repo_name
stringlengths 7
91
| path
stringlengths 8
658
| copies
stringclasses 125
values | size
stringlengths 3
6
| content
stringlengths 118
674k
| license
stringclasses 15
values | hash
stringlengths 32
32
| line_mean
float64 6.09
99.2
| line_max
int64 17
995
| alpha_frac
float64 0.3
0.9
| ratio
float64 2
9.18
| autogenerated
bool 1
class | config_or_test
bool 2
classes | has_no_keywords
bool 2
classes | has_few_assignments
bool 1
class |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
jay18001/brickkit-ios | Source/Layout/BrickLayout.swift | 1 | 4825 | //
// BrickLayout.swift
// BrickApp
//
// Created by Ruben Cagnie on 5/30/16.
// Copyright © 2016 Wayfair. All rights reserved.
//
import Foundation
internal typealias OnAttributesUpdatedHandler = (_ attributes: BrickLayoutAttributes, _ oldFrame: CGRect?) -> Void
public protocol BrickLayout: class {
var widthRatio: CGFloat { get set }
var behaviors: Set<BrickLayoutBehavior> { get set }
var contentSize: CGSize { get }
var zIndexBehavior: BrickLayoutZIndexBehavior { get set }
var maxZIndex: Int { get }
var scrollDirection: UICollectionViewScrollDirection { get set }
weak var dataSource: BrickLayoutDataSource? { get set }
weak var delegate: BrickLayoutDelegate? { get set }
weak var hideBehaviorDataSource: HideBehaviorDataSource? { get set }
var appearBehavior: BrickAppearBehavior? { get set }
}
public enum BrickLayoutType {
case brick
case section(sectionIndex: Int)
}
open class BrickLayoutAttributes: UICollectionViewLayoutAttributes {
// These properties are intentially unwrapper, because otherwise we needed to override every initializer
/// The calculated frame before any behaviors (behaviors can change the frame of an attribute)
open internal(set) var originalFrame: CGRect!
/// Brick Identifier
internal var identifier: String!
// Flag that indicates if the size of this attribute is an estimate
internal var isEstimateSize = true
/// Flag that keeps track if the zIndex has been set manually. This is to prevent that the `setAutoZIndex` will override the zIndex
fileprivate var fixedZIndex: Bool = false
/// zIndex
open override var zIndex: Int {
didSet {
fixedZIndex = true
}
}
/// Set a zIndex that is calculated automatically. If the zIndex was set manually, the given zIndex will be ignored
///
/// - Parameter zIndex: The zIndex that is intended to be set
func setAutoZIndex(_ zIndex: Int) {
if !fixedZIndex {
self.zIndex = zIndex
self.fixedZIndex = false
}
}
/// Copy the attributes with all custom attributes. This is needed as UICollectionView will make copies of the attributes for height calculation etc
open override func copy(with zone: NSZone?) -> Any {
let any = super.copy(with: zone)
(any as? BrickLayoutAttributes)?.originalFrame = originalFrame
(any as? BrickLayoutAttributes)?.identifier = identifier
(any as? BrickLayoutAttributes)?.isEstimateSize = isEstimateSize
(any as? BrickLayoutAttributes)?.fixedZIndex = fixedZIndex
return any
}
}
extension BrickLayoutAttributes {
open override var description: String {
return super.description + " originalFrame: \(originalFrame); identifier: \(identifier)"
}
}
public protocol BrickLayoutDataSource: class {
func brickLayout(_ layout: BrickLayout, widthForItemAt indexPath: IndexPath, totalWidth: CGFloat, widthRatio: CGFloat, startingAt origin: CGFloat) -> CGFloat
func brickLayout(_ layout: BrickLayout, estimatedHeightForItemAt indexPath: IndexPath, containedIn width: CGFloat) -> CGFloat
func brickLayout(_ layout: BrickLayout, edgeInsetsFor section: Int) -> UIEdgeInsets
func brickLayout(_ layout: BrickLayout, insetFor section: Int) -> CGFloat
func brickLayout(_ layout: BrickLayout, isAlignRowHeightsFor section: Int) -> Bool
func brickLayout(_ layout: BrickLayout, alignmentFor section: Int) -> BrickAlignment
func brickLayout(_ layout: BrickLayout, brickLayoutTypeForItemAt indexPath: IndexPath) -> BrickLayoutType
func brickLayout(_ layout: BrickLayout, identifierFor indexPath: IndexPath) -> String
func brickLayout(_ layout: BrickLayout, indexPathFor section: Int) -> IndexPath?
func brickLayout(_ layout: BrickLayout, isEstimatedHeightFor indexPath: IndexPath) -> Bool
func brickLayout(_ layout: BrickLayout, isItemHiddenAt indexPath: IndexPath) -> Bool
}
extension BrickLayoutDataSource {
public func brickLayout(_ layout: BrickLayout, indexPathFor section: Int) -> IndexPath? {
return nil
}
func brickLayout(_ layout: BrickLayout, isEstimatedHeightFor indexPath: IndexPath) -> Bool {
return true
}
func brickLayout(_ layout: BrickLayout, isAlignRowHeightsFor section: Int) -> Bool {
return false
}
public func brickLayout(_ layout: BrickLayout, alignmentFor section: Int) -> BrickAlignment {
return BrickAlignment(horizontal: .left, vertical: .top)
}
public func brickLayout(_ layout: BrickLayout, isItemHiddenAt indexPath: IndexPath) -> Bool {
return false
}
}
public protocol BrickLayoutDelegate: class {
func brickLayout(_ layout: BrickLayout, didUpdateHeightForItemAtIndexPath indexPath: IndexPath)
}
| apache-2.0 | 5e5ca04b3616d401bc38ea06979b6f65 | 37.903226 | 161 | 0.719735 | 4.804781 | false | false | false | false |
ontouchstart/swift3-playground | Learn to Code 2.playgroundbook/Contents/Sources/WorldNodeIdentifier.swift | 2 | 1346 | //
// WorldNodeIdentifier.swift
//
// Copyright (c) 2016 Apple Inc. All Rights Reserved.
//
import SceneKit
public enum WorldNodeIdentifier: String {
case block = "Block"
case stair = "Stair"
case wall = "Wall"
case water = "Water"
case item = "Item"
case `switch` = "Switch"
case portal = "Portal"
case platformLock = "PlatformLock"
case platform = "Platform"
case startMarker = "StartMarker"
case randomNode = "RandomNode"
case actor = "Actor"
static var allIdentifiers: [WorldNodeIdentifier] {
return [
.block, .stair, .wall, .water,
.item, .switch,
.portal, .platformLock, .platform,
.startMarker, .actor, .randomNode
]
}
func makeNode() -> SCNNode {
let scnNode = SCNNode()
scnNode.name = self.rawValue
return scnNode
}
}
extension WorldNodeIdentifier {
// Used to calculate solution in GridSolutions.swift.
static var goals: [WorldNodeIdentifier] {
return [ .item, .switch ]
}
}
// MARK: GridNodeIdentifier
// Important nodes pertaining to the construction of the grid
let GridNodeName = "GridNode"
let CameraHandleName = "CameraHandle"
let CameraNodeName = "camera"
let DirectionalLightName = "directional"
let ActorStartMarker = "ActorStartMarker"
| mit | e3489e2c348ade041e165595a64f4e7f | 24.396226 | 61 | 0.639673 | 4.005952 | false | false | false | false |
HeMet/AppCoordinatorExample | CoordinatorExample/Coordinator.swift | 1 | 3246 | //
// Coordinator.swift
// CoordinatorExample
//
// Created by Evgeniy Gubin on 12.03.17.
// Copyright © 2017 Eugene Gubin. All rights reserved.
//
import UIKit
/*
Основной блок для организации приложения.
Вместе с другими компонентами образует древовидную структуру.
*/
protocol Component: class {
typealias Callback = (Component) -> Void
var identifier: String { get }
weak var parent: Component? { get set }
var children: [String: Component] { get set }
func start(completion: Callback?)
func stop(completion: Callback?)
func startChild(_ coordinator: Component, completion: Callback?)
func stopChild(identifier: String, completion: Callback?)
func childFinished(identifier: String)
}
/*
Координатор - это компонент, который координирует работу
представления (View) и модели (Model)
*/
protocol Coordinator: Component {
var sceneViewController: UIViewController { get }
}
/*
Отображающий компонент - это компонент, который может отобразить
координаторы. При этом он сам может являться координатором,
но не обязательно.
*/
protocol PresentingComponent: Component {
func present(childCoordinator: Coordinator)
func dismiss(childCoordinator: Coordinator)
}
typealias PresentingCoordinator = PresentingComponent & Coordinator
extension Component {
var identifier: String { return String(describing: type(of: self)) }
func startChild(_ coordinator: Component, completion: Callback?) {
children[coordinator.identifier] = coordinator
coordinator.parent = self
coordinator.start(completion: completion)
}
func stopChild(identifier: String, completion: Callback?) {
guard let child = children[identifier] else {
fatalError("Child coordinator with identifier \(identifier) not found.")
}
child.stop { [weak self] coordinator in
coordinator.parent = nil
self?.children[identifier] = nil
completion?(coordinator)
}
}
func childFinished(identifier: String) {
stopChild(identifier: identifier, completion: nil)
}
}
extension Coordinator {
var parentCoordinator: Coordinator? {
return parent as? Coordinator
}
}
extension PresentingComponent {
func presentChild(_ coordinator: Coordinator, completion: Callback? = nil) {
startChild(coordinator) { [weak self] child in
self?.present(childCoordinator: coordinator)
completion?(child)
}
}
func dismissChild(identifier: String, completion: Callback? = nil) {
stopChild(identifier: identifier) { [weak self] child in
guard let childCoordinator = child as? Coordinator else {
fatalError("\(type(of:self)) tried to dismiss not a Coordinator")
}
self?.dismiss(childCoordinator: childCoordinator)
completion?(child)
}
}
}
| mit | 9c65e1fcc9659b8dede49297df29a49a | 28.376238 | 84 | 0.671722 | 4.220484 | false | false | false | false |
dreamsxin/swift | stdlib/public/core/FlatMap.swift | 2 | 4227 | //===--- FlatMap.swift ----------------------------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2016 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See http://swift.org/LICENSE.txt for license information
// See http://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
extension LazySequenceProtocol {
/// Returns the concatenated results of mapping `transform` over
/// `self`. Equivalent to
///
/// self.map(transform).flatten()
///
/// - Complexity: O(1)
public func flatMap<SegmentOfResult : Sequence>(
_ transform: (Elements.Iterator.Element) -> SegmentOfResult
) -> LazySequence<
FlattenSequence<LazyMapSequence<Elements, SegmentOfResult>>> {
return self.map(transform).flatten()
}
/// Returns a `LazyMapSequence` containing the concatenated non-nil
/// results of mapping transform over this `Sequence`.
///
/// Use this method to receive only nonoptional values when your
/// transformation produces an optional value.
///
/// - Parameter transform: A closure that accepts an element of this
/// sequence as its argument and returns an optional value.
public func flatMap<ElementOfResult>(
_ transform: (Elements.Iterator.Element) -> ElementOfResult?
) -> LazyMapSequence<
LazyFilterSequence<
LazyMapSequence<Elements, ElementOfResult?>>,
ElementOfResult
> {
return self.map(transform).filter { $0 != nil }.map { $0! }
}
}
extension LazyCollectionProtocol {
/// Returns the concatenated results of mapping `transform` over
/// `self`. Equivalent to
///
/// self.map(transform).flatten()
///
/// - Complexity: O(1)
public func flatMap<SegmentOfResult : Collection>(
_ transform: (Elements.Iterator.Element) -> SegmentOfResult
) -> LazyCollection<
FlattenCollection<
LazyMapCollection<Elements, SegmentOfResult>>
> {
return self.map(transform).flatten()
}
/// Returns a `LazyMapCollection` containing the concatenated non-nil
/// results of mapping transform over this collection.
///
/// Use this method to receive only nonoptional values when your
/// transformation produces an optional value.
///
/// - Parameter transform: A closure that accepts an element of this
/// collection as its argument and returns an optional value.
public func flatMap<ElementOfResult>(
_ transform: (Elements.Iterator.Element) -> ElementOfResult?
) -> LazyMapCollection<
LazyFilterCollection<
LazyMapCollection<Elements, ElementOfResult?>>,
ElementOfResult
> {
return self.map(transform).filter { $0 != nil }.map { $0! }
}
}
extension LazyCollectionProtocol
where
Self : BidirectionalCollection,
Elements : BidirectionalCollection
{
/// Returns the concatenated results of mapping `transform` over
/// `self`. Equivalent to
///
/// self.map(transform).flatten()
///
/// - Complexity: O(1)
public func flatMap<SegmentOfResult : Collection>(
_ transform: (Elements.Iterator.Element) -> SegmentOfResult
) -> LazyCollection<
FlattenBidirectionalCollection<
LazyMapBidirectionalCollection<Elements, SegmentOfResult>>>
where SegmentOfResult : BidirectionalCollection {
return self.map(transform).flatten()
}
/// Returns a `LazyMapBidirectionalCollection` containing the concatenated non-nil
/// results of mapping transform over this collection.
///
/// Use this method to receive only nonoptional values when your
/// transformation produces an optional value.
///
/// - Parameter transform: A closure that accepts an element of this
/// collection as its argument and returns an optional value.
public func flatMap<ElementOfResult>(
_ transform: (Elements.Iterator.Element) -> ElementOfResult?
) -> LazyMapBidirectionalCollection<
LazyFilterBidirectionalCollection<
LazyMapBidirectionalCollection<Elements, ElementOfResult?>>,
ElementOfResult
> {
return self.map(transform).filter { $0 != nil }.map { $0! }
}
}
| apache-2.0 | 8b4f2d1ad2638d4cd1e2df5aa94b1d1d | 34.822034 | 84 | 0.682754 | 5.014235 | false | false | false | false |
swernimo/iOS | iOS Networking with Swift/OnTheMap/OnTheMap/NetworkHelper.swift | 1 | 1141 | //
// NetworkHelper.swift
// OnTheMap
//
// Created by Sean Wernimont on 2/7/16.
// Copyright © 2016 Just One Guy. All rights reserved.
//
//code taken from http://stackoverflow.com/questions/25623272/how-to-use-scnetworkreachability-in-swift/25623647#25623647
import Foundation
import SystemConfiguration
public class NetworkHelper {
class func isConnectedToNetwork() -> Bool {
var zeroAddress = sockaddr_in()
zeroAddress.sin_len = UInt8(sizeofValue(zeroAddress))
zeroAddress.sin_family = sa_family_t(AF_INET)
guard let defaultRouteReachability = withUnsafePointer(&zeroAddress, {
SCNetworkReachabilityCreateWithAddress(nil, UnsafePointer($0))
}) else {
return false
}
var flags : SCNetworkReachabilityFlags = []
if !SCNetworkReachabilityGetFlags(defaultRouteReachability, &flags) {
return false
}
let isReachable = flags.contains(.Reachable)
let needsConnection = flags.contains(.ConnectionRequired)
return (isReachable && !needsConnection)
}
}
| mit | 6368ba7acb71c7a24cfa40bfdf08809c | 29 | 121 | 0.657018 | 4.892704 | false | false | false | false |
vrutberg/VRPicker | VRPicker/Classes/VRPickerConfiguration.swift | 1 | 2354 | //
// VRPickerConfiguration.swift
// Pods
//
// Created by Viktor Rutberg on 2016-12-17.
//
//
import Foundation
public enum GradientPosition {
case above
case below
}
public protocol VRPickerItem: CustomStringConvertible {}
public struct VRPickerConfiguration<T: VRPickerItem> {
let items: [T]
let defaultSelectedIndex: Int
let selectedFont: UIFont
let selectedFontColor: UIColor
let nonSelectedFont: UIFont
let nonSelectedFontColor: UIColor
let selectionRadiusInPercent: Double
let selectionBackgroundColor: UIColor
let gradientColors: [UIColor]
let gradientWidthInPercent: Double
let gradientPosition: GradientPosition
let itemWidth: Int
let sliderVelocityCoefficient: Double
public init(items: [T],
defaultSelectedIndex: Int = 0,
selectedFont: UIFont = UIFont.boldSystemFont(ofSize: 20),
selectedFontColor: UIColor = .white,
nonSelectedFont: UIFont = UIFont.systemFont(ofSize: 14),
nonSelectedFontColor: UIColor = .black,
selectionRadiusInPercent: Double = 0.3,
selectionBackgroundColor: UIColor = .green,
gradientColors: [UIColor] = [UIColor.white.withAlphaComponent(0.8),
UIColor.white.withAlphaComponent(0)],
gradientWidthInPercent: Double = 0.4,
gradientPosition: GradientPosition = .above,
itemWidth: Int = 100,
sliderVelocityCoefficient: Double = 60) {
self.items = items
self.defaultSelectedIndex = defaultSelectedIndex
self.selectedFont = selectedFont
self.selectedFontColor = selectedFontColor
self.nonSelectedFont = nonSelectedFont
self.nonSelectedFontColor = nonSelectedFontColor
self.selectionRadiusInPercent = selectionRadiusInPercent
self.selectionBackgroundColor = selectionBackgroundColor
self.gradientColors = gradientColors
self.gradientWidthInPercent = gradientWidthInPercent
self.gradientPosition = gradientPosition
self.itemWidth = itemWidth
self.sliderVelocityCoefficient = sliderVelocityCoefficient
}
internal var itemsAsStrings: [String] {
return items.map { $0.description }
}
}
| mit | 5d5a8750cdd35ab5c5c0a488715c7984 | 29.571429 | 83 | 0.668649 | 5.362187 | false | false | false | false |
Diego5529/quaddro-mm | AprendendoSwift/AprendendoSwift-parte7-funcoesclosures.playground/Contents.swift | 3 | 1061 | //: Quaddro - noun: a place where people can learn
//Função que retorna a área do quadrado
func quadrado(lado: Float)->Float{
return lado * lado
}
//Função que retorna a área do Cubo
func cubo(lado: Float)->Float{
return lado * lado * lado
}
//Criando nossa função que recebe uma closure
func media(ladoA: Float, ladoB: Float, forma: (Float -> Float))->Float{
return (forma(ladoA) + forma(ladoB)) / 2
}
//Utilizando nossas funções.
media(10, 20, cubo)
media(2, 4, quadrado)
media(2, 4, {(valor: Float)-> Float in
return valor * valor
})
media(2, 4, {$0 * $0})
media(2, 4){$0 * $0}
//Criamos um array
let numeros: [Int] = [10, 32, 1, 15, 40, 10329, 198, 947]
//Filtramos apenas os valores pares dentro do array numerosPares
var numerosPares = numeros.filter({(valor) in valor % 2 == 0})
numerosPares
var somaImpar = numeros.filter({$0 % 2 != 0})
.reduce(0, combine: {$0 + $1})
somaImpar
var novosPares = numeros.filter({$0 % 2 != 0})
.map({$0 + 1})
novosPares
| apache-2.0 | 29432570141a71a683035a380acb8441 | 18.109091 | 71 | 0.616556 | 2.751309 | false | false | false | false |
tkremenek/swift | benchmark/single-source/Hash.swift | 20 | 18547 | //===--- Hash.swift -------------------------------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2017 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
// Derived from the C samples in:
// Source: http://en.wikipedia.org/wiki/MD5 and
// http://en.wikipedia.org/wiki/SHA-1
import TestsUtils
public let HashTest = BenchmarkInfo(
name: "HashTest",
runFunction: run_HashTest,
tags: [.validation, .algorithm],
legacyFactor: 10)
class Hash {
/// C'tor.
init(_ bs: Int) {
blocksize = bs
messageLength = 0
dataLength = 0
assert(blocksize <= 64, "Invalid block size")
}
/// Add the bytes in \p Msg to the hash.
func update(_ Msg: String) {
for c in Msg.unicodeScalars {
data[dataLength] = UInt8(ascii: c)
dataLength += 1
messageLength += 1
if dataLength == blocksize { hash() }
}
}
/// Add the bytes in \p Msg to the hash.
func update(_ Msg: [UInt8]) {
for c in Msg {
data[dataLength] = c
dataLength += 1
messageLength += 1
if dataLength == blocksize { hash() }
}
}
/// \returns the hash of the data that was updated.
func digest() -> String {
fillBlock()
hash()
let x = hashState()
return x
}
// private:
// Hash state:
final var messageLength: Int = 0
final var dataLength: Int = 0
final var data = [UInt8](repeating: 0, count: 64)
final var blocksize: Int
/// Hash the internal data.
func hash() {
fatalError("Pure virtual")
}
/// \returns a string representation of the state.
func hashState() -> String {
fatalError("Pure virtual")
}
func hashStateFast(_ Res: inout [UInt8]) {
fatalError("Pure virtual")
}
/// Blow the data to fill the block.
func fillBlock() {
fatalError("Pure virtual")
}
var HexTbl = ["0","1","2","3","4","5","6","7","8","9","a","b","c","d","e","f"]
final
var HexTblFast : [UInt8] = [48,49,50,51,52,53,54,55,56,57,97,98,99,100,101,102]
/// Convert a 4-byte integer to a hex string.
final
func toHex(_ In: UInt32) -> String {
var In = In
var Res = ""
for _ in 0..<8 {
Res = HexTbl[Int(In & 0xF)] + Res
In = In >> 4
}
return Res
}
final
func toHexFast(_ In: UInt32, _ Res: inout Array<UInt8>, _ Index : Int) {
var In = In
for i in 0..<4 {
// Convert one byte each iteration.
Res[Index + 2*i] = HexTblFast[Int(In >> 4) & 0xF]
Res[Index + 2*i + 1] = HexTblFast[Int(In & 0xF)]
In = In >> 8
}
}
/// Left-rotate \p x by \p c.
final
func rol(_ x: UInt32, _ c: UInt32) -> UInt32 {
return x &<< c | x &>> (32 &- c)
}
/// Right-rotate \p x by \p c.
final
func ror(_ x: UInt32, _ c: UInt32) -> UInt32 {
return x &>> c | x &<< (32 &- c)
}
}
final
class MD5 : Hash {
// Integer part of the sines of integers (in radians) * 2^32.
let k : [UInt32] = [0xd76aa478, 0xe8c7b756, 0x242070db, 0xc1bdceee ,
0xf57c0faf, 0x4787c62a, 0xa8304613, 0xfd469501 ,
0x698098d8, 0x8b44f7af, 0xffff5bb1, 0x895cd7be ,
0x6b901122, 0xfd987193, 0xa679438e, 0x49b40821 ,
0xf61e2562, 0xc040b340, 0x265e5a51, 0xe9b6c7aa ,
0xd62f105d, 0x02441453, 0xd8a1e681, 0xe7d3fbc8 ,
0x21e1cde6, 0xc33707d6, 0xf4d50d87, 0x455a14ed ,
0xa9e3e905, 0xfcefa3f8, 0x676f02d9, 0x8d2a4c8a ,
0xfffa3942, 0x8771f681, 0x6d9d6122, 0xfde5380c ,
0xa4beea44, 0x4bdecfa9, 0xf6bb4b60, 0xbebfbc70 ,
0x289b7ec6, 0xeaa127fa, 0xd4ef3085, 0x04881d05 ,
0xd9d4d039, 0xe6db99e5, 0x1fa27cf8, 0xc4ac5665 ,
0xf4292244, 0x432aff97, 0xab9423a7, 0xfc93a039 ,
0x655b59c3, 0x8f0ccc92, 0xffeff47d, 0x85845dd1 ,
0x6fa87e4f, 0xfe2ce6e0, 0xa3014314, 0x4e0811a1 ,
0xf7537e82, 0xbd3af235, 0x2ad7d2bb, 0xeb86d391 ]
// Per-round shift amounts
let r : [UInt32] = [7, 12, 17, 22, 7, 12, 17, 22, 7, 12, 17, 22, 7, 12, 17, 22,
5, 9, 14, 20, 5, 9, 14, 20, 5, 9, 14, 20, 5, 9, 14, 20,
4, 11, 16, 23, 4, 11, 16, 23, 4, 11, 16, 23, 4, 11, 16, 23,
6, 10, 15, 21, 6, 10, 15, 21, 6, 10, 15, 21, 6, 10, 15, 21]
// State
var h0: UInt32 = 0
var h1: UInt32 = 0
var h2: UInt32 = 0
var h3: UInt32 = 0
init() {
super.init(64)
reset()
}
func reset() {
// Set the initial values.
h0 = 0x67452301
h1 = 0xefcdab89
h2 = 0x98badcfe
h3 = 0x10325476
messageLength = 0
dataLength = 0
}
func appendBytes(_ Val: Int, _ Message: inout Array<UInt8>, _ Offset : Int) {
Message[Offset] = UInt8(truncatingIfNeeded: Val)
Message[Offset + 1] = UInt8(truncatingIfNeeded: Val >> 8)
Message[Offset + 2] = UInt8(truncatingIfNeeded: Val >> 16)
Message[Offset + 3] = UInt8(truncatingIfNeeded: Val >> 24)
}
override
func fillBlock() {
// Pre-processing:
// Append "1" bit to message
// Append "0" bits until message length in bits -> 448 (mod 512)
// Append length mod (2^64) to message
var new_len = messageLength + 1
while (new_len % (512/8) != 448/8) {
new_len += 1
}
// Append the "1" bit - most significant bit is "first"
data[dataLength] = UInt8(0x80)
dataLength += 1
// Append "0" bits
for _ in 0..<(new_len - (messageLength + 1)) {
if dataLength == blocksize { hash() }
data[dataLength] = UInt8(0)
dataLength += 1
}
// Append the len in bits at the end of the buffer.
// initial_len>>29 == initial_len*8>>32, but avoids overflow.
appendBytes(messageLength * 8, &data, dataLength)
appendBytes(messageLength>>29 * 8, &data, dataLength+4)
dataLength += 8
}
func toUInt32(_ Message: Array<UInt8>, _ Offset: Int) -> UInt32 {
let first = UInt32(Message[Offset + 0])
let second = UInt32(Message[Offset + 1]) << 8
let third = UInt32(Message[Offset + 2]) << 16
let fourth = UInt32(Message[Offset + 3]) << 24
return first | second | third | fourth
}
var w = [UInt32](repeating: 0, count: 16)
override
func hash() {
assert(dataLength == blocksize, "Invalid block size")
// Break chunk into sixteen 32-bit words w[j], 0 ≤ j ≤ 15
for i in 0..<16 {
w[i] = toUInt32(data, i*4)
}
// We don't need the original data anymore.
dataLength = 0
var a = h0
var b = h1
var c = h2
var d = h3
var f, g: UInt32
// Main loop:
for i in 0..<64 {
if i < 16 {
f = (b & c) | ((~b) & d)
g = UInt32(i)
} else if i < 32 {
f = (d & b) | ((~d) & c)
g = UInt32(5*i + 1) % 16
} else if i < 48 {
f = b ^ c ^ d
g = UInt32(3*i + 5) % 16
} else {
f = c ^ (b | (~d))
g = UInt32(7*i) % 16
}
let temp = d
d = c
c = b
b = b &+ rol(a &+ f &+ k[i] &+ w[Int(g)], r[i])
a = temp
}
// Add this chunk's hash to result so far:
h0 = a &+ h0
h1 = b &+ h1
h2 = c &+ h2
h3 = d &+ h3
}
func reverseBytes(_ In: UInt32) -> UInt32 {
let B0 = (In >> 0 ) & 0xFF
let B1 = (In >> 8 ) & 0xFF
let B2 = (In >> 16) & 0xFF
let B3 = (In >> 24) & 0xFF
return (B0 << 24) | (B1 << 16) | (B2 << 8) | B3
}
override
func hashState() -> String {
var S = ""
for h in [h0, h1, h2, h3] {
S += toHex(reverseBytes(h))
}
return S
}
override
func hashStateFast(_ Res: inout [UInt8]) {
#if !NO_RANGE
var Idx: Int = 0
for h in [h0, h1, h2, h3] {
toHexFast(h, &Res, Idx)
Idx += 8
}
#else
toHexFast(h0, &Res, 0)
toHexFast(h1, &Res, 8)
toHexFast(h2, &Res, 16)
toHexFast(h3, &Res, 24)
#endif
}
}
class SHA1 : Hash {
// State
var h0: UInt32 = 0
var h1: UInt32 = 0
var h2: UInt32 = 0
var h3: UInt32 = 0
var h4: UInt32 = 0
init() {
super.init(64)
reset()
}
func reset() {
// Set the initial values.
h0 = 0x67452301
h1 = 0xEFCDAB89
h2 = 0x98BADCFE
h3 = 0x10325476
h4 = 0xC3D2E1F0
messageLength = 0
dataLength = 0
}
override
func fillBlock() {
// Append a 1 to the message.
data[dataLength] = UInt8(0x80)
dataLength += 1
var new_len = messageLength + 1
while ((new_len % 64) != 56) {
new_len += 1
}
for _ in 0..<new_len - (messageLength + 1) {
if dataLength == blocksize { hash() }
data[dataLength] = UInt8(0x0)
dataLength += 1
}
// Append the original message length as 64bit big endian:
let len_in_bits = Int64(messageLength)*8
for i in 0..<(8 as Int64) {
let val = (len_in_bits >> ((7-i)*8)) & 0xFF
data[dataLength] = UInt8(val)
dataLength += 1
}
}
override
func hash() {
assert(dataLength == blocksize, "Invalid block size")
// Init the "W" buffer.
var w = [UInt32](repeating: 0, count: 80)
// Convert the Byte array to UInt32 array.
var word: UInt32 = 0
for i in 0..<64 {
word = word << 8
word = word &+ UInt32(data[i])
if i%4 == 3 { w[i/4] = word; word = 0 }
}
// Init the rest of the "W" buffer.
for t in 16..<80 {
// splitting into 2 subexpressions to help typechecker
let lhs = w[t-3] ^ w[t-8]
let rhs = w[t-14] ^ w[t-16]
w[t] = rol(lhs ^ rhs, 1)
}
dataLength = 0
var A = h0
var B = h1
var C = h2
var D = h3
var E = h4
var K: UInt32, F: UInt32
for t in 0..<80 {
if t < 20 {
K = 0x5a827999
F = (B & C) | ((B ^ 0xFFFFFFFF) & D)
} else if t < 40 {
K = 0x6ed9eba1
F = B ^ C ^ D
} else if t < 60 {
K = 0x8f1bbcdc
F = (B & C) | (B & D) | (C & D)
} else {
K = 0xca62c1d6
F = B ^ C ^ D
}
let Temp: UInt32 = rol(A,5) &+ F &+ E &+ w[t] &+ K
E = D
D = C
C = rol(B,30)
B = A
A = Temp
}
h0 = h0 &+ A
h1 = h1 &+ B
h2 = h2 &+ C
h3 = h3 &+ D
h4 = h4 &+ E
}
override
func hashState() -> String {
var Res: String = ""
for state in [h0, h1, h2, h3, h4] {
Res += toHex(state)
}
return Res
}
}
class SHA256 : Hash {
// State
var h0: UInt32 = 0
var h1: UInt32 = 0
var h2: UInt32 = 0
var h3: UInt32 = 0
var h4: UInt32 = 0
var h5: UInt32 = 0
var h6: UInt32 = 0
var h7: UInt32 = 0
let k : [UInt32] = [
0x428a2f98, 0x71374491, 0xb5c0fbcf, 0xe9b5dba5, 0x3956c25b, 0x59f111f1, 0x923f82a4, 0xab1c5ed5,
0xd807aa98, 0x12835b01, 0x243185be, 0x550c7dc3, 0x72be5d74, 0x80deb1fe, 0x9bdc06a7, 0xc19bf174,
0xe49b69c1, 0xefbe4786, 0x0fc19dc6, 0x240ca1cc, 0x2de92c6f, 0x4a7484aa, 0x5cb0a9dc, 0x76f988da,
0x983e5152, 0xa831c66d, 0xb00327c8, 0xbf597fc7, 0xc6e00bf3, 0xd5a79147, 0x06ca6351, 0x14292967,
0x27b70a85, 0x2e1b2138, 0x4d2c6dfc, 0x53380d13, 0x650a7354, 0x766a0abb, 0x81c2c92e, 0x92722c85,
0xa2bfe8a1, 0xa81a664b, 0xc24b8b70, 0xc76c51a3, 0xd192e819, 0xd6990624, 0xf40e3585, 0x106aa070,
0x19a4c116, 0x1e376c08, 0x2748774c, 0x34b0bcb5, 0x391c0cb3, 0x4ed8aa4a, 0x5b9cca4f, 0x682e6ff3,
0x748f82ee, 0x78a5636f, 0x84c87814, 0x8cc70208, 0x90befffa, 0xa4506ceb, 0xbef9a3f7, 0xc67178f2]
init() {
super.init(64)
reset()
}
func reset() {
// Set the initial values.
h0 = 0x6a09e667
h1 = 0xbb67ae85
h2 = 0x3c6ef372
h3 = 0xa54ff53a
h4 = 0x510e527f
h5 = 0x9b05688c
h6 = 0x1f83d9ab
h7 = 0x5be0cd19
messageLength = 0
dataLength = 0
}
override
func fillBlock() {
// Append a 1 to the message.
data[dataLength] = UInt8(0x80)
dataLength += 1
var new_len = messageLength + 1
while ((new_len % 64) != 56) {
new_len += 1
}
for _ in 0..<new_len - (messageLength+1) {
if dataLength == blocksize { hash() }
data[dataLength] = UInt8(0)
dataLength += 1
}
// Append the original message length as 64bit big endian:
let len_in_bits = Int64(messageLength)*8
for i in 0..<(8 as Int64) {
let val = (len_in_bits >> ((7-i)*8)) & 0xFF
data[dataLength] = UInt8(val)
dataLength += 1
}
}
override
func hash() {
assert(dataLength == blocksize, "Invalid block size")
// Init the "W" buffer.
var w = [UInt32](repeating: 0, count: 64)
// Convert the Byte array to UInt32 array.
var word: UInt32 = 0
for i in 0..<64 {
word = word << 8
word = word &+ UInt32(data[i])
if i%4 == 3 { w[i/4] = word; word = 0 }
}
// Init the rest of the "W" buffer.
for i in 16..<64 {
let s0 = ror(w[i-15], 7) ^ ror(w[i-15], 18) ^ (w[i-15] >> 3)
let s1 = ror(w[i-2], 17) ^ ror(w[i-2], 19) ^ (w[i-2] >> 10)
w[i] = w[i-16] &+ s0 &+ w[i-7] &+ s1
}
dataLength = 0
var a = h0
var b = h1
var c = h2
var d = h3
var e = h4
var f = h5
var g = h6
var h = h7
for i in 0..<64 {
let S1 = ror(e, 6) ^ ror(e, 11) ^ ror(e, 25)
let ch = (e & f) ^ ((~e) & g)
let temp1 = h &+ S1 &+ ch &+ k[i] &+ w[i]
let S0 = ror(a, 2) ^ ror(a, 13) ^ ror(a, 22)
let maj = (a & b) ^ (a & c) ^ (b & c)
let temp2 = S0 &+ maj
h = g
g = f
f = e
e = d &+ temp1
d = c
c = b
b = a
a = temp1 &+ temp2
}
h0 = h0 &+ a
h1 = h1 &+ b
h2 = h2 &+ c
h3 = h3 &+ d
h4 = h4 &+ e
h5 = h5 &+ f
h6 = h6 &+ g
h7 = h7 &+ h
}
override
func hashState() -> String {
var Res: String = ""
for state in [h0, h1, h2, h3, h4, h5, h6, h7] {
Res += toHex(state)
}
return Res
}
}
func == (lhs: [UInt8], rhs: [UInt8]) -> Bool {
if lhs.count != rhs.count { return false }
for idx in 0..<lhs.count {
if lhs[idx] != rhs[idx] { return false }
}
return true
}
@inline(never)
public func run_HashTest(_ N: Int) {
let TestMD5 = ["" : "d41d8cd98f00b204e9800998ecf8427e",
"The quick brown fox jumps over the lazy dog." : "e4d909c290d0fb1ca068ffaddf22cbd0",
"The quick brown fox jumps over the lazy cog." : "68aa5deab43e4df2b5e1f80190477fb0"]
let TestSHA1 = ["" : "da39a3ee5e6b4b0d3255bfef95601890afd80709",
"The quick brown fox jumps over the lazy dog" : "2fd4e1c67a2d28fced849ee1bb76e7391b93eb12",
"The quick brown fox jumps over the lazy cog" : "de9f2c7fd25e1b3afad3e85a0bd17d9b100db4b3"]
let TestSHA256 = ["" : "e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855",
"The quick brown fox jumps over the lazy dog" : "d7a8fbb307d7809469ca9abcb0082e4f8d5651e46d3cdb762d02d0bf37c9e592",
"The quick brown fox jumps over the lazy dog." : "ef537f25c895bfa782526529a9b63d97aa631564d5d789c2b765448c8635fb6c"]
let size = 50
for _ in 1...N {
// Check for precomputed values.
let MD = MD5()
for (K, V) in TestMD5 {
MD.update(K)
CheckResults(MD.digest() == V)
MD.reset()
}
// Check that we don't crash on large strings.
var S: String = ""
for _ in 1...size {
S += "a"
MD.reset()
MD.update(S)
}
// Check that the order in which we push values does not change the result.
MD.reset()
var L: String = ""
for _ in 1...size {
L += "a"
MD.update("a")
}
let MD2 = MD5()
MD2.update(L)
CheckResults(MD.digest() == MD2.digest())
// Test the famous MD5 collision from 2009: http://www.mscs.dal.ca/~selinger/md5collision/
let Src1 : [UInt8] =
[0xd1, 0x31, 0xdd, 0x02, 0xc5, 0xe6, 0xee, 0xc4, 0x69, 0x3d, 0x9a, 0x06, 0x98, 0xaf, 0xf9, 0x5c, 0x2f, 0xca, 0xb5, 0x87, 0x12, 0x46, 0x7e, 0xab, 0x40, 0x04, 0x58, 0x3e, 0xb8, 0xfb, 0x7f, 0x89,
0x55, 0xad, 0x34, 0x06, 0x09, 0xf4, 0xb3, 0x02, 0x83, 0xe4, 0x88, 0x83, 0x25, 0x71, 0x41, 0x5a, 0x08, 0x51, 0x25, 0xe8, 0xf7, 0xcd, 0xc9, 0x9f, 0xd9, 0x1d, 0xbd, 0xf2, 0x80, 0x37, 0x3c, 0x5b,
0xd8, 0x82, 0x3e, 0x31, 0x56, 0x34, 0x8f, 0x5b, 0xae, 0x6d, 0xac, 0xd4, 0x36, 0xc9, 0x19, 0xc6, 0xdd, 0x53, 0xe2, 0xb4, 0x87, 0xda, 0x03, 0xfd, 0x02, 0x39, 0x63, 0x06, 0xd2, 0x48, 0xcd, 0xa0,
0xe9, 0x9f, 0x33, 0x42, 0x0f, 0x57, 0x7e, 0xe8, 0xce, 0x54, 0xb6, 0x70, 0x80, 0xa8, 0x0d, 0x1e, 0xc6, 0x98, 0x21, 0xbc, 0xb6, 0xa8, 0x83, 0x93, 0x96, 0xf9, 0x65, 0x2b, 0x6f, 0xf7, 0x2a, 0x70]
let Src2 : [UInt8] =
[0xd1, 0x31, 0xdd, 0x02, 0xc5, 0xe6, 0xee, 0xc4, 0x69, 0x3d, 0x9a, 0x06, 0x98, 0xaf, 0xf9, 0x5c, 0x2f, 0xca, 0xb5, 0x07, 0x12, 0x46, 0x7e, 0xab, 0x40, 0x04, 0x58, 0x3e, 0xb8, 0xfb, 0x7f, 0x89,
0x55, 0xad, 0x34, 0x06, 0x09, 0xf4, 0xb3, 0x02, 0x83, 0xe4, 0x88, 0x83, 0x25, 0xf1, 0x41, 0x5a, 0x08, 0x51, 0x25, 0xe8, 0xf7, 0xcd, 0xc9, 0x9f, 0xd9, 0x1d, 0xbd, 0x72, 0x80, 0x37, 0x3c, 0x5b,
0xd8, 0x82, 0x3e, 0x31, 0x56, 0x34, 0x8f, 0x5b, 0xae, 0x6d, 0xac, 0xd4, 0x36, 0xc9, 0x19, 0xc6, 0xdd, 0x53, 0xe2, 0x34, 0x87, 0xda, 0x03, 0xfd, 0x02, 0x39, 0x63, 0x06, 0xd2, 0x48, 0xcd, 0xa0,
0xe9, 0x9f, 0x33, 0x42, 0x0f, 0x57, 0x7e, 0xe8, 0xce, 0x54, 0xb6, 0x70, 0x80, 0x28, 0x0d, 0x1e, 0xc6, 0x98, 0x21, 0xbc, 0xb6, 0xa8, 0x83, 0x93, 0x96, 0xf9, 0x65, 0xab, 0x6f, 0xf7, 0x2a, 0x70]
let H1 = MD5()
let H2 = MD5()
H1.update(Src1)
H2.update(Src2)
let A1 = H1.digest()
let A2 = H2.digest()
CheckResults(A1 == A2)
CheckResults(A1 == "79054025255fb1a26e4bc422aef54eb4")
H1.reset()
H2.reset()
let SH = SHA1()
let SH256 = SHA256()
for (K, V) in TestSHA1 {
SH.update(K)
CheckResults(SH.digest() == V)
SH.reset()
}
for (K, V) in TestSHA256 {
SH256.update(K)
CheckResults(SH256.digest() == V)
SH256.reset()
}
// Check that we don't crash on large strings.
S = ""
for _ in 1...size {
S += "a"
SH.reset()
SH.update(S)
}
// Check that the order in which we push values does not change the result.
SH.reset()
L = ""
for _ in 1...size {
L += "a"
SH.update("a")
}
let SH2 = SHA1()
SH2.update(L)
CheckResults(SH.digest() == SH2.digest())
}
}
| apache-2.0 | 54585e909bdf36de26bbef2c35e7d5b1 | 26.634873 | 196 | 0.551205 | 2.605818 | false | false | false | false |
devpunk/velvet_room | Source/View/Abstract/Generic/VMenu.swift | 1 | 1059 | import UIKit
final class VMenu:UIView
{
weak var collectionView:UICollectionView!
private(set) weak var controller:ControllerParent!
var cellSize:CGSize?
let kDeselectTime:TimeInterval = 0.3
private let kBorderHeight:CGFloat = 1
init(controller:ControllerParent)
{
super.init(frame:CGRect.zero)
clipsToBounds = true
backgroundColor = UIColor.white
translatesAutoresizingMaskIntoConstraints = false
self.controller = controller
let border:VBorder = VBorder(
colour:UIColor.colourBackgroundDark.withAlphaComponent(0.2))
addSubview(border)
NSLayoutConstraint.topToTop(
view:border,
toView:self)
NSLayoutConstraint.height(
view:border,
constant:kBorderHeight)
NSLayoutConstraint.equalsHorizontal(
view:border,
toView:self)
factoryCollection()
}
required init?(coder:NSCoder)
{
return nil
}
}
| mit | cde2b13dbb1d9f1ff3ad2a6546f668e3 | 24.829268 | 72 | 0.618508 | 5.375635 | false | false | false | false |
ddaguro/clintonconcord | OIMApp/Controls/Spring/DesignableTextView.swift | 1 | 2597 | // The MIT License (MIT)
//
// Copyright (c) 2015 Meng To ([email protected])
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
import UIKit
@IBDesignable public class DesignableTextView: SpringTextView {
@IBInspectable public var borderColor: UIColor = UIColor.clearColor() {
didSet {
layer.borderColor = borderColor.CGColor
}
}
@IBInspectable public var borderWidth: CGFloat = 0 {
didSet {
layer.borderWidth = borderWidth
}
}
@IBInspectable public var cornerRadius: CGFloat = 0 {
didSet {
layer.cornerRadius = cornerRadius
}
}
@IBInspectable public var containerInset: CGFloat = 0 {
didSet {
contentInset = UIEdgeInsetsMake(-4, -4, -4, -4)
}
}
@IBInspectable public var lineHeight: CGFloat = 1.5 {
didSet {
var font = UIFont(name: self.font!.fontName, size: self.font!.pointSize)
var text = self.text
var paragraphStyle = NSMutableParagraphStyle()
paragraphStyle.lineSpacing = lineHeight
var attributedString = NSMutableAttributedString(string: text!)
attributedString.addAttribute(NSParagraphStyleAttributeName, value: paragraphStyle, range: NSMakeRange(0, attributedString.length))
attributedString.addAttribute(NSFontAttributeName, value: font!, range: NSMakeRange(0, attributedString.length))
self.attributedText = attributedString
}
}
}
| mit | ed82fde09464176b635037f2a95ff5f6 | 37.761194 | 143 | 0.67886 | 5.142574 | false | false | false | false |
looopTools/irisPause | irisPause/settings_handling.swift | 1 | 2858 | //
// settings_handling.swift
// irisPause
//
// Copyright (C) 2015 Lars Nielsen
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <http://www.gnu.org/licenses/>.
import Foundation
class settings_handling: NSObject {
var default_timeout: Int = 15
var default_work_period: Int = 15
var timeout_time: Int = 0
var work_period: Int = 0
var settings = UserDefaults.standard
static let settings_handler = settings_handling()
func load_settings() {
if Array(settings.dictionaryRepresentation().keys).contains("timeout") && get_timeout_time() != 0 {
timeout_time = get_timeout_time()
}else {
timeout_time = default_timeout
set_timeout_time(timeout_time)
}
if Array(settings.dictionaryRepresentation().keys).contains("work_period") {
work_period = get_work_period()
} else {
work_period = default_work_period
set_work_period(work_period)
}
}
func set_timeout_time(_ time:Int) {
settings.set(time, forKey: "timeout")
}
func get_timeout_time() -> Int {
let timeout = settings.integer(forKey: "timeout")
if timeout >= 0 {
return timeout
} else {
return -1
}
}
func set_work_period(_ time:Int) {
settings.set(time, forKey: "work_period")
}
func get_work_period() -> Int {
let work_period = settings.integer(forKey: "work_period")
if work_period >= 0 {
return work_period
} else {
return -1
}
}
func set_enable_extend_work_period(_ enable:Bool) {
settings.set(enable, forKey: "enable_extend")
}
func get_enable_extend_work_period() -> Bool {
let enable_extend = settings.bool(forKey: "enable_extend")
return enable_extend
}
// Needs testing to check if it returns a string
func set_localised_language(_ language:String) {
settings.set(language, forKey: "local_lang")
}
func get_localised_language() -> String {
let localised_language = settings.string(forKey: "local_lang")
return localised_language!
}
}
| gpl-3.0 | abaf905d90bf4afeaa9d33692a419bfe | 27.58 | 107 | 0.605668 | 4.202941 | false | false | false | false |
AlexeyTyurenkov/NBUStats | NBUStatProject/NBUStat/Modules/Government budget Module /GovermentBudgetModuleBuilder.swift | 1 | 1040 | //
// GovermentBudgetModuleBuilder.swift
// FinStat Ukraine
//
// Created by Aleksey Tyurenkov on 2/27/18.
// Copyright © 2018 Oleksii Tiurenkov. All rights reserved.
//
import Foundation
import UIKit
class GovermentBudgetModuleBuilder: ModuleBuilderProtocol
{
func viewController() -> UIViewController {
let storyBoard = UIStoryboard(name: "CurrencyRates", bundle: nil)
let initialController = storyBoard.instantiateInitialViewController()
if let navigationController = initialController as? UINavigationController
{
if let controller = navigationController.topViewController as? CurrencyViewController
{
controller.presenter = GovermentBudgetPresenter(date: Calendar.current.date(byAdding: .year, value: -1, to: Date()) ?? Date())
}
}
return initialController ?? UIViewController()
}
var moduleName: String
{
return NSLocalizedString("Державні фінанси", comment: "")
}
}
| mit | 4ae5bcae4873b02b6b12e6de61f1d9e6 | 29.117647 | 142 | 0.675781 | 4.740741 | false | false | false | false |
spitzgoby/Tunits | Example/Tunits/TunitsViewController.swift | 1 | 5519 | //
// TunitsViewController.swift
// Tunits
//
// Created by Tom on 9/5/15.
// Copyright (c) 2015 CocoaPods. All rights reserved.
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
//
import UIKit
import Tunits
class TunitsViewController: UIViewController, UITableViewDelegate, UITableViewDataSource {
// MARK: - Properties and Lifecycle
@IBOutlet weak var tableView : UITableView?
weak var datePicker : UIDatePicker?
lazy var dateFormatter : NSDateFormatter = {
var tempFormatter = NSDateFormatter()
tempFormatter.dateFormat = NSDateFormatter.dateFormatFromTemplate("MMMM dd, yyyy j:mm", options: 0, locale: NSLocale.autoupdatingCurrentLocale())
return tempFormatter
}()
var timeUnits : [[NSDate]]?
override func viewDidLoad() {
super.viewDidLoad()
self.tableView!.delegate = self
self.tableView!.dataSource = self
self.tableView!.translatesAutoresizingMaskIntoConstraints = false
}
// MARK: - UI Events
func dateChanged(sender:UIDatePicker) {
let tunits = TimeUnit()
self.timeUnits = [
tunits.monthsOfYear(sender.date),
tunits.daysOfMonth(sender.date),
tunits.hoursOfDay(sender.date),
tunits.minutesOfHour(sender.date),
]
self.tableView!.reloadData()
}
// MARK: - Table View
let kNumberOfSections = 5
let kDatePickerSection = 0
let kMonthsOfYearSection = 1
let kDaysOfMonthSection = 2
let kHoursOfDaySection = 3
let kMinutesOfHourSection = 4
let kDatePickerCellHeight : CGFloat = 216.0
let kTimeUnitCellHeight : CGFloat = 44.0
let kDatePickerCellIdentifier = "datePickerCell"
let kTimeUnitCellIdentifier = "timeUnitCell"
func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return kNumberOfSections
}
func tableView(tableView: UITableView, heightForRowAtIndexPath indexPath: NSIndexPath) -> CGFloat {
if indexPath.section == kDatePickerSection {
return kDatePickerCellHeight
} else {
return kTimeUnitCellHeight
}
}
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
if section == kDatePickerSection {
return 1
} else {
if let timeUnits = self.timeUnits {
return timeUnits[section - 1].count
} else {
return 0
}
}
}
func tableView(tableView: UITableView, titleForHeaderInSection section: Int) -> String? {
switch section {
case kMonthsOfYearSection:
return "Months Of Year"
case kDaysOfMonthSection:
return "Days Of Month"
case kHoursOfDaySection:
return "Hours Of Day"
case kMinutesOfHourSection:
return "Minutes Of Hour"
default:
return nil
}
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
if indexPath.section == kDatePickerSection {
return self.datePickerCell()
} else {
return self.timeUnitCell(indexPath)
}
}
private func datePickerCell() -> UITableViewCell {
let datePickerCell : TunitsDatePickerCell = self.tableView!.dequeueReusableCellWithIdentifier(kDatePickerCellIdentifier) as! TunitsDatePickerCell
if self.datePicker == nil {
self.datePicker = datePickerCell.datePicker!
self.datePicker!.addTarget(self, action: "dateChanged:", forControlEvents: .ValueChanged)
self.datePicker!.date = NSDate()
}
return datePickerCell
}
private func timeUnitCell(indexPath:NSIndexPath) -> UITableViewCell {
let timeUnitCell = self.tableView!.dequeueReusableCellWithIdentifier(kTimeUnitCellIdentifier)!
timeUnitCell.textLabel!.text = self.titleForTimeUnitCell(indexPath)
return timeUnitCell
}
private func titleForTimeUnitCell(indexPath:NSIndexPath) -> String {
if let timeUnits = self.timeUnits {
return self.dateFormatter.stringFromDate(timeUnits[indexPath.section - 1][indexPath.row])
} else {
return ""
}
}
}
| mit | 77aa475c8a41511a4916b2fd1177792a | 34.152866 | 153 | 0.650299 | 5.216446 | false | false | false | false |
stephentyrone/swift | test/IRGen/prespecialized-metadata/struct-inmodule-1argument-3distinct_use.swift | 3 | 6959 | // RUN: %swift -prespecialize-generic-metadata -target %module-target-future -emit-ir %s | %FileCheck %s -DINT=i%target-ptrsize -DALIGNMENT=%target-alignment
// REQUIRES: OS=macosx || OS=ios || OS=tvos || OS=watchos || OS=linux-gnu
// UNSUPPORTED: CPU=i386 && OS=ios
// UNSUPPORTED: CPU=armv7 && OS=ios
// UNSUPPORTED: CPU=armv7s && OS=ios
// CHECK: @"$s4main5ValueVySSGWV" = linkonce_odr hidden constant %swift.vwtable {
// CHECK-SAME: i8* bitcast ({{[^@]*}}@"$s4main5ValueVySSGwCP{{[^@]* to i8\*}}),
// CHECK-SAME: i8* bitcast ({{[^@]*}}@"$s4main5ValueVySSGwxx{{[^@]* to i8\*}}),
// CHECK-SAME: i8* bitcast ({{[^@]*}}@"$s4main5ValueVySSGwcp{{[^@]* to i8\*}}),
// CHECK-SAME: i8* bitcast ({{[^@]*}}@"$s4main5ValueVySSGwca{{[^@]* to i8\*}}),
// CHECK-SAME: i8* bitcast ({{[^@]*}}@__swift_memcpy{{[^[:space:]]+ to i8\*}}),
// CHECK-SAME: i8* bitcast ({{[^@]*}}@"$s4main5ValueVySSGwta{{[^@]* to i8\*}}),
// CHECK-SAME: i8* bitcast ({{[^@]*}}@"$s4main5ValueVySSGwet{{[^@]* to i8\*}}),
// CHECK-SAME: i8* bitcast ({{[^@]*}}@"$s4main5ValueVySSGwst{{[^@]* to i8\*}}),
// CHECK-SAME: [[INT]] {{[0-9]+}},
// CHECK-SAME: [[INT]] {{[0-9]+}},
// CHECK-SAME: i32 {{[0-9]+}},
// CHECK-SAME: i32 {{[0-9]+}}
// CHECK-SAME: },
// NOTE: ignore COMDAT on PE/COFF targets
// CHECK-SAME: align [[ALIGNMENT]]
// CHECK: @"$s4main5ValueVySSGMf" = linkonce_odr hidden constant {{.+}}$s4main5ValueVMn{{.+}} to %swift.type_descriptor*), %swift.type* @"$sSSN", i32 0{{(, \[4 x i8\] zeroinitializer)?}}, i64 3 }>, align [[ALIGNMENT]]
// CHECK: @"$s4main5ValueVySdGMf" = linkonce_odr hidden constant <{
// CHECK-SAME: i8**,
// CHECK-SAME: [[INT]],
// CHECK-SAME: %swift.type_descriptor*,
// CHECK-SAME: %swift.type*,
// CHECK-SAME: i32{{(, \[4 x i8\])?}},
// CHECK-SAME: i64
// CHECK-SAME: }> <{
// i8** @"$sBi64_WV",
// i8** getelementptr inbounds (%swift.vwtable, %swift.vwtable* @"$s4main5ValueVySdGWV", i32 0, i32 0),
// CHECK-SAME: [[INT]] 512,
// CHECK-SAME: %swift.type_descriptor* bitcast ({{.+}}$s4main5ValueVMn{{.+}} to %swift.type_descriptor*),
// CHECK-SAME: %swift.type* @"$sSdN",
// CHECK-SAME: i32 0{{(, \[4 x i8\] zeroinitializer)?}},
// CHECK-SAME: i64 3
// CHECK-SAME: }>, align [[ALIGNMENT]]
// CHECK: @"$s4main5ValueVySiGMf" = linkonce_odr hidden constant <{
// CHECK-SAME: i8**,
// CHECK-SAME: [[INT]],
// CHECK-SAME: %swift.type_descriptor*,
// CHECK-SAME: %swift.type*,
// CHECK-SAME: i32{{(, \[4 x i8\])?}},
// CHECK-SAME: i64
// CHECK-SAME: }> <{
// i8** @"$sB[[INT]]_WV",
// i8** getelementptr inbounds (%swift.vwtable, %swift.vwtable* @"$s4main5ValueVySiGWV", i32 0, i32 0),
// CHECK-SAME: [[INT]] 512,
// CHECK-SAME: %swift.type_descriptor* bitcast ({{.+}}$s4main5ValueVMn{{.+}} to %swift.type_descriptor*),
// CHECK-SAME: %swift.type* @"$sSiN",
// CHECK-SAME: i32 0{{(, \[4 x i8\] zeroinitializer)?}},
// CHECK-SAME: i64 3
// CHECK-SAME: }>, align [[ALIGNMENT]]
struct Value<First> {
let first: First
}
@inline(never)
func consume<T>(_ t: T) {
withExtendedLifetime(t) { t in
}
}
// CHECK: define hidden swiftcc void @"$s4main4doityyF"() #{{[0-9]+}} {
// CHECK: call swiftcc void @"$s4main7consumeyyxlF"(%swift.opaque* noalias nocapture %{{[0-9]+}}, %swift.type* getelementptr inbounds (%swift.full_type, %swift.full_type* bitcast (<{ i8**, [[INT]], %swift.type_descriptor*, %swift.type*, i32{{(, \[4 x i8\])?}}, i64 }>* @"$s4main5ValueVySiGMf" to %swift.full_type*), i32 0, i32 1))
// CHECK: call swiftcc void @"$s4main7consumeyyxlF"(%swift.opaque* noalias nocapture %{{[0-9]+}}, %swift.type* getelementptr inbounds (%swift.full_type, %swift.full_type* bitcast (<{ i8**, [[INT]], %swift.type_descriptor*, %swift.type*, i32{{(, \[4 x i8\])?}}, i64 }>* @"$s4main5ValueVySdGMf" to %swift.full_type*), i32 0, i32 1))
// CHECK: call swiftcc void @"$s4main7consumeyyxlF"(%swift.opaque* noalias nocapture %{{[0-9]+}}, %swift.type* getelementptr inbounds (%swift.full_type, %swift.full_type* bitcast (<{ i8**, [[INT]], %swift.type_descriptor*, %swift.type*, i32{{(, \[4 x i8\])?}}, i64 }>* @"$s4main5ValueVySSGMf" to %swift.full_type*), i32 0, i32 1))
// CHECK: }
func doit() {
consume( Value(first: 13) )
consume( Value(first: 13.0) )
consume( Value(first: "13") )
}
doit()
// CHECK: ; Function Attrs: noinline nounwind readnone
// CHECK: define hidden swiftcc %swift.metadata_response @"$s4main5ValueVMa"([[INT]] %0, %swift.type* %1) #{{[0-9]+}} {
// CHECK: entry:
// CHECK: [[ERASED_TYPE:%[0-9]+]] = bitcast %swift.type* %1 to i8*
// CHECK: br label %[[TYPE_COMPARISON_1:[0-9]+]]
// CHECK: [[TYPE_COMPARISON_1]]:
// CHECK: [[EQUAL_TYPE_1:%[0-9]+]] = icmp eq i8* bitcast (%swift.type* @"$sSiN" to i8*), [[ERASED_TYPE]]
// CHECK: [[EQUAL_TYPES_1:%[0-9]+]] = and i1 true, [[EQUAL_TYPE_1]]
// CHECK: br i1 [[EQUAL_TYPES_1]], label %[[EXIT_PRESPECIALIZED_1:[0-9]+]], label %[[TYPE_COMPARISON_2:[0-9]+]]
// CHECK: [[TYPE_COMPARISON_2]]:
// CHECK: [[EQUAL_TYPE_2:%[0-9]+]] = icmp eq i8* bitcast (%swift.type* @"$sSdN" to i8*), [[ERASED_TYPE]]
// CHECK: [[EQUAL_TYPES_2:%[0-9]+]] = and i1 true, [[EQUAL_TYPE_2]]
// CHECK: br i1 [[EQUAL_TYPES_2]], label %[[EXIT_PRESPECIALIZED_2:[0-9]+]], label %[[TYPE_COMPARISON_3:[0-9]+]]
// CHECK: [[TYPE_COMPARISON_3]]:
// CHECK: [[EQUAL_TYPE_3:%[0-9]+]] = icmp eq i8* bitcast (%swift.type* @"$sSSN" to i8*), [[ERASED_TYPE]]
// CHECK: [[EQUAL_TYPES_3:%[0-9]+]] = and i1 true, [[EQUAL_TYPE_3]]
// CHECK: br i1 [[EQUAL_TYPES_3]], label %[[EXIT_PRESPECIALIZED_3:[0-9]+]], label %[[EXIT_NORMAL:[0-9]+]]
// CHECK: [[EXIT_PRESPECIALIZED_1]]:
// CHECK: ret %swift.metadata_response { %swift.type* getelementptr inbounds (%swift.full_type, %swift.full_type* bitcast (<{ i8**, [[INT]], %swift.type_descriptor*, %swift.type*, i32{{(, \[4 x i8\])?}}, i64 }>* @"$s4main5ValueVySiGMf" to %swift.full_type*), i32 0, i32 1), [[INT]] 0 }
// CHECK: [[EXIT_PRESPECIALIZED_2]]:
// CHECK: ret %swift.metadata_response { %swift.type* getelementptr inbounds (%swift.full_type, %swift.full_type* bitcast (<{ i8**, [[INT]], %swift.type_descriptor*, %swift.type*, i32{{(, \[4 x i8\])?}}, i64 }>* @"$s4main5ValueVySdGMf" to %swift.full_type*), i32 0, i32 1), [[INT]] 0 }
// CHECK: [[EXIT_PRESPECIALIZED_3]]:
// CHECK: ret %swift.metadata_response { %swift.type* getelementptr inbounds (%swift.full_type, %swift.full_type* bitcast (<{ i8**, [[INT]], %swift.type_descriptor*, %swift.type*, i32{{(, \[4 x i8\])?}}, i64 }>* @"$s4main5ValueVySSGMf" to %swift.full_type*), i32 0, i32 1), [[INT]] 0 }
// CHECK: [[EXIT_NORMAL]]:
// CHECK: {{%[0-9]+}} = call swiftcc %swift.metadata_response @__swift_instantiateGenericMetadata([[INT]] %0, i8* [[ERASED_TYPE]], i8* undef, i8* undef, %swift.type_descriptor* bitcast ({{.+}}$s4main5ValueVMn{{.+}} to %swift.type_descriptor*)) #{{[0-9]+}}
// CHECK: ret %swift.metadata_response {{%[0-9]+}}
// CHECK: }
| apache-2.0 | 8ffa551c7c8c8a6657607a1a894aaf02 | 62.844037 | 332 | 0.594051 | 2.979024 | false | false | false | false |
yunzixun/V2ex-Swift | View/MemberTopicCell.swift | 1 | 5218 | //
// MemberTopicCell.swift
// V2ex-Swift
//
// Created by huangfeng on 2/1/16.
// Copyright © 2016 Fin. All rights reserved.
//
import UIKit
class MemberTopicCell: UITableViewCell {
/// 日期 和 最后发送人
var dateAndLastPostUserLabel: UILabel = {
let dateAndLastPostUserLabel = UILabel();
dateAndLastPostUserLabel.textColor=V2EXColor.colors.v2_TopicListDateColor;
dateAndLastPostUserLabel.font=v2Font(12);
return dateAndLastPostUserLabel
}()
/// 评论数量
var replyCountLabel: UILabel = {
let replyCountLabel = UILabel()
replyCountLabel.textColor = V2EXColor.colors.v2_TopicListDateColor
replyCountLabel.font = v2Font(12)
return replyCountLabel
}()
var replyCountIconImageView: UIImageView = {
let replyCountIconImageView = UIImageView(image: UIImage(named: "reply_n"))
replyCountIconImageView.contentMode = .scaleAspectFit
return replyCountIconImageView
}()
/// 节点
var nodeNameLabel: UILabel = {
let nodeNameLabel = UILabel();
nodeNameLabel.textColor = V2EXColor.colors.v2_TopicListDateColor
nodeNameLabel.font = v2Font(11)
nodeNameLabel.backgroundColor = V2EXColor.colors.v2_NodeBackgroundColor
nodeNameLabel.layer.cornerRadius=2;
nodeNameLabel.clipsToBounds = true
return nodeNameLabel
}()
/// 帖子标题
var topicTitleLabel: UILabel = {
let topicTitleLabel=V2SpacingLabel();
topicTitleLabel.textColor=V2EXColor.colors.v2_TopicListTitleColor;
topicTitleLabel.font=v2Font(15);
topicTitleLabel.numberOfLines=0;
topicTitleLabel.preferredMaxLayoutWidth=SCREEN_WIDTH-24;
return topicTitleLabel
}()
/// 装上面定义的那些元素的容器
var contentPanel:UIView = {
let contentPanel = UIView();
contentPanel.backgroundColor = V2EXColor.colors.v2_CellWhiteBackgroundColor
return contentPanel
}()
override init(style: UITableViewCellStyle, reuseIdentifier: String?) {
super.init(style: style, reuseIdentifier: reuseIdentifier);
self.setup();
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
func setup()->Void{
self.selectionStyle = .none
self.backgroundColor = V2EXColor.colors.v2_backgroundColor
self.contentView .addSubview(self.contentPanel);
self.contentPanel.addSubview(self.dateAndLastPostUserLabel);
self.contentPanel.addSubview(self.replyCountLabel);
self.contentPanel.addSubview(self.replyCountIconImageView);
self.contentPanel.addSubview(self.nodeNameLabel)
self.contentPanel.addSubview(self.topicTitleLabel);
self.setupLayout()
self.dateAndLastPostUserLabel.backgroundColor = self.contentPanel.backgroundColor
self.replyCountLabel.backgroundColor = self.contentPanel.backgroundColor
self.replyCountIconImageView.backgroundColor = self.contentPanel.backgroundColor
self.topicTitleLabel.backgroundColor = self.contentPanel.backgroundColor
}
func setupLayout(){
self.contentPanel.snp.makeConstraints{ (make) -> Void in
make.top.left.right.equalTo(self.contentView);
}
self.dateAndLastPostUserLabel.snp.makeConstraints{ (make) -> Void in
make.top.equalTo(self.contentPanel).offset(12);
make.left.equalTo(self.contentPanel).offset(12);
}
self.replyCountLabel.snp.makeConstraints{ (make) -> Void in
make.centerY.equalTo(self.dateAndLastPostUserLabel);
make.right.equalTo(self.contentPanel).offset(-12);
}
self.replyCountIconImageView.snp.makeConstraints{ (make) -> Void in
make.centerY.equalTo(self.replyCountLabel);
make.width.height.equalTo(18);
make.right.equalTo(self.replyCountLabel.snp.left).offset(-2);
}
self.nodeNameLabel.snp.makeConstraints{ (make) -> Void in
make.centerY.equalTo(self.replyCountLabel);
make.right.equalTo(self.replyCountIconImageView.snp.left).offset(-4)
make.bottom.equalTo(self.replyCountLabel).offset(1);
make.top.equalTo(self.replyCountLabel).offset(-1);
}
self.topicTitleLabel.snp.makeConstraints{ (make) -> Void in
make.top.equalTo(self.dateAndLastPostUserLabel.snp.bottom).offset(12);
make.left.equalTo(self.dateAndLastPostUserLabel);
make.right.equalTo(self.contentPanel).offset(-12);
}
self.contentPanel.snp.makeConstraints{ (make) -> Void in
make.bottom.equalTo(self.topicTitleLabel.snp.bottom).offset(12);
make.bottom.equalTo(self.contentView).offset(SEPARATOR_HEIGHT * -1);
}
}
func bind(_ model:MemberTopicsModel){
self.dateAndLastPostUserLabel.text = model.date
self.topicTitleLabel.text = model.topicTitle;
self.replyCountLabel.text = model.replies;
if let node = model.nodeName{
self.nodeNameLabel.text = " " + node + " "
}
}
}
| mit | 477b0eb1f5742a4073c7bc93b8b5cc8d | 38.653846 | 89 | 0.672939 | 4.510061 | false | false | false | false |
PopcornTimeTV/PopcornTimeTV | PopcornTime/Extensions/MPMediaItemProperty+Extension.swift | 1 | 283 |
import Foundation
public let MPMediaItemPropertySummary = "summary"
public let MPMediaItemPropertyShow = "show"
public let MPMediaItemPropertyEpisode = "episode"
public let MPMediaItemPropertySeason = "season"
public let MPMediaItemPropertyBackgroundArtwork = "backgroundArtwork"
| gpl-3.0 | d46e24e3f1053fd747987d5be00595b2 | 30.444444 | 69 | 0.840989 | 5.339623 | false | false | false | false |
PopcornTimeTV/PopcornTimeTV | PopcornTime/UI/Shared/Main View Controllers/WatchlistViewController.swift | 1 | 2569 |
import UIKit
import PopcornKit
class WatchlistViewController: MainViewController {
override func viewDidLoad() {
super.viewDidLoad()
collectionViewController.paginated = false
}
override func viewWillAppear(_ animated: Bool) {
load(page: -1) // Refresh watchlsit
super.viewWillAppear(animated)
}
override func load(page: Int) {
let group = DispatchGroup()
group.enter()
self.collectionViewController.dataSources = [WatchlistManager<Movie>.movie.getWatchlist { [unowned self] (updated) in
self.collectionViewController.dataSources[0] = updated.sorted(by: {$0.title < $1.title})
self.collectionViewController.collectionView?.reloadData()
self.collectionViewController.collectionView?.collectionViewLayout.invalidateLayout()
}.sorted(by: {$0.title < $1.title})]
self.collectionViewController.dataSources.append(WatchlistManager<Show>.show.getWatchlist { [unowned self] (updated) in
self.collectionViewController.dataSources[1] = updated.sorted(by: {$0.title < $1.title})
self.collectionViewController.collectionView?.reloadData()
self.collectionViewController.collectionView?.collectionViewLayout.invalidateLayout()
}.sorted(by: {$0.title < $1.title}))
self.collectionViewController.collectionView?.reloadData()
self.collectionViewController.collectionView?.collectionViewLayout.invalidateLayout()
}
override func collectionView(_ collectionView: UICollectionView, titleForHeaderInSection section: Int) -> String? {
if section == 0 {
return "Movies".localized
} else if section == 1 {
return "Shows".localized
}
return nil
}
override func collectionView(_ collectionView: UICollectionView, insetForSectionAt section: Int) -> UIEdgeInsets? {
let isTv = UIDevice.current.userInterfaceIdiom == .tv
return isTv ? UIEdgeInsets(top: 60, left: 90, bottom: 0, right: 90) : UIEdgeInsets(top: 5, left: 15, bottom: 15, right: 15)
}
override func collectionView(isEmptyForUnknownReason collectionView: UICollectionView) {
if let background: ErrorBackgroundView = .fromNib() {
background.setUpView(title: "Watchlist Empty".localized, description: "Try adding movies or shows to your watchlist.".localized)
collectionView.backgroundView = background
}
}
}
| gpl-3.0 | d37069a0517c6bb08d4d29312ce52d7c | 41.816667 | 140 | 0.66485 | 5.179435 | false | false | false | false |
GenerallyHelpfulSoftware/Scalar2D | SVG/Sources/SVGElements.swift | 1 | 2290 | //
// SVGElements.swift
// Scalar2D
//
// The MIT License (MIT)
// Copyright (c) 2016-2019 Generally Helpful Software
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
//
//
// Created by Glenn Howes on 3/20/18.
// Copyright © 2018-2019 Generally Helpful Software. All rights reserved.
//
import Foundation
#if os(iOS) || os(tvOS) || os(OSX)
import CoreGraphics
public typealias NativePath = CGPath
public typealias NativeImage = CGImage
public typealias NativeGradient = CGGradient
public typealias NativeAffineTransform = CGAffineTransform
#else // put whatever is appropriate here
public typealias NativePath = Any
public typealias NativeImage = Any
public typealias NativeGradient = Any
public typealias NativeAffineTransform = Any
#endif
//protocol SVGElement
//{
// var identifier : String? {get}
// var className : String? {get}
//}
//
//protocol SVGContainer : SVGElement
//{
// var children : [SVGElement]? {get}
//}
//
//struct SVGRoot : SVGContainer
//{
// public var children: [SVGElement]?
//}
//
//struct SVGDefinitions : SVGContainer
//{
// public var children: [SVGElement]?
//}
//
//struct SVGGroup : SVGContainer
//{
//
//}
//
//struct SVGImage : SVGElement
//{
//
//}
| mit | 6ffd3613bc1ac4a658d70eb16ad4e157 | 28.346154 | 81 | 0.716907 | 4.161818 | false | false | false | false |
dfortuna/theCraic3 | theCraic3/Main/Map/EventPreferences.swift | 1 | 16143 | //
// EventPreferences.swift
// The Craic
//
// Created by Denis Fortuna on 1/7/17.
// Copyright © 2017 Denis. All rights reserved.
//
import Foundation
import Firebase
import CoreLocation
protocol EventPreferencesDelegate: class {
func handleEventsResult(sender: EventPreferences)
}
class EventPreferences: NSObject, CLLocationManagerDelegate {
var refEvents: DatabaseReference = Database.database().reference().child("events")
var refTags: DatabaseReference = Database.database().reference().child("tags")
var refUsers: DatabaseReference = Database.database().reference().child("users")
var eventToMap = [Event]() {
didSet {
DispatchQueue.main.async {
self.delegate?.handleEventsResult(sender: self)
}
}
}
var foundTags = true
var foundCategory = true
var foundpriceRange = true
var foundGender = true
var isPublicEvent = false
var removeFollingFriendsIDs = [String]()
var removeFriendsEventsIDs = [String : String]()
var removeEventsIDs = [String]()
weak var delegate: EventPreferencesDelegate?
let date = Date()
func findEventsCoordinatesFromSettings(userID: String?, userLocation: CLLocation?, tags: [String]?, category: String, price: String, distance: Double, gender: String, friends: Bool) {
eventToMap.removeAll()
if friends {
//friends events should always pass through "public Event" filter in EventChild func
isPublicEvent = true
fetchFollingFriends(userID: userID!,
userLocation: userLocation,
tags: tags,
category: category,
price: price,
distance: distance,
gender: gender,
friends: true)
removeFriendsObservers(userID: userID!)
} else {
refEvents.observe(.childAdded, with: { (snapshot) in
self.removeEventsIDs.append(snapshot.key)
self.eventChilds(snapshot: snapshot,
userLocation: userLocation,
tags: tags,
category: category,
price: price,
distance: distance,
gender: gender,
isFriendEvent: false)
}, withCancel: { (error) in
print("error fetching from events data base")
})
}
}
//function reads event childs
func eventChilds(snapshot: DataSnapshot, userLocation: CLLocation?, tags: [String]?, category: String, price: String, distance: Double, gender: String, isFriendEvent: Bool) {
var newEvent = Event()
if let eventData = snapshot.value as? [String: AnyObject] {
newEvent.eventID = snapshot.key
let eventDateFormat = newEvent.eventDate.getDateAndTimeDate()
if eventDateFormat == Date() {
//Check if isPublicPost has a valid value and if its true
if let isPublicData = eventData["isPublicPost"] as? Bool {
if isPublicData {
isPublicEvent = isPublicData
}
}
//events will pass this if public or from friends
var checkEvent = false
if isFriendEvent {
checkEvent = true
} else {
if isPublicEvent {
checkEvent = true
}
}
if checkEvent {
let locationData = eventData["Location"] as! [String: String] ?? ["":""]
newEvent.eventAddress = locationData["Address"] ?? ""
newEvent.eventLatitude = locationData["Latitude"] ?? ""
newEvent.eventLongitude = locationData["Longitude"] ?? ""
let eventLat = CLLocationDegrees(newEvent.eventLatitude)
let eventLon = CLLocationDegrees(newEvent.eventLongitude)
let eventCLLocation = CLLocation(latitude: eventLat!, longitude: eventLon!)
if let userLoc = userLocation {
let distanceInMeters = eventCLLocation.distance(from: userLoc)
let distanceInKM = distanceInMeters / 1000
//Distance Filter:
if (distanceInKM < distance) || (distanceInKM == distance) {
//Tags filter
if let searchingTags = tags {
if !searchingTags.isEmpty {
self.foundTags = false
if let tagsData = eventData["Tags"] as? [String: String] {
for (_, dBTag) in tagsData {
self.foundTags = false
for tag in searchingTags {
if dBTag == tag {
self.foundTags = true
break
}
}
if self.foundTags{
break
}
}
}
}
}
if self.foundTags {
//Category filter
if (category != "-- Select --") && (category != "" ) {
self.foundCategory = false
if let categoryData = eventData["Category"] as? String {
if category == categoryData {
newEvent.eventCategory = categoryData
self.foundCategory = true
}
}
}
if self.foundCategory {
//price filter
if (price != "-- Select --") && (price != "") {
self.foundpriceRange = false
if let priceData = eventData["price"] as? String {
if price == priceData {
self.foundpriceRange = true
}
}
}
if self.foundpriceRange {
//Gender Filter
if (gender != "Men & Women") && (gender != "" ) {
self.foundGender = false
if let attendeeData = eventData["Attendees"] as? [String : Any]{
if let aMasc = attendeeData["Attendee_Masc"] as? Int,
let aFem = attendeeData["Attendee_Fem"] as? Int {
switch gender {
case "Men":
if aMasc > aFem {
self.foundGender = true
}
case "Women":
if aFem > aMasc {
self.foundGender = true
}
default: break
}
}
}
}
if self.foundGender {
newEvent.eventCategory = eventData["Category"] as? String ?? ""
newEvent.eventDescription = eventData["Description"] as? String ?? ""
newEvent.eventName = eventData["Event Name"] as? String ?? ""
newEvent.eventHost = eventData["Host"] as? String ?? ""
newEvent.eventPictures = eventData["Pictures"] as? [String: String] ?? ["":""]
newEvent.eventprice = eventData["price"] as? String ?? " "
newEvent.eventTags = eventData["Tags"] as? [String :String] ?? [:]
let locationData = eventData["Location"] as? [String: String] ?? ["":""]
newEvent.eventAddress = locationData["Address"] ?? ""
newEvent.eventLatitude = locationData["Latitude"] ?? ""
newEvent.eventLongitude = locationData["Longitude"] ?? ""
var attendeeData = eventData["Attendees"] as? [String : Any] ?? [:]
let aMasc = attendeeData["Attendee_Masc"] as? Int ?? 0
let aFem = attendeeData["Attendee_Fem"] as? Int ?? 0
let aCodData = attendeeData["Attendees COD"] as? [String : String] ?? ["":""]
newEvent.eventAttendees = Array(aCodData.keys)
newEvent.eventAttendeesFem = aFem
newEvent.eventAttendeesMasc = aMasc
eventToMap.append(newEvent)
// self.removeFirebaseObservers(following: removeFollingFriendsIDs,
// friendsEvents: removeFriendsEventsIDs,
// events: removeEventsIDs,
// userID: <#T##String#>)
}
}
}
}
}
}
}
} else {
print("***Data menor EventPrefereces ****")
print("eventDateFormat \(eventDateFormat)")
print("today \(Date())")
}
foundTags = true
foundCategory = true
foundpriceRange = true
foundGender = true
isPublicEvent = false
}
}
func removeFirebaseObservers(following:[String], friendsEvents:[String: String], events:[String], userID: String){
for (friendID, eventID) in friendsEvents {
refUsers.child(friendID).child("myEvents").child(eventID).removeAllObservers()
}
for eventID in events {
refEvents.child(eventID).removeAllObservers()
}
}
func removeFriendsObservers(userID: String) {
// for followingFriendID in following {
// refUsers.child(userID).child("Friends").child(followingFriendID).removeAllObservers()
// }
}
func fetchFollingFriends (userID: String, userLocation: CLLocation?, tags: [String]?, category: String, price: String, distance: Double, gender: String, friends: Bool) {
//Fetch friends that user is following
refUsers.child(userID).child("Friends").observe(.childAdded, with: { (snapshot) in
if let friendData = snapshot.value as? [String: Bool]{
let friendID = snapshot.key
self.removeFollingFriendsIDs.append(friendID)
let followingFriend = friendData["Following"] //as? Bool ?? false
if followingFriend! {
self.checkIfAllowedByFriend(userID: userID,
friendID: friendID,
userLocation: userLocation,
tags: tags,
category: category,
price: price,
distance: distance,
gender: gender,
friends: true)
}
}
}, withCancel: nil)
}
func checkIfAllowedByFriend(userID: String, friendID: String, userLocation: CLLocation?, tags: [String]?, category: String, price: String, distance: Double, gender: String, friends: Bool) {
//Check if user's friend permit user to follow him/her
refUsers.child(friendID).child("Friends").child(userID).observeSingleEvent(of: .childAdded, with: { (snapshot) in
if snapshot.key == "Allowed to Follow" {
if let friendData = snapshot.value as? Bool{
if friendData{
self.fetchFriendsEvents(friend: friendID,
userId: userID,
userLocation: userLocation,
tags: tags,
category: category,
price: price,
distance: distance,
gender: gender,
friends: true)
}
}
}
}) { (error) in
print("error checkIfAllowedByFriend \(error)")
}
}
func fetchFriendsEvents(friend: String, userId: String, userLocation: CLLocation?, tags: [String]?, category: String, price: String, distance: Double, gender: String, friends: Bool) {
refUsers.child(friend).child("myEvents").observe(.childAdded, with: { (snapshot) in
let eventID = snapshot.key
self.removeFriendsEventsIDs[friend] = eventID
self.refEvents.child(eventID).observeSingleEvent(of: .value, with: { (snapshot2) in
self.eventChilds(snapshot: snapshot2,
userLocation: userLocation,
tags: tags,
category: category,
price: price,
distance: distance,
gender: gender,
isFriendEvent: true)
}, withCancel: { (error) in
print("error fetching from events data base")
})
}) { (error) in
print("error fetchFriendsEvents \(error)")
}
}
}
| apache-2.0 | 543f2be6520bbceeeda6ea9c0b20a3ab | 49.44375 | 193 | 0.404163 | 6.686827 | false | false | false | false |
GrfxGuru/CodeNotesForiOS | Code Notes/UserSettingsViewController.swift | 1 | 3597 | //
// applicationUserSettingsViewController.swift
// Code Notes
//
// Created by Peter Witham on 1/21/17.
// Copyright © 2017 Peter Witham. All rights reserved.
//
import UIKit
import CoreData
class UserSettingsViewController: UIViewController {
@IBOutlet weak var swConfirmNoteDeletion: UISwitch!
@IBOutlet weak var swPasteReplace: UISwitch!
@IBOutlet weak var myWebsite: UILabel!
@IBOutlet weak var lblEvergreenURL: UILabel!
@IBOutlet weak var swDarkTheme: UISwitch!
@IBOutlet weak var btnRemoveAllNotes: UIButton!
@IBOutlet weak var btnManageLanguages: UIButton!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
let displayDeleteAlert = UserDefaults.standard.bool(forKey: "confirmNoteDeletion")
swConfirmNoteDeletion.isOn = displayDeleteAlert
let pasteReplace = UserDefaults.standard.bool(forKey: "pasteReplace")
swPasteReplace.isOn = pasteReplace
let darkTheme = UserDefaults.standard.bool(forKey: "darkTheme")
swDarkTheme.isOn = darkTheme
navigationItem.leftBarButtonItem = splitViewController?.displayModeButtonItem
self.view.backgroundColor = Theme.viewBackgroundColor
let orientation = UIApplication.shared.statusBarOrientation
if orientation.isPortrait {
self.splitViewController?.preferredDisplayMode = .primaryHidden
}
btnRemoveAllNotes.setTitleColor(UIColor.white, for: .normal)
btnManageLanguages.setTitleColor(UIColor.white, for: .normal)
btnManageLanguages.backgroundColor = UIColor.lightGray
btnRemoveAllNotes.backgroundColor = UIColor.lightGray
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// MARK: - Segues
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "saveSettings" {
UserDefaults.standard.set(swConfirmNoteDeletion.isOn, forKey: "confirmNoteDeletion")
UserDefaults.standard.set(swPasteReplace.isOn, forKey: "pasteReplace")
UserDefaults.standard.set(swDarkTheme.isOn, forKey: "darkTheme")
} else if segue.identifier == "cancelButton" {
}
}
@IBAction func btnResetDatabase(_ sender: UIButton) {
let fetchRequest = NSFetchRequest<NSFetchRequestResult>(entityName: "NoteRecord")
let deleteRequest = NSBatchDeleteRequest(fetchRequest: fetchRequest)
do {
try AppConfiguration.context.execute(deleteRequest)
} catch let error as NSError {
print(error)
}
let navVC: UINavigationController = (self.splitViewController!.viewControllers[0] as? UINavigationController)!
let sectionsVC: MasterViewController = (navVC.topViewController as? MasterViewController)!
sectionsVC.getData()
sectionsVC.tableView.reloadData()
}
@IBAction func swDarkTheme(_ sender: UISwitch) {
if swDarkTheme.isOn {
Theme.darkTheme()
} else {
Theme.defaultTheme()
}
splitViewController?.loadView()
}
/*
// MARK: - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
// Get the new view controller using segue.destinationViewController.
// Pass the selected object to the new view controller.
}
*/
}
| mit | 016ae1bda2dd61576a5b3d4135f9ae6a | 37.666667 | 118 | 0.696329 | 5.100709 | false | false | false | false |
justindarc/firefox-ios | XCUITests/BookmarkingTests.swift | 1 | 9815 | /* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
import XCTest
let url_1 = "test-example.html"
let url_2 = ["url": "test-mozilla-org.html", "bookmarkLabel": "Internet for people, not profit — Mozilla"]
let urlLabelExample_3 = "Example Domain"
let url_3 = "localhost:\(serverPort)/test-fixture/test-example.html"
let urlLabelExample_4 = "Example Login Page 2"
let url_4 = "test-password-2.html"
class BookmarkingTests: BaseTestCase {
private func bookmark() {
navigator.goto(PageOptionsMenu)
waitForExistence(app.tables.cells["Bookmark This Page"])
app.tables.cells["Bookmark This Page"].tap()
navigator.nowAt(BrowserTab)
}
private func unbookmark() {
navigator.goto(PageOptionsMenu)
waitForExistence(app.tables.cells["Remove Bookmark"])
app.cells["Remove Bookmark"].tap()
navigator.nowAt(BrowserTab)
}
private func checkBookmarked() {
navigator.goto(PageOptionsMenu)
waitForExistence(app.tables.cells["Remove Bookmark"])
if iPad() {
app.otherElements["PopoverDismissRegion"].tap()
navigator.nowAt(BrowserTab)
} else {
navigator.goto(BrowserTab)
}
}
private func checkUnbookmarked() {
navigator.goto(PageOptionsMenu)
waitForExistence(app.tables.cells["Bookmark This Page"])
if iPad() {
app.otherElements["PopoverDismissRegion"].tap()
navigator.nowAt(BrowserTab)
} else {
navigator.goto(BrowserTab)
}
}
func testBookmarkingUI() {
// Go to a webpage, and add to bookmarks, check it's added
navigator.openURL(path(forTestPage: url_1))
navigator.nowAt(BrowserTab)
waitForTabsButton()
bookmark()
waitForTabsButton()
checkBookmarked()
// Load a different page on a new tab, check it's not bookmarked
navigator.openNewURL(urlString: path(forTestPage: url_2["url"]!))
navigator.nowAt(BrowserTab)
waitForTabsButton()
checkUnbookmarked()
// Go back, check it's still bookmarked, check it's on bookmarks home panel
waitForTabsButton()
navigator.goto(TabTray)
app.collectionViews.cells["Example Domain"].tap()
navigator.nowAt(BrowserTab)
waitForTabsButton()
checkBookmarked()
// Open it, then unbookmark it, and check it's no longer on bookmarks home panel
unbookmark()
waitForTabsButton()
checkUnbookmarked()
}
private func checkEmptyBookmarkList() {
let list = app.tables["Bookmarks List"].cells.count
XCTAssertEqual(list, 0, "There should not be any entry in the bookmarks list")
}
private func checkItemInBookmarkList() {
waitForExistence(app.tables["Bookmarks List"])
let list = app.tables["Bookmarks List"].cells.count
XCTAssertEqual(list, 1, "There should be an entry in the bookmarks list")
XCTAssertTrue(app.tables["Bookmarks List"].staticTexts[url_2["bookmarkLabel"]!].exists)
}
func testAccessBookmarksFromContextMenu() {
//Add a bookmark
navigator.openURL(path(forTestPage: url_2["url"]!))
waitUntilPageLoad()
navigator.nowAt(BrowserTab)
waitForExistence(app.buttons["TabLocationView.pageOptionsButton"], timeout: 10)
bookmark()
//There should be a bookmark
navigator.goto(MobileBookmarks)
checkItemInBookmarkList()
}
func testRecentBookmarks() {
// Verify that there are only 4 cells without recent bookmarks
navigator.goto(LibraryPanel_Bookmarks)
XCTAssertEqual(app.tables["Bookmarks List"].cells.count, 4)
//Add a bookmark
navigator.openURL(url_3)
waitForTabsButton()
bookmark()
// Check if it shows in recent bookmarks
navigator.goto(LibraryPanel_Bookmarks)
waitForExistence(app.otherElements["Recent Bookmarks"])
waitForExistence(app.staticTexts[urlLabelExample_3])
XCTAssertEqual(app.tables["Bookmarks List"].cells.count, 5)
// Add another
navigator.openURL(path(forTestPage: url_4))
waitForTabsButton()
bookmark()
// Check if it shows in recent bookmarks
navigator.goto(LibraryPanel_Bookmarks)
waitForExistence(app.otherElements["Recent Bookmarks"])
waitForExistence(app.staticTexts[urlLabelExample_4])
XCTAssertEqual(app.tables["Bookmarks List"].cells.count, 6)
// Click a recent bookmark and make sure it navigates. Disabled because of issue 5038 not opening recent bookmarks when tapped
app.tables["Bookmarks List"].cells.element(boundBy: 5).tap()
waitForExistence(app.textFields["url"], timeout: 6)
waitForValueContains(app.textFields["url"], value: url_3)
}
// Smoketest
func testBookmarksAwesomeBar() {
navigator.goto(URLBarOpen)
typeOnSearchBar(text: "www.ebay")
waitForExistence(app.tables["SiteTable"])
waitForExistence(app.buttons["www.ebay.com"])
XCTAssertTrue(app.buttons["www.ebay.com"].exists)
typeOnSearchBar(text: ".com")
typeOnSearchBar(text: "\r")
navigator.nowAt(BrowserTab)
//Clear text and enter new url
waitForTabsButton()
navigator.performAction(Action.OpenNewTabFromTabTray)
navigator.goto(URLBarOpen)
typeOnSearchBar(text: "http://www.olx.ro")
// Site table existes but is empty
waitForExistence(app.tables["SiteTable"])
XCTAssertEqual(app.tables["SiteTable"].cells.count, 0)
typeOnSearchBar(text: "\r")
navigator.nowAt(BrowserTab)
// Add page to bookmarks
waitForTabsButton()
bookmark()
// Now the site should be suggested
navigator.performAction(Action.AcceptClearPrivateData)
navigator.goto(BrowserTab)
navigator.goto(URLBarOpen)
typeOnSearchBar(text: "olx.ro")
waitForExistence(app.tables["SiteTable"])
waitForExistence(app.buttons["olx.ro"])
XCTAssertNotEqual(app.tables["SiteTable"].cells.count, 0)
}
func testAddBookmark() {
addNewBookmark()
// Verify that clicking on bookmark opens the website
app.tables["Bookmarks List"].cells.element(boundBy: 0).tap()
waitForExistence(app.textFields["url"], timeout: 5)
}
func testAddNewFolder() {
navigator.goto(MobileBookmarks)
navigator.goto(MobileBookmarksAdd)
navigator.performAction(Action.AddNewFolder)
waitForExistence(app.navigationBars["New Folder"])
// XCTAssertFalse(app.buttons["Save"].isEnabled), is this a bug allowing empty folder name?
app.tables["SiteTable"].cells.textFields.element(boundBy: 0).tap()
app.tables["SiteTable"].cells.textFields.element(boundBy: 0).typeText("Test Folder")
app.buttons["Save"].tap()
app.buttons["Done"].tap()
checkItemsInBookmarksList(items: 1)
navigator.nowAt(MobileBookmarks)
// Now remove the folder
navigator.performAction(Action.RemoveItemMobileBookmarks)
waitForExistence(app.buttons["Delete"])
navigator.performAction(Action.ConfirmRemoveItemMobileBookmarks)
checkItemsInBookmarksList(items: 0)
}
func testAddNewMarker() {
navigator.goto(MobileBookmarks)
navigator.goto(MobileBookmarksAdd)
navigator.performAction(Action.AddNewSeparator)
app.buttons["Done"].tap()
checkItemsInBookmarksList(items: 1)
// Remove it
navigator.nowAt(MobileBookmarks)
navigator.performAction(Action.RemoveItemMobileBookmarks)
waitForExistence(app.buttons["Delete"])
navigator.performAction(Action.ConfirmRemoveItemMobileBookmarks)
checkItemsInBookmarksList(items: 0)
}
func testDeleteBookmarkSwiping() {
addNewBookmark()
// Remove by swiping
app.tables["Bookmarks List"].staticTexts["BBC"].swipeLeft()
app.buttons["Delete"].tap()
checkItemsInBookmarksList(items: 0)
}
func testDeleteBookmarkContextMenu() {
addNewBookmark()
// Remove by long press and select option from context menu
app.tables.staticTexts.element(boundBy: 0).press(forDuration: 1)
waitForExistence(app.tables["Context Menu"])
app.tables["Context Menu"].cells["action_bookmark_remove"].tap()
checkItemsInBookmarksList(items: 0)
}
private func addNewBookmark() {
navigator.goto(MobileBookmarksAdd)
navigator.performAction(Action.AddNewBookmark)
waitForExistence(app.navigationBars["New Bookmark"], timeout: 3)
// Enter the bookmarks details
app.tables["SiteTable"].cells.textFields.element(boundBy: 0).tap()
app.tables["SiteTable"].cells.textFields.element(boundBy: 0).typeText("BBC")
app.tables["SiteTable"].cells.textFields["https://"].tap()
app.tables["SiteTable"].cells.textFields["https://"].typeText("bbc.com")
navigator.performAction(Action.SaveCreatedBookmark)
app.buttons["Done"].tap()
checkItemsInBookmarksList(items: 1)
}
private func checkItemsInBookmarksList(items: Int) {
waitForExistence(app.tables["Bookmarks List"], timeout: 3)
XCTAssertEqual(app.tables["Bookmarks List"].cells.count, items)
}
private func typeOnSearchBar(text: String) {
waitForExistence(app.textFields["address"])
app.textFields["address"].typeText(text)
}
}
| mpl-2.0 | 3f38144a3da855cbece7f45776ffcf0a | 37.182879 | 134 | 0.659941 | 4.670633 | false | true | false | false |
DarrenKong/firefox-ios | Client/Frontend/Browser/SearchEngines.swift | 1 | 9572 | /* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
import Foundation
import Shared
import Storage
import XCGLogger
private let log = Logger.browserLogger
private let OrderedEngineNames = "search.orderedEngineNames"
private let DisabledEngineNames = "search.disabledEngineNames"
private let ShowSearchSuggestionsOptIn = "search.suggestions.showOptIn"
private let ShowSearchSuggestions = "search.suggestions.show"
private let customSearchEnginesFileName = "customEngines.plist"
/**
* Manage a set of Open Search engines.
*
* The search engines are ordered. Individual search engines can be enabled and disabled. The
* first search engine is distinguished and labeled the "default" search engine; it can never be
* disabled. Search suggestions should always be sourced from the default search engine.
*
* Two additional bits of information are maintained: whether the user should be shown "opt-in to
* search suggestions" UI, and whether search suggestions are enabled.
*
* Consumers will almost always use `defaultEngine` if they want a single search engine, and
* `quickSearchEngines()` if they want a list of enabled quick search engines (possibly empty,
* since the default engine is never included in the list of enabled quick search engines, and
* it is possible to disable every non-default quick search engine).
*
* The search engines are backed by a write-through cache into a ProfilePrefs instance. This class
* is not thread-safe -- you should only access it on a single thread (usually, the main thread)!
*/
class SearchEngines {
fileprivate let prefs: Prefs
fileprivate let fileAccessor: FileAccessor
init(prefs: Prefs, files: FileAccessor) {
self.prefs = prefs
// By default, show search suggestions
self.shouldShowSearchSuggestions = prefs.boolForKey(ShowSearchSuggestions) ?? true
self.fileAccessor = files
self.disabledEngineNames = getDisabledEngineNames()
self.orderedEngines = getOrderedEngines()
}
var defaultEngine: OpenSearchEngine {
get {
return self.orderedEngines[0]
}
set(defaultEngine) {
// The default engine is always enabled.
self.enableEngine(defaultEngine)
// The default engine is always first in the list.
var orderedEngines = self.orderedEngines.filter { engine in engine.shortName != defaultEngine.shortName }
orderedEngines.insert(defaultEngine, at: 0)
self.orderedEngines = orderedEngines
}
}
func isEngineDefault(_ engine: OpenSearchEngine) -> Bool {
return defaultEngine.shortName == engine.shortName
}
// The keys of this dictionary are used as a set.
fileprivate var disabledEngineNames: [String: Bool]! {
didSet {
self.prefs.setObject(Array(self.disabledEngineNames.keys), forKey: DisabledEngineNames)
}
}
var orderedEngines: [OpenSearchEngine]! {
didSet {
self.prefs.setObject(self.orderedEngines.map { $0.shortName }, forKey: OrderedEngineNames)
}
}
var quickSearchEngines: [OpenSearchEngine]! {
get {
return self.orderedEngines.filter({ (engine) in !self.isEngineDefault(engine) && self.isEngineEnabled(engine) })
}
}
var shouldShowSearchSuggestions: Bool {
didSet {
self.prefs.setObject(shouldShowSearchSuggestions, forKey: ShowSearchSuggestions)
}
}
func isEngineEnabled(_ engine: OpenSearchEngine) -> Bool {
return disabledEngineNames.index(forKey: engine.shortName) == nil
}
func enableEngine(_ engine: OpenSearchEngine) {
disabledEngineNames.removeValue(forKey: engine.shortName)
}
func disableEngine(_ engine: OpenSearchEngine) {
if isEngineDefault(engine) {
// Can't disable default engine.
return
}
disabledEngineNames[engine.shortName] = true
}
func deleteCustomEngine(_ engine: OpenSearchEngine) {
// We can't delete a preinstalled engine or an engine that is currently the default.
if !engine.isCustomEngine || isEngineDefault(engine) {
return
}
customEngines.remove(at: customEngines.index(of: engine)!)
saveCustomEngines()
orderedEngines = getOrderedEngines()
}
/// Adds an engine to the front of the search engines list.
func addSearchEngine(_ engine: OpenSearchEngine) {
customEngines.append(engine)
orderedEngines.insert(engine, at: 1)
saveCustomEngines()
}
func queryForSearchURL(_ url: URL?) -> String? {
for engine in orderedEngines {
guard let searchTerm = engine.queryForSearchURL(url) else { continue }
return searchTerm
}
return nil
}
fileprivate func getDisabledEngineNames() -> [String: Bool] {
if let disabledEngineNames = self.prefs.stringArrayForKey(DisabledEngineNames) {
var disabledEngineDict = [String: Bool]()
for engineName in disabledEngineNames {
disabledEngineDict[engineName] = true
}
return disabledEngineDict
} else {
return [String: Bool]()
}
}
fileprivate func customEngineFilePath() -> String {
let profilePath = try! self.fileAccessor.getAndEnsureDirectory() as NSString
return profilePath.appendingPathComponent(customSearchEnginesFileName)
}
fileprivate lazy var customEngines: [OpenSearchEngine] = {
return NSKeyedUnarchiver.unarchiveObject(withFile: self.customEngineFilePath()) as? [OpenSearchEngine] ?? []
}()
fileprivate func saveCustomEngines() {
NSKeyedArchiver.archiveRootObject(customEngines, toFile: self.customEngineFilePath())
}
/// Return all possible language identifiers in the order of most specific to least specific.
/// For example, zh-Hans-CN will return [zh-Hans-CN, zh-CN, zh].
class func possibilitiesForLanguageIdentifier(_ languageIdentifier: String) -> [String] {
var possibilities: [String] = []
let components = languageIdentifier.components(separatedBy: "-")
possibilities.append(languageIdentifier)
if components.count == 3, let first = components.first, let last = components.last {
possibilities.append("\(first)-\(last)")
}
if components.count >= 2, let first = components.first {
possibilities.append("\(first)")
}
return possibilities
}
/// Get all bundled (not custom) search engines, with the default search engine first,
/// but the others in no particular order.
class func getUnorderedBundledEnginesFor(locale: Locale) -> [OpenSearchEngine] {
let languageIdentifier = locale.identifier
let region = locale.regionCode ?? "US"
let parser = OpenSearchParser(pluginMode: true)
guard let pluginDirectory = Bundle.main.resourceURL?.appendingPathComponent("SearchPlugins") else {
assertionFailure("Search plugins not found. Check bundle")
return []
}
guard let defaultSearchPrefs = DefaultSearchPrefs(with: pluginDirectory.appendingPathComponent("list.json")) else {
assertionFailure("Failed to parse List.json")
return []
}
let possibilities = possibilitiesForLanguageIdentifier(languageIdentifier)
let engineNames = defaultSearchPrefs.visibleDefaultEngines(for: possibilities, and: region)
let defaultEngineName = defaultSearchPrefs.searchDefault(for: possibilities, and: region)
assert(engineNames.count > 0, "No search engines")
return engineNames.map({ (name: $0, path: pluginDirectory.appendingPathComponent("\($0).xml").path) })
.filter({ FileManager.default.fileExists(atPath: $0.path) })
.flatMap({ parser.parse($0.path, engineID: $0.name) })
.sorted { e, _ in e.shortName == defaultEngineName }
}
/// Get all known search engines, possibly as ordered by the user.
fileprivate func getOrderedEngines() -> [OpenSearchEngine] {
let unorderedEngines = customEngines + SearchEngines.getUnorderedBundledEnginesFor(locale: Locale.current)
// might not work to change the default.
guard let orderedEngineNames = prefs.stringArrayForKey(OrderedEngineNames) else {
// We haven't persisted the engine order, so return whatever order we got from disk.
return unorderedEngines
}
// We have a persisted order of engines, so try to use that order.
// We may have found engines that weren't persisted in the ordered list
// (if the user changed locales or added a new engine); these engines
// will be appended to the end of the list.
return unorderedEngines.sorted { engine1, engine2 in
let index1 = orderedEngineNames.index(of: engine1.shortName)
let index2 = orderedEngineNames.index(of: engine2.shortName)
if index1 == nil && index2 == nil {
return engine1.shortName < engine2.shortName
}
// nil < N for all non-nil values of N.
if index1 == nil || index2 == nil {
return index1 ?? -1 > index2 ?? -1
}
return index1! < index2!
}
}
}
| mpl-2.0 | ead015e286b097bbe31f1815ee56d89e | 40.081545 | 124 | 0.671646 | 4.858883 | false | false | false | false |
MoooveOn/Advanced-Frameworks | PreviewLivePhoto/LivePreview/LivePreview/LivePhotoViewController.swift | 1 | 2276 | //
// LivePhotoViewController.swift
// LivePreview
//
// Created by Pavel Selivanov on 04.07.17.
// Copyright © 2017 Pavel Selivanov. All rights reserved.
//
import UIKit
import Photos
import PhotosUI
class LivePhotoViewController: UIViewController {
var photoView: PHLivePhotoView!
var livePhotoAsset: PHAsset?
override func viewDidLoad() {
super.viewDidLoad()
photoView = PHLivePhotoView(frame: self.view.bounds)
photoView.contentMode = .scaleAspectFit
self.view.addSubview(photoView)
if self.traitCollection.forceTouchCapability == .unavailable {
let playbarButton = UIBarButtonItem(barButtonSystemItem: .play, target: self, action: #selector(LivePhotoViewController.playAnimation))
self.navigationItem.rightBarButtonItem = playbarButton
}
}
func playAnimation() {
photoView.startPlayback(with: .full)
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
configurateView()
}
func configurateView() {
if let photoAsset = livePhotoAsset {
//PHImageManager.default().requestImage(for: <#T##PHAsset#>, targetSize: <#T##CGSize#>, contentMode: <#T##PHImageContentMode#>, options: , resultHandler: <#T##(UIImage?, [AnyHashable : Any]?) -> Void#>)
PHImageManager.default().requestLivePhoto(for: photoAsset, targetSize: photoView.frame.size, contentMode: PHImageContentMode.aspectFit, options: nil, resultHandler: { (photo: PHLivePhoto?, info: [AnyHashable : Any]?) in
if let livePhoto = photo {
self.photoView.livePhoto = livePhoto
//next setting only for live photo
self.photoView.startPlayback(with: PHLivePhotoViewPlaybackStyle.hint)
//Usage metadata
let geoCoder = CLGeocoder()
geoCoder.reverseGeocodeLocation(photoAsset.location!, completionHandler: { (placemarks: [CLPlacemark]?,error: Error?) in
self.navigationItem.title = placemarks?.first?.locality
})
}
})
}
}
}
| mit | 6768cb86640a62b0301e944072a743ce | 35.693548 | 231 | 0.618901 | 5.365566 | false | false | false | false |
WhisperSystems/Signal-iOS | Signal/src/ViewControllers/ContactsPicker.swift | 1 | 15520 | //
// Copyright (c) 2019 Open Whisper Systems. All rights reserved.
//
// Originally based on EPContacts
//
// Created by Prabaharan Elangovan on 12/10/15.
// Parts Copyright © 2015 Prabaharan Elangovan. All rights reserved
import UIKit
import Contacts
import SignalServiceKit
@objc
public protocol ContactsPickerDelegate: class {
func contactsPicker(_: ContactsPicker, contactFetchDidFail error: NSError)
func contactsPickerDidCancel(_: ContactsPicker)
func contactsPicker(_: ContactsPicker, didSelectContact contact: Contact)
func contactsPicker(_: ContactsPicker, didSelectMultipleContacts contacts: [Contact])
func contactsPicker(_: ContactsPicker, shouldSelectContact contact: Contact) -> Bool
}
@objc
public enum SubtitleCellValue: Int {
case phoneNumber, email, none
}
@objc
public class ContactsPicker: OWSViewController, UITableViewDelegate, UITableViewDataSource, UISearchBarDelegate {
var tableView: UITableView!
var searchBar: UISearchBar!
// MARK: - Properties
private let contactCellReuseIdentifier = "contactCellReuseIdentifier"
private var contactsManager: OWSContactsManager {
return Environment.shared.contactsManager
}
// HACK: Though we don't have an input accessory view, the VC we are presented above (ConversationVC) does.
// If the app is backgrounded and then foregrounded, when OWSWindowManager calls mainWindow.makeKeyAndVisible
// the ConversationVC's inputAccessoryView will appear *above* us unless we'd previously become first responder.
override public var canBecomeFirstResponder: Bool {
Logger.debug("")
return true
}
override public func becomeFirstResponder() -> Bool {
Logger.debug("")
return super.becomeFirstResponder()
}
override public func resignFirstResponder() -> Bool {
Logger.debug("")
return super.resignFirstResponder()
}
private let collation = UILocalizedIndexedCollation.current()
public var collationForTests: UILocalizedIndexedCollation {
get {
return collation
}
}
private let contactStore = CNContactStore()
// Data Source State
private lazy var sections = [[CNContact]]()
private lazy var filteredSections = [[CNContact]]()
private lazy var selectedContacts = [Contact]()
// Configuration
@objc
public weak var contactsPickerDelegate: ContactsPickerDelegate?
private let subtitleCellType: SubtitleCellValue
private let allowsMultipleSelection: Bool
private let allowedContactKeys: [CNKeyDescriptor] = ContactsFrameworkContactStoreAdaptee.allowedContactKeys
// MARK: - Initializers
@objc
required public init(allowsMultipleSelection: Bool, subtitleCellType: SubtitleCellValue) {
self.allowsMultipleSelection = allowsMultipleSelection
self.subtitleCellType = subtitleCellType
super.init(nibName: nil, bundle: nil)
}
required public init?(coder aDecoder: NSCoder) {
notImplemented()
}
// MARK: - Lifecycle Methods
override public func loadView() {
self.view = UIView()
let tableView = UITableView()
self.tableView = tableView
view.addSubview(tableView)
tableView.autoPinEdge(toSuperviewEdge: .top)
tableView.autoPinEdge(toSuperviewEdge: .bottom)
tableView.autoPinEdge(toSuperviewSafeArea: .leading)
tableView.autoPinEdge(toSuperviewSafeArea: .trailing)
tableView.delegate = self
tableView.dataSource = self
let searchBar = OWSSearchBar()
self.searchBar = searchBar
searchBar.delegate = self
searchBar.sizeToFit()
tableView.tableHeaderView = searchBar
}
override open func viewDidLoad() {
super.viewDidLoad()
searchBar.placeholder = NSLocalizedString("INVITE_FRIENDS_PICKER_SEARCHBAR_PLACEHOLDER", comment: "Search")
// Auto size cells for dynamic type
tableView.estimatedRowHeight = 60.0
tableView.rowHeight = UITableView.automaticDimension
tableView.estimatedRowHeight = 60
tableView.allowsMultipleSelection = allowsMultipleSelection
tableView.separatorInset = UIEdgeInsets(top: 0, left: ContactCell.kSeparatorHInset, bottom: 0, right: 16)
registerContactCell()
initializeBarButtons()
reloadContacts()
updateSearchResults(searchText: "")
NotificationCenter.default.addObserver(self, selector: #selector(self.didChangePreferredContentSize), name: UIContentSizeCategory.didChangeNotification, object: nil)
}
public override func traitCollectionDidChange(_ previousTraitCollection: UITraitCollection?) {
super.traitCollectionDidChange(previousTraitCollection)
view.backgroundColor = Theme.backgroundColor
tableView.backgroundColor = Theme.backgroundColor
tableView.separatorColor = Theme.cellSeparatorColor
}
@objc
public func didChangePreferredContentSize() {
self.tableView.reloadData()
}
private func initializeBarButtons() {
let cancelButton = UIBarButtonItem(barButtonSystemItem: .cancel, target: self, action: #selector(onTouchCancelButton))
self.navigationItem.leftBarButtonItem = cancelButton
if allowsMultipleSelection {
let doneButton = UIBarButtonItem(barButtonSystemItem: .done, target: self, action: #selector(onTouchDoneButton))
self.navigationItem.rightBarButtonItem = doneButton
}
}
private func registerContactCell() {
tableView.register(ContactCell.self, forCellReuseIdentifier: contactCellReuseIdentifier)
}
// MARK: - Contact Operations
private func reloadContacts() {
getContacts( onError: { error in
Logger.error("failed to reload contacts with error:\(error)")
})
}
private func getContacts(onError errorHandler: @escaping (_ error: Error) -> Void) {
switch CNContactStore.authorizationStatus(for: CNEntityType.contacts) {
case CNAuthorizationStatus.denied, CNAuthorizationStatus.restricted:
let title = NSLocalizedString("INVITE_FLOW_REQUIRES_CONTACT_ACCESS_TITLE", comment: "Alert title when contacts disabled while trying to invite contacts to signal")
let body = NSLocalizedString("INVITE_FLOW_REQUIRES_CONTACT_ACCESS_BODY", comment: "Alert body when contacts disabled while trying to invite contacts to signal")
let alert = UIAlertController(title: title, message: body, preferredStyle: .alert)
let dismissText = CommonStrings.cancelButton
let cancelAction = UIAlertAction(title: dismissText, style: .cancel, handler: { _ in
let error = NSError(domain: "contactsPickerErrorDomain", code: 1, userInfo: [NSLocalizedDescriptionKey: "No Contacts Access"])
self.contactsPickerDelegate?.contactsPicker(self, contactFetchDidFail: error)
errorHandler(error)
})
alert.addAction(cancelAction)
let settingsText = CommonStrings.openSettingsButton
let openSettingsAction = UIAlertAction(title: settingsText, style: .default, handler: { (_) in
UIApplication.shared.openSystemSettings()
})
alert.addAction(openSettingsAction)
self.presentAlert(alert)
case CNAuthorizationStatus.notDetermined:
//This case means the user is prompted for the first time for allowing contacts
contactStore.requestAccess(for: CNEntityType.contacts) { (granted, error) -> Void in
//At this point an alert is provided to the user to provide access to contacts. This will get invoked if a user responds to the alert
if granted {
self.getContacts(onError: errorHandler)
} else {
errorHandler(error!)
}
}
case CNAuthorizationStatus.authorized:
//Authorization granted by user for this app.
var contacts = [CNContact]()
do {
let contactFetchRequest = CNContactFetchRequest(keysToFetch: allowedContactKeys)
contactFetchRequest.sortOrder = .userDefault
try contactStore.enumerateContacts(with: contactFetchRequest) { (contact, _) -> Void in
contacts.append(contact)
}
self.sections = collatedContacts(contacts)
} catch let error as NSError {
Logger.error("Failed to fetch contacts with error:\(error)")
}
@unknown default:
errorHandler(OWSAssertionError("Unexpected enum value"))
break
}
}
func collatedContacts(_ contacts: [CNContact]) -> [[CNContact]] {
let selector: Selector = #selector(getter: CNContact.nameForCollating)
var collated = Array(repeating: [CNContact](), count: collation.sectionTitles.count)
for contact in contacts {
let sectionNumber = collation.section(for: contact, collationStringSelector: selector)
collated[sectionNumber].append(contact)
}
return collated
}
// MARK: - Table View DataSource
open func numberOfSections(in tableView: UITableView) -> Int {
return self.collation.sectionTitles.count
}
open func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
let dataSource = filteredSections
guard section < dataSource.count else {
return 0
}
return dataSource[section].count
}
// MARK: - Table View Delegates
open func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
guard let cell = tableView.dequeueReusableCell(withIdentifier: contactCellReuseIdentifier, for: indexPath) as? ContactCell else {
owsFailDebug("cell had unexpected type")
return UITableViewCell()
}
let dataSource = filteredSections
let cnContact = dataSource[indexPath.section][indexPath.row]
let contact = Contact(systemContact: cnContact)
cell.configure(contact: contact, subtitleType: subtitleCellType, showsWhenSelected: self.allowsMultipleSelection, contactsManager: self.contactsManager)
let isSelected = selectedContacts.contains(where: { $0.uniqueId == contact.uniqueId })
cell.isSelected = isSelected
// Make sure we preserve selection across tableView.reloadData which happens when toggling between
// search controller
if (isSelected) {
self.tableView.selectRow(at: indexPath, animated: false, scrollPosition: .none)
} else {
self.tableView.deselectRow(at: indexPath, animated: false)
}
return cell
}
open func tableView(_ tableView: UITableView, didDeselectRowAt indexPath: IndexPath) {
let cell = tableView.cellForRow(at: indexPath) as! ContactCell
let deselectedContact = cell.contact!
selectedContacts = selectedContacts.filter {
return $0.uniqueId != deselectedContact.uniqueId
}
}
open func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
Logger.verbose("")
let cell = tableView.cellForRow(at: indexPath) as! ContactCell
let selectedContact = cell.contact!
guard (contactsPickerDelegate == nil || contactsPickerDelegate!.contactsPicker(self, shouldSelectContact: selectedContact)) else {
self.tableView.deselectRow(at: indexPath, animated: false)
return
}
selectedContacts.append(selectedContact)
if !allowsMultipleSelection {
// Single selection code
self.contactsPickerDelegate?.contactsPicker(self, didSelectContact: selectedContact)
}
}
open func tableView(_ tableView: UITableView, sectionForSectionIndexTitle title: String, at index: Int) -> Int {
return collation.section(forSectionIndexTitle: index)
}
open func sectionIndexTitles(for tableView: UITableView) -> [String]? {
return collation.sectionIndexTitles
}
open func tableView(_ tableView: UITableView, titleForHeaderInSection section: Int) -> String? {
let dataSource = filteredSections
guard section < dataSource.count else {
return nil
}
// Don't show empty sections
if dataSource[section].count > 0 {
guard section < collation.sectionTitles.count else {
return nil
}
return collation.sectionTitles[section]
} else {
return nil
}
}
public func scrollViewDidScroll(_ scrollView: UIScrollView) {
searchBar.resignFirstResponder()
}
// MARK: - Button Actions
@objc func onTouchCancelButton() {
contactsPickerDelegate?.contactsPickerDidCancel(self)
}
@objc func onTouchDoneButton() {
contactsPickerDelegate?.contactsPicker(self, didSelectMultipleContacts: selectedContacts)
}
// MARK: - Search Actions
open func searchBar(_ searchBar: UISearchBar, textDidChange searchText: String) {
updateSearchResults(searchText: searchText)
}
public func searchBarSearchButtonClicked(_ searchBar: UISearchBar) {
searchBar.resignFirstResponder()
}
open func updateSearchResults(searchText: String) {
let predicate: NSPredicate
if searchText.isEmpty {
filteredSections = sections
} else {
do {
predicate = CNContact.predicateForContacts(matchingName: searchText)
let filteredContacts = try contactStore.unifiedContacts(matching: predicate, keysToFetch: allowedContactKeys)
filteredSections = collatedContacts(filteredContacts)
} catch let error as NSError {
Logger.error("updating search results failed with error: \(error)")
}
}
self.tableView.reloadData()
}
}
let ContactSortOrder = computeSortOrder()
func computeSortOrder() -> CNContactSortOrder {
let comparator = CNContact.comparator(forNameSortOrder: .userDefault)
let contact0 = CNMutableContact()
contact0.givenName = "A"
contact0.familyName = "Z"
let contact1 = CNMutableContact()
contact1.givenName = "Z"
contact1.familyName = "A"
let result = comparator(contact0, contact1)
if result == .orderedAscending {
return .givenName
} else {
return .familyName
}
}
fileprivate extension CNContact {
/**
* Sorting Key used by collation
*/
@objc var nameForCollating: String {
get {
if self.familyName.isEmpty && self.givenName.isEmpty {
return self.emailAddresses.first?.value as String? ?? ""
}
let compositeName: String
if ContactSortOrder == .familyName {
compositeName = "\(self.familyName) \(self.givenName)"
} else {
compositeName = "\(self.givenName) \(self.familyName)"
}
return compositeName.trimmingCharacters(in: CharacterSet.whitespacesAndNewlines)
}
}
}
| gpl-3.0 | 4d7199c6364743895fc1c587133661c0 | 36.038186 | 179 | 0.666473 | 5.692957 | false | false | false | false |
citysite102/kapi-kaffeine | kapi-kaffeine/kapi-kaffeine/KPCheckBoxAnimationGenerator.swift | 1 | 7075 | //
// KPCheckBoxAnimationGenerator.swift
// kapi-kaffeine
//
// Created by YU CHONKAO on 2017/5/5.
// Copyright © 2017年 kapi-kaffeine. All rights reserved.
//
import UIKit
class KPCheckBoxAnimationGenerator {
//----------------------------
// MARK: - Properties
//----------------------------
// The duration of animations that are generated by the animation manager.
var animationDuration: TimeInterval = 0.2
// The frame rate for certian keyframe animations.
fileprivate var frameRate: CGFloat = 60.0
//----------------------------
// MARK: - Quick Animations
//----------------------------
final func quickAnimation(_ key: String, reverse: Bool) -> CABasicAnimation {
let animation = CABasicAnimation(keyPath: key)
// Set the start and end.
if !reverse {
animation.fromValue = 0.0
animation.toValue = 1.0
animation.timingFunction = CAMediaTimingFunction(name: kCAMediaTimingFunctionEaseIn)
} else {
animation.fromValue = 1.0
animation.toValue = 0.0
animation.beginTime = CACurrentMediaTime() + (animationDuration * 0.9)
animation.timingFunction = CAMediaTimingFunction(name: kCAMediaTimingFunctionEaseOut)
}
// Set animation properties.
animation.duration = animationDuration / 10.0
animation.isRemovedOnCompletion = false
animation.fillMode = kCAFillModeForwards
return animation
}
/**
Creates an animation that either quickly fades a layer in or out.
- note: Mainly used to smooth out the start and end of various animations.
- parameter reverse: The direction of the animation.
- returns: A `CABasicAnimation` that animates the opacity property.
*/
final func quickOpacityAnimation(_ reverse: Bool) -> CABasicAnimation {
return quickAnimation("opacity", reverse: reverse)
}
/**
Creates an animation that either quickly changes the line width of a layer from 0% to 100%.
- note: Mainly used to smooth out the start and end of various animations.
- parameter reverse: The direction of the animation.
- returns: A `CABasicAnimation` that animates the opacity property.
*/
final func quickLineWidthAnimation(_ width: CGFloat, reverse: Bool) -> CABasicAnimation {
let animation = quickAnimation("lineWidth", reverse: reverse)
// Set the start and end.
if !reverse {
animation.toValue = width
} else {
animation.fromValue = width
}
return animation
}
//----------------------------
// MARK: - Animation Component Generation
//----------------------------
final func animation(_ key: String, reverse: Bool) -> CABasicAnimation {
let animation = CABasicAnimation(keyPath: key)
// Set the start and end.
if !reverse {
animation.fromValue = 0.0
animation.toValue = 1.0
} else {
animation.fromValue = 1.0
animation.toValue = 0.0
}
// Set animation properties.
animation.duration = animationDuration
animation.isRemovedOnCompletion = false
animation.fillMode = kCAFillModeForwards
animation.timingFunction = CAMediaTimingFunction(name: kCAMediaTimingFunctionEaseInEaseOut)
return animation
}
/**
Creates an animation that animates the stroke property.
- parameter reverse: The direction of the animation.
- returns: A `CABasicAnimation` that animates the stroke property.
*/
final func strokeAnimation(_ reverse: Bool) -> CABasicAnimation {
return animation("strokeEnd", reverse: reverse)
}
/**
Creates an animation that animates the opacity property.
- parameter reverse: The direction of the animation.
- returns: A `CABasicAnimation` that animates the opacity property.
*/
final func opacityAnimation(_ reverse: Bool) -> CABasicAnimation {
return animation("opacity", reverse: reverse)
}
/**
Creates an animation that animates between two `UIBezierPath`s.
- parameter fromPath: The start path.
- parameter toPath: The end path.
- returns: A `CABasicAnimation` that animates a path between the `fromPath` and `toPath`.
*/
final func morphAnimation(_ fromPath: UIBezierPath?, toPath: UIBezierPath?) -> CABasicAnimation {
let animation = CABasicAnimation(keyPath: "path")
// Set the start and end.
animation.fromValue = fromPath?.cgPath
animation.toValue = toPath?.cgPath
// Set animation properties.
animation.duration = animationDuration
animation.timingFunction = CAMediaTimingFunction(name: kCAMediaTimingFunctionEaseInEaseOut)
animation.fillMode = kCAFillModeForwards
animation.isRemovedOnCompletion = false
return animation
}
/**
Creates an animation that animates between a filled an unfilled box.
- parameter numberOfBounces: The number of bounces in the animation.
- parameter amplitude: The distance of the bounce.
- parameter reverse: The direction of the animation.
- returns: A `CAKeyframeAnimation` that animates a change in fill.
*/
final func fillAnimation(_ numberOfBounces: Int, amplitude: CGFloat, reverse: Bool) -> CAKeyframeAnimation {
var values = [CATransform3D]()
var keyTimes = [Float]()
// Add the start scale
if !reverse {
values.append(CATransform3DMakeScale(0.0, 0.0, 0.0))
} else {
values.append(CATransform3DMakeScale(1.0, 1.0, 1.0))
}
keyTimes.append(0.0)
// Add the bounces.
if numberOfBounces > 0 {
for i in 1...numberOfBounces {
let scale = i % 2 == 1 ? (1.0 + (amplitude / CGFloat(i))) : (1.0 - (amplitude / CGFloat(i)))
let time = (Float(i) * 1.0) / Float(numberOfBounces + 1)
values.append(CATransform3DMakeScale(scale, scale, scale))
keyTimes.append(time)
}
}
// Add the end scale.
if !reverse {
values.append(CATransform3DMakeScale(1.0, 1.0, 1.0))
} else {
values.append(CATransform3DMakeScale(0.0001, 0.0001, 0.0001))
}
keyTimes.append(1.0)
// Create the animation.
let animation = CAKeyframeAnimation(keyPath: "transform")
animation.values = values.map({ NSValue(caTransform3D: $0) })
animation.keyTimes = keyTimes.map({ NSNumber(value: $0 as Float) })
animation.isRemovedOnCompletion = false
animation.fillMode = kCAFillModeForwards
animation.duration = animationDuration
animation.timingFunction = CAMediaTimingFunction(name: kCAMediaTimingFunctionEaseInEaseOut)
return animation
}
}
| mit | d2330aca6d8c61c285891c9bfafd86c7 | 37.021505 | 112 | 0.616516 | 5.223043 | false | false | false | false |
modocache/swift | benchmark/single-source/AngryPhonebook.swift | 7 | 1925 | //===--- AngryPhonebook.swift ---------------------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2016 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See http://swift.org/LICENSE.txt for license information
// See http://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
// This test is based on single-source/Phonebook, with
// to test uppercase and lowercase ASCII string fast paths.
import TestsUtils
import Foundation
var words = [
"James", "John", "Robert", "Michael", "William", "David", "Richard", "Joseph",
"Charles", "Thomas", "Christopher", "Daniel", "Matthew", "Donald", "Anthony",
"Paul", "Mark", "George", "Steven", "Kenneth", "Andrew", "Edward", "Brian",
"Joshua", "Kevin", "Ronald", "Timothy", "Jason", "Jeffrey", "Gary", "Ryan",
"Nicholas", "Eric", "Stephen", "Jacob", "Larry", "Frank", "Jonathan", "Scott",
"Justin", "Raymond", "Brandon", "Gregory", "Samuel", "Patrick", "Benjamin",
"Jack", "Dennis", "Jerry", "Alexander", "Tyler", "Douglas", "Henry", "Peter",
"Walter", "Aaron", "Jose", "Adam", "Harold", "Zachary", "Nathan", "Carl",
"Kyle", "Arthur", "Gerald", "Lawrence", "Roger", "Albert", "Keith", "Jeremy",
"Terry", "Joe", "Sean", "Willie", "Jesse", "Ralph", "Billy", "Austin", "Bruce",
"Christian", "Roy", "Bryan", "Eugene", "Louis", "Harry", "Wayne", "Ethan",
"Jordan", "Russell", "Alan", "Philip", "Randy", "Juan", "Howard", "Vincent",
"Bobby", "Dylan", "Johnny", "Phillip", "Craig"]
@inline(never)
public func run_AngryPhonebook(_ N: Int) {
// Permute the names.
for _ in 1...N {
for firstname in words {
for lastname in words {
_ = (firstname.uppercased(), lastname.lowercased())
}
}
}
}
| apache-2.0 | 66ecd521a7bc97a4b3879798870b79b1 | 43.767442 | 81 | 0.583896 | 3.279387 | false | true | false | false |
AlbertXYZ/HDCP | HDCP/HDCP/HDCoreDataManager.swift | 1 | 3485 | //
// CoreDataManager.swift
// HDCP
//
// Created by 徐琰璋 on 16/1/5.
// Copyright © 2016年 batonsoft. All rights reserved.
//
import Foundation
import CoreData
open class HDCoreDataManager: NSObject {
/**
* 单利模式
*/
open static let sharedInstance = HDCoreDataManager()
// class var shareInstance: HDCoreDataManager {
//
// struct Singleton {
// static var instance: HDCoreDataManager?
// static var token: dispatch_once_t = 0
// }
//
// dispatch_once(&Singleton.token) {
// Singleton.instance = HDCoreDataManager()
// }
//
// return Singleton.instance!
// }
/**
* 应用程序Docment目录的NSURL类型
*/
lazy var applicationDocumentsDirectory: URL = {
let urls = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask)
return urls[urls.count - 1]
}()
/**
* 被管理的对象模型 -> 加载数据模型
*/
lazy var managedObjectModel: NSManagedObjectModel = {
let modelURL = Bundle.main.url(forResource: "HDCP", withExtension: "momd")!
return NSManagedObjectModel(contentsOf: modelURL)!
}()
/**
* 持久化存储协调者 -> 连接数据库
*/
lazy var persistentStoreCoordinator: NSPersistentStoreCoordinator = {
let coordinator = NSPersistentStoreCoordinator(managedObjectModel: self.managedObjectModel)
let url = self.applicationDocumentsDirectory.appendingPathComponent("SingleViewCoreData.sqlite")
var failureReason = "There was an error creating or loading the application's saved data."
// 这里是添加的部分,名如其意,当我们需要自动版本迁移时,我们需要在addPersistentStoreWithType方法中设置如下options
let options = [NSInferMappingModelAutomaticallyOption: true, NSMigratePersistentStoresAutomaticallyOption: true]
do {
try coordinator.addPersistentStore(ofType: NSSQLiteStoreType, configurationName: nil, at: url, options: options)
} catch {
var dict = [String: AnyObject]()
dict[NSLocalizedDescriptionKey] = "Failed to initialize the application's saved data" as AnyObject?
dict[NSLocalizedFailureReasonErrorKey] = failureReason as AnyObject?
dict[NSUnderlyingErrorKey] = error as NSError
let wrappedError = NSError(domain: "YOUR_ERROR_DOMAIN", code: 9999, userInfo: dict)
NSLog("Unresolved error \(wrappedError), \(wrappedError.userInfo)")
abort()
}
return coordinator
}()
/**
* 被管理的对象上下文 -> 对数据进行添、删、改、查等
*/
lazy var managedObjectContext: NSManagedObjectContext = {
let coordinator = self.persistentStoreCoordinator
var managedObjectContext = NSManagedObjectContext(concurrencyType: .mainQueueConcurrencyType)
managedObjectContext.persistentStoreCoordinator = coordinator
return managedObjectContext
}()
// MARK: - Core Data Saving support
/**
* 保存数据
*/
func saveContext () {
if managedObjectContext.hasChanges {
do {
try managedObjectContext.save()
} catch {
let nserror = error as NSError
NSLog("Unresolved error \(nserror), \(nserror.userInfo)")
abort()
}
}
}
}
| mit | a4a73b09b2ceac0a3c77394988ada717 | 28.423423 | 124 | 0.636252 | 4.971081 | false | false | false | false |
ibm-bluemix-mobile-services/bms-samples-ios-bluelist | bluelist-swift/bluelist-swift/ListTableViewController.swift | 1 | 20185 | // Copyright 2014, 2015 IBM Corp. All Rights Reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
import UIKit
class ListTableViewController: UITableViewController, UITextFieldDelegate, CDTReplicatorDelegate{
let DATATYPE_FIELD = "@datatype"
let DATATYPE_VALUE = "TodoItem"
let NAME_FIELD = "name"
let PRIORITY_FIELD = "priority"
//Priority Images
var highImage = UIImage(named: "priorityHigh.png")
var mediumImage = UIImage(named: "priorityMedium.png")
var lowImage = UIImage(named: "priorityLow.png")
@IBOutlet var segmentFilter: UISegmentedControl!
@IBOutlet weak var settingsButton: UIBarButtonItem!
//Intialize some list items
var itemList: [CDTDocumentRevision] = []
var filteredListItems = [CDTDocumentRevision]()
var idTracker = 0
// Cloud sync properties
var dbName:String!
var datastore: CDTDatastore!
var datastoreManager:CDTDatastoreManager!
var remotedatastoreurl: NSURL!
var cloudantHttpInterceptor:CDTHTTPInterceptor!
var replicatorFactory: CDTReplicatorFactory!
var pullReplication: CDTPullReplication!
var pullReplicator: CDTReplicator!
var pushReplication: CDTPushReplication!
var pushReplicator: CDTReplicator!
var doingPullReplication: Bool!
//logger
let logger = IMFLogger(forName: "BlueList")
//MARK: - ViewController functions
override func viewDidLoad() {
super.viewDidLoad()
// Setting up the refresh control
settingsButton.enabled = false
self.refreshControl = UIRefreshControl()
self.refreshControl?.addTarget(self, action: Selector("handleRefreshAction") , forControlEvents: UIControlEvents.ValueChanged)
self.refreshControl?.beginRefreshing()
self.setupIMFDatabase()
//Logging
self.logger.logInfoWithMessages("this is a info test log in ListTableViewController:viewDidLoad")
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
//MARK: - Data Management
func setupIMFDatabase() {
var error:NSError?
var encryptionEnabled:Bool = false
let configurationPath = NSBundle.mainBundle().pathForResource("bluelist", ofType: "plist")
let configuration = NSDictionary(contentsOfFile: configurationPath!)
let encryptionPassword = configuration?["encryptionPassword"] as! String
if(encryptionPassword.isEmpty){
encryptionEnabled = false
}
else {
encryptionEnabled = true
self.dbName = self.dbName + "secure"
}
// Create DatastoreManager
let fileManager = NSFileManager()
let documentDir = fileManager.URLsForDirectory(.DocumentDirectory, inDomains: .UserDomainMask).last
let storeUrl = documentDir?.URLByAppendingPathComponent("bluelistdir", isDirectory: true)
let exists = fileManager.fileExistsAtPath((storeUrl?.path)!)
if(!exists){
do{
try fileManager.createDirectoryAtURL(storeUrl!, withIntermediateDirectories: true, attributes: nil)
}catch let error as NSError{
let alert:UIAlertView = UIAlertView(title: "Error", message: "Could not create CDTDatastoreManager with directory \(storeUrl?.absoluteString). Error: \(error)", delegate:self , cancelButtonTitle: "Okay")
alert.show()
return
}
}
do{
self.datastoreManager = try CDTDatastoreManager(directory: storeUrl?.path)
}catch let error as NSError{
let alert:UIAlertView = UIAlertView(title: "Error", message: "Could not create CDTDatastoreManager with directory \(storeUrl?.absoluteString). Error: \(error)", delegate:self , cancelButtonTitle: "Okay")
alert.show()
return
}
//CDTEncryptionKeyProvider used for encrypting local datastore
var keyProvider:CDTEncryptionKeyProvider!
//create a local data store. Encrypt the local store if the setting is enabled
if(encryptionEnabled){
//Initialize the key provider
keyProvider = CDTEncryptionKeychainProvider(password: encryptionPassword, forIdentifier:"bluelist")
NSLog("%@", "Attempting to create an encrypted local data store")
do {
//Initialize the encrypted store
self.datastore = try self.datastoreManager.datastoreNamed(self.dbName, withEncryptionKeyProvider: keyProvider)
} catch let error1 as NSError {
error = error1
self.datastore = nil
}
}
else{
NSLog("%@","Attempting to create a local data store")
do {
self.datastore = try self.datastoreManager.datastoreNamed(self.dbName)
} catch let error1 as NSError {
error = error1
self.datastore = nil
}
}
if ((error) != nil) {
NSLog("%@", "Could not create local data store with name " + dbName)
if(encryptionEnabled){
let alert:UIAlertView = UIAlertView(title: "Error", message: "Could not create an encrypted local store with credentials provided. Check the encryptionPassword in the bluelist.plist file.", delegate:self , cancelButtonTitle: "Okay")
alert.show()
return
}
}
else{
NSLog("%@", "Local data store create successfully: " + dbName)
}
// Setup required indexes for Query
self.datastore.ensureIndexed([DATATYPE_FIELD], withName: "datatypeindex")
if (!IBM_SYNC_ENABLE) {
self.listItems({ () -> Void in
self.logger.logDebugWithMessages("Done refreshing we are not using cloud")
self.refreshControl?.endRefreshing()
})
return
}
self.replicatorFactory = CDTReplicatorFactory(datastoreManager: self.datastoreManager)
self.pullReplication = CDTPullReplication(source: self.remotedatastoreurl, target: self.datastore)
self.pullReplication.addInterceptor(self.cloudantHttpInterceptor)
self.pushReplication = CDTPushReplication(source: self.datastore, target: self.remotedatastoreurl)
self.pushReplication.addInterceptor(self.cloudantHttpInterceptor)
self.pullItems()
}
func listItems(cb:()->Void) {
logger.logDebugWithMessages("listItems called")
let resultSet:CDTQResultSet = self.datastore.find([DATATYPE_FIELD : DATATYPE_VALUE])
var results:[CDTDocumentRevision] = Array()
resultSet.enumerateObjectsUsingBlock { (rev, idx, stop) -> Void in
results.append(rev)
}
self.itemList = results
self.reloadLocalTableData()
cb()
}
func createItem(item: CDTMutableDocumentRevision) {
do{
try self.datastore.createDocumentFromRevision(item)
self.listItems({ () -> Void in
self.logger.logInfoWithMessages("Item succesfuly created")
})
}catch let error as NSError{
self.logger.logErrorWithMessages("createItem failed with error \(error.description)")
}
}
func updateItem(item: CDTMutableDocumentRevision) {
do{
try self.datastore.updateDocumentFromRevision(item)
self.listItems({ () -> Void in
self.logger.logInfoWithMessages("Item successfully updated")
})
}catch let error as NSError{
self.logger.logErrorWithMessages("updateItem failed with error \(error.description)")
}
}
func deleteItem(item: CDTDocumentRevision) {
do{
try self.datastore.deleteDocumentFromRevision(item)
self.listItems({ () -> Void in
self.logger.logInfoWithMessages("Item successfully deleted")
})
}catch let error as NSError{
self.logger.logErrorWithMessages("deleteItem failed with error \(error)")
}
}
// MARK: - Cloud Sync
func pullItems() {
var error:NSError?
do {
self.pullReplicator = try self.replicatorFactory.oneWay(self.pullReplication)
} catch let error1 as NSError {
error = error1
self.pullReplicator = nil
}
if(error != nil){
self.logger.logErrorWithMessages("Error creating oneWay pullReplicator \(error)")
}
self.pullReplicator.delegate = self
self.doingPullReplication = true
self.refreshControl?.attributedTitle = NSAttributedString(string: "Pull Items from Cloudant")
error = nil
print("Replicating data with NoSQL Database on the cloud")
do {
try self.pullReplicator.start()
} catch let error1 as NSError {
error = error1
}
if(error != nil){
self.logger.logErrorWithMessages("Error starting pullReplicator \(error)")
}
}
func pushItems() {
var error:NSError?
do {
self.pushReplicator = try self.replicatorFactory.oneWay(self.pushReplication)
} catch let error1 as NSError {
error = error1
self.pushReplicator = nil
}
if(error != nil){
self.logger.logErrorWithMessages("Error creating oneWay pullReplicator \(error)")
}
self.pushReplicator.delegate = self
self.doingPullReplication = false
//update UI in main thread
dispatch_async(dispatch_get_main_queue()) {
self.refreshControl?.attributedTitle = NSAttributedString(string: "Pushing Items to Cloudant")
}
error = nil
do {
try self.pushReplicator.start()
} catch let error1 as NSError {
error = error1
}
if(error != nil){
self.logger.logErrorWithMessages("Error starting pushReplicator \(error)")
}
}
// MARK: - CDTReplicator delegate methods
/**
* Called when the replicator changes state.
*/
func replicatorDidChangeState(replicator: CDTReplicator!) {
self.logger.logInfoWithMessages("replicatorDidChangeState \(CDTReplicator.stringForReplicatorState(replicator.state))")
}
/**
* Called whenever the replicator changes progress
*/
func replicatorDidChangeProgress(replicator: CDTReplicator!) {
self.logger.logInfoWithMessages("replicatorDidChangeProgress \(CDTReplicator.stringForReplicatorState(replicator.state))")
}
/**
* Called when a state transition to COMPLETE or STOPPED is
* completed.
*/
func replicatorDidComplete(replicator: CDTReplicator!) {
self.logger.logInfoWithMessages("replicatorDidComplete \(CDTReplicator.stringForReplicatorState(replicator.state))")
if self.doingPullReplication! {
//done doing pull, lets start push
self.pushItems()
} else {
//doing push, push is done read items from local data store and end the refresh UI
self.listItems({ () -> Void in
self.logger.logDebugWithMessages("Done refreshing table after replication")
//update UI in main thread
dispatch_async(dispatch_get_main_queue()) {
self.refreshControl?.attributedTitle = NSAttributedString(string: " ")
self.refreshControl?.endRefreshing()
self.settingsButton.enabled = true
}
})
}
}
/**
* Called when a state transition to ERROR is completed.
*/
func replicatorDidError(replicator: CDTReplicator!, info: NSError!) {
self.refreshControl?.attributedTitle = NSAttributedString(string: "Error replicating with Cloudant")
self.logger.logErrorWithMessages("replicatorDidError \(info)")
self.listItems({ () -> Void in
print("")
self.refreshControl?.endRefreshing()
})
}
// MARK: - Table view data source
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
// Return the number of sections.
return 2
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
// Return the number of rows in the section.
if section == 0 {
return self.filteredListItems.count
} else {
return 1
}
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
if indexPath.section == 0 {
let cell = tableView.dequeueReusableCellWithIdentifier("ItemCell", forIndexPath: indexPath)
let item = self.filteredListItems[indexPath.row] as CDTDocumentRevision
// Configure the cell
cell.imageView?.image = self.getPriorityImage(item.body()[PRIORITY_FIELD]!.integerValue)
let textField = cell.contentView.viewWithTag(3) as! UITextField
textField.hidden = false
textField.text = item.body()[NAME_FIELD]! as? String
cell.contentView.tag = 0
return cell
} else {
let cell = tableView.dequeueReusableCellWithIdentifier("AddCell", forIndexPath: indexPath)
cell.contentView.tag = 1
return cell
}
}
override func tableView(tableView: UITableView, canEditRowAtIndexPath indexPath: NSIndexPath) -> Bool {
return indexPath.section == 0
}
// Override to support editing the table view.
override func tableView(tableView: UITableView, commitEditingStyle editingStyle: UITableViewCellEditingStyle, forRowAtIndexPath indexPath: NSIndexPath) {
if editingStyle == .Delete {
//Delete the row from the data source
self.deleteItem(self.filteredListItems[indexPath.row])
self.filteredListItems.removeAtIndex(indexPath.row)
tableView.deleteRowsAtIndexPaths([indexPath], withRowAnimation: .Fade)
}
}
override func tableView(tableView: UITableView, didSelectRowAtIndexPath indexPath: NSIndexPath) {
if indexPath.section == 0 {
let cell = tableView.cellForRowAtIndexPath(indexPath)
self.changePriorityForCell(cell!)
}
}
// MARK: - Handle Priority Indicators
func changePriorityForCell(cell: UITableViewCell){
let indexPath = self.tableView.indexPathForCell(cell)
let item = (self.filteredListItems[indexPath!.row] as CDTDocumentRevision).mutableCopy()
let selectedPriority = item.body()[PRIORITY_FIELD]!.integerValue
let newPriority = self.getNextPriority(selectedPriority)
item.body()[PRIORITY_FIELD] = NSNumber(integer: newPriority)
//update UI in main thread
dispatch_async(dispatch_get_main_queue()) {
cell.imageView?.image = self.getPriorityImage(newPriority)
self.updateItem(item)
}
}
func getNextPriority(currentPriority: Int) -> Int {
var newPriority: Int
switch currentPriority {
case 2:
newPriority = 0
case 1:
newPriority = 2
default:
newPriority = 1
}
return newPriority
}
func getPriorityImage (priority: Int) -> UIImage {
var resultImage : UIImage
switch priority {
case 2:
resultImage = self.highImage!
case 1:
resultImage = self.mediumImage!
default:
resultImage = self.lowImage!
}
return resultImage
}
func getPriorityForString(priorityString: String = "") -> Int {
var priority : Int
switch priorityString {
case "All":
priority = 0
case "Medium":
priority = 1
case "High":
priority = 2
default:
priority = 0
}
return priority
}
// MARK: Custom filtering
func filterContentForPriority(scope: String = "All") {
let priority = self.getPriorityForString(scope)
if(priority == 1 || priority == 2){
self.filteredListItems = self.itemList.filter({ (item: CDTDocumentRevision) -> Bool in
if item.body()[PRIORITY_FIELD]!.integerValue == priority {
return true
} else {
return false
}
})
} else {
//don't filter select all
self.filteredListItems.removeAll(keepCapacity: false)
self.filteredListItems = self.itemList
}
}
@IBAction func filterTable(sender: UISegmentedControl){
self.reloadLocalTableData()
}
// MARK: textField delegate functions
func textFieldShouldReturn(textField: UITextField) -> Bool {
self.handleTextFields(textField)
return true
}
func handleTextFields(textField: UITextField) {
if textField.superview?.tag == 1 && textField.text!.isEmpty == false {
self.addItemFromtextField(textField)
} else {
self.updateItemFromtextField(textField)
}
textField.resignFirstResponder()
}
func updateItemFromtextField(textField: UITextField) {
let cell = textField.superview?.superview as! UITableViewCell
let indexPath = self.tableView.indexPathForCell(cell)
let item = self.filteredListItems[indexPath!.row].mutableCopy()
item.body()[NAME_FIELD] = textField.text!
self.updateItem(item)
}
func addItemFromtextField(textField: UITextField) {
let priority = self.getPriorityForString(self.segmentFilter.titleForSegmentAtIndex(self.segmentFilter.selectedSegmentIndex)!)
let name = textField.text
let item = CDTMutableDocumentRevision()
item.setBody([DATATYPE_FIELD : DATATYPE_VALUE, NAME_FIELD : name!, PRIORITY_FIELD : NSNumber(integer: priority)])
self.createItem(item)
textField.text = ""
}
func reloadLocalTableData() {
self.filterContentForPriority(self.segmentFilter.titleForSegmentAtIndex(self.segmentFilter.selectedSegmentIndex)!)
self.filteredListItems.sortInPlace { (item1: CDTDocumentRevision, item2: CDTDocumentRevision) -> Bool in
return item1.body()[NAME_FIELD]!.localizedCaseInsensitiveCompare(item2.body()[NAME_FIELD]! as! String) == .OrderedAscending
}
if self.tableView != nil {
dispatch_async(dispatch_get_main_queue()) {
self.tableView.reloadData()
}
}
}
func handleRefreshAction()
{
if (IBM_SYNC_ENABLE) {
self.pullItems()
} else {
self.listItems({ () -> Void in
self.logger.logDebugWithMessages("Done refreshing table in handleRefreshAction")
self.refreshControl?.endRefreshing()
})
}
}
}
| apache-2.0 | 64f85f52164a8f129b738f7edb2fe92d | 35.109123 | 248 | 0.616844 | 5.210377 | false | false | false | false |
SJTBA/Scrape | Sources/XMLParserOptions.swift | 1 | 6838 | //
// XMLParserOptions.swift
// Scrape
//
// Created by Sergej Jaskiewicz on 10.09.16.
//
//
import CLibxml2
/// This is the set of XML parser options that can be passed down
/// to the `XMLDocument` initializers.
public struct XMLParserOptions: OptionSet {
/// The corresponding value of the raw type.
///
/// A new instance initialized with `rawValue` will be equivalent to this
/// instance. For example:
///
/// ```swift
/// enum PaperSize: String {
/// case A4, A5, Letter, Legal
/// }
///
/// let selectedSize = PaperSize.Letter
/// print(selectedSize.rawValue)
/// // Prints "Letter"
///
/// print(selectedSize == PaperSize(rawValue: selectedSize.rawValue)!)
/// // Prints "true"
/// ```
public var rawValue: UInt = 0
/// Creates a new option set from the given raw value.
///
/// This initializer always succeeds, even if the value passed as `rawValue`
/// exceeds the static properties declared as part of the option set. This
/// example creates an instance of `ShippingOptions` with a raw value beyond
/// the highest element, with a bit mask that effectively contains all the
/// declared static members.
///
/// ```swift
/// let extraOptions = ShippingOptions(rawValue: 255)
/// print(extraOptions.isStrictSuperset(of: .all))
/// // Prints "true"
/// ```
///
/// - Parameter rawValue: The raw value of the option set to create. Each bit
/// of `rawValue` potentially represents an element of the option set,
/// though raw values may include bits that are not defined as distinct
/// values of the `OptionSet` type.
public init(rawValue: UInt) {
self.rawValue = rawValue
}
private init(_ opt: xmlParserOption) {
rawValue = UInt(opt.rawValue)
}
/// Default parsing option.
///
/// Equivalent to `[.recoverOnErrors, .noError, .noWarning]`.
public static let `default`: XMLParserOptions = [.recoverOnErrors, .noError, .noWarning]
/// Creates a new instance of `XMLParserOptions` initialized with zero raw value.
public static var allZeros: XMLParserOptions {
return .init(rawValue: 0)
}
/// Strict parsing. Equivalent to `.allZeros`.
public static let strict = XMLParserOptions.allZeros
/// Recover on errors.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_RECOVER`
public static let recoverOnErrors = XMLParserOptions(XML_PARSE_RECOVER)
/// Substitute entities.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_NOENT`
public static let substituteEntities = XMLParserOptions(XML_PARSE_NOENT)
/// Load the external subset.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_DTDLOAD`
public static let loadExternalSubset = XMLParserOptions(XML_PARSE_DTDLOAD)
/// Default DTD attributes.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_DTDATTR`
public static let defaultDTDAttributes = XMLParserOptions(XML_PARSE_DTDATTR)
/// Validate with the DTD.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_DTDVALID`
public static let validateWithDTD = XMLParserOptions(XML_PARSE_DTDVALID)
/// Suppress error reports.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_NOERROR`
public static let noError = XMLParserOptions(XML_PARSE_NOERROR)
/// Suppress warning reports.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_NOWARNING`
public static let noWarning = XMLParserOptions(XML_PARSE_NOWARNING)
/// Pedantic error reporting.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_PEDANTIC`
public static let pedantic = XMLParserOptions(XML_PARSE_PEDANTIC)
/// Remove blank nodes.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_NOBLANKS`
public static let noBlankNodes = XMLParserOptions(XML_PARSE_NOBLANKS)
/// Use the SAX1 interface internally.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_SAX1`
public static let useSAX1 = XMLParserOptions(XML_PARSE_SAX1)
/// Implement XInclude substitition.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_XINCLUDE`
public static let xInclude = XMLParserOptions(XML_PARSE_XINCLUDE)
/// Forbid network access.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_NONET`
public static let forbidNetworkAccess = XMLParserOptions(XML_PARSE_NONET)
/// Do not reuse the context dictionary.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_NODICT`
public static let noContextDictionary = XMLParserOptions(XML_PARSE_NODICT)
/// Remove redundant namespaces declarations.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_NSCLEAN`
public static let cleanNamespace = XMLParserOptions(XML_PARSE_NSCLEAN)
/// Merge CDATA as text nodes.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_NOCDATA`
public static let noCDATA = XMLParserOptions(XML_PARSE_NOCDATA)
/// Do not generate XINCLUDE START/END nodes.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_NOXINCNODE`
public static let noXIncludeNodes = XMLParserOptions(XML_PARSE_NOXINCNODE)
/// Compact small text nodes; no modification of the tree allowed afterwards
/// (will possibly crash if you try to modify the tree).
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_COMPACT`
public static let compact = XMLParserOptions(XML_PARSE_COMPACT)
/// Parse using XML-1.0 before update 5.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_OLD10`
public static let old10 = XMLParserOptions(XML_PARSE_OLD10)
/// Do not fixup XINCLUDE xml:base uris.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_NOBASEFIX`
public static let noBasefix = XMLParserOptions(XML_PARSE_NOBASEFIX)
/// Relax any hardcoded limit from the parser.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_HUGE`
public static let huge = XMLParserOptions(XML_PARSE_HUGE)
/// Parse using SAX2 interface before 2.7.0.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_OLDSAX`
public static let oldSAX = XMLParserOptions(XML_PARSE_OLDSAX)
/// Ignore internal document encoding hint.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_IGNORE_ENC`
public static let ignoreEncoding = XMLParserOptions(XML_PARSE_IGNORE_ENC)
/// Store big lines numbers in text PSVI field.
///
/// **Corresponding libxml2 enum case**: `XML_PARSE_BIG_LINES`
public static let bigLines = XMLParserOptions(XML_PARSE_BIG_LINES)
}
| mit | 1edcb5bb951850efb0fb3698e379cbbe | 35.179894 | 92 | 0.654431 | 4.197667 | false | false | false | false |
SwiftKit/Reactant | Tests/Configuration/ConfigurationTest.swift | 2 | 2138 | //
// ConfigurationTest.swift
// Reactant
//
// Created by Filip Dolnik on 26.02.17.
// Copyright © 2017 Brightify. All rights reserved.
//
import Quick
import Nimble
import Reactant
private typealias Configuration = Reactant.Configuration
class ConfigurationTest: QuickSpec {
override func spec() {
describe("Configuration") {
describe("init") {
it("copies configurations preserving last value") {
let configuration1 = Configuration()
configuration1.set(Properties.int, to: 1)
let configuration2 = Configuration()
configuration2.set(Properties.int, to: 2)
let configuration = Configuration(copy: configuration1, configuration2)
expect(configuration.get(valueFor: Properties.int)) == 2
expect(configuration.get(valueFor: Properties.string)) == ""
}
}
describe("get/set") {
it("gets and sets value for property") {
let configuration = Configuration()
configuration.set(Properties.int, to: 1)
configuration.set(Properties.string, to: "A")
expect(configuration.get(valueFor: Properties.int)) == 1
expect(configuration.get(valueFor: Properties.string)) == "A"
}
}
describe("get") {
it("returns Property.defaultValue if not set") {
let configuration = Configuration()
expect(configuration.get(valueFor: Properties.int)) == 0
expect(configuration.get(valueFor: Properties.string)) == ""
}
}
}
}
func apiTest() {
_ = Configuration.global
}
private struct Properties {
static let int = Property<Int>(defaultValue: 0)
static let string = Property<String>(defaultValue: "")
}
}
| mit | 24d39ada6851d0e0eda0d8854d2b7665 | 32.920635 | 91 | 0.518952 | 5.536269 | false | true | false | false |
CodeMozart/MHzSinaWeibo-Swift- | SinaWeibo(stroyboard)/SinaWeibo/Classes/Main/OAuthViewController.swift | 1 | 5256 | //
// OAuthViewController.swift
// SinaWeibo
//
// Created by Minghe on 11/10/15.
// Copyright © 2015 Stanford swift. All rights reserved.
//
import UIKit
import SVProgressHUD
class OAuthViewController: UIViewController {
@IBOutlet weak var webView: UIWebView!
override func viewDidLoad() {
super.viewDidLoad()
// 1.加载登陆界面
loadPage()
}
/// 加载登陆界面
private func loadPage() {
let str = "https://api.weibo.com/oauth2/authorize?client_id=\(MHz_App_Key)&redirect_uri=\(MHz_Redirect_URI)"
guard let url = NSURL(string: str) else {
return
}
let request = NSURLRequest(URL: url)
webView.loadRequest(request)
}
// MARK: - 内部控制方法 ------------------------------
@IBAction func closeBtnClick(sender: AnyObject) {
dismissViewControllerAnimated(true, completion: nil)
}
/// 自定义填充账号密码
@IBAction func fillBtnClick(sender: AnyObject) {
let js = "document.getElementById('userId').value = '[email protected]';"+"document.getElementById('passwd').value = '19910115qxmhz';"
webView.stringByEvaluatingJavaScriptFromString(js)
}
}
extension OAuthViewController: UIWebViewDelegate
{
func webViewDidStartLoad(webView: UIWebView) {
// 显示提醒,提醒用户正在加载登陆界面
SVProgressHUD.showInfoWithStatus("正在加载……", maskType: .Black)
}
func webViewDidFinishLoad(webView: UIWebView) {
// 关闭提醒
SVProgressHUD.dismiss()
}
// webView每次请求一个新的地址都会调用该方法,返回true代表允许访问,返回false代表不允许访问
/*
如果是新浪的界面:https://api.weibo.com/
授权成功:http://www.520it.com/?code=87ed79fd39c8cfe5c55a4b4cf4e2c0d5
授权失败:http://www.520it.com/?error_uri=%2Foauth2%2Fauthorize&error=access_denied&error_description=user%20denied%20your%20request.&error_code=21330
*/
func webView(webView: UIWebView, shouldStartLoadWithRequest request: NSURLRequest, navigationType: UIWebViewNavigationType) -> Bool {
// 1.判断是否是授权回调地址,如果不是就允许继续跳转
guard let urlStr = request.URL?.absoluteString where urlStr.hasPrefix(MHz_Redirect_URI) else
{
MHzLog("api.weibo.com")
return true
}
// 2.判断是否授权成功
let code = "code="
guard urlStr.containsString(code) else
{
// 授权失败
MHzLog("error_uri")
return false
}
// 3.授权成功
// .query获取的是?号后面的字符串
if let temp = request.URL?.query
{
// 截取code=后面的字符串
let codeStr = temp.substringFromIndex(code.endIndex)
// 利用RequestToken换取AccessToken
loadAccessToken(codeStr)
}
return false
}
/// 根据RequestToken换取AccessToken
private func loadAccessToken(codeStr: String) {
NetworkTools.shareInstance.loadAccessToken(codeStr) { (dict, error) -> () in
// 0.进行安全校验
if let _ = error {
SVProgressHUD.showErrorWithStatus("换取AccessToken错误", maskType: SVProgressHUDMaskType.Black)
return
}
guard let info = dict else {
SVProgressHUD.showErrorWithStatus("服务器返回的数据为空", maskType: SVProgressHUDMaskType.Black)
return
}
// 1.将授权信息转换为模型
let userAccount = UserAccount(dict: info)
// 2.根据授权信息获取用户信息
self.loadUserInfo(userAccount)
}
}
/// 获取用户信息(姓名&头像)
private func loadUserInfo(account: UserAccount) {
NetworkTools.shareInstance.loadUserInfo(account) { (dict, error) -> () in
// 0.进行安全校验
if let _ = error {
SVProgressHUD.showErrorWithStatus("换取AccessToken错误", maskType: SVProgressHUDMaskType.Black)
return
}
guard let info = dict else {
SVProgressHUD.showErrorWithStatus("服务器返回的数据为空", maskType: SVProgressHUDMaskType.Black)
return
}
// 1.获取到的用户信息
account.screen_name = info["screen_name"] as? String
account.avatar_large = info["avatar_large"] as? String
// 2.保存用户授权信息
account.saveAccount()
// 3.切换到欢迎页面
// 发送通知,通知AppDelegate切换根控制器
NSNotificationCenter.defaultCenter().postNotificationName(MHzRootViewControllerChanged, object: self, userInfo: ["message": true])
}
}
}
| mit | b80841c9966b7397ef155acaf9946c8d | 27.065868 | 153 | 0.569234 | 4.677645 | false | false | false | false |
raymondshadow/SwiftDemo | SwiftApp/BaseTest/BaseTest/collection/GeneratorDemo.swift | 1 | 461 | //
// GeneratorDemo.swift
// BaseTest
//
// Created by wuyp on 2017/6/20.
// Copyright © 2017年 raymond. All rights reserved.
//
import Foundation
class GeneratorObject: Sequence, IteratorProtocol {
var count: Int = 0
func next() -> Int? {
if count == 0 {
return nil
} else {
defer {count -= 1}
return count
}
}
lazy var name: String = {
return "jack"
}()
}
| apache-2.0 | 4876a4bcce022d37b8855c0899b7e587 | 15.962963 | 51 | 0.521834 | 3.91453 | false | false | false | false |
jamalping/XPUtil | XPUtil/Foundation/Dictionary+Extension.swift | 1 | 2314 | //
// Dictionary+Extension.swift
// XPUtilExample
//
// Created by xyj on 2017/11/2.
// Copyright © 2017年 xyj. All rights reserved.
//
import Foundation
public extension Dictionary {
/// 获取一个随机值
func random() -> Value? {
return Array(values).random
}
/// 是否存在该key
func has(_ key: Key) -> Bool {
return index(forKey: key) != nil
}
/// 将不存在的键值对加进来。已存在的进行值得更新
func union(_ dictionaries: Dictionary...) -> Dictionary {
var result = self
dictionaries.forEach { (dictionary) -> Void in
dictionary.forEach { (key, value) -> Void in
result[key] = value
}
}
return result
}
///
func difference(_ dictionaries: [Key: Value]...) -> [Key: Value] {
var result = self
for dictionary in dictionaries {
for (key, value) in dictionary {
if result.has(key) && result[key] == value as! _OptionalNilComparisonType {
result.removeValue(forKey: key)
}
}
}
return result
}
/// 转换字典键值对的类型
func map<K, V>(_ map: (Key, Value) -> (K, V)) -> [K: V] {
var mapped: [K: V] = [:]
forEach {
let (_key, _value) = map($0, $1)
mapped[_key] = _value
}
return mapped
}
/// 将JSONString转换成Dictionary
static func constructFromJSON (json: String) -> Dictionary? {
if let data = (try? JSONSerialization.jsonObject(
with: json.data(using: String.Encoding.utf8,
allowLossyConversion: true)!,
options: JSONSerialization.ReadingOptions.mutableContainers)) as? Dictionary {
return data
} else {
return nil
}
}
/// 转换成JSON
func formatJSON() -> String? {
if let jsonData = try? JSONSerialization.data(withJSONObject: self, options: JSONSerialization.WritingOptions()) {
let jsonStr = String(data: jsonData, encoding: String.Encoding(rawValue: String.Encoding.utf8.rawValue))
return String(jsonStr ?? "")
}
return nil
}
}
| mit | a686920a34937818f18df323df869391 | 27.320513 | 122 | 0.532368 | 4.435743 | false | false | false | false |
chelseafarley/SwiftTextFields | SwiftTextFieldsSample/SwiftTextFields/SwiftTextFields/NumericTextField.swift | 1 | 2698 | //
// NumericTextField.swift
// SwiftTextFieldsSample
//
// Created by Chelsea Farley on 8/06/15.
// Copyright (c) 2015 Trip Wire. All rights reserved.
//
import UIKit
public class NumericTextField: UIValidationTextField, UITextFieldDelegate {
override init(frame: CGRect) {
super.init(frame: frame)
self.delegate = self
self.keyboardType = UIKeyboardType.NumberPad
}
convenience public init(bounds: CGRect, overlayLabelBounds: CGRect, textColor: UIColor, textAlignment : NSTextAlignment,
message: String, font: UIFont, textXMargin: CGFloat) {
self.init(frame: bounds)
self.overlayView = UIOverlayLabel(bounds: overlayLabelBounds, textColor: textColor,
textAlignment: textAlignment, message: message, font: font, textXMargin: textXMargin)
}
convenience public init(bounds: CGRect, overlayLabelBounds: CGRect, textColor: UIColor, textAlignment : NSTextAlignment,
message: String, font: UIFont) {
self.init(frame: bounds)
self.overlayView = UIOverlayLabel(bounds: overlayLabelBounds, textColor: textColor,
textAlignment: textAlignment, message: message, font: font)
}
convenience public init(bounds: CGRect, overlayView: UIView) {
self.init(frame: bounds)
self.overlayView = overlayView
}
// Default if using story board
required public init(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
self.delegate = self
self.keyboardType = UIKeyboardType.NumberPad
var bounds = CGRect(x: 0, y: 0, width: 150, height: 40)
var textColor = UIColor(red: 0, green: 122/255, blue: 1, alpha: 1)
var font = UIFont.systemFontOfSize(10)
self.overlayView = UIOverlayLabel(bounds: bounds, textColor: textColor,
textAlignment: NSTextAlignment.Right, message: "Please enter a numeric value", font: font)
}
public func textField(textField: UITextField, shouldChangeCharactersInRange range: NSRange, replacementString string: String) -> Bool {
// Get the attempted new string by replacing the new characters in the
// appropriate range
let newString = (textField.text as NSString).stringByReplacingCharactersInRange(range, withString: string)
if (count(newString) > 0) {
let scanner: NSScanner = NSScanner(string: newString)
isValid = scanner.scanDecimal(nil) && scanner.atEnd
showOrHideOverlay()
return isValid
} else {
isValid = false
showOrHideOverlay()
return true
}
}
} | cc0-1.0 | 10096f026b984b32bad467291d66bfb5 | 39.283582 | 139 | 0.657153 | 4.835125 | false | false | false | false |
oisdk/Using-Protocols-to-Build-a-very-Generic-Deque | Deque.playground/Sources/Source.swift | 1 | 15504 | // MARK: Definition
/**
A [Deque](https://en.wikipedia.org/wiki/Double-ended_queue) is a data structure comprised
of two queues. This implementation has a front queue, which is reversed, and a back queue,
which is not. Operations at either end of the Deque have the same complexity as operations
on the end of either queue.
Three structs conform to the `DequeType` protocol: `Deque`, `DequeSlice`, and
`ContiguousDeque`. These correspond to the standard library array types.
*/
public protocol DequeType :
MutableSliceable,
RangeReplaceableCollectionType,
CustomDebugStringConvertible,
ArrayLiteralConvertible {
/// The type that represents the queues.
typealias Container : RangeReplaceableCollectionType, MutableSliceable
/// The front queue. It is stored in reverse.
var front: Container { get set }
/// The back queue.
var back : Container { get set }
/// Constructs an empty `Deque`
init()
}
// MARK: DebugDescription
extension DequeType {
/// A textual representation of `self`, suitable for debugging.
public var debugDescription: String {
let fStr = front.reverse().map{String(reflecting: $0)}.joinWithSeparator(", ")
let bStr = back.map{String(reflecting: $0)}.joinWithSeparator(", ")
return "[" + fStr + " | " + bStr + "]"
}
}
// MARK: Initializers
extension DequeType {
public init(balancedF: Container, balancedB: Container) {
self.init()
front = balancedF
back = balancedB
}
/// Construct from a collection
public init<
C : CollectionType where
C.Index : RandomAccessIndexType,
C.SubSequence.Generator.Element == Container.Generator.Element,
C.Index.Distance == Container.Index.Distance
>(col: C) {
self.init()
let mid = col.count / 2
let midInd = col.startIndex.advancedBy(mid)
front.reserveCapacity(mid)
back.reserveCapacity(mid.successor())
front.appendContentsOf(col[col.startIndex..<midInd].reverse())
back.appendContentsOf(col[midInd..<col.endIndex])
}
}
extension DequeType where Container.Index.Distance == Int {
/// Create an instance containing `elements`.
public init(arrayLiteral elements: Container.Generator.Element...) {
self.init(col: elements)
}
/// Initialise from a sequence.
public init<
S : SequenceType where
S.Generator.Element == Container.Generator.Element
>(_ seq: S) {
self.init(col: Array(seq))
}
}
// MARK: Indexing
public enum IndexLocation<I> {
case Front(I), Back(I)
}
extension IndexLocation : CustomDebugStringConvertible {
public var debugDescription: String {
switch self {
case let .Front(i): return "Front: \(i)"
case let .Back(i): return "Back: \(i)"
}
}
}
extension DequeType where
Container.Index : RandomAccessIndexType,
Container.Index.Distance : ForwardIndexType {
public func translate(i: Container.Index.Distance) -> IndexLocation<Container.Index> {
return i < front.count ?
.Front(front.endIndex.predecessor().advancedBy(-i)) :
.Back(back.startIndex.advancedBy(i - front.count))
}
/**
The position of the first element in a non-empty `Deque`.
In an empty `Deque`, `startIndex == endIndex`.
*/
public var startIndex: Container.Index.Distance { return 0 }
/**
The `Deque`'s "past the end" position.
`endIndex` is not a valid argument to `subscript`, and is always reachable from
`startIndex` by zero or more applications of `successor()`.
*/
public var endIndex : Container.Index.Distance { return front.count + back.count }
public subscript(i: Container.Index.Distance) -> Container.Generator.Element {
get {
switch translate(i) {
case let .Front(i): return front[i]
case let .Back(i): return back[i]
}
} set {
switch translate(i) {
case let .Front(i): front[i] = newValue
case let .Back(i): back[i] = newValue
}
}
}
}
// MARK: Index Ranges
public enum IndexRangeLocation<I : ForwardIndexType> {
case Front(Range<I>), Over(Range<I>, Range<I>), Back(Range<I>), Between
}
extension IndexRangeLocation : CustomDebugStringConvertible {
public var debugDescription: String {
switch self {
case let .Front(i): return "Front: \(i)"
case let .Back(i): return "Back: \(i)"
case let .Over(f, b): return "Over: \(f), \(b)"
case .Between: return "Between"
}
}
}
extension DequeType where
Container.Index : RandomAccessIndexType,
Container.Index.Distance : BidirectionalIndexType {
public func translate
(i: Range<Container.Index.Distance>)
-> IndexRangeLocation<Container.Index> {
if i.endIndex <= front.count {
let s = front.endIndex.advancedBy(-i.endIndex)
if s == front.startIndex && i.isEmpty { return .Between }
let e = front.endIndex.advancedBy(-i.startIndex)
return .Front(s..<e)
}
if i.startIndex >= front.count {
let s = back.startIndex.advancedBy(i.startIndex - front.count)
let e = back.startIndex.advancedBy(i.endIndex - front.count)
return .Back(s..<e)
}
let f = front.startIndex..<front.endIndex.advancedBy(-i.startIndex)
let b = back.startIndex..<back.startIndex.advancedBy(i.endIndex - front.count)
return .Over(f, b)
}
}
extension DequeType where
Container.Index : RandomAccessIndexType,
Container.Index.Distance : BidirectionalIndexType,
SubSequence : DequeType,
SubSequence.Container == Container.SubSequence,
SubSequence.Generator.Element == Container.Generator.Element {
public subscript(idxs: Range<Container.Index.Distance>) -> SubSequence {
set { for (index, value) in zip(idxs, newValue) { self[index] = value } }
get {
switch translate(idxs) {
case .Between: return SubSequence()
case let .Over(f, b):
return SubSequence( balancedF: front[f], balancedB: back[b] )
case let .Front(i):
if i.isEmpty { return SubSequence() }
return SubSequence(
balancedF: front[i.startIndex.successor()..<i.endIndex],
balancedB: front[i.startIndex...i.startIndex]
)
case let .Back(i):
if i.isEmpty { return SubSequence() }
return SubSequence(
balancedF: back[i.startIndex..<i.startIndex.successor()],
balancedB: back[i.startIndex.successor()..<i.endIndex]
)
}
}
}
}
// MARK: Balance
public enum Balance {
case FrontEmpty, BackEmpty, Balanced
}
extension DequeType {
public var balance: Balance {
let (f, b) = (front.count, back.count)
if f == 0 {
if b > 1 {
return .FrontEmpty
}
} else if b == 0 {
if f > 1 {
return .BackEmpty
}
}
return .Balanced
}
public var isBalanced: Bool {
return balance == .Balanced
}
}
extension DequeType where Container.Index : BidirectionalIndexType {
public mutating func check() {
switch balance {
case .FrontEmpty:
let newBack = back.removeLast()
front.reserveCapacity(back.count)
front.replaceRange(front.indices, with: back.reverse())
back.replaceRange(back.indices, with: [newBack])
case .BackEmpty:
let newFront = front.removeLast()
back.reserveCapacity(front.count)
back.replaceRange(back.indices, with: front.reverse())
front.replaceRange(front.indices, with: [newFront])
case .Balanced: return
}
}
}
// MARK: ReserveCapacity
extension DequeType {
/**
Reserve enough space to store `minimumCapacity` elements.
- Postcondition: `capacity >= minimumCapacity` and the `Deque` has mutable
contiguous storage.
- Complexity: O(`count`).
*/
public mutating func reserveCapacity(n: Container.Index.Distance) {
front.reserveCapacity(n / 2)
back.reserveCapacity(n / 2)
}
}
// MARK: ReplaceRange
extension DequeType where
Container.Index : RandomAccessIndexType,
Container.Index.Distance : BidirectionalIndexType {
/**
Replace the given `subRange` of elements with `newElements`.
Invalidates all indices with respect to `self`.
- Complexity: O(`subRange.count`) if `subRange.endIndex == self.endIndex` and
`isEmpty(newElements)`, O(`self.count + newElements.count`) otherwise.
*/
public mutating func replaceRange<
C : CollectionType where C.Generator.Element == Container.Generator.Element
>(subRange: Range<Container.Index.Distance>, with newElements: C) {
defer { check() }
switch translate(subRange) {
case .Between:
back.replaceRange(back.startIndex..<back.startIndex, with: newElements)
case let .Front(r):
front.replaceRange(r, with: newElements.reverse())
case let .Back(r):
back.replaceRange(r, with: newElements)
case let .Over(f, b):
front.removeRange(f)
back.replaceRange(b, with: newElements)
}
}
}
extension RangeReplaceableCollectionType where Index : BidirectionalIndexType {
public mutating func popLast() -> Generator.Element? {
return isEmpty ? nil : removeLast()
}
}
// MARK: StackLike
extension DequeType where Container.Index : BidirectionalIndexType {
/**
If `!self.isEmpty`, remove the first element and return it, otherwise return `nil`.
- Complexity: Amortized O(1)
*/
public mutating func popFirst() -> Container.Generator.Element? {
defer { check() }
return front.popLast() ?? back.popLast()
}
/**
If `!self.isEmpty`, remove the last element and return it, otherwise return `nil`.
- Complexity: Amortized O(1)
*/
public mutating func popLast() -> Container.Generator.Element? {
defer { check() }
return back.popLast() ?? front.popLast()
}
}
// MARK: SequenceType
/// :nodoc:
public struct DequeGenerator<
Container : RangeReplaceableCollectionType where
Container.Index : BidirectionalIndexType
> : GeneratorType {
public let front, back: Container
public var i: Container.Index
public var onBack: Bool
/**
Advance to the next element and return it, or `nil` if no next element exists.
- Requires: `next()` has not been applied to a copy of `self`.
*/
public mutating func next() -> Container.Generator.Element? {
if onBack { return i == back.endIndex ? nil : back[i++] }
if i == front.startIndex { onBack = true; return next() }
return front[--i]
}
}
extension DequeType where Container.Index : BidirectionalIndexType {
/**
Return a `DequeGenerator` over the elements of this `Deque`.
- Complexity: O(1)
*/
public func generate() -> DequeGenerator<Container> {
return DequeGenerator(front: front, back: back, i: front.endIndex, onBack: false)
}
}
// MARK: Rotations
extension DequeType where Container.Index : BidirectionalIndexType {
/**
Removes an element from the end of `self`, and prepends it to `self`
```swift
var deque: Deque = [1, 2, 3, 4, 5]
deque.rotateRight()
// deque == [5, 1, 2, 3, 4]
```
- Complexity: Amortized O(1)
*/
public mutating func rotateRight() {
if back.isEmpty { return }
front.append(back.removeLast())
check()
}
/**
Removes an element from the start of `self`, and appends it to `self`
```swift
var deque: Deque = [1, 2, 3, 4, 5]
deque.rotateLeft()
// deque == [2, 3, 4, 5, 1]
```
- Complexity: Amortized O(1)
*/
public mutating func rotateLeft() {
if front.isEmpty { return }
back.append(front.removeLast())
check()
}
/**
Removes an element from the end of `self`, and prepends `x` to `self`
```swift
var deque: Deque = [1, 2, 3, 4, 5]
deque.rotateRight(0)
// deque == [0, 1, 2, 3, 4]
```
- Complexity: Amortized O(1)
*/
public mutating func rotateRight(x: Container.Generator.Element) {
back.popLast() ?? front.popLast()
front.append(x)
check()
}
/**
Removes an element from the start of `self`, and appends `x` to `self`
```swift
var deque: Deque = [1, 2, 3, 4, 5]
deque.rotateLeft(0)
// deque == [2, 3, 4, 5, 0]
```
- Complexity: Amortized O(1)
*/
public mutating func rotateLeft(x: Container.Generator.Element) {
front.popLast() ?? back.popLast()
back.append(x)
check()
}
}
// MARK: Reverse
extension DequeType {
/**
Return a `Deque` containing the elements of `self` in reverse order.
*/
public func reverse() -> Self {
return Self(balancedF: back, balancedB: front)
}
}
// MARK: -ending
extension DequeType where Container.Index : BidirectionalIndexType {
/**
Prepend `x` to `self`.
The index of the new element is `self.startIndex`.
- Complexity: Amortized O(1).
*/
public mutating func prepend(x: Container.Generator.Element) {
front.append(x)
check()
}
/**
Prepend the elements of `newElements` to `self`.
- Complexity: O(*length of result*).
*/
public mutating func prextend<
S : SequenceType where
S.Generator.Element == Container.Generator.Element
>(x: S) {
front.appendContentsOf(x.reverse())
check()
}
}
// MARK: Underestimate Count
extension DequeType {
/**
Return a value less than or equal to the number of elements in `self`,
**nondestructively**.
*/
public func underestimateCount() -> Int {
return front.underestimateCount() + back.underestimateCount()
}
}
// MARK: Structs
/**
A [Deque](https://en.wikipedia.org/wiki/Double-ended_queue) is a data structure comprised
of two queues. This implementation has a front queue, which is a reversed array, and a
back queue, which is an array. Operations at either end of the Deque have the same
complexity as operations on the end of either array.
*/
public struct Deque<Element> : DequeType {
/// The front and back queues. The front queue is stored in reverse.
public var front, back: [Element]
public typealias SubSequence = DequeSlice<Element>
/// Initialise an empty `Deque`
public init() { (front, back) = ([], []) }
}
/**
A [Deque](https://en.wikipedia.org/wiki/Double-ended_queue) is a data structure comprised
of two queues. This implementation has a front queue, which is a reversed ArraySlice, and
a back queue, which is an ArraySlice. Operations at either end of the Deque have the same
complexity as operations on the end of either ArraySlice.
Because an `ArraySlice` presents a view onto the storage of some larger array even after
the original array's lifetime ends, storing the slice may prolong the lifetime of elements
that are no longer accessible, which can manifest as apparent memory and object leakage.
To prevent this effect, use `DequeSlice` only for transient computation.
*/
public struct DequeSlice<Element> : DequeType {
/// The front and back queues. The front queue is stored in reverse
public var front, back: ArraySlice<Element>
public typealias SubSequence = DequeSlice
/// Initialise an empty `DequeSlice`
public init() { (front, back) = ([], []) }
}
/**
A [Deque](https://en.wikipedia.org/wiki/Double-ended_queue) is a data structure comprised
of two queues. This implementation has a front queue, which is a reversed ContiguousArray,
and a back queue, which is a ContiguousArray. Operations at either end of the Deque have
the same complexity as operations on the end of either ContiguousArray.
*/
public struct ContiguousDeque<Element> : DequeType {
/// The front and back queues. The front queue is stored in reverse
public var front, back: ContiguousArray<Element>
public typealias SubSequence = DequeSlice<Element>
/// Initialise an empty `ContiguousDeque`
public init() { (front, back) = ([], []) }
} | mit | 08175862e31489945735dd22389b9fb9 | 28.477186 | 90 | 0.673955 | 4.056515 | false | false | false | false |
roosmaa/Octowire-iOS | Octowire/EventModel.swift | 1 | 2750 | //
// EventModel.swift
// Octowire
//
// Created by Mart Roosmaa on 25/02/2017.
// Copyright © 2017 Mart Roosmaa. All rights reserved.
//
import Foundation
import ObjectMapper
import RxDataSources
class EventModel: StaticMappable {
var id: String!
var type: String!
var createdAt: Date?
var repoId: Int64?
var repoName: String?
var actorId: Int64?
var actorUsername: String?
var actorAvatarUrl: URL?
static func objectForMapping(map: Map) -> BaseMappable? {
guard let type: String = map["type"].value() else {
return nil
}
switch type {
case "CreateEvent": return CreateEventModel()
case "ForkEvent": return ForkEventModel()
case "WatchEvent": return WatchEventModel()
case "PullRequestEvent": return PullRequestEventModel()
default: return EventModel()
}
}
init() {
}
func mapping(map: Map) {
self.id <- map["id"]
self.type <- map["type"]
self.createdAt <- (map["created_at"], ISO8601DateTransform())
self.repoId <- map["repo.id"]
self.repoName <- map["repo.name"]
self.actorId <- map["actor.id"]
self.actorUsername <- map["actor.login"]
self.actorAvatarUrl <- (map["actor.avatar_url"], URLTransform())
}
}
class CreateEventModel: EventModel {
enum RefType: String {
case repository = "repository"
case brand = "branch"
case tag = "tag"
}
var refType: RefType?
override func mapping(map: Map) {
super.mapping(map: map)
self.refType <- map["payload.ref_type"]
}
}
class ForkEventModel: EventModel {
var forkRepoName: String?
override func mapping(map: Map) {
super.mapping(map: map)
self.forkRepoName <- map["payload.forkee.name"]
}
}
class WatchEventModel: EventModel {
override func mapping(map: Map) {
super.mapping(map: map)
}
}
class PullRequestEventModel: EventModel {
enum ActionKind: String {
case assigned = "assigned"
case unassigned = "unassigned"
case reviewRequested = "review_requested"
case reviewRequestRemoved = "review_request_removed"
case labeled = "labeled"
case unlabeled = "unlabeled"
case opened = "opened"
case edited = "edited"
case closed = "closed"
case reopened = "reopened"
}
var action: ActionKind?
var pullRequestNumber: Int64?
override func mapping(map: Map) {
super.mapping(map: map)
self.action <- map["payload.action"]
self.pullRequestNumber <- map["payload.number"]
}
}
| mit | fe4af3c46de3efcd98f1c76b960f808d | 23.765766 | 72 | 0.593307 | 4.18417 | false | false | false | false |
petester42/SwiftCharts | Examples/Examples/Examples/GroupedAndStackedBarsExample.swift | 4 | 8764 | //
// GroupedAndStackedBarsExample.swift
// Examples
//
// Created by ischuetz on 20/05/15.
// Copyright (c) 2015 ivanschuetz. All rights reserved.
//
import UIKit
class GroupedAndStackedBarsExample: UIViewController {
private var chart: Chart?
private let dirSelectorHeight: CGFloat = 50
private func barsChart(#horizontal: Bool) -> Chart {
let labelSettings = ChartLabelSettings(font: ExamplesDefaults.labelFont)
let groupsData: [(title: String, bars: [(start: CGFloat, quantities: [Double])])] = [
("A", [
(0,
[-20, -5, -10]
),
(0,
[10, 20, 30]
),
(0,
[30, 14, 5]
)
]),
("B", [
(0,
[-10, -15, -5]
),
(0,
[30, 25, 40]
),
(0,
[25, 40, 10]
)
]),
("C", [
(0,
[-15, -30, -10]
),
(0,
[-10, -10, -5]
),
(0,
[15, 30, 10]
)
]),
("D", [
(0,
[-20, -10, -10]
),
(0,
[30, 15, 27]
),
(0,
[8, 10, 25]
)
])
]
let frameColors = [UIColor.redColor().colorWithAlphaComponent(0.6), UIColor.blueColor().colorWithAlphaComponent(0.6), UIColor.greenColor().colorWithAlphaComponent(0.6)]
let groups: [ChartPointsBarGroup<ChartStackedBarModel>] = Array(enumerate(groupsData)).map {index, entry in
let constant = ChartAxisValueFloat(CGFloat(index))
let bars: [ChartStackedBarModel] = Array(enumerate(entry.bars)).map {index, bars in
let items = Array(enumerate(bars.quantities)).map {index, quantity in
ChartStackedBarItemModel(quantity, frameColors[index])
}
return ChartStackedBarModel(constant: constant, start: ChartAxisValueFloat(bars.start), items: items)
}
return ChartPointsBarGroup(constant: constant, bars: bars)
}
let (axisValues1: [ChartAxisValue], axisValues2: [ChartAxisValue]) = (
Array(stride(from: -60, through: 100, by: 20)).map {ChartAxisValueFloat($0, labelSettings: labelSettings)},
[ChartAxisValueString(order: -1)] +
Array(enumerate(groupsData)).map {index, tuple in ChartAxisValueString(tuple.0, order: index, labelSettings: labelSettings)} +
[ChartAxisValueString(order: groupsData.count)]
)
let (xValues, yValues) = horizontal ? (axisValues1, axisValues2) : (axisValues2, axisValues1)
let xModel = ChartAxisModel(axisValues: xValues, axisTitleLabel: ChartAxisLabel(text: "Axis title", settings: labelSettings))
let yModel = ChartAxisModel(axisValues: yValues, axisTitleLabel: ChartAxisLabel(text: "Axis title", settings: labelSettings.defaultVertical()))
let frame = ExamplesDefaults.chartFrame(self.view.bounds)
let chartFrame = self.chart?.frame ?? CGRectMake(frame.origin.x, frame.origin.y, frame.size.width, frame.size.height - self.dirSelectorHeight)
let coordsSpace = ChartCoordsSpaceLeftBottomSingleAxis(chartSettings: ExamplesDefaults.chartSettings, chartFrame: chartFrame, xModel: xModel, yModel: yModel)
let (xAxis, yAxis, innerFrame) = (coordsSpace.xAxis, coordsSpace.yAxis, coordsSpace.chartInnerFrame)
let groupsLayer = ChartGroupedStackedBarsLayer(xAxis: xAxis, yAxis: yAxis, innerFrame: innerFrame, groups: groups, horizontal: horizontal, barSpacing: 2, groupSpacing: 30, animDuration: 0.5)
var settings = ChartGuideLinesLayerSettings(linesColor: UIColor.blackColor(), linesWidth: ExamplesDefaults.guidelinesWidth)
let guidelinesLayer = ChartGuideLinesLayer(xAxis: xAxis, yAxis: yAxis, innerFrame: innerFrame, axis: horizontal ? .X : .Y, settings: settings)
let dummyZeroChartPoint = ChartPoint(x: ChartAxisValueFloat(0), y: ChartAxisValueFloat(0))
let zeroGuidelineLayer = ChartPointsViewsLayer(xAxis: xAxis, yAxis: yAxis, innerFrame: innerFrame, chartPoints: [dummyZeroChartPoint], viewGenerator: {(chartPointModel, layer, chart) -> UIView? in
let width: CGFloat = 2
let viewFrame: CGRect = {
if horizontal {
return CGRectMake(chartPointModel.screenLoc.x - width / 2, innerFrame.origin.y, width, innerFrame.size.height)
} else {
return CGRectMake(innerFrame.origin.x, chartPointModel.screenLoc.y - width / 2, innerFrame.size.width, width)
}
}()
let v = UIView(frame: viewFrame)
v.backgroundColor = UIColor.blackColor()
return v
})
return Chart(
frame: chartFrame,
layers: [
xAxis,
yAxis,
guidelinesLayer,
groupsLayer,
zeroGuidelineLayer
]
)
}
private func showChart(#horizontal: Bool) {
self.chart?.clearView()
let chart = self.barsChart(horizontal: horizontal)
self.view.addSubview(chart.view)
self.chart = chart
}
override func viewDidLoad() {
self.showChart(horizontal: false)
if let chart = self.chart {
let dirSelector = DirSelector(frame: CGRectMake(0, chart.frame.origin.y + chart.frame.size.height, self.view.frame.size.width, self.dirSelectorHeight), controller: self)
self.view.addSubview(dirSelector)
}
}
class DirSelector: UIView {
let horizontal: UIButton
let vertical: UIButton
weak var controller: GroupedAndStackedBarsExample?
private let buttonDirs: [UIButton : Bool]
init(frame: CGRect, controller: GroupedAndStackedBarsExample) {
self.controller = controller
self.horizontal = UIButton()
self.horizontal.setTitle("Horizontal", forState: .Normal)
self.vertical = UIButton()
self.vertical.setTitle("Vertical", forState: .Normal)
self.buttonDirs = [self.horizontal : true, self.vertical : false]
super.init(frame: frame)
self.addSubview(self.horizontal)
self.addSubview(self.vertical)
for button in [self.horizontal, self.vertical] {
button.titleLabel?.font = ExamplesDefaults.fontWithSize(14)
button.setTitleColor(UIColor.blueColor(), forState: .Normal)
button.addTarget(self, action: "buttonTapped:", forControlEvents: .TouchUpInside)
}
}
func buttonTapped(sender: UIButton) {
let horizontal = sender == self.horizontal ? true : false
controller?.showChart(horizontal: horizontal)
}
override func didMoveToSuperview() {
let views = [self.horizontal, self.vertical]
for v in views {
v.setTranslatesAutoresizingMaskIntoConstraints(false)
}
let namedViews = Array(enumerate(views)).map{index, view in
("v\(index)", view)
}
let viewsDict = namedViews.reduce(Dictionary<String, UIView>()) {(var u, tuple) in
u[tuple.0] = tuple.1
return u
}
let buttonsSpace: CGFloat = Env.iPad ? 20 : 10
let hConstraintStr = namedViews.reduce("H:|") {str, tuple in
"\(str)-(\(buttonsSpace))-[\(tuple.0)]"
}
let vConstraits = namedViews.flatMap {NSLayoutConstraint.constraintsWithVisualFormat("V:|[\($0.0)]", options: NSLayoutFormatOptions.allZeros, metrics: nil, views: viewsDict)}
self.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat(hConstraintStr, options: NSLayoutFormatOptions.allZeros, metrics: nil, views: viewsDict)
+ vConstraits)
}
required init(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
}
| apache-2.0 | 41eeb6691660ab37c86c0b9521de192c | 39.953271 | 204 | 0.552716 | 5.110204 | false | false | false | false |
averagedude2992/Taper-2016 | Taper-2016/ViewController.swift | 1 | 1834 | //
// ViewController.swift
// Taper-2016
//
// Created by Briano Santos on 8/24/16.
// Copyright © 2016 FairSkiesTechnologies. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
//Properties
var maxTaps = 0
var currentTaps = 0
//Outlets
@ IBOutlet weak var logoImg: UIImageView!
@ IBOutlet weak var howManyTapsTxt: UITextField!
@ IBOutlet weak var playBtn: UIButton!
@ IBOutlet weak var tapBtn: UIButton!
@ IBOutlet weak var tapsLbl: UILabel!
@ IBAction func onCoinBtnPressed(sender: UIButton!) {
currentTaps += 1
updateTapsLbl()
if isGameOver() {
restartGame()
}
}
@ IBAction func onPlayButtonPressed(sender: UIButton!) {
if howManyTapsTxt.text != nil && howManyTapsTxt.text != "" {
logoImg.hidden = true
playBtn.hidden = true
howManyTapsTxt.hidden = true
tapBtn.hidden = false
tapsLbl.hidden = false
maxTaps = Int(howManyTapsTxt.text!)!
currentTaps = 0
updateTapsLbl()
}
}
func restartGame() {
maxTaps = 0
howManyTapsTxt.text = ""
logoImg.hidden = false
playBtn.hidden = false
howManyTapsTxt.hidden = false
tapBtn.hidden = true
tapsLbl.hidden = true
}
func isGameOver() -> Bool {
if currentTaps >= maxTaps {
return true
} else {
return false
}
}
//DRY (do not repeate yourself coding prenciple//
func updateTapsLbl() {
tapsLbl.text = "\(currentTaps) Taps"
}
}
| mit | 52d35e1a5a5d7eeaebf32c3a597547c3 | 20.313953 | 68 | 0.52264 | 4.927419 | false | false | false | false |
qingtianbuyu/Mono | Moon/Classes/Main/Model/MNMode.swift | 1 | 670 | //
// MNMode.swift
// Moon
//
// Created by YKing on 16/6/4.
// Copyright © 2016年 YKing. All rights reserved.
//
import UIKit
class MNMode: MNBanner {
var group: MNGroup?
var campaign: MNRefCampaign?
override init(dict: [String: AnyObject]) {
super.init(dict: dict)
setValuesForKeys(dict)
}
override func setValue(_ value: Any?, forKey key: String) {
if key == "group" {
group = MNGroup(dict: (value as! [String: AnyObject]))
return
}
if key == "campaign" {
campaign = MNRefCampaign(dict: (value as! [String: AnyObject]))
return
}
super.setValue(value, forKey: key)
}
}
| mit | caed450d53f32ad6e9beb0c2186c98ce | 17.527778 | 69 | 0.596702 | 3.529101 | false | false | false | false |
sstanic/Shopfred | Shopfred/Shopfred/View Controller/GenericSelectionViewController.swift | 1 | 7157 | //
// GenericSelectionViewController.swift
// Shopfred
//
// Created by Sascha Stanic on 7/1/17.
// Copyright © 2017 Sascha Stanic. All rights reserved.
//
import UIKit
import CoreData
/**
Shows a list of core data entities.
These entities are managed by the view controller:
- *Unit*
- *Category*
- *Context*
The entity type is defined by the generic type
parameter of the class.
*/
class GenericSelectionViewController<T: NSManagedObject>: UIViewController, UITableViewDataSource, UITableViewDelegate {
// MARK: - Private Attributes
private var setSelection: ((_ o: T) -> Void)!
private var myTableView: UITableView!
private var myLabel: UILabel!
private var myStackView: UIStackView!
private var myToolBar: UIToolbar!
private var list = [ManagedObjectName]()
private var info: String!
private var selectedName: String?
// MARK: - Initializer
convenience init(setSelection: @escaping (_ o: T) -> Void) {
self.init(nibName: nil, bundle: nil)
self.setSelection = setSelection
self.info = ""
self.selectedName = ""
}
convenience init(info: String, setSelection: @escaping (_ o: T) -> Void) {
self.init(setSelection: setSelection)
self.info = info
self.selectedName = ""
}
convenience init(info: String, selectedName: String?, setSelection: @escaping (_ o: T) -> Void) {
self.init(info: info, setSelection: setSelection)
self.selectedName = selectedName
}
// MARK: - Lifecycle
override func viewDidLoad() {
super.viewDidLoad()
self.createElementList()
self.initializeView()
self.setStyle()
}
override func viewWillAppear(_ animated: Bool) {
self.myTableView.reloadData()
if let navController = self.navigationController {
navController.isToolbarHidden = false
}
}
override func viewWillDisappear(_ animated: Bool) {
if let navController = self.navigationController {
navController.isToolbarHidden = true
}
}
// MARK: - Styling
private func setStyle() {
self.myTableView.style(style: ViewStyle.TableListView.standard)
self.myTableView.separatorColor = UIColor.clear
self.myTableView.contentInset = UIEdgeInsets(top: 8, left: 0, bottom: 0, right: 0)
self.myLabel.style(style: TextStyle.title)
self.view.style(style: ViewStyle.TableListView.standard)
}
// MARK: - Initialize View
private func createElementList() {
let shoppingSpace = DataStore.sharedInstance().shoppingStore.shoppingSpace
let request: NSFetchRequest = T.fetchRequest()
let predicate = NSPredicate(format: "shoppingSpace.id == %@", shoppingSpace?.id ?? "")
request.predicate = predicate
do {
self.list = try DataStore.sharedInstance().stack.context.fetch(request) as! [ManagedObjectName]
}
catch {
print("fetch request for \(T.self) failed.")
}
}
private func initializeView() {
self.myStackView = UIStackView()
self.myStackView.translatesAutoresizingMaskIntoConstraints=false
self.view.addSubview(myStackView)
self.view.addConstraints(NSLayoutConstraint.constraints(withVisualFormat: "V:|-64-[stackView]-0-|", options: NSLayoutFormatOptions.alignAllLeading, metrics: nil, views: ["stackView":self.myStackView]))
self.view.addConstraints(NSLayoutConstraint.constraints(withVisualFormat: "H:|-0-[stackView]-0-|", options: NSLayoutFormatOptions.alignAllLeading, metrics: nil, views: ["stackView":self.myStackView]))
self.myStackView.axis = .vertical
self.myStackView.alignment = .fill
self.myStackView.distribution = .fill
self.myStackView.spacing = 5
self.myLabel = UILabel()
self.myLabel.text = info
self.myLabel.textAlignment = .center
self.myLabel.backgroundColor = UIColor.groupTableViewBackground
let height : CGFloat = 40.0
let heightConstraint = NSLayoutConstraint(item: self.myLabel, attribute: NSLayoutAttribute.height, relatedBy: NSLayoutRelation.equal, toItem: nil, attribute: NSLayoutAttribute.notAnAttribute, multiplier: 1, constant: height)
self.myStackView.addArrangedSubview(self.myLabel)
NSLayoutConstraint.activate([heightConstraint])
// tableView
self.myTableView = UITableView()
self.myTableView.register(GenericSelectionTableViewCell.self, forCellReuseIdentifier: "genericSelectionTableViewCell")
self.myTableView.dataSource = self
self.myTableView.delegate = self
self.myStackView.addArrangedSubview(self.myTableView)
var items = [UIBarButtonItem]()
items.append(UIBarButtonItem(title: "Manage entries", style: .plain, target: self, action: #selector(navigateToSettings)))
self.toolbarItems = items
}
// MARK: - Navigation
@objc private func navigateToSettings() {
let vc = UIStoryboard(name:"Main", bundle:nil).instantiateViewController(withIdentifier: "SettingsListViewController") as! SettingsListViewController
vc.type = T.self
vc.titleText = "Manage entries"
if let navController = self.navigationController {
navController.pushViewController(vc, animated: true)
}
}
private func navigateBack() {
if let navController = self.navigationController {
navController.popViewController(animated: true)
}
else {
Utils.showAlert(self, alertMessage: "Sorry, something went wrong. Please restart the app.", completion: nil)
}
}
// MARK: - UITableViewDataSource, UITableViewDelegate
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return self.list.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "genericSelectionTableViewCell", for: indexPath) as! GenericSelectionTableViewCell
let selectionItem = self.list[indexPath.row]
if let txtLbl = cell.textLabel {
txtLbl.text = selectionItem.name
}
if (selectionItem.name == self.selectedName) {
cell.accessoryType = .checkmark
}
cell.setStyle()
return cell
}
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
self.setSelection(list[indexPath.row] as! T)
DataStore.sharedInstance().saveContext() { success, error in
// completion parameters not used
self.navigateBack()
}
}
}
| mit | 89bc96226a35eecb90e1c1bc9b9940ff | 31.976959 | 232 | 0.635551 | 5.219548 | false | false | false | false |
brentdax/swift | validation-test/Sema/type_checker_perf/slow/rdar23429943.swift | 4 | 264 | // RUN: %target-typecheck-verify-swift -solver-expression-time-threshold=1
// REQUIRES: tools-release,no_asserts
let _ = [0].reduce([Int]()) {
// expected-error@-1 {{reasonable time}}
return $0.count == 0 && ($1 == 0 || $1 == 2 || $1 == 3) ? [] : $0 + [$1]
}
| apache-2.0 | 2e41afb3303b42c2a686f15058f0ea60 | 36.714286 | 74 | 0.568182 | 2.869565 | false | false | false | false |
volodg/iAsync.async | Lib/AsyncsOwner.swift | 1 | 2433 | //
// AsyncsOwner.swift
// iAsync_async
//
// Created by Vladimir Gorbenko on 19.06.14.
// Copyright (c) 2014 EmbeddedSources. All rights reserved.
//
import Foundation
import iAsync_utils
import ReactiveKit
final public class AsyncsOwner {
private final class ActiveLoaderData {
var handler: AsyncHandler?
func clear() {
handler = nil
}
}
private var loaders = [ActiveLoaderData]()
let task: AsyncHandlerTask
public init(task: AsyncHandlerTask) {
self.task = task
}
public func ownedAsync<Value>(loader: AsyncTypes<Value, NSError>.Async) -> AsyncTypes<Value, NSError>.Async {
return { [weak self] (
progressCallback: AsyncProgressCallback?,
finishCallback : AsyncTypes<Value, NSError>.DidFinishAsyncCallback?) -> AsyncHandler in
guard let self_ = self else {
finishCallback?(result: .Failure(AsyncInterruptedError()))
return jStubHandlerAsyncBlock
}
let loaderData = ActiveLoaderData()
self_.loaders.append(loaderData)
let finishCallbackWrapper = { (result: Result<Value, NSError>) -> () in
if let self_ = self {
for (index, _) in self_.loaders.enumerate() {
if self_.loaders[index] === loaderData {
self_.loaders.removeAtIndex(index)
break
}
}
}
finishCallback?(result: result)
loaderData.clear()
}
loaderData.handler = loader(
progressCallback: progressCallback,
finishCallback : finishCallbackWrapper)
return { (task: AsyncHandlerTask) -> () in
guard let self_ = self, loaderIndex = self_.loaders.indexOf( { $0 === loaderData } ) else { return }
self_.loaders.removeAtIndex(loaderIndex)
loaderData.handler?(task: task)
loaderData.clear()
}
}
}
public func handleAll(task: AsyncHandlerTask) {
let tmpLoaders = loaders
loaders.removeAll(keepCapacity: false)
for (_, element) in tmpLoaders.enumerate() {
element.handler?(task: task)
}
}
deinit {
handleAll(self.task)
}
}
| mit | cb8494c28bddc050c808af392e66ffc9 | 25.16129 | 116 | 0.549938 | 4.955193 | false | false | false | false |
karivalkama/Agricola-Scripture-Editor | TranslationEditor/PostCommentVC.swift | 1 | 5480 | //
// PostCommentVC.swift
// TranslationEditor
//
// Created by Mikko Hilpinen on 25.1.2017.
// Copyright © 2017 SIL. All rights reserved.
//
import UIKit
class PostCommentVC: UIViewController, UITextViewDelegate
{
// OUTLETS ------------
@IBOutlet weak var allContentStackView: SquishableStackView!
@IBOutlet weak var commentTextView: UITextView!
@IBOutlet weak var postButton: BasicButton!
@IBOutlet weak var originalCommentTextView: UITextView!
@IBOutlet weak var originalCommentLabel: UILabel!
@IBOutlet weak var verseTable: UITableView!
@IBOutlet weak var titleLabel: UILabel!
@IBOutlet weak var originalCommentTitleLabel: UILabel!
@IBOutlet weak var contentView: KeyboardReactiveView!
@IBOutlet weak var contentTopConstraint: NSLayoutConstraint!
@IBOutlet weak var contentBottomConstraint: NSLayoutConstraint!
// ATTRIBUTES --------
private var configured = false
private var selectedComment: NotesPost!
private var targetThread: NotesThread!
// Title (eg. English:) + paragraph
private var associatedParagraphData: [(String, Paragraph)]!
private var verseTableDS: VerseTableDS?
// INIT ----------------
override func viewDidLoad()
{
super.viewDidLoad()
// Sets up the components
postButton.isEnabled = false
commentTextView.delegate = self
titleLabel.text = targetThread.name
// Sets up the verse table
verseTable.register(UINib(nibName: "VerseDataCell", bundle: nil), forCellReuseIdentifier: VerseCell.identifier)
verseTable.estimatedRowHeight = 240
verseTable.rowHeight = UITableViewAutomaticDimension
do
{
guard let targetParagraph = try Paragraph.get(targetThread.originalTargetParagraphId) else
{
print("ERROR: Could not find the targeted paragraph for the comment")
return
}
verseTableDS = VerseTableDS(originalParagraph: targetParagraph, resourceData: associatedParagraphData)
verseTableDS?.targetVerseIndex = targetThread.targetVerseIndex
verseTable.dataSource = verseTableDS
}
catch
{
print("ERROR: Failed to read paragraph data from the database. \(error)")
}
// The contents are slightly different when editing an existing comment
let isEditing = selectedComment.creatorId == Session.instance.avatarId
do
{
var originalComment: NotesPost?
if isEditing
{
if let originalCommentId = selectedComment.originalCommentId
{
originalComment = try NotesPost.get(originalCommentId)
}
commentTextView.text = selectedComment.content
}
else
{
originalComment = selectedComment
}
var originalCommentWriter = NSLocalizedString("Someone", comment: "A placeholder for comment author title. Used when no user name is available")
if let originalCommentCreatorId = originalComment?.creatorId, let originalCreator = try Avatar.get(originalCommentCreatorId)
{
originalCommentWriter = originalCreator.name
}
originalCommentTitleLabel.text = "\(originalCommentWriter) \(NSLocalizedString("wrote", comment: "a portion of a comment's title. Something like 'this and this wrote:'")):"
originalCommentTextView.text = originalComment?.content
}
catch
{
print("ERROR: Failed to read database data. \(error)")
}
contentView.configure(mainView: view, elements: [commentTextView, postButton], topConstraint: contentTopConstraint, bottomConstraint: contentBottomConstraint, style: .squish, squishedElements: [allContentStackView])
}
override func viewDidAppear(_ animated: Bool)
{
super.viewDidAppear(animated)
contentView.startKeyboardListening()
}
override func viewDidDisappear(_ animated: Bool)
{
super.viewDidDisappear(animated)
contentView.endKeyboardListening()
}
// IB ACTIONS ---------
@IBAction func postButtonPressed(_ sender: Any)
{
// Creates a new post instance and saves it to the database
do
{
// Again, the functionality is slightly different when editing
let avatarId = Session.instance.avatarId!
let isEditing = avatarId == selectedComment.creatorId
let newText: String = commentTextView.text
if isEditing
{
// Modifies the existing comment
if newText != selectedComment.content
{
selectedComment.content = newText
try selectedComment.push()
}
}
else
{
try NotesPost(threadId: targetThread.idString, creatorId: avatarId, content: newText, originalCommentId: selectedComment.idString).push()
}
// If the thread was already marked as resolved, it is reopened
if targetThread.isResolved
{
try targetThread.setResolved(false)
}
dismiss(animated: true, completion: nil)
}
catch
{
print("ERROR: Failed to save the post to the database \(error)")
}
}
@IBAction func cancelButtonPressed(_ sender: Any)
{
dismiss(animated: true, completion: nil)
}
@IBAction func backgroundTapped(_ sender: Any)
{
dismiss(animated: true, completion: nil)
}
@IBAction func commentLabelTapped(_ sender: Any)
{
commentTextView.becomeFirstResponder()
}
// IMPLEMENTED METHODS ----
func textViewDidChange(_ textView: UITextView)
{
postButton.isEnabled = !textView.text.isEmpty
}
// OTHER METHODS ---------
func configure(thread: NotesThread, selectedComment: NotesPost, associatedParagraphData: [(String, Paragraph)])
{
self.configured = true
self.targetThread = thread
self.selectedComment = selectedComment
self.associatedParagraphData = associatedParagraphData
}
}
| mit | a7e08df7ed99de55e97499edf6f0a6c7 | 26.532663 | 217 | 0.730973 | 4.163374 | false | false | false | false |
Hypercubesoft/HCFramework | HCFramework/Extensions/HCViewController.swift | 1 | 13110 | //
// HCViewController.swift
//
// Created by Hypercube on 9/20/17.
// Copyright © 2017 Hypercube. All rights reserved.
//
import UIKit
/// Completion handler without parameters
public typealias EmptyCompletionHandler = () -> Void
/// Completion handler with one numerical parameter
public typealias CompletionNumberHandler = (CGFloat) -> Void
/// Completion handler with two numerical parameters
public typealias CompletionTwoNumbersHandler = (CGFloat, CGFloat) -> Void
// MARK: - UIViewController extension
extension UIViewController
{
// MARK: - Set up navigation bar
/// Hide navigation bar
open func hcHideNavigationBar()
{
self.hcSetNavigationBar(hidden: true)
}
/// Show navigation bar
open func hcShowNavigationBar()
{
self.hcSetNavigationBar(hidden: false)
}
/// Clear navigation stack
open func hcClearNavigationStack()
{
self.navigationController?.hcClearAllPreviousPages()
}
/// Hide or show navigation bar
///
/// - Parameter hidden: Defines if navigation bar is hidden or not
open func hcSetNavigationBar(hidden:Bool)
{
self.navigationController?.isNavigationBarHidden = hidden
}
/// Generate empty navigation bar icon
///
/// - Returns: Generated empty navigation bar icon
open func generateEmptyNavigationBarIcon() -> UIImage
{
func getImageWithColor(color: UIColor, size: CGSize) -> UIImage
{
let rect = CGRect(x: 0, y: 0, width: size.width, height: size.height)
UIGraphicsBeginImageContextWithOptions(size, false, 0)
color.setFill()
UIRectFill(rect)
let image: UIImage = UIGraphicsGetImageFromCurrentImageContext()!
UIGraphicsEndImageContext()
return image
}
let scale = UIScreen.main.scale
return getImageWithColor(color: .clear, size: CGSize(width: 25.0*scale, height: 25.0*scale))
}
/// Set navigation bar background color
///
/// - Parameter backgroundColor: Navigation bar background color
open func hcSetNavigationbarBackgroundColor(backgroundColor:UIColor?)
{
if let backgroundColor = backgroundColor
{
self.navigationController?.navigationBar.barTintColor = backgroundColor
self.navigationController?.navigationBar.backgroundColor = backgroundColor
}
}
/// Set navigation bar button items
///
/// - Parameters:
/// - leftIcon: Left icon image for the navigaton bar
/// - leftAction: Left action for the navigaton bar
/// - rightIcon: Right icon image for the navigaton bar
/// - rightAction: Right action for the navigaton bar
open func hcSetNavigationBar(leftIcon:UIImage? = nil, leftAction:Selector?, rightIcon:UIImage? = nil, rightAction:Selector?)
{
self.hcShowNavigationBar()
self.navigationController?.navigationBar.isTranslucent = false
self.navigationItem.leftBarButtonItem = UIBarButtonItem(image: leftIcon ?? self.generateEmptyNavigationBarIcon(), style: .plain, target: self, action: leftAction)
self.navigationItem.rightBarButtonItem = UIBarButtonItem(image: rightIcon ?? self.generateEmptyNavigationBarIcon(), style: .plain, target: self, action: rightAction)
}
/// Set navigation bar title and navigation bar button items
///
/// - Parameters:
/// - backgroundColor: Navigation bar background color
/// - title: Navigation bar title
/// - font: Navigation bar title font
/// - titleColor: Navigation bar title color
/// - leftIcon: Left icon image for the navigaton bar
/// - leftAction: Left action for the navigaton bar
/// - rightIcon: Right icon image for the navigaton bar
/// - rightAction: Right action for the navigaton bar
open func hcSetNavigationBar(backgroundColor:UIColor? = nil, title:String = "", font:UIFont? = nil, titleColor:UIColor? = nil, leftIcon:UIImage? = nil, leftAction:Selector? = nil, rightIcon:UIImage? = nil, rightAction:Selector? = nil)
{
self.hcSetNavigationbarBackgroundColor(backgroundColor: backgroundColor)
if let font = font
{
let headerLabel = UILabel()
headerLabel.frame.size = CGSize(width: UIScreen.main.bounds.width, height: 33.0)
headerLabel.textAlignment = .center
headerLabel.text = title
headerLabel.font = font
headerLabel.sizeToFit()
if let titleColor = titleColor
{
headerLabel.textColor = titleColor
}
self.navigationItem.titleView = headerLabel
}
else
{
self.navigationItem.title = title
}
self.hcSetNavigationBar(leftIcon: leftIcon, leftAction: leftAction, rightIcon: rightIcon, rightAction: rightAction)
}
/// Set navigation bar header image and navigation bar button items
///
/// - Parameters:
/// - backgroundColor: Navigation bar background color
/// - headerImage: Navigation bar header image
/// - leftIcon: Left icon image for the navigaton bar
/// - leftAction: Left action for the navigaton bar
/// - rightIcon: Right icon image for the navigaton bar
/// - rightAction: Right action for the navigaton bar
open func hcSetNavigationBar(backgroundColor:UIColor? = nil, headerImage:UIImage? = nil, leftIcon:UIImage? = nil, leftAction:Selector? = nil, rightIcon:UIImage? = nil, rightAction:Selector? = nil)
{
self.hcSetNavigationbarBackgroundColor(backgroundColor: backgroundColor)
self.navigationController?.navigationBar.setValue(true, forKey: "hidesShadow")
if let headerImage = headerImage
{
let headerIV = UIImageView(image: headerImage)
self.navigationItem.titleView = headerIV
}
self.hcSetNavigationBar(leftIcon: leftIcon, leftAction: leftAction, rightIcon: rightIcon, rightAction: rightAction)
}
/// Set search bar inside navigation bar and navigation bar button items
///
/// - Parameters:
/// - backgroundColor: Navigation bar background color
/// - searchBar: Search bar which should be places inside navigation bar
/// - leftIcon: Left icon image for the navigaton bar
/// - leftAction: Left action for the navigaton bar
/// - rightIcon: Right icon image for the navigaton bar
/// - rightAction: Right action for the navigaton bar
open func hcSetNavigationBar(backgroundColor:UIColor? = nil, searchBar:UISearchBar, leftIcon:UIImage? = nil, leftAction:Selector? = nil, rightIcon:UIImage? = nil, rightAction:Selector? = nil)
{
self.hcSetNavigationbarBackgroundColor(backgroundColor: backgroundColor)
if #available(iOS 11.0, *) {
if let navigationBarHeight = self.navigationController?.navigationBar.frame.height
{
searchBar.heightAnchor.constraint(equalToConstant: navigationBarHeight).isActive = true
}
}
self.navigationItem.titleView = searchBar
self.hcSetNavigationBar(leftIcon: leftIcon, leftAction: leftAction, rightIcon: rightIcon, rightAction: rightAction)
}
// MARK: - Handle keyboard events
/// Struct with associated keys for attaching handles as attributes to UIViewController inside extension
private struct AssociatedKeys {
/// Associated key string for handler which handles event when keyboard will appear
static var AppearHandler = "AppearHandler"
/// Associated key string for handler which handles event when keyboard will disappear
static var HideHandler = "HideHandler"
}
/// Handler which handles event when keyboard will appear
private var appearHandler : CompletionTwoNumbersHandler {
get {
if let value = objc_getAssociatedObject(self, &AssociatedKeys.AppearHandler) as? CompletionTwoNumbersHandler
{
return value
}
return { _,_ in }
}
set {
objc_setAssociatedObject(self, &AssociatedKeys.AppearHandler, newValue, .OBJC_ASSOCIATION_RETAIN_NONATOMIC)
}
}
/// Handler which handles event when keyboard will disappear
private var hideHandler : EmptyCompletionHandler {
get {
if let value = objc_getAssociatedObject(self, &AssociatedKeys.HideHandler) as? EmptyCompletionHandler
{
return value
}
return { }
}
set {
objc_setAssociatedObject(self, &AssociatedKeys.HideHandler, newValue, .OBJC_ASSOCIATION_RETAIN_NONATOMIC)
}
}
/// Allows you to close the keyboard on a tap anywhere within the controller.
open func hcHideKeyboardWhenTappedAround()
{
let tap: UITapGestureRecognizer = UITapGestureRecognizer(target: self, action: #selector(UIViewController.hcDismissKeyboard))
tap.cancelsTouchesInView = false
view.addGestureRecognizer(tap)
}
/// Close the keyboard
@objc open func hcDismissKeyboard()
{
view.endEditing(true)
}
/// Change constraints constant based on keyboard size or based on calculated value
///
/// - Parameters:
/// - constraints: Array of constraint which contant value should be changed animated if it is required to avoid keyboard appearing above a text field or text view. Constraints should be set to move a view in right direction when keyboard appears or dissapears (for example, if a view should be pushed up when keyboard appears, increasing constraints constant should support moving that view up, and vice versa)
/// - changeConstraintConstantExactlyBasedOnMoveValue: Boolean value which indicates if
/// - additionalOffset: Additional offset if we don't want a keyboard to be sticked to a text field or to a text view
open func hcChangeConstraintsConstantBasedOnKeyboardAnimatingPosition(constraints:NSLayoutConstraint..., changeConstraintConstantExactlyBasedOnMoveValue:Bool = false, additionalOffset:CGFloat = 0.0)
{
self.hcSetKeyboardListener(onAppear: { moveValue, keyboardHeight in
for constraint in constraints
{
constraint.constant = changeConstraintConstantExactlyBasedOnMoveValue ? moveValue + additionalOffset : keyboardHeight + additionalOffset
}
UIView.animate(withDuration: 0.3, animations: {
self.view.layoutIfNeeded()
})
}, onHide: {
for constraint in constraints
{
constraint.constant = 0.0
}
UIView.animate(withDuration: 0.3, animations: {
self.view.layoutIfNeeded()
})
})
}
/// Set keyboard listener in order to handle appearing and disappearing keyboard
///
/// - Parameters:
/// - onAppear: Handler for handling event when keyboard will appear
/// - onHide: Handler for handling event when keyboard will disappear
open func hcSetKeyboardListener(onAppear:@escaping CompletionTwoNumbersHandler, onHide:@escaping EmptyCompletionHandler)
{
self.appearHandler = onAppear
self.hideHandler = onHide
NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillAppear(_:)), name: UIResponder.keyboardWillShowNotification, object: nil)
NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillDisappear), name: UIResponder.keyboardWillHideNotification, object: nil)
}
/// Handdle event when keyboard will appear
///
/// - Parameter notification: Notification which has some information about keyboard, for example keyboard size
@objc private func keyboardWillAppear(_ notification:NSNotification) {
//Do something here
if let keyboardFrame: NSValue = notification.userInfo?[UIResponder.keyboardFrameEndUserInfoKey] as? NSValue
{
let keyboardRectangle = keyboardFrame.cgRectValue
let keyboardHeight = keyboardRectangle.height
if let firstResponder = UIView.hcFindFirstResponder(inView: self.view)
{
if let firstResponderSuperview = firstResponder.superview
{
let firstResponderBottom = firstResponderSuperview.convert(firstResponder.frame.origin, to: self.view).y + firstResponder.frame.size.height
let move = firstResponderBottom + keyboardHeight < self.view.frame.size.height ? 0.0 : firstResponderBottom + keyboardHeight - self.view.frame.size.height
self.appearHandler(move, keyboardHeight)
}
}
}
}
/// Handdle event when keyboard will disappear
@objc private func keyboardWillDisappear() {
//Do something here
hideHandler()
}
}
| mit | 64c1f8655ee018e3d26bdcb04a3680b0 | 42.407285 | 417 | 0.666412 | 5.228959 | false | false | false | false |
airbnb/lottie-ios | Sources/Private/Model/ShapeItems/Rectangle.swift | 3 | 2404 | //
// Rectangle.swift
// lottie-swift
//
// Created by Brandon Withrow on 1/8/19.
//
import Foundation
final class Rectangle: ShapeItem {
// MARK: Lifecycle
required init(from decoder: Decoder) throws {
let container = try decoder.container(keyedBy: Rectangle.CodingKeys.self)
direction = try container.decodeIfPresent(PathDirection.self, forKey: .direction) ?? .clockwise
position = try container.decode(KeyframeGroup<LottieVector3D>.self, forKey: .position)
size = try container.decode(KeyframeGroup<LottieVector3D>.self, forKey: .size)
cornerRadius = try container.decode(KeyframeGroup<LottieVector1D>.self, forKey: .cornerRadius)
try super.init(from: decoder)
}
required init(dictionary: [String: Any]) throws {
if
let directionRawType = dictionary[CodingKeys.direction.rawValue] as? Int,
let direction = PathDirection(rawValue: directionRawType)
{
self.direction = direction
} else {
direction = .clockwise
}
let positionDictionary: [String: Any] = try dictionary.value(for: CodingKeys.position)
position = try KeyframeGroup<LottieVector3D>(dictionary: positionDictionary)
let sizeDictionary: [String: Any] = try dictionary.value(for: CodingKeys.size)
size = try KeyframeGroup<LottieVector3D>(dictionary: sizeDictionary)
let cornerRadiusDictionary: [String: Any] = try dictionary.value(for: CodingKeys.cornerRadius)
cornerRadius = try KeyframeGroup<LottieVector1D>(dictionary: cornerRadiusDictionary)
try super.init(dictionary: dictionary)
}
// MARK: Internal
/// The direction of the rect.
let direction: PathDirection
/// The position
let position: KeyframeGroup<LottieVector3D>
/// The size
let size: KeyframeGroup<LottieVector3D>
/// The Corner radius of the rectangle
let cornerRadius: KeyframeGroup<LottieVector1D>
override func encode(to encoder: Encoder) throws {
try super.encode(to: encoder)
var container = encoder.container(keyedBy: CodingKeys.self)
try container.encode(direction, forKey: .direction)
try container.encode(position, forKey: .position)
try container.encode(size, forKey: .size)
try container.encode(cornerRadius, forKey: .cornerRadius)
}
// MARK: Private
private enum CodingKeys: String, CodingKey {
case direction = "d"
case position = "p"
case size = "s"
case cornerRadius = "r"
}
}
| apache-2.0 | 92a6ec1b77b58a32741b04bf94817a6d | 32.388889 | 99 | 0.725458 | 4.217544 | false | false | false | false |
cwoloszynski/FluentCustomFieldExample | Tests/FluentTestTests/XCTAssertExtensions.swift | 1 | 722 | //
// XCTAssertExtensions.swift
// GotYourBackServer
//
// Created by Charlie Woloszynski on 11/23/16.
//
//
import XCTest
func XCTAssertThrows<T>(_ expression: @autoclosure () throws -> T, _ message: String = "", file: StaticString = #file, line: UInt = #line) {
do {
let _ = try expression()
XCTAssert(false, "No error to catch! - \(message)", file: file, line: line)
} catch {
}
}
func XCTAssertNoThrow<T>(_ expression: @autoclosure () throws -> T, _ message: String = "", file: StaticString = #file, line: UInt = #line) {
do {
let _ = try expression()
} catch let error {
XCTAssert(false, "Caught error: \(error) - \(message)", file: file, line: line)
}
}
| mit | fbb8079410d435724444e22487591dbf | 27.88 | 141 | 0.599723 | 3.55665 | false | false | false | false |
niunaruto/DeDaoAppSwift | DeDaoSwift/Pods/ReactiveSwift/Sources/UnidirectionalBinding.swift | 1 | 6102 | import Foundation
import Dispatch
import enum Result.NoError
precedencegroup BindingPrecedence {
associativity: right
// Binds tighter than assignment but looser than everything else
higherThan: AssignmentPrecedence
}
infix operator <~ : BindingPrecedence
/// Describes a source which can be bound.
public protocol BindingSourceProtocol {
associatedtype Value
associatedtype Error: Swift.Error
/// Observe the binding source by sending any events to the given observer.
@discardableResult
func observe(_ observer: Observer<Value, Error>, during lifetime: Lifetime) -> Disposable?
}
extension Signal: BindingSourceProtocol {
@discardableResult
public func observe(_ observer: Observer, during lifetime: Lifetime) -> Disposable? {
return self.take(during: lifetime).observe(observer)
}
}
extension SignalProducer: BindingSourceProtocol {
@discardableResult
public func observe(_ observer: ProducedSignal.Observer, during lifetime: Lifetime) -> Disposable? {
var disposable: Disposable!
self
.take(during: lifetime)
.startWithSignal { signal, signalDisposable in
disposable = signalDisposable
signal.observe(observer)
}
return disposable
}
}
/// Describes a target which can be bound.
public protocol BindingTargetProtocol: class {
associatedtype Value
/// The lifetime of `self`. The binding operators use this to determine when
/// the binding should be torn down.
var lifetime: Lifetime { get }
/// Consume a value from the binding.
func consume(_ value: Value)
}
/// Binds a source to a target, updating the target's value to the latest
/// value sent by the source.
///
/// - note: The binding will automatically terminate when the target is
/// deinitialized, or when the source sends a `completed` event.
///
/// ````
/// let property = MutableProperty(0)
/// let signal = Signal({ /* do some work after some time */ })
/// property <~ signal
/// ````
///
/// ````
/// let property = MutableProperty(0)
/// let signal = Signal({ /* do some work after some time */ })
/// let disposable = property <~ signal
/// ...
/// // Terminates binding before property dealloc or signal's
/// // `completed` event.
/// disposable.dispose()
/// ````
///
/// - parameters:
/// - target: A target to be bond to.
/// - source: A source to bind.
///
/// - returns: A disposable that can be used to terminate binding before the
/// deinitialization of the target or the source's `completed`
/// event.
@discardableResult
public func <~
<Target: BindingTargetProtocol, Source: BindingSourceProtocol>
(target: Target, source: Source) -> Disposable?
where Source.Value == Target.Value, Source.Error == NoError
{
// Alter the semantics of `BindingTarget` to not require it to be retained.
// This is done here--and not in a separate function--so that all variants
// of `<~` can get this behavior.
let observer: Observer<Target.Value, NoError>
if let target = target as? BindingTarget<Target.Value> {
observer = Observer(value: { [setter = target.setter] in setter($0) })
} else {
observer = Observer(value: { [weak target] in target?.consume($0) })
}
return source.observe(observer, during: target.lifetime)
}
/// Binds a source to a target, updating the target's value to the latest
/// value sent by the source.
///
/// - note: The binding will automatically terminate when the target is
/// deinitialized, or when the source sends a `completed` event.
///
/// ````
/// let property = MutableProperty(0)
/// let signal = Signal({ /* do some work after some time */ })
/// property <~ signal
/// ````
///
/// ````
/// let property = MutableProperty(0)
/// let signal = Signal({ /* do some work after some time */ })
/// let disposable = property <~ signal
/// ...
/// // Terminates binding before property dealloc or signal's
/// // `completed` event.
/// disposable.dispose()
/// ````
///
/// - parameters:
/// - target: A target to be bond to.
/// - source: A source to bind.
///
/// - returns: A disposable that can be used to terminate binding before the
/// deinitialization of the target or the source's `completed`
/// event.
@discardableResult
public func <~
<Target: BindingTargetProtocol, Source: BindingSourceProtocol>
(target: Target, source: Source) -> Disposable?
where Target.Value: OptionalProtocol, Source.Value == Target.Value.Wrapped, Source.Error == NoError
{
// Alter the semantics of `BindingTarget` to not require it to be retained.
// This is done here--and not in a separate function--so that all variants
// of `<~` can get this behavior.
let observer: Observer<Source.Value, NoError>
if let target = target as? BindingTarget<Target.Value> {
observer = Observer(value: { [setter = target.setter] in setter(Target.Value(reconstructing: $0)) })
} else {
observer = Observer(value: { [weak target] in target?.consume(Target.Value(reconstructing: $0)) })
}
return source.observe(observer, during: target.lifetime)
}
/// A binding target that can be used with the `<~` operator.
public final class BindingTarget<Value>: BindingTargetProtocol {
public let lifetime: Lifetime
fileprivate let setter: (Value) -> Void
/// Creates a binding target.
///
/// - parameters:
/// - lifetime: The expected lifetime of any bindings towards `self`.
/// - setter: The action to consume values.
public init(lifetime: Lifetime, setter: @escaping (Value) -> Void) {
self.setter = setter
self.lifetime = lifetime
}
/// Creates a binding target which consumes values on the specified scheduler.
///
/// - parameters:
/// - scheduler: The scheduler on which the `setter` consumes the values.
/// - lifetime: The expected lifetime of any bindings towards `self`.
/// - setter: The action to consume values.
public convenience init(on scheduler: SchedulerProtocol, lifetime: Lifetime, setter: @escaping (Value) -> Void) {
let setter: (Value) -> Void = { value in
scheduler.schedule {
setter(value)
}
}
self.init(lifetime: lifetime, setter: setter)
}
public func consume(_ value: Value) {
setter(value)
}
}
| mit | 635f4f6a188ac238f144b77fb6bdbc22 | 31.457447 | 114 | 0.695182 | 3.975244 | false | false | false | false |
alexsosn/ConvNetSwift | ConvNetSwift/convnet-swift/Util.swift | 1 | 2366 | import Foundation
import GameplayKit
// Random number utilities
class RandUtils {
static var return_v = false
static var v_val = 0.0
static func random_js() -> Double {
return drand48()
// should be [0 .. 1)
}
static func randf(_ a: Double, _ b: Double) -> Double {
return random_js()*(b-a)+a
}
static func randi(_ a: Int, _ b: Int) -> Int {
return Int(floor(random_js()))*(b-a)+a
}
private static let gaussDistribution = GKGaussianDistribution(randomSource: GKRandomSource(), mean:0, deviation: 1)
static func randn(_ mu: Double, std: Double) -> Double {
return (Double(gaussDistribution.nextUniform()) + mu) * std
}
}
// Array utilities
struct ArrayUtils {
static func zerosInt(_ n: Int) -> [Int] {
return [Int](repeating: 0, count: n)
}
static func zerosDouble(_ n: Int) -> [Double] {
return [Double](repeating: 0.0, count: n)
}
static func zerosBool(_ n: Int) -> [Bool] {
return [Bool](repeating: false, count: n)
}
static func arrUnique(_ arr: [Int]) -> [Int] {
return Array(Set(arr))
}
}
// return max and min of a given non-empty array.
struct Maxmin {
var maxi: Int
var maxv: Double
var mini: Int
var minv: Double
var dv: Double
}
func maxmin(_ w: [Double]) -> Maxmin? {
guard (w.count > 0),
let maxv = w.max(),
let maxi = w.index(of: maxv),
let minv = w.min(),
let mini = w.index(of: minv)
else {
return nil
}
return Maxmin(maxi: maxi, maxv: maxv, mini: mini, minv: minv, dv: maxv-minv)
}
// create random permutation of numbers, in range [0...n-1]
func randomPermutation(_ n: Int) -> [Int]{
let dist = GKShuffledDistribution(lowestValue: 0, highestValue: n-1)
return (0..<n).map{_ in dist.nextInt()}
}
// sample from list lst according to probabilities in list probs
// the two lists are of same size, and probs adds up to 1
func weightedSample(_ lst: [Double], probs: [Double]) -> Double? {
let p = RandUtils.randf(0, 1.0)
var cumprob = 0.0
let n=lst.count
for k in 0 ..< n {
cumprob += probs[k]
if p < cumprob { return lst[k] }
}
return nil
}
struct TimeUtils {
static func getNanoseconds() {
}
}
| mit | dac8cb310c0de2f4e8538f2dddd49f5e | 23.391753 | 119 | 0.575655 | 3.424023 | false | false | false | false |
loudnate/Loop | Learn/Lessons/TimeInRangeLesson.swift | 1 | 6674 | //
// LessonPlayground.swift
// Learn
//
// Copyright © 2019 LoopKit Authors. All rights reserved.
//
import Foundation
import LoopCore
import LoopKit
import LoopKitUI
import LoopUI
import HealthKit
import os.log
final class TimeInRangeLesson: Lesson {
let title = NSLocalizedString("Time in Range", comment: "Lesson title")
let subtitle = NSLocalizedString("Computes the percentage of glucose measurements within a specified range", comment: "Lesson subtitle")
let configurationSections: [LessonSectionProviding]
private let dataManager: DataManager
private let glucoseUnit: HKUnit
private let glucoseFormatter = QuantityFormatter()
private let dateIntervalEntry: DateIntervalEntry
private let rangeEntry: QuantityRangeEntry
init(dataManager: DataManager) {
self.dataManager = dataManager
self.glucoseUnit = dataManager.glucoseStore.preferredUnit ?? .milligramsPerDeciliter
glucoseFormatter.setPreferredNumberFormatter(for: glucoseUnit)
dateIntervalEntry = DateIntervalEntry(
end: Date(),
weeks: 2
)
rangeEntry = QuantityRangeEntry.glucoseRange(
minValue: HKQuantity(unit: .milligramsPerDeciliter, doubleValue: 80),
maxValue: HKQuantity(unit: .milligramsPerDeciliter, doubleValue: 160),
quantityFormatter: glucoseFormatter,
unit: glucoseUnit)
self.configurationSections = [
dateIntervalEntry,
rangeEntry
]
}
func execute(completion: @escaping ([LessonSectionProviding]) -> Void) {
guard let dates = dateIntervalEntry.dateInterval, let closedRange = rangeEntry.closedRange else {
// TODO: Cleaner error presentation
completion([LessonSection(headerTitle: "Error: Please fill out all fields", footerTitle: nil, cells: [])])
return
}
let calculator = TimeInRangeCalculator(dataManager: dataManager, dates: dates, range: closedRange)
calculator.execute { result in
switch result {
case .failure(let error):
completion([
LessonSection(cells: [TextCell(text: String(describing: error))])
])
case .success(let resultsByDay):
guard resultsByDay.count > 0 else {
completion([
LessonSection(cells: [TextCell(text: NSLocalizedString("No data available", comment: "Lesson result text for no data"))])
])
return
}
let dateFormatter = DateIntervalFormatter(dateStyle: .short, timeStyle: .none)
let numberFormatter = NumberFormatter()
numberFormatter.numberStyle = .percent
var aggregator = TimeInRangeAggregator()
resultsByDay.forEach({ (pair) in
aggregator.add(percentInRange: pair.value, for: pair.key)
})
completion([
TimesInRangeSection(
ranges: aggregator.results.map { [$0.range:$0.value] } ?? [:],
dateFormatter: dateFormatter,
numberFormatter: numberFormatter
),
TimesInRangeSection(
ranges: resultsByDay,
dateFormatter: dateFormatter,
numberFormatter: numberFormatter
)
])
}
}
}
}
class TimesInRangeSection: LessonSectionProviding {
let cells: [LessonCellProviding]
init(ranges: [DateInterval: Double], dateFormatter: DateIntervalFormatter, numberFormatter: NumberFormatter) {
cells = ranges.sorted(by: { $0.0 < $1.0 }).map { pair -> LessonCellProviding in
DatesAndNumberCell(date: pair.key, value: NSNumber(value: pair.value), dateFormatter: dateFormatter, numberFormatter: numberFormatter)
}
}
}
struct TimeInRangeAggregator {
private var count = 0
private var sum: Double = 0
var allDates: DateInterval?
var averagePercentInRange: Double? {
guard count > 0 else {
return nil
}
return sum / Double(count)
}
var results: (range: DateInterval, value: Double)? {
guard let allDates = allDates, let averagePercentInRange = averagePercentInRange else {
return nil
}
return (range: allDates, value: averagePercentInRange)
}
mutating func add(percentInRange: Double, for dates: DateInterval) {
sum += percentInRange
count += 1
if let allDates = self.allDates {
self.allDates = DateInterval(start: min(allDates.start, dates.start), end: max(allDates.end, dates.end))
} else {
self.allDates = dates
}
}
}
/// Time-in-range, e.g. "2 weeks starting on March 5"
private class TimeInRangeCalculator {
let calculator: DayCalculator<[DateInterval: Double]>
let range: ClosedRange<HKQuantity>
private let log: OSLog
private let unit = HKUnit.milligramsPerDeciliter
init(dataManager: DataManager, dates: DateInterval, range: ClosedRange<HKQuantity>) {
self.calculator = DayCalculator(dataManager: dataManager, dates: dates, initial: [:])
self.range = range
log = OSLog(category: String(describing: type(of: self)))
}
func execute(completion: @escaping (_ result: Result<[DateInterval: Double]>) -> Void) {
os_log(.default, log: log, "Computing Time in range from %{public}@ between %{public}@", String(describing: calculator.dates), String(describing: range))
calculator.execute(calculator: { (dataManager, day, results, completion) in
os_log(.default, log: self.log, "Fetching samples in %{public}@", String(describing: day))
dataManager.glucoseStore.getGlucoseSamples(start: day.start, end: day.end) { (result) in
switch result {
case .failure(let error):
os_log(.error, log: self.log, "Failed to fetch samples: %{public}@", String(describing: error))
completion(error)
case .success(let samples):
if let timeInRange = samples.proportion(where: { self.range.contains($0.quantity) }) {
_ = results.mutate({ (results) in
results[day] = timeInRange
})
}
completion(nil)
}
}
}, completion: completion)
}
}
| apache-2.0 | a81c284b75733c5ff35603c5297a5f4c | 34.121053 | 161 | 0.604676 | 5.188958 | false | false | false | false |
andrebocchini/SwiftChattyOSX | Pods/SwiftChatty/SwiftChatty/Requests/Messages/DeleteMessageRequest.swift | 1 | 698 | //
// DeleteMessageRequest.swift
// SwiftChatty
//
// Created by Andre Bocchini on 1/24/16.
// Copyright © 2016 Andre Bocchini. All rights reserved.
//
import Alamofire
/// - SeeAlso: http://winchatty.com/v2/readme#_Toc421451695
public struct DeleteMessageRequest: Request {
public let endpoint: ApiEndpoint = .DeleteMessage
public let account: Account
public let httpMethod: Alamofire.Method = .POST
public var parameters: [String : AnyObject] = [:]
public init(withAccount account: Account, messageId: Int, folder: Mailbox) {
self.account = account
self.parameters["messageId"] = messageId
self.parameters["folder"] = folder.rawValue
}
}
| mit | a4fe8c96e38179890f19694e13a5db35 | 26.88 | 80 | 0.695839 | 4.198795 | false | false | false | false |
JaSpa/swift | test/SILOptimizer/cast_folding_objc_no_foundation.swift | 4 | 2989 | // RUN: %target-swift-frontend(mock-sdk: %clang-importer-sdk) -Xllvm -new-mangling-for-tests -O -emit-sil %s | %FileCheck %s
// REQUIRES: objc_interop
// TODO: Update optimizer for id-as-Any changes.
// Note: no 'import Foundation'
struct PlainStruct {}
// CHECK-LABEL: sil hidden [noinline] @_T031cast_folding_objc_no_foundation23testAnyObjectToArrayIntSbs0gH0_pFTf4g_n
// CHECK: bb0(%0 : $AnyObject):
// CHECK: [[SOURCE:%.*]] = alloc_stack $AnyObject
// CHECK: [[TARGET:%.*]] = alloc_stack $Array<Int>
// CHECK: checked_cast_addr_br take_always AnyObject in [[SOURCE]] : $*AnyObject to Array<Int> in [[TARGET]] : $*Array<Int>, bb1, bb2
@inline(never)
func testAnyObjectToArrayInt(_ a: AnyObject) -> Bool {
return a is [Int]
}
// CHECK-LABEL: sil hidden [noinline] @_T031cast_folding_objc_no_foundation26testAnyObjectToArrayStringSbs0gH0_pFTf4g_n
// CHECK: bb0(%0 : $AnyObject):
// CHECK: [[SOURCE:%.*]] = alloc_stack $AnyObject
// CHECK: [[TARGET:%.*]] = alloc_stack $Array<String>
// CHECK: checked_cast_addr_br take_always AnyObject in [[SOURCE]] : $*AnyObject to Array<String> in [[TARGET]] : $*Array<String>, bb1, bb2
@inline(never)
func testAnyObjectToArrayString(_ a: AnyObject) -> Bool {
return a is [String]
}
// CHECK-LABEL: sil hidden [noinline] @_T031cast_folding_objc_no_foundation30testAnyObjectToArrayNotBridged{{.*}}
// CHECK: bb0(%0 : $AnyObject):
// CHECK: [[SOURCE:%.*]] = alloc_stack $AnyObject
// CHECK: [[TARGET:%.*]] = alloc_stack $Array<PlainStruct>
// CHECK: checked_cast_addr_br take_always AnyObject in [[SOURCE]] : $*AnyObject to Array<PlainStruct> in [[TARGET]] : $*Array<PlainStruct>, bb1, bb2
@inline(never)
func testAnyObjectToArrayNotBridged(_ a: AnyObject) -> Bool {
return a is [PlainStruct]
}
// CHECK-LABEL: sil hidden [noinline] @_T031cast_folding_objc_no_foundation25testAnyObjectToDictionarySbs0gH0_pFTf4g_n
// CHECK: bb0(%0 : $AnyObject):
// CHECK: [[SOURCE:%.*]] = alloc_stack $AnyObject
// CHECK: [[TARGET:%.*]] = alloc_stack $Dictionary<Int, String>
// CHECK: checked_cast_addr_br take_always AnyObject in [[SOURCE]] : $*AnyObject to Dictionary<Int, String> in [[TARGET]] : $*Dictionary<Int, String>, bb1, bb2
@inline(never)
func testAnyObjectToDictionary(_ a: AnyObject) -> Bool {
return a is [Int: String]
}
// CHECK-LABEL: sil hidden [noinline] @_T031cast_folding_objc_no_foundation21testAnyObjectToStringSbs0gH0_pFTf4g_n
// CHECK: bb0(%0 : $AnyObject):
// CHECK: [[SOURCE:%.*]] = alloc_stack $AnyObject
// CHECK: [[TARGET:%.*]] = alloc_stack $String
// CHECK: checked_cast_addr_br take_always AnyObject in [[SOURCE]] : $*AnyObject to String in [[TARGET]] : $*String, bb1, bb2
@inline(never)
func testAnyObjectToString(_ a: AnyObject) -> Bool {
return a is String
}
class SomeObject {}
print(testAnyObjectToArrayInt(SomeObject()))
print(testAnyObjectToArrayString(SomeObject()))
print(testAnyObjectToArrayNotBridged(SomeObject()))
print(testAnyObjectToDictionary(SomeObject()))
print(testAnyObjectToString(SomeObject()))
| apache-2.0 | 7198860a15fd60449fdc5345abc7f028 | 44.287879 | 159 | 0.710271 | 3.423826 | false | true | false | false |
scinfu/SwiftSoup | Sources/Element.swift | 1 | 48253 | //
// Element.swift
// SwifSoup
//
// Created by Nabil Chatbi on 29/09/16.
// Copyright © 2016 Nabil Chatbi.. All rights reserved.
//
import Foundation
open class Element: Node {
var _tag: Tag
private static let classString = "class"
private static let emptyString = ""
private static let idString = "id"
private static let rootString = "#root"
//private static let classSplit : Pattern = Pattern("\\s+")
private static let classSplit = "\\s+"
/**
* Create a new, standalone Element. (Standalone in that is has no parent.)
*
* @param tag tag of this element
* @param baseUri the base URI
* @param attributes initial attributes
* @see #appendChild(Node)
* @see #appendElement(String)
*/
public init(_ tag: Tag, _ baseUri: String, _ attributes: Attributes) {
self._tag = tag
super.init(baseUri, attributes)
}
/**
* Create a new Element from a tag and a base URI.
*
* @param tag element tag
* @param baseUri the base URI of this element. It is acceptable for the base URI to be an empty
* string, but not null.
* @see Tag#valueOf(String, ParseSettings)
*/
public init(_ tag: Tag, _ baseUri: String) {
self._tag = tag
super.init(baseUri, Attributes())
}
open override func nodeName() -> String {
return _tag.getName()
}
/**
* Get the name of the tag for this element. E.g. {@code div}
*
* @return the tag name
*/
open func tagName() -> String {
return _tag.getName()
}
open func tagNameNormal() -> String {
return _tag.getNameNormal()
}
/**
* Change the tag of this element. For example, convert a {@code <span>} to a {@code <div>} with
* {@code el.tagName("div")}.
*
* @param tagName new tag name for this element
* @return this element, for chaining
*/
@discardableResult
public func tagName(_ tagName: String)throws->Element {
try Validate.notEmpty(string: tagName, msg: "Tag name must not be empty.")
_tag = try Tag.valueOf(tagName, ParseSettings.preserveCase) // preserve the requested tag case
return self
}
/**
* Get the Tag for this element.
*
* @return the tag object
*/
open func tag() -> Tag {
return _tag
}
/**
* Test if this element is a block-level element. (E.g. {@code <div> == true} or an inline element
* {@code <p> == false}).
*
* @return true if block, false if not (and thus inline)
*/
open func isBlock() -> Bool {
return _tag.isBlock()
}
/**
* Get the {@code id} attribute of this element.
*
* @return The id attribute, if present, or an empty string if not.
*/
open func id() -> String {
guard let attributes = attributes else {return Element.emptyString}
do {
return try attributes.getIgnoreCase(key: Element.idString)
} catch {}
return Element.emptyString
}
/**
* Set an attribute value on this element. If this element already has an attribute with the
* key, its value is updated; otherwise, a new attribute is added.
*
* @return this element
*/
@discardableResult
open override func attr(_ attributeKey: String, _ attributeValue: String)throws->Element {
try super.attr(attributeKey, attributeValue)
return self
}
/**
* Set a boolean attribute value on this element. Setting to <code>true</code> sets the attribute value to "" and
* marks the attribute as boolean so no value is written out. Setting to <code>false</code> removes the attribute
* with the same key if it exists.
*
* @param attributeKey the attribute key
* @param attributeValue the attribute value
*
* @return this element
*/
@discardableResult
open func attr(_ attributeKey: String, _ attributeValue: Bool)throws->Element {
try attributes?.put(attributeKey, attributeValue)
return self
}
/**
* Get this element's HTML5 custom data attributes. Each attribute in the element that has a key
* starting with "data-" is included the dataset.
* <p>
* E.g., the element {@code <div data-package="SwiftSoup" data-language="Java" class="group">...} has the dataset
* {@code package=SwiftSoup, language=java}.
* <p>
* This map is a filtered view of the element's attribute map. Changes to one map (add, remove, update) are reflected
* in the other map.
* <p>
* You can find elements that have data attributes using the {@code [^data-]} attribute key prefix selector.
* @return a map of {@code key=value} custom data attributes.
*/
open func dataset()->Dictionary<String, String> {
return attributes!.dataset()
}
open override func parent() -> Element? {
return parentNode as? Element
}
/**
* Get this element's parent and ancestors, up to the document root.
* @return this element's stack of parents, closest first.
*/
open func parents() -> Elements {
let parents: Elements = Elements()
Element.accumulateParents(self, parents)
return parents
}
private static func accumulateParents(_ el: Element, _ parents: Elements) {
let parent: Element? = el.parent()
if (parent != nil && !(parent!.tagName() == Element.rootString)) {
parents.add(parent!)
accumulateParents(parent!, parents)
}
}
/**
* Get a child element of this element, by its 0-based index number.
* <p>
* Note that an element can have both mixed Nodes and Elements as children. This method inspects
* a filtered list of children that are elements, and the index is based on that filtered list.
* </p>
*
* @param index the index number of the element to retrieve
* @return the child element, if it exists, otherwise throws an {@code IndexOutOfBoundsException}
* @see #childNode(int)
*/
open func child(_ index: Int) -> Element {
return children().get(index)
}
/**
* Get this element's child elements.
* <p>
* This is effectively a filter on {@link #childNodes()} to get Element nodes.
* </p>
* @return child elements. If this element has no children, returns an
* empty list.
* @see #childNodes()
*/
open func children() -> Elements {
// create on the fly rather than maintaining two lists. if gets slow, memoize, and mark dirty on change
var elements = Array<Element>()
for node in childNodes {
if let n = node as? Element {
elements.append(n)
}
}
return Elements(elements)
}
/**
* Get this element's child text nodes. The list is unmodifiable but the text nodes may be manipulated.
* <p>
* This is effectively a filter on {@link #childNodes()} to get Text nodes.
* @return child text nodes. If this element has no text nodes, returns an
* empty list.
* </p>
* For example, with the input HTML: {@code <p>One <span>Two</span> Three <br> Four</p>} with the {@code p} element selected:
* <ul>
* <li>{@code p.text()} = {@code "One Two Three Four"}</li>
* <li>{@code p.ownText()} = {@code "One Three Four"}</li>
* <li>{@code p.children()} = {@code Elements[<span>, <br>]}</li>
* <li>{@code p.childNodes()} = {@code List<Node>["One ", <span>, " Three ", <br>, " Four"]}</li>
* <li>{@code p.textNodes()} = {@code List<TextNode>["One ", " Three ", " Four"]}</li>
* </ul>
*/
open func textNodes()->Array<TextNode> {
var textNodes = Array<TextNode>()
for node in childNodes {
if let n = node as? TextNode {
textNodes.append(n)
}
}
return textNodes
}
/**
* Get this element's child data nodes. The list is unmodifiable but the data nodes may be manipulated.
* <p>
* This is effectively a filter on {@link #childNodes()} to get Data nodes.
* </p>
* @return child data nodes. If this element has no data nodes, returns an
* empty list.
* @see #data()
*/
open func dataNodes()->Array<DataNode> {
var dataNodes = Array<DataNode>()
for node in childNodes {
if let n = node as? DataNode {
dataNodes.append(n)
}
}
return dataNodes
}
/**
* Find elements that match the {@link CssSelector} CSS query, with this element as the starting context. Matched elements
* may include this element, or any of its children.
* <p>
* This method is generally more powerful to use than the DOM-type {@code getElementBy*} methods, because
* multiple filters can be combined, e.g.:
* </p>
* <ul>
* <li>{@code el.select("a[href]")} - finds links ({@code a} tags with {@code href} attributes)
* <li>{@code el.select("a[href*=example.com]")} - finds links pointing to example.com (loosely)
* </ul>
* <p>
* See the query syntax documentation in {@link CssSelector}.
* </p>
*
* @param cssQuery a {@link CssSelector} CSS-like query
* @return elements that match the query (empty if none match)
* @see CssSelector
* @throws CssSelector.SelectorParseException (unchecked) on an invalid CSS query.
*/
public func select(_ cssQuery: String)throws->Elements {
return try CssSelector.select(cssQuery, self)
}
/**
* Check if this element matches the given {@link CssSelector} CSS query.
* @param cssQuery a {@link CssSelector} CSS query
* @return if this element matches the query
*/
public func iS(_ cssQuery: String)throws->Bool {
return try iS(QueryParser.parse(cssQuery))
}
/**
* Check if this element matches the given {@link CssSelector} CSS query.
* @param cssQuery a {@link CssSelector} CSS query
* @return if this element matches the query
*/
public func iS(_ evaluator: Evaluator)throws->Bool {
guard let od = self.ownerDocument() else {
return false
}
return try evaluator.matches(od, self)
}
/**
* Add a node child node to this element.
*
* @param child node to add.
* @return this element, so that you can add more child nodes or elements.
*/
@discardableResult
public func appendChild(_ child: Node)throws->Element {
// was - Node#addChildren(child). short-circuits an array create and a loop.
try reparentChild(child)
ensureChildNodes()
childNodes.append(child)
child.setSiblingIndex(childNodes.count - 1)
return self
}
/**
* Add a node to the start of this element's children.
*
* @param child node to add.
* @return this element, so that you can add more child nodes or elements.
*/
@discardableResult
public func prependChild(_ child: Node)throws->Element {
try addChildren(0, child)
return self
}
/**
* Inserts the given child nodes into this element at the specified index. Current nodes will be shifted to the
* right. The inserted nodes will be moved from their current parent. To prevent moving, copy the nodes first.
*
* @param index 0-based index to insert children at. Specify {@code 0} to insert at the start, {@code -1} at the
* end
* @param children child nodes to insert
* @return this element, for chaining.
*/
@discardableResult
public func insertChildren(_ index: Int, _ children: Array<Node>)throws->Element {
//Validate.notNull(children, "Children collection to be inserted must not be null.")
var index = index
let currentSize: Int = childNodeSize()
if (index < 0) { index += currentSize + 1} // roll around
try Validate.isTrue(val: index >= 0 && index <= currentSize, msg: "Insert position out of bounds.")
try addChildren(index, children)
return self
}
/**
* Create a new element by tag name, and add it as the last child.
*
* @param tagName the name of the tag (e.g. {@code div}).
* @return the new element, to allow you to add content to it, e.g.:
* {@code parent.appendElement("h1").attr("id", "header").text("Welcome")}
*/
@discardableResult
public func appendElement(_ tagName: String)throws->Element {
let child: Element = Element(try Tag.valueOf(tagName), getBaseUri())
try appendChild(child)
return child
}
/**
* Create a new element by tag name, and add it as the first child.
*
* @param tagName the name of the tag (e.g. {@code div}).
* @return the new element, to allow you to add content to it, e.g.:
* {@code parent.prependElement("h1").attr("id", "header").text("Welcome")}
*/
@discardableResult
public func prependElement(_ tagName: String)throws->Element {
let child: Element = Element(try Tag.valueOf(tagName), getBaseUri())
try prependChild(child)
return child
}
/**
* Create and append a new TextNode to this element.
*
* @param text the unencoded text to add
* @return this element
*/
@discardableResult
public func appendText(_ text: String)throws->Element {
let node: TextNode = TextNode(text, getBaseUri())
try appendChild(node)
return self
}
/**
* Create and prepend a new TextNode to this element.
*
* @param text the unencoded text to add
* @return this element
*/
@discardableResult
public func prependText(_ text: String)throws->Element {
let node: TextNode = TextNode(text, getBaseUri())
try prependChild(node)
return self
}
/**
* Add inner HTML to this element. The supplied HTML will be parsed, and each node appended to the end of the children.
* @param html HTML to add inside this element, after the existing HTML
* @return this element
* @see #html(String)
*/
@discardableResult
public func append(_ html: String)throws->Element {
let nodes: Array<Node> = try Parser.parseFragment(html, self, getBaseUri())
try addChildren(nodes)
return self
}
/**
* Add inner HTML into this element. The supplied HTML will be parsed, and each node prepended to the start of the element's children.
* @param html HTML to add inside this element, before the existing HTML
* @return this element
* @see #html(String)
*/
@discardableResult
public func prepend(_ html: String)throws->Element {
let nodes: Array<Node> = try Parser.parseFragment(html, self, getBaseUri())
try addChildren(0, nodes)
return self
}
/**
* Insert the specified HTML into the DOM before this element (as a preceding sibling).
*
* @param html HTML to add before this element
* @return this element, for chaining
* @see #after(String)
*/
@discardableResult
open override func before(_ html: String)throws->Element {
return try super.before(html) as! Element
}
/**
* Insert the specified node into the DOM before this node (as a preceding sibling).
* @param node to add before this element
* @return this Element, for chaining
* @see #after(Node)
*/
@discardableResult
open override func before(_ node: Node)throws->Element {
return try super.before(node) as! Element
}
/**
* Insert the specified HTML into the DOM after this element (as a following sibling).
*
* @param html HTML to add after this element
* @return this element, for chaining
* @see #before(String)
*/
@discardableResult
open override func after(_ html: String)throws->Element {
return try super.after(html) as! Element
}
/**
* Insert the specified node into the DOM after this node (as a following sibling).
* @param node to add after this element
* @return this element, for chaining
* @see #before(Node)
*/
open override func after(_ node: Node)throws->Element {
return try super.after(node) as! Element
}
/**
* Remove all of the element's child nodes. Any attributes are left as-is.
* @return this element
*/
@discardableResult
public func empty() -> Element {
childNodes.removeAll()
return self
}
/**
* Wrap the supplied HTML around this element.
*
* @param html HTML to wrap around this element, e.g. {@code <div class="head"></div>}. Can be arbitrarily deep.
* @return this element, for chaining.
*/
@discardableResult
open override func wrap(_ html: String)throws->Element {
return try super.wrap(html) as! Element
}
/**
* Get a CSS selector that will uniquely select this element.
* <p>
* If the element has an ID, returns #id;
* otherwise returns the parent (if any) CSS selector, followed by {@literal '>'},
* followed by a unique selector for the element (tag.class.class:nth-child(n)).
* </p>
*
* @return the CSS Path that can be used to retrieve the element in a selector.
*/
public func cssSelector()throws->String {
let elementId = id()
if (elementId.count > 0) {
return "#" + elementId
}
// Translate HTML namespace ns:tag to CSS namespace syntax ns|tag
let tagName: String = self.tagName().replacingOccurrences(of: ":", with: "|")
var selector: String = tagName
let cl = try classNames()
let classes: String = cl.joined(separator: ".")
if (classes.count > 0) {
selector.append(".")
selector.append(classes)
}
if (parent() == nil || ((parent() as? Document) != nil)) // don't add Document to selector, as will always have a html node
{
return selector
}
selector.insert(contentsOf: " > ", at: selector.startIndex)
if (try parent()!.select(selector).array().count > 1) {
selector.append(":nth-child(\(try elementSiblingIndex() + 1))")
}
return try parent()!.cssSelector() + (selector)
}
/**
* Get sibling elements. If the element has no sibling elements, returns an empty list. An element is not a sibling
* of itself, so will not be included in the returned list.
* @return sibling elements
*/
public func siblingElements() -> Elements {
if (parentNode == nil) {return Elements()}
let elements: Array<Element>? = parent()?.children().array()
let siblings: Elements = Elements()
if let elements = elements {
for el: Element in elements {
if (el != self) {
siblings.add(el)
}
}
}
return siblings
}
/**
* Gets the next sibling element of this element. E.g., if a {@code div} contains two {@code p}s,
* the {@code nextElementSibling} of the first {@code p} is the second {@code p}.
* <p>
* This is similar to {@link #nextSibling()}, but specifically finds only Elements
* </p>
* @return the next element, or null if there is no next element
* @see #previousElementSibling()
*/
public func nextElementSibling()throws->Element? {
if (parentNode == nil) {return nil}
let siblings: Array<Element>? = parent()?.children().array()
let index: Int? = try Element.indexInList(self, siblings)
try Validate.notNull(obj: index)
if let siblings = siblings {
if (siblings.count > index!+1) {
return siblings[index!+1]
} else {
return nil}
}
return nil
}
/**
* Gets the previous element sibling of this element.
* @return the previous element, or null if there is no previous element
* @see #nextElementSibling()
*/
public func previousElementSibling()throws->Element? {
if (parentNode == nil) {return nil}
let siblings: Array<Element>? = parent()?.children().array()
let index: Int? = try Element.indexInList(self, siblings)
try Validate.notNull(obj: index)
if (index! > 0) {
return siblings?[index!-1]
} else {
return nil
}
}
/**
* Gets the first element sibling of this element.
* @return the first sibling that is an element (aka the parent's first element child)
*/
public func firstElementSibling() -> Element? {
// todo: should firstSibling() exclude this?
let siblings: Array<Element>? = parent()?.children().array()
return (siblings != nil && siblings!.count > 1) ? siblings![0] : nil
}
/*
* Get the list index of this element in its element sibling list. I.e. if this is the first element
* sibling, returns 0.
* @return position in element sibling list
*/
public func elementSiblingIndex()throws->Int {
if (parent() == nil) {return 0}
let x = try Element.indexInList(self, parent()?.children().array())
return x == nil ? 0 : x!
}
/**
* Gets the last element sibling of this element
* @return the last sibling that is an element (aka the parent's last element child)
*/
public func lastElementSibling() -> Element? {
let siblings: Array<Element>? = parent()?.children().array()
return (siblings != nil && siblings!.count > 1) ? siblings![siblings!.count - 1] : nil
}
private static func indexInList(_ search: Element, _ elements: Array<Element>?)throws->Int? {
try Validate.notNull(obj: elements)
if let elements = elements {
for i in 0..<elements.count {
let element: Element = elements[i]
if (element == search) {
return i
}
}
}
return nil
}
// DOM type methods
/**
* Finds elements, including and recursively under this element, with the specified tag name.
* @param tagName The tag name to search for (case insensitively).
* @return a matching unmodifiable list of elements. Will be empty if this element and none of its children match.
*/
public func getElementsByTag(_ tagName: String)throws->Elements {
try Validate.notEmpty(string: tagName)
let tagName = tagName.lowercased().trim()
return try Collector.collect(Evaluator.Tag(tagName), self)
}
/**
* Find an element by ID, including or under this element.
* <p>
* Note that this finds the first matching ID, starting with this element. If you search down from a different
* starting point, it is possible to find a different element by ID. For unique element by ID within a Document,
* use {@link Document#getElementById(String)}
* @param id The ID to search for.
* @return The first matching element by ID, starting with this element, or null if none found.
*/
public func getElementById(_ id: String)throws->Element? {
try Validate.notEmpty(string: id)
let elements: Elements = try Collector.collect(Evaluator.Id(id), self)
if (elements.array().count > 0) {
return elements.get(0)
} else {
return nil
}
}
/**
* Find elements that have this class, including or under this element. Case insensitive.
* <p>
* Elements can have multiple classes (e.g. {@code <div class="header round first">}. This method
* checks each class, so you can find the above with {@code el.getElementsByClass("header")}.
*
* @param className the name of the class to search for.
* @return elements with the supplied class name, empty if none
* @see #hasClass(String)
* @see #classNames()
*/
public func getElementsByClass(_ className: String)throws->Elements {
try Validate.notEmpty(string: className)
return try Collector.collect(Evaluator.Class(className), self)
}
/**
* Find elements that have a named attribute set. Case insensitive.
*
* @param key name of the attribute, e.g. {@code href}
* @return elements that have this attribute, empty if none
*/
public func getElementsByAttribute(_ key: String)throws->Elements {
try Validate.notEmpty(string: key)
let key = key.trim()
return try Collector.collect(Evaluator.Attribute(key), self)
}
/**
* Find elements that have an attribute name starting with the supplied prefix. Use {@code data-} to find elements
* that have HTML5 datasets.
* @param keyPrefix name prefix of the attribute e.g. {@code data-}
* @return elements that have attribute names that start with with the prefix, empty if none.
*/
public func getElementsByAttributeStarting(_ keyPrefix: String)throws->Elements {
try Validate.notEmpty(string: keyPrefix)
let keyPrefix = keyPrefix.trim()
return try Collector.collect(Evaluator.AttributeStarting(keyPrefix), self)
}
/**
* Find elements that have an attribute with the specific value. Case insensitive.
*
* @param key name of the attribute
* @param value value of the attribute
* @return elements that have this attribute with this value, empty if none
*/
public func getElementsByAttributeValue(_ key: String, _ value: String)throws->Elements {
return try Collector.collect(Evaluator.AttributeWithValue(key, value), self)
}
/**
* Find elements that either do not have this attribute, or have it with a different value. Case insensitive.
*
* @param key name of the attribute
* @param value value of the attribute
* @return elements that do not have a matching attribute
*/
public func getElementsByAttributeValueNot(_ key: String, _ value: String)throws->Elements {
return try Collector.collect(Evaluator.AttributeWithValueNot(key, value), self)
}
/**
* Find elements that have attributes that start with the value prefix. Case insensitive.
*
* @param key name of the attribute
* @param valuePrefix start of attribute value
* @return elements that have attributes that start with the value prefix
*/
public func getElementsByAttributeValueStarting(_ key: String, _ valuePrefix: String)throws->Elements {
return try Collector.collect(Evaluator.AttributeWithValueStarting(key, valuePrefix), self)
}
/**
* Find elements that have attributes that end with the value suffix. Case insensitive.
*
* @param key name of the attribute
* @param valueSuffix end of the attribute value
* @return elements that have attributes that end with the value suffix
*/
public func getElementsByAttributeValueEnding(_ key: String, _ valueSuffix: String)throws->Elements {
return try Collector.collect(Evaluator.AttributeWithValueEnding(key, valueSuffix), self)
}
/**
* Find elements that have attributes whose value contains the match string. Case insensitive.
*
* @param key name of the attribute
* @param match substring of value to search for
* @return elements that have attributes containing this text
*/
public func getElementsByAttributeValueContaining(_ key: String, _ match: String)throws->Elements {
return try Collector.collect(Evaluator.AttributeWithValueContaining(key, match), self)
}
/**
* Find elements that have attributes whose values match the supplied regular expression.
* @param key name of the attribute
* @param pattern compiled regular expression to match against attribute values
* @return elements that have attributes matching this regular expression
*/
public func getElementsByAttributeValueMatching(_ key: String, _ pattern: Pattern)throws->Elements {
return try Collector.collect(Evaluator.AttributeWithValueMatching(key, pattern), self)
}
/**
* Find elements that have attributes whose values match the supplied regular expression.
* @param key name of the attribute
* @param regex regular expression to match against attribute values. You can use <a href="http://java.sun.com/docs/books/tutorial/essential/regex/pattern.html#embedded">embedded flags</a> (such as (?i) and (?m) to control regex options.
* @return elements that have attributes matching this regular expression
*/
public func getElementsByAttributeValueMatching(_ key: String, _ regex: String)throws->Elements {
var pattern: Pattern
do {
pattern = Pattern.compile(regex)
try pattern.validate()
} catch {
throw Exception.Error(type: ExceptionType.IllegalArgumentException, Message: "Pattern syntax error: \(regex)")
}
return try getElementsByAttributeValueMatching(key, pattern)
}
/**
* Find elements whose sibling index is less than the supplied index.
* @param index 0-based index
* @return elements less than index
*/
public func getElementsByIndexLessThan(_ index: Int)throws->Elements {
return try Collector.collect(Evaluator.IndexLessThan(index), self)
}
/**
* Find elements whose sibling index is greater than the supplied index.
* @param index 0-based index
* @return elements greater than index
*/
public func getElementsByIndexGreaterThan(_ index: Int)throws->Elements {
return try Collector.collect(Evaluator.IndexGreaterThan(index), self)
}
/**
* Find elements whose sibling index is equal to the supplied index.
* @param index 0-based index
* @return elements equal to index
*/
public func getElementsByIndexEquals(_ index: Int)throws->Elements {
return try Collector.collect(Evaluator.IndexEquals(index), self)
}
/**
* Find elements that contain the specified string. The search is case insensitive. The text may appear directly
* in the element, or in any of its descendants.
* @param searchText to look for in the element's text
* @return elements that contain the string, case insensitive.
* @see Element#text()
*/
public func getElementsContainingText(_ searchText: String)throws->Elements {
return try Collector.collect(Evaluator.ContainsText(searchText), self)
}
/**
* Find elements that directly contain the specified string. The search is case insensitive. The text must appear directly
* in the element, not in any of its descendants.
* @param searchText to look for in the element's own text
* @return elements that contain the string, case insensitive.
* @see Element#ownText()
*/
public func getElementsContainingOwnText(_ searchText: String)throws->Elements {
return try Collector.collect(Evaluator.ContainsOwnText(searchText), self)
}
/**
* Find elements whose text matches the supplied regular expression.
* @param pattern regular expression to match text against
* @return elements matching the supplied regular expression.
* @see Element#text()
*/
public func getElementsMatchingText(_ pattern: Pattern)throws->Elements {
return try Collector.collect(Evaluator.Matches(pattern), self)
}
/**
* Find elements whose text matches the supplied regular expression.
* @param regex regular expression to match text against. You can use <a href="http://java.sun.com/docs/books/tutorial/essential/regex/pattern.html#embedded">embedded flags</a> (such as (?i) and (?m) to control regex options.
* @return elements matching the supplied regular expression.
* @see Element#text()
*/
public func getElementsMatchingText(_ regex: String)throws->Elements {
let pattern: Pattern
do {
pattern = Pattern.compile(regex)
try pattern.validate()
} catch {
throw Exception.Error(type: ExceptionType.IllegalArgumentException, Message: "Pattern syntax error: \(regex)")
}
return try getElementsMatchingText(pattern)
}
/**
* Find elements whose own text matches the supplied regular expression.
* @param pattern regular expression to match text against
* @return elements matching the supplied regular expression.
* @see Element#ownText()
*/
public func getElementsMatchingOwnText(_ pattern: Pattern)throws->Elements {
return try Collector.collect(Evaluator.MatchesOwn(pattern), self)
}
/**
* Find elements whose text matches the supplied regular expression.
* @param regex regular expression to match text against. You can use <a href="http://java.sun.com/docs/books/tutorial/essential/regex/pattern.html#embedded">embedded flags</a> (such as (?i) and (?m) to control regex options.
* @return elements matching the supplied regular expression.
* @see Element#ownText()
*/
public func getElementsMatchingOwnText(_ regex: String)throws->Elements {
let pattern: Pattern
do {
pattern = Pattern.compile(regex)
try pattern.validate()
} catch {
throw Exception.Error(type: ExceptionType.IllegalArgumentException, Message: "Pattern syntax error: \(regex)")
}
return try getElementsMatchingOwnText(pattern)
}
/**
* Find all elements under this element (including self, and children of children).
*
* @return all elements
*/
public func getAllElements()throws->Elements {
return try Collector.collect(Evaluator.AllElements(), self)
}
/**
* Gets the combined text of this element and all its children. Whitespace is normalized and trimmed.
* <p>
* For example, given HTML {@code <p>Hello <b>there</b> now! </p>}, {@code p.text()} returns {@code "Hello there now!"}
*
* @return unencoded text, or empty string if none.
* @see #ownText()
* @see #textNodes()
*/
class textNodeVisitor: NodeVisitor {
let accum: StringBuilder
let trimAndNormaliseWhitespace: Bool
init(_ accum: StringBuilder, trimAndNormaliseWhitespace: Bool) {
self.accum = accum
self.trimAndNormaliseWhitespace = trimAndNormaliseWhitespace
}
public func head(_ node: Node, _ depth: Int) {
if let textNode = (node as? TextNode) {
if trimAndNormaliseWhitespace {
Element.appendNormalisedText(accum, textNode)
} else {
accum.append(textNode.getWholeText())
}
} else if let element = (node as? Element) {
if !accum.isEmpty &&
(element.isBlock() || element._tag.getName() == "br") &&
!TextNode.lastCharIsWhitespace(accum) {
accum.append(" ")
}
}
}
public func tail(_ node: Node, _ depth: Int) {
}
}
public func text(trimAndNormaliseWhitespace: Bool = true)throws->String {
let accum: StringBuilder = StringBuilder()
try NodeTraversor(textNodeVisitor(accum, trimAndNormaliseWhitespace: trimAndNormaliseWhitespace)).traverse(self)
let text = accum.toString()
if trimAndNormaliseWhitespace {
return text.trim()
}
return text
}
/**
* Gets the text owned by this element only; does not get the combined text of all children.
* <p>
* For example, given HTML {@code <p>Hello <b>there</b> now!</p>}, {@code p.ownText()} returns {@code "Hello now!"},
* whereas {@code p.text()} returns {@code "Hello there now!"}.
* Note that the text within the {@code b} element is not returned, as it is not a direct child of the {@code p} element.
*
* @return unencoded text, or empty string if none.
* @see #text()
* @see #textNodes()
*/
public func ownText() -> String {
let sb: StringBuilder = StringBuilder()
ownText(sb)
return sb.toString().trim()
}
private func ownText(_ accum: StringBuilder) {
for child: Node in childNodes {
if let textNode = (child as? TextNode) {
Element.appendNormalisedText(accum, textNode)
} else if let child = (child as? Element) {
Element.appendWhitespaceIfBr(child, accum)
}
}
}
private static func appendNormalisedText(_ accum: StringBuilder, _ textNode: TextNode) {
let text: String = textNode.getWholeText()
if (Element.preserveWhitespace(textNode.parentNode)) {
accum.append(text)
} else {
StringUtil.appendNormalisedWhitespace(accum, string: text, stripLeading: TextNode.lastCharIsWhitespace(accum))
}
}
private static func appendWhitespaceIfBr(_ element: Element, _ accum: StringBuilder) {
if (element._tag.getName() == "br" && !TextNode.lastCharIsWhitespace(accum)) {
accum.append(" ")
}
}
static func preserveWhitespace(_ node: Node?) -> Bool {
// looks only at this element and one level up, to prevent recursion & needless stack searches
if let element = (node as? Element) {
return element._tag.preserveWhitespace() || element.parent() != nil && element.parent()!._tag.preserveWhitespace()
}
return false
}
/**
* Set the text of this element. Any existing contents (text or elements) will be cleared
* @param text unencoded text
* @return this element
*/
@discardableResult
public func text(_ text: String)throws->Element {
empty()
let textNode: TextNode = TextNode(text, baseUri)
try appendChild(textNode)
return self
}
/**
Test if this element has any text content (that is not just whitespace).
@return true if element has non-blank text content.
*/
public func hasText() -> Bool {
for child: Node in childNodes {
if let textNode = (child as? TextNode) {
if (!textNode.isBlank()) {
return true
}
} else if let el = (child as? Element) {
if (el.hasText()) {
return true
}
}
}
return false
}
/**
* Get the combined data of this element. Data is e.g. the inside of a {@code script} tag.
* @return the data, or empty string if none
*
* @see #dataNodes()
*/
public func data() -> String {
let sb: StringBuilder = StringBuilder()
for childNode: Node in childNodes {
if let data = (childNode as? DataNode) {
sb.append(data.getWholeData())
} else if let element = (childNode as? Element) {
let elementData: String = element.data()
sb.append(elementData)
}
}
return sb.toString()
}
/**
* Gets the literal value of this element's "class" attribute, which may include multiple class names, space
* separated. (E.g. on <code><div class="header gray"></code> returns, "<code>header gray</code>")
* @return The literal class attribute, or <b>empty string</b> if no class attribute set.
*/
public func className()throws->String {
return try attr(Element.classString).trim()
}
/**
* Get all of the element's class names. E.g. on element {@code <div class="header gray">},
* returns a set of two elements {@code "header", "gray"}. Note that modifications to this set are not pushed to
* the backing {@code class} attribute; use the {@link #classNames(java.util.Set)} method to persist them.
* @return set of classnames, empty if no class attribute
*/
public func classNames()throws->OrderedSet<String> {
let fitted = try className().replaceAll(of: Element.classSplit, with: " ", options: .caseInsensitive)
let names: [String] = fitted.components(separatedBy: " ")
let classNames: OrderedSet<String> = OrderedSet(sequence: names)
classNames.remove(Element.emptyString) // if classNames() was empty, would include an empty class
return classNames
}
/**
Set the element's {@code class} attribute to the supplied class names.
@param classNames set of classes
@return this element, for chaining
*/
@discardableResult
public func classNames(_ classNames: OrderedSet<String>)throws->Element {
try attributes?.put(Element.classString, StringUtil.join(classNames, sep: " "))
return self
}
/**
* Tests if this element has a class. Case insensitive.
* @param className name of class to check for
* @return true if it does, false if not
*/
// performance sensitive
public func hasClass(_ className: String) -> Bool {
let classAtt: String? = attributes?.get(key: Element.classString)
let len: Int = (classAtt != nil) ? classAtt!.count : 0
let wantLen: Int = className.count
if (len == 0 || len < wantLen) {
return false
}
let classAttr = classAtt!
// if both lengths are equal, only need compare the className with the attribute
if (len == wantLen) {
return className.equalsIgnoreCase(string: classAttr)
}
// otherwise, scan for whitespace and compare regions (with no string or arraylist allocations)
var inClass: Bool = false
var start: Int = 0
for i in 0..<len {
if (classAttr.charAt(i).isWhitespace) {
if (inClass) {
// white space ends a class name, compare it with the requested one, ignore case
if (i - start == wantLen && classAttr.regionMatches(ignoreCase: true, selfOffset: start,
other: className, otherOffset: 0,
targetLength: wantLen)) {
return true
}
inClass = false
}
} else {
if (!inClass) {
// we're in a class name : keep the start of the substring
inClass = true
start = i
}
}
}
// check the last entry
if (inClass && len - start == wantLen) {
return classAttr.regionMatches(ignoreCase: true, selfOffset: start,
other: className, otherOffset: 0, targetLength: wantLen)
}
return false
}
/**
Add a class name to this element's {@code class} attribute.
@param className class name to add
@return this element
*/
@discardableResult
public func addClass(_ className: String)throws->Element {
let classes: OrderedSet<String> = try classNames()
classes.append(className)
try classNames(classes)
return self
}
/**
Remove a class name from this element's {@code class} attribute.
@param className class name to remove
@return this element
*/
@discardableResult
public func removeClass(_ className: String)throws->Element {
let classes: OrderedSet<String> = try classNames()
classes.remove(className)
try classNames(classes)
return self
}
/**
Toggle a class name on this element's {@code class} attribute: if present, remove it; otherwise add it.
@param className class name to toggle
@return this element
*/
@discardableResult
public func toggleClass(_ className: String)throws->Element {
let classes: OrderedSet<String> = try classNames()
if (classes.contains(className)) {classes.remove(className)
} else {
classes.append(className)
}
try classNames(classes)
return self
}
/**
* Get the value of a form element (input, textarea, etc).
* @return the value of the form element, or empty string if not set.
*/
public func val()throws->String {
if (tagName()=="textarea") {
return try text()
} else {
return try attr("value")
}
}
/**
* Set the value of a form element (input, textarea, etc).
* @param value value to set
* @return this element (for chaining)
*/
@discardableResult
public func val(_ value: String)throws->Element {
if (tagName() == "textarea") {
try text(value)
} else {
try attr("value", value)
}
return self
}
override func outerHtmlHead(_ accum: StringBuilder, _ depth: Int, _ out: OutputSettings)throws {
if (out.prettyPrint() && (_tag.formatAsBlock() || (parent() != nil && parent()!.tag().formatAsBlock()) || out.outline())) {
if !accum.isEmpty {
indent(accum, depth, out)
}
}
accum
.append("<")
.append(tagName())
try attributes?.html(accum: accum, out: out)
// selfclosing includes unknown tags, isEmpty defines tags that are always empty
if (childNodes.isEmpty && _tag.isSelfClosing()) {
if (out.syntax() == OutputSettings.Syntax.html && _tag.isEmpty()) {
accum.append(">")
} else {
accum.append(" />") // <img> in html, <img /> in xml
}
} else {
accum.append(">")
}
}
override func outerHtmlTail(_ accum: StringBuilder, _ depth: Int, _ out: OutputSettings) {
if (!(childNodes.isEmpty && _tag.isSelfClosing())) {
if (out.prettyPrint() && (!childNodes.isEmpty && (
_tag.formatAsBlock() || (out.outline() && (childNodes.count>1 || (childNodes.count==1 && !(((childNodes[0] as? TextNode) != nil)))))
))) {
indent(accum, depth, out)
}
accum.append("</").append(tagName()).append(">")
}
}
/**
* Retrieves the element's inner HTML. E.g. on a {@code <div>} with one empty {@code <p>}, would return
* {@code <p></p>}. (Whereas {@link #outerHtml()} would return {@code <div><p></p></div>}.)
*
* @return String of HTML.
* @see #outerHtml()
*/
public func html()throws->String {
let accum: StringBuilder = StringBuilder()
try html2(accum)
return getOutputSettings().prettyPrint() ? accum.toString().trim() : accum.toString()
}
private func html2(_ accum: StringBuilder)throws {
for node in childNodes {
try node.outerHtml(accum)
}
}
/**
* {@inheritDoc}
*/
open override func html(_ appendable: StringBuilder)throws->StringBuilder {
for node in childNodes {
try node.outerHtml(appendable)
}
return appendable
}
/**
* Set this element's inner HTML. Clears the existing HTML first.
* @param html HTML to parse and set into this element
* @return this element
* @see #append(String)
*/
@discardableResult
public func html(_ html: String)throws->Element {
empty()
try append(html)
return self
}
public override func copy(with zone: NSZone? = nil) -> Any {
let clone = Element(_tag, baseUri!, attributes!)
return copy(clone: clone)
}
public override func copy(parent: Node?) -> Node {
let clone = Element(_tag, baseUri!, attributes!)
return copy(clone: clone, parent: parent)
}
public override func copy(clone: Node, parent: Node?) -> Node {
return super.copy(clone: clone, parent: parent)
}
override public func hash(into hasher: inout Hasher) {
super.hash(into: &hasher)
hasher.combine(_tag)
}
}
| mit | 4825e56f546122ff446bf2cf6cd81066 | 35.889908 | 241 | 0.614089 | 4.434111 | false | false | false | false |
wuwen1030/ViewControllerTransitionDemo | ViewControllerTransition/HomeTableViewController.swift | 1 | 916 | //
// HomeTableViewController.swift
// ViewControllerTransition
//
// Created by Ben on 15/7/20.
// Copyright (c) 2015年 X-Team. All rights reserved.
//
import UIKit
class HomeTableViewController: UITableViewController {
let modalTransition = ModalTrainsition(animationType: ModalAnimationType.simple)
override func viewDidLoad() {
super.viewDidLoad()
}
@IBAction func modalControllerDismiss(sender: UIStoryboardSegue) {
}
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
if segue.identifier == "modalViewController" {
let destinationViewController = segue.destinationViewController as! UIViewController
destinationViewController.transitioningDelegate = modalTransition
modalTransition.interactiveDismissAnimator.wireToViewController(destinationViewController)
}
}
}
| mit | d09d94490d83094df22c888da83971ed | 29.466667 | 102 | 0.719912 | 5.7125 | false | false | false | false |
alskipp/Monoid | Monoid/Types/Down.swift | 1 | 1214 | // Copyright © 2016 Al Skipp. All rights reserved.
/*
A simple type that inverts the usual comparison order of a type.
For example:
Down(2) < Down(1) // true
It can be used to sort in reverse order:
records.sortBy(comparing { Down($0.height) })
Which is equivalent to:
records.sort { $0.height > $1.height }
It might be more useful here (compare by height in descending order):
records.sortBy { x, y in
comparing(x, y) { Down($0.height) } <>
comparing(x, y) { $0.weight } <>
comparing(x, y) { $0.age }
}
Which is equivalent to the following (where it's easy to miss the flipped 'x' and 'y')
records.sortBy { x, y in
comparing(y, x) { $0.height } <>
comparing(x, y) { $0.weight } <>
comparing(x, y) { $0.age }
}
*/
public struct Down<T: Orderable> {
public let value: T
public init (_ v: T) { value = v }
}
extension Down: Equatable, Comparable, Orderable {}
public func == <T: Equatable>(x: Down<T>, y: Down<T>) -> Bool {
return x.value == y.value
}
public func < <T: Comparable>(x: Down<T>, y: Down<T>) -> Bool {
return y.value < x.value
}
extension Down: CustomStringConvertible {
public var description: String {
return "Down(\(value))"
}
}
| mit | 4267895ca6115c89685688f837d57d84 | 22.326923 | 86 | 0.631492 | 3.142487 | false | false | false | false |
dsoisson/SwiftLearning | SwiftLearning.playground/Pages/Inheritance.xcplaygroundpage/Contents.swift | 3 | 19498 | /*:
[Table of Contents](@first) | [Previous](@previous) | [Next](@next)
- - -
# Inheritance
* callout(Session Overview): When you think of inheritance, you probably think of material wealth that your parents or grandparents pass along to you when they die. Or possibly the genes you inherited from your parents and grandparents. Those aspects also ring true in object oriented programming. Inheritance is a technique in sharing and extending code within your programs and is an attribute of languages that support object oriented programming or *OOP*, such as Swift.
*/
import Foundation
/*:
## What Is It?
In the context of OOP, inheritance means that a class can inherit properties and methods from a superclass. The class that inherited the properties and methods is called the subclass. Swift only has the capability of single inheritance, meaning that a Swift class can only inherit from one superclass. Also, inheritance only applies to reference types, a.k.a classes.
*/
/*:
## The Base Class
Classes that don’t inherit from any other class is called the base class. Unlike most OOP languages, Swift classes don’t inherit from a universal base class like *Object*. Classes you define without specifying a superclass automatically become base classes for you to subclass.
*/
class Father {
var name: String
var height: Float
var hairColor: String
var eyeColor: String
init(name: String, height: Float, hairColor: String, eyeColor: String) {
self.name = name
self.height = height
self.hairColor = hairColor
self.eyeColor = eyeColor
}
func sayHello() -> String {
return "Hello! My name is \(name)."
}
var description: String {
return "I'm \(height) inches, with \(hairColor) hair and \(eyeColor) eyes"
}
}
let steve = Father(name: "Steve", height: 76, hairColor: "Blond", eyeColor: "Blue")
print(steve.sayHello())
print(steve.description)
/*:
Above we created a `Father` class. This is the base class for all subclasses of a `Father` class. We have also created an instance of the `Father` class providing the necessary arguments to initialize the `Father` class correctly.
*/
/*:
## The Subclass
Having a class inherit from another class, or subclassing and superclass is done with the colon `:` directly after the class name and then the class you want to inherit after the colon. Once a class is defined as having subclassing another class, the subclass can immediately access the visible properties and methods (type and instance). The subclass can also have new properties and methods.
*/
class Son: Father {
var age: Int?
}
let joe = Son(name: "Joe", height: 67, hairColor: "Blond", eyeColor: "Green")
joe.age = 18
print(joe.sayHello())
print(joe.description)
/*:
Above we have created the subclass `Son`. The `Son` class inherited all the properties and methods from the `Father` class. `Son` also defined a new property `age`. Notice when we created an instance of `Son` we are able to call the method `sayHello()` and access the computed property`description`.
*/
/*:
## Accessing superclass initializers, properties, methods and subscripts
The subclass can access properties and methods from the superclass by using the keyword `super` in front of the property or method you want to access. The `super` keyword can also be used with initializers to delegate initialization to the superclass to initialize stored properties of the superclass.
*/
class Mother {
var name: String
var height: Float
var hairColor: String
var eyeColor: String
init(name: String, height: Float, hairColor: String, eyeColor: String) {
self.name = name
self.height = height
self.hairColor = hairColor
self.eyeColor = eyeColor
}
func sayHello() -> String {
return "Hello! My name is \(name)."
}
var description: String {
return "I'm \(height) inches, with \(hairColor) hair and \(eyeColor) eyes"
}
}
class Daughter: Mother {
var age: Int
init(age: Int, name: String, height: Float, hairColor: String, eyeColor: String) {
self.age = age
super.init(name: name, height: height, hairColor: hairColor, eyeColor: eyeColor)
}
func sayHi() -> String {
let hello = sayHello()
let description = super.description
return "\(hello) I'm the daughter. \(description)"
}
}
let amelia = Daughter(age: 18, name: "Amelia", height: 61, hairColor: "Brown", eyeColor: "Brown")
print(amelia.sayHello())
print(amelia.sayHi())
/*:
Above we create a base class `Mother` and a subclass `Daughter`. The `Daughter` class inherits all the properties and methods from `Mother` and can call methods and access properties by using the `super` keyword. The `Daughter` class leverages the superclass’s initializer and the property `description` within the initializer and `sayHi()` method.
*/
/*:
## Overriding
A subclass can change the implementation of a property and method (both type and instance) or a subscript that is inherited from a superclass by *overriding* the implementation inherited. This is done with the `override` keyword in front of the property, method or subscript you want to override.
*/
/*:
### Overriding Methods
Overriding a method (either instance or type) is done by placing the `override` keyword before the function definition. If you override a method that is not defined in the superclass, you will receive a compiler error. When you override a method, it’s the implementation of the subclass that gets executed.
*/
class Grandson: Son {
override func sayHello() -> String {
return "\(super.sayHello()) I'm the grandson."
}
}
let grandson = Grandson(name: "Sam", height: 48, hairColor: "Blond", eyeColor: "Green")
print(grandson.sayHello())
// below is a function that accepts a Father as a parameter. A Grandson is acceptable because a Grandson is a subclass of a Father
func sayHello(father: Father) {
print(father.sayHello())
}
sayHello(grandson)
/*:
Above we have created a new class `Grandson` inheriting from the `Son` class. We want the `sayHello` method of the `Grandson` class to provide a different implementation. We do this by overriding the `sayHello` method defined in the base class `Father`.
*/
/*:
### Overriding Properties
Overriding a property (either instance or type) is similar to overriding a function; you also place the override keyword before the property definition. It doesn’t matter if the superclass’s property is a stored property or computed property. You can override a property in a subclass as long as the name and type are the same as in the superclass. You can override a property’s get and set methods as well as the property observers.
*/
class Granddaughter: Daughter {
// Overriding Property Getters and Setters
override var height: Float {
get {
return super.height / 12
}
set {
super.height = newValue * 12
}
}
// Overriding Property Observers
override var age: Int {
willSet {
print("age will be \(newValue)")
}
didSet {
print("age was \(oldValue) and is now \(age)")
}
}
override var description: String {
return "I'm \(self.height) feet, with \(hairColor) hair and \(eyeColor) eyes"
}
}
let granddaughter = Granddaughter(age: 5, name: "Olivia", height: 44, hairColor: "Brown", eyeColor: "Blue")
granddaughter.height = 5
granddaughter.age = 6
print(granddaughter.description)
/*:
Above we have created a new class `Granddaughter` inheriting from the `Daughter` class. We override the `height` property by providing custom implementations of the `get` and `set` property methods. We also override the `age` property and provide property observers. Finally we override the `description` computed property printing out a different string than what is implemented in the `Mother` class.
*/
/*:
## Inheritance and Initialization
When inheriting properties, especially stored properties, from a superclass, you not only need to initialize store properties within your subclass, you also need to initialize properties in the superclass. You can require an initializer to be present in a subclass with the `require` keyword preceding the custom initializer. The required custom initializer forces all subclasses to implement the superclass’s required custom initializer.
*/
class Lightsaber {
var color: String
var length: Int?
required init(color: String) {
self.color = color
}
convenience init(color: String, length: Int) {
self.init(color: color)
self.length = length
}
func hitType() -> String {
return "Hit"
}
var description: String {
return "Lightsaber"
}
}
class JediLightsaber: Lightsaber {
required init(color: String) {
super.init(color: color) // delegating to the superclass
}
override func hitType() -> String {
return "Smack"
}
}
/*:
Above we have created a `Lightsaber` class and have made the `init(color: String)` initializer required for all subclasses. We have also created a convenience initializer that also accepts length. The subclass `JediLightsaber` must also implement the `init(color: String)`. The `JediLightsaber` also overrides the method `hitType() -> String`
*/
/*:
## Preventing Overrides
To prevent a class from being overridden, use the final keyword preceding the class keyword in the class definition. You can also prevent a specific property, method, or subscript from being overridden with the `final` keyword.
*/
class SithLightsaber: Lightsaber {
required init(color: String) {
super.init(color: color)
}
final override func hitType() -> String {
return "Slam"
}
}
final class DoubleEdged: SithLightsaber {
// this will cause a compiler error
// override func hitType() -> String {
//
// return "Bang"
// }
}
/*:
Above have create two new classes. `SithLightsaber`, inherits from `Lightsaber`, and `DoubleEdged` inherits from `SithLightsaber`. The `hitType() -> String` method is overridden in but `SithLightsaber` prevents all subclass of `SithLightsaber` to override `hitType() -> String`. `DoubleEdged` will never be a superclass because of the `final` keyword in the the class definition.
*/
/*:
## Type Casting
When working with class hierarchies that you have created in your program or provided to you from a framework, it’s often necessary to check if a class can become a specific type and perform type casting to make the object that your are working with become a valid type in the hierarchy.
*/
class JediTrainerLightsaber: JediLightsaber {
override var description: String {
return "\(color) Trainer \(super.description)"
}
}
class SithTrainerLightsaber: SithLightsaber {
override var description: String {
return "\(color) Trainer \(super.description)"
}
}
let jediTrainer = JediTrainerLightsaber(color: "Blue")
let sithTrainer = SithTrainerLightsaber(color: "Red")
var trainers = [Lightsaber]()
trainers.append(jediTrainer)
trainers.append(sithTrainer)
/*:
Above we have created two new classes `JediTrainerLightsaber` and `SithTrainerLightsaber`. We store instances of these two classes in an array storing instances of `Lightsaber`. This is allowable because our instances of `JediTrainerLightsaber` and `SithTrainerLightsaber` have the same base class `Lightsaber`.
*/
/*:
### Checking the type
You can check the type of an instance of a class by using the type check operator `is`. The `is` keyword checks if a class is of a certain subclass. The type check operator will return `true` if the class can be casted as the subclass and `false` if the class is not in the class hierarchy.
*/
for trainer in trainers {
if trainer is JediTrainerLightsaber {
print("Jedi \(trainer.color) Trainer Lightsaber")
} else if trainer is SithTrainerLightsaber {
print("Sith \(trainer.color) Trainer Lightsaber")
}
}
/*:
Above we iterate over the `trainers` array and use the type check operator `is` to print the correct string for each `trainer`.
*/
/*:
### Downcasting to get the type
To actually cast a class as a subclass, downcasting, use the type cast operator `as` with either a question mark `?` or exclamation point `!`. Use `as?` if you are unsure if the class belongs in the class hierarchy, resulting in a `nil` if the class is not in the hierarchy. Conversely, use `as!` to force the type casting. If the class is not in the hierarchy, you will receive a runtime error.
*/
for trainer in trainers {
if let jediTrainer = trainer as? JediTrainerLightsaber {
print("\(jediTrainer.hitType()) \(jediTrainer.description)")
}
if trainer is SithTrainerLightsaber {
let sithTrainer = trainer as! SithTrainerLightsaber
print("\(sithTrainer.hitType()) \(sithTrainer.description)")
}
}
/*:
Above we iterate over the `trainers` array and use the type cast operator to print the correct string for each `trainer`. The first `if` uses optional binding with the type cast operator `as?` for optional downcasting. The second `if` uses the type check operator and then the `as!` type cast operator to force the downcasting.
*/
/*:
### Type Casting for Any and AnyObject
Some frameworks that you will leverage within your program store an array of non specified types and indicate so with either using `typealias` of `AnyObject` or `Any`. `AnyObject` marks the type or collection of types as instances of classes. `Any` marks the type of collection of any thing, including tuples and function type. You can use the `is`, type check operator or `as`, type cast operator to determine what it is you are actually working with.
*/
var anyObjects = [AnyObject]()
anyObjects.append(steve) // instance of Father
anyObjects.append(joe) // instance of Son, subclass of Father
anyObjects.append(amelia) // instance of Daughter, subclass of Mother
anyObjects.append(jediTrainer) // instance of JediTrainerLightsaber
anyObjects.append(sithTrainer) // instance of SithTrainerLightsaber
anyObjects.append("A random String")// instance of String
anyObjects.append(100) // instance of Int
for anyObject in anyObjects {
switch anyObject {
case let son as Son:
print("I'm \(son.name), an instance of Son")
case let father as Father:
print("I'm \(father.name), an instance of Father")
case is Daughter:
print("I'm an instance of Daughter")
case let jediLightsaber as JediTrainerLightsaber:
print("I'm \(jediLightsaber.color), an instance of JediTrainerLightsaber")
case let sithLightsaber as SithTrainerLightsaber:
print("I'm \(sithLightsaber.color), an instance of SithTrainerLightsaber")
case let someString as String:
print("I'm a String with a value of \(someString)")
case let someInt as Int:
print("I'm an Int with a value of \(someInt)")
default:
print("I'm something strange")
}
}
/*:
Above we create an array that can store any instance of a class by using the typealias `AnyObject`. We add instances of `Father`, `Son`, `Daughter`, `JediTrainerLightsaber`, `SithTrainerLightsaber` and instances of a `String` and `Int`. We then iterate over the array and by leveraging the `switch-case` statement match on the correct type using `is` and `as` and print the correct string.
*/
var anyThings = [Any]()
for anyObject in anyObjects {
anyThings.append(anyObject)
}
anyThings.append((name: "Matt", age: 33))
func twice(num: Int) -> Int {
return num * 2
}
anyThings.append(twice)
for anyThing in anyThings {
switch anyThing {
case let child as Father:
print("I'm an instance of Father")
case let child as Mother:
print("I'm an instance of Mother")
case let lightsaber as Lightsaber:
print("I'm an instance of Lightsaber")
case is String:
print("I'm a String")
case is Int:
print("I'm a Int")
case let (name, age) as (String, Int):
print("I'm a tuple with name: \(name), age: \(age)")
case let twice as (num: Int) -> Int:
let amount = 4
print("I'm a closure that can twice something like \(amount) * 2 = \(twice(num: amount))")
default:
print("I'm something strange")
}
}
/*:
Above we do something similar to storing instances of `AnyObject`, here we store an array of `Any`, representing any type, such as tuples of function types. Again we iterate and use the `switch-case` statement to match on a type and print the correct string.
*/
/*:
- - -
* callout(Exercise): Build upon your `Dog` and `Cat` classes in the previous exercise by applying inheritance. Create a superclass that both `Dog` and `Cat` can inherit from. Modify your `Owner` class to hold a collection of pets. Iterate over your pets and print what sound the pet makes.
**Constraints:**
- Create a new class as the superclass to `Dog` and `Cat`
- The superclass needs to have a method of `makeSound()`
- The subclasses need to override the `makeSound()` method and print the appropriate sound
- The `pets` property needs to store both `Dog` and `Cat` instances
* callout(Checkpoint): At this point, you have learned how to leverage inheritance to share and reuse code and how to access the properties, methods and subscripts of a superclass. Overriding a superclass provide a subclass with having it’s custom implementation of a property, method or subscript. You can prevent a class, property, method or subscript from being overridden with he `final` keyword. `AnyObject` and `Any` are used to mark a type or collection of types as unspecified types while using the type check operator and type cast operator to convert the type type into a type you are interested in.
**Keywords to remember:**
- `super` = To access a superclass's properties, methods or subscripts
- `override` = Custom implementation within the subclass of a superclass's properties, methods or subscripts
- `required` = An initializer that all subclasses must implement
- `final` = A class, property or method that cannot be overridden
- `is` = Type check operator to check if an instance is of a specific type
- `as` = Type cast operator that will downcast to the subclass type. Use `as?` is casting can fail; use `as!` if downcast will always succeed
- `AnyObject` = Represents an instance of any class type
- `Any` = Represents an instance of any type, including function types and tuples
* callout(Supporting Materials): Chapters and sections from the Guide and Vidoes from WWDC
- [Guide: Inheritance](https://developer.apple.com/library/ios/documentation/Swift/Conceptual/Swift_Programming_Language/Inheritance.html)
- [Guide: Type Casting](https://developer.apple.com/library/ios/documentation/Swift/Conceptual/Swift_Programming_Language/TypeCasting.html)
- [Video: Intermediate Swift](https://developer.apple.com/videos/play/wwdc2014-403/)
- - -
[Table of Contents](@first) | [Previous](@previous) | [Next](@next)
*/
| mit | bd85ccc7ab2d4598305c3b3cc20506fc | 42.574944 | 610 | 0.707824 | 4.188817 | false | false | false | false |
iCodeForever/ifanr | ifanr/ifanr/Views/HomeView/HomeHeaderView.swift | 1 | 9367 | //
// HomeHeaderView.swift
// ifanr
//
// Created by 梁亦明 on 16/7/2.
// Copyright © 2016年 ifanrOrg. All rights reserved.
//
import UIKit
class HomeHeaderView: UIView {
override init(frame: CGRect) {
super.init(frame: frame)
// 添加3个UIImageVIew到scrollview
contentScrollView.addSubview(currentItem)
contentScrollView.addSubview(lastItem)
contentScrollView.addSubview(nextItem)
addSubview(contentScrollView)
addSubview(pageControl)
addSubview(tagImageView)
// 添加点击事件
let tap = UITapGestureRecognizer(target: self, action: #selector(HomeHeaderView.currentItemTap))
currentItem.addGestureRecognizer(tap)
}
convenience init(frame: CGRect, modelArray: [CommonModel]!) {
self.init(frame: frame)
self.modelArray = modelArray
pageControl.numberOfPage = self.modelArray.count
// 默认显示第一张图片
self.indexOfCurrentImage = 0
// 设置uiimageview位置
self.setScrollViewOfImage()
contentScrollView.setContentOffset(CGPoint(x: self.width, y: 0), animated: false)
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
//MARK: --------------------------- Event or Action --------------------------
@objc fileprivate func currentItemTap() {
if let callBack = callBack {
callBack(indexOfCurrentImage)
}
}
func currentItemDidClick(_ callBack: CurrentItemTapCallBack?) {
self.callBack = callBack
}
//MARK: --------------------------- Private Methods --------------------------
typealias CurrentItemTapCallBack = (_ index: Int) -> Void
fileprivate var callBack: CurrentItemTapCallBack?
/// 定时器,定时轮播图片
fileprivate var timer : Timer?
/**
得到上一张图片的下标
- parameter index: 当前图片下标位置
- returns: 上一个图片标位置
*/
fileprivate func getLastImageIndex(indexOfCurrentImage index: Int) -> Int {
let tempIndex = index - 1
if tempIndex == -1 {
return self.modelArray.count - 1
} else {
return tempIndex
}
}
/**
得到下一张图片的下标
- parameter index: 当前图片下标位置
- returns: 下一个图片下标位置
*/
fileprivate func getNextImageIndex(indexOfCurrentImage index: Int) -> Int {
let tempIndex = index + 1
return tempIndex < self.modelArray.count ? tempIndex : 0
}
/// 移除定时器
fileprivate func removeTimer () {
self.timer?.invalidate()
self.timer = nil
}
/// 启动定时器
fileprivate func addTimer() {
if self.timer == nil {
self.timer = Timer.scheduledTimer(timeInterval: 4.0, target: self, selector: #selector(HomeHeaderView.timerAction), userInfo: nil, repeats: true)
RunLoop.current.add((self.timer!), forMode: RunLoopMode.commonModes)
}
}
//事件触发方法
@objc fileprivate func timerAction() {
contentScrollView.setContentOffset(CGPoint(x: self.width*2, y: 0), animated: true)
}
/**
重新设置scrollview的图片
*/
fileprivate func setScrollViewOfImage() {
// 获取当前模型数据
let currentModel = self.modelArray[self.indexOfCurrentImage]
self.currentItem.imageURL = currentModel.image
self.currentItem.title = currentModel.title
self.currentItem.date = "\(currentModel.category) | \(Date.getCommonExpressionOfDate(currentModel.pubDate))"
// 获取下一张图片的模型
let nextImageModel = self.modelArray[self.getNextImageIndex(indexOfCurrentImage: self.indexOfCurrentImage)]
self.nextItem.imageURL = nextImageModel.image
self.nextItem.title = nextImageModel.title
self.nextItem.date = "\(nextImageModel.category) | \(Date.getCommonExpressionOfDate(nextImageModel.pubDate))"
// 获取上衣张图片的模型
let lastImageModel = self.modelArray[self.getLastImageIndex(indexOfCurrentImage: self.indexOfCurrentImage)]
self.lastItem.imageURL = lastImageModel.image
self.lastItem.title = lastImageModel.title
self.lastItem.date = "\(lastImageModel.category) | \(Date.getCommonExpressionOfDate(lastImageModel.pubDate)))"
}
//MARK: --------------------------- Getter and Setter --------------------------
fileprivate lazy var contentScrollView: UIScrollView = {
let contentScrollView = UIScrollView(frame: CGRect(x: 0, y: 0, width: self.width, height: self.height-45))
contentScrollView.isUserInteractionEnabled = false
contentScrollView.bounces = false
contentScrollView.isPagingEnabled = true
contentScrollView.showsHorizontalScrollIndicator = false
contentScrollView.contentSize = CGSize(width: self.width * 3, height: 0)
contentScrollView.delegate = self
return contentScrollView
}()
/// 监听图片数组的变化,如果有变化立即刷新轮转图中显示的图片
var modelArray: [CommonModel]! {
willSet {
self.modelArray = newValue
}
/**
* 如果数据源改变,则需要改变scrollView、分页指示器的数量
*/
didSet {
if modelArray.count<=0 {
return
}
contentScrollView.isUserInteractionEnabled = true
contentScrollView.isScrollEnabled = !(modelArray.count == 1)
pageControl.numberOfPage = self.modelArray.count
setScrollViewOfImage()
contentScrollView.setContentOffset(CGPoint(x: self.width, y: 0), animated: false)
tagImageView.isHidden = false
// 启动定时器
self.addTimer()
}
}
/// 监听显示的第几张图片,来更新分页指示器
var indexOfCurrentImage: Int! = 0{
didSet {
self.pageControl.currentPage = indexOfCurrentImage
}
}
/// 当前显示的View
fileprivate lazy var currentItem: HomeHeaderItem = {
var currentItem: HomeHeaderItem = HomeHeaderItem()
currentItem.frame = CGRect(x: self.width, y: 0, width: self.width, height: self.contentScrollView.height)
return currentItem
}()
/// 上一个显示的View
fileprivate lazy var lastItem: HomeHeaderItem = {
var lastItem: HomeHeaderItem = HomeHeaderItem()
lastItem.frame = CGRect(x: 0, y: 0, width: self.width, height: self.contentScrollView.height)
return lastItem
}()
/// 下一个显示的View
fileprivate lazy var nextItem: HomeHeaderItem = {
var nextItem: HomeHeaderItem = HomeHeaderItem()
nextItem.frame = CGRect(x: self.width * 2, y: 0, width: self.width, height: self.contentScrollView.height)
return nextItem
}()
fileprivate lazy var pageControl: HomePageControl = {
var pageControl = HomePageControl(frame: CGRect(x: UIConstant.UI_MARGIN_10, y: self.contentScrollView.height-35, width: self.width, height: 20))
return pageControl
}()
/// 最下面那张图片
fileprivate lazy var tagImageView: UIImageView = {
var tagImageView = UIImageView(frame: CGRect(x: 0, y: 0, width: UIConstant.SCREEN_WIDTH, height: 25))
tagImageView.isHidden = true
tagImageView.center = CGPoint(x: self.center.x, y: self.height-13)
tagImageView.image = UIImage(imageLiteralResourceName: "tag_latest_press")
tagImageView.contentMode = UIViewContentMode.scaleAspectFit
return tagImageView
}()
}
extension HomeHeaderView: UIScrollViewDelegate {
/**
* 开始拖拽的时候调用
*/
func scrollViewWillBeginDragging(_ scrollView: UIScrollView) {
// 停止定时器(一旦定时器停止了,就不能再使用)
self.removeTimer()
}
/**
* 停止拖拽的时候调用
*/
func scrollViewDidEndDragging(_ scrollView: UIScrollView, willDecelerate decelerate: Bool) {
//如果用户手动拖动到了一个整数页的位置就不会发生滑动了 所以需要判断手动调用滑动停止滑动方法
if !decelerate {
self.scrollViewDidEndDecelerating(scrollView)
}
self.addTimer()
}
func scrollViewDidEndDecelerating(_ scrollView: UIScrollView) {
let offset = scrollView.contentOffset.x
if offset == 0 {
self.indexOfCurrentImage = self.getLastImageIndex(indexOfCurrentImage: self.indexOfCurrentImage)
} else if offset == self.frame.size.width * 2 {
self.indexOfCurrentImage = self.getNextImageIndex(indexOfCurrentImage: self.indexOfCurrentImage)
}
// 重新布局图片
self.setScrollViewOfImage()
//布局后把contentOffset设为中间
scrollView.setContentOffset(CGPoint(x: self.width, y: 0), animated: false)
}
func scrollViewDidEndScrollingAnimation(_ scrollView: UIScrollView) {
self.scrollViewDidEndDecelerating(scrollView)
}
}
| mit | 1480138d0c344c4c86cba715a0496020 | 34.794239 | 157 | 0.63049 | 4.792287 | false | false | false | false |
easyui/EZPlayer | EZPlayerExample/EZPlayerExample/ParamsViewController.swift | 1 | 2840 | //
// ParamsViewController.swift
// EZPlayerExample
//
// Created by yangjun zhu on 2016/12/28.
// Copyright © 2016年 yangjun zhu. All rights reserved.
//
import UIKit
import EZPlayer
class ParamsViewController: UIViewController {
@IBOutlet weak var dlView: UIView!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func playButtonAction(_ sender: Any) {
// MediaManager.sharedInstance.playEmbeddedVideo(url: URL(string: "http://gslb.miaopai.com/stream/kPzSuadRd2ipEo82jk9~sA__.mp4")!, embeddedContentView: self.dlView)
// MediaManager.sharedInstance.playEmbeddedVideo(url: URL(string: "http://baobab.wdjcdn.com/14571455324031.mp4")!, embeddedContentView: self.dlView)
MediaManager.sharedInstance.playEmbeddedVideo(url:URL.Test.localMP4_1, embeddedContentView: self.dlView)
// MediaManager.sharedInstance.playEmbeddedVideo(url:Bundle.main.url(forResource: "testVideo", withExtension: "m3u8")!, embeddedContentView: self.dlView)
// MediaManager.sharedInstance.playEmbeddedVideo(url: URL(string: "http://www.streambox.fr/playlists/x36xhzz/url_6/193039199_mp4_h264_aac_hq_7.m3u8")!, embeddedContentView: self.dlView)
// MediaManager.sharedInstance.playEmbeddedVideo(url: URL(string: "http://baobab.wdjcdn.com/1455968234865481297704.mp4")!, embeddedContentView: self.dlView)
// MediaManager.sharedInstance.playEmbeddedVideo(url: URL(string: "http://172.16.1.113/packager/media1/hls/chicago.mp4.m3u8")!, embeddedContentView: self.dlView)
}
// MARK: - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "ParamsEmbedTableViewController"{
let vc = segue.destination as! ParamsEmbedTableViewController
vc.paramsViewController = self
}
}
override var shouldAutorotate : Bool {
return true
}
override var supportedInterfaceOrientations : UIInterfaceOrientationMask {
if UIDevice.current.userInterfaceIdiom == .pad {
return .landscape
}else {
return .portrait
}
}
override var preferredInterfaceOrientationForPresentation: UIInterfaceOrientation{
if UIDevice.current.userInterfaceIdiom == .pad {
return .landscapeRight
}else {
return .portrait
}
}
override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) {
self.view.endEditing(true)
}
}
| mit | 2d113c36695a31838fc02f2713c62ffe | 34.4625 | 192 | 0.697568 | 4.253373 | false | false | false | false |
netguru/inbbbox-ios | Inbbbox/Source Files/Networking/PageableComponentSerializer.swift | 1 | 2527 | //
// PageableComponentSerializer.swift
// Inbbbox
//
// Created by Patryk Kaczmarek on 04/02/16.
// Copyright © 2016 Netguru Sp. z o.o. All rights reserved.
//
import Foundation
class PageableComponentSerializer {
/// Provides next page component for given query.
///
/// - parameter query: Query which the next page component should be created for.
/// - parameter receivedHeader: Received response's header.
///
/// - returns: Component for next page.
class func nextPageableComponentWithSentQuery(_ query: Query,
receivedHeader header: [String: AnyObject]) -> PageableComponent? {
return stringComponentFromLinkHeader(header, withName: "next")?.url?.pageableComponentFromQuery(query)
}
/// Provides previous page component for given query.
///
/// - parameter query: Query which the previous page component should be created for.
/// - parameter receivedHeader: Received response's header.
///
/// - returns: Component for previous page.
class func previousPageableComponentWithSentQuery(_ query: Query, receivedHeader header: [String: AnyObject])
-> PageableComponent? {
return stringComponentFromLinkHeader(header, withName: "prev")?.url?.pageableComponentFromQuery(query)
}
}
private extension PageableComponentSerializer {
class func stringComponentFromLinkHeader(_ header: [String: AnyObject], withName name: String) -> String? {
let link = header["Link"] as? String
let linkHeaderComponents = link?.components(separatedBy: ",")
return linkHeaderComponents?.filter { $0.range(of: name) != nil }.first
}
}
private extension String {
var url: URL? {
guard let leftBracket = self.range(of: "<"), let rightBracket = self.range(of: ">") else {
return nil
}
let range: Range<String.Index> = self.index(leftBracket.lowerBound, offsetBy: 1) ..< self.index(rightBracket.upperBound, offsetBy: -1)
let urlString = substring(with: range)
return URL(string: urlString)
}
}
private extension URL {
func pageableComponentFromQuery(_ requestQuery: Query) -> PageableComponent? {
if let substringEndIndex = path.range(of: requestQuery.service.version)?.upperBound {
let subPath = path.substring(from: substringEndIndex)
return PageableComponent(path: subPath, query: query)
}
return nil
}
}
| gpl-3.0 | fc4bdf9c14b04273ab66f3224f7a374a | 35.085714 | 142 | 0.658749 | 4.601093 | false | false | false | false |
svedm/SVSlider | SVSlider/SVSlider.swift | 1 | 11261 | //
// SVSliderView.swift
// SVSlider
//
// Created by Svetoslav Karasev on 30.08.16.
// Copyright © 2016 svedm. All rights reserved.
//
import UIKit
@IBDesignable class SVSlider: UIControl {
// MARK: - Private Variables
private var slider: UIView = UIView()
private var sliderWidthConstraint: NSLayoutConstraint!
private var sliderPositionConstraint: NSLayoutConstraint!
private var sliderHorizontalPaddingConstraints: [NSLayoutConstraint]!
private var sliderVerticalPaddingConstraints: [NSLayoutConstraint]!
private var slideInLabel: UILabel = UILabel()
private var slideOutLabel: UILabel = UILabel()
private var slideOutView: UIView = UIView()
private var imageView: UIImageView = UIImageView()
private var shouldSlide: Bool = false
// MARK: - Public Variables
private(set) var progress: Float = 0.0 {
didSet {
slideOutView.alpha = CGFloat(progress)
}
}
// MARK: - IBInspectable Variables
@IBInspectable var sliderColor: UIColor = UIColor.lightGrayColor() {
didSet {
slider.backgroundColor = sliderColor
setNeedsLayout()
}
}
@IBInspectable var sliderPadding: CGFloat = 2 {
didSet {
for constraint in sliderHorizontalPaddingConstraints {
constraint.constant = sliderPadding
}
for constraint in sliderVerticalPaddingConstraints {
constraint.constant = sliderPadding
}
sliderPositionConstraint.constant = sliderPadding
setNeedsLayout()
}
}
@IBInspectable var sliderShadowColor: UIColor = UIColor.blackColor() {
didSet {
slider.layer.shadowColor = sliderShadowColor.CGColor
setNeedsLayout()
}
}
@IBInspectable var sliderShadowOpacity: Float = 0.5 {
didSet {
slider.layer.shadowOpacity = sliderShadowOpacity
setNeedsLayout()
}
}
@IBInspectable var sliderShadowOffset: CGPoint = CGPoint(x: 0, y: 2) {
didSet {
slider.layer.shadowOffset = CGSize(width: sliderShadowOffset.x, height: sliderShadowOffset.y)
setNeedsLayout()
}
}
@IBInspectable var sliderShadowRadius: CGFloat = 2 {
didSet {
slider.layer.shadowRadius = sliderShadowRadius
setNeedsLayout()
}
}
@IBInspectable var slideInTextColor: UIColor = UIColor.blackColor() {
didSet {
slideInLabel.textColor = slideInTextColor
setNeedsLayout()
}
}
@IBInspectable var slideOutTextColor: UIColor = UIColor.blackColor() {
didSet {
slideOutLabel.textColor = slideOutTextColor
setNeedsLayout()
}
}
@IBInspectable var cornerRadius: CGFloat = 0 {
didSet {
layer.cornerRadius = cornerRadius
slider.layer.cornerRadius = cornerRadius
slideOutView.layer.cornerRadius = cornerRadius
setNeedsLayout()
}
}
@IBInspectable var slideInText: String = "" {
didSet {
slideInLabel.text = slideInText
setNeedsLayout()
}
}
@IBInspectable var slideOutText: String = "" {
didSet {
slideOutLabel.text = slideOutText
setNeedsLayout()
}
}
@IBInspectable var sliderWidth: CGFloat = 50.0 {
didSet {
sliderWidthConstraint.constant = sliderWidth
setNeedsLayout()
}
}
@IBInspectable var sliderImage: UIImage? = nil {
didSet {
imageView.image = sliderImage
setNeedsLayout()
}
}
@IBInspectable var sliderBackImage: UIImage? = nil
@IBInspectable var sliderImageContentMode: UIViewContentMode = .Center {
didSet {
imageView.contentMode = sliderImageContentMode
setNeedsLayout()
}
}
@IBInspectable var slideInFont: UIFont = UIFont.systemFontOfSize(15) {
didSet {
slideInLabel.font = slideInFont
setNeedsLayout()
}
}
@IBInspectable var slideOutFont: UIFont = UIFont.systemFontOfSize(15) {
didSet {
slideOutLabel.font = slideOutFont
setNeedsLayout()
}
}
@IBInspectable var slideOutColor: UIColor = UIColor.greenColor() {
didSet {
slideOutView.backgroundColor = slideOutColor
setNeedsLayout()
}
}
// MARK: - Initializers
override init(frame: CGRect) {
super.init(frame: frame)
setup()
}
required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
setup()
}
// MARK: - Private Methods
private func addVisualConstraints(vertical: String, horizontal: String, view: UIView, toView: UIView) {
let verticalConstraints = NSLayoutConstraint.constraintsWithVisualFormat(vertical, options: [], metrics: nil, views: ["view": view])
let horizontalConstraints = NSLayoutConstraint.constraintsWithVisualFormat(horizontal, options: [], metrics: nil, views: ["view": view])
addConstraints(verticalConstraints)
addConstraints(horizontalConstraints)
}
private func addSlideInLabel() {
// Add slideIn label
slideInLabel.translatesAutoresizingMaskIntoConstraints = false
slideInLabel.textAlignment = .Center
slideInLabel.textColor = slideInTextColor
slideInLabel.font = slideInFont
addSubview(slideInLabel)
addVisualConstraints("V:|[view]|", horizontal: "H:|[view]|", view: slideInLabel, toView: self)
}
private func addSlideOutView() {
slideOutView.translatesAutoresizingMaskIntoConstraints = false
slideOutView.backgroundColor = slideOutColor
slideOutView.alpha = 0
slideOutView.layer.cornerRadius = cornerRadius
addSubview(slideOutView)
addVisualConstraints("V:|[view]|", horizontal: "H:|[view]|", view: slideOutView, toView: self)
// Add slideOut label to slideOutView
slideOutLabel.translatesAutoresizingMaskIntoConstraints = false
slideOutLabel.textAlignment = .Center
slideOutLabel.textColor = slideOutTextColor
slideOutLabel.font = slideOutFont
slideOutView.addSubview(slideOutLabel)
addVisualConstraints("V:|[view]|", horizontal: "H:|[view]|", view: slideOutLabel, toView: slideOutView)
}
private func addSlider() {
// Create Slider
slider.translatesAutoresizingMaskIntoConstraints = false
slider.backgroundColor = sliderColor
slider.layer.cornerRadius = cornerRadius
slider.layer.masksToBounds = false
slider.layer.shadowOffset = CGSize(width: sliderShadowOffset.x, height: sliderShadowOffset.y)
slider.layer.shadowRadius = sliderShadowRadius
slider.layer.shadowOpacity = sliderShadowOpacity
slider.layer.shadowColor = sliderShadowColor.CGColor
addSubview(slider)
sliderHorizontalPaddingConstraints = NSLayoutConstraint.constraintsWithVisualFormat("H:[view]->=padding-|", options: [], metrics: ["padding": sliderPadding], views: ["view": slider])
sliderVerticalPaddingConstraints = NSLayoutConstraint.constraintsWithVisualFormat("V:|-padding-[view]-padding-|", options: [], metrics: ["padding": sliderPadding], views: ["view": slider])
addConstraints(sliderVerticalPaddingConstraints)
addConstraints(sliderHorizontalPaddingConstraints)
sliderWidthConstraint = NSLayoutConstraint(item: slider, attribute: .Width, relatedBy: .Equal, toItem: nil, attribute: .NotAnAttribute, multiplier: 1, constant: sliderWidth)
sliderPositionConstraint = NSLayoutConstraint(item: slider, attribute: .Leading, relatedBy: .Equal, toItem: self, attribute: .Leading, multiplier: 1, constant: sliderPadding)
addConstraint(sliderPositionConstraint)
slider.addConstraint(sliderWidthConstraint)
// ImageView for optional slider image
imageView.translatesAutoresizingMaskIntoConstraints = false
slider.addSubview(imageView)
imageView.contentMode = sliderImageContentMode
imageView.image = sliderImage
addVisualConstraints("V:|[view]|", horizontal: "H:|[view]|", view: imageView, toView: slider)
}
private func setup() {
// Apply the custom slider styling
layer.cornerRadius = cornerRadius
layer.masksToBounds = true
// Add views
addSlideInLabel()
addSlideOutView()
addSlider()
// Add pan gesture to slide the slider view
let pan = UIPanGestureRecognizer(target: self, action: #selector(panGesture))
addGestureRecognizer(pan)
}
// MARK: - Public Methods
func reset() {
progress = 0
sliderWidthConstraint.constant = sliderWidth
sliderPositionConstraint.constant = sliderPadding
setNeedsUpdateConstraints()
layoutIfNeeded()
}
private func changeSliderViewsState(success: Bool) {
imageView.image = success ? sliderBackImage : sliderImage
slideInLabel.hidden = success
slideOutLabel.hidden = !success
}
func panGesture(recognizer: UIPanGestureRecognizer) {
let x = recognizer.locationInView(self).x
let lastPosition = bounds.size.width - sliderWidth - sliderPadding
let startPosition = sliderPadding
switch recognizer.state {
case .Began:
sendActionsForControlEvents(.EditingDidBegin)
shouldSlide = x >= sliderPositionConstraint.constant - sliderPadding
&& x <= sliderPositionConstraint.constant + sliderWidth + sliderPadding
slideInLabel.hidden = progress > 0.5
slideOutLabel.hidden = progress < 0.5
case .Changed:
guard shouldSlide && x >= startPosition && x < lastPosition else { return }
sliderPositionConstraint.constant = x
progress = Float(x / bounds.size.width)
sendActionsForControlEvents(.ValueChanged)
case .Ended: fallthrough
case .Cancelled:
guard shouldSlide else { return }
progress = Float(x / bounds.size.width)
// If we are more than 50% through the swipe and moving the the right direction
let success = progress > 0.5 && recognizer.velocityInView(self).x > -1
let finalX = success ? lastPosition : startPosition
progress = success ? 1 : 0
changeSliderViewsState(success)
sliderPositionConstraint.constant = finalX
setNeedsUpdateConstraints()
UIView.animateWithDuration(0.25, animations: {
self.layoutIfNeeded()
}) { _ in
if success {
if #available(iOS 9.0, *) {
self.sendActionsForControlEvents(.PrimaryActionTriggered)
}
self.sendActionsForControlEvents(.EditingDidEnd)
} else {
self.sendActionsForControlEvents(.TouchCancel)
}
}
default: break
}
}
}
| mit | 98dbe1251f6f3c24dba9b306e88adad4 | 34.632911 | 196 | 0.641385 | 5.514202 | false | false | false | false |
juliand665/LeagueKit | Sources/LeagueKit/Model/Dynamic API/Match.swift | 1 | 3775 | // Created by Julian Dunskus
import Foundation
public struct MatchList: Codable {
public var startIndex: Int
public var endIndex: Int
public var totalGames: Int
public var matches: [MatchReference]
}
public struct MatchReference: Codable {
public var matchID: Int
public var time: Date
public var championID: Int
public var season: Int
public var queue: Queue
public var lane: Lane
public var role: Role
private enum CodingKeys: String, CodingKey {
case matchID = "gameId"
case time = "timestamp"
case championID = "champion"
case season
case queue
case lane
case role
}
}
public struct Player: Codable {
public var summonerName: String
public var summonerID: Int?
public var accountID: Int
public var profileIconID: Int
public var matchHistoryPath: String
private enum CodingKeys: String, CodingKey {
case summonerName
case summonerID = "summonerId"
case accountID = "accountId"
case profileIconID = "profileIcon"
case matchHistoryPath = "matchHistoryUri"
}
}
public struct ParticipantIdentity: Codable {
public var participantID: Int
public var player: Player
private enum CodingKeys: String, CodingKey {
case participantID = "participantId"
case player
}
}
public struct Participant: Codable {
public var participantID: Int
public var teamID: Int
public var championID: Int
private enum CodingKeys: String, CodingKey {
case participantID = "participantId"
case teamID = "teamId"
case championID = "championId"
}
}
public struct Ban: Codable {
public var championID: Int
public var pickTurn: Int
private enum CodingKeys: String, CodingKey {
case championID = "championId"
case pickTurn
}
}
public enum Outcome: String, Codable {
case victory = "Win"
case defeat = "Fail"
}
public struct TeamStats: Codable {
public var teamID: Int
public var outcome: Outcome
public var bans: [Ban]
public var gotFirstBlood: Bool
public var towerKillCount: Int
public var gotFirstTower: Bool
public var dragonKillCount: Int
public var gotFirstDragon: Bool
public var inhibitorKillCount: Int
public var gotFirstInhibitor: Bool
public var riftHeraldKillCount: Int
public var gotFirstRiftHerald: Bool
public var baronKillCount: Int
public var gotFirstBaron: Bool
public var vilemawKillCount: Int
public var dominionScore: Int
private enum CodingKeys: String, CodingKey {
case teamID = "teamId"
case outcome = "win"
case bans = "bans"
case gotFirstBlood = "firstBlood"
case towerKillCount = "towerKills"
case gotFirstTower = "firstTower"
case dragonKillCount = "dragonKills"
case gotFirstDragon = "firstDragon"
case inhibitorKillCount = "inhibitorKills"
case gotFirstInhibitor = "firstInhibitor"
case riftHeraldKillCount = "riftHeraldKills"
case gotFirstRiftHerald = "firstRiftHerald"
case baronKillCount = "baronKills"
case gotFirstBaron = "firstBaron"
case vilemawKillCount = "vilemawKills"
case dominionScore = "dominionVictoryScore"
}
}
public typealias MatchID = ID<Match>
public struct Match: Codable {
public var matchID: Int
public var startTime: Date
public var duration: TimeInterval // technically an Int, but this is more natural
public var season: Season
public var queue: Queue
public var mode: GameMode
public var type: GameType
public var map: Map
public var participantIdentities: [ParticipantIdentity]
public var participants: [Participant]
public var teamStats: [TeamStats]
private enum CodingKeys: String, CodingKey {
case matchID = "gameId"
case startTime = "gameCreation"
case duration = "gameDuration"
case season = "seasonId"
case queue = "queueId"
case type = "gameType"
case mode = "gameMode"
case map = "mapId"
case participantIdentities
case participants
case teamStats = "teams"
}
}
| mit | fe4e1f4cb63c40f90ec4482d936c054e | 24.166667 | 82 | 0.755497 | 3.453797 | false | false | false | false |
tbkka/swift-protobuf | Sources/SwiftProtobuf/FieldTypes.swift | 3 | 19791 | // Sources/SwiftProtobuf/FieldTypes.swift - Proto data types
//
// Copyright (c) 2014 - 2016 Apple Inc. and the project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See LICENSE.txt for license information:
// https://github.com/apple/swift-protobuf/blob/master/LICENSE.txt
//
// -----------------------------------------------------------------------------
///
/// Serialization/deserialization support for each proto field type.
///
/// Note that we cannot just extend the standard Int32, etc, types
/// with serialization information since proto language supports
/// distinct types (with different codings) that use the same
/// in-memory representation. For example, proto "sint32" and
/// "sfixed32" both are represented in-memory as Int32.
///
/// These types are used generically and also passed into
/// various coding/decoding functions to provide type-specific
/// information.
///
// -----------------------------------------------------------------------------
import Foundation
// Note: The protobuf- and JSON-specific methods here are defined
// in ProtobufTypeAdditions.swift and JSONTypeAdditions.swift
public protocol FieldType {
// The Swift type used to store data for this field. For example,
// proto "sint32" fields use Swift "Int32" type.
associatedtype BaseType: Hashable
// The default value for this field type before it has been set.
// This is also used, for example, when JSON decodes a "null"
// value for a field.
static var proto3DefaultValue: BaseType { get }
// Generic reflector methods for looking up the correct
// encoding/decoding for extension fields, map keys, and map
// values.
static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws
static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws
static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws
static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws
static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws
}
///
/// Marker protocol for types that can be used as map keys
///
public protocol MapKeyType: FieldType {
}
///
/// Marker Protocol for types that can be used as map values.
///
public protocol MapValueType: FieldType {
}
//
// We have a struct for every basic proto field type which provides
// serialization/deserialization support as static methods.
//
///
/// Float traits
///
public struct ProtobufFloat: FieldType, MapValueType {
public typealias BaseType = Float
public static var proto3DefaultValue: Float {return 0.0}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularFloatField(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedFloatField(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularFloatField(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedFloatField(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitPackedFloatField(value: value, fieldNumber: fieldNumber)
}
}
///
/// Double
///
public struct ProtobufDouble: FieldType, MapValueType {
public typealias BaseType = Double
public static var proto3DefaultValue: Double {return 0.0}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularDoubleField(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedDoubleField(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularDoubleField(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedDoubleField(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitPackedDoubleField(value: value, fieldNumber: fieldNumber)
}
}
///
/// Int32
///
public struct ProtobufInt32: FieldType, MapKeyType, MapValueType {
public typealias BaseType = Int32
public static var proto3DefaultValue: Int32 {return 0}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularInt32Field(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedInt32Field(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularInt32Field(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedInt32Field(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitPackedInt32Field(value: value, fieldNumber: fieldNumber)
}
}
///
/// Int64
///
public struct ProtobufInt64: FieldType, MapKeyType, MapValueType {
public typealias BaseType = Int64
public static var proto3DefaultValue: Int64 {return 0}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularInt64Field(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedInt64Field(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularInt64Field(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedInt64Field(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitPackedInt64Field(value: value, fieldNumber: fieldNumber)
}
}
///
/// UInt32
///
public struct ProtobufUInt32: FieldType, MapKeyType, MapValueType {
public typealias BaseType = UInt32
public static var proto3DefaultValue: UInt32 {return 0}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularUInt32Field(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedUInt32Field(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularUInt32Field(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedUInt32Field(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitPackedUInt32Field(value: value, fieldNumber: fieldNumber)
}
}
///
/// UInt64
///
public struct ProtobufUInt64: FieldType, MapKeyType, MapValueType {
public typealias BaseType = UInt64
public static var proto3DefaultValue: UInt64 {return 0}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularUInt64Field(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedUInt64Field(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularUInt64Field(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedUInt64Field(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitPackedUInt64Field(value: value, fieldNumber: fieldNumber)
}
}
///
/// SInt32
///
public struct ProtobufSInt32: FieldType, MapKeyType, MapValueType {
public typealias BaseType = Int32
public static var proto3DefaultValue: Int32 {return 0}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularSInt32Field(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedSInt32Field(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularSInt32Field(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedSInt32Field(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitPackedSInt32Field(value: value, fieldNumber: fieldNumber)
}
}
///
/// SInt64
///
public struct ProtobufSInt64: FieldType, MapKeyType, MapValueType {
public typealias BaseType = Int64
public static var proto3DefaultValue: Int64 {return 0}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularSInt64Field(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedSInt64Field(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularSInt64Field(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedSInt64Field(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitPackedSInt64Field(value: value, fieldNumber: fieldNumber)
}
}
///
/// Fixed32
///
public struct ProtobufFixed32: FieldType, MapKeyType, MapValueType {
public typealias BaseType = UInt32
public static var proto3DefaultValue: UInt32 {return 0}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularFixed32Field(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedFixed32Field(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularFixed32Field(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedFixed32Field(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitPackedFixed32Field(value: value, fieldNumber: fieldNumber)
}
}
///
/// Fixed64
///
public struct ProtobufFixed64: FieldType, MapKeyType, MapValueType {
public typealias BaseType = UInt64
public static var proto3DefaultValue: UInt64 {return 0}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularFixed64Field(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedFixed64Field(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularFixed64Field(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedFixed64Field(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitPackedFixed64Field(value: value, fieldNumber: fieldNumber)
}
}
///
/// SFixed32
///
public struct ProtobufSFixed32: FieldType, MapKeyType, MapValueType {
public typealias BaseType = Int32
public static var proto3DefaultValue: Int32 {return 0}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularSFixed32Field(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedSFixed32Field(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularSFixed32Field(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedSFixed32Field(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitPackedSFixed32Field(value: value, fieldNumber: fieldNumber)
}
}
///
/// SFixed64
///
public struct ProtobufSFixed64: FieldType, MapKeyType, MapValueType {
public typealias BaseType = Int64
public static var proto3DefaultValue: Int64 {return 0}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularSFixed64Field(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedSFixed64Field(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularSFixed64Field(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedSFixed64Field(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitPackedSFixed64Field(value: value, fieldNumber: fieldNumber)
}
}
///
/// Bool
///
public struct ProtobufBool: FieldType, MapKeyType, MapValueType {
public typealias BaseType = Bool
public static var proto3DefaultValue: Bool {return false}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularBoolField(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedBoolField(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularBoolField(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedBoolField(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitPackedBoolField(value: value, fieldNumber: fieldNumber)
}
}
///
/// String
///
public struct ProtobufString: FieldType, MapKeyType, MapValueType {
public typealias BaseType = String
public static var proto3DefaultValue: String {return String()}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularStringField(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedStringField(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularStringField(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedStringField(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
assert(false)
}
}
///
/// Bytes
///
public struct ProtobufBytes: FieldType, MapValueType {
public typealias BaseType = Data
public static var proto3DefaultValue: Data {return Data()}
public static func decodeSingular<D: Decoder>(value: inout BaseType?, from decoder: inout D) throws {
try decoder.decodeSingularBytesField(value: &value)
}
public static func decodeRepeated<D: Decoder>(value: inout [BaseType], from decoder: inout D) throws {
try decoder.decodeRepeatedBytesField(value: &value)
}
public static func visitSingular<V: Visitor>(value: BaseType, fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitSingularBytesField(value: value, fieldNumber: fieldNumber)
}
public static func visitRepeated<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
try visitor.visitRepeatedBytesField(value: value, fieldNumber: fieldNumber)
}
public static func visitPacked<V: Visitor>(value: [BaseType], fieldNumber: Int, with visitor: inout V) throws {
assert(false)
}
}
| apache-2.0 | a71d7515c2a55bb7ab9a56a543425f1d | 47.036408 | 117 | 0.720681 | 4.552795 | false | false | false | false |
blockchain/My-Wallet-V3-iOS | Modules/FeatureAccountPicker/UI/Model/AccountPickerRowPaymentMethod.swift | 1 | 957 | // Copyright © Blockchain Luxembourg S.A. All rights reserved.
import Errors
import SwiftUI
extension AccountPickerRow {
public struct PaymentMethod: Equatable {
// MARK: - Internal properties
let id: AnyHashable
let block: Bool
let ux: UX.Dialog?
var title: String
var description: String
var badgeView: Image?
var badgeBackground: Color
// MARK: - Init
public init(
id: AnyHashable,
block: Bool = false,
ux: UX.Dialog? = nil,
title: String,
description: String,
badgeView: Image?,
badgeBackground: Color
) {
self.id = id
self.ux = ux
self.block = block
self.title = title
self.description = description
self.badgeView = badgeView
self.badgeBackground = badgeBackground
}
}
}
| lgpl-3.0 | 457bbc2911817083226b9d2d83b030e9 | 22.9 | 62 | 0.538703 | 5.167568 | false | false | false | false |
marty-suzuki/QiitaWithFluxSample | QiitaWithFluxSample/Source/Search/Model/SearchTopDataSource.swift | 1 | 2552 | //
// SearchTopDataSource.swift
// QiitaWithFluxSample
//
// Created by marty-suzuki on 2017/04/16.
// Copyright © 2017年 marty-suzuki. All rights reserved.
//
import UIKit
import RxSwift
final class SearchTopDataSource: NSObject {
let viewModel: SearchTopViewModel
let selectedIndexPath: Observable<IndexPath>
fileprivate let _selectedIndexPath = PublishSubject<IndexPath>()
fileprivate var canShowLoadingView: Bool {
return viewModel.hasNext.value && !viewModel.items.value.isEmpty && viewModel.lastItemsRequest.value != nil
}
init(viewModel: SearchTopViewModel) {
self.viewModel = viewModel
self.selectedIndexPath = _selectedIndexPath
}
func configure(with tableView: UITableView) {
tableView.register(SearchTopViewCell.self)
tableView.dataSource = self
tableView.delegate = self
tableView.rowHeight = UITableViewAutomaticDimension
tableView.estimatedRowHeight = 100
}
}
extension SearchTopDataSource: UITableViewDataSource {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return viewModel.items.value.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(SearchTopViewCell.self, for: indexPath)
let item = viewModel.items.value[indexPath.row]
cell.configure(with: item)
return cell
}
func tableView(_ tableView: UITableView, viewForFooterInSection section: Int) -> UIView? {
if canShowLoadingView {
let view = LoadingView(indicatorStyle: .gray)
view.isHidden = false
return view
}
return nil
}
}
extension SearchTopDataSource: UITableViewDelegate {
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return UITableViewAutomaticDimension
}
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
tableView.deselectRow(at: indexPath, animated: false)
_selectedIndexPath.onNext(indexPath)
}
func tableView(_ tableView: UITableView, heightForHeaderInSection section: Int) -> CGFloat {
return .leastNormalMagnitude
}
func tableView(_ tableView: UITableView, heightForFooterInSection section: Int) -> CGFloat {
if canShowLoadingView {
return 44
}
return .leastNormalMagnitude
}
}
| mit | e23b1de65f43b575c6af7cde4ff568e4 | 31.679487 | 115 | 0.688505 | 5.389006 | false | false | false | false |
blockchain/My-Wallet-V3-iOS | Modules/Platform/Sources/PlatformUIKit/Components/AccountPicker/Header/AccountPickerHeaderBuilder.swift | 1 | 1734 | // Copyright © Blockchain Luxembourg S.A. All rights reserved.
import UIKit
public protocol AccountPickerHeaderViewAPI: UIView {
var searchBar: UISearchBar? { get }
}
public struct AccountPickerHeaderBuilder {
private let headerType: AccountPickerHeaderType
public init(headerType: AccountPickerHeaderType) {
self.headerType = headerType
}
var isAlwaysVisible: Bool {
switch headerType {
case .none:
return false
case .simple(let model):
return model.searchable
case .default(let model):
return model.searchable
}
}
public var defaultHeight: CGFloat {
switch headerType {
case .none:
return 0
case .default(let model):
return model.height
case .simple(let model):
return model.height
}
}
public func headerView(fittingWidth width: CGFloat, customHeight: CGFloat?) -> AccountPickerHeaderViewAPI? {
let height = customHeight ?? defaultHeight
let frame = CGRect(x: 0, y: 0, width: width, height: height)
switch headerType {
case .none:
return nil
case .default(let model):
let view = AccountPickerHeaderView(frame: frame)
view.model = model
return view
case .simple(let model):
let view = AccountPickerSimpleHeaderView(frame: frame)
view.model = model
return view
}
}
}
extension AccountPickerHeaderBuilder: HeaderBuilder {
public func view(fittingWidth width: CGFloat, customHeight: CGFloat?) -> UIView? {
headerView(fittingWidth: width, customHeight: customHeight)
}
}
| lgpl-3.0 | 9b39a194546eaa96182637ee73b9a443 | 27.409836 | 112 | 0.620312 | 4.89548 | false | false | false | false |
Gitliming/Demoes | Demoes/Demoes/CustomAnimationNav/NavigateAnimation.swift | 1 | 2771 | //
// NavigateAnimation.swift
// Dispersive switch
//
// Created by xpming on 16/6/8.
// Copyright © 2016年 xpming. All rights reserved.
//
import UIKit
class NavigateAnimation: NSObject, UIViewControllerAnimatedTransitioning, CAAnimationDelegate {
var transitionContext:UIViewControllerContextTransitioning?
var isPush:Bool = false
var imageRect:CGRect?
func transitionDuration(using transitionContext: UIViewControllerContextTransitioning?) -> TimeInterval {
return 1.0
}
func animateTransition(using transitionContext: UIViewControllerContextTransitioning) {
self.transitionContext = transitionContext
pushOrPopAnimation()
}
func pushOrPopAnimation(){
let x = imageRect!.origin.x + (imageRect?.size.width)! / 2
let y = imageRect!.origin.y + (imageRect?.size.height)! / 2
let radiu = sqrt(x * x + y * y)
var origionPath:UIBezierPath?
var finalPath:UIBezierPath?
if isPush == true {
origionPath = UIBezierPath(ovalIn: CGRect(x: x, y: y, width: 0, height: 0))
finalPath = UIBezierPath(ovalIn: CGRect(x: x, y: y, width: 0, height: 0).insetBy(dx: -radiu, dy: -radiu))
}else{
origionPath = UIBezierPath(ovalIn: CGRect(x: x, y: y, width: 0, height: 0).insetBy(dx: -radiu, dy: -radiu))
finalPath = UIBezierPath(ovalIn: CGRect(x: x, y: y, width: 0, height: 0))
}
let toVc = transitionContext?.viewController(forKey: UITransitionContextViewControllerKey.to)
let fromVc = transitionContext?.viewController(forKey: UITransitionContextViewControllerKey.from)
let contentView = transitionContext?.containerView
let animation = CABasicAnimation(keyPath: "path")
animation.duration = 0.3
animation.fromValue = origionPath!.cgPath
animation.toValue = finalPath!.cgPath
animation.delegate = self
let layer = CAShapeLayer()
layer.path = finalPath!.cgPath
layer.add(animation, forKey: "path")
if isPush == true {
contentView?.addSubview((toVc?.view)!)
toVc?.view.layer.mask = layer
}else{
fromVc?.view.layer.mask = layer
contentView?.addSubview((toVc?.view)!)
contentView?.addSubview((fromVc?.view)!)
}
}
func animationDidStop(_ anim: CAAnimation, finished flag: Bool) {
transitionContext?.completeTransition(true)
transitionContext?.viewController(forKey: UITransitionContextViewControllerKey.from)?.view.layer.mask = nil
transitionContext?.viewController(forKey: UITransitionContextViewControllerKey.to)?.view.layer.mask = nil
if !isPush {
}
}
}
| apache-2.0 | 1140b80b3f2f568dee0cb01560bdb30e | 38.542857 | 115 | 0.653902 | 4.715503 | false | false | false | false |
NordicSemiconductor/IOS-nRF-Toolbox | nRF Toolbox/Profiles/HeartRateMonitor/HeartRateMonitorTableViewController.swift | 1 | 4068 | /*
* Copyright (c) 2020, Nordic Semiconductor
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without modification,
* are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright notice, this
* list of conditions and the following disclaimer in the documentation and/or
* other materials provided with the distribution.
*
* 3. Neither the name of the copyright holder nor the names of its contributors may
* be used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
* IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT,
* INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
* NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
* PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
import UIKit
import CoreBluetooth
private extension Identifier where Value == Section {
static let sensorLocation: Identifier<Section> = "SensorLocation"
static let heartRateValue: Identifier<Section> = "HeartRateValue"
static let heartRateChart: Identifier<Section> = "HeartRateChart"
static let chartSection: Identifier<Section> = "ChartSection"
}
class HeartRateMonitorTableViewController: PeripheralTableViewController {
private let locationSection = SernsorLocationSection(id: .sensorLocation)
private let instantaneousHeartRateSection = HeartRateSection(id: .heartRateValue)
private var chartSection = HeartRateChartSection(id: .chartSection)
#if RAND
var randomizer = Randomizer(top: 120, bottom: 60, value: 80, delta: 2)
#endif
override var peripheralDescription: PeripheralDescription { .heartRateSensor }
override var navigationTitle: String { "Heart Rate" }
override var internalSections: [Section] { [instantaneousHeartRateSection, locationSection, chartSection] }
override func viewDidLoad() {
super.viewDidLoad()
tableView.register(LinearChartTableViewCell.self, forCellReuseIdentifier: "LinearChartTableViewCell")
}
override func didUpdateValue(for characteristic: CBCharacteristic) {
switch characteristic.uuid {
case CBUUID.Characteristics.HeartRate.measurement:
let data: HeartRateMeasurementCharacteristic
#if RAND
data = HeartRateMeasurementCharacteristic(value: randomizer.next()!)
#else
do {
data = try HeartRateMeasurementCharacteristic(with: characteristic.value!, date: Date())
} catch let error {
displayErrorAlert(error: error)
return
}
#endif
instantaneousHeartRateSection.update(with: data)
chartSection.update(with: data)
tableView.reloadData()
case CBUUID.Characteristics.HeartRate.location:
guard let value = characteristic.value else { return }
do {
let bodySensorCharacteristic = try BodySensorLocationCharacteristic(with: value)
self.locationSection.update(with: bodySensorCharacteristic)
tableView.reloadData()
} catch let error {
displayErrorAlert(error: error)
}
default:
super.didUpdateValue(for: characteristic)
}
}
}
| bsd-3-clause | 1c8baa1ffe9d5eab93be92c8d29b4e86 | 43.703297 | 111 | 0.716814 | 5.097744 | false | false | false | false |
Incipia/Goalie | Goalie/OnboardingTransitionManager.swift | 1 | 2294 | //
// OnboardingTransitionManager.swift
// Goalie
//
// Created by Gregory Klein on 1/13/16.
// Copyright © 2016 Incipia. All rights reserved.
//
import UIKit
class OnboardingTransitionManager: NSObject, UIViewControllerAnimatedTransitioning, UIViewControllerTransitioningDelegate
{
// MARK: - UIViewControllerAnimatedTransitioning protocol methods
func animateTransition(using transitionContext: UIViewControllerContextTransitioning)
{
let container = transitionContext.containerView
let fromController = transitionContext.viewController(forKey: UITransitionContextViewControllerKey.from) as! OnboardingViewController
let toController = transitionContext.viewController(forKey: UITransitionContextViewControllerKey.to) as! MainTasksViewController
container.addSubview(toController.view)
let whiteView = UIView()
whiteView.frame = toController.view.bounds
whiteView.backgroundColor = UIColor.white
container.addSubview(whiteView)
container.addSubview(fromController.view)
let duration = transitionDuration(using: transitionContext)
UIView.animate(withDuration: duration * 0.5, delay: 0, options: UIViewAnimationOptions.curveEaseIn, animations: { () -> Void in
fromController.view.alpha = 0
fromController.view.transform = CGAffineTransform(scaleX: 5, y: 5)
}, completion: { finished in
UIView.animate(withDuration: duration * 0.5, delay: 0, options: UIViewAnimationOptions.curveEaseOut, animations: { () -> Void in
whiteView.alpha = 0
}, completion: { (finished) -> Void in
transitionContext.completeTransition(true)
})
})
}
func transitionDuration(using transitionContext: UIViewControllerContextTransitioning?) -> TimeInterval {
return 0.6
}
// MARK: - UIViewControllerTransitioningDelegate protocol methods
func animationController(forPresented presented: UIViewController, presenting: UIViewController, source: UIViewController) -> UIViewControllerAnimatedTransitioning? {
return self
}
func animationController(forDismissed dismissed: UIViewController) -> UIViewControllerAnimatedTransitioning? {
return self
}
}
| apache-2.0 | c7355c4b11b27e928ff676b05f5bd7f6 | 40.690909 | 169 | 0.727867 | 6.002618 | false | false | false | false |
LeeShiYoung/LSYWeibo | LSYWeiBo/PictureSelector/PictureButtonCollectionViewCell.swift | 1 | 2182 | //
// PictureButtonCollectionViewCell.swift
// PictureSelector
//
// Created by 李世洋 on 16/5/30.
// Copyright © 2016年 李世洋. All rights reserved.
//
import UIKit
protocol PictureButtonCellDelegate: NSObjectProtocol {
func showPictureSelector(cell: PictureButtonCollectionViewCell?)
func removeBtnClick(tag: Int)
}
class PictureButtonCollectionViewCell: UICollectionViewCell {
weak var delegate: PictureButtonCellDelegate?
@IBOutlet weak var selectedImage: UIImageView!
@IBOutlet weak var removeButton: UIButton!
var picture: Pictures? {
didSet{
selectedImage.image = picture?.image
picture?.isRemove == false ? isRemoveBtn() : unRemoveBtn()
}
}
func isRemoveBtn() {
removeButton.hidden = true
removeButton.enabled = false
}
func unRemoveBtn() {
removeButton.hidden = false
removeButton.enabled = true
}
@IBAction func removeBtnClick(sender: UIButton) {
delegate?.removeBtnClick(sender.tag - 3000)
}
@objc private func selectedBtnClick(sender: UIButton) {
delegate?.showPictureSelector(self)
}
override func awakeFromNib() {
super.awakeFromNib()
selectedImage.userInteractionEnabled = true
let tapGes = UITapGestureRecognizer(target: self, action: .selectedBtnClick)
selectedImage.addGestureRecognizer(tapGes)
}
}
class PictureSelectorFlowLayout: UICollectionViewFlowLayout {
override func prepareLayout() {
super.prepareLayout()
let margin:CGFloat = 5
let screenMargin:CGFloat = 20
sectionInset = UIEdgeInsets(top: 10, left: screenMargin, bottom: 10, right: screenMargin)
let w = collectionView?.frame.size.width
let size = (w! - (margin*2 + screenMargin*2)) / 3
itemSize = CGSize(width: size, height: size)
minimumLineSpacing = margin
minimumInteritemSpacing = margin
}
}
private extension Selector {
static let selectedBtnClick = #selector(PictureButtonCollectionViewCell.selectedBtnClick(_:))
} | artistic-2.0 | 7c008bdbe603ef653d6733c8813c3579 | 26.443038 | 98 | 0.658976 | 5.135071 | false | false | false | false |
joneshq/MessagePack.swift | MessagePackTests/Source/Matchers.swift | 2 | 990 | import Nimble
func beContainedIn<S: SequenceType, T: Equatable where S.Generator.Element == T>(sequence: S) -> MatcherFunc<T> {
return MatcherFunc { actualExpression, failureMessage in
failureMessage.postfixMessage = "beContainedIn <\(stringify(sequence))>"
if let value = actualExpression.evaluate() {
return contains(sequence, value)
} else {
return false
}
}
}
func beContainedIn<S: SequenceType, L: BooleanType>(sequence: S, predicate: (S.Generator.Element, S.Generator.Element) -> L) -> MatcherFunc<S.Generator.Element> {
return MatcherFunc { actualExpression, failureMessage in
failureMessage.postfixMessage = "beContainedIn <\(stringify(sequence))>"
if let value = actualExpression.evaluate() {
return contains(sequence) { (element: S.Generator.Element) -> L in
return predicate(value, element)
}
} else {
return false
}
}
}
| mit | 601248c9ccec67080fbbf0b0ffad4d83 | 38.6 | 162 | 0.640404 | 4.805825 | false | false | false | false |
jmtrachy/poc-game | ProofOfConceptGame/CircleService.swift | 1 | 2817 | //
// CircleService.swift
// ProofOfConceptGame
//
// Created by James Trachy on 6/22/14.
// Copyright (c) 2014 James Trachy. All rights reserved.
//
import Foundation
class CircleService {
var circles: [Circle];
var circleCallCounter = 0;
let canvasSize = (200, 200);
init () {
circles = [Circle]()
//createCenteredCircle()
}
func createCenteredCircle() {
// figure out x and y position in this method or have it passed in
createCircle(100, yPos: 100)
}
func createCircle(xPos: Int, yPos: Int) -> Circle {
let circle = Circle(x: xPos, y: yPos)
circles.append(circle)
return circle
}
func createRandomCircle() -> Circle {
var validPoint = false
var newX: Int
var newY: Int
var attempts = 0
repeat {
newX = Int(arc4random_uniform(320))
newY = Int(arc4random_uniform(568))
validPoint = isNewCircleValid(newX, yPos: newY)
} while (!validPoint && attempts++ < 100)
return createCircle(newX, yPos: newY)
}
func isNewCircleValid(xPos: Int, yPos: Int) -> Bool {
/*
var overlapsExistingCircle = false
var counter = 0
while !overlapsExistingCircle && counter < circles.count {
var c = circles[counter++]
let xDifference = abs(xPos - c.xCoord)
let yDifference = abs(yPos - c.yCoord)
let distanceFromCenters = sqrt((Double) (xDifference * xDifference + yDifference * yDifference))
let combinedCircleSize = (Double) (2 * c.getMaxRadius())
if distanceFromCenters < combinedCircleSize {
overlapsExistingCircle = true
println("Couldn't create cirlce at point (\(xPos), \(yPos))")
}
}
return !overlapsExistingCircle
*/
return true
}
func advanceGame() {
deleteMaxCircles()
growCircles()
if circleCallCounter++ == 5 {
circleCallCounter = 0
createRandomCircle()
}
}
func deleteMaxCircles() {
/*
var recordsToDelete = Int[]()
var counter = 0
for c in circles {
if c.currentRadius == c.maxRadius {
recordsToDelete.append(counter)
}
counter++
}
recordsToDelete = recordsToDelete.reverse()
for i in recordsToDelete {
circles.removeAtIndex(i);
}
*/
}
func growCircles() {
/*
for c in circles {
if c.currentRadius < c.maxRadius {
c.currentRadius = c.currentRadius + 0.25;
}
}
*/
}
}
| apache-2.0 | b11dd626fbf61a652753860b0754ded6 | 25.083333 | 108 | 0.532481 | 4.536232 | false | false | false | false |
xuzhuoxi/SearchKit | Source/cs/cache/IDCache.swift | 1 | 2201 | //
// IDCache.swift
// SearchKit
//
// Created by 许灼溪 on 16/1/4.
//
//
import Foundation
/**
*
* @author xuzhuoxi
*
*/
class IDCache: IDCacheProtocol, CacheInitProtocol {
fileprivate(set) var _cacheName: String!
var key2IDs: Dictionary<String, [String]>!
var cacheName: String {
return _cacheName
}
var keysSize: Int {
return nil == key2IDs ? 0 : key2IDs!.count
}
final func initCache(_ cacheName: String, _ valueCodingInstance: ValueCodingStrategyProtocol?, _ initialCapacity: UInt) {
self._cacheName = cacheName
self.key2IDs = Dictionary<String, [String]>(minimumCapacity: Int(initialCapacity))
}
final func supplyData(_ data: String) {
if let resource = Resource.getResourceByData(data) {
supplyResource(resource)
}
}
final func supplyData(_ key: String, value: String) {
tryCacheKeyValue(key, value)
}
final func supplyResource(_ resource: Resource) {
let size = resource.size
for i in 0 ..< size {
tryCacheKeyValue(resource.getKey(i), resource.getValue(i))
}
}
final func supplyResource(_ url: URL) {
if let resource = Resource.getResource(url.path) {
supplyResource(resource)
}
}
final func isKey(_ key: String) -> Bool {
return key2IDs.has(key)
}
final func getIDs(_ key: String) -> [String]? {
return key2IDs[key]
}
final func toString() ->String {
return "\(_cacheName)\n\(key2IDs)"
}
/**
* 尝试缓存一对键值对<br>
* 先检查有效性,不通过则忽略<br>
*
* @param resourceKey
* 键
* @param resourceValue
* 值
* @return 缓存成功true,否则false.
*/
func tryCacheKeyValue(_ key: String, _ value: String){
//子类实现
}
static func createWeightCache(_ cacheName: String, resource: Resource) ->IDCacheProtocol {
let rs = IDCacheImpl()
rs.initCache(cacheName, nil, UInt(resource.size))
rs.supplyResource(resource)
return rs;
}
}
| mit | 26aafb97e9666a7ec893c1ddd9b21dae | 23.425287 | 125 | 0.576941 | 3.986867 | false | false | false | false |
JohnSansoucie/MyProject2 | BlueCapKit/External/SimpleFutures/ExecutionContext.swift | 1 | 1869 | //
// ExecutionContext.swift
// BlueCapKit
//
// Created by Troy Stribling on 12/3/14.
// Copyright (c) 2014 gnos.us. All rights reserved.
//
import Foundation
public protocol ExecutionContext {
func execute(task:Void->Void)
}
public class QueueContext : ExecutionContext {
public class var main : QueueContext {
struct Static {
static let instance = QueueContext(queue:Queue.main)
}
return Static.instance
}
public class var global: QueueContext {
struct Static {
static let instance : QueueContext = QueueContext(queue:Queue.global)
}
return Static.instance
}
let queue:Queue
public init(queue:Queue) {
self.queue = queue
}
public func execute(task:Void -> Void) {
queue.async(task)
}
}
public struct Queue {
public static let main = Queue(dispatch_get_main_queue());
public static let global = Queue(dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0))
internal static let simpleFutures = Queue("us.gnos.simpleFutures")
internal static let simpleFutureStreams = Queue("us.gnos.simpleFutureStreams")
var queue: dispatch_queue_t
public init(_ queueName:String) {
self.queue = dispatch_queue_create(queueName, DISPATCH_QUEUE_SERIAL)
}
public init(_ queue:dispatch_queue_t) {
self.queue = queue
}
public func sync(block:Void -> Void) {
dispatch_sync(self.queue, block)
}
public func sync<T>(block:Void -> T) -> T {
var result:T!
dispatch_sync(self.queue, {
result = block();
});
return result;
}
public func async(block:dispatch_block_t) {
dispatch_async(self.queue, block);
}
}
| mit | e76b3322c2c91a1f1174dc2b72fab2e4 | 22.074074 | 110 | 0.598716 | 4.2 | false | false | false | false |
turbolent/regex | Sources/Regex/Instruction.swift | 1 | 5486 | import Foundation
/// Matches a pattern, compiled into instructions, against a sequence of values.
///
/// An implementation of Thompson's Virtual Machine-based regular expression engine, as described
/// in great detail by Russ Cox in "Regular Expression Matching: the Virtual Machine Approach"
/// (see http://swtch.com/~rsc/regexp/regexp2.html), generalized to arbitrary instructions,
/// based on simple effects
///
public indirect enum Instruction<Value, Matcher, Keyer, Result>
where Matcher: Regex.Matcher, Matcher.Value == Value,
Keyer: Regex.Keyer, Keyer.Value == Value
{
case end
case accept(Result)
case split([Instruction])
case match(Matcher, Instruction)
case skip(Instruction)
case atEnd(Instruction)
case lookup(Keyer, [Keyer.Key: Instruction])
public enum Location {
case next
case current
}
public enum Effect {
case resume(Location, [Instruction])
case result(Result)
case end
}
public init(instructions: [Instruction]) {
if instructions.isEmpty {
self = .end
return
}
if let instruction = instructions.first, instructions.count == 1 {
self = instruction
return
}
self = .split(instructions)
}
public func evaluate(value: Value?) -> Effect {
switch self {
case .end:
return .end
case let .accept(result):
return .result(result)
case let .match(matcher, next):
guard
let value = value,
matcher.match(value: value)
else {
return .end
}
return .resume(.current, [next])
case let .lookup(keyer, table):
guard
let value = value,
let instruction = table[keyer.key(for: value)]
else {
return .end
}
return .resume(.current, [instruction])
case let .split(instructions):
return .resume(.current, instructions)
case let .atEnd(next):
guard value == nil else {
return .end
}
return .resume(.current, [next])
case let .skip(next):
return .resume(.next, [next])
}
}
public func match<S: Sequence>(_ values: S, greedy: Bool = true)
-> [MatchResult<Result>]
where S.Element == Value
{
var currentThreads: [Instruction] = []
var newThreads: [Instruction] = []
currentThreads.append(self)
// NOTE: can't use for-loop, as last iteration needs to be value == nil
var valueIterator = values.makeIterator()
var nextValue = valueIterator.next()
var valueIndex = 0
var results: [MatchResult<Result>] = []
while !currentThreads.isEmpty {
let value = nextValue
nextValue = valueIterator.next()
// NOTE: can't use for-loop, as additions to currentThreads in body should be observed
var threadIndex = 0
while threadIndex < currentThreads.count {
defer { threadIndex += 1 }
let instruction = currentThreads[threadIndex]
switch instruction.evaluate(value: value) {
case let .resume(.next, instructions):
newThreads.append(contentsOf: instructions)
case let .resume(.current, instructions):
currentThreads.append(contentsOf: instructions)
case let .result(result):
results.append(
MatchResult(
result: result,
length: valueIndex
)
)
case .end:
break
}
}
if !greedy && !results.isEmpty {
return results
}
if value == nil {
return results
}
swap(¤tThreads, &newThreads)
newThreads.removeAll()
valueIndex += 1
}
return results
}
}
extension Instruction: Equatable
where Matcher: Equatable,
Keyer: Equatable,
Result: Equatable {}
extension Instruction: Hashable
where Matcher: Hashable,
Keyer: Hashable,
Result: Hashable {}
public extension Instruction
where Value: Equatable,
Matcher == EquatableMatcher<Value>
{
static func atom(_ expected: Value, _ next: Instruction) -> Instruction {
return .match(EquatableMatcher(expected: expected), .skip(next))
}
}
public extension Instruction
where Keyer == HashableKeyer<Value>
{
static func lookup(_ table: [Keyer.Value: Instruction]) -> Instruction {
return .lookup(HashableKeyer(), table)
}
}
public extension Instruction
where Keyer == AnyHashableKeyer<Value>
{
static func lookup(_ table: [AnyHashable: Instruction]) -> Instruction {
return .lookup(AnyHashableKeyer(), table)
}
}
public typealias HashableInstruction<Value, Result> =
Instruction<Value, EquatableMatcher<Value>, HashableKeyer<Value>, Result>
where Value: Hashable
public typealias AnyHashableInstruction<Value, Result> =
Instruction<Value, EquatableMatcher<Value>, AnyHashableKeyer<Value>, Result>
where Value: Hashable
| mit | b124448c795b5a1bda47f5b34f42910e | 26.989796 | 98 | 0.570361 | 4.937894 | false | false | false | false |
adding/iOS-BaseArch | iOS-BaseArch/iOS-BaseArch/AFDateExtension.swift | 1 | 18910 | //
// AFDateExtension.swift
//
// Version 2.0.0
//
// Created by Melvin Rivera on 7/15/14.
// Copyright (c) 2014. All rights reserved.
//
import Foundation
enum DateFormat {
case ISO8601, DotNet, RSS, AltRSS
case Custom(String)
}
extension NSDate {
// MARK: Intervals In Seconds
private class func minuteInSeconds() -> Double { return 60 }
private class func hourInSeconds() -> Double { return 3600 }
private class func dayInSeconds() -> Double { return 86400 }
private class func weekInSeconds() -> Double { return 604800 }
private class func yearInSeconds() -> Double { return 31556926 }
// MARK: Components
private class func componentFlags() -> NSCalendarUnit { return NSCalendarUnit.CalendarUnitYear | NSCalendarUnit.CalendarUnitMonth | NSCalendarUnit.CalendarUnitDay | NSCalendarUnit.CalendarUnitWeekOfYear | NSCalendarUnit.CalendarUnitHour | NSCalendarUnit.CalendarUnitMinute | NSCalendarUnit.CalendarUnitSecond | NSCalendarUnit.CalendarUnitWeekday | NSCalendarUnit.CalendarUnitWeekdayOrdinal | NSCalendarUnit.CalendarUnitWeekOfYear }
private class func components(#fromDate: NSDate) -> NSDateComponents! {
return NSCalendar.currentCalendar().components(NSDate.componentFlags(), fromDate: fromDate)
}
private func components() -> NSDateComponents {
return NSDate.components(fromDate: self)!
}
// MARK: Date From String
convenience init(fromString string: String, format:DateFormat)
{
if string.isEmpty {
self.init()
return
}
let string = string as NSString
switch format {
case .DotNet:
// Expects "/Date(1268123281843)/"
let startIndex = string.rangeOfString("(").location + 1
let endIndex = string.rangeOfString(")").location
let range = NSRange(location: startIndex, length: endIndex-startIndex)
let milliseconds = (string.substringWithRange(range) as NSString).longLongValue
let interval = NSTimeInterval(milliseconds / 1000)
self.init(timeIntervalSince1970: interval)
case .ISO8601:
var s = string
if string.hasSuffix(" 00:00") {
s = s.substringToIndex(s.length-6) + "GMT"
} else if string.hasSuffix("Z") {
s = s.substringToIndex(s.length-1) + "GMT"
}
struct Static {
static var formatter: NSDateFormatter? = nil
static var token: dispatch_once_t = 0;
}
dispatch_once(&Static.token) {
Static.formatter = NSDateFormatter();
Static.formatter?.dateFormat = "yyyy-MM-dd'T'HH:mm:ssZZZ"
}
if let date = Static.formatter!.dateFromString(string as String) {
self.init(timeInterval:0, sinceDate:date)
} else {
self.init()
}
case .RSS:
var s = string
if string.hasSuffix("Z") {
s = s.substringToIndex(s.length-1) + "GMT"
}
struct Static {
static var formatter: NSDateFormatter? = nil
static var token: dispatch_once_t = 0;
}
dispatch_once(&Static.token) {
Static.formatter = NSDateFormatter();
Static.formatter?.dateFormat = "EEE, d MMM yyyy HH:mm:ss ZZZ"
}
if let date = Static.formatter!.dateFromString(string as String) {
self.init(timeInterval:0, sinceDate:date)
} else {
self.init()
}
case .AltRSS:
var s = string
if string.hasSuffix("Z") {
s = s.substringToIndex(s.length-1) + "GMT"
}
struct Static {
static var formatter: NSDateFormatter? = nil
static var token: dispatch_once_t = 0;
}
dispatch_once(&Static.token) {
Static.formatter = NSDateFormatter();
Static.formatter?.dateFormat = "d MMM yyyy HH:mm:ss ZZZ"
}
if let date = Static.formatter!.dateFromString(string as String) {
self.init(timeInterval:0, sinceDate:date)
} else {
self.init()
}
case .Custom(let dateFormat):
struct Static {
static var formatter: NSDateFormatter? = nil
static var token: dispatch_once_t = 0;
}
dispatch_once(&Static.token) {
Static.formatter = NSDateFormatter();
Static.formatter?.dateFormat = dateFormat
}
if let date = Static.formatter!.dateFromString(string as String) {
self.init(timeInterval:0, sinceDate:date)
} else {
self.init()
}
}
}
// MARK: Comparing Dates
func isEqualToDateIgnoringTime(date: NSDate) -> Bool
{
let comp1 = NSDate.components(fromDate: self)
let comp2 = NSDate.components(fromDate: date)
return ((comp1.year == comp2.year) && (comp1.month == comp2.month) && (comp1.day == comp2.day))
}
func isToday() -> Bool
{
return self.isEqualToDateIgnoringTime(NSDate())
}
func isTomorrow() -> Bool
{
return self.isEqualToDateIgnoringTime(NSDate().dateByAddingDays(1))
}
func isYesterday() -> Bool
{
return self.isEqualToDateIgnoringTime(NSDate().dateBySubtractingDays(1))
}
func isSameWeekAsDate(date: NSDate) -> Bool
{
let comp1 = NSDate.components(fromDate: self)
let comp2 = NSDate.components(fromDate: date)
// Must be same week. 12/31 and 1/1 will both be week "1" if they are in the same week
if comp1.weekOfYear != comp2.weekOfYear {
return false
}
// Must have a time interval under 1 week
return abs(self.timeIntervalSinceDate(date)) < NSDate.weekInSeconds()
}
func isThisWeek() -> Bool
{
return self.isSameWeekAsDate(NSDate())
}
func isNextWeek() -> Bool
{
let interval: NSTimeInterval = NSDate().timeIntervalSinceReferenceDate + NSDate.weekInSeconds()
let date = NSDate(timeIntervalSinceReferenceDate: interval)
return self.isSameWeekAsDate(date)
}
func isLastWeek() -> Bool
{
let interval: NSTimeInterval = NSDate().timeIntervalSinceReferenceDate - NSDate.weekInSeconds()
let date = NSDate(timeIntervalSinceReferenceDate: interval)
return self.isSameWeekAsDate(date)
}
func isSameYearAsDate(date: NSDate) -> Bool
{
let comp1 = NSDate.components(fromDate: self)
let comp2 = NSDate.components(fromDate: date)
return (comp1.year == comp2.year)
}
func isThisYear() -> Bool
{
return self.isSameYearAsDate(NSDate())
}
func isNextYear() -> Bool
{
let comp1 = NSDate.components(fromDate: self)
let comp2 = NSDate.components(fromDate: NSDate())
return (comp1.year == comp2.year + 1)
}
func isLastYear() -> Bool
{
let comp1 = NSDate.components(fromDate: self)
let comp2 = NSDate.components(fromDate: NSDate())
return (comp1.year == comp2.year - 1)
}
func isEarlierThanDate(date: NSDate) -> Bool
{
return self.earlierDate(date) == self
}
func isLaterThanDate(date: NSDate) -> Bool
{
return self.laterDate(date) == self
}
// MARK: Adjusting Dates
func dateByAddingDays(days: Int) -> NSDate
{
let interval: NSTimeInterval = self.timeIntervalSinceReferenceDate + NSDate.dayInSeconds() * Double(days)
return NSDate(timeIntervalSinceReferenceDate: interval)
}
func dateBySubtractingDays(days: Int) -> NSDate
{
let interval: NSTimeInterval = self.timeIntervalSinceReferenceDate - NSDate.dayInSeconds() * Double(days)
return NSDate(timeIntervalSinceReferenceDate: interval)
}
func dateByAddingHours(hours: Int) -> NSDate
{
let interval: NSTimeInterval = self.timeIntervalSinceReferenceDate + NSDate.hourInSeconds() * Double(hours)
return NSDate(timeIntervalSinceReferenceDate: interval)
}
func dateBySubtractingHours(hours: Int) -> NSDate
{
let interval: NSTimeInterval = self.timeIntervalSinceReferenceDate - NSDate.hourInSeconds() * Double(hours)
return NSDate(timeIntervalSinceReferenceDate: interval)
}
func dateByAddingMinutes(minutes: Int) -> NSDate
{
let interval: NSTimeInterval = self.timeIntervalSinceReferenceDate + NSDate.minuteInSeconds() * Double(minutes)
return NSDate(timeIntervalSinceReferenceDate: interval)
}
func dateBySubtractingMinutes(minutes: Int) -> NSDate
{
let interval: NSTimeInterval = self.timeIntervalSinceReferenceDate - NSDate.minuteInSeconds() * Double(minutes)
return NSDate(timeIntervalSinceReferenceDate: interval)
}
func dateAtStartOfDay() -> NSDate
{
var components = self.components()
components.hour = 0
components.minute = 0
components.second = 0
return NSCalendar.currentCalendar().dateFromComponents(components)!
}
func dateAtEndOfDay() -> NSDate
{
var components = self.components()
components.hour = 23
components.minute = 59
components.second = 59
return NSCalendar.currentCalendar().dateFromComponents(components)!
}
func dateAtStartOfWeek() -> NSDate
{
let flags :NSCalendarUnit = NSCalendarUnit.CalendarUnitYear | NSCalendarUnit.CalendarUnitMonth | NSCalendarUnit.CalendarUnitWeekOfYear | NSCalendarUnit.CalendarUnitWeekday
var components = NSCalendar.currentCalendar().components(flags, fromDate: self)
components.weekday = NSCalendar.currentCalendar().firstWeekday
components.hour = 0
components.minute = 0
components.second = 0
return NSCalendar.currentCalendar().dateFromComponents(components)!
}
func dateAtEndOfWeek() -> NSDate
{
let flags :NSCalendarUnit = NSCalendarUnit.CalendarUnitYear | NSCalendarUnit.CalendarUnitMonth | NSCalendarUnit.CalendarUnitWeekOfYear | NSCalendarUnit.CalendarUnitWeekday
var components = NSCalendar.currentCalendar().components(flags, fromDate: self)
components.weekday = NSCalendar.currentCalendar().firstWeekday + 7
components.hour = 0
components.minute = 0
components.second = 0
return NSCalendar.currentCalendar().dateFromComponents(components)!
}
// MARK: Retrieving Intervals
func minutesAfterDate(date: NSDate) -> Int
{
let interval = self.timeIntervalSinceDate(date)
return Int(interval / NSDate.minuteInSeconds())
}
func minutesBeforeDate(date: NSDate) -> Int
{
let interval = date.timeIntervalSinceDate(self)
return Int(interval / NSDate.minuteInSeconds())
}
func hoursAfterDate(date: NSDate) -> Int
{
let interval = self.timeIntervalSinceDate(date)
return Int(interval / NSDate.hourInSeconds())
}
func hoursBeforeDate(date: NSDate) -> Int
{
let interval = date.timeIntervalSinceDate(self)
return Int(interval / NSDate.hourInSeconds())
}
func daysAfterDate(date: NSDate) -> Int
{
let interval = self.timeIntervalSinceDate(date)
return Int(interval / NSDate.dayInSeconds())
}
func daysBeforeDate(date: NSDate) -> Int
{
let interval = date.timeIntervalSinceDate(self)
return Int(interval / NSDate.dayInSeconds())
}
// MARK: Decomposing Dates
func nearestHour () -> Int {
let halfHour = NSDate.minuteInSeconds() * 30
var interval = self.timeIntervalSinceReferenceDate
if self.seconds() < 30 {
interval -= halfHour
} else {
interval += halfHour
}
let date = NSDate(timeIntervalSinceReferenceDate: interval)
return date.hour()
}
func year () -> Int { return self.components().year }
func month () -> Int { return self.components().month }
func week () -> Int { return self.components().weekOfYear }
func day () -> Int { return self.components().day }
func hour () -> Int { return self.components().hour }
func minute () -> Int { return self.components().minute }
func seconds () -> Int { return self.components().second }
func weekday () -> Int { return self.components().weekday }
func nthWeekday () -> Int { return self.components().weekdayOrdinal } //// e.g. 2nd Tuesday of the month is 2
func monthDays () -> Int { return NSCalendar.currentCalendar().rangeOfUnit(NSCalendarUnit.CalendarUnitDay, inUnit: NSCalendarUnit.CalendarUnitMonth, forDate: self).length }
func firstDayOfWeek () -> Int {
let distanceToStartOfWeek = NSDate.dayInSeconds() * Double(self.components().weekday - 1)
let interval: NSTimeInterval = self.timeIntervalSinceReferenceDate - distanceToStartOfWeek
return NSDate(timeIntervalSinceReferenceDate: interval).day()
}
func lastDayOfWeek () -> Int {
let distanceToStartOfWeek = NSDate.dayInSeconds() * Double(self.components().weekday - 1)
let distanceToEndOfWeek = NSDate.dayInSeconds() * Double(7)
let interval: NSTimeInterval = self.timeIntervalSinceReferenceDate - distanceToStartOfWeek + distanceToEndOfWeek
return NSDate(timeIntervalSinceReferenceDate: interval).day()
}
func isWeekday() -> Bool {
return !self.isWeekend()
}
func isWeekend() -> Bool {
let range = NSCalendar.currentCalendar().maximumRangeOfUnit(NSCalendarUnit.CalendarUnitWeekday)
return (self.weekday() == range.location || self.weekday() == range.length)
}
// MARK: To String
func toString() -> String {
return self.toString(dateStyle: .ShortStyle, timeStyle: .ShortStyle, doesRelativeDateFormatting: false)
}
func toString(#format: DateFormat) -> String
{
var dateFormat: String
switch format {
case .DotNet:
let offset = NSTimeZone.defaultTimeZone().secondsFromGMT / 3600
let nowMillis = 1000 * self.timeIntervalSince1970
return "/Date(\(nowMillis)\(offset))/"
case .ISO8601:
dateFormat = "yyyy-MM-dd'T'HH:mm:ssZ"
case .RSS:
dateFormat = "EEE, d MMM yyyy HH:mm:ss ZZZ"
case .AltRSS:
dateFormat = "d MMM yyyy HH:mm:ss ZZZ"
case .Custom(let string):
dateFormat = string
}
let formatter = NSDateFormatter()
formatter.dateFormat = dateFormat
return formatter.stringFromDate(self)
}
func toString(#dateStyle: NSDateFormatterStyle, timeStyle: NSDateFormatterStyle, doesRelativeDateFormatting: Bool = false) -> String
{
let formatter = NSDateFormatter()
formatter.dateStyle = dateStyle
formatter.timeStyle = timeStyle
formatter.doesRelativeDateFormatting = doesRelativeDateFormatting
return formatter.stringFromDate(self)
}
func relativeTimeToString() -> String
{
let time = self.timeIntervalSince1970
let now = NSDate().timeIntervalSince1970
let seconds = now - time
let minutes = round(seconds/60)
let hours = round(minutes/60)
let days = round(hours/24)
if seconds < 10 {
return NSLocalizedString("just now", comment: "Show the relative time from a date")
} else if seconds < 60 {
let relativeTime = NSLocalizedString("%.f seconds ago", comment: "Show the relative time from a date")
return String(format: relativeTime, seconds)
}
if minutes < 60 {
if minutes == 1 {
return NSLocalizedString("1 minute ago", comment: "Show the relative time from a date")
} else {
let relativeTime = NSLocalizedString("%.f minutes ago", comment: "Show the relative time from a date")
return String(format: relativeTime, minutes)
}
}
if hours < 24 {
if hours == 1 {
return NSLocalizedString("1 hour ago", comment: "Show the relative time from a date")
} else {
let relativeTime = NSLocalizedString("%.f hours ago", comment: "Show the relative time from a date")
return String(format: relativeTime, hours)
}
}
if days < 7 {
if days == 1 {
return NSLocalizedString("1 day ago", comment: "Show the relative time from a date")
} else {
let relativeTime = NSLocalizedString("%.f days ago", comment: "Show the relative time from a date")
return String(format: relativeTime, days)
}
}
return self.toString()
}
func weekdayToString() -> String {
let formatter = NSDateFormatter()
return formatter.weekdaySymbols[self.weekday()-1] as! String
}
func shortWeekdayToString() -> String {
let formatter = NSDateFormatter()
return formatter.shortWeekdaySymbols[self.weekday()-1] as! String
}
func veryShortWeekdayToString() -> String {
let formatter = NSDateFormatter()
return formatter.veryShortWeekdaySymbols[self.weekday()-1] as! String
}
func monthToString() -> String {
let formatter = NSDateFormatter()
return formatter.monthSymbols[self.month()-1] as! String
}
func shortMonthToString() -> String {
let formatter = NSDateFormatter()
return formatter.shortMonthSymbols[self.month()-1] as! String
}
func veryShortMonthToString() -> String {
let formatter = NSDateFormatter()
return formatter.veryShortMonthSymbols[self.month()-1] as! String
}
}
| gpl-2.0 | cbdcedef9224ca84fd86f38ae138d35b | 35.718447 | 436 | 0.597303 | 5.179403 | false | false | false | false |
sindresorhus/refined-github | safari/Shared (App)/ViewController.swift | 1 | 2715 | import WebKit
#if os(iOS)
import UIKit
typealias XViewController = UIViewController
#elseif os(macOS)
import Cocoa
import SafariServices
typealias XViewController = NSViewController
#endif
let extensionBundleIdentifier = "com.sindresorhus.Refined-GitHub.Extension"
@MainActor
final class ViewController: XViewController, WKNavigationDelegate, WKScriptMessageHandler {
@IBOutlet var webView: WKWebView!
override func viewDidLoad() {
super.viewDidLoad()
webView.navigationDelegate = self
#if os(iOS)
webView.scrollView.isScrollEnabled = false
#endif
#if os(macOS)
webView.drawsBackground = false
#endif
webView.configuration.userContentController.add(self, name: "controller")
webView.loadFileURL(Bundle.main.url(forResource: "Main", withExtension: "html")!, allowingReadAccessTo: Bundle.main.resourceURL!)
#if os(macOS)
DispatchQueue.main.async { [self] in
view.window?.titlebarAppearsTransparent = true
}
#endif
}
func webView(_ webView: WKWebView, didFinish navigation: WKNavigation!) {
// TODO: Use `callAsyncJavaScript` when targeting macOS 12.
#if os(iOS)
webView.evaluateJavaScript("show('ios')")
#elseif os(macOS)
webView.evaluateJavaScript("show('mac')")
Task {
do {
let state = try await SFSafariExtensionManager.stateOfSafariExtension(withIdentifier: extensionBundleIdentifier)
if #available(macOS 12, iOS 15, *) {
_ = try await webView.callAsyncJavaScript("show('mac', isEnabled)", arguments: ["isEnabled": state.isEnabled], contentWorld: .page)
} else {
_ = try await webView.evaluateJavaScript("show('mac', \(state.isEnabled)); 0") // The `0` works around a bug in `evaluateJavaScript`.
}
} catch {
_ = await MainActor.run { // Required since `presentError` is not yet annotated with `@MainActor`.
DispatchQueue.main.async {
NSApp.presentError(error)
}
}
}
}
#endif
}
func userContentController(_ userContentController: WKUserContentController, didReceive message: WKScriptMessage) {
#if os(macOS)
guard let action = message.body as? String else {
return
}
if action == "open-help" {
NSWorkspace.shared.open(URL(string: "https://github.com/refined-github/refined-github/issues/4216#issuecomment-817097886")!)
return
}
guard action == "open-preferences" else {
return;
}
Task {
do {
try await SFSafariApplication.showPreferencesForExtension(withIdentifier: extensionBundleIdentifier)
NSApplication.shared.terminate(nil)
} catch {
_ = await MainActor.run { // Required since `presentError` is not yet annotated with `@MainActor`.
DispatchQueue.main.async {
NSApp.presentError(error)
}
}
}
}
#endif
}
}
| mit | 2be8a66b0ac941b627194a30ceb38da5 | 26.704082 | 138 | 0.720074 | 3.845609 | false | false | false | false |
TENDIGI/LocaleKit | Pod/Locale.swift | 1 | 5316 | //
// Locale.swift
// Pods
//
// Created by Nick Lee on 1/26/16.
//
//
import Foundation
import zipzap
private let languageComponents = Foundation.Locale.components(fromIdentifier: Foundation.Locale.current.identifier)
private let languageCode = languageComponents[NSLocale.Key.languageCode.rawValue] ?? Constants.BaseLocaleName
private let countryCode = languageComponents[NSLocale.Key.countryCode.rawValue]
private let scriptCode = languageComponents[NSLocale.Key.scriptCode.rawValue]
@objc public final class Locale: NSObject {
// MARK: Singleton
public static let sharedInstance = Locale()
/// The device's language code
public static let lc = languageCode
/// The device's country code
public static let cc = countryCode
// MARK: Private Properties
fileprivate typealias LocalizationMap = [String : AnyObject]
fileprivate let fileManager = FileManager.default
fileprivate var localizations: [String : AnyObject] = [
Constants.BaseLocaleName : Dictionary<String, AnyObject>() as AnyObject
]
fileprivate var activeLocalization: LocalizationMap {
let c1 = [languageCode, scriptCode].flatMap({ $0 }).joined(separator: "-")
let fullComponentString = [c1, countryCode].flatMap({ $0 }).joined(separator: "_")
if let loc = localizations[fullComponentString] as? LocalizationMap {
return loc
}
else if let script = scriptCode, let loc = localizations[languageCode + "-" + script] as? LocalizationMap {
return loc
}
else if let country = countryCode, let loc = localizations[languageCode + "_" + country] as? LocalizationMap {
return loc
}
else if let loc = localizations[languageCode] as? LocalizationMap {
return loc
}
else if let loc = localizations[Constants.BaseLocaleName] as? LocalizationMap {
return loc
}
return [:]
}
fileprivate let mutex = DispatchSemaphore(value: 1)
// MARK: Initialization
fileprivate override init() {
super.init()
}
// MARK: Loading
public func load(_ bundleURL: URL) throws {
mutex.wait(timeout: DispatchTime.distantFuture)
defer { mutex.signal() }
let archive: ZZArchive = try {
let cachedPath = Constants.CachedLocalizationArchivePath
let cachedURL = URL(fileURLWithPath: cachedPath)
if let cached = try? ZZArchive(url: cachedURL) {
return cached
}
return try ZZArchive(url: bundleURL)
}()
let entries = archive.entries.filter { $0.fileName.hasSuffix(".json") }
let localizationEntries = entries.filter { $0.fileName.hasPrefix(Constants.LocalizationDirectoryName) }
try loadLocalizations(localizationEntries)
}
fileprivate func loadLocalizations(_ entries: [ZZArchiveEntry]) throws {
let data = try entries.flatMap { entry -> (URL, Data)? in
let url = URL(fileURLWithPath: entry.fileName)
let data = try entry.newData()
return (url, data)
}
let jsonObjects = try data.flatMap { (url, data) -> (URL, LocalizationMap)? in
guard let json = (try? JSONSerialization.jsonObject(with: data, options: [])) as? LocalizationMap else {
throw LocaleKitError.failedToParseJSON(path: url.absoluteString)
}
return (url, json)
}
let locales = jsonObjects.flatMap { (url, json) -> (String, LocalizationMap)? in
let components = url.pathComponents
guard components.count >= 2 else {
return nil
}
let directory = components[components.count - 2]
return (directory, json)
}
for (locale, data) in locales {
let target = (localizations[locale] as? LocalizationMap) ?? (locale == Constants.BaseLocaleName ? [:] : localizations[Constants.BaseLocaleName] as? LocalizationMap) ?? [:]
let merged = deepMerge(target, data)
localizations[locale] = merged as AnyObject
}
}
public class func activeLocaleEqualsCode(_ identifier: String) -> Bool {
let localeIdentifier = (Foundation.Locale.current as NSLocale).object(forKey: NSLocale.Key.identifier) as! String
return localeIdentifier == identifier
}
public class func activeLanguageEqualsCode(_ identifier: String) -> Bool {
return identifier == languageCode
}
public class func activeCountryEqualsCode(_ identifier: String) -> Bool {
return identifier == countryCode
}
// MARK: Localizations
public class func group(_ group: Any) -> LPath {
return LPath(components: [String(describing: group)])
}
internal func traverse(_ components: [String]) -> AnyObject? {
mutex.wait(timeout: DispatchTime.distantFuture)
defer { mutex.signal() }
var comps = components
var currentValue: AnyObject? = activeLocalization as AnyObject?
while comps.count > 0 {
let component = comps.removeFirst()
currentValue = (currentValue as? LocalizationMap)?[component]
}
return currentValue
}
}
| mit | 28d249f5f1ea71473acad9506779cf66 | 34.677852 | 183 | 0.638074 | 4.949721 | false | false | false | false |
brentsimmons/Evergreen | Shared/Extensions/IconImage.swift | 1 | 5475 | //
// IconImage.swift
// NetNewsWire
//
// Created by Maurice Parker on 11/5/19.
// Copyright © 2019 Ranchero Software. All rights reserved.
//
#if os(macOS)
import AppKit
#else
import UIKit
#endif
import RSCore
final class IconImage {
lazy var isDark: Bool = {
return image.isDark()
}()
lazy var isBright: Bool = {
return image.isBright()
}()
let image: RSImage
let isSymbol: Bool
let isBackgroundSupressed: Bool
let preferredColor: CGColor?
init(_ image: RSImage, isSymbol: Bool = false, isBackgroundSupressed: Bool = false, preferredColor: CGColor? = nil) {
self.image = image
self.isSymbol = isSymbol
self.preferredColor = preferredColor
self.isBackgroundSupressed = isBackgroundSupressed
}
}
#if os(macOS)
extension NSImage {
func isDark() -> Bool {
return self.cgImage(forProposedRect: nil, context: nil, hints: nil)?.isDark() ?? false
}
func isBright() -> Bool {
return self.cgImage(forProposedRect: nil, context: nil, hints: nil)?.isBright() ?? false
}
}
#else
extension UIImage {
func isDark() -> Bool {
return self.cgImage?.isDark() ?? false
}
func isBright() -> Bool {
return self.cgImage?.isBright() ?? false
}
}
#endif
fileprivate enum ImageLuminanceType {
case regular, bright, dark
}
extension CGImage {
func isBright() -> Bool {
guard let luminanceType = getLuminanceType() else {
return false
}
return luminanceType == .bright
}
func isDark() -> Bool {
guard let luminanceType = getLuminanceType() else {
return false
}
return luminanceType == .dark
}
fileprivate func getLuminanceType() -> ImageLuminanceType? {
// This has been rewritten with information from https://christianselig.com/2021/04/efficient-average-color/
// First, resize the image. We do this for two reasons, 1) less pixels to deal with means faster
// calculation and a resized image still has the "gist" of the colors, and 2) the image we're dealing
// with may come in any of a variety of color formats (CMYK, ARGB, RGBA, etc.) which complicates things,
// and redrawing it normalizes that into a base color format we can deal with.
// 40x40 is a good size to resize to still preserve quite a bit of detail but not have too many pixels
// to deal with. Aspect ratio is irrelevant for just finding average color.
let size = CGSize(width: 40, height: 40)
let width = Int(size.width)
let height = Int(size.height)
let totalPixels = width * height
let colorSpace = CGColorSpaceCreateDeviceRGB()
// ARGB format
let bitmapInfo: UInt32 = CGBitmapInfo.byteOrder32Little.rawValue | CGImageAlphaInfo.premultipliedFirst.rawValue
// 8 bits for each color channel, we're doing ARGB so 32 bits (4 bytes) total, and thus if the image is n pixels wide,
// and has 4 bytes per pixel, the total bytes per row is 4n. That gives us 2^8 = 256 color variations for each RGB channel
// or 256 * 256 * 256 = ~16.7M color options in total. That seems like a lot, but lots of HDR movies are in 10 bit, which
// is (2^10)^3 = 1 billion color options!
guard let context = CGContext(data: nil, width: width, height: height, bitsPerComponent: 8, bytesPerRow: width * 4, space: colorSpace, bitmapInfo: bitmapInfo) else { return nil }
// Draw our resized image
context.draw(self, in: CGRect(origin: .zero, size: size))
guard let pixelBuffer = context.data else { return nil }
// Bind the pixel buffer's memory location to a pointer we can use/access
let pointer = pixelBuffer.bindMemory(to: UInt32.self, capacity: width * height)
var totalLuminance = 0.0
// Column of pixels in image
for x in 0 ..< width {
// Row of pixels in image
for y in 0 ..< height {
// To get the pixel location just think of the image as a grid of pixels, but stored as one long row
// rather than columns and rows, so for instance to map the pixel from the grid in the 15th row and 3
// columns in to our "long row", we'd offset ourselves 15 times the width in pixels of the image, and
// then offset by the amount of columns
let pixel = pointer[(y * width) + x]
let r = red(for: pixel)
let g = green(for: pixel)
let b = blue(for: pixel)
let luminance = (0.299 * Double(r) + 0.587 * Double(g) + 0.114 * Double(b))
totalLuminance += luminance
}
}
let avgLuminance = totalLuminance / Double(totalPixels)
if totalLuminance == 0 || avgLuminance < 40 {
return .dark
} else if avgLuminance > 180 {
return .bright
} else {
return .regular
}
}
private func red(for pixelData: UInt32) -> UInt8 {
return UInt8((pixelData >> 16) & 255)
}
private func green(for pixelData: UInt32) -> UInt8 {
return UInt8((pixelData >> 8) & 255)
}
private func blue(for pixelData: UInt32) -> UInt8 {
return UInt8((pixelData >> 0) & 255)
}
}
enum IconSize: Int, CaseIterable {
case small = 1
case medium = 2
case large = 3
private static let smallDimension = CGFloat(integerLiteral: 24)
private static let mediumDimension = CGFloat(integerLiteral: 36)
private static let largeDimension = CGFloat(integerLiteral: 48)
var size: CGSize {
switch self {
case .small:
return CGSize(width: IconSize.smallDimension, height: IconSize.smallDimension)
case .medium:
return CGSize(width: IconSize.mediumDimension, height: IconSize.mediumDimension)
case .large:
return CGSize(width: IconSize.largeDimension, height: IconSize.largeDimension)
}
}
}
| mit | 43be2029417bad252f09fbe35f97e00d | 28.589189 | 180 | 0.693825 | 3.573107 | false | false | false | false |
mperovic/my41 | my41/Classes/Debuger.swift | 1 | 3856 | //
// Debuger.swift
// my41
//
// Created by Miroslav Perovic on 9/21/14.
// Copyright (c) 2014 iPera. All rights reserved.
//
import Foundation
import Cocoa
class DebugWindowController: NSWindowController {
}
// MARK: - SpitView
class DebugSplitViewController: NSSplitViewController {
override func viewWillAppear() {
let menuVC = self.splitViewItems[0].viewController as! DebugMenuViewController
let containerVC = self.splitViewItems[1].viewController as! DebugContainerViewController
menuVC.debugContainerViewController = containerVC
self.view.addConstraint(
NSLayoutConstraint(
item: self.splitViewItems[0].viewController.view,
attribute: NSLayoutConstraint.Attribute.width,
relatedBy: NSLayoutConstraint.Relation.equal,
toItem: nil,
attribute: NSLayoutConstraint.Attribute.notAnAttribute,
multiplier: 0,
constant: 194
)
)
self.view.addConstraint(
NSLayoutConstraint(
item: self.splitViewItems[0].viewController.view,
attribute: NSLayoutConstraint.Attribute.height,
relatedBy: NSLayoutConstraint.Relation.equal,
toItem: nil,
attribute: NSLayoutConstraint.Attribute.notAnAttribute,
multiplier: 0,
constant: 379
)
)
self.view.addConstraint(
NSLayoutConstraint(
item: self.splitViewItems[1].viewController.view,
attribute: NSLayoutConstraint.Attribute.width,
relatedBy: NSLayoutConstraint.Relation.equal,
toItem: nil,
attribute: NSLayoutConstraint.Attribute.notAnAttribute,
multiplier: 0,
constant: 710
)
)
self.view.addConstraint(
NSLayoutConstraint(
item: self.splitViewItems[1].viewController.view,
attribute: NSLayoutConstraint.Attribute.height,
relatedBy: NSLayoutConstraint.Relation.equal,
toItem: nil,
attribute: NSLayoutConstraint.Attribute.notAnAttribute,
multiplier: 0,
constant: 379
)
)
}
}
class DebugContainerViewController: NSViewController {
var debugCPUViewController: DebugCPUViewController?
var debugMemoryViewController: DebugMemoryViewController?
override func prepare(for segue: NSStoryboardSegue, sender: Any?) {
let segid = segue.identifier ?? "(none)"
if segid == "showCPUView" {
debugCPUViewController = segue.destinationController as? DebugCPUViewController
debugCPUViewController?.debugContainerViewController = self
}
if segid == "showMemoryView" {
debugMemoryViewController = segue.destinationController as? DebugMemoryViewController
debugMemoryViewController?.debugContainerViewController = self
}
}
override func viewDidLoad() {
loadCPUViewController()
let defaults = UserDefaults.standard
TRACE = defaults.integer(forKey: "traceActive")
if TRACE == 0 {
debugCPUViewController?.traceSwitch.state = NSControl.StateValue.off
} else {
debugCPUViewController?.traceSwitch.state = NSControl.StateValue.on
}
}
func loadCPUViewController() {
self.performSegue(withIdentifier: "showCPUView", sender: self)
}
func loadMemoryViewController() {
self.performSegue(withIdentifier: "showMemoryView", sender: self)
}
}
//MARK: -
class DebugerSegue: NSStoryboardSegue {
override func perform() {
let source = self.sourceController as! NSViewController
let destination = self.destinationController as! NSViewController
if source.view.subviews.count > 0 {
let aView: AnyObject = source.view.subviews[0]
if aView is NSView {
aView.removeFromSuperview()
}
}
let dView = destination.view
source.view.addSubview(dView)
source.view.addConstraints(
NSLayoutConstraint.constraints(
withVisualFormat: "H:|[dView]|",
options: [],
metrics: nil,
views: ["dView": dView]
)
)
source.view.addConstraints(
NSLayoutConstraint.constraints(
withVisualFormat: "V:|[dView]|",
options: [],
metrics: nil,
views: ["dView": dView]
)
)
}
}
| bsd-3-clause | 1bb7ccda9afdce4e4c5910bcdb07becd | 25.777778 | 90 | 0.740405 | 4.037696 | false | false | false | false |
shiwwgis/MyDiaryForEvernote | MyDiraryForEvernote/Base/BaseFunction.swift | 1 | 3310 | //
// BaseFunction.swift
// MyDiaryForEvernote
//
// Created by shiweiwei on 16/2/26.
// Copyright © 2016年 shiww. All rights reserved.
//
import Foundation
class BaseFunction:NSObject
{
//根据城市获取天气状态
static func getCityWeather(city: String)->String {
let url = "http://apis.baidu.com/heweather/weather/free"
let spacelessString = city.stringByAddingPercentEncodingWithAllowedCharacters(NSCharacterSet.URLQueryAllowedCharacterSet());
//city.stringByAddingPercentEscapesUsingEncoding(NSUTF8StringEncoding);
let httpArg = "city="+spacelessString!;
var strWeather:String="";
let req = NSMutableURLRequest(URL: NSURL(string: url + "?" + httpArg)!)
req.timeoutInterval = 6
req.HTTPMethod = "GET"
req.addValue("4a21ed362d7f05ac4ae1604665de9a9d", forHTTPHeaderField: "apikey")
// print(req);
let session=NSURLSession.sharedSession();
let semaphore = dispatch_semaphore_create(0)
let task=session.dataTaskWithRequest(req)
{
(data, response, error) -> Void in
// let res = response as! NSHTTPURLResponse;
// print(res.statusCode)
if error != nil {
// print("请求失败")
}
if data != nil
{
//处理JSON
let json = JSON(data: data!);
// print(json);
let result=json["HeWeather data service 3.0",0,"status"].string!;
if result=="ok"
{
strWeather=json["HeWeather data service 3.0",0,"basic","city"].string!;//城市名
strWeather=strWeather+":"+json["HeWeather data service 3.0",0,"now","cond","txt"].string!;//实况天气
if json["HeWeather data service 3.0",0,"aqi","city","pm25"].string != nil
{
strWeather=strWeather+" PM2.5:"+json["HeWeather data service 3.0",0,"aqi","city","pm25"].string!;//PM2.5
}
}
}
dispatch_semaphore_signal(semaphore);
}
//使用resume方法启动任务
task.resume()
dispatch_semaphore_wait(semaphore, DISPATCH_TIME_FOREVER)
return strWeather;
}
//生成日期+天气的字符串
static func getDiaryTitle(cityname:String="Beijing")->String
{
var strDiaryTitle:String="";
let dateFormatter=NSDateFormatter();
dateFormatter.dateFormat="YYYY-MM-dd EEEE "
strDiaryTitle="\(dateFormatter.stringFromDate(NSDate()))";
strDiaryTitle=strDiaryTitle+BaseFunction.getCityWeather(cityname);
return strDiaryTitle;
}
//返回国际化字符串
static func getIntenetString(key:String)->String{
let string=NSLocalizedString(key,comment:"");
// print("key=\(key),string=\(string)");
return string;
}
}
| mit | 3dd94d3f42a2ce06a52f864645bab406 | 30.772277 | 139 | 0.521035 | 4.726068 | false | false | false | false |
JakeLin/IBAnimatable | IBAnimatable/IBAnimatableTests/TestProtocols/CornerDesignableTests.swift | 2 | 3304 | //
// CornerDesignableTests.swift
// IBAnimatable
//
// Created by Steven on 5/12/17.
// Copyright © 2017 IBAnimatable. All rights reserved.
//
import XCTest
@testable import IBAnimatable
// MARK: - CornerDesignableTests Protocol
protocol CornerDesignableTests: class {
associatedtype Element
var element: Element { get set }
func testCornerRadius()
func test_cornerSides()
}
// MARK: - Universal Tests
extension CornerDesignableTests where Element: StringCornerDesignable {
func _test_cornerSides() {
element._cornerSides = "topLeft"
XCTAssertEqual(element.cornerSides, .topLeft)
element._cornerSides = "topRight"
XCTAssertEqual(element.cornerSides, .topRight)
element._cornerSides = "bottomLeft"
XCTAssertEqual(element.cornerSides, .bottomLeft)
element._cornerSides = "bottomRight"
XCTAssertEqual(element.cornerSides, .bottomRight)
element._cornerSides = "allSides"
XCTAssertEqual(element.cornerSides, .allSides)
element._cornerSides = ""
XCTAssertEqual(element.cornerSides, .allSides)
}
}
// MARK: - UIView Tests
extension CornerDesignableTests where Element: UIView, Element: CornerDesignable {
func _testCornerRadius() {
element.cornerRadius = 3
element.cornerSides = .allSides
testRadiusPath(for: .allCorners)
element.cornerSides = [.bottomLeft, .bottomRight, .topLeft]
testRadiusPath(for: [.bottomLeft, .bottomRight, .topLeft])
}
private func testRadiusPath(for sides: UIRectCorner) {
let mask = element.layer.mask as? CAShapeLayer
if sides == .allCorners {
XCTAssertNil(mask)
} else {
XCTAssertEqual(mask?.frame, CGRect(origin: .zero, size: element.bounds.size))
XCTAssertEqual(mask?.name, "cornerSideLayer")
let cornerRadii = CGSize(width: element.cornerRadius, height: element.cornerRadius)
let corners: UIRectCorner = sides
let mockPath = UIBezierPath(roundedRect: element.bounds, byRoundingCorners: corners, cornerRadii: cornerRadii).cgPath
XCTAssertEqual(mask?.path, mockPath)
}
}
}
// MARK: - UICollectionViewCell Tests
extension CornerDesignableTests where Element: UICollectionViewCell, Element: CornerDesignable {
func _testCornerRadius() {
element.cornerRadius = -1
XCTAssertFalse(element.contentView.layer.masksToBounds)
element.cornerRadius = 3.0
XCTAssertNil(element.layer.mask)
XCTAssertEqual(element.layer.cornerRadius, 0.0)
element.cornerSides = .allSides
testRadiusPath(for: .allCorners)
element.cornerSides = [.bottomLeft, .bottomRight, .topLeft]
testRadiusPath(for: [.bottomLeft, .bottomRight, .topLeft])
}
private func testRadiusPath(for sides: UIRectCorner) {
let mask = element.contentView.layer.mask as? CAShapeLayer
if sides == .allCorners {
XCTAssertNil(mask)
} else {
XCTAssertEqual(mask?.frame, CGRect(origin: .zero, size: element.contentView.bounds.size))
XCTAssertEqual(mask?.name, "cornerSideLayer")
let cornerRadii = CGSize(width: element.cornerRadius, height: element.cornerRadius)
let corners: UIRectCorner = sides
let mockPath = UIBezierPath(roundedRect: element.contentView.bounds, byRoundingCorners: corners, cornerRadii: cornerRadii).cgPath
XCTAssertEqual(mask?.path, mockPath)
}
}
}
| mit | b12d55436b6ffa75b8bbbd8365f2cb64 | 30.457143 | 135 | 0.729337 | 4.35752 | false | true | false | false |
Incipia/Elemental | Elemental/Classes/Cells/TextFieldInputElementCell.swift | 1 | 9056 | //
// TextInputElementCell.swift
// Elemental
//
// Created by Gregory Klein on 2/23/17.
// Copyright © 2017 Incipia. All rights reserved.
//
import UIKit
class TextFieldInputElementCell: BindableElementCell {
@IBOutlet private var _label: UILabel!
@IBOutlet private var _detailLabel: UILabel!
@IBOutlet private var _detailLabelVerticalSpaceConstraint: NSLayoutConstraint!
@IBOutlet private var _nameLabelVerticalSpaceConstraint: NSLayoutConstraint!
@IBOutlet fileprivate var _textField: InsetTextField!
@IBOutlet private var _textFieldHeightConstraint: NSLayoutConstraint!
@IBOutlet fileprivate var _horizontalConstraints: [NSLayoutConstraint]!
@IBOutlet fileprivate var _verticalConstraints: [NSLayoutConstraint]!
fileprivate var _readyToUpdateConstraints: Bool = false
fileprivate var _action: InputElementAction?
fileprivate var _isEnabled: Bool {
get { return _textField.isEnabled }
set {
if !newValue, _textField.isFirstResponder {
_textField.resignFirstResponder()
}
_textField.isEnabled = newValue
}
}
override class var bindableKeys: [BindableElementKey] { return [.text, .doubleValue, .isEnabled] }
override func awakeFromNib() {
super.awakeFromNib()
_textField.addTarget(self, action: #selector(_textChanged), for: .editingChanged)
_textField.delegate = self
// the constraints installed in the xib are activated sometime after awakeFromNib() and configure(with:) get called,
// so activating uninstalled constraints before then causes conflicts
DispatchQueue.main.async {
self._readyToUpdateConstraints = true
self.setNeedsUpdateConstraints()
}
}
override func configure(with component: Elemental) {
super.configure(with: component)
guard let element = component as? TextFieldInputElement else { fatalError() }
let content = element.content
let style = element.configuration
let action = element.action
_label.text = content.name
_detailLabel.text = content.detail
_label.font = style.nameStyle.font
_textField.font = style.inputStyle.font
_detailLabel.font = style.detailStyle?.font
_label.textColor = style.nameStyle.color
_textField.textColor = style.inputStyle.color
_detailLabel.textColor = style.detailStyle?.color
_textField.backgroundColor = style.inputBackgroundColor
_textField.keyboardType = style.keyboardStyle.type
_textField.keyboardAppearance = style.keyboardStyle.appearance
_textField.isSecureTextEntry = style.keyboardStyle.isSecureTextEntry
_textField.autocapitalizationType = style.keyboardStyle.autocapitalizationType
_textField.autocorrectionType = style.keyboardStyle.autocorrectionType
_textField.returnKeyType = style.keyboardStyle.returnKeyType
_textField.tintColor = style.inputTintColor ?? style.inputStyle.color
_textField.isEnabled = element.configuration.isEnabled
if let placeholder = content.placeholder, let placeholderStyle = style.placeholderStyle {
let attrs: [String : AnyHashable] = [
NSFontAttributeName : placeholderStyle.font,
NSForegroundColorAttributeName : placeholderStyle.color
]
let attrPlaceholder = NSAttributedString(string: placeholder, attributes: attrs)
_textField.attributedPlaceholder = attrPlaceholder
} else {
_textField.placeholder = nil
}
_detailLabelVerticalSpaceConstraint.constant = content.detail != nil ? 10.0 : 0.0
_nameLabelVerticalSpaceConstraint.constant = content.name != "" ? 10.0 : 0.0
_textFieldHeightConstraint.constant = style.inputHeight
_textField.textAlignment = style.layoutDirection == .vertical ? .left : .right
_action = action
}
override class func intrinsicContentSize(for element: Elemental, constrainedSize size: CGSize) -> CGSize {
let width = size.width
guard let element = element as? TextFieldInputElement else { fatalError() }
let content = element.content
let style = element.configuration
var nameHeight: CGFloat
switch style.layoutDirection {
case .horizontal: nameHeight = 0
case .vertical: nameHeight = content.name != "" ? content.name.heightWithConstrainedWidth(width: width, font: style.nameStyle.font) : 0
}
if style.layoutDirection == .horizontal {
nameHeight = 0
}
let namePadding: CGFloat = nameHeight != 0 ? 10.0 : 0.0
var detailHeight: CGFloat = 0
if let detail = content.detail, let detailFont = style.detailStyle?.font {
detailHeight = detail.heightWithConstrainedWidth(width: width, font: detailFont)
}
let detailPadding: CGFloat = content.detail != nil ? 10.0 : 0.0
let totalHeight = nameHeight + detailHeight + detailPadding + namePadding + style.inputHeight
return CGSize(width: width, height: totalHeight)
}
override func value(for key: BindableElementKey) -> Any? {
switch key {
case .text: return _textField.text ?? ""
case .isEnabled: return _isEnabled
default: fatalError("\(type(of: self)) cannot retrieve value for \(key))")
}
}
override func setOwn(value: inout Any?, for key: BindableElementKey) throws {
switch key {
case .text:
guard value == nil || value is String else { throw key.kvTypeError(value: value) }
_textField.text = value as? String ?? ""
case .doubleValue, .intValue: _textField.text = "\(value ?? "")"
case .isEnabled:
guard let validValue = value as? Bool else { throw key.kvTypeError(value: value) }
_isEnabled = validValue
default: fatalError("\(type(of: self)) cannot set value for \(key))")
}
}
override func updateConstraints() {
guard _readyToUpdateConstraints, let layoutDirection = element?.elementalConfig.layoutDirection else {
super.updateConstraints()
return
}
switch layoutDirection {
case .horizontal:
NSLayoutConstraint.deactivate(_verticalConstraints)
NSLayoutConstraint.activate(_horizontalConstraints)
case .vertical:
NSLayoutConstraint.deactivate(_horizontalConstraints)
NSLayoutConstraint.activate(_verticalConstraints)
}
super.updateConstraints()
}
override func prepareForReuse() {
_action = nil
super.prepareForReuse()
}
public func startEditing() {
_textField.becomeFirstResponder()
}
}
extension TextFieldInputElementCell {
@objc fileprivate func _textChanged() {
trySetBoundValue(_textField.text, for: .text)
if _textField.text == "" {
trySetBoundValue(Double(0), for: .doubleValue)
trySetBoundValue(0, for: .intValue)
} else {
trySetBoundValue(Double(_textField.text!), for: .doubleValue)
trySetBoundValue(Int(_textField.text!), for: .intValue)
}
}
}
extension TextFieldInputElementCell: UITextFieldDelegate {
func textFieldShouldBeginEditing(_ textField: UITextField) -> Bool {
guard let action = _action else { return true }
let nextState: InputElementState = action(.unfocused, .focused) ?? .focused
textField.inputView = nextState == .unfocused ? UIView() : nil
return true
}
func textFieldDidBeginEditing(_ textField: UITextField) {
guard textField.inputView == nil else {
DispatchQueue.main.async {
textField.resignFirstResponder()
}
return
}
guard let action = _action else { return }
let nextState = action(.focused, nil)
if nextState == .unfocused {
DispatchQueue.main.async {
textField.resignFirstResponder()
}
}
}
func textFieldShouldEndEditing(_ textField: UITextField) -> Bool {
guard textField.inputView == nil, let action = _action else { return true }
let nextState: InputElementState = action(.focused, .unfocused) ?? .unfocused
return nextState.shouldEndEditing
}
func textFieldDidEndEditing(_ textField: UITextField) {
defer {
if textField.inputView != nil {
textField.inputView = nil
}
}
guard let action = _action else { return }
let proposedNextState: InputElementState? = textField.inputView == nil ? nil : .unfocused
let nextState = action(.unfocused, proposedNextState)
if nextState == .focused {
DispatchQueue.main.async {
textField.becomeFirstResponder()
}
}
}
}
extension InputElementState {
var shouldBeginEditing: Bool {
switch self {
case .focused: return true
case .unfocused: return false
}
}
var shouldEndEditing: Bool {
switch self {
case .focused: return false
case .unfocused: return true
}
}
}
| mit | ad48ffcd6d702dc090b7d7ca95b0d888 | 36.110656 | 141 | 0.669244 | 5.011068 | false | false | false | false |
bradhilton/Table | Pods/DeviceKit/Source/Device.generated.swift | 1 | 26979 | //===----------------------------------------------------------------------===//
//
// This source file is part of the DeviceKit open source project
//
// Copyright © 2014 - 2017 Dennis Weissmann and the DeviceKit project authors
//
// License: https://github.com/dennisweissmann/DeviceKit/blob/master/LICENSE
// Contributors: https://github.com/dennisweissmann/DeviceKit#contributors
//
//===----------------------------------------------------------------------===//
import UIKit
// MARK: - Device
/// This enum is a value-type wrapper and extension of
/// [`UIDevice`](https://developer.apple.com/library/ios/documentation/UIKit/Reference/UIDevice_Class/).
///
/// Usage:
///
/// let device = Device()
///
/// print(device) // prints, for example, "iPhone 6 Plus"
///
/// if device == .iPhone6Plus {
/// // Do something
/// } else {
/// // Do something else
/// }
///
/// ...
///
/// if device.batteryState == .full || device.batteryState >= .charging(75) {
/// print("Your battery is happy! 😊")
/// }
///
/// ...
///
/// if device.batteryLevel >= 50 {
/// install_iOS()
/// } else {
/// showError()
/// }
///
public enum Device {
#if os(iOS)
/// Device is an [iPod Touch (5th generation)](https://support.apple.com/kb/SP657)
///
/// 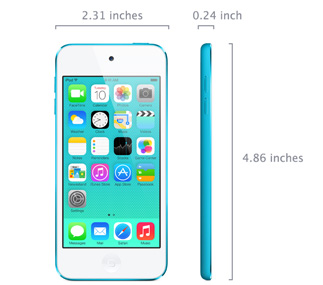
case iPodTouch5
/// Device is an [iPod Touch (6th generation)](https://support.apple.com/kb/SP720)
///
/// 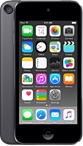
case iPodTouch6
/// Device is an [iPhone 4](https://support.apple.com/kb/SP587)
///
/// 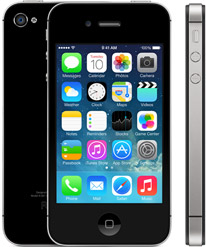
case iPhone4
/// Device is an [iPhone 4s](https://support.apple.com/kb/SP643)
///
/// 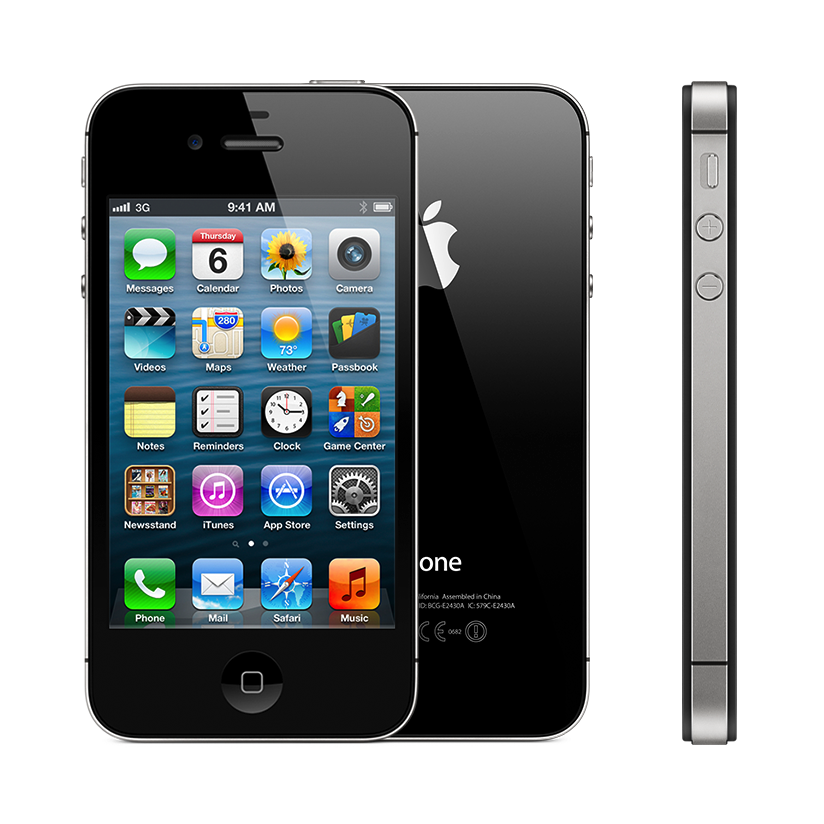
case iPhone4s
/// Device is an [iPhone 5](https://support.apple.com/kb/SP655)
///
/// 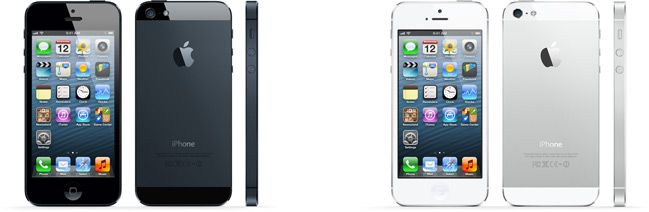
case iPhone5
/// Device is an [iPhone 5c](https://support.apple.com/kb/SP684)
///
/// 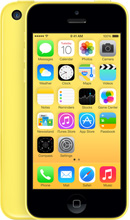
case iPhone5c
/// Device is an [iPhone 5s](https://support.apple.com/kb/SP685)
///
/// 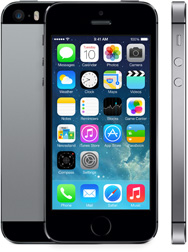
case iPhone5s
/// Device is an [iPhone 6](https://support.apple.com/kb/SP705)
///
/// 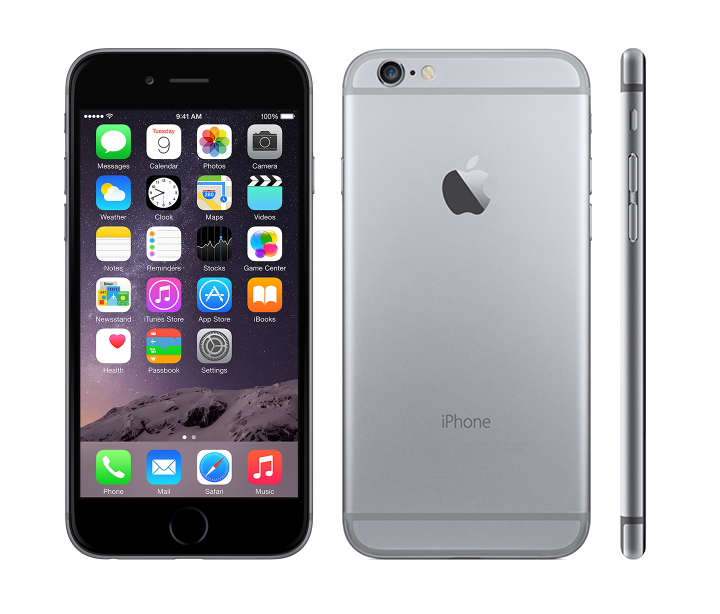
case iPhone6
/// Device is an [iPhone 6 Plus](https://support.apple.com/kb/SP706)
///
/// 
case iPhone6Plus
/// Device is an [iPhone 6s](https://support.apple.com/kb/SP726)
///
/// 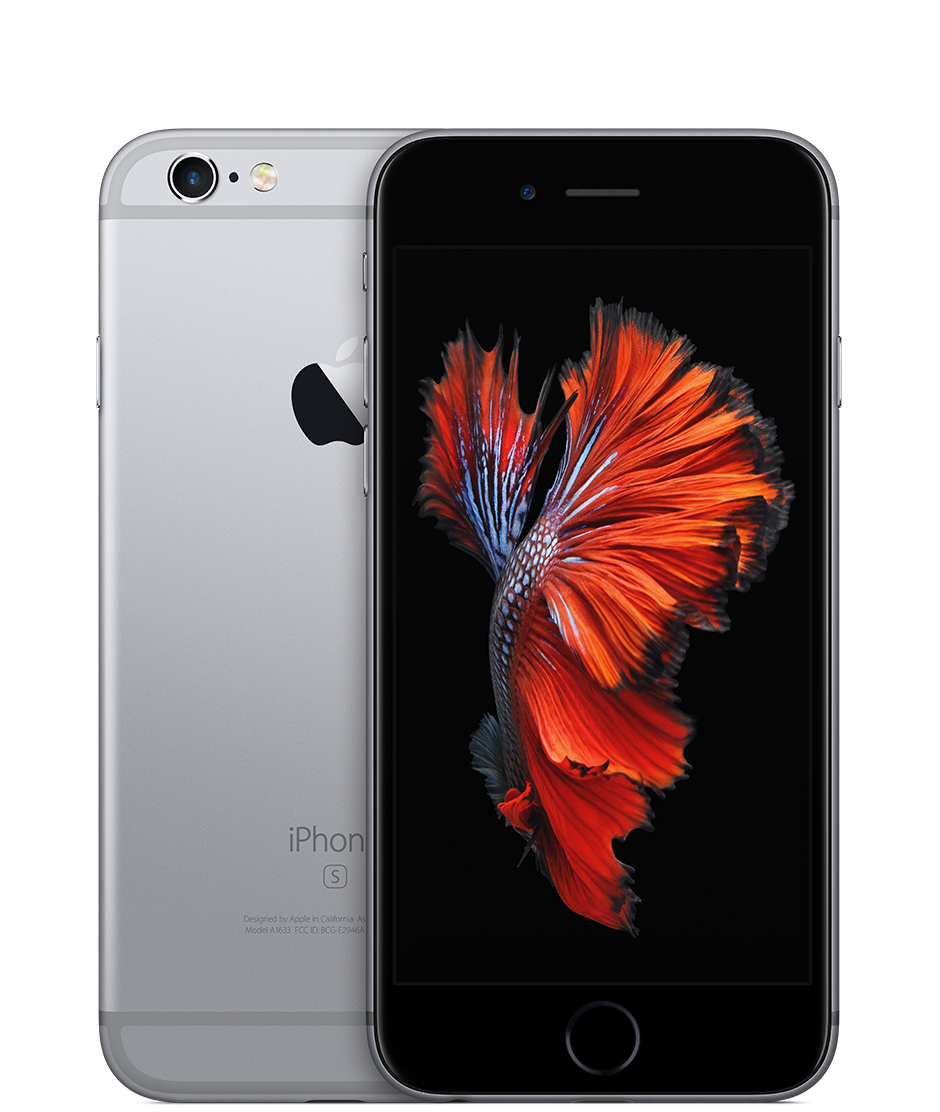
case iPhone6s
/// Device is an [iPhone 6s Plus](https://support.apple.com/kb/SP727)
///
/// 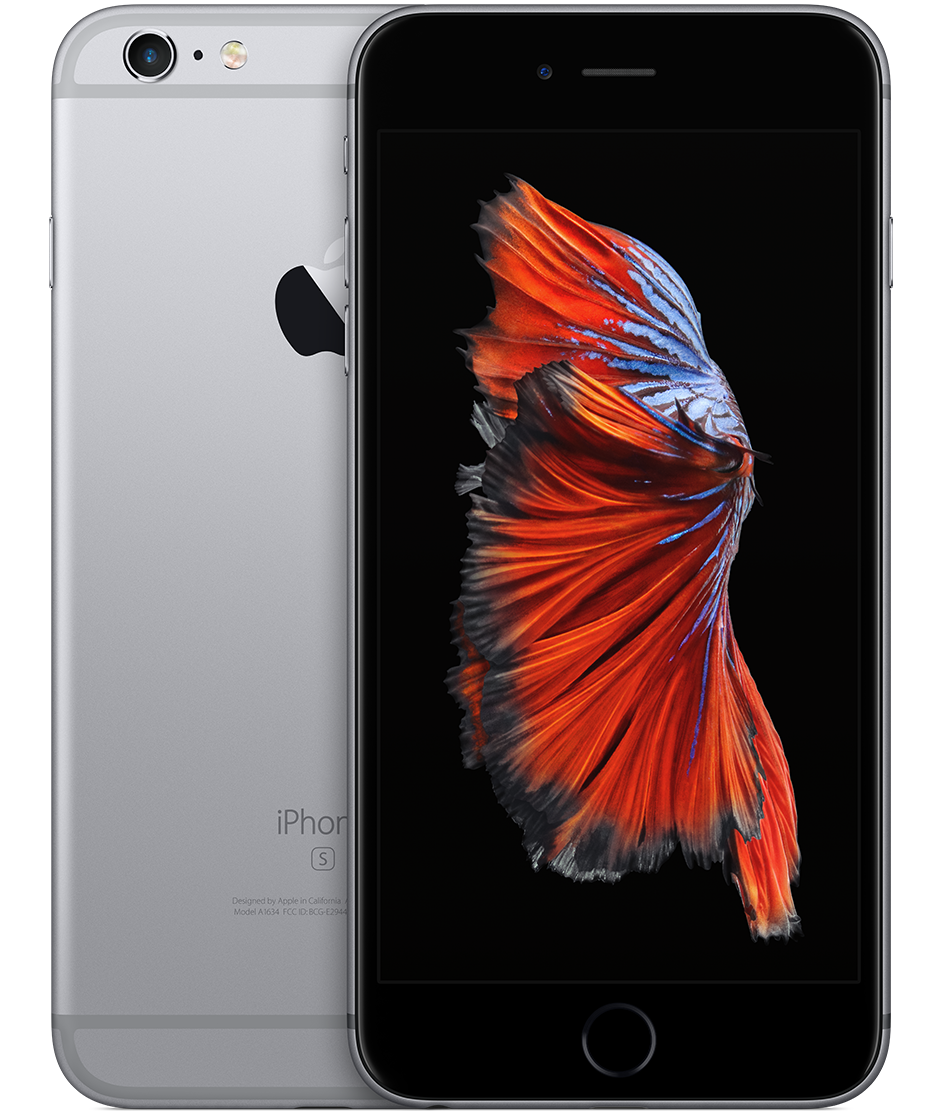
case iPhone6sPlus
/// Device is an [iPhone 7](https://support.apple.com/kb/SP743)
///
/// 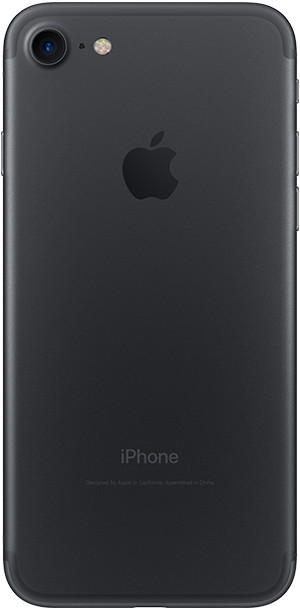
case iPhone7
/// Device is an [iPhone 7 Plus](https://support.apple.com/kb/SP744)
///
/// 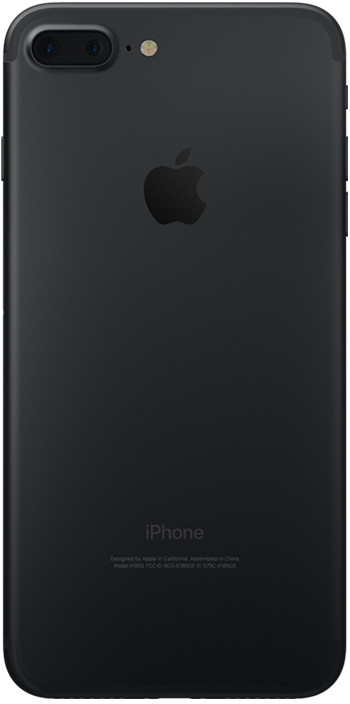
case iPhone7Plus
/// Device is an [iPhone SE](https://support.apple.com/kb/SP738)
///
/// 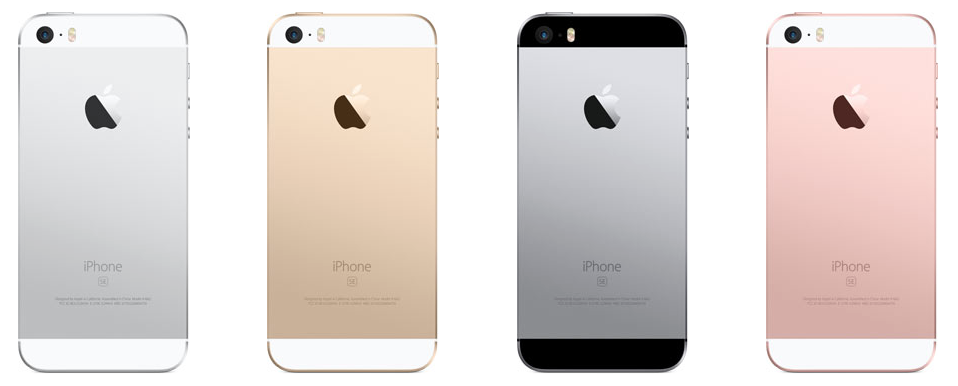
case iPhoneSE
/// Device is an [iPhone 8](https://support.apple.com/kb/SP767)
///
/// 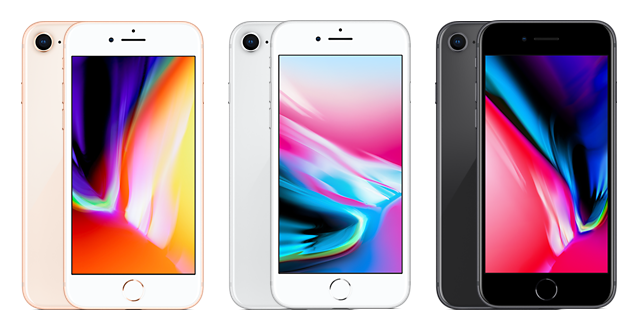
case iPhone8
/// Device is an [iPhone 8 Plus](https://support.apple.com/kb/SP768)
///
/// 
case iPhone8Plus
/// Device is an [iPhone X](https://support.apple.com/kb/SP770)
///
/// 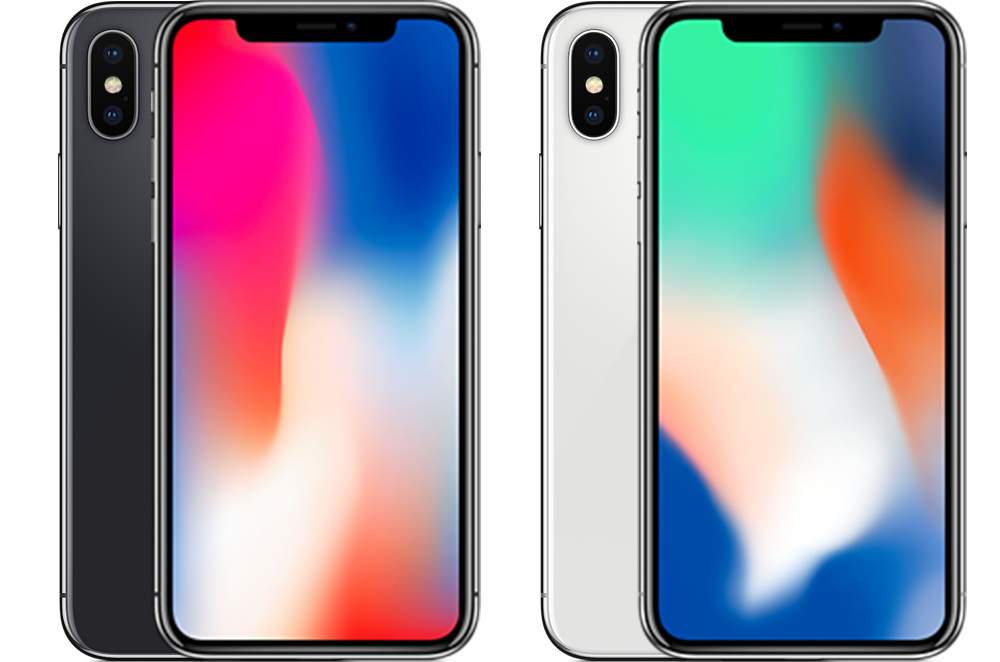
case iPhoneX
/// Device is an [iPad 2](https://support.apple.com/kb/SP622)
///
/// 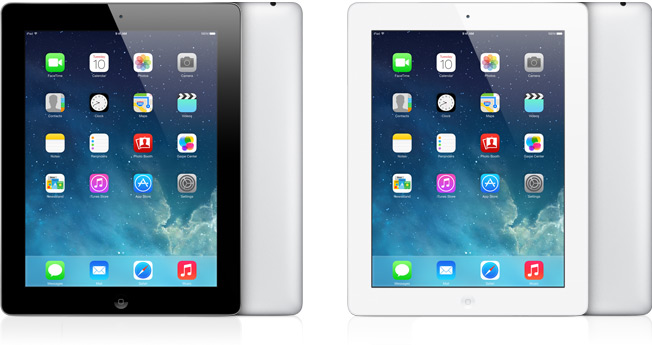
case iPad2
/// Device is an [iPad (3rd generation)](https://support.apple.com/kb/SP647)
///
/// 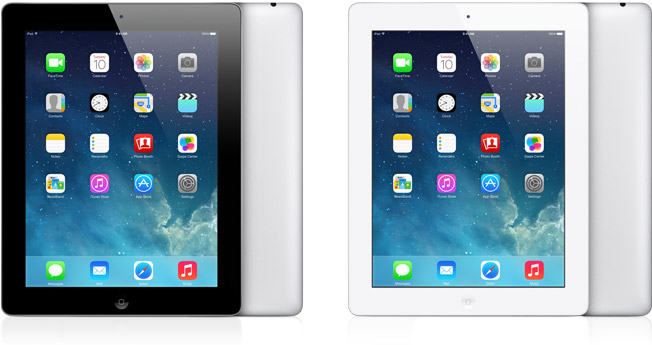
case iPad3
/// Device is an [iPad (4th generation)](https://support.apple.com/kb/SP662)
///
/// 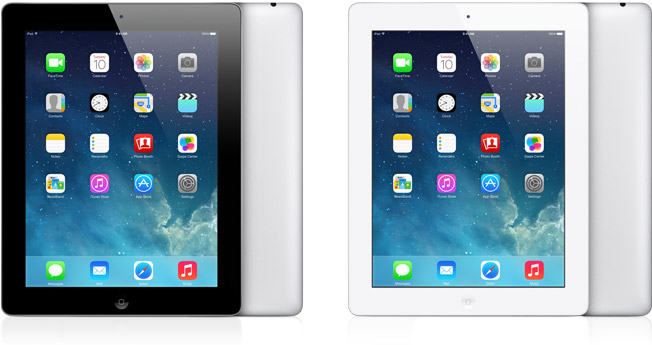
case iPad4
/// Device is an [iPad Air](https://support.apple.com/kb/SP692)
///
/// 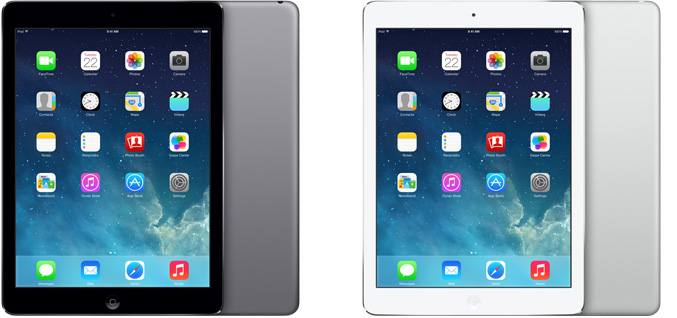
case iPadAir
/// Device is an [iPad Air 2](https://support.apple.com/kb/SP708)
///
/// 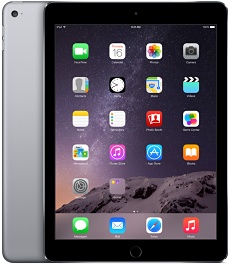
case iPadAir2
/// Device is an [iPad 5](https://support.apple.com/kb/SP751)
///
/// 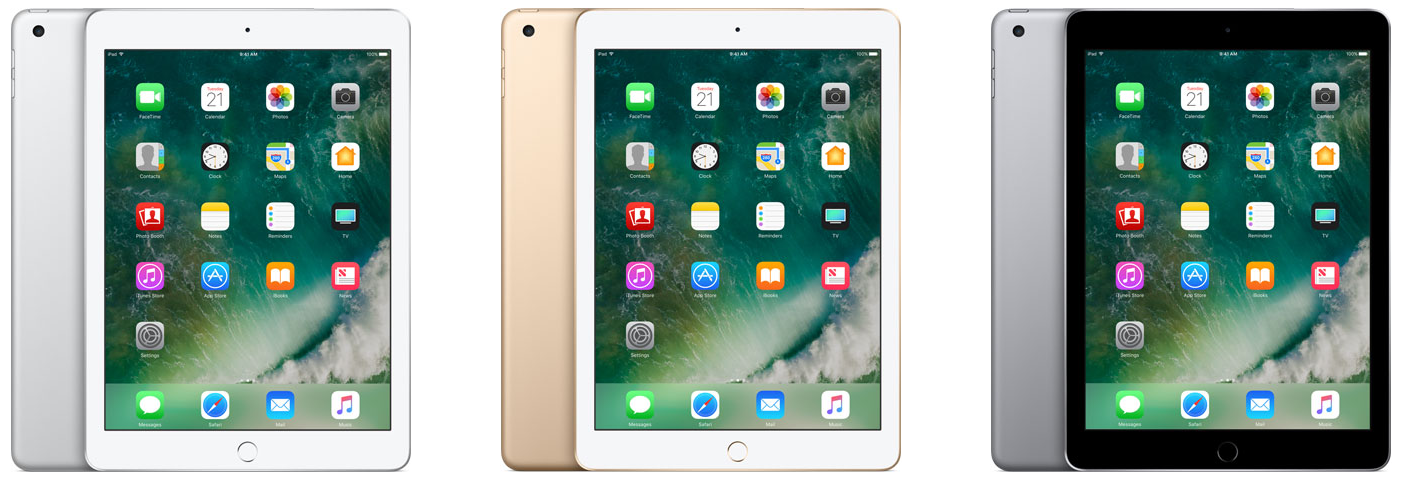
case iPad5
/// Device is an [iPad Mini](https://support.apple.com/kb/SP661)
///
/// 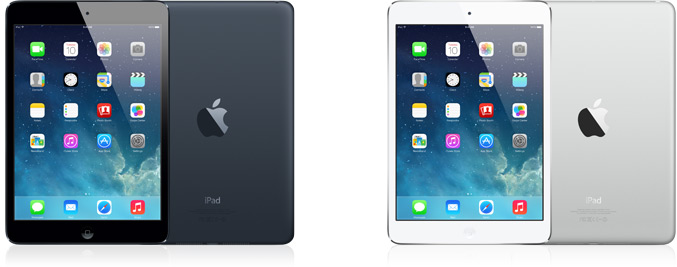
case iPadMini
/// Device is an [iPad Mini 2](https://support.apple.com/kb/SP693)
///
/// 
case iPadMini2
/// Device is an [iPad Mini 3](https://support.apple.com/kb/SP709)
///
/// 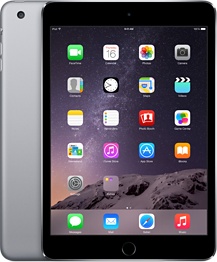
case iPadMini3
/// Device is an [iPad Mini 4](https://support.apple.com/kb/SP725)
///
/// 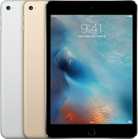
case iPadMini4
/// Device is an [iPad Pro](https://support.apple.com/kb/SP739)
///
/// 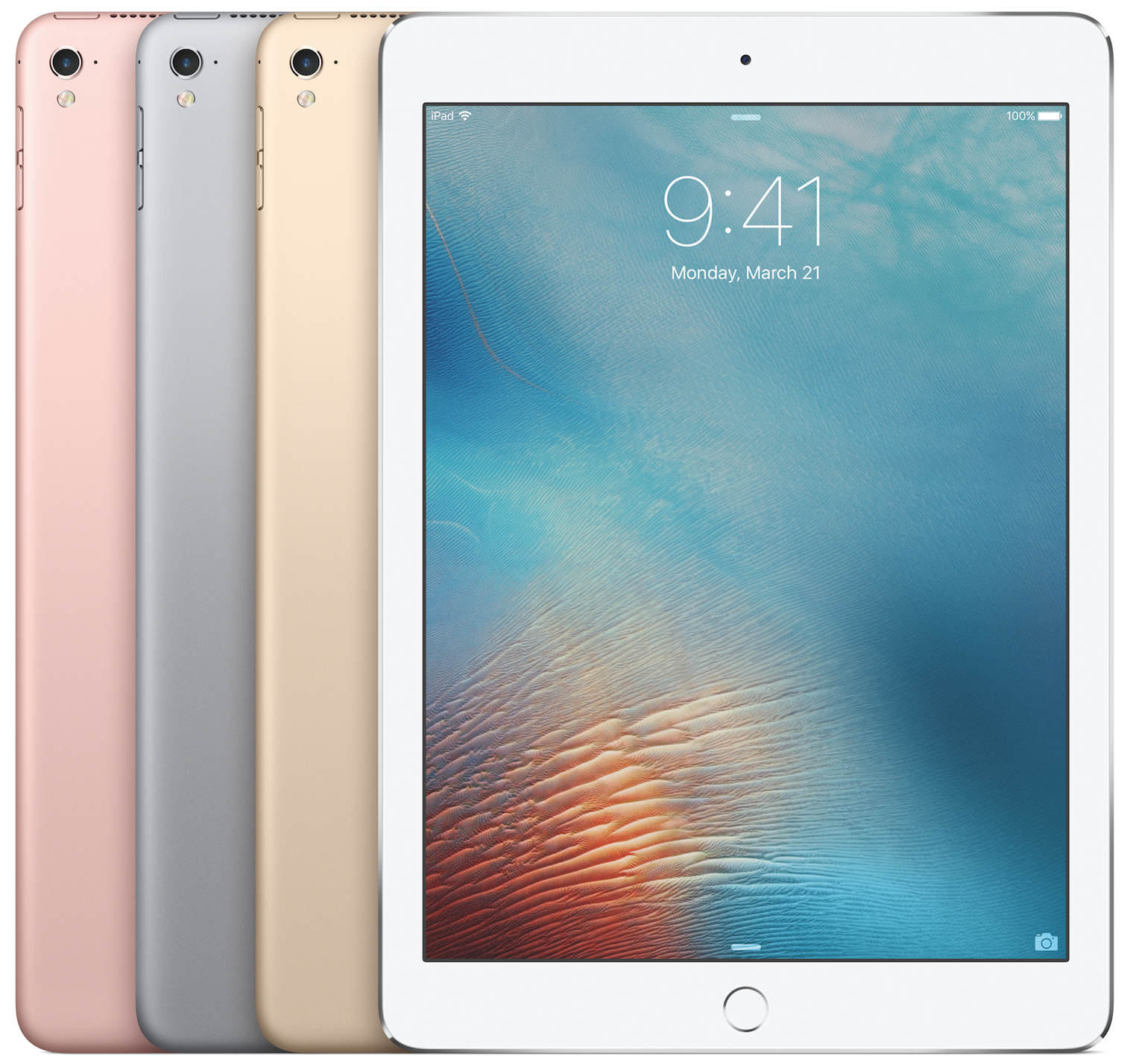
case iPadPro9Inch
/// Device is an [iPad Pro](https://support.apple.com/kb/sp723)
///
/// 
case iPadPro12Inch
/// Device is an [iPad Pro](https://support.apple.com/kb/SP761)
///
/// 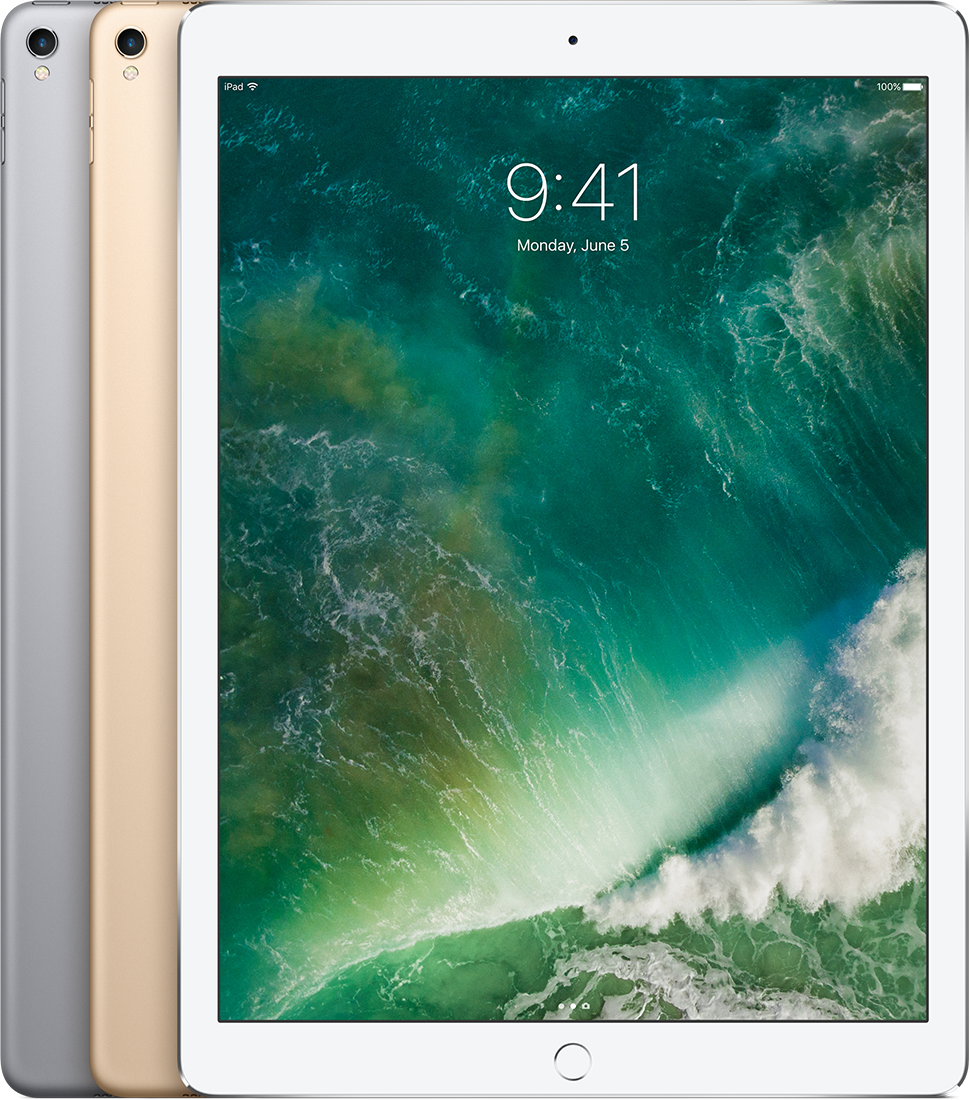
case iPadPro12Inch2
/// Device is an [iPad Pro 10.5](https://support.apple.com/kb/SP762)
///
/// 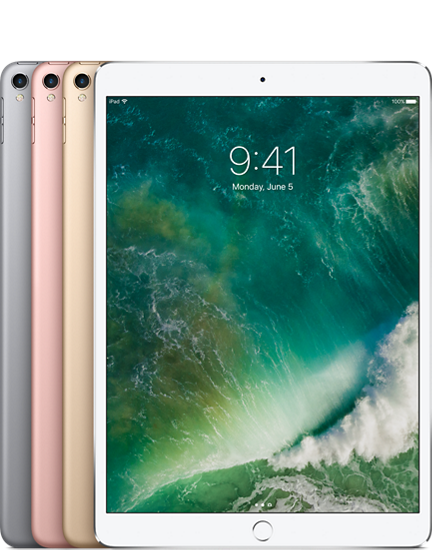
case iPadPro10Inch
#elseif os(tvOS)
/// Device is an [Apple TV](http://www.apple.com/tv/)
///
/// 
case appleTV4
#endif
/// Device is [Simulator](https://developer.apple.com/library/ios/documentation/IDEs/Conceptual/iOS_Simulator_Guide/Introduction/Introduction.html)
///
/// 
indirect case simulator(Device)
/// Device is not yet known (implemented)
/// You can still use this enum as before but the description equals the identifier (you can get multiple identifiers for the same product class
/// (e.g. "iPhone6,1" or "iPhone 6,2" do both mean "iPhone 5s"))
case unknown(String)
/// Initializes a `Device` representing the current device this software runs on.
public init() {
self = Device.mapToDevice(identifier: Device.identifier)
}
/// Gets the identifier from the system, such as "iPhone7,1".
public static var identifier: String {
var systemInfo = utsname()
uname(&systemInfo)
let mirror = Mirror(reflecting: systemInfo.machine)
let identifier = mirror.children.reduce("") { identifier, element in
guard let value = element.value as? Int8, value != 0 else { return identifier }
return identifier + String(UnicodeScalar(UInt8(value)))
}
return identifier
}
/// Maps an identifier to a Device. If the identifier can not be mapped to an existing device, `UnknownDevice(identifier)` is returned.
///
/// - parameter identifier: The device identifier, e.g. "iPhone7,1". Can be obtained from `Device.identifier`.
///
/// - returns: An initialized `Device`.
public static func mapToDevice(identifier: String) -> Device { // swiftlint:disable:this cyclomatic_complexity
#if os(iOS)
switch identifier {
case "iPod5,1": return iPodTouch5
case "iPod7,1": return iPodTouch6
case "iPhone3,1", "iPhone3,2", "iPhone3,3": return iPhone4
case "iPhone4,1": return iPhone4s
case "iPhone5,1", "iPhone5,2": return iPhone5
case "iPhone5,3", "iPhone5,4": return iPhone5c
case "iPhone6,1", "iPhone6,2": return iPhone5s
case "iPhone7,2": return iPhone6
case "iPhone7,1": return iPhone6Plus
case "iPhone8,1": return iPhone6s
case "iPhone8,2": return iPhone6sPlus
case "iPhone9,1", "iPhone9,3": return iPhone7
case "iPhone9,2", "iPhone9,4": return iPhone7Plus
case "iPhone8,4": return iPhoneSE
case "iPhone10,4": return iPhone8
case "iPhone10,5": return iPhone8Plus
case "iPhone10,3": return iPhoneX
case "iPad2,1", "iPad2,2", "iPad2,3", "iPad2,4": return iPad2
case "iPad3,1", "iPad3,2", "iPad3,3": return iPad3
case "iPad3,4", "iPad3,5", "iPad3,6": return iPad4
case "iPad4,1", "iPad4,2", "iPad4,3": return iPadAir
case "iPad5,3", "iPad5,4": return iPadAir2
case "iPad6,11", "iPad6,12": return iPad5
case "iPad2,5", "iPad2,6", "iPad2,7": return iPadMini
case "iPad4,4", "iPad4,5", "iPad4,6": return iPadMini2
case "iPad4,7", "iPad4,8", "iPad4,9": return iPadMini3
case "iPad5,1", "iPad5,2": return iPadMini4
case "iPad6,3", "iPad6,4": return iPadPro9Inch
case "iPad6,7", "iPad6,8": return iPadPro12Inch
case "iPad7,1", "iPad7,2": return iPadPro12Inch2
case "iPad7,3", "iPad7,4": return iPadPro10Inch
case "i386", "x86_64": return simulator(mapToDevice(identifier: ProcessInfo().environment["SIMULATOR_MODEL_IDENTIFIER"] ?? "iOS"))
default: return unknown(identifier)
}
#elseif os(tvOS)
switch identifier {
case "AppleTV5,3": return appleTV4
case "i386", "x86_64": return simulator(mapToDevice(identifier: ProcessInfo().environment["SIMULATOR_MODEL_IDENTIFIER"] ?? "tvOS"))
default: return unknown(identifier)
}
#endif
}
#if os(iOS)
/// All iPods
public static var allPods: [Device] {
return [.iPodTouch5, .iPodTouch6]
}
/// All iPhones
public static var allPhones: [Device] {
return [.iPhone4, .iPhone4s, .iPhone5, .iPhone5c, .iPhone5s, .iPhone6, .iPhone6Plus, .iPhone6s, .iPhone6sPlus, .iPhone7, .iPhone7Plus, .iPhoneSE, .iPhone8, .iPhone8Plus, .iPhoneX]
}
/// All iPads
public static var allPads: [Device] {
return [.iPad2, .iPad3, .iPad4, .iPadAir, .iPadAir2, .iPad5, .iPadMini, .iPadMini2, .iPadMini3, .iPadMini4, .iPadPro9Inch, .iPadPro12Inch, .iPadPro12Inch2, .iPadPro10Inch]
}
/// All simulator iPods
public static var allSimulatorPods: [Device] {
return allPods.map(Device.simulator)
}
/// All simulator iPhones
public static var allSimulatorPhones: [Device] {
return allPhones.map(Device.simulator)
}
/// All simulator iPads
public static var allSimulatorPads: [Device] {
return allPads.map(Device.simulator)
}
/// Returns whether the device is an iPod (real or simulator)
public var isPod: Bool {
return isOneOf(Device.allPods) || isOneOf(Device.allSimulatorPods)
}
/// Returns whether the device is an iPhone (real or simulator)
public var isPhone: Bool {
return isOneOf(Device.allPhones) || isOneOf(Device.allSimulatorPhones) || UIDevice.current.userInterfaceIdiom == .phone
}
/// Returns whether the device is an iPad (real or simulator)
public var isPad: Bool {
return isOneOf(Device.allPads) || isOneOf(Device.allSimulatorPads) || UIDevice.current.userInterfaceIdiom == .pad
}
/// Returns whether the device is any of the simulator
/// Useful when there is a need to check and skip running a portion of code (location request or others)
public var isSimulator: Bool {
return isOneOf(Device.allSimulators)
}
public var isZoomed: Bool {
if Int(UIScreen.main.scale.rounded()) == 3 {
// Plus-sized
return UIScreen.main.nativeScale > 2.7
} else {
return UIScreen.main.nativeScale > UIScreen.main.scale
}
}
/// Returns diagonal screen length in inches
public var diagonal: Double {
switch self {
case .iPodTouch5: return 4
case .iPodTouch6: return 4
case .iPhone4: return 3.5
case .iPhone4s: return 3.5
case .iPhone5: return 4
case .iPhone5c: return 4
case .iPhone5s: return 4
case .iPhone6: return 4.7
case .iPhone6Plus: return 5.5
case .iPhone6s: return 4.7
case .iPhone6sPlus: return 5.5
case .iPhone7: return 4.7
case .iPhone7Plus: return 5.5
case .iPhoneSE: return 4
case .iPhone8: return 4.7
case .iPhone8Plus: return 5.5
case .iPhoneX: return 5.8
case .iPad2: return 9.7
case .iPad3: return 9.7
case .iPad4: return 9.7
case .iPadAir: return 9.7
case .iPadAir2: return 9.7
case .iPad5: return 9.7
case .iPadMini: return 7.9
case .iPadMini2: return 7.9
case .iPadMini3: return 7.9
case .iPadMini4: return 7.9
case .iPadPro9Inch: return 9.7
case .iPadPro12Inch: return 12.9
case .iPadPro12Inch2: return 12.9
case .iPadPro10Inch: return 10.5
case .simulator(let model): return model.diagonal
case .unknown: return -1
}
}
/// Returns screen ratio as a tuple
public var screenRatio: (width: Double, height: Double) {
switch self {
case .iPodTouch5: return (width: 9, height: 16)
case .iPodTouch6: return (width: 9, height: 16)
case .iPhone4: return (width: 2, height: 3)
case .iPhone4s: return (width: 2, height: 3)
case .iPhone5: return (width: 9, height: 16)
case .iPhone5c: return (width: 9, height: 16)
case .iPhone5s: return (width: 9, height: 16)
case .iPhone6: return (width: 9, height: 16)
case .iPhone6Plus: return (width: 9, height: 16)
case .iPhone6s: return (width: 9, height: 16)
case .iPhone6sPlus: return (width: 9, height: 16)
case .iPhone7: return (width: 9, height: 16)
case .iPhone7Plus: return (width: 9, height: 16)
case .iPhoneSE: return (width: 9, height: 16)
case .iPhone8: return (width: 9, height: 16)
case .iPhone8Plus: return (width: 9, height: 16)
case .iPhoneX: return (width: 9, height: 19.5)
case .iPad2: return (width: 3, height: 4)
case .iPad3: return (width: 3, height: 4)
case .iPad4: return (width: 3, height: 4)
case .iPadAir: return (width: 3, height: 4)
case .iPadAir2: return (width: 3, height: 4)
case .iPad5: return (width: 3, height: 4)
case .iPadMini: return (width: 3, height: 4)
case .iPadMini2: return (width: 3, height: 4)
case .iPadMini3: return (width: 3, height: 4)
case .iPadMini4: return (width: 3, height: 4)
case .iPadPro9Inch: return (width: 3, height: 4)
case .iPadPro12Inch: return (width: 3, height: 4)
case .iPadPro12Inch2: return (width: 3, height: 4)
case .iPadPro10Inch: return (width: 3, height: 4)
case .simulator(let model): return model.screenRatio
case .unknown: return (width: -1, height: -1)
}
}
#elseif os(tvOS)
/// All TVs
public static var allTVs: [Device] {
return [.appleTV4]
}
/// All simulator TVs
public static var allSimulatorTVs: [Device] {
return allTVs.map(Device.simulator)
}
#endif
/// All real devices (i.e. all devices except for all simulators)
public static var allRealDevices: [Device] {
#if os(iOS)
return allPods + allPhones + allPads
#elseif os(tvOS)
return allTVs
#endif
}
/// All simulators
public static var allSimulators: [Device] {
return allRealDevices.map(Device.simulator)
}
/**
This method saves you in many cases from the need of updating your code with every new device.
Most uses for an enum like this are the following:
```
switch Device() {
case .iPodTouch5, .iPodTouch6: callMethodOnIPods()
case .iPhone4, iPhone4s, .iPhone5, .iPhone5s, .iPhone6, .iPhone6Plus, .iPhone6s, .iPhone6sPlus, .iPhone7, .iPhone7Plus, .iPhoneSE, .iPhone8, .iPhone8Plus, .iPhoneX: callMethodOnIPhones()
case .iPad2, .iPad3, .iPad4, .iPadAir, .iPadAir2, .iPadMini, .iPadMini2, .iPadMini3, .iPadMini4, .iPadPro: callMethodOnIPads()
default: break
}
```
This code can now be replaced with
```
let device = Device()
if device.isOneOf(Device.allPods) {
callMethodOnIPods()
} else if device.isOneOf(Device.allPhones) {
callMethodOnIPhones()
} else if device.isOneOf(Device.allPads) {
callMethodOnIPads()
}
```
- parameter devices: An array of devices.
- returns: Returns whether the current device is one of the passed in ones.
*/
public func isOneOf(_ devices: [Device]) -> Bool {
return devices.contains(self)
}
/// The name identifying the device (e.g. "Dennis' iPhone").
public var name: String {
return UIDevice.current.name
}
/// The name of the operating system running on the device represented by the receiver (e.g. "iOS" or "tvOS").
public var systemName: String {
return UIDevice.current.systemName
}
/// The current version of the operating system (e.g. 8.4 or 9.2).
public var systemVersion: String {
return UIDevice.current.systemVersion
}
/// The model of the device (e.g. "iPhone" or "iPod Touch").
public var model: String {
return UIDevice.current.model
}
/// The model of the device as a localized string.
public var localizedModel: String {
return UIDevice.current.localizedModel
}
/// PPI (Pixels per Inch) on the current device's screen (if applicable). When the device is not applicable this property returns nil.
public var ppi: Int? {
#if os(iOS)
switch self {
case .iPodTouch5: return 326
case .iPodTouch6: return 326
case .iPhone4: return 326
case .iPhone4s: return 326
case .iPhone5: return 326
case .iPhone5c: return 326
case .iPhone5s: return 326
case .iPhone6: return 326
case .iPhone6Plus: return 401
case .iPhone6s: return 326
case .iPhone6sPlus: return 401
case .iPhone7: return 326
case .iPhone7Plus: return 401
case .iPhoneSE: return 326
case .iPhone8: return 326
case .iPhone8Plus: return 401
case .iPhoneX: return 458
case .iPad2: return 132
case .iPad3: return 264
case .iPad4: return 264
case .iPadAir: return 264
case .iPadAir2: return 264
case .iPad5: return 264
case .iPadMini: return 163
case .iPadMini2: return 326
case .iPadMini3: return 326
case .iPadMini4: return 326
case .iPadPro9Inch: return 264
case .iPadPro12Inch: return 264
case .iPadPro12Inch2: return 264
case .iPadPro10Inch: return 264
case .simulator(let model): return model.ppi
case .unknown: return nil
}
#elseif os(tvOS)
return nil
#endif
}
}
// MARK: - CustomStringConvertible
extension Device: CustomStringConvertible {
/// A textual representation of the device.
public var description: String {
#if os(iOS)
switch self {
case .iPodTouch5: return "iPod Touch 5"
case .iPodTouch6: return "iPod Touch 6"
case .iPhone4: return "iPhone 4"
case .iPhone4s: return "iPhone 4s"
case .iPhone5: return "iPhone 5"
case .iPhone5c: return "iPhone 5c"
case .iPhone5s: return "iPhone 5s"
case .iPhone6: return "iPhone 6"
case .iPhone6Plus: return "iPhone 6 Plus"
case .iPhone6s: return "iPhone 6s"
case .iPhone6sPlus: return "iPhone 6s Plus"
case .iPhone7: return "iPhone 7"
case .iPhone7Plus: return "iPhone 7 Plus"
case .iPhoneSE: return "iPhone SE"
case .iPhone8: return "iPhone 8"
case .iPhone8Plus: return "iPhone 8 Plus"
case .iPhoneX: return "iPhone X"
case .iPad2: return "iPad 2"
case .iPad3: return "iPad 3"
case .iPad4: return "iPad 4"
case .iPadAir: return "iPad Air"
case .iPadAir2: return "iPad Air 2"
case .iPad5: return "iPad 5"
case .iPadMini: return "iPad Mini"
case .iPadMini2: return "iPad Mini 2"
case .iPadMini3: return "iPad Mini 3"
case .iPadMini4: return "iPad Mini 4"
case .iPadPro9Inch: return "iPad Pro (9.7-inch)"
case .iPadPro12Inch: return "iPad Pro (12.9-inch)"
case .iPadPro12Inch2: return "iPad Pro (12.9-inch) 2"
case .iPadPro10Inch: return "iPad Pro (10.5-inch)"
case .simulator(let model): return "Simulator (\(model))"
case .unknown(let identifier): return identifier
}
#elseif os(tvOS)
switch self {
case .appleTV4: return "Apple TV 4"
case .simulator(let model): return "Simulator (\(model))"
case .unknown(let identifier): return identifier
}
#endif
}
}
// MARK: - Equatable
extension Device: Equatable {
/// Compares two devices
///
/// - parameter lhs: A device.
/// - parameter rhs: Another device.
///
/// - returns: `true` iff the underlying identifier is the same.
public static func == (lhs: Device, rhs: Device) -> Bool {
return lhs.description == rhs.description
}
}
#if os(iOS)
// MARK: - Battery
extension Device {
/**
This enum describes the state of the battery.
- Full: The device is plugged into power and the battery is 100% charged or the device is the iOS Simulator.
- Charging: The device is plugged into power and the battery is less than 100% charged.
- Unplugged: The device is not plugged into power; the battery is discharging.
*/
public enum BatteryState: CustomStringConvertible, Equatable {
/// The device is plugged into power and the battery is 100% charged or the device is the iOS Simulator.
case full
/// The device is plugged into power and the battery is less than 100% charged.
/// The associated value is in percent (0-100).
case charging(Int)
/// The device is not plugged into power; the battery is discharging.
/// The associated value is in percent (0-100).
case unplugged(Int)
fileprivate init() {
UIDevice.current.isBatteryMonitoringEnabled = true
let batteryLevel = Int(round(UIDevice.current.batteryLevel * 100)) // round() is actually not needed anymore since -[batteryLevel] seems to always return a two-digit precision number
// but maybe that changes in the future.
switch UIDevice.current.batteryState {
case .charging: self = .charging(batteryLevel)
case .full: self = .full
case .unplugged:self = .unplugged(batteryLevel)
case .unknown: self = .full // Should never happen since `batteryMonitoring` is enabled.
}
UIDevice.current.isBatteryMonitoringEnabled = false
}
/// Provides a textual representation of the battery state.
/// Examples:
/// ```
/// Battery level: 90%, device is plugged in.
/// Battery level: 100 % (Full), device is plugged in.
/// Battery level: \(batteryLevel)%, device is unplugged.
/// ```
public var description: String {
switch self {
case .charging(let batteryLevel): return "Battery level: \(batteryLevel)%, device is plugged in."
case .full: return "Battery level: 100 % (Full), device is plugged in."
case .unplugged(let batteryLevel): return "Battery level: \(batteryLevel)%, device is unplugged."
}
}
}
/// The state of the battery
public var batteryState: BatteryState {
return BatteryState()
}
/// Battery level ranges from 0 (fully discharged) to 100 (100% charged).
public var batteryLevel: Int {
switch BatteryState() {
case .charging(let value): return value
case .full: return 100
case .unplugged(let value): return value
}
}
}
// MARK: - Device.Batterystate: Comparable
extension Device.BatteryState: Comparable {
/// Tells if two battery states are equal.
///
/// - parameter lhs: A battery state.
/// - parameter rhs: Another battery state.
///
/// - returns: `true` iff they are equal, otherwise `false`
public static func == (lhs: Device.BatteryState, rhs: Device.BatteryState) -> Bool {
return lhs.description == rhs.description
}
/// Compares two battery states.
///
/// - parameter lhs: A battery state.
/// - parameter rhs: Another battery state.
///
/// - returns: `true` if rhs is `.Full`, `false` when lhs is `.Full` otherwise their battery level is compared.
public static func < (lhs: Device.BatteryState, rhs: Device.BatteryState) -> Bool {
switch (lhs, rhs) {
case (.full, _): return false // return false (even if both are `.Full` -> they are equal)
case (_, .full): return true // lhs is *not* `.Full`, rhs is
case (.charging(let lhsLevel), .charging(let rhsLevel)): return lhsLevel < rhsLevel
case (.charging(let lhsLevel), .unplugged(let rhsLevel)): return lhsLevel < rhsLevel
case (.unplugged(let lhsLevel), .charging(let rhsLevel)): return lhsLevel < rhsLevel
case (.unplugged(let lhsLevel), .unplugged(let rhsLevel)): return lhsLevel < rhsLevel
default: return false // compiler won't compile without it, though it cannot happen
}
}
}
#endif
| mit | 3ad6eb995431ef36708f384c11dcb2f0 | 38.322157 | 190 | 0.647859 | 3.550744 | false | false | false | false |
OctMon/OMExtension | OMExtension/OMExtension/Source/Foundation/OMString.swift | 1 | 26933 | //
// OMString.swift
// OMExtension
//
// The MIT License (MIT)
//
// Copyright (c) 2016 OctMon
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
import Foundation
#if !os(macOS)
import UIKit
#endif
// MARK: - convert
public extension String {
var omToFloat: Float? {
if let num = NumberFormatter().number(from: self) {
return num.floatValue
}
return nil
}
var omToFloatValue: Float {
return omToFloat ?? 0
}
var omToDouble: Double? {
if let num = NumberFormatter().number(from: self) {
return num.doubleValue
}
return nil
}
var omToDoubleValue: Double {
return omToDouble ?? 0.0
}
var omToInt: Int? {
if let num = NumberFormatter().number(from: self) {
return num.intValue
}
return nil
}
var omToIntValue: Int {
return omToInt ?? 0
}
var omToBoolValue: Bool {
return ["true", "y", "t", "yes", "1"].contains { self.caseInsensitiveCompare($0) == .orderedSame }
}
/// Date object from "yyyy-MM-dd" formatted string
var omToDate: Date? {
let selfLowercased = self.omTrimming.lowercased()
let formatter = DateFormatter()
formatter.timeZone = TimeZone.current
formatter.dateFormat = "yyyy-MM-dd"
return formatter.date(from: selfLowercased)
}
/// Date object from "yyyy-MM-dd HH:mm:ss" formatted string.
var omToDateTime: Date? {
let selfLowercased = self.omTrimming.lowercased()
let formatter = DateFormatter()
formatter.timeZone = TimeZone.current
formatter.dateFormat = "yyyy-MM-dd HH:mm:ss"
return formatter.date(from: selfLowercased)
}
var omToJson: Any? {
if let data = data(using: String.Encoding.utf8) {
let json = try? JSONSerialization.jsonObject(with: data)
if let json = json {
return json
}
}
return nil
}
var omToURLParameters: [String: Any]? {
// 截取是否有参数
guard let urlComponents = URLComponents(string: self), let queryItems = urlComponents.queryItems else {
return nil
}
var parameters = [String: Any]()
queryItems.forEach({ (item) in
if let existValue = parameters[item.name], let value = item.value {
if var existValue = existValue as? [Any] {
existValue.append(value)
} else {
parameters[item.name] = [existValue, value]
}
} else {
parameters[item.name] = item.value
}
})
return parameters
}
}
// MARK: - base64
// https://github.com/Reza-Rg/Base64-Swift-Extension/blob/master/Base64.swift
public extension String {
var omBase64Decoded: String? {
guard let decodedData = Data(base64Encoded: self) else {
return nil
}
return String(data: decodedData, encoding: .utf8)
}
var omBase64Encoded: String? {
let plainData = self.data(using: .utf8)
return plainData?.base64EncodedString()
}
}
// MARK: - valid
public extension String {
func omIsRegex(_ regex: String) -> Bool {
let regExPredicate: NSPredicate = NSPredicate(format: "SELF MATCHES %@", regex)
return regExPredicate.evaluate(with: self.lowercased())
}
/// 纯数字验证
var omIsNumber: Bool { return omIsRegex("^[0-9]*$") }
/// 邮箱验证
var omIsEmail: Bool { return omIsRegex("^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$") }
/// 手机号验证
var omIsMobile: Bool { return omIsRegex("^(0|86|17951)?(13[0-9]|14[0-9]|15[0-9]|16[0-9]|17[0-9]|18[0-9]|19[0-9])[0-9]{8}$") }
/// 电话号码验证
var omIsPhoneTelephone: Bool { return omIsRegex("([\\d]{7,25}(?!\\d))|((\\d{3,4})-(\\d{7,8}))|((\\d{3,4})-(\\d{7,8})-(\\d{1,4}))") }
/// URL网址验证
var omIsURL: Bool { return omIsHttpUrl || omIsHttpsUrl }
/// URL网址验证
var omIsHttpsUrl: Bool {
guard lowercased().hasPrefix("https://") else {
return false
}
return URL(string: self) != nil
}
/// URL网址验证
var omIsHttpUrl: Bool {
guard lowercased().hasPrefix("http://") else {
return false
}
return URL(string: self) != nil
}
/// IP地址验证
var omIsIP: Bool {
if omIsRegex("^(\\d{1,3})\\.(\\d{1,3})\\.(\\d{1,3})\\.(\\d{1,3})$") {
for string in self.components(separatedBy: ".") {
if string.omToIntValue > 255 {
return false
}
}
return true
} else {
return false
}
}
var omIsIncludeUppercaseLetterCharact: Bool {
if let regular = try? NSRegularExpression(pattern: "[A-Z]") {
return regular.numberOfMatches(in: self, options: .reportProgress, range: NSMakeRange(0, count)) > 0
}
return false
}
var omIsIncludeLowercaseLetterCharact: Bool {
if let regular = try? NSRegularExpression(pattern: "[a-z]") {
return regular.numberOfMatches(in: self, options: .reportProgress, range: NSMakeRange(0, count)) > 0
}
return false
}
var omIsIncludeSpecialCharact: Bool {
guard let range = rangeOfCharacter(from: CharacterSet(charactersIn: "~¥#&*<>《》()[]{}【】^@/£¤¥|§¨「」『』¢¬ ̄~@#¥&*()——+|《》$_€")) else {
return false
}
if range.isEmpty {
return false
}
return true
}
/// 身份证号码验证
var omIsIDCard: Bool {
if count != 18 {
return false
}
let mmdd = "(((0[13578]|1[02])(0[1-9]|[12][0-9]|3[01]))|((0[469]|11)(0[1-9]|[12][0-9]|30))|(02(0[1-9]|[1][0-9]|2[0-8])))"
let leapMmdd = "0229"
let year = "(19|20)[0-9]{2}"
let leapYear = "(19|20)(0[48]|[2468][048]|[13579][26])"
let yearMmdd = year + mmdd
let leapyearMmdd = leapYear + leapMmdd
let yyyyMmdd = "((\(yearMmdd))|(\(leapyearMmdd))|(20000229))"
let area = "(1[1-5]|2[1-3]|3[1-7]|4[1-6]|5[0-4]|6[1-5]|82|[7-9]1)[0-9]{4}"
let regex = "\(area)\(yyyyMmdd)[0-9]{3}[0-9Xx]"
let predicate = NSPredicate(format: "SELF MATCHES %@", regex)
if predicate.evaluate(with: self) == false {
return false
}
let chars = uppercased().map { return String($0) }
let c1 = chars[7].omToInt!
let c2 = (chars[6].omToInt! + chars[16].omToInt!) * 2
let c3 = chars[9].omToInt! * 3
let c4 = (chars[5].omToInt! + chars[15].omToInt!) * 4
let c5 = (chars[3].omToInt! + chars[13].omToInt!) * 5
let c6 = chars[8].omToInt! * 6
let c7 = (chars[0].omToInt! + chars[10].omToInt!) * 7
let c8 = (chars[4].omToInt! + chars[14].omToInt!) * 8
let c9 = (chars[1].omToInt! + chars[11].omToInt!) * 9
let c10 = (chars[2].omToInt! + chars[12].omToInt!) * 10
let summary: Int = c1 + c2 + c3 + c4 + c5 + c6 + c7 + c8 + c9 + c10
let remainder = summary % 11
let checkString = "10X98765432"
let checkBit = checkString.map { return String($0) }[remainder]
return (checkBit == chars.last)
}
}
public extension String {
/// 密码验证
///
/// - Parameters:
/// - min: 最小长度
/// - max: 最大长大
/// - includeCase: 必须包含大小写
/// - Returns: 检测密码必须包含大写字母、小写字母、数字
func omIsPasswordRegulation(min: Int = 6, max: Int = 16, includeCase: Bool = true) -> Bool {
var casesensitive = omIsIncludeLowercaseLetterCharact || omIsIncludeUppercaseLetterCharact
if includeCase {
casesensitive = omIsIncludeLowercaseLetterCharact && omIsIncludeUppercaseLetterCharact
}
guard count >= min && count <= max && !omIsNumber && !omIsIncludeSpecialCharact && casesensitive else {
return false
}
return true
}
}
// MARK: - common
public extension String {
var omExtractURL: [URL] {
var urls: [URL] = []
let detector: NSDataDetector?
do {
detector = try NSDataDetector(types: NSTextCheckingResult.CheckingType.link.rawValue)
} catch _ as NSError {
detector = nil
}
let text = self
if let detector = detector {
detector.enumerateMatches(in: text, options: [], range: NSRange(location: 0, length: text.count), using: {
(result: NSTextCheckingResult?, flags: NSRegularExpression.MatchingFlags, stop: UnsafeMutablePointer<ObjCBool>) -> Void in
if let result = result, let url = result.url {
urls.append(url)
}
})
}
return urls
}
#if os(iOS)
func omCopyToPasteboard() {
UIPasteboard.general.string = self
}
func omHeight(_ width: CGFloat, font: UIFont, lineBreakMode: NSLineBreakMode? = nil) -> CGFloat {
var attrib: [NSAttributedStringKey: Any] = [.font: font]
if lineBreakMode != nil {
let paragraphStyle = NSMutableParagraphStyle()
paragraphStyle.lineBreakMode = lineBreakMode!
attrib.updateValue(paragraphStyle, forKey: .paragraphStyle)
}
let size = CGSize(width: width, height: CGFloat(Double.greatestFiniteMagnitude))
return ceil((self as NSString).boundingRect(with: size, options: NSStringDrawingOptions.usesLineFragmentOrigin, attributes: attrib, context: nil).height)
}
#endif
mutating func omTrim() {
self = omTrimming
}
var omTrimming: String {
return trimmingCharacters(in: CharacterSet.whitespacesAndNewlines)
}
var omTrimmingWithoutSpacesAndNewLines: String {
return replacingOccurrences(of: " ", with: "").replacingOccurrences(of: "\n", with: "")
}
mutating func omReverse() {
self = omReversed
}
var omReversed: String {
return String(reversed())
}
var omIsContainEmoji: Bool {
// http://stackoverflow.com/questions/30757193/find-out-if-character-in-string-is-emoji
for scalar in unicodeScalars {
switch scalar.value {
case 0x3030, 0x00AE, 0x00A9, // Special Characters
0x1D000...0x1F77F, // Emoticons
0x2100...0x27BF, // Misc symbols and Dingbats
0xFE00...0xFE0F, // Variation Selectors
0x1F900...0x1F9FF: // Supplemental Symbols and Pictographs
return true
default:
continue
}
}
return false
}
func omSplit(_ separator: String) -> [String] {
return components(separatedBy: separator).filter({
return !$0.omTrimming.isEmpty
})
}
func omContain(_ subStirng: String, caseSensitive: Bool = true) -> Bool {
if !caseSensitive {
return range(of: subStirng, options: .caseInsensitive) != nil
}
return range(of: subStirng) != nil
}
func omCount(of subString: String, caseSensitive: Bool = true) -> Int {
if !caseSensitive {
return lowercased().components(separatedBy: subString).count - 1
}
return components(separatedBy: subString).count - 1
}
func omHasPrefix(_ prefix: String, caseSensitive: Bool = true) -> Bool {
if !caseSensitive {
return lowercased().hasPrefix(prefix.lowercased())
}
return hasPrefix(prefix)
}
func omHasSuffix(_ suffix: String, caseSensitive: Bool = true) -> Bool {
if !caseSensitive {
return lowercased().hasSuffix(suffix.lowercased())
}
return hasSuffix(suffix)
}
func omJoinSeparator(_ separator: String) -> String {
return map({ "\($0)" }).joined(separator: separator)
}
mutating func omJoinedSeparatored(_ separator: String) {
self = omJoinSeparator(separator)
}
/// 字符串截取
///
/// - Parameters:
/// - from: 从下标from开始截取
/// - to: 截取多少位
mutating func omSubString(from: Int = 0, to: Int) {
let fromIndex = index(startIndex, offsetBy: from)
let toIndex = index(fromIndex, offsetBy: to)
self = String(self[fromIndex..<toIndex])
}
func omGetRanges(_ searchString: String) -> [NSRange] {
var start = 0
var ranges: [NSRange] = []
while true {
let range = (self as NSString).range(of: searchString, options: NSString.CompareOptions.literal, range: NSRange(location: start, length: (self as NSString).length - start))
if range.location == NSNotFound {
break
} else {
ranges.append(range)
start = range.location + range.length
}
}
return ranges
}
#if !os(macOS)
func omGetAttributes(color: [(color: UIColor, subString: String?)]? = nil, font: [(font: UIFont, subString: String?)]? = nil, underlineStyle: [String]? = nil, strikethroughStyle: [String]? = nil, lineSpacing: CGFloat? = nil) -> NSMutableAttributedString {
let mutableAttributedString = NSMutableAttributedString(string: self)
color?.forEach { (color, subString) in
if let string = subString , string.count > 0 {
for range in omGetRanges(string) {
mutableAttributedString.addAttribute(.foregroundColor, value: color, range: range)
}
} else {
mutableAttributedString.addAttribute(.foregroundColor, value: color, range: NSRange(location: 0, length: count))
}
}
font?.forEach { (font, subString) in
if let string = subString , string.count > 0 {
for range in omGetRanges(string) {
mutableAttributedString.addAttribute(.font, value: font, range: range)
}
} else {
mutableAttributedString.addAttribute(.font, value: font, range: NSRange(location: 0, length: count))
}
}
underlineStyle?.forEach { (subString) in
if subString.count > 0 {
for range in omGetRanges(subString) {
mutableAttributedString.addAttribute(.underlineStyle, value: NSUnderlineStyle.styleSingle.rawValue, range: range)
}
}
}
strikethroughStyle?.forEach { (subString) in
if subString.count > 0 {
for range in omGetRanges(subString) {
mutableAttributedString.addAttribute(.strikethroughStyle, value: NSNumber(value: NSUnderlineStyle.styleSingle.rawValue as Int), range: range)
}
}
}
if let lineSpacing = lineSpacing {
let style = NSMutableParagraphStyle()
style.lineSpacing = lineSpacing
mutableAttributedString.addAttribute(.paragraphStyle, value: style, range: NSRange(location: 0, length: count))
}
return mutableAttributedString
}
#endif
}
// MARK: - MD5 https://github.com/krzyzanowskim/CryptoSwift
public extension String {
var omMD5: String {
if let data = self.data(using: .utf8, allowLossyConversion: true) {
let message = data.withUnsafeBytes { bytes -> [UInt8] in
return Array(UnsafeBufferPointer(start: bytes, count: data.count))
}
let MD5Calculator = MD5(message)
let MD5Data = MD5Calculator.calculate()
let MD5String = NSMutableString()
for c in MD5Data {
MD5String.appendFormat("%02x", c)
}
return MD5String as String
} else {
return self
}
}
}
/** array of bytes, little-endian representation */
func arrayOfBytes<T>(_ value: T, length: Int? = nil) -> [UInt8] {
let totalBytes = length ?? (MemoryLayout<T>.size * 8)
let valuePointer = UnsafeMutablePointer<T>.allocate(capacity: 1)
valuePointer.pointee = value
let bytes = valuePointer.withMemoryRebound(to: UInt8.self, capacity: totalBytes) { (bytesPointer) -> [UInt8] in
var bytes = [UInt8](repeating: 0, count: totalBytes)
for j in 0..<min(MemoryLayout<T>.size, totalBytes) {
bytes[totalBytes - 1 - j] = (bytesPointer + j).pointee
}
return bytes
}
#if swift(>=4.1)
valuePointer.deinitialize(count: 1)
valuePointer.deallocate()
#else
valuePointer.deinitialize()
valuePointer.deallocate(capacity: 1)
#endif
return bytes
}
extension Int {
/** Array of bytes with optional padding (little-endian) */
func bytes(_ totalBytes: Int = MemoryLayout<Int>.size) -> [UInt8] {
return arrayOfBytes(self, length: totalBytes)
}
}
extension NSMutableData {
/** Convenient way to append bytes */
@objc func appendBytes(_ arrayOfBytes: [UInt8]) {
append(arrayOfBytes, length: arrayOfBytes.count)
}
}
protocol HashProtocol {
var message: Array<UInt8> { get }
/** Common part for hash calculation. Prepare header data. */
func prepare(_ len: Int) -> Array<UInt8>
}
extension HashProtocol {
func prepare(_ len: Int) -> Array<UInt8> {
var tmpMessage = message
// Step 1. Append Padding Bits
tmpMessage.append(0x80) // append one bit (UInt8 with one bit) to message
// append "0" bit until message length in bits ≡ 448 (mod 512)
var msgLength = tmpMessage.count
var counter = 0
while msgLength % len != (len - 8) {
counter += 1
msgLength += 1
}
tmpMessage += Array<UInt8>(repeating: 0, count: counter)
return tmpMessage
}
}
func toUInt32Array(_ slice: ArraySlice<UInt8>) -> Array<UInt32> {
var result = Array<UInt32>()
result.reserveCapacity(16)
for idx in stride(from: slice.startIndex, to: slice.endIndex, by: MemoryLayout<UInt32>.size) {
let d0 = UInt32(slice[idx.advanced(by: 3)]) << 24
let d1 = UInt32(slice[idx.advanced(by: 2)]) << 16
let d2 = UInt32(slice[idx.advanced(by: 1)]) << 8
let d3 = UInt32(slice[idx])
let val: UInt32 = d0 | d1 | d2 | d3
result.append(val)
}
return result
}
struct BytesIterator: IteratorProtocol {
let chunkSize: Int
let data: [UInt8]
init(chunkSize: Int, data: [UInt8]) {
self.chunkSize = chunkSize
self.data = data
}
var offset = 0
mutating func next() -> ArraySlice<UInt8>? {
let end = min(chunkSize, data.count - offset)
let result = data[offset..<offset + end]
offset += result.count
return result.count > 0 ? result : nil
}
}
struct BytesSequence: Sequence {
let chunkSize: Int
let data: [UInt8]
func makeIterator() -> BytesIterator {
return BytesIterator(chunkSize: chunkSize, data: data)
}
}
func rotateLeft(_ value: UInt32, bits: UInt32) -> UInt32 {
return ((value << bits) & 0xFFFFFFFF) | (value >> (32 - bits))
}
class MD5: HashProtocol {
static let size = 16 // 128 / 8
let message: [UInt8]
init (_ message: [UInt8]) {
self.message = message
}
/** specifies the per-round shift amounts */
private let shifts: [UInt32] = [7, 12, 17, 22, 7, 12, 17, 22, 7, 12, 17, 22, 7, 12, 17, 22,
5, 9, 14, 20, 5, 9, 14, 20, 5, 9, 14, 20, 5, 9, 14, 20,
4, 11, 16, 23, 4, 11, 16, 23, 4, 11, 16, 23, 4, 11, 16, 23,
6, 10, 15, 21, 6, 10, 15, 21, 6, 10, 15, 21, 6, 10, 15, 21]
/** binary integer part of the sines of integers (Radians) */
private let sines: [UInt32] = [0xd76aa478, 0xe8c7b756, 0x242070db, 0xc1bdceee,
0xf57c0faf, 0x4787c62a, 0xa8304613, 0xfd469501,
0x698098d8, 0x8b44f7af, 0xffff5bb1, 0x895cd7be,
0x6b901122, 0xfd987193, 0xa679438e, 0x49b40821,
0xf61e2562, 0xc040b340, 0x265e5a51, 0xe9b6c7aa,
0xd62f105d, 0x02441453, 0xd8a1e681, 0xe7d3fbc8,
0x21e1cde6, 0xc33707d6, 0xf4d50d87, 0x455a14ed,
0xa9e3e905, 0xfcefa3f8, 0x676f02d9, 0x8d2a4c8a,
0xfffa3942, 0x8771f681, 0x6d9d6122, 0xfde5380c,
0xa4beea44, 0x4bdecfa9, 0xf6bb4b60, 0xbebfbc70,
0x289b7ec6, 0xeaa127fa, 0xd4ef3085, 0x4881d05,
0xd9d4d039, 0xe6db99e5, 0x1fa27cf8, 0xc4ac5665,
0xf4292244, 0x432aff97, 0xab9423a7, 0xfc93a039,
0x655b59c3, 0x8f0ccc92, 0xffeff47d, 0x85845dd1,
0x6fa87e4f, 0xfe2ce6e0, 0xa3014314, 0x4e0811a1,
0xf7537e82, 0xbd3af235, 0x2ad7d2bb, 0xeb86d391]
private let hashes: [UInt32] = [0x67452301, 0xefcdab89, 0x98badcfe, 0x10325476]
func calculate() -> [UInt8] {
var tmpMessage = prepare(64)
tmpMessage.reserveCapacity(tmpMessage.count + 4)
// hash values
var hh = hashes
// Step 2. Append Length a 64-bit representation of lengthInBits
let lengthInBits = (message.count * 8)
let lengthBytes = lengthInBits.bytes(64 / 8)
tmpMessage += lengthBytes.reversed()
// Process the message in successive 512-bit chunks:
let chunkSizeBytes = 512 / 8 // 64
for chunk in BytesSequence(chunkSize: chunkSizeBytes, data: tmpMessage) {
// break chunk into sixteen 32-bit words M[j], 0 ≤ j ≤ 15
var M = toUInt32Array(chunk)
assert(M.count == 16, "Invalid array")
// Initialize hash value for this chunk:
var A: UInt32 = hh[0]
var B: UInt32 = hh[1]
var C: UInt32 = hh[2]
var D: UInt32 = hh[3]
var dTemp: UInt32 = 0
// Main loop
for j in 0 ..< sines.count {
var g = 0
var F: UInt32 = 0
switch j {
case 0...15:
F = (B & C) | ((~B) & D)
g = j
break
case 16...31:
F = (D & B) | (~D & C)
g = (5 * j + 1) % 16
break
case 32...47:
F = B ^ C ^ D
g = (3 * j + 5) % 16
break
case 48...63:
F = C ^ (B | (~D))
g = (7 * j) % 16
break
default:
break
}
dTemp = D
D = C
C = B
B = B &+ rotateLeft((A &+ F &+ sines[j] &+ M[g]), bits: shifts[j])
A = dTemp
}
hh[0] = hh[0] &+ A
hh[1] = hh[1] &+ B
hh[2] = hh[2] &+ C
hh[3] = hh[3] &+ D
}
var result = [UInt8]()
result.reserveCapacity(hh.count / 4)
hh.forEach {
let itemLE = $0.littleEndian
result += [UInt8(itemLE & 0xff), UInt8((itemLE >> 8) & 0xff), UInt8((itemLE >> 16) & 0xff), UInt8((itemLE >> 24) & 0xff)]
}
return result
}
}
| mit | 55df6018dfed215cfdd915f20d0e1094 | 29.865741 | 259 | 0.516162 | 4.251235 | false | false | false | false |
codeswimmer/SwiftCommon | SwiftCommon/SwiftCommon/Source/Extensions/UIView+Geometry.swift | 1 | 2845 | //
// UIView+Geometry.swift
// SwiftCommon
//
// Created by Keith Ermel on 7/2/14.
// Copyright (c) 2014 Keith Ermel. All rights reserved.
//
import UIKit
public extension CGPoint {
public func toNSValue() -> NSValue {return NSValue(CGPoint: self)}
public static func from(value: NSValue) -> CGPoint {return value.CGPointValue()}
/** TODO:
While this is possible, I prefer returning a new CGPoint, thus maintaining
immutability;
There may be cases, though, were this might be useful so I'm leaving it here
for now.
// public mutating func fromNSValue(value: NSValue) {self = value.CGPointValue()}
*/
}
public extension UIView {
public func centerAsNSValue() -> NSValue {return center.toNSValue()}
public func centerFrom(value: NSValue) {center = CGPoint.from(value)}
public func positionAtTopLeftOfSuperview() {
center = _G.point(frame.width / 2.0, frame.height / 2.0)
}
public func positionAtBottomRight(parent: UIView) {
center = _G.point(
(parent.frame.maxX - parent.frame.origin.x) - frame.width / 2.0,
(parent.frame.maxY - parent.frame.origin.y) - frame.height / 2.0)
}
public func positionAtBottomRightOfSuperview() {
if let sv = superview {
center = _G.point(
(sv.frame.maxX - sv.frame.origin.x) - frame.width / 2.0,
(sv.frame.maxY - sv.frame.origin.y) - frame.height / 2.0)
}
}
public func positionAtBottomRightOfView(view: UIView) {
center = _G.point(
(view.frame.maxX - view.frame.origin.x) - frame.width / 2.0,
(view.frame.maxY - view.frame.origin.y) - frame.height / 2.0)
}
public func moveToBottomRightOfFrame(rightOfFrame: CGRect) {
let r = rightOfFrame
let x: CGFloat = r.origin.x + (r.width - frame.width)
let y: CGFloat = r.origin.y + (r.height - frame.height)
frame = _G.rect(x, y, frame.width, frame.height)
}
public func positionOffscreenTop() {
let y = -(UIScreen.mainScreen().bounds.size.height / 2.0)
frame = _G.rect(frame.origin.x, y, frame.width, frame.height)
}
public func positionOffscreenBottom() {
let y = UIScreen.mainScreen().bounds.size.height + (bounds.height / 2.0)
frame = _G.rect(frame.origin.x, y, frame.width, frame.height)
}
public func positionOffscreenLeft() {
let x = -(UIScreen.mainScreen().bounds.size.width / 2.0)
frame = _G.rect(x, frame.origin.y, frame.width, frame.height)
}
public func positionOffscreenRight() {
let x = UIScreen.mainScreen().bounds.size.width + (bounds.width / 2.0)
frame = _G.rect(x, frame.origin.y, frame.width, frame.height)
}
} | mit | 240e51554f2371083231a8cb6d4d1cb9 | 33.707317 | 84 | 0.610545 | 3.685233 | false | false | false | false |
i-schuetz/SwiftCharts | SwiftCharts/Convenience/LineChart.swift | 4 | 2823 | //
// LineChart.swift
// Examples
//
// Created by ischuetz on 19/07/15.
// Copyright (c) 2015 ivanschuetz. All rights reserved.
//
import UIKit
open class LineChart: Chart {
public typealias ChartLine = (chartPoints: [(Double, Double)], color: UIColor)
// Initializer for single line
public convenience init(frame: CGRect, chartConfig: ChartConfigXY, xTitle: String, yTitle: String, line: ChartLine) {
self.init(frame: frame, chartConfig: chartConfig, xTitle: xTitle, yTitle: yTitle, lines: [line])
}
// Initializer for multiple lines
public init(frame: CGRect, chartConfig: ChartConfigXY, xTitle: String, yTitle: String, lines: [ChartLine]) {
let xValues = stride(from: chartConfig.xAxisConfig.from, through: chartConfig.xAxisConfig.to, by: chartConfig.xAxisConfig.by).map{ChartAxisValueDouble($0)}
let yValues = stride(from: chartConfig.yAxisConfig.from, through: chartConfig.yAxisConfig.to, by: chartConfig.yAxisConfig.by).map{ChartAxisValueDouble($0)}
let xModel = ChartAxisModel(axisValues: xValues, axisTitleLabel: ChartAxisLabel(text: xTitle, settings: chartConfig.xAxisLabelSettings))
let yModel = ChartAxisModel(axisValues: yValues, axisTitleLabel: ChartAxisLabel(text: yTitle, settings: chartConfig.yAxisLabelSettings.defaultVertical()))
let coordsSpace = ChartCoordsSpaceLeftBottomSingleAxis(chartSettings: chartConfig.chartSettings, chartFrame: frame, xModel: xModel, yModel: yModel)
let (xAxisLayer, yAxisLayer, innerFrame) = (coordsSpace.xAxisLayer, coordsSpace.yAxisLayer, coordsSpace.chartInnerFrame)
let lineLayers: [ChartLayer] = lines.map {line in
let chartPoints = line.chartPoints.map {chartPointScalar in
ChartPoint(x: ChartAxisValueDouble(chartPointScalar.0), y: ChartAxisValueDouble(chartPointScalar.1))
}
let lineModel = ChartLineModel(chartPoints: chartPoints, lineColor: line.color, animDuration: 0.5, animDelay: 0)
return ChartPointsLineLayer(xAxis: xAxisLayer.axis, yAxis: yAxisLayer.axis, lineModels: [lineModel])
}
let guidelinesLayer = GuidelinesDefaultLayerGenerator.generateOpt(xAxisLayer: xAxisLayer, yAxisLayer: yAxisLayer, guidelinesConfig: chartConfig.guidelinesConfig)
let view = ChartBaseView(frame: frame)
let layers: [ChartLayer] = [xAxisLayer, yAxisLayer] + (guidelinesLayer.map{[$0]} ?? []) + lineLayers
super.init(
view: view,
innerFrame: innerFrame,
settings: chartConfig.chartSettings,
layers: layers
)
}
public required init(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
| apache-2.0 | 5711ca1c0613c885952e7f82e991239b | 48.526316 | 169 | 0.692171 | 4.875648 | false | true | false | false |
benjaminsnorris/SharedHelpers | Sources/UIColor+Hex.swift | 1 | 1487 | /*
| _ ____ ____ _
| | |‾| ⚈ |-| ⚈ |‾| |
| | | ‾‾‾‾| |‾‾‾‾ | |
| ‾ ‾ ‾
*/
import UIKit
public extension UIColor {
enum InputError: Error {
case unableToScanHexValue
}
convenience init(hex: Int, alpha: CGFloat = 1.0) {
let red = CGFloat((hex >> 16) & 0xFF)/255.0
let green = CGFloat((hex >> 8) & 0xFF)/255.0
let blue = CGFloat((hex) & 0xFF)/255.0
self.init(red: red, green: green, blue: blue, alpha: alpha)
}
convenience init(hexString: String, alpha: CGFloat = 1.0) throws {
var hexValue: UInt32 = 0
guard Scanner(string: hexString).scanHexInt32(&hexValue) else {
self.init() // Must init or we get EXEC_BAD_ACCESS
throw InputError.unableToScanHexValue
}
self.init(hex: Int(hexValue), alpha: alpha)
}
var hexString: String {
var red: CGFloat = 0, green: CGFloat = 0, blue: CGFloat = 0, alpha: CGFloat = 0
getRed(&red, green: &green, blue: &blue, alpha: &alpha)
red = CGFloat(roundf(Float(red) * 255.0))
green = CGFloat(roundf(Float(green) * 255.0))
blue = CGFloat(roundf(Float(blue) * 255.0))
alpha = CGFloat(roundf(Float(alpha) * 255.0))
// Ignore alpha for now
let hex: UInt = (UInt(red) << 16) | (UInt(green) << 8) | (UInt(blue))
return String(format: "%06x", hex)
}
}
| mit | 73ace86ad743d8c649c19086d15c0e96 | 30 | 87 | 0.52162 | 3.545012 | false | false | false | false |
hq7781/MoneyBook | MoneyBook/Model/Event/EventService.swift | 1 | 2861 | //
// EventService.swift
// MoneyBook
//
// Created by HongQuan on 2017/05/03.
// Copyright © 2017年 Roan.Hong. All rights reserved.
//
import Foundation
/// Manage for the events.
class EventService: NSObject {
/// Collection of userNames.
var userList: Array<String> {
get {
return self.eventCache.userList
}
}
/// Dictionary of event collection classified by userName.
var eventsByUser: Dictionary<String, Array<Event>> {
get {
return self.eventCache.eventsByUser
}
}
/// Factory of a data access objects.
private let daoFactory: DAOFactory
/// Manager for the event data.
private var eventCache: EventCache!
/// Initialize the instance.
///
/// - Parameter daoFactory: Factory of a data access objects.
init(daoFactory: DAOFactory) {
self.daoFactory = daoFactory
super.init()
if let dao = self.daoFactory.eventDAO() {
dao.create()
self.eventCache = EventCache(events: dao.read())
}
}
/// Add the new event.
///
/// - Parameter event: event data.
/// - Returns: "true" if successful.
func add(event: Event) -> Bool {
if let dao = self.daoFactory.eventDAO(),
let newEvent = dao.add(eventType: event.eventType,
eventCategory: event.eventCategory,
eventSubCategory: event.eventSubCategory,
eventAccountType: event.eventAccountType,
eventMemo: event.eventMemo,
eventPayment: event.eventPayment,
currencyType: event.currencyType,
userName: event.userName,
recodedDate: event.recodedDate,
modifiedDate: event.modifiedDate) {
return self.eventCache.add(event: newEvent)
}
return false
}
/// Remove the event.
///
/// - Parameter event: Event data.
/// - Returns: "true" if successful.
func remove(event: Event) -> Bool {
if let dao = self.daoFactory.eventDAO(), dao.remove(eventId: event.eventId) {
return self.eventCache.remove(event: event)
}
return false
}
/// Update the event.
///
/// - Parameter oldEvent: New event data.
/// - Parameter newBook: Old book data.
/// - Returns: "true" if successful.
func update(oldEvent: Event, newEvent: Event) -> Bool {
if let dao = self.daoFactory.eventDAO(), dao.update(event: newEvent) {
return self.eventCache.update(oldEvent: oldEvent, newEvent: newEvent)
}
return false
}
}
| mit | eaf21d8f769e6779bf67596bd4217061 | 30.755556 | 85 | 0.546186 | 4.602254 | false | false | false | false |
seei/instapaper-ios-sdk | InstapaperSDK/ISCredentialStore.swift | 1 | 1931 | //
// InstapaperSimpleAPIStore.swift
// InstapaperSDK
//
// Created by Sei Kataoka on 7/2/14.
// Copyright (c) 2014 Sei Kataoka. All rights reserved.
//
import Foundation
class ISCredentialStore {
let serviceName = "InstapaperSDK"
func get() -> (username: String?, password: String?) {
let username: AnyObject? = getValueForKey("username")
let password: AnyObject? = getValueForKey("password")
return (username as? String, password as? String)
}
func save(username: String?, password: String?) {
self.setValue(username, key: "username")
self.setValue(password, key: "password")
}
func clear() {
self.setValue(nil, key: "username")
self.setValue(nil, key: "password")
}
func getValueForKey(key: String) -> (key: AnyObject?) {
// TODO: Use TARGET_IPHONE_SIMULATOR when it become available in Swift.
if IPHONE_SIMULATOR == 1 {
return NSUserDefaults.standardUserDefaults().objectForKey("\(serviceName).\(key)")
} else {
return SSKeychain.passwordForService(serviceName, account: key)
}
}
func setValue(value: String?, key: String) {
if value {
// TODO: Use TARGET_IPHONE_SIMULATOR when it become available in Swift.
if IPHONE_SIMULATOR == 1 {
NSUserDefaults.standardUserDefaults().setObject(value, forKey: "\(serviceName).\(key)")
} else {
SSKeychain.setPassword(value, forService: serviceName, account: key)
}
} else {
// TODO: Use TARGET_IPHONE_SIMULATOR when it become available in Swift.
if IPHONE_SIMULATOR == 1 {
NSUserDefaults.standardUserDefaults().removeObjectForKey("\(serviceName).\(key)")
} else {
SSKeychain.deletePasswordForService(serviceName, account: key)
}
}
}
}
| mit | 04fec8fa4bd64413dd8f2872c93dfa95 | 31.728814 | 103 | 0.606939 | 4.501166 | false | false | false | false |
ndagrawal/MovieFeed | MovieFeed/DVDCollectionViewCell.swift | 1 | 981 |
//
// DVDCollectionViewCell.swift
// MovieFeed
//
// Created by Nilesh Agrawal on 9/17/15.
// Copyright © 2015 Nilesh Agrawal. All rights reserved.
//
import UIKit
class DVDCollectionViewCell: UICollectionViewCell {
@IBOutlet weak var DVDImage:UIImageView!
@IBOutlet weak var DVDName:UILabel!
@IBOutlet weak var DVDTime:UILabel!
@IBOutlet weak var DVDRating:UILabel!
@IBOutlet weak var DVDPercentage:UILabel!
@IBOutlet weak var DVDCasts:UILabel!
func setUpCellUI(){
self.layer.borderColor=UIColor.grayColor().CGColor
self.layer.borderWidth=1.0
}
func setDVDCell(dvd:DVD){
setUpCellUI()
let url:NSURL = NSURL(string:dvd.DVDImageURL)!
DVDImage.setImageWithURL(url)
DVDName.text = dvd.DVDName
DVDTime.text = dvd.DVDMinute
DVDRating.text = dvd.DVDRating
DVDPercentage.text = dvd.DVDPercentage
DVDCasts.text = dvd.DVDcasts
}
}
| mit | ca171538562535263872237fc3030db2 | 23.5 | 58 | 0.663265 | 3.935743 | false | false | false | false |
Molbie/Outlaw | Tests/OutlawTests/TypesForTests.swift | 1 | 2002 | //
// Types.swift
// Outlaw
//
// Created by Brian Mullen on 11/15/16.
// Copyright © 2016 Molbie LLC. All rights reserved.
//
import Foundation
import Outlaw
enum DayOfWeek: Int {
case sunday
case monday
case tuesday
case wednesday
case thursday
case friday
case saturday
}
struct Address: Hashable {
var street: String
var city: String
func hash(into hasher: inout Hasher) {
hasher.combine(street)
hasher.combine(city)
}
}
struct Address2 {
var street: String
var city: String
}
func ==(lhs: Address, rhs: Address) -> Bool {
return lhs.street == rhs.street && lhs.city == rhs.city
}
struct Person: Hashable {
var firstName: String
var lastName: String
var address: Address?
func hash(into hasher: inout Hasher) {
hasher.combine(firstName)
hasher.combine(lastName)
if let address = address {
hasher.combine(address)
}
}
}
struct Person2 {
var firstName: String
var lastName: String
var address: Address2?
}
func ==(lhs: Person, rhs: Person) -> Bool {
return lhs.firstName == rhs.firstName && lhs.lastName == rhs.lastName && lhs.address == rhs.address
}
protocol AddressContext {}
protocol PersonContext {}
protocol PersonContextIncludeAddress: PersonContext, AddressContext {}
class SomeAddressContext: AddressContext {}
class SomePersonContextExcludeAddress: PersonContext {}
class SomePersonContextIncludeAddress: PersonContextIncludeAddress {}
class NewObjectContext: PersonContextIncludeAddress {
func newPerson() -> Person {
return Person(firstName: "First", lastName: "Last", address: nil)
}
func newPerson2() -> Person2 {
return Person2(firstName: "First", lastName: "Last", address: nil)
}
func newAddress() -> Address {
return Address(street: "Street", city: "City")
}
func newAddress2() -> Address2 {
return Address2(street: "Street", city: "City")
}
}
| mit | bb57123034ad219440d3e07e220a2b45 | 23.402439 | 103 | 0.661669 | 4.092025 | false | false | false | false |
superlopuh/SuperGraph | SuperGraph/SGDOTWriter.swift | 1 | 1702 | //
// SGDOTWriter.swift
// SuperGraph
//
// Created by Alexandre Lopoukhine on 17/04/2015.
// Copyright (c) 2015 bluetatami. All rights reserved.
//
import Foundation
public class SGDOTWriter {
public static func writeGraph<N, E: Hashable>(graph: SGGraph<N,E>, withName name: String, toFile outputFileAddress: NSURL) {
// If folder doesn't exist, create one
if let folderPath = outputFileAddress.URLByDeletingLastPathComponent?.path {
// check if element is a directory
var isDirectory: ObjCBool = ObjCBool(false)
// Mutates isDirectory pointer
NSFileManager.defaultManager().fileExistsAtPath(folderPath, isDirectory: &isDirectory)
if !isDirectory {
var error: NSError?
NSFileManager.defaultManager().createDirectoryAtPath(folderPath, withIntermediateDirectories: false, attributes: nil, error: &error)
}
}
// Create new .dot file
if let dotFilePath = outputFileAddress.path {
let dotData = dotDescriptionOfGraph(graph, withName: name).dataUsingEncoding(NSUTF8StringEncoding, allowLossyConversion: false)
NSFileManager.defaultManager().createFileAtPath(dotFilePath, contents: dotData, attributes: nil)
}
}
public static func dotDescriptionOfGraph<N, E: Hashable>(graph: SGGraph<N,E>, withName name: String) -> String {
var dotDescription = "digraph \(name) {"
for edge in graph.edges {
dotDescription += "\n\t\(edge.nodeStart.label) -> \(edge.nodeEnd.label);"
}
return dotDescription + "\n}\n"
}
}
| mit | 68c52d205142e6cf64a9840ccd28654b | 38.581395 | 148 | 0.636898 | 4.780899 | false | false | false | false |
EagleKing/SwiftStudy | 枚举.playground/Contents.swift | 1 | 3878 | //: Playground - noun: a place where people can play
import UIKit
enum CompassPoint
{
case North
case South
case East
case West
}
enum Planet
{
case Mercury,Venus,Earth,Mars,Jupiter,Saturn,Uranus,Neptune
}
//每一个枚举定义了一个全新的类型**************************
var directionToHead = CompassPoint.West//接下来赋值的时候可以被推断类型
//简短赋值
directionToHead = .East
//使用Switch语句匹配单个枚举值***************************
directionToHead = .South
switch directionToHead
{
case .North:
print("north")
case .South:
print("south")
case .West:
print("west")
//case .East:
// print("east")
default://不需要匹配每个枚举成员时,使用default覆盖未明确处理的分支
print("no safe place for human!")
}
//关联值***************************************
enum Barcode
{
case UPCA(Int,Int,Int,Int)//定义了可以存储的关联值的类型
case QRCode(String)
}
var productBarcode = Barcode.UPCA(8, 85909, 51226, 3)
productBarcode = .QRCode("ABCDREFMKJDKDKDKD")//赋值过一次之后自动判断类型
switch productBarcode
{
//case .UPCA(let numberSystem,let manufacturer,let product,let check):
//所有关联值被提起为常量或者变量,即可简写
case let .UPCA(numberSystem,manufacturer,product,check):
print("UPCA")
case .QRCode(let productCode)://提取为常量
print("QR code\(productCode)")
}
//原始值****************************************************************
enum ASCIIControlCharacter:Character
{//枚举成员可以被默认值(原始值)预填充,这些默认值类型必须相同
//默认值(原始值)始终不变
case Tab = "\t"
case LineFeed = "\n"
case CarriageReturn = "\r"
}
//原始值的隐式赋值***************************
//不需要显示地为每一个枚举成员设置原始值,swift将会自动为你赋值
enum name:Int
{
case mercy = 1,mike,eagle,bingo
}
//当使用字符串作为枚举类型的原始值时,每个枚举成员的隐式原始值为该枚举成员的名称。
enum cityName:String
{
case SuZhou,GuangZhou,Beijing,Nanjing
}
let jiangsuCity = cityName.SuZhou.rawValue;
let erathsOrder = name.mercy.rawValue
//使用原始值初始化枚举实例(枚举使用了原始值,才会有一个初始化方法)
let possibleName = name(rawValue: 2)//创建一个枚举实例子,返回可选值
let positionToFind = 3
if let someName = name(rawValue: positionToFind)
{
switch someName
{
case .mike:
print("yes he is!")
default:
print("Not a safe place for humans")
}
}else
{
print("no this name!")
}
//递归枚举************************************************************
//indirect 间接
//indirect关键字加在枚举类型开头表示枚举成员都是可以递归的
enum ArithmeticExpression
{
case Number(Int)
indirect case Addition(ArithmeticExpression,ArithmeticExpression)
indirect case Multiplication(ArithmeticExpression,ArithmeticExpression)
}
func evaluate(expression:ArithmeticExpression) -> Int
{
switch expression
{
case .Number(let value):
return value
case .Addition(let left, let right)://加法
return evaluate(left) + evaluate(right)
case .Multiplication(let left, let right)://乘法
return evaluate(left) * evaluate(right)
}
}
let five = ArithmeticExpression.Number(5)
let four = ArithmeticExpression.Number(4)
let sum = ArithmeticExpression.Addition(five, four)
let product = ArithmeticExpression.Multiplication(sum, ArithmeticExpression.Number(2))
print(evaluate(product))
| mit | dd00d1a9c70cb0b2c45e4c4267781eb1 | 21.466165 | 86 | 0.664324 | 2.776952 | false | false | false | false |
aatalyk/swift-algorithm-club | AVL Tree/AVLTree.swift | 2 | 10989 | // The MIT License (MIT)
// Copyright (c) 2016 Mike Taghavi (mitghi[at]me.com)
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
public class TreeNode<Key: Comparable, Payload> {
public typealias Node = TreeNode<Key, Payload>
var payload: Payload?
fileprivate var key: Key
internal var leftChild: Node?
internal var rightChild: Node?
fileprivate var height: Int
fileprivate weak var parent: Node?
public init(key: Key, payload: Payload?, leftChild: Node?, rightChild: Node?, parent: Node?, height: Int) {
self.key = key
self.payload = payload
self.leftChild = leftChild
self.rightChild = rightChild
self.parent = parent
self.height = height
self.leftChild?.parent = self
self.rightChild?.parent = self
}
public convenience init(key: Key, payload: Payload?) {
self.init(key: key, payload: payload, leftChild: nil, rightChild: nil, parent: nil, height: 1)
}
public convenience init(key: Key) {
self.init(key: key, payload: nil)
}
var isRoot: Bool {
return parent == nil
}
var isLeaf: Bool {
return rightChild == nil && leftChild == nil
}
var isLeftChild: Bool {
return parent?.leftChild === self
}
var isRightChild: Bool {
return parent?.rightChild === self
}
var hasLeftChild: Bool {
return leftChild != nil
}
var hasRightChild: Bool {
return rightChild != nil
}
var hasAnyChild: Bool {
return leftChild != nil || rightChild != nil
}
var hasBothChildren: Bool {
return leftChild != nil && rightChild != nil
}
}
// MARK: - The AVL tree
open class AVLTree<Key: Comparable, Payload> {
public typealias Node = TreeNode<Key, Payload>
fileprivate(set) var root: Node?
fileprivate(set) var size = 0
public init() { }
}
// MARK: - Searching
extension TreeNode {
public func minimum() -> TreeNode? {
if let leftChild = self.leftChild {
return leftChild.minimum()
}
return self
}
public func maximum() -> TreeNode? {
if let rightChild = self.rightChild {
return rightChild.maximum()
}
return self
}
}
extension AVLTree {
subscript(key: Key) -> Payload? {
get { return search(input: key) }
set { insert(key: key, payload: newValue) }
}
public func search(input: Key) -> Payload? {
if let result = search(key: input, node: root) {
return result.payload
} else {
return nil
}
}
fileprivate func search(key: Key, node: Node?) -> Node? {
if let node = node {
if key == node.key {
return node
} else if key < node.key {
return search(key: key, node: node.leftChild)
} else {
return search(key: key, node: node.rightChild)
}
}
return nil
}
}
// MARK: - Inserting new items
extension AVLTree {
public func insert(key: Key, payload: Payload? = nil) {
if let root = root {
insert(input: key, payload: payload, node: root)
} else {
root = Node(key: key, payload: payload)
}
size += 1
}
private func insert(input: Key, payload: Payload?, node: Node) {
if input < node.key {
if let child = node.leftChild {
insert(input: input, payload: payload, node: child)
} else {
let child = Node(key: input, payload: payload, leftChild: nil, rightChild: nil, parent: node, height: 1)
node.leftChild = child
balance(node: child)
}
} else {
if let child = node.rightChild {
insert(input: input, payload: payload, node: child)
} else {
let child = Node(key: input, payload: payload, leftChild: nil, rightChild: nil, parent: node, height: 1)
node.rightChild = child
balance(node: child)
}
}
}
}
// MARK: - Balancing tree
extension AVLTree {
fileprivate func updateHeightUpwards(node: Node?) {
if let node = node {
let lHeight = node.leftChild?.height ?? 0
let rHeight = node.rightChild?.height ?? 0
node.height = max(lHeight, rHeight) + 1
updateHeightUpwards(node: node.parent)
}
}
fileprivate func lrDifference(node: Node?) -> Int {
let lHeight = node?.leftChild?.height ?? 0
let rHeight = node?.rightChild?.height ?? 0
return lHeight - rHeight
}
fileprivate func balance(node: Node?) {
guard let node = node else {
return
}
updateHeightUpwards(node: node.leftChild)
updateHeightUpwards(node: node.rightChild)
var nodes = [Node?](repeating: nil, count: 3)
var subtrees = [Node?](repeating: nil, count: 4)
let nodeParent = node.parent
let lrFactor = lrDifference(node: node)
if lrFactor > 1 {
// left-left or left-right
if lrDifference(node: node.leftChild) > 0 {
// left-left
nodes[0] = node
nodes[2] = node.leftChild
nodes[1] = nodes[2]?.leftChild
subtrees[0] = nodes[1]?.leftChild
subtrees[1] = nodes[1]?.rightChild
subtrees[2] = nodes[2]?.rightChild
subtrees[3] = nodes[0]?.rightChild
} else {
// left-right
nodes[0] = node
nodes[1] = node.leftChild
nodes[2] = nodes[1]?.rightChild
subtrees[0] = nodes[1]?.leftChild
subtrees[1] = nodes[2]?.leftChild
subtrees[2] = nodes[2]?.rightChild
subtrees[3] = nodes[0]?.rightChild
}
} else if lrFactor < -1 {
// right-left or right-right
if lrDifference(node: node.rightChild) < 0 {
// right-right
nodes[1] = node
nodes[2] = node.rightChild
nodes[0] = nodes[2]?.rightChild
subtrees[0] = nodes[1]?.leftChild
subtrees[1] = nodes[2]?.leftChild
subtrees[2] = nodes[0]?.leftChild
subtrees[3] = nodes[0]?.rightChild
} else {
// right-left
nodes[1] = node
nodes[0] = node.rightChild
nodes[2] = nodes[0]?.leftChild
subtrees[0] = nodes[1]?.leftChild
subtrees[1] = nodes[2]?.leftChild
subtrees[2] = nodes[2]?.rightChild
subtrees[3] = nodes[0]?.rightChild
}
} else {
// Don't need to balance 'node', go for parent
balance(node: node.parent)
return
}
// nodes[2] is always the head
if node.isRoot {
root = nodes[2]
root?.parent = nil
} else if node.isLeftChild {
nodeParent?.leftChild = nodes[2]
nodes[2]?.parent = nodeParent
} else if node.isRightChild {
nodeParent?.rightChild = nodes[2]
nodes[2]?.parent = nodeParent
}
nodes[2]?.leftChild = nodes[1]
nodes[1]?.parent = nodes[2]
nodes[2]?.rightChild = nodes[0]
nodes[0]?.parent = nodes[2]
nodes[1]?.leftChild = subtrees[0]
subtrees[0]?.parent = nodes[1]
nodes[1]?.rightChild = subtrees[1]
subtrees[1]?.parent = nodes[1]
nodes[0]?.leftChild = subtrees[2]
subtrees[2]?.parent = nodes[0]
nodes[0]?.rightChild = subtrees[3]
subtrees[3]?.parent = nodes[0]
updateHeightUpwards(node: nodes[1]) // Update height from left
updateHeightUpwards(node: nodes[0]) // Update height from right
balance(node: nodes[2]?.parent)
}
}
// MARK: - Displaying tree
extension AVLTree {
fileprivate func display(node: Node?, level: Int) {
if let node = node {
display(node: node.rightChild, level: level + 1)
print("")
if node.isRoot {
print("Root -> ", terminator: "")
}
for _ in 0..<level {
print(" ", terminator: "")
}
print("(\(node.key):\(node.height))", terminator: "")
display(node: node.leftChild, level: level + 1)
}
}
public func display(node: Node) {
display(node: node, level: 0)
print("")
}
}
// MARK: - Delete node
extension AVLTree {
public func delete(key: Key) {
if size == 1 {
root = nil
size -= 1
} else if let node = search(key: key, node: root) {
delete(node: node)
size -= 1
}
}
private func delete(node: Node) {
if node.isLeaf {
// Just remove and balance up
if let parent = node.parent {
guard node.isLeftChild || node.isRightChild else {
// just in case
fatalError("Error: tree is invalid.")
}
if node.isLeftChild {
parent.leftChild = nil
} else if node.isRightChild {
parent.rightChild = nil
}
balance(node: parent)
} else {
// at root
root = nil
}
} else {
// Handle stem cases
if let replacement = node.leftChild?.maximum() , replacement !== node {
node.key = replacement.key
node.payload = replacement.payload
delete(node: replacement)
} else if let replacement = node.rightChild?.minimum() , replacement !== node {
node.key = replacement.key
node.payload = replacement.payload
delete(node: replacement)
}
}
}
}
// MARK: - Debugging
extension TreeNode: CustomDebugStringConvertible {
public var debugDescription: String {
var s = "key: \(key), payload: \(payload), height: \(height)"
if let parent = parent {
s += ", parent: \(parent.key)"
}
if let left = leftChild {
s += ", left = [" + left.debugDescription + "]"
}
if let right = rightChild {
s += ", right = [" + right.debugDescription + "]"
}
return s
}
}
extension AVLTree: CustomDebugStringConvertible {
public var debugDescription: String {
if let root = root {
return root.debugDescription
} else {
return "[]"
}
}
}
extension TreeNode: CustomStringConvertible {
public var description: String {
var s = ""
if let left = leftChild {
s += "(\(left.description)) <- "
}
s += "\(key)"
if let right = rightChild {
s += " -> (\(right.description))"
}
return s
}
}
extension AVLTree: CustomStringConvertible {
public var description: String {
if let root = root {
return root.description
} else {
return "[]"
}
}
}
| mit | 59f7f3953c9df22be7a0e08beabfbedf | 25.289474 | 112 | 0.609155 | 3.967148 | false | false | false | false |
mercadopago/px-ios | MercadoPagoSDK/MercadoPagoSDKTests/PXDeviceSizeTests.swift | 1 | 2046 |
import XCTest
@testable import MercadoPagoSDKV4
class PXDeviceSizeTests: XCTestCase {
let iPhone12ProMax: CGFloat = 926
let iPhone12Pro: CGFloat = 844
let iPhone12: CGFloat = 844
let iPhone12mini: CGFloat = 812
let iPhone11ProMax: CGFloat = 896
let iPhone11Pro: CGFloat = 812
let iPhone11: CGFloat = 896
let iPhoneXSMax: CGFloat = 896
let iPhoneXS: CGFloat = 812
let iPhoneXR: CGFloat = 896
let iPhoneX: CGFloat = 812
let iPhone8Plus: CGFloat = 736
let iPhone8: CGFloat = 667
let iPhone7Plus: CGFloat = 736
let iPhone7: CGFloat = 667
let iPhone6sPlus: CGFloat = 736
let iPhone6s: CGFloat = 667
let iPhone6Plus: CGFloat = 736
let iPhone6: CGFloat = 667
let iPhoneSE2: CGFloat = 667
let iPhoneSE1: CGFloat = 568
func testiPhoneSE1stGen () {
let deviceSize = PXDeviceSize.getDeviceSize(deviceHeight: iPhoneSE1)
XCTAssertEqual(deviceSize, PXDeviceSize.Sizes.small)
}
func testiPhoneSE2stGen () {
let deviceSize = PXDeviceSize.getDeviceSize(deviceHeight: iPhoneSE2)
XCTAssertEqual(deviceSize, PXDeviceSize.Sizes.regular)
}
func testiPhone7 () {
let deviceSize = PXDeviceSize.getDeviceSize(deviceHeight: iPhone7)
XCTAssertEqual(deviceSize, PXDeviceSize.Sizes.regular)
}
func testiPhone8Plus () {
let deviceSize = PXDeviceSize.getDeviceSize(deviceHeight: iPhone8Plus)
XCTAssertEqual(deviceSize, PXDeviceSize.Sizes.regular)
}
func testiPhoneX () {
let deviceSize = PXDeviceSize.getDeviceSize(deviceHeight: iPhoneX)
XCTAssertEqual(deviceSize, PXDeviceSize.Sizes.large)
}
func testiPhoneXR () {
let deviceSize = PXDeviceSize.getDeviceSize(deviceHeight: iPhoneXR)
XCTAssertEqual(deviceSize, PXDeviceSize.Sizes.extraLarge)
}
func testiPhone12ProMax () {
let deviceSize = PXDeviceSize.getDeviceSize(deviceHeight: iPhone12ProMax)
XCTAssertEqual(deviceSize, PXDeviceSize.Sizes.extraLarge)
}
}
| mit | 7c032afd6f4a5e5f9c74eaf32043f6e2 | 28.652174 | 81 | 0.701369 | 4.043478 | false | true | false | false |
webelectric/AspirinKit | AspirinKit/Sources/Math/Angle.swift | 1 | 4912 | //
// Angle.swift
// AspirinKit
//
// Copyright © 2015-2017 The Web Electric Corp. All rights reserved.
//
//
import Foundation
public let π = Float(Double.pi)
public func==(lhs:Angle, rhs:Angle) -> Bool {
return lhs.sign == rhs.sign && lhs.deg == rhs.deg && lhs.min == rhs.min && lhs.sec == rhs.sec
}
public func+(lhs:Angle, rhs:Angle) -> Angle {
return lhs.add(rhs)
}
public func-(lhs:Angle, rhs:Angle) -> Angle {
return lhs.subtract(rhs)
}
public class Angle : CustomStringConvertible {
public var sign:Int = 1 {
didSet {
assert(sign == -1 || sign == 1, "Sign must be either 1 or -1")
}
}
public var deg:UInt = 0
public var min:UInt = 0 {
didSet {
assert(min < 60, "Minutes must be less than 60 (value passed=\(min), to add beyond that, check the add(seconds, minutes, degrees) function")
}
}
public var sec:UInt = 0 {
didSet {
assert(sec < 60, "Seconds must be less than 60 (value passed=\(sec), to add beyond that, check the function self.add(seconds, minutes, degrees)")
}
}
//this constructor supports overflows, so for example a call like
//add(seconds:61, minutes:1) will turn into adding 1 second and 2 minutes
//it's also possible to specify a sign to the values, which will result in the
//"correct" assignment
public init(degrees d:Int = 0, minutes m:Int = 0, seconds s:Int = 0) {
var degValue = d
var minValue = m
var secValue = s
if secValue < 0 || secValue > 59 {
//with this calculation, for example 0' -59'' would turn into -1' 1''
//the next if() statement would carry the negative over to the degrees
let sign = secValue < 0 ? -1 : 1
secValue = abs(secValue)
minValue += (secValue / 60) * sign //minutes 'overflow' from seconds
secValue = (secValue % 60)
}
if minValue < 0 || minValue > 59 {
let sign = minValue < 0 ? -1 : 1
minValue = abs(minValue)
degValue += (minValue / 60) * sign //degrees 'overflow' from minutes
minValue = (minValue % 60)
}
self.sign = degValue >= 0 ? 1 : -1
degValue = abs(degValue)
self.deg = UInt(degValue)
self.min = UInt(minValue)
self.sec = UInt(secValue)
}
public init(degrees d:Float) {
self.degrees = d
}
public init(radians r:Float) {
self.radians = r
}
public convenience init(degrees d:Double) {
self.init(degrees:Float(d))
}
public convenience init(radians r:Double) {
self.init(radians:Float(r))
}
public var degrees:Float {
get {
let val:Float = Float(deg) + (Float(min)/60) + (Float(sec)/3600)
return val * Float(sign)
}
set {
self.sign = newValue < 0 ? -1 : 1
let absNewValue = abs(newValue)
let degVal:Float = floor(absNewValue)
self.deg = UInt(degVal)
let decimals:Float = (absNewValue - degVal)
if decimals > 0 {
let m:Float = decimals*60
self.min = UInt(floor(m))
let s:Float = abs((decimals - (m/60)) * 3600) // it's possible to get results like -0.001etc due to Float calculation innacuracies
self.sec = UInt(floor(s))
}
else {
self.min = 0
self.sec = 0
}
}
}
public let degreesPerRadian:Float = (Float(Double.pi * 2)/Float(360))
public var radians:Float {
get {
return self.degrees * self.degreesPerRadian
}
set {
self.degrees = newValue/self.degreesPerRadian
}
}
public var description: String {
return "Angle: \(deg)°\(min)'\(sec)'' = \(degrees)° = \(radians) radians"
}
public func subtract(_ angle:Angle) -> Angle {
return Angle(degrees: self.degrees-angle.degrees)
}
public func subtract(degrees d:Int = 0, minutes m:Int = 0, seconds s:Int = 0) -> Angle {
return self.subtract(Angle(degrees: d, minutes: m, seconds: s))
}
//this function supports overflows, so for example a call like
//add(seconds:61, minutes:1) will turn into adding 1 second and 2 minutes
public func add(_ angle:Angle) -> Angle {
return Angle(degrees: self.degrees+angle.degrees)
}
//this function supports overflows, so for example a call like
//add(seconds:61, minutes:1) will turn into adding 1 second and 2 minutes
public func add(degrees d:Int = 0, minutes m:Int = 0, seconds s:Int = 0) -> Angle {
return self.add(Angle(degrees: d, minutes: m, seconds: s))
}
}
| mit | f90dbb982cd6ee459e00c3dd890624ce | 31.078431 | 157 | 0.555623 | 3.929544 | false | false | false | false |
taniadsouza/SlideMenuControllerSwift | Source/SlideMenuController.swift | 5 | 35192 | //
// SlideMenuController.swift
//
// Created by Yuji Hato on 12/3/14.
//
import Foundation
import UIKit
public struct SlideMenuOptions {
public static var leftViewWidth: CGFloat = 270.0
public static var leftBezelWidth: CGFloat = 16.0
public static var contentViewScale: CGFloat = 0.96
public static var contentViewOpacity: CGFloat = 0.5
public static var shadowOpacity: CGFloat = 0.0
public static var shadowRadius: CGFloat = 0.0
public static var shadowOffset: CGSize = CGSizeMake(0,0)
public static var panFromBezel: Bool = true
public static var animationDuration: CGFloat = 0.4
public static var rightViewWidth: CGFloat = 270.0
public static var rightBezelWidth: CGFloat = 16.0
public static var rightPanFromBezel: Bool = true
public static var hideStatusBar: Bool = true
public static var pointOfNoReturnWidth: CGFloat = 44.0
public static var opacityViewBackgroundColor: UIColor = UIColor.blackColor()
}
public class SlideMenuController: UIViewController, UIGestureRecognizerDelegate {
public enum SlideAction {
case Open
case Close
}
public enum TrackAction {
case TapOpen
case TapClose
case FlickOpen
case FlickClose
}
struct PanInfo {
var action: SlideAction
var shouldBounce: Bool
var velocity: CGFloat
}
public var opacityView = UIView()
public var mainContainerView = UIView()
public var leftContainerView = UIView()
public var rightContainerView = UIView()
public var mainViewController: UIViewController?
public var leftViewController: UIViewController?
public var leftPanGesture: UIPanGestureRecognizer?
public var leftTapGetsture: UITapGestureRecognizer?
public var rightViewController: UIViewController?
public var rightPanGesture: UIPanGestureRecognizer?
public var rightTapGesture: UITapGestureRecognizer?
public required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
public override init(nibName nibNameOrNil: String?, bundle nibBundleOrNil: NSBundle?) {
super.init(nibName: nibNameOrNil, bundle: nibBundleOrNil)
}
public convenience init(mainViewController: UIViewController, leftMenuViewController: UIViewController) {
self.init()
self.mainViewController = mainViewController
leftViewController = leftMenuViewController
initView()
}
public convenience init(mainViewController: UIViewController, rightMenuViewController: UIViewController) {
self.init()
self.mainViewController = mainViewController
rightViewController = rightMenuViewController
initView()
}
public convenience init(mainViewController: UIViewController, leftMenuViewController: UIViewController, rightMenuViewController: UIViewController) {
self.init()
self.mainViewController = mainViewController
leftViewController = leftMenuViewController
rightViewController = rightMenuViewController
initView()
}
deinit { }
func initView() {
mainContainerView = UIView(frame: view.bounds)
mainContainerView.backgroundColor = UIColor.clearColor()
mainContainerView.autoresizingMask = [.FlexibleHeight, .FlexibleWidth]
view.insertSubview(mainContainerView, atIndex: 0)
var opacityframe: CGRect = view.bounds
let opacityOffset: CGFloat = 0
opacityframe.origin.y = opacityframe.origin.y + opacityOffset
opacityframe.size.height = opacityframe.size.height - opacityOffset
opacityView = UIView(frame: opacityframe)
opacityView.backgroundColor = SlideMenuOptions.opacityViewBackgroundColor
opacityView.autoresizingMask = [UIViewAutoresizing.FlexibleHeight, UIViewAutoresizing.FlexibleWidth]
opacityView.layer.opacity = 0.0
view.insertSubview(opacityView, atIndex: 1)
var leftFrame: CGRect = view.bounds
leftFrame.size.width = SlideMenuOptions.leftViewWidth
leftFrame.origin.x = leftMinOrigin();
let leftOffset: CGFloat = 0
leftFrame.origin.y = leftFrame.origin.y + leftOffset
leftFrame.size.height = leftFrame.size.height - leftOffset
leftContainerView = UIView(frame: leftFrame)
leftContainerView.backgroundColor = UIColor.clearColor()
leftContainerView.autoresizingMask = UIViewAutoresizing.FlexibleHeight
view.insertSubview(leftContainerView, atIndex: 2)
var rightFrame: CGRect = view.bounds
rightFrame.size.width = SlideMenuOptions.rightViewWidth
rightFrame.origin.x = rightMinOrigin()
let rightOffset: CGFloat = 0
rightFrame.origin.y = rightFrame.origin.y + rightOffset;
rightFrame.size.height = rightFrame.size.height - rightOffset
rightContainerView = UIView(frame: rightFrame)
rightContainerView.backgroundColor = UIColor.clearColor()
rightContainerView.autoresizingMask = UIViewAutoresizing.FlexibleHeight
view.insertSubview(rightContainerView, atIndex: 3)
addLeftGestures()
addRightGestures()
}
public override func viewWillTransitionToSize(size: CGSize, withTransitionCoordinator coordinator: UIViewControllerTransitionCoordinator) {
super.viewWillTransitionToSize(size, withTransitionCoordinator: coordinator)
mainContainerView.transform = CGAffineTransformMakeScale(1.0, 1.0)
leftContainerView.hidden = true
rightContainerView.hidden = true
coordinator.animateAlongsideTransition(nil, completion: { (context: UIViewControllerTransitionCoordinatorContext!) -> Void in
self.closeLeftNonAnimation()
self.closeRightNonAnimation()
self.leftContainerView.hidden = false
self.rightContainerView.hidden = false
if self.leftPanGesture != nil && self.leftPanGesture != nil {
self.removeLeftGestures()
self.addLeftGestures()
}
if self.rightPanGesture != nil && self.rightPanGesture != nil {
self.removeRightGestures()
self.addRightGestures()
}
})
}
public override func viewDidLoad() {
super.viewDidLoad()
edgesForExtendedLayout = UIRectEdge.None
}
public override func viewWillLayoutSubviews() {
// topLayoutGuideの値が確定するこのタイミングで各種ViewControllerをセットする
setUpViewController(mainContainerView, targetViewController: mainViewController)
setUpViewController(leftContainerView, targetViewController: leftViewController)
setUpViewController(rightContainerView, targetViewController: rightViewController)
}
public override func openLeft() {
setOpenWindowLevel()
//leftViewControllerのviewWillAppearを呼ぶため
leftViewController?.beginAppearanceTransition(isLeftHidden(), animated: true)
openLeftWithVelocity(0.0)
track(.TapOpen)
}
public override func openRight() {
setOpenWindowLevel()
//menuViewControllerのviewWillAppearを呼ぶため
rightViewController?.beginAppearanceTransition(isRightHidden(), animated: true)
openRightWithVelocity(0.0)
}
public override func closeLeft() {
leftViewController?.beginAppearanceTransition(isLeftHidden(), animated: true)
closeLeftWithVelocity(0.0)
setCloseWindowLebel()
}
public override func closeRight() {
rightViewController?.beginAppearanceTransition(isRightHidden(), animated: true)
closeRightWithVelocity(0.0)
setCloseWindowLebel()
}
public func addLeftGestures() {
if (leftViewController != nil) {
if leftPanGesture == nil {
leftPanGesture = UIPanGestureRecognizer(target: self, action: "handleLeftPanGesture:")
leftPanGesture!.delegate = self
view.addGestureRecognizer(leftPanGesture!)
}
if leftTapGetsture == nil {
leftTapGetsture = UITapGestureRecognizer(target: self, action: "toggleLeft")
leftTapGetsture!.delegate = self
view.addGestureRecognizer(leftTapGetsture!)
}
}
}
public func addRightGestures() {
if (rightViewController != nil) {
if rightPanGesture == nil {
rightPanGesture = UIPanGestureRecognizer(target: self, action: "handleRightPanGesture:")
rightPanGesture!.delegate = self
view.addGestureRecognizer(rightPanGesture!)
}
if rightTapGesture == nil {
rightTapGesture = UITapGestureRecognizer(target: self, action: "toggleRight")
rightTapGesture!.delegate = self
view.addGestureRecognizer(rightTapGesture!)
}
}
}
public func removeLeftGestures() {
if leftPanGesture != nil {
view.removeGestureRecognizer(leftPanGesture!)
leftPanGesture = nil
}
if leftTapGetsture != nil {
view.removeGestureRecognizer(leftTapGetsture!)
leftTapGetsture = nil
}
}
public func removeRightGestures() {
if rightPanGesture != nil {
view.removeGestureRecognizer(rightPanGesture!)
rightPanGesture = nil
}
if rightTapGesture != nil {
view.removeGestureRecognizer(rightTapGesture!)
rightTapGesture = nil
}
}
public func isTagetViewController() -> Bool {
// Function to determine the target ViewController
// Please to override it if necessary
return true
}
public func track(trackAction: TrackAction) {
// function is for tracking
// Please to override it if necessary
}
struct LeftPanState {
static var frameAtStartOfPan: CGRect = CGRectZero
static var startPointOfPan: CGPoint = CGPointZero
static var wasOpenAtStartOfPan: Bool = false
static var wasHiddenAtStartOfPan: Bool = false
}
func handleLeftPanGesture(panGesture: UIPanGestureRecognizer) {
if !isTagetViewController() {
return
}
if isRightOpen() {
return
}
switch panGesture.state {
case UIGestureRecognizerState.Began:
LeftPanState.frameAtStartOfPan = leftContainerView.frame
LeftPanState.startPointOfPan = panGesture.locationInView(view)
LeftPanState.wasOpenAtStartOfPan = isLeftOpen()
LeftPanState.wasHiddenAtStartOfPan = isLeftHidden()
leftViewController?.beginAppearanceTransition(LeftPanState.wasHiddenAtStartOfPan, animated: true)
addShadowToView(leftContainerView)
setOpenWindowLevel()
case UIGestureRecognizerState.Changed:
let translation: CGPoint = panGesture.translationInView(panGesture.view!)
leftContainerView.frame = applyLeftTranslation(translation, toFrame: LeftPanState.frameAtStartOfPan)
applyLeftOpacity()
applyLeftContentViewScale()
case UIGestureRecognizerState.Ended:
let velocity:CGPoint = panGesture.velocityInView(panGesture.view)
let panInfo: PanInfo = panLeftResultInfoForVelocity(velocity)
if panInfo.action == .Open {
if !LeftPanState.wasHiddenAtStartOfPan {
leftViewController?.beginAppearanceTransition(true, animated: true)
}
openLeftWithVelocity(panInfo.velocity)
track(.FlickOpen)
} else {
if LeftPanState.wasHiddenAtStartOfPan {
leftViewController?.beginAppearanceTransition(false, animated: true)
}
closeLeftWithVelocity(panInfo.velocity)
setCloseWindowLebel()
track(.FlickClose)
}
default:
break
}
}
struct RightPanState {
static var frameAtStartOfPan: CGRect = CGRectZero
static var startPointOfPan: CGPoint = CGPointZero
static var wasOpenAtStartOfPan: Bool = false
static var wasHiddenAtStartOfPan: Bool = false
}
func handleRightPanGesture(panGesture: UIPanGestureRecognizer) {
if !isTagetViewController() {
return
}
if isLeftOpen() {
return
}
switch panGesture.state {
case UIGestureRecognizerState.Began:
RightPanState.frameAtStartOfPan = rightContainerView.frame
RightPanState.startPointOfPan = panGesture.locationInView(view)
RightPanState.wasOpenAtStartOfPan = isRightOpen()
RightPanState.wasHiddenAtStartOfPan = isRightHidden()
rightViewController?.beginAppearanceTransition(RightPanState.wasHiddenAtStartOfPan, animated: true)
addShadowToView(rightContainerView)
setOpenWindowLevel()
case UIGestureRecognizerState.Changed:
let translation: CGPoint = panGesture.translationInView(panGesture.view!)
rightContainerView.frame = applyRightTranslation(translation, toFrame: RightPanState.frameAtStartOfPan)
applyRightOpacity()
applyRightContentViewScale()
case UIGestureRecognizerState.Ended:
let velocity: CGPoint = panGesture.velocityInView(panGesture.view)
let panInfo: PanInfo = panRightResultInfoForVelocity(velocity)
if panInfo.action == .Open {
if !RightPanState.wasHiddenAtStartOfPan {
rightViewController?.beginAppearanceTransition(true, animated: true)
}
openRightWithVelocity(panInfo.velocity)
} else {
if RightPanState.wasHiddenAtStartOfPan {
rightViewController?.beginAppearanceTransition(false, animated: true)
}
closeRightWithVelocity(panInfo.velocity)
setCloseWindowLebel()
}
default:
break
}
}
public func openLeftWithVelocity(velocity: CGFloat) {
let xOrigin: CGFloat = leftContainerView.frame.origin.x
let finalXOrigin: CGFloat = 0.0
var frame = leftContainerView.frame;
frame.origin.x = finalXOrigin;
var duration: NSTimeInterval = Double(SlideMenuOptions.animationDuration)
if velocity != 0.0 {
duration = Double(fabs(xOrigin - finalXOrigin) / velocity)
duration = Double(fmax(0.1, fmin(1.0, duration)))
}
addShadowToView(leftContainerView)
UIView.animateWithDuration(duration, delay: 0.0, options: UIViewAnimationOptions.CurveEaseInOut, animations: { [weak self]() -> Void in
if let strongSelf = self {
strongSelf.leftContainerView.frame = frame
strongSelf.opacityView.layer.opacity = Float(SlideMenuOptions.contentViewOpacity)
strongSelf.mainContainerView.transform = CGAffineTransformMakeScale(SlideMenuOptions.contentViewScale, SlideMenuOptions.contentViewScale)
}
}) { [weak self](Bool) -> Void in
if let strongSelf = self {
strongSelf.disableContentInteraction()
strongSelf.leftViewController?.endAppearanceTransition()
}
}
}
public func openRightWithVelocity(velocity: CGFloat) {
let xOrigin: CGFloat = rightContainerView.frame.origin.x
// CGFloat finalXOrigin = SlideMenuOptions.rightViewOverlapWidth;
let finalXOrigin: CGFloat = CGRectGetWidth(view.bounds) - rightContainerView.frame.size.width
var frame = rightContainerView.frame
frame.origin.x = finalXOrigin
var duration: NSTimeInterval = Double(SlideMenuOptions.animationDuration)
if velocity != 0.0 {
duration = Double(fabs(xOrigin - CGRectGetWidth(view.bounds)) / velocity)
duration = Double(fmax(0.1, fmin(1.0, duration)))
}
addShadowToView(rightContainerView)
UIView.animateWithDuration(duration, delay: 0.0, options: UIViewAnimationOptions.CurveEaseInOut, animations: { [weak self]() -> Void in
if let strongSelf = self {
strongSelf.rightContainerView.frame = frame
strongSelf.opacityView.layer.opacity = Float(SlideMenuOptions.contentViewOpacity)
strongSelf.mainContainerView.transform = CGAffineTransformMakeScale(SlideMenuOptions.contentViewScale, SlideMenuOptions.contentViewScale)
}
}) { [weak self](Bool) -> Void in
if let strongSelf = self {
strongSelf.disableContentInteraction()
strongSelf.rightViewController?.endAppearanceTransition()
}
}
}
public func closeLeftWithVelocity(velocity: CGFloat) {
let xOrigin: CGFloat = leftContainerView.frame.origin.x
let finalXOrigin: CGFloat = leftMinOrigin()
var frame: CGRect = leftContainerView.frame;
frame.origin.x = finalXOrigin
var duration: NSTimeInterval = Double(SlideMenuOptions.animationDuration)
if velocity != 0.0 {
duration = Double(fabs(xOrigin - finalXOrigin) / velocity)
duration = Double(fmax(0.1, fmin(1.0, duration)))
}
UIView.animateWithDuration(duration, delay: 0.0, options: UIViewAnimationOptions.CurveEaseInOut, animations: { [weak self]() -> Void in
if let strongSelf = self {
strongSelf.leftContainerView.frame = frame
strongSelf.opacityView.layer.opacity = 0.0
strongSelf.mainContainerView.transform = CGAffineTransformMakeScale(1.0, 1.0)
}
}) { [weak self](Bool) -> Void in
if let strongSelf = self {
strongSelf.removeShadow(strongSelf.leftContainerView)
strongSelf.enableContentInteraction()
strongSelf.leftViewController?.endAppearanceTransition()
}
}
}
public func closeRightWithVelocity(velocity: CGFloat) {
let xOrigin: CGFloat = rightContainerView.frame.origin.x
let finalXOrigin: CGFloat = CGRectGetWidth(view.bounds)
var frame: CGRect = rightContainerView.frame
frame.origin.x = finalXOrigin
var duration: NSTimeInterval = Double(SlideMenuOptions.animationDuration)
if velocity != 0.0 {
duration = Double(fabs(xOrigin - CGRectGetWidth(view.bounds)) / velocity)
duration = Double(fmax(0.1, fmin(1.0, duration)))
}
UIView.animateWithDuration(duration, delay: 0.0, options: UIViewAnimationOptions.CurveEaseInOut, animations: { [weak self]() -> Void in
if let strongSelf = self {
strongSelf.rightContainerView.frame = frame
strongSelf.opacityView.layer.opacity = 0.0
strongSelf.mainContainerView.transform = CGAffineTransformMakeScale(1.0, 1.0)
}
}) { [weak self](Bool) -> Void in
if let strongSelf = self {
strongSelf.removeShadow(strongSelf.rightContainerView)
strongSelf.enableContentInteraction()
strongSelf.rightViewController?.endAppearanceTransition()
}
}
}
public override func toggleLeft() {
if isLeftOpen() {
closeLeft()
setCloseWindowLebel()
// closeMenuはメニュータップ時にも呼ばれるため、closeタップのトラッキングはここに入れる
track(.TapClose)
} else {
openLeft()
}
}
public func isLeftOpen() -> Bool {
return leftContainerView.frame.origin.x == 0.0
}
public func isLeftHidden() -> Bool {
return leftContainerView.frame.origin.x <= leftMinOrigin()
}
public override func toggleRight() {
if isRightOpen() {
closeRight()
setCloseWindowLebel()
} else {
openRight()
}
}
public func isRightOpen() -> Bool {
return rightContainerView.frame.origin.x == CGRectGetWidth(view.bounds) - rightContainerView.frame.size.width
}
public func isRightHidden() -> Bool {
return rightContainerView.frame.origin.x >= CGRectGetWidth(view.bounds)
}
public func changeMainViewController(mainViewController: UIViewController, close: Bool) {
removeViewController(self.mainViewController)
self.mainViewController = mainViewController
setUpViewController(mainContainerView, targetViewController: mainViewController)
if (close) {
closeLeft()
closeRight()
}
}
public func changeLeftViewController(leftViewController: UIViewController, closeLeft:Bool) {
removeViewController(self.leftViewController)
self.leftViewController = leftViewController
setUpViewController(leftContainerView, targetViewController: leftViewController)
if closeLeft {
self.closeLeft()
}
}
public func changeRightViewController(rightViewController: UIViewController, closeRight:Bool) {
removeViewController(self.rightViewController)
self.rightViewController = rightViewController;
setUpViewController(rightContainerView, targetViewController: rightViewController)
if closeRight {
self.closeRight()
}
}
private func leftMinOrigin() -> CGFloat {
return -SlideMenuOptions.leftViewWidth
}
private func rightMinOrigin() -> CGFloat {
return CGRectGetWidth(view.bounds)
}
private func panLeftResultInfoForVelocity(velocity: CGPoint) -> PanInfo {
let thresholdVelocity: CGFloat = 1000.0
let pointOfNoReturn: CGFloat = CGFloat(floor(leftMinOrigin())) + SlideMenuOptions.pointOfNoReturnWidth
let leftOrigin: CGFloat = leftContainerView.frame.origin.x
var panInfo: PanInfo = PanInfo(action: .Close, shouldBounce: false, velocity: 0.0)
panInfo.action = leftOrigin <= pointOfNoReturn ? .Close : .Open;
if velocity.x >= thresholdVelocity {
panInfo.action = .Open
panInfo.velocity = velocity.x
} else if velocity.x <= (-1.0 * thresholdVelocity) {
panInfo.action = .Close
panInfo.velocity = velocity.x
}
return panInfo
}
private func panRightResultInfoForVelocity(velocity: CGPoint) -> PanInfo {
let thresholdVelocity: CGFloat = -1000.0
let pointOfNoReturn: CGFloat = CGFloat(floor(CGRectGetWidth(view.bounds)) - SlideMenuOptions.pointOfNoReturnWidth)
let rightOrigin: CGFloat = rightContainerView.frame.origin.x
var panInfo: PanInfo = PanInfo(action: .Close, shouldBounce: false, velocity: 0.0)
panInfo.action = rightOrigin >= pointOfNoReturn ? .Close : .Open
if velocity.x <= thresholdVelocity {
panInfo.action = .Open
panInfo.velocity = velocity.x
} else if (velocity.x >= (-1.0 * thresholdVelocity)) {
panInfo.action = .Close
panInfo.velocity = velocity.x
}
return panInfo
}
private func applyLeftTranslation(translation: CGPoint, toFrame:CGRect) -> CGRect {
var newOrigin: CGFloat = toFrame.origin.x
newOrigin += translation.x
let minOrigin: CGFloat = leftMinOrigin()
let maxOrigin: CGFloat = 0.0
var newFrame: CGRect = toFrame
if newOrigin < minOrigin {
newOrigin = minOrigin
} else if newOrigin > maxOrigin {
newOrigin = maxOrigin
}
newFrame.origin.x = newOrigin
return newFrame
}
private func applyRightTranslation(translation: CGPoint, toFrame: CGRect) -> CGRect {
var newOrigin: CGFloat = toFrame.origin.x
newOrigin += translation.x
let minOrigin: CGFloat = rightMinOrigin()
let maxOrigin: CGFloat = rightMinOrigin() - rightContainerView.frame.size.width
var newFrame: CGRect = toFrame
if newOrigin > minOrigin {
newOrigin = minOrigin
} else if newOrigin < maxOrigin {
newOrigin = maxOrigin
}
newFrame.origin.x = newOrigin
return newFrame
}
private func getOpenedLeftRatio() -> CGFloat {
let width: CGFloat = leftContainerView.frame.size.width
let currentPosition: CGFloat = leftContainerView.frame.origin.x - leftMinOrigin()
return currentPosition / width
}
private func getOpenedRightRatio() -> CGFloat {
let width: CGFloat = rightContainerView.frame.size.width
let currentPosition: CGFloat = rightContainerView.frame.origin.x
return -(currentPosition - CGRectGetWidth(view.bounds)) / width
}
private func applyLeftOpacity() {
let openedLeftRatio: CGFloat = getOpenedLeftRatio()
let opacity: CGFloat = SlideMenuOptions.contentViewOpacity * openedLeftRatio
opacityView.layer.opacity = Float(opacity)
}
private func applyRightOpacity() {
let openedRightRatio: CGFloat = getOpenedRightRatio()
let opacity: CGFloat = SlideMenuOptions.contentViewOpacity * openedRightRatio
opacityView.layer.opacity = Float(opacity)
}
private func applyLeftContentViewScale() {
let openedLeftRatio: CGFloat = getOpenedLeftRatio()
let scale: CGFloat = 1.0 - ((1.0 - SlideMenuOptions.contentViewScale) * openedLeftRatio);
mainContainerView.transform = CGAffineTransformMakeScale(scale, scale)
}
private func applyRightContentViewScale() {
let openedRightRatio: CGFloat = getOpenedRightRatio()
let scale: CGFloat = 1.0 - ((1.0 - SlideMenuOptions.contentViewScale) * openedRightRatio)
mainContainerView.transform = CGAffineTransformMakeScale(scale, scale)
}
private func addShadowToView(targetContainerView: UIView) {
targetContainerView.layer.masksToBounds = false
targetContainerView.layer.shadowOffset = SlideMenuOptions.shadowOffset
targetContainerView.layer.shadowOpacity = Float(SlideMenuOptions.shadowOpacity)
targetContainerView.layer.shadowRadius = SlideMenuOptions.shadowRadius
targetContainerView.layer.shadowPath = UIBezierPath(rect: targetContainerView.bounds).CGPath
}
private func removeShadow(targetContainerView: UIView) {
targetContainerView.layer.masksToBounds = true
mainContainerView.layer.opacity = 1.0
}
private func removeContentOpacity() {
opacityView.layer.opacity = 0.0
}
private func addContentOpacity() {
opacityView.layer.opacity = Float(SlideMenuOptions.contentViewOpacity)
}
private func disableContentInteraction() {
mainContainerView.userInteractionEnabled = false
}
private func enableContentInteraction() {
mainContainerView.userInteractionEnabled = true
}
private func setOpenWindowLevel() {
if (SlideMenuOptions.hideStatusBar) {
dispatch_async(dispatch_get_main_queue(), {
if let window = UIApplication.sharedApplication().keyWindow {
window.windowLevel = UIWindowLevelStatusBar + 1
}
})
}
}
private func setCloseWindowLebel() {
if (SlideMenuOptions.hideStatusBar) {
dispatch_async(dispatch_get_main_queue(), {
if let window = UIApplication.sharedApplication().keyWindow {
window.windowLevel = UIWindowLevelNormal
}
})
}
}
private func setUpViewController(targetView: UIView, targetViewController: UIViewController?) {
if let viewController = targetViewController {
addChildViewController(viewController)
viewController.view.frame = targetView.bounds
targetView.addSubview(viewController.view)
viewController.didMoveToParentViewController(self)
}
}
private func removeViewController(viewController: UIViewController?) {
if let _viewController = viewController {
_viewController.willMoveToParentViewController(nil)
_viewController.view.removeFromSuperview()
_viewController.removeFromParentViewController()
}
}
public func closeLeftNonAnimation(){
setCloseWindowLebel()
let finalXOrigin: CGFloat = leftMinOrigin()
var frame: CGRect = leftContainerView.frame;
frame.origin.x = finalXOrigin
leftContainerView.frame = frame
opacityView.layer.opacity = 0.0
mainContainerView.transform = CGAffineTransformMakeScale(1.0, 1.0)
removeShadow(leftContainerView)
enableContentInteraction()
}
public func closeRightNonAnimation(){
setCloseWindowLebel()
let finalXOrigin: CGFloat = CGRectGetWidth(view.bounds)
var frame: CGRect = rightContainerView.frame
frame.origin.x = finalXOrigin
rightContainerView.frame = frame
opacityView.layer.opacity = 0.0
mainContainerView.transform = CGAffineTransformMakeScale(1.0, 1.0)
removeShadow(rightContainerView)
enableContentInteraction()
}
//pragma mark – UIGestureRecognizerDelegate
public func gestureRecognizer(gestureRecognizer: UIGestureRecognizer, shouldReceiveTouch touch: UITouch) -> Bool {
let point: CGPoint = touch.locationInView(view)
if gestureRecognizer == leftPanGesture {
return slideLeftForGestureRecognizer(gestureRecognizer, point: point)
} else if gestureRecognizer == rightPanGesture {
return slideRightViewForGestureRecognizer(gestureRecognizer, withTouchPoint: point)
} else if gestureRecognizer == leftTapGetsture {
return isLeftOpen() && !isPointContainedWithinLeftRect(point)
} else if gestureRecognizer == rightTapGesture {
return isRightOpen() && !isPointContainedWithinRightRect(point)
}
return true
}
private func slideLeftForGestureRecognizer( gesture: UIGestureRecognizer, point:CGPoint) -> Bool{
return isLeftOpen() || SlideMenuOptions.panFromBezel && isLeftPointContainedWithinBezelRect(point)
}
private func isLeftPointContainedWithinBezelRect(point: CGPoint) -> Bool{
var leftBezelRect: CGRect = CGRectZero
var tempRect: CGRect = CGRectZero
let bezelWidth: CGFloat = SlideMenuOptions.leftBezelWidth
CGRectDivide(view.bounds, &leftBezelRect, &tempRect, bezelWidth, CGRectEdge.MinXEdge)
return CGRectContainsPoint(leftBezelRect, point)
}
private func isPointContainedWithinLeftRect(point: CGPoint) -> Bool {
return CGRectContainsPoint(leftContainerView.frame, point)
}
private func slideRightViewForGestureRecognizer(gesture: UIGestureRecognizer, withTouchPoint point: CGPoint) -> Bool {
return isRightOpen() || SlideMenuOptions.rightPanFromBezel && isRightPointContainedWithinBezelRect(point)
}
private func isRightPointContainedWithinBezelRect(point: CGPoint) -> Bool {
var rightBezelRect: CGRect = CGRectZero
var tempRect: CGRect = CGRectZero
let bezelWidth: CGFloat = CGRectGetWidth(view.bounds) - SlideMenuOptions.rightBezelWidth
CGRectDivide(view.bounds, &tempRect, &rightBezelRect, bezelWidth, CGRectEdge.MinXEdge)
return CGRectContainsPoint(rightBezelRect, point)
}
private func isPointContainedWithinRightRect(point: CGPoint) -> Bool {
return CGRectContainsPoint(rightContainerView.frame, point)
}
}
extension UIViewController {
public func slideMenuController() -> SlideMenuController? {
var viewController: UIViewController? = self
while viewController != nil {
if viewController is SlideMenuController {
return viewController as? SlideMenuController
}
viewController = viewController?.parentViewController
}
return nil;
}
public func addLeftBarButtonWithImage(buttonImage: UIImage) {
let leftButton: UIBarButtonItem = UIBarButtonItem(image: buttonImage, style: UIBarButtonItemStyle.Plain, target: self, action: "toggleLeft")
navigationItem.leftBarButtonItem = leftButton;
}
public func addRightBarButtonWithImage(buttonImage: UIImage) {
let rightButton: UIBarButtonItem = UIBarButtonItem(image: buttonImage, style: UIBarButtonItemStyle.Plain, target: self, action: "toggleRight")
navigationItem.rightBarButtonItem = rightButton;
}
public func toggleLeft() {
slideMenuController()?.toggleLeft()
}
public func toggleRight() {
slideMenuController()?.toggleRight()
}
public func openLeft() {
slideMenuController()?.openLeft()
}
public func openRight() {
slideMenuController()?.openRight() }
public func closeLeft() {
slideMenuController()?.closeLeft()
}
public func closeRight() {
slideMenuController()?.closeRight()
}
// Please specify if you want menu gesuture give priority to than targetScrollView
public func addPriorityToMenuGesuture(targetScrollView: UIScrollView) {
guard let slideControlelr = slideMenuController(), let recognizers = slideControlelr.view.gestureRecognizers else {
return
}
for recognizer in recognizers where recognizer is UIPanGestureRecognizer {
targetScrollView.panGestureRecognizer.requireGestureRecognizerToFail(recognizer)
}
}
}
| mit | 40a87d0a9f54bf25a8f78cf857c5e7ab | 37.389923 | 153 | 0.644165 | 6.230004 | false | false | false | false |
Shaquu/SwiftProjectsPub | Shopping List/Shopping List/ViewController.swift | 1 | 5133 | //
// ViewController.swift
// Shopping List
//
// Created by Tadeusz Wyrzykowski on 04.11.2016.
// Copyright © 2016 Tadeusz Wyrzykowski. All rights reserved.
//
import UIKit
import CoreData
class ViewController: UIViewController, UITableViewDataSource, UITableViewDelegate, UITextFieldDelegate {
var items = [NSManagedObject]()
@IBOutlet var textField: UITextField!
@IBOutlet var tableView: UITableView!
func textFieldShouldReturn(_ textField: UITextField) -> Bool {
addItem()
return true
}
func addItem() {
let newItem = textField.text
let trimmedItem = newItem?.trimmingCharacters(in: .whitespacesAndNewlines)
if (trimmedItem?.characters.count)! > 0 {
self.saveItem(trimmedItem!, marked: false)
tableView.reloadData()
//let range = NSMakeRange(0, self.tableView.numberOfSections + 1)
//let sections = NSIndexSet(indexesIn: range)
//self.tableView.reloadSections(sections as IndexSet, with: .automatic)
}
textField.text = ""
textField.resignFirstResponder()
}
func saveItem(_ item: String, marked: Bool) {
let context = (UIApplication.shared.delegate as! AppDelegate).persistentContainer.viewContext
let entity = NSEntityDescription.entity(forEntityName: "Entity", in: context)
let newItem = NSManagedObject(entity: entity!, insertInto: context)
newItem.setValue(item, forKey: "item")
newItem.setValue(marked, forKey: "marked")
items.append(newItem)
do {
try context.save()
} catch let error as NSError {
print("Could not save \(error), \(error.userInfo)")
}
}
func deleteItem(_ at: Int) {
let context = (UIApplication.shared.delegate as! AppDelegate).persistentContainer.viewContext
context.delete(items[at])
items.remove(at: at)
do {
try context.save()
} catch let error as NSError {
print("Could not save \(error), \(error.userInfo)")
}
}
func modifyItem(_ at: Int, item: String, marked: Bool) {
let context = (UIApplication.shared.delegate as! AppDelegate).persistentContainer.viewContext
items[at].setValue(item, forKey: "item")
items[at].setValue(marked, forKey: "marked")
do {
try context.save()
} catch let error as NSError {
print("Could not save \(error), \(error.userInfo)")
}
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
let context = (UIApplication.shared.delegate as! AppDelegate).persistentContainer.viewContext
let fetchRequest = NSFetchRequest<NSFetchRequestResult>(entityName: "Entity")
do {
let results = try context.fetch(fetchRequest)
items = results as! [NSManagedObject]
} catch let error as NSError {
print("Could not fetch \(error), \(error.userInfo)")
}
}
override func viewDidLoad() {
super.viewDidLoad()
self.textField.delegate = self
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
items.removeAll()
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return items.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath)
let item = items[indexPath.row]
cell.textLabel?.text = item.value(forKey: "item") as! String?
let marked = item.value(forKey: "marked") as! Bool?
if marked == true {
cell.accessoryType = .checkmark
cell.tintColor = .green
} else {
cell.accessoryType = .none
}
return cell
}
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
let selectedRow = tableView.cellForRow(at: indexPath)
var isMarked = false;
if selectedRow?.accessoryType != UITableViewCellAccessoryType.checkmark {
selectedRow?.accessoryType = .checkmark
selectedRow?.tintColor = .green
isMarked = true
} else {
selectedRow?.accessoryType = .none
}
self.modifyItem(indexPath.row, item: (selectedRow?.textLabel?.text)!, marked: isMarked)
}
func tableView(_ tableView: UITableView, commit editingStyle: UITableViewCellEditingStyle, forRowAt indexPath: IndexPath) {
let deletedRow = tableView.cellForRow(at: indexPath)
if editingStyle == .delete {
self.deleteItem(indexPath.row)
tableView.deleteRows(at: [indexPath], with: .automatic)
deletedRow?.accessoryType = .none
}
}
}
| gpl-3.0 | d8c2da7a3ed31d9cf2208e1609bf4bf3 | 31.897436 | 127 | 0.606196 | 5.199595 | false | false | false | false |
TakeScoop/SwiftyRSA | Source/SwiftyRSA+ObjC.swift | 1 | 9792 | //
// SwiftyRSA+ObjC.swift
// SwiftyRSA
//
// Created by Lois Di Qual on 3/6/17.
// Copyright © 2017 Scoop. All rights reserved.
//
import Foundation
/// This files allows the ObjC runtime to access SwiftyRSA classes while keeping the swift code Swiftyish.
/// Things like protocol extensions or throwing and returning booleans are not well supported by ObjC, so instead
/// of giving access to the Swift classes directly, we're wrapping then their `_objc_*` counterpart.
/// They are exposed under the same name to the ObjC runtime, and all methods are present – they're just delegated
/// to the wrapped swift value.
private protocol ObjcBridgeable {
associatedtype SwiftType
var swiftValue: SwiftType { get }
init(swiftValue: SwiftType)
}
// MARK: – SwiftyRSA
@objc(KeyPair)
public class _objc_KeyPair: NSObject { // swiftlint:disable:this type_name
@objc public let privateKey: _objc_PrivateKey
@objc public let publicKey: _objc_PublicKey
init(privateKey: _objc_PrivateKey, publicKey: _objc_PublicKey) {
self.privateKey = privateKey
self.publicKey = publicKey
}
}
@objc(SwiftyRSA)
public class _objc_SwiftyRSA: NSObject { // swiftlint:disable:this type_name
@available(iOS 10.0, watchOS 3.0, tvOS 10.0, *)
@objc public class func generateRSAKeyPair(sizeInBits size: Int) throws -> _objc_KeyPair {
let (privateKey, publicKey) = try SwiftyRSA.generateRSAKeyPair(sizeInBits: size)
return _objc_KeyPair(
privateKey: _objc_PrivateKey(swiftValue: privateKey),
publicKey: _objc_PublicKey(swiftValue: publicKey)
)
}
}
// MARK: - PublicKey
@objc(PublicKey)
public class _objc_PublicKey: NSObject, Key, ObjcBridgeable { // swiftlint:disable:this type_name
fileprivate let swiftValue: PublicKey
@objc public var reference: SecKey {
return swiftValue.reference
}
@objc public var originalData: Data? {
return swiftValue.originalData
}
@objc public func pemString() throws -> String {
return try swiftValue.pemString()
}
@objc public func data() throws -> Data {
return try swiftValue.data()
}
@objc public func base64String() throws -> String {
return try swiftValue.base64String()
}
required public init(swiftValue: PublicKey) {
self.swiftValue = swiftValue
}
@objc required public init(data: Data) throws {
self.swiftValue = try PublicKey(data: data)
}
@objc public required init(reference: SecKey) throws {
self.swiftValue = try PublicKey(reference: reference)
}
@objc public required init(base64Encoded base64String: String) throws {
self.swiftValue = try PublicKey(base64Encoded: base64String)
}
@objc public required init(pemEncoded pemString: String) throws {
self.swiftValue = try PublicKey(pemEncoded: pemString)
}
@objc public required init(pemNamed pemName: String, in bundle: Bundle) throws {
self.swiftValue = try PublicKey(pemNamed: pemName, in: bundle)
}
@objc public required init(derNamed derName: String, in bundle: Bundle) throws {
self.swiftValue = try PublicKey(derNamed: derName, in: bundle)
}
@objc public static func publicKeys(pemEncoded pemString: String) -> [_objc_PublicKey] {
return PublicKey.publicKeys(pemEncoded: pemString).map { _objc_PublicKey(swiftValue: $0) }
}
}
// MARK: - PrivateKey
@objc(PrivateKey)
public class _objc_PrivateKey: NSObject, Key, ObjcBridgeable { // swiftlint:disable:this type_name
fileprivate let swiftValue: PrivateKey
@objc public var reference: SecKey {
return swiftValue.reference
}
@objc public var originalData: Data? {
return swiftValue.originalData
}
@objc public func pemString() throws -> String {
return try swiftValue.pemString()
}
@objc public func data() throws -> Data {
return try swiftValue.data()
}
@objc public func base64String() throws -> String {
return try swiftValue.base64String()
}
public required init(swiftValue: PrivateKey) {
self.swiftValue = swiftValue
}
@objc public required init(data: Data) throws {
self.swiftValue = try PrivateKey(data: data)
}
@objc public required init(reference: SecKey) throws {
self.swiftValue = try PrivateKey(reference: reference)
}
@objc public required init(base64Encoded base64String: String) throws {
self.swiftValue = try PrivateKey(base64Encoded: base64String)
}
@objc public required init(pemEncoded pemString: String) throws {
self.swiftValue = try PrivateKey(pemEncoded: pemString)
}
@objc public required init(pemNamed pemName: String, in bundle: Bundle) throws {
self.swiftValue = try PrivateKey(pemNamed: pemName, in: bundle)
}
@objc public required init(derNamed derName: String, in bundle: Bundle) throws {
self.swiftValue = try PrivateKey(derNamed: derName, in: bundle)
}
}
// MARK: - VerificationResult
@objc(VerificationResult)
public class _objc_VerificationResult: NSObject { // swiftlint:disable:this type_name
@objc public let isSuccessful: Bool
init(isSuccessful: Bool) {
self.isSuccessful = isSuccessful
}
}
// MARK: - ClearMessage
@objc(ClearMessage)
public class _objc_ClearMessage: NSObject, Message, ObjcBridgeable { // swiftlint:disable:this type_name
fileprivate let swiftValue: ClearMessage
@objc public var base64String: String {
return swiftValue.base64String
}
@objc public var data: Data {
return swiftValue.data
}
public required init(swiftValue: ClearMessage) {
self.swiftValue = swiftValue
}
@objc public required init(data: Data) {
self.swiftValue = ClearMessage(data: data)
}
@objc public required init(string: String, using rawEncoding: UInt) throws {
let encoding = String.Encoding(rawValue: rawEncoding)
self.swiftValue = try ClearMessage(string: string, using: encoding)
}
@objc public required init(base64Encoded base64String: String) throws {
self.swiftValue = try ClearMessage(base64Encoded: base64String)
}
@objc public func string(encoding rawEncoding: UInt) throws -> String {
let encoding = String.Encoding(rawValue: rawEncoding)
return try swiftValue.string(encoding: encoding)
}
@objc public func encrypted(with key: _objc_PublicKey, padding: Padding) throws -> _objc_EncryptedMessage {
let encryptedMessage = try swiftValue.encrypted(with: key.swiftValue, padding: padding)
return _objc_EncryptedMessage(swiftValue: encryptedMessage)
}
@objc public func signed(with key: _objc_PrivateKey, digestType: _objc_Signature.DigestType) throws -> _objc_Signature {
let signature = try swiftValue.signed(with: key.swiftValue, digestType: digestType.swiftValue)
return _objc_Signature(swiftValue: signature)
}
@objc public func verify(with key: _objc_PublicKey, signature: _objc_Signature, digestType: _objc_Signature.DigestType) throws -> _objc_VerificationResult {
let isSuccessful = try swiftValue.verify(with: key.swiftValue, signature: signature.swiftValue, digestType: digestType.swiftValue)
return _objc_VerificationResult(isSuccessful: isSuccessful)
}
}
// MARK: - EncryptedMessage
@objc(EncryptedMessage)
public class _objc_EncryptedMessage: NSObject, Message, ObjcBridgeable { // swiftlint:disable:this type_name
fileprivate let swiftValue: EncryptedMessage
@objc public var base64String: String {
return swiftValue.base64String
}
@objc public var data: Data {
return swiftValue.data
}
public required init(swiftValue: EncryptedMessage) {
self.swiftValue = swiftValue
}
@objc public required init(data: Data) {
self.swiftValue = EncryptedMessage(data: data)
}
@objc public required init(base64Encoded base64String: String) throws {
self.swiftValue = try EncryptedMessage(base64Encoded: base64String)
}
@objc public func decrypted(with key: _objc_PrivateKey, padding: Padding) throws -> _objc_ClearMessage {
let clearMessage = try swiftValue.decrypted(with: key.swiftValue, padding: padding)
return _objc_ClearMessage(swiftValue: clearMessage)
}
}
// MARK: - Signature
@objc(Signature)
public class _objc_Signature: NSObject, ObjcBridgeable { // swiftlint:disable:this type_name
@objc
public enum DigestType: Int {
case sha1
case sha224
case sha256
case sha384
case sha512
fileprivate var swiftValue: Signature.DigestType {
switch self {
case .sha1: return .sha1
case .sha224: return .sha224
case .sha256: return .sha256
case .sha384: return .sha384
case .sha512: return .sha512
}
}
}
fileprivate let swiftValue: Signature
@objc public var base64String: String {
return swiftValue.base64String
}
@objc public var data: Data {
return swiftValue.data
}
public required init(swiftValue: Signature) {
self.swiftValue = swiftValue
}
@objc public init(data: Data) {
self.swiftValue = Signature(data: data)
}
@objc public required init(base64Encoded base64String: String) throws {
self.swiftValue = try Signature(base64Encoded: base64String)
}
}
| mit | e4dc4ea424d53ce20738f7e88b7e7422 | 30.879479 | 160 | 0.669459 | 4.45674 | false | false | false | false |
ios-ximen/DYZB | 斗鱼直播/斗鱼直播/Classes/Main/Models/AnchorGroup.swift | 1 | 937 | //
// AnchorGroup.swift
// 斗鱼直播
//
// Created by niujinfeng on 2017/6/30.
// Copyright © 2017年 niujinfeng. All rights reserved.
//
import UIKit
class AnchorGroup: BaseGameModel {
///该组中对应的房间信息
var room_list : [[String : NSObject]]?{
didSet{
guard let room_list = room_list else { return }
for dict in room_list {
anchors.append(AnchorModel(dict : dict))
}
}
}
lazy var anchors : [AnchorModel] = [AnchorModel]()
///组显示的图标
var icon_name: String = "home_header_normal"
/*
override func setValue(_ value: Any?, forKey key: String) {
if key == "room_list"{
if let dataArray = value as? [[String: NSObject]]{
for dict in dataArray {
anchors.append(AnchorModel(dict:dict))
}
}
}
}
*/
}
| mit | 42e9c7d6329f027019cfcf00a76297ba | 23.162162 | 64 | 0.524609 | 4.045249 | false | false | false | false |
fdstevex/swift-mysql | MySQLResult.swift | 1 | 2464 | //
// MySQLResult.swift
// swiftmysql
//
// Created by Steve Tibbett on 2015-12-15.
// Copyright © 2015 Fall Day Software Inc. All rights reserved.
//
import Foundation
// TODO: Make this iterable
class MySQLResult {
let mysql_res: UnsafeMutablePointer<MYSQL_RES>
let numFields: Int
let fields: UnsafeMutablePointer<MYSQL_FIELD>
init(mysql_res: UnsafeMutablePointer<MYSQL_RES>) {
self.mysql_res = mysql_res
self.numFields = Int(mysql_num_fields(mysql_res))
self.fields = mysql_fetch_fields(mysql_res)
}
deinit {
mysql_free_result(mysql_res)
}
func fetchRow() throws -> Dictionary<String, Any?>? {
let mysql_row = mysql_fetch_row(mysql_res)
if mysql_row == nil {
return nil
}
var dict = Dictionary<String, Any?>()
// Populate the row dictionary
for fieldIdx in 0..<numFields {
let rowBytes = mysql_row[fieldIdx]
let field = MySQLField(mysql_field: fields[fieldIdx])
let stringValue = String.fromCString(rowBytes) ?? ""
switch (field.type) {
case MYSQL_TYPE_VAR_STRING:
dict[field.name] = stringValue
case MYSQL_TYPE_LONGLONG:
let int64value = Int64(stringValue)
dict[field.name] = int64value
case MYSQL_TYPE_DATETIME:
let dateFmt = NSDateFormatter()
dateFmt.timeZone = NSTimeZone.defaultTimeZone()
dateFmt.dateFormat = "yyyy-MM-dd HH:mm:ss"
dateFmt.locale = NSLocale(localeIdentifier: "en_us")
dict[field.name] = dateFmt.dateFromString(stringValue)!
case MYSQL_TYPE_BLOB:
let data = row_data(rowBytes, fieldIndex: fieldIdx)
dict[field.name] = data
default:
throw MySQLConnection.MySQLError.UnsupportedTypeInResult(fieldName: field.name, type: field.type)
}
}
return dict
}
func row_data(bytes: UnsafeMutablePointer<Int8>, fieldIndex: Int) -> NSData {
let lengths = UnsafeMutablePointer<UInt64>(mysql_fetch_lengths(mysql_res))
let fieldLength = Int(lengths[fieldIndex])
let data = NSData(bytes: bytes, length: fieldLength)
return data
}
}
| apache-2.0 | b22f19b0d06c88723a6818abd76d84ad | 32.283784 | 117 | 0.568006 | 4.390374 | false | false | false | false |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.