repo_name
stringlengths 7
91
| path
stringlengths 8
658
| copies
stringclasses 125
values | size
stringlengths 3
6
| content
stringlengths 118
674k
| license
stringclasses 15
values | hash
stringlengths 32
32
| line_mean
float64 6.09
99.2
| line_max
int64 17
995
| alpha_frac
float64 0.3
0.9
| ratio
float64 2
9.18
| autogenerated
bool 1
class | config_or_test
bool 2
classes | has_no_keywords
bool 2
classes | has_few_assignments
bool 1
class |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
valitovaza/NewsAP | NewsAPTests/Article/ArticleTests.swift | 1 | 4385 | import XCTest
@testable import NewsAP
class ArticleTests: XCTestCase, JsonTestable {
// MARK: - Parameters & Constants
private let author = "Napier Lopez"
private let title = "Leak: Fitbit’s new smartwatch and headphones"
private let desc = "To no one's surprise, Fitbit appears to be preparing its first proper smartwatch to take on the Apple Wa..."
private let url = "https://thenextweb.com/gadgets/2017/05/01/heres-first-look-fitbits-new-smartwatch-headphones/"
private let urlToImage = "https://cdn0.tnwcdn.com/wp-content/blogs.dir/1/files/2017/05/084f67a7daa653343a69ff34b72636f6.jpg"
private let publishedAt = "2017-05-01T23:21:20Z"
private var validDict = [String: Any]()
private let testArticle = Article(author: "testauthor",
title: "testtitle",
desc: "testdesc",
url: "http://google.com",
urlToImage: "testurlToImage",
publishedAt: "testpublishedAt")
// MARK: - Test variables
private var sut: Article!
// MARK: - Set up and tear down
override func setUp() {
super.setUp()
validDict = ["author": author,
"title": title,
"description": desc,
"url": url,
"urlToImage": urlToImage,
"publishedAt": publishedAt]
sut = Article(validDict)
}
override func tearDown() {
sut = nil
super.tearDown()
}
// MARK: - Tests
func testAuthorField() {
validateField(TestField(Article.authorKey, actualField: sut.author, expectedField: author))
}
func testTitleField() {
validateField(TestField(Article.titleKey, actualField: sut.title, expectedField: title))
}
func testDescriptionField() {
validateField(TestField(Article.descriptionKey, actualField: sut.desc, expectedField: desc))
}
func testUrlField() {
validateField(TestField(Article.urlKey, actualField: sut.url, expectedField: url))
}
func testUrlToImageField() {
validateField(TestField(Article.urlToImageKey, actualField: sut.urlToImage, expectedField: urlToImage))
}
func testPublishedAtField() {
validateField(TestField(Article.publishedAtKey, actualField: sut.publishedAt, expectedField: publishedAt))
}
func testDate() {
let date = dateFormatter.date(from: sut.publishedAt)!
XCTAssertEqual(calendar.component(.hour, from: date), 23)
XCTAssertEqual(calendar.component(.minute, from: date), 21)
XCTAssertEqual(calendar.component(.second, from: date), 20)
}
private var dateFormatter: DateFormatter {
let dateFormat = Article.dateFormat
let formatter = DateFormatter()
formatter.dateFormat = dateFormat
formatter.locale = Locale(identifier: enLocaleIdentifier)
formatter.timeZone = TimeZone(secondsFromGMT: 0)
return formatter
}
private var calendar: Calendar {
var calendar = Calendar.current
calendar.timeZone = dateFormatter.timeZone
calendar.locale = dateFormatter.locale
return calendar
}
func testEncodeMustProvideConvertableDictionary() {
let article = Article(validDict)
if let dict = article?.encode() {
let tArticle = Article(dict)
compareArticles(l: article, r: tArticle)
}else{
XCTFail("Invalid dictionary produced")
}
}
private func compareArticles(l: Article?, r: Article?) {
XCTAssertEqual(l?.author, r?.author)
XCTAssertEqual(l?.title, r?.title)
XCTAssertEqual(l?.desc, r?.desc)
XCTAssertEqual(l?.url, r?.url)
XCTAssertEqual(l?.urlToImage, r?.urlToImage)
XCTAssertEqual(l?.publishedAt, r?.publishedAt)
}
func testCompareArticles() {
XCTAssertEqual(sut, sut)
XCTAssertNotEqual(testArticle, sut)
}
// MARK: - Auxiliary methods
private func validateField<F>(_ field: TestField<F>) where F: Equatable {
tstField(field, type: Article.self, validDict: validDict)
}
}
extension Article: DictionaryInitable{}
| mit | f36fad7bfb17a290872a7166f2938dc8 | 35.525 | 132 | 0.614419 | 4.589529 | false | true | false | false |
GraphKit/MaterialKit | Sources/iOS/Card.swift | 1 | 8023 | /*
* Copyright (C) 2015 - 2016, Daniel Dahan and CosmicMind, Inc. <http://cosmicmind.com>.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* * Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* * Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* * Neither the name of CosmicMind nor the names of its
* contributors may be used to endorse or promote products derived from
* this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
import UIKit
open class Card: PulseView {
/// A container view for subviews.
open let container = UIView()
@IBInspectable
open override var cornerRadiusPreset: CornerRadiusPreset {
didSet {
container.cornerRadiusPreset = cornerRadiusPreset
}
}
@IBInspectable
open override var cornerRadius: CGFloat {
didSet {
container.cornerRadius = cornerRadius
}
}
open override var shapePreset: ShapePreset {
didSet {
container.shapePreset = shapePreset
}
}
@IBInspectable
open override var backgroundColor: UIColor? {
didSet {
container.backgroundColor = backgroundColor
}
}
/// A reference to the toolbar.
@IBInspectable
open var toolbar: Toolbar? {
didSet {
oldValue?.removeFromSuperview()
if let v = toolbar {
container.addSubview(v)
}
layoutSubviews()
}
}
/// A preset wrapper around toolbarEdgeInsets.
open var toolbarEdgeInsetsPreset = EdgeInsetsPreset.none {
didSet {
toolbarEdgeInsets = EdgeInsetsPresetToValue(preset: toolbarEdgeInsetsPreset)
}
}
/// A reference to toolbarEdgeInsets.
@IBInspectable
open var toolbarEdgeInsets = EdgeInsets.zero {
didSet {
layoutSubviews()
}
}
/// A reference to the contentView.
@IBInspectable
open var contentView: UIView? {
didSet {
oldValue?.removeFromSuperview()
if let v = contentView {
v.clipsToBounds = true
container.addSubview(v)
}
layoutSubviews()
}
}
/// A preset wrapper around contentViewEdgeInsets.
open var contentViewEdgeInsetsPreset = EdgeInsetsPreset.none {
didSet {
contentViewEdgeInsets = EdgeInsetsPresetToValue(preset: contentViewEdgeInsetsPreset)
}
}
/// A reference to contentViewEdgeInsets.
@IBInspectable
open var contentViewEdgeInsets = EdgeInsets.zero {
didSet {
layoutSubviews()
}
}
/// A reference to the bottomBar.
@IBInspectable
open var bottomBar: Bar? {
didSet {
oldValue?.removeFromSuperview()
if let v = bottomBar {
container.addSubview(v)
}
layoutSubviews()
}
}
/// A preset wrapper around bottomBarEdgeInsets.
open var bottomBarEdgeInsetsPreset = EdgeInsetsPreset.none {
didSet {
bottomBarEdgeInsets = EdgeInsetsPresetToValue(preset: bottomBarEdgeInsetsPreset)
}
}
/// A reference to bottomBarEdgeInsets.
@IBInspectable
open var bottomBarEdgeInsets = EdgeInsets.zero {
didSet {
layoutSubviews()
}
}
/**
An initializer that accepts a NSCoder.
- Parameter coder aDecoder: A NSCoder.
*/
public required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
/**
An initializer that accepts a CGRect.
- Parameter frame: A CGRect.
*/
public override init(frame: CGRect) {
super.init(frame: frame)
}
/// A convenience initializer.
public convenience init() {
self.init(frame: .zero)
}
/**
A convenience initiazlier.
- Parameter toolbar: An optional Toolbar.
- Parameter contentView: An optional UIView.
- Parameter bottomBar: An optional Bar.
*/
public convenience init?(toolbar: Toolbar?, contentView: UIView?, bottomBar: Bar?) {
self.init(frame: .zero)
prepareProperties(toolbar: toolbar, contentView: contentView, bottomBar: bottomBar)
}
open override func layoutSubviews() {
super.layoutSubviews()
container.width = width
reload()
}
/// Reloads the layout.
open func reload() {
var h: CGFloat = 0
if let v = toolbar {
h = prepare(view: v, with: toolbarEdgeInsets, from: h)
}
if let v = contentView {
h = prepare(view: v, with: contentViewEdgeInsets, from: h)
}
if let v = bottomBar {
h = prepare(view: v, with: bottomBarEdgeInsets, from: h)
}
container.height = h
height = h
}
/**
Prepares the view instance when intialized. When subclassing,
it is recommended to override the prepare method
to initialize property values and other setup operations.
The super.prepare method should always be called immediately
when subclassing.
*/
open override func prepare() {
super.prepare()
depthPreset = .depth1
pulseAnimation = .none
cornerRadiusPreset = .cornerRadius1
prepareContainer()
}
/**
Prepare the view size from a given top position.
- Parameter view: A UIView.
- Parameter edge insets: An EdgeInsets.
- Parameter from top: A CGFloat.
- Returns: A CGFloat.
*/
@discardableResult
open func prepare(view: UIView, with insets: EdgeInsets, from top: CGFloat) -> CGFloat {
let y = insets.top + top
view.y = y
view.x = insets.left
let w = container.width - insets.left - insets.right
var h = view.height
if 0 == h || nil != view as? UILabel {
(view as? UILabel)?.sizeToFit()
h = view.sizeThatFits(CGSize(width: w, height: CGFloat.greatestFiniteMagnitude)).height
}
view.width = w
view.height = h
return y + h + insets.bottom
}
/**
A preparation method that sets the base UI elements.
- Parameter toolbar: An optional Toolbar.
- Parameter contentView: An optional UIView.
- Parameter bottomBar: An optional Bar.
*/
internal func prepareProperties(toolbar: Toolbar?, contentView: UIView?, bottomBar: Bar?) {
self.toolbar = toolbar
self.contentView = contentView
self.bottomBar = bottomBar
}
/// Prepares the container.
private func prepareContainer() {
container.clipsToBounds = true
addSubview(container)
}
}
| agpl-3.0 | a76feceedaed3d85dc30a8c10c5a99d2 | 29.390152 | 99 | 0.619344 | 5.152858 | false | false | false | false |
luzefeng/MLSwiftBasic | MLSwiftBasic/Classes/Category/MB+UIColor.swift | 11 | 716 | // github: https://github.com/MakeZL/MLSwiftBasic
// author: @email <[email protected]>
//
// MB+UIColor.swift
// MakeBolo
//
// Created by 张磊 on 15/6/23.
// Copyright (c) 2015年 MakeZL. All rights reserved.
//
import UIKit
extension UIColor{
convenience init(rgba :String) {
var scanner = NSScanner(string: rgba)
var hexNum = 0 as UInt32
if (scanner.scanHexInt(&hexNum)){
var r = (hexNum >> 16) & 0xFF
var g = (hexNum >> 8) & 0xFF
var b = (hexNum) & 0xFF
self.init(red: CGFloat(r)/255.0, green: CGFloat(g)/255.0, blue: CGFloat(b)/255.0, alpha: 1.0)
}else{
self.init()
}
}
} | mit | 11e31fb8c3cf0bc5f9959442043c7a7c | 24.392857 | 105 | 0.542254 | 3.227273 | false | false | false | false |
SASAbus/SASAbus-ios | SASAbus/Util/DeviceUtils.swift | 1 | 626 | import Foundation
import UIKit
class DeviceUtils {
static func getModel() -> String {
var systemInfo = utsname()
uname(&systemInfo)
let machineMirror = Mirror(reflecting: systemInfo.machine)
let identifier = machineMirror.children.reduce("") { identifier, element in
guard let value = element.value as? Int8, value != 0 else { return identifier }
return identifier + String(UnicodeScalar(UInt8(value)))
}
return identifier
}
static func getIdentifier() -> String {
return UIDevice.current.identifierForVendor!.uuidString
}
}
| gpl-3.0 | 01b10d9110c82b90bb7a890f22b703ef | 27.454545 | 91 | 0.65016 | 5.131148 | false | false | false | false |
saeta/penguin | Sources/PenguinCSV/CSVReader.swift | 1 | 2693 | // Copyright 2020 Penguin Authors
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
import Foundation
public class CSVReader: Sequence {
public init(file filename: String, fileManager: FileManager = FileManager.default) throws {
guard fileManager.isReadableFile(atPath: filename) else {
throw CSVErrors.invalidFile(filename: filename)
}
guard let data = fileManager.contents(atPath: filename) else {
throw CSVErrors.invalidFile(filename: filename)
}
// TODO: support more efficient processing here.
guard let str = String(data: data, encoding: .utf8) else {
throw CSVErrors.invalidFormat(filename: filename)
}
self.metadata = try? Self.sniffMetadata(contents: str)
self.parser = CSVRowParser(str.makeIterator(), delimiter: metadata?.separator ?? ",")
}
public init(contents: String) throws {
self.metadata = try? Self.sniffMetadata(contents: contents)
self.parser = CSVRowParser(contents.makeIterator(), delimiter: metadata?.separator ?? ",")
}
public func readAll() -> [[String]] {
var rows = [[String]]()
for row in parser {
rows.append(row)
}
return rows
}
public typealias Element = [String]
public func makeIterator() -> CSVReaderIterator {
if self.metadata?.hasHeaderRow ?? false {
_ = parser.next() // Strip off the header row.
}
return CSVReaderIterator(self)
}
private static func sniffMetadata(contents: String) throws -> CSVGuess {
var str = contents
return try str.withUTF8 { str in
let first100Kb = UnsafeBufferPointer<UInt8>(
start: str.baseAddress, count: Swift.min(str.count, 100_000))
return try sniffCSV(buffer: first100Kb)
}
}
fileprivate var parser: CSVRowParser<String.Iterator>
public let metadata: CSVGuess?
}
public struct CSVReaderIterator: IteratorProtocol {
public typealias Element = [String]
public mutating func next() -> [String]? {
return reader.parser.next()
}
fileprivate init(_ reader: CSVReader) {
self.reader = reader
}
private var reader: CSVReader
}
enum CSVErrors: Error {
case invalidFile(filename: String)
case invalidFormat(filename: String)
}
| apache-2.0 | e4b8085e5082c7be916c1efb3e1e5ea0 | 31.059524 | 94 | 0.70479 | 4.234277 | false | false | false | false |
brave/browser-ios | brave/src/webview/WebViewScripting.swift | 2 | 5075 | /* This Source Code Form is subject to the terms of the Mozilla Public License, v. 2.0. If a copy of the MPL was not distributed with this file, You can obtain one at http://mozilla.org/MPL/2.0/. */
import Foundation
func hashString (_ obj: AnyObject) -> String {
return String(UInt(bitPattern: ObjectIdentifier(obj)))
}
class LegacyUserContentController
{
var scriptHandlersMainFrame = [String:WKScriptMessageHandler]()
var scriptHandlersSubFrames = [String:WKScriptMessageHandler]()
var scripts:[WKUserScript] = []
weak var webView: BraveWebView?
func addScriptMessageHandler(_ scriptMessageHandler: WKScriptMessageHandler, name: String) {
scriptHandlersMainFrame[name] = scriptMessageHandler
}
func removeScriptMessageHandler(_ name: String) {
scriptHandlersMainFrame.removeValue(forKey: name)
scriptHandlersSubFrames.removeValue(forKey: name)
}
func addUserScript(_ script:WKUserScript) {
var mainFrameOnly = true
if !script.isForMainFrameOnly {
// Only contextMenu injection to subframes for now,
// whitelist this explicitly, don't just inject scripts willy-nilly into frames without
// careful consideration. For instance, there are security implications with password management in frames
mainFrameOnly = false
}
scripts.append(WKUserScript(source: script.source, injectionTime: script.injectionTime, forMainFrameOnly: mainFrameOnly))
}
init(_ webView: BraveWebView) {
self.webView = webView
}
static var jsPageHasBlankTargets:String = {
let path = Bundle.main.path(forResource: "BlankTargetDetector", ofType: "js")!
let source = try! NSString(contentsOfFile: path, encoding: String.Encoding.utf8.rawValue) as String
return source
}()
func injectIntoMain() {
guard let webView = webView else { return }
let result = webView.stringByEvaluatingJavaScript(from: "window.hasOwnProperty('__firefox__')")
if result == "true" {
// already injected into this context
return
}
// use tap detection until this returns false/
// on page start reset enableBlankTargetTapDetection, then set it off when page loaded
webView.blankTargetLinkDetectionOn = true
if webView.stringByEvaluatingJavaScript(from: LegacyUserContentController.jsPageHasBlankTargets) != "true" {
// no _blank
webView.blankTargetLinkDetectionOn = false
}
let js = LegacyJSContext()
js.windowOpenOverride(webView, context:nil)
for (name, handler) in scriptHandlersMainFrame {
js.installHandler(for: webView, handlerName: name, handler:handler)
}
for script in scripts {
webView.stringByEvaluatingJavaScript(from: script.source)
}
}
func injectFingerprintProtection() {
guard let webView = webView,
let handler = scriptHandlersMainFrame[FingerprintingProtection.scriptMessageHandlerName()!] else { return }
let js = LegacyJSContext()
js.installHandler(for: webView, handlerName: FingerprintingProtection.scriptMessageHandlerName(), handler:handler)
webView.stringByEvaluatingJavaScript(from: FingerprintingProtection.script)
let frames = js.findNewFrames(for: webView, withFrameContexts: nil)
for ctx in frames! {
js.installHandler(forContext: ctx, handlerName: FingerprintingProtection.scriptMessageHandlerName(), handler:handler, webView:webView)
js.call(onContext: ctx, script: FingerprintingProtection.script)
}
}
func injectIntoSubFrame() {
let js = LegacyJSContext()
let contexts = js.findNewFrames(for: webView, withFrameContexts: webView?.knownFrameContexts)
for ctx in contexts! {
js.windowOpenOverride(webView, context:ctx)
webView?.knownFrameContexts.insert((ctx as AnyObject).hash as! NSObject)
for (name, handler) in scriptHandlersSubFrames {
js.installHandler(forContext: ctx, handlerName: name, handler:handler, webView:webView)
}
for script in scripts {
if !script.isForMainFrameOnly {
js.call(onContext: ctx, script: script.source)
}
}
}
}
static func injectJsIntoAllFrames(_ webView: BraveWebView, script: String) {
webView.stringByEvaluatingJavaScript(from: script)
let js = LegacyJSContext()
let contexts = js.findNewFrames(for: webView, withFrameContexts: nil)
for ctx in contexts! {
js.call(onContext: ctx, script: script)
}
}
func injectJsIntoPage() {
injectIntoMain()
injectIntoSubFrame()
}
}
class BraveWebViewConfiguration
{
let userContentController: LegacyUserContentController
init(webView: BraveWebView) {
userContentController = LegacyUserContentController(webView)
}
}
| mpl-2.0 | 38466d51ab7859e2d29fae07b6f3f663 | 37.157895 | 198 | 0.673892 | 4.927184 | false | false | false | false |
manavgabhawala/swift | test/stdlib/Renames.swift | 4 | 48267 | // RUN: %target-typecheck-verify-swift
func _Algorithm<I : IteratorProtocol, S : Sequence>(i: I, s: S) {
func fn1(_: EnumerateGenerator<I>) {} // expected-error {{'EnumerateGenerator' has been renamed to 'EnumeratedIterator'}} {{15-33=EnumeratedIterator}} {{none}}
func fn2(_: EnumerateSequence<S>) {} // expected-error {{'EnumerateSequence' has been renamed to 'EnumeratedSequence'}} {{15-32=EnumeratedSequence}} {{none}}
_ = EnumeratedIterator(i) // expected-error {{use the 'enumerated()' method on the sequence}} {{none}}
_ = EnumeratedSequence(s) // expected-error {{use the 'enumerated()' method on the sequence}} {{none}}
}
func _Arrays<T>(e: T) {
// _ = ContiguousArray(count: 1, repeatedValue: e) // xpected-error {{Please use init(repeating:count:) instead}} {{none}}
// _ = ArraySlice(count: 1, repeatedValue: e) // xpected-error {{Please use init(repeating:count:) instead}} {{none}}
// _ = Array(count: 1, repeatedValue: e) // xpected-error {{Please use init(repeating:count:) instead}} {{none}}
// The actual error is: {{argument 'repeatedValue' must precede argument 'count'}}
var a = ContiguousArray<T>()
_ = a.removeAtIndex(0) // expected-error {{'removeAtIndex' has been renamed to 'remove(at:)'}} {{9-22=remove}} {{23-23=at: }} {{none}}
_ = a.replaceRange(0..<1, with: []) // expected-error {{'replaceRange(_:with:)' has been renamed to 'replaceSubrange(_:with:)'}} {{9-21=replaceSubrange}} {{none}}
_ = a.appendContentsOf([]) // expected-error {{'appendContentsOf' has been renamed to 'append(contentsOf:)'}} {{9-25=append}} {{26-26=contentsOf: }} {{none}}
var b = ArraySlice<T>()
_ = b.removeAtIndex(0) // expected-error {{'removeAtIndex' has been renamed to 'remove(at:)'}} {{9-22=remove}} {{23-23=at: }} {{none}}
_ = b.replaceRange(0..<1, with: []) // expected-error {{'replaceRange(_:with:)' has been renamed to 'replaceSubrange(_:with:)'}} {{9-21=replaceSubrange}} {{none}}
_ = b.appendContentsOf([]) // expected-error {{'appendContentsOf' has been renamed to 'append(contentsOf:)'}} {{9-25=append}} {{26-26=contentsOf: }} {{none}}
var c = Array<T>()
_ = c.removeAtIndex(0) // expected-error {{'removeAtIndex' has been renamed to 'remove(at:)'}} {{9-22=remove}} {{23-23=at: }} {{none}}
_ = c.replaceRange(0..<1, with: []) // expected-error {{'replaceRange(_:with:)' has been renamed to 'replaceSubrange(_:with:)'}} {{9-21=replaceSubrange}} {{none}}
_ = c.appendContentsOf([]) // expected-error {{'appendContentsOf' has been renamed to 'append(contentsOf:)'}} {{9-25=append}} {{26-26=contentsOf: }} {{none}}
}
func _Builtin(o: AnyObject, oo: AnyObject?) {
_ = unsafeAddressOf(o) // expected-error {{Removed in Swift 3. Use Unmanaged.passUnretained(x).toOpaque() instead.}} {{none}}
_ = unsafeAddress(of: o) // expected-error {{Removed in Swift 3. Use Unmanaged.passUnretained(x).toOpaque() instead.}} {{none}}
_ = unsafeUnwrap(oo) // expected-error {{Removed in Swift 3. Please use Optional.unsafelyUnwrapped instead.}} {{none}}
}
func _CString() {
_ = String.fromCString([]) // expected-error {{'fromCString' is unavailable: Please use String.init?(validatingUTF8:) instead. Note that it no longer accepts NULL as a valid input. Also consider using String(cString:), that will attempt to repair ill-formed code units.}} {{none}}
_ = String.fromCStringRepairingIllFormedUTF8([]) // expected-error {{'fromCStringRepairingIllFormedUTF8' is unavailable: Please use String.init(cString:) instead. Note that it no longer accepts NULL as a valid input. See also String.decodeCString if you need more control.}} {{none}}
}
func _CTypes<T>(x: Unmanaged<T>) {
func fn(_: COpaquePointer) {} // expected-error {{'COpaquePointer' has been renamed to 'OpaquePointer'}} {{14-28=OpaquePointer}} {{none}}
_ = OpaquePointer(bitPattern: x) // expected-error {{'init(bitPattern:)' is unavailable: use 'Unmanaged.toOpaque()' instead}} {{none}}
}
func _ClosedRange(x: ClosedRange<Int>) {
_ = x.startIndex // expected-error {{'startIndex' has been renamed to 'lowerBound'}} {{9-19=lowerBound}} {{none}}
_ = x.endIndex // expected-error {{'endIndex' has been renamed to 'upperBound'}} {{9-17=upperBound}} {{none}}
}
func _Collection() {
func fn(a: Bit) {} // expected-error {{'Bit' is unavailable: Bit enum has been removed. Please use Int instead.}} {{none}}
func fn<T>(b: IndexingGenerator<T>) {} // expected-error {{'IndexingGenerator' has been renamed to 'IndexingIterator'}} {{17-34=IndexingIterator}} {{none}}
func fn<T : CollectionType>(c: T) {} // expected-error {{'CollectionType' has been renamed to 'Collection'}} {{15-29=Collection}} {{none}}
func fn<T>(d: PermutationGenerator<T, T>) {} // expected-error {{'PermutationGenerator' is unavailable: PermutationGenerator has been removed in Swift 3}}
}
func _Collection<C : Collection>(c: C) {
func fn<T : Collection, U>(_: T, _: U) where T.Generator == U {} // expected-error {{'Generator' has been renamed to 'Iterator'}} {{50-59=Iterator}} {{none}}
_ = c.generate() // expected-error {{'generate()' has been renamed to 'makeIterator()'}} {{9-17=makeIterator}} {{none}}
_ = c.underestimateCount() // expected-error {{'underestimateCount()' has been replaced by 'underestimatedCount'}} {{9-27=underestimatedCount}} {{27-29=}} {{none}}
_ = c.split(1) { _ in return true} // expected-error {{split(maxSplits:omittingEmptySubsequences:whereSeparator:) instead}} {{none}}
}
func _Collection<C : Collection, E>(c: C, e: E) where C.Iterator.Element: Equatable, C.Iterator.Element == E {
_ = c.split(e) // expected-error {{'split(_:maxSplit:allowEmptySlices:)' is unavailable: Please use split(separator:maxSplits:omittingEmptySubsequences:) instead}} {{none}}
}
func _CollectionAlgorithms<C : MutableCollection, I>(c: C, i: I) where C : RandomAccessCollection, C.Index == I {
var c = c
_ = c.partition(i..<i) { _, _ in true } // expected-error {{slice the collection using the range, and call partition(by:)}} {{none}}
c.sortInPlace { _, _ in true } // expected-error {{'sortInPlace' has been renamed to 'sort(by:)'}} {{5-16=sort}} {{none}}
_ = c.partition { _, _ in true } // expected-error {{call partition(by:)}} {{none}}
}
func _CollectionAlgorithms<C : MutableCollection, I>(c: C, i: I) where C : RandomAccessCollection, C.Iterator.Element : Comparable, C.Index == I {
var c = c
_ = c.partition() // expected-error {{call partition(by:)}} {{none}}
_ = c.partition(i..<i) // expected-error {{slice the collection using the range, and call partition(by:)}} {{none}}
c.sortInPlace() // expected-error {{'sortInPlace()' has been renamed to 'sort()'}} {{5-16=sort}} {{none}}
}
func _CollectionAlgorithms<C : Collection, E>(c: C, e: E) where C.Iterator.Element : Equatable, C.Iterator.Element == E {
_ = c.indexOf(e) // expected-error {{'indexOf' has been renamed to 'index(of:)'}} {{9-16=index}} {{17-17=of: }} {{none}}
}
func _CollectionAlgorithms<C : Collection>(c: C) {
_ = c.indexOf { _ in true } // expected-error {{'indexOf' has been renamed to 'index(where:)'}} {{9-16=index}} {{none}}
}
func _CollectionAlgorithms<C : Sequence>(c: C) {
_ = c.sort { _, _ in true } // expected-error {{'sort' has been renamed to 'sorted(by:)'}} {{9-13=sorted}} {{none}}
_ = c.sort({ _, _ in true }) // expected-error {{'sort' has been renamed to 'sorted(by:)'}} {{9-13=sorted}} {{14-14=by: }} {{none}}
}
func _CollectionAlgorithms<C : Sequence>(c: C) where C.Iterator.Element : Comparable {
_ = c.sort() // expected-error {{'sort()' has been renamed to 'sorted()'}} {{9-13=sorted}} {{none}}
}
func _CollectionAlgorithms<C : MutableCollection>(c: C) {
_ = c.sort { _, _ in true } // expected-error {{'sort' has been renamed to 'sorted(by:)'}} {{9-13=sorted}} {{none}}
_ = c.sort({ _, _ in true }) // expected-error {{'sort' has been renamed to 'sorted(by:)'}} {{9-13=sorted}} {{14-14=by: }} {{none}}
}
func _CollectionAlgorithms<C : MutableCollection>(c: C) where C.Iterator.Element : Comparable {
_ = c.sort() // expected-error {{'sort()' has been renamed to 'sorted()'}} {{9-13=sorted}} {{none}}
var a: [Int] = [1,2,3]
var _: [Int] = a.sort() // expected-error {{'sort()' has been renamed to 'sorted()'}} {{20-24=sorted}} {{none}}
var _: [Int] = a.sort { _, _ in true } // expected-error {{'sort' has been renamed to 'sorted(by:)'}} {{20-24=sorted}} {{none}}
var _: [Int] = a.sort({ _, _ in true }) // expected-error {{'sort' has been renamed to 'sorted(by:)'}} {{20-24=sorted}} {{25-25=by: }} {{none}}
_ = a.sort() // OK, in `Void`able context, `sort()` is a renamed `sortInPlace()`.
_ = a.sort { _, _ in true } // OK, `Void`able context, `sort(by:)` is a renamed `sortInPlace(_:)`.
}
func _CollectionOfOne<T>(i: IteratorOverOne<T>) {
func fn(_: GeneratorOfOne<T>) {} // expected-error {{'GeneratorOfOne' has been renamed to 'IteratorOverOne'}} {{14-28=IteratorOverOne}} {{none}}
_ = i.generate() // expected-error {{'generate()' has been renamed to 'makeIterator()'}} {{9-17=makeIterator}} {{none}}
}
func _CompilerProtocols() {
func fn(_: BooleanType) {} // expected-error {{'BooleanType' has been renamed to 'Bool'}} {{14-25=Bool}} {{none}}
}
func _EmptyCollection<T>(i: EmptyIterator<T>) {
func fn(_: EmptyGenerator<T>) {} // expected-error {{'EmptyGenerator' has been renamed to 'EmptyIterator'}} {{14-28=EmptyIterator}} {{none}}
_ = i.generate() // expected-error {{'generate()' has been renamed to 'makeIterator()'}} {{9-17=makeIterator}} {{none}}
}
func _ErrorType() {
func fn(_: ErrorType) {} // expected-error {{'ErrorType' has been renamed to 'Error'}} {{14-23=Error}}
}
func _ExistentialCollection<T>(i: AnyIterator<T>) {
func fn1<T>(_: AnyGenerator<T>) {} // expected-error {{'AnyGenerator' has been renamed to 'AnyIterator'}} {{18-30=AnyIterator}} {{none}}
func fn2<T : AnyCollectionType>(_: T) {} // expected-error {{'AnyCollectionType' has been renamed to '_AnyCollectionProtocol'}} {{16-33=_AnyCollectionProtocol}} {{none}}
func fn3(_: AnyForwardIndex) {} // expected-error {{'AnyForwardIndex' has been renamed to 'AnyIndex'}} {{15-30=AnyIndex}} {{none}}
func fn4(_: AnyBidirectionalIndex) {} // expected-error {{'AnyBidirectionalIndex' has been renamed to 'AnyIndex'}} {{15-36=AnyIndex}} {{none}}
func fn5(_: AnyRandomAccessIndex) {} // expected-error {{'AnyRandomAccessIndex' has been renamed to 'AnyIndex'}} {{15-35=AnyIndex}} {{none}}
_ = anyGenerator(i) // expected-error {{'anyGenerator' has been replaced by 'AnyIterator.init(_:)'}} {{7-19=AnyIterator}} {{none}}
_ = anyGenerator { i.next() } // expected-error {{'anyGenerator' has been replaced by 'AnyIterator.init(_:)'}} {{7-19=AnyIterator}} {{none}}
}
func _ExistentialCollection<T>(s: AnySequence<T>) {
_ = s.underestimateCount() // expected-error {{'underestimateCount()' has been replaced by 'underestimatedCount'}} {{9-27=underestimatedCount}} {{27-29=}} {{none}}
}
func _ExistentialCollection<T>(c: AnyCollection<T>) {
_ = c.underestimateCount() // expected-error {{'underestimateCount()' has been replaced by 'underestimatedCount'}} {{9-27=underestimatedCount}} {{27-29=}} {{none}}
}
func _ExistentialCollection<T>(c: AnyBidirectionalCollection<T>) {
_ = c.underestimateCount() // expected-error {{'underestimateCount()' has been replaced by 'underestimatedCount'}} {{9-27=underestimatedCount}} {{27-29=}} {{none}}
}
func _ExistentialCollection<T>(c: AnyRandomAccessCollection<T>) {
_ = c.underestimateCount() // expected-error {{'underestimateCount()' has been replaced by 'underestimatedCount'}} {{9-27=underestimatedCount}} {{27-29=}} {{none}}
}
func _ExistentialCollection<C : _AnyCollectionProtocol>(c: C) {
_ = c.generate() // expected-error {{'generate()' has been renamed to 'makeIterator()'}} {{9-17=makeIterator}} {{none}}
}
func _Filter() {
func fn<T>(_: LazyFilterGenerator<T>) {} // expected-error {{'LazyFilterGenerator' has been renamed to 'LazyFilterIterator'}} {{17-36=LazyFilterIterator}} {{none}}
}
func _Filter<I : IteratorProtocol>(i: I) {
_ = LazyFilterIterator(i) { _ in true } // expected-error {{'init(_:whereElementsSatisfy:)' is unavailable: use '.lazy.filter' on the sequence}}
}
func _Filter<S : Sequence>(s: S) {
_ = LazyFilterSequence(s) { _ in true } // expected-error {{'init(_:whereElementsSatisfy:)' is unavailable: use '.lazy.filter' on the sequence}}
}
func _Filter<S>(s: LazyFilterSequence<S>) {
_ = s.generate() // expected-error {{'generate()' has been renamed to 'makeIterator()'}} {{9-17=makeIterator}} {{none}}
}
func _Filter<C : Collection>(c: C) {
_ = LazyFilterCollection(c) { _ in true} // expected-error {{'init(_:whereElementsSatisfy:)' is unavailable: use '.lazy.filter' on the collection}}
}
func _Filter<C>(c: LazyFilterCollection<C>) {
_ = c.generate() // expected-error {{'generate()' has been renamed to 'makeIterator()'}} {{9-17=makeIterator}} {{none}}
}
func _FixedPoint() {
var i: Int = 0
var u: UInt = 0
i++ // expected-error {{'++' is unavailable: it has been removed in Swift 3}} {{4-6= += 1}} {{none}}
++i // expected-error {{'++' is unavailable: it has been removed in Swift 3}} {{3-5=}} {{6-6= += 1}} {{none}}
i-- // expected-error {{'--' is unavailable: it has been removed in Swift 3}} {{4-6= -= 1}} {{none}}
--i // expected-error {{'--' is unavailable: it has been removed in Swift 3}} {{3-5=}} {{6-6= -= 1}} {{none}}
u++ // expected-error {{'++' is unavailable: it has been removed in Swift 3}} {{4-6= += 1}} {{none}}
++u // expected-error {{'++' is unavailable: it has been removed in Swift 3}} {{3-5=}} {{6-6= += 1}} {{none}}
u-- // expected-error {{'--' is unavailable: it has been removed in Swift 3}} {{4-6= -= 1}} {{none}}
--u // expected-error {{'--' is unavailable: it has been removed in Swift 3}} {{3-5=}} {{6-6= -= 1}} {{none}}
func fn1<T: IntegerType>(i: T) {} // expected-error {{'IntegerType' has been renamed to 'Integer'}} {{15-26=Integer}} {{none}}
func fn2<T: SignedIntegerType>(i: T) {} // expected-error {{'SignedIntegerType' has been renamed to 'SignedInteger'}} {{15-32=SignedInteger}} {{none}}
func fn3<T: UnsignedIntegerType>(i: T) {} // expected-error {{'UnsignedIntegerType' has been renamed to 'UnsignedInteger'}} {{15-34=UnsignedInteger}} {{none}}
}
func _Flatten() {
func fn<T>(i: FlattenGenerator<T>) {} // expected-error {{'FlattenGenerator' has been renamed to 'FlattenIterator'}} {{17-33=FlattenIterator}} {{none}}
}
func _Flatten<T>(s: FlattenSequence<T>) {
_ = s.generate() // expected-error {{'generate()' has been renamed to 'makeIterator()'}} {{9-17=makeIterator}} {{none}}
}
func _Flatten<T>(c: FlattenCollection<T>) {
_ = c.underestimateCount() // expected-error {{'underestimateCount()' has been replaced by 'underestimatedCount'}} {{9-27=underestimatedCount}} {{27-29=}} {{none}}
}
func _Flatten<T>(c: FlattenBidirectionalCollection<T>) {
_ = c.underestimateCount() // expected-error {{'underestimateCount()' has been replaced by 'underestimatedCount'}} {{9-27=underestimatedCount}} {{27-29=}} {{none}}
}
func _FloatingPoint() {
func fn<F : FloatingPointType>(f: F) {} // expected-error {{'FloatingPointType' has been renamed to 'FloatingPoint'}} {{15-32=FloatingPoint}} {{none}}
}
func _FloatingPoint<F : BinaryFloatingPoint>(f: F) {
_ = f.isSignaling // expected-error {{'isSignaling' has been renamed to 'isSignalingNaN'}} {{9-20=isSignalingNaN}} {{none}}
}
func _FloatingPointTypes() {
var x: Float = 1, y: Float = 1
// FIXME: isSignMinus -> sign is OK? different type.
_ = x.isSignMinus // expected-error {{'isSignMinus' has been renamed to 'sign'}} {{9-20=sign}} {{none}}
_ = x % y // expected-error {{'%' is unavailable: Use truncatingRemainder instead}} {{none}}
x %= y // expected-error {{'%=' is unavailable: Use formTruncatingRemainder instead}} {{none}}
++x // expected-error {{'++' is unavailable: it has been removed in Swift 3}} {{3-5=}} {{6-6= += 1}} {{none}}
--x // expected-error {{'--' is unavailable: it has been removed in Swift 3}} {{3-5=}} {{6-6= -= 1}} {{none}}
x++ // expected-error {{'++' is unavailable: it has been removed in Swift 3}} {{4-6= += 1}} {{none}}
x-- // expected-error {{'--' is unavailable: it has been removed in Swift 3}} {{4-6= -= 1}} {{none}}
}
func _HashedCollection<T>(x: Set<T>, i: Set<T>.Index, e: T) {
var x = x
_ = x.removeAtIndex(i) // expected-error {{'removeAtIndex' has been renamed to 'remove(at:)'}} {{9-22=remove}} {{23-23=at: }} {{none}}
_ = x.generate() // expected-error {{'generate()' has been renamed to 'makeIterator()'}} {{9-17=makeIterator}} {{none}}
_ = x.indexOf(e) // expected-error {{'indexOf' has been renamed to 'index(of:)'}} {{9-16=index}} {{17-17=of: }} {{none}}
}
func _HashedCollection<K, V>(x: Dictionary<K, V>, i: Dictionary<K, V>.Index, k: K) {
var x = x
_ = x.removeAtIndex(i) // expected-error {{'removeAtIndex' has been renamed to 'remove(at:)'}} {{9-22=remove}} {{23-23=at: }} {{none}}
_ = x.indexForKey(k) // expected-error {{'indexForKey' has been renamed to 'index(forKey:)'}} {{9-20=index}} {{21-21=forKey: }} {{none}}
_ = x.removeValueForKey(k) // expected-error {{'removeValueForKey' has been renamed to 'removeValue(forKey:)'}} {{9-26=removeValue}} {{27-27=forKey: }} {{none}}
_ = x.generate() // expected-error {{'generate()' has been renamed to 'makeIterator()'}} {{9-17=makeIterator}} {{none}}
}
func _ImplicitlyUnwrappedOptional<T>(x: ImplicitlyUnwrappedOptional<T>) {
_ = ImplicitlyUnwrappedOptional<T>() // expected-error {{'init()' is unavailable: Please use nil literal instead.}} {{none}}
_ = ImplicitlyUnwrappedOptional<T>.map(x)() { _ in true } // expected-error {{'map' is unavailable: Has been removed in Swift 3.}}
_ = ImplicitlyUnwrappedOptional<T>.flatMap(x)() { _ in true } // expected-error {{'flatMap' is unavailable: Has been removed in Swift 3.}}
// FIXME: No way to call map and flatMap as method?
// _ = (x as ImplicitlyUnwrappedOptional).map { _ in true } // xpected-error {{}} {{none}}
// _ = (x as ImplicitlyUnwrappedOptional).flatMap { _ in true } // xpected-error {{}} {{none}}
}
func _Index<T : _Incrementable>(i: T) {
var i = i
--i // expected-error {{'--' is unavailable: it has been removed in Swift 3}} {{3-5=}} {{6-6= = i.predecessor()}} {{none}}
i-- // expected-error {{'--' is unavailable: it has been removed in Swift 3}} {{4-6= = i.predecessor()}} {{none}}
++i // expected-error {{'++' is unavailable: it has been removed in Swift 3}} {{3-5=}} {{6-6= = i.successor()}} {{none}}
i++ // expected-error {{'++' is unavailable: it has been removed in Swift 3}} {{4-6= = i.successor()}} {{none}}
}
func _Index() {
func fn1<T : ForwardIndexType>(_: T) {} // expected-error {{'ForwardIndexType' has been renamed to 'Comparable'}} {{16-32=Comparable}} {{none}}
func fn2<T : BidirectionalIndexType>(_: T) {} // expected-error {{'BidirectionalIndexType' has been renamed to 'Comparable'}} {{16-38=Comparable}} {{none}}
func fn3<T : RandomAccessIndexType>(_: T) {} // expected-error {{'RandomAccessIndexType' has been renamed to 'Strideable'}} {{16-37=Strideable}} {{none}}
}
func _InputStream() {
_ = readLine(stripNewline: true) // expected-error {{'readLine(stripNewline:)' has been renamed to 'readLine(strippingNewline:)'}} {{7-15=readLine}} {{16-28=strippingNewline}} {{none}}
_ = readLine() // ok
}
func _IntegerArithmetic() {
func fn1<T : IntegerArithmeticType>(_: T) {} // expected-error {{'IntegerArithmeticType' has been renamed to 'IntegerArithmetic'}} {{16-37=IntegerArithmetic}} {{none}}
func fn2<T : SignedNumberType>(_: T) {} // expected-error {{'SignedNumberType' has been renamed to 'SignedNumber'}} {{16-32=SignedNumber}} {{none}}
}
func _Join() {
func fn<T>(_: JoinGenerator<T>) {} // expected-error {{'JoinGenerator' has been renamed to 'JoinedIterator'}} {{17-30=JoinedIterator}} {{none}}
}
func _Join<T>(s: JoinedSequence<T>) {
_ = s.generate() // expected-error {{'generate()' has been renamed to 'makeIterator()'}} {{9-17=makeIterator}} {{none}}
}
func _Join<S : Sequence>(s: S) where S.Iterator.Element : Sequence {
_ = s.joinWithSeparator(s) // expected-error {{'joinWithSeparator' has been renamed to 'joined(separator:)'}} {{9-26=joined}} {{27-27=separator: }} {{none}}
}
func _LazyCollection() {
func fn<T : LazyCollectionType>(_: T) {} // expected-error {{'LazyCollectionType' has been renamed to 'LazyCollectionProtocol'}} {{15-33=LazyCollectionProtocol}} {{none}}
}
func _LazySequence() {
func fn<T : LazySequenceType>(_: T) {} // expected-error {{'LazySequenceType' has been renamed to 'LazySequenceProtocol'}} {{15-31=LazySequenceProtocol}} {{none}}
}
func _LazySequence<S : LazySequenceProtocol>(s: S) {
_ = s.array // expected-error {{'array' is unavailable: Please use Array initializer instead.}} {{none}}
}
func _LifetimeManager<T>(x: T) {
var x = x
_ = withUnsafeMutablePointer(&x) { _ in } // expected-error {{'withUnsafeMutablePointer' has been renamed to 'withUnsafeMutablePointer(to:_:)'}} {{7-31=withUnsafeMutablePointer}} {{32-32=to: }} {{none}}
_ = withUnsafeMutablePointers(&x, &x) { _, _ in } // expected-error {{'withUnsafeMutablePointers' is unavailable: use nested withUnsafeMutablePointer(to:_:) instead}} {{none}}
_ = withUnsafeMutablePointers(&x, &x, &x) { _, _, _ in } // expected-error {{'withUnsafeMutablePointers' is unavailable: use nested withUnsafeMutablePointer(to:_:) instead}} {{none}}
_ = withUnsafePointer(&x) { _ in } // expected-error {{'withUnsafePointer' has been renamed to 'withUnsafePointer(to:_:)'}} {7-24=withUnsafePointer}} {{25-25=to: }} {{none}}
_ = withUnsafePointers(&x, &x) { _, _ in } // expected-error {{'withUnsafePointers' is unavailable: use nested withUnsafePointer(to:_:) instead}} {{none}}
_ = withUnsafePointers(&x, &x, &x) { _, _, _ in } // expected-error {{'withUnsafePointers' is unavailable: use nested withUnsafePointer(to:_:) instead}} {{none}}
}
func _ManagedBuffer<H, E>(x: ManagedBufferPointer<H, E>, h: H, bc: AnyClass) {
_ = x.allocatedElementCount // expected-error {{'allocatedElementCount' has been renamed to 'capacity'}} {{9-30=capacity}} {{none}}
_ = ManagedBuffer<H, E>.create(1) { _ in h } // expected-error {{'create(_:initialValue:)' has been renamed to 'create(minimumCapacity:makingHeaderWith:)'}} {{27-33=create}} {{34-34=minimumCapacity: }} {{none}}
_ = ManagedBuffer<H, E>.create(1, initialValue: { _ in h }) // expected-error {{'create(_:initialValue:)' has been renamed to 'create(minimumCapacity:makingHeaderWith:)'}} {{27-33=create}} {{34-34=minimumCapacity: }} {{37-49=makingHeaderWith}} {{none}}
_ = ManagedBufferPointer<H, E>(bufferClass: bc, minimumCapacity: 1, initialValue: { _, _ in h }) // expected-error {{'init(bufferClass:minimumCapacity:initialValue:)' has been renamed to 'init(bufferClass:minimumCapacity:makingHeaderWith:)'}} {{71-83=makingHeaderWith}} {{none}}
_ = ManagedBufferPointer<H, E>(bufferClass: bc, minimumCapacity: 1) { _, _ in h } // OK
func fn(_: ManagedProtoBuffer<H, E>) {} // expected-error {{'ManagedProtoBuffer' has been renamed to 'ManagedBuffer'}} {{14-32=ManagedBuffer}} {{none}}
}
func _Map() {
func fn<B, E>(_: LazyMapGenerator<B, E>) {} // expected-error {{'LazyMapGenerator' has been renamed to 'LazyMapIterator'}} {{20-36=LazyMapIterator}} {{none}}
}
func _Map<S : Sequence>(s: S) {
_ = LazyMapSequence(s) { _ in true } // expected-error {{'init(_:transform:)' is unavailable: use '.lazy.map' on the sequence}} {{none}}
}
func _Map<C : Collection>(c: C) {
_ = LazyMapCollection(c) { _ in true } // expected-error {{'init(_:transform:)' is unavailable: use '.lazy.map' on the collection}} {{none}}
}
func _MemoryLayout<T>(t: T) {
_ = sizeof(T.self) // expected-error {{'sizeof' is unavailable: use MemoryLayout<T>.size instead.}} {{7-14=MemoryLayout<}} {{15-21=>.size}} {{none}}
_ = alignof(T.self) // expected-error {{'alignof' is unavailable: use MemoryLayout<T>.alignment instead.}} {{7-15=MemoryLayout<}} {{16-22=>.alignment}} {{none}}
_ = strideof(T.self) // expected-error {{'strideof' is unavailable: use MemoryLayout<T>.stride instead.}} {{7-16=MemoryLayout<}} {{17-23=>.stride}} {{none}}
_ = sizeofValue(t) // expected-error {{'sizeofValue' has been replaced by 'MemoryLayout.size(ofValue:)'}} {{7-18=MemoryLayout.size}} {{19-19=ofValue: }} {{none}}
_ = alignofValue(t) // expected-error {{'alignofValue' has been replaced by 'MemoryLayout.alignment(ofValue:)'}} {{7-19=MemoryLayout.alignment}} {{20-20=ofValue: }} {{none}}
_ = strideofValue(t) // expected-error {{'strideofValue' has been replaced by 'MemoryLayout.stride(ofValue:)'}} {{7-20=MemoryLayout.stride}} {{21-21=ofValue: }} {{none}}
}
func _Mirror() {
func fn<M : MirrorPathType>(_: M) {} // expected-error {{'MirrorPathType' has been renamed to 'MirrorPath'}} {{15-29=MirrorPath}} {{none}}
}
func _MutableCollection() {
func fn1<C : MutableCollectionType>(_: C) {} // expected-error {{'MutableCollectionType' has been renamed to 'MutableCollection'}} {{16-37=MutableCollection}} {{none}}
func fn2<C : MutableSliceable>(_: C) {} // expected-error {{'MutableSliceable' is unavailable: Please use 'Collection where SubSequence : MutableCollection'}} {{none}}
}
func _OptionSet() {
func fn<O : OptionSetType>(_: O) {} // expected-error {{'OptionSetType' has been renamed to 'OptionSet'}} {{15-28=OptionSet}} {{none}}
}
func _Optional<T>(x: T) {
_ = Optional<T>.None // expected-error {{'None' has been renamed to 'none'}} {{19-23=none}} {{none}}
_ = Optional<T>.Some(x) // expected-error {{'Some' has been renamed to 'some'}} {{19-23=some}} {{none}}
}
func _TextOutputStream() {
func fn<S : OutputStreamType>(_: S) {} // expected-error {{'OutputStreamType' has been renamed to 'TextOutputStream'}} {{15-31=TextOutputStream}} {{none}}
}
func _TextOutputStream<S : TextOutputStreamable, O : TextOutputStream>(s: S, o: O) {
var o = o
s.writeTo(&o) // expected-error {{'writeTo' has been renamed to 'write(to:)'}} {{5-12=write}} {{13-13=to: }} {{none}}
}
func _Policy() {
func fn<O : BitwiseOperationsType>(_: O) {} // expected-error {{'BitwiseOperationsType' has been renamed to 'BitwiseOperations'}} {{15-36=BitwiseOperations}} {{none}}
}
func _Print<T, O : TextOutputStream>(x: T, out: O) {
var out = out
print(x, toStream: &out) // expected-error {{'print(_:separator:terminator:toStream:)' has been renamed to 'print(_:separator:terminator:to:)'}} {{3-8=print}} {{12-20=to}} {{none}}
print(x, x, separator: "/", toStream: &out) // expected-error {{'print(_:separator:terminator:toStream:)' has been renamed to 'print(_:separator:terminator:to:)'}} {{3-8=print}} {{31-39=to}} {{none}}
print(terminator: "|", toStream: &out) // expected-error {{'print(_:separator:terminator:toStream:)' has been renamed to 'print(_:separator:terminator:to:)'}} {{3-8=print}} {{26-34=to}} {{none}}
print(x, separator: "*", terminator: "$", toStream: &out) // expected-error {{'print(_:separator:terminator:toStream:)' has been renamed to 'print(_:separator:terminator:to:)'}} {{3-8=print}} {{45-53=to}} {{none}}
debugPrint(x, toStream: &out) // expected-error {{'debugPrint(_:separator:terminator:toStream:)' has been renamed to 'debugPrint(_:separator:terminator:to:)'}} {{3-13=debugPrint}} {{17-25=to}} {{none}}
}
func _Print<T>(x: T) {
print(x, appendNewline: true) // expected-error {{'print(_:appendNewline:)' is unavailable: Please use 'terminator: ""' instead of 'appendNewline: false': 'print((...), terminator: "")'}} {{none}}
debugPrint(x, appendNewline: true) // expected-error {{'debugPrint(_:appendNewline:)' is unavailable: Please use 'terminator: ""' instead of 'appendNewline: false': 'debugPrint((...), terminator: "")'}} {{none}}
}
func _Print<T, O : TextOutputStream>(x: T, o: O) {
// FIXME: Not working due to <rdar://22101775>
//var o = o
//print(x, &o) // xpected-error {{}} {{none}}
//debugPrint(x, &o) // xpected-error {{}} {{none}}
//print(x, &o, appendNewline: true) // xpected-error {{}} {{none}}
//debugPrint(x, &o, appendNewline: true) // xpected-error {{}} {{none}}
}
func _Range() {
func fn1<B>(_: RangeGenerator<B>) {} // expected-error {{'RangeGenerator' has been renamed to 'IndexingIterator'}} {{18-32=IndexingIterator}} {{none}}
func fn2<I : IntervalType>(_: I) {} // expected-error {{'IntervalType' is unavailable: IntervalType has been removed in Swift 3. Use ranges instead.}} {{none}}
func fn3<B>(_: HalfOpenInterval<B>) {} // expected-error {{'HalfOpenInterval' has been renamed to 'Range'}} {{18-34=Range}} {{none}}
func fn4<B>(_: ClosedInterval<B>) {} // expected-error {{'ClosedInterval' has been renamed to 'ClosedRange'}} {{18-32=ClosedRange}} {{none}}
}
func _Range<T>(r: Range<T>) {
_ = r.startIndex // expected-error {{'startIndex' has been renamed to 'lowerBound'}} {{9-19=lowerBound}} {{none}}
_ = r.endIndex // expected-error {{'endIndex' has been renamed to 'upperBound'}} {{9-17=upperBound}} {{none}}
}
func _Range<T>(r: ClosedRange<T>) {
_ = r.clamp(r) // expected-error {{'clamp' is unavailable: Call clamped(to:) and swap the argument and the receiver. For example, x.clamp(y) becomes y.clamped(to: x) in Swift 3.}} {{none}}
}
func _Range<T>(r: CountableClosedRange<T>) {
_ = r.clamp(r) // expected-error {{'clamp' is unavailable: Call clamped(to:) and swap the argument and the receiver. For example, x.clamp(y) becomes y.clamped(to: x) in Swift 3.}} {{none}}
}
func _RangeReplaceableCollection() {
func fn<I : RangeReplaceableCollectionType>(_: I) {} // expected-error {{'RangeReplaceableCollectionType' has been renamed to 'RangeReplaceableCollection'}} {{15-45=RangeReplaceableCollection}} {{none}}
}
func _RangeReplaceableCollection<C : RangeReplaceableCollection>(c: C, i: C.Index) {
var c = c
c.replaceRange(i..<i, with: []) // expected-error {{'replaceRange(_:with:)' has been renamed to 'replaceSubrange(_:with:)'}} {{5-17=replaceSubrange}} {{none}}
_ = c.removeAtIndex(i) // expected-error {{'removeAtIndex' has been renamed to 'remove(at:)'}} {{9-22=remove}} {{23-23=at: }} {{none}}
c.removeRange(i..<i) // expected-error {{'removeRange' has been renamed to 'removeSubrange'}} {{5-16=removeSubrange}} {{none}}
c.appendContentsOf([]) // expected-error {{'appendContentsOf' has been renamed to 'append(contentsOf:)'}} {{5-21=append}} {{22-22=contentsOf: }} {{none}}
c.insertContentsOf(c, at: i) // expected-error {{'insertContentsOf(_:at:)' has been renamed to 'insert(contentsOf:at:)'}} {{5-21=insert}} {{22-22=contentsOf: }} {{none}}
}
func _Reflection(x: ObjectIdentifier) {
_ = x.uintValue // expected-error {{'uintValue' is unavailable: use the 'UInt(_:)' initializer}} {{none}}
}
func _Repeat() {
func fn<E>(_: Repeat<E>) {} // expected-error {{'Repeat' has been renamed to 'Repeated'}} {{17-23=Repeated}} {{none}}
}
func _Repeat<E>(e: E) {
_ = Repeated(count: 0, repeatedValue: e) // expected-error {{'init(count:repeatedValue:)' is unavailable: Please use repeatElement(_:count:) function instead}} {{none}}
}
func _Reverse<C : BidirectionalCollection>(c: C) {
_ = ReverseCollection(c) // expected-error {{'ReverseCollection' has been renamed to 'ReversedCollection'}} {{7-24=ReversedCollection}} {{none}}
_ = ReversedCollection(c) // expected-error {{'init' has been replaced by instance method 'BidirectionalCollection.reversed()'}} {{7-25=c.reversed}} {{26-27=}} {{none}}
_ = c.reverse() // expected-error {{'reverse()' has been renamed to 'reversed()'}} {{9-16=reversed}} {{none}}
}
func _Reverse<C : RandomAccessCollection>(c: C) {
_ = ReverseRandomAccessCollection(c) // expected-error {{'ReverseRandomAccessCollection' has been renamed to 'ReversedRandomAccessCollection'}} {{7-36=ReversedRandomAccessCollection}} {{none}}
_ = ReversedRandomAccessCollection(c) // expected-error {{'init' has been replaced by instance method 'RandomAccessCollection.reversed()'}} {{7-37=c.reversed}} {{38-39=}} {{none}}
_ = c.reverse() // expected-error {{'reverse()' has been renamed to 'reversed()'}} {{9-16=reversed}} {{none}}
}
func _Reverse<C : LazyCollectionProtocol>(c: C) where C : BidirectionalCollection, C.Elements : BidirectionalCollection {
_ = c.reverse() // expected-error {{'reverse()' has been renamed to 'reversed()'}} {{9-16=reversed}} {{none}}
}
func _Reverse<C : LazyCollectionProtocol>(c: C) where C : RandomAccessCollection, C.Elements : RandomAccessCollection {
_ = c.reverse() // expected-error {{'reverse()' has been renamed to 'reversed()'}} {{9-16=reversed}} {{none}}
}
func _Sequence() {
func fn1<G : GeneratorType>(_: G) {} // expected-error {{'GeneratorType' has been renamed to 'IteratorProtocol'}} {{16-29=IteratorProtocol}} {{none}}
func fn2<S : SequenceType>(_: S) {} // expected-error {{'SequenceType' has been renamed to 'Sequence'}} {{16-28=Sequence}} {{none}}
func fn3<I : IteratorProtocol>(_: GeneratorSequence<I>) {} // expected-error {{'GeneratorSequence' has been renamed to 'IteratorSequence'}} {{37-54=IteratorSequence}} {{none}}
}
func _Sequence<S : Sequence>(s: S) {
_ = s.generate() // expected-error {{'generate()' has been renamed to 'makeIterator()'}} {{9-17=makeIterator}} {{none}}
_ = s.underestimateCount() // expected-error {{'underestimateCount()' has been replaced by 'underestimatedCount'}} {{9-27=underestimatedCount}} {{27-29=}} {{none}}
_ = s.split(1, allowEmptySlices: true) { _ in true } // expected-error {{'split(_:allowEmptySlices:isSeparator:)' is unavailable: call 'split(maxSplits:omittingEmptySubsequences:whereSeparator:)' and invert the 'allowEmptySlices' argument}} {{none}}
}
func _Sequence<S : Sequence>(s: S, e: S.Iterator.Element) where S.Iterator.Element : Equatable {
_ = s.split(e, maxSplit: 1, allowEmptySlices: true) // expected-error {{'split(_:maxSplit:allowEmptySlices:)' is unavailable: call 'split(separator:maxSplits:omittingEmptySubsequences:)' and invert the 'allowEmptySlices' argument}} {{none}}
}
func _SequenceAlgorithms<S : Sequence>(x: S) {
_ = x.enumerate() // expected-error {{'enumerate()' has been renamed to 'enumerated()'}} {{9-18=enumerated}} {{none}}
_ = x.minElement { _, _ in true } // expected-error {{'minElement' has been renamed to 'min(by:)'}} {{9-19=min}} {{none}}
_ = x.maxElement { _, _ in true } // expected-error {{'maxElement' has been renamed to 'max(by:)'}} {{9-19=max}} {{none}}
_ = x.reverse() // expected-error {{'reverse()' has been renamed to 'reversed()'}} {{9-16=reversed}} {{none}}
_ = x.startsWith([]) { _ in true } // expected-error {{'startsWith(_:isEquivalent:)' has been renamed to 'starts(with:by:)'}} {{9-19=starts}} {{20-20=with: }} {{none}}
_ = x.elementsEqual([], isEquivalent: { _, _ in true }) // expected-error {{'elementsEqual(_:isEquivalent:)' has been renamed to 'elementsEqual(_:by:)'}} {{9-22=elementsEqual}} {{27-39=by}} {{none}}
_ = x.elementsEqual([]) { _, _ in true } // OK
_ = x.lexicographicalCompare([]) { _, _ in true } // expected-error {{'lexicographicalCompare(_:isOrderedBefore:)' has been renamed to 'lexicographicallyPrecedes(_:by:)'}} {{9-31=lexicographicallyPrecedes}}{{none}}
_ = x.contains({ _ in true }) // expected-error {{'contains' has been renamed to 'contains(where:)'}} {{9-17=contains}} {{18-18=where: }} {{none}}
_ = x.contains { _ in true } // OK
_ = x.reduce(1, combine: { _, _ in 1 }) // expected-error {{'reduce(_:combine:)' has been renamed to 'reduce(_:_:)'}} {{9-15=reduce}} {{19-28=}} {{none}}
_ = x.reduce(1) { _, _ in 1 } // OK
}
func _SequenceAlgorithms<S : Sequence>(x: S) where S.Iterator.Element : Comparable {
_ = x.minElement() // expected-error {{'minElement()' has been renamed to 'min()'}} {{9-19=min}} {{none}}
_ = x.maxElement() // expected-error {{'maxElement()' has been renamed to 'max()'}} {{9-19=max}} {{none}}
_ = x.startsWith([]) // expected-error {{'startsWith' has been renamed to 'starts(with:)'}} {{9-19=starts}} {{20-20=with: }} {{none}}
_ = x.lexicographicalCompare([]) // expected-error {{'lexicographicalCompare' has been renamed to 'lexicographicallyPrecedes'}} {{9-31=lexicographicallyPrecedes}}{{none}}
}
func _SetAlgebra() {
func fn<S : SetAlgebraType>(_: S) {} // expected-error {{'SetAlgebraType' has been renamed to 'SetAlgebra'}} {{15-29=SetAlgebra}} {{none}}
}
func _SetAlgebra<S : SetAlgebra>(s: S) {
var s = s
_ = s.intersect(s) // expected-error {{'intersect' has been renamed to 'intersection(_:)'}} {{9-18=intersection}} {{none}}
_ = s.exclusiveOr(s) // expected-error {{'exclusiveOr' has been renamed to 'symmetricDifference(_:)'}} {{9-20=symmetricDifference}} {{none}}
s.unionInPlace(s) // expected-error {{'unionInPlace' has been renamed to 'formUnion(_:)'}} {{5-17=formUnion}} {{none}}
s.intersectInPlace(s) // expected-error {{'intersectInPlace' has been renamed to 'formIntersection(_:)'}} {{5-21=formIntersection}} {{none}}
s.exclusiveOrInPlace(s) // expected-error {{'exclusiveOrInPlace' has been renamed to 'formSymmetricDifference(_:)'}} {{5-23=formSymmetricDifference}} {{none}}
_ = s.isSubsetOf(s) // expected-error {{'isSubsetOf' has been renamed to 'isSubset(of:)'}} {{9-19=isSubset}} {{20-20=of: }} {{none}}
_ = s.isDisjointWith(s) // expected-error {{'isDisjointWith' has been renamed to 'isDisjoint(with:)'}} {{9-23=isDisjoint}} {{24-24=with: }} {{none}}
s.subtractInPlace(s) // expected-error {{'subtractInPlace' has been renamed to 'subtract(_:)'}} {{5-20=subtract}} {{none}}
_ = s.isStrictSupersetOf(s) // expected-error {{'isStrictSupersetOf' has been renamed to 'isStrictSuperset(of:)'}} {{9-27=isStrictSuperset}} {{28-28=of: }} {{none}}
_ = s.isStrictSubsetOf(s) // expected-error {{'isStrictSubsetOf' has been renamed to 'isStrictSubset(of:)'}} {{9-25=isStrictSubset}} {{26-26=of: }} {{none}}
}
func _StaticString(x: StaticString) {
_ = x.byteSize // expected-error {{'byteSize' has been renamed to 'utf8CodeUnitCount'}} {{9-17=utf8CodeUnitCount}} {{none}}
_ = x.stringValue // expected-error {{'stringValue' is unavailable: use the 'String(_:)' initializer}} {{none}}
}
func _Stride<T : Strideable>(x: T, d: T.Stride) {
func fn1<T>(_: StrideToGenerator<T>) {} // expected-error {{'StrideToGenerator' has been renamed to 'StrideToIterator'}} {{18-35=StrideToIterator}} {{none}}
func fn2<T>(_: StrideThroughGenerator<T>) {} // expected-error {{'StrideThroughGenerator' has been renamed to 'StrideThroughIterator'}} {{18-40=StrideThroughIterator}} {{none}}
_ = x.stride(to: x, by: d) // expected-error {{'stride(to:by:)' is unavailable: Use stride(from:to:by:) free function instead}} {{none}}
_ = x.stride(through: x, by: d) // expected-error {{'stride(through:by:)' is unavailable: Use stride(from:through:by:) free function instead}}
}
func _String<S, C>(x: String, s: S, c: C, i: String.Index)
where S : Sequence, S.Iterator.Element == Character, C : Collection, C.Iterator.Element == Character {
var x = x
x.appendContentsOf(x) // expected-error {{'appendContentsOf' has been renamed to 'append(_:)'}} {{5-21=append}} {{none}}
x.appendContentsOf(s) // expected-error {{'appendContentsOf' has been renamed to 'append(contentsOf:)'}} {{5-21=append}} {{22-22=contentsOf: }} {{none}}
x.insertContentsOf(c, at: i) // expected-error {{'insertContentsOf(_:at:)' has been renamed to 'insert(contentsOf:at:)'}} {{5-21=insert}} {{22-22=contentsOf: }} {{none}}
x.replaceRange(i..<i, with: c) // expected-error {{'replaceRange(_:with:)' has been renamed to 'replaceSubrange'}} {{5-17=replaceSubrange}} {{none}}
x.replaceRange(i..<i, with: x) // expected-error {{'replaceRange(_:with:)' has been renamed to 'replaceSubrange'}} {{5-17=replaceSubrange}} {{none}}
_ = x.removeAtIndex(i) // expected-error {{'removeAtIndex' has been renamed to 'remove(at:)'}} {{9-22=remove}} {{23-23=at: }} {{none}}
x.removeRange(i..<i) // expected-error {{'removeRange' has been renamed to 'removeSubrange'}} {{5-16=removeSubrange}} {{none}}
_ = x.lowercaseString // expected-error {{'lowercaseString' has been renamed to 'lowercased()'}} {{9-24=lowercased()}} {{none}}
_ = x.uppercaseString // expected-error {{'uppercaseString' has been renamed to 'uppercased()'}} {{9-24=uppercased()}} {{none}}
// FIXME: SR-1649 <rdar://problem/26563343>; We should suggest to add '()'
}
func _String<S : Sequence>(s: S, sep: String) where S.Iterator.Element == String {
_ = s.joinWithSeparator(sep) // expected-error {{'joinWithSeparator' has been renamed to 'joined(separator:)'}} {{9-26=joined}} {{27-27=separator: }} {{none}}
}
func _StringCharacterView<S, C>(x: String.CharacterView, s: S, c: C, i: String.CharacterView.Index)
where S : Sequence, S.Iterator.Element == Character, C : Collection, C.Iterator.Element == Character {
var x = x
x.replaceRange(i..<i, with: c) // expected-error {{'replaceRange(_:with:)' has been renamed to 'replaceSubrange'}} {{5-17=replaceSubrange}} {{none}}
x.appendContentsOf(s) // expected-error {{'appendContentsOf' has been renamed to 'append(contentsOf:)'}} {{5-21=append}} {{22-22=contentsOf: }} {{none}}
}
func _StringAppend(s: inout String, u: UnicodeScalar) {
s.append(u) // expected-error {{'append' is unavailable: Replaced by append(_: String)}} {{none}}
}
func _StringLegacy(c: Character, u: UnicodeScalar) {
_ = String(count: 1, repeatedValue: c) // expected-error {{'init(count:repeatedValue:)' is unavailable: Renamed to init(repeating:count:) and reordered parameters}} {{none}}
_ = String(count: 1, repeatedValue: u) // expected-error {{'init(count:repeatedValue:)' is unavailable: Renamed to init(repeating:count:) and reordered parameters}} {{none}}
_ = String(repeating: c, count: 1) // expected-error {{'init(repeating:count:)' is unavailable: Replaced by init(repeating: String, count: Int)}} {{none}}
_ = String(repeating: u, count: 1) // expected-error {{'init(repeating:count:)' is unavailable: Replaced by init(repeating: String, count: Int)}} {{none}}
}
func _Unicode<C : UnicodeCodec>(s: UnicodeScalar, c: C.Type, out: (C.CodeUnit) -> Void) {
func fn<T : UnicodeCodecType>(_: T) {} // expected-error {{'UnicodeCodecType' has been renamed to 'UnicodeCodec'}} {{15-31=UnicodeCodec}} {{none}}
c.encode(s, output: out) // expected-error {{encode(_:output:)' has been renamed to 'encode(_:into:)}} {{5-11=encode}} {{15-21=into}} {{none}}
c.encode(s) { _ in } // OK
UTF8.encode(s, output: { _ in }) // expected-error {{'encode(_:output:)' has been renamed to 'encode(_:into:)'}} {{8-14=encode}} {{18-24=into}} {{none}}
UTF16.encode(s, output: { _ in }) // expected-error {{'encode(_:output:)' has been renamed to 'encode(_:into:)'}} {{9-15=encode}} {{19-25=into}} {{none}}
UTF32.encode(s, output: { _ in }) // expected-error {{'encode(_:output:)' has been renamed to 'encode(_:into:)'}} {{9-15=encode}} {{19-25=into}} {{none}}
}
func _Unicode<I : IteratorProtocol, E : UnicodeCodec>(i: I, e: E.Type) where I.Element == E.CodeUnit {
_ = transcode(e, e, i, { _ in }, stopOnError: true) // expected-error {{'transcode(_:_:_:_:stopOnError:)' is unavailable: use 'transcode(_:from:to:stoppingOnError:into:)'}} {{none}}
_ = UTF16.measure(e, input: i, repairIllFormedSequences: true) // expected-error {{'measure(_:input:repairIllFormedSequences:)' is unavailable: use 'transcodedLength(of:decodedAs:repairingIllFormedSequences:)'}} {{none}}
}
func _UnicodeScalar(s: UnicodeScalar) {
_ = UnicodeScalar() // expected-error {{'init()' is unavailable: use 'UnicodeScalar(0)'}} {{none}}
_ = s.escape(asASCII: true) // expected-error {{'escape(asASCII:)' has been renamed to 'escaped(asASCII:)'}} {{9-15=escaped}} {{none}}
}
func _Unmanaged<T>(x: Unmanaged<T>, p: OpaquePointer) {
_ = Unmanaged<T>.fromOpaque(p) // expected-error {{'fromOpaque' is unavailable: use 'fromOpaque(_: UnsafeRawPointer)' instead}} {{none}}
let _: OpaquePointer = x.toOpaque() // expected-error {{'toOpaque()' is unavailable: use 'toOpaque() -> UnsafeRawPointer' instead}} {{none}}
}
func _UnsafeBufferPointer() {
func fn<T>(x: UnsafeBufferPointerGenerator<T>) {} // expected-error {{'UnsafeBufferPointerGenerator' has been renamed to 'UnsafeBufferPointerIterator'}} {{17-45=UnsafeBufferPointerIterator}} {{none}}
}
func _UnsafePointer<T>(x: UnsafePointer<T>) {
_ = UnsafePointer<T>.Memory.self // expected-error {{'Memory' has been renamed to 'Pointee'}} {{24-30=Pointee}} {{none}}
_ = UnsafePointer<T>() // expected-error {{'init()' is unavailable: use 'nil' literal}} {{none}}
_ = x.memory // expected-error {{'memory' has been renamed to 'pointee'}} {{9-15=pointee}} {{none}}
}
func _UnsafePointer<T>(x: UnsafeMutablePointer<T>, e: T) {
var x = x
_ = UnsafeMutablePointer<T>.Memory.self // expected-error {{'Memory' has been renamed to 'Pointee'}} {{31-37=Pointee}} {{none}}
_ = UnsafeMutablePointer<T>() // expected-error {{'init()' is unavailable: use 'nil' literal}} {{none}}
_ = x.memory // expected-error {{'memory' has been renamed to 'pointee'}} {{9-15=pointee}} {{none}}
_ = UnsafeMutablePointer<T>.alloc(1) // expected-error {{'alloc' has been renamed to 'allocate(capacity:)'}} {{31-36=allocate}} {{37-37=capacity: }} {{none}}
x.dealloc(1) // expected-error {{'dealloc' has been renamed to 'deallocate(capacity:)'}} {{5-12=deallocate}} {{13-13=capacity: }} {{none}}
x.memory = e // expected-error {{'memory' has been renamed to 'pointee'}} {{5-11=pointee}} {{none}}
x.initialize(e) // expected-error {{'initialize' has been renamed to 'initialize(to:)'}} {{5-15=initialize}} {{16-16=to: }} {{none}}
x.destroy() // expected-error {{'destroy()' has been renamed to 'deinitialize(count:)'}} {{5-12=deinitialize}} {{none}}
x.destroy(1) // expected-error {{'destroy' has been renamed to 'deinitialize(count:)'}} {{5-12=deinitialize}} {{13-13=count: }} {{none}}
x.initialize(with: e) // expected-error {{'initialize(with:count:)' has been renamed to 'initialize(to:count:)'}} {{5-15=initialize}} {{16-20=to}} {{none}}
let ptr1 = UnsafeMutablePointer<T>(allocatingCapacity: 1) // expected-error {{'init(allocatingCapacity:)' is unavailable: use 'UnsafeMutablePointer.allocate(capacity:)'}} {{none}}
ptr1.initialize(with: e, count: 1) // expected-error {{'initialize(with:count:)' has been renamed to 'initialize(to:count:)'}} {{8-18=initialize}} {{19-23=to}} {{none}}
let ptr2 = UnsafeMutablePointer<T>.allocate(capacity: 1)
ptr2.initializeFrom(ptr1, count: 1) // expected-error {{'initializeFrom(_:count:)' has been renamed to 'initialize(from:count:)'}} {{8-22=initialize}} {{23-23=from: }} {{none}}
ptr1.assignFrom(ptr2, count: 1) // expected-error {{'assignFrom(_:count:)' has been renamed to 'assign(from:count:)'}} {{8-18=assign}} {{19-19=from: }} {{none}}
ptr2.assignBackwardFrom(ptr1, count: 1) // expected-error {{'assignBackwardFrom(_:count:)' has been renamed to 'assign(from:count:)'}} {{8-26=assign}} {{27-27=from: }} {{none}}
ptr1.moveAssignFrom(ptr2, count: 1) // expected-error {{'moveAssignFrom(_:count:)' has been renamed to 'moveAssign(from:count:)'}} {{8-22=moveAssign}} {{23-23=from: }} {{none}}
ptr2.moveInitializeFrom(ptr1, count: 1) // expected-error {{'moveInitializeFrom(_:count:)' has been renamed to 'moveInitialize(from:count:)'}} {{8-26=moveInitialize}} {{27-27=from: }} {{none}}
ptr1.moveInitializeBackwardFrom(ptr1, count: 1) // expected-error {{'moveInitializeBackwardFrom(_:count:)' has been renamed to 'moveInitialize(from:count:)'}} {{8-34=moveInitialize}} {{35-35=from: }} {{none}}
ptr1.deinitialize(count:1)
ptr1.deallocateCapacity(1) // expected-error {{'deallocateCapacity' has been renamed to 'deallocate(capacity:)'}} {{8-26=deallocate}} {{27-27=capacity: }} {{none}}
ptr2.deallocate(capacity: 1)
}
func _UnsafePointer<T, C : Collection>(x: UnsafeMutablePointer<T>, c: C) where C.Iterator.Element == T {
x.initializeFrom(c) // expected-error {{'initializeFrom' has been renamed to 'initialize(from:)'}}
}
func _VarArgs() {
func fn1(_: CVarArgType) {} // expected-error {{'CVarArgType' has been renamed to 'CVarArg'}} {{15-26=CVarArg}}{{none}}
func fn2(_: VaListBuilder) {} // expected-error {{'VaListBuilder' is unavailable}} {{none}}
}
func _Zip<S1 : Sequence, S2: Sequence>(s1: S1, s2: S2) {
_ = Zip2Sequence(s1, s2) // expected-error {{use zip(_:_:) free function instead}} {{none}}
_ = Zip2Sequence<S1, S2>.Generator.self // expected-error {{'Generator' has been renamed to 'Iterator'}} {{28-37=Iterator}} {{none}}
}
| apache-2.0 | 0b3a5cdde8d993388e6b59dfac627ad6 | 76.724638 | 285 | 0.660948 | 3.679729 | false | false | false | false |
DylanModesitt/Verb | Verb/Verb/Event.swift | 1 | 2472 | //
// Event.swift
// noun
//
// Created by dcm on 12/23/15.
// Copyright © 2015 noun. All rights reserved.
//
import Foundation
import Parse
/*
@brief Create a new event given the desired name and the noun it belongs to
@init This method begins creates a new event object
@param name The name of the event to be created
@return The event PFObject created
*/
class Event {
/* The heart of Verb */
let event = PFObject(className: "Event")
var eventName: String
var numberOfMembers: Int
var upvotes: Int
var timeOfEvent: String
var isPrivate: Bool
var downvotes: Int
var nounParentObjectId: String
init(givenName: String, timeOfEvent: String, isPrivate: Bool, userCreatingEvent: PFUser, nounParentObjectId: String) throws {
self.eventName = givenName
self.timeOfEvent = timeOfEvent
self.isPrivate = isPrivate
self.numberOfMembers = 1
self.upvotes = 0
self.downvotes = 0
self.nounParentObjectId = nounParentObjectId
// Initialize the properties of the object
event["name"] = eventName
event["numberOfMembers"] = numberOfMembers
event["upvotes"] = upvotes
event["downvotes"] = downvotes
// Contact parent (noun the event is under) and save it to the parent. Otherwise, abandon the object.
do {
try ServerRequests().updateArray("Noun", objectID: nounParentObjectId, key: "events", valueToUpdate: event)
try ServerRequests().changeKeyByOne("Noun", objectID: nounParentObjectId, keyToIncrement: "numberOfEvents", increment: true)
} catch {
try event.delete()
throw errors.failureToAddEventToNoun
}
// TODO- Ad users and such. Users have a relationship with events
// Attempt to save directly. If not, save the object to the server eventually
do {
try event.save()
} catch {
event.saveEventually()
throw errors.failureToSaveData
}
}
// @returns The event PFObject
func getEvent() -> PFObject {
return event
}
// @returns the event's objectId
func getEventObjectID() -> String? {
if let _ = event.objectId {
return event.objectId!
} else {
return nil
}
}
}
| apache-2.0 | a6ea10c9e09abdac419fd37649ae9fd8 | 26.455556 | 136 | 0.600162 | 4.697719 | false | false | false | false |
limsangjin12/Hero | Sources/HeroTargetState.swift | 4 | 4138 | // The MIT License (MIT)
//
// Copyright (c) 2016 Luke Zhao <[email protected]>
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
import UIKit
public enum HeroSnapshotType {
/// Will optimize for different type of views
/// For custom views or views with masking, .optimizedDefault might create snapshots
/// that appear differently than the actual view.
/// In that case, use .normal or .slowRender to disable the optimization
case optimized
/// snapshotView(afterScreenUpdates:)
case normal
/// layer.render(in: currentContext)
case layerRender
/// will not create snapshot. animate the view directly.
/// This will mess up the view hierarchy, therefore, view controllers have to rebuild
/// its view structure after the transition finishes
case noSnapshot
}
public enum HeroCoordinateSpace {
case global
case local
case sameParent
}
public struct HeroTargetState {
class HeroTargetStateWrapper {
var state: HeroTargetState
init(state: HeroTargetState) {
self.state = state
}
}
internal var beginState: HeroTargetStateWrapper?
public var beginStateIfMatched: [HeroModifier]?
public var position: CGPoint?
public var size: CGSize?
public var transform: CATransform3D?
public var opacity: Float?
public var cornerRadius: CGFloat?
public var backgroundColor: CGColor?
public var zPosition: CGFloat?
public var contentsRect: CGRect?
public var contentsScale: CGFloat?
public var borderWidth: CGFloat?
public var borderColor: CGColor?
public var shadowColor: CGColor?
public var shadowOpacity: Float?
public var shadowOffset: CGSize?
public var shadowRadius: CGFloat?
public var shadowPath: CGPath?
public var masksToBounds: Bool?
public var displayShadow: Bool = true
public var overlay: (color: CGColor, opacity: CGFloat)?
public var spring: (CGFloat, CGFloat)?
public var delay: TimeInterval = 0
public var duration: TimeInterval?
public var timingFunction: CAMediaTimingFunction?
public var arc: CGFloat?
public var source: String?
public var cascade: (TimeInterval, CascadeDirection, Bool)?
public var ignoreSubviewModifiers: Bool?
public var coordinateSpace: HeroCoordinateSpace?
public var useScaleBasedSizeChange: Bool?
public var snapshotType: HeroSnapshotType?
public var nonFade: Bool = false
public var forceAnimate: Bool = false
public var custom: [String:Any]?
init(modifiers: [HeroModifier]) {
append(contentsOf: modifiers)
}
public mutating func append(_ modifier: HeroModifier) {
modifier.apply(&self)
}
public mutating func append(contentsOf modifiers: [HeroModifier]) {
for modifier in modifiers {
modifier.apply(&self)
}
}
/**
- Returns: custom item for a specific key
*/
public subscript(key: String) -> Any? {
get {
return custom?[key]
}
set {
if custom == nil {
custom = [:]
}
custom![key] = newValue
}
}
}
extension HeroTargetState: ExpressibleByArrayLiteral {
public init(arrayLiteral elements: HeroModifier...) {
append(contentsOf: elements)
}
}
| mit | f0bba7efaadd8768144bb7e61b582886 | 29.426471 | 87 | 0.732479 | 4.602892 | false | false | false | false |
kousun12/RxSwift | RxCocoa/Common/CocoaUnits/ControlProperty.swift | 5 | 2665 | //
// ControlProperty.swift
// Rx
//
// Created by Krunoslav Zaher on 8/28/15.
// Copyright © 2015 Krunoslav Zaher. All rights reserved.
//
import Foundation
#if !RX_NO_MODULE
import RxSwift
#endif
/**
Protocol that enables extension of `ControlProperty`.
*/
public protocol ControlPropertyType : ObservableType, ObserverType {
/**
- returns: `ControlProperty` interface
*/
func asControlProperty() -> ControlProperty<E>
}
/**
Unit for `Observable`/`ObservableType` that represents property of UI element.
It's properties are:
- it never fails
- `shareReplay(1)` behavior
- it's stateful, upon subscription (calling subscribe) last element is immediatelly replayed if it was produced
- it will `Complete` sequence on control being deallocated
- it never errors out
- it delivers events on `MainScheduler.sharedInstance`
*/
public struct ControlProperty<PropertyType> : ControlPropertyType {
public typealias E = PropertyType
let source: Observable<PropertyType>
let observer: AnyObserver<PropertyType>
init(source: Observable<PropertyType>, observer: AnyObserver<PropertyType>) {
self.source = source.subscribeOn(ConcurrentMainScheduler.sharedInstance)
self.observer = observer
}
/**
Subscribes an observer to control property values.
- parameter observer: Observer to subscribe to property values.
- returns: Disposable object that can be used to unsubscribe the observer from receiving control property values.
*/
public func subscribe<O : ObserverType where O.E == E>(observer: O) -> Disposable {
return self.source.subscribe(observer)
}
/**
- returns: `Observable` interface.
*/
@warn_unused_result(message="http://git.io/rxs.uo")
public func asObservable() -> Observable<E> {
return self.source
}
/**
- returns: `ControlProperty` interface.
*/
@warn_unused_result(message="http://git.io/rxs.uo")
public func asControlProperty() -> ControlProperty<E> {
return self
}
/**
Binds event to user interface.
- In case next element is received, it is being set to control value.
- In case error is received, DEBUG buids raise fatal error, RELEASE builds log event to standard output.
- In case sequence completes, nothing happens.
*/
public func on(event: Event<E>) {
switch event {
case .Error(let error):
bindingErrorToInterface(error)
case .Next:
self.observer.on(event)
case .Completed:
self.observer.on(event)
}
}
} | mit | e3a199046009709ed10c19639756f8cf | 28.285714 | 119 | 0.665541 | 4.665499 | false | false | false | false |
tardieu/swift | stdlib/public/core/String.swift | 6 | 30400 | //===----------------------------------------------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2017 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
import SwiftShims
// FIXME: complexity documentation for most of methods on String is ought to be
// qualified with "amortized" at least, as Characters are variable-length.
/// A Unicode string value.
///
/// A string is a series of characters, such as `"Swift"`. Strings in Swift are
/// Unicode correct, locale insensitive, and designed to be efficient. The
/// `String` type bridges with the Objective-C class `NSString` and offers
/// interoperability with C functions that works with strings.
///
/// You can create new strings using string literals or string interpolations.
/// A string literal is a series of characters enclosed in quotes.
///
/// let greeting = "Welcome!"
///
/// String interpolations are string literals that evaluate any included
/// expressions and convert the results to string form. String interpolations
/// are an easy way to build a string from multiple pieces. Wrap each
/// expression in a string interpolation in parentheses, prefixed by a
/// backslash.
///
/// let name = "Rosa"
/// let personalizedGreeting = "Welcome, \(name)!"
///
/// let price = 2
/// let number = 3
/// let cookiePrice = "\(number) cookies: $\(price * number)."
///
/// Combine strings using the concatenation operator (`+`).
///
/// let longerGreeting = greeting + " We're glad you're here!"
/// print(longerGreeting)
/// // Prints "Welcome! We're glad you're here!"
///
/// Modifying and Comparing Strings
/// ===============================
///
/// Strings always have value semantics. Modifying a copy of a string leaves
/// the original unaffected.
///
/// var otherGreeting = greeting
/// otherGreeting += " Have a nice time!"
/// print(otherGreeting)
/// // Prints "Welcome! Have a nice time!"
///
/// print(greeting)
/// // Prints "Welcome!"
///
/// Comparing strings for equality using the equal-to operator (`==`) or a
/// relational operator (like `<` and `>=`) is always performed using the
/// Unicode canonical representation. This means that different
/// representations of a string compare as being equal.
///
/// let cafe1 = "Cafe\u{301}"
/// let cafe2 = "Café"
/// print(cafe1 == cafe2)
/// // Prints "true"
///
/// The Unicode code point `"\u{301}"` modifies the preceding character to
/// include an accent, so `"e\u{301}"` has the same canonical representation
/// as the single Unicode code point `"é"`.
///
/// Basic string operations are not sensitive to locale settings. This ensures
/// that string comparisons and other operations always have a single, stable
/// result, allowing strings to be used as keys in `Dictionary` instances and
/// for other purposes.
///
/// Representing Strings: Views
/// ===========================
///
/// A string is not itself a collection. Instead, it has properties that
/// present its contents as meaningful collections. Each of these collections
/// is a particular type of *view* of the string's visible and data
/// representation.
///
/// To demonstrate the different views available for every string, the
/// following examples use this `String` instance:
///
/// let cafe = "Cafe\u{301} du 🌍"
/// print(cafe)
/// // Prints "Café du 🌍"
///
/// Character View
/// --------------
///
/// A string's `characters` property is a collection of *extended grapheme
/// clusters*, which approximate human-readable characters. Many individual
/// characters, such as "é", "김", and "🇮🇳", can be made up of multiple Unicode
/// code points. These code points are combined by Unicode's boundary
/// algorithms into extended grapheme clusters, represented by Swift's
/// `Character` type. Each element of the `characters` view is represented by
/// a `Character` instance.
///
/// print(cafe.characters.count)
/// // Prints "9"
/// print(Array(cafe.characters))
/// // Prints "["C", "a", "f", "é", " ", "d", "u", " ", "🌍"]"
///
/// Each visible character in the `cafe` string is a separate element of the
/// `characters` view.
///
/// Unicode Scalar View
/// -------------------
///
/// A string's `unicodeScalars` property is a collection of Unicode scalar
/// values, the 21-bit codes that are the basic unit of Unicode. Each scalar
/// value is represented by a `UnicodeScalar` instance and is equivalent to a
/// UTF-32 code unit.
///
/// print(cafe.unicodeScalars.count)
/// // Prints "10"
/// print(Array(cafe.unicodeScalars))
/// // Prints "["C", "a", "f", "e", "\u{0301}", " ", "d", "u", " ", "\u{0001F30D}"]"
/// print(cafe.unicodeScalars.map { $0.value })
/// // Prints "[67, 97, 102, 101, 769, 32, 100, 117, 32, 127757]"
///
/// The `unicodeScalars` view's elements comprise each Unicode scalar value in
/// the `cafe` string. In particular, because `cafe` was declared using the
/// decomposed form of the `"é"` character, `unicodeScalars` contains the code
/// points for both the letter `"e"` (101) and the accent character `"´"`
/// (769).
///
/// UTF-16 View
/// -----------
///
/// A string's `utf16` property is a collection of UTF-16 code units, the
/// 16-bit encoding form of the string's Unicode scalar values. Each code unit
/// is stored as a `UInt16` instance.
///
/// print(cafe.utf16.count)
/// // Prints "11"
/// print(Array(cafe.utf16))
/// // Prints "[67, 97, 102, 101, 769, 32, 100, 117, 32, 55356, 57101]"
///
/// The elements of the `utf16` view are the code units for the string when
/// encoded in UTF-16.
///
/// The elements of this collection match those accessed through indexed
/// `NSString` APIs.
///
/// let nscafe = cafe as NSString
/// print(nscafe.length)
/// // Prints "11"
/// print(nscafe.character(at: 3))
/// // Prints "101"
///
/// UTF-8 View
/// ----------
///
/// A string's `utf8` property is a collection of UTF-8 code units, the 8-bit
/// encoding form of the string's Unicode scalar values. Each code unit is
/// stored as a `UInt8` instance.
///
/// print(cafe.utf8.count)
/// // Prints "14"
/// print(Array(cafe.utf8))
/// // Prints "[67, 97, 102, 101, 204, 129, 32, 100, 117, 32, 240, 159, 140, 141]"
///
/// The elements of the `utf8` view are the code units for the string when
/// encoded in UTF-8. This representation matches the one used when `String`
/// instances are passed to C APIs.
///
/// let cLength = strlen(cafe)
/// print(cLength)
/// // Prints "14"
///
/// Counting the Length of a String
/// ===============================
///
/// When you need to know the length of a string, you must first consider what
/// you'll use the length for. Are you measuring the number of characters that
/// will be displayed on the screen, or are you measuring the amount of
/// storage needed for the string in a particular encoding? A single string
/// can have greatly differing lengths when measured by its different views.
///
/// For example, an ASCII character like the capital letter *A* is represented
/// by a single element in each of its four views. The Unicode scalar value of
/// *A* is `65`, which is small enough to fit in a single code unit in both
/// UTF-16 and UTF-8.
///
/// let capitalA = "A"
/// print(capitalA.characters.count)
/// // Prints "1"
/// print(capitalA.unicodeScalars.count)
/// // Prints "1"
/// print(capitalA.utf16.count)
/// // Prints "1"
/// print(capitalA.utf8.count)
/// // Prints "1"
///
/// On the other hand, an emoji flag character is constructed from a pair of
/// Unicode scalars values, like `"\u{1F1F5}"` and `"\u{1F1F7}"`. Each of
/// these scalar values, in turn, is too large to fit into a single UTF-16 or
/// UTF-8 code unit. As a result, each view of the string `"🇵🇷"` reports a
/// different length.
///
/// let flag = "🇵🇷"
/// print(flag.characters.count)
/// // Prints "1"
/// print(flag.unicodeScalars.count)
/// // Prints "2"
/// print(flag.utf16.count)
/// // Prints "4"
/// print(flag.utf8.count)
/// // Prints "8"
///
/// To check whether a string is empty, use its `isEmpty` property instead
/// of comparing the length of one of the views to `0`. Unlike `isEmpty`,
/// calculating a view's `count` property requires iterating through the
/// elements of the string.
///
/// Accessing String View Elements
/// ==============================
///
/// To find individual elements of a string, use the appropriate view for your
/// task. For example, to retrieve the first word of a longer string, you can
/// search the `characters` view for a space and then create a new string from
/// a prefix of the `characters` view up to that point.
///
/// let name = "Marie Curie"
/// let firstSpace = name.characters.index(of: " ")!
/// let firstName = String(name.characters.prefix(upTo: firstSpace))
/// print(firstName)
/// // Prints "Marie"
///
/// You can convert an index into one of a string's views to an index into
/// another view.
///
/// let firstSpaceUTF8 = firstSpace.samePosition(in: name.utf8)
/// print(Array(name.utf8.prefix(upTo: firstSpaceUTF8)))
/// // Prints "[77, 97, 114, 105, 101]"
///
/// Performance Optimizations
/// =========================
///
/// Although strings in Swift have value semantics, strings use a copy-on-write
/// strategy to store their data in a buffer. This buffer can then be shared
/// by different copies of a string. A string's data is only copied lazily,
/// upon mutation, when more than one string instance is using the same
/// buffer. Therefore, the first in any sequence of mutating operations may
/// cost O(*n*) time and space.
///
/// When a string's contiguous storage fills up, a new buffer must be allocated
/// and data must be moved to the new storage. String buffers use an
/// exponential growth strategy that makes appending to a string a constant
/// time operation when averaged over many append operations.
///
/// Bridging between String and NSString
/// ====================================
///
/// Any `String` instance can be bridged to `NSString` using the type-cast
/// operator (`as`), and any `String` instance that originates in Objective-C
/// may use an `NSString` instance as its storage. Because any arbitrary
/// subclass of `NSString` can become a `String` instance, there are no
/// guarantees about representation or efficiency when a `String` instance is
/// backed by `NSString` storage. Because `NSString` is immutable, it is just
/// as though the storage was shared by a copy: The first in any sequence of
/// mutating operations causes elements to be copied into unique, contiguous
/// storage which may cost O(*n*) time and space, where *n* is the length of
/// the string's encoded representation (or more, if the underlying `NSString`
/// has unusual performance characteristics).
///
/// For more information about the Unicode terms used in this discussion, see
/// the [Unicode.org glossary][glossary]. In particular, this discussion
/// mentions [extended grapheme clusters][clusters],
/// [Unicode scalar values][scalars], and [canonical equivalence][equivalence].
///
/// [glossary]: http://www.unicode.org/glossary/
/// [clusters]: http://www.unicode.org/glossary/#extended_grapheme_cluster
/// [scalars]: http://www.unicode.org/glossary/#unicode_scalar_value
/// [equivalence]: http://www.unicode.org/glossary/#canonical_equivalent
///
/// - SeeAlso: `String.CharacterView`, `String.UnicodeScalarView`,
/// `String.UTF16View`, `String.UTF8View`
@_fixed_layout
public struct String {
/// Creates an empty string.
public init() {
_core = _StringCore()
}
public // @testable
init(_ _core: _StringCore) {
self._core = _core
}
public // @testable
var _core: _StringCore
}
extension String {
public // @testable
static func _fromWellFormedCodeUnitSequence<Encoding, Input>(
_ encoding: Encoding.Type, input: Input
) -> String
where
Encoding: UnicodeCodec,
Input: Collection,
Input.Iterator.Element == Encoding.CodeUnit {
return String._fromCodeUnitSequence(encoding, input: input)!
}
public // @testable
static func _fromCodeUnitSequence<Encoding, Input>(
_ encoding: Encoding.Type, input: Input
) -> String?
where
Encoding: UnicodeCodec,
Input: Collection,
Input.Iterator.Element == Encoding.CodeUnit {
let (stringBufferOptional, _) =
_StringBuffer.fromCodeUnits(input, encoding: encoding,
repairIllFormedSequences: false)
return stringBufferOptional.map { String(_storage: $0) }
}
public // @testable
static func _fromCodeUnitSequenceWithRepair<Encoding, Input>(
_ encoding: Encoding.Type, input: Input
) -> (String, hadError: Bool)
where
Encoding: UnicodeCodec,
Input: Collection,
Input.Iterator.Element == Encoding.CodeUnit {
let (stringBuffer, hadError) =
_StringBuffer.fromCodeUnits(input, encoding: encoding,
repairIllFormedSequences: true)
return (String(_storage: stringBuffer!), hadError)
}
}
extension String : _ExpressibleByBuiltinUnicodeScalarLiteral {
@effects(readonly)
public // @testable
init(_builtinUnicodeScalarLiteral value: Builtin.Int32) {
self = String._fromWellFormedCodeUnitSequence(
UTF32.self, input: CollectionOfOne(UInt32(value)))
}
}
extension String : ExpressibleByUnicodeScalarLiteral {
/// Creates an instance initialized to the given Unicode scalar value.
///
/// Do not call this initializer directly. It may be used by the compiler when
/// you initialize a string using a string literal that contains a single
/// Unicode scalar value.
public init(unicodeScalarLiteral value: String) {
self = value
}
}
extension String : _ExpressibleByBuiltinExtendedGraphemeClusterLiteral {
@effects(readonly)
@_semantics("string.makeUTF8")
public init(
_builtinExtendedGraphemeClusterLiteral start: Builtin.RawPointer,
utf8CodeUnitCount: Builtin.Word,
isASCII: Builtin.Int1) {
self = String._fromWellFormedCodeUnitSequence(
UTF8.self,
input: UnsafeBufferPointer(
start: UnsafeMutablePointer<UTF8.CodeUnit>(start),
count: Int(utf8CodeUnitCount)))
}
}
extension String : ExpressibleByExtendedGraphemeClusterLiteral {
/// Creates an instance initialized to the given extended grapheme cluster
/// literal.
///
/// Do not call this initializer directly. It may be used by the compiler when
/// you initialize a string using a string literal containing a single
/// extended grapheme cluster.
public init(extendedGraphemeClusterLiteral value: String) {
self = value
}
}
extension String : _ExpressibleByBuiltinUTF16StringLiteral {
@effects(readonly)
@_semantics("string.makeUTF16")
public init(
_builtinUTF16StringLiteral start: Builtin.RawPointer,
utf16CodeUnitCount: Builtin.Word
) {
self = String(
_StringCore(
baseAddress: UnsafeMutableRawPointer(start),
count: Int(utf16CodeUnitCount),
elementShift: 1,
hasCocoaBuffer: false,
owner: nil))
}
}
extension String : _ExpressibleByBuiltinStringLiteral {
@effects(readonly)
@_semantics("string.makeUTF8")
public init(
_builtinStringLiteral start: Builtin.RawPointer,
utf8CodeUnitCount: Builtin.Word,
isASCII: Builtin.Int1) {
if Bool(isASCII) {
self = String(
_StringCore(
baseAddress: UnsafeMutableRawPointer(start),
count: Int(utf8CodeUnitCount),
elementShift: 0,
hasCocoaBuffer: false,
owner: nil))
}
else {
self = String._fromWellFormedCodeUnitSequence(
UTF8.self,
input: UnsafeBufferPointer(
start: UnsafeMutablePointer<UTF8.CodeUnit>(start),
count: Int(utf8CodeUnitCount)))
}
}
}
extension String : ExpressibleByStringLiteral {
/// Creates an instance initialized to the given string value.
///
/// Do not call this initializer directly. It is used by the compiler when you
/// initialize a string using a string literal. For example:
///
/// let nextStop = "Clark & Lake"
///
/// This assignment to the `nextStop` constant calls this string literal
/// initializer behind the scenes.
public init(stringLiteral value: String) {
self = value
}
}
extension String : CustomDebugStringConvertible {
/// A representation of the string that is suitable for debugging.
public var debugDescription: String {
var result = "\""
for us in self.unicodeScalars {
result += us.escaped(asASCII: false)
}
result += "\""
return result
}
}
extension String {
/// Returns the number of code units occupied by this string
/// in the given encoding.
func _encodedLength<
Encoding: UnicodeCodec
>(_ encoding: Encoding.Type) -> Int {
var codeUnitCount = 0
self._encode(encoding, into: { _ in codeUnitCount += 1 })
return codeUnitCount
}
// FIXME: this function does not handle the case when a wrapped NSString
// contains unpaired surrogates. Fix this before exposing this function as a
// public API. But it is unclear if it is valid to have such an NSString in
// the first place. If it is not, we should not be crashing in an obscure
// way -- add a test for that.
// Related: <rdar://problem/17340917> Please document how NSString interacts
// with unpaired surrogates
func _encode<
Encoding: UnicodeCodec
>(
_ encoding: Encoding.Type,
into processCodeUnit: (Encoding.CodeUnit) -> Void
) {
return _core.encode(encoding, into: processCodeUnit)
}
}
// Support for copy-on-write
extension String {
/// Appends the given string to this string.
///
/// The following example builds a customized greeting by using the
/// `append(_:)` method:
///
/// var greeting = "Hello, "
/// if let name = getUserName() {
/// greeting.append(name)
/// } else {
/// greeting.append("friend")
/// }
/// print(greeting)
/// // Prints "Hello, friend"
///
/// - Parameter other: Another string.
public mutating func append(_ other: String) {
_core.append(other._core)
}
/// Appends the given Unicode scalar to the string.
///
/// - Parameter x: A Unicode scalar value.
///
/// - Complexity: Appending a Unicode scalar to a string averages to O(1)
/// over many additions.
@available(*, unavailable, message: "Replaced by append(_: String)")
public mutating func append(_ x: UnicodeScalar) {
Builtin.unreachable()
}
public // SPI(Foundation)
init(_storage: _StringBuffer) {
_core = _StringCore(_storage)
}
}
extension String {
@effects(readonly)
@_semantics("string.concat")
public static func + (lhs: String, rhs: String) -> String {
if lhs.isEmpty {
return rhs
}
var lhs = lhs
lhs._core.append(rhs._core)
return lhs
}
// String append
public static func += (lhs: inout String, rhs: String) {
if lhs.isEmpty {
lhs = rhs
}
else {
lhs._core.append(rhs._core)
}
}
/// Constructs a `String` in `resultStorage` containing the given UTF-8.
///
/// Low-level construction interface used by introspection
/// implementation in the runtime library.
@_silgen_name("swift_stringFromUTF8InRawMemory")
public // COMPILER_INTRINSIC
static func _fromUTF8InRawMemory(
_ resultStorage: UnsafeMutablePointer<String>,
start: UnsafeMutablePointer<UTF8.CodeUnit>,
utf8CodeUnitCount: Int
) {
resultStorage.initialize(to:
String._fromWellFormedCodeUnitSequence(
UTF8.self,
input: UnsafeBufferPointer(start: start, count: utf8CodeUnitCount)))
}
}
extension Sequence where Iterator.Element == String {
/// Returns a new string by concatenating the elements of the sequence,
/// adding the given separator between each element.
///
/// The following example shows how an array of strings can be joined to a
/// single, comma-separated string:
///
/// let cast = ["Vivien", "Marlon", "Kim", "Karl"]
/// let list = cast.joined(separator: ", ")
/// print(list)
/// // Prints "Vivien, Marlon, Kim, Karl"
///
/// - Parameter separator: A string to insert between each of the elements
/// in this sequence. The default separator is an empty string.
/// - Returns: A single, concatenated string.
public func joined(separator: String = "") -> String {
var result = ""
// FIXME(performance): this code assumes UTF-16 in-memory representation.
// It should be switched to low-level APIs.
let separatorSize = separator.utf16.count
let reservation = self._preprocessingPass {
() -> Int in
var r = 0
for chunk in self {
// FIXME(performance): this code assumes UTF-16 in-memory representation.
// It should be switched to low-level APIs.
r += separatorSize + chunk.utf16.count
}
return r - separatorSize
}
if let n = reservation {
result.reserveCapacity(n)
}
if separatorSize == 0 {
for x in self {
result.append(x)
}
return result
}
var iter = makeIterator()
if let first = iter.next() {
result.append(first)
while let next = iter.next() {
result.append(separator)
result.append(next)
}
}
return result
}
}
#if _runtime(_ObjC)
@_silgen_name("swift_stdlib_NSStringLowercaseString")
func _stdlib_NSStringLowercaseString(_ str: AnyObject) -> _CocoaString
@_silgen_name("swift_stdlib_NSStringUppercaseString")
func _stdlib_NSStringUppercaseString(_ str: AnyObject) -> _CocoaString
#else
internal func _nativeUnicodeLowercaseString(_ str: String) -> String {
var buffer = _StringBuffer(
capacity: str._core.count, initialSize: str._core.count, elementWidth: 2)
// Allocation of a StringBuffer requires binding the memory to the correct
// encoding type.
let dest = buffer.start.bindMemory(
to: UTF16.CodeUnit.self, capacity: str._core.count)
// Try to write it out to the same length.
let z = _swift_stdlib_unicode_strToLower(
dest, Int32(str._core.count),
str._core.startUTF16, Int32(str._core.count))
let correctSize = Int(z)
// If more space is needed, do it again with the correct buffer size.
if correctSize != str._core.count {
buffer = _StringBuffer(
capacity: correctSize, initialSize: correctSize, elementWidth: 2)
let dest = buffer.start.bindMemory(
to: UTF16.CodeUnit.self, capacity: str._core.count)
_swift_stdlib_unicode_strToLower(
dest, Int32(correctSize), str._core.startUTF16, Int32(str._core.count))
}
return String(_storage: buffer)
}
internal func _nativeUnicodeUppercaseString(_ str: String) -> String {
var buffer = _StringBuffer(
capacity: str._core.count, initialSize: str._core.count, elementWidth: 2)
// Allocation of a StringBuffer requires binding the memory to the correct
// encoding type.
let dest = buffer.start.bindMemory(
to: UTF16.CodeUnit.self, capacity: str._core.count)
// Try to write it out to the same length.
let z = _swift_stdlib_unicode_strToUpper(
dest, Int32(str._core.count),
str._core.startUTF16, Int32(str._core.count))
let correctSize = Int(z)
// If more space is needed, do it again with the correct buffer size.
if correctSize != str._core.count {
buffer = _StringBuffer(
capacity: correctSize, initialSize: correctSize, elementWidth: 2)
let dest = buffer.start.bindMemory(
to: UTF16.CodeUnit.self, capacity: str._core.count)
_swift_stdlib_unicode_strToUpper(
dest, Int32(correctSize), str._core.startUTF16, Int32(str._core.count))
}
return String(_storage: buffer)
}
#endif
// Unicode algorithms
extension String {
// FIXME: implement case folding without relying on Foundation.
// <rdar://problem/17550602> [unicode] Implement case folding
/// A "table" for which ASCII characters need to be upper cased.
/// To determine which bit corresponds to which ASCII character, subtract 1
/// from the ASCII value of that character and divide by 2. The bit is set iff
/// that character is a lower case character.
internal var _asciiLowerCaseTable: UInt64 {
@inline(__always)
get {
return 0b0001_1111_1111_1111_0000_0000_0000_0000_0000_0000_0000_0000_0000_0000_0000_0000
}
}
/// The same table for upper case characters.
internal var _asciiUpperCaseTable: UInt64 {
@inline(__always)
get {
return 0b0000_0000_0000_0000_0001_1111_1111_1111_0000_0000_0000_0000_0000_0000_0000_0000
}
}
/// Returns a lowercase version of the string.
///
/// Here's an example of transforming a string to all lowercase letters.
///
/// let cafe = "Café 🍵"
/// print(cafe.lowercased())
/// // Prints "café 🍵"
///
/// - Returns: A lowercase copy of the string.
///
/// - Complexity: O(*n*)
public func lowercased() -> String {
if let asciiBuffer = self._core.asciiBuffer {
let count = asciiBuffer.count
let source = asciiBuffer.baseAddress!
let buffer = _StringBuffer(
capacity: count, initialSize: count, elementWidth: 1)
let dest = buffer.start
for i in 0..<count {
// For each character in the string, we lookup if it should be shifted
// in our ascii table, then we return 0x20 if it should, 0x0 if not.
// This code is equivalent to:
// switch source[i] {
// case let x where (x >= 0x41 && x <= 0x5a):
// dest[i] = x &+ 0x20
// case let x:
// dest[i] = x
// }
let value = source[i]
let isUpper =
_asciiUpperCaseTable >>
UInt64(((value &- 1) & 0b0111_1111) >> 1)
let add = (isUpper & 0x1) << 5
// Since we are left with either 0x0 or 0x20, we can safely truncate to
// a UInt8 and add to our ASCII value (this will not overflow numbers in
// the ASCII range).
dest.storeBytes(of: value &+ UInt8(truncatingBitPattern: add),
toByteOffset: i, as: UInt8.self)
}
return String(_storage: buffer)
}
#if _runtime(_ObjC)
return _cocoaStringToSwiftString_NonASCII(
_stdlib_NSStringLowercaseString(self._bridgeToObjectiveCImpl()))
#else
return _nativeUnicodeLowercaseString(self)
#endif
}
/// Returns an uppercase version of the string.
///
/// The following example transforms a string to uppercase letters:
///
/// let cafe = "Café 🍵"
/// print(cafe.uppercased())
/// // Prints "CAFÉ 🍵"
///
/// - Returns: An uppercase copy of the string.
///
/// - Complexity: O(*n*)
public func uppercased() -> String {
if let asciiBuffer = self._core.asciiBuffer {
let count = asciiBuffer.count
let source = asciiBuffer.baseAddress!
let buffer = _StringBuffer(
capacity: count, initialSize: count, elementWidth: 1)
let dest = buffer.start
for i in 0..<count {
// See the comment above in lowercaseString.
let value = source[i]
let isLower =
_asciiLowerCaseTable >>
UInt64(((value &- 1) & 0b0111_1111) >> 1)
let add = (isLower & 0x1) << 5
dest.storeBytes(of: value &- UInt8(truncatingBitPattern: add),
toByteOffset: i, as: UInt8.self)
}
return String(_storage: buffer)
}
#if _runtime(_ObjC)
return _cocoaStringToSwiftString_NonASCII(
_stdlib_NSStringUppercaseString(self._bridgeToObjectiveCImpl()))
#else
return _nativeUnicodeUppercaseString(self)
#endif
}
/// Creates an instance from the description of a given
/// `LosslessStringConvertible` instance.
public init<T : LosslessStringConvertible>(_ value: T) {
self = value.description
}
}
extension String : CustomStringConvertible {
public var description: String {
return self
}
}
extension String : LosslessStringConvertible {
public init?(_ description: String) {
self = description
}
}
extension String {
@available(*, unavailable, renamed: "append(_:)")
public mutating func appendContentsOf(_ other: String) {
Builtin.unreachable()
}
@available(*, unavailable, renamed: "append(contentsOf:)")
public mutating func appendContentsOf<S : Sequence>(_ newElements: S)
where S.Iterator.Element == Character {
Builtin.unreachable()
}
@available(*, unavailable, renamed: "insert(contentsOf:at:)")
public mutating func insertContentsOf<S : Collection>(
_ newElements: S, at i: Index
) where S.Iterator.Element == Character {
Builtin.unreachable()
}
@available(*, unavailable, renamed: "replaceSubrange")
public mutating func replaceRange<C : Collection>(
_ subRange: Range<Index>, with newElements: C
) where C.Iterator.Element == Character {
Builtin.unreachable()
}
@available(*, unavailable, renamed: "replaceSubrange")
public mutating func replaceRange(
_ subRange: Range<Index>, with newElements: String
) {
Builtin.unreachable()
}
@available(*, unavailable, renamed: "remove(at:)")
public mutating func removeAtIndex(_ i: Index) -> Character {
Builtin.unreachable()
}
@available(*, unavailable, renamed: "removeSubrange")
public mutating func removeRange(_ subRange: Range<Index>) {
Builtin.unreachable()
}
@available(*, unavailable, renamed: "lowercased()")
public var lowercaseString: String {
Builtin.unreachable()
}
@available(*, unavailable, renamed: "uppercased()")
public var uppercaseString: String {
Builtin.unreachable()
}
@available(*, unavailable, renamed: "init(describing:)")
public init<T>(_: T) {
Builtin.unreachable()
}
}
extension Sequence where Iterator.Element == String {
@available(*, unavailable, renamed: "joined(separator:)")
public func joinWithSeparator(_ separator: String) -> String {
Builtin.unreachable()
}
}
| apache-2.0 | 4f163971e8fefcba145dffc0c6e344d1 | 33.137233 | 94 | 0.658363 | 4.16011 | false | false | false | false |
bwhiteley/EnumTable | EnumTable/UIImage.swift | 1 | 524 | //
// UIImage.swift
// EnumTable
//
// Created by Bart Whiteley on 6/17/15.
// Copyright © 2015 whiteley.org. All rights reserved.
//
import UIKit
extension UIImage {
enum AssetIdentifier:String {
case Facebook = "facebook"
case Twitter = "twitter"
case Instagram = "instagram"
case Gear = "gear"
case Phone = "phone"
case User = "user"
}
convenience init!(assetIdentifier: AssetIdentifier) {
self.init(named: assetIdentifier.rawValue)
}
}
| mit | 3c6a22146f8268c8bfea9b9f1ccf4976 | 20.791667 | 57 | 0.613767 | 3.992366 | false | false | false | false |
ryet231ere/DouYuSwift | douyu/douyu/Classes/Main/ViewModel/BaseViewModel.swift | 1 | 1279 | //
// BaseViewModel.swift
// douyu
//
// Created by 练锦波 on 2017/3/9.
// Copyright © 2017年 练锦波. All rights reserved.
//
import UIKit
class BaseViewModel {
lazy var anchorGroups : [AnchorGroup] = [AnchorGroup]()
}
extension BaseViewModel {
func loadAnchorData(isGroupData : Bool, URLString : String, parameters : [String : Any]? = nil, finishedCallback : @escaping () -> ()) {
NetworkTools.requestData(.get, URLString: URLString, parameters: parameters) { (result) in
// 1.获取数据
guard let resultDict = result as? [String : Any] else { return }
guard let dataArray = resultDict["data"] as? [[String : Any]] else { return }
if isGroupData {
// 2.字典转模型
for dict in dataArray {
self.anchorGroups.append(AnchorGroup(dict: dict))
}
} else {
let group = AnchorGroup()
for dict in dataArray {
group.anchors.append(ANchorModel(dict: dict))
}
self.anchorGroups.append(group)
}
finishedCallback()
}
}
}
| mit | 77a4157338c678ddb6b7bc1b756fd091 | 28.666667 | 140 | 0.510433 | 5.065041 | false | false | false | false |
lipka/JSON | Tests/JSONTests/Post.swift | 2 | 500 | import Foundation
import JSON
struct Post: Equatable {
enum State: String {
case draft
case published
}
let title: String
let author: User
let state: State
static func == (lhs: Post, rhs: Post) -> Bool {
return lhs.title == rhs.title && lhs.author == rhs.author && lhs.state == rhs.state
}
}
extension Post: JSONDeserializable {
init(json: JSON) throws {
title = try json.decode(key: "title")
author = try json.decode(key: "author")
state = try json.decode(key: "state")
}
}
| mit | c5141f5003e91b37dad5e1e4e9413476 | 19 | 85 | 0.67 | 3.08642 | false | false | false | false |
hchhatbar/SAHC_iOS_App | SAHC/SAHC/CoreDataManager.swift | 1 | 5769 | //
// CoreDataManager.swift
// SAHC
//
// Created by Hemen Chhatbar on 10/26/15.
// Copyright (c) 2015 AppForCause. All rights reserved.
//
/*
Singleton controller to manage the main Core Data stack for the application. It vends a persistent store coordinator, the managed object model, and a URL for the persistent store.
*/
import Foundation
import CoreData
class CoreDataManager {
private struct Constants{
static let applicationDocumentsDirectoryName = "com.appforcause.sahc"
static let mainStoreFileName = "sahc.storedata"
static let errorDomain = "CoreDataManager"
}
class var sharedManager: CoreDataManager{
struct Singleton{
static let coreDataManager = CoreDataManager()
}
return Singleton.coreDataManager
}
/// The managed object model for the application.
// Schema for the application
lazy var managedObjectModel:NSManagedObjectModel = {
// This property is not optional. It is a fatal error for the application not to be able to find and load its model.
let modelURL = NSBundle.mainBundle().URLForResource("DB", withExtension: "momd")!
return NSManagedObjectModel(contentsOfURL: modelURL)!
}()
lazy var applicationDocumentsDirectory: NSURL = {
// The directory the application uses to store the Core Data store file. This code uses a directory named "com.AppForCause.TestCoreData" in the application's documents Application Support directory.
let urls = NSFileManager.defaultManager().URLsForDirectory(.DocumentDirectory, inDomains: .UserDomainMask)
return urls[urls.count-1]
}()
lazy var persistentStoreCoordinator: NSPersistentStoreCoordinator? = {
// The persistent store coordinator for the application. This implementation creates and return a coordinator, having added the store for the application to it. This property is optional since there are legitimate error conditions that could cause the creation of the store to fail.
// Create the coordinator and store
var coordinator: NSPersistentStoreCoordinator? = NSPersistentStoreCoordinator(managedObjectModel: self.managedObjectModel)
let url = self.applicationDocumentsDirectory.URLByAppendingPathComponent("sahc.sqlite")
var error: NSError? = nil
var failureReason = "There was an error creating or loading the application's saved data."
do {
try coordinator!.addPersistentStoreWithType(NSSQLiteStoreType, configuration: nil, URL: url, options: nil)
} catch _ {
}
// if coordinator!.addPersistentStoreWithType(NSSQLiteStoreType, configuration: nil, URL: url, options: nil) == nil {
// coordinator = nil
// // Report any error we got.
// var dict = [String: AnyObject]()
// dict[NSLocalizedDescriptionKey] = "Failed to initialize the application's saved data"
// dict[NSLocalizedFailureReasonErrorKey] = failureReason
// dict[NSUnderlyingErrorKey] = error
// error = NSError(domain: "YOUR_ERROR_DOMAIN", code: 9999, userInfo: dict)
// // Replace this with code to handle the error appropriately.
// // abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
// NSLog("Unresolved error \(error), \(error!.userInfo)")
// abort()
// }
return coordinator
}()
lazy var managedObjectContext: NSManagedObjectContext? = {
// Returns the managed object context for the application (which is already bound to the persistent store coordinator for the application.) This property is optional since there are legitimate error conditions that could cause the creation of the context to fail.
let coordinator = self.persistentStoreCoordinator
if coordinator == nil {
return nil
}
var managedObjectContext = NSManagedObjectContext()
managedObjectContext.persistentStoreCoordinator = coordinator
return managedObjectContext
}()
lazy var mainContext : NSManagedObjectContext? = {
var mainContext = NSManagedObjectContext (concurrencyType: .MainQueueConcurrencyType)
mainContext.parentContext = self.managedObjectContext
mainContext.mergePolicy = NSMergeByPropertyObjectTrumpMergePolicy
return mainContext;
}()
// Worker context
func newWorkerContext() -> NSManagedObjectContext {
let workerContext = NSManagedObjectContext(concurrencyType:.PrivateQueueConcurrencyType)
workerContext.parentContext = self.mainContext
workerContext.mergePolicy = NSMergeByPropertyObjectTrumpMergePolicy
return workerContext;
}
func saveContext () {
if let moc = self.managedObjectContext {
//let error: NSError? = nil
//if moc.hasChanges {
do {
try moc.save()
// Replace this implementation with code to handle the error appropriately.
// abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
//NSLog("Unresolved error \(error), \(error!.userInfo)")
//abort()
} catch _ {
print("Save failed")
}
//}
}
}
} | mit | 49f486b0b05f212e5e3b3bd5c351e34e | 43.384615 | 290 | 0.65904 | 5.874745 | false | false | false | false |
andersio/ReactiveCocoa | ReactiveCocoaTests/Swift/PropertySpec.swift | 1 | 40894 | //
// PropertySpec.swift
// ReactiveCocoa
//
// Created by Justin Spahr-Summers on 2015-01-23.
// Copyright (c) 2015 GitHub. All rights reserved.
//
import Result
import Nimble
import Quick
import ReactiveCocoa
private let initialPropertyValue = "InitialValue"
private let subsequentPropertyValue = "SubsequentValue"
private let finalPropertyValue = "FinalValue"
private let initialOtherPropertyValue = "InitialOtherValue"
private let subsequentOtherPropertyValue = "SubsequentOtherValue"
private let finalOtherPropertyValue = "FinalOtherValue"
class PropertySpec: QuickSpec {
override func spec() {
describe("ConstantProperty") {
it("should have the value given at initialization") {
let constantProperty = ConstantProperty(initialPropertyValue)
expect(constantProperty.value) == initialPropertyValue
}
it("should yield a signal that interrupts observers without emitting any value.") {
let constantProperty = ConstantProperty(initialPropertyValue)
var signalInterrupted = false
var hasUnexpectedEventsEmitted = false
constantProperty.signal.observe { event in
switch event {
case .Interrupted:
signalInterrupted = true
case .Next, .Failed, .Completed:
hasUnexpectedEventsEmitted = true
}
}
expect(signalInterrupted) == true
expect(hasUnexpectedEventsEmitted) == false
}
it("should yield a producer that sends the current value then completes") {
let constantProperty = ConstantProperty(initialPropertyValue)
var sentValue: String?
var signalCompleted = false
constantProperty.producer.start { event in
switch event {
case let .Next(value):
sentValue = value
case .Completed:
signalCompleted = true
case .Failed, .Interrupted:
break
}
}
expect(sentValue) == initialPropertyValue
expect(signalCompleted) == true
}
}
describe("MutableProperty") {
it("should have the value given at initialization") {
let mutableProperty = MutableProperty(initialPropertyValue)
expect(mutableProperty.value) == initialPropertyValue
}
it("should yield a producer that sends the current value then all changes") {
let mutableProperty = MutableProperty(initialPropertyValue)
var sentValue: String?
mutableProperty.producer.startWithNext { sentValue = $0 }
expect(sentValue) == initialPropertyValue
mutableProperty.value = subsequentPropertyValue
expect(sentValue) == subsequentPropertyValue
mutableProperty.value = finalPropertyValue
expect(sentValue) == finalPropertyValue
}
it("should yield a producer that sends the current value then all changes, even if the value actually remains unchanged") {
let mutableProperty = MutableProperty(initialPropertyValue)
var count = 0
mutableProperty.producer.startWithNext { _ in count = count + 1 }
expect(count) == 1
mutableProperty.value = initialPropertyValue
expect(count) == 2
mutableProperty.value = initialPropertyValue
expect(count) == 3
}
it("should yield a signal that emits subsequent changes to the value") {
let mutableProperty = MutableProperty(initialPropertyValue)
var sentValue: String?
mutableProperty.signal.observeNext { sentValue = $0 }
expect(sentValue).to(beNil())
mutableProperty.value = subsequentPropertyValue
expect(sentValue) == subsequentPropertyValue
mutableProperty.value = finalPropertyValue
expect(sentValue) == finalPropertyValue
}
it("should yield a signal that emits subsequent changes to the value, even if the value actually remains unchanged") {
let mutableProperty = MutableProperty(initialPropertyValue)
var count = 0
mutableProperty.signal.observeNext { _ in count = count + 1 }
expect(count) == 0
mutableProperty.value = initialPropertyValue
expect(count) == 1
mutableProperty.value = initialPropertyValue
expect(count) == 2
}
it("should complete its producer when deallocated") {
var mutableProperty: MutableProperty? = MutableProperty(initialPropertyValue)
var producerCompleted = false
mutableProperty!.producer.startWithCompleted { producerCompleted = true }
mutableProperty = nil
expect(producerCompleted) == true
}
it("should complete its signal when deallocated") {
var mutableProperty: MutableProperty? = MutableProperty(initialPropertyValue)
var signalCompleted = false
mutableProperty!.signal.observeCompleted { signalCompleted = true }
mutableProperty = nil
expect(signalCompleted) == true
}
it("should yield a producer which emits the latest value and complete even if the property is deallocated") {
var mutableProperty: MutableProperty? = MutableProperty(initialPropertyValue)
let producer = mutableProperty!.producer
var producerCompleted = false
var hasUnanticipatedEvent = false
var latestValue = mutableProperty?.value
mutableProperty!.value = subsequentPropertyValue
mutableProperty = nil
producer.start { event in
switch event {
case let .Next(value):
latestValue = value
case .Completed:
producerCompleted = true
case .Interrupted, .Failed:
hasUnanticipatedEvent = true
}
}
expect(hasUnanticipatedEvent) == false
expect(producerCompleted) == true
expect(latestValue) == subsequentPropertyValue
}
it("should modify the value atomically") {
let property = MutableProperty(initialPropertyValue)
expect(property.modify({ _ in subsequentPropertyValue })) == initialPropertyValue
expect(property.value) == subsequentPropertyValue
}
it("should modify the value atomically and subsquently send out a Next event with the new value") {
let property = MutableProperty(initialPropertyValue)
var value: String?
property.producer.startWithNext {
value = $0
}
expect(value) == initialPropertyValue
expect(property.modify({ _ in subsequentPropertyValue })) == initialPropertyValue
expect(property.value) == subsequentPropertyValue
expect(value) == subsequentPropertyValue
}
it("should swap the value atomically") {
let property = MutableProperty(initialPropertyValue)
expect(property.swap(subsequentPropertyValue)) == initialPropertyValue
expect(property.value) == subsequentPropertyValue
}
it("should swap the value atomically and subsquently send out a Next event with the new value") {
let property = MutableProperty(initialPropertyValue)
var value: String?
property.producer.startWithNext {
value = $0
}
expect(value) == initialPropertyValue
expect(property.swap(subsequentPropertyValue)) == initialPropertyValue
expect(property.value) == subsequentPropertyValue
expect(value) == subsequentPropertyValue
}
it("should perform an action with the value") {
let property = MutableProperty(initialPropertyValue)
let result: Bool = property.withValue { $0.isEmpty }
expect(result) == false
expect(property.value) == initialPropertyValue
}
it("should not deadlock on recursive value access") {
let (producer, observer) = SignalProducer<Int, NoError>.pipe()
let property = MutableProperty(0)
var value: Int?
property <~ producer
property.producer.startWithNext { _ in
value = property.value
}
observer.sendNext(10)
expect(value) == 10
}
it("should not deadlock on recursive value access with a closure") {
let (producer, observer) = SignalProducer<Int, NoError>.pipe()
let property = MutableProperty(0)
var value: Int?
property <~ producer
property.producer.startWithNext { _ in
value = property.withValue { $0 + 1 }
}
observer.sendNext(10)
expect(value) == 11
}
it("should not deadlock on recursive observation") {
let property = MutableProperty(0)
var value: Int?
property.producer.startWithNext { _ in
property.producer.startWithNext { x in value = x }
}
expect(value) == 0
property.value = 1
expect(value) == 1
}
it("should not deadlock on recursive ABA observation") {
let propertyA = MutableProperty(0)
let propertyB = MutableProperty(0)
var value: Int?
propertyA.producer.startWithNext { _ in
propertyB.producer.startWithNext { _ in
propertyA.producer.startWithNext { x in value = x }
}
}
expect(value) == 0
propertyA.value = 1
expect(value) == 1
}
}
describe("AnyProperty") {
describe("from a PropertyType") {
it("should pass through behaviors of the input property") {
let constantProperty = ConstantProperty(initialPropertyValue)
let property = AnyProperty(constantProperty)
var sentValue: String?
var signalSentValue: String?
var producerCompleted = false
var signalInterrupted = false
property.producer.start { event in
switch event {
case let .Next(value):
sentValue = value
case .Completed:
producerCompleted = true
case .Failed, .Interrupted:
break
}
}
property.signal.observe { event in
switch event {
case let .Next(value):
signalSentValue = value
case .Interrupted:
signalInterrupted = true
case .Failed, .Completed:
break
}
}
expect(sentValue) == initialPropertyValue
expect(signalSentValue).to(beNil())
expect(producerCompleted) == true
expect(signalInterrupted) == true
}
describe("transformed properties") {
it("should have the latest value available before sending any value") {
var latestValue: Int!
let property = MutableProperty(1)
let mappedProperty = property.map { $0 + 1 }
mappedProperty.producer.startWithNext { _ in latestValue = mappedProperty.value }
expect(latestValue) == 2
property.value = 2
expect(latestValue) == 3
property.value = 3
expect(latestValue) == 4
}
it("should retain its source property") {
var property = Optional(MutableProperty(1))
weak var weakProperty = property
var firstMappedProperty = Optional(property!.map { $0 + 1 })
var secondMappedProperty = Optional(firstMappedProperty!.map { $0 + 2 })
_ = secondMappedProperty
property = nil
expect(weakProperty).toNot(beNil())
firstMappedProperty = nil
expect(weakProperty).toNot(beNil())
secondMappedProperty = nil
expect(weakProperty).to(beNil())
}
describe("signal lifetime and producer lifetime") {
it("should return a producer and a signal which respect the lifetime of the source property instead of the read-only view itself") {
var signalCompleted = 0
var producerCompleted = 0
var property = Optional(MutableProperty(1))
var firstMappedProperty = Optional(property!.map { $0 + 1 })
var secondMappedProperty = Optional(firstMappedProperty!.map { $0 + 2 })
var thirdMappedProperty = Optional(secondMappedProperty!.map { $0 + 2 })
firstMappedProperty!.signal.observeCompleted { signalCompleted += 1 }
secondMappedProperty!.signal.observeCompleted { signalCompleted += 1 }
thirdMappedProperty!.signal.observeCompleted { signalCompleted += 1 }
firstMappedProperty!.producer.startWithCompleted { producerCompleted += 1 }
secondMappedProperty!.producer.startWithCompleted { producerCompleted += 1 }
thirdMappedProperty!.producer.startWithCompleted { producerCompleted += 1 }
firstMappedProperty = nil
expect(signalCompleted) == 0
expect(producerCompleted) == 0
secondMappedProperty = nil
expect(signalCompleted) == 0
expect(producerCompleted) == 0
property = nil
expect(signalCompleted) == 0
expect(producerCompleted) == 0
thirdMappedProperty = nil
expect(signalCompleted) == 3
expect(producerCompleted) == 3
}
}
}
}
describe("from a value and SignalProducer") {
it("should initially take on the supplied value") {
let property = AnyProperty(
initialValue: initialPropertyValue,
producer: SignalProducer.never)
expect(property.value) == initialPropertyValue
}
it("should take on each value sent on the producer") {
let property = AnyProperty(
initialValue: initialPropertyValue,
producer: SignalProducer(value: subsequentPropertyValue))
expect(property.value) == subsequentPropertyValue
}
}
describe("from a value and Signal") {
it("should initially take on the supplied value, then values sent on the signal") {
let (signal, observer) = Signal<String, NoError>.pipe()
let property = AnyProperty(
initialValue: initialPropertyValue,
signal: signal)
expect(property.value) == initialPropertyValue
observer.sendNext(subsequentPropertyValue)
expect(property.value) == subsequentPropertyValue
}
}
}
describe("PropertyType") {
describe("map") {
it("should transform the current value and all subsequent values") {
let property = MutableProperty(1)
let mappedProperty = property
.map { $0 + 1 }
expect(mappedProperty.value) == 2
property.value = 2
expect(mappedProperty.value) == 3
}
}
describe("combineLatest") {
var property: MutableProperty<String>!
var otherProperty: MutableProperty<String>!
beforeEach {
property = MutableProperty(initialPropertyValue)
otherProperty = MutableProperty(initialOtherPropertyValue)
}
it("should forward the latest values from both inputs") {
let combinedProperty = property.combineLatestWith(otherProperty)
var latest: (String, String)?
combinedProperty.signal.observeNext { latest = $0 }
property.value = subsequentPropertyValue
expect(latest?.0) == subsequentPropertyValue
expect(latest?.1) == initialOtherPropertyValue
// is there a better way to test tuples?
otherProperty.value = subsequentOtherPropertyValue
expect(latest?.0) == subsequentPropertyValue
expect(latest?.1) == subsequentOtherPropertyValue
property.value = finalPropertyValue
expect(latest?.0) == finalPropertyValue
expect(latest?.1) == subsequentOtherPropertyValue
}
it("should complete when the source properties are deinitialized") {
var completed = false
var combinedProperty = Optional(property.combineLatestWith(otherProperty))
combinedProperty!.signal.observeCompleted { completed = true }
combinedProperty = nil
expect(completed) == false
property = nil
expect(completed) == false
otherProperty = nil
expect(completed) == true
}
}
describe("zip") {
var property: MutableProperty<String>!
var otherProperty: MutableProperty<String>!
beforeEach {
property = MutableProperty(initialPropertyValue)
otherProperty = MutableProperty(initialOtherPropertyValue)
}
it("should combine pairs") {
var result: [String] = []
let zippedProperty = property.zipWith(otherProperty)
zippedProperty.producer.startWithNext { (left, right) in result.append("\(left)\(right)") }
let firstResult = [ "\(initialPropertyValue)\(initialOtherPropertyValue)" ]
let secondResult = firstResult + [ "\(subsequentPropertyValue)\(subsequentOtherPropertyValue)" ]
let thirdResult = secondResult + [ "\(finalPropertyValue)\(finalOtherPropertyValue)" ]
let finalResult = thirdResult + [ "\(initialPropertyValue)\(initialOtherPropertyValue)" ]
expect(result) == firstResult
property.value = subsequentPropertyValue
expect(result) == firstResult
otherProperty.value = subsequentOtherPropertyValue
expect(result) == secondResult
property.value = finalPropertyValue
otherProperty.value = finalOtherPropertyValue
expect(result) == thirdResult
property.value = initialPropertyValue
expect(result) == thirdResult
property.value = subsequentPropertyValue
expect(result) == thirdResult
otherProperty.value = initialOtherPropertyValue
expect(result) == finalResult
}
it("should complete its producer only when the source properties are deinitialized") {
var result: [String] = []
var completed = false
var zippedProperty = Optional(property.zipWith(otherProperty))
zippedProperty!.producer.start { event in
switch event {
case let .Next(left, right):
result.append("\(left)\(right)")
case .Completed:
completed = true
default:
break
}
}
expect(completed) == false
expect(result) == [ "\(initialPropertyValue)\(initialOtherPropertyValue)" ]
property.value = subsequentPropertyValue
expect(result) == [ "\(initialPropertyValue)\(initialOtherPropertyValue)" ]
zippedProperty = nil
expect(completed) == false
property = nil
otherProperty = nil
expect(completed) == true
}
}
describe("unary operators") {
var property: MutableProperty<String>!
beforeEach {
property = MutableProperty(initialPropertyValue)
}
describe("combinePrevious") {
it("should pack the current value and the previous value a tuple") {
let transformedProperty = property.combinePrevious(initialPropertyValue)
expect(transformedProperty.value.0) == initialPropertyValue
expect(transformedProperty.value.1) == initialPropertyValue
property.value = subsequentPropertyValue
expect(transformedProperty.value.0) == initialPropertyValue
expect(transformedProperty.value.1) == subsequentPropertyValue
property.value = finalPropertyValue
expect(transformedProperty.value.0) == subsequentPropertyValue
expect(transformedProperty.value.1) == finalPropertyValue
}
it("should complete its producer only when the source property is deinitialized") {
var result: (String, String)?
var completed = false
var transformedProperty = Optional(property.combinePrevious(initialPropertyValue))
transformedProperty!.producer.start { event in
switch event {
case let .Next(tuple):
result = tuple
case .Completed:
completed = true
default:
break
}
}
expect(result?.0) == initialPropertyValue
expect(result?.1) == initialPropertyValue
property.value = subsequentPropertyValue
expect(result?.0) == initialPropertyValue
expect(result?.1) == subsequentPropertyValue
transformedProperty = nil
expect(completed) == false
property = nil
expect(completed) == true
}
}
describe("skipRepeats") {
it("should not emit events for subsequent equatable values that are the same as the current value") {
let transformedProperty = property.skipRepeats()
var counter = 0
transformedProperty.signal.observeNext { _ in
counter += 1
}
property.value = initialPropertyValue
property.value = initialPropertyValue
property.value = initialPropertyValue
expect(counter) == 0
property.value = subsequentPropertyValue
property.value = subsequentPropertyValue
property.value = subsequentPropertyValue
expect(counter) == 1
property.value = finalPropertyValue
property.value = initialPropertyValue
property.value = subsequentPropertyValue
expect(counter) == 4
}
it("should not emit events for subsequent values that are regarded as the same as the current value by the supplied closure") {
var counter = 0
let transformedProperty = property.skipRepeats { _, newValue in newValue == initialPropertyValue }
transformedProperty.signal.observeNext { _ in
counter += 1
}
property.value = initialPropertyValue
expect(counter) == 0
property.value = subsequentPropertyValue
expect(counter) == 1
property.value = finalPropertyValue
expect(counter) == 2
property.value = initialPropertyValue
expect(counter) == 2
}
it("should complete its producer only when the source property is deinitialized") {
var counter = 0
var completed = false
var transformedProperty = Optional(property.skipRepeats())
transformedProperty!.producer.start { event in
switch event {
case .Next:
counter += 1
case .Completed:
completed = true
default:
break
}
}
expect(counter) == 1
property.value = initialPropertyValue
expect(counter) == 1
transformedProperty = nil
expect(completed) == false
property = nil
expect(completed) == true
}
}
describe("uniqueValues") {
it("should emit hashable values that have not been emited before") {
let transformedProperty = property.uniqueValues()
var counter = 0
transformedProperty.signal.observeNext { _ in
counter += 1
}
property.value = initialPropertyValue
expect(counter) == 0
property.value = subsequentPropertyValue
property.value = subsequentPropertyValue
expect(counter) == 1
property.value = finalPropertyValue
property.value = initialPropertyValue
property.value = subsequentPropertyValue
expect(counter) == 2
}
it("should emit only the values of which the computed identity have not been captured before") {
let transformedProperty = property.uniqueValues { _ in 0 }
var counter = 0
transformedProperty.signal.observeNext { _ in
counter += 1
}
property.value = initialPropertyValue
property.value = subsequentPropertyValue
property.value = finalPropertyValue
expect(counter) == 0
}
it("should complete its producer only when the source property is deinitialized") {
var counter = 0
var completed = false
var transformedProperty = Optional(property.uniqueValues())
transformedProperty!.producer.start { event in
switch event {
case .Next:
counter += 1
case .Completed:
completed = true
default:
break
}
}
expect(counter) == 1
property.value = initialPropertyValue
expect(counter) == 1
transformedProperty = nil
expect(completed) == false
property = nil
expect(completed) == true
}
}
}
describe("flattening") {
describe("flatten") {
describe("FlattenStrategy.Concat") {
it("should concatenate the values as the inner property is replaced and deinitialized") {
var firstProperty = Optional(MutableProperty(0))
var secondProperty = Optional(MutableProperty(10))
var thirdProperty = Optional(MutableProperty(20))
var outerProperty = Optional(MutableProperty(firstProperty!))
var receivedValues: [Int] = []
var errored = false
var completed = false
var flattenedProperty = Optional(outerProperty!.flatten(.Concat))
flattenedProperty!.producer.start { event in
switch event {
case let .Next(value):
receivedValues.append(value)
case .Completed:
completed = true
case .Failed:
errored = true
case .Interrupted:
break
}
}
expect(receivedValues) == [ 0 ]
outerProperty!.value = secondProperty!
secondProperty!.value = 11
outerProperty!.value = thirdProperty!
thirdProperty!.value = 21
expect(receivedValues) == [ 0 ]
expect(completed) == false
secondProperty!.value = 12
thirdProperty!.value = 22
expect(receivedValues) == [ 0 ]
expect(completed) == false
firstProperty = nil
expect(receivedValues) == [ 0, 12 ]
expect(completed) == false
secondProperty = nil
expect(receivedValues) == [ 0, 12, 22 ]
expect(completed) == false
outerProperty = nil
expect(completed) == false
thirdProperty = nil
expect(completed) == false
flattenedProperty = nil
expect(completed) == true
expect(errored) == false
}
}
describe("FlattenStrategy.Merge") {
it("should merge the values of all inner properties") {
var firstProperty = Optional(MutableProperty(0))
var secondProperty = Optional(MutableProperty(10))
var thirdProperty = Optional(MutableProperty(20))
var outerProperty = Optional(MutableProperty(firstProperty!))
var receivedValues: [Int] = []
var errored = false
var completed = false
var flattenedProperty = Optional(outerProperty!.flatten(.Merge))
flattenedProperty!.producer.start { event in
switch event {
case let .Next(value):
receivedValues.append(value)
case .Completed:
completed = true
case .Failed:
errored = true
case .Interrupted:
break
}
}
expect(receivedValues) == [ 0 ]
outerProperty!.value = secondProperty!
secondProperty!.value = 11
outerProperty!.value = thirdProperty!
thirdProperty!.value = 21
expect(receivedValues) == [ 0, 10, 11, 20, 21 ]
expect(completed) == false
secondProperty!.value = 12
thirdProperty!.value = 22
expect(receivedValues) == [ 0, 10, 11, 20, 21, 12, 22 ]
expect(completed) == false
firstProperty = nil
expect(receivedValues) == [ 0, 10, 11, 20, 21, 12, 22 ]
expect(completed) == false
secondProperty = nil
expect(receivedValues) == [ 0, 10, 11, 20, 21, 12, 22 ]
expect(completed) == false
outerProperty = nil
expect(completed) == false
thirdProperty = nil
expect(completed) == false
flattenedProperty = nil
expect(completed) == true
expect(errored) == false
}
}
describe("FlattenStrategy.Latest") {
it("should forward values from the latest inner property") {
let firstProperty = Optional(MutableProperty(0))
var secondProperty = Optional(MutableProperty(10))
var thirdProperty = Optional(MutableProperty(20))
var outerProperty = Optional(MutableProperty(firstProperty!))
var receivedValues: [Int] = []
var errored = false
var completed = false
outerProperty!.flatten(.Latest).producer.start { event in
switch event {
case let .Next(value):
receivedValues.append(value)
case .Completed:
completed = true
case .Failed:
errored = true
case .Interrupted:
break
}
}
expect(receivedValues) == [ 0 ]
outerProperty!.value = secondProperty!
secondProperty!.value = 11
outerProperty!.value = thirdProperty!
thirdProperty!.value = 21
expect(receivedValues) == [ 0, 10, 11, 20, 21 ]
expect(errored) == false
expect(completed) == false
secondProperty!.value = 12
secondProperty = nil
thirdProperty!.value = 22
thirdProperty = nil
expect(receivedValues) == [ 0, 10, 11, 20, 21, 22 ]
expect(errored) == false
expect(completed) == false
outerProperty = nil
expect(errored) == false
expect(completed) == true
}
it("should release the old properties when switched or deallocated") {
var firstProperty = Optional(MutableProperty(0))
var secondProperty = Optional(MutableProperty(10))
var thirdProperty = Optional(MutableProperty(20))
weak var weakFirstProperty = firstProperty
weak var weakSecondProperty = secondProperty
weak var weakThirdProperty = thirdProperty
var outerProperty = Optional(MutableProperty(firstProperty!))
var flattened = Optional(outerProperty!.flatten(.Latest))
var errored = false
var completed = false
flattened!.producer.start { event in
switch event {
case .Completed:
completed = true
case .Failed:
errored = true
case .Interrupted, .Next:
break
}
}
firstProperty = nil
outerProperty!.value = secondProperty!
expect(weakFirstProperty).to(beNil())
secondProperty = nil
outerProperty!.value = thirdProperty!
expect(weakSecondProperty).to(beNil())
thirdProperty = nil
outerProperty = nil
flattened = nil
expect(weakThirdProperty).to(beNil())
expect(errored) == false
expect(completed) == true
}
}
}
describe("flatMap") {
describe("PropertyFlattenStrategy.Latest") {
it("should forward values from the latest inner transformed property") {
let firstProperty = Optional(MutableProperty(0))
var secondProperty = Optional(MutableProperty(10))
var thirdProperty = Optional(MutableProperty(20))
var outerProperty = Optional(MutableProperty(firstProperty!))
var receivedValues: [String] = []
var errored = false
var completed = false
outerProperty!.flatMap(.Latest) { $0.map { "\($0)" } }.producer.start { event in
switch event {
case let .Next(value):
receivedValues.append(value)
case .Completed:
completed = true
case .Failed:
errored = true
case .Interrupted:
break
}
}
expect(receivedValues) == [ "0" ]
outerProperty!.value = secondProperty!
secondProperty!.value = 11
outerProperty!.value = thirdProperty!
thirdProperty!.value = 21
expect(receivedValues) == [ "0", "10", "11", "20", "21" ]
expect(errored) == false
expect(completed) == false
secondProperty!.value = 12
secondProperty = nil
thirdProperty!.value = 22
thirdProperty = nil
expect(receivedValues) == [ "0", "10", "11", "20", "21", "22" ]
expect(errored) == false
expect(completed) == false
outerProperty = nil
expect(errored) == false
expect(completed) == true
}
}
}
}
}
describe("DynamicProperty") {
var object: ObservableObject!
var property: DynamicProperty<Int>!
let propertyValue: () -> Int? = {
if let value: AnyObject = property?.value {
return value as? Int
} else {
return nil
}
}
beforeEach {
object = ObservableObject()
expect(object.rac_value) == 0
property = DynamicProperty<Int>(object: object, keyPath: "rac_value")
}
afterEach {
object = nil
}
it("should read the underlying object") {
expect(propertyValue()) == 0
object.rac_value = 1
expect(propertyValue()) == 1
}
it("should write the underlying object") {
property.value = 1
expect(object.rac_value) == 1
expect(propertyValue()) == 1
}
it("should yield a producer that sends the current value and then the changes for the key path of the underlying object") {
var values: [Int] = []
property.producer.startWithNext { value in
expect(value).notTo(beNil())
values.append(value!)
}
expect(values) == [ 0 ]
property.value = 1
expect(values) == [ 0, 1 ]
object.rac_value = 2
expect(values) == [ 0, 1, 2 ]
}
it("should yield a producer that sends the current value and then the changes for the key path of the underlying object, even if the value actually remains unchanged") {
var values: [Int] = []
property.producer.startWithNext { value in
expect(value).notTo(beNil())
values.append(value!)
}
expect(values) == [ 0 ]
property.value = 0
expect(values) == [ 0, 0 ]
object.rac_value = 0
expect(values) == [ 0, 0, 0 ]
}
it("should yield a signal that emits subsequent values for the key path of the underlying object") {
var values: [Int] = []
property.signal.observeNext { value in
expect(value).notTo(beNil())
values.append(value!)
}
expect(values) == []
property.value = 1
expect(values) == [ 1 ]
object.rac_value = 2
expect(values) == [ 1, 2 ]
}
it("should yield a signal that emits subsequent values for the key path of the underlying object, even if the value actually remains unchanged") {
var values: [Int] = []
property.signal.observeNext { value in
expect(value).notTo(beNil())
values.append(value!)
}
expect(values) == []
property.value = 0
expect(values) == [ 0 ]
object.rac_value = 0
expect(values) == [ 0, 0 ]
}
it("should have a completed producer when the underlying object deallocates") {
var completed = false
property = {
// Use a closure so this object has a shorter lifetime.
let object = ObservableObject()
let property = DynamicProperty<Int>(object: object, keyPath: "rac_value")
property.producer.startWithCompleted {
completed = true
}
expect(completed) == false
expect(property.value).notTo(beNil())
return property
}()
expect(completed).toEventually(beTruthy())
expect(property.value).to(beNil())
}
it("should have a completed signal when the underlying object deallocates") {
var completed = false
property = {
// Use a closure so this object has a shorter lifetime.
let object = ObservableObject()
let property = DynamicProperty<Int>(object: object, keyPath: "rac_value")
property.signal.observeCompleted {
completed = true
}
expect(completed) == false
expect(property.value).notTo(beNil())
return property
}()
expect(completed).toEventually(beTruthy())
expect(property.value).to(beNil())
}
it("should retain property while DynamicProperty's underlying object is retained"){
weak var dynamicProperty: DynamicProperty<Int>? = property
property = nil
expect(dynamicProperty).toNot(beNil())
object = nil
expect(dynamicProperty).to(beNil())
}
it("should support un-bridged reference types") {
let dynamicProperty = DynamicProperty<UnbridgedObject>(object: object, keyPath: "rac_reference")
dynamicProperty.value = UnbridgedObject("foo")
expect(object.rac_reference.value) == "foo"
}
}
describe("binding") {
describe("from a Signal") {
it("should update the property with values sent from the signal") {
let (signal, observer) = Signal<String, NoError>.pipe()
let mutableProperty = MutableProperty(initialPropertyValue)
mutableProperty <~ signal
// Verify that the binding hasn't changed the property value:
expect(mutableProperty.value) == initialPropertyValue
observer.sendNext(subsequentPropertyValue)
expect(mutableProperty.value) == subsequentPropertyValue
}
it("should tear down the binding when disposed") {
let (signal, observer) = Signal<String, NoError>.pipe()
let mutableProperty = MutableProperty(initialPropertyValue)
let bindingDisposable = mutableProperty <~ signal
bindingDisposable.dispose()
observer.sendNext(subsequentPropertyValue)
expect(mutableProperty.value) == initialPropertyValue
}
it("should tear down the binding when bound signal is completed") {
let (signal, observer) = Signal<String, NoError>.pipe()
let mutableProperty = MutableProperty(initialPropertyValue)
let bindingDisposable = mutableProperty <~ signal
expect(bindingDisposable.disposed) == false
observer.sendCompleted()
expect(bindingDisposable.disposed) == true
}
it("should tear down the binding when the property deallocates") {
let (signal, _) = Signal<String, NoError>.pipe()
var mutableProperty: MutableProperty<String>? = MutableProperty(initialPropertyValue)
let bindingDisposable = mutableProperty! <~ signal
mutableProperty = nil
expect(bindingDisposable.disposed) == true
}
}
describe("from a SignalProducer") {
it("should start a signal and update the property with its values") {
let signalValues = [initialPropertyValue, subsequentPropertyValue]
let signalProducer = SignalProducer<String, NoError>(values: signalValues)
let mutableProperty = MutableProperty(initialPropertyValue)
mutableProperty <~ signalProducer
expect(mutableProperty.value) == signalValues.last!
}
it("should tear down the binding when disposed") {
let signalValues = [initialPropertyValue, subsequentPropertyValue]
let signalProducer = SignalProducer<String, NoError>(values: signalValues)
let mutableProperty = MutableProperty(initialPropertyValue)
let disposable = mutableProperty <~ signalProducer
disposable.dispose()
// TODO: Assert binding was torn down?
}
it("should tear down the binding when bound signal is completed") {
let (signalProducer, observer) = SignalProducer<String, NoError>.pipe()
let mutableProperty = MutableProperty(initialPropertyValue)
mutableProperty <~ signalProducer
observer.sendCompleted()
// TODO: Assert binding was torn down?
}
it("should tear down the binding when the property deallocates") {
let signalValues = [initialPropertyValue, subsequentPropertyValue]
let signalProducer = SignalProducer<String, NoError>(values: signalValues)
var mutableProperty: MutableProperty<String>? = MutableProperty(initialPropertyValue)
let disposable = mutableProperty! <~ signalProducer
mutableProperty = nil
expect(disposable.disposed) == true
}
}
describe("from another property") {
it("should take the source property's current value") {
let sourceProperty = ConstantProperty(initialPropertyValue)
let destinationProperty = MutableProperty("")
destinationProperty <~ sourceProperty.producer
expect(destinationProperty.value) == initialPropertyValue
}
it("should update with changes to the source property's value") {
let sourceProperty = MutableProperty(initialPropertyValue)
let destinationProperty = MutableProperty("")
destinationProperty <~ sourceProperty.producer
sourceProperty.value = subsequentPropertyValue
expect(destinationProperty.value) == subsequentPropertyValue
}
it("should tear down the binding when disposed") {
let sourceProperty = MutableProperty(initialPropertyValue)
let destinationProperty = MutableProperty("")
let bindingDisposable = destinationProperty <~ sourceProperty.producer
bindingDisposable.dispose()
sourceProperty.value = subsequentPropertyValue
expect(destinationProperty.value) == initialPropertyValue
}
it("should tear down the binding when the source property deallocates") {
var sourceProperty: MutableProperty<String>? = MutableProperty(initialPropertyValue)
let destinationProperty = MutableProperty("")
destinationProperty <~ sourceProperty!.producer
sourceProperty = nil
// TODO: Assert binding was torn down?
}
it("should tear down the binding when the destination property deallocates") {
let sourceProperty = MutableProperty(initialPropertyValue)
var destinationProperty: MutableProperty<String>? = MutableProperty("")
let bindingDisposable = destinationProperty! <~ sourceProperty.producer
destinationProperty = nil
expect(bindingDisposable.disposed) == true
}
}
describe("to a dynamic property") {
var object: ObservableObject!
var property: DynamicProperty<Int>!
beforeEach {
object = ObservableObject()
expect(object.rac_value) == 0
property = DynamicProperty<Int>(object: object, keyPath: "rac_value")
}
afterEach {
object = nil
}
it("should bridge values sent on a signal to Objective-C") {
let (signal, observer) = Signal<Int, NoError>.pipe()
property <~ signal
observer.sendNext(1)
expect(object.rac_value) == 1
}
it("should bridge values sent on a signal producer to Objective-C") {
let producer = SignalProducer<Int, NoError>(value: 1)
property <~ producer
expect(object.rac_value) == 1
}
it("should bridge values from a source property to Objective-C") {
let source = MutableProperty(1)
property <~ source
expect(object.rac_value) == 1
}
}
}
}
}
private class ObservableObject: NSObject {
dynamic var rac_value: Int = 0
dynamic var rac_reference: UnbridgedObject = UnbridgedObject("")
}
private class UnbridgedObject: NSObject {
let value: String
init(_ value: String) {
self.value = value
}
}
| mit | 93aaaf53a96095297da6f7259cc5f34e | 27.778325 | 172 | 0.6678 | 4.369017 | false | false | false | false |
bmichotte/HSTracker | HSTracker/UIs/Preferences/Splashscreen.swift | 3 | 1280 | /*
* This file is part of the HSTracker package.
* (c) Benjamin Michotte <[email protected]>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*
* Created on 13/02/16.
*/
import Cocoa
class Splashscreen: NSWindowController {
@IBOutlet weak var information: NSTextField!
@IBOutlet weak var progressBar: NSProgressIndicator!
func display(_ str: String, indeterminate: Bool) {
DispatchQueue.main.async { [weak self] in
self?.information.stringValue = str
self?.progressBar.isIndeterminate = indeterminate
}
}
func display(_ str: String, total: Double) {
DispatchQueue.main.async { [weak self] in
self?.progressBar.isIndeterminate = false
self?.information.stringValue = str
self?.progressBar.maxValue = total
self?.progressBar.doubleValue = 0
}
}
func increment(_ str: String? = nil) {
// UI should be adjusted on the main thread
DispatchQueue.main.async { [weak self] in
self?.progressBar.increment(by: 1)
if let str = str {
self?.information.stringValue = str
}
}
}
}
| mit | 7cbaf8b76e2e6fe9d714d4296394e42b | 28.767442 | 74 | 0.61875 | 4.688645 | false | false | false | false |
lorentey/swift | test/Constraints/overload.swift | 3 | 6201 | // RUN: %target-typecheck-verify-swift
func markUsed<T>(_ t: T) {}
func f0(_: Float) -> Float {}
// expected-note@-1 {{candidate expects value of type 'Float' for parameter #1}}
func f0(_: Int) -> Int {}
// expected-note@-1 {{candidate expects value of type 'Int' for parameter #1}}
func f1(_: Int) {}
func identity<T>(_: T) -> T {}
func f2<T>(_: T) -> T {}
// FIXME: Fun things happen when we make this T, U!
func f2<T>(_: T, _: T) -> (T, T) { }
struct X {}
var x : X
var i : Int
var f : Float
_ = f0(i)
_ = f0(1.0)
_ = f0(1)
f1(f0(1))
f1(identity(1))
f0(x) // expected-error{{no exact matches in call to global function 'f0'}}
_ = f + 1
_ = f2(i)
_ = f2((i, f))
class A {
init() {}
}
class B : A {
override init() { super.init() }
}
class C : B {
override init() { super.init() }
}
func bar(_ b: B) -> Int {} // #1
func bar(_ a: A) -> Float {} // #2
var barResult = bar(C()) // selects #1, which is more specialized
i = barResult // make sure we got #1
f = bar(C()) // selects #2 because of context
// Overload resolution for constructors
protocol P1 { }
struct X1a : P1 { }
struct X1b {
init(x : X1a) { }
init<T : P1>(x : T) { }
}
X1b(x: X1a()) // expected-warning{{unused}}
// Overload resolution for subscript operators.
class X2a { }
class X2b : X2a { }
class X2c : X2b { }
struct X2d {
subscript (index : X2a) -> Int {
return 5
}
subscript (index : X2b) -> Int {
return 7
}
func foo(_ x : X2c) -> Int {
return self[x]
}
}
// Invalid declarations
// FIXME: Suppress the diagnostic for the call below, because the invalid
// declaration would have matched.
func f3(_ x: Intthingy) -> Int { } // expected-error{{use of undeclared type 'Intthingy'}}
func f3(_ x: Float) -> Float { }
f3(i) // expected-error{{cannot convert value of type 'Int' to expected argument type 'Float'}}
func f4(_ i: Wonka) { } // expected-error{{use of undeclared type 'Wonka'}}
func f4(_ j: Wibble) { } // expected-error{{use of undeclared type 'Wibble'}}
f4(5)
func f1() {
var c : Class // expected-error{{use of undeclared type 'Class'}}
markUsed(c.x) // make sure error does not cascade here
}
// We don't provide return-type sensitivity unless there is context.
func f5(_ i: Int) -> A { return A() } // expected-note{{candidate}}
func f5(_ i: Int) -> B { return B() } // expected-note{{candidate}}
f5(5) // expected-error{{ambiguous use of 'f5'}}
struct HasX1aProperty {
func write(_: X1a) {}
func write(_: P1) {}
var prop = X1a()
func test() {
write(prop) // no error, not ambiguous
}
}
// rdar://problem/16554496
@available(*, unavailable)
func availTest(_ x: Int) {}
func availTest(_ x: Any) { markUsed("this one") }
func doAvailTest(_ x: Int) {
availTest(x)
}
// rdar://problem/20886179
func test20886179(_ handlers: [(Int) -> Void], buttonIndex: Int) {
handlers[buttonIndex](buttonIndex)
}
// The problem here is that the call has a contextual result type incompatible
// with *all* overload set candidates. This is not an ambiguity.
func overloaded_identity(_ a : Int) -> Int {}
func overloaded_identity(_ b : Float) -> Float {}
func test_contextual_result_1() {
return overloaded_identity() // expected-error {{cannot invoke 'overloaded_identity' with no arguments}}
// expected-note @-1 {{overloads for 'overloaded_identity' exist with these partially matching parameter lists: (Float), (Int)}}
}
func test_contextual_result_2() {
return overloaded_identity(1) // expected-error {{unexpected non-void return value in void function}}
}
// rdar://problem/24128153
struct X0 {
init(_ i: Any.Type) { }
init?(_ i: Any.Type, _ names: String...) { }
}
let x0 = X0(Int.self)
let x0check: X0 = x0 // okay: chooses first initializer
struct X1 {
init?(_ i: Any.Type) { }
init(_ i: Any.Type, _ names: String...) { }
}
let x1 = X1(Int.self)
let x1check: X1 = x1 // expected-error{{value of optional type 'X1?' must be unwrapped}}
// expected-note@-1{{coalesce}}
// expected-note@-2{{force-unwrap}}
struct X2 {
init?(_ i: Any.Type) { }
init(_ i: Any.Type, a: Int = 0) { }
init(_ i: Any.Type, a: Int = 0, b: Int = 0) { }
init(_ i: Any.Type, a: Int = 0, c: Int = 0) { }
}
let x2 = X2(Int.self)
let x2check: X2 = x2 // expected-error{{value of optional type 'X2?' must be unwrapped}}
// expected-note@-1{{coalesce}}
// expected-note@-2{{force-unwrap}}
// rdar://problem/28051973
struct R_28051973 {
mutating func f(_ i: Int) {}
@available(*, deprecated, message: "deprecated")
func f(_ f: Float) {}
}
let r28051973: Int = 42
R_28051973().f(r28051973) // expected-error {{cannot use mutating member on immutable value: function call returns immutable value}}
// Fix for CSDiag vs CSSolver disagreement on what constitutes a
// valid overload.
func overloadedMethod(n: Int) {} // expected-note {{'overloadedMethod(n:)' declared here}}
func overloadedMethod<T>() {}
// expected-error@-1 {{generic parameter 'T' is not used in function signature}}
overloadedMethod()
// expected-error@-1 {{missing argument for parameter 'n' in call}}
// Ensure we select the overload of '??' returning T? rather than T.
func SR3817(_ d: [String : Any], _ s: String, _ t: String) -> Any {
if let r = d[s] ?? d[t] {
return r
} else {
return 0
}
}
// Overloading with mismatched labels.
func f6<T>(foo: T) { }
func f6<T: P1>(bar: T) { }
struct X6 {
init<T>(foo: T) { }
init<T: P1>(bar: T) { }
}
func test_f6() {
let _: (X1a) -> Void = f6
let _: (X1a) -> X6 = X6.init
}
func curry<LHS, RHS, R>(_ f: @escaping (LHS, RHS) -> R) -> (LHS) -> (RHS) -> R {
return { lhs in { rhs in f(lhs, rhs) } }
}
// We need to have an alternative version of this to ensure that there's an overload disjunction created.
func curry<F, S, T, R>(_ f: @escaping (F, S, T) -> R) -> (F) -> (S) -> (T) -> R {
return { fst in { snd in { thd in f(fst, snd, thd) } } }
}
// Ensure that we consider these unambiguous
let _ = curry(+)(1)
let _ = [0].reduce(0, +)
let _ = curry(+)("string vs. pointer")
func autoclosure1<T>(_: T, _: @autoclosure () -> X) { }
func autoclosure1<T>(_: [T], _: X) { }
func test_autoclosure1(ia: [Int]) {
autoclosure1(ia, X()) // okay: resolves to the second function
}
| apache-2.0 | 52d4db1ef1b25f67fb3ff9d16564393b | 24.945607 | 132 | 0.620706 | 2.961318 | false | false | false | false |
lorentey/swift | test/Serialization/autolinking.swift | 11 | 3904 | // RUN: %empty-directory(%t)
// RUN: %target-swift-frontend -emit-module -parse-stdlib -o %t -module-name someModule -module-link-name module %S/../Inputs/empty.swift
// RUN: %target-swift-frontend -disable-autolinking-runtime-compatibility-dynamic-replacements -runtime-compatibility-version none -emit-ir -lmagic %s -I %t > %t/out.txt
// RUN: %FileCheck %s < %t/out.txt
// RUN: %FileCheck -check-prefix=NO-FORCE-LOAD %s < %t/out.txt
// RUN: %empty-directory(%t/someModule.framework/Modules/someModule.swiftmodule)
// RUN: mv %t/someModule.swiftmodule %t/someModule.framework/Modules/someModule.swiftmodule/%target-swiftmodule-name
// RUN: %target-swift-frontend -disable-autolinking-runtime-compatibility-dynamic-replacements -runtime-compatibility-version none -emit-ir -lmagic %s -F %t > %t/framework.txt
// RUN: %FileCheck -check-prefix=FRAMEWORK %s < %t/framework.txt
// RUN: %FileCheck -check-prefix=NO-FORCE-LOAD %s < %t/framework.txt
// RUN: %target-swift-frontend -emit-module -parse-stdlib -o %t -module-name someModule -module-link-name module %S/../Inputs/empty.swift -autolink-force-load
// RUN: %target-swift-frontend -runtime-compatibility-version none -emit-ir -lmagic %s -I %t > %t/force-load.txt
// RUN: %FileCheck %s < %t/force-load.txt
// RUN: %FileCheck -check-prefix FORCE-LOAD-CLIENT -check-prefix FORCE-LOAD-CLIENT-%target-object-format %s < %t/force-load.txt
// RUN: %target-swift-frontend -runtime-compatibility-version none -emit-ir -debugger-support %s -I %t > %t/force-load.txt
// RUN: %FileCheck -check-prefix NO-FORCE-LOAD-CLIENT %s < %t/force-load.txt
// RUN: %target-swift-frontend -disable-autolinking-runtime-compatibility-dynamic-replacements -runtime-compatibility-version none -emit-ir -parse-stdlib -module-name someModule -module-link-name module %S/../Inputs/empty.swift | %FileCheck --check-prefix=NO-FORCE-LOAD %s
// RUN: %target-swift-frontend -runtime-compatibility-version none -emit-ir -parse-stdlib -module-name someModule -module-link-name module %S/../Inputs/empty.swift -autolink-force-load | %FileCheck --check-prefix=FORCE-LOAD %s
// RUN: %target-swift-frontend -runtime-compatibility-version none -emit-ir -parse-stdlib -module-name someModule -module-link-name 0module %S/../Inputs/empty.swift -autolink-force-load | %FileCheck --check-prefix=FORCE-LOAD-HEX %s
// Linux uses a different autolinking mechanism, based on
// swift-autolink-extract. This file tests the Darwin mechanism.
// UNSUPPORTED: autolink-extract
import someModule
// CHECK: !llvm.linker.options = !{
// CHECK-DAG: !{{[0-9]+}} = !{!{{"-lmagic"|"/DEFAULTLIB:magic.lib"}}}
// CHECK-DAG: !{{[0-9]+}} = !{!{{"-lmodule"|"/DEFAULTLIB:module.lib"}}}
// FRAMEWORK: !llvm.linker.options = !{
// FRAMEWORK-DAG: !{{[0-9]+}} = !{!{{"-lmagic"|"/DEFAULTLIB:magic.lib"}}}
// FRAMEWORK-DAG: !{{[0-9]+}} = !{!{{"-lmodule"|"/DEFAULTLIB:module.lib"}}}
// FRAMEWORK-DAG: !{{[0-9]+}} = !{!"-framework", !"someModule"}
// NO-FORCE-LOAD-NOT: FORCE_LOAD
// NO-FORCE-LOAD-NOT -lmodule
// NO-FORCE-LOAD-NOT -lmagic
// FORCE-LOAD: define{{( dllexport)?}} void @"_swift_FORCE_LOAD_$_module"() {{(comdat )?}}{
// FORCE-LOAD: ret void
// FORCE-LOAD: }
// FORCE-LOAD-HEX: define{{( dllexport)?}} void @"_swift_FORCE_LOAD_$306d6f64756c65"() {{(comdat )?}}{
// FORCE-LOAD-HEX: ret void
// FORCE-LOAD-HEX: }
// NO-FORCE-LOAD-CLIENT-NOT: FORCE_LOAD
// FORCE-LOAD-CLIENT: @"_swift_FORCE_LOAD_$_module_$_autolinking" = weak_odr hidden constant void ()* @"_swift_FORCE_LOAD_$_module"
// FORCE-LOAD-CLIENT: @llvm.used = appending global [{{[0-9]+}} x i8*] [
// FORCE-LOAD-CLIENT: i8* bitcast (void ()** @"_swift_FORCE_LOAD_$_module_$_autolinking" to i8*)
// FORCE-LOAD-CLIENT: ], section "llvm.metadata"
// FORCE-LOAD-CLIENT-MACHO: declare extern_weak {{(dllimport )?}}void @"_swift_FORCE_LOAD_$_module"()
// FORCE-LOAD-CLIENT-COFF: declare extern {{(dllimport )?}}void @"_swift_FORCE_LOAD_$_module"()
| apache-2.0 | 4c6cb8e00618ade0da7e56644ac980f6 | 67.491228 | 272 | 0.702613 | 3.24792 | false | false | false | false |
rene-dohan/CS-IOS | Renetik/Renetik/Classes/Core/Classes/Controller/CSNavigationHidingByKeyboardController.swift | 1 | 1403 | //
// Renetik
//
// Created by Rene Dohan on 7/9/19.
//
import RenetikObjc
public class CSNavigationHidingByKeyboardController: CSViewController {
private var navigationBarHidden = false
private let keyboardManager = CSKeyboardObserverController()
@discardableResult
public override func construct(_ parent: UIViewController) -> Self {
super.construct(parent).asViewLess()
keyboardManager.construct(self, onKeyboardChange)
return self
}
private func onKeyboardChange(keyboardHeight: CGFloat) {
if keyboardHeight > 0 { hideNavigationBar() } else { showNavigationBar() }
}
public func hideNavigationBar() {
if navigationBarHidden { return }
animate(duration: 0.5) {
navigation.navigationBar.bottom = UIApplication.statusBarHeight
navigation.last!.view.margin(top: navigation.navigationBar.bottom)
}
navigation.navigationBar.fadeOut(duration: 0.7)
navigationBarHidden = true
}
public func showNavigationBar() {
if !navigationBarHidden { return }
animate(duration: 0.5) {
navigation.navigationBar.top = UIApplication.shared.statusBarFrame.height
navigation.last!.view.margin(top: navigation.navigationBar.bottom)
}
navigation.navigationBar.fadeIn(duration: 0.7)
navigationBarHidden = false
}
}
| mit | 3d4dccf6a823646f33da112d3f2d3ee6 | 30.886364 | 85 | 0.683535 | 5.139194 | false | false | false | false |
vgatto/protobuf-swift | src/ProtocolBuffers/runtime-pb-swift/CodedInputStream.swift | 2 | 20465 | // Protocol Buffers for Swift
//
// Copyright 2014 Alexey Khohklov(AlexeyXo).
// Copyright 2008 Google Inc.
//
// Licensed under the Apache License, Version 2.0 (the "License")
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
import Foundation
let DEFAULT_RECURSION_LIMIT:Int32 = 64
let DEFAULT_SIZE_LIMIT:Int32 = 64 << 20 // 64MB
let BUFFER_SIZE:Int32 = 4096
public class CodedInputStream
{
public var buffer:NSMutableData
private var input:NSInputStream!
private var bufferSize:Int32 = 0
private var bufferSizeAfterLimit:Int32 = 0
private var bufferPos:Int32 = 0
private var lastTag:Int32 = 0
private var totalBytesRetired:Int32 = 0
private var currentLimit:Int32 = 0
private var recursionDepth:Int32 = 0
private var recursionLimit:Int32 = 0
private var sizeLimit:Int32 = 0
public init (data aData:NSData)
{
buffer = NSMutableData(data: aData)
bufferSize = Int32(buffer.length)
currentLimit = INT_MAX
recursionLimit = DEFAULT_RECURSION_LIMIT
sizeLimit = DEFAULT_SIZE_LIMIT
}
public init (inputStream aInputStream:NSInputStream)
{
buffer = NSMutableData(length: Int(BUFFER_SIZE))!
bufferSize = 0
input = aInputStream
input!.open()
//
currentLimit = INT_MAX
recursionLimit = DEFAULT_RECURSION_LIMIT
sizeLimit = DEFAULT_SIZE_LIMIT
}
private func isAtEnd() ->Bool {
return ((bufferPos == bufferSize) && !refillBuffer(false))
}
private func refillBuffer(mustSucceed:Bool) -> Bool
{
if bufferPos < bufferSize
{
NSException(name:"IllegalState", reason:"refillBuffer called when buffer wasn't empty.", userInfo: nil).raise()
}
if (totalBytesRetired + bufferSize == currentLimit) {
if (mustSucceed) {
NSException(name:"InvalidProtocolBuffer:", reason:"truncatedMessage", userInfo: nil).raise()
}
else
{
return false
}
}
totalBytesRetired += bufferSize
bufferPos = 0
bufferSize = 0
if input != nil
{
var pointer = UnsafeMutablePointer<UInt8>(buffer.mutableBytes)
bufferSize = Int32(input!.read(pointer, maxLength:buffer.length))
}
if bufferSize <= 0
{
bufferSize = 0
if mustSucceed
{
NSException(name:"InvalidProtocolBuffer:", reason:"truncatedMessage", userInfo: nil).raise()
}
else
{
return false
}
}
else
{
recomputeBufferSizeAfterLimit()
var totalBytesRead:Int32 = totalBytesRetired + bufferSize + bufferSizeAfterLimit
if (totalBytesRead > sizeLimit || totalBytesRead < 0)
{
NSException(name:"InvalidProtocolBuffer:", reason:"sizeLimitExceeded", userInfo: nil).raise()
}
return true
}
return false
}
public func readRawData(size:Int32) -> NSData {
if (size < 0) {
NSException(name:"InvalidProtocolBuffer", reason:"negativeSize", userInfo: nil).raise()
}
if (totalBytesRetired + bufferPos + size > currentLimit) {
skipRawData(currentLimit - totalBytesRetired - bufferPos)
NSException(name:"InvalidProtocolBuffer", reason:"truncatedMessage", userInfo: nil).raise()
}
if (size <= bufferSize - bufferPos) {
var pointer = UnsafePointer<UInt8>(buffer.bytes)
var data = NSData(bytes: pointer + Int(bufferPos), length: Int(size))
bufferPos += size
return data
}
else if (size < BUFFER_SIZE) {
var bytes = NSMutableData(length: Int(size))!
var pos:Int32 = bufferSize - bufferPos
memcpy(bytes.mutableBytes, buffer.mutableBytes + Int(bufferPos), Int(pos))
bufferPos = bufferSize
refillBuffer(true)
while (size - pos > bufferSize)
{
memcpy(bytes.mutableBytes + Int(pos), buffer.mutableBytes, Int(bufferSize))
pos += bufferSize
bufferPos = bufferSize
refillBuffer(true)
}
memcpy(bytes.mutableBytes + Int(pos), buffer.mutableBytes, Int(size - pos))
bufferPos = size - pos
return bytes
}
else
{
var originalBufferPos:Int32 = bufferPos
var originalBufferSize:Int32 = bufferSize
totalBytesRetired += bufferSize
bufferPos = 0
bufferSize = 0
var sizeLeft:Int32 = size - (originalBufferSize - originalBufferPos)
var chunks:Array<NSData> = Array<NSData>()
while (sizeLeft > 0) {
var chunk = NSMutableData(length:Int(min(sizeLeft, BUFFER_SIZE)))!
var pos:Int = 0
while (pos < chunk.length) {
var n:Int = 0
if input != nil {
var pointer = UnsafeMutablePointer<UInt8>(chunk.mutableBytes)
n = input!.read(pointer + Int(pos), maxLength:chunk.length - Int(pos))
}
if (n <= 0) {
NSException(name:"InvalidProtocolBuffer", reason:"truncatedMessage", userInfo: nil).raise()
}
totalBytesRetired += n
pos += n
}
sizeLeft -= chunk.length
chunks.append(chunk)
}
var bytes = NSMutableData(length:Int(size))!
var pos:Int = originalBufferSize - originalBufferPos
memcpy(bytes.mutableBytes, buffer.mutableBytes + Int(originalBufferPos), pos)
for chunk in chunks
{
memcpy(bytes.mutableBytes + pos, chunk.bytes, chunk.length)
pos += chunk.length
}
return bytes
}
}
public func skipRawData(var size:Int32)
{
if (size < 0) {
NSException(name:"InvalidProtocolBuffer", reason:"negativeSize", userInfo: nil).raise()
}
if (totalBytesRetired + bufferPos + size > currentLimit) {
skipRawData(currentLimit - totalBytesRetired - bufferPos)
NSException(name:"InvalidProtocolBuffer", reason:"truncatedMessage", userInfo: nil).raise()
}
if (size <= (bufferSize - bufferPos)) {
bufferPos += size
}
else
{
var pos:Int32 = bufferSize - bufferPos
totalBytesRetired += pos
bufferPos = 0
bufferSize = 0
while (pos < size) {
var data = NSMutableData(length: Int(size - pos))!
var n:Int = 0
if input == nil
{
n = -1
}
else
{
var pointer = UnsafeMutablePointer<UInt8>(data.mutableBytes)
n = input!.read(pointer, maxLength:Int(size - pos))
}
if (n <= 0) {
NSException(name:"InvalidProtocolBuffer", reason:"truncatedMessage", userInfo: nil).raise()
}
pos += n
totalBytesRetired += n
}
}
}
public func readRawLittleEndian32() -> Int32
{
var b1:Int8 = readRawByte()
var b2:Int8 = readRawByte()
var b3:Int8 = readRawByte()
var b4:Int8 = readRawByte()
var result:Int32 = (Int32(b1) & 0xff)
result |= ((Int32(b2) & 0xff) << 8)
result |= ((Int32(b3) & 0xff) << 16)
result |= ((Int32(b4) & 0xff) << 24)
return result
}
public func readRawLittleEndian64() -> Int64
{
var b1:Int8 = readRawByte()
var b2:Int8 = readRawByte()
var b3:Int8 = readRawByte()
var b4:Int8 = readRawByte()
var b5:Int8 = readRawByte()
var b6:Int8 = readRawByte()
var b7:Int8 = readRawByte()
var b8:Int8 = readRawByte()
var result:Int64 = (Int64(b1) & 0xff)
result |= ((Int64(b2) & 0xff) << 8)
result |= ((Int64(b3) & 0xff) << 16)
result |= ((Int64(b4) & 0xff) << 24)
result |= ((Int64(b5) & 0xff) << 32)
result |= ((Int64(b6) & 0xff) << 40)
result |= ((Int64(b7) & 0xff) << 48)
result |= ((Int64(b8) & 0xff) << 56)
return result
}
public func readTag()->Int32
{
if (isAtEnd())
{
lastTag = 0
return 0
}
var tag = lastTag
lastTag = readRawVarint32()
if lastTag == 0
{
NSException(name:"InvalidProtocolBuffer", reason:"Invalid Tag: after tag \(tag)", userInfo: nil).raise()
}
return lastTag
}
public func checkLastTagWas(value:Int32)
{
if lastTag != value
{
NSException(name:"InvalidProtocolBuffer", reason:"Invalid Tag: last tag \(lastTag)", userInfo: nil).raise()
}
}
public func skipField(tag:Int32) -> Bool
{
var wireFormat = WireFormat.wireFormatGetTagWireType(tag)
if (WireFormat(rawValue:wireFormat) == WireFormat.WireFormatVarint)
{
readInt32()
return true
}
else if (WireFormat(rawValue:wireFormat) == WireFormat.WireFormatFixed64)
{
readRawLittleEndian64()
return true
}
else if (WireFormat(rawValue:wireFormat) == WireFormat.WireFormatLengthDelimited)
{
skipRawData(readRawVarint32())
return true
}
else if (WireFormat(rawValue:wireFormat) == WireFormat.WireFormatStartGroup)
{
skipMessage()
checkLastTagWas(WireFormat.WireFormatEndGroup.wireFormatMakeTag(WireFormat.wireFormatGetTagFieldNumber(tag)))
return true
}
else if (WireFormat(rawValue:wireFormat) == WireFormat.WireFormatEndGroup)
{
return false
}
else if (WireFormat(rawValue:wireFormat) == WireFormat.WireFormatFixed32)
{
readRawLittleEndian32()
return true
}
else
{
NSException(name:"InvalidProtocolBuffer", reason:"Invalid Wire Type", userInfo: nil).raise()
}
return false
}
private func skipMessage()
{
while (true)
{
var tag:Int32 = readTag()
if tag == 0 || !skipField(tag)
{
break
}
}
}
public func readDouble() -> Double
{
var convert:Int64 = readRawLittleEndian64()
var result:Double = 0
WireFormat.convertTypes(convertValue: convert, retValue: &result)
return result
}
public func readFloat() -> Float
{
var convert:Int32 = readRawLittleEndian32()
var result:Float = 0
WireFormat.convertTypes(convertValue: convert, retValue: &result)
return result
}
public func readUInt64() -> UInt64
{
var retvalue:UInt64 = 0
WireFormat.convertTypes(convertValue: readRawVarint64(), retValue: &retvalue)
return retvalue
}
public func readInt64() -> Int64
{
return readRawVarint64()
}
public func readInt32() -> Int32
{
return readRawVarint32()
}
public func readFixed64() -> UInt64
{
var retvalue:UInt64 = 0
WireFormat.convertTypes(convertValue: readRawLittleEndian64(), retValue: &retvalue)
return retvalue
}
public func readFixed32() -> UInt32
{
var retvalue:UInt32 = 0
WireFormat.convertTypes(convertValue: readRawLittleEndian32(), retValue: &retvalue)
return retvalue
}
public func readBool() ->Bool
{
return readRawVarint32() != 0
}
public func readRawByte() -> Int8
{
if (bufferPos == bufferSize)
{
refillBuffer(true)
}
var pointer = UnsafeMutablePointer<Int8>(buffer.mutableBytes)
var res = pointer[Int(bufferPos++)]
return res
}
public func readRawVarint32() -> Int32
{
var tmp : Int8 = readRawByte();
if (tmp >= 0) {
return Int32(tmp);
}
var result : Int32 = Int32(tmp) & 0x7f;
tmp = readRawByte()
if (tmp >= 0) {
result |= Int32(tmp) << 7;
} else {
result |= (Int32(tmp) & 0x7f) << 7;
tmp = readRawByte()
if (tmp >= 0) {
result |= Int32(tmp) << 14;
} else {
result |= (Int32(tmp) & 0x7f) << 14;
tmp = readRawByte()
if (tmp >= 0) {
result |= Int32(tmp) << 21;
} else {
result |= (Int32(tmp) & 0x7f) << 21;
tmp = readRawByte()
result |= (Int32(tmp) << 28);
if (tmp < 0) {
// Discard upper 32 bits.
for (var i : Int = 0; i < 5; i++) {
if (readRawByte() >= 0) {
return result;
}
}
NSException(name:"InvalidProtocolBuffer", reason:"malformedVarint", userInfo: nil).raise()
}
}
}
}
return result;
}
public func readRawVarint64() -> Int64
{
var shift:Int64 = 0
var result:Int64 = 0
while (shift < 64) {
var b = readRawByte()
result |= (Int64(b & 0x7F) << shift)
if ((Int32(b) & 0x80) == 0) {
return result
}
shift += 7
}
NSException(name:"InvalidProtocolBuffer", reason:"malformedVarint", userInfo: nil).raise()
return 0
}
public func readString() -> String
{
var size:Int32 = readRawVarint32()
if (size <= (bufferSize - bufferPos) && size > 0)
{
var result:String = NSString(bytes: (buffer.mutableBytes + Int(bufferPos)), length: Int(size), encoding: NSUTF8StringEncoding)! as String
bufferPos += size
return result
}
else
{
let data = readRawData(size)
return NSString(data: data, encoding: NSUTF8StringEncoding)! as String
}
}
public func readData() -> NSData
{
let size = readRawVarint32()
if (size < bufferSize - bufferPos && size > 0)
{
var data = NSData(bytes: buffer.bytes + Int(bufferPos), length: Int(size))
bufferPos += size
return data
}
else
{
return readRawData(size)
}
}
public func readUInt32() -> UInt32
{
var value:Int32 = readRawVarint32()
var retvalue:UInt32 = 0
WireFormat.convertTypes(convertValue: value, retValue: &retvalue)
return retvalue
}
public func readEnum() ->Int32 {
return readRawVarint32()
}
public func readSFixed32() ->Int32
{
return readRawLittleEndian32()
}
public func readSFixed64() ->Int64
{
return readRawLittleEndian64()
}
public func readSInt32() ->Int32 {
return WireFormat.decodeZigZag32(readRawVarint32())
}
public func readSInt64() ->Int64
{
return WireFormat.decodeZigZag64(readRawVarint64())
}
public func setRecursionLimit(limit:Int32) -> Int32 {
if (limit < 0) {
NSException(name:"IllegalArgument", reason:"Recursion limit cannot be negative", userInfo: nil).raise()
}
var oldLimit:Int32 = recursionLimit
recursionLimit = limit
return oldLimit
}
public func setSizeLimit(limit:Int32) -> Int32
{
if (limit < 0) {
NSException(name:"IllegalArgument", reason:" Size limit cannot be negative", userInfo: nil).raise()
}
var oldLimit:Int32 = sizeLimit
sizeLimit = limit
return oldLimit
}
private func resetSizeCounter()
{
totalBytesRetired = 0
}
private func recomputeBufferSizeAfterLimit()
{
bufferSize += bufferSizeAfterLimit
var bufferEnd:Int32 = totalBytesRetired + bufferSize
if (bufferEnd > currentLimit)
{
bufferSizeAfterLimit = bufferEnd - currentLimit
bufferSize -= bufferSizeAfterLimit
}
else
{
bufferSizeAfterLimit = 0
}
}
public func pushLimit(var byteLimit:Int32) -> Int32
{
if (byteLimit < 0)
{
NSException(name:"InvalidProtocolBuffer", reason:"negativeSize", userInfo: nil).raise()
}
byteLimit += totalBytesRetired + bufferPos
var oldLimit = currentLimit
if (byteLimit > oldLimit) {
NSException(name:"InvalidProtocolBuffer", reason:"truncatedMessage", userInfo: nil).raise()
}
currentLimit = byteLimit
recomputeBufferSizeAfterLimit()
return oldLimit
}
public func popLimit(oldLimit:Int32)
{
currentLimit = oldLimit
recomputeBufferSizeAfterLimit()
}
public func bytesUntilLimit() ->Int32
{
if (currentLimit == INT_MAX)
{
return -1
}
var currentAbsolutePosition:Int32 = totalBytesRetired + bufferPos
return currentLimit - currentAbsolutePosition
}
public func readGroup(fieldNumber:Int32, builder:MessageBuilder, extensionRegistry:ExtensionRegistry)
{
if (recursionDepth >= recursionLimit) {
NSException(name:"InvalidProtocolBuffer", reason:"Recursion Limit Exceeded", userInfo: nil).raise()
}
++recursionDepth
builder.mergeFromCodedInputStream(self, extensionRegistry:extensionRegistry)
checkLastTagWas(WireFormat.WireFormatEndGroup.wireFormatMakeTag(fieldNumber))
--recursionDepth
}
public func readUnknownGroup(fieldNumber:Int32, builder:UnknownFieldSet.Builder)
{
if (recursionDepth >= recursionLimit) {
NSException(name:"InvalidProtocolBuffer", reason:"Recursion Limit Exceeded", userInfo: nil).raise()
}
++recursionDepth
builder.mergeFromCodedInputStream(self)
checkLastTagWas(WireFormat.WireFormatEndGroup.wireFormatMakeTag(fieldNumber))
--recursionDepth
}
public func readMessage(builder:MessageBuilder, extensionRegistry:ExtensionRegistry) {
var length = readRawVarint32()
if (recursionDepth >= recursionLimit) {
NSException(name:"InvalidProtocolBuffer", reason:"Recursion Limit Exceeded", userInfo: nil).raise()
}
var oldLimit = pushLimit(length)
++recursionDepth
builder.mergeFromCodedInputStream(self, extensionRegistry:extensionRegistry)
checkLastTagWas(0)
--recursionDepth
popLimit(oldLimit)
}
}
| apache-2.0 | a4fd9f09070dd427ae44ab546442a9f5 | 29.774436 | 150 | 0.536477 | 4.831209 | false | false | false | false |
titoi2/IchigoJamSerialConsole | IchigoJamSerialConsole/Controllers/FileLoadViewController.swift | 1 | 3431 | //
// FileLoadViewController.swift
// IchigoJamSerialConsole
//
// Created by titoi2 on 2015/04/11.
// Copyright (c) 2015年 titoi2. All rights reserved.
//
import Cocoa
class FileLoadViewController: NSViewController {
@IBOutlet weak var infoLabel: NSTextField!
@IBOutlet weak var progressIndicator:NSProgressIndicator!
let serialPortManager = ORSSerialPortManager.sharedSerialPortManager()
var serialPort: ORSSerialPort?
var fileUrl:NSURL? = nil
let serialManager = IJCSerialManager.sharedInstance
var loadStop:Bool = false
override func viewDidLoad() {
super.viewDidLoad()
// Do view setup here.
}
override func viewDidAppear() {
progressIndicator.startAnimation(self)
let panel:NSOpenPanel = NSOpenPanel()
panel.beginWithCompletionHandler { [unowned self] (result:Int) -> Void in
if result == NSFileHandlingPanelOKButton {
self.fileUrl = panel.URLs[0] as? NSURL
let queue = dispatch_queue_create("queueFileLoad", DISPATCH_QUEUE_SERIAL)
dispatch_async(queue, {
self.load()
dispatch_sync(dispatch_get_main_queue(), {
}
)
})
} else {
self.dismissViewController(self)
}
}
}
override func viewDidDisappear() {
}
func load() {
if fileUrl == nil {
return
}
let theDoc = fileUrl!
var err: NSError?;
let data = NSData(contentsOfURL: theDoc,
options: NSDataReadingOptions.DataReadingMappedIfSafe, error: &err)
if let nerr = err {
NSLog("FILE READ ERROR:\(nerr.localizedDescription)")
return
}
if let ndata = data {
self.loadData2Ichigojam(ndata)
}
}
// IchigoJamにファイルデータをプログラムとして転送する
func loadData2Ichigojam(data:NSData) {
let count:Int! = data.length
var buf = [UInt8](count: count, repeatedValue: 0)
data.getBytes(&buf, length: count)
// プログラム停止、ESC送信
serialManager.sendByte(ICHIGOJAM_KEY_ESC)
NSThread.sleepForTimeInterval(0.05)
serialManager.sendString("CLS \u{0000A}")
NSThread.sleepForTimeInterval(0.05)
// NEWで既存プログラム消去
serialManager.sendString("NEW \u{0000A}")
NSThread.sleepForTimeInterval(0.05)
progressIndicator.maxValue = Double(buf.count)
loadStop = false
for var i=0; i < buf.count; i++ {
if loadStop {
break
}
progressIndicator.incrementBy(1.0)
let d = buf[i]
if d == 13 {
// CRを除去
continue
}
serialManager.sendByte(d)
NSThread.sleepForTimeInterval(0.02)
}
// 一応、最後に改行
if !loadStop {
serialManager.sendString("\u{0000A}")
}
dismissViewController(self)
}
@IBAction func pushCancelButton(sender: NSButton) {
loadStop = true
dismissViewController(self)
}
}
| mit | fc5813e959115a66e8336af6e2b9604c | 27.177966 | 89 | 0.547068 | 4.618056 | false | false | false | false |
cmoulton/grokSwiftREST_v1.2 | grokSwiftREST/GistRouter.swift | 1 | 2993 | //
// GistRouter.swift
// grokSwiftREST
//
// Created by Christina Moulton on 2016-04-02.
// Copyright © 2016 Teak Mobile Inc. All rights reserved.
//
import Foundation
import Alamofire
enum GistRouter: URLRequestConvertible {
static let baseURLString:String = "https://api.github.com/"
case GetPublic() // GET https://api.github.com/gists/public
case GetMyStarred() // GET https://api.github.com/gists/starred
case GetMine() // GET https://api.github.com/gists
case IsStarred(String) // GET https://api.github.com/gists/\(gistId)/star
case GetAtPath(String) // GET at given path
case Star(String) // PUT https://api.github.com/gists/\(gistId)/star
case Unstar(String) // DELETE https://api.github.com/gists/\(gistId)/star
case Delete(String) // DELETE https://api.github.com/gists/\(gistId)
case Create([String: AnyObject]) // POST https://api.github.com/gists
var URLRequest: NSMutableURLRequest {
var method: Alamofire.Method {
switch self {
case .GetPublic, .GetAtPath, .GetMine, .GetMyStarred, .IsStarred:
return .GET
case .Star:
return .PUT
case .Unstar, .Delete:
return .DELETE
case .Create:
return .POST
}
}
let url:NSURL = {
// build up and return the URL for each endpoint
let relativePath:String?
switch self {
case .GetAtPath(let path):
// already have the full URL, so just return it
return NSURL(string: path)!
case .GetPublic():
relativePath = "gists/public"
case .GetMyStarred:
relativePath = "gists/starred"
case .GetMine():
relativePath = "gists"
case .IsStarred(let id):
relativePath = "gists/\(id)/star"
case .Star(let id):
relativePath = "gists/\(id)/star"
case .Unstar(let id):
relativePath = "gists/\(id)/star"
case .Delete(let id):
relativePath = "gists/\(id)"
case .Create:
relativePath = "gists"
}
var URL = NSURL(string: GistRouter.baseURLString)!
if let relativePath = relativePath {
URL = URL.URLByAppendingPathComponent(relativePath)
}
return URL
}()
let params: ([String: AnyObject]?) = {
switch self {
case .GetPublic, .GetAtPath, .GetMyStarred, .GetMine, .IsStarred, .Star, .Unstar, .Delete:
return nil
case .Create(let params):
return params
}
}()
let URLRequest = NSMutableURLRequest(URL: url)
// Set OAuth token if we have one
if let token = GitHubAPIManager.sharedInstance.OAuthToken {
URLRequest.setValue("token \(token)", forHTTPHeaderField: "Authorization")
}
let encoding = Alamofire.ParameterEncoding.JSON
let (encodedRequest, _) = encoding.encode(URLRequest, parameters: params)
encodedRequest.HTTPMethod = method.rawValue
return encodedRequest
}
}
| mit | a72d499397c9cbe85b17fa4ba451914d | 30.829787 | 98 | 0.61865 | 4.214085 | false | false | false | false |
patrick-ogrady/godseye-ios | sample/SampleBroadcaster-Swift/SampleBroadcaster-Swift/popularTableViewController.swift | 1 | 6576 | //
// popularTableViewController.swift
// SampleBroadcaster-Swift
//
// Created by Patrick O'Grady on 9/8/15.
// Copyright © 2015 videocore. All rights reserved.
//
import UIKit
import CoreLocation
class popularTableViewController: UITableViewController, CLLocationManagerDelegate {
var events:NSMutableArray = NSMutableArray()
var selectedEvent:PFObject!
var locationManager: CLLocationManager!
override func viewDidLoad() {
super.viewDidLoad()
self.navigationController?.navigationBar.barStyle = UIBarStyle.BlackTranslucent
// self.tabBarController!.tabBar.barStyle = UIBarStyle.BlackTranslucent
tableView.registerNib(UINib(nibName: "eventsCell", bundle: nil), forCellReuseIdentifier: "eventsCell")
tableView.rowHeight = 150
// Uncomment the following line to preserve selection between presentations
// self.clearsSelectionOnViewWillAppear = false
// Uncomment the following line to display an Edit button in the navigation bar for this view controller.
// self.navigationItem.rightBarButtonItem = self.editButtonItem()
var query = PFQuery(className: "events")
// let then = NSDate(timeIntervalSinceNow: -3)
// query.whereKey("createdAt", greaterThanOrEqualTo:then)
query.orderByDescending("views")
// query.limit = 4
query.findObjectsInBackground().continueWithBlock({ (task: BFTask!) -> AnyObject! in
if(task.result != nil) {
self.events.removeAllObjects()
self.events.addObjectsFromArray(task.result as! [AnyObject])
}
dispatch_async(dispatch_get_main_queue(), { () -> Void in
self.tableView.reloadData()
})
return task
})
//update location
locationManager = CLLocationManager()
locationManager.delegate = self
locationManager.desiredAccuracy = kCLLocationAccuracyBest
locationManager.requestWhenInUseAuthorization()
locationManager.startUpdatingLocation()
}
func locationManager(manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
print(locations)
var mostRecentLocation = locations[0]
var recentLocation = PFGeoPoint(latitude: mostRecentLocation.coordinate.latitude, longitude: mostRecentLocation.coordinate.longitude)
var currentUser = PFUser.currentUser()!
currentUser["recentLocation"] = recentLocation
currentUser.saveInBackground()
manager.stopUpdatingLocation()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// MARK: - Table view data source
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
// #warning Incomplete implementation, return the number of sections
return 1
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
// #warning Incomplete implementation, return the number of rows
print(self.events.count)
return self.events.count
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("eventsCell", forIndexPath: indexPath) as! eventsTableViewCell
var object = self.events.objectAtIndex(indexPath.row) as! PFObject
cell.eventTitle.text = " " + (object["name"] as! String) + " "
cell.eventTitle.numberOfLines = 0
cell.eventTitle.sizeToFit()
var file = object["image"] as! PFFile
cell.eventImage.image = UIImage(data: file.getData()!)
// cell.newsdeskTitle.text = object["name"] as! String
// cell.eventImage.image = UIImage(named: "Arab-Israeli-Conflict.jpg")
//
// cell.textLabel?.text = object["name"] as! String
var views = object["views"] as! Int
cell.viewsText.text = " Views: " + String(views) + " "
return cell
}
override func tableView(tableView: UITableView, didSelectRowAtIndexPath indexPath: NSIndexPath) {
self.selectedEvent = self.events.objectAtIndex(indexPath.row) as! PFObject
self.performSegueWithIdentifier("viewFeeds", sender: nil)
}
/*
// Override to support conditional editing of the table view.
override func tableView(tableView: UITableView, canEditRowAtIndexPath indexPath: NSIndexPath) -> Bool {
// Return false if you do not want the specified item to be editable.
return true
}
*/
/*
// Override to support editing the table view.
override func tableView(tableView: UITableView, commitEditingStyle editingStyle: UITableViewCellEditingStyle, forRowAtIndexPath indexPath: NSIndexPath) {
if editingStyle == .Delete {
// Delete the row from the data source
tableView.deleteRowsAtIndexPaths([indexPath], withRowAnimation: .Fade)
} else if editingStyle == .Insert {
// Create a new instance of the appropriate class, insert it into the array, and add a new row to the table view
}
}
*/
/*
// Override to support rearranging the table view.
override func tableView(tableView: UITableView, moveRowAtIndexPath fromIndexPath: NSIndexPath, toIndexPath: NSIndexPath) {
}
*/
/*
// Override to support conditional rearranging of the table view.
override func tableView(tableView: UITableView, canMoveRowAtIndexPath indexPath: NSIndexPath) -> Bool {
// Return false if you do not want the item to be re-orderable.
return true
}
*/
// MARK: - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
// Get the new view controller using segue.destinationViewController.
// Pass the selected object to the new view controller.
if(segue.identifier == "viewFeeds") {
var destination = segue.destinationViewController as! feedsTabViewController
destination.eventObject = selectedEvent
}
}
}
| mit | 20cd3ad7af9666895ce9d827bca47c1e | 35.126374 | 157 | 0.657034 | 5.557904 | false | false | false | false |
khizkhiz/swift | stdlib/public/SDK/WatchKit/WatchKit.swift | 2 | 1500 | //===----------------------------------------------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2016 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See http://swift.org/LICENSE.txt for license information
// See http://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
@_exported import WatchKit
import Foundation
@available(iOS 8.2, *)
extension WatchKitErrorCode : _BridgedNSError {
public static var _nsErrorDomain: String { return WatchKitErrorDomain }
}
@available(iOS, introduced=8.2)
extension WKInterfaceController {
// Swift convenience type (class) method for
// reloadRootControllersWithNames:contexts: that takes an array of tuples
public class func reloadRootControllers(
namesAndContexts: [(name: String, context: AnyObject)]
) {
WKInterfaceController.reloadRootControllers(
withNames: namesAndContexts.map { $0.name },
contexts: namesAndContexts.map { $0.context })
}
// Swift convenience method for presentControllerWithNames:contexts: that
// takes an array of tuples
public func presentController(
namesAndContexts: [(name: String, context: AnyObject)]
) {
self.present(
withNames: namesAndContexts.map { $0.name },
contexts: namesAndContexts.map { $0.context })
}
}
| apache-2.0 | 523d8f08a4fcf4453564d890f928a653 | 33.883721 | 80 | 0.656 | 4.6875 | false | false | false | false |
xwu/swift | test/Driver/linker.swift | 3 | 25667 | // Must be able to run xcrun-return-self.sh
// REQUIRES: shell
// REQUIRES: rdar65281056
// FIXME: When this is turned on, please move the test from linker-library-with-space.swift
// to this file and remove that file.
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-macosx10.9 %s 2>&1 > %t.simple.txt
// RUN: %FileCheck %s < %t.simple.txt
// RUN: %FileCheck -check-prefix SIMPLE %s < %t.simple.txt
// RUN: not %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-macosx10.9 -static-stdlib %s 2>&1 | %FileCheck -check-prefix=SIMPLE_STATIC %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-ios7.1-simulator %s 2>&1 > %t.simple.txt
// RUN: %FileCheck -check-prefix IOS_SIMPLE %s < %t.simple.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-tvos9.0-simulator %s 2>&1 > %t.simple.txt
// RUN: %FileCheck -check-prefix tvOS_SIMPLE %s < %t.simple.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target i386-apple-watchos2.0-simulator %s 2>&1 > %t.simple.txt
// RUN: %FileCheck -check-prefix watchOS_SIMPLE %s < %t.simple.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-unknown-linux-gnu -Ffoo -Fsystem car -F cdr -framework bar -Lbaz -lboo -Xlinker -undefined %s 2>&1 > %t.linux.txt
// RUN: %FileCheck -check-prefix LINUX-x86_64 %s < %t.linux.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target armv6-unknown-linux-gnueabihf -Ffoo -Fsystem car -F cdr -framework bar -Lbaz -lboo -Xlinker -undefined %s 2>&1 > %t.linux.txt
// RUN: %FileCheck -check-prefix LINUX-armv6 %s < %t.linux.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target armv7-unknown-linux-gnueabihf -Ffoo -Fsystem car -F cdr -framework bar -Lbaz -lboo -Xlinker -undefined %s 2>&1 > %t.linux.txt
// RUN: %FileCheck -check-prefix LINUX-armv7 %s < %t.linux.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target thumbv7-unknown-linux-gnueabihf -Ffoo -Fsystem car -F cdr -framework bar -Lbaz -lboo -Xlinker -undefined %s 2>&1 > %t.linux.txt
// RUN: %FileCheck -check-prefix LINUX-thumbv7 %s < %t.linux.txt
// RUN: %swiftc_driver_plain -driver-print-jobs -target armv7-unknown-linux-androideabi -Ffoo -Fsystem car -F cdr -framework bar -Lbaz -lboo -Xlinker -undefined %s 2>&1 > %t.android.txt
// RUN: %FileCheck -check-prefix ANDROID-armv7 %s < %t.android.txt
// RUN: %FileCheck -check-prefix ANDROID-armv7-NEGATIVE %s < %t.android.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-unknown-windows-cygnus -Ffoo -Fsystem car -F cdr -framework bar -Lbaz -lboo -Xlinker -undefined %s 2>&1 > %t.cygwin.txt
// RUN: %FileCheck -check-prefix CYGWIN-x86_64 %s < %t.cygwin.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-unknown-windows-msvc -Ffoo -Fsystem car -F cdr -framework bar -Lbaz -lboo -Xlinker -undefined %s 2>&1 > %t.windows.txt
// RUN: %FileCheck -check-prefix WINDOWS-x86_64 %s < %t.windows.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target amd64-unknown-openbsd -Ffoo -Fsystem car -F cdr -framework bar -Lbaz -lboo -Xlinker -undefined %s 2>&1 > %t.openbsd.txt
// RUN: %FileCheck -check-prefix OPENBSD-amd64 %s < %t.openbsd.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -emit-library -target x86_64-unknown-linux-gnu %s -Lbar -o dynlib.out 2>&1 > %t.linux.dynlib.txt
// RUN: %FileCheck -check-prefix LINUX_DYNLIB-x86_64 %s < %t.linux.dynlib.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -emit-library -target x86_64-apple-macosx10.9.1 %s -sdk %S/../Inputs/clang-importer-sdk -lfoo -framework bar -Lbaz -Fgarply -Fsystem car -F cdr -Xlinker -undefined -Xlinker dynamic_lookup -o sdk.out 2>&1 > %t.complex.txt
// RUN: %FileCheck %s < %t.complex.txt
// RUN: %FileCheck -check-prefix COMPLEX %s < %t.complex.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-ios7.1-simulator -Xlinker -rpath -Xlinker customrpath -L foo %s 2>&1 > %t.simple.txt
// RUN: %FileCheck -check-prefix IOS-linker-order %s < %t.simple.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target armv7-unknown-linux-gnueabihf -Xlinker -rpath -Xlinker customrpath -L foo %s 2>&1 > %t.linux.txt
// RUN: %FileCheck -check-prefix LINUX-linker-order %s < %t.linux.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-unknown-linux-gnu -Xclang-linker -foo -Xclang-linker foopath %s 2>&1 > %t.linux.txt
// RUN: %FileCheck -check-prefix LINUX-clang-linker-order %s < %t.linux.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-unknown-windows-msvc -Xclang-linker -foo -Xclang-linker foopath %s 2>&1 > %t.windows.txt
// RUN: %FileCheck -check-prefix WINDOWS-clang-linker-order %s < %t.windows.txt
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target wasm32-unknown-wasi -Xclang-linker -flag -Xclang-linker arg %s 2>&1 | %FileCheck -check-prefix WASI-clang-linker-order %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-macosx10.9 -g %s | %FileCheck -check-prefix DEBUG %s
// RUN: %swiftc_driver_plain -driver-print-jobs -target x86_64-unknown-linux-gnu -toolchain-stdlib-rpath %s 2>&1 | %FileCheck -check-prefix LINUX-STDLIB-RPATH %s
// RUN: %swiftc_driver_plain -driver-print-jobs -target x86_64-unknown-linux-gnu -no-toolchain-stdlib-rpath %s 2>&1 | %FileCheck -check-prefix LINUX-NO-STDLIB-RPATH %s
// RUN: %swiftc_driver_plain -driver-print-jobs -target armv7-unknown-linux-androideabi -toolchain-stdlib-rpath %s 2>&1 | %FileCheck -check-prefix ANDROID-STDLIB-RPATH %s
// RUN: %swiftc_driver_plain -driver-print-jobs -target armv7-unknown-linux-androideabi -no-toolchain-stdlib-rpath %s 2>&1 | %FileCheck -check-prefix ANDROID-NO-STDLIB-RPATH %s
// RUN: %empty-directory(%t)
// RUN: touch %t/a.o
// RUN: touch %t/a.swiftmodule
// RUN: touch %t/b.o
// RUN: touch %t/b.swiftmodule
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-macosx10.9 %s %t/a.o %t/a.swiftmodule %t/b.o %t/b.swiftmodule -o linker | %FileCheck -check-prefix LINK-SWIFTMODULES %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-macosx10.10 %s > %t.simple-macosx10.10.txt
// RUN: %FileCheck %s < %t.simple-macosx10.10.txt
// RUN: %FileCheck -check-prefix SIMPLE %s < %t.simple-macosx10.10.txt
// RUN: %empty-directory(%t)
// RUN: echo "int dummy;" >%t/a.cpp
// RUN: cc -c %t/a.cpp -o %t/a.o
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-macosx10.9 %s %t/a.o -o linker 2>&1 | %FileCheck -check-prefix COMPILE_AND_LINK %s
// RUN: %swiftc_driver -sdk "" -save-temps -driver-print-jobs -target x86_64-apple-macosx10.9 %s %t/a.o -driver-filelist-threshold=0 -o linker 2>&1 | tee %t/forFilelistCapture | %FileCheck -check-prefix FILELIST %s
// Extract filelist name and check it out
// RUN: tail -1 %t/forFilelistCapture | sed 's/.*-filelist //' | sed 's/ .*//' >%t/filelistName
// RUN: %FileCheck -check-prefix FILELIST-CONTENTS %s < `cat %t/filelistName`
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-macosx10.9 -emit-library %s -module-name LINKER | %FileCheck -check-prefix INFERRED_NAME_DARWIN %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-unknown-linux-gnu -emit-library %s -module-name LINKER | %FileCheck -check-prefix INFERRED_NAME_LINUX %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-unknown-windows-cygnus -emit-library %s -module-name LINKER | %FileCheck -check-prefix INFERRED_NAME_WINDOWS %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-unknown-windows-msvc -emit-library %s -module-name LINKER | %FileCheck -check-prefix INFERRED_NAME_WINDOWS %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target wasm32-unknown-wasi -emit-library %s -module-name LINKER | %FileCheck -check-prefix INFERRED_NAME_WASI %s
// Here we specify an output file name using '-o'. For ease of writing these
// tests, we happen to specify the same file name as is inferred in the
// INFERRED_NAMED_DARWIN tests above: 'libLINKER.dylib'.
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-macosx10.9 -emit-library %s -o libLINKER.dylib | %FileCheck -check-prefix INFERRED_NAME_DARWIN %s
// On Darwin, when C++ interop is turned on, we link against libc++ explicitly
// regardless of whether -experimental-cxx-stdlib is specified or not. So also
// run a test where C++ interop is turned off to make sure we don't link
// against libc++ in this case.
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-ios7.1 %s 2>&1 | %FileCheck -check-prefix IOS-no-cxx-interop %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-ios7.1 -enable-experimental-cxx-interop %s 2>&1 | %FileCheck -check-prefix IOS-cxx-interop-libcxx %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-ios7.1 -enable-experimental-cxx-interop -experimental-cxx-stdlib libc++ %s 2>&1 | %FileCheck -check-prefix IOS-cxx-interop-libcxx %s
// RUN: not %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-ios7.1 -enable-experimental-cxx-interop -experimental-cxx-stdlib libstdc++ %s 2>&1 | %FileCheck -check-prefix IOS-cxx-interop-libstdcxx %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-unknown-linux-gnu -enable-experimental-cxx-interop %s 2>&1 | %FileCheck -check-prefix LINUX-cxx-interop %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-unknown-linux-gnu -enable-experimental-cxx-interop -experimental-cxx-stdlib libc++ %s 2>&1 | %FileCheck -check-prefix LINUX-cxx-interop-libcxx %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-unknown-windows-msvc -enable-experimental-cxx-interop %s 2>&1 | %FileCheck -check-prefix WINDOWS-cxx-interop %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-unknown-windows-msvc -enable-experimental-cxx-interop -experimental-cxx-stdlib libc++ %s 2>&1 | %FileCheck -check-prefix WINDOWS-cxx-interop-libcxx %s
// Check reading the SDKSettings.json from an SDK
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-macosx10.9 -sdk %S/Inputs/MacOSX10.15.versioned.sdk %s 2>&1 | %FileCheck -check-prefix MACOS_10_15 %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-macosx10.9 -sdk %S/Inputs/MacOSX10.15.4.versioned.sdk %s 2>&1 | %FileCheck -check-prefix MACOS_10_15_4 %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-macosx10.9 -sdk %S/Inputs/MacOSX10.15.sdk %s 2>&1 | %FileCheck -check-prefix MACOS_UNVERSIONED %s
// Check arm64 macOS first deployment version adjustment.
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target arm64-apple-macosx10.15.1 %s 2>&1 | %FileCheck -check-prefix MACOS_11_0 %s
// Check x86 macOS 11 deployment version adjustment is gone.
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target x86_64-apple-macosx11.0 %s 2>&1 | %FileCheck -check-prefix MACOS_11_0 %s
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target arm64-apple-macosx11.0 %s 2>&1 | %FileCheck -check-prefix MACOS_11_0 %s
// Check arm64 simulators first deployment version adjustment.
// RUN: %swiftc_driver -sdk "" -driver-print-jobs -target arm64-apple-ios13.0-simulator %s 2>&1 | %FileCheck -check-prefix ARM64_IOS_SIMULATOR_LINKER %s
// MACOS_10_15: -platform_version macos 10.9.0 10.15.0
// MACOS_10_15_4: -platform_version macos 10.9.0 10.15.4
// MACOS_11_0: -platform_version macos 11.0.0
// MACOS_UNVERSIONED: -platform_version macos 10.9.0 0.0.0
// X86_64_WATCHOS_SIM_LINKER: -platform_version watchos-simulator 7.0.0
// ARM64_IOS_SIMULATOR_LINKER: -platform_version ios-simulator 14.0.0
// There are more RUN lines further down in the file.
// CHECK: swift
// CHECK: -o [[OBJECTFILE:.*]]
// CHECK-NEXT: {{(bin/)?}}ld{{"? }}
// CHECK-DAG: [[OBJECTFILE]]
// CHECK-DAG: -L [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)macosx]]
// CHECK-DAG: -rpath [[STDLIB_PATH]]
// CHECK-DAG: -lSystem
// CHECK-DAG: -arch x86_64
// CHECK: -o {{[^ ]+}}
// SIMPLE: {{(bin/)?}}ld{{"? }}
// SIMPLE-NOT: -syslibroot
// SIMPLE: -platform_version macos 10.{{[0-9]+}}.{{[0-9]+}} 0.0.0
// SIMPLE-NOT: -syslibroot
// SIMPLE: -o linker
// SIMPLE_STATIC: error: -static-stdlib is no longer supported on Apple platforms
// IOS_SIMPLE: swift
// IOS_SIMPLE: -o [[OBJECTFILE:.*]]
// IOS_SIMPLE: {{(bin/)?}}ld{{"? }}
// IOS_SIMPLE-DAG: [[OBJECTFILE]]
// IOS_SIMPLE-DAG: -L {{[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)iphonesimulator}}
// IOS_SIMPLE-DAG: -lSystem
// IOS_SIMPLE-DAG: -arch x86_64
// IOS_SIMPLE-DAG: -platform_version ios-simulator 7.1.{{[0-9]+}} 0.0.0
// IOS_SIMPLE: -o linker
// tvOS_SIMPLE: swift
// tvOS_SIMPLE: -o [[OBJECTFILE:.*]]
// tvOS_SIMPLE: {{(bin/)?}}ld{{"? }}
// tvOS_SIMPLE-DAG: [[OBJECTFILE]]
// tvOS_SIMPLE-DAG: -L {{[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)appletvsimulator}}
// tvOS_SIMPLE-DAG: -lSystem
// tvOS_SIMPLE-DAG: -arch x86_64
// tvOS_SIMPLE-DAG: -platform_version tvos-simulator 9.0.{{[0-9]+}} 0.0.0
// tvOS_SIMPLE: -o linker
// watchOS_SIMPLE: swift
// watchOS_SIMPLE: -o [[OBJECTFILE:.*]]
// watchOS_SIMPLE: {{(bin/)?}}ld{{"? }}
// watchOS_SIMPLE-DAG: [[OBJECTFILE]]
// watchOS_SIMPLE-DAG: -L {{[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)watchsimulator}}
// watchOS_SIMPLE-DAG: -lSystem
// watchOS_SIMPLE-DAG: -arch i386
// watchOS_SIMPLE-DAG: -platform_version watchos-simulator 2.0.{{[0-9]+}} 0.0.0
// watchOS_SIMPLE: -o linker
// LINUX-x86_64: swift
// LINUX-x86_64: -o [[OBJECTFILE:.*]]
// LINUX-x86_64: clang{{(\.exe)?"? }}
// LINUX-x86_64-DAG: -pie
// LINUX-x86_64-DAG: [[OBJECTFILE]]
// LINUX-x86_64-DAG: -lswiftCore
// LINUX-x86_64-DAG: -L [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)]]
// LINUX-x86_64-DAG: -Xlinker -rpath -Xlinker [[STDLIB_PATH]]
// LINUX-x86_64-DAG: -F foo -iframework car -F cdr
// LINUX-x86_64-DAG: -framework bar
// LINUX-x86_64-DAG: -L baz
// LINUX-x86_64-DAG: -lboo
// LINUX-x86_64-DAG: -Xlinker -undefined
// LINUX-x86_64: -o linker
// LINUX-armv6: swift
// LINUX-armv6: -o [[OBJECTFILE:.*]]
// LINUX-armv6: clang{{(\.exe)?"? }}
// LINUX-armv6-DAG: -pie
// LINUX-armv6-DAG: [[OBJECTFILE]]
// LINUX-armv6-DAG: -lswiftCore
// LINUX-armv6-DAG: -L [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)]]
// LINUX-armv6-DAG: -target armv6-unknown-linux-gnueabihf
// LINUX-armv6-DAG: -Xlinker -rpath -Xlinker [[STDLIB_PATH]]
// LINUX-armv6-DAG: -F foo -iframework car -F cdr
// LINUX-armv6-DAG: -framework bar
// LINUX-armv6-DAG: -L baz
// LINUX-armv6-DAG: -lboo
// LINUX-armv6-DAG: -Xlinker -undefined
// LINUX-armv6: -o linker
// LINUX-armv7: swift
// LINUX-armv7: -o [[OBJECTFILE:.*]]
// LINUX-armv7: clang{{(\.exe)?"? }}
// LINUX-armv7-DAG: -pie
// LINUX-armv7-DAG: [[OBJECTFILE]]
// LINUX-armv7-DAG: -lswiftCore
// LINUX-armv7-DAG: -L [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)]]
// LINUX-armv7-DAG: -target armv7-unknown-linux-gnueabihf
// LINUX-armv7-DAG: -Xlinker -rpath -Xlinker [[STDLIB_PATH]]
// LINUX-armv7-DAG: -F foo -iframework car -F cdr
// LINUX-armv7-DAG: -framework bar
// LINUX-armv7-DAG: -L baz
// LINUX-armv7-DAG: -lboo
// LINUX-armv7-DAG: -Xlinker -undefined
// LINUX-armv7: -o linker
// LINUX-thumbv7: swift
// LINUX-thumbv7: -o [[OBJECTFILE:.*]]
// LINUX-thumbv7: clang{{(\.exe)?"? }}
// LINUX-thumbv7-DAG: -pie
// LINUX-thumbv7-DAG: [[OBJECTFILE]]
// LINUX-thumbv7-DAG: -lswiftCore
// LINUX-thumbv7-DAG: -L [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)]]
// LINUX-thumbv7-DAG: -target thumbv7-unknown-linux-gnueabihf
// LINUX-thumbv7-DAG: -Xlinker -rpath -Xlinker [[STDLIB_PATH]]
// LINUX-thumbv7-DAG: -F foo -iframework car -F cdr
// LINUX-thumbv7-DAG: -framework bar
// LINUX-thumbv7-DAG: -L baz
// LINUX-thumbv7-DAG: -lboo
// LINUX-thumbv7-DAG: -Xlinker -undefined
// LINUX-thumbv7: -o linker
// ANDROID-armv7: swift
// ANDROID-armv7: -o [[OBJECTFILE:.*]]
// ANDROID-armv7: clang{{(\.exe)?"? }}
// ANDROID-armv7-DAG: -pie
// ANDROID-armv7-DAG: [[OBJECTFILE]]
// ANDROID-armv7-DAG: -lswiftCore
// ANDROID-armv7-DAG: -L [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift]]
// ANDROID-armv7-DAG: -target armv7-unknown-linux-androideabi
// ANDROID-armv7-DAG: -F foo -iframework car -F cdr
// ANDROID-armv7-DAG: -framework bar
// ANDROID-armv7-DAG: -L baz
// ANDROID-armv7-DAG: -lboo
// ANDROID-armv7-DAG: -Xlinker -undefined
// ANDROID-armv7: -o linker
// ANDROID-armv7-NEGATIVE-NOT: -Xlinker -rpath
// CYGWIN-x86_64: swift
// CYGWIN-x86_64: -o [[OBJECTFILE:.*]]
// CYGWIN-x86_64: clang{{(\.exe)?"? }}
// CYGWIN-x86_64-DAG: [[OBJECTFILE]]
// CYGWIN-x86_64-DAG: -lswiftCore
// CYGWIN-x86_64-DAG: -L [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift]]
// CYGWIN-x86_64-DAG: -Xlinker -rpath -Xlinker [[STDLIB_PATH]]
// CYGWIN-x86_64-DAG: -F foo -iframework car -F cdr
// CYGWIN-x86_64-DAG: -framework bar
// CYGWIN-x86_64-DAG: -L baz
// CYGWIN-x86_64-DAG: -lboo
// CYGWIN-x86_64-DAG: -Xlinker -undefined
// CYGWIN-x86_64: -o linker
// WINDOWS-x86_64: swift
// WINDOWS-x86_64: -o [[OBJECTFILE:.*]]
// WINDOWS-x86_64: clang{{(\.exe)?"? }}
// WINDOWS-x86_64-DAG: [[OBJECTFILE]]
// WINDOWS-x86_64-DAG: -L [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)windows(/|\\\\)x86_64]]
// WINDOWS-x86_64-DAG: -F foo -iframework car -F cdr
// WINDOWS-x86_64-DAG: -framework bar
// WINDOWS-x86_64-DAG: -L baz
// WINDOWS-x86_64-DAG: -lboo
// WINDOWS-x86_64-DAG: -Xlinker -undefined
// WINDOWS-x86_64: -o linker
// OPENBSD-amd64: swift
// OPENBSD-amd64: -o [[OBJECTFILE:.*]]
// OPENBSD-amd64: clang
// OPENBSD-amd64-DAG: -fuse-ld=lld
// OPENBSD-amd64-DAG: [[OBJECTFILE]]
// OPENBSD-amd64-DAG: -lswiftCore
// OPENBSD-amd64-DAG: -L [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)]]
// OPENBSD-amd64-DAG: -Xlinker -rpath -Xlinker [[STDLIB_PATH]]
// OPENBSD-amd64-DAG: -F foo -iframework car -F cdr
// OPENBSD-amd64-DAG: -framework bar
// OPENBSD-amd64-DAG: -L baz
// OPENBSD-amd64-DAG: -lboo
// OPENBSD-amd64-DAG: -Xlinker -undefined
// OPENBSD-amd64: -o linker
// COMPLEX: {{(bin/)?}}ld{{"? }}
// COMPLEX-DAG: -dylib
// COMPLEX-DAG: -syslibroot {{.*}}/Inputs/clang-importer-sdk
// COMPLEX-DAG: -lfoo
// COMPLEX-DAG: -framework bar
// COMPLEX-DAG: -L baz
// COMPLEX-DAG: -F garply -F car -F cdr
// COMPLEX-DAG: -undefined dynamic_lookup
// COMPLEX-DAG: -platform_version macos 10.9.1 0.0.0
// COMPLEX: -o sdk.out
// LINUX_DYNLIB-x86_64: swift
// LINUX_DYNLIB-x86_64: -o [[OBJECTFILE:.*]]
// LINUX_DYNLIB-x86_64: -o {{"?}}[[AUTOLINKFILE:.*]]
// LINUX_DYNLIB-x86_64: clang{{(\.exe)?"? }}
// LINUX_DYNLIB-x86_64-DAG: -shared
// LINUX_DYNLIB-x86_64-DAG: -fuse-ld=gold
// LINUX_DYNLIB-x86_64-NOT: -pie
// LINUX_DYNLIB-x86_64-DAG: -Xlinker -rpath -Xlinker [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)linux]]
// LINUX_DYNLIB-x86_64: [[STDLIB_PATH]]{{/|\\\\}}x86_64{{/|\\\\}}swiftrt.o
// LINUX_DYNLIB-x86_64-DAG: [[OBJECTFILE]]
// LINUX_DYNLIB-x86_64-DAG: @[[AUTOLINKFILE]]
// LINUX_DYNLIB-x86_64-DAG: [[STDLIB_PATH]]
// LINUX_DYNLIB-x86_64-DAG: -lswiftCore
// LINUX_DYNLIB-x86_64-DAG: -L bar
// LINUX_DYNLIB-x86_64: -o dynlib.out
// IOS-linker-order: swift
// IOS-linker-order: -o [[OBJECTFILE:.*]]
// IOS-linker-order: {{(bin/)?}}ld{{"? }}
// IOS-linker-order: -rpath [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)iphonesimulator]]
// IOS-linker-order: -L foo
// IOS-linker-order: -rpath customrpath
// IOS-linker-order: -o {{.*}}
// LINUX-linker-order: swift
// LINUX-linker-order: -o [[OBJECTFILE:.*]]
// LINUX-linker-order: clang{{(\.exe)?"? }}
// LINUX-linker-order: -Xlinker -rpath -Xlinker {{[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)linux}}
// LINUX-linker-order: -L foo
// LINUX-linker-order: -Xlinker -rpath -Xlinker customrpath
// LINUX-linker-order: -o {{.*}}
// LINUX-clang-linker-order: swift
// LINUX-clang-linker-order: -o [[OBJECTFILE:.*]]
// LINUX-clang-linker-order: clang{{"? }}
// LINUX-clang-linker-order: -foo foopath
// LINUX-clang-linker-order: -o {{.*}}
// WINDOWS-clang-linker-order: swift
// WINDOWS-clang-linker-order: -o [[OBJECTFILE:.*]]
// WINDOWS-clang-linker-order: clang{{"? }}
// WINDOWS-clang-linker-order: -foo foopath
// WINDOWS-clang-linker-order: -o {{.*}}
// WASI-clang-linker-order: swift
// WASI-clang-linker-order: -o [[OBJECTFILE:.*]]
// WASI-clang-linker-order: clang{{"? }}
// WASI-clang-linker-order: -flag arg
// WASI-clang-linker-order: -o {{.*}}
// DEBUG: bin{{/|\\\\}}swift{{c?(\.EXE)?}}
// DEBUG-NEXT: bin{{/|\\\\}}swift{{c?(\.EXE)?}}
// DEBUG-NEXT: {{(bin/)?}}ld{{"? }}
// DEBUG: -add_ast_path {{.*(/|\\\\)[^/]+}}.swiftmodule
// DEBUG: -o linker
// DEBUG-NEXT: bin{{/|\\\\}}dsymutil
// DEBUG: linker
// DEBUG: -o linker.dSYM
// LINK-SWIFTMODULES: bin{{/|\\\\}}swift{{c?(\.EXE)?}}
// LINK-SWIFTMODULES-NEXT: {{(bin/)?}}ld{{"? }}
// LINK-SWIFTMODULES-SAME: -add_ast_path {{.*}}/a.swiftmodule
// LINK-SWIFTMODULES-SAME: -add_ast_path {{.*}}/b.swiftmodule
// LINK-SWIFTMODULES-SAME: -o linker
// COMPILE_AND_LINK: bin{{/|\\\\}}swift{{c?(\.EXE)?}}
// COMPILE_AND_LINK-NOT: /a.o
// COMPILE_AND_LINK: linker.swift
// COMPILE_AND_LINK-NOT: /a.o
// COMPILE_AND_LINK-NEXT: {{(bin/)?}}ld{{"? }}
// COMPILE_AND_LINK-DAG: /a.o
// COMPILE_AND_LINK-DAG: .o
// COMPILE_AND_LINK: -o linker
// FILELIST: {{(bin/)?}}ld{{"? }}
// FILELIST-NOT: .o{{"? }}
// FILELIST: -filelist {{"?[^-]}}
// FILELIST-NOT: .o{{"? }}
// FILELIST: -o linker
// FILELIST-CONTENTS: /linker-{{.*}}.o
// FILELIST-CONTENTS: /a.o
// INFERRED_NAME_DARWIN: bin{{/|\\\\}}swift{{c?(\.EXE)?}}
// INFERRED_NAME_DARWIN: -module-name LINKER
// INFERRED_NAME_DARWIN: {{(bin/)?}}ld{{"? }}
// INFERRED_NAME_DARWIN: -o libLINKER.dylib
// INFERRED_NAME_LINUX: -o libLINKER.so
// INFERRED_NAME_WINDOWS: -o LINKER.dll
// INFERRED_NAME_WASI: -o libLINKER.so
// Instead of a single "NOT" check for this run, we would really want to check
// for all of the driver arguments that we _do_ expect, and then use an
// --implicit-check-not to check that -lc++ doesn't occur.
// However, --implicit-check-not has a bug where it fails to flag the
// unexpected text when it occurs after text matched by a CHECK-DAG; see
// https://bugs.llvm.org/show_bug.cgi?id=45629
// For this reason, we use a single "NOT" check for the time being here.
// The same consideration applies to the Linux and Windows cases below.
// IOS-no-cxx-interop-NOT: -lc++
// IOS-cxx-interop-libcxx: swift
// IOS-cxx-interop-libcxx-DAG: -enable-cxx-interop
// IOS-cxx-interop-libcxx-DAG: -o [[OBJECTFILE:.*]]
// IOS-cxx-interop-libcxx: {{(bin/)?}}ld{{"? }}
// IOS-cxx-interop-libcxx-DAG: [[OBJECTFILE]]
// IOS-cxx-interop-libcxx-DAG: -lc++
// IOS-cxx-interop-libcxx: -o linker
// IOS-cxx-interop-libstdcxx: error: The only C++ standard library supported on Apple platforms is libc++
// LINUX-cxx-interop-NOT: -stdlib
// LINUX-cxx-interop-libcxx: swift
// LINUX-cxx-interop-libcxx-DAG: -enable-cxx-interop
// LINUX-cxx-interop-libcxx-DAG: -o [[OBJECTFILE:.*]]
// LINUX-cxx-interop-libcxx: clang++{{(\.exe)?"? }}
// LINUX-cxx-interop-libcxx-DAG: [[OBJECTFILE]]
// LINUX-cxx-interop-libcxx-DAG: -stdlib=libc++
// LINUX-cxx-interop-libcxx: -o linker
// WINDOWS-cxx-interop-NOT: -stdlib
// WINDOWS-cxx-interop-libcxx: swift
// WINDOWS-cxx-interop-libcxx-DAG: -enable-cxx-interop
// WINDOWS-cxx-interop-libcxx-DAG: -o [[OBJECTFILE:.*]]
// WINDOWS-cxx-interop-libcxx: clang++{{(\.exe)?"? }}
// WINDOWS-cxx-interop-libcxx-DAG: [[OBJECTFILE]]
// WINDOWS-cxx-interop-libcxx-DAG: -stdlib=libc++
// WINDOWS-cxx-interop-libcxx: -o linker
// Test ld detection. We use hard links to make sure
// the Swift driver really thinks it's been moved.
// RUN: rm -rf %t
// RUN: %empty-directory(%t/DISTINCTIVE-PATH/usr/bin)
// RUN: touch %t/DISTINCTIVE-PATH/usr/bin/ld
// RUN: chmod +x %t/DISTINCTIVE-PATH/usr/bin/ld
// RUN: %hardlink-or-copy(from: %swift_frontend_plain, to: %t/DISTINCTIVE-PATH/usr/bin/swiftc)
// RUN: %t/DISTINCTIVE-PATH/usr/bin/swiftc -target x86_64-apple-macosx10.9 %s -### | %FileCheck -check-prefix=RELATIVE-LINKER %s
// RELATIVE-LINKER: {{/|\\\\}}DISTINCTIVE-PATH{{/|\\\\}}usr{{/|\\\\}}bin{{/|\\\\}}swift
// RELATIVE-LINKER: {{/|\\\\}}DISTINCTIVE-PATH{{/|\\\\}}usr{{/|\\\\}}bin{{/|\\\\}}ld
// RELATIVE-LINKER: -o {{[^ ]+}}
// Also test arclite detection. This uses xcrun to find arclite when it's not
// next to Swift.
// RUN: %empty-directory(%t/ANOTHER-DISTINCTIVE-PATH/usr/bin)
// RUN: %empty-directory(%t/ANOTHER-DISTINCTIVE-PATH/usr/lib/arc)
// RUN: cp %S/Inputs/xcrun-return-self.sh %t/ANOTHER-DISTINCTIVE-PATH/usr/bin/xcrun
// RUN: env PATH=%t/ANOTHER-DISTINCTIVE-PATH/usr/bin %t/DISTINCTIVE-PATH/usr/bin/swiftc -target x86_64-apple-macosx10.9 %s -### | %FileCheck -check-prefix=XCRUN_ARCLITE %s
// XCRUN_ARCLITE: bin{{/|\\\\}}ld
// XCRUN_ARCLITE: {{/|\\\\}}ANOTHER-DISTINCTIVE-PATH{{/|\\\\}}usr{{/|\\\\}}lib{{/|\\\\}}arc{{/|\\\\}}libarclite_macosx.a
// XCRUN_ARCLITE: -o {{[^ ]+}}
// RUN: %empty-directory(%t/DISTINCTIVE-PATH/usr/lib/arc)
// RUN: env PATH=%t/ANOTHER-DISTINCTIVE-PATH/usr/bin %t/DISTINCTIVE-PATH/usr/bin/swiftc -target x86_64-apple-macosx10.9 %s -### | %FileCheck -check-prefix=RELATIVE_ARCLITE %s
// RELATIVE_ARCLITE: bin{{/|\\\\}}ld
// RELATIVE_ARCLITE: {{/|\\\\}}DISTINCTIVE-PATH{{/|\\\\}}usr{{/|\\\\}}lib{{/|\\\\}}arc{{/|\\\\}}libarclite_macosx.a
// RELATIVE_ARCLITE: -o {{[^ ]+}}
// LINUX-STDLIB-RPATH: -Xlinker -rpath -Xlinker [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)linux]]
// LINUX-NO-STDLIB-RPATH-NOT: -Xlinker -rpath -Xlinker [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)linux]]
// ANDROID-STDLIB-RPATH: -Xlinker -rpath -Xlinker [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)android]]
// ANDROID-NO-STDLIB-RPATH-NOT: -Xlinker -rpath -Xlinker [[STDLIB_PATH:[^ ]+(/|\\\\)lib(/|\\\\)swift(/|\\\\)android]]
// Clean up the test executable because hard links are expensive.
// RUN: rm -rf %t/DISTINCTIVE-PATH/usr/bin/swiftc
| apache-2.0 | 293f19420dd5e3e181bb7bb986c6a603 | 47.519849 | 270 | 0.665952 | 2.895645 | false | false | false | false |
shaowei-su/swiftmi-app | swiftmi/swiftmi/PostDetailController.swift | 4 | 11713 | //
// PostDetailController.swift
// swiftmi
//
// Created by yangyin on 15/4/4.
// Copyright (c) 2015年 swiftmi. All rights reserved.
//
import UIKit
import Alamofire
import SwiftyJSON
import Kingfisher
class PostDetailController: UIViewController,UIScrollViewDelegate,UIWebViewDelegate,UITextViewDelegate {
@IBOutlet weak var webView: UIWebView!
@IBOutlet weak var inputWrapView: UIView!
@IBOutlet weak var inputReply: UITextView!
var article:AnyObject?
var postId:Int?
var postDetail:JSON = nil
var keyboardShow = false
override func viewDidLoad() {
super.viewDidLoad()
var center: NSNotificationCenter = NSNotificationCenter.defaultCenter()
center.addObserver(self, selector: "keyboardWillShow:", name: UIKeyboardWillShowNotification, object: nil)
center.addObserver(self, selector: "keyboardWillHide:", name: UIKeyboardWillHideNotification, object: nil)
//center.addObserver(self, selector:"keyboardWillChangeFrame:", name:UIKeyboardWillChangeFrameNotification,object:nil)
self.setViews()
self.inputReply.layer.borderWidth = 1
self.inputReply.layer.borderColor = UIColor(red: 0.85, green: 0.85, blue:0.85, alpha: 0.9).CGColor
// Keyboard stuff.
// Do any additional setup after loading the view.
}
override func viewDidAppear(animated: Bool) {
self.userActivity = NSUserActivity(activityType: "com.swiftmi.handoff.view-web")
self.userActivity?.title = "view article on mac"
self.userActivity?.webpageURL = NSURL(string: ServiceApi.getTopicShareDetail(article!.valueForKey("postId") as! Int))
self.userActivity?.becomeCurrent()
}
override func viewWillDisappear(animated: Bool) {
NSNotificationCenter.defaultCenter().removeObserver(self, name: UIKeyboardWillShowNotification, object: nil)
NSNotificationCenter.defaultCenter().removeObserver(self, name: UIKeyboardWillHideNotification, object: nil)
self.userActivity?.invalidate()
}
func keyboardWillShow(notification: NSNotification) {
var info:NSDictionary = notification.userInfo!
let duration = (info[UIKeyboardAnimationDurationUserInfoKey] as! NSNumber).doubleValue
var beginKeyboardRect = (info[UIKeyboardFrameBeginUserInfoKey] as! NSValue).CGRectValue()
var endKeyboardRect = (info[UIKeyboardFrameEndUserInfoKey] as! NSValue).CGRectValue()
var yOffset = endKeyboardRect.origin.y - beginKeyboardRect.origin.y
var frame = self.view.frame
frame.origin.y = -endKeyboardRect.height
var inputW = self.inputWrapView.frame
var newFrame = CGRectMake(inputW.origin.x, inputW.origin.y-80, inputW.width, inputW.height+90)
UIView.animateWithDuration(duration, delay: 0, options: UIViewAnimationOptions.CurveEaseInOut, animations: {
self.webView.stringByEvaluatingJavaScriptFromString("$('body').css({'padding-top':'\(endKeyboardRect.height)px'});")
for constraint in self.inputWrapView.constraints() {
if constraint.firstAttribute == NSLayoutAttribute.Height {
var inputWrapContraint = constraint as? NSLayoutConstraint
inputWrapContraint?.constant = 80
// self.inputWrapView.updateConstraintsIfNeeded()
break;
}
}
self.view.frame = frame
}, completion: nil)
}
func keyboardWillHide(notification: NSNotification) {
var info:NSDictionary = notification.userInfo!
var keyboardSize = (info[UIKeyboardFrameEndUserInfoKey] as! NSValue).CGRectValue()
var keyboardHeight:CGFloat = keyboardSize.height
let duration = (info[UIKeyboardAnimationDurationUserInfoKey] as! NSNumber).doubleValue
var frame = self.view.frame
frame.origin.y = 0 // keyboardHeight
UIView.animateWithDuration(duration, delay: 0, options: UIViewAnimationOptions.CurveEaseInOut, animations: {
self.webView.stringByEvaluatingJavaScriptFromString("$('body').css({'padding-top':'0px'});")
self.view.frame = frame
for constraint in self.inputWrapView.constraints() {
if constraint.firstAttribute == NSLayoutAttribute.Height {
var inputWrapContraint = constraint as? NSLayoutConstraint
inputWrapContraint?.constant = 50
// self.inputWrapView.updateConstraintsIfNeeded()
break;
}
}
}, completion: nil)
}
private func GetLoadData() -> JSON {
if postDetail != nil {
return self.postDetail
}
var json:JSON = ["comments":[]]
json["topic"] = JSON(self.article!)
return json
}
func loadData(){
Alamofire.request(Router.TopicDetail(topicId: (article!.valueForKey("postId") as! Int))).responseJSON{
(_,_,json,error) in
if(error != nil){
var alert = UIAlertView(title: "网络异常", message: "请检查网络设置", delegate: nil, cancelButtonTitle: "确定")
alert.show()
}
else {
var result = JSON(json!)
if result["isSuc"].boolValue {
self.postDetail = result["result"]
}
}
var path=NSBundle.mainBundle().pathForResource("article", ofType: "html")
var url=NSURL.fileURLWithPath(path!)
var request = NSURLRequest(URL:url!)
dispatch_async(dispatch_get_main_queue()) {
self.inputWrapView.hidden = false
self.webView.loadRequest(request)
}
}
}
func setViews(){
self.view.backgroundColor=UIColor.whiteColor()
self.webView.backgroundColor=UIColor.clearColor()
self.inputReply.resignFirstResponder()
self.webView.delegate=self
self.webView.scrollView.delegate=self
// self.inputView
self.startLoading()
self.inputWrapView.hidden = true
self.title="主题贴"
self.loadData()
}
@IBAction func replyClick(sender: AnyObject) {
var msg = inputReply.text;
inputReply.text = "";
if msg != nil {
var postId = article!.valueForKey("postId") as! Int
let params:[String:AnyObject] = ["postId":postId,"content":msg]
Alamofire.request(Router.TopicComment(parameters: params)).responseJSON{
(_,_,json,error) in
if error != nil {
self.notice("网络异常", type: NoticeType.error, autoClear: true)
return
}
var result = JSON(json!)
if result["isSuc"].boolValue {
self.notice("评论成功!", type: NoticeType.success, autoClear: true)
self.webView.stringByEvaluatingJavaScriptFromString("article.addComment("+result["result"].rawString()!+");")
} else {
self.notice("评论失败!", type: NoticeType.error, autoClear: true)
}
}
}
}
func startLoading(){
self.pleaseWait()
self.webView.hidden=true
}
func stopLoading(){
self.webView.hidden=false
self.clearAllNotice()
}
func webView(webView: UIWebView, shouldStartLoadWithRequest request: NSURLRequest, navigationType: UIWebViewNavigationType) -> Bool
{
var reqUrl=request.URL!.absoluteString
var params = reqUrl!.componentsSeparatedByString("://")
dispatch_async(dispatch_get_main_queue(),{
if(params.count>=2){
if(params[0].compare("html")==NSComparisonResult.OrderedSame && params[1].compare("docready") == NSComparisonResult.OrderedSame ){
var data = self.GetLoadData()
self.webView.stringByEvaluatingJavaScriptFromString("article.render("+data.rawString()!+");")
}
else if(params[0].compare("html")==NSComparisonResult.OrderedSame && params[1].compare("contentready")==NSComparisonResult.OrderedSame){
//doc content ok
self.stopLoading()
}
else if params[0].compare("http") == NSComparisonResult.OrderedSame || params[0].compare("https") == NSComparisonResult.OrderedSame {
var webViewController:WebViewController = Utility.GetViewController("webViewController")
webViewController.webUrl = reqUrl
self.presentViewController(webViewController, animated: true, completion: nil)
}
}
})
if params[0].compare("http") == NSComparisonResult.OrderedSame || params[0].compare("https") == NSComparisonResult.OrderedSame {
return false
}
return true;
}
func webViewDidStartLoad(webView: UIWebView)
{
}
func webViewDidFinishLoad(webView: UIWebView)
{
}
func webView(webView: UIWebView, didFailLoadWithError error: NSError)
{
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func shareClick(sender: AnyObject) {
share()
}
private func share() {
var data = GetLoadData()
var title = data["topic"]["title"].stringValue
var url = ServiceApi.getTopicShareDetail(data["topic"]["postId"].intValue)
var desc = data["topic"]["desc"].stringValue
var img = self.webView.stringByEvaluatingJavaScriptFromString("article.getShareImage()")
Utility.share(title, desc: desc, imgUrl: img, linkUrl: url)
}
// MARK: - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
// Get the new view controller using segue.destinationViewController.
// Pass the selected object to the new view controller.
}
override func viewDidDisappear(animated: Bool) {
super.viewDidDisappear(animated)
self.clearAllNotice()
}
}
| mit | ded6869ec7244d59809e79321491f00f | 31.196133 | 152 | 0.557872 | 5.789866 | false | false | false | false |
lfaoro/Cast | Carthage/Checkouts/RxSwift/RxExample/RxExample/Examples/TableView/TableViewController.swift | 1 | 6635 | //
// TableViewController.swift
// RxExample
//
// Created by carlos on 26/5/15.
// Copyright (c) 2015 Krunoslav Zaher. All rights reserved.
//
import UIKit
#if !RX_NO_MODULE
import RxSwift
import RxCocoa
#endif
class TableViewController: ViewController, UITableViewDelegate {
@IBOutlet weak var tableView: UITableView!
var disposeBag = DisposeBag()
let users = Variable([User]())
let favoriteUsers = Variable([User]())
var allSections: [SectionModel<String, User>] = []
let dataSource = RxTableViewSectionedReloadDataSource<SectionModel<String, User>>()
typealias Section = SectionModel<String, User>
override func viewDidLoad() {
super.viewDidLoad()
self.navigationItem.rightBarButtonItem = self.editButtonItem()
let allUsers = combineLatest(favoriteUsers, users) { favoriteUsers, users in
return [
SectionModel(model: "Favorite Users", items: favoriteUsers),
SectionModel(model: "Normal Users", items: users)
]
}
// This is for demonstration purposes of UITableViewDelegate/DataSource
// only, try to not do something like this in your app
allUsers
.subscribeNext { [unowned self] n in
self.allSections = n
}
.addDisposableTo(disposeBag)
dataSource.cellFactory = { (tv, ip, user: User) in
let cell = tv.dequeueReusableCellWithIdentifier("Cell")!
cell.textLabel?.text = user.firstName + " " + user.lastName
return cell
}
dataSource.titleForHeaderInSection = { [unowned dataSource] sectionIndex in
return dataSource.sectionAtIndex(sectionIndex).model
}
// reactive data source
allUsers
.subscribe(tableView, withReactiveDataSource: dataSource)
.addDisposableTo(disposeBag)
// customization using delegate
// RxTableViewDelegateBridge will forward correct messages
tableView.rx_setDelegate(self)
.addDisposableTo(disposeBag)
tableView.rx_itemSelected
.subscribeNext { [unowned self] indexPath in
self.showDetailsForUserAtIndexPath(indexPath)
}
.addDisposableTo(disposeBag)
tableView.rx_itemDeleted
.subscribeNext { [unowned self] indexPath in
self.removeUser(indexPath)
}
.addDisposableTo(disposeBag)
tableView.rx_itemMoved
.subscribeNext { [unowned self] (s, d) in
self.moveUserFrom(s, to: d)
}
.addDisposableTo(disposeBag)
// Rx content offset
tableView.rx_contentOffset
.subscribeNext { co in
print("Content offset from Rx observer \(co)")
}
RandomUserAPI.sharedAPI.getExampleUserResultSet()
.subscribeNext { [unowned self] array in
self.users.sendNext(array)
}
.addDisposableTo(disposeBag)
favoriteUsers.sendNext([User(firstName: "Super", lastName: "Man", imageURL: "http://nerdreactor.com/wp-content/uploads/2015/02/Superman1.jpg")])
}
override func setEditing(editing: Bool, animated: Bool) {
super.setEditing(editing, animated: animated)
tableView.editing = editing
}
// MARK: Table view delegate ;)
func tableView(tableView: UITableView, viewForHeaderInSection section: Int) -> UIView? {
let title = dataSource.sectionAtIndex(section)
let label = UILabel(frame: CGRect.zero)
// hacky I know :)
label.text = " \(title)"
label.textColor = UIColor.whiteColor()
label.backgroundColor = UIColor.darkGrayColor()
label.alpha = 0.9
return label
}
func tableView(tableView: UITableView, heightForHeaderInSection section: Int) -> CGFloat {
return 40
}
func scrollViewDidScroll(scrollView: UIScrollView) {
print("Content offset from delegate \(scrollView.contentOffset)")
}
// MARK: Navigation
private func showDetailsForUserAtIndexPath(indexPath: NSIndexPath) {
let sb = UIStoryboard(name: "Main", bundle: NSBundle(identifier: "RxExample-iOS"))
let vc = sb.instantiateViewControllerWithIdentifier("DetailViewController") as! DetailViewController
vc.user = getUser(indexPath)
self.navigationController?.pushViewController(vc, animated: true)
}
// MARK: Work over Variable
func getUser(indexPath: NSIndexPath) -> User {
var array: [User]
switch indexPath.section {
case 0:
array = favoriteUsers.value
case 1:
array = users.value
default:
fatalError("Section out of range")
}
return array[indexPath.row]
}
func moveUserFrom(from: NSIndexPath, to: NSIndexPath) {
var user: User
var fromArray: [User]
var toArray: [User]
switch from.section {
case 0:
fromArray = favoriteUsers.value
user = fromArray.removeAtIndex(from.row)
favoriteUsers.sendNext(fromArray)
case 1:
fromArray = users.value
user = fromArray.removeAtIndex(from.row)
users.sendNext(fromArray)
default:
fatalError("Section out of range")
}
switch to.section {
case 0:
toArray = favoriteUsers.value
toArray.insert(user, atIndex: to.row)
favoriteUsers.sendNext(toArray)
case 1:
toArray = users.value
toArray.insert(user, atIndex: to.row)
users.sendNext(toArray)
default:
fatalError("Section out of range")
}
}
func addUser(user: User) {
var array = users.value
array.append(user)
users.sendNext(array)
}
func removeUser(indexPath: NSIndexPath) {
var array: [User]
switch indexPath.section {
case 0:
array = favoriteUsers.value
array.removeAtIndex(indexPath.row)
favoriteUsers.sendNext(array)
case 1:
array = users.value
array.removeAtIndex(indexPath.row)
users.sendNext(array)
default:
fatalError("Section out of range")
}
}
}
| mit | b413c34ec2dfdac5e21bd3c7e2b74076 | 30.445498 | 152 | 0.588696 | 5.274245 | false | false | false | false |
Czajnikowski/TrainTrippin | Pods/RxCocoa/RxCocoa/iOS/UIBarButtonItem+Rx.swift | 3 | 2086 | //
// UIBarButtonItem+Rx.swift
// RxCocoa
//
// Created by Daniel Tartaglia on 5/31/15.
// Copyright © 2015 Krunoslav Zaher. All rights reserved.
//
#if os(iOS) || os(tvOS)
import UIKit
#if !RX_NO_MODULE
import RxSwift
#endif
var rx_tap_key: UInt8 = 0
extension Reactive where Base: UIBarButtonItem {
/**
Bindable sink for `enabled` property.
*/
public var enabled: UIBindingObserver<Base, Bool> {
return UIBindingObserver(UIElement: self.base) { UIElement, value in
UIElement.isEnabled = value
}
}
/**
Reactive wrapper for target action pattern on `self`.
*/
public var tap: ControlEvent<Void> {
let source = lazyInstanceObservable(&rx_tap_key) { () -> Observable<Void> in
Observable.create { [weak control = self.base] observer in
guard let control = control else {
observer.on(.completed)
return Disposables.create()
}
let target = BarButtonItemTarget(barButtonItem: control) {
observer.on(.next())
}
return target
}
.takeUntil(self.deallocated)
.share()
}
return ControlEvent(events: source)
}
}
@objc
class BarButtonItemTarget: RxTarget {
typealias Callback = () -> Void
weak var barButtonItem: UIBarButtonItem?
var callback: Callback!
init(barButtonItem: UIBarButtonItem, callback: @escaping () -> Void) {
self.barButtonItem = barButtonItem
self.callback = callback
super.init()
barButtonItem.target = self
barButtonItem.action = #selector(BarButtonItemTarget.action(_:))
}
override func dispose() {
super.dispose()
#if DEBUG
MainScheduler.ensureExecutingOnScheduler()
#endif
barButtonItem?.target = nil
barButtonItem?.action = nil
callback = nil
}
func action(_ sender: AnyObject) {
callback()
}
}
#endif
| mit | d992c2de6d7a06be4eee734f6a47b76a | 23.244186 | 84 | 0.580336 | 4.815242 | false | false | false | false |
spark/photon-tinker-ios | Photon-Tinker/Global/ParticleUtils.swift | 1 | 3855 | //
// ParticleUtils.swift
// Particle
//
// Created by Ido Kleinman on 6/29/16.
// Copyright (c) 2019 Particle. All rights reserved.
//
import Foundation
extension NSNotification.Name {
public static let ParticleDeviceSystemEvent: NSNotification.Name = NSNotification.Name(rawValue: "io.particle.event.ParticleDeviceSystemEvent")
}
class ParticleUtils: NSObject {
static var particleCyanColor = UIColor(rgb: 0x00ADEF)
static var particleAlmostWhiteColor = UIColor(rgb: 0xF7F7F7)
static var particleDarkGrayColor = UIColor(rgb: 0x333333)
static var particleGrayColor = UIColor(rgb: 0x777777)
static var particleLightGrayColor = UIColor(rgb: 0xC7C7C7)
static var particlePomegranateColor = UIColor(rgb: 0xC0392B)
static var particleEmeraldColor = UIColor(rgb: 0x2ECC71)
static var particleRegularFont = UIFont(name: "Gotham-book", size: 16.0)!
static var particleBoldFont = UIFont(name: "Gotham-medium", size: 16.0)!
@objc class func shouldDisplayTutorialForViewController(_ vc : UIViewController) -> Bool {
let prefs = UserDefaults.standard
let defaultsKeyName = "Tutorial"
let dictKeyName = String(describing: type(of: vc))
if let onceDict = prefs.dictionary(forKey: defaultsKeyName) {
let keyExists = onceDict[dictKeyName] != nil
if keyExists {
return false
} else {
return true
}
} else {
return true
}
}
@objc class func setTutorialWasDisplayedForViewController(_ vc : UIViewController) {
let prefs = UserDefaults.standard
let defaultsKeyName = "Tutorial"
let dictKeyName = String(describing: type(of: vc))
if var onceDict = prefs.dictionary(forKey: defaultsKeyName) {
onceDict[dictKeyName] = true
prefs.set(onceDict, forKey: defaultsKeyName)
} else {
prefs.set([dictKeyName : true], forKey: defaultsKeyName)
}
}
class func resetTutorialWasDisplayed() {
let prefs = UserDefaults.standard
let keyName = "Tutorial"
prefs.removeObject(forKey: keyName)
}
@objc class func animateOnlineIndicatorImageView(_ imageView: UIImageView, online: Bool, flashing: Bool) {
DispatchQueue.main.async(execute: {
imageView.image = UIImage(named: "ImgCircle")!.withRenderingMode(UIImage.RenderingMode.alwaysTemplate)
if flashing {
imageView.tintColor = UIColor(rgb: 0xB31983) // Flashing purple
imageView.alpha = 1
UIView.animate(withDuration: 0.12, delay: 0, options: [.autoreverse, .repeat], animations: {
imageView.alpha = 0
}, completion: nil)
} else if online {
imageView.tintColor = UIColor(rgb: 0x00AEEF) // ParticleCyan
if imageView.alpha == 1 {
// print ("1-->0")
UIView.animate(withDuration: 2.5, delay: 0, options: [.autoreverse, .repeat], animations: {
imageView.alpha = 0.15
}, completion: nil)
} else {
// print ("0-->1")
imageView.alpha = 0.15
UIView.animate(withDuration: 2.5, delay: 0, options: [.autoreverse, .repeat], animations: {
imageView.alpha = 1
}, completion: nil)
}
} else {
imageView.tintColor = UIColor(rgb: 0xD9D8D6) // ParticleGray
imageView.alpha = 1
imageView.layer.removeAllAnimations()
}
})
}
}
| apache-2.0 | 1dd6dc17773628722e9f8c4edbc7f869 | 35.367925 | 148 | 0.578988 | 4.695493 | false | false | false | false |
davecom/DKAsyncImageView | DKAsyncImageView.swift | 1 | 11517 | //
// DKAsyncImageView.swift
// The MIT License (MIT)
//
// Copyright (c) 2014-2017 David Kopec
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
import Foundation
import Cocoa
/// A Swift subclass of NSImageView for loading remote images asynchronously.
open class DKAsyncImageView: NSImageView, URLSessionDelegate, URLSessionDownloadDelegate {
public enum DownloadTaskResponse {
case success(_ image: Data)
case failure(_ error: Error?)
}
fileprivate var networkSession: URLSession {
return currentNetworkSession ??
URLSession.init(configuration:URLSessionConfiguration.default, delegate: self, delegateQueue: OperationQueue.main)
}
fileprivate var currentNetworkSession: URLSession?
fileprivate var imageURLDownloadTask: URLSessionDownloadTask?
fileprivate var imageDownloadData: Data?
fileprivate var errorImage: NSImage?
fileprivate var spinningWheel: NSProgressIndicator?
fileprivate var trackingArea: NSTrackingArea?
var isLoadingImage: Bool = false
var userDidCancel: Bool = false
var didFailLoadingImage: Bool = false
var completionHandler: ((Data?, Error?) -> Void)?
fileprivate var attemptCompletionHandler: (DownloadTaskResponse) -> Void? { return respondToDownloadAttempt(withResponse:) }
fileprivate var downloadTaskAttemptLimit: Int = 0
fileprivate var downloadTaskAttempts: Int = 0
fileprivate var toolTipWhileLoading: String?
fileprivate var toolTipWhenFinished: String?
fileprivate var toolTipWhenFinishedWithError: String?
deinit {
cancelDownload()
}
/// Grab an image form a URL and asynchronously load it into the image view
///
/// - parameter url: A String representing the URL of the image.
/// - parameter placeHolderImage: an optional NSImage to temporarily display while the image is downloading
/// - parameter errorImage: an optional NSImage that displays if the download fails.
/// - parameter usesSpinningWheel: A Bool that determines whether or not a spinning wheel indicator displays during download
/// - parameter completion: A block to be executed when the download task finishes. The block takes two optional parameters: Data and Error and has no return value.
open func downloadImageFromURL(_ url: String, placeHolderImage:NSImage? = nil, errorImage:NSImage? = nil, usesSpinningWheel: Bool = false, allowedAttempts: Int = 0, completion: ((Data?, Error?) -> Void)? = nil) {
cancelDownload()
completionHandler = completion
downloadTaskAttemptLimit = allowedAttempts
isLoadingImage = true
didFailLoadingImage = false
userDidCancel = false
image = placeHolderImage
self.errorImage = errorImage
imageDownloadData = NSMutableData() as Data
guard let URL = URL(string: url) else {
isLoadingImage = false
NSLog("Error: malformed URL passed to downloadImageFromURL")
return
}
imageURLDownloadTask = networkSession.downloadTask(with: URL)
imageURLDownloadTask?.resume()
if usesSpinningWheel {
if self.frame.size.height >= 64 && self.frame.size.width >= 64 {
spinningWheel = NSProgressIndicator()
if let spinningWheel = spinningWheel {
addSubview(spinningWheel)
spinningWheel.style = NSProgressIndicator.Style.spinning
spinningWheel.isDisplayedWhenStopped = false
spinningWheel.frame = NSMakeRect(self.frame.size.width * 0.5 - 16, self.frame.size.height * 0.5 - 16, 32, 32)
spinningWheel.controlSize = NSControl.ControlSize.regular
spinningWheel.startAnimation(self)
}
} else if (self.frame.size.height < 64 && self.frame.size.height >= 16) && (self.frame.size.width < 64 && self.frame.size.width >= 16) {
spinningWheel = NSProgressIndicator()
if let spinningWheel = spinningWheel {
addSubview(spinningWheel)
spinningWheel.style = NSProgressIndicator.Style.spinning
spinningWheel.isDisplayedWhenStopped = false
spinningWheel.frame = NSMakeRect(self.frame.size.width * 0.5 - 8, self.frame.size.height * 0.5 - 8, 16, 16)
spinningWheel.controlSize = NSControl.ControlSize.small
spinningWheel.startAnimation(self)
}
}
}
}
/// Cancel the download
open func cancelDownload() {
userDidCancel = true
isLoadingImage = false
didFailLoadingImage = false
if let networkSession = currentNetworkSession {
networkSession.invalidateAndCancel()
}
resetForNewTask()
image = nil
}
fileprivate func resetForNewTask() {
imageDownloadData = nil
imageURLDownloadTask = nil
errorImage = nil
downloadTaskAttempts = 0
spinningWheel?.stopAnimation(self)
spinningWheel?.removeFromSuperview()
}
fileprivate func failureReset() {
isLoadingImage = false
didFailLoadingImage = true
userDidCancel = false
networkSession.finishTasksAndInvalidate()
image = errorImage
resetForNewTask()
}
// MARK: Intermediate completion handler
open func respondToDownloadAttempt(withResponse response: DownloadTaskResponse) {
switch response {
case .success(let data):
Swift.print("Image download task successful with URL.")
isLoadingImage = false
networkSession.finishTasksAndInvalidate()
resetForNewTask()
completionHandler?(data, nil)
return
case .failure(let error):
if downloadTaskAttempts >= downloadTaskAttemptLimit {
Swift.print("Image download task exceeded retry attempts.")
image = errorImage
completionHandler?(nil, error)
failureReset()
return
}
downloadTaskAttempts += 1
Swift.print("Image download task retrying attempt \(downloadTaskAttempts) / \(downloadTaskAttemptLimit).")
}
guard let url = imageURLDownloadTask?.originalRequest?.url else {
NSLog("Error: malformed URL passed to downloadImageFromURL internal retry.")
return
}
imageURLDownloadTask = networkSession.downloadTask(with: url)
imageURLDownloadTask?.resume()
}
//MARK: NSURLSessionDownloadTask Delegate
open func urlSession(_ session: URLSession, downloadTask: URLSessionDownloadTask, didFinishDownloadingTo location: URL) {
imageDownloadData = try? Data.init(contentsOf: location)
}
//MARK: NSURLSessionTask Delegate
open func urlSession(_ session: URLSession, task: URLSessionTask, didCompleteWithError error: Error?) {
guard error == nil else {
if (error as NSError?)?.code == NSURLErrorCancelled {
return
}
Swift.print(error!.localizedDescription)
attemptCompletionHandler(.failure(error))
return
}
didFailLoadingImage = false
userDidCancel = false
guard let data = imageDownloadData else {
Swift.print("Image data not downloaded correctly.")
attemptCompletionHandler(.failure(error))
return;
}
guard let img: NSImage = NSImage(data: data) else {
Swift.print("Error forming image from data.")
attemptCompletionHandler(.failure(error))
return;
}
image = img
attemptCompletionHandler(.success(data))
}
//MARK: Tooltips
/// Set tooltips for loading, finished, and error states
///
/// - parameter ttip1: The tool tip to show while loading
/// - parameter whenFinished: The tool tip that shows after the image downloaded.
/// - parameter andWhenFinishedwithError: The tool tip that shows when an error occurs.
open func setToolTipWhileLoading(_ ttip1: String?, whenFinished ttip2:String?, andWhenFinishedWithError ttip3: String?) {
toolTipWhileLoading = ttip1
toolTipWhenFinished = ttip2
toolTipWhenFinishedWithError = ttip3
}
/// Remove all tooltips
open func deleteToolTips() {
toolTip = nil
toolTipWhileLoading = nil
toolTipWhenFinished = nil
toolTipWhenFinishedWithError = nil
}
override open func mouseEntered(with theEvent: NSEvent) {
if !userDidCancel { // the user didn't cancel the operation so show the tooltips
if isLoadingImage {
if let toolTipWhileLoading = toolTipWhileLoading {
toolTip = toolTipWhileLoading
} else {
toolTip = nil
}
}
else if didFailLoadingImage { //connection failed
if let toolTipWhenFinishedWithError = toolTipWhenFinishedWithError {
toolTip = toolTipWhenFinishedWithError
} else {
toolTip = nil
}
}
else if !isLoadingImage { // it's not loading image
if let toolTipWhenFinished = toolTipWhenFinished {
toolTip = toolTipWhenFinished
} else {
toolTip = nil
}
}
}
}
override open func updateTrackingAreas() {
if let trackingArea = trackingArea {
removeTrackingArea(trackingArea)
}
let opts: NSTrackingArea.Options = NSTrackingArea.Options(rawValue: NSTrackingArea.Options.mouseEnteredAndExited.rawValue | NSTrackingArea.Options.activeAlways.rawValue)
trackingArea = NSTrackingArea(rect: self.bounds, options: opts, owner: self, userInfo: nil)
if let trackingArea = trackingArea {
self.addTrackingArea(trackingArea)
}
}
}
| mit | f5aece5c4c4d17e036a536d1fa9d0e3c | 38.307167 | 216 | 0.631501 | 5.585354 | false | false | false | false |
iOSWizards/AwesomeMedia | Example/Pods/AwesomeCore/AwesomeCore/Classes/Model/UserProfile.swift | 1 | 8034 | //
// UserProfile.swift
// AwesomeCore
//
// Created by Antonio da Silva on 04/09/2017.
//
import Foundation
public struct UserProfile: Codable, Equatable {
public let id: String?
public let uid: String?
public let email: String
public let firstName: String?
public let lastName: String?
public let currentSignInAt: Date?
public let lastSignInAt: Date?
public let lastSignInIp: String?
public let enrollmentsCount: Int?
public let signInCount: Int?
public let createdAt: Date?
public let updatedAt: Date?
public let lastReadCourse: ACCourse?
public let lang: String?
public let country: String?
public let location: String?
public let timezone: String?
public let role: String?
public let avatarUrl: String?
public var name: String {
var name = ""
if let firstName = firstName, firstName.count > 0 {
name.append(firstName)
}
if let lastName = lastName, lastName.count > 0 {
name.append(" \(lastName)")
}
return name
}
init(
id: String?,
uid: String?,
email: String,
firstName: String?,
lastName: String?,
currentSignInAt: Date?,
lastSignInAt: Date?,
lastSignInIp: String,
enrollmentsCount: Int?,
signInCount: Int?,
createdAt: Date?,
updatedAt: Date?,
lastReadCourse: ACCourse?,
lang: String?,
country: String?,
location: String?,
timezone: String?,
role: String?,
avatarUrl: String?) {
self.id = id
self.uid = uid
self.email = email
self.firstName = firstName
self.lastName = lastName
self.currentSignInAt = currentSignInAt
self.lastSignInAt = lastSignInAt
self.lastSignInIp = lastSignInIp
self.enrollmentsCount = enrollmentsCount
self.signInCount = signInCount
self.createdAt = createdAt
self.updatedAt = updatedAt
self.lastReadCourse = lastReadCourse
self.lang = lang
self.country = country
self.location = location
self.timezone = timezone
self.role = role
self.avatarUrl = avatarUrl
}
init(
email: String,
firstName: String?,
lastName: String?,
currentSignInAt: Date?,
lastSignInAt: Date?,
lastSignInIp: String,
enrollmentsCount: Int?,
signInCount: Int?,
createdAt: Date?,
updatedAt: Date?,
lastReadCourse: ACCourse?) {
self.id = nil
self.uid = nil
self.email = email
self.firstName = firstName
self.lastName = lastName
self.currentSignInAt = currentSignInAt
self.lastSignInAt = lastSignInAt
self.lastSignInIp = lastSignInIp
self.enrollmentsCount = enrollmentsCount
self.signInCount = signInCount
self.createdAt = createdAt
self.updatedAt = updatedAt
self.lastReadCourse = lastReadCourse
self.lang = nil
self.country = nil
self.location = nil
self.timezone = nil
self.role = nil
self.avatarUrl = nil
}
}
// MARK: - JSON Key
public struct UserProfileDataKey: Codable {
public let data: UserProfileKey
}
public struct UserProfileKey: Codable {
public let profile: UserProfile?
}
public struct HomeUserProfile: Codable {
public let id: Int?
public let uid: String?
public let email: String?
public let photo: String?
public let firstName: String?
public let lastName: String?
public let phone: String?
public let language: String?
public let countryName: String?
public let gender: String?
public let profession: String?
public let industry: String?
public let languageName: String?
public let country: String?
public let city: String?
public let location: String?
public let dateOfBirth: String?
public let timezone: String?
public let needsToCreatePassword: Bool?
public let facebookId: String?
public let title: String?
public let shortBio: String?
public let discoverable: Bool?
public let ageGroup: String?
public let website: String?
public let linkedin: String?
public let facebook: String?
public let twitter: String?
public let tags: [String]?
public let metaTags: [String]?
public let eventTags: [Int?]?
private let eventRegistrationsPriv: [EventRegistrationKey]?
public var name: String {
var name = ""
if let firstName = firstName, firstName.count > 0 {
name.append(firstName)
}
if let lastName = lastName, lastName.count > 0 {
name.append(" \(lastName)")
}
return name
}
public var eventRegistrations: [EventRegistration] {
if let eventRegistrationsPriv = eventRegistrationsPriv {
return eventRegistrationsPriv.map { registration in
registration.eventRegistration
}
}
return []
}
}
public struct HomeUserProfileDataKey: Codable {
public let user: HomeUserProfile
}
extension HomeUserProfile {
private enum CodingKeys: String, CodingKey {
case id
case uid
case email
case photo = "profile_photo_url"
case firstName = "first_name"
case lastName = "last_name"
case phone
case language = "lang"
case countryName = "country_name"
case dateOfBirth = "date_of_birth"
case gender
case profession
case industry
case languageName = "language_name"
case country
case city
case location
case timezone = "timezone_identifier"
case needsToCreatePassword = "need_to_create_password"
case facebookId = "facebook_id"
case title
case shortBio = "bio"
case discoverable
case ageGroup = "enrolment_group"
case website
case linkedin = "linked_in"
case facebook
case twitter
case tags
case metaTags = "meta_tags"
case eventTags = "event_tags"
case eventRegistrationsPriv = "event_registrations"
}
}
// MARK: - Equatable
extension UserProfile {
public static func ==(lhs: UserProfile, rhs: UserProfile) -> Bool {
if lhs.id != rhs.id {
return false
}
if lhs.uid != rhs.uid {
return false
}
if lhs.email != rhs.email {
return false
}
if lhs.firstName != rhs.firstName {
return false
}
if lhs.lastName != rhs.lastName {
return false
}
if lhs.currentSignInAt != rhs.currentSignInAt {
return false
}
if lhs.lastSignInAt != rhs.lastSignInAt {
return false
}
if lhs.lastSignInIp != rhs.lastSignInIp {
return false
}
if lhs.enrollmentsCount != rhs.enrollmentsCount {
return false
}
if lhs.signInCount != rhs.signInCount {
return false
}
if lhs.createdAt != rhs.createdAt {
return false
}
if lhs.updatedAt != rhs.updatedAt {
return false
}
if lhs.lastReadCourse != rhs.lastReadCourse {
return false
}
if lhs.lang != rhs.lang {
return false
}
if lhs.country != rhs.country {
return false
}
if lhs.location != rhs.location {
return false
}
if lhs.timezone != rhs.timezone {
return false
}
if lhs.role != rhs.role {
return false
}
if lhs.avatarUrl != rhs.avatarUrl {
return false
}
return true
}
}
| mit | b64a16d1969ebebc5a02f71f799ffb15 | 25.69103 | 71 | 0.580408 | 4.734237 | false | false | false | false |
abiaoLHB/LHBWeiBo-Swift | LHBWeibo/LHBWeibo/MainWibo/Home/HomeModel/StatusViewModel.swift | 1 | 3578 | //
// StatusViewModel.swift
// LHBWeibo
//
// Created by LHB on 16/8/19.
// Copyright © 2016年 LHB. All rights reserved.
//
import UIKit
//视图模型:对某一个模型进行封装,这里是对statusModel进行封装
class StatusViewModel: NSObject {
//MARK: - 定义属性
var status : StatusModel?
//属性处理属性,像source属性,新浪返回source不能直接展示"source": "<a href="http://weibo.com" rel="nofollow">新浪微博</a>",所以得用另一属性属性处理,上面做属性监听
var sourceText : String?
var creatAtText : String?
//用户模型里的两个属性
var verifiedImage : UIImage?
var vipImage : UIImage?
//额外属性:目的是简化一些获取值的长度
var profileURL : NSURL? ///处理头像
var cellHeight : CGFloat = 0 //cell高度
var picUrls : [NSURL] = [NSURL]() //处理微博配图,初始化数组
// 自定义构造函数,要想创建statusViewModel,必须传进来一个要封装的model,否则没意义
init(status : StatusModel) {
//把传进来的model给属性赋值
self.status = status
//对微博来源进行处理
//nil值校验 where相当于&&。。。。这里就不能用guard了
// guard let source = source where source != "" else{
// return
// }
//1、处理来源
if let source = status.source where source != "" {
//从哪里截取
let startIndex = (source as NSString).rangeOfString(">").location + 1
//截取多长
let lengh = (source as NSString).rangeOfString("</").location - startIndex
//截取
sourceText = (source as NSString).substringWithRange(NSRange(location: startIndex, length: lengh))
}
//2、处理时间
if let creatAt = status.created_at {
creatAtText = NSDate.createDateString(creatAt)
}
//3、处理认证 如果??前面的值为空,就取-1
let verifiedType = status.user?.verified_type ?? -1
switch verifiedType {
case 0://个人认证
verifiedImage = UIImage(named: "avatar_vip")
case 2,3,5://企业认证
verifiedImage = UIImage(named: "avatar_enterprise_vip")
case 220://微博达人
verifiedImage = UIImage(named: "avatar_grassroot")
default:
verifiedImage = nil
}
// 4、处理会员等级
let mbrank = status.user?.mbrank ?? 0
if mbrank > 0 && mbrank <= 6 {
vipImage = UIImage(named: "common_icon_membership_level\(mbrank)")
}
//5 、处理头像url
let profiledUrlStr = status.user?.profile_image_url ?? ""
profileURL = NSURL(string: profiledUrlStr)
//6、处理配图数据
//获取配图数组
let picURLDicts = status.pic_urls!.count != 0 ? status.pic_urls : status.retweeted_status?.pic_urls
if let picURLDicts = picURLDicts {
//遍历配图字典数组
for picURLDict in picURLDicts {
guard let picURLStr = picURLDict["thumbnail_pic"] else{
continue
}
print("picURLStr:\(picURLStr)")
picUrls.append(NSURL(string: picURLStr)!)
}
}
}
}
| apache-2.0 | 61c2e5de2793eba2713a088e15fdaad2 | 24.341667 | 122 | 0.534035 | 4.043883 | false | false | false | false |
jackyheart/MovingHelper | MovingHelper/ModelControllers/TaskLoader.swift | 1 | 1434 | //
// TaskLoader.swift
// MovingHelper
//
// Created by Ellen Shapiro on 6/7/15.
// Copyright (c) 2015 Razeware. All rights reserved.
//
import UIKit
/*
Struct to load tasks from JSON.
*/
public struct TaskLoader {
static func loadSavedTasksFromJSONFile(fileName: FileName) -> [Task]? {
let path = fileName.jsonFileName().pathInDocumentsDirectory()
if let data = NSData(contentsOfFile: path) {
return tasksFromData(data)
} else {
return nil
}
}
/**
- returns: The stock moving tasks included with the app.
*/
public static func loadStockTasks() -> [Task] {
if let path = NSBundle.mainBundle()
.pathForResource(FileName.StockTasks.rawValue, ofType: "json"),
data = NSData(contentsOfFile: path),
tasks = tasksFromData(data) {
return tasks
}
//Fall through case
NSLog("Tasks did not load!")
return [Task]()
}
private static func tasksFromData(data: NSData) -> [Task]? {
let error = NSErrorPointer()
do {
if let arrayOfTaskDictionaries = try NSJSONSerialization.JSONObjectWithData(data, options: []) as? [NSDictionary] {
return Task.tasksFromArrayOfJSONDictionaries(arrayOfTaskDictionaries)
} else {
NSLog("Error loading data: " + error.debugDescription)
return nil
}
} catch {
print("error: \(error)\n")
}
return nil
}
} | mit | 639f6d0942f3b556a3425d74173673d5 | 22.916667 | 123 | 0.627615 | 4.345455 | false | false | false | false |
victorchee/VCWaitingView | VCWaitingView/VCWaitingView/VCWaitingView.swift | 1 | 4588 | //
// VCWaitingView.swift
// VCWaitingView
//
// Created by qihaijun on 9/9/15.
// Copyright (c) 2015 VictorChee. All rights reserved.
//
import UIKit
class VCWaitingView: UIView {
var size = CGSize(width: 100.0, height: 100.0)
var backgroundInset = UIEdgeInsets(top: 10, left: 10, bottom: 10, right: 10)
private var transformScale: CGFloat = 0.000001
private var rotationTransform = CGAffineTransformIdentity
private var activityIndicatorView: UIActivityIndicatorView!
override init(frame: CGRect) {
super.init(frame: frame)
backgroundColor = UIColor.purpleColor()
alpha = 0 // Make it invisible for now
activityIndicatorView = UIActivityIndicatorView(activityIndicatorStyle: UIActivityIndicatorViewStyle.WhiteLarge)
activityIndicatorView.center = center
activityIndicatorView.color = UIColor.orangeColor()
activityIndicatorView.hidesWhenStopped = false
addSubview(activityIndicatorView)
registerNotifications()
}
deinit {
unregisterNotifications()
}
required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
convenience init(addToView: UIView) {
self.init(frame: addToView.bounds)
addToView.addSubview(self)
}
override func layoutSubviews() {
super.layoutSubviews()
// Entirely cover the parent view
if let parent = superview {
frame = CGRect(x: backgroundInset.left, y: backgroundInset.top, width: parent.bounds.width - backgroundInset.left - backgroundInset.right, height: parent.bounds.height - backgroundInset.top - backgroundInset.bottom)
}
activityIndicatorView.frame = CGRectMake((frame.width - activityIndicatorView.frame.width)/2.0, (frame.height - activityIndicatorView.frame.height)/2.0, activityIndicatorView.frame.width, activityIndicatorView.frame.height)
}
override func drawRect(rect: CGRect) {
if let context = UIGraphicsGetCurrentContext() {
UIGraphicsPushContext(context)
CGContextSetGrayFillColor(context, 0, 0.8)
// Draw rounded HUD background rect
let boxRect = CGRect(x: (bounds.width - size.width) / 2.0, y: (bounds.height - size.height) / 2.0, width: size.width, height: size.height)
let bezierPath = UIBezierPath(roundedRect: boxRect, cornerRadius: 7.0)
CGContextAddPath(context, bezierPath.CGPath)
CGContextFillPath(context)
UIGraphicsPopContext()
}
}
func show(animated: Bool) {
transform = CGAffineTransformConcat(rotationTransform, CGAffineTransformMakeScale(transformScale, transformScale))
if animated {
[UIView .animateWithDuration(0.25, delay: 0, options: UIViewAnimationOptions.CurveEaseInOut, animations: { () -> Void in
self.transform = self.rotationTransform
self.alpha = 1.0
}, completion: { (finished) -> Void in
})];
} else {
alpha = 1.0
}
activityIndicatorView.startAnimating()
}
func hide(animated: Bool) {
activityIndicatorView.stopAnimating()
if animated {
[UIView .animateWithDuration(0.25, delay: 0, options: UIViewAnimationOptions.CurveEaseInOut, animations: { () -> Void in
self.transform = CGAffineTransformConcat(self.rotationTransform, CGAffineTransformMakeScale(self.transformScale, self.transformScale))
self.alpha = 0.02
}, completion: { (finished) -> Void in
self.done()
})]
} else {
alpha = 0
done()
}
}
private func done() {
NSObject.cancelPreviousPerformRequestsWithTarget(self)
alpha = 0
}
// MARK - Notification
private func registerNotifications() {
NSNotificationCenter.defaultCenter().addObserver(self, selector: "statusBarOrientationDidChange:", name: UIApplicationDidChangeStatusBarOrientationNotification, object: nil)
}
private func unregisterNotifications() {
NSNotificationCenter.defaultCenter().removeObserver(self, name: UIApplicationDidChangeStatusBarOrientationNotification, object: nil)
}
func statusBarOrientationDidChange(notification: NSNotification) {
if let _ = self.superview {
setNeedsLayout()
setNeedsDisplay()
}
}
}
| mit | b70ea807a726ab47046c5fd9201108a2 | 36.300813 | 231 | 0.643854 | 5.304046 | false | false | false | false |
mohsinalimat/TKSubmitTransition | SubmitTransition/Classes/TransitionSubmitButton.swift | 9 | 3283 | //
// TransitionSubmitButton.swift
// SubmitTransition
//
// Created by Takuya Okamoto on 2015/08/06.
// Copyright (c) 2015年 Uniface. All rights reserved.
//
import Foundation
import UIKit
public class TKTransitionSubmitButton : UIButton, UIViewControllerTransitioningDelegate {
public var didEndFinishAnimation : (()->())? = nil
let pink = UIColor(red:0.992157, green: 0.215686, blue: 0.403922, alpha: 1)
let darkPink = UIColor(red:0.798012, green: 0.171076, blue: 0.321758, alpha: 1)
let springGoEase = CAMediaTimingFunction(controlPoints: 0.45,-0.36,0.44,0.92)
let shrinkCurve = CAMediaTimingFunction(name: kCAMediaTimingFunctionLinear)
let expandCurve = CAMediaTimingFunction(controlPoints: 0.95,0.02,1,0.05)
let shrinkDuration: CFTimeInterval = 0.1
let spiner: SpinerLayer!
public override init(frame: CGRect) {
self.spiner = SpinerLayer(frame: frame)
super.init(frame: frame)
self.backgroundColor = pink
self.layer.cornerRadius = self.frame.height / 2
self.clipsToBounds = true
self.layer.addSublayer(self.spiner)
}
override public var highlighted: Bool {
didSet {
if (highlighted) {
self.backgroundColor = darkPink
}
else {
self.backgroundColor = pink
}
}
}
public required init(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
public func startLoadingAnimation() {
self.titleLabel?.hidden = true
self.shrink()
NSTimer.schedule(delay: shrinkDuration - 0.25) { timer in
self.spiner.animation()
}
}
public func startFinishAnimation(completion:(()->())?) {
self.didEndFinishAnimation = completion
self.expand()
self.spiner.stopAnimation()
}
public override func animationDidStop(anim: CAAnimation!, finished flag: Bool) {
let a = anim as! CABasicAnimation
if a.keyPath == "transform.scale" {
didEndFinishAnimation?()
NSTimer.schedule(delay: 1) { timer in
self.returnToOriginalState()
}
}
}
func returnToOriginalState() {
self.layer.removeAllAnimations()
self.titleLabel?.hidden = false
}
func shrink() {
let shrinkAnim = CABasicAnimation(keyPath: "bounds.size.width")
shrinkAnim.fromValue = frame.width
shrinkAnim.toValue = frame.height
shrinkAnim.duration = shrinkDuration
shrinkAnim.timingFunction = shrinkCurve
shrinkAnim.fillMode = kCAFillModeForwards
shrinkAnim.removedOnCompletion = false
layer.addAnimation(shrinkAnim, forKey: shrinkAnim.keyPath)
}
func expand() {
let expandAnim = CABasicAnimation(keyPath: "transform.scale")
expandAnim.fromValue = 1.0
expandAnim.toValue = 26.0
expandAnim.timingFunction = expandCurve
expandAnim.duration = 0.3
expandAnim.delegate = self
expandAnim.fillMode = kCAFillModeForwards
expandAnim.removedOnCompletion = false
layer.addAnimation(expandAnim, forKey: expandAnim.keyPath)
}
}
| mit | 174c2511fa1cab284a9c8ef1b4e2e749 | 31.166667 | 89 | 0.635172 | 4.576011 | false | false | false | false |
samantharachelb/todolist | Pods/OctoKit.swift/OctoKit/Milestone.swift | 1 | 1635 | import Foundation
@objc open class Milestone: NSObject {
open var url: URL?
open var htmlURL: URL?
open var labelsURL: URL?
open var id: Int
open var number: Int?
open var state: Openness?
open var title: String?
open var milestoneDescription: String?
open var creator: User?
open var openIssues: Int?
open var closedIssues: Int?
open var createdAt: Date?
open var updatedAt: Date?
open var closedAt: Date?
open var dueOn: Date?
public init?(_ json: [String: AnyObject]) {
if let id = json["id"] as? Int {
if let urlString = json["html_url"] as? String, let url = URL(string: urlString) {
htmlURL = url
}
if let urlString = json["labels_url"] as? String, let url = URL(string: urlString) {
labelsURL = url
}
self.id = id
number = json["number"] as? Int
state = Openness(rawValue: json["state"] as? String ?? "")
title = json["title"] as? String
milestoneDescription = json["description"] as? String
creator = User(json["creator"] as? [String: AnyObject] ?? [:])
openIssues = json["open_issues"] as? Int
closedIssues = json["closed_issues"] as? Int
createdAt = Time.rfc3339Date(json["created_at"] as? String)
updatedAt = Time.rfc3339Date(json["updated_at"] as? String)
closedAt = Time.rfc3339Date(json["closed_at"] as? String)
dueOn = Time.rfc3339Date(json["due_on"] as? String)
} else {
id = -1
}
}
}
| mit | 39af9715d3f922c429301a4b1d887253 | 36.159091 | 96 | 0.565138 | 4.057072 | false | false | false | false |
huangboju/Moots | UICollectionViewLayout/SectionReactor-master/Sources/SectionReactor/Empty.swift | 1 | 1229 | #if os(iOS) || os(tvOS)
import UIKit
public extension UICollectionView {
func emptyCell(for indexPath: IndexPath) -> UICollectionViewCell {
let identifier = "SectionReactor.UICollectionView.emptyCell"
self.register(UICollectionViewCell.self, forCellWithReuseIdentifier: identifier)
let cell = self.dequeueReusableCell(withReuseIdentifier: identifier, for: indexPath)
cell.isHidden = true
return cell
}
func emptyView(for indexPath: IndexPath, kind: String) -> UICollectionReusableView {
let identifier = "SectionReactor.UICollectionView.emptyView"
self.register(UICollectionReusableView.self, forSupplementaryViewOfKind: kind, withReuseIdentifier: identifier)
let view = self.dequeueReusableSupplementaryView(ofKind: kind, withReuseIdentifier: identifier, for: indexPath)
view.isHidden = true
return view
}
}
public extension UITableView {
func emptyCell(for indexPath: IndexPath) -> UITableViewCell {
let identifier = "SectionReactor.UITableView.emptyCell"
self.register(UITableViewCell.self, forCellReuseIdentifier: identifier)
let cell = self.dequeueReusableCell(withIdentifier: identifier, for: indexPath)
cell.isHidden = true
return cell
}
}
#endif
| mit | 2b5d01adf8943e7e0b9ba93a38410d1f | 38.645161 | 115 | 0.7738 | 5.142259 | false | false | false | false |
kkolli/MathGame | client/GameViewController.swift | 1 | 10660 | //
// GameViewController.swift
// Speedy
//
// Created by Tyler Levine on 1/27/15.
// Copyright (c) 2015 Krishna Kolli. All rights reserved.
//
// NS Timer Reference- http://ios-blog.co.uk/tutorials/swift-nstimer-tutorial-lets-create-a-counter-application/
import SpriteKit
import UIKit
import Alamofire
import Crashlytics
class GameViewController : UIViewController, SKPhysicsContactDelegate {
// @IBOutlet weak var GameTimerLabel: UILabel!
// @IBOutlet weak var GameScoreLabel: UILabel!
// @IBOutlet weak var GameTargetNumLabel: UILabel!
var user : FBGraphUser!
var appDelegate:AppDelegate!
var timer: Timer!
var game_start_time = 60 // TODO - modify this somehow later
//var score = 0
//var targetNumber: Int?
let TIME_DEBUG = false
var scene: GameScene?
var boardController: BoardController?
var numTargetNumbersMatched:Int!
override func viewDidLoad() {
super.viewDidLoad();
// UNCOMMENT THIS TO TEST CRASHLYTICS
// Crashlytics.sharedInstance().crash()
appDelegate = UIApplication.sharedApplication().delegate as AppDelegate
self.navigationController?.navigationBarHidden = true
self.tabBarController?.tabBar.hidden = true
self.user = appDelegate.user
println("In Game View controller")
// start the counter to go!
timer = Timer(duration: game_start_time, {(elapsedTime: Int) -> () in
if self.timer.getTime() <= 0 {
//self.GameTimerLabel.text = "done"
//self.postScore(self.GameScoreLabel.text!)
self.performSegueToSummary()
} else {
if self.TIME_DEBUG {
println("time printout: " + String(self.timer.getTime()))
}
if self.boardController != nil {
self.boardController?.setTimeInHeader(self.timer.getTime())
}
//self.GameTimerLabel.text = self.timer.convertIntToTime(self.timer.getTime())
}
})
numTargetNumbersMatched = 0
//GameTimerLabel.text = timer.convertIntToTime(self.timer.getTime())
//GameScoreLabel.text = String(score)
scene = GameScene(size: view.frame.size)
boardController = BoardController(scene: scene!, mode: .SINGLE)
boardController!.notifyScoreChanged = updateScoreAndTime
//scene!.boardController = boardController
//updateTargetNumber()
// Configure the view.
let skView = self.view as SKView
//skView.showsFPS = true
//skView.showsNodeCount = true
//skView.showsPhysics = true
/* Sprite Kit applies additional optimizations to improve rendering performance */
skView.ignoresSiblingOrder = false
/* Set the scale mode to scale to fit the window */
scene!.scaleMode = .AspectFill
//scene!.scoreHandler = handleMerge
scene!.physicsWorld.contactDelegate = self
skView.presentScene(scene)
timer.start()
let tracker = GAI.sharedInstance().defaultTracker
tracker.set(kGAIScreenName, value: "singleplayer")
tracker.send(GAIDictionaryBuilder.createScreenView().build())
}
func updateScoreAndTime(){
if numTargetNumbersMatched > 0 {
timer.addTime(timer.getExtraTimeSub())
} else {
timer.addTime(timer.getExtraTimeFirst())
}
numTargetNumbersMatched!++
}
func postScore(score:String){
var uri = "http://mathisspeedy.herokuapp.com/HighScores/" + user.objectID
let parameters = [
"score": score
]
Alamofire.request(.POST, uri, parameters: parameters, encoding: .JSON)
}
/*
func updateTargetNumber(){
if targetNumber != nil{
let numberCircleList = boardController!.circleList.filter{$0 is NumberCircle}
let numberList = numberCircleList.map{($0 as NumberCircle).number!}
targetNumber = boardController!.randomNumbers.generateTarget(numberList)
}else{
targetNumber = boardController!.randomNumbers.generateTarget()
}
GameTargetNumLabel.text = String(targetNumber!)
}
*/
/*
this takes something like 0 and turns it into 00:00
and if it takes something like 60 -> 01:00
if it takes 170 -> 02:10
func convertIntToTime(secondsPassed: Int) -> String {
var count = game_max_time - secondsPassed
let seconds_per_minute = 60
var minutes = count / seconds_per_minute
var seconds = count % seconds_per_minute
var minute_display = "", second_display = ""
if (minutes >= 10) {
minute_display = String(minutes)
} else {
minute_display = "0" + String(minutes)
}
if (seconds >= 10) {
second_display = String(seconds)
} else {
second_display = "0" + String(seconds)
}
if (TIME_DEBUG) {
println("seconds: \(seconds)" + " second display : " + second_display)
println("displaying: " + minute_display + ":" + second_display)
}
return minute_display + ":" + second_display
}
*/
func didBeginContact(contact: SKPhysicsContact) {
var numberBody: SKPhysicsBody
var opBody: SKPhysicsBody
//A neccessary check to prevent contacts from throwing runtime errors
if !(contact.bodyA.node != nil && contact.bodyB.node != nil && contact.bodyA.node!.parent != nil && contact.bodyB.node!.parent != nil) {
return
}
if contact.bodyA.node! is NumberCircle{
numberBody = contact.bodyA
if contact.bodyB.node! is OperatorCircle{
opBody = contact.bodyB
let numberNode = numberBody.node! as NumberCircle
let opNode = opBody.node! as OperatorCircle
if !numberNode.hasNeighbor() && !opNode.hasNeighbor() {
if scene!.releaseNumber != nil && scene!.releaseOperator != nil{
println("NO")
return
}
numberNode.setNeighbor(opNode)
opNode.setNeighbor(numberNode)
let joint = scene!.createBestJoint(contact.contactPoint, nodeA: numberNode, nodeB: opNode)
scene!.physicsWorld.addJoint(joint)
scene!.currentJoint = joint
scene!.joinedNodeA = numberNode
scene!.joinedNodeB = opNode
}else{
if let leftNumberCircle = opNode.neighbor as? NumberCircle {
let opCircle = opNode
boardController!.handleMerge(leftNumberCircle, rightNumberCircle: numberNode, opCircle: opCircle)
}
}
}else{
return
}
}else if contact.bodyA.node! is OperatorCircle{
opBody = contact.bodyA
if contact.bodyB.node! is NumberCircle{
numberBody = contact.bodyB
let numberNode = numberBody.node! as NumberCircle
let opNode = opBody.node! as OperatorCircle
// all nodes touching together have no neighbors (1st contact)
if numberNode.hasNeighbor() == false && opNode.hasNeighbor() == false{
if scene!.releaseNumber != nil && scene!.releaseOperator != nil{
return
}
numberNode.setNeighbor(opNode)
opNode.setNeighbor(numberNode)
let joint = scene!.createBestJoint(contact.contactPoint, nodeA: numberNode, nodeB: opNode)
scene!.physicsWorld.addJoint(joint)
scene!.currentJoint = joint
scene!.joinedNodeA = numberNode
scene!.joinedNodeB = opNode
}else{
// if hitting all 3
if let leftNumberCircle = opNode.neighbor as? NumberCircle {
let opCircle = opNode
boardController!.handleMerge(leftNumberCircle, rightNumberCircle: numberNode, opCircle: opCircle)
}
}
}else{
return
}
}
}
//TODO: Refactor mergeNodes and handleMerge together
/*
func mergeNodes(leftNumberCircle: NumberCircle, rightNumberCircle: NumberCircle, opCircle: OperatorCircle){
let (result, removeNode) = boardController!.handleMerge(leftNumberCircle, rightNumberCircle: rightNumberCircle, opCircle: opCircle)
/*
let op1Upgrade = leftNumberCircle.upgrade
let op2Upgrade = rightNumberCircle.upgrade
*/
if removeNode {
//rightNumberCircle.removeFromParent()
//boardController!.nodeRemoved(rightNumberCircle.boardPos!)
} else {
rightNumberCircle.setNumber(result)
rightNumberCircle.neighbor = nil
}
leftNumberCircle.removeFromParent()
opCircle.removeFromParent()
}
*/
func didEndContact(contact: SKPhysicsContact) {}
//func didEndContact(contact: SKPhysicsContact) {}
func performSegueToSummary() {
self.performSegueWithIdentifier("segueToSummary", sender: nil)
}
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject!) {
println("preparing for segue!!")
if segue.identifier == "segueToSummary" {
println("performing segue to summary")
let vc = segue.destinationViewController as SummaryViewController
vc.operatorsUsed = boardController!.operatorsUsed
vc.score = boardController!.score
vc.numTargetNumbersMatched = numTargetNumbersMatched
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning();
}
override func shouldAutorotate() -> Bool {
return false
}
override func supportedInterfaceOrientations() -> Int {
return Int(UIInterfaceOrientationMask.Portrait.rawValue)
}
}
| gpl-2.0 | d9c3c479a9866dcf98d83f5ec89bb0cc | 35.632302 | 144 | 0.575797 | 5.10292 | false | false | false | false |
lfaoro/Cast | Cast/GistClient.swift | 1 | 4149 | //
// Created by Leonardo on 18/07/2015.
// Copyright © 2015 Leonardo Faoro. All rights reserved.
//
import Cocoa
import SwiftyJSON
import RxSwift
import RxCocoa
public enum ConnectionError: ErrorType {
case InvalidData(String), NoResponse(String), NotAuthenticated(String), StatusCode(Int)
}
/**
GistClient: is a wrapper around the gist.github.com service API.
An instance of GistClient allows you login via OAuth with your GitHub account or remain anonymous.
You may create new gists as anonymous
but you may modify a gist only if you're logged into the service.
*/
public class GistClient {
//MARK:- Properties
private static let defaultURL = NSURL(string: "https://api.github.com/gists")!
private let gistAPIURL: NSURL
private var gistID: String? {
get {
let userDefaults = NSUserDefaults.standardUserDefaults()
if OAuthClient.getToken() != nil {
return userDefaults.stringForKey("gistID")
} else {
userDefaults.removeObjectForKey("gistID")
return nil
}
}
set {
if OAuthClient.getToken() != nil {
let userDefaults = NSUserDefaults.standardUserDefaults()
userDefaults.setObject(newValue, forKey: "gistID")
}
}
}
//MARK:- Initialisation
public init(baseURL: NSURL = defaultURL) {
self.gistAPIURL = baseURL
}
/**
Used for Unit Tests purposes only, this API doesn't support any other service
except `gist.github.com`
*/
public convenience init?(baseURLString: String) {
if let baseURL = NSURL(string: baseURLString) {
self.init(baseURL: baseURL)
} else {
print(__FUNCTION__)
print("URL not conforming to RFCs 1808, 1738, and 2732 formats")
return nil
}
}
//MARK:- Public API
/**
- returns: `true` if a Gist has already been created otherwise `false`
*/
public var gistCreated: Bool {
let userDefaults = NSUserDefaults.standardUserDefaults()
guard let _ = userDefaults.stringForKey("gistID") else { return false }
return true
}
/**
setGist: creates or updates a gist on the *gist.github.com* service
based on the value of `gistID`.
- returns: an `Observable` object containing the URL link of the created gist -
for more information checkout
[RxSwift](https://github.com/ReactiveX/RxSwift)
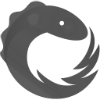
*/
public func setGist(content content: String, updateGist: Bool = false,
isPublic: Bool = false, fileName: String = "Casted.swift") // defaults
-> Observable<NSURL> {
return create { stream in
let githubHTTPBody = [
"description": "Generated with Cast (cast.lfaoro.com)",
"public": isPublic,
"files": [fileName: ["content": content]],
]
var request: NSMutableURLRequest
if let gistID = self.gistID where updateGist {
let updateURL = self.gistAPIURL.URLByAppendingPathComponent(gistID)
request = NSMutableURLRequest(URL: updateURL)
request.HTTPMethod = "PATCH"
} else {
request = NSMutableURLRequest(URL: self.gistAPIURL)
request.HTTPMethod = "POST"
}
request.HTTPBody = try! JSON(githubHTTPBody).rawData()
request.addValue("application/vnd.github.v3+json", forHTTPHeaderField: "Accept")
if let token = OAuthClient.getToken() {
request.addValue("token \(token)", forHTTPHeaderField: "Authorization")
}
let session = NSURLSession.sharedSession()
let task = session.dataTaskWithRequest(request) { (data, response, error) in
if let data = data, response = response as? NSHTTPURLResponse {
if !((200..<300) ~= response.statusCode) {
sendError(stream, ConnectionError.StatusCode(response.statusCode))
print(response)
}
let jsonData = JSON(data: data)
if let gistURL = jsonData["html_url"].URL, gistID = jsonData["id"].string {
self.gistID = gistID
sendNext(stream, gistURL)
sendCompleted(stream)
} else {
sendError(stream, ConnectionError.InvalidData(
"Unable to read data received from \(self.gistAPIURL)"))
}
} else {
sendError(stream, ConnectionError.NoResponse(error!.localizedDescription))
}
}
task.resume()
return NopDisposable.instance
}
}
}
| mit | abafbc32434e49d937cd60cab0521fd8 | 26.111111 | 98 | 0.694069 | 3.830102 | false | false | false | false |
tardieu/swift | benchmark/utils/TestsUtils.swift | 5 | 1812 | //===--- TestsUtils.swift -------------------------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2017 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
import Darwin
// Linear function shift register.
//
// This is just to drive benchmarks. I don't make any claim about its
// strength. According to Wikipedia, it has the maximal period for a
// 32-bit register.
struct LFSR {
// Set the register to some seed that I pulled out of a hat.
var lfsr : UInt32 = 0xb78978e7
mutating func shift() {
lfsr = (lfsr >> 1) ^ (UInt32(bitPattern: -Int32((lfsr & 1))) & 0xD0000001)
}
mutating func randInt() -> Int64 {
var result : UInt32 = 0
for _ in 0..<32 {
result = (result << 1) | (lfsr & 1)
shift()
}
return Int64(bitPattern: UInt64(result))
}
}
var lfsrRandomGenerator = LFSR()
// Start the generator from the beginning
public func SRand() {
lfsrRandomGenerator = LFSR()
}
public func Random() -> Int64 {
return lfsrRandomGenerator.randInt()
}
public func CheckResults(_ res: Bool, _ message: String = "") {
if res {
return
}
print(message)
abort()
}
public func False() -> Bool { return false }
/// This is a dummy protocol to test the speed of our protocol dispatch.
public protocol SomeProtocol { func getValue() -> Int }
struct MyStruct : SomeProtocol {
init() {}
func getValue() -> Int { return 1 }
}
public func someProtocolFactory() -> SomeProtocol { return MyStruct() }
| apache-2.0 | 81dbeed1e03f2602d51b653bc64b49c6 | 26.876923 | 80 | 0.625828 | 4.053691 | false | false | false | false |
HabitRPG/habitrpg-ios | Habitica API Client/Habitica API Client/Models/Group/APIGroupInvitation.swift | 1 | 1122 | //
// APIGroupInvitation.swift
// Habitica API Client
//
// Created by Phillip Thelen on 22.06.18.
// Copyright © 2018 HabitRPG Inc. All rights reserved.
//
import Foundation
import Habitica_Models
struct APIGroupInvitationHelper: Decodable {
var guilds: [APIGroupInvitation]?
var parties: [APIGroupInvitation]?
}
class APIGroupInvitation: GroupInvitationProtocol, Decodable {
var id: String?
var name: String?
var inviterID: String?
var isPartyInvitation: Bool = false
var isPublicGuild: Bool = false
enum CodingKeys: String, CodingKey {
case id
case name
case inviterID = "inviter"
case isPublicGuild = "publicGuild"
}
public required init(from decoder: Decoder) throws {
let values = try decoder.container(keyedBy: CodingKeys.self)
id = try? values.decode(String.self, forKey: .id)
name = try? values.decode(String.self, forKey: .name)
inviterID = try? values.decode(String.self, forKey: .inviterID)
isPublicGuild = (try? values.decode(Bool.self, forKey: .isPublicGuild)) ?? false
}
}
| gpl-3.0 | dd332a8cd6a4e844f924c754eaea8f6d | 28.5 | 88 | 0.67529 | 3.961131 | false | false | false | false |
milseman/swift | test/SILGen/global_resilience.swift | 8 | 3483 | // RUN: %empty-directory(%t)
// RUN: %target-swift-frontend -emit-module -enable-resilience -emit-module-path=%t/resilient_global.swiftmodule -module-name=resilient_global %S/../Inputs/resilient_global.swift
// RUN: %target-swift-frontend -I %t -emit-silgen -enable-resilience -parse-as-library %s | %FileCheck %s
// RUN: %target-swift-frontend -I %t -emit-sil -O -enable-resilience -parse-as-library %s | %FileCheck --check-prefix=CHECK-OPT %s
import resilient_global
public struct MyEmptyStruct {}
// CHECK-LABEL: sil_global @_T017global_resilience13myEmptyGlobalAA02MyD6StructVv : $MyEmptyStruct
public var myEmptyGlobal = MyEmptyStruct()
// CHECK-LABEL: sil_global @_T017global_resilience19myFixedLayoutGlobalAA13MyEmptyStructVv : $MyEmptyStruct
@_fixed_layout public var myFixedLayoutGlobal = MyEmptyStruct()
// Mutable addressor for resilient global (should not be public?)
// CHECK-LABEL: sil [global_init] @_T017global_resilience13myEmptyGlobalAA02MyD6StructVfau : $@convention(thin) () -> Builtin.RawPointer
// CHECK: global_addr @_T017global_resilience13myEmptyGlobalAA02MyD6StructVv
// CHECK: return
// Synthesized getter and setter for our resilient global variable
// CHECK-LABEL: sil @_T017global_resilience13myEmptyGlobalAA02MyD6StructVfg
// CHECK: function_ref @_T017global_resilience13myEmptyGlobalAA02MyD6StructVfau
// CHECK: return
// CHECK-LABEL: sil @_T017global_resilience13myEmptyGlobalAA02MyD6StructVfs
// CHECK: function_ref @_T017global_resilience13myEmptyGlobalAA02MyD6StructVfau
// CHECK: return
// Mutable addressor for fixed-layout global
// CHECK-LABEL: sil [global_init] @_T017global_resilience19myFixedLayoutGlobalAA13MyEmptyStructVfau
// CHECK: global_addr @_T017global_resilience19myFixedLayoutGlobalAA13MyEmptyStructVv
// CHECK: return
// CHECK-OPT-LABEL: sil private @globalinit_{{.*}}_func0
// CHECK-OPT: alloc_global @_T017global_resilience19myFixedLayoutGlobalAA13MyEmptyStructVv
// CHECK-OPT: return
// CHECK-OPT-LABEL: sil [global_init] @_T017global_resilience19myFixedLayoutGlobalAA13MyEmptyStructVfau
// CHECK-OPT: global_addr @_T017global_resilience19myFixedLayoutGlobalAA13MyEmptyStructVv
// CHECK-OPT: function_ref @globalinit_{{.*}}_func0
// CHECK-OPT: return
// Accessing resilient global from our resilience domain --
// call the addressor directly
// CHECK-LABEL: sil @_T017global_resilience16getMyEmptyGlobalAA0dE6StructVyF
// CHECK: function_ref @_T017global_resilience13myEmptyGlobalAA02MyD6StructVfau
// CHECK: return
public func getMyEmptyGlobal() -> MyEmptyStruct {
return myEmptyGlobal
}
// Accessing resilient global from a different resilience domain --
// access it with accessors
// CHECK-LABEL: sil @_T017global_resilience14getEmptyGlobal010resilient_A00D15ResilientStructVyF
// CHECK: function_ref @_T016resilient_global11emptyGlobalAA20EmptyResilientStructVfg
// CHECK: return
public func getEmptyGlobal() -> EmptyResilientStruct {
return emptyGlobal
}
// Accessing fixed-layout global from a different resilience domain --
// call the addressor directly
// CHECK-LABEL: sil @_T017global_resilience20getFixedLayoutGlobal010resilient_A020EmptyResilientStructVyF
// CHECK: function_ref @_T016resilient_global17fixedLayoutGlobalAA20EmptyResilientStructVfau
// CHECK: return
public func getFixedLayoutGlobal() -> EmptyResilientStruct {
return fixedLayoutGlobal
}
| apache-2.0 | fc26af36a410dec682c6ae91b4c58ea9 | 44.233766 | 178 | 0.771748 | 4.201448 | false | false | false | false |
blockchain/My-Wallet-V3-iOS | Modules/FeatureCardIssuing/Sources/FeatureCardIssuingData/Repositories/CardRepository.swift | 1 | 5613 | // Copyright © Blockchain Luxembourg S.A. All rights reserved.
import Combine
import Errors
import FeatureCardIssuingDomain
import Foundation
import NetworkKit
import ToolKit
final class CardRepository: CardRepositoryAPI {
private static let marqetaPath = "/marqeta-card/#/"
private struct AccountKey: Hashable {
let id: String
}
private let client: CardClientAPI
private let userInfoProvider: UserInfoProviderAPI
private let baseCardHelperUrl: String
private let cachedCardValue: CachedValueNew<
String,
[Card],
NabuNetworkError
>
private let cachedAccountValue: CachedValueNew<
AccountKey,
AccountCurrency,
NabuNetworkError
>
private let accountCache: AnyCache<AccountKey, AccountCurrency>
private let cardCache: AnyCache<String, [Card]>
init(
client: CardClientAPI,
userInfoProvider: UserInfoProviderAPI,
baseCardHelperUrl: String
) {
self.client = client
self.userInfoProvider = userInfoProvider
self.baseCardHelperUrl = baseCardHelperUrl
let cardCache: AnyCache<String, [Card]> = InMemoryCache(
configuration: .onLoginLogout(),
refreshControl: PerpetualCacheRefreshControl()
).eraseToAnyCache()
cachedCardValue = CachedValueNew(
cache: cardCache,
fetch: { _ in
client.fetchCards()
}
)
self.cardCache = cardCache
let accountCache: AnyCache<AccountKey, AccountCurrency> = InMemoryCache(
configuration: .onLoginLogout(),
refreshControl: PerpetualCacheRefreshControl()
).eraseToAnyCache()
cachedAccountValue = CachedValueNew(
cache: accountCache,
fetch: { accountKey in
client.fetchLinkedAccount(with: accountKey.id)
}
)
self.accountCache = accountCache
}
func orderCard(
product: Product,
at address: Card.Address,
with ssn: String
) -> AnyPublisher<Card, NabuNetworkError> {
client
.orderCard(
with: .init(productCode: product.productCode, deliveryAddress: address, ssn: ssn)
)
.handleEvents(receiveOutput: { [weak self] _ in
self?.cachedCardValue.invalidateCache()
})
.eraseToAnyPublisher()
}
func fetchCards() -> AnyPublisher<[Card], NabuNetworkError> {
cachedCardValue.get(key: #file)
}
func fetchCard(with id: String) -> AnyPublisher<Card, NabuNetworkError> {
client.fetchCard(with: id)
}
func delete(card: Card) -> AnyPublisher<Card, NabuNetworkError> {
client
.deleteCard(with: card.id)
.handleEvents(receiveOutput: { [weak self] _ in
self?.cachedCardValue.invalidateCache()
}, receiveCompletion: { [weak self] _ in
self?.cachedCardValue.invalidateCache()
})
.eraseToAnyPublisher()
}
func helperUrl(for card: Card) -> AnyPublisher<URL, NabuNetworkError> {
let baseCardHelperUrl = baseCardHelperUrl
return client
.generateSensitiveDetailsToken(with: card.id)
.combineLatest(userInfoProvider.fullName)
.map { token, fullName in
Self.buildCardHelperUrl(
with: baseCardHelperUrl,
token: token,
for: card,
with: fullName
)
}
.eraseToAnyPublisher()
}
func generatePinToken(for card: Card) -> AnyPublisher<String, NabuNetworkError> {
client.generatePinToken(with: card.id)
}
func fetchLinkedAccount(for card: Card) -> AnyPublisher<AccountCurrency, NabuNetworkError> {
cachedAccountValue.get(key: AccountKey(id: card.id))
}
func update(account: AccountBalance, for card: Card) -> AnyPublisher<AccountCurrency, NabuNetworkError> {
client
.updateAccount(
with: AccountCurrency(accountCurrency: account.balance.symbol),
for: card.id
)
.flatMap { [accountCache] accountCurrency in
accountCache
.set(accountCurrency, for: AccountKey(id: card.id))
.replaceOutput(with: accountCurrency)
}
.eraseToAnyPublisher()
}
func eligibleAccounts(for card: Card) -> AnyPublisher<[AccountBalance], NabuNetworkError> {
client.eligibleAccounts(for: card.id)
}
func lock(card: Card) -> AnyPublisher<Card, NabuNetworkError> {
client
.lock(cardId: card.id)
.handleEvents(receiveOutput: { [weak self] _ in
self?.cachedCardValue.invalidateCache()
})
.eraseToAnyPublisher()
}
func unlock(card: Card) -> AnyPublisher<Card, NabuNetworkError> {
client
.unlock(cardId: card.id)
.handleEvents(receiveOutput: { [weak self] _ in
self?.cachedCardValue.invalidateCache()
})
.eraseToAnyPublisher()
}
private static func buildCardHelperUrl(
with base: String,
token: String,
for card: Card,
with name: String
) -> URL {
let nameParam = name.addingPercentEncoding(withAllowedCharacters: .urlPathAllowed) ?? "-"
return URL(
string: "\(base)\(Self.marqetaPath)\(token)/\(card.last4)/\(nameParam)"
)!
}
}
| lgpl-3.0 | da61277f5fa32b93412540868ace832b | 30.005525 | 109 | 0.599252 | 4.905594 | false | false | false | false |
borglab/SwiftFusion | Sources/SwiftFusion/Optimizers/CGLS.swift | 1 | 2583 | // Copyright 2019 The SwiftFusion Authors. All Rights Reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
import _Differentiation
import PenguinStructures
/// Conjugate Gradient Least Squares (CGLS) optimizer.
///
/// An optimizer that implements CGLS second order optimizer
public struct GenericCGLS {
/// The set of steps taken.
public var step: Int = 0
public var precision: Double = 1e-10
public var max_iteration: Int = 400
/// Constructor
public init(precision p: Double = 1e-10, max_iteration maxiter: Int = 400) {
precision = p
max_iteration = maxiter
}
/// Optimize the Gaussian Factor Graph with a initial estimate
/// Reference: Bjorck96book_numerical-methods-for-least-squares-problems
/// Page 289, Algorithm 7.4.1
public mutating func optimize(gfg: GaussianFactorGraph, initial x: inout VariableAssignments) {
startTimer("cgls")
defer { stopTimer("cgls") }
step += 1
var r = (-1) * gfg.errorVectors(at: x) // r(0) = b - A * x(0), the residual
var p = gfg.errorVectors_linearComponent_adjoint(r) // p(0) = s(0) = A^T * r(0), residual in value space
var s = p // residual of normal equations
var gamma = s.squaredNorm // γ(0) = ||s(0)||^2
while step < max_iteration && gamma > precision {
incrementCounter("cgls step")
// print("[CGLS ] residual = \(r.squaredNorm), true = \(gfg.errorVectors(at: x).squaredNorm)")
let q = gfg.errorVectors_linearComponent(at: p) // q(k) = A * p(k)
let alpha: Double = gamma / q.squaredNorm // α(k) = γ(k)/||q(k)||^2
x = x + (alpha * p) // x(k+1) = x(k) + α(k) * p(k)
r = r + (-alpha) * q // r(k+1) = r(k) - α(k) * q(k)
s = gfg.errorVectors_linearComponent_adjoint(r) // s(k+1) = A.T * r(k+1)
let gamma_next = s.squaredNorm // γ(k+1) = ||s(k+1)||^2
let beta: Double = gamma_next/gamma // β(k) = γ(k+1)/γ(k)
gamma = gamma_next
p = s + beta * p // p(k+1) = s(k+1) + β(k) * p(k)
if (alpha * p).squaredNorm < precision {
break
}
step += 1
}
}
}
| apache-2.0 | 4592fccd324d0050b2ad069e84e7880a | 37.402985 | 108 | 0.636222 | 3.273537 | false | false | false | false |
Great-Li-Xin/Xcode | MORE/hangge_756/hangge_756/ysocket/MyTcpSocketServer.swift | 1 | 4918 | //
// MyTcpSocketServer.swift
// hangge_756
//
// Created by hangge on 2016/11/21.
// Copyright © 2016年 hangge. All rights reserved.
//
import UIKit
//服务器端口
var serverport = 8080
//客户端管理类(便于服务端管理所有连接的客户端)
class ChatUser:NSObject{
var tcpClient:TCPClient?
var username:String=""
var socketServer:MyTcpSocketServer?
//解析收到的消息
func readMsg()->[String:Any]?{
//read 4 byte int as type
if let data=self.tcpClient!.read(4){
if data.count==4{
let ndata=NSData(bytes: data, length: data.count)
var len:Int32=0
ndata.getBytes(&len, length: data.count)
if let buff=self.tcpClient!.read(Int(len)){
let msgd = Data(bytes: buff, count: buff.count)
let msgi = (try! JSONSerialization.jsonObject(with: msgd,
options: .mutableContainers)) as! [String:Any]
return msgi
}
}
}
return nil
}
//循环接收消息
func messageloop(){
while true{
if let msg=self.readMsg(){
self.processMsg(msg: msg)
}else{
self.removeme()
break
}
}
}
//处理收到的消息
func processMsg(msg:[String:Any]){
if msg["cmd"] as! String=="nickname"{
self.username=msg["nickname"] as! String
}
self.socketServer!.processUserMsg(user: self, msg: msg)
}
//发送消息
func sendMsg(msg:[String:Any]){
//消息数据包
let jsondata=try? JSONSerialization.data(withJSONObject: msg, options:
JSONSerialization.WritingOptions.prettyPrinted)
//消息数据包大小
var len:Int32=Int32(jsondata!.count)
//消息包头数据
var data = Data(bytes: &len, count: 4)
//包头后面添加正真的消息数据包
data.append(jsondata!)
//发送合并后的数据包
_ = self.tcpClient!.send(data: data)
}
//移除该客户端
func removeme(){
self.socketServer!.removeUser(u: self)
}
//关闭连接
func kill(){
_ = self.tcpClient!.close()
}
}
//服务端类
class MyTcpSocketServer: NSObject {
var clients:[ChatUser]=[]
var server:TCPServer=TCPServer(addr: "127.0.0.1", port: serverport)
var serverRuning:Bool=false
//启动服务
func start() {
_ = server.listen()
self.serverRuning=true
DispatchQueue.global(qos: .background).async {
while self.serverRuning{
let client=self.server.accept()
if let c=client{
DispatchQueue.global(qos: .background).async {
self.handleClient(c: c)
}
}
}
}
self.log(msg: "server started...")
}
//停止服务
func stop() {
self.serverRuning=false
_ = self.server.close()
//forth close all client socket
for c:ChatUser in self.clients{
c.kill()
}
self.log(msg: "server stoped...")
}
//处理连接的客户端
func handleClient(c:TCPClient){
self.log(msg: "new client from:"+c.addr)
let u=ChatUser()
u.tcpClient=c
clients.append(u)
u.socketServer=self
u.messageloop()
}
//处理各消息命令
func processUserMsg(user:ChatUser, msg:[String:Any]){
self.log(msg: "\(user.username)[\(user.tcpClient!.addr)]cmd:"+(msg["cmd"] as! String))
//boardcast message
var msgtosend=[String:String]()
let cmd = msg["cmd"] as! String
if cmd=="nickname"{
msgtosend["cmd"]="join"
msgtosend["nickname"]=user.username
msgtosend["addr"]=user.tcpClient!.addr
}else if(cmd=="msg"){
msgtosend["cmd"]="msg"
msgtosend["from"]=user.username
msgtosend["content"]=(msg["content"] as! String)
}else if(cmd=="leave"){
msgtosend["cmd"]="leave"
msgtosend["nickname"]=user.username
msgtosend["addr"]=user.tcpClient!.addr
}
for user:ChatUser in self.clients{
//if u~=user{
user.sendMsg(msg: msgtosend)
//}
}
}
//移除用户
func removeUser(u:ChatUser){
self.log(msg: "remove user\(u.tcpClient!.addr)")
if let possibleIndex=self.clients.index(of: u){
self.clients.remove(at: possibleIndex)
self.processUserMsg(user: u, msg: ["cmd":"leave"])
}
}
//日志打印
func log(msg:String){
print(msg)
}
}
| mit | b5e4fd9d505ca85c857d863dcb5868a6 | 26.128655 | 94 | 0.520371 | 3.856193 | false | false | false | false |
cwwise/CWWeChat | CWWeChat/ChatModule/CWInput/ChatToolBar/CWChatToolBar.swift | 2 | 13500 | //
// CWChatToolBar.swift
// CWWeChat
//
// Created by chenwei on 2017/4/1.
// Copyright © 2017年 cwcoder. All rights reserved.
//
import UIKit
import YYText
/// toolBar代理事件
public protocol CWChatToolBarDelegate: NSObjectProtocol {
func chatToolBar(_ chatToolBar: CWChatToolBar, voiceButtonPressed select: Bool, keyBoardState change:Bool)
func chatToolBar(_ chatToolBar: CWChatToolBar, emoticonButtonPressed select: Bool, keyBoardState change:Bool)
func chatToolBar(_ chatToolBar: CWChatToolBar, moreButtonPressed select: Bool, keyBoardState change:Bool)
///发送文字
func chatToolBar(_ chatToolBar: CWChatToolBar, sendText text: String)
}
public class CWChatToolBar: UIView {
weak var delegate: CWChatToolBarDelegate?
/// 临时记录输入的textView
var currentText: String?
var previousTextViewHeight: CGFloat = 36
// MARK: 属性
/// 输入框
lazy var inputTextView: CWInputTextView = {
let inputTextView = CWInputTextView(frame:CGRect.zero)
inputTextView.delegate = self
return inputTextView
}()
/// 表情按钮
lazy var emoticonButton: UIButton = {
let emoticonButton = UIButton(type: .custom)
emoticonButton.autoresizingMask = [.flexibleTopMargin]
emoticonButton.setNormalImage(self.kEmojiImage, highlighted:self.kEmojiImageHL)
emoticonButton.addTarget(self, action: #selector(handelEmotionClick(_:)), for: .touchDown)
return emoticonButton
}()
/// 文字和录音切换
lazy var voiceButton: UIButton = {
let voiceButton = UIButton(type: .custom)
voiceButton.autoresizingMask = [.flexibleTopMargin]
voiceButton.setNormalImage(self.kVoiceImage, highlighted:self.kVoiceImageHL)
voiceButton.addTarget(self, action: #selector(handelVoiceClick(_:)), for: .touchDown)
return voiceButton
}()
///更多按钮
lazy var moreButton: UIButton = {
let moreButton = UIButton(type: .custom)
moreButton.autoresizingMask = [.flexibleTopMargin]
moreButton.setNormalImage(self.kMoreImage, highlighted:self.kMoreImageHL)
moreButton.addTarget(self, action: #selector(handelMoreClick(_:)), for: .touchDown)
return moreButton
}()
/// 录音按钮
lazy var recordButton: CWRecordButton = {
let recordButton = CWRecordButton(frame: CGRect.zero)
recordButton.tag = 103
return recordButton
}()
var allowVoice: Bool = true
var allowFaceView: Bool = true
var allowMoreView: Bool = true
var voiceSelected: Bool {
return self.voiceButton.isSelected
}
var faceSelected: Bool {
return self.emoticonButton.isSelected
}
var moreSelected: Bool {
return self.moreButton.isSelected
}
//按钮的图片
var kVoiceImage:UIImage = CWAsset.Chat_toolbar_voice.image
var kVoiceImageHL:UIImage = CWAsset.Chat_toolbar_voice_HL.image
var kEmojiImage:UIImage = CWAsset.Chat_toolbar_emotion.image
var kEmojiImageHL:UIImage = CWAsset.Chat_toolbar_emotion_HL.image
//图片名称待修改
var kMoreImage:UIImage = CWAsset.Chat_toolbar_more.image
var kMoreImageHL:UIImage = CWAsset.Chat_toolbar_more_HL.image
var kKeyboardImage:UIImage = CWAsset.Chat_toolbar_keyboard.image
var kKeyboardImageHL:UIImage = CWAsset.Chat_toolbar_keyboard_HL.image
//MARK: 初始化
override init(frame: CGRect) {
super.init(frame: frame)
setupUI()
}
func setupUI() {
self.autoresizingMask = [UIViewAutoresizing.flexibleWidth,UIViewAutoresizing.flexibleTopMargin]
self.backgroundColor = UIColor.white
addSubview(self.voiceButton)
addSubview(self.emoticonButton)
addSubview(self.moreButton)
addSubview(self.recordButton)
addSubview(self.inputTextView)
setupFrame()
self.kvoController.observe(self.inputTextView, keyPath: "contentSize", options: .new, action: #selector(layoutAndAnimateTextView))
setupTopLine()
}
func setupFrame() {
let toolBarHeight = self.height
let kItem: CGFloat = 42
let buttonSize = CGSize(width: kItem, height: kChatToolBarHeight)
if self.allowVoice {
let origin = CGPoint(x: 0, y: toolBarHeight-buttonSize.height)
voiceButton.frame = CGRect(origin: origin, size: buttonSize)
} else {
voiceButton.frame = CGRect.zero
}
if self.allowMoreView {
let origin = CGPoint(x: kScreenWidth-buttonSize.width, y: toolBarHeight-buttonSize.height)
moreButton.frame = CGRect(origin: origin, size: buttonSize)
} else {
moreButton.frame = CGRect.zero
}
if self.allowFaceView {
let origin = CGPoint(x: kScreenWidth-buttonSize.width*2, y: toolBarHeight-buttonSize.height)
emoticonButton.frame = CGRect(origin: origin, size: buttonSize)
} else {
emoticonButton.frame = CGRect.zero
}
var textViewX = voiceButton.right
var textViewWidth = emoticonButton.left - voiceButton.right
if textViewX == 0 {
textViewX = 8
textViewWidth -= textViewX
}
let height = self.textViewLineHeight()
inputTextView.frame = CGRect(x: textViewX, y: (kChatToolBarHeight - height)/2.0,
width: textViewWidth, height: height)
recordButton.frame = inputTextView.frame
}
func setupTopLine() {
let line = UIView()
line.width = self.width
line.height = YYTextCGFloatFromPixel(1)
line.backgroundColor = UIColor(hex: "#e9e9e9")
line.autoresizingMask = .flexibleWidth
self.addSubview(line)
}
public func setTextViewContent(_ content: String) {
self.currentText = content
self.inputTextView.text = content
}
public func clearTextViewContent() {
self.currentText = ""
self.inputTextView.text = ""
}
func beginInputing() {
self.inputTextView.becomeFirstResponder()
}
func endInputing() {
if voiceButton.isSelected {
return
}
voiceButton.isSelected = false
emoticonButton.isSelected = false
moreButton.isSelected = false
}
func prepareForBeginComment() {
}
func prepareForEndComment() {
}
@objc func handelVoiceClick(_ sender: UIButton) {
self.voiceButton.isSelected = !self.voiceButton.isSelected
self.emoticonButton.isSelected = false
self.moreButton.isSelected = false
var keyBoardChanged = true
if sender.isSelected {
if inputTextView.isFirstResponder == false {
keyBoardChanged = false
}
self.adjustTextViewContentSize()
self.inputTextView.resignFirstResponder()
} else {
self.resumeTextViewContentSize()
self.inputTextView.becomeFirstResponder()
}
UIView.animate(withDuration: 0.2) {
self.recordButton.isHidden = !sender.isSelected
self.inputTextView.isHidden = sender.isSelected
}
self.delegate?.chatToolBar(self, voiceButtonPressed: sender.isSelected, keyBoardState: keyBoardChanged)
}
@objc func handelEmotionClick(_ sender: UIButton) {
self.emoticonButton.isSelected = !self.emoticonButton.isSelected
self.voiceButton.isSelected = false
self.moreButton.isSelected = false
var keyBoardChanged = true
if sender.isSelected {
if inputTextView.isFirstResponder == false {
keyBoardChanged = false
}
self.inputTextView.resignFirstResponder()
} else {
self.inputTextView.becomeFirstResponder()
}
self.resumeTextViewContentSize()
UIView.animate(withDuration: 0.2) {
self.recordButton.isHidden = true
self.inputTextView.isHidden = false
}
self.delegate?.chatToolBar(self, emoticonButtonPressed: sender.isSelected, keyBoardState: keyBoardChanged)
}
@objc func handelMoreClick(_ sender: UIButton) {
self.moreButton.isSelected = !self.moreButton.isSelected
self.voiceButton.isSelected = false
self.emoticonButton.isSelected = false
var keyBoardChanged = true
if sender.isSelected {
if inputTextView.isFirstResponder == false {
keyBoardChanged = false
}
self.inputTextView.resignFirstResponder()
} else {
self.inputTextView.becomeFirstResponder()
}
self.resumeTextViewContentSize()
UIView.animate(withDuration: 0.2) {
self.recordButton.isHidden = true
self.inputTextView.isHidden = false
}
self.delegate?.chatToolBar(self, moreButtonPressed: sender.isSelected, keyBoardState: keyBoardChanged)
}
// MARK: 功能
func resumeTextViewContentSize() {
self.inputTextView.text = self.currentText
}
func adjustTextViewContentSize() {
self.currentText = self.inputTextView.text
self.inputTextView.text = ""
inputTextView.contentSize = CGSize(width: inputTextView.width,
height: self.textViewLineHeight())
}
// MARK: 高度变化
@objc func layoutAndAnimateTextView() {
let maxHeight = self.textViewLineHeight() * 4
let contentHeight = ceil(inputTextView.sizeThatFits(inputTextView.size).height)
let isShrinking = contentHeight < self.previousTextViewHeight
var changeInHeight = contentHeight - self.previousTextViewHeight
let result = self.previousTextViewHeight == maxHeight || inputTextView.text.characters.count == 0
if !isShrinking && result {
changeInHeight = 0
} else {
changeInHeight = min(changeInHeight, maxHeight-self.previousTextViewHeight)
}
if changeInHeight != 0 {
UIView.animate(withDuration: 0.25, animations: {
if !isShrinking {
self.adjustTextViewHeightBy(changeInHeight)
}
let inputViewFrame = self.frame
self.frame = CGRect(x: 0,
y: inputViewFrame.minY - changeInHeight,
width: inputViewFrame.width,
height: inputViewFrame.height + changeInHeight)
if isShrinking {
self.adjustTextViewHeightBy(changeInHeight)
}
})
self.previousTextViewHeight = min(contentHeight, maxHeight)
}
if self.previousTextViewHeight == maxHeight {
DispatchQueueDelay(0.01, task: {
let bottomOffset = CGPoint(x: 0.0, y: contentHeight - self.inputTextView.bounds.height)
self.inputTextView.setContentOffset(bottomOffset, animated: true)
})
}
}
func adjustTextViewHeightBy(_ changeInHeight: CGFloat) {
let prevFrame = self.inputTextView.frame
let numLines = self.inputTextView.numberOfLinesOfText()
self.inputTextView.frame = CGRect(x: prevFrame.minX,
y: prevFrame.minY,
width: prevFrame.width,
height: prevFrame.height + changeInHeight)
if numLines > 6 {
self.inputTextView.contentInset = UIEdgeInsetsMake(4, 0, 4, 0)
} else {
self.inputTextView.contentInset = UIEdgeInsetsMake(0, 0, 0, 0)
}
if numLines > 6 {
}
}
//MARK: 计算高度
func textViewLineHeight() -> CGFloat {
return 36.0
}
required public init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
// MARK: - UITextViewDelegate
extension CWChatToolBar: UITextViewDelegate {
// 开始编辑的时候
public func textViewShouldBeginEditing(_ textView: UITextView) -> Bool {
voiceButton.isSelected = false
emoticonButton.isSelected = false
moreButton.isSelected = false
return true
}
public func textViewDidEndEditing(_ textView: UITextView) {
textView.resignFirstResponder()
}
public func textView(_ textView: UITextView, shouldChangeTextIn range: NSRange, replacementText text: String) -> Bool {
if text == "\n" {
sendCurrentTextViewText()
return false
}
return true
}
public func textViewDidChange(_ textView: UITextView) {
self.currentText = textView.text
}
///发送文字
func sendCurrentTextViewText() {
delegate?.chatToolBar(self, sendText: self.inputTextView.text)
self.currentText = ""
}
}
| mit | fc156d4efb4077d5d01f4cfa6f6111f0 | 31.793612 | 138 | 0.610399 | 4.982083 | false | false | false | false |
uasys/swift | stdlib/public/SDK/Dispatch/Time.swift | 1 | 7701 |
//===----------------------------------------------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2017 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
import _SwiftDispatchOverlayShims
public struct DispatchTime : Comparable {
private static let timebaseInfo: mach_timebase_info_data_t = {
var info = mach_timebase_info_data_t(numer: 1, denom: 1)
mach_timebase_info(&info)
return info
}()
public let rawValue: dispatch_time_t
public static func now() -> DispatchTime {
let t = __dispatch_time(0, 0)
return DispatchTime(rawValue: t)
}
public static let distantFuture = DispatchTime(rawValue: ~0)
fileprivate init(rawValue: dispatch_time_t) {
self.rawValue = rawValue
}
/// Creates a `DispatchTime` relative to the system clock that
/// ticks since boot.
///
/// - Parameters:
/// - uptimeNanoseconds: The number of nanoseconds since boot, excluding
/// time the system spent asleep
/// - Returns: A new `DispatchTime`
/// - Discussion: This clock is the same as the value returned by
/// `mach_absolute_time` when converted into nanoseconds.
/// On some platforms, the nanosecond value is rounded up to a
/// multiple of the Mach timebase, using the conversion factors
/// returned by `mach_timebase_info()`. The nanosecond equivalent
/// of the rounded result can be obtained by reading the
/// `uptimeNanoseconds` property.
/// Note that `DispatchTime(uptimeNanoseconds: 0)` is
/// equivalent to `DispatchTime.now()`, that is, its value
/// represents the number of nanoseconds since boot (excluding
/// system sleep time), not zero nanoseconds since boot.
public init(uptimeNanoseconds: UInt64) {
var rawValue = uptimeNanoseconds
if (DispatchTime.timebaseInfo.numer != DispatchTime.timebaseInfo.denom) {
rawValue = (rawValue * UInt64(DispatchTime.timebaseInfo.denom)
+ UInt64(DispatchTime.timebaseInfo.numer - 1)) / UInt64(DispatchTime.timebaseInfo.numer)
}
self.rawValue = dispatch_time_t(rawValue)
}
public var uptimeNanoseconds: UInt64 {
var result = self.rawValue
if (DispatchTime.timebaseInfo.numer != DispatchTime.timebaseInfo.denom) {
result = result * UInt64(DispatchTime.timebaseInfo.numer) / UInt64(DispatchTime.timebaseInfo.denom)
}
return result
}
}
extension DispatchTime {
public static func < (a: DispatchTime, b: DispatchTime) -> Bool {
return a.rawValue < b.rawValue
}
public static func ==(a: DispatchTime, b: DispatchTime) -> Bool {
return a.rawValue == b.rawValue
}
}
public struct DispatchWallTime : Comparable {
public let rawValue: dispatch_time_t
public static func now() -> DispatchWallTime {
return DispatchWallTime(rawValue: __dispatch_walltime(nil, 0))
}
public static let distantFuture = DispatchWallTime(rawValue: ~0)
fileprivate init(rawValue: dispatch_time_t) {
self.rawValue = rawValue
}
public init(timespec: timespec) {
var t = timespec
self.rawValue = __dispatch_walltime(&t, 0)
}
}
extension DispatchWallTime {
public static func <(a: DispatchWallTime, b: DispatchWallTime) -> Bool {
let negativeOne: dispatch_time_t = ~0
if b.rawValue == negativeOne {
return a.rawValue != negativeOne
} else if a.rawValue == negativeOne {
return false
}
return -Int64(bitPattern: a.rawValue) < -Int64(bitPattern: b.rawValue)
}
public static func ==(a: DispatchWallTime, b: DispatchWallTime) -> Bool {
return a.rawValue == b.rawValue
}
}
// Returns m1 * m2, clamped to the range [Int64.min, Int64.max].
// Because of the way this function is used, we can always assume
// that m2 > 0.
private func clampedInt64Product(_ m1: Int64, _ m2: Int64) -> Int64 {
assert(m2 > 0, "multiplier must be positive")
let (result, overflow) = m1.multipliedReportingOverflow(by: m2)
if overflow {
return m1 > 0 ? Int64.max : Int64.min
}
return result
}
// Returns its argument clamped to the range [Int64.min, Int64.max].
private func toInt64Clamped(_ value: Double) -> Int64 {
if value.isNaN { return Int64.max }
if value >= Double(Int64.max) { return Int64.max }
if value <= Double(Int64.min) { return Int64.min }
return Int64(value)
}
/// Represents a time interval that can be used as an offset from a `DispatchTime`
/// or `DispatchWallTime`.
///
/// For example:
/// let inOneSecond = DispatchTime.now() + DispatchTimeInterval.seconds(1)
///
/// If the requested time interval is larger then the internal representation
/// permits, the result of adding it to a `DispatchTime` or `DispatchWallTime`
/// is `DispatchTime.distantFuture` and `DispatchWallTime.distantFuture`
/// respectively. Such time intervals compare as equal:
///
/// let t1 = DispatchTimeInterval.seconds(Int.max)
/// let t2 = DispatchTimeInterval.milliseconds(Int.max)
/// let result = t1 == t2 // true
public enum DispatchTimeInterval : Equatable {
case seconds(Int)
case milliseconds(Int)
case microseconds(Int)
case nanoseconds(Int)
@_downgrade_exhaustivity_check
case never
internal var rawValue: Int64 {
switch self {
case .seconds(let s): return clampedInt64Product(Int64(s), Int64(NSEC_PER_SEC))
case .milliseconds(let ms): return clampedInt64Product(Int64(ms), Int64(NSEC_PER_MSEC))
case .microseconds(let us): return clampedInt64Product(Int64(us), Int64(NSEC_PER_USEC))
case .nanoseconds(let ns): return Int64(ns)
case .never: return Int64.max
}
}
public static func ==(lhs: DispatchTimeInterval, rhs: DispatchTimeInterval) -> Bool {
switch (lhs, rhs) {
case (.never, .never): return true
case (.never, _): return false
case (_, .never): return false
default: return lhs.rawValue == rhs.rawValue
}
}
}
public func +(time: DispatchTime, interval: DispatchTimeInterval) -> DispatchTime {
let t = __dispatch_time(time.rawValue, interval.rawValue)
return DispatchTime(rawValue: t)
}
public func -(time: DispatchTime, interval: DispatchTimeInterval) -> DispatchTime {
let t = __dispatch_time(time.rawValue, -interval.rawValue)
return DispatchTime(rawValue: t)
}
public func +(time: DispatchTime, seconds: Double) -> DispatchTime {
let t = __dispatch_time(time.rawValue, toInt64Clamped(seconds * Double(NSEC_PER_SEC)));
return DispatchTime(rawValue: t)
}
public func -(time: DispatchTime, seconds: Double) -> DispatchTime {
let t = __dispatch_time(time.rawValue, toInt64Clamped(-seconds * Double(NSEC_PER_SEC)));
return DispatchTime(rawValue: t)
}
public func +(time: DispatchWallTime, interval: DispatchTimeInterval) -> DispatchWallTime {
let t = __dispatch_time(time.rawValue, interval.rawValue)
return DispatchWallTime(rawValue: t)
}
public func -(time: DispatchWallTime, interval: DispatchTimeInterval) -> DispatchWallTime {
let t = __dispatch_time(time.rawValue, -interval.rawValue)
return DispatchWallTime(rawValue: t)
}
public func +(time: DispatchWallTime, seconds: Double) -> DispatchWallTime {
let t = __dispatch_time(time.rawValue, toInt64Clamped(seconds * Double(NSEC_PER_SEC)));
return DispatchWallTime(rawValue: t)
}
public func -(time: DispatchWallTime, seconds: Double) -> DispatchWallTime {
let t = __dispatch_time(time.rawValue, toInt64Clamped(-seconds * Double(NSEC_PER_SEC)));
return DispatchWallTime(rawValue: t)
}
| apache-2.0 | e9455b59a04708c33a538f2e9b5b19bb | 34.325688 | 102 | 0.694196 | 3.818047 | false | false | false | false |
apple/swift | stdlib/private/StdlibCollectionUnittest/CheckSequenceInstance.swift | 21 | 6649 | //===----------------------------------------------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2020 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
import StdlibUnittest
// Generate two overloads: one for Array (which will get
// picked up when the caller passes a literal), and another that
// accepts any appropriate Collection type.
public func checkIterator<
Expected: Collection, I : IteratorProtocol
>(
_ expected: Expected,
_ iterator: I,
_ message: @autoclosure () -> String = "",
stackTrace: SourceLocStack = SourceLocStack(),
showFrame: Bool = true,
file: String = #file, line: UInt = #line,
resiliencyChecks: CollectionMisuseResiliencyChecks = .all,
sameValue: (Expected.Element, Expected.Element) -> Bool
) where I.Element == Expected.Element {
// Copying a `IteratorProtocol` is allowed.
var mutableGen = iterator
var actual: [Expected.Element] = []
while let e = mutableGen.next() {
actual.append(e)
}
expectEqualSequence(
expected, actual, message(),
stackTrace: stackTrace.pushIf(showFrame, file: file, line: line), sameValue: sameValue)
// Having returned `.None` once, a `IteratorProtocol` should not generate
// more elements.
for _ in 0..<10 {
expectNil(mutableGen.next(), message(),
stackTrace: stackTrace.pushIf(showFrame, file: file, line: line))
}
}
public func checkIterator<
Expected: Collection, I : IteratorProtocol
>(
_ expected: Expected,
_ iterator: I,
_ message: @autoclosure () -> String = "",
stackTrace: SourceLocStack = SourceLocStack(),
showFrame: Bool = true,
file: String = #file, line: UInt = #line,
resiliencyChecks: CollectionMisuseResiliencyChecks = .all
) where I.Element == Expected.Element, Expected.Element : Equatable {
checkIterator(
expected, iterator, message(),
stackTrace: stackTrace.pushIf(showFrame, file: file, line: line), showFrame: false,
resiliencyChecks: resiliencyChecks
) { $0 == $1 }
}
public func checkSequence<
Expected: Collection, S : Sequence
>(
_ expected: Expected,
_ sequence: S,
_ message: @autoclosure () -> String = "",
stackTrace: SourceLocStack = SourceLocStack(),
showFrame: Bool = true,
file: String = #file, line: UInt = #line,
resiliencyChecks: CollectionMisuseResiliencyChecks = .all,
sameValue: (Expected.Element, Expected.Element) -> Bool
) where S.Element == Expected.Element {
let expectedCount: Int = expected.count
checkIterator(
expected, sequence.makeIterator(), message(),
stackTrace: stackTrace.pushIf(showFrame, file: file, line: line),
resiliencyChecks: resiliencyChecks,
sameValue: sameValue)
expectGE(
expectedCount, sequence.underestimatedCount, message(),
stackTrace: stackTrace.pushIf(showFrame, file: file, line: line))
}
public func checkSequence<
Expected: Collection, S : Sequence
>(
_ expected: Expected,
_ sequence: S,
_ message: @autoclosure () -> String = "",
stackTrace: SourceLocStack = SourceLocStack(),
showFrame: Bool = true,
file: String = #file, line: UInt = #line,
resiliencyChecks: CollectionMisuseResiliencyChecks = .all
) where
S.Element == Expected.Element,
S.Element : Equatable {
checkSequence(
expected, sequence, message(),
stackTrace: stackTrace.pushIf(showFrame, file: file, line: line), showFrame: false,
resiliencyChecks: resiliencyChecks
) { $0 == $1 }
}
public func checkIterator<
Element, I : IteratorProtocol
>(
_ expected: Array<Element>,
_ iterator: I,
_ message: @autoclosure () -> String = "",
stackTrace: SourceLocStack = SourceLocStack(),
showFrame: Bool = true,
file: String = #file, line: UInt = #line,
resiliencyChecks: CollectionMisuseResiliencyChecks = .all,
sameValue: (Element, Element) -> Bool
) where I.Element == Element {
// Copying a `IteratorProtocol` is allowed.
var mutableGen = iterator
var actual: [Element] = []
while let e = mutableGen.next() {
actual.append(e)
}
expectEqualSequence(
expected, actual, message(),
stackTrace: stackTrace.pushIf(showFrame, file: file, line: line), sameValue: sameValue)
// Having returned `.None` once, a `IteratorProtocol` should not generate
// more elements.
for _ in 0..<10 {
expectNil(mutableGen.next(), message(),
stackTrace: stackTrace.pushIf(showFrame, file: file, line: line))
}
}
public func checkIterator<
Element, I : IteratorProtocol
>(
_ expected: Array<Element>,
_ iterator: I,
_ message: @autoclosure () -> String = "",
stackTrace: SourceLocStack = SourceLocStack(),
showFrame: Bool = true,
file: String = #file, line: UInt = #line,
resiliencyChecks: CollectionMisuseResiliencyChecks = .all
) where I.Element == Element, Element : Equatable {
checkIterator(
expected, iterator, message(),
stackTrace: stackTrace.pushIf(showFrame, file: file, line: line), showFrame: false,
resiliencyChecks: resiliencyChecks
) { $0 == $1 }
}
public func checkSequence<
Element, S : Sequence
>(
_ expected: Array<Element>,
_ sequence: S,
_ message: @autoclosure () -> String = "",
stackTrace: SourceLocStack = SourceLocStack(),
showFrame: Bool = true,
file: String = #file, line: UInt = #line,
resiliencyChecks: CollectionMisuseResiliencyChecks = .all,
sameValue: (Element, Element) -> Bool
) where S.Element == Element {
let expectedCount: Int = expected.count
checkIterator(
expected, sequence.makeIterator(), message(),
stackTrace: stackTrace.pushIf(showFrame, file: file, line: line),
resiliencyChecks: resiliencyChecks,
sameValue: sameValue)
expectGE(
expectedCount, sequence.underestimatedCount, message(),
stackTrace: stackTrace.pushIf(showFrame, file: file, line: line))
}
public func checkSequence<
Element, S : Sequence
>(
_ expected: Array<Element>,
_ sequence: S,
_ message: @autoclosure () -> String = "",
stackTrace: SourceLocStack = SourceLocStack(),
showFrame: Bool = true,
file: String = #file, line: UInt = #line,
resiliencyChecks: CollectionMisuseResiliencyChecks = .all
) where
S.Element == Element,
S.Element : Equatable {
checkSequence(
expected, sequence, message(),
stackTrace: stackTrace.pushIf(showFrame, file: file, line: line), showFrame: false,
resiliencyChecks: resiliencyChecks
) { $0 == $1 }
}
| apache-2.0 | 1616c0196c8c31bf8b518c69e67e82e8 | 31.434146 | 91 | 0.682358 | 4.374342 | false | false | false | false |
AndrewBennet/readinglist | ReadingList_UnitTests/Extensions.swift | 1 | 660 | import Foundation
import CoreData
extension NSPersistentContainer {
/**
Creates a NSPersistentContainer with a single in memory store description.
*/
convenience init(inMemoryStoreWithName name: String) {
self.init(name: name)
self.persistentStoreDescriptions = [ {
let description = NSPersistentStoreDescription()
description.shouldInferMappingModelAutomatically = false
description.shouldMigrateStoreAutomatically = false
description.type = NSInMemoryStoreType
description.shouldAddStoreAsynchronously = false
return description
}()]
}
}
| gpl-3.0 | 96b7fb409cce665f1007401be43e8145 | 32 | 79 | 0.686364 | 6.346154 | false | false | false | false |
algolia/algoliasearch-client-swift | Sources/AlgoliaSearchClient/Models/Data structures/SingleOrList.swift | 1 | 1028 | //
// SingleOrList.swift
//
//
// Created by Vladislav Fitc on 07/07/2020.
//
import Foundation
public enum SingleOrList<T> {
case single(T)
case list([T])
}
extension SingleOrList: Equatable where T: Equatable {
public static func == (lhs: SingleOrList<T>, rhs: SingleOrList<T>) -> Bool {
switch (lhs, rhs) {
case (.single(let left), .single(let right)):
return left == right
case (.list(let left), .list(let right)):
return left == right
default:
return false
}
}
}
extension SingleOrList: Encodable where T: Encodable {
public func encode(to encoder: Encoder) throws {
switch self {
case .single(let value):
try value.encode(to: encoder)
case .list(let list):
try list.encode(to: encoder)
}
}
}
extension SingleOrList: Decodable where T: Decodable {
public init(from decoder: Decoder) throws {
if let list = try? [T](from: decoder) {
self = .list(list)
} else {
self = .single(try T(from: decoder))
}
}
}
| mit | af4283902f68184f943f95ef73879898 | 18.769231 | 78 | 0.620623 | 3.426667 | false | false | false | false |
AnthonyMDev/Nimble | Sources/Nimble/Matchers/BeLogical.swift | 47 | 4993 | import Foundation
extension Int8: ExpressibleByBooleanLiteral {
public init(booleanLiteral value: Bool) {
self = NSNumber(value: value).int8Value
}
}
extension UInt8: ExpressibleByBooleanLiteral {
public init(booleanLiteral value: Bool) {
self = NSNumber(value: value).uint8Value
}
}
extension Int16: ExpressibleByBooleanLiteral {
public init(booleanLiteral value: Bool) {
self = NSNumber(value: value).int16Value
}
}
extension UInt16: ExpressibleByBooleanLiteral {
public init(booleanLiteral value: Bool) {
self = NSNumber(value: value).uint16Value
}
}
extension Int32: ExpressibleByBooleanLiteral {
public init(booleanLiteral value: Bool) {
self = NSNumber(value: value).int32Value
}
}
extension UInt32: ExpressibleByBooleanLiteral {
public init(booleanLiteral value: Bool) {
self = NSNumber(value: value).uint32Value
}
}
extension Int64: ExpressibleByBooleanLiteral {
public init(booleanLiteral value: Bool) {
self = NSNumber(value: value).int64Value
}
}
extension UInt64: ExpressibleByBooleanLiteral {
public init(booleanLiteral value: Bool) {
self = NSNumber(value: value).uint64Value
}
}
extension Float: ExpressibleByBooleanLiteral {
public init(booleanLiteral value: Bool) {
self = NSNumber(value: value).floatValue
}
}
extension Double: ExpressibleByBooleanLiteral {
public init(booleanLiteral value: Bool) {
self = NSNumber(value: value).doubleValue
}
}
extension Int: ExpressibleByBooleanLiteral {
public init(booleanLiteral value: Bool) {
self = NSNumber(value: value).intValue
}
}
extension UInt: ExpressibleByBooleanLiteral {
public init(booleanLiteral value: Bool) {
self = NSNumber(value: value).uintValue
}
}
internal func rename<T>(_ matcher: Predicate<T>, failureMessage message: ExpectationMessage) -> Predicate<T> {
return Predicate { actualExpression in
let result = try matcher.satisfies(actualExpression)
return PredicateResult(status: result.status, message: message)
}.requireNonNil
}
// MARK: beTrue() / beFalse()
/// A Nimble matcher that succeeds when the actual value is exactly true.
/// This matcher will not match against nils.
public func beTrue() -> Predicate<Bool> {
return rename(equal(true), failureMessage: .expectedActualValueTo("be true"))
}
/// A Nimble matcher that succeeds when the actual value is exactly false.
/// This matcher will not match against nils.
public func beFalse() -> Predicate<Bool> {
return rename(equal(false), failureMessage: .expectedActualValueTo("be false"))
}
// MARK: beTruthy() / beFalsy()
/// A Nimble matcher that succeeds when the actual value is not logically false.
public func beTruthy<T: ExpressibleByBooleanLiteral & Equatable>() -> Predicate<T> {
return Predicate.simpleNilable("be truthy") { actualExpression in
let actualValue = try actualExpression.evaluate()
if let actualValue = actualValue {
return PredicateStatus(bool: actualValue == (true as T))
}
return PredicateStatus(bool: actualValue != nil)
}
}
/// A Nimble matcher that succeeds when the actual value is logically false.
/// This matcher will match against nils.
public func beFalsy<T: ExpressibleByBooleanLiteral & Equatable>() -> Predicate<T> {
return Predicate.simpleNilable("be falsy") { actualExpression in
let actualValue = try actualExpression.evaluate()
if let actualValue = actualValue {
return PredicateStatus(bool: actualValue == (false as T))
}
return PredicateStatus(bool: actualValue == nil)
}
}
#if canImport(Darwin)
extension NMBObjCMatcher {
@objc public class func beTruthyMatcher() -> NMBMatcher {
return NMBPredicate { actualExpression in
let expr = actualExpression.cast { ($0 as? NSNumber)?.boolValue ?? false }
return try beTruthy().satisfies(expr).toObjectiveC()
}
}
@objc public class func beFalsyMatcher() -> NMBMatcher {
return NMBPredicate { actualExpression in
let expr = actualExpression.cast { ($0 as? NSNumber)?.boolValue ?? false }
return try beFalsy().satisfies(expr).toObjectiveC()
}
}
@objc public class func beTrueMatcher() -> NMBMatcher {
return NMBPredicate { actualExpression in
let expr = actualExpression.cast { ($0 as? NSNumber)?.boolValue ?? false }
return try beTrue().satisfies(expr).toObjectiveC()
}
}
@objc public class func beFalseMatcher() -> NMBMatcher {
return NMBPredicate { actualExpression in
let expr = actualExpression.cast { value -> Bool? in
guard let value = value else { return nil }
return (value as? NSNumber)?.boolValue ?? false
}
return try beFalse().satisfies(expr).toObjectiveC()
}
}
}
#endif
| apache-2.0 | 71bca5829cb42da312b539f702f80f45 | 31.422078 | 110 | 0.680152 | 4.833495 | false | false | false | false |
BradLarson/GPUImage2 | framework/Source/Operations/Histogram.swift | 1 | 4866 | #if canImport(OpenGL)
import OpenGL.GL3
#endif
#if canImport(OpenGLES)
import OpenGLES
#endif
#if canImport(COpenGLES)
import COpenGLES.gles2
#endif
#if canImport(COpenGL)
import COpenGL
#endif
/* Unlike other filters, this one uses a grid of GL_POINTs to sample the incoming image in a grid. A custom vertex shader reads the color in the texture at its position
and outputs a bin position in the final histogram as the vertex position. That point is then written into the image of the histogram using translucent pixels.
The degree of translucency is controlled by the scalingFactor, which lets you adjust the dynamic range of the histogram. The histogram can only be generated for one
color channel or luminance value at a time.
This is based on this implementation: http://www.shaderwrangler.com/publications/histogram/histogram_cameraready.pdf
Or at least that's how it would work if iOS could read from textures in a vertex shader, which it can't. Therefore, I read the texture data down from the
incoming frame and process the texture colors as vertices.
*/
public enum HistogramType {
case red
case blue
case green
case luminance
case rgb
}
public class Histogram: BasicOperation {
public var downsamplingFactor:UInt = 16
var shader2:ShaderProgram? = nil
var shader3:ShaderProgram? = nil
public init(type:HistogramType) {
switch type {
case .red: super.init(vertexShader:HistogramRedSamplingVertexShader, fragmentShader:HistogramAccumulationFragmentShader, numberOfInputs:1)
case .blue: super.init(vertexShader:HistogramBlueSamplingVertexShader, fragmentShader:HistogramAccumulationFragmentShader, numberOfInputs:1)
case .green: super.init(vertexShader:HistogramGreenSamplingVertexShader, fragmentShader:HistogramAccumulationFragmentShader, numberOfInputs:1)
case .luminance: super.init(vertexShader:HistogramLuminanceSamplingVertexShader, fragmentShader:HistogramAccumulationFragmentShader, numberOfInputs:1)
case .rgb:
super.init(vertexShader:HistogramRedSamplingVertexShader, fragmentShader:HistogramAccumulationFragmentShader, numberOfInputs:1)
shader2 = crashOnShaderCompileFailure("Histogram"){try sharedImageProcessingContext.programForVertexShader(HistogramGreenSamplingVertexShader, fragmentShader:HistogramAccumulationFragmentShader)}
shader3 = crashOnShaderCompileFailure("Histogram"){try sharedImageProcessingContext.programForVertexShader(HistogramBlueSamplingVertexShader, fragmentShader:HistogramAccumulationFragmentShader)}
}
}
override func renderFrame() {
let inputSize = sizeOfInitialStageBasedOnFramebuffer(inputFramebuffers[0]!)
let inputByteSize = Int(inputSize.width * inputSize.height * 4)
let data = UnsafeMutablePointer<UInt8>.allocate(capacity:inputByteSize)
glReadPixels(0, 0, inputSize.width, inputSize.height, GLenum(GL_RGBA), GLenum(GL_UNSIGNED_BYTE), data)
renderFramebuffer = sharedImageProcessingContext.framebufferCache.requestFramebufferWithProperties(orientation:.portrait, size:GLSize(width:256, height:3), stencil:mask != nil)
releaseIncomingFramebuffers()
renderFramebuffer.activateFramebufferForRendering()
clearFramebufferWithColor(Color.black)
enableAdditiveBlending()
shader.use()
guard let positionAttribute = shader.attributeIndex("position") else { fatalError("A position attribute was missing from the shader program during rendering.") }
glVertexAttribPointer(positionAttribute, 4, GLenum(GL_UNSIGNED_BYTE), 0, (GLint(downsamplingFactor) - 1) * 4, data)
glDrawArrays(GLenum(GL_POINTS), 0, inputSize.width * inputSize.height / GLint(downsamplingFactor))
if let shader2 = shader2 {
shader2.use()
guard let positionAttribute2 = shader.attributeIndex("position") else { fatalError("A position attribute was missing from the shader program during rendering.") }
glVertexAttribPointer(positionAttribute2, 4, GLenum(GL_UNSIGNED_BYTE), 0, (GLint(downsamplingFactor) - 1) * 4, data)
glDrawArrays(GLenum(GL_POINTS), 0, inputSize.width * inputSize.height / GLint(downsamplingFactor))
}
if let shader3 = shader3 {
shader3.use()
guard let positionAttribute3 = shader.attributeIndex("position") else { fatalError("A position attribute was missing from the shader program during rendering.") }
glVertexAttribPointer(positionAttribute3, 4, GLenum(GL_UNSIGNED_BYTE), 0, (GLint(downsamplingFactor) - 1) * 4, data)
glDrawArrays(GLenum(GL_POINTS), 0, inputSize.width * inputSize.height / GLint(downsamplingFactor))
}
disableBlending()
data.deallocate()
}
}
| bsd-3-clause | fa97c5a600a0af148928a78156160f6a | 51.891304 | 211 | 0.745787 | 4.841791 | false | false | false | false |
poetmountain/MotionMachine | Sources/ValueAssistants/ValueAssistantGroup.swift | 1 | 8433 | //
// ValueAssistantGroup.swift
// MotionMachine
//
// Created by Brett Walker on 5/18/16.
// Copyright © 2016-2018 Poet & Mountain, LLC. All rights reserved.
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
//
import Foundation
/// The `ValueAssistantGroup` class enables multiple `ValueAssistant` objects to be attached to a single motion class.
public class ValueAssistantGroup : ValueAssistant {
public var additive: Bool = false {
didSet {
for index in 0 ..< assistants.count {
assistants[index].additive = additive
}
}
}
public var additiveWeighting: Double = 1.0 {
didSet {
// constrain weighting to range of 0.0 - 1.0
additiveWeighting = max(min(additiveWeighting, 1.0), 0.0)
for index in 0 ..< assistants.count {
assistants[index].additiveWeighting = additiveWeighting
}
}
}
private(set) public var assistants: [ValueAssistant] = []
/**
* Initializer.
*
* - parameters:
* - assistants: An optional array of `ValueAssistant` objects to which the ValueAssistantGroup should delegate `ValueAssistant` method calls.
*/
public convenience init(assistants: [ValueAssistant]? = []) {
self.init()
if let unwrapped_assists = assistants {
self.assistants = unwrapped_assists
}
}
public required init() {}
// MARK: Public Methods
/**
* Adds a `ValueAssistant` to the group.
*
* - parameter assistant: A `ValueAssistant` object.
* - note: The added assistant will be assigned the same values for `additive` and `additiveWeighting` as this group's values.
* - seealso: additive, additiveWeighting
*/
public func add(_ assistant: ValueAssistant) {
var assist = assistant
assist.additive = additive
assist.additiveWeighting = additiveWeighting
assistants.append(assist)
}
// MARK: ValueAssistant methods
public func generateProperties(targetObject target: AnyObject, propertyStates: PropertyStates) throws -> [PropertyData] {
var properties: [PropertyData] = []
for assistant in assistants {
if (assistant.supports(propertyStates.end as AnyObject)) {
if let generated = try? assistant.generateProperties(targetObject: target, propertyStates: propertyStates) {
properties += generated
break
}
}
}
return properties
}
public func retrieveValue(inObject object: Any, keyPath path: String) -> Double? {
var retrieved_value: Double?
for assistant in assistants {
if (assistant.supports(object as AnyObject)) {
if let retrieved = try? assistant.retrieveValue(inObject: object, keyPath: path) {
retrieved_value = retrieved
break
}
}
}
if (retrieved_value == nil) {
let path_value = (object as AnyObject).value(forKeyPath: path)!
// cast numeric value to a double
retrieved_value = MotionSupport.cast(path_value as AnyObject)
let components = path.components(separatedBy: ".")
let first_component = components.first!
let child_object = (object as AnyObject).value(forKey: first_component)
if let unwrapped_child = child_object as AnyObject? {
if (acceptsKeypath(unwrapped_child)) {
}
}
}
return retrieved_value
}
public func updateValue(inObject object: Any, newValues: Dictionary<String, Double>) -> NSObject? {
guard newValues.count > 0 else { return nil }
var new_parent_value:NSObject?
for assistant in assistants {
if (assistant.supports(object as AnyObject)) {
new_parent_value = assistant.updateValue(inObject: object, newValues: newValues)
break
}
}
return new_parent_value
}
public func retrieveCurrentObjectValue(forProperty property: PropertyData) -> Double? {
guard let unwrapped_target = property.target else { return nil }
var current_value: Double?
for assistant in assistants {
if (assistant.supports(unwrapped_target)) {
current_value = assistant.retrieveCurrentObjectValue(forProperty: property)
break
}
}
return current_value
}
public func calculateValue(forProperty property: PropertyData, newValue: Double) -> NSObject? {
guard let unwrapped_target = property.target else { return nil }
var new_prop: NSObject? = NSNumber.init(value: property.current)
// this code path will execute if the object passed in was an NSValue
// as such we must replace the value object directly
if ((property.targetObject == nil || property.targetObject === unwrapped_target) && unwrapped_target is NSValue) {
for assistant in assistants {
if (assistant.supports(unwrapped_target)) {
new_prop = assistant.calculateValue(forProperty: property, newValue: newValue)
if (new_prop != nil) { break }
}
}
return new_prop
}
if (property.targetObject != nil) {
// we have a normal object whose property is being changed
for assistant in assistants {
if (assistant.supports(unwrapped_target)) {
new_prop = assistant.calculateValue(forProperty: property, newValue: newValue)
break
}
}
return new_prop
} else {
// we have no base object as it's not a NSValue, so find assistant that supports target
// this will typically be a UIColor
for assistant in assistants {
if (assistant.supports(unwrapped_target)) {
new_prop = assistant.calculateValue(forProperty: property, newValue: newValue)
break
}
}
}
return new_prop
}
public func supports(_ object: AnyObject) -> Bool {
var is_supported: Bool = false
for assistant in assistants {
is_supported = assistant.supports(object)
if (is_supported) { break }
}
return is_supported
}
public func acceptsKeypath(_ object: AnyObject) -> Bool {
var accepts = true
for assistant in assistants {
if (!assistant.acceptsKeypath(object) && assistant.supports(object)) {
accepts = false
break
}
}
return accepts
}
}
| mit | 446a826d63aebd5b809b4fc0b908985b | 32.19685 | 151 | 0.574241 | 5.192118 | false | false | false | false |
zhiquan911/chance_btc_wallet | chance_btc_wallet/chance_btc_wallet/service/BlockchainRemoteService.swift | 1 | 13831 | //
// BlockchainRemoteService.swift
// Chance_wallet
//
// Created by Chance on 16/3/1.
// Copyright © 2016年 Chance. All rights reserved.
//
import UIKit
import Alamofire
import SwiftyJSON
class BlockchainRemoteService: RemoteService {
var apiUrl: String {
let network = CHWalletWrapper.selectedBlockchainNetwork
switch network {
case .main:
return "https://blockchain.info/"
case .test:
return "https://testnet.blockchain.info/"
}
}
/// 全局唯一实例
static let sharedInstance: BlockchainRemoteService = {
let instance = BlockchainRemoteService()
return instance
}()
//MARK: - 重载标准的接口方法
func userBalance(address: String, callback: @escaping (MessageModule, UserBalance) -> Void) {
let params: [String: Any] = [
"address": address
]
var url = apiUrl + "rawaddr"
url.append("/\(address)")
self.sendJsonRequest(url, method: .get, parameters: params) {
(json, isCache) -> Void in
let message = MessageModule(json: json["resMsg"])
let data = json["datas"]
let userBalance = UserBalance()
if data.exists() {
// "addrStr": "16VvieA7tLFZx5aRb5q8bJTZuV378A8pWv",
// "balance": 0,
// "balanceSat": 0,
// "totalReceived": 0.0726,
// "totalReceivedSat": 7260000,
// "totalSent": 0.0726,
// "totalSentSat": 7260000,
// "unconfirmedBalance": 0,
// "unconfirmedBalanceSat": 0,
// "unconfirmedTxApperances": 0,
// "txApperances": 9,
userBalance.currencyType = .BTC
userBalance.address = data["address"].stringValue
userBalance.balanceSat = data["final_balance"].intValue
userBalance.totalReceivedSat = data["total_received"].intValue
userBalance.totalSentSat = data["total_sent"].intValue
userBalance.txApperances = data["n_tx"].intValue
}
callback(message, userBalance)
}
}
func userTransactions(address: String, from: String, to: String, limit: String, callback: @escaping (MessageModule, String, UserBalance?, [UserTransaction], PageModule?) -> Void) {
let params: [String: Any] = [
"address": address
]
var url = apiUrl + "rawaddr"
url.append("/\(address)?offset=\(from)&limit=\(limit)")
self.sendJsonRequest(url, method: .get, parameters: params) {
(json, isCache) -> Void in
let message = MessageModule(json: json["resMsg"])
let data = json["datas"]
var userTransactions = [UserTransaction]()
//用户余额
let userBalance = UserBalance()
if data.exists() {
let items = data["txs"].arrayValue
for dic in items {
// "ver":1,
// "inputs":[
// {
// "sequence":4294967295,
// "prev_out":{
// "spent":true,
// "tx_index":129980108,
// "type":0,
// "addr":"1HvgRTi2CmaSHUkfWUCAqkYjF7AiBohzbB",
// "value":10000,
// "n":0,
// "script":"76a914b9a8f753a620aa27836db34e28a3582cbd25c71588ac"
// },
// "script":"483045022100a260961b93d3b283ef76c161a81f30911ee313dfadd10fe455bcdaf65c42107602204f6824ac4e95c3b2a81de493e0e7521a1af202e2bcaa22b44b5a3fb0b4e2cf6c012103306e60b8c65a7b1338ae632a9b78fd627477dcc8e4d02a547df034a974144fe9"
// },
// {
// "sequence":4294967295,
// "prev_out":{
// "spent":true,
// "tx_index":129817786,
// "type":0,
// "addr":"1HvgRTi2CmaSHUkfWUCAqkYjF7AiBohzbB",
// "value":10000,
// "n":0,
// "script":"76a914b9a8f753a620aa27836db34e28a3582cbd25c71588ac"
// },
// "script":"473044022068c10112ae2dd16ea02a0930df17456e3fec05f28b77d5912723850d9a1c2b16022075ceff91b598a70785f33f39953cde5336c0d6693b8a5059f9dc0e2e88adf82e012103306e60b8c65a7b1338ae632a9b78fd627477dcc8e4d02a547df034a974144fe9"
// },
// {
// "sequence":4294967295,
// "prev_out":{
// "spent":true,
// "tx_index":129816887,
// "type":0,
// "addr":"1HvgRTi2CmaSHUkfWUCAqkYjF7AiBohzbB",
// "value":10000,
// "n":0,
// "script":"76a914b9a8f753a620aa27836db34e28a3582cbd25c71588ac"
// },
// "script":"483045022100b9b000e11ce3b2641084f277c1837694ae30384ca99a4eaaf4cd986025dae373022007cfd399c5271d1f37331dc238cb72247b4191c56cd131971c29eae8c0bf65f1012103306e60b8c65a7b1338ae632a9b78fd627477dcc8e4d02a547df034a974144fe9"
// }
// ],
//
// "out":[
// {
// "spent":true,
// "tx_index":129980308,
// "type":0,
// "addr":"1L8WssQRCrDKRSF5MwUb2E4HhGzkVTP93U",
// "value":20000,
// "n":0,
// "script":"76a914d1d63de21e37c2845b9c134edd02a74881a53d1e88ac"
// }
// ],
// "lock_time":0,
// "result":0,
// "size":487,
// "time":1455932732,
// "tx_index":129980308,
// "vin_sz":3,
// "hash":"d756d527db180e646189daf667dfca4d3127607fb790c2542652d9809816b23e",
// "vout_sz":1
// "block_height":399242,
// "relayed_by":"37.205.10.140",
let tx = UserTransaction()
tx.txid = dic["hash"].stringValue
tx.version = dic["ver"].intValue
tx.locktime = dic["lock_time"].intValue
tx.blockHeight = dic["block_height"].intValue
if tx.blockHeight > 0 {
tx.confirmations = 1
} else {
tx.confirmations = 0
}
tx.blocktime = dic["time"].intValue
tx.size = dic["size"].intValue
//封装交易输入输出的单元
let vins = dic["inputs"].arrayValue
for vin in vins {
let txin = TransactionUnit()
txin.address = vin["prev_out"]["addr"].stringValue
txin.value = BTCAmount(vin["prev_out"]["value"].intValue)
tx.vinTxs.append(txin)
}
let vouts = dic["out"].arrayValue
for vout in vouts {
let txout = TransactionUnit()
txout.address = vout["addr"].stringValue
txout.value = BTCAmount(vout["value"].intValue)
tx.voutTxs.append(txout)
}
userTransactions.append(tx)
}
//更新余额字段到实例中
userBalance.currencyType = .BTC
userBalance.address = data["address"].stringValue
userBalance.balanceSat = data["final_balance"].intValue
userBalance.totalReceivedSat = data["total_received"].intValue
userBalance.totalSentSat = data["total_sent"].intValue
userBalance.txApperances = data["n_tx"].intValue
}
callback(message, address, userBalance, userTransactions, nil)
}
}
/**
获取用户未花交易记录
- parameter address:
- parameter callback:
*/
func userUnspentTransactions(address: String,
callback: @escaping (MessageModule, [UnspentTransaction]) -> Void) {
let params: [String: Any] = [
"active": address
]
let url = apiUrl + "unspent"
self.sendJsonRequest(url, parameters: params) {
(json, isCache) -> Void in
let message = MessageModule(json: json["resMsg"])
let data = json["datas"]
let items = data["unspent_outputs"].arrayValue
var unspentUserTransactions = [UnspentTransaction]()
for dic in items {
// "tx_hash":"0387d2b856a4e4d69ceb8b73e13d5d03a44419b19f00ee7b30f8afbf57df033f",
// "tx_hash_big_endian":"3f03df57bfaff8307bee009fb11944a4035d3de1738beb9cd6e4a456b8d28703",
// "tx_index":132619844,
// "tx_output_n": 1,
// "script":"76a914b9a8f753a620aa27836db34e28a3582cbd25c71588ac",
// "value": 90000,
// "value_hex": "015f90",
// "confirmations":0
let tx = UnspentTransaction()
tx.txid = dic["tx_hash_big_endian"].stringValue
// tx.address = dic["address"].stringValue
tx.vout = dic["tx_output_n"].intValue
tx.timestamp = dic["ts"].intValue
tx.confirmations = dic["confirmations"].intValue
tx.scriptPubKey = dic["script"].stringValue
tx.amount = BTCAmount(dic["value"].intValue)
unspentUserTransactions.append(tx)
}
callback(message, unspentUserTransactions)
}
}
/**
广播交易信息
- parameter transactionHexString: 交易数据16进制字节流
- parameter callback: 返回交易id
*/
func sendTransaction(transactionHexString: String, callback: @escaping (MessageModule, String) -> Void) {
let params: [String: Any] = [
"tx": transactionHexString
]
let url = apiUrl + "pushtx"
self.sendJsonRequest(url, parameters: params, responseDataType: .string) {
(json, isCache) -> Void in
let message: MessageModule
let data = json["datas"]
if let result = data.rawString(), result == "Transaction Submitted\n" {
message = MessageModule(
code: "\(ApiResultCode.Success.rawValue)",
message: "success")
} else {
message = MessageModule(
code: "\(ApiResultCode.ErrorTips.rawValue)",
message: json.rawString()!)
}
callback(message, "")
}
}
/// 价格行情
func ticker(callback: @escaping (MessageModule, [String: WorldTicker]) -> Void) {
let params: [String: Any] = [String: Any]()
let url = apiUrl + "ticker"
self.sendJsonRequest(url, method: .get, parameters: params) {
(json, isCache) -> Void in
let message = MessageModule(json: json["resMsg"])
let data = json["datas"]
var tickers = [String: WorldTicker]()
if data.exists() {
let map = data.dictionaryValue
let keys = map.keys
for cur in keys {
let obj = WorldTicker(json: map[cur]!, currency: "BTC", legalCurrency: cur)
tickers[cur] = obj
}
}
callback(message, tickers)
}
}
}
| mit | 42b4d67c89e8ddcdfba92a2f5d57d376 | 44.155116 | 267 | 0.432612 | 4.556111 | false | false | false | false |
rain2540/RGAppTools | Sources/Extensions/UIKit/UIViewController+RGAppTools.swift | 1 | 2728 | //
// UIViewController+RGAppTools.swift
// RGAppTools
//
// Created by RAIN on 2017/8/16.
// Copyright © 2017年 Smartech. All rights reserved.
//
import UIKit
extension RGAppTools where Base: UIViewController {
/// 获取位于最上层的视图
public var topViewController: UIViewController? {
UIViewController.rat.topViewController(of: base)
}
/// 获取当前屏幕显示的 view controller
public static var topViewControllerOfKeyWindowRoot: UIViewController? {
guard let rootVC = UIApplication.shared.keyWindow?.rootViewController else { return nil }
let topVC = topViewController(of: rootVC)
return topVC
}
/// 获取 root view controller 的最上层视图
/// - Parameter rootViewController: 需要获取 top view controller 的根视图
/// - Returns: 获取到的 最上层视图
public static func topViewController(of rootViewController: UIViewController) -> UIViewController? {
var rootVC = rootViewController
var topVC: UIViewController? = nil
if rootVC.presentedViewController != nil {
// 根视图是被 present 出来的
rootVC = rootVC.presentedViewController!
}
if rootVC is UITabBarController {
// 根视图为 UITabBarController
let tabBarController = rootVC as! UITabBarController
topVC = topViewController(of: tabBarController.selectedViewController!)
} else if rootVC is UINavigationController {
// 根视图为 UINavigationController
let navigationController = rootVC as! UINavigationController
topVC = topViewController(of: navigationController.visibleViewController!)
} else {
// 根视图非导航类
topVC = rootVC
}
return topVC
}
/// 返回到指定页面
/// - Parameters:
/// - targetVC: 指定的页面
/// - animated: 是否开启动画
/// - Returns: 能否返回
public func pop(to targetVC: UIViewController.Type, animated: Bool = true) -> Bool {
var isSuccess = false
if let navigationController = base.navigationController {
for controller in navigationController.viewControllers where controller.isKind(of: targetVC) {
navigationController.popToViewController(controller, animated: animated)
isSuccess = true
break
}
}
return isSuccess
}
/// 返回到上一个页面 或 根视图
/// - Parameters:
/// - isPopToRoot: 是否返回根视图
/// - animated: 是否开启动画
public func popViewController(isPopToRoot: Bool = false, animated: Bool = true) {
if isPopToRoot {
base.navigationController?.popToRootViewController(animated: animated)
} else {
base.navigationController?.popViewController(animated: animated)
}
}
}
| mpl-2.0 | c84eb70263b465cb225b532192197460 | 29.45122 | 102 | 0.704045 | 4.693609 | false | false | false | false |
Quick/Nimble | Tests/NimbleTests/PollingTest.swift | 1 | 11075 | #if !os(WASI)
import Dispatch
import CoreFoundation
import Foundation
import XCTest
import Nimble
// swiftlint:disable:next type_body_length
final class PollingTest: XCTestCase {
class Error: Swift.Error {}
let errorToThrow = Error()
private func doThrowError() throws -> Int {
throw errorToThrow
}
func testToEventuallyPositiveMatches() {
var value = 0
deferToMainQueue { value = 1 }
expect { value }.toEventually(equal(1))
deferToMainQueue { value = 0 }
expect { value }.toEventuallyNot(equal(1))
}
func testToEventuallyNegativeMatches() {
let value = 0
failsWithErrorMessage("expected to eventually not equal <0>, got <0>") {
expect { value }.toEventuallyNot(equal(0))
}
failsWithErrorMessage("expected to eventually equal <1>, got <0>") {
expect { value }.toEventually(equal(1))
}
failsWithErrorMessage("unexpected error thrown: <\(errorToThrow)>") {
expect { try self.doThrowError() }.toEventually(equal(1))
}
failsWithErrorMessage("unexpected error thrown: <\(errorToThrow)>") {
expect { try self.doThrowError() }.toEventuallyNot(equal(0))
}
}
func testToEventuallySyncCase() {
expect(1).toEventually(equal(1), timeout: .seconds(300))
}
func testToEventuallyWithCustomDefaultTimeout() {
AsyncDefaults.timeout = .seconds(2)
defer {
AsyncDefaults.timeout = .seconds(1)
}
var value = 0
let sleepThenSetValueTo: (Int) -> Void = { newValue in
Thread.sleep(forTimeInterval: 1.1)
value = newValue
}
var asyncOperation: () -> Void = { sleepThenSetValueTo(1) }
DispatchQueue.global().async(execute: asyncOperation)
expect { value }.toEventually(equal(1))
asyncOperation = { sleepThenSetValueTo(0) }
DispatchQueue.global().async(execute: asyncOperation)
expect { value }.toEventuallyNot(equal(1))
}
func testWaitUntilWithCustomDefaultsTimeout() {
AsyncDefaults.timeout = .seconds(3)
defer {
AsyncDefaults.timeout = .seconds(1)
}
waitUntil { done in
Thread.sleep(forTimeInterval: 2.8)
done()
}
}
func testWaitUntilPositiveMatches() {
waitUntil { done in
done()
}
waitUntil { done in
deferToMainQueue {
done()
}
}
}
func testWaitUntilTimesOutIfNotCalled() {
failsWithErrorMessage("Waited more than 1.0 second") {
waitUntil(timeout: .seconds(1)) { _ in return }
}
}
func testWaitUntilTimesOutWhenExceedingItsTime() {
var waiting = true
failsWithErrorMessage("Waited more than 0.01 seconds") {
waitUntil(timeout: .milliseconds(10)) { done in
let asyncOperation: () -> Void = {
Thread.sleep(forTimeInterval: 0.1)
done()
waiting = false
}
DispatchQueue.global().async(execute: asyncOperation)
}
}
// "clear" runloop to ensure this test doesn't poison other tests
repeat {
RunLoop.main.run(until: Date().addingTimeInterval(0.2))
} while(waiting)
}
func testWaitUntilNegativeMatches() {
failsWithErrorMessage("expected to equal <2>, got <1>") {
waitUntil { done in
Thread.sleep(forTimeInterval: 0.1)
expect(1).to(equal(2))
done()
}
}
}
func testWaitUntilDetectsStalledMainThreadActivity() {
let msg = "-waitUntil() timed out but was unable to run the timeout handler because the main thread is unresponsive (0.5 seconds is allow after the wait times out). Conditions that may cause this include processing blocking IO on the main thread, calls to sleep(), deadlocks, and synchronous IPC. Nimble forcefully stopped run loop which may cause future failures in test run."
failsWithErrorMessage(msg) {
waitUntil(timeout: .seconds(1)) { done in
Thread.sleep(forTimeInterval: 3.0)
done()
}
}
}
func testCombiningAsyncWaitUntilAndToEventuallyIsNotAllowed() {
// Currently we are unable to catch Objective-C exceptions when built by the Swift Package Manager
#if !SWIFT_PACKAGE
let referenceLine = #line + 10
let msg = """
Unexpected exception raised: Nested async expectations are not allowed to avoid creating flaky tests.
The call to
\texpect(...).toEventually(...) at \(#file):\(referenceLine + 7)
triggered this exception because
\twaitUntil(...) at \(#file):\(referenceLine + 1)
is currently managing the main run loop.
"""
failsWithErrorMessage(msg) { // reference line
waitUntil(timeout: .seconds(2)) { done in
var protected: Int = 0
DispatchQueue.main.async {
protected = 1
}
expect(protected).toEventually(equal(1))
done()
}
}
#endif
}
func testWaitUntilErrorsIfDoneIsCalledMultipleTimes() {
failsWithErrorMessage("waitUntil(..) expects its completion closure to be only called once") {
waitUntil { done in
deferToMainQueue {
done()
done()
}
}
}
}
func testWaitUntilDoesNotCompleteBeforeRunLoopIsWaiting() {
#if canImport(Darwin)
// This verifies the fix for a race condition in which `done()` is
// called asynchronously on a background thread after the main thread checks
// for completion, but prior to `RunLoop.current.run(mode:before:)` being called.
// This race condition resulted in the RunLoop locking up.
var failed = false
let timeoutQueue = DispatchQueue(label: "Nimble.waitUntilTest.timeout", qos: .background)
let timer = DispatchSource.makeTimerSource(flags: .strict, queue: timeoutQueue)
timer.schedule(
deadline: DispatchTime.now() + 5,
repeating: .never,
leeway: .milliseconds(1)
)
timer.setEventHandler {
failed = true
fail("Timed out: Main RunLoop stalled.")
CFRunLoopStop(CFRunLoopGetMain())
}
timer.resume()
for index in 0..<100 {
if failed { break }
waitUntil(line: UInt(index)) { done in
DispatchQueue(label: "Nimble.waitUntilTest.\(index)").async {
done()
}
}
}
timer.cancel()
#endif // canImport(Darwin)
}
func testWaitUntilAllowsInBackgroundThread() {
#if !SWIFT_PACKAGE
var executedAsyncBlock: Bool = false
let asyncOperation: () -> Void = {
expect {
waitUntil { done in done() }
}.toNot(raiseException(named: "InvalidNimbleAPIUsage"))
executedAsyncBlock = true
}
DispatchQueue.global().async(execute: asyncOperation)
expect(executedAsyncBlock).toEventually(beTruthy())
#endif
}
func testToEventuallyAllowsInBackgroundThread() {
#if !SWIFT_PACKAGE
var executedAsyncBlock: Bool = false
let asyncOperation: () -> Void = {
expect {
expect(1).toEventually(equal(1))
}.toNot(raiseException(named: "InvalidNimbleAPIUsage"))
executedAsyncBlock = true
}
DispatchQueue.global().async(execute: asyncOperation)
expect(executedAsyncBlock).toEventually(beTruthy())
#endif
}
final class ClassUnderTest {
var deinitCalled: (() -> Void)?
var count = 0
deinit { deinitCalled?() }
}
func testSubjectUnderTestIsReleasedFromMemory() {
var subject: ClassUnderTest? = ClassUnderTest()
if let sub = subject {
expect(sub.count).toEventually(equal(0), timeout: .milliseconds(100))
expect(sub.count).toEventuallyNot(equal(1), timeout: .milliseconds(100))
}
waitUntil(timeout: .milliseconds(500)) { done in
subject?.deinitCalled = {
done()
}
deferToMainQueue { subject = nil }
}
}
func testToNeverPositiveMatches() {
var value = 0
deferToMainQueue { value = 1 }
expect { value }.toNever(beGreaterThan(1))
deferToMainQueue { value = 0 }
expect { value }.neverTo(beGreaterThan(1))
}
func testToNeverNegativeMatches() {
var value = 0
failsWithErrorMessage("expected to never equal <0>, got <0>") {
expect { value }.toNever(equal(0))
}
failsWithErrorMessage("expected to never equal <0>, got <0>") {
expect { value }.neverTo(equal(0))
}
failsWithErrorMessage("expected to never equal <1>, got <1>") {
deferToMainQueue { value = 1 }
expect { value }.toNever(equal(1))
}
failsWithErrorMessage("expected to never equal <1>, got <1>") {
deferToMainQueue { value = 1 }
expect { value }.neverTo(equal(1))
}
failsWithErrorMessage("unexpected error thrown: <\(errorToThrow)>") {
expect { try self.doThrowError() }.toNever(equal(0))
}
failsWithErrorMessage("unexpected error thrown: <\(errorToThrow)>") {
expect { try self.doThrowError() }.neverTo(equal(0))
}
}
func testToAlwaysPositiveMatches() {
var value = 1
deferToMainQueue { value = 2 }
expect { value }.toAlways(beGreaterThan(0))
deferToMainQueue { value = 2 }
expect { value }.alwaysTo(beGreaterThan(1))
}
func testToAlwaysNegativeMatches() {
var value = 1
failsWithErrorMessage("expected to always equal <0>, got <1>") {
expect { value }.toAlways(equal(0))
}
failsWithErrorMessage("expected to always equal <0>, got <1>") {
expect { value }.alwaysTo(equal(0))
}
failsWithErrorMessage("expected to always equal <1>, got <0>") {
deferToMainQueue { value = 0 }
expect { value }.toAlways(equal(1))
}
failsWithErrorMessage("expected to always equal <1>, got <0>") {
deferToMainQueue { value = 0 }
expect { value }.alwaysTo(equal(1))
}
failsWithErrorMessage("unexpected error thrown: <\(errorToThrow)>") {
expect { try self.doThrowError() }.toAlways(equal(0))
}
failsWithErrorMessage("unexpected error thrown: <\(errorToThrow)>") {
expect { try self.doThrowError() }.alwaysTo(equal(0))
}
}
}
#endif // #if !os(WASI)
| apache-2.0 | fa9144f24df402e4306e261e38d4bb79 | 32.662614 | 385 | 0.57833 | 4.993237 | false | true | false | false |
Minecodecraft/50DaysOfSwift | Day 12 - LrcShareTool/LrcShareTool/LrcShareTool/MCLrcDecoder.swift | 1 | 1186 | //
// MCLrcDecoder.swift
// LrcShareTool
//
// Created by Minecode on 2017/12/5.
// Copyright © 2017年 Minecode. All rights reserved.
//
import UIKit
class MCLrcDecoder: NSObject {
// 存储歌词的数组
lazy var lrcArray = [String]()
// 存储歌词时间的数组
lazy var timeArray = [String]()
// 歌词文件名
var fileName = ""
public func loadLrc(fileName: String) -> NSString {
let filePath = Bundle.main.path(forResource: fileName, ofType: "lrc")
let data = NSData.init(contentsOfFile: filePath!)
return NSString(data: data! as Data, encoding: String.Encoding.utf8.rawValue)!
}
func decodeLrc(lrcString: NSString) {
var sepArray = lrcString.components(separatedBy: "[")
var lineArray = [String]()
for i in 0..<sepArray.count {
if sepArray[i].count > 0 {
lineArray = sepArray[i].components(separatedBy: "]");
if (lineArray[0] != "\n") {
self.timeArray.append(lineArray[0])
self.lrcArray.append(lineArray.count>1 ? lineArray[1] : "")
}
}
}
}
}
| mit | b1d4d6400a4dcfada8f013807e13d970 | 27.525 | 86 | 0.567923 | 3.816054 | false | false | false | false |
sammyd/Concurrency-VideoSeries | projects/005_CancellingOperations/005_ChallengeComplete/Cancellation.playground/Contents.swift | 1 | 2462 | import Foundation
//: # Operation Cancellation
//: It's important to make sure that individual operations respect the `cancelled` property, and therefore can be cancelled.
//:
//: However, in addition to cancelling a single operation you can cancel all the operations on a queue. This is especially helpful when you have a collection of operations all working towards a single goal - either splitting up an operation to run in parallel or a dependency graph of operations acting as a pipeline.
//:
//: In this challenge you will achieve the same as in the demo, but by using lots of the following single-shot `SumOperation`s:
class SumOperation: NSOperation {
let inputPair: (Int, Int)
var output: Int?
init(input: (Int, Int)) {
inputPair = input
super.init()
}
override func main() {
if cancelled { return }
output = slowAdd(inputPair)
}
}
//: `GroupAdd` is a vanilla class that manages an operation queue and multiple `SumOperation`s to calculate the sum of all the pairs in the input array.
class GroupAdd {
let queue = NSOperationQueue()
let appendQueue = NSOperationQueue()
var outputArray = [(Int, Int, Int)]()
init(input: [(Int, Int)]) {
queue.suspended = true
queue.maxConcurrentOperationCount = 2
appendQueue.maxConcurrentOperationCount = 1
generateOperations(input)
}
func generateOperations(numberArray: [(Int, Int)]) {
for pair in numberArray {
let operation = SumOperation(input: pair)
operation.completionBlock = {
guard let result = operation.output else { return }
appendQueue.addOperationWithBlock {
self.outputArray.append((pair.0, pair.1, result))
}
}
queue.addOperation(operation)
}
}
func start() {
// TODO: implement this
queue.suspended = false
}
func cancel() {
// TODO: implement this
queue.cancelAllOperations()
}
func wait() {
queue.waitUntilAllOperationsAreFinished()
}
}
//: Input array
let numberArray = [(1,2), (3,4), (5,6), (7,8), (9,10)]
//: Create the group add
let groupAdd = GroupAdd(input: numberArray)
//: Start the operation and
startClock()
groupAdd.start()
//: __TODO:__ Try uncommenting this call to `cancel()` to check that you've implemented it correctly. You should see the `stopClock()` result reduce, as should the length of the output.
groupAdd.cancel()
groupAdd.wait()
stopClock()
//: Inspect the results
groupAdd.outputArray
| mit | b9ac0c029142ace860b040d2fcdf1e78 | 28.309524 | 317 | 0.693745 | 4.252159 | false | false | false | false |
IBM-MIL/IBM-Ready-App-for-Retail | iOS/ReadyAppRetail/Summit WatchKit Extension/Controllers/InterfaceController.swift | 1 | 1778 | /*
Licensed Materials - Property of IBM
© Copyright IBM Corporation 2015. All Rights Reserved.
*/
import WatchKit
import Foundation
class InterfaceController: WKInterfaceController {
@IBOutlet weak var listLabel: WKInterfaceLabel!
@IBOutlet weak var storeLabel: WKInterfaceLabel!
@IBOutlet weak var salesLabel: WKInterfaceLabel!
override func awakeWithContext(context: AnyObject?) {
super.awakeWithContext(context)
}
override func willActivate() {
// This method is called when watch view controller is about to be visible to user
super.willActivate()
print("WatchKit App is open")
let font = UIFont.systemFontOfSize(12)
let attirbuteData = [NSFontAttributeName : font]
var labelText = NSAttributedString(string: "View Lists", attributes: attirbuteData)
self.listLabel.setAttributedText(labelText)
labelText = NSAttributedString(string: "Locate Store", attributes: attirbuteData)
self.storeLabel.setAttributedText(labelText)
labelText = NSAttributedString(string: "Current Sales", attributes: attirbuteData)
self.salesLabel.setAttributedText(labelText)
}
override func didDeactivate() {
// This method is called when watch view controller is no longer visible
super.didDeactivate()
}
@IBAction func viewLists() {
self.pushControllerWithName("ListOverviewInterface", context: nil)
}
@IBAction func requestData() {
let request = ProductDataRequest(productId: "0000000002")
ParentAppDataManager.sharedInstance.execute(request, retry: true, result: { (resultDictionary) -> () in
print("REsults: \(resultDictionary)")
})
}
}
| epl-1.0 | db9c0119a928b2f425536c0aadc68816 | 31.309091 | 111 | 0.687113 | 5.106322 | false | false | false | false |
JovannyEspinal/FirebaseJokes-iOS | FirebaseJokes/JokeCellTableViewCell.swift | 1 | 2718 | //
// JokeCellTableViewCell.swift
// FirebaseJokes
//
// Created by Matthew Maher on 1/23/16.
// Copyright © 2016 Matt Maher. All rights reserved.
//
import UIKit
import Firebase
class JokeCellTableViewCell: UITableViewCell {
@IBOutlet weak var jokeText: UITextView!
@IBOutlet weak var usernameLabel: UILabel!
@IBOutlet weak var totalVotesLabel: UILabel!
@IBOutlet weak var thumbVoteImage: UIImageView!
var joke: Joke!
var voteRef: Firebase!
override func awakeFromNib() {
super.awakeFromNib()
// UITapGestureRecognizer
let tap = UITapGestureRecognizer(target: self, action: "voteTapped:")
tap.numberOfTapsRequired = 1
thumbVoteImage.addGestureRecognizer(tap)
thumbVoteImage.userInteractionEnabled = true
}
func configureCell(joke: Joke) {
self.joke = joke
// Set the labesl and textView
jokeText.text = joke.jokeText
totalVotesLabel.text = "Total votes: \(joke.jokeVotes)"
usernameLabel.text = joke.username
//Set votes as a child of the current user in Firebase and save the joke's key in votes as a boolean
voteRef = DataService.dataService.CURRENT_USER_REF.childByAppendingPath("votes").childByAppendingPath(joke.jokeKey)
//observeSingleEventOfType() listens for the thumb to be tapped, by any user, on any device
voteRef.observeEventType(.Value, withBlock: { snapshot in
// Set the thumb image
if let thumbsUpDown = snapshot.value as? NSNull {
//Current user hasn't voted for the joke...yet
print(thumbsUpDown)
} else {
// Current user voted for the joke!
self.thumbVoteImage.image = UIImage(named: "thumb-up")
}
})
}
func voteTapped(sender: UITapGestureRecognizer) {
//observeSingleEventOfType listens for a tap by the current user/
voteRef.observeSingleEventOfType(.Value, withBlock: { snapshot in
if let thumbsUpDown = snapshot.value as? NSNull {
print(thumbsUpDown)
self.thumbVoteImage.image = UIImage(named: "thumb-down")
//addSubtractVote(), in Joke.swift handles the vote
self.joke.addSubtractVote(true)
} else {
self.thumbVoteImage.image = UIImage(named: "thumb-up")
self.joke.addSubtractVote(false)
self.voteRef.removeValue()
}
})
}
}
| mit | 66c4cf60f6e4c50c8b1250eb686f7983 | 31.345238 | 123 | 0.590357 | 5.06903 | false | false | false | false |
baottran/nSURE | nSURE/PFCustomerList.swift | 1 | 5023 | //
// PFCustomerList.swift
// nSURE
//
// Created by Bao on 6/23/15.
// Copyright (c) 2015 Sprout Designs. All rights reserved.
//
import UIKit
protocol CustomerSelectionDelegate: class {
func customerSelected(newCustomer: PFObject)
}
class PFCustomerList: PFQueryTableViewController, UISearchBarDelegate {
// ========================
// Mark: = Setup
// ========================
@IBOutlet weak var searchBar: UISearchBar!
var customers: [Customer]?
weak var delegate: CustomerSelectionDelegate?
weak var intakeDelegate: CustomerSelectionDelegate?
override func viewDidAppear(animated: Bool) {
tableView.reloadData()
searchBar.delegate = self
}
// ========================
// Mark: - Search Bar Delegate Methods
// ========================
func searchBarTextDidEndEditing(searchBar: UISearchBar) {
// Dismiss the keyboard
// Force reload of table data
searchBar.resignFirstResponder()
self.loadObjects()
}
func searchBar(searchBar: UISearchBar, textDidChange searchText: String) {
self.loadObjects()
}
func searchBarSearchButtonClicked(searchBar: UISearchBar) {
// Dismiss the keyboard
// Force reload of table data
searchBar.resignFirstResponder()
self.loadObjects()
}
func searchBarCancelButtonClicked(searchBar: UISearchBar) {
// Clear any search criteria
searchBar.text = ""
// Dismiss the keyboard
searchBar.resignFirstResponder()
// Force reload of table data
self.loadObjects()
}
// ========================
// Mark: - Initialise the PFQueryTable tableview
// ========================
override init(style: UITableViewStyle, className: String!) {
super.init(style: style, className: className)
}
required init(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)!
// Configure the PFQueryTableView
self.parseClassName = "Customer"
self.textKey = "firstName"
self.pullToRefreshEnabled = true
self.paginationEnabled = false
title = "Customers"
}
// ========================
// Define the query that will provide the data for the table view
// ========================
override func queryForTable() -> PFQuery {
let query = PFQuery(className: "Customer")
if searchBar.text != "" {
query.whereKey("searchString", containsString: searchBar.text)
print("searching", terminator: "")
}
query.orderByAscending("lastName")
return query
}
// ========================
// Mark: - Tableview Methods
// ========================
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath, object: PFObject?) -> PFTableViewCell {
var cell = tableView.dequeueReusableCellWithIdentifier("CustomerItem") as! PFTableViewCell!
if cell == nil {
cell = PFTableViewCell(style: UITableViewCellStyle.Default, reuseIdentifier: "CustomerItem")
}
if let firstName = object?["firstName"] as? String {
if let lastName = object?["lastName"] as? String {
cell?.textLabel?.text = "\(lastName), \(firstName)"
}
}
if let defaultPhone = object?["defaultPhone"] as? PFObject
{
queryForPhoneNumber(defaultPhone.objectId!, cell: cell)
}
return cell
}
override func tableView(tableView: UITableView, didSelectRowAtIndexPath indexPath: NSIndexPath){
tableView.deselectRowAtIndexPath(indexPath, animated: true)
if let customer = objects as? [PFObject]{
// self.performSegueWithIdentifier("ViewCustDetail", sender: nil)
self.delegate?.customerSelected(customer[indexPath.row])
self.intakeDelegate?.customerSelected(customer[indexPath.row])
} else {
print("object not converted", terminator: "")
}
}
// ========================
// Mark: - Cell Load Helpers
// ========================
func queryForPhoneNumber(objID: String, cell: PFTableViewCell){
let query = PFQuery(className: "Phone")
query.getObjectInBackgroundWithId(objID){ result, error in
if (result != nil) {
if let phone = result as PFObject? {
if let phoneNumber = phone["number"] as? String {
cell.detailTextLabel?.text = phoneNumber
}
}
} else {
print("error", terminator: "")
}
}
}
}
| mit | 78680c859f2c820dbbe2c4ce2f007f2b | 27.867816 | 137 | 0.541509 | 5.707955 | false | false | false | false |
Eonil/EditorLegacy | Modules/EditorFileTreeNavigationFeature/EditorFileTreeNavigationFeature/FileNavigating/FileSystemMonitor3.swift | 1 | 3207 | ////
//// FileSystemMonitor3.swift
//// Editor
////
//// Created by Hoon H. on 2015/01/13.
//// Copyright (c) 2015 Eonil. All rights reserved.
////
//
//import Foundation
//import EonilDispatch
//import EonilFileSystemEvents
//import Precompilation
//
//
//
//
//
//
///// This starts monitoring automatically when created, and stops when deallocated.
///// This notifies all events immediately on the specified GCD queue.
//final class FileSystemMonitor3 {
// /// We don't need to know what happens because whatever it happens it might not be exist at point of receive
// /// due to nature of asynchronous notification.
// init(monitoringRootURL:NSURL, callback:(NSURL)->()) {
// assert(NSThread.currentThread() == NSThread.mainThread())
//
//// let sm = SuspensionManager()
//// sm.callback = callback
//
// self.queue = Queue(label: "FileSystemMonitor2/EventStreamQueue", attribute: Queue.Attribute.Serial)
// self.stream = EonilFileSystemEventStream(callback: { (events:[AnyObject]!) -> Void in
// async(Queue.main, {
// for e1 in events as [EonilFileSystemEvent] {
// let isDir = (e1.flag & EonilFileSystemEventFlag.ItemIsDir) == EonilFileSystemEventFlag.ItemIsDir
// let u1 = NSURL(fileURLWithPath: e1.path, isDirectory: isDir)!
//
// callback(u1)
//// sm.routeEvent(u1)
// }
// })
// }, pathsToWatch: [monitoringRootURL.path!], latency:1, watchRoot: false, queue: rawObjectOf(queue))
//
//// self.susman = sm
// }
//
//// var isPending:Bool {
//// get {
//// return susman.isPending
//// }
//// }
////
//// /// Suspends file-system monitoring event callback dispatch until you call `resume`.
//// /// Any events occured during suspension will be queued and dispatched at resume.
//// func suspendEventCallbackDispatch() {
//// susman.suspendEventCallbackDispatch()
//// }
//// func resumeEventCallbackDispatch() {
//// susman.resumeEventCallbackDispatch()
//// }
//
// ////
//
//// private let susman:SuspensionManager
//
// private let queue:Queue
// private let stream:EonilFileSystemEventStream
//}
//
//
//
//
//
//
//
//
//
//
//
//
//
//
////private final class SuspensionManager {
//// init() {
//// }
////
//// func routeEvent(u:NSURL) {
//// if is_pending {
//// pending_events.append(u)
//// } else {
//// callback!(u)
//// }
//// }
////
////
////
//// var isPending:Bool {
//// get {
//// return is_pending
//// }
//// }
////
//// /// Suspends file-system monitoring event callback dispatch until you call `resume`.
//// /// Any events occured during suspension will be queued and dispatched at resume.
//// func suspendEventCallbackDispatch() {
//// precondition(is_pending == false)
//// Debug.log("FS monitoring suspended")
////
//// is_pending = true
//// }
//// func resumeEventCallbackDispatch() {
//// precondition(is_pending == true)
//// Debug.log("FS monitoring resumed")
////
//// pending_events.map { [unowned self] u in
//// self.callback!(u)
//// }
//// pending_events = []
//// is_pending = false
//// }
////
//// ////
////
//// private var pending_events = [] as [NSURL]
//// private var is_pending = false
////
//// private var callback:((NSURL)->())?
////}
//
//
//
//
| mit | 51822cd315a972758d6d873c036ad389 | 23.480916 | 111 | 0.622077 | 3.159606 | false | false | false | false |
64characters/Telephone | Domain/NullSystemAudioDevice.swift | 1 | 935 | //
// NullSystemAudioDevice.swift
// Telephone
//
// Copyright © 2008-2016 Alexey Kuznetsov
// Copyright © 2016-2022 64 Characters
//
// Telephone is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// Telephone is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
public struct NullSystemAudioDevice: SystemAudioDevice {
public let identifier = -1
public let uniqueIdentifier = ""
public let name = ""
public let inputs = 0
public let outputs = 0
public let isBuiltIn = false
public let isNil = true
public init() {}
}
| gpl-3.0 | e629f747996e4a40e93b95fdd31449d1 | 31.172414 | 72 | 0.714898 | 4.299539 | false | false | false | false |
binarylevel/Tinylog-iOS | Tinylog/Views/Cells/TLITextFieldCell.swift | 1 | 3409 | //
// TLITextFieldCell.swift
// Tinylog
//
// Created by Spiros Gerokostas on 18/10/15.
// Copyright © 2015 Spiros Gerokostas. All rights reserved.
//
import UIKit
class TLITextFieldCell: UITableViewCell, UITextFieldDelegate, TLITextFieldCellDelegate {
var textField:TLITextField?
var indexPath:NSIndexPath?
var delegate:TLITextFieldCellDelegate?
override init(style: UITableViewCellStyle, reuseIdentifier: String?) {
super.init(style: style, reuseIdentifier: reuseIdentifier)
self.backgroundColor = UIColor.clearColor()
textField = TLITextField(frame: CGRectZero)
textField?.clearsOnBeginEditing = false
textField?.clearButtonMode = UITextFieldViewMode.WhileEditing
textField?.textAlignment = NSTextAlignment.Left
textField?.contentVerticalAlignment = UIControlContentVerticalAlignment.Center
textField?.keyboardAppearance = UIKeyboardAppearance.Light
textField?.adjustsFontSizeToFitWidth = true
textField?.delegate = self
textField?.font = UIFont.regularFontWithSize(17.0)
textField?.textColor = UIColor.tinylogTextColor()
textField?.clearButtonEdgeInsets = UIEdgeInsetsMake(0.0, 0.0, 0.0, -20.0)
self.contentView.addSubview(textField!)
}
required init(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
deinit {
delegate = nil
textField?.resignFirstResponder()
}
override func layoutSubviews() {
super.layoutSubviews()
let frame:CGRect = self.contentView.frame
if textField?.text != nil || textField?.placeholder != nil {
textField?.hidden = false
textField?.frame = CGRectMake(frame.origin.x + 16.0, frame.origin.y, frame.size.width, frame.size.height)
textField?.autocapitalizationType = UITextAutocapitalizationType.None
}
self.setNeedsDisplay()
}
func textFieldShouldReturn(textField: UITextField) -> Bool {
let value = delegate?.shouldReturnForIndexPath!(indexPath!, value: textField.text!)
if value != nil {
textField.resignFirstResponder()
}
return value!
}
func textField(textField: UITextField, shouldChangeCharactersInRange range: NSRange, replacementString string: String) -> Bool {
var textString:NSString = textField.text!
textString = textString.stringByReplacingCharactersInRange(range, withString: string)
delegate?.updateTextLabelAtIndexPath!(indexPath!, value: textString as String)
return true
}
func textFieldShouldClear(textField: UITextField) -> Bool {
delegate?.updateTextLabelAtIndexPath!(indexPath!, value: textField.text!)
return true
}
func textFieldShouldBeginEditing(textField: UITextField) -> Bool {
delegate?.textFieldShouldBeginEditing!(textField)
return true
}
func textFieldShouldEndEditing(textField: UITextField) -> Bool {
delegate?.textFieldShouldEndEditing!(textField)
return true
}
func textFieldDidBeginEditing(textField: UITextField) {
delegate?.textFieldDidBeginEditing!(textField)
}
func textFieldDidEndEditing(textField: UITextField) {
delegate?.textFieldDidEndEditing!(textField)
}
}
| mit | 64e28a6486f40c9d6d884d0eeb459ece | 34.873684 | 132 | 0.682805 | 5.633058 | false | false | false | false |
wordpress-mobile/WordPress-Aztec-iOS | Aztec/Classes/Converters/ElementsToAttributedString/Implementations/VideoElementConverter.swift | 2 | 3714 | import UIKit
/// Provides a representation for `<video>` element.
///
class VideoElementConverter: AttachmentElementConverter {
// MARK: - AttachmentElementConverter
typealias AttachmentType = VideoAttachment
func convert(
_ element: ElementNode,
inheriting attributes: [NSAttributedString.Key: Any],
contentSerializer serialize: ContentSerializer) -> (attachment: VideoAttachment, string: NSAttributedString) {
let attachment = self.attachment(for: element)
let intrinsicRepresentation = NSAttributedString(attachment: attachment, attributes: attributes)
let serialization = serialize(element, intrinsicRepresentation, attributes, false)
return (attachment, serialization)
}
// MARK: - Attachment Creation
func attachment(for element: ElementNode) -> VideoAttachment {
var extraAttributes = [Attribute]()
for attribute in element.attributes {
if let value = attribute.value.toString() {
extraAttributes[attribute.name] = .string(value)
}
}
let srcURL: URL?
let srcAttribute = element.attributes.first(where: { $0.name == "src" })
if let urlString = srcAttribute?.value.toString() {
srcURL = URL(string: urlString)
extraAttributes.remove(named: "src")
} else {
srcURL = nil
}
let posterURL: URL?
let posterAttribute = element.attributes.first(where: { $0.name == "poster" })
if let urlString = posterAttribute?.value.toString() {
posterURL = URL(string: urlString)
extraAttributes.remove(named: "poster")
} else {
posterURL = nil
}
let sources = extractSources(from: element)
let attachment = VideoAttachment(identifier: UUID().uuidString, srcURL: srcURL, posterURL: posterURL, sources: sources)
attachment.extraAttributes = extraAttributes
return attachment
}
/// This method search for source elements on the children of the video element and return an array of VideoSource with the corresponding information.<#Description#>
///
/// - Parameter element: the video element to search for sources
/// - Returns: an array with the source information found
///
private func extractSources(from element: ElementNode) -> [VideoSource] {
var children = element.children
//search for source subelements
let sources = searchSources(in: element)
children.removeAll(where: { $0 is ElementNode && $0.name == "source"})
element.children = children
return sources
}
/// This method recursively searches for source elements on the children of element provided and return an array of VideoSource with the corresponding information.
///
/// - Parameter element: the element to search for sources
/// - Returns: an array with the source information found
///
private func searchSources(in element: ElementNode) -> [VideoSource] {
var sources:[VideoSource] = []
for node in element.children {
guard let sourceElement = node as? ElementNode, sourceElement.name == "source" else {
continue
}
let src = sourceElement.attributes.first(where: { $0.name == "src"} )?.value.toString()
let type = sourceElement.attributes.first(where: { $0.name == "type"} )?.value.toString()
sources.append(VideoSource(src: src, type: type) )
sources.append(contentsOf: searchSources(in: sourceElement))
}
return sources
}
}
| gpl-2.0 | fb13332a56a19ecbcb39a3c4d0ba1490 | 36.515152 | 169 | 0.637588 | 5.087671 | false | false | false | false |
ra1028/OwnKit | OwnKit/Util/DynamicDefaults.swift | 1 | 2194 | //
// DynamicDefaults.swift
// OwnKit
//
// Created by Ryo Aoyama on 3/3/16.
// Copyright © 2016 Ryo Aoyama. All rights reserved.
//
import Foundation
public class DynamicDefaults: NSObject {
let userDefaults = NSUserDefaults.standardUserDefaults()
public override init() {
super.init()
registerDefaults()
setupProperty()
addObserver()
}
deinit {
removeObserver()
}
}
extension DynamicDefaults {
override public func observeValueForKeyPath(keyPath: String?, ofObject object: AnyObject?, change: [String : AnyObject]?, context: UnsafeMutablePointer<Void>) {
if let keyPath = keyPath {
if let value = change?["new"] where !(value is NSNull) {
userDefaults.setObject(value is NSCoding ? NSKeyedArchiver.archivedDataWithRootObject(value) : value, forKey: storeKey(keyPath))
}else{
userDefaults.removeObjectForKey(storeKey(keyPath))
}
userDefaults.synchronize()
}
}
}
private extension DynamicDefaults {
func storeKey(propertyName: String) -> String{
return "\(self.dynamicType)_\(propertyName)"
}
func registerDefaults() {
var dic = [String: AnyObject]()
propertyNames.forEach {
dic[storeKey($0)] = valueForKey($0)
}
userDefaults.registerDefaults(dic)
}
func setupProperty() {
propertyNames.forEach {
let value = userDefaults.objectForKey(storeKey($0))
if let data = value as? NSData, decodedValue = NSKeyedUnarchiver.unarchiveObjectWithData(data){
setValue(decodedValue, forKey: $0)
}else{
setValue(value, forKey: $0)
}
}
}
func addObserver() {
propertyNames.forEach {
addObserver(self, forKeyPath: $0, options: .New, context: nil)
}
}
func removeObserver() {
propertyNames.forEach {
removeObserver(self, forKeyPath: $0)
}
}
var propertyNames: [String] {
return Mirror(reflecting: self).children.flatMap { $0.label }
}
} | mit | 2fada27a96fd4e4bfe55756128745d3e | 26.772152 | 164 | 0.594163 | 4.90604 | false | false | false | false |
KoheiHayakawa/KHATableViewWithSeamlessScrollingHeaderView | KHATableViewWithSeamlessScrollingHeaderView/KHATableViewWithSeamlessScrollingHeaderViewController.swift | 1 | 8103 | //
// KHATableViewWithSeamlessScrollingHeaderViewController.swift
// KHATableViewWithSeamlessScrollingHeaderView
//
// Created by Kohei Hayakawa on 12/24/15.
// Copyright © 2015 Kohei Hayakawa. All rights reserved.
//
import UIKit
public protocol KHATableViewWithSeamlessScrollingHeaderViewDataSource {
func headerViewInView(view: KHATableViewWithSeamlessScrollingHeaderViewController) -> UIView
}
public class KHATableViewWithSeamlessScrollingHeaderViewController: UIViewController, UITableViewDelegate, UITableViewDataSource, KHATableViewWithSeamlessScrollingHeaderViewDataSource {
public var tableView: UITableView!
public var headerView = UIView(frame: CGRect(x: 0, y: 0, width: 0, height: 0))
public var navigationBarBackgroundColor = UIColor.whiteColor()
public var navigationBarTitleColor = UIColor.blackColor()
public var navigationBarShadowColor = UIColor.lightGrayColor()
public var statusBarStyle = UIApplication.sharedApplication().statusBarStyle
private let previousStatusBarStyle = UIApplication.sharedApplication().statusBarStyle
override public func viewDidLoad() {
headerView = headerViewInView(self)
super.viewDidLoad()
let statusBarHeight = UIApplication.sharedApplication().statusBarFrame.height
let navBarHeight = self.navigationController?.navigationBar.frame.size.height ?? 0
let headerViewHeight = headerView.frame.size.height
navigationController?.setNavigationBarHidden(false, animated: false)
navigationController?.navigationBar.tintColor = UIColor.blackColor()
headerView.translatesAutoresizingMaskIntoConstraints = false
tableView = UITableView(frame: self.view.frame, style: .Plain)
tableView.contentInset = UIEdgeInsetsMake(headerView.frame.size.height, 0, 0, 0)
tableView.scrollIndicatorInsets = UIEdgeInsetsMake(headerView.frame.size.height, 0, 0, 0)
tableView.translatesAutoresizingMaskIntoConstraints = false
tableView.registerClass(UITableViewCell.self, forCellReuseIdentifier: "id")
tableView.dataSource = self
tableView.delegate = self
view.addSubview(tableView)
view.addSubview(headerView)
view.addConstraints([
NSLayoutConstraint(
item: tableView,
attribute: .Left,
relatedBy: .Equal,
toItem: view,
attribute: .Left,
multiplier: 1,
constant: 0),
NSLayoutConstraint(
item: tableView,
attribute: .Right,
relatedBy: .Equal,
toItem: view,
attribute: .Right,
multiplier: 1,
constant: 0),
NSLayoutConstraint(
item: tableView,
attribute: .Top,
relatedBy: .Equal,
toItem: view,
attribute: .Top,
multiplier: 1,
constant: 0),
NSLayoutConstraint(
item: tableView,
attribute: .Bottom,
relatedBy: .Equal,
toItem: view,
attribute: .Bottom,
multiplier: 1,
constant: 0)]
)
view.addConstraints([
NSLayoutConstraint(
item: headerView,
attribute: .Left,
relatedBy: .Equal,
toItem: view,
attribute: .Left,
multiplier: 1,
constant: 0),
NSLayoutConstraint(
item: headerView,
attribute: .Right,
relatedBy: .Equal,
toItem: view,
attribute: .Right,
multiplier: 1,
constant: 0),
NSLayoutConstraint(
item: headerView,
attribute: .Top,
relatedBy: .Equal,
toItem: view,
attribute: .Top,
multiplier: 1,
constant: statusBarHeight+navBarHeight),
NSLayoutConstraint(
item: headerView,
attribute: .Height,
relatedBy: .Equal,
toItem: nil,
attribute: .NotAnAttribute,
multiplier: 1,
constant: headerViewHeight)]
)
}
override public func viewWillAppear(animated: Bool) {
UIApplication.sharedApplication().statusBarStyle = statusBarStyle
}
override public func viewWillDisappear(animated: Bool) {
self.navigationController?.setNavigationBarHidden(true, animated: true)
UIApplication.sharedApplication().statusBarStyle = previousStatusBarStyle
}
override public func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
public
func headerViewInView(view: KHATableViewWithSeamlessScrollingHeaderViewController) -> UIView {
return headerView
}
// MARK: - UIScrollViewDelegate
public func scrollViewDidScroll(scrollView: UIScrollView) {
let statusBarHeight = UIApplication.sharedApplication().statusBarFrame.height
let navBarHeight = self.navigationController?.navigationBar.frame.size.height ?? 0
let headerViewHeight = headerView.bounds.size.height
let tableViewOriginY = statusBarHeight + navBarHeight + headerViewHeight
if tableView.contentOffset.y + tableViewOriginY <= 0 {
headerView.frame = CGRectMake(headerView.bounds.origin.x,
statusBarHeight + navBarHeight,
headerView.bounds.size.width,
headerView.bounds.size.height)
setColorWithAlpha(0.0)
} else {
headerView.frame = CGRectMake(headerView.bounds.origin.x,
-(tableView.contentOffset.y + headerViewHeight),
headerView.bounds.size.width,
headerViewHeight)
let alpha = (tableView.contentOffset.y + tableViewOriginY) / tableViewOriginY
setColorWithAlpha(alpha)
}
}
// MARK: - Color handler
/*! Seemlessly transparenting three colors of navbar background, navbar shadow and navbar title
*/
private func setColorWithAlpha(alpha: CGFloat) {
let alphaForNavBar = 8 * (alpha - 0.5)
headerView.alpha = 1 - 3 * alpha
self.navigationController?.navigationBar.setBackgroundImage(self.colorImage(navigationBarBackgroundColor.colorWithAlphaComponent(alphaForNavBar), size: CGSize(width: 1, height: 1)), forBarMetrics: .Default)
self.navigationController?.navigationBar.shadowImage = self.colorImage(navigationBarShadowColor.colorWithAlphaComponent(alphaForNavBar), size: CGSize(width: 1, height: 1))
self.navigationController?.navigationBar.titleTextAttributes = [NSForegroundColorAttributeName: navigationBarTitleColor.colorWithAlphaComponent(alphaForNavBar)]
}
/*! Create UIImage from UIColor
*/
private func colorImage(color: UIColor, size: CGSize) -> UIImage {
UIGraphicsBeginImageContext(size)
let rect = CGRect(origin: CGPointZero, size: size)
let context = UIGraphicsGetCurrentContext()
CGContextSetFillColorWithColor(context, color.CGColor)
CGContextFillRect(context, rect)
let image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return image
}
// MARK: - UITableViewDataSource
public func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 0
}
public func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("id", forIndexPath: indexPath)
return cell
}
} | mit | 7d463141ced180ebd80c625f68d26695 | 39.113861 | 214 | 0.630585 | 5.931186 | false | false | false | false |
liushuaikobe/fargo | fargo/GooglFargoTask.swift | 1 | 1393 | //
// GooglFargoTask.swift
// fargo
//
// Created by Shuai Liu on 16/1/8.
// Copyright © 2016年 vars.me. All rights reserved.
//
import Foundation
public class GooglFargoTask: FargoTask {
let api_key: String
public init(_ apiKey: String) {
api_key = apiKey
}
override func apiURL() -> String {
return "https://www.googleapis.com/urlshortener/v1/url?key=\(api_key)"
}
override func httpMethod() -> FargoHTTPMethod {
return .POST
}
override func postData(url: String?) -> NSData? {
if let param = url {
let body = ["longUrl": param]
do {
return try NSJSONSerialization.dataWithJSONObject(body, options: [])
} catch {
return nil
}
}
return nil
}
override func parseResult(data: NSData?) -> String? {
if let result = data {
do {
if let json = try NSJSONSerialization.JSONObjectWithData(result, options: []) as? [String: String] {
return json["id"]
} else {
return nil
}
} catch {
return nil
}
}
return nil
}
override func headers() -> [String : String] {
return ["Content-Type": "application/json"]
}
} | gpl-3.0 | d85534b6d3c6c46d4ccc9cfad6313802 | 23.403509 | 116 | 0.502878 | 4.512987 | false | false | false | false |
thehorbach/flickrSearch | FlickrSearch/FlickrFiles/Flickr.swift | 1 | 5927 | /**
* Copyright (c) 2016 Razeware LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
import UIKit
let apiKey = "426749f766377c88df06ae819e5faf4a"
class Flickr {
let processingQueue = OperationQueue()
func searchFlickrForTerm(_ searchTerm: String, completion : @escaping (_ results: FlickrSearchResults?, _ error : NSError?) -> Void){
guard let searchURL = flickrSearchURLForSearchTerm(searchTerm) else {
let APIError = NSError(domain: "FlickrSearch", code: 0, userInfo: [NSLocalizedFailureReasonErrorKey:"Unknown API response"])
completion(nil, APIError)
return
}
let searchRequest = URLRequest(url: searchURL)
URLSession.shared.dataTask(with: searchRequest, completionHandler: { (data, response, error) in
if let _ = error {
let APIError = NSError(domain: "FlickrSearch", code: 0, userInfo: [NSLocalizedFailureReasonErrorKey:"Unknown API response"])
OperationQueue.main.addOperation({
completion(nil, APIError)
})
return
}
guard let _ = response as? HTTPURLResponse,
let data = data else {
let APIError = NSError(domain: "FlickrSearch", code: 0, userInfo: [NSLocalizedFailureReasonErrorKey:"Unknown API response"])
OperationQueue.main.addOperation({
completion(nil, APIError)
})
return
}
do {
guard let resultsDictionary = try JSONSerialization.jsonObject(with: data, options: JSONSerialization.ReadingOptions(rawValue: 0)) as? [String: AnyObject],
let stat = resultsDictionary["stat"] as? String else {
let APIError = NSError(domain: "FlickrSearch", code: 0, userInfo: [NSLocalizedFailureReasonErrorKey:"Unknown API response"])
OperationQueue.main.addOperation({
completion(nil, APIError)
})
return
}
switch (stat) {
case "ok":
print("Results processed OK")
case "fail":
if let message = resultsDictionary["message"] {
let APIError = NSError(domain: "FlickrSearch", code: 0, userInfo: [NSLocalizedFailureReasonErrorKey:message])
OperationQueue.main.addOperation({
completion(nil, APIError)
})
}
let APIError = NSError(domain: "FlickrSearch", code: 0, userInfo: nil)
OperationQueue.main.addOperation({
completion(nil, APIError)
})
return
default:
let APIError = NSError(domain: "FlickrSearch", code: 0, userInfo: [NSLocalizedFailureReasonErrorKey:"Unknown API response"])
OperationQueue.main.addOperation({
completion(nil, APIError)
})
return
}
guard let photosContainer = resultsDictionary["photos"] as? [String: AnyObject], let photosReceived = photosContainer["photo"] as? [[String: AnyObject]] else {
let APIError = NSError(domain: "FlickrSearch", code: 0, userInfo: [NSLocalizedFailureReasonErrorKey:"Unknown API response"])
OperationQueue.main.addOperation({
completion(nil, APIError)
})
return
}
var flickrPhotos = [FlickrPhoto]()
for photoObject in photosReceived {
guard let photoID = photoObject["id"] as? String,
let farm = photoObject["farm"] as? Int ,
let server = photoObject["server"] as? String ,
let secret = photoObject["secret"] as? String else {
break
}
let flickrPhoto = FlickrPhoto(photoID: photoID, farm: farm, server: server, secret: secret)
guard let url = flickrPhoto.flickrImageURL(),
let imageData = try? Data(contentsOf: url as URL) else {
break
}
if let image = UIImage(data: imageData) {
flickrPhoto.thumbnail = image
flickrPhotos.append(flickrPhoto)
}
}
OperationQueue.main.addOperation({
completion(FlickrSearchResults(searchTerm: searchTerm, searchResults: flickrPhotos), nil)
})
} catch _ {
completion(nil, nil)
return
}
}) .resume()
}
fileprivate func flickrSearchURLForSearchTerm(_ searchTerm:String) -> URL? {
guard let escapedTerm = searchTerm.addingPercentEncoding(withAllowedCharacters: CharacterSet.alphanumerics) else {
return nil
}
let URLString = "https://api.flickr.com/services/rest/?method=flickr.photos.search&api_key=\(apiKey)&text=\(escapedTerm)&per_page=20&format=json&nojsoncallback=1"
guard let url = URL(string:URLString) else {
return nil
}
return url
}
}
| mit | 5b6b1b083e206ee29359de162ef5ab32 | 36.512658 | 167 | 0.629661 | 5.091924 | false | false | false | false |
iceman201/NZAirQuality | NZAirQuality/View/Weather/WeatherHeaderViewCell.swift | 1 | 1923 | //
// WeatherHeaderViewCell.swift
// NZAirQuality
//
// Created by Liguo Jiao on 27/07/17.
// Copyright © 2017 Liguo Jiao. All rights reserved.
//
import UIKit
class WeatherHeaderViewCell: UITableViewCell {
@IBOutlet weak var weatherStatusImage: UIImageView!
@IBOutlet weak var conponentImage: UIImageView!
@IBOutlet weak var montainImage: UIImageView!
@IBOutlet weak var weatherInfoSection: WeatherInfoView!
@IBOutlet weak var tempture: UILabel!
@IBOutlet weak var location: UILabel!
@IBOutlet weak var weatherStatus: UILabel!
var weatherData: Weather?
override func awakeFromNib() {
super.awakeFromNib()
self.selectionStyle = .none
self.backgroundColor = NZABackgroundColor
self.weatherStatus.adjustsFontSizeToFitWidth = true
self.weatherStatus.minimumScaleFactor = 0.2
self.weatherStatus.numberOfLines = 0
self.tempture.text = "25℃"
tempture.backgroundColor = UIColor.clear
tempture.textColor = UIColor.white
tempture.textAlignment = .center
tempture.numberOfLines = 1
tempture.layer.shadowColor = UIColor.white.cgColor
tempture.layer.shadowOffset = CGSize(width: 3, height: 3)
tempture.layer.shadowOpacity = 0.8
tempture.layer.shadowRadius = 6
}
func loadContent() {
if let city = weatherData?.data.channel.location?.city, let _ = weatherData?.data.channel.location?.country {
location.text = "\(city)"
}
if let weatherCondition = weatherData?.data.channel.item?.currentCondition?.text {
weatherStatus.text = weatherCondition
}
if let temp = weatherData?.data.channel.item?.currentCondition?.temp, let tempF = Float(temp) {
tempture.text = String(format: "%.0f℃", fahrenheitToCelsius(feh: tempF))
}
}
}
| mit | ef75bbff037a09cec4b58c862760f948 | 32.649123 | 117 | 0.660063 | 4.512941 | false | false | false | false |
ivanbruel/SwipeIt | SwipeIt/ViewModels/Link/Items/LinkItemImageViewModel.swift | 1 | 2156 | //
// LinkItemImageViewModel.swift
// Reddit
//
// Created by Ivan Bruel on 09/05/16.
// Copyright © 2016 Faber Ventures. All rights reserved.
//
import Foundation
import RxSwift
import Kingfisher
// MARK: Properties and initializer
class LinkItemImageViewModel: LinkItemViewModel {
// MARK: Constants
private static let defaultImageSize = CGSize(width: 4, height: 3)
// MARK: Private Properties
private var prefetcher: Prefetcher? = nil
private let _imageSize: Variable<CGSize>
// MARK: Public Properties
let imageURL: NSURL?
let indicator: String?
let overlay: String?
var imageSize: Observable<CGSize> {
return _imageSize.asObservable()
}
override init(user: User, accessToken: AccessToken, link: Link, showSubreddit: Bool) {
imageURL = link.imageURL
indicator = LinkItemImageViewModel.indicatorFromLink(link)
overlay = LinkItemImageViewModel.overlayFromLink(link)
_imageSize = Variable(link.imageSize ?? LinkItemImageViewModel.defaultImageSize)
super.init(user: user, accessToken: accessToken, link: link, showSubreddit: showSubreddit)
}
deinit {
prefetcher?.stop()
}
// MARK: API
func setImageSize(imageSize: CGSize) {
_imageSize.value = imageSize
}
override func preloadData() {
guard let imageURL = imageURL else { return }
prefetcher = Prefetcher(imageURL: imageURL) { [weak self] image in
guard let image = image else { return }
self?._imageSize.value = image.size
}
prefetcher?.start()
}
}
// MARK: Helpers
extension LinkItemImageViewModel {
private class func indicatorFromLink(link: Link) -> String? {
if link.type == .GIF {
return tr(.LinkIndicatorGIF)
} else if link.type == .Album {
return tr(.LinkIndicatorAlbum)
} else if link.isSpoiler {
return tr(.LinkIndicatorSpoiler)
} else if link.nsfw == true {
return tr(.LinkIndicatorNSFW)
}
return nil
}
private class func overlayFromLink(link: Link) -> String? {
if link.nsfw == true {
return tr(.LinkIndicatorNSFW)
} else if link.isSpoiler {
return tr(.LinkIndicatorSpoiler)
}
return nil
}
}
| mit | a09be0264f37ff4e554dca6c53e39869 | 24.963855 | 94 | 0.692343 | 4.120459 | false | false | false | false |
rudkx/swift | test/Generics/rdar65263302.swift | 1 | 971 | // RUN: %target-swift-frontend -typecheck -debug-generic-signatures %s -requirement-machine-inferred-signatures=verify 2>&1 | %FileCheck %s
// RUN: %target-swift-frontend -verify -emit-ir -requirement-machine-inferred-signatures=verify %s
public protocol P {
associatedtype Element
}
public class C<O: P>: P {
public typealias Element = O.Element
}
// CHECK: Generic signature: <T, O, E where T : C<E>, O : P, E : P, O.[P]Element == E.[P]Element>
public func toe1<T, O, E>(_: T, _: O, _: E, _: T.Element)
where T : P, // expected-warning {{redundant conformance constraint 'T' : 'P'}}
O : P,
O.Element == T.Element,
T : C<E> {} // expected-note {{conformance constraint 'T' : 'P' implied here}}
// CHECK: Generic signature: <T, O, E where T : C<E>, O : P, E : P, O.[P]Element == E.[P]Element>
public func toe2<T, O, E>(_: T, _: O, _: E, _: T.Element)
where O : P,
O.Element == T.Element,
T : C<E> {}
| apache-2.0 | c3379d41fc6ff3ec2fbcdabb671ea5d9 | 39.458333 | 139 | 0.594233 | 3.072785 | false | false | false | false |
briceZhao/ZXRefresh | ZXRefreshExample/ZXRefreshExample/QQStyleRefresh/QQRefreshHeader.swift | 1 | 3135 | //
// QQRefreshHeader.swift
// ZXRefreshExample
//
// Created by briceZhao on 2017/8/17.
// Copyright © 2017年 chengyue. All rights reserved.
//
import UIKit
class QQRefreshHeader: ZXRefreshHeader, ZXRefreshHeaderDelegate {
let control: QQStylePullingIndicator
open let textLabel: UILabel = UILabel(frame: CGRect(x: 0,y: 0,width: 120,height: 40))
open let imageView: UIImageView = UIImageView()
override public init(frame: CGRect) {
control = QQStylePullingIndicator(frame: frame)
super.init(frame: frame)
self.autoresizingMask = .flexibleWidth
self.backgroundColor = UIColor.white
imageView.sizeToFit()
imageView.frame = CGRect(x: 0, y: 0, width: 20, height: 20)
textLabel.font = UIFont.systemFont(ofSize: 15)
textLabel.textAlignment = .center
textLabel.textColor = UIColor.darkGray
addSubview(control)
addSubview(textLabel)
addSubview(imageView)
}
required public init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
open override func layoutSubviews() {
super.layoutSubviews()
control.frame = self.bounds
let totalHegiht = self.contentHeight()
imageView.center = CGPoint(x: frame.width/2 - 40 - 40, y: totalHegiht * 0.75)
textLabel.center = CGPoint(x: frame.size.width/2, y: totalHegiht * 0.75)
}
public override func refreshingHoldHeight() -> CGFloat {
return 40.0
}
// MARK: ZXRefreshHeaderDelegate
func contentHeight() -> CGFloat {
return 80.0
}
func toNormalState() {
self.control.isHidden = false
self.imageView.isHidden = true
self.textLabel.isHidden = true
}
func toRefreshingState() {
self.control.animating = true
}
func toPullingState() {
}
func changePullingPercent(percent: CGFloat) {
self.control.animating = false
if percent > 0.5 && percent <= 1.0{
self.control.progress = (percent - 0.5)/0.5
}else if percent <= 0.5{
self.control.progress = 0.0
}else{
self.control.progress = 1.0
}
}
func toWillRefreshState() {
}
func willBeginEndRefershing(isSuccess: Bool) {
if isSuccess {
self.control.isHidden = true
imageView.isHidden = false
textLabel.isHidden = false
textLabel.text = ZXHeaderString.refreshSuccess
imageView.image = UIImage(named: "success", in: Bundle(for: ZXRefreshHeader.self), compatibleWith: nil)
} else {
self.control.isHidden = true
imageView.isHidden = false
textLabel.isHidden = false
textLabel.text = ZXHeaderString.refreshFailure
imageView.image = UIImage(named: "failure", in: Bundle(for: ZXRefreshHeader.self), compatibleWith: nil)
}
}
func willCompleteEndRefreshing() {
}
}
| mit | bc82edb7be9c0a7bea787ce5ed063ef2 | 27.472727 | 115 | 0.59834 | 4.512968 | false | false | false | false |
yonaskolb/SwagGen | Templates/Swift/Sources/APIService.swift | 1 | 1164 | {% include "Includes/Header.stencil" %}
public struct APIService<ResponseType: APIResponseValue> {
public let id: String
public let tag: String
public let method: String
public let path: String
public let hasBody: Bool
public let isUpload: Bool
public let securityRequirements: [SecurityRequirement]
public init(id: String, tag: String = "", method:String, path:String, hasBody: Bool, isUpload: Bool = false, securityRequirements: [SecurityRequirement] = []) {
self.id = id
self.tag = tag
self.method = method
self.path = path
self.hasBody = hasBody
self.isUpload = isUpload
self.securityRequirements = securityRequirements
}
}
extension APIService: CustomStringConvertible {
public var name: String {
return "\(tag.isEmpty ? "" : "\(tag).")\(id)"
}
public var description: String {
return "\(name): \(method) \(path)"
}
}
public struct SecurityRequirement {
public let type: String
public let scopes: [String]
public init(type: String, scopes: [String]) {
self.type = type
self.scopes = scopes
}
}
| mit | 6884281506bc12e40a8983ebe2aefc95 | 25.454545 | 164 | 0.640034 | 4.442748 | false | false | false | false |
JGiola/swift | test/Concurrency/flow_isolation_nonstrict.swift | 1 | 813 | // RUN: %target-swift-frontend -strict-concurrency=targeted -swift-version 5 -parse-as-library -emit-sil -verify %s
@_nonSendable
class NonSendableType {
var x: Int = 0
func f() {}
}
// rdar://94699928 - don't emit sendable diagnostics in non-'complete' mode
// for deinits of actor or global-actor isolated types
@available(SwiftStdlib 5.1, *)
@MainActor class AwesomeUIView {}
@available(SwiftStdlib 5.1, *)
class CheckDeinitFromClass: AwesomeUIView {
var ns: NonSendableType?
deinit {
ns?.f()
ns = nil
}
}
@available(SwiftStdlib 5.1, *)
actor CheckDeinitFromActor {
var ns: NonSendableType?
deinit {
ns?.f()
ns = nil
}
}
@MainActor class X {
var ns: NonSendableType = NonSendableType()
nonisolated func something() { }
deinit {
something()
print(ns)
}
}
| apache-2.0 | 3165268d51bd9db2d533ff73f1ba4232 | 18.357143 | 115 | 0.677737 | 3.43038 | false | false | false | false |
adrfer/swift | test/SILGen/downcast_reabstraction.swift | 2 | 1268 | // RUN: %target-swift-frontend -emit-silgen %s | FileCheck %s
// CHECK-LABEL: sil hidden @_TF22downcast_reabstraction19condFunctionFromAnyFP_T_
// CHECK: checked_cast_addr_br take_always protocol<> in [[IN:%.*]] : $*protocol<> to () -> () in [[OUT:%.*]] : $*@callee_owned (@out (), @in ()) -> (), [[YES:bb[0-9]+]], [[NO:bb[0-9]+]]
// CHECK: [[YES]]:
// CHECK: [[ORIG_VAL:%.*]] = load [[OUT]]
// CHECK: [[REABSTRACT:%.*]] = function_ref @_TTRXFo_iT__iT__XFo__dT__
// CHECK: [[SUBST_VAL:%.*]] = partial_apply [[REABSTRACT]]([[ORIG_VAL]])
func condFunctionFromAny(x: Any) {
if let f = x as? () -> () {
f()
}
}
// CHECK-LABEL: sil hidden @_TF22downcast_reabstraction21uncondFunctionFromAnyFP_T_ : $@convention(thin) (@in protocol<>) -> () {
// CHECK: unconditional_checked_cast_addr take_always protocol<> in [[IN:%.*]] : $*protocol<> to () -> () in [[OUT:%.*]] : $*@callee_owned (@out (), @in ()) -> ()
// CHECK: [[ORIG_VAL:%.*]] = load [[OUT]]
// CHECK: [[REABSTRACT:%.*]] = function_ref @_TTRXFo_iT__iT__XFo__dT__
// CHECK: [[SUBST_VAL:%.*]] = partial_apply [[REABSTRACT]]([[ORIG_VAL]])
// CHECK: apply [[SUBST_VAL]]()
func uncondFunctionFromAny(x: Any) {
(x as! () -> ())()
}
| apache-2.0 | 45c95ac4f47e5835c8291df1e483aec1 | 51.833333 | 194 | 0.5347 | 3.242967 | false | false | false | false |
thierrybucco/Eureka | Eureka.playground/Contents.swift | 2 | 5439 | //: **Eureka Playground** - let us walk you through Eureka! cool features, we will show how to
//: easily create powerful forms using Eureka!
//: It allows us to create complex dynamic table view forms and obviously simple static table view. It's ideal for data entry task or settings pages.
import UIKit
import XCPlayground
import PlaygroundSupport
//: Start by importing Eureka module
import Eureka
//: Any **Eureka** form must extend from `FromViewController`
let formController = FormViewController()
PlaygroundPage.current.liveView = formController.view
let b = [Int]()
b.last
let f = Form()
f.last
//: ## Operators
//: ### +++
//: Adds a Section to a Form when the left operator is a Form
let form = Form() +++ Section()
//: When both operators are a Section it creates a new Form containing both Section and return the created form.
let form2 = Section("First") +++ Section("Second") +++ Section("Third")
//: Notice that you don't need to add parenthesis in the above expresion, +++ operator is left associative so it will create a form containing the 2 first sections and then append last Section (Third) to the created form.
//: form and form2 don't have any row and are not useful at all. Let's add some rows.
//: ### +++
//: Can be used to append a row to a form without having to create a Section to contain the row. The form section is implicitly created as a result of the +++ operator.
formController.form +++ TextRow("Text")
//: it can also be used to append a Section like this:
formController.form +++ Section()
//: ### <<<
//: Can be used to append rows to a section.
formController.form.last! <<< SwitchRow("Switch") { $0.title = "Switch"; $0.value = true }
//: it can also be used to create a section and append rows to it. Let's use that to add a new section to the form
formController.form +++ PhoneRow("Phone") { $0.title = "Phone"}
<<< IntRow("IntRow") { $0.title = "Int"; $0.value = 5 }
formController.view
//: # Callbacks
//: Rows might have several closures associated to be called at different events. Let's see them one by one. Sadly, we can not interact with it in our playground.
//: ### onChange callback
//: Will be called when the value of a row changes. Lots of things can be done with this feature. Let's create a new section for the new rows:
formController.form +++ Section("Callbacks") <<< SwitchRow("scr1") { $0.title = "Switch to turn red"; $0.value = false }
.onChange({ row in
if row.value == true {
row.cell.backgroundColor = .red
} else {
row.cell.backgroundColor = .black
}
})
//: Now when we change the value of this row its background color will change to red. Try that by (un)commenting the following line:
formController.view
formController.form.last?.last?.baseValue = true
formController.view
//: Notice that we set the `baseValue` attribute because we did not downcast the result of `formController.form.last?.last`. It is essentially the same as the `value` attribute
//: ### cellSetup and cellUpdate callbacks
//: The cellSetup will be called when the cell of this row is configured (just once at the beginning)
//: and the cellUpdate will be called when it is updated (each time it reappears on screen). Here you should define the appearance of the cell
formController.form.last! <<< SegmentedRow<String>("Segments") { $0.title = "Choose an animal"; $0.value = "🐼"; $0.options = ["🐼", "🐶", "🐻"]}.cellSetup({ cell, _ in
cell.backgroundColor = .red
}).cellUpdate({ (cell, _) -> Void in
cell.textLabel?.textColor = .yellow
})
//: ### onSelection and onPresent callbacks
//: OnSelection will be called when this row is selected (tapped by the user). It might be useful for certain rows instead of the onChange callback.
//: OnPresent will be called when a row that presents another view controller is tapped. It might be useful to set up the presented view controller.
//: We can not try them out in the playground but they can be set just like the others.
formController.form.last! <<< SegmentedRow<String>("Segments2") { $0.title = "Choose an animal"; $0.value = "🐼"; $0.options = ["🐼", "🐶", "🐻"]
}.onCellSelection { cell, row in
print("\(cell) for \(row) got selected")
}
formController.view
//: ### Hiding rows
//: We can hide rows by defining conditions that will tell if they should appear on screen or not. Let's create a row that will hide when the previous one is "🐼".
formController.form.last! <<< LabelRow("Confirm") {
$0.title = "Are you sure you do not want the 🐼?"
$0.hidden = "$Segments2 == '🐼'"
}
//: Now let's see how this works:
formController.view
formController.form.rowBy(tag: "Segments2")?.baseValue = "🐶"
formController.view
//: We can do the same using functions. Functions are specially useful for more complicated conditions.
//: This applies when the value of the row we depend on is not compatible with NSPredicates (which is not the current case, but anyway).
formController.form.last! <<< LabelRow("Confirm2") {
$0.title = "Well chosen!!"
$0.hidden = Condition.function(["Segments2"]) { form in
if let r: SegmentedRow<String> = form.rowBy(tag: "Segments2") {
return r.value != "🐼"
}
return true
}
}
//: Now let's see how this works:
formController.view
formController.form.rowBy(tag: "Segments2")?.baseValue = "🐼"
formController.view
| mit | d2b3b1bfbcde1bc7c0c57adaaaaa287b | 43.975 | 221 | 0.696313 | 4.064006 | false | false | false | false |
CallMeMrAlex/DYTV | DYTV-AlexanderZ-Swift/DYTV-AlexanderZ-Swift/Classes/LiveVideo(直播页)/Controller/LiveVideoViewController.swift | 1 | 2052 | ////
//// LiveVideoViewController.swift
//// DYTV-AlexanderZ-Swift
////
//// Created by Alexander Zou on 16/10/12.
//// Copyright © 2016年 Alexander Zou. All rights reserved.
////
//
//import UIKit
//import IJKMediaFramework
//
//class LiveVideoViewController: UIViewController {
//
// var player : IJKFFMoviePlayerController!
//
// override func viewDidLoad() {
// super.viewDidLoad()
//
// self.view.backgroundColor = UIColor.blueColor()
//
// let options = IJKFFOptions.optionsByDefault()
//
// let url = NSURL(string: "rtmp://live.hkstv.hk.lxdns.com/live/hks")
//
// let player = IJKFFMoviePlayerController(contentURL: url, withOptions: options)
//
// let autoresize = UIViewAutoresizing.FlexibleWidth.rawValue |
// UIViewAutoresizing.FlexibleHeight.rawValue
//
// player.view.autoresizingMask = UIViewAutoresizing(rawValue: autoresize)
//
// player.view.frame = self.view.bounds
// player.scalingMode = .AspectFit
// player.shouldAutoplay = true
//
// self.view.autoresizesSubviews = true
// self.view.addSubview(player.view)
// self.player = player
//
//
// }
//
// override func viewWillAppear(animated: Bool) {
// self.player.prepareToPlay()
// }
//
// override func viewWillDisappear(animated: Bool) {
// self.player.shutdown()
// }
//
// override func didReceiveMemoryWarning() {
// super.didReceiveMemoryWarning()
// // Dispose of any resources that can be recreated.
// }
//
//
// /*
// // MARK: - Navigation
//
// // In a storyboard-based application, you will often want to do a little preparation before navigation
// override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
// // Get the new view controller using segue.destinationViewController.
// // Pass the selected object to the new view controller.
// }
// */
//
//}
| mit | 5afc522f43592a0f4c14459e1718e2a8 | 29.58209 | 108 | 0.611518 | 4.049407 | false | false | false | false |
rundfunk47/pest | pest/Array+Sort.swift | 1 | 1102 | //
// Array+Sort.swift
// pest
//
// Created by Narek M on 14/03/16.
// Copyright © 2016 Narek M. All rights reserved.
//
import Foundation
extension Array {
// generously stolen from:
// http://stackoverflow.com/questions/26678362/how-do-i-insert-an-element-at-the-correct-position-into-a-sorted-array-in-swift
private func insertionIndexOf(elem: Element, isOrderedBefore: (Element, Element) -> Bool) -> Int {
var lo = 0
var hi = self.count - 1
while lo <= hi {
let mid = (lo + hi)/2
if isOrderedBefore(self[mid], elem) {
lo = mid + 1
} else if isOrderedBefore(elem, self[mid]) {
hi = mid - 1
} else {
return mid // found at position mid
}
}
return lo // not found, would be inserted at position lo
}
mutating func insertSorted(elem: Element, isOrderedBefore: (Element, Element) -> Bool) {
let index = self.insertionIndexOf(elem, isOrderedBefore: isOrderedBefore)
self.insert(elem, atIndex: index)
}
} | gpl-3.0 | 877ec34e4ac2f160c67108f37a58db85 | 31.411765 | 130 | 0.585831 | 3.946237 | false | false | false | false |
arcturus/magnet-client | ios/History.swift | 2 | 1088 | //
// History.swift
// magnet
//
// This class manages the high level access to the history
// of urls that the user has found.
//
// Created by Francisco Jordano on 19/07/2016.
//
import Foundation
class History {
private static var sharedInstance: History!
private var db: HistorySQLite
private init() {
db = HistorySQLite()
}
class func getInstance() -> History {
// Synchronized
let lockQueue = dispatch_queue_create("com.mozilla.magnet.history", nil)
dispatch_sync(lockQueue) {
if (sharedInstance == nil) {
sharedInstance = History()
}
}
return sharedInstance
}
func record(url: String) {
let recent = getRecent(url)
if (recent != nil) {
db.updateLastSeen(recent.id)
return
}
db.insert(url)
}
func getRecent(url: String) -> HistoryRecord! {
let earlyDate = NSCalendar.currentCalendar().dateByAddingUnit(
.Hour,
value: -1,
toDate: NSDate(),
options: [])
return db.getSince(url, sinceDate: earlyDate!)
}
func clear() {
db.clear()
}
}
| mpl-2.0 | 1035d198a3426da047d935d57553c928 | 19.923077 | 76 | 0.624081 | 3.927798 | false | false | false | false |
mumuda/Swift_Weibo | weibo/weibo/Classes/Discover/DiscoverTableViewController.swift | 1 | 3235 | //
// DiscoverTableViewController.swift
// weibo
//
// Created by ldj on 16/5/26.
// Copyright © 2016年 ldj. All rights reserved.
//
import UIKit
class DiscoverTableViewController: BaseTableViewController {
override func viewDidLoad() {
super.viewDidLoad()
// 1.如果没有登陆,就设置未登陆界面的信息
if userLogin {
}else
{
visitorView?.setupVisitorInfo(false, imageName: "visitordiscover_image_message", message: "登录后,最新、最热微博尽在掌握,不再会与实事潮流擦肩而过")
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// MARK: - Table view data source
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
// #warning Incomplete implementation, return the number of sections
return 0
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
// #warning Incomplete implementation, return the number of rows
return 0
}
/*
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("reuseIdentifier", forIndexPath: indexPath)
// Configure the cell...
return cell
}
*/
/*
// Override to support conditional editing of the table view.
override func tableView(tableView: UITableView, canEditRowAtIndexPath indexPath: NSIndexPath) -> Bool {
// Return false if you do not want the specified item to be editable.
return true
}
*/
/*
// Override to support editing the table view.
override func tableView(tableView: UITableView, commitEditingStyle editingStyle: UITableViewCellEditingStyle, forRowAtIndexPath indexPath: NSIndexPath) {
if editingStyle == .Delete {
// Delete the row from the data source
tableView.deleteRowsAtIndexPaths([indexPath], withRowAnimation: .Fade)
} else if editingStyle == .Insert {
// Create a new instance of the appropriate class, insert it into the array, and add a new row to the table view
}
}
*/
/*
// Override to support rearranging the table view.
override func tableView(tableView: UITableView, moveRowAtIndexPath fromIndexPath: NSIndexPath, toIndexPath: NSIndexPath) {
}
*/
/*
// Override to support conditional rearranging of the table view.
override func tableView(tableView: UITableView, canMoveRowAtIndexPath indexPath: NSIndexPath) -> Bool {
// Return false if you do not want the item to be re-orderable.
return true
}
*/
/*
// MARK: - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
// Get the new view controller using segue.destinationViewController.
// Pass the selected object to the new view controller.
}
*/
}
| mit | 5ff1ed8eff64ebbc93e65208bea31d3b | 31.371134 | 157 | 0.672293 | 5.304054 | false | false | false | false |
ChrisAU/Papyrus | Papyrus/Drawables/ScoreDrawable.swift | 2 | 899 | //
// ScoreDrawable.swift
// Papyrus
//
// Created by Chris Nevin on 4/07/2016.
// Copyright © 2016 CJNevin. All rights reserved.
//
import UIKit
struct ScoreDrawable : Drawable {
var shader: Shader
private let rect: CGRect
private let text: String
private let font: UIFont
init(text: String, highlighted: Bool, myTurn: Bool, rect: CGRect) {
self.shader = ScoreShader(highlighted: highlighted)
self.text = text
self.rect = rect
self.font = UIFont.systemFont(ofSize: 13, weight: myTurn ? UIFont.Weight.semibold : UIFont.Weight.light)
}
func draw(renderer: Renderer) {
let attrText = NSAttributedString(string: text, attributes: [NSAttributedString.Key.font: font])
let attrRect = rect.centeredRect(forSize: attrText.size())
renderer.draw(text: attrText, rect: attrRect, shader: shader)
}
}
| mit | ed9bd0d06646565570307ee841cd70c0 | 28.933333 | 112 | 0.663697 | 3.973451 | false | false | false | false |
mathewsheets/UIKitExtensions | UIKitExtensions/UIKitExtensions/UIImage+Extension.swift | 1 | 6476 | //
// UIImage+Extensions.swift
// ImageOperators
//
// Created by Mathew Sheets on 4/21/16.
// Copyright © 2016 Mathew Sheets. All rights reserved.
//
import UIKit
public extension UIImage {
enum Direction {
case horizontal
case vertical
}
convenience init(direction: Direction, images: [UIImage]) {
var image: UIImage
switch direction {
case .horizontal:
image = UIImage.combineHorizontally(images)
case .vertical:
image = UIImage.combineVertically(images)
}
self.init(cgImage: image.cgImage!, scale: UIScreen.main.scale, orientation: UIImage.Orientation.up)
}
convenience init(direction: Direction, image: UIImage, percentage: Float, mirrored: Bool = false) {
var newImage: UIImage
var orientation: UIImage.Orientation!
switch direction {
case .horizontal:
newImage = UIImage.cropHorizontally(image, percentage: percentage, mirrored: mirrored)
orientation = mirrored ? .upMirrored : .up
case .vertical:
newImage = UIImage.cropVertically(image, percentage: percentage, mirrored: mirrored)
orientation = mirrored ? .down : .up
}
self.init(cgImage: newImage.cgImage!, scale: UIScreen.main.scale, orientation: orientation)
}
func flip(_ direction: Direction) -> UIImage {
return UIImage(cgImage: self.cgImage!, scale: UIScreen.main.scale, orientation: direction == .horizontal ? .upMirrored : .down)
}
static func cropHorizontally(_ image: UIImage, percentage: Float, mirrored: Bool = false) -> UIImage {
var goodPercentage = percentage
if percentage <= 0.0 {
goodPercentage = 0.000000000000001
} else if percentage >= 1.0 {
goodPercentage = 0.999999999999999
}
let newImageSize = CGSize(width: image.size.width * CGFloat(goodPercentage), height: image.size.height)
UIGraphicsBeginImageContextWithOptions(newImageSize, false, UIScreen.main.scale)
image.draw(at: CGPoint(x: 0, y: 0))
let newImage = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
if mirrored {
return UIImage(cgImage: newImage!.cgImage!, scale: UIScreen.main.scale, orientation: .upMirrored)
}
return newImage!
}
static func combineHorizontally(_ images: [UIImage]) -> UIImage {
var width = CGFloat(0)
for image in images {
width += image.size.width
}
let height = images[0].size.height
let size = CGSize(width: width, height: height)
UIGraphicsBeginImageContextWithOptions(size, false, UIScreen.main.scale)
var offset = CGFloat(0)
for image in images {
let width = image.size.width
image.draw(in: CGRect(x: offset, y: 0, width: width, height: height))
offset += width
}
let image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return image!
}
static func cropVertically(_ image: UIImage, percentage: Float, mirrored: Bool = false) -> UIImage {
var goodPercentage = percentage
if percentage <= 0.0 {
goodPercentage = 0.000000000000001
} else if percentage >= 1.0 {
goodPercentage = 0.999999999999999
}
let newImageSize = CGSize(width: image.size.width, height: image.size.height * CGFloat(goodPercentage))
UIGraphicsBeginImageContextWithOptions(newImageSize, false, UIScreen.main.scale)
image.draw(at: CGPoint(x: 0, y: 0))
let newImage = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
if mirrored {
return UIImage(cgImage: newImage!.cgImage!, scale: UIScreen.main.scale, orientation: .down)
}
return newImage!
}
static func combineVertically(_ images: [UIImage]) -> UIImage {
let width = images[0].size.width
var height = CGFloat(0)
for image in images {
height += image.size.height
}
UIGraphicsBeginImageContextWithOptions(CGSize(width: width, height: height), false, UIScreen.main.scale)
var offset = CGFloat(0)
for image in images {
let height = image.size.height
image.draw(in: CGRect(x: 0, y: offset, width: width, height: height))
offset += height
}
let image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return image!
}
}
precedencegroup CropPrecedence {
associativity: left
}
precedencegroup CombinePrecedence {
associativity: left
higherThan: CropPrecedence
}
// image -* 0.5 = crop image horizontally 50%
infix operator -* : CropPrecedence
public func -* (left: UIImage, right: Float) -> UIImage {
return UIImage(direction: .horizontal, image: left, percentage: right)
}
// image -*~ 0.5 = crop image horizontally 50% then mirror
infix operator -*~ : CropPrecedence
public func -*~ (left: UIImage, right: Float) -> UIImage {
return UIImage(direction: .horizontal, image: left, percentage: right, mirrored: true)
}
// image |* 0.5 = crop image vertically 50%
infix operator |* : CropPrecedence
public func |* (left: UIImage, right: Float) -> UIImage {
return UIImage(direction: .vertical, image: left, percentage: right)
}
// image |*~ 0.5 = crop image vertically 50% then mirror
infix operator |*~ : CropPrecedence
public func |*~ (left: UIImage, right: Float) -> UIImage {
return UIImage(direction: .vertical, image: left, percentage: right, mirrored: true)
}
// (image <- image) <- image = combine two images horizontally
infix operator <- : CombinePrecedence
public func <- (left: UIImage, right: UIImage) -> UIImage {
return UIImage(direction: .horizontal, images: [left, right])
}
// (image ^- image) ^- image = combine two images vertically
infix operator ^- : CombinePrecedence
public func ^- (left: UIImage, right: UIImage) -> UIImage {
return UIImage(direction: .vertical, images: [left, right])
}
| mit | 653ddf3ed5aaabe60c78a21706f63e32 | 30.896552 | 135 | 0.619305 | 4.806978 | false | false | false | false |
thomas-mcdonald/SoundTop | SoundTop/STSoundCloudPlaylist.swift | 1 | 2455 | // STSoundCloudPlaylist.swift
//
// Copyright (c) 2015 Thomas McDonald
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions are met:
//
// Redistributions of source code must retain the above copyright notice, this
// list of conditions and the following disclaimer. Redistributions in binary
// form must reproduce the above copyright notice, this list of conditions and
// the following disclaimer in the documentation and/or other materials
// provided with the distribution. Neither the name of the nor the names of
// its contributors may be used to endorse or promote products derived from
// this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
// AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
// ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE
// LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
// CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
// SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
// INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
// CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
// ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
// POSSIBILITY OF SUCH DAMAGE.
import Cocoa
class STSoundCloudPlaylist: NSObject {
private let clientId = "027aa73b22641da241a74cfdd3c5210b"
func fetchPlaylist(playlistURL: NSString, success: (STPlaylist) -> ()) {
let url = NSURL(string: resolverURL(playlistURL) as String)
let request = NSURLRequest(URL: url!)
NSURLConnection.sendAsynchronousRequest(request, queue: NSOperationQueue.mainQueue(), completionHandler: { (response: NSURLResponse?, data: NSData?, error: NSError?) in
// hit callback
let playlist: STPlaylist? = STPlaylist(playlistData: data!)
if(playlist == nil) {
// failure callback
} else {
success(playlist!)
}
})
}
private func resolverURL(playlistURL: NSString) -> NSString {
return "https://api.soundcloud.com/resolve.json?url=\(playlistURL)&client_id=\(clientId)"
}
}
| bsd-3-clause | 41b1ed0bec12cb089b7bfd377acbfa74 | 46.211538 | 176 | 0.727495 | 4.739382 | false | false | false | false |
asalom/Cocoa-Design-Patterns-in-Swift | DesignPatterns/DesignPatterns/Hide Complexity/Facade/Facade.swift | 1 | 1033 |
//
// Facade.swift
// DesignPatterns
//
// Created by Alex Salom on 12/11/15.
// Copyright © 2015 Alex Salom © alexsalom.es. All rights reserved.
//
import Foundation
class CPU {
var freezed = false
var executed = false
func freeze() {
self.freezed = true
}
func execute() {
self.executed = true
}
}
class Memory {
var loaded = false
func load() {
self.loaded = true
}
}
class HardDrive {
var booted = false
func boot() {
self.booted = true
}
}
class Computer {
private var processor: CPU = CPU()
private var ram: Memory = Memory()
private var harddrive: HardDrive = HardDrive()
var started: Bool {
return self.processor.freezed &&
self.processor.executed &&
self.ram.loaded &&
self.harddrive.booted
}
func start() {
self.processor.freeze()
self.ram.load()
self.harddrive.boot()
self.processor.execute()
}
} | mit | 6ca0922afe428f4fa9e71a071170fffc | 16.491525 | 68 | 0.556741 | 3.87594 | false | false | false | false |
testpress/ios-app | ios-app/UI/MoreOptionsViewController.swift | 1 | 2102 | //
// MoreOptionsViewController.swift
// ios-app
//
// Copyright © 2017 Testpress. All rights reserved.
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
//
import UIKit
class MoreOptionsViewController: UIViewController {
@IBAction func rateUs() {
if let url = URL(string: Constants.APP_STORE_LINK),
UIApplication.shared.canOpenURL(url) {
if #available(iOS 10, *) {
UIApplication.shared.open(url, options: [:])
} else {
UIApplication.shared.openURL(url)
}
}
}
@IBAction func shareApp() {
let textToShare = [ Constants.APP_SHARE_MESSAGE ]
let activityViewController =
UIActivityViewController(activityItems: textToShare, applicationActivities: nil)
activityViewController.popoverPresentationController?.sourceView = view
activityViewController.excludedActivityTypes = [ UIActivity.ActivityType.airDrop ]
present(activityViewController, animated: true, completion: nil)
}
}
| mit | 6f652904f1652f47f679fc4e49d2ddf4 | 40.196078 | 92 | 0.701571 | 4.775 | false | false | false | false |
michael-lehew/swift-corelibs-foundation | Foundation/NSHost.swift | 1 | 3787 | // This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2016 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See http://swift.org/LICENSE.txt for license information
// See http://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
#if os(OSX) || os(iOS)
import Darwin
#elseif os(Linux) || CYGWIN
import Glibc
#endif
import CoreFoundation
open class Host: NSObject {
enum ResolveType {
case name
case address
case current
}
internal var _info: String?
internal var _type: ResolveType
internal var _resolved = false
internal var _names = [String]()
internal var _addresses = [String]()
internal init(_ info: String?, _ type: ResolveType) {
_info = info
_type = type
}
static internal let _current = Host(nil, .current)
open class func current() -> Host {
return _current
}
public convenience init(name: String?) {
self.init(name, .name)
}
public convenience init(address: String) {
self.init(address, .address)
}
open func isEqual(to aHost: Host) -> Bool {
return false
}
internal func _resolveCurrent() {
// TODO: cannot access getifaddrs here...
}
internal func _resolve() {
if _resolved {
return
}
if let info = _info {
var flags: Int32 = 0
switch (_type) {
case .name:
flags = AI_PASSIVE | AI_CANONNAME
break
case .address:
flags = AI_PASSIVE | AI_CANONNAME | AI_NUMERICHOST
break
case .current:
_resolveCurrent()
return
}
var hints = addrinfo()
hints.ai_family = PF_UNSPEC
hints.ai_socktype = _CF_SOCK_STREAM()
hints.ai_flags = flags
var res0: UnsafeMutablePointer<addrinfo>? = nil
let r = getaddrinfo(info, nil, &hints, &res0)
if r != 0 {
return
}
var res: UnsafeMutablePointer<addrinfo>? = res0
while res != nil {
let info = res!.pointee
let family = info.ai_family
if family != AF_INET && family != AF_INET6 {
res = info.ai_next
continue
}
let sa_len: socklen_t = socklen_t((family == AF_INET6) ? MemoryLayout<sockaddr_in6>.size : MemoryLayout<sockaddr_in>.size)
let lookupInfo = { (content: inout [String], flags: Int32) in
let hname = UnsafeMutablePointer<Int8>.allocate(capacity: 1024)
if (getnameinfo(info.ai_addr, sa_len, hname, 1024, nil, 0, flags) == 0) {
content.append(String(describing: hname))
}
hname.deinitialize()
hname.deallocate(capacity: 1024)
}
lookupInfo(&_addresses, NI_NUMERICHOST)
lookupInfo(&_names, NI_NAMEREQD)
lookupInfo(&_names, NI_NOFQDN|NI_NAMEREQD)
res = info.ai_next
}
freeaddrinfo(res0)
}
}
open var name: String? {
return names.first
}
open var names: [String] {
_resolve()
return _names
}
open var address: String? {
return addresses.first
}
open var addresses: [String] {
_resolve()
return _addresses
}
open var localizedName: String? {
return nil
}
}
| apache-2.0 | 0cd8888d44bb3fc7fd398634ce867416 | 27.051852 | 138 | 0.518352 | 4.568154 | false | false | false | false |
rogeruiz/MacPin | modules/MacPin/iOS/WebViewControllerIOS.swift | 2 | 3310 | /// MacPin WebViewControllerIOS
///
/// WebViewController subclass for iOS
import UIKit
import WebKit
@objc class WebViewControllerIOS: WebViewController {
//var modalPresentationStyle = .OverFullScreen
//var modalTransitionStyle = .CrossDissolve // .CoverVertical | .CrossDissolve | .FlipHorizontal
override func viewDidLoad() {
super.viewDidLoad()
//view.wantsLayer = true //use CALayer to coalesce views
//^ default=true: https://github.com/WebKit/webkit/blob/master/Source/WebKit2/UIProcess/API/mac/WKView.mm#L3641
view.autoresizingMask = .FlexibleHeight | .FlexibleWidth
}
override func viewWillAppear(animated: Bool) { // window popping up with this tab already selected & showing
super.viewWillAppear(animated) // or tab switching into view as current selection
}
//override func removeFromParentViewController() { super.removeFromParentViewController() }
deinit { warn("\(reflect(self).summary)") }
//override func closeTab() { dismissViewControllerAnimated(true, completion: nil); super() }
/*
override func viewWillTransitionToSize(size: CGSize, withTransitionCoordinator coordinator: UIViewControllerTransitionCoordinator) {
warn()
super.viewWillTransitionToSize(size, withTransitionCoordinator: coordinator)
view.frame.size = size
view.layoutIfNeeded()
}
*/
}
extension WebViewControllerIOS { // AppGUI funcs
func shareButtonClicked(sender: AnyObject?) {
warn()
// http://nshipster.com/uiactivityviewcontroller/
if let url = webview.URL, title = webview.title {
let activityViewController = UIActivityViewController(activityItems: [title, url], applicationActivities: nil)
presentViewController(activityViewController, animated: true, completion: nil)
}
}
func askToOpenURL(url: NSURL) {
if UIApplication.sharedApplication().canOpenURL(url) {
let alerter = UIAlertController(title: "Open non-browser URL", message: url.description, preferredStyle: .ActionSheet)
let OK = UIAlertAction(title: "OK", style: .Default) { (_) in UIApplication.sharedApplication().openURL(url) }
alerter.addAction(OK)
let Cancel = UIAlertAction(title: "Cancel", style: .Cancel) { (_) in }
alerter.addAction(Cancel)
presentViewController(alerter, animated: true, completion: nil)
} else {
// FIXME: complain
}
}
/*
func print(sender: AnyObject?) {
if ([UIPrintInteractionController isPrintingAvailable])
{
UIPrintInfo *pi = [UIPrintInfo printInfo];
pi.outputType = UIPrintInfoOutputGeneral;
pi.jobName = @"Print";
pi.orientation = UIPrintInfoOrientationPortrait;
pi.duplex = UIPrintInfoDuplexLongEdge;
UIPrintInteractionController *pic = [UIPrintInteractionController sharedPrintController];
pic.printInfo = pi;
pic.showsPageRange = YES;
pic.printFormatter = self.webView.viewPrintFormatter;
[pic presentFromBarButtonItem:barButton animated:YES completionHandler:^(UIPrintInteractionController *printInteractionController, BOOL completed, NSError *error) {
if(error)
NSLog(@"handle error here");
else
NSLog(@"success");
}];
}
}
//FIXME: support new scaling https://github.com/WebKit/webkit/commit/b40b702baeb28a497d29d814332fbb12a2e25d03
func zoomIn() { webview.magnification += 0.2 }
func zoomOut() { webview.magnification -= 0.2 }
*/
}
| gpl-3.0 | b83f582d990a7d99afd5b9378dba7685 | 35.777778 | 168 | 0.745619 | 4.221939 | false | false | false | false |
garygriswold/Bible.js | Plugins/AudioPlayer/src/ios_oldApp/AudioPlayer/AudioAnalytics.swift | 2 | 5045 | //
// AudioAnalytics.swift
// AudioPlayer
//
// Created by Gary Griswold on 8/25/17.
// Copyright © 2017 ShortSands. All rights reserved.
//
import CoreMedia
import UIKit
#if USE_FRAMEWORK
import AWS
#endif
class AudioAnalytics {
var dictionary = [String: String]()
let dateFormatter = DateFormatter()
let mediaSource: String
let mediaId: String
let languageId: String
let textVersion: String
let silLang: String
let sessionId: String
// Pass following from play to playEnd
var timeStarted: Date
var startingPosition: Float64
init(mediaSource: String,
mediaId: String,
languageId: String,
textVersion: String,
silLang: String) {
self.mediaSource = mediaSource
self.mediaId = mediaId
self.languageId = languageId
self.textVersion = textVersion
self.silLang = silLang
let analyticsSessionId = AudioAnalyticsSessionId()
self.sessionId = analyticsSessionId.getSessionId()
self.timeStarted = Date()
self.startingPosition = 0.0
// ISO8601 DateFormatter (ios 10.3 has a function for this)
self.dateFormatter.locale = Locale(identifier: "en_US_POSIX")
self.dateFormatter.dateFormat = "yyyy-MM-dd'T'HH:mm:ssZZZZZ"
self.dateFormatter.timeZone = TimeZone(secondsFromGMT: 0)
}
deinit {
print("***** Deinit AudioAnalytics *****")
}
func playStarted(item: String, position: CMTime) -> Void {
self.dictionary.removeAll()
self.dictionary["sessionId"] = self.sessionId
self.dictionary["mediaSource"] = self.mediaSource
self.dictionary["mediaId"] = self.mediaId
self.dictionary["languageId"] = self.languageId
self.dictionary["textVersion"] = self.textVersion
self.dictionary["silLang"] = self.silLang
let locale = Locale.current
self.dictionary["language"] = locale.languageCode
self.dictionary["country"] = locale.regionCode
self.dictionary["locale"] = locale.identifier
let device = UIDevice.current
self.dictionary["deviceType"] = "mobile"
self.dictionary["deviceFamily"] = "Apple"
self.dictionary["deviceName"] = device.model
self.dictionary["deviceOS"] = "ios"
print("system name \(device.systemName)")
self.dictionary["osVersion"] = device.systemVersion
let bundle = Bundle.main
let info = bundle.infoDictionary
self.dictionary["appName"] = info?["CFBundleIdentifier"] as? String
self.dictionary["appVersion"] = info?["CFBundleShortVersionString"] as? String
self.timeStarted = Date()
self.dictionary["timeStarted"] = dateFormatter.string(from: self.timeStarted)
self.dictionary["isStreaming"] = "true" // should this be 1 instead
self.dictionary["startingItem"] = item
self.startingPosition = CMTimeGetSeconds(position)
self.dictionary["startingPosition"] = String(round(self.startingPosition * 1000) / 1000)
AwsS3Manager.findSS().uploadAnalytics(sessionId: self.sessionId,
timestamp: self.dictionary["timeStarted"]! + "-B",
prefix: "AudioBegV1",
dictionary: self.dictionary, complete: { (error) in
if let err = error {
print("Error in upload analytics \(err)")
}
})
}
func playEnded(item: String, position: CMTime) {
print("INSIDE PLAY END \(position)")
self.dictionary.removeAll()
self.dictionary["sessionId"] = self.sessionId
self.dictionary["timeStarted"] = dateFormatter.string(from: self.timeStarted)
let timeCompleted = Date()
self.dictionary["timeCompleted"] = dateFormatter.string(from: timeCompleted)
let duration: TimeInterval = timeCompleted.timeIntervalSince(self.timeStarted)
self.dictionary["elapsedTime"] = String(round(duration * 1000) / 1000)
self.dictionary["endingItem"] = item
let endingPosition = CMTimeGetSeconds(position)
self.dictionary["endingPosition"] = String(round(endingPosition * 1000) / 1000)
AwsS3Manager.findSS().uploadAnalytics(sessionId: self.sessionId,
timestamp: self.dictionary["timeCompleted"]! + "-E",
prefix: "AudioEndV1",
dictionary: self.dictionary,
complete: { (error) in
if let err = error {
print("Error in upload of analytics \(err)")
}
})
}
}
| mit | 48555b9eccb6089ba8b7117c68dacfc3 | 38.100775 | 96 | 0.577914 | 5.028913 | false | false | false | false |
Neku/easy-hodl | ios/CryptoSaver/Carthage/Checkouts/QRCodeReader.swift/Sources/QRCodeReader.swift | 3 | 12175 | /*
* QRCodeReader.swift
*
* Copyright 2014-present Yannick Loriot.
* http://yannickloriot.com
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*
*/
import UIKit
import AVFoundation
/// Reader object base on the `AVCaptureDevice` to read / scan 1D and 2D codes.
public final class QRCodeReader: NSObject, AVCaptureMetadataOutputObjectsDelegate {
var defaultDevice: AVCaptureDevice = .defaultDevice(withMediaType: AVMediaTypeVideo)
var frontDevice: AVCaptureDevice? = {
if #available(iOS 10, *) {
return AVCaptureDevice.defaultDevice(withDeviceType: .builtInWideAngleCamera, mediaType: AVMediaTypeVideo, position: .front)
}
else {
for device in AVCaptureDevice.devices(withMediaType: AVMediaTypeVideo) {
if let _device = device as? AVCaptureDevice , _device.position == AVCaptureDevicePosition.front {
return _device
}
}
}
return nil
}()
lazy var defaultDeviceInput: AVCaptureDeviceInput? = {
return try? AVCaptureDeviceInput(device: self.defaultDevice)
}()
lazy var frontDeviceInput: AVCaptureDeviceInput? = {
if let _frontDevice = self.frontDevice {
return try? AVCaptureDeviceInput(device: _frontDevice)
}
return nil
}()
public var metadataOutput = AVCaptureMetadataOutput()
var session = AVCaptureSession()
// MARK: - Managing the Properties
/// CALayer that you use to display video as it is being captured by an input device.
public lazy var previewLayer: AVCaptureVideoPreviewLayer = {
return AVCaptureVideoPreviewLayer(session: self.session)
}()
/// An array of strings identifying the types of metadata objects to process.
public let metadataObjectTypes: [String]
// MARK: - Managing the Code Discovery
/// Flag to know whether the scanner should stop scanning when a code is found.
public var stopScanningWhenCodeIsFound: Bool = true
/// Block is executed when a metadata object is found.
public var didFindCode: ((QRCodeReaderResult) -> Void)?
/// Block is executed when a found metadata object string could not be decoded.
public var didFailDecoding: ((Void) -> Void)?
// MARK: - Creating the Code Reade
/**
Initializes the code reader with the QRCode metadata type object.
*/
public convenience override init() {
self.init(metadataObjectTypes: [AVMetadataObjectTypeQRCode], captureDevicePosition: .back)
}
/**
Initializes the code reader with an array of metadata object types, and the default initial capture position
- parameter metadataObjectTypes: An array of strings identifying the types of metadata objects to process.
*/
public convenience init(metadataObjectTypes types: [String]) {
self.init(metadataObjectTypes: types, captureDevicePosition: .back)
}
/**
Initializes the code reader with the starting capture device position, and the default array of metadata object types
- parameter captureDevicePosition: The capture position to use on start of scanning
*/
public convenience init(captureDevicePosition position: AVCaptureDevicePosition) {
self.init(metadataObjectTypes: [AVMetadataObjectTypeQRCode], captureDevicePosition: position)
}
/**
Initializes the code reader with an array of metadata object types.
- parameter metadataObjectTypes: An array of strings identifying the types of metadata objects to process.
- parameter captureDevicePosition: The Camera to use on start of scanning.
*/
public init(metadataObjectTypes types: [String], captureDevicePosition: AVCaptureDevicePosition) {
metadataObjectTypes = types
super.init()
configureDefaultComponents(withCaptureDevicePosition: captureDevicePosition)
}
// MARK: - Initializing the AV Components
private func configureDefaultComponents(withCaptureDevicePosition: AVCaptureDevicePosition) {
session.addOutput(metadataOutput)
switch withCaptureDevicePosition {
case .front:
if let _frontDeviceInput = frontDeviceInput {
session.addInput(_frontDeviceInput)
}
case .back, .unspecified:
if let _defaultDeviceInput = defaultDeviceInput {
session.addInput(_defaultDeviceInput)
}
}
metadataOutput.setMetadataObjectsDelegate(self, queue: DispatchQueue.main)
metadataOutput.metadataObjectTypes = metadataObjectTypes
previewLayer.videoGravity = AVLayerVideoGravityResizeAspectFill
}
/// Switch between the back and the front camera.
@discardableResult
public func switchDeviceInput() -> AVCaptureDeviceInput? {
if let _frontDeviceInput = frontDeviceInput {
session.beginConfiguration()
if let _currentInput = session.inputs.first as? AVCaptureDeviceInput {
session.removeInput(_currentInput)
let newDeviceInput = (_currentInput.device.position == .front) ? defaultDeviceInput : _frontDeviceInput
session.addInput(newDeviceInput)
}
session.commitConfiguration()
}
return session.inputs.first as? AVCaptureDeviceInput
}
// MARK: - Controlling Reader
/**
Starts scanning the codes.
*Notes: if `stopScanningWhenCodeIsFound` is sets to true (default behaviour), each time the scanner found a code it calls the `stopScanning` method.*
*/
public func startScanning() {
if !session.isRunning {
session.startRunning()
}
}
/// Stops scanning the codes.
public func stopScanning() {
if session.isRunning {
session.stopRunning()
}
}
/**
Indicates whether the session is currently running.
The value of this property is a Bool indicating whether the receiver is running.
Clients can key value observe the value of this property to be notified when
the session automatically starts or stops running.
*/
public var isRunning: Bool {
return session.isRunning
}
/**
Indicates whether a front device is available.
- returns: true whether the device has a front device.
*/
public var hasFrontDevice: Bool {
return frontDevice != nil
}
/**
Indicates whether the torch is available.
- returns: true if a torch is available.
*/
public var isTorchAvailable: Bool {
return defaultDevice.isTorchAvailable
}
/**
Toggles torch on the default device.
*/
public func toggleTorch() {
do {
try defaultDevice.lockForConfiguration()
let current = defaultDevice.torchMode
defaultDevice.torchMode = AVCaptureTorchMode.on == current ? .off : .on
defaultDevice.unlockForConfiguration()
}
catch _ { }
}
// MARK: - Managing the Orientation
/**
Returns the video orientation corresponding to the given device orientation.
- parameter orientation: The orientation of the app's user interface.
- parameter supportedOrientations: The supported orientations of the application.
- parameter fallbackOrientation: The video orientation if the device orientation is FaceUp or FaceDown.
*/
public class func videoOrientation(deviceOrientation orientation: UIDeviceOrientation, withSupportedOrientations supportedOrientations: UIInterfaceOrientationMask, fallbackOrientation: AVCaptureVideoOrientation? = nil) -> AVCaptureVideoOrientation {
let result: AVCaptureVideoOrientation
switch (orientation, fallbackOrientation) {
case (.landscapeLeft, _):
result = .landscapeRight
case (.landscapeRight, _):
result = .landscapeLeft
case (.portrait, _):
result = .portrait
case (.portraitUpsideDown, _):
result = .portraitUpsideDown
case (_, .some(let orientation)):
result = orientation
default:
result = .portrait
}
if supportedOrientations.contains(orientationMask(videoOrientation: result)) {
return result
}
else if let orientation = fallbackOrientation , supportedOrientations.contains(orientationMask(videoOrientation: orientation)) {
return orientation
}
else if supportedOrientations.contains(.portrait) {
return .portrait
}
else if supportedOrientations.contains(.landscapeLeft) {
return .landscapeLeft
}
else if supportedOrientations.contains(.landscapeRight) {
return .landscapeRight
}
else {
return .portraitUpsideDown
}
}
class func orientationMask(videoOrientation orientation: AVCaptureVideoOrientation) -> UIInterfaceOrientationMask {
switch orientation {
case .landscapeLeft:
return .landscapeLeft
case .landscapeRight:
return .landscapeRight
case .portrait:
return .portrait
case .portraitUpsideDown:
return .portraitUpsideDown
}
}
// MARK: - Checking the Reader Availabilities
/**
Checks whether the reader is available.
- returns: A boolean value that indicates whether the reader is available.
*/
public class func isAvailable() -> Bool {
let captureDevice = AVCaptureDevice.defaultDevice(withMediaType: AVMediaTypeVideo)
return (try? AVCaptureDeviceInput(device: captureDevice)) != nil
}
/**
Checks and return whether the given metadata object types are supported by the current device.
- parameter metadataTypes: An array of strings identifying the types of metadata objects to check.
- returns: A boolean value that indicates whether the device supports the given metadata object types.
*/
public class func supportsMetadataObjectTypes(_ metadataTypes: [String]? = nil) throws -> Bool {
let captureDevice = AVCaptureDevice.defaultDevice(withMediaType: AVMediaTypeVideo)
let deviceInput = try AVCaptureDeviceInput(device: captureDevice)
let output = AVCaptureMetadataOutput()
let session = AVCaptureSession()
session.addInput(deviceInput)
session.addOutput(output)
var metadataObjectTypes = metadataTypes
if metadataObjectTypes == nil || metadataObjectTypes?.count == 0 {
// Check the QRCode metadata object type by default
metadataObjectTypes = [AVMetadataObjectTypeQRCode]
}
for metadataObjectType in metadataObjectTypes! {
if !output.availableMetadataObjectTypes.contains(where: { $0 as! String == metadataObjectType }) {
return false
}
}
return true
}
// MARK: - AVCaptureMetadataOutputObjects Delegate Methods
public func captureOutput(_ captureOutput: AVCaptureOutput!, didOutputMetadataObjects metadataObjects: [Any]!, from connection: AVCaptureConnection!) {
for current in metadataObjects {
if let _readableCodeObject = current as? AVMetadataMachineReadableCodeObject {
if _readableCodeObject.stringValue != nil {
if metadataObjectTypes.contains(_readableCodeObject.type) {
if let sVal = _readableCodeObject.stringValue {
if stopScanningWhenCodeIsFound {
stopScanning()
}
let scannedResult = QRCodeReaderResult(value: sVal, metadataType:_readableCodeObject.type)
DispatchQueue.main.async(execute: { [weak self] in
self?.didFindCode?(scannedResult)
})
}
}
}
else {
didFailDecoding?()
}
}
}
}
}
| mit | 8f530e7125996d2c579a3bc0221fdaf9 | 32.632597 | 251 | 0.719343 | 5.354002 | false | false | false | false |
cuzv/ExtensionKit | Sources/Extension/UIDevice+Extension.swift | 1 | 2279 | //
// UIDevice+Extension.swift
// Copyright (c) 2015-2016 Red Rain (http://mochxiao.com).
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
//
import UIKit
public extension UIDevice {
fileprivate static let _currentDevice = UIDevice.current
public class var sysVersion: String {
return _currentDevice.systemVersion
}
public class var majorVersion: Int {
return Int(UIDevice.sysVersion.components(separatedBy: ".").first!)!
}
public class var iOS7x: Bool {
return majorVersion >= 7
}
public class var iOS8x: Bool {
return majorVersion >= 8
}
public class var iOS9x: Bool {
return majorVersion >= 9
}
public class var iOS10x: Bool {
return majorVersion >= 10
}
public class var iOS11x: Bool {
return majorVersion >= 11
}
fileprivate static func deviceOrientation(_ result: (UIDeviceOrientation) -> ()) {
if !_currentDevice.isGeneratingDeviceOrientationNotifications {
_currentDevice.beginGeneratingDeviceOrientationNotifications()
}
result(_currentDevice.orientation)
_currentDevice.endGeneratingDeviceOrientationNotifications()
}
}
| mit | 3f7ee92062b7c4f916df9049b77d38d4 | 34.609375 | 86 | 0.698552 | 4.838641 | false | false | false | false |
nathanlanza/CoordinatorKit | Source/UIWindow+CoordinatorKit.swift | 1 | 1435 | import UIKit
//MARK: - Swizzling
fileprivate let swizzling: (UIWindow.Type) -> () = { window in
let originalSelector = #selector(window.makeKeyAndVisible)
let swizzledSelector = #selector(window.ck_makeKeyAndVisible)
let originalMethod = class_getInstanceMethod(window, originalSelector)
let swizzledMethod = class_getInstanceMethod(window, swizzledSelector)
method_exchangeImplementations(originalMethod!, swizzledMethod!)
}
extension UIWindow {
@objc func ck_makeKeyAndVisible() {
guard rootCoordinator != nil else { fatalError("You must set a rootCoordinator before making the UIWindow key and visible") }
ck_makeKeyAndVisible()
}
open class func performSwizzling() {
guard self === UIWindow.self else { return }
swizzling(self)
}
}
// MARK: - Associated Objects
extension UIWindow {
private struct AssociatedKeys {
static var rootCoordinator = "ck_rootCoordinator"
}
public var rootCoordinator: Coordinator? {
get {
return objc_getAssociatedObject(self, &AssociatedKeys.rootCoordinator) as? Coordinator
}
set {
if let coordinator = newValue {
objc_setAssociatedObject(self, &AssociatedKeys.rootCoordinator, coordinator, .OBJC_ASSOCIATION_RETAIN_NONATOMIC)
}
rootViewController = newValue?.viewController
}
}
}
| mit | e5de6abc581455290050a709aa74fa73 | 31.613636 | 133 | 0.673171 | 5.354478 | false | false | false | false |
bangslosan/CVCalendar | CVCalendar/CVDate.swift | 5 | 1074 | //
// CVDate.swift
// CVCalendar
//
// Created by Мак-ПК on 12/31/14.
// Copyright (c) 2014 GameApp. All rights reserved.
//
import UIKit
class CVDate: NSObject {
private let date: NSDate?
let year: Int?
let month: Int?
let week: Int?
let day: Int?
init(date: NSDate) {
super.init()
let calendarManager = CVCalendarManager.sharedManager
self.date = date
self.year = calendarManager.dateRange(date).year
self.month = calendarManager.dateRange(date).month
self.day = calendarManager.dateRange(date).day
}
init(day: Int, month: Int, week: Int, year: Int) {
super.init()
self.year = year
self.month = month
self.week = week
self.day = day
}
func description() -> String {
let dateFormatter = NSDateFormatter()
dateFormatter.dateFormat = "MMMM"
let month = dateFormatter.stringFromDate(self.date!)
return "\(month), \(self.year!)"
}
}
| mit | 85cb301e4cde0272ccfc5a9d51bb1282 | 21.744681 | 61 | 0.565014 | 4.258964 | false | false | false | false |
kickstarter/ios-oss | KsApi/models/ProjectAndBackingEnvelope.swift | 1 | 1079 | import Foundation
import ReactiveSwift
public struct ProjectAndBackingEnvelope: Equatable {
public var project: Project
public var backing: Backing
}
// MARK: - GraphQL Adapters
extension ProjectAndBackingEnvelope {
static func envelopeProducer(
from data: GraphAPI.FetchBackingQuery.Data
) -> SignalProducer<ProjectAndBackingEnvelope, ErrorEnvelope> {
let addOns = data.backing?.addOns?.nodes?
.compactMap { $0 }
.compactMap { $0.fragments.rewardFragment }
.compactMap { Reward.reward(from: $0) }
guard
let backingFragment = data.backing?.fragments.backingFragment,
let projectFragment = data.backing?.fragments.backingFragment.project?.fragments.projectFragment,
let backing = Backing.backing(from: backingFragment, addOns: addOns),
let project = Project.project(from: projectFragment, backing: backing, currentUserChosenCurrency: nil)
else {
return SignalProducer(error: .couldNotParseJSON)
}
return SignalProducer(value: ProjectAndBackingEnvelope(project: project, backing: backing))
}
}
| apache-2.0 | 2ed43211bc424f99524f5edba0a566b7 | 33.806452 | 108 | 0.745134 | 4.495833 | false | false | false | false |
mlgoogle/viossvc | viossvc/Scenes/Home/MyInformationVC.swift | 1 | 12280 | //
// MyClientVC.swift
// viossvc
//
// Created by macbook air on 17/3/15.
// Copyright © 2017年 com.yundian. All rights reserved.
//
import Foundation
import XCGLogger
import SVProgressHUD
import MJRefresh
class MyInformationVC: UIViewController,UITableViewDelegate,UITableViewDataSource {
var table:UITableView?
var timer:NSTimer?
//订单消息列表单个消息
var orders = [MyMessageListStatusModel]()
var isFirstTime = true
//请求行数
var pageCount = 0
//视图是否刷新
var isRefresh:Bool = false
var allDataDict:[String : Array<MyMessageListStatusModel>] = Dictionary()
var dateArray:[String] = Array()
var activityList = [MyMessageListStatusModel]()
var activityListDict:[String : Array<MyMessageListStatusModel>] = Dictionary()
var activityListArray:[String] = Array()
var deleArray = [MyMessageListStatusModel]()
let header:MJRefreshStateHeader = MJRefreshStateHeader()
let footer:MJRefreshAutoStateFooter = MJRefreshAutoStateFooter()
override func viewDidLoad() {
super.viewDidLoad()
view.backgroundColor = UIColor.init(hexString: "#ffffff")
initTableView()
//加载页面请求活动页面
AppAPIHelper.userAPI().getActivityList({ [weak self](response) in
if let models = response as? [MyMessageListStatusModel]{
for ele in models{
ele.timestamp = ele.campaign_time
}
self?.orders += models
}
}) { (error) in
}
let btn : UIButton = UIButton.init(type: .Custom)
btn.setTitle("", forState: .Normal)
btn.setBackgroundImage(UIImage.init(named: "return"), forState: .Normal)
btn.addTarget(self, action: #selector(popself), forControlEvents: .TouchUpInside)
btn.frame = CGRect.init(x: 0, y: 0, width: 12, height: 20)
navigationItem.leftBarButtonItem = UIBarButtonItem(customView: btn)
}
func popself() {
navigationController?.popViewControllerAnimated(true)
}
override func viewWillAppear(animated: Bool) {
super.viewWillAppear(animated)
if isRefresh {
}else{
header.performSelector(#selector(MJRefreshHeader.beginRefreshing), withObject: nil, afterDelay: 0.5)
}
}
//MARK: -- tableView
func initTableView() {
table = UITableView(frame: CGRectZero, style: .Grouped)
table?.backgroundColor = UIColor.init(decR: 241, decG: 242, decB: 243, a: 1)
table?.autoresizingMask = [.FlexibleHeight, .FlexibleWidth]
table?.delegate = self
table?.dataSource = self
table?.estimatedRowHeight = 80
table?.rowHeight = UITableViewAutomaticDimension
table?.separatorStyle = .SingleLine
table?.registerClass(MyInformationCell.self, forCellReuseIdentifier: "MyInformationCell")
view.addSubview(table!)
table?.snp_makeConstraints(closure: { (make) in
make.left.equalTo(view)
make.top.equalTo(view)
make.right.equalTo(view)
make.bottom.equalTo(view)
})
header.setRefreshingTarget(self, refreshingAction: #selector(headerRefresh))
table?.mj_header = header
footer.hidden = true
footer.setRefreshingTarget(self, refreshingAction: #selector(footerRefresh))
table?.mj_footer = footer
}
//下拉刷新
func headerRefresh() {
footer.state = .Idle
pageCount = 0
let req = OrderListRequestModel()
// req.uid_ = CurrentUser.uid_
AppAPIHelper.userAPI().orderListSum(req, complete: { [weak self](response) in
if response != nil {
self!.footer.hidden = false
}
if let models = response as? [MyMessageListStatusModel]{
self?.orders.removeAll()
AppAPIHelper.userAPI().getActivityList({ [weak self](response) in
if let models = response as? [MyMessageListStatusModel]{
for ele in models{
ele.timestamp = ele.campaign_time
}
self?.orders += models
}
}) { (error) in
}
for ele in models{
ele.timestamp = ele.order_time
}
self?.orders += models
//进行排序
self!.setupDataWithModels((self?.orders)!)
self?.endRefresh()
}
if self?.orders.count < 10{
self?.noMoreData()
}
}) { [weak self](error) in
self?.endRefresh()
}
timer = NSTimer.scheduledTimerWithTimeInterval(5, target: self, selector: #selector(endRefresh), userInfo: nil, repeats: false)
NSRunLoop.mainRunLoop().addTimer(timer!, forMode: NSRunLoopCommonModes)
}
//上拉刷新
func footerRefresh() {
pageCount += 1
let req = OrderListRequestModel()
req.page_num = pageCount
AppAPIHelper.userAPI().orderListSum(req, complete: { [weak self](response) in
if let models = response as? [MyMessageListStatusModel]{
for ele in models{
ele.timestamp = ele.order_time
}
self?.orders += models
self!.setupDataWithModels((self?.orders)!)
self?.endRefresh()
}
else{
self?.noMoreData()
}
}) { [weak self](error) in
self?.endRefresh()
}
timer = NSTimer.scheduledTimerWithTimeInterval(5, target: self, selector: #selector(endRefresh), userInfo: nil, repeats: false)
NSRunLoop.mainRunLoop().addTimer(timer!, forMode: NSRunLoopCommonModes)
}
//结束刷新
func endRefresh() {
isFirstTime = false
if header.state == .Refreshing {
header.endRefreshing()
}
if footer.state == .Refreshing {
footer.endRefreshing()
}
if timer != nil {
timer?.invalidate()
timer = nil
}
table?.reloadData()
}
func noMoreData() {
endRefresh()
footer.state = .NoMoreData
footer.setTitle("没有更多信息", forState: .NoMoreData)
}
//数据分组处理
func setupDataWithModels(models:[MyMessageListStatusModel]){
self.allDataDict.removeAll()
self.dateArray.removeAll()
//将传入的模型根据timestamp进行降序
if self.orders.count == 0 || self.orders.count == 1 || self.orders.count == 2{
}
else{
for i in 0...(self.orders.count - 2) {
for j in 0...(self.orders.count - i - 2){
// let str1 = models[j].timestamp! as NSString
// let str2 = self.orders[j + 1].timestamp! as NSString
// let result = str1.compare(str2 as String, options: NSStringCompareOptions.NumericSearch)
if self.orders[j].timestamp < self.orders[j + 1].timestamp {
//交换位置
let temp = self.orders[j]
self.orders[j] = self.orders[j + 1]
self.orders[j + 1] = temp
}
}
}
}
let dateFormatter = NSDateFormatter()
var dateString: String?
for model in self.orders{
dateFormatter.dateFormat = "yyyy-MM-dd HH:mm:ss"
let date = dateFormatter.dateFromString(model.timestamp!)
if date == nil {
continue
}
else{
dateFormatter.dateFormat = "MM"
dateString = dateFormatter.stringFromDate(date!)
}
/*
- 判断 model 对应的分组 是否已经有当天数据信息
- 如果已经有信息则直接将model 插入当天信息array
- 反之,创建当天分组array 插入数据
*/
if dateArray.contains(dateString!){
allDataDict[dateString!]?.append(model)
}
else{
var list:[MyMessageListStatusModel] = Array()
list.append(model)
dateArray.append(dateString!)
allDataDict[dateString!] = list
}
}
}
//tableViewDelegate
func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return dateArray.count
}
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
let array = allDataDict[dateArray[section]]
return array == nil ? 0 : array!.count
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("MyInformationCell", forIndexPath: indexPath) as! MyInformationCell
let array = allDataDict[dateArray[indexPath.section]]
if array![indexPath.row].campaign_time != nil {
cell.activityList(array![indexPath.row])
}
else{
cell.updeat(array![indexPath.row])
}
return cell
}
func tableView(tableView: UITableView, heightForRowAtIndexPath indexPath: NSIndexPath) -> CGFloat {
return 65
}
//MARK: -- 返回组标题索引
func tableView(tableView: UITableView, viewForHeaderInSection section: Int) -> UIView? {
var NYR: [String] = Array()
let sumView = UIView(frame: CGRect(x: 0, y: 0, width: UIScreen.mainScreen().bounds.width, height: 34))
let label = UILabel()
label.textColor = UIColor.init(red: 102/255.0, green: 102/255.0, blue: 102/255.0, alpha: 1)
label.font = UIFont.systemFontOfSize(13)
sumView.addSubview(label)
label.snp_makeConstraints { (make) in
make.centerX.equalTo(sumView)
make.height.equalTo(13)
make.top.equalTo(sumView).offset(10)
make.bottom.equalTo(sumView.snp_bottom).offset(-10)
}
if section == 0 {
let date = NSDate()
let timeFormatter = NSDateFormatter()
timeFormatter.dateFormat = "MM'at' HH:mm:ss.SSS"
let strNowTime = timeFormatter.stringFromDate(date) as String
let timeArray = strNowTime.componentsSeparatedByString(".")
NYR = timeArray[0].componentsSeparatedByString("at")
if NYR[0] == dateArray[0] {
label.text = "最近30天内的订单消息"
return sumView
}
}
label.text = dateArray[section] + "月"
label.textColor = UIColor.init(red: 102/255.0, green: 102/255.0, blue: 102/255.0, alpha: 1)
label.font = UIFont.systemFontOfSize(13)
return sumView
}
//组头高
func tableView(tableView: UITableView, heightForHeaderInSection section: Int) -> CGFloat {
return 34
}
//组尾高
func tableView(tableView: UITableView, heightForFooterInSection section: Int) -> CGFloat {
return 0.01
}
func tableView(tableView: UITableView, didSelectRowAtIndexPath indexPath: NSIndexPath) {
let array = allDataDict[dateArray[indexPath.section]]
if array![indexPath.row].campaign_time != nil {
let vc = ActivityVC()
vc.isRefresh = { ()->() in
self.isRefresh = true
}
navigationController?.pushViewController(vc, animated: true)
}
}
deinit {
NSNotificationCenter.defaultCenter().removeObserver(self)
if timer != nil {
timer?.invalidate()
timer = nil
}
}
}
| apache-2.0 | cd8d333346442924cd984f96c4784efa | 34.049708 | 135 | 0.556603 | 4.920772 | false | false | false | false |
longjianjiang/Drizzling | Drizzling/Drizzling/Extension/LJLocationManager.swift | 1 | 2608 | //
// LJLocationManager.swift
// Drizzling
//
// Created by longjianjiang on 2017/10/19.
// Copyright © 2017年 Jiang. All rights reserved.
//
import UIKit
import MapKit
protocol LJLocationManagerAuthorizationDelegate: class {
func locationManager(_ manager: LJLocationManager, userDidChooseLocation status: CLAuthorizationStatus)
}
protocol LJLocationManagerGetLocationDelegate: class {
func locationManager(_ manager: LJLocationManager, current city: String)
}
class LJLocationManager: NSObject {
static let shared = LJLocationManager()
weak var authorizationDelegate: LJLocationManagerAuthorizationDelegate?
weak var getLocationDelegate: LJLocationManagerGetLocationDelegate?
var locationManager: CLLocationManager!
lazy var geocoder: CLGeocoder = {
return CLGeocoder()
}()
override init() {
super.init()
locationManager = CLLocationManager.init()
locationManager.delegate = self
}
public func isLocationServiceEnable() -> Bool {
if CLLocationManager.locationServicesEnabled() {
let authStatus = CLLocationManager.authorizationStatus()
switch authStatus {
case .authorizedAlways, .authorizedWhenInUse:
return true
default:
return false
}
}
return false
}
public func askLocation() {
if CLLocationManager.locationServicesEnabled() {
locationManager.requestWhenInUseAuthorization()
}
}
public func startUseLocation() {
locationManager.startUpdatingLocation()
}
}
extension LJLocationManager: CLLocationManagerDelegate {
func locationManager(_ manager: CLLocationManager, didChangeAuthorization status: CLAuthorizationStatus) {
authorizationDelegate?.locationManager(self, userDidChooseLocation: status)
}
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
locationManager.stopUpdatingLocation()
guard let currentLocation = locations.last else { return }
geocoder.reverseGeocodeLocation(currentLocation) { (places: [CLPlacemark]?, error: Error?) in
if error == nil { // normal condition
guard let pl = places?.first else { return }
if let city = pl.locality {
self.getLocationDelegate?.locationManager(self, current: city)
}
} else {
print("reverse geocode location error: \(error)")
}
}
}
}
| apache-2.0 | 6c20841694555103572f167f8d6e4881 | 31.160494 | 110 | 0.66334 | 5.814732 | false | false | false | false |
younata/RSSClient | TethysKit/Services/Account/CredentialService.swift | 1 | 2654 | import Result
import CBGPromise
import FutureHTTP
import SwiftKeychainWrapper
protocol CredentialService {
func credentials() -> Future<Result<[Credential], TethysError>>
func store(credential: Credential) -> Future<Result<Void, TethysError>>
func delete(credential: Credential) -> Future<Result<Void, TethysError>>
}
private let credentialKey = "credentials"
struct KeychainCredentialService: CredentialService {
let keychain: KeychainWrapper
func credentials() -> Future<Result<[Credential], TethysError>> {
guard let data = self.keychain.data(forKey: credentialKey) else {
return Promise<Result<[Credential], TethysError>>.resolved(.success([]))
}
do {
let results = try JSONDecoder().decode([Credential].self, from: data)
return Promise<Result<[Credential], TethysError>>.resolved(.success(results))
} catch let error {
dump(error)
return Promise<Result<[Credential], TethysError>>.resolved(.failure(.database(.unknown)))
}
}
func store(credential: Credential) -> Future<Result<Void, TethysError>> {
return self.credentials().map { result -> Result<Void, TethysError> in
switch result {
case .failure(let error):
return .failure(error)
case .success(var credentials):
credentials.removeAll(where: { $0.accountId == credential.accountId })
credentials.append(credential)
do {
let data = try JSONEncoder().encode(credentials)
_ = self.keychain.set(data, forKey: credentialKey)
return .success(Void())
} catch let error {
dump(error)
return .failure(.database(.unknown))
}
}
}
}
func delete(credential: Credential) -> Future<Result<Void, TethysError>> {
return self.credentials().map { result -> Result<Void, TethysError> in
switch result {
case .failure(let error):
return .failure(error)
case .success(var credentials):
credentials.removeAll(where: { $0.accountId == credential.accountId })
do {
let data = try JSONEncoder().encode(credentials)
_ = self.keychain.set(data, forKey: credentialKey)
return .success(Void())
} catch let error {
dump(error)
return .failure(.database(.unknown))
}
}
}
}
}
| mit | a92e9d4cda2277cdfacc3a730e7f5f04 | 36.914286 | 101 | 0.571967 | 5.214145 | false | false | false | false |
OneBusAway/onebusaway-iphone | Carthage/Checkouts/SwiftEntryKit/Source/MessageViews/Notes/EKXStatusBarMessageView.swift | 3 | 1425 | //
// EKXStatusBarMessageView.swift
// SwiftEntryKit
//
// Created by Daniel Huri on 4/19/18.
// Copyright (c) 2018 [email protected]. All rights reserved.
//
import UIKit
import QuickLayout
public class EKXStatusBarMessageView: UIView {
// MARK: Props
private let leadingLabel = UILabel()
private let trailingLabel = UILabel()
// MARK: Setup
public init(leading: EKProperty.LabelContent, trailing: EKProperty.LabelContent) {
super.init(frame: UIScreen.main.bounds)
setup(leading: leading, trailing: trailing)
}
public required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
private func setup(leading: EKProperty.LabelContent, trailing: EKProperty.LabelContent) {
clipsToBounds = true
set(.height, of: UIApplication.shared.statusBarFrame.maxY)
addSubview(leadingLabel)
leadingLabel.content = leading
leadingLabel.layoutToSuperview(axis: .vertically)
leadingLabel.layoutToSuperview(.leading)
leadingLabel.layoutToSuperview(.width, ratio: 0.26)
addSubview(trailingLabel)
trailingLabel.content = trailing
trailingLabel.layoutToSuperview(axis: .vertically)
trailingLabel.layoutToSuperview(.trailing)
trailingLabel.layoutToSuperview(.width, ratio: 0.26)
}
}
| apache-2.0 | 11d585815048640517d0ee47caacdb7a | 29.319149 | 93 | 0.671579 | 4.672131 | false | false | false | false |
pyromobile/nubomedia-ouatclient-src | ios/LiveTales/uoat/UserModel.swift | 2 | 10992 | //
// UserModel.swift
// uoat
//
// Created by Pyro User on 8/8/16.
// Copyright © 2016 Zed. All rights reserved.
//
import Foundation
class UserModel
{
class func login(userName:String, password:String, onLogin:(isOK:Bool,userId:String,nick:String,code:String)->Void)
{
KuasarsCore.loginWithAppInternalToken(password, userId:userName){ (response:KuasarsResponse!, error:KuasarsError!) -> Void in
if( ( error ) != nil )
{
print( "Error from kuasars: \(error.description)" )
onLogin( isOK:false, userId:"", nick:"", code:"" )
}
else
{
print( "User logged Ok!" )
let info = response.contentObjects[0] as! NSDictionary
let id:String = info["userId"] as! String
UserModel.loadUserEntities( id, callback:{ (isOk, nick, code) in
if( isOk )
{
onLogin( isOK:true, userId:id, nick:nick, code:code )
}
else
{
onLogin( isOK:false, userId:"", nick:"", code:"" )
}
})
}
}
}
class func signup(userName:String, password:String, secretCode:String, onSignup:(isOK:Bool,userId:String)->Void)
{
let user = KuasarsUser( internalToken:password, andInternalIdentifier: userName )
user.fullName = userName
user.custom = ["code":secretCode]
user.save { ( response:KuasarsResponse!, error:KuasarsError! ) -> Void in
if( error != nil )
{
print( "Error from kuasars: \(error.description)" )
onSignup( isOK:false, userId:"" )
}
else
{
print( "User created Ok!" )
KuasarsCore.loginWithAppInternalToken(password, userId: userName) { (response:KuasarsResponse!, error:KuasarsError!) -> Void in
if( ( error ) != nil )
{
//Utils.alertMessage( self, title:"Error", message:"User or password wrong!" )
print( "Error from kuasars: \(error.description)" )
onSignup( isOK:false, userId:"" )
}
else
{
print( "User logged Ok!" )
let info = response.contentObjects[0] as! NSDictionary
let id:String = info["userId"] as! String
//self.user.setProfile( id, name:userName, password:password )
//self.createUserEntities()
UserModel.createUserEntities( id, nick:"Guest",code:secretCode, callback: { (isOk) in
if( isOk )
{
onSignup( isOK:true, userId:id )
}
else
{
onSignup( isOK:false, userId:"" )
}
})
}
}
}
}
}
class func logout()
{
KuasarsCore.logout { (response:KuasarsResponse!, error:KuasarsError!) in
//No hacemos nada.
}
}
class func updateNick(userId:String, nick:String, uniqueCode:String, onUpdateNick:(isOK:Bool)->Void)
{
let entity = ["id":"pub_\(userId)","nick":nick,"logged":true,"code":uniqueCode]
let usersEntity = KuasarsEntity( type:"users", customData:entity as [NSObject : AnyObject], expirationDate:0, occEnabled:false )
let acl = KuasarsPermissions()
acl.setReadPermissions( KuasarsReadPermissionALL, usersList:nil, groupList:nil )
usersEntity.setPermissions( acl )
usersEntity.replace({ (response:KuasarsResponse!, error:KuasarsError!) -> Void in
if( error != nil )
{
print( "Error from kuasars: \(error.description)" )
//Utils.alertMessage( self, title: "Error", message: "Nick name wasn't changed!" )
onUpdateNick( isOK:false )
}
else
{
print( "Update users entity Ok!" )
//Utils.alertMessage( self, title: "Info", message: "Nick name was changed correctly!" )
onUpdateNick( isOK:true )
}
})
}
class func loadUserFriends(userId:String,callback:(friends:[(id:String,nick:String)]?)->Void)
{
let criteria:[String:AnyObject] = ["me":"pub_\(userId)"]
let query = KuasarsQuery( criteria, retrievingFields: nil ) as KuasarsQuery
KuasarsEntity.query( query, entityType: "friends", occEnabled: false, completion:{ (response:KuasarsResponse!, error:KuasarsError!) -> Void in
if( error != nil )
{
print( "Error from kuasars: \(error.description)" )
callback( friends:nil )
}
else
{
if( response.statusCode == 200 )
{
let batch = KuasarsBatch()
let entities = response.contentObjects as! [KuasarsEntity]
for entity:KuasarsEntity in entities
{
let friendId:String = (entity.customData!["friend"])! as! String
print("Amigo encontrado:\(friendId)")
let criteria:[String:AnyObject] = ["id":"\(friendId)"]
let query = KuasarsQuery( criteria, retrievingFields: nil ) as KuasarsQuery
let request:KuasarsRequest = KuasarsServices.queryEntities( query, type:"users", occEnabled:false )
batch.addRequest(request)
}
batch.performRequests({ (response:KuasarsResponse!, error:KuasarsError!) in
if( error != nil )
{
print( "Error from kuasars: \(error.description)" )
callback(friends:nil)
}
else
{
var friends:[(id:String,nick:String)] = [(id:String,nick:String)]()
let responses = response.contentObjects as! [NSDictionary]
for index in 0 ..< responses.count
{
let rsp:NSDictionary = responses[index] as NSDictionary
let body:[NSDictionary] = rsp["body"] as! [NSDictionary]
let friendNick = body[0]["nick"] as! String
let id:String = entities[index].customData["friend"] as! String
friends.append( (id:id, nick:friendNick) )
}
callback(friends: friends)
}
})
}
else
{
//No results!
callback(friends:[])
}
}
})
}
/*=============================================================*/
/* Private Section */
/*=============================================================*/
private class func loadUserEntities(userId:String, callback:(isOk:Bool,nick:String,code:String)->Void)
{
//load user's public profile.
KuasarsEntity.getWithType( "users", entityID: "pub_\(userId)", occEnabled: false) { (response:KuasarsResponse!, error:KuasarsError!) -> Void in
if( error != nil )
{
print( "Error from kuasars: \(error.description)" )
callback( isOk:false, nick:"", code:"" )
}
else
{
let entity = response.contentObjects[0] as! KuasarsEntity
let nick:String = entity.customData!["nick"]! as! String
let code:String = entity.customData!["code"]! as! String
//load user's friend group.
callback( isOk:true, nick:nick, code:code )
}
}
}
private class func createUserEntities(userId:String,nick:String,code:String,callback:(isOk:Bool)->Void)
{
//creates user's public profile.
let entity = ["id":"pub_\(userId)","nick":nick,"logged":true, "code":code]
let usersEntity = KuasarsEntity( type:"users", customData:entity as [NSObject : AnyObject], expirationDate:0, occEnabled:false )
let acl = KuasarsPermissions()
acl.setReadPermissions( KuasarsReadPermissionALL, usersList:nil, groupList:nil )
usersEntity.setPermissions( acl )
usersEntity.save { ( response:KuasarsResponse!, error:KuasarsError! ) -> Void in
if( error != nil )
{
print( "Error from kuasars: \(error.description)" )
callback( isOk:false )
}
else
{
//creates user's friends group.
let entity = ["id":"grp_\(userId)","friends":[]]
let friendsEntity = KuasarsEntity( type:"friends", customData:entity as [NSObject:AnyObject], expirationDate:0, occEnabled:false )
let acl = KuasarsPermissions()
acl.setReadPermissions( KuasarsReadPermissionALL, usersList: nil, groupList: nil )
friendsEntity.setPermissions( acl )
friendsEntity.save({ ( response:KuasarsResponse!, error:KuasarsError! ) -> Void in
if( error != nil )
{
print( "Error from kuasars: \(error.description)" )
callback( isOk:false )
}
else
{
//let mainViewController = self.storyboard?.instantiateViewControllerWithIdentifier( "mainViewController" ) as? ViewController;
//mainViewController?.user = self.user
//self.presentViewController( mainViewController!, animated: true, completion: nil )
/*
self.dismissViewControllerAnimated( true, completion:{[unowned self] in
self.delegate?.onLoginSignupReady()
})
*/
callback( isOk:true )
}
})
}
}
}
} | apache-2.0 | f131eaa6697c8ab3ea0879ea1591999a | 43.144578 | 151 | 0.470294 | 5.345817 | false | false | false | false |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.