text
stringlengths 2
100k
| meta
dict |
---|---|
using System;
namespace GoodAI.BasicNodes.DyBM
{
public class MyRandom
{
static Random rand = new Random();
public double NextDouble(float mean, float stdDev)
{
//these are uniform(0,1) random doubles
double u1 = (float)rand.NextDouble();
double u2 = (float)rand.NextDouble();
//random normal(0,1)
double randStdNormal = Math.Sqrt(-2.0 * Math.Log(u1)) * Math.Sin(2.0 * Math.PI * u2);
//random normal(mean,stdDev^2)
return mean + stdDev * randStdNormal;
}
}
}
| {
"pile_set_name": "Github"
} |
package gen
import (
"fmt"
"github.com/Aptomi/aptomi/pkg/config"
"github.com/Aptomi/aptomi/pkg/lang"
"github.com/Aptomi/aptomi/pkg/plugin/k8s"
log "github.com/sirupsen/logrus"
"github.com/spf13/cobra"
"gopkg.in/yaml.v2"
"k8s.io/client-go/tools/clientcmd"
"k8s.io/client-go/tools/clientcmd/api"
)
func newClusterCommand(_ *config.Client) *cobra.Command {
var sourceContext, clusterName, defaultNamespace string
var local bool
cmd := &cobra.Command{
Use: "cluster",
Short: "gen cluster",
Long: "gen cluster long",
Run: func(cmd *cobra.Command, args []string) {
if !local && len(sourceContext) == 0 {
log.Fatalf("context should be specified")
}
if local && len(sourceContext) > 0 {
log.Fatalf("one of local or context could be specified")
}
var clusterConfig *k8s.ClusterConfig
var err error
if local {
if len(clusterName) == 0 {
clusterName = "local"
}
clusterConfig = &k8s.ClusterConfig{Local: true, DefaultNamespace: "default"}
} else {
if len(clusterName) == 0 {
clusterName = sourceContext
}
clusterConfig, err = handleKubeConfigCluster(sourceContext)
}
if err != nil {
panic(err)
}
if len(defaultNamespace) > 0 {
clusterConfig.DefaultNamespace = defaultNamespace
}
cluster := lang.Cluster{
TypeKind: lang.TypeCluster.GetTypeKind(),
Metadata: lang.Metadata{
Name: clusterName,
Namespace: "system",
},
Type: "kubernetes",
Config: clusterConfig,
}
log.Infof("Generating cluster: %s", clusterName)
data, err := yaml.Marshal(cluster)
if err != nil {
panic(fmt.Sprintf("error while marshaling generated cluster: %s", err))
}
fmt.Println(string(data))
},
}
cmd.Flags().BoolVarP(&local, "local", "l", false, "Build Aptomi cluster with local kubernetes")
cmd.Flags().StringVarP(&sourceContext, "context", "c", "", "Context in kubeconfig to be used for Aptomi cluster creation (run 'kubectl config get-contexts' to get list of available contexts and clusters")
cmd.Flags().StringVarP(&defaultNamespace, "default-namespace", "N", "", "Set default k8s namespace for all deployments into this cluster")
cmd.Flags().StringVarP(&clusterName, "name", "n", "", "Name of the Aptomi cluster to create")
return cmd
}
func handleKubeConfigCluster(sourceContext string) (*k8s.ClusterConfig, error) {
kubeConfig, err := buildTempKubeConfigWith(sourceContext)
if err != nil {
return nil, fmt.Errorf("error while building temp kube config with context %s: %s", sourceContext, err)
}
clusterConfig := &k8s.ClusterConfig{
KubeConfig: kubeConfig,
}
return clusterConfig, err
}
func buildTempKubeConfigWith(sourceContext string) (*interface{}, error) {
rawConf, err := getKubeConfig()
if err != nil {
return nil, err
}
newConfig := api.NewConfig()
newConfig.CurrentContext = sourceContext
context, exist := rawConf.Contexts[sourceContext]
if !exist {
return nil, fmt.Errorf("requested context not found: %s", sourceContext)
}
newConfig.Contexts[sourceContext] = context
if newConfig.Clusters[context.Cluster], exist = rawConf.Clusters[context.Cluster]; !exist {
return nil, fmt.Errorf("requested cluster (from specified context) not found: %s", context.Cluster)
}
if newConfig.AuthInfos[context.AuthInfo], exist = rawConf.AuthInfos[context.AuthInfo]; !exist {
return nil, fmt.Errorf("requested auth info (user from specified context) not found: %s", context.AuthInfo)
}
content, err := clientcmd.Write(*newConfig)
if err != nil {
return nil, fmt.Errorf("error while marshaling temp kubeconfig: %s", err)
}
kubeConfig := new(interface{})
err = yaml.Unmarshal(content, kubeConfig)
if err != nil {
return nil, fmt.Errorf("error while unmarshaling temp kubeconfig: %s", err)
}
return kubeConfig, err
}
func getKubeConfig() (*api.Config, error) {
rules := clientcmd.NewDefaultClientConfigLoadingRules()
overrides := &clientcmd.ConfigOverrides{}
conf := clientcmd.NewNonInteractiveDeferredLoadingClientConfig(rules, overrides)
rawConf, err := conf.RawConfig()
if err != nil {
return nil, fmt.Errorf("error while getting raw kube config: %s", err)
}
return &rawConf, err
}
| {
"pile_set_name": "Github"
} |
package healthcheck
import (
"bytes"
"testing"
"github.com/mitchellh/cli"
"github.com/spiffe/go-spiffe/v2/proto/spiffe/workload"
common_cli "github.com/spiffe/spire/pkg/common/cli"
"github.com/spiffe/spire/test/fakes/fakeworkloadapi"
"github.com/stretchr/testify/suite"
"google.golang.org/grpc/codes"
"google.golang.org/grpc/status"
)
func TestHealthCheck(t *testing.T) {
suite.Run(t, new(HealthCheckSuite))
}
type HealthCheckSuite struct {
suite.Suite
stdin *bytes.Buffer
stdout *bytes.Buffer
stderr *bytes.Buffer
cmd cli.Command
}
func (s *HealthCheckSuite) SetupTest() {
s.stdin = new(bytes.Buffer)
s.stdout = new(bytes.Buffer)
s.stderr = new(bytes.Buffer)
s.cmd = newHealthCheckCommand(&common_cli.Env{
Stdin: s.stdin,
Stdout: s.stdout,
Stderr: s.stderr,
})
}
func (s *HealthCheckSuite) TestSynopsis() {
s.Equal("Determines agent health status", s.cmd.Synopsis())
}
func (s *HealthCheckSuite) TestHelp() {
s.Equal("", s.cmd.Help())
s.Equal(`Usage of health:
-shallow
Perform a less stringent health check
-socketPath string
Path to Workload API socket (default "/tmp/agent.sock")
-verbose
Print verbose information
`, s.stderr.String(), "stderr")
}
func (s *HealthCheckSuite) TestBadFlags() {
code := s.cmd.Run([]string{"-badflag"})
s.NotEqual(0, code, "exit code")
s.Equal("", s.stdout.String(), "stdout")
s.Equal(`flag provided but not defined: -badflag
Usage of health:
-shallow
Perform a less stringent health check
-socketPath string
Path to Workload API socket (default "/tmp/agent.sock")
-verbose
Print verbose information
`, s.stderr.String(), "stderr")
}
func (s *HealthCheckSuite) TestFailsOnUnavailable() {
code := s.cmd.Run([]string{"--socketPath", "doesnotexist.sock"})
s.NotEqual(0, code, "exit code")
s.Equal("", s.stdout.String(), "stdout")
s.Equal("Agent is unavailable.\n", s.stderr.String(), "stderr")
}
func (s *HealthCheckSuite) TestFailsOnUnavailableVerbose() {
code := s.cmd.Run([]string{"--socketPath", "doesnotexist.sock", "--verbose"})
s.NotEqual(0, code, "exit code")
s.Equal(`Contacting Workload API...
Workload API returned rpc error: code = Unavailable desc = connection error: desc = "transport: Error while dialing dial unix doesnotexist.sock: connect: no such file or directory"
`, s.stdout.String(), "stdout")
s.Equal("Agent is unavailable.\n", s.stderr.String(), "stderr")
}
func (s *HealthCheckSuite) TestSucceedsOnPermissionDenied() {
w := s.makeFailedWorkloadAPI(status.Error(codes.PermissionDenied, "permission denied"))
code := s.cmd.Run([]string{"--socketPath", w.Addr().Name})
s.Equal(0, code, "exit code")
s.Equal("Agent is healthy.\n", s.stdout.String(), "stdout")
s.Equal("", s.stderr.String(), "stderr")
}
func (s *HealthCheckSuite) TestSucceedsOnUnknown() {
w := s.makeFailedWorkloadAPI(status.Error(codes.Unknown, "unknown"))
code := s.cmd.Run([]string{"--socketPath", w.Addr().Name})
s.Equal(0, code, "exit code")
s.Equal("Agent is healthy.\n", s.stdout.String(), "stdout")
s.Equal("", s.stderr.String(), "stderr")
}
func (s *HealthCheckSuite) TestSucceedsOnGoodResponse() {
w := s.makeGoodWorkloadAPI()
code := s.cmd.Run([]string{"--socketPath", w.Addr().Name})
s.Equal(0, code, "exit code")
s.Equal("Agent is healthy.\n", s.stdout.String(), "stdout")
s.Equal("", s.stderr.String(), "stderr")
}
func (s *HealthCheckSuite) TestSucceedsOnGoodResponseVerbose() {
w := s.makeGoodWorkloadAPI()
code := s.cmd.Run([]string{"--socketPath", w.Addr().Name, "--verbose"})
s.Equal(0, code, "exit code")
s.Equal(`Contacting Workload API...
SVID received over Workload API.
Agent is healthy.
`, s.stdout.String(), "stdout")
s.Equal("", s.stderr.String(), "stderr")
}
func (s *HealthCheckSuite) makeFailedWorkloadAPI(err error) *fakeworkloadapi.WorkloadAPI {
return fakeworkloadapi.New(s.T(), fakeworkloadapi.FetchX509SVIDErrorOnce(err))
}
func (s *HealthCheckSuite) makeGoodWorkloadAPI() *fakeworkloadapi.WorkloadAPI {
return fakeworkloadapi.New(s.T(), fakeworkloadapi.FetchX509SVIDResponses(&workload.X509SVIDResponse{}))
}
| {
"pile_set_name": "Github"
} |
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<startup>
<supportedRuntime version="v4.0" sku=".NETFramework,Version=v4.5.2" />
</startup>
</configuration> | {
"pile_set_name": "Github"
} |
//======== (C) Copyright 2002 Charles G. Cleveland All rights reserved. =========
//
// The copyright to the contents herein is the property of Charles G. Cleveland.
// The contents may be used and/or copied only with the written permission of
// Charles G. Cleveland, or in accordance with the terms and conditions stipulated in
// the agreement/contract under which the contents have been supplied.
//
// Purpose:
//
// $Workfile: hldm.h $
// $Date: 2002/08/02 21:42:34 $
//
//-------------------------------------------------------------------------------
// $Log: hldm.h,v $
// Revision 1.4 2002/08/02 21:42:34 Flayra
// - Allow ability to control how often a ricochet sound plays
//
// Revision 1.3 2002/07/08 16:17:46 Flayra
// - Reworked bullet firing to add random spread (bug #236)
//
//===============================================================================
#ifndef HLDM_H
#define HLDM_H
#include "common/pmtrace.h"
void EV_HLDM_GunshotDecalTrace( pmtrace_t *pTrace, char *decalName, int inChanceOfSound = 1);
void EV_HLDM_DecalGunshot( pmtrace_t *pTrace, int iBulletType, int inChanceOfSound = 1);
int EV_HLDM_CheckTracer( int idx, float *vecSrc, float *end, float *forward, float *right, int iBulletType, int iTracerFreq, int *tracerCount );
void EV_HLDM_FireBullets( int idx, float *forward, float *right, float *up, int cShots, float *vecSrc, float *vecDirShooting, float flDistance, int iBulletType, int iTracerFreq, int *tracerCount, float flSpreadX, float flSpreadY );
void EV_HLDM_FireBulletsPlayer( int idx, float *forward, float *right, float *up, int cShots, float *vecSrc, float *vecDirShooting, float flDistance, int iBulletType, int iTracerFreq, int *tracerCount, Vector& inSpread, int inRandomSeed);
#endif | {
"pile_set_name": "Github"
} |
---
title: StorSimple 8000 シリーズ デバイスへの Update 5.1 のインストール | Microsoft Docs
description: StorSimple 8000 シリーズ デバイスに StorSimple 8000 シリーズの Update 5.1 をインストールする方法について説明します。
services: storsimple
documentationcenter: NA
author: twooley
ms.assetid: ''
ms.service: storsimple
ms.devlang: NA
ms.topic: how-to
ms.tgt_pltfrm: NA
ms.workload: TBD
ms.date: 03/05/2020
ms.author: twooley
ms.openlocfilehash: f9cc5181d6cc29ee4b3c2373dbbc91d6290fbe6e
ms.sourcegitcommit: 9c3cfbe2bee467d0e6966c2bfdeddbe039cad029
ms.translationtype: HT
ms.contentlocale: ja-JP
ms.lasthandoff: 08/24/2020
ms.locfileid: "88782772"
---
# <a name="install-update-51-on-your-storsimple-device"></a>StorSimple デバイスへの Update 5.1 のインストール
## <a name="overview"></a>概要
このチュートリアルでは、Update 5.1 より前のソフトウェア バージョンを実行している StorSimple デバイスに、Azure portal 経由で Update 5.1 をインストールする方法について説明します。 <!--The hotfix method is used when you are trying to install Update 5.1 on a device running pre-Update 3 versions. The hotfix method is also used when a gateway is configured on a network interface other than DATA 0 of the StorSimple device and you are trying to update from a pre-Update 1 software version.-->
Update 5.1 には、中断なしのセキュリティ更新プログラムが含まれています。 中断なしまたは通常の更新プログラムは、Azure portal を使用して適用できます <!--or by the hotfix method-->。
> [!IMPORTANT]
>
> * Update 5.1 は必須の更新プログラムであり、すぐにインストールする必要があります。 詳細については、[Update 5.1 リリース ノート](storsimple-update51-release-notes.md)に関する記事を参照してください。
> * インストールの前に、ハードウェアの状態とネットワーク接続の点からデバイスの正常性を判断するための手動と自動の一連の事前チェックが行われます。 これらの事前チェックは、Azure portal から更新プログラムを適用する場合にのみ実行されます。
> * 修正プログラムによる方法を使用してインストールする場合は、[Microsoft サポート](mailto:[email protected])にお問い合わせください。
<!--
> * We strongly recommend that when updating a device running versions prior to Update 3, you install the updates using hotfix method. If you encounter any issues, [log a support ticket](storsimple-8000-contact-microsoft-support.md).
> * We recommend that you install the software and other regular updates via the Azure portal. You should only go to the Windows PowerShell interface of the device (to install updates) if the pre-update gateway check fails in the portal. Depending upon the version you are updating from, the updates may take 4 hours (or greater) to install. The maintenance mode updates must be installed through the Windows PowerShell interface of the device. As maintenance mode updates are disruptive updates, these result in a down time for your device.
> * If running the optional StorSimple Snapshot Manager, ensure that you have upgraded your Snapshot Manager version to Update 5.1 prior to updating the device.
-->
[!INCLUDE [storsimple-preparing-for-update](../../includes/storsimple-preparing-for-updates.md)]
## <a name="install-update-51-through-the-azure-portal"></a>Azure portal を使用して Update 5.1 をインストールする
デバイスを [Update 5.1](storsimple-update51-release-notes.md) に更新するには、次の手順を実行します。
> [!NOTE]
> Microsoft はデバイスから追加の診断情報を取得します。 その結果、Microsoft の運用チームが問題のあるデバイスを識別したときに、デバイスから情報を収集して問題を診断する能力が向上します。
#### <a name="to-install-an-update-from-the-azure-portal"></a>Azure ポータルから 更新プログラムをインストールするには
1. StorSimple サービス ページでデバイスを選択します。

2. **[デバイスの設定]** > **[デバイスの更新プログラム]** の順に移動します。
![[デバイスの更新プログラム] をクリック](./media/storsimple-8000-install-update-51/update2.png)
3. 新しい更新プログラムが利用できる場合は、通知が表示されます。 または、 **[デバイスの更新プログラム]** ブレードで **[更新プログラムのスキャン]** をクリックします。 利用可能な更新プログラムをスキャンするジョブが作成されます。 ジョブが正常に完了すると、その旨が通知されます。
![[デバイスの更新プログラム] をクリック](./media/storsimple-8000-install-update-51/update3.png)
4. 更新プログラムをデバイスに適用する前に、リリース ノートを確認することをお勧めします。 **[更新プログラムのインストール]** をクリックすると、更新プログラムが適用されます。 **[定期更新プログラムの確認]** ブレードで、更新プログラムを適用する前に完了する必要のある前提条件を確認します。 デバイスを更新する準備ができたことを示すチェック ボックスをオンにし、 **[インストール]** をクリックします。
![[デバイスの更新プログラム] をクリック](./media/storsimple-8000-install-update-51/update4.png)
5. 一連の前提条件のチェックが開始されます。 これらのチェックは次のとおりです。
* **コントローラーの正常性チェック** では、両方のデバイス コントローラーが正常であり、オンラインであることを確認します。
* **ハードウェア コンポーネントの正常性チェック** では、StorSimple デバイスのすべてのハードウェア コンポーネントが正常であることを確認します。
* **DATA 0 チェック** では、デバイスで DATA 0 が有効であることを確認します。 このインターフェイスが有効でない場合は、有効にしてから再試行する必要があります。
すべてのチェックが正常に完了した場合にのみ、更新プログラムがダウンロードされてインストールされます。 チェックが実行中のときは通知されます。 事前チェックに失敗した場合、失敗の理由が表示されます。 それらの問題を解決してから操作をやり直してください。 これらの問題に自分で対処できない場合、Microsoft サポートに連絡することが必要になる場合があります。
7. 事前チェックが正常に完了したら、更新ジョブが作成されます。 更新ジョブが正常に作成されると、通知されます。

その後、更新プログラムがデバイスに適用されます。
9. 更新の完了には数時間かかります。 更新ジョブを選択し、 **[詳細]** をクリックすると、ジョブの詳細をいつでも表示できます。

**[デバイスの設定]、[ジョブ]** の順に移動して、更新ジョブの進行状況を監視することもできます。 **[ジョブ]** ブレードで、更新の進行状況を確認できます。

10. ジョブが完了したら、 **[デバイスの設定]、[デバイスの更新プログラム]** の順に移動します。 ソフトウェアのバージョンが更新されています。
デバイスで **StorSimple 8000 Series Update 5.1 (6.3.9600.17885)** が実行されていることを確認します。 **[最終更新日]** が変更されています。
<!-- 5.1 - KB 4542887-->
<!--You will now see that the Maintenance mode updates are available (this message might continue to be displayed for up to 24 hours after you install the updates). The steps to install maintenance mode update are detailed in the next section.
[!INCLUDE [storsimple-8000-install-maintenance-mode-updates](../../includes/storsimple-8000-install-maintenance-mode-updates.md)]
## Install Update 5.1 as a hotfix
The software versions that can be upgraded using the hotfix method are:
* Update 0.1, 0.2, 0.3
* Update 1, 1.1, 1.2
* Update 2, 2.1, 2.2
* Update 3, 3.1
* Update 4
* Update 5
> [!NOTE]
> The recommended method to install Update 5.1 is through the Azure portal when trying to update from Update 3 and later version. When updating a device running versions prior to Update 3, use this procedure. You can also use this procedure if you fail the gateway check when trying to install the updates through the Azure portal. The check fails when you have a gateway assigned to a non-DATA 0 network interface and your device is running a software version earlier than Update 1.
The hotfix method involves the following three steps:
1. Download the hotfixes from the Microsoft Update Catalog.
2. Install and verify the regular mode hotfixes.
3. Install and verify the maintenance mode hotfix.
#### Download updates for your device
You must download and install the following hotfixes in the prescribed order and the suggested folders:
| Order | KB | Description | Update type | Install time |Install in folder|
| --- | --- | --- | --- | --- | --- |
| 1. |KB4037264 |Software update<br> Download both _HcsSoftwareUpdate.exe_ and _CisMSDAgent.exe_ |Regular <br></br>Non-disruptive |~ 25 mins |FirstOrderUpdate|
If updating from a device running Update 4, you only need to install the OS cumulative updates as second order updates.
| Order | KB | Description | Update type | Install time |Install in folder|
| --- | --- | --- | --- | --- | --- |
| 2A. |KB4025336 |OS cumulative updates package <br> Download Windows Server 2012 R2 version |Regular <br></br>Non-disruptive |- |SecondOrderUpdate|
If installing from a device running Update 3 or earlier, install the following in addition to the cumulative updates.
| Order | KB | Description | Update type | Install time |Install in folder|
| --- | --- | --- | --- | --- | --- |
| 2B. |KB4011841 <br> KB4011842 |LSI driver and firmware updates <br> USM firmware update (version 3.38) |Regular <br></br>Non-disruptive |~ 3 hrs <br> (includes 2A. + 2B. + 2C.)|SecondOrderUpdate|
| 2C. |KB3139398 <br> KB3142030 <br> KB3108381 <br> KB3153704 <br> KB3174644 <br> KB3139914 |OS security updates package <br> Download Windows Server 2012 R2 version |Regular <br></br>Non-disruptive |- |SecondOrderUpdate|
| 2D. |KB3146621 <br> KB3103616 <br> KB3121261 <br> KB3123538 |OS updates package <br> Download Windows Server 2012 R2 version |Regular <br></br>Non-disruptive |- |SecondOrderUpdate|
You may also need to install disk firmware updates on top of all the updates shown in the preceding tables. You can verify whether you need the disk firmware updates by running the `Get-HcsFirmwareVersion` cmdlet. If you are running these firmware versions: `XMGJ`, `XGEG`, `KZ50`, `F6C2`, `VR08`, `N003`, `0107`, then you do not need to install these updates.
| Order | KB | Description | Update type | Install time | Install in folder|
| --- | --- | --- | --- | --- | --- |
| 3. |KB4037263 |Disk firmware |Maintenance <br></br>Disruptive |~ 30 mins | ThirdOrderUpdate |
<br></br>
> [!IMPORTANT]
> * If updating from Update 4, the total install time is close to 4 hours.
> * Before using this procedure to apply the update, make sure that both the device controllers are online and all the hardware components are healthy.
Perform the following steps to download and install the hotfixes.
[!INCLUDE [storsimple-install-update5-hotfix](../../includes/storsimple-install-update5-hotfix.md)]
-->
<!--
[!INCLUDE [storsimple-8000-install-troubleshooting](../../includes/storsimple-8000-install-troubleshooting.md)]
-->
## <a name="next-steps"></a>次のステップ
詳しくは、[Update 5.1 リリース](storsimple-update51-release-notes.md)に関するページをご覧ください。
| {
"pile_set_name": "Github"
} |
// RUN: %clang_cc1 %s -fcxx-exceptions -fexceptions -fsyntax-only -verify -fblocks -std=c++11 -Wunreachable-code-aggressive -Wno-unused-value -Wno-tautological-compare
int &halt() __attribute__((noreturn));
int &live();
int dead();
int liveti() throw(int);
int (*livetip)() throw(int);
int test1() {
try {
live();
} catch (int i) {
live();
}
return 1;
}
void test2() {
try {
live();
} catch (int i) {
live();
}
try {
liveti();
} catch (int i) {
live();
}
try {
livetip();
} catch (int i) {
live();
}
throw 1;
dead(); // expected-warning {{will never be executed}}
}
void test3() {
halt()
--; // expected-warning {{will never be executed}}
// FIXME: The unreachable part is just the '?', but really all of this
// code is unreachable and shouldn't be separately reported.
halt() // expected-warning {{will never be executed}}
?
dead() : dead();
live(),
float
(halt()); // expected-warning {{will never be executed}}
}
void test4() {
struct S {
int mem;
} s;
S &foor();
halt(), foor()// expected-warning {{will never be executed}}
.mem;
}
void test5() {
struct S {
int mem;
} s;
S &foonr() __attribute__((noreturn));
foonr()
.mem; // expected-warning {{will never be executed}}
}
void test6() {
struct S {
~S() { }
S(int i) { }
};
live(),
S
(halt()); // expected-warning {{will never be executed}}
}
// Don't warn about unreachable code in template instantiations, as
// they may only be unreachable in that specific instantiation.
void isUnreachable();
template <typename T> void test_unreachable_templates() {
T::foo();
isUnreachable(); // no-warning
}
struct TestUnreachableA {
static void foo() __attribute__((noreturn));
};
struct TestUnreachableB {
static void foo();
};
void test_unreachable_templates_harness() {
test_unreachable_templates<TestUnreachableA>();
test_unreachable_templates<TestUnreachableB>();
}
// Do warn about explict template specializations, as they represent
// actual concrete functions that somebody wrote.
template <typename T> void funcToSpecialize() {}
template <> void funcToSpecialize<int>() {
halt();
dead(); // expected-warning {{will never be executed}}
}
// Handle 'try' code dominating a dead return.
enum PR19040_test_return_t
{ PR19040_TEST_FAILURE };
namespace PR19040_libtest
{
class A {
public:
~A ();
};
}
PR19040_test_return_t PR19040_fn1 ()
{
try
{
throw PR19040_libtest::A ();
} catch (...)
{
return PR19040_TEST_FAILURE;
}
return PR19040_TEST_FAILURE; // expected-warning {{will never be executed}}
}
__attribute__((noreturn))
void raze();
namespace std {
template<typename T> struct basic_string {
basic_string(const T* x) {}
~basic_string() {};
};
typedef basic_string<char> string;
}
std::string testStr() {
raze();
return ""; // expected-warning {{'return' will never be executed}}
}
std::string testStrWarn(const char *s) {
raze();
return s; // expected-warning {{will never be executed}}
}
bool testBool() {
raze();
return true; // expected-warning {{'return' will never be executed}}
}
static const bool ConditionVar = 1;
int test_global_as_conditionVariable() {
if (ConditionVar)
return 1;
return 0; // no-warning
}
// Handle unreachable temporary destructors.
class A {
public:
A();
~A();
};
__attribute__((noreturn))
void raze(const A& x);
void test_with_unreachable_tmp_dtors(int x) {
raze(x ? A() : A()); // no-warning
}
// Test sizeof - sizeof in enum declaration.
enum { BrownCow = sizeof(long) - sizeof(char) };
enum { CowBrown = 8 - 1 };
int test_enum_sizeof_arithmetic() {
if (BrownCow)
return 1;
return 2;
}
int test_enum_arithmetic() {
if (CowBrown)
return 1;
return 2; // expected-warning {{never be executed}}
}
int test_arithmetic() {
if (8 -1)
return 1;
return 2; // expected-warning {{never be executed}}
}
int test_treat_const_bool_local_as_config_value() {
const bool controlValue = false;
if (!controlValue)
return 1;
test_treat_const_bool_local_as_config_value(); // no-warning
return 0;
}
int test_treat_non_const_bool_local_as_non_config_value() {
bool controlValue = false;
if (!controlValue)
return 1;
// There is no warning here because 'controlValue' isn't really
// a control value at all. The CFG will not treat this
// branch as unreachable.
test_treat_non_const_bool_local_as_non_config_value(); // no-warning
return 0;
}
void test_do_while(int x) {
// Handle trivial expressions with
// implicit casts to bool.
do {
break;
} while (0); // no-warning
}
class Frobozz {
public:
Frobozz(int x);
~Frobozz();
};
Frobozz test_return_object(int flag) {
return Frobozz(flag);
return Frobozz(42); // expected-warning {{'return' will never be executed}}
}
Frobozz test_return_object_control_flow(int flag) {
return Frobozz(flag);
return Frobozz(flag ? 42 : 24); // expected-warning {{code will never be executed}}
}
void somethingToCall();
static constexpr bool isConstExprConfigValue() { return true; }
int test_const_expr_config_value() {
if (isConstExprConfigValue()) {
somethingToCall();
return 0;
}
somethingToCall(); // no-warning
return 1;
}
int test_const_expr_config_value_2() {
if (!isConstExprConfigValue()) {
somethingToCall(); // no-warning
return 0;
}
somethingToCall();
return 1;
}
class Frodo {
public:
static const bool aHobbit = true;
};
void test_static_class_var() {
if (Frodo::aHobbit)
somethingToCall();
else
somethingToCall(); // no-warning
}
void test_static_class_var(Frodo &F) {
if (F.aHobbit)
somethingToCall();
else
somethingToCall(); // no-warning
}
void test_unreachable_for_null_increment() {
for (unsigned i = 0; i < 10 ; ) // no-warning
break;
}
void test_unreachable_forrange_increment() {
int x[10] = { 0 };
for (auto i : x) { // expected-warning {{loop will run at most once (loop increment never executed)}}
break;
}
}
void calledFun() {}
// Test "silencing" with parentheses.
void test_with_paren_silencing(int x) {
if (false) calledFun(); // expected-warning {{will never be executed}} expected-note {{silence by adding parentheses to mark code as explicitly dead}}
if ((false)) calledFun(); // no-warning
if (true) // expected-note {{silence by adding parentheses to mark code as explicitly dead}}
calledFun();
else
calledFun(); // expected-warning {{will never be executed}}
if ((true))
calledFun();
else
calledFun(); // no-warning
if (!true) // expected-note {{silence by adding parentheses to mark code as explicitly dead}}
calledFun(); // expected-warning {{code will never be executed}}
else
calledFun();
if ((!true))
calledFun(); // no-warning
else
calledFun();
if (!(true))
calledFun(); // no-warning
else
calledFun();
}
void test_with_paren_silencing_impcast(int x) {
if (0) calledFun(); // expected-warning {{will never be executed}} expected-note {{silence by adding parentheses to mark code as explicitly dead}}
if ((0)) calledFun(); // no-warning
if (1) // expected-note {{silence by adding parentheses to mark code as explicitly dead}}
calledFun();
else
calledFun(); // expected-warning {{will never be executed}}
if ((1))
calledFun();
else
calledFun(); // no-warning
if (!1) // expected-note {{silence by adding parentheses to mark code as explicitly dead}}
calledFun(); // expected-warning {{code will never be executed}}
else
calledFun();
if ((!1))
calledFun(); // no-warning
else
calledFun();
if (!(1))
calledFun(); // no-warning
else
calledFun();
}
void tautological_compare(bool x, int y) {
if (x > 10) // expected-note {{silence}}
calledFun(); // expected-warning {{will never be executed}}
if (10 < x) // expected-note {{silence}}
calledFun(); // expected-warning {{will never be executed}}
if (x == 10) // expected-note {{silence}}
calledFun(); // expected-warning {{will never be executed}}
if (x < 10) // expected-note {{silence}}
calledFun();
else
calledFun(); // expected-warning {{will never be executed}}
if (10 > x) // expected-note {{silence}}
calledFun();
else
calledFun(); // expected-warning {{will never be executed}}
if (x != 10) // expected-note {{silence}}
calledFun();
else
calledFun(); // expected-warning {{will never be executed}}
if (y != 5 && y == 5) // expected-note {{silence}}
calledFun(); // expected-warning {{will never be executed}}
if (y > 5 && y < 4) // expected-note {{silence}}
calledFun(); // expected-warning {{will never be executed}}
if (y < 10 || y > 5) // expected-note {{silence}}
calledFun();
else
calledFun(); // expected-warning {{will never be executed}}
// TODO: Extend warning to the following code:
if (x < -1)
calledFun();
if (x == -1)
calledFun();
if (x != -1)
calledFun();
else
calledFun();
if (-1 > x)
calledFun();
else
calledFun();
if (y == -1 && y != -1)
calledFun();
}
| {
"pile_set_name": "Github"
} |
//------------------------------------------------------------
// Copyright (c) Microsoft Corporation. All rights reserved.
//------------------------------------------------------------
#pragma warning disable 1634, 1691
namespace System.Workflow.Activities
{
using System;
using System.Collections;
using System.Collections.Generic;
using System.ComponentModel;
using System.Globalization;
using System.Reflection;
using System.ServiceModel;
internal sealed class ContractType : Type, ICloneable
{
private static readonly char[] elementDecorators = new char[] { '[', '*', '&' };
private static readonly char[] nameSeparators = new char[] { '.', '+' };
private Attribute[] attributes = null;
private ConstructorInfo[] constructors = null;
private EventInfo[] events = null;
private FieldInfo[] fields = null;
private string fullName;
private Guid guid = Guid.Empty;
private MethodInfo[] methods = null;
private string name;
private Type[] nestedTypes = Type.EmptyTypes;
private PropertyInfo[] properties = null;
private TypeAttributes typeAttributes;
internal ContractType(string name)
{
if (string.IsNullOrEmpty(name))
{
throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(
"name", SR2.GetString(SR2.Error_ArgumentValueNullOrEmptyString));
}
this.fullName = name;
this.name = this.fullName;
// detect first bracket, any name seperators after it are part of a generic parameter...
int idx = name.IndexOf('[');
// Get the name after the last dot
if (idx != -1)
{
idx = this.name.Substring(0, idx).LastIndexOfAny(nameSeparators);
}
else
{
idx = this.name.LastIndexOfAny(nameSeparators);
}
if (idx != -1)
{
this.name = this.fullName.Substring(idx + 1);
}
this.typeAttributes = TypeAttributes.Interface |
TypeAttributes.Sealed |
TypeAttributes.Public |
TypeAttributes.Abstract;
this.attributes = new Attribute[] { new ServiceContractAttribute() };
this.methods = new MethodInfo[0];
}
public override Assembly Assembly
{
get
{
return null;
}
}
public override string AssemblyQualifiedName
{
get
{
return this.FullName;
}
}
public override Type BaseType
{
get
{
return null;
}
}
public override Type DeclaringType
{
get
{
return null;
}
}
public override string FullName
{
get
{
return this.fullName;
}
}
public override Guid GUID
{
get
{
if (this.guid == Guid.Empty)
{
this.guid = Guid.NewGuid();
}
return this.guid;
}
}
public override Module Module
{
get
{
return null;
}
}
public override string Name
{
get
{
return this.name;
}
}
public override string Namespace
{
get
{
if (this.fullName == Name)
{
return string.Empty;
}
return this.fullName.Substring(0, this.fullName.Length - Name.Length - 1);
}
}
public override RuntimeTypeHandle TypeHandle
{
get
{
#pragma warning suppress 56503
throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(
new NotImplementedException(SR2.GetString(SR2.Error_RuntimeNotSupported)));
}
}
public override Type UnderlyingSystemType
{
get
{
return this;
}
}
public object Clone()
{
return this;
}
public override bool Equals(object obj)
{
if (obj == null)
{
return false;
}
ContractType contract = obj as ContractType;
if (contract == null)
{
return false;
}
if (string.Compare(this.AssemblyQualifiedName, contract.AssemblyQualifiedName, StringComparison.Ordinal) != 0 ||
this.methods.Length != contract.methods.Length)
{
return false;
}
foreach (MethodInfo methodInfo in this.methods)
{
if (this.GetMemberHelper<MethodInfo>(BindingFlags.Public | BindingFlags.Instance,
new MemberSignature(methodInfo),
ref contract.methods) == null)
{
return false;
}
}
return true;
}
public override int GetArrayRank()
{
throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(
SR2.GetString(SR2.Error_CurrentTypeNotAnArray));
}
public override ConstructorInfo[] GetConstructors(BindingFlags bindingAttr)
{
return GetMembersHelper<ConstructorInfo>(bindingAttr, ref this.constructors, false);
}
public override object[] GetCustomAttributes(bool inherit)
{
return GetCustomAttributes(typeof(object), inherit);
}
public override object[] GetCustomAttributes(Type attributeType, bool inherit)
{
if (attributeType == null)
{
throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("attributeType");
}
return ServiceOperationHelpers.GetCustomAttributes(attributeType, this.attributes);
}
public override MemberInfo[] GetDefaultMembers()
{
// Get all of the custom attributes
DefaultMemberAttribute attr = null;
for (Type t = this; t != null; t = t.BaseType)
{
object[] attrs = GetCustomAttributes(typeof(DefaultMemberAttribute), false);
if (attrs != null && attrs.Length > 0)
{
attr = attrs[0] as DefaultMemberAttribute;
}
if (attr != null)
{
break;
}
}
if (attr == null)
{
return new MemberInfo[0];
}
String defaultMember = attr.MemberName;
MemberInfo[] members = GetMember(defaultMember);
if (members == null)
{
members = new MemberInfo[0];
}
return members;
}
public override Type GetElementType()
{
return null;
}
public override EventInfo GetEvent(string name, BindingFlags bindingAttr)
{
return GetMemberHelper<EventInfo>(bindingAttr, new MemberSignature(name, null, null), ref this.events);
}
public override EventInfo[] GetEvents(BindingFlags bindingAttr)
{
return GetMembersHelper<EventInfo>(bindingAttr, ref this.events, true);
}
public override FieldInfo GetField(string name, BindingFlags bindingAttr)
{
return GetMemberHelper<FieldInfo>(bindingAttr, new MemberSignature(name, null, null), ref this.fields);
}
public override FieldInfo[] GetFields(BindingFlags bindingAttr)
{
return GetMembersHelper<FieldInfo>(bindingAttr, ref this.fields, true);
}
public override int GetHashCode()
{
return this.name.GetHashCode();
}
public override Type GetInterface(string name, bool ignoreCase)
{
if (string.IsNullOrEmpty(name))
{
throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(
"name", SR2.GetString(SR2.Error_ArgumentValueNullOrEmptyString));
}
if (string.Compare(this.name, name, StringComparison.Ordinal) == 0)
{
return this;
}
return null;
}
public override Type[] GetInterfaces()
{
return Type.EmptyTypes;
}
public override MemberInfo[] GetMember(string name, MemberTypes type, BindingFlags bindingAttr)
{
List<MemberInfo> members = new List<MemberInfo>();
// Methods
if ((type & MemberTypes.Method) != 0)
{
members.AddRange(GetMembersHelper<MethodInfo>(bindingAttr, new MemberSignature(name, null, null), ref this.methods));
}
// Constructors
if ((type & MemberTypes.Constructor) != 0)
{
members.AddRange(GetMembersHelper<ConstructorInfo>(bindingAttr, new MemberSignature(name, null, null), ref this.constructors));
}
// Properties
if ((type & MemberTypes.Property) != 0)
{
members.AddRange(GetMembersHelper<PropertyInfo>(bindingAttr, new MemberSignature(name, null, null), ref this.properties));
}
// Events
if ((type & MemberTypes.Event) != 0)
{
members.AddRange(GetMembersHelper<EventInfo>(bindingAttr, new MemberSignature(name, null, null), ref this.events));
}
// Fields
if ((type & MemberTypes.Field) != 0)
{
members.AddRange(GetMembersHelper<FieldInfo>(bindingAttr, new MemberSignature(name, null, null), ref this.fields));
}
// Nested types
if ((type & MemberTypes.NestedType) != 0)
{
members.AddRange(GetMembersHelper<Type>(bindingAttr, new MemberSignature(name, null, null), ref this.nestedTypes));
}
return members.ToArray();
}
public override MemberInfo[] GetMembers(BindingFlags bindingAttr)
{
ArrayList members = new ArrayList();
members.AddRange(GetMethods(bindingAttr));
members.AddRange(GetProperties(bindingAttr));
members.AddRange(GetEvents(bindingAttr));
members.AddRange(GetFields(bindingAttr));
members.AddRange(GetNestedTypes(bindingAttr));
return (MemberInfo[])members.ToArray(typeof(MemberInfo));
}
public override MethodInfo[] GetMethods(BindingFlags bindingAttr)
{
return GetMembersHelper<MethodInfo>(bindingAttr, ref this.methods, true);
}
public override Type GetNestedType(string name, BindingFlags bindingAttr)
{
return null;
}
public override Type[] GetNestedTypes(BindingFlags bindingAttr)
{
return Type.EmptyTypes;
}
public override PropertyInfo[] GetProperties(BindingFlags bindingAttr)
{
return GetMembersHelper<PropertyInfo>(bindingAttr, ref this.properties, true);
}
public override object InvokeMember(string name, BindingFlags bindingFlags, Binder binder, object target, object[] providedArgs, ParameterModifier[] modifiers, CultureInfo culture, string[] namedParams)
{
throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(
new NotImplementedException(SR2.GetString(SR2.Error_RuntimeNotSupported)));
}
public override bool IsAssignableFrom(Type type)
{
if (type == null)
{
return false;
}
if (!(type is ContractType))
{
return false;
}
return (this.Equals((Object)type));
}
public override bool IsDefined(Type attributeType, bool inherit)
{
if (attributeType == null)
{
throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("attributeType");
}
return ServiceOperationHelpers.IsDefined(attributeType, attributes);
}
public override bool IsSubclassOf(Type type)
{
return false;
}
public override Type MakeByRefType()
{
return this;
}
public override string ToString()
{
return this.FullName;
}
internal void AddMethod(ContractMethodInfo methodInfo)
{
if (methodInfo == null)
{
throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("methodInfo");
}
MemberSignature signature = new MemberSignature(methodInfo);
if (this.GetMemberHelper<MethodInfo>(BindingFlags.Public | BindingFlags.Instance,
signature,
ref this.methods) != null)
{
return;
}
else
{
List<MethodInfo> localMethods = new List<MethodInfo>();
if (this.methods != null)
{
localMethods.AddRange(this.methods);
}
localMethods.Add(methodInfo);
this.methods = new MethodInfo[localMethods.Count];
localMethods.CopyTo(this.methods);
}
}
protected override TypeAttributes GetAttributeFlagsImpl()
{
return typeAttributes;
}
protected override ConstructorInfo GetConstructorImpl(BindingFlags bindingAttr, Binder binder, CallingConventions callConvention, Type[] types, ParameterModifier[] modifiers)
{
return GetMemberHelper<ConstructorInfo>(bindingAttr, new MemberSignature(null, types, null), ref this.constructors);
}
protected override MethodInfo GetMethodImpl(string name, BindingFlags bindingAttr, Binder binder, CallingConventions callConvention, Type[] types, ParameterModifier[] modifiers)
{
return GetMemberHelper<MethodInfo>(bindingAttr, new MemberSignature(name, types, null), ref this.methods);
}
protected override PropertyInfo GetPropertyImpl(String name, BindingFlags bindingAttr, Binder binder, Type returnType, Type[] types, ParameterModifier[] modifiers)
{
return GetMemberHelper<PropertyInfo>(bindingAttr, new MemberSignature(name, types, null), ref this.properties);
}
protected override bool HasElementTypeImpl()
{
int elementCharPosition = Name.LastIndexOfAny(elementDecorators);
return (elementCharPosition != -1);
}
protected override bool IsArrayImpl()
{
int elementCharPosition = Name.LastIndexOfAny(elementDecorators);
if ((elementCharPosition != -1) && (Name[elementCharPosition] == '['))
{
return true;
}
return false;
}
protected override bool IsByRefImpl()
{
return false;
}
protected override bool IsCOMObjectImpl()
{
return false;
}
protected override bool IsContextfulImpl()
{
return false;
}
protected override bool IsMarshalByRefImpl()
{
return false;
}
protected override bool IsPointerImpl()
{
return (this.name[this.name.Length - 1] == '*');
}
protected override bool IsPrimitiveImpl()
{
return false;
}
private bool FilterMember(MemberInfo memberInfo, BindingFlags bindingFlags)
{
bool isPublic = false;
bool isStatic = false;
if (this.IsInterface)
{
isPublic = true;
isStatic = false;
}
else if (memberInfo is MethodBase)
{
isPublic = (memberInfo as MethodBase).IsPublic;
isStatic = (memberInfo as MethodBase).IsStatic;
}
else if (memberInfo is FieldInfo)
{
isPublic = (memberInfo as FieldInfo).IsPublic;
isStatic = (memberInfo as FieldInfo).IsStatic;
}
else if (memberInfo is PropertyInfo)
{
// Property public\static attributes can be fetched using the accessors
PropertyInfo propertyInfo = memberInfo as PropertyInfo;
MethodInfo accessorMethod = null;
if (propertyInfo.CanRead)
{
accessorMethod = propertyInfo.GetGetMethod(true);
}
else
{
accessorMethod = propertyInfo.GetSetMethod(true);
}
if (accessorMethod != null)
{
isPublic = accessorMethod.IsPublic;
isStatic = accessorMethod.IsStatic;
}
}
else if (memberInfo is Type)
{
isPublic = (memberInfo as Type).IsPublic || (memberInfo as Type).IsNestedPublic;
// No static check.
return ((((isPublic) && ((bindingFlags & BindingFlags.Public) != 0)) || ((!isPublic) && ((bindingFlags & BindingFlags.NonPublic) != 0))));
}
return ((((isPublic) && ((bindingFlags & BindingFlags.Public) != 0)) || ((!isPublic) && ((bindingFlags & BindingFlags.NonPublic) != 0))) && (((isStatic) && ((bindingFlags & BindingFlags.Static) != 0)) || ((!isStatic) && ((bindingFlags & BindingFlags.Instance) != 0))));
}
//private MemberInfo[] GetBaseMembers(Type type, Type baseType, BindingFlags bindingAttr)
//{
// MemberInfo[] members = null;
// if (type == typeof(PropertyInfo))
// {
// members = baseType.GetProperties(bindingAttr);
// }
// else if (type == typeof(EventInfo))
// {
// members = baseType.GetEvents(bindingAttr);
// }
// else if (type == typeof(ConstructorInfo))
// {
// members = baseType.GetConstructors(bindingAttr);
// }
// else if (type == typeof(MethodInfo))
// {
// members = baseType.GetMethods(bindingAttr);
// }
// else if (type == typeof(FieldInfo))
// {
// members = baseType.GetFields(bindingAttr);
// }
// else if (type == typeof(Type))
// {
// members = baseType.GetNestedTypes(bindingAttr);
// }
// return members;
//}
// generic method that implements all GetXXX methods
private T GetMemberHelper<T>(BindingFlags bindingAttr, MemberSignature memberSignature, ref T[] members)
where T : MemberInfo
{
if (members != null)
{
// search the local type
foreach (T memberInfo in members)
{
MemberSignature candididateMemberSignature = new MemberSignature(memberInfo);
if (candididateMemberSignature.FilterSignature(memberSignature) && FilterMember(memberInfo, bindingAttr))
{
return memberInfo;
}
}
}
return null;
}
// generic method that implements all GetXXXs methods
private T[] GetMembersHelper<T>(BindingFlags bindingAttr, ref T[] members, bool searchBase)
where T : MemberInfo
{
Dictionary<MemberSignature, T> membersDictionary = new Dictionary<MemberSignature, T>();
if (members != null)
{
// get local properties
foreach (T memberInfo in members)
{
MemberSignature memberSignature = new MemberSignature(memberInfo);
if ((FilterMember(memberInfo, bindingAttr)) && (!membersDictionary.ContainsKey(memberSignature)))
{
membersDictionary.Add(new MemberSignature(memberInfo), memberInfo);
}
}
}
if (searchBase && (bindingAttr & BindingFlags.DeclaredOnly) == 0)
{
// FlattenHierarchy is required to return static members from base classes.
if ((bindingAttr & BindingFlags.FlattenHierarchy) == 0)
{
bindingAttr &= ~BindingFlags.Static;
}
// Type baseType = BaseType;
// if (baseType != null)
// {
// T[] baseMembers = GetBaseMembers(typeof(T), baseType, bindingAttr) as T[];
// foreach (T memberInfo in baseMembers)
// {
// // We should not return private members from base classes. Note: Generics requires us to use "as".
// if ((memberInfo is FieldInfo && (memberInfo as FieldInfo).IsPrivate) || (memberInfo is MethodBase && (memberInfo as MethodBase).IsPrivate) || (memberInfo is Type && (memberInfo as Type).IsNestedPrivate))
// {
// continue;
// }
// // verify a member with this signature was not already created
// MemberSignature memberSignature = new MemberSignature(memberInfo);
// if (!membersDictionary.ContainsKey(memberSignature))
// {
// membersDictionary.Add(memberSignature, memberInfo);
// }
// }
// }
}
List<T> memberCollection = new List<T>(membersDictionary.Values);
return memberCollection.ToArray();
}
private T[] GetMembersHelper<T>(BindingFlags bindingAttr, MemberSignature memberSignature, ref T[] members)
where T : MemberInfo
{
List<T> memberCandidates = new List<T>();
foreach (T memberInfo in this.GetMembersHelper<T>(bindingAttr, ref members, true))
{
MemberSignature candididateMemberSignature = new MemberSignature(memberInfo);
if (candididateMemberSignature.FilterSignature(memberSignature))
{
memberCandidates.Add(memberInfo);
}
}
return memberCandidates.ToArray();
}
internal class MemberSignature
{
private string name = null;
private Type[] parameters = null;
private Type returnType = null;
internal MemberSignature(MemberInfo memberInfo)
{
this.name = memberInfo.Name;
if (memberInfo is MethodBase)
{
List<Type> typeCollection = new List<Type>();
// method/constructor arguments
foreach (ParameterInfo parameterInfo in (memberInfo as MethodBase).GetParameters())
{
typeCollection.Add(parameterInfo.ParameterType);
}
this.parameters = typeCollection.ToArray();
if (memberInfo is MethodInfo)
{
this.returnType = ((MethodInfo)memberInfo).ReturnType;
}
}
else if (memberInfo is PropertyInfo)
{
PropertyInfo propertyInfo = memberInfo as PropertyInfo;
List<Type> typeCollection = new List<Type>();
// indexer arguments
foreach (ParameterInfo parameterInfo in propertyInfo.GetIndexParameters())
{
typeCollection.Add(parameterInfo.ParameterType);
}
this.parameters = typeCollection.ToArray();
// return type for property
this.returnType = propertyInfo.PropertyType;
}
}
internal MemberSignature(string name, Type[] parameters, Type returnType)
{
this.name = name;
this.returnType = returnType;
if (parameters != null)
{
this.parameters = (Type[])parameters.Clone();
}
}
public string Name
{
get
{
return name;
}
}
public Type[] Parameters
{
get
{
if (parameters == null)
{
return null;
}
return (Type[])parameters.Clone();
}
}
public Type ReturnType
{
get
{
return returnType;
}
}
public override bool Equals(object obj)
{
MemberSignature memberSignature = obj as MemberSignature;
if ((memberSignature == null) ||
(this.name != memberSignature.Name) ||
(this.returnType != memberSignature.ReturnType))
{
return false;
}
if ((this.Parameters == null) && (memberSignature.Parameters != null) ||
(this.Parameters != null) && (memberSignature.Parameters == null))
{
return false;
}
if (this.Parameters != null)
{
if (this.parameters.Length != memberSignature.parameters.Length)
{
return false;
}
for (int loop = 0; loop < this.parameters.Length; loop++)
{
if (this.parameters[loop] != memberSignature.parameters[loop])
{
return false;
}
}
}
return true;
}
// this method will filter using a mask signautre. only non-null mask members are used to filter
// the signature, the rest are ignored
public bool FilterSignature(MemberSignature maskSignature)
{
if (maskSignature == null)
{
throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("maskSignature");
}
if (((maskSignature.Name != null) && (this.name != maskSignature.name)) ||
((maskSignature.returnType != null) && (this.returnType != maskSignature.returnType)))
{
return false;
}
if (maskSignature.parameters != null)
{
if (this.parameters == null)
{
return false;
}
if (this.parameters.Length != maskSignature.parameters.Length)
{
return false;
}
for (int loop = 0; loop < this.parameters.Length; loop++)
{
if (!this.parameters[loop].Equals(maskSignature.parameters[loop]))
{
return false;
}
}
}
return true;
}
public override int GetHashCode()
{
return ToString().GetHashCode();
}
public override string ToString()
{
string str = string.Empty;
if (returnType != null)
{
str = returnType.FullName + " ";
}
if (name != null && name.Length != 0)
{
str += name;
}
if (parameters != null && parameters.Length > 0)
{
str += "(";
for (int i = 0; i < parameters.Length; i++)
{
if (i > 0)
{
str += ", ";
}
if (parameters[i] != null)
{
if (parameters[i].GetType() != null && parameters[i].GetType().IsByRef)
{
str += "ref ";
}
str += parameters[i].FullName;
}
}
str += ")";
}
return str;
}
}
}
}
| {
"pile_set_name": "Github"
} |
using System;
using System.Diagnostics;
using System.IO;
using System.Linq;
using System.Windows.Forms;
using Microsoft.Win32;
using Nexus.Client.Settings;
using Nexus.Client.UI;
using Nexus.Client.Util;
using Nexus.Client.Games.Steam;
using System.Xml;
namespace Nexus.Client.Games.NoMansSky
{
/// <summary>
/// The base game mode factory that provides the commond functionality for
/// factories that build game modes for NoMansSky based games.
/// </summary>
public class NoMansSkyGameModeFactory : IGameModeFactory
{
private readonly IGameModeDescriptor m_gmdGameModeDescriptor = null;
#region Properties
/// <summary>
/// Gets the application's environement info.
/// </summary>
/// <value>The application's environement info.</value>
protected IEnvironmentInfo EnvironmentInfo { get; private set; }
/// <summary>
/// Gets the descriptor of the game mode that this factory builds.
/// </summary>
/// <value>The descriptor of the game mode that this factory builds.</value>
public IGameModeDescriptor GameModeDescriptor
{
get
{
return m_gmdGameModeDescriptor;
}
}
#endregion
#region Constructors
/// <summary>
/// A simple consturctor that initializes the object with the given values.
/// </summary>
/// <param name="p_eifEnvironmentInfo">The application's environement info.</param>
public NoMansSkyGameModeFactory(IEnvironmentInfo p_eifEnvironmentInfo)
{
EnvironmentInfo = p_eifEnvironmentInfo;
m_gmdGameModeDescriptor = new NoMansSkyGameModeDescriptor(p_eifEnvironmentInfo);
}
#endregion
/// <summary>
/// Gets the path where mod files should be installed.
/// </summary>
/// <returns>The path where mod files should be installed, or
/// <c>null</c> if the path could not be determined.</returns>
public string GetInstallationPath()
{
string strValue = SteamInstallationPathDetector.Instance.GetSteamInstallationPath("275850", "No Man's Sky", "NMS.exe");
return strValue;
}
/// <summary>
/// Gets the path where mod files should be installed.
/// </summary>
/// <remarks>
/// This method uses the given path to the installed game
/// to determine the installaiton path for mods.
/// </remarks>
/// <returns>The path where mod files should be installed, or
/// <c>null</c> if the path could be be determined.</returns>
public string GetInstallationPath(string p_strGameInstallPath)
{
return p_strGameInstallPath;
}
/// <summary>
/// Gets the path to the game executable.
/// </summary>
/// <returns>The path to the game executable, or
/// <c>null</c> if the path could not be determined.</returns>
public string GetExecutablePath(string p_strPath)
{
return p_strPath;
}
/// <summary>
/// Builds the game mode.
/// </summary>
/// <param name="p_futFileUtility">The file utility class to be used by the game mode.</param>
/// <param name="p_imsWarning">The resultant warning resultant from the creation of the game mode.
/// <c>null</c> if there are no warnings.</param>
/// <returns>The game mode.</returns>
public IGameMode BuildGameMode(FileUtil p_futFileUtility, out ViewMessage p_imsWarning)
{
if (EnvironmentInfo.Settings.CustomGameModeSettings[GameModeDescriptor.ModeId] == null)
EnvironmentInfo.Settings.CustomGameModeSettings[GameModeDescriptor.ModeId] = new PerGameModeSettings<object>();
if (!EnvironmentInfo.Settings.CustomGameModeSettings[GameModeDescriptor.ModeId].ContainsKey("AskAboutReadOnlySettingsFiles"))
{
EnvironmentInfo.Settings.CustomGameModeSettings[GameModeDescriptor.ModeId]["AskAboutReadOnlySettingsFiles"] = true;
EnvironmentInfo.Settings.CustomGameModeSettings[GameModeDescriptor.ModeId]["UnReadOnlySettingsFiles"] = true;
EnvironmentInfo.Settings.Save();
}
NoMansSkyGameMode gmdGameMode = InstantiateGameMode(p_futFileUtility);
p_imsWarning = null;
return gmdGameMode;
}
/// <summary>
/// Instantiates the game mode.
/// </summary>
/// <param name="p_futFileUtility">The file utility class to be used by the game mode.</param>
/// <returns>The game mode for which this is a factory.</returns>
protected NoMansSkyGameMode InstantiateGameMode(FileUtil p_futFileUtility)
{
return new NoMansSkyGameMode(EnvironmentInfo, p_futFileUtility);
}
/// <summary>
/// Performs the initial setup for the game mode being created.
/// </summary>
/// <param name="p_dlgShowView">The delegate to use to display a view.</param>
/// <param name="p_dlgShowMessage">The delegate to use to display a message.</param>
/// <returns><c>true</c> if the setup completed successfully;
/// <c>false</c> otherwise.</returns>
public bool PerformInitialSetup(ShowViewDelegate p_dlgShowView, ShowMessageDelegate p_dlgShowMessage)
{
if (EnvironmentInfo.Settings.CustomGameModeSettings[GameModeDescriptor.ModeId] == null)
EnvironmentInfo.Settings.CustomGameModeSettings[GameModeDescriptor.ModeId] = new PerGameModeSettings<object>();
NoMansSkySetupVM vmlSetup = new NoMansSkySetupVM(EnvironmentInfo, GameModeDescriptor);
SetupForm frmSetup = new SetupForm(vmlSetup);
if (((DialogResult)p_dlgShowView(frmSetup, true)) == DialogResult.Cancel)
return false;
return vmlSetup.Save();
}
/// <summary>
/// Performs the initializtion for the game mode being created.
/// </summary>
/// <param name="p_dlgShowView">The delegate to use to display a view.</param>
/// <param name="p_dlgShowMessage">The delegate to use to display a message.</param>
/// <returns><c>true</c> if the setup completed successfully;
/// <c>false</c> otherwise.</returns>
public bool PerformInitialization(ShowViewDelegate p_dlgShowView, ShowMessageDelegate p_dlgShowMessage)
{
return true;
}
}
}
| {
"pile_set_name": "Github"
} |
&FORCE_EVAL
METHOD Quickstep
&DFT
&QS
METHOD PM6
&SE
&END
&END QS
&SCF
MAX_SCF 0
&END SCF
&END DFT
&SUBSYS
&CELL
#Mn_2 O_3 & Ia(-3)-T_h^7 #206 (bde) & D5_3 & cI80 & Dachs, Zf Kristall. 107, 37
A -4.70000000 4.70000000 4.70000000
B 4.70000000 -4.70000000 4.70000000
C 4.70000000 4.70000000 -4.70000000
&END CELL
&COORD
SCALED
Fe 0.50000000 0.50000000 0.50000000 2.35000000 2.35000000 2.35000000
Fe 0.50000000 0.00000000 0.00000000 -2.35000000 2.35000000 2.35000000
Fe 0.00000000 0.50000000 0.00000000 2.35000000 -2.35000000 2.35000000
Fe 0.00000000 0.00000000 0.50000000 2.35000000 2.35000000 -2.35000000
Mn 0.25000000 0.21560000 -0.03440000 -0.32336000 0.00000000 2.35000000
Mn -0.25000000 0.28440000 -0.46560000 0.32336000 -4.70000000 2.35000000
Mn -0.03440000 0.25000000 0.21560000 2.35000000 -0.32336000 0.00000000
Mn -0.46560000 -0.25000000 0.28440000 2.35000000 0.32336000 -4.70000000
Mn 0.21560000 -0.03440000 0.25000000 0.00000000 2.35000000 -0.32336000
Mn 0.28440000 -0.46560000 -0.25000000 -4.70000000 2.35000000 0.32336000
Mn -0.25000000 -0.21560000 0.03440000 0.32336000 0.00000000 -2.35000000
Mn 0.25000000 -0.28440000 0.46560000 -0.32336000 4.70000000 -2.35000000
Mn 0.03440000 -0.25000000 -0.21560000 -2.35000000 0.32336000 0.00000000
Mn 0.46560000 0.25000000 -0.28440000 -2.35000000 -0.32336000 4.70000000
Mn -0.21560000 0.03440000 -0.25000000 0.00000000 -2.35000000 0.32336000
Mn -0.28440000 0.46560000 0.25000000 4.70000000 -2.35000000 -0.32336000
O 0.22500000 0.46300000 0.43800000 3.17720000 0.94000000 1.17500000
O -0.47500000 -0.21300000 0.06200000 1.52280000 -0.94000000 -3.52500000
O -0.02500000 0.03700000 0.26200000 1.52280000 0.94000000 -1.17500000
O 0.27500000 -0.28700000 0.23800000 -1.52280000 3.76000000 -1.17500000
O 0.43800000 0.22500000 0.46300000 1.17500000 3.17720000 0.94000000
O 0.06200000 -0.47500000 -0.21300000 -3.52500000 1.52280000 -0.94000000
O 0.26200000 -0.02500000 0.03700000 -1.17500000 1.52280000 0.94000000
O 0.23800000 0.27500000 -0.28700000 -1.17500000 -1.52280000 3.76000000
O 0.46300000 0.43800000 0.22500000 0.94000000 1.17500000 3.17720000
O -0.21300000 0.06200000 -0.47500000 -0.94000000 -3.52500000 1.52280000
O 0.03700000 0.26200000 -0.02500000 0.94000000 -1.17500000 1.52280000
O -0.28700000 0.23800000 0.27500000 3.76000000 -1.17500000 -1.52280000
O -0.22500000 -0.46300000 -0.43800000 -3.17720000 -0.94000000 -1.17500000
O 0.47500000 0.21300000 -0.06200000 -1.52280000 0.94000000 3.52500000
O 0.02500000 -0.03700000 -0.26200000 -1.52280000 -0.94000000 1.17500000
O -0.27500000 0.28700000 -0.23800000 1.52280000 -3.76000000 1.17500000
O -0.43800000 -0.22500000 -0.46300000 -1.17500000 -3.17720000 -0.94000000
O -0.06200000 0.47500000 0.21300000 3.52500000 -1.52280000 0.94000000
O -0.26200000 0.02500000 -0.03700000 1.17500000 -1.52280000 -0.94000000
O -0.23800000 -0.27500000 0.28700000 1.17500000 1.52280000 -3.76000000
O -0.46300000 -0.43800000 -0.22500000 -0.94000000 -1.17500000 -3.17720000
O 0.21300000 -0.06200000 0.47500000 0.94000000 3.52500000 -1.52280000
O -0.03700000 -0.26200000 0.02500000 -0.94000000 1.17500000 -1.52280000
O 0.28700000 -0.23800000 -0.27500000 -3.76000000 1.17500000 1.52280000
&END COORD
&PRINT
&SYMMETRY
CHECK_SYMMETRY m<3>
&END
&END
&TOPOLOGY
CONNECTIVITY OFF
&END
&END SUBSYS
&END FORCE_EVAL
&GLOBAL
PROJECT c_29_bixbyite
RUN_TYPE ENERGY
&END GLOBAL
| {
"pile_set_name": "Github"
} |
import * as ts from "typescript";
import * as Lint from "tslint";
import * as utils from "tsutils/typeguard/2.8";
import * as Ignore from "./shared/ignore";
import {
createInvalidNode,
CheckNodeResult,
createCheckNodeRule
} from "./shared/check-node";
type Options = Ignore.IgnoreLocalOption & Ignore.IgnoreOption;
// tslint:disable-next-line:variable-name
export const Rule = createCheckNodeRule(
Ignore.checkNodeWithIgnore(checkNode),
"Unexpected let, use const instead."
);
function checkNode(
node: ts.Node,
ctx: Lint.WalkContext<Options>
): CheckNodeResult {
const results = [
checkVariableStatement(node, ctx),
checkForStatements(node, ctx)
];
return {
invalidNodes: results.reduce(
(merged, result) => [...merged, ...result.invalidNodes],
[]
),
skipChildren: results.some(result => result.skipChildren === true)
};
}
function checkVariableStatement(
node: ts.Node,
ctx: Lint.WalkContext<Options>
): CheckNodeResult {
if (utils.isVariableStatement(node)) {
return checkDeclarationList(node.declarationList, ctx);
}
return { invalidNodes: [] };
}
function checkForStatements(
node: ts.Node,
ctx: Lint.WalkContext<Options>
): CheckNodeResult {
if (
(utils.isForStatement(node) ||
utils.isForInStatement(node) ||
utils.isForOfStatement(node)) &&
node.initializer &&
utils.isVariableDeclarationList(node.initializer) &&
Lint.isNodeFlagSet(node.initializer, ts.NodeFlags.Let)
) {
return checkDeclarationList(node.initializer, ctx);
}
return { invalidNodes: [] };
}
function checkDeclarationList(
declarationList: ts.VariableDeclarationList,
ctx: Lint.WalkContext<Options>
): CheckNodeResult {
if (Lint.isNodeFlagSet(declarationList, ts.NodeFlags.Let)) {
// It is a let declaration, now check each variable that is declared
const invalidVariableDeclarationNodes = [];
// If the declaration list contains multiple variables, eg. let x = 0, y = 1, mutableZ = 3; then
// we should only provide one fix for the list even if two variables are invalid.
// NOTE: When we have a mix of allowed and disallowed variables in the same DeclarationList
// there is no sure way to know if we should do a fix or not, eg. if ignore-prefix=mutable
// and the list is "let x, mutableZ", then "x" is invalid but "mutableZ" is valid, should we change
// "let" to "const" or not? For now we change to const if at least one variable is invalid.
let addFix = true;
for (const variableDeclarationNode of declarationList.declarations) {
if (
!Ignore.shouldIgnore(
variableDeclarationNode,
ctx.options,
ctx.sourceFile
)
) {
invalidVariableDeclarationNodes.push(
createInvalidNode(
variableDeclarationNode,
addFix
? [
new Lint.Replacement(
declarationList.getStart(ctx.sourceFile),
"let".length,
"const"
)
]
: []
)
);
addFix = false;
}
}
return { invalidNodes: invalidVariableDeclarationNodes };
}
return { invalidNodes: [] };
}
| {
"pile_set_name": "Github"
} |
require(['GlobalShortcutsHelp'], function (gs) {
'use strict';
function init() {
$('.global-shortcuts-help-entry-point').on('click', gs.open);
}
$(init);
});
| {
"pile_set_name": "Github"
} |
using System;
using System.Reflection;
using FluentAssertions;
using Newtonsoft.Json.Linq;
using Xunit;
namespace Serilog.Sinks.Elasticsearch.Tests.Templating
{
public class SendsTemplateTests : ElasticsearchSinkTestsBase
{
private readonly Tuple<Uri, string> _templatePut;
public SendsTemplateTests()
{
_options.AutoRegisterTemplate = true;
var loggerConfig = new LoggerConfiguration()
.MinimumLevel.Debug()
.Enrich.WithMachineName()
.WriteTo.ColoredConsole()
.WriteTo.Elasticsearch(_options);
var logger = loggerConfig.CreateLogger();
using (logger as IDisposable)
{
logger.Error("Test exception. Should not contain an embedded exception object.");
}
this._seenHttpPosts.Should().NotBeNullOrEmpty().And.HaveCount(1);
this._seenHttpPuts.Should().NotBeNullOrEmpty().And.HaveCount(1);
_templatePut = this._seenHttpPuts[0];
}
[Fact]
public void ShouldRegisterTheCorrectTemplateOnRegistration()
{
var method = typeof(SendsTemplateTests).GetMethod(nameof(ShouldRegisterTheCorrectTemplateOnRegistration));
JsonEquals(_templatePut.Item2, method, "template");
}
[Fact]
public void TemplatePutToCorrectUrl()
{
var uri = _templatePut.Item1;
uri.AbsolutePath.Should().Be("/_template/serilog-events-template");
}
protected void JsonEquals(string json, MethodBase method, string fileName = null)
{
#if DOTNETCORE
var assembly = typeof(SendsTemplateTests).GetTypeInfo().Assembly;
#else
var assembly = Assembly.GetExecutingAssembly();
#endif
var expected = TestDataHelper.ReadEmbeddedResource(assembly, "template.json");
var nJson = JObject.Parse(json);
var nOtherJson = JObject.Parse(expected);
var equals = JToken.DeepEquals(nJson, nOtherJson);
if (equals) return;
expected.Should().BeEquivalentTo(json);
}
}
} | {
"pile_set_name": "Github"
} |
// Sandstorm - Personal Cloud Sandbox
// Copyright (c) 2016 Sandstorm Development Group, Inc. and contributors
// All rights reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
"use strict";
var crypto = require("crypto");
var utils = require("../utils"),
short_wait = utils.short_wait,
medium_wait = utils.medium_wait,
long_wait = utils.long_wait;
var COLLECTIONS_APP_ID = "s3u2xgmqwznz2n3apf30sm3gw1d85y029enw5pymx734cnk5n78h";
var COLLECTIONS_PACKAGE_ID = "e9408a7c077f7a9baeb9c02f0437ae40";
var COLLECTIONS_PACKAGE_URL = "https://sandstorm.io/apps/david/collections3.spk";
module.exports = {};
function setGrainTitle(browser, collectionTitle) {
return browser
.waitForElementVisible("#grainTitle", short_wait)
.click("#grainTitle")
.setAlertText(collectionTitle)
.acceptAlert()
.grainFrame()
.waitForElementVisible("button[title='add description']", short_wait)
.click("button[title='add description']")
.waitForElementVisible("form.description-row>input[type=text]", short_wait)
.setValue("form.description-row>input[type=text]", "This is " + collectionTitle)
.click("form.description-row>button")
.frame(null)
}
function powerboxCardSelector(grainId) {
return ".popup.request .candidate-cards .powerbox-card button[data-card-id=grain-" + grainId + "]";
}
module.exports["Test Collections"] = function (browser) {
// Prepend 'A' so that the default handle is valid.
var devNameAlice = "A" + crypto.randomBytes(10).toString("hex");
var devNameBob = "A" + crypto.randomBytes(10).toString("hex");
var devNameCarol = "A" + crypto.randomBytes(10).toString("hex");
browser
.loginDevAccount(devNameBob)
.executeAsync(function (done) {
done(Meteor.userId());
}, [], function (result) {
var bobAccountId = result.value;
browser.execute("window.Meteor.logout()")
.loginDevAccount(devNameCarol)
.executeAsync(function (done) {
done(Meteor.userId());
}, [], function (result) {
var carolAccountId = result.value;
browser.execute("window.Meteor.logout()")
.init()
.loginDevAccount(devNameAlice)
.installApp(COLLECTIONS_PACKAGE_URL, COLLECTIONS_PACKAGE_ID, COLLECTIONS_APP_ID);
browser = setGrainTitle(browser, "Collection A");
browser.executeAsync(function (bobAccountId, done) {
// Share Collection A to Bob.
var grainId = Grains.findOne()._id;
Meteor.call("newApiToken", { accountId: Meteor.userId() },
grainId, "petname", { allAccess: null },
{ user: { accountId: bobAccountId, title: "Collection A", } },
function(error, result) {
done({ error: error, grainId: grainId, });
});
}, [bobAccountId], function (result) {
var grainIdA = result.value.grainId;
browser.assert.equal(!result.value.error, true);
browser.newGrain(COLLECTIONS_APP_ID, function (grainIdB) {
browser = setGrainTitle(browser, "Collection B");
browser.newGrain(COLLECTIONS_APP_ID, function (grainIdC) {
browser = setGrainTitle(browser, "Collection C");
browser = browser
.url(browser.launch_url + "/grain/" + grainIdA)
.grainFrame()
.waitForElementVisible("table.grain-list-table>tbody>tr.add-grain>td>button", medium_wait)
.click("table.grain-list-table>tbody>tr.add-grain>td>button")
.frame(null)
.waitForElementVisible(powerboxCardSelector(grainIdB), short_wait)
.click(powerboxCardSelector(grainIdB))
// Add with 'editor' permissions.
.waitForElementVisible(".popup.request .selected-card>form input[value='0']", short_wait)
.click(".popup.request .selected-card>form input[value='0']")
.click(".popup.request .selected-card>form button.connect-button")
.grainFrame()
.waitForElementVisible("table.grain-list-table>tbody>tr.add-grain>td>button", short_wait)
.click("table.grain-list-table>tbody>tr.add-grain>td>button")
.frame(null)
.waitForElementVisible(powerboxCardSelector(grainIdC), short_wait)
.click(powerboxCardSelector(grainIdC))
// Add with 'viewer' permissions.
.waitForElementVisible(".popup.request .selected-card>form input[value='1']", short_wait)
.click(".popup.request .selected-card>form input[value='1']")
.click(".popup.request .selected-card>form button.connect-button")
.grainFrame()
.waitForElementVisible("table.grain-list-table>tbody tr:nth-child(3).grain", short_wait)
.click("table.grain-list-table>tbody tr:nth-child(3).grain .click-to-go")
.frame(null)
.grainFrame(grainIdC)
.waitForElementVisible(".description-row p", short_wait)
.assert.containsText(".description-row p", "This is Collection C")
.waitForElementVisible(".description-row button.description-button", short_wait)
.frame(null)
.execute("window.Meteor.logout()")
// Log in as Bob
.loginDevAccount(devNameBob)
.url(browser.launch_url + "/grain/" + grainIdA)
.grainFrame()
.waitForElementVisible(".description-row p", short_wait)
.assert.containsText(".description-row p", "This is Collection A")
.waitForElementVisible(".description-row button.description-button", short_wait)
.waitForElementVisible("table.grain-list-table>tbody>tr.add-grain>td>button", short_wait)
.waitForElementVisible("table.grain-list-table>tbody tr:nth-child(2).grain", short_wait)
.assert.containsText("table.grain-list-table>tbody tr:nth-child(2).grain td>button",
"Collection B")
.click("table.grain-list-table>tbody tr:nth-child(2).grain .click-to-go")
.frame(null)
.grainFrame(grainIdB)
.waitForElementVisible(".description-row p", short_wait)
.assert.containsText(".description-row p", "This is Collection B")
.waitForElementVisible(".description-row button.description-button", short_wait)
// As Bob, add collection A to collection B, creating a cycle of references.
.waitForElementVisible("table.grain-list-table>tbody>tr.add-grain>td>button", short_wait)
.click("table.grain-list-table>tbody>tr.add-grain>td>button")
.frame(null)
.waitForElementVisible(powerboxCardSelector(grainIdA), short_wait)
.click(powerboxCardSelector(grainIdA))
// Add with 'viewer' permissions.
.waitForElementVisible(".popup.request .selected-card>form input[value='1']", short_wait)
.click(".popup.request .selected-card>form input[value='1']")
.click(".popup.request .selected-card>form button.connect-button")
// Navigate back to collection A by clicking on it in collection B.
.grainFrame()
.waitForElementVisible("table.grain-list-table>tbody tr:nth-child(2).grain", short_wait)
.click("table.grain-list-table>tbody tr:nth-child(2).grain .click-to-go")
.frame(null)
.grainFrame(grainIdA)
.waitForElementVisible("table.grain-list-table>tbody>tr.add-grain>td>button", short_wait)
.waitForElementVisible("table.grain-list-table>tbody tr:nth-child(3).grain", short_wait)
.assert.containsText("table.grain-list-table>tbody tr:nth-child(3).grain td>button",
"Collection C")
.click("table.grain-list-table>tbody tr:nth-child(3).grain .click-to-go")
.frame(null)
.grainFrame(grainIdC)
.waitForElementVisible(".description-row p", short_wait)
.assert.containsText(".description-row p", "This is Collection C")
.assert.elementNotPresent(".description-row button.description-button")
.frame(null)
.executeAsync(function (carolAccountId, grainIdB, done) {
// As Bob, share Collection B to Carol.
Meteor.call("newApiToken", { accountId: Meteor.userId() },
grainIdB, "petname", { allAccess: null },
{ user: { accountId: carolAccountId, title: "Collection B", } },
function(error, result) {
done({ error: error });
});
}, [carolAccountId, grainIdB], function (result) {
browser.assert.equal(!result.value.error, true);
browser
.execute("window.Meteor.logout()")
// Log in as Carol
.loginDevAccount(devNameCarol)
.url(browser.launch_url + "/grain/" + grainIdB)
.grainFrame()
.waitForElementVisible(".description-row p", short_wait)
.assert.containsText(".description-row p", "This is Collection B")
.waitForElementVisible(".description-row button.description-button", short_wait)
.waitForElementVisible("table.grain-list-table>tbody tr:nth-child(2).grain",
short_wait)
.assert.containsText("table.grain-list-table>tbody tr:nth-child(2).grain td>button",
"Collection A")
.click("table.grain-list-table>tbody tr:nth-child(2).grain .click-to-go")
.grainFrame(grainIdA)
.waitForElementVisible(".description-row p", short_wait)
.assert.containsText(".description-row p", "This is Collection A")
// Carol does not have edit permissions.
.assert.elementNotPresent(".description-row button.description-button")
.frame(null)
.execute("window.Meteor.logout()")
// Log back in as Alice
.loginDevAccount(devNameAlice)
.url(browser.launch_url + "/grain/" + grainIdA)
.disableGuidedTour()
.grainFrame()
// Unlink collection B from collection A.
.waitForElementVisible("table.grain-list-table>tbody tr:nth-child(3).grain",
short_wait)
.waitForElementVisible("table.grain-list-table>tbody tr:nth-child(2).grain",
short_wait)
.assert.containsText("table.grain-list-table>tbody tr:nth-child(2).grain td>button",
"Collection B")
.click("table.grain-list-table>tbody tr:nth-child(2).grain td>input[type=checkbox]")
.waitForElementVisible(".bulk-action-buttons>button[title='unlink selected grains']",
short_wait)
.click(".bulk-action-buttons>button[title='unlink selected grains']")
.frame(null)
.execute("window.Meteor.logout()")
// Log back in as Carol. Check that she can no longer access collection A.
.loginDevAccount(devNameCarol)
// Add some characters onto the end of the URL because otherwise we trigger
// the grain-tab restore logic and tabs open for both Collection A and Collection B.
.url(browser.launch_url + "/grain/" + grainIdA + "/#")
.waitForElementVisible(".grain-interstitial.request-access", medium_wait)
.end();
});
});
});
});
});
});
};
module.exports["Test collections anonymous user"] = function (browser) {
browser = browser
.init()
.loginDevAccount()
.installApp(COLLECTIONS_PACKAGE_URL, COLLECTIONS_PACKAGE_ID, COLLECTIONS_APP_ID);
browser = setGrainTitle(browser, "Collection A");
browser.executeAsync(function (done) {
var grainId = Grains.findOne()._id;
Meteor.call("newApiToken", { accountId: Meteor.userId() },
grainId, "petname", { allAccess: null },
{ webkey: { forSharing: true }, },
function(error, result) {
done({ error: error, grainId: grainId, token: (result || {}).token });
});
}, [], function (result) {
var grainIdA = result.value.grainId;
var tokenA = result.value.token;
browser.assert.equal(!result.value.error, true);
browser.newGrain(COLLECTIONS_APP_ID, function (grainIdB) {
browser = setGrainTitle(browser, "Collection B");
browser
.url(browser.launch_url + "/grain/" + grainIdA)
.grainFrame()
.waitForElementVisible("table.grain-list-table>tbody>tr.add-grain>td>button", medium_wait)
.click("table.grain-list-table>tbody>tr.add-grain>td>button")
.frame(null)
.waitForElementVisible(powerboxCardSelector(grainIdB), short_wait)
.click(powerboxCardSelector(grainIdB))
// Add with 'editor' permissions.
.waitForElementVisible(".popup.request .selected-card>form input[value='0']", short_wait)
.click(".popup.request .selected-card>form input[value='0']")
.click(".popup.request .selected-card>form button.connect-button")
// Visit token A anonymously. The link should still work.
.frame(null)
.execute("window.Meteor.logout()")
.url(browser.launch_url + "/shared/" + tokenA)
.waitForElementVisible(".popup.login button.close-popup", short_wait)
.click(".popup.login button.close-popup")
.grainFrame()
.waitForElementVisible(".description-row p", short_wait)
.assert.containsText(".description-row p", "This is Collection A")
.waitForElementVisible(".description-row button.description-button", short_wait)
.waitForElementVisible("table.grain-list-table>tbody tr:nth-child(1).grain", short_wait)
.assert.containsText("table.grain-list-table>tbody tr:nth-child(1).grain td>button",
"Collection B")
.click("table.grain-list-table>tbody tr:nth-child(1).grain .click-to-go")
.frame(null)
.grainFrame(grainIdB)
.waitForElementVisible(".description-row p", short_wait)
.assert.containsText(".description-row p", "This is Collection B")
.waitForElementVisible(".description-row button.description-button", short_wait)
.frame(null)
.url(function (sharedUrlGrainB) {
browser.loginDevAccount()
.url(sharedUrlGrainB.value)
.waitForElementVisible(".grain-interstitial button.reveal-identity-button", short_wait)
.click(".grain-interstitial button.reveal-identity-button")
.grainFrame(grainIdB)
.end();
});
});
});
}
| {
"pile_set_name": "Github"
} |
// Copyright (c) 2012-2015 Ugorji Nwoke. All rights reserved.
// Use of this source code is governed by a MIT license found in the LICENSE file.
/*
MSGPACK
Msgpack-c implementation powers the c, c++, python, ruby, etc libraries.
We need to maintain compatibility with it and how it encodes integer values
without caring about the type.
For compatibility with behaviour of msgpack-c reference implementation:
- Go intX (>0) and uintX
IS ENCODED AS
msgpack +ve fixnum, unsigned
- Go intX (<0)
IS ENCODED AS
msgpack -ve fixnum, signed
*/
package codec
import (
"fmt"
"io"
"math"
"net/rpc"
"reflect"
)
const (
mpPosFixNumMin byte = 0x00
mpPosFixNumMax = 0x7f
mpFixMapMin = 0x80
mpFixMapMax = 0x8f
mpFixArrayMin = 0x90
mpFixArrayMax = 0x9f
mpFixStrMin = 0xa0
mpFixStrMax = 0xbf
mpNil = 0xc0
_ = 0xc1
mpFalse = 0xc2
mpTrue = 0xc3
mpFloat = 0xca
mpDouble = 0xcb
mpUint8 = 0xcc
mpUint16 = 0xcd
mpUint32 = 0xce
mpUint64 = 0xcf
mpInt8 = 0xd0
mpInt16 = 0xd1
mpInt32 = 0xd2
mpInt64 = 0xd3
// extensions below
mpBin8 = 0xc4
mpBin16 = 0xc5
mpBin32 = 0xc6
mpExt8 = 0xc7
mpExt16 = 0xc8
mpExt32 = 0xc9
mpFixExt1 = 0xd4
mpFixExt2 = 0xd5
mpFixExt4 = 0xd6
mpFixExt8 = 0xd7
mpFixExt16 = 0xd8
mpStr8 = 0xd9 // new
mpStr16 = 0xda
mpStr32 = 0xdb
mpArray16 = 0xdc
mpArray32 = 0xdd
mpMap16 = 0xde
mpMap32 = 0xdf
mpNegFixNumMin = 0xe0
mpNegFixNumMax = 0xff
)
// MsgpackSpecRpcMultiArgs is a special type which signifies to the MsgpackSpecRpcCodec
// that the backend RPC service takes multiple arguments, which have been arranged
// in sequence in the slice.
//
// The Codec then passes it AS-IS to the rpc service (without wrapping it in an
// array of 1 element).
type MsgpackSpecRpcMultiArgs []interface{}
// A MsgpackContainer type specifies the different types of msgpackContainers.
type msgpackContainerType struct {
fixCutoff int
bFixMin, b8, b16, b32 byte
hasFixMin, has8, has8Always bool
}
var (
msgpackContainerStr = msgpackContainerType{32, mpFixStrMin, mpStr8, mpStr16, mpStr32, true, true, false}
msgpackContainerBin = msgpackContainerType{0, 0, mpBin8, mpBin16, mpBin32, false, true, true}
msgpackContainerList = msgpackContainerType{16, mpFixArrayMin, 0, mpArray16, mpArray32, true, false, false}
msgpackContainerMap = msgpackContainerType{16, mpFixMapMin, 0, mpMap16, mpMap32, true, false, false}
)
//---------------------------------------------
type msgpackEncDriver struct {
noBuiltInTypes
encNoSeparator
e *Encoder
w encWriter
h *MsgpackHandle
x [8]byte
}
func (e *msgpackEncDriver) EncodeNil() {
e.w.writen1(mpNil)
}
func (e *msgpackEncDriver) EncodeInt(i int64) {
if i >= 0 {
e.EncodeUint(uint64(i))
} else if i >= -32 {
e.w.writen1(byte(i))
} else if i >= math.MinInt8 {
e.w.writen2(mpInt8, byte(i))
} else if i >= math.MinInt16 {
e.w.writen1(mpInt16)
bigenHelper{e.x[:2], e.w}.writeUint16(uint16(i))
} else if i >= math.MinInt32 {
e.w.writen1(mpInt32)
bigenHelper{e.x[:4], e.w}.writeUint32(uint32(i))
} else {
e.w.writen1(mpInt64)
bigenHelper{e.x[:8], e.w}.writeUint64(uint64(i))
}
}
func (e *msgpackEncDriver) EncodeUint(i uint64) {
if i <= math.MaxInt8 {
e.w.writen1(byte(i))
} else if i <= math.MaxUint8 {
e.w.writen2(mpUint8, byte(i))
} else if i <= math.MaxUint16 {
e.w.writen1(mpUint16)
bigenHelper{e.x[:2], e.w}.writeUint16(uint16(i))
} else if i <= math.MaxUint32 {
e.w.writen1(mpUint32)
bigenHelper{e.x[:4], e.w}.writeUint32(uint32(i))
} else {
e.w.writen1(mpUint64)
bigenHelper{e.x[:8], e.w}.writeUint64(uint64(i))
}
}
func (e *msgpackEncDriver) EncodeBool(b bool) {
if b {
e.w.writen1(mpTrue)
} else {
e.w.writen1(mpFalse)
}
}
func (e *msgpackEncDriver) EncodeFloat32(f float32) {
e.w.writen1(mpFloat)
bigenHelper{e.x[:4], e.w}.writeUint32(math.Float32bits(f))
}
func (e *msgpackEncDriver) EncodeFloat64(f float64) {
e.w.writen1(mpDouble)
bigenHelper{e.x[:8], e.w}.writeUint64(math.Float64bits(f))
}
func (e *msgpackEncDriver) EncodeExt(v interface{}, xtag uint64, ext Ext, _ *Encoder) {
bs := ext.WriteExt(v)
if bs == nil {
e.EncodeNil()
return
}
if e.h.WriteExt {
e.encodeExtPreamble(uint8(xtag), len(bs))
e.w.writeb(bs)
} else {
e.EncodeStringBytes(c_RAW, bs)
}
}
func (e *msgpackEncDriver) EncodeRawExt(re *RawExt, _ *Encoder) {
e.encodeExtPreamble(uint8(re.Tag), len(re.Data))
e.w.writeb(re.Data)
}
func (e *msgpackEncDriver) encodeExtPreamble(xtag byte, l int) {
if l == 1 {
e.w.writen2(mpFixExt1, xtag)
} else if l == 2 {
e.w.writen2(mpFixExt2, xtag)
} else if l == 4 {
e.w.writen2(mpFixExt4, xtag)
} else if l == 8 {
e.w.writen2(mpFixExt8, xtag)
} else if l == 16 {
e.w.writen2(mpFixExt16, xtag)
} else if l < 256 {
e.w.writen2(mpExt8, byte(l))
e.w.writen1(xtag)
} else if l < 65536 {
e.w.writen1(mpExt16)
bigenHelper{e.x[:2], e.w}.writeUint16(uint16(l))
e.w.writen1(xtag)
} else {
e.w.writen1(mpExt32)
bigenHelper{e.x[:4], e.w}.writeUint32(uint32(l))
e.w.writen1(xtag)
}
}
func (e *msgpackEncDriver) EncodeArrayStart(length int) {
e.writeContainerLen(msgpackContainerList, length)
}
func (e *msgpackEncDriver) EncodeMapStart(length int) {
e.writeContainerLen(msgpackContainerMap, length)
}
func (e *msgpackEncDriver) EncodeString(c charEncoding, s string) {
if c == c_RAW && e.h.WriteExt {
e.writeContainerLen(msgpackContainerBin, len(s))
} else {
e.writeContainerLen(msgpackContainerStr, len(s))
}
if len(s) > 0 {
e.w.writestr(s)
}
}
func (e *msgpackEncDriver) EncodeSymbol(v string) {
e.EncodeString(c_UTF8, v)
}
func (e *msgpackEncDriver) EncodeStringBytes(c charEncoding, bs []byte) {
if c == c_RAW && e.h.WriteExt {
e.writeContainerLen(msgpackContainerBin, len(bs))
} else {
e.writeContainerLen(msgpackContainerStr, len(bs))
}
if len(bs) > 0 {
e.w.writeb(bs)
}
}
func (e *msgpackEncDriver) writeContainerLen(ct msgpackContainerType, l int) {
if ct.hasFixMin && l < ct.fixCutoff {
e.w.writen1(ct.bFixMin | byte(l))
} else if ct.has8 && l < 256 && (ct.has8Always || e.h.WriteExt) {
e.w.writen2(ct.b8, uint8(l))
} else if l < 65536 {
e.w.writen1(ct.b16)
bigenHelper{e.x[:2], e.w}.writeUint16(uint16(l))
} else {
e.w.writen1(ct.b32)
bigenHelper{e.x[:4], e.w}.writeUint32(uint32(l))
}
}
//---------------------------------------------
type msgpackDecDriver struct {
d *Decoder
r decReader // *Decoder decReader decReaderT
h *MsgpackHandle
b [scratchByteArrayLen]byte
bd byte
bdRead bool
br bool // bytes reader
noBuiltInTypes
noStreamingCodec
decNoSeparator
}
// Note: This returns either a primitive (int, bool, etc) for non-containers,
// or a containerType, or a specific type denoting nil or extension.
// It is called when a nil interface{} is passed, leaving it up to the DecDriver
// to introspect the stream and decide how best to decode.
// It deciphers the value by looking at the stream first.
func (d *msgpackDecDriver) DecodeNaked() {
if !d.bdRead {
d.readNextBd()
}
bd := d.bd
n := &d.d.n
var decodeFurther bool
switch bd {
case mpNil:
n.v = valueTypeNil
d.bdRead = false
case mpFalse:
n.v = valueTypeBool
n.b = false
case mpTrue:
n.v = valueTypeBool
n.b = true
case mpFloat:
n.v = valueTypeFloat
n.f = float64(math.Float32frombits(bigen.Uint32(d.r.readx(4))))
case mpDouble:
n.v = valueTypeFloat
n.f = math.Float64frombits(bigen.Uint64(d.r.readx(8)))
case mpUint8:
n.v = valueTypeUint
n.u = uint64(d.r.readn1())
case mpUint16:
n.v = valueTypeUint
n.u = uint64(bigen.Uint16(d.r.readx(2)))
case mpUint32:
n.v = valueTypeUint
n.u = uint64(bigen.Uint32(d.r.readx(4)))
case mpUint64:
n.v = valueTypeUint
n.u = uint64(bigen.Uint64(d.r.readx(8)))
case mpInt8:
n.v = valueTypeInt
n.i = int64(int8(d.r.readn1()))
case mpInt16:
n.v = valueTypeInt
n.i = int64(int16(bigen.Uint16(d.r.readx(2))))
case mpInt32:
n.v = valueTypeInt
n.i = int64(int32(bigen.Uint32(d.r.readx(4))))
case mpInt64:
n.v = valueTypeInt
n.i = int64(int64(bigen.Uint64(d.r.readx(8))))
default:
switch {
case bd >= mpPosFixNumMin && bd <= mpPosFixNumMax:
// positive fixnum (always signed)
n.v = valueTypeInt
n.i = int64(int8(bd))
case bd >= mpNegFixNumMin && bd <= mpNegFixNumMax:
// negative fixnum
n.v = valueTypeInt
n.i = int64(int8(bd))
case bd == mpStr8, bd == mpStr16, bd == mpStr32, bd >= mpFixStrMin && bd <= mpFixStrMax:
if d.h.RawToString {
n.v = valueTypeString
n.s = d.DecodeString()
} else {
n.v = valueTypeBytes
n.l = d.DecodeBytes(nil, false, false)
}
case bd == mpBin8, bd == mpBin16, bd == mpBin32:
n.v = valueTypeBytes
n.l = d.DecodeBytes(nil, false, false)
case bd == mpArray16, bd == mpArray32, bd >= mpFixArrayMin && bd <= mpFixArrayMax:
n.v = valueTypeArray
decodeFurther = true
case bd == mpMap16, bd == mpMap32, bd >= mpFixMapMin && bd <= mpFixMapMax:
n.v = valueTypeMap
decodeFurther = true
case bd >= mpFixExt1 && bd <= mpFixExt16, bd >= mpExt8 && bd <= mpExt32:
n.v = valueTypeExt
clen := d.readExtLen()
n.u = uint64(d.r.readn1())
n.l = d.r.readx(clen)
default:
d.d.errorf("Nil-Deciphered DecodeValue: %s: hex: %x, dec: %d", msgBadDesc, bd, bd)
}
}
if !decodeFurther {
d.bdRead = false
}
if n.v == valueTypeUint && d.h.SignedInteger {
n.v = valueTypeInt
n.i = int64(n.v)
}
return
}
// int can be decoded from msgpack type: intXXX or uintXXX
func (d *msgpackDecDriver) DecodeInt(bitsize uint8) (i int64) {
if !d.bdRead {
d.readNextBd()
}
switch d.bd {
case mpUint8:
i = int64(uint64(d.r.readn1()))
case mpUint16:
i = int64(uint64(bigen.Uint16(d.r.readx(2))))
case mpUint32:
i = int64(uint64(bigen.Uint32(d.r.readx(4))))
case mpUint64:
i = int64(bigen.Uint64(d.r.readx(8)))
case mpInt8:
i = int64(int8(d.r.readn1()))
case mpInt16:
i = int64(int16(bigen.Uint16(d.r.readx(2))))
case mpInt32:
i = int64(int32(bigen.Uint32(d.r.readx(4))))
case mpInt64:
i = int64(bigen.Uint64(d.r.readx(8)))
default:
switch {
case d.bd >= mpPosFixNumMin && d.bd <= mpPosFixNumMax:
i = int64(int8(d.bd))
case d.bd >= mpNegFixNumMin && d.bd <= mpNegFixNumMax:
i = int64(int8(d.bd))
default:
d.d.errorf("Unhandled single-byte unsigned integer value: %s: %x", msgBadDesc, d.bd)
return
}
}
// check overflow (logic adapted from std pkg reflect/value.go OverflowUint()
if bitsize > 0 {
if trunc := (i << (64 - bitsize)) >> (64 - bitsize); i != trunc {
d.d.errorf("Overflow int value: %v", i)
return
}
}
d.bdRead = false
return
}
// uint can be decoded from msgpack type: intXXX or uintXXX
func (d *msgpackDecDriver) DecodeUint(bitsize uint8) (ui uint64) {
if !d.bdRead {
d.readNextBd()
}
switch d.bd {
case mpUint8:
ui = uint64(d.r.readn1())
case mpUint16:
ui = uint64(bigen.Uint16(d.r.readx(2)))
case mpUint32:
ui = uint64(bigen.Uint32(d.r.readx(4)))
case mpUint64:
ui = bigen.Uint64(d.r.readx(8))
case mpInt8:
if i := int64(int8(d.r.readn1())); i >= 0 {
ui = uint64(i)
} else {
d.d.errorf("Assigning negative signed value: %v, to unsigned type", i)
return
}
case mpInt16:
if i := int64(int16(bigen.Uint16(d.r.readx(2)))); i >= 0 {
ui = uint64(i)
} else {
d.d.errorf("Assigning negative signed value: %v, to unsigned type", i)
return
}
case mpInt32:
if i := int64(int32(bigen.Uint32(d.r.readx(4)))); i >= 0 {
ui = uint64(i)
} else {
d.d.errorf("Assigning negative signed value: %v, to unsigned type", i)
return
}
case mpInt64:
if i := int64(bigen.Uint64(d.r.readx(8))); i >= 0 {
ui = uint64(i)
} else {
d.d.errorf("Assigning negative signed value: %v, to unsigned type", i)
return
}
default:
switch {
case d.bd >= mpPosFixNumMin && d.bd <= mpPosFixNumMax:
ui = uint64(d.bd)
case d.bd >= mpNegFixNumMin && d.bd <= mpNegFixNumMax:
d.d.errorf("Assigning negative signed value: %v, to unsigned type", int(d.bd))
return
default:
d.d.errorf("Unhandled single-byte unsigned integer value: %s: %x", msgBadDesc, d.bd)
return
}
}
// check overflow (logic adapted from std pkg reflect/value.go OverflowUint()
if bitsize > 0 {
if trunc := (ui << (64 - bitsize)) >> (64 - bitsize); ui != trunc {
d.d.errorf("Overflow uint value: %v", ui)
return
}
}
d.bdRead = false
return
}
// float can either be decoded from msgpack type: float, double or intX
func (d *msgpackDecDriver) DecodeFloat(chkOverflow32 bool) (f float64) {
if !d.bdRead {
d.readNextBd()
}
if d.bd == mpFloat {
f = float64(math.Float32frombits(bigen.Uint32(d.r.readx(4))))
} else if d.bd == mpDouble {
f = math.Float64frombits(bigen.Uint64(d.r.readx(8)))
} else {
f = float64(d.DecodeInt(0))
}
if chkOverflow32 && chkOvf.Float32(f) {
d.d.errorf("msgpack: float32 overflow: %v", f)
return
}
d.bdRead = false
return
}
// bool can be decoded from bool, fixnum 0 or 1.
func (d *msgpackDecDriver) DecodeBool() (b bool) {
if !d.bdRead {
d.readNextBd()
}
if d.bd == mpFalse || d.bd == 0 {
// b = false
} else if d.bd == mpTrue || d.bd == 1 {
b = true
} else {
d.d.errorf("Invalid single-byte value for bool: %s: %x", msgBadDesc, d.bd)
return
}
d.bdRead = false
return
}
func (d *msgpackDecDriver) DecodeBytes(bs []byte, isstring, zerocopy bool) (bsOut []byte) {
if !d.bdRead {
d.readNextBd()
}
var clen int
// ignore isstring. Expect that the bytes may be found from msgpackContainerStr or msgpackContainerBin
if bd := d.bd; bd == mpBin8 || bd == mpBin16 || bd == mpBin32 {
clen = d.readContainerLen(msgpackContainerBin)
} else {
clen = d.readContainerLen(msgpackContainerStr)
}
// println("DecodeBytes: clen: ", clen)
d.bdRead = false
// bytes may be nil, so handle it. if nil, clen=-1.
if clen < 0 {
return nil
}
if zerocopy {
if d.br {
return d.r.readx(clen)
} else if len(bs) == 0 {
bs = d.b[:]
}
}
return decByteSlice(d.r, clen, bs)
}
func (d *msgpackDecDriver) DecodeString() (s string) {
return string(d.DecodeBytes(d.b[:], true, true))
}
func (d *msgpackDecDriver) readNextBd() {
d.bd = d.r.readn1()
d.bdRead = true
}
func (d *msgpackDecDriver) ContainerType() (vt valueType) {
bd := d.bd
if bd == mpNil {
return valueTypeNil
} else if bd == mpBin8 || bd == mpBin16 || bd == mpBin32 ||
(!d.h.RawToString &&
(bd == mpStr8 || bd == mpStr16 || bd == mpStr32 || (bd >= mpFixStrMin && bd <= mpFixStrMax))) {
return valueTypeBytes
} else if d.h.RawToString &&
(bd == mpStr8 || bd == mpStr16 || bd == mpStr32 || (bd >= mpFixStrMin && bd <= mpFixStrMax)) {
return valueTypeString
} else if bd == mpArray16 || bd == mpArray32 || (bd >= mpFixArrayMin && bd <= mpFixArrayMax) {
return valueTypeArray
} else if bd == mpMap16 || bd == mpMap32 || (bd >= mpFixMapMin && bd <= mpFixMapMax) {
return valueTypeMap
} else {
// d.d.errorf("isContainerType: unsupported parameter: %v", vt)
}
return valueTypeUnset
}
func (d *msgpackDecDriver) TryDecodeAsNil() (v bool) {
if !d.bdRead {
d.readNextBd()
}
if d.bd == mpNil {
d.bdRead = false
v = true
}
return
}
func (d *msgpackDecDriver) readContainerLen(ct msgpackContainerType) (clen int) {
bd := d.bd
if bd == mpNil {
clen = -1 // to represent nil
} else if bd == ct.b8 {
clen = int(d.r.readn1())
} else if bd == ct.b16 {
clen = int(bigen.Uint16(d.r.readx(2)))
} else if bd == ct.b32 {
clen = int(bigen.Uint32(d.r.readx(4)))
} else if (ct.bFixMin & bd) == ct.bFixMin {
clen = int(ct.bFixMin ^ bd)
} else {
d.d.errorf("readContainerLen: %s: hex: %x, decimal: %d", msgBadDesc, bd, bd)
return
}
d.bdRead = false
return
}
func (d *msgpackDecDriver) ReadMapStart() int {
return d.readContainerLen(msgpackContainerMap)
}
func (d *msgpackDecDriver) ReadArrayStart() int {
return d.readContainerLen(msgpackContainerList)
}
func (d *msgpackDecDriver) readExtLen() (clen int) {
switch d.bd {
case mpNil:
clen = -1 // to represent nil
case mpFixExt1:
clen = 1
case mpFixExt2:
clen = 2
case mpFixExt4:
clen = 4
case mpFixExt8:
clen = 8
case mpFixExt16:
clen = 16
case mpExt8:
clen = int(d.r.readn1())
case mpExt16:
clen = int(bigen.Uint16(d.r.readx(2)))
case mpExt32:
clen = int(bigen.Uint32(d.r.readx(4)))
default:
d.d.errorf("decoding ext bytes: found unexpected byte: %x", d.bd)
return
}
return
}
func (d *msgpackDecDriver) DecodeExt(rv interface{}, xtag uint64, ext Ext) (realxtag uint64) {
if xtag > 0xff {
d.d.errorf("decodeExt: tag must be <= 0xff; got: %v", xtag)
return
}
realxtag1, xbs := d.decodeExtV(ext != nil, uint8(xtag))
realxtag = uint64(realxtag1)
if ext == nil {
re := rv.(*RawExt)
re.Tag = realxtag
re.Data = detachZeroCopyBytes(d.br, re.Data, xbs)
} else {
ext.ReadExt(rv, xbs)
}
return
}
func (d *msgpackDecDriver) decodeExtV(verifyTag bool, tag byte) (xtag byte, xbs []byte) {
if !d.bdRead {
d.readNextBd()
}
xbd := d.bd
if xbd == mpBin8 || xbd == mpBin16 || xbd == mpBin32 {
xbs = d.DecodeBytes(nil, false, true)
} else if xbd == mpStr8 || xbd == mpStr16 || xbd == mpStr32 ||
(xbd >= mpFixStrMin && xbd <= mpFixStrMax) {
xbs = d.DecodeBytes(nil, true, true)
} else {
clen := d.readExtLen()
xtag = d.r.readn1()
if verifyTag && xtag != tag {
d.d.errorf("Wrong extension tag. Got %b. Expecting: %v", xtag, tag)
return
}
xbs = d.r.readx(clen)
}
d.bdRead = false
return
}
//--------------------------------------------------
//MsgpackHandle is a Handle for the Msgpack Schema-Free Encoding Format.
type MsgpackHandle struct {
BasicHandle
// RawToString controls how raw bytes are decoded into a nil interface{}.
RawToString bool
// WriteExt flag supports encoding configured extensions with extension tags.
// It also controls whether other elements of the new spec are encoded (ie Str8).
//
// With WriteExt=false, configured extensions are serialized as raw bytes
// and Str8 is not encoded.
//
// A stream can still be decoded into a typed value, provided an appropriate value
// is provided, but the type cannot be inferred from the stream. If no appropriate
// type is provided (e.g. decoding into a nil interface{}), you get back
// a []byte or string based on the setting of RawToString.
WriteExt bool
binaryEncodingType
}
func (h *MsgpackHandle) SetBytesExt(rt reflect.Type, tag uint64, ext BytesExt) (err error) {
return h.SetExt(rt, tag, &setExtWrapper{b: ext})
}
func (h *MsgpackHandle) newEncDriver(e *Encoder) encDriver {
return &msgpackEncDriver{e: e, w: e.w, h: h}
}
func (h *MsgpackHandle) newDecDriver(d *Decoder) decDriver {
return &msgpackDecDriver{d: d, r: d.r, h: h, br: d.bytes}
}
func (e *msgpackEncDriver) reset() {
e.w = e.e.w
}
func (d *msgpackDecDriver) reset() {
d.r = d.d.r
}
//--------------------------------------------------
type msgpackSpecRpcCodec struct {
rpcCodec
}
// /////////////// Spec RPC Codec ///////////////////
func (c *msgpackSpecRpcCodec) WriteRequest(r *rpc.Request, body interface{}) error {
// WriteRequest can write to both a Go service, and other services that do
// not abide by the 1 argument rule of a Go service.
// We discriminate based on if the body is a MsgpackSpecRpcMultiArgs
var bodyArr []interface{}
if m, ok := body.(MsgpackSpecRpcMultiArgs); ok {
bodyArr = ([]interface{})(m)
} else {
bodyArr = []interface{}{body}
}
r2 := []interface{}{0, uint32(r.Seq), r.ServiceMethod, bodyArr}
return c.write(r2, nil, false, true)
}
func (c *msgpackSpecRpcCodec) WriteResponse(r *rpc.Response, body interface{}) error {
var moe interface{}
if r.Error != "" {
moe = r.Error
}
if moe != nil && body != nil {
body = nil
}
r2 := []interface{}{1, uint32(r.Seq), moe, body}
return c.write(r2, nil, false, true)
}
func (c *msgpackSpecRpcCodec) ReadResponseHeader(r *rpc.Response) error {
return c.parseCustomHeader(1, &r.Seq, &r.Error)
}
func (c *msgpackSpecRpcCodec) ReadRequestHeader(r *rpc.Request) error {
return c.parseCustomHeader(0, &r.Seq, &r.ServiceMethod)
}
func (c *msgpackSpecRpcCodec) ReadRequestBody(body interface{}) error {
if body == nil { // read and discard
return c.read(nil)
}
bodyArr := []interface{}{body}
return c.read(&bodyArr)
}
func (c *msgpackSpecRpcCodec) parseCustomHeader(expectTypeByte byte, msgid *uint64, methodOrError *string) (err error) {
if c.isClosed() {
return io.EOF
}
// We read the response header by hand
// so that the body can be decoded on its own from the stream at a later time.
const fia byte = 0x94 //four item array descriptor value
// Not sure why the panic of EOF is swallowed above.
// if bs1 := c.dec.r.readn1(); bs1 != fia {
// err = fmt.Errorf("Unexpected value for array descriptor: Expecting %v. Received %v", fia, bs1)
// return
// }
var b byte
b, err = c.br.ReadByte()
if err != nil {
return
}
if b != fia {
err = fmt.Errorf("Unexpected value for array descriptor: Expecting %v. Received %v", fia, b)
return
}
if err = c.read(&b); err != nil {
return
}
if b != expectTypeByte {
err = fmt.Errorf("Unexpected byte descriptor in header. Expecting %v. Received %v", expectTypeByte, b)
return
}
if err = c.read(msgid); err != nil {
return
}
if err = c.read(methodOrError); err != nil {
return
}
return
}
//--------------------------------------------------
// msgpackSpecRpc is the implementation of Rpc that uses custom communication protocol
// as defined in the msgpack spec at https://github.com/msgpack-rpc/msgpack-rpc/blob/master/spec.md
type msgpackSpecRpc struct{}
// MsgpackSpecRpc implements Rpc using the communication protocol defined in
// the msgpack spec at https://github.com/msgpack-rpc/msgpack-rpc/blob/master/spec.md .
// Its methods (ServerCodec and ClientCodec) return values that implement RpcCodecBuffered.
var MsgpackSpecRpc msgpackSpecRpc
func (x msgpackSpecRpc) ServerCodec(conn io.ReadWriteCloser, h Handle) rpc.ServerCodec {
return &msgpackSpecRpcCodec{newRPCCodec(conn, h)}
}
func (x msgpackSpecRpc) ClientCodec(conn io.ReadWriteCloser, h Handle) rpc.ClientCodec {
return &msgpackSpecRpcCodec{newRPCCodec(conn, h)}
}
var _ decDriver = (*msgpackDecDriver)(nil)
var _ encDriver = (*msgpackEncDriver)(nil)
| {
"pile_set_name": "Github"
} |
define( [
"./core",
"./core/toType",
"./var/rcheckableType",
"./var/isFunction",
"./core/init",
"./traversing", // filter
"./attributes/prop"
], function( jQuery, toType, rcheckableType, isFunction ) {
"use strict";
var
rbracket = /\[\]$/,
rCRLF = /\r?\n/g,
rsubmitterTypes = /^(?:submit|button|image|reset|file)$/i,
rsubmittable = /^(?:input|select|textarea|keygen)/i;
function buildParams( prefix, obj, traditional, add ) {
var name;
if ( Array.isArray( obj ) ) {
// Serialize array item.
jQuery.each( obj, function( i, v ) {
if ( traditional || rbracket.test( prefix ) ) {
// Treat each array item as a scalar.
add( prefix, v );
} else {
// Item is non-scalar (array or object), encode its numeric index.
buildParams(
prefix + "[" + ( typeof v === "object" && v != null ? i : "" ) + "]",
v,
traditional,
add
);
}
} );
} else if ( !traditional && toType( obj ) === "object" ) {
// Serialize object item.
for ( name in obj ) {
buildParams( prefix + "[" + name + "]", obj[ name ], traditional, add );
}
} else {
// Serialize scalar item.
add( prefix, obj );
}
}
// Serialize an array of form elements or a set of
// key/values into a query string
jQuery.param = function( a, traditional ) {
var prefix,
s = [],
add = function( key, valueOrFunction ) {
// If value is a function, invoke it and use its return value
var value = isFunction( valueOrFunction ) ?
valueOrFunction() :
valueOrFunction;
s[ s.length ] = encodeURIComponent( key ) + "=" +
encodeURIComponent( value == null ? "" : value );
};
if ( a == null ) {
return "";
}
// If an array was passed in, assume that it is an array of form elements.
if ( Array.isArray( a ) || ( a.jquery && !jQuery.isPlainObject( a ) ) ) {
// Serialize the form elements
jQuery.each( a, function() {
add( this.name, this.value );
} );
} else {
// If traditional, encode the "old" way (the way 1.3.2 or older
// did it), otherwise encode params recursively.
for ( prefix in a ) {
buildParams( prefix, a[ prefix ], traditional, add );
}
}
// Return the resulting serialization
return s.join( "&" );
};
jQuery.fn.extend( {
serialize: function() {
return jQuery.param( this.serializeArray() );
},
serializeArray: function() {
return this.map( function() {
// Can add propHook for "elements" to filter or add form elements
var elements = jQuery.prop( this, "elements" );
return elements ? jQuery.makeArray( elements ) : this;
} )
.filter( function() {
var type = this.type;
// Use .is( ":disabled" ) so that fieldset[disabled] works
return this.name && !jQuery( this ).is( ":disabled" ) &&
rsubmittable.test( this.nodeName ) && !rsubmitterTypes.test( type ) &&
( this.checked || !rcheckableType.test( type ) );
} )
.map( function( i, elem ) {
var val = jQuery( this ).val();
if ( val == null ) {
return null;
}
if ( Array.isArray( val ) ) {
return jQuery.map( val, function( val ) {
return { name: elem.name, value: val.replace( rCRLF, "\r\n" ) };
} );
}
return { name: elem.name, value: val.replace( rCRLF, "\r\n" ) };
} ).get();
}
} );
return jQuery;
} );
| {
"pile_set_name": "Github"
} |
#pragma once
#include <util/generic/vector.h>
#include <util/generic/stroka.h>
#include "synchain.h"
#include "factfields.h"
#include "factgroup.h"
#include "tomitaitemsholder.h"
#include "normalization.h"
typedef ymap< Stroka, yvector<CFactFields> > CFactSubsets;
class CCommonGrammarInterpretation: public CTomitaItemsHolder
{
public:
CCommonGrammarInterpretation(const CWordVector& Words, const CWorkGrammar& gram,
yvector<CWordSequence*>& foundFacts, const yvector<SWordHomonymNum>& clauseWords,
CReferenceSearcher* RefSearcher, size_t factCountConstraint = 128);
~CCommonGrammarInterpretation();
virtual void Run(bool allowAmbiguity=false);
bool AddFactField(SReduceConstraints* rReduceConstraints) const;
void AddTextFactField(CFactSynGroup* pItem, const fact_field_reference_t& rFieldRef) const;
void AddTextFactFieldValue(CTextWS& ws, const fact_field_descr_t fieldDescr) const;
void AddFioFactField(CFactSynGroup* pItem, const fact_field_reference_t& rFieldRef) const;
void AddDateFactField(CFactSynGroup* pItem, const fact_field_reference_t& rFieldRef) const;
void AddBoolFactField(CFactSynGroup* pItem, const fact_field_reference_t& rFieldRef) const;
void GetFioWSForFactField(CFactSynGroup* pItem, const fact_field_reference_t& rFieldRef, CFioWS& newFioWS) const;
void AddCommonFactField(CFactSynGroup* pItem, const fact_field_reference_t& rFieldRef, CWordsPair& rValueWS) const;
//ф-ция берет заданное поле из уже построенного другой грамматикой факта
bool TryCutOutFactField(CFactSynGroup* pItem, const fact_field_reference_t& rFieldRef) const;
//ф-ция разбивает все можество фактов на подмножества по имени факта
void DivideFactsSetIntoSubsets(CFactSubsets& rFactSubsets, const yvector<CFactFields>& vec_facts) const;
void AddWordsInfo(const CFactSynGroup* rItem, CWordSequence& fact_group, bool bArtificial = false) const;
void AddFioFromFactToSequence(CFactSynGroup* pNewGroup);
void AddWordsInfoToCompanyPostSequence(EWordSequenceType e_WSType, int iWStart, int iWEnd, CWordSequence& fdo_froup);
virtual bool MergeFactGroups(yvector<CFactFields>& vec_facts1, const yvector<CFactFields>& vec_facts2,
const TGrammarBunch& child_grammems, CWordSequenceWithAntecedent::EEllipses eEllipseType);
bool MergeEqualNameFacts(yvector<CFactFields>& vec_facts1,
yvector<CFactFields>& vec_facts2) const;
using CTomitaItemsHolder::CreateNewItem; // to avoid hiding of CTomitaItemsHolder::CreateNewItem(size_t, size_t)
virtual CInputItem* CreateNewItem(size_t SymbolNo, const CRuleAgreement& agreements,
size_t iFirstItemNo, size_t iLastItemNo,
size_t iSynMainItemNo, yvector<CInputItem*>& children);
void CheckTextFieldAlgorithms(CFactsWS* pFacts);
void MergeTwoFacts(CFactFields& rFact1, CFactFields& rFact2) const;
void MiddleInterpretation(yvector<CFactFields>& facts, const yvector<CInputItem*>& children) const;
void MiddleInterpretationExchangeMerge(yvector<CFactFields>& facts, const Stroka& rKeyFactName) const;
bool InterpretationExchangeMerge(CFactFields& fact1, CFactFields& fact2) const;
CWordSequenceWithAntecedent::EEllipses HasGroupEllipse(CFactSynGroup* pNewGroup) const;
//ограничение на кол-во фактов в предложении
void CheckFactCountConstraintInSent();
//(если несклоняемая или ненайденная в морфологии фамилия - одиночная или содержит только инициалы) и (должность стоит в косвенном падеже),
//то не строить факт
bool CheckConstraintForLonelyFIO(yvector<CFactFields>& facts);
void SetGenFlag(CFactFields& occur);
protected:
void AdjustChildHomonyms(CFactSynGroup* pFactGroup);
void RefillFactFields(CFactSynGroup* pFactGroup);
bool RefillFioFactField(CFactSynGroup* pFactGroup, Stroka factName, Stroka factField, CFioWS& value);
bool RefillTextFactField(CFactSynGroup* pFactGroup, Stroka factName, Stroka factField, CTextWS& value);
bool IsFactIntersection(yvector<CFactFields>& vec_facts1, yvector<CFactFields>& vec_facts2) const;
void SimpleFactsMerge(yvector<CFactFields>& vec_facts1, const yvector<CFactFields>& vec_facts2);
bool IsEqualCoordinationMembers(const yvector<CFactFields>& vec_facts1, const yvector<CFactFields>& vec_facts2) const;
bool IsOneToMany(const yvector<CFactFields>& vec_facts1, const yvector<CFactFields>& vec_facts2) const;
void BuildFactWS(const COccurrence& occur, CWordSequence* pFactSequence);
void LogRule(size_t SymbolNo, size_t iFirstItemNo, size_t iLastItemNo, size_t iSynMainItemNo,
const yvector<CInputItem*>& children, const Stroka& msg = Stroka()) const;
Stroka GetAgreementStr(const SRuleExternalInformationAndAgreements& agr, ECharset encoding) const;
bool FillFactFieldFromFactWS(const fact_field_reference_t& fact_field, const CFactsWS* factWS, yvector<CFactFields>& newFacts) const;
bool HasAllNecessaryFields(CFactFields& fact, const CWordsPair& artificialPair) const;
bool CheckNecessaryFields(yvector<CFactFields>& newFacts, const CWordsPair& artificialPair) const;
bool TrimTree(CFactsWS* pFactWordSequence, CFactSynGroup* pGroup, yset<int>& excludedWords, CWordsPair& newPair) const;
bool IsTrimChild(const yvector<CInputItem*>& rChildren) const;
void ComparePair(int& iL, int& iR, const CWordsPair* rSPair) const;
void FieldsConcatenation(CFactFields& rFact1, CFactFields& rFact2, const Stroka& sFieldName, EFactFieldType eFieldType) const;
bool CheckUnwantedStatusAlg(const CFactFields& rStatusFact) const;
bool CheckShortNameAlg(const CFactFields& rShortNameFact) const;
void CheckDelPronounAlg(CFactFields& rPronounFact);
void CheckQuotedOrgNormAlg(CFactFields& rOrgFact);
void CheckNotNorm(CFactFields& rFact);
void CheckCapitalized(CFactFields& rFact);
void CheckCommonAlg(CFactFields& rFact, EFactAlgorithm eAlg, yvector<CTextWS*>& rTWS);
void NormalizeSequence(CTextWS* sequence, const CFactSynGroup* parent, const TGramBitSet& grammems) const;
void ReplaceLemmaAlways(CTextWS* sequence, const CFactSynGroup* parent) const;
void NormalizeFacts(CFactsWS* pFactWordSequence, const CFactSynGroup* parent);
void NormalizeFact(CFactFields& fact, const CFactSynGroup* parent) const;
void NormalizeText(CTextWS* pWS, const CFactSynGroup* parent, const fact_field_descr_t& field) const;
void NormalizeDate(CWordSequence* ws) const;
void NormalizeFio(CFioWS* fioWS, const CFactSynGroup* parent) const;
protected:
CNormalization m_InterpNorm;
//игнорируя покрытие, выбирает из двух построенных графов A и B, пересечение слов которых не пусто,
//граф, левая граница которого находится ближе к началу предложения
//(т.е. нужен для эмпирического правила выбора левостороннего ветвления ФДО фактов);
//для этого в языке вводится помета при правилах:
//A -> X {left_dominant};
//B -> Y {right_recessive};
//из примера: "...президента Юкос М. Ходорковского, корреспондента аналитического журнала "Эксперт"..."
//будет выбран ФДО факт "президента Юкос М. Ходорковского"
yset<CWordsPair> m_LeftPriorityOrder;
//сейчас, если существуют в грамматике два правила с одинаковыми левыми частями
//X -> A B {trim};
//X -> C D;
//процедура trim будет применена ко всем выбранным лучшими деревьям, в которые вошел нетерминал X,
//несмотря на то, что trim приписан только первому правилу,
//в будущем, если будет нужно, можно сделать тоньше и отслеживать правило, участвовавшее в построении дерева, а не X
yset<size_t> m_TrimTree;
private:
size_t m_FactCountConstraint;
bool m_bLog;
TOutputStream* m_pLogStream;
const CFactSynGroup* m_CurrentFactGroup;
public:
yvector<CWordSequence*>& m_FoundFacts;
CReferenceSearcher* m_pReferenceSearcher;
};
| {
"pile_set_name": "Github"
} |
<?xml version="1.0" encoding="UTF-8"?>
<!-- Foundation -->
<window type="window" id="1119">
<defaultcontrol always="true">9501</defaultcontrol>
<controls>
<include content="DefHubWindow">
<param name="list" value="skinshortcuts-group-x1119" />
</include>
</controls>
</window>
| {
"pile_set_name": "Github"
} |
<?php
class CoverageNamespacedFunctionTest extends PHPUnit_Framework_TestCase
{
/**
* @covers foo\func()
*/
public function testFunc()
{
foo\func();
}
}
| {
"pile_set_name": "Github"
} |
#第二次课总结:
##编程中为什么会有丰富化的数据格式?
+ 编程操作的对象是多种多样的,如名字、数值、属性、关系等。
+ 数据格式有数值、字符串、布尔、null和undefined、对象、数组、函数等。
+ 我们编程的目的是处理数据,得到结果。这个过程需要时间,而多样的数据格式可供选择与使用,可以缩短时间,节省大量内存,提高运算的效率。
+ 丰富的数据格式因为拆分的细,所以使用很灵活。即使碰到复杂的数据格式,也可以用基础类型通过搭积木的方式组合出来。
+ 多样的数据格式方便理解应用。多人协同工作的时候有标准可依、互相之间底层共识相同,大大提高协同工作效率。
###个人静态网页
https://sam-tiao.github.io/diaozhicong/ | {
"pile_set_name": "Github"
} |
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netcoreapp3.0</TargetFramework>
<RuntimeFrameworkVersion>3.0.3</RuntimeFrameworkVersion>
<LangVersion>8.0</LangVersion>
<AssemblyName>AgileObjects.AgileMapper.UnitTests.NetCore3</AssemblyName>
<RootNamespace>AgileObjects.AgileMapper.UnitTests.NetCore3</RootNamespace>
<TreatWarningsAsErrors>true</TreatWarningsAsErrors>
<WarningsAsErrors></WarningsAsErrors>
<NoWarn>0649;1701;1702</NoWarn>
<GenerateRuntimeConfigurationFiles>true</GenerateRuntimeConfigurationFiles>
<IsPackable>false</IsPackable>
</PropertyGroup>
<PropertyGroup>
<DefineConstants>$(DefineConstants);NET_STANDARD;NET_STANDARD_2;TRACE;FEATURE_SERIALIZATION;FEATURE_DYNAMIC;FEATURE_DYNAMIC_ROOT_SOURCE;FEATURE_ISET;FEATURE_STRINGSPLIT_OPTIONS</DefineConstants>
</PropertyGroup>
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Debug|AnyCPU'">
<DefineConstants>$(DefineConstants);DEBUG</DefineConstants>
</PropertyGroup>
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Release|AnyCPU'">
<DefineConstants>$(DefineConstants);RELEASE</DefineConstants>
</PropertyGroup>
<ItemGroup>
<Compile Include="..\AgileMapper.UnitTests\**\*.cs" Exclude="..\AgileMapper.UnitTests\obj\**\*.cs;..\AgileMapper.UnitTests\Properties\*.cs;..\AgileMapper.UnitTests\WhenUsingPartialTrust.cs;">
<Link>%(RecursiveDir)%(Filename)%(Extension)</Link>
</Compile>
</ItemGroup>
<ItemGroup>
<PackageReference Include="Microsoft.Extensions.DependencyInjection" Version="3.0.3" />
<PackageReference Include="Microsoft.Extensions.Primitives" Version="3.0.3" />
<PackageReference Include="Microsoft.NET.Test.Sdk" Version="16.5.0" />
<PackageReference Include="System.Data.Common" Version="4.3.0" />
<PackageReference Include="xunit" Version="2.4.1" />
<PackageReference Include="xunit.runner.visualstudio" Version="2.4.3">
<PrivateAssets>all</PrivateAssets>
<IncludeAssets>runtime; build; native; contentfiles; analyzers</IncludeAssets>
</PackageReference>
</ItemGroup>
<ItemGroup>
<ProjectReference Include="..\AgileMapper.UnitTests.Common\AgileMapper.UnitTests.Common.csproj" />
<ProjectReference Include="..\AgileMapper.UnitTests.MoreTestClasses\AgileMapper.UnitTests.MoreTestClasses.csproj" />
</ItemGroup>
</Project>
| {
"pile_set_name": "Github"
} |
function UnityProgress (dom, args) {
this.progress = 0.0;
this.message = "";
this.dom = dom;
var parent = dom.parentNode;
var background = document.createElement("div");
if (args.backgroundcolor)
background.style.background = "#"+args.backgroundcolor;
else
background.style.background = "#ffffff";
background.style.position = "absolute";
parent.appendChild(background);
this.background = background;
var logoImage = document.createElement("img");
if (args.logoimage)
logoImage.src = args.logoimage;
else
logoImage.src = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAHoAAAAoCAYAAAAxH+4YAAAAGXRFWHRTb2Z0d2FyZQBBZG9iZSBJbWFnZVJlYWR5ccllPAAADIxJREFUeNrsXAtUlHUWv/MChhnQ4aUDIggC8vI1KZKuYam5KVumuJ2go6cS02x7mFYm5qNt4WRKbZtp57TVHsvHOZ21UmKBFRFkBUkNeSMLgoDyhhlmeMyw9/7ho48ENGRgZOee84fv/fr97+/+7v3+3wi6urqAZxbYgrFNxeaCTQhmu5/MgO0GtmJs6djauRUCHtDu2B7HpjA/rzFhDdhOYivlA+2L7Y+meLU6nU5o0OsF1jKZ3ozdkOwYtjwx/pFiW2lS4Gq1wvPnzzuXlZV5NDY1uctlsivrIyOvmDEbkhG2peKemGwxmleiVqtFP1+5Yl9QUOBy69Ytt6bmJhdc5qBuUUOrVgsuSmUbbmYGemjGdBcB7T0aZ8/Pz7e9evXqxPLycncE1+fmzZuK1tZWQVtbG3R0dACFFJFICAKBEFrUarecnBwbf3//FjNuQzJvAtpxJM/4988/n5afXzC/pvaWo0atsSSPbW/vFocCgYD9Fwm7xb5eb0DA9Qh0i/RiZqbSDPSQbTwBLRqps2HMlX733XfLNRqNXK9HbYW4dhm6QG/QM1AtJBIYP348eTB0Yy5g/5HGIS8vbxIuKDRjNiSzEo/k2Q59+qmquaVF3qbTgcFgAJFYDCi0YMKECeDm7g4B/gGQ/p90yMjIAIlYAgb0ZpFIjNt2QUVFxaTOzk6BWCzuMuP2281oQNfX10vSz593pWmJRGK4ceOGdWpa2gKKuy4uLuDj4wPe3t6sEchOTk5QXFwMH370EesE7R1tMG4cendzM+iRymtqayYUFhbK/fz8zPRtKkBXVt6wemfnO6uqqqumEiWTwJqAQD737LMM2MmTJ4O9gwNYWVn9UtJBcA8cOADNzU0sRgcEBEBoaCjs27ePebXeYLBOTEiYjEDnmMKDq6ystEpNTXXm5tesWVPyfwV0XV2dZMfbO1YXFRd76pCiCWQHB3uIjIyERQ8/POB+8fHxkJycDNZSKWhxv9VhqyEkZBF8cvAggt+MOYIFoCCj0qxJAE0gR0dHP8MDercpAy0cbpDf2LZtNaZOnlqmpttALpfDjh1Rg4Lc2NgIu3btgqqqKigtK4OZM2fCsmW/R+oex2herdEAxmeoqq5WIpWL4T4x8vrLly8rxpRHYx5s+eabb65BdexBntzY1Ag2chvYvXs3PDwIyGQxMTFMgLHUSiSCjRs3gqWlJZufNWsWfPPNNyBD0WZjY2OflZWlCFm0qMaUAUb2WnHx4kUVTS9fvjwRO27amACaQN6yZcsqvDmP2ro6aEIPdXR0hD/HvgtLliwZdN/S0lI4/NlnIESADZhyPYtxfMGCBb3rFy9eDBYWFizFwiZOTEycZApAo9ZoIBD7W1dbW2tyL4buGWi8KYv1658PO5Oc7EklSzIC+VOMrX94/PE77h8VFQX12DnIk11dXWH79u191k+bNo0JuOzsbBBjynXhwgV3XHxptB8cemmDKXjqiADd1NQkjggPX5OQlORJ3kiVLWdnZ/jqq6/uSNdk6enpcOz4cZBaWwPF9E1I2e7u7n0zfVTmDzzwAAPawtKiC70ojxS6UDiwvHj11VcXl5WVKWk6ODg4d+vWrVncuoSEBOXBgwcXc/PffvvtP/jLnnrqqTRklMojR474Y3z1wDRRgYylxFy/6oknnkjdsGFD7kDHef/991V4T36oNZx5ok315JNPetC0m5tbVU/hqN9r4yw8PDwMn4cVdz3DoejF90LX69auXZV05oynEAEWI71SfZpAwQcEGEvZPJW2BPwduTInevDJkycZYFQl+x3S9aYXX+z3XETfX375JcybOzf36aefvuNN04PEkMAerr+/f5/tGxoapNy6/pYheApU04p+7lf5xRdfhAYFBVWRN/d3HMwOrH69DJ1BQY0DGsWp9lfb9AGaOhDqHD9uHjvdiVHz6JqaGouIiIjVqWlpUwk2qnCRNxNop+PiGIC/AAvsxUQ3xj2g94BN3iqTWbPy55bXXgNbW9t+zxcSEgIBgYFq7P3n7OztOwbz5ns1DpT+DFNFKwTCA4HO6m89Xr8OGamEPJq2pWWYOTQoFAoaBEBsR3RfeerUKU6feJAyx+U67hgpKSm9ncDX1zeXv27Egda2toradLp2omuxRALk0dADtLhnxApN84EV9DTOqwXdFTPcTsQ6wnjFwPqFFLhELBbR/iNRAiVw0JOyVq1alevk5KRFKo3kOgB57UD79dBwFlL1M5zX0nH27t3bJ5a/9957DdzxKETw6RsFrT9PBwxbEWZIQCvs7Nr/9skn8ai0O8+np0/nQCWqDnnoIXB3c2Mxl/Nc7gUFz8lZB7mI9I40C3rMkakqtnDhwl86A8/OpqRAXkGBdP/+/Y88umzZ18YG+syZMx/1uV/0yME8fQhCLvfs2bPzabqoqEjJLaecm0IEL1bnjCrQmM/qPTw8NDHR0f9+6623BBcyMwMJIBJJldVVsGfPHpg1cybo2tq6d+i63QnRMyHuxzjY8MIL6LEyOJeaykTc2rVrb9v2XMo5GvMEufn5Xh/Gxvq9/Morufdz3fmxxx7L4YBGkUke/ENPfO6lbRJ/w0Xb91QZk8lkem8fH/W+Dz5ImDtnTg6JK6LY/5aUwOaXXoLikmusyMGaXH5bs8T4vGJFKDy69FHo7Ohg+yJLUIzscx6qiF2+chmsMZYTYyQmJfnAfW5LliypovDAxf3jx4979Hh0L9Aoaoe11HtPqobAnjJlSmvsgQM/Bs2dm01gy21soKCwECIinoGCgoLB6QS9euvrr4O9vT0rihCN70cK59v169fZcisrKXvZERwUVAJjwLy8vEp4aSYDmK+2SR+YVK2bwJ7s5qbdu2dPkmr27GwRiqtxCDYB9Nzzz0NJyeC4BE6fDitXrmQvP2zQ048fO8ZeV/LiJWg0Gibc0Os7ZqtUN8cC0CqVqoQXm/0OHTrkx6dtSuFMCmg+jb+7d2/SjOnTr5Jn29jIGY2vX7+elTkHrQ3jNt5eXmxao2mFv378MXDjzZPPnmXeTsd0cHCow47R+FuuTa1WS7lpephHjx6dbwpAU+EFO66OS+ni4+NVxqLtYQOaA3uql5caBdq/fKdNyxUysG2hsKgINm7aBOXl5QPuSwMPSIR1Yromt5HBD99/TxUlNkiwCMOAFOM5DViY7OpaqVQq7yhQpFJp7zYkeijdCQ4OfgOBDvt1QcMYxj9/YmLigqioqPlUraNiSB82CwzsBZR/XcNN28MKdG/MRjX+l+jo+EA//58JHCqC5Ofnd23evJmVMUlc9WdhYWEwLygIhVkn897Dnx2GtLQ0aGhsBAl6NOXaDz74YPHdFEswI6jkz9ND5AoYI1QHL+EXWU6dOrWYOhxStPNA9M3P4Yebtu+51j1AbzZQ6rVt27bkjIyMvJ7iSRfGWXFkZORS9EhbX19fmDFjBnsF6enpyYormLIBdQZKt6gAk/1zNsTGxrIBgwJcb2VpqZ4XHFx9N9dABYqcnBwPvpf0PMBcSm1OnDih4l4jGsOoAFJZWangUih+iZQ/HxoaWoIsc1uObYxrok9ydhnjwAisiAbzMaB7iiA7d+6cF5+Q8EhnezsYMAbTiE96MzUdBRkNNqBxZB9jfI6Li0MvloC+U8+UOeXoyokTS458/fVRhULRcbfXQAWIwsJCxWgN8+EPNxrsGhYtWvQnriATExNzmNIvk/doPo3z56mYsm7duuyMzMxZOp3OztBlwBjcDpcuXYKMzAwgtW5npwCFwg4saNABdgSJBC+vq7tGPsXdveK3gNzjHUahwbs1KnjcqZNR3OZAJtYxBsjDHqMHPRHSL4m1iPDweI8pU7LlMlktAYhUz2jb2tqaKe7y8uu9hVImvGnsN3YKVNs3YAza6dOn/Y1N20b16P4M0wnD6rCw0qVLl1Zgni376aefnIqKiuizHGV9fb2jVqezFQjF3SVTAVc5FdB+LXPmzLk1FoGmHJpfGjUm0JQKjIgipTdPjo6O7UTBE5XKttkqVUNra2txXW2tRUVFhYw+u8nNy5tUffOmsqWlxQ5jvCUxgYuz8/WAwMDmsQbySNE2WjuJsRdwYuJo3jCJNq1WK8T/QgReVIUi5tq1a7b5eXkOV3Ny3FChl25/++1LxnwPPcatmoCmMT8LTeWKSLR1dHQI29vahDps6pYWFl4mubpqJRKJ+XOcoVkKAS3DiZdhlL+RNpvRjD5V/ZC4UIPtn+bnMWaNsNXwf6zGE9sKMP9YzVgxqh/Q4DT2KlDQz89PUdmO+/kps91/xv38VBrwfn7qfwIMAHktq8bcYvfUAAAAAElFTkSuQmCC";
logoImage.style.position = "absolute";
parent.appendChild(logoImage);
this.logoImage = logoImage;
var progressFrame = document.createElement("img");
if (args.progressframeimage)
progressFrame.src = args.progressframeimage;
else
progressFrame.src = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAIAAAAGCAYAAADpJ08yAAAAGXRFWHRTb2Z0d2FyZQBBZG9iZSBJbWFnZVJlYWR5ccllPAAAAB5JREFUeNpi/P//fwMDEDAxQAEeBmNoaOhNEAMgwADBCwVgaIqyZQAAAABJRU5ErkJggg==";
progressFrame.style.position = "absolute";
parent.appendChild(progressFrame);
this.progressFrame = progressFrame;
var progressBar = document.createElement("img");
if (args.progressbarimage)
progressBar.src = args.progressbarimage;
else
progressBar.src = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAHoAAAAGCAIAAACD2x0JAAAAGXRFWHRTb2Z0d2FyZQBBZG9iZSBJbWFnZVJlYWR5ccllPAAAACVJREFUeNpiNDQ0ZBgF9AJMo0EwGtyjwT0KRoN7NLhHAT4AEGAAK8kAn2rIe4AAAAAASUVORK5CYII=";
progressBar.style.position = "absolute";
parent.appendChild(progressBar);
this.progressBar = progressBar;
var messageArea = document.createElement("p");
messageArea.style.position = "absolute";
parent.appendChild(messageArea);
this.messageArea = messageArea;
this.SetProgress = function (progress) {
if (this.progress < progress)
this.progress = progress;
this.messageArea.style.display = "none";
this.progressFrame.style.display = "inline";
this.progressBar.style.display = "inline";
this.Update();
}
this.SetMessage = function (message) {
this.message = message;
this.background.style.display = "inline";
this.logoImage.style.display = "inline";
this.progressFrame.style.display = "none";
this.progressBar.style.display = "none";
this.Update();
}
this.Clear = function() {
this.background.style.display = "none";
this.logoImage.style.display = "none";
this.progressFrame.style.display = "none";
this.progressBar.style.display = "none";
}
this.Update = function() {
this.background.style.top = this.dom.offsetTop + 'px';
this.background.style.left = this.dom.offsetLeft + 'px';
this.background.style.width = this.dom.offsetWidth + 'px';
this.background.style.height = this.dom.offsetHeight + 'px';
var logoImg = new Image();
logoImg.src = this.logoImage.src;
var progressFrameImg = new Image();
progressFrameImg.src = this.progressFrame.src;
this.logoImage.style.top = this.dom.offsetTop + (this.dom.offsetHeight * 0.5 - logoImg.height * 0.5) + 'px';
this.logoImage.style.left = this.dom.offsetLeft + (this.dom.offsetWidth * 0.5 - logoImg.width * 0.5) + 'px';
this.logoImage.style.width = logoImg.width+'px';
this.logoImage.style.height = logoImg.height+'px';
this.progressFrame.style.top = this.dom.offsetTop + (this.dom.offsetHeight * 0.5 + logoImg.height * 0.5 + 10) + 'px';
this.progressFrame.style.left = this.dom.offsetLeft + (this.dom.offsetWidth * 0.5 - progressFrameImg.width * 0.5) + 'px';
this.progressFrame.width = progressFrameImg.width;
this.progressFrame.height = progressFrameImg.height;
this.progressBar.style.top = this.progressFrame.style.top;
this.progressBar.style.left = this.progressFrame.style.left;
this.progressBar.width = progressFrameImg.width * Math.min(this.progress, 1);
this.progressBar.height = progressFrameImg.height;
this.messageArea.style.top = this.progressFrame.style.top;
this.messageArea.style.left = 0;
this.messageArea.style.width = '100%';
this.messageArea.style.textAlign = 'center';
this.messageArea.innerHTML = this.message;
}
this.Update ();
} | {
"pile_set_name": "Github"
} |
#!/usr/bin/env bash
set -euo pipefail
IFS=$'\n\t'
cd "$(dirname "$0")/.."
source scripts/utils.sh
if [[ -x "$(command -v clang-format-9)" ]]; then
CF=clang-format-9
else
CF=clang-format
clang-format --version | grep " 9." > /dev/null || ( print_warning "WARNING: MediaElch requires clang-format version 9")
fi
print_important "Format all source files using ${CF}"
find src -type f \( -name "*.cpp" -o -name "*.h" -o -name "*.hpp" \) -exec ${CF} -i -style=file {} \+
print_important "Format all test files using ${CF}"
find test -type f \( -name "*.cpp" -o -name "*.h" -o -name "*.hpp" \) -exec ${CF} -i -style=file {} \+
print_success "Done"
| {
"pile_set_name": "Github"
} |
/// <reference path="modernizr-2.6.2.js" />
/// <reference path="jquery-1.9.1.js" />
/// <reference path="knockout-2.1.0.debug.js" />
/// <reference path="q.js" />
/// <reference path="toastr.js" />
/// <reference path="require.js" />
/// <reference path="breeze.debug.js" />
| {
"pile_set_name": "Github"
} |
/*
Copyright The Kubernetes Authors.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
// Code generated by client-gen. DO NOT EDIT.
package fake
import (
"context"
v1beta1 "k8s.io/api/authentication/v1beta1"
v1 "k8s.io/apimachinery/pkg/apis/meta/v1"
schema "k8s.io/apimachinery/pkg/runtime/schema"
testing "k8s.io/client-go/testing"
)
// FakeTokenReviews implements TokenReviewInterface
type FakeTokenReviews struct {
Fake *FakeAuthenticationV1beta1
}
var tokenreviewsResource = schema.GroupVersionResource{Group: "authentication.k8s.io", Version: "v1beta1", Resource: "tokenreviews"}
var tokenreviewsKind = schema.GroupVersionKind{Group: "authentication.k8s.io", Version: "v1beta1", Kind: "TokenReview"}
// Create takes the representation of a tokenReview and creates it. Returns the server's representation of the tokenReview, and an error, if there is any.
func (c *FakeTokenReviews) Create(ctx context.Context, tokenReview *v1beta1.TokenReview, opts v1.CreateOptions) (result *v1beta1.TokenReview, err error) {
obj, err := c.Fake.
Invokes(testing.NewRootCreateAction(tokenreviewsResource, tokenReview), &v1beta1.TokenReview{})
if obj == nil {
return nil, err
}
return obj.(*v1beta1.TokenReview), err
}
| {
"pile_set_name": "Github"
} |
[Icon Data]
DisplayName=New
DisplayName[af]=Nuut
DisplayName[am]=አዲስ
DisplayName[ar]=جديد
DisplayName[az]=Yeni
DisplayName[be]=Новы
DisplayName[be@latin]=Novy
DisplayName[bg]=Ново
DisplayName[bn]=নতুন
DisplayName[bn_IN]=নতুন
DisplayName[bs]=Novo
DisplayName[ca]=Nou
DisplayName[cs]=Nové
DisplayName[cy]=Newydd
DisplayName[da]=Ny
DisplayName[de]=Neu
DisplayName[dz]=གསརཔ།
DisplayName[el]=Νέο
DisplayName[en_CA]=New
DisplayName[en_GB]=New
DisplayName[es]=Nuevo
DisplayName[et]=Uus
DisplayName[eu]=Berria
DisplayName[fa]=جدید
DisplayName[fi]=Uusi
DisplayName[fr]=Nouveau
DisplayName[fur]=Gnûf
DisplayName[ga]=Nua
DisplayName[gl]=Novo
DisplayName[gu]=નવું
DisplayName[he]=חדש
DisplayName[hi]=नया
DisplayName[hr]=Novi
DisplayName[hu]=Új
DisplayName[id]=Baru
DisplayName[is]=Nýtt
DisplayName[it]=Nuovo
DisplayName[ja]=新規
DisplayName[ka]=ახალი
DisplayName[kn]=ಹೊಸ
DisplayName[ko]=새 것
DisplayName[li]=Nuuj
DisplayName[lt]=Nauja
DisplayName[lv]=Jauns
DisplayName[mg]=Vaovao
DisplayName[mk]=Ново
DisplayName[ml]=പുതിയ
DisplayName[mn]=Шинэ
DisplayName[mr]=नविन
DisplayName[ms]=Baru
DisplayName[nb]=Ny
DisplayName[ne]=नयाँ
DisplayName[nl]=Nieuw
DisplayName[nn]=Ny
DisplayName[oc]=Novèl
DisplayName[or]=ନୂତନ
DisplayName[pa]=ਨਵਾਂ
DisplayName[pl]=Nowy
DisplayName[pt]=Novo
DisplayName[pt_BR]=Novo
DisplayName[ro]=Nou
DisplayName[ru]=Новый
DisplayName[rw]=Gishya
DisplayName[si]=නව
DisplayName[sk]=Nový
DisplayName[sl]=Novo
DisplayName[sq]=E re
DisplayName[sr]=Ново
DisplayName[sr@Latn]=Novo
DisplayName[sv]=Ny
DisplayName[ta]=புதிய
DisplayName[te]=కొత్త
DisplayName[th]=ใหม่
DisplayName[tk]=Täze
DisplayName[tr]=Yeni
DisplayName[uk]=Нове
DisplayName[vi]=Mới
DisplayName[wa]=Novea
DisplayName[xh]=Entsha
DisplayName[zh_CN]=新
DisplayName[zh_HK]=嶄新
DisplayName[zh_TW]=嶄新
| {
"pile_set_name": "Github"
} |
.\" Automatically generated by Pod::Man 2.27 (Pod::Simple 3.28)
.\"
.\" Standard preamble:
.\" ========================================================================
.de Sp \" Vertical space (when we can't use .PP)
.if t .sp .5v
.if n .sp
..
.de Vb \" Begin verbatim text
.ft CW
.nf
.ne \\$1
..
.de Ve \" End verbatim text
.ft R
.fi
..
.\" Set up some character translations and predefined strings. \*(-- will
.\" give an unbreakable dash, \*(PI will give pi, \*(L" will give a left
.\" double quote, and \*(R" will give a right double quote. \*(C+ will
.\" give a nicer C++. Capital omega is used to do unbreakable dashes and
.\" therefore won't be available. \*(C` and \*(C' expand to `' in nroff,
.\" nothing in troff, for use with C<>.
.tr \(*W-
.ds C+ C\v'-.1v'\h'-1p'\s-2+\h'-1p'+\s0\v'.1v'\h'-1p'
.ie n \{\
. ds -- \(*W-
. ds PI pi
. if (\n(.H=4u)&(1m=24u) .ds -- \(*W\h'-12u'\(*W\h'-12u'-\" diablo 10 pitch
. if (\n(.H=4u)&(1m=20u) .ds -- \(*W\h'-12u'\(*W\h'-8u'-\" diablo 12 pitch
. ds L" ""
. ds R" ""
. ds C` ""
. ds C' ""
'br\}
.el\{\
. ds -- \|\(em\|
. ds PI \(*p
. ds L" ``
. ds R" ''
. ds C`
. ds C'
'br\}
.\"
.\" Escape single quotes in literal strings from groff's Unicode transform.
.ie \n(.g .ds Aq \(aq
.el .ds Aq '
.\"
.\" If the F register is turned on, we'll generate index entries on stderr for
.\" titles (.TH), headers (.SH), subsections (.SS), items (.Ip), and index
.\" entries marked with X<> in POD. Of course, you'll have to process the
.\" output yourself in some meaningful fashion.
.\"
.\" Avoid warning from groff about undefined register 'F'.
.de IX
..
.nr rF 0
.if \n(.g .if rF .nr rF 1
.if (\n(rF:(\n(.g==0)) \{
. if \nF \{
. de IX
. tm Index:\\$1\t\\n%\t"\\$2"
..
. if !\nF==2 \{
. nr % 0
. nr F 2
. \}
. \}
.\}
.rr rF
.\"
.\" Accent mark definitions (@(#)ms.acc 1.5 88/02/08 SMI; from UCB 4.2).
.\" Fear. Run. Save yourself. No user-serviceable parts.
. \" fudge factors for nroff and troff
.if n \{\
. ds #H 0
. ds #V .8m
. ds #F .3m
. ds #[ \f1
. ds #] \fP
.\}
.if t \{\
. ds #H ((1u-(\\\\n(.fu%2u))*.13m)
. ds #V .6m
. ds #F 0
. ds #[ \&
. ds #] \&
.\}
. \" simple accents for nroff and troff
.if n \{\
. ds ' \&
. ds ` \&
. ds ^ \&
. ds , \&
. ds ~ ~
. ds /
.\}
.if t \{\
. ds ' \\k:\h'-(\\n(.wu*8/10-\*(#H)'\'\h"|\\n:u"
. ds ` \\k:\h'-(\\n(.wu*8/10-\*(#H)'\`\h'|\\n:u'
. ds ^ \\k:\h'-(\\n(.wu*10/11-\*(#H)'^\h'|\\n:u'
. ds , \\k:\h'-(\\n(.wu*8/10)',\h'|\\n:u'
. ds ~ \\k:\h'-(\\n(.wu-\*(#H-.1m)'~\h'|\\n:u'
. ds / \\k:\h'-(\\n(.wu*8/10-\*(#H)'\z\(sl\h'|\\n:u'
.\}
. \" troff and (daisy-wheel) nroff accents
.ds : \\k:\h'-(\\n(.wu*8/10-\*(#H+.1m+\*(#F)'\v'-\*(#V'\z.\h'.2m+\*(#F'.\h'|\\n:u'\v'\*(#V'
.ds 8 \h'\*(#H'\(*b\h'-\*(#H'
.ds o \\k:\h'-(\\n(.wu+\w'\(de'u-\*(#H)/2u'\v'-.3n'\*(#[\z\(de\v'.3n'\h'|\\n:u'\*(#]
.ds d- \h'\*(#H'\(pd\h'-\w'~'u'\v'-.25m'\f2\(hy\fP\v'.25m'\h'-\*(#H'
.ds D- D\\k:\h'-\w'D'u'\v'-.11m'\z\(hy\v'.11m'\h'|\\n:u'
.ds th \*(#[\v'.3m'\s+1I\s-1\v'-.3m'\h'-(\w'I'u*2/3)'\s-1o\s+1\*(#]
.ds Th \*(#[\s+2I\s-2\h'-\w'I'u*3/5'\v'-.3m'o\v'.3m'\*(#]
.ds ae a\h'-(\w'a'u*4/10)'e
.ds Ae A\h'-(\w'A'u*4/10)'E
. \" corrections for vroff
.if v .ds ~ \\k:\h'-(\\n(.wu*9/10-\*(#H)'\s-2\u~\d\s+2\h'|\\n:u'
.if v .ds ^ \\k:\h'-(\\n(.wu*10/11-\*(#H)'\v'-.4m'^\v'.4m'\h'|\\n:u'
. \" for low resolution devices (crt and lpr)
.if \n(.H>23 .if \n(.V>19 \
\{\
. ds : e
. ds 8 ss
. ds o a
. ds d- d\h'-1'\(ga
. ds D- D\h'-1'\(hy
. ds th \o'bp'
. ds Th \o'LP'
. ds ae ae
. ds Ae AE
.\}
.rm #[ #] #H #V #F C
.\" ========================================================================
.\"
.IX Title "SSL_session_reused 3"
.TH SSL_session_reused 3 "2017-12-07" "0.9.8y" "OpenSSL"
.\" For nroff, turn off justification. Always turn off hyphenation; it makes
.\" way too many mistakes in technical documents.
.if n .ad l
.nh
.SH "NAME"
SSL_session_reused \- query whether a reused session was negotiated during handshake
.SH "SYNOPSIS"
.IX Header "SYNOPSIS"
.Vb 1
\& #include <openssl/ssl.h>
\&
\& int SSL_session_reused(SSL *ssl);
.Ve
.SH "DESCRIPTION"
.IX Header "DESCRIPTION"
Query, whether a reused session was negotiated during the handshake.
.SH "NOTES"
.IX Header "NOTES"
During the negotiation, a client can propose to reuse a session. The server
then looks up the session in its cache. If both client and server agree
on the session, it will be reused and a flag is being set that can be
queried by the application.
.SH "RETURN VALUES"
.IX Header "RETURN VALUES"
The following return values can occur:
.IP "0" 4
A new session was negotiated.
.IP "1" 4
.IX Item "1"
A session was reused.
.SH "SEE ALSO"
.IX Header "SEE ALSO"
\&\fIssl\fR\|(3), \fISSL_set_session\fR\|(3),
\&\fISSL_CTX_set_session_cache_mode\fR\|(3)
| {
"pile_set_name": "Github"
} |
## \file
## \ingroup tutorial_pyroot_legacy
## \notebook
## This macro displays the Tree data structures
##
## \macro_image
## \macro_code
##
## \author Wim Lavrijsen
from ROOT import TCanvas, TPaveLabel, TPaveText, TPavesText, TText
from ROOT import TArrow, TLine
from ROOT import gROOT, gBenchmark
#gROOT.Reset()
c1 = TCanvas('c1','Tree Data Structure',200,10,750,940)
c1.Range(0,-0.1,1,1.15)
gBenchmark.Start('tree')
branchcolor = 26
leafcolor = 30
basketcolor = 42
offsetcolor = 43
#title = TPaveLabel(.3,1.05,.8,1.13,c1.GetTitle())
title = TPaveLabel(.3,1.05,.8,1.13,'Tree Data Structure')
title.SetFillColor(16)
title.Draw()
tree = TPaveText(.01,.75,.15,1.00)
tree.SetFillColor(18)
tree.SetTextAlign(12)
tnt = tree.AddText('Tree')
tnt.SetTextAlign(22)
tnt.SetTextSize(0.030)
tree.AddText('fScanField')
tree.AddText('fMaxEventLoop')
tree.AddText('fMaxVirtualSize')
tree.AddText('fEntries')
tree.AddText('fDimension')
tree.AddText('fSelectedRows')
tree.Draw()
farm = TPavesText(.01,1.02,.15,1.1,9,'tr')
tfarm = farm.AddText('CHAIN')
tfarm.SetTextSize(0.024)
farm.AddText('Collection')
farm.AddText('of Trees')
farm.Draw()
link = TLine(.15,.92,.80,.92)
link.SetLineWidth(2)
link.SetLineColor(1)
link.Draw()
link.DrawLine(.21,.87,.21,.275)
link.DrawLine(.23,.87,.23,.375)
link.DrawLine(.25,.87,.25,.775)
link.DrawLine(.41,.25,.41,-.025)
link.DrawLine(.43,.25,.43,.075)
link.DrawLine(.45,.25,.45,.175)
branch0 = TPaveLabel(.20,.87,.35,.97,'Branch 0')
branch0.SetTextSize(0.35)
branch0.SetFillColor(branchcolor)
branch0.Draw()
branch1 = TPaveLabel(.40,.87,.55,.97,'Branch 1')
branch1.SetTextSize(0.35)
branch1.SetFillColor(branchcolor)
branch1.Draw()
branch2 = TPaveLabel(.60,.87,.75,.97,'Branch 2')
branch2.SetTextSize(0.35)
branch2.SetFillColor(branchcolor)
branch2.Draw()
branch3 = TPaveLabel(.80,.87,.95,.97,'Branch 3')
branch3.SetTextSize(0.35)
branch3.SetFillColor(branchcolor)
branch3.Draw()
leaf0 = TPaveLabel(.4,.75,.5,.8,'Leaf 0')
leaf0.SetFillColor(leafcolor)
leaf0.Draw()
leaf1 = TPaveLabel(.6,.75,.7,.8,'Leaf 1')
leaf1.SetFillColor(leafcolor)
leaf1.Draw()
leaf2 = TPaveLabel(.8,.75,.9,.8,'Leaf 2')
leaf2.SetFillColor(leafcolor)
leaf2.Draw()
firstevent = TPaveText(.4,.35,.9,.4)
firstevent.AddText('First event of each basket')
firstevent.AddText('Array of fMaxBaskets Integers')
firstevent.SetFillColor(basketcolor)
firstevent.Draw()
basket0 = TPaveLabel(.4,.25,.5,.3,'Basket 0')
basket0.SetFillColor(basketcolor)
basket0.Draw()
basket1 = TPaveLabel(.6,.25,.7,.3,'Basket 1')
basket1.SetFillColor(basketcolor)
basket1.Draw()
basket2 = TPaveLabel(.8,.25,.9,.3,'Basket 2')
basket2.SetFillColor(basketcolor)
basket2.Draw()
offset = TPaveText(.55,.15,.9,.2)
offset.AddText('Offset of events in fBuffer')
offset.AddText('Array of fEventOffsetLen Integers')
offset.AddText('(if variable length structure)')
offset.SetFillColor(offsetcolor)
offset.Draw()
buffer = TPaveText(.55,.05,.9,.1)
buffer.AddText('Basket buffer')
buffer.AddText('Array of fBasketSize chars')
buffer.SetFillColor(offsetcolor)
buffer.Draw()
zipbuffer = TPaveText(.55,-.05,.75,.0)
zipbuffer.AddText('Basket compressed buffer')
zipbuffer.AddText('(if compression)')
zipbuffer.SetFillColor(offsetcolor)
zipbuffer.Draw()
ar1 = TArrow()
ar1.SetLineWidth(2)
ar1.SetLineColor(1)
ar1.SetFillStyle(1001)
ar1.SetFillColor(1)
ar1.DrawArrow(.21,.275,.39,.275,0.015,'|>')
ar1.DrawArrow(.23,.375,.39,.375,0.015,'|>')
ar1.DrawArrow(.25,.775,.39,.775,0.015,'|>')
ar1.DrawArrow(.50,.775,.59,.775,0.015,'|>')
ar1.DrawArrow(.70,.775,.79,.775,0.015,'|>')
ar1.DrawArrow(.50,.275,.59,.275,0.015,'|>')
ar1.DrawArrow(.70,.275,.79,.275,0.015,'|>')
ar1.DrawArrow(.45,.175,.54,.175,0.015,'|>')
ar1.DrawArrow(.43,.075,.54,.075,0.015,'|>')
ar1.DrawArrow(.41,-.025,.54,-.025,0.015,'|>')
ldot = TLine(.95,.92,.99,.92)
ldot.SetLineStyle(3)
ldot.Draw()
ldot.DrawLine(.9,.775,.99,.775)
ldot.DrawLine(.9,.275,.99,.275)
ldot.DrawLine(.55,.05,.55,0)
ldot.DrawLine(.9,.05,.75,0)
pname = TText(.46,.21,'fEventOffset')
pname.SetTextFont(72)
pname.SetTextSize(0.018)
pname.Draw()
pname.DrawText(.44,.11,'fBuffer')
pname.DrawText(.42,.01,'fZipBuffer')
pname.DrawText(.26,.81,'fLeaves = TObjArray of TLeaf')
pname.DrawText(.24,.40,'fBasketEvent')
pname.DrawText(.22,.31,'fBaskets = TObjArray of TBasket')
pname.DrawText(.20,1.0,'fBranches = TObjArray of TBranch')
ntleaf = TPaveText(0.30,.42,.62,.7)
ntleaf.SetTextSize(0.014)
ntleaf.SetFillColor(leafcolor)
ntleaf.SetTextAlign(12)
ntleaf.AddText('fLen: number of fixed elements')
ntleaf.AddText('fLenType: number of bytes of data type')
ntleaf.AddText('fOffset: relative to Leaf0-fAddress')
ntleaf.AddText('fNbytesIO: number of bytes used for I/O')
ntleaf.AddText('fIsPointer: True if pointer')
ntleaf.AddText('fIsRange: True if leaf has a range')
ntleaf.AddText('fIsUnsigned: True if unsigned')
ntleaf.AddText('*fLeafCount: points to Leaf counter')
ntleaf.AddText(' ')
ntleaf.AddLine(0,0,0,0)
ntleaf.AddText('fName = Leaf name')
ntleaf.AddText('fTitle = Leaf type (see Type codes)')
ntleaf.Draw()
type = TPaveText(.65,.42,.95,.7)
type.SetTextAlign(12)
type.SetFillColor(leafcolor)
type.AddText(' ')
type.AddText('C : a character string')
type.AddText('B : an 8 bit signed integer')
type.AddText('b : an 8 bit unsigned integer')
type.AddText('S : a 16 bit signed short integer')
type.AddText('s : a 16 bit unsigned short integer')
type.AddText('I : a 32 bit signed integer')
type.AddText('i : a 32 bit unsigned integer')
type.AddText('F : a 32 bit floating point')
type.AddText('D : a 64 bit floating point')
type.AddText('TXXXX : a class name TXXXX')
type.Draw()
typecode = TPaveLabel(.7,.68,.9,.72,'fType codes')
typecode.SetFillColor(leafcolor)
typecode.Draw()
ldot.DrawLine(.4,.75,.30,.7)
ldot.DrawLine(.5,.75,.62,.7)
ntbasket = TPaveText(0.02,-0.07,0.35,.25)
ntbasket.SetFillColor(basketcolor)
ntbasket.SetTextSize(0.014)
ntbasket.SetTextAlign(12)
ntbasket.AddText('fNbytes: Size of compressed Basket')
ntbasket.AddText('fObjLen: Size of uncompressed Basket')
ntbasket.AddText('fDatime: Date/Time when written to store')
ntbasket.AddText('fKeylen: Number of bytes for the key')
ntbasket.AddText('fCycle : Cycle number')
ntbasket.AddText('fSeekKey: Pointer to Basket on file')
ntbasket.AddText('fSeekPdir: Pointer to directory on file')
ntbasket.AddText("fClassName: 'TBasket'")
ntbasket.AddText('fName: Branch name')
ntbasket.AddText('fTitle: Tree name')
ntbasket.AddText(' ')
ntbasket.AddLine(0,0,0,0)
ntbasket.AddText('fNevBuf: Number of events in Basket')
ntbasket.AddText('fLast: pointer to last used byte in Basket')
ntbasket.Draw()
ldot.DrawLine(.4,.3,0.02,0.25)
ldot.DrawLine(.5,.25,0.35,-.07)
ldot.DrawLine(.5,.3,0.35,0.25)
ntbranch = TPaveText(0.02,0.40,0.18,0.68)
ntbranch.SetFillColor(branchcolor)
ntbranch.SetTextSize(0.015)
ntbranch.SetTextAlign(12)
ntbranch.AddText('fBasketSize')
ntbranch.AddText('fEventOffsetLen')
ntbranch.AddText('fMaxBaskets')
ntbranch.AddText('fEntries')
ntbranch.AddText('fAddress of Leaf0')
ntbranch.AddText(' ')
ntbranch.AddLine(0,0,0,0)
ntbranch.AddText('fName: Branchname')
ntbranch.AddText('fTitle: leaflist')
ntbranch.Draw()
ldot.DrawLine(.2,.97,.02,.68)
ldot.DrawLine(.35,.97,.18,.68)
ldot.DrawLine(.35,.87,.18,.40)
basketstore = TPavesText(.8,-0.088,0.952,-0.0035,7,'tr')
basketstore.SetFillColor(28)
basketstore.AddText('Baskets')
basketstore.AddText('Stores')
basketstore.Draw()
c1.Update()
gBenchmark.Show('tree')
| {
"pile_set_name": "Github"
} |
// Copyright 2013-2015 Bowery, Inc.
package prompt
import (
"os"
"syscall"
"unsafe"
)
// Flags to control the terminals mode.
const (
echoInputFlag = 0x0004
insertModeFlag = 0x0020
lineInputFlag = 0x0002
mouseInputFlag = 0x0010
processedInputFlag = 0x0001
windowInputFlag = 0x0008
)
// Error number returned for an invalid handle.
const errnoInvalidHandle = 0x6
var (
kernel = syscall.NewLazyDLL("kernel32.dll")
getConsoleScreenBufferInfo = kernel.NewProc("GetConsoleScreenBufferInfo")
setConsoleMode = kernel.NewProc("SetConsoleMode")
)
// consoleScreenBufferInfo contains various fields for the terminal.
type consoleScreenBufferInfo struct {
size coord
cursorPosition coord
attributes uint16
window smallRect
maximumWindowSize coord
}
// coord contains coords for positioning.
type coord struct {
x int16
y int16
}
// smallRect contains positions for the window edges.
type smallRect struct {
left int16
top int16
right int16
bottom int16
}
// terminalSize retrieves the cols/rows for the terminal connected to out.
func terminalSize(out *os.File) (int, int, error) {
csbi := new(consoleScreenBufferInfo)
ret, _, err := getConsoleScreenBufferInfo.Call(out.Fd(), uintptr(unsafe.Pointer(csbi)))
if ret == 0 {
return 0, 0, err
}
// Results are always off by one.
cols := csbi.window.right - csbi.window.left + 1
rows := csbi.window.bottom - csbi.window.top + 1
return int(cols), int(rows), nil
}
// isNotTerminal checks if an error is related to the input not being a terminal.
func isNotTerminal(err error) bool {
errno, ok := err.(syscall.Errno)
return ok && errno == errnoInvalidHandle
}
// terminal contains the private fields for a Windows terminal.
type terminal struct {
supportsEditing bool
fd uintptr
origMode uint32
}
// newTerminal creates a terminal and sets it to raw input mode.
func newTerminal(in *os.File) (*terminal, error) {
term := &terminal{fd: in.Fd()}
err := syscall.GetConsoleMode(syscall.Handle(term.fd), &term.origMode)
if err != nil {
return term, nil
}
mode := term.origMode
term.supportsEditing = true
// Set new mode flags.
mode &^= (echoInputFlag | insertModeFlag | lineInputFlag | mouseInputFlag |
processedInputFlag | windowInputFlag)
ret, _, err := setConsoleMode.Call(term.fd, uintptr(mode))
if ret == 0 {
return nil, err
}
return term, nil
}
// Close disables the terminals raw input.
func (term *terminal) Close() error {
if term.supportsEditing {
ret, _, err := setConsoleMode.Call(term.fd, uintptr(term.origMode))
if ret == 0 {
return err
}
}
return nil
}
| {
"pile_set_name": "Github"
} |
Android Accordion View
======================
Example
-------
* git pull
* import to eclipse
* properties->android uncheck is library
* run as android application
See [main.xml](https://github.com/hamsterready/android-accordion-view/blob/master/res/layout/main.xml).
Screenshot
----------
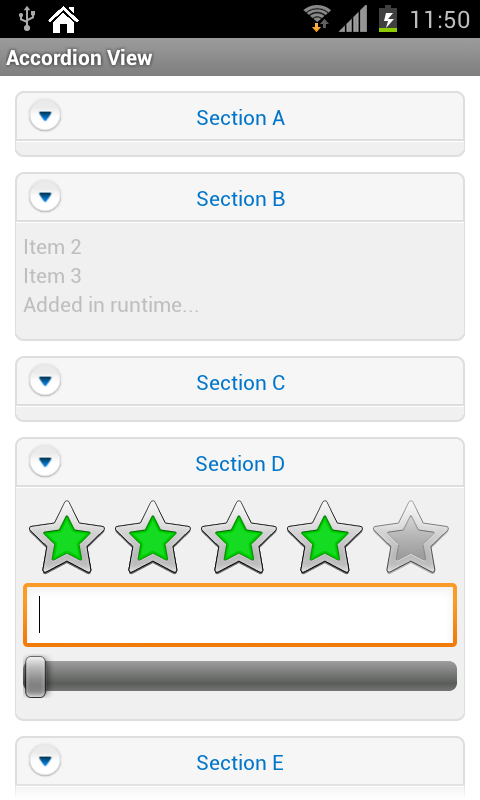
License
-------
The MIT License
Copyright (c) 2011 Sentaca Poland sp. z o.o. / http://sentaca.com/
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
| {
"pile_set_name": "Github"
} |
# coding=utf-8
"""
The Batch Webhooks API Endpoint
Documentation: https://developer.mailchimp.com/documentation/mailchimp/reference/batch-webhooks/
"""
from __future__ import unicode_literals
from mailchimp3.baseapi import BaseApi
class BatchWebhooks(BaseApi):
"""
Manage webhooks for batch operations.
"""
def __init__(self, *args, **kwargs):
"""
Initialize the endpoint
"""
super(BatchWebhooks, self).__init__(*args, **kwargs)
self.endpoint = 'batch-webhooks'
self.batch_webhook_id = None
def create(self, data):
"""
Configure a webhook that will fire whenever any batch request
completes processing.
:param data: The request body parameters
:type data: :py:class:`dict`
data = {
"url": string*
}
"""
if 'url' not in data:
raise KeyError('The batch webhook must have a valid url')
response = self._mc_client._post(url=self._build_path(), data=data)
if response is not None:
self.batch_webhook_id = response['id']
else:
self.batch_webhook_id = None
return response
def all(self, get_all=False, **queryparams):
"""
Get all webhooks that have been configured for batches.
:param get_all: Should the query get all results
:type get_all: :py:class:`bool`
:param queryparams: The query string parameters
queryparams['fields'] = []
queryparams['exclude_fields'] = []
queryparams['count'] = integer
queryparams['offset'] = integer
"""
self.batch_webhook_id = None
if get_all:
return self._iterate(url=self._build_path(), **queryparams)
else:
return self._mc_client._get(url=self._build_path(), **queryparams)
def get(self, batch_webhook_id, **queryparams):
"""
Get information about a specific batch webhook.
:param batch_webhook_id: The unique id for the batch webhook.
:type batch_webhook_id: :py:class:`str`
:param queryparams: The query string parameters
queryparams['fields'] = []
queryparams['exclude_fields'] = []
"""
self.batch_webhook_id = batch_webhook_id
return self._mc_client._get(url=self._build_path(batch_webhook_id), **queryparams)
def update(self, batch_webhook_id, data):
"""
Update a webhook that will fire whenever any batch request completes
processing.
:param batch_webhook_id: The unique id for the batch webhook.
:type batch_webhook_id: :py:class:`str`
:param data: The request body parameters
:type data: :py:class:`dict`
data = {
"url": string*
}
"""
self.batch_webhook_id = batch_webhook_id
if 'url' not in data:
raise KeyError('The batch webhook must have a valid url')
return self._mc_client._patch(url=self._build_path(batch_webhook_id), data=data)
def delete(self, batch_webhook_id):
"""
Remove a batch webhook. Webhooks will no longer be sent to the given
URL.
:param batch_webhook_id: The unique id for the batch webhook.
:type batch_webhook_id: :py:class:`str`
"""
self.batch_webhook_id = batch_webhook_id
return self._mc_client._delete(url=self._build_path(batch_webhook_id))
| {
"pile_set_name": "Github"
} |
package com.itcast.ay05
/**
* ClassName:`19.排序`
* Description:
*/
fun main(args: Array<String>) {
val list = listOf("z","b","d")
// 正序排序 b d z
println(list.sorted())
// 倒序排序
println(list.sortedDescending())
// 按字段排序
val list1 = listOf(Person("林青霞",50),Person("张曼玉",30),Person("柳岩",70))
// list1.sorted()
val list3 = list1.sortedBy { it.age }
// println(list3)
val list4 = list1.sortedByDescending { it.age }
println(list4)
}
data class Person(var name:String,var age:Int) | {
"pile_set_name": "Github"
} |
#include <iostream>
#include <sophus/test_macros.hpp>
#include <sophus/formatstring.hpp>
namespace Sophus {
namespace {
bool testFormatString() {
bool passed = true;
SOPHUS_TEST_EQUAL(passed, details::FormatString(), std::string());
std::string test_str = "Hello World!";
SOPHUS_TEST_EQUAL(passed, details::FormatString(test_str.c_str()), test_str);
SOPHUS_TEST_EQUAL(passed, details::FormatString("Number: %", 5),
std::string("Number: 5"));
SOPHUS_TEST_EQUAL(passed,
details::FormatString("Real: % msg %", 1.5, test_str),
std::string("Real: 1.5 msg Hello World!"));
SOPHUS_TEST_EQUAL(passed,
details::FormatString(
"vec: %", Eigen::Vector3f(0.f, 1.f, 1.5f).transpose()),
std::string("vec: 0 1 1.5"));
SOPHUS_TEST_EQUAL(
passed, details::FormatString("Number: %", 1, 2),
std::string("Number: 1\nFormat-Warning: There are 1 args unused."));
return passed;
}
bool testSmokeDetails() {
bool passed = true;
std::cout << details::pretty(4.2) << std::endl;
std::cout << details::pretty(Vector2f(1, 2)) << std::endl;
bool dummy = true;
details::testFailed(dummy, "dummyFunc", "dummyFile", 99,
"This is just a pratice alarm!");
SOPHUS_TEST_EQUAL(passed, dummy, false);
double val = transpose(42.0);
SOPHUS_TEST_EQUAL(passed, val, 42.0);
Matrix<float, 1, 2> row = transpose(Vector2f(1, 7));
Matrix<float, 1, 2> expected_row(1, 7);
SOPHUS_TEST_EQUAL(passed, row, expected_row);
optional<int> opt(nullopt);
SOPHUS_TEST(passed, !opt);
return passed;
}
void runAll() {
std::cerr << "Common tests:" << std::endl;
bool passed = testFormatString();
passed &= testSmokeDetails();
processTestResult(passed);
}
} // namespace
} // namespace Sophus
int main() { Sophus::runAll(); }
| {
"pile_set_name": "Github"
} |
/**
******************************************************************************
* @file stm32f10x_tim.h
* @author MCD Application Team
* @version V3.5.0
* @date 11-March-2011
* @brief This file contains all the functions prototypes for the TIM firmware
* library.
******************************************************************************
* @attention
*
* THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS
* WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE
* TIME. AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY
* DIRECT, INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING
* FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE
* CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS.
*
* <h2><center>© COPYRIGHT 2011 STMicroelectronics</center></h2>
******************************************************************************
*/
/* Define to prevent recursive inclusion -------------------------------------*/
#ifndef __STM32F10x_TIM_H
#define __STM32F10x_TIM_H
#ifdef __cplusplus
extern "C" {
#endif
/* Includes ------------------------------------------------------------------*/
#include "stm32f10x.h"
/** @addtogroup STM32F10x_StdPeriph_Driver
* @{
*/
/** @addtogroup TIM
* @{
*/
/** @defgroup TIM_Exported_Types
* @{
*/
/**
* @brief TIM Time Base Init structure definition
* @note This structure is used with all TIMx except for TIM6 and TIM7.
*/
typedef struct
{
uint16_t TIM_Prescaler; /*!< Specifies the prescaler value used to divide the TIM clock.
This parameter can be a number between 0x0000 and 0xFFFF */
uint16_t TIM_CounterMode; /*!< Specifies the counter mode.
This parameter can be a value of @ref TIM_Counter_Mode */
uint16_t TIM_Period; /*!< Specifies the period value to be loaded into the active
Auto-Reload Register at the next update event.
This parameter must be a number between 0x0000 and 0xFFFF. */
uint16_t TIM_ClockDivision; /*!< Specifies the clock division.
This parameter can be a value of @ref TIM_Clock_Division_CKD */
uint8_t TIM_RepetitionCounter; /*!< Specifies the repetition counter value. Each time the RCR downcounter
reaches zero, an update event is generated and counting restarts
from the RCR value (N).
This means in PWM mode that (N+1) corresponds to:
- the number of PWM periods in edge-aligned mode
- the number of half PWM period in center-aligned mode
This parameter must be a number between 0x00 and 0xFF.
@note This parameter is valid only for TIM1 and TIM8. */
} TIM_TimeBaseInitTypeDef;
/**
* @brief TIM Output Compare Init structure definition
*/
typedef struct
{
uint16_t TIM_OCMode; /*!< Specifies the TIM mode.
This parameter can be a value of @ref TIM_Output_Compare_and_PWM_modes */
uint16_t TIM_OutputState; /*!< Specifies the TIM Output Compare state.
This parameter can be a value of @ref TIM_Output_Compare_state */
uint16_t TIM_OutputNState; /*!< Specifies the TIM complementary Output Compare state.
This parameter can be a value of @ref TIM_Output_Compare_N_state
@note This parameter is valid only for TIM1 and TIM8. */
uint16_t TIM_Pulse; /*!< Specifies the pulse value to be loaded into the Capture Compare Register.
This parameter can be a number between 0x0000 and 0xFFFF */
uint16_t TIM_OCPolarity; /*!< Specifies the output polarity.
This parameter can be a value of @ref TIM_Output_Compare_Polarity */
uint16_t TIM_OCNPolarity; /*!< Specifies the complementary output polarity.
This parameter can be a value of @ref TIM_Output_Compare_N_Polarity
@note This parameter is valid only for TIM1 and TIM8. */
uint16_t TIM_OCIdleState; /*!< Specifies the TIM Output Compare pin state during Idle state.
This parameter can be a value of @ref TIM_Output_Compare_Idle_State
@note This parameter is valid only for TIM1 and TIM8. */
uint16_t TIM_OCNIdleState; /*!< Specifies the TIM Output Compare pin state during Idle state.
This parameter can be a value of @ref TIM_Output_Compare_N_Idle_State
@note This parameter is valid only for TIM1 and TIM8. */
} TIM_OCInitTypeDef;
/**
* @brief TIM Input Capture Init structure definition
*/
typedef struct
{
uint16_t TIM_Channel; /*!< Specifies the TIM channel.
This parameter can be a value of @ref TIM_Channel */
uint16_t TIM_ICPolarity; /*!< Specifies the active edge of the input signal.
This parameter can be a value of @ref TIM_Input_Capture_Polarity */
uint16_t TIM_ICSelection; /*!< Specifies the input.
This parameter can be a value of @ref TIM_Input_Capture_Selection */
uint16_t TIM_ICPrescaler; /*!< Specifies the Input Capture Prescaler.
This parameter can be a value of @ref TIM_Input_Capture_Prescaler */
uint16_t TIM_ICFilter; /*!< Specifies the input capture filter.
This parameter can be a number between 0x0 and 0xF */
} TIM_ICInitTypeDef;
/**
* @brief BDTR structure definition
* @note This structure is used only with TIM1 and TIM8.
*/
typedef struct
{
uint16_t TIM_OSSRState; /*!< Specifies the Off-State selection used in Run mode.
This parameter can be a value of @ref OSSR_Off_State_Selection_for_Run_mode_state */
uint16_t TIM_OSSIState; /*!< Specifies the Off-State used in Idle state.
This parameter can be a value of @ref OSSI_Off_State_Selection_for_Idle_mode_state */
uint16_t TIM_LOCKLevel; /*!< Specifies the LOCK level parameters.
This parameter can be a value of @ref Lock_level */
uint16_t TIM_DeadTime; /*!< Specifies the delay time between the switching-off and the
switching-on of the outputs.
This parameter can be a number between 0x00 and 0xFF */
uint16_t TIM_Break; /*!< Specifies whether the TIM Break input is enabled or not.
This parameter can be a value of @ref Break_Input_enable_disable */
uint16_t TIM_BreakPolarity; /*!< Specifies the TIM Break Input pin polarity.
This parameter can be a value of @ref Break_Polarity */
uint16_t TIM_AutomaticOutput; /*!< Specifies whether the TIM Automatic Output feature is enabled or not.
This parameter can be a value of @ref TIM_AOE_Bit_Set_Reset */
} TIM_BDTRInitTypeDef;
/** @defgroup TIM_Exported_constants
* @{
*/
#define IS_TIM_ALL_PERIPH(PERIPH) (((PERIPH) == TIM1) || \
((PERIPH) == TIM2) || \
((PERIPH) == TIM3) || \
((PERIPH) == TIM4) || \
((PERIPH) == TIM5) || \
((PERIPH) == TIM6) || \
((PERIPH) == TIM7) || \
((PERIPH) == TIM8) || \
((PERIPH) == TIM9) || \
((PERIPH) == TIM10)|| \
((PERIPH) == TIM11)|| \
((PERIPH) == TIM12)|| \
((PERIPH) == TIM13)|| \
((PERIPH) == TIM14)|| \
((PERIPH) == TIM15)|| \
((PERIPH) == TIM16)|| \
((PERIPH) == TIM17))
/* LIST1: TIM 1 and 8 */
#define IS_TIM_LIST1_PERIPH(PERIPH) (((PERIPH) == TIM1) || \
((PERIPH) == TIM8))
/* LIST2: TIM 1, 8, 15 16 and 17 */
#define IS_TIM_LIST2_PERIPH(PERIPH) (((PERIPH) == TIM1) || \
((PERIPH) == TIM8) || \
((PERIPH) == TIM15)|| \
((PERIPH) == TIM16)|| \
((PERIPH) == TIM17))
/* LIST3: TIM 1, 2, 3, 4, 5 and 8 */
#define IS_TIM_LIST3_PERIPH(PERIPH) (((PERIPH) == TIM1) || \
((PERIPH) == TIM2) || \
((PERIPH) == TIM3) || \
((PERIPH) == TIM4) || \
((PERIPH) == TIM5) || \
((PERIPH) == TIM8))
/* LIST4: TIM 1, 2, 3, 4, 5, 8, 15, 16 and 17 */
#define IS_TIM_LIST4_PERIPH(PERIPH) (((PERIPH) == TIM1) || \
((PERIPH) == TIM2) || \
((PERIPH) == TIM3) || \
((PERIPH) == TIM4) || \
((PERIPH) == TIM5) || \
((PERIPH) == TIM8) || \
((PERIPH) == TIM15)|| \
((PERIPH) == TIM16)|| \
((PERIPH) == TIM17))
/* LIST5: TIM 1, 2, 3, 4, 5, 8 and 15 */
#define IS_TIM_LIST5_PERIPH(PERIPH) (((PERIPH) == TIM1) || \
((PERIPH) == TIM2) || \
((PERIPH) == TIM3) || \
((PERIPH) == TIM4) || \
((PERIPH) == TIM5) || \
((PERIPH) == TIM8) || \
((PERIPH) == TIM15))
/* LIST6: TIM 1, 2, 3, 4, 5, 8, 9, 12 and 15 */
#define IS_TIM_LIST6_PERIPH(PERIPH) (((PERIPH) == TIM1) || \
((PERIPH) == TIM2) || \
((PERIPH) == TIM3) || \
((PERIPH) == TIM4) || \
((PERIPH) == TIM5) || \
((PERIPH) == TIM8) || \
((PERIPH) == TIM9) || \
((PERIPH) == TIM12)|| \
((PERIPH) == TIM15))
/* LIST7: TIM 1, 2, 3, 4, 5, 6, 7, 8, 9, 12 and 15 */
#define IS_TIM_LIST7_PERIPH(PERIPH) (((PERIPH) == TIM1) || \
((PERIPH) == TIM2) || \
((PERIPH) == TIM3) || \
((PERIPH) == TIM4) || \
((PERIPH) == TIM5) || \
((PERIPH) == TIM6) || \
((PERIPH) == TIM7) || \
((PERIPH) == TIM8) || \
((PERIPH) == TIM9) || \
((PERIPH) == TIM12)|| \
((PERIPH) == TIM15))
/* LIST8: TIM 1, 2, 3, 4, 5, 8, 9, 10, 11, 12, 13, 14, 15, 16 and 17 */
#define IS_TIM_LIST8_PERIPH(PERIPH) (((PERIPH) == TIM1) || \
((PERIPH) == TIM2) || \
((PERIPH) == TIM3) || \
((PERIPH) == TIM4) || \
((PERIPH) == TIM5) || \
((PERIPH) == TIM8) || \
((PERIPH) == TIM9) || \
((PERIPH) == TIM10)|| \
((PERIPH) == TIM11)|| \
((PERIPH) == TIM12)|| \
((PERIPH) == TIM13)|| \
((PERIPH) == TIM14)|| \
((PERIPH) == TIM15)|| \
((PERIPH) == TIM16)|| \
((PERIPH) == TIM17))
/* LIST9: TIM 1, 2, 3, 4, 5, 6, 7, 8, 15, 16, and 17 */
#define IS_TIM_LIST9_PERIPH(PERIPH) (((PERIPH) == TIM1) || \
((PERIPH) == TIM2) || \
((PERIPH) == TIM3) || \
((PERIPH) == TIM4) || \
((PERIPH) == TIM5) || \
((PERIPH) == TIM6) || \
((PERIPH) == TIM7) || \
((PERIPH) == TIM8) || \
((PERIPH) == TIM15)|| \
((PERIPH) == TIM16)|| \
((PERIPH) == TIM17))
/**
* @}
*/
/** @defgroup TIM_Output_Compare_and_PWM_modes
* @{
*/
#define TIM_OCMode_Timing ((uint16_t)0x0000)
#define TIM_OCMode_Active ((uint16_t)0x0010)
#define TIM_OCMode_Inactive ((uint16_t)0x0020)
#define TIM_OCMode_Toggle ((uint16_t)0x0030)
#define TIM_OCMode_PWM1 ((uint16_t)0x0060)
#define TIM_OCMode_PWM2 ((uint16_t)0x0070)
#define IS_TIM_OC_MODE(MODE) (((MODE) == TIM_OCMode_Timing) || \
((MODE) == TIM_OCMode_Active) || \
((MODE) == TIM_OCMode_Inactive) || \
((MODE) == TIM_OCMode_Toggle)|| \
((MODE) == TIM_OCMode_PWM1) || \
((MODE) == TIM_OCMode_PWM2))
#define IS_TIM_OCM(MODE) (((MODE) == TIM_OCMode_Timing) || \
((MODE) == TIM_OCMode_Active) || \
((MODE) == TIM_OCMode_Inactive) || \
((MODE) == TIM_OCMode_Toggle)|| \
((MODE) == TIM_OCMode_PWM1) || \
((MODE) == TIM_OCMode_PWM2) || \
((MODE) == TIM_ForcedAction_Active) || \
((MODE) == TIM_ForcedAction_InActive))
/**
* @}
*/
/** @defgroup TIM_One_Pulse_Mode
* @{
*/
#define TIM_OPMode_Single ((uint16_t)0x0008)
#define TIM_OPMode_Repetitive ((uint16_t)0x0000)
#define IS_TIM_OPM_MODE(MODE) (((MODE) == TIM_OPMode_Single) || \
((MODE) == TIM_OPMode_Repetitive))
/**
* @}
*/
/** @defgroup TIM_Channel
* @{
*/
#define TIM_Channel_1 ((uint16_t)0x0000)
#define TIM_Channel_2 ((uint16_t)0x0004)
#define TIM_Channel_3 ((uint16_t)0x0008)
#define TIM_Channel_4 ((uint16_t)0x000C)
#define IS_TIM_CHANNEL(CHANNEL) (((CHANNEL) == TIM_Channel_1) || \
((CHANNEL) == TIM_Channel_2) || \
((CHANNEL) == TIM_Channel_3) || \
((CHANNEL) == TIM_Channel_4))
#define IS_TIM_PWMI_CHANNEL(CHANNEL) (((CHANNEL) == TIM_Channel_1) || \
((CHANNEL) == TIM_Channel_2))
#define IS_TIM_COMPLEMENTARY_CHANNEL(CHANNEL) (((CHANNEL) == TIM_Channel_1) || \
((CHANNEL) == TIM_Channel_2) || \
((CHANNEL) == TIM_Channel_3))
/**
* @}
*/
/** @defgroup TIM_Clock_Division_CKD
* @{
*/
#define TIM_CKD_DIV1 ((uint16_t)0x0000)
#define TIM_CKD_DIV2 ((uint16_t)0x0100)
#define TIM_CKD_DIV4 ((uint16_t)0x0200)
#define IS_TIM_CKD_DIV(DIV) (((DIV) == TIM_CKD_DIV1) || \
((DIV) == TIM_CKD_DIV2) || \
((DIV) == TIM_CKD_DIV4))
/**
* @}
*/
/** @defgroup TIM_Counter_Mode
* @{
*/
#define TIM_CounterMode_Up ((uint16_t)0x0000)
#define TIM_CounterMode_Down ((uint16_t)0x0010)
#define TIM_CounterMode_CenterAligned1 ((uint16_t)0x0020)
#define TIM_CounterMode_CenterAligned2 ((uint16_t)0x0040)
#define TIM_CounterMode_CenterAligned3 ((uint16_t)0x0060)
#define IS_TIM_COUNTER_MODE(MODE) (((MODE) == TIM_CounterMode_Up) || \
((MODE) == TIM_CounterMode_Down) || \
((MODE) == TIM_CounterMode_CenterAligned1) || \
((MODE) == TIM_CounterMode_CenterAligned2) || \
((MODE) == TIM_CounterMode_CenterAligned3))
/**
* @}
*/
/** @defgroup TIM_Output_Compare_Polarity
* @{
*/
#define TIM_OCPolarity_High ((uint16_t)0x0000)
#define TIM_OCPolarity_Low ((uint16_t)0x0002)
#define IS_TIM_OC_POLARITY(POLARITY) (((POLARITY) == TIM_OCPolarity_High) || \
((POLARITY) == TIM_OCPolarity_Low))
/**
* @}
*/
/** @defgroup TIM_Output_Compare_N_Polarity
* @{
*/
#define TIM_OCNPolarity_High ((uint16_t)0x0000)
#define TIM_OCNPolarity_Low ((uint16_t)0x0008)
#define IS_TIM_OCN_POLARITY(POLARITY) (((POLARITY) == TIM_OCNPolarity_High) || \
((POLARITY) == TIM_OCNPolarity_Low))
/**
* @}
*/
/** @defgroup TIM_Output_Compare_state
* @{
*/
#define TIM_OutputState_Disable ((uint16_t)0x0000)
#define TIM_OutputState_Enable ((uint16_t)0x0001)
#define IS_TIM_OUTPUT_STATE(STATE) (((STATE) == TIM_OutputState_Disable) || \
((STATE) == TIM_OutputState_Enable))
/**
* @}
*/
/** @defgroup TIM_Output_Compare_N_state
* @{
*/
#define TIM_OutputNState_Disable ((uint16_t)0x0000)
#define TIM_OutputNState_Enable ((uint16_t)0x0004)
#define IS_TIM_OUTPUTN_STATE(STATE) (((STATE) == TIM_OutputNState_Disable) || \
((STATE) == TIM_OutputNState_Enable))
/**
* @}
*/
/** @defgroup TIM_Capture_Compare_state
* @{
*/
#define TIM_CCx_Enable ((uint16_t)0x0001)
#define TIM_CCx_Disable ((uint16_t)0x0000)
#define IS_TIM_CCX(CCX) (((CCX) == TIM_CCx_Enable) || \
((CCX) == TIM_CCx_Disable))
/**
* @}
*/
/** @defgroup TIM_Capture_Compare_N_state
* @{
*/
#define TIM_CCxN_Enable ((uint16_t)0x0004)
#define TIM_CCxN_Disable ((uint16_t)0x0000)
#define IS_TIM_CCXN(CCXN) (((CCXN) == TIM_CCxN_Enable) || \
((CCXN) == TIM_CCxN_Disable))
/**
* @}
*/
/** @defgroup Break_Input_enable_disable
* @{
*/
#define TIM_Break_Enable ((uint16_t)0x1000)
#define TIM_Break_Disable ((uint16_t)0x0000)
#define IS_TIM_BREAK_STATE(STATE) (((STATE) == TIM_Break_Enable) || \
((STATE) == TIM_Break_Disable))
/**
* @}
*/
/** @defgroup Break_Polarity
* @{
*/
#define TIM_BreakPolarity_Low ((uint16_t)0x0000)
#define TIM_BreakPolarity_High ((uint16_t)0x2000)
#define IS_TIM_BREAK_POLARITY(POLARITY) (((POLARITY) == TIM_BreakPolarity_Low) || \
((POLARITY) == TIM_BreakPolarity_High))
/**
* @}
*/
/** @defgroup TIM_AOE_Bit_Set_Reset
* @{
*/
#define TIM_AutomaticOutput_Enable ((uint16_t)0x4000)
#define TIM_AutomaticOutput_Disable ((uint16_t)0x0000)
#define IS_TIM_AUTOMATIC_OUTPUT_STATE(STATE) (((STATE) == TIM_AutomaticOutput_Enable) || \
((STATE) == TIM_AutomaticOutput_Disable))
/**
* @}
*/
/** @defgroup Lock_level
* @{
*/
#define TIM_LOCKLevel_OFF ((uint16_t)0x0000)
#define TIM_LOCKLevel_1 ((uint16_t)0x0100)
#define TIM_LOCKLevel_2 ((uint16_t)0x0200)
#define TIM_LOCKLevel_3 ((uint16_t)0x0300)
#define IS_TIM_LOCK_LEVEL(LEVEL) (((LEVEL) == TIM_LOCKLevel_OFF) || \
((LEVEL) == TIM_LOCKLevel_1) || \
((LEVEL) == TIM_LOCKLevel_2) || \
((LEVEL) == TIM_LOCKLevel_3))
/**
* @}
*/
/** @defgroup OSSI_Off_State_Selection_for_Idle_mode_state
* @{
*/
#define TIM_OSSIState_Enable ((uint16_t)0x0400)
#define TIM_OSSIState_Disable ((uint16_t)0x0000)
#define IS_TIM_OSSI_STATE(STATE) (((STATE) == TIM_OSSIState_Enable) || \
((STATE) == TIM_OSSIState_Disable))
/**
* @}
*/
/** @defgroup OSSR_Off_State_Selection_for_Run_mode_state
* @{
*/
#define TIM_OSSRState_Enable ((uint16_t)0x0800)
#define TIM_OSSRState_Disable ((uint16_t)0x0000)
#define IS_TIM_OSSR_STATE(STATE) (((STATE) == TIM_OSSRState_Enable) || \
((STATE) == TIM_OSSRState_Disable))
/**
* @}
*/
/** @defgroup TIM_Output_Compare_Idle_State
* @{
*/
#define TIM_OCIdleState_Set ((uint16_t)0x0100)
#define TIM_OCIdleState_Reset ((uint16_t)0x0000)
#define IS_TIM_OCIDLE_STATE(STATE) (((STATE) == TIM_OCIdleState_Set) || \
((STATE) == TIM_OCIdleState_Reset))
/**
* @}
*/
/** @defgroup TIM_Output_Compare_N_Idle_State
* @{
*/
#define TIM_OCNIdleState_Set ((uint16_t)0x0200)
#define TIM_OCNIdleState_Reset ((uint16_t)0x0000)
#define IS_TIM_OCNIDLE_STATE(STATE) (((STATE) == TIM_OCNIdleState_Set) || \
((STATE) == TIM_OCNIdleState_Reset))
/**
* @}
*/
/** @defgroup TIM_Input_Capture_Polarity
* @{
*/
#define TIM_ICPolarity_Rising ((uint16_t)0x0000)
#define TIM_ICPolarity_Falling ((uint16_t)0x0002)
#define TIM_ICPolarity_BothEdge ((uint16_t)0x000A)
#define IS_TIM_IC_POLARITY(POLARITY) (((POLARITY) == TIM_ICPolarity_Rising) || \
((POLARITY) == TIM_ICPolarity_Falling))
#define IS_TIM_IC_POLARITY_LITE(POLARITY) (((POLARITY) == TIM_ICPolarity_Rising) || \
((POLARITY) == TIM_ICPolarity_Falling)|| \
((POLARITY) == TIM_ICPolarity_BothEdge))
/**
* @}
*/
/** @defgroup TIM_Input_Capture_Selection
* @{
*/
#define TIM_ICSelection_DirectTI ((uint16_t)0x0001) /*!< TIM Input 1, 2, 3 or 4 is selected to be
connected to IC1, IC2, IC3 or IC4, respectively */
#define TIM_ICSelection_IndirectTI ((uint16_t)0x0002) /*!< TIM Input 1, 2, 3 or 4 is selected to be
connected to IC2, IC1, IC4 or IC3, respectively. */
#define TIM_ICSelection_TRC ((uint16_t)0x0003) /*!< TIM Input 1, 2, 3 or 4 is selected to be connected to TRC. */
#define IS_TIM_IC_SELECTION(SELECTION) (((SELECTION) == TIM_ICSelection_DirectTI) || \
((SELECTION) == TIM_ICSelection_IndirectTI) || \
((SELECTION) == TIM_ICSelection_TRC))
/**
* @}
*/
/** @defgroup TIM_Input_Capture_Prescaler
* @{
*/
#define TIM_ICPSC_DIV1 ((uint16_t)0x0000) /*!< Capture performed each time an edge is detected on the capture input. */
#define TIM_ICPSC_DIV2 ((uint16_t)0x0004) /*!< Capture performed once every 2 events. */
#define TIM_ICPSC_DIV4 ((uint16_t)0x0008) /*!< Capture performed once every 4 events. */
#define TIM_ICPSC_DIV8 ((uint16_t)0x000C) /*!< Capture performed once every 8 events. */
#define IS_TIM_IC_PRESCALER(PRESCALER) (((PRESCALER) == TIM_ICPSC_DIV1) || \
((PRESCALER) == TIM_ICPSC_DIV2) || \
((PRESCALER) == TIM_ICPSC_DIV4) || \
((PRESCALER) == TIM_ICPSC_DIV8))
/**
* @}
*/
/** @defgroup TIM_interrupt_sources
* @{
*/
#define TIM_IT_Update ((uint16_t)0x0001)
#define TIM_IT_CC1 ((uint16_t)0x0002)
#define TIM_IT_CC2 ((uint16_t)0x0004)
#define TIM_IT_CC3 ((uint16_t)0x0008)
#define TIM_IT_CC4 ((uint16_t)0x0010)
#define TIM_IT_COM ((uint16_t)0x0020)
#define TIM_IT_Trigger ((uint16_t)0x0040)
#define TIM_IT_Break ((uint16_t)0x0080)
#define IS_TIM_IT(IT) ((((IT) & (uint16_t)0xFF00) == 0x0000) && ((IT) != 0x0000))
#define IS_TIM_GET_IT(IT) (((IT) == TIM_IT_Update) || \
((IT) == TIM_IT_CC1) || \
((IT) == TIM_IT_CC2) || \
((IT) == TIM_IT_CC3) || \
((IT) == TIM_IT_CC4) || \
((IT) == TIM_IT_COM) || \
((IT) == TIM_IT_Trigger) || \
((IT) == TIM_IT_Break))
/**
* @}
*/
/** @defgroup TIM_DMA_Base_address
* @{
*/
#define TIM_DMABase_CR1 ((uint16_t)0x0000)
#define TIM_DMABase_CR2 ((uint16_t)0x0001)
#define TIM_DMABase_SMCR ((uint16_t)0x0002)
#define TIM_DMABase_DIER ((uint16_t)0x0003)
#define TIM_DMABase_SR ((uint16_t)0x0004)
#define TIM_DMABase_EGR ((uint16_t)0x0005)
#define TIM_DMABase_CCMR1 ((uint16_t)0x0006)
#define TIM_DMABase_CCMR2 ((uint16_t)0x0007)
#define TIM_DMABase_CCER ((uint16_t)0x0008)
#define TIM_DMABase_CNT ((uint16_t)0x0009)
#define TIM_DMABase_PSC ((uint16_t)0x000A)
#define TIM_DMABase_ARR ((uint16_t)0x000B)
#define TIM_DMABase_RCR ((uint16_t)0x000C)
#define TIM_DMABase_CCR1 ((uint16_t)0x000D)
#define TIM_DMABase_CCR2 ((uint16_t)0x000E)
#define TIM_DMABase_CCR3 ((uint16_t)0x000F)
#define TIM_DMABase_CCR4 ((uint16_t)0x0010)
#define TIM_DMABase_BDTR ((uint16_t)0x0011)
#define TIM_DMABase_DCR ((uint16_t)0x0012)
#define IS_TIM_DMA_BASE(BASE) (((BASE) == TIM_DMABase_CR1) || \
((BASE) == TIM_DMABase_CR2) || \
((BASE) == TIM_DMABase_SMCR) || \
((BASE) == TIM_DMABase_DIER) || \
((BASE) == TIM_DMABase_SR) || \
((BASE) == TIM_DMABase_EGR) || \
((BASE) == TIM_DMABase_CCMR1) || \
((BASE) == TIM_DMABase_CCMR2) || \
((BASE) == TIM_DMABase_CCER) || \
((BASE) == TIM_DMABase_CNT) || \
((BASE) == TIM_DMABase_PSC) || \
((BASE) == TIM_DMABase_ARR) || \
((BASE) == TIM_DMABase_RCR) || \
((BASE) == TIM_DMABase_CCR1) || \
((BASE) == TIM_DMABase_CCR2) || \
((BASE) == TIM_DMABase_CCR3) || \
((BASE) == TIM_DMABase_CCR4) || \
((BASE) == TIM_DMABase_BDTR) || \
((BASE) == TIM_DMABase_DCR))
/**
* @}
*/
/** @defgroup TIM_DMA_Burst_Length
* @{
*/
#define TIM_DMABurstLength_1Transfer ((uint16_t)0x0000)
#define TIM_DMABurstLength_2Transfers ((uint16_t)0x0100)
#define TIM_DMABurstLength_3Transfers ((uint16_t)0x0200)
#define TIM_DMABurstLength_4Transfers ((uint16_t)0x0300)
#define TIM_DMABurstLength_5Transfers ((uint16_t)0x0400)
#define TIM_DMABurstLength_6Transfers ((uint16_t)0x0500)
#define TIM_DMABurstLength_7Transfers ((uint16_t)0x0600)
#define TIM_DMABurstLength_8Transfers ((uint16_t)0x0700)
#define TIM_DMABurstLength_9Transfers ((uint16_t)0x0800)
#define TIM_DMABurstLength_10Transfers ((uint16_t)0x0900)
#define TIM_DMABurstLength_11Transfers ((uint16_t)0x0A00)
#define TIM_DMABurstLength_12Transfers ((uint16_t)0x0B00)
#define TIM_DMABurstLength_13Transfers ((uint16_t)0x0C00)
#define TIM_DMABurstLength_14Transfers ((uint16_t)0x0D00)
#define TIM_DMABurstLength_15Transfers ((uint16_t)0x0E00)
#define TIM_DMABurstLength_16Transfers ((uint16_t)0x0F00)
#define TIM_DMABurstLength_17Transfers ((uint16_t)0x1000)
#define TIM_DMABurstLength_18Transfers ((uint16_t)0x1100)
#define IS_TIM_DMA_LENGTH(LENGTH) (((LENGTH) == TIM_DMABurstLength_1Transfer) || \
((LENGTH) == TIM_DMABurstLength_2Transfers) || \
((LENGTH) == TIM_DMABurstLength_3Transfers) || \
((LENGTH) == TIM_DMABurstLength_4Transfers) || \
((LENGTH) == TIM_DMABurstLength_5Transfers) || \
((LENGTH) == TIM_DMABurstLength_6Transfers) || \
((LENGTH) == TIM_DMABurstLength_7Transfers) || \
((LENGTH) == TIM_DMABurstLength_8Transfers) || \
((LENGTH) == TIM_DMABurstLength_9Transfers) || \
((LENGTH) == TIM_DMABurstLength_10Transfers) || \
((LENGTH) == TIM_DMABurstLength_11Transfers) || \
((LENGTH) == TIM_DMABurstLength_12Transfers) || \
((LENGTH) == TIM_DMABurstLength_13Transfers) || \
((LENGTH) == TIM_DMABurstLength_14Transfers) || \
((LENGTH) == TIM_DMABurstLength_15Transfers) || \
((LENGTH) == TIM_DMABurstLength_16Transfers) || \
((LENGTH) == TIM_DMABurstLength_17Transfers) || \
((LENGTH) == TIM_DMABurstLength_18Transfers))
/**
* @}
*/
/** @defgroup TIM_DMA_sources
* @{
*/
#define TIM_DMA_Update ((uint16_t)0x0100)
#define TIM_DMA_CC1 ((uint16_t)0x0200)
#define TIM_DMA_CC2 ((uint16_t)0x0400)
#define TIM_DMA_CC3 ((uint16_t)0x0800)
#define TIM_DMA_CC4 ((uint16_t)0x1000)
#define TIM_DMA_COM ((uint16_t)0x2000)
#define TIM_DMA_Trigger ((uint16_t)0x4000)
#define IS_TIM_DMA_SOURCE(SOURCE) ((((SOURCE) & (uint16_t)0x80FF) == 0x0000) && ((SOURCE) != 0x0000))
/**
* @}
*/
/** @defgroup TIM_External_Trigger_Prescaler
* @{
*/
#define TIM_ExtTRGPSC_OFF ((uint16_t)0x0000)
#define TIM_ExtTRGPSC_DIV2 ((uint16_t)0x1000)
#define TIM_ExtTRGPSC_DIV4 ((uint16_t)0x2000)
#define TIM_ExtTRGPSC_DIV8 ((uint16_t)0x3000)
#define IS_TIM_EXT_PRESCALER(PRESCALER) (((PRESCALER) == TIM_ExtTRGPSC_OFF) || \
((PRESCALER) == TIM_ExtTRGPSC_DIV2) || \
((PRESCALER) == TIM_ExtTRGPSC_DIV4) || \
((PRESCALER) == TIM_ExtTRGPSC_DIV8))
/**
* @}
*/
/** @defgroup TIM_Internal_Trigger_Selection
* @{
*/
#define TIM_TS_ITR0 ((uint16_t)0x0000)
#define TIM_TS_ITR1 ((uint16_t)0x0010)
#define TIM_TS_ITR2 ((uint16_t)0x0020)
#define TIM_TS_ITR3 ((uint16_t)0x0030)
#define TIM_TS_TI1F_ED ((uint16_t)0x0040)
#define TIM_TS_TI1FP1 ((uint16_t)0x0050)
#define TIM_TS_TI2FP2 ((uint16_t)0x0060)
#define TIM_TS_ETRF ((uint16_t)0x0070)
#define IS_TIM_TRIGGER_SELECTION(SELECTION) (((SELECTION) == TIM_TS_ITR0) || \
((SELECTION) == TIM_TS_ITR1) || \
((SELECTION) == TIM_TS_ITR2) || \
((SELECTION) == TIM_TS_ITR3) || \
((SELECTION) == TIM_TS_TI1F_ED) || \
((SELECTION) == TIM_TS_TI1FP1) || \
((SELECTION) == TIM_TS_TI2FP2) || \
((SELECTION) == TIM_TS_ETRF))
#define IS_TIM_INTERNAL_TRIGGER_SELECTION(SELECTION) (((SELECTION) == TIM_TS_ITR0) || \
((SELECTION) == TIM_TS_ITR1) || \
((SELECTION) == TIM_TS_ITR2) || \
((SELECTION) == TIM_TS_ITR3))
/**
* @}
*/
/** @defgroup TIM_TIx_External_Clock_Source
* @{
*/
#define TIM_TIxExternalCLK1Source_TI1 ((uint16_t)0x0050)
#define TIM_TIxExternalCLK1Source_TI2 ((uint16_t)0x0060)
#define TIM_TIxExternalCLK1Source_TI1ED ((uint16_t)0x0040)
#define IS_TIM_TIXCLK_SOURCE(SOURCE) (((SOURCE) == TIM_TIxExternalCLK1Source_TI1) || \
((SOURCE) == TIM_TIxExternalCLK1Source_TI2) || \
((SOURCE) == TIM_TIxExternalCLK1Source_TI1ED))
/**
* @}
*/
/** @defgroup TIM_External_Trigger_Polarity
* @{
*/
#define TIM_ExtTRGPolarity_Inverted ((uint16_t)0x8000)
#define TIM_ExtTRGPolarity_NonInverted ((uint16_t)0x0000)
#define IS_TIM_EXT_POLARITY(POLARITY) (((POLARITY) == TIM_ExtTRGPolarity_Inverted) || \
((POLARITY) == TIM_ExtTRGPolarity_NonInverted))
/**
* @}
*/
/** @defgroup TIM_Prescaler_Reload_Mode
* @{
*/
#define TIM_PSCReloadMode_Update ((uint16_t)0x0000)
#define TIM_PSCReloadMode_Immediate ((uint16_t)0x0001)
#define IS_TIM_PRESCALER_RELOAD(RELOAD) (((RELOAD) == TIM_PSCReloadMode_Update) || \
((RELOAD) == TIM_PSCReloadMode_Immediate))
/**
* @}
*/
/** @defgroup TIM_Forced_Action
* @{
*/
#define TIM_ForcedAction_Active ((uint16_t)0x0050)
#define TIM_ForcedAction_InActive ((uint16_t)0x0040)
#define IS_TIM_FORCED_ACTION(ACTION) (((ACTION) == TIM_ForcedAction_Active) || \
((ACTION) == TIM_ForcedAction_InActive))
/**
* @}
*/
/** @defgroup TIM_Encoder_Mode
* @{
*/
#define TIM_EncoderMode_TI1 ((uint16_t)0x0001)
#define TIM_EncoderMode_TI2 ((uint16_t)0x0002)
#define TIM_EncoderMode_TI12 ((uint16_t)0x0003)
#define IS_TIM_ENCODER_MODE(MODE) (((MODE) == TIM_EncoderMode_TI1) || \
((MODE) == TIM_EncoderMode_TI2) || \
((MODE) == TIM_EncoderMode_TI12))
/**
* @}
*/
/** @defgroup TIM_Event_Source
* @{
*/
#define TIM_EventSource_Update ((uint16_t)0x0001)
#define TIM_EventSource_CC1 ((uint16_t)0x0002)
#define TIM_EventSource_CC2 ((uint16_t)0x0004)
#define TIM_EventSource_CC3 ((uint16_t)0x0008)
#define TIM_EventSource_CC4 ((uint16_t)0x0010)
#define TIM_EventSource_COM ((uint16_t)0x0020)
#define TIM_EventSource_Trigger ((uint16_t)0x0040)
#define TIM_EventSource_Break ((uint16_t)0x0080)
#define IS_TIM_EVENT_SOURCE(SOURCE) ((((SOURCE) & (uint16_t)0xFF00) == 0x0000) && ((SOURCE) != 0x0000))
/**
* @}
*/
/** @defgroup TIM_Update_Source
* @{
*/
#define TIM_UpdateSource_Global ((uint16_t)0x0000) /*!< Source of update is the counter overflow/underflow
or the setting of UG bit, or an update generation
through the slave mode controller. */
#define TIM_UpdateSource_Regular ((uint16_t)0x0001) /*!< Source of update is counter overflow/underflow. */
#define IS_TIM_UPDATE_SOURCE(SOURCE) (((SOURCE) == TIM_UpdateSource_Global) || \
((SOURCE) == TIM_UpdateSource_Regular))
/**
* @}
*/
/** @defgroup TIM_Output_Compare_Preload_State
* @{
*/
#define TIM_OCPreload_Enable ((uint16_t)0x0008)
#define TIM_OCPreload_Disable ((uint16_t)0x0000)
#define IS_TIM_OCPRELOAD_STATE(STATE) (((STATE) == TIM_OCPreload_Enable) || \
((STATE) == TIM_OCPreload_Disable))
/**
* @}
*/
/** @defgroup TIM_Output_Compare_Fast_State
* @{
*/
#define TIM_OCFast_Enable ((uint16_t)0x0004)
#define TIM_OCFast_Disable ((uint16_t)0x0000)
#define IS_TIM_OCFAST_STATE(STATE) (((STATE) == TIM_OCFast_Enable) || \
((STATE) == TIM_OCFast_Disable))
/**
* @}
*/
/** @defgroup TIM_Output_Compare_Clear_State
* @{
*/
#define TIM_OCClear_Enable ((uint16_t)0x0080)
#define TIM_OCClear_Disable ((uint16_t)0x0000)
#define IS_TIM_OCCLEAR_STATE(STATE) (((STATE) == TIM_OCClear_Enable) || \
((STATE) == TIM_OCClear_Disable))
/**
* @}
*/
/** @defgroup TIM_Trigger_Output_Source
* @{
*/
#define TIM_TRGOSource_Reset ((uint16_t)0x0000)
#define TIM_TRGOSource_Enable ((uint16_t)0x0010)
#define TIM_TRGOSource_Update ((uint16_t)0x0020)
#define TIM_TRGOSource_OC1 ((uint16_t)0x0030)
#define TIM_TRGOSource_OC1Ref ((uint16_t)0x0040)
#define TIM_TRGOSource_OC2Ref ((uint16_t)0x0050)
#define TIM_TRGOSource_OC3Ref ((uint16_t)0x0060)
#define TIM_TRGOSource_OC4Ref ((uint16_t)0x0070)
#define IS_TIM_TRGO_SOURCE(SOURCE) (((SOURCE) == TIM_TRGOSource_Reset) || \
((SOURCE) == TIM_TRGOSource_Enable) || \
((SOURCE) == TIM_TRGOSource_Update) || \
((SOURCE) == TIM_TRGOSource_OC1) || \
((SOURCE) == TIM_TRGOSource_OC1Ref) || \
((SOURCE) == TIM_TRGOSource_OC2Ref) || \
((SOURCE) == TIM_TRGOSource_OC3Ref) || \
((SOURCE) == TIM_TRGOSource_OC4Ref))
/**
* @}
*/
/** @defgroup TIM_Slave_Mode
* @{
*/
#define TIM_SlaveMode_Reset ((uint16_t)0x0004)
#define TIM_SlaveMode_Gated ((uint16_t)0x0005)
#define TIM_SlaveMode_Trigger ((uint16_t)0x0006)
#define TIM_SlaveMode_External1 ((uint16_t)0x0007)
#define IS_TIM_SLAVE_MODE(MODE) (((MODE) == TIM_SlaveMode_Reset) || \
((MODE) == TIM_SlaveMode_Gated) || \
((MODE) == TIM_SlaveMode_Trigger) || \
((MODE) == TIM_SlaveMode_External1))
/**
* @}
*/
/** @defgroup TIM_Master_Slave_Mode
* @{
*/
#define TIM_MasterSlaveMode_Enable ((uint16_t)0x0080)
#define TIM_MasterSlaveMode_Disable ((uint16_t)0x0000)
#define IS_TIM_MSM_STATE(STATE) (((STATE) == TIM_MasterSlaveMode_Enable) || \
((STATE) == TIM_MasterSlaveMode_Disable))
/**
* @}
*/
/** @defgroup TIM_Flags
* @{
*/
#define TIM_FLAG_Update ((uint16_t)0x0001)
#define TIM_FLAG_CC1 ((uint16_t)0x0002)
#define TIM_FLAG_CC2 ((uint16_t)0x0004)
#define TIM_FLAG_CC3 ((uint16_t)0x0008)
#define TIM_FLAG_CC4 ((uint16_t)0x0010)
#define TIM_FLAG_COM ((uint16_t)0x0020)
#define TIM_FLAG_Trigger ((uint16_t)0x0040)
#define TIM_FLAG_Break ((uint16_t)0x0080)
#define TIM_FLAG_CC1OF ((uint16_t)0x0200)
#define TIM_FLAG_CC2OF ((uint16_t)0x0400)
#define TIM_FLAG_CC3OF ((uint16_t)0x0800)
#define TIM_FLAG_CC4OF ((uint16_t)0x1000)
#define IS_TIM_GET_FLAG(FLAG) (((FLAG) == TIM_FLAG_Update) || \
((FLAG) == TIM_FLAG_CC1) || \
((FLAG) == TIM_FLAG_CC2) || \
((FLAG) == TIM_FLAG_CC3) || \
((FLAG) == TIM_FLAG_CC4) || \
((FLAG) == TIM_FLAG_COM) || \
((FLAG) == TIM_FLAG_Trigger) || \
((FLAG) == TIM_FLAG_Break) || \
((FLAG) == TIM_FLAG_CC1OF) || \
((FLAG) == TIM_FLAG_CC2OF) || \
((FLAG) == TIM_FLAG_CC3OF) || \
((FLAG) == TIM_FLAG_CC4OF))
#define IS_TIM_CLEAR_FLAG(TIM_FLAG) ((((TIM_FLAG) & (uint16_t)0xE100) == 0x0000) && ((TIM_FLAG) != 0x0000))
/**
* @}
*/
/** @defgroup TIM_Input_Capture_Filer_Value
* @{
*/
#define IS_TIM_IC_FILTER(ICFILTER) ((ICFILTER) <= 0xF)
/**
* @}
*/
/** @defgroup TIM_External_Trigger_Filter
* @{
*/
#define IS_TIM_EXT_FILTER(EXTFILTER) ((EXTFILTER) <= 0xF)
/**
* @}
*/
/** @defgroup TIM_Legacy
* @{
*/
#define TIM_DMABurstLength_1Byte TIM_DMABurstLength_1Transfer
#define TIM_DMABurstLength_2Bytes TIM_DMABurstLength_2Transfers
#define TIM_DMABurstLength_3Bytes TIM_DMABurstLength_3Transfers
#define TIM_DMABurstLength_4Bytes TIM_DMABurstLength_4Transfers
#define TIM_DMABurstLength_5Bytes TIM_DMABurstLength_5Transfers
#define TIM_DMABurstLength_6Bytes TIM_DMABurstLength_6Transfers
#define TIM_DMABurstLength_7Bytes TIM_DMABurstLength_7Transfers
#define TIM_DMABurstLength_8Bytes TIM_DMABurstLength_8Transfers
#define TIM_DMABurstLength_9Bytes TIM_DMABurstLength_9Transfers
#define TIM_DMABurstLength_10Bytes TIM_DMABurstLength_10Transfers
#define TIM_DMABurstLength_11Bytes TIM_DMABurstLength_11Transfers
#define TIM_DMABurstLength_12Bytes TIM_DMABurstLength_12Transfers
#define TIM_DMABurstLength_13Bytes TIM_DMABurstLength_13Transfers
#define TIM_DMABurstLength_14Bytes TIM_DMABurstLength_14Transfers
#define TIM_DMABurstLength_15Bytes TIM_DMABurstLength_15Transfers
#define TIM_DMABurstLength_16Bytes TIM_DMABurstLength_16Transfers
#define TIM_DMABurstLength_17Bytes TIM_DMABurstLength_17Transfers
#define TIM_DMABurstLength_18Bytes TIM_DMABurstLength_18Transfers
/**
* @}
*/
/**
* @}
*/
/** @defgroup TIM_Exported_Macros
* @{
*/
/**
* @}
*/
/** @defgroup TIM_Exported_Functions
* @{
*/
void TIM_DeInit(TIM_TypeDef* TIMx);
void TIM_TimeBaseInit(TIM_TypeDef* TIMx, TIM_TimeBaseInitTypeDef* TIM_TimeBaseInitStruct);
void TIM_OC1Init(TIM_TypeDef* TIMx, TIM_OCInitTypeDef* TIM_OCInitStruct);
void TIM_OC2Init(TIM_TypeDef* TIMx, TIM_OCInitTypeDef* TIM_OCInitStruct);
void TIM_OC3Init(TIM_TypeDef* TIMx, TIM_OCInitTypeDef* TIM_OCInitStruct);
void TIM_OC4Init(TIM_TypeDef* TIMx, TIM_OCInitTypeDef* TIM_OCInitStruct);
void TIM_ICInit(TIM_TypeDef* TIMx, TIM_ICInitTypeDef* TIM_ICInitStruct);
void TIM_PWMIConfig(TIM_TypeDef* TIMx, TIM_ICInitTypeDef* TIM_ICInitStruct);
void TIM_BDTRConfig(TIM_TypeDef* TIMx, TIM_BDTRInitTypeDef *TIM_BDTRInitStruct);
void TIM_TimeBaseStructInit(TIM_TimeBaseInitTypeDef* TIM_TimeBaseInitStruct);
void TIM_OCStructInit(TIM_OCInitTypeDef* TIM_OCInitStruct);
void TIM_ICStructInit(TIM_ICInitTypeDef* TIM_ICInitStruct);
void TIM_BDTRStructInit(TIM_BDTRInitTypeDef* TIM_BDTRInitStruct);
void TIM_Cmd(TIM_TypeDef* TIMx, FunctionalState NewState);
void TIM_CtrlPWMOutputs(TIM_TypeDef* TIMx, FunctionalState NewState);
void TIM_ITConfig(TIM_TypeDef* TIMx, uint16_t TIM_IT, FunctionalState NewState);
void TIM_GenerateEvent(TIM_TypeDef* TIMx, uint16_t TIM_EventSource);
void TIM_DMAConfig(TIM_TypeDef* TIMx, uint16_t TIM_DMABase, uint16_t TIM_DMABurstLength);
void TIM_DMACmd(TIM_TypeDef* TIMx, uint16_t TIM_DMASource, FunctionalState NewState);
void TIM_InternalClockConfig(TIM_TypeDef* TIMx);
void TIM_ITRxExternalClockConfig(TIM_TypeDef* TIMx, uint16_t TIM_InputTriggerSource);
void TIM_TIxExternalClockConfig(TIM_TypeDef* TIMx, uint16_t TIM_TIxExternalCLKSource,
uint16_t TIM_ICPolarity, uint16_t ICFilter);
void TIM_ETRClockMode1Config(TIM_TypeDef* TIMx, uint16_t TIM_ExtTRGPrescaler, uint16_t TIM_ExtTRGPolarity,
uint16_t ExtTRGFilter);
void TIM_ETRClockMode2Config(TIM_TypeDef* TIMx, uint16_t TIM_ExtTRGPrescaler,
uint16_t TIM_ExtTRGPolarity, uint16_t ExtTRGFilter);
void TIM_ETRConfig(TIM_TypeDef* TIMx, uint16_t TIM_ExtTRGPrescaler, uint16_t TIM_ExtTRGPolarity,
uint16_t ExtTRGFilter);
void TIM_PrescalerConfig(TIM_TypeDef* TIMx, uint16_t Prescaler, uint16_t TIM_PSCReloadMode);
void TIM_CounterModeConfig(TIM_TypeDef* TIMx, uint16_t TIM_CounterMode);
void TIM_SelectInputTrigger(TIM_TypeDef* TIMx, uint16_t TIM_InputTriggerSource);
void TIM_EncoderInterfaceConfig(TIM_TypeDef* TIMx, uint16_t TIM_EncoderMode,
uint16_t TIM_IC1Polarity, uint16_t TIM_IC2Polarity);
void TIM_ForcedOC1Config(TIM_TypeDef* TIMx, uint16_t TIM_ForcedAction);
void TIM_ForcedOC2Config(TIM_TypeDef* TIMx, uint16_t TIM_ForcedAction);
void TIM_ForcedOC3Config(TIM_TypeDef* TIMx, uint16_t TIM_ForcedAction);
void TIM_ForcedOC4Config(TIM_TypeDef* TIMx, uint16_t TIM_ForcedAction);
void TIM_ARRPreloadConfig(TIM_TypeDef* TIMx, FunctionalState NewState);
void TIM_SelectCOM(TIM_TypeDef* TIMx, FunctionalState NewState);
void TIM_SelectCCDMA(TIM_TypeDef* TIMx, FunctionalState NewState);
void TIM_CCPreloadControl(TIM_TypeDef* TIMx, FunctionalState NewState);
void TIM_OC1PreloadConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPreload);
void TIM_OC2PreloadConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPreload);
void TIM_OC3PreloadConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPreload);
void TIM_OC4PreloadConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPreload);
void TIM_OC1FastConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCFast);
void TIM_OC2FastConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCFast);
void TIM_OC3FastConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCFast);
void TIM_OC4FastConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCFast);
void TIM_ClearOC1Ref(TIM_TypeDef* TIMx, uint16_t TIM_OCClear);
void TIM_ClearOC2Ref(TIM_TypeDef* TIMx, uint16_t TIM_OCClear);
void TIM_ClearOC3Ref(TIM_TypeDef* TIMx, uint16_t TIM_OCClear);
void TIM_ClearOC4Ref(TIM_TypeDef* TIMx, uint16_t TIM_OCClear);
void TIM_OC1PolarityConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPolarity);
void TIM_OC1NPolarityConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCNPolarity);
void TIM_OC2PolarityConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPolarity);
void TIM_OC2NPolarityConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCNPolarity);
void TIM_OC3PolarityConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPolarity);
void TIM_OC3NPolarityConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCNPolarity);
void TIM_OC4PolarityConfig(TIM_TypeDef* TIMx, uint16_t TIM_OCPolarity);
void TIM_CCxCmd(TIM_TypeDef* TIMx, uint16_t TIM_Channel, uint16_t TIM_CCx);
void TIM_CCxNCmd(TIM_TypeDef* TIMx, uint16_t TIM_Channel, uint16_t TIM_CCxN);
void TIM_SelectOCxM(TIM_TypeDef* TIMx, uint16_t TIM_Channel, uint16_t TIM_OCMode);
void TIM_UpdateDisableConfig(TIM_TypeDef* TIMx, FunctionalState NewState);
void TIM_UpdateRequestConfig(TIM_TypeDef* TIMx, uint16_t TIM_UpdateSource);
void TIM_SelectHallSensor(TIM_TypeDef* TIMx, FunctionalState NewState);
void TIM_SelectOnePulseMode(TIM_TypeDef* TIMx, uint16_t TIM_OPMode);
void TIM_SelectOutputTrigger(TIM_TypeDef* TIMx, uint16_t TIM_TRGOSource);
void TIM_SelectSlaveMode(TIM_TypeDef* TIMx, uint16_t TIM_SlaveMode);
void TIM_SelectMasterSlaveMode(TIM_TypeDef* TIMx, uint16_t TIM_MasterSlaveMode);
void TIM_SetCounter(TIM_TypeDef* TIMx, uint16_t Counter);
void TIM_SetAutoreload(TIM_TypeDef* TIMx, uint16_t Autoreload);
void TIM_SetCompare1(TIM_TypeDef* TIMx, uint16_t Compare1);
void TIM_SetCompare2(TIM_TypeDef* TIMx, uint16_t Compare2);
void TIM_SetCompare3(TIM_TypeDef* TIMx, uint16_t Compare3);
void TIM_SetCompare4(TIM_TypeDef* TIMx, uint16_t Compare4);
void TIM_SetIC1Prescaler(TIM_TypeDef* TIMx, uint16_t TIM_ICPSC);
void TIM_SetIC2Prescaler(TIM_TypeDef* TIMx, uint16_t TIM_ICPSC);
void TIM_SetIC3Prescaler(TIM_TypeDef* TIMx, uint16_t TIM_ICPSC);
void TIM_SetIC4Prescaler(TIM_TypeDef* TIMx, uint16_t TIM_ICPSC);
void TIM_SetClockDivision(TIM_TypeDef* TIMx, uint16_t TIM_CKD);
uint16_t TIM_GetCapture1(TIM_TypeDef* TIMx);
uint16_t TIM_GetCapture2(TIM_TypeDef* TIMx);
uint16_t TIM_GetCapture3(TIM_TypeDef* TIMx);
uint16_t TIM_GetCapture4(TIM_TypeDef* TIMx);
uint16_t TIM_GetCounter(TIM_TypeDef* TIMx);
uint16_t TIM_GetPrescaler(TIM_TypeDef* TIMx);
FlagStatus TIM_GetFlagStatus(TIM_TypeDef* TIMx, uint16_t TIM_FLAG);
void TIM_ClearFlag(TIM_TypeDef* TIMx, uint16_t TIM_FLAG);
ITStatus TIM_GetITStatus(TIM_TypeDef* TIMx, uint16_t TIM_IT);
void TIM_ClearITPendingBit(TIM_TypeDef* TIMx, uint16_t TIM_IT);
#ifdef __cplusplus
}
#endif
#endif /*__STM32F10x_TIM_H */
/**
* @}
*/
/**
* @}
*/
/**
* @}
*/
/******************* (C) COPYRIGHT 2011 STMicroelectronics *****END OF FILE****/
| {
"pile_set_name": "Github"
} |
// Copyright 2006-2008 the V8 project authors. All rights reserved.
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions are
// met:
//
// * Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
// * Redistributions in binary form must reproduce the above
// copyright notice, this list of conditions and the following
// disclaimer in the documentation and/or other materials provided
// with the distribution.
// * Neither the name of Google Inc. nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
// "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
// LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
// A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
// OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
// SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
// LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
// DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
// THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
// OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
#ifndef GAY_SHORTEST_H_
#define GAY_SHORTEST_H_
namespace v8 {
namespace internal {
struct PrecomputedShortest {
double v;
const char* representation;
int decimal_point;
};
Vector<const PrecomputedShortest> PrecomputedShortestRepresentations();
} } // namespace v8::internal
#endif // GAY_SHORTEST_H_
| {
"pile_set_name": "Github"
} |
// go run linux/mksysnum.go -Wall -Werror -static -I/tmp/include /tmp/include/asm/unistd.h
// Code generated by the command above; see README.md. DO NOT EDIT.
// +build ppc64le,linux
package unix
const (
SYS_RESTART_SYSCALL = 0
SYS_EXIT = 1
SYS_FORK = 2
SYS_READ = 3
SYS_WRITE = 4
SYS_OPEN = 5
SYS_CLOSE = 6
SYS_WAITPID = 7
SYS_CREAT = 8
SYS_LINK = 9
SYS_UNLINK = 10
SYS_EXECVE = 11
SYS_CHDIR = 12
SYS_TIME = 13
SYS_MKNOD = 14
SYS_CHMOD = 15
SYS_LCHOWN = 16
SYS_BREAK = 17
SYS_OLDSTAT = 18
SYS_LSEEK = 19
SYS_GETPID = 20
SYS_MOUNT = 21
SYS_UMOUNT = 22
SYS_SETUID = 23
SYS_GETUID = 24
SYS_STIME = 25
SYS_PTRACE = 26
SYS_ALARM = 27
SYS_OLDFSTAT = 28
SYS_PAUSE = 29
SYS_UTIME = 30
SYS_STTY = 31
SYS_GTTY = 32
SYS_ACCESS = 33
SYS_NICE = 34
SYS_FTIME = 35
SYS_SYNC = 36
SYS_KILL = 37
SYS_RENAME = 38
SYS_MKDIR = 39
SYS_RMDIR = 40
SYS_DUP = 41
SYS_PIPE = 42
SYS_TIMES = 43
SYS_PROF = 44
SYS_BRK = 45
SYS_SETGID = 46
SYS_GETGID = 47
SYS_SIGNAL = 48
SYS_GETEUID = 49
SYS_GETEGID = 50
SYS_ACCT = 51
SYS_UMOUNT2 = 52
SYS_LOCK = 53
SYS_IOCTL = 54
SYS_FCNTL = 55
SYS_MPX = 56
SYS_SETPGID = 57
SYS_ULIMIT = 58
SYS_OLDOLDUNAME = 59
SYS_UMASK = 60
SYS_CHROOT = 61
SYS_USTAT = 62
SYS_DUP2 = 63
SYS_GETPPID = 64
SYS_GETPGRP = 65
SYS_SETSID = 66
SYS_SIGACTION = 67
SYS_SGETMASK = 68
SYS_SSETMASK = 69
SYS_SETREUID = 70
SYS_SETREGID = 71
SYS_SIGSUSPEND = 72
SYS_SIGPENDING = 73
SYS_SETHOSTNAME = 74
SYS_SETRLIMIT = 75
SYS_GETRLIMIT = 76
SYS_GETRUSAGE = 77
SYS_GETTIMEOFDAY = 78
SYS_SETTIMEOFDAY = 79
SYS_GETGROUPS = 80
SYS_SETGROUPS = 81
SYS_SELECT = 82
SYS_SYMLINK = 83
SYS_OLDLSTAT = 84
SYS_READLINK = 85
SYS_USELIB = 86
SYS_SWAPON = 87
SYS_REBOOT = 88
SYS_READDIR = 89
SYS_MMAP = 90
SYS_MUNMAP = 91
SYS_TRUNCATE = 92
SYS_FTRUNCATE = 93
SYS_FCHMOD = 94
SYS_FCHOWN = 95
SYS_GETPRIORITY = 96
SYS_SETPRIORITY = 97
SYS_PROFIL = 98
SYS_STATFS = 99
SYS_FSTATFS = 100
SYS_IOPERM = 101
SYS_SOCKETCALL = 102
SYS_SYSLOG = 103
SYS_SETITIMER = 104
SYS_GETITIMER = 105
SYS_STAT = 106
SYS_LSTAT = 107
SYS_FSTAT = 108
SYS_OLDUNAME = 109
SYS_IOPL = 110
SYS_VHANGUP = 111
SYS_IDLE = 112
SYS_VM86 = 113
SYS_WAIT4 = 114
SYS_SWAPOFF = 115
SYS_SYSINFO = 116
SYS_IPC = 117
SYS_FSYNC = 118
SYS_SIGRETURN = 119
SYS_CLONE = 120
SYS_SETDOMAINNAME = 121
SYS_UNAME = 122
SYS_MODIFY_LDT = 123
SYS_ADJTIMEX = 124
SYS_MPROTECT = 125
SYS_SIGPROCMASK = 126
SYS_CREATE_MODULE = 127
SYS_INIT_MODULE = 128
SYS_DELETE_MODULE = 129
SYS_GET_KERNEL_SYMS = 130
SYS_QUOTACTL = 131
SYS_GETPGID = 132
SYS_FCHDIR = 133
SYS_BDFLUSH = 134
SYS_SYSFS = 135
SYS_PERSONALITY = 136
SYS_AFS_SYSCALL = 137
SYS_SETFSUID = 138
SYS_SETFSGID = 139
SYS__LLSEEK = 140
SYS_GETDENTS = 141
SYS__NEWSELECT = 142
SYS_FLOCK = 143
SYS_MSYNC = 144
SYS_READV = 145
SYS_WRITEV = 146
SYS_GETSID = 147
SYS_FDATASYNC = 148
SYS__SYSCTL = 149
SYS_MLOCK = 150
SYS_MUNLOCK = 151
SYS_MLOCKALL = 152
SYS_MUNLOCKALL = 153
SYS_SCHED_SETPARAM = 154
SYS_SCHED_GETPARAM = 155
SYS_SCHED_SETSCHEDULER = 156
SYS_SCHED_GETSCHEDULER = 157
SYS_SCHED_YIELD = 158
SYS_SCHED_GET_PRIORITY_MAX = 159
SYS_SCHED_GET_PRIORITY_MIN = 160
SYS_SCHED_RR_GET_INTERVAL = 161
SYS_NANOSLEEP = 162
SYS_MREMAP = 163
SYS_SETRESUID = 164
SYS_GETRESUID = 165
SYS_QUERY_MODULE = 166
SYS_POLL = 167
SYS_NFSSERVCTL = 168
SYS_SETRESGID = 169
SYS_GETRESGID = 170
SYS_PRCTL = 171
SYS_RT_SIGRETURN = 172
SYS_RT_SIGACTION = 173
SYS_RT_SIGPROCMASK = 174
SYS_RT_SIGPENDING = 175
SYS_RT_SIGTIMEDWAIT = 176
SYS_RT_SIGQUEUEINFO = 177
SYS_RT_SIGSUSPEND = 178
SYS_PREAD64 = 179
SYS_PWRITE64 = 180
SYS_CHOWN = 181
SYS_GETCWD = 182
SYS_CAPGET = 183
SYS_CAPSET = 184
SYS_SIGALTSTACK = 185
SYS_SENDFILE = 186
SYS_GETPMSG = 187
SYS_PUTPMSG = 188
SYS_VFORK = 189
SYS_UGETRLIMIT = 190
SYS_READAHEAD = 191
SYS_PCICONFIG_READ = 198
SYS_PCICONFIG_WRITE = 199
SYS_PCICONFIG_IOBASE = 200
SYS_MULTIPLEXER = 201
SYS_GETDENTS64 = 202
SYS_PIVOT_ROOT = 203
SYS_MADVISE = 205
SYS_MINCORE = 206
SYS_GETTID = 207
SYS_TKILL = 208
SYS_SETXATTR = 209
SYS_LSETXATTR = 210
SYS_FSETXATTR = 211
SYS_GETXATTR = 212
SYS_LGETXATTR = 213
SYS_FGETXATTR = 214
SYS_LISTXATTR = 215
SYS_LLISTXATTR = 216
SYS_FLISTXATTR = 217
SYS_REMOVEXATTR = 218
SYS_LREMOVEXATTR = 219
SYS_FREMOVEXATTR = 220
SYS_FUTEX = 221
SYS_SCHED_SETAFFINITY = 222
SYS_SCHED_GETAFFINITY = 223
SYS_TUXCALL = 225
SYS_IO_SETUP = 227
SYS_IO_DESTROY = 228
SYS_IO_GETEVENTS = 229
SYS_IO_SUBMIT = 230
SYS_IO_CANCEL = 231
SYS_SET_TID_ADDRESS = 232
SYS_FADVISE64 = 233
SYS_EXIT_GROUP = 234
SYS_LOOKUP_DCOOKIE = 235
SYS_EPOLL_CREATE = 236
SYS_EPOLL_CTL = 237
SYS_EPOLL_WAIT = 238
SYS_REMAP_FILE_PAGES = 239
SYS_TIMER_CREATE = 240
SYS_TIMER_SETTIME = 241
SYS_TIMER_GETTIME = 242
SYS_TIMER_GETOVERRUN = 243
SYS_TIMER_DELETE = 244
SYS_CLOCK_SETTIME = 245
SYS_CLOCK_GETTIME = 246
SYS_CLOCK_GETRES = 247
SYS_CLOCK_NANOSLEEP = 248
SYS_SWAPCONTEXT = 249
SYS_TGKILL = 250
SYS_UTIMES = 251
SYS_STATFS64 = 252
SYS_FSTATFS64 = 253
SYS_RTAS = 255
SYS_SYS_DEBUG_SETCONTEXT = 256
SYS_MIGRATE_PAGES = 258
SYS_MBIND = 259
SYS_GET_MEMPOLICY = 260
SYS_SET_MEMPOLICY = 261
SYS_MQ_OPEN = 262
SYS_MQ_UNLINK = 263
SYS_MQ_TIMEDSEND = 264
SYS_MQ_TIMEDRECEIVE = 265
SYS_MQ_NOTIFY = 266
SYS_MQ_GETSETATTR = 267
SYS_KEXEC_LOAD = 268
SYS_ADD_KEY = 269
SYS_REQUEST_KEY = 270
SYS_KEYCTL = 271
SYS_WAITID = 272
SYS_IOPRIO_SET = 273
SYS_IOPRIO_GET = 274
SYS_INOTIFY_INIT = 275
SYS_INOTIFY_ADD_WATCH = 276
SYS_INOTIFY_RM_WATCH = 277
SYS_SPU_RUN = 278
SYS_SPU_CREATE = 279
SYS_PSELECT6 = 280
SYS_PPOLL = 281
SYS_UNSHARE = 282
SYS_SPLICE = 283
SYS_TEE = 284
SYS_VMSPLICE = 285
SYS_OPENAT = 286
SYS_MKDIRAT = 287
SYS_MKNODAT = 288
SYS_FCHOWNAT = 289
SYS_FUTIMESAT = 290
SYS_NEWFSTATAT = 291
SYS_UNLINKAT = 292
SYS_RENAMEAT = 293
SYS_LINKAT = 294
SYS_SYMLINKAT = 295
SYS_READLINKAT = 296
SYS_FCHMODAT = 297
SYS_FACCESSAT = 298
SYS_GET_ROBUST_LIST = 299
SYS_SET_ROBUST_LIST = 300
SYS_MOVE_PAGES = 301
SYS_GETCPU = 302
SYS_EPOLL_PWAIT = 303
SYS_UTIMENSAT = 304
SYS_SIGNALFD = 305
SYS_TIMERFD_CREATE = 306
SYS_EVENTFD = 307
SYS_SYNC_FILE_RANGE2 = 308
SYS_FALLOCATE = 309
SYS_SUBPAGE_PROT = 310
SYS_TIMERFD_SETTIME = 311
SYS_TIMERFD_GETTIME = 312
SYS_SIGNALFD4 = 313
SYS_EVENTFD2 = 314
SYS_EPOLL_CREATE1 = 315
SYS_DUP3 = 316
SYS_PIPE2 = 317
SYS_INOTIFY_INIT1 = 318
SYS_PERF_EVENT_OPEN = 319
SYS_PREADV = 320
SYS_PWRITEV = 321
SYS_RT_TGSIGQUEUEINFO = 322
SYS_FANOTIFY_INIT = 323
SYS_FANOTIFY_MARK = 324
SYS_PRLIMIT64 = 325
SYS_SOCKET = 326
SYS_BIND = 327
SYS_CONNECT = 328
SYS_LISTEN = 329
SYS_ACCEPT = 330
SYS_GETSOCKNAME = 331
SYS_GETPEERNAME = 332
SYS_SOCKETPAIR = 333
SYS_SEND = 334
SYS_SENDTO = 335
SYS_RECV = 336
SYS_RECVFROM = 337
SYS_SHUTDOWN = 338
SYS_SETSOCKOPT = 339
SYS_GETSOCKOPT = 340
SYS_SENDMSG = 341
SYS_RECVMSG = 342
SYS_RECVMMSG = 343
SYS_ACCEPT4 = 344
SYS_NAME_TO_HANDLE_AT = 345
SYS_OPEN_BY_HANDLE_AT = 346
SYS_CLOCK_ADJTIME = 347
SYS_SYNCFS = 348
SYS_SENDMMSG = 349
SYS_SETNS = 350
SYS_PROCESS_VM_READV = 351
SYS_PROCESS_VM_WRITEV = 352
SYS_FINIT_MODULE = 353
SYS_KCMP = 354
SYS_SCHED_SETATTR = 355
SYS_SCHED_GETATTR = 356
SYS_RENAMEAT2 = 357
SYS_SECCOMP = 358
SYS_GETRANDOM = 359
SYS_MEMFD_CREATE = 360
SYS_BPF = 361
SYS_EXECVEAT = 362
SYS_SWITCH_ENDIAN = 363
SYS_USERFAULTFD = 364
SYS_MEMBARRIER = 365
SYS_MLOCK2 = 378
SYS_COPY_FILE_RANGE = 379
SYS_PREADV2 = 380
SYS_PWRITEV2 = 381
SYS_KEXEC_FILE_LOAD = 382
SYS_STATX = 383
SYS_PKEY_ALLOC = 384
SYS_PKEY_FREE = 385
SYS_PKEY_MPROTECT = 386
SYS_RSEQ = 387
SYS_IO_PGETEVENTS = 388
SYS_SEMTIMEDOP = 392
SYS_SEMGET = 393
SYS_SEMCTL = 394
SYS_SHMGET = 395
SYS_SHMCTL = 396
SYS_SHMAT = 397
SYS_SHMDT = 398
SYS_MSGGET = 399
SYS_MSGSND = 400
SYS_MSGRCV = 401
SYS_MSGCTL = 402
SYS_PIDFD_SEND_SIGNAL = 424
SYS_IO_URING_SETUP = 425
SYS_IO_URING_ENTER = 426
SYS_IO_URING_REGISTER = 427
SYS_OPEN_TREE = 428
SYS_MOVE_MOUNT = 429
SYS_FSOPEN = 430
SYS_FSCONFIG = 431
SYS_FSMOUNT = 432
SYS_FSPICK = 433
SYS_PIDFD_OPEN = 434
SYS_CLONE3 = 435
SYS_OPENAT2 = 437
SYS_PIDFD_GETFD = 438
)
| {
"pile_set_name": "Github"
} |
# Since we rely on paths relative to the makefile location, abort if make isn't being run from there.
$(if $(findstring /,$(MAKEFILE_LIST)),$(error Please only invoke this makefile from the directory it resides in))
# The files that need updating when incrementing the version number.
VERSIONED_FILES := *.js *.json README*
# Add the local npm packages' bin folder to the PATH, so that `make` can find them, when invoked directly.
# Note that rather than using `$(npm bin)` the 'node_modules/.bin' path component is hard-coded, so that invocation works even from an environment
# where npm is (temporarily) unavailable due to having deactivated an nvm instance loaded into the calling shell in order to avoid interference with tests.
export PATH := $(shell printf '%s' "$$PWD/node_modules/.bin:$$PATH")
UTILS := semver
# Make sure that all required utilities can be located.
UTIL_CHECK := $(or $(shell PATH="$(PATH)" which $(UTILS) >/dev/null && echo 'ok'),$(error Did you forget to run `npm install` after cloning the repo? At least one of the required supporting utilities not found: $(UTILS)))
# Default target (by virtue of being the first non '.'-prefixed in the file).
.PHONY: _no-target-specified
_no-target-specified:
$(error Please specify the target to make - `make list` shows targets. Alternatively, use `npm test` to run the default tests; `npm run` shows all tests)
# Lists all targets defined in this makefile.
.PHONY: list
list:
@$(MAKE) -pRrn : -f $(MAKEFILE_LIST) 2>/dev/null | awk -v RS= -F: '/^# File/,/^# Finished Make data base/ {if ($$1 !~ "^[#.]") {print $$1}}' | command grep -v -e '^[^[:alnum:]]' -e '^$@$$command ' | sort
# All-tests target: invokes the specified test suites for ALL shells defined in $(SHELLS).
.PHONY: test
test:
@npm test
.PHONY: _ensure-tag
_ensure-tag:
ifndef TAG
$(error Please invoke with `make TAG=<new-version> release`, where <new-version> is either an increment specifier (patch, minor, major, prepatch, preminor, premajor, prerelease), or an explicit major.minor.patch version number)
endif
CHANGELOG_ERROR = $(error No CHANGELOG specified)
.PHONY: _ensure-changelog
_ensure-changelog:
@ (git status -sb --porcelain | command grep -E '^( M|[MA] ) CHANGELOG.md' > /dev/null) || (echo no CHANGELOG.md specified && exit 2)
# Ensures that the git workspace is clean.
.PHONY: _ensure-clean
_ensure-clean:
@[ -z "$$((git status --porcelain --untracked-files=no || echo err) | command grep -v 'CHANGELOG.md')" ] || { echo "Workspace is not clean; please commit changes first." >&2; exit 2; }
# Makes a release; invoke with `make TAG=<versionOrIncrementSpec> release`.
.PHONY: release
release: _ensure-tag _ensure-changelog _ensure-clean
@old_ver=`git describe --abbrev=0 --tags --match 'v[0-9]*.[0-9]*.[0-9]*'` || { echo "Failed to determine current version." >&2; exit 1; }; old_ver=$${old_ver#v}; \
new_ver=`echo "$(TAG)" | sed 's/^v//'`; new_ver=$${new_ver:-patch}; \
if printf "$$new_ver" | command grep -q '^[0-9]'; then \
semver "$$new_ver" >/dev/null || { echo 'Invalid version number specified: $(TAG) - must be major.minor.patch' >&2; exit 2; }; \
semver -r "> $$old_ver" "$$new_ver" >/dev/null || { echo 'Invalid version number specified: $(TAG) - must be HIGHER than current one.' >&2; exit 2; } \
else \
new_ver=`semver -i "$$new_ver" "$$old_ver"` || { echo 'Invalid version-increment specifier: $(TAG)' >&2; exit 2; } \
fi; \
printf "=== Bumping version **$$old_ver** to **$$new_ver** before committing and tagging:\n=== TYPE 'proceed' TO PROCEED, anything else to abort: " && read response && [ "$$response" = 'proceed' ] || { echo 'Aborted.' >&2; exit 2; }; \
replace "$$old_ver" "$$new_ver" -- $(VERSIONED_FILES) && \
git commit -m "v$$new_ver" $(VERSIONED_FILES) CHANGELOG.md && \
git tag -a -m "v$$new_ver" "v$$new_ver"
| {
"pile_set_name": "Github"
} |
// Copyright (c) 2016, 2018, 2019, Oracle and/or its affiliates. All rights reserved.
// Code generated. DO NOT EDIT.
// Key Management Service API
//
// API for managing and performing operations with keys and vaults.
//
package keymanagement
import (
"github.com/oracle/oci-go-sdk/common"
)
// EncryptDataDetails The representation of EncryptDataDetails
type EncryptDataDetails struct {
// The OCID of the key to encrypt with.
KeyId *string `mandatory:"true" json:"keyId"`
// The plaintext data to encrypt.
Plaintext *string `mandatory:"true" json:"plaintext"`
// Information that can be used to provide an encryption context for the
// encrypted data. The length of the string representation of the associatedData
// must be fewer than 4096 characters.
AssociatedData map[string]string `mandatory:"false" json:"associatedData"`
// Information that can be used to provide context for audit logging. It is a map that contains any addtional
// data the users may have and will be added to the audit logs (if audit logging is enabled)
LoggingContext map[string]string `mandatory:"false" json:"loggingContext"`
}
func (m EncryptDataDetails) String() string {
return common.PointerString(m)
}
| {
"pile_set_name": "Github"
} |
/** @file
EFI Shell protocol as defined in the UEFI Shell 2.0 specification including errata.
(C) Copyright 2014 Hewlett-Packard Development Company, L.P.<BR>
Copyright (c) 2006 - 2015, Intel Corporation. All rights reserved.<BR>
This program and the accompanying materials
are licensed and made available under the terms and conditions of the BSD License
which accompanies this distribution. The full text of the license may be found at
http://opensource.org/licenses/bsd-license.php
THE PROGRAM IS DISTRIBUTED UNDER THE BSD LICENSE ON AN "AS IS" BASIS,
WITHOUT WARRANTIES OR REPRESENTATIONS OF ANY KIND, EITHER EXPRESS OR IMPLIED.
**/
#ifndef __EFI_SHELL_PROTOCOL__
#define __EFI_SHELL_PROTOCOL__
#include <ShellBase.h>
#include <Guid/FileInfo.h>
#define EFI_SHELL_PROTOCOL_GUID \
{ \
0x6302d008, 0x7f9b, 0x4f30, { 0x87, 0xac, 0x60, 0xc9, 0xfe, 0xf5, 0xda, 0x4e } \
}
// replaced EFI_LIST_ENTRY with LIST_ENTRY for simplicity.
// they are identical outside of the name.
typedef struct {
LIST_ENTRY Link; ///< Linked list members.
EFI_STATUS Status; ///< Status of opening the file. Valid only if Handle != NULL.
CONST CHAR16 *FullName; ///< Fully qualified filename.
CONST CHAR16 *FileName; ///< name of this file.
SHELL_FILE_HANDLE Handle; ///< Handle for interacting with the opened file or NULL if closed.
EFI_FILE_INFO *Info; ///< Pointer to the FileInfo struct for this file or NULL.
} EFI_SHELL_FILE_INFO;
/**
Returns whether any script files are currently being processed.
@retval TRUE There is at least one script file active.
@retval FALSE No script files are active now.
**/
typedef
BOOLEAN
(EFIAPI *EFI_SHELL_BATCH_IS_ACTIVE) (
VOID
);
/**
Closes the file handle.
This function closes a specified file handle. All 'dirty' cached file data is
flushed to the device, and the file is closed. In all cases, the handle is
closed.
@param[in] FileHandle The file handle to be closed.
@retval EFI_SUCCESS The file closed sucessfully.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_CLOSE_FILE)(
IN SHELL_FILE_HANDLE FileHandle
);
/**
Creates a file or directory by name.
This function creates an empty new file or directory with the specified attributes and
returns the new file's handle. If the file already exists and is read-only, then
EFI_INVALID_PARAMETER will be returned.
If the file already existed, it is truncated and its attributes updated. If the file is
created successfully, the FileHandle is the file's handle, else, the FileHandle is NULL.
If the file name begins with >v, then the file handle which is returned refers to the
shell environment variable with the specified name. If the shell environment variable
already exists and is non-volatile then EFI_INVALID_PARAMETER is returned.
@param[in] FileName Pointer to NULL-terminated file path.
@param[in] FileAttribs The new file's attrbiutes. The different attributes are
described in EFI_FILE_PROTOCOL.Open().
@param[out] FileHandle On return, points to the created file handle or directory's handle.
@retval EFI_SUCCESS The file was opened. FileHandle points to the new file's handle.
@retval EFI_INVALID_PARAMETER One of the parameters has an invalid value.
@retval EFI_UNSUPPORTED The file path could not be opened.
@retval EFI_NOT_FOUND The specified file could not be found on the device, or could not
file the file system on the device.
@retval EFI_NO_MEDIA The device has no medium.
@retval EFI_MEDIA_CHANGED The device has a different medium in it or the medium is no
longer supported.
@retval EFI_DEVICE_ERROR The device reported an error or can't get the file path according
the DirName.
@retval EFI_VOLUME_CORRUPTED The file system structures are corrupted.
@retval EFI_WRITE_PROTECTED An attempt was made to create a file, or open a file for write
when the media is write-protected.
@retval EFI_ACCESS_DENIED The service denied access to the file.
@retval EFI_OUT_OF_RESOURCES Not enough resources were available to open the file.
@retval EFI_VOLUME_FULL The volume is full.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_CREATE_FILE)(
IN CONST CHAR16 *FileName,
IN UINT64 FileAttribs,
OUT SHELL_FILE_HANDLE *FileHandle
);
/**
Deletes the file specified by the file handle.
This function closes and deletes a file. In all cases, the file handle is closed. If the file
cannot be deleted, the warning code EFI_WARN_DELETE_FAILURE is returned, but the
handle is still closed.
@param[in] FileHandle The file handle to delete.
@retval EFI_SUCCESS The file was closed and deleted and the handle was closed.
@retval EFI_WARN_DELETE_FAILURE The handle was closed but the file was not deleted.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_DELETE_FILE)(
IN SHELL_FILE_HANDLE FileHandle
);
/**
Deletes the file specified by the file name.
This function deletes a file.
@param[in] FileName Points to the NULL-terminated file name.
@retval EFI_SUCCESS The file was deleted.
@retval EFI_WARN_DELETE_FAILURE The handle was closed but the file was not deleted.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_DELETE_FILE_BY_NAME)(
IN CONST CHAR16 *FileName
);
/**
Disables the page break output mode.
**/
typedef
VOID
(EFIAPI *EFI_SHELL_DISABLE_PAGE_BREAK) (
VOID
);
/**
Enables the page break output mode.
**/
typedef
VOID
(EFIAPI *EFI_SHELL_ENABLE_PAGE_BREAK) (
VOID
);
/**
Execute the command line.
This function creates a nested instance of the shell and executes the specified
command (CommandLine) with the specified environment (Environment). Upon return,
the status code returned by the specified command is placed in StatusCode.
If Environment is NULL, then the current environment is used and all changes made
by the commands executed will be reflected in the current environment. If the
Environment is non-NULL, then the changes made will be discarded.
The CommandLine is executed from the current working directory on the current
device.
@param[in] ParentImageHandle A handle of the image that is executing the specified
command line.
@param[in] CommandLine Points to the NULL-terminated UCS-2 encoded string
containing the command line. If NULL then the command-
line will be empty.
@param[in] Environment Points to a NULL-terminated array of environment
variables with the format 'x=y', where x is the
environment variable name and y is the value. If this
is NULL, then the current shell environment is used.
@param[out] ErrorCode Points to the status code returned by the command.
@retval EFI_SUCCESS The command executed successfully. The status code
returned by the command is pointed to by StatusCode.
@retval EFI_INVALID_PARAMETER The parameters are invalid.
@retval EFI_OUT_OF_RESOURCES Out of resources.
@retval EFI_UNSUPPORTED Nested shell invocations are not allowed.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_EXECUTE) (
IN EFI_HANDLE *ParentImageHandle,
IN CHAR16 *CommandLine OPTIONAL,
IN CHAR16 **Environment OPTIONAL,
OUT EFI_STATUS *StatusCode OPTIONAL
);
/**
Find files that match a specified pattern.
This function searches for all files and directories that match the specified
FilePattern. The FilePattern can contain wild-card characters. The resulting file
information is placed in the file list FileList.
The files in the file list are not opened. The OpenMode field is set to 0 and the FileInfo
field is set to NULL.
@param[in] FilePattern Points to a NULL-terminated shell file path, including wildcards.
@param[out] FileList On return, points to the start of a file list containing the names
of all matching files or else points to NULL if no matching files
were found.
@retval EFI_SUCCESS Files found.
@retval EFI_NOT_FOUND No files found.
@retval EFI_NO_MEDIA The device has no media.
@retval EFI_DEVICE_ERROR The device reported an error.
@retval EFI_VOLUME_CORRUPTED The file system structures are corrupted.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_FIND_FILES)(
IN CONST CHAR16 *FilePattern,
OUT EFI_SHELL_FILE_INFO **FileList
);
/**
Find all files in a specified directory.
@param[in] FileDirHandle Handle of the directory to search.
@param[out] FileList On return, points to the list of files in the directory
or NULL if there are no files in the directory.
@retval EFI_SUCCESS File information was returned successfully.
@retval EFI_VOLUME_CORRUPTED The file system structures have been corrupted.
@retval EFI_DEVICE_ERROR The device reported an error.
@retval EFI_NO_MEDIA The device media is not present.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_FIND_FILES_IN_DIR)(
IN SHELL_FILE_HANDLE FileDirHandle,
OUT EFI_SHELL_FILE_INFO **FileList
);
/**
Flushes data back to a device.
This function flushes all modified data associated with a file to a device.
@param[in] FileHandle The handle of the file to flush.
@retval EFI_SUCCESS The data was flushed.
@retval EFI_NO_MEDIA The device has no medium.
@retval EFI_DEVICE_ERROR The device reported an error.
@retval EFI_VOLUME_CORRUPTED The file system structures are corrupted.
@retval EFI_WRITE_PROTECTED The file or medium is write-protected.
@retval EFI_ACCESS_DENIED The file was opened read-only.
@retval EFI_VOLUME_FULL The volume is full.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_FLUSH_FILE)(
IN SHELL_FILE_HANDLE FileHandle
);
/**
Frees the file list.
This function cleans up the file list and any related data structures. It has no
impact on the files themselves.
@param[in] FileList The file list to free. Type EFI_SHELL_FILE_INFO is
defined in OpenFileList().
@retval EFI_SUCCESS Free the file list successfully.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_FREE_FILE_LIST) (
IN EFI_SHELL_FILE_INFO **FileList
);
/**
Returns the current directory on the specified device.
If FileSystemMapping is NULL, it returns the current working directory. If the
FileSystemMapping is not NULL, it returns the current directory associated with the
FileSystemMapping. In both cases, the returned name includes the file system
mapping (i.e. fs0:\current-dir).
Note that the current directory string should exclude the tailing backslash character.
@param[in] FileSystemMapping A pointer to the file system mapping. If NULL,
then the current working directory is returned.
@retval !=NULL The current directory.
@retval NULL Current directory does not exist.
**/
typedef
CONST CHAR16 *
(EFIAPI *EFI_SHELL_GET_CUR_DIR) (
IN CONST CHAR16 *FileSystemMapping OPTIONAL
);
typedef UINT32 EFI_SHELL_DEVICE_NAME_FLAGS;
#define EFI_DEVICE_NAME_USE_COMPONENT_NAME 0x00000001
#define EFI_DEVICE_NAME_USE_DEVICE_PATH 0x00000002
/**
Gets the name of the device specified by the device handle.
This function gets the user-readable name of the device specified by the device
handle. If no user-readable name could be generated, then *BestDeviceName will be
NULL and EFI_NOT_FOUND will be returned.
If EFI_DEVICE_NAME_USE_COMPONENT_NAME is set, then the function will return the
device's name using the EFI_COMPONENT_NAME2_PROTOCOL, if present on
DeviceHandle.
If EFI_DEVICE_NAME_USE_DEVICE_PATH is set, then the function will return the
device's name using the EFI_DEVICE_PATH_PROTOCOL, if present on DeviceHandle.
If both EFI_DEVICE_NAME_USE_COMPONENT_NAME and
EFI_DEVICE_NAME_USE_DEVICE_PATH are set, then
EFI_DEVICE_NAME_USE_COMPONENT_NAME will have higher priority.
@param[in] DeviceHandle The handle of the device.
@param[in] Flags Determines the possible sources of component names.
@param[in] Language A pointer to the language specified for the device
name, in the same format as described in the UEFI
specification, Appendix M.
@param[out] BestDeviceName On return, points to the callee-allocated NULL-
terminated name of the device. If no device name
could be found, points to NULL. The name must be
freed by the caller...
@retval EFI_SUCCESS Get the name successfully.
@retval EFI_NOT_FOUND Fail to get the device name.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_GET_DEVICE_NAME) (
IN EFI_HANDLE DeviceHandle,
IN EFI_SHELL_DEVICE_NAME_FLAGS Flags,
IN CHAR8 *Language,
OUT CHAR16 **BestDeviceName
);
/**
Gets the device path from the mapping.
This function gets the device path associated with a mapping.
@param[in] Mapping A pointer to the mapping
@retval !=NULL Pointer to the device path that corresponds to the
device mapping. The returned pointer does not need
to be freed.
@retval NULL There is no device path associated with the
specified mapping.
**/
typedef
CONST EFI_DEVICE_PATH_PROTOCOL *
(EFIAPI *EFI_SHELL_GET_DEVICE_PATH_FROM_MAP) (
IN CONST CHAR16 *Mapping
);
/**
Converts a file system style name to a device path.
This function converts a file system style name to a device path, by replacing any
mapping references to the associated device path.
@param[in] Path The pointer to the path.
@return The pointer of the file path. The file path is callee
allocated and should be freed by the caller.
**/
typedef
EFI_DEVICE_PATH_PROTOCOL *
(EFIAPI *EFI_SHELL_GET_DEVICE_PATH_FROM_FILE_PATH) (
IN CONST CHAR16 *Path
);
/**
Gets either a single or list of environment variables.
If name is not NULL then this function returns the current value of the specified
environment variable.
If Name is NULL than a list of all environment variable names is returned. Each a
NULL terminated string with a double NULL terminating the list.
@param[in] Name A pointer to the environment variable name. If
Name is NULL, then the function will return all
of the defined shell environment variables. In
the case where multiple environment variables are
being returned, each variable will be terminated by
a NULL, and the list will be terminated by a double
NULL.
@return A pointer to the returned string.
The returned pointer does not need to be freed by the caller.
@retval NULL The environment variable doesn't exist or there are
no environment variables.
**/
typedef
CONST CHAR16 *
(EFIAPI *EFI_SHELL_GET_ENV) (
IN CONST CHAR16 *Name OPTIONAL
);
/**
Gets the environment variable and Attributes, or list of environment variables. Can be
used instead of GetEnv().
This function returns the current value of the specified environment variable and
the Attributes. If no variable name was specified, then all of the known
variables will be returned.
@param[in] Name A pointer to the environment variable name. If Name is NULL,
then the function will return all of the defined shell
environment variables. In the case where multiple environment
variables are being returned, each variable will be terminated
by a NULL, and the list will be terminated by a double NULL.
@param[out] Attributes If not NULL, a pointer to the returned attributes bitmask for
the environment variable. In the case where Name is NULL, and
multiple environment variables are being returned, Attributes
is undefined.
@retval NULL The environment variable doesn't exist.
@return The environment variable's value. The returned pointer does not
need to be freed by the caller.
**/
typedef
CONST CHAR16 *
(EFIAPI *EFI_SHELL_GET_ENV_EX) (
IN CONST CHAR16 *Name,
OUT UINT32 *Attributes OPTIONAL
);
/**
Gets the file information from an open file handle.
This function allocates a buffer to store the file's information. It's the caller's
responsibility to free the buffer.
@param[in] FileHandle A File Handle.
@retval NULL Cannot get the file info.
@return A pointer to a buffer with file information.
**/
typedef
EFI_FILE_INFO *
(EFIAPI *EFI_SHELL_GET_FILE_INFO)(
IN SHELL_FILE_HANDLE FileHandle
);
/**
Converts a device path to a file system-style path.
This function converts a device path to a file system path by replacing part, or all, of
the device path with the file-system mapping. If there are more than one application
file system mappings, the one that most closely matches Path will be used.
@param[in] Path The pointer to the device path.
@return The pointer of the NULL-terminated file path. The path
is callee-allocated and should be freed by the caller.
**/
typedef
CHAR16 *
(EFIAPI *EFI_SHELL_GET_FILE_PATH_FROM_DEVICE_PATH) (
IN CONST EFI_DEVICE_PATH_PROTOCOL *Path
);
/**
Gets a file's current position.
This function returns the current file position for the file handle. For directories, the
current file position has no meaning outside of the file system driver and as such, the
operation is not supported.
@param[in] FileHandle The file handle on which to get the current position.
@param[out] Position Byte position from the start of the file.
@retval EFI_SUCCESS Data was accessed.
@retval EFI_UNSUPPORTED The request is not valid on open directories.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_GET_FILE_POSITION)(
IN SHELL_FILE_HANDLE FileHandle,
OUT UINT64 *Position
);
/**
Gets the size of a file.
This function returns the size of the file specified by FileHandle.
@param[in] FileHandle The handle of the file.
@param[out] Size The size of this file.
@retval EFI_SUCCESS Get the file's size.
@retval EFI_DEVICE_ERROR Can't access the file.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_GET_FILE_SIZE)(
IN SHELL_FILE_HANDLE FileHandle,
OUT UINT64 *Size
);
/**
Get the GUID value from a human readable name.
If GuidName is a known GUID name, then update Guid to have the correct value for
that GUID.
This function is only available when the major and minor versions in the
EfiShellProtocol are greater than or equal to 2 and 1, respectively.
@param[in] GuidName A pointer to the localized name for the GUID being queried.
@param[out] Guid A pointer to the GUID structure to be filled in.
@retval EFI_SUCCESS The operation was successful.
@retval EFI_INVALID_PARAMETER Guid was NULL.
@retval EFI_INVALID_PARAMETER GuidName was NULL.
@retval EFI_NOT_FOUND GuidName is not a known GUID Name.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_GET_GUID_FROM_NAME)(
IN CONST CHAR16 *GuidName,
OUT EFI_GUID *Guid
);
/**
Get the human readable name for a GUID from the value.
If Guid is assigned a name, then update *GuidName to point to the name. The callee
should not modify the value.
This function is only available when the major and minor versions in the
EfiShellProtocol are greater than or equal to 2 and 1, respectively.
@param[in] Guid A pointer to the GUID being queried.
@param[out] GuidName A pointer to a pointer the localized to name for the GUID being requested
@retval EFI_SUCCESS The operation was successful.
@retval EFI_INVALID_PARAMETER Guid was NULL.
@retval EFI_INVALID_PARAMETER GuidName was NULL.
@retval EFI_NOT_FOUND Guid is not assigned a name.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_GET_GUID_NAME)(
IN CONST EFI_GUID *Guid,
OUT CONST CHAR16 **GuidName
);
/**
Return help information about a specific command.
This function returns the help information for the specified command. The help text
can be internal to the shell or can be from a UEFI Shell manual page.
If Sections is specified, then each section name listed will be compared in a casesensitive
manner, to the section names described in Appendix B. If the section exists,
it will be appended to the returned help text. If the section does not exist, no
information will be returned. If Sections is NULL, then all help text information
available will be returned.
@param[in] Command Points to the NULL-terminated UEFI Shell command name.
@param[in] Sections Points to the NULL-terminated comma-delimited
section names to return. If NULL, then all
sections will be returned.
@param[out] HelpText On return, points to a callee-allocated buffer
containing all specified help text.
@retval EFI_SUCCESS The help text was returned.
@retval EFI_OUT_OF_RESOURCES The necessary buffer could not be allocated to hold the
returned help text.
@retval EFI_INVALID_PARAMETER HelpText is NULL.
@retval EFI_NOT_FOUND There is no help text available for Command.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_GET_HELP_TEXT) (
IN CONST CHAR16 *Command,
IN CONST CHAR16 *Sections OPTIONAL,
OUT CHAR16 **HelpText
);
/**
Gets the mapping(s) that most closely matches the device path.
This function gets the mapping which corresponds to the device path *DevicePath. If
there is no exact match, then the mapping which most closely matches *DevicePath
is returned, and *DevicePath is updated to point to the remaining portion of the
device path. If there is an exact match, the mapping is returned and *DevicePath
points to the end-of-device-path node.
If there are multiple map names they will be semi-colon seperated in the
NULL-terminated string.
@param[in, out] DevicePath On entry, points to a device path pointer. On
exit, updates the pointer to point to the
portion of the device path after the mapping.
@retval NULL No mapping was found.
@retval !=NULL Pointer to NULL-terminated mapping. The buffer
is callee allocated and should be freed by the caller.
**/
typedef
CONST CHAR16 *
(EFIAPI *EFI_SHELL_GET_MAP_FROM_DEVICE_PATH) (
IN OUT EFI_DEVICE_PATH_PROTOCOL **DevicePath
);
/**
Gets the enable status of the page break output mode.
User can use this function to determine current page break mode.
@retval TRUE The page break output mode is enabled.
@retval FALSE The page break output mode is disabled.
**/
typedef
BOOLEAN
(EFIAPI *EFI_SHELL_GET_PAGE_BREAK) (
VOID
);
/**
Judges whether the active shell is the root shell.
This function makes the user to know that whether the active Shell is the root shell.
@retval TRUE The active Shell is the root Shell.
@retval FALSE The active Shell is NOT the root Shell.
**/
typedef
BOOLEAN
(EFIAPI *EFI_SHELL_IS_ROOT_SHELL) (
VOID
);
/**
Opens a file or a directory by file name.
This function opens the specified file in the specified OpenMode and returns a file
handle.
If the file name begins with '>v', then the file handle which is returned refers to the
shell environment variable with the specified name. If the shell environment variable
exists, is non-volatile and the OpenMode indicates EFI_FILE_MODE_WRITE, then
EFI_INVALID_PARAMETER is returned.
If the file name is '>i', then the file handle which is returned refers to the standard
input. If the OpenMode indicates EFI_FILE_MODE_WRITE, then EFI_INVALID_PARAMETER
is returned.
If the file name is '>o', then the file handle which is returned refers to the standard
output. If the OpenMode indicates EFI_FILE_MODE_READ, then EFI_INVALID_PARAMETER
is returned.
If the file name is '>e', then the file handle which is returned refers to the standard
error. If the OpenMode indicates EFI_FILE_MODE_READ, then EFI_INVALID_PARAMETER
is returned.
If the file name is 'NUL', then the file handle that is returned refers to the standard NUL
file. If the OpenMode indicates EFI_FILE_MODE_READ, then EFI_INVALID_PARAMETER is
returned.
If return EFI_SUCCESS, the FileHandle is the opened file's handle, else, the
FileHandle is NULL.
@param[in] FileName Points to the NULL-terminated UCS-2 encoded file name.
@param[out] FileHandle On return, points to the file handle.
@param[in] OpenMode File open mode. Either EFI_FILE_MODE_READ or
EFI_FILE_MODE_WRITE from section 12.4 of the UEFI
Specification.
@retval EFI_SUCCESS The file was opened. FileHandle has the opened file's handle.
@retval EFI_INVALID_PARAMETER One of the parameters has an invalid value. FileHandle is NULL.
@retval EFI_UNSUPPORTED Could not open the file path. FileHandle is NULL.
@retval EFI_NOT_FOUND The specified file could not be found on the device or the file
system could not be found on the device. FileHandle is NULL.
@retval EFI_NO_MEDIA The device has no medium. FileHandle is NULL.
@retval EFI_MEDIA_CHANGED The device has a different medium in it or the medium is no
longer supported. FileHandle is NULL.
@retval EFI_DEVICE_ERROR The device reported an error or can't get the file path according
the FileName. FileHandle is NULL.
@retval EFI_VOLUME_CORRUPTED The file system structures are corrupted. FileHandle is NULL.
@retval EFI_WRITE_PROTECTED An attempt was made to create a file, or open a file for write
when the media is write-protected. FileHandle is NULL.
@retval EFI_ACCESS_DENIED The service denied access to the file. FileHandle is NULL.
@retval EFI_OUT_OF_RESOURCES Not enough resources were available to open the file. FileHandle
is NULL.
@retval EFI_VOLUME_FULL The volume is full. FileHandle is NULL.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_OPEN_FILE_BY_NAME) (
IN CONST CHAR16 *FileName,
OUT SHELL_FILE_HANDLE *FileHandle,
IN UINT64 OpenMode
);
/**
Opens the files that match the path specified.
This function opens all of the files specified by Path. Wildcards are processed
according to the rules specified in UEFI Shell 2.0 spec section 3.7.1. Each
matching file has an EFI_SHELL_FILE_INFO structure created in a linked list.
@param[in] Path A pointer to the path string.
@param[in] OpenMode Specifies the mode used to open each file, EFI_FILE_MODE_READ or
EFI_FILE_MODE_WRITE.
@param[in, out] FileList Points to the start of a list of files opened.
@retval EFI_SUCCESS Create the file list successfully.
@return Can't create the file list.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_OPEN_FILE_LIST) (
IN CHAR16 *Path,
IN UINT64 OpenMode,
IN OUT EFI_SHELL_FILE_INFO **FileList
);
/**
Opens the root directory of a device.
This function opens the root directory of a device and returns a file handle to it.
@param[in] DevicePath Points to the device path corresponding to the device where the
EFI_SIMPLE_FILE_SYSTEM_PROTOCOL is installed.
@param[out] FileHandle On exit, points to the file handle corresponding to the root directory on the
device.
@retval EFI_SUCCESS Root opened successfully.
@retval EFI_NOT_FOUND EFI_SIMPLE_FILE_SYSTEM could not be found or the root directory
could not be opened.
@retval EFI_VOLUME_CORRUPTED The data structures in the volume were corrupted.
@retval EFI_DEVICE_ERROR The device had an error.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_OPEN_ROOT)(
IN EFI_DEVICE_PATH_PROTOCOL *DevicePath,
OUT SHELL_FILE_HANDLE *FileHandle
);
/**
Opens the root directory of a device on a handle.
This function opens the root directory of a device and returns a file handle to it.
@param[in] DeviceHandle The handle of the device that contains the volume.
@param[out] FileHandle On exit, points to the file handle corresponding to the root directory on the
device.
@retval EFI_SUCCESS Root opened successfully.
@retval EFI_NOT_FOUND EFI_SIMPLE_FILE_SYSTEM could not be found or the root directory
could not be opened.
@retval EFI_VOLUME_CORRUPTED The data structures in the volume were corrupted.
@retval EFI_DEVICE_ERROR The device had an error.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_OPEN_ROOT_BY_HANDLE)(
IN EFI_HANDLE DeviceHandle,
OUT SHELL_FILE_HANDLE *FileHandle
);
/**
Reads data from the file.
If FileHandle is not a directory, the function reads the requested number of bytes
from the file at the file's current position and returns them in Buffer. If the read goes
beyond the end of the file, the read length is truncated to the end of the file. The file's
current position is increased by the number of bytes returned.
If FileHandle is a directory, then an error is returned.
@param[in] FileHandle The opened file handle for read.
@param[in] ReadSize On input, the size of Buffer, in bytes. On output, the amount of data read.
@param[in, out] Buffer The buffer in which data is read.
@retval EFI_SUCCESS Data was read.
@retval EFI_NO_MEDIA The device has no media.
@retval EFI_DEVICE_ERROR The device reported an error.
@retval EFI_VOLUME_CORRUPTED The file system structures are corrupted.
@retval EFI_BUFFER_TO_SMALL Buffer is too small. ReadSize contains required size.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_READ_FILE) (
IN SHELL_FILE_HANDLE FileHandle,
IN OUT UINTN *ReadSize,
IN OUT VOID *Buffer
);
/**
Register a GUID and a localized human readable name for it.
If Guid is not assigned a name, then assign GuidName to Guid. This list of GUID
names must be used whenever a shell command outputs GUID information.
This function is only available when the major and minor versions in the
EfiShellProtocol are greater than or equal to 2 and 1, respectively.
@param[in] Guid A pointer to the GUID being registered.
@param[in] GuidName A pointer to the localized name for the GUID being registered.
@retval EFI_SUCCESS The operation was successful.
@retval EFI_INVALID_PARAMETER Guid was NULL.
@retval EFI_INVALID_PARAMETER GuidName was NULL.
@retval EFI_ACCESS_DENIED Guid already is assigned a name.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_REGISTER_GUID_NAME)(
IN CONST EFI_GUID *Guid,
IN CONST CHAR16 *GuidName
);
/**
Deletes the duplicate file names files in the given file list.
@param[in] FileList A pointer to the first entry in the file list.
@retval EFI_SUCCESS Always success.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_REMOVE_DUP_IN_FILE_LIST) (
IN EFI_SHELL_FILE_INFO **FileList
);
/**
Changes a shell command alias.
This function creates an alias for a shell command.
@param[in] Command Points to the NULL-terminated shell command or existing alias.
@param[in] Alias Points to the NULL-terminated alias for the shell command. If this is NULL, and
Command refers to an alias, that alias will be deleted.
@param[in] Replace If TRUE and the alias already exists, then the existing alias will be replaced. If
FALSE and the alias already exists, then the existing alias is unchanged and
EFI_ACCESS_DENIED is returned.
@param[in] Volatile if TRUE the Alias being set will be stored in a volatile fashion. if FALSE the
Alias being set will be stored in a non-volatile fashion.
@retval EFI_SUCCESS Alias created or deleted successfully.
@retval EFI_ACCESS_DENIED The alias is a built-in alias or already existed and Replace was set to
FALSE.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_SET_ALIAS)(
IN CONST CHAR16 *Command,
IN CONST CHAR16 *Alias,
IN BOOLEAN Replace,
IN BOOLEAN Volatile
);
/**
This function returns the command associated with a alias or a list of all
alias'.
@param[in] Alias Points to the NULL-terminated shell alias.
If this parameter is NULL, then all
aliases will be returned in ReturnedData.
@param[out] Volatile Upon return of a single command if TRUE indicates
this is stored in a volatile fashion. FALSE otherwise.
@return If Alias is not NULL, it will return a pointer to
the NULL-terminated command for that alias.
If Alias is NULL, ReturnedData points to a ';'
delimited list of alias (e.g.
ReturnedData = "dir;del;copy;mfp") that is NULL-terminated.
@retval NULL An error ocurred.
@retval NULL Alias was not a valid Alias.
**/
typedef
CONST CHAR16 *
(EFIAPI *EFI_SHELL_GET_ALIAS)(
IN CONST CHAR16 *Alias,
OUT BOOLEAN *Volatile OPTIONAL
);
/**
Changes the current directory on the specified device.
If the FileSystem is NULL, and the directory Dir does not contain a file system's
mapped name, this function changes the current working directory. If FileSystem is
NULL and the directory Dir contains a mapped name, then the current file system and
the current directory on that file system are changed.
If FileSystem is not NULL, and Dir is NULL, then this changes the current working file
system.
If FileSystem is not NULL and Dir is not NULL, then this function changes the current
directory on the specified file system.
If the current working directory or the current working file system is changed then the
%cwd% environment variable will be updated.
@param[in] FileSystem A pointer to the file system's mapped name. If NULL, then the current working
directory is changed.
@param[in] Dir Points to the NULL-terminated directory on the device specified by FileSystem.
@retval NULL Current directory does not exist.
@return The current directory.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_SET_CUR_DIR) (
IN CONST CHAR16 *FileSystem OPTIONAL,
IN CONST CHAR16 *Dir
);
/**
Sets the environment variable.
This function changes the current value of the specified environment variable. If the
environment variable exists and the Value is an empty string, then the environment
variable is deleted. If the environment variable exists and the Value is not an empty
string, then the value of the environment variable is changed. If the environment
variable does not exist and the Value is an empty string, there is no action. If the
environment variable does not exist and the Value is a non-empty string, then the
environment variable is created and assigned the specified value.
For a description of volatile and non-volatile environment variables, see UEFI Shell
2.0 specification section 3.6.1.
@param[in] Name Points to the NULL-terminated environment variable name.
@param[in] Value Points to the NULL-terminated environment variable value. If the value is an
empty string then the environment variable is deleted.
@param[in] Volatile Indicates whether the variable is non-volatile (FALSE) or volatile (TRUE).
@retval EFI_SUCCESS The environment variable was successfully updated.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_SET_ENV) (
IN CONST CHAR16 *Name,
IN CONST CHAR16 *Value,
IN BOOLEAN Volatile
);
/**
Sets the file information to an opened file handle.
This function changes file information. All file information in the EFI_FILE_INFO
struct will be updated to the passed in data.
@param[in] FileHandle A file handle.
@param[in] FileInfo Points to new file information.
@retval EFI_SUCCESS The information was set.
@retval EFI_NO_MEDIA The device has no medium.
@retval EFI_DEVICE_ERROR The device reported an error.
@retval EFI_VOLUME_CORRUPTED The file system structures are corrupted.
@retval EFI_WRITE_PROTECTED The file or medium is write-protected.
@retval EFI_ACCESS_DENIED The file was opened read-only.
@retval EFI_VOLUME_FULL The volume is full.
@retval EFI_BAD_BUFFER_SIZE BufferSize is smaller than the size of EFI_FILE_INFO.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_SET_FILE_INFO)(
IN SHELL_FILE_HANDLE FileHandle,
IN CONST EFI_FILE_INFO *FileInfo
);
/**
Sets a file's current position.
This function sets the current file position for the handle to the position supplied. With
the exception of seeking to position 0xFFFFFFFFFFFFFFFF, only absolute positioning is
supported, and seeking past the end of the file is allowed (a subsequent write would
grow the file). Seeking to position 0xFFFFFFFFFFFFFFFF causes the current position
to be set to the end of the file.
@param[in] FileHandle The file handle on which requested position will be set.
@param[in] Position Byte position from the start of the file.
@retval EFI_SUCCESS Data was written.
@retval EFI_UNSUPPORTED The seek request for nonzero is not valid on open directories.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_SET_FILE_POSITION)(
IN SHELL_FILE_HANDLE FileHandle,
IN UINT64 Position
);
/**
This function creates a mapping for a device path.
@param[in] DevicePath Points to the device path. If this is NULL and Mapping points to a valid mapping,
then the mapping will be deleted.
@param[in] Mapping Points to the NULL-terminated mapping for the device path.
@retval EFI_SUCCESS Mapping created or deleted successfully.
@retval EFI_NO_MAPPING There is no handle that corresponds exactly to DevicePath. See the
boot service function LocateDevicePath().
@retval EFI_ACCESS_DENIED The mapping is a built-in alias.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_SET_MAP)(
IN CONST EFI_DEVICE_PATH_PROTOCOL *DevicePath,
IN CONST CHAR16 *Mapping
);
/**
Writes data to the file.
This function writes the specified number of bytes to the file at the current file position.
The current file position is advanced the actual number of bytes written, which is
returned in BufferSize. Partial writes only occur when there has been a data error
during the write attempt (such as "volume space full"). The file automatically grows to
hold the data, if required.
Direct writes to opened directories are not supported.
@param[in] FileHandle The opened file handle for writing.
@param[in, out] BufferSize On input, size of Buffer.
@param[in] Buffer The buffer in which data to write.
@retval EFI_SUCCESS Data was written.
@retval EFI_UNSUPPORTED Writes to open directory are not supported.
@retval EFI_NO_MEDIA The device has no media.
@retval EFI_DEVICE_ERROR The device reported an error.
@retval EFI_VOLUME_CORRUPTED The file system structures are corrupted.
@retval EFI_WRITE_PROTECTED The device is write-protected.
@retval EFI_ACCESS_DENIED The file was open for read only.
@retval EFI_VOLUME_FULL The volume is full.
**/
typedef
EFI_STATUS
(EFIAPI *EFI_SHELL_WRITE_FILE)(
IN SHELL_FILE_HANDLE FileHandle,
IN OUT UINTN *BufferSize,
IN VOID *Buffer
);
//
// EFI_SHELL_PROTOCOL has been updated since UEFI Shell Spec 2.0
// Usage of this protocol will require version checking before attempting
// to use any new members. There is no need to check the version for
// members that existed in UEFI Shell Spec 2.0.
//
// Update below for any future UEFI Shell spec changes to this protocol.
//
// Check EFI_SHELL_PROTOCOL MajorVersion and MinorVersion:
// if ((2 == gEfiShellProtocol->MajorVersion) &&
// (0 == gEfiShellProtocol->MinorVersion)) {
// //
// // Cannot call:
// // RegisterGuidName - UEFI Shell 2.1
// // GetGuidName - UEFI Shell 2.1
// // GetGuidFromName - UEFI Shell 2.1
// // GetEnvEx - UEFI Shell 2.1
// //
// } else {
// //
// // Can use all members
// //
// }
//
typedef struct _EFI_SHELL_PROTOCOL {
EFI_SHELL_EXECUTE Execute;
EFI_SHELL_GET_ENV GetEnv;
EFI_SHELL_SET_ENV SetEnv;
EFI_SHELL_GET_ALIAS GetAlias;
EFI_SHELL_SET_ALIAS SetAlias;
EFI_SHELL_GET_HELP_TEXT GetHelpText;
EFI_SHELL_GET_DEVICE_PATH_FROM_MAP GetDevicePathFromMap;
EFI_SHELL_GET_MAP_FROM_DEVICE_PATH GetMapFromDevicePath;
EFI_SHELL_GET_DEVICE_PATH_FROM_FILE_PATH GetDevicePathFromFilePath;
EFI_SHELL_GET_FILE_PATH_FROM_DEVICE_PATH GetFilePathFromDevicePath;
EFI_SHELL_SET_MAP SetMap;
EFI_SHELL_GET_CUR_DIR GetCurDir;
EFI_SHELL_SET_CUR_DIR SetCurDir;
EFI_SHELL_OPEN_FILE_LIST OpenFileList;
EFI_SHELL_FREE_FILE_LIST FreeFileList;
EFI_SHELL_REMOVE_DUP_IN_FILE_LIST RemoveDupInFileList;
EFI_SHELL_BATCH_IS_ACTIVE BatchIsActive;
EFI_SHELL_IS_ROOT_SHELL IsRootShell;
EFI_SHELL_ENABLE_PAGE_BREAK EnablePageBreak;
EFI_SHELL_DISABLE_PAGE_BREAK DisablePageBreak;
EFI_SHELL_GET_PAGE_BREAK GetPageBreak;
EFI_SHELL_GET_DEVICE_NAME GetDeviceName;
EFI_SHELL_GET_FILE_INFO GetFileInfo;
EFI_SHELL_SET_FILE_INFO SetFileInfo;
EFI_SHELL_OPEN_FILE_BY_NAME OpenFileByName;
EFI_SHELL_CLOSE_FILE CloseFile;
EFI_SHELL_CREATE_FILE CreateFile;
EFI_SHELL_READ_FILE ReadFile;
EFI_SHELL_WRITE_FILE WriteFile;
EFI_SHELL_DELETE_FILE DeleteFile;
EFI_SHELL_DELETE_FILE_BY_NAME DeleteFileByName;
EFI_SHELL_GET_FILE_POSITION GetFilePosition;
EFI_SHELL_SET_FILE_POSITION SetFilePosition;
EFI_SHELL_FLUSH_FILE FlushFile;
EFI_SHELL_FIND_FILES FindFiles;
EFI_SHELL_FIND_FILES_IN_DIR FindFilesInDir;
EFI_SHELL_GET_FILE_SIZE GetFileSize;
EFI_SHELL_OPEN_ROOT OpenRoot;
EFI_SHELL_OPEN_ROOT_BY_HANDLE OpenRootByHandle;
EFI_EVENT ExecutionBreak;
UINT32 MajorVersion;
UINT32 MinorVersion;
// Added for Shell 2.1
EFI_SHELL_REGISTER_GUID_NAME RegisterGuidName;
EFI_SHELL_GET_GUID_NAME GetGuidName;
EFI_SHELL_GET_GUID_FROM_NAME GetGuidFromName;
EFI_SHELL_GET_ENV_EX GetEnvEx;
} EFI_SHELL_PROTOCOL;
extern EFI_GUID gEfiShellProtocolGuid;
enum ShellVersion {
SHELL_MAJOR_VERSION = 2,
SHELL_MINOR_VERSION = 2
};
#endif
| {
"pile_set_name": "Github"
} |
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using NLog;
using YiSha.Util.Extension;
namespace YiSha.Util
{
public class LogHelper
{
private static readonly Logger log = LogManager.GetLogger(string.Empty);
public static void Trace(object msg, Exception ex = null)
{
if (ex == null)
{
log.Trace(msg.ParseToString());
}
else
{
log.Trace(msg + GetExceptionMessage(ex));
}
}
public static void Debug(object msg, Exception ex = null)
{
if (ex == null)
{
log.Debug(msg.ParseToString());
}
else
{
log.Debug(msg + GetExceptionMessage(ex));
}
}
public static void Info(object msg, Exception ex = null)
{
if (ex == null)
{
log.Info(msg.ParseToString());
}
else
{
log.Info(msg + GetExceptionMessage(ex));
}
}
public static void Warn(object msg, Exception ex = null)
{
if (ex == null)
{
log.Warn(msg.ParseToString());
}
else
{
log.Warn(msg + GetExceptionMessage(ex));
}
}
public static void Error(object msg, Exception ex = null)
{
if (ex == null)
{
log.Error(msg.ParseToString());
}
else
{
log.Error(msg + GetExceptionMessage(ex));
}
}
public static void Error(Exception ex)
{
if (ex != null)
{
log.Error(GetExceptionMessage(ex));
}
}
public static void Fatal(object msg, Exception ex = null)
{
if (ex == null)
{
log.Fatal(msg.ParseToString());
}
else
{
log.Fatal(msg + GetExceptionMessage(ex));
}
}
public static void Fatal(Exception ex)
{
if (ex != null)
{
log.Fatal(GetExceptionMessage(ex));
}
}
private static string GetExceptionMessage(Exception ex)
{
string message = string.Empty;
if (ex != null)
{
message += ex.Message;
message += Environment.NewLine;
Exception originalException = ex.GetOriginalException();
if (originalException != null)
{
if (originalException.Message != ex.Message)
{
message += originalException.Message;
message += Environment.NewLine;
}
}
message += ex.StackTrace;
message += Environment.NewLine;
}
return message;
}
}
}
| {
"pile_set_name": "Github"
} |
{
"$schema": "../../../../testbot.schema",
"$kind": "Microsoft.AdaptiveDialog",
"recognizer": {
"$kind": "Microsoft.RegexRecognizer",
"intents": [
{
"intent": "CreateMeetingIntent",
"pattern": "(?i)create meeting"
},
{
"intent": "HelpIntent",
"pattern": "(?i)help"
},
{
"intent": "ShowNextPageIntent",
"pattern": "(?i)next page meeting"
},
{
"intent": "UpdateMeetingIntent",
"pattern": "(?i)update meeting"
},
{
"intent": "AcceptMeetingIntent",
"pattern": "(?i)accept meeting"
},
{
"intent": "DeclineMeetingIntent",
"pattern": "(?i)decline meeting"
}
]
},
"triggers": [
{
"$kind": "Microsoft.OnBeginDialog",
"actions": [
"GetDisplayMeetings",
{
"$kind": "Microsoft.SendActivity",
"activity": "${ShowMeetingSummaryResponse(user)}"
},
{
"$kind": "Microsoft.IfCondition",
"condition": "count(user.meetings) > 0",
"actions": [
{
"$kind": "Microsoft.SendActivity",
"activity": "${ShowMeetingList(user)}"
},
{
"$kind": "Microsoft.TextInput",
"prompt": "${ChooseReadMeetingList(user)}",
"property": "dialog.choice"
},
{
"$kind": "Microsoft.IfCondition",
"condition": "dialog.choice == '1'",
"actions": [
{
"$kind": "Microsoft.SetProperty",
"property": "user.focusedMeeting",
"value": "=user.meetings[0]"
}
]
},
{
"$kind": "Microsoft.IfCondition",
"condition": "dialog.choice == '2'",
"actions": [
{
"$kind": "Microsoft.SetProperty",
"property": "user.focusedMeeting",
"value": "=user.meetings[1]"
}
]
},
{
"$kind": "Microsoft.IfCondition",
"condition": "dialog.choice == '3'",
"actions": [
{
"$kind": "Microsoft.SetProperty",
"property": "user.focusedMeeting",
"value": "=user.meetings[2]"
}
]
},
{
"$kind": "Microsoft.SendActivity",
"activity": "${ReadFocusedMeeting(user)}"
},
{
"$kind": "Microsoft.SendActivity",
"activity": "${ShowMeetingCard(user.focusedMeeting)}"
},
{
"$kind": "Microsoft.ConfirmInput",
"property": "dialog.confirmed",
"prompt": "You can say 'update meeting' or 'decline meeting' or 'accept meeting' to do action on this meeting.",
"alwaysPrompt": true
}
]
}
]
},
{
"$kind": "Microsoft.OnIntent",
"intent": "UpdateMeetingIntent",
"actions": [
"UpdateMeeting"
]
},
{
"$kind": "Microsoft.OnIntent",
"intent": "AcceptMeetingIntent",
"actions": [
"AcceptMeeting"
]
},
{
"$kind": "Microsoft.OnIntent",
"intent": "DeclineMeetingIntent",
"actions": [
"DeclineMeeting"
]
}
]
} | {
"pile_set_name": "Github"
} |
# OE has been triggered.
# there should be no %pass shunts on either side and an active tunnel
ipsec trafficstatus
ipsec shuntstatus
../../pluto/bin/ipsec-look.sh
| {
"pile_set_name": "Github"
} |
// Emacs style mode select -*- C++ -*-
//-----------------------------------------------------------------------------
//
// Copyright (C) 2013 James Haley et al.
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see http://www.gnu.org/licenses/
//
//--------------------------------------------------------------------------
//
// DESCRIPTION:
// Created by the sound utility written by Dave Taylor.
// Kept as a sample, DOOM2 sounds. Frozen.
//
// haleyjd: erm... ::lights a fire to warm it up::
//
//-----------------------------------------------------------------------------
#ifndef __SOUNDS__
#define __SOUNDS__
#include "doomtype.h"
#include "m_dllist.h"
//
// SoundFX struct.
//
// haleyjd 06/12/08: origin subchannels
typedef enum
{
CHAN_ALL = -1, // to S_StopSound, this means stop everything
CHAN_AUTO,
CHAN_WEAPON,
CHAN_VOICE,
CHAN_ITEM,
CHAN_BODY,
CHAN_SLOT5,
CHAN_SLOT6,
CHAN_SLOT7,
NUMSCHANNELS
} schannel_e;
// haleyjd 03/27/11: sound flags
enum
{
SFXF_PREFIX = 0x00000001, // lumpname should be prefixed with "DS"
SFXF_NOPCSOUND = 0x00000002, // sound won't play under PC speaker emulation
SFXF_EDF = 0x00000004, // sfxinfo was born via EDF
SFXF_SNDINFO = 0x00000008, // sfxinfo was born via SNDINFO lump
SFXF_WAD = 0x00000010 // sfxinfo was born as an implicit wad sound
};
struct sfxinfo_t
{
// Sfx singularity (only one at a time)
enum
{
sg_none,
sg_itemup,
sg_wpnup,
sg_oof,
sg_getpow
};
// haleyjd 09/23/06: pitch variance types
enum
{
pitch_none, // none: always at normal pitch.
pitch_doom, // normal variance for DOOM v1.1
pitch_doomsaw, // variance for DOOM chainsaw
pitch_heretic, // normal variance for Heretic
pitch_hticamb, // variance for Heretic ambient sounds
};
char name[9]; // haleyjd: up to 8-character lump name
char pcslump[9]; // haleyjd: explicitly provided PC speaker effect lump
int singularity; // killough 12/98: implement separate classes of singularity
int priority; // Sfx priority
int pitch; // pitch if a link
int volume; // volume if a link
int pitch_type; // haleyjd 09/23/06: pitch variance type
int skinsound; // sf: skin sound number to use in place
int subchannel; // haleyjd 06/12/08: origin subchannels - default = CHAN_AUTO.
unsigned int flags; // haleyjd 03/27/11: sound effect flags
// haleyjd 07/13/05: sound attenuation properties now customizable
// on a per-sound basis to allow differing behaviors.
int clipping_dist; // distance when sound is clipped entirely
int close_dist; // distance when sound is at maximum volume
// links to other sound definitions
sfxinfo_t *link; // referenced sound if a link
sfxinfo_t *alias; // haleyjd 09/24/06: referenced sound if an alias
sfxinfo_t **randomsounds; // haleyjd 05/12/09: random sounds
int numrandomsounds;
// haleyjd 04/23/08: additional caching data
void *data; // sound data
int length; // lump length
unsigned int alen; // length of converted sound pointed to by data
// this is checked every second to see if sound
// can be thrown out (if 0, then decrement, if -1,
// then throw out, if > 0, then it is in use)
int usefulness;
// haleyjd: EDF mnemonic
char mnemonic[129];
char *lfn; // alison: long file name
char *pcslfn; // alison: long file name for PC speaker sound
// haleyjd 09/03/03: revised for dynamic EDF sounds
DLListItem<sfxinfo_t> numlinks; // haleyjd 04/13/08: numeric hash links
sfxinfo_t *next; // next in mnemonic hash chain
int dehackednum; // dehacked number
};
//
// MusicInfo struct.
//
struct musicinfo_t
{
// up to 6-character name
const char *name;
// haleyjd 04/10/11: whether to apply prefix or not
bool prefix;
// lump number of music
// int lumpnum;
// music data
void *data;
// music handle once registered
int handle;
// sf: for hashing
musicinfo_t *next;
};
// the complete set of sound effects
// haleyjd 11/05/03: made dynamic with EDF
extern int NUMSFX;
// the complete set of music
extern musicinfo_t S_music[];
// heretic music
extern musicinfo_t H_music[];
// haleyjd: clever indirection for heretic maps
extern int H_Mus_Matrix[6][9];
//
// Identifiers for all music in game.
//
typedef enum {
mus_None,
mus_e1m1,
mus_e1m2,
mus_e1m3,
mus_e1m4,
mus_e1m5,
mus_e1m6,
mus_e1m7,
mus_e1m8,
mus_e1m9,
mus_e2m1,
mus_e2m2,
mus_e2m3,
mus_e2m4,
mus_e2m5,
mus_e2m6,
mus_e2m7,
mus_e2m8,
mus_e2m9,
mus_e3m1,
mus_e3m2,
mus_e3m3,
mus_e3m4,
mus_e3m5,
mus_e3m6,
mus_e3m7,
mus_e3m8,
mus_e3m9,
mus_inter,
mus_intro,
mus_bunny,
mus_victor,
mus_introa,
mus_runnin,
mus_stalks,
mus_countd,
mus_betwee,
mus_doom,
mus_the_da,
mus_shawn,
mus_ddtblu,
mus_in_cit,
mus_dead,
mus_stlks2,
mus_theda2,
mus_doom2,
mus_ddtbl2,
mus_runni2,
mus_dead2,
mus_stlks3,
mus_romero,
mus_shawn2,
mus_messag,
mus_count2,
mus_ddtbl3,
mus_ampie,
mus_theda3,
mus_adrian,
mus_messg2,
mus_romer2,
mus_tense,
mus_shawn3,
mus_openin,
mus_evil,
mus_ultima,
mus_read_m,
mus_dm2ttl,
mus_dm2int,
NUMMUSIC
} doom_musicenum_t;
// haleyjd: heretic music
typedef enum
{
hmus_None,
hmus_e1m1,
hmus_e1m2,
hmus_e1m3,
hmus_e1m4,
hmus_e1m5,
hmus_e1m6,
hmus_e1m7,
hmus_e1m8,
hmus_e1m9,
hmus_e2m1,
hmus_e2m2,
hmus_e2m3,
hmus_e2m4,
hmus_e2m6,
hmus_e2m7,
hmus_e2m8,
hmus_e2m9,
hmus_e3m2,
hmus_e3m3,
hmus_titl,
hmus_intr,
hmus_cptd,
NUMHTICMUSIC
} htic_musicenum_t;
//
// Identifiers for all sfx in game.
//
typedef enum {
sfx_None,
sfx_pistol,
sfx_shotgn,
sfx_sgcock,
sfx_dshtgn,
sfx_dbopn,
sfx_dbcls,
sfx_dbload,
sfx_plasma,
sfx_bfg,
sfx_sawup,
sfx_sawidl,
sfx_sawful,
sfx_sawhit,
sfx_rlaunc,
sfx_rxplod,
sfx_firsht,
sfx_firxpl,
sfx_pstart,
sfx_pstop,
sfx_doropn,
sfx_dorcls,
sfx_stnmov,
sfx_swtchn,
sfx_swtchx,
sfx_plpain,
sfx_dmpain,
sfx_popain,
sfx_vipain,
sfx_mnpain,
sfx_pepain,
sfx_slop,
sfx_itemup,
sfx_wpnup,
sfx_oof,
sfx_telept,
sfx_posit1,
sfx_posit2,
sfx_posit3,
sfx_bgsit1,
sfx_bgsit2,
sfx_sgtsit,
sfx_cacsit,
sfx_brssit,
sfx_cybsit,
sfx_spisit,
sfx_bspsit,
sfx_kntsit,
sfx_vilsit,
sfx_mansit,
sfx_pesit,
sfx_sklatk,
sfx_sgtatk,
sfx_skepch,
sfx_vilatk,
sfx_claw,
sfx_skeswg,
sfx_pldeth,
sfx_pdiehi,
sfx_podth1,
sfx_podth2,
sfx_podth3,
sfx_bgdth1,
sfx_bgdth2,
sfx_sgtdth,
sfx_cacdth,
sfx_skldth,
sfx_brsdth,
sfx_cybdth,
sfx_spidth,
sfx_bspdth,
sfx_vildth,
sfx_kntdth,
sfx_pedth,
sfx_skedth,
sfx_posact,
sfx_bgact,
sfx_dmact,
sfx_bspact,
sfx_bspwlk,
sfx_vilact,
sfx_noway,
sfx_barexp,
sfx_punch,
sfx_hoof,
sfx_metal,
sfx_chgun,
sfx_tink,
sfx_bdopn,
sfx_bdcls,
sfx_itmbk,
sfx_flame,
sfx_flamst,
sfx_getpow,
sfx_bospit,
sfx_boscub,
sfx_bossit,
sfx_bospn,
sfx_bosdth,
sfx_manatk,
sfx_mandth,
sfx_sssit,
sfx_ssdth,
sfx_keenpn,
sfx_keendt,
sfx_skeact,
sfx_skesit,
sfx_skeatk,
sfx_radio,
// killough 11/98: dog sounds
sfx_dgsit,
sfx_dgatk,
sfx_dgact,
sfx_dgdth,
sfx_dgpain,
// haleyjd: Eternity Engine sounds
sfx_eefly, // buzzing flies
sfx_gloop, // water terrain sound
sfx_thundr, // global lightning sound effect
sfx_muck, // swamp terrain sound
sfx_plfeet, // player feet hit
sfx_plfall, // player falling scream
sfx_fallht, // player fall hit
sfx_burn, // lava terrain sound
sfx_eehtsz, // heat sizzle
sfx_eedrip, // drip
sfx_quake2, // earthquake
sfx_plwdth, // player wimpy death
sfx_jump,
// haleyjd 10/08/02: heretic sounds
sfx_gldhit = 300,
sfx_htelept,
sfx_chat,
sfx_keyup,
sfx_hitemup,
sfx_mumsit,
sfx_mumat1,
sfx_mumat2,
sfx_mumpai,
sfx_mumdth,
sfx_mumhed,
sfx_clksit,
sfx_clkatk,
sfx_clkpai,
sfx_clkdth,
sfx_clkact,
sfx_wizsit,
sfx_wizatk,
sfx_wizdth,
sfx_wizact,
sfx_wizpai,
sfx_sorzap,
sfx_sorrise,
sfx_sorsit,
sfx_soratk,
sfx_sorpai,
sfx_soract,
sfx_sordsph,
sfx_sordexp,
sfx_sordbon,
sfx_wind,
sfx_waterfl,
sfx_podexp,
sfx_newpod,
sfx_kgtsit,
sfx_kgtatk,
sfx_kgtat2,
sfx_kgtdth,
sfx_kgtpai,
sfx_hrnhit,
sfx_bstsit,
sfx_bstatk,
sfx_bstpai,
sfx_bstdth,
sfx_bstact,
sfx_snksit,
sfx_snkatk,
sfx_snkpai,
sfx_snkdth,
sfx_snkact,
sfx_hdoropn,
sfx_hdorcls,
sfx_hswitch,
sfx_hpstart,
sfx_hpstop,
sfx_hstnmov,
sfx_sbtpai,
sfx_sbtdth,
sfx_sbtact,
sfx_lobhit,
sfx_minsit,
sfx_minat1,
sfx_minat2,
sfx_minpai,
sfx_mindth,
sfx_minact,
sfx_stfpow,
sfx_phohit,
sfx_hedsit,
sfx_hedat1,
sfx_hedat2,
sfx_hedat3,
sfx_heddth,
sfx_hedact,
sfx_hedpai,
sfx_impsit,
sfx_impat1,
sfx_impat2,
sfx_imppai,
sfx_impdth,
sfx_htamb1,
sfx_htamb2,
sfx_htamb3,
sfx_htamb4,
sfx_htamb5,
sfx_htamb6,
sfx_htamb7,
sfx_htamb8,
sfx_htamb9,
sfx_htamb10,
sfx_htamb11,
sfx_htdormov,
sfx_hplroof,
sfx_hplrpai,
sfx_hplrdth,
sfx_hgibdth,
sfx_hplrwdth,
sfx_hplrcdth,
sfx_hstfhit,
sfx_hchicatk,
sfx_hhrnsht,
sfx_hphosht,
sfx_hwpnup,
sfx_hrespawn,
sfx_artiup,
sfx_artiuse,
sfx_blssht,
sfx_bowsht,
sfx_gntful,
sfx_gnthit,
sfx_gntpow,
sfx_gntact,
sfx_gntuse,
sfx_stfcrk,
sfx_lobsht,
sfx_bounce,
sfx_phopow,
sfx_blshit,
sfx_hnoway,
// haleyjd 11/05/03: NUMSFX is a variable now
// NUMSFX
} sfxenum_t;
#endif
//----------------------------------------------------------------------------
//
// $Log: sounds.h,v $
// Revision 1.3 1998/05/03 22:44:30 killough
// beautification
//
// Revision 1.2 1998/01/26 19:27:53 phares
// First rev with no ^Ms
//
// Revision 1.1.1.1 1998/01/19 14:03:03 rand
// Lee's Jan 19 sources
//
//----------------------------------------------------------------------------
| {
"pile_set_name": "Github"
} |
{
"title": "Predefined Symbols (Marker)",
"callback": "initMap",
"libraries": [],
"version": "weekly",
"tag": "marker_symbol_predefined",
"name": "marker-symbol-predefined"
}
| {
"pile_set_name": "Github"
} |
/*
drbd_req.c
This file is part of DRBD by Philipp Reisner and Lars Ellenberg.
Copyright (C) 2001-2008, LINBIT Information Technologies GmbH.
Copyright (C) 1999-2008, Philipp Reisner <[email protected]>.
Copyright (C) 2002-2008, Lars Ellenberg <[email protected]>.
drbd is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; either version 2, or (at your option)
any later version.
drbd is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with drbd; see the file COPYING. If not, write to
the Free Software Foundation, 675 Mass Ave, Cambridge, MA 02139, USA.
*/
#include <linux/module.h>
#include <linux/slab.h>
#include <linux/drbd.h>
#include "drbd_int.h"
#include "drbd_req.h"
/* Update disk stats at start of I/O request */
static void _drbd_start_io_acct(struct drbd_conf *mdev, struct drbd_request *req, struct bio *bio)
{
const int rw = bio_data_dir(bio);
int cpu;
cpu = part_stat_lock();
part_stat_inc(cpu, &mdev->vdisk->part0, ios[rw]);
part_stat_add(cpu, &mdev->vdisk->part0, sectors[rw], bio_sectors(bio));
part_inc_in_flight(&mdev->vdisk->part0, rw);
part_stat_unlock();
}
/* Update disk stats when completing request upwards */
static void _drbd_end_io_acct(struct drbd_conf *mdev, struct drbd_request *req)
{
int rw = bio_data_dir(req->master_bio);
unsigned long duration = jiffies - req->start_time;
int cpu;
cpu = part_stat_lock();
part_stat_add(cpu, &mdev->vdisk->part0, ticks[rw], duration);
part_round_stats(cpu, &mdev->vdisk->part0);
part_dec_in_flight(&mdev->vdisk->part0, rw);
part_stat_unlock();
}
static void _req_is_done(struct drbd_conf *mdev, struct drbd_request *req, const int rw)
{
const unsigned long s = req->rq_state;
/* if it was a write, we may have to set the corresponding
* bit(s) out-of-sync first. If it had a local part, we need to
* release the reference to the activity log. */
if (rw == WRITE) {
/* remove it from the transfer log.
* well, only if it had been there in the first
* place... if it had not (local only or conflicting
* and never sent), it should still be "empty" as
* initialized in drbd_req_new(), so we can list_del() it
* here unconditionally */
list_del(&req->tl_requests);
/* Set out-of-sync unless both OK flags are set
* (local only or remote failed).
* Other places where we set out-of-sync:
* READ with local io-error */
if (!(s & RQ_NET_OK) || !(s & RQ_LOCAL_OK))
drbd_set_out_of_sync(mdev, req->sector, req->size);
if ((s & RQ_NET_OK) && (s & RQ_LOCAL_OK) && (s & RQ_NET_SIS))
drbd_set_in_sync(mdev, req->sector, req->size);
/* one might be tempted to move the drbd_al_complete_io
* to the local io completion callback drbd_endio_pri.
* but, if this was a mirror write, we may only
* drbd_al_complete_io after this is RQ_NET_DONE,
* otherwise the extent could be dropped from the al
* before it has actually been written on the peer.
* if we crash before our peer knows about the request,
* but after the extent has been dropped from the al,
* we would forget to resync the corresponding extent.
*/
if (s & RQ_LOCAL_MASK) {
if (get_ldev_if_state(mdev, D_FAILED)) {
drbd_al_complete_io(mdev, req->sector);
put_ldev(mdev);
} else if (__ratelimit(&drbd_ratelimit_state)) {
dev_warn(DEV, "Should have called drbd_al_complete_io(, %llu), "
"but my Disk seems to have failed :(\n",
(unsigned long long) req->sector);
}
}
}
/* if it was a local io error, we want to notify our
* peer about that, and see if we need to
* detach the disk and stuff.
* to avoid allocating some special work
* struct, reuse the request. */
/* THINK
* why do we do this not when we detect the error,
* but delay it until it is "done", i.e. possibly
* until the next barrier ack? */
if (rw == WRITE &&
((s & RQ_LOCAL_MASK) && !(s & RQ_LOCAL_OK))) {
if (!(req->w.list.next == LIST_POISON1 ||
list_empty(&req->w.list))) {
/* DEBUG ASSERT only; if this triggers, we
* probably corrupt the worker list here */
dev_err(DEV, "req->w.list.next = %p\n", req->w.list.next);
dev_err(DEV, "req->w.list.prev = %p\n", req->w.list.prev);
}
req->w.cb = w_io_error;
drbd_queue_work(&mdev->data.work, &req->w);
/* drbd_req_free() is done in w_io_error */
} else {
drbd_req_free(req);
}
}
static void queue_barrier(struct drbd_conf *mdev)
{
struct drbd_tl_epoch *b;
/* We are within the req_lock. Once we queued the barrier for sending,
* we set the CREATE_BARRIER bit. It is cleared as soon as a new
* barrier/epoch object is added. This is the only place this bit is
* set. It indicates that the barrier for this epoch is already queued,
* and no new epoch has been created yet. */
if (test_bit(CREATE_BARRIER, &mdev->flags))
return;
b = mdev->newest_tle;
b->w.cb = w_send_barrier;
/* inc_ap_pending done here, so we won't
* get imbalanced on connection loss.
* dec_ap_pending will be done in got_BarrierAck
* or (on connection loss) in tl_clear. */
inc_ap_pending(mdev);
drbd_queue_work(&mdev->data.work, &b->w);
set_bit(CREATE_BARRIER, &mdev->flags);
}
static void _about_to_complete_local_write(struct drbd_conf *mdev,
struct drbd_request *req)
{
const unsigned long s = req->rq_state;
struct drbd_request *i;
struct drbd_epoch_entry *e;
struct hlist_node *n;
struct hlist_head *slot;
/* before we can signal completion to the upper layers,
* we may need to close the current epoch */
if (mdev->state.conn >= C_CONNECTED &&
req->epoch == mdev->newest_tle->br_number)
queue_barrier(mdev);
/* we need to do the conflict detection stuff,
* if we have the ee_hash (two_primaries) and
* this has been on the network */
if ((s & RQ_NET_DONE) && mdev->ee_hash != NULL) {
const sector_t sector = req->sector;
const int size = req->size;
/* ASSERT:
* there must be no conflicting requests, since
* they must have been failed on the spot */
#define OVERLAPS overlaps(sector, size, i->sector, i->size)
slot = tl_hash_slot(mdev, sector);
hlist_for_each_entry(i, n, slot, colision) {
if (OVERLAPS) {
dev_alert(DEV, "LOGIC BUG: completed: %p %llus +%u; "
"other: %p %llus +%u\n",
req, (unsigned long long)sector, size,
i, (unsigned long long)i->sector, i->size);
}
}
/* maybe "wake" those conflicting epoch entries
* that wait for this request to finish.
*
* currently, there can be only _one_ such ee
* (well, or some more, which would be pending
* P_DISCARD_ACK not yet sent by the asender...),
* since we block the receiver thread upon the
* first conflict detection, which will wait on
* misc_wait. maybe we want to assert that?
*
* anyways, if we found one,
* we just have to do a wake_up. */
#undef OVERLAPS
#define OVERLAPS overlaps(sector, size, e->sector, e->size)
slot = ee_hash_slot(mdev, req->sector);
hlist_for_each_entry(e, n, slot, colision) {
if (OVERLAPS) {
wake_up(&mdev->misc_wait);
break;
}
}
}
#undef OVERLAPS
}
void complete_master_bio(struct drbd_conf *mdev,
struct bio_and_error *m)
{
bio_endio(m->bio, m->error);
dec_ap_bio(mdev);
}
/* Helper for __req_mod().
* Set m->bio to the master bio, if it is fit to be completed,
* or leave it alone (it is initialized to NULL in __req_mod),
* if it has already been completed, or cannot be completed yet.
* If m->bio is set, the error status to be returned is placed in m->error.
*/
void _req_may_be_done(struct drbd_request *req, struct bio_and_error *m)
{
const unsigned long s = req->rq_state;
struct drbd_conf *mdev = req->mdev;
/* only WRITES may end up here without a master bio (on barrier ack) */
int rw = req->master_bio ? bio_data_dir(req->master_bio) : WRITE;
/* we must not complete the master bio, while it is
* still being processed by _drbd_send_zc_bio (drbd_send_dblock)
* not yet acknowledged by the peer
* not yet completed by the local io subsystem
* these flags may get cleared in any order by
* the worker,
* the receiver,
* the bio_endio completion callbacks.
*/
if (s & RQ_NET_QUEUED)
return;
if (s & RQ_NET_PENDING)
return;
if (s & RQ_LOCAL_PENDING)
return;
if (req->master_bio) {
/* this is data_received (remote read)
* or protocol C P_WRITE_ACK
* or protocol B P_RECV_ACK
* or protocol A "handed_over_to_network" (SendAck)
* or canceled or failed,
* or killed from the transfer log due to connection loss.
*/
/*
* figure out whether to report success or failure.
*
* report success when at least one of the operations succeeded.
* or, to put the other way,
* only report failure, when both operations failed.
*
* what to do about the failures is handled elsewhere.
* what we need to do here is just: complete the master_bio.
*
* local completion error, if any, has been stored as ERR_PTR
* in private_bio within drbd_endio_pri.
*/
int ok = (s & RQ_LOCAL_OK) || (s & RQ_NET_OK);
int error = PTR_ERR(req->private_bio);
/* remove the request from the conflict detection
* respective block_id verification hash */
if (!hlist_unhashed(&req->colision))
hlist_del(&req->colision);
else
D_ASSERT((s & RQ_NET_MASK) == 0);
/* for writes we need to do some extra housekeeping */
if (rw == WRITE)
_about_to_complete_local_write(mdev, req);
/* Update disk stats */
_drbd_end_io_acct(mdev, req);
m->error = ok ? 0 : (error ?: -EIO);
m->bio = req->master_bio;
req->master_bio = NULL;
}
if ((s & RQ_NET_MASK) == 0 || (s & RQ_NET_DONE)) {
/* this is disconnected (local only) operation,
* or protocol C P_WRITE_ACK,
* or protocol A or B P_BARRIER_ACK,
* or killed from the transfer log due to connection loss. */
_req_is_done(mdev, req, rw);
}
/* else: network part and not DONE yet. that is
* protocol A or B, barrier ack still pending... */
}
/*
* checks whether there was an overlapping request
* or ee already registered.
*
* if so, return 1, in which case this request is completed on the spot,
* without ever being submitted or send.
*
* return 0 if it is ok to submit this request.
*
* NOTE:
* paranoia: assume something above us is broken, and issues different write
* requests for the same block simultaneously...
*
* To ensure these won't be reordered differently on both nodes, resulting in
* diverging data sets, we discard the later one(s). Not that this is supposed
* to happen, but this is the rationale why we also have to check for
* conflicting requests with local origin, and why we have to do so regardless
* of whether we allowed multiple primaries.
*
* BTW, in case we only have one primary, the ee_hash is empty anyways, and the
* second hlist_for_each_entry becomes a noop. This is even simpler than to
* grab a reference on the net_conf, and check for the two_primaries flag...
*/
static int _req_conflicts(struct drbd_request *req)
{
struct drbd_conf *mdev = req->mdev;
const sector_t sector = req->sector;
const int size = req->size;
struct drbd_request *i;
struct drbd_epoch_entry *e;
struct hlist_node *n;
struct hlist_head *slot;
D_ASSERT(hlist_unhashed(&req->colision));
if (!get_net_conf(mdev))
return 0;
/* BUG_ON */
ERR_IF (mdev->tl_hash_s == 0)
goto out_no_conflict;
BUG_ON(mdev->tl_hash == NULL);
#define OVERLAPS overlaps(i->sector, i->size, sector, size)
slot = tl_hash_slot(mdev, sector);
hlist_for_each_entry(i, n, slot, colision) {
if (OVERLAPS) {
dev_alert(DEV, "%s[%u] Concurrent local write detected! "
"[DISCARD L] new: %llus +%u; "
"pending: %llus +%u\n",
current->comm, current->pid,
(unsigned long long)sector, size,
(unsigned long long)i->sector, i->size);
goto out_conflict;
}
}
if (mdev->ee_hash_s) {
/* now, check for overlapping requests with remote origin */
BUG_ON(mdev->ee_hash == NULL);
#undef OVERLAPS
#define OVERLAPS overlaps(e->sector, e->size, sector, size)
slot = ee_hash_slot(mdev, sector);
hlist_for_each_entry(e, n, slot, colision) {
if (OVERLAPS) {
dev_alert(DEV, "%s[%u] Concurrent remote write detected!"
" [DISCARD L] new: %llus +%u; "
"pending: %llus +%u\n",
current->comm, current->pid,
(unsigned long long)sector, size,
(unsigned long long)e->sector, e->size);
goto out_conflict;
}
}
}
#undef OVERLAPS
out_no_conflict:
/* this is like it should be, and what we expected.
* our users do behave after all... */
put_net_conf(mdev);
return 0;
out_conflict:
put_net_conf(mdev);
return 1;
}
/* obviously this could be coded as many single functions
* instead of one huge switch,
* or by putting the code directly in the respective locations
* (as it has been before).
*
* but having it this way
* enforces that it is all in this one place, where it is easier to audit,
* it makes it obvious that whatever "event" "happens" to a request should
* happen "atomically" within the req_lock,
* and it enforces that we have to think in a very structured manner
* about the "events" that may happen to a request during its life time ...
*/
void __req_mod(struct drbd_request *req, enum drbd_req_event what,
struct bio_and_error *m)
{
struct drbd_conf *mdev = req->mdev;
m->bio = NULL;
switch (what) {
default:
dev_err(DEV, "LOGIC BUG in %s:%u\n", __FILE__ , __LINE__);
break;
/* does not happen...
* initialization done in drbd_req_new
case created:
break;
*/
case to_be_send: /* via network */
/* reached via drbd_make_request_common
* and from w_read_retry_remote */
D_ASSERT(!(req->rq_state & RQ_NET_MASK));
req->rq_state |= RQ_NET_PENDING;
inc_ap_pending(mdev);
break;
case to_be_submitted: /* locally */
/* reached via drbd_make_request_common */
D_ASSERT(!(req->rq_state & RQ_LOCAL_MASK));
req->rq_state |= RQ_LOCAL_PENDING;
break;
case completed_ok:
if (bio_data_dir(req->master_bio) == WRITE)
mdev->writ_cnt += req->size>>9;
else
mdev->read_cnt += req->size>>9;
req->rq_state |= (RQ_LOCAL_COMPLETED|RQ_LOCAL_OK);
req->rq_state &= ~RQ_LOCAL_PENDING;
_req_may_be_done(req, m);
put_ldev(mdev);
break;
case write_completed_with_error:
req->rq_state |= RQ_LOCAL_COMPLETED;
req->rq_state &= ~RQ_LOCAL_PENDING;
dev_alert(DEV, "Local WRITE failed sec=%llus size=%u\n",
(unsigned long long)req->sector, req->size);
/* and now: check how to handle local io error. */
__drbd_chk_io_error(mdev, FALSE);
_req_may_be_done(req, m);
put_ldev(mdev);
break;
case read_ahead_completed_with_error:
/* it is legal to fail READA */
req->rq_state |= RQ_LOCAL_COMPLETED;
req->rq_state &= ~RQ_LOCAL_PENDING;
_req_may_be_done(req, m);
put_ldev(mdev);
break;
case read_completed_with_error:
drbd_set_out_of_sync(mdev, req->sector, req->size);
req->rq_state |= RQ_LOCAL_COMPLETED;
req->rq_state &= ~RQ_LOCAL_PENDING;
dev_alert(DEV, "Local READ failed sec=%llus size=%u\n",
(unsigned long long)req->sector, req->size);
/* _req_mod(req,to_be_send); oops, recursion... */
D_ASSERT(!(req->rq_state & RQ_NET_MASK));
req->rq_state |= RQ_NET_PENDING;
inc_ap_pending(mdev);
__drbd_chk_io_error(mdev, FALSE);
put_ldev(mdev);
/* NOTE: if we have no connection,
* or know the peer has no good data either,
* then we don't actually need to "queue_for_net_read",
* but we do so anyways, since the drbd_io_error()
* and the potential state change to "Diskless"
* needs to be done from process context */
/* fall through: _req_mod(req,queue_for_net_read); */
case queue_for_net_read:
/* READ or READA, and
* no local disk,
* or target area marked as invalid,
* or just got an io-error. */
/* from drbd_make_request_common
* or from bio_endio during read io-error recovery */
/* so we can verify the handle in the answer packet
* corresponding hlist_del is in _req_may_be_done() */
hlist_add_head(&req->colision, ar_hash_slot(mdev, req->sector));
set_bit(UNPLUG_REMOTE, &mdev->flags);
D_ASSERT(req->rq_state & RQ_NET_PENDING);
req->rq_state |= RQ_NET_QUEUED;
req->w.cb = (req->rq_state & RQ_LOCAL_MASK)
? w_read_retry_remote
: w_send_read_req;
drbd_queue_work(&mdev->data.work, &req->w);
break;
case queue_for_net_write:
/* assert something? */
/* from drbd_make_request_common only */
hlist_add_head(&req->colision, tl_hash_slot(mdev, req->sector));
/* corresponding hlist_del is in _req_may_be_done() */
/* NOTE
* In case the req ended up on the transfer log before being
* queued on the worker, it could lead to this request being
* missed during cleanup after connection loss.
* So we have to do both operations here,
* within the same lock that protects the transfer log.
*
* _req_add_to_epoch(req); this has to be after the
* _maybe_start_new_epoch(req); which happened in
* drbd_make_request_common, because we now may set the bit
* again ourselves to close the current epoch.
*
* Add req to the (now) current epoch (barrier). */
/* otherwise we may lose an unplug, which may cause some remote
* io-scheduler timeout to expire, increasing maximum latency,
* hurting performance. */
set_bit(UNPLUG_REMOTE, &mdev->flags);
/* see drbd_make_request_common,
* just after it grabs the req_lock */
D_ASSERT(test_bit(CREATE_BARRIER, &mdev->flags) == 0);
req->epoch = mdev->newest_tle->br_number;
list_add_tail(&req->tl_requests,
&mdev->newest_tle->requests);
/* increment size of current epoch */
mdev->newest_tle->n_req++;
/* queue work item to send data */
D_ASSERT(req->rq_state & RQ_NET_PENDING);
req->rq_state |= RQ_NET_QUEUED;
req->w.cb = w_send_dblock;
drbd_queue_work(&mdev->data.work, &req->w);
/* close the epoch, in case it outgrew the limit */
if (mdev->newest_tle->n_req >= mdev->net_conf->max_epoch_size)
queue_barrier(mdev);
break;
case send_canceled:
/* treat it the same */
case send_failed:
/* real cleanup will be done from tl_clear. just update flags
* so it is no longer marked as on the worker queue */
req->rq_state &= ~RQ_NET_QUEUED;
/* if we did it right, tl_clear should be scheduled only after
* this, so this should not be necessary! */
_req_may_be_done(req, m);
break;
case handed_over_to_network:
/* assert something? */
if (bio_data_dir(req->master_bio) == WRITE &&
mdev->net_conf->wire_protocol == DRBD_PROT_A) {
/* this is what is dangerous about protocol A:
* pretend it was successfully written on the peer. */
if (req->rq_state & RQ_NET_PENDING) {
dec_ap_pending(mdev);
req->rq_state &= ~RQ_NET_PENDING;
req->rq_state |= RQ_NET_OK;
} /* else: neg-ack was faster... */
/* it is still not yet RQ_NET_DONE until the
* corresponding epoch barrier got acked as well,
* so we know what to dirty on connection loss */
}
req->rq_state &= ~RQ_NET_QUEUED;
req->rq_state |= RQ_NET_SENT;
/* because _drbd_send_zc_bio could sleep, and may want to
* dereference the bio even after the "write_acked_by_peer" and
* "completed_ok" events came in, once we return from
* _drbd_send_zc_bio (drbd_send_dblock), we have to check
* whether it is done already, and end it. */
_req_may_be_done(req, m);
break;
case connection_lost_while_pending:
/* transfer log cleanup after connection loss */
/* assert something? */
if (req->rq_state & RQ_NET_PENDING)
dec_ap_pending(mdev);
req->rq_state &= ~(RQ_NET_OK|RQ_NET_PENDING);
req->rq_state |= RQ_NET_DONE;
/* if it is still queued, we may not complete it here.
* it will be canceled soon. */
if (!(req->rq_state & RQ_NET_QUEUED))
_req_may_be_done(req, m);
break;
case write_acked_by_peer_and_sis:
req->rq_state |= RQ_NET_SIS;
case conflict_discarded_by_peer:
/* for discarded conflicting writes of multiple primaries,
* there is no need to keep anything in the tl, potential
* node crashes are covered by the activity log. */
if (what == conflict_discarded_by_peer)
dev_alert(DEV, "Got DiscardAck packet %llus +%u!"
" DRBD is not a random data generator!\n",
(unsigned long long)req->sector, req->size);
req->rq_state |= RQ_NET_DONE;
/* fall through */
case write_acked_by_peer:
/* protocol C; successfully written on peer.
* Nothing to do here.
* We want to keep the tl in place for all protocols, to cater
* for volatile write-back caches on lower level devices.
*
* A barrier request is expected to have forced all prior
* requests onto stable storage, so completion of a barrier
* request could set NET_DONE right here, and not wait for the
* P_BARRIER_ACK, but that is an unnecessary optimization. */
/* this makes it effectively the same as for: */
case recv_acked_by_peer:
/* protocol B; pretends to be successfully written on peer.
* see also notes above in handed_over_to_network about
* protocol != C */
req->rq_state |= RQ_NET_OK;
D_ASSERT(req->rq_state & RQ_NET_PENDING);
dec_ap_pending(mdev);
req->rq_state &= ~RQ_NET_PENDING;
_req_may_be_done(req, m);
break;
case neg_acked:
/* assert something? */
if (req->rq_state & RQ_NET_PENDING)
dec_ap_pending(mdev);
req->rq_state &= ~(RQ_NET_OK|RQ_NET_PENDING);
req->rq_state |= RQ_NET_DONE;
_req_may_be_done(req, m);
/* else: done by handed_over_to_network */
break;
case barrier_acked:
if (req->rq_state & RQ_NET_PENDING) {
/* barrier came in before all requests have been acked.
* this is bad, because if the connection is lost now,
* we won't be able to clean them up... */
dev_err(DEV, "FIXME (barrier_acked but pending)\n");
list_move(&req->tl_requests, &mdev->out_of_sequence_requests);
}
D_ASSERT(req->rq_state & RQ_NET_SENT);
req->rq_state |= RQ_NET_DONE;
_req_may_be_done(req, m);
break;
case data_received:
D_ASSERT(req->rq_state & RQ_NET_PENDING);
dec_ap_pending(mdev);
req->rq_state &= ~RQ_NET_PENDING;
req->rq_state |= (RQ_NET_OK|RQ_NET_DONE);
_req_may_be_done(req, m);
break;
};
}
/* we may do a local read if:
* - we are consistent (of course),
* - or we are generally inconsistent,
* BUT we are still/already IN SYNC for this area.
* since size may be bigger than BM_BLOCK_SIZE,
* we may need to check several bits.
*/
static int drbd_may_do_local_read(struct drbd_conf *mdev, sector_t sector, int size)
{
unsigned long sbnr, ebnr;
sector_t esector, nr_sectors;
if (mdev->state.disk == D_UP_TO_DATE)
return 1;
if (mdev->state.disk >= D_OUTDATED)
return 0;
if (mdev->state.disk < D_INCONSISTENT)
return 0;
/* state.disk == D_INCONSISTENT We will have a look at the BitMap */
nr_sectors = drbd_get_capacity(mdev->this_bdev);
esector = sector + (size >> 9) - 1;
D_ASSERT(sector < nr_sectors);
D_ASSERT(esector < nr_sectors);
sbnr = BM_SECT_TO_BIT(sector);
ebnr = BM_SECT_TO_BIT(esector);
return 0 == drbd_bm_count_bits(mdev, sbnr, ebnr);
}
static int drbd_make_request_common(struct drbd_conf *mdev, struct bio *bio)
{
const int rw = bio_rw(bio);
const int size = bio->bi_size;
const sector_t sector = bio->bi_sector;
struct drbd_tl_epoch *b = NULL;
struct drbd_request *req;
int local, remote;
int err = -EIO;
/* allocate outside of all locks; */
req = drbd_req_new(mdev, bio);
if (!req) {
dec_ap_bio(mdev);
/* only pass the error to the upper layers.
* if user cannot handle io errors, that's not our business. */
dev_err(DEV, "could not kmalloc() req\n");
bio_endio(bio, -ENOMEM);
return 0;
}
local = get_ldev(mdev);
if (!local) {
bio_put(req->private_bio); /* or we get a bio leak */
req->private_bio = NULL;
}
if (rw == WRITE) {
remote = 1;
} else {
/* READ || READA */
if (local) {
if (!drbd_may_do_local_read(mdev, sector, size)) {
/* we could kick the syncer to
* sync this extent asap, wait for
* it, then continue locally.
* Or just issue the request remotely.
*/
local = 0;
bio_put(req->private_bio);
req->private_bio = NULL;
put_ldev(mdev);
}
}
remote = !local && mdev->state.pdsk >= D_UP_TO_DATE;
}
/* If we have a disk, but a READA request is mapped to remote,
* we are R_PRIMARY, D_INCONSISTENT, SyncTarget.
* Just fail that READA request right here.
*
* THINK: maybe fail all READA when not local?
* or make this configurable...
* if network is slow, READA won't do any good.
*/
if (rw == READA && mdev->state.disk >= D_INCONSISTENT && !local) {
err = -EWOULDBLOCK;
goto fail_and_free_req;
}
/* For WRITES going to the local disk, grab a reference on the target
* extent. This waits for any resync activity in the corresponding
* resync extent to finish, and, if necessary, pulls in the target
* extent into the activity log, which involves further disk io because
* of transactional on-disk meta data updates. */
if (rw == WRITE && local)
drbd_al_begin_io(mdev, sector);
remote = remote && (mdev->state.pdsk == D_UP_TO_DATE ||
(mdev->state.pdsk == D_INCONSISTENT &&
mdev->state.conn >= C_CONNECTED));
if (!(local || remote)) {
dev_err(DEV, "IO ERROR: neither local nor remote disk\n");
goto fail_free_complete;
}
/* For WRITE request, we have to make sure that we have an
* unused_spare_tle, in case we need to start a new epoch.
* I try to be smart and avoid to pre-allocate always "just in case",
* but there is a race between testing the bit and pointer outside the
* spinlock, and grabbing the spinlock.
* if we lost that race, we retry. */
if (rw == WRITE && remote &&
mdev->unused_spare_tle == NULL &&
test_bit(CREATE_BARRIER, &mdev->flags)) {
allocate_barrier:
b = kmalloc(sizeof(struct drbd_tl_epoch), GFP_NOIO);
if (!b) {
dev_err(DEV, "Failed to alloc barrier.\n");
err = -ENOMEM;
goto fail_free_complete;
}
}
/* GOOD, everything prepared, grab the spin_lock */
spin_lock_irq(&mdev->req_lock);
if (remote) {
remote = (mdev->state.pdsk == D_UP_TO_DATE ||
(mdev->state.pdsk == D_INCONSISTENT &&
mdev->state.conn >= C_CONNECTED));
if (!remote)
dev_warn(DEV, "lost connection while grabbing the req_lock!\n");
if (!(local || remote)) {
dev_err(DEV, "IO ERROR: neither local nor remote disk\n");
spin_unlock_irq(&mdev->req_lock);
goto fail_free_complete;
}
}
if (b && mdev->unused_spare_tle == NULL) {
mdev->unused_spare_tle = b;
b = NULL;
}
if (rw == WRITE && remote &&
mdev->unused_spare_tle == NULL &&
test_bit(CREATE_BARRIER, &mdev->flags)) {
/* someone closed the current epoch
* while we were grabbing the spinlock */
spin_unlock_irq(&mdev->req_lock);
goto allocate_barrier;
}
/* Update disk stats */
_drbd_start_io_acct(mdev, req, bio);
/* _maybe_start_new_epoch(mdev);
* If we need to generate a write barrier packet, we have to add the
* new epoch (barrier) object, and queue the barrier packet for sending,
* and queue the req's data after it _within the same lock_, otherwise
* we have race conditions were the reorder domains could be mixed up.
*
* Even read requests may start a new epoch and queue the corresponding
* barrier packet. To get the write ordering right, we only have to
* make sure that, if this is a write request and it triggered a
* barrier packet, this request is queued within the same spinlock. */
if (remote && mdev->unused_spare_tle &&
test_and_clear_bit(CREATE_BARRIER, &mdev->flags)) {
_tl_add_barrier(mdev, mdev->unused_spare_tle);
mdev->unused_spare_tle = NULL;
} else {
D_ASSERT(!(remote && rw == WRITE &&
test_bit(CREATE_BARRIER, &mdev->flags)));
}
/* NOTE
* Actually, 'local' may be wrong here already, since we may have failed
* to write to the meta data, and may become wrong anytime because of
* local io-error for some other request, which would lead to us
* "detaching" the local disk.
*
* 'remote' may become wrong any time because the network could fail.
*
* This is a harmless race condition, though, since it is handled
* correctly at the appropriate places; so it just defers the failure
* of the respective operation.
*/
/* mark them early for readability.
* this just sets some state flags. */
if (remote)
_req_mod(req, to_be_send);
if (local)
_req_mod(req, to_be_submitted);
/* check this request on the collision detection hash tables.
* if we have a conflict, just complete it here.
* THINK do we want to check reads, too? (I don't think so...) */
if (rw == WRITE && _req_conflicts(req)) {
/* this is a conflicting request.
* even though it may have been only _partially_
* overlapping with one of the currently pending requests,
* without even submitting or sending it, we will
* pretend that it was successfully served right now.
*/
if (local) {
bio_put(req->private_bio);
req->private_bio = NULL;
drbd_al_complete_io(mdev, req->sector);
put_ldev(mdev);
local = 0;
}
if (remote)
dec_ap_pending(mdev);
_drbd_end_io_acct(mdev, req);
/* THINK: do we want to fail it (-EIO), or pretend success? */
bio_endio(req->master_bio, 0);
req->master_bio = NULL;
dec_ap_bio(mdev);
drbd_req_free(req);
remote = 0;
}
/* NOTE remote first: to get the concurrent write detection right,
* we must register the request before start of local IO. */
if (remote) {
/* either WRITE and C_CONNECTED,
* or READ, and no local disk,
* or READ, but not in sync.
*/
_req_mod(req, (rw == WRITE)
? queue_for_net_write
: queue_for_net_read);
}
spin_unlock_irq(&mdev->req_lock);
kfree(b); /* if someone else has beaten us to it... */
if (local) {
req->private_bio->bi_bdev = mdev->ldev->backing_bdev;
if (FAULT_ACTIVE(mdev, rw == WRITE ? DRBD_FAULT_DT_WR
: rw == READ ? DRBD_FAULT_DT_RD
: DRBD_FAULT_DT_RA))
bio_endio(req->private_bio, -EIO);
else
generic_make_request(req->private_bio);
}
/* we need to plug ALWAYS since we possibly need to kick lo_dev.
* we plug after submit, so we won't miss an unplug event */
drbd_plug_device(mdev);
return 0;
fail_free_complete:
if (rw == WRITE && local)
drbd_al_complete_io(mdev, sector);
fail_and_free_req:
if (local) {
bio_put(req->private_bio);
req->private_bio = NULL;
put_ldev(mdev);
}
bio_endio(bio, err);
drbd_req_free(req);
dec_ap_bio(mdev);
kfree(b);
return 0;
}
/* helper function for drbd_make_request
* if we can determine just by the mdev (state) that this request will fail,
* return 1
* otherwise return 0
*/
static int drbd_fail_request_early(struct drbd_conf *mdev, int is_write)
{
/* Unconfigured */
if (mdev->state.conn == C_DISCONNECTING &&
mdev->state.disk == D_DISKLESS)
return 1;
if (mdev->state.role != R_PRIMARY &&
(!allow_oos || is_write)) {
if (__ratelimit(&drbd_ratelimit_state)) {
dev_err(DEV, "Process %s[%u] tried to %s; "
"since we are not in Primary state, "
"we cannot allow this\n",
current->comm, current->pid,
is_write ? "WRITE" : "READ");
}
return 1;
}
/*
* Paranoia: we might have been primary, but sync target, or
* even diskless, then lost the connection.
* This should have been handled (panic? suspend?) somewhere
* else. But maybe it was not, so check again here.
* Caution: as long as we do not have a read/write lock on mdev,
* to serialize state changes, this is racy, since we may lose
* the connection *after* we test for the cstate.
*/
if (mdev->state.disk < D_UP_TO_DATE && mdev->state.pdsk < D_UP_TO_DATE) {
if (__ratelimit(&drbd_ratelimit_state))
dev_err(DEV, "Sorry, I have no access to good data anymore.\n");
return 1;
}
return 0;
}
int drbd_make_request_26(struct request_queue *q, struct bio *bio)
{
unsigned int s_enr, e_enr;
struct drbd_conf *mdev = (struct drbd_conf *) q->queuedata;
if (drbd_fail_request_early(mdev, bio_data_dir(bio) & WRITE)) {
bio_endio(bio, -EPERM);
return 0;
}
/* Reject barrier requests if we know the underlying device does
* not support them.
* XXX: Need to get this info from peer as well some how so we
* XXX: reject if EITHER side/data/metadata area does not support them.
*
* because of those XXX, this is not yet enabled,
* i.e. in drbd_init_set_defaults we set the NO_BARRIER_SUPP bit.
*/
if (unlikely(bio_rw_flagged(bio, BIO_RW_BARRIER) && test_bit(NO_BARRIER_SUPP, &mdev->flags))) {
/* dev_warn(DEV, "Rejecting barrier request as underlying device does not support\n"); */
bio_endio(bio, -EOPNOTSUPP);
return 0;
}
/*
* what we "blindly" assume:
*/
D_ASSERT(bio->bi_size > 0);
D_ASSERT((bio->bi_size & 0x1ff) == 0);
D_ASSERT(bio->bi_idx == 0);
/* to make some things easier, force alignment of requests within the
* granularity of our hash tables */
s_enr = bio->bi_sector >> HT_SHIFT;
e_enr = (bio->bi_sector+(bio->bi_size>>9)-1) >> HT_SHIFT;
if (likely(s_enr == e_enr)) {
inc_ap_bio(mdev, 1);
return drbd_make_request_common(mdev, bio);
}
/* can this bio be split generically?
* Maybe add our own split-arbitrary-bios function. */
if (bio->bi_vcnt != 1 || bio->bi_idx != 0 || bio->bi_size > DRBD_MAX_SEGMENT_SIZE) {
/* rather error out here than BUG in bio_split */
dev_err(DEV, "bio would need to, but cannot, be split: "
"(vcnt=%u,idx=%u,size=%u,sector=%llu)\n",
bio->bi_vcnt, bio->bi_idx, bio->bi_size,
(unsigned long long)bio->bi_sector);
bio_endio(bio, -EINVAL);
} else {
/* This bio crosses some boundary, so we have to split it. */
struct bio_pair *bp;
/* works for the "do not cross hash slot boundaries" case
* e.g. sector 262269, size 4096
* s_enr = 262269 >> 6 = 4097
* e_enr = (262269+8-1) >> 6 = 4098
* HT_SHIFT = 6
* sps = 64, mask = 63
* first_sectors = 64 - (262269 & 63) = 3
*/
const sector_t sect = bio->bi_sector;
const int sps = 1 << HT_SHIFT; /* sectors per slot */
const int mask = sps - 1;
const sector_t first_sectors = sps - (sect & mask);
bp = bio_split(bio,
#if LINUX_VERSION_CODE < KERNEL_VERSION(2,6,28)
bio_split_pool,
#endif
first_sectors);
/* we need to get a "reference count" (ap_bio_cnt)
* to avoid races with the disconnect/reconnect/suspend code.
* In case we need to split the bio here, we need to get two references
* atomically, otherwise we might deadlock when trying to submit the
* second one! */
inc_ap_bio(mdev, 2);
D_ASSERT(e_enr == s_enr + 1);
drbd_make_request_common(mdev, &bp->bio1);
drbd_make_request_common(mdev, &bp->bio2);
bio_pair_release(bp);
}
return 0;
}
/* This is called by bio_add_page(). With this function we reduce
* the number of BIOs that span over multiple DRBD_MAX_SEGMENT_SIZEs
* units (was AL_EXTENTs).
*
* we do the calculation within the lower 32bit of the byte offsets,
* since we don't care for actual offset, but only check whether it
* would cross "activity log extent" boundaries.
*
* As long as the BIO is empty we have to allow at least one bvec,
* regardless of size and offset. so the resulting bio may still
* cross extent boundaries. those are dealt with (bio_split) in
* drbd_make_request_26.
*/
int drbd_merge_bvec(struct request_queue *q, struct bvec_merge_data *bvm, struct bio_vec *bvec)
{
struct drbd_conf *mdev = (struct drbd_conf *) q->queuedata;
unsigned int bio_offset =
(unsigned int)bvm->bi_sector << 9; /* 32 bit */
unsigned int bio_size = bvm->bi_size;
int limit, backing_limit;
limit = DRBD_MAX_SEGMENT_SIZE
- ((bio_offset & (DRBD_MAX_SEGMENT_SIZE-1)) + bio_size);
if (limit < 0)
limit = 0;
if (bio_size == 0) {
if (limit <= bvec->bv_len)
limit = bvec->bv_len;
} else if (limit && get_ldev(mdev)) {
struct request_queue * const b =
mdev->ldev->backing_bdev->bd_disk->queue;
if (b->merge_bvec_fn && mdev->ldev->dc.use_bmbv) {
backing_limit = b->merge_bvec_fn(b, bvm, bvec);
limit = min(limit, backing_limit);
}
put_ldev(mdev);
}
return limit;
}
| {
"pile_set_name": "Github"
} |
<test-metadata>
<benchmark-version>1.2</benchmark-version>
<category>sqli</category>
<test-number>01877</test-number>
<vulnerability>false</vulnerability>
<cwe>89</cwe>
</test-metadata>
| {
"pile_set_name": "Github"
} |
/*
Copyright 2015 The Kubernetes Authors.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
// Package streaming implements encoder and decoder for streams
// of runtime.Objects over io.Writer/Readers.
package streaming
import (
"bytes"
"fmt"
"io"
"k8s.io/apimachinery/pkg/runtime"
"k8s.io/apimachinery/pkg/runtime/schema"
)
// Encoder is a runtime.Encoder on a stream.
type Encoder interface {
// Encode will write the provided object to the stream or return an error. It obeys the same
// contract as runtime.VersionedEncoder.
Encode(obj runtime.Object) error
}
// Decoder is a runtime.Decoder from a stream.
type Decoder interface {
// Decode will return io.EOF when no more objects are available.
Decode(defaults *schema.GroupVersionKind, into runtime.Object) (runtime.Object, *schema.GroupVersionKind, error)
// Close closes the underlying stream.
Close() error
}
// Serializer is a factory for creating encoders and decoders that work over streams.
type Serializer interface {
NewEncoder(w io.Writer) Encoder
NewDecoder(r io.ReadCloser) Decoder
}
type decoder struct {
reader io.ReadCloser
decoder runtime.Decoder
buf []byte
maxBytes int
resetRead bool
}
// NewDecoder creates a streaming decoder that reads object chunks from r and decodes them with d.
// The reader is expected to return ErrShortRead if the provided buffer is not large enough to read
// an entire object.
func NewDecoder(r io.ReadCloser, d runtime.Decoder) Decoder {
return &decoder{
reader: r,
decoder: d,
buf: make([]byte, 1024),
maxBytes: 16 * 1024 * 1024,
}
}
var ErrObjectTooLarge = fmt.Errorf("object to decode was longer than maximum allowed size")
// Decode reads the next object from the stream and decodes it.
func (d *decoder) Decode(defaults *schema.GroupVersionKind, into runtime.Object) (runtime.Object, *schema.GroupVersionKind, error) {
base := 0
for {
n, err := d.reader.Read(d.buf[base:])
if err == io.ErrShortBuffer {
if n == 0 {
return nil, nil, fmt.Errorf("got short buffer with n=0, base=%d, cap=%d", base, cap(d.buf))
}
if d.resetRead {
continue
}
// double the buffer size up to maxBytes
if len(d.buf) < d.maxBytes {
base += n
d.buf = append(d.buf, make([]byte, len(d.buf))...)
continue
}
// must read the rest of the frame (until we stop getting ErrShortBuffer)
d.resetRead = true
base = 0
return nil, nil, ErrObjectTooLarge
}
if err != nil {
return nil, nil, err
}
if d.resetRead {
// now that we have drained the large read, continue
d.resetRead = false
continue
}
base += n
break
}
return d.decoder.Decode(d.buf[:base], defaults, into)
}
func (d *decoder) Close() error {
return d.reader.Close()
}
type encoder struct {
writer io.Writer
encoder runtime.Encoder
buf *bytes.Buffer
}
// NewEncoder returns a new streaming encoder.
func NewEncoder(w io.Writer, e runtime.Encoder) Encoder {
return &encoder{
writer: w,
encoder: e,
buf: &bytes.Buffer{},
}
}
// Encode writes the provided object to the nested writer.
func (e *encoder) Encode(obj runtime.Object) error {
if err := e.encoder.Encode(obj, e.buf); err != nil {
return err
}
_, err := e.writer.Write(e.buf.Bytes())
e.buf.Reset()
return err
}
| {
"pile_set_name": "Github"
} |
#
# Author:: Daniel DeLeo (<[email protected]>)
# Copyright:: Copyright (c) Chef Software Inc.
# License:: Apache License, Version 2.0
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
class Chef
class RunList
class RunListItem
QUALIFIED_RECIPE = /^recipe\[([^\]@]+)(@([0-9]+(\.[0-9]+){1,2}))?\]$/.freeze
QUALIFIED_ROLE = /^role\[([^\]]+)\]$/.freeze
VERSIONED_UNQUALIFIED_RECIPE = /^([^@]+)(@([0-9]+(\.[0-9]+){1,2}))$/.freeze
FALSE_FRIEND = /[\[\]]/.freeze
attr_reader :name, :type, :version
def initialize(item)
@version = nil
case item
when Hash
assert_hash_is_valid_run_list_item!(item)
@type = (item["type"] || item[:type]).to_sym
@name = item["name"] || item[:name]
if item.key?("version") || item.key?(:version)
@version = item["version"] || item[:version]
end
when String
if match = QUALIFIED_RECIPE.match(item)
# recipe[recipe_name]
# recipe[[email protected]]
@type = :recipe
@name = match[1]
@version = match[3] if match[3]
elsif match = QUALIFIED_ROLE.match(item)
# role[role_name]
@type = :role
@name = match[1]
elsif match = VERSIONED_UNQUALIFIED_RECIPE.match(item)
# [email protected]
@type = :recipe
@name = match[1]
@version = match[3] if match[3]
elsif match = FALSE_FRIEND.match(item)
# Recipe[recipe_name]
# roles[role_name]
name = match[1]
raise ArgumentError, "Unable to create #{self.class} from #{item.class}:#{item.inspect}: must be recipe[#{name}] or role[#{name}]"
else
# recipe_name
@type = :recipe
@name = item
end
else
raise ArgumentError, "Unable to create #{self.class} from #{item.class}:#{item.inspect}: must be a Hash or String"
end
end
def to_s
"#{@type}[#{@name}#{@version ? "@#{@version}" : ""}]"
end
def role?
@type == :role
end
def recipe?
@type == :recipe
end
def ==(other)
if other.is_a?(String)
to_s == other.to_s
else
other.respond_to?(:type) && other.respond_to?(:name) && other.respond_to?(:version) && other.type == @type && other.name == @name && other.version == @version
end
end
def assert_hash_is_valid_run_list_item!(item)
unless (item.key?("type") || item.key?(:type)) && (item.key?("name") || item.key?(:name))
raise ArgumentError, "Initializing a #{self.class} from a hash requires that it have a 'type' and 'name' key"
end
end
end
end
end
| {
"pile_set_name": "Github"
} |
<!DOCTYPE html>
<html lang="en">
<script src="http://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<!-- d3 is used by histogram.js
<script src="http://d3js.org/d3.v3.min.js" charset="utf-8"></script>
-->
<script src="https://raw.githubusercontent.com/nnnick/Chart.js/master/Chart.js"></script>
<script src="script.js"></script>
<script src="histogram.js"></script>
<head>
<meta content="text/html; charset=utf-8" http-equiv="Content-Type">
<meta charset="utf-8">
<meta content="width=device-width" name="viewport">
<link href="/style.css" rel="stylesheet">
<title>((( - PRODUCTION -))) Guestbook</title>
</head>
<body>
<TABLE>
<TR>
<TD>
<div id="k8petstore-entries">
<p>Waiting for database connection...This will get overwritten...</p>
</div>
</TD>
<TD>
<div id="header">
<h1>k8-bps.</h1>
</div><br>
<div>
<p><h2 id="k8petstore-host-address"></h2></p>
<p><a href="/env">/env</a>
<a href="/info">/info</a></p>
</div>
</TD>
</TR>
<TR >
<TD colspan="2">
<canvas id="myChart" width="2000" height="600"></canvas>
</TD>
</TR>
</TABLE>
</body>
</html>
| {
"pile_set_name": "Github"
} |
#include <QtScript/QScriptEngine>
#include <QtScript/QScriptContext>
#include <QtScript/QScriptValue>
#include <QtCore/QStringList>
#include <QtCore/QDebug>
#include <qmetaobject.h>
#include <qhttp.h>
#include <QVariant>
#include <qauthenticator.h>
#include <qbytearray.h>
#include <qcoreevent.h>
#include <qhttp.h>
#include <qiodevice.h>
#include <qlist.h>
#include <qnetworkproxy.h>
#include <qobject.h>
#include <qsslerror.h>
#include <qtcpsocket.h>
#include "qtscriptshell_QHttp.h"
static const char * const qtscript_QHttp_function_names[] = {
"QHttp"
// static
// prototype
, "bytesAvailable"
, "clearPendingRequests"
, "close"
, "currentDestinationDevice"
, "currentId"
, "currentRequest"
, "currentSourceDevice"
, "error"
, "errorString"
, "get"
, "hasPendingRequests"
, "head"
, "lastResponse"
, "post"
, "read"
, "readAll"
, "request"
, "setHost"
, "setProxy"
, "setSocket"
, "setUser"
, "state"
, "toString"
};
static const char * const qtscript_QHttp_function_signatures[] = {
"QObject parent\nString hostname, ConnectionMode mode, unsigned short port, QObject parent\nString hostname, unsigned short port, QObject parent"
// static
// prototype
, ""
, ""
, ""
, ""
, ""
, ""
, ""
, ""
, ""
, "String path, QIODevice to"
, ""
, "String path"
, ""
, "String path, QIODevice data, QIODevice to\nString path, QByteArray data, QIODevice to"
, "char data, qint64 maxlen"
, ""
, "QHttpRequestHeader header, QIODevice device, QIODevice to\nQHttpRequestHeader header, QByteArray data, QIODevice to"
, "String hostname, ConnectionMode mode, unsigned short port\nString hostname, unsigned short port"
, "QNetworkProxy proxy\nString host, int port, String username, String password"
, "QTcpSocket socket"
, "String username, String password"
, ""
""
};
static const int qtscript_QHttp_function_lengths[] = {
4
// static
// prototype
, 0
, 0
, 0
, 0
, 0
, 0
, 0
, 0
, 0
, 2
, 0
, 1
, 0
, 3
, 2
, 0
, 3
, 3
, 4
, 1
, 2
, 0
, 0
};
static QScriptValue qtscript_QHttp_throw_ambiguity_error_helper(
QScriptContext *context, const char *functionName, const char *signatures)
{
QStringList lines = QString::fromLatin1(signatures).split(QLatin1Char('\n'));
QStringList fullSignatures;
for (int i = 0; i < lines.size(); ++i)
fullSignatures.append(QString::fromLatin1("%0(%1)").arg(functionName).arg(lines.at(i)));
return context->throwError(QString::fromLatin1("QHttp::%0(): could not find a function match; candidates are:\n%1")
.arg(functionName).arg(fullSignatures.join(QLatin1String("\n"))));
}
Q_DECLARE_METATYPE(QHttp*)
Q_DECLARE_METATYPE(QtScriptShell_QHttp*)
Q_DECLARE_METATYPE(QHttp::Error)
Q_DECLARE_METATYPE(QHttp::ConnectionMode)
Q_DECLARE_METATYPE(QHttp::State)
Q_DECLARE_METATYPE(QIODevice*)
Q_DECLARE_METATYPE(QHttpRequestHeader)
Q_DECLARE_METATYPE(QHttpResponseHeader)
Q_DECLARE_METATYPE(char*)
Q_DECLARE_METATYPE(QNetworkProxy)
Q_DECLARE_METATYPE(QTcpSocket*)
static QScriptValue qtscript_create_enum_class_helper(
QScriptEngine *engine,
QScriptEngine::FunctionSignature construct,
QScriptEngine::FunctionSignature valueOf,
QScriptEngine::FunctionSignature toString)
{
QScriptValue proto = engine->newObject();
proto.setProperty(QString::fromLatin1("valueOf"),
engine->newFunction(valueOf), QScriptValue::SkipInEnumeration);
proto.setProperty(QString::fromLatin1("toString"),
engine->newFunction(toString), QScriptValue::SkipInEnumeration);
return engine->newFunction(construct, proto, 1);
}
//
// QHttp::Error
//
static const QHttp::Error qtscript_QHttp_Error_values[] = {
QHttp::NoError
, QHttp::UnknownError
, QHttp::HostNotFound
, QHttp::ConnectionRefused
, QHttp::UnexpectedClose
, QHttp::InvalidResponseHeader
, QHttp::WrongContentLength
, QHttp::Aborted
, QHttp::AuthenticationRequiredError
, QHttp::ProxyAuthenticationRequiredError
};
static const char * const qtscript_QHttp_Error_keys[] = {
"NoError"
, "UnknownError"
, "HostNotFound"
, "ConnectionRefused"
, "UnexpectedClose"
, "InvalidResponseHeader"
, "WrongContentLength"
, "Aborted"
, "AuthenticationRequiredError"
, "ProxyAuthenticationRequiredError"
};
static QString qtscript_QHttp_Error_toStringHelper(QHttp::Error value)
{
if ((value >= QHttp::NoError) && (value <= QHttp::ProxyAuthenticationRequiredError))
return qtscript_QHttp_Error_keys[static_cast<int>(value)-static_cast<int>(QHttp::NoError)];
return QString();
}
static QScriptValue qtscript_QHttp_Error_toScriptValue(QScriptEngine *engine, const QHttp::Error &value)
{
QScriptValue clazz = engine->globalObject().property(QString::fromLatin1("QHttp"));
return clazz.property(qtscript_QHttp_Error_toStringHelper(value));
}
static void qtscript_QHttp_Error_fromScriptValue(const QScriptValue &value, QHttp::Error &out)
{
out = qvariant_cast<QHttp::Error>(value.toVariant());
}
static QScriptValue qtscript_construct_QHttp_Error(QScriptContext *context, QScriptEngine *engine)
{
int arg = context->argument(0).toInt32();
if ((arg >= QHttp::NoError) && (arg <= QHttp::ProxyAuthenticationRequiredError))
return qScriptValueFromValue(engine, static_cast<QHttp::Error>(arg));
return context->throwError(QString::fromLatin1("Error(): invalid enum value (%0)").arg(arg));
}
static QScriptValue qtscript_QHttp_Error_valueOf(QScriptContext *context, QScriptEngine *engine)
{
QHttp::Error value = qscriptvalue_cast<QHttp::Error>(context->thisObject());
return QScriptValue(engine, static_cast<int>(value));
}
static QScriptValue qtscript_QHttp_Error_toString(QScriptContext *context, QScriptEngine *engine)
{
QHttp::Error value = qscriptvalue_cast<QHttp::Error>(context->thisObject());
return QScriptValue(engine, qtscript_QHttp_Error_toStringHelper(value));
}
static QScriptValue qtscript_create_QHttp_Error_class(QScriptEngine *engine, QScriptValue &clazz)
{
QScriptValue ctor = qtscript_create_enum_class_helper(
engine, qtscript_construct_QHttp_Error,
qtscript_QHttp_Error_valueOf, qtscript_QHttp_Error_toString);
qScriptRegisterMetaType<QHttp::Error>(engine, qtscript_QHttp_Error_toScriptValue,
qtscript_QHttp_Error_fromScriptValue, ctor.property(QString::fromLatin1("prototype")));
for (int i = 0; i < 10; ++i) {
clazz.setProperty(QString::fromLatin1(qtscript_QHttp_Error_keys[i]),
engine->newVariant(qVariantFromValue(qtscript_QHttp_Error_values[i])),
QScriptValue::ReadOnly | QScriptValue::Undeletable);
}
return ctor;
}
//
// QHttp::ConnectionMode
//
static const QHttp::ConnectionMode qtscript_QHttp_ConnectionMode_values[] = {
QHttp::ConnectionModeHttp
, QHttp::ConnectionModeHttps
};
static const char * const qtscript_QHttp_ConnectionMode_keys[] = {
"ConnectionModeHttp"
, "ConnectionModeHttps"
};
static QString qtscript_QHttp_ConnectionMode_toStringHelper(QHttp::ConnectionMode value)
{
if ((value >= QHttp::ConnectionModeHttp) && (value <= QHttp::ConnectionModeHttps))
return qtscript_QHttp_ConnectionMode_keys[static_cast<int>(value)-static_cast<int>(QHttp::ConnectionModeHttp)];
return QString();
}
static QScriptValue qtscript_QHttp_ConnectionMode_toScriptValue(QScriptEngine *engine, const QHttp::ConnectionMode &value)
{
QScriptValue clazz = engine->globalObject().property(QString::fromLatin1("QHttp"));
return clazz.property(qtscript_QHttp_ConnectionMode_toStringHelper(value));
}
static void qtscript_QHttp_ConnectionMode_fromScriptValue(const QScriptValue &value, QHttp::ConnectionMode &out)
{
out = qvariant_cast<QHttp::ConnectionMode>(value.toVariant());
}
static QScriptValue qtscript_construct_QHttp_ConnectionMode(QScriptContext *context, QScriptEngine *engine)
{
int arg = context->argument(0).toInt32();
if ((arg >= QHttp::ConnectionModeHttp) && (arg <= QHttp::ConnectionModeHttps))
return qScriptValueFromValue(engine, static_cast<QHttp::ConnectionMode>(arg));
return context->throwError(QString::fromLatin1("ConnectionMode(): invalid enum value (%0)").arg(arg));
}
static QScriptValue qtscript_QHttp_ConnectionMode_valueOf(QScriptContext *context, QScriptEngine *engine)
{
QHttp::ConnectionMode value = qscriptvalue_cast<QHttp::ConnectionMode>(context->thisObject());
return QScriptValue(engine, static_cast<int>(value));
}
static QScriptValue qtscript_QHttp_ConnectionMode_toString(QScriptContext *context, QScriptEngine *engine)
{
QHttp::ConnectionMode value = qscriptvalue_cast<QHttp::ConnectionMode>(context->thisObject());
return QScriptValue(engine, qtscript_QHttp_ConnectionMode_toStringHelper(value));
}
static QScriptValue qtscript_create_QHttp_ConnectionMode_class(QScriptEngine *engine, QScriptValue &clazz)
{
QScriptValue ctor = qtscript_create_enum_class_helper(
engine, qtscript_construct_QHttp_ConnectionMode,
qtscript_QHttp_ConnectionMode_valueOf, qtscript_QHttp_ConnectionMode_toString);
qScriptRegisterMetaType<QHttp::ConnectionMode>(engine, qtscript_QHttp_ConnectionMode_toScriptValue,
qtscript_QHttp_ConnectionMode_fromScriptValue, ctor.property(QString::fromLatin1("prototype")));
for (int i = 0; i < 2; ++i) {
clazz.setProperty(QString::fromLatin1(qtscript_QHttp_ConnectionMode_keys[i]),
engine->newVariant(qVariantFromValue(qtscript_QHttp_ConnectionMode_values[i])),
QScriptValue::ReadOnly | QScriptValue::Undeletable);
}
return ctor;
}
//
// QHttp::State
//
static const QHttp::State qtscript_QHttp_State_values[] = {
QHttp::Unconnected
, QHttp::HostLookup
, QHttp::Connecting
, QHttp::Sending
, QHttp::Reading
, QHttp::Connected
, QHttp::Closing
};
static const char * const qtscript_QHttp_State_keys[] = {
"Unconnected"
, "HostLookup"
, "Connecting"
, "Sending"
, "Reading"
, "Connected"
, "Closing"
};
static QString qtscript_QHttp_State_toStringHelper(QHttp::State value)
{
if ((value >= QHttp::Unconnected) && (value <= QHttp::Closing))
return qtscript_QHttp_State_keys[static_cast<int>(value)-static_cast<int>(QHttp::Unconnected)];
return QString();
}
static QScriptValue qtscript_QHttp_State_toScriptValue(QScriptEngine *engine, const QHttp::State &value)
{
QScriptValue clazz = engine->globalObject().property(QString::fromLatin1("QHttp"));
return clazz.property(qtscript_QHttp_State_toStringHelper(value));
}
static void qtscript_QHttp_State_fromScriptValue(const QScriptValue &value, QHttp::State &out)
{
out = qvariant_cast<QHttp::State>(value.toVariant());
}
static QScriptValue qtscript_construct_QHttp_State(QScriptContext *context, QScriptEngine *engine)
{
int arg = context->argument(0).toInt32();
if ((arg >= QHttp::Unconnected) && (arg <= QHttp::Closing))
return qScriptValueFromValue(engine, static_cast<QHttp::State>(arg));
return context->throwError(QString::fromLatin1("State(): invalid enum value (%0)").arg(arg));
}
static QScriptValue qtscript_QHttp_State_valueOf(QScriptContext *context, QScriptEngine *engine)
{
QHttp::State value = qscriptvalue_cast<QHttp::State>(context->thisObject());
return QScriptValue(engine, static_cast<int>(value));
}
static QScriptValue qtscript_QHttp_State_toString(QScriptContext *context, QScriptEngine *engine)
{
QHttp::State value = qscriptvalue_cast<QHttp::State>(context->thisObject());
return QScriptValue(engine, qtscript_QHttp_State_toStringHelper(value));
}
static QScriptValue qtscript_create_QHttp_State_class(QScriptEngine *engine, QScriptValue &clazz)
{
QScriptValue ctor = qtscript_create_enum_class_helper(
engine, qtscript_construct_QHttp_State,
qtscript_QHttp_State_valueOf, qtscript_QHttp_State_toString);
qScriptRegisterMetaType<QHttp::State>(engine, qtscript_QHttp_State_toScriptValue,
qtscript_QHttp_State_fromScriptValue, ctor.property(QString::fromLatin1("prototype")));
for (int i = 0; i < 7; ++i) {
clazz.setProperty(QString::fromLatin1(qtscript_QHttp_State_keys[i]),
engine->newVariant(qVariantFromValue(qtscript_QHttp_State_values[i])),
QScriptValue::ReadOnly | QScriptValue::Undeletable);
}
return ctor;
}
//
// QHttp
//
static QScriptValue qtscript_QHttp_prototype_call(QScriptContext *context, QScriptEngine *)
{
#if QT_VERSION > 0x040400
Q_ASSERT(context->callee().isFunction());
uint _id = context->callee().data().toUInt32();
#else
uint _id;
if (context->callee().isFunction())
_id = context->callee().data().toUInt32();
else
_id = 0xBABE0000 + 22;
#endif
Q_ASSERT((_id & 0xFFFF0000) == 0xBABE0000);
_id &= 0x0000FFFF;
QHttp* _q_self = qscriptvalue_cast<QHttp*>(context->thisObject());
if (!_q_self) {
return context->throwError(QScriptContext::TypeError,
QString::fromLatin1("QHttp.%0(): this object is not a QHttp")
.arg(qtscript_QHttp_function_names[_id+1]));
}
switch (_id) {
case 0:
if (context->argumentCount() == 0) {
qint64 _q_result = _q_self->bytesAvailable();
return qScriptValueFromValue(context->engine(), _q_result);
}
break;
case 1:
if (context->argumentCount() == 0) {
_q_self->clearPendingRequests();
return context->engine()->undefinedValue();
}
break;
case 2:
if (context->argumentCount() == 0) {
int _q_result = _q_self->close();
return QScriptValue(context->engine(), _q_result);
}
break;
case 3:
if (context->argumentCount() == 0) {
QIODevice* _q_result = _q_self->currentDestinationDevice();
return qScriptValueFromValue(context->engine(), _q_result);
}
break;
case 4:
if (context->argumentCount() == 0) {
int _q_result = _q_self->currentId();
return QScriptValue(context->engine(), _q_result);
}
break;
case 5:
if (context->argumentCount() == 0) {
QHttpRequestHeader _q_result = _q_self->currentRequest();
return qScriptValueFromValue(context->engine(), _q_result);
}
break;
case 6:
if (context->argumentCount() == 0) {
QIODevice* _q_result = _q_self->currentSourceDevice();
return qScriptValueFromValue(context->engine(), _q_result);
}
break;
case 7:
if (context->argumentCount() == 0) {
QHttp::Error _q_result = _q_self->error();
return qScriptValueFromValue(context->engine(), _q_result);
}
break;
case 8:
if (context->argumentCount() == 0) {
QString _q_result = _q_self->errorString();
return QScriptValue(context->engine(), _q_result);
}
break;
case 9:
if (context->argumentCount() == 1) {
QString _q_arg0 = context->argument(0).toString();
int _q_result = _q_self->get(_q_arg0);
return QScriptValue(context->engine(), _q_result);
}
if (context->argumentCount() == 2) {
QString _q_arg0 = context->argument(0).toString();
QIODevice* _q_arg1 = qscriptvalue_cast<QIODevice*>(context->argument(1));
int _q_result = _q_self->get(_q_arg0, _q_arg1);
return QScriptValue(context->engine(), _q_result);
}
break;
case 10:
if (context->argumentCount() == 0) {
bool _q_result = _q_self->hasPendingRequests();
return QScriptValue(context->engine(), _q_result);
}
break;
case 11:
if (context->argumentCount() == 1) {
QString _q_arg0 = context->argument(0).toString();
int _q_result = _q_self->head(_q_arg0);
return QScriptValue(context->engine(), _q_result);
}
break;
case 12:
if (context->argumentCount() == 0) {
QHttpResponseHeader _q_result = _q_self->lastResponse();
return qScriptValueFromValue(context->engine(), _q_result);
}
break;
case 13:
if (context->argumentCount() == 2) {
if (context->argument(0).isString()
&& qscriptvalue_cast<QIODevice*>(context->argument(1))) {
QString _q_arg0 = context->argument(0).toString();
QIODevice* _q_arg1 = qscriptvalue_cast<QIODevice*>(context->argument(1));
int _q_result = _q_self->post(_q_arg0, _q_arg1);
return QScriptValue(context->engine(), _q_result);
} else if (context->argument(0).isString()
&& (qMetaTypeId<QByteArray>() == context->argument(1).toVariant().userType())) {
QString _q_arg0 = context->argument(0).toString();
QByteArray _q_arg1 = qscriptvalue_cast<QByteArray>(context->argument(1));
int _q_result = _q_self->post(_q_arg0, _q_arg1);
return QScriptValue(context->engine(), _q_result);
}
}
if (context->argumentCount() == 3) {
if (context->argument(0).isString()
&& qscriptvalue_cast<QIODevice*>(context->argument(1))
&& qscriptvalue_cast<QIODevice*>(context->argument(2))) {
QString _q_arg0 = context->argument(0).toString();
QIODevice* _q_arg1 = qscriptvalue_cast<QIODevice*>(context->argument(1));
QIODevice* _q_arg2 = qscriptvalue_cast<QIODevice*>(context->argument(2));
int _q_result = _q_self->post(_q_arg0, _q_arg1, _q_arg2);
return QScriptValue(context->engine(), _q_result);
} else if (context->argument(0).isString()
&& (qMetaTypeId<QByteArray>() == context->argument(1).toVariant().userType())
&& qscriptvalue_cast<QIODevice*>(context->argument(2))) {
QString _q_arg0 = context->argument(0).toString();
QByteArray _q_arg1 = qscriptvalue_cast<QByteArray>(context->argument(1));
QIODevice* _q_arg2 = qscriptvalue_cast<QIODevice*>(context->argument(2));
int _q_result = _q_self->post(_q_arg0, _q_arg1, _q_arg2);
return QScriptValue(context->engine(), _q_result);
}
}
break;
case 14:
if (context->argumentCount() == 2) {
char* _q_arg0 = qscriptvalue_cast<char*>(context->argument(0));
qint64 _q_arg1 = qscriptvalue_cast<qint64>(context->argument(1));
qint64 _q_result = _q_self->read(_q_arg0, _q_arg1);
return qScriptValueFromValue(context->engine(), _q_result);
}
break;
case 15:
if (context->argumentCount() == 0) {
QByteArray _q_result = _q_self->readAll();
return qScriptValueFromValue(context->engine(), _q_result);
}
break;
case 16:
if (context->argumentCount() == 1) {
QHttpRequestHeader _q_arg0 = qscriptvalue_cast<QHttpRequestHeader>(context->argument(0));
int _q_result = _q_self->request(_q_arg0);
return QScriptValue(context->engine(), _q_result);
}
if (context->argumentCount() == 2) {
if ((qMetaTypeId<QHttpRequestHeader>() == context->argument(0).toVariant().userType())
&& qscriptvalue_cast<QIODevice*>(context->argument(1))) {
QHttpRequestHeader _q_arg0 = qscriptvalue_cast<QHttpRequestHeader>(context->argument(0));
QIODevice* _q_arg1 = qscriptvalue_cast<QIODevice*>(context->argument(1));
int _q_result = _q_self->request(_q_arg0, _q_arg1);
return QScriptValue(context->engine(), _q_result);
} else if ((qMetaTypeId<QHttpRequestHeader>() == context->argument(0).toVariant().userType())
&& (qMetaTypeId<QByteArray>() == context->argument(1).toVariant().userType())) {
QHttpRequestHeader _q_arg0 = qscriptvalue_cast<QHttpRequestHeader>(context->argument(0));
QByteArray _q_arg1 = qscriptvalue_cast<QByteArray>(context->argument(1));
int _q_result = _q_self->request(_q_arg0, _q_arg1);
return QScriptValue(context->engine(), _q_result);
}
}
if (context->argumentCount() == 3) {
if ((qMetaTypeId<QHttpRequestHeader>() == context->argument(0).toVariant().userType())
&& qscriptvalue_cast<QIODevice*>(context->argument(1))
&& qscriptvalue_cast<QIODevice*>(context->argument(2))) {
QHttpRequestHeader _q_arg0 = qscriptvalue_cast<QHttpRequestHeader>(context->argument(0));
QIODevice* _q_arg1 = qscriptvalue_cast<QIODevice*>(context->argument(1));
QIODevice* _q_arg2 = qscriptvalue_cast<QIODevice*>(context->argument(2));
int _q_result = _q_self->request(_q_arg0, _q_arg1, _q_arg2);
return QScriptValue(context->engine(), _q_result);
} else if ((qMetaTypeId<QHttpRequestHeader>() == context->argument(0).toVariant().userType())
&& (qMetaTypeId<QByteArray>() == context->argument(1).toVariant().userType())
&& qscriptvalue_cast<QIODevice*>(context->argument(2))) {
QHttpRequestHeader _q_arg0 = qscriptvalue_cast<QHttpRequestHeader>(context->argument(0));
QByteArray _q_arg1 = qscriptvalue_cast<QByteArray>(context->argument(1));
QIODevice* _q_arg2 = qscriptvalue_cast<QIODevice*>(context->argument(2));
int _q_result = _q_self->request(_q_arg0, _q_arg1, _q_arg2);
return QScriptValue(context->engine(), _q_result);
}
}
break;
case 17:
if (context->argumentCount() == 1) {
QString _q_arg0 = context->argument(0).toString();
int _q_result = _q_self->setHost(_q_arg0);
return QScriptValue(context->engine(), _q_result);
}
if (context->argumentCount() == 2) {
if (context->argument(0).isString()
&& (qMetaTypeId<QHttp::ConnectionMode>() == context->argument(1).toVariant().userType())) {
QString _q_arg0 = context->argument(0).toString();
QHttp::ConnectionMode _q_arg1 = qscriptvalue_cast<QHttp::ConnectionMode>(context->argument(1));
int _q_result = _q_self->setHost(_q_arg0, _q_arg1);
return QScriptValue(context->engine(), _q_result);
} else if (context->argument(0).isString()
&& context->argument(1).isNumber()) {
QString _q_arg0 = context->argument(0).toString();
unsigned short _q_arg1 = qscriptvalue_cast<unsigned short>(context->argument(1));
int _q_result = _q_self->setHost(_q_arg0, _q_arg1);
return QScriptValue(context->engine(), _q_result);
}
}
if (context->argumentCount() == 3) {
QString _q_arg0 = context->argument(0).toString();
QHttp::ConnectionMode _q_arg1 = qscriptvalue_cast<QHttp::ConnectionMode>(context->argument(1));
unsigned short _q_arg2 = qscriptvalue_cast<unsigned short>(context->argument(2));
int _q_result = _q_self->setHost(_q_arg0, _q_arg1, _q_arg2);
return QScriptValue(context->engine(), _q_result);
}
break;
case 18:
if (context->argumentCount() == 1) {
QNetworkProxy _q_arg0 = qscriptvalue_cast<QNetworkProxy>(context->argument(0));
int _q_result = _q_self->setProxy(_q_arg0);
return QScriptValue(context->engine(), _q_result);
}
if (context->argumentCount() == 2) {
QString _q_arg0 = context->argument(0).toString();
int _q_arg1 = context->argument(1).toInt32();
int _q_result = _q_self->setProxy(_q_arg0, _q_arg1);
return QScriptValue(context->engine(), _q_result);
}
if (context->argumentCount() == 3) {
QString _q_arg0 = context->argument(0).toString();
int _q_arg1 = context->argument(1).toInt32();
QString _q_arg2 = context->argument(2).toString();
int _q_result = _q_self->setProxy(_q_arg0, _q_arg1, _q_arg2);
return QScriptValue(context->engine(), _q_result);
}
if (context->argumentCount() == 4) {
QString _q_arg0 = context->argument(0).toString();
int _q_arg1 = context->argument(1).toInt32();
QString _q_arg2 = context->argument(2).toString();
QString _q_arg3 = context->argument(3).toString();
int _q_result = _q_self->setProxy(_q_arg0, _q_arg1, _q_arg2, _q_arg3);
return QScriptValue(context->engine(), _q_result);
}
break;
case 19:
if (context->argumentCount() == 1) {
QTcpSocket* _q_arg0 = qscriptvalue_cast<QTcpSocket*>(context->argument(0));
int _q_result = _q_self->setSocket(_q_arg0);
return QScriptValue(context->engine(), _q_result);
}
break;
case 20:
if (context->argumentCount() == 1) {
QString _q_arg0 = context->argument(0).toString();
int _q_result = _q_self->setUser(_q_arg0);
return QScriptValue(context->engine(), _q_result);
}
if (context->argumentCount() == 2) {
QString _q_arg0 = context->argument(0).toString();
QString _q_arg1 = context->argument(1).toString();
int _q_result = _q_self->setUser(_q_arg0, _q_arg1);
return QScriptValue(context->engine(), _q_result);
}
break;
case 21:
if (context->argumentCount() == 0) {
QHttp::State _q_result = _q_self->state();
return qScriptValueFromValue(context->engine(), _q_result);
}
break;
case 22: {
QString result = QString::fromLatin1("QHttp");
return QScriptValue(context->engine(), result);
}
default:
Q_ASSERT(false);
}
return qtscript_QHttp_throw_ambiguity_error_helper(context,
qtscript_QHttp_function_names[_id+1],
qtscript_QHttp_function_signatures[_id+1]);
}
static QScriptValue qtscript_QHttp_static_call(QScriptContext *context, QScriptEngine *)
{
uint _id = context->callee().data().toUInt32();
Q_ASSERT((_id & 0xFFFF0000) == 0xBABE0000);
_id &= 0x0000FFFF;
switch (_id) {
case 0:
if (context->thisObject().strictlyEquals(context->engine()->globalObject())) {
return context->throwError(QString::fromLatin1("QHttp(): Did you forget to construct with 'new'?"));
}
if (context->argumentCount() == 0) {
QtScriptShell_QHttp* _q_cpp_result = new QtScriptShell_QHttp();
QScriptValue _q_result = context->engine()->newQObject(context->thisObject(), (QHttp*)_q_cpp_result, QScriptEngine::AutoOwnership);
_q_cpp_result->__qtscript_self = _q_result;
return _q_result;
} else if (context->argumentCount() == 1) {
if (context->argument(0).isQObject()) {
QObject* _q_arg0 = context->argument(0).toQObject();
QtScriptShell_QHttp* _q_cpp_result = new QtScriptShell_QHttp(_q_arg0);
QScriptValue _q_result = context->engine()->newQObject(context->thisObject(), (QHttp*)_q_cpp_result, QScriptEngine::AutoOwnership);
_q_cpp_result->__qtscript_self = _q_result;
return _q_result;
} else if (context->argument(0).isString()) {
QString _q_arg0 = context->argument(0).toString();
QtScriptShell_QHttp* _q_cpp_result = new QtScriptShell_QHttp(_q_arg0);
QScriptValue _q_result = context->engine()->newQObject(context->thisObject(), (QHttp*)_q_cpp_result, QScriptEngine::AutoOwnership);
_q_cpp_result->__qtscript_self = _q_result;
return _q_result;
}
} else if (context->argumentCount() == 2) {
if (context->argument(0).isString()
&& (qMetaTypeId<QHttp::ConnectionMode>() == context->argument(1).toVariant().userType())) {
QString _q_arg0 = context->argument(0).toString();
QHttp::ConnectionMode _q_arg1 = qscriptvalue_cast<QHttp::ConnectionMode>(context->argument(1));
QtScriptShell_QHttp* _q_cpp_result = new QtScriptShell_QHttp(_q_arg0, _q_arg1);
QScriptValue _q_result = context->engine()->newQObject(context->thisObject(), (QHttp*)_q_cpp_result, QScriptEngine::AutoOwnership);
_q_cpp_result->__qtscript_self = _q_result;
return _q_result;
} else if (context->argument(0).isString()
&& context->argument(1).isNumber()) {
QString _q_arg0 = context->argument(0).toString();
unsigned short _q_arg1 = qscriptvalue_cast<unsigned short>(context->argument(1));
QtScriptShell_QHttp* _q_cpp_result = new QtScriptShell_QHttp(_q_arg0, _q_arg1);
QScriptValue _q_result = context->engine()->newQObject(context->thisObject(), (QHttp*)_q_cpp_result, QScriptEngine::AutoOwnership);
_q_cpp_result->__qtscript_self = _q_result;
return _q_result;
}
} else if (context->argumentCount() == 3) {
if (context->argument(0).isString()
&& (qMetaTypeId<QHttp::ConnectionMode>() == context->argument(1).toVariant().userType())
&& context->argument(2).isNumber()) {
QString _q_arg0 = context->argument(0).toString();
QHttp::ConnectionMode _q_arg1 = qscriptvalue_cast<QHttp::ConnectionMode>(context->argument(1));
unsigned short _q_arg2 = qscriptvalue_cast<unsigned short>(context->argument(2));
QtScriptShell_QHttp* _q_cpp_result = new QtScriptShell_QHttp(_q_arg0, _q_arg1, _q_arg2);
QScriptValue _q_result = context->engine()->newQObject(context->thisObject(), (QHttp*)_q_cpp_result, QScriptEngine::AutoOwnership);
_q_cpp_result->__qtscript_self = _q_result;
return _q_result;
} else if (context->argument(0).isString()
&& context->argument(1).isNumber()
&& context->argument(2).isQObject()) {
QString _q_arg0 = context->argument(0).toString();
unsigned short _q_arg1 = qscriptvalue_cast<unsigned short>(context->argument(1));
QObject* _q_arg2 = context->argument(2).toQObject();
QtScriptShell_QHttp* _q_cpp_result = new QtScriptShell_QHttp(_q_arg0, _q_arg1, _q_arg2);
QScriptValue _q_result = context->engine()->newQObject(context->thisObject(), (QHttp*)_q_cpp_result, QScriptEngine::AutoOwnership);
_q_cpp_result->__qtscript_self = _q_result;
return _q_result;
}
} else if (context->argumentCount() == 4) {
QString _q_arg0 = context->argument(0).toString();
QHttp::ConnectionMode _q_arg1 = qscriptvalue_cast<QHttp::ConnectionMode>(context->argument(1));
unsigned short _q_arg2 = qscriptvalue_cast<unsigned short>(context->argument(2));
QObject* _q_arg3 = context->argument(3).toQObject();
QtScriptShell_QHttp* _q_cpp_result = new QtScriptShell_QHttp(_q_arg0, _q_arg1, _q_arg2, _q_arg3);
QScriptValue _q_result = context->engine()->newQObject(context->thisObject(), (QHttp*)_q_cpp_result, QScriptEngine::AutoOwnership);
_q_cpp_result->__qtscript_self = _q_result;
return _q_result;
}
break;
default:
Q_ASSERT(false);
}
return qtscript_QHttp_throw_ambiguity_error_helper(context,
qtscript_QHttp_function_names[_id],
qtscript_QHttp_function_signatures[_id]);
}
static QScriptValue qtscript_QHttp_toScriptValue(QScriptEngine *engine, QHttp* const &in)
{
return engine->newQObject(in, QScriptEngine::QtOwnership, QScriptEngine::PreferExistingWrapperObject);
}
static void qtscript_QHttp_fromScriptValue(const QScriptValue &value, QHttp* &out)
{
out = qobject_cast<QHttp*>(value.toQObject());
}
QScriptValue qtscript_create_QHttp_class(QScriptEngine *engine)
{
engine->setDefaultPrototype(qMetaTypeId<QHttp*>(), QScriptValue());
QScriptValue proto = engine->newVariant(qVariantFromValue((QHttp*)0));
proto.setPrototype(engine->defaultPrototype(qMetaTypeId<QObject*>()));
for (int i = 0; i < 23; ++i) {
QScriptValue fun = engine->newFunction(qtscript_QHttp_prototype_call, qtscript_QHttp_function_lengths[i+1]);
fun.setData(QScriptValue(engine, uint(0xBABE0000 + i)));
proto.setProperty(QString::fromLatin1(qtscript_QHttp_function_names[i+1]),
fun, QScriptValue::SkipInEnumeration);
}
qScriptRegisterMetaType<QHttp*>(engine, qtscript_QHttp_toScriptValue,
qtscript_QHttp_fromScriptValue, proto);
QScriptValue ctor = engine->newFunction(qtscript_QHttp_static_call, proto, qtscript_QHttp_function_lengths[0]);
ctor.setData(QScriptValue(engine, uint(0xBABE0000 + 0)));
ctor.setProperty(QString::fromLatin1("Error"),
qtscript_create_QHttp_Error_class(engine, ctor));
ctor.setProperty(QString::fromLatin1("ConnectionMode"),
qtscript_create_QHttp_ConnectionMode_class(engine, ctor));
ctor.setProperty(QString::fromLatin1("State"),
qtscript_create_QHttp_State_class(engine, ctor));
return ctor;
}
| {
"pile_set_name": "Github"
} |
// hat_yaw00_lev2_p43.mh:
#ifndef stasm_hat_yaw00_lev2_p43_mh
#define stasm_hat_yaw00_lev2_p43_mh
namespace stasm {
// tasm -V1 /b/stasm/train/conf/tasm_muct77.conf
// static const int EYEMOUTH_DIST = 100;
// static const int FACESCALE = 1
// static const int PYRSCALE = 1
// static const double SIGMOIDSCALE = 0
// static const double PYR_RATIO = 2;
// static const int NEGTRAIN_SEED = 2013;
// static const int HAT_PATCH_WIDTH = 19;
// static const int HAT_PATCH_WIDTH_ADJ = -6;
// static const int GRIDHEIGHT = 4;
// static const int GRIDWIDTH = 5;
// static const int BINS_PER_HIST = 8;
// static const double WINDOW_SCALE = 0.5;
static double hatfit_yaw00_lev2_p43(const double* const d) // d has 160 elements
{
const double intercept = 0.00648366;
const double coef[160] =
{
-0.0152163, -0.00938193, -0.0190419, -0.0687831, -0.0468449, -0.0880119, 0.0812563, -0.00542163,
-0.0303208, 0.00806224, 0.0623734, 0.0413201, -0.00380485, -0.0133008, -0.00241608, -0.0323745,
-0.0738325, -0.0242139, 0.0190181, 0.0157716, 0.0080979, 0.00166531, -0.0480585, -0.0434921,
-0.0302065, -0.0234743, -0.0184186, 0.0244355, 0.0155824, 0.0468844, 0.0080336, 0.00117218,
-0.0331888, -0.0584217, -0.0338365, -0.026523, -0.0119799, 0.0596711, 0.0115523, -0.0122433,
-0.0458657, -0.0397486, 0.00973897, 0.0146185, 0.069101, -0.0167322, 0.0669437, 0.0339211,
0.00604615, 0.00201924, -0.0142197, -0.0430294, -0.00720905, -0.0326489, -0.0359383, 0.0285836,
0.0545388, 0.0379828, 0.0598856, 0.0112929, -0.0060677, -0.00368719, 0.0259153, 0.0502069,
0.0226217, 0.0012846, 0.0148117, -0.0016945, -0.000385082, 0.00444569, 0.0713966, 0.090965,
0.0507963, -0.00174389, -0.0463333, 0.00418675, 0.0053341, -0.0537939, -0.0550999, 0.0945129,
0.113893, -0.0139617, 0.0316123, 0.0529792, 0.0495666, 0.0340494, -0.039953, 0.0260375,
0.00641793, 0.0377733, -0.0625308, -0.0868844, -0.048856, 0.0186374, -0.0411507, -0.0667005,
0.0029778, 0.0232979, 0.0265188, 0.0512988, 0.0191031, 0.00196293, -0.0345508, -0.0318195,
0.00777352, 0.0336489, 0.0340635, 0.0523148, 0.03348, 0.0224716, 0.0471509, -0.0111932,
0.0163388, 0.0360007, -0.0550825, -0.0319889, 0.00674877, 0.0246315, 0.0166669, -0.00832142,
-0.0104868, -0.0427722, 0.0921329, 0.0220887, -0.0355666, -0.0185576, -0.00916089, -0.0092353,
-0.0317364, 0.0305874, 0.00675156, -0.0249873, 0.01326, 0.0109879, -0.016378, 0.00128751,
-0.0210845, -0.0114771, -0.00718837, -0.00888316, -0.0110957, 0.0127997, 0.0179059, 0.0174434,
-0.0127198, -0.0120589, -0.0196548, -0.0179925, -0.071387, 0.00189062, -0.0285034, 0.0140863,
-0.0971454, 0.0467506, -0.00717021, -0.0347361, -0.0281356, -0.0441394, -0.0500878, -0.0092695
};
return linmod(d, intercept, coef, 160);
}
static const HatDescMod hat_yaw00_lev2_p43(hatfit_yaw00_lev2_p43);
} // namespace stasm
#endif // stasm_hat_yaw00_lev2_p43_mh
| {
"pile_set_name": "Github"
} |
### WinUI 3
To build Fluent experiences on Windows using WinUI controls, please see our [WinUI controls documentation](https://docs.microsoft.com/en-us/windows/uwp/design/controls-and-patterns/).
### Fluent UI React Native
To build Fluent experiences on Windows using Fluent UI React Native, please see our [Cross-platform Controls page](#/controls/crossplatform).
| {
"pile_set_name": "Github"
} |
/**
* Copyright 2013-2020 the original author or authors from the JHipster project.
*
* This file is part of the JHipster project, see http://www.jhipster.tech/
* for more information.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
const Options = {
DTO: 'dto',
SERVICE: 'service',
PAGINATION: 'pagination',
MICROSERVICE: 'microservice',
SEARCH: 'search',
ANGULAR_SUFFIX: 'angularSuffix',
CLIENT_ROOT_FOLDER: 'clientRootFolder'
};
const optionNames = Object.values(Options);
const Values = {
[Options.DTO]: { MAPSTRUCT: 'mapstruct' },
[Options.SERVICE]: { SERVICE_CLASS: 'serviceClass', SERVICE_IMPL: 'serviceImpl' },
[Options.PAGINATION]: {
PAGINATION: 'pagination',
'INFINITE-SCROLL': 'infinite-scroll'
},
[Options.SEARCH]: { ELASTIC_SEARCH: 'elasticsearch', COUCHBASE: 'couchbase' }
};
function forEach(passedFunction) {
if (!passedFunction) {
throw new Error('A function has to be passed to loop over the binary options.');
}
optionNames.forEach(passedFunction);
}
function exists(passedOption, passedValue) {
return (
!Object.values(Options).includes(passedOption) ||
Object.values(Options).some(
option =>
passedOption === option &&
(passedOption === Options.MICROSERVICE ||
passedOption === Options.ANGULAR_SUFFIX ||
passedOption === Options.CLIENT_ROOT_FOLDER ||
Object.values(Values[option]).includes(passedValue))
)
);
}
module.exports = {
Options,
Values,
exists,
forEach
};
| {
"pile_set_name": "Github"
} |
DROP TABLE IF EXISTS DECIMAL_6_1;
DROP TABLE IF EXISTS DECIMAL_6_2;
DROP TABLE IF EXISTS DECIMAL_6_3;
CREATE TABLE DECIMAL_6_1(key decimal(10,5), value int)
ROW FORMAT DELIMITED
FIELDS TERMINATED BY ' '
STORED AS TEXTFILE;
CREATE TABLE DECIMAL_6_2(key decimal(17,4), value int)
ROW FORMAT DELIMITED
FIELDS TERMINATED BY ' '
STORED AS TEXTFILE;
LOAD DATA LOCAL INPATH '../../data/files/kv9.txt' INTO TABLE DECIMAL_6_1;
LOAD DATA LOCAL INPATH '../../data/files/kv9.txt' INTO TABLE DECIMAL_6_2;
SELECT T.key from (
SELECT key, value from DECIMAL_6_1
UNION ALL
SELECT key, value from DECIMAL_6_2
) T order by T.key;
CREATE TABLE DECIMAL_6_3 AS SELECT key + 5.5 AS k, value * 11 AS v from DECIMAL_6_1 ORDER BY v;
desc DECIMAL_6_3;
| {
"pile_set_name": "Github"
} |
package com.xiaolyuh.service.impl;
import com.github.pagehelper.Page;
import com.github.pagehelper.PageHelper;
import com.xiaolyuh.domain.mapper.PersonMapper;
import com.xiaolyuh.domain.model.Person;
import com.xiaolyuh.service.PersonService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import java.util.List;
import java.util.concurrent.atomic.AtomicInteger;
/**
* Created by yuhao.wang on 2017/6/19.
*/
@Service
public class PersonServiceImpl implements PersonService {
@Autowired
private PersonMapper personMapper;
public static AtomicInteger atomicInteger = new AtomicInteger();
@Override
public List<Person> findAll() {
return personMapper.findAll();
}
@Override
public Page<Person> findByPage(int pageNo, int pageSize) {
PageHelper.startPage(pageNo, pageSize);
return personMapper.findByPage();
}
@Override
@Transactional(rollbackFor = Exception.class)
public void insert(Person person) {
personMapper.insert(person);
}
@Override
@Transactional(rollbackFor = Exception.class)
public int updateAge(long id) {
int result = personMapper.updateAge(id);
int i = atomicInteger.getAndIncrement();
if (i > 990) {
System.out.println(i);
}
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
}
return result;
}
}
| {
"pile_set_name": "Github"
} |
<refentry xmlns="http://docbook.org/ns/docbook"
xmlns:xlink="http://www.w3.org/1999/xlink"
xmlns:xi="http://www.w3.org/2001/XInclude"
xmlns:src="http://nwalsh.com/xmlns/litprog/fragment"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform"
version="5.0" xml:id="ulink.footnotes">
<refmeta>
<refentrytitle>ulink.footnotes</refentrytitle>
<refmiscinfo class="other" otherclass="datatype">boolean</refmiscinfo>
</refmeta>
<refnamediv>
<refname>ulink.footnotes</refname>
<refpurpose>Generate footnotes for <tag>ulink</tag>s?</refpurpose>
</refnamediv>
<refsynopsisdiv>
<src:fragment xml:id="ulink.footnotes.frag">
<xsl:param name="ulink.footnotes" select="0"/>
</src:fragment>
</refsynopsisdiv>
<refsection><info><title>Description</title></info>
<para>If non-zero, and if <parameter>ulink.show</parameter> also is non-zero,
the URL of each <tag>ulink</tag> will appear as a footnote.</para>
<note><para>DocBook 5 does not have an <tag>ulink</tag> element. When processing
DocBoook 5 documents, <parameter>ulink.footnotes</parameter> applies to all inline
elements that are marked up with <tag class="attribute">xlink:href</tag> attributes
that point to external resources.</para>
</note>
</refsection>
</refentry>
| {
"pile_set_name": "Github"
} |
// Copyright 2014 The Go Authors. All rights reserved.
// Use of this source code is governed by a BSD-style
// license that can be found in the LICENSE file.
// +build !darwin,!freebsd,!linux,!solaris
package ipv4
import (
"net"
"golang.org/x/net/internal/socket"
)
func (so *sockOpt) setGroupReq(c *socket.Conn, ifi *net.Interface, grp net.IP) error {
return errNotImplemented
}
func (so *sockOpt) setGroupSourceReq(c *socket.Conn, ifi *net.Interface, grp, src net.IP) error {
return errNotImplemented
}
| {
"pile_set_name": "Github"
} |
#define _GNU_SOURCE
#include <errno.h>
#include <sched.h>
#include "syscall.h"
#include "atomic.h"
#ifdef VDSO_GETCPU_SYM
static void *volatile vdso_func;
typedef long (*getcpu_f)(unsigned *, unsigned *, void *);
static long getcpu_init(unsigned *cpu, unsigned *node, void *unused)
{
void *p = __vdsosym(VDSO_GETCPU_VER, VDSO_GETCPU_SYM);
getcpu_f f = (getcpu_f)p;
a_cas_p(&vdso_func, (void *)getcpu_init, p);
return f ? f(cpu, node, unused) : -ENOSYS;
}
static void *volatile vdso_func = (void *)getcpu_init;
#endif
int sched_getcpu(void)
{
int r;
unsigned cpu;
#ifdef VDSO_GETCPU_SYM
getcpu_f f = (getcpu_f)vdso_func;
if (f) {
r = f(&cpu, 0, 0);
if (!r) return cpu;
if (r != -ENOSYS) return __syscall_ret(r);
}
#endif
r = __syscall(SYS_getcpu, &cpu, 0, 0);
if (!r) return cpu;
return __syscall_ret(r);
}
| {
"pile_set_name": "Github"
} |
//Copyright (c) ServiceStack, Inc. All Rights Reserved.
//License: https://raw.github.com/ServiceStack/ServiceStack/master/license.txt
using System;
using System.Collections;
using System.Collections.Generic;
namespace ServiceStack.Data
{
public interface IEntityStore : IDisposable
{
T GetById<T>(object id);
IList<T> GetByIds<T>(ICollection ids);
T Store<T>(T entity);
void StoreAll<TEntity>(IEnumerable<TEntity> entities);
void Delete<T>(T entity);
void DeleteById<T>(object id);
void DeleteByIds<T>(ICollection ids);
void DeleteAll<TEntity>();
}
} | {
"pile_set_name": "Github"
} |
test_noargs
Example program is called without arguments so that default range
[0..9] is used.
test_2_to_2000
Example program is called with "2" and "2000" as arguments.
test_overflow
Example program is called with "0" and "100000" as arguments. The
resulting sum is too large to be stored as an int variable.
| {
"pile_set_name": "Github"
} |
---
layout : blocks/working-session
title : Top 10 2017 - Call for Data and Weightings Discussion
type : workshop
track : Owasp Top 10 2017
technology :
related-to :
status : done
when-day : Mon
when-time : PM-1
location : Room-2
organizers : Dave Wichers, Andrew Van Der Stock
participants : Amani Altarawneh, Chris Cooper, Christian DeHoyos, Daniel Miessler, Erez Yalon, Jason Li, Jonas vanalderweireldt, Kevin Greene, Nuno Loureiro, Sandor Lenart, Tiago Mendo, Tiffany Long, Torsten Gigler
outcomes : mapped
---
## Why
## What
What sort of data does the Top 10 need, and where/who do we ask for it, and how do we weight the various types of responses (see Brian Glas' blog for "tools augmented with humans" vs "humans with augmented tooling").
This weightings of data will help define the approach in OWASP Top 10 2017 RC2, and also be used in the 2020 and 2023 OWASP Top 10. I want the community to drive this discussion.
## Outcomes
### Synopsis and Takeaways
We talked about how data was collected and the process by which it was analysed. For Top 10 2017, there was an open call for data, but it wasn't widely reported nor sufficiently pushed once open. This possibly resulted in fewer responses than in a perfect world. There was a lot of discussion around the process such as "if we use data scientists, can we use the existing data?", and "should we re-open the data collection?"
It was incredibly valuable discussion, and struck good pragmatic balance. We want to drive a release this year, but RC2 will not come out this week, so we will not be running editing / creating sessions this week, but will instead work on collecting some more data.
The outcomes from this session were:
- A data collection process and timeline will be published to the wiki to make sure everyone knows how data is collected and analysed, and when the next data call will be held. Some of this will appear in the text, probably an appendix to make sure that our process is transparent and open.
- Andrew van der Stock will work on a process with Foundation staff to ensure that we can maximise publicity for the next data call round in 2019. There was suggestion to keep it open all the time, but this was felt to be unworkable without a data scientist to volunteer. For smaller consultancies, obtaining this data is already difficult, and we don't want people to be overly burdened by the data call.
- A data call extension will be pushed out for interested parties. Andrew will take care of this on Tuesday 12 June, 2017. As long as data is roughly in the same Excel format as the existing data and provided by the end of July, It ought to be possible to use it.
- Dave Wichers will reach out to Brian Glas for feedback for tomorrow morning's data weighting session.
- For 2020, we will try to find data scientists to help us to improve our data methodology and analysis, so we can at least ensure that data drives inclusion for the non-forward looking data.
- Ordering will never be strictly data order; to provide continuity, there is a decision (which will now be documented) that if A1 ... A3 in 2010 are the same in 2017 but in a slightly different order, those will retain a previous order. This helps folks map results year on year and prevents massive upheaval between years.
- Feedback obtained from the OWASP Top 10 mail list will end up in Git Hub tomorrow as issues. For feedback sent privately to Dave, Andrew will reach out to these individuals to ask permission to create issues at GitHub. This will help with project transparency. From now on, if you have feedback, please provide it at GitHub: https://github.com/OWASP/Top10/issues
## Who
The target audience for this Working Session is:
- OWASP Top 10 2017 Track participants
## References
---
## Working materials
| {
"pile_set_name": "Github"
} |
# Copyright 2018/2019 The RLgraph authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
from __future__ import absolute_import, division, print_function
from rlgraph import get_backend
from rlgraph.components.component import Component
from rlgraph.utils.decorators import rlgraph_api
from rlgraph.utils.ops import DataOpDict, DataOpTuple, FLATTEN_SCOPE_PREFIX
class ContainerMerger(Component):
"""
Merges incoming items into one FlattenedDataOp.
"""
def __init__(self, *input_names_or_num_items, **kwargs):
"""
Args:
*input_names_or_num_items (Union[str,int]): List of the names of the different inputs in the
order they will be passed into the `merge` API-method in the returned merged Dict.
Or the number of items in the Tuple to be merged.
Example:
input_names_or_num_items = ["A", "B"]
- merge(Dict(c=1, d=2), Tuple(3, 4))
- returned value: Dict(A=Dict(c=1, d=2), B=Tuple(3, 4))
input_names_or_num_items = 3: 3 items will be merged into a Tuple.
Keyword Args:
merge_tuples_into_one (bool): Whether to merge incoming DataOpTuples into one single DataOpTuple.
If True: tupleA + tupleB -> (tupleA[0] + tupleA[1] + tupleA[...] + tupleB[0] + tupleB[1] ...).
If False: tupleA + tupleB -> (tupleA + tupleB).
is_tuple (bool): Whether we should merge a tuple.
"""
self.merge_tuples_into_one = kwargs.pop("merge_tuples_into_one", False)
self.is_tuple = kwargs.pop("is_tuple", self.merge_tuples_into_one)
super(ContainerMerger, self).__init__(scope=kwargs.pop("scope", "container-merger"), **kwargs)
self.dict_keys = None
if len(input_names_or_num_items) == 1 and isinstance(input_names_or_num_items[0], int):
self.is_tuple = True
else:
# and not re.search(r'/', i)
# or some of them have '/' characters in them, which are not allowed
assert all(isinstance(i, str) for i in input_names_or_num_items), \
"ERROR: Not all input names of DictMerger Component '{}' are strings.".format(self.global_scope)
self.dict_keys = input_names_or_num_items
def check_input_spaces(self, input_spaces, action_space=None):
spaces = []
idx = 0
while True:
key = "inputs[{}]".format(idx)
if key not in input_spaces:
break
spaces.append(input_spaces[key])
idx += 1
# If Tuple -> Incoming inputs could be of any number.
if self.dict_keys:
len_ = len(self.dict_keys)
assert len(spaces) == len_,\
"ERROR: Number of incoming Spaces ({}) does not match number of given `dict_keys` ({}) in" \
"ContainerMerger Component '{}'!".format(len(spaces), len_, self.global_scope)
@rlgraph_api
def _graph_fn_merge(self, *inputs):
"""
Merges the inputs into a single DataOpDict OR DataOpTuple with the flat keys given in `self.dict_keys`.
Args:
*inputs (FlattenedDataOp): The input items to be merged into a ContainerDataOp.
Returns:
ContainerDataOp: The DataOpDict or DataOpTuple as a merger of all *inputs.
"""
if self.is_tuple is True:
ret = []
for op in inputs:
# Merge single items inside a DataOpTuple into resulting tuple.
if self.merge_tuples_into_one and isinstance(op, DataOpTuple):
ret.extend(list(op))
# Strict by-input merging.
else:
ret.append(op)
return DataOpTuple(ret)
else:
ret = DataOpDict()
for i, op in enumerate(inputs):
if get_backend() == "pytorch" and self.execution_mode == "define_by_run":
ret[FLATTEN_SCOPE_PREFIX + self.dict_keys[i]] = op
else:
ret[self.dict_keys[i]] = op
return ret
| {
"pile_set_name": "Github"
} |
// RUN: %clang_cc1 -fsyntax-only -verify %s
#include "Inputs/cuda.h"
// expected-no-diagnostics
// Check that we can handle gnu_inline functions when compiling in CUDA mode.
void foo();
inline __attribute__((gnu_inline)) void bar() { foo(); }
| {
"pile_set_name": "Github"
} |
// Copyright 2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
// SPDX-License-Identifier: Apache-2.0
package software.aws.toolkits.jetbrains.services.lambda.nodejs
import com.intellij.openapi.roots.ProjectFileIndex
import com.intellij.openapi.vfs.VfsUtilCore
import com.intellij.testFramework.runInEdtAndWait
import org.assertj.core.api.Assertions.assertThat
import org.junit.Rule
import org.junit.Test
import software.aws.toolkits.jetbrains.utils.rules.NodeJsCodeInsightTestFixtureRule
import software.aws.toolkits.jetbrains.utils.rules.addLambdaHandler
import software.aws.toolkits.jetbrains.utils.rules.addPackageJsonFile
class NodeJsHelperTest {
@Rule
@JvmField
val projectRule = NodeJsCodeInsightTestFixtureRule()
@Test
fun inferSourceRoot_noPackageJsonReturnsContentRoot() {
val element = projectRule.fixture.addLambdaHandler(
subPath = "foo/bar",
fileName = "app.js",
handlerName = "someHandler"
)
runInEdtAndWait {
val contentRoot = ProjectFileIndex.getInstance(projectRule.project).getContentRootForFile(element.containingFile.virtualFile)
val sourceRoot = inferSourceRoot(projectRule.project, element.containingFile.virtualFile)
assertThat(contentRoot).isEqualTo(sourceRoot)
}
}
@Test
fun inferSourceRoot_packageJsonInSubFolder() {
val element = projectRule.fixture.addLambdaHandler(
subPath = "foo/bar",
fileName = "app.js",
handlerName = "someHandler"
)
projectRule.fixture.addPackageJsonFile(
subPath = "foo"
)
runInEdtAndWait {
val contentRoot = ProjectFileIndex.getInstance(projectRule.project).getContentRootForFile(element.containingFile.virtualFile)
val sourceRoot = inferSourceRoot(projectRule.project, element.containingFile.virtualFile)
assertThat(VfsUtilCore.findRelativeFile("foo", contentRoot)).isEqualTo(sourceRoot)
}
}
@Test
fun inferSourceRoot_packageJsonInRootFolder() {
val element = projectRule.fixture.addLambdaHandler(
subPath = "foo/bar",
fileName = "app.js",
handlerName = "someHandler"
)
projectRule.fixture.addPackageJsonFile(
subPath = "."
)
runInEdtAndWait {
val contentRoot = ProjectFileIndex.getInstance(projectRule.project).getContentRootForFile(element.containingFile.virtualFile)
val sourceRoot = inferSourceRoot(projectRule.project, element.containingFile.virtualFile)
assertThat(contentRoot).isEqualTo(sourceRoot)
}
}
}
| {
"pile_set_name": "Github"
} |
/*
* This file is part of mpv.
*
* mpv is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* mpv is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with mpv. If not, see <http://www.gnu.org/licenses/>.
*/
#include "config.h"
#include "ao.h"
#include "internal.h"
#include "audio/format.h"
#include "osdep/timer.h"
#include "options/m_option.h"
#include "common/msg.h"
#include "ao_coreaudio_utils.h"
#include "ao_coreaudio_chmap.h"
#import <AudioUnit/AudioUnit.h>
#import <CoreAudio/CoreAudioTypes.h>
#import <AudioToolbox/AudioToolbox.h>
#import <AVFoundation/AVFoundation.h>
#import <mach/mach_time.h>
struct priv {
AudioUnit audio_unit;
double device_latency;
};
static OSStatus render_cb_lpcm(void *ctx, AudioUnitRenderActionFlags *aflags,
const AudioTimeStamp *ts, UInt32 bus,
UInt32 frames, AudioBufferList *buffer_list)
{
struct ao *ao = ctx;
struct priv *p = ao->priv;
void *planes[MP_NUM_CHANNELS] = {0};
for (int n = 0; n < ao->num_planes; n++)
planes[n] = buffer_list->mBuffers[n].mData;
int64_t end = mp_time_us();
end += p->device_latency * 1e6;
end += ca_get_latency(ts) + ca_frames_to_us(ao, frames);
ao_read_data(ao, planes, frames, end);
return noErr;
}
static bool init_audiounit(struct ao *ao)
{
AudioStreamBasicDescription asbd;
OSStatus err;
uint32_t size;
struct priv *p = ao->priv;
AVAudioSession *instance = AVAudioSession.sharedInstance;
AVAudioSessionPortDescription *port = nil;
NSInteger maxChannels = instance.maximumOutputNumberOfChannels;
NSInteger prefChannels = MIN(maxChannels, ao->channels.num);
[instance setCategory:AVAudioSessionCategoryPlayback error:nil];
[instance setMode:AVAudioSessionModeMoviePlayback error:nil];
[instance setActive:YES error:nil];
[instance setPreferredOutputNumberOfChannels:prefChannels error:nil];
AudioComponentDescription desc = (AudioComponentDescription) {
.componentType = kAudioUnitType_Output,
.componentSubType = kAudioUnitSubType_RemoteIO,
.componentManufacturer = kAudioUnitManufacturer_Apple,
.componentFlags = 0,
.componentFlagsMask = 0,
};
AudioComponent comp = AudioComponentFindNext(NULL, &desc);
if (comp == NULL) {
MP_ERR(ao, "unable to find audio component\n");
goto coreaudio_error;
}
err = AudioComponentInstanceNew(comp, &(p->audio_unit));
CHECK_CA_ERROR("unable to open audio component");
err = AudioUnitInitialize(p->audio_unit);
CHECK_CA_ERROR_L(coreaudio_error_component,
"unable to initialize audio unit");
if (af_fmt_is_spdif(ao->format) || instance.outputNumberOfChannels <= 2) {
ao->channels = (struct mp_chmap)MP_CHMAP_INIT_STEREO;
} else {
port = instance.currentRoute.outputs.firstObject;
if (port.channels.count == 2 &&
port.channels[0].channelLabel == kAudioChannelLabel_Unknown) {
// Special case when using an HDMI adapter. The iOS device will
// perform SPDIF conversion for us, so send all available channels
// using the AC3 mapping.
ao->channels = (struct mp_chmap)MP_CHMAP6(FL, FC, FR, SL, SR, LFE);
} else {
ao->channels.num = (uint8_t)port.channels.count;
for (AVAudioSessionChannelDescription *ch in port.channels) {
ao->channels.speaker[ch.channelNumber - 1] =
ca_label_to_mp_speaker_id(ch.channelLabel);
}
}
}
ca_fill_asbd(ao, &asbd);
size = sizeof(AudioStreamBasicDescription);
err = AudioUnitSetProperty(p->audio_unit,
kAudioUnitProperty_StreamFormat,
kAudioUnitScope_Input, 0, &asbd, size);
CHECK_CA_ERROR_L(coreaudio_error_audiounit,
"unable to set the input format on the audio unit");
AURenderCallbackStruct render_cb = (AURenderCallbackStruct) {
.inputProc = render_cb_lpcm,
.inputProcRefCon = ao,
};
err = AudioUnitSetProperty(p->audio_unit,
kAudioUnitProperty_SetRenderCallback,
kAudioUnitScope_Input, 0, &render_cb,
sizeof(AURenderCallbackStruct));
CHECK_CA_ERROR_L(coreaudio_error_audiounit,
"unable to set render callback on audio unit");
return true;
coreaudio_error_audiounit:
AudioUnitUninitialize(p->audio_unit);
coreaudio_error_component:
AudioComponentInstanceDispose(p->audio_unit);
coreaudio_error:
return false;
}
static void stop(struct ao *ao)
{
struct priv *p = ao->priv;
OSStatus err = AudioOutputUnitStop(p->audio_unit);
CHECK_CA_WARN("can't stop audio unit");
}
static void start(struct ao *ao)
{
struct priv *p = ao->priv;
AVAudioSession *instance = AVAudioSession.sharedInstance;
p->device_latency = [instance outputLatency] + [instance IOBufferDuration];
OSStatus err = AudioOutputUnitStart(p->audio_unit);
CHECK_CA_WARN("can't start audio unit");
}
static void uninit(struct ao *ao)
{
struct priv *p = ao->priv;
AudioOutputUnitStop(p->audio_unit);
AudioUnitUninitialize(p->audio_unit);
AudioComponentInstanceDispose(p->audio_unit);
[AVAudioSession.sharedInstance
setActive:NO
withOptions:AVAudioSessionSetActiveOptionNotifyOthersOnDeactivation
error:nil];
}
static int init(struct ao *ao)
{
if (!init_audiounit(ao))
goto coreaudio_error;
return CONTROL_OK;
coreaudio_error:
return CONTROL_ERROR;
}
#define OPT_BASE_STRUCT struct priv
const struct ao_driver audio_out_audiounit = {
.description = "AudioUnit (iOS)",
.name = "audiounit",
.uninit = uninit,
.init = init,
.reset = stop,
.start = start,
.priv_size = sizeof(struct priv),
};
| {
"pile_set_name": "Github"
} |
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/net_cellular"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.pencilbox.netknight.view.NetCellular">
<LinearLayout
android:id="@+id/topchart"
android:layout_width="match_parent"
android:layout_height="match_parent">
<com.github.mikephil.charting.charts.LineChart
android:id="@+id/celluar_chart"
android:layout_width="match_parent"
android:layout_height="match_parent">
</com.github.mikephil.charting.charts.LineChart>
</LinearLayout>
</RelativeLayout>
| {
"pile_set_name": "Github"
} |
(:name howm
:website "http://howm.sourceforge.jp/"
:description "Write fragmentarily and read collectively."
:type http-tar
:options ("xzf")
:url "http://howm.sourceforge.jp/a/howm-1.4.4.tar.gz"
:build `(("./configure" ,(concat "--with-emacs=" el-get-emacs)) ("make")))
| {
"pile_set_name": "Github"
} |
0.7.1 / 2015-04-20
==================
* prevent extraordinary long inputs (@evilpacket)
* Fixed broken readme link
0.7.0 / 2014-11-24
==================
* add time abbreviations, updated tests and readme for the new units
* fix example in the readme.
* add LICENSE file
0.6.2 / 2013-12-05
==================
* Adding repository section to package.json to suppress warning from NPM.
0.6.1 / 2013-05-10
==================
* fix singularization [visionmedia]
0.6.0 / 2013-03-15
==================
* fix minutes
0.5.1 / 2013-02-24
==================
* add component namespace
0.5.0 / 2012-11-09
==================
* add short formatting as default and .long option
* add .license property to component.json
* add version to component.json
0.4.0 / 2012-10-22
==================
* add rounding to fix crazy decimals
0.3.0 / 2012-09-07
==================
* fix `ms(<String>)` [visionmedia]
0.2.0 / 2012-09-03
==================
* add component.json [visionmedia]
* add days support [visionmedia]
* add hours support [visionmedia]
* add minutes support [visionmedia]
* add seconds support [visionmedia]
* add ms string support [visionmedia]
* refactor tests to facilitate ms(number) [visionmedia]
0.1.0 / 2012-03-07
==================
* Initial release
| {
"pile_set_name": "Github"
} |
/**
* SyntaxHighlighter
* http://alexgorbatchev.com/
*
* SyntaxHighlighter is donationware. If you are using it, please donate.
* http://alexgorbatchev.com/wiki/SyntaxHighlighter:Donate
*
* @version
* 2.0.320 (May 03 2009)
*
* @copyright
* Copyright (C) 2004-2009 Alex Gorbatchev.
*
* @license
* This file is part of SyntaxHighlighter.
*
* SyntaxHighlighter is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* SyntaxHighlighter is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with SyntaxHighlighter. If not, see <http://www.gnu.org/copyleft/lesser.html>.
*/
SyntaxHighlighter.brushes.Perl = function()
{
// Contributed by David Simmons-Duffin and Marty Kube
var funcs =
'abs accept alarm atan2 bind binmode chdir chmod chomp chop chown chr ' +
'chroot close closedir connect cos crypt defined delete each endgrent ' +
'endhostent endnetent endprotoent endpwent endservent eof exec exists ' +
'exp fcntl fileno flock fork format formline getc getgrent getgrgid ' +
'getgrnam gethostbyaddr gethostbyname gethostent getlogin getnetbyaddr ' +
'getnetbyname getnetent getpeername getpgrp getppid getpriority ' +
'getprotobyname getprotobynumber getprotoent getpwent getpwnam getpwuid ' +
'getservbyname getservbyport getservent getsockname getsockopt glob ' +
'gmtime grep hex index int ioctl join keys kill lc lcfirst length link ' +
'listen localtime lock log lstat map mkdir msgctl msgget msgrcv msgsnd ' +
'oct open opendir ord pack pipe pop pos print printf prototype push ' +
'quotemeta rand read readdir readline readlink readpipe recv rename ' +
'reset reverse rewinddir rindex rmdir scalar seek seekdir select semctl ' +
'semget semop send setgrent sethostent setnetent setpgrp setpriority ' +
'setprotoent setpwent setservent setsockopt shift shmctl shmget shmread ' +
'shmwrite shutdown sin sleep socket socketpair sort splice split sprintf ' +
'sqrt srand stat study substr symlink syscall sysopen sysread sysseek ' +
'system syswrite tell telldir time times tr truncate uc ucfirst umask ' +
'undef unlink unpack unshift utime values vec wait waitpid warn write';
var keywords =
'bless caller continue dbmclose dbmopen die do dump else elsif eval exit ' +
'for foreach goto if import last local my next no our package redo ref ' +
'require return sub tie tied unless untie until use wantarray while';
this.regexList = [
{ regex: new RegExp('#[^!].*$', 'gm'), css: 'comments' },
{ regex: new RegExp('^\\s*#!.*$', 'gm'), css: 'preprocessor' }, // shebang
{ regex: SyntaxHighlighter.regexLib.doubleQuotedString, css: 'string' },
{ regex: SyntaxHighlighter.regexLib.singleQuotedString, css: 'string' },
{ regex: new RegExp('(\\$|@|%)\\w+', 'g'), css: 'variable' },
{ regex: new RegExp(this.getKeywords(funcs), 'gmi'), css: 'functions' },
{ regex: new RegExp(this.getKeywords(keywords), 'gm'), css: 'keyword' }
];
this.forHtmlScript(SyntaxHighlighter.regexLib.phpScriptTags);
}
SyntaxHighlighter.brushes.Perl.prototype = new SyntaxHighlighter.Highlighter();
SyntaxHighlighter.brushes.Perl.aliases = ['perl', 'Perl', 'pl']; | {
"pile_set_name": "Github"
} |
module com.networknt.monadresult {
exports com.networknt.monad;
requires com.networknt.status;
requires com.networknt.config;
requires com.networknt.utility;
requires java.management;
requires org.slf4j;
requires jdk.unsupported;
} | {
"pile_set_name": "Github"
} |
# Use public servers from the pool.ntp.org project.
server {{ groups['chrony'][0] }} iburst
# Ignor source level
stratumweight 0
# Record the rate at which the system clock gains/losses time.
driftfile /var/lib/chrony/drift
# Allow the system clock to be stepped in the first three updates
# if its offset is larger than 1 second.
makestep 1.0 3
# Enable kernel synchronization of the real-time clock (RTC).
rtcsync
# Enable hardware timestamping on all interfaces that support it.
#hwtimestamp *
# Increase the minimum number of selectable sources required to adjust
# the system clock.
#minsources 2
# Allow NTP client access from local network.
allow {{ local_network }}
#
bindcmdaddress 127.0.0.1
bindcmdaddress ::1
# Serve time even if not synchronized to a time source.
#local stratum 10
# Specify file containing keys for NTP authentication.
keyfile /etc/chrony.keys
# Specify directory for log files.
logdir /var/log/chrony
# Select which information is logged.
#log measurements statistics tracking
#
logchange 1
| {
"pile_set_name": "Github"
} |
import { Convert } from "../ExtensionMethods";
import { DateTime } from "../DateTime";
import { EwsServiceJsonReader } from "../Core/EwsServiceJsonReader";
import { ExchangeService } from "../Core/ExchangeService";
import { ExtendedPropertyCollection } from "../ComplexProperties/ExtendedPropertyCollection";
import { FailedSearchMailbox } from "./FailedSearchMailbox";
import { Importance } from "../Enumerations/Importance";
import { ItemId } from "../ComplexProperties/ItemId";
import { KeywordStatisticsSearchResult } from "./KeywordStatisticsSearchResult";
import { MailboxQuery } from "./MailboxQuery";
import { MailboxSearchLocation } from "../Enumerations/MailboxSearchLocation";
import { MailboxSearchScope } from "./MailboxSearchScope";
import { MailboxStatisticsItem } from "./MailboxStatisticsItem";
import { PreviewItemMailbox } from "./PreviewItemMailbox";
import { SearchPreviewItem } from "./SearchPreviewItem";
import { SearchRefinerItem } from "./SearchRefinerItem";
import { SearchResultType } from "../Enumerations/SearchResultType";
import { XmlElementNames } from "../Core/XmlElementNames";
/**
* Represents search mailbox result.
*
* @sealed
*/
export class SearchMailboxesResult {
/**
* Search queries
*/
SearchQueries: MailboxQuery[] = null;
/**
* Result type
*/
ResultType: SearchResultType = SearchResultType.StatisticsOnly;
/**
* Item count
*/
ItemCount: number = 0;
/**
* Total size
* [CLSCompliant(false)]
*/
Size: number = 0;
/**
* Page item count
*/
PageItemCount: number = 0;
/**
* Total page item size
* [CLSCompliant(false)]
*/
PageItemSize: number = 0;
/**
* Keyword statistics search result
*/
KeywordStats: KeywordStatisticsSearchResult[] = null;
/**
* Search preview items
*/
PreviewItems: SearchPreviewItem[] = null;
/**
* Failed mailboxes
*/
FailedMailboxes: FailedSearchMailbox[] = null;
/**
* Refiners
*/
Refiners: SearchRefinerItem[] = null;
/**
* Mailbox statistics
*/
MailboxStats: MailboxStatisticsItem[] = null;
/**
* Get collection of recipients
*
* @param {any} jsObject Json Object converted from XML.
* @return {string[]} Array of recipients
*/
private static GetRecipients(jsObject: any): string[] {
let recipients: string[] = EwsServiceJsonReader.ReadAsArray(jsObject, XmlElementNames.SmtpAddress)
return recipients.length === 0 ? null : recipients;
}
/**
* Load extended properties from XML.
*
* @param {any} jsObject Json Object converted from XML.
* @param {ExchangeService} service The service.
* @return {ExtendedPropertyCollection} Extended properties collection
*/
private static LoadExtendedPropertiesXmlJsObject(jsObject: any, service: ExchangeService): ExtendedPropertyCollection {
let extendedProperties: ExtendedPropertyCollection = new ExtendedPropertyCollection();
for (let extendedProperty of EwsServiceJsonReader.ReadAsArray(jsObject, XmlElementNames.ExtendedProperty)) {
extendedProperties.LoadFromXmlJsObject(extendedProperty, service);
}
return extendedProperties.Count === 0 ? null : extendedProperties;
}
/**
* Loads service object from XML.
*
* @param {any} jsObject Json Object converted from XML.
* @param {ExchangeService} service The service.
* @return {SearchMailboxesResult} Search result object
*/
static LoadFromXmlJsObject(jsObject: any, service: ExchangeService): SearchMailboxesResult {
let searchResult: SearchMailboxesResult = new SearchMailboxesResult();
if (jsObject[XmlElementNames.SearchQueries]) {
searchResult.SearchQueries = [];
for (let searchQuery of EwsServiceJsonReader.ReadAsArray(jsObject[XmlElementNames.SearchQueries], XmlElementNames.SearchQuery)) {
let query: string = searchQuery[XmlElementNames.Query];
let mailboxSearchScopes: MailboxSearchScope[] = [];
if (searchQuery[XmlElementNames.MailboxSearchScopes]) {
for (let mailboxSearchScope of EwsServiceJsonReader.ReadAsArray(searchQuery[XmlElementNames.MailboxSearchScopes], XmlElementNames.MailboxSearchScope)) {
let mailbox: string = mailboxSearchScope[XmlElementNames.Mailbox];
let searchScope: MailboxSearchLocation = MailboxSearchLocation[<string>mailboxSearchScope[XmlElementNames.SearchScope]];
mailboxSearchScopes.push(new MailboxSearchScope(mailbox, searchScope));
}
}
searchResult.SearchQueries.push(new MailboxQuery(query, mailboxSearchScopes));
}
}
if (jsObject[XmlElementNames.ResultType]) {
searchResult.ResultType = SearchResultType[<string>jsObject[XmlElementNames.ResultType]]
}
if (jsObject[XmlElementNames.ItemCount]) {
searchResult.ItemCount = Convert.toNumber(jsObject[XmlElementNames.ItemCount]);
}
if (jsObject[XmlElementNames.Size]) {
searchResult.Size = Convert.toNumber(jsObject[XmlElementNames.Size]);
}
if (jsObject[XmlElementNames.PageItemCount]) {
searchResult.PageItemCount = Convert.toNumber(jsObject[XmlElementNames.PageItemCount]);
}
if (jsObject[XmlElementNames.PageItemSize]) {
searchResult.PageItemSize = Convert.toNumber(jsObject[XmlElementNames.PageItemSize]);
}
if (jsObject[XmlElementNames.KeywordStats]) {
searchResult.KeywordStats = this.LoadKeywordStatsXmlJsObject(jsObject[XmlElementNames.KeywordStats]);
}
if (jsObject[XmlElementNames.Items]) {
searchResult.PreviewItems = this.LoadPreviewItemsXmlJsObject(jsObject[XmlElementNames.Items], service);
}
if (jsObject[XmlElementNames.FailedMailboxes]) {
searchResult.FailedMailboxes = FailedSearchMailbox.LoadFromXmlJsObject(jsObject[XmlElementNames.FailedMailboxes], service);
}
if (jsObject[XmlElementNames.Refiners]) {
let refiners: SearchRefinerItem[] = [];
for (let refiner of EwsServiceJsonReader.ReadAsArray(jsObject[XmlElementNames.Refiners], XmlElementNames.Refiner)) {
refiners.push(SearchRefinerItem.LoadFromXmlJsObject(refiner, service));
}
if (refiners.length > 0) {
searchResult.Refiners = refiners;
}
}
if (jsObject[XmlElementNames.MailboxStats]) {
let mailboxStats: MailboxStatisticsItem[] = [];
for (let mailboxStat of EwsServiceJsonReader.ReadAsArray(jsObject[XmlElementNames.MailboxStats], XmlElementNames.MailboxStat)) {
mailboxStats.push(MailboxStatisticsItem.LoadFromXmlJsObject(mailboxStat, service));
}
if (mailboxStats.length > 0) {
searchResult.MailboxStats = mailboxStats;
}
}
return searchResult;
}
/**
* Load keyword stats from XML.
*
* @param {any} jsObject Json Object converted from XML.
* @return {KeywordStatisticsSearchResult[]} Array of keyword statistics
*/
private static LoadKeywordStatsXmlJsObject(jsObject: any): KeywordStatisticsSearchResult[] {
let keywordStats: KeywordStatisticsSearchResult[] = [];
for (let keywordStatObj of EwsServiceJsonReader.ReadAsArray(jsObject, XmlElementNames.KeywordStat)) {
let keywordStat: KeywordStatisticsSearchResult = new KeywordStatisticsSearchResult();
keywordStat.Keyword = jsObject[XmlElementNames.Keyword];
keywordStat.ItemHits = Convert.toNumber(jsObject[XmlElementNames.ItemHits]);
keywordStat.Size = Convert.toNumber(jsObject[XmlElementNames.Size]);
keywordStats.push(keywordStat);
}
return keywordStats.length === 0 ? null : keywordStats;
}
/**
* Load preview items from XML.
*
* @param {any} jsObject Json Object converted from XML.
* @param {ExchangeService} service The service.
* @return {SearchPreviewItem[]} Array of preview items
*/
private static LoadPreviewItemsXmlJsObject(jsObject: any, service: ExchangeService): SearchPreviewItem[] {
let previewItems: SearchPreviewItem[] = [];
for (let searchPreviewItem of EwsServiceJsonReader.ReadAsArray(jsObject, XmlElementNames.SearchPreviewItem)) {
let previewItem: SearchPreviewItem = new SearchPreviewItem();
if (searchPreviewItem[XmlElementNames.Id]) {
previewItem.Id = new ItemId();
previewItem.Id.LoadFromXmlJsObject(searchPreviewItem[XmlElementNames.Id], service);
}
if (searchPreviewItem[XmlElementNames.ParentId]) {
previewItem.ParentId = new ItemId();
previewItem.ParentId.LoadFromXmlJsObject(searchPreviewItem[XmlElementNames.ParentId], service);
}
if (searchPreviewItem[XmlElementNames.Mailbox]) {
previewItem.Mailbox = new PreviewItemMailbox();
previewItem.Mailbox.MailboxId = searchPreviewItem[XmlElementNames.Mailbox][XmlElementNames.MailboxId];
previewItem.Mailbox.PrimarySmtpAddress = searchPreviewItem[XmlElementNames.Mailbox][XmlElementNames.PrimarySmtpAddress];
}
//missing in official repo
if (searchPreviewItem[XmlElementNames.ItemClass]) {
previewItem.ItemClass = searchPreviewItem[XmlElementNames.ItemClass];
}
if (searchPreviewItem[XmlElementNames.UniqueHash]) {
previewItem.UniqueHash = searchPreviewItem[XmlElementNames.UniqueHash];
}
if (searchPreviewItem[XmlElementNames.SortValue]) {
previewItem.SortValue = searchPreviewItem[XmlElementNames.SortValue];
}
if (searchPreviewItem[XmlElementNames.OwaLink]) {
previewItem.OwaLink = searchPreviewItem[XmlElementNames.OwaLink];
}
if (searchPreviewItem[XmlElementNames.Sender]) {
previewItem.Sender = searchPreviewItem[XmlElementNames.Sender];
}
if (searchPreviewItem[XmlElementNames.ToRecipients]) {
previewItem.ToRecipients = this.GetRecipients(searchPreviewItem[XmlElementNames.ToRecipients]);
}
if (searchPreviewItem[XmlElementNames.CcRecipients]) {
previewItem.CcRecipients = this.GetRecipients(searchPreviewItem[XmlElementNames.CcRecipients]);
}
if (searchPreviewItem[XmlElementNames.BccRecipients]) {
previewItem.BccRecipients = this.GetRecipients(searchPreviewItem[XmlElementNames.BccRecipients]);
}
if (searchPreviewItem[XmlElementNames.CreatedTime]) {
previewItem.CreatedTime = DateTime.Parse(searchPreviewItem[XmlElementNames.CreatedTime]);
}
if (searchPreviewItem[XmlElementNames.ReceivedTime]) {
previewItem.ReceivedTime = DateTime.Parse(searchPreviewItem[XmlElementNames.ReceivedTime]);
}
if (searchPreviewItem[XmlElementNames.SentTime]) {
previewItem.SentTime = DateTime.Parse(searchPreviewItem[XmlElementNames.SentTime]);
}
if (searchPreviewItem[XmlElementNames.Subject]) {
previewItem.Subject = searchPreviewItem[XmlElementNames.Subject];
}
if (searchPreviewItem[XmlElementNames.Preview]) {
previewItem.Preview = searchPreviewItem[XmlElementNames.Preview];
}
if (searchPreviewItem[XmlElementNames.Size]) {
previewItem.Size = Convert.toNumber(searchPreviewItem[XmlElementNames.Size]);
}
if (searchPreviewItem[XmlElementNames.Importance]) {
previewItem.Importance = Importance[<string>searchPreviewItem[XmlElementNames.Importance]];
}
if (searchPreviewItem[XmlElementNames.Read]) {
previewItem.Read = Convert.toBool(searchPreviewItem[XmlElementNames.Read]);
}
if (searchPreviewItem[XmlElementNames.HasAttachment]) {
previewItem.HasAttachment = Convert.toBool(searchPreviewItem[XmlElementNames.HasAttachment]);
}
if (searchPreviewItem[XmlElementNames.ExtendedProperties]) {
previewItem.ExtendedProperties = this.LoadExtendedPropertiesXmlJsObject(searchPreviewItem[XmlElementNames.ExtendedProperties], service);
}
previewItems.push(previewItem);
}
return previewItems.length === 0 ? null : previewItems;
}
} | {
"pile_set_name": "Github"
} |
/*
Copyright (C) 2012 Sebastian Herbord. All rights reserved.
This file is part of Mod Organizer.
Mod Organizer is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
Mod Organizer is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with Mod Organizer. If not, see <http://www.gnu.org/licenses/>.
*/
#ifndef OVERWRITEINFODIALOG_H
#define OVERWRITEINFODIALOG_H
#include "modinfo.h"
#include <QDialog>
#include <QFileSystemModel>
namespace Ui {
class OverwriteInfoDialog;
}
class OverwriteFileSystemModel : public QFileSystemModel
{
Q_OBJECT;
public:
OverwriteFileSystemModel(QObject *parent)
: QFileSystemModel(parent), m_RegularColumnCount(0) {}
virtual int columnCount(const QModelIndex &parent) const {
m_RegularColumnCount = QFileSystemModel::columnCount(parent);
return m_RegularColumnCount;
}
virtual QVariant headerData(int section, Qt::Orientation orientation, int role) const {
if ((orientation == Qt::Horizontal) &&
(section >= m_RegularColumnCount)) {
if (role == Qt::DisplayRole) {
return tr("Overwrites");
} else {
return QVariant();
}
} else {
return QFileSystemModel::headerData(section, orientation, role);
}
}
virtual QVariant data(const QModelIndex &index, int role) const {
if (index.column() == m_RegularColumnCount + 0) {
if (role == Qt::DisplayRole) {
return tr("not implemented");
} else {
return QVariant();
}
} else {
return QFileSystemModel::data(index, role);
}
}
private:
mutable int m_RegularColumnCount;
};
class OverwriteInfoDialog : public QDialog
{
Q_OBJECT
public:
explicit OverwriteInfoDialog(ModInfo::Ptr modInfo, QWidget *parent = 0);
~OverwriteInfoDialog();
ModInfo::Ptr modInfo() const { return m_ModInfo; }
// saves geometry
//
void done(int r) override;
void setModInfo(ModInfo::Ptr modInfo);
protected:
// restores geometry
//
void showEvent(QShowEvent* e) override;
private:
void openFile(const QModelIndex &index);
bool recursiveDelete(const QModelIndex &index);
void deleteFile(const QModelIndex &index);
private slots:
void delete_activated();
void deleteTriggered();
void renameTriggered();
void openTriggered();
void createDirectoryTriggered();
void on_explorerButton_clicked();
void on_filesView_customContextMenuRequested(const QPoint &pos);
private:
Ui::OverwriteInfoDialog *ui;
QFileSystemModel *m_FileSystemModel;
QModelIndexList m_FileSelection;
QAction *m_DeleteAction;
QAction *m_RenameAction;
QAction *m_OpenAction;
QAction *m_NewFolderAction;
ModInfo::Ptr m_ModInfo;
};
#endif // OVERWRITEINFODIALOG_H
| {
"pile_set_name": "Github"
} |
package org.robolectric.shadows;
import static org.robolectric.shadow.api.Shadow.directlyOn;
import android.os.Message;
import android.os.Messenger;
import android.os.RemoteException;
import org.robolectric.annotation.Implementation;
import org.robolectric.annotation.Implements;
import org.robolectric.annotation.RealObject;
import org.robolectric.annotation.Resetter;
@Implements(Messenger.class)
public class ShadowMessenger {
private static Message lastMessageSent = null;
/** Returns the last {@link Message} sent, or {@code null} if there isn't any message sent. */
public static Message getLastMessageSent() {
return lastMessageSent;
}
@RealObject private Messenger messenger;
@Implementation
protected void send(Message message) throws RemoteException {
lastMessageSent = Message.obtain(message);
directlyOn(messenger, Messenger.class).send(message);
}
@Resetter
public static void reset() {
lastMessageSent = null;
}
}
| {
"pile_set_name": "Github"
} |
.. currentmodule:: PyQt5.QtWidgets
QKeyEventTransition
-------------------
.. class:: QKeyEventTransition
`C++ documentation <https://doc.qt.io/qt-5/qkeyeventtransition.html>`_
| {
"pile_set_name": "Github"
} |
encode_krb5_ldap_seqof_key_data:
[Sequence/Sequence Of]
. [0] [Integer] 1
. [1] [Integer] 1
. [2] [Integer] 42
. [3] [Integer] 14
. [4] [Sequence/Sequence Of]
. . [Sequence/Sequence Of]
. . . [0] [Sequence/Sequence Of]
. . . . [0] [Integer] 0
. . . . [1] [Octet String] "salt0"
. . . [1] [Sequence/Sequence Of]
. . . . [0] [Integer] 2
. . . . [1] [Octet String] "key0"
. . [Sequence/Sequence Of]
. . . [0] [Sequence/Sequence Of]
. . . . [0] [Integer] 1
. . . . [1] [Octet String] "salt1"
. . . [1] [Sequence/Sequence Of]
. . . . [0] [Integer] 2
. . . . [1] [Octet String] "key1"
. . [Sequence/Sequence Of]
. . . [0] [Sequence/Sequence Of]
. . . . [0] [Integer] 2
. . . . [1] [Octet String] "salt2"
. . . [1] [Sequence/Sequence Of]
. . . . [0] [Integer] 2
. . . . [1] [Octet String] "key2"
| {
"pile_set_name": "Github"
} |
package au.com.dius.pact.provider.junit
import au.com.dius.pact.core.model.Pact
import au.com.dius.pact.core.model.ProviderState
import au.com.dius.pact.core.model.Request
import au.com.dius.pact.core.model.RequestResponseInteraction
import au.com.dius.pact.core.model.RequestResponsePact
import au.com.dius.pact.core.model.Response
import au.com.dius.pact.provider.junitsupport.IgnoreNoPactsToVerify
import au.com.dius.pact.provider.junitsupport.Provider
import au.com.dius.pact.provider.junitsupport.loader.PactFilter
import au.com.dius.pact.provider.junitsupport.loader.PactFolder
import au.com.dius.pact.provider.junitsupport.target.Target
import au.com.dius.pact.provider.junitsupport.target.TestTarget
import org.junit.runner.notification.RunNotifier
import org.junit.runners.model.InitializationError
import spock.lang.Specification
@SuppressWarnings('UnnecessaryGetter')
class FilteredPactRunnerSpec extends Specification {
private List<Pact> pacts
private au.com.dius.pact.core.model.Consumer consumer, consumer2
private au.com.dius.pact.core.model.Provider provider
private List<RequestResponseInteraction> interactions, interactions2
@Provider('myAwesomeService')
@PactFolder('pacts')
@PactFilter('State 1')
@IgnoreNoPactsToVerify
class TestClass {
@TestTarget
Target target
}
@Provider('myAwesomeService')
@PactFolder('pacts')
@PactFilter('')
class TestClassEmptyFilter {
@TestTarget
Target target
}
@Provider('myAwesomeService')
@PactFolder('pacts')
@PactFilter(['', '', ''])
class TestClassEmptyFilters {
@TestTarget
Target target
}
@Provider('myAwesomeService')
@PactFolder('pacts')
@PactFilter('')
class TestClassNoFilterAnnotations {
@TestTarget
Target target
}
@Provider('myAwesomeService')
@PactFolder('pacts')
@PactFilter(['State 1', 'State 3'])
@IgnoreNoPactsToVerify
class TestMultipleStatesClass {
@TestTarget
Target target
}
@Provider('myAwesomeService')
@PactFolder('pacts')
@PactFilter('State \\d+')
@IgnoreNoPactsToVerify
class TestRegexClass {
@TestTarget
Target target
}
@Provider('myAwesomeService')
@PactFolder('pacts')
@PactFilter(['State 6'])
class TestFilterOutAllPactsClass {
@TestTarget
Target target
}
@Provider('myAwesomeService')
@PactFolder('pacts')
@PactFilter(['State 6'])
@IgnoreNoPactsToVerify
class TestFilterOutAllPactsIgnoreNoPactsToVerifyClass {
@TestTarget
Target target
}
def setup() {
consumer = new au.com.dius.pact.core.model.Consumer('Consumer 1')
consumer2 = new au.com.dius.pact.core.model.Consumer('Consumer 2')
provider = new au.com.dius.pact.core.model.Provider('myAwesomeService')
interactions = [
new RequestResponseInteraction('Req 1', [
new ProviderState('State 1')
], new Request(), new Response()),
new RequestResponseInteraction('Req 2', [
new ProviderState('State 1'),
new ProviderState('State 2')
], new Request(), new Response())
]
interactions2 = [
new RequestResponseInteraction('Req 3', [
new ProviderState('State 3')
], new Request(), new Response()),
new RequestResponseInteraction('Req 4', [
new ProviderState('State X')
], new Request(), new Response())
]
pacts = [
new RequestResponsePact(provider, consumer, interactions),
new RequestResponsePact(provider, consumer2, interactions2)
]
}
def 'handles a test class with no filter annotations'() {
given:
PactRunner pactRunner = new PactRunner(TestClassNoFilterAnnotations)
when:
def result = pactRunner.filterPacts(pacts)
then:
result.is pacts
}
def 'handles a test class with an empty filter annotation'() {
given:
PactRunner pactRunner = new PactRunner(TestClassEmptyFilter)
PactRunner pactRunner2 = new PactRunner(TestClassEmptyFilters)
when:
def result = pactRunner.filterPacts(pacts)
def result2 = pactRunner2.filterPacts(pacts)
then:
result.is pacts
result2.is pacts
}
def 'filters the interactions by provider state'() {
given:
PactRunner pactRunner = new PactRunner(TestClass)
when:
def result = pactRunner.filterPacts(pacts)
then:
result.size() == 1
result*.interactions*.description.flatten() == ['Req 1', 'Req 2']
result[0].isNotFiltered()
!result[0].isFiltered()
}
def 'filters the interactions correctly when given multiple provider states'() {
given:
PactRunner pactRunner = new PactRunner(TestMultipleStatesClass)
when:
def result = pactRunner.filterPacts(pacts)
then:
result.size() == 2
result*.interactions*.description.flatten() == ['Req 1', 'Req 2', 'Req 3']
result[0].isNotFiltered()
!result[0].isFiltered()
!result[1].isNotFiltered()
result[1].isFiltered()
}
def 'filters the interactions correctly when given a regex'() {
given:
PactRunner pactRunner = new PactRunner(TestRegexClass)
when:
def result = pactRunner.filterPacts(pacts)
then:
result.size() == 2
result*.interactions*.description.flatten() == ['Req 1', 'Req 2', 'Req 3']
result[0].isNotFiltered()
!result[0].isFiltered()
!result[1].isNotFiltered()
result[1].isFiltered()
}
@SuppressWarnings('UnusedObject')
def 'Throws an initialisation error if all pacts are filtered out'() {
when:
new PactRunner(TestFilterOutAllPactsClass).run(new RunNotifier())
then:
thrown(InitializationError)
}
@SuppressWarnings('UnusedObject')
def 'Does not throw an initialisation error if all pacts are filtered out but @IgnoreNoPactsToVerify is present'() {
when:
new PactRunner(TestFilterOutAllPactsIgnoreNoPactsToVerifyClass)
then:
notThrown(InitializationError)
}
}
| {
"pile_set_name": "Github"
} |
/*
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS HEADER.
*
* Copyright (c) 1997-2011 Oracle and/or its affiliates. All rights reserved.
*
* The contents of this file are subject to the terms of either the GNU
* General Public License Version 2 only ("GPL") or the Common Development
* and Distribution License("CDDL") (collectively, the "License"). You
* may not use this file except in compliance with the License. You can
* obtain a copy of the License at
* https://glassfish.dev.java.net/public/CDDL+GPL_1_1.html
* or packager/legal/LICENSE.txt. See the License for the specific
* language governing permissions and limitations under the License.
*
* When distributing the software, include this License Header Notice in each
* file and include the License file at packager/legal/LICENSE.txt.
*
* GPL Classpath Exception:
* Oracle designates this particular file as subject to the "Classpath"
* exception as provided by Oracle in the GPL Version 2 section of the License
* file that accompanied this code.
*
* Modifications:
* If applicable, add the following below the License Header, with the fields
* enclosed by brackets [] replaced by your own identifying information:
* "Portions Copyright [year] [name of copyright owner]"
*
* Contributor(s):
* If you wish your version of this file to be governed by only the CDDL or
* only the GPL Version 2, indicate your decision by adding "[Contributor]
* elects to include this software in this distribution under the [CDDL or GPL
* Version 2] license." If you don't indicate a single choice of license, a
* recipient has the option to distribute your version of this file under
* either the CDDL, the GPL Version 2 or to extend the choice of license to
* its licensees as provided above. However, if you add GPL Version 2 code
* and therefore, elected the GPL Version 2 license, then the option applies
* only if the new code is made subject to such option by the copyright
* holder.
*/
// Portions Copyright [2018] [Payara Foundation and/or its affiliates]
package com.sun.enterprise.security.jauth;
import java.util.*;
import java.lang.reflect.Method;
import java.lang.reflect.InvocationTargetException;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.security.auth.login.AppConfigurationEntry;
/**
* Shared logic from Client and ServerAuthContext reside here.
*/
final class AuthContext {
static final String INIT = "initialize";
static final String DISPOSE_SUBJECT = "disposeSubject";
static final String SECURE_REQUEST = "secureRequest";
static final String VALIDATE_RESPONSE = "validateResponse";
static final String VALIDATE_REQUEST = "validateRequest";
static final String SECURE_RESPONSE = "secureResponse";
// managesSessions method is implemented by looking for
// corresponding option value in module configuration
static final String MANAGES_SESSIONS = "managesSessions";
static final String MANAGES_SESSIONS_OPTION = "managessessions";
private ConfigFile.Entry[] entries;
private Logger logger;
AuthContext(ConfigFile.Entry[] entries,
Logger logger) throws AuthException {
this.entries = entries;
this.logger = logger;
}
/**
* Invoke modules according to configuration
*/
Object[] invoke(final String methodName, final Object[] args)
throws AuthException {
// invoke modules in a doPrivileged
final Object rValues[] = new Object[entries.length];
try {
java.security.AccessController.doPrivileged(new java.security.PrivilegedExceptionAction() {
@Override
public Object run() throws AuthException {
invokePriv(methodName, args, rValues);
return null;
}
});
} catch (java.security.PrivilegedActionException pae) {
if (pae.getException() instanceof AuthException) {
throw (AuthException) pae.getException();
} else {
AuthException ae = new AuthException();
ae.initCause(pae.getException());
throw ae;
}
}
return rValues;
}
void invokePriv(String methodName, Object[] args, Object[] rValues)
throws AuthException {
// special treatment for managesSessions until the module
// interface can be extended.
if (methodName.equals(AuthContext.MANAGES_SESSIONS)) {
for (int i = 0; i < entries.length; i++) {
Map options = entries[i].getOptions();
String mS = (String) options.get(AuthContext.MANAGES_SESSIONS_OPTION);
rValues[i] = Boolean.valueOf(mS);
}
return;
}
boolean success = false;
AuthException firstRequiredError = null;
AuthException firstError = null;
// XXX no way to reverse module invocation
for (int i = 0; i < entries.length; i++) {
// get initialized module instance
Object module = entries[i].module;
// invoke the module
try {
Method[] mArray = module.getClass().getMethods();
for (int j = 0; j < mArray.length; j++) {
if (mArray[j].getName().equals(methodName)) {
// invoke module
rValues[i] = mArray[j].invoke(module, args);
// success -
// return if SUFFICIENT and no previous REQUIRED errors
if (firstRequiredError == null &&
entries[i].getControlFlag() == AppConfigurationEntry.LoginModuleControlFlag.SUFFICIENT) {
if (logger != null && logger.isLoggable(Level.FINE)) {
logger.fine(entries[i].getLoginModuleName() +
"." +
methodName +
" SUFFICIENT success");
}
return;
}
if (logger != null && logger.isLoggable(Level.FINE)) {
logger.fine(entries[i].getLoginModuleName() +
"." +
methodName +
" success");
}
success = true;
break;
}
}
if (!success) {
// PLEASE NOTE:
// this exception will be thrown if any module
// in the context does not support the method.
NoSuchMethodException nsme = new NoSuchMethodException("module " +
module.getClass().getName() +
" does not implement " +
methodName);
AuthException ae = new AuthException();
ae.initCause(nsme);
throw ae;
}
} catch (IllegalAccessException iae) {
AuthException ae = new AuthException();
ae.initCause(iae);
throw ae;
} catch (InvocationTargetException ite) {
// failure cases
AuthException ae;
if (ite.getCause() instanceof AuthException) {
ae = (AuthException) ite.getCause();
} else {
ae = new AuthException();
ae.initCause(ite.getCause());
}
if (entries[i].getControlFlag() == AppConfigurationEntry.LoginModuleControlFlag.REQUISITE) {
if (logger != null && logger.isLoggable(Level.FINE)) {
logger.fine(entries[i].getLoginModuleName() +
"." +
methodName +
" REQUISITE failure");
}
// immediately throw exception
if (firstRequiredError != null) {
throw firstRequiredError;
} else {
throw ae;
}
} else if (entries[i].getControlFlag() == AppConfigurationEntry.LoginModuleControlFlag.REQUIRED) {
if (logger != null && logger.isLoggable(Level.FINE)) {
logger.fine(entries[i].getLoginModuleName() +
"." +
methodName +
" REQUIRED failure");
}
// save exception and continue
if (firstRequiredError == null) {
firstRequiredError = ae;
}
} else {
if (logger != null && logger.isLoggable(Level.FINE)) {
logger.fine(entries[i].getLoginModuleName() +
"." +
methodName +
" OPTIONAL failure");
}
// save exception and continue
if (firstError == null) {
firstError = ae;
}
}
}
}
// done invoking entire stack of modules
if (firstRequiredError != null) {
throw firstRequiredError;
} else if (firstError != null && !success) {
throw firstError;
}
// if no errors, return gracefully
if (logger != null && logger.isLoggable(Level.FINE)) {
logger.fine("overall " + methodName + " success");
}
}
}
| {
"pile_set_name": "Github"
} |
import {
ChangeDetectionStrategy,
Component,
Input,
OnDestroy,
OnInit,
Output,
} from '@angular/core';
import { RxState } from '@rx-angular/state';
import { distinctUntilKeyChanged, map, startWith, switchMap, tap } from 'rxjs/operators';
import {
ListServerItem,
ListService,
} from '../../../data-access/list-resource';
import { interval, Subject, Subscription } from 'rxjs';
export interface DemoBasicsItem {
id: string;
name: string;
}
interface ComponentState {
refreshInterval: number;
list: DemoBasicsItem[];
listExpanded: boolean;
}
const initComponentState = {
refreshInterval: 10000,
listExpanded: false,
list: [],
};
@Component({
selector: 'output-bindings-solution',
template: `
<h3>
Output Bindings
</h3>
<mat-expansion-panel
*ngIf="model$ | async as vm"
(expandedChange)="listExpandedChanges.next($event)"
[expanded]="vm.listExpanded"
>
<mat-expansion-panel-header class="list">
<mat-progress-bar *ngIf="false" [mode]="'query'"></mat-progress-bar>
<mat-panel-title>
List
</mat-panel-title>
<mat-panel-description>
<span
>{{ (storeList$ | async)?.length }} Repositories Updated every:
{{ vm.refreshInterval }} ms
</span>
</mat-panel-description>
</mat-expansion-panel-header>
<button
mat-raised-button
color="primary"
(click)="onRefreshClicks($event)"
>
Refresh List
</button>
<ng-container *ngIf="storeList$ | async as list">
<div *ngIf="list?.length; else noList">
<mat-list>
<mat-list-item *ngFor="let item of list">
{{ item.name }}
</mat-list-item>
</mat-list>
</div>
</ng-container>
<ng-template #noList>
<mat-card>No list given!</mat-card>
</ng-template>
</mat-expansion-panel>
`,
changeDetection: ChangeDetectionStrategy.OnPush,
})
export class OutputBindingsSolution extends RxState<ComponentState>
implements OnInit, OnDestroy {
model$ = this.select();
intervalSubscription = new Subscription();
listExpandedChanges = new Subject<boolean>();
storeList$ = this.listService.list$.pipe(
map(this.parseListItems),
startWith(initComponentState.list)
);
@Input()
set refreshInterval(refreshInterval: number) {
if (refreshInterval > 4000) {
this.set({refreshInterval});
this.resetRefreshTick();
}
}
listExpanded: boolean = initComponentState.listExpanded;
@Output()
listExpandedChange = this.$.pipe(distinctUntilKeyChanged('listExpanded'), map(s => s.listExpanded));
constructor(private listService: ListService) {
super();
this.set(initComponentState);
this.connect('listExpanded', this.listExpandedChanges)
}
ngOnDestroy(): void {
this.intervalSubscription.unsubscribe();
}
ngOnInit(): void {
this.resetRefreshTick();
}
resetRefreshTick() {
this.intervalSubscription.unsubscribe();
this.intervalSubscription = interval(this.get('refreshInterval'))
.pipe(tap((_) => this.listService.refetchList()))
.subscribe();
}
onRefreshClicks(event) {
this.listService.refetchList();
}
parseListItems(l: ListServerItem[]): DemoBasicsItem[] {
return l.map(({ id, name }) => ({ id, name }));
}
}
| {
"pile_set_name": "Github"
} |
#include <stdio.h>
int main()
{
int map[256], i;
for (i = 0; i < 256; i++)
map[i] = i;
for (i = 0; i < 26; i++)
map['A'+i] = map['a'+i] = "22233344455566677778889999"[i];
while ((i = getchar()) != EOF)
putchar(map[i]);
return 0;
}
| {
"pile_set_name": "Github"
} |
Nevada is a state in the Western, Mountain West, and Southwestern regions of the United States .
| {
"pile_set_name": "Github"
} |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Telerik.Windows.Controls;
namespace CustomizingScatterPoints
{
public class ViewModel : ViewModelBase
{
private List<ChartData> data;
public ViewModel()
{
this.Data = this.GetData();
}
public List<ChartData> Data
{
get
{
return this.data;
}
set
{
if (this.data != value)
{
this.data = value;
this.OnPropertyChanged("Data");
}
}
}
private List<ChartData> GetData()
{
List<ChartData> data = new List<ChartData>();
data.Add(new ChartData(0.1, 100));
data.Add(new ChartData(0.1, 101));
data.Add(new ChartData(11, 106));
data.Add(new ChartData(101, 104));
data.Add(new ChartData(101, 108));
return data;
}
}
} | {
"pile_set_name": "Github"
} |
function arrowFunctionBodyToCase( j, test, body ) {
if ( body.type === 'BlockStatement' ) {
return j.switchCase( test, [ body ] );
}
return j.switchCase( test, [ j.returnStatement( body ) ] );
}
function getCases( j, handlerMap ) {
let hasPersistence = false;
const cases = handlerMap.properties.map( ( actionNode ) => {
const test = actionNode.computed
? actionNode.key
: j.literal( actionNode.key.name || String( actionNode.key.value ) );
const fn = actionNode.value;
if (
test.type === 'Identifier' &&
( test.name === 'SERIALIZE' || test.name === 'DESERIALIZE' )
) {
hasPersistence = true;
}
if (
test.type === 'Literal' &&
( test.value === 'SERIALIZE' || test.value === 'DESERIALIZE' )
) {
hasPersistence = true;
}
// If it's an arrow function without parameters, just return the body.
if ( fn.type === 'ArrowFunctionExpression' && fn.params.length === 0 ) {
return arrowFunctionBodyToCase( j, test, fn.body );
}
// If it's an arrow function with the right parameter names, just return the body.
if (
fn.type === 'ArrowFunctionExpression' &&
fn.params[ 0 ].name === 'state' &&
( fn.params.length === 1 || ( fn.params.length === 2 && fn.params[ 1 ].name === 'action' ) )
) {
return arrowFunctionBodyToCase( j, test, fn.body );
}
// If it's an arrow function with a deconstructed action, do magic.
if (
fn.type === 'ArrowFunctionExpression' &&
fn.params[ 0 ].name === 'state' &&
fn.params.length === 2 &&
fn.params[ 1 ].type === 'ObjectPattern'
) {
const declaration = j.variableDeclaration( 'const', [
j.variableDeclarator( fn.params[ 1 ], j.identifier( 'action' ) ),
] );
const prevBody =
fn.body.type === 'BlockStatement' ? fn.body.body : [ j.returnStatement( fn.body ) ];
const body = j.blockStatement( [ declaration, ...prevBody ] );
return arrowFunctionBodyToCase( j, test, body );
}
return j.switchCase( test, [
j.returnStatement(
j.callExpression( actionNode.value, [ j.identifier( 'state' ), j.identifier( 'action' ) ] )
),
] );
} );
return { cases, hasPersistence };
}
function handlePersistence( j, createReducerPath, newNode ) {
const parent = createReducerPath.parentPath;
const grandParentValue =
parent &&
parent.parentPath.value &&
parent.parentPath.value.length === 1 &&
parent.parentPath.value[ 0 ];
const greatGrandParent =
grandParentValue && parent && parent.parentPath && parent.parentPath.parentPath;
if (
parent &&
grandParentValue &&
greatGrandParent &&
parent.value.type === 'VariableDeclarator' &&
grandParentValue.type === 'VariableDeclarator' &&
greatGrandParent.value.type === 'VariableDeclaration'
) {
const varName = parent.value.id.name;
const persistenceNode = j.expressionStatement(
j.assignmentExpression(
'=',
j.memberExpression(
j.identifier( varName ),
j.identifier( 'hasCustomPersistence' ),
false
),
j.literal( true )
)
);
if ( greatGrandParent.parentPath.value.type === 'ExportNamedDeclaration' ) {
// Handle `export const reducer = ...` case.
greatGrandParent.parentPath.insertAfter( persistenceNode );
} else {
// Handle `const reducer = ...` case.
greatGrandParent.insertAfter( persistenceNode );
}
} else if ( parent && parent.value.type === 'AssignmentExpression' ) {
const persistenceNode = j.expressionStatement(
j.assignmentExpression(
'=',
j.memberExpression( parent.value.left, j.identifier( 'hasCustomPersistence' ), false ),
j.literal( true )
)
);
parent.parentPath.insertAfter( persistenceNode );
} else {
newNode.comments = newNode.comments || [];
newNode.comments.push( j.commentLine( ' TODO: HANDLE PERSISTENCE', true, false ) );
}
return newNode;
}
export default function transformer( file, api ) {
const j = api.jscodeshift;
const root = j( file.source );
let usedWithoutPersistence = false;
// Handle createReducer
root
.find(
j.CallExpression,
( node ) => node.callee.type === 'Identifier' && node.callee.name === 'createReducer'
)
.forEach( ( createReducerPath ) => {
if ( createReducerPath.value.arguments.length !== 2 ) {
throw new Error( 'Unable to translate createReducer' );
}
const [ defaultState, handlerMap ] = createReducerPath.value.arguments;
const { cases, hasPersistence } = getCases( j, handlerMap );
let newNode = j.arrowFunctionExpression(
[ j.assignmentPattern( j.identifier( 'state' ), defaultState ), j.identifier( 'action' ) ],
j.blockStatement( [
j.switchStatement(
j.memberExpression( j.identifier( 'action' ), j.identifier( 'type' ) ),
cases
),
j.returnStatement( j.identifier( 'state' ) ),
] )
);
if ( hasPersistence ) {
newNode = handlePersistence( j, createReducerPath, newNode );
} else {
usedWithoutPersistence = true;
newNode = j.callExpression( j.identifier( 'withoutPersistence' ), [ newNode ] );
}
createReducerPath.replace( newNode );
} );
// Handle createReducerWithValidation
root
.find(
j.CallExpression,
( node ) =>
node.callee.type === 'Identifier' && node.callee.name === 'createReducerWithValidation'
)
.forEach( ( createReducerPath ) => {
if ( createReducerPath.value.arguments.length !== 3 ) {
throw new Error( 'Unable to translate createReducerWithValidation' );
}
const [ defaultState, handlerMap, schema ] = createReducerPath.value.arguments;
const { cases } = getCases( j, handlerMap );
const newNode = j.callExpression( j.identifier( 'withSchemaValidation' ), [
schema,
j.arrowFunctionExpression(
[
j.assignmentPattern( j.identifier( 'state' ), defaultState ),
j.identifier( 'action' ),
],
j.blockStatement( [
j.switchStatement(
j.memberExpression( j.identifier( 'action' ), j.identifier( 'type' ) ),
cases
),
j.returnStatement( j.identifier( 'state' ) ),
] )
),
] );
createReducerPath.replace( newNode );
} );
// Handle imports.
root
.find(
j.ImportDeclaration,
( node ) =>
node.specifiers &&
node.specifiers.some(
( s ) =>
s &&
s.imported &&
( s.imported.name === 'createReducer' ||
s.imported.name === 'createReducerWithValidation' )
)
)
.forEach( ( nodePath ) => {
const filtered = nodePath.value.specifiers.filter(
( s ) =>
s.imported.name !== 'createReducer' && s.imported.name !== 'createReducerWithValidation'
);
if (
nodePath.value.specifiers.find( ( s ) => s.imported.name === 'createReducerWithValidation' )
) {
if ( ! filtered.find( ( s ) => s.imported.name === 'withSchemaValidation' ) ) {
filtered.push(
j.importSpecifier(
j.identifier( 'withSchemaValidation' ),
j.identifier( 'withSchemaValidation' )
)
);
}
}
if ( usedWithoutPersistence ) {
if ( ! filtered.find( ( s ) => s.imported.name === 'withoutPersistence' ) ) {
filtered.push(
j.importSpecifier(
j.identifier( 'withoutPersistence' ),
j.identifier( 'withoutPersistence' )
)
);
}
}
if ( filtered.length === 0 ) {
const { comments } = nodePath.node;
const { parentPath } = nodePath;
const nextNode = parentPath.value[ nodePath.name + 1 ];
j( nodePath ).remove();
nextNode.comments = comments;
} else {
nodePath.value.specifiers = filtered;
}
} );
return root.toSource();
}
| {
"pile_set_name": "Github"
} |
/*
* Copyright 2002-2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*/
package com.phoenixnap.oss.ramlplugin.raml2code.rules.spring;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestHeader;
import org.springframework.web.bind.annotation.RequestParam;
import com.phoenixnap.oss.ramlplugin.raml2code.data.ApiActionMetadata;
import com.phoenixnap.oss.ramlplugin.raml2code.data.ApiParameterMetadata;
import com.phoenixnap.oss.ramlplugin.raml2code.helpers.CodeModelHelper;
import com.sun.codemodel.JAnnotationUse;
import com.sun.codemodel.JClass;
import com.sun.codemodel.JVar;
/**
* Overrides method parameters set by {@link SpringMethodParamsRule}.
*
*
* @author Aleksandar Stojsavljevic ([email protected])
* @since 2.0.4
*/
public class SpringFeignClientMethodParamsRule extends SpringMethodParamsRule {
private static final List<String> ANNOTATIONS_TO_OVERRIDE = new ArrayList<String>();
static {
ANNOTATIONS_TO_OVERRIDE.add(RequestParam.class.getName());
ANNOTATIONS_TO_OVERRIDE.add(RequestHeader.class.getName());
ANNOTATIONS_TO_OVERRIDE.add(PathVariable.class.getName());
}
@Override
protected JVar paramQueryForm(ApiParameterMetadata paramMetaData, CodeModelHelper.JExtMethod generatableType,
ApiActionMetadata endpointMetadata) {
JVar paramQueryForm = super.paramQueryForm(paramMetaData, generatableType, endpointMetadata);
// name of request/header/path parameter needs to be set for feign
// client even when it matches method parameter name
// if name is already set this will not override it
Collection<JAnnotationUse> annotations = paramQueryForm.annotations();
for (JAnnotationUse annotation : annotations) {
JClass annotationClass = annotation.getAnnotationClass();
if (ANNOTATIONS_TO_OVERRIDE.contains(annotationClass.fullName())) {
annotation.param("name", paramMetaData.getName());
}
}
return paramQueryForm;
}
}
| {
"pile_set_name": "Github"
} |
package:
name: conda-build-test-extra-metadata
version: 1.0
test:
requires:
- pyyaml
extra:
custom: metadata
however: {we: want}
| {
"pile_set_name": "Github"
} |
// mksyscall_aix_ppc64.pl -aix -tags aix,ppc64 syscall_aix.go syscall_aix_ppc64.go
// Code generated by the command above; see README.md. DO NOT EDIT.
// +build aix,ppc64
// +build gccgo
package unix
/*
#include <stdint.h>
int utimes(uintptr_t, uintptr_t);
int utimensat(int, uintptr_t, uintptr_t, int);
int getcwd(uintptr_t, size_t);
int accept(int, uintptr_t, uintptr_t);
int getdirent(int, uintptr_t, size_t);
int wait4(int, uintptr_t, int, uintptr_t);
int ioctl(int, int, uintptr_t);
int fcntl(uintptr_t, int, uintptr_t);
int acct(uintptr_t);
int chdir(uintptr_t);
int chroot(uintptr_t);
int close(int);
int dup(int);
void exit(int);
int faccessat(int, uintptr_t, unsigned int, int);
int fchdir(int);
int fchmod(int, unsigned int);
int fchmodat(int, uintptr_t, unsigned int, int);
int fchownat(int, uintptr_t, int, int, int);
int fdatasync(int);
int fsync(int);
int getpgid(int);
int getpgrp();
int getpid();
int getppid();
int getpriority(int, int);
int getrusage(int, uintptr_t);
int getsid(int);
int kill(int, int);
int syslog(int, uintptr_t, size_t);
int mkdir(int, uintptr_t, unsigned int);
int mkdirat(int, uintptr_t, unsigned int);
int mkfifo(uintptr_t, unsigned int);
int mknod(uintptr_t, unsigned int, int);
int mknodat(int, uintptr_t, unsigned int, int);
int nanosleep(uintptr_t, uintptr_t);
int open64(uintptr_t, int, unsigned int);
int openat(int, uintptr_t, int, unsigned int);
int read(int, uintptr_t, size_t);
int readlink(uintptr_t, uintptr_t, size_t);
int renameat(int, uintptr_t, int, uintptr_t);
int setdomainname(uintptr_t, size_t);
int sethostname(uintptr_t, size_t);
int setpgid(int, int);
int setsid();
int settimeofday(uintptr_t);
int setuid(int);
int setgid(int);
int setpriority(int, int, int);
int statx(int, uintptr_t, int, int, uintptr_t);
int sync();
uintptr_t times(uintptr_t);
int umask(int);
int uname(uintptr_t);
int unlink(uintptr_t);
int unlinkat(int, uintptr_t, int);
int ustat(int, uintptr_t);
int write(int, uintptr_t, size_t);
int dup2(int, int);
int posix_fadvise64(int, long long, long long, int);
int fchown(int, int, int);
int fstat(int, uintptr_t);
int fstatat(int, uintptr_t, uintptr_t, int);
int fstatfs(int, uintptr_t);
int ftruncate(int, long long);
int getegid();
int geteuid();
int getgid();
int getuid();
int lchown(uintptr_t, int, int);
int listen(int, int);
int lstat(uintptr_t, uintptr_t);
int pause();
int pread64(int, uintptr_t, size_t, long long);
int pwrite64(int, uintptr_t, size_t, long long);
int pselect(int, uintptr_t, uintptr_t, uintptr_t, uintptr_t, uintptr_t);
int setregid(int, int);
int setreuid(int, int);
int shutdown(int, int);
long long splice(int, uintptr_t, int, uintptr_t, int, int);
int stat(uintptr_t, uintptr_t);
int statfs(uintptr_t, uintptr_t);
int truncate(uintptr_t, long long);
int bind(int, uintptr_t, uintptr_t);
int connect(int, uintptr_t, uintptr_t);
int getgroups(int, uintptr_t);
int setgroups(int, uintptr_t);
int getsockopt(int, int, int, uintptr_t, uintptr_t);
int setsockopt(int, int, int, uintptr_t, uintptr_t);
int socket(int, int, int);
int socketpair(int, int, int, uintptr_t);
int getpeername(int, uintptr_t, uintptr_t);
int getsockname(int, uintptr_t, uintptr_t);
int recvfrom(int, uintptr_t, size_t, int, uintptr_t, uintptr_t);
int sendto(int, uintptr_t, size_t, int, uintptr_t, uintptr_t);
int recvmsg(int, uintptr_t, int);
int sendmsg(int, uintptr_t, int);
int munmap(uintptr_t, uintptr_t);
int madvise(uintptr_t, size_t, int);
int mprotect(uintptr_t, size_t, int);
int mlock(uintptr_t, size_t);
int mlockall(int);
int msync(uintptr_t, size_t, int);
int munlock(uintptr_t, size_t);
int munlockall();
int pipe(uintptr_t);
int poll(uintptr_t, int, int);
int gettimeofday(uintptr_t, uintptr_t);
int time(uintptr_t);
int utime(uintptr_t, uintptr_t);
int getrlimit(int, uintptr_t);
int setrlimit(int, uintptr_t);
long long lseek(int, long long, int);
uintptr_t mmap64(uintptr_t, uintptr_t, int, int, int, long long);
*/
import "C"
import (
"syscall"
)
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callutimes(_p0 uintptr, times uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.utimes(C.uintptr_t(_p0), C.uintptr_t(times)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callutimensat(dirfd int, _p0 uintptr, times uintptr, flag int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.utimensat(C.int(dirfd), C.uintptr_t(_p0), C.uintptr_t(times), C.int(flag)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetcwd(_p0 uintptr, _lenp0 int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getcwd(C.uintptr_t(_p0), C.size_t(_lenp0)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callaccept(s int, rsa uintptr, addrlen uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.accept(C.int(s), C.uintptr_t(rsa), C.uintptr_t(addrlen)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetdirent(fd int, _p0 uintptr, _lenp0 int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getdirent(C.int(fd), C.uintptr_t(_p0), C.size_t(_lenp0)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callwait4(pid int, status uintptr, options int, rusage uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.wait4(C.int(pid), C.uintptr_t(status), C.int(options), C.uintptr_t(rusage)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callioctl(fd int, req int, arg uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.ioctl(C.int(fd), C.int(req), C.uintptr_t(arg)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callfcntl(fd uintptr, cmd int, arg uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.fcntl(C.uintptr_t(fd), C.int(cmd), C.uintptr_t(arg)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callacct(_p0 uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.acct(C.uintptr_t(_p0)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callchdir(_p0 uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.chdir(C.uintptr_t(_p0)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callchroot(_p0 uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.chroot(C.uintptr_t(_p0)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callclose(fd int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.close(C.int(fd)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func calldup(oldfd int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.dup(C.int(oldfd)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callexit(code int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.exit(C.int(code)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callfaccessat(dirfd int, _p0 uintptr, mode uint32, flags int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.faccessat(C.int(dirfd), C.uintptr_t(_p0), C.uint(mode), C.int(flags)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callfchdir(fd int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.fchdir(C.int(fd)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callfchmod(fd int, mode uint32) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.fchmod(C.int(fd), C.uint(mode)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callfchmodat(dirfd int, _p0 uintptr, mode uint32, flags int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.fchmodat(C.int(dirfd), C.uintptr_t(_p0), C.uint(mode), C.int(flags)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callfchownat(dirfd int, _p0 uintptr, uid int, gid int, flags int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.fchownat(C.int(dirfd), C.uintptr_t(_p0), C.int(uid), C.int(gid), C.int(flags)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callfdatasync(fd int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.fdatasync(C.int(fd)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callfsync(fd int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.fsync(C.int(fd)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetpgid(pid int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getpgid(C.int(pid)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetpgrp() (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getpgrp())
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetpid() (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getpid())
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetppid() (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getppid())
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetpriority(which int, who int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getpriority(C.int(which), C.int(who)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetrusage(who int, rusage uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getrusage(C.int(who), C.uintptr_t(rusage)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetsid(pid int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getsid(C.int(pid)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callkill(pid int, sig int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.kill(C.int(pid), C.int(sig)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsyslog(typ int, _p0 uintptr, _lenp0 int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.syslog(C.int(typ), C.uintptr_t(_p0), C.size_t(_lenp0)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callmkdir(dirfd int, _p0 uintptr, mode uint32) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.mkdir(C.int(dirfd), C.uintptr_t(_p0), C.uint(mode)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callmkdirat(dirfd int, _p0 uintptr, mode uint32) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.mkdirat(C.int(dirfd), C.uintptr_t(_p0), C.uint(mode)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callmkfifo(_p0 uintptr, mode uint32) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.mkfifo(C.uintptr_t(_p0), C.uint(mode)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callmknod(_p0 uintptr, mode uint32, dev int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.mknod(C.uintptr_t(_p0), C.uint(mode), C.int(dev)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callmknodat(dirfd int, _p0 uintptr, mode uint32, dev int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.mknodat(C.int(dirfd), C.uintptr_t(_p0), C.uint(mode), C.int(dev)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callnanosleep(time uintptr, leftover uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.nanosleep(C.uintptr_t(time), C.uintptr_t(leftover)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callopen64(_p0 uintptr, mode int, perm uint32) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.open64(C.uintptr_t(_p0), C.int(mode), C.uint(perm)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callopenat(dirfd int, _p0 uintptr, flags int, mode uint32) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.openat(C.int(dirfd), C.uintptr_t(_p0), C.int(flags), C.uint(mode)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callread(fd int, _p0 uintptr, _lenp0 int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.read(C.int(fd), C.uintptr_t(_p0), C.size_t(_lenp0)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callreadlink(_p0 uintptr, _p1 uintptr, _lenp1 int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.readlink(C.uintptr_t(_p0), C.uintptr_t(_p1), C.size_t(_lenp1)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callrenameat(olddirfd int, _p0 uintptr, newdirfd int, _p1 uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.renameat(C.int(olddirfd), C.uintptr_t(_p0), C.int(newdirfd), C.uintptr_t(_p1)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsetdomainname(_p0 uintptr, _lenp0 int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.setdomainname(C.uintptr_t(_p0), C.size_t(_lenp0)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsethostname(_p0 uintptr, _lenp0 int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.sethostname(C.uintptr_t(_p0), C.size_t(_lenp0)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsetpgid(pid int, pgid int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.setpgid(C.int(pid), C.int(pgid)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsetsid() (r1 uintptr, e1 Errno) {
r1 = uintptr(C.setsid())
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsettimeofday(tv uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.settimeofday(C.uintptr_t(tv)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsetuid(uid int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.setuid(C.int(uid)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsetgid(uid int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.setgid(C.int(uid)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsetpriority(which int, who int, prio int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.setpriority(C.int(which), C.int(who), C.int(prio)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callstatx(dirfd int, _p0 uintptr, flags int, mask int, stat uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.statx(C.int(dirfd), C.uintptr_t(_p0), C.int(flags), C.int(mask), C.uintptr_t(stat)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsync() (r1 uintptr, e1 Errno) {
r1 = uintptr(C.sync())
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func calltimes(tms uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.times(C.uintptr_t(tms)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callumask(mask int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.umask(C.int(mask)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func calluname(buf uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.uname(C.uintptr_t(buf)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callunlink(_p0 uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.unlink(C.uintptr_t(_p0)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callunlinkat(dirfd int, _p0 uintptr, flags int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.unlinkat(C.int(dirfd), C.uintptr_t(_p0), C.int(flags)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callustat(dev int, ubuf uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.ustat(C.int(dev), C.uintptr_t(ubuf)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callwrite(fd int, _p0 uintptr, _lenp0 int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.write(C.int(fd), C.uintptr_t(_p0), C.size_t(_lenp0)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func calldup2(oldfd int, newfd int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.dup2(C.int(oldfd), C.int(newfd)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callposix_fadvise64(fd int, offset int64, length int64, advice int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.posix_fadvise64(C.int(fd), C.longlong(offset), C.longlong(length), C.int(advice)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callfchown(fd int, uid int, gid int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.fchown(C.int(fd), C.int(uid), C.int(gid)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callfstat(fd int, stat uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.fstat(C.int(fd), C.uintptr_t(stat)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callfstatat(dirfd int, _p0 uintptr, stat uintptr, flags int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.fstatat(C.int(dirfd), C.uintptr_t(_p0), C.uintptr_t(stat), C.int(flags)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callfstatfs(fd int, buf uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.fstatfs(C.int(fd), C.uintptr_t(buf)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callftruncate(fd int, length int64) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.ftruncate(C.int(fd), C.longlong(length)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetegid() (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getegid())
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgeteuid() (r1 uintptr, e1 Errno) {
r1 = uintptr(C.geteuid())
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetgid() (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getgid())
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetuid() (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getuid())
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func calllchown(_p0 uintptr, uid int, gid int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.lchown(C.uintptr_t(_p0), C.int(uid), C.int(gid)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func calllisten(s int, n int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.listen(C.int(s), C.int(n)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func calllstat(_p0 uintptr, stat uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.lstat(C.uintptr_t(_p0), C.uintptr_t(stat)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callpause() (r1 uintptr, e1 Errno) {
r1 = uintptr(C.pause())
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callpread64(fd int, _p0 uintptr, _lenp0 int, offset int64) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.pread64(C.int(fd), C.uintptr_t(_p0), C.size_t(_lenp0), C.longlong(offset)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callpwrite64(fd int, _p0 uintptr, _lenp0 int, offset int64) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.pwrite64(C.int(fd), C.uintptr_t(_p0), C.size_t(_lenp0), C.longlong(offset)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callpselect(nfd int, r uintptr, w uintptr, e uintptr, timeout uintptr, sigmask uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.pselect(C.int(nfd), C.uintptr_t(r), C.uintptr_t(w), C.uintptr_t(e), C.uintptr_t(timeout), C.uintptr_t(sigmask)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsetregid(rgid int, egid int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.setregid(C.int(rgid), C.int(egid)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsetreuid(ruid int, euid int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.setreuid(C.int(ruid), C.int(euid)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callshutdown(fd int, how int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.shutdown(C.int(fd), C.int(how)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsplice(rfd int, roff uintptr, wfd int, woff uintptr, len int, flags int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.splice(C.int(rfd), C.uintptr_t(roff), C.int(wfd), C.uintptr_t(woff), C.int(len), C.int(flags)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callstat(_p0 uintptr, stat uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.stat(C.uintptr_t(_p0), C.uintptr_t(stat)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callstatfs(_p0 uintptr, buf uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.statfs(C.uintptr_t(_p0), C.uintptr_t(buf)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func calltruncate(_p0 uintptr, length int64) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.truncate(C.uintptr_t(_p0), C.longlong(length)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callbind(s int, addr uintptr, addrlen uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.bind(C.int(s), C.uintptr_t(addr), C.uintptr_t(addrlen)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callconnect(s int, addr uintptr, addrlen uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.connect(C.int(s), C.uintptr_t(addr), C.uintptr_t(addrlen)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetgroups(n int, list uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getgroups(C.int(n), C.uintptr_t(list)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsetgroups(n int, list uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.setgroups(C.int(n), C.uintptr_t(list)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetsockopt(s int, level int, name int, val uintptr, vallen uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getsockopt(C.int(s), C.int(level), C.int(name), C.uintptr_t(val), C.uintptr_t(vallen)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsetsockopt(s int, level int, name int, val uintptr, vallen uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.setsockopt(C.int(s), C.int(level), C.int(name), C.uintptr_t(val), C.uintptr_t(vallen)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsocket(domain int, typ int, proto int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.socket(C.int(domain), C.int(typ), C.int(proto)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsocketpair(domain int, typ int, proto int, fd uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.socketpair(C.int(domain), C.int(typ), C.int(proto), C.uintptr_t(fd)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetpeername(fd int, rsa uintptr, addrlen uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getpeername(C.int(fd), C.uintptr_t(rsa), C.uintptr_t(addrlen)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetsockname(fd int, rsa uintptr, addrlen uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getsockname(C.int(fd), C.uintptr_t(rsa), C.uintptr_t(addrlen)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callrecvfrom(fd int, _p0 uintptr, _lenp0 int, flags int, from uintptr, fromlen uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.recvfrom(C.int(fd), C.uintptr_t(_p0), C.size_t(_lenp0), C.int(flags), C.uintptr_t(from), C.uintptr_t(fromlen)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsendto(s int, _p0 uintptr, _lenp0 int, flags int, to uintptr, addrlen uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.sendto(C.int(s), C.uintptr_t(_p0), C.size_t(_lenp0), C.int(flags), C.uintptr_t(to), C.uintptr_t(addrlen)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callrecvmsg(s int, msg uintptr, flags int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.recvmsg(C.int(s), C.uintptr_t(msg), C.int(flags)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsendmsg(s int, msg uintptr, flags int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.sendmsg(C.int(s), C.uintptr_t(msg), C.int(flags)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callmunmap(addr uintptr, length uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.munmap(C.uintptr_t(addr), C.uintptr_t(length)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callmadvise(_p0 uintptr, _lenp0 int, advice int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.madvise(C.uintptr_t(_p0), C.size_t(_lenp0), C.int(advice)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callmprotect(_p0 uintptr, _lenp0 int, prot int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.mprotect(C.uintptr_t(_p0), C.size_t(_lenp0), C.int(prot)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callmlock(_p0 uintptr, _lenp0 int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.mlock(C.uintptr_t(_p0), C.size_t(_lenp0)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callmlockall(flags int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.mlockall(C.int(flags)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callmsync(_p0 uintptr, _lenp0 int, flags int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.msync(C.uintptr_t(_p0), C.size_t(_lenp0), C.int(flags)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callmunlock(_p0 uintptr, _lenp0 int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.munlock(C.uintptr_t(_p0), C.size_t(_lenp0)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callmunlockall() (r1 uintptr, e1 Errno) {
r1 = uintptr(C.munlockall())
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callpipe(p uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.pipe(C.uintptr_t(p)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callpoll(fds uintptr, nfds int, timeout int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.poll(C.uintptr_t(fds), C.int(nfds), C.int(timeout)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgettimeofday(tv uintptr, tzp uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.gettimeofday(C.uintptr_t(tv), C.uintptr_t(tzp)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func calltime(t uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.time(C.uintptr_t(t)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callutime(_p0 uintptr, buf uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.utime(C.uintptr_t(_p0), C.uintptr_t(buf)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callgetrlimit(resource int, rlim uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.getrlimit(C.int(resource), C.uintptr_t(rlim)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callsetrlimit(resource int, rlim uintptr) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.setrlimit(C.int(resource), C.uintptr_t(rlim)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func calllseek(fd int, offset int64, whence int) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.lseek(C.int(fd), C.longlong(offset), C.int(whence)))
e1 = syscall.GetErrno()
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func callmmap64(addr uintptr, length uintptr, prot int, flags int, fd int, offset int64) (r1 uintptr, e1 Errno) {
r1 = uintptr(C.mmap64(C.uintptr_t(addr), C.uintptr_t(length), C.int(prot), C.int(flags), C.int(fd), C.longlong(offset)))
e1 = syscall.GetErrno()
return
}
| {
"pile_set_name": "Github"
} |
#!{{pkgPathFor "core/bash"}}/bin/bash
set -e
exec 2>&1
# Call the script to block until user accepts the MLSA via the package's config
{{pkgPathFor "chef/mlsa"}}/bin/accept {{cfg.mlsa.accept}}
pg-helper ensure-service-database chef_license_control_service
# Run the License Control gRPC server
exec license-control-service serve --config {{pkg.svc_config_path}}/config.toml
| {
"pile_set_name": "Github"
} |
#include <linux/bio.h>
void dummy(struct bio *bio)
{
bio->bi_status = BLK_STS_IOERR;
bio_endio(bio);
}
| {
"pile_set_name": "Github"
} |
package io.buoyant.namer
import com.twitter.finagle.Namer
/** For better java compatibility */
abstract class JNamer extends Namer
| {
"pile_set_name": "Github"
} |
#pragma once
enum LocationServiceStatus
{
kLocationServiceStopped,
kLocationServiceInitializing,
kLocationServiceRunning,
kLocationServiceFailed
};
class LocationService
{
public:
static void SetDesiredAccuracy(float val);
static float GetDesiredAccuracy();
static void SetDistanceFilter(float val);
static float GetDistanceFilter();
static bool IsServiceEnabledByUser();
static void StartUpdatingLocation();
static void StopUpdatingLocation();
static void SetHeadingUpdatesEnabled(bool enabled);
static bool IsHeadingUpdatesEnabled();
static LocationServiceStatus GetLocationStatus();
static LocationServiceStatus GetHeadingStatus();
static bool IsHeadingAvailable();
};
#if UNITY_TVOS_SIMULATOR_FAKE_REMOTE
void ReportSimulatedRemoteButtonPress(UIPressType type);
void ReportSimulatedRemoteButtonRelease(UIPressType type);
void ReportSimulatedRemoteTouchesBegan(UIView* view, NSSet* touches);
void ReportSimulatedRemoteTouchesMoved(UIView* view, NSSet* touches);
void ReportSimulatedRemoteTouchesEnded(UIView* view, NSSet* touches);
#endif
| {
"pile_set_name": "Github"
} |
# Translation of Odoo Server.
# This file contains the translation of the following modules:
# * partner_identification
#
# Translators:
# OCA Transbot <[email protected]>, 2017
# Giuliano Lotta <[email protected]>, 2017
msgid ""
msgstr ""
"Project-Id-Version: Odoo Server 10.0\n"
"Report-Msgid-Bugs-To: \n"
"POT-Creation-Date: 2017-11-21 01:49+0000\n"
"PO-Revision-Date: 2018-12-24 17:58+0000\n"
"Last-Translator: Sergio Zanchetta <[email protected]>\n"
"Language-Team: Italian (https://www.transifex.com/oca/teams/23907/it/)\n"
"Language: it\n"
"MIME-Version: 1.0\n"
"Content-Type: text/plain; charset=UTF-8\n"
"Content-Transfer-Encoding: \n"
"Plural-Forms: nplurals=2; plural=n != 1;\n"
"X-Generator: Weblate 3.3\n"
#. module: partner_identification
#: code:addons/partner_identification/models/res_partner_id_category.py:0
#, python-format
msgid ""
"\n"
"# Python code. Use failed = True to specify that the id number is not "
"valid.\n"
"# You can use the following variables :\n"
"# - self: browse_record of the current ID Category browse_record\n"
"# - id_number: browse_record of ID number to validate"
msgstr ""
"\n"
"# Codice Python. Usare failed = True per specificare che il numero "
"documento non è valido.\n"
"# È possibile utilizzare le seguenti variabili :\n"
"# - self: browse_record della categoria documento corrente browse_record\n"
"# - id_number: browse_record del numero documento da validare"
#. module: partner_identification
#: code:addons/partner_identification/models/res_partner_id_category.py:0
#, python-format
msgid "%s is not a valid %s identifier"
msgstr "%s non è un identificatore %s valido"
#. module: partner_identification
#: model:ir.model.fields,help:partner_identification.field_res_partner_id_category__code
msgid "Abbreviation or acronym of this ID type. For example, 'driver_license'"
msgstr ""
"Abbreviazione o acronimo per questo tipo di documento. Per esempio, "
"\"driver_license\""
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_category__active
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__active
msgid "Active"
msgstr "Attivo"
#. module: partner_identification
#: model:ir.model.fields,help:partner_identification.field_res_partner_id_number__partner_issued_id
msgid ""
"Another partner, who issued this ID. For example, Traffic National "
"Institution"
msgstr ""
"Un altro partner, che ha emesso questo documento. Per esempio, "
"Motorizzazione Civile"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__category_id
msgid "Category"
msgstr "Categoria"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_category__code
msgid "Code"
msgstr "Codice"
#. module: partner_identification
#: model:ir.model,name:partner_identification.model_res_partner
msgid "Contact"
msgstr "Contatto"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_category__create_uid
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__create_uid
msgid "Created by"
msgstr "Creato da"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_category__create_date
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__create_date
msgid "Created on"
msgstr "Creato il"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_category__display_name
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__display_name
msgid "Display Name"
msgstr "Nome visualizzato"
#. module: partner_identification
#: code:addons/partner_identification/models/res_partner_id_category.py:0
#, python-format
msgid ""
"Error when evaluating the id_category validation code::\n"
" %s \n"
"(%s)"
msgstr ""
"Errore durante l'esame del codice di convalida id_category.:\n"
" %s \n"
"(%s)"
#. module: partner_identification
#: model:ir.model.fields,help:partner_identification.field_res_partner_id_number__valid_until
msgid ""
"Expiration date. For example, date when person needs to renew his driver "
"license, 21/10/2019"
msgstr ""
"Data di scadenza. Per esempio, data in cui la persona deve rinnovare la sua "
"patente di guida, 21/10/2019"
#. module: partner_identification
#: model:ir.model.fields.selection,name:partner_identification.selection__res_partner_id_number__status__close
msgid "Expired"
msgstr "Scaduto"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_category__id
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__id
msgid "ID"
msgstr "Documento"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__name
msgid "ID Number"
msgstr "Numero documento"
#. module: partner_identification
#: model_terms:ir.ui.view,arch_db:partner_identification.view_partner_form
msgid "ID Numbers"
msgstr "Numeri documenti"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_category__name
msgid "ID name"
msgstr "Nome documento"
#. module: partner_identification
#: model:ir.model.fields,help:partner_identification.field_res_partner_id_number__category_id
msgid "ID type defined in configuration. For example, Driver License"
msgstr ""
"Tipo di documento definito nella configurazione. Per esempio, patente di "
"guida"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner__id_numbers
#: model:ir.model.fields,field_description:partner_identification.field_res_users__id_numbers
msgid "Identification Numbers"
msgstr "Numeri di identificazione"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__partner_issued_id
msgid "Issued by"
msgstr "Emesso da"
#. module: partner_identification
#: model:ir.model.fields,help:partner_identification.field_res_partner_id_number__date_issued
msgid ""
"Issued date. For example, date when person approved his driving exam, "
"21/10/2009"
msgstr ""
"Data di emissione. Per esempio, data in cui la persona ha superato l'esame "
"di guida 21/10/2009"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__date_issued
msgid "Issued on"
msgstr "Emesso il"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_category____last_update
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number____last_update
msgid "Last Modified on"
msgstr "Ultima modifica il"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_category__write_uid
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__write_uid
msgid "Last Updated by"
msgstr "Ultimo aggiornamento il"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_category__write_date
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__write_date
msgid "Last Updated on"
msgstr "Ultimo aggiornamento di"
#. module: partner_identification
#: model:ir.model.fields,help:partner_identification.field_res_partner_id_category__name
msgid "Name of this ID type. For example, 'Driver License'"
msgstr "Nome del tipo di documento. Per esempio, \"Patente di guida\""
#. module: partner_identification
#: model:ir.model.fields.selection,name:partner_identification.selection__res_partner_id_number__status__draft
msgid "New"
msgstr "Nuovo"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__comment
#: model_terms:ir.ui.view,arch_db:partner_identification.view_partner_id_numbers_form
msgid "Notes"
msgstr "Note"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__partner_id
msgid "Partner"
msgstr "Partner"
#. module: partner_identification
#: model:ir.actions.act_window,name:partner_identification.action_partner_id_category
#: model:ir.ui.menu,name:partner_identification.menu_partner_id_category
msgid "Partner ID Categories"
msgstr "Categorie documenti del partner"
#. module: partner_identification
#: model:ir.model,name:partner_identification.model_res_partner_id_category
#, fuzzy
msgid "Partner ID Category"
msgstr "Categorie documenti del partner"
#. module: partner_identification
#: model:ir.model,name:partner_identification.model_res_partner_id_number
#, fuzzy
msgid "Partner ID Number"
msgstr "Numeri documenti del partner"
#. module: partner_identification
#: model:ir.actions.act_window,name:partner_identification.action_partner_id_numbers_form
#: model_terms:ir.ui.view,arch_db:partner_identification.view_partner_id_numbers_form
#: model_terms:ir.ui.view,arch_db:partner_identification.view_partner_id_numbers_tree
msgid "Partner ID Numbers"
msgstr "Numeri documenti del partner"
#. module: partner_identification
#: model_terms:ir.ui.view,arch_db:partner_identification.view_partner_id_category_form
#: model_terms:ir.ui.view,arch_db:partner_identification.view_partner_id_category_tree
msgid "Partner Identification Categories"
msgstr "Categorie di identificazione del partner"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__place_issuance
msgid "Place of Issuance"
msgstr "Luogo di emissione"
#. module: partner_identification
#: model:ir.model.fields,help:partner_identification.field_res_partner_id_category__validation_code
msgid "Python code called to validate an id number."
msgstr "Codice Python richiamato per validare un numero documento."
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_category__validation_code
msgid "Python validation code"
msgstr "Codice di validazione Python"
#. module: partner_identification
#: model:ir.model.fields.selection,name:partner_identification.selection__res_partner_id_number__status__open
msgid "Running"
msgstr "Valido"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__status
msgid "Status"
msgstr "Stato"
#. module: partner_identification
#: model:ir.model.fields,help:partner_identification.field_res_partner_id_number__name
msgid "The ID itself. For example, Driver License number of this person"
msgstr ""
"L'identificativo stesso. Per esempio, il numero della patente di guida di "
"questa persona"
#. module: partner_identification
#: model:ir.model.fields,help:partner_identification.field_res_partner_id_number__place_issuance
msgid ""
"The place where the ID has been issued. For example the country for "
"passports and visa"
msgstr ""
"Il luogo di emissione del documento. Per esempio, la nazione del passaporto "
"e del visto"
#. module: partner_identification
#: code:addons/partner_identification/models/res_partner.py:0
#, python-format
msgid ""
"This %s has multiple IDs of this type (%s), so a write via the %s field is "
"not possible. In order to fix this, please use the IDs tab."
msgstr ""
#. module: partner_identification
#: model:ir.model.fields.selection,name:partner_identification.selection__res_partner_id_number__status__pending
msgid "To Renew"
msgstr "Da rinnovare"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__valid_from
msgid "Valid from"
msgstr "Valido da"
#. module: partner_identification
#: model:ir.model.fields,field_description:partner_identification.field_res_partner_id_number__valid_until
msgid "Valid until"
msgstr "Valido fino a"
#. module: partner_identification
#: model:ir.model.fields,help:partner_identification.field_res_partner_id_number__valid_from
msgid "Validation period stating date."
msgstr "Data di inizio periodo di validità."
#~ msgid "res.partner.id_category"
#~ msgstr "res.partner.id_category"
#~ msgid "res.partner.id_number"
#~ msgstr "res.partner.id_number"
| {
"pile_set_name": "Github"
} |
---
layout: base
title: 'Statistics of obl in UD_Moksha-JR'
udver: '2'
---
## Treebank Statistics: UD_Moksha-JR: Relations: `obl`
This relation is universal.
There are 3 language-specific subtypes of `obl`: <tt><a href="mdf_jr-dep-obl-agent.html">obl:agent</a></tt>, <tt><a href="mdf_jr-dep-obl-lmod.html">obl:lmod</a></tt>, <tt><a href="mdf_jr-dep-obl-tmod.html">obl:tmod</a></tt>.
46 nodes (4%) are attached to their parents as `obl`.
24 instances of `obl` (52%) are left-to-right (parent precedes child).
Average distance between parent and child is 1.71739130434783.
The following 6 pairs of parts of speech are connected with `obl`: <tt><a href="mdf_jr-pos-VERB.html">VERB</a></tt>-<tt><a href="mdf_jr-pos-NOUN.html">NOUN</a></tt> (30; 65% instances), <tt><a href="mdf_jr-pos-ADJ.html">ADJ</a></tt>-<tt><a href="mdf_jr-pos-NOUN.html">NOUN</a></tt> (6; 13% instances), <tt><a href="mdf_jr-pos-VERB.html">VERB</a></tt>-<tt><a href="mdf_jr-pos-PRON.html">PRON</a></tt> (5; 11% instances), <tt><a href="mdf_jr-pos-NOUN.html">NOUN</a></tt>-<tt><a href="mdf_jr-pos-NOUN.html">NOUN</a></tt> (2; 4% instances), <tt><a href="mdf_jr-pos-VERB.html">VERB</a></tt>-<tt><a href="mdf_jr-pos-ADP.html">ADP</a></tt> (2; 4% instances), <tt><a href="mdf_jr-pos-VERB.html">VERB</a></tt>-<tt><a href="mdf_jr-pos-PROPN.html">PROPN</a></tt> (1; 2% instances).
~~~ conllu
# visual-style 5 bgColor:blue
# visual-style 5 fgColor:white
# visual-style 6 bgColor:blue
# visual-style 6 fgColor:white
# visual-style 6 5 obl color:blue
1 Лётчикне лётчик NOUN N Case=Nom|Definite=Def|Number=Plur 6 nsubj _ SpaceAfter=No
2 , , PUNCT CLB _ 3 punct _ _
3 улема улема PART Pcle _ 6 advmod:mmod _ SpaceAfter=No
4 , , PUNCT CLB _ 3 punct _ _
5 кядьса кядь NOUN N Case=Ine|Definite=Ind|Number=Plur,Sing 6 obl _ _
6 токсесазь токсемс VERB V Mood=Ind|Number[obj]=Plur|Number[subj]=Plur|Person[obj]=3|Person[subj]=3|Tense=Pres|Valency=2 0 root _ _
7 коволнятнень ковол NOUN N Case=Gen|Definite=Def|Derivation=Dimin|Number=Plur 6 obj _ SpaceAfter=No
8 . . PUNCT CLB _ 6 punct _ _
~~~
~~~ conllu
# visual-style 4 bgColor:blue
# visual-style 4 fgColor:white
# visual-style 6 bgColor:blue
# visual-style 6 fgColor:white
# visual-style 6 4 obl color:blue
1 Ну ну INTJ Interj _ 6 discourse _ SpaceAfter=No
2 , , PUNCT CLB _ 6 punct _ _
3 кли кли ADV Adv _ 4 advmod _ _
4 онцтон он NOUN N Case=Ela|Number=Plur,Sing|Number[psor]=Sing|Person[psor]=1 6 obl _ SpaceAfter=No
5 , , PUNCT CLB _ 4 punct _ _
6 пара пара ADJ A Case=Nom|Definite=Ind|Number=Sing 0 root _ SpaceAfter=No
7 : : PUNCT CLB _ 11 punct _ _
8 гулянять гуляня NOUN N Case=Gen|Definite=Def|Number=Sing 9 nmod _ _
9 пацяняц паця NOUN N Case=Nom|Derivation=Dimin|Number=Sing|Number[psor]=Sing|Person[psor]=3 11 obj _ _
10 апак апак AUX Aux Polarity=Neg 11 aux:neg _ _
11 синтть синдемс VERB V Connegative=Yes|Valency=2 6 csubj _ SpaceAfter=No
12 . . PUNCT CLB _ 6 punct _ _
~~~
~~~ conllu
# visual-style 4 bgColor:blue
# visual-style 4 fgColor:white
# visual-style 3 bgColor:blue
# visual-style 3 fgColor:white
# visual-style 3 4 obl color:blue
1 ― ― PUNCT PUNCT _ 3 punct _ _
2 Терешкова Терешкова PROPN N Case=Nom|Definite=Ind|NameType=Sur|Number=Sing 3 nsubj _ _
3 панчсь панжемс VERB V Mood=Ind|Number[subj]=Sing|Person[subj]=3|Tense=Past|Valency=2 0 root _ _
4 теень мон PRON Pron Case=Dat|Number=Sing|Person=1|PronType=Prs|Variant=Short 3 obl _ _
5 ки ки NOUN N Case=Nom|Definite=Ind|Number=Sing 3 obj _ SpaceAfter=No
6 . . PUNCT CLB _ 3 punct _ _
~~~
| {
"pile_set_name": "Github"
} |
# Sourcegraph development documentation
This documentation is for developers contributing to Sourcegraph itself.
Sourcegraph development is open source at [github.com/sourcegraph/sourcegraph](https://github.com/sourcegraph/sourcegraph).
### Project links
- [Repository](https://github.com/sourcegraph/sourcegraph)
- [Issue tracker](https://github.com/sourcegraph/sourcegraph/issues)
### Technical
- [Quickstart](local_development.md)
- [Documentation guidelines](documentation.md)
- [Tech stack](tech_stack.md)
- [Architecture](architecture/index.md)
- [Developing the web clients](web/index.md)
- [Developing the web app](web/web_app.md)
- [Developing the code host integrations](code_host_integrations.md)
- [Developing the GraphQL API](graphql_api.md)
- [Developing indexed search](zoekt.md)
- [Developing campaigns](campaigns_development.md)
- [Developing code intelligence](codeintel/index.md)
- [Using PostgreSQL](postgresql.md)
- [Testing](testing.md)
- [Go style guide](https://about.sourcegraph.com/handbook/engineering/languages/go)
- [TypeScript style guide](https://about.sourcegraph.com/handbook/engineering/languages/typescript)
- [Code reviews](https://about.sourcegraph.com/handbook/engineering/code_reviews)
- [Renovate updates](renovate.md)
- [Telemetry](telemetry.md)
- [Observability](observability.md)
- [Phabricator/Gitolite documentation](phabricator_gitolite.md)
### Other
- [Open source FAQ](https://about.sourcegraph.com/community/faq)
- [Code of conduct](https://about.sourcegraph.com/community/code_of_conduct)
| {
"pile_set_name": "Github"
} |
<?xml version="1.0" encoding="utf-8"?>
<examples>
<example>
<code>Add-AWSLoggingListener -Name MyAWSLogs -LogFilePath c:\logs\aws.txt</code>
<description>Attaches a listener for the source 'Amazon', matching responses from all services for the current script or shell. Log output will be written to the specified file (the folder path must exist). Multiple listeners for different namespaces can be active at a time. By default only error responses are logged.</description>
</example>
<example>
<code>Add-AWSLoggingListener -Name MyS3Logs -LogFilePath c:\logs\s3.txt -Source Amazon.S3</code>
<description>Attaches a listener for the source 'Amazon.S3'. Responses matching only this namespace will be logged to the specified file (the folder path must exist). Multiple listeners for different namespaces can be active at a time. By default only error responses are logged.</description>
</example>
</examples> | {
"pile_set_name": "Github"
} |
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en">
<head>
<meta http-equiv="content-type" content="text/html; charset=utf-8" />
<meta name="generator" content="JsDoc Toolkit" />
<title>JsDoc Reference - Guacamole.ChainedTunnel</title>
<style type="text/css">
/* default.css */
body
{
font: 12px "Lucida Grande", Tahoma, Arial, Helvetica, sans-serif;
width: 800px;
}
.header
{
clear: both;
background-color: #ccc;
padding: 8px;
}
h1
{
font-size: 150%;
font-weight: bold;
padding: 0;
margin: 1em 0 0 .3em;
}
hr
{
border: none 0;
border-top: 1px solid #7F8FB1;
height: 1px;
}
pre.code
{
display: block;
padding: 8px;
border: 1px dashed #ccc;
}
#index
{
margin-top: 24px;
float: left;
width: 160px;
position: absolute;
left: 8px;
background-color: #F3F3F3;
padding: 8px;
}
#content
{
margin-left: 190px;
width: 600px;
}
.classList
{
list-style-type: none;
padding: 0;
margin: 0 0 0 8px;
font-family: arial, sans-serif;
font-size: 1em;
overflow: auto;
}
.classList li
{
padding: 0;
margin: 0 0 8px 0;
}
.summaryTable { width: 100%; }
h1.classTitle
{
font-size:170%;
line-height:130%;
}
h2 { font-size: 110%; }
caption, div.sectionTitle
{
background-color: #7F8FB1;
color: #fff;
font-size:130%;
text-align: left;
padding: 2px 6px 2px 6px;
border: 1px #7F8FB1 solid;
}
div.sectionTitle { margin-bottom: 8px; }
.summaryTable thead { display: none; }
.summaryTable td
{
vertical-align: top;
padding: 4px;
border-bottom: 1px #7F8FB1 solid;
border-right: 1px #7F8FB1 solid;
}
/*col#summaryAttributes {}*/
.summaryTable td.attributes
{
border-left: 1px #7F8FB1 solid;
width: 140px;
text-align: right;
}
td.attributes, .fixedFont
{
line-height: 15px;
color: #002EBE;
font-family: "Courier New",Courier,monospace;
font-size: 13px;
}
.summaryTable td.nameDescription
{
text-align: left;
font-size: 13px;
line-height: 15px;
}
.summaryTable td.nameDescription, .description
{
line-height: 15px;
padding: 4px;
padding-left: 4px;
}
.summaryTable { margin-bottom: 8px; }
ul.inheritsList
{
list-style: square;
margin-left: 20px;
padding-left: 0;
}
.detailList {
margin-left: 20px;
line-height: 15px;
}
.detailList dt { margin-left: 20px; }
.detailList .heading
{
font-weight: bold;
padding-bottom: 6px;
margin-left: 0;
}
.light, td.attributes, .light a:link, .light a:visited
{
color: #777;
font-style: italic;
}
.fineprint
{
text-align: right;
font-size: 10px;
}
</style>
</head>
<body>
<!-- ============================== header ================================= -->
<!-- begin static/header.html -->
<div id="header">
</div>
<!-- end static/header.html -->
<!-- ============================== classes index ============================ -->
<div id="index">
<!-- begin publish.classesIndex -->
<div align="center"><a href="../index.html">Class Index</a>
| <a href="../files.html">File Index</a></div>
<hr />
<h2>Classes</h2>
<ul class="classList">
<li><i><a href="../symbols/_global_.html">_global_</a></i></li>
<li><a href="../symbols/Guacamole.ArrayBufferReader.html">Guacamole.ArrayBufferReader</a></li>
<li><a href="../symbols/Guacamole.ArrayBufferWriter.html">Guacamole.ArrayBufferWriter</a></li>
<li><a href="../symbols/Guacamole.AudioChannel.html">Guacamole.AudioChannel</a></li>
<li><a href="../symbols/Guacamole.AudioChannel.Packet.html">Guacamole.AudioChannel.Packet</a></li>
<li><a href="../symbols/Guacamole.BlobReader.html">Guacamole.BlobReader</a></li>
<li><a href="../symbols/Guacamole.ChainedTunnel.html">Guacamole.ChainedTunnel</a></li>
<li><a href="../symbols/Guacamole.Client.html">Guacamole.Client</a></li>
<li><a href="../symbols/Guacamole.Display.html">Guacamole.Display</a></li>
<li><a href="../symbols/Guacamole.Display.VisibleLayer.html">Guacamole.Display.VisibleLayer</a></li>
<li><a href="../symbols/Guacamole.HTTPTunnel.html">Guacamole.HTTPTunnel</a></li>
<li><a href="../symbols/Guacamole.InputStream.html">Guacamole.InputStream</a></li>
<li><a href="../symbols/Guacamole.IntegerPool.html">Guacamole.IntegerPool</a></li>
<li><a href="../symbols/Guacamole.Keyboard.html">Guacamole.Keyboard</a></li>
<li><a href="../symbols/Guacamole.Keyboard.ModifierState.html">Guacamole.Keyboard.ModifierState</a></li>
<li><a href="../symbols/Guacamole.Layer.html">Guacamole.Layer</a></li>
<li><a href="../symbols/Guacamole.Layer.Pixel.html">Guacamole.Layer.Pixel</a></li>
<li><a href="../symbols/Guacamole.Mouse.html">Guacamole.Mouse</a></li>
<li><a href="../symbols/Guacamole.Mouse.State.html">Guacamole.Mouse.State</a></li>
<li><a href="../symbols/Guacamole.Mouse.Touchpad.html">Guacamole.Mouse.Touchpad</a></li>
<li><a href="../symbols/Guacamole.Mouse.Touchscreen.html">Guacamole.Mouse.Touchscreen</a></li>
<li><a href="../symbols/Guacamole.OnScreenKeyboard.html">Guacamole.OnScreenKeyboard</a></li>
<li><a href="../symbols/Guacamole.OnScreenKeyboard.Cap.html">Guacamole.OnScreenKeyboard.Cap</a></li>
<li><a href="../symbols/Guacamole.OnScreenKeyboard.Key.html">Guacamole.OnScreenKeyboard.Key</a></li>
<li><a href="../symbols/Guacamole.OutputStream.html">Guacamole.OutputStream</a></li>
<li><a href="../symbols/Guacamole.Parser.html">Guacamole.Parser</a></li>
<li><a href="../symbols/Guacamole.StringReader.html">Guacamole.StringReader</a></li>
<li><a href="../symbols/Guacamole.StringWriter.html">Guacamole.StringWriter</a></li>
<li><a href="../symbols/Guacamole.Tunnel.html">Guacamole.Tunnel</a></li>
<li><a href="../symbols/Guacamole.WebSocketTunnel.html">Guacamole.WebSocketTunnel</a></li>
</ul>
<hr />
<!-- end publish.classesIndex -->
</div>
<div id="content">
<!-- ============================== class title ============================ -->
<h1 class="classTitle">
Class Guacamole.ChainedTunnel
</h1>
<!-- ============================== class summary ========================== -->
<p class="description">
<br />Extends
<a href="../symbols/Guacamole.Tunnel.html">Guacamole.Tunnel</a>.<br />
<br /><i>Defined in: </i> <a href="../symbols/src/src_main_webapp_modules_Tunnel.js.html">Tunnel.js</a>.
</p>
<!-- ============================== constructor summary ==================== -->
<table class="summaryTable" cellspacing="0" summary="A summary of the constructor documented in the class Guacamole.ChainedTunnel.">
<caption>Class Summary</caption>
<thead>
<tr>
<th scope="col">Constructor Attributes</th>
<th scope="col">Constructor Name and Description</th>
</tr>
</thead>
<tbody>
<tr>
<td class="attributes"> </td>
<td class="nameDescription" >
<div class="fixedFont">
<b><a href="../symbols/Guacamole.ChainedTunnel.html#constructor">Guacamole.ChainedTunnel</a></b>(tunnel_chain)
</div>
<div class="description">Guacamole Tunnel which cycles between all specified tunnels until
no tunnels are left.</div>
</td>
</tr>
</tbody>
</table>
<!-- ============================== properties summary ===================== -->
<dl class="inheritsList">
<dt>Fields borrowed from class <a href="../symbols/Guacamole.Tunnel.html">Guacamole.Tunnel</a>: </dt><dd><a href="../symbols/Guacamole.Tunnel.html#receiveTimeout">receiveTimeout</a>, <a href="../symbols/Guacamole.Tunnel.html#state">state</a></dd>
</dl>
<!-- ============================== methods summary ======================== -->
<dl class="inheritsList">
<dt>Methods borrowed from class <a href="../symbols/Guacamole.Tunnel.html">Guacamole.Tunnel</a>: </dt><dd><a href="../symbols/Guacamole.Tunnel.html#connect">connect</a>, <a href="../symbols/Guacamole.Tunnel.html#disconnect">disconnect</a>, <a href="../symbols/Guacamole.Tunnel.html#sendMessage">sendMessage</a></dd>
</dl>
<!-- ============================== events summary ======================== -->
<dl class="inheritsList">
<dt>Events borrowed from class <a href="../symbols/Guacamole.Tunnel.html">Guacamole.Tunnel</a>: </dt><dd><a href="../symbols/Guacamole.Tunnel.html#event:onerror">onerror</a>, <a href="../symbols/Guacamole.Tunnel.html#event:oninstruction">oninstruction</a>, <a href="../symbols/Guacamole.Tunnel.html#event:onstatechange">onstatechange</a></dd>
</dl>
<!-- ============================== constructor details ==================== -->
<div class="details"><a name="constructor"> </a>
<div class="sectionTitle">
Class Detail
</div>
<div class="fixedFont">
<b>Guacamole.ChainedTunnel</b>(tunnel_chain)
</div>
<div class="description">
Guacamole Tunnel which cycles between all specified tunnels until
no tunnels are left. Another tunnel is used if an error occurs but
no instructions have been received. If an instruction has been
received, or no tunnels remain, the error is passed directly out
through the onerror handler (if defined).
</div>
<dl class="detailList">
<dt class="heading">Parameters:</dt>
<dt>
<span class="light fixedFont">{...}</span> <b>tunnel_chain</b>
</dt>
<dd>The tunnels to use, in order of priority.</dd>
</dl>
</div>
<!-- ============================== field details ========================== -->
<!-- ============================== method details ========================= -->
<!-- ============================== event details ========================= -->
<hr />
</div>
<!-- ============================== footer ================================= -->
<div class="fineprint" style="clear:both">
Documentation generated by <a href="http://code.google.com/p/jsdoc-toolkit/" target="_blank">JsDoc Toolkit</a> 2.4.0 on Fri May 23 2014 12:26:58 GMT-0700 (PDT)
</div>
<!-- Google Analytics -->
<script type="text/javascript">
(function(i,s,o,g,r,a,m){i['GoogleAnalyticsObject']=r;i[r]=i[r]||function(){
(i[r].q=i[r].q||[]).push(arguments)},i[r].l=1*new Date();a=s.createElement(o),
m=s.getElementsByTagName(o)[0];a.async=1;a.src=g;m.parentNode.insertBefore(a,m)
})(window,document,'script','//www.google-analytics.com/analytics.js','ga');
ga('create', 'UA-75289145-1', 'auto');
ga('send', 'pageview');
</script>
<!-- End Google Analytics -->
</body>
</html>
| {
"pile_set_name": "Github"
} |
// #include <ogg/ogg.h> (FIXME)
int main() {
}
| {
"pile_set_name": "Github"
} |
#!/usr/bin/env bash
# --build: Build images before starting containers.
# --abort-on-container-exit: Stops all containers if any container is stopped
docker-compose -f 'docker-compose.test.yml' -p ci up --build --abort-on-container-exit
exit $(docker wait ci_express-mongoose-es6-rest-api_1)
| {
"pile_set_name": "Github"
} |
%YAML 1.1
%TAG !u! tag:unity3d.com,2011:
--- !u!159 &1
EditorSettings:
m_ObjectHideFlags: 0
serializedVersion: 7
m_ExternalVersionControlSupport: Visible Meta Files
m_SerializationMode: 2
m_LineEndingsForNewScripts: 1
m_DefaultBehaviorMode: 0
m_SpritePackerMode: 0
m_SpritePackerPaddingPower: 1
m_EtcTextureCompressorBehavior: 1
m_EtcTextureFastCompressor: 1
m_EtcTextureNormalCompressor: 2
m_EtcTextureBestCompressor: 4
m_ProjectGenerationIncludedExtensions: txt;xml;fnt;cd
m_ProjectGenerationRootNamespace:
m_UserGeneratedProjectSuffix:
m_CollabEditorSettings:
inProgressEnabled: 1
m_EnableTextureStreamingInPlayMode: 1
| {
"pile_set_name": "Github"
} |
fileFormatVersion: 2
guid: 5930d7d2e705ea74ca30ee6652eb4571
timeCreated: 1454362609
licenseType: Store
AudioImporter:
serializedVersion: 6
defaultSettings:
loadType: 0
sampleRateSetting: 0
sampleRateOverride: 44100
compressionFormat: 0
quality: 1
conversionMode: 0
platformSettingOverrides: {}
forceToMono: 0
normalize: 1
preloadAudioData: 1
loadInBackground: 0
3D: 1
userData:
assetBundleName:
assetBundleVariant:
| {
"pile_set_name": "Github"
} |
/**
* The contents of this file are subject to the license and copyright
* detailed in the LICENSE and NOTICE files at the root of the source
* tree and available online at
*
* http://www.dspace.org/license/
*/
package org.dspace.workflow;
import java.io.IOException;
import java.sql.SQLException;
import java.util.List;
import org.dspace.authorize.AuthorizeException;
import org.dspace.content.Collection;
import org.dspace.content.WorkspaceItem;
import org.dspace.core.Context;
import org.dspace.eperson.EPerson;
import org.dspace.eperson.Group;
import org.dspace.xmlworkflow.WorkflowConfigurationException;
/**
* Service interface class for the WorkflowService framework.
* All WorkflowServices service classes should implement this class since it offers some basic methods which all
* Workflows
* are required to have.
*
* @param <T> some implementation of workflow item.
* @author kevinvandevelde at atmire.com
*/
public interface WorkflowService<T extends WorkflowItem> {
/**
* startWorkflow() begins a workflow - in a single transaction do away with
* the PersonalWorkspace entry and turn it into a WorkflowItem.
*
* @param context The relevant DSpace Context.
* @param wsi The WorkspaceItem to convert to a workflow item
* @return The resulting workflow item
* @throws SQLException An exception that provides information on a database access error or other errors.
* @throws AuthorizeException Exception indicating the current user of the context does not have permission
* to perform a particular action.
* @throws IOException A general class of exceptions produced by failed or interrupted I/O operations.
* @throws WorkflowException if workflow error
*/
public T start(Context context, WorkspaceItem wsi)
throws SQLException, AuthorizeException, IOException, WorkflowException;
/**
* startWithoutNotify() starts the workflow normally, but disables
* notifications (useful for large imports,) for the first workflow step -
* subsequent notifications happen normally
*
* @param c The relevant DSpace Context.
* @param wsi workspace item
* @return the resulting workflow item.
* @throws SQLException An exception that provides information on a database access error or other errors.
* @throws AuthorizeException Exception indicating the current user of the context does not have permission
* to perform a particular action.
* @throws IOException A general class of exceptions produced by failed or interrupted I/O operations.
* @throws WorkflowException if workflow error
*/
public T startWithoutNotify(Context c, WorkspaceItem wsi)
throws SQLException, AuthorizeException, IOException, WorkflowException;
/**
* abort() aborts a workflow, completely deleting it (administrator do this)
* (it will basically do a reject from any state - the item ends up back in
* the user's PersonalWorkspace
*
* @param c The relevant DSpace Context.
* @param wi WorkflowItem to operate on
* @param e EPerson doing the operation
* @return workspace item returned to workspace
* @throws SQLException An exception that provides information on a database access error or other errors.
* @throws AuthorizeException Exception indicating the current user of the context does not have permission
* to perform a particular action.
* @throws IOException A general class of exceptions produced by failed or interrupted I/O operations.
*/
public WorkspaceItem abort(Context c, T wi, EPerson e) throws SQLException, AuthorizeException, IOException;
public WorkspaceItem sendWorkflowItemBackSubmission(Context c, T workflowItem, EPerson e, String provenance,
String rejection_message)
throws SQLException, AuthorizeException, IOException;
public String getMyDSpaceLink();
public void deleteCollection(Context context, Collection collection)
throws SQLException, IOException, AuthorizeException;
public List<String> getEPersonDeleteConstraints(Context context, EPerson ePerson) throws SQLException;
public Group getWorkflowRoleGroup(Context context, Collection collection, String roleName, Group roleGroup)
throws SQLException, IOException, WorkflowConfigurationException, AuthorizeException, WorkflowException;
/**
* This method will create the workflowRoleGroup for a collection and the given rolename
* @param context The relevant DSpace context
* @param collection The collection
* @param roleName The rolename
* @return The created Group
* @throws AuthorizeException If something goes wrong
* @throws SQLException If something goes wrong
* @throws IOException If something goes wrong
* @throws WorkflowConfigurationException If something goes wrong
*/
public Group createWorkflowRoleGroup(Context context, Collection collection, String roleName)
throws AuthorizeException, SQLException, IOException, WorkflowConfigurationException;
public List<String> getFlywayMigrationLocations();
}
| {
"pile_set_name": "Github"
} |
Subsets and Splits