repo_name
stringlengths 7
91
| path
stringlengths 8
658
| copies
stringclasses 125
values | size
stringlengths 3
6
| content
stringlengths 118
674k
| license
stringclasses 15
values | hash
stringlengths 32
32
| line_mean
float64 6.09
99.2
| line_max
int64 17
995
| alpha_frac
float64 0.3
0.9
| ratio
float64 2
9.18
| autogenerated
bool 1
class | config_or_test
bool 2
classes | has_no_keywords
bool 2
classes | has_few_assignments
bool 1
class |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
icapps/swiftGenericWebService | Sources/Service/Service.swift | 3 | 7170 | //
// Service.swift
// Pods
//
// Created by Stijn Willems on 20/04/2017.
//
//
import Foundation
/*:
Create a service instance to perform all kinds of requests. You can choose to use Service or its subclasses:
1. ServiceHandler -> can be used for a single type and single handler
2. ServicQueue -> can be used when you want to fire requests in paralel but want to know when all are done.
All subclasses use this class to perform the requests.
*/
open class Service {
open var call: Call
open var autoStart: Bool
public let session: FaroURLSession = FaroURLSession.shared()
/**
Init a servcie instance to perform calls
- Parameters:
- call: points to the request you want to perform
- autoStart: from the call a task is made. This task is returned by the perform function. The task is started automatically unless you set autoStart to no.
- configuration: describes the base url to from a request with from the provided call.
*/
public init(call: Call, autoStart: Bool = true) {
self.autoStart = autoStart
self.call = call
}
// MARK: Error
/// Prints the error and throws it
/// Possible to override this to have custom behaviour for your app.
open func handleError(_ error: Error) {
print(error)
}
}
// MARK: - Perform Call and decode
extension Service {
/*: Gets a model(s) from the service and decodes it using native `Decodable` protocol. To do this it asks the URLSession to provide a task.
This task can be:
1. DownloadTask for httpMethod GET (will finish in the background)
2. DownloadTask for httpMethod PUT, POST, PATCH (will finish in the background) but if you Provide `Service.NoResponseData` as an expected return type the upload does not expect data returned
* It is a bit wierd but otherwise response data should be there
3. DataTask for all others (NO finish in the background)
Provide a type, that can be an array, to decode the data received from the service into type 'M'
- parameter type: Generic type to decode the returend data to. If service returns no response data use type `Service.NoResponseData`
- parameter complete: Is an inner function that is returned and that can throw. Why this is can be read on blog https://appventure.me/2015/06/19/swift-try-catch-asynchronous-closures/
*/
@discardableResult
open func perform<M>(_ type: M.Type, complete: @escaping(_ inner: @escaping () throws -> (M)) -> Void) -> URLSessionTask where M: Decodable {
let call = self.call
let config = self.session.backendConfiguration
let request = call.request(with: config)
var task: URLSessionTask!
let urlSession = FaroURLSession.shared().urlSession
task = urlSession.downloadTask(with: request)
session.tasksDone[task] = { [weak self] (data, response, error) in
guard let `self` = self else {return}
let error = raisesServiceError(data: data, urlResponse: response, error: error, for: request)
guard error == nil else {
self.handleError(error!)
complete { throw error! }
return
}
guard type.self != Service.NoResponseData.self else {
complete {
// Constructing a no data data with an empty response
let data = """
{}
""".data(using: .utf8)!
return try config.decoder.decode(M.self, from: data)
}
return
}
guard let returnData = data else {
let error = ServiceError.invalidResponseData(data, call: call)
self.handleError(error)
complete { throw error }
return
}
complete {
do {
return try config.decoder.decode(M.self, from: returnData)
} catch let error as DecodingError {
let error = ServiceError.decodingError(error, inData: returnData, call: call)
self.handleError(error)
throw error
}
}
}
guard autoStart else {
return task
}
task.resume()
return task
}
// MARK: - Update model instead of create
/*: Simular to norma perform but the properties on the provided model will be updated.
- parameter model: The model object whose properties will be updated with the json from the service.
- parameter complete: Is an inner function that is returned and that can throw. Why this is can be read on blog https://appventure.me/2015/06/19/swift-try-catch-asynchronous-closures/
*/
open func performUpdate<M>(model: M, complete: @escaping(@escaping () throws -> ()) -> Void) -> URLSessionTask? where M: Decodable & Updatable {
let task = perform(M.self) { (resultFunction) in
complete {
let serviceModel = try resultFunction()
try model.update(serviceModel)
return
}
}
return task
}
/*: Simular to norma perform but the properties on the provided model will be updated.
- parameter model: The array with model objects whose properties will be updated with the json from the service.
- parameter complete: Is an inner function that is returned and that can throw. Why this is can be read on blog https://appventure.me/2015/06/19/swift-try-catch-asynchronous-closures/
*/
open func performUpdate<M>(array: [M], complete: @escaping(@escaping () throws -> ()) -> Void) -> URLSessionTask? where M: Decodable & Updatable {
let task = perform([M].self) { (resultFunction) in
complete {
var serviceModels = Set(try resultFunction())
try array.forEach { element in
try element.update(array: Array(serviceModels))
serviceModels.remove(element)
}
return
}
}
return task
}
// MARK - No response data Type
/// Use this type for `perform` when service returns no data
public struct NoResponseData: Decodable {
}
}
// MARK: - Internal error functions
func raisesServiceError(data: Data?, urlResponse: URLResponse?, error: Error?, for request: URLRequest) -> Error? {
guard error == nil else {
return error
}
guard let httpResponse = urlResponse as? HTTPURLResponse else {
let returnError = ServiceError.networkError(0, data: data, request: request)
return returnError
}
let statusCode = httpResponse.statusCode
guard statusCode < 400 else {
let returnError = ServiceError.networkError(statusCode, data: data, request: request)
return returnError
}
guard 200...204 ~= statusCode else {
let returnError = ServiceError.networkError(statusCode, data: data, request: request)
return returnError
}
return nil
}
| mit | 166f92c0f48dd555642f7890ad26bcec | 36.150259 | 200 | 0.623013 | 4.770459 | false | false | false | false |
rain2540/Play-with-Algorithms-in-Swift | LeetCode/01-Array.playground/Pages/Merge Sorted Array.xcplaygroundpage/Contents.swift | 1 | 2313 | //: [Previous](@previous)
import Foundation
//: ## Merge Sorted Array
//:
//: Given two sorted integer arrays A and B, merge B into A as one sorted array.
//:
//: Note: You may assume that A has enough space (size that is greater or equal to m + n) to hold additional elements from B. The number of elements initialized in A and B are m and n respectively.
func merge(sortedB: [Int], into sortedA: inout [Int]) {
var i = sortedA.count + sortedB.count - 1
var j = sortedA.count - 1
var k = sortedB.count - 1
sortedA.append(contentsOf: sortedB)
while i >= 0 {
if j >= 0 && k >= 0 {
if sortedA[j] > sortedB[k] {
sortedA[i] = sortedA[j]
j -= 1
} else {
sortedA[i] = sortedB[k]
k -= 1
}
} else if j >= 0 {
sortedA[i] = sortedA[j]
j -= 1
} else if k >= 0 {
sortedA[i] = sortedB[k]
k -= 1
}
i -= 1
}
}
var a = [1, 3, 5]
let b = [2, 4, 6]
merge(sortedB: b, into: &a)
print(a)
//: Another implementation (with return value)
func merged(sortedA: [Int], sortedB: [Int]) -> [Int] {
var i = sortedA.count + sortedB.count - 1
var j = sortedA.count - 1
var k = sortedB.count - 1
var res = Array<Int>(repeating: 0, count: sortedA.count + sortedB.count)
while i >= 0 {
if j >= 0 && k >= 0 {
if sortedA[j] > sortedB[k] {
res[i] = sortedA[j]
j -= 1
} else {
res[i] = sortedB[k]
k -= 1
}
} else if j >= 0 {
res[i] = sortedA[j]
j -= 1
} else if k >= 0 {
res[i] = sortedB[k]
k -= 1
}
i -= 1
}
return res
}
let c = [1, 3, 5]
let d = [2, 4, 6]
print(merged(sortedA: c, sortedB: d))
//: Update
func merge(b: [Int], into a: inout [Int]) {
let c = b.sorted()
a.sort()
merge(sortedB: c, into: &a)
}
var e = [1, 5, 3]
let f = [4, 6, 2]
merge(b: f, into: &e)
print(e)
func merged(a: [Int], b: [Int]) -> [Int] {
let c = a.sorted()
let d = b.sorted()
return merged(sortedA: c, sortedB: d)
}
let g = [1, 5, 3]
let h = [4, 6, 2]
print(merged(a: g, b: h))
//: [Next](@next)
| mit | af9d2a63a7be648b0a8bc3d37b8a4b2e | 21.028571 | 197 | 0.475573 | 3.039422 | false | false | false | false |
StachkaConf/ios-app | StachkaIOS/Pods/RectangleDissolve/RectangleDissolve/Classes/TempoCounter.swift | 2 | 1422 | //
// TempoCounter.swift
// GithubItunesViewer
//
// Created by MIKHAIL RAKHMANOV on 12.02.17.
// Copyright © 2017 m.rakhmanov. All rights reserved.
//
import Foundation
import Foundation
import QuartzCore
enum BeatStep: Int {
case fourth = 4
case eighth = 8
case sixteenth = 16
case thirtyTwo = 32
}
typealias Handler = () -> ()
class TempoCounter {
fileprivate var displayLink: CADisplayLink?
fileprivate let frameRate = 60
fileprivate var internalCounter = 0
var tempo = 60.0
var beatStep = BeatStep.fourth
var handler: Handler?
fileprivate var nextTickLength: Int {
return Int(Double(frameRate) / (tempo / 60.0 * Double(beatStep.rawValue) / 4.0))
}
init() {
displayLink = CADisplayLink(target: self, selector: #selector(fire))
if #available(iOS 10.0, *) {
displayLink?.preferredFramesPerSecond = frameRate
}
displayLink?.add(to: RunLoop.main, forMode: .commonModes)
displayLink?.isPaused = true
}
deinit {
displayLink?.remove(from: RunLoop.main, forMode: .commonModes)
}
func start() {
displayLink?.isPaused = false
}
func stop() {
displayLink?.isPaused = true
}
@objc func fire() {
internalCounter += 1
if internalCounter >= nextTickLength {
internalCounter = 0
handler?()
}
}
}
| mit | 4f3e60adfb23233f3d18d359ead97e78 | 20.861538 | 88 | 0.617875 | 4.216617 | false | false | false | false |
mbrandonw/project-euler-swift-playgrounds | 5/project-euler-5.playground/section-1.swift | 1 | 4183 | import Foundation
/*========================================================
================= Problem #6 ===========================
========================================================
https://projecteuler.net/problem=5
2520 is the smallest number that can be divided by each of the numbers from 1 to 10 without any remainder.
What is the smallest positive number that is evenly divisible by all of the numbers from 1 to 20?
========================================================
========================================================
======================================================== */
/**
Naive solution:
The playground has trouble with plugging in 20, so
use at your own risk.
*/
func naiveEuler5 (n: Int) -> Int {
var answer = 0
while true {
answer += n
var allDivisible = true
for d in 2..<n {
if answer % d != 0 {
allDivisible = false
break
}
}
if allDivisible {
return answer
}
}
}
/**
Smarter solution:
The problem is essentially asking for the least common multiple
of 1 through 20. The easy way to do this is probably to factor
each of the numbers (since they are so small) and gather all
of their prime factors. We can also use the observation that
lcm(1, 2, ..., 20) = lcm(10, 11, ..., 20)
since all of the numbers in the first half of the range [1, 20]
appear as factors in the numbers in the second half of the range.
*/
// Truncates a sequence by using a predicate.
func takeWhile <S: SequenceType> (p: S.Generator.Element -> Bool) -> S -> [S.Generator.Element] {
return {sequence in
var taken: [S.Generator.Element] = []
for s in sequence {
if p(s) {
taken.append(s)
} else {
break
}
}
return taken
}
}
// A sequence to generate prime numbers.
struct PrimeSequence : SequenceType {
func generate() -> GeneratorOf<Int> {
var knownPrimes: [Int] = []
return GeneratorOf<Int>() {
if let lastPrime = knownPrimes.last {
var possiblePrime = lastPrime+1
while true {
let sqrtPossiblePrime = Int(sqrtf(Float(possiblePrime)))
var somePrimeDivides = false
for prime in knownPrimes {
// Sieve of Eratosthenes
if prime > sqrtPossiblePrime {
break
}
if possiblePrime % prime == 0 {
somePrimeDivides = true
break
}
}
if somePrimeDivides {
possiblePrime++
} else {
break
}
}
knownPrimes.append(possiblePrime)
return possiblePrime
} else {
knownPrimes.append(2)
return 2
}
}
}
}
// Easy integer exponentiation
infix operator ** {}
func ** (lhs: Int, rhs: Int) -> Int {
return Int(pow(Float(lhs), Float(rhs)))
}
// Returns a dictionary mapping primes to powers in an
// integer's factorization.
func primeFactorization (n: Int) -> [Int:Int] {
let primes = takeWhile { $0 <= n } (PrimeSequence())
let sqrtN = Int(sqrtf(Float(n)))
return primes.reduce([Int:Int]()) { accum, prime in
var r = accum
for power in 1...n {
if n % (prime ** power) != 0 {
if power != 1 {
r[prime] = power-1
}
break
}
}
return r
}
}
func smartEuler5 (n: Int) -> Int {
let a = Int(n/2) + 1
// Prime/power factors of LCM of 11-20
let factors = Array(a...n)
.map { primeFactorization($0) }
.reduce([Int:Int]()) { accum, factorization in
var r = accum
for (prime, power) in factorization {
if let currentPower = accum[prime] {
r[prime] = max(power, currentPower)
} else {
r[prime] = power
}
}
return r
}
return Array(factors.keys).reduce (1) { accum, prime in accum * (prime ** factors[prime]!) }
}
smartEuler5(10)
naiveEuler5(10)
smartEuler5(20)
//naiveEuler5(20) // <-- takes too long, use at your own risk
// Let's go crazy and solve the problem for 42! This
// is the biggest number we can plug into `smartEuler5`
// before we get an integer overflow
smartEuler5(42)
"done"
| mit | c1fe00a56d4b9bbd7ce3b38a821c4462 | 22.902857 | 107 | 0.545063 | 4.057226 | false | false | false | false |
Inspirato/SwiftPhotoGallery | Example/SwiftPhotoGallery/MainCollectionViewController.swift | 1 | 3885 | //
// MainCollectionViewController.swift
// SwiftPhotoGallery
//
// Created by Justin Vallely on 8/1/17.
// Copyright © 2017 CocoaPods. All rights reserved.
//
import UIKit
import SwiftPhotoGallery
private let reuseIdentifier = "Cell"
class MainCollectionViewController: UICollectionViewController {
let imageNames = ["image1.jpeg", "image2.jpeg", "image3.jpeg"]
let imageTitles = ["Image 1", "Image 2", "Image 3"]
var index: Int = 0
override func viewDidLoad() {
super.viewDidLoad()
}
// MARK: UICollectionViewDataSource
override func numberOfSections(in collectionView: UICollectionView) -> Int {
return 1
}
override func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return 3
}
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: reuseIdentifier, for: indexPath) as! MainCollectionViewCell
cell.imageView.image = UIImage(named: imageNames[indexPath.item])
return cell
}
// MARK: UICollectionViewDelegate
override func collectionView(_ collectionView: UICollectionView, didSelectItemAt indexPath: IndexPath) {
index = indexPath.item
let gallery = SwiftPhotoGallery(delegate: self, dataSource: self)
gallery.backgroundColor = UIColor.black
gallery.pageIndicatorTintColor = UIColor.gray.withAlphaComponent(0.5)
gallery.currentPageIndicatorTintColor = UIColor(red: 0.0, green: 0.66, blue: 0.875, alpha: 1.0)
gallery.hidePageControl = false
gallery.modalPresentationStyle = .custom
gallery.transitioningDelegate = self
/// Load the first page like this:
// present(gallery, animated: true, completion: nil)
/// Or load on a specific page like this:
present(gallery, animated: true, completion: { () -> Void in
gallery.currentPage = self.index
})
}
}
// MARK: SwiftPhotoGalleryDataSource Methods
extension MainCollectionViewController: SwiftPhotoGalleryDataSource {
func numberOfImagesInGallery(gallery: SwiftPhotoGallery) -> Int {
return imageNames.count
}
func imageInGallery(gallery: SwiftPhotoGallery, forIndex: Int) -> UIImage? {
return UIImage(named: imageNames[forIndex])
}
}
// MARK: SwiftPhotoGalleryDelegate Methods
extension MainCollectionViewController: SwiftPhotoGalleryDelegate {
func galleryDidTapToClose(gallery: SwiftPhotoGallery) {
self.index = gallery.currentPage
dismiss(animated: true, completion: nil)
}
}
// MARK: UIViewControllerTransitioningDelegate Methods
extension MainCollectionViewController: UIViewControllerTransitioningDelegate {
func presentationController(forPresented presented: UIViewController, presenting: UIViewController?, source: UIViewController) -> UIPresentationController? {
return DimmingPresentationController(presentedViewController: presented, presenting: presenting)
}
func animationController(forPresented presented: UIViewController, presenting: UIViewController, source: UIViewController) -> UIViewControllerAnimatedTransitioning? {
guard let selectedCellFrame = self.collectionView?.cellForItem(at: IndexPath(item: index, section: 0))?.frame else { return nil }
return PresentingAnimator(pageIndex: index, originFrame: selectedCellFrame)
}
func animationController(forDismissed dismissed: UIViewController) -> UIViewControllerAnimatedTransitioning? {
guard let returnCellFrame = self.collectionView?.cellForItem(at: IndexPath(item: index, section: 0))?.frame else { return nil }
return DismissingAnimator(pageIndex: index, finalFrame: returnCellFrame)
}
}
| apache-2.0 | ace2a1e30eadcd1589a8c97f5192e863 | 34.633028 | 170 | 0.73172 | 5.372061 | false | false | false | false |
mluedke2/snowshoe-swift | Pod/Classes/SnowShoeDateTransform.swift | 1 | 819 | //
// SnowShoeDateTransform.swift
// Pods
//
// Created by Matt Luedke on 8/25/15.
//
//
import Foundation
import ObjectMapper
public class SnowShoeDateTransform: TransformType {
let dateFormat = "yyyy-MM-dd HH:mm:ss.SSSSSS" // "2015-03-24 11:27:33.014149"
public init() {}
public func transformFromJSON(value: AnyObject?) -> NSDate? {
if let stringValue = value as? String {
let dateFormatter = NSDateFormatter()
dateFormatter.dateFormat = dateFormat
return dateFormatter.dateFromString(stringValue)
}
return nil
}
public func transformToJSON(value: NSDate?) -> String? {
if let date = value {
let dateFormatter = NSDateFormatter()
dateFormatter.dateFormat = dateFormat
return dateFormatter.stringFromDate(date)
}
return nil
}
}
| mit | 535546a2cf9dfd858bfa576f7b411ac1 | 22.4 | 79 | 0.680098 | 4.265625 | false | false | false | false |
dhardiman/Fetch | Tests/FetchTests/FetchCombineTests.swift | 1 | 6255 | //
// FetchCombineTests.swift
// Fetch-iOS
//
// Created by David Hardiman on 21/06/2019.
// Copyright © 2019 David Hardiman. All rights reserved.
//
#if canImport(Combine)
import Combine
@testable import Fetch
import Nimble
import OHHTTPStubs
import XCTest
extension FetchTests {
func testItIsPossibleToMakeAGetRequestUsingAPublisher() {
guard #available(OSX 10.15, iOS 13.0, tvOS 13.0, watchOS 6.0, *, *) else {
// @available attribute doesn't seem to work for tests.
// I assume XCTest can't read the attribute, which would make sense.
return
}
stubRequest(passingTest: { $0.url! == testURL && $0.httpMethod == "GET" })
let publisher = session!.publisher(for: basicRequest, errorParser: nil) as AnyPublisher<TestResponse, Error>
let exp = expectation(description: "get request")
let sub = publisher.sink(receiveCompletion: { _ in }, receiveValue: {
expect($0.name).to(equal("test name"))
expect($0.desc).to(equal("test desc"))
exp.fulfill()
})
waitForExpectations(timeout: 10.0, handler: nil)
print("\(sub.hashValue)") // Need to keep a reference to avoid the subscriber being disposed immediately, this is to silence the warning
}
func testSessionErrorsAreReturnedUsingAPublisher() {
guard #available(OSX 10.15, iOS 13.0, tvOS 13.0, watchOS 6.0, *, *) else { return }
let testError = NSError(domain: NSURLErrorDomain, code: NSURLErrorCancelled, userInfo: nil)
HTTPStubs.stubRequests(passingTest: { (request) -> Bool in
return request.url! == testURL && request.httpMethod == "GET"
}, withStubResponse: { (_) -> HTTPStubsResponse in
return HTTPStubsResponse(error: testError)
})
let publisher = session!.publisher(for: basicRequest, errorParser: nil) as AnyPublisher<TestResponse, Error>
let exp = expectation(description: "get request")
let sub = publisher.sink(receiveCompletion: {
switch $0 {
case .finished:
fail("Expected an error")
case .failure(let error as NSError):
expect(error.code).to(equal(testError.code))
expect(error.domain).to(equal(testError.domain))
}
exp.fulfill()
}, receiveValue: { _ in })
waitForExpectations(timeout: 1.0, handler: nil)
print("\(sub.hashValue)")
}
func testItReturnsParsedErrorsCorrectlyUsingAPublisher() {
guard #available(OSX 10.15, iOS 13.0, tvOS 13.0, watchOS 6.0, *, *) else { return }
stubRequest(statusCode: 400) { (request) -> Bool in
return request.url! == testURL && request.httpMethod == "GET"
}
let exp = expectation(description: "get request")
let publisher = session!.publisher(for: basicRequest, errorParser: CustomError.self) as AnyPublisher<NoDataResponse, Error>
var receivedError: Error?
let sub = publisher.sink(receiveCompletion: {
switch $0 {
case .finished:
fail("Expected an error")
case .failure(let error):
receivedError = error
}
exp.fulfill()
}, receiveValue: { _ in })
waitForExpectations(timeout: 1.0, handler: nil)
let customError = receivedError as! CustomError // swiftlint:disable:this force_cast
expect(customError).to(equal(CustomError.error))
print("\(sub.hashValue)")
}
func testItIncrementsTheActivityMonitorCorrectly() {
guard #available(OSX 10.15, iOS 13.0, tvOS 13.0, watchOS 6.0, *, *) else { return }
let stubMonitor = SessionActivityMonitor(initialValue: 0, isAsynchronous: false)
stubRequest(passingTest: { $0.url! == testURL && $0.httpMethod == "GET" })
var isCurrentlyActive: Bool = false
SessionActivityMonitor.sessionActivityChanged = {
isCurrentlyActive = $0
}
session.activityMonitor = stubMonitor
let publisher = session!.publisher(for: basicRequest, errorParser: nil) as AnyPublisher<TestResponse, Error>
expect(isCurrentlyActive).to(beTrue())
let exp = expectation(description: "get request")
let sub = publisher.sink(receiveCompletion: { _ in }, receiveValue: { _ in
exp.fulfill()
})
waitForExpectations(timeout: 1.0, handler: nil)
expect(isCurrentlyActive).to(beFalse())
print("\(sub.hashValue)")
}
func testItDecrementsTheActivityMonitorCorrectlyOnSessionErrors() {
guard #available(OSX 10.15, iOS 13.0, tvOS 13.0, watchOS 6.0, *, *) else { return }
let stubMonitor = SessionActivityMonitor(initialValue: 0, isAsynchronous: false)
session.activityMonitor = stubMonitor
let testError = NSError(domain: NSURLErrorDomain, code: NSURLErrorCancelled, userInfo: nil)
HTTPStubs.stubRequests(passingTest: { (request) -> Bool in
return request.url! == testURL && request.httpMethod == "GET"
}, withStubResponse: { (_) -> HTTPStubsResponse in
return HTTPStubsResponse(error: testError)
})
var isCurrentlyActive: Bool = false
SessionActivityMonitor.sessionActivityChanged = {
isCurrentlyActive = $0
}
let publisher = session!.publisher(for: basicRequest, errorParser: nil) as AnyPublisher<TestResponse, Error>
let exp = expectation(description: "get request")
let sub = publisher.sink(receiveCompletion: { _ in
exp.fulfill()
}, receiveValue: { _ in })
waitForExpectations(timeout: 1.0, handler: nil)
expect(isCurrentlyActive).to(beFalse())
print("\(sub.hashValue)")
}
}
#endif
| mit | 9a0a9c29fe4db175e54e907f8237644f | 48.244094 | 148 | 0.587304 | 4.912804 | false | true | false | false |
AlphaJian/PaperCalculator | PaperCalculator/PaperCalculator/Utility/DataManager.swift | 1 | 6882 | //
// DataManager.swift
// PaperCalculator
//
// Created by Jian Zhang on 9/28/16.
// Copyright © 2016 apple. All rights reserved.
//
import UIKit
class DataManager: NSObject {
var papersArray = [PaperModel]()
var paperModel = PaperModel()
var paperNum = 1
var paperModelTemp = PaperModel()
//statistics
var numOf90plus = 0
var numOf80plus = 0
var numOf70plus = 0
var numOf60plus = 0
var numOf60off = 0
var bestArr = [PaperModel]()
var lastArr = [PaperModel]()
static let shareManager = DataManager()
func createSectionQuestion(numberOfQuestions : Int, score : Float, style : QuestionStyle){
var arr = [CellQuestionModel]()
for i in 0 ... numberOfQuestions - 1 {
let qModel = CellQuestionModel()
qModel.questionNo = i + 1
qModel.score = score
qModel.realScore = score
if qModel.score >= 5 {
qModel.questionStyle = QuestionStyle.multiScore
} else {
qModel.questionStyle = style
}
arr.append(qModel)
}
let sectionModel = SectionQuestionModel()
sectionModel.cellQuestionArr = arr
sectionModel.sectionScore = 0
sectionModel.editStatus = QuestionStatus.none
sectionModel.preSettingQuesScore = Int(score)
if paperModel.sectionQuestionArr == nil
{
paperModel.sectionQuestionArr = [SectionQuestionModel]()
}
paperModel.sectionQuestionArr.append(sectionModel)
// paperModel.totalScore = paperModel.totalScore + sectionModel.sectionScore
}
func editCellQuestion(cellModel : CellQuestionModel, indexPath : IndexPath)
{
let section = paperModel.sectionQuestionArr[indexPath.section]
let oldScore = section.cellQuestionArr[indexPath.row].realScore!
section.cellQuestionArr[indexPath.row] = cellModel
section.sectionScore = section.sectionScore - oldScore + cellModel.realScore
paperModel.sectionQuestionArr[indexPath.section] = section
paperModel.totalScore = paperModel.totalScore - oldScore + cellModel.realScore
getGrade()
}
func getTotalScore(){
if paperModel.sectionQuestionArr.count > 0{
for i in 0...paperModel.sectionQuestionArr.count - 1 {
for j in 0...paperModel.sectionQuestionArr[i].cellQuestionArr.count - 1 {
paperModel.sectionQuestionArr[i].sectionScore = paperModel.sectionQuestionArr[i].sectionScore + (paperModel.sectionQuestionArr[i].cellQuestionArr[j] as CellQuestionModel).score
}
paperModel.totalScore = paperModel.totalScore + paperModel.sectionQuestionArr[i].sectionScore
getGrade()
}
}
}
func getGrade() {
if paperModel.totalScore >= 80 {
paperModel.grade = "优秀"
} else if paperModel.totalScore >= 70 {
paperModel.grade = "良好"
} else if paperModel.totalScore >= 60 {
paperModel.grade = "及格"
} else {
paperModel.grade = "需努力"
}
}
func getStatistics() {
if papersArray.count > 0 {
numOf90plus = 0
numOf80plus = 0
numOf70plus = 0
numOf60plus = 0
numOf60off = 0
for i in 0...papersArray.count - 1 {
if papersArray[i].totalScore >= 90 {
numOf90plus = numOf90plus + 1
} else if papersArray[i].totalScore >= 80 {
numOf80plus = numOf80plus + 1
} else if papersArray[i].totalScore >= 70 {
numOf70plus = numOf70plus + 1
} else if papersArray[i].totalScore >= 60 {
numOf60plus = numOf60plus + 1
} else {
numOf60off = numOf60off + 1
}
}
}
}
// for i in 0...papersArray.count - 1 {
// for j in 0...papersArray[i].sectionQuestionArr.count - 1 {
// for k in 0...papersArray[i].sectionQuestionArr[j].cellQuestionArr.count - 1 {
// var sumOfQuestionScore = 0
// var sumOfRealScore = 0
// sumOfQuestionScore = sumOfQuestionScore.papersArray[i].sectionQuestionArr[j].cellQuestionArr[k].score
//
// }
//
//
// }
// }
func getQuestionStates (section: Int, row: Int) -> (score: Float, realScore: Float, faultNum: Int) {
var sumOfQuestionScore : Float = 0
var sumOfRealScore : Float = 0
var faultNum = 0
for i in 0...papersArray.count - 1 {
let paper = papersArray[i]
if section != paper.sectionQuestionArr.count {
let section = paper.sectionQuestionArr[section]
let question = section.cellQuestionArr[row]
sumOfQuestionScore = sumOfQuestionScore + question.score
sumOfRealScore = sumOfRealScore + question.realScore
if question.score != question.realScore {
faultNum = faultNum + 1
}
}
}
return (sumOfQuestionScore, sumOfRealScore, faultNum)
}
func getRank () {
var bestMark : Float = 0
var lastMark : Float = 100
for i in 0...papersArray.count - 1{
papersArray[i].name = "\(i+1)号"
if papersArray[i].totalScore > bestMark {
bestArr.removeAll()
bestMark = papersArray[i].totalScore
bestArr.append(papersArray[i])
} else if papersArray[i].totalScore == bestMark {
bestArr.append(papersArray[i])
}
if papersArray[i].totalScore < lastMark {
lastArr.removeAll()
lastMark = papersArray[i].totalScore
lastArr.append(papersArray[i])
} else if papersArray[i].totalScore == lastMark {
lastArr.append(papersArray[i])
}
}
}
func removeSectionQuestion(sectionNum : Int){
paperModel.sectionQuestionArr.remove(at: sectionNum)
}
func mockData(){
createSectionQuestion(numberOfQuestions: 5, score: 2, style: .yesOrNo)
// createSectionQuestion(numberOfQuestions: 10, score: 4, style: .yesOrNo)
// createSectionQuestion(numberOfQuestions: 4, score: 5, style: .yesOrNo)
// createSectionQuestion(numberOfQuestions: 3, score: 15, style: .multiScore)
}
}
| gpl-3.0 | af2a11067145a5aaddab0268fccc1754 | 31.211268 | 189 | 0.556916 | 4.574 | false | false | false | false |
iosprogrammingwithswift/iosprogrammingwithswift | 04_Swift2015_Final.playground/Pages/10_Properties.xcplaygroundpage/Contents.swift | 1 | 5216 | //: [Previous](@previous) | [Next](@next)
//: Stored Properties
struct FixedLengthRange {
var firstValue: Int
let length: Int
}
var rangeOfThreeItems = FixedLengthRange(firstValue: 0, length: 3)
// the range represents integer values 0, 1, and 2
rangeOfThreeItems.firstValue = 6
// the range now represents integer values 6, 7, and 8
//: Stored Properties of Constant Structure Instances
let rangeOfFourItems = FixedLengthRange(firstValue: 0, length: 4)
// this range represents integer values 0, 1, 2, and 3
//rangeOfFourItems.firstValue = 6
// this will report an error, even though firstValue is a variable property
//: Lazy Stored Properties
//: You must always declare a lazy property as a variable (with the var keyword)
class DataImporter {
/*
DataImporter is a class to import data from an external file.
The class is assumed to take a non-trivial amount of time to initialize.
*/
var fileName = "data.txt"
// the DataImporter class would provide data importing functionality here
}
class DataManager {
lazy var importer = DataImporter()
var data = [String]()
// the DataManager class would provide data management functionality here
}
let manager = DataManager()
manager.data.append("Some data")
manager.data.append("Some more data")
// the DataImporter instance for the importer property has not yet been created
print(manager.importer.fileName)
//: Computed Properties
struct Point {
var x = 0.0, y = 0.0
}
struct Size {
var width = 0.0, height = 0.0
}
struct Rect {
var origin = Point()
var size = Size()
var center: Point {
get {
let centerX = origin.x + (size.width / 2)
let centerY = origin.y + (size.height / 2)
return Point(x: centerX, y: centerY)
}
set(newCenter) {
origin.x = newCenter.x - (size.width / 2)
origin.y = newCenter.y - (size.height / 2)
}
}
}
var square = Rect(origin: Point(x: 0.0, y: 0.0),
size: Size(width: 10.0, height: 10.0))
let initialSquareCenter = square.center
square.center = Point(x: 15.0, y: 15.0)
print("square.origin is now at (\(square.origin.x), \(square.origin.y))")
// prints "square.origin is now at (10.0, 10.0)"
//: Shorthand setter
struct AlternativeRect {
var origin = Point()
var size = Size()
var center: Point {
get {
let centerX = origin.x + (size.width / 2)
let centerY = origin.y + (size.height / 2)
return Point(x: centerX, y: centerY)
}
set {
origin.x = newValue.x - (size.width / 2)
origin.y = newValue.y - (size.height / 2)
}
}
}
//: Read-Only Computed Properties
//: You must declare computed properties—including read-only computed properties—as variable properties with the var keyword, because their value is not fixed.
struct Cuboid {
var width = 0.0, height = 0.0, depth = 0.0
var volume: Double {
return width * height * depth
}
}
let fourByFiveByTwo = Cuboid(width: 4.0, height: 5.0, depth: 2.0)
print("the volume of fourByFiveByTwo is \(fourByFiveByTwo.volume)")
// prints "the volume of fourByFiveByTwo is 40.0"
//: Property Observers
class StepCounter {
var totalSteps: Int = 0 {
willSet(newTotalSteps) {
print("About to set totalSteps to \(newTotalSteps)")
}
didSet {
if totalSteps > oldValue {
print("Added \(totalSteps - oldValue) steps")
}
}
}
}
let stepCounter = StepCounter()
stepCounter.totalSteps = 200
// About to set totalSteps to 200
// Added 200 steps
stepCounter.totalSteps = 360
// About to set totalSteps to 360
// Added 160 steps
stepCounter.totalSteps = 896
// About to set totalSteps to 896
// Added 536 steps
//: static
struct AudioChannel {
static let thresholdLevel = 10
static var maxInputLevelForAllChannels = 0
var currentLevel: Int = 0 {
didSet {
if currentLevel > AudioChannel.thresholdLevel {
// cap the new audio level to the threshold level
currentLevel = AudioChannel.thresholdLevel
}
if currentLevel > AudioChannel.maxInputLevelForAllChannels {
// store this as the new overall maximum input level
AudioChannel.maxInputLevelForAllChannels = currentLevel
}
}
}
}
var leftChannel = AudioChannel()
var rightChannel = AudioChannel()
leftChannel.currentLevel = 7
print(leftChannel.currentLevel)
// prints "7"
print(AudioChannel.maxInputLevelForAllChannels)
// prints "7"
rightChannel.currentLevel = 11
print(rightChannel.currentLevel)
// prints "10"
print(AudioChannel.maxInputLevelForAllChannels)
// prints "10"
/*:
largely Based of [Apple's Swift Language Guide: Properties](https://developer.apple.com/library/ios/documentation/Swift/Conceptual/Swift_Programming_Language/Properties.html#//apple_ref/doc/uid/TP40014097-CH14-ID254 ) & [Apple's A Swift Tour](https://developer.apple.com/library/ios/documentation/Swift/Conceptual/Swift_Programming_Language/GuidedTour.html#//apple_ref/doc/uid/TP40014097-CH2-ID1 )
*/
//: [Previous](@previous) | [Next](@next)
| mit | 4348ebb5aa907ee3b97f6ecd25c7cc35 | 30.39759 | 397 | 0.665196 | 3.907046 | false | false | false | false |
nathawes/swift | test/SourceKit/Indexing/index_operators.swift | 9 | 497 | // RUN: %sourcekitd-test -req=index %s -- -Xfrontend -serialize-diagnostics-path -Xfrontend %t.dia %s | %sed_clean > %t.response
// RUN: %diff -u %s.response %t.response
class ClassA {
init(){}
}
func +(lhs: ClassA, rhs: ClassA) -> ClassA {
return lhs
}
struct StructB {
func method() {
let a = ClassA()
let b = a + a
let c = StructB()
let d = c - c
}
public static func -(lhs: StructB, rhs: StructB) -> StructB {
return lhs
}
}
| apache-2.0 | 89dce0214207781939c349c08a98e0aa | 20.608696 | 128 | 0.555332 | 3.248366 | false | false | false | false |
vkaramov/VKSplitViewController | SwiftDemo/SwiftDemo/SDDetailViewController.swift | 1 | 1104 | //
// SDDetailViewController.swift
// SwiftDemo
//
// Created by Viacheslav Karamov on 05.07.15.
// Copyright (c) 2015 Viacheslav Karamov. All rights reserved.
//
import UIKit
class SDDetailViewController: UIViewController
{
static let kNavigationStoryboardId = "DetailNavigationController";
private var splitController : VKSplitViewController?;
@IBOutlet private var colorView : UIView?;
override func viewDidLoad()
{
super.viewDidLoad();
splitController = UIApplication.sharedApplication().delegate?.window??.rootViewController as? VKSplitViewController;
}
func setBackgroundColor(color : UIColor)
{
colorView?.backgroundColor = color;
}
@IBAction func menuBarTapped(sender : UIBarButtonItem?)
{
if let splitController = splitController
{
let visible = splitController.masterViewControllerVisible;
self.navigationItem.leftBarButtonItem?.title? = visible ? "Show" : "Hide";
splitController.masterViewControllerVisible = !visible;
}
}
}
| mit | 242059050895497b28554fcaeadf29d7 | 27.307692 | 124 | 0.677536 | 5.158879 | false | false | false | false |
Swift-Squirrel/Squirrel | Sources/SquirrelConfig/Config.swift | 1 | 8154 | //
// Config.swift
// Micros
//
// Created by Filip Klembara on 6/27/17.
//
//
import Foundation
import PathKit
import SwiftyBeaver
import Yams
/// Squirrel config shared instance
public let squirrelConfig = Config.sharedInstance
/// Squirrel config class
public class Config {
private var _serverRoot: Path = Path().absolute()
private let _webRoot: Path
private let _cache: Path
private var _port: UInt16 = 8000
private let _isAllowedDirBrowsing: Bool
private let _logDir: Path
private let _logFile: Path
private let _resourcesDir: Path
private let _viewsDir: Path
private let _storageViews: Path
private let _configFile: Path?
private let _publicStorage: Path
/// Maximum pending connections
public let maximumPendingConnections: Int
/// Sotrage directory
public let storage: Path
/// Symlink to public storage
private let publicStorageSymlink: Path
/// Session directory
public let session: Path
/// Domain name or IP
public let domain: String
/// Logger
public let log = SwiftyBeaver.self
/// Console log
public let consoleLog: ConsoleDestination
/// File log
public let fileLog: FileDestination
private let logFileName = "server.log"
/// Log file destination
public var logFile: Path {
return _logFile
}
/// Server root destination
public var serverRoot: Path {
return _serverRoot
}
/// Webroot destination
public var webRoot: Path {
return _webRoot
}
/// Cache destination
public var cache: Path {
return _cache
}
/// HTTP Requests port
public var port: UInt16 {
return _port
}
/// If is allowed directory browsing
public var isAllowedDirBrowsing: Bool {
return _isAllowedDirBrowsing
}
/// Storage views destination
public var storageViews: Path {
return _storageViews
}
/// Views *.nut* destination
public var views: Path {
return _viewsDir
}
/// Public storage destination
public var publicStorage: Path {
return _publicStorage
}
/// Shared instance
public static let sharedInstance = Config()
private static func getEnviromentVariable(name: String) -> String? {
let start = name.index(after: name.startIndex)
let key = String(name[start..<name.endIndex])
return ProcessInfo.processInfo.environment[key]
}
private static func getStringVariable(from node: Node?) -> String? {
guard let string = node?.string else {
return nil
}
if string.first == "$" {
return getEnviromentVariable(name: string)
}
return string
}
private static func getIntVariable(from node: Node?) -> Int? {
guard let node = node else {
return nil
}
if let string = node.string, string.first == "$" {
guard let value = getEnviromentVariable(name: string) else {
return nil
}
return Int(value)
}
return node.int
}
// swiftlint:disable cyclomatic_complexity
// swiftlint:disable function_body_length
private init() {
let configFile: Path = {
let p1 = Path().absolute() + ".squirrel.yaml"
if p1.exists {
return p1
}
return Path().absolute() + ".squirrel.yml"
}()
var domainConfig = "127.0.0.1"
var maxPendingConnectionsConfig = 50
if configFile.exists {
_configFile = configFile
do {
let content: String = try configFile.read()
guard let yaml = try compose(yaml: content) else {
throw ConfigError(kind: .yamlSyntax)
}
if let serv = yaml["server"] {
if let serverRoot = Config.getStringVariable(from: serv["serverRoot"]) {
let sr = Path(serverRoot).absolute()
if sr.exists {
_serverRoot = sr
} else {
print(sr.string + " does not exists using default server root")
}
}
if let port = Config.getIntVariable(from: serv["port"]) {
_port = UInt16(port)
}
if let dom = Config.getStringVariable(from: serv["domain"]) {
domainConfig = dom
}
if let maxPending = Config.getIntVariable(from: serv["max_pending"]) {
maxPendingConnectionsConfig = maxPending
}
}
} catch {
print("config.yaml is not valid, using default values")
}
} else {
_configFile = nil
}
maximumPendingConnections = maxPendingConnectionsConfig
domain = domainConfig
_webRoot = _serverRoot + "Public"
publicStorageSymlink = _webRoot + "Storage"
storage = _serverRoot + "Storage"
_cache = storage + "Cache"
_publicStorage = storage + "Public"
_logDir = storage + "Logs"
session = storage + "Sessions"
_logFile = _logDir + logFileName
_resourcesDir = _serverRoot + "Resources"
_viewsDir = _resourcesDir + "NutViews"
_storageViews = storage + "Fruits"
_isAllowedDirBrowsing = false
consoleLog = ConsoleDestination()
fileLog = FileDestination()
initLog()
createDirectories()
if !(publicStorageSymlink.exists && publicStorageSymlink.isSymlink) {
guard (try? publicStorageSymlink.symlink(publicStorage)) != nil else {
fatalError("Could not create symlink to \(publicStorage)")
}
}
}
// swiftlint:enable cyclomatic_complexity
// swiftlint:enable function_body_length
/// Get database config from config file
///
/// - Returns: dabase config data
/// - Throws: Yaml error, File read error
public func getDBData() throws -> DBCredentials {
guard let configFile = _configFile else {
throw ConfigError(kind: .missingConfigFile)
}
let content: String = try configFile.read()
guard let yaml = try Yams.load(yaml: content) as? [String: Any] else {
throw ConfigError(kind: .yamlSyntax)
}
guard let dbDataYaml = yaml["MongoDB"] as? [String: Any] else {
throw ConfigError(kind: .missingDBConfig)
}
guard let host = dbDataYaml["host"] as? String else {
throw ConfigError(kind: .missingValue(for: "host"))
}
let dbname = (dbDataYaml["dbname"] as? String) ?? "squirrel"
let port = (dbDataYaml["port"] as? Int) ?? 27017
let user: DBCredentials.UserCredentails?
if let username = dbDataYaml["username"] as? String,
let password = dbDataYaml["password"] as? String {
user = DBCredentials.UserCredentails(username: username, password: password)
} else {
user = nil
}
return DBCredentials(user: user, dbname: dbname, host: host, port: port)
}
private func initLog() {
consoleLog.minLevel = .debug
#if !Xcode
consoleLog.useTerminalColors = true
#endif
fileLog.logFileURL = URL(fileURLWithPath: logFile.description)
log.addDestination(consoleLog)
log.addDestination(fileLog)
}
private func createDir(path dir: Path) {
guard !dir.exists else {
return
}
try? dir.mkpath()
log.info("creating folder: \(dir.string)")
}
private func createDirectories() {
createDir(path: _logDir)
createDir(path: serverRoot)
createDir(path: webRoot)
createDir(path: storageViews)
createDir(path: views)
createDir(path: cache)
createDir(path: publicStorage)
createDir(path: session)
}
}
| apache-2.0 | 941f483612e9bfe297f1d1aff20a66c1 | 29.539326 | 92 | 0.575914 | 4.724218 | false | true | false | false |
mscline/BarGraph | LifespanPlanning/BarGraphColumnCell.swift | 1 | 1705 | //
// BarGraphColumnCell.swift
// Lifespan
//
// Created by Monsoon Co on 6/3/15.
// Copyright (c) 2015 Monsoon Co. All rights reserved.
//
import UIKit
class BarGraphColumnCell: UICollectionViewCell {
var title = ""
var label = UILabel(frame: CGRectMake(0, 0, 0, 0))
var bottomLabel = UILabel(frame: CGRectMake(0, 0, 0, 0))
override init(frame: CGRect) {
super.init(frame: frame)
setup()
}
required init(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
abort() // will be created programmatically
}
func setup(){
backgroundColor = UIColor.clearColor()
label.backgroundColor = UIColor.clearColor()
bottomLabel.backgroundColor = UIColor.clearColor()
label.frame = CGRectMake(0, 0, self.frame.size.width, self.frame.size.height)
label.textAlignment = NSTextAlignment.Center
self.contentView.addSubview(label)
// add label 2
bottomLabel.frame = CGRectMake(0, self.frame.size.height - 14, self.frame.size.width, 14)
bottomLabel.textAlignment = NSTextAlignment.Center
bottomLabel.numberOfLines = 2
bottomLabel.lineBreakMode = NSLineBreakMode.ByCharWrapping
contentView.addSubview(bottomLabel)
// bottomLabel.backgroundColor = UIColor.purpleColor()
}
override func layoutSubviews() {
super.layoutSubviews()
print("in")
label.frame = CGRectMake(0, 0, self.frame.size.width, self.frame.size.height)
bottomLabel = UILabel(frame: CGRectMake(0, self.frame.size.height - 14, self.frame.size.width, 14))
}
}
| mit | 47eaa3f9a806f2ab238071307c37d66f | 28.396552 | 107 | 0.631672 | 4.451697 | false | false | false | false |
miletliyusuf/EasyZoom | Pod/Classes/EasyZoom.swift | 1 | 9997 | /**
This class provides easy zooming for UIImageViews
*/
import UIKit
import Foundation
import ImageIO
public class EasyZoom: NSObject,UIScrollViewDelegate {
//Instant imageView and ScrollView which will show your image and will make them zoom.
var instantImageView:UIImageView?
var instantScrollView:UIScrollView = UIScrollView()
var instantImage:UIImage?
var instantView:UIView?
public var mainView = UIView()
var doubleTapRecognizer:UITapGestureRecognizer
var singleTapRecognizer:UITapGestureRecognizer
var panGesture:UIPanGestureRecognizer
var touchBack:UITapGestureRecognizer
var didDoubleTapped:Bool = false
public var fadeOutAnimationTime:NSTimeInterval?
public init(imageView:UIImageView, image:UIImage, superView: UIView) {
instantView = superView
instantImageView = imageView
instantImageView?.userInteractionEnabled = true
instantImage = image
doubleTapRecognizer = UITapGestureRecognizer()
singleTapRecognizer = UITapGestureRecognizer()
panGesture = UIPanGestureRecognizer()
touchBack = UITapGestureRecognizer()
//scroll visible
instantScrollView.showsHorizontalScrollIndicator = false
instantScrollView.showsVerticalScrollIndicator = false
}
/**
This public function adds zoom for imageViews.
@param imageView: UIImageView - The imageView where you can place zoom
@param image: UIImage - The image of imageView
@param superView: UIView - The superView which gonna cover zoomed imageView
*/
public func zoomForImageView() -> Void {
//delegating of instant scrollView
instantScrollView.delegate = self
instantImage = cropTheImage(instantImage!, i_width: instantView!.frame.size.width, i_height: instantView!.frame.size.height)
//setting instant scrollView frame to user imageView
instantScrollView.frame = instantView!.frame
// instantImageView.frame = CGRect(origin: CGPoint(x: 0, y: 0), size: instantImage.size)
//setting constraints
instantScrollView.contentSize = instantImage!.size
setTheConstraints(instantImageView!, superView: instantScrollView, hConstraints: "|-[subview]", vConstraints: "|-[subview]")
setTheConstraints(instantScrollView, superView: instantView!, hConstraints: "|-[subview]-|", vConstraints: "|-[subview]-|")
instantImageView!.contentMode = .ScaleAspectFit
instantImageView!.image = instantImage
//setting tap gesture recognizer
doubleTapRecognizer = UITapGestureRecognizer(target: self, action: "didDoubleTapped:")
doubleTapRecognizer.numberOfTapsRequired = 2
doubleTapRecognizer.numberOfTouchesRequired = 1
instantImageView!.addGestureRecognizer(doubleTapRecognizer)
//didDoubleTapped = true
//setting minumum and maximum zoom scale
setTheZoomScale()
//centerizing content
centerizeContent(instantScrollView, imageView: instantImageView!)
//adding tap gesture recognizer
singleTouchToNewView()
}
/**
This function makes image view to return its default view format.
*/
func returnDefaultState() {
instantImage = cropTheImage(instantImage!, i_width: instantView!.frame.size.width, i_height: instantView!.frame.size.height)
UIView.animateWithDuration(fadeOutAnimationTime!, animations: { () -> Void in
self.instantScrollView.frame = self.instantView!.frame
self.instantImageView!.frame = CGRect(origin: CGPoint(x: 0, y: 0), size: self.instantImage!.size)
})
// //setting instant scrollView frame to user imageView
// instantScrollView.frame = instantView!.frame
// instantImageView!.frame = CGRect(origin: CGPoint(x: 0, y: 0), size: instantImage!.size)
// //setting constraints
instantScrollView.contentSize = instantImage!.size
setTheConstraints(instantImageView!, superView: instantScrollView, hConstraints: "|-[subview]", vConstraints: "|-[subview]")
instantImageView!.contentMode = .ScaleAspectFit
// //centerizing content
centerizeContent(instantScrollView, imageView: instantImageView!)
}
func setTheZoomScale() {
let scrollViewFrame = instantScrollView.frame
let scaleWidth = scrollViewFrame.size.width / instantScrollView.contentSize.width
let scaleHeight = scrollViewFrame.size.height / instantScrollView.contentSize.height
let minScale = min(scaleWidth, scaleHeight)
instantScrollView.minimumZoomScale = minScale
instantScrollView.maximumZoomScale = 10.0
instantScrollView.zoomScale = minScale
}
func setTheConstraints(subview:UIView, superView:UIView,hConstraints:String,vConstraints:String) {
let views:Dictionary = ["subview":subview]
let imageViewSize = instantScrollView.frame.size
let imageViewSizesMetric = ["height":imageViewSize.height,"width":imageViewSize.width]
subview.setTranslatesAutoresizingMaskIntoConstraints(false)
superView.addSubview(subview)
let vCons:Array = NSLayoutConstraint.constraintsWithVisualFormat("V:\(vConstraints)", options: NSLayoutFormatOptions(0), metrics: imageViewSizesMetric, views: views)
let hCons:Array = NSLayoutConstraint.constraintsWithVisualFormat("H:\(hConstraints)", options: NSLayoutFormatOptions(0), metrics: imageViewSizesMetric, views: views)
superView.addConstraints(vCons)
superView.addConstraints(hCons)
}
/**
This is for single tap tap to new view which has same features with the normal one
*/
public func singleTouchToNewView() {
singleTapRecognizer = UITapGestureRecognizer(target: self, action: "singleTouchHandler")
singleTapRecognizer.requireGestureRecognizerToFail(doubleTapRecognizer)
instantScrollView.addGestureRecognizer(singleTapRecognizer)
}
func singleTouchHandler() {
let screenSize:CGRect = UIScreen.mainScreen().bounds.standardizedRect
var fullSizeView = UIView(frame: screenSize)
//setting constraints of new full size view
self.setTheConstraints(fullSizeView, superView: mainView, hConstraints: "|-0-[subview]-0-|", vConstraints: "|-0-[subview]-0-|")
self.setTheConstraints(instantScrollView, superView: fullSizeView, hConstraints: "|-[subview]-|", vConstraints: "|-[subview]-|")
self.setTheConstraints(instantImageView!, superView: instantScrollView, hConstraints: "|-[subview]", vConstraints: "|-[subview]")
instantScrollView.setNeedsLayout()
instantScrollView.setNeedsDisplay()
//adding pan recognizer
panGesture = UIPanGestureRecognizer(target: self, action: "panToFullScreen:")
touchBack = UITapGestureRecognizer(target: self, action: "touchBackHandler:")
instantScrollView.addGestureRecognizer(panGesture)
instantScrollView.addGestureRecognizer(touchBack)
touchBack.requireGestureRecognizerToFail(doubleTapRecognizer)
}
/**
Pan gesture recognizer selector function.
@param recognizer - UIPanGestureRecognizer
*/
func panToFullScreen(recognizer:UIPanGestureRecognizer) {
let screenBounds:CGRect = UIScreen.mainScreen().bounds
let constantFrame:CGRect = instantImageView!.frame
let translation = recognizer.translationInView(instantView!)
switch (recognizer.state) {
case UIGestureRecognizerState.Began:
println("pan has begun")
break;
case UIGestureRecognizerState.Ended:
returnDefaultState()
instantScrollView.removeGestureRecognizer(panGesture)
instantScrollView.removeGestureRecognizer(touchBack)
println("pan is ended")
break;
case UIGestureRecognizerState.Changed:
println("pan is changed")
if let view = recognizer.view {
view.center = CGPoint(x: view.center.x + translation.x , y: view.center.y + translation.y)
}
recognizer.setTranslation(CGPointZero, inView: instantView)
break;
case UIGestureRecognizerState.Failed:
println("failed pan");
break;
default:
println("cancelled pan")
break;
}
}
func touchBackHandler(recognizer:UITapGestureRecognizer) {
returnDefaultState()
instantScrollView.removeGestureRecognizer(panGesture)
instantScrollView.removeGestureRecognizer(touchBack)
}
func cropTheImage(sourceImage:UIImage, i_width:CGFloat, i_height:CGFloat) -> UIImage {
var newHeight = i_width
var newWidth = i_height
UIGraphicsBeginImageContext(CGSizeMake(newWidth, newHeight))
sourceImage.drawInRect(CGRectMake(0, 0, newWidth, newHeight))
var newImage:UIImage = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return newImage
}
/**
Centerizing content.
@param scrollView - UIScrollView
@param imageView - UIImageView
*/
func centerizeContent(scrollView:UIScrollView, imageView:UIImageView) {
let boundSize = scrollView.bounds.size
var contentsFrame = imageView.frame
if contentsFrame.size.width < boundSize.width {
contentsFrame.origin.x = (boundSize.width - contentsFrame.size.width) / 2.0
}
else {
contentsFrame.origin.x = 0.0
}
if contentsFrame.size.height < boundSize.height {
contentsFrame.origin.y = (boundSize.height - contentsFrame.size.height) / 2.0
}
else {
contentsFrame.origin.y = 0.0
}
imageView.frame = contentsFrame
}
/**
NSNotification handler method. This methods recognize user tap area and zoom over there.
@param recognizer - UITapGestureRecognizer
*/
func didDoubleTapped (recognizer:UITapGestureRecognizer) {
if (didDoubleTapped == true) {
let pointInView = recognizer.locationInView(instantImageView)
var newZoomScale = instantScrollView.zoomScale * 3.5
newZoomScale = min(newZoomScale, instantScrollView.maximumZoomScale)
let scrollViewSize = instantScrollView.bounds.size
let w = scrollViewSize.width / newZoomScale
let h = scrollViewSize.height / newZoomScale
let x = pointInView.x - (w / 2.0)
let y = pointInView.y - (h / 2.0)
let rectToZoomTo = CGRectMake(x,y, w, h)
instantScrollView.zoomToRect(rectToZoomTo, animated: true)
didDoubleTapped = false
}
else {
instantScrollView.zoomScale = 0;
didDoubleTapped = true
}
}
public func scrollViewDidZoom(scrollView: UIScrollView) {
centerizeContent(instantScrollView, imageView: instantImageView!)
}
public func viewForZoomingInScrollView(scrollView: UIScrollView) -> UIView? {
return instantImageView
}
}
| mit | 50a34d63a8983d88cdb6efad6b4298ce | 34.200704 | 167 | 0.770931 | 4.398152 | false | false | false | false |
TheTekton/Malibu | MalibuTests/Specs/Helpers/ETagStorageSpec.swift | 1 | 1616 | @testable import Malibu
import Quick
import Nimble
class ETagStorageSpec: QuickSpec {
override func spec() {
describe("ETagStorage") {
let filePath = ETagStorage.path
let value = "value", key = "key"
var storage: ETagStorage!
let fileManager = NSFileManager.defaultManager()
beforeEach {
storage = ETagStorage()
}
afterEach {
do {
try fileManager.removeItemAtPath(filePath)
} catch {}
}
describe("#addValue:forKey:save") {
it("adds value to the dictionary") {
storage.add(value, forKey: key)
expect(storage.get(key)).to(equal(value))
storage.reload()
expect(storage.get(key)).to(equal(value))
}
}
describe("#get") {
context("key exists") {
it("returns a value") {
storage.add(value, forKey: key)
expect(storage.get(key)).to(equal(value))
}
}
context("key does not exist") {
it("returns nil") {
expect(storage.get(key)).to(beNil())
}
}
}
describe("#save") {
it("saves dictionary to the file") {
storage.add("value", forKey: "key")
storage.save()
let exists = NSFileManager.defaultManager().fileExistsAtPath(filePath)
expect(exists).to(beTrue())
}
}
describe("#reload") {
it("reloads values") {
storage.add(value, forKey: key)
storage.reload()
expect(storage.get(key)).to(equal(value))
}
}
}
}
}
| mit | d7bfe8bfd846245a52f37b2177dfefd3 | 21.760563 | 80 | 0.528465 | 4.379404 | false | false | false | false |
PGSSoft/AutoMate | AutoMate/Locators/KeyboardLocator.swift | 1 | 1451 | //
// KeyboardLocator.swift
// AutoMate
//
// Created by Bartosz Janda on 03.03.2017.
// Copyright © 2017 PGS Software. All rights reserved.
//
import Foundation
/// iOS keyboard locators.
///
/// Contains known set of locators used by the system keyboard on the iOS.
///
/// - `shift`: Shift key.
/// - `delete`: Delete key.
/// - `more`: More, digits key.
/// - `dictation`: Dictation key.
/// - `return`: Return key.
/// - `go`: Go key.
/// - `google`: Google key.
/// - `join`: Join key.
/// - `next`: Next key.
/// - `route`: Route key.
/// - `search`: Search key.
/// - `send`: Send key.
/// - `yahoo`: Yahoo key.
/// - `done`: Done key.
/// - `emergencyCall`: Emergency call key
public enum KeyboardLocator: String, Locator {
/// Shift key.
case shift
/// Delete key.
case delete
/// More, digits key.
case more
/// Dictation key.
case dictation
/// Return key.
case `return` = "Return"
/// Go key.
case go = "Go" // swiftlint:disable:this identifier_name
/// Google key.
case google = "Google"
/// Join key.
case join = "Join"
/// Next key.
case next = "Next"
/// Route key.
case route = "Route"
/// Search key.
case search = "Search"
/// Send key.
case send = "Send"
/// Yahoo key.
case yahoo = "Yahoo"
/// Done key.
case done = "Done"
/// Emergency call key
case emergencyCall = "Emergency call"
}
| mit | 1339a4a439c3ef55201b481e91b44063 | 18.078947 | 74 | 0.563448 | 3.477218 | false | false | false | false |
PlanTeam/BSON | Sources/BSON/Document/Document+Validation.swift | 1 | 10411 | import NIO
public struct ValidationResult {
public internal(set) var errorPosition: Int?
public let reason: String?
public let key: String?
public var isValid: Bool {
return errorPosition == nil && reason == nil
}
static func valid() -> ValidationResult {
return ValidationResult(errorPosition: nil, reason: nil, key: nil)
}
}
fileprivate extension String {
static let notEnoughBytesForValue = "A value identifier was found, but the associated value could not be parsed"
static let notEnoughBytesForDocumentHeader = "Not enough bytes were remaining to parse a Document header"
static let notEnoughBytesForDocument = "There were not enough bytes left to match the length in the document header"
static let trailingBytes = "After parsing the Document, trailing bytes were found"
static let invalidBoolean = "A value other than 0x00 or 0x01 was used to represent a boolean"
static let incorrectDocumentTermination = "The 0x00 byte was not found where it should have been terminating a Document"
}
extension Document {
/// Validates the given `buffer` as a Document
static func validate(buffer: ByteBuffer, asArray validateAsArray: Bool) -> ValidationResult {
var currentIndex = 0
func errorFound(reason: String, key: String? = nil) -> ValidationResult {
return ValidationResult(errorPosition: currentIndex, reason: reason, key: key)
}
/// Moves the readerIndex by `amount` if at least that many bytes are present
/// Returns `false` if the operation was unsuccessful
func has(_ amount: Int) -> Bool {
guard buffer.readableBytes > currentIndex + amount else {
return false
}
currentIndex += amount
return true
}
func hasString() -> Bool {
guard let stringLengthWithNull = buffer.getInteger(at: currentIndex, endianness: .little, as: Int32.self) else {
return false
}
guard stringLengthWithNull >= 1 else {
return false
}
currentIndex += 4
// check if string content present
guard buffer.getString(at: currentIndex, length: Int(stringLengthWithNull) &- 1) != nil else {
return false
}
currentIndex += Int(stringLengthWithNull)
guard buffer.getInteger(at: currentIndex - 1, endianness: .little, as: UInt8.self) == 0 else {
return false
}
return true
}
/// Moves the reader index past the CString and returns `true` if a CString (null terminated string) is present
func hasCString() -> Bool {
guard let length = buffer.firstRelativeIndexOf(startingAt: currentIndex) else {
return false
}
currentIndex += length + 1
return true
}
func validateDocument(array: Bool) -> ValidationResult {
guard let documentLength = buffer.getInteger(at: currentIndex, endianness: .little, as: Int32.self) else {
return errorFound(reason: .notEnoughBytesForDocumentHeader)
}
guard documentLength > 0 else {
return errorFound(reason: "Negative subdocument length")
}
guard let subBuffer = buffer.getSlice(at: currentIndex, length: Int(documentLength)) else {
return errorFound(reason: .notEnoughBytesForValue)
}
var recursiveValidation = Document.validate(buffer: subBuffer, asArray: array)
currentIndex += Int(documentLength)
guard recursiveValidation.isValid else {
if let errorPosition = recursiveValidation.errorPosition {
recursiveValidation.errorPosition = errorPosition + currentIndex
}
return recursiveValidation
}
return .valid()
}
// Extract the document header
guard let numberOfBytesFromHeader = buffer.getInteger(at: currentIndex, endianness: .little, as: Int32.self) else {
return errorFound(reason: .notEnoughBytesForDocumentHeader)
}
currentIndex += 4
// Validate that the number of bytes is correct
guard Int(numberOfBytesFromHeader) == buffer.readableBytes else { // +4 because the header is already parsed
return errorFound(reason: .notEnoughBytesForDocument)
}
// Validate document contents
while buffer.readableBytes > 1 {
guard let typeId = buffer.getInteger(at: currentIndex, endianness: .little, as: UInt8.self) else {
return errorFound(reason: .notEnoughBytesForValue)
}
guard let typeIdentifier = TypeIdentifier(rawValue: typeId) else {
if typeId == 0x00, currentIndex + 1 == Int(numberOfBytesFromHeader) {
return .valid()
}
return errorFound(reason: "The type identifier \(typeId) is not valid")
}
currentIndex += 1
/// Check for key
guard let keyLengthWithoutNull = buffer.firstRelativeIndexOf(startingAt: currentIndex) else {
return errorFound(reason: "Could not parse the element key")
}
let key = buffer.getString(at: currentIndex, length: keyLengthWithoutNull)
currentIndex += keyLengthWithoutNull + 1
// if validateAsArray {
// guard key == "\(currentIndex)" else {
// return errorFound(reason: "The document should be an array, but the element key does not have the expected value of \(currentIndex)", key: key)
// }
// }
switch typeIdentifier {
case .double:
guard has(8) else {
return errorFound(reason: .notEnoughBytesForValue, key: key)
}
case .string:
guard hasString() else {
return errorFound(reason: .notEnoughBytesForValue, key: key)
}
case .document, .array:
let result = validateDocument(array: typeIdentifier == .array)
guard result.isValid else {
return result
}
case .binary:
guard let numberOfBytes = buffer.getInteger(at: currentIndex, endianness: .little, as: Int32.self) else {
return errorFound(reason: .notEnoughBytesForValue, key: key)
}
currentIndex += 4
// Binary type
guard buffer.getInteger(at: currentIndex, endianness: .little, as: UInt8.self) != nil else {
return errorFound(reason: .notEnoughBytesForValue, key: key)
}
currentIndex += 1
guard has(Int(numberOfBytes)) else {
return errorFound(reason: .notEnoughBytesForValue, key: key)
}
case .objectId:
guard has(12) else {
return errorFound(reason: .notEnoughBytesForValue, key: key)
}
case .boolean:
guard let value = buffer.getInteger(at: currentIndex, endianness: .little, as: UInt8.self) else {
return errorFound(reason: .notEnoughBytesForValue, key: key)
}
currentIndex += 1
guard value == 0x00 || value == 0x01 else {
return errorFound(reason: .invalidBoolean, key: key)
}
case .datetime, .timestamp, .int64:
guard has(8) else {
return errorFound(reason: .notEnoughBytesForValue, key: key)
}
case .null, .minKey, .maxKey:
// no data
break
case .regex:
guard hasCString() && hasCString() else {
return errorFound(reason: "The regular expression is malformed", key: key)
}
case .javascript:
guard hasString() else {
return errorFound(reason: "Could not parse JavascriptCode string", key: key)
}
case .javascriptWithScope:
guard let size = buffer.getInteger(at: currentIndex, endianness: .little, as: Int32.self) else {
return errorFound(reason: .notEnoughBytesForValue, key: key)
}
let finalOffset = currentIndex + Int(size)
currentIndex += 4
guard hasString() else {
return errorFound(reason: "Could not parse JavascriptCode (with scope) string", key: key)
}
// validate scope document
let result = validateDocument(array: false)
guard result.isValid else {
return result
}
guard currentIndex == finalOffset else {
return errorFound(reason: .notEnoughBytesForValue, key: key)
}
case .int32:
guard has(4) else {
return errorFound(reason: .notEnoughBytesForValue, key: key)
}
case .decimal128:
guard has(16) else {
return errorFound(reason: .notEnoughBytesForValue, key: key)
}
}
}
// Check the terminator
guard buffer.getInteger(at: currentIndex, endianness: .little, as: UInt8.self) == 0 else {
return errorFound(reason: .incorrectDocumentTermination)
}
return .valid()
}
/// Validates this document's technical correctness
///
/// If `validatingRecursively` is `true` the subdocuments will be traversed, too
public func validate() -> ValidationResult {
return Document.validate(buffer: storage, asArray: self.isArray)
}
}
| mit | 2853621c56b79663c5d666af2ef793c3 | 39.352713 | 165 | 0.556046 | 5.311735 | false | false | false | false |
AttilaTheFun/SwaggerParser | Sources/ArrayItem.swift | 1 | 1664 |
public struct ArrayItem {
/// Metadata about the array type including the number of items and their uniqueness.
public let metadata: ArrayMetadata
/// Describes the type of items in the array.
public let items: Items
/// Determines the format of the array if type array is used. Possible values are:
/// csv - comma separated values foo,bar.
/// ssv - space separated values foo bar.
/// tsv - tab separated values foo\tbar.
/// pipes - pipe separated values foo|bar.
/// Default value is csv.
public let collectionFormat: CollectionFormat
}
struct ArrayItemBuilder: Codable {
let metadataBuilder: ArrayMetadataBuilder
let itemsBuilder: ItemsBuilder
let collectionFormat: CollectionFormat
enum CodingKeys: String, CodingKey {
case itemsBuilder = "items"
case collectionFormat
}
init(from decoder: Decoder) throws {
let values = try decoder.container(keyedBy: CodingKeys.self)
self.metadataBuilder = try ArrayMetadataBuilder(from: decoder)
self.itemsBuilder = try values.decode(ItemsBuilder.self, forKey: .itemsBuilder)
self.collectionFormat = try values.decodeIfPresent(CollectionFormat.self,
forKey: .collectionFormat) ?? .csv
}
}
extension ArrayItemBuilder: Builder {
typealias Building = ArrayItem
func build(_ swagger: SwaggerBuilder) throws -> ArrayItem {
return ArrayItem(
metadata: try self.metadataBuilder.build(swagger),
items: try self.itemsBuilder.build(swagger),
collectionFormat: self.collectionFormat)
}
}
| mit | 29d9cd28a9bea3b8ab680caccd2d28cc | 34.404255 | 93 | 0.67488 | 4.809249 | false | false | false | false |
malaonline/iOS | mala-ios/Common/Support/MalaDataCenter.swift | 1 | 2347 | //
// MalaDataCenter.swift
// mala-ios
//
// Created by 王新宇 on 3/3/16.
// Copyright © 2016 Mala Online. All rights reserved.
//
import UIKit
/// 获取第一个可用的优惠券对象
///
/// - parameter coupons: 优惠券模型数组
func getFirstUnusedCoupon(_ coupons: [CouponModel]) -> CouponModel? {
for coupon in coupons {
if coupon.status == .unused {
return coupon
}
}
return nil
}
/// 根据时间戳(优惠券有效期)判断优惠券是否过期
///
/// - parameter timeStamp: 有效期时间戳
///
/// - returns: Bool
func couponIsExpired(_ timeStamp: TimeInterval) -> Bool {
let date = Date(timeIntervalSince1970: timeStamp)
let result = Date().compare(date)
switch result {
// 有效期大于当前时间,未过期
case .orderedAscending:
return false
// 时间相同,已过期(考虑到后续操作所消耗的时间)
case .orderedSame:
return true
// 当前时间大于有效期,已过期
case .orderedDescending:
return true
}
}
/// 根据支付方式获取AppURLScheme
///
/// - parameter channel: 支付手段
///
/// - returns: URLScheme
func getURLScheme(_ channel: MalaPaymentChannel) -> String {
switch channel {
case .Alipay:
return MalaAppURLScheme.Alipay.rawValue
case .Wechat:
return MalaAppURLScheme.Wechat.rawValue
default:
return ""
}
}
/// Generate a dictionary and insert the data by first letter in mandarin latin
///
/// - Parameter models: regions
/// - Returns: regions data grouped by first letter in mandarin latin
func handleRegion(_ models: [BaseObjectModel]) -> (models: [[BaseObjectModel]], titles: [String]) {
var dict: [String: [BaseObjectModel]] = [:]
for model in models {
let letter: String = model.firstLetter
if let _ = dict[letter] {
dict[letter]?.append(model)
}else {
dict[letter] = [model]
}
}
let array: [[BaseObjectModel]] = dict.values.enumerated().map{ $0.element }.sorted{ $0.0.first?.firstLetter < $0.1.first?.firstLetter }
let strings: [String] = array.map{ $0.first?.firstLetter ?? "#" }.sorted{ $0.0 < $0.1 }.map{ $0.uppercased() }
return (array, strings)
}
| mit | 0c5fd091b5fd32966675f8f79710d19e | 24.309524 | 139 | 0.605833 | 3.703833 | false | false | false | false |
nixzhu/MonkeyKing | Sources/MonkeyKing/Networking.swift | 1 | 21558 |
import UIKit
class Networking {
static let shared = Networking()
private let session = URLSession.shared
typealias NetworkingResponseHandler = ([String: Any]?, URLResponse?, Error?) -> Void
enum Method: String {
case get = "GET"
case post = "POST"
}
enum ParameterEncoding {
case url
case urlEncodedInURL
case json
func encode(_ request: URLRequest, parameters: [String: Any]?) -> URLRequest {
guard let parameters = parameters else {
return request
}
guard let httpMethod = request.httpMethod else {
return request
}
guard let url = request.url else {
return request
}
var mutableURLRequest = request
switch self {
case .url, .urlEncodedInURL:
func query(_ parameters: [String: Any]) -> String {
var components: [(String, String)] = []
for key in parameters.keys.sorted(by: <) {
let value = parameters[key]!
components += queryComponents(key, value)
}
return (components.map { "\($0)=\($1)" } as [String]).joined(separator: "&")
}
func encodesParametersInURL(_ method: Method) -> Bool {
switch self {
case .urlEncodedInURL:
return true
default:
break
}
switch method {
case .get:
return true
default:
return false
}
}
if let method = Method(rawValue: httpMethod), encodesParametersInURL(method) {
if var urlComponents = URLComponents(url: url, resolvingAgainstBaseURL: false) {
let percentEncodedQuery = (urlComponents.percentEncodedQuery.map { $0 + "&" } ?? "") + query(parameters)
urlComponents.percentEncodedQuery = percentEncodedQuery
mutableURLRequest.url = urlComponents.url
}
} else {
if mutableURLRequest.value(forHTTPHeaderField: "Content-Type") == nil {
mutableURLRequest.setValue(
"application/x-www-form-urlencoded; charset=utf-8",
forHTTPHeaderField: "Content-Type"
)
}
mutableURLRequest.httpBody = query(parameters).data(
using: .utf8,
allowLossyConversion: false
)
}
case .json:
do {
let data = try JSONSerialization.data(withJSONObject: parameters)
mutableURLRequest.setValue("application/json; charset=UTF-8", forHTTPHeaderField: "Content-Type")
mutableURLRequest.setValue("application/json", forHTTPHeaderField: "X-Accept")
mutableURLRequest.httpBody = data
} catch {
print("error: \(error)")
}
}
return mutableURLRequest
}
func queryComponents(_ key: String, _ value: Any) -> [(String, String)] {
var components: [(String, String)] = []
if let dictionary = value as? [String: Any] {
for (nestedKey, value) in dictionary {
components += queryComponents("\(key)[\(nestedKey)]", value)
}
} else if let array = value as? [AnyObject] {
for value in array {
components += queryComponents("\(key)[]", value)
}
} else {
components.append((escape(key), escape("\(value)")))
}
return components
}
func escape(_ string: String) -> String {
let generalDelimitersToEncode = ":#[]@" // does not include "?" or "/" due to RFC 3986 - Section 3.4
let subDelimitersToEncode = "!$&'()*+,;="
var allowedCharacterSet = CharacterSet.urlQueryAllowed
allowedCharacterSet.remove(charactersIn: generalDelimitersToEncode + subDelimitersToEncode)
var escaped = ""
if #available(iOS 8.3, *) {
escaped = string.addingPercentEncoding(withAllowedCharacters: allowedCharacterSet) ?? string
} else {
let batchSize = 50
var index = string.startIndex
while index != string.endIndex {
let startIndex = index
let endIndex = string.index(index, offsetBy: batchSize, limitedBy: string.endIndex) ?? startIndex
let substring = string[startIndex ..< endIndex]
escaped += (substring.addingPercentEncoding(withAllowedCharacters: allowedCharacterSet as CharacterSet) ?? String(substring))
index = endIndex
}
}
return escaped
}
}
func request(_ urlString: String, method: Method, parameters: [String: Any]? = nil, encoding: ParameterEncoding = .url, headers: [String: String]? = nil, completionHandler: @escaping ([String: Any]?, URLResponse?, Error?) -> Void) {
guard let url = URL(string: urlString) else {
return
}
var mutableURLRequest = URLRequest(url: url)
mutableURLRequest.httpMethod = method.rawValue
if let headers = headers {
for (headerField, headerValue) in headers {
mutableURLRequest.setValue(headerValue, forHTTPHeaderField: headerField)
}
}
let request = encoding.encode(mutableURLRequest, parameters: parameters)
let task = session.dataTask(with: request) { data, response, error in
var json: [String: Any]?
defer {
DispatchQueue.main.async {
completionHandler(json, response, error as Error?)
}
}
if let httpResponse = response as? HTTPURLResponse,
let data = data,
httpResponse.url!.absoluteString.contains("api.twitter.com") {
let contentType = httpResponse.allHeaderFields["Content-Type"] as? String
if contentType == nil || contentType!.contains("application/json") == false {
let responseText = String(data: data, encoding: .utf8)
// TWITTER SUCKS. API WILL RETURN <application/html>
// oauth_token=sample&oauth_token_secret=sample&oauth_callback_confirmed=true
json = responseText?.queryStringParameters
return
}
}
guard let validData = data,
let jsonData = validData.monkeyking_json else {
print("requst fail: JSON could not be serialized because input data was nil.")
return
}
json = jsonData
}
task.resume()
}
func upload(_ urlString: String, parameters: [String: Any], headers: [String: String]? = nil, completionHandler: @escaping ([String: Any]?, URLResponse?, Error?) -> Void) {
let tuple = urlRequestWithComponents(urlString, parameters: parameters)
guard let request = tuple.request, let data = tuple.data else {
return
}
var mutableURLRequest = request
if let headers = headers {
for (headerField, headerValue) in headers {
mutableURLRequest.setValue(headerValue, forHTTPHeaderField: headerField)
}
}
let uploadTask = session.uploadTask(with: mutableURLRequest, from: data) { data, response, error in
var json: [String: Any]?
defer {
DispatchQueue.main.async {
completionHandler(json, response, error as Error?)
}
}
guard let validData = data,
let jsonData = validData.monkeyking_json else {
print("upload fail: JSON could not be serialized because input data was nil.")
return
}
json = jsonData
}
uploadTask.resume()
}
func urlRequestWithComponents(_ urlString: String, parameters: [String: Any], encoding: ParameterEncoding = .url) -> (request: URLRequest?, data: Data?) {
guard let url = URL(string: urlString) else {
return (nil, nil)
}
var mutableURLRequest = URLRequest(url: url)
mutableURLRequest.httpMethod = Method.post.rawValue
let boundaryConstant = "NET-POST-boundary-\(arc4random())-\(arc4random())"
let contentType = "multipart/form-data;boundary=" + boundaryConstant
mutableURLRequest.setValue(contentType, forHTTPHeaderField: "Content-Type")
var uploadData = Data()
// add parameters
for (key, value) in parameters {
guard let encodeBoundaryData = "\r\n--\(boundaryConstant)\r\n".data(using: .utf8) else {
return (nil, nil)
}
uploadData.append(encodeBoundaryData)
if let imageData = value as? Data {
let filename = arc4random()
let filenameClause = "filename=\"\(filename)\""
let contentDispositionString = "Content-Disposition: form-data; name=\"\(key)\";\(filenameClause)\r\n"
let contentDispositionData = contentDispositionString.data(using: .utf8)
uploadData.append(contentDispositionData!)
// append content type
let contentTypeString = "Content-Type: image/JPEG\r\n\r\n"
guard let contentTypeData = contentTypeString.data(using: .utf8) else {
return (nil, nil)
}
uploadData.append(contentTypeData)
uploadData.append(imageData)
} else {
guard let encodeDispositionData = "Content-Disposition: form-data; name=\"\(key)\"\r\n\r\n\(value)".data(using: .utf8) else {
return (nil, nil)
}
uploadData.append(encodeDispositionData)
}
}
uploadData.append("\r\n--\(boundaryConstant)--\r\n".data(using: .utf8)!)
return (encoding.encode(mutableURLRequest, parameters: nil), uploadData)
}
func authorizationHeader(for method: Method, urlString: String, appID: String, appKey: String, accessToken: String?, accessTokenSecret: String?, parameters: [String: Any]?, isMediaUpload: Bool) -> String {
var authorizationParameters = [String: Any]()
authorizationParameters["oauth_version"] = "1.0"
authorizationParameters["oauth_signature_method"] = "HMAC-SHA1"
authorizationParameters["oauth_consumer_key"] = appID
authorizationParameters["oauth_timestamp"] = String(Int(Date().timeIntervalSince1970))
authorizationParameters["oauth_nonce"] = UUID().uuidString
if let accessToken = accessToken {
authorizationParameters["oauth_token"] = accessToken
}
if let parameters = parameters {
for (key, value) in parameters where key.hasPrefix("oauth_") {
authorizationParameters.updateValue(value, forKey: key)
}
}
var finalParameters = authorizationParameters
if isMediaUpload {
if let parameters = parameters {
for (k, v) in parameters {
finalParameters[k] = v
}
}
}
authorizationParameters["oauth_signature"] = oauthSignature(for: method, urlString: urlString, parameters: finalParameters, appKey: appKey, accessTokenSecret: accessTokenSecret)
let authorizationParameterComponents = authorizationParameters.urlEncodedQueryString(using: .utf8).components(separatedBy: "&").sorted()
var headerComponents = [String]()
for component in authorizationParameterComponents {
let subcomponent = component.components(separatedBy: "=")
if subcomponent.count == 2 {
headerComponents.append("\(subcomponent[0])=\"\(subcomponent[1])\"")
}
}
return "OAuth " + headerComponents.joined(separator: ", ")
}
func oauthSignature(for method: Method, urlString: String, parameters: [String: Any], appKey: String, accessTokenSecret tokenSecret: String?) -> String {
let tokenSecret = tokenSecret?.urlEncodedString() ?? ""
let encodedConsumerSecret = appKey.urlEncodedString()
let signingKey = "\(encodedConsumerSecret)&\(tokenSecret)"
let parameterComponents = parameters.urlEncodedQueryString(using: .utf8).components(separatedBy: "&").sorted()
let parameterString = parameterComponents.joined(separator: "&")
let encodedParameterString = parameterString.urlEncodedString()
let encodedURL = urlString.urlEncodedString()
let signatureBaseString = "\(method.rawValue)&\(encodedURL)&\(encodedParameterString)"
let key = signingKey.data(using: .utf8)!
let msg = signatureBaseString.data(using: .utf8)!
let sha1 = HMAC.sha1(key: key, message: msg)!
return sha1.base64EncodedString(options: [])
}
}
// MARK: URLEncode
extension Dictionary {
func urlEncodedQueryString(using encoding: String.Encoding) -> String {
var parts = [String]()
for (key, value) in self {
let keyString = "\(key)".urlEncodedString()
let valueString = "\(value)".urlEncodedString(keyString == "status")
let query: String = "\(keyString)=\(valueString)"
parts.append(query)
}
return parts.joined(separator: "&")
}
}
extension String {
var queryStringParameters: [String: String] {
var parameters = [String: String]()
let scanner = Scanner(string: self)
var key: NSString?
var value: NSString?
while !scanner.isAtEnd {
key = nil
scanner.scanUpTo("=", into: &key)
scanner.scanString("=", into: nil)
value = nil
scanner.scanUpTo("&", into: &value)
scanner.scanString("&", into: nil)
if let key = key as String?, let value = value as String? {
parameters.updateValue(value, forKey: key)
}
}
return parameters
}
func urlEncodedString(_ encodeAll: Bool = false) -> String {
var allowedCharacterSet: CharacterSet = .urlQueryAllowed
allowedCharacterSet.remove(charactersIn: "\n:#/?@!$&'()*+,;=")
if !encodeAll {
allowedCharacterSet.insert(charactersIn: "[]")
}
return addingPercentEncoding(withAllowedCharacters: allowedCharacterSet)!
}
}
public struct HMAC {
static func sha1(key: Data, message: Data) -> Data? {
var key = key.rawBytes
let message = message.rawBytes
// key
if key.count > 64 {
key = SHA1(message: Data(bytes: key)).calculate().rawBytes
}
if key.count < 64 {
key = key + [UInt8](repeating: 0, count: 64 - key.count)
}
var opad = [UInt8](repeating: 0x5C, count: 64)
for (idx, _) in key.enumerated() {
opad[idx] = key[idx] ^ opad[idx]
}
var ipad = [UInt8](repeating: 0x36, count: 64)
for (idx, _) in key.enumerated() {
ipad[idx] = key[idx] ^ ipad[idx]
}
let ipadAndMessageHash = SHA1(message: Data(bytes: ipad + message)).calculate().rawBytes
let finalHash = SHA1(message: Data(bytes: opad + ipadAndMessageHash)).calculate().rawBytes
let mac = finalHash
return mac.withUnsafeBufferPointer { Data($0) }
}
}
// MARK: SHA1
struct SHA1 {
var message: Data
/** Common part for hash calculation. Prepare header data. */
func prepare(_ len: Int = 64) -> Data {
var tmpMessage: Data = message
// Step 1. Append Padding Bits
tmpMessage.append([0x80]) // append one bit (Byte with one bit) to message
// append "0" bit until message length in bits ≡ 448 (mod 512)
while tmpMessage.count % len != (len - 8) {
tmpMessage.append([0x00])
}
return tmpMessage
}
func calculate() -> Data {
// var tmpMessage = self.prepare()
let len = 64
let h: [UInt32] = [0x6745_2301, 0xEFCD_AB89, 0x98BA_DCFE, 0x1032_5476, 0xC3D2_E1F0]
var tmpMessage: Data = message
// Step 1. Append Padding Bits
tmpMessage.append([0x80]) // append one bit (Byte with one bit) to message
// append "0" bit until message length in bits ≡ 448 (mod 512)
while tmpMessage.count % len != (len - 8) {
tmpMessage.append([0x00])
}
// hash values
var hh = h
// append message length, in a 64-bit big-endian integer. So now the message length is a multiple of 512 bits.
tmpMessage.append((message.count * 8).bytes(64 / 8))
// Process the message in successive 512-bit chunks:
let chunkSizeBytes = 512 / 8 // 64
var leftMessageBytes = tmpMessage.count
var i = 0
while i < tmpMessage.count {
let chunk = tmpMessage.subdata(in: i ..< i + min(chunkSizeBytes, leftMessageBytes))
// break chunk into sixteen 32-bit words M[j], 0 ≤ j ≤ 15, big-endian
// Extend the sixteen 32-bit words into eighty 32-bit words:
var M = [UInt32](repeating: 0, count: 80)
for x in 0 ..< M.count {
switch x {
case 0 ... 15:
var le: UInt32 = 0
let range = NSRange(location: x * MemoryLayout<UInt32>.size, length: MemoryLayout<UInt32>.size)
(chunk as NSData).getBytes(&le, range: range)
M[x] = le.bigEndian
default:
M[x] = rotateLeft(M[x - 3] ^ M[x - 8] ^ M[x - 14] ^ M[x - 16], n: 1)
}
}
var A = hh[0], B = hh[1], C = hh[2], D = hh[3], E = hh[4]
// Main loop
for j in 0 ... 79 {
var f: UInt32 = 0
var k: UInt32 = 0
switch j {
case 0 ... 19:
f = (B & C) | ((~B) & D)
k = 0x5A82_7999
case 20 ... 39:
f = B ^ C ^ D
k = 0x6ED9_EBA1
case 40 ... 59:
f = (B & C) | (B & D) | (C & D)
k = 0x8F1B_BCDC
case 60 ... 79:
f = B ^ C ^ D
k = 0xCA62_C1D6
default:
break
}
let temp = (rotateLeft(A, n: 5) &+ f &+ E &+ M[j] &+ k) & 0xFFFF_FFFF
E = D
D = C
C = rotateLeft(B, n: 30)
B = A
A = temp
}
hh[0] = (hh[0] &+ A) & 0xFFFF_FFFF
hh[1] = (hh[1] &+ B) & 0xFFFF_FFFF
hh[2] = (hh[2] &+ C) & 0xFFFF_FFFF
hh[3] = (hh[3] &+ D) & 0xFFFF_FFFF
hh[4] = (hh[4] &+ E) & 0xFFFF_FFFF
i = i + chunkSizeBytes
leftMessageBytes -= chunkSizeBytes
}
// Produce the final hash value (big-endian) as a 160 bit number:
let mutableBuff = NSMutableData()
hh.forEach {
var i = $0.bigEndian
mutableBuff.append(&i, length: MemoryLayout<UInt32>.size)
}
return mutableBuff as Data
}
}
func arrayOfBytes<T>(_ value: T, length: Int? = nil) -> [UInt8] {
let totalBytes = length ?? (MemoryLayout<T>.size * 8)
let valuePointer = UnsafeMutablePointer<T>.allocate(capacity: 1)
valuePointer.pointee = value
let bytesPointer = valuePointer.withMemoryRebound(to: UInt8.self, capacity: 1) { $0 }
var bytes = [UInt8](repeating: 0, count: totalBytes)
for j in 0 ..< min(MemoryLayout<T>.size, totalBytes) {
bytes[totalBytes - 1 - j] = (bytesPointer + j).pointee
}
valuePointer.deinitialize(count: totalBytes)
valuePointer.deallocate()
return bytes
}
func rotateLeft(_ v: UInt16, n: UInt16) -> UInt16 {
return ((v << n) & 0xFFFF) | (v >> (16 - n))
}
func rotateLeft(_ v: UInt32, n: UInt32) -> UInt32 {
return ((v << n) & 0xFFFF_FFFF) | (v >> (32 - n))
}
func rotateLeft(_ x: UInt64, n: UInt64) -> UInt64 {
return (x << n) | (x >> (64 - n))
}
extension Int {
public func bytes(_ totalBytes: Int = MemoryLayout<Int>.size) -> [UInt8] {
return arrayOfBytes(self, length: totalBytes)
}
}
extension Data {
var rawBytes: [UInt8] {
let count = self.count / MemoryLayout<UInt8>.size
var bytesArray = [UInt8](repeating: 0, count: count)
(self as NSData).getBytes(&bytesArray, length: count * MemoryLayout<UInt8>.size)
return bytesArray
}
init(bytes: [UInt8]) {
self = bytes.withUnsafeBufferPointer { Data($0) }
}
mutating func append(_ bytes: [UInt8]) {
bytes.withUnsafeBufferPointer {
append($0)
}
}
}
| mit | f955bf9a38177d8afce24ece66351512 | 41.089844 | 236 | 0.547378 | 4.859076 | false | false | false | false |
spritekitbook/spritekitbook-swift | Chapter 14/Start/SpaceRunner/SpaceRunner/GameSettings.swift | 6 | 1740 | //
// GameSettings.swift
// SpaceRunner
//
// Created by Jeremy Novak on 9/12/16.
// Copyright © 2016 Spritekit Book. All rights reserved.
//
import Foundation
class GameSettings {
static let sharedInstance = GameSettings()
// MARK: - Private class constants
private let defaults = UserDefaults.standard
private let keyFirstRun = "FirstRun"
private let keyBestScore = "BestScore"
private let keyBestStars = "BestStars"
private let keyBestStreak = "BestStreak"
// MARK: - Init
init() {
if defaults.object(forKey: keyFirstRun) == nil {
firstLaunch()
}
}
// MARK: - Private methods
private func firstLaunch() {
defaults.set(0, forKey: keyBestScore)
defaults.set(0, forKey: keyBestStars)
defaults.set(0, forKey: keyBestStreak)
defaults.set(false, forKey: keyFirstRun)
defaults.synchronize()
}
// MARK: - Public saving methods
func saveBestScore(score: Int) {
defaults.set(score, forKey: keyBestScore)
defaults.synchronize()
}
func saveBestStars(stars: Int) {
defaults.set(stars, forKey: keyBestStars)
defaults.synchronize()
}
func saveBestStreak(streak: Int) {
defaults.set(streak, forKey: keyBestStreak)
defaults.synchronize()
}
// MARK: - Public retrieving methods
func getBestScore() -> Int {
return defaults.integer(forKey: keyBestScore)
}
func getBestStars() -> Int {
return defaults.integer(forKey: keyBestStars)
}
func getBestStreak() -> Int {
return defaults.integer(forKey: keyBestStreak)
}
}
| apache-2.0 | 4374618028c4bd982c917327eed20c98 | 23.842857 | 57 | 0.608971 | 4.293827 | false | false | false | false |
hoowang/WKRefreshDemo | RefreshToolkit/Views/WKPureRefreshFooter.swift | 1 | 1876 | //
// WKPureRefreshFooter.swift
// WKRefreshDemo
//
// Created by hooge on 16/5/5.
// Copyright © 2016年 hooge. All rights reserved.
//
// 这是一个纯洁的上拉刷新组件 仅仅显示一个指示器
import UIKit
public class WKPureRefreshFooter: WKRefreshFooter {
private let activityView = UIActivityIndicatorView(activityIndicatorStyle:.Gray)
override func initialize() {
super.initialize()
self.addSubview(self.activityView)
}
override func placeSubViews() {
super.placeSubViews()
let activityWH:CGFloat = 30.0
let activityX = (self.wk_Width - activityWH) * 0.5
let activityY = (self.wk_Height - activityWH) * 0.5
self.activityView.frame = CGRectMake(activityX, activityY, activityWH, activityWH)
}
override func loadAnimators() {
self.activityView.startAnimating()
}
override func unloadAnimators() {
self.activityView.stopAnimating()
}
public override func completeRefresh() {
super.completeRefresh()
self.activityView.stopAnimating()
}
}
extension WKPureRefreshFooter{
public override class func refreshFooterFor(
scrollView:UIScrollView, callback:()->(Void)) -> (WKRefreshControl){
let header = WKPureRefreshFooter()
header.callbackMode = .ClosureMode
header.callback = callback
scrollView.addSubview(header)
return header
}
public override class func refreshFooterFor(
scrollView:UIScrollView, target:AnyObject, action:Selector) -> (WKRefreshControl){
let refreshHeader = WKPureRefreshFooter()
refreshHeader.callbackMode = .SelectorMode
refreshHeader.targetObject = target
refreshHeader.selector = action
scrollView.addSubview(refreshHeader)
return refreshHeader
}
} | mit | 9900cd62f36575e650d377f132c40c83 | 28.047619 | 90 | 0.672499 | 4.665816 | false | false | false | false |
CosmicMind/MaterialKit | Sources/iOS/Color.swift | 1 | 27796 | /*
* Copyright (C) 2015 - 2018, Daniel Dahan and CosmicMind, Inc. <http://cosmicmind.com>.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* * Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* * Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* * Neither the name of CosmicMind nor the names of its
* contributors may be used to endorse or promote products derived from
* this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
import UIKit
@objc(ColorPalette)
public protocol ColorPalette {
/// Material color code: 50
static var lighten5: UIColor { get }
/// Material color code: 100
static var lighten4: UIColor { get }
/// Material color code: 200
static var lighten3: UIColor { get }
/// Material color code: 300
static var lighten2: UIColor { get }
/// Material color code: 400
static var lighten1: UIColor { get }
/// Material color code: 500
static var base: UIColor { get }
/// Material color code: 600
static var darken1: UIColor { get }
/// Material color code: 700
static var darken2: UIColor { get }
/// Material color code: 800
static var darken3: UIColor { get }
/// Material color code: 900
static var darken4: UIColor { get }
/// Material color code: A100
@objc
optional static var accent1: UIColor { get }
/// Material color code: A200
@objc
optional static var accent2: UIColor { get }
/// Material color code: A400
@objc
optional static var accent3: UIColor { get }
/// Material color code: A700
@objc
optional static var accent4: UIColor { get }
}
open class Color: UIColor {
// dark text
open class darkText {
open static let primary = Color.black.withAlphaComponent(0.87)
open static let secondary = Color.black.withAlphaComponent(0.54)
open static let others = Color.black.withAlphaComponent(0.38)
open static let dividers = Color.black.withAlphaComponent(0.12)
}
// light text
open class lightText {
open static let primary = Color.white
open static let secondary = Color.white.withAlphaComponent(0.7)
open static let others = Color.white.withAlphaComponent(0.5)
open static let dividers = Color.white.withAlphaComponent(0.12)
}
// red
open class red: ColorPalette {
open static let lighten5 = UIColor(red: 255/255, green: 235/255, blue: 238/255, alpha: 1)
open static let lighten4 = UIColor(red: 255/255, green: 205/255, blue: 210/255, alpha: 1)
open static let lighten3 = UIColor(red: 239/255, green: 154/255, blue: 154/255, alpha: 1)
open static let lighten2 = UIColor(red: 229/255, green: 115/255, blue: 115/255, alpha: 1)
open static let lighten1 = UIColor(red: 229/255, green: 83/255, blue: 80/255, alpha: 1)
open static let base = UIColor(red: 244/255, green: 67/255, blue: 54/255, alpha: 1)
open static let darken1 = UIColor(red: 229/255, green: 57/255, blue: 53/255, alpha: 1)
open static let darken2 = UIColor(red: 211/255, green: 47/255, blue: 47/255, alpha: 1)
open static let darken3 = UIColor(red: 198/255, green: 40/255, blue: 40/255, alpha: 1)
open static let darken4 = UIColor(red: 183/255, green: 28/255, blue: 28/255, alpha: 1)
open static let accent1 = UIColor(red: 255/255, green: 138/255, blue: 128/255, alpha: 1)
open static let accent2 = UIColor(red: 255/255, green: 82/255, blue: 82/255, alpha: 1)
open static let accent3 = UIColor(red: 255/255, green: 23/255, blue: 68/255, alpha: 1)
open static let accent4 = UIColor(red: 213/255, green: 0/255, blue: 0/255, alpha: 1)
}
// pink
open class pink: ColorPalette {
open static let lighten5 = UIColor(red: 252/255, green: 228/255, blue: 236/255, alpha: 1)
open static let lighten4 = UIColor(red: 248/255, green: 187/255, blue: 208/255, alpha: 1)
open static let lighten3 = UIColor(red: 244/255, green: 143/255, blue: 177/255, alpha: 1)
open static let lighten2 = UIColor(red: 240/255, green: 98/255, blue: 146/255, alpha: 1)
open static let lighten1 = UIColor(red: 236/255, green: 64/255, blue: 122/255, alpha: 1)
open static let base = UIColor(red: 233/255, green: 30/255, blue: 99/255, alpha: 1)
open static let darken1 = UIColor(red: 216/255, green: 27/255, blue: 96/255, alpha: 1)
open static let darken2 = UIColor(red: 194/255, green: 24/255, blue: 91/255, alpha: 1)
open static let darken3 = UIColor(red: 173/255, green: 20/255, blue: 87/255, alpha: 1)
open static let darken4 = UIColor(red: 136/255, green: 14/255, blue: 79/255, alpha: 1)
open static let accent1 = UIColor(red: 255/255, green: 128/255, blue: 171/255, alpha: 1)
open static let accent2 = UIColor(red: 255/255, green: 64/255, blue: 129/255, alpha: 1)
open static let accent3 = UIColor(red: 245/255, green: 0/255, blue: 87/255, alpha: 1)
open static let accent4 = UIColor(red: 197/255, green: 17/255, blue: 98/255, alpha: 1)
}
// purple
open class purple: ColorPalette {
open static let lighten5 = UIColor(red: 243/255, green: 229/255, blue: 245/255, alpha: 1)
open static let lighten4 = UIColor(red: 225/255, green: 190/255, blue: 231/255, alpha: 1)
open static let lighten3 = UIColor(red: 206/255, green: 147/255, blue: 216/255, alpha: 1)
open static let lighten2 = UIColor(red: 186/255, green: 104/255, blue: 200/255, alpha: 1)
open static let lighten1 = UIColor(red: 171/255, green: 71/255, blue: 188/255, alpha: 1)
open static let base = UIColor(red: 156/255, green: 39/255, blue: 176/255, alpha: 1)
open static let darken1 = UIColor(red: 142/255, green: 36/255, blue: 170/255, alpha: 1)
open static let darken2 = UIColor(red: 123/255, green: 31/255, blue: 162/255, alpha: 1)
open static let darken3 = UIColor(red: 106/255, green: 27/255, blue: 154/255, alpha: 1)
open static let darken4 = UIColor(red: 74/255, green: 20/255, blue: 140/255, alpha: 1)
open static let accent1 = UIColor(red: 234/255, green: 128/255, blue: 252/255, alpha: 1)
open static let accent2 = UIColor(red: 224/255, green: 64/255, blue: 251/255, alpha: 1)
open static let accent3 = UIColor(red: 213/255, green: 0/255, blue: 249/255, alpha: 1)
open static let accent4 = UIColor(red: 170/255, green: 0/255, blue: 255/255, alpha: 1)
}
// deepPurple
open class deepPurple: ColorPalette {
open static let lighten5 = UIColor(red: 237/255, green: 231/255, blue: 246/255, alpha: 1)
open static let lighten4 = UIColor(red: 209/255, green: 196/255, blue: 233/255, alpha: 1)
open static let lighten3 = UIColor(red: 179/255, green: 157/255, blue: 219/255, alpha: 1)
open static let lighten2 = UIColor(red: 149/255, green: 117/255, blue: 205/255, alpha: 1)
open static let lighten1 = UIColor(red: 126/255, green: 87/255, blue: 194/255, alpha: 1)
open static let base = UIColor(red: 103/255, green: 58/255, blue: 183/255, alpha: 1)
open static let darken1 = UIColor(red: 94/255, green: 53/255, blue: 177/255, alpha: 1)
open static let darken2 = UIColor(red: 81/255, green: 45/255, blue: 168/255, alpha: 1)
open static let darken3 = UIColor(red: 69/255, green: 39/255, blue: 160/255, alpha: 1)
open static let darken4 = UIColor(red: 49/255, green: 27/255, blue: 146/255, alpha: 1)
open static let accent1 = UIColor(red: 179/255, green: 136/255, blue: 255/255, alpha: 1)
open static let accent2 = UIColor(red: 124/255, green: 77/255, blue: 255/255, alpha: 1)
open static let accent3 = UIColor(red: 101/255, green: 31/255, blue: 255/255, alpha: 1)
open static let accent4 = UIColor(red: 98/255, green: 0/255, blue: 234/255, alpha: 1)
}
// indigo
open class indigo: ColorPalette {
open static let lighten5 = UIColor(red: 232/255, green: 234/255, blue: 246/255, alpha: 1)
open static let lighten4 = UIColor(red: 197/255, green: 202/255, blue: 233/255, alpha: 1)
open static let lighten3 = UIColor(red: 159/255, green: 168/255, blue: 218/255, alpha: 1)
open static let lighten2 = UIColor(red: 121/255, green: 134/255, blue: 203/255, alpha: 1)
open static let lighten1 = UIColor(red: 92/255, green: 107/255, blue: 192/255, alpha: 1)
open static let base = UIColor(red: 63/255, green: 81/255, blue: 181/255, alpha: 1)
open static let darken1 = UIColor(red: 57/255, green: 73/255, blue: 171/255, alpha: 1)
open static let darken2 = UIColor(red: 48/255, green: 63/255, blue: 159/255, alpha: 1)
open static let darken3 = UIColor(red: 40/255, green: 53/255, blue: 147/255, alpha: 1)
open static let darken4 = UIColor(red: 26/255, green: 35/255, blue: 126/255, alpha: 1)
open static let accent1 = UIColor(red: 140/255, green: 158/255, blue: 255/255, alpha: 1)
open static let accent2 = UIColor(red: 83/255, green: 109/255, blue: 254/255, alpha: 1)
open static let accent3 = UIColor(red: 61/255, green: 90/255, blue: 254/255, alpha: 1)
open static let accent4 = UIColor(red: 48/255, green: 79/255, blue: 254/255, alpha: 1)
}
// blue
open class blue: ColorPalette {
open static let lighten5 = UIColor(red: 227/255, green: 242/255, blue: 253/255, alpha: 1)
open static let lighten4 = UIColor(red: 187/255, green: 222/255, blue: 251/255, alpha: 1)
open static let lighten3 = UIColor(red: 144/255, green: 202/255, blue: 249/255, alpha: 1)
open static let lighten2 = UIColor(red: 100/255, green: 181/255, blue: 246/255, alpha: 1)
open static let lighten1 = UIColor(red: 66/255, green: 165/255, blue: 245/255, alpha: 1)
open static let base = UIColor(red: 33/255, green: 150/255, blue: 243/255, alpha: 1)
open static let darken1 = UIColor(red: 30/255, green: 136/255, blue: 229/255, alpha: 1)
open static let darken2 = UIColor(red: 25/255, green: 118/255, blue: 210/255, alpha: 1)
open static let darken3 = UIColor(red: 21/255, green: 101/255, blue: 192/255, alpha: 1)
open static let darken4 = UIColor(red: 13/255, green: 71/255, blue: 161/255, alpha: 1)
open static let accent1 = UIColor(red: 130/255, green: 177/255, blue: 255/255, alpha: 1)
open static let accent2 = UIColor(red: 68/255, green: 138/255, blue: 255/255, alpha: 1)
open static let accent3 = UIColor(red: 41/255, green: 121/255, blue: 255/255, alpha: 1)
open static let accent4 = UIColor(red: 41/255, green: 98/255, blue: 255/255, alpha: 1)
}
// light blue
open class lightBlue: ColorPalette {
open static let lighten5 = UIColor(red: 225/255, green: 245/255, blue: 254/255, alpha: 1)
open static let lighten4 = UIColor(red: 179/255, green: 229/255, blue: 252/255, alpha: 1)
open static let lighten3 = UIColor(red: 129/255, green: 212/255, blue: 250/255, alpha: 1)
open static let lighten2 = UIColor(red: 79/255, green: 195/255, blue: 247/255, alpha: 1)
open static let lighten1 = UIColor(red: 41/255, green: 182/255, blue: 246/255, alpha: 1)
open static let base = UIColor(red: 3/255, green: 169/255, blue: 244/255, alpha: 1)
open static let darken1 = UIColor(red: 3/255, green: 155/255, blue: 229/255, alpha: 1)
open static let darken2 = UIColor(red: 2/255, green: 136/255, blue: 209/255, alpha: 1)
open static let darken3 = UIColor(red: 2/255, green: 119/255, blue: 189/255, alpha: 1)
open static let darken4 = UIColor(red: 1/255, green: 87/255, blue: 155/255, alpha: 1)
open static let accent1 = UIColor(red: 128/255, green: 216/255, blue: 255/255, alpha: 1)
open static let accent2 = UIColor(red: 64/255, green: 196/255, blue: 255/255, alpha: 1)
open static let accent3 = UIColor(red: 0/255, green: 176/255, blue: 255/255, alpha: 1)
open static let accent4 = UIColor(red: 0/255, green: 145/255, blue: 234/255, alpha: 1)
}
// cyan
open class cyan: ColorPalette {
open static let lighten5 = UIColor(red: 224/255, green: 247/255, blue: 250/255, alpha: 1)
open static let lighten4 = UIColor(red: 178/255, green: 235/255, blue: 242/255, alpha: 1)
open static let lighten3 = UIColor(red: 128/255, green: 222/255, blue: 234/255, alpha: 1)
open static let lighten2 = UIColor(red: 77/255, green: 208/255, blue: 225/255, alpha: 1)
open static let lighten1 = UIColor(red: 38/255, green: 198/255, blue: 218/255, alpha: 1)
open static let base = UIColor(red: 0/255, green: 188/255, blue: 212/255, alpha: 1)
open static let darken1 = UIColor(red: 0/255, green: 172/255, blue: 193/255, alpha: 1)
open static let darken2 = UIColor(red: 0/255, green: 151/255, blue: 167/255, alpha: 1)
open static let darken3 = UIColor(red: 0/255, green: 131/255, blue: 143/255, alpha: 1)
open static let darken4 = UIColor(red: 0/255, green: 96/255, blue: 100/255, alpha: 1)
open static let accent1 = UIColor(red: 132/255, green: 255/255, blue: 255/255, alpha: 1)
open static let accent2 = UIColor(red: 24/255, green: 255/255, blue: 255/255, alpha: 1)
open static let accent3 = UIColor(red: 0/255, green: 229/255, blue: 255/255, alpha: 1)
open static let accent4 = UIColor(red: 0/255, green: 184/255, blue: 212/255, alpha: 1)
}
// teal
open class teal: ColorPalette {
open static let lighten5 = UIColor(red: 224/255, green: 242/255, blue: 241/255, alpha: 1)
open static let lighten4 = UIColor(red: 178/255, green: 223/255, blue: 219/255, alpha: 1)
open static let lighten3 = UIColor(red: 128/255, green: 203/255, blue: 196/255, alpha: 1)
open static let lighten2 = UIColor(red: 77/255, green: 182/255, blue: 172/255, alpha: 1)
open static let lighten1 = UIColor(red: 38/255, green: 166/255, blue: 154/255, alpha: 1)
open static let base = UIColor(red: 0/255, green: 150/255, blue: 136/255, alpha: 1)
open static let darken1 = UIColor(red: 0/255, green: 137/255, blue: 123/255, alpha: 1)
open static let darken2 = UIColor(red: 0/255, green: 121/255, blue: 107/255, alpha: 1)
open static let darken3 = UIColor(red: 0/255, green: 105/255, blue: 92/255, alpha: 1)
open static let darken4 = UIColor(red: 0/255, green: 77/255, blue: 64/255, alpha: 1)
open static let accent1 = UIColor(red: 167/255, green: 255/255, blue: 235/255, alpha: 1)
open static let accent2 = UIColor(red: 100/255, green: 255/255, blue: 218/255, alpha: 1)
open static let accent3 = UIColor(red: 29/255, green: 233/255, blue: 182/255, alpha: 1)
open static let accent4 = UIColor(red: 0/255, green: 191/255, blue: 165/255, alpha: 1)
}
// green
open class green: ColorPalette {
open static let lighten5 = UIColor(red: 232/255, green: 245/255, blue: 233/255, alpha: 1)
open static let lighten4 = UIColor(red: 200/255, green: 230/255, blue: 201/255, alpha: 1)
open static let lighten3 = UIColor(red: 165/255, green: 214/255, blue: 167/255, alpha: 1)
open static let lighten2 = UIColor(red: 129/255, green: 199/255, blue: 132/255, alpha: 1)
open static let lighten1 = UIColor(red: 102/255, green: 187/255, blue: 106/255, alpha: 1)
open static let base = UIColor(red: 76/255, green: 175/255, blue: 80/255, alpha: 1)
open static let darken1 = UIColor(red: 67/255, green: 160/255, blue: 71/255, alpha: 1)
open static let darken2 = UIColor(red: 56/255, green: 142/255, blue: 60/255, alpha: 1)
open static let darken3 = UIColor(red: 46/255, green: 125/255, blue: 50/255, alpha: 1)
open static let darken4 = UIColor(red: 27/255, green: 94/255, blue: 32/255, alpha: 1)
open static let accent1 = UIColor(red: 185/255, green: 246/255, blue: 202/255, alpha: 1)
open static let accent2 = UIColor(red: 105/255, green: 240/255, blue: 174/255, alpha: 1)
open static let accent3 = UIColor(red: 0/255, green: 230/255, blue: 118/255, alpha: 1)
open static let accent4 = UIColor(red: 0/255, green: 200/255, blue: 83/255, alpha: 1)
}
// light green
open class lightGreen: ColorPalette {
open static let lighten5 = UIColor(red: 241/255, green: 248/255, blue: 233/255, alpha: 1)
open static let lighten4 = UIColor(red: 220/255, green: 237/255, blue: 200/255, alpha: 1)
open static let lighten3 = UIColor(red: 197/255, green: 225/255, blue: 165/255, alpha: 1)
open static let lighten2 = UIColor(red: 174/255, green: 213/255, blue: 129/255, alpha: 1)
open static let lighten1 = UIColor(red: 156/255, green: 204/255, blue: 101/255, alpha: 1)
open static let base = UIColor(red: 139/255, green: 195/255, blue: 74/255, alpha: 1)
open static let darken1 = UIColor(red: 124/255, green: 179/255, blue: 66/255, alpha: 1)
open static let darken2 = UIColor(red: 104/255, green: 159/255, blue: 56/255, alpha: 1)
open static let darken3 = UIColor(red: 85/255, green: 139/255, blue: 47/255, alpha: 1)
open static let darken4 = UIColor(red: 51/255, green: 105/255, blue: 30/255, alpha: 1)
open static let accent1 = UIColor(red: 204/255, green: 255/255, blue: 144/255, alpha: 1)
open static let accent2 = UIColor(red: 178/255, green: 255/255, blue: 89/255, alpha: 1)
open static let accent3 = UIColor(red: 118/255, green: 255/255, blue: 3/255, alpha: 1)
open static let accent4 = UIColor(red: 100/255, green: 221/255, blue: 23/255, alpha: 1)
}
// lime
open class lime: ColorPalette {
open static let lighten5 = UIColor(red: 249/255, green: 251/255, blue: 231/255, alpha: 1)
open static let lighten4 = UIColor(red: 240/255, green: 244/255, blue: 195/255, alpha: 1)
open static let lighten3 = UIColor(red: 230/255, green: 238/255, blue: 156/255, alpha: 1)
open static let lighten2 = UIColor(red: 220/255, green: 231/255, blue: 117/255, alpha: 1)
open static let lighten1 = UIColor(red: 212/255, green: 225/255, blue: 87/255, alpha: 1)
open static let base = UIColor(red: 205/255, green: 220/255, blue: 57/255, alpha: 1)
open static let darken1 = UIColor(red: 192/255, green: 202/255, blue: 51/255, alpha: 1)
open static let darken2 = UIColor(red: 175/255, green: 180/255, blue: 43/255, alpha: 1)
open static let darken3 = UIColor(red: 158/255, green: 157/255, blue: 36/255, alpha: 1)
open static let darken4 = UIColor(red: 130/255, green: 119/255, blue: 23/255, alpha: 1)
open static let accent1 = UIColor(red: 244/255, green: 255/255, blue: 129/255, alpha: 1)
open static let accent2 = UIColor(red: 238/255, green: 255/255, blue: 65/255, alpha: 1)
open static let accent3 = UIColor(red: 198/255, green: 255/255, blue: 0/255, alpha: 1)
open static let accent4 = UIColor(red: 174/255, green: 234/255, blue: 0/255, alpha: 1)
}
// yellow
open class yellow: ColorPalette {
open static let lighten5 = UIColor(red: 255/255, green: 253/255, blue: 231/255, alpha: 1)
open static let lighten4 = UIColor(red: 255/255, green: 249/255, blue: 196/255, alpha: 1)
open static let lighten3 = UIColor(red: 255/255, green: 245/255, blue: 157/255, alpha: 1)
open static let lighten2 = UIColor(red: 255/255, green: 241/255, blue: 118/255, alpha: 1)
open static let lighten1 = UIColor(red: 255/255, green: 238/255, blue: 88/255, alpha: 1)
open static let base = UIColor(red: 255/255, green: 235/255, blue: 59/255, alpha: 1)
open static let darken1 = UIColor(red: 253/255, green: 216/255, blue: 53/255, alpha: 1)
open static let darken2 = UIColor(red: 251/255, green: 192/255, blue: 45/255, alpha: 1)
open static let darken3 = UIColor(red: 249/255, green: 168/255, blue: 37/255, alpha: 1)
open static let darken4 = UIColor(red: 245/255, green: 127/255, blue: 23/255, alpha: 1)
open static let accent1 = UIColor(red: 255/255, green: 255/255, blue: 141/255, alpha: 1)
open static let accent2 = UIColor(red: 255/255, green: 255/255, blue: 0/255, alpha: 1)
open static let accent3 = UIColor(red: 255/255, green: 234/255, blue: 0/255, alpha: 1)
open static let accent4 = UIColor(red: 255/255, green: 214/255, blue: 0/255, alpha: 1)
}
// amber
open class amber: ColorPalette {
open static let lighten5 = UIColor(red: 255/255, green: 248/255, blue: 225/255, alpha: 1)
open static let lighten4 = UIColor(red: 255/255, green: 236/255, blue: 179/255, alpha: 1)
open static let lighten3 = UIColor(red: 255/255, green: 224/255, blue: 130/255, alpha: 1)
open static let lighten2 = UIColor(red: 255/255, green: 213/255, blue: 79/255, alpha: 1)
open static let lighten1 = UIColor(red: 255/255, green: 202/255, blue: 40/255, alpha: 1)
open static let base = UIColor(red: 255/255, green: 193/255, blue: 7/255, alpha: 1)
open static let darken1 = UIColor(red: 255/255, green: 179/255, blue: 0/255, alpha: 1)
open static let darken2 = UIColor(red: 255/255, green: 160/255, blue: 0/255, alpha: 1)
open static let darken3 = UIColor(red: 255/255, green: 143/255, blue: 0/255, alpha: 1)
open static let darken4 = UIColor(red: 255/255, green: 111/255, blue: 0/255, alpha: 1)
open static let accent1 = UIColor(red: 255/255, green: 229/255, blue: 127/255, alpha: 1)
open static let accent2 = UIColor(red: 255/255, green: 215/255, blue: 64/255, alpha: 1)
open static let accent3 = UIColor(red: 255/255, green: 196/255, blue: 0/255, alpha: 1)
open static let accent4 = UIColor(red: 255/255, green: 171/255, blue: 0/255, alpha: 1)
}
// orange
open class orange: ColorPalette {
open static let lighten5 = UIColor(red: 255/255, green: 243/255, blue: 224/255, alpha: 1)
open static let lighten4 = UIColor(red: 255/255, green: 224/255, blue: 178/255, alpha: 1)
open static let lighten3 = UIColor(red: 255/255, green: 204/255, blue: 128/255, alpha: 1)
open static let lighten2 = UIColor(red: 255/255, green: 183/255, blue: 77/255, alpha: 1)
open static let lighten1 = UIColor(red: 255/255, green: 167/255, blue: 38/255, alpha: 1)
open static let base = UIColor(red: 255/255, green: 152/255, blue: 0/255, alpha: 1)
open static let darken1 = UIColor(red: 251/255, green: 140/255, blue: 0/255, alpha: 1)
open static let darken2 = UIColor(red: 245/255, green: 124/255, blue: 0/255, alpha: 1)
open static let darken3 = UIColor(red: 239/255, green: 108/255, blue: 0/255, alpha: 1)
open static let darken4 = UIColor(red: 230/255, green: 81/255, blue: 0/255, alpha: 1)
open static let accent1 = UIColor(red: 255/255, green: 209/255, blue: 128/255, alpha: 1)
open static let accent2 = UIColor(red: 255/255, green: 171/255, blue: 64/255, alpha: 1)
open static let accent3 = UIColor(red: 255/255, green: 145/255, blue: 0/255, alpha: 1)
open static let accent4 = UIColor(red: 255/255, green: 109/255, blue: 0/255, alpha: 1)
}
// deep orange
open class deepOrange: ColorPalette {
open static let lighten5 = UIColor(red: 251/255, green: 233/255, blue: 231/255, alpha: 1)
open static let lighten4 = UIColor(red: 255/255, green: 204/255, blue: 188/255, alpha: 1)
open static let lighten3 = UIColor(red: 255/255, green: 171/255, blue: 145/255, alpha: 1)
open static let lighten2 = UIColor(red: 255/255, green: 138/255, blue: 101/255, alpha: 1)
open static let lighten1 = UIColor(red: 255/255, green: 112/255, blue: 67/255, alpha: 1)
open static let base = UIColor(red: 255/255, green: 87/255, blue: 34/255, alpha: 1)
open static let darken1 = UIColor(red: 244/255, green: 81/255, blue: 30/255, alpha: 1)
open static let darken2 = UIColor(red: 230/255, green: 74/255, blue: 25/255, alpha: 1)
open static let darken3 = UIColor(red: 216/255, green: 67/255, blue: 21/255, alpha: 1)
open static let darken4 = UIColor(red: 191/255, green: 54/255, blue: 12/255, alpha: 1)
open static let accent1 = UIColor(red: 255/255, green: 158/255, blue: 128/255, alpha: 1)
open static let accent2 = UIColor(red: 255/255, green: 110/255, blue: 64/255, alpha: 1)
open static let accent3 = UIColor(red: 255/255, green: 61/255, blue: 0/255, alpha: 1)
open static let accent4 = UIColor(red: 221/255, green: 44/255, blue: 0/255, alpha: 1)
}
// brown
open class brown: ColorPalette {
open static let lighten5 = UIColor(red: 239/255, green: 235/255, blue: 233/255, alpha: 1)
open static let lighten4 = UIColor(red: 215/255, green: 204/255, blue: 200/255, alpha: 1)
open static let lighten3 = UIColor(red: 188/255, green: 170/255, blue: 164/255, alpha: 1)
open static let lighten2 = UIColor(red: 161/255, green: 136/255, blue: 127/255, alpha: 1)
open static let lighten1 = UIColor(red: 141/255, green: 110/255, blue: 99/255, alpha: 1)
open static let base = UIColor(red: 121/255, green: 85/255, blue: 72/255, alpha: 1)
open static let darken1 = UIColor(red: 109/255, green: 76/255, blue: 65/255, alpha: 1)
open static let darken2 = UIColor(red: 93/255, green: 64/255, blue: 55/255, alpha: 1)
open static let darken3 = UIColor(red: 78/255, green: 52/255, blue: 46/255, alpha: 1)
open static let darken4 = UIColor(red: 62/255, green: 39/255, blue: 35/255, alpha: 1)
}
// grey
open class grey: ColorPalette {
open static let lighten5 = UIColor(red: 250/255, green: 250/255, blue: 250/255, alpha: 1)
open static let lighten4 = UIColor(red: 245/255, green: 245/255, blue: 245/255, alpha: 1)
open static let lighten3 = UIColor(red: 238/255, green: 238/255, blue: 238/255, alpha: 1)
open static let lighten2 = UIColor(red: 224/255, green: 224/255, blue: 224/255, alpha: 1)
open static let lighten1 = UIColor(red: 189/255, green: 189/255, blue: 189/255, alpha: 1)
open static let base = UIColor(red: 158/255, green: 158/255, blue: 158/255, alpha: 1)
open static let darken1 = UIColor(red: 117/255, green: 117/255, blue: 117/255, alpha: 1)
open static let darken2 = UIColor(red: 97/255, green: 97/255, blue: 97/255, alpha: 1)
open static let darken3 = UIColor(red: 66/255, green: 66/255, blue: 66/255, alpha: 1)
open static let darken4 = UIColor(red: 33/255, green: 33/255, blue: 33/255, alpha: 1)
}
// blue grey
open class blueGrey: ColorPalette {
open static let lighten5 = UIColor(red: 236/255, green: 239/255, blue: 241/255, alpha: 1)
open static let lighten4 = UIColor(red: 207/255, green: 216/255, blue: 220/255, alpha: 1)
open static let lighten3 = UIColor(red: 176/255, green: 190/255, blue: 197/255, alpha: 1)
open static let lighten2 = UIColor(red: 144/255, green: 164/255, blue: 174/255, alpha: 1)
open static let lighten1 = UIColor(red: 120/255, green: 144/255, blue: 156/255, alpha: 1)
open static let base = UIColor(red: 96/255, green: 125/255, blue: 139/255, alpha: 1)
open static let darken1 = UIColor(red: 84/255, green: 110/255, blue: 122/255, alpha: 1)
open static let darken2 = UIColor(red: 69/255, green: 90/255, blue: 100/255, alpha: 1)
open static let darken3 = UIColor(red: 55/255, green: 71/255, blue: 79/255, alpha: 1)
open static let darken4 = UIColor(red: 38/255, green: 50/255, blue: 56/255, alpha: 1)
}
}
| bsd-3-clause | 8ac9eba3305ff5be2c9aec2bd6c54caf | 65.023753 | 93 | 0.677544 | 3.134769 | false | false | false | false |
gongmingqm10/DriftBook | DriftReading/DriftReading/DiscoveryViewController.swift | 1 | 2286 | //
// DiscoveryViewController.swift
// DriftReading
//
// Created by Ming Gong on 7/7/15.
// Copyright © 2015 gongmingqm10. All rights reserved.
//
import UIKit
class DiscoveryViewController: UITableViewController {
let driftAPI = DriftAPI()
@IBOutlet var booksTableView: UITableView!
var books: [Book] = []
let TYPE_DRIFTING = "drifting"
let TYPE_READING = "reading"
var selectedBook: Book?
var currentType: String?
override func viewDidLoad() {
currentType = TYPE_DRIFTING
}
override func viewDidAppear(animated: Bool) {
loadBooksByType()
}
private func loadBooksByType() {
driftAPI.getBooks(currentType!, success: { (books) -> Void in
self.books = books
self.booksTableView.reloadData()
}) { (error) -> Void in
print(error.description)
}
}
@IBAction func switchSegment(sender: UISegmentedControl) {
currentType = sender.selectedSegmentIndex == 0 ? TYPE_DRIFTING : TYPE_READING
loadBooksByType()
}
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
if segue.identifier == "BookDetailSegue" {
let bookDetailController = segue.destinationViewController as! BookDetailViewController
bookDetailController.bookId = selectedBook!.id
}
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return books.count
}
override func tableView(tableView: UITableView, didSelectRowAtIndexPath indexPath: NSIndexPath) {
self.selectedBook = books[indexPath.row]
self.performSegueWithIdentifier("BookDetailSegue", sender: self)
}
override func tableView(tableView: UITableView, heightForRowAtIndexPath indexPath: NSIndexPath) -> CGFloat {
return 150
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = booksTableView.dequeueReusableCellWithIdentifier("BookTableCell", forIndexPath: indexPath) as! BookTableCell
let book = books[indexPath.row]
cell.populate(book)
return cell
}
}
| mit | 5e2c82ad0fb0d01953d2c43e3ee39609 | 30.30137 | 127 | 0.661269 | 4.989083 | false | false | false | false |
lyle-luan/firefox-ios | Storage/Storage/Bookmarks.swift | 2 | 8899 | /* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
import Foundation
import UIKit
public struct ShareItem {
public let url: String
public let title: String?
public init(url: String, title: String?) {
self.url = url
self.title = title
}
}
public protocol ShareToDestination {
func shareItem(item: ShareItem)
}
public struct BookmarkRoots {
// These are stolen from Fennec's BrowserContract.
static let MOBILE_FOLDER_GUID = "mobile"
static let PLACES_FOLDER_GUID = "places"
static let MENU_FOLDER_GUID = "menu"
static let TAGS_FOLDER_GUID = "tags"
static let TOOLBAR_FOLDER_GUID = "toolbar"
static let UNFILED_FOLDER_GUID = "unfiled"
static let FAKE_DESKTOP_FOLDER_GUID = "desktop"
static let PINNED_FOLDER_GUID = "pinned"
}
/**
* The immutable base interface for bookmarks and folders.
*/
@objc public protocol BookmarkNode {
var id: String { get }
var title: String { get }
var icon: UIImage { get }
}
/**
* An immutable item representing a bookmark.
*
* To modify this, issue changes against the backing store and get an updated model.
*/
public class BookmarkItem: BookmarkNode {
public let id: String
public let url: String!
public let title: String
var _icon: UIImage?
public var icon: UIImage {
if (self._icon != nil) {
return self._icon!
}
return UIImage(named: "leaf.png")!
}
public init(id: String, title: String, url: String) {
self.id = id
self.title = title
self.url = url
}
}
/**
* A folder is an immutable abstraction over a named
* thing that can return its child nodes by index.
*/
@objc public protocol BookmarkFolder: BookmarkNode {
var count: Int { get }
subscript(index: Int) -> BookmarkNode { get }
}
/**
* A folder that contains an array of children.
*/
public class MemoryBookmarkFolder: BookmarkFolder, SequenceType {
public let id: String
public let title: String
let children: [BookmarkNode]
public init(id: String, title: String, children: [BookmarkNode]) {
self.id = id;
self.title = title
self.children = children
}
public struct BookmarkNodeGenerator: GeneratorType {
typealias Element = BookmarkNode
let children: [BookmarkNode]
var index: Int = 0
init(children: [BookmarkNode]) {
self.children = children
}
public mutating func next() -> BookmarkNode? {
return index < children.count ? children[index++] : nil
}
}
public var icon: UIImage {
return UIImage(named: "bookmark_folder_closed")!
}
init(id: String, name: String, children: [BookmarkNode]) {
self.id = id
self.title = name
self.children = children
}
public var count: Int {
return children.count
}
public subscript(index: Int) -> BookmarkNode {
get {
return children[index]
}
}
public func generate() -> BookmarkNodeGenerator {
return BookmarkNodeGenerator(children: self.children)
}
/**
* Return a new immutable folder that's just like this one,
* but also contains the new items.
*/
func append(items: [BookmarkNode]) -> MemoryBookmarkFolder {
if (items.isEmpty) {
return self
}
return MemoryBookmarkFolder(id: self.id, name: self.title, children: self.children + items)
}
}
/**
* A model is a snapshot of the bookmarks store, suitable for backing a table view.
*
* Navigation through the folder hierarchy produces a sequence of models.
*
* Changes to the backing store implicitly invalidates a subset of models.
*
* 'Refresh' means requesting a new model from the store.
*/
public class BookmarksModel {
let modelFactory: BookmarksModelFactory
public let current: BookmarkFolder
public init(modelFactory: BookmarksModelFactory, root: BookmarkFolder) {
self.modelFactory = modelFactory
self.current = root
}
/**
* Produce a new model rooted at the appropriate folder. Fails if the folder doesn't exist.
*/
public func selectFolder(folder: BookmarkFolder, success: (BookmarksModel) -> (), failure: (Any) -> ()) {
modelFactory.modelForFolder(folder, success: success, failure: failure)
}
/**
* Produce a new model rooted at the appropriate folder. Fails if the folder doesn't exist.
*/
public func selectFolder(guid: String, success: (BookmarksModel) -> (), failure: (Any) -> ()) {
modelFactory.modelForFolder(guid, success: success, failure: failure)
}
/**
* Produce a new model rooted at the base of the hierarchy. Should never fail.
*/
public func selectRoot(success: (BookmarksModel) -> (), failure: (Any) -> ()) {
modelFactory.modelForRoot(success, failure: failure)
}
/**
* Produce a new model rooted at the same place as this model. Can fail if
* the folder has been deleted from the backing store.
*/
public func reloadData(success: (BookmarksModel) -> (), failure: (Any) -> ()) {
modelFactory.modelForFolder(current, success: success, failure: failure)
}
}
public class MemoryBookmarksSink: ShareToDestination {
var queue: [BookmarkNode] = []
public init() { }
public func shareItem(item: ShareItem) {
let title = item.title == nil ? "Untitled" : item.title!
func exists(e: BookmarkNode) -> Bool {
if let bookmark = e as? BookmarkItem {
return bookmark.url == item.url;
}
return false;
}
// Don't create duplicates.
if (!contains(queue, exists)) {
queue.append(BookmarkItem(id: NSUUID().UUIDString, title: title, url: item.url))
}
}
}
public protocol BookmarksModelFactory {
func modelForFolder(folder: BookmarkFolder, success: (BookmarksModel) -> (), failure: (Any) -> ())
func modelForFolder(guid: String, success: (BookmarksModel) -> (), failure: (Any) -> ())
func modelForRoot(success: (BookmarksModel) -> (), failure: (Any) -> ())
// Whenever async construction is necessary, we fall into a pattern of needing
// a placeholder that behaves correctly for the period between kickoff and set.
var nullModel: BookmarksModel { get }
}
/**
* A trivial offline model factory that represents a simple hierarchy.
*/
public class MockMemoryBookmarksStore: BookmarksModelFactory, ShareToDestination {
let mobile: MemoryBookmarkFolder
let root: MemoryBookmarkFolder
var unsorted: MemoryBookmarkFolder
let sink: MemoryBookmarksSink
public init() {
var res = [BookmarkItem]()
for i in 0...10 {
res.append(BookmarkItem(id: NSUUID().UUIDString, title: "Title \(i)", url: "http://www.example.com/\(i)"))
}
mobile = MemoryBookmarkFolder(id: BookmarkRoots.MOBILE_FOLDER_GUID, name: "Mobile Bookmarks", children: res)
unsorted = MemoryBookmarkFolder(id: BookmarkRoots.UNFILED_FOLDER_GUID, name: "Unsorted Bookmarks", children: [])
sink = MemoryBookmarksSink()
root = MemoryBookmarkFolder(id: BookmarkRoots.PLACES_FOLDER_GUID, name: "Root", children: [mobile, unsorted])
}
public func modelForFolder(folder: BookmarkFolder, success: (BookmarksModel) -> (), failure: (Any) -> ()) {
self.modelForFolder(folder.id, success, failure)
}
public func modelForFolder(guid: String, success: (BookmarksModel) -> (), failure: (Any) -> ()) {
var m: BookmarkFolder
switch (guid) {
case BookmarkRoots.MOBILE_FOLDER_GUID:
// Transparently merges in any queued items.
m = self.mobile.append(self.sink.queue)
break;
case BookmarkRoots.PLACES_FOLDER_GUID:
m = self.root
break;
case BookmarkRoots.UNFILED_FOLDER_GUID:
m = self.unsorted
break;
default:
failure("No such folder.")
return
}
success(BookmarksModel(modelFactory: self, root: m))
}
public func modelForRoot(success: (BookmarksModel) -> (), failure: (Any) -> ()) {
success(BookmarksModel(modelFactory: self, root: self.root))
}
/**
* This class could return the full data immediately. We don't, because real DB-backed code won't.
*/
public var nullModel: BookmarksModel {
let f = MemoryBookmarkFolder(id: BookmarkRoots.PLACES_FOLDER_GUID, name: "Root", children: [])
return BookmarksModel(modelFactory: self, root: f)
}
public func shareItem(item: ShareItem) {
self.sink.shareItem(item)
}
}
| mpl-2.0 | f44af5077a1d4bd040b090d07c4213f4 | 30.115385 | 120 | 0.639173 | 4.303191 | false | false | false | false |
ryanorendorff/tablescope | tablescope/date.swift | 1 | 724 | //
// date.swift
// TableScope
//
// Created by Ryan Orendorff on 29/4/15.
// Copyright (c) 2015 cellscope. All rights reserved.
//
import Foundation
// Allows NSDate to be compared using the >, ==, etc operators. It makes
// the code much more readable versus determining whether the order of
// the dates is ascending or descending.
public func ==(lhs: NSDate, rhs: NSDate) -> Bool {
// Naïve equality that uses string comparison rather than resolving equivalent selectors
return lhs.isEqualToDate(rhs)
}
extension NSDate : Comparable {}
public func <(lhs: NSDate, rhs: NSDate) -> Bool {
if lhs.compare(rhs) == .OrderedAscending {
return true
} else {
return false
}
} | gpl-3.0 | 1cfd03b264c5203e27fd787831d2cf10 | 21.625 | 92 | 0.674965 | 3.994475 | false | false | false | false |
remirobert/go-mobile-twitter-stream | TwitterStream/Interactors/Implementations/TwitterStreamInteractor.swift | 1 | 1165 | //
// TwitterStreamService.swift
// TwitterStream
//
// Created by Remi Robert on 11/08/2017.
// Copyright © 2017 Remi Robert. All rights reserved.
//
import Twitter
class TwitterStreamInteractor: NSObject, StreamInteractor {
fileprivate let config: TwitterConfiguration
fileprivate var client: TwitterTwitter!
weak var delegate: StreamInteractorDelegate?
init(config: TwitterConfiguration = PlistConfiguration()) {
self.config = config
let streamConfig = TwitterNewConfig(config.consumer,
config.consumerSecret,
config.token,
config.tokenSecret)
super.init()
client = TwitterNewTitter(self, streamConfig)
}
}
extension TwitterStreamInteractor {
func start() {
client.startRead(config.track)
}
}
extension TwitterStreamInteractor: TwitterTwitterCallbackProtocol {
func getContent(_ response: TwitterTweet!) {
let tweet = response.toTweet()
DispatchQueue.main.async {
self.delegate?.didRead(tweet: tweet)
}
}
}
| mit | 806b66e94a9db1491fa31cd7e034c418 | 27.390244 | 67 | 0.623711 | 4.932203 | false | true | false | false |
therealbnut/swift | stdlib/public/SDK/Foundation/URL.swift | 1 | 62119 | //===----------------------------------------------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2017 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
@_exported import Foundation // Clang module
/// Keys used in the result of `URLResourceValues.thumbnailDictionary`.
@available(OSX 10.10, iOS 8.0, *)
public struct URLThumbnailSizeKey : RawRepresentable, Hashable {
public typealias RawValue = String
public init(rawValue: RawValue) { self.rawValue = rawValue }
private(set) public var rawValue: RawValue
/// Key for a 1024 x 1024 thumbnail image.
static public let none: URLThumbnailSizeKey = URLThumbnailSizeKey(rawValue: URLThumbnailDictionaryItem.NSThumbnail1024x1024SizeKey.rawValue)
public var hashValue: Int {
return rawValue.hashValue
}
}
/**
URLs to file system resources support the properties defined below. Note that not all property values will exist for all file system URLs. For example, if a file is located on a volume that does not support creation dates, it is valid to request the creation date property, but the returned value will be nil, and no error will be generated.
Only the fields requested by the keys you pass into the `URL` function to receive this value will be populated. The others will return `nil` regardless of the underlying property on the file system.
As a convenience, volume resource values can be requested from any file system URL. The value returned will reflect the property value for the volume on which the resource is located.
*/
public struct URLResourceValues {
fileprivate var _values: [URLResourceKey: Any]
fileprivate var _keys: Set<URLResourceKey>
public init() {
_values = [:]
_keys = []
}
fileprivate init(keys: Set<URLResourceKey>, values: [URLResourceKey: Any]) {
_values = values
_keys = keys
}
private func contains(_ key: URLResourceKey) -> Bool {
return _keys.contains(key)
}
private func _get<T>(_ key : URLResourceKey) -> T? {
return _values[key] as? T
}
private func _get(_ key : URLResourceKey) -> Bool? {
return (_values[key] as? NSNumber)?.boolValue
}
private func _get(_ key: URLResourceKey) -> Int? {
return (_values[key] as? NSNumber)?.intValue
}
private mutating func _set(_ key : URLResourceKey, newValue : Any?) {
_keys.insert(key)
_values[key] = newValue
}
private mutating func _set(_ key : URLResourceKey, newValue : String?) {
_keys.insert(key)
_values[key] = newValue as NSString?
}
private mutating func _set(_ key : URLResourceKey, newValue : [String]?) {
_keys.insert(key)
_values[key] = newValue as NSObject?
}
private mutating func _set(_ key : URLResourceKey, newValue : Date?) {
_keys.insert(key)
_values[key] = newValue as NSDate?
}
private mutating func _set(_ key : URLResourceKey, newValue : URL?) {
_keys.insert(key)
_values[key] = newValue as NSURL?
}
private mutating func _set(_ key : URLResourceKey, newValue : Bool?) {
_keys.insert(key)
if let value = newValue {
_values[key] = NSNumber(value: value)
} else {
_values[key] = nil
}
}
private mutating func _set(_ key : URLResourceKey, newValue : Int?) {
_keys.insert(key)
if let value = newValue {
_values[key] = NSNumber(value: value)
} else {
_values[key] = nil
}
}
/// A loosely-typed dictionary containing all keys and values.
///
/// If you have set temporary keys or non-standard keys, you can find them in here.
public var allValues : [URLResourceKey : Any] {
return _values
}
/// The resource name provided by the file system.
public var name: String? {
get { return _get(.nameKey) }
set { _set(.nameKey, newValue: newValue) }
}
/// Localized or extension-hidden name as displayed to users.
public var localizedName: String? { return _get(.localizedNameKey) }
/// True for regular files.
public var isRegularFile: Bool? { return _get(.isRegularFileKey) }
/// True for directories.
public var isDirectory: Bool? { return _get(.isDirectoryKey) }
/// True for symlinks.
public var isSymbolicLink: Bool? { return _get(.isSymbolicLinkKey) }
/// True for the root directory of a volume.
public var isVolume: Bool? { return _get(.isVolumeKey) }
/// True for packaged directories.
///
/// - note: You can only set or clear this property on directories; if you try to set this property on non-directory objects, the property is ignored. If the directory is a package for some other reason (extension type, etc), setting this property to false will have no effect.
public var isPackage: Bool? {
get { return _get(.isPackageKey) }
set { _set(.isPackageKey, newValue: newValue) }
}
/// True if resource is an application.
@available(OSX 10.11, iOS 9.0, *)
public var isApplication: Bool? { return _get(.isApplicationKey) }
#if os(OSX)
/// True if the resource is scriptable. Only applies to applications.
@available(OSX 10.11, *)
public var applicationIsScriptable: Bool? { return _get(.applicationIsScriptableKey) }
#endif
/// True for system-immutable resources.
public var isSystemImmutable: Bool? { return _get(.isSystemImmutableKey) }
/// True for user-immutable resources
public var isUserImmutable: Bool? {
get { return _get(.isUserImmutableKey) }
set { _set(.isUserImmutableKey, newValue: newValue) }
}
/// True for resources normally not displayed to users.
///
/// - note: If the resource is a hidden because its name starts with a period, setting this property to false will not change the property.
public var isHidden: Bool? {
get { return _get(.isHiddenKey) }
set { _set(.isHiddenKey, newValue: newValue) }
}
/// True for resources whose filename extension is removed from the localized name property.
public var hasHiddenExtension: Bool? {
get { return _get(.hasHiddenExtensionKey) }
set { _set(.hasHiddenExtensionKey, newValue: newValue) }
}
/// The date the resource was created.
public var creationDate: Date? {
get { return _get(.creationDateKey) }
set { _set(.creationDateKey, newValue: newValue) }
}
/// The date the resource was last accessed.
public var contentAccessDate: Date? {
get { return _get(.contentAccessDateKey) }
set { _set(.contentAccessDateKey, newValue: newValue) }
}
/// The time the resource content was last modified.
public var contentModificationDate: Date? {
get { return _get(.contentModificationDateKey) }
set { _set(.contentModificationDateKey, newValue: newValue) }
}
/// The time the resource's attributes were last modified.
public var attributeModificationDate: Date? { return _get(.attributeModificationDateKey) }
/// Number of hard links to the resource.
public var linkCount: Int? { return _get(.linkCountKey) }
/// The resource's parent directory, if any.
public var parentDirectory: URL? { return _get(.parentDirectoryURLKey) }
/// URL of the volume on which the resource is stored.
public var volume: URL? { return _get(.volumeURLKey) }
/// Uniform type identifier (UTI) for the resource.
public var typeIdentifier: String? { return _get(.typeIdentifierKey) }
/// User-visible type or "kind" description.
public var localizedTypeDescription: String? { return _get(.localizedTypeDescriptionKey) }
/// The label number assigned to the resource.
public var labelNumber: Int? {
get { return _get(.labelNumberKey) }
set { _set(.labelNumberKey, newValue: newValue) }
}
/// The user-visible label text.
public var localizedLabel: String? {
get { return _get(.localizedLabelKey) }
}
/// An identifier which can be used to compare two file system objects for equality using `isEqual`.
///
/// Two object identifiers are equal if they have the same file system path or if the paths are linked to same inode on the same file system. This identifier is not persistent across system restarts.
public var fileResourceIdentifier: (NSCopying & NSCoding & NSSecureCoding & NSObjectProtocol)? { return _get(.fileResourceIdentifierKey) }
/// An identifier that can be used to identify the volume the file system object is on.
///
/// Other objects on the same volume will have the same volume identifier and can be compared using for equality using `isEqual`. This identifier is not persistent across system restarts.
public var volumeIdentifier: (NSCopying & NSCoding & NSSecureCoding & NSObjectProtocol)? { return _get(.volumeIdentifierKey) }
/// The optimal block size when reading or writing this file's data, or nil if not available.
public var preferredIOBlockSize: Int? { return _get(.preferredIOBlockSizeKey) }
/// True if this process (as determined by EUID) can read the resource.
public var isReadable: Bool? { return _get(.isReadableKey) }
/// True if this process (as determined by EUID) can write to the resource.
public var isWritable: Bool? { return _get(.isWritableKey) }
/// True if this process (as determined by EUID) can execute a file resource or search a directory resource.
public var isExecutable: Bool? { return _get(.isExecutableKey) }
/// The file system object's security information encapsulated in a FileSecurity object.
public var fileSecurity: NSFileSecurity? {
get { return _get(.fileSecurityKey) }
set { _set(.fileSecurityKey, newValue: newValue) }
}
/// True if resource should be excluded from backups, false otherwise.
///
/// This property is only useful for excluding cache and other application support files which are not needed in a backup. Some operations commonly made to user documents will cause this property to be reset to false and so this property should not be used on user documents.
public var isExcludedFromBackup: Bool? {
get { return _get(.isExcludedFromBackupKey) }
set { _set(.isExcludedFromBackupKey, newValue: newValue) }
}
#if os(OSX)
/// The array of Tag names.
public var tagNames: [String]? { return _get(.tagNamesKey) }
#endif
/// The URL's path as a file system path.
public var path: String? { return _get(.pathKey) }
/// The URL's path as a canonical absolute file system path.
@available(OSX 10.12, iOS 10.0, tvOS 10.0, watchOS 3.0, *)
public var canonicalPath: String? { return _get(.canonicalPathKey) }
/// True if this URL is a file system trigger directory. Traversing or opening a file system trigger will cause an attempt to mount a file system on the trigger directory.
public var isMountTrigger: Bool? { return _get(.isMountTriggerKey) }
/// An opaque generation identifier which can be compared using `==` to determine if the data in a document has been modified.
///
/// For URLs which refer to the same file inode, the generation identifier will change when the data in the file's data fork is changed (changes to extended attributes or other file system metadata do not change the generation identifier). For URLs which refer to the same directory inode, the generation identifier will change when direct children of that directory are added, removed or renamed (changes to the data of the direct children of that directory will not change the generation identifier). The generation identifier is persistent across system restarts. The generation identifier is tied to a specific document on a specific volume and is not transferred when the document is copied to another volume. This property is not supported by all volumes.
@available(OSX 10.10, iOS 8.0, *)
public var generationIdentifier: (NSCopying & NSCoding & NSSecureCoding & NSObjectProtocol)? { return _get(.generationIdentifierKey) }
/// The document identifier -- a value assigned by the kernel to a document (which can be either a file or directory) and is used to identify the document regardless of where it gets moved on a volume.
///
/// The document identifier survives "safe save" operations; i.e it is sticky to the path it was assigned to (`replaceItem(at:,withItemAt:,backupItemName:,options:,resultingItem:) throws` is the preferred safe-save API). The document identifier is persistent across system restarts. The document identifier is not transferred when the file is copied. Document identifiers are only unique within a single volume. This property is not supported by all volumes.
@available(OSX 10.10, iOS 8.0, *)
public var documentIdentifier: Int? { return _get(.documentIdentifierKey) }
/// The date the resource was created, or renamed into or within its parent directory. Note that inconsistent behavior may be observed when this attribute is requested on hard-linked items. This property is not supported by all volumes.
@available(OSX 10.10, iOS 8.0, *)
public var addedToDirectoryDate: Date? { return _get(.addedToDirectoryDateKey) }
#if os(OSX)
/// The quarantine properties as defined in LSQuarantine.h. To remove quarantine information from a file, pass `nil` as the value when setting this property.
@available(OSX 10.10, *)
public var quarantineProperties: [String : Any]? {
get {
// If a caller has caused us to stash NSNull in the dictionary (via set), make sure to return nil instead of NSNull
if let isNull = _values[.quarantinePropertiesKey] as? NSNull {
return nil
} else {
return _values[.quarantinePropertiesKey] as? [String : Any]
}
}
set {
if let v = newValue {
_set(.quarantinePropertiesKey, newValue: newValue as NSObject?)
} else {
// Set to NSNull, a special case for deleting quarantine properties
_set(.quarantinePropertiesKey, newValue: NSNull())
}
}
}
#endif
/// Returns the file system object type.
public var fileResourceType: URLFileResourceType? { return _get(.fileResourceTypeKey) }
/// The user-visible volume format.
public var volumeLocalizedFormatDescription : String? { return _get(.volumeLocalizedFormatDescriptionKey) }
/// Total volume capacity in bytes.
public var volumeTotalCapacity : Int? { return _get(.volumeTotalCapacityKey) }
/// Total free space in bytes.
public var volumeAvailableCapacity : Int? { return _get(.volumeAvailableCapacityKey) }
/// Total number of resources on the volume.
public var volumeResourceCount : Int? { return _get(.volumeResourceCountKey) }
/// true if the volume format supports persistent object identifiers and can look up file system objects by their IDs.
public var volumeSupportsPersistentIDs : Bool? { return _get(.volumeSupportsPersistentIDsKey) }
/// true if the volume format supports symbolic links.
public var volumeSupportsSymbolicLinks : Bool? { return _get(.volumeSupportsSymbolicLinksKey) }
/// true if the volume format supports hard links.
public var volumeSupportsHardLinks : Bool? { return _get(.volumeSupportsHardLinksKey) }
/// true if the volume format supports a journal used to speed recovery in case of unplanned restart (such as a power outage or crash). This does not necessarily mean the volume is actively using a journal.
public var volumeSupportsJournaling : Bool? { return _get(.volumeSupportsJournalingKey) }
/// true if the volume is currently using a journal for speedy recovery after an unplanned restart.
public var volumeIsJournaling : Bool? { return _get(.volumeIsJournalingKey) }
/// true if the volume format supports sparse files, that is, files which can have 'holes' that have never been written to, and thus do not consume space on disk. A sparse file may have an allocated size on disk that is less than its logical length.
public var volumeSupportsSparseFiles : Bool? { return _get(.volumeSupportsSparseFilesKey) }
/// For security reasons, parts of a file (runs) that have never been written to must appear to contain zeroes. true if the volume keeps track of allocated but unwritten runs of a file so that it can substitute zeroes without actually writing zeroes to the media.
public var volumeSupportsZeroRuns : Bool? { return _get(.volumeSupportsZeroRunsKey) }
/// true if the volume format treats upper and lower case characters in file and directory names as different. Otherwise an upper case character is equivalent to a lower case character, and you can't have two names that differ solely in the case of the characters.
public var volumeSupportsCaseSensitiveNames : Bool? { return _get(.volumeSupportsCaseSensitiveNamesKey) }
/// true if the volume format preserves the case of file and directory names. Otherwise the volume may change the case of some characters (typically making them all upper or all lower case).
public var volumeSupportsCasePreservedNames : Bool? { return _get(.volumeSupportsCasePreservedNamesKey) }
/// true if the volume supports reliable storage of times for the root directory.
public var volumeSupportsRootDirectoryDates : Bool? { return _get(.volumeSupportsRootDirectoryDatesKey) }
/// true if the volume supports returning volume size values (`volumeTotalCapacity` and `volumeAvailableCapacity`).
public var volumeSupportsVolumeSizes : Bool? { return _get(.volumeSupportsVolumeSizesKey) }
/// true if the volume can be renamed.
public var volumeSupportsRenaming : Bool? { return _get(.volumeSupportsRenamingKey) }
/// true if the volume implements whole-file flock(2) style advisory locks, and the O_EXLOCK and O_SHLOCK flags of the open(2) call.
public var volumeSupportsAdvisoryFileLocking : Bool? { return _get(.volumeSupportsAdvisoryFileLockingKey) }
/// true if the volume implements extended security (ACLs).
public var volumeSupportsExtendedSecurity : Bool? { return _get(.volumeSupportsExtendedSecurityKey) }
/// true if the volume should be visible via the GUI (i.e., appear on the Desktop as a separate volume).
public var volumeIsBrowsable : Bool? { return _get(.volumeIsBrowsableKey) }
/// The largest file size (in bytes) supported by this file system, or nil if this cannot be determined.
public var volumeMaximumFileSize : Int? { return _get(.volumeMaximumFileSizeKey) }
/// true if the volume's media is ejectable from the drive mechanism under software control.
public var volumeIsEjectable : Bool? { return _get(.volumeIsEjectableKey) }
/// true if the volume's media is removable from the drive mechanism.
public var volumeIsRemovable : Bool? { return _get(.volumeIsRemovableKey) }
/// true if the volume's device is connected to an internal bus, false if connected to an external bus, or nil if not available.
public var volumeIsInternal : Bool? { return _get(.volumeIsInternalKey) }
/// true if the volume is automounted. Note: do not mistake this with the functionality provided by kCFURLVolumeSupportsBrowsingKey.
public var volumeIsAutomounted : Bool? { return _get(.volumeIsAutomountedKey) }
/// true if the volume is stored on a local device.
public var volumeIsLocal : Bool? { return _get(.volumeIsLocalKey) }
/// true if the volume is read-only.
public var volumeIsReadOnly : Bool? { return _get(.volumeIsReadOnlyKey) }
/// The volume's creation date, or nil if this cannot be determined.
public var volumeCreationDate : Date? { return _get(.volumeCreationDateKey) }
/// The `URL` needed to remount a network volume, or nil if not available.
public var volumeURLForRemounting : URL? { return _get(.volumeURLForRemountingKey) }
/// The volume's persistent `UUID` as a string, or nil if a persistent `UUID` is not available for the volume.
public var volumeUUIDString : String? { return _get(.volumeUUIDStringKey) }
/// The name of the volume
public var volumeName : String? {
get { return _get(.volumeNameKey) }
set { _set(.volumeNameKey, newValue: newValue) }
}
/// The user-presentable name of the volume
public var volumeLocalizedName : String? { return _get(.volumeLocalizedNameKey) }
/// true if the volume is encrypted.
@available(OSX 10.12, iOS 10.0, tvOS 10.0, watchOS 3.0, *)
public var volumeIsEncrypted : Bool? { return _get(.volumeIsEncryptedKey) }
/// true if the volume is the root filesystem.
@available(OSX 10.12, iOS 10.0, tvOS 10.0, watchOS 3.0, *)
public var volumeIsRootFileSystem : Bool? { return _get(.volumeIsRootFileSystemKey) }
/// true if the volume supports transparent decompression of compressed files using decmpfs.
@available(OSX 10.12, iOS 10.0, tvOS 10.0, watchOS 3.0, *)
public var volumeSupportsCompression : Bool? { return _get(.volumeSupportsCompressionKey) }
/// true if this item is synced to the cloud, false if it is only a local file.
public var isUbiquitousItem : Bool? { return _get(.isUbiquitousItemKey) }
/// true if this item has conflicts outstanding.
public var ubiquitousItemHasUnresolvedConflicts : Bool? { return _get(.ubiquitousItemHasUnresolvedConflictsKey) }
/// true if data is being downloaded for this item.
public var ubiquitousItemIsDownloading : Bool? { return _get(.ubiquitousItemIsDownloadingKey) }
/// true if there is data present in the cloud for this item.
public var ubiquitousItemIsUploaded : Bool? { return _get(.ubiquitousItemIsUploadedKey) }
/// true if data is being uploaded for this item.
public var ubiquitousItemIsUploading : Bool? { return _get(.ubiquitousItemIsUploadingKey) }
/// returns the download status of this item.
public var ubiquitousItemDownloadingStatus : URLUbiquitousItemDownloadingStatus? { return _get(.ubiquitousItemDownloadingStatusKey) }
/// returns the error when downloading the item from iCloud failed, see the NSUbiquitousFile section in FoundationErrors.h
public var ubiquitousItemDownloadingError : NSError? { return _get(.ubiquitousItemDownloadingErrorKey) }
/// returns the error when uploading the item to iCloud failed, see the NSUbiquitousFile section in FoundationErrors.h
public var ubiquitousItemUploadingError : NSError? { return _get(.ubiquitousItemUploadingErrorKey) }
/// returns whether a download of this item has already been requested with an API like `startDownloadingUbiquitousItem(at:) throws`.
@available(OSX 10.10, iOS 8.0, *)
public var ubiquitousItemDownloadRequested : Bool? { return _get(.ubiquitousItemDownloadRequestedKey) }
/// returns the name of this item's container as displayed to users.
@available(OSX 10.10, iOS 8.0, *)
public var ubiquitousItemContainerDisplayName : String? { return _get(.ubiquitousItemContainerDisplayNameKey) }
#if !os(OSX)
/// The protection level for this file
@available(iOS 9.0, *)
public var fileProtection : URLFileProtection? { return _get(.fileProtectionKey) }
#endif
/// Total file size in bytes
///
/// - note: Only applicable to regular files.
public var fileSize : Int? { return _get(.fileSizeKey) }
/// Total size allocated on disk for the file in bytes (number of blocks times block size)
///
/// - note: Only applicable to regular files.
public var fileAllocatedSize : Int? { return _get(.fileAllocatedSizeKey) }
/// Total displayable size of the file in bytes (this may include space used by metadata), or nil if not available.
///
/// - note: Only applicable to regular files.
public var totalFileSize : Int? { return _get(.totalFileSizeKey) }
/// Total allocated size of the file in bytes (this may include space used by metadata), or nil if not available. This can be less than the value returned by `totalFileSize` if the resource is compressed.
///
/// - note: Only applicable to regular files.
public var totalFileAllocatedSize : Int? { return _get(.totalFileAllocatedSizeKey) }
/// true if the resource is a Finder alias file or a symlink, false otherwise
///
/// - note: Only applicable to regular files.
public var isAliasFile : Bool? { return _get(.isAliasFileKey) }
}
/**
A URL is a type that can potentially contain the location of a resource on a remote server, the path of a local file on disk, or even an arbitrary piece of encoded data.
You can construct URLs and access their parts. For URLs that represent local files, you can also manipulate properties of those files directly, such as changing the file's last modification date. Finally, you can pass URLs to other APIs to retrieve the contents of those URLs. For example, you can use the URLSession classes to access the contents of remote resources, as described in URL Session Programming Guide.
URLs are the preferred way to refer to local files. Most objects that read data from or write data to a file have methods that accept a URL instead of a pathname as the file reference. For example, you can get the contents of a local file URL as `String` by calling `func init(contentsOf:encoding) throws`, or as a `Data` by calling `func init(contentsOf:options) throws`.
*/
public struct URL : ReferenceConvertible, Equatable {
public typealias ReferenceType = NSURL
fileprivate var _url : NSURL
public typealias BookmarkResolutionOptions = NSURL.BookmarkResolutionOptions
public typealias BookmarkCreationOptions = NSURL.BookmarkCreationOptions
/// Initialize with string.
///
/// Returns `nil` if a `URL` cannot be formed with the string (for example, if the string contains characters that are illegal in a URL, or is an empty string).
public init?(string: String) {
guard !string.isEmpty else { return nil }
if let inner = NSURL(string: string) {
_url = URL._converted(from: inner)
} else {
return nil
}
}
/// Initialize with string, relative to another URL.
///
/// Returns `nil` if a `URL` cannot be formed with the string (for example, if the string contains characters that are illegal in a URL, or is an empty string).
public init?(string: String, relativeTo url: URL?) {
guard !string.isEmpty else { return nil }
if let inner = NSURL(string: string, relativeTo: url) {
_url = URL._converted(from: inner)
} else {
return nil
}
}
/// Initializes a newly created file URL referencing the local file or directory at path, relative to a base URL.
///
/// If an empty string is used for the path, then the path is assumed to be ".".
/// - note: This function avoids an extra file system access to check if the file URL is a directory. You should use it if you know the answer already.
@available(OSX 10.11, iOS 9.0, *)
public init(fileURLWithPath path: String, isDirectory: Bool, relativeTo base: URL?) {
_url = URL._converted(from: NSURL(fileURLWithPath: path.isEmpty ? "." : path, isDirectory: isDirectory, relativeTo: base))
}
/// Initializes a newly created file URL referencing the local file or directory at path, relative to a base URL.
///
/// If an empty string is used for the path, then the path is assumed to be ".".
@available(OSX 10.11, iOS 9.0, *)
public init(fileURLWithPath path: String, relativeTo base: URL?) {
_url = URL._converted(from: NSURL(fileURLWithPath: path.isEmpty ? "." : path, relativeTo: base))
}
/// Initializes a newly created file URL referencing the local file or directory at path.
///
/// If an empty string is used for the path, then the path is assumed to be ".".
/// - note: This function avoids an extra file system access to check if the file URL is a directory. You should use it if you know the answer already.
public init(fileURLWithPath path: String, isDirectory: Bool) {
_url = URL._converted(from: NSURL(fileURLWithPath: path.isEmpty ? "." : path, isDirectory: isDirectory))
}
/// Initializes a newly created file URL referencing the local file or directory at path.
///
/// If an empty string is used for the path, then the path is assumed to be ".".
public init(fileURLWithPath path: String) {
_url = URL._converted(from: NSURL(fileURLWithPath: path.isEmpty ? "." : path))
}
/// Initializes a newly created URL using the contents of the given data, relative to a base URL.
///
/// If the data representation is not a legal URL string as ASCII bytes, the URL object may not behave as expected. If the URL cannot be formed then this will return nil.
@available(OSX 10.11, iOS 9.0, *)
public init?(dataRepresentation: Data, relativeTo url: URL?, isAbsolute: Bool = false) {
guard dataRepresentation.count > 0 else { return nil }
if isAbsolute {
_url = URL._converted(from: NSURL(absoluteURLWithDataRepresentation: dataRepresentation, relativeTo: url))
} else {
_url = URL._converted(from: NSURL(dataRepresentation: dataRepresentation, relativeTo: url))
}
}
/// Initializes a URL that refers to a location specified by resolving bookmark data.
public init?(resolvingBookmarkData data: Data, options: BookmarkResolutionOptions = [], relativeTo url: URL? = nil, bookmarkDataIsStale: inout Bool) throws {
var stale : ObjCBool = false
_url = URL._converted(from: try NSURL(resolvingBookmarkData: data, options: options, relativeTo: url, bookmarkDataIsStale: &stale))
bookmarkDataIsStale = stale.boolValue
}
/// Creates and initializes an NSURL that refers to the location specified by resolving the alias file at url. If the url argument does not refer to an alias file as defined by the NSURLIsAliasFileKey property, the NSURL returned is the same as url argument. This method fails and returns nil if the url argument is unreachable, or if the original file or directory could not be located or is not reachable, or if the original file or directory is on a volume that could not be located or mounted. The URLBookmarkResolutionWithSecurityScope option is not supported by this method.
@available(OSX 10.10, iOS 8.0, *)
public init(resolvingAliasFileAt url: URL, options: BookmarkResolutionOptions = []) throws {
self.init(reference: try NSURL(resolvingAliasFileAt: url, options: options))
}
/// Initializes a newly created URL referencing the local file or directory at the file system representation of the path. File system representation is a null-terminated C string with canonical UTF-8 encoding.
public init(fileURLWithFileSystemRepresentation path: UnsafePointer<Int8>, isDirectory: Bool, relativeTo baseURL: URL?) {
_url = URL._converted(from: NSURL(fileURLWithFileSystemRepresentation: path, isDirectory: isDirectory, relativeTo: baseURL))
}
public var hashValue: Int {
return _url.hash
}
// MARK: -
/// Returns the data representation of the URL's relativeString.
///
/// If the URL was initialized with `init?(dataRepresentation:relativeTo:isAbsolute:)`, the data representation returned are the same bytes as those used at initialization; otherwise, the data representation returned are the bytes of the `relativeString` encoded with UTF8 string encoding.
@available(OSX 10.11, iOS 9.0, *)
public var dataRepresentation: Data {
return _url.dataRepresentation
}
// MARK: -
// Future implementation note:
// NSURL (really CFURL, which provides its implementation) has quite a few quirks in its processing of some more esoteric (and some not so esoteric) strings. We would like to move much of this over to the more modern NSURLComponents, but binary compat concerns have made this difficult.
// Hopefully soon, we can replace some of the below delegation to NSURL with delegation to NSURLComponents instead. It cannot be done piecemeal, because otherwise we will get inconsistent results from the API.
/// Returns the absolute string for the URL.
public var absoluteString: String {
if let string = _url.absoluteString {
return string
} else {
// This should never fail for non-file reference URLs
return ""
}
}
/// The relative portion of a URL.
///
/// If `baseURL` is nil, or if the receiver is itself absolute, this is the same as `absoluteString`.
public var relativeString: String {
return _url.relativeString
}
/// Returns the base URL.
///
/// If the URL is itself absolute, then this value is nil.
public var baseURL: URL? {
return _url.baseURL
}
/// Returns the absolute URL.
///
/// If the URL is itself absolute, this will return self.
public var absoluteURL: URL {
if let url = _url.absoluteURL {
return url
} else {
// This should never fail for non-file reference URLs
return self
}
}
// MARK: -
/// Returns the scheme of the URL.
public var scheme: String? {
return _url.scheme
}
/// Returns true if the scheme is `file:`.
public var isFileURL: Bool {
return _url.isFileURL
}
// This thing was never really part of the URL specs
@available(*, unavailable, message: "Use `path`, `query`, and `fragment` instead")
public var resourceSpecifier: String {
fatalError()
}
/// If the URL conforms to RFC 1808 (the most common form of URL), returns the host component of the URL; otherwise it returns nil.
///
/// - note: This function will resolve against the base `URL`.
public var host: String? {
return _url.host
}
/// If the URL conforms to RFC 1808 (the most common form of URL), returns the port component of the URL; otherwise it returns nil.
///
/// - note: This function will resolve against the base `URL`.
public var port: Int? {
return _url.port?.intValue
}
/// If the URL conforms to RFC 1808 (the most common form of URL), returns the user component of the URL; otherwise it returns nil.
///
/// - note: This function will resolve against the base `URL`.
public var user: String? {
return _url.user
}
/// If the URL conforms to RFC 1808 (the most common form of URL), returns the password component of the URL; otherwise it returns nil.
///
/// - note: This function will resolve against the base `URL`.
public var password: String? {
return _url.password
}
/// If the URL conforms to RFC 1808 (the most common form of URL), returns the path component of the URL; otherwise it returns an empty string.
///
/// If the URL contains a parameter string, it is appended to the path with a `;`.
/// - note: This function will resolve against the base `URL`.
/// - returns: The path, or an empty string if the URL has an empty path.
public var path: String {
if let parameterString = _url.parameterString {
if let path = _url.path {
return path + ";" + parameterString
} else {
return ";" + parameterString
}
} else if let path = _url.path {
return path
} else {
return ""
}
}
/// If the URL conforms to RFC 1808 (the most common form of URL), returns the relative path of the URL; otherwise it returns nil.
///
/// This is the same as path if baseURL is nil.
/// If the URL contains a parameter string, it is appended to the path with a `;`.
///
/// - note: This function will resolve against the base `URL`.
/// - returns: The relative path, or an empty string if the URL has an empty path.
public var relativePath: String {
if let parameterString = _url.parameterString {
if let path = _url.relativePath {
return path + ";" + parameterString
} else {
return ";" + parameterString
}
} else if let path = _url.relativePath {
return path
} else {
return ""
}
}
/// If the URL conforms to RFC 1808 (the most common form of URL), returns the fragment component of the URL; otherwise it returns nil.
///
/// - note: This function will resolve against the base `URL`.
public var fragment: String? {
return _url.fragment
}
@available(*, unavailable, message: "use the 'path' property")
public var parameterString: String? {
fatalError()
}
/// If the URL conforms to RFC 1808 (the most common form of URL), returns the query of the URL; otherwise it returns nil.
///
/// - note: This function will resolve against the base `URL`.
public var query: String? {
return _url.query
}
/// Returns true if the URL path represents a directory.
@available(OSX 10.11, iOS 9.0, *)
public var hasDirectoryPath: Bool {
return _url.hasDirectoryPath
}
/// Passes the URL's path in file system representation to `block`.
///
/// File system representation is a null-terminated C string with canonical UTF-8 encoding.
/// - note: The pointer is not valid outside the context of the block.
@available(OSX 10.9, iOS 7.0, *)
public func withUnsafeFileSystemRepresentation<ResultType>(_ block: (UnsafePointer<Int8>?) throws -> ResultType) rethrows -> ResultType {
return try block(_url.fileSystemRepresentation)
}
// MARK: -
// MARK: Path manipulation
/// Returns the path components of the URL, or an empty array if the path is an empty string.
public var pathComponents: [String] {
// In accordance with our above change to never return a nil path, here we return an empty array.
return _url.pathComponents ?? []
}
/// Returns the last path component of the URL, or an empty string if the path is an empty string.
public var lastPathComponent: String {
return _url.lastPathComponent ?? ""
}
/// Returns the path extension of the URL, or an empty string if the path is an empty string.
public var pathExtension: String {
return _url.pathExtension ?? ""
}
/// Returns a URL constructed by appending the given path component to self.
///
/// - parameter pathComponent: The path component to add.
/// - parameter isDirectory: If `true`, then a trailing `/` is added to the resulting path.
public func appendingPathComponent(_ pathComponent: String, isDirectory: Bool) -> URL {
if let result = _url.appendingPathComponent(pathComponent, isDirectory: isDirectory) {
return result
} else {
// Now we need to do something more expensive
if var c = URLComponents(url: self, resolvingAgainstBaseURL: true) {
c.path = (c.path as NSString).appendingPathComponent(pathComponent)
if let result = c.url {
return result
} else {
// Couldn't get url from components
// Ultimate fallback:
return self
}
} else {
return self
}
}
}
/// Returns a URL constructed by appending the given path component to self.
///
/// - note: This function performs a file system operation to determine if the path component is a directory. If so, it will append a trailing `/`. If you know in advance that the path component is a directory or not, then use `func appendingPathComponent(_:isDirectory:)`.
/// - parameter pathComponent: The path component to add.
public func appendingPathComponent(_ pathComponent: String) -> URL {
if let result = _url.appendingPathComponent(pathComponent) {
return result
} else {
// Now we need to do something more expensive
if var c = URLComponents(url: self, resolvingAgainstBaseURL: true) {
c.path = (c.path as NSString).appendingPathComponent(pathComponent)
if let result = c.url {
return result
} else {
// Couldn't get url from components
// Ultimate fallback:
return self
}
} else {
// Ultimate fallback:
return self
}
}
}
/// Returns a URL constructed by removing the last path component of self.
///
/// This function may either remove a path component or append `/..`.
///
/// If the URL has an empty path (e.g., `http://www.example.com`), then this function will return the URL unchanged.
public func deletingLastPathComponent() -> URL {
// This is a slight behavior change from NSURL, but better than returning "http://www.example.com../".
if path.isEmpty {
return self
}
if let result = _url.deletingLastPathComponent.map({ URL(reference: $0 as NSURL) }) {
return result
} else {
return self
}
}
/// Returns a URL constructed by appending the given path extension to self.
///
/// If the URL has an empty path (e.g., `http://www.example.com`), then this function will return the URL unchanged.
///
/// Certain special characters (for example, Unicode Right-To-Left marks) cannot be used as path extensions. If any of those are contained in `pathExtension`, the function will return the URL unchanged.
/// - parameter pathExtension: The extension to append.
public func appendingPathExtension(_ pathExtension: String) -> URL {
if path.isEmpty {
return self
}
if let result = _url.appendingPathExtension(pathExtension) {
return result
} else {
return self
}
}
/// Returns a URL constructed by removing any path extension.
///
/// If the URL has an empty path (e.g., `http://www.example.com`), then this function will return the URL unchanged.
public func deletingPathExtension() -> URL {
if path.isEmpty {
return self
}
if let result = _url.deletingPathExtension.map({ URL(reference: $0 as NSURL) }) {
return result
} else {
return self
}
}
/// Appends a path component to the URL.
///
/// - parameter pathComponent: The path component to add.
/// - parameter isDirectory: Use `true` if the resulting path is a directory.
public mutating func appendPathComponent(_ pathComponent: String, isDirectory: Bool) {
self = appendingPathComponent(pathComponent, isDirectory: isDirectory)
}
/// Appends a path component to the URL.
///
/// - note: This function performs a file system operation to determine if the path component is a directory. If so, it will append a trailing `/`. If you know in advance that the path component is a directory or not, then use `func appendingPathComponent(_:isDirectory:)`.
/// - parameter pathComponent: The path component to add.
public mutating func appendPathComponent(_ pathComponent: String) {
self = appendingPathComponent(pathComponent)
}
/// Appends the given path extension to self.
///
/// If the URL has an empty path (e.g., `http://www.example.com`), then this function will do nothing.
/// Certain special characters (for example, Unicode Right-To-Left marks) cannot be used as path extensions. If any of those are contained in `pathExtension`, the function will return the URL unchanged.
/// - parameter pathExtension: The extension to append.
public mutating func appendPathExtension(_ pathExtension: String) {
self = appendingPathExtension(pathExtension)
}
/// Returns a URL constructed by removing the last path component of self.
///
/// This function may either remove a path component or append `/..`.
///
/// If the URL has an empty path (e.g., `http://www.example.com`), then this function will do nothing.
public mutating func deleteLastPathComponent() {
self = deletingLastPathComponent()
}
/// Returns a URL constructed by removing any path extension.
///
/// If the URL has an empty path (e.g., `http://www.example.com`), then this function will do nothing.
public mutating func deletePathExtension() {
self = deletingPathExtension()
}
/// Returns a `URL` with any instances of ".." or "." removed from its path.
public var standardized : URL {
// The NSURL API can only return nil in case of file reference URL, which we should not be
if let result = _url.standardized.map({ URL(reference: $0 as NSURL) }) {
return result
} else {
return self
}
}
/// Standardizes the path of a file URL.
///
/// If the `isFileURL` is false, this method does nothing.
public mutating func standardize() {
self = self.standardized
}
/// Standardizes the path of a file URL.
///
/// If the `isFileURL` is false, this method returns `self`.
public var standardizedFileURL : URL {
// NSURL should not return nil here unless this is a file reference URL, which should be impossible
if let result = _url.standardizingPath.map({ URL(reference: $0 as NSURL) }) {
return result
} else {
return self
}
}
/// Resolves any symlinks in the path of a file URL.
///
/// If the `isFileURL` is false, this method returns `self`.
public func resolvingSymlinksInPath() -> URL {
// NSURL should not return nil here unless this is a file reference URL, which should be impossible
if let result = _url.resolvingSymlinksInPath.map({ URL(reference: $0 as NSURL) }) {
return result
} else {
return self
}
}
/// Resolves any symlinks in the path of a file URL.
///
/// If the `isFileURL` is false, this method does nothing.
public mutating func resolveSymlinksInPath() {
self = self.resolvingSymlinksInPath()
}
// MARK: - Reachability
/// Returns whether the URL's resource exists and is reachable.
///
/// This method synchronously checks if the resource's backing store is reachable. Checking reachability is appropriate when making decisions that do not require other immediate operations on the resource, e.g. periodic maintenance of UI state that depends on the existence of a specific document. When performing operations such as opening a file or copying resource properties, it is more efficient to simply try the operation and handle failures. This method is currently applicable only to URLs for file system resources. For other URL types, `false` is returned.
public func checkResourceIsReachable() throws -> Bool {
var error : NSError?
let result = _url.checkResourceIsReachableAndReturnError(&error)
if let e = error {
throw e
} else {
return result
}
}
/// Returns whether the promised item URL's resource exists and is reachable.
///
/// This method synchronously checks if the resource's backing store is reachable. Checking reachability is appropriate when making decisions that do not require other immediate operations on the resource, e.g. periodic maintenance of UI state that depends on the existence of a specific document. When performing operations such as opening a file or copying resource properties, it is more efficient to simply try the operation and handle failures. This method is currently applicable only to URLs for file system resources. For other URL types, `false` is returned.
@available(OSX 10.10, iOS 8.0, *)
public func checkPromisedItemIsReachable() throws -> Bool {
var error : NSError?
let result = _url.checkPromisedItemIsReachableAndReturnError(&error)
if let e = error {
throw e
} else {
return result
}
}
// MARK: - Resource Values
/// Sets the resource value identified by a given resource key.
///
/// This method writes the new resource values out to the backing store. Attempts to set a read-only resource property or to set a resource property not supported by the resource are ignored and are not considered errors. This method is currently applicable only to URLs for file system resources.
///
/// `URLResourceValues` keeps track of which of its properties have been set. Those values are the ones used by this function to determine which properties to write.
public mutating func setResourceValues(_ values: URLResourceValues) throws {
try _url.setResourceValues(values._values)
}
/// Return a collection of resource values identified by the given resource keys.
///
/// This method first checks if the URL object already caches the resource value. If so, it returns the cached resource value to the caller. If not, then this method synchronously obtains the resource value from the backing store, adds the resource value to the URL object's cache, and returns the resource value to the caller. The type of the resource value varies by resource property (see resource key definitions). If this method does not throw and the resulting value in the `URLResourceValues` is populated with nil, it means the resource property is not available for the specified resource and no errors occurred when determining the resource property was not available. This method is currently applicable only to URLs for file system resources.
///
/// When this function is used from the main thread, resource values cached by the URL (except those added as temporary properties) are removed the next time the main thread's run loop runs. `func removeCachedResourceValue(forKey:)` and `func removeAllCachedResourceValues()` also may be used to remove cached resource values.
///
/// Only the values for the keys specified in `keys` will be populated.
public func resourceValues(forKeys keys: Set<URLResourceKey>) throws -> URLResourceValues {
return URLResourceValues(keys: keys, values: try _url.resourceValues(forKeys: Array(keys)))
}
/// Sets a temporary resource value on the URL object.
///
/// Temporary resource values are for client use. Temporary resource values exist only in memory and are never written to the resource's backing store. Once set, a temporary resource value can be copied from the URL object with `func resourceValues(forKeys:)`. The values are stored in the loosely-typed `allValues` dictionary property.
///
/// To remove a temporary resource value from the URL object, use `func removeCachedResourceValue(forKey:)`. Care should be taken to ensure the key that identifies a temporary resource value is unique and does not conflict with system defined keys (using reverse domain name notation in your temporary resource value keys is recommended). This method is currently applicable only to URLs for file system resources.
public mutating func setTemporaryResourceValue(_ value : Any, forKey key: URLResourceKey) {
_url.setTemporaryResourceValue(value, forKey: key)
}
/// Removes all cached resource values and all temporary resource values from the URL object.
///
/// This method is currently applicable only to URLs for file system resources.
public mutating func removeAllCachedResourceValues() {
_url.removeAllCachedResourceValues()
}
/// Removes the cached resource value identified by a given resource value key from the URL object.
///
/// Removing a cached resource value may remove other cached resource values because some resource values are cached as a set of values, and because some resource values depend on other resource values (temporary resource values have no dependencies). This method is currently applicable only to URLs for file system resources.
public mutating func removeCachedResourceValue(forKey key: URLResourceKey) {
_url.removeCachedResourceValue(forKey: key)
}
/// Get resource values from URLs of 'promised' items.
///
/// A promised item is not guaranteed to have its contents in the file system until you use `FileCoordinator` to perform a coordinated read on its URL, which causes the contents to be downloaded or otherwise generated. Promised item URLs are returned by various APIs, including currently:
/// NSMetadataQueryUbiquitousDataScope
/// NSMetadataQueryUbiquitousDocumentsScope
/// A `FilePresenter` presenting the contents of the directory located by -URLForUbiquitousContainerIdentifier: or a subdirectory thereof
///
/// The following methods behave identically to their similarly named methods above (`func resourceValues(forKeys:)`, etc.), except that they allow you to get resource values and check for presence regardless of whether the promised item's contents currently exist at the URL. You must use these APIs instead of the normal URL resource value APIs if and only if any of the following are true:
/// You are using a URL that you know came directly from one of the above APIs
/// You are inside the accessor block of a coordinated read or write that used NSFileCoordinatorReadingImmediatelyAvailableMetadataOnly, NSFileCoordinatorWritingForDeleting, NSFileCoordinatorWritingForMoving, or NSFileCoordinatorWritingContentIndependentMetadataOnly
///
/// Most of the URL resource value keys will work with these APIs. However, there are some that are tied to the item's contents that will not work, such as `contentAccessDateKey` or `generationIdentifierKey`. If one of these keys is used, the method will return a `URLResourceValues` value, but the value for that property will be nil.
@available(OSX 10.10, iOS 8.0, *)
public func promisedItemResourceValues(forKeys keys: Set<URLResourceKey>) throws -> URLResourceValues {
return URLResourceValues(keys: keys, values: try _url.promisedItemResourceValues(forKeys: Array(keys)))
}
@available(*, unavailable, message: "Use struct URLResourceValues and URL.setResourceValues(_:) instead")
public func setResourceValue(_ value: AnyObject?, forKey key: URLResourceKey) throws {
fatalError()
}
@available(*, unavailable, message: "Use struct URLResourceValues and URL.setResourceValues(_:) instead")
public func setResourceValues(_ keyedValues: [URLResourceKey : AnyObject]) throws {
fatalError()
}
@available(*, unavailable, message: "Use struct URLResourceValues and URL.setResourceValues(_:) instead")
public func getResourceValue(_ value: AutoreleasingUnsafeMutablePointer<AnyObject?>, forKey key: URLResourceKey) throws {
fatalError()
}
// MARK: - Bookmarks and Alias Files
/// Returns bookmark data for the URL, created with specified options and resource values.
public func bookmarkData(options: BookmarkCreationOptions = [], includingResourceValuesForKeys keys: Set<URLResourceKey>? = nil, relativeTo url: URL? = nil) throws -> Data {
let result = try _url.bookmarkData(options: options, includingResourceValuesForKeys: keys.flatMap { Array($0) }, relativeTo: url)
return result as Data
}
/// Returns the resource values for properties identified by a specified array of keys contained in specified bookmark data. If the result dictionary does not contain a resource value for one or more of the requested resource keys, it means those resource properties are not available in the bookmark data.
public static func resourceValues(forKeys keys: Set<URLResourceKey>, fromBookmarkData data: Data) -> URLResourceValues? {
return NSURL.resourceValues(forKeys: Array(keys), fromBookmarkData: data).map { URLResourceValues(keys: keys, values: $0) }
}
/// Creates an alias file on disk at a specified location with specified bookmark data. bookmarkData must have been created with the URLBookmarkCreationSuitableForBookmarkFile option. bookmarkFileURL must either refer to an existing file (which will be overwritten), or to location in an existing directory.
public static func writeBookmarkData(_ data : Data, to url: URL) throws {
// Options are unused
try NSURL.writeBookmarkData(data, to: url, options: 0)
}
/// Initializes and returns bookmark data derived from an alias file pointed to by a specified URL. If bookmarkFileURL refers to an alias file created prior to OS X v10.6 that contains Alias Manager information but no bookmark data, this method synthesizes bookmark data for the file.
public static func bookmarkData(withContentsOf url: URL) throws -> Data {
let result = try NSURL.bookmarkData(withContentsOf: url)
return result as Data
}
/// Given an NSURL created by resolving a bookmark data created with security scope, make the resource referenced by the url accessible to the process. When access to this resource is no longer needed the client must call stopAccessingSecurityScopedResource. Each call to startAccessingSecurityScopedResource must be balanced with a call to stopAccessingSecurityScopedResource (Note: this is not reference counted).
@available(OSX 10.7, iOS 8.0, *)
public func startAccessingSecurityScopedResource() -> Bool {
return _url.startAccessingSecurityScopedResource()
}
/// Revokes the access granted to the url by a prior successful call to startAccessingSecurityScopedResource.
@available(OSX 10.7, iOS 8.0, *)
public func stopAccessingSecurityScopedResource() {
_url.stopAccessingSecurityScopedResource()
}
// MARK: - Bridging Support
/// We must not store an NSURL without running it through this function. This makes sure that we do not hold a file reference URL, which changes the nullability of many NSURL functions.
private static func _converted(from url: NSURL) -> NSURL {
// Future readers: file reference URL here is not the same as playgrounds "file reference"
if url.isFileReferenceURL() {
// Convert to a file path URL, or use an invalid scheme
return (url.filePathURL ?? URL(string: "com-apple-unresolvable-file-reference-url:")!) as NSURL
} else {
return url
}
}
fileprivate init(reference: NSURL) {
_url = URL._converted(from: reference).copy() as! NSURL
}
private var reference : NSURL {
return _url
}
public static func ==(lhs: URL, rhs: URL) -> Bool {
return lhs.reference.isEqual(rhs.reference)
}
}
extension URL : _ObjectiveCBridgeable {
@_semantics("convertToObjectiveC")
public func _bridgeToObjectiveC() -> NSURL {
return _url
}
public static func _forceBridgeFromObjectiveC(_ source: NSURL, result: inout URL?) {
if !_conditionallyBridgeFromObjectiveC(source, result: &result) {
fatalError("Unable to bridge \(_ObjectiveCType.self) to \(self)")
}
}
public static func _conditionallyBridgeFromObjectiveC(_ source: NSURL, result: inout URL?) -> Bool {
result = URL(reference: source)
return true
}
public static func _unconditionallyBridgeFromObjectiveC(_ source: NSURL?) -> URL {
var result: URL?
_forceBridgeFromObjectiveC(source!, result: &result)
return result!
}
}
extension URL : CustomStringConvertible, CustomDebugStringConvertible {
public var description: String {
return _url.description
}
public var debugDescription: String {
return _url.debugDescription
}
}
extension NSURL : _HasCustomAnyHashableRepresentation {
// Must be @nonobjc to avoid infinite recursion during bridging.
@nonobjc
public func _toCustomAnyHashable() -> AnyHashable? {
return AnyHashable(self as URL)
}
}
extension URL : CustomPlaygroundQuickLookable {
public var customPlaygroundQuickLook: PlaygroundQuickLook {
return .url(absoluteString)
}
}
//===----------------------------------------------------------------------===//
// File references, for playgrounds.
//===----------------------------------------------------------------------===//
extension URL : _ExpressibleByFileReferenceLiteral {
public init(fileReferenceLiteralResourceName name: String) {
self = Bundle.main.url(forResource: name, withExtension: nil)!
}
}
public typealias _FileReferenceLiteralType = URL
| apache-2.0 | d77bb5c2da3a2d001ae347846871aaa6 | 50.636741 | 765 | 0.681563 | 4.991081 | false | false | false | false |
aliceatlas/daybreak | Classes/SBWindowController.swift | 1 | 2301 | /*
SBWindowController.swift
Copyright (c) 2014, Alice Atlas
Copyright (c) 2010, Atsushi Jike
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
1. Redistributions of source code must retain the above copyright notice, this
list of conditions and the following disclaimer.
2. Redistributions in binary form must reproduce the above copyright notice,
this list of conditions and the following disclaimer in the documentation
and/or other materials provided with the distribution.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR
ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
class SBWindowController: NSWindowController {
var viewSize: NSSize
init(viewSize inViewSize: NSSize) {
var frameRect = NSZeroRect
var window: NSWindow?
let screen = NSScreen.screens()![0]
let visibleRect = screen.visibleFrame
viewSize = inViewSize
frameRect.size.width = viewSize.width + 20 * 2
frameRect.size.height = viewSize.height < visibleRect.size.height ? viewSize.height : visibleRect.size.height
frameRect.origin.y = visibleRect.origin.y + (visibleRect.size.height - frameRect.size.height)
frameRect.origin.x = visibleRect.origin.x
window = NSWindow(contentRect: frameRect, styleMask: (NSTitledWindowMask | NSClosableWindowMask | NSResizableWindowMask), backing: .Buffered, defer: true)
super.init(window: window!)
window!.center()
}
required init(coder: NSCoder) {
fatalError("NSCoding not supported")
}
} | bsd-2-clause | 2709085d4252cfc88e636c95f8db561e | 45.04 | 162 | 0.757931 | 4.754132 | false | false | false | false |
buyiyang/iosstar | iOSStar/Scenes/Discover/Controllers/VoiceHistoryVC.swift | 3 | 8049 | //
// VoiceHistoryVC.swift
// iOSStar
//
// Created by mu on 2017/8/16.
// Copyright © 2017年 YunDian. All rights reserved.
//
import UIKit
import SVProgressHUD
class VoiceHistoryCell: OEZTableViewCell {
@IBOutlet weak var voiceBtn: UIButton!
@IBOutlet weak var voiceIcon: UIImageView!
@IBOutlet weak var voiceCountLabel: UILabel!
@IBOutlet var title: UILabel!
@IBOutlet weak var contentLabel: UILabel!
@IBOutlet weak var nameLab: UILabel!
@IBOutlet var status: UILabel!
@IBOutlet var timeLabel: UILabel!
@IBOutlet var headImg: UIImageView!
@IBOutlet var voiceImg: UIImageView!
override func awakeFromNib() {
}
func voiceDidClick(_ sender : UIButton){
didSelectRowAction(3, data: nil)
}
override func update(_ data: Any!) {
if let response = data as? UserAskDetailList{
contentLabel.text = response.uask
voiceBtn.addTarget(self, action: #selector(voiceDidClick(_:)), for: .touchUpInside)
timeLabel.text = Date.yt_convertDateStrWithTimestempWithSecond(Int(response.ask_t), format: "YYYY-MM-dd")
let titleTip = response.answer_t == 0 ? "点击播放(未回复)" : "点击播放"
let attr = NSMutableAttributedString.init(string: titleTip)
title.attributedText = attr
voiceCountLabel.text = "听过\(response.s_total)"
if !response.isplay{
voiceImg.image = UIImage.init(named: String.init(format: "listion"))
}
if response.isall == 1{
if response.answer_t == 0{
status.text = "明星未回复"
}else{
status.text = "已定制"
}
StartModel.getStartName(startCode: response.starcode) { [weak self](response) in
if let star = response as? StartModel {
self?.headImg.kf.setImage(with: URL(string:ShareDataModel.share().qiniuHeader + star.pic_url_tail))
self?.nameLab.text = star.name
}
}
}
}
}
}
class VoiceHistoryVC: BasePageListTableViewController ,OEZTableViewDelegate,PLPlayerDelegate{
@IBOutlet weak var titlesView: UIView!
@IBOutlet weak var closeButton: UIButton!
@IBOutlet weak var openButton: UIButton!
var selectRow = 99999999
var voiceimg: UIImageView!
var time = 1
var isplayIng = false
var type = true
var starModel: StarSortListModel = StarSortListModel()
private var selectedButton: UIButton?
override func viewDidLoad() {
super.viewDidLoad()
title = "历史定制"
tableView.rowHeight = UITableViewAutomaticDimension
tableView.estimatedRowHeight = 200
titleViewButtonAction(openButton)
}
@IBAction func titleViewButtonAction(_ sender: UIButton) {
self.selectedButton?.isSelected = false
if voiceimg != nil{
PLPlayerHelper.shared().doChanggeStatus(4)
self.voiceimg.image = UIImage.init(named: String.init(format: "listion"))
}
if PLPlayerHelper.shared().player.isPlaying{
PLPlayerHelper.shared().player.stop()
}
self.selectedButton?.backgroundColor = UIColor.clear
sender.backgroundColor = UIColor(hexString: "ffffff")
self.selectedButton = sender
if self.dataSource?.count != 0{
self.dataSource?.removeAll()
}
if selectedButton == closeButton{
type = false
}else{
type = true
}
self.didRequest(1)
}
override func didRequest(_ pageIndex: Int) {
let model = UserAskRequestModel()
model.aType = 2
model.starcode = starModel.symbol
model.pos = pageIndex == 1 ? 1 : dataSource?.count ?? 0
model.pType = type ? 1 : 0
AppAPIHelper.discoverAPI().useraskQuestion(requestModel: model, complete: { [weak self](result) in
if let response = result as? UserAskList {
for model in response.circle_list!{
model.calculateCellHeight()
}
self?.didRequestComplete(response.circle_list as AnyObject )
self?.tableView.reloadData()
}
}) { (error) in
self.didRequestComplete(nil )
}
}
override func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
if let model = dataSource?[indexPath.row] as? UserAskDetailList{
return model.cellHeight
}
return 200
}
func tableView(_ tableView: UITableView!, rowAt indexPath: IndexPath!, didAction action: Int, data: Any!)
{
if voiceimg != nil{
self.voiceimg.image = UIImage.init(named: String.init(format: "listion"))
}
if PLPlayerHelper.shared().player.isPlaying{
PLPlayerHelper.shared().player.stop()
}
if let cell = tableView.cellForRow(at: indexPath) as? VoiceHistoryCell{
voiceimg = cell.voiceImg
}
if let model = self.dataSource?[indexPath.row] as? UserAskDetailList{
if model.answer_t == 0{
SVProgressHUD.showErrorMessage(ErrorMessage: "明星未回复", ForDuration: 2, completion: nil)
return
}
let url = URL(string: ShareDataModel.share().qiniuHeader + model.sanswer)
model.isplay = true
PLPlayerHelper.shared().player.play(with: url)
PLPlayerHelper.shared().resultBlock = { [weak self] (result) in
if let status = result as? PLPlayerStatus{
if status == .statusStopped{
if let arr = self?.dataSource?[0] as? Array<AnyObject>{
if let model = arr[(self?.selectRow)!] as? UserAskDetailList{
model.isplay = false
}
}
PLPlayerHelper.shared().doChanggeStatus(4)
self?.voiceimg.image = UIImage.init(named: String.init(format: "listion"))
}
if status == .statusPaused{
if let arr = self?.dataSource?[0] as? Array<AnyObject>{
if let model = arr[(self?.selectRow)!] as? UserAskDetailList{
model.isplay = false
}
}
PLPlayerHelper.shared().doChanggeStatus(4)
self?.voiceimg.image = UIImage.init(named: String.init(format: "listion"))
}
if status == .statusPreparing{
PLPlayerHelper.shared().doChanggeStatus(0)
PLPlayerHelper.shared().resultCountDown = {[weak self] (result) in
if let response = result as? Int{
self?.voiceimg.image = UIImage.init(named: String.init(format: "voice_%d",response))
}
}
}
if status == .statusError{
PLPlayerHelper.shared().doChanggeStatus(4)
self?.voiceimg.image = UIImage.init(named: String.init(format: "listion"))
}
}
}
}
}
override func tableView(_ tableView: UITableView, cellIdentifierForRowAtIndexPath indexPath: IndexPath) -> String? {
let model = dataSource?[indexPath.row] as! UserAskDetailList
if model.isall == 1{
return "VoiceALLCell"
}
return VoiceQuestionCell.className()
}
}
| gpl-3.0 | b56ab8b19efa9c7bfb90651682c241e0 | 36.650943 | 123 | 0.54673 | 4.933251 | false | false | false | false |
shinobicontrols/iOS8-LevellingUp | LevellingUp/HorseRaceController.swift | 1 | 2569 | //
// Copyright 2014 Scott Logic
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
import Foundation
import UIKit
public struct Horse {
public var horseView: UIView
var startConstraint: NSLayoutConstraint
var finishConstraint: NSLayoutConstraint?
init(horseView: UIView, startConstraint: NSLayoutConstraint, finishLineOffset:CGFloat) {
self.horseView = horseView
self.startConstraint = startConstraint
self.finishConstraint = NSLayoutConstraint(item: horseView, attribute: .Right, relatedBy: .Equal, toItem: horseView.superview, attribute: .Right, multiplier: 1, constant: finishLineOffset)
}
}
public class TwoHorseRaceController {
var horses: [Horse]
init(horses: [Horse]) {
self.horses = horses
srand48(time(nil));
}
public func reset() {
for horse in horses {
updateContraintsToStartOfRace(horse)
horse.horseView.layoutIfNeeded()
}
}
public func startRace(maxDuration: NSTimeInterval, horseCrossedLineCallback: ((Horse) -> Void)?) {
for horse in horses {
// Generate a random time
let duration = maxDuration / 2.0 * (1 + drand48())
// Perform the animation
UIView.animateWithDuration(duration, delay: 0, options: .CurveEaseIn,
animations: {
self.updateConstraintsToEndOfRace(horse)
horse.horseView.layoutIfNeeded()
}, completion: { _ in
if let callback = horseCrossedLineCallback {
callback(horse)
}
})
}
}
func updateConstraintsToEndOfRace(horse: Horse) {
horse.startConstraint.active = false
horse.finishConstraint?.active = true
}
func updateContraintsToStartOfRace(horse: Horse) {
horse.finishConstraint?.active = false
horse.startConstraint.active = true
}
public func someKindOfAsyncMethod(completionHandler: () -> ()) {
dispatch_async(dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0), {
sleep(3)
dispatch_async(dispatch_get_main_queue(), {
completionHandler()})
})
}
}
| apache-2.0 | 8938a19bc04e043604b392c33b1c7b2e | 28.872093 | 192 | 0.693655 | 4.303183 | false | false | false | false |
AnRanScheme/magiGlobe | magi/magiGlobe/Pods/RxCocoa/RxCocoa/Traits/SharedSequence/SharedSequence.swift | 61 | 8307 | //
// SharedSequence.swift
// RxCocoa
//
// Created by Krunoslav Zaher on 8/27/15.
// Copyright © 2015 Krunoslav Zaher. All rights reserved.
//
#if !RX_NO_MODULE
import RxSwift
#endif
/**
Trait that represents observable sequence that shares computation resources with following properties:
- it never fails
- it delivers events on `SharingStrategy.scheduler`
- sharing strategy is customizable using `SharingStrategy.share` behavior
`SharedSequence<Element>` can be considered a builder pattern for observable sequences that share computation resources.
To find out more about units and how to use them, please visit `Documentation/Traits.md`.
*/
public struct SharedSequence<S: SharingStrategyProtocol, Element> : SharedSequenceConvertibleType {
public typealias E = Element
public typealias SharingStrategy = S
let _source: Observable<E>
init(_ source: Observable<E>) {
self._source = S.share(source)
}
init(raw: Observable<E>) {
self._source = raw
}
#if EXPANDABLE_SHARED_SEQUENCE
/**
This method is extension hook in case this unit needs to extended from outside the library.
By defining `EXPANDABLE_SHARED_SEQUENCE` one agrees that it's up to him to ensure shared sequence
properties are preserved after extension.
*/
public static func createUnsafe<O: ObservableType>(source: O) -> SharedSequence<S, O.E> {
return SharedSequence<S, O.E>(raw: source.asObservable())
}
#endif
/**
- returns: Built observable sequence.
*/
public func asObservable() -> Observable<E> {
return _source
}
/**
- returns: `self`
*/
public func asSharedSequence() -> SharedSequence<SharingStrategy, E> {
return self
}
}
/**
Different `SharedSequence` sharing strategies must conform to this protocol.
*/
public protocol SharingStrategyProtocol {
/**
Scheduled on which all sequence events will be delivered.
*/
static var scheduler: SchedulerType { get }
/**
Computation resources sharing strategy for multiple sequence observers.
E.g. One can choose `shareReplayWhenConnected`, `shareReplay` or `share`
as sequence event sharing strategies, but also do something more exotic, like
implementing promises or lazy loading chains.
*/
static func share<E>(_ source: Observable<E>) -> Observable<E>
}
/**
A type that can be converted to `SharedSequence`.
*/
public protocol SharedSequenceConvertibleType : ObservableConvertibleType {
associatedtype SharingStrategy: SharingStrategyProtocol
/**
Converts self to `SharedSequence`.
*/
func asSharedSequence() -> SharedSequence<SharingStrategy, E>
}
extension SharedSequenceConvertibleType {
public func asObservable() -> Observable<E> {
return asSharedSequence().asObservable()
}
}
extension SharedSequence {
/**
Returns an empty observable sequence, using the specified scheduler to send out the single `Completed` message.
- returns: An observable sequence with no elements.
*/
public static func empty() -> SharedSequence<S, E> {
return SharedSequence(raw: Observable.empty().subscribeOn(S.scheduler))
}
/**
Returns a non-terminating observable sequence, which can be used to denote an infinite duration.
- returns: An observable sequence whose observers will never get called.
*/
public static func never() -> SharedSequence<S, E> {
return SharedSequence(raw: Observable.never())
}
/**
Returns an observable sequence that contains a single element.
- parameter element: Single element in the resulting observable sequence.
- returns: An observable sequence containing the single specified element.
*/
public static func just(_ element: E) -> SharedSequence<S, E> {
return SharedSequence(raw: Observable.just(element).subscribeOn(S.scheduler))
}
/**
Returns an observable sequence that invokes the specified factory function whenever a new observer subscribes.
- parameter observableFactory: Observable factory function to invoke for each observer that subscribes to the resulting sequence.
- returns: An observable sequence whose observers trigger an invocation of the given observable factory function.
*/
public static func deferred(_ observableFactory: @escaping () -> SharedSequence<S, E>)
-> SharedSequence<S, E> {
return SharedSequence(Observable.deferred { observableFactory().asObservable() })
}
/**
This method creates a new Observable instance with a variable number of elements.
- seealso: [from operator on reactivex.io](http://reactivex.io/documentation/operators/from.html)
- parameter elements: Elements to generate.
- returns: The observable sequence whose elements are pulled from the given arguments.
*/
public static func of(_ elements: E ...) -> SharedSequence<S, E> {
let source = Observable.from(elements, scheduler: S.scheduler)
return SharedSequence(raw: source)
}
}
extension SharedSequence {
/**
This method converts an array to an observable sequence.
- seealso: [from operator on reactivex.io](http://reactivex.io/documentation/operators/from.html)
- returns: The observable sequence whose elements are pulled from the given enumerable sequence.
*/
public static func from(_ array: [E]) -> SharedSequence<S, E> {
let source = Observable.from(array, scheduler: S.scheduler)
return SharedSequence(raw: source)
}
/**
This method converts a sequence to an observable sequence.
- seealso: [from operator on reactivex.io](http://reactivex.io/documentation/operators/from.html)
- returns: The observable sequence whose elements are pulled from the given enumerable sequence.
*/
public static func from<S: Sequence>(_ sequence: S) -> SharedSequence<SharingStrategy, E> where S.Iterator.Element == E {
let source = Observable.from(sequence, scheduler: SharingStrategy.scheduler)
return SharedSequence(raw: source)
}
/**
This method converts a optional to an observable sequence.
- seealso: [from operator on reactivex.io](http://reactivex.io/documentation/operators/from.html)
- parameter optional: Optional element in the resulting observable sequence.
- returns: An observable sequence containing the wrapped value or not from given optional.
*/
public static func from(optional: E?) -> SharedSequence<S, E> {
let source = Observable.from(optional: optional, scheduler: S.scheduler)
return SharedSequence(raw: source)
}
}
extension SharedSequence where Element : SignedInteger {
/**
Returns an observable sequence that produces a value after each period, using the specified scheduler to run timers and to send out observer messages.
- seealso: [interval operator on reactivex.io](http://reactivex.io/documentation/operators/interval.html)
- parameter period: Period for producing the values in the resulting sequence.
- returns: An observable sequence that produces a value after each period.
*/
public static func interval(_ period: RxTimeInterval)
-> SharedSequence<S, E> {
return SharedSequence(Observable.interval(period, scheduler: S.scheduler))
}
}
// MARK: timer
extension SharedSequence where Element: SignedInteger {
/**
Returns an observable sequence that periodically produces a value after the specified initial relative due time has elapsed, using the specified scheduler to run timers.
- seealso: [timer operator on reactivex.io](http://reactivex.io/documentation/operators/timer.html)
- parameter dueTime: Relative time at which to produce the first value.
- parameter period: Period to produce subsequent values.
- returns: An observable sequence that produces a value after due time has elapsed and then each period.
*/
public static func timer(_ dueTime: RxTimeInterval, period: RxTimeInterval)
-> SharedSequence<S, E> {
return SharedSequence(Observable.timer(dueTime, period: period, scheduler: S.scheduler))
}
}
| mit | 29f6fdcf671c847fe7497bdd5f3805c8 | 34.95671 | 174 | 0.703347 | 4.955847 | false | false | false | false |
Vluxe/Swift-API-Project | Client/Client/NewGuitarController.swift | 1 | 2363 | //
// NewGuitarController.swift
// Client
//
// Created by Dalton Cherry on 11/4/14.
// Copyright (c) 2014 vluxe. All rights reserved.
//
import UIKit
protocol NewDelegate {
func didCreateGuitar(guitar: Guitar)
}
class NewGuitarController: UIViewController {
var delegate: NewDelegate?
let colors = ["Black", "Red", "Blue", "Green", "White"]
@IBOutlet weak var nameTextField: UITextField!
@IBOutlet weak var brandTextField: UITextField!
@IBOutlet weak var yearTextField: UITextField!
@IBOutlet weak var priceTextField: UITextField!
@IBOutlet weak var saveButton: UIBarButtonItem!
@IBOutlet weak var pickerWheel: UIPickerView!
//cancel creating a new guitar
@IBAction func CloseModal(sender: UIBarButtonItem) {
self.dismissViewControllerAnimated(true, completion: nil)
}
//save the guitar
@IBAction func Save(sender: UIBarButtonItem) {
let color = colors[pickerWheel.selectedRowInComponent(0)]
var price = 200
if let p = priceTextField.text.toInt() {
price = p
}
let guitar = Guitar(name: nameTextField.text, brand: brandTextField.text, year: yearTextField.text, price: price, color: color, imageUrl: "")
self.delegate?.didCreateGuitar(guitar)
self.dismissViewControllerAnimated(true, completion: nil)
}
//MARK - Picker Wheel Delegate
func textFieldShouldReturn(textField: UITextField) -> Bool {
textField.resignFirstResponder()
if textField.tag != 3 {
let tag = textField.tag+1
let txtField = self.view.viewWithTag(tag) as? UITextField
if let field = txtField {
field.becomeFirstResponder()
saveButton.enabled = true
}
}
return true
}
//MARK - Picker Wheel Delegate
func numberOfComponentsInPickerView(pickerView: UIPickerView) -> Int {
return 1
}
func pickerView(pickerView: UIPickerView, numberOfRowsInComponent component: Int) -> Int {
return colors.count
}
func pickerView(pickerView: UIPickerView,rowHeightForComponent component: Int) -> CGFloat {
return 30
}
func pickerView(pickerView: UIPickerView, titleForRow row: Int, forComponent component: Int) -> String! {
return colors[row]
}
}
| apache-2.0 | 65f80ab8dbb32249c76e13818c7d63e1 | 32.28169 | 149 | 0.657215 | 4.852156 | false | false | false | false |
etiennemartin/jugglez | Jugglez/GameScores.swift | 1 | 10026 | //
// GameScores.swift
// Jugglez
//
// Created by Etienne Martin on 2015-01-22.
// Copyright (c) 2015 Etienne Martin. All rights reserved.
//
import Foundation
import GameKit
// NSCoding keys
private let kEasyHighScoreKey = "easy_high_score_key"
private let kMediumHighScoreKey = "medium_high_score_key"
private let kHardHighScoreKey = "hard_high_score_key"
private let kExpertHighScoreKey = "expert_high_score_key"
private let kGameScoreFileName = "gameScores"
// Game Center Leaderboard Ids
private let kEasyModeLeaderboardId = "com.jugglez.mode.easy"
private let kMediumModeLeaderboardId = "com.jugglez.mode.medium"
private let kHardModeLeaderboardId = "com.jugglez.mode.hard"
private let kExpertModeLeaderboardId = "com.jugglez.mode.expert"
public class GameScores: NSObject, NSCoding, GameCenterManagerDelegate {
// MARK: Singleton access
public class var sharedInstance: GameScores {
struct InstanceStruct {
static var instanceToken: dispatch_once_t = 0
static var instance: GameScores? = nil
}
// Load the data from file at start up.
dispatch_once(&InstanceStruct.instanceToken) {
GameCenterManager.sharedInstance.authenticateLocalUser()
let filePath = GameScores.filePath()
let data = try? NSData(contentsOfFile: filePath, options: NSDataReadingOptions.DataReadingMappedIfSafe)
if data != nil {
print("Loading high scores from archive...")
InstanceStruct.instance = NSKeyedUnarchiver.unarchiveObjectWithData(data!) as! GameScores?
} else {
print("Creating new high scores...")
InstanceStruct.instance = GameScores()
}
GameCenterManager.sharedInstance.delegate = InstanceStruct.instance
}
return InstanceStruct.instance!
}
// MARK: Class impl
override init() {
super.init()
}
public required init(coder aDecoder: NSCoder) {
super.init()
// Load from Local Archive
_easyHighScore = aDecoder.decodeInt64ForKey(kEasyHighScoreKey)
_mediumHighScore = aDecoder.decodeInt64ForKey(kMediumHighScoreKey)
_hardHighScore = aDecoder.decodeInt64ForKey(kHardHighScoreKey)
_expertHighScore = aDecoder.decodeInt64ForKey(kExpertHighScoreKey)
}
public func encodeWithCoder(coder: NSCoder) {
coder.encodeInt64(easyHighScore, forKey: kEasyHighScoreKey)
coder.encodeInt64(mediumHighScore, forKey: kMediumHighScoreKey)
coder.encodeInt64(hardHighScore, forKey: kHardHighScoreKey)
coder.encodeInt64(expertHighScore, forKey: kExpertHighScoreKey)
}
// Set the high score for a mode if it's higher than the current one
public func setScoreForMode(mode: GameMode, score: Int64) {
switch mode {
case GameMode.Easy:
easyHighScore = max(easyHighScore, score)
break
case GameMode.Medium:
mediumHighScore = max(mediumHighScore, score)
break
case GameMode.Hard:
hardHighScore = max(hardHighScore, score)
break
case GameMode.Expert:
expertHighScore = max(expertHighScore, score)
break
default:
break
}
}
public func getScoreForMode(mode: GameMode) -> Int64 {
switch mode {
case GameMode.Easy:
return easyHighScore
case GameMode.Medium:
return mediumHighScore
case GameMode.Hard:
return hardHighScore
case GameMode.Expert:
return expertHighScore
default:
return 0
}
}
public func isRecordNewForMode(mode: GameMode) -> Bool {
switch mode {
case GameMode.Easy:
return _newEasyScore
case GameMode.Medium:
return _newMediumScore
case GameMode.Hard:
return _newHardScore
case GameMode.Expert:
return _newExpertScore
default:
return false
}
}
// High Scores
public var easyHighScore: Int64 {
get {
return _easyHighScore
}
set(value) {
if _easyHighScore < value {
_newEasyScore = true
reportGameCenterScore(value, identifier: kEasyModeLeaderboardId)
}
_easyHighScore = value
}
}
public var mediumHighScore: Int64 {
get {
return _mediumHighScore
}
set(value) {
if _mediumHighScore < value {
_newMediumScore = true
reportGameCenterScore(value, identifier: kMediumModeLeaderboardId)
}
_mediumHighScore = value
}
}
public var hardHighScore: Int64 {
get {
return _hardHighScore
}
set (value) {
if _hardHighScore < value {
_newHardScore = true
reportGameCenterScore(value, identifier: kHardModeLeaderboardId)
}
_hardHighScore = value
}
}
public var expertHighScore: Int64 {
get {
return _expertHighScore
}
set (value) {
if _expertHighScore < value {
_newExpertScore = true
reportGameCenterScore(value, identifier: kExpertModeLeaderboardId)
}
_expertHighScore = value
}
}
public func clearHighScoreFlags() {
_newEasyScore = false
_newMediumScore = false
_newHardScore = false
_newExpertScore = false
}
// Manually disable Game Center integration
public func disableGameCenter() {
_gameCenterEnabled = false
}
public func resetScores() {
easyHighScore = 0
mediumHighScore = 0
hardHighScore = 0
expertHighScore = 0
save()
}
// Store data
public func save() {
let encodedData: NSData = NSKeyedArchiver .archivedDataWithRootObject(self)
encodedData.writeToFile(GameScores.filePath(), atomically: true)
}
public func reportGameCenterScore(score: Int64, identifier: String) {
if _gameCenterEnabled == false {
return
}
if GameCenterManager.sharedInstance.enabled == true {
GameCenterManager.sharedInstance.reportScore(score, identifier: identifier)
}
}
// Generate a path for the game scores file
private class func filePath() -> String {
let documentsPath = NSSearchPathForDirectoriesInDomains(.DocumentDirectory, .UserDomainMask, true)[0]
return NSURL(fileURLWithPath: documentsPath).URLByAppendingPathComponent(kGameScoreFileName).path!
}
private func reloadLeaderboards() {
if GameCenterManager.sharedInstance.enabled == false {
return
}
GameCenterManager.sharedInstance.reloadHighScoresForIdentity(kEasyModeLeaderboardId)
GameCenterManager.sharedInstance.reloadHighScoresForIdentity(kMediumModeLeaderboardId)
GameCenterManager.sharedInstance.reloadHighScoresForIdentity(kHardModeLeaderboardId)
GameCenterManager.sharedInstance.reloadHighScoresForIdentity(kExpertModeLeaderboardId)
}
// MARK: GameCenterManagerDelegate methods
internal func processGameCenterAuth(error: NSError?) {
if error == nil {
reloadLeaderboards()
} else {
print("Failed to authenticate with game center err:"+error.debugDescription)
}
}
internal func scoreReported(leaderboardId: String, error: NSError?) {
if error != nil {
print("Failed to report "+leaderboardId+" score. err="+error!.debugDescription)
}
}
internal func reloadScoresComplete(leaderBoard: GKLeaderboard, error: NSError?) {
var oldScore: Int64 = 0
var curScore: Int64 = 0
var gcScore: Int64 = 0
if leaderBoard.localPlayerScore != nil {
gcScore = leaderBoard.localPlayerScore!.value
}
if leaderBoard.identifier == kEasyModeLeaderboardId {
oldScore = _easyHighScore
_easyHighScore = max(oldScore, gcScore)
curScore = _easyHighScore
} else if leaderBoard.identifier == kMediumModeLeaderboardId {
oldScore = _mediumHighScore
_mediumHighScore = max(oldScore, gcScore)
curScore = _mediumHighScore
} else if leaderBoard.identifier == kHardModeLeaderboardId {
oldScore = _hardHighScore
_hardHighScore = max(oldScore, gcScore)
curScore = _hardHighScore
} else if leaderBoard.identifier == kExpertModeLeaderboardId {
oldScore = _expertHighScore
_expertHighScore = max(oldScore, gcScore)
curScore = _expertHighScore
}
// Update Game Center with local score if it has changed (offline)
if curScore != gcScore && GameCenterManager.sharedInstance.enabled == true {
GameCenterManager.sharedInstance.reportScore(curScore, identifier: leaderBoard.identifier!)
}
}
internal func achievementSubmitted(achievement: GKAchievement?, error: NSError?) {
// Reserved for future use
}
internal func achievementResetResult(error: NSError?) {
// Reserved for future use
}
internal func mappedPlayerIDToPlayer(player: GKPlayer?, error: NSError?) {
// Reserved for future use
}
// MARK: Private members
private var _newEasyScore: Bool = false
private var _newMediumScore: Bool = false
private var _newHardScore: Bool = false
private var _newExpertScore: Bool = false
private var _easyHighScore: Int64 = 0
private var _mediumHighScore: Int64 = 0
private var _hardHighScore: Int64 = 0
private var _expertHighScore: Int64 = 0
private var _gameCenterEnabled: Bool = true
}
| mit | 3761528918d97133d10458782a7b96ec | 31.341935 | 115 | 0.632954 | 4.843478 | false | false | false | false |
djfink-carglass/analytics-ios | AnalyticsTests/HTTPClientTest.swift | 1 | 7762 | //
// HTTPClientTest.swift
// Analytics
//
// Created by Tony Xiao on 9/16/16.
// Copyright © 2016 Segment. All rights reserved.
//
import Quick
import Nimble
import Nocilla
import Analytics
class HTTPClientTest: QuickSpec {
override func spec() {
var client: SEGHTTPClient!
beforeEach {
LSNocilla.sharedInstance().start()
client = SEGHTTPClient(requestFactory: nil)
}
afterEach {
LSNocilla.sharedInstance().clearStubs()
LSNocilla.sharedInstance().stop()
}
describe("defaultRequestFactory") {
it("preserves url") {
let factory = SEGHTTPClient.defaultRequestFactory()
let url = URL(string: "https://api.segment.io/v1/batch")
let request = factory(url!)
expect(request.url) == url
}
}
describe("settingsForWriteKey") {
it("succeeds for 2xx response") {
_ = stubRequest("GET", "https://cdn-settings.segment.com/v1/projects/foo/settings" as NSString)!
.withHeaders(["Accept-Encoding" : "gzip" ])!
.andReturn(200)!
.withHeaders(["Content-Type" : "application/json"])!
.withBody("{\"integrations\":{\"Segment.io\":{\"apiKey\":\"foo\"}},\"plan\":{\"track\":{}}}" as NSString)
var done = false
let task = client.settings(forWriteKey: "foo", completionHandler: { success, settings in
expect(success) == true
expect((settings as? NSDictionary)) == [
"integrations": [
"Segment.io": [
"apiKey":"foo"
]
],
"plan":[
"track": [:]
]
] as NSDictionary
done = true
})
expect(task.state).toEventually(equal(URLSessionTask.State.completed))
expect(done).toEventually(beTrue())
}
it("fails for non 2xx response") {
_ = stubRequest("GET", "https://cdn-settings.segment.com/v1/projects/foo/settings" as NSString)!
.withHeaders(["Accept-Encoding" : "gzip" ])!
.andReturn(400)!
.withHeaders(["Content-Type" : "application/json" ])!
.withBody("{\"integrations\":{\"Segment.io\":{\"apiKey\":\"foo\"}},\"plan\":{\"track\":{}}}" as NSString)
var done = false
client.settings(forWriteKey: "foo", completionHandler: { success, settings in
expect(success) == false
expect(settings).to(beNil())
done = true
})
expect(done).toEventually(beTrue())
}
it("fails for json error") {
_ = stubRequest("GET", "https://cdn-settings.segment.com/v1/projects/foo/settings" as NSString)!
.withHeaders(["Accept-Encoding":"gzip"])!
.andReturn(200)!
.withHeaders(["Content-Type":"application/json"])!
.withBody("{\"integrations" as NSString)
var done = false
client.settings(forWriteKey: "foo", completionHandler: { success, settings in
expect(success) == false
expect(settings).to(beNil())
done = true
})
expect(done).toEventually(beTrue())
}
}
describe("upload") {
it("does not ask to retry for json error") {
// Dates cannot be serialized as is so the json serialzation will fail.
let batch = [[ "foo" : NSDate() ] ]
var done = false
let task = client.upload(batch, forWriteKey: "bar") { retry in
expect(retry) == false
done = true
}
expect(task).to(beNil())
expect(done).toEventually(beTrue())
}
let batch = [["type":"track", "event":"foo"]]
it("does not ask to retry for 2xx response") {
_ = stubRequest("POST", "https://api.segment.io/v1/batch" as NSString)!
.withJsonGzippedBody(batch as AnyObject)
.withWriteKey("bar")
.andReturn(200)
var done = false
let task = client.upload(batch, forWriteKey: "bar") { retry in
expect(retry) == false
done = true
}
expect(done).toEventually(beTrue())
expect(task.state).toEventually(equal(URLSessionTask.State.completed))
}
it("asks to retry for 3xx response") {
_ = stubRequest("POST", "https://api.segment.io/v1/batch" as NSString)!
.withJsonGzippedBody(batch as AnyObject)
.withWriteKey("bar")
.andReturn(304)
var done = false
let task = client.upload(batch, forWriteKey: "bar") { retry in
expect(retry) == true
done = true
}
expect(done).toEventually(beTrue())
expect(task.state).toEventually(equal(URLSessionTask.State.completed))
}
it("does not ask to retry for 4xx response") {
_ = stubRequest("POST", "https://api.segment.io/v1/batch" as NSString)!
.withJsonGzippedBody(batch as AnyObject)
.withWriteKey("bar")
.andReturn(401)
var done = false
let task = client.upload(batch, forWriteKey: "bar") { retry in
expect(retry) == false
done = true
}
expect(done).toEventually(beTrue())
expect(task.state).toEventually(equal(URLSessionTask.State.completed))
}
it("asks to retry for 5xx response") {
_ = stubRequest("POST", "https://api.segment.io/v1/batch" as NSString)!
.withJsonGzippedBody(batch as AnyObject)
.withWriteKey("bar")
.andReturn(504)
var done = false
let task = client.upload(batch, forWriteKey: "bar") { retry in
expect(retry) == true
done = true
}
expect(done).toEventually(beTrue())
expect(task.state).toEventually(equal(URLSessionTask.State.completed))
}
}
describe("attribution") {
it("fails for json error") {
let device = [
// Dates cannot be serialized as is so the json serialzation will fail.
"sentAt": NSDate(),
]
var done = false
let task = client.attribution(withWriteKey: "bar", forDevice: device) { success, properties in
expect(success) == false
done = true
}
expect(task).to(beNil())
expect(done).toEventually(beTrue())
}
let context: [String: Any] = [
"os": [
"name": "iPhone OS",
"version" : "8.1.3",
],
"ip": "8.8.8.8",
]
it("succeeds for 2xx response") {
_ = stubRequest("POST", "https://mobile-service.segment.com/v1/attribution" as NSString)!
.withWriteKey("foo")
.andReturn(200)!
.withBody("{\"provider\": \"mock\"}" as NSString)
var done = false
let task = client.attribution(withWriteKey: "foo", forDevice: context) { success, properties in
expect(success) == true
expect(properties as? [String: String]) == [
"provider": "mock"
]
done = true
}
expect(task.state).toEventually(equal(URLSessionTask.State.completed))
expect(done).toEventually(beTrue())
}
it("fails for non 2xx response") {
_ = stubRequest("POST", "https://mobile-service.segment.com/v1/attribution" as NSString)!
.withWriteKey("foo")
.andReturn(404)!
.withBody("not found" as NSString)
var done = false
let task = client.attribution(withWriteKey: "foo", forDevice: context) { success, properties in
expect(success) == false
expect(properties).to(beNil())
done = true
}
expect(task.state).toEventually(equal(URLSessionTask.State.completed))
expect(done).toEventually(beTrue())
}
}
}
}
| mit | f1127adce8a4a5004f02f7651ed9908c | 33.340708 | 115 | 0.560881 | 4.350336 | false | false | false | false |
lnds/9d9l | desafio2/swift/Sources/api.swift | 1 | 1473 | import Foundation
enum ApiResult {
case Error(String, String) // city, error
case Weather(String,Double,Double,Double,String) // city, temp, max, min,conditions
}
func httpGet(city:String, url:String) -> ApiResult {
if let myURL = URL(string: url) {
for _ in 1...10 {
do {
let xmlDoc = try XMLDocument(contentsOf: myURL, options:0)
if let root = xmlDoc.rootElement() {
let cityName = root.elements(forName:"city")[0].attribute(forName:"name")!.stringValue!
let temp = (root.elements(forName:"temperature")[0].attribute(forName:"value")!.stringValue! as NSString).doubleValue
let max = (root.elements(forName:"temperature")[0].attribute(forName:"max")!.stringValue! as NSString).doubleValue
let min = (root.elements(forName:"temperature")[0].attribute(forName:"min")!.stringValue! as NSString).doubleValue
let weatherConds = root.elements(forName:"weather")[0].attribute(forName:"value")!.stringValue!
return ApiResult.Weather(cityName, temp, max, min, weatherConds)
}
} catch { /* DO NOTHING, and is good */ }
usleep(100000)
}
}
return ApiResult.Error(city, "no pudo leer url")
}
func makeUrl(city: String, apiKey: String) -> String {
return "http://api.openweathermap.org/data/2.5/weather?q=" + city + "&mode=xml&units=metric&appid=" + apiKey + "&lang=sp"
}
func callApi(city: String, apiKey: String) -> ApiResult {
let url = makeUrl(city:city, apiKey:apiKey)
return httpGet(city:city, url:url)
}
| mit | b73511d634557b8c704c4aae7ee83cab | 39.916667 | 122 | 0.693822 | 3.370709 | false | false | false | false |
iOS-mamu/SS | P/Library/Eureka/Eureka.playground/Contents.swift | 1 | 6112 | //: **Eureka Playground** - let us walk you through Eureka! cool features, we will show how to
//: easily create powerful forms using Eureka!
//: It allows us to create complex dynamic table view forms and obviously simple static table view. It's ideal for data entry task or settings pages.
import UIKit
import XCPlayground
//: Start by importing Eureka module
import Eureka
//: Any **Eureka** form must extend from `FromViewController`
let formController = FormViewController()
XCPlaygroundPage.currentPage.liveView = formController.view
//: ## Operators
//: ### +++
//: Adds a Section to a Form when the left operator is a Form
let form = Form() +++ Section()
//: When both operators are a Section it creates a new Form containing both Section and return the created form.
let form2 = Section("First") +++ Section("Second") +++ Section("Third")
//: Notice that you don't need to add parenthesis in the above expresion, +++ operator is left associative so it will create a form containing the 2 first sections and then append last Section (Third) to the created form.
//: form and form2 don't have any row and are not useful at all. Let's add some rows.
//: ### +++
//: Can be used to append a row to a form without having to create a Section to contain the row. The form section is implicitly created as a result of the +++ operator.
formController.form +++ TextRow("Text")
//: it can also be used to append a Section like this:
formController.form +++ Section()
//: ### <<<
//: Can be used to append rows to a section.
formController.form.last! <<< SwitchRow("Switch") { $0.title = "Switch"; $0.value = true }
//: it can also be used to create a section and append rows to it. Let's use that to add a new section to the form
formController.form +++ PhoneRow("Phone"){ $0.title = "Phone"}
<<< IntRow("IntRow"){ $0.title = "Int"; $0.value = 5 }
formController.view
//: # Callbacks
//: Rows might have several closures associated to be called at different events. Let's see them one by one. Sadly, we can not interact with it in our playground.
//: ### onChange callback
//: Will be called when the value of a row changes. Lots of things can be done with this feature. Let's create a new section for the new rows:
formController.form +++ Section("Callbacks") <<< SwitchRow("scr1"){ $0.title = "Switch to turn red"; $0.value = false }
.onChange({ row in
if row.value == true {
row.cell.backgroundColor = .redColor()
}
else {
row.cell.backgroundColor = .blackColor()
}
})
//: Now when we change the value of this row its background color will change to red. Try that by (un)commenting the following line:
formController.view
formController.form.last?.last?.baseValue = true
formController.view
//: Notice that we set the `baseValue` attribute because we did not downcast the result of `formController.form.last?.last`. It is essentially the same as the `value` attribute
//: ### cellSetup and cellUpdate callbacks
//: The cellSetup will be called when the cell of this row is configured (just once at the beginning) and the cellUpdate will be called when it is updated (each time it reappears on screen). Here you should define the appearance of the cell
formController.form.last! <<< SegmentedRow<String>("Segments") { $0.title = "Choose an animal"; $0.value = "🐼"; $0.options = ["🐼", "🐶", "🐻"]}.cellSetup({ cell, row in
cell.backgroundColor = .redColor()
}).cellUpdate({ (cell, row) -> () in
cell.textLabel?.textColor = .yellowColor()
})
//: ### onSelection and onPresent callbacks
//: OnSelection will be called when this row is selected (tapped by the user). It might be useful for certain rows instead of the onChange callback.
//: OnPresent will be called when a row that presents another view controller is tapped. It might be useful to set up the presented view controller.
//: We can not try them out in the playground but they can be set just like the others.
formController.form.last! <<< SegmentedRow<String>("Segments2") { $0.title = "Choose an animal"; $0.value = "🐼"; $0.options = ["🐼", "🐶", "🐻"]
}.onCellSelection{ cell, row in
print("\(cell) for \(row) got selected")
}
formController.view
//: ### Hiding rows
//: We can hide rows by defining conditions that will tell if they should appear on screen or not. Let's create a row that will hide when the previous one is "🐼".
formController.form.last! <<< LabelRow("Confirm") {
$0.title = "Are you sure you do not want the 🐼?"
$0.hidden = "$Segments2 == '🐼'"
}
//: Now let's see how this works:
formController.view
formController.form.rowByTag("Segments2")?.baseValue = "🐶"
formController.view
//: We can do the same using functions. Functions are specially useful for more complicated conditions. This applies when the value of the row we depend on is not compatible with NSPredicates (which is not the current case, but anyway).
formController.form.last! <<< LabelRow("Confirm2") {
$0.title = "Well chosen!!"
$0.hidden = Condition.Function(["Segments2"]){ form in
if let r:SegmentedRow<String> = form.rowByTag("Segments2") {
return r.value != "🐼"
}
return true
}
}
//: Now let's see how this works:
formController.view
formController.form.rowByTag("Segments2")?.baseValue = "🐼"
formController.view
| mit | f0dc6b06f5e98fc58c47c4d1d27bfcba | 50.880342 | 705 | 0.615815 | 4.676425 | false | false | false | false |
Clean-Swift/CleanStore | CleanStore/Scenes/CreateOrder/CreateOrderViewController.swift | 1 | 11820 | //
// CreateOrderViewController.swift
// CleanStore
//
// Created by Raymond Law on 2/12/19.
// Copyright (c) 2019 Clean Swift LLC. All rights reserved.
//
// This file was generated by the Clean Swift Xcode Templates so
// you can apply clean architecture to your iOS and Mac projects,
// see http://clean-swift.com
//
import UIKit
protocol CreateOrderDisplayLogic: AnyObject
{
func displayExpirationDate(viewModel: CreateOrder.FormatExpirationDate.ViewModel)
func displayCreatedOrder(viewModel: CreateOrder.CreateOrder.ViewModel)
func displayOrderToEdit(viewModel: CreateOrder.EditOrder.ViewModel)
func displayUpdatedOrder(viewModel: CreateOrder.UpdateOrder.ViewModel)
}
class CreateOrderViewController: UITableViewController, CreateOrderDisplayLogic, UITextFieldDelegate, UIPickerViewDataSource, UIPickerViewDelegate
{
var interactor: CreateOrderBusinessLogic?
var router: (NSObjectProtocol & CreateOrderRoutingLogic & CreateOrderDataPassing)?
// MARK: Object lifecycle
override init(nibName nibNameOrNil: String?, bundle nibBundleOrNil: Bundle?)
{
super.init(nibName: nibNameOrNil, bundle: nibBundleOrNil)
setup()
}
required init?(coder aDecoder: NSCoder)
{
super.init(coder: aDecoder)
setup()
}
// MARK: Setup
private func setup()
{
let viewController = self
let interactor = CreateOrderInteractor()
let presenter = CreateOrderPresenter()
let router = CreateOrderRouter()
viewController.interactor = interactor
viewController.router = router
interactor.presenter = presenter
presenter.viewController = viewController
router.viewController = viewController
router.dataStore = interactor
}
// MARK: Routing
override func prepare(for segue: UIStoryboardSegue, sender: Any?)
{
if let scene = segue.identifier {
let selector = NSSelectorFromString("routeTo\(scene)WithSegue:")
if let router = router, router.responds(to: selector) {
router.perform(selector, with: segue)
}
}
}
// MARK: View lifecycle
override func viewDidLoad()
{
super.viewDidLoad()
configurePickers()
showOrderToEdit()
}
func configurePickers()
{
shippingMethodTextField.inputView = shippingMethodPicker
expirationDateTextField.inputView = expirationDatePicker
}
// MARK: Text fields
@IBOutlet var textFields: [UITextField]!
func textFieldShouldReturn(_ textField: UITextField) -> Bool
{
textField.resignFirstResponder()
if let index = textFields.firstIndex(of: textField) {
if index < textFields.count - 1 {
let nextTextField = textFields[index + 1]
nextTextField.becomeFirstResponder()
}
}
return true
}
override func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath)
{
if let cell = tableView.cellForRow(at: indexPath) {
for textField in textFields {
if textField.isDescendant(of: cell) {
textField.becomeFirstResponder()
}
}
}
}
// MARK: - Shipping method
@IBOutlet weak var shippingMethodTextField: UITextField!
@IBOutlet var shippingMethodPicker: UIPickerView!
func numberOfComponents(in pickerView: UIPickerView) -> Int
{
return 1
}
func pickerView(_ pickerView: UIPickerView, numberOfRowsInComponent component: Int) -> Int
{
return interactor?.shippingMethods.count ?? 0
}
func pickerView(_ pickerView: UIPickerView, titleForRow row: Int, forComponent component: Int) -> String?
{
return interactor?.shippingMethods[row]
}
func pickerView(_ pickerView: UIPickerView, didSelectRow row: Int, inComponent component: Int)
{
shippingMethodTextField.text = interactor?.shippingMethods[row]
}
// MARK: - Expiration date
@IBOutlet weak var expirationDateTextField: UITextField!
@IBOutlet var expirationDatePicker: UIDatePicker!
@IBAction func expirationDatePickerValueChanged(_ sender: Any)
{
let date = expirationDatePicker.date
let request = CreateOrder.FormatExpirationDate.Request(date: date)
interactor?.formatExpirationDate(request: request)
}
func displayExpirationDate(viewModel: CreateOrder.FormatExpirationDate.ViewModel)
{
let date = viewModel.date
expirationDateTextField.text = date
}
// MARK: - Create order
// MARK: Contact info
@IBOutlet weak var firstNameTextField: UITextField!
@IBOutlet weak var lastNameTextField: UITextField!
@IBOutlet weak var phoneTextField: UITextField!
@IBOutlet weak var emailTextField: UITextField!
// MARK: Payment info
@IBOutlet weak var billingAddressStreet1TextField: UITextField!
@IBOutlet weak var billingAddressStreet2TextField: UITextField!
@IBOutlet weak var billingAddressCityTextField: UITextField!
@IBOutlet weak var billingAddressStateTextField: UITextField!
@IBOutlet weak var billingAddressZIPTextField: UITextField!
@IBOutlet weak var creditCardNumberTextField: UITextField!
@IBOutlet weak var ccvTextField: UITextField!
// MARK: Shipping info
@IBOutlet weak var shipmentAddressStreet1TextField: UITextField!
@IBOutlet weak var shipmentAddressStreet2TextField: UITextField!
@IBOutlet weak var shipmentAddressCityTextField: UITextField!
@IBOutlet weak var shipmentAddressStateTextField: UITextField!
@IBOutlet weak var shipmentAddressZIPTextField: UITextField!
@IBAction func saveButtonTapped(_ sender: Any)
{
// MARK: Contact info
let firstName = firstNameTextField.text!
let lastName = lastNameTextField.text!
let phone = phoneTextField.text!
let email = emailTextField.text!
// MARK: Payment info
let billingAddressStreet1 = billingAddressStreet1TextField.text!
let billingAddressStreet2 = billingAddressStreet2TextField.text!
let billingAddressCity = billingAddressCityTextField.text!
let billingAddressState = billingAddressStateTextField.text!
let billingAddressZIP = billingAddressZIPTextField.text!
let paymentMethodCreditCardNumber = creditCardNumberTextField.text!
let paymentMethodCVV = ccvTextField.text!
let paymentMethodExpirationDate = expirationDatePicker.date
let paymentMethodExpirationDateString = ""
// MARK: Shipping info
let shipmentAddressStreet1 = shipmentAddressStreet1TextField.text!
let shipmentAddressStreet2 = shipmentAddressStreet2TextField.text!
let shipmentAddressCity = shipmentAddressCityTextField.text!
let shipmentAddressState = shipmentAddressStateTextField.text!
let shipmentAddressZIP = shipmentAddressZIPTextField.text!
let shipmentMethodSpeed = shippingMethodPicker.selectedRow(inComponent: 0)
let shipmentMethodSpeedString = ""
// MARK: Misc
var id: String? = nil
var date = Date()
var total = NSDecimalNumber.notANumber
if let orderToEdit = interactor?.orderToEdit {
id = orderToEdit.id
date = orderToEdit.date
total = orderToEdit.total
let request = CreateOrder.UpdateOrder.Request(orderFormFields: CreateOrder.OrderFormFields(firstName: firstName, lastName: lastName, phone: phone, email: email, billingAddressStreet1: billingAddressStreet1, billingAddressStreet2: billingAddressStreet2, billingAddressCity: billingAddressCity, billingAddressState: billingAddressState, billingAddressZIP: billingAddressZIP, paymentMethodCreditCardNumber: paymentMethodCreditCardNumber, paymentMethodCVV: paymentMethodCVV, paymentMethodExpirationDate: paymentMethodExpirationDate, paymentMethodExpirationDateString: paymentMethodExpirationDateString, shipmentAddressStreet1: shipmentAddressStreet1, shipmentAddressStreet2: shipmentAddressStreet2, shipmentAddressCity: shipmentAddressCity, shipmentAddressState: shipmentAddressState, shipmentAddressZIP: shipmentAddressZIP, shipmentMethodSpeed: shipmentMethodSpeed, shipmentMethodSpeedString: shipmentMethodSpeedString, id: id, date: date, total: total))
interactor?.updateOrder(request: request)
} else {
let request = CreateOrder.CreateOrder.Request(orderFormFields: CreateOrder.OrderFormFields(firstName: firstName, lastName: lastName, phone: phone, email: email, billingAddressStreet1: billingAddressStreet1, billingAddressStreet2: billingAddressStreet2, billingAddressCity: billingAddressCity, billingAddressState: billingAddressState, billingAddressZIP: billingAddressZIP, paymentMethodCreditCardNumber: paymentMethodCreditCardNumber, paymentMethodCVV: paymentMethodCVV, paymentMethodExpirationDate: paymentMethodExpirationDate, paymentMethodExpirationDateString: paymentMethodExpirationDateString, shipmentAddressStreet1: shipmentAddressStreet1, shipmentAddressStreet2: shipmentAddressStreet2, shipmentAddressCity: shipmentAddressCity, shipmentAddressState: shipmentAddressState, shipmentAddressZIP: shipmentAddressZIP, shipmentMethodSpeed: shipmentMethodSpeed, shipmentMethodSpeedString: shipmentMethodSpeedString, id: id, date: date, total: total))
interactor?.createOrder(request: request)
}
}
func displayCreatedOrder(viewModel: CreateOrder.CreateOrder.ViewModel)
{
if viewModel.order != nil {
router?.routeToListOrders(segue: nil)
} else {
showOrderFailureAlert(title: "Failed to create order", message: "Please correct your order and submit again.")
}
}
// MARK: - Edit order
func showOrderToEdit()
{
let request = CreateOrder.EditOrder.Request()
interactor?.showOrderToEdit(request: request)
}
func displayOrderToEdit(viewModel: CreateOrder.EditOrder.ViewModel)
{
let orderFormFields = viewModel.orderFormFields
firstNameTextField.text = orderFormFields.firstName
lastNameTextField.text = orderFormFields.lastName
phoneTextField.text = orderFormFields.phone
emailTextField.text = orderFormFields.email
billingAddressStreet1TextField.text = orderFormFields.billingAddressStreet1
billingAddressStreet2TextField.text = orderFormFields.billingAddressStreet2
billingAddressCityTextField.text = orderFormFields.billingAddressCity
billingAddressStateTextField.text = orderFormFields.billingAddressState
billingAddressZIPTextField.text = orderFormFields.billingAddressZIP
creditCardNumberTextField.text = orderFormFields.paymentMethodCreditCardNumber
ccvTextField.text = orderFormFields.paymentMethodCVV
shipmentAddressStreet1TextField.text = orderFormFields.shipmentAddressStreet1
shipmentAddressStreet2TextField.text = orderFormFields.shipmentAddressStreet2
shipmentAddressCityTextField.text = orderFormFields.shipmentAddressCity
shipmentAddressStateTextField.text = orderFormFields.shipmentAddressState
shipmentAddressZIPTextField.text = orderFormFields.shipmentAddressZIP
shippingMethodPicker.selectRow(orderFormFields.shipmentMethodSpeed, inComponent: 0, animated: true)
shippingMethodTextField.text = orderFormFields.shipmentMethodSpeedString
expirationDatePicker.date = orderFormFields.paymentMethodExpirationDate
expirationDateTextField.text = orderFormFields.paymentMethodExpirationDateString
}
// MARK: - Update order
func displayUpdatedOrder(viewModel: CreateOrder.UpdateOrder.ViewModel)
{
if viewModel.order != nil {
router?.routeToShowOrder(segue: nil)
} else {
showOrderFailureAlert(title: "Failed to update order", message: "Please correct your order and submit again.")
}
}
// MARK: Error handling
private func showOrderFailureAlert(title: String, message: String)
{
let alertController = UIAlertController(title: title, message: message, preferredStyle: .alert)
let alertAction = UIAlertAction(title: "OK", style: .default, handler: nil)
alertController.addAction(alertAction)
showDetailViewController(alertController, sender: nil)
}
}
| mit | 9d1ea110c921c6940afe2bef71d8dfa9 | 39.204082 | 957 | 0.772504 | 5.188762 | false | false | false | false |
KrishMunot/swift | test/DebugInfo/bbentry-location.swift | 2 | 1064 | // REQUIRES: OS=ios
// REQUIRES: objc_interop
// RUN: %target-swift-frontend -emit-ir -g %s -o - | FileCheck %s
import UIKit
@available(iOS, introduced=8.0)
class ActionViewController
{
var imageView: UIImageView!
func viewDidLoad(_ inputItems: [AnyObject]) {
for item: AnyObject in inputItems {
let inputItem = item as! NSExtensionItem
for provider: AnyObject in inputItem.attachments! {
let itemProvider = provider as! NSItemProvider
// CHECK: load {{.*}}selector
// CHECK:; <label>{{.*}} ; preds = %{{[0-9]+}}
// CHECK: @rt_swift_allocObject({{.*}}, !dbg ![[DBG:[0-9]+]]
// Test that the location is reset at the entry of a new basic block.
// CHECK: ![[DBG]] = {{.*}}line: 0
if itemProvider.hasItemConformingToTypeIdentifier("") {
weak var weakImageView = self.imageView
itemProvider.loadItem(forTypeIdentifier: "", options: nil,
completionHandler: { (image, error) in
if let imageView = weakImageView {
}
})
}
}
}
}
}
| apache-2.0 | e9b60f9119bf14cef764884e2499bfe1 | 33.322581 | 77 | 0.606203 | 4.360656 | false | false | false | false |
qds-hoi/suracare | suracare/suracare/App/Helpers/rSConstant.swift | 1 | 671 | //
// rSConstant.swift
// suracare
//
// Created by hoi on 7/21/16.
// Copyright © 2016 Sugar Rain. All rights reserved.
//
import Foundation
struct rSConstant {
let baseReference: String = "https://sugarrain.firebaseio.com"
}
struct Text {
struct Dialogues {
static let errorTitle = "Whoops!"
static let errorMessage = "Something went wrong."
static let okayText = "Okay"
}
}
struct rSFont {
struct Helvetica {
static let system: String = "Helvetica"
static let bold: String = "Helvetica-Bold"
static let thin: String = "Helvetica-Thin"
static let light: String = "Helvetica-Light"
}
} | mit | 5b757b5ac3f91d5027db3eea59392778 | 21.366667 | 66 | 0.637313 | 3.828571 | false | false | false | false |
Bartlebys/Bartleby | Bartleby.xOS/bsfs/Flocker.swift | 1 | 36669 | //
// Flocker.swift
// BartlebyKit
//
// Created by Benoit Pereira da silva on 05/11/2016.
//
//
import Foundation
enum FlockerError:Error{
case unableToAccessToContainer(at:String,message:String)
}
/*
Binary Format specs
--------
data -> the Nodes binary
--------
footer -> serialized crypted and compressed Container
--------
8Bytes for one UInt64 -> gives the footer size
--------
*/
struct Flocker{
/// The file manager is used on the utility queue
fileprivate let _fileManager:FileManager
fileprivate var _key:String=""
// The Crypto Helper
fileprivate let _cryptoHelper:CryptoHelper
/// Designated Initializer
///
/// - Parameters:
/// - fileManager: the file manager to use on the Flocker Queue
/// - cryptoKey: the key used for crypto 32 char min
/// - cryptoSalt: the salt
/// - keySize: the key size
init(fileManager:FileManager,cryptoKey:String,cryptoSalt:String,keySize:KeySize = .s128bits) {
self._fileManager=fileManager
self._key=cryptoKey
self._cryptoHelper=CryptoHelper(salt: cryptoSalt,keySize:keySize)
}
// MARK: - Flocking
/// Flocks the files means you transform all the files to a single file
/// By using this method the FileReference Authorization will apply to any Node
///
/// - Parameters:
/// - folderReference: the reference to folder to flock
/// - path: the destination path
func flockFolder(folderReference:FileReference,
destination path:String,
progression:@escaping((Progression)->()),
success:@escaping (BytesStats)->(),
failure:@escaping (Container,String)->())->(){
self._flock(filesIn: folderReference.absolutePath,
flockFilePath: path,
authorized:folderReference.authorized,
chunkMaxSize: folderReference.chunkMaxSize,
compress: folderReference.compressed,
encrypt: folderReference.crypted,
progression: progression,
success: success,
failure: failure)
}
/// Breaks recursively any file, folder, alias from a given folder path into block and adds theme to flock
/// - The hard stuff is done Asynchronously on a the Utility queue
/// - we use an Autorelease pool to lower the memory foot print
/// - closures are called on the Main thread
fileprivate func _flock( filesIn path:String,
flockFilePath:String,
authorized:[String],
chunkMaxSize:Int=10*MB,
compress:Bool=true,
encrypt:Bool=true,
progression:@escaping((Progression)->()),
success:@escaping (BytesStats)->(),
failure:@escaping (Container,String)->()){
var stats=BytesStats(name: "Flocking: \(path)")
let container=Container()
let box=Box()
container.boxes.append(box)
Async.utility{
try? self._fileManager.removeItem(atPath: flockFilePath)
let r = self._fileManager.createFile(atPath: flockFilePath, contents: nil, attributes: nil)
if let flockFileHandle = FileHandle(forWritingAtPath: flockFilePath){
var folderPath=path
var paths=[String]()
let progressionState=Progression()
progressionState.quietChanges{
progressionState.totalTaskCount=0
progressionState.currentTaskIndex=0
progressionState.externalIdentifier=path
progressionState.message=""
}
var failuresMessages=[String]()
let fm:FileManager = self._fileManager
var isDirectory:ObjCBool=false
if fm.fileExists(atPath: path, isDirectory: &isDirectory){
if isDirectory.boolValue{
/// Directory scanning
if let rootURL=URL(string: path){
let keys:[URLResourceKey]=[ URLResourceKey.fileSizeKey,
URLResourceKey.fileResourceTypeKey,
URLResourceKey.attributeModificationDateKey,
URLResourceKey.pathKey,
URLResourceKey.isRegularFileKey,
URLResourceKey.isDirectoryKey,
URLResourceKey.isAliasFileKey,
URLResourceKey.isSymbolicLinkKey ]
let options: FileManager.DirectoryEnumerationOptions = []//.skipsHiddenFiles TODO to be verified
let enumerator=fm.enumerator(at: rootURL, includingPropertiesForKeys: keys, options: options, errorHandler: { (URL, error) -> Bool in
return false
})
while let url:URL = enumerator?.nextObject() as? URL {
let set:Set=Set(keys)
if let r:URLResourceValues = try? url.resourceValues(forKeys:set){
if r.isRegularFile == true || r.isDirectory==true || r.isAliasFile==true{
let path:String=r.path!.replacingOccurrences(of: path, with: "")
paths.append(path)
}
}
}
}else{
// Close the flockFileHandle
flockFileHandle.closeFile()
syncOnMain{
failure(container,NSLocalizedString("Invalid URL", tableName:"system", comment: "Invalid URL")+" \(path)")
}
}
}else{
// Append one file
let pathURL=URL(fileURLWithPath: path)
folderPath=pathURL.deletingLastPathComponent().path
paths.append("/"+pathURL.lastPathComponent)
}
}else{
syncOnMain{
failure(container,NSLocalizedString("Unexisting path: ", tableName:"system", comment: "Unexisting path: ")+flockFilePath)
}
}
progressionState.totalTaskCount += paths.count
let pathNb=paths.count
var counter=0
// Relays the progression
func __onProgression(message:String, incrementGlobalCounter:Bool=true){
if incrementGlobalCounter{
counter += 1
}
progressionState.quietChanges{
progressionState.currentTaskIndex=counter
progressionState.message=message
}
progressionState.currentPercentProgress=Double(counter)*Double(100)/Double(progressionState.totalTaskCount)
syncOnMain{
// Relay the progression
progression(progressionState)
}
}
for relativePath in paths{
self._append(folderPath: folderPath,
relativePath:relativePath,
writeHandle: flockFileHandle,
container: container,
authorized:authorized,
chunkMaxSize:chunkMaxSize,
compress:compress,
encrypt:encrypt,
stats:stats,
progression: { (subProgression) in
__onProgression(message: subProgression.message,incrementGlobalCounter: false)
}, success: {
__onProgression(message: "Flocked: \(relativePath)")
if counter == pathNb{
defer{
// Close the flockFileHandle
flockFileHandle.closeFile()
}
do{
try self._writeContainerIntoFlock(handle: flockFileHandle, container: container)
}catch{
failuresMessages.append("_writeContainerIntoFlock \(error)")
}
if failuresMessages.count==0{
// it is a success
success(stats)
}else{
// Reduce the errors
failure(container,failuresMessages.reduce("Errors: ", { (r, s) -> String in
return r + " \(s)"
}))
}
}
}, failure: { (message) in
counter += 1
failuresMessages.append(message)
})
}
}else{
syncOnMain{
failure(container,NSLocalizedString("Invalid Path", tableName:"system", comment: "Invalid Path")+" \(path)")
}
}
}
}
/// Appends a file a folder or an alias to flock file
/// IMPORTANT! this method Must be called on the utility Queue
/// - Parameters:
/// - folderPath: the base folder path
/// - relativePath: the relative path
/// - writeHandle: the flock file handle
/// - container: the container (flock informations)
/// - authorized: the authorized list
/// - chunkMaxSize: the chunk or block max size
/// - compress: should we compress ?
/// - encrypt: should we encrypt?
/// - progression: the progression closure
/// - success: the success closure
/// - failure: the failure closure
fileprivate func _append( folderPath:String,
relativePath:String,
writeHandle:FileHandle,
container:Container,
authorized:[String],
chunkMaxSize:Int=10*MB,
compress:Bool=true,
encrypt:Bool=true,
stats:BytesStats,
progression:@escaping((Progression)->()),
success:@escaping ()->(),
failure:@escaping (String)->() )->(){
let filePath=folderPath+relativePath
let node=Node()
node.relativePath=relativePath
container.boxes[0].declaresOwnership(of: node)
node.compressedBlocks=compress
node.cryptedBlocks=encrypt
node.authorized=authorized
if self._isPathValid(filePath){
if let attributes:[FileAttributeKey : Any] = try? self._fileManager.attributesOfItem(atPath: filePath){
if let type=attributes[FileAttributeKey.type] as? FileAttributeType{
if (URL(fileURLWithPath: filePath).isAnAlias){
// It is an alias
var aliasDestinationPath:String=Default.NO_PATH
do{
aliasDestinationPath = try self._resolveAlias(at:filePath)
}catch{
glog("Alias resolution error for path \(filePath) s\(error)", file: #file, function: #function, line: #line, category: Default.LOG_DEFAULT, decorative: false)
}
node.nature=Node.Nature.alias
node.proxyPath=aliasDestinationPath
container.nodes.append(node)
syncOnMain{
success()
}
}else if type==FileAttributeType.typeRegular{
// It is a file
node.nature=Node.Nature.file
if let fileHandle=FileHandle(forReadingAtPath:filePath ){
// We Can't guess what will Happen
// But we want a guarantee the handle will be closed
defer{
fileHandle.closeFile()
}
// determine the length
let _=fileHandle.seekToEndOfFile()
let length=fileHandle.offsetInFile
// reposition to 0
fileHandle.seek(toFileOffset: 0)
let maxSize:UInt64 = UInt64(chunkMaxSize)
let n:UInt64 = length / maxSize
var rest:UInt64 = length % maxSize
var nb:UInt64=0
if rest>0 && length >= maxSize{
nb += n
}
if length < maxSize{
rest = length
}
var offset:UInt64=0
var position:UInt64=0
var counter=0
let progressionState=Progression()
progressionState.quietChanges{
progressionState.totalTaskCount=Int(nb)
progressionState.currentTaskIndex=0
progressionState.externalIdentifier=filePath
progressionState.message=""
}
do {
for i in 0 ... nb{
// We try to reduce the memory usage
// To the footprint of a Chunk + Derivated Data.
try autoreleasepool{
fileHandle.seek(toFileOffset: position)
offset = (i==nb ? rest : maxSize)
position += offset
// Read the block data
var data=fileHandle.readData(ofLength: Int(offset))
// Store the original sha1 (independent from compression and encryption)
let sha1=data.sha1
if compress{
data = try data.compress(algorithm: .lz4)
}
if encrypt{
data = try self._cryptoHelper.encryptData(data,useKey:self._key)
}
// Store the current write position before adding the new data
let writePosition=Int(writeHandle.offsetInFile)
// How much data will we write ?
let lengthOfAddedData:Int=data.count
// Write the data to flock File
writeHandle.write(data)
// Consign the processing
stats.consign(numberOfBytes:UInt(offset),compressedBytes: UInt(lengthOfAddedData))
// Create the related block
let block=Block()
block.startsAt = writePosition
block.size = lengthOfAddedData
block.digest=sha1
block.rank=counter
node.declaresOwnership(of: block)
block.compressed=compress
block.crypted=encrypt
node.addBlock(block)
container.blocks.append(block)
counter+=1
syncOnMain{
progressionState.currentTaskIndex=counter
progressionState.message="\(i) \(filePath): <\( block.startsAt), \(block.startsAt+block.size)> + \(block.size) Bytes"
progression(progressionState)
}
}
}
container.nodes.append(node)
syncOnMain{
success()
}
}catch{
syncOnMain{
failure("\(error)")
}
}
}else{
syncOnMain{
failure(NSLocalizedString("Enable to create Reading file Handle", tableName:"system", comment: "Enable to create Reading file Handle")+" \(filePath)")
}
}
}else if type==FileAttributeType.typeDirectory{
node.nature=Node.Nature.folder
container.nodes.append(node)
syncOnMain{
success()
}
}
}
}else{
syncOnMain{
failure(NSLocalizedString("Unable to extract attributes at path:", tableName:"system", comment: "Unable to extract attributes at path:")+" \(filePath)")
}
}
}else{
syncOnMain{
failure(NSLocalizedString("Invalid file at path:", tableName:"system", comment: "Unexisting file at path:")+" \(filePath)")
}
}
}
/// Appends a file a folder or an alias to flock file
/// IMPORTANT! this method Must be called on the utility Queue
///
/// - Parameters:
/// - handle: the flock file handle
/// - container: the container to be written
fileprivate func _writeContainerIntoFlock( handle: FileHandle,
container: Container)throws->(){
try autoreleasepool { () -> Void in
var data = try JSON.encoder.encode(container)
data = try data.compress(algorithm: .lz4)
data = try self._cryptoHelper.encryptData(data,useKey:self._key)
var cryptedSize:UInt64=UInt64(data.count)
let intSize=MemoryLayout<UInt64>.size
// Write the serialized container
handle.write(data)
// Write its size
let sizeData=Data(bytes:&cryptedSize,count:intSize)
handle.write(sizeData)
}
}
// MARK: - UnFlocking
/// Transforms a .flk to a file tree
///
/// - Parameters:
/// - flockedFile: the flock
/// - destination: the folder path
/// - progression:
/// - success:
/// - failure:
func unFlock(flockedFile:String
,to destination:String
,progression:@escaping((Progression)->()),
success:@escaping (BytesStats)->(),
failure:@escaping(_ message:String)->()){
do{
let container = try self.containerFrom(flockedFile: flockedFile)
Async.utility{
if let fileHandle=FileHandle(forReadingAtPath:flockedFile ){
let progressionState=Progression()
progressionState.quietChanges{
progressionState.totalTaskCount=container.nodes.count
progressionState.currentTaskIndex=0
progressionState.externalIdentifier=flockedFile
progressionState.message=""
}
let stats=BytesStats(name: "Unflocking: \(flockedFile)")
var failuresMessages=[String]()
var counter=0
// Relays the progression
func __onProgression(message:String, incrementGlobalCounter:Bool=true){
if incrementGlobalCounter{
counter += 1
}
progressionState.quietChanges{
progressionState.currentTaskIndex=counter
progressionState.message=message
}
progressionState.currentPercentProgress=Double(counter)*Double(100)/Double(progressionState.totalTaskCount)
syncOnMain{
// Relay the progression
progression(progressionState)
}
}
for node in container.nodes{
let blocks:[Block]=container.blocks
// Consecutive blocks are faster to assemble
let sortedBlocks=blocks.sorted(by: { (lb, rb) -> Bool in
return lb.rank < rb.rank
})
self._assembleNode(node: node,
blocks: sortedBlocks,
flockFileHandle:fileHandle,
assemblyFolderPath: destination,
stats:stats,
progression: {
(subProgression) in
__onProgression(message:subProgression.message,incrementGlobalCounter: false)
}, success: {
__onProgression(message:"Unflocked: \(node.relativePath)",incrementGlobalCounter: true)
if counter==container.nodes.count{
syncOnMain{
if failuresMessages.count==0{
// it is a success
success(stats)
}else{
// Reduce the errors
failure(failuresMessages.reduce("Errors: ", { (r, s) -> String in
return r + " \(s)"
}))
}
}
}
}, failure: { (createdPaths,message) in
__onProgression(message:"Unflocking error: \(message)",incrementGlobalCounter: true)
failuresMessages.append(message)
})
}
}else{
syncOnMain{
failure(NSLocalizedString("Enable to create Reading file Handle", tableName:"system", comment: "Enable to create Reading file Handle")+" \(flockedFile)")
}
}
}
}catch{
failure(NSLocalizedString("Container error", tableName:"system", comment: "Container Error") + " \(error)")
}
}
/// Returns the deserialized container from the flock file
///
/// - Parameter flockedFile: the flocked file
/// - Returns: the Container for potential random access
func containerFrom(flockedFile:String)throws->Container{
let group=AsyncGroup()
var container:Container?
var error:Error?=nil
group.utility {
if let fileHandle=FileHandle(forReadingAtPath:flockedFile ){
// We Can't guess what will Happen
// But we want a guarantee the handle will be closed
defer{
fileHandle.closeFile()
}
/*
Binary Format specs
--------
data -> the Nodes binary
--------
footer -> serialized crypted and compressed Container
--------
8Bytes for one (UInt64) -> gives the footer size
--------
*/
do{
let _=fileHandle.seekToEndOfFile()
let l=fileHandle.offsetInFile
let intSize=MemoryLayout<UInt64>.size
// Go to size position
let sizePosition=UInt64(l)-UInt64(intSize)
fileHandle.seek(toFileOffset:sizePosition)
let footerSizeData=fileHandle.readData(ofLength: intSize)
let footerSize:UInt64=footerSizeData.withUnsafeBytes { $0.pointee }
/// Go to footer position
let footerPosition=UInt64(l)-(UInt64(intSize)+footerSize)
fileHandle.seek(toFileOffset:footerPosition)
var data=fileHandle.readData(ofLength: Int(footerSize))
data = try self._cryptoHelper.decryptData(data,useKey: self._key)
data = try data.decompress(algorithm: .lz4)
container = try JSON.decoder.decode(Container.self, from: data)
}catch let e{
error=e
}
}else{
error=FlockerError.unableToAccessToContainer(at:flockedFile,message:"Unable to create Handle")
}
}
group.wait()
if error != nil{
throw error!
}
if let container = container{
return container
}else{
throw FlockerError.unableToAccessToContainer(at:flockedFile,message: "Container is void")
}
}
/// Assembles the node
/// IMPORTANT! this method Must be called on the utility Queue
/// - Parameters:
/// - node: the node
/// - blocks: the blocks
/// - assemblyFolderPath: the destination folder where the chunks will be joined (or assembled)
/// - progression: the progression closure
/// - success: the success closure
/// - failure: the failure closure with to created paths (to be able to roll back)
public func _assembleNode( node:Node,
blocks:[Block],
flockFileHandle:FileHandle,
assemblyFolderPath:String,
stats:BytesStats,
progression:@escaping((Progression)->()),
success:@escaping ()->(),
failure:@escaping (_ createdPaths:[String],_ message:String)->()){
var failuresMessages=[String]()
var createdPaths=[String]()
let decrypt=node.cryptedBlocks
let decompress=node.compressedBlocks
let progressionState=Progression()
progressionState.quietChanges{
progressionState.totalTaskCount=blocks.count
progressionState.currentTaskIndex=0
progressionState.externalIdentifier=""
progressionState.message=""
}
var counter=0
let destinationFile=assemblyFolderPath+node.relativePath
let nodeNature=node.nature
// Sub func for normalized progression and finalization handling
func __progressWithPath(_ path:String,error:Bool=false,message:String=""){
counter += 1
if (!error){
createdPaths.append(path)
}
progressionState.quietChanges{
progressionState.message = (message == "") ? path : message
progressionState.currentTaskIndex=counter
}
progressionState.currentPercentProgress=Double(counter)*Double(100)/Double(progressionState.totalTaskCount)
// Relay the progression
syncOnMain{
progression(progressionState)
}
if counter>=blocks.count{
syncOnMain{
if failuresMessages.count==0{
// it is a success
success()
}else{
let messages=failuresMessages.reduce("Errors: ", { (r, s) -> String in
return r + " \(s)"
})
// Reduce the errors
failure(createdPaths, messages)
}
}
}
}
if nodeNature == .file{
do{
let folderPath=(destinationFile as NSString).deletingLastPathComponent
try self._fileManager.createDirectory(atPath: folderPath, withIntermediateDirectories: true, attributes: nil)
self._fileManager.createFile(atPath: destinationFile, contents: nil, attributes: nil)
if let writeFileHandler = FileHandle(forWritingAtPath:destinationFile ){
// We Can't guess what will Happen
// But we want a guarantee the handle will be closed
defer{
writeFileHandler.closeFile()
}
writeFileHandler.seek(toFileOffset: 0)
for block in blocks{
autoreleasepool{
do{
let startsAt=UInt64(block.startsAt)
let size=block.size
flockFileHandle.seek(toFileOffset: startsAt)
var data = flockFileHandle.readData(ofLength: size)
if decrypt{
data = try self._cryptoHelper.decryptData(data,useKey: self._key)
}
if decompress{
data = try data.decompress(algorithm: .lz4)
}
let decompressDataSize=data.count
writeFileHandler.write(data)
stats.consign(numberOfBytes: UInt(decompressDataSize),compressedBytes: UInt(size))
let message="\(block.rank) \(destinationFile): <\( block.startsAt), \(block.startsAt+block.size)> + \(block.size) Bytes"
__progressWithPath(destinationFile,error: false,message:message )
}catch{
failuresMessages.append("\(error)")
__progressWithPath(destinationFile,error:true)
}
}
}
}else{
failuresMessages.append("Enable to create file Handle \(destinationFile)")
__progressWithPath(destinationFile,error:true)
}
}catch{
failuresMessages.append("\(error)")
__progressWithPath(destinationFile,error:true)
}
}else if nodeNature == .folder{
do{
if !self._fileManager.fileExists(atPath: destinationFile){
try self._fileManager.createDirectory(atPath: destinationFile, withIntermediateDirectories: true, attributes: nil)
}
__progressWithPath(destinationFile)
}catch{
failuresMessages.append("\(error)")
__progressWithPath(destinationFile,error:true)
}
}else if nodeNature == .alias{
do{
if self._fileManager.fileExists(atPath: destinationFile){
try? self._fileManager.removeItem(atPath: destinationFile)
}
try self._fileManager.createSymbolicLink(atPath: destinationFile, withDestinationPath: node.proxyPath!)
__progressWithPath(destinationFile)
}catch{
failuresMessages.append("\(error)")
__progressWithPath(destinationFile,error:true)
}
}
}
// MARK: - Random Access TODO
// MARK: Read Access
func extractNode(node:Node,from container:Container, of flockedFilePath:String, destinationPath:String){
}
// MARK: Write Access
// LOGICAL action bytes are not removed.
func delete(node:Node,from container:Container, of flockedFilePath:String, destinationPath:String)->String{
return ""
}
func add(fileReference:FileReference,from container:Container, of flockedFilePath:String,to relativePath:String){
}
// MARK: Utility
// Remove the holes
func compact(container:Container, of flockedFilePath:String){
// TODO
}
// MARK: - Private implementation details
/// Test the validity of a path
/// 1# We test the existence of the path.
/// 2# Some typeSymbolicLink may point to themselves and then be considerated as inexistent
/// We consider those symlinks as valid entries (those symlink are generally in frameworks QA required)
///
/// - Parameter path: the path
/// - Returns: true if the path is valid
fileprivate func _isPathValid(_ path:String)->Bool{
if self._fileManager.fileExists(atPath:path){
return true
}
if let attributes:[FileAttributeKey : Any] = try? self._fileManager.attributesOfItem(atPath: path){
if let type=attributes[FileAttributeKey.type] as? FileAttributeType{
if type == .typeSymbolicLink{
return true
}
}
}
return false
}
fileprivate func _resolveAlias(at path:String) throws -> String {
let pathURL=URL(fileURLWithPath: path)
let original = try URL(resolvingAliasFileAt: pathURL, options:[])
return original.path
}
}
| apache-2.0 | 1968af8f44b018777e60a82f81e80ce9 | 44.495037 | 186 | 0.453653 | 6.439937 | false | false | false | false |
OpenCraft/OCHuds | OCHuds/Classes/AnimatedView.swift | 1 | 996 | //
// AnimatedView.swift
// Pods
//
// Created by Giovane Berny Possebon on 16/08/16.
//
//
import UIKit
internal class AnimatedView: HudView {
var imageView: UIImageView
var images: [UIImage] = []
init(withViewController viewController: UIViewController, images: [UIImage], backgroundColor: UIColor, animationDuration: Double) {
self.imageView = UIImageView()
imageView.animationDuration = animationDuration
imageView.animationImages = images
super.init(withViewController: viewController)
centerView.addSubview(imageView)
centerView.backgroundColor = backgroundColor
imageView.centerSuperview(withSize: CGSize(width: 40, height: 40))
imageView.backgroundColor = UIColor.clear
imageView.contentMode = .scaleAspectFit
imageView.startAnimating()
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
| mit | 30ed102a906720a12a9f0e34654d85cd | 27.457143 | 135 | 0.677711 | 4.98 | false | false | false | false |
mitochrome/complex-gestures-demo | apps/GestureRecognizer/Carthage/Checkouts/swift-protobuf/Sources/protoc-gen-swift/FieldGenerator.swift | 3 | 3549 | // Sources/protoc-gen-swift/FieldGenerator.swift - Base class for Field Generators
//
// Copyright (c) 2014 - 2017 Apple Inc. and the project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See LICENSE.txt for license information:
// https://github.com/apple/swift-protobuf/blob/master/LICENSE.txt
//
// -----------------------------------------------------------------------------
///
/// Code generation for the private storage class used inside copy-on-write
/// messages.
///
// -----------------------------------------------------------------------------
import Foundation
import PluginLibrary
import SwiftProtobuf
/// Interface for field generators.
protocol FieldGenerator {
var number: Int { get }
/// Name mapping entry for the field.
var fieldMapNames: String { get }
/// Generate the interface for this field, this is includes any extra methods (has/clear).
func generateInterface(printer: inout CodePrinter)
/// Generate any additional storage needed for this field.
func generateStorage(printer: inout CodePrinter)
/// Generate the line to copy this field during a _StorageClass clone.
func generateStorageClassClone(printer: inout CodePrinter)
/// Generate the case and decoder invoke needed for this field.
func generateDecodeFieldCase(printer: inout CodePrinter)
/// Generate the support for traversing this field.
func generateTraverse(printer: inout CodePrinter)
/// Generate support for comparing this field's value.
/// The generated code should return false in the current scope if the field's don't match.
func generateFieldComparison(printer: inout CodePrinter)
/// Generate any support needed to ensure required fields are set.
/// The generated code should return false the field isn't set.
func generateRequiredFieldCheck(printer: inout CodePrinter)
/// Generate any support needed to this field's value is initialized.
/// The generated code should return false if it isn't set.
func generateIsInitializedCheck(printer: inout CodePrinter)
}
/// Simple base class for FieldGenerators that also provides fieldMapNames.
class FieldGeneratorBase {
let number: Int
let fieldDescriptor: FieldDescriptor
var fieldMapNames: String {
// Protobuf Text uses the unqualified group name for the field
// name instead of the field name provided by protoc. As far
// as I can tell, no one uses the fieldname provided by protoc,
// so let's just put the field name that Protobuf Text
// actually uses here.
let protoName: String
if fieldDescriptor.type == .group {
protoName = fieldDescriptor.messageType.name
} else {
protoName = fieldDescriptor.name
}
let jsonName = fieldDescriptor.jsonName ?? protoName
if jsonName == protoName {
/// The proto and JSON names are identical:
return ".same(proto: \"\(protoName)\")"
} else {
let libraryGeneratedJsonName = NamingUtils.toJsonFieldName(protoName)
if jsonName == libraryGeneratedJsonName {
/// The library will generate the same thing protoc gave, so
/// we can let the library recompute this:
return ".standard(proto: \"\(protoName)\")"
} else {
/// The library's generation didn't match, so specify this explicitly.
return ".unique(proto: \"\(protoName)\", json: \"\(jsonName)\")"
}
}
}
init(descriptor: FieldDescriptor) {
precondition(!descriptor.isExtension)
number = Int(descriptor.number)
fieldDescriptor = descriptor
}
}
| mit | eadfa21ff0b902999b1aaf77d0feb866 | 35.96875 | 93 | 0.689208 | 4.828571 | false | false | false | false |
aschwaighofer/swift | test/Driver/Dependencies/fail-chained-fine.swift | 2 | 6984 | /// a ==> bad ==> c ==> d | b --> bad --> e ==> f
// RUN: %empty-directory(%t)
// RUN: cp -r %S/Inputs/fail-chained-fine/* %t
// RUN: touch -t 201401240005 %t/*
// RUN: cd %t && %swiftc_driver -c -driver-use-frontend-path "%{python};%S/Inputs/update-dependencies.py" -output-file-map %t/output.json -incremental -driver-always-rebuild-dependents ./a.swift ./b.swift ./c.swift ./d.swift ./e.swift ./f.swift ./bad.swift -module-name main -j1 -v 2>&1 | %FileCheck -check-prefix=CHECK-FIRST %s
// RUN: %FileCheck -check-prefix=CHECK-RECORD-CLEAN %s < %t/main~buildrecord.swiftdeps
// CHECK-FIRST-NOT: warning
// CHECK-FIRST: Handled a.swift
// CHECK-FIRST: Handled b.swift
// CHECK-FIRST: Handled c.swift
// CHECK-FIRST: Handled d.swift
// CHECK-FIRST: Handled e.swift
// CHECK-FIRST: Handled f.swift
// CHECK-FIRST: Handled bad.swift
// CHECK-RECORD-CLEAN-DAG: "./a.swift": [
// CHECK-RECORD-CLEAN-DAG: "./b.swift": [
// CHECK-RECORD-CLEAN-DAG: "./c.swift": [
// CHECK-RECORD-CLEAN-DAG: "./d.swift": [
// CHECK-RECORD-CLEAN-DAG: "./e.swift": [
// CHECK-RECORD-CLEAN-DAG: "./f.swift": [
// CHECK-RECORD-CLEAN-DAG: "./bad.swift": [
// RUN: touch -t 201401240006 %t/a.swift
// RUN: cd %t && not %swiftc_driver -c -driver-use-frontend-path "%{python};%S/Inputs/update-dependencies-bad.py" -output-file-map %t/output.json -incremental -driver-always-rebuild-dependents ./a.swift ./bad.swift ./b.swift ./c.swift ./d.swift ./e.swift ./f.swift -module-name main -j1 -v > %t/a.txt 2>&1
// RUN: %FileCheck -check-prefix=CHECK-A %s < %t/a.txt
// RUN: %FileCheck -check-prefix=NEGATIVE-A %s < %t/a.txt
// RUN: %FileCheck -check-prefix=CHECK-RECORD-A %s < %t/main~buildrecord.swiftdeps
// CHECK-A: Handled a.swift
// CHECK-A: Handled bad.swift
// NEGATIVE-A-NOT: Handled b.swift
// NEGATIVE-A-NOT: Handled c.swift
// NEGATIVE-A-NOT: Handled d.swift
// NEGATIVE-A-NOT: Handled e.swift
// NEGATIVE-A-NOT: Handled f.swift
// CHECK-RECORD-A-DAG: "./a.swift": [
// CHECK-RECORD-A-DAG: "./b.swift": [
// CHECK-RECORD-A-DAG: "./c.swift": !private [
// CHECK-RECORD-A-DAG: "./d.swift": !private [
// CHECK-RECORD-A-DAG: "./e.swift": !private [
// CHECK-RECORD-A-DAG: "./f.swift": [
// CHECK-RECORD-A-DAG: "./bad.swift": !private [
// RUN: cd %t && %swiftc_driver -c -driver-use-frontend-path "%{python};%S/Inputs/update-dependencies.py" -output-file-map %t/output.json -incremental -driver-always-rebuild-dependents ./a.swift ./b.swift ./c.swift ./d.swift ./e.swift ./f.swift ./bad.swift -module-name main -j1 -v > %t/a2.txt 2>&1
// RUN: %FileCheck -check-prefix=CHECK-A2 %s < %t/a2.txt
// RUN: %FileCheck -check-prefix=NEGATIVE-A2 %s < %t/a2.txt
// RUN: %FileCheck -check-prefix=CHECK-RECORD-CLEAN %s < %t/main~buildrecord.swiftdeps
// CHECK-A2-DAG: Handled c.swift
// CHECK-A2-DAG: Handled d.swift
// CHECK-A2-DAG: Handled e.swift
// CHECK-A2-DAG: Handled bad.swift
// NEGATIVE-A2-NOT: Handled a.swift
// NEGATIVE-A2-NOT: Handled b.swift
// NEGATIVE-A2-NOT: Handled f.swift
// RUN: %empty-directory(%t)
// RUN: cp -r %S/Inputs/fail-chained-fine/* %t
// RUN: touch -t 201401240005 %t/*
// RUN: cd %t && %swiftc_driver -c -driver-use-frontend-path "%{python};%S/Inputs/update-dependencies.py" -output-file-map %t/output.json -incremental -driver-always-rebuild-dependents ./a.swift ./b.swift ./c.swift ./d.swift ./e.swift ./f.swift ./bad.swift -module-name main -j1 -v 2>&1 | %FileCheck -check-prefix=CHECK-FIRST %s
// RUN: touch -t 201401240006 %t/b.swift
// RUN: cd %t && not %swiftc_driver -c -driver-use-frontend-path "%{python};%S/Inputs/update-dependencies-bad.py" -output-file-map %t/output.json -incremental -driver-always-rebuild-dependents ./a.swift ./b.swift ./c.swift ./d.swift ./e.swift ./f.swift ./bad.swift -module-name main -j1 -v > %t/b.txt 2>&1
// RUN: %FileCheck -check-prefix=CHECK-B %s < %t/b.txt
// RUN: %FileCheck -check-prefix=NEGATIVE-B %s < %t/b.txt
// RUN: %FileCheck -check-prefix=CHECK-RECORD-B %s < %t/main~buildrecord.swiftdeps
// CHECK-B: Handled b.swift
// CHECK-B: Handled bad.swift
// NEGATIVE-B-NOT: Handled a.swift
// NEGATIVE-B-NOT: Handled c.swift
// NEGATIVE-B-NOT: Handled d.swift
// NEGATIVE-B-NOT: Handled e.swift
// NEGATIVE-B-NOT: Handled f.swift
// CHECK-RECORD-B-DAG: "./a.swift": [
// CHECK-RECORD-B-DAG: "./b.swift": [
// CHECK-RECORD-B-DAG: "./c.swift": [
// CHECK-RECORD-B-DAG: "./d.swift": [
// CHECK-RECORD-B-DAG: "./e.swift": [
// CHECK-RECORD-B-DAG: "./f.swift": [
// CHECK-RECORD-B-DAG: "./bad.swift": !private [
// RUN: cd %t && %swiftc_driver -c -driver-use-frontend-path "%{python};%S/Inputs/update-dependencies.py" -output-file-map %t/output.json -incremental -driver-always-rebuild-dependents ./a.swift ./b.swift ./c.swift ./d.swift ./e.swift ./f.swift ./bad.swift -module-name main -j1 -v > %t/b2.txt 2>&1
// RUN: %FileCheck -check-prefix=CHECK-B2 %s < %t/b2.txt
// RUN: %FileCheck -check-prefix=NEGATIVE-B2 %s < %t/b2.txt
// RUN: %FileCheck -check-prefix=CHECK-RECORD-CLEAN %s < %t/main~buildrecord.swiftdeps
// CHECK-B2-DAG: Handled bad.swift
// NEGATIVE-B2-NOT: Handled a.swift
// NEGATIVE-B2-NOT: Handled b.swift
// NEGATIVE-B2-NOT: Handled c.swift
// NEGATIVE-B2-NOT: Handled d.swift
// NEGATIVE-B2-NOT: Handled e.swift
// NEGATIVE-B2-NOT: Handled f.swift
// RUN: %empty-directory(%t)
// RUN: cp -r %S/Inputs/fail-chained-fine/* %t
// RUN: touch -t 201401240005 %t/*
// RUN: cd %t && %swiftc_driver -c -driver-use-frontend-path "%{python};%S/Inputs/update-dependencies.py" -output-file-map %t/output.json -incremental -driver-always-rebuild-dependents ./a.swift ./b.swift ./c.swift ./d.swift ./e.swift ./f.swift ./bad.swift -module-name main -j1 -v 2>&1 | %FileCheck -check-prefix=CHECK-FIRST %s
// RUN: touch -t 201401240006 %t/bad.swift
// RUN: cd %t && not %swiftc_driver -c -driver-use-frontend-path "%{python};%S/Inputs/update-dependencies-bad.py" -output-file-map %t/output.json -incremental -driver-always-rebuild-dependents ./bad.swift ./a.swift ./b.swift ./c.swift ./d.swift ./e.swift ./f.swift -module-name main -j1 -v > %t/bad.txt 2>&1
// RUN: %FileCheck -check-prefix=CHECK-BAD %s < %t/bad.txt
// RUN: %FileCheck -check-prefix=NEGATIVE-BAD %s < %t/bad.txt
// RUN: %FileCheck -check-prefix=CHECK-RECORD-A %s < %t/main~buildrecord.swiftdeps
// CHECK-BAD: Handled bad.swift
// NEGATIVE-BAD-NOT: Handled a.swift
// NEGATIVE-BAD-NOT: Handled b.swift
// NEGATIVE-BAD-NOT: Handled c.swift
// NEGATIVE-BAD-NOT: Handled d.swift
// NEGATIVE-BAD-NOT: Handled e.swift
// NEGATIVE-BAD-NOT: Handled f.swift
// RUN: cd %t && %swiftc_driver -c -driver-use-frontend-path "%{python};%S/Inputs/update-dependencies.py" -output-file-map %t/output.json -incremental -driver-always-rebuild-dependents ./a.swift ./b.swift ./c.swift ./d.swift ./e.swift ./f.swift ./bad.swift -module-name main -j1 -v > %t/bad2.txt 2>&1
// RUN: %FileCheck -check-prefix=CHECK-A2 %s < %t/bad2.txt
// RUN: %FileCheck -check-prefix=NEGATIVE-A2 %s < %t/bad2.txt
// RUN: %FileCheck -check-prefix=CHECK-RECORD-CLEAN %s < %t/main~buildrecord.swiftdeps
| apache-2.0 | d92ef5a97f96098a71cbc08970fbcc21 | 53.139535 | 329 | 0.675687 | 2.783579 | false | false | false | false |
28stephlim/HeartbeatAnalysis-master | VoiceMemos/Controller/OutputViewController.swift | 1 | 7737 | ////
//// OutputViewController.swift
//// VoiceMemos
////
//// Created by Jordan O'Callaghan on 10/09/2016.
//// Copyright © 2016 Zhouqi Mo. All rights reserved.
////
//
//import UIKit
//import FDWaveformView
//import Foundation
//
//class OutputViewController: UIViewController {
//
// @IBOutlet weak var Diagnosis1: UILabel!
// @IBOutlet weak var Diagnosis2: UILabel!
// @IBOutlet weak var Diagnosis3: UILabel!
// @IBOutlet weak var Diagnosis4: UILabel!
// @IBOutlet weak var Diagnosis5: UILabel!
// @IBOutlet weak var Diagnosis6: UILabel!
// @IBOutlet weak var Diagnosis7: UILabel!
//
// @IBOutlet weak var waveform: FDWaveformView!
// var inputURL: NSURL!
// var supine = ["Apex Supine Early Sys Mur", "Apex Supine Holo Sys Mur", "Apex Supine Late Sys Mur", "Apex Supine Mid Sys Click", "Apex Supine Mid Sys Mur", "Apex Supine Single S1 S2", "Apex Supine Split S1"]
// var diagnosisname = String ()
//
//
// override func viewDidLoad() {
// super.viewDidLoad()
//
// // Do any additional setup after loading the view.
// }
//
// override func didReceiveMemoryWarning() {
// super.didReceiveMemoryWarning()
// // Dispose of any resources that can be recreated.
// }
//
// //preparing data for 2nd VC
// override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
// let VC2 : ConditionViewController = segue.destinationViewController as! ConditionViewController
// VC2.highname = diagnosisname
//
// }
//
//
//
// override func viewDidAppear(animated: Bool) {
//
// self.Diagnosis1.text = ""
// self.Diagnosis2.text = ""
// self.Diagnosis3.text = ""
// self.Diagnosis4.text = ""
// self.Diagnosis5.text = ""
// self.Diagnosis6.text = ""
// self.Diagnosis7.text = ""
//
// self.waveform.audioURL = inputURL
// self.waveform.progressSamples = 0
// self.waveform.doesAllowScroll = true
// self.waveform.doesAllowStretch = true
// self.waveform.doesAllowScrubbing = false
// self.waveform.wavesColor = UIColor.blueColor()
//
//
//
// /*
// self.Diagnosis3.text = supine[0] //+" \(match variable)"
// self.Diagnosis4.text = supine[1]
// */
//
// compareHeartbeat()
// }
//
//
//
// func compareHeartbeat(){
//
//
// let alertController = UIAlertController(title: "Select Measurement Type", message: "Press one of these buttons", preferredStyle: .Alert)
// //We add buttons to the alert controller by creating UIAlertActions:
//
// let actionSupine = UIAlertAction(title: "Apex Supine",
// style: .Default)
// {(alertController: UIAlertAction!)->Void in
//
// self.signalCompare(self.supine)
//
// }
//
//
// alertController.addAction(actionSupine)
//
// let actionDucubitus = UIAlertAction(title: "Apex Left Ducubitus",
// style: .Default)
// {(alertController: UIAlertAction!)->Void in
//
// self.signalCompare(self.supine)
// }
//
// alertController.addAction(actionDucubitus)
//
// let actionAortic = UIAlertAction(title: "Aortic",
// style: .Default)
// {(alertController: UIAlertAction!)->Void in
//
// self.signalCompare(self.supine)
// }
//
// alertController.addAction(actionAortic)
//
// let actionPulmonic = UIAlertAction(title: "Pulmonic",
// style: .Default)
// {(alertController: UIAlertAction!)->Void in
//
// self.signalCompare(self.supine)
// }
//
// alertController.addAction(actionPulmonic)
//
// self.presentViewController(alertController, animated: true, completion: nil)
//
//
// }
//
//
// func signalCompare(type: [String]){
//
// var detective = LBAudioDetectiveNew()
// var bundle = NSBundle.mainBundle()
// var matchArray = [Float32]()
//
//
// dispatch_async(dispatch_get_main_queue(), {() -> Void in
//
// (type as NSArray).enumerateObjectsUsingBlock({(sequenceType: Any, idx: Int, stop: UnsafeMutablePointer<ObjCBool>) -> Void in
//
// if let str = sequenceType as? String {
//
// var sequenceURL = bundle.URLForResource(str, withExtension: "caf")!
//
// var match: Float32 = 0.0
// LBAudioDetectiveCompareAudioURLs(detective, self.inputURL, sequenceURL, 0, &match)
// print("Match = \(match)")
// matchArray.append(match)
// }
// })
//
// var maxMatch = matchArray.maxElement()
// var maxLocationIndex = matchArray.indexOf(maxMatch!)
// var maxLocationInt = matchArray.startIndex.distanceTo(maxLocationIndex!)
// var typeSize = type.count
//
//
// self.Diagnosis1.text = type[0] + ": \(matchArray[0])"
// self.Diagnosis2.text = type[1] + ": \(matchArray[1])"
// self.Diagnosis3.text = type[2] + ": \(matchArray[2])"
// self.Diagnosis4.text = type[3] + ": \(matchArray[3])"
// if (typeSize>4){
// self.Diagnosis5.text = type[4] + ": \(matchArray[4])"
// self.Diagnosis6.text = type[5] + ": \(matchArray[5])"
// if (typeSize>6){ // These if statements make sure the data shows properly since we have
// self.Diagnosis7.text = type[6] + ": \(matchArray[6])" //different amounts of audio files for each category
//
// }
// }
// self.diagnosisname = type[maxLocationIndex!]
//
// switch maxLocationInt {
// case 0:
// self.Diagnosis1.textColor = UIColor.redColor()
// case 1:
// self.Diagnosis2.textColor = UIColor.redColor()
// case 2:
// self.Diagnosis3.textColor = UIColor.redColor()
// case 3:
// self.Diagnosis4.textColor = UIColor.redColor()
// case 4:
// self.Diagnosis5.textColor = UIColor.redColor()
// case 5:
// self.Diagnosis6.textColor = UIColor.redColor()
// case 6:
// self.Diagnosis7.textColor = UIColor.redColor()
// default:
// print("error has occurred changing text colour") //This probably wont happen
// }
//
// //let diagnosisAlert = UIAlertController(title: "Diagnosis", message: "\(type[maxLocation!])", preferredStyle: .Alert)
// //let okButton = UIAlertAction(title: "OK", style: .Default){(diagnosisAlert: UIAlertAction!)->Void in }
// //diagnosisAlert.addAction(okButton)
// //self.presentViewController(diagnosisAlert, animated: true, completion: nil)
//
//
// })
// }
//} | mit | 71fd01655058072ad6b2c4051578431b | 38.274112 | 212 | 0.512797 | 4.255226 | false | false | false | false |
kyungmin/pigeon | Pigeon/Pigeon/LaunchViewController.swift | 1 | 4015 | //
// LaunchViewController.swift
// Pigeon
//
// Created by Wanting Huang on 3/21/15.
// Copyright (c) 2015 Kyungmin Kim. All rights reserved.
//
import UIKit
class LaunchViewController: UIViewController {
@IBOutlet weak var pigeonImageView: UIImageView!
@IBOutlet weak var postcardImageView: UIImageView!
@IBOutlet weak var pigeonText: UIImageView!
override func viewDidLoad() {
super.viewDidLoad()
// now create a bezier path that defines our curve
// the animation function needs the curve defined as a CGPath
// but these are more difficult to work with, so instead
// we'll create a UIBezierPath, and then create a
// CGPath from the bezier when we need it
let pathPigeon = UIBezierPath()
pathPigeon.moveToPoint(CGPoint(x: -10,y: 53))
pathPigeon.addCurveToPoint(CGPoint(x: 350, y: 70), controlPoint1: CGPoint(x: 180, y: 130), controlPoint2: CGPoint(x: 200, y: 150))
let pathPostcard = UIBezierPath()
pathPostcard.moveToPoint(CGPoint(x: -10,y: 78))
pathPostcard.addCurveToPoint(CGPoint(x: 160, y:430 ), controlPoint1: CGPoint(x: 110, y: 260), controlPoint2: CGPoint(x: 150, y: 100))
// create a new CAKeyframeAnimation that animates the objects position
let animPigeon = CAKeyframeAnimation(keyPath: "position")
let animPostcard = CAKeyframeAnimation(keyPath: "position")
// set the animations path to our bezier curve
animPigeon.path = pathPigeon.CGPath
animPostcard.path = pathPostcard.CGPath
// set some more parameters for the animation
// this rotation mode means that our object will rotate so that it's parallel to whatever point it is currently on the curve
animPigeon.rotationMode = kCAAnimationRotateAuto
//animPigeon.repeatCount = Float.infinity
animPigeon.duration = 2.0
animPigeon.timingFunctions = [CAMediaTimingFunction(name: kCAMediaTimingFunctionEaseInEaseOut)]
//animPostcard.rotationMode = kCAAnimationRotateAuto
//animPostcard.repeatCount = Float.infinity
animPostcard.duration = 3.0
animPostcard.timingFunctions = [CAMediaTimingFunction(name: kCAMediaTimingFunctionEaseInEaseOut)]
// we add the animation to the squares 'layer' property
pigeonImageView.layer.addAnimation(animPigeon, forKey: "animate position along path")
postcardImageView.layer.addAnimation(animPostcard, forKey: "animate position along path")
UIView.animateWithDuration(0.5, delay: 3, usingSpringWithDamping: 0.7, initialSpringVelocity: 10, options: nil, animations: {
//self.postcardImageView.frame.origin.y + 10
}, completion: { finished in
// any code entered here will be applied
// once the animation has completed
})
UIView.animateWithDuration(0.5, delay: 2.5, options: nil, animations: { () -> Void in
self.pigeonText.alpha = 0.99
}) { finished in
UIView.animateWithDuration(1.5, animations: { () -> Void in
self.pigeonText.alpha = 1
}) { finished in
self.performSegueWithIdentifier("frontContainerSegue", sender: self)
}
}
// Do any additional setup after loading the view.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
/*
// MARK: - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
// Get the new view controller using segue.destinationViewController.
// Pass the selected object to the new view controller.
}
*/
}
| mit | 4d5ccac7c5284070864014f4cee2f762 | 41.263158 | 141 | 0.652802 | 5.012484 | false | false | false | false |
dennisweissmann/Basics | Source/Device.generated.swift | 1 | 80253 | //===----------------------------------------------------------------------===//
//
// This source file is part of the DeviceKit open source project
//
// Copyright © 2014 - 2018 Dennis Weissmann and the DeviceKit project authors
//
// License: https://github.com/dennisweissmann/DeviceKit/blob/master/LICENSE
// Contributors: https://github.com/dennisweissmann/DeviceKit#contributors
//
//===----------------------------------------------------------------------===//
#if os(watchOS)
import WatchKit
#else
import UIKit
#endif
// MARK: Device
/// This enum is a value-type wrapper and extension of
/// [`UIDevice`](https://developer.apple.com/library/ios/documentation/UIKit/Reference/UIDevice_Class/).
///
/// Usage:
///
/// let device = Device.current
///
/// print(device) // prints, for example, "iPhone 6 Plus"
///
/// if device == .iPhone6Plus {
/// // Do something
/// } else {
/// // Do something else
/// }
///
/// ...
///
/// if device.batteryState == .full || device.batteryState >= .charging(75) {
/// print("Your battery is happy! 😊")
/// }
///
/// ...
///
/// if device.batteryLevel >= 50 {
/// install_iOS()
/// } else {
/// showError()
/// }
///
public enum Device {
#if os(iOS)
/// Device is an [iPod touch (5th generation)](https://support.apple.com/kb/SP657)
///
/// 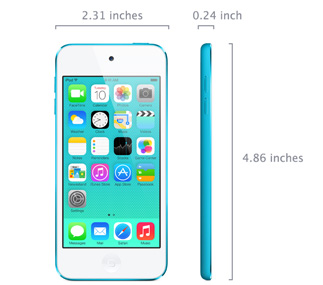
case iPodTouch5
/// Device is an [iPod touch (6th generation)](https://support.apple.com/kb/SP720)
///
/// 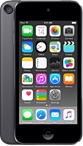
case iPodTouch6
/// Device is an [iPod touch (7th generation)](https://support.apple.com/kb/SP796)
///
/// 
case iPodTouch7
/// Device is an [iPhone 4](https://support.apple.com/kb/SP587)
///
/// 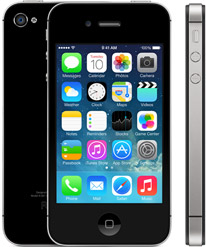
case iPhone4
/// Device is an [iPhone 4s](https://support.apple.com/kb/SP643)
///
/// 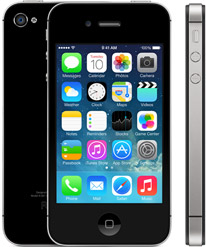
case iPhone4s
/// Device is an [iPhone 5](https://support.apple.com/kb/SP655)
///
/// 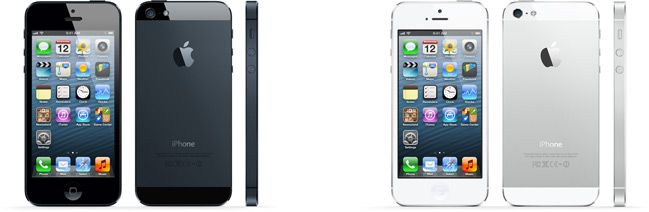
case iPhone5
/// Device is an [iPhone 5c](https://support.apple.com/kb/SP684)
///
/// 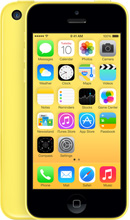
case iPhone5c
/// Device is an [iPhone 5s](https://support.apple.com/kb/SP685)
///
/// 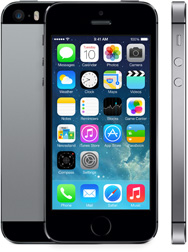
case iPhone5s
/// Device is an [iPhone 6](https://support.apple.com/kb/SP705)
///
/// 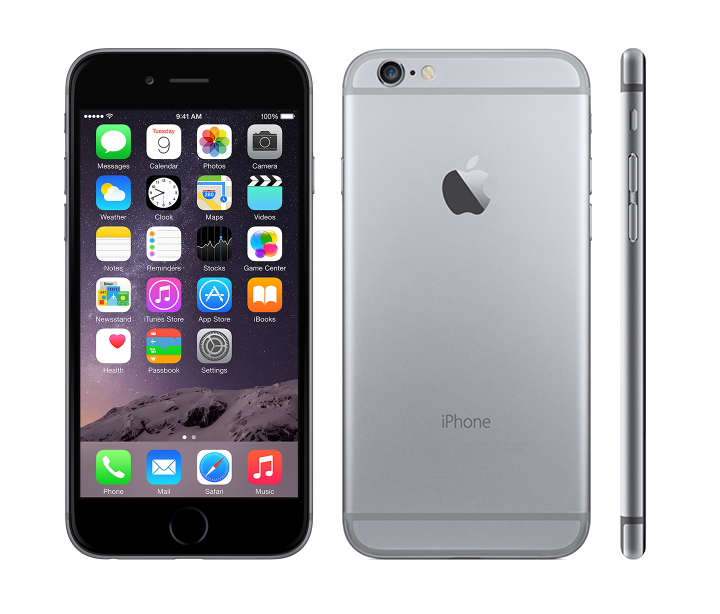
case iPhone6
/// Device is an [iPhone 6 Plus](https://support.apple.com/kb/SP706)
///
/// 
case iPhone6Plus
/// Device is an [iPhone 6s](https://support.apple.com/kb/SP726)
///
/// 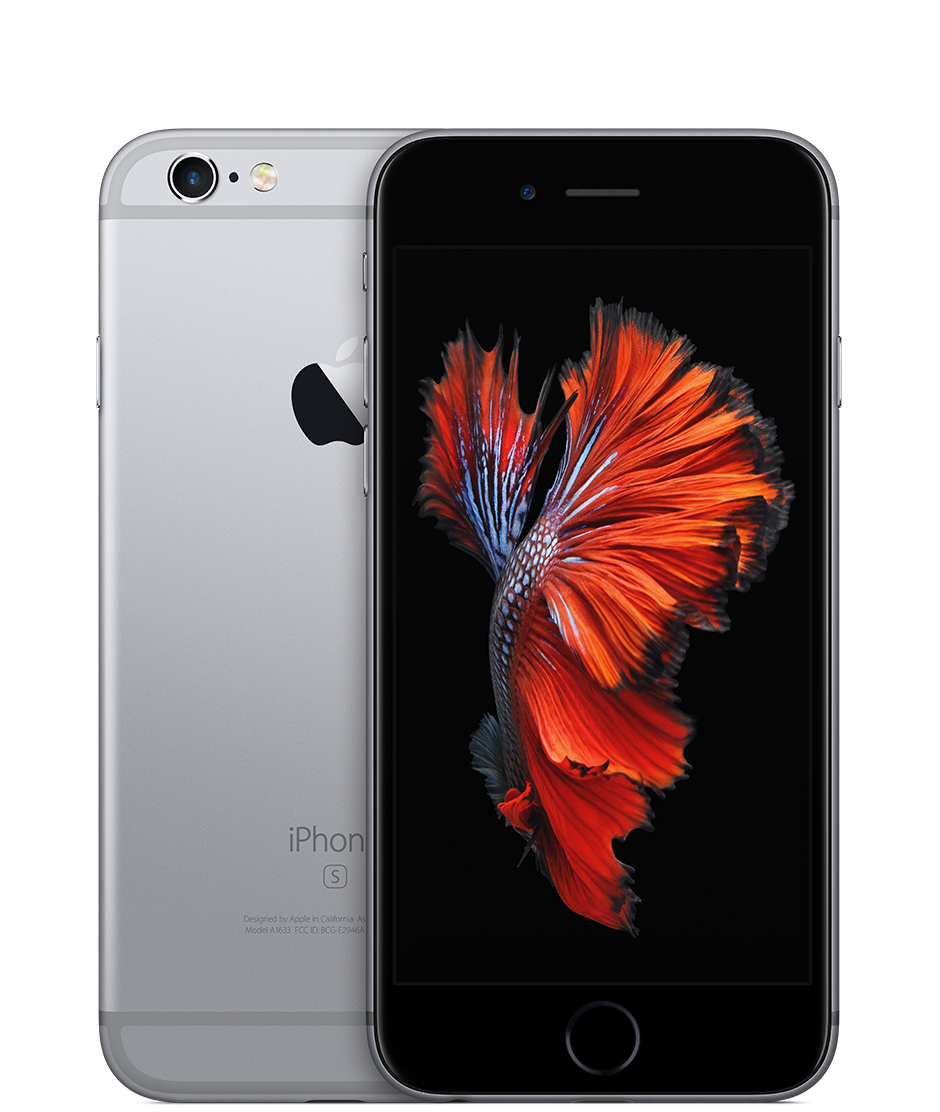
case iPhone6s
/// Device is an [iPhone 6s Plus](https://support.apple.com/kb/SP727)
///
/// 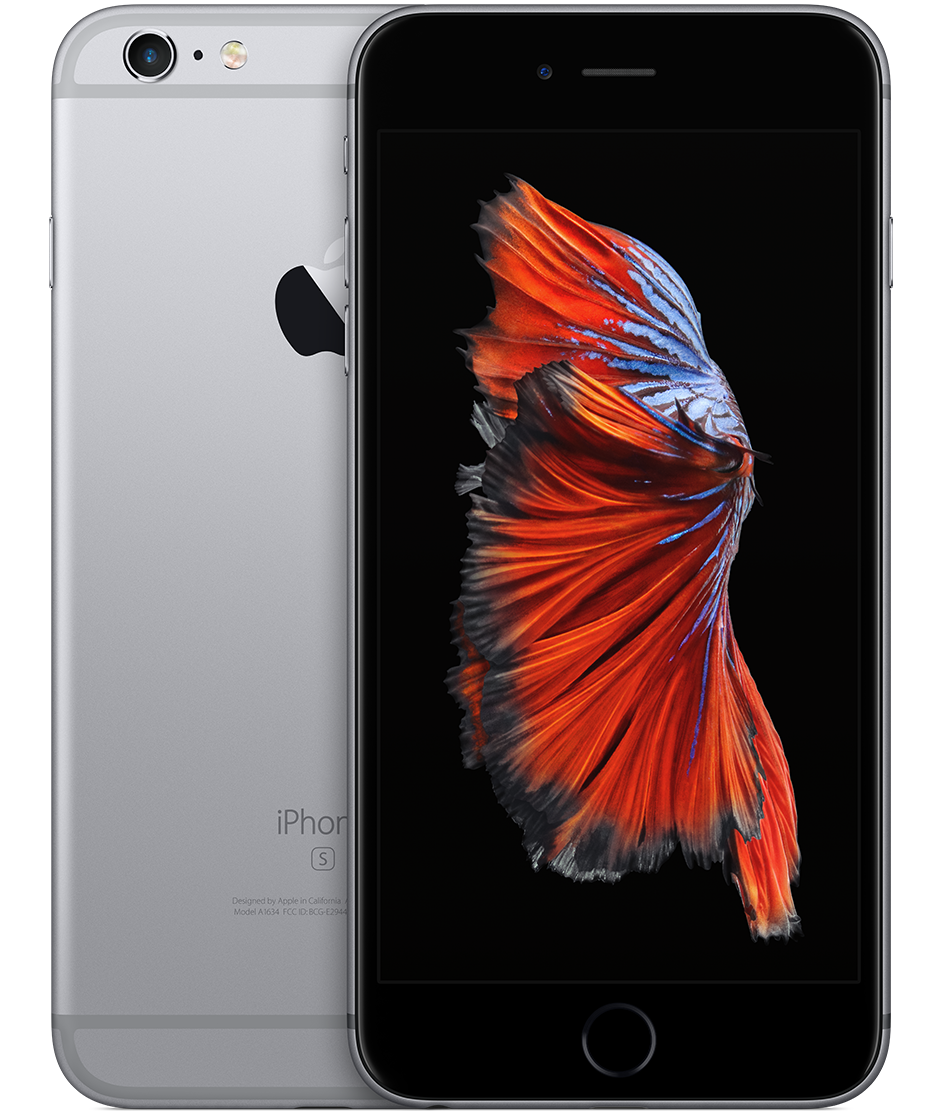
case iPhone6sPlus
/// Device is an [iPhone 7](https://support.apple.com/kb/SP743)
///
/// 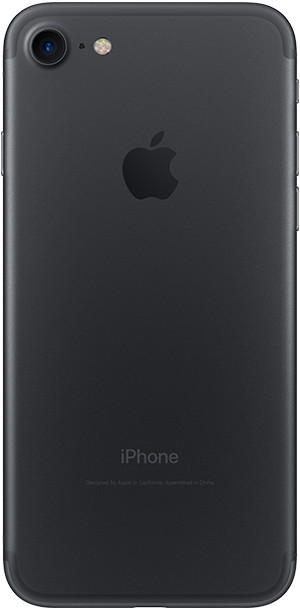
case iPhone7
/// Device is an [iPhone 7 Plus](https://support.apple.com/kb/SP744)
///
/// 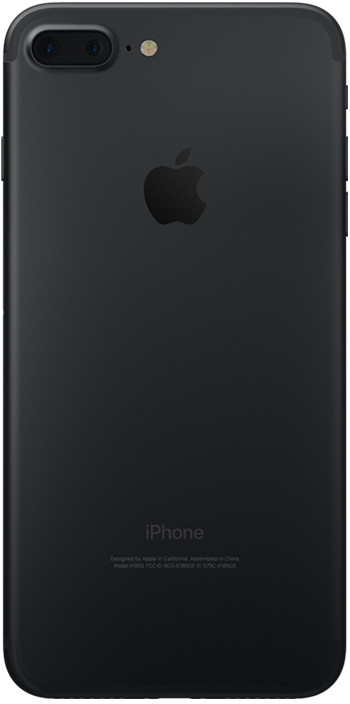
case iPhone7Plus
/// Device is an [iPhone SE](https://support.apple.com/kb/SP738)
///
/// 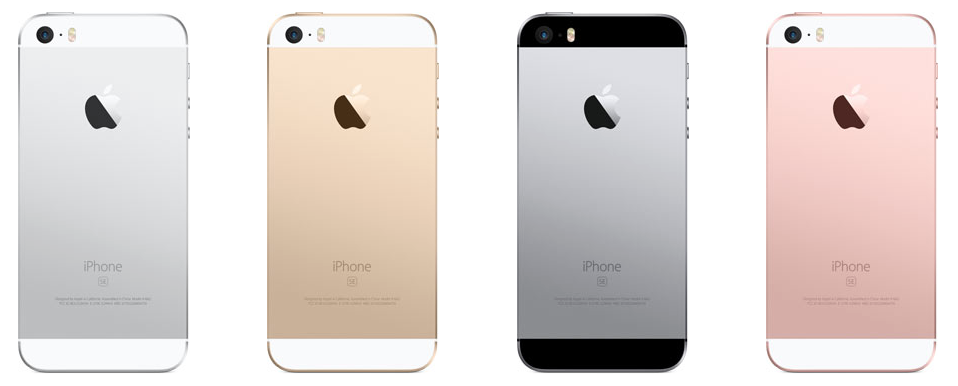
case iPhoneSE
/// Device is an [iPhone 8](https://support.apple.com/kb/SP767)
///
/// 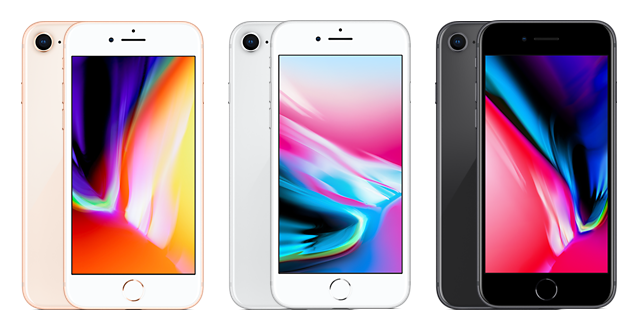
case iPhone8
/// Device is an [iPhone 8 Plus](https://support.apple.com/kb/SP768)
///
/// 
case iPhone8Plus
/// Device is an [iPhone X](https://support.apple.com/kb/SP770)
///
/// 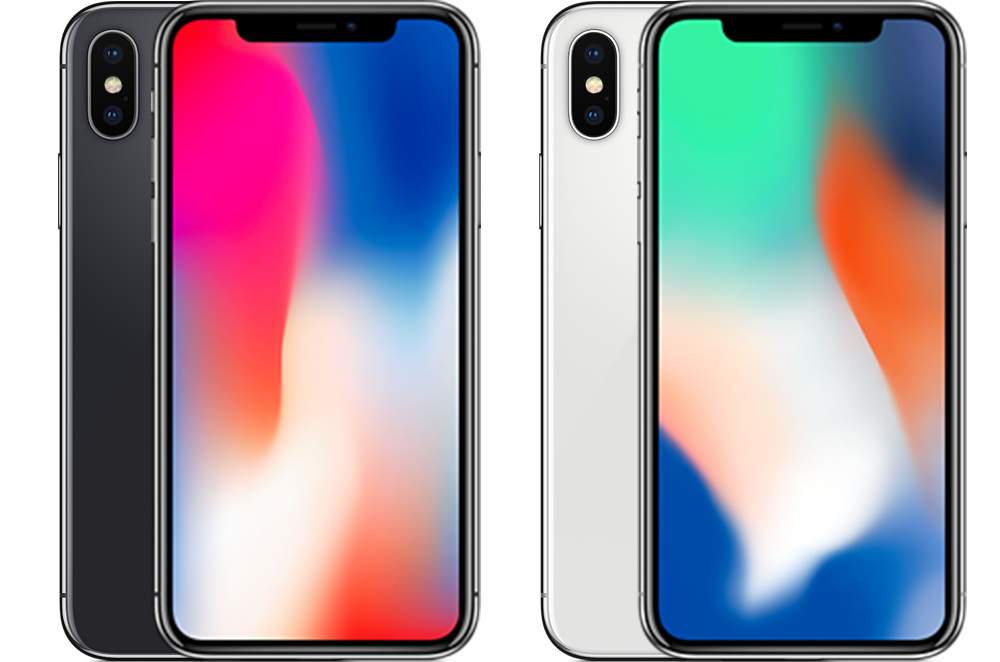
case iPhoneX
/// Device is an [iPhone Xs](https://support.apple.com/kb/SP779)
///
/// 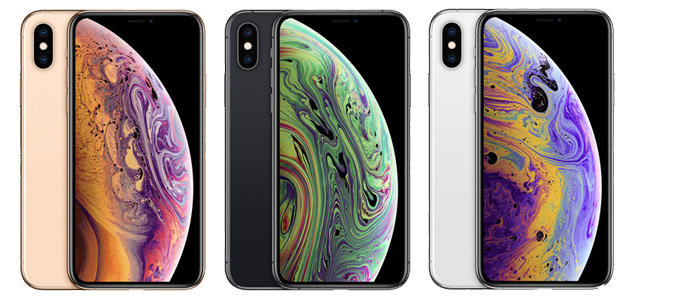
case iPhoneXS
/// Device is an [iPhone Xs Max](https://support.apple.com/kb/SP780)
///
/// 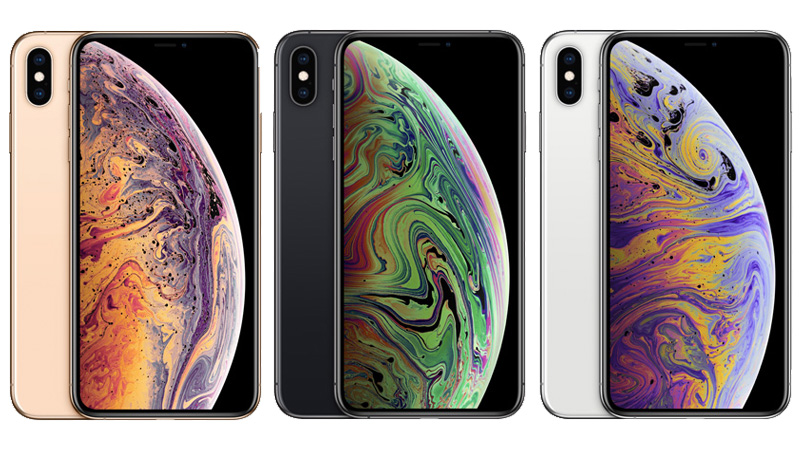
case iPhoneXSMax
/// Device is an [iPhone Xʀ](https://support.apple.com/kb/SP781)
///
/// 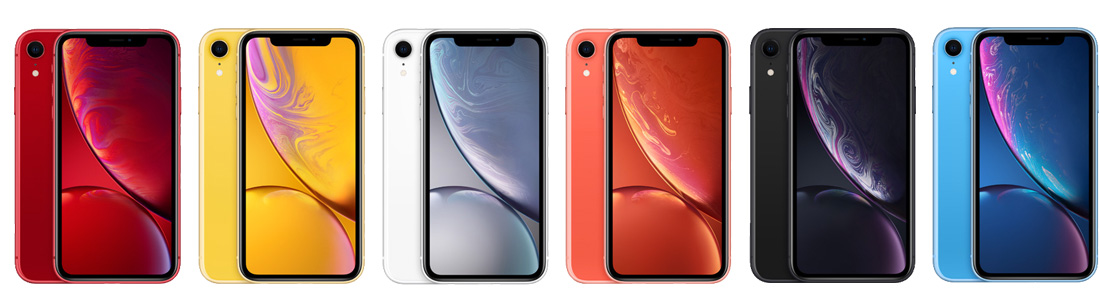
case iPhoneXR
/// Device is an [iPhone 11](https://support.apple.com/kb/SP804)
///
/// 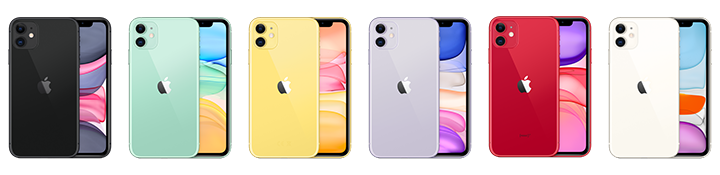
case iPhone11
/// Device is an [iPhone 11 Pro](https://support.apple.com/kb/SP805)
///
/// 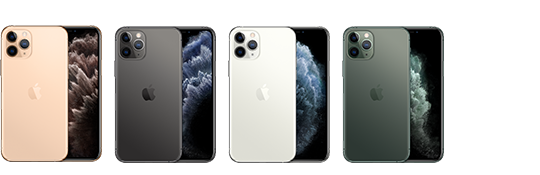
case iPhone11Pro
/// Device is an [iPhone 11 Pro Max](https://support.apple.com/kb/SP806)
///
/// 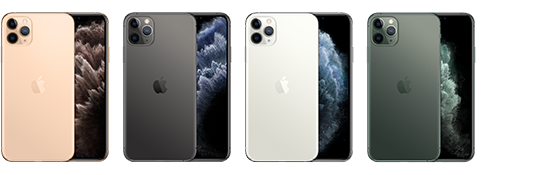
case iPhone11ProMax
/// Device is an [iPhone SE (2nd generation)](https://support.apple.com/kb/SP820)
///
/// 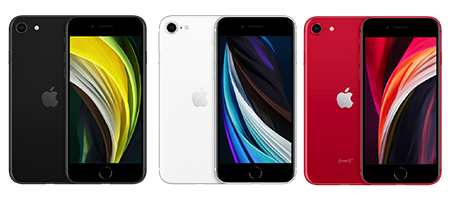
case iPhoneSE2
/// Device is an [iPhone 12](https://support.apple.com/kb/SP830)
///
/// 
case iPhone12
/// Device is an [iPhone 12 mini](https://support.apple.com/kb/SP829)
///
/// 
case iPhone12Mini
/// Device is an [iPhone 12 Pro](https://support.apple.com/kb/SP831)
///
/// 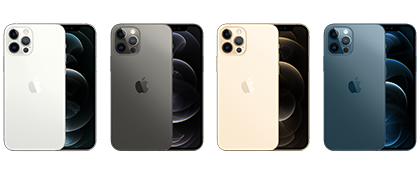
case iPhone12Pro
/// Device is an [iPhone 12 Pro Max](https://support.apple.com/kb/SP832)
///
/// 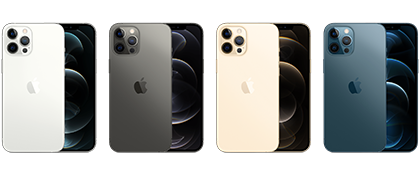
case iPhone12ProMax
/// Device is an [iPhone 13](https://support.apple.com/kb/TODO)
///
/// 
case iPhone13
/// Device is an [iPhone 13 mini](https://support.apple.com/kb/TODO)
///
/// 
case iPhone13Mini
/// Device is an [iPhone 13 Pro](https://support.apple.com/kb/TODO)
///
/// 
case iPhone13Pro
/// Device is an [iPhone 13 Pro Max](https://support.apple.com/kb/TODO)
///
/// 
case iPhone13ProMax
/// Device is an [iPad 2](https://support.apple.com/kb/SP622)
///
/// 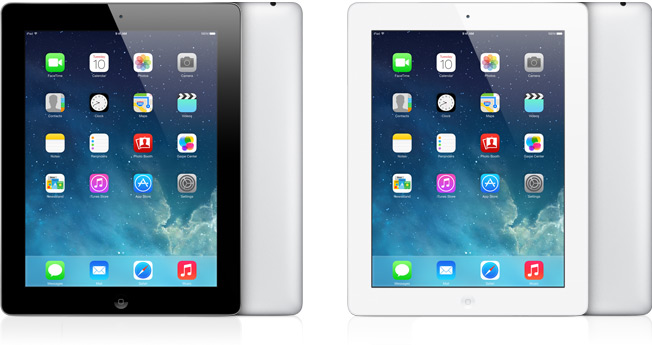
case iPad2
/// Device is an [iPad (3rd generation)](https://support.apple.com/kb/SP647)
///
/// 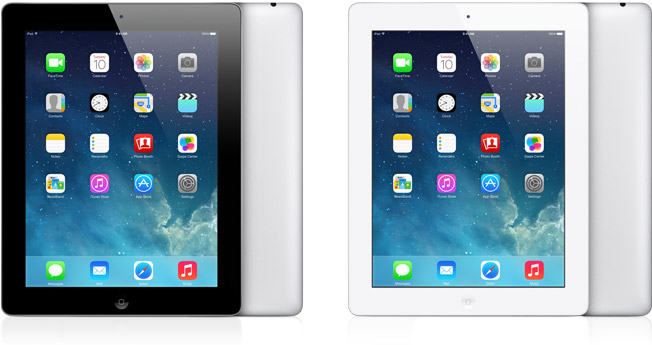
case iPad3
/// Device is an [iPad (4th generation)](https://support.apple.com/kb/SP662)
///
/// 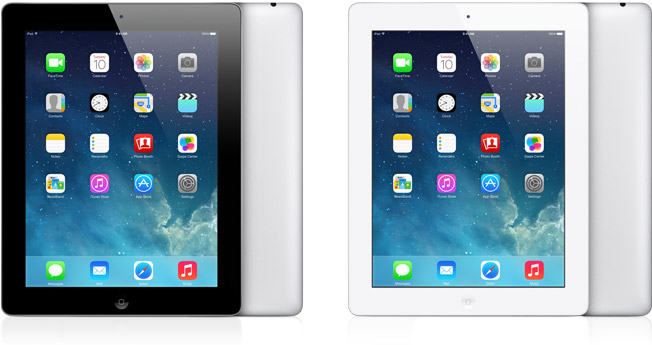
case iPad4
/// Device is an [iPad Air](https://support.apple.com/kb/SP692)
///
/// 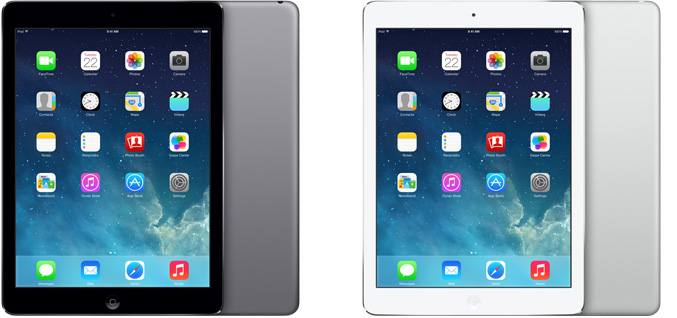
case iPadAir
/// Device is an [iPad Air 2](https://support.apple.com/kb/SP708)
///
/// 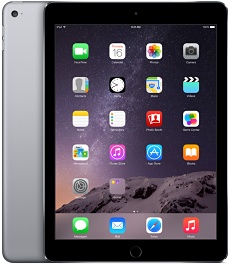
case iPadAir2
/// Device is an [iPad (5th generation)](https://support.apple.com/kb/SP751)
///
/// 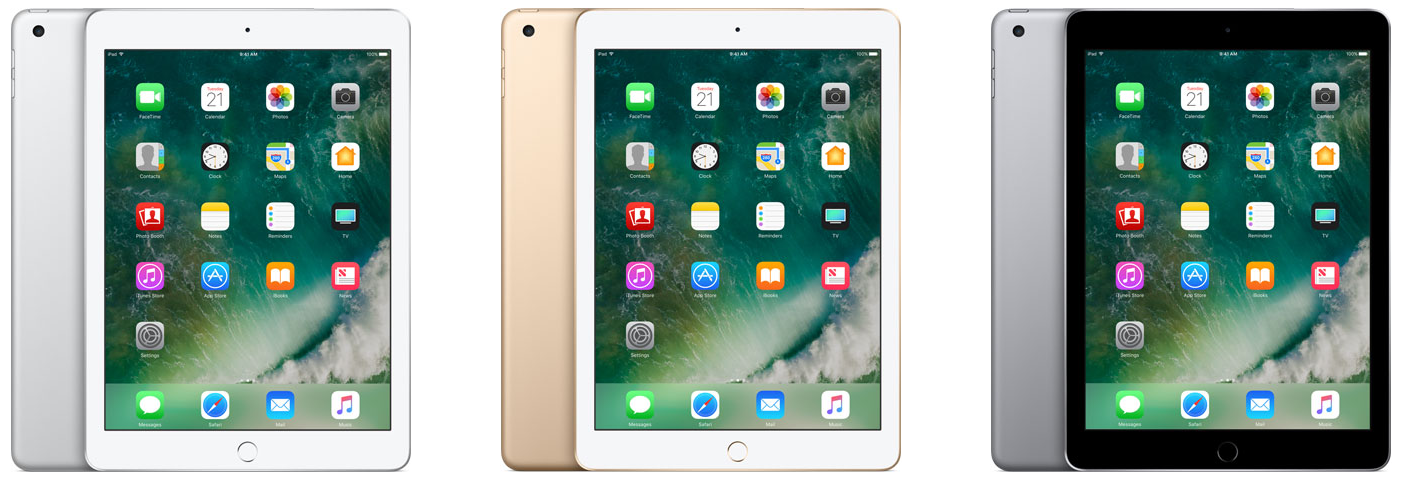
case iPad5
/// Device is an [iPad (6th generation)](https://support.apple.com/kb/SP774)
///
/// 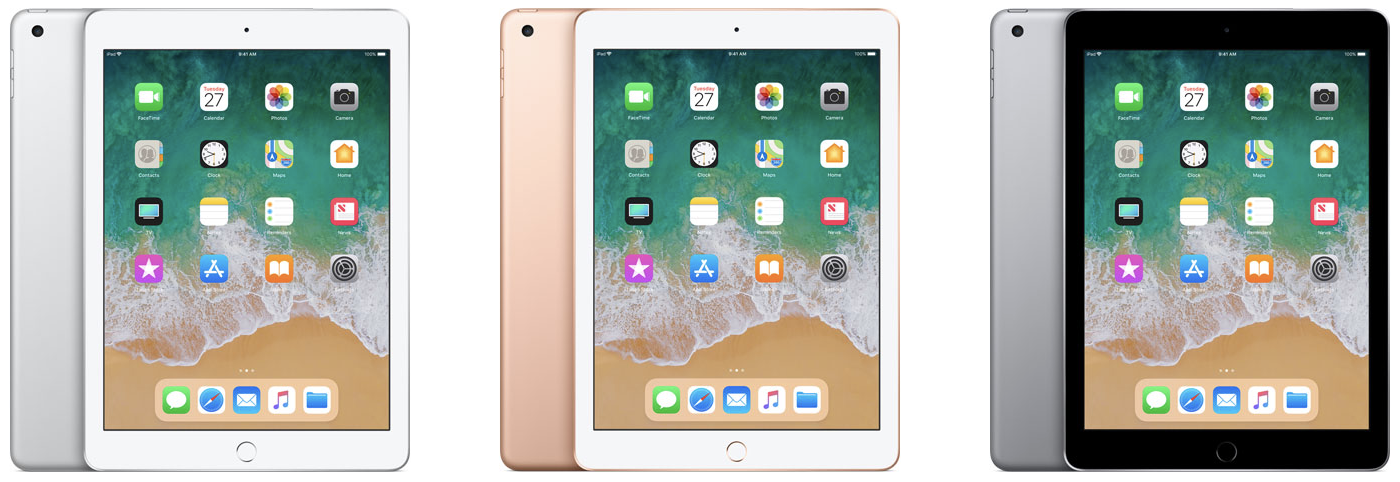
case iPad6
/// Device is an [iPad Air (3rd generation)](https://support.apple.com/kb/SP787)
///
/// 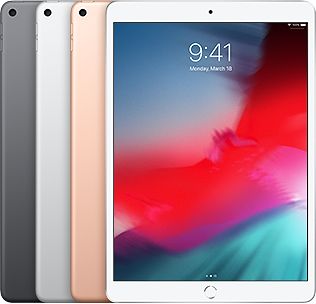
case iPadAir3
/// Device is an [iPad (7th generation)](https://support.apple.com/kb/SP807)
///
/// 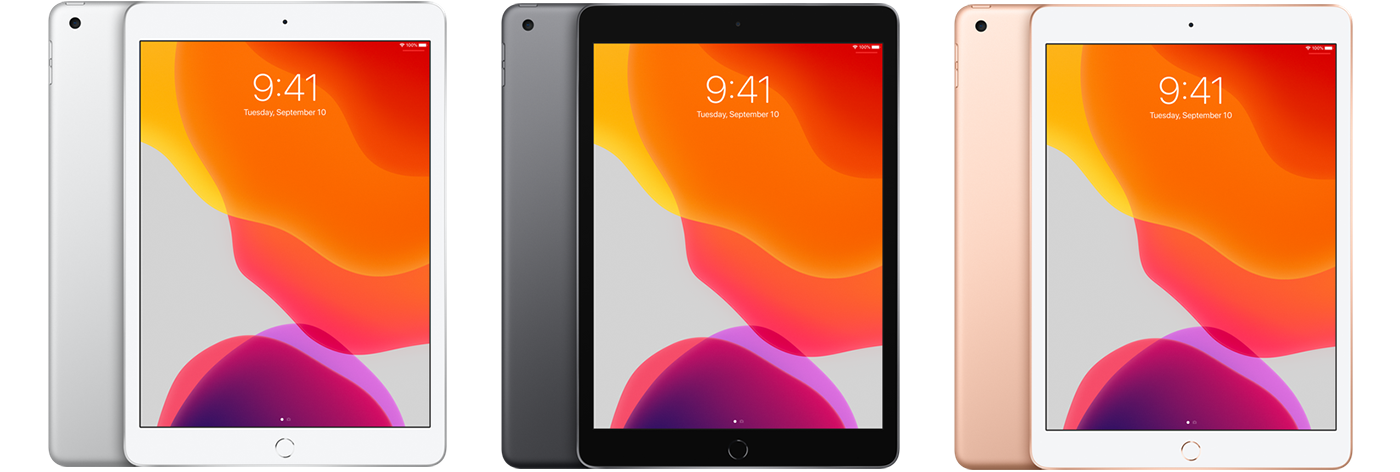
case iPad7
/// Device is an [iPad (8th generation)](https://support.apple.com/kb/SP822)
///
/// 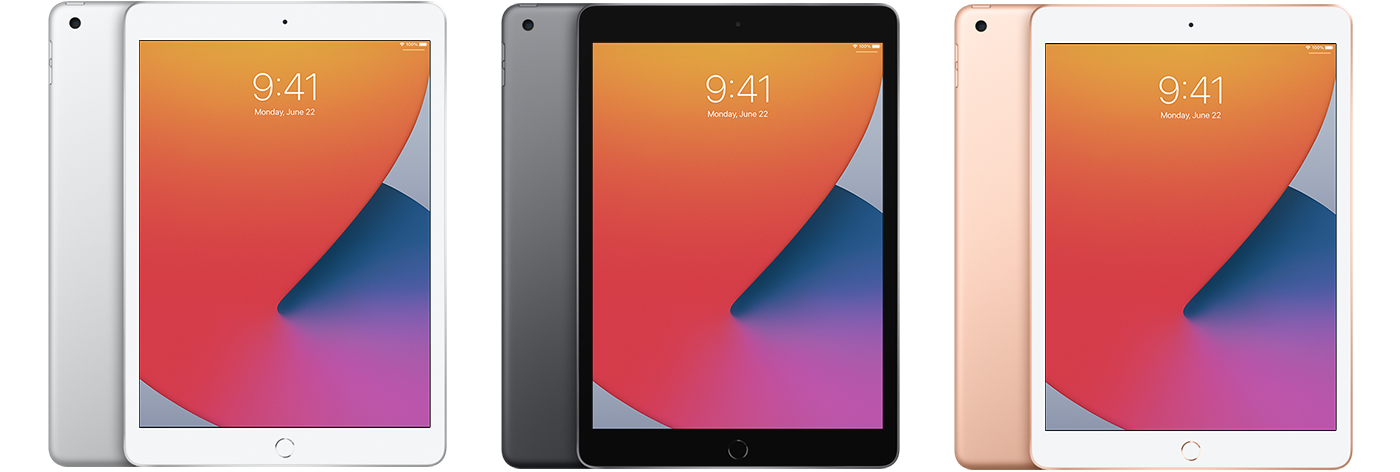
case iPad8
/// Device is an [iPad (9th generation)](https://support.apple.com/kb/TODO)
///
/// 
case iPad9
/// Device is an [iPad Air (4th generation)](https://support.apple.com/kb/SP828)
///
/// 
case iPadAir4
/// Device is an [iPad Mini](https://support.apple.com/kb/SP661)
///
/// 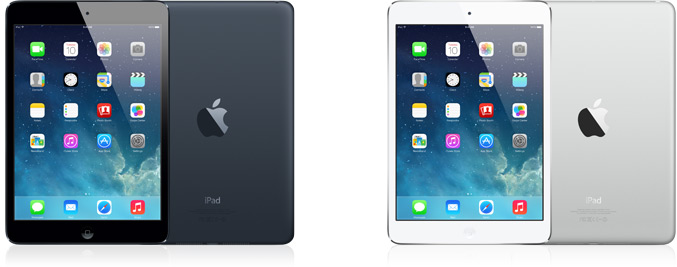
case iPadMini
/// Device is an [iPad Mini 2](https://support.apple.com/kb/SP693)
///
/// 
case iPadMini2
/// Device is an [iPad Mini 3](https://support.apple.com/kb/SP709)
///
/// 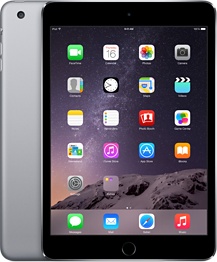
case iPadMini3
/// Device is an [iPad Mini 4](https://support.apple.com/kb/SP725)
///
/// 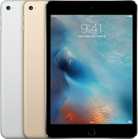
case iPadMini4
/// Device is an [iPad Mini (5th generation)](https://support.apple.com/kb/SP788)
///
/// 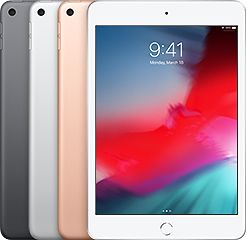
case iPadMini5
/// Device is an [iPad Mini (6th generation)](https://support.apple.com/kb/TODO)
///
/// 
case iPadMini6
/// Device is an [iPad Pro 9.7-inch](https://support.apple.com/kb/SP739)
///
/// 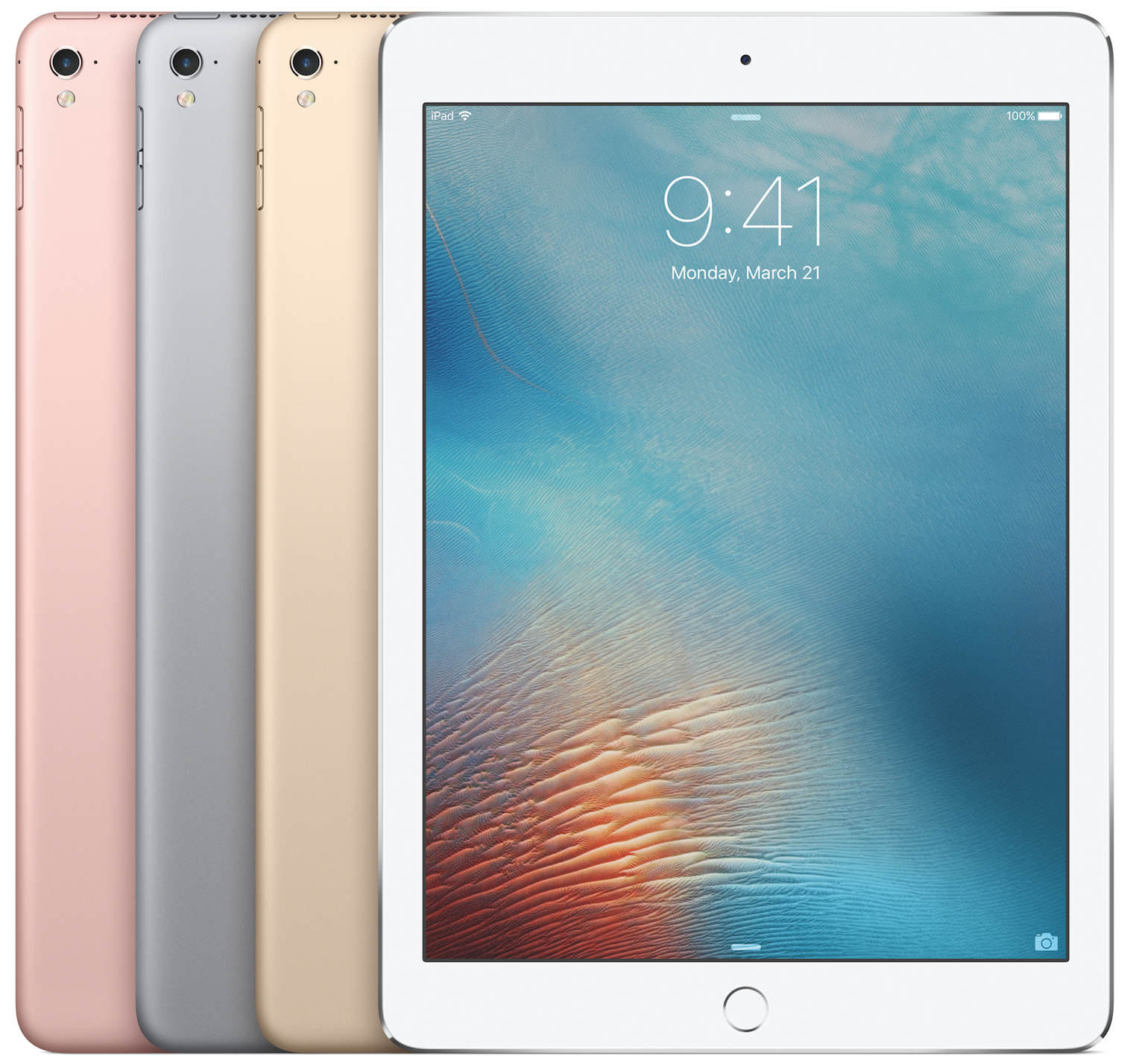
case iPadPro9Inch
/// Device is an [iPad Pro 12-inch](https://support.apple.com/kb/SP723)
///
/// 
case iPadPro12Inch
/// Device is an [iPad Pro 12-inch (2nd generation)](https://support.apple.com/kb/SP761)
///
/// 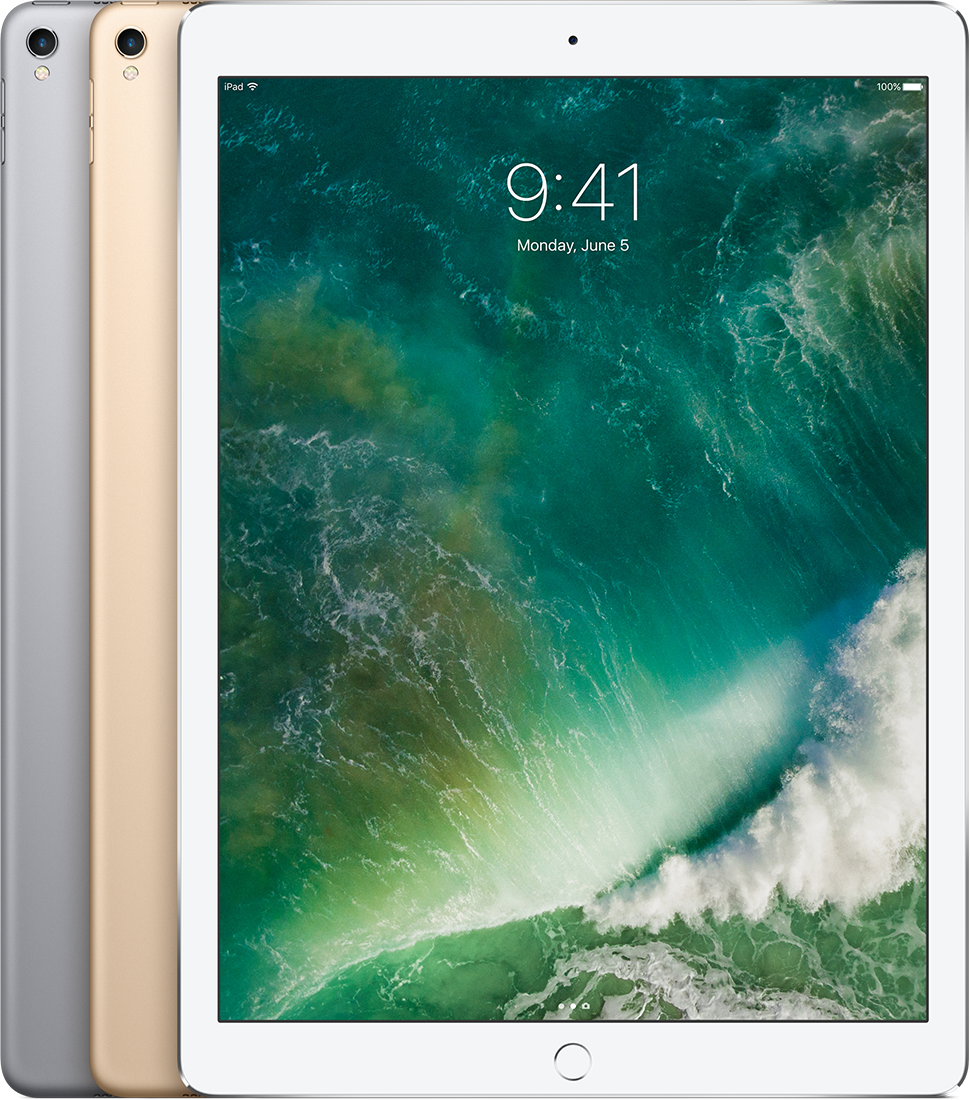
case iPadPro12Inch2
/// Device is an [iPad Pro 10.5-inch](https://support.apple.com/kb/SP762)
///
/// 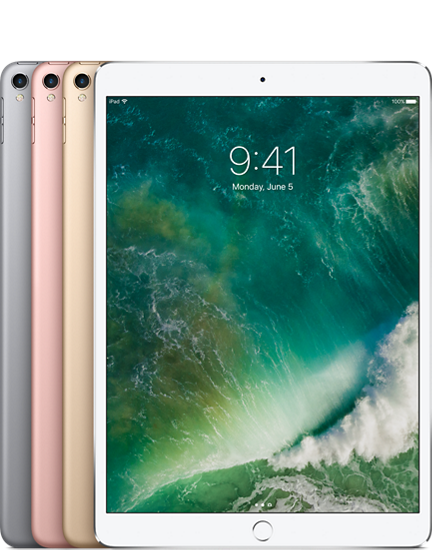
case iPadPro10Inch
/// Device is an [iPad Pro 11-inch](https://support.apple.com/kb/SP784)
///
/// 
case iPadPro11Inch
/// Device is an [iPad Pro 12.9-inch (3rd generation)](https://support.apple.com/kb/SP785)
///
/// 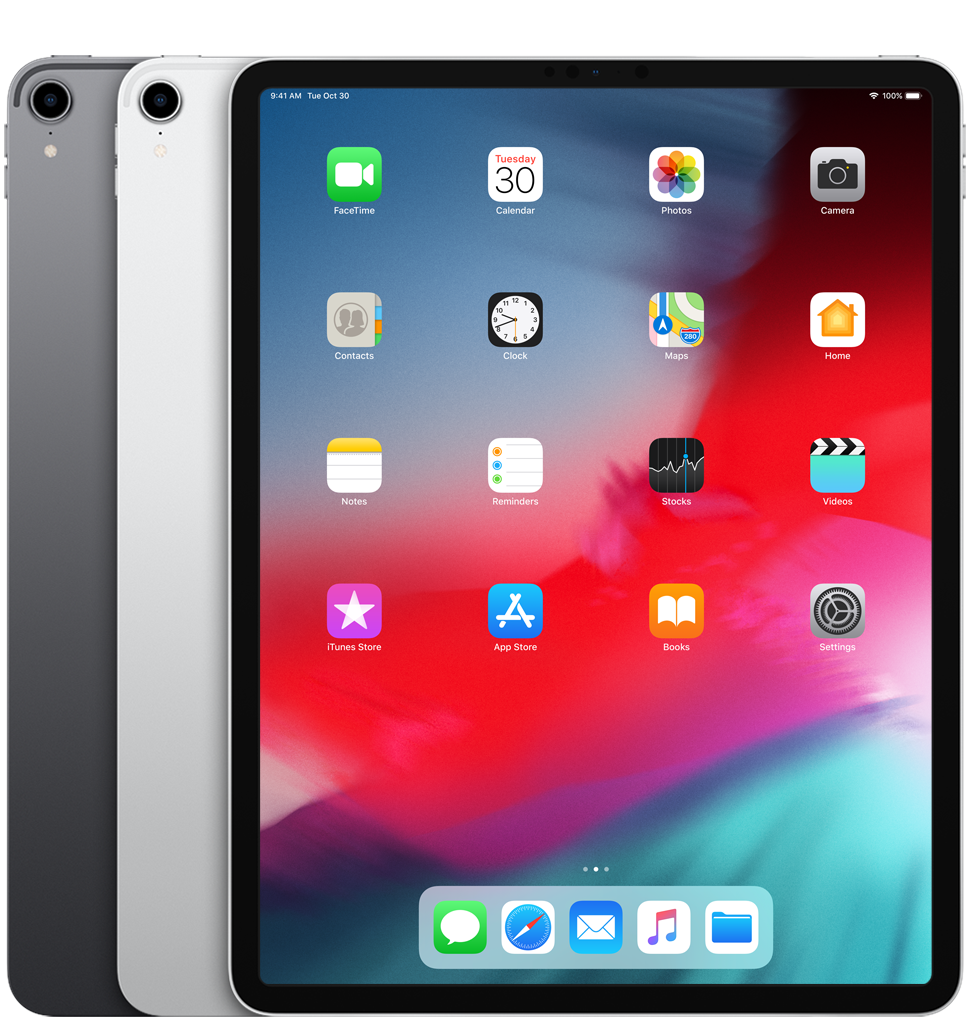
case iPadPro12Inch3
/// Device is an [iPad Pro 11-inch (2nd generation)](https://support.apple.com/kb/SP814)
///
/// 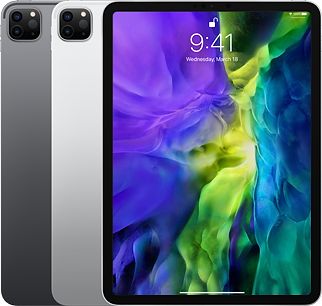
case iPadPro11Inch2
/// Device is an [iPad Pro 12.9-inch (4th generation)](https://support.apple.com/kb/SP815)
///
/// 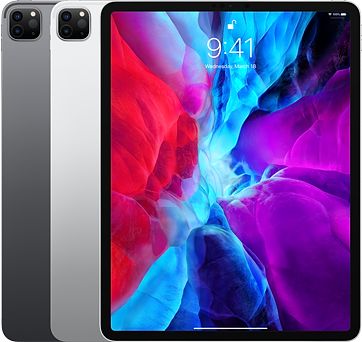
case iPadPro12Inch4
/// Device is an [iPad Pro 11-inch (3rd generation)](https://support.apple.com/kb/TODO)
///
/// 
case iPadPro11Inch3
/// Device is an [iPad Pro 12.9-inch (5th generation)](https://support.apple.com/kb/TODO)
///
/// 
case iPadPro12Inch5
/// Device is a [HomePod](https://support.apple.com/kb/SP773)
///
/// 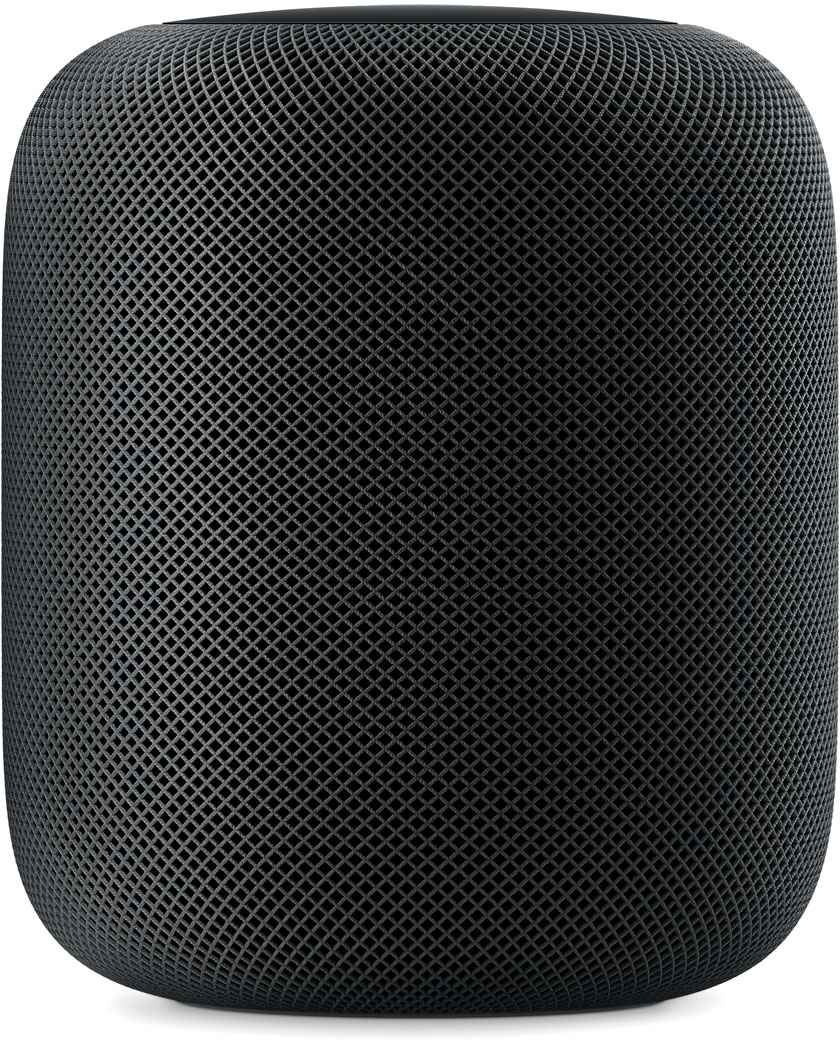
case homePod
#elseif os(tvOS)
/// Device is an [Apple TV HD](https://support.apple.com/kb/SP724) (Previously Apple TV (4th generation))
///
/// 
case appleTVHD
/// Device is an [Apple TV 4K](https://support.apple.com/kb/SP769)
///
/// 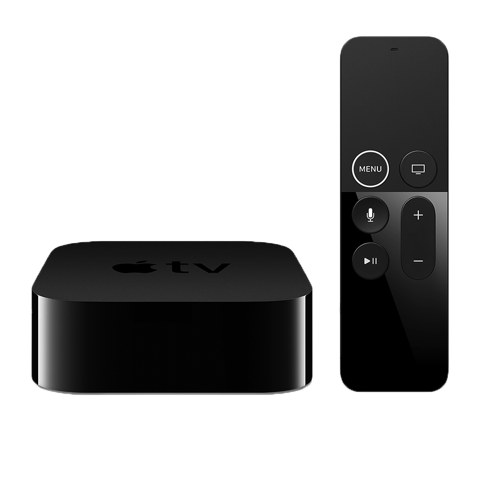
case appleTV4K
/// Device is an [Apple TV 4K (2nd generation)](https://support.apple.com/kb/TODO)
///
/// 
case appleTV4K2
#elseif os(watchOS)
/// Device is an [Apple Watch (1st generation)](https://support.apple.com/kb/SP735)
///
/// 
case appleWatchSeries0_38mm
/// Device is an [Apple Watch (1st generation)](https://support.apple.com/kb/SP735)
///
/// 
case appleWatchSeries0_42mm
/// Device is an [Apple Watch Series 1](https://support.apple.com/kb/SP745)
///
/// 
case appleWatchSeries1_38mm
/// Device is an [Apple Watch Series 1](https://support.apple.com/kb/SP745)
///
/// 
case appleWatchSeries1_42mm
/// Device is an [Apple Watch Series 2](https://support.apple.com/kb/SP746)
///
/// 
case appleWatchSeries2_38mm
/// Device is an [Apple Watch Series 2](https://support.apple.com/kb/SP746)
///
/// 
case appleWatchSeries2_42mm
/// Device is an [Apple Watch Series 3](https://support.apple.com/kb/SP766)
///
/// 
case appleWatchSeries3_38mm
/// Device is an [Apple Watch Series 3](https://support.apple.com/kb/SP766)
///
/// 
case appleWatchSeries3_42mm
/// Device is an [Apple Watch Series 4](https://support.apple.com/kb/SP778)
///
/// 
case appleWatchSeries4_40mm
/// Device is an [Apple Watch Series 4](https://support.apple.com/kb/SP778)
///
/// 
case appleWatchSeries4_44mm
/// Device is an [Apple Watch Series 5](https://support.apple.com/kb/SP808)
///
/// 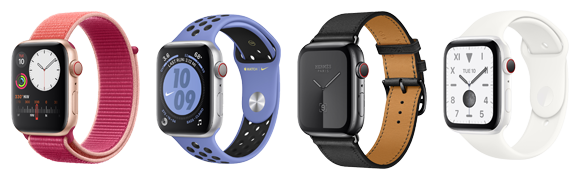
case appleWatchSeries5_40mm
/// Device is an [Apple Watch Series 5](https://support.apple.com/kb/SP808)
///
/// 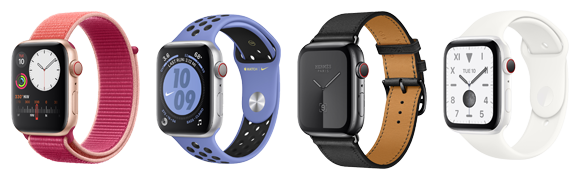
case appleWatchSeries5_44mm
/// Device is an [Apple Watch Series 6](https://support.apple.com/kb/SP826)
///
/// 
case appleWatchSeries6_40mm
/// Device is an [Apple Watch Series 6](https://support.apple.com/kb/SP826)
///
/// 
case appleWatchSeries6_44mm
/// Device is an [Apple Watch SE](https://support.apple.com/kb/SP827)
///
/// 
case appleWatchSE_40mm
/// Device is an [Apple Watch SE](https://support.apple.com/kb/SP827)
///
/// 
case appleWatchSE_44mm
#endif
/// Device is [Simulator](https://developer.apple.com/library/ios/documentation/IDEs/Conceptual/iOS_Simulator_Guide/Introduction/Introduction.html)
///
/// 
indirect case simulator(Device)
/// Device is not yet known (implemented)
/// You can still use this enum as before but the description equals the identifier (you can get multiple identifiers for the same product class
/// (e.g. "iPhone6,1" or "iPhone 6,2" do both mean "iPhone 5s"))
case unknown(String)
/// Returns a `Device` representing the current device this software runs on.
public static var current: Device {
return Device.mapToDevice(identifier: Device.identifier)
}
/// Gets the identifier from the system, such as "iPhone7,1".
public static var identifier: String = {
var systemInfo = utsname()
uname(&systemInfo)
let mirror = Mirror(reflecting: systemInfo.machine)
let identifier = mirror.children.reduce("") { identifier, element in
guard let value = element.value as? Int8, value != 0 else { return identifier }
return identifier + String(UnicodeScalar(UInt8(value)))
}
return identifier
}()
/// Maps an identifier to a Device. If the identifier can not be mapped to an existing device, `UnknownDevice(identifier)` is returned.
///
/// - parameter identifier: The device identifier, e.g. "iPhone7,1". Can be obtained from `Device.identifier`.
///
/// - returns: An initialized `Device`.
public static func mapToDevice(identifier: String) -> Device { // swiftlint:disable:this cyclomatic_complexity function_body_length
#if os(iOS)
switch identifier {
case "iPod5,1": return iPodTouch5
case "iPod7,1": return iPodTouch6
case "iPod9,1": return iPodTouch7
case "iPhone3,1", "iPhone3,2", "iPhone3,3": return iPhone4
case "iPhone4,1": return iPhone4s
case "iPhone5,1", "iPhone5,2": return iPhone5
case "iPhone5,3", "iPhone5,4": return iPhone5c
case "iPhone6,1", "iPhone6,2": return iPhone5s
case "iPhone7,2": return iPhone6
case "iPhone7,1": return iPhone6Plus
case "iPhone8,1": return iPhone6s
case "iPhone8,2": return iPhone6sPlus
case "iPhone9,1", "iPhone9,3": return iPhone7
case "iPhone9,2", "iPhone9,4": return iPhone7Plus
case "iPhone8,4": return iPhoneSE
case "iPhone10,1", "iPhone10,4": return iPhone8
case "iPhone10,2", "iPhone10,5": return iPhone8Plus
case "iPhone10,3", "iPhone10,6": return iPhoneX
case "iPhone11,2": return iPhoneXS
case "iPhone11,4", "iPhone11,6": return iPhoneXSMax
case "iPhone11,8": return iPhoneXR
case "iPhone12,1": return iPhone11
case "iPhone12,3": return iPhone11Pro
case "iPhone12,5": return iPhone11ProMax
case "iPhone12,8": return iPhoneSE2
case "iPhone13,2": return iPhone12
case "iPhone13,1": return iPhone12Mini
case "iPhone13,3": return iPhone12Pro
case "iPhone13,4": return iPhone12ProMax
case "iPhone14,5": return iPhone13
case "iPhone14,4": return iPhone13Mini
case "iPhone14,2": return iPhone13Pro
case "iPhone14,3": return iPhone13ProMax
case "iPad2,1", "iPad2,2", "iPad2,3", "iPad2,4": return iPad2
case "iPad3,1", "iPad3,2", "iPad3,3": return iPad3
case "iPad3,4", "iPad3,5", "iPad3,6": return iPad4
case "iPad4,1", "iPad4,2", "iPad4,3": return iPadAir
case "iPad5,3", "iPad5,4": return iPadAir2
case "iPad6,11", "iPad6,12": return iPad5
case "iPad7,5", "iPad7,6": return iPad6
case "iPad11,3", "iPad11,4": return iPadAir3
case "iPad7,11", "iPad7,12": return iPad7
case "iPad11,6", "iPad11,7": return iPad8
case "iPad12,1", "iPad12,2": return iPad9
case "iPad13,1", "iPad13,2": return iPadAir4
case "iPad2,5", "iPad2,6", "iPad2,7": return iPadMini
case "iPad4,4", "iPad4,5", "iPad4,6": return iPadMini2
case "iPad4,7", "iPad4,8", "iPad4,9": return iPadMini3
case "iPad5,1", "iPad5,2": return iPadMini4
case "iPad11,1", "iPad11,2": return iPadMini5
case "iPad14,1", "iPad14,2": return iPadMini6
case "iPad6,3", "iPad6,4": return iPadPro9Inch
case "iPad6,7", "iPad6,8": return iPadPro12Inch
case "iPad7,1", "iPad7,2": return iPadPro12Inch2
case "iPad7,3", "iPad7,4": return iPadPro10Inch
case "iPad8,1", "iPad8,2", "iPad8,3", "iPad8,4": return iPadPro11Inch
case "iPad8,5", "iPad8,6", "iPad8,7", "iPad8,8": return iPadPro12Inch3
case "iPad8,9", "iPad8,10": return iPadPro11Inch2
case "iPad8,11", "iPad8,12": return iPadPro12Inch4
case "iPad13,4", "iPad13,5", "iPad13,6", "iPad13,7": return iPadPro11Inch3
case "iPad13,8", "iPad13,9", "iPad13,10", "iPad13,11": return iPadPro12Inch5
case "AudioAccessory1,1": return homePod
case "i386", "x86_64", "arm64": return simulator(mapToDevice(identifier: ProcessInfo().environment["SIMULATOR_MODEL_IDENTIFIER"] ?? "iOS"))
default: return unknown(identifier)
}
#elseif os(tvOS)
switch identifier {
case "AppleTV5,3": return appleTVHD
case "AppleTV6,2": return appleTV4K
case "AppleTV11,1": return appleTV4K2
case "i386", "x86_64", "arm64": return simulator(mapToDevice(identifier: ProcessInfo().environment["SIMULATOR_MODEL_IDENTIFIER"] ?? "tvOS"))
default: return unknown(identifier)
}
#elseif os(watchOS)
switch identifier {
case "Watch1,1": return appleWatchSeries0_38mm
case "Watch1,2": return appleWatchSeries0_42mm
case "Watch2,6": return appleWatchSeries1_38mm
case "Watch2,7": return appleWatchSeries1_42mm
case "Watch2,3": return appleWatchSeries2_38mm
case "Watch2,4": return appleWatchSeries2_42mm
case "Watch3,1", "Watch3,3": return appleWatchSeries3_38mm
case "Watch3,2", "Watch3,4": return appleWatchSeries3_42mm
case "Watch4,1", "Watch4,3": return appleWatchSeries4_40mm
case "Watch4,2", "Watch4,4": return appleWatchSeries4_44mm
case "Watch5,1", "Watch5,3": return appleWatchSeries5_40mm
case "Watch5,2", "Watch5,4": return appleWatchSeries5_44mm
case "Watch6,1", "Watch6,3": return appleWatchSeries6_40mm
case "Watch6,2", "Watch6,4": return appleWatchSeries6_44mm
case "Watch5,9", "Watch5,11": return appleWatchSE_40mm
case "Watch5,10", "Watch5,12": return appleWatchSE_44mm
case "i386", "x86_64", "arm64": return simulator(mapToDevice(identifier: ProcessInfo().environment["SIMULATOR_MODEL_IDENTIFIER"] ?? "watchOS"))
default: return unknown(identifier)
}
#endif
}
/// Get the real device from a device.
/// If the device is a an iPhone8Plus simulator this function returns .iPhone8Plus (the real device).
/// If the parameter is a real device, this function returns just that passed parameter.
///
/// - parameter device: A device.
///
/// - returns: the underlying device If the `device` is a `simulator`,
/// otherwise return the `device`.
public static func realDevice(from device: Device) -> Device {
if case let .simulator(model) = device {
return model
}
return device
}
#if os(iOS) || os(watchOS)
/// Returns diagonal screen length in inches
public var diagonal: Double {
#if os(iOS)
switch self {
case .iPodTouch5: return 4
case .iPodTouch6: return 4
case .iPodTouch7: return 4
case .iPhone4: return 3.5
case .iPhone4s: return 3.5
case .iPhone5: return 4
case .iPhone5c: return 4
case .iPhone5s: return 4
case .iPhone6: return 4.7
case .iPhone6Plus: return 5.5
case .iPhone6s: return 4.7
case .iPhone6sPlus: return 5.5
case .iPhone7: return 4.7
case .iPhone7Plus: return 5.5
case .iPhoneSE: return 4
case .iPhone8: return 4.7
case .iPhone8Plus: return 5.5
case .iPhoneX: return 5.8
case .iPhoneXS: return 5.8
case .iPhoneXSMax: return 6.5
case .iPhoneXR: return 6.1
case .iPhone11: return 6.1
case .iPhone11Pro: return 5.8
case .iPhone11ProMax: return 6.5
case .iPhoneSE2: return 4.7
case .iPhone12: return 6.1
case .iPhone12Mini: return 5.4
case .iPhone12Pro: return 6.1
case .iPhone12ProMax: return 6.7
case .iPhone13: return 6.1
case .iPhone13Mini: return 5.4
case .iPhone13Pro: return 6.1
case .iPhone13ProMax: return 6.7
case .iPad2: return 9.7
case .iPad3: return 9.7
case .iPad4: return 9.7
case .iPadAir: return 9.7
case .iPadAir2: return 9.7
case .iPad5: return 9.7
case .iPad6: return 9.7
case .iPadAir3: return 10.5
case .iPad7: return 10.2
case .iPad8: return 10.2
case .iPad9: return 10.2
case .iPadAir4: return 10.9
case .iPadMini: return 7.9
case .iPadMini2: return 7.9
case .iPadMini3: return 7.9
case .iPadMini4: return 7.9
case .iPadMini5: return 7.9
case .iPadMini6: return 7.9
case .iPadPro9Inch: return 9.7
case .iPadPro12Inch: return 12.9
case .iPadPro12Inch2: return 12.9
case .iPadPro10Inch: return 10.5
case .iPadPro11Inch: return 11.0
case .iPadPro12Inch3: return 12.9
case .iPadPro11Inch2: return 11.0
case .iPadPro12Inch4: return 12.9
case .iPadPro11Inch3: return 11.0
case .iPadPro12Inch5: return 12.9
case .homePod: return -1
case .simulator(let model): return model.diagonal
case .unknown: return -1
}
#elseif os(watchOS)
switch self {
case .appleWatchSeries0_38mm: return 1.5
case .appleWatchSeries0_42mm: return 1.6
case .appleWatchSeries1_38mm: return 1.5
case .appleWatchSeries1_42mm: return 1.6
case .appleWatchSeries2_38mm: return 1.5
case .appleWatchSeries2_42mm: return 1.6
case .appleWatchSeries3_38mm: return 1.5
case .appleWatchSeries3_42mm: return 1.6
case .appleWatchSeries4_40mm: return 1.8
case .appleWatchSeries4_44mm: return 2.0
case .appleWatchSeries5_40mm: return 1.8
case .appleWatchSeries5_44mm: return 2.0
case .appleWatchSeries6_40mm: return 1.8
case .appleWatchSeries6_44mm: return 2.0
case .appleWatchSE_40mm: return 1.8
case .appleWatchSE_44mm: return 2.0
case .simulator(let model): return model.diagonal
case .unknown: return -1
}
#endif
}
#endif
/// Returns screen ratio as a tuple
public var screenRatio: (width: Double, height: Double) {
#if os(iOS)
switch self {
case .iPodTouch5: return (width: 9, height: 16)
case .iPodTouch6: return (width: 9, height: 16)
case .iPodTouch7: return (width: 9, height: 16)
case .iPhone4: return (width: 2, height: 3)
case .iPhone4s: return (width: 2, height: 3)
case .iPhone5: return (width: 9, height: 16)
case .iPhone5c: return (width: 9, height: 16)
case .iPhone5s: return (width: 9, height: 16)
case .iPhone6: return (width: 9, height: 16)
case .iPhone6Plus: return (width: 9, height: 16)
case .iPhone6s: return (width: 9, height: 16)
case .iPhone6sPlus: return (width: 9, height: 16)
case .iPhone7: return (width: 9, height: 16)
case .iPhone7Plus: return (width: 9, height: 16)
case .iPhoneSE: return (width: 9, height: 16)
case .iPhone8: return (width: 9, height: 16)
case .iPhone8Plus: return (width: 9, height: 16)
case .iPhoneX: return (width: 9, height: 19.5)
case .iPhoneXS: return (width: 9, height: 19.5)
case .iPhoneXSMax: return (width: 9, height: 19.5)
case .iPhoneXR: return (width: 9, height: 19.5)
case .iPhone11: return (width: 9, height: 19.5)
case .iPhone11Pro: return (width: 9, height: 19.5)
case .iPhone11ProMax: return (width: 9, height: 19.5)
case .iPhoneSE2: return (width: 9, height: 16)
case .iPhone12: return (width: 9, height: 19.5)
case .iPhone12Mini: return (width: 9, height: 19.5)
case .iPhone12Pro: return (width: 9, height: 19.5)
case .iPhone12ProMax: return (width: 9, height: 19.5)
case .iPhone13: return (width: 9, height: 19.5)
case .iPhone13Mini: return (width: 9, height: 19.5)
case .iPhone13Pro: return (width: 9, height: 19.5)
case .iPhone13ProMax: return (width: 9, height: 19.5)
case .iPad2: return (width: 3, height: 4)
case .iPad3: return (width: 3, height: 4)
case .iPad4: return (width: 3, height: 4)
case .iPadAir: return (width: 3, height: 4)
case .iPadAir2: return (width: 3, height: 4)
case .iPad5: return (width: 3, height: 4)
case .iPad6: return (width: 3, height: 4)
case .iPadAir3: return (width: 3, height: 4)
case .iPad7: return (width: 3, height: 4)
case .iPad8: return (width: 3, height: 4)
case .iPad9: return (width: 3, height: 4)
case .iPadAir4: return (width: 41, height: 59)
case .iPadMini: return (width: 3, height: 4)
case .iPadMini2: return (width: 3, height: 4)
case .iPadMini3: return (width: 3, height: 4)
case .iPadMini4: return (width: 3, height: 4)
case .iPadMini5: return (width: 3, height: 4)
case .iPadMini6: return (width: 3, height: 4)
case .iPadPro9Inch: return (width: 3, height: 4)
case .iPadPro12Inch: return (width: 3, height: 4)
case .iPadPro12Inch2: return (width: 3, height: 4)
case .iPadPro10Inch: return (width: 3, height: 4)
case .iPadPro11Inch: return (width: 139, height: 199)
case .iPadPro12Inch3: return (width: 512, height: 683)
case .iPadPro11Inch2: return (width: 139, height: 199)
case .iPadPro12Inch4: return (width: 512, height: 683)
case .iPadPro11Inch3: return (width: 139, height: 199)
case .iPadPro12Inch5: return (width: 512, height: 683)
case .homePod: return (width: 4, height: 5)
case .simulator(let model): return model.screenRatio
case .unknown: return (width: -1, height: -1)
}
#elseif os(watchOS)
switch self {
case .appleWatchSeries0_38mm: return (width: 4, height: 5)
case .appleWatchSeries0_42mm: return (width: 4, height: 5)
case .appleWatchSeries1_38mm: return (width: 4, height: 5)
case .appleWatchSeries1_42mm: return (width: 4, height: 5)
case .appleWatchSeries2_38mm: return (width: 4, height: 5)
case .appleWatchSeries2_42mm: return (width: 4, height: 5)
case .appleWatchSeries3_38mm: return (width: 4, height: 5)
case .appleWatchSeries3_42mm: return (width: 4, height: 5)
case .appleWatchSeries4_40mm: return (width: 4, height: 5)
case .appleWatchSeries4_44mm: return (width: 4, height: 5)
case .appleWatchSeries5_40mm: return (width: 4, height: 5)
case .appleWatchSeries5_44mm: return (width: 4, height: 5)
case .appleWatchSeries6_40mm: return (width: 4, height: 5)
case .appleWatchSeries6_44mm: return (width: 4, height: 5)
case .appleWatchSE_40mm: return (width: 4, height: 5)
case .appleWatchSE_44mm: return (width: 4, height: 5)
case .simulator(let model): return model.screenRatio
case .unknown: return (width: -1, height: -1)
}
#elseif os(tvOS)
return (width: -1, height: -1)
#endif
}
#if os(iOS)
/// All iPods
public static var allPods: [Device] {
return [.iPodTouch5, .iPodTouch6, .iPodTouch7]
}
/// All iPhones
public static var allPhones: [Device] {
return [.iPhone4, .iPhone4s, .iPhone5, .iPhone5c, .iPhone5s, .iPhone6, .iPhone6Plus, .iPhone6s, .iPhone6sPlus, .iPhone7, .iPhone7Plus, .iPhoneSE, .iPhone8, .iPhone8Plus, .iPhoneX, .iPhoneXS, .iPhoneXSMax, .iPhoneXR, .iPhone11, .iPhone11Pro, .iPhone11ProMax, .iPhoneSE2, .iPhone12, .iPhone12Mini, .iPhone12Pro, .iPhone12ProMax, .iPhone13, .iPhone13Mini, .iPhone13Pro, .iPhone13ProMax]
}
/// All iPads
public static var allPads: [Device] {
return [.iPad2, .iPad3, .iPad4, .iPadAir, .iPadAir2, .iPad5, .iPad6, .iPadAir3, .iPad7, .iPad8, .iPad9, .iPadAir4, .iPadMini, .iPadMini2, .iPadMini3, .iPadMini4, .iPadMini5, .iPadMini6, .iPadPro9Inch, .iPadPro12Inch, .iPadPro12Inch2, .iPadPro10Inch, .iPadPro11Inch, .iPadPro12Inch3, .iPadPro11Inch2, .iPadPro12Inch4, .iPadPro11Inch3, .iPadPro12Inch5]
}
/// All X-Series Devices
@available(*, deprecated, renamed: "allDevicesWithSensorHousing")
public static var allXSeriesDevices: [Device] {
return [.iPhoneX, .iPhoneXS, .iPhoneXSMax, .iPhoneXR, .iPhone11, .iPhone11Pro, .iPhone11ProMax, .iPhone12, .iPhone12Mini, .iPhone12Pro, .iPhone12ProMax, .iPhone13, .iPhone13Mini, .iPhone13Pro, .iPhone13ProMax]
}
/// All Plus and Max-Sized Devices
public static var allPlusSizedDevices: [Device] {
return [.iPhone6Plus, .iPhone6sPlus, .iPhone7Plus, .iPhone8Plus, .iPhoneXSMax, .iPhone11ProMax, .iPhone12ProMax, .iPhone13ProMax]
}
/// All Pro Devices
public static var allProDevices: [Device] {
return [.iPhone11Pro, .iPhone11ProMax, .iPhone12Pro, .iPhone12ProMax, .iPhone13Pro, .iPhone13ProMax, .iPadPro9Inch, .iPadPro12Inch, .iPadPro12Inch2, .iPadPro10Inch, .iPadPro11Inch, .iPadPro12Inch3, .iPadPro11Inch2, .iPadPro12Inch4, .iPadPro11Inch3, .iPadPro12Inch5]
}
/// All mini Devices
public static var allMiniDevices: [Device] {
return [.iPadMini, .iPadMini2, .iPadMini3, .iPadMini4, .iPadMini5, .iPadMini6]
}
/// All simulator iPods
public static var allSimulatorPods: [Device] {
return allPods.map(Device.simulator)
}
/// All simulator iPhones
public static var allSimulatorPhones: [Device] {
return allPhones.map(Device.simulator)
}
/// All simulator iPads
public static var allSimulatorPads: [Device] {
return allPads.map(Device.simulator)
}
/// All simulator iPad mini
public static var allSimulatorMiniDevices: [Device] {
return allMiniDevices.map(Device.simulator)
}
/// All simulator X series Devices
@available(*, deprecated, renamed: "allSimulatorDevicesWithSensorHousing")
public static var allSimulatorXSeriesDevices: [Device] {
return allDevicesWithSensorHousing.map(Device.simulator)
}
/// All simulator Plus and Max-Sized Devices
public static var allSimulatorPlusSizedDevices: [Device] {
return allPlusSizedDevices.map(Device.simulator)
}
/// All simulator Pro Devices
public static var allSimulatorProDevices: [Device] {
return allProDevices.map(Device.simulator)
}
/// Returns whether the device is an iPod (real or simulator)
public var isPod: Bool {
return isOneOf(Device.allPods) || isOneOf(Device.allSimulatorPods)
}
/// Returns whether the device is an iPhone (real or simulator)
public var isPhone: Bool {
return (isOneOf(Device.allPhones)
|| isOneOf(Device.allSimulatorPhones)
|| (UIDevice.current.userInterfaceIdiom == .phone && isCurrent)) && !isPod
}
/// Returns whether the device is an iPad (real or simulator)
public var isPad: Bool {
return isOneOf(Device.allPads)
|| isOneOf(Device.allSimulatorPads)
|| (UIDevice.current.userInterfaceIdiom == .pad && isCurrent)
}
/// Returns whether the device is any of the simulator
/// Useful when there is a need to check and skip running a portion of code (location request or others)
public var isSimulator: Bool {
return isOneOf(Device.allSimulators)
}
/// If this device is a simulator return the underlying device,
/// otherwise return `self`.
public var realDevice: Device {
return Device.realDevice(from: self)
}
public var isZoomed: Bool? {
guard isCurrent else { return nil }
if Int(UIScreen.main.scale.rounded()) == 3 {
// Plus-sized
return UIScreen.main.nativeScale > 2.7 && UIScreen.main.nativeScale < 3
} else {
return UIScreen.main.nativeScale > UIScreen.main.scale
}
}
/// All Touch ID Capable Devices
public static var allTouchIDCapableDevices: [Device] {
return [.iPhone5s, .iPhone6, .iPhone6Plus, .iPhone6s, .iPhone6sPlus, .iPhone7, .iPhone7Plus, .iPhoneSE, .iPhone8, .iPhone8Plus, .iPhoneSE2, .iPadAir2, .iPad5, .iPad6, .iPadAir3, .iPad7, .iPad8, .iPad9, .iPadAir4, .iPadMini3, .iPadMini4, .iPadMini5, .iPadMini6, .iPadPro9Inch, .iPadPro12Inch, .iPadPro12Inch2, .iPadPro10Inch]
}
/// All Face ID Capable Devices
public static var allFaceIDCapableDevices: [Device] {
return [.iPhoneX, .iPhoneXS, .iPhoneXSMax, .iPhoneXR, .iPhone11, .iPhone11Pro, .iPhone11ProMax, .iPhone12, .iPhone12Mini, .iPhone12Pro, .iPhone12ProMax, .iPhone13, .iPhone13Mini, .iPhone13Pro, .iPhone13ProMax, .iPadPro11Inch, .iPadPro12Inch3, .iPadPro11Inch2, .iPadPro12Inch4, .iPadPro11Inch3, .iPadPro12Inch5]
}
/// All Devices with Touch ID or Face ID
public static var allBiometricAuthenticationCapableDevices: [Device] {
return [.iPhone5s, .iPhone6, .iPhone6Plus, .iPhone6s, .iPhone6sPlus, .iPhone7, .iPhone7Plus, .iPhoneSE, .iPhone8, .iPhone8Plus, .iPhoneX, .iPhoneXS, .iPhoneXSMax, .iPhoneXR, .iPhone11, .iPhone11Pro, .iPhone11ProMax, .iPhoneSE2, .iPhone12, .iPhone12Mini, .iPhone12Pro, .iPhone12ProMax, .iPhone13, .iPhone13Mini, .iPhone13Pro, .iPhone13ProMax, .iPadAir2, .iPad5, .iPad6, .iPadAir3, .iPad7, .iPad8, .iPad9, .iPadAir4, .iPadMini3, .iPadMini4, .iPadMini5, .iPadMini6, .iPadPro9Inch, .iPadPro12Inch, .iPadPro12Inch2, .iPadPro10Inch, .iPadPro11Inch, .iPadPro12Inch3, .iPadPro11Inch2, .iPadPro12Inch4, .iPadPro11Inch3, .iPadPro12Inch5]
}
/// Returns whether or not the device has Touch ID
public var isTouchIDCapable: Bool {
return isOneOf(Device.allTouchIDCapableDevices) || isOneOf(Device.allTouchIDCapableDevices.map(Device.simulator))
}
/// Returns whether or not the device has Face ID
public var isFaceIDCapable: Bool {
return isOneOf(Device.allFaceIDCapableDevices) || isOneOf(Device.allFaceIDCapableDevices.map(Device.simulator))
}
/// Returns whether or not the device has any biometric sensor (i.e. Touch ID or Face ID)
public var hasBiometricSensor: Bool {
return isTouchIDCapable || isFaceIDCapable
}
/// All devices that feature a sensor housing in the screen
public static var allDevicesWithSensorHousing: [Device] {
return [.iPhoneX, .iPhoneXS, .iPhoneXSMax, .iPhoneXR, .iPhone11, .iPhone11Pro, .iPhone11ProMax, .iPhone12, .iPhone12Mini, .iPhone12Pro, .iPhone12ProMax, .iPhone13, .iPhone13Mini, .iPhone13Pro, .iPhone13ProMax]
}
/// All simulator devices that feature a sensor housing in the screen
public static var allSimulatorDevicesWithSensorHousing: [Device] {
return allDevicesWithSensorHousing.map(Device.simulator)
}
/// Returns whether or not the device has a sensor housing
public var hasSensorHousing: Bool {
return isOneOf(Device.allDevicesWithSensorHousing) || isOneOf(Device.allDevicesWithSensorHousing.map(Device.simulator))
}
/// All devices that feature a screen with rounded corners.
public static var allDevicesWithRoundedDisplayCorners: [Device] {
return [.iPhoneX, .iPhoneXS, .iPhoneXSMax, .iPhoneXR, .iPhone11, .iPhone11Pro, .iPhone11ProMax, .iPhone12, .iPhone12Mini, .iPhone12Pro, .iPhone12ProMax, .iPhone13, .iPhone13Mini, .iPhone13Pro, .iPhone13ProMax, .iPadAir4, .iPadMini6, .iPadPro11Inch, .iPadPro12Inch3, .iPadPro11Inch2, .iPadPro12Inch4, .iPadPro11Inch3, .iPadPro12Inch5]
}
/// Returns whether or not the device has a screen with rounded corners.
public var hasRoundedDisplayCorners: Bool {
return isOneOf(Device.allDevicesWithRoundedDisplayCorners) || isOneOf(Device.allDevicesWithRoundedDisplayCorners.map(Device.simulator))
}
/// All devices that have 3D Touch support.
public static var allDevicesWith3dTouchSupport: [Device] {
return [.iPhone6s, .iPhone6sPlus, .iPhone7, .iPhone7Plus, .iPhone8, .iPhone8Plus, .iPhoneX, .iPhoneXS, .iPhoneXSMax]
}
/// Returns whether or not the device has 3D Touch support.
public var has3dTouchSupport: Bool {
return isOneOf(Device.allDevicesWith3dTouchSupport) || isOneOf(Device.allDevicesWith3dTouchSupport.map(Device.simulator))
}
/// All devices that support wireless charging.
public static var allDevicesWithWirelessChargingSupport: [Device] {
return [.iPhone8, .iPhone8Plus, .iPhoneX, .iPhoneXS, .iPhoneXSMax, .iPhoneXR, .iPhone11, .iPhone11Pro, .iPhone11ProMax, .iPhoneSE2, .iPhone12, .iPhone12Mini, .iPhone12Pro, .iPhone12ProMax, .iPhone13, .iPhone13Mini, .iPhone13Pro, .iPhone13ProMax]
}
/// Returns whether or not the device supports wireless charging.
public var supportsWirelessCharging: Bool {
return isOneOf(Device.allDevicesWithWirelessChargingSupport) || isOneOf(Device.allDevicesWithWirelessChargingSupport.map(Device.simulator))
}
/// All devices that have a LiDAR sensor.
public static var allDevicesWithALidarSensor: [Device] {
return [.iPhone12Pro, .iPhone12ProMax, .iPhone13Pro, .iPhone13ProMax, .iPadPro11Inch2, .iPadPro12Inch4, .iPadPro11Inch3, .iPadPro12Inch5]
}
/// Returns whether or not the device has a LiDAR sensor.
public var hasLidarSensor: Bool {
return isOneOf(Device.allDevicesWithALidarSensor) || isOneOf(Device.allDevicesWithALidarSensor.map(Device.simulator))
}
#elseif os(tvOS)
/// All TVs
public static var allTVs: [Device] {
return [.appleTVHD, .appleTV4K, .appleTV4K2]
}
/// All simulator TVs
public static var allSimulatorTVs: [Device] {
return allTVs.map(Device.simulator)
}
#elseif os(watchOS)
/// All Watches
public static var allWatches: [Device] {
return [.appleWatchSeries0_38mm, .appleWatchSeries0_42mm, .appleWatchSeries1_38mm, .appleWatchSeries1_42mm, .appleWatchSeries2_38mm, .appleWatchSeries2_42mm, .appleWatchSeries3_38mm, .appleWatchSeries3_42mm, .appleWatchSeries4_40mm, .appleWatchSeries4_44mm, .appleWatchSeries5_40mm, .appleWatchSeries5_44mm, .appleWatchSeries6_40mm, .appleWatchSeries6_44mm, .appleWatchSE_40mm, .appleWatchSE_44mm]
}
/// All simulator Watches
public static var allSimulatorWatches: [Device] {
return allWatches.map(Device.simulator)
}
/// All watches that have Force Touch support.
public static var allWatchesWithForceTouchSupport: [Device] {
return [.appleWatchSeries0_38mm, .appleWatchSeries0_42mm, .appleWatchSeries1_38mm, .appleWatchSeries1_42mm, .appleWatchSeries2_38mm, .appleWatchSeries2_42mm, .appleWatchSeries3_38mm, .appleWatchSeries3_42mm, .appleWatchSeries4_40mm, .appleWatchSeries4_44mm, .appleWatchSeries5_40mm, .appleWatchSeries5_44mm]
}
/// Returns whether or not the device has Force Touch support.
public var hasForceTouchSupport: Bool {
return isOneOf(Device.allWatchesWithForceTouchSupport) || isOneOf(Device.allWatchesWithForceTouchSupport.map(Device.simulator))
}
#endif
/// All real devices (i.e. all devices except for all simulators)
public static var allRealDevices: [Device] {
#if os(iOS)
return allPods + allPhones + allPads
#elseif os(tvOS)
return allTVs
#elseif os(watchOS)
return allWatches
#endif
}
/// All simulators
public static var allSimulators: [Device] {
return allRealDevices.map(Device.simulator)
}
/**
This method saves you in many cases from the need of updating your code with every new device.
Most uses for an enum like this are the following:
```
switch Device.current {
case .iPodTouch5, .iPodTouch6: callMethodOnIPods()
case .iPhone4, iPhone4s, .iPhone5, .iPhone5s, .iPhone6, .iPhone6Plus, .iPhone6s, .iPhone6sPlus, .iPhone7, .iPhone7Plus, .iPhoneSE, .iPhone8, .iPhone8Plus, .iPhoneX: callMethodOnIPhones()
case .iPad2, .iPad3, .iPad4, .iPadAir, .iPadAir2, .iPadMini, .iPadMini2, .iPadMini3, .iPadMini4, .iPadPro: callMethodOnIPads()
default: break
}
```
This code can now be replaced with
```
let device = Device.current
if device.isOneOf(Device.allPods) {
callMethodOnIPods()
} else if device.isOneOf(Device.allPhones) {
callMethodOnIPhones()
} else if device.isOneOf(Device.allPads) {
callMethodOnIPads()
}
```
- parameter devices: An array of devices.
- returns: Returns whether the current device is one of the passed in ones.
*/
public func isOneOf(_ devices: [Device]) -> Bool {
return devices.contains(self)
}
// MARK: Current Device
/// Whether or not the current device is the current device.
private var isCurrent: Bool {
return self == Device.current
}
/// The name identifying the device (e.g. "Dennis' iPhone").
public var name: String? {
guard isCurrent else { return nil }
#if os(watchOS)
return WKInterfaceDevice.current().name
#else
return UIDevice.current.name
#endif
}
/// The name of the operating system running on the device represented by the receiver (e.g. "iOS" or "tvOS").
public var systemName: String? {
guard isCurrent else { return nil }
#if os(watchOS)
return WKInterfaceDevice.current().systemName
#else
return UIDevice.current.systemName
#endif
}
/// The current version of the operating system (e.g. 8.4 or 9.2).
public var systemVersion: String? {
guard isCurrent else { return nil }
#if os(watchOS)
return WKInterfaceDevice.current().systemVersion
#else
return UIDevice.current.systemVersion
#endif
}
/// The model of the device (e.g. "iPhone" or "iPod Touch").
public var model: String? {
guard isCurrent else { return nil }
#if os(watchOS)
return WKInterfaceDevice.current().model
#else
return UIDevice.current.model
#endif
}
/// The model of the device as a localized string.
public var localizedModel: String? {
guard isCurrent else { return nil }
#if os(watchOS)
return WKInterfaceDevice.current().localizedModel
#else
return UIDevice.current.localizedModel
#endif
}
/// PPI (Pixels per Inch) on the current device's screen (if applicable). When the device is not applicable this property returns nil.
public var ppi: Int? {
#if os(iOS)
switch self {
case .iPodTouch5: return 326
case .iPodTouch6: return 326
case .iPodTouch7: return 326
case .iPhone4: return 326
case .iPhone4s: return 326
case .iPhone5: return 326
case .iPhone5c: return 326
case .iPhone5s: return 326
case .iPhone6: return 326
case .iPhone6Plus: return 401
case .iPhone6s: return 326
case .iPhone6sPlus: return 401
case .iPhone7: return 326
case .iPhone7Plus: return 401
case .iPhoneSE: return 326
case .iPhone8: return 326
case .iPhone8Plus: return 401
case .iPhoneX: return 458
case .iPhoneXS: return 458
case .iPhoneXSMax: return 458
case .iPhoneXR: return 326
case .iPhone11: return 326
case .iPhone11Pro: return 458
case .iPhone11ProMax: return 458
case .iPhoneSE2: return 326
case .iPhone12: return 460
case .iPhone12Mini: return 476
case .iPhone12Pro: return 460
case .iPhone12ProMax: return 458
case .iPhone13: return 460
case .iPhone13Mini: return 476
case .iPhone13Pro: return 460
case .iPhone13ProMax: return 458
case .iPad2: return 132
case .iPad3: return 264
case .iPad4: return 264
case .iPadAir: return 264
case .iPadAir2: return 264
case .iPad5: return 264
case .iPad6: return 264
case .iPadAir3: return 264
case .iPad7: return 264
case .iPad8: return 264
case .iPad9: return 264
case .iPadAir4: return 264
case .iPadMini: return 163
case .iPadMini2: return 326
case .iPadMini3: return 326
case .iPadMini4: return 326
case .iPadMini5: return 326
case .iPadMini6: return 326
case .iPadPro9Inch: return 264
case .iPadPro12Inch: return 264
case .iPadPro12Inch2: return 264
case .iPadPro10Inch: return 264
case .iPadPro11Inch: return 264
case .iPadPro12Inch3: return 264
case .iPadPro11Inch2: return 264
case .iPadPro12Inch4: return 264
case .iPadPro11Inch3: return 264
case .iPadPro12Inch5: return 264
case .homePod: return -1
case .simulator(let model): return model.ppi
case .unknown: return nil
}
#elseif os(watchOS)
switch self {
case .appleWatchSeries0_38mm: return 290
case .appleWatchSeries0_42mm: return 303
case .appleWatchSeries1_38mm: return 290
case .appleWatchSeries1_42mm: return 303
case .appleWatchSeries2_38mm: return 290
case .appleWatchSeries2_42mm: return 303
case .appleWatchSeries3_38mm: return 290
case .appleWatchSeries3_42mm: return 303
case .appleWatchSeries4_40mm: return 326
case .appleWatchSeries4_44mm: return 326
case .appleWatchSeries5_40mm: return 326
case .appleWatchSeries5_44mm: return 326
case .appleWatchSeries6_40mm: return 326
case .appleWatchSeries6_44mm: return 326
case .appleWatchSE_40mm: return 326
case .appleWatchSE_44mm: return 326
case .simulator(let model): return model.ppi
case .unknown: return nil
}
#elseif os(tvOS)
return nil
#endif
}
/// True when a Guided Access session is currently active; otherwise, false.
public var isGuidedAccessSessionActive: Bool {
#if os(iOS)
#if swift(>=4.2)
return UIAccessibility.isGuidedAccessEnabled
#else
return UIAccessibilityIsGuidedAccessEnabled()
#endif
#else
return false
#endif
}
/// The brightness level of the screen.
public var screenBrightness: Int {
#if os(iOS)
return Int(UIScreen.main.brightness * 100)
#else
return 100
#endif
}
}
// MARK: CustomStringConvertible
extension Device: CustomStringConvertible {
/// A textual representation of the device.
public var description: String {
#if os(iOS)
switch self {
case .iPodTouch5: return "iPod touch (5th generation)"
case .iPodTouch6: return "iPod touch (6th generation)"
case .iPodTouch7: return "iPod touch (7th generation)"
case .iPhone4: return "iPhone 4"
case .iPhone4s: return "iPhone 4s"
case .iPhone5: return "iPhone 5"
case .iPhone5c: return "iPhone 5c"
case .iPhone5s: return "iPhone 5s"
case .iPhone6: return "iPhone 6"
case .iPhone6Plus: return "iPhone 6 Plus"
case .iPhone6s: return "iPhone 6s"
case .iPhone6sPlus: return "iPhone 6s Plus"
case .iPhone7: return "iPhone 7"
case .iPhone7Plus: return "iPhone 7 Plus"
case .iPhoneSE: return "iPhone SE"
case .iPhone8: return "iPhone 8"
case .iPhone8Plus: return "iPhone 8 Plus"
case .iPhoneX: return "iPhone X"
case .iPhoneXS: return "iPhone Xs"
case .iPhoneXSMax: return "iPhone Xs Max"
case .iPhoneXR: return "iPhone Xʀ"
case .iPhone11: return "iPhone 11"
case .iPhone11Pro: return "iPhone 11 Pro"
case .iPhone11ProMax: return "iPhone 11 Pro Max"
case .iPhoneSE2: return "iPhone SE (2nd generation)"
case .iPhone12: return "iPhone 12"
case .iPhone12Mini: return "iPhone 12 mini"
case .iPhone12Pro: return "iPhone 12 Pro"
case .iPhone12ProMax: return "iPhone 12 Pro Max"
case .iPhone13: return "iPhone 13"
case .iPhone13Mini: return "iPhone 13 mini"
case .iPhone13Pro: return "iPhone 13 Pro"
case .iPhone13ProMax: return "iPhone 13 Pro Max"
case .iPad2: return "iPad 2"
case .iPad3: return "iPad (3rd generation)"
case .iPad4: return "iPad (4th generation)"
case .iPadAir: return "iPad Air"
case .iPadAir2: return "iPad Air 2"
case .iPad5: return "iPad (5th generation)"
case .iPad6: return "iPad (6th generation)"
case .iPadAir3: return "iPad Air (3rd generation)"
case .iPad7: return "iPad (7th generation)"
case .iPad8: return "iPad (8th generation)"
case .iPad9: return "iPad (9th generation)"
case .iPadAir4: return "iPad Air (4th generation)"
case .iPadMini: return "iPad Mini"
case .iPadMini2: return "iPad Mini 2"
case .iPadMini3: return "iPad Mini 3"
case .iPadMini4: return "iPad Mini 4"
case .iPadMini5: return "iPad Mini (5th generation)"
case .iPadMini6: return "iPad Mini (6th generation)"
case .iPadPro9Inch: return "iPad Pro (9.7-inch)"
case .iPadPro12Inch: return "iPad Pro (12.9-inch)"
case .iPadPro12Inch2: return "iPad Pro (12.9-inch) (2nd generation)"
case .iPadPro10Inch: return "iPad Pro (10.5-inch)"
case .iPadPro11Inch: return "iPad Pro (11-inch)"
case .iPadPro12Inch3: return "iPad Pro (12.9-inch) (3rd generation)"
case .iPadPro11Inch2: return "iPad Pro (11-inch) (2nd generation)"
case .iPadPro12Inch4: return "iPad Pro (12.9-inch) (4th generation)"
case .iPadPro11Inch3: return "iPad Pro (11-inch) (3rd generation)"
case .iPadPro12Inch5: return "iPad Pro (12.9-inch) (5th generation)"
case .homePod: return "HomePod"
case .simulator(let model): return "Simulator (\(model.description))"
case .unknown(let identifier): return identifier
}
#elseif os(watchOS)
switch self {
case .appleWatchSeries0_38mm: return "Apple Watch (1st generation) 38mm"
case .appleWatchSeries0_42mm: return "Apple Watch (1st generation) 42mm"
case .appleWatchSeries1_38mm: return "Apple Watch Series 1 38mm"
case .appleWatchSeries1_42mm: return "Apple Watch Series 1 42mm"
case .appleWatchSeries2_38mm: return "Apple Watch Series 2 38mm"
case .appleWatchSeries2_42mm: return "Apple Watch Series 2 42mm"
case .appleWatchSeries3_38mm: return "Apple Watch Series 3 38mm"
case .appleWatchSeries3_42mm: return "Apple Watch Series 3 42mm"
case .appleWatchSeries4_40mm: return "Apple Watch Series 4 40mm"
case .appleWatchSeries4_44mm: return "Apple Watch Series 4 44mm"
case .appleWatchSeries5_40mm: return "Apple Watch Series 5 40mm"
case .appleWatchSeries5_44mm: return "Apple Watch Series 5 44mm"
case .appleWatchSeries6_40mm: return "Apple Watch Series 6 40mm"
case .appleWatchSeries6_44mm: return "Apple Watch Series 6 44mm"
case .appleWatchSE_40mm: return "Apple Watch SE 40mm"
case .appleWatchSE_44mm: return "Apple Watch SE 44mm"
case .simulator(let model): return "Simulator (\(model.description))"
case .unknown(let identifier): return identifier
}
#elseif os(tvOS)
switch self {
case .appleTVHD: return "Apple TV HD"
case .appleTV4K: return "Apple TV 4K"
case .appleTV4K2: return "Apple TV 4K (2nd generation)"
case .simulator(let model): return "Simulator (\(model.description))"
case .unknown(let identifier): return identifier
}
#endif
}
/// A safe version of `description`.
/// Example:
/// Device.iPhoneXR.description: iPhone Xʀ
/// Device.iPhoneXR.safeDescription: iPhone XR
public var safeDescription: String {
#if os(iOS)
switch self {
case .iPodTouch5: return "iPod touch (5th generation)"
case .iPodTouch6: return "iPod touch (6th generation)"
case .iPodTouch7: return "iPod touch (7th generation)"
case .iPhone4: return "iPhone 4"
case .iPhone4s: return "iPhone 4s"
case .iPhone5: return "iPhone 5"
case .iPhone5c: return "iPhone 5c"
case .iPhone5s: return "iPhone 5s"
case .iPhone6: return "iPhone 6"
case .iPhone6Plus: return "iPhone 6 Plus"
case .iPhone6s: return "iPhone 6s"
case .iPhone6sPlus: return "iPhone 6s Plus"
case .iPhone7: return "iPhone 7"
case .iPhone7Plus: return "iPhone 7 Plus"
case .iPhoneSE: return "iPhone SE"
case .iPhone8: return "iPhone 8"
case .iPhone8Plus: return "iPhone 8 Plus"
case .iPhoneX: return "iPhone X"
case .iPhoneXS: return "iPhone XS"
case .iPhoneXSMax: return "iPhone XS Max"
case .iPhoneXR: return "iPhone XR"
case .iPhone11: return "iPhone 11"
case .iPhone11Pro: return "iPhone 11 Pro"
case .iPhone11ProMax: return "iPhone 11 Pro Max"
case .iPhoneSE2: return "iPhone SE (2nd generation)"
case .iPhone12: return "iPhone 12"
case .iPhone12Mini: return "iPhone 12 mini"
case .iPhone12Pro: return "iPhone 12 Pro"
case .iPhone12ProMax: return "iPhone 12 Pro Max"
case .iPhone13: return "iPhone 13"
case .iPhone13Mini: return "iPhone 13 mini"
case .iPhone13Pro: return "iPhone 13 Pro"
case .iPhone13ProMax: return "iPhone 13 Pro Max"
case .iPad2: return "iPad 2"
case .iPad3: return "iPad (3rd generation)"
case .iPad4: return "iPad (4th generation)"
case .iPadAir: return "iPad Air"
case .iPadAir2: return "iPad Air 2"
case .iPad5: return "iPad (5th generation)"
case .iPad6: return "iPad (6th generation)"
case .iPadAir3: return "iPad Air (3rd generation)"
case .iPad7: return "iPad (7th generation)"
case .iPad8: return "iPad (8th generation)"
case .iPad9: return "iPad (9th generation)"
case .iPadAir4: return "iPad Air (4th generation)"
case .iPadMini: return "iPad Mini"
case .iPadMini2: return "iPad Mini 2"
case .iPadMini3: return "iPad Mini 3"
case .iPadMini4: return "iPad Mini 4"
case .iPadMini5: return "iPad Mini (5th generation)"
case .iPadMini6: return "iPad Mini (6th generation)"
case .iPadPro9Inch: return "iPad Pro (9.7-inch)"
case .iPadPro12Inch: return "iPad Pro (12.9-inch)"
case .iPadPro12Inch2: return "iPad Pro (12.9-inch) (2nd generation)"
case .iPadPro10Inch: return "iPad Pro (10.5-inch)"
case .iPadPro11Inch: return "iPad Pro (11-inch)"
case .iPadPro12Inch3: return "iPad Pro (12.9-inch) (3rd generation)"
case .iPadPro11Inch2: return "iPad Pro (11-inch) (2nd generation)"
case .iPadPro12Inch4: return "iPad Pro (12.9-inch) (4th generation)"
case .iPadPro11Inch3: return "iPad Pro (11-inch) (3rd generation)"
case .iPadPro12Inch5: return "iPad Pro (12.9-inch) (5th generation)"
case .homePod: return "HomePod"
case .simulator(let model): return "Simulator (\(model.safeDescription))"
case .unknown(let identifier): return identifier
}
#elseif os(watchOS)
switch self {
case .appleWatchSeries0_38mm: return "Apple Watch (1st generation) 38mm"
case .appleWatchSeries0_42mm: return "Apple Watch (1st generation) 42mm"
case .appleWatchSeries1_38mm: return "Apple Watch Series 1 38mm"
case .appleWatchSeries1_42mm: return "Apple Watch Series 1 42mm"
case .appleWatchSeries2_38mm: return "Apple Watch Series 2 38mm"
case .appleWatchSeries2_42mm: return "Apple Watch Series 2 42mm"
case .appleWatchSeries3_38mm: return "Apple Watch Series 3 38mm"
case .appleWatchSeries3_42mm: return "Apple Watch Series 3 42mm"
case .appleWatchSeries4_40mm: return "Apple Watch Series 4 40mm"
case .appleWatchSeries4_44mm: return "Apple Watch Series 4 44mm"
case .appleWatchSeries5_40mm: return "Apple Watch Series 5 40mm"
case .appleWatchSeries5_44mm: return "Apple Watch Series 5 44mm"
case .appleWatchSeries6_40mm: return "Apple Watch Series 6 40mm"
case .appleWatchSeries6_44mm: return "Apple Watch Series 6 44mm"
case .appleWatchSE_40mm: return "Apple Watch SE 40mm"
case .appleWatchSE_44mm: return "Apple Watch SE 44mm"
case .simulator(let model): return "Simulator (\(model.safeDescription))"
case .unknown(let identifier): return identifier
}
#elseif os(tvOS)
switch self {
case .appleTVHD: return "Apple TV HD"
case .appleTV4K: return "Apple TV 4K"
case .appleTV4K2: return "Apple TV 4K (2nd generation)"
case .simulator(let model): return "Simulator (\(model.safeDescription))"
case .unknown(let identifier): return identifier
}
#endif
}
}
// MARK: Equatable
extension Device: Equatable {
/// Compares two devices
///
/// - parameter lhs: A device.
/// - parameter rhs: Another device.
///
/// - returns: `true` iff the underlying identifier is the same.
public static func == (lhs: Device, rhs: Device) -> Bool {
return lhs.description == rhs.description
}
}
// MARK: Battery
#if os(iOS) || os(watchOS)
@available(iOS 8.0, watchOS 4.0, *)
extension Device {
/**
This enum describes the state of the battery.
- Full: The device is plugged into power and the battery is 100% charged or the device is the iOS Simulator.
- Charging: The device is plugged into power and the battery is less than 100% charged.
- Unplugged: The device is not plugged into power; the battery is discharging.
*/
public enum BatteryState: CustomStringConvertible, Equatable {
/// The device is plugged into power and the battery is 100% charged or the device is the iOS Simulator.
case full
/// The device is plugged into power and the battery is less than 100% charged.
/// The associated value is in percent (0-100).
case charging(Int)
/// The device is not plugged into power; the battery is discharging.
/// The associated value is in percent (0-100).
case unplugged(Int)
#if os(iOS)
fileprivate init() {
let wasBatteryMonitoringEnabled = UIDevice.current.isBatteryMonitoringEnabled
UIDevice.current.isBatteryMonitoringEnabled = true
let batteryLevel = Int(round(UIDevice.current.batteryLevel * 100)) // round() is actually not needed anymore since -[batteryLevel] seems to always return a two-digit precision number
// but maybe that changes in the future.
switch UIDevice.current.batteryState {
case .charging: self = .charging(batteryLevel)
case .full: self = .full
case .unplugged: self = .unplugged(batteryLevel)
case .unknown: self = .full // Should never happen since `batteryMonitoring` is enabled.
@unknown default:
self = .full // To cover any future additions for which DeviceKit might not have updated yet.
}
UIDevice.current.isBatteryMonitoringEnabled = wasBatteryMonitoringEnabled
}
#elseif os(watchOS)
fileprivate init() {
let wasBatteryMonitoringEnabled = WKInterfaceDevice.current().isBatteryMonitoringEnabled
WKInterfaceDevice.current().isBatteryMonitoringEnabled = true
let batteryLevel = Int(round(WKInterfaceDevice.current().batteryLevel * 100)) // round() is actually not needed anymore since -[batteryLevel] seems to always return a two-digit precision number
// but maybe that changes in the future.
switch WKInterfaceDevice.current().batteryState {
case .charging: self = .charging(batteryLevel)
case .full: self = .full
case .unplugged: self = .unplugged(batteryLevel)
case .unknown: self = .full // Should never happen since `batteryMonitoring` is enabled.
@unknown default:
self = .full // To cover any future additions for which DeviceKit might not have updated yet.
}
WKInterfaceDevice.current().isBatteryMonitoringEnabled = wasBatteryMonitoringEnabled
}
#endif
/// The user enabled Low Power mode
public var lowPowerMode: Bool {
return ProcessInfo.processInfo.isLowPowerModeEnabled
}
/// Provides a textual representation of the battery state.
/// Examples:
/// ```
/// Battery level: 90%, device is plugged in.
/// Battery level: 100 % (Full), device is plugged in.
/// Battery level: \(batteryLevel)%, device is unplugged.
/// ```
public var description: String {
switch self {
case .charging(let batteryLevel): return "Battery level: \(batteryLevel)%, device is plugged in."
case .full: return "Battery level: 100 % (Full), device is plugged in."
case .unplugged(let batteryLevel): return "Battery level: \(batteryLevel)%, device is unplugged."
}
}
}
/// The state of the battery
public var batteryState: BatteryState? {
guard isCurrent else { return nil }
return BatteryState()
}
/// Battery level ranges from 0 (fully discharged) to 100 (100% charged).
public var batteryLevel: Int? {
guard isCurrent else { return nil }
switch BatteryState() {
case .charging(let value): return value
case .full: return 100
case .unplugged(let value): return value
}
}
}
#endif
// MARK: Device.Batterystate: Comparable
#if os(iOS) || os(watchOS)
@available(iOS 8.0, watchOS 4.0, *)
extension Device.BatteryState: Comparable {
/// Tells if two battery states are equal.
///
/// - parameter lhs: A battery state.
/// - parameter rhs: Another battery state.
///
/// - returns: `true` iff they are equal, otherwise `false`
public static func == (lhs: Device.BatteryState, rhs: Device.BatteryState) -> Bool {
return lhs.description == rhs.description
}
/// Compares two battery states.
///
/// - parameter lhs: A battery state.
/// - parameter rhs: Another battery state.
///
/// - returns: `true` if rhs is `.Full`, `false` when lhs is `.Full` otherwise their battery level is compared.
public static func < (lhs: Device.BatteryState, rhs: Device.BatteryState) -> Bool {
switch (lhs, rhs) {
case (.full, _): return false // return false (even if both are `.Full` -> they are equal)
case (_, .full): return true // lhs is *not* `.Full`, rhs is
case let (.charging(lhsLevel), .charging(rhsLevel)): return lhsLevel < rhsLevel
case let (.charging(lhsLevel), .unplugged(rhsLevel)): return lhsLevel < rhsLevel
case let (.unplugged(lhsLevel), .charging(rhsLevel)): return lhsLevel < rhsLevel
case let (.unplugged(lhsLevel), .unplugged(rhsLevel)): return lhsLevel < rhsLevel
default: return false // compiler won't compile without it, though it cannot happen
}
}
}
#endif
#if os(iOS)
extension Device {
// MARK: Orientation
/**
This enum describes the state of the orientation.
- Landscape: The device is in Landscape Orientation
- Portrait: The device is in Portrait Orientation
*/
public enum Orientation {
case landscape
case portrait
}
public var orientation: Orientation {
if UIDevice.current.orientation.isLandscape {
return .landscape
} else {
return .portrait
}
}
}
#endif
#if os(iOS)
// MARK: DiskSpace
extension Device {
/// Return the root url
///
/// - returns: the NSHomeDirectory() url
private static let rootURL = URL(fileURLWithPath: NSHomeDirectory())
/// The volume’s total capacity in bytes.
public static var volumeTotalCapacity: Int? {
return (try? Device.rootURL.resourceValues(forKeys: [.volumeTotalCapacityKey]))?.volumeTotalCapacity
}
/// The volume’s available capacity in bytes.
public static var volumeAvailableCapacity: Int? {
return (try? rootURL.resourceValues(forKeys: [.volumeAvailableCapacityKey]))?.volumeAvailableCapacity
}
/// The volume’s available capacity in bytes for storing important resources.
@available(iOS 11.0, *)
public static var volumeAvailableCapacityForImportantUsage: Int64? {
return (try? rootURL.resourceValues(forKeys: [.volumeAvailableCapacityForImportantUsageKey]))?.volumeAvailableCapacityForImportantUsage
}
/// The volume’s available capacity in bytes for storing nonessential resources.
@available(iOS 11.0, *)
public static var volumeAvailableCapacityForOpportunisticUsage: Int64? { //swiftlint:disable:this identifier_name
return (try? rootURL.resourceValues(forKeys: [.volumeAvailableCapacityForOpportunisticUsageKey]))?.volumeAvailableCapacityForOpportunisticUsage
}
/// All volumes capacity information in bytes.
@available(iOS 11.0, *)
public static var volumes: [URLResourceKey: Int64]? {
do {
let values = try rootURL.resourceValues(forKeys: [.volumeAvailableCapacityForImportantUsageKey,
.volumeAvailableCapacityKey,
.volumeAvailableCapacityForOpportunisticUsageKey,
.volumeTotalCapacityKey
])
return values.allValues.mapValues {
if let int = $0 as? Int64 {
return int
}
if let int = $0 as? Int {
return Int64(int)
}
return 0
}
} catch {
return nil
}
}
}
#endif
#if os(iOS)
// MARK: Apple Pencil
extension Device {
/**
This option set describes the current Apple Pencils
- firstGeneration: 1st Generation Apple Pencil
- secondGeneration: 2nd Generation Apple Pencil
*/
public struct ApplePencilSupport: OptionSet {
public var rawValue: UInt
public init(rawValue: UInt) {
self.rawValue = rawValue
}
public static let firstGeneration = ApplePencilSupport(rawValue: 0x01)
public static let secondGeneration = ApplePencilSupport(rawValue: 0x02)
}
/// All Apple Pencil Capable Devices
public static var allApplePencilCapableDevices: [Device] {
return [.iPad6, .iPadAir3, .iPad7, .iPad8, .iPad9, .iPadAir4, .iPadMini5, .iPadMini6, .iPadPro9Inch, .iPadPro12Inch, .iPadPro12Inch2, .iPadPro10Inch, .iPadPro11Inch, .iPadPro12Inch3, .iPadPro11Inch2, .iPadPro12Inch4, .iPadPro11Inch3, .iPadPro12Inch5]
}
/// Returns supported version of the Apple Pencil
public var applePencilSupport: ApplePencilSupport {
switch self {
case .iPad6: return .firstGeneration
case .iPadAir3: return .firstGeneration
case .iPad7: return .firstGeneration
case .iPad8: return .firstGeneration
case .iPad9: return .firstGeneration
case .iPadMini5: return .firstGeneration
case .iPadPro9Inch: return .firstGeneration
case .iPadPro12Inch: return .firstGeneration
case .iPadPro12Inch2: return .firstGeneration
case .iPadPro10Inch: return .firstGeneration
case .iPadAir4: return .secondGeneration
case .iPadMini6: return .secondGeneration
case .iPadPro11Inch: return .secondGeneration
case .iPadPro12Inch3: return .secondGeneration
case .iPadPro11Inch2: return .secondGeneration
case .iPadPro12Inch4: return .secondGeneration
case .iPadPro11Inch3: return .secondGeneration
case .iPadPro12Inch5: return .secondGeneration
case .simulator(let model): return model.applePencilSupport
default: return []
}
}
}
#endif
#if os(iOS)
// MARK: Cameras
extension Device {
public enum CameraType {
@available(*, deprecated, renamed: "wide")
case normal
case wide
case telephoto
case ultraWide
}
/// Returns an array of the types of cameras the device has
public var cameras: [CameraType] {
switch self {
case .iPodTouch5: return [.wide]
case .iPodTouch6: return [.wide]
case .iPodTouch7: return [.wide]
case .iPhone4: return [.wide]
case .iPhone4s: return [.wide]
case .iPhone5: return [.wide]
case .iPhone5c: return [.wide]
case .iPhone5s: return [.wide]
case .iPhone6: return [.wide]
case .iPhone6Plus: return [.wide]
case .iPhone6s: return [.wide]
case .iPhone6sPlus: return [.wide]
case .iPhone7: return [.wide]
case .iPhoneSE: return [.wide]
case .iPhone8: return [.wide]
case .iPhoneXR: return [.wide]
case .iPhoneSE2: return [.wide]
case .iPad2: return [.wide]
case .iPad3: return [.wide]
case .iPad4: return [.wide]
case .iPadAir: return [.wide]
case .iPadAir2: return [.wide]
case .iPad5: return [.wide]
case .iPad6: return [.wide]
case .iPadAir3: return [.wide]
case .iPad7: return [.wide]
case .iPad8: return [.wide]
case .iPad9: return [.wide]
case .iPadAir4: return [.wide]
case .iPadMini: return [.wide]
case .iPadMini2: return [.wide]
case .iPadMini3: return [.wide]
case .iPadMini4: return [.wide]
case .iPadMini5: return [.wide]
case .iPadMini6: return [.wide]
case .iPadPro9Inch: return [.wide]
case .iPadPro12Inch: return [.wide]
case .iPadPro12Inch2: return [.wide]
case .iPadPro10Inch: return [.wide]
case .iPadPro11Inch: return [.wide]
case .iPadPro12Inch3: return [.wide]
case .iPhone7Plus: return [.wide, .telephoto]
case .iPhone8Plus: return [.wide, .telephoto]
case .iPhoneX: return [.wide, .telephoto]
case .iPhoneXS: return [.wide, .telephoto]
case .iPhoneXSMax: return [.wide, .telephoto]
case .iPhone11: return [.wide, .ultraWide]
case .iPhone12: return [.wide, .ultraWide]
case .iPhone12Mini: return [.wide, .ultraWide]
case .iPhone13: return [.wide, .ultraWide]
case .iPhone13Mini: return [.wide, .ultraWide]
case .iPadPro11Inch2: return [.wide, .ultraWide]
case .iPadPro12Inch4: return [.wide, .ultraWide]
case .iPadPro11Inch3: return [.wide, .ultraWide]
case .iPadPro12Inch5: return [.wide, .ultraWide]
case .iPhone11Pro: return [.wide, .telephoto, .ultraWide]
case .iPhone11ProMax: return [.wide, .telephoto, .ultraWide]
case .iPhone12Pro: return [.wide, .telephoto, .ultraWide]
case .iPhone12ProMax: return [.wide, .telephoto, .ultraWide]
case .iPhone13Pro: return [.wide, .telephoto, .ultraWide]
case .iPhone13ProMax: return [.wide, .telephoto, .ultraWide]
default: return []
}
}
/// All devices that feature a camera
public static var allDevicesWithCamera: [Device] {
return [.iPodTouch5, .iPodTouch6, .iPodTouch7, .iPhone4, .iPhone4s, .iPhone5, .iPhone5c, .iPhone5s, .iPhone6, .iPhone6Plus, .iPhone6s, .iPhone6sPlus, .iPhone7, .iPhone7Plus, .iPhoneSE, .iPhone8, .iPhone8Plus, .iPhoneX, .iPhoneXS, .iPhoneXSMax, .iPhoneXR, .iPhone11, .iPhone11Pro, .iPhone11ProMax, .iPhoneSE2, .iPhone12, .iPhone12Mini, .iPhone12Pro, .iPhone12ProMax, .iPhone13, .iPhone13Mini, .iPhone13Pro, .iPhone13ProMax, .iPad2, .iPad3, .iPad4, .iPadAir, .iPadAir2, .iPad5, .iPad6, .iPadAir3, .iPad7, .iPad8, .iPad9, .iPadAir4, .iPadMini, .iPadMini2, .iPadMini3, .iPadMini4, .iPadMini5, .iPadMini6, .iPadPro9Inch, .iPadPro12Inch, .iPadPro12Inch2, .iPadPro10Inch, .iPadPro11Inch, .iPadPro12Inch3, .iPadPro11Inch2, .iPadPro12Inch4, .iPadPro11Inch3, .iPadPro12Inch5]
}
/// All devices that feature a normal camera
@available(*, deprecated, renamed: "allDevicesWithWideCamera")
public static var allDevicesWithNormalCamera: [Device] {
return Device.allDevicesWithWideCamera
}
/// All devices that feature a wide camera
public static var allDevicesWithWideCamera: [Device] {
return [.iPodTouch5, .iPodTouch6, .iPodTouch7, .iPhone4, .iPhone4s, .iPhone5, .iPhone5c, .iPhone5s, .iPhone6, .iPhone6Plus, .iPhone6s, .iPhone6sPlus, .iPhone7, .iPhone7Plus, .iPhoneSE, .iPhone8, .iPhone8Plus, .iPhoneX, .iPhoneXS, .iPhoneXSMax, .iPhoneXR, .iPhone11, .iPhone11Pro, .iPhone11ProMax, .iPhoneSE2, .iPhone12, .iPhone12Mini, .iPhone12Pro, .iPhone12ProMax, .iPhone13, .iPhone13Mini, .iPhone13Pro, .iPhone13ProMax, .iPad2, .iPad3, .iPad4, .iPadAir, .iPadAir2, .iPad5, .iPad6, .iPadAir3, .iPad7, .iPad8, .iPad9, .iPadAir4, .iPadMini, .iPadMini2, .iPadMini3, .iPadMini4, .iPadMini5, .iPadMini6, .iPadPro9Inch, .iPadPro12Inch, .iPadPro12Inch2, .iPadPro10Inch, .iPadPro11Inch, .iPadPro12Inch3, .iPadPro11Inch2, .iPadPro12Inch4, .iPadPro11Inch3, .iPadPro12Inch5]
}
/// All devices that feature a telephoto camera
public static var allDevicesWithTelephotoCamera: [Device] {
return [.iPhone7Plus, .iPhone8Plus, .iPhoneX, .iPhoneXS, .iPhoneXSMax, .iPhone11Pro, .iPhone11ProMax, .iPhone12Pro, .iPhone12ProMax, .iPhone13Pro, .iPhone13ProMax]
}
/// All devices that feature an ultra wide camera
public static var allDevicesWithUltraWideCamera: [Device] {
return [.iPhone11, .iPhone11Pro, .iPhone11ProMax, .iPhone12, .iPhone12Mini, .iPhone12Pro, .iPhone12ProMax, .iPhone13, .iPhone13Mini, .iPhone13Pro, .iPhone13ProMax, .iPadPro11Inch2, .iPadPro12Inch4, .iPadPro11Inch3, .iPadPro12Inch5]
}
/// Returns whether or not the current device has a camera
public var hasCamera: Bool {
return !self.cameras.isEmpty
}
/// Returns whether or not the current device has a normal camera
@available(*, deprecated, renamed: "hasWideCamera")
public var hasNormalCamera: Bool {
return self.hasWideCamera
}
/// Returns whether or not the current device has a wide camera
public var hasWideCamera: Bool {
return self.cameras.contains(.wide)
}
/// Returns whether or not the current device has a telephoto camera
public var hasTelephotoCamera: Bool {
return self.cameras.contains(.telephoto)
}
/// Returns whether or not the current device has an ultra wide camera
public var hasUltraWideCamera: Bool {
return self.cameras.contains(.ultraWide)
}
}
#endif
| mit | 53fedd3f6df2311f099a0bd883102f53 | 43.750697 | 769 | 0.675042 | 3.494839 | false | false | false | false |
natan/Toucan | Source/Toucan.swift | 1 | 26758 | // Toucan.swift
//
// Copyright (c) 2014 Gavin Bunney, Bunney Apps (http://bunney.net.au)
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
import UIKit
import CoreGraphics
/**
Toucan - Fabulous Image Processing in Swift.
The Toucan class provides two methods of interaction - either through an instance, wrapping an single image,
or through the static functions, providing an image for each invocation.
This allows for some flexible usage. Using static methods when you need a single operation:
let resizedImage = Toucan.resize(myImage, size: CGSize(width: 100, height: 150))
Or create an instance for easy method chaining:
let resizedAndMaskedImage = Toucan(withImage: myImage).resize(CGSize(width: 100, height: 150)).maskWithEllipse().image
*/
public class Toucan : NSObject {
public var image : UIImage
public init(image withImage: UIImage) {
self.image = withImage
}
// MARK: - Resize
/**
Resize the contained image to the specified size. Depending on what fitMode is supplied, the image
may be clipped, cropped or scaled. @see documentation on FitMode.
The current image on this toucan instance is replaced with the resized image.
- parameter size: Size to resize the image to
- parameter fitMode: How to handle the image resizing process
- returns: Self, allowing method chaining
*/
public func resize(size: CGSize, fitMode: Toucan.Resize.FitMode = .Clip) -> Toucan {
self.image = Toucan.Resize.resizeImage(self.image, size: size, fitMode: fitMode)
return self
}
/**
Resize the contained image to the specified size by resizing the image to fit
within the width and height boundaries without cropping or scaling the image.
The current image on this toucan instance is replaced with the resized image.
- parameter size: Size to resize the image to
- returns: Self, allowing method chaining
*/
@objc
public func resizeByClipping(size: CGSize) -> Toucan {
self.image = Toucan.Resize.resizeImage(self.image, size: size, fitMode: .Clip)
return self
}
/**
Resize the contained image to the specified size by resizing the image to fill the
width and height boundaries and crops any excess image data.
The resulting image will match the width and height constraints without scaling the image.
The current image on this toucan instance is replaced with the resized image.
- parameter size: Size to resize the image to
- returns: Self, allowing method chaining
*/
@objc
public func resizeByCropping(size: CGSize) -> Toucan {
self.image = Toucan.Resize.resizeImage(self.image, size: size, fitMode: .Crop)
return self
}
/**
Resize the contained image to the specified size by scaling the image to fit the
constraining dimensions exactly.
The current image on this toucan instance is replaced with the resized image.
- parameter size: Size to resize the image to
- returns: Self, allowing method chaining
*/
@objc
public func resizeByScaling(size: CGSize) -> Toucan {
self.image = Toucan.Resize.resizeImage(self.image, size: size, fitMode: .Scale)
return self
}
/**
Container struct for all things Resize related
*/
public struct Resize {
/**
FitMode drives the resizing process to determine what to do with an image to
make it fit the given size bounds.
- Clip: Resizes the image to fit within the width and height boundaries without cropping or scaling the image.
- Crop: Resizes the image to fill the width and height boundaries and crops any excess image data.
- Scale: Scales the image to fit the constraining dimensions exactly.
*/
public enum FitMode {
/**
Resizes the image to fit within the width and height boundaries without cropping or scaling the image.
The resulting image is assured to match one of the constraining dimensions, while
the other dimension is altered to maintain the same aspect ratio of the input image.
*/
case Clip
/**
Resizes the image to fill the width and height boundaries and crops any excess image data.
The resulting image will match the width and height constraints without scaling the image.
*/
case Crop
/**
Scales the image to fit the constraining dimensions exactly.
*/
case Scale
}
/**
Resize an image to the specified size. Depending on what fitMode is supplied, the image
may be clipped, cropped or scaled. @see documentation on FitMode.
- parameter image: Image to Resize
- parameter size: Size to resize the image to
- parameter fitMode: How to handle the image resizing process
- returns: Resized image
*/
public static func resizeImage(image: UIImage, size: CGSize, fitMode: FitMode = .Clip) -> UIImage {
let imgRef = Util.CGImageWithCorrectOrientation(image)
let originalWidth = CGFloat(CGImageGetWidth(imgRef))
let originalHeight = CGFloat(CGImageGetHeight(imgRef))
let widthRatio = size.width / originalWidth
let heightRatio = size.height / originalHeight
let scaleRatio = widthRatio > heightRatio ? widthRatio : heightRatio
let resizedImageBounds = CGRect(x: 0, y: 0, width: round(originalWidth * scaleRatio), height: round(originalHeight * scaleRatio))
let resizedImage = Util.drawImageInBounds(image, bounds: resizedImageBounds)
switch (fitMode) {
case .Clip:
return resizedImage
case .Crop:
let croppedRect = CGRect(x: (resizedImage.size.width - size.width) / 2,
y: (resizedImage.size.height - size.height) / 2,
width: size.width, height: size.height)
return Util.croppedImageWithRect(resizedImage, rect: croppedRect)
case .Scale:
return Util.drawImageInBounds(resizedImage, bounds: CGRect(x: 0, y: 0, width: size.width, height: size.height))
}
}
}
// MARK: - Mask
/**
Mask the contained image with another image mask.
Note that the areas in the original image that correspond to the black areas of the mask
show through in the resulting image. The areas that correspond to the white areas of
the mask aren’t painted. The areas that correspond to the gray areas in the mask are painted
using an intermediate alpha value that’s equal to 1 minus the image mask sample value.
- parameter maskImage: Image Mask to apply to the Image
- returns: Self, allowing method chaining
*/
public func maskWithImage(#maskImage : UIImage) -> Toucan {
self.image = Toucan.Mask.maskImageWithImage(self.image, maskImage: maskImage)
return self
}
/**
Mask the contained image with an ellipse.
Allows specifying an additional border to draw on the clipped image.
For a circle, ensure the image width and height are equal!
- parameter borderWidth: Optional width of the border to apply - default 0
- parameter borderColor: Optional color of the border - default White
- returns: Self, allowing method chaining
*/
public func maskWithEllipse(borderWidth: CGFloat = 0, borderColor: UIColor = UIColor.whiteColor()) -> Toucan {
self.image = Toucan.Mask.maskImageWithEllipse(self.image, borderWidth: borderWidth, borderColor: borderColor)
return self
}
/**
Mask the contained image with a path (UIBezierPath) that will be scaled to fit the image.
- parameter path: UIBezierPath to mask the image
- returns: Self, allowing method chaining
*/
public func maskWithPath(#path: UIBezierPath) -> Toucan {
self.image = Toucan.Mask.maskImageWithPath(self.image, path: path)
return self
}
/**
Mask the contained image with a path (UIBezierPath) which is provided via a closure.
- parameter path: closure that returns a UIBezierPath. Using a closure allows the user to provide the path after knowing the size of the image
- returns: Self, allowing method chaining
*/
public func maskWithPathClosure(#path: (rect: CGRect) -> (UIBezierPath)) -> Toucan {
self.image = Toucan.Mask.maskImageWithPathClosure(self.image, pathInRect: path)
return self
}
/**
Mask the contained image with a rounded rectangle border.
Allows specifying an additional border to draw on the clipped image.
- parameter cornerRadius: Radius of the rounded rect corners
- parameter borderWidth: Optional width of border to apply - default 0
- parameter borderColor: Optional color of the border - default White
- returns: Self, allowing method chaining
*/
public func maskWithRoundedRect(#cornerRadius: CGFloat, borderWidth: CGFloat = 0, borderColor: UIColor = UIColor.whiteColor()) -> Toucan {
self.image = Toucan.Mask.maskImageWithRoundedRect(self.image, cornerRadius: cornerRadius, borderWidth: borderWidth, borderColor: borderColor)
return self
}
/**
Container struct for all things Mask related
*/
public struct Mask {
/**
Mask the given image with another image mask.
Note that the areas in the original image that correspond to the black areas of the mask
show through in the resulting image. The areas that correspond to the white areas of
the mask aren’t painted. The areas that correspond to the gray areas in the mask are painted
using an intermediate alpha value that’s equal to 1 minus the image mask sample value.
- parameter image: Image to apply the mask to
- parameter maskImage: Image Mask to apply to the Image
- returns: Masked image
*/
public static func maskImageWithImage(image: UIImage, maskImage: UIImage) -> UIImage {
let imgRef = Util.CGImageWithCorrectOrientation(image)
let maskRef = maskImage.CGImage
let mask = CGImageMaskCreate(CGImageGetWidth(maskRef),
CGImageGetHeight(maskRef),
CGImageGetBitsPerComponent(maskRef),
CGImageGetBitsPerPixel(maskRef),
CGImageGetBytesPerRow(maskRef),
CGImageGetDataProvider(maskRef), nil, false);
let masked = CGImageCreateWithMask(imgRef, mask);
return Util.drawImageWithClosure(size: image.size) { (size: CGSize, context: CGContext) -> () in
// need to flip the transform matrix, CoreGraphics has (0,0) in lower left when drawing image
CGContextScaleCTM(context, 1, -1)
CGContextTranslateCTM(context, 0, -size.height)
CGContextDrawImage(context, CGRect(x: 0, y: 0, width: size.width, height: size.height), masked);
}
}
/**
Mask the given image with an ellipse.
Allows specifying an additional border to draw on the clipped image.
For a circle, ensure the image width and height are equal!
- parameter image: Image to apply the mask to
- parameter borderWidth: Optional width of the border to apply - default 0
- parameter borderColor: Optional color of the border - default White
- returns: Masked image
*/
public static func maskImageWithEllipse(image: UIImage,
borderWidth: CGFloat = 0, borderColor: UIColor = UIColor.whiteColor()) -> UIImage {
let imgRef = Util.CGImageWithCorrectOrientation(image)
let size = CGSize(width: CGFloat(CGImageGetWidth(imgRef)) / image.scale, height: CGFloat(CGImageGetHeight(imgRef)) / image.scale)
return Util.drawImageWithClosure(size: size) { (size: CGSize, context: CGContext) -> () in
let rect = CGRect(x: 0, y: 0, width: size.width, height: size.height)
CGContextAddEllipseInRect(context, rect)
CGContextClip(context)
image.drawInRect(rect)
if (borderWidth > 0) {
CGContextSetStrokeColorWithColor(context, borderColor.CGColor);
CGContextSetLineWidth(context, borderWidth);
CGContextAddEllipseInRect(context, CGRect(x: borderWidth / 2,
y: borderWidth / 2,
width: size.width - borderWidth,
height: size.height - borderWidth));
CGContextStrokePath(context);
}
}
}
/**
Mask the given image with a path(UIBezierPath) that will be scaled to fit the image.
- parameter image: Image to apply the mask to
- parameter path: UIBezierPath to make as the mask
- returns: Masked image
*/
public static func maskImageWithPath(image: UIImage,
path: UIBezierPath) -> UIImage {
let imgRef = Util.CGImageWithCorrectOrientation(image)
let size = CGSize(width: CGFloat(CGImageGetWidth(imgRef)) / image.scale, height: CGFloat(CGImageGetHeight(imgRef)) / image.scale)
return Util.drawImageWithClosure(size: size) { (size: CGSize, context: CGContext) -> () in
let boundSize = path.bounds.size
let pathRatio = boundSize.width / boundSize.height
let imageRatio = size.width / size.height
if pathRatio > imageRatio {
//scale based on width
let scale = size.width / boundSize.width
path.applyTransform(CGAffineTransformMakeScale(scale, scale))
path.applyTransform(CGAffineTransformMakeTranslation(0, (size.height - path.bounds.height) / 2.0))
} else {
//scale based on height
let scale = size.height / boundSize.height
path.applyTransform(CGAffineTransformMakeScale(scale, scale))
path.applyTransform(CGAffineTransformMakeTranslation((size.width - path.bounds.width) / 2.0, 0))
}
let newBounds = path.bounds
let rect = CGRect(x: 0, y: 0, width: size.width, height: size.height)
CGContextAddPath(context, path.CGPath)
CGContextClip(context)
image.drawInRect(rect)
}
}
/**
Mask the given image with a path(UIBezierPath) provided via a closure. This allows the user to get the size of the image before computing their path variable.
- parameter image: Image to apply the mask to
- parameter path: UIBezierPath to make as the mask
- returns: Masked image
*/
public static func maskImageWithPathClosure(image: UIImage,
pathInRect:(rect: CGRect) -> (UIBezierPath)) -> UIImage {
let imgRef = Util.CGImageWithCorrectOrientation(image)
let size = CGSize(width: CGFloat(CGImageGetWidth(imgRef)) / image.scale, height: CGFloat(CGImageGetHeight(imgRef)) / image.scale)
return maskImageWithPath(image, path: pathInRect(rect: CGRectMake(0, 0, size.width, size.height)))
}
/**
Mask the given image with a rounded rectangle border.
Allows specifying an additional border to draw on the clipped image.
- parameter image: Image to apply the mask to
- parameter cornerRadius: Radius of the rounded rect corners
- parameter borderWidth: Optional width of border to apply - default 0
- parameter borderColor: Optional color of the border - default White
- returns: Masked image
*/
public static func maskImageWithRoundedRect(image: UIImage, cornerRadius: CGFloat,
borderWidth: CGFloat = 0, borderColor: UIColor = UIColor.whiteColor()) -> UIImage {
let imgRef = Util.CGImageWithCorrectOrientation(image)
let size = CGSize(width: CGFloat(CGImageGetWidth(imgRef)) / image.scale, height: CGFloat(CGImageGetHeight(imgRef)) / image.scale)
return Util.drawImageWithClosure(size: size) { (size: CGSize, context: CGContext) -> () in
let rect = CGRect(x: 0, y: 0, width: size.width, height: size.height)
UIBezierPath(roundedRect:rect, cornerRadius: cornerRadius).addClip()
image.drawInRect(rect)
if (borderWidth > 0) {
CGContextSetStrokeColorWithColor(context, borderColor.CGColor);
CGContextSetLineWidth(context, borderWidth);
let borderRect = CGRect(x: 0, y: 0,
width: size.width, height: size.height)
let borderPath = UIBezierPath(roundedRect: borderRect, cornerRadius: cornerRadius)
borderPath.lineWidth = borderWidth * 2
borderPath.stroke()
}
}
}
}
// MARK: - Layer
/**
Overlay an image ontop of the current image.
- parameter image: Image to be on the bottom layer
- parameter overlayImage: Image to be on the top layer, i.e. drawn on top of image
- parameter overlayFrame: Frame of the overlay image
- returns: Self, allowing method chaining
*/
public func layerWithOverlayImage(overlayImage: UIImage, overlayFrame: CGRect) -> Toucan {
self.image = Toucan.Layer.overlayImage(self.image, overlayImage:overlayImage, overlayFrame:overlayFrame)
return self
}
/**
Container struct for all things Layer related
*/
public struct Layer {
/**
Overlay the given image into a new layout ontop of the image.
- parameter image: Image to be on the bottom layer
- parameter overlayImage: Image to be on the top layer, i.e. drawn on top of image
- parameter overlayFrame: Frame of the overlay image
- returns: Masked image
*/
public static func overlayImage(image: UIImage, overlayImage: UIImage, overlayFrame: CGRect) -> UIImage {
let imgRef = Util.CGImageWithCorrectOrientation(image)
let size = CGSize(width: CGFloat(CGImageGetWidth(imgRef)) / image.scale, height: CGFloat(CGImageGetHeight(imgRef)) / image.scale)
return Util.drawImageWithClosure(size: size) { (size: CGSize, context: CGContext) -> () in
let rect = CGRect(x: 0, y: 0, width: size.width, height: size.height)
image.drawInRect(rect)
overlayImage.drawInRect(overlayFrame);
}
}
}
/**
Container struct for internally used utility functions.
*/
internal struct Util {
/**
Get the CGImage of the image with the orientation fixed up based on EXF data.
This helps to normalise input images to always be the correct orientation when performing
other core graphics tasks on the image.
- parameter image: Image to create CGImageRef for
- returns: CGImageRef with rotated/transformed image context
*/
static func CGImageWithCorrectOrientation(image : UIImage) -> CGImageRef {
if (image.imageOrientation == UIImageOrientation.Up) {
return image.CGImage!
}
var transform : CGAffineTransform = CGAffineTransformIdentity;
switch (image.imageOrientation) {
case UIImageOrientation.Right, UIImageOrientation.RightMirrored:
transform = CGAffineTransformTranslate(transform, 0, image.size.height)
transform = CGAffineTransformRotate(transform, CGFloat(-1.0 * M_PI_2))
break
case UIImageOrientation.Left, UIImageOrientation.LeftMirrored:
transform = CGAffineTransformTranslate(transform, image.size.width, 0)
transform = CGAffineTransformRotate(transform, CGFloat(M_PI_2))
break
case UIImageOrientation.Down, UIImageOrientation.DownMirrored:
transform = CGAffineTransformTranslate(transform, image.size.width, image.size.height)
transform = CGAffineTransformRotate(transform, CGFloat(M_PI))
break
default:
break
}
switch (image.imageOrientation) {
case UIImageOrientation.RightMirrored, UIImageOrientation.LeftMirrored:
transform = CGAffineTransformTranslate(transform, image.size.height, 0);
transform = CGAffineTransformScale(transform, -1, 1);
break
case UIImageOrientation.DownMirrored, UIImageOrientation.UpMirrored:
transform = CGAffineTransformTranslate(transform, image.size.width, 0);
transform = CGAffineTransformScale(transform, -1, 1);
break
default:
break
}
var context : CGContextRef = CGBitmapContextCreate(nil, Int(image.size.width), Int(image.size.height),
CGImageGetBitsPerComponent(image.CGImage), 0,
CGImageGetColorSpace(image.CGImage),
CGImageGetBitmapInfo(image.CGImage));
CGContextConcatCTM(context, transform);
switch (image.imageOrientation) {
case UIImageOrientation.Left, UIImageOrientation.LeftMirrored,
UIImageOrientation.Right, UIImageOrientation.RightMirrored:
CGContextDrawImage(context, CGRectMake(0, 0, image.size.height, image.size.width), image.CGImage);
break;
default:
CGContextDrawImage(context, CGRectMake(0, 0, image.size.width, image.size.height), image.CGImage);
break;
}
let cgImage = CGBitmapContextCreateImage(context);
return cgImage!;
}
/**
Draw the image within the given bounds (i.e. resizes)
- parameter image: Image to draw within the given bounds
- parameter bounds: Bounds to draw the image within
- returns: Resized image within bounds
*/
static func drawImageInBounds(image: UIImage, bounds : CGRect) -> UIImage {
return drawImageWithClosure(size: bounds.size) { (size: CGSize, context: CGContext) -> () in
image.drawInRect(bounds)
};
}
/**
Crap the image within the given rect (i.e. resizes and crops)
- parameter image: Image to clip within the given rect bounds
- parameter rect: Bounds to draw the image within
- returns: Resized and cropped image
*/
static func croppedImageWithRect(image: UIImage, rect: CGRect) -> UIImage {
return drawImageWithClosure(size: rect.size) { (size: CGSize, context: CGContext) -> () in
let drawRect = CGRectMake(-rect.origin.x, -rect.origin.y, image.size.width, image.size.height)
CGContextClipToRect(context, CGRectMake(0, 0, rect.size.width, rect.size.height))
image.drawInRect(drawRect)
};
}
/**
Closure wrapper around image context - setting up, ending and grabbing the image from the context.
- parameter size: Size of the graphics context to create
- parameter closure: Closure of magic to run in a new context
- returns: Image pulled from the end of the closure
*/
static func drawImageWithClosure(#size: CGSize!, closure: (size: CGSize, context: CGContext) -> ()) -> UIImage {
UIGraphicsBeginImageContextWithOptions(size, false, 0)
closure(size: size, context: UIGraphicsGetCurrentContext())
let image : UIImage = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return image
}
}
} | mit | 97853df7e4652954fe5e732be6c74e55 | 43.659432 | 166 | 0.608374 | 5.481557 | false | false | false | false |
jhaigler94/cs4720-iOS | Pensieve/Pensieve/MapViewController.swift | 1 | 2575 | //
// TypesTableViewController.swift
// Feed Me
//
/*
* Copyright (c) 2015 Razeware LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
//
// MapViewController.swift
// Pensieve
//
// Created by Jennifer Ruth Haigler on 11/22/15.
// Copyright © 2015 University of Virginia. All rights reserved.
//
import UIKit
class MapViewController: UIViewController {
@IBOutlet weak var mapView: GMSMapView!
//@IBOutlet weak var mapCenterPinImage: UIImageView!
//@IBOutlet weak var pinImageVerticalConstraint: NSLayoutConstraint!
var searchedTypes = ["bakery", "bar", "cafe", "grocery_or_supermarket", "restaurant"]
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
if segue.identifier == "Types Segue" {
let navigationController = segue.destinationViewController as! UINavigationController
let controller = navigationController.topViewController as! TypesTableViewController
controller.selectedTypes = searchedTypes
controller.delegate = self
}
}
}
// MARK: - TypesTableViewControllerDelegate
extension MapViewController: TypesTableViewControllerDelegate {
func typesController(controller: TypesTableViewController, didSelectTypes types: [String]) {
searchedTypes = controller.selectedTypes.sort()
dismissViewControllerAnimated(true, completion: nil)
}
} | apache-2.0 | 6335a253dbfe3d0dd3bd1c0d93ff55ca | 37.432836 | 97 | 0.737762 | 4.865784 | false | false | false | false |
ewhitley/CDAKit | CDAKit/health-data-standards/lib/models/qrda/CDAKQRDAAuthor.swift | 1 | 1868 | //
// CDAKQRDAAuthor.swift
// CDAKit
//
// Created by Eric Whitley on 2/16/16.
// Copyright © 2016 Eric Whitley. All rights reserved.
//
import Foundation
import Mustache
public class CDAKQRDAAuthor {
public var time = NSDate()
public var ids: [CDAKCDAIdentifier] = []
public var addresses: [CDAKAddress] = []
public var telecoms: [CDAKTelecom] = []
public var person: CDAKPerson?
public var device: CDAKQRDADevice?
public var organization: CDAKOrganization?
}
extension CDAKQRDAAuthor: CustomStringConvertible {
public var description: String {
return "CDAKQRDAAuthor => time:\(time), ids:\(ids), addresses:\(addresses), telecoms:\(telecoms), person:\(person), device:\(device), organization: \(organization)"
}
}
extension CDAKQRDAAuthor: MustacheBoxable {
var boxedValues: [String:MustacheBox] {
return [
"time" : Box(time.timeIntervalSince1970),
"ids" : Box(ids),
"addresses" : Box(addresses),
"telecoms" : Box(telecoms),
"person" : Box(person),
"device" : Box(device),
"organization" : Box(organization)
]
}
public var mustacheBox: MustacheBox {
return Box(boxedValues)
}
}
extension CDAKQRDAAuthor: CDAKJSONExportable {
public var jsonDict: [String: AnyObject] {
var dict: [String: AnyObject] = [:]
dict["time"] = time.description
if ids.count > 0 {
dict["ids"] = ids.map({$0.jsonDict})
}
if addresses.count > 0 {
dict["addresses"] = addresses.map({$0.jsonDict})
}
if telecoms.count > 0 {
dict["telecoms"] = telecoms.map({$0.jsonDict})
}
if let person = person {
dict["person"] = person.jsonDict
}
if let device = device {
dict["device"] = device.jsonDict
}
if let organization = organization {
dict["organization"] = organization.jsonDict
}
return dict
}
}
| mit | ac16a0adc168d72e54b1b8ed1b7cbf99 | 25.671429 | 168 | 0.644349 | 3.771717 | false | false | false | false |
pushy-me/pushy-demo-ios | PushyDemo/ViewController.swift | 1 | 2610 | //
// ViewController.swift
// PushyDemo
//
// Created by Pushy on 10/18/16.
// Copyright © 2016 Pushy. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
// MARK: Properties
@IBOutlet weak var instructions: UILabel!
@IBOutlet weak var deviceToken: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
// Check for persisted device token
if let deviceToken = UserDefaults.standard.string(forKey: "pushyToken") {
// Update UI to display device token
onRegistrationSuccess(deviceToken)
}
// Listen for registration result notification
NotificationCenter.default.addObserver(self, selector: #selector(onRegistrationResult), name: Notification.Name("registrationResult"), object: nil)
}
@objc func onRegistrationResult(notification: Notification) {
// Get registration result dictionary from notification
guard let registrationResult = notification.userInfo else {
return
}
// Registration error?
if let error = registrationResult["error"] {
// Print error to console
print("Registration failed: \(error)")
// Update UI to reflect failure
self.deviceToken.text = "Registration failed"
self.instructions.text = "(restart app to try again)"
// Create an alert dialog with the error message
let alert = UIAlertController(title: "Registration Error", message: "\(error)", preferredStyle: UIAlertControllerStyle.actionSheet)
// Add an action button
alert.addAction(UIAlertAction(title: "OK", style: UIAlertActionStyle.default, handler: nil))
// Show the alert dialog
self.present(alert, animated: true, completion: nil)
}
// Registration success?
else if let deviceToken = registrationResult["deviceToken"] as? String {
// Update UI to display device token
onRegistrationSuccess(deviceToken)
}
}
func onRegistrationSuccess(_ deviceToken: String) {
// Already displaying this device token?
if (self.deviceToken.text! == deviceToken){
return
}
// Print device token to console
print("Pushy device token: \(deviceToken)")
// Update UI to display device token
self.deviceToken.text = deviceToken
self.instructions.text = "(copy from console)"
}
}
| apache-2.0 | c5a0f02cc6b6f03619f46c63407a4e40 | 34.256757 | 155 | 0.614795 | 5.435417 | false | false | false | false |
takuran/UsingCocoaPodsInSwift | UsingCocoaPodsInSwift/MemoViewController.swift | 1 | 4290 | //
// MemoViewController.swift
// UsingCocoaPodsInSwift
//
// Created by Naoyuki Takura on 2014/09/02.
// Copyright (c) 2014年 Naoyuki Takura. All rights reserved.
//
import Foundation
import UIKit
class MemoViewController: UITableViewController {
@IBOutlet weak var addButton: UIBarButtonItem!
private var contents:[(Int32, String!, NSDate!)] = []
override func viewDidLoad() {
super.viewDidLoad()
//get all contents
let dataManager: DataManager = DataManager.sharedInstance()
dataManager.open()
contents = dataManager.allContents()
//for refreshcontrol
let refreshControl = UIRefreshControl()
refreshControl.addTarget(self, action: Selector("onRefreshTable"), forControlEvents: .ValueChanged)
self.refreshControl = refreshControl
}
deinit {
DataManager.sharedInstance().close()
}
@IBAction func addButtonClicked(sender: AnyObject) {
NSLog("add button clicked.")
//show alert view
createAlertController()
}
//for refresh
func onRefreshTable() {
self.refreshControl?.beginRefreshing()
refreshTable()
self.refreshControl?.endRefreshing();
}
private func refreshTable() {
self.contents = DataManager.sharedInstance().allContents()
self.tableView.reloadData()
}
//Alert View
private func createAlertController() {
//create alert controller
let alertController = UIAlertController(title: "enter new memo", message: "", preferredStyle: .Alert)
//add text field
alertController.addTextFieldWithConfigurationHandler { (testField) -> Void in
//
testField.placeholder = "enter your memo"
}
//create actions
//ok
let okAction = UIAlertAction(title: "ok", style: .Default) { action in
//TODO
if let textField = alertController.textFields?[0] as? UITextField {
//got text
NSLog("test : \(textField.text)")
if textField.text.utf16Count > 0 {
//add to database
let dataManager = DataManager.sharedInstance()
if dataManager.createNewContent(textField.text) {
//success
//refresh
self.refreshTable()
}
}
}
}
//cancel
let cancelAction = UIAlertAction(title: "cancel", style: .Cancel, handler: nil)
//add actions to controller
alertController.addAction(okAction)
alertController.addAction(cancelAction)
//show alertController(ios8)
presentViewController(alertController, animated: true, completion: nil)
}
//tableview delegate
//tableview datasource
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return 1
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return contents.count
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
var cell = tableView.dequeueReusableCellWithIdentifier("cell", forIndexPath: indexPath) as UITableViewCell
//contents
var content = contents[indexPath.row]
cell.textLabel?.text = content.1
cell.tag = Int(content.0)
return cell
}
override func tableView(tableView: UITableView, canEditRowAtIndexPath indexPath: NSIndexPath) -> Bool {
return true;
}
override func tableView(tableView: UITableView, commitEditingStyle editingStyle: UITableViewCellEditingStyle, forRowAtIndexPath indexPath: NSIndexPath) {
if editingStyle == .Delete {
let cell = self.tableView.cellForRowAtIndexPath(indexPath)
if let index = cell?.tag {
if DataManager.sharedInstance().deleteRecord(index) {
//success
refreshTable()
}
}
}
}
}
| mit | b27de78eec465f528e9aed5f3271a158 | 29.628571 | 157 | 0.597715 | 5.755705 | false | false | false | false |
exyte/Macaw | Source/views/MacawZoom.swift | 1 | 4380 | //
// MacawZoom.swift
// Macaw
//
// Created by Yuri Strot on 4/5/19.
// Copyright © 2019 Exyte. All rights reserved.
//
import Foundation
#if os(iOS)
import UIKit
#elseif os(OSX)
import AppKit
#endif
open class MacawZoom {
private unowned let view: MacawView
private var onChange: ((Transform) -> Void)?
private var touches = [TouchData]()
private var zoomData = ZoomData()
private var trackMove = false
private var trackScale = false
private var trackRotate = false
init(view: MacawView) {
self.view = view
}
open func enable(move: Bool = true, scale: Bool = true, rotate: Bool = false) {
trackMove = move
trackScale = scale
trackRotate = rotate
if scale || rotate {
#if os(iOS)
view.isMultipleTouchEnabled = true
#endif
}
}
open func disable() {
trackMove = false
trackScale = false
trackRotate = false
}
open func set(offset: Size? = nil, scale: Double? = nil, angle: Double? = nil) {
let o = offset ?? zoomData.offset
let s = scale ?? zoomData.scale
let a = angle ?? zoomData.angle
zoomData = ZoomData(offset: o, scale: s, angle: a)
onChange?(zoomData.transform())
}
func initialize(onChange: @escaping (Transform) -> Void) {
self.onChange = onChange
}
func touchesBegan(_ touches: Set<MTouch>) {
zoomData = getNewZoom()
self.touches = self.touches.map { TouchData(touch: $0.touch, in: view) }
self.touches.append(contentsOf: touches.map { TouchData(touch: $0, in: view) })
}
func touchesMoved(_ touches: Set<MTouch>) {
let zoom = cleanTouches() ?? getNewZoom()
onChange?(zoom.transform())
}
func touchesEnded(_ touches: Set<MTouch>) {
cleanTouches()
}
@discardableResult private func cleanTouches() -> ZoomData? {
let newTouches = touches.filter { $0.touch.phase.rawValue < MTouch.Phase.ended.rawValue }
if newTouches.count != touches.count {
zoomData = getNewZoom()
touches = newTouches.map { TouchData(touch: $0.touch, in: view) }
return zoomData
}
return nil
}
private func getNewZoom() -> ZoomData {
if !trackMove && !trackScale && !trackRotate {
return zoomData
}
if touches.isEmpty || (touches.count == 1 && !trackMove) {
return zoomData
}
let s1 = touches[0].point
let e1 = touches[0].current(in: view)
if touches.count == 1 {
return zoomData.move(delta: e1 - s1)
}
let s2 = touches[1].point
let e2 = touches[1].current(in: view)
let scale = trackScale ? e1.distance(to: e2) / s1.distance(to: s2) : 1
let a = trackRotate ? (e1 - e2).angle() - (s1 - s2).angle() : 0
return ZoomData(offset: .zero, scale: scale, angle: a).combine(with: zoomData)
}
}
fileprivate class ZoomData {
let offset: Size
let scale: Double
let angle: Double
init(offset: Size = Size.zero, scale: Double = 1, angle: Double = 0) {
self.offset = offset
self.scale = scale
self.angle = angle
}
func transform() -> Transform {
return Transform.move(dx: offset.w, dy: offset.h).scale(sx: scale, sy: scale).rotate(angle: angle)
}
func move(delta: Size) -> ZoomData {
return ZoomData(offset: offset + delta, scale: scale, angle: angle)
}
func combine(with: ZoomData) -> ZoomData {
let sina = sin(angle)
let cosa = cos(angle)
let w = offset.w + scale * (cosa * with.offset.w - sina * with.offset.h)
let h = offset.h + scale * (sina * with.offset.w + cosa * with.offset.h)
let s = scale * with.scale
let a = angle + with.angle
return ZoomData(offset: Size(w: w, h: h), scale: s, angle: a)
}
}
fileprivate class TouchData {
let touch: MTouch
let point: Point
convenience init(touch: MTouch, in view: MacawView) {
self.init(touch: touch, point: touch.location(in: view).toMacaw())
}
init(touch: MTouch, point: Point) {
self.touch = touch
self.point = point
}
func current(in view: MacawView) -> Point {
return touch.location(in: view).toMacaw()
}
}
| mit | 5c2fafe43a14c104ef216342034125bd | 26.89172 | 106 | 0.58438 | 3.717317 | false | false | false | false |
milseman/swift | stdlib/public/core/KeyPath.swift | 4 | 82695 | //===----------------------------------------------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2017 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
import SwiftShims
@_transparent
internal func _abstract(
methodName: StaticString = #function,
file: StaticString = #file, line: UInt = #line
) -> Never {
#if INTERNAL_CHECKS_ENABLED
_fatalErrorMessage("abstract method", methodName, file: file, line: line,
flags: _fatalErrorFlags())
#else
_conditionallyUnreachable()
#endif
}
// MARK: Type-erased abstract base classes
/// A type-erased key path, from any root type to any resulting value type.
public class AnyKeyPath: Hashable, _AppendKeyPath {
/// The root type for this key path.
@_inlineable
public static var rootType: Any.Type {
return _rootAndValueType.root
}
/// The value type for this key path.
@_inlineable
public static var valueType: Any.Type {
return _rootAndValueType.value
}
internal final var _kvcKeyPathStringPtr: UnsafePointer<CChar>?
final public var hashValue: Int {
var hash = 0
withBuffer {
var buffer = $0
while true {
let (component, type) = buffer.next()
hash ^= _mixInt(component.value.hashValue)
if let type = type {
hash ^= _mixInt(unsafeBitCast(type, to: Int.self))
} else {
break
}
}
}
return hash
}
public static func ==(a: AnyKeyPath, b: AnyKeyPath) -> Bool {
// Fast-path identical objects
if a === b {
return true
}
// Short-circuit differently-typed key paths
if type(of: a) != type(of: b) {
return false
}
return a.withBuffer {
var aBuffer = $0
return b.withBuffer {
var bBuffer = $0
// Two equivalent key paths should have the same reference prefix
if aBuffer.hasReferencePrefix != bBuffer.hasReferencePrefix {
return false
}
while true {
let (aComponent, aType) = aBuffer.next()
let (bComponent, bType) = bBuffer.next()
if aComponent.header.endOfReferencePrefix
!= bComponent.header.endOfReferencePrefix
|| aComponent.value != bComponent.value
|| aType != bType {
return false
}
if aType == nil {
return true
}
}
}
}
}
// SPI for the Foundation overlay to allow interop with KVC keypath-based
// APIs.
public var _kvcKeyPathString: String? {
guard let ptr = _kvcKeyPathStringPtr else { return nil }
return String(validatingUTF8: ptr)
}
// MARK: Implementation details
// Prevent normal initialization. We use tail allocation via
// allocWithTailElems().
internal init() {
_sanityCheckFailure("use _create(...)")
}
// internal-with-availability
public class var _rootAndValueType: (root: Any.Type, value: Any.Type) {
_abstract()
}
public // @testable
static func _create(
capacityInBytes bytes: Int,
initializedBy body: (UnsafeMutableRawBufferPointer) -> Void
) -> Self {
_sanityCheck(bytes > 0 && bytes % 4 == 0,
"capacity must be multiple of 4 bytes")
let result = Builtin.allocWithTailElems_1(self, (bytes/4)._builtinWordValue,
Int32.self)
result._kvcKeyPathStringPtr = nil
let base = UnsafeMutableRawPointer(Builtin.projectTailElems(result,
Int32.self))
body(UnsafeMutableRawBufferPointer(start: base, count: bytes))
return result
}
func withBuffer<T>(_ f: (KeyPathBuffer) throws -> T) rethrows -> T {
defer { _fixLifetime(self) }
let base = UnsafeRawPointer(Builtin.projectTailElems(self, Int32.self))
return try f(KeyPathBuffer(base: base))
}
}
/// A partially type-erased key path, from a concrete root type to any
/// resulting value type.
public class PartialKeyPath<Root>: AnyKeyPath { }
// MARK: Concrete implementations
internal enum KeyPathKind { case readOnly, value, reference }
/// A key path from a specific root type to a specific resulting value type.
public class KeyPath<Root, Value>: PartialKeyPath<Root> {
public typealias _Root = Root
public typealias _Value = Value
public final override class var _rootAndValueType: (
root: Any.Type,
value: Any.Type
) {
return (Root.self, Value.self)
}
// MARK: Implementation
typealias Kind = KeyPathKind
class var kind: Kind { return .readOnly }
static func appendedType<AppendedValue>(
with t: KeyPath<Value, AppendedValue>.Type
) -> KeyPath<Root, AppendedValue>.Type {
let resultKind: Kind
switch (self.kind, t.kind) {
case (_, .reference):
resultKind = .reference
case (let x, .value):
resultKind = x
default:
resultKind = .readOnly
}
switch resultKind {
case .readOnly:
return KeyPath<Root, AppendedValue>.self
case .value:
return WritableKeyPath.self
case .reference:
return ReferenceWritableKeyPath.self
}
}
final func projectReadOnly(from root: Root) -> Value {
// TODO: For perf, we could use a local growable buffer instead of Any
var curBase: Any = root
return withBuffer {
var buffer = $0
while true {
let (rawComponent, optNextType) = buffer.next()
let valueType = optNextType ?? Value.self
let isLast = optNextType == nil
func project<CurValue>(_ base: CurValue) -> Value? {
func project2<NewValue>(_: NewValue.Type) -> Value? {
switch rawComponent.projectReadOnly(base,
to: NewValue.self, endingWith: Value.self) {
case .continue(let newBase):
if isLast {
_sanityCheck(NewValue.self == Value.self,
"key path does not terminate in correct type")
return unsafeBitCast(newBase, to: Value.self)
} else {
curBase = newBase
return nil
}
case .break(let result):
return result
}
}
return _openExistential(valueType, do: project2)
}
if let result = _openExistential(curBase, do: project) {
return result
}
}
}
}
deinit {
withBuffer { $0.destroy() }
}
}
/// A key path that supports reading from and writing to the resulting value.
public class WritableKeyPath<Root, Value>: KeyPath<Root, Value> {
// MARK: Implementation detail
override class var kind: Kind { return .value }
// `base` is assumed to be undergoing a formal access for the duration of the
// call, so must not be mutated by an alias
func projectMutableAddress(from base: UnsafePointer<Root>)
-> (pointer: UnsafeMutablePointer<Value>, owner: AnyObject?) {
var p = UnsafeRawPointer(base)
var type: Any.Type = Root.self
var keepAlive: AnyObject?
return withBuffer {
var buffer = $0
_sanityCheck(!buffer.hasReferencePrefix,
"WritableKeyPath should not have a reference prefix")
while true {
let (rawComponent, optNextType) = buffer.next()
let nextType = optNextType ?? Value.self
func project<CurValue>(_: CurValue.Type) {
func project2<NewValue>(_: NewValue.Type) {
p = rawComponent.projectMutableAddress(p,
from: CurValue.self,
to: NewValue.self,
isRoot: p == UnsafeRawPointer(base),
keepAlive: &keepAlive)
}
_openExistential(nextType, do: project2)
}
_openExistential(type, do: project)
if optNextType == nil { break }
type = nextType
}
// TODO: With coroutines, it would be better to yield here, so that
// we don't need the hack of the keepAlive reference to manage closing
// accesses.
let typedPointer = p.assumingMemoryBound(to: Value.self)
return (pointer: UnsafeMutablePointer(mutating: typedPointer),
owner: keepAlive)
}
}
}
/// A key path that supports reading from and writing to the resulting value
/// with reference semantics.
public class ReferenceWritableKeyPath<Root, Value>: WritableKeyPath<Root, Value> {
// MARK: Implementation detail
final override class var kind: Kind { return .reference }
final override func projectMutableAddress(from base: UnsafePointer<Root>)
-> (pointer: UnsafeMutablePointer<Value>, owner: AnyObject?) {
// Since we're a ReferenceWritableKeyPath, we know we don't mutate the base in
// practice.
return projectMutableAddress(from: base.pointee)
}
final func projectMutableAddress(from origBase: Root)
-> (pointer: UnsafeMutablePointer<Value>, owner: AnyObject?) {
var keepAlive: AnyObject?
var address: UnsafeMutablePointer<Value> = withBuffer {
var buffer = $0
// Project out the reference prefix.
var base: Any = origBase
while buffer.hasReferencePrefix {
let (rawComponent, optNextType) = buffer.next()
_sanityCheck(optNextType != nil,
"reference prefix should not go to end of buffer")
let nextType = optNextType.unsafelyUnwrapped
func project<NewValue>(_: NewValue.Type) -> Any {
func project2<CurValue>(_ base: CurValue) -> Any {
return rawComponent.projectReadOnly(
base, to: NewValue.self, endingWith: Value.self)
.assumingContinue
}
return _openExistential(base, do: project2)
}
base = _openExistential(nextType, do: project)
}
// Start formal access to the mutable value, based on the final base
// value.
func formalMutation<MutationRoot>(_ base: MutationRoot)
-> UnsafeMutablePointer<Value> {
var base2 = base
return withUnsafeBytes(of: &base2) { baseBytes in
var p = baseBytes.baseAddress.unsafelyUnwrapped
var curType: Any.Type = MutationRoot.self
while true {
let (rawComponent, optNextType) = buffer.next()
let nextType = optNextType ?? Value.self
func project<CurValue>(_: CurValue.Type) {
func project2<NewValue>(_: NewValue.Type) {
p = rawComponent.projectMutableAddress(p,
from: CurValue.self,
to: NewValue.self,
isRoot: p == baseBytes.baseAddress,
keepAlive: &keepAlive)
}
_openExistential(nextType, do: project2)
}
_openExistential(curType, do: project)
if optNextType == nil { break }
curType = nextType
}
let typedPointer = p.assumingMemoryBound(to: Value.self)
return UnsafeMutablePointer(mutating: typedPointer)
}
}
return _openExistential(base, do: formalMutation)
}
return (address, keepAlive)
}
}
// MARK: Implementation details
internal enum KeyPathComponentKind {
/// The keypath projects within the storage of the outer value, like a
/// stored property in a struct.
case `struct`
/// The keypath projects from the referenced pointer, like a
/// stored property in a class.
case `class`
/// The keypath projects using a getter/setter pair.
case computed
/// The keypath optional-chains, returning nil immediately if the input is
/// nil, or else proceeding by projecting the value inside.
case optionalChain
/// The keypath optional-forces, trapping if the input is
/// nil, or else proceeding by projecting the value inside.
case optionalForce
/// The keypath wraps a value in an optional.
case optionalWrap
}
internal struct ComputedPropertyID: Hashable {
var value: Int
var isStoredProperty: Bool
var isTableOffset: Bool
static func ==(x: ComputedPropertyID, y: ComputedPropertyID) -> Bool {
return x.value == y.value
&& x.isStoredProperty == y.isStoredProperty
&& x.isTableOffset == x.isTableOffset
}
var hashValue: Int {
var hash = 0
hash ^= _mixInt(value)
hash ^= _mixInt(isStoredProperty ? 13 : 17)
hash ^= _mixInt(isTableOffset ? 19 : 23)
return hash
}
}
internal struct ComputedArgumentWitnesses {
typealias Destroy = @convention(thin)
(_ instanceArguments: UnsafeMutableRawPointer, _ size: Int) -> ()
typealias Copy = @convention(thin)
(_ srcInstanceArguments: UnsafeRawPointer,
_ destInstanceArguments: UnsafeMutableRawPointer,
_ size: Int) -> ()
typealias Equals = @convention(thin)
(_ xInstanceArguments: UnsafeRawPointer,
_ yInstanceArguments: UnsafeRawPointer,
_ size: Int) -> Bool
typealias Hash = @convention(thin)
(_ instanceArguments: UnsafeRawPointer,
_ size: Int) -> Int
let destroy: Destroy?
let copy: Copy
let equals: Equals
let hash: Hash
}
internal enum KeyPathComponent: Hashable {
struct ArgumentRef {
var data: UnsafeRawBufferPointer
var witnesses: UnsafePointer<ComputedArgumentWitnesses>
}
/// The keypath projects within the storage of the outer value, like a
/// stored property in a struct.
case `struct`(offset: Int)
/// The keypath projects from the referenced pointer, like a
/// stored property in a class.
case `class`(offset: Int)
/// The keypath projects using a getter.
case get(id: ComputedPropertyID,
get: UnsafeRawPointer, argument: ArgumentRef?)
/// The keypath projects using a getter/setter pair. The setter can mutate
/// the base value in-place.
case mutatingGetSet(id: ComputedPropertyID,
get: UnsafeRawPointer, set: UnsafeRawPointer,
argument: ArgumentRef?)
/// The keypath projects using a getter/setter pair that does not mutate its
/// base.
case nonmutatingGetSet(id: ComputedPropertyID,
get: UnsafeRawPointer, set: UnsafeRawPointer,
argument: ArgumentRef?)
/// The keypath optional-chains, returning nil immediately if the input is
/// nil, or else proceeding by projecting the value inside.
case optionalChain
/// The keypath optional-forces, trapping if the input is
/// nil, or else proceeding by projecting the value inside.
case optionalForce
/// The keypath wraps a value in an optional.
case optionalWrap
static func ==(a: KeyPathComponent, b: KeyPathComponent) -> Bool {
switch (a, b) {
case (.struct(offset: let a), .struct(offset: let b)),
(.class (offset: let a), .class (offset: let b)):
return a == b
case (.optionalChain, .optionalChain),
(.optionalForce, .optionalForce),
(.optionalWrap, .optionalWrap):
return true
case (.get(id: let id1, get: _, argument: let argument1),
.get(id: let id2, get: _, argument: let argument2)),
(.mutatingGetSet(id: let id1, get: _, set: _, argument: let argument1),
.mutatingGetSet(id: let id2, get: _, set: _, argument: let argument2)),
(.nonmutatingGetSet(id: let id1, get: _, set: _, argument: let argument1),
.nonmutatingGetSet(id: let id2, get: _, set: _, argument: let argument2)):
if id1 != id2 {
return false
}
if let arg1 = argument1, let arg2 = argument2 {
// TODO: Sizes may differ if one key path was formed in a context
// capturing generic arguments and one wasn't.
_sanityCheck(arg1.data.count == arg2.data.count)
return arg1.witnesses.pointee.equals(
arg1.data.baseAddress.unsafelyUnwrapped,
arg2.data.baseAddress.unsafelyUnwrapped,
arg1.data.count)
}
// If only one component has arguments, that should indicate that the
// only arguments in that component were generic captures and therefore
// not affecting equality.
return true
case (.struct, _),
(.class, _),
(.optionalChain, _),
(.optionalForce, _),
(.optionalWrap, _),
(.get, _),
(.mutatingGetSet, _),
(.nonmutatingGetSet, _):
return false
}
}
var hashValue: Int {
var hash: Int = 0
func mixHashFromArgument(_ argument: KeyPathComponent.ArgumentRef?) {
if let argument = argument {
let addedHash = argument.witnesses.pointee.hash(
argument.data.baseAddress.unsafelyUnwrapped,
argument.data.count)
// Returning 0 indicates that the arguments should not impact the
// hash value of the overall key path.
if addedHash != 0 {
hash ^= _mixInt(addedHash)
}
}
}
switch self {
case .struct(offset: let a):
hash ^= _mixInt(0)
hash ^= _mixInt(a)
case .class(offset: let b):
hash ^= _mixInt(1)
hash ^= _mixInt(b)
case .optionalChain:
hash ^= _mixInt(2)
case .optionalForce:
hash ^= _mixInt(3)
case .optionalWrap:
hash ^= _mixInt(4)
case .get(id: let id, get: _, argument: let argument):
hash ^= _mixInt(5)
hash ^= _mixInt(id.hashValue)
mixHashFromArgument(argument)
case .mutatingGetSet(id: let id, get: _, set: _, argument: let argument):
hash ^= _mixInt(6)
hash ^= _mixInt(id.hashValue)
mixHashFromArgument(argument)
case .nonmutatingGetSet(id: let id, get: _, set: _, argument: let argument):
hash ^= _mixInt(7)
hash ^= _mixInt(id.hashValue)
mixHashFromArgument(argument)
}
return hash
}
}
// A class that maintains ownership of another object while a mutable projection
// into it is underway.
internal final class ClassHolder {
let previous: AnyObject?
let instance: AnyObject
init(previous: AnyObject?, instance: AnyObject) {
self.previous = previous
self.instance = instance
}
}
// A class that triggers writeback to a pointer when destroyed.
internal final class MutatingWritebackBuffer<CurValue, NewValue> {
let previous: AnyObject?
let base: UnsafeMutablePointer<CurValue>
let set: @convention(thin) (NewValue, inout CurValue, UnsafeRawPointer) -> ()
let argument: UnsafeRawPointer
var value: NewValue
deinit {
set(value, &base.pointee, argument)
}
init(previous: AnyObject?,
base: UnsafeMutablePointer<CurValue>,
set: @escaping @convention(thin) (NewValue, inout CurValue, UnsafeRawPointer) -> (),
argument: UnsafeRawPointer,
value: NewValue) {
self.previous = previous
self.base = base
self.set = set
self.argument = argument
self.value = value
}
}
// A class that triggers writeback to a non-mutated value when destroyed.
internal final class NonmutatingWritebackBuffer<CurValue, NewValue> {
let previous: AnyObject?
let base: CurValue
let set: @convention(thin) (NewValue, CurValue, UnsafeRawPointer) -> ()
let argument: UnsafeRawPointer
var value: NewValue
deinit {
set(value, base, argument)
}
init(previous: AnyObject?,
base: CurValue,
set: @escaping @convention(thin) (NewValue, CurValue, UnsafeRawPointer) -> (),
argument: UnsafeRawPointer,
value: NewValue) {
self.previous = previous
self.base = base
self.set = set
self.argument = argument
self.value = value
}
}
internal struct RawKeyPathComponent {
var header: Header
var body: UnsafeRawBufferPointer
struct Header {
static var payloadMask: UInt32 {
return _SwiftKeyPathComponentHeader_PayloadMask
}
static var discriminatorMask: UInt32 {
return _SwiftKeyPathComponentHeader_DiscriminatorMask
}
static var discriminatorShift: UInt32 {
return _SwiftKeyPathComponentHeader_DiscriminatorShift
}
static var structTag: UInt32 {
return _SwiftKeyPathComponentHeader_StructTag
}
static var computedTag: UInt32 {
return _SwiftKeyPathComponentHeader_ComputedTag
}
static var classTag: UInt32 {
return _SwiftKeyPathComponentHeader_ClassTag
}
static var optionalTag: UInt32 {
return _SwiftKeyPathComponentHeader_OptionalTag
}
static var optionalChainPayload: UInt32 {
return _SwiftKeyPathComponentHeader_OptionalChainPayload
}
static var optionalWrapPayload: UInt32 {
return _SwiftKeyPathComponentHeader_OptionalWrapPayload
}
static var optionalForcePayload: UInt32 {
return _SwiftKeyPathComponentHeader_OptionalForcePayload
}
static var endOfReferencePrefixFlag: UInt32 {
return _SwiftKeyPathComponentHeader_EndOfReferencePrefixFlag
}
static var outOfLineOffsetPayload: UInt32 {
return _SwiftKeyPathComponentHeader_OutOfLineOffsetPayload
}
static var unresolvedFieldOffsetPayload: UInt32 {
return _SwiftKeyPathComponentHeader_UnresolvedFieldOffsetPayload
}
static var unresolvedIndirectOffsetPayload: UInt32 {
return _SwiftKeyPathComponentHeader_UnresolvedIndirectOffsetPayload
}
static var computedMutatingFlag: UInt32 {
return _SwiftKeyPathComponentHeader_ComputedMutatingFlag
}
static var computedSettableFlag: UInt32 {
return _SwiftKeyPathComponentHeader_ComputedSettableFlag
}
static var computedIDByStoredPropertyFlag: UInt32 {
return _SwiftKeyPathComponentHeader_ComputedIDByStoredPropertyFlag
}
static var computedIDByVTableOffsetFlag: UInt32 {
return _SwiftKeyPathComponentHeader_ComputedIDByVTableOffsetFlag
}
static var computedHasArgumentsFlag: UInt32 {
return _SwiftKeyPathComponentHeader_ComputedHasArgumentsFlag
}
static var computedIDResolutionMask: UInt32 {
return _SwiftKeyPathComponentHeader_ComputedIDResolutionMask
}
static var computedIDResolved: UInt32 {
return _SwiftKeyPathComponentHeader_ComputedIDResolved
}
static var computedIDUnresolvedIndirectPointer: UInt32 {
return _SwiftKeyPathComponentHeader_ComputedIDUnresolvedIndirectPointer
}
var _value: UInt32
var discriminator: UInt32 {
return (_value & Header.discriminatorMask) >> Header.discriminatorShift
}
var payload: UInt32 {
get {
return _value & Header.payloadMask
}
set {
_sanityCheck(newValue & Header.payloadMask == newValue,
"payload too big")
_value = _value & ~Header.payloadMask | newValue
}
}
var endOfReferencePrefix: Bool {
get {
return _value & Header.endOfReferencePrefixFlag != 0
}
set {
if newValue {
_value |= Header.endOfReferencePrefixFlag
} else {
_value &= ~Header.endOfReferencePrefixFlag
}
}
}
var kind: KeyPathComponentKind {
switch (discriminator, payload) {
case (Header.structTag, _):
return .struct
case (Header.classTag, _):
return .class
case (Header.computedTag, _):
return .computed
case (Header.optionalTag, Header.optionalChainPayload):
return .optionalChain
case (Header.optionalTag, Header.optionalWrapPayload):
return .optionalWrap
case (Header.optionalTag, Header.optionalForcePayload):
return .optionalForce
default:
_sanityCheckFailure("invalid header")
}
}
// The component header is 4 bytes, but may be followed by an aligned
// pointer field for some kinds of component, forcing padding.
static var pointerAlignmentSkew: Int {
return MemoryLayout<Int>.size - MemoryLayout<Int32>.size
}
}
var bodySize: Int {
switch header.kind {
case .struct, .class:
if header.payload == Header.payloadMask { return 4 } // overflowed
return 0
case .optionalChain, .optionalForce, .optionalWrap:
return 0
case .computed:
let ptrSize = MemoryLayout<Int>.size
// align to pointer, minimum two pointers for id and get
var total = Header.pointerAlignmentSkew + ptrSize * 2
// additional word for a setter
if header.payload & Header.computedSettableFlag != 0 {
total += ptrSize
}
// include the argument size
if header.payload & Header.computedHasArgumentsFlag != 0 {
// two words for argument header: size, witnesses
total += ptrSize * 2
// size of argument area
total += _computedArgumentSize
}
return total
}
}
var _structOrClassOffset: Int {
_sanityCheck(header.kind == .struct || header.kind == .class,
"no offset for this kind")
// An offset too large to fit inline is represented by a signal and stored
// in the body.
if header.payload == Header.outOfLineOffsetPayload {
// Offset overflowed into body
_sanityCheck(body.count >= MemoryLayout<UInt32>.size,
"component not big enough")
return Int(body.load(as: UInt32.self))
}
return Int(header.payload)
}
var _computedIDValue: Int {
_sanityCheck(header.kind == .computed,
"not a computed property")
return body.load(fromByteOffset: Header.pointerAlignmentSkew,
as: Int.self)
}
var _computedID: ComputedPropertyID {
let payload = header.payload
return ComputedPropertyID(
value: _computedIDValue,
isStoredProperty: payload & Header.computedIDByStoredPropertyFlag != 0,
isTableOffset: payload & Header.computedIDByVTableOffsetFlag != 0)
}
var _computedGetter: UnsafeRawPointer {
_sanityCheck(header.kind == .computed,
"not a computed property")
return body.load(
fromByteOffset: Header.pointerAlignmentSkew + MemoryLayout<Int>.size,
as: UnsafeRawPointer.self)
}
var _computedSetter: UnsafeRawPointer {
_sanityCheck(header.kind == .computed,
"not a computed property")
_sanityCheck(header.payload & Header.computedSettableFlag != 0,
"not a settable property")
return body.load(
fromByteOffset: Header.pointerAlignmentSkew + MemoryLayout<Int>.size * 2,
as: UnsafeRawPointer.self)
}
typealias ComputedArgumentLayoutFn = @convention(thin)
(_ patternArguments: UnsafeRawPointer) -> (size: Int, alignmentMask: Int)
typealias ComputedArgumentInitializerFn = @convention(thin)
(_ patternArguments: UnsafeRawPointer,
_ instanceArguments: UnsafeMutableRawPointer) -> ()
var _computedArgumentHeaderPointer: UnsafeRawPointer {
_sanityCheck(header.kind == .computed,
"not a computed property")
_sanityCheck(header.payload & Header.computedHasArgumentsFlag != 0,
"no arguments")
return body.baseAddress.unsafelyUnwrapped
+ Header.pointerAlignmentSkew
+ MemoryLayout<Int>.size *
(header.payload & Header.computedSettableFlag != 0 ? 3 : 2)
}
var _computedArgumentSize: Int {
return _computedArgumentHeaderPointer.load(as: Int.self)
}
var _computedArgumentWitnesses: UnsafePointer<ComputedArgumentWitnesses> {
return _computedArgumentHeaderPointer.load(
fromByteOffset: MemoryLayout<Int>.size,
as: UnsafePointer<ComputedArgumentWitnesses>.self)
}
var _computedArguments: UnsafeRawPointer {
return _computedArgumentHeaderPointer + MemoryLayout<Int>.size * 2
}
var _computedMutableArguments: UnsafeMutableRawPointer {
return UnsafeMutableRawPointer(mutating: _computedArguments)
}
var value: KeyPathComponent {
switch header.kind {
case .struct:
return .struct(offset: _structOrClassOffset)
case .class:
return .class(offset: _structOrClassOffset)
case .optionalChain:
return .optionalChain
case .optionalForce:
return .optionalForce
case .optionalWrap:
return .optionalWrap
case .computed:
let isSettable = header.payload & Header.computedSettableFlag != 0
let isMutating = header.payload & Header.computedMutatingFlag != 0
let id = _computedID
let get = _computedGetter
// Argument value is unused if there are no arguments, so pick something
// likely to already be in a register as a default.
let argument: KeyPathComponent.ArgumentRef?
if header.payload & Header.computedHasArgumentsFlag != 0 {
argument = KeyPathComponent.ArgumentRef(
data: UnsafeRawBufferPointer(start: _computedArguments,
count: _computedArgumentSize),
witnesses: _computedArgumentWitnesses)
} else {
argument = nil
}
switch (isSettable, isMutating) {
case (false, false):
return .get(id: id, get: get, argument: argument)
case (true, false):
return .nonmutatingGetSet(id: id,
get: get,
set: _computedSetter,
argument: argument)
case (true, true):
return .mutatingGetSet(id: id,
get: get,
set: _computedSetter,
argument: argument)
case (false, true):
_sanityCheckFailure("impossible")
}
}
}
func destroy() {
switch header.kind {
case .struct,
.class,
.optionalChain,
.optionalForce,
.optionalWrap:
// trivial
break
case .computed:
// Run destructor, if any
if header.payload & Header.computedHasArgumentsFlag != 0,
let destructor = _computedArgumentWitnesses.pointee.destroy {
destructor(_computedMutableArguments, _computedArgumentSize)
}
}
}
func clone(into buffer: inout UnsafeMutableRawBufferPointer,
endOfReferencePrefix: Bool) {
var newHeader = header
newHeader.endOfReferencePrefix = endOfReferencePrefix
var componentSize = MemoryLayout<Header>.size
buffer.storeBytes(of: newHeader, as: Header.self)
switch header.kind {
case .struct,
.class:
if header.payload == Header.outOfLineOffsetPayload {
let overflowOffset = body.load(as: UInt32.self)
buffer.storeBytes(of: overflowOffset, toByteOffset: 4,
as: UInt32.self)
componentSize += 4
}
case .optionalChain,
.optionalForce,
.optionalWrap:
break
case .computed:
// Fields are pointer-aligned after the header
componentSize += Header.pointerAlignmentSkew
// TODO: nontrivial arguments need to be copied by value witness
buffer.storeBytes(of: _computedIDValue,
toByteOffset: MemoryLayout<Int>.size,
as: Int.self)
buffer.storeBytes(of: _computedGetter,
toByteOffset: 2 * MemoryLayout<Int>.size,
as: UnsafeRawPointer.self)
var addedSize = MemoryLayout<Int>.size * 2
if header.payload & Header.computedSettableFlag != 0 {
buffer.storeBytes(of: _computedSetter,
toByteOffset: MemoryLayout<Int>.size * 3,
as: UnsafeRawPointer.self)
addedSize += MemoryLayout<Int>.size
}
if header.payload & Header.computedHasArgumentsFlag != 0 {
let argumentSize = _computedArgumentSize
buffer.storeBytes(of: argumentSize,
toByteOffset: addedSize + MemoryLayout<Int>.size,
as: Int.self)
buffer.storeBytes(of: _computedArgumentWitnesses,
toByteOffset: addedSize + MemoryLayout<Int>.size * 2,
as: UnsafePointer<ComputedArgumentWitnesses>.self)
_computedArgumentWitnesses.pointee.copy(
_computedArguments,
buffer.baseAddress.unsafelyUnwrapped + addedSize
+ MemoryLayout<Int>.size * 3,
argumentSize)
addedSize += MemoryLayout<Int>.size * 2 + argumentSize
}
componentSize += addedSize
}
buffer = UnsafeMutableRawBufferPointer(
start: buffer.baseAddress.unsafelyUnwrapped + componentSize,
count: buffer.count - componentSize)
}
enum ProjectionResult<NewValue, LeafValue> {
/// Continue projecting the key path with the given new value.
case `continue`(NewValue)
/// Stop projecting the key path and use the given value as the final
/// result of the projection.
case `break`(LeafValue)
var assumingContinue: NewValue {
switch self {
case .continue(let x):
return x
case .break:
_sanityCheckFailure("should not have stopped key path projection")
}
}
}
func projectReadOnly<CurValue, NewValue, LeafValue>(
_ base: CurValue,
to: NewValue.Type,
endingWith: LeafValue.Type
) -> ProjectionResult<NewValue, LeafValue> {
switch value {
case .struct(let offset):
var base2 = base
return .continue(withUnsafeBytes(of: &base2) {
let p = $0.baseAddress.unsafelyUnwrapped.advanced(by: offset)
// The contents of the struct should be well-typed, so we can assume
// typed memory here.
return p.assumingMemoryBound(to: NewValue.self).pointee
})
case .class(let offset):
_sanityCheck(CurValue.self is AnyObject.Type,
"base is not a class")
let baseObj = unsafeBitCast(base, to: AnyObject.self)
let basePtr = UnsafeRawPointer(Builtin.bridgeToRawPointer(baseObj))
defer { _fixLifetime(baseObj) }
return .continue(basePtr.advanced(by: offset)
.assumingMemoryBound(to: NewValue.self)
.pointee)
case .get(id: _, get: let rawGet, argument: let argument),
.mutatingGetSet(id: _, get: let rawGet, set: _, argument: let argument),
.nonmutatingGetSet(id: _, get: let rawGet, set: _, argument: let argument):
typealias Getter
= @convention(thin) (CurValue, UnsafeRawPointer) -> NewValue
let get = unsafeBitCast(rawGet, to: Getter.self)
return .continue(get(base, argument?.data.baseAddress ?? rawGet))
case .optionalChain:
// TODO: IUO shouldn't be a first class type
_sanityCheck(CurValue.self == Optional<NewValue>.self
|| CurValue.self == ImplicitlyUnwrappedOptional<NewValue>.self,
"should be unwrapping optional value")
_sanityCheck(_isOptional(LeafValue.self),
"leaf result should be optional")
if let baseValue = unsafeBitCast(base, to: Optional<NewValue>.self) {
return .continue(baseValue)
} else {
// TODO: A more efficient way of getting the `none` representation
// of a dynamically-optional type...
return .break((Optional<()>.none as Any) as! LeafValue)
}
case .optionalForce:
// TODO: IUO shouldn't be a first class type
_sanityCheck(CurValue.self == Optional<NewValue>.self
|| CurValue.self == ImplicitlyUnwrappedOptional<NewValue>.self,
"should be unwrapping optional value")
return .continue(unsafeBitCast(base, to: Optional<NewValue>.self)!)
case .optionalWrap:
// TODO: IUO shouldn't be a first class type
_sanityCheck(NewValue.self == Optional<CurValue>.self
|| CurValue.self == ImplicitlyUnwrappedOptional<CurValue>.self,
"should be wrapping optional value")
return .continue(
unsafeBitCast(base as Optional<CurValue>, to: NewValue.self))
}
}
func projectMutableAddress<CurValue, NewValue>(
_ base: UnsafeRawPointer,
from _: CurValue.Type,
to _: NewValue.Type,
isRoot: Bool,
keepAlive: inout AnyObject?
) -> UnsafeRawPointer {
switch value {
case .struct(let offset):
return base.advanced(by: offset)
case .class(let offset):
// A class dereference should only occur at the root of a mutation,
// since otherwise it would be part of the reference prefix.
_sanityCheck(isRoot,
"class component should not appear in the middle of mutation")
// AnyObject memory can alias any class reference memory, so we can
// assume type here
let object = base.assumingMemoryBound(to: AnyObject.self).pointee
// The base ought to be kept alive for the duration of the derived access
keepAlive = keepAlive == nil
? object
: ClassHolder(previous: keepAlive, instance: object)
return UnsafeRawPointer(Builtin.bridgeToRawPointer(object))
.advanced(by: offset)
case .mutatingGetSet(id: _, get: let rawGet, set: let rawSet,
argument: let argument):
typealias Getter
= @convention(thin) (CurValue, UnsafeRawPointer) -> NewValue
typealias Setter
= @convention(thin) (NewValue, inout CurValue, UnsafeRawPointer) -> ()
let get = unsafeBitCast(rawGet, to: Getter.self)
let set = unsafeBitCast(rawSet, to: Setter.self)
let baseTyped = UnsafeMutablePointer(
mutating: base.assumingMemoryBound(to: CurValue.self))
let argValue = argument?.data.baseAddress ?? rawGet
let writeback = MutatingWritebackBuffer(previous: keepAlive,
base: baseTyped,
set: set,
argument: argValue,
value: get(baseTyped.pointee, argValue))
keepAlive = writeback
// A maximally-abstracted, final, stored class property should have
// a stable address.
return UnsafeRawPointer(Builtin.addressof(&writeback.value))
case .nonmutatingGetSet(id: _, get: let rawGet, set: let rawSet,
argument: let argument):
// A nonmutating property should only occur at the root of a mutation,
// since otherwise it would be part of the reference prefix.
_sanityCheck(isRoot,
"nonmutating component should not appear in the middle of mutation")
typealias Getter
= @convention(thin) (CurValue, UnsafeRawPointer) -> NewValue
typealias Setter
= @convention(thin) (NewValue, CurValue, UnsafeRawPointer) -> ()
let get = unsafeBitCast(rawGet, to: Getter.self)
let set = unsafeBitCast(rawSet, to: Setter.self)
let baseValue = base.assumingMemoryBound(to: CurValue.self).pointee
let argValue = argument?.data.baseAddress ?? rawGet
let writeback = NonmutatingWritebackBuffer(previous: keepAlive,
base: baseValue,
set: set,
argument: argValue,
value: get(baseValue, argValue))
keepAlive = writeback
// A maximally-abstracted, final, stored class property should have
// a stable address.
return UnsafeRawPointer(Builtin.addressof(&writeback.value))
case .optionalForce:
// TODO: ImplicitlyUnwrappedOptional should not be a first-class type
_sanityCheck(CurValue.self == Optional<NewValue>.self
|| CurValue.self == ImplicitlyUnwrappedOptional<NewValue>.self,
"should be unwrapping an optional value")
// Optional's layout happens to always put the payload at the start
// address of the Optional value itself, if a value is present at all.
let baseOptionalPointer
= base.assumingMemoryBound(to: Optional<NewValue>.self)
// Assert that a value exists
_ = baseOptionalPointer.pointee!
return base
case .optionalChain, .optionalWrap, .get:
_sanityCheckFailure("not a mutable key path component")
}
}
}
internal struct KeyPathBuffer {
var data: UnsafeRawBufferPointer
var trivial: Bool
var hasReferencePrefix: Bool
var mutableData: UnsafeMutableRawBufferPointer {
return UnsafeMutableRawBufferPointer(mutating: data)
}
struct Header {
var _value: UInt32
static var sizeMask: UInt32 {
return _SwiftKeyPathBufferHeader_SizeMask
}
static var reservedMask: UInt32 {
return _SwiftKeyPathBufferHeader_ReservedMask
}
static var trivialFlag: UInt32 {
return _SwiftKeyPathBufferHeader_TrivialFlag
}
static var hasReferencePrefixFlag: UInt32 {
return _SwiftKeyPathBufferHeader_HasReferencePrefixFlag
}
init(size: Int, trivial: Bool, hasReferencePrefix: Bool) {
_sanityCheck(size <= Int(Header.sizeMask), "key path too big")
_value = UInt32(size)
| (trivial ? Header.trivialFlag : 0)
| (hasReferencePrefix ? Header.hasReferencePrefixFlag : 0)
}
var size: Int { return Int(_value & Header.sizeMask) }
var trivial: Bool { return _value & Header.trivialFlag != 0 }
var hasReferencePrefix: Bool {
get {
return _value & Header.hasReferencePrefixFlag != 0
}
set {
if newValue {
_value |= Header.hasReferencePrefixFlag
} else {
_value &= ~Header.hasReferencePrefixFlag
}
}
}
// In a key path pattern, the "trivial" flag is used to indicate
// "instantiable in-line"
var instantiableInLine: Bool {
return trivial
}
func validateReservedBits() {
_precondition(_value & Header.reservedMask == 0,
"reserved bits set to an unexpected bit pattern")
}
}
init(base: UnsafeRawPointer) {
let header = base.load(as: Header.self)
data = UnsafeRawBufferPointer(
start: base + MemoryLayout<Int>.size,
count: header.size)
trivial = header.trivial
hasReferencePrefix = header.hasReferencePrefix
}
func destroy() {
// Short-circuit if nothing in the object requires destruction.
if trivial { return }
var bufferToDestroy = self
while true {
let (component, type) = bufferToDestroy.next()
component.destroy()
guard let _ = type else { break }
}
}
mutating func next() -> (RawKeyPathComponent, Any.Type?) {
let header = pop(RawKeyPathComponent.Header.self)
// Track if this is the last component of the reference prefix.
if header.endOfReferencePrefix {
_sanityCheck(self.hasReferencePrefix,
"beginMutation marker in non-reference-writable key path?")
self.hasReferencePrefix = false
}
var component = RawKeyPathComponent(header: header, body: data)
// Shrinkwrap the component buffer size.
let size = component.bodySize
component.body = UnsafeRawBufferPointer(start: component.body.baseAddress,
count: size)
_ = popRaw(size: size, alignment: 1)
// fetch type, which is in the buffer unless it's the final component
let nextType: Any.Type?
if data.count == 0 {
nextType = nil
} else {
nextType = pop(Any.Type.self)
}
return (component, nextType)
}
mutating func pop<T>(_ type: T.Type) -> T {
_sanityCheck(_isPOD(T.self), "should be POD")
let raw = popRaw(size: MemoryLayout<T>.size,
alignment: MemoryLayout<T>.alignment)
let resultBuf = UnsafeMutablePointer<T>.allocate(capacity: 1)
_memcpy(dest: resultBuf,
src: UnsafeMutableRawPointer(mutating: raw.baseAddress.unsafelyUnwrapped),
size: UInt(MemoryLayout<T>.size))
let result = resultBuf.pointee
resultBuf.deallocate(capacity: 1)
return result
}
mutating func popRaw(size: Int, alignment: Int) -> UnsafeRawBufferPointer {
var baseAddress = data.baseAddress.unsafelyUnwrapped
var misalignment = Int(bitPattern: baseAddress) % alignment
if misalignment != 0 {
misalignment = alignment - misalignment
baseAddress += misalignment
}
let result = UnsafeRawBufferPointer(start: baseAddress, count: size)
data = UnsafeRawBufferPointer(
start: baseAddress + size,
count: data.count - size - misalignment
)
return result
}
}
// MARK: Library intrinsics for projecting key paths.
@_inlineable
public // COMPILER_INTRINSIC
func _projectKeyPathPartial<Root>(
root: Root,
keyPath: PartialKeyPath<Root>
) -> Any {
func open<Value>(_: Value.Type) -> Any {
return _projectKeyPathReadOnly(root: root,
keyPath: unsafeDowncast(keyPath, to: KeyPath<Root, Value>.self))
}
return _openExistential(type(of: keyPath).valueType, do: open)
}
@_inlineable
public // COMPILER_INTRINSIC
func _projectKeyPathAny<RootValue>(
root: RootValue,
keyPath: AnyKeyPath
) -> Any? {
let (keyPathRoot, keyPathValue) = type(of: keyPath)._rootAndValueType
func openRoot<KeyPathRoot>(_: KeyPathRoot.Type) -> Any? {
guard let rootForKeyPath = root as? KeyPathRoot else {
return nil
}
func openValue<Value>(_: Value.Type) -> Any {
return _projectKeyPathReadOnly(root: rootForKeyPath,
keyPath: unsafeDowncast(keyPath, to: KeyPath<KeyPathRoot, Value>.self))
}
return _openExistential(keyPathValue, do: openValue)
}
return _openExistential(keyPathRoot, do: openRoot)
}
public // COMPILER_INTRINSIC
func _projectKeyPathReadOnly<Root, Value>(
root: Root,
keyPath: KeyPath<Root, Value>
) -> Value {
return keyPath.projectReadOnly(from: root)
}
public // COMPILER_INTRINSIC
func _projectKeyPathWritable<Root, Value>(
root: UnsafeMutablePointer<Root>,
keyPath: WritableKeyPath<Root, Value>
) -> (UnsafeMutablePointer<Value>, AnyObject?) {
return keyPath.projectMutableAddress(from: root)
}
public // COMPILER_INTRINSIC
func _projectKeyPathReferenceWritable<Root, Value>(
root: Root,
keyPath: ReferenceWritableKeyPath<Root, Value>
) -> (UnsafeMutablePointer<Value>, AnyObject?) {
return keyPath.projectMutableAddress(from: root)
}
// MARK: Appending type system
// FIXME(ABI): The type relationships between KeyPath append operands are tricky
// and don't interact well with our overriding rules. Hack things by injecting
// a bunch of `appending` overloads as protocol extensions so they aren't
// constrained by being overrides, and so that we can use exact-type constraints
// on `Self` to prevent dynamically-typed methods from being inherited by
// statically-typed key paths.
/// This protocol is an implementation detail of key path expressions; do not
/// use it directly.
@_show_in_interface
public protocol _AppendKeyPath {}
extension _AppendKeyPath where Self == AnyKeyPath {
/// Returns a new key path created by appending the given key path to this
/// one.
///
/// Use this method to extend this key path to the value type of another key
/// path. Appending the key path passed as `path` is successful only if the
/// root type for `path` matches this key path's value type. This example
/// creates key paths from `Array<Int>` to `String` and from `String` to
/// `Int`, and then tries appending each to the other:
///
/// let arrayDescription: AnyKeyPath = \Array<Int>.description
/// let stringLength: AnyKeyPath = \String.count
///
/// // Creates a key path from `Array<Int>` to `Int`
/// let arrayDescriptionLength = arrayDescription.appending(path: stringLength)
///
/// let invalidKeyPath = stringLength.appending(path: arrayDescription)
/// // invalidKeyPath == nil
///
/// The second call to `appending(path:)` returns `nil`
/// because the root type of `arrayDescription`, `Array<Int>`, does not
/// match the value type of `stringLength`, `Int`.
///
/// - Parameter path: The key path to append.
/// - Returns: A key path from the root of this key path and the value type
/// of `path`, if `path` can be appended. If `path` can't be appended,
/// returns `nil`.
public func appending(path: AnyKeyPath) -> AnyKeyPath? {
return _tryToAppendKeyPaths(root: self, leaf: path)
}
}
extension _AppendKeyPath /* where Self == PartialKeyPath<T> */ {
/// Returns a new key path created by appending the given key path to this
/// one.
///
/// Use this method to extend this key path to the value type of another key
/// path. Appending the key path passed as `path` is successful only if the
/// root type for `path` matches this key path's value type. This example
/// creates key paths from `Array<Int>` to `String` and from `String` to
/// `Int`, and then tries appending each to the other:
///
/// let arrayDescription: PartialKeyPath<Array<Int>> = \.description
/// let stringLength: PartialKeyPath<String> = \.count
///
/// // Creates a key path from `Array<Int>` to `Int`
/// let arrayDescriptionLength = arrayDescription.appending(path: stringLength)
///
/// let invalidKeyPath = stringLength.appending(path: arrayDescription)
/// // invalidKeyPath == nil
///
/// The second call to `appending(path:)` returns `nil`
/// because the root type of `arrayDescription`, `Array<Int>`, does not
/// match the value type of `stringLength`, `Int`.
///
/// - Parameter path: The key path to append.
/// - Returns: A key path from the root of this key path and the value type
/// of `path`, if `path` can be appended. If `path` can't be appended,
/// returns `nil`.
public func appending<Root>(path: AnyKeyPath) -> PartialKeyPath<Root>?
where Self == PartialKeyPath<Root> {
return _tryToAppendKeyPaths(root: self, leaf: path)
}
/// Returns a new key path created by appending the given key path to this
/// one.
///
/// Use this method to extend this key path to the value type of another key
/// path. Appending the key path passed as `path` is successful only if the
/// root type for `path` matches this key path's value type. This example
/// creates a key path from `Array<Int>` to `String`, and then tries
/// appending compatible and incompatible key paths:
///
/// let arrayDescription: PartialKeyPath<Array<Int>> = \.description
///
/// // Creates a key path from `Array<Int>` to `Int`
/// let arrayDescriptionLength = arrayDescription.appending(path: \String.count)
///
/// let invalidKeyPath = arrayDescription.appending(path: \Double.isZero)
/// // invalidKeyPath == nil
///
/// The second call to `appending(path:)` returns `nil` because the root type
/// of the `path` parameter, `Double`, does not match the value type of
/// `arrayDescription`, `String`.
///
/// - Parameter path: The key path to append.
/// - Returns: A key path from the root of this key path to the the value type
/// of `path`, if `path` can be appended. If `path` can't be appended,
/// returns `nil`.
public func appending<Root, AppendedRoot, AppendedValue>(
path: KeyPath<AppendedRoot, AppendedValue>
) -> KeyPath<Root, AppendedValue>?
where Self == PartialKeyPath<Root> {
return _tryToAppendKeyPaths(root: self, leaf: path)
}
/// Returns a new key path created by appending the given key path to this
/// one.
///
/// Use this method to extend this key path to the value type of another key
/// path. Appending the key path passed as `path` is successful only if the
/// root type for `path` matches this key path's value type.
///
/// - Parameter path: The reference writeable key path to append.
/// - Returns: A key path from the root of this key path to the the value type
/// of `path`, if `path` can be appended. If `path` can't be appended,
/// returns `nil`.
public func appending<Root, AppendedRoot, AppendedValue>(
path: ReferenceWritableKeyPath<AppendedRoot, AppendedValue>
) -> ReferenceWritableKeyPath<Root, AppendedValue>?
where Self == PartialKeyPath<Root> {
return _tryToAppendKeyPaths(root: self, leaf: path)
}
}
extension _AppendKeyPath /* where Self == KeyPath<T,U> */ {
/// Returns a new key path created by appending the given key path to this
/// one.
///
/// Use this method to extend this key path to the value type of another key
/// path. Calling `appending(path:)` results in the same key path as if the
/// given key path had been specified using dot notation. In the following
/// example, `keyPath1` and `keyPath2` are equivalent:
///
/// let arrayDescription = \Array<Int>.description
/// let keyPath1 = arrayDescription.appending(path: \String.count)
///
/// let keyPath2 = \Array<Int>.description.count
///
/// - Parameter path: The key path to append.
/// - Returns: A key path from the root of this key path to the value type of
/// `path`.
public func appending<Root, Value, AppendedValue>(
path: KeyPath<Value, AppendedValue>
) -> KeyPath<Root, AppendedValue>
where Self: KeyPath<Root, Value> {
return _appendingKeyPaths(root: self, leaf: path)
}
/* TODO
public func appending<Root, Value, Leaf>(
path: Leaf,
// FIXME: Satisfy "Value generic param not used in signature" constraint
_: Value.Type = Value.self
) -> PartialKeyPath<Root>?
where Self: KeyPath<Root, Value>, Leaf == AnyKeyPath {
return _tryToAppendKeyPaths(root: self, leaf: path)
}
*/
/// Returns a new key path created by appending the given key path to this
/// one.
///
/// Use this method to extend this key path to the value type of another key
/// path. Calling `appending(path:)` results in the same key path as if the
/// given key path had been specified using dot notation.
///
/// - Parameter path: The key path to append.
/// - Returns: A key path from the root of this key path to the value type of
/// `path`.
public func appending<Root, Value, AppendedValue>(
path: ReferenceWritableKeyPath<Value, AppendedValue>
) -> ReferenceWritableKeyPath<Root, AppendedValue>
where Self == KeyPath<Root, Value> {
return _appendingKeyPaths(root: self, leaf: path)
}
}
extension _AppendKeyPath /* where Self == WritableKeyPath<T,U> */ {
/// Returns a new key path created by appending the given key path to this
/// one.
///
/// Use this method to extend this key path to the value type of another key
/// path. Calling `appending(path:)` results in the same key path as if the
/// given key path had been specified using dot notation.
///
/// - Parameter path: The key path to append.
/// - Returns: A key path from the root of this key path to the value type of
/// `path`.
public func appending<Root, Value, AppendedValue>(
path: WritableKeyPath<Value, AppendedValue>
) -> WritableKeyPath<Root, AppendedValue>
where Self == WritableKeyPath<Root, Value> {
return _appendingKeyPaths(root: self, leaf: path)
}
/// Returns a new key path created by appending the given key path to this
/// one.
///
/// Use this method to extend this key path to the value type of another key
/// path. Calling `appending(path:)` results in the same key path as if the
/// given key path had been specified using dot notation.
///
/// - Parameter path: The key path to append.
/// - Returns: A key path from the root of this key path to the value type of
/// `path`.
public func appending<Root, Value, AppendedValue>(
path: ReferenceWritableKeyPath<Value, AppendedValue>
) -> ReferenceWritableKeyPath<Root, AppendedValue>
where Self == WritableKeyPath<Root, Value> {
return _appendingKeyPaths(root: self, leaf: path)
}
}
extension _AppendKeyPath /* where Self == ReferenceWritableKeyPath<T,U> */ {
/// Returns a new key path created by appending the given key path to this
/// one.
///
/// Use this method to extend this key path to the value type of another key
/// path. Calling `appending(path:)` results in the same key path as if the
/// given key path had been specified using dot notation.
///
/// - Parameter path: The key path to append.
/// - Returns: A key path from the root of this key path to the value type of
/// `path`.
public func appending<Root, Value, AppendedValue>(
path: WritableKeyPath<Value, AppendedValue>
) -> ReferenceWritableKeyPath<Root, AppendedValue>
where Self == ReferenceWritableKeyPath<Root, Value> {
return _appendingKeyPaths(root: self, leaf: path)
}
}
// internal-with-availability
public func _tryToAppendKeyPaths<Result: AnyKeyPath>(
root: AnyKeyPath,
leaf: AnyKeyPath
) -> Result? {
let (rootRoot, rootValue) = type(of: root)._rootAndValueType
let (leafRoot, leafValue) = type(of: leaf)._rootAndValueType
if rootValue != leafRoot {
return nil
}
func open<Root>(_: Root.Type) -> Result {
func open2<Value>(_: Value.Type) -> Result {
func open3<AppendedValue>(_: AppendedValue.Type) -> Result {
let typedRoot = unsafeDowncast(root, to: KeyPath<Root, Value>.self)
let typedLeaf = unsafeDowncast(leaf,
to: KeyPath<Value, AppendedValue>.self)
let result = _appendingKeyPaths(root: typedRoot, leaf: typedLeaf)
return unsafeDowncast(result, to: Result.self)
}
return _openExistential(leafValue, do: open3)
}
return _openExistential(rootValue, do: open2)
}
return _openExistential(rootRoot, do: open)
}
// internal-with-availability
public func _appendingKeyPaths<
Root, Value, AppendedValue,
Result: KeyPath<Root, AppendedValue>
>(
root: KeyPath<Root, Value>,
leaf: KeyPath<Value, AppendedValue>
) -> Result {
let resultTy = type(of: root).appendedType(with: type(of: leaf))
return root.withBuffer {
var rootBuffer = $0
return leaf.withBuffer {
var leafBuffer = $0
// Reserve room for the appended KVC string, if both key paths are
// KVC-compatible.
let appendedKVCLength: Int, rootKVCLength: Int, leafKVCLength: Int
if let rootPtr = root._kvcKeyPathStringPtr,
let leafPtr = leaf._kvcKeyPathStringPtr {
rootKVCLength = Int(_swift_stdlib_strlen(rootPtr))
leafKVCLength = Int(_swift_stdlib_strlen(leafPtr))
// root + "." + leaf
appendedKVCLength = rootKVCLength + 1 + leafKVCLength
} else {
rootKVCLength = 0
leafKVCLength = 0
appendedKVCLength = 0
}
// Result buffer has room for both key paths' components, plus the
// header, plus space for the middle type.
// Align up the root so that we can put the component type after it.
let alignMask = MemoryLayout<Int>.alignment - 1
let rootSize = (rootBuffer.data.count + alignMask) & ~alignMask
let resultSize = rootSize + leafBuffer.data.count
+ 2 * MemoryLayout<Int>.size
// Tail-allocate space for the KVC string.
let totalResultSize = (resultSize + appendedKVCLength + 3) & ~3
var kvcStringBuffer: UnsafeMutableRawPointer? = nil
let result = resultTy._create(capacityInBytes: totalResultSize) {
var destBuffer = $0
// Remember where the tail-allocated KVC string buffer begins.
if appendedKVCLength > 0 {
kvcStringBuffer = destBuffer.baseAddress.unsafelyUnwrapped
.advanced(by: resultSize)
destBuffer = .init(start: destBuffer.baseAddress,
count: resultSize)
}
func pushRaw(size: Int, alignment: Int)
-> UnsafeMutableRawBufferPointer {
var baseAddress = destBuffer.baseAddress.unsafelyUnwrapped
var misalign = Int(bitPattern: baseAddress) % alignment
if misalign != 0 {
misalign = alignment - misalign
baseAddress = baseAddress.advanced(by: misalign)
}
let result = UnsafeMutableRawBufferPointer(
start: baseAddress,
count: size)
destBuffer = UnsafeMutableRawBufferPointer(
start: baseAddress + size,
count: destBuffer.count - size - misalign)
return result
}
func push<T>(_ value: T) {
let buf = pushRaw(size: MemoryLayout<T>.size,
alignment: MemoryLayout<T>.alignment)
buf.storeBytes(of: value, as: T.self)
}
// Save space for the header.
let leafIsReferenceWritable = type(of: leaf).kind == .reference
let header = KeyPathBuffer.Header(
size: resultSize - MemoryLayout<Int>.size,
trivial: rootBuffer.trivial && leafBuffer.trivial,
hasReferencePrefix: rootBuffer.hasReferencePrefix
|| leafIsReferenceWritable
)
push(header)
// Start the components at pointer alignment
_ = pushRaw(size: RawKeyPathComponent.Header.pointerAlignmentSkew,
alignment: 4)
let leafHasReferencePrefix = leafBuffer.hasReferencePrefix
// Clone the root components into the buffer.
while true {
let (component, type) = rootBuffer.next()
let isLast = type == nil
// If the leaf appended path has a reference prefix, then the
// entire root is part of the reference prefix.
let endOfReferencePrefix: Bool
if leafHasReferencePrefix {
endOfReferencePrefix = false
} else if isLast && leafIsReferenceWritable {
endOfReferencePrefix = true
} else {
endOfReferencePrefix = component.header.endOfReferencePrefix
}
component.clone(
into: &destBuffer,
endOfReferencePrefix: endOfReferencePrefix)
if let type = type {
push(type)
} else {
// Insert our endpoint type between the root and leaf components.
push(Value.self as Any.Type)
break
}
}
// Clone the leaf components into the buffer.
while true {
let (component, type) = leafBuffer.next()
component.clone(
into: &destBuffer,
endOfReferencePrefix: component.header.endOfReferencePrefix)
if let type = type {
push(type)
} else {
break
}
}
_sanityCheck(destBuffer.count == 0,
"did not fill entire result buffer")
}
// Build the KVC string if there is one.
if let kvcStringBuffer = kvcStringBuffer {
let rootPtr = root._kvcKeyPathStringPtr.unsafelyUnwrapped
let leafPtr = leaf._kvcKeyPathStringPtr.unsafelyUnwrapped
_memcpy(dest: kvcStringBuffer,
src: UnsafeMutableRawPointer(mutating: rootPtr),
size: UInt(rootKVCLength))
kvcStringBuffer.advanced(by: rootKVCLength)
.storeBytes(of: 0x2E /* '.' */, as: CChar.self)
_memcpy(dest: kvcStringBuffer.advanced(by: rootKVCLength + 1),
src: UnsafeMutableRawPointer(mutating: leafPtr),
size: UInt(leafKVCLength))
result._kvcKeyPathStringPtr =
UnsafePointer(kvcStringBuffer.assumingMemoryBound(to: CChar.self))
kvcStringBuffer.advanced(by: rootKVCLength + leafKVCLength + 1)
.storeBytes(of: 0 /* '\0' */, as: CChar.self)
}
return unsafeDowncast(result, to: Result.self)
}
}
}
// The distance in bytes from the address point of a KeyPath object to its
// buffer header. Includes the size of the Swift heap object header and the
// pointer to the KVC string.
internal var keyPathObjectHeaderSize: Int {
return MemoryLayout<HeapObject>.size + MemoryLayout<Int>.size
}
// Runtime entry point to instantiate a key path object.
@_cdecl("swift_getKeyPath")
public func _swift_getKeyPath(pattern: UnsafeMutableRawPointer,
arguments: UnsafeRawPointer)
-> UnsafeRawPointer {
// The key path pattern is laid out like a key path object, with a few
// modifications:
// - Instead of the two-word object header with isa and refcount, two
// pointers to metadata accessors are provided for the root and leaf
// value types of the key path.
// - The header reuses the "trivial" bit to mean "instantiable in-line",
// meaning that the key path described by this pattern has no contextually
// dependent parts (no dependence on generic parameters, subscript indexes,
// etc.), so it can be set up as a global object once. (The resulting
// global object will itself always have the "trivial" bit set, since it
// never needs to be destroyed.)
// - Components may have unresolved forms that require instantiation.
// - Type metadata pointers are unresolved, and instead
// point to accessor functions that instantiate the metadata.
//
// The pattern never precomputes the capabilities of the key path (readonly/
// writable/reference-writable), nor does it encode the reference prefix.
// These are resolved dynamically, so that they always reflect the dynamic
// capability of the properties involved.
let oncePtr = pattern
let patternPtr = pattern.advanced(by: MemoryLayout<Int>.size)
let bufferPtr = patternPtr.advanced(by: keyPathObjectHeaderSize)
// If the pattern is instantiable in-line, do a dispatch_once to
// initialize it. (The resulting object will still have the
// "trivial" bit set, since a global object never needs destruction.)
let bufferHeader = bufferPtr.load(as: KeyPathBuffer.Header.self)
bufferHeader.validateReservedBits()
if bufferHeader.instantiableInLine {
Builtin.onceWithContext(oncePtr._rawValue, _getKeyPath_instantiateInline,
patternPtr._rawValue)
// Return the instantiated object at +1.
// TODO: This will be unnecessary once we support global objects with inert
// refcounting.
let object = Unmanaged<AnyKeyPath>.fromOpaque(patternPtr)
_ = object.retain()
return UnsafeRawPointer(patternPtr)
}
// Otherwise, instantiate a new key path object modeled on the pattern.
return _getKeyPath_instantiatedOutOfLine(patternPtr, arguments)
}
internal func _getKeyPath_instantiatedOutOfLine(
_ pattern: UnsafeRawPointer,
_ arguments: UnsafeRawPointer)
-> UnsafeRawPointer {
// Do a pass to determine the class of the key path we'll be instantiating
// and how much space we'll need for it.
let (keyPathClass, rootType, size, alignmentMask)
= _getKeyPathClassAndInstanceSizeFromPattern(pattern, arguments)
_sanityCheck(alignmentMask < MemoryLayout<Int>.alignment,
"overalignment not implemented")
// Allocate the instance.
let instance = keyPathClass._create(capacityInBytes: size) { instanceData in
// Instantiate the pattern into the instance.
let patternBufferPtr = pattern.advanced(by: keyPathObjectHeaderSize)
let patternBuffer = KeyPathBuffer(base: patternBufferPtr)
_instantiateKeyPathBuffer(patternBuffer, instanceData, rootType, arguments)
}
// Take the KVC string from the pattern.
let kvcStringPtr = pattern.advanced(by: MemoryLayout<HeapObject>.size)
instance._kvcKeyPathStringPtr = kvcStringPtr
.load(as: Optional<UnsafePointer<CChar>>.self)
// Hand it off at +1.
return UnsafeRawPointer(Unmanaged.passRetained(instance).toOpaque())
}
internal func _getKeyPath_instantiateInline(
_ objectRawPtr: Builtin.RawPointer
) {
let objectPtr = UnsafeMutableRawPointer(objectRawPtr)
// Do a pass to determine the class of the key path we'll be instantiating
// and how much space we'll need for it.
// The pattern argument doesn't matter since an in-place pattern should never
// have arguments.
let (keyPathClass, rootType, instantiatedSize, alignmentMask)
= _getKeyPathClassAndInstanceSizeFromPattern(objectPtr, objectPtr)
_sanityCheck(alignmentMask < MemoryLayout<Int>.alignment,
"overalignment not implemented")
let bufferPtr = objectPtr.advanced(by: keyPathObjectHeaderSize)
let buffer = KeyPathBuffer(base: bufferPtr)
let totalSize = buffer.data.count + MemoryLayout<Int>.size
let bufferData = UnsafeMutableRawBufferPointer(
start: bufferPtr,
count: instantiatedSize)
// TODO: Eventually, we'll need to handle cases where the instantiated
// key path has a larger size than the pattern (because it involves
// resilient types, for example), and fall back to out-of-place instantiation
// when that happens.
_sanityCheck(instantiatedSize <= totalSize,
"size-increasing in-place instantiation not implemented")
// Instantiate the pattern in place.
_instantiateKeyPathBuffer(buffer, bufferData, rootType, bufferPtr)
_swift_instantiateInertHeapObject(objectPtr,
unsafeBitCast(keyPathClass, to: OpaquePointer.self))
}
internal typealias MetadataAccessor =
@convention(c) (UnsafeRawPointer) -> UnsafeRawPointer
internal func _getKeyPathClassAndInstanceSizeFromPattern(
_ pattern: UnsafeRawPointer,
_ arguments: UnsafeRawPointer
) -> (
keyPathClass: AnyKeyPath.Type,
rootType: Any.Type,
size: Int,
alignmentMask: Int
) {
// Resolve the root and leaf types.
let rootAccessor = pattern.load(as: MetadataAccessor.self)
let leafAccessor = pattern.load(fromByteOffset: MemoryLayout<Int>.size,
as: MetadataAccessor.self)
let root = unsafeBitCast(rootAccessor(arguments), to: Any.Type.self)
let leaf = unsafeBitCast(leafAccessor(arguments), to: Any.Type.self)
// Scan the pattern to figure out the dynamic capability of the key path.
// Start off assuming the key path is writable.
var capability: KeyPathKind = .value
let bufferPtr = pattern.advanced(by: keyPathObjectHeaderSize)
var buffer = KeyPathBuffer(base: bufferPtr)
var size = buffer.data.count + MemoryLayout<Int>.size
var alignmentMask = MemoryLayout<Int>.alignment - 1
scanComponents: while true {
let header = buffer.pop(RawKeyPathComponent.Header.self)
func popOffset() {
if header.payload == RawKeyPathComponent.Header.unresolvedFieldOffsetPayload
|| header.payload == RawKeyPathComponent.Header.outOfLineOffsetPayload {
_ = buffer.pop(UInt32.self)
}
if header.payload == RawKeyPathComponent.Header.unresolvedIndirectOffsetPayload {
_ = buffer.pop(Int.self)
// On 64-bit systems the pointer to the ivar offset variable is
// pointer-sized and -aligned, but the resulting offset ought to be
// 32 bits only and fit into padding between the 4-byte header and
// pointer-aligned type word. We don't need this space after
// instantiation.
if MemoryLayout<Int>.size == 8 {
size -= MemoryLayout<UnsafeRawPointer>.size
}
}
}
switch header.kind {
case .struct:
// No effect on the capability.
// TODO: we should dynamically prevent "let" properties from being
// reassigned.
popOffset()
case .class:
// The rest of the key path could be reference-writable.
// TODO: we should dynamically prevent "let" properties from being
// reassigned.
capability = .reference
popOffset()
case .computed:
let settable =
header.payload & RawKeyPathComponent.Header.computedSettableFlag != 0
let mutating =
header.payload & RawKeyPathComponent.Header.computedMutatingFlag != 0
let hasArguments =
header.payload & RawKeyPathComponent.Header.computedHasArgumentsFlag != 0
switch (settable, mutating) {
case (false, false):
// If the property is get-only, the capability becomes read-only, unless
// we get another reference-writable component.
capability = .readOnly
case (true, false):
capability = .reference
case (true, true):
// Writable if the base is. No effect.
break
case (false, true):
_sanityCheckFailure("unpossible")
}
_ = buffer.popRaw(size: MemoryLayout<Int>.size * (settable ? 3 : 2),
alignment: MemoryLayout<Int>.alignment)
// Get the instantiated size and alignment of the argument payload
// by asking the layout function to compute it for our given argument
// file.
if hasArguments {
let getLayoutRaw =
buffer.pop(UnsafeRawPointer.self)
let _ /*witnesses*/ = buffer.pop(UnsafeRawPointer.self)
let _ /*initializer*/ = buffer.pop(UnsafeRawPointer.self)
let getLayout = unsafeBitCast(getLayoutRaw,
to: RawKeyPathComponent.ComputedArgumentLayoutFn.self)
let (addedSize, addedAlignmentMask) = getLayout(arguments)
// TODO: Handle over-aligned values
_sanityCheck(addedAlignmentMask < MemoryLayout<Int>.alignment,
"overaligned computed property element not supported")
// Argument payload replaces the space taken by the initializer
// function pointer in the pattern.
size += (addedSize + alignmentMask) & ~alignmentMask
- MemoryLayout<Int>.size
}
case .optionalChain,
.optionalWrap:
// Chaining always renders the whole key path read-only.
capability = .readOnly
break scanComponents
case .optionalForce:
// No effect.
break
}
// Break if this is the last component.
if buffer.data.count == 0 { break }
// Pop the type accessor reference.
_ = buffer.popRaw(size: MemoryLayout<Int>.size,
alignment: MemoryLayout<Int>.alignment)
}
// Grab the class object for the key path type we'll end up with.
func openRoot<Root>(_: Root.Type) -> AnyKeyPath.Type {
func openLeaf<Leaf>(_: Leaf.Type) -> AnyKeyPath.Type {
switch capability {
case .readOnly:
return KeyPath<Root, Leaf>.self
case .value:
return WritableKeyPath<Root, Leaf>.self
case .reference:
return ReferenceWritableKeyPath<Root, Leaf>.self
}
}
return _openExistential(leaf, do: openLeaf)
}
let classTy = _openExistential(root, do: openRoot)
return (keyPathClass: classTy, rootType: root,
size: size, alignmentMask: alignmentMask)
}
internal func _instantiateKeyPathBuffer(
_ origPatternBuffer: KeyPathBuffer,
_ origDestData: UnsafeMutableRawBufferPointer,
_ rootType: Any.Type,
_ arguments: UnsafeRawPointer
) {
// NB: patternBuffer and destData alias when the pattern is instantiable
// in-line. Therefore, do not read from patternBuffer after the same position
// in destData has been written to.
var patternBuffer = origPatternBuffer
let destHeaderPtr = origDestData.baseAddress.unsafelyUnwrapped
var destData = UnsafeMutableRawBufferPointer(
start: destHeaderPtr.advanced(by: MemoryLayout<Int>.size),
count: origDestData.count - MemoryLayout<Int>.size)
func pushDest<T>(_ value: T) {
_sanityCheck(_isPOD(T.self))
var value2 = value
let size = MemoryLayout<T>.size
let alignment = MemoryLayout<T>.alignment
var baseAddress = destData.baseAddress.unsafelyUnwrapped
var misalign = Int(bitPattern: baseAddress) % alignment
if misalign != 0 {
misalign = alignment - misalign
baseAddress = baseAddress.advanced(by: misalign)
}
_memcpy(dest: baseAddress, src: &value2,
size: UInt(size))
destData = UnsafeMutableRawBufferPointer(
start: baseAddress + size,
count: destData.count - size - misalign)
}
// Track the triviality of the resulting object data.
var isTrivial = true
// Track where the reference prefix begins.
var endOfReferencePrefixComponent: UnsafeMutableRawPointer? = nil
var previousComponentAddr: UnsafeMutableRawPointer? = nil
// Instantiate components that need it.
var base: Any.Type = rootType
// Some pattern forms are pessimistically larger than what we need in the
// instantiated key path. Keep track of this.
while true {
let componentAddr = destData.baseAddress.unsafelyUnwrapped
let header = patternBuffer.pop(RawKeyPathComponent.Header.self)
func tryToResolveOffset() {
if header.payload == RawKeyPathComponent.Header.unresolvedFieldOffsetPayload {
// Look up offset in type metadata. The value in the pattern is the
// offset within the metadata object.
let metadataPtr = unsafeBitCast(base, to: UnsafeRawPointer.self)
let offsetOfOffset = patternBuffer.pop(UInt32.self)
let offset = metadataPtr.load(fromByteOffset: Int(offsetOfOffset),
as: UInt32.self)
// Rewrite the header for a resolved offset.
var newHeader = header
newHeader.payload = RawKeyPathComponent.Header.outOfLineOffsetPayload
pushDest(newHeader)
pushDest(offset)
return
}
if header.payload == RawKeyPathComponent.Header.unresolvedIndirectOffsetPayload {
// Look up offset in the indirectly-referenced variable we have a
// pointer.
let offsetVar = patternBuffer.pop(UnsafeRawPointer.self)
let offsetValue = UInt32(offsetVar.load(as: UInt.self))
// Rewrite the header for a resolved offset.
var newHeader = header
newHeader.payload = RawKeyPathComponent.Header.outOfLineOffsetPayload
pushDest(newHeader)
pushDest(offsetValue)
return
}
// Otherwise, just transfer the pre-resolved component.
pushDest(header)
if header.payload == RawKeyPathComponent.Header.outOfLineOffsetPayload {
let offset = patternBuffer.pop(UInt32.self)
pushDest(offset)
}
}
switch header.kind {
case .struct:
// The offset may need to be resolved dynamically.
tryToResolveOffset()
case .class:
// Crossing a class can end the reference prefix, and makes the following
// key path potentially reference-writable.
endOfReferencePrefixComponent = previousComponentAddr
// The offset may need to be resolved dynamically.
tryToResolveOffset()
case .optionalChain,
.optionalWrap,
.optionalForce:
// No instantiation necessary.
pushDest(header)
break
case .computed:
// A nonmutating settable property can end the reference prefix and
// makes the following key path potentially reference-writable.
if header.payload & RawKeyPathComponent.Header.computedSettableFlag != 0
&& header.payload & RawKeyPathComponent.Header.computedMutatingFlag == 0 {
endOfReferencePrefixComponent = previousComponentAddr
}
// The ID may need resolution if the property is keyed by a selector.
var newHeader = header
var id = patternBuffer.pop(Int.self)
switch header.payload
& RawKeyPathComponent.Header.computedIDResolutionMask {
case RawKeyPathComponent.Header.computedIDResolved:
// Nothing to do.
break
case RawKeyPathComponent.Header.computedIDUnresolvedIndirectPointer:
// The value in the pattern is a pointer to the actual unique word-sized
// value in memory.
let idPtr = UnsafeRawPointer(bitPattern: id).unsafelyUnwrapped
id = idPtr.load(as: Int.self)
default:
_sanityCheckFailure("unpossible")
}
newHeader.payload &= ~RawKeyPathComponent.Header.computedIDResolutionMask
pushDest(newHeader)
pushDest(id)
// Carry over the accessors.
let getter = patternBuffer.pop(UnsafeRawPointer.self)
pushDest(getter)
if header.payload & RawKeyPathComponent.Header.computedSettableFlag != 0{
let setter = patternBuffer.pop(UnsafeRawPointer.self)
pushDest(setter)
}
// Carry over the arguments.
if header.payload
& RawKeyPathComponent.Header.computedHasArgumentsFlag != 0 {
let getLayoutRaw = patternBuffer.pop(UnsafeRawPointer.self)
let getLayout = unsafeBitCast(getLayoutRaw,
to: RawKeyPathComponent.ComputedArgumentLayoutFn.self)
let witnesses = patternBuffer.pop(
UnsafePointer<ComputedArgumentWitnesses>.self)
if let _ = witnesses.pointee.destroy {
isTrivial = false
}
let initializerRaw = patternBuffer.pop(UnsafeRawPointer.self)
let initializer = unsafeBitCast(initializerRaw,
to: RawKeyPathComponent.ComputedArgumentInitializerFn.self)
let (size, alignmentMask) = getLayout(arguments)
_sanityCheck(alignmentMask < MemoryLayout<Int>.alignment,
"overaligned computed arguments not implemented yet")
// The real buffer stride will be rounded up to alignment.
let stride = (size + alignmentMask) & ~alignmentMask
pushDest(stride)
pushDest(witnesses)
_sanityCheck(Int(bitPattern: destData.baseAddress) & alignmentMask == 0,
"argument destination not aligned")
initializer(arguments, destData.baseAddress.unsafelyUnwrapped)
destData = UnsafeMutableRawBufferPointer(
start: destData.baseAddress.unsafelyUnwrapped + stride,
count: destData.count - stride)
}
}
// Break if this is the last component.
if patternBuffer.data.count == 0 { break }
// Resolve the component type.
let componentTyAccessor = patternBuffer.pop(MetadataAccessor.self)
base = unsafeBitCast(componentTyAccessor(arguments), to: Any.Type.self)
pushDest(base)
previousComponentAddr = componentAddr
}
// We should have traversed both buffers.
_sanityCheck(patternBuffer.data.isEmpty && destData.count == 0)
// Write out the header.
let destHeader = KeyPathBuffer.Header(
size: origDestData.count - MemoryLayout<Int>.size,
trivial: isTrivial,
hasReferencePrefix: endOfReferencePrefixComponent != nil)
destHeaderPtr.storeBytes(of: destHeader, as: KeyPathBuffer.Header.self)
// Mark the reference prefix if there is one.
if let endOfReferencePrefixComponent = endOfReferencePrefixComponent {
var componentHeader = endOfReferencePrefixComponent
.load(as: RawKeyPathComponent.Header.self)
componentHeader.endOfReferencePrefix = true
endOfReferencePrefixComponent.storeBytes(of: componentHeader,
as: RawKeyPathComponent.Header.self)
}
}
| apache-2.0 | e0df404ff01a8b69d27aec46e59bdb94 | 35.917411 | 91 | 0.65513 | 4.781163 | false | false | false | false |
krishnagawade/Core-Data-Helper | Notes-Swift/Notes-Swift/NotesListTableViewController.swift | 1 | 7791 | //
// NotesListTableViewController.swift
// Notes-Swift
//
// Created by Dion Larson on 11/13/14.
// Copyright (c) 2014 MakeSchool. All rights reserved.
//
import UIKit
import CoreData
class NotesListTableViewController: UITableViewController, NSFetchedResultsControllerDelegate
{
//MARK: - Class member
@IBOutlet weak var addBtn: UIBarButtonItem!
var isChangeNote : Bool! = false
//MARK: View lify cycle
override func viewDidLoad() {
super.viewDidLoad()
addBtn?.target = self
addBtn?.action = "addNote"
var error: NSError? = nil
do {
try fetchedResultsController.performFetch()
} catch let error1 as NSError {
error = error1
}
// Uncomment the following line to preserve selection between presentations
// self.clearsSelectionOnViewWillAsppear = false
// Uncomment the following line to display an Edit button in the navigation bar for this view controller.
// self.navigationItem.rightBarButtonItem = self.editButtonItem()
}
override func viewWillAppear(animated: Bool) {
super.viewWillAppear(animated)
tableView.reloadData()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// MARK: - Table view data source
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
// Return the number of rows in the section.
return fetchedResultsController.fetchedObjects!.count
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("NotesCell", forIndexPath: indexPath)
self.configureCellAtIndexPath(cell, indexPath: indexPath)
return cell
}
func configureCellAtIndexPath(cell: UITableViewCell, indexPath: NSIndexPath)
{
let note : Note! = fetchedResultsController.fetchedObjects![indexPath.row] as! Note
if note.title?.isEmpty != nil
{
cell.textLabel?.text = note.title;
}
else
{
cell.textLabel?.text = note.detail;
}
}
override func tableView(tableView: UITableView, commitEditingStyle editingStyle: UITableViewCellEditingStyle, forRowAtIndexPath indexPath: NSIndexPath)
{
if editingStyle == UITableViewCellEditingStyle.Delete
{
if let note = self.fetchedResultsController.fetchedObjects![indexPath.row] as? Note
{
NSThread.currentThread().context().deleteObject(note)
LocalStorage.saveThreadsContext()
}
}
}
override func tableView(tableView: UITableView, didSelectRowAtIndexPath indexPath: NSIndexPath) {
self.performSegueWithIdentifier(VC_CONSTANT.VC_NoteDetailViewController, sender: fetchedResultsController.fetchedObjects![indexPath.row])
}
//MARK: FetchController
lazy var fetchedResultsController: NSFetchedResultsController =
{
let frc = NSFetchedResultsController(
fetchRequest: Note.fetchRequest(),
managedObjectContext: NSThread.currentThread().context(),
sectionNameKeyPath: nil,
cacheName: nil)
frc.delegate = self
return frc
}()
//MARK: FetchController delegate
/* Notifies the delegate that section and object changes are about to be processed and notifications will be sent. Enables NSFetchedResultsController change tracking.
Clients utilizing a UITableView may prepare for a batch of updates by responding to this method with -beginUpdates
*/
func controllerWillChangeContent(controller: NSFetchedResultsController)
{
self.tableView.beginUpdates()
}
/* Notifies the delegate that a fetched object has been changed due to an add, remove, move, or update. Enables NSFetchedResultsController change tracking.
controller - controller instance that noticed the change on its fetched objects
anObject - changed object
indexPath - indexPath of changed object (nil for inserts)
type - indicates if the change was an insert, delete, move, or update
newIndexPath - the destination path for inserted or moved objects, nil otherwise
Changes are reported with the following heuristics:
On Adds and Removes, only the Added/Removed object is reported. It's assumed that all objects that come after the affected object are also moved, but these moves are not reported.
The Move object is reported when the changed attribute on the object is one of the sort descriptors used in the fetch request. An update of the object is assumed in this case, but no separate update message is sent to the delegate.
The Update object is reported when an object's state changes, and the changed attributes aren't part of the sort keys.
*/
func controller(controller: NSFetchedResultsController, didChangeObject anObject: AnyObject, atIndexPath indexPath: NSIndexPath?, forChangeType type: NSFetchedResultsChangeType, newIndexPath: NSIndexPath?)
{
switch(type)
{
case NSFetchedResultsChangeType.Insert:
if let insertIndexPath = newIndexPath
{
isChangeNote = true
self.tableView.insertRowsAtIndexPaths([insertIndexPath], withRowAnimation: UITableViewRowAnimation.Right)
}
case NSFetchedResultsChangeType.Delete:
if let insertIndexPath = indexPath
{
self.tableView.deleteRowsAtIndexPaths([insertIndexPath], withRowAnimation: UITableViewRowAnimation.Automatic)
}
case NSFetchedResultsChangeType.Update:
self.configureCellAtIndexPath(self.tableView(tableView, cellForRowAtIndexPath: indexPath!), indexPath: indexPath!)
default:
print("");
}
}
/* Notifies the delegate that all section and object changes have been sent. Enables NSFetchedResultsController change tracking.
Providing an empty implementation will enable change tracking if you do not care about the individual callbacks.
*/
func controllerDidChangeContent(controller: NSFetchedResultsController)
{
self.tableView.endUpdates()
if !isChangeNote
{
self.tableView.reloadData()
}
}
//MARK: - Navigation
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?)
{
if segue.identifier == VC_CONSTANT.VC_NoteDetailViewController
{
let vc : NoteDetailViewController = segue.destinationViewController as! NoteDetailViewController
vc.noteHandler = NoteHandler()
vc.noteHandler!.notes = self.fetchedResultsController.fetchedObjects
vc.noteHandler!.currentNote = sender as? Note
if sender != nil
{
let notes : NSArray = self.fetchedResultsController.fetchedObjects!
vc.noteHandler?.currentIndex = notes.indexOfObject(sender!)
}
}
}
//MARK: - Other Functionality
func addNote()
{
self.performSegueWithIdentifier(VC_CONSTANT.VC_NoteDetailViewController, sender: nil)
}
}
| unlicense | ad2f64a05e29eb5d8e58ec3793d4e6a5 | 35.069444 | 236 | 0.652419 | 6.086719 | false | false | false | false |
USAssignmentWarehouse/EnglishNow | EnglishNow/Model/HomeTimeline/Comment.swift | 1 | 645 | //
// Comment.swift
// EnglishNow
//
// Created by Nha T.Tran on 6/20/17.
// Copyright © 2017 IceTeaViet. All rights reserved.
//
import Foundation
class Comment {
var userId: String?
var username: String?
var avatar: UIImage?
var content: String?
var commentId:String?
var time: TimeInterval?
init(userId: String, username: String ,avatar: UIImage, content: String ,commentId:String , time: TimeInterval) {
self.userId = userId
self.username = username
self.avatar = avatar
self.content = content
self.commentId = commentId
self.time = time
}
}
| apache-2.0 | 1682f7485c1094e9b20dead6b7d76645 | 20.466667 | 117 | 0.630435 | 4.050314 | false | false | false | false |
matthewpurcell/firefox-ios | Client/Frontend/Browser/SearchEngines.swift | 1 | 11510 | /* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
import Foundation
import Shared
private let OrderedEngineNames = "search.orderedEngineNames"
private let DisabledEngineNames = "search.disabledEngineNames"
private let ShowSearchSuggestionsOptIn = "search.suggestions.showOptIn"
private let ShowSearchSuggestions = "search.suggestions.show"
/**
* Manage a set of Open Search engines.
*
* The search engines are ordered. Individual search engines can be enabled and disabled. The
* first search engine is distinguished and labeled the "default" search engine; it can never be
* disabled. Search suggestions should always be sourced from the default search engine.
*
* Two additional bits of information are maintained: whether the user should be shown "opt-in to
* search suggestions" UI, and whether search suggestions are enabled.
*
* Consumers will almost always use `defaultEngine` if they want a single search engine, and
* `quickSearchEngines()` if they want a list of enabled quick search engines (possibly empty,
* since the default engine is never included in the list of enabled quick search engines, and
* it is possible to disable every non-default quick search engine).
*
* The search engines are backed by a write-through cache into a ProfilePrefs instance. This class
* is not thread-safe -- you should only access it on a single thread (usually, the main thread)!
*/
class SearchEngines {
let prefs: Prefs
init(prefs: Prefs) {
self.prefs = prefs
// By default, show search suggestions opt-in and don't show search suggestions automatically.
self.shouldShowSearchSuggestionsOptIn = prefs.boolForKey(ShowSearchSuggestionsOptIn) ?? true
self.shouldShowSearchSuggestions = prefs.boolForKey(ShowSearchSuggestions) ?? false
self.disabledEngineNames = getDisabledEngineNames()
self.orderedEngines = getOrderedEngines()
NSNotificationCenter.defaultCenter().addObserver(self, selector: "SELdidResetPrompt:", name: "SearchEnginesPromptReset", object: nil)
}
deinit {
NSNotificationCenter.defaultCenter().removeObserver(self)
}
var defaultEngine: OpenSearchEngine {
get {
return self.orderedEngines[0]
}
set(defaultEngine) {
// The default engine is always enabled.
self.enableEngine(defaultEngine)
// The default engine is always first in the list.
var orderedEngines = self.orderedEngines.filter({ engine in engine.shortName != defaultEngine.shortName })
orderedEngines.insert(defaultEngine, atIndex: 0)
self.orderedEngines = orderedEngines
}
}
@objc
func SELdidResetPrompt(notification: NSNotification) {
self.shouldShowSearchSuggestionsOptIn = true
self.shouldShowSearchSuggestions = false
}
func isEngineDefault(engine: OpenSearchEngine) -> Bool {
return defaultEngine.shortName == engine.shortName
}
// The keys of this dictionary are used as a set.
private var disabledEngineNames: [String: Bool]! {
didSet {
self.prefs.setObject(Array(self.disabledEngineNames.keys), forKey: DisabledEngineNames)
}
}
var orderedEngines: [OpenSearchEngine]! {
didSet {
self.prefs.setObject(self.orderedEngines.map({ (engine) in engine.shortName }), forKey: OrderedEngineNames)
}
}
var quickSearchEngines: [OpenSearchEngine]! {
get {
return self.orderedEngines.filter({ (engine) in
!self.isEngineDefault(engine) && self.isEngineEnabled(engine) })
}
}
var shouldShowSearchSuggestionsOptIn: Bool {
didSet {
self.prefs.setObject(shouldShowSearchSuggestionsOptIn, forKey: ShowSearchSuggestionsOptIn)
}
}
var shouldShowSearchSuggestions: Bool {
didSet {
self.prefs.setObject(shouldShowSearchSuggestions, forKey: ShowSearchSuggestions)
}
}
func isEngineEnabled(engine: OpenSearchEngine) -> Bool {
return disabledEngineNames.indexForKey(engine.shortName) == nil
}
func enableEngine(engine: OpenSearchEngine) {
disabledEngineNames.removeValueForKey(engine.shortName)
}
func disableEngine(engine: OpenSearchEngine) {
if isEngineDefault(engine) {
// Can't disable default engine.
return
}
disabledEngineNames[engine.shortName] = true
}
func queryForSearchURL(url: NSURL?) -> String? {
for engine in orderedEngines {
guard let searchTerm = engine.queryForSearchURL(url) else { continue }
return searchTerm
}
return nil
}
private func getDisabledEngineNames() -> [String: Bool] {
if let disabledEngineNames = self.prefs.stringArrayForKey(DisabledEngineNames) {
var disabledEngineDict = [String: Bool]()
for engineName in disabledEngineNames {
disabledEngineDict[engineName] = true
}
return disabledEngineDict
} else {
return [String: Bool]()
}
}
/// Return all possible paths for a language identifier in the order of most specific to least specific.
/// For example, zh-Hans-CN with a default of en will return [zh-Hans-CN, zh-CN, zh, en]. The fallback
/// identifier must be a known one that is guaranteed to exist in the SearchPlugins directory.
class func directoriesForLanguageIdentifier(languageIdentifier: String, basePath: NSString, fallbackIdentifier: String) -> [String] {
var directories = [String]()
let components = languageIdentifier.componentsSeparatedByString("-")
if components.count == 1 {
// zh
directories.append(languageIdentifier)
} else if components.count == 2 {
// zh-CN
directories.append(languageIdentifier)
directories.append(components[0])
} else if components.count == 3 {
directories.append(languageIdentifier)
directories.append(components[0] + "-" + components[2])
directories.append(components[0])
}
if !directories.contains(fallbackIdentifier) {
directories.append(fallbackIdentifier)
}
return directories.map { (path) -> String in
return basePath.stringByAppendingPathComponent(path)
}
}
// Return the language identifier to be used for the search engine selection. This returns the first
// identifier from preferredLanguages and takes into account that on iOS 8, zh-Hans-CN is returned as
// zh-Hans. In that case it returns the longer form zh-Hans-CN. Same for traditional Chinese.
//
// These exceptions can go away when we drop iOS 8 or when we start using a better mechanism for search
// engine selection that is not based on language identifier.
class func languageIdentifierForSearchEngines() -> String {
let languageIdentifier = NSLocale.preferredLanguages().first!
switch languageIdentifier {
case "zh-Hans":
return "zh-Hans-CN"
case "zh-Hant":
return "zh-Hant-TW"
default:
return languageIdentifier
}
}
// Get all known search engines, with the default search engine first, but the others in no
// particular order.
class func getUnorderedEngines() -> [OpenSearchEngine] {
let pluginBasePath: NSString = (NSBundle.mainBundle().resourcePath! as NSString).stringByAppendingPathComponent("SearchPlugins")
let languageIdentifier = languageIdentifierForSearchEngines()
let fallbackDirectory: NSString = pluginBasePath.stringByAppendingPathComponent("en")
var directory: String?
for path in directoriesForLanguageIdentifier(languageIdentifier, basePath: pluginBasePath, fallbackIdentifier: "en") {
if NSFileManager.defaultManager().fileExistsAtPath(path) {
directory = path
break
}
}
// This cannot happen if we include the fallback, but if it does we return no engines at all
guard let searchDirectory = directory else {
return []
}
let index = (searchDirectory as NSString).stringByAppendingPathComponent("list.txt")
let listFile = try? String(contentsOfFile: index, encoding: NSUTF8StringEncoding)
assert(listFile != nil, "Read the list of search engines")
let engineNames = listFile!
.stringByTrimmingCharactersInSet(NSCharacterSet.newlineCharacterSet())
.componentsSeparatedByCharactersInSet(NSCharacterSet.newlineCharacterSet())
var engines = [OpenSearchEngine]()
let parser = OpenSearchParser(pluginMode: true)
for engineName in engineNames {
// Search the current localized search plugins directory for the search engine.
// If it doesn't exist, fall back to English.
var fullPath = (searchDirectory as NSString).stringByAppendingPathComponent("\(engineName).xml")
if !NSFileManager.defaultManager().fileExistsAtPath(fullPath) {
fullPath = fallbackDirectory.stringByAppendingPathComponent("\(engineName).xml")
}
assert(NSFileManager.defaultManager().fileExistsAtPath(fullPath), "\(fullPath) exists")
let engine = parser.parse(fullPath)
assert(engine != nil, "Engine at \(fullPath) successfully parsed")
engines.append(engine!)
}
let defaultEngineFile = (searchDirectory as NSString).stringByAppendingPathComponent("default.txt")
let defaultEngineName = try? String(contentsOfFile: defaultEngineFile, encoding: NSUTF8StringEncoding).stringByTrimmingCharactersInSet(NSCharacterSet.whitespaceAndNewlineCharacterSet())
return engines.sort({ e, _ in e.shortName == defaultEngineName })
}
// Get all known search engines, possibly as ordered by the user.
private func getOrderedEngines() -> [OpenSearchEngine] {
let unorderedEngines = SearchEngines.getUnorderedEngines()
if let orderedEngineNames = prefs.stringArrayForKey(OrderedEngineNames) {
// We have a persisted order of engines, so try to use that order.
// We may have found engines that weren't persisted in the ordered list
// (if the user changed locales or added a new engine); these engines
// will be appended to the end of the list.
return unorderedEngines.sort { engine1, engine2 in
let index1 = orderedEngineNames.indexOf(engine1.shortName)
let index2 = orderedEngineNames.indexOf(engine2.shortName)
if index1 == nil && index2 == nil {
return engine1.shortName < engine2.shortName
}
// nil < N for all non-nil values of N.
if index1 == nil || index2 == nil {
return index1 > index2
}
return index1 < index2
}
} else {
// We haven't persisted the engine order, so return whatever order we got from disk.
return unorderedEngines
}
}
}
| mpl-2.0 | 12922b725843bb9c55ce1a87c461cebf | 42.108614 | 193 | 0.666638 | 5.17071 | false | false | false | false |
colemancda/Seam | SeamDemo/SeamDemo/CoreDataStack.swift | 1 | 4188 | //
// CoreDataStack.swift
// SeamDemo
//
// Created by Nofel Mahmood on 12/08/2015.
// Copyright © 2015 CloudKitSpace. All rights reserved.
//
import UIKit
import CoreData
// import Seam
class CoreDataStack: NSObject {
static let defaultStack = CoreDataStack()
var seamStore: SMStore?
// MARK: - Core Data stack
lazy var applicationDocumentsDirectory: NSURL = {
// The directory the application uses to store the Core Data store file. This code uses a directory named "com.CloudKitSpace.SeamDemo" in the application's documents Application Support directory.
let urls = NSFileManager.defaultManager().URLsForDirectory(.DocumentDirectory, inDomains: .UserDomainMask)
return urls[urls.count-1]
}()
lazy var managedObjectModel: NSManagedObjectModel = {
// The managed object model for the application. This property is not optional. It is a fatal error for the application not to be able to find and load its model.
let modelURL = NSBundle.mainBundle().URLForResource("SeamDemo", withExtension: "momd")!
return NSManagedObjectModel(contentsOfURL: modelURL)!
}()
lazy var persistentStoreCoordinator: NSPersistentStoreCoordinator = {
// The persistent store coordinator for the application. This implementation creates and returns a coordinator, having added the store for the application to it. This property is optional since there are legitimate error conditions that could cause the creation of the store to fail.
// Create the coordinator and store
let coordinator = NSPersistentStoreCoordinator(managedObjectModel: self.managedObjectModel)
let url = self.applicationDocumentsDirectory.URLByAppendingPathComponent("SingleViewCoreData.sqlite")
var failureReason = "There was an error creating or loading the application's saved data."
do {
self.seamStore = try coordinator.addPersistentStoreWithType(SeamStoreType, configuration: nil, URL: url, options: nil) as? SMStore
} catch {
// Report any error we got.
var dict = [String: AnyObject]()
dict[NSLocalizedDescriptionKey] = "Failed to initialize the application's saved data"
dict[NSLocalizedFailureReasonErrorKey] = failureReason
dict[NSUnderlyingErrorKey] = error as NSError
let wrappedError = NSError(domain: "YOUR_ERROR_DOMAIN", code: 9999, userInfo: dict)
// Replace this with code to handle the error appropriately.
// abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
NSLog("Unresolved error \(wrappedError), \(wrappedError.userInfo)")
abort()
}
return coordinator
}()
lazy var managedObjectContext: NSManagedObjectContext = {
// Returns the managed object context for the application (which is already bound to the persistent store coordinator for the application.) This property is optional since there are legitimate error conditions that could cause the creation of the context to fail.
let coordinator = self.persistentStoreCoordinator
var managedObjectContext = NSManagedObjectContext(concurrencyType: .MainQueueConcurrencyType)
managedObjectContext.persistentStoreCoordinator = coordinator
return managedObjectContext
}()
// MARK: - Core Data Saving support
func saveContext () {
if managedObjectContext.hasChanges {
do {
try managedObjectContext.save()
} catch {
// Replace this implementation with code to handle the error appropriately.
// abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
let nserror = error as NSError
NSLog("Unresolved error \(nserror), \(nserror.userInfo)")
abort()
}
}
}
}
| mit | 41b0768f2b9fa4c9048c220a992d36a0 | 51.3375 | 291 | 0.691904 | 5.831476 | false | false | false | false |
apple/swift-argument-parser | Tests/ArgumentParserPackageManagerTests/PackageManager/Config.swift | 1 | 1674 | //===----------------------------------------------------------*- swift -*-===//
//
// This source file is part of the Swift Argument Parser open source project
//
// Copyright (c) 2020 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
//
//===----------------------------------------------------------------------===//
import ArgumentParser
extension Package {
/// Manipulate configuration of the package
struct Config: ParsableCommand {}
}
extension Package.Config {
public static var configuration = CommandConfiguration(
subcommands: [GetMirror.self, SetMirror.self, UnsetMirror.self])
/// Print mirror configuration for the given package dependency
struct GetMirror: ParsableCommand {
@OptionGroup()
var options: Options
@Option(name: .customLong("package-url"), help: "The package dependency URL")
var packageURL: String
}
/// Set a mirror for a dependency
struct SetMirror: ParsableCommand {
@OptionGroup()
var options: Options
@Option(name: .customLong("mirror-url"), help: "The mirror URL")
var mirrorURL: String
@Option(name: .customLong("package-url"), help: "The package dependency URL")
var packageURL: String
}
/// Remove an existing mirror
struct UnsetMirror: ParsableCommand {
@OptionGroup()
var options: Options
@Option(name: .customLong("mirror-url"), help: "The mirror URL")
var mirrorURL: String
@Option(name: .customLong("package-url"), help: "The package dependency URL")
var packageURL: String
}
}
| apache-2.0 | 363222356af41e8cca8bca4b6352a6d7 | 29.436364 | 81 | 0.635603 | 4.796562 | false | true | false | false |
bourdakos1/Visual-Recognition-Tool | iOS/Visual Recognition/ClassifyViewController.swift | 1 | 25645 | //
// ClassifyViewController.swift
// Visual Recognition
//
// Created by Nicholas Bourdakos on 7/17/17.
// Copyright © 2017 Nicholas Bourdakos. All rights reserved.
//
import UIKit
import AVFoundation
import Alamofire
class ClassifyViewController: CameraViewController, AKPickerViewDelegate, AKPickerViewDataSource {
// Blurred effect for the API key form.
let blurredEffectView = UIVisualEffectView(effect: UIBlurEffect(style: .dark))
// Demo API key constant.
let VISION_API_KEY: String
@IBOutlet var tempImageView: UIImageView!
// All the buttons.
@IBOutlet var classifiersButton: UIButton!
@IBOutlet var retakeButton: UIButton!
@IBOutlet var apiKeyDoneButton: UIButton!
@IBOutlet var apiKeySubmit: UIButton!
@IBOutlet var apiKeyLogOut: UIButton!
@IBOutlet var apiKeyTextField: UITextField!
@IBOutlet var hintTextView: UITextView!
@IBOutlet var pickerView: AKPickerView!
// Init with the demo API key.
required init?(coder aDecoder: NSCoder) {
var keys: NSDictionary?
if let path = Bundle.main.path(forResource: "Keys", ofType: "plist") {
keys = NSDictionary(contentsOfFile: path)
}
VISION_API_KEY = (keys?["VISION_API_KEY"] as? String)!
super.init(coder: aDecoder)
}
override open func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
if let drawer = self.parent as? PulleyViewController {
// Look in user defaults to see if we have a real key.
var apiKeyText = UserDefaults.standard.string(forKey: "api_key")
if apiKeyText == nil || apiKeyText == "" {
// If we don't have a key set the text of the bar to "API Key".
apiKeyText = "🔑 API Key"
} else {
// If we do have a key, obscure it.
apiKeyText = obscureKey(key: apiKeyText!)
}
let apiKey2 = UIButton()
// Set the title to be the size of the navigation bar so we can click anywhere.
apiKey2.frame.size.width = (drawer.navigationController?.navigationBar.frame.width)!
apiKey2.frame.size.height = (drawer.navigationController?.navigationBar.frame.height)!
// Style the API text.
apiKey2.layer.shadowOffset = CGSize(width: 0, height: 1)
apiKey2.layer.shadowOpacity = 0.2
apiKey2.layer.shadowRadius = 5
apiKey2.layer.masksToBounds = false
apiKey2.titleLabel?.font = UIFont.boldSystemFont(ofSize: 17)
apiKey2.setAttributedTitle(NSAttributedString(string: apiKeyText!, attributes: [NSForegroundColorAttributeName : UIColor.white, NSStrokeColorAttributeName : UIColor(red: 0.6, green: 0.6, blue: 0.6, alpha: 1.0), NSStrokeWidthAttributeName : -0.5]), for: .normal)
drawer.navigationItem.titleView = apiKey2
let recognizer = UITapGestureRecognizer(target: self, action: #selector(addApiKey))
drawer.navigationItem.titleView?.isUserInteractionEnabled = true
drawer.navigationItem.titleView?.addGestureRecognizer(recognizer)
}
loadClassifiers()
}
func loadClassifiers() {
print("loading classifiers")
let readyClassifiers = classifiers.filter({ $0.status == .ready })
for (index, item) in readyClassifiers.enumerated() {
if item.classifierId == UserDefaults.standard.string(forKey: "classifier_id") {
select = index
} else if item.classifierId == String() && item.name == UserDefaults.standard.string(forKey: "classifier_id") {
select = index
}
}
if select >= classifiers.count {
pickerView.selectItem(classifiers.count - 1)
} else if select >= 0 {
pickerView.selectItem(select)
}
// Load from Watson.
let apiKey = UserDefaults.standard.string(forKey: "api_key")
// Just reset it if its not the same.
if apiKey == nil && !classifiers.first!.isEqual(Classifier.defaults.first!) && classifiers.count != Classifier.defaults.count {
classifiers = []
classifiers.append(contentsOf: Classifier.defaults)
pickerView.selectItem(0)
pickerView.reloadData()
return
} else if apiKey == nil {
return
}
if classifiers.count <= 0 {
classifiers.append(Classifier(name: "Loading..."))
pickerView.selectItem(0)
pickerView.reloadData()
}
let url = "https://gateway-a.watsonplatform.net/visual-recognition/api/v3/classifiers"
let params = [
"api_key": apiKey ?? "none",
"version": "2016-05-20",
"verbose": "true"
]
Alamofire.request(url, parameters: params).validate().responseJSON { response in
switch response.result {
case .success:
if let json = response.result.value as? [String : Any] {
if let classifiersJSON = json["classifiers"] as? [Any] {
// Build classifiers from json.
var classifiers = [Classifier]()
for classifierJSON in classifiersJSON {
let classifier = Classifier(json: classifierJSON)!
classifiers.append(classifier)
}
classifiers = classifiers.sorted(by: { $0.created > $1.created })
classifiers.append(contentsOf: Classifier.defaults)
// If the count and head are the same nothing was deleted or added.
if !(self.classifiers.first!.isEqual(classifiers.first!)
&& self.classifiers.count == classifiers.count) {
self.classifiers = classifiers
if self.select >= self.classifiers.count {
self.pickerView.selectItem(self.classifiers.count - 1)
}
self.pickerView.reloadData()
if self.select >= 0 {
self.pickerView.selectItem(self.select)
}
}
}
}
case .failure(let error):
print(error)
}
}
}
var classifiers = [Classifier]()
var select = -1
func numberOfItemsInPickerView(_ pickerView: AKPickerView) -> Int {
return classifiers.filter({ $0.status == .ready }).count
}
func pickerView(_ pickerView: AKPickerView, titleForItem item: Int) -> String {
let readyClassifier = classifiers.filter({ $0.status == .ready })[item]
if readyClassifier.classifierId == UserDefaults.standard.string(forKey: "classifier_id") {
select = item
} else if readyClassifier.classifierId == String() && readyClassifier.name == UserDefaults.standard.string(forKey: "classifier_id") {
select = item
}
return classifiers.filter({ $0.status == .ready })[item].name
}
func pickerView(_ pickerView: AKPickerView, didSelectItem item: Int) {
// This should be safe because the picker only shows ready classifiers.
let readyClassifier = classifiers.filter({ $0.status == .ready })[item]
if readyClassifier.classifierId == String() {
UserDefaults.standard.set(readyClassifier.name, forKey: "classifier_id")
} else {
UserDefaults.standard.set(readyClassifier.classifierId, forKey: "classifier_id")
}
}
override func viewDidLoad() {
super.viewDidLoad()
// Set up PickerView.
pickerView.delegate = self
pickerView.dataSource = self
pickerView.interitemSpacing = CGFloat(25.0)
pickerView.pickerViewStyle = .flat
pickerView.maskDisabled = true
pickerView.font = UIFont.boldSystemFont(ofSize: 14)
pickerView.highlightedFont = UIFont.boldSystemFont(ofSize: 14)
pickerView.highlightedTextColor = UIColor.white
pickerView.textColor = UIColor(red: 1, green: 1, blue: 1, alpha: 0.6)
if classifiers.count <= 0 {
classifiers.append(Classifier(name: "Loading..."))
}
pickerView.reloadData()
// Give the API TextField styles and a stroke.
apiKeyTextField.attributedPlaceholder = NSAttributedString(string: "API Key", attributes: [NSForegroundColorAttributeName: UIColor(red: 1, green: 1, blue: 1, alpha: 0.5)])
apiKeyTextField.setLeftPaddingPoints(20)
apiKeyTextField.setRightPaddingPoints(50)
// Retake just resets the UI.
retake()
// Create and hide the blur effect.
blurredEffectView.frame = CGRect(x: 0, y: 0, width: view.bounds.width, height: view.bounds.height)
view.addSubview(blurredEffectView)
blurredEffectView.isHidden = true
// Bring all the API key views to the front of the blur effect
view.bringSubview(toFront: apiKeyTextField)
view.bringSubview(toFront: apiKeyDoneButton)
view.bringSubview(toFront: apiKeySubmit)
view.bringSubview(toFront: apiKeyLogOut)
view.bringSubview(toFront: hintTextView)
}
// Delegate for QR Codes.
override func captureOutput(_ captureOutput: AVCaptureOutput!, didOutputMetadataObjects metadataObjects: [Any]!, from connection: AVCaptureConnection!) {
// Check if the metadataObjects array is not nil and it contains at least one object.
if metadataObjects == nil || metadataObjects.count == 0 {
print("No QR code is detected")
return
}
// Get the metadata object.
let metadataObj = metadataObjects[0] as! AVMetadataMachineReadableCodeObject
guard let qrCode = metadataObj.stringValue else {
return
}
print(qrCode)
testKey(key: qrCode)
}
override func captured(image: UIImage) {
var apiKey = UserDefaults.standard.string(forKey: "api_key")
if apiKey == nil || apiKey == "" {
apiKey = VISION_API_KEY
}
let reducedImage = image.resized(toWidth: 300)!
let classifierId = UserDefaults.standard.string(forKey: "classifier_id")?.lowercased() ?? "default"
if classifierId == "face detection" {
detectFaces(key: apiKey!, image: reducedImage)
} else {
classify(key: apiKey!, id: classifierId, image: reducedImage)
}
// Set the screen to our captured photo.
tempImageView.image = image
tempImageView.isHidden = false
photoButton.isHidden = true
cameraButton.isHidden = true
retakeButton.isHidden = false
classifiersButton.isHidden = true
pickerView.isHidden = true
if let drawer = self.parent as? PulleyViewController {
drawer.navigationController?.navigationBar.isHidden = true
}
// Show an activity indicator while its loading.
let alert = UIAlertController(title: nil, message: "Please wait...", preferredStyle: .alert)
alert.view.tintColor = UIColor.black
let loadingIndicator: UIActivityIndicatorView = UIActivityIndicatorView(frame: CGRect(x: 10, y: 5, width: 50, height: 50)) as UIActivityIndicatorView
loadingIndicator.hidesWhenStopped = true
loadingIndicator.activityIndicatorViewStyle = UIActivityIndicatorViewStyle.gray
loadingIndicator.startAnimating()
alert.view.addSubview(loadingIndicator)
present(alert, animated: true, completion: nil)
}
func detectFaces(key: String, image: UIImage) {
let url = URL(string: "https://gateway-a.watsonplatform.net/visual-recognition/api/v3/detect_faces")!
let urlRequest = URLRequest(url: url)
let parameters: Parameters = [
"api_key": key,
"version": "2016-05-20"
]
do {
let encodedURLRequest = try URLEncoding.queryString.encode(urlRequest, with: parameters)
Alamofire.upload(UIImageJPEGRepresentation(image, 0.4)!, to: encodedURLRequest.url!).responseJSON { response in
// Start parsing json, throw error popup if it messed up.
guard let json = response.result.value as? [String: Any],
let images = json["images"] as? [Any],
let image = images.first as? [String: Any],
let faces = image["faces"] as? [Any] else {
print("Error: No faces found.")
self.retake()
let alert = UIAlertController(title: nil, message: "No faces found.", preferredStyle: .alert)
let cancelAction = UIAlertAction(title: "Okay", style: .cancel) { action in
print("cancel")
}
alert.addAction(cancelAction)
self.dismiss(animated: true, completion: {
self.present(alert, animated: true, completion: nil)
})
return
}
var myNewData = [FaceResult]()
let scale = self.tempImageView.frame.width / 300
print(scale)
for case let faceResult in faces {
let face = FaceResult(json: faceResult)!
myNewData.append(face)
let view = UIView()
view.frame.size.width = face.location.width * scale
view.frame.size.height = face.location.height * scale
view.frame.origin.x = face.location.left * scale
view.frame.origin.y = face.location.top * scale
view.layer.borderWidth = 5
view.layer.borderColor = view.tintColor.cgColor
view.clipsToBounds = false
self.tempImageView.addSubview(view)
}
print(myNewData)
self.dismiss(animated: false, completion: nil)
}
} catch {
print("Error: \(error)")
}
}
func classify(key: String, id: String, image: UIImage) {
let url = URL(string: "https://gateway-a.watsonplatform.net/visual-recognition/api/v3/classify")!
let urlRequest = URLRequest(url: url)
let parameters: Parameters = [
"api_key": key,
"version": "2016-05-20",
"threshold": "0",
"classifier_ids": "\(id)"
]
do {
let encodedURLRequest = try URLEncoding.queryString.encode(urlRequest, with: parameters)
Alamofire.upload(UIImageJPEGRepresentation(image, 0.4)!, to: encodedURLRequest.url!).responseJSON { response in
// Start parsing json, throw error popup if it messed up.
guard let json = response.result.value as? [String: Any],
let images = json["images"] as? [Any],
let image = images.first as? [String: Any],
let classifiers = image["classifiers"] as? [Any],
let classifier = classifiers.first as? [String: Any],
let classes = classifier["classes"] as? [Any] else {
print("Error: No classes returned.")
self.retake()
let alert = UIAlertController(title: nil, message: "No classes found.", preferredStyle: .alert)
let cancelAction = UIAlertAction(title: "Okay", style: .cancel) { action in
print("cancel")
}
alert.addAction(cancelAction)
self.dismiss(animated: true, completion: {
self.present(alert, animated: true, completion: nil)
})
return
}
var myNewData = [ClassResult]()
for case let classResult in classes {
myNewData.append(ClassResult(json: classResult)!)
}
// Sort data by score and reload table.
myNewData = myNewData.sorted(by: { $0.score > $1.score })
self.push(data: myNewData)
}
} catch {
print("Error: \(error)")
}
}
// Convenience method for pushing data to the TableView.
func push(data: [ClassResult]) {
getTableController { tableController, drawer in
tableController.classes = data
self.dismiss(animated: false, completion: nil)
drawer.setDrawerPosition(position: .partiallyRevealed, animated: true)
}
}
// Convenience method for reloading the TableView.
func getTableController(run: @escaping (_ tableController: ResultsTableViewController, _ drawer: PulleyViewController) -> Void) {
if let drawer = self.parent as? PulleyViewController {
if let tableController = drawer.drawerContentViewController as? ResultsTableViewController {
DispatchQueue.main.async {
run(tableController, drawer)
tableController.tableView.reloadData()
}
}
}
}
// Convenience method for obscuring the API key.
func obscureKey(key: String) -> String {
let a = key[key.index(key.startIndex, offsetBy: 0)]
let start = key.index(key.endIndex, offsetBy: -3)
let end = key.index(key.endIndex, offsetBy: 0)
let b = key[Range(start ..< end)]
return "🔑 \(a)•••••••••••••••••••••••••••••••••••••\(b)"
}
// Verify the API entered or scanned.
func testKey(key: String) {
// Escape if they are already logged in with this key.
if key == UserDefaults.standard.string(forKey: "api_key") {
return
}
apiKeyDone()
let alert = UIAlertController(title: nil, message: "Please wait...", preferredStyle: .alert)
alert.view.tintColor = UIColor.black
let loadingIndicator: UIActivityIndicatorView = UIActivityIndicatorView(frame: CGRect(x: 10, y: 5, width: 50, height: 50)) as UIActivityIndicatorView
loadingIndicator.hidesWhenStopped = true
loadingIndicator.activityIndicatorViewStyle = UIActivityIndicatorViewStyle.gray
loadingIndicator.startAnimating()
alert.view.addSubview(loadingIndicator)
present(alert, animated: true, completion: nil)
let url = "https://gateway-a.watsonplatform.net/visual-recognition/api"
let params = [
"api_key": key,
"version": "2016-05-20"
]
Alamofire.request(url, parameters: params).responseJSON { response in
if let json = response.result.value as? [String : Any] {
if json["statusInfo"] as! String == "invalid-api-key" {
print("Ivalid api key!")
let alert = UIAlertController(title: nil, message: "Invalid api key.", preferredStyle: .alert)
let cancelAction = UIAlertAction(title: "Okay", style: .cancel) { action in
print("cancel")
}
alert.addAction(cancelAction)
self.dismiss(animated: true, completion: {
self.present(alert, animated: true, completion: nil)
})
return
}
}
print("Key set!")
self.dismiss(animated: true, completion: nil)
UserDefaults.standard.set(key, forKey: "api_key")
self.loadClassifiers()
let key = self.obscureKey(key: key)
if let drawer = self.parent as? PulleyViewController {
(drawer.navigationItem.titleView as! UIButton).setAttributedTitle(NSAttributedString(string: key, attributes: [NSForegroundColorAttributeName : UIColor.white, NSStrokeColorAttributeName : UIColor(red: 0.6, green: 0.6, blue: 0.6, alpha: 1.0), NSStrokeWidthAttributeName : -0.5]), for: .normal)
}
}
}
@IBAction func unwindToVC(segue: UIStoryboardSegue) {
}
func addApiKey() {
if let drawer = self.parent as? PulleyViewController {
drawer.navigationController?.navigationBar.isHidden = true
}
blurredEffectView.isHidden = false
apiKeyTextField.isHidden = false
apiKeyDoneButton.isHidden = false
apiKeySubmit.isHidden = false
apiKeyLogOut.isHidden = false
hintTextView.isHidden = false
// If the key isn't set disable the logout button.
apiKeyLogOut.isEnabled = UserDefaults.standard.string(forKey: "api_key") != nil
apiKeyTextField.becomeFirstResponder()
}
@IBAction func apiKeyDone() {
if let drawer = self.parent as? PulleyViewController {
drawer.navigationController?.navigationBar.isHidden = false
}
apiKeyTextField.isHidden = true
apiKeyDoneButton.isHidden = true
apiKeySubmit.isHidden = true
apiKeyLogOut.isHidden = true
hintTextView.isHidden = true
blurredEffectView.isHidden = true
view.endEditing(true)
apiKeyTextField.text = ""
}
@IBAction func submitApiKey() {
let key = apiKeyTextField.text
testKey(key: key!)
}
@IBAction func logOut() {
apiKeyDone()
UserDefaults.standard.set(nil, forKey: "api_key")
if let drawer = self.parent as? PulleyViewController {
(drawer.navigationItem.titleView as! UIButton).setAttributedTitle(NSAttributedString(string: "🔑 API Key", attributes: [NSForegroundColorAttributeName : UIColor.white, NSStrokeColorAttributeName : UIColor(red: 0.6, green: 0.6, blue: 0.6, alpha: 1.0), NSStrokeWidthAttributeName : -0.5]), for: .normal)
}
loadClassifiers()
}
@IBAction func retake() {
tempImageView.subviews.forEach({ $0.removeFromSuperview() })
tempImageView.isHidden = true
photoButton.isHidden = false
cameraButton.isHidden = false
retakeButton.isHidden = true
classifiersButton.isHidden = false
pickerView.isHidden = false
if let drawer = self.parent as? PulleyViewController {
drawer.navigationController?.navigationBar.isHidden = false
}
getTableController { tableController, drawer in
drawer.setDrawerPosition(position: .closed, animated: true)
tableController.classes = []
}
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "showClassifiers",
let destination = segue.destination as? ClassifiersTableViewController {
destination.classifiers = self.classifiers
}
}
}
extension UIImage {
func resized(withPercentage percentage: CGFloat) -> UIImage? {
let canvasSize = CGSize(width: size.width * percentage, height: size.height * percentage)
UIGraphicsBeginImageContextWithOptions(canvasSize, false, scale)
defer { UIGraphicsEndImageContext() }
draw(in: CGRect(origin: .zero, size: canvasSize))
return UIGraphicsGetImageFromCurrentImageContext()
}
func resized(toWidth width: CGFloat) -> UIImage? {
let canvasSize = CGSize(width: width, height: CGFloat(ceil(width/size.width * size.height)))
UIGraphicsBeginImageContextWithOptions(canvasSize, false, scale)
defer { UIGraphicsEndImageContext() }
draw(in: CGRect(origin: .zero, size: canvasSize))
return UIGraphicsGetImageFromCurrentImageContext()
}
}
extension UITextField {
func setLeftPaddingPoints(_ amount:CGFloat){
let paddingView = UIView(frame: CGRect(x: 0, y: 0, width: amount, height: self.frame.size.height))
self.leftView = paddingView
self.leftViewMode = .always
}
func setRightPaddingPoints(_ amount:CGFloat) {
let paddingView = UIView(frame: CGRect(x: 0, y: 0, width: amount, height: self.frame.size.height))
self.rightView = paddingView
self.rightViewMode = .always
}
}
| mit | 5190a110c6dc8e4fbcaa6aab12f18ed4 | 41.110379 | 312 | 0.572122 | 5.158628 | false | false | false | false |
blg-andreasbraun/ProcedureKit | Tests/Cloud/CKFetchRecordChangesOperationTests.swift | 2 | 9153 | //
// ProcedureKit
//
// Copyright © 2016 ProcedureKit. All rights reserved.
//
import XCTest
import CloudKit
import ProcedureKit
import TestingProcedureKit
@testable import ProcedureKitCloud
class TestCKFetchRecordChangesOperation: TestCKDatabaseOperation, CKFetchRecordChangesOperationProtocol, AssociatedErrorProtocol {
typealias AssociatedError = FetchRecordChangesError<ServerChangeToken>
var token: String?
var data: Data?
var error: Error?
var recordZoneID: RecordZoneID = "zone-id"
var recordChangedBlock: ((Record) -> Void)? = nil
var recordWithIDWasDeletedBlock: ((RecordID) -> Void)? = nil
var fetchRecordChangesCompletionBlock: ((ServerChangeToken?, Data?, Error?) -> Void)? = nil
init(token: String? = "new-token", data: Data? = nil, error: Error? = nil) {
self.token = token
self.data = data
self.error = error
super.init()
}
override func main() {
fetchRecordChangesCompletionBlock?(token, data, error)
}
}
class CKFetchRecordChangesOperationTests: CKProcedureTestCase {
var target: TestCKFetchRecordChangesOperation!
var operation: CKProcedure<TestCKFetchRecordChangesOperation>!
override func setUp() {
super.setUp()
target = TestCKFetchRecordChangesOperation()
operation = CKProcedure(operation: target)
}
func test__set_get__recordZoneID() {
let recordZoneID: String = "I'm a record zone ID"
operation.recordZoneID = recordZoneID
XCTAssertEqual(operation.recordZoneID, recordZoneID)
XCTAssertEqual(target.recordZoneID, recordZoneID)
}
func test__set_get__recordChangedBlock() {
var setByBlock = false
let block: (String) -> Void = { record in
setByBlock = true
}
operation.recordChangedBlock = block
XCTAssertNotNil(operation.recordChangedBlock)
target.recordChangedBlock?("I'm a record")
XCTAssertTrue(setByBlock)
}
func test__set_get__recordWithIDWasDeletedBlock() {
var setByBlock = false
let block: (String) -> Void = { recordID in
setByBlock = true
}
operation.recordWithIDWasDeletedBlock = block
XCTAssertNotNil(operation.recordWithIDWasDeletedBlock)
target.recordWithIDWasDeletedBlock?("I'm a record ID")
XCTAssertTrue(setByBlock)
}
func test__success_without_completion_block() {
wait(for: operation)
XCTAssertProcedureFinishedWithoutErrors(operation)
}
func test__success_with_completion_block() {
var didExecuteBlock = false
operation.setFetchRecordChangesCompletionBlock { _, _ in
didExecuteBlock = true
}
wait(for: operation)
XCTAssertProcedureFinishedWithoutErrors(operation)
XCTAssertTrue(didExecuteBlock)
}
func test__error_without_completion_block() {
target.error = TestError()
wait(for: operation)
XCTAssertProcedureFinishedWithoutErrors(operation)
}
func test__error_with_completion_block() {
var didExecuteBlock = false
operation.setFetchRecordChangesCompletionBlock { _, _ in
didExecuteBlock = true
}
target.error = TestError()
wait(for: operation)
XCTAssertProcedureFinishedWithErrors(operation, count: 1)
XCTAssertFalse(didExecuteBlock)
}
}
class CloudKitProcedureFetchRecordChangesOperationTests: CKProcedureTestCase {
var cloudkit: CloudKitProcedure<TestCKFetchRecordChangesOperation>!
var setByRecordChangedBlock: TestCKFetchRecordChangesOperation.Record!
var setByRecordWithIDWasDeletedBlock: TestCKFetchRecordChangesOperation.RecordID!
override func setUp() {
super.setUp()
cloudkit = CloudKitProcedure(strategy: .immediate) { TestCKFetchRecordChangesOperation() }
cloudkit.container = container
cloudkit.previousServerChangeToken = token
cloudkit.resultsLimit = 10
cloudkit.recordZoneID = "a record zone id"
cloudkit.recordChangedBlock = { [unowned self] record in
self.setByRecordChangedBlock = record
}
cloudkit.recordWithIDWasDeletedBlock = { [unowned self] recordId in
self.setByRecordWithIDWasDeletedBlock = recordId
}
}
func test__set_get__errorHandlers() {
cloudkit.set(errorHandlers: [.internalError: cloudkit.passthroughSuggestedErrorHandler])
XCTAssertEqual(cloudkit.errorHandlers.count, 1)
XCTAssertNotNil(cloudkit.errorHandlers[.internalError])
}
func test__set_get_container() {
cloudkit.container = "I'm a different container!"
XCTAssertEqual(cloudkit.container, "I'm a different container!")
}
func test__set_get_previousServerChangeToken() {
cloudkit.previousServerChangeToken = "I'm a different token!"
XCTAssertEqual(cloudkit.previousServerChangeToken, "I'm a different token!")
}
func test__set_get_resultsLimit() {
cloudkit.resultsLimit = 20
XCTAssertEqual(cloudkit.resultsLimit, 20)
}
func test__set_get_recordZoneID() {
cloudkit.recordZoneID = "a record zone id"
XCTAssertEqual(cloudkit.recordZoneID, "a record zone id")
}
func test__set_get_recordChangedBlock() {
XCTAssertNotNil(cloudkit.recordChangedBlock)
cloudkit.recordChangedBlock?("a record")
XCTAssertEqual(setByRecordChangedBlock, "a record")
}
func test__set_get_recordWithIDWasDeletedBlock() {
XCTAssertNotNil(cloudkit.recordWithIDWasDeletedBlock)
cloudkit.recordWithIDWasDeletedBlock?("a record id")
XCTAssertEqual(setByRecordWithIDWasDeletedBlock, "a record id")
}
func test__cancellation() {
cloudkit.cancel()
wait(for: cloudkit)
XCTAssertProcedureCancelledWithoutErrors(cloudkit)
}
func test__success_without_completion_block_set() {
wait(for: cloudkit)
XCTAssertProcedureFinishedWithoutErrors(cloudkit)
}
func test__success_with_completion_block_set() {
var didExecuteBlock = false
cloudkit.setFetchRecordChangesCompletionBlock { _ in
didExecuteBlock = true
}
wait(for: cloudkit)
XCTAssertProcedureFinishedWithoutErrors(cloudkit)
XCTAssertTrue(didExecuteBlock)
}
func test__error_without_completion_block_set() {
cloudkit = CloudKitProcedure(strategy: .immediate) {
let operation = TestCKFetchRecordChangesOperation()
operation.error = NSError(domain: CKErrorDomain, code: CKError.internalError.rawValue, userInfo: nil)
return operation
}
wait(for: cloudkit)
XCTAssertProcedureFinishedWithoutErrors(cloudkit)
}
func test__error_with_completion_block_set() {
cloudkit = CloudKitProcedure(strategy: .immediate) {
let operation = TestCKFetchRecordChangesOperation()
operation.error = NSError(domain: CKErrorDomain, code: CKError.internalError.rawValue, userInfo: nil)
return operation
}
var didExecuteBlock = false
cloudkit.setFetchRecordChangesCompletionBlock { _ in
didExecuteBlock = true
}
wait(for: cloudkit)
XCTAssertProcedureFinishedWithErrors(cloudkit, count: 1)
XCTAssertFalse(didExecuteBlock)
}
func test__error_which_retries_using_retry_after_key() {
var shouldError = true
cloudkit = CloudKitProcedure(strategy: .immediate) {
let op = TestCKFetchRecordChangesOperation()
if shouldError {
let userInfo = [CKErrorRetryAfterKey: NSNumber(value: 0.001)]
op.error = NSError(domain: CKErrorDomain, code: CKError.Code.serviceUnavailable.rawValue, userInfo: userInfo)
shouldError = false
}
return op
}
var didExecuteBlock = false
cloudkit.setFetchRecordChangesCompletionBlock { _ in didExecuteBlock = true }
wait(for: cloudkit)
XCTAssertProcedureFinishedWithoutErrors(cloudkit)
XCTAssertTrue(didExecuteBlock)
}
func test__error_which_retries_using_custom_handler() {
var shouldError = true
cloudkit = CloudKitProcedure(strategy: .immediate) {
let op = TestCKFetchRecordChangesOperation()
if shouldError {
op.error = NSError(domain: CKErrorDomain, code: CKError.Code.limitExceeded.rawValue, userInfo: nil)
shouldError = false
}
return op
}
var didRunCustomHandler = false
cloudkit.set(errorHandlerForCode: .limitExceeded) { _, _, _, suggestion in
didRunCustomHandler = true
return suggestion
}
var didExecuteBlock = false
cloudkit.setFetchRecordChangesCompletionBlock { _ in didExecuteBlock = true }
wait(for: cloudkit)
XCTAssertProcedureFinishedWithoutErrors(cloudkit)
XCTAssertTrue(didExecuteBlock)
XCTAssertTrue(didRunCustomHandler)
}
}
| mit | af1e03ea33b9eccc12e5b51c9d78f9e0 | 34.2 | 130 | 0.673077 | 5.211845 | false | true | false | false |
skedgo/tripkit-ios | Sources/TripKit/search/TKModeImageBuilder.swift | 1 | 1360 | //
// TKModeImageBuilder.swift
// TripKit
//
// Created by Adrian Schönig on 13.05.19.
// Copyright © 2019 SkedGo Pty Ltd. All rights reserved.
//
import Foundation
@objc
public class TKModeImageFactory: NSObject {
@objc(sharedInstance)
public static let shared = TKModeImageFactory()
private let cache = NSCache<NSString, TKImage>()
private override init() {
super.init()
}
@objc(imageForModeInfo:)
public func image(for modeInfo: TKModeInfo) -> TKImage? {
guard let imageIdentifier = modeInfo.imageIdentifier else { return nil }
if let existing = cache.object(forKey: imageIdentifier as NSString) {
return existing
} else {
guard let generated = buildImage(for: modeInfo) else { return nil }
cache.setObject(generated, forKey: imageIdentifier as NSString)
return generated
}
}
private func buildImage(for modeInfo: TKModeInfo) -> TKImage? {
guard let overlayImage = modeInfo.image else { return nil }
#if os(iOS) || os(tvOS)
return TKImageBuilder.drawCircularImage(insideOverlay: overlayImage, background: #colorLiteral(red: 0.795085609, green: 0.8003450036, blue: 0.7956672311, alpha: 1))
#elseif os(OSX)
return nil
#endif
}
}
fileprivate extension TKModeInfo {
var imageIdentifier: String? {
return localImageName
}
}
| apache-2.0 | f23533ec15c6411b5d2fa574e642a99c | 23.690909 | 168 | 0.689985 | 4.102719 | false | false | false | false |
alusev/EGFormValidator | Classes/UITextField+maxLength.swift | 1 | 1432 | //
// UITextField+maxLength.swift
// EGFormValidator
//
// Created by Evgeny Gushchin on 19/12/16.
// Copyright © 2016 Evgeny Gushchin. All rights reserved.
//
import UIKit
/// The variable holds an array of maxlength values of all `UITextFields`
private var maxLengths = [UITextField: Int]()
/// The extension adds MaxLength validator
extension UITextField {
/// Defines a maximum characters number allow for the textfield
@IBInspectable public var maxLength: Int {
get {
guard let length = maxLengths[self] else {
return Int.max
}
return length
}
set {
maxLengths[self] = newValue
addTarget(
self,
action: #selector(limitLength),
for: UIControl.Event.editingChanged
)
}
}
/**
The method to be called on `UIControlEvents.editingChanged` event
- Parameter textField: A textfield beeing edited
*/
@objc func limitLength(textField: UITextField) {
guard let prospectiveText = textField.text, prospectiveText.count > maxLength else {
return
}
let selection = selectedTextRange
let index = prospectiveText.index(prospectiveText.startIndex, offsetBy: maxLength)
text = String(prospectiveText[..<index])
selectedTextRange = selection
}
}
| mit | 4b514a01502409c2042f5d7323f6ec85 | 27.058824 | 92 | 0.610063 | 4.834459 | false | false | false | false |
globelabs/globe-connect-swift | Sources/Request.swift | 1 | 1704 | import Foundation
public struct Request {
typealias RequestCallback = ((Data?, Any?, Any?) -> ())
func get(
url: String,
payload: String? = nil,
headers: [String: String]?,
callback: @escaping RequestCallback
) {
// build out the request
var request = URLRequest(url: URL(string: url)!)
// set the request method
request.httpMethod = "GET"
// check if there are given headers
if headers != nil {
// loop
for (key, value) in headers! {
request.setValue(value, forHTTPHeaderField: key)
}
}
// send the request
let task = URLSession.shared.dataTask(with: request) { data, response, error in
callback(data, response, error)
}
task.resume();
}
func post(
url: String,
payload: String,
headers: [String: String]?,
callback: @escaping RequestCallback
) {
// build out the request
var request = URLRequest(url: URL(string: url)!)
// set the request method
request.httpMethod = "POST"
// check if there are given headers
if headers != nil {
// loop
for (key, value) in headers! {
request.setValue(value, forHTTPHeaderField: key)
}
}
// set the request body and convert the data to UTF-8
request.httpBody = payload.data(using: .utf8)
let task = URLSession.shared.dataTask(with: request) { data, response, error in
// return whatever
callback(data, response, error)
}
task.resume();
}
}
| mit | d570dd75ee934e80faa2bcbf5172c7b0 | 25.625 | 87 | 0.539319 | 4.707182 | false | false | false | false |
asavihay/PBROrbs-iOS10-SceneKit | PBROrbs/GameViewController.swift | 1 | 3453 | //
// GameViewController.swift
// PBROrbs
//
// Created by Avihay Assouline on 23/07/2016.
// Copyright © 2016 MediumBlog. All rights reserved.
//
import UIKit
import QuartzCore
import SceneKit
class GameViewController: UIViewController {
let materialPrefixes : [String] = ["bamboo-wood-semigloss",
"oakfloor2",
"scuffed-plastic",
"rustediron-streaks"];
override func viewDidLoad() {
super.viewDidLoad()
// create a new scene
let scene = SCNScene(named: "sphere.obj")!
// select the sphere node - As we know we only loaded one object
// we select the first item on the children list
let sphereNode = scene.rootNode.childNodes[0]
// create and add a camera to the scene
let cameraNode = SCNNode()
cameraNode.camera = SCNCamera()
scene.rootNode.addChildNode(cameraNode)
// place the camera
cameraNode.position = SCNVector3(x: 0, y: 0, z: 15)
let material = sphereNode.geometry?.firstMaterial
// Declare that you intend to work in PBR shading mode
// Note that this requires iOS 10 and up
material?.lightingModel = SCNMaterial.LightingModel.physicallyBased
// Setup the material maps for your object
let materialFilePrefix = materialPrefixes[0];
material?.diffuse.contents = UIImage(named: "\(materialFilePrefix)-albedo.png")
material?.roughness.contents = UIImage(named: "\(materialFilePrefix)-roughness.png")
material?.metalness.contents = UIImage(named: "\(materialFilePrefix)-metal.png")
material?.normal.contents = UIImage(named: "\(materialFilePrefix)-normal.png")
// Setup background - This will be the beautiful blurred background
// that assist the user understand the 3D envirnoment
let bg = UIImage(named: "sphericalBlurred.png")
scene.background.contents = bg;
// Setup Image Based Lighting (IBL) map
let env = UIImage(named: "spherical.jpg")
scene.lightingEnvironment.contents = env
scene.lightingEnvironment.intensity = 2.0
// retrieve the SCNView
let scnView = self.view as! SCNView
// set the scene to the view
scnView.scene = scene
// allows the user to manipulate the camera
scnView.allowsCameraControl = true
/*
* The following was not a part of my blog post but are pretty easy to understand:
* To make the Orb cool, we'll add rotation animation to it
*/
sphereNode.runAction(SCNAction.repeatForever(SCNAction.rotateBy(x: 1, y: 1, z: 1, duration: 10)))
}
override var shouldAutorotate: Bool {
return true
}
override var prefersStatusBarHidden: Bool {
return true
}
override var supportedInterfaceOrientations: UIInterfaceOrientationMask {
if UIDevice.current.userInterfaceIdiom == .phone {
return .allButUpsideDown
} else {
return .all
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Release any cached data, images, etc that aren't in use.
}
}
| mit | e02960e72a4940aa55a1e668c61413ce | 33.178218 | 105 | 0.602549 | 5.03207 | false | false | false | false |
shajrawi/swift | test/SILGen/fixed_layout_attribute.swift | 1 | 2743 | // RUN: %target-swift-emit-silgen -parse-as-library %s | %FileCheck %s --check-prefix=FRAGILE --check-prefix=CHECK
// RUN: %target-swift-emit-silgen -enable-library-evolution -parse-as-library %s | %FileCheck %s --check-prefix=RESILIENT --check-prefix=CHECK
// RUN: %target-swift-emit-silgen -parse-as-library -enable-testing %s
// RUN: %target-swift-emit-silgen -parse-as-library -enable-testing -enable-library-evolution %s
public let global = 0
struct InternalStruct {
var storedProperty = global
}
// CHECK-LABEL: sil hidden [transparent] [ossa] @$s22fixed_layout_attribute14InternalStructV14storedPropertySivpfi : $@convention(thin) () -> Int
//
// ... okay to directly reference the addressor here:
// CHECK: function_ref @$s22fixed_layout_attribute6globalSivau
// CHECK: return
public struct NonFixedStruct {
public var storedProperty = global
}
// FRAGILE-LABEL: sil [transparent] [ossa] @$s22fixed_layout_attribute14NonFixedStructV14storedPropertySivpfi : $@convention(thin) () -> Int
// RESILIENT-LABEL: sil hidden [transparent] [ossa] @$s22fixed_layout_attribute14NonFixedStructV14storedPropertySivpfi : $@convention(thin) () -> Int
//
// ... okay to directly reference the addressor here:
// CHECK: function_ref @$s22fixed_layout_attribute6globalSivau
// CHECK: return
@_fixed_layout
public struct FixedStruct {
public var storedProperty = global
}
// CHECK-LABEL: sil non_abi [transparent] [serialized] [ossa] @$s22fixed_layout_attribute11FixedStructV14storedPropertySivpfi : $@convention(thin) () -> Int
//
// ... a fragile build can still reference the addressor:
// FRAGILE: function_ref @$s22fixed_layout_attribute6globalSivau
// ... a resilient build has to use the getter because the addressor
// is not public, and the initializer is serialized:
// RESILIENT: function_ref @$s22fixed_layout_attribute6globalSivg
// CHECK: return
// This would crash with -enable-testing
private let privateGlobal = 0
struct AnotherInternalStruct {
var storedProperty = privateGlobal
}
// Static properties in fixed-layout type is still resilient
@_fixed_layout
public struct HasStaticProperty {
public static var staticProperty: Int = 0
}
// CHECK-LABEL: sil [ossa] @$s22fixed_layout_attribute18usesStaticPropertyyyF : $@convention(thin) () -> ()
// CHECK: function_ref @$s22fixed_layout_attribute17HasStaticPropertyV06staticF0Sivau : $@convention(thin) () -> Builtin.RawPointer
// CHECK: return
public func usesStaticProperty() {
_ = HasStaticProperty.staticProperty
}
// CHECK-LABEL: sil [serialized] [ossa] @$s22fixed_layout_attribute27usesStaticPropertyInlinableyyF : $@convention(thin) () -> ()
@inlinable
public func usesStaticPropertyInlinable() {
_ = HasStaticProperty.staticProperty
}
| apache-2.0 | 0570327d87b30f062d39d5b27546ddbb | 37.097222 | 156 | 0.752461 | 3.731973 | false | false | false | false |
OpsLabJPL/MarsImagesIOS | Pods/EFInternetIndicator/EFInternetIndicator/Classes/InternetViewIndicator.swift | 1 | 2883 | import Foundation
import SwiftMessages
extension String {
var localized: String {
return NSLocalizedString(self, tableName: nil, bundle: Bundle.main, value: "", comment: "")
}
}
public class InternetViewIndicator {
private var _reachability: Reachability?
private var status:MessageView
init(backgroundColor:UIColor = UIColor.red, style: MessageView.Layout = .statusLine, textColor:UIColor = UIColor.white, message:String = "Please, check your internet connection", remoteHostName: String = "apple.com") {
status = MessageView.viewFromNib(layout: style)
self.initializer(backgroundColor: backgroundColor, style: style, textColor: textColor, message: message, remoteHostName: remoteHostName, hideButton: true)
}
private func initializer(backgroundColor:UIColor = UIColor.red, style: MessageView.Layout = .statusLine, textColor:UIColor = UIColor.white, message:String = "Please, check your internet connection", remoteHostName: String = "apple.com", hideButton:Bool = true) {
status.button?.isHidden = hideButton
status.iconLabel?.text = "❌"
//TO DO: Refactor and make this parameters
status.iconImageView?.isHidden = true
status.titleLabel?.isHidden = true
status.backgroundView.backgroundColor = backgroundColor
status.bodyLabel?.textColor = textColor
status.configureContent(body: NSLocalizedString(message, comment: "internet failure"))
self.setupReachability(remoteHostName)
self.startNotifier()
}
func setupReachability(_ hostName: String?) {
let reachability = hostName == nil ? Reachability() : Reachability(hostname: hostName!)
self._reachability = reachability
NotificationCenter.default.addObserver(self, selector: #selector(InternetViewIndicator.reachabilityChanged(_:)), name: NSNotification.Name.reachabilityChanged, object: reachability)
}
@objc func reachabilityChanged(_ note: Notification) {
let reachability = note.object as! Reachability
var statusConfig = SwiftMessages.defaultConfig
statusConfig.duration = .forever
statusConfig.presentationContext = .window(windowLevel: UIWindow.Level.statusBar)
if reachability.connection != .none {
SwiftMessages.hide()
} else {
SwiftMessages.show(config: statusConfig, view: status)
}
}
func startNotifier() {
do {
try _reachability?.startNotifier()
} catch {
return
}
}
func stopNotifier() {
_reachability?.stopNotifier()
NotificationCenter.default.removeObserver(self, name: .reachabilityChanged, object: nil)
_reachability = nil
}
}
| apache-2.0 | 2622397b34e43f7cee7aee06b25f9e12 | 35.935897 | 266 | 0.661576 | 5.395131 | false | true | false | false |
vooooo/CP_Yelp | Yelp/YelpClient.swift | 1 | 3319 | //
// YelpClient.swift
// Yelp
//
// Created by Timothy Lee on 9/19/14.
// Copyright (c) 2014 Timothy Lee. All rights reserved.
//
import UIKit
// You can register for Yelp API keys here: http://www.yelp.com/developers/manage_api_keys
let yelpConsumerKey = "A6DqflqHBgUjcYrHCVgTmQ"
let yelpConsumerSecret = "kVlUSzt7fdt6tyHzDeE22GzTJqc"
let yelpToken = "fF-2c6DAgEbKnevDk4NPgRM_u4flaTeo"
let yelpTokenSecret = "GqXo1ya9hAov7V3NxRBKHcuLSyU"
enum YelpSortMode: Int {
case BestMatched = 0, Distance, HighestRated
}
class YelpClient: BDBOAuth1RequestOperationManager {
var accessToken: String!
var accessSecret: String!
class var sharedInstance : YelpClient {
struct Static {
static var token : dispatch_once_t = 0
static var instance : YelpClient? = nil
}
dispatch_once(&Static.token) {
Static.instance = YelpClient(consumerKey: yelpConsumerKey, consumerSecret: yelpConsumerSecret, accessToken: yelpToken, accessSecret: yelpTokenSecret)
}
return Static.instance!
}
required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
init(consumerKey key: String!, consumerSecret secret: String!, accessToken: String!, accessSecret: String!) {
self.accessToken = accessToken
self.accessSecret = accessSecret
let baseUrl = NSURL(string: "https://api.yelp.com/v2/")
super.init(baseURL: baseUrl, consumerKey: key, consumerSecret: secret);
let token = BDBOAuth1Credential(token: accessToken, secret: accessSecret, expiration: nil)
self.requestSerializer.saveAccessToken(token)
}
func searchWithTerm(term: String, completion: ([Business]!, NSError!) -> Void) -> AFHTTPRequestOperation {
return searchWithTerm(term, sort: nil, categories: nil, deals: nil, distance: nil, completion: completion)
}
func searchWithTerm(term: String, sort: YelpSortMode?, categories: [String]?, deals: Bool?, distance: Int?, completion: ([Business]!, NSError!) -> Void) -> AFHTTPRequestOperation {
// For additional parameters, see http://www.yelp.com/developers/documentation/v2/search_api
// Default the location to San Francisco
var parameters: [String : AnyObject] = ["term": term, "ll": "37.785771,-122.406165"]
if sort != nil {
parameters["sort"] = sort!.rawValue
}
if categories != nil && categories!.count > 0 {
parameters["category_filter"] = (categories!).joinWithSeparator(",")
}
if deals != nil {
parameters["deals_filter"] = deals!
}
if distance != nil {
parameters["radius_filter"] = distance!
}
print(parameters)
return self.GET("search", parameters: parameters, success: { (operation: AFHTTPRequestOperation!, response: AnyObject!) -> Void in
let dictionaries = response["businesses"] as? [NSDictionary]
if dictionaries != nil {
completion(Business.businesses(array: dictionaries!), nil)
}
}, failure: { (operation: AFHTTPRequestOperation!, error: NSError!) -> Void in
completion(nil, error)
})
}
}
| mit | ce0b4fefb1481dde879fbfb465096c1b | 36.715909 | 184 | 0.636336 | 4.293661 | false | false | false | false |
roambotics/swift | validation-test/Sema/issue-60958.swift | 2 | 1090 | // RUN: %target-swift-frontend -emit-silgen %s | %FileCheck %s
// https://github.com/apple/swift/issues/60958
// Make sure that `makeIterator` witness is picked over the non-witness.
struct S: Sequence {
private var _storage: [UInt8] = []
func makeIterator() -> Array<UInt8>.Iterator {
_storage.makeIterator()
}
typealias Element = UInt8
class Iterator: IteratorProtocol {
func next() -> UInt8? { 0 }
typealias Element = UInt8
}
func makeIterator() -> Iterator {
Iterator()
}
}
extension S {
// CHECK: sil hidden [ossa] @$s4main1SV1fyyF
func f() {
for elt in self {
// CHECK: [[ITERATOR_VAR:%.*]] = project_box {{.*}} : ${ var S.Iterator }, 0
// CHECK: [[MAKE_ITERATOR_REF:%.*]] = function_ref @$s4main1SV12makeIteratorAC0C0CyF : $@convention(method) (@guaranteed S) -> @owned S.Iterator
// CHECK-NEXT: [[ITERATOR:%.*]] = apply [[MAKE_ITERATOR_REF]](%0) : $@convention(method) (@guaranteed S) -> @owned S.Iterator
// CHECK-NEXT: store [[ITERATOR]] to [init] [[ITERATOR_VAR]] : $*S.Iterator
print(elt)
}
}
}
| apache-2.0 | 87fad531907b5a16acb74a0871ce1c6d | 29.277778 | 150 | 0.621101 | 3.516129 | false | false | false | false |
Lweek/Formulary | Formulary/UIKit Extensions/UIControlEventAcion.swift | 1 | 1182 | //
// UIControlEventAction.swift
// Formulary
//
// Created by Fabian Canas on 8/9/15.
// Copyright (c) 2015 Fabian Canas. All rights reserved.
//
import UIKit
private let ActionTargetControlKey :UnsafePointer<Void> = UnsafePointer<Void>()
/// Allows attaching a closure to a UIControlEvent on a UIControl
class ActionTarget {
let control: UIControl
let closure: (UIControl) -> Void
init(control: UIControl, controlEvents: UIControlEvents = .ValueChanged, action: (UIControl) -> Void) {
self.control = control
closure = action
control.addTarget(self, action: Selector("action:"), forControlEvents: controlEvents)
objc_setAssociatedObject(control, ActionTargetControlKey, self, objc_AssociationPolicy(OBJC_ASSOCIATION_RETAIN_NONATOMIC))
}
@objc func action(sender: UIControl) {
closure(sender)
}
class func clear(control :UIControl, controlEvents :UIControlEvents) {
if let target = objc_getAssociatedObject(control, ActionTargetControlKey) as? ActionTarget {
control.removeTarget(target, action: Selector("action:"), forControlEvents: controlEvents)
}
}
}
| mit | 06b96be943f0fc9e7b0de4d18f764f47 | 33.764706 | 130 | 0.699662 | 4.635294 | false | false | false | false |
jopamer/swift | test/Interpreter/SDK/mixed_mode_class_with_missing_properties.swift | 1 | 2135 | // RUN: %empty-directory(%t)
// RUN: cp %s %t/main.swift
// RUN: %target-build-swift -whole-module-optimization -emit-module-path %t/UsingObjCStuff.swiftmodule -c -o %t/UsingObjCStuff.o -module-name UsingObjCStuff -I %t -I %S/Inputs/mixed_mode -swift-version 5 -parse-as-library %S/Inputs/mixed_mode/UsingObjCStuff.swift
// RUN: %target-build-swift -o %t/a.out.v4 -I %t -I %S/Inputs/mixed_mode -module-name main -swift-version 4 %t/main.swift %t/UsingObjCStuff.o
// RUN: %target-build-swift -o %t/a.out.v5 -I %t -I %S/Inputs/mixed_mode -module-name main -swift-version 5 %t/main.swift %t/UsingObjCStuff.o
// RUN: %target-run %t/a.out.v4 | %FileCheck %s
// RUN: %target-run %t/a.out.v5 | %FileCheck %s
// REQUIRES: objc_interop
// REQUIRES: executable_test
import UsingObjCStuff
print("Let's go") // CHECK: Let's go
let holder = ButtHolder()
holder.x += 17
print(holder.x) // CHECK-NEXT: 17
holder.x += 38
print(holder.x) // CHECK-NEXT: 55
holder.z += "hello"
print(holder.z) // CHECK-NEXT: hello
holder.z += " world"
print(holder.z) // CHECK-NEXT: hello world
holder.virtual() // CHECK-NEXT: 55 [:] hello world
class SubButtHolder: ButtHolder {
final var w: Double = 0
override func virtual() {
print("~* ", terminator: "")
super.virtual()
print(" \(w) *~")
}
func subVirtual() {
print("~* \(x) ~*~ \(z) ~*~ \(w) *~")
}
}
class SubSubButtHolder: SubButtHolder {
override func virtual() {
print("!!! ", terminator: "")
super.virtual()
print(" !!!")
}
override func subVirtual() {
print("!!! ", terminator: "")
super.subVirtual()
print(" !!!")
}
}
@inline(never)
func exerciseSubHolder(with subHolder: SubButtHolder) {
subHolder.x = 679
subHolder.z = "goodbye folks"
subHolder.w = 90.5
subHolder.virtual() // CHECK-NEXT: !!! ~* 679 [:] goodbye folks
// CHECK-NEXT: 90.5 *~
// CHECK-NEXT: !!!
subHolder.subVirtual() // CHECK-NEXT: !!! ~* 679 ~*~ goodbye folks ~*~ 90.5 *~
// CHECK-NEXT: !!!
}
exerciseSubHolder(with: SubSubButtHolder())
print("OK that's it") // CHECK-NEXT: OK that's it
| apache-2.0 | b2a5b94fa01476bbef23ac4586c0bd5a | 29.070423 | 263 | 0.621546 | 3.116788 | false | false | false | false |
OpenKitten/BSON | Sources/BSON/Codable/Encoding/BSONEncoder.swift | 1 | 14226 | import Foundation
private enum BSONEncoderError: Error {
case encodableNotDocument
}
/// An object that encodes instances of `Encodable` types as BSON Documents.
public final class BSONEncoder {
// MARK: Encoding
/// Creates a new, reusable encoder with the given strategies
public init(strategies: BSONEncoderStrategies = .default) {
self.strategies = strategies
}
/// Returns the BSON-encoded representation of the value you supply
///
/// If there's a problem encoding the value you supply, this method throws an error based on the type of problem:
///
/// - The value fails to encode, or contains a nested value that fails to encode—this method throws the corresponding error.
/// - The value can't be encoded as a BSON array or BSON object—this method throws the invalidValue error.
public func encode(_ value: Encodable) throws -> Document {
let encoder = _BSONEncoder(strategies: self.strategies, userInfo: self.userInfo)
try value.encode(to: encoder)
guard encoder.target == .document else {
throw BSONEncoderError.encodableNotDocument
}
return encoder.document
}
/// Returns the BSON-encoded representation of the value you supply
///
/// If there's a problem encoding the value you supply, this method throws an error based on the type of problem:
///
/// - The value fails to encode, or contains a nested value that fails to encode—this method throws the corresponding error.
public func encodePrimitive(_ value: Encodable) throws -> Primitive? {
let encoder = _BSONEncoder(
strategies: self.strategies,
userInfo: self.userInfo
)
try value.encode(to: encoder)
guard let target = encoder.target else {
return nil
}
switch target {
case .primitive:
return encoder.primitive
case .document:
return encoder.document
}
}
// MARK: Configuration
/// Configures the behavior of the BSON Encoder. See the documentation on `BSONEncoderStrategies` for details.
public var strategies: BSONEncoderStrategies
/// Contextual user-provided information for use during encoding.
public var userInfo: [CodingUserInfoKey: Any] = [:]
}
fileprivate final class _BSONEncoder: Encoder, AnyBSONEncoder {
enum Target {
case document
case primitive
}
var target: Target?
var document: Document! {
didSet {
writer?(document)
}
}
var primitive: Primitive! {
didSet {
writer?(primitive)
}
}
// MARK: Configuration
let strategies: BSONEncoderStrategies
var codingPath: [CodingKey]
var writer: ((Primitive?) -> ())?
var userInfo: [CodingUserInfoKey: Any]
// MARK: Initialization
init(strategies: BSONEncoderStrategies, codingPath: [CodingKey] = [], userInfo: [CodingUserInfoKey: Any]) {
self.strategies = strategies
self.codingPath = codingPath
self.userInfo = userInfo
self.target = nil
}
func container<Key>(keyedBy type: Key.Type) -> KeyedEncodingContainer<Key> where Key: CodingKey {
self.target = .document
self.document = Document()
let container = _BSONKeyedEncodingContainer<Key>(
encoder: self,
codingPath: codingPath
)
return KeyedEncodingContainer(container)
}
func unkeyedContainer() -> UnkeyedEncodingContainer {
self.target = .document
self.document = Document()
return _BSONUnkeyedEncodingContainer(
encoder: self,
codingPath: codingPath
)
}
func singleValueContainer() -> SingleValueEncodingContainer {
self.target = .primitive
return _BSONSingleValueEncodingContainer(
encoder: self,
codingPath: codingPath
)
}
// MARK: Encoding
func encode(document: Document) throws {
self.document = document
}
subscript(key: CodingKey) -> Primitive? {
get {
return self.document[converted(key.stringValue)]
}
set {
self.document[converted(key.stringValue)] = newValue
}
}
func converted(_ key: String) -> String {
// TODO: Implement key strategies
return key
}
func makePrimitive(_ value: UInt64) throws -> Primitive {
switch strategies.unsignedIntegerEncodingStrategy {
case .int64:
guard value <= UInt64(Int.max) else {
let debugDescription = "Cannot encode \(value) as Int in BSON, because it is too large. You can use BSONEncodingStrategies.UnsignedIntegerEncodingStrategy.string to encode the integer as a String."
throw EncodingError.invalidValue(
value,
EncodingError.Context(
codingPath: codingPath,
debugDescription: debugDescription
)
)
}
return _BSON64BitInteger(value)
case .string:
return "\(value)"
}
}
func nestedEncoder(forKey key: CodingKey) -> _BSONEncoder {
let encoder = _BSONEncoder(
strategies: strategies,
codingPath: codingPath + [key],
userInfo: userInfo
)
encoder.writer = { primitive in
self[key] = primitive
}
return encoder
}
}
fileprivate struct _BSONKeyedEncodingContainer<Key: CodingKey> : KeyedEncodingContainerProtocol {
var encoder: _BSONEncoder
var codingPath: [CodingKey]
init(encoder: _BSONEncoder, codingPath: [CodingKey]) {
self.encoder = encoder
self.codingPath = codingPath
}
// MARK: KeyedEncodingContainerProtocol
mutating func encodeNil(forKey key: Key) throws {
switch encoder.strategies.keyedNilEncodingStrategy {
case .null:
encoder.document[encoder.converted(key.stringValue)] = BSON.Null()
case .omitted:
return
}
}
mutating func encode(_ value: Bool, forKey key: Key) throws {
encoder[key] = value
}
mutating func encode(_ value: String, forKey key: Key) throws {
encoder[key] = value
}
mutating func encode(_ value: Double, forKey key: Key) throws {
encoder[key] = value
}
mutating func encode(_ value: Float, forKey key: Key) throws {
encoder[key] = Double(value)
}
mutating func encode(_ value: Int, forKey key: Key) throws {
encoder[key] = _BSON64BitInteger(value)
}
mutating func encode(_ value: Int8, forKey key: Key) throws {
encoder[key] = Int32(value)
}
mutating func encode(_ value: Int16, forKey key: Key) throws {
encoder[key] = Int32(value)
}
mutating func encode(_ value: Int32, forKey key: Key) throws {
encoder[key] = value
}
mutating func encode(_ value: Int64, forKey key: Key) throws {
encoder[key] = _BSON64BitInteger(value)
}
mutating func encode(_ value: UInt, forKey key: Key) throws {
encoder[key] = try encoder.makePrimitive(UInt64(value))
}
mutating func encode(_ value: UInt8, forKey key: Key) throws {
encoder[key] = try encoder.makePrimitive(UInt64(value))
}
mutating func encode(_ value: UInt16, forKey key: Key) throws {
encoder[key] = try encoder.makePrimitive(UInt64(value))
}
mutating func encode(_ value: UInt32, forKey key: Key) throws {
encoder[key] = try encoder.makePrimitive(UInt64(value))
}
mutating func encode(_ value: UInt64, forKey key: Key) throws {
encoder[key] = try encoder.makePrimitive(value)
}
mutating func encode<T>(_ value: T, forKey key: Key) throws where T: Encodable {
switch value {
case let primitive as Primitive:
encoder[key] = primitive
default:
let nestedEncoder = encoder.nestedEncoder(forKey: key)
try value.encode(to: nestedEncoder)
}
}
mutating func nestedContainer<NestedKey>(keyedBy keyType: NestedKey.Type, forKey key: Key) -> KeyedEncodingContainer<NestedKey> where NestedKey: CodingKey {
let nestedEncoder = encoder.nestedEncoder(forKey: key)
return nestedEncoder.container(keyedBy: NestedKey.self)
}
mutating func nestedUnkeyedContainer(forKey key: Key) -> UnkeyedEncodingContainer {
let nestedEncoder = encoder.nestedEncoder(forKey: key)
return nestedEncoder.unkeyedContainer()
}
mutating func superEncoder() -> Encoder {
return encoder.nestedEncoder(forKey: BSONKey.super)
}
mutating func superEncoder(forKey key: Key) -> Encoder {
return encoder.nestedEncoder(forKey: key)
}
}
fileprivate struct _BSONUnkeyedEncodingContainer: UnkeyedEncodingContainer {
var count: Int {
return encoder.document.count
}
var encoder: _BSONEncoder
var codingPath: [CodingKey]
init(encoder: _BSONEncoder, codingPath: [CodingKey]) {
self.encoder = encoder
self.codingPath = codingPath
encoder.document = Document(isArray: true)
}
// MARK: UnkeyedEncodingContainerProtocol
mutating func encodeNil() throws {
encoder.document.append(BSON.Null())
}
mutating func encode(_ value: Bool) throws {
encoder.document.append(value)
}
mutating func encode(_ value: String) throws {
encoder.document.append(value)
}
mutating func encode(_ value: Double) throws {
encoder.document.append(value)
}
mutating func encode(_ value: Float) throws {
encoder.document.append(Double(value))
}
mutating func encode(_ value: Int) throws {
encoder.document.append(_BSON64BitInteger(value))
}
mutating func encode(_ value: Int8) throws {
encoder.document.append(Int32(value))
}
mutating func encode(_ value: Int16) throws {
encoder.document.append(Int32(value))
}
mutating func encode(_ value: Int32) throws {
encoder.document.append(value)
}
mutating func encode(_ value: Int64) throws {
encoder.document.append(_BSON64BitInteger(value))
}
mutating func encode(_ value: UInt) throws {
encoder.document.append(try encoder.makePrimitive(UInt64(value)))
}
mutating func encode(_ value: UInt8) throws {
encoder.document.append(try encoder.makePrimitive(UInt64(value)))
}
mutating func encode(_ value: UInt16) throws {
encoder.document.append(try encoder.makePrimitive(UInt64(value)))
}
mutating func encode(_ value: UInt32) throws {
encoder.document.append(try encoder.makePrimitive(UInt64(value)))
}
mutating func encode(_ value: UInt64) throws {
encoder.document.append(try encoder.makePrimitive(value))
}
mutating func encode<T>(_ value: T) throws where T: Encodable {
switch value {
case let primitive as Primitive:
encoder.document.append(primitive)
default:
let nestedEncoder = makeNestedEncoder()
try value.encode(to: nestedEncoder)
}
}
func makeNestedEncoder() -> _BSONEncoder {
let index = encoder.document.count
return encoder.nestedEncoder(forKey: BSONKey(stringValue: "\(index)", intValue: index))
}
mutating func nestedContainer<NestedKey>(keyedBy keyType: NestedKey.Type) -> KeyedEncodingContainer<NestedKey> where NestedKey: CodingKey {
return makeNestedEncoder().container(keyedBy: NestedKey.self)
}
mutating func nestedUnkeyedContainer() -> UnkeyedEncodingContainer {
return makeNestedEncoder().unkeyedContainer()
}
mutating func superEncoder() -> Encoder {
return encoder
}
}
fileprivate struct _BSONSingleValueEncodingContainer: SingleValueEncodingContainer, AnySingleValueBSONEncodingContainer {
var codingPath: [CodingKey]
var encoder: _BSONEncoder
init(encoder: _BSONEncoder, codingPath: [CodingKey]) {
self.encoder = encoder
self.codingPath = codingPath
}
mutating func encodeNil() throws {
encoder.primitive = nil
}
mutating func encode(_ value: Bool) throws {
encoder.primitive = value
}
mutating func encode(_ value: String) throws {
encoder.primitive = value
}
mutating func encode(_ value: Double) throws {
encoder.primitive = value
}
mutating func encode(_ value: Float) throws {
encoder.primitive = Double(value)
}
mutating func encode(_ value: Int) throws {
encoder.primitive = _BSON64BitInteger(value)
}
mutating func encode(_ value: Int8) throws {
encoder.primitive = Int32(value)
}
mutating func encode(_ value: Int16) throws {
encoder.primitive = Int32(value)
}
mutating func encode(_ value: Int32) throws {
encoder.primitive = value
}
mutating func encode(_ value: Int64) throws {
encoder.primitive = _BSON64BitInteger(value)
}
mutating func encode(_ value: UInt) throws {
encoder.primitive = try encoder.makePrimitive(UInt64(value))
}
mutating func encode(_ value: UInt8) throws {
encoder.primitive = try encoder.makePrimitive(UInt64(value))
}
mutating func encode(_ value: UInt16) throws {
encoder.primitive = try encoder.makePrimitive(UInt64(value))
}
mutating func encode(_ value: UInt32) throws {
encoder.primitive = try encoder.makePrimitive(UInt64(value))
}
mutating func encode(_ value: UInt64) throws {
encoder.primitive = try encoder.makePrimitive(value)
}
mutating func encode(primitive: Primitive) throws {
encoder.primitive = primitive
}
mutating func encode<T>(_ value: T) throws where T: Encodable {
switch value {
case let primitive as Primitive:
encoder.primitive = primitive
default:
try value.encode(to: encoder)
}
}
}
| mit | 9a702e641a9716ae055fd7dc86570f25 | 28.319588 | 213 | 0.63692 | 4.751086 | false | false | false | false |
Jan0707/SwiftColorArt | SwiftColorArt/Classes/SwiftColorArt.swift | 1 | 15415 | import Foundation
import UIKit
import CoreGraphics
/// SWColorArt
///
/// A class to analyze an UIImage and get it's basic color usage
///
public class SWColorArt {
var minimunColorCount: Int
var image: UIImage
public var backgroundColor: UIColor?
public var primaryColor: UIColor?
public var secondaryColor: UIColor?
public var detailColor: UIColor?
var border: Border
let analyzedBackgroundColor: String = "analyzedBackgroundColor"
let analyzedPrimaryColor: String = "analyzedPrimaryColor"
let analyzedSecondaryColor: String = "analyzedSecondaryColor"
let analyzedDetailColor: String = "analyzedDetailColor"
public convenience init(inputImage: UIImage) {
let sampleSize: CGSize = CGSize(width: 128, height: 128)
self.init(inputImage: inputImage, imageSampleSize: sampleSize, minimunColorCount: 0)
}
public init(inputImage: UIImage, imageSampleSize: CGSize, minimunColorCount: Int) {
self.minimunColorCount = minimunColorCount
self.image = SWColorArt.resizeImage(inputImage, targetSize: imageSampleSize)
let rect = CGRect(x: 0, y: 0, width: self.image.size.width, height: self.image.size.height)
border = Border(rect: rect, width: 3, top: true, right: true, bottom: true, left: true)
self.processImage()
}
private func processImage() {
let storage = Storage()
if storage.doesCacheExistForImage(self.image) {
let storedColors = storage.loadColorsForImage(self.image)
self.backgroundColor = storedColors.backgroundColor
self.primaryColor = storedColors.primaryColor
self.secondaryColor = storedColors.secondaryColor
self.detailColor = storedColors.detailColor
} else {
var colors: [String: UIColor] = self.analyzeImage(self.image)
self.backgroundColor = colors[self.analyzedBackgroundColor]!
self.primaryColor = colors[self.analyzedPrimaryColor]!
self.secondaryColor = colors[self.analyzedSecondaryColor]!
self.detailColor = colors[self.analyzedDetailColor]!
let storedColors = Colors(backgroundColor: self.backgroundColor!, primaryColor: self.primaryColor!, secondaryColor: self.secondaryColor!, detailColor: self.detailColor!)
storage.storeColorsForImage(storedColors, image: self.image)
}
}
private func analyzeImage(_ inputImage: UIImage) -> [String: UIColor] {
var imageColors: [CountedColor] = []
var backgroundColor: UIColor = self.findEdgeColorInImage(inputImage, colors: &imageColors);
var primaryColor: UnsafeMutablePointer<UIColor>? = nil
var secondaryColor: UnsafeMutablePointer<UIColor>? = nil
var detailColor: UnsafeMutablePointer<UIColor>? = nil
let darkBackground: Bool = backgroundColor.sca_isDarkColor()
self.findTextColors(imageColors, primaryColor: &primaryColor, secondaryColor: &secondaryColor, detailColor: &detailColor, backgroundColor: &backgroundColor)
var dict: Dictionary = Dictionary<String, UIColor>()
dict[self.analyzedBackgroundColor] = backgroundColor
if primaryColor == nil {
if darkBackground {
dict[self.analyzedPrimaryColor] = UIColor.white()
} else {
dict[self.analyzedPrimaryColor] = UIColor.black()
}
} else {
dict[self.analyzedPrimaryColor] = primaryColor?.pointee
}
if secondaryColor == nil {
if darkBackground {
dict[self.analyzedSecondaryColor] = UIColor.white()
} else {
dict[self.analyzedSecondaryColor] = UIColor.black()
}
} else {
dict[self.analyzedSecondaryColor] = secondaryColor?.pointee
}
if detailColor == nil {
if darkBackground {
dict[self.analyzedDetailColor] = UIColor.white()
} else {
dict[self.analyzedDetailColor] = UIColor.black()
}
} else {
dict[self.analyzedDetailColor] = detailColor?.pointee
}
return dict
}
private func findEdgeColorInImage(_ inputImage: UIImage, colors: inout [CountedColor]) -> UIColor {
let imageRep: CGImage = image.cgImage!;
let width: Int = imageRep.width
let height: Int = imageRep.height
let context: CGContext = self.createBitmapContextFromImage(image.cgImage!)
let rect: CGRect = CGRect(x: CGFloat(0), y: CGFloat(0), width: CGFloat(width), height: CGFloat(height))
context.draw(in: rect, image: image.cgImage!);
let imageColors: CountedSet = CountedSet(capacity: Int(width * height))
let edgeColors: CountedSet = CountedSet(capacity: Int(height))
let data = context.data
let dataType = UnsafeMutablePointer<UInt8>(data)
for y in 0...height-1 {
for x in 0...width-1 {
let offset:Int = Int(4 * (x + y * width))
let alpha = CGFloat((dataType?[offset])!) / 255.0
let red = CGFloat((dataType?[offset+1])!) / 255.0
let green = CGFloat((dataType?[offset+2])!) / 255.0
let blue = CGFloat((dataType?[offset+3])!) / 255.0
let color:UIColor = UIColor(red: red, green: green, blue: blue, alpha: alpha)
let point = CGPoint(x: Int(x), y: Int(y))
if border.isPointInBorder(point) {
edgeColors.add(color)
} else {
imageColors.add(color)
}
}
}
let imageEnumerator: NSEnumerator = imageColors.objectEnumerator()
while let curColor = imageEnumerator.nextObject() as? UIColor
{
let colorCount: Int = edgeColors.count(for: curColor)
if colorCount >= minimunColorCount {
let container: CountedColor = CountedColor(color: curColor, count: colorCount)
colors.append(container)
}
}
let enumerator: NSEnumerator = edgeColors.objectEnumerator()
let sortedColors: NSMutableArray = []
while let curColor = enumerator.nextObject() as? UIColor
{
let colorCount: Int = edgeColors.count(for: curColor)
if colorCount <= minimunColorCount {
continue
}
let container: CountedColor = CountedColor(color: curColor, count: colorCount)
sortedColors.add(container)
}
// TODO: Use swifts sorting capabilities for this...
let finalColors: Array = sortedColors.sortedArray(using: #selector(CountedColor.compare(_:)))
var proposedEdgeColor: CountedColor?
if finalColors.count > 0 {
proposedEdgeColor = finalColors[0] as? CountedColor
if proposedEdgeColor!.color.sca_isBlackOrWhite() {
for i in 1...finalColors.count - 1 {
let nextProposedColor: CountedColor = finalColors[i] as! CountedColor
if Double( nextProposedColor.count / proposedEdgeColor!.count) > 0.4 { // make sure the second choice color is 40% as common as the first choice
if nextProposedColor.color.sca_isBlackOrWhite() {
proposedEdgeColor = nextProposedColor;
break;
}
} else {
// reached color threshold less than 40% of the original proposed edge color so bail
break;
}
}
}
}
if proposedEdgeColor == nil {
return UIColor.white()
}
return proposedEdgeColor!.color;
}
private func findTextColors(_ imageColors: [CountedColor], primaryColor: inout UnsafeMutablePointer<UIColor>?,
secondaryColor: inout UnsafeMutablePointer<UIColor>?, detailColor: inout UnsafeMutablePointer<UIColor>?,
backgroundColor: inout UIColor) {
var curColor: UIColor
let sortedColors: NSMutableArray = NSMutableArray(capacity: imageColors.count)
let findDarkTextColor: Bool = !backgroundColor.sca_isDarkColor()
if (imageColors.count > 0) {
for index in 0...imageColors.count-1 {
let countedColor: CountedColor = imageColors[index]
let curColor: UIColor = countedColor.color.sca_colorWithMinimumSaturation(0.15)
if curColor.sca_isDarkColor() == findDarkTextColor {
let colorCount: Int = countedColor.count;
//if ( colorCount <= 2 ) // prevent using random colors, threshold should be based on input image size
// continue;
let container: CountedColor = CountedColor(color: curColor, count: colorCount)
sortedColors.add(container)
}
}
}
var finalSortedColors: Array = sortedColors.sortedArray(using: #selector(CountedColor.compare(_:)))
if finalSortedColors.count > 0 {
for index in 0...finalSortedColors.count-1 {
let curContainer: CountedColor = finalSortedColors[index] as! CountedColor
curColor = curContainer.color;
if primaryColor == nil {
if curColor.sca_isContrastingColor(backgroundColor) {
primaryColor = UnsafeMutablePointer<UIColor>(calloc(1, sizeof(UIColor)))
primaryColor?.pointee = curColor;
}
} else if secondaryColor == nil {
if !(primaryColor?.pointee.sca_isDistinct(curColor))! || !curColor.sca_isContrastingColor(backgroundColor) {
continue;
}
secondaryColor = UnsafeMutablePointer<UIColor>(calloc(1, sizeof(UIColor)))
secondaryColor?.pointee = curColor;
} else if detailColor == nil {
if
!(secondaryColor?.pointee.sca_isDistinct(curColor))! ||
!(primaryColor?.pointee.sca_isDistinct(curColor))! ||
!curColor.sca_isContrastingColor(backgroundColor) {
continue;
}
detailColor = UnsafeMutablePointer<UIColor>(calloc(1, sizeof(UIColor)))
detailColor?.pointee = curColor;
break;
}
}
}
}
private func createBitmapContextFromImage(_ inImage: CGImage) -> CGContext {
let pixelsWide: Int = inImage.width
let pixelsHigh: Int = inImage.height
let bitmapBytesPerRow: Int = Int(pixelsWide * 4)
let bitmapByteCount: Int = Int(pixelsHigh) * bitmapBytesPerRow
let colorSpace: CGColorSpace = CGColorSpaceCreateDeviceRGB()
let bitmapData: UnsafeMutablePointer<Void> = malloc(bitmapByteCount)
let bitmapInfo = CGBitmapInfo(rawValue: CGImageAlphaInfo.premultipliedFirst.rawValue)
let context: CGContext = CGContext(data: bitmapData, width: pixelsWide, height: pixelsHigh, bitsPerComponent: 8, bytesPerRow: bitmapBytesPerRow, space: colorSpace, bitmapInfo: bitmapInfo.rawValue)!
return context
}
class func resizeImage(_ image: UIImage, targetSize: CGSize) -> UIImage {
let size: CGSize = image.size
let widthRatio: CGFloat = targetSize.width / image.size.width
let heightRatio: CGFloat = targetSize.height / image.size.height
var newSize: CGSize
if widthRatio > heightRatio {
newSize = CGSize(width: size.width * heightRatio, height: size.height * heightRatio)
} else {
newSize = CGSize(width: size.width * widthRatio, height: size.height * widthRatio)
}
let rect: CGRect = CGRect(x: 0, y: 0, width: newSize.width, height: newSize.height)
UIGraphicsBeginImageContextWithOptions(newSize, false, 1.0)
image.draw(in: rect)
let newImage: UIImage = UIGraphicsGetImageFromCurrentImageContext()!
UIGraphicsEndImageContext()
return newImage
}
}
//MARK: Additional classes and necessary extensions
/// CountedColor
///
/// A class to keep track of times a color appears
///
public class CountedColor: NSObject {
let color: UIColor
let count: Int
init (color: UIColor, count: Int) {
self.color = color;
self.count = count;
super.init()
}
func compare(_ object: CountedColor) -> ComparisonResult {
if self.count < object.count {
return ComparisonResult.orderedDescending
} else if self.count == object.count {
return ComparisonResult.orderedSame
}
return ComparisonResult.orderedAscending
}
}
extension UIColor {
public func sca_isDarkColor() -> Bool {
let convertedColor: UIColor = self
var r: CGFloat = CGFloat()
var g: CGFloat = CGFloat()
var b: CGFloat = CGFloat()
var a: CGFloat = CGFloat()
convertedColor.getRed(&r, green: &g, blue: &b, alpha: &a)
let lum: CGFloat = 0.2126 * r + 0.7152 * g + 0.0722 * b
if lum < 0.5 {
return true;
}
return false;
}
public func sca_isBlackOrWhite() -> Bool {
let tempColor: UIColor = self
var r: CGFloat = CGFloat()
var g: CGFloat = CGFloat()
var b: CGFloat = CGFloat()
var a: CGFloat = CGFloat()
tempColor.getRed(&r, green: &g, blue: &b, alpha: &a)
if r > 0.91 && g > 0.91 && b > 0.91 {
return true
}
if r < 0.09 && g < 0.09 && b < 0.09 {
return true
}
return false
}
public func sca_colorWithMinimumSaturation(_ minSaturation: CGFloat) -> UIColor {
let tempColor: UIColor = self
var hue: CGFloat = CGFloat(0.0)
var saturation: CGFloat = CGFloat(0.0)
var brightness: CGFloat = CGFloat(0.0);
var alpha: CGFloat = CGFloat(0.0);
tempColor.getHue(&hue, saturation: &saturation, brightness: &brightness, alpha: &alpha)
if saturation < minSaturation {
return UIColor(hue: hue, saturation: saturation, brightness: brightness, alpha: alpha)
}
return self;
}
public func sca_isContrastingColor(_ color: UIColor) -> Bool {
let backgroundColor: UIColor = self;
let foregroundColor: UIColor = color;
var br: CGFloat = CGFloat(0)
var bg: CGFloat = CGFloat(0)
var bb: CGFloat = CGFloat(0)
var ba: CGFloat = CGFloat(0)
var fr: CGFloat = CGFloat(0)
var fg: CGFloat = CGFloat(0)
var fb: CGFloat = CGFloat(0)
var fa: CGFloat = CGFloat(0)
backgroundColor.getRed(&br, green: &bg, blue: &bb, alpha: &ba)
foregroundColor.getRed(&fr, green: &fg, blue: &fb, alpha: &fa)
let bLum: CGFloat = CGFloat(0.2126 * br + 0.7152 * bg + 0.0722 * bb)
let fLum: CGFloat = CGFloat(0.2126 * fr + 0.7152 * fg + 0.0722 * fb)
var contrast: CGFloat = CGFloat(0);
if bLum > fLum {
contrast = (bLum + 0.05) / (fLum + 0.05);
} else {
contrast = (fLum + 0.05) / (bLum + 0.05);
}
return contrast > 1.8
}
public func sca_isDistinct(_ compareColor: UIColor) -> Bool {
let convertedColor: UIColor = self;
let convertedCompareColor: UIColor = compareColor;
var r: CGFloat = CGFloat(0)
var g: CGFloat = CGFloat(0)
var b: CGFloat = CGFloat(0)
var a: CGFloat = CGFloat(0)
var r1: CGFloat = CGFloat(0)
var g1: CGFloat = CGFloat(0)
var b1: CGFloat = CGFloat(0)
var a1: CGFloat = CGFloat(0)
convertedColor.getRed(&r, green: &g, blue: &b, alpha: &a)
convertedCompareColor.getRed(&r1, green: &g1, blue: &b1, alpha: &a1)
let threshold:CGFloat = CGFloat(0.15)
if fabs(r - r1) > threshold || fabs(g - g1) > threshold || fabs(b - b1) > threshold || fabs(a - a1) > threshold {
// check for grays, prevent multiple gray colors
if fabs(r - g) < 0.03 && fabs(r - b) < 0.03 {
if fabs(r1 - g1) < 0.03 && fabs(r1 - b1) < 0.03 {
return false
}
}
return true
}
return false
}
}
| mit | 7b184752f88b81b8c1528957195f79b8 | 31.452632 | 201 | 0.640415 | 4.344701 | false | false | false | false |
bhyzy/Ripped | Ripped/Ripped/UI/ExerciseResultsView.swift | 1 | 1618 | //
// ExerciseResultsView.swift
// Ripped
//
// Created by Bartlomiej Hyzy on 12/29/16.
// Copyright © 2016 Bartlomiej Hyzy. All rights reserved.
//
import UIKit
class ExerciseResultsView: UIView {
// MARK: - Properties
@IBOutlet private weak var titleLabel: UILabel!
@IBOutlet private weak var commentLabel: UILabel!
@IBOutlet private weak var resultsStackView: UIStackView!
// MARK: - Initialization
static func create() -> ExerciseResultsView {
return Bundle.main.loadNibNamed("ExerciseResultsView", owner: nil, options: nil)?.first as! ExerciseResultsView
}
// MARK: - Overriden
override func awakeFromNib() {
super.awakeFromNib()
clearContents()
}
// MARK: - Internal
func configure(withTitle title: String, results: [String], comment: String?) {
titleLabel.text = title
commentLabel.text = comment
results.map { resultLabel(withText: $0) }.forEach {
resultsStackView.addArrangedSubview($0)
}
}
// MARK: - Private
private func clearContents() {
titleLabel.text = nil
commentLabel.text = nil
resultsStackView.arrangedSubviews.forEach {
$0.removeFromSuperview()
}
}
private func resultLabel(withText text: String) -> UILabel {
let label = UILabel()
label.text = text
label.font = UIFont.systemFont(ofSize: 15)
label.textColor = #colorLiteral(red: 0.370555222, green: 0.3705646992, blue: 0.3705595732, alpha: 1)
return label
}
}
| mit | 65f06e77fbd982e58713728c613302d3 | 25.95 | 119 | 0.624613 | 4.454545 | false | false | false | false |
uny/SwiftDate | ExampleProject/ExampleProject/ViewController.swift | 6 | 707 | //
// ViewController.swift
// ExampleProject
//
// Created by Mark Norgren on 5/21/15.
//
//
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let date = "2015-06-17T09:10:00.000Z".toDate(format: DateFormat.ISO8601)
var d = NSDate()-1.hour
var abb = d.toRelativeString(abbreviated: true, maxUnits: 3)
println("data: \(abb)")
//
// let w = NSDate()-1.month
// print("Prev month: \(w)")
//
// var w2 = NSDate().add("month",value:-1)
// print(date)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
| mit | ec0b74f064e338f97c0eea3f82e7cabf | 18.638889 | 74 | 0.626591 | 3.319249 | false | false | false | false |
fgengine/quickly | Quickly/Keyboard/QKeyboard.swift | 1 | 5206 | //
// Quickly
//
public protocol IQKeyboardObserver {
func willShowKeyboard(_ keyboard: QKeyboard, animationInfo: QKeyboardAnimationInfo)
func didShowKeyboard(_ keyboard: QKeyboard, animationInfo: QKeyboardAnimationInfo)
func willHideKeyboard(_ keyboard: QKeyboard, animationInfo: QKeyboardAnimationInfo)
func didHideKeyboard(_ keyboard: QKeyboard, animationInfo: QKeyboardAnimationInfo)
}
public final class QKeyboardAnimationInfo {
public let beginFrame: CGRect
public let endFrame: CGRect
public let duration: TimeInterval
public let curve: UIView.AnimationCurve
public let local: Bool
public init?(_ userInfo: [ AnyHashable: Any ]) {
guard
let beginFrameValue = userInfo[UIResponder.keyboardFrameBeginUserInfoKey] as? NSValue,
let endFrameValue = userInfo[UIResponder.keyboardFrameEndUserInfoKey] as? NSValue,
let durationValue = userInfo[UIResponder.keyboardAnimationDurationUserInfoKey] as? NSNumber,
let curveValue = userInfo[UIResponder.keyboardAnimationCurveUserInfoKey] as? NSNumber,
let localValue = userInfo[UIResponder.keyboardIsLocalUserInfoKey] as? NSNumber
else { return nil }
self.beginFrame = beginFrameValue.cgRectValue
self.endFrame = endFrameValue.cgRectValue
self.duration = TimeInterval(durationValue.doubleValue)
self.curve = UIView.AnimationCurve(rawValue: curveValue.intValue) ?? .easeInOut
self.local = localValue.boolValue
}
public func animationOptions(_ base: UIView.AnimationOptions) -> UIView.AnimationOptions {
var options: UIView.AnimationOptions = base
switch self.curve {
case .linear: options.insert(.curveLinear)
case .easeIn: options.insert(.curveEaseIn)
case .easeOut: options.insert(.curveEaseOut)
default: options.insert(.curveEaseInOut)
}
return options
}
}
public final class QKeyboard {
private var _observer: QObserver< IQKeyboardObserver >
public init() {
self._observer = QObserver< IQKeyboardObserver >()
self._start()
}
deinit {
self._stop()
}
public func add(observer: IQKeyboardObserver, priority: UInt) {
self._observer.add(observer, priority: priority)
}
public func remove(observer: IQKeyboardObserver) {
self._observer.remove(observer)
}
private func _start() {
NotificationCenter.default.addObserver(self, selector: #selector(self._willShow(_:)), name: UIResponder.keyboardWillShowNotification, object: nil)
NotificationCenter.default.addObserver(self, selector: #selector(self._didShow(_:)), name: UIResponder.keyboardDidShowNotification, object: nil)
NotificationCenter.default.addObserver(self, selector: #selector(self._willHide(_:)), name: UIResponder.keyboardWillHideNotification, object: nil)
NotificationCenter.default.addObserver(self, selector: #selector(self._didHide(_:)), name: UIResponder.keyboardDidHideNotification, object: nil)
}
private func _stop() {
NotificationCenter.default.removeObserver(self, name: UIResponder.keyboardWillShowNotification, object: nil)
NotificationCenter.default.removeObserver(self, name: UIResponder.keyboardDidShowNotification, object: nil)
NotificationCenter.default.removeObserver(self, name: UIResponder.keyboardWillHideNotification, object: nil)
NotificationCenter.default.removeObserver(self, name: UIResponder.keyboardDidHideNotification, object: nil)
}
}
extension QKeyboard {
@objc
private func _willShow(_ notification: Notification) {
guard
let notificationInfo = notification.userInfo,
let animationInfo = QKeyboardAnimationInfo(notificationInfo)
else { return }
self._observer.notify({ (observer: IQKeyboardObserver) in
observer.willShowKeyboard(self, animationInfo: animationInfo)
})
}
@objc
private func _didShow(_ notification: Notification) {
guard
let notificationInfo = notification.userInfo,
let animationInfo = QKeyboardAnimationInfo(notificationInfo)
else { return }
self._observer.notify({ (observer: IQKeyboardObserver) in
observer.didShowKeyboard(self, animationInfo: animationInfo)
})
}
@objc
private func _willHide(_ notification: Notification) {
guard
let notificationInfo = notification.userInfo,
let animationInfo = QKeyboardAnimationInfo(notificationInfo)
else { return }
self._observer.notify({ (observer: IQKeyboardObserver) in
observer.willHideKeyboard(self, animationInfo: animationInfo)
})
}
@objc
private func _didHide(_ notification: Notification) {
guard
let notificationInfo = notification.userInfo,
let animationInfo = QKeyboardAnimationInfo(notificationInfo)
else { return }
self._observer.notify({ (observer: IQKeyboardObserver) in
observer.didHideKeyboard(self, animationInfo: animationInfo)
})
}
}
| mit | f622d0acf871343a8c93dcb8c7fc6a82 | 37.850746 | 154 | 0.695928 | 5.258586 | false | false | false | false |
audiokit/AudioKit | Tests/AudioKitTests/Node Tests/Effects Tests/Filter Tests/KorgLowPassFilterTests.swift | 2 | 1810 | // Copyright AudioKit. All Rights Reserved. Revision History at http://github.com/AudioKit/AudioKit/
import AudioKit
import XCTest
class KorgLowPassFilterTests: XCTestCase {
func testCutoffFrequency() {
let engine = AudioEngine()
let input = Oscillator()
engine.output = KorgLowPassFilter(input, cutoffFrequency: 500)
input.start()
let audio = engine.startTest(totalDuration: 1.0)
audio.append(engine.render(duration: 1.0))
testMD5(audio)
}
func testDefault() {
let engine = AudioEngine()
let input = Oscillator()
engine.output = KorgLowPassFilter(input)
input.start()
let audio = engine.startTest(totalDuration: 1.0)
audio.append(engine.render(duration: 1.0))
testMD5(audio)
}
func testParameters() {
let engine = AudioEngine()
let input = Oscillator()
engine.output = KorgLowPassFilter(input, cutoffFrequency: 500, resonance: 0.5, saturation: 1)
input.start()
let audio = engine.startTest(totalDuration: 1.0)
audio.append(engine.render(duration: 1.0))
testMD5(audio)
}
func testResonance() {
let engine = AudioEngine()
let input = Oscillator()
engine.output = KorgLowPassFilter(input, resonance: 0.5)
input.start()
let audio = engine.startTest(totalDuration: 1.0)
audio.append(engine.render(duration: 1.0))
testMD5(audio)
}
func testSaturation() {
let engine = AudioEngine()
let input = Oscillator()
engine.output = KorgLowPassFilter(input, saturation: 1)
input.start()
let audio = engine.startTest(totalDuration: 1.0)
audio.append(engine.render(duration: 1.0))
testMD5(audio)
}
}
| mit | 1dd44408b5c89345f84781a593d2e3a5 | 30.754386 | 101 | 0.629282 | 4.2891 | false | true | false | false |
RxSwiftCommunity/RxGRDB | Tests/RxGRDBTests/ValueObservationTests.swift | 1 | 13006 | import XCTest
import GRDB
import RxSwift
import RxGRDB
private struct Player: Codable, FetchableRecord, PersistableRecord {
var id: Int64
var name: String
var score: Int?
static func createTable(_ db: Database) throws {
try db.create(table: "player") { t in
t.autoIncrementedPrimaryKey("id")
t.column("name", .text).notNull()
t.column("score", .integer)
}
}
}
class ValueObservationTests : XCTestCase {
// MARK: - Default Scheduler
func testDefaultSchedulerChangesNotifications() throws {
func setUp<Writer: DatabaseWriter>(_ writer: Writer) throws -> Writer {
try writer.write(Player.createTable)
return writer
}
func test(writer: DatabaseWriter) throws {
let disposeBag = DisposeBag()
try withExtendedLifetime(disposeBag) {
let testSubject = ReplaySubject<Int>.createUnbounded()
ValueObservation
.tracking(Player.fetchCount)
.rx.observe(in: writer)
.subscribe(testSubject)
.disposed(by: disposeBag)
try writer.writeWithoutTransaction { db in
try Player(id: 1, name: "Arthur", score: 1000).insert(db)
try db.inTransaction {
try Player(id: 2, name: "Barbara", score: 750).insert(db)
try Player(id: 3, name: "Craig", score: 500).insert(db)
return .commit
}
}
let expectedElements = [0, 1, 3]
if writer is DatabaseQueue {
let elements = try testSubject
.take(expectedElements.count)
.toBlocking(timeout: 1).toArray()
XCTAssertEqual(elements, expectedElements)
} else {
let elements = try testSubject
.take(until: { $0 == expectedElements.last }, behavior: .inclusive)
.toBlocking(timeout: 1).toArray()
assertValueObservationRecordingMatch(recorded: elements, expected: expectedElements)
}
}
}
try Test(test)
.run { try setUp(DatabaseQueue()) }
.runAtTemporaryDatabasePath { try setUp(DatabaseQueue(path: $0)) }
.runAtTemporaryDatabasePath { try setUp(DatabasePool(path: $0)) }
}
func testDefaultSchedulerFirstValueIsEmittedAsynchronously() throws {
func setUp<Writer: DatabaseWriter>(_ writer: Writer) throws -> Writer {
try writer.write(Player.createTable)
return writer
}
func test(writer: DatabaseWriter) throws {
let disposeBag = DisposeBag()
withExtendedLifetime(disposeBag) {
let expectation = self.expectation(description: "")
let semaphore = DispatchSemaphore(value: 0)
ValueObservation
.tracking(Player.fetchCount)
.rx.observe(in: writer)
.subscribe(onNext: { _ in
semaphore.wait()
expectation.fulfill()
})
.disposed(by: disposeBag)
semaphore.signal()
waitForExpectations(timeout: 1, handler: nil)
}
}
try Test(test)
.run { try setUp(DatabaseQueue()) }
.runAtTemporaryDatabasePath { try setUp(DatabaseQueue(path: $0)) }
.runAtTemporaryDatabasePath { try setUp(DatabasePool(path: $0)) }
}
func testDefaultSchedulerError() throws {
func test(writer: DatabaseWriter) throws {
let observable = ValueObservation
.tracking { try $0.execute(sql: "THIS IS NOT SQL") }
.rx.observe(in: writer)
let result = observable.toBlocking().materialize()
switch result {
case .completed:
XCTFail("Expected error")
case let .failed(elements: _, error: error):
XCTAssertNotNil(error as? DatabaseError)
}
}
try Test(test)
.run { try DatabaseQueue() }
.runAtTemporaryDatabasePath { try DatabaseQueue(path: $0) }
.runAtTemporaryDatabasePath { try DatabasePool(path: $0) }
}
// MARK: - Immediate Scheduler
func testImmediateSchedulerChangesNotifications() throws {
func setUp<Writer: DatabaseWriter>(_ writer: Writer) throws -> Writer {
try writer.write(Player.createTable)
return writer
}
func test(writer: DatabaseWriter) throws {
let disposeBag = DisposeBag()
try withExtendedLifetime(disposeBag) {
let testSubject = ReplaySubject<Int>.createUnbounded()
ValueObservation
.tracking(Player.fetchCount)
.rx.observe(in: writer, scheduling: .immediate)
.subscribe(testSubject)
.disposed(by: disposeBag)
try writer.writeWithoutTransaction { db in
try Player(id: 1, name: "Arthur", score: 1000).insert(db)
try db.inTransaction {
try Player(id: 2, name: "Barbara", score: 750).insert(db)
try Player(id: 3, name: "Craig", score: 500).insert(db)
return .commit
}
}
let expectedElements = [0, 1, 3]
if writer is DatabaseQueue {
let elements = try testSubject
.take(expectedElements.count)
.toBlocking(timeout: 1).toArray()
XCTAssertEqual(elements, expectedElements)
} else {
let elements = try testSubject
.take(until: { $0 == expectedElements.last }, behavior: .inclusive)
.toBlocking(timeout: 1).toArray()
assertValueObservationRecordingMatch(recorded: elements, expected: expectedElements)
}
}
}
try Test(test)
.run { try setUp(DatabaseQueue()) }
.runAtTemporaryDatabasePath { try setUp(DatabaseQueue(path: $0)) }
.runAtTemporaryDatabasePath { try setUp(DatabasePool(path: $0)) }
}
func testImmediateSchedulerEmitsFirstValueSynchronously() throws {
func setUp<Writer: DatabaseWriter>(_ writer: Writer) throws -> Writer {
try writer.write(Player.createTable)
return writer
}
func test(writer: DatabaseWriter) throws {
let disposeBag = DisposeBag()
withExtendedLifetime(disposeBag) {
let semaphore = DispatchSemaphore(value: 0)
ValueObservation
.tracking(Player.fetchCount)
.rx.observe(in: writer, scheduling: .immediate)
.subscribe(onNext: { _ in
semaphore.signal()
})
.disposed(by: disposeBag)
semaphore.wait()
}
}
try Test(test)
.run { try setUp(DatabaseQueue()) }
.runAtTemporaryDatabasePath { try setUp(DatabaseQueue(path: $0)) }
.runAtTemporaryDatabasePath { try setUp(DatabasePool(path: $0)) }
}
func testImmediateSchedulerError() throws {
func test(writer: DatabaseWriter) throws {
let observable = ValueObservation
.tracking { try $0.execute(sql: "THIS IS NOT SQL") }
.rx.observe(in: writer, scheduling: .immediate)
let result = observable.toBlocking().materialize()
switch result {
case .completed:
XCTFail("Expected error")
case let .failed(elements: _, error: error):
XCTAssertNotNil(error as? DatabaseError)
}
}
try Test(test)
.run { try DatabaseQueue() }
.runAtTemporaryDatabasePath { try DatabaseQueue(path: $0) }
.runAtTemporaryDatabasePath { try DatabasePool(path: $0) }
}
func testIssue780() throws {
func test(dbPool: DatabasePool) throws {
struct Entity: Codable, FetchableRecord, PersistableRecord, Equatable {
var id: Int64
var name: String
}
try dbPool.write { db in
try db.create(table: "entity") { t in
t.autoIncrementedPrimaryKey("id")
t.column("name", .text)
}
}
let observation = ValueObservation.tracking(Entity.fetchAll)
let entities = try dbPool.rx
.write { db in try Entity(id: 1, name: "foo").insert(db) }
.asCompletable()
.andThen(observation.rx.observe(in: dbPool, scheduling: .immediate))
.take(1)
.toBlocking(timeout: 1)
.single()
XCTAssertEqual(entities, [Entity(id: 1, name: "foo")])
}
try Test(test).runAtTemporaryDatabasePath { try DatabasePool(path: $0) }
}
// MARK: - Utils
/// This test checks the fundamental promise of ValueObservation by
/// comparing recorded values with expected values.
///
/// Recorded values match the expected values if and only if:
///
/// - The last recorded value is the last expected value
/// - Recorded values are in the same order as expected values
///
/// However, both missing and repeated values are allowed - with the only
/// exception of the last expected value which can not be missed.
///
/// For example, if the expected values are [0, 1], then the following
/// recorded values match:
///
/// - `[0, 1]` (identical values)
/// - `[1]` (missing value but the last one)
/// - `[0, 0, 1, 1]` (repeated value)
///
/// However the following recorded values don't match, and fail the test:
///
/// - `[1, 0]` (wrong order)
/// - `[0]` (missing last value)
/// - `[]` (missing last value)
/// - `[0, 1, 2]` (unexpected value)
/// - `[1, 0, 1]` (unexpected value)
func assertValueObservationRecordingMatch<Value>(
recorded recordedValues: [Value],
expected expectedValues: [Value],
_ message: @autoclosure () -> String = "",
file: StaticString = #file,
line: UInt = #line)
where Value: Equatable
{
_assertValueObservationRecordingMatch(
recorded: recordedValues,
expected: expectedValues,
// Last value can't be missed
allowMissingLastValue: false,
message(), file: file, line: line)
}
private func _assertValueObservationRecordingMatch<R, E>(
recorded recordedValues: R,
expected expectedValues: E,
allowMissingLastValue: Bool,
_ message: @autoclosure () -> String = "",
file: StaticString = #file,
line: UInt = #line)
where
R: BidirectionalCollection,
E: BidirectionalCollection,
R.Element == E.Element,
R.Element: Equatable
{
guard let value = expectedValues.last else {
if !recordedValues.isEmpty {
XCTFail("unexpected recorded prefix \(Array(recordedValues)) - \(message())", file: file, line: line)
}
return
}
let recordedSuffix = recordedValues.reversed().prefix(while: { $0 == value })
let expectedSuffix = expectedValues.reversed().prefix(while: { $0 == value })
if !allowMissingLastValue {
// Both missing and repeated values are allowed in the recorded values.
// This is because of asynchronous DatabasePool observations.
if recordedSuffix.isEmpty {
XCTFail("missing expected value \(value) - \(message())", file: file, line: line)
}
}
let remainingRecordedValues = recordedValues.prefix(recordedValues.count - recordedSuffix.count)
let remainingExpectedValues = expectedValues.prefix(expectedValues.count - expectedSuffix.count)
_assertValueObservationRecordingMatch(
recorded: remainingRecordedValues,
expected: remainingExpectedValues,
// Other values can be missed
allowMissingLastValue: true,
message(), file: file, line: line)
}
}
| mit | 9385d03e9bb99e2abe9f793630cb1f8e | 38.895706 | 117 | 0.538982 | 5.352263 | false | true | false | false |
LucianoPAlmeida/SwifterSwift | Sources/Extensions/UIKit/UIAlertControllerExtensions.swift | 2 | 4069 | //
// UIAlertControllerExtensions.swift
// SwifterSwift
//
// Created by Omar Albeik on 8/23/16.
// Copyright © 2016 SwifterSwift
//
#if os(iOS)
import UIKit
import AudioToolbox
// MARK: - Methods
public extension UIAlertController {
/// SwifterSwift: Present alert view controller in the current view controller.
///
/// - Parameters:
/// - animated: set true to animate presentation of alert controller (default is true).
/// - vibrate: set true to vibrate the device while presenting the alert (default is false).
/// - completion: an optional completion handler to be called after presenting alert controller (default is nil).
public func show(animated: Bool = true, vibrate: Bool = false, completion: (() -> Void)? = nil) {
UIApplication.shared.keyWindow?.rootViewController?.present(self, animated: animated, completion: completion)
if vibrate {
AudioServicesPlayAlertSound(kSystemSoundID_Vibrate)
}
}
/// SwifterSwift: Add an action to Alert
///
/// - Parameters:
/// - title: action title
/// - style: action style (default is UIAlertActionStyle.default)
/// - isEnabled: isEnabled status for action (default is true)
/// - handler: optional action handler to be called when button is tapped (default is nil)
/// - Returns: action created by this method
@discardableResult public func addAction(title: String, style: UIAlertActionStyle = .default, isEnabled: Bool = true, handler: ((UIAlertAction) -> Void)? = nil) -> UIAlertAction {
let action = UIAlertAction(title: title, style: style, handler: handler)
action.isEnabled = isEnabled
addAction(action)
return action
}
/// SwifterSwift: Add a text field to Alert
///
/// - Parameters:
/// - text: text field text (default is nil)
/// - placeholder: text field placeholder text (default is nil)
/// - editingChangedTarget: an optional target for text field's editingChanged
/// - editingChangedSelector: an optional selector for text field's editingChanged
public func addTextField(text: String? = nil, placeholder: String? = nil, editingChangedTarget: Any?, editingChangedSelector: Selector?) {
addTextField { tf in
tf.text = text
tf.placeholder = placeholder
if let target = editingChangedTarget, let selector = editingChangedSelector {
tf.addTarget(target, action: selector, for: .editingChanged)
}
}
}
}
// MARK: - Initializers
public extension UIAlertController {
/// SwifterSwift: Create new alert view controller with default OK action.
///
/// - Parameters:
/// - title: alert controller's title.
/// - message: alert controller's message (default is nil).
/// - defaultActionButtonTitle: default action button title (default is "OK")
/// - tintColor: alert controller's tint color (default is nil)
public convenience init(title: String, message: String? = nil, defaultActionButtonTitle: String = "OK", tintColor: UIColor? = nil) {
self.init(title: title, message: message, preferredStyle: .alert)
let defaultAction = UIAlertAction(title: defaultActionButtonTitle, style: .default, handler: nil)
addAction(defaultAction)
if let color = tintColor {
view.tintColor = color
}
}
/// SwifterSwift: Create new error alert view controller from Error with default OK action.
///
/// - Parameters:
/// - title: alert controller's title (default is "Error").
/// - error: error to set alert controller's message to it's localizedDescription.
/// - defaultActionButtonTitle: default action button title (default is "OK")
/// - tintColor: alert controller's tint color (default is nil)
public convenience init(title: String = "Error", error: Error, defaultActionButtonTitle: String = "OK", preferredStyle: UIAlertControllerStyle = .alert, tintColor: UIColor? = nil) {
self.init(title: title, message: error.localizedDescription, preferredStyle: preferredStyle)
let defaultAction = UIAlertAction(title: defaultActionButtonTitle, style: .default, handler: nil)
addAction(defaultAction)
if let color = tintColor {
view.tintColor = color
}
}
}
#endif
| mit | e6dd63a86774caa50c3b01c04286d243 | 40.090909 | 182 | 0.719518 | 4.134146 | false | false | false | false |
FahimF/FloatLabelFields | FloatLabelFields/FloatLabelTextView.swift | 1 | 5869 | //
// FloatLabelTextView.swift
// FloatLabelFields
//
// Created by Fahim Farook on 28/11/14.
// Copyright (c) 2014 RookSoft Ltd. All rights reserved.
//
import UIKit
@IBDesignable class FloatLabelTextView: UITextView {
let animationDuration = 0.3
let placeholderTextColor = UIColor.lightGray.withAlphaComponent(0.65)
fileprivate var isIB = false
fileprivate var title = UILabel()
fileprivate var hintLabel = UILabel()
fileprivate var initialTopInset:CGFloat = 0
// MARK:- Properties
override var accessibilityLabel:String? {
get {
if text.isEmpty {
return title.text!
} else {
return text
}
}
set {
}
}
var titleFont:UIFont = UIFont.systemFont(ofSize: 12.0) {
didSet {
title.font = titleFont
}
}
@IBInspectable var hint:String = "" {
didSet {
title.text = hint
title.sizeToFit()
var r = title.frame
r.size.width = frame.size.width
title.frame = r
hintLabel.text = hint
hintLabel.sizeToFit()
}
}
@IBInspectable var hintYPadding:CGFloat = 0.0 {
didSet {
adjustTopTextInset()
}
}
@IBInspectable var titleYPadding:CGFloat = 0.0 {
didSet {
var r = title.frame
r.origin.y = titleYPadding
title.frame = r
}
}
@IBInspectable var titleTextColour:UIColor = UIColor.gray {
didSet {
if !isFirstResponder {
title.textColor = titleTextColour
}
}
}
@IBInspectable var titleActiveTextColour:UIColor = UIColor.cyan {
didSet {
if isFirstResponder {
title.textColor = titleActiveTextColour
}
}
}
// MARK:- Init
required init?(coder aDecoder:NSCoder) {
super.init(coder:aDecoder)
setup()
}
override init(frame:CGRect, textContainer:NSTextContainer?) {
super.init(frame:frame, textContainer:textContainer)
setup()
}
deinit {
if !isIB {
let nc = NotificationCenter.default
nc.removeObserver(self, name:NSNotification.Name.UITextViewTextDidChange, object:self)
nc.removeObserver(self, name:NSNotification.Name.UITextViewTextDidBeginEditing, object:self)
nc.removeObserver(self, name:NSNotification.Name.UITextViewTextDidEndEditing, object:self)
}
}
// MARK:- Overrides
override func prepareForInterfaceBuilder() {
isIB = true
setup()
}
override func layoutSubviews() {
super.layoutSubviews()
adjustTopTextInset()
hintLabel.alpha = text.isEmpty ? 1.0 : 0.0
let r = textRect()
hintLabel.frame = CGRect(x:r.origin.x, y:r.origin.y, width:hintLabel.frame.size.width, height:hintLabel.frame.size.height)
setTitlePositionForTextAlignment()
let isResp = isFirstResponder
if isResp && !text.isEmpty {
title.textColor = titleActiveTextColour
} else {
title.textColor = titleTextColour
}
// Should we show or hide the title label?
if text.isEmpty {
// Hide
hideTitle(isResp)
} else {
// Show
showTitle(isResp)
}
}
// MARK:- Private Methods
fileprivate func setup() {
initialTopInset = textContainerInset.top
textContainer.lineFragmentPadding = 0.0
titleActiveTextColour = tintColor
// Placeholder label
hintLabel.font = font
hintLabel.text = hint
hintLabel.numberOfLines = 0
hintLabel.lineBreakMode = NSLineBreakMode.byWordWrapping
hintLabel.backgroundColor = UIColor.clear
hintLabel.textColor = placeholderTextColor
insertSubview(hintLabel, at:0)
// Set up title label
title.alpha = 0.0
title.font = titleFont
title.textColor = titleTextColour
title.backgroundColor = backgroundColor
if !hint.isEmpty {
title.text = hint
title.sizeToFit()
}
self.addSubview(title)
// Observers
if !isIB {
let nc = NotificationCenter.default
nc.addObserver(self, selector:#selector(UIView.layoutSubviews), name:NSNotification.Name.UITextViewTextDidChange, object:self)
nc.addObserver(self, selector:#selector(UIView.layoutSubviews), name:NSNotification.Name.UITextViewTextDidBeginEditing, object:self)
nc.addObserver(self, selector:#selector(UIView.layoutSubviews), name:NSNotification.Name.UITextViewTextDidEndEditing, object:self)
}
}
fileprivate func adjustTopTextInset() {
var inset = textContainerInset
inset.top = initialTopInset + title.font.lineHeight + hintYPadding
textContainerInset = inset
}
fileprivate func textRect()->CGRect {
var r = UIEdgeInsetsInsetRect(bounds, contentInset)
r.origin.x += textContainer.lineFragmentPadding
r.origin.y += textContainerInset.top
return r.integral
}
fileprivate func setTitlePositionForTextAlignment() {
var titleLabelX = textRect().origin.x
var placeholderX = titleLabelX
if textAlignment == NSTextAlignment.center {
titleLabelX = (frame.size.width - title.frame.size.width) * 0.5
placeholderX = (frame.size.width - hintLabel.frame.size.width) * 0.5
} else if textAlignment == NSTextAlignment.right {
titleLabelX = frame.size.width - title.frame.size.width
placeholderX = frame.size.width - hintLabel.frame.size.width
}
var r = title.frame
r.origin.x = titleLabelX
title.frame = r
r = hintLabel.frame
r.origin.x = placeholderX
hintLabel.frame = r
}
fileprivate func showTitle(_ animated:Bool) {
let dur = animated ? animationDuration : 0
UIView.animate(withDuration: dur, delay:0, options: [UIViewAnimationOptions.beginFromCurrentState, UIViewAnimationOptions.curveEaseOut], animations:{
// Animation
self.title.alpha = 1.0
var r = self.title.frame
r.origin.y = self.titleYPadding + self.contentOffset.y
self.title.frame = r
}, completion:nil)
}
fileprivate func hideTitle(_ animated:Bool) {
let dur = animated ? animationDuration : 0
UIView.animate(withDuration: dur, delay:0, options: [UIViewAnimationOptions.beginFromCurrentState, UIViewAnimationOptions.curveEaseIn], animations:{
// Animation
self.title.alpha = 0.0
var r = self.title.frame
r.origin.y = self.title.font.lineHeight + self.hintYPadding
self.title.frame = r
}, completion:nil)
}
}
| mit | 3f09508a40b13cbf5a10f437ab63c308 | 26.553991 | 151 | 0.725848 | 3.629561 | false | false | false | false |
brocktonpoint/CawBoxKit | CawBoxKit/UIColor.swift | 1 | 2777 | /*
The MIT License (MIT)
Copyright (c) 2015 CawBox
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
*/
import UIKit
extension UIColor {
public convenience init (RGB red: Int, green: Int, blue: Int, alpha: Int) {
self.init (
red: CGFloat(red) / 255,
green: CGFloat(green) / 255,
blue: CGFloat(blue) / 255,
alpha: CGFloat(alpha) / 255
)
}
public convenience init (hex: String) {
var correctedString = hex.trimmingCharacters (in: NSCharacterSet.alphanumerics().inverted)
if correctedString.characters.count == 6 {
correctedString += "FF"
}
let scanner = NSScanner (string: correctedString)
var result: UInt32 = 0
scanner.scanHexInt32 (&result)
self.init (
red: CGFloat (result & 0xFF000000) / 255.0,
green: CGFloat (result & 0x00FF0000) / 255.0,
blue: CGFloat (result & 0x0000FF00) / 255.0,
alpha: CGFloat (result & 0x000000FF) / 255.0
)
}
}
public func ==(lhs: UIColor, rhs: UIColor) -> Bool {
var lhsRgba = (r: CGFloat (0), g: CGFloat (0), b: CGFloat (0), a: CGFloat (0))
lhs.getRed (
&lhsRgba.r,
green: &lhsRgba.g,
blue: &lhsRgba.b,
alpha: &lhsRgba.a
)
var rhsRgba = (r: CGFloat (0), g: CGFloat (0), b: CGFloat (0), a: CGFloat (0))
rhs.getRed (
&rhsRgba.r,
green: &rhsRgba.g,
blue: &rhsRgba.b,
alpha: &rhsRgba.a
)
return round (lhsRgba.r * 255) == round (rhsRgba.r * 255)
&& round (lhsRgba.g * 255) == round (rhsRgba.g * 255)
&& round (lhsRgba.b * 255) == round (rhsRgba.b * 255)
&& round (lhsRgba.a * 255) == round (rhsRgba.a * 255)
}
| mit | 2df985c64623661c6556d57c90025af7 | 34.602564 | 98 | 0.635218 | 3.830345 | false | false | false | false |
appsembler/edx-app-ios | Source/CourseHandoutsViewController.swift | 1 | 4499 | //
// CourseHandoutsViewController.swift
// edX
//
// Created by Ehmad Zubair Chughtai on 26/06/2015.
// Copyright (c) 2015 edX. All rights reserved.
//
import UIKit
public class CourseHandoutsViewController: OfflineSupportViewController, UIWebViewDelegate {
public typealias Environment = protocol<DataManagerProvider, NetworkManagerProvider, ReachabilityProvider, OEXAnalyticsProvider>
let courseID : String
let environment : Environment
let webView : UIWebView
let loadController : LoadStateViewController
let handouts : BackedStream<String> = BackedStream()
init(environment : Environment, courseID : String) {
self.environment = environment
self.courseID = courseID
self.webView = UIWebView()
self.loadController = LoadStateViewController()
super.init(env: environment)
addListener()
}
required public init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
override public func viewDidLoad() {
super.viewDidLoad()
loadController.setupInController(self, contentView: webView)
addSubviews()
setConstraints()
setStyles()
webView.delegate = self
loadHandouts()
}
public override func viewWillAppear(animated: Bool) {
super.viewWillAppear(animated)
environment.analytics.trackScreenWithName(OEXAnalyticsScreenHandouts, courseID: courseID, value: nil)
}
override func reloadViewData() {
loadHandouts()
}
private func addSubviews() {
view.addSubview(webView)
}
private func setConstraints() {
webView.snp_makeConstraints { (make) -> Void in
make.edges.equalTo(self.view)
}
}
private func setStyles() {
self.view.backgroundColor = OEXStyles.sharedStyles().standardBackgroundColor()
self.navigationItem.title = Strings.courseHandouts
}
private func streamForCourse(course : OEXCourse) -> Stream<String>? {
if let access = course.courseware_access where !access.has_access {
return Stream<String>(error: OEXCoursewareAccessError(coursewareAccess: access, displayInfo: course.start_display_info))
}
else {
let request = CourseInfoAPI.getHandoutsForCourseWithID(courseID, overrideURL: course.course_handouts)
let loader = self.environment.networkManager.streamForRequest(request, persistResponse: true)
return loader
}
}
private func loadHandouts() {
if !handouts.active {
loadController.state = .Initial
let courseStream = self.environment.dataManager.enrollmentManager.streamForCourseWithID(courseID)
let handoutStream = courseStream.transform {[weak self] enrollment in
return self?.streamForCourse(enrollment.course) ?? Stream<String>(error : NSError.oex_courseContentLoadError())
}
self.handouts.backWithStream(handoutStream)
}
}
private func addListener() {
handouts.listen(self, success: { [weak self] courseHandouts in
if let
displayHTML = OEXStyles.sharedStyles().styleHTMLContent(courseHandouts, stylesheet: "handouts-announcements"),
apiHostUrl = OEXConfig.sharedConfig().apiHostURL()
{
self?.webView.loadHTMLString(displayHTML, baseURL: apiHostUrl)
self?.loadController.state = .Loaded
}
else {
self?.loadController.state = LoadState.failed()
}
}, failure: {[weak self] error in
self?.loadController.state = LoadState.failed(error)
} )
}
override public func updateViewConstraints() {
loadController.insets = UIEdgeInsets(top: self.topLayoutGuide.length, left: 0, bottom: self.bottomLayoutGuide.length, right: 0)
super.updateViewConstraints()
}
//MARK: UIWebView delegate
public func webView(webView: UIWebView, shouldStartLoadWithRequest request: NSURLRequest, navigationType: UIWebViewNavigationType) -> Bool {
if (navigationType != UIWebViewNavigationType.Other) {
if let URL = request.URL {
UIApplication.sharedApplication().openURL(URL)
return false
}
}
return true
}
}
| apache-2.0 | ee7b1c66832e015722b72c6548d0b16d | 34.425197 | 144 | 0.644143 | 5.368735 | false | false | false | false |
TG908/iOS | TUM Campus App/LecturesTableViewController.swift | 1 | 3376 | //
// LecturesTableViewController.swift
// TUM Campus App
//
// Created by Mathias Quintero on 12/10/15.
// Copyright © 2015 LS1 TUM. All rights reserved.
//
import Sweeft
import UIKit
class LecturesTableViewController: UITableViewController, TumDataReceiver, DetailViewDelegate, DetailView {
var lectures = [(String,[DataElement])]()
var delegate: DetailViewDelegate?
var currentLecture: DataElement?
func dataManager() -> TumDataManager {
return delegate?.dataManager() ?? TumDataManager(user: nil)
}
func receiveData(_ data: [DataElement]) {
lectures.removeAll()
let semesters = data.reduce([String](), { (array, element) in
if let lecture = element as? Lecture {
if !array.contains(lecture.semester) {
var newArray = array
newArray.append(lecture.semester)
return newArray
}
}
return array
})
for semester in semesters {
let lecturesInSemester = data.filter { (element: DataElement) in
if let lecture = element as? Lecture {
return lecture.semester == semester
}
return false
}
lectures.append((semester,lecturesInSemester))
}
tableView.reloadData()
}
}
extension LecturesTableViewController {
override func viewDidLoad() {
super.viewDidLoad()
delegate?.dataManager().getLectures(self)
tableView.estimatedRowHeight = tableView.rowHeight
tableView.rowHeight = UITableViewAutomaticDimension
tableView.tableFooterView = UIView(frame: CGRect.zero)
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if let mvc = segue.destination as? LectureDetailsTableViewController {
mvc.lecture = currentLecture
mvc.delegate = self
}
}
}
extension LecturesTableViewController {
override func numberOfSections(in tableView: UITableView) -> Int {
return lectures.count
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return lectures[section].1.count
}
override func tableView(_ tableView: UITableView, titleForHeaderInSection section: Int) -> String? {
return lectures[section].0
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let item = lectures[indexPath.section].1[indexPath.row]
let cell = tableView.dequeueReusableCell(withIdentifier: item.getCellIdentifier()) as? CardTableViewCell ?? CardTableViewCell()
cell.setElement(item)
return cell
}
override func tableView(_ tableView: UITableView, willDisplayHeaderView view: UIView, forSection section: Int) {
if let header = view as? UITableViewHeaderFooterView {
header.contentView.backgroundColor = Constants.tumBlue
header.textLabel?.textColor = UIColor.white
}
}
override func tableView(_ tableView: UITableView, willSelectRowAt indexPath: IndexPath) -> IndexPath? {
currentLecture = lectures[indexPath.section].1[indexPath.row]
return indexPath
}
}
| gpl-3.0 | 730a144ac06eb2323b3d3e56471d2fb1 | 32.088235 | 135 | 0.637037 | 5.200308 | false | false | false | false |
lwg123/swift_LWGWB | LWGWB/LWGWB/classes/Home/HomeModel/StatusViewModel.swift | 1 | 2676 | //
// StatusViewModel.swift
// LWGWB
//
// Created by weiguang on 2017/4/11.
// Copyright © 2017年 weiguang. All rights reserved.
//
import UIKit
class StatusViewModel: NSObject {
// MARK: - 定义属性
var status : Status?
// MARK: - 对数据处理属性
var sourceText: String? // 处理来源
var createAtText: String? // 处理创建时间
var verfiedImage : UIImage? // 处理用户认证图标
var vipImage : UIImage? // 处理用户会员等级
var profileURL : URL? // 处理用户头像的地址
var picURLs : [URL] = [URL]() // 处理微博配图的数据
// MARK: - 自定义构造函数
init(status: Status) {
self.status = status
// 1.对微博来源处理
if let source = status.source, source != "" {
// 1.1.获取起始位置和截取的长度
let startIndex = (source as NSString).range(of: ">").location + 1
let length = (source as NSString).range(of: "</").location - startIndex
// 1.2.截取字符串
sourceText = (source as NSString).substring(with: NSRange(location: startIndex, length: length))
}
// 2. 处理时间
if let created_at = status.created_at {
//对时间处理
createAtText = Date.createDateString(created_at)
}
// 3.处理认证
let verified_type = status.user?.verified_type ?? -1
switch verified_type {
case 0:
verfiedImage = UIImage(named: "avatar_vip")
case 2, 3, 5:
verfiedImage = UIImage(named: "avatar_enterprise_vip")
case 220:
verfiedImage = UIImage(named: "avatar_grassroot")
default:
verfiedImage = nil
}
// 4. 处理会员等级
let mbrank = status.user?.mbrank ?? 0
if mbrank > 0 && mbrank <= 6 {
vipImage = UIImage(named: "common_icon_membership_level\(mbrank)")
}
// 5.处理头像url
let profileURLStr = status.user?.profile_image_url ?? ""
profileURL = URL(string: profileURLStr)
// 6. 处理微博图片
let picURLDicts = status.pic_urls!.count != 0 ? status.pic_urls : status.retweeted_status?.pic_urls
if let picURLDicts = picURLDicts {
for picURLDict in picURLDicts{
guard let picURLString = picURLDict["thumbnail_pic"] else {
continue
}
picURLs.append(URL(string: picURLString)!)
}
}
}
}
| mit | 43d24a03bf9f0a13d1d83fecbda0bfe3 | 29.848101 | 108 | 0.52975 | 4.151618 | false | false | false | false |
nguyenantinhbk77/practice-swift | Tutorials/Developing_iOS_Apps_With_Swift/lesson8/lesson8/Album.swift | 3 | 664 | import Foundation
/**
Album Model
*/
class Album{
var title: String?
var price: String?
var thumbnailImageURL: String?
var largeImageURL: String?
var itemURL: String?
var artistURL: String?
var collectionId: Int?
init(name: String!, price: String!, thumbnailImageURL: String!, largeImageURL: String!, itemURL: String!, artistURL: String!, collectionId:Int?) {
self.title = name
self.price = price
self.thumbnailImageURL = thumbnailImageURL
self.largeImageURL = largeImageURL
self.itemURL = itemURL
self.artistURL = artistURL
self.collectionId = collectionId
}
} | mit | 3ee72d452bafb3a5d3876cbfcd131190 | 25.6 | 150 | 0.658133 | 4.611111 | false | false | false | false |
wireapp/wire-ios-data-model | Source/ManagedObjectContext/NSPersistentStore+Metadata.swift | 1 | 5078 | //
// Wire
// Copyright (C) 2016 Wire Swiss GmbH
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see http://www.gnu.org/licenses/.
//
import Foundation
// MARK: - Public accessors
@objc(ZMPersistableMetadata) public protocol PersistableInMetadata: NSObjectProtocol {}
extension NSString: PersistableInMetadata {}
extension NSNumber: PersistableInMetadata {}
extension NSDate: PersistableInMetadata {}
extension NSData: PersistableInMetadata {}
public protocol SwiftPersistableInMetadata {}
extension String: SwiftPersistableInMetadata {}
extension Date: SwiftPersistableInMetadata {}
extension Data: SwiftPersistableInMetadata {}
extension Array: SwiftPersistableInMetadata {}
extension Int: SwiftPersistableInMetadata {}
// TODO: Swift 4
// extension Array where Element == SwiftPersistableInMetadata: SwiftPersistableInMetadata {}
extension NSManagedObjectContext {
@objc(setPersistentStoreMetadata:forKey:) public func setPersistentStoreMetadata(_ persistable: PersistableInMetadata?, key: String) {
self.setPersistentStoreMetadata(data: persistable, key: key)
}
public func setPersistentStoreMetadata(_ data: SwiftPersistableInMetadata?, key: String) {
self.setPersistentStoreMetadata(data: data, key: key)
}
public func setPersistentStoreMetadata<T: SwiftPersistableInMetadata>(array: [T], key: String) {
self.setPersistentStoreMetadata(data: array as NSArray, key: key)
}
}
// MARK: - Internal setters/getters
private let metadataKey = "ZMMetadataKey"
private let metadataKeysToRemove = "ZMMetadataKeysToRemove"
extension NSManagedObjectContext {
/// Non-persisted store metadata
@objc internal var nonCommittedMetadata: NSMutableDictionary {
return self.userInfo[metadataKey] as? NSMutableDictionary ?? NSMutableDictionary()
}
/// Non-persisted deleted metadata (need to keep around to know what to remove
/// from the store when persisting)
@objc internal var nonCommittedDeletedMetadataKeys: Set<String> {
return self.userInfo[metadataKeysToRemove] as? Set<String> ?? Set<String>()
}
/// Discard non commited store metadata
fileprivate func discardNonCommitedMetadata() {
self.userInfo[metadataKeysToRemove] = [String]()
self.userInfo[metadataKey] = [String: Any]()
}
/// Persist in-memory metadata to persistent store
@objc func makeMetadataPersistent() -> Bool {
guard nonCommittedMetadata.count > 0 || nonCommittedDeletedMetadataKeys.count > 0 else { return false }
let store = self.persistentStoreCoordinator!.persistentStores.first!
var storedMetadata = self.persistentStoreCoordinator!.metadata(for: store)
// remove keys
self.nonCommittedDeletedMetadataKeys.forEach { storedMetadata.removeValue(forKey: $0) }
// set keys
for (key, value) in self.nonCommittedMetadata {
guard let stringKey = key as? String else {
fatal("Wrong key in nonCommittedMetadata: \(key), value is \(value)")
}
storedMetadata[stringKey] = value
}
self.persistentStoreCoordinator?.setMetadata(storedMetadata, for: store)
self.discardNonCommitedMetadata()
return true
}
/// Remove key from list of keys that will be deleted next time
/// the metadata is persisted to disk
fileprivate func removeFromNonCommittedDeteledMetadataKeys(key: String) {
var deletedKeys = self.nonCommittedDeletedMetadataKeys
deletedKeys.remove(key)
self.userInfo[metadataKeysToRemove] = deletedKeys
}
/// Adds a key to the list of keys to be deleted next time the metadata is persisted to disk
fileprivate func addNonCommittedDeletedMetadataKey(key: String) {
var deletedKeys = self.nonCommittedDeletedMetadataKeys
deletedKeys.insert(key)
self.userInfo[metadataKeysToRemove] = deletedKeys
}
/// Set a value in the metadata for the store. The value won't be persisted until the metadata is persisted
fileprivate func setPersistentStoreMetadata(data: Any?, key: String) {
let metadata = self.nonCommittedMetadata
if let data = data {
self.removeFromNonCommittedDeteledMetadataKeys(key: key)
metadata.setObject(data, forKey: key as NSCopying)
} else {
self.addNonCommittedDeletedMetadataKey(key: key)
metadata.removeObject(forKey: key)
}
self.userInfo[metadataKey] = metadata
}
}
| gpl-3.0 | d4aec5633b48ac9e3efb60549c71d024 | 37.763359 | 138 | 0.724498 | 4.750234 | false | false | false | false |
benmatselby/sugarcrmcandybar | sugarcrmcandybarTests/SugarCRM/Record/RecordContactTest.swift | 1 | 1195 | //
// RecordContactTest.swift
// sugarcandybar
//
// Created by Ben Selby on 22/10/2016.
// Copyright © 2016 Ben Selby. All rights reserved.
//
import XCTest
@testable import sugarcrmcandybar
class RecordContactTest: XCTestCase {
let jsonStructure:JSON = [
"id": "007-001-002-006",
"first_name": "James",
"last_name": "Bond",
"description": "Shaken, not stirred",
"_module": "Contacts",
]
func testGetIdReturnsIdSetInDictionary() {
let record = RecordContact(rawRecord: self.jsonStructure)
XCTAssertEqual("007-001-002-006", record.getId())
}
func testGetNameReturnsNameSetInDictionary() {
let record = RecordContact(rawRecord: self.jsonStructure)
XCTAssertEqual("James Bond", record.getName())
}
func testGetDescriptionReturnsDescriptionSetInDictionary() {
let record = RecordContact(rawRecord: self.jsonStructure)
XCTAssertEqual("Shaken, not stirred", record.getDescription())
}
func testGetModuleReturnModuleSetInDictionary() {
let record = RecordContact(rawRecord: self.jsonStructure)
XCTAssertEqual("Contacts", record.getModule())
}
}
| mit | 283e32fa629a22f201d2ee27ffec1d85 | 28.121951 | 70 | 0.673367 | 4.033784 | false | true | false | false |
DanielCech/Vapor-Catalogue | Sources/App/Models/User.swift | 1 | 7218 | import Auth
import Foundation
import HTTP
import Vapor
import VaporJWT
struct Email: ValidationSuite, Validatable {
let value: String
public static func validate(input value: Email) throws {
try Vapor.Email.validate(input: value.value)
}
}
struct Username: ValidationSuite, Validatable {
let value: String
public static func validate(input value: Username) throws {
let evaluation = OnlyAlphanumeric.self
&& Count.min(2)
&& Count.max(50)
try evaluation.validate(input: value.value)
}
}
public final class User: Model {
typealias Secret = String
typealias Salt = String
struct Constants {
static let email = "email"
static let id = "id"
static let username = "username"
static let lastPasswordUpdate = "last_password_update"
static let salt = "salt"
static let secret = "secret"
static let token = "token" // Added by Dan
}
public var exists = false
public var id: Node?
var email: Email
var username: Username
fileprivate var secret: Secret
fileprivate var salt: Salt
fileprivate var lastPasswordUpdate: Date
convenience init(email: Valid<Email>, username: Valid<Username>, salt: Salt, secret: Secret) {
self.init(email: email,
username: username,
salt: salt,
secret: secret,
lastPasswordUpdate: Date())
}
init(email: Valid<Email>,
username: Valid<Username>,
salt: Salt,
secret: Secret,
lastPasswordUpdate: Date) {
self.email = email.value
self.lastPasswordUpdate = lastPasswordUpdate
self.username = username.value
self.salt = salt
self.secret = secret
}
// NodeInitializable
public init(node: Node, in context: Context) throws {
email = Email(value: try node.extract(Constants.email))
id = try node.extract(Constants.id)
username = Username(value: try node.extract(Constants.username))
lastPasswordUpdate = try node.extract(Constants.lastPasswordUpdate,
transform: Date.init(timeIntervalSince1970:))
salt = try node.extract(Constants.salt)
secret = try node.extract(Constants.secret)
}
static func find(byEmail email: Email) throws -> User? {
return try User.query().filter(Constants.email, email.value).first()
}
static func find(byToken token: String) throws -> User? {
return try User.query().filter(Constants.token, token).first()
}
static func getUserIDFromAuthorizationHeader(request: Request) throws -> Int {
guard var token = request.auth.header?.bearer?.string else {
throw Abort.custom(status: .badRequest, message: "Missing token")
}
// token = token.replacingOccurrences(of: "Bearer ", with: "")
print("Token: \(token)")
let jwt3 = try JWT(token: token)
let isValid = try jwt3.verifySignatureWith(HS256(key: "default-key"))
print("JWT: payload", jwt3.payload)
// if !isValid {
// throw Abort.custom(status: .unauthorized, message: "Invalid token")
// }
if let userId = jwt3.payload["userId"]?.int {
return userId
}
else {
throw Abort.custom(status: .unauthorized, message: "Invalid user ID")
}
}
}
// ResponseRepresentable
extension User {
public func makeResponse() throws -> Response {
return try JSON([
Constants.username: .string(username.value),
Constants.email: .string(email.value)])
.makeResponse()
}
}
// NodeRepresentable
extension User {
public func makeNode(context: Context) throws -> Node {
return try Node(node: [
Constants.email: email.value,
Constants.id: id,
Constants.username: username.value,
Constants.lastPasswordUpdate: lastPasswordUpdate.timeIntervalSince1970,
Constants.salt: salt,
Constants.secret: secret
])
}
}
// Preparation
extension User {
public static func prepare(_ database: Database) throws {
try database.create(entity) { users in
users.id()
users.double(Constants.lastPasswordUpdate)
users.string(Constants.email)
users.string(Constants.username)
users.string(Constants.salt)
users.string(Constants.secret)
}
}
public static func revert(_ database: Database) throws {
try database.delete(entity)
}
}
extension User: Auth.User {
public static func authenticate(credentials: Credentials) throws -> Auth.User {
switch credentials {
case let authenticatedUserCredentials as AuthenticatedUserCredentials:
return try authenticatedUserCredentials.user()
case let userCredentials as UserCredentials:
return try userCredentials.user()
default:
let type = type(of: credentials)
throw Abort.custom(status: .forbidden, message: "Unsupported credential type: \(type).")
}
}
public static func register(credentials: Credentials) throws -> Auth.User {
throw Abort.custom(status: .notImplemented, message: "")
}
}
extension User {
func update(hashedPassword: HashedPassword, now: Date) {
lastPasswordUpdate = now
salt = hashedPassword.salt
secret = hashedPassword.secret
}
}
extension User: Storable {
public var node: Node {
return [Constants.id: Node(id?.int ?? -1),
Constants.lastPasswordUpdate: Node(Int(lastPasswordUpdate.timeIntervalSince1970))]
}
}
extension Request {
func user() throws -> User {
guard let user = try auth.user() as? User else {
throw Abort.custom(status: .badRequest, message: "Invalid user type.")
}
return user
}
}
extension UserCredentials {
fileprivate func user() throws -> User {
guard
let user = try User.find(byEmail: email),
try hashPassword(using: user.salt).secret == user.secret else {
throw Abort.custom(status: .badRequest,
message: "User not found or incorrect password")
}
return user
}
}
extension AuthenticatedUserCredentials {
fileprivate func user() throws -> User {
guard let user = try User.find(id) else {
throw Abort.custom(status: .badRequest, message: "User not found")
}
// guard Int(user.lastPasswordUpdate.timeIntervalSince1970) <= Int(lastPasswordUpdate) else {
// throw Abort.custom(status: .forbidden, message: "Incorrect password")
// }
return user
}
}
extension User {
func albums() -> [Album] {
do {
return try children(nil, Album.self).all()
} catch {
print("Problem")
}
return []
}
}
| mit | 809661072b006bd5b6434c42d7230d25 | 29.714894 | 100 | 0.599889 | 4.638817 | false | false | false | false |
vermont42/Conjugar | Conjugar/QuizVC.swift | 1 | 6543 | //
// QuizVC.swift
// Conjugar
//
// Created by Joshua Adams on 6/18/17.
// Copyright © 2017 Josh Adams. All rights reserved.
//
import UIKit
class QuizVC: UIViewController, UITextFieldDelegate, QuizDelegate {
static let englishTitle = "Quiz"
var quizView: QuizUIV {
if let castedView = view as? QuizUIV {
return castedView
} else {
fatalError(fatalCastMessage(view: QuizUIV.self))
}
}
override func loadView() {
let quizView: QuizUIV
quizView = QuizUIV(frame: UIScreen.main.bounds)
quizView.startRestartButton.addTarget(self, action: #selector(startRestart), for: .touchUpInside)
quizView.quitButton.addTarget(self, action: #selector(quit), for: .touchUpInside)
navigationItem.titleView = UILabel.titleLabel(title: Localizations.Quiz.localizedTitle)
quizView.conjugationField.delegate = self
view = quizView
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
Current.quiz.delegate = self
switch Current.quiz.quizState {
case .notStarted, .finished:
quizView.hideInProgressUI()
quizView.startRestartButton.setTitle(Localizations.Quiz.start, for: .normal)
case .inProgress:
quizView.showInProgressUI()
quizView.startRestartButton.setTitle(Localizations.Quiz.restart, for: .normal)
let verb = Current.quiz.verb
quizView.verb.text = verb
let translationResult = Conjugator.shared.conjugate(infinitive: verb, tense: .translation, personNumber: .none)
switch translationResult {
case let .success(value):
quizView.translation.text = value
default:
fatalError("translation not found.")
}
quizView.tenseLabel.text = Current.quiz.tense.displayName
quizView.pronoun.text = Current.quiz.currentPersonNumber.pronoun
quizView.score.text = String(Current.quiz.score)
quizView.progress.text = String(Current.quiz.currentQuestionIndex + 1) + " / " + String(Current.quiz.questionCount)
}
quizView.startRestartButton.pulsate()
authenticate()
Current.analytics.recordVisitation(viewController: "\(QuizVC.self)")
}
private func authenticate() {
if !Current.gameCenter.isAuthenticated && Current.settings.userRejectedGameCenter {
if !Current.settings.didShowGameCenterDialog {
showGameCenterDialog()
} else {
Current.gameCenter.authenticate(onViewController: self, completion: nil)
}
}
}
private func showGameCenterDialog() {
Current.settings.didShowGameCenterDialog = true
let gameCenterController = UIAlertController(title: Localizations.Quiz.gameCenter, message: Localizations.Quiz.gameCenterMessage, preferredStyle: UIAlertController.Style.alert)
let noAction = UIAlertAction(title: Localizations.Quiz.no, style: UIAlertAction.Style.destructive) { _ in
SoundPlayer.play(.sadTrombone)
Current.settings.userRejectedGameCenter = true
}
gameCenterController.addAction(noAction)
let yesAction = UIAlertAction(title: Localizations.Quiz.yes, style: UIAlertAction.Style.default) { _ in
Current.gameCenter.authenticate(onViewController: self, completion: nil)
}
gameCenterController.addAction(yesAction)
present(gameCenterController, animated: true, completion: nil)
}
@objc func startRestart() {
SoundPlayer.play(.gun)
Current.quiz.start()
quizView.startRestartButton.setTitle(Localizations.Quiz.restart, for: .normal)
[quizView.lastLabel, quizView.correctLabel, quizView.last, quizView.correct].forEach {
$0.isHidden = true
}
quizView.showInProgressUI()
quizView.startRestartButton.pulsate()
quizView.conjugationField.becomeFirstResponder()
quizView.quitButton.isHidden = false
Current.analytics.recordQuizStart()
}
@objc func quit() {
Current.quiz.quit()
SoundPlayer.play(.sadTrombone)
resetUI()
Current.analytics.recordQuizQuit(currentQuestionIndex: Current.quiz.currentQuestionIndex, score: Current.quiz.score)
}
func scoreDidChange(newScore: Int) {
quizView.score.text = String(newScore)
}
func timeDidChange(newTime: Int) {
quizView.elapsed.text = newTime.timeString
}
func progressDidChange(current: Int, total: Int) {
quizView.progress.text = String(current + 1) + " / " + String(total)
}
func questionDidChange(verb: String, tense: Tense, personNumber: PersonNumber) {
quizView.verb.text = verb
let translationResult = Conjugator.shared.conjugate(infinitive: verb, tense: .translation, personNumber: .none)
switch translationResult {
case let .success(value):
quizView.translation.text = value
default:
fatalError()
}
quizView.tenseLabel.text = Localizations.Quiz.tense + ": " + tense.displayName
quizView.pronoun.text = personNumber.pronoun
quizView.conjugationField.becomeFirstResponder()
}
func quizDidFinish() {
SoundPlayer.playRandomApplause()
let resultsVC = ResultsVC()
navigationController?.pushViewController(resultsVC, animated: true)
resetUI()
Current.gameCenter.showLeaderboard()
Current.analytics.recordQuizCompletion(score: Current.quiz.score)
}
private func resetUI() {
quizView.hideInProgressUI()
quizView.conjugationField.text = ""
quizView.conjugationField.resignFirstResponder()
quizView.startRestartButton.setTitle(Localizations.Quiz.start, for: .normal)
quizView.quitButton.isHidden = true
}
func textFieldShouldReturn(_ textField: UITextField) -> Bool {
guard let text = quizView.conjugationField.text else { return false }
guard text != "" else { return false }
quizView.conjugationField.resignFirstResponder()
let (result, correctConjugation) = Current.quiz.process(proposedAnswer: text)
quizView.conjugationField.text = nil
switch result {
case .totalMatch:
SoundPlayer.play(.chime)
case .partialMatch:
SoundPlayer.play(.chirp)
case .noMatch:
SoundPlayer.play(.buzz)
}
if let correctConjugation = correctConjugation, Current.quiz.quizState == .inProgress {
[quizView.lastLabel, quizView.last, quizView.correctLabel, quizView.correct].forEach {
$0.isHidden = false
}
quizView.last.attributedText = text.coloredString(color: Colors.blue)
quizView.correct.attributedText = correctConjugation.conjugatedString
} else {
[quizView.lastLabel, quizView.last, quizView.correctLabel, quizView.correct].forEach {
$0.isHidden = true
}
}
return true
}
}
| agpl-3.0 | 74378d7fe54858bcece1945b971d4c45 | 35.960452 | 180 | 0.722868 | 4.161578 | false | false | false | false |
gringoireDM/LNZWeakCollection | LNZWeakCollection/Classes/WeakCollection.swift | 1 | 3475 | //
// LNZWeakCollection.swift
// LNZWeakCollection
//
// Created by Giuseppe Lanza on 22/03/17.
// Copyright © 2017 Giuseppe Lanza. All rights reserved.
//
import Foundation
public struct WeakCollection<T: AnyObject>: Sequence, IteratorProtocol {
private let queue = DispatchQueue(label: "read-write", qos: DispatchQoS.background, attributes: DispatchQueue.Attributes.concurrent)
private var weakReferecesContainers: [WeakContainer<T>] = [WeakContainer<T>]()
private var currentIndex: Int = 0
///The count of nonnil objects stored in the array
public var count: Int { return weakReferences.count }
///All the nonnil objects in the collection
public var weakReferences: [T] { return weakReferecesContainers.compactMap({ return $0.weakReference }) }
public init(){}
/**
Add an object to the collection. A weak reference to this object will
be stored in the collection.
- parameter object: The object to be stored in the collection. If this
object is deallocated it will be removed from the collection. This collection
does not have strong references to the object.
*/
public mutating func add(object: T) {
cleanup()
queue.sync(flags: .barrier) {
guard weakReferecesContainers.filter({ $0.weakReference === object}).count == 0 else { return }
let container = WeakContainer(weakReference: object)
weakReferecesContainers.append(container)
}
}
/**
Remove an object from the stack, if the object is actually in the collection.
Else this method will produce no results.
- parameter object: The object to be removed.
*/
@discardableResult public mutating func remove(object: T)-> T? {
cleanup()
return queue.sync(flags: .barrier) {
guard let index = weakReferecesContainers.firstIndex(where: { $0.weakReference === object }) else { return nil }
return weakReferecesContainers.remove(at: index).weakReference
}
}
/**
Execute a closure for each weak object still alive. This method will cause the cleanup
of the collection if needed.
- parameter closure: The closure to be executed. Just nonnil objects will be executed.
*/
public mutating func execute(_ closure: ((_ object: T) throws -> Void)) rethrows {
cleanup()
try queue.sync(flags: .barrier) {
try weakReferecesContainers.forEach { (weakContainer) in
guard let weakReference = weakContainer.weakReference else { return }
try closure(weakReference)
}
}
}
///Clean all the weak objects that are now nil
mutating func cleanup() {
queue.sync(flags: .barrier) {
while let index = weakReferecesContainers.firstIndex(where: { $0.weakReference == nil }) {
weakReferecesContainers.remove(at: index)
if index < currentIndex {
currentIndex -= 1
}
}
}
}
//MARK: IteratorProtocol and Sequence conformance
public mutating func next() -> T? {
cleanup()
return queue.sync(flags: .barrier) {
guard count > 0 && currentIndex < count else { return nil }
let object = weakReferences[currentIndex]
currentIndex += 1
return object
}
}
}
| mit | 8c878df9a98f52f7ab791cc24fb5e6d1 | 35.568421 | 136 | 0.627519 | 4.720109 | false | false | false | false |
ontouchstart/swift3-playground | ontouchstart/ontouchstart.playgroundbook/Contents/Chapters/Chapter1.playgroundchapter/Pages/Page3.playgroundpage/LiveView.swift | 1 | 355 | import UIKit
import PlaygroundSupport
let frame = CGRect(x: 0, y:0, width: 400, height: 300)
let label = UILabel(frame: frame)
label.text = "ontouchstart"
label.textColor = .blue()
label.textAlignment = .center
label.font = UIFont.boldSystemFont(ofSize: 60)
var view = UIView(frame: frame)
view.addSubview(label)
PlaygroundPage.current.liveView = view
| mit | 0a78889bfb7db80de312bb3881f47729 | 24.357143 | 54 | 0.757746 | 3.413462 | false | false | false | false |
mshhmzh/firefox-ios | Storage/ThirdParty/SwiftData.swift | 3 | 39822 | /* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this file,
* You can obtain one at http://mozilla.org/MPL/2.0/. */
/*
* This is a heavily modified version of SwiftData.swift by Ryan Fowler
* This has been enhanced to support custom files, correct binding, versioning,
* and a streaming results via Cursors. The API has also been changed to use NSError, Cursors, and
* to force callers to request a connection before executing commands. Database creation helpers, savepoint
* helpers, image support, and other features have been removed.
*/
// SwiftData.swift
//
// Copyright (c) 2014 Ryan Fowler
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
import Foundation
import UIKit
import Shared
import XCGLogger
private let DatabaseBusyTimeout: Int32 = 3 * 1000
private let log = Logger.syncLogger
/**
* Handle to a SQLite database.
* Each instance holds a single connection that is shared across all queries.
*/
public class SwiftData {
let filename: String
static var EnableWAL = true
static var EnableForeignKeys = true
/// Used to keep track of the corrupted databases we've logged.
static var corruptionLogsWritten = Set<String>()
/// Used for testing.
static var ReuseConnections = true
/// For thread-safe access to the shared connection.
private let sharedConnectionQueue: dispatch_queue_t
/// Shared connection to this database.
private var sharedConnection: ConcreteSQLiteDBConnection?
private var key: String? = nil
private var prevKey: String? = nil
init(filename: String, key: String? = nil, prevKey: String? = nil) {
self.filename = filename
self.sharedConnectionQueue = dispatch_queue_create("SwiftData queue: \(filename)", DISPATCH_QUEUE_SERIAL)
// Ensure that multi-thread mode is enabled by default.
// See https://www.sqlite.org/threadsafe.html
assert(sqlite3_threadsafe() == 2)
self.key = key
self.prevKey = prevKey
}
private func getSharedConnection() -> ConcreteSQLiteDBConnection? {
var connection: ConcreteSQLiteDBConnection?
dispatch_sync(sharedConnectionQueue) {
if self.sharedConnection == nil {
log.debug(">>> Creating shared SQLiteDBConnection for \(self.filename) on thread \(NSThread.currentThread()).")
self.sharedConnection = ConcreteSQLiteDBConnection(filename: self.filename, flags: SwiftData.Flags.ReadWriteCreate.toSQL(), key: self.key, prevKey: self.prevKey)
}
connection = self.sharedConnection
}
return connection
}
/**
* The real meat of all the execute methods. This is used internally to open and
* close a database connection and run a block of code inside it.
*/
func withConnection(flags: SwiftData.Flags, synchronous: Bool=true, cb: (db: SQLiteDBConnection) -> NSError?) -> NSError? {
let conn: ConcreteSQLiteDBConnection?
if SwiftData.ReuseConnections {
conn = getSharedConnection()
} else {
log.debug(">>> Creating non-shared SQLiteDBConnection for \(self.filename) on thread \(NSThread.currentThread()).")
conn = ConcreteSQLiteDBConnection(filename: filename, flags: flags.toSQL(), key: self.key, prevKey: self.prevKey)
}
guard let connection = conn else {
// Run the callback with a fake failed connection.
// Yeah, that means we have an error return value but we still run the callback.
let failed = FailedSQLiteDBConnection()
let queue = self.sharedConnectionQueue
let noConnection = NSError(domain: "mozilla", code: 0, userInfo: [NSLocalizedDescriptionKey: "Could not create a connection"])
if synchronous {
var error: NSError? = nil
dispatch_sync(queue) {
error = cb(db: failed)
}
return error ?? noConnection
}
dispatch_async(queue) {
cb(db: failed)
}
return noConnection
}
if synchronous {
var error: NSError? = nil
dispatch_sync(connection.queue) {
error = cb(db: connection)
}
return error
}
dispatch_async(connection.queue) {
cb(db: connection)
}
return nil
}
func transaction(transactionClosure: (db: SQLiteDBConnection) -> Bool) -> NSError? {
return self.transaction(synchronous: true, transactionClosure: transactionClosure)
}
/**
* Helper for opening a connection, starting a transaction, and then running a block of code inside it.
* The code block can return true if the transaction should be committed. False if we should roll back.
*/
func transaction(synchronous synchronous: Bool=true, transactionClosure: (db: SQLiteDBConnection) -> Bool) -> NSError? {
return withConnection(SwiftData.Flags.ReadWriteCreate, synchronous: synchronous) { db in
if let err = db.executeChange("BEGIN EXCLUSIVE") {
log.warning("BEGIN EXCLUSIVE failed.")
return err
}
if transactionClosure(db: db) {
log.verbose("Op in transaction succeeded. Committing.")
if let err = db.executeChange("COMMIT") {
log.error("COMMIT failed. Rolling back.")
db.executeChange("ROLLBACK")
return err
}
} else {
log.debug("Op in transaction failed. Rolling back.")
if let err = db.executeChange("ROLLBACK") {
return err
}
}
return nil
}
}
// Don't use this unless you know what you're doing. The deinitializer
// should be used to achieve refcounting semantics.
func forceClose() {
self.sharedConnection = nil
}
public enum Flags {
case ReadOnly
case ReadWrite
case ReadWriteCreate
private func toSQL() -> Int32 {
switch self {
case .ReadOnly:
return SQLITE_OPEN_READONLY
case .ReadWrite:
return SQLITE_OPEN_READWRITE
case .ReadWriteCreate:
return SQLITE_OPEN_READWRITE | SQLITE_OPEN_CREATE
}
}
}
}
/**
* Wrapper class for a SQLite statement.
* This class helps manage the statement lifecycle. By holding a reference to the SQL connection, we ensure
* the connection is never deinitialized while the statement is active. This class is responsible for
* finalizing the SQL statement once it goes out of scope.
*/
private class SQLiteDBStatement {
var pointer: COpaquePointer = nil
private let connection: ConcreteSQLiteDBConnection
init(connection: ConcreteSQLiteDBConnection, query: String, args: [AnyObject?]?) throws {
self.connection = connection
let status = sqlite3_prepare_v2(connection.sqliteDB, query, -1, &pointer, nil)
if status != SQLITE_OK {
throw connection.createErr("During: SQL Prepare \(query)", status: Int(status))
}
if let args = args,
let bindError = bind(args) {
throw bindError
}
}
/// Binds arguments to the statement.
private func bind(objects: [AnyObject?]) -> NSError? {
let count = Int(sqlite3_bind_parameter_count(pointer))
if (count < objects.count) {
return connection.createErr("During: Bind", status: 202)
}
if (count > objects.count) {
return connection.createErr("During: Bind", status: 201)
}
for (index, obj) in objects.enumerate() {
var status: Int32 = SQLITE_OK
// Doubles also pass obj as Int, so order is important here.
if obj is Double {
status = sqlite3_bind_double(pointer, Int32(index+1), obj as! Double)
} else if obj is Int {
status = sqlite3_bind_int(pointer, Int32(index+1), Int32(obj as! Int))
} else if obj is Bool {
status = sqlite3_bind_int(pointer, Int32(index+1), (obj as! Bool) ? 1 : 0)
} else if obj is String {
typealias CFunction = @convention(c) (UnsafeMutablePointer<()>) -> Void
let transient = unsafeBitCast(-1, CFunction.self)
status = sqlite3_bind_text(pointer, Int32(index+1), (obj as! String).cStringUsingEncoding(NSUTF8StringEncoding)!, -1, transient)
} else if obj is NSData {
status = sqlite3_bind_blob(pointer, Int32(index+1), (obj as! NSData).bytes, -1, nil)
} else if obj is NSDate {
let timestamp = (obj as! NSDate).timeIntervalSince1970
status = sqlite3_bind_double(pointer, Int32(index+1), timestamp)
} else if obj === nil {
status = sqlite3_bind_null(pointer, Int32(index+1))
}
if status != SQLITE_OK {
return connection.createErr("During: Bind", status: Int(status))
}
}
return nil
}
func close() {
if nil != self.pointer {
sqlite3_finalize(self.pointer)
self.pointer = nil
}
}
deinit {
if nil != self.pointer {
sqlite3_finalize(self.pointer)
}
}
}
protocol SQLiteDBConnection {
var lastInsertedRowID: Int { get }
var numberOfRowsModified: Int { get }
func executeChange(sqlStr: String) -> NSError?
func executeChange(sqlStr: String, withArgs args: [AnyObject?]?) -> NSError?
func executeQuery<T>(sqlStr: String, factory: ((SDRow) -> T)) -> Cursor<T>
func executeQuery<T>(sqlStr: String, factory: ((SDRow) -> T), withArgs args: [AnyObject?]?) -> Cursor<T>
func executeQueryUnsafe<T>(sqlStr: String, factory: ((SDRow) -> T), withArgs args: [AnyObject?]?) -> Cursor<T>
func interrupt()
func checkpoint()
func checkpoint(mode: Int32)
func vacuum() -> NSError?
}
// Represents a failure to open.
class FailedSQLiteDBConnection: SQLiteDBConnection {
private func fail(str: String) -> NSError {
return NSError(domain: "mozilla", code: 0, userInfo: [NSLocalizedDescriptionKey: str])
}
var lastInsertedRowID: Int { return 0 }
var numberOfRowsModified: Int { return 0 }
func executeChange(sqlStr: String) -> NSError? {
return self.fail("Non-open connection; can't execute change.")
}
func executeChange(sqlStr: String, withArgs args: [AnyObject?]?) -> NSError? {
return self.fail("Non-open connection; can't execute change.")
}
func executeQuery<T>(sqlStr: String, factory: ((SDRow) -> T)) -> Cursor<T> {
return self.executeQuery(sqlStr, factory: factory, withArgs: nil)
}
func executeQuery<T>(sqlStr: String, factory: ((SDRow) -> T), withArgs args: [AnyObject?]?) -> Cursor<T> {
return Cursor<T>(err: self.fail("Non-open connection; can't execute query."))
}
func executeQueryUnsafe<T>(sqlStr: String, factory: ((SDRow) -> T), withArgs args: [AnyObject?]?) -> Cursor<T> {
return Cursor<T>(err: self.fail("Non-open connection; can't execute query."))
}
func interrupt() {}
func checkpoint() {}
func checkpoint(mode: Int32) {}
func vacuum() -> NSError? {
return self.fail("Non-open connection; can't vacuum.")
}
}
public class ConcreteSQLiteDBConnection: SQLiteDBConnection {
private var sqliteDB: COpaquePointer = nil
private let filename: String
private let debug_enabled = false
private let queue: dispatch_queue_t
public var version: Int {
get {
return pragma("user_version", factory: IntFactory) ?? 0
}
set {
executeChange("PRAGMA user_version = \(newValue)")
}
}
private func setKey(key: String?) -> NSError? {
sqlite3_key(sqliteDB, key ?? "", Int32((key ?? "").characters.count))
let cursor = executeQuery("SELECT count(*) FROM sqlite_master;", factory: IntFactory, withArgs: nil)
if cursor.status != .Success {
return NSError(domain: "mozilla", code: 0, userInfo: [NSLocalizedDescriptionKey: "Invalid key"])
}
return nil
}
private func reKey(oldKey: String?, newKey: String?) -> NSError? {
sqlite3_key(sqliteDB, oldKey ?? "", Int32((oldKey ?? "").characters.count))
sqlite3_rekey(sqliteDB, newKey ?? "", Int32((newKey ?? "").characters.count))
// Check that the new key actually works
sqlite3_key(sqliteDB, newKey ?? "", Int32((newKey ?? "").characters.count))
let cursor = executeQuery("SELECT count(*) FROM sqlite_master;", factory: IntFactory, withArgs: nil)
if cursor.status != .Success {
return NSError(domain: "mozilla", code: 0, userInfo: [NSLocalizedDescriptionKey: "Rekey failed"])
}
return nil
}
func interrupt() {
log.debug("Interrupt")
sqlite3_interrupt(sqliteDB)
}
private func pragma<T: Equatable>(pragma: String, expected: T?, factory: SDRow -> T, message: String) throws {
let cursorResult = self.pragma(pragma, factory: factory)
if cursorResult != expected {
log.error("\(message): \(cursorResult), \(expected)")
throw NSError(domain: "mozilla", code: 0, userInfo: [NSLocalizedDescriptionKey: "PRAGMA didn't return expected output: \(message)."])
}
}
private func pragma<T>(pragma: String, factory: SDRow -> T) -> T? {
let cursor = executeQueryUnsafe("PRAGMA \(pragma)", factory: factory, withArgs: nil)
defer { cursor.close() }
return cursor[0]
}
private func prepareShared() {
if SwiftData.EnableForeignKeys {
pragma("foreign_keys=ON", factory: IntFactory)
}
// Retry queries before returning locked errors.
sqlite3_busy_timeout(self.sqliteDB, DatabaseBusyTimeout)
}
private func prepareEncrypted(flags: Int32, key: String?, prevKey: String? = nil) throws {
// Setting the key needs to be the first thing done with the database.
if let _ = setKey(key) {
if let err = closeCustomConnection(immediately: true) {
log.error("Couldn't close connection: \(err). Failing to open.")
throw err
}
if let err = openWithFlags(flags) {
throw err
}
if let err = reKey(prevKey, newKey: key) {
log.error("Unable to encrypt database")
throw err
}
}
if SwiftData.EnableWAL {
log.info("Enabling WAL mode.")
try pragma("journal_mode=WAL", expected: "wal",
factory: StringFactory, message: "WAL journal mode set")
}
self.prepareShared()
}
private func prepareCleartext() throws {
// If we just created the DB -- i.e., no tables have been created yet -- then
// we can set the page size right now and save a vacuum.
//
// For where these values come from, see Bug 1213623.
//
// Note that sqlcipher uses cipher_page_size instead, but we don't set that
// because it needs to be set from day one.
let desiredPageSize = 32 * 1024
pragma("page_size=\(desiredPageSize)", factory: IntFactory)
let currentPageSize = pragma("page_size", factory: IntFactory)
// This has to be done without WAL, so we always hop into rollback/delete journal mode.
if currentPageSize != desiredPageSize {
try pragma("journal_mode=DELETE", expected: "delete",
factory: StringFactory, message: "delete journal mode set")
try pragma("page_size=\(desiredPageSize)", expected: nil,
factory: IntFactory, message: "Page size set")
log.info("Vacuuming to alter database page size from \(currentPageSize) to \(desiredPageSize).")
if let err = self.vacuum() {
log.error("Vacuuming failed: \(err).")
} else {
log.debug("Vacuuming succeeded.")
}
}
if SwiftData.EnableWAL {
log.info("Enabling WAL mode.")
let desiredPagesPerJournal = 16
let desiredCheckpointSize = desiredPagesPerJournal * desiredPageSize
let desiredJournalSizeLimit = 3 * desiredCheckpointSize
/*
* With whole-module-optimization enabled in Xcode 7.2 and 7.2.1, the
* compiler seems to eagerly discard these queries if they're simply
* inlined, causing a crash in `pragma`.
*
* Hackily hold on to them.
*/
let journalModeQuery = "journal_mode=WAL"
let autoCheckpointQuery = "wal_autocheckpoint=\(desiredPagesPerJournal)"
let journalSizeQuery = "journal_size_limit=\(desiredJournalSizeLimit)"
try withExtendedLifetime(journalModeQuery, {
try pragma(journalModeQuery, expected: "wal",
factory: StringFactory, message: "WAL journal mode set")
})
try withExtendedLifetime(autoCheckpointQuery, {
try pragma(autoCheckpointQuery, expected: desiredPagesPerJournal,
factory: IntFactory, message: "WAL autocheckpoint set")
})
try withExtendedLifetime(journalSizeQuery, {
try pragma(journalSizeQuery, expected: desiredJournalSizeLimit,
factory: IntFactory, message: "WAL journal size limit set")
})
}
self.prepareShared()
}
init?(filename: String, flags: Int32, key: String? = nil, prevKey: String? = nil) {
log.debug("Opening connection to \(filename).")
self.filename = filename
self.queue = dispatch_queue_create("SQLite connection: \(filename)", DISPATCH_QUEUE_SERIAL)
if let failure = openWithFlags(flags) {
log.warning("Opening connection to \(filename) failed: \(failure).")
return nil
}
if key == nil && prevKey == nil {
do {
try self.prepareCleartext()
} catch {
return nil
}
} else {
do {
try self.prepareEncrypted(flags, key: key, prevKey: prevKey)
} catch {
return nil
}
}
}
deinit {
log.debug("deinit: closing connection on thread \(NSThread.currentThread()).")
dispatch_sync(self.queue) {
self.closeCustomConnection()
}
}
public var lastInsertedRowID: Int {
return Int(sqlite3_last_insert_rowid(sqliteDB))
}
public var numberOfRowsModified: Int {
return Int(sqlite3_changes(sqliteDB))
}
func checkpoint() {
self.checkpoint(SQLITE_CHECKPOINT_FULL)
}
/**
* Blindly attempts a WAL checkpoint on all attached databases.
*/
func checkpoint(mode: Int32) {
guard sqliteDB != nil else {
log.warning("Trying to checkpoint a nil DB!")
return
}
log.debug("Running WAL checkpoint on \(self.filename) on thread \(NSThread.currentThread()).")
sqlite3_wal_checkpoint_v2(sqliteDB, nil, mode, nil, nil)
log.debug("WAL checkpoint done on \(self.filename).")
}
func vacuum() -> NSError? {
return self.executeChange("VACUUM")
}
/// Creates an error from a sqlite status. Will print to the console if debug_enabled is set.
/// Do not call this unless you're going to return this error.
private func createErr(description: String, status: Int) -> NSError {
var msg = SDError.errorMessageFromCode(status)
if (debug_enabled) {
log.debug("SwiftData Error -> \(description)")
log.debug(" -> Code: \(status) - \(msg)")
}
if let errMsg = String.fromCString(sqlite3_errmsg(sqliteDB)) {
msg += " " + errMsg
if (debug_enabled) {
log.debug(" -> Details: \(errMsg)")
}
}
return NSError(domain: "org.mozilla", code: status, userInfo: [NSLocalizedDescriptionKey: msg])
}
/// Open the connection. This is called when the db is created. You should not call it yourself.
private func openWithFlags(flags: Int32) -> NSError? {
let status = sqlite3_open_v2(filename.cStringUsingEncoding(NSUTF8StringEncoding)!, &sqliteDB, flags, nil)
if status != SQLITE_OK {
return createErr("During: Opening Database with Flags", status: Int(status))
}
return nil
}
/// Closes a connection. This is called via deinit. Do not call this yourself.
private func closeCustomConnection(immediately immediately: Bool=false) -> NSError? {
log.debug("Closing custom connection for \(self.filename) on \(NSThread.currentThread()).")
// TODO: add a lock here?
let db = self.sqliteDB
self.sqliteDB = nil
// Don't bother trying to call sqlite3_close multiple times.
guard db != nil else {
log.warning("Connection was nil.")
return nil
}
let status = immediately ? sqlite3_close(db) : sqlite3_close_v2(db)
// Note that if we use sqlite3_close_v2, this will still return SQLITE_OK even if
// there are outstanding prepared statements
if status != SQLITE_OK {
log.error("Got status \(status) while attempting to close.")
return createErr("During: closing database with flags", status: Int(status))
}
log.debug("Closed \(self.filename).")
return nil
}
public func executeChange(sqlStr: String) -> NSError? {
return self.executeChange(sqlStr, withArgs: nil)
}
/// Executes a change on the database.
public func executeChange(sqlStr: String, withArgs args: [AnyObject?]?) -> NSError? {
var error: NSError?
let statement: SQLiteDBStatement?
do {
statement = try SQLiteDBStatement(connection: self, query: sqlStr, args: args)
} catch let error1 as NSError {
error = error1
statement = nil
log.error("SQL error: \(error1.localizedDescription) for SQL \(sqlStr).")
}
// Close, not reset -- this isn't going to be reused.
defer { statement?.close() }
if let error = error {
// Special case: Write additional info to the database log in the case of a database corruption.
if error.code == Int(SQLITE_CORRUPT) {
writeCorruptionInfoForDBNamed(filename, toLogger: Logger.corruptLogger)
}
log.error("SQL error: \(error.localizedDescription) for SQL \(sqlStr).")
return error
}
let status = sqlite3_step(statement!.pointer)
if status != SQLITE_DONE && status != SQLITE_OK {
error = createErr("During: SQL Step \(sqlStr)", status: Int(status))
}
return error
}
func executeQuery<T>(sqlStr: String, factory: ((SDRow) -> T)) -> Cursor<T> {
return self.executeQuery(sqlStr, factory: factory, withArgs: nil)
}
/// Queries the database.
/// Returns a cursor pre-filled with the complete result set.
func executeQuery<T>(sqlStr: String, factory: ((SDRow) -> T), withArgs args: [AnyObject?]?) -> Cursor<T> {
var error: NSError?
let statement: SQLiteDBStatement?
do {
statement = try SQLiteDBStatement(connection: self, query: sqlStr, args: args)
} catch let error1 as NSError {
error = error1
statement = nil
}
// Close, not reset -- this isn't going to be reused, and the FilledSQLiteCursor
// consumes everything.
defer { statement?.close() }
if let error = error {
// Special case: Write additional info to the database log in the case of a database corruption.
if error.code == Int(SQLITE_CORRUPT) {
writeCorruptionInfoForDBNamed(filename, toLogger: Logger.corruptLogger)
}
log.error("SQL error: \(error.localizedDescription).")
return Cursor<T>(err: error)
}
return FilledSQLiteCursor<T>(statement: statement!, factory: factory)
}
func writeCorruptionInfoForDBNamed(dbFilename: String, toLogger logger: XCGLogger) {
dispatch_sync(dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0)) {
guard !SwiftData.corruptionLogsWritten.contains(dbFilename) else { return }
logger.error("Corrupt DB detected! DB filename: \(dbFilename)")
let dbFileSize = ("file://\(dbFilename)".asURL)?.allocatedFileSize() ?? 0
logger.error("DB file size: \(dbFileSize) bytes")
logger.error("Integrity check:")
let messages = self.executeQueryUnsafe("PRAGMA integrity_check", factory: StringFactory, withArgs: nil)
defer { messages.close() }
if messages.status == CursorStatus.Success {
for message in messages {
logger.error(message)
}
logger.error("----")
} else {
logger.error("Couldn't run integrity check: \(messages.statusMessage).")
}
// Write call stack.
logger.error("Call stack: ")
for message in NSThread.callStackSymbols() {
logger.error(" >> \(message)")
}
logger.error("----")
// Write open file handles.
let openDescriptors = FSUtils.openFileDescriptors()
logger.error("Open file descriptors: ")
for (k, v) in openDescriptors {
logger.error(" \(k): \(v)")
}
logger.error("----")
SwiftData.corruptionLogsWritten.insert(dbFilename)
}
}
/**
* Queries the database.
* Returns a live cursor that holds the query statement and database connection.
* Instances of this class *must not* leak outside of the connection queue!
*/
func executeQueryUnsafe<T>(sqlStr: String, factory: ((SDRow) -> T), withArgs args: [AnyObject?]?) -> Cursor<T> {
var error: NSError?
let statement: SQLiteDBStatement?
do {
statement = try SQLiteDBStatement(connection: self, query: sqlStr, args: args)
} catch let error1 as NSError {
error = error1
statement = nil
}
if let error = error {
return Cursor(err: error)
}
return LiveSQLiteCursor(statement: statement!, factory: factory)
}
}
/// Helper for queries that return a single integer result.
func IntFactory(row: SDRow) -> Int {
return row[0] as! Int
}
/// Helper for queries that return a single String result.
func StringFactory(row: SDRow) -> String {
return row[0] as! String
}
/// Wrapper around a statement for getting data from a row. This provides accessors for subscript indexing
/// and a generator for iterating over columns.
class SDRow: SequenceType {
// The sqlite statement this row came from.
private let statement: SQLiteDBStatement
// The columns of this database. The indices of these are assumed to match the indices
// of the statement.
private let columnNames: [String]
private init(statement: SQLiteDBStatement, columns: [String]) {
self.statement = statement
self.columnNames = columns
}
// Return the value at this index in the row
private func getValue(index: Int) -> AnyObject? {
let i = Int32(index)
let type = sqlite3_column_type(statement.pointer, i)
var ret: AnyObject? = nil
switch type {
case SQLITE_NULL, SQLITE_INTEGER:
ret = NSNumber(longLong: sqlite3_column_int64(statement.pointer, i))
case SQLITE_TEXT:
let text = UnsafePointer<Int8>(sqlite3_column_text(statement.pointer, i))
ret = String.fromCString(text)
case SQLITE_BLOB:
let blob = sqlite3_column_blob(statement.pointer, i)
if blob != nil {
let size = sqlite3_column_bytes(statement.pointer, i)
ret = NSData(bytes: blob, length: Int(size))
}
case SQLITE_FLOAT:
ret = Double(sqlite3_column_double(statement.pointer, i))
default:
log.warning("SwiftData Warning -> Column: \(index) is of an unrecognized type, returning nil")
}
return ret
}
// Accessor getting column 'key' in the row
subscript(key: Int) -> AnyObject? {
return getValue(key)
}
// Accessor getting a named column in the row. This (currently) depends on
// the columns array passed into this Row to find the correct index.
subscript(key: String) -> AnyObject? {
get {
if let index = columnNames.indexOf(key) {
return getValue(index)
}
return nil
}
}
// Allow iterating through the row. This is currently broken.
func generate() -> AnyGenerator<Any> {
let nextIndex = 0
return AnyGenerator() {
// This crashes the compiler. Yay!
if (nextIndex < self.columnNames.count) {
return nil // self.getValue(nextIndex)
}
return nil
}
}
}
/// Helper for pretty printing SQL (and other custom) error codes.
private struct SDError {
private static func errorMessageFromCode(errorCode: Int) -> String {
switch errorCode {
case -1:
return "No error"
// SQLite error codes and descriptions as per: http://www.sqlite.org/c3ref/c_abort.html
case 0:
return "Successful result"
case 1:
return "SQL error or missing database"
case 2:
return "Internal logic error in SQLite"
case 3:
return "Access permission denied"
case 4:
return "Callback routine requested an abort"
case 5:
return "The database file is busy"
case 6:
return "A table in the database is locked"
case 7:
return "A malloc() failed"
case 8:
return "Attempt to write a readonly database"
case 9:
return "Operation terminated by sqlite3_interrupt()"
case 10:
return "Some kind of disk I/O error occurred"
case 11:
return "The database disk image is malformed"
case 12:
return "Unknown opcode in sqlite3_file_control()"
case 13:
return "Insertion failed because database is full"
case 14:
return "Unable to open the database file"
case 15:
return "Database lock protocol error"
case 16:
return "Database is empty"
case 17:
return "The database schema changed"
case 18:
return "String or BLOB exceeds size limit"
case 19:
return "Abort due to constraint violation"
case 20:
return "Data type mismatch"
case 21:
return "Library used incorrectly"
case 22:
return "Uses OS features not supported on host"
case 23:
return "Authorization denied"
case 24:
return "Auxiliary database format error"
case 25:
return "2nd parameter to sqlite3_bind out of range"
case 26:
return "File opened that is not a database file"
case 27:
return "Notifications from sqlite3_log()"
case 28:
return "Warnings from sqlite3_log()"
case 100:
return "sqlite3_step() has another row ready"
case 101:
return "sqlite3_step() has finished executing"
// Custom SwiftData errors
// Binding errors
case 201:
return "Not enough objects to bind provided"
case 202:
return "Too many objects to bind provided"
// Custom connection errors
case 301:
return "A custom connection is already open"
case 302:
return "Cannot open a custom connection inside a transaction"
case 303:
return "Cannot open a custom connection inside a savepoint"
case 304:
return "A custom connection is not currently open"
case 305:
return "Cannot close a custom connection inside a transaction"
case 306:
return "Cannot close a custom connection inside a savepoint"
// Index and table errors
case 401:
return "At least one column name must be provided"
case 402:
return "Error extracting index names from sqlite_master"
case 403:
return "Error extracting table names from sqlite_master"
// Transaction and savepoint errors
case 501:
return "Cannot begin a transaction within a savepoint"
case 502:
return "Cannot begin a transaction within another transaction"
// Unknown error
default:
return "Unknown error"
}
}
}
/// Provides access to the result set returned by a database query.
/// The entire result set is cached, so this does not retain a reference
/// to the statement or the database connection.
private class FilledSQLiteCursor<T>: ArrayCursor<T> {
private init(statement: SQLiteDBStatement, factory: (SDRow) -> T) {
var status = CursorStatus.Success
var statusMessage = ""
let data = FilledSQLiteCursor.getValues(statement, factory: factory, status: &status, statusMessage: &statusMessage)
super.init(data: data, status: status, statusMessage: statusMessage)
}
/// Return an array with the set of results and release the statement.
private class func getValues(statement: SQLiteDBStatement, factory: (SDRow) -> T, inout status: CursorStatus, inout statusMessage: String) -> [T] {
var rows = [T]()
var count = 0
status = CursorStatus.Success
statusMessage = "Success"
var columns = [String]()
let columnCount = sqlite3_column_count(statement.pointer)
for i in 0..<columnCount {
let columnName = String.fromCString(sqlite3_column_name(statement.pointer, i))!
columns.append(columnName)
}
while true {
let sqlStatus = sqlite3_step(statement.pointer)
if sqlStatus != SQLITE_ROW {
if sqlStatus != SQLITE_DONE {
// NOTE: By setting our status to failure here, we'll report our count as zero,
// regardless of how far we've read at this point.
status = CursorStatus.Failure
statusMessage = SDError.errorMessageFromCode(Int(sqlStatus))
}
break
}
count += 1
let row = SDRow(statement: statement, columns: columns)
let result = factory(row)
rows.append(result)
}
return rows
}
}
/// Wrapper around a statement to help with iterating through the results.
private class LiveSQLiteCursor<T>: Cursor<T> {
private var statement: SQLiteDBStatement!
// Function for generating objects of type T from a row.
private let factory: (SDRow) -> T
// Status of the previous fetch request.
private var sqlStatus: Int32 = 0
// Number of rows in the database
// XXX - When Cursor becomes an interface, this should be a normal property, but right now
// we can't override the Cursor getter for count with a stored property.
private var _count: Int = 0
override var count: Int {
get {
if status != .Success {
return 0
}
return _count
}
}
private var position: Int = -1 {
didSet {
// If we're already there, shortcut out.
if (oldValue == position) {
return
}
var stepStart = oldValue
// If we're currently somewhere in the list after this position
// we'll have to jump back to the start.
if (position < oldValue) {
sqlite3_reset(self.statement.pointer)
stepStart = -1
}
// Now step up through the list to the requested position
for _ in stepStart..<position {
sqlStatus = sqlite3_step(self.statement.pointer)
}
}
}
init(statement: SQLiteDBStatement, factory: (SDRow) -> T) {
self.factory = factory
self.statement = statement
// The only way I know to get a count. Walk through the entire statement to see how many rows there are.
var count = 0
self.sqlStatus = sqlite3_step(statement.pointer)
while self.sqlStatus != SQLITE_DONE {
count += 1
self.sqlStatus = sqlite3_step(statement.pointer)
}
sqlite3_reset(statement.pointer)
self._count = count
super.init(status: .Success, msg: "success")
}
// Helper for finding all the column names in this statement.
private lazy var columns: [String] = {
// This untangles all of the columns and values for this row when its created
let columnCount = sqlite3_column_count(self.statement.pointer)
var columns = [String]()
for i: Int32 in 0 ..< columnCount {
let columnName = String.fromCString(sqlite3_column_name(self.statement.pointer, i))!
columns.append(columnName)
}
return columns
}()
override subscript(index: Int) -> T? {
get {
if status != .Success {
return nil
}
self.position = index
if self.sqlStatus != SQLITE_ROW {
return nil
}
let row = SDRow(statement: statement, columns: self.columns)
return self.factory(row)
}
}
override func close() {
statement = nil
super.close()
}
}
| mpl-2.0 | 7ae8195d4ccb537ff670f5690e31d703 | 36.286517 | 177 | 0.599794 | 4.768531 | false | false | false | false |
ThumbWorks/i-meditated | Pods/RxTests/RxTests/Event+Equatable.swift | 4 | 1192 | //
// Event+Equatable.swift
// Rx
//
// Created by Krunoslav Zaher on 12/19/15.
// Copyright © 2015 Krunoslav Zaher. All rights reserved.
//
import Foundation
import RxSwift
/**
Compares two events. They are equal if they are both the same member of `Event` enumeration.
In case `Error` events are being compared, they are equal in case their `NSError` representations are equal (domain and code)
and their string representations are equal.
*/
public func == <Element: Equatable>(lhs: Event<Element>, rhs: Event<Element>) -> Bool {
switch (lhs, rhs) {
case (.completed, .completed): return true
case (.error(let e1), .error(let e2)):
// if the references are equal, then it's the same object
if (lhs as AnyObject) === (rhs as AnyObject) {
return true
}
#if os(Linux)
return "\(e1)" == "\(e2)"
#else
let error1 = e1 as NSError
let error2 = e2 as NSError
return error1.domain == error2.domain
&& error1.code == error2.code
&& "\(e1)" == "\(e2)"
#endif
case (.next(let v1), .next(let v2)): return v1 == v2
default: return false
}
}
| mit | 8f81527c6701ebabc396ecfe4335e2fc | 28.775 | 125 | 0.602015 | 3.805112 | false | false | false | false |
OctoberHammer/CoreDataCollViewFRC | Pods/PMSuperButton/Sources/PMSuperButton.swift | 1 | 9739 | //
// PMSuperButton.swift
// PMSuperButton
//
// Created by Paolo Musolino on 14/06/17.
// Copyright © 2017 PMSuperButton. All rights reserved.
//
import UIKit
@IBDesignable
open class PMSuperButton: UIButton {
//MARK: - General Appearance
@IBInspectable open var borderColor: UIColor = UIColor.clear{
didSet{
self.layer.borderColor = borderColor.cgColor
}
}
@IBInspectable open var borderWidth: CGFloat = 0{
didSet{
self.layer.borderWidth = borderWidth
}
}
@IBInspectable open var cornerRadius: CGFloat = 0{
didSet{
self.layer.cornerRadius = cornerRadius
if let gradientLayer = gradient {
gradientLayer.cornerRadius = cornerRadius
}
}
}
@IBInspectable open var shadowColor: UIColor = UIColor.clear{
didSet{
self.layer.shadowColor = shadowColor.cgColor
}
}
@IBInspectable open var shadowOpacity: Float = 0{
didSet{
self.layer.shadowOpacity = shadowOpacity
}
}
@IBInspectable open var shadowOffset: CGSize = CGSize.zero{
didSet{
self.layer.shadowOffset = shadowOffset
}
}
@IBInspectable open var shadowRadius: CGFloat = 0{
didSet{
self.layer.shadowRadius = shadowRadius
}
}
@IBInspectable open var gradientEnabled: Bool = false{
didSet{
setupGradient()
}
}
//MARK: - Gradient Background
@IBInspectable open var gradientStartColor: UIColor = UIColor.clear{
didSet{
setupGradient()
}
}
@IBInspectable open var gradientEndColor: UIColor = UIColor.clear{
didSet{
setupGradient()
}
}
@IBInspectable open var gradientHorizontal: Bool = false{
didSet{
setupGradient()
}
}
var gradient: CAGradientLayer?
func setupGradient(){
guard gradientEnabled != false else{
return
}
gradient?.removeFromSuperlayer()
gradient = CAGradientLayer()
guard let gradient = gradient else { return }
gradient.frame = self.layer.bounds
gradient.colors = [gradientStartColor.cgColor, gradientEndColor.cgColor]
gradient.startPoint = CGPoint(x: 0, y: 0)
gradient.endPoint = gradientHorizontal ? CGPoint(x: 1, y: 0) : CGPoint(x: 0, y: 1)
gradient.cornerRadius = self.cornerRadius
self.layer.insertSublayer(gradient, below: self.imageView?.layer)
}
//MARK: - Animations
@IBInspectable open var animatedScaleWhenHighlighted: CGFloat = 1.0
@IBInspectable open var animatedScaleDurationWhenHightlighted: Double = 0.2
override open var isHighlighted: Bool {
didSet {
guard animatedScaleWhenHighlighted != 1.0 else {
return
}
if isHighlighted{
UIView.animate(withDuration: animatedScaleDurationWhenHightlighted, animations: {
self.transform = CGAffineTransform(scaleX: self.animatedScaleWhenHighlighted, y: self.animatedScaleWhenHighlighted)
})
}
else{
UIView.animate(withDuration: animatedScaleDurationWhenHightlighted, animations: {
self.transform = CGAffineTransform.identity
})
}
}
}
@IBInspectable open var animatedScaleWhenSelected: CGFloat = 1.0
@IBInspectable open var animatedScaleDurationWhenSelected: Double = 0.2
override open var isSelected: Bool{
didSet {
guard animatedScaleWhenSelected != 1.0 else {
return
}
UIView.animate(withDuration: animatedScaleDurationWhenSelected, animations: {
self.transform = CGAffineTransform(scaleX: self.animatedScaleWhenSelected, y: self.animatedScaleWhenSelected)
}) { (finished) in
UIView.animate(withDuration: self.animatedScaleDurationWhenSelected, animations: {
self.transform = CGAffineTransform.identity
})
}
}
}
//MARK: - Ripple button
@IBInspectable open var ripple: Bool = false{
didSet{
self.clipsToBounds = true
}
}
@IBInspectable open var rippleColor: UIColor = UIColor(white: 1.0, alpha: 0.3)
@IBInspectable open var rippleSpeed: Double = 1.0
//MARK: - Checkbox
@IBInspectable open var checkboxButton: Bool = false{
didSet{
if checkboxButton == true{
self.setImage(uncheckedImage, for: .normal)
self.setImage(checkedImage, for: .selected)
self.addTarget(self, action: #selector(buttonChecked), for: .touchUpInside)
}
}
}
@IBInspectable open var checkedImage: UIImage?
@IBInspectable open var uncheckedImage: UIImage?
@objc func buttonChecked(sender:AnyObject){
self.isSelected = !self.isSelected
}
//MARK: - Image
///Image UIButton content mode
@IBInspectable open var imageViewContentMode: Int = UIViewContentMode.scaleToFill.rawValue{
didSet{
imageView?.contentMode = UIViewContentMode(rawValue: imageViewContentMode) ?? .scaleToFill
}
}
@IBInspectable open var imageAlpha: CGFloat = 1.0 {
didSet {
if let imageView = imageView {
imageView.alpha = imageAlpha
}
}
}
//MARK: - Action Closure
private var action: (() -> Void)?
open func touchUpInside(action: (() -> Void)? = nil){
self.action = action
}
@objc func tapped(sender: PMSuperButton) {
self.action?()
}
//MARK: - Loading
let indicator: UIActivityIndicatorView = UIActivityIndicatorView(activityIndicatorStyle: UIActivityIndicatorViewStyle.gray)
/**
Show a loader inside the button, and enable or disable user interection while loading
*/
open func showLoader(userInteraction: Bool = true){
guard self.subviews.contains(indicator) == false else {
return
}
self.isUserInteractionEnabled = userInteraction
indicator.isUserInteractionEnabled = false
indicator.center = CGPoint(x: self.bounds.size.width/2, y: self.bounds.size.height/2)
UIView.transition(with: self, duration: 0.5, options: .curveEaseOut, animations: {
self.titleLabel?.alpha = 0.0
self.imageAlpha = 0.0
}) { (finished) in
self.addSubview(self.indicator)
self.indicator.startAnimating()
}
}
open func hideLoader(){
guard self.subviews.contains(indicator) == true else {
return
}
self.isUserInteractionEnabled = true
self.indicator.stopAnimating()
self.indicator.removeFromSuperview()
UIView.transition(with: self, duration: 0.5, options: .curveEaseIn, animations: {
self.titleLabel?.alpha = 1.0
self.imageAlpha = 1.0
}) { (finished) in
}
}
//MARK: - Interface Builder Methods
override open func layoutSubviews() {
super.layoutSubviews()
gradient?.frame = self.layer.bounds
self.imageView?.alpha = imageAlpha
self.addTarget(self, action: #selector(tapped), for: .touchUpInside)
}
override open func prepareForInterfaceBuilder() {
}
}
extension PMSuperButton: CAAnimationDelegate{
//MARK: Material touch animation for ripple button
open override func beginTracking(_ touch: UITouch, with event: UIEvent?) -> Bool {
guard ripple == true else {
return true
}
let tapLocation = touch.location(in: self)
let aLayer = CALayer()
aLayer.backgroundColor = rippleColor.cgColor
let initialSize: CGFloat = 20.0
aLayer.frame = CGRect(x: 0, y: 0, width: initialSize, height: initialSize)
aLayer.cornerRadius = initialSize/2
aLayer.masksToBounds = true
aLayer.position = tapLocation
self.layer.insertSublayer(aLayer, below: self.titleLabel?.layer)
// Create a basic animation changing the transform.scale value
let animation = CABasicAnimation(keyPath: "transform.scale")
// Set the initial and the final values+
animation.toValue = 10.5 * max(self.frame.size.width, self.frame.size.height) / initialSize
// Set duration
animation.duration = rippleSpeed
// Set animation to be consistent on completion
animation.isRemovedOnCompletion = false
animation.fillMode = kCAFillModeForwards
// Add animation to the view's layer
let fade = CAKeyframeAnimation(keyPath: "opacity")
fade.values = [1.0, 1.0, 0.5, 0.5, 0.0]
fade.duration = 0.5
let animGroup = CAAnimationGroup()
animGroup.duration = 0.5
animGroup.delegate = self
animGroup.animations = [animation, fade]
animGroup.setValue(aLayer, forKey: "animationLayer")
aLayer.add(animGroup, forKey: "scale")
return true
}
public func animationDidStop(_ anim: CAAnimation, finished flag: Bool) {
let layer: CALayer? = anim.value(forKeyPath: "animationLayer") as? CALayer
if layer != nil{
layer?.removeAnimation(forKey: "scale")
layer?.removeFromSuperlayer()
}
}
}
| mit | 4f6e2e7dabb546e6d4f64d913f732395 | 31.898649 | 135 | 0.602588 | 5.090434 | false | false | false | false |
aapierce0/MatrixClient | MatrixClient/ImageProvider.swift | 1 | 2867 | /*
Copyright 2017 Avery Pierce
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
import Foundation
import SwiftMatrixSDK
struct UnknownError : Error {
var localizedDescription: String {
return "error object was unexpectedly nil"
}
}
/// Downloads images and provides them.
class ImageProvider {
var semaphores: [URL: DispatchSemaphore] = [:]
var cache: [URL: NSImage] = [:]
func semaphore(for url: URL) -> DispatchSemaphore {
if let semaphore = self.semaphores[url] { return semaphore }
let newSemaphore = DispatchSemaphore(value: 1)
self.semaphores[url] = newSemaphore
return newSemaphore
}
func cachedImage(for url: URL) -> NSImage? {
return cache[url]
}
func image(for url: URL, completion: @escaping (_ response: MXResponse<NSImage>) -> Void) {
// Get the semaphore for this url
let semaphore = self.semaphore(for: url)
// This operation needs to be performed on a background thread
let queue = DispatchQueue(label: "Image Provider")
queue.async {
// Wait until any downloads are complete
semaphore.wait()
// If the image already exists in the cache, return it.
if let image = self.cache[url] {
completion(.success(image))
semaphore.signal()
return
}
URLSession.shared.dataTask(with: url) { (data, response, error) in
// The request is complete, so make sure to signal
defer { semaphore.signal() }
// Create a result object from the URLSession response
let result: MXResponse<NSImage>
if let data = data, let image = NSImage(data: data) {
self.cache[url] = image
result = .success(image)
} else if let error = error {
result = .failure(error)
} else {
result = .failure(UnknownError())
}
// Perform the completion block on the main thread.
DispatchQueue.main.async { completion(result) }
}.resume()
}
}
}
| apache-2.0 | 1b5e85fbdb987e172293851f81d1050d | 32.337209 | 95 | 0.574468 | 5.065371 | false | false | false | false |
diejmon/KanbanizeAPI | Tests/KanbanizeAPITests/APIKey.swift | 1 | 326 | import Foundation
internal let subdomain = env("subdomain")
internal let apiKey = env("apiKey")
internal let validEmail = env("validEmail")
internal let validPassword = env("validPassword")
internal let testTaskID = env("testTaskID")
private func env(_ name: String) -> String {
return ProcessInfo().environment[name]!
}
| mit | a1e8a7bfd8aca80b9f07b10af3848319 | 26.166667 | 49 | 0.748466 | 4.024691 | false | true | false | false |
cozkurt/coframework | COFramework/COFramework/Swift/Components/UIDynamics/UIBehaviourHelper.swift | 1 | 8447 | //
// UIBehaviourHelper.swift
// FuzFuz
//
// Created by Cenker Ozkurt on 01/06/20.
// Copyright © 2019 Cenker Ozkurt, Inc. All rights reserved.
//
import UIKit
import Foundation
import ObjectMapper
open class UIBehaviourHelper {
var settings: UIBehaviourModel?
//
// MARK: - sharedInstance for singleton access
//
public static let sharedInstance: UIBehaviourHelper = UIBehaviourHelper()
init() {
self.loadModels("UIBehaviours")
}
/**
loadFlowModels load predefined modals and applies to
flowModels array.
- parameters:
- fileName: json array for Flow events.
*/
fileprivate func loadModels(_ fileName: String?) {
guard let fileName = fileName else {
Logger.sharedInstance.LogInfo("fileName is missing")
return
}
if let jsonString = try? FileLoader.loadFile(fileName: fileName) {
let mappable = Mapper<UIBehaviourModel>()
if let models = mappable.map(JSONString: jsonString) {
self.settings = models
}
Logger.sharedInstance.LogInfo("File: \(fileName) loaded")
} else {
Logger.sharedInstance.LogError("File: \(fileName) NOT loaded")
}
}
func viewsFromTags(_ parentView: UIView, tags: String) -> [UIView] {
var views: [UIView] = []
for tagString in tags.components(separatedBy: ",") {
if let tag = Int(tagString), let view = parentView.viewWithTag(Int(tag)) {
views.append(view)
}
}
return views
}
func updateItemState(_ parentView: UIView?, tag: Int) {
guard let parentView = parentView, let view = parentView.viewWithTag(tag) else {
return
}
UIBehaviourFactory.sharedInstance.updateItemState(parentView, view: view)
}
func setupKingNodeDynamics(_ parentView: UIView?, tag: Int) {
guard let parentView = parentView, let view = parentView.viewWithTag(tag), let settings = self.settings, let density = settings.kingDensity else {
return
}
let params = UIBehaviourItemsParams(allowsRotation: false, density: CGFloat(density), elasticity: 0, friction: 1, resistance: 1, angularResistance: 0)
UIBehaviourFactory.sharedInstance.dynamicItemBehavior(parentView, views: [view], params: params)
}
func setUpNodeDynamics(_ parentView: UIView?, tag: Int) {
guard let parentView = parentView, let view = parentView.viewWithTag(tag), let settings = self.settings, let density = settings.nodeDensity else {
return
}
let params = UIBehaviourItemsParams(allowsRotation: false, density: CGFloat(density), elasticity: 1.0, friction: 1, resistance: 1, angularResistance: 0)
UIBehaviourFactory.sharedInstance.dynamicItemBehavior(parentView, views: [view], params: params)
}
func setUpCollision(_ parentView: UIView?, barrierViewTags: String, tags: String) {
guard let parentView = parentView else { return }
UIBehaviourFactory.sharedInstance.collisionBehavior(parentView, views: self.viewsFromTags(parentView, tags: tags), barrierViews: self.viewsFromTags(parentView, tags: barrierViewTags), delegate: nil)
}
func setUpGravity(_ parentView: UIView?, tags: String) {
guard let parentView = parentView else { return }
let param = UIBehaviourGravityParams(angle: 0, magnitude: 1, gravityDirection: CGPoint(x: 0, y: 0.5))
UIBehaviourFactory.sharedInstance.gravityBehavior(parentView, views: self.viewsFromTags(parentView, tags: tags), params: param)
}
func setUpRadialGravityField(_ parentView: UIView?, centerView: UIView, tags: String, strength: CGFloat) {
guard let parentView = parentView else { return }
let param = UIBehaviourFieldParams(region: 300, strength: strength, falloff: 1, minimumRadius: 10)
UIBehaviourFactory.sharedInstance.radialGravityFieldBehavior(parentView, centerView: centerView, orbitingViews: self.viewsFromTags(parentView, tags: tags), params: param)
}
func setUpVortexField(_ parentView: UIView?, centerView: UIView, tags: String) {
guard let parentView = parentView else { return }
let param = UIBehaviourVortexFieldParams(region: 200, strength: 1, position: centerView.center)
UIBehaviourFactory.sharedInstance.vortexFieldBehavior(parentView, centerView: centerView, orbitingViews: self.viewsFromTags(parentView, tags: tags), params: param)
}
func attachToTag(_ parentView: UIView?, fromTag: Int, toTag: Int, length: Int) {
guard let parentView = parentView, let settings = self.settings, let damping = settings.attachDamping else { return }
let params = UIBehaviourAttachmentParams(frequency: 1.0, damping: CGFloat(damping), length: CGFloat(length), anchorPoint: CGPoint(x: 0, y: 0), attachedToTag: toTag, attachedFromTag: fromTag)
UIBehaviourFactory.sharedInstance.attachmentBehavior(parentView, params: params)
}
func snapToPoint(_ parentView: UIView?, tags: String, point: CGPoint) {
guard let parentView = parentView, let settings = self.settings, let damping = settings.snapDamping else {
return
}
for tagString in tags.components(separatedBy: ",") {
guard let tag = Int(tagString), let view = parentView.viewWithTag(Int(tag)) else {
continue
}
// remove prevous snap behaviour
self.removeBehaviour(parentView, tag: tag, behaviourType: UIBehaviourType.Snap)
let params = UIBehaviourSnapParams(damping: CGFloat(damping), snapToPoint: point)
UIBehaviourFactory.sharedInstance.snapBehavior(parentView, view: view, params: params)
UIBehaviourFactory.sharedInstance.updateItemState(parentView, view: view)
}
}
func randomPush(_ parentView: UIView?, tags: String) {
guard let parentView = parentView else { return }
for tagString in tags.components(separatedBy: ",") {
guard let tag = Int(tagString), let view = parentView.viewWithTag(Int(tag)) else {
continue
}
let params = UIBehaviourPushParams(angle: CGFloat(arc4random_uniform(360)), magnitude: CGFloat(10), pushDirection: CGPoint(x: -10 + CGFloat(arc4random_uniform(10)), y: -10 + CGFloat(arc4random_uniform(10))), mode: .instantaneous)
UIBehaviourFactory.sharedInstance.pushBehavior(parentView, views: [view], params: params)
}
}
func removeBehaviour(_ parentView: UIView?, tag: Int, behaviourType: UIBehaviourType) {
guard let parentView = parentView, let view = parentView.viewWithTag(tag) else {
return
}
UIBehaviourFactory.sharedInstance.removeBehaviour(parentView, view: view, behaviourType: behaviourType)
}
func removeAllBehaviours(_ parentView: UIView?, tag: Int) {
guard let parentView = parentView, let view = parentView.viewWithTag(tag) else {
return
}
UIBehaviourFactory.sharedInstance.removeBehaviour(parentView, view: view, behaviourType: UIBehaviourType.RadialGravityField)
UIBehaviourFactory.sharedInstance.removeBehaviour(parentView, view: view, behaviourType: UIBehaviourType.VortexField)
UIBehaviourFactory.sharedInstance.removeBehaviour(parentView, view: view, behaviourType: UIBehaviourType.Attachment)
UIBehaviourFactory.sharedInstance.removeBehaviour(parentView, view: view, behaviourType: UIBehaviourType.Collision)
UIBehaviourFactory.sharedInstance.removeBehaviour(parentView, view: view, behaviourType: UIBehaviourType.Dynamic)
UIBehaviourFactory.sharedInstance.removeBehaviour(parentView, view: view, behaviourType: UIBehaviourType.Gravity)
UIBehaviourFactory.sharedInstance.removeBehaviour(parentView, view: view, behaviourType: UIBehaviourType.Push)
UIBehaviourFactory.sharedInstance.removeBehaviour(parentView, view: view, behaviourType: UIBehaviourType.Snap)
}
}
| gpl-3.0 | 16daf6f2de5f321ee2344617980fe9b5 | 43.687831 | 241 | 0.662799 | 4.823529 | false | false | false | false |
alexktchen/rescue.iOS | Rescue/StorageService.swift | 1 | 3834 | //
// StorageService.swift
// Rescue
//
// Created by Alex Chen on 2015/2/8.
// Copyright (c) 2015年 KKAwesome. All rights reserved.
//
import Foundation
import UIKit
class StorageService{
var tableBlobBlobs: MSTable?
var tableContainers: MSTable?
init(){
let client = MSClient(applicationURLString: "https://recuse-mobile-service.azure-mobile.net/", withApplicationKey: "oTghGlBNZdBTAqCUbrBfLIKrEnXHXJ26")
self.tableBlobBlobs = client.getTable("BlobBlobs")
self.tableContainers = client.getTable("BlobContainers")
// loadData()
//loadContainer()
//uploadImage()
}
func containerExistAndCreate(userId: String, completion: (isExist: Bool)-> Void){
let containerName = "container=\(userId)"
self.tableContainers?.readWithQueryString(containerName, completion: {(results:[AnyObject]!, totalCount: Int!, error: NSError!) -> Void in
if(totalCount == -1){
completion(isExist: false)
}
else{
completion(isExist: true)
}
})
}
func insertContainers(userId: String, completion: (name: String)-> Void){
let item: NSDictionary = ["containerName":"\(userId)"]
let params: NSDictionary = ["isPublic": "\(NSNumber(bool: true))"]
self.tableContainers?.insert(item, parameters: params, completion: {
(results:[NSObject : AnyObject]!, error: NSError!) -> Void in
var json = JSON(results)
println(json)
})
}
func uploadImage(image:UIImage, hud: MBProgressHUD , completion: (url: String) -> Void){
let data: NSData = UIImagePNGRepresentation(image)
let item: NSDictionary = NSDictionary()
let dateFormatter = NSDateFormatter()
dateFormatter.dateStyle = .LongStyle
dateFormatter.timeStyle = NSDateFormatterStyle.NoStyle
dateFormatter.dateFormat = "yyyyMMddhhmmsss"
let fileName = dateFormatter.stringFromDate(NSDate())
let params: NSDictionary = ["containerName":"qqq","blobName":"\(fileName)"]
self.tableBlobBlobs?.insert(item, parameters: params, completion: { (results:[NSObject : AnyObject]!, error: NSError!) -> Void in
if(error == nil){
var json = JSON(results)
let sasUrl = json["sasUrl"].string
let request = NSMutableURLRequest(URL: NSURL(string: sasUrl!)!)
request.setValue("image/jpeg", forHTTPHeaderField: "Content-Type")
request.HTTPMethod = "PUT"
upload(request, data).progress(closure: {
(bytesWritten, totalBytesWritten, totalBytesExpectedToWrite) -> Void in
println(totalBytesWritten)
hud.progress = Float(totalBytesWritten)/Float(totalBytesExpectedToWrite)
}).responseJSON { (request, response, json, error) in
if(error == nil){
let strUrl = "http://recusemobilestorage.blob.core.windows.net/qqq/" + fileName
completion(url: strUrl)
println(request)
println(json)
}
else{
println(error)
}
}
}
else{
println(error)
}
})
}
}
| mit | fe1f7f2ffd1965fed99d09f4c7cb120f | 29.903226 | 158 | 0.517484 | 5.427762 | false | false | false | false |
kstaring/swift | validation-test/compiler_crashers_fixed/01501-swift-diagnosticengine-flushactivediagnostic.swift | 11 | 2316 | // This source file is part of the Swift.org open source project
// Copyright (c) 2014 - 2016 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See http://swift.org/LICENSE.txt for license information
// See http://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
// RUN: not %target-swift-frontend %s -parse
}
func b([T]
let start = j> Any) {
var b {
func i("[1)
b[]
struct Q<A
}
typealias R = A, "foo")
func a
protocol A {
return S<(AnyObject, g<T> {
}
return "foobar")
}
protocol a {
func a")
override init(c {
}(t: AnyObject> Any) -> {
}
class a : $0))
typealias f : a())
var b where B = b: A, i : B? = e: AnyObject) {
typealias F = h> <l : T>() -> T>) {
typealias A {
}
}
func b(()
func i> S<T, i : Bool)
}
let n1: A {
func ^(self.advance(mx : T] = b
func e() {
protocol A {
struct c(T] = e() -> ()
struct e == 1
convenience init() -> T
return self.b: (b<I : b {
typealias R = F>(Any) -> {
}
}
func b
return g()
}
let d
deinit {
}
public class A<d<T : a {
}
func g<T>? = c
}
protocol a : ({
S.b {
}
b: [T> String {
var b {
}
var a(b((Range(.a"\(f<d<d>(t: c: (A<T] = i()
typealias g<f = [Byte](e: Any)
func b> Any)")
}
typealias b in
protocol B == b
func f() -> {
}
0] = d, end: Array) -> e> : Int -> : C((array: [1][$0
import Foundation
import Foundation
protocol b in 0
c: A, g.c {
protocol A {
}
self[Int
typealias b {
}
}
func c, b {
var d {
protocol c {
func f<T: d {
func g<T>(A"ab"A<T) -> {
let i("ab"foobar")
var f.A<T> T>(Range<(T) {
extension String {
func c, a(m: A<T {
}
class d>: A, (Any)(a<C> [T> A = { _, i> {
}
}
}
}
struct S(Any) -> Any, Any) -> Void>()
}
func i: A.f : [$0) -> S : a {
struct d
}
return !.d {
}
f = T>
func g(t: A, A<f : c)()
switch x in return self.c(Any) {
func c<T, range.e : Int = []
}
assert() -> S {
class A {
convenience init(1, i<T>(self, range.dynamicType.c where h.c, range.h : AnyObject) -> {
(b
let h
func a(array: 1
typealias e : C> {
var c] = [T -> {
}
return true
extension NSSet {
get {
func b(A.d) in x }
print(1
class A : Array<T>() {
}
return S) {
}
typealias b = a<b, "cd"ab""cd")
class A.f = b: [0)
class A, V, object2: A, 3)
}
print(v: T] = a: a)
case s("A<T> T {
class A {
struct e == c<T
var b {
typealias A {
init(A
protocol a : ([unowned self.<d
b: Int], A, g = ")
}
}
| apache-2.0 | c4fee032b33742f28c7e63e6c421bf17 | 15.083333 | 87 | 0.575561 | 2.390093 | false | false | false | false |
carabina/AmazonS3RequestManager | AmazonS3RequestManager/AmazonS3ACL.swift | 1 | 10556 | //
// AmazonS3ACL.swift
// AmazonS3RequestManager
//
// Created by Anthony Miller. 2015.
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
import Foundation
/// MARK: - Constants
private let AmazonS3PredefinedACLHeaderKey = "x-amz-acl"
/**
MARK: - AmazonS3ACL Protocol
An object conforming to the `AmazonS3ACL` protocol describes an access control list (ACL) that can be used by `AmazonS3RequestManager` to set the ACL Headers for a request.
*/
public protocol AmazonS3ACL {
/**
This method should be implemented to set the ACL headers for the object.
*/
func setACLHeaders(inout forRequest request: NSMutableURLRequest)
}
/**
MARK: - Predefined (Canned) ACLs.
A list of predefined, or canned, ACLs recognized by the Amazon S3 service.
:see: For more information on Predefined ACLs, see "http://docs.aws.amazon.com/AmazonS3/latest/dev/acl-overview.html#canned-acl"
- Private: Owner gets full control. No one else has access rights. This is the default ACL for a new bucket/object. Applies to buckets and objects.
- Public: Owner gets full control. All other users (anonymous or authenticated) get READ and WRITE access. Granting this on a bucket is generally not recommended. Applies to buckets and objects.
- PublicReadOnly: Owner gets full control. All other users (anonymous or authenticated) get READ access. Applies to buckets and objects.
- AuthenticatedReadOnly: Owner gets full control. All authenticated users get READ access. Applies to buckets and objects.
- BucketOwnerReadOnly: Object owner gets full control. Bucket owner gets READ access. Applies to objects only; if you specify this canned ACL when creating a bucket, Amazon S3 ignores it.
- BucketOwnerFullControl: Both the object owner and the bucket owner get full control over the object. Applies to objects only; if you specify this canned ACL when creating a bucket, Amazon S3 ignores it.
- LogDeliveryWrite: The `LogDelivery` group gets WRITE and READ_ACP permissions on the bucket.
:see: For more information on logs, see "http://docs.aws.amazon.com/AmazonS3/latest/dev/ServerLogs.html"
*/
public enum AmazonS3PredefinedACL: String, AmazonS3ACL {
case Private = "private",
Public = "public-read-write",
PublicReadOnly = "public-read",
AuthenticatedReadOnly = "authenticated-read",
BucketOwnerReadOnly = "bucket-owner-read",
BucketOwnerFullControl = "bucket-owner-full-control",
LogDeliveryWrite = "log-delivery-write"
public func setACLHeaders(inout forRequest request: NSMutableURLRequest) {
request.addValue(self.rawValue, forHTTPHeaderField: AmazonS3PredefinedACLHeaderKey)
}
}
/**
MARK: - ACL Permissions
The list of permission types for the Amazon S3 Service
:see: For more information on the access allowed by each permission, see "http://docs.aws.amazon.com/AmazonS3/latest/dev/acl-overview.html#permissions"
- Read: Allows grantee to list the objects in the bucket or read the object data and its metadata
- Write: Allows grantee to create, overwrite, and delete any object in the bucket.
:note: This permission applies only to buckets.
- ReadACL: Allows grantee to read the ACL for the bucket or object
- WriteACL: Allows grantee to write the ACL for the bucket or object
- FullControl: Allows grantee the `Read`, `Write`, `ReadACL`, and `WriteACL` permissions on the bucket or object
*/
public enum AmazonS3ACLPermission {
case Read,
Write,
ReadACL,
WriteACL,
FullControl
var valueForRequestBody: String {
switch (self) {
case .Read:
return "READ"
case .Write:
return "WRITE"
case .ReadACL:
return "READ_ACP"
case .WriteACL:
return "WRITE_ACP"
case .FullControl:
return "FULL_CONTROL"
}
}
var requestHeaderFieldKey: String {
switch (self) {
case .Read:
return "x-amz-grant-read"
case .Write:
return "x-amz-grant-write"
case .ReadACL:
return "x-amz-grant-read-acp"
case .WriteACL:
return "x-amz-grant-write-acp"
case .FullControl:
return "x-amz-grant-full-control"
}
}
}
/**
MARK: - ACL Grantees
Defines a grantee to assign to a permission.
A grantee can be an AWS account or one of the predefined Amazon S3 groups. You grant permission to an AWS account by the email address or the canonical user ID.
:note: If you provide an email in your grant request, Amazon S3 finds the canonical user ID for that account and adds it to the ACL. The resulting ACLs will always contain the canonical user ID for the AWS account, not the AWS account's email address.
:see: For more information on Amazon S3 Service grantees, see "http://docs.aws.amazon.com/AmazonS3/latest/dev/acl-overview.html#specifying-grantee"
- AuthenticatedUsers: This group represents all AWS accounts. Access permission to this group allows any AWS account to access the resource. However, all requests must be signed (authenticated).
- AllUsers: Access permission to this group allows anyone to access the resource. The requests can be signed (authenticated) or unsigned (anonymous). Unsigned requests omit the Authentication header in the request.
- LogDeliveryGroup: WRITE permission on a bucket enables this group to write server access logs to the bucket.
:see: For more information on the log delivery group, see "http://docs.aws.amazon.com/AmazonS3/latest/dev/ServerLogs.html"
- EmailAddress: A grantee for the AWS account with the given email address.
- UserID: A grantee for the AWS account with the given canonical user ID.
*/
public enum AmazonS3ACLGrantee: Hashable {
case AuthenticatedUsers,
AllUsers,
LogDeliveryGroup,
EmailAddress(String),
UserID(String)
var requestHeaderFieldValue: String {
switch (self) {
case .AuthenticatedUsers:
return "uri=\"http://acs.amazonaws.com/groups/global/AuthenticatedUsers\""
case .AllUsers:
return "uri=\"http://acs.amazonaws.com/groups/global/AllUsers\""
case .LogDeliveryGroup:
return "uri=\"http://acs.amazonaws.com/groups/s3/LogDelivery\""
case .EmailAddress(let email):
return "emailAddress=\"\(email)\""
case .UserID(let id):
return "id=\"\(id)\""
}
}
public var hashValue: Int {
get {
return requestHeaderFieldValue.hashValue
}
}
}
public func ==(lhs: AmazonS3ACLGrantee, rhs: AmazonS3ACLGrantee) -> Bool {
return lhs.hashValue == rhs.hashValue
}
/**
MARK: - ACL Permission Grants
An `AmazonS3PermissionGrant` represents a grant for a single permission to a list of grantees.
*/
public struct AmazonS3ACLPermissionGrant: AmazonS3ACL, Hashable {
/**
Creates a grant with the given permission for a `Set` of grantees
:param: permission The `AmazonS3ACLPermission` to set for the `grantees`
:param: grantees The `Set` of `AmazonS3ACLGrantees` to set the permission for
:returns: An grant for the given permission and grantees
*/
public init(permission: AmazonS3ACLPermission, grantees: Set<AmazonS3ACLGrantee>) {
self.permission = permission
self.grantees = grantees
}
/**
Creates a grant with the given permission for a single grantee
:param: permission The `AmazonS3ACLPermission` to set for the `grantee`
:param: grantees The single `AmazonS3ACLGrantees` to set the permission for
:returns: An grant for the given permission and grantees
*/
public init(permission: AmazonS3ACLPermission, grantee: AmazonS3ACLGrantee) {
self.permission = permission
self.grantees = [grantee]
}
/// The permission for the grant
private(set) public var permission: AmazonS3ACLPermission
/// The set of grantees for the grant
private(set) public var grantees: Set<AmazonS3ACLGrantee>
public func setACLHeaders(inout forRequest request: NSMutableURLRequest) {
let granteeList = join(", ", granteeStrings())
request.addValue(granteeList, forHTTPHeaderField: permission.requestHeaderFieldKey)
}
private func granteeStrings() -> [String] {
var strings: [String] = []
for grantee in grantees {
strings.append(grantee.requestHeaderFieldValue)
}
return strings
}
public var hashValue: Int {
get {
return permission.hashValue
}
}
}
public func ==(lhs: AmazonS3ACLPermissionGrant, rhs: AmazonS3ACLPermissionGrant) -> Bool {
return lhs.permission == rhs.permission
}
/**
MARK: - Custom ACLs
An `AmazonS3CustomACL` contains an array of `AmazonS3ACLPermissionGrant`s and can be used to create a custom access control list (ACL).
:note: The Amazon S3 Service accepts a maximum of 100 permission grants per bucket/object.
*/
public struct AmazonS3CustomACL: AmazonS3ACL {
/**
The set of `AmazonS3ACLPermissionGrants` to use for the access control list
:note: Only one `AmazonS3PermissionGrant` can be added to the set for each `AmazonS3ACLPermission` type. Each permission may map to multiple grantees.
*/
public var grants: Set<AmazonS3ACLPermissionGrant>
/**
Initializes an `AmazonS3CustomACL` with a given array of `AmazonS3PermissionGrant`s.
:param: grant The grants for the custom ACL
:returns: An `AmazonS3CustomACL` with the given grants
*/
public init(grants: Set<AmazonS3ACLPermissionGrant>) {
self.grants = grants
}
public func setACLHeaders(inout forRequest request: NSMutableURLRequest) {
for grant in grants {
grant.setACLHeaders(forRequest: &request)
}
}
} | mit | a216e5c5603bb84ec284de24b1a9e537 | 34.426174 | 251 | 0.730296 | 4.120219 | false | false | false | false |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.