0748"], ["0749"], ["0750"], ["0751"], ["0752"], ["0753"], ["0754"], ["0755"], ["0756"], ["0757"], ["0758"], ["0759"], ["0800"], ["0801"], ["0802"], ["0803"], ["0804"], ["0805"], ["0806"], ["0807"], ["0808"], ["0809"], ["0810"], ["0811"], ["0812"], ["0813"], ["0814"], ["0815"], ["0816"], ["0817"], ["0818"], ["0819"], ["0820"], ["0821"], ["0822"], ["0823"], ["0824"], ["0825"], ["0826"], ["0827"], ["0828"], ["0829"], ["0830"], ["0831"], ["0832"], ["0833"], ["0834"], ["0835"], ["0836"], ["0837"], ["0838"], ["0839"], ["0840"], ["0841"], ["0842"], ["0843"], ["0844"], ["0845"], ["0846"], ["0847"], ["0848"], ["0849"], ["0850"], ["0851"], ["0852"], ["0853"], ["0854"], ["0855"], ["0856"], ["0857"], ["0858"], ["0859"], ["0900"], ["0901"], ["0902"], ["0903"], ["0904"], ["0905"], ["0906"], ["0907"], ["0908"], ["0909"], ["0910"], ["0911"], ["0912"], ["0913"], ["0914"], ["0915"], ["0916"], ["0917"], ["0918"], ["0919"], ["0920"], ["0921"], ["0922"], ["0923"], ["0924"], ["0925"], ["0926"], ["0927"], ["0928"], ["0929"], ["0930"], ["0931"], ["0932"], ["0933"], ["0934"], ["0935"], ["0936"], ["0937"], ["0938"], ["0939"], ["0940"], ["0941"], ["0942"], ["0943"], ["0944"], ["0945"], ["0946"], ["0947"], ["0948"], ["0949"], ["0950"], ["0951"], ["0952"], ["0953"], ["0954"], ["0955"], ["0956"], ["0957"], ["0958"], ["0959"], ["1000"], ["1001"], ["1002"], ["1003"], ["1004"], ["1005"], ["1006"], ["1007"], ["1008"], ["1009"], ["1010"], ["1011"], ["1012"], ["1013"], ["1014"], ["1015"], ["1016"], ["1017"], ["1018"], ["1019"], ["1020"], ["1021"], ["1022"], ["1023"], ["1024"], ["1025"], ["1026"], ["1027"], ["1028"], ["1029"], ["1030"], ["1031"], ["1032"], ["1033"], ["1034"], ["1035"], ["1036"], ["1037"], ["1038"], ["1039"], ["1040"], ["1041"], ["1042"], ["1043"], ["1044"], ["1045"], ["1046"], ["1047"], ["1048"], ["1049"], ["1050"], ["1051"], ["1052"], ["1053"], ["1054"], ["1055"], ["1056"], ["1057"], ["1058"], ["1059"], ["1100"], ["1101"], ["1102"], ["1103"], ["1104"], ["1105"], ["1106"], ["1107"], ["1108"], ["1109"], ["1110"], ["1111"], ["1112"], ["1113"], ["1114"], ["1115"], ["1116"], ["1117"], ["1118"], ["1119"], ["1120"], ["1121"], ["1122"], ["1123"], ["1124"], ["1125"], ["1126"], ["1127"], ["1128"], ["1129"], ["1130"], ["1131"], ["1132"], ["1133"], ["1134"], ["1135"], ["1136"], ["1137"], ["1138"], ["1139"], ["1140"], ["1141"], ["1142"], ["1143"], ["1144"], ["1145"], ["1146"], ["1147"], ["1148"], ["1149"], ["1150"], ["1151"], ["1152"], ["1153"], ["1154"], ["1155"], ["1156"], ["1157"], ["1158"], ["1159"], ["1200"], ["1201"], ["1202"], ["1203"], ["1204"], ["1205"], ["1206"], ["1207"], ["1208"], ["1209"], ["1210"], ["1211"], ["1212"], ["1213"], ["1214"], ["1215"], ["1216"], ["1217"], ["1218"], ["1219"], ["1220"], ["1221"], ["1222"], ["1223"], ["1224"], ["1225"], ["1226"], ["1227"], ["1228"], ["1229"], ["1230"], ["1231"], ["1232"], ["1233"], ["1234"], ["1235"], ["1236"], ["1237"], ["1238"], ["1239"], ["1240"], ["1241"], ["1242"], ["1243"], ["1244"], ["1245"], ["1246"], ["1247"], ["1248"], ["1249"], ["1250"], ["1251"], ["1252"], ["1253"], ["1254"], ["1255"], ["1256"], ["1257"], ["1258"], ["1259"], ["1300"], ["1301"], ["1302"], ["1303"], ["1304"], ["1305"], ["1306"], ["1307"], ["1308"], ["1309"], ["1310"], ["1311"], ["1312"], ["1313"], ["1314"], ["1315"], ["1316"], ["1317"], ["1318"], ["1319"], ["1320"], ["1321"], ["1322"], ["1323"], ["1324"], ["1325"], ["1326"], ["1327"], ["1328"], ["1329"], ["1330"], ["1331"], ["1332"], ["1333"], ["1334"], ["1335"], ["1336"], ["1337"], ["1338"], ["1339"], ["1340"], ["1341"], ["1342"], ["1343"], ["1344"], ["1345"], ["1346"], ["1347"], ["1348"], ["1349"], ["1350"], ["1351"], ["1352"], ["1353"], ["1354"], ["1355"], ["1356"], ["1357"], ["1358"], ["1359"], ["1400"], ["1401"], ["1402"], ["1403"], ["1404"], ["1405"], ["1406"], ["1407"], ["1408"], ["1409"], ["1410"], ["1411"], ["1412"], ["1413"], ["1414"], ["1415"], ["1416"], ["1417"], ["1418"], ["1419"], ["1420"], ["1421"], ["1422"], ["1423"], ["1424"], ["1425"], ["1426"], ["1427"], ["1428"], ["1429"], ["1430"], ["1431"], ["1432"], ["1433"], ["1434"], ["1435"], ["1436"], ["1437"], ["1438"], ["1439"], ["1440"], ["1441"], ["1442"], ["1443"], ["1444"], ["1445"], ["1446"], ["1447"], ["1448"], ["1449"], ["1450"], ["1451"], ["1452"], ["1453"], ["1454"], ["1455"], ["1456"], ["1457"], ["1458"], ["1459"], ["1500"], ["1501"], ["1502"], ["1503"], ["1504"], ["1505"], ["1506"], ["1507"], ["1508"], ["1509"], ["1510"], ["1511"], ["1512"], ["1513"], ["1514"], ["1515"], ["1516"], ["1517"], ["1518"], ["1519"], ["1520"], ["1521"], ["1522"], ["1523"], ["1524"], ["1525"], ["1526"], ["1527"], ["1528"], ["1529"], ["1530"], ["1531"], ["1532"], ["1533"], ["1534"], ["1535"], ["1536"], ["1537"], ["1538"], ["1539"], ["1540"], ["1541"], ["1542"], ["1543"], ["1544"], ["1545"], ["1546"], ["1547"], ["1548"], ["1549"], ["1550"], ["1551"], ["1552"], ["1553"], ["1554"], ["1555"], ["1556"], ["1557"], ["1558"], ["1559"], ["1600"], ["1601"], ["1602"], ["1603"], ["1604"], ["1605"], ["1606"], ["1607"], ["1608"], ["1609"], ["1610"], ["1611"], ["1612"], ["1613"], ["1614"], ["1615"], ["1616"], ["1617"], ["1618"], ["1619"], ["1620"], ["1621"], ["1622"], ["1623"], ["1624"], ["1625"], ["1626"], ["1627"], ["1628"], ["1629"], ["1630"], ["1631"], ["1632"], ["1633"], ["1634"], ["1635"], ["1636"], ["1637"], ["1638"], ["1639"], ["1640"], ["1641"], ["1642"], ["1643"], ["1644"], ["1645"], ["1646"], ["1647"], ["1648"], ["1649"], ["1650"], ["1651"], ["1652"], ["1653"], ["1654"], ["1655"], ["1656"], ["1657"], ["1658"], ["1659"], ["1700"], ["1701"], ["1702"], ["1703"], ["1704"], ["1705"], ["1706"], ["1707"], ["1708"], ["1709"], ["1710"], ["1711"], ["1712"], ["1713"], ["1714"], ["1715"], ["1716"], ["1717"], ["1718"], ["1719"], ["1720"], ["1721"], ["1722"], ["1723"], ["1724"], ["1725"], ["1726"], ["1727"], ["1728"], ["1729"], ["1730"], ["1731"], ["1732"], ["1733"], ["1734"], ["1735"], ["1736"], ["1737"], ["1738"], ["1739"], ["1740"], ["1741"], ["1742"], ["1743"], ["1744"], ["1745"], ["1746"], ["1747"], ["1748"], ["1749"], ["1750"], ["1751"], ["1752"], ["1753"], ["1754"], ["1755"], ["1756"], ["1757"], ["1758"], ["1759"], ["1800"], ["1801"], ["1802"], ["1803"], ["1804"], ["1805"], ["1806"], ["1807"], ["1808"], ["1809"], ["1810"], ["1811"], ["1812"], ["1813"], ["1814"], ["1815"], ["1816"], ["1817"], ["1818"], ["1819"], ["1820"], ["1821"], ["1822"], ["1823"], ["1824"], ["1825"], ["1826"], ["1827"], ["1828"], ["1829"], ["1830"], ["1831"], ["1832"], ["1833"], ["1834"], ["1835"], ["1836"], ["1837"], ["1838"], ["1839"], ["1840"], ["1841"], ["1842"], ["1843"], ["1844"], ["1845"], ["1846"], ["1847"], ["1848"], ["1849"], ["1850"], ["1851"], ["1852"], ["1853"], ["1854"], ["1855"], ["1856"], ["1857"], ["1858"], ["1859"], ["1900"], ["1901"], ["1902"], ["1903"], ["1904"], ["1905"], ["1906"], ["1907"], ["1908"], ["1909"], ["1910"], ["1911"], ["1912"], ["1913"], ["1914"], ["1915"], ["1916"], ["1917"], ["1918"], ["1919"], ["1920"], ["1921"], ["1922"], ["1923"], ["1924"], ["1925"], ["1926"], ["1927"], ["1928"], ["1929"], ["1930"], ["1931"], ["1932"], ["1933"], ["1934"], ["1935"], ["1936"], ["1937"], ["1938"], ["1939"], ["1940"], ["1941"], ["1942"], ["1943"], ["1944"], ["1945"], ["1946"], ["1947"], ["1948"], ["1949"], ["1950"], ["1951"], ["1952"], ["1953"], ["1954"], ["1955"], ["1956"], ["1957"], ["1958"], ["1959"], ["2000"], ["2001"], ["2002"], ["2003"], ["2004"], ["2005"], ["2006"], ["2007"], ["2008"], ["2009"], ["2010"], ["2011"], ["2012"], ["2013"], ["2014"], ["2015"], ["2016"], ["2017"], ["2018"], ["2019"], ["2020"], ["2021"], ["2022"], ["2023"], ["2024"], ["2025"], ["2026"], ["2027"], ["2028"], ["2029"], ["2030"], ["2031"], ["2032"], ["2033"], ["2034"], ["2035"], ["2036"], ["2037"], ["2038"], ["2039"], ["2040"], ["2041"], ["2042"], ["2043"], ["2044"], ["2045"], ["2046"], ["2047"], ["2048"], ["2049"], ["2050"], ["2051"], ["2052"], ["2053"], ["2054"], ["2055"], ["2056"], ["2057"], ["2058"], ["2059"], ["2100"], ["2101"], ["2102"], ["2103"], ["2104"], ["2105"], ["2106"], ["2107"], ["2108"], ["2109"], ["2110"], ["2111"], ["2112"], ["2113"], ["2114"], ["2115"], ["2116"], ["2117"], ["2118"], ["2119"], ["2120"], ["2121"], ["2122"], ["2123"], ["2124"], ["2125"], ["2126"], ["2127"], ["2128"], ["2129"], ["2130"], ["2131"], ["2132"], ["2133"], ["2134"], ["2135"], ["2136"], ["2137"], ["2138"], ["2139"], ["2140"], ["2141"], ["2142"], ["2143"], ["2144"], ["2145"], ["2146"], ["2147"], ["2148"], ["2149"], ["2150"], ["2151"], ["2152"], ["2153"], ["2154"], ["2155"], ["2156"], ["2157"], ["2158"], ["2159"], ["2200"], ["2201"], ["2202"], ["2203"], ["2204"], ["2205"], ["2206"], ["2207"], ["2208"], ["2209"], ["2210"], ["2211"], ["2212"], ["2213"], ["2214"], ["2215"], ["2216"], ["2217"], ["2218"], ["2219"], ["2220"], ["2221"], ["2222"], ["2223"], ["2224"], ["2225"], ["2226"], ["2227"], ["2228"], ["2229"], ["2230"], ["2231"], ["2232"], ["2233"], ["2234"], ["2235"], ["2236"], ["2237"], ["2238"], ["2239"], ["2240"], ["2241"], ["2242"], ["2243"], ["2244"], ["2245"], ["2246"], ["2247"], ["2248"], ["2249"], ["2250"], ["2251"], ["2252"], ["2253"], ["2254"], ["2255"], ["2256"], ["2257"], ["2258"], ["2259"], ["2300"], ["2301"], ["2302"], ["2303"], ["2304"], ["2305"], ["2306"], ["2307"], ["2308"], ["2309"], ["2310"], ["2311"], ["2312"], ["2313"], ["2314"], ["2315"], ["2316"], ["2317"], ["2318"], ["2319"], ["2320"], ["2321"], ["2322"], ["2323"], ["2324"], ["2325"], ["2326"], ["2327"], ["2328"], ["2329"], ["2330"], ["2331"], ["2332"], ["2333"], ["2334"], ["2335"], ["2336"], ["2337"], ["2338"], ["2339"], ["2340"], ["2341"], ["2342"], ["2343"], ["2344"], ["2345"], ["2346"], ["2347"], ["2348"], ["2349"], ["2350"], ["2351"], ["2352"], ["2353"], ["2354"], ["2355"], ["2356"], ["2357"], ["2358"], ["2359"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,070 |
def to24hourtime(hour, minute, period):
|
fbebbb7b8bd7aca588e7ea9600f48796 | UNKNOWN | You have a grid with `$m$` rows and `$n$` columns. Return the number of unique ways that start from the top-left corner and go to the bottom-right corner. You are only allowed to move right and down.
For example, in the below grid of `$2$` rows and `$3$` columns, there are `$10$` unique paths:
```
o----o----o----o
| | | |
o----o----o----o
| | | |
o----o----o----o
```
**Note:** there are random tests for grids up to 1000 x 1000 in most languages, so a naive solution will not work.
---
*Hint: use mathematical permutation and combination* | ["from math import factorial as f\nnumber_of_routes=lambda m,n:f(m+n)//(f(m)*f(n))", "import sys\n\nsys.setrecursionlimit(1200)\n\ncache = {}\n\ndef number_of_routes(m, n):\n if m == 0 or n == 0: return 1\n if m > n: return number_of_routes(n, m)\n result = cache.get((m,n))\n if result is None:\n cache[m,n] = result = number_of_routes(m-1, n) + number_of_routes(m, n-1)\n return result", "from operator import mul\nfrom functools import reduce\n\ndef choose(n, p):\n if (p > n): return 0\n if (p > n - p): p = n - p\n return reduce(mul, range((n-p+1), n+1), 1) // reduce( mul, range(1,p+1), 1)\ndef number_of_routes(m, n):\n return choose(m + n, n)"] | {"fn_name": "number_of_routes", "inputs": [[1, 1], [5, 1], [3, 4], [5, 6], [10, 10], [100, 3], [123, 456]], "outputs": [[2], [6], [35], [462], [184756], [176851], [448843261729071620474858205566477025894357385375655014634306680560209909590802545266425906052279365647506075241055256064119806400]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 686 |
def number_of_routes(m, n):
|
8b6c463823b6f0a62a95399051ef85cf | UNKNOWN | Complete the solution so that it returns true if it contains any duplicate argument values. Any number of arguments may be passed into the function.
The array values passed in will only be strings or numbers. The only valid return values are `true` and `false`.
Examples:
```
solution(1, 2, 3) --> false
solution(1, 2, 3, 2) --> true
solution('1', '2', '3', '2') --> true
``` | ["def solution(*args):\n return len(args) != len(set(args))", "def solution(*args):\n print (args)\n result = {}\n for x in args:\n if x in result:\n return True\n else :\n result[x] = 1\n return False\n", "def solution(*args):\n return len(args) > len(set(args))", "def solution(*args):\n return len(set(args)) != len(args)", "def solution(*lst):\n return not len(set(lst))==len(lst)", "def solution(*args):\n s = set()\n for x in args:\n if x in s:\n return 1\n s.add(x)\n return 0", "def solution(*args):\n return True if [x for x in args if args.count(x) > 1] else False", "def solution(x=None, *args):\n args = args + (x,)\n tam1 = len(args)\n tam2 = len(set(args))\n if tam1 == 0: return False\n if tam1 == 1:\n if 1 in args: return True\n else: return False\n elif tam1 != tam2: return True\n else: return False", "def solution(*args):\n # your code here\n l = list(args)\n s = set(l)\n return len(s)!=len(l)", "solution = lambda *args: len(set(args)) < len(args)\n"] | {"fn_name": "solution", "inputs": [[1, 2, 3, 1, 2], [1, 1], [1, 0], ["a", "b"], ["a", "b", "a"], [1, 2, 42, 3, 4, 5, 42], ["a", "b", "c", "d"], ["a", "b", "c", "c"], ["a", "b", "c", "d", "e", "f", "f", "b"]], "outputs": [[true], [true], [false], [false], [true], [true], [false], [true], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,097 |
def solution(*args):
|
1b1d3813cbc0263b6e2b1ce70a8a3c68 | UNKNOWN | The number ```89``` is the first integer with more than one digit that fulfills the property partially introduced in the title of this kata.
What's the use of saying "Eureka"? Because this sum gives the same number.
In effect: ```89 = 8^1 + 9^2```
The next number in having this property is ```135```.
See this property again: ```135 = 1^1 + 3^2 + 5^3```
We need a function to collect these numbers, that may receive two integers ```a```, ```b``` that defines the range ```[a, b]``` (inclusive) and outputs a list of the sorted numbers in the range that fulfills the property described above.
Let's see some cases:
```python
sum_dig_pow(1, 10) == [1, 2, 3, 4, 5, 6, 7, 8, 9]
sum_dig_pow(1, 100) == [1, 2, 3, 4, 5, 6, 7, 8, 9, 89]
```
If there are no numbers of this kind in the range [a, b] the function should output an empty list.
```python
sum_dig_pow(90, 100) == []
```
Enjoy it!! | ["def dig_pow(n):\n return sum(int(x)**y for y,x in enumerate(str(n), 1))\n\ndef sum_dig_pow(a, b): \n return [x for x in range(a,b + 1) if x == dig_pow(x)]", "def sum_dig_pow(a, b):\n return [x for x in range(a, b+1) if sum(int(d)**i for i, d in enumerate(str(x), 1)) == x]", "def sum_dig_pow(a, b): # range(a, b + 1) will be studied by the function\n res = []\n for number in range(a, b+1):\n digits = [int(i) for i in str(number)]\n s = 0\n for idx, val in enumerate(digits):\n s += val ** (idx + 1)\n if s == number:\n res.append(number)\n return res", "def sum_dig_pow(a, b): # range(a, b + 1) will be studied by the function\n return [i for i in range(a,b+1) if i == sum(int(x)**(j+1) for j,x in enumerate(str(i)))]", "def sum_dig_pow(a, b):\n l = []\n for i in range(a,b+1):\n k = 0\n p = str(i)\n for j in range(len(p)):\n k += int(p[j]) ** (j+1)\n if k == i:\n l.append(i)\n return l", "def sum_dig_pow(a, b):\n ans = []\n while a <= b:\n if sum(int(j) ** k for j,k in zip(str(a),range(1,len(str(a)) + 1))) == a:\n ans += [a]\n a += 1\n return ans", "def sum_dig_pow(a, b): # range(a, b) will be studied by the function\n lis=[]\n for i in range(a,b+1):\n temp=str(i)\n su=0\n for l in range(0,len(temp)):\n su+=int(temp[l:l+1])**(l+1)\n if su==i:\n lis.append(i)\n return lis"] | {"fn_name": "sum_dig_pow", "inputs": [[1, 100], [10, 89], [10, 100], [90, 100], [90, 150], [50, 150], [10, 150], [89, 135]], "outputs": [[[1, 2, 3, 4, 5, 6, 7, 8, 9, 89]], [[89]], [[89]], [[]], [[135]], [[89, 135]], [[89, 135]], [[89, 135]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,459 |
def sum_dig_pow(a, b):
|
401bcda047952411522673f368064bd3 | UNKNOWN | Your task is to create a new implementation of `modpow` so that it computes `(x^y)%n` for large `y`. The problem with the current implementation is that the output of `Math.pow` is so large on our inputs that it won't fit in a 64-bit float.
You're also going to need to be efficient, because we'll be testing some pretty big numbers. | ["def power_mod(b, e, m):\n res, b = 1, b % m\n while e > 0:\n if e & 1: res = res * b % m\n e >>= 1\n b = b * b % m\n return res", "def power_mod(base, exponent, modulus):\n if base is 0: return 0\n if exponent is 0: return 0\n base = base % modulus\n result = 1\n while (exponent > 0):\n if (exponent % 2 == 1):\n result = (result * base) % modulus\n exponent = exponent >> 1\n base = (base * base) % modulus\n return result", "def power_mod(x, a, n):\n ret = 1\n x = x % n\n while a:\n (a, r) = divmod(a, 2)\n if r:\n ret = ret * x % n\n x = x ** 2 % n\n return ret\n", "def rests(n, b):\n rests = []\n while n:\n n, r = divmod(n, b)\n rests.append(r)\n return rests\n\n# k-ary LR method (~800 ms)\ndef power_mod(x, y, n, k=5):\n base, table, res = 2 << (k-1), [1], 1\n for i in range(1, base):\n table.append(x*table[i-1] % n)\n for rest in reversed(rests(y, base)):\n for _ in range(k): res = res*res % n\n if rest: res = res*table[rest] % n\n return res", "def power_mod(a, b, n):\n res = 1\n while b:\n if b % 2:\n res = res * a % n\n a = a * a % n\n b = b // 2\n return res", "from math import floor\ndef power_mod(base, exponent, modulus):\n if (base < 0) or (exponent < 0) or (modulus < 1):\n return -1\n result = 1\n while exponent > 0:\n if (exponent % 2) == 1:\n result = (result * base) % modulus\n base = (base * base) % modulus\n exponent = floor(exponent / 2)\n return result", "def power_mod(x, y, n):\n x = x%n\n b = bin(y)[2:]\n l = len(b)\n res = [0]*l\n for i in range(l):\n res[-i-1] = x\n x *= x\n x = x%n\n prod = 1\n for i in range(l):\n if b[-i-1] == '1':\n prod *= res[-i-1]\n prod = prod%n\n return prod", "def power_mod(b, e, m):\n r, b = 1, b % m\n while e > 0:\n if e % 2 == 1: r = (r * b) % m\n e, b = e >> 1, (b * b) % m\n return r"] | {"fn_name": "power_mod", "inputs": [[11, 10, 300], [11, 100000, 49], [5, 100000000, 19], [2, 3, 5], [4, 12, 3], [200, 3000, 10], [8132, 21302, 5], [9, 193125, 37], [13, 81230123, 22], [29, 1013293125, 492], [31, 902938423012, 1023]], "outputs": [[1], [32], [5], [3], [1], [0], [4], [26], [19], [161], [961]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,116 |
def power_mod(b, e, m):
|
7578819889855af53b11d07a5df7a88c | UNKNOWN | In the wake of the npm's `left-pad` debacle, you decide to write a new super padding method that superceds the functionality of `left-pad`. Your version will provide the same functionality, but will additionally add right, and justified padding of string -- the `super_pad`.
Your function `super_pad` should take three arguments: the string `string`, the width of the final string `width`, and a fill character `fill`. However, the fill character can be enriched with a format string resulting in different padding strategies. If `fill` begins with `'<'` the string is padded on the left with the remaining fill string and if `fill` begins with `'>'` the string is padded on the right. Finally, if `fill` begins with `'^'` the string is padded on the left and the right, where the left padding is always greater or equal to the right padding. The `fill` string can contain more than a single char, of course.
Some examples to clarify the inner workings:
- `super_pad("test", 10)` returns " test"
- `super_pad("test", 10, "x")` returns `"xxxxxxtest"`
- `super_pad("test", 10, "xO")` returns `"xOxOxOtest"`
- `super_pad("test", 10, "xO-")` returns `"xO-xO-test"`
- `super_pad("some other test", 10, "nope")` returns `"other test"`
- `super_pad("some other test", 10, "> ")` returns `"some other"`
- `super_pad("test", 7, ">nope")` returns `"testnop"`
- `super_pad("test", 7, "^more complex")` returns `"motestm"`
- `super_pad("test", 7, "")` returns `"test"`
The `super_pad` method always returns a string of length `width` if possible. We expect the `width` to be positive (including 0) and the fill could be also an empty string. | ["def super_pad(string, width, fill=\" \"):\n if fill.startswith('>'):\n return (string + width * fill[1:])[:width]\n elif fill.startswith('^'):\n pad = (width * fill[1:])[:max(0, width - len(string) + 1) // 2]\n return (pad + string + pad)[:width]\n else:\n if fill.startswith('<'): fill = fill[1:]\n return (width * fill)[:max(0, width - len(string))] + string[max(0, len(string) - width):]", "def super_pad(s, width, fill=\" \"):\n padding = width - len(s)\n if width == 0: return ''\n \n def _left_pad():\n if padding >= 0:\n return (fill * padding)[:padding] + s\n return s[-width:]\n \n def _right_pad():\n if padding >= 0:\n return s + (fill * padding)[:padding]\n return s[:width]\n \n def _center_pad():\n right = padding // 2\n left = padding - right\n if padding >= 0:\n return (fill * left)[:left] + s + (fill * right)[:right]\n return s[-left:right]\n \n if fill and fill[0] == '>':\n fill = fill[1:]\n return _right_pad()\n if fill and fill[0] == '^':\n fill = fill[1:]\n return _center_pad()\n if fill and fill[0] == '<':\n fill = fill[1:]\n return _left_pad()", "def super_pad(string, width, fill=\" \"):\n if not fill or fill in '<^>':\n return string\n \n fill_len = width - len(string)\n \n # check padding mode\n if fill[0] == '>':\n l_pad, r_pad = 0, fill_len\n elif fill[0] == '^':\n l_pad, r_pad = (fill_len + 1) // 2, fill_len // 2\n else:\n l_pad, r_pad = fill_len, 0\n \n # if width < string length\n if fill_len < 0:\n return string[-l_pad if l_pad else None : r_pad if r_pad else None]\n \n # adjust the fill string\n if fill[0] in '<^>':\n fill = fill[1:]\n fill *= fill_len // len(fill) + 1\n \n return fill[:l_pad] + string + fill[:r_pad]", "def super_pad(string, width, fill=\" \"):\n if not fill: return string\n needed = width - len(string)\n \n if fill[0] == \">\":\n if needed < 0: return string[:needed]\n return string + (fill[1:] * needed)[:needed]\n elif fill[0] == \"^\":\n filler = fill[1:] * needed\n return (filler[:needed // 2 + needed % 2] + string + filler)[:width]\n else:\n if fill[0] == \"<\": fill = fill[1:]\n if needed < 0: return string[-needed:]\n return (fill * needed)[:needed] + string\n", "def super_pad(s,w,f=\" \"):\n if not f:return s\n if not w:return \"\"\n if f[0]=='>':return (s+f[1:]*w)[:w]\n if f[0]=='^':\n l=r=(f[1:]*w)[:(w-len(s))//2+(1 if (w-len(s))&1 else 0)]\n return (l+s+r)[:w]\n return (((f[1:] if f[0]=='<' else f)*w)[:w-len(s)]+s)[-w:]", "def super_pad(string, width, fill=\" \"):\n str_len = len(string)\n ###### RIGHT\n if fill.find('>')+1:\n fill = fill[1:]\n fill_len = len(fill)\n to_fill_len = width - str_len\n if width < str_len: # For any: fill_len\n retval = string[0:width]\n # For all below: width >= str_len\n elif fill_len == 0:\n retval = string\n elif fill_len == 1:\n RHS_to_fill = width - str_len\n retval = string + fill*RHS_to_fill\n elif fill_len > 1:\n chunks = int(to_fill_len / fill_len)\n parts = to_fill_len % fill_len\n total_len = (chunks*fill_len+parts) # DEBUG\n retvalb = string + (fill*(chunks+1))[0:total_len]\n retval = retvalb\n else:\n retval = 'RIGHT: Oops'\n ##### CENTER\n elif fill.find('^')+1:\n fill = fill[1:]\n fill_len = len(fill)\n to_fill_len = int((width - str_len) / 2)\n LHS_to_fill_len = to_fill_len + (width - str_len) % 2\n RHS_to_fill_len = to_fill_len\n if width < str_len: # For any: fill_len\n retval = string[0:width]\n # For all below: width >= str_len\n elif fill_len == 0:\n retval = string\n else:\n LHS_chunks = int(LHS_to_fill_len / fill_len)\n LHS_parts = LHS_to_fill_len % fill_len\n LHS_total_len = (LHS_chunks*fill_len+LHS_parts)\n RHS_chunks = int(RHS_to_fill_len / fill_len)\n RHS_parts = RHS_to_fill_len % fill_len\n RHS_total_len = (RHS_chunks*fill_len+RHS_parts)\n retval = (fill*(LHS_chunks+1))[0:LHS_total_len] + string + (fill*(RHS_chunks+1))[0:RHS_total_len]\n ###### LEFT\n else:\n if fill.find('<')+1:fill = fill[1:]\n fill_len = len(fill)\n if width < str_len: # For any: fill_len\n retval = string[str_len-width:]\n # For all below: width >= str_len\n elif fill_len == 0:\n retval = string\n elif fill_len == 1:\n LHS_to_fill = width - str_len\n retval = fill*LHS_to_fill + string\n elif fill_len > 1:\n LHS_to_fill = width - str_len\n fill_count = int(LHS_to_fill / fill_len)\n fill_count_rem = LHS_to_fill % fill_len\n retval = fill*fill_count + fill[0:fill_count_rem] +string\n else:\n retval = 'Left_TEST_Left'\n return retval\n", "def super_pad(string, width, fill=\" \"):\n if width == 0:\n return ''\n diff = width - len(string)\n f = fill\n if diff >= 0:\n if len(f) > 0 and f[0] in ['<', '^', '>']:\n if f[0] == '<':\n f = fill[1:]\n return (f*width)[:width-len(string)] + string\n elif f[0] == '^':\n f = fill[1:]\n a = (width-len(string))//2\n b = width-len(string)-a\n return (f*width)[:b] + string + (f*width)[:a]\n elif f[0] == '>':\n f = fill[1:]\n return string + (f*width)[:width-len(string)]\n else:\n return (f*width)[:width-len(string)] + string\n else:\n if f[0] == '<':\n f = fill[1:]\n return string[len(string)-width:]\n elif f[0] == '^':\n f = fill[1:]\n a = abs(width-len(string))//2\n b = abs(width-len(string))-a\n return string[:a] + string[:-b]\n elif f[0] == '>':\n f = fill[1:]\n return string[:width-len(string)]\n else:\n return string[len(string)-width:]", "def super_pad(string, width, fill=\" \"):\n if fill == '':\n return string\n if width == 0:\n return ''\n diff = width-len(string)\n if diff <= 0:\n if fill[0] == '>':\n return string[:width]\n elif fill[0] == '^':\n a = abs(diff)//2\n b = abs(diff)-a\n if a == 0 and diff == -1:\n return string[:-1]\n return string[b:] + string[:-a]\n elif fill[0] == '<':\n fill = fill[1:]\n return string[-width:]\n else:\n if fill[0] == '>':\n fill = fill[1:]\n if len(fill) > diff:\n return string + fill[:diff]\n else:\n ans, i = '', 0\n while i < diff:\n ans += fill\n if len(ans) >= diff:\n ans = ans[:diff]\n break\n i += 1\n return string + ans \n elif fill[0] == '^':\n fill = fill[1:]\n a = diff//2\n b = diff-a\n if len(fill) > diff:\n return fill[:b] + string + fill[:a]\n else:\n ans1, i, ans2 = '', 0, ''\n while i < b:\n ans1 += fill\n ans2 += fill\n if len(ans2) >= diff//2 :\n ans1 = ans1[:a]\n if len(ans1) >= diff-len(ans2):\n ans2 = ans2[:b]\n break\n i += 1\n return ans2 + string + ans1\n elif fill[0] == '<':\n fill = fill[1:]\n if len(fill) > diff:\n return fill[:diff] + string\n else:\n ans, i = '', 0\n while i < diff:\n ans += fill\n if len(ans) >= diff:\n ans = ans[:diff]\n break\n i += 1\n return ans + string\n else:\n if len(fill) > diff:\n return fill[:diff] + string\n else:\n ans, i = '', 0\n while i < diff:\n ans += fill\n if len(ans) >= diff:\n ans = ans[:diff]\n break\n i += 1\n return ans + string", "def super_pad(string, width, fill=\" \"):\n if not width:\n return ''\n if not fill:\n return string\n direction = fill[0]\n if direction in \"<^>\":\n fill = fill[1:]\n else:\n direction = \"<\"\n \n if direction == \"<\":\n return ((fill*width)[:max(0,width-len(string))]+string)[-width:]\n elif direction == \">\":\n return (string+fill*width)[:width]\n else:\n left = (width - len(string) + 1) // 2\n right = (width - len(string) ) // 2\n if right>=0:\n left_padding = (fill*width)[:left]\n right_padding = (fill*width)[:right]\n return left_padding + string + right_padding\n else:\n return string[-left:right]\n \n \n \n \n return string", "from itertools import chain, islice, cycle\n\npadding = lambda fill, size: islice(cycle(fill), size)\n\ndef super_pad(string, width, fill=\" \"):\n if not width: return ''\n if not fill: return string[:width]\n size = width - len(string)\n if size <= 0: return string[:width] if fill[0] in '>^' else string[-width:]\n if fill[0] == '>': return string + ''.join(padding(fill[1:], size))\n if fill[0] == '^': return ''.join(padding(fill[1:], (size + 1) >> 1)) + string + ''.join(padding(fill[1:], size >> 1))\n if fill[0] == '<': fill = fill[1:]\n return ''.join(padding(fill, size)) + string"] | {"fn_name": "super_pad", "inputs": [["test", 10], ["test", 10, "x"], ["test", 10, "xO"], ["test", 10, "xO-"], ["some other test", 10, "nope"], ["some other test", 10, "> "], ["test", 7, ">nope"], ["test", 7, "^more complex"], ["test", 7, ""], ["test", 10, "< "], ["test", 3], ["test", 3, "> "], ["test", 10, ""], ["test", 10, ">"], ["test", 10, "^"], ["test", 0, "> "], ["test", 0, "^ "], ["test", 0, "< "]], "outputs": [[" test"], ["xxxxxxtest"], ["xOxOxOtest"], ["xO-xO-test"], ["other test"], ["some other"], ["testnop"], ["motestm"], ["test"], [" test"], ["est"], ["tes"], ["test"], ["test"], ["test"], [""], [""], [""]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 10,374 |
def super_pad(string, width, fill=" "):
|
c741c4661886657244b5e04f164f4212 | UNKNOWN | We want to know the index of the vowels in a given word, for example, there are two vowels in the word super (the second and fourth letters).
So given a string "super", we should return a list of [2, 4].
Some examples:
Mmmm => []
Super => [2,4]
Apple => [1,5]
YoMama -> [1,2,4,6]
**NOTES:**
* Vowels in this context refers to: a e i o u y (including upper case)
* This is indexed from `[1..n]` (not zero indexed!) | ["def vowel_indices(word):\n return [i for i,x in enumerate(word,1) if x.lower() in 'aeiouy']", "def vowel_indices(word):\n return [i+1 for i,c in enumerate(word.lower()) if c in 'aeiouy']", "def vowel_indices(word):\n return [index for index, value in enumerate(word.lower(), 1) if value in 'aeyuio']", "VOWELS = set(\"aeiuoy\")\n\ndef vowel_indices(word):\n return [i for i,v in enumerate(word.lower(),1) if v in VOWELS]", "def vowel_indices(word):\n vowels = frozenset(('A','E','I','O','U','Y','a','e','i','o','u','y'));\n idx_list = []\n for idx, letter in enumerate(word):\n if (letter in vowels):\n idx_list.append(idx+1)\n return idx_list", "def vowel_indices(word):\n return [i + 1 for i, v in enumerate(word) if v.lower() in \"aeiouy\"]", "def vowel_indices(word):\n return [i+1 for i, c in enumerate(word) if c.lower() in 'aeiouy']", "def vowel_indices(word):\n word = word.lower()\n vowels = ['a', 'e', 'i', 'o', 'u', 'y']\n lst = []\n for index in range(len(word)):\n if word[index] in vowels:\n lst.append(index + 1)\n return lst", "def vowel_indices(word):\n word = word.lower()\n vowel_lst = ['a', 'e', 'i', 'o', 'u', 'y']\n new_lst = []\n for index in range(len(word)):\n if word[index] in vowel_lst:\n new_lst.append(index + 1)\n return new_lst", "is_vowel = set(\"aeiouyAEIOUY\").__contains__\n\ndef vowel_indices(word):\n return [i for i,c in enumerate(word, 1) if is_vowel(c)]", "def vowel_indices(words):\n word = words.lower()\n vowels = []\n i = 0\n for letter in word:\n i += 1\n if letter == \"a\" or letter == \"e\" or letter == \"i\" or letter == \"o\" or letter == \"u\" or letter == \"y\":\n vowels.append(i)\n return vowels", "def vowel_indices(word):\n # your code here\n result = []\n for _ in range(len(word)):\n if word[_].lower() in (\"aeiouy\"):\n result.append(_ + 1)\n return result", "vowel_indices=lambda n:[i+1 for i,j in enumerate(n) if j.lower() in \"aeiouy\"]", "def vowel_indices(word):\n word = word.lower()\n vowels = ['a','e','i','o','u','y']\n vowel_indicies = []\n for i in range (len(word)):\n if word[i] in vowels:\n vowel_indicies.append(i + 1)\n else:\n pass\n return vowel_indicies\n \n", "def vowel_indices(word):\n return([count for count, char in enumerate(word, 1) if char.lower() in [\"a\",\"e\",\"i\",\"o\",\"u\",\"y\"]])\n \n \n", "vowels = set('aeiouyAEIOUY')\n\ndef vowel_indices(word):\n return [i + 1 for i, c in enumerate(word) if c in vowels]", "def vowel_indices(word):\n return [i+1 for i, c in enumerate(word) if c in set('aeiouyAEIOUY')]", "def vowel_indices(word):\n return [i + 1 for (i, x) in enumerate(word.lower()) if x in 'aeiouy']", "def vowel_indices(word):\n vowels = ['a', 'e', 'i', 'o', 'u', 'y'] \n return [i+1 for i in range(0, len(word)) if word[i].lower() in vowels]", "def vowel_indices(word):\n return [i for i, c in enumerate(word.lower(), 1) if c in \"aeiouy\"]", "def vowel_indices(word):\n return [i+1 for i in range(len(word)) if word[i].lower() in ['a', 'e', 'i', 'o', 'u', 'y']]", "def vowel_indices(word):\n return [\n i+1 for i, letter in enumerate(word.lower())\n if letter in 'aeiouy'\n ]", "def vowel_indices(word):\n return([i+1 for i,lett in list(enumerate(word.lower())) if lett in \"aeiouy\"])", "def vowel_indices(word):\n return [i + 1 for i, z in enumerate(word) if z in ['a', 'e', 'i', 'o', 'u', 'y', 'A', 'E', 'I', 'O', 'U', 'Y']]", "vowel_indices=lambda w: [i+1 for i,k in enumerate(w) if k.lower() in \"aeiouy\"]", "def vowel_indices(word):\n vowels = ['a','e','i','o','u','y']\n ret = []\n ind = 1\n for z in word:\n if z.lower() in vowels:\n ret.append(ind)\n ind += 1 \n return ret", "def vowel_indices(word):\n vowels = \"aeiouyAEIOUY\"\n result = [] \n \n for i in range(0,len(word)):\n if word[i] in vowels:\n result.append(i+1)\n \n return result", "def vowel_indices(word):\n retVal = [] # return list\n vow = ['a', 'e', 'i', 'o', 'u', 'y'] # vowel list\n word = word.lower() # make word all lowercase\n i = 1 # init position counter\n \n # loop through word and check for vowel\n for letter in word:\n # test for vowel\n if letter in vow:\n retVal.append(i) # append to return list\n i += 1 # inc position counter\n return retVal # return answer", "import re\n\ndef vowel_indices(word):\n iter = re.finditer(r\"[aeiouy]\", word.lower())\n indices = [m.start(0)+1 for m in iter]\n return indices", "def vowel_indices(word):\n new_list=[]\n vowels=\"a\",\"e\",\"i\",\"o\",\"u\",\"A\",\"E\",\"I\",\"O\",\"U\",\"y\",\"Y\"\n for i in range(len(word)):\n if word[i] in vowels:\n new_list.append(i+1)\n return new_list", "def vowel_indices(word):\n return [loop + 1 for loop, i in enumerate(word) if i in 'aeiouyAEIOUY']", "def vowel_indices(word):\n word = word.lower()\n res = []\n for i in range(len(word)):\n if word[i] in \"aeiouy\":\n res.append(i + 1)\n return res", "def vowel_indices(word):\n l = []\n for i, letter in enumerate(word):\n if letter in ['a', 'e', 'i', 'o', 'u', 'y', 'A', 'E', 'I', 'O', 'U', 'Y']:\n l.append(i + 1)\n return l", "def vowel_indices(word):\n vowels = { 'a', 'e', 'i', 'o', 'u', 'y' }\n return [i for i, letter in enumerate(word.lower(), start=1) if letter in vowels]", "def vowel_indices(word):\n vowels = \"aeuioyAEUIO\"\n l=[]\n for i in range(len(word)):\n if word[i] in vowels:\n l.append(i+1)\n \n return l", "def vowel_indices(word):\n out=[]\n for i,s in enumerate(word):\n if s.lower() in \"aeiouy\":\n out.append(i+1)\n return out", "vowel=\"aeiouy\"\ndef vowel_indices(word):\n ans=[]\n for i in range(len(word)):\n if word[i].lower() in vowel:\n ans.append(i+1)\n return ans", "def vowel_indices(word):\n vowels = ['a','e','i','o','u', 'y']\n return [char + 1 for char in range(0,len(word)) if word[char].lower() in vowels]\n", "def vowel_indices(word):\n ans = []\n c = 1\n for i in [char for char in word.lower()]:\n if i in 'aeiouy':\n ans.append(c)\n c += 1\n return ans", "def vowel_indices(word):\n return [i+1 for i,n in enumerate(word.lower()) if n in ['a','e','i','o','u','y']]", "def vowel_indices(word):\n i = 0\n positions = []\n vowels = ['a', 'e', 'i', 'o', 'u', 'y', 'A', 'E', 'I', 'O', 'U', 'Y']\n for letter in word:\n if letter in vowels:\n positions.append(word.index(letter, i) + 1)\n i += 1\n return positions", "def vowel_indices(word):\n index = 1\n res = []\n for i in word.lower():\n if i in \"aeiouy\":\n res.append(index)\n index += 1\n return res\n", "def vowel_indices(w):\n return [i+1 for i, c in enumerate(w) if c in \"aeiouyAEIOUY\"]", "def vowel_indices(word):\n return [i+1 for i in range(len(word)) if word[i].lower() in 'aeioyu']", "def vowel_indices(word):\n pw = []\n vowles = \"aeiouy\"\n for i in range(len(word)):\n if word[i].lower() in vowles:\n pw.append(i+1)\n return pw", "def vowel_indices(word):\n # your code here\n import re\n return [index+1 for index, element in enumerate(word.lower()) if re.match('[aeiouy]', element)]", "def vowel_indices(word):\n res = []\n for idx, letter in enumerate(word.lower(), 1):\n if letter in 'aeiouy':\n res.append(idx)\n return res", "def vowel_indices(word):\n word = word.lower()\n vowels = \"aeiouy\"\n l = []\n sum = 1\n for i in word:\n if i in vowels:\n l.append(sum)\n sum = sum + 1\n else:\n sum = sum + 1\n \n return l\n", "def vowel_indices(word):\n vowels=list()\n test='aeiouyAEIOUY'\n for i in range(0,len(word)):\n if test.find(word[i])>=0:\n vowels.append(i+1)\n return vowels", "VOWELS = 'aeiouyAEIOUY'\n\ndef vowel_indices(word):\n return [i + 1 for i, l in enumerate(word) if l in VOWELS]", "def vowel_indices(word):\n # your code here\n count = []\n words = word.lower()\n \n for i in range(len(words)): \n if words[i] in \"aeiouy\": \n count.append(i+1) \n \n return count\n \n \n\n", "def vowel_indices(w):\n return [x[0]+1 for x in enumerate(w.lower()) if x[1] in 'aeiouy']", "def vowel_indices(word):\n \n vowels = ['a','A','e','E','i','I','o','O','u','U','y','Y']\n letters = list(word)\n answer = list()\n \n i = 0\n \n while i < len(letters):\n if letters[i] in vowels:\n answer.append(i + 1)\n i = i + 1\n return answer", "def vowel_indices(word):\n vowels = \"aeiouy\"\n list = []\n counter = 0\n word = word.lower()\n for letter in word:\n counter += 1\n if letter in vowels:\n list.append(counter)\n return list", "def vowel_indices(word):\n\n return [i+1 for i in range(0,len(word)) if word.lower()[i] in 'aeiouy']", "def vowel_indices(word):\n vowel = [\"a\", \"e\", \"i\", \"o\", \"u\", \"y\", \"A\", \"E\", \"I\", \"O\", \"U\", \"Y\"]\n count = 1\n lst = []\n for letter in word:\n if letter in vowel:\n \n lst.append(count)\n count += 1\n return lst", "def vowel_indices(word):\n retval=[]\n word=word.lower()\n for counter, value in enumerate(word):\n if value== \"a\":\n retval.append(counter+1)\n if value== \"e\":\n retval.append(counter+1)\n if value== \"i\":\n retval.append(counter+1)\n if value== \"o\":\n retval.append(counter+1)\n if value== \"u\":\n retval.append(counter+1)\n if value== \"y\":\n retval.append(counter+1)\n return retval\n", "def vowel_indices(word):\n vowels = [\"a\", \"e\", \"i\", \"o\", \"u\", \"y\"]\n index_list = []\n for indx, letter in enumerate(word.lower()):\n if letter in vowels:\n index_list.append(indx + 1)\n return index_list\n", "def vowel_indices(word):\n vowels =['a','e','i','o','u','y']\n return [ i for i,x in enumerate(word.lower(),start =1) if x in vowels]\n \n\nprint((vowel_indices(\"Apple\")))\n\n # your code here\n", "def vowel_indices(word):\n vowels = ['a','e','i','o','u','y']\n return [i + 1 for i, c in enumerate(list(word)) if c.lower() in vowels]\n", "import re\n\ndef vowel_indices(word):\n return [k+1 for k, l in enumerate(word) if bool(re.match('[aeuioy]', l, re.I))]", "def vowel_indices(word):\n return [word.find(c,i)+1 for i,c in enumerate(word) if c.lower() in 'aeiouy']", "def vowel_indices(word):\n return [i for i, ch in enumerate(word.lower(), 1) if ch in 'aeiouy']", "def vowel_indices(word):\n res=[]\n for i in range(len(word)):\n if word.lower()[i] in 'aeiouy':\n res.append(i+1)\n return res\n", "def vowel_indices(word):\n vowels = 'aeiouyAEIOUY'\n return [i for i, l in enumerate(word, start=1) if l in vowels]", "def vowel_indices(word):\n word = word.lower()\n vowels = ['a', 'e', 'i', 'o', 'u', 'y']\n a = len(word)\n n=[]\n for i in range(a):\n if word[i] in vowels:\n #i = i+1\n n.append(i+1)\n return(n)", "def vowel_indices(word):\n return [idx for idx, i in enumerate(word, start=1) if i.lower() in 'aeiouy']", "import unittest\n\nVOWEL = {'a', 'e', 'i', 'o', 'u', 'y'}\n\n\ndef vowel_indices(word):\n return [index for index, ele in enumerate(word, 1) if ele.lower() in VOWEL]\n \n \nclass TestVowelIndices(unittest.TestCase):\n def test_vowel_indices(self):\n word = 'Super'\n actual = vowel_indices(word)\n self.assertEqual(actual, [2, 4])\n", "\ndef vowel_indices(word):\n res = []\n for i, char in enumerate(word):\n if char in 'aeiouyAEIOUY':\n res.append(i+1)\n return res", "def vowel_indices(word):\n vowels = 'aeiouyAEIOUY'\n count = 0\n vowel_list = []\n for x in word:\n count += 1\n if x in vowels:\n vowel_list.append(count)\n return(vowel_list)", "def vowel_indices(word):\n # your code here\n li = []\n vowel = [\"a\",\"A\",\"e\",\"E\",\"i\",\"I\",\"o\",\"O\",\"u\",\"U\",\"y\",\"Y\"]\n for i in range(len(word)):\n for j in range(len(vowel)):\n if word[i] == vowel[j]:\n li.append(i+1)\n return li", "def vowel_indices(word):\n return [a+1 for (a,c) in enumerate(word) if c in 'aeiouyAEIOUY']", "def vowel_indices(word):\n output = []\n for i in range(0,len(word)):\n if word[i] in'aeiouyAEIOUY':\n output.append(i+1)\n return output", "def vowel_indices(word):\n n = 0\n a = []\n for i in word:\n n = n+1\n if i.lower() in ['a','e','i','o','u','y']:\n a.append(n)\n return a\n", "def vowel_indices(word):\n lst = [i+1 for i in range(0, len(word)) if word[i] in 'aeiouyAEIOUY']\n return lst", "def vowel_indices(word):\n word = word.lower()\n indexes = []\n vowels = ['a', 'e', 'i', 'o', 'u', 'y']\n for i in range(0, len(word)):\n if word[i] in vowels:\n indexes.append(i + 1)\n return indexes\n # your code here\n", "def vowel_indices(word):\n word = word.lower()\n \n new_list = []\n \n vow = ['a', 'o', 'e', 'i', 'u', 'y']\n for x in range(len(word)):\n if word[x] in vow:\n new_list.append(x+1)\n return new_list", "def vowel_indices(word):\n word=word.lower()\n word=list(word)\n vowel=['a','e','i','o','u','y']\n result=[]\n for i,j in enumerate(word):\n if j in vowel:\n result.append(i+1)\n return result", "def vowel_indices(word):\n word = word.lower()\n a = [\"a\", \"e\", \"i\", \"o\", \"u\", \"y\"]\n res = []\n count = 1\n for i in word: \n if i in a:\n res.append(count)\n count += 1\n return res", "def vowel_indices(word):\n return [(index+1) for index, ele in enumerate(word.lower()) if ele in 'aeiouy']", "def vowel_indices(word):\n word = word.lower()\n vowels = ['a','e','i','o','u','y']\n return [i+1 for i in range(len(word)) if word[i] in vowels ]\n", "def vowel_indices(word):\n location = []\n for letter in range(len(word)):\n if word[letter] in \"aeiouyAEIOUY\":\n location.append(letter + 1)\n return location\n", "def vowel_indices(word):\n word = word.casefold()\n found = 'aeiouy'\n return [i+1 for i in range(len(word)) if word[i] in found]", "def vowel_indices(word):\n return [p for p, c in enumerate(word.lower(), 1) if c in 'aeiouy']", "def vowel_indices(word):\n vowels = \"aeiouyAEIOUY\"\n result = [i+1 for i, char in enumerate(word) if char in vowels]\n return result", "L = 'aeiouyAEIOUY'\nvowel_indices = lambda s: [i + 1 for i, c in enumerate(s) if c in L]", "def vowel_indices(word):\n l = []\n for num in range(len(word)):\n if word[num] in \"aeiouyAEIOUY\":\n l.append(num + 1)\n return l", "def vowel_indices(word):\n res = []\n vowels = [\"a\",\"o\",\"u\",\"i\",\"e\",\"y\"]\n for i in range(len(word)):\n if word[i].lower() in vowels:\n res.append(i+1)\n return res\n", "def vowel_indices(word):\n l=[]\n for i in range(len(word)):\n if word[i] in 'aeiouAEIOUyY':\n l.append(i+1)\n return l", "def vowel_indices(word):\n return [i + 1 for i, c in enumerate(word) if c in \"aAeEiIoOuUyY\"]\n", "def vowel_indices(word):\n vowels = ['a','e','i','o','u','y']\n return [x+1 for x, y in enumerate(word) if y.lower() in vowels]", "def vowel_indices(word):\n word = word.lower()\n vowels_lst = [\"a\", \"e\", \"i\", \"o\", \"u\", \"y\"]\n index_lst = []\n for i in range(len(word)):\n if word[i] in vowels_lst:\n index_lst.append(i + 1)\n return index_lst", "def vowel_indices(word):\n vowel = \"aeiouyAEIOUY\"\n\n return [x + 1 for x in range(len(word)) if word[x] in vowel]", "def vowel_indices(word):\n return [i for i,x in enumerate(word,1) if x in 'AEIOUYaeiouy']", "def vowel_indices(word):\n retVal = []\n checkList = \"aeiouy\"\n for i, x in enumerate(word.lower()):\n print((\"Looking at \", x))\n try:\n l = checkList.index(x)\n retVal.append(i+1)\n print((\"think this is a vowel\",x))\n except ValueError:\n print((\"discarded\",x)) \n return retVal\n \n\n\n# word= list(word)\n# retVal=[]\n# for x in word:\n# x=x.lower()\n# if x==\"a\" or x==\"e\" or x==\"i\" or x==\"o\" or x==\"u\" or x==\"y\":\n# print(\"think this is a vowel\",x)\n# retVal.append(word.index(x)+1)\n# print(x)\n# else:\n# print(\"discarded\",x)\n# return retVal\n", "def vowel_indices(word):\n return [i + 1 for i, x in enumerate(list(word)) if x in 'aeiouyAEIOUY']", "def vowel_indices(word: str):\n i = []\n word = word.lower()\n word = list(word)\n for l in 'aeiouy':\n while l in word:\n i.append(word.index(l) + 1)\n word[i[-1]-1] = ' '\n return sorted(i)", "def vowel_indices(word):\n r = []\n for i, c in enumerate(word):\n if c in 'aeiouyAEIOUY':\n r.append(i + 1)\n return r\n # Flez\n", "def vowel_indices(word):\n return [i+1 for i, a in enumerate(word.lower()) if a in (list('aeiouy'))]", "def vowel_indices(word):\n return [c+1 for c,i in enumerate(word.lower()) if i in 'aeiouy']"] | {"fn_name": "vowel_indices", "inputs": [["mmm"], ["apple"], ["super"], ["orange"], ["grapes"], ["supercalifragilisticexpialidocious"], ["123456"], ["crIssUm"], ["Implied"], ["rIc"], ["UNDISARMED"], ["bialy"], ["stumpknocker"], ["narboonnee"], ["carlstadt"], ["ephodee"], ["spicery"], ["oftenness"], ["bewept"], ["capsized"]], "outputs": [[[]], [[1, 5]], [[2, 4]], [[1, 3, 6]], [[3, 5]], [[2, 4, 7, 9, 12, 14, 16, 19, 21, 24, 25, 27, 29, 31, 32, 33]], [[]], [[3, 6]], [[1, 5, 6]], [[2]], [[1, 4, 6, 9]], [[2, 3, 5]], [[3, 8, 11]], [[2, 5, 6, 9, 10]], [[2, 7]], [[1, 4, 6, 7]], [[3, 5, 7]], [[1, 4, 7]], [[2, 4]], [[2, 5, 7]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 17,980 |
def vowel_indices(word):
|
7b6d8d17a2d172051651e12a7a4c7814 | UNKNOWN | ## Enough is enough!
Alice and Bob were on a holiday. Both of them took many pictures of the places they've been, and now they want to show Charlie their entire collection. However, Charlie doesn't like these sessions, since the motive usually repeats. He isn't fond of seeing the Eiffel tower 40 times. He tells them that he will only sit during the session if they show the same motive at most N times. Luckily, Alice and Bob are able to encode the motive as a number. Can you help them to remove numbers such that their list contains each number only up to N times, without changing the order?
## Task
Given a list lst and a number N, create a new list that contains each number of lst at most N times without reordering. For example if N = 2, and the input is [1,2,3,1,2,1,2,3], you take [1,2,3,1,2], drop the next [1,2] since this would lead to 1 and 2 being in the result 3 times, and then take 3, which leads to [1,2,3,1,2,3].
~~~if:nasm
## NASM notes
Write the output numbers into the `out` parameter, and return its length.
The input array will contain only integers between 1 and 50 inclusive. Use it to your advantage.
~~~
~~~if:c
For C:
* Assign the return array length to the pointer parameter `*szout`.
* Do not mutate the input array.
~~~
## Example
```python
delete_nth ([1,1,1,1],2) # return [1,1]
delete_nth ([20,37,20,21],1) # return [20,37,21]
``` | ["def delete_nth(order,max_e):\n ans = []\n for o in order:\n if ans.count(o) < max_e: ans.append(o)\n return ans\n", "def delete_nth(order, max_e):\n d = {}\n res = []\n for item in order:\n n = d.get(item, 0)\n if n < max_e:\n res.append(item)\n d[item] = n+1\n return res", "def delete_nth(order,max_e):\n answer = []\n for x in order:\n if answer.count(x) < max_e:\n answer.append(x)\n \n return answer", "def delete_nth(order,max_e):\n return [o for i,o in enumerate(order) if order[:i].count(o)<max_e ] # yes!", "from collections import defaultdict\n\ndef delete_nth(order,max_e):\n dct = defaultdict(int)\n res = []\n for i in order:\n dct[i] += 1\n if dct[i] <= max_e:\n res.append(i)\n return res", "from collections import Counter\n\ndef delete_nth(order, max_e):\n c = Counter()\n result = []\n for element in order:\n if c[element] < max_e:\n c[element] += 1\n result.append(element)\n return result", "def delete_nth(order,max_e):\n return [order[i] for i in range(len(order)) if order[0:i+1].count(order[i]) <= max_e]", "def delete_nth(xs, max_count):\n ret=[]\n counts={x:0 for x in xs}\n for x in xs:\n counts[x]+=1\n if counts[x]<=max_count:\n ret.append(x)\n return ret", "from collections import defaultdict\n\ndef delete_nth(order,max_e):\n count = defaultdict(int)\n ret = []\n for x in order:\n count[x] += 1\n if count[x] <= max_e:\n ret.append(x)\n return ret"] | {"fn_name": "delete_nth", "inputs": [[[20, 37, 20, 21], 1], [[1, 1, 3, 3, 7, 2, 2, 2, 2], 3], [[1, 2, 3, 1, 1, 2, 1, 2, 3, 3, 2, 4, 5, 3, 1], 3], [[1, 1, 1, 1, 1], 5], [[], 5]], "outputs": [[[20, 37, 21]], [[1, 1, 3, 3, 7, 2, 2, 2]], [[1, 2, 3, 1, 1, 2, 2, 3, 3, 4, 5]], [[1, 1, 1, 1, 1]], [[]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,611 |
def delete_nth(order,max_e):
|
bc1a6cbca5e7769920f0fe692ea4292f | UNKNOWN | ## Task
Given a positive integer `n`, calculate the following sum:
```
n + n/2 + n/4 + n/8 + ...
```
All elements of the sum are the results of integer division.
## Example
```
25 => 25 + 12 + 6 + 3 + 1 = 47
``` | ["def halving_sum(n): \n s=0\n while n: \n s+=n ; n>>=1\n return s", "def halving_sum(n): \n if n == 1:\n return 1\n else:\n return n + halving_sum(n//2)", "def halving_sum(n): \n return n and n + halving_sum(n>>1)", "def halving_sum(n):\n result = n\n \n while n >= 1:\n n //= 2\n result += n\n \n return result", "import math\ndef halving_sum(n): \n return sum(n//(2**x) for x in range(int(math.log2(n))+1))", "def halving_sum(n):\n return n + halving_sum(n // 2) if n else 0", "def halving_sum(n): \n L=[n]\n for i in range(1,n+1):\n a = n//(2**i)\n if a not in L:\n L.append(a)\n return sum(L)", "def halving_sum(n):\n r = 0\n while n:\n r += n\n n >>= 1\n return r", "def halving_sum(n):\n if n == 1:\n return 1\n else:\n return n + halving_sum(int(n/2))", "def halving_sum(n): \n divisor = 1\n addends = []\n addend = n // divisor\n while addend > 0:\n addends.append(addend)\n divisor *= 2\n addend = n // divisor\n return sum(addends)", "def halving_sum(n): \n # your code here\n re = n\n while n > 1:\n n = n//2\n re += n\n return re", "def halving_sum(n): \n return n + halving_sum(n >> 1) if n > 1 else n", "def halving_sum(n):\n\n b = n // 2\n c = []\n c.append(n)\n c.append(b)\n\n\n for i in range(0,n):\n\n b = b // 2\n c.append(b)\n\n return sum(c)", "def halving_sum(n):\n total = 0\n \n while True:\n if n == 0:\n break\n else:\n total += (n)\n n = n // 2\n \n return total", "def halving_sum(n):\n if n < 1:\n return 0\n else:\n return n + halving_sum (n // 2)\n", "def halving_sum(n): \n return 1 if n<2 else n + halving_sum((int)(n/2))\n \n", "def halving_sum(n): \n sum = 0\n while n != 1:\n sum = sum + n\n n = n // 2\n return sum+1", "def halving_sum(n):\n result = n\n\n if n < 1:\n return n\n else:\n while n > 1:\n result += n // 2\n n = n // 2\n return result\n\nprint((halving_sum(25)))\n", "def halving_sum(n): \n if n > 1 :\n n = n + halving_sum(n//2)\n return n", "def halving_sum(n): \n result = 0\n\n while n:\n result += n\n n //= 2\n\n return result", "def halving_sum(n): \n return 1 if n<=1 else n + halving_sum(n//2)", "def halving_sum(n): \n return sum(n//2**i for i in range(14))", "def halving_sum(n): \n return n if n <= 1 else n + halving_sum(n >> 1)", "def halving_sum(n): \n total = n\n while n > 0:\n n //= 2\n total += n\n return total", "def halving_sum(n): \n return n if n == 1 else n + halving_sum(n // 2)", "def square_gen(n):\n x = 1\n while x <= n:\n yield x\n x *= 2\n \ndef halving_sum(n):\n return sum([int(n/i) for i in square_gen(n)])", "import math\ndef halving_sum(n):\n counter = 0\n div = n\n result = 0\n while div >= 1:\n div = math.floor(n/2**counter)\n counter += 1\n result += div\n return result\n \n", "def halving_sum(n): \n return sum(n >> i for i in range(15))", "def halving_sum(n): \n cnt = 2\n s = n\n \n while n//cnt > 0:\n s += n//cnt\n cnt *= 2\n\n return s", "def halving_sum(n): \n # your code here\n total = n\n while n > 0:\n n = n//2\n total += n\n return total\n pass", "def halving_sum(n): \n return 1 if n//1 == 1 else n//1 + halving_sum((n//1)/2)\n", "def halving_sum(n): \n sum = 1\n while (n != 1):\n sum+=n\n n = n//2\n return (sum)", "def halving_sum(n): \n my_list = []\n while n:\n my_list.append(n)\n n = n//2\n return sum(my_list)", "import math\ndef halving_sum(n): \n return sum([ n // 2 ** i for i in range(math.ceil(math.log(n, 2)))]) if n > 1 else 1", "def halving_sum(n): \n if n//2 < 1:\n return n\n if n//2 == 1:\n return n + 1\n return n + halving_sum(n//2)", "def halving_sum(n):\n add = n\n while n>1:\n n=n//2\n add += n\n \n return add", "def halving_sum(n): \n return n if n <= 1 else halving_sum(n//2) + n", "def halving_sum(n):\n summa = 0\n while n != 1:\n summa += n\n n = n // 2 \n return summa + 1", "def halving_sum(n): \n sum = 0\n exp = 0\n while(n // (2 ** exp)):\n sum += (n // (2 ** exp))\n exp += 1\n return sum", "def halving_sum(n):\n result = n\n denom = 2\n while n // denom > 0:\n result += n // denom\n denom *= 2\n return result", "def halving_sum(n): \n tot = 0\n while n != 1:\n tot += n\n n = n // 2\n return tot + 1\n", "def halving_sum(n): \n m = n\n summ = 0\n count = 1\n while n // count >= 1:\n summ += n // count\n count *= 2\n return summ\n", "import math\n\ndef halving_sum(n):\n half = n/2\n if half <= 1:\n return math.ceil(half)\n else:\n return math.floor(n + halving_sum(half))", "def halving_sum(n): \n sum = n\n while n > 1:\n n //= 2 # n=n//2\n sum += n\n return sum", "def halving_sum(n): \n hold = 0\n x = 1\n while n//x > 0:\n hold += n//x\n x *= 2\n return hold", "import math\ndef halving_sum(n):\n if n < 1:\n return 0\n elif n == 1:\n return 1\n return math.floor(n) + halving_sum(n/2)", "def halving_sum(n):\n res=n\n while n != 1:\n n = int(n/2)\n res+=n\n \n return res", "def halving_sum(n): \n # your code here\n print(n)\n i = 1\n suma = 0\n resultado = 0\n while i < n:\n #print(i)\n suma = n/i\n print((int(suma)))\n resultado = resultado + int(suma)\n #print(int(resultado))\n #print(i)\n i *= 2\n #print(i)\n if(int(resultado) == 0):\n resultado = 1\n return(int(resultado))\n else:\n print((int(resultado)))\n return(int(resultado))\n", "def halving_sum(n): \n a = 1\n b = 0\n while n//a != 1:\n b = b + n//a\n a = a*2\n return b + 1", "def halving_sum(n): \n sum = 0\n while n>=1:\n sum += n\n n >>= 1\n return sum", "def halving_sum(n): \n sum = n\n while True:\n if n//2==0: break\n else : n = n//2\n sum += n\n return sum", "def halving_sum(n): \n a = [n]\n b = n\n while n > 1:\n c = n//2\n a.append(c)\n b += c\n n = c\n return b", "from itertools import takewhile, count\n\ndef halving_sum(n):\n return sum(takewhile(lambda x: x >= 1, map(lambda y: n//2**y, count())))", "def halving_sum(n): \n sm=0\n while n>0 :\n sm+=n ; n//=2\n return sm", "def halving_sum(n):\n start = n\n total = 0\n while start >= 1:\n total += start\n start = int(start / 2)\n return total", "def halving_sum(n): \n if n<=1: return n\n return n + halving_sum(n//2)", "import math\ndef halving_sum(n):\n ne = 1\n while n > 1:\n ne += n\n n = n/2\n n = math.floor(n)\n return ne", "def halving_sum(n): \n k = n\n t = n\n while k > 0:\n k = int(k /2)\n t+=k\n return t", "def halving_sum(n): \n suma = 0\n sumando = n\n while sumando >= 1:\n suma = suma+ sumando\n sumando = sumando //2\n return suma", "def halving_sum(n):\n i, sum = 2, n\n while(i <= n):\n sum += n//i\n i += i\n return sum", "def halving_sum(n):\n summed = 0\n x = 1\n while n // x > 0:\n summed += n // x\n x *= 2\n\n return summed\n", "def halving_sum(n): \n sum = 0\n if n >= 1:\n sum += n + halving_sum(n//2)\n return sum\n \n", "def halving_sum(n): \n a=[]\n for i in range(n):\n a.append(n)\n n=int(n/2)\n if int(n) >= 1:\n pass\n else:\n break\n return sum(a)", "def halving_sum(n): \n result = 0\n while n > 0:\n result += n\n n = int(n / 2)\n return result", "def halving_sum(n): \n if n > 1:\n n += halving_sum(n//2)\n return n\n", "def halving_sum(n): \n r=n\n while(n>0):\n n//=2\n r+=n\n return r", "def halving_sum(n): \n running_total = 0\n while (n > 0):\n running_total += n\n n = n//2\n return running_total\n", "def halving_sum(n): \n div = 2\n total = n\n while n//div:\n total += n//div\n div *= 2\n return total", "def halving_sum(n):\n sum = n\n divide = n\n while divide // 2:\n divide = divide // 2\n sum += divide\n return sum\n", "def halving_sum(n): \n sm = 0\n i = 0\n while n // 2**i > 0:\n sm += n // 2**i\n i += 1\n return sm\n", "def halving_sum(number): \n\n n = number\n\n while number != 1:\n number = number//2\n n += number\n\n return n", "def halving_sum(n): \n sum_list = []\n sum_int = 0\n sum_list.append(int(n))\n while(n != 1):\n sum_list.append(int(n)//2)\n n = int(n)//2\n for q in range(len(sum_list)):\n sum_int = sum_int + sum_list[q]\n return sum_int", "def halving_sum(n): \n l=0\n while n!=1:\n l+=n\n n=n//2\n return l+1", "def halving_sum(n): \n output = 0\n while n > 0:\n output += n\n n //= 2\n return output", "def halving_sum(n): \n half = 0\n while n > 1:\n half += n\n n = n//2\n return half +1", "def halving_sum(n): \n i = 0\n x = 0\n while True:\n if n // 2 ** i > 0:\n x = x + n // 2 ** i\n i = i + 1\n else:\n break\n return x", "def halving_sum(n): \n if n == 1:\n return n\n else:\n print(n)\n return n + halving_sum(n//2)", "def halving_sum(n):\n return 1 if n == 1 else sum([n//(2**i) for i in range(0, n//2) if n//(2**i) >=1])", "import math\ndef halving_sum(n):\n return 1 if n == 1 else sum([math.floor(n/2**i) for i in range(0,math.floor(n/2)) if math.floor(n/2**i) >= 1])", "def halving_sum(n): \n m = 0\n while n >= 1:\n m += n\n n //= 2\n return m", "def halving_sum(n):\n sums=[]\n nums=n\n while nums>0:\n sums.append(nums)\n nums=int(nums/2)\n if nums<=0:\n break\n return sum(sums)", "def halving_sum(n): \n # your code here\n b=1\n top=0\n while b<=n:\n top+=int(n/b)\n b*=2\n return top", "def halving_sum(n): \n res = n\n while n != 1:\n n = n//2\n res += n\n return res", "def halving_sum(n):\n total = n\n divisor = 2\n while n // divisor > 0:\n if n // divisor == 1:\n total += 1\n break\n else:\n total += (n // divisor)\n divisor *= 2\n return(total)", "def halving_sum(n): \n # your code here\n res=0\n while n>1:\n res=res+n\n n=n//2\n return res+1", "def halving_sum(n):\n c=0\n while n>=1:\n c=c+n\n n=n//2\n return c", "from itertools import count, takewhile\n\ndef halving_sum(n): \n g = (2**j for j in count(0))\n g = takewhile(lambda x: n >= x, g)\n return sum(n // i for i in g)\n", "import unittest\n\n\ndef halving_sum(n):\n temp = []\n while True:\n if n == 1:\n break\n temp.append(n)\n n = n // 2\n return sum(temp) + 1\n \n \nclass TestHalvingSum(unittest.TestCase):\n def test_halving_sum_when_given_n_is_25(self):\n n = 25\n actual = halving_sum(n)\n self.assertEqual(actual, 47)\n\n def test_halving_sum_when_given_n_is_127(self):\n n = 127\n actual = halving_sum(n)\n self.assertEqual(actual, 247)\n", "import math\n\ndef halving_sum(n): \n a = 0\n d = 1\n res = 0\n while a != 1:\n a = math.floor(n / d)\n d *= 2\n res += a\n return res", "def halving_sum(n): \n delit = 2\n sum = n\n while n > 0:\n n = n//delit\n delit*2\n sum+=n\n return sum", "import math\ndef halving_sum(n):\n accum = 0\n while n > 0:\n accum += n\n n = math.floor(n/2)\n return accum\n", "from math import trunc\ndef halving_sum(n): \n result = divided = n\n divisor = 1\n while divided > 1:\n divisor *= 2\n divided = n // divisor\n result += divided\n return result", "def halving_sum(n): \n \n x = n \n \n while n != 1:\n x += n//2\n n //= 2\n \n return x", "def halving_sum(n): \n a = 0\n while n >= 1:\n a += n\n n *= 1/2\n n = int(n)\n return a", "def halving_sum(n): \n\n number = n\n while number > 1:\n n += int(number/2)\n number /= 2\n return n", "import math\ndef halving_sum(n): \n # your code here\n number = n\n \n while number >= 1:\n n += (math.floor(number/2))\n number /= 2\n \n return n", "import math\n\ndef halving_sum(n): \n score = 0\n\n while (n >= 1):\n score += n\n\n n = math.floor((n * 1) / 2)\n \n return score", "def halving_sum(n):\n total_sum = n\n\n while n != 1:\n n = n // 2\n total_sum += n\n \n return total_sum\n", "def halving_sum(n): \n answer = 0\n while n > 0:\n answer += n\n n = n // 2\n return answer", "def halving_sum(n):\n \n x = 2\n sol = n\n \n while (n//x)>0:\n sol = sol + n//x\n x*=2\n \n return sol"] | {"fn_name": "halving_sum", "inputs": [[25], [127], [38], [1], [320], [13], [15], [47], [101], [257]], "outputs": [[47], [247], [73], [1], [638], [23], [26], [89], [198], [512]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 13,810 |
def halving_sum(n):
|
63a4307734e9b2f72c1939f8eaef3637 | UNKNOWN | Two red beads are placed between every two blue beads. There are N blue beads. After looking at the arrangement below work out the number of red beads.
@
@@
@
@@
@
@@
@
@@
@
@@
@
Implement count_red_beads(n) (in PHP count_red_beads($n); in Java, Javascript, TypeScript, C, C++ countRedBeads(n)) so that it returns the number of red beads.
If there are less than 2 blue beads return 0. | ["def count_red_beads(nb):\n return max(0, 2 * (nb - 1) )", "def count_red_beads(n_blue):\n return (n_blue - 1) * 2 if n_blue >= 2 else 0\n", "def count_red_beads(n):\n return max(0, 2 * (n-1))\n #maximum value -> two for every two beads times number of blue beads - 1\n", "def count_red_beads(n):\n return 2*(n - 1) if n > 1 else 0", "count_red_beads=lambda b:b and b*2-2", "def count_red_beads(n):\n return 2*max(n-1,0)", "def count_red_beads(n):\n if n >= 2:\n red = n+(n-2)\n return red\n else: \n return 0", "def count_red_beads(N_blue):\n return (N_blue-2)*2+2 if N_blue >2 else 0", "def count_red_beads(N_blue):\n return max(N_blue - 1, 0) * 2", "def count_red_beads(n_blue):\n return 2 * (n_blue - 1) if n_blue > 1 else 0", "def count_red_beads(n):\n return max(0, (n-1) * 2)", "def count_red_beads(n):\n return (n-1)*2 if n > 1 else 0", "def count_red_beads(n):\n return (n-1) * 2 if n else 0", "def count_red_beads(N_blue):\n return 2*(N_blue - 1) if N_blue >= 2 else 0", "def count_red_beads(N_b):\n return 0 if N_b == 0 else (N_b - 1) * 2", "def count_red_beads(N_blue):\n return (N_blue - 1) * 2 if N_blue >= 2 else 0", "count_red_beads = lambda n: n and (n - 1) * 2", "def count_red_beads(N_blue):\n return N_blue and (N_blue-1)*2", "def count_red_beads(n):\n return 0 if n == 0 else n*2 - 2", "def count_red_beads(n):\n return n and n*2-2", "count_red_beads=lambda b:(b>1)*(b-1)*2", "count_red_beads=lambda n:max(n-1,0)*2", "def count_red_beads(n):\n if n < 2:\n return 0\n elif n == 2:\n return 2\n else:\n return ((n-2) * 2) +2", "def count_red_beads(n):\n return (n-1)*(n>0)*2", "import math\n\ndef count_red_beads(n):\n\n if n<2:\n \n return 0\n \n else:\n \n if n%2!=0:\n \n g = math.floor(n/2)\n \n d = g*4\n \n return d\n \n if n%2==0:\n \n g = math.floor(n/2)\n \n d = g*4\n \n return d-2\n", "def count_red_beads(n):\n if (n != 0):\n return 2 * (n - 1)\n return 0", "\ndef count_red_beads(n):\n lista = []\n if n < 2:\n return 0\n for i in range(1,n):\n lista.append(i*2)\n return lista[-1]\n \n", "def count_red_beads(n):\n return 2*n-2*(n>0)", "def count_red_beads(n):\n return (max(1,n)-1)*2 ", "def count_red_beads(n):\n return (n - 1) * 2 if n else n", "def count_red_beads(n):\n if n<=1:\n return 0\n return 2*(n-1)", "def count_red_beads(n):\n if n < 2:\n return 0\n if n == 2:\n return 2\n else:\n return n*2 - 2", "def count_red_beads(n):\n if n == 0:\n return 0\n else:\n output = (n - 1) * 2\n \n return output", "def count_red_beads(n):\n if n == 0 or n == 1:\n return 0\n else:\n return (n - 1) * 2", "count_red_beads = lambda n: max(0, 2 * (n - 1))", "def count_red_beads(n):\n return 0 if n < 2 else 2 * ((3*n-1)//3) ", "def count_red_beads(n):\n return [0, (n-1)*2][n > 2]", "def count_red_beads(n):\n if n==1 or n==0:\n return 0\n return (n-1)*2", "count_red_beads = lambda n: 0 if n in [0,1] else (n-1)*2", "def count_red_beads(n):\n return range(0,10001,2)[n-1 if n>0 else n]", "def count_red_beads(n):\n if n-2<0:\n return 0\n else:\n y= n*2\n z= y-2\n return(z)\n", "def count_red_beads(n):\n return max(0, n - 1 << 1)", "def count_red_beads(n):\n if n-3<0:\n return 0\n else:\n y= n*2\n z= y-2\n return(z)", "def count_red_beads(n):\n counter = 0\n if n < 2:\n return counter\n else:\n for x in range(1, n):\n counter +=2\n return counter", "def count_red_beads(n):\n if n>=2:\n return (2*n)-2\n return 0", "def count_red_beads(n):\n if n <= 0:\n return 0\n\n return (n - 1) * 2", "def count_red_beads(n):\n if n < 2:\n return 0\n red = (n - 1) * 2\n return red", "count_red_beads = lambda n: (n - 1) * 2 if n else 0", "def count_red_beads(n):\n if n < 2:\n return 0\n elif n % 2 == 0:\n return (n - 1) * 2\n else:\n return (n * 2) - 2", "def count_red_beads(n):\n if n < 2:\n r = 0\n else:\n r = (n*2) - 2\n return(r)", "def count_red_beads(n):\n if n <= 1:\n return 0\n elif n > 1:\n return (n*2)-2\n", "def count_red_beads(n):\n red_beads = 0\n if n < 2:\n return 0\n red_beads = (n-1) * 2\n return red_beads\n \n", "def count_red_beads(n):\n return 0 if n-1 < 2 else(n - 1) * 2", "def count_red_beads(n):\n return 0 if n < 2 else 2 if n == 2 else (n-1)*2", "def count_red_beads(n):\n return (n - 1) * 2 if n > 1 else 0\n # Flez\n", "def count_red_beads(blue):\n if blue <= 1:\n return 0\n return (blue - 1) * 2", "def count_red_beads(n):\n if n < 1:\n return 0\n else:\n return n + (n-2)", "def count_red_beads(n):\n if n < 2:\n return 0\n else:\n count = 2\n for i in range(2, n):\n count += 2\n\n return count", "def count_red_beads(n):\n while n > 0:\n return (n *2) - 2\n return 0", "def count_red_beads(n):\n if n >= 2:\n x = n -2\n result = n + x\n return result\n else:\n return 0", "def count_red_beads(n):\n count = 0\n if n < 2:\n return 0\n for x in range(1,n):\n count += 2\n return count", "def count_red_beads(n):\n return 'rr'.join(['b' for b in range(n)]).count('r')", "def count_red_beads(n):\n return (max(n,1)-1) * 2", "def count_red_beads(n):\n res=0\n for i in range(1,n):\n res+=2\n return res", "def count_red_beads(n):\n if n < 2:\n return 0\n else:\n y = (n * 2) - 2\n return y", "def count_red_beads(n):\n if n > 2:\n return 2*n-2\n else:\n return 0", "def count_red_beads(n):\n if n < 2:\n return 0\n \n r = n * 2 - 2\n return r", "def count_red_beads(n):\n if n < 2:\n return 0\n else:\n n -= 1\n output = n * 2\n return output", "def count_red_beads(n):\n return (n-1)*2 if n>1 else 0 #I solved this Kata on [28-Nov-2019] ^_^ [11:33 PM]...#Hussam'sCodingDiary", "def count_red_beads(n):\n numOfRed = 0\n if n < 2:\n return 0\n else:\n numOfRed += n * 2 - 2\n return numOfRed", "def count_red_beads(n):\n if n < 2:\n return 0\n elif n >= 2:\n return 2*(n-1)", "def count_red_beads(n):\n r = 2\n if n < 2:\n return 0\n for x in range(2, n):\n r += 2\n return r", "def count_red_beads(n):\n if (n - 1) * 2 < 0:\n return 0\n return (n - 1) * 2", "count_red_beads = lambda n: max(0, (n-1)*2)", "def count_red_beads(n):\n count = 0\n if n < 2:\n return count\n for i in range(2, n+1):\n count += 2\n return count", "count_red_beads = lambda n: (n-1)*2*(n>0)", "def count_red_beads(n):\n if n == 0:\n return 0\n else:\n return ((n*2)-2)", "def count_red_beads(n):\n if n < 2:\n return 0\n else: \n p = (n*2)-2\n return p", "def count_red_beads(x):\n if x>1:\n return(2*(x-1))\n else:\n return(0)", "def count_red_beads(n):\n if n == 2:\n return 0\n elif n>0:\n return (n-1)*2\n return 0\n", "def count_red_beads(n):\n return [0, (n-1)*2][n>0]", "def count_red_beads(n):\n return (n + n) - 2 if n > 0 else 0", "count_red_beads = lambda n: (n - (1 if n else 0)) * 2", "count_red_beads = lambda n: (0, (n - 1) * 2)[bool(n)]", "def count_red_beads(n):\n if n > 1:\n red = 2*n - 2\n return red\n else:\n return 0", "def count_red_beads(n):\n if n < 2:\n return 0\n else:\n red_beds = n * 2 - 2\n return red_beds", "def count_red_beads(n):\n return n and (n-1)*2", "def count_red_beads(n):\n if n < 2:\n return 0\n else:\n n -= 1\n return 2 * n", "def count_red_beads(n):\n if n == 0 or n == 1:\n return 0\n return (n-1)*2", "def count_red_beads(n):\n if n > 1:\n return n * 2 - 2\n elif n == 1:\n return 0\n elif n < 1:\n return 0", "def count_red_beads(n):\n if n==1 or n==0:\n return 0\n elif n%2!=0:\n return (n-1)*2\n else:\n return(n*2)-2", "def count_red_beads(n):\n a = -2\n for i in range(n):\n a += 2\n return (a if a > 0 else 0)", "def count_red_beads(n):\n if n < 2:\n return 0\n if n > 2:\n red = (n-1)*2\n return red", "def count_red_beads(n):\n if n<2:\n return 0\n else:\n i =0\n RedCount = -2\n while(i<n):\n RedCount+=2\n i+=1\n return RedCount\n", "def count_red_beads(n):\n beads = 0\n if n < 2:\n return 0\n else: \n for i in range(n-1):\n beads += 2 \n return beads ", "def count_red_beads(n):\n ecount = 2\n ocount = 4\n oplst1 = []\n \n if n < 2:\n return 0\n else:\n for i in range(2, n+1):\n if i % 2 == 0:\n oplst1.append(ecount)\n ecount += 4\n else:\n oplst1.append(ocount)\n ocount += 4\n \n return oplst1[-1]", "def count_red_beads(n):\n if n == 2:\n return n\n elif n > 2:\n return n * 2 - 2\n else:\n return 0", "def count_red_beads(n):\n beads = ''\n for i in range(n-1):\n beads += 'B'\n beads += 'RR'\n beads += 'B'\n# print(beads)\n return beads.count('R')", "def count_red_beads(n):\n return ((n or 1)-1)*2"] | {"fn_name": "count_red_beads", "inputs": [[0], [1], [3], [5]], "outputs": [[0], [0], [4], [8]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 9,826 |
def count_red_beads(n):
|
01ee8730452d20100f7b65c458bc9a4f | UNKNOWN | Hector the hacker has stolen some information, but it is encrypted. In order to decrypt it, he needs to write a function that will generate a decryption key from the encryption key which he stole (it is in hexadecimal). To do this, he has to determine the two prime factors `P` and `Q` of the encyption key, and return the product `(P-1) * (Q-1)`.
**Note:** the primes used are < 10^(5)
## Examples
For example if the encryption key is `"47b"`, it is 1147 in decimal. This factors to 31\*37, so the key Hector needs is 1080 (= 30\*36).
More examples:
* input: `"2533"`, result: 9328 (primes: 89, 107)
* input: `"1ba9"`, result: 6912 (primes: 73, 97) | ["def find_key(key):\n n = int(key, 16)\n return next((k - 1) * ((n // k) - 1) for k in range(2, int(n**0.5)+1) if n % k == 0)\n", "def find_key(enc):\n n = int(enc,16)\n for i in range(2,int(n**.5)+1):\n if not n%i:\n return (i-1)*(n//i-1)\n", "def prime_factors(n):\n s, a = [], 2\n while a * a <= n:\n while n % a == 0:\n s.append(a)\n n //= a\n if a >= n: return s\n a += 1\n if n > 1: s.append(n)\n return s\n \ndef find_key(encryption_key):\n a, b = prime_factors(int(encryption_key, 16))\n return (a - 1) * (b - 1)", "def find_key(key):\n n = int(key, 16)\n a, b = factor(n)\n return (a - 1) * (b - 1)\n\ndef factor(n):\n for i in range(2, n):\n if n % i == 0:\n return i, int(n/i)", "def find_key(encryption_key):\n n = int(encryption_key, 16)\n i = next(i for i in range(2, int(n**0.5)+1) if n % i == 0)\n return ((n//i) - 1) * (i - 1)", "def find_key(encryption_key):\n product = int(encryption_key, 16)\n for p1 in range(2, int(product ** 0.5) + 1):\n if product % p1 == 0:\n p2 = product // p1\n return (p1 - 1) * (p2 - 1)\n", "import math\n\ndef find_key(key):\n n=int(key,16)\n p=[d for d in range(2,int(math.sqrt(n))) if n%d==0]\n return (p[0]-1)*(n//p[0]-1)", "def find_key(encryption_key):\n is_prime=lambda p:all(p%i for i in range(3,int(p**0.5)+1,2)) and (p==2 or p%2)\n n=int(encryption_key,16)\n if n%2==0 and is_prime(n//2):return n//2-1\n for i in range(3,n+1,2):\n if n%i==0 and is_prime(i) and is_prime(n//i):\n return (i-1)*(n//i-1)"] | {"fn_name": "find_key", "inputs": [["47b"], ["2533"], ["1ba9"]], "outputs": [[1080], [9328], [6912]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,661 |
def find_key(key):
|
2dd33152b633365e46bfed5bb4a0024c | UNKNOWN | # Task
John was in math class and got bored, so he decided to fold some origami from a rectangular `a × b` sheet of paper (`a > b`). His first step is to make a square piece of paper from the initial rectangular piece of paper by folding the sheet along the bisector of the right angle and cutting off the excess part.
After moving the square piece of paper aside, John wanted to make even more squares! He took the remaining (`a-b`) × `b` strip of paper and went on with the process until he was left with a square piece of paper.
Your task is to determine how many square pieces of paper John can make.
# Example:
For: `a = 2, b = 1`, the output should be `2`.
Given `a = 2` and `b = 1`, John can fold a `1 × 1` then another `1 × 1`.
So the answer is `2`.
For: `a = 10, b = 7`, the output should be `6`.
We are given `a = 10` and `b = 7`. The following is the order of squares John folds: `7 × 7, 3 × 3, 3 × 3, 1 × 1, 1 × 1, and 1 × 1`.
Here are pictures for the example cases.
# Input/Output
- `[input]` integer `a`
`2 ≤ a ≤ 1000`
- `[input]` integer `b`
`1 ≤ b < a ≤ 1000`
- `[output]` an integer
The maximum number of squares. | ["def folding(a,b):\n squares = 1\n while a != b:\n squares += 1\n b, a = sorted((a - b, b))\n return squares", "def folding(a,b):\n count = 0\n while a > 0 and b > 0:\n a, b, count = max(a-b, b), min(a-b, b), count+1\n return count", "def folding(a, b):\n return 0 if b == 0 else a // b + folding(b, a % b)", "from itertools import count\n\ndef folding(a, b):\n for c in count(0):\n if a == 1: return c + b\n if b == 1: return c + a\n if a == b: return c + 1\n a, b = abs(a-b), min(a, b)", "import sys\nsys.setrecursionlimit(1500)\ndef folding(a,b, t = 1):\n if a == b:\n return t\n else:\n t += 1\n return folding(min(a,b), abs(a-b), t)", "def folding(a, b, c=0): \n return folding(b, a % b, c + a // b) if b != 0 else c", "def folding(a,b):\n #coding and coding..\n n=1\n while a > b:\n a_new = a-b\n a = max(a_new,b)\n b = min(a_new,b)\n n+=1\n return n\n", "def folding(a,b):\n if a%b==0:\n return int(a/b)\n elif a>b:\n return folding(b,a%b)+int(a/b)\n elif b>a:\n return folding(a,b%a)+int(b/a)\n", "def folding(a,b):\n count = 0\n while a != b:\n if a < b: a, b = b, a\n a = a - b\n count += 1\n return count + 1", "def folding(a,b):\n n = 1\n while a != b:\n n += 1\n a, b = abs(a-b), min(a,b)\n return n"] | {"fn_name": "folding", "inputs": [[2, 1], [10, 7], [3, 1], [4, 1], [3, 2], [4, 2], [1000, 700], [1000, 999]], "outputs": [[2], [6], [3], [4], [3], [2], [6], [1000]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,428 |
def folding(a,b):
|
ce211889465ea60dab2940dcb39842aa | UNKNOWN | Create a function that will return true if all numbers in the sequence follow the same counting pattern. If the sequence of numbers does not follow the same pattern, the function should return false.
Sequences will be presented in an array of varied length. Each array will have a minimum of 3 numbers in it.
The sequences are all simple and will not step up in varying increments such as a Fibonacci sequence.
JavaScript examples: | ["def validate_sequence(seq):\n return len({a - b for a, b in zip(seq, seq[1:])}) == 1", "def validate_sequence(sequence):\n return sequence == list(range(sequence[0], sequence[-1] + 1, sequence[1] - sequence[0]))\n", "def validate_sequence(s):\n return sum(s) == (s[0] + s[-1]) * len(s) /2", "def validate_sequence(sequence):\n print(sequence)\n d=sequence[1]-sequence[0]\n for i in range(2,len(sequence)):\n if sequence[i] - sequence[i-1] != d: return False\n return True", "def validate_sequence(sequence):\n step = sequence[1] - sequence[0]\n return all(b-a == step for a, b in zip(sequence, sequence[1:]))", "def validate_sequence(sequence):\n return len(set([n - p for p, n in zip(sequence, sequence[1:])])) == 1", "validate_sequence=lambda a:eval('=='.join([str(x) for x in [a[i]-a[i+1] for i in range(len(a)-1)]]))", "def validate_sequence(sequence):\n increment = sequence[1] - sequence[0]\n return all(y - x == increment for x, y in zip(sequence, sequence[1:]))\n", "def validate_sequence(sequence):\n i = sequence[1] - sequence[0]\n l = list(range(min(sequence),max(sequence)+1,i))\n return l == sequence", "def validate_sequence(seq):\n diff = seq[1] - seq[0]\n for i,n in enumerate(seq[:-1]):\n if seq[i + 1] - n != diff:\n return False\n return True"] | {"fn_name": "validate_sequence", "inputs": [[[1, 2, 3, 4, 5, 8, 7, 8, 9]], [[2, 8, 6, 7, 4, 3, 1, 5, 9]], [[1, 2, 3, 4, 5, 6, 7, 8, 9]], [[0, 1, 2, 3, 4, 5, 6, 7, 8]], [[1, 3, 5, 7, 9, 11, 13, 15]], [[1, 3, 5, 7, 8, 12, 14, 16]], [[0, 1, 1, 2, 3, 5, 8, 13, 21, 34]], [[0, 2, 4, 6, 8]], [[2, 4, 6, 8, 10]], [[2, 4, 6, 8, 10, 12, 14, 16, 18, 20]], [[2, 4, 6, 8, 10, 12, 14, 16, 18, 22]], [[2, 4, 6, 8, 10, 12, 13, 16, 18, 20]], [[3, 7, 9]], [[3, 6, 9]], [[3, 6, 8]], [[50, 100, 200, 400, 800]], [[50, 100, 150, 200, 250]], [[100, 200, 300, 400, 500, 600]]], "outputs": [[false], [false], [true], [true], [true], [false], [false], [true], [true], [true], [false], [false], [false], [true], [false], [false], [true], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,344 |
def validate_sequence(sequence):
|
205a08f2545d81b2650b3c605ec52aa4 | UNKNOWN | # Introduction
The ragbaby cipher is a substitution cipher that encodes/decodes a text using a keyed alphabet and their position in the plaintext word they are a part of.
To encrypt the text `This is an example.` with the key `cipher`, first construct a keyed alphabet:
```
c i p h e r a b d f g j k l m n o q s t u v w x y z
```
Then, number the letters in the text as follows:
```
T h i s i s a n e x a m p l e .
1 2 3 4 1 2 1 2 1 2 3 4 5 6 7
```
To obtain the encoded text, replace each character of the word with the letter in the keyed alphabet the corresponding number of places to the right of it (wrapping if necessary).
Non-alphabetic characters are preserved to mark word boundaries.
Our ciphertext is then `Urew pu bq rzfsbtj.`
# Task
Wirate functions `encode` and `decode` which accept 2 parameters:
- `text` - string - a text to encode/decode
- `key` - string - a key
# Notes
- handle lower and upper case in `text` string
- `key` consists of only lowercase characters | ["from string import ascii_lowercase as aLow\nimport re\n\ndef rotateWord(w, alpha, dct, d):\n lst = []\n for i,c in enumerate(w.lower(), 1):\n transChar = alpha[ (dct[c] + i*d) % 26 ]\n if w[i-1].isupper(): transChar = transChar.upper()\n lst.append(transChar)\n return ''.join(lst)\n\ndef encode(text, key, d=1):\n remains, alpha = set(aLow), []\n for c in key+aLow:\n if c in remains:\n remains.remove(c)\n alpha.append(c)\n alpha = ''.join(alpha)\n dct = {c:i for i,c in enumerate(alpha)}\n return re.sub(r'[a-zA-Z]+', lambda m: rotateWord(m.group(),alpha,dct,d), text)\n \ndef decode(text, key):\n return encode(text, key, -1)", "import re\nfrom collections import OrderedDict\nfrom string import ascii_lowercase\n\ndef encode(text, key, mul=1):\n i2c = ''.join(OrderedDict.fromkeys(key)) + ''.join(c for c in ascii_lowercase if c not in key)\n c2i = {c: i for i, c in enumerate(i2c)}\n return re.sub(\n '[a-z]+',\n lambda m: ''.join((str.upper if c.isupper() else str)(i2c[(c2i[c.lower()] + i * mul) % 26])\n for i, c in enumerate(m.group(), 1)),\n text,\n flags=re.I\n )\n\ndef decode(text, key):\n return encode(text, key, -1)", "import re\nfrom string import ascii_lowercase as alphabet\n\ndef keyed_alphabet(key):\n char2idx, idx2char = {}, {}\n for letter in key + alphabet:\n if letter not in char2idx:\n char2idx[letter] = len(char2idx)\n idx2char[len(idx2char)] = letter\n return char2idx, idx2char\n\ndef ragbaby(mode, text, key):\n char2idx, idx2char = keyed_alphabet(key)\n cipher_word = lambda match: ''.join(\n [str.upper, str.lower][letter.islower()](idx2char[(char2idx[letter.lower()] + idx * mode) % len(alphabet)])\n for idx, letter in enumerate(match.group(), 1))\n return re.sub('[{}]+'.format(alphabet), cipher_word, text, flags=re.I)\n\nencode, decode = lambda *args: ragbaby(1, *args), lambda *args: ragbaby(-1, *args)", "from string import ascii_lowercase as aLow\nimport re\n\ndef rotateWord(w, alpha, dct, d):\n return ''.join( (str.lower if w[i-1].islower() else str.upper)(alpha[ (dct[c]+i*d) % 26 ]) for i,c in enumerate(w.lower(), 1) )\n\ndef encode(text, key, d=1):\n s = set(aLow)\n alpha = ''.join(s.remove(c) or c for c in key+aLow if c in s)\n dct = {c:i for i,c in enumerate(alpha)}\n return re.sub(r'[a-zA-Z]+', lambda m: rotateWord(m.group(),alpha,dct,d), text)\n \ndef decode(text, key):\n return encode(text, key, -1)", "def encode(text, key):\n \n string = \"abcdefghijklmnopqrstuvwxyz\"\n string_me = key+string\n periods = []\n spaces = []\n commas = []\n \n string_me = list(dict.fromkeys(string_me))\n\n \n for x in range(0, len(text)):\n if text[x] == \".\":\n periods.append(x)\n elif text[x] == \",\":\n commas.append(x)\n elif text[x] == \" \":\n spaces.append(x)\n \n text = text.replace(\".\", \" \").replace(\",\", \" \").split() \n \n word = 0\n \n new_word = []\n \n while word < len(text):\n \n for x in range(0, len(text[word])):\n \n if text[word][x].isupper():\n \n if string_me.index(text[word][x].lower())+x+1 >= 26:\n \n number = string_me.index(text[word][x].lower())+x+1\n \n while number >= 26:\n number -= 26\n \n new_word.append(string_me[number].upper())\n else:\n new_word.append(string_me[string_me.index(text[word][x].lower())+x+1].upper())\n \n else:\n \n if string_me.index(text[word][x])+x+1 >= 26:\n \n number = string_me.index(text[word][x].lower())+x+1\n \n while number >= 26:\n number -= 26\n \n new_word.append(string_me[number])\n else:\n new_word.append(string_me[string_me.index(text[word][x].lower())+x+1])\n \n text[word] = \"\".join(new_word)\n new_word = []\n word += 1\n \n \n text = list(\"\".join(text))\n \n newwww = periods+spaces+commas\n \n newwww.sort()\n\n if newwww:\n for x in newwww:\n text.insert(x, \" \")\n \n for x in periods:\n text[x] = \".\"\n for x in commas:\n text[x] = \",\"\n \n return(\"\".join(text))\n\n \ndef decode(text, key):\n \n string = \"abcdefghijklmnopqrstuvwxyz\"\n string_me = key+string\n periods = []\n spaces = []\n commas = []\n \n string_me = list(dict.fromkeys(string_me))\n \n for x in range(0, len(text)):\n if text[x] == \".\":\n periods.append(x)\n elif text[x] == \",\":\n commas.append(x)\n elif text[x] == \" \":\n spaces.append(x)\n \n text = text.replace(\".\", \" \").replace(\",\", \" \").split() \n \n word = 0\n \n new_word = []\n \n while word < len(text):\n \n for x in range(0, len(text[word])):\n \n if text[word][x].isupper():\n \n\n \n if string_me.index(text[word][x].lower())-x-1 >= 26:\n \n number = string_me.index(text[word][x].lower())-x-1\n \n while number >= 26:\n number -= 26\n \n new_word.append(string_me[number].upper())\n \n elif string_me.index(text[word][x].lower())-x-1 < 0:\n \n number = string_me.index(text[word][x].lower())-x-1\n \n while number < 0:\n number += 26\n \n new_word.append(string_me[number].upper())\n else:\n new_word.append(string_me[string_me.index(text[word][x].lower())-x-1].upper())\n \n else:\n\n\n \n if string_me.index(text[word][x])-x-1 >= 26:\n \n number = string_me.index(text[word][x].lower())-x-1\n \n while number >= 26:\n number -= 26\n new_word.append(string_me[number]) \n elif string_me.index(text[word][x].lower())-x-1 < 0:\n \n number = string_me.index(text[word][x].lower())-x-1\n \n while number < 0:\n number += 26\n \n new_word.append(string_me[number])\n else:\n new_word.append(string_me[string_me.index(text[word][x].lower())-x-1])\n \n text[word] = \"\".join(new_word)\n new_word = []\n word += 1\n \n \n text = list(\"\".join(text))\n \n newwww = periods+spaces+commas\n \n newwww.sort()\n\n if newwww:\n for x in newwww:\n text.insert(x, \" \")\n \n for x in periods:\n text[x] = \".\"\n for x in commas:\n text[x] = \",\"\n \n return(\"\".join(text))\n", "from string import ascii_lowercase as AL\nimport re\n\ndef f(op):\n def g(s, key):\n a = \"\".join(dict.fromkeys(key + AL))\n d = {x: i for i, x in enumerate(a)}\n def f(s):\n s, r = s[0], []\n for i, x in enumerate(s, 1):\n y = a[(op(d[x.lower()], i)) % len(a)]\n if x.isupper():\n y = y.upper()\n r.append(y)\n return \"\".join(r)\n return re.sub(r\"\\w+\", f, s)\n return g\n\nencode = f(int.__add__)\ndecode = f(int.__sub__)", "def encode(text, key):\n k=''\n for c in key+'abcdefghijklmnopqrstuvwxyz':\n if c not in k:\n k+=c\n r=''\n i=1\n for c in text:\n if c.isalpha():\n j=(k.index(c.lower())+i)%26\n if c.isupper():\n r+=k[j].upper()\n else:\n r+=k[j]\n i+=1\n else:\n r+=c\n i=1\n return r\n \ndef decode(text, key):\n k=''\n for c in key+'abcdefghijklmnopqrstuvwxyz':\n if c not in k:\n k+=c\n r=''\n i=1\n for c in text:\n if c.isalpha():\n j=(k.index(c.lower())-i)%26\n if c.isupper():\n r+=k[j].upper()\n else:\n r+=k[j]\n i+=1\n else:\n r+=c\n i=1\n return r\n return text", "def encode(text, key): return cipher(text, key, 1)\n\n\ndef decode(text, key): return cipher(text, key, -1)\n\n\ndef cipher(text, key, mode):\n U = tuple(dict.fromkeys(key.upper() + 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'))\n L = tuple(dict.fromkeys(key.lower() + 'abcdefghijklmnopqrstuvwxyz'))\n\n output = ''\n i = mode\n for x in text:\n if x in U:\n output += U[(U.index(x) + i) % 26]\n i += mode\n elif x in L:\n output += L[(L.index(x) + i) % 26]\n i += mode\n else:\n output += x\n i = mode\n return output", "from string import ascii_lowercase\nfrom operator import add, sub\nfrom itertools import starmap\nfrom re import sub as rsub\n\ndef change(text, key, o):\n tmp = dict.fromkeys(key)\n cipher = ''.join(tmp) + ''.join(c for c in ascii_lowercase if c not in tmp)\n D = {c:i for i,c in enumerate(cipher)}\n a = lambda i,c: cipher[o(D[c], i) % 26]\n b = lambda i,c: cipher[o(D[c.lower()], i) % 26].upper()\n f = lambda i,c: a(i, c) if c.islower() else b(i, c) if c.isupper() else c\n g = lambda w: ''.join(starmap(f, enumerate(w.group(), 1)))\n return rsub(r\"[a-zA-z]+\", g, text)\n\ndef encode(text, key):\n return change(text, key, add)\n \ndef decode(text, key):\n return change(text, key, sub)", "def encode(msg,k):\n k = ''.join(dict.fromkeys(k)) + ''.join(i for i in 'abcdefghijklmnopqrstuvwxyz' if i not in k)\n r,i = '',1\n for s in msg:\n x = s.lower()\n if x in k:\n t = k[(k.find(x)+i)%26]\n r += t.upper() if s.isupper() else t\n i += 1\n else:\n r += s\n i = 1\n return r\n\n \n \ndef decode(msg,k): \n k = ''.join(dict.fromkeys(k)) + ''.join(i for i in 'abcdefghijklmnopqrstuvwxyz' if i not in k)\n r,i = '',1\n for s in msg:\n x = s.lower()\n if x in k:\n t = k[(k.find(x)-i)%26]\n r += t.upper() if s.isupper() else t\n i += 1\n else:\n r += s\n i = 1\n return r"] | {"fn_name": "encode", "inputs": [["cipher", "cipher"], ["cipher", "cccciiiiippphheeeeerrrrr"]], "outputs": [["ihrbfj"], ["ihrbfj"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 11,211 |
def encode(text, key):
|
f93243a4d1928c6a64995b36b036a77c | UNKNOWN | Your colleagues have been good enough(?) to buy you a birthday gift. Even though it is your birthday and not theirs, they have decided to play pass the parcel with it so that everyone has an even chance of winning. There are multiple presents, and you will receive one, but not all are nice... One even explodes and covers you in soil... strange office. To make up for this one present is a dog! Happy days! (do not buy dogs as presents, and if you do, never wrap them).
Depending on the number of passes in the game (y), and the present you unwrap (x), return as follows:
x == goodpresent --> return x with num of passes added to each charCode (turn to charCode, add y to each, turn back)
x == crap || x == empty --> return string sorted alphabetically
x == bang --> return string turned to char codes, each code reduced by number of passes and summed to a single figure
x == badpresent --> return 'Take this back!'
x == dog, return 'pass out from excitement y times' (where y is the value given for y). | ["_RESULTS = {\n 'goodpresent': lambda y: ''.join(chr(ord(c) + y) for c in 'goodpresent'),\n 'crap': lambda y: 'acpr',\n 'empty': lambda y: 'empty',\n 'bang': lambda y: str(sum(ord(c) - y for c in 'bang')),\n 'badpresent': lambda y: 'Take this back!',\n 'dog': lambda y: 'pass out from excitement {} times'.format(y)\n}\n\npresent = lambda x, y: _RESULTS[x](y)", "def present(x,y):\n if x == 'badpresent':\n return 'Take this back!'\n if x == 'goodpresent':\n return ''.join(chr(ord(i)+y) for i in x)\n if x == 'crap' or x == 'empty':\n return ''.join(sorted(x))\n if x == 'bang':\n return str(sum([ord(i) - y for i in x]))\n if x == 'dog':\n return \"pass out from excitement {} times\".format(y)", "present=lambda x,y:['Take this back!',''.join(chr(ord(e)+y) for e in x),'acpr',str(sum(ord(e)-y for e in x)),'pass out from excitement {} times'.format(y),x]['doangp'.index(x[2])]", "def present(x,y):\n if x==\"goodpresent\":return \"\".join(chr(ord(c)+y) for c in x)\n elif x in {\"crap\",\"empty\"}:return \"\".join(sorted(x))\n elif x==\"bang\":return str(sum(ord(c)-y for c in x))\n elif x==\"badpresent\":return \"Take this back!\"\n elif x==\"dog\":return f\"pass out from excitement {y} times\"", "def present(x,y):\n if x == 'badpresent': return 'Take this back!'\n if x == 'goodpresent': return ''.join(chr(ord(i)+y) for i in x)\n if x == 'crap': return 'acpr'\n if x == 'bang': return str(sum(ord(i)-y for i in x))\n if x == 'dog': return f'pass out from excitement {y} times'\n if x == 'empty': return x", "def present(x,y):\n if x == \"badpresent\":\n return \"Take this back!\"\n if x == \"goodpresent\":\n return \"\".join([chr(ord(el) + y) for el in x ])\n if x == \"crap\" or x == \"empty\":\n return \"\".join(sorted(x))\n if x == \"bang\":\n return str(sum([ord(el) - y for el in x]))\n if x == \"dog\":\n return f'pass out from excitement {y} times'", "def present(x,y):\n if x == \"empty\":\n return \"empty\"\n if x == \"crap\":\n return \"\".join(sorted(x))\n if x == \"badpresent\":\n return \"Take this back!\"\n if x == \"dog\":\n return f\"pass out from excitement {y} times\"\n if x == \"bang\":\n return str(sum([ord(el)-y for el in x]))\n if x == \"goodpresent\":\n return \"\".join([chr(ord(el)+y)for el in x])\n", "def present(x,y):\n if x == '' or x == 'empty':\n return 'empty'\n elif x == 'crap':\n return 'acpr'\n elif x == 'badpresent':\n return 'Take this back!'\n elif x == 'dog':\n return 'pass out from excitement ' + str(y) + ' times'\n elif x == 'bang':\n output = 0\n for char in list(x):\n output += ord(char)-y\n return str(output)\n elif x == 'goodpresent':\n output = ''\n for char in list(x):\n output += chr(ord(char)+y)\n return output\n else:\n return 'lol'", "def present(present, passes):\n if present == \"goodpresent\":\n return \"\".join(chr(ord(c) + passes) for c in present)\n elif present == \"crap\" or present == \"\":\n return \"\".join(sorted(present))\n elif present == \"bang\":\n return str(sum(map(ord, present)) - passes * len(present))\n elif present == \"badpresent\":\n return \"Take this back!\"\n elif present == \"dog\":\n return f\"pass out from excitement {passes} times\"\n else:\n return \"empty\"", "def present(x,y):\n if x == 'badpresent': return \"Take this back!\"\n elif x == 'dog': return f'pass out from excitement {y} times'\n elif x in ['crap', 'empty']: return ''.join(sorted(x))\n elif x == 'goodpresent': return ''.join(map(lambda a: chr(ord(a) + y), x))\n else: return str(sum(map(lambda a: ord(a) - y, x)))"] | {"fn_name": "present", "inputs": [["badpresent", 3], ["goodpresent", 9], ["crap", 10], ["bang", 27], ["dog", 23]], "outputs": [["Take this back!"], ["pxxmy{n|nw}"], ["acpr"], ["300"], ["pass out from excitement 23 times"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,908 |
def present(x,y):
|
90b9611bd524209596bf50b1c02a0090 | UNKNOWN | # Let's watch a parade!
## Brief
You're going to watch a parade, but you only care about one of the groups marching. The parade passes through the street where your house is. Your house is at number `location` of the street. Write a function `parade_time` that will tell you the times when you need to appear to see all appearences of that group.
## Specifications
You'll be given:
* A `list` of `string`s containing `groups` in the parade, in order of appearance. A group may appear multiple times. You want to see all the parts of your favorite group.
* An positive `integer` with the `location` on the parade route where you'll be watching.
* An positive `integer` with the `speed` of the parade
* A `string` with the `pref`ferred group you'd like to see
You need to return the time(s) you need to be at the parade to see your favorite group as a `list` of `integer`s.
It's possible the group won't be at your `location` at an exact `time`. In that case, just be sure to get there right before it passes (i.e. the largest integer `time` before the float passes the `position`).
## Example
```python
groups = ['A','B','C','D','E','F']
location = 3
speed = 2
pref = 'C'
v
Locations: |0|1|2|3|4|5|6|7|8|9|...
F E D C B A | | time = 0
> > F E D C B A | | time = 1
> > F E D C B|A| time = 2
> > F E D|C|B A time = 3
^
parade_time(['A','B','C','D','E','F'], 3, 2,'C']) == [3]
``` | ["def parade_time(groups, location, speed, pref):\n return [c // speed for c, p in enumerate(groups, 1 + location) if p == pref]", "def parade_time(groups, location, speed, pref):\n result = []\n for i in range(1,len(groups)+1):\n if groups[i-1] == pref:\n result.append((location+i) // speed)\n return result", "def parade_time(groups, location, speed, pref):\n return [(location + pos + 1) // speed for pos in [i for i, g in enumerate(groups) if g == pref]]", "def parade_time(groups, location, speed, pref):\n return [(location + i) // speed for i, g in enumerate(groups, 1) if g == pref]", "def parade_time(groups, location, speed, pref):\n return [(location + i + 1) // speed for i, group in enumerate(groups) if group == pref]", "def parade_time(groups, location, speed, pref):\n return [int((1 + location + i) / speed) for i, g in enumerate(groups) if g == pref]\n", "def parade_time(groups, location, speed, pref):\n result = []\n for i, x in enumerate(groups):\n if x == pref:\n result.append((location + i + 1) // speed)\n return result"] | {"fn_name": "parade_time", "inputs": [[["a", "b", "c", "d", "e", "f"], 3, 2, "c"], [["c", "b", "c", "d", "e", "f"], 3, 2, "c"], [["a", "b", "c", "d", "e", "f"], 3, 2, "g"], [["a", "b", "c", "c", "e", "c"], 7, 1, "c"], [["a", "b", "c", "d", "e", "f"], 1, 2, "b"], [["a", "b", "c", "d", "e", "b"], 1, 2, "b"]], "outputs": [[[3]], [[2, 3]], [[]], [[10, 11, 13]], [[1]], [[1, 3]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,117 |
def parade_time(groups, location, speed, pref):
|
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.