index
int64 0
0
| repo_id
stringlengths 16
181
| file_path
stringlengths 28
270
| content
stringlengths 1
11.6M
| __index_level_0__
int64 0
10k
|
---|---|---|---|---|
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/creating-themed-components/creating-themed-components.md | # Creating themed components
<p class="description">Learn how to create fully custom components that accept your app's theme.</p>
## Introduction
Material UI provides a powerful theming feature that lets you add your own components to the theme and treat them as if they're built-in components.
If you are building a component library on top of Material UI, you can follow the step-by-step guide below to create a custom component that is themeable across multiple projects.
Alternatively, you can use the provided [template](#template) as a starting point for your component.
:::info
You don't need to connect your component to the theme if you are only using it in a single project.
:::
## Step-by-step guide
This guide will walk you through how to build this statistics component, which accepts the app's theme as though it were a built-in Material UI component:
{{"demo": "StatComponent.js", "hideToolbar": true}}
### 1. Create the component slots
Slots let you customize each individual element of the component by targeting its respective name in the [theme's styleOverrides](/material-ui/customization/theme-components/#theme-style-overrides) and [theme's variants](/material-ui/customization/theme-components/#creating-new-component-variants).
This statistics component is composed of three slots:
- `root`: the container of the component
- `value`: the number of the statistics
- `unit`: the unit or description of the statistics
:::success
Though you can give these slots any names you prefer, we recommend using `root` for the outermost container element for consistency with the rest of the library.
:::
{{"demo": "StatSlots.js", "hideToolbar": true}}
Use the `styled` API with `name` and `slot` parameters to create the slots, as shown below:
```js
import * as React from 'react';
import { styled } from '@mui/material/styles';
const StatRoot = styled('div', {
name: 'MuiStat', // The component name
slot: 'root', // The slot name
})(({ theme }) => ({
display: 'flex',
flexDirection: 'column',
gap: theme.spacing(0.5),
padding: theme.spacing(3, 4),
backgroundColor: theme.palette.background.paper,
borderRadius: theme.shape.borderRadius,
boxShadow: theme.shadows[2],
letterSpacing: '-0.025em',
fontWeight: 600,
}));
const StatValue = styled('div', {
name: 'MuiStat',
slot: 'value',
})(({ theme }) => ({
...theme.typography.h3,
}));
const StatUnit = styled('div', {
name: 'MuiStat',
slot: 'unit',
})(({ theme }) => ({
...theme.typography.body2,
color: theme.palette.text.secondary,
}));
```
### 2. Create the component
Assemble the component using the slots created in the previous step:
```js
// /path/to/Stat.js
import * as React from 'react';
const StatRoot = styled('div', {
name: 'MuiStat',
slot: 'root',
})(…);
const StatValue = styled('div', {
name: 'MuiStat',
slot: 'value',
})(…);
const StatUnit = styled('div', {
name: 'MuiStat',
slot: 'unit',
})(…);
const Stat = React.forwardRef(function Stat(props, ref) {
const { value, unit, ...other } = props;
return (
<StatRoot ref={ref} {...other}>
<StatValue>{value}</StatValue>
<StatUnit>{unit}</StatUnit>
</StatRoot>
);
});
export default Stat;
```
At this point, you'll be able to apply the theme to the `Stat` component like this:
```js
import { createTheme } from '@mui/material/styles';
const theme = createTheme({
components: {
// the component name defined in the `name` parameter
// of the `styled` API
MuiStat: {
styleOverrides: {
// the slot name defined in the `slot` and `overridesResolver` parameters
// of the `styled` API
root: {
backgroundColor: '#121212',
},
value: {
color: '#fff',
},
unit: {
color: '#888',
},
},
},
},
});
```
### 3. Style the slot with ownerState
When you need to style the slot-based props or internal state, wrap them in the `ownerState` object and pass it to each slot as a prop.
The `ownerState` is a special name that will not spread to the DOM via the `styled` API.
Add a `variant` prop to the `Stat` component and use it to style the `root` slot, as shown below:
```diff
const Stat = React.forwardRef(function Stat(props, ref) {
+ const { value, unit, variant, ...other } = props;
+
+ const ownerState = { ...props, variant };
return (
- <StatRoot ref={ref} {...other}>
- <StatValue>{value}</StatValue>
- <StatUnit>{unit}</StatUnit>
- </StatRoot>
+ <StatRoot ref={ref} ownerState={ownerState} {...other}>
+ <StatValue ownerState={ownerState}>{value}</StatValue>
+ <StatUnit ownerState={ownerState}>{unit}</StatUnit>
+ </StatRoot>
);
});
```
Then you can read `ownerState` in the slot to style it based on the `variant` prop.
```diff
const StatRoot = styled('div', {
name: 'MuiStat',
slot: 'root',
- })(({ theme }) => ({
+ })(({ theme, ownerState }) => ({
display: 'flex',
flexDirection: 'column',
gap: theme.spacing(0.5),
padding: theme.spacing(3, 4),
backgroundColor: theme.palette.background.paper,
borderRadius: theme.shape.borderRadius,
boxShadow: theme.shadows[2],
letterSpacing: '-0.025em',
fontWeight: 600,
+ ...ownerState.variant === 'outlined' && {
+ border: `2px solid ${theme.palette.divider}`,
+ },
}));
```
### 4. Support theme default props
To customize your component's default props for different projects, you need to use the `useThemeProps` API.
```diff
+ import { useThemeProps } from '@mui/material/styles';
- const Stat = React.forwardRef(function Stat(props, ref) {
+ const Stat = React.forwardRef(function Stat(inProps, ref) {
+ const props = useThemeProps({ props: inProps, name: 'MuiStat' });
const { value, unit, ...other } = props;
return (
<StatRoot ref={ref} {...other}>
<StatValue>{value}</StatValue>
<StatUnit>{unit}</StatUnit>
</StatRoot>
);
});
```
Then you can customize the default props of your component like this:
```js
import { createTheme } from '@mui/material/styles';
const theme = createTheme({
components: {
MuiStat: {
defaultProps: {
variant: 'outlined',
},
},
},
});
```
## TypeScript
If you use TypeScript, you must create interfaces for the component props and ownerState:
```js
interface StatProps {
value: number | string;
unit: string;
variant?: 'outlined';
}
interface StatOwnerState extends StatProps {
// …key value pairs for the internal state that you want to style the slot
// but don't want to expose to the users
}
```
Then you can use them in the component and slots.
```js
const StatRoot = styled('div', {
name: 'MuiStat',
slot: 'root',
})<{ ownerState: StatOwnerState }>(({ theme, ownerState }) => ({
display: 'flex',
flexDirection: 'column',
gap: theme.spacing(0.5),
padding: theme.spacing(3, 4),
backgroundColor: theme.palette.background.paper,
borderRadius: theme.shape.borderRadius,
boxShadow: theme.shadows[2],
letterSpacing: '-0.025em',
fontWeight: 600,
// typed-safe access to the `variant` prop
...(ownerState.variant === 'outlined' && {
border: `2px solid ${theme.palette.divider}`,
boxShadow: 'none',
}),
}));
// …do the same for other slots
const Stat = React.forwardRef<HTMLDivElement, StatProps>(function Stat(inProps, ref) {
const props = useThemeProps({ props: inProps, name: 'MuiStat' });
const { value, unit, variant, ...other } = props;
const ownerState = { ...props, variant };
return (
<StatRoot ref={ref} ownerState={ownerState} {...other}>
<StatValue ownerState={ownerState}>{value}</StatValue>
<StatUnit ownerState={ownerState}>{unit}</StatUnit>
</StatRoot>
);
});
```
Finally, add the Stat component to the theme types.
```ts
import {
ComponentsOverrides,
ComponentsVariants,
Theme as MuiTheme,
} from '@mui/material/styles';
import { StatProps } from 'path/to/Stat';
type Theme = Omit<MuiTheme, 'components'>;
declare module '@mui/material/styles' {
interface ComponentNameToClassKey {
MuiStat: 'root' | 'value' | 'unit';
}
interface ComponentsPropsList {
MuiStat: Partial<StatProps>;
}
interface Components {
MuiStat?: {
defaultProps?: ComponentsPropsList['MuiStat'];
styleOverrides?: ComponentsOverrides<Theme>['MuiStat'];
variants?: ComponentsVariants['MuiStat'];
};
}
}
```
---
## Template
This template is the final product of the step-by-step guide above, demonstrating how to build a custom component that can be styled with the theme as if it was a built-in component.
{{"demo": "StatFullTemplate.js", "defaultCodeOpen": true}}
| 3,600 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/EmotionCSS.js | /* eslint-disable react/react-in-jsx-scope -- Unaware of jsxImportSource */
/** @jsxImportSource @emotion/react */
import { css } from '@emotion/react';
import Slider from '@mui/material/Slider';
import Box from '@mui/material/Box';
export default function EmotionCSS() {
return (
<Box sx={{ width: 300 }}>
<Slider defaultValue={30} />
<Slider
defaultValue={30}
css={css`
color: #20b2aa;
:hover {
color: #2e8b57;
}
`}
/>
</Box>
);
}
| 3,601 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/EmotionCSS.tsx | /* eslint-disable react/react-in-jsx-scope -- Unaware of jsxImportSource */
/** @jsxImportSource @emotion/react */
import { css } from '@emotion/react';
import Slider from '@mui/material/Slider';
import Box from '@mui/material/Box';
export default function EmotionCSS() {
return (
<Box sx={{ width: 300 }}>
<Slider defaultValue={30} />
<Slider
defaultValue={30}
css={css`
color: #20b2aa;
:hover {
color: #2e8b57;
}
`}
/>
</Box>
);
}
| 3,602 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/EmotionCSS.tsx.preview | <Slider defaultValue={30} />
<Slider
defaultValue={30}
css={css`
color: #20b2aa;
:hover {
color: #2e8b57;
}
`}
/> | 3,603 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/StyledComponents.js | import * as React from 'react';
import { styled } from '@mui/material/styles';
import Slider from '@mui/material/Slider';
import Box from '@mui/material/Box';
const SliderCustomized = styled(Slider)`
color: #20b2aa;
:hover {
color: #2e8b57;
}
`;
export default function StyledComponents() {
return (
<Box sx={{ width: 300 }}>
<Slider defaultValue={30} />
<SliderCustomized defaultValue={30} />
</Box>
);
}
| 3,604 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/StyledComponents.tsx | import * as React from 'react';
import { styled } from '@mui/material/styles';
import Slider from '@mui/material/Slider';
import Box from '@mui/material/Box';
const SliderCustomized = styled(Slider)`
color: #20b2aa;
:hover {
color: #2e8b57;
}
`;
export default function StyledComponents() {
return (
<Box sx={{ width: 300 }}>
<Slider defaultValue={30} />
<SliderCustomized defaultValue={30} />
</Box>
);
}
| 3,605 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/StyledComponents.tsx.preview | <Slider defaultValue={30} />
<SliderCustomized defaultValue={30} /> | 3,606 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/StyledComponentsDeep.js | import * as React from 'react';
import { styled } from '@mui/material/styles';
import Slider from '@mui/material/Slider';
import Box from '@mui/material/Box';
const CustomizedSlider = styled(Slider)`
color: #20b2aa;
:hover {
color: #2e8b57;
}
& .MuiSlider-thumb {
border-radius: 1px;
}
`;
export default function StyledComponentsDeep() {
return (
<Box sx={{ width: 300 }}>
<Slider defaultValue={30} />
<CustomizedSlider defaultValue={30} />
</Box>
);
}
| 3,607 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/StyledComponentsDeep.tsx | import * as React from 'react';
import { styled } from '@mui/material/styles';
import Slider from '@mui/material/Slider';
import Box from '@mui/material/Box';
const CustomizedSlider = styled(Slider)`
color: #20b2aa;
:hover {
color: #2e8b57;
}
& .MuiSlider-thumb {
border-radius: 1px;
}
`;
export default function StyledComponentsDeep() {
return (
<Box sx={{ width: 300 }}>
<Slider defaultValue={30} />
<CustomizedSlider defaultValue={30} />
</Box>
);
}
| 3,608 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/StyledComponentsDeep.tsx.preview | <Slider defaultValue={30} />
<CustomizedSlider defaultValue={30} /> | 3,609 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/StyledComponentsPortal.js | import * as React from 'react';
import { styled } from '@mui/material/styles';
import Button from '@mui/material/Button';
import Tooltip from '@mui/material/Tooltip';
const StyledTooltip = styled(({ className, ...props }) => (
<Tooltip {...props} classes={{ popper: className }} />
))`
& .MuiTooltip-tooltip {
background: navy;
}
`;
export default function StyledComponentsPortal() {
return (
<StyledTooltip title="I am navy">
<Button variant="contained" color="primary">
Styled tooltip
</Button>
</StyledTooltip>
);
}
| 3,610 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/StyledComponentsPortal.tsx | import * as React from 'react';
import { styled } from '@mui/material/styles';
import Button from '@mui/material/Button';
import Tooltip, { TooltipProps } from '@mui/material/Tooltip';
const StyledTooltip = styled(({ className, ...props }: TooltipProps) => (
<Tooltip {...props} classes={{ popper: className }} />
))`
& .MuiTooltip-tooltip {
background: navy;
}
`;
export default function StyledComponentsPortal() {
return (
<StyledTooltip title="I am navy">
<Button variant="contained" color="primary">
Styled tooltip
</Button>
</StyledTooltip>
);
}
| 3,611 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/StyledComponentsPortal.tsx.preview | <StyledTooltip title="I am navy">
<Button variant="contained" color="primary">
Styled tooltip
</Button>
</StyledTooltip> | 3,612 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/StyledComponentsTheme.js | import * as React from 'react';
import { createTheme, styled, ThemeProvider, darken } from '@mui/material/styles';
import Slider from '@mui/material/Slider';
import Box from '@mui/material/Box';
const customTheme = createTheme({
palette: {
primary: {
main: '#20b2aa',
},
},
});
const CustomizedSlider = styled(Slider)(
({ theme }) => `
color: ${theme.palette.primary.main};
:hover {
color: ${darken(theme.palette.primary.main, 0.2)};
}
`,
);
export default function StyledComponentsTheme() {
return (
<Box sx={{ width: 300 }}>
<ThemeProvider theme={customTheme}>
<CustomizedSlider defaultValue={30} />
</ThemeProvider>
</Box>
);
}
| 3,613 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/StyledComponentsTheme.tsx | import * as React from 'react';
import { createTheme, styled, ThemeProvider, darken } from '@mui/material/styles';
import Slider from '@mui/material/Slider';
import Box from '@mui/material/Box';
const customTheme = createTheme({
palette: {
primary: {
main: '#20b2aa',
},
},
});
const CustomizedSlider = styled(Slider)(
({ theme }) => `
color: ${theme.palette.primary.main};
:hover {
color: ${darken(theme.palette.primary.main, 0.2)};
}
`,
);
export default function StyledComponentsTheme() {
return (
<Box sx={{ width: 300 }}>
<ThemeProvider theme={customTheme}>
<CustomizedSlider defaultValue={30} />
</ThemeProvider>
</Box>
);
}
| 3,614 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/StyledComponentsTheme.tsx.preview | <ThemeProvider theme={customTheme}>
<CustomizedSlider defaultValue={30} />
</ThemeProvider> | 3,615 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/interoperability/interoperability.md | # Style library interoperability
<p class="description">While you can use the Emotion-based styling solution provided by Material UI, you can also use the one you already know, from plain CSS to styled-components.</p>
This guide aims to document the most popular alternatives,
but you should find that the principles applied here can be adapted to other libraries.
There are examples for the following styling solutions:
- [Plain CSS](#plain-css)
- [Global CSS](#global-css)
- [Styled Components](#styled-components)
- [CSS Modules](#css-modules)
- [Emotion](#emotion)
- [Tailwind CSS](#tailwind-css)
- [~~JSS~~ TSS](#jss-tss)
## Plain CSS
Nothing fancy, just plain CSS.
{{"demo": "StyledComponents.js", "hideToolbar": true}}
[](https://codesandbox.io/s/plain-css-fdue7)
**PlainCssSlider.css**
```css
.slider {
color: #20b2aa;
}
.slider:hover {
color: #2e8b57;
}
```
**PlainCssSlider.js**
```jsx
import * as React from 'react';
import Slider from '@mui/material/Slider';
import './PlainCssSlider.css';
export default function PlainCssSlider() {
return (
<div>
<Slider defaultValue={30} />
<Slider defaultValue={30} className="slider" />
</div>
);
}
```
### CSS injection order ⚠️
**Note:** Most CSS-in-JS solutions inject their styles at the bottom of the HTML `<head>`, which gives Material UI precedence over your custom styles. To remove the need for **!important**, you need to change the CSS injection order. Here's a demo of how it can be done in Material UI:
```jsx
import * as React from 'react';
import { StyledEngineProvider } from '@mui/material/styles';
export default function GlobalCssPriority() {
return (
<StyledEngineProvider injectFirst>
{/* Your component tree. Now you can override Material UI's styles. */}
</StyledEngineProvider>
);
}
```
**Note:** If you are using Emotion and have a custom cache in your app, that one will override the one coming from Material UI. In order for the injection order to still be correct, you need to add the prepend option. Here is an example:
```jsx
import * as React from 'react';
import { CacheProvider } from '@emotion/react';
import createCache from '@emotion/cache';
const cache = createCache({
key: 'css',
prepend: true,
});
export default function PlainCssPriority() {
return (
<CacheProvider value={cache}>
{/* Your component tree. Now you can override Material UI's styles. */}
</CacheProvider>
);
}
```
**Note:** If you are using styled-components and have `StyleSheetManager` with a custom `target`, make sure that the target is the first element in the HTML `<head>`. If you are curious to see how it can be done, you can take a look on the [`StyledEngineProvider`](https://github.com/mui/material-ui/blob/-/packages/mui-styled-engine-sc/src/StyledEngineProvider/StyledEngineProvider.js) implementation in the `@mui/styled-engine-sc` package.
### Deeper elements
If you attempt to style the Slider,
you will likely need to affect some of the Slider's child elements, for example the thumb.
In Material UI, all child elements have an increased specificity of 2: `.parent .child {}`. When writing overrides, you need to do the same.
The following examples override the slider's `thumb` style in addition to the custom styles on the slider itself.
{{"demo": "StyledComponentsDeep.js", "hideToolbar": true}}
**PlainCssSliderDeep1.css**
```css
.slider {
color: #20b2aa;
}
.slider:hover {
color: #2e8b57;
}
.slider .MuiSlider-thumb {
border-radius: 1px;
}
```
**PlainCssSliderDeep1.js**
```jsx
import * as React from 'react';
import Slider from '@mui/material/Slider';
import './PlainCssSliderDeep1.css';
export default function PlainCssSliderDeep1() {
return (
<div>
<Slider defaultValue={30} />
<Slider defaultValue={30} className="slider" />
</div>
);
}
```
The above demo relies on the [default `className` values](/system/styles/advanced/), but you can provide your own class name with the `slotProps` API.
**PlainCssSliderDeep2.css**
```css
.slider {
color: #20b2aa;
}
.slider:hover {
color: #2e8b57;
}
.slider .thumb {
border-radius: 1px;
}
```
**PlainCssSliderDeep2.js**
```jsx
import * as React from 'react';
import Slider from '@mui/material/Slider';
import './PlainCssSliderDeep2.css';
export default function PlainCssSliderDeep2() {
return (
<div>
<Slider defaultValue={30} />
<Slider
defaultValue={30}
className="slider"
slotProps={{ thumb: { className: 'thumb' } }}
/>
</div>
);
}
```
## Global CSS
Explicitly providing the class names to the component is too much effort?
[You can target the class names generated by Material UI](/system/styles/advanced/).
[](https://codesandbox.io/s/global-classnames-dho8k)
**GlobalCssSlider.css**
```css
.MuiSlider-root {
color: #20b2aa;
}
.MuiSlider-root:hover {
color: #2e8b57;
}
```
**GlobalCssSlider.js**
```jsx
import * as React from 'react';
import Slider from '@mui/material/Slider';
import './GlobalCssSlider.css';
export default function GlobalCssSlider() {
return <Slider defaultValue={30} />;
}
```
### CSS injection order ⚠️
**Note:** Most CSS-in-JS solutions inject their styles at the bottom of the HTML `<head>`, which gives Material UI precedence over your custom styles. To remove the need for **!important**, you need to change the CSS injection order. Here's a demo of how it can be done in Material UI:
```jsx
import * as React from 'react';
import { StyledEngineProvider } from '@mui/material/styles';
export default function GlobalCssPriority() {
return (
<StyledEngineProvider injectFirst>
{/* Your component tree. Now you can override Material UI's styles. */}
</StyledEngineProvider>
);
}
```
**Note:** If you are using Emotion and have a custom cache in your app, that one will override the one coming from Material UI. In order for the injection order to still be correct, you need to add the prepend option. Here is an example:
```jsx
import * as React from 'react';
import { CacheProvider } from '@emotion/react';
import createCache from '@emotion/cache';
const cache = createCache({
key: 'css',
prepend: true,
});
export default function GlobalCssPriority() {
return (
<CacheProvider value={cache}>
{/* Your component tree. Now you can override Material UI's styles. */}
</CacheProvider>
);
}
```
**Note:** If you are using styled-components and have `StyleSheetManager` with a custom `target`, make sure that the target is the first element in the HTML `<head>`. If you are curious to see how it can be done, you can take a look on the [`StyledEngineProvider`](https://github.com/mui/material-ui/blob/-/packages/mui-styled-engine-sc/src/StyledEngineProvider/StyledEngineProvider.js) implementation in the `@mui/styled-engine-sc` package.
### Deeper elements
If you attempt to style the Slider,
you will likely need to affect some of the Slider's child elements, for example the thumb.
In Material UI, all child elements have an increased specificity of 2: `.parent .child {}`. When writing overrides, you need to do the same.
The following example overrides the slider's `thumb` style in addition to the custom styles on the slider itself.
{{"demo": "StyledComponentsDeep.js", "hideToolbar": true}}
**GlobalCssSliderDeep.css**
```css
.MuiSlider-root {
color: #20b2aa;
}
.MuiSlider-root:hover {
color: #2e8b57;
}
.MuiSlider-root .MuiSlider-thumb {
border-radius: 1px;
}
```
**GlobalCssSliderDeep.js**
```jsx
import * as React from 'react';
import Slider from '@mui/material/Slider';
import './GlobalCssSliderDeep.css';
export default function GlobalCssSliderDeep() {
return <Slider defaultValue={30} />;
}
```
## Styled Components


### Change the default styled engine
By default, Material UI components come with Emotion as their style engine.
If, however, you would like to use styled-components, you can configure your app by following the [styled-components guide](/material-ui/guides/styled-components/) or starting with one of the example projects:
<!-- #default-branch-switch -->
- [Create React App with styled-components](https://github.com/mui/material-ui/tree/master/examples/material-ui-cra-styled-components)
- [Create React App with styled-components and TypeScript](https://github.com/mui/material-ui/tree/master/examples/material-ui-cra-styled-components-ts)
Following this approach reduces the bundle size, and removes the need to configure the CSS injection order.
After the style engine is configured properly, you can use the [`styled()`](/system/styled/) utility
from `@mui/material/styles` and have direct access to the theme.
{{"demo": "StyledComponents.js", "hideToolbar": true}}
[](https://codesandbox.io/s/styled-components-interoperability-w9z9d)
```jsx
import * as React from 'react';
import Slider from '@mui/material/Slider';
import { styled } from '@mui/material/styles';
const CustomizedSlider = styled(Slider)`
color: #20b2aa;
:hover {
color: #2e8b57;
}
`;
export default function StyledComponents() {
return <CustomizedSlider defaultValue={30} />;
}
```
### Deeper elements
If you attempt to style the Slider,
you will likely need to affect some of the Slider's child elements, for example the thumb.
In Material UI, all child elements have an increased specificity of 2: `.parent .child {}`. When writing overrides, you need to do the same.
The following examples override the slider's `thumb` style in addition to the custom styles on the slider itself.
{{"demo": "StyledComponentsDeep.js", "defaultCodeOpen": true}}
The above demo relies on the [default `className` values](/system/styles/advanced/), but you can provide your own class name with the `slotProps` API.
```jsx
import * as React from 'react';
import { styled } from '@mui/material/styles';
import Slider from '@mui/material/Slider';
const CustomizedSlider = styled((props) => (
<Slider slotProps={{ thumb: { className: 'thumb' } }} {...props} />
))`
color: #20b2aa;
:hover {
color: #2e8b57;
}
& .thumb {
border-radius: 1px;
}
`;
export default function StyledComponentsDeep2() {
return (
<div>
<Slider defaultValue={30} />
<CustomizedSlider defaultValue={30} />
</div>
);
}
```
### Theme
By using the Material UI theme provider, the theme will be available in the theme context
of the styled engine too (Emotion or styled-components, depending on your configuration).
:::warning
If you are already using a custom theme with styled-components or Emotion,
it might not be compatible with Material UI's theme specification. If it's not
compatible, you need to render Material UI's ThemeProvider first. This will
ensure the theme structures are isolated. This is ideal for the progressive adoption
of Material UI's components in the codebase.
:::
You are encouraged to share the same theme object between Material UI and the rest of your project.
```jsx
const CustomizedSlider = styled(Slider)(
({ theme }) => `
color: ${theme.palette.primary.main};
:hover {
color: ${darken(theme.palette.primary.main, 0.2)};
}
`,
);
```
{{"demo": "StyledComponentsTheme.js"}}
### Portals
The [Portal](/material-ui/react-portal/) provides a first-class way to render children into a DOM node that exists outside the DOM hierarchy of the parent component.
Because of the way styled-components scopes its CSS, you may run into issues where styling is not applied.
For example, if you attempt to style the `tooltip` generated by the [Tooltip](/material-ui/react-tooltip/) component,
you will need to pass along the `className` property to the element being rendered outside of it's DOM hierarchy.
The following example shows a workaround:
```jsx
import * as React from 'react';
import { styled } from '@mui/material/styles';
import Button from '@mui/material/Button';
import Tooltip from '@mui/material/Tooltip';
const StyledTooltip = styled(({ className, ...props }) => (
<Tooltip {...props} classes={{ popper: className }} />
))`
& .MuiTooltip-tooltip {
background: navy;
}
`;
```
{{"demo": "StyledComponentsPortal.js"}}
## CSS Modules

It's hard to know the market share of [this styling solution](https://github.com/css-modules/css-modules) as it's dependent on the
bundling solution people are using.
{{"demo": "StyledComponents.js", "hideToolbar": true}}
[](https://codesandbox.io/s/css-modules-nuyg8)
**CssModulesSlider.module.css**
```css
.slider {
color: #20b2aa;
}
.slider:hover {
color: #2e8b57;
}
```
**CssModulesSlider.js**
```jsx
import * as React from 'react';
import Slider from '@mui/material/Slider';
// webpack, parcel or else will inject the CSS into the page
import styles from './CssModulesSlider.module.css';
export default function CssModulesSlider() {
return (
<div>
<Slider defaultValue={30} />
<Slider defaultValue={30} className={styles.slider} />
</div>
);
}
```
### CSS injection order ⚠️
**Note:** Most CSS-in-JS solutions inject their styles at the bottom of the HTML `<head>`, which gives Material UI precedence over your custom styles. To remove the need for **!important**, you need to change the CSS injection order. Here's a demo of how it can be done in Material UI:
```jsx
import * as React from 'react';
import { StyledEngineProvider } from '@mui/material/styles';
export default function GlobalCssPriority() {
return (
<StyledEngineProvider injectFirst>
{/* Your component tree. Now you can override Material UI's styles. */}
</StyledEngineProvider>
);
}
```
**Note:** If you are using Emotion and have a custom cache in your app, that one will override the one coming from Material UI. In order for the injection order to still be correct, you need to add the prepend option. Here is an example:
```jsx
import * as React from 'react';
import { CacheProvider } from '@emotion/react';
import createCache from '@emotion/cache';
const cache = createCache({
key: 'css',
prepend: true,
});
export default function CssModulesPriority() {
return (
<CacheProvider value={cache}>
{/* Your component tree. Now you can override Material UI's styles. */}
</CacheProvider>
);
}
```
**Note:** If you are using styled-components and have `StyleSheetManager` with a custom `target`, make sure that the target is the first element in the HTML `<head>`. If you are curious to see how it can be done, you can take a look on the [`StyledEngineProvider`](https://github.com/mui/material-ui/blob/-/packages/mui-styled-engine-sc/src/StyledEngineProvider/StyledEngineProvider.js) implementation in the `@mui/styled-engine-sc` package.
### Deeper elements
If you attempt to style the Slider,
you will likely need to affect some of the Slider's child elements, for example the thumb.
In Material UI, all child elements have an increased specificity of 2: `.parent .child {}`. When writing overrides, you need to do the same.
It's important to keep in mind that CSS Modules adds an unique id to each class, and that id won't be present on the Material UI provided children class. To escape from that, CSS Modules provides a functionality, the `:global` selector.
The following examples override the slider's `thumb` style in addition to the custom styles on the slider itself.
{{"demo": "StyledComponentsDeep.js", "hideToolbar": true}}
**CssModulesSliderDeep1.module.css**
```css
.slider {
color: #20b2aa;
}
.slider:hover {
color: #2e8b57;
}
.slider :global .MuiSlider-thumb {
border-radius: 1px;
}
```
**CssModulesSliderDeep1.js**
```jsx
import * as React from 'react';
// webpack, parcel or else will inject the CSS into the page
import styles from './CssModulesSliderDeep1.module.css';
import Slider from '@mui/material/Slider';
export default function CssModulesSliderDeep1() {
return (
<div>
<Slider defaultValue={30} />
<Slider defaultValue={30} className={styles.slider} />
</div>
);
}
```
The above demo relies on the [default `className` values](/system/styles/advanced/), but you can provide your own class name with the `slotProps` API.
**CssModulesSliderDeep2.module.css**
```css
.slider {
color: #20b2aa;
}
.slider:hover {
color: #2e8b57;
}
.slider .thumb {
border-radius: 1px;
}
```
**CssModulesSliderDeep2.js**
```jsx
import * as React from 'react';
// webpack, parcel or else will inject the CSS into the page
import styles from './CssModulesSliderDeep2.module.css';
import Slider from '@mui/material/Slider';
export default function CssModulesSliderDeep2() {
return (
<div>
<Slider defaultValue={30} />
<Slider
defaultValue={30}
className={styles.slider}
slotProps={{ thumb: { className: styles.thumb } }}
/>
</div>
);
}
```
## Emotion


### The `css` prop
Emotion's **css()** method works seamlessly with Material UI.
{{"demo": "EmotionCSS.js", "defaultCodeOpen": true}}
### Theme
It works exactly like styled components. You can [use the same guide](/material-ui/guides/interoperability/#styled-components).
### The `styled()` API
It works exactly like styled components. You can [use the same guide](/material-ui/guides/interoperability/#styled-components).
## Tailwind CSS


### Setup
If you are used to Tailwind CSS and want to use it together with the Material UI components, you can start by cloning the [Tailwind CSS](https://github.com/mui/material-ui/tree/master/examples/material-ui-cra-tailwind-ts) example project.
If you use a different framework, or already have set up your project, follow these steps:
1. Add Tailwind CSS to your project, following the instructions in https://tailwindcss.com/docs/installation.
2. Remove [Tailwind CSS's preflight](https://tailwindcss.com/docs/preflight) style so it can use the Material UI's preflight instead ([CssBaseline](/material-ui/react-css-baseline/)).
**tailwind.config.js**
```diff
module.exports = {
+ corePlugins: {
+ preflight: false,
+ },
};
```
3. Add the `important` option, using the id of your app wrapper. For example, `#__next` for Next.js and `"#root"` for CRA:
**tailwind.config.js**
```diff
module.exports = {
content: [
"./src/**/*.{js,jsx,ts,tsx}",
],
+ important: '#root',
theme: {
extend: {},
},
plugins: [],
}
```
Most of the CSS used by Material UI has as specificity of 1, hence this `important` property is unnecessary.
However, in a few edge cases, Material UI uses nested CSS selectors that win over Tailwind CSS.
Use this step to help ensure that the [deeper elements](#deeper-elements-5) can always be customized using Tailwind's utility classes.
More details on this option can be found here https://tailwindcss.com/docs/configuration#selector-strategy
4. Fix the CSS injection order. Most CSS-in-JS solutions inject their styles at the bottom of the HTML `<head>`, which gives Material UI precedence over Tailwind CSS. To reduce the need for the `important` property, you need to change the CSS injection order. Here's a demo of how it can be done in Material UI:
```jsx
import * as React from 'react';
import { StyledEngineProvider } from '@mui/material/styles';
export default function GlobalCssPriority() {
return (
<StyledEngineProvider injectFirst>
{/* Your component tree. Now you can override Material UI's styles. */}
</StyledEngineProvider>
);
}
```
**Note:** If you are using Emotion and have a custom cache in your app, it will override the one coming from Material UI. In order for the injection order to still be correct, you need to add the prepend option. Here is an example:
```jsx
import * as React from 'react';
import { CacheProvider } from '@emotion/react';
import createCache from '@emotion/cache';
const cache = createCache({
key: 'css',
prepend: true,
});
export default function PlainCssPriority() {
return (
<CacheProvider value={cache}>
{/* Your component tree. Now you can override Material UI's styles. */}
</CacheProvider>
);
}
```
**Note:** If you are using styled-components and have `StyleSheetManager` with a custom `target`, make sure that the target is the first element in the HTML `<head>`. If you are curious to see how it can be done, you can take a look at the [`StyledEngineProvider`](https://github.com/mui/material-ui/blob/-/packages/mui-styled-engine-sc/src/StyledEngineProvider/StyledEngineProvider.js) implementation in the `@mui/styled-engine-sc` package.
5. Change the target container for `Portal`-related elements so that they are injected under the main app wrapper that was used in step 3 for setting up the `important` option in the Tailwind config.
```jsx
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
const theme = createTheme({
components: {
MuiPopover: {
defaultProps: {
container: rootElement,
},
},
MuiPopper: {
defaultProps: {
container: rootElement,
},
},
MuiDialog: {
defaultProps: {
container: rootElement,
},
},
MuiModal: {
defaultProps: {
container: rootElement,
},
},
},
});
root.render(
<StyledEngineProvider injectFirst>
<ThemeProvider theme={theme}>
<App />
</ThemeProvider>
</StyledEngineProvider>;
);
```
### Usage
Now it's all set up and you can start using Tailwind CSS on the Material UI components!
{{"demo": "StyledComponents.js", "hideToolbar": true}}
[](https://stackblitz.com/edit/github-ndkshy?file=pages%2Findex.tsx)
**index.tsx**
```jsx
import * as React from 'react';
import Slider from '@mui/material/Slider';
export default function App() {
return (
<div>
<Slider defaultValue={30} />
<Slider defaultValue={30} className="text-teal-600" />
</div>
);
}
```
### Deeper elements
If you attempt to style the Slider, for example, you'll likely want to customize its child elements.
This example showcases how to override the Slider's `thumb` style.
{{"demo": "StyledComponentsDeep.js", "hideToolbar": true}}
**SliderThumbOverrides.tsx**
```jsx
import * as React from 'react';
import Slider from '@mui/material/Slider';
export default function SliderThumbOverrides() {
return (
<div>
<Slider defaultValue={30} />
<Slider
defaultValue={30}
className="text-teal-600"
slotProps={{ thumb: { className: 'rounded-sm' } }}
/>
</div>
);
}
```
### Styling pseudo states
If you want to style a component's pseudo-state, you can use the appropriate key in the `classes` prop.
Here is an example of how you can style the Slider's active state:
**SliderPseudoStateOverrides.tsx**
```jsx
import * as React from 'react';
import Slider from '@mui/material/Slider';
export default function SliderThumbOverrides() {
return <Slider defaultValue={30} classes={{ active: 'shadow-none' }} />;
}
```
## ~~JSS~~ TSS
[JSS](https://cssinjs.org/) itself is no longer supported in Material UI, however,
if you like the hook-based API (`makeStyles` → `useStyles`) that [`react-jss`](https://codesandbox.io/s/j3l06yyqpw) was offering you can opt for [`tss-react`](https://github.com/garronej/tss-react).
[TSS](https://docs.tss-react.dev) integrates well with Material UI and provide a better
TypeScript support than JSS.
:::info
If you are updating from `@material-ui/core` (v4) to `@mui/material` (v5), check out the [tss-react section](/material-ui/migration/migrating-from-jss/#2-use-tss-react) of the Migration guide.
:::
```tsx
import { render } from 'react-dom';
import { CacheProvider } from '@emotion/react';
import createCache from '@emotion/cache';
import { ThemeProvider } from '@mui/material/styles';
export const muiCache = createCache({
key: 'mui',
prepend: true,
});
//NOTE: Don't use <StyledEngineProvider injectFirst/>
render(
<CacheProvider value={muiCache}>
<ThemeProvider theme={myTheme}>
<Root />
</ThemeProvider>
</CacheProvider>,
document.getElementById('root'),
);
```
Now you can simply
`import { makeStyles, withStyles } from 'tss-react/mui'`.
The theme object that will be passed to your callbacks functions will be the one you
get with
`import { useTheme } from '@mui/material/styles'`.
If you want to take controls over what the `theme` object should be,
you can re-export `makeStyles` and `withStyles` from a file called, for example, `makesStyles.ts`:
```ts
import { useTheme } from '@mui/material/styles';
//WARNING: tss-react require TypeScript v4.4 or newer. If you can't update use:
//import { createMakeAndWithStyles } from "tss-react/compat";
import { createMakeAndWithStyles } from 'tss-react';
export const { makeStyles, withStyles } = createMakeAndWithStyles({
useTheme,
/*
OR, if you have extended the default mui theme adding your own custom properties:
Let's assume the myTheme object that you provide to the <ThemeProvider /> is of
type MyTheme then you'll write:
*/
//"useTheme": useTheme as (()=> MyTheme)
});
```
Then, the library is used like this:
```tsx
import { makeStyles } from 'tss-react/mui';
export function MyComponent(props: Props) {
const { className } = props;
const [color, setColor] = useState<'red' | 'blue'>('red');
const { classes, cx } = useStyles({ color });
//Thanks to cx, className will take priority over classes.root
return <span className={cx(classes.root, className)}>hello world</span>;
}
const useStyles = makeStyles<{ color: 'red' | 'blue' }>()((theme, { color }) => ({
root: {
color,
'&:hover': {
backgroundColor: theme.palette.primary.main,
},
},
}));
```
For info on how to setup SSR or anything else, please refer to [the TSS documentation](https://github.com/garronej/tss-react).
:::info
There is [an ESLint plugin](https://docs.tss-react.dev/detecting-unused-classes) for detecting unused classes.
:::
:::warning
**Keep `@emotion/styled` as a dependency of your project**. Even if you never use it explicitly,
it's a peer dependency of `@mui/material`.
:::
| 3,616 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/localization/Locales.js | import * as React from 'react';
import TablePagination from '@mui/material/TablePagination';
import Autocomplete from '@mui/material/Autocomplete';
import Box from '@mui/material/Box';
import TextField from '@mui/material/TextField';
import { createTheme, ThemeProvider, useTheme } from '@mui/material/styles';
import * as locales from '@mui/material/locale';
export default function Locales() {
const [locale, setLocale] = React.useState('zhCN');
const theme = useTheme();
const themeWithLocale = React.useMemo(
() => createTheme(theme, locales[locale]),
[locale, theme],
);
return (
<Box sx={{ width: '100%' }}>
<ThemeProvider theme={themeWithLocale}>
<Autocomplete
options={Object.keys(locales)}
getOptionLabel={(key) => `${key.substring(0, 2)}-${key.substring(2, 4)}`}
style={{ width: 300 }}
value={locale}
disableClearable
onChange={(event, newValue) => {
setLocale(newValue);
}}
renderInput={(params) => (
<TextField {...params} label="Locale" fullWidth />
)}
/>
<TablePagination
count={2000}
rowsPerPage={10}
page={1}
component="div"
onPageChange={() => {}}
/>
</ThemeProvider>
</Box>
);
}
| 3,617 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/localization/Locales.tsx | import * as React from 'react';
import TablePagination from '@mui/material/TablePagination';
import Autocomplete from '@mui/material/Autocomplete';
import Box from '@mui/material/Box';
import TextField from '@mui/material/TextField';
import { createTheme, ThemeProvider, useTheme } from '@mui/material/styles';
import * as locales from '@mui/material/locale';
type SupportedLocales = keyof typeof locales;
export default function Locales() {
const [locale, setLocale] = React.useState<SupportedLocales>('zhCN');
const theme = useTheme();
const themeWithLocale = React.useMemo(
() => createTheme(theme, locales[locale]),
[locale, theme],
);
return (
<Box sx={{ width: '100%' }}>
<ThemeProvider theme={themeWithLocale}>
<Autocomplete
options={Object.keys(locales)}
getOptionLabel={(key) => `${key.substring(0, 2)}-${key.substring(2, 4)}`}
style={{ width: 300 }}
value={locale}
disableClearable
onChange={(event: any, newValue: string | null) => {
setLocale(newValue as SupportedLocales);
}}
renderInput={(params) => (
<TextField {...params} label="Locale" fullWidth />
)}
/>
<TablePagination
count={2000}
rowsPerPage={10}
page={1}
component="div"
onPageChange={() => {}}
/>
</ThemeProvider>
</Box>
);
}
| 3,618 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/localization/localization.md | # Localization
<p class="description">Localization (also referred to as "l10n") is the process of adapting a product or content to a specific locale or market.</p>
The default locale of Material UI is English (United States). If you want to use other locales, follow the instructions below.
## Locale text
Use the theme to configure the locale text globally:
```jsx
import { createTheme, ThemeProvider } from '@mui/material/styles';
import { zhCN } from '@mui/material/locale';
const theme = createTheme(
{
palette: {
primary: { main: '#1976d2' },
},
},
zhCN,
);
<ThemeProvider theme={theme}>
<App />
</ThemeProvider>;
```
### Example
{{"demo": "Locales.js"}}
:::warning
The [Data Grid and Data Grid Pro](/x/react-data-grid/) components have their own [localization](/x/react-data-grid/localization/).
:::
### Supported locales
| Locale | BCP 47 language tag | Import name |
| :---------------------- | :------------------ | :---------- |
| Amharic | am-ET | `amET` |
| Arabic (Egypt) | ar-EG | `arEG` |
| Arabic (Saudi Arabia) | ar-SA | `arSA` |
| Arabic (Sudan) | ar-SD | `arSD` |
| Armenian | hy-AM | `hyAM` |
| Azerbaijani | az-AZ | `azAZ` |
| Bangla | bn-BD | `bnBD` |
| Bulgarian | bg-BG | `bgBG` |
| Catalan | ca-ES | `caES` |
| Chinese (Hong Kong) | zh-HK | `zhHK` |
| Chinese (Simplified) | zh-CN | `zhCN` |
| Chinese (Taiwan) | zh-TW | `zhTW` |
| Croatian | hr-HR | `hrHR` |
| Czech | cs-CZ | `csCZ` |
| Danish | da-DK | `daDK` |
| Dutch | nl-NL | `nlNL` |
| English (United States) | en-US | `enUS` |
| Estonian | et-EE | `etEE` |
| Finnish | fi-FI | `fiFI` |
| French | fr-FR | `frFR` |
| German | de-DE | `deDE` |
| Greek | el-GR | `elGR` |
| Hebrew | he-IL | `heIL` |
| Hindi | hi-IN | `hiIN` |
| Hungarian | hu-HU | `huHU` |
| Icelandic | is-IS | `isIS` |
| Indonesian | id-ID | `idID` |
| Italian | it-IT | `itIT` |
| Japanese | ja-JP | `jaJP` |
| Khmer | kh-KH | `khKH` |
| Kazakh | kk-KZ | `kkKZ` |
| Korean | ko-KR | `koKR` |
| Kurdish (Central) | ku-CKB | `kuCKB` |
| Macedonian | mk-MK | `mkMK` |
| Myanmar | my-MY | `myMY` |
| Malay | ms-MS | `msMS` |
| Nepali | ne-NP | `neNP` |
| Norwegian (bokmål) | nb-NO | `nbNO` |
| Norwegian (nynorsk) | nn-NO | `nnNO` |
| Persian | fa-IR | `faIR` |
| Polish | pl-PL | `plPL` |
| Portuguese | pt-PT | `ptPT` |
| Portuguese (Brazil) | pt-BR | `ptBR` |
| Romanian | ro-RO | `roRO` |
| Russian | ru-RU | `ruRU` |
| Serbian | sr-RS | `srRS` |
| Sinhalese | si-LK | `siLK` |
| Slovak | sk-SK | `skSK` |
| Spanish | es-ES | `esES` |
| Swedish | sv-SE | `svSE` |
| Thai | th-TH | `thTH` |
| Turkish | tr-TR | `trTR` |
| Tagalog | tl-TL | `tlTL` |
| Ukrainian | uk-UA | `ukUA` |
| Urdu (Pakistan) | ur-PK | `urPK` |
| Vietnamese | vi-VN | `viVN` |
<!-- #default-branch-switch -->
You can [find the source](https://github.com/mui/material-ui/blob/master/packages/mui-material/src/locale/index.ts) in the GitHub repository.
To create your own translation, or to customize the English text, copy this file to your project, make any changes needed and import the locale from there.
Please do consider contributing new translations back to Material UI by opening a pull request.
However, Material UI aims to support the [100 most common](https://en.wikipedia.org/wiki/List_of_languages_by_number_of_native_speakers) [locales](https://www.ethnologue.com/guides/ethnologue200), we might not accept contributions for locales that are not frequently used, for instance `gl-ES` that has "only" 2.5 million native speakers.
## RTL Support
Right-to-left languages such as Arabic, Persian, or Hebrew are supported.
Follow [this guide](/material-ui/guides/right-to-left/) to use them.
| 3,619 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/minimizing-bundle-size/minimizing-bundle-size.md | # Minimizing bundle size
<p class="description">Learn more about the tools you can leverage to reduce the bundle size.</p>
## Bundle size matters
Material UI's maintainers take bundle size very seriously. Size snapshots are taken
on every commit for every package and critical parts of those packages ([view the latest snapshot](/size-snapshot/)).
Combined with [dangerJS](https://danger.systems/js/) we can inspect
[detailed bundle size changes](https://github.com/mui/material-ui/pull/14638#issuecomment-466658459) on every Pull Request.
## When and how to use tree-shaking?
Tree-shaking Material UI works out of the box in modern frameworks.
Material UI exposes its full API on the top-level `@mui` imports.
If you're using ES6 modules and a bundler that supports tree-shaking ([`webpack` >= 2.x](https://webpack.js.org/guides/tree-shaking/), [`parcel` with a flag](https://en.parceljs.org/cli.html#enable-experimental-scope-hoisting/tree-shaking-support)) you can safely use named imports and still get an optimized bundle size automatically:
```js
import { Button, TextField } from '@mui/material';
```
⚠️ The following instructions are only needed if you want to optimize your development startup times or if you are using an older bundler
that doesn't support tree-shaking.
## Development environment
Development bundles can contain the full library which can lead to **slower startup times**.
This is especially noticeable if you use named imports from `@mui/icons-material`, which can be up to six times slower than the default import.
For example, between the following two imports, the first (named) can be significantly slower than the second (default):
```js
// 🐌 Named
import { Delete } from '@mui/icons-material';
```
```js
// 🚀 Default
import Delete from '@mui/icons-material/Delete';
```
If this is an issue for you, you have two options:
### Option one: use path imports
You can use path imports to avoid pulling in unused modules.
For instance, use:
```js
// 🚀 Fast
import Button from '@mui/material/Button';
import TextField from '@mui/material/TextField';
```
instead of top-level imports (without a Babel plugin):
```js
import { Button, TextField } from '@mui/material';
```
This is the option we document in all the demos since it requires no configuration.
It is encouraged for library authors that are extending the components.
Head to [Option 2](#option-two-use-a-babel-plugin) for the approach that yields the best DX and UX.
While importing directly in this manner doesn't use the exports in [the main file of `@mui/material`](https://unpkg.com/@mui/material), this file can serve as a handy reference as to which modules are public.
Be aware that we only support first and second-level imports.
Anything deeper is considered private and can cause issues, such as module duplication in your bundle.
```js
// ✅ OK
import { Add as AddIcon } from '@mui/icons-material';
import { Tabs } from '@mui/material';
// ^^^^^^^^ 1st or top-level
// ✅ OK
import AddIcon from '@mui/icons-material/Add';
import Tabs from '@mui/material/Tabs';
// ^^^^ 2nd level
// ❌ NOT OK
import TabIndicator from '@mui/material/Tabs/TabIndicator';
// ^^^^^^^^^^^^ 3rd level
```
If you're using `eslint` you can catch problematic imports with the [`no-restricted-imports` rule](https://eslint.org/docs/latest/rules/no-restricted-imports). The following `.eslintrc` configuration will highlight problematic imports from `@mui` packages:
```json
{
"rules": {
"no-restricted-imports": [
"error",
{
"patterns": ["@mui/*/*/*"]
}
]
}
}
```
### Option two: use a Babel plugin
This option provides the best user experience and developer experience:
- UX: The Babel plugin enables top-level tree-shaking even if your bundler doesn't support it.
- DX: The Babel plugin makes startup time in dev mode as fast as Option 1.
- DX: This syntax reduces the duplication of code, requiring only a single import for multiple modules.
Overall, the code is easier to read, and you are less likely to make a mistake when importing a new module.
```js
import { Button, TextField } from '@mui/material';
```
However, you need to apply the two following steps correctly.
#### 1. Configure Babel
Pick one of the following plugins:
- [babel-plugin-import](https://github.com/umijs/babel-plugin-import) with the following configuration:
`yarn add -D babel-plugin-import`
Create a `.babelrc.js` file in the root directory of your project:
```js
const plugins = [
[
'babel-plugin-import',
{
libraryName: '@mui/material',
libraryDirectory: '',
camel2DashComponentName: false,
},
'core',
],
[
'babel-plugin-import',
{
libraryName: '@mui/icons-material',
libraryDirectory: '',
camel2DashComponentName: false,
},
'icons',
],
];
module.exports = { plugins };
```
- [babel-plugin-direct-import](https://github.com/avocadowastaken/babel-plugin-direct-import) with the following configuration:
`yarn add -D babel-plugin-direct-import`
Create a `.babelrc.js` file in the root directory of your project:
```js
const plugins = [
[
'babel-plugin-direct-import',
{ modules: ['@mui/material', '@mui/icons-material'] },
],
];
module.exports = { plugins };
```
If you are using Create React App, you will need to use a couple of projects that let you use `.babelrc` configuration, without ejecting.
`yarn add -D react-app-rewired customize-cra`
Create a `config-overrides.js` file in the root directory:
```js
/* config-overrides.js */
/* eslint-disable react-hooks/rules-of-hooks */
const { useBabelRc, override } = require('customize-cra');
module.exports = override(useBabelRc());
```
If you wish, `babel-plugin-import` can be configured through `config-overrides.js` instead of `.babelrc` by using this [configuration](https://github.com/arackaf/customize-cra/blob/master/api.md#fixbabelimportslibraryname-options).
Modify your `package.json` commands:
```diff
"scripts": {
- "start": "react-scripts start",
- "build": "react-scripts build",
- "test": "react-scripts test",
+ "start": "react-app-rewired start",
+ "build": "react-app-rewired build",
+ "test": "react-app-rewired test",
"eject": "react-scripts eject"
}
```
Enjoy significantly faster start times.
#### 2. Convert all your imports
Finally, you can convert your existing codebase to this option with this [top-level-imports codemod](https://www.npmjs.com/package/@mui/codemod#top-level-imports).
It will perform the following diffs:
```diff
-import Button from '@mui/material/Button';
-import TextField from '@mui/material/TextField';
+import { Button, TextField } from '@mui/material';
```
## Available bundles
### Default bundle
The packages published on npm are **transpiled** with [Babel](https://github.com/babel/babel), optimized for performance with the [supported platforms](/material-ui/getting-started/supported-platforms/).
Custom bundles are also available:
- [Modern bundle](#modern-bundle)
- [Legacy bundle](#legacy-bundle)
### How to use custom bundles?
:::error
You are strongly discouraged to:
- Import from any of the custom bundles directly. Do not do this:
```js
import { Button } from '@mui/material/legacy';
```
You have no guarantee that the dependencies also use legacy or modern bundles, leading to module duplication in your JavaScript files.
- Import from any of the undocumented files or folders. Do not do this:
```js
import { Button } from '@mui/material/esm';
```
You have no guarantee that these imports will continue to work from one version to the next.
:::
A great way to use these bundles is to configure bundler aliases, for example with [Webpack's `resolve.alias`](https://webpack.js.org/configuration/resolve/#resolvealias):
```js
{
resolve: {
alias: {
'@mui/material': '@mui/material/legacy',
'@mui/styled-engine': '@mui/styled-engine/legacy',
'@mui/system': '@mui/system/legacy',
'@mui/base': '@mui/base/legacy',
'@mui/utils': '@mui/utils/legacy',
'@mui/lab': '@mui/lab/legacy',
}
}
}
```
### Modern bundle
The modern bundle can be found under the [`/modern` folder](https://unpkg.com/@mui/material/modern/).
It targets the latest released versions of evergreen browsers (Chrome, Firefox, Safari, Edge).
This can be used to make separate bundles targeting different browsers.
### Legacy bundle
If you need to support IE 11 you cannot use the default or modern bundle without transpilation.
However, you can use the legacy bundle found under the [`/legacy` folder](https://unpkg.com/@mui/material/legacy/).
You don't need any additional polyfills.
| 3,620 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/next-js-app-router/next-js-app-router.md | # Next.js App Router
<p class="description">Learn how to use Material UI with the Next.js App Router.</p>
## Example
Starting fresh on a new App Router-based project?
Jump right into the code with [this example: Material UI - Next.js App Router in TypeScript](https://github.com/mui/material-ui/tree/master/examples/material-ui-nextjs-ts).
## Next.js and React Server Components
The Next.js App Router implements React Server Components, [an upcoming feature for React](https://github.com/reactjs/rfcs/blob/main/text/0227-server-module-conventions.md).
To support the App Router, the components and hooks from Material UI that need access to browser APIs are exported with the `"use client"` directive.
:::warning
React Server Components is not the same concept as server-side rendering (SSR).
So-called Client Components are still server-rendered to HTML.
For more details, see [this explanation](https://github.com/reactwg/server-components/discussions/4) of Client Components and SSR from the React Working Group.
:::
## Using Material UI with the default theme
If you're using the default theme, you can add Material UI components to Next.js routing files such as `layout.js` or `page.js` (which are Server Components by default) without any additional configuration, as shown below:
<!-- TODO: investigate whether it still needs an explicit <head/> to prevent FOUC https://github.com/mui/material-ui/issues/34905#issuecomment-1332040656 -->
```jsx
// app/layout.js - no directives needed
export default function RootLayout(props) {
const { children } = props;
return (
<html lang="en">
<body>{children}</body>
</html>
)
}
// app/page.js - no directives needed
import Box from '@mui/material/Box';
import Card from '@mui/material/Card';
import Container from '@mui/material/Container';
import Typography from '@mui/material/Typography';
export default function Home() {
return (
<main>
<Container>
<Box>
<Card>
<Typography variant="h2">Hello World ~</Typography>
</Card>
</Box>
</Container>
</main>
);
}
```
## Using Material UI with a custom theme
### Theme Registry
To set up the theme context, create a custom `ThemeRegistry` component that combines the Emotion `CacheProvider`, the Material UI `ThemeProvider`, and the `useServerInsertedHTML` hook from `next/navigation` as follows:
```tsx
// app/ThemeRegistry.tsx
'use client';
import createCache from '@emotion/cache';
import { useServerInsertedHTML } from 'next/navigation';
import { CacheProvider } from '@emotion/react';
import { ThemeProvider } from '@mui/material/styles';
import CssBaseline from '@mui/material/CssBaseline';
import theme from '/path/to/your/theme';
// This implementation is from emotion-js
// https://github.com/emotion-js/emotion/issues/2928#issuecomment-1319747902
export default function ThemeRegistry(props) {
const { options, children } = props;
const [{ cache, flush }] = React.useState(() => {
const cache = createCache(options);
cache.compat = true;
const prevInsert = cache.insert;
let inserted: string[] = [];
cache.insert = (...args) => {
const serialized = args[1];
if (cache.inserted[serialized.name] === undefined) {
inserted.push(serialized.name);
}
return prevInsert(...args);
};
const flush = () => {
const prevInserted = inserted;
inserted = [];
return prevInserted;
};
return { cache, flush };
});
useServerInsertedHTML(() => {
const names = flush();
if (names.length === 0) {
return null;
}
let styles = '';
for (const name of names) {
styles += cache.inserted[name];
}
return (
<style
key={cache.key}
data-emotion={`${cache.key} ${names.join(' ')}`}
dangerouslySetInnerHTML={{
__html: styles,
}}
/>
);
});
return (
<CacheProvider value={cache}>
<ThemeProvider theme={theme}>
<CssBaseline />
{children}
</ThemeProvider>
</CacheProvider>
);
}
// app/layout.js
export default function RootLayout(props) {
const { children } = props;
return (
<html lang="en">
<body>
<ThemeRegistry options={{ key: 'mui' }}>{children}</ThemeRegistry>
</body>
</html>
);
}
```
### CSS injection order
<!-- https://github.com/emotion-js/emotion/issues/3059 -->
By default, Emotion injects Material UI styles at the bottom of the HTML `<head>`, which gives them precedence over custom styles—for example if you are customizing Material UI with CSS modules, Tailwind CSS, or even plain CSS.
Emotion provides the `prepend: true` option for `createCache` to reverse the injection order, so custom styles can override Material UI styles without using `!important`.
Currently, `prepend` does not work reliably with the App Router, but you can work around it by wrapping Emotion styles in a CSS `@layer` with a modification to the snippet above:
```diff
useServerInsertedHTML(() => {
const names = flush();
if (names.length === 0) {
return null;
}
let styles = '';
for (const name of names) {
styles += cache.inserted[name];
}
return (
<style
key={cache.key}
data-emotion={`${cache.key} ${names.join(' ')}`}
dangerouslySetInnerHTML={{
- __html: styles,
+ __html: options.prepend ? `@layer emotion {${styles}}` : styles,
}}
/>
);
});
```
## Props serialization
Props passed from Server Components—for example `page.js` or other routing files—must be [serializable](https://nextjs.org/docs/app/building-your-application/rendering/composition-patterns#passing-props-from-server-to-client-components-serialization).
:::success
This works without any additional directives:
```tsx
// app/page.tsx
import Container from '@mui/material/Container';
import Typography from '@mui/material/Typography';
export default function Page() {
return (
<Container>
<Typography variant="h2">Hello World</Typography>
</Container>
);
}
```
:::
:::error
This _doesn't work_ because the Button's click handler is **non-serializable**:
```tsx
// app/page.tsx
import Container from '@mui/material/Container';
import Button from '@mui/material/Button';
export default function Page() {
return (
<Container>
{/* Next.js won't render this button without 'use-client' */}
<Button
onClick={() => {
console.log('handle click');
}}
>
Submit
</Button>
</Container>
);
}
```
Instead, the Next.js team recommend moving components like these ["down the tree"](https://nextjs.org/docs/app/building-your-application/rendering/composition-patterns#moving-client-components-down-the-tree) to avoid this issue and improve overall performance.
:::
| 3,621 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/pickers-migration/pickers-migration.md | # Migration from @material-ui/pickers
<p class="description"><code>@material-ui/pickers</code> was moved to the <code>@mui/lab</code>.</p>
:::success
**Only use this migration guide if you need to use Date and Time pickers from `@mui/lab`.**
The components are available in alpha version in the lab between `v5.0.0-alpha.30` and `v5.0.0-alpha.89` inclusively.
They won't receive any new feature of bug fixes and won't be compatible with future major releases of MUI packages.
If you want to use the **stable version** of those components, please have a look at the new [MUI X packages](/x/react-date-pickers/getting-started/) `@mui/x-date-pickers` and `@mui/x-date-pickers-pro`.
To migrate from `@mui/lab` to `@mui/x-date-pickers` you can follow the dedicated [migration guide](/x/migration/migration-pickers-lab/).
:::
:::warning
**The date picker components were rewritten**. In most places, the logic was rewritten from scratch, so it isn't possible to maintain the whole list of changes. Here's an overview of the most important concepts that were changed. If you are going to upgrade, the easiest way might be to go through each picker usage in your codebase, and rewrite them one at a time. Don't forget to run your tests after each!
:::
This guide is an overview of the core concepts that were changed from pickers v3.2.10.
## Installation
You need to install the `@mui/lab` package if it's not already installed.
⚠️ Make sure you have installed a version between `v5.0.0-alpha.30` and `v5.0.0-alpha.89` inclusively.
:::warning
The pickers are no longer available in `@mui/lab` starting `v5.0.0-alpha.90`.
Please refer to the information on top of the page to use the latest pickers components.
:::
## Imports
The `keyboard` version of pickers is no longer published. All versions of mobile and desktop pickers implement keyboard input for accessibility.
```diff
-import { KeyboardDatePicker } from '@material-ui/pickers';
+import DatePicker from '@mui/lab/DatePicker';
-<KeyboardDatePicker />
+<DatePicker />
```
Also, instead of providing a `variant` prop, these were moved to different imports, meaning that your bundle won't include `Dialog` if you are using only the desktop picker.
- `<DesktopDatePicker />` – Only desktop view.
- `<MobileDatePicker />` – Only mobile view.
- `<DatePicker />` – Mobile or Desktop view according to the user **pointer** preference.
- `<StaticDatePicker />` – The picker view itself, without input or any other wrapper.
```diff
-import { DatePicker } from '@material-ui/pickers';
+import DesktopDatePicker from '@mui/lab/DesktopDatePicker';
-<DatePicker variant="inline" />
+<DesktopDatePicker />
```
The same convention applies to `TimePicker` – `<DesktopTimePicker>` and `<MobileTimePicker />`.
## MuiPickersUtilsProvider
The `MuiPickersUtilsProvider` was removed in favor of `LocalizationProvider`. Also, pickers do not require you to install date-io adapters manually. Everything is included with the `lab`.
❌ Before:
```js
import AdapterDateFns from '@date-io/date-fns';
import { MuiPickersUtilsProvider } from '@material-ui/pickers';
```
✅ After:
```jsx
import AdapterDateFns from '@mui/lab/AdapterDateFns';
import LocalizationProvider from '@mui/lab/LocalizationProvider';
function App() {
return (
<LocalizationProvider dateAdapter={AdapterDateFns}>
...
</LocalizationProvider>
)
);
```
## Render input
We introduced a new **required** `renderInput` prop. This simplifies using non-Material UI text field input components.
```jsx
<DatePicker renderInput={(props) => <TextField {...props} />} />
<TimePicker renderInput={(props) => <TextField {...props} />} />
```
Previously, props were spread on the `<TextField />` component. From now on you will need to use the new `renderInput` prop to provide these:
```diff
<DatePicker
- label="Date"
- helperText="Something"
+ renderInput={props => <TextField label="Date" helperText="Something" /> }
/>
```
## State management
The state/value management logic for pickers was rewritten from scratch. Pickers will now call the `onChange` prop when each view of the date picker ends is completed. The `onError` handler is also completely different. Triple-check your pickers with forms integration, because form-integration issues can be subtle.
## No required mask
Mask is no longer required. Also, if your provided mask is not valid, pickers will just ignore the mask, and allow arbitrary input.
```jsx
<DatePicker
mask="mm"
value={new Date()}
onChange={console.log}
renderInput={(props) => (
<TextField {...props} helperText="invalid mask" />
)}
/>
<DatePicker
value={new Date()}
onChange={console.log}
renderInput={(props) => (
<TextField {...props} helperText="valid mask" />
)}
/>
```
## And many more
```diff
<DatePicker
- format="DD-MM-YYYY"
+ inputFormat="DD-MM-YYYY"
```
There are many changes, be careful, make sure your tests, and build passes.
In the event you have an advanced usage of the date picker, it will likely be simpler to rewrite it.
:::success
In case you are considering your picker component rewrite, consider using the latest [MUI X packages](/x/react-date-pickers/getting-started/).
:::
Please open a pull request to improve the guide if you notice an opportunity for doing such.
| 3,622 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/responsive-ui/responsive-ui.md | # Responsive UI
<p class="description">Material Design layouts encourage consistency across platforms, environments, and screen sizes by using uniform elements and spacing.</p>
[Responsive layouts](https://m2.material.io/design/layout/responsive-layout-grid.html) in Material Design adapt to any possible screen size.
We provide the following helpers to make the UI responsive:
- [Grid](/material-ui/react-grid/): The Material Design responsive layout grid adapts to screen size and orientation, ensuring consistency across layouts.
- [Container](/material-ui/react-container/): The container centers your content horizontally. It's the most basic layout element.
- [Breakpoints](/material-ui/customization/breakpoints/): API that enables the use of breakpoints in a wide variety of contexts.
- [useMediaQuery](/material-ui/react-use-media-query/): This is a CSS media query hook for React. It listens for matches to a CSS media query.
| 3,623 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/right-to-left/Direction.js | import * as React from 'react';
import { createTheme, ThemeProvider } from '@mui/material/styles';
import TextField from '@mui/material/TextField';
import rtlPlugin from 'stylis-plugin-rtl';
import { prefixer } from 'stylis';
import { CacheProvider } from '@emotion/react';
import createCache from '@emotion/cache';
const theme = createTheme({
direction: 'rtl', // Both here and <body dir="rtl">
});
// Create rtl cache
const cacheRtl = createCache({
key: 'muirtl',
stylisPlugins: [prefixer, rtlPlugin],
});
export default function Direction() {
return (
<CacheProvider value={cacheRtl}>
<ThemeProvider theme={theme}>
<div dir="rtl">
<TextField label="Name" variant="standard" />
<input type="text" placeholder="Name" />
</div>
</ThemeProvider>
</CacheProvider>
);
}
| 3,624 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/right-to-left/Direction.tsx | import * as React from 'react';
import { createTheme, ThemeProvider } from '@mui/material/styles';
import TextField from '@mui/material/TextField';
import rtlPlugin from 'stylis-plugin-rtl';
import { prefixer } from 'stylis';
import { CacheProvider } from '@emotion/react';
import createCache from '@emotion/cache';
const theme = createTheme({
direction: 'rtl', // Both here and <body dir="rtl">
});
// Create rtl cache
const cacheRtl = createCache({
key: 'muirtl',
stylisPlugins: [prefixer, rtlPlugin],
});
export default function Direction() {
return (
<CacheProvider value={cacheRtl}>
<ThemeProvider theme={theme}>
<div dir="rtl">
<TextField label="Name" variant="standard" />
<input type="text" placeholder="Name" />
</div>
</ThemeProvider>
</CacheProvider>
);
}
| 3,625 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/right-to-left/Direction.tsx.preview | <CacheProvider value={cacheRtl}>
<ThemeProvider theme={theme}>
<div dir="rtl">
<TextField label="Name" variant="standard" />
<input type="text" placeholder="Name" />
</div>
</ThemeProvider>
</CacheProvider> | 3,626 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/right-to-left/RtlOptOutStylis.js | import * as React from 'react';
import { prefixer } from 'stylis';
import rtlPlugin from 'stylis-plugin-rtl';
import { CacheProvider } from '@emotion/react';
import createCache from '@emotion/cache';
import { styled } from '@mui/material/styles';
import FormControlLabel from '@mui/material/FormControlLabel';
import Switch from '@mui/material/Switch';
const Root = styled('div')((props) => ({
width: '100%',
marginTop: props.theme?.spacing(6),
}));
const AffectedText = styled('div')`
text-align: left;
`;
const UnaffectedText = styled('div')`
/* @noflip */
text-align: left;
`;
const rtlCache = createCache({
key: 'muirtl',
stylisPlugins: [prefixer, rtlPlugin],
});
const ltrCache = createCache({
key: 'mui',
});
export default function RtlOptOutStylis() {
const [rtl, setRtl] = React.useState(false);
const handleChange = () => {
setRtl(!rtl);
};
return (
<React.Fragment>
<div>
<FormControlLabel
control={<Switch checked={rtl} onChange={handleChange} />}
label="RTL"
/>
</div>
<CacheProvider value={rtl ? rtlCache : ltrCache}>
<Root {...(rtl ? { dir: 'rtl' } : {})}>
<AffectedText>Affected</AffectedText>
<UnaffectedText>Unaffected</UnaffectedText>
</Root>
</CacheProvider>
</React.Fragment>
);
}
| 3,627 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/right-to-left/RtlOptOutStylis.tsx | import * as React from 'react';
import { prefixer } from 'stylis';
import rtlPlugin from 'stylis-plugin-rtl';
import { CacheProvider } from '@emotion/react';
import createCache from '@emotion/cache';
import { styled } from '@mui/material/styles';
import FormControlLabel from '@mui/material/FormControlLabel';
import Switch from '@mui/material/Switch';
const Root = styled('div')((props) => ({
width: '100%',
marginTop: props.theme?.spacing(6),
}));
const AffectedText = styled('div')`
text-align: left;
`;
const UnaffectedText = styled('div')`
/* @noflip */
text-align: left;
`;
const rtlCache = createCache({
key: 'muirtl',
stylisPlugins: [prefixer, rtlPlugin],
});
const ltrCache = createCache({
key: 'mui',
});
export default function RtlOptOutStylis() {
const [rtl, setRtl] = React.useState(false);
const handleChange = () => {
setRtl(!rtl);
};
return (
<React.Fragment>
<div>
<FormControlLabel
control={<Switch checked={rtl} onChange={handleChange} />}
label="RTL"
/>
</div>
<CacheProvider value={rtl ? rtlCache : ltrCache}>
<Root {...(rtl ? { dir: 'rtl' } : {})}>
<AffectedText>Affected</AffectedText>
<UnaffectedText>Unaffected</UnaffectedText>
</Root>
</CacheProvider>
</React.Fragment>
);
}
| 3,628 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/right-to-left/RtlOptOutStylis.tsx.preview | <React.Fragment>
<div>
<FormControlLabel
control={<Switch checked={rtl} onChange={handleChange} />}
label="RTL"
/>
</div>
<CacheProvider value={rtl ? rtlCache : ltrCache}>
<Root {...(rtl ? { dir: 'rtl' } : {})}>
<AffectedText>Affected</AffectedText>
<UnaffectedText>Unaffected</UnaffectedText>
</Root>
</CacheProvider>
</React.Fragment> | 3,629 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/right-to-left/right-to-left.md | # Right-to-left
<p class="description">Right-to-left languages such as Arabic, Persian, or Hebrew are supported. To change the direction of Material UI components you must follow the following steps.</p>
## Steps
### 1. HTML
Make sure the `dir` attribute is set on the `html` tag, otherwise native components will break:
```html
<html dir="rtl"></html>
```
If you need to change the direction of the text at runtime, but React does not control the root HTML element, you may use the JS API:
```js
document.dir = 'rtl';
```
As an alternative to the above, you can also wrap your application (or part of it) in an element with the `dir` attribute.
This, however, will not work correctly with portaled elements, such as Dialogs, as they will render outside of the element with the `dir` attribute.
To fix the portaled components, add an explicit `dir` attribute to them:
```jsx
<Dialog dir="rtl">
<MyComponent />
</Dialog>
```
### 2. Theme
Set the direction in your custom theme:
```js
import { createTheme } from '@mui/material/styles';
const theme = createTheme({
direction: 'rtl',
});
```
### 3. Install the rtl plugin
When using either `emotion` or `styled-components`, you need [`stylis-plugin-rtl`](https://github.com/styled-components/stylis-plugin-rtl) to flip the styles.
```bash
npm install stylis stylis-plugin-rtl
```
:::warning
Only Emotion is compatible with version 2 of the plugin.
styled-components requires version 1.
If you're using [styled-components instead of Emotion](/material-ui/guides/styled-components/), make sure to install the correct version.
:::
In case you are using `jss` (up to v4) or with the legacy `@mui/styles` package, you need [`jss-rtl`](https://github.com/alitaheri/jss-rtl) to flip the styles.
```bash
npm install jss-rtl
```
Having installed the plugin in your project, Material UI components still require it to be loaded by the style engine instance that you use. Find bellow guides on how you can load it.
### 4. Load the rtl plugin
#### 4.1 Emotion
If you use Emotion as your style engine, you should create a new cache instance that uses the `stylis-plugin-rtl` (the default `prefixer` plugin must also be included in order to retain vendor prefixing) and provide that on the top of your application tree.
The [CacheProvider](https://emotion.sh/docs/cache-provider) component enables this:
```jsx
import rtlPlugin from 'stylis-plugin-rtl';
import { CacheProvider } from '@emotion/react';
import createCache from '@emotion/cache';
import { prefixer } from 'stylis';
// Create rtl cache
const cacheRtl = createCache({
key: 'muirtl',
stylisPlugins: [prefixer, rtlPlugin],
});
function RTL(props) {
return <CacheProvider value={cacheRtl}>{props.children}</CacheProvider>;
}
```
#### 4.2 styled-components
If you use `styled-components` as your style engine, you can use the [StyleSheetManager](https://styled-components.com/docs/api#stylesheetmanager) and provide the stylis-plugin-rtl as an item in the `stylisPlugins` property:
```jsx
import { StyleSheetManager } from 'styled-components';
import rtlPlugin from 'stylis-plugin-rtl';
function RTL(props) {
return (
<StyleSheetManager stylisPlugins={[rtlPlugin]}>
{props.children}
</StyleSheetManager>
);
}
```
#### 4.3 JSS
After installing the plugin in your project, you need to configure the JSS instance to load it.
The next step is to make the new JSS instance available to all the components in the component tree.
The [`StylesProvider`](/system/styles/api/#stylesprovider) component enables this:
```jsx
import { create } from 'jss';
import rtl from 'jss-rtl';
import { StylesProvider, jssPreset } from '@mui/styles';
// Configure JSS
const jss = create({
plugins: [...jssPreset().plugins, rtl()],
});
function RTL(props) {
return <StylesProvider jss={jss}>{props.children}</StylesProvider>;
}
```
For more information on the plugin, head to the [plugin README](https://github.com/alitaheri/jss-rtl).
**Note**: Internally, withStyles is using this JSS plugin when `direction: 'rtl'` is set on the theme.
## Demo
_Use the direction toggle button on the top right corner to flip the whole documentation_
{{"demo": "Direction.js"}}
## Opting out of rtl transformation
### Emotion & styled-components
You have to use the template literal syntax and add the `/* @noflip */` directive before the rule or property for which you want to disable right-to-left styles.
```jsx
const AffectedText = styled('div')`
text-align: left;
`;
const UnaffectedText = styled('div')`
/* @noflip */
text-align: left;
`;
```
{{"demo": "RtlOptOutStylis.js", "hideToolbar": true}}
### JSS
If you want to prevent a specific rule-set from being affected by the `rtl` transformation you can add `flip: false` at the beginning.
```jsx
const useStyles = makeStyles(
(theme) => ({
affected: {
textAlign: 'right',
},
unaffected: {
flip: false,
textAlign: 'right',
},
}),
{ defaultTheme },
);
```
| 3,630 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/ButtonDemo.js | import * as React from 'react';
import Button from '@mui/material/Button';
export default function ButtonDemo() {
return (
<Button href="/" variant="contained">
Link
</Button>
);
}
| 3,631 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/ButtonDemo.tsx | import * as React from 'react';
import Button from '@mui/material/Button';
export default function ButtonDemo() {
return (
<Button href="/" variant="contained">
Link
</Button>
);
}
| 3,632 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/ButtonDemo.tsx.preview | <Button href="/" variant="contained">
Link
</Button> | 3,633 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/ButtonRouter.js | import * as React from 'react';
import PropTypes from 'prop-types';
import { Link as RouterLink, MemoryRouter } from 'react-router-dom';
import { StaticRouter } from 'react-router-dom/server';
import Button from '@mui/material/Button';
const LinkBehavior = React.forwardRef((props, ref) => (
<RouterLink ref={ref} to="/" {...props} role={undefined} />
));
function Router(props) {
const { children } = props;
if (typeof window === 'undefined') {
return <StaticRouter location="/">{children}</StaticRouter>;
}
return <MemoryRouter>{children}</MemoryRouter>;
}
Router.propTypes = {
children: PropTypes.node,
};
export default function ButtonRouter() {
return (
<div>
<Router>
<Button component={RouterLink} to="/">
With prop forwarding
</Button>
<br />
<Button component={LinkBehavior}>With inlining</Button>
</Router>
</div>
);
}
| 3,634 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/ButtonRouter.tsx | import * as React from 'react';
import {
Link as RouterLink,
LinkProps as RouterLinkProps,
MemoryRouter,
} from 'react-router-dom';
import { StaticRouter } from 'react-router-dom/server';
import Button from '@mui/material/Button';
const LinkBehavior = React.forwardRef<any, Omit<RouterLinkProps, 'to'>>(
(props, ref) => <RouterLink ref={ref} to="/" {...props} role={undefined} />,
);
function Router(props: { children?: React.ReactNode }) {
const { children } = props;
if (typeof window === 'undefined') {
return <StaticRouter location="/">{children}</StaticRouter>;
}
return <MemoryRouter>{children}</MemoryRouter>;
}
export default function ButtonRouter() {
return (
<div>
<Router>
<Button component={RouterLink} to="/">
With prop forwarding
</Button>
<br />
<Button component={LinkBehavior}>With inlining</Button>
</Router>
</div>
);
}
| 3,635 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/ButtonRouter.tsx.preview | <Router>
<Button component={RouterLink} to="/">
With prop forwarding
</Button>
<br />
<Button component={LinkBehavior}>With inlining</Button>
</Router> | 3,636 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/LinkDemo.js | import * as React from 'react';
import Link from '@mui/material/Link';
import Box from '@mui/material/Box';
export default function LinkDemo() {
return (
<Box sx={{ typography: 'body1' }}>
<Link href="/">Link</Link>
</Box>
);
}
| 3,637 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/LinkDemo.tsx | import * as React from 'react';
import Link from '@mui/material/Link';
import Box from '@mui/material/Box';
export default function LinkDemo() {
return (
<Box sx={{ typography: 'body1' }}>
<Link href="/">Link</Link>
</Box>
);
}
| 3,638 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/LinkDemo.tsx.preview | <Link href="/">Link</Link> | 3,639 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/LinkRouter.js | /* eslint-disable jsx-a11y/anchor-is-valid */
import * as React from 'react';
import PropTypes from 'prop-types';
import { Link as RouterLink, MemoryRouter } from 'react-router-dom';
import { StaticRouter } from 'react-router-dom/server';
import Link from '@mui/material/Link';
import Box from '@mui/material/Box';
const LinkBehavior = React.forwardRef((props, ref) => (
<RouterLink ref={ref} to="/material-ui/getting-started/installation/" {...props} />
));
function Router(props) {
const { children } = props;
if (typeof window === 'undefined') {
return <StaticRouter location="/">{children}</StaticRouter>;
}
return <MemoryRouter>{children}</MemoryRouter>;
}
Router.propTypes = {
children: PropTypes.node,
};
export default function LinkRouter() {
return (
<Box sx={{ typography: 'body1' }}>
<Router>
<Link component={RouterLink} to="/">
With prop forwarding
</Link>
<br />
<Link component={LinkBehavior}>Without prop forwarding</Link>
</Router>
</Box>
);
}
| 3,640 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/LinkRouter.tsx | /* eslint-disable jsx-a11y/anchor-is-valid */
import * as React from 'react';
import {
Link as RouterLink,
LinkProps as RouterLinkProps,
MemoryRouter,
} from 'react-router-dom';
import { StaticRouter } from 'react-router-dom/server';
import Link from '@mui/material/Link';
import Box from '@mui/material/Box';
const LinkBehavior = React.forwardRef<any, Omit<RouterLinkProps, 'to'>>(
(props, ref) => (
<RouterLink
ref={ref}
to="/material-ui/getting-started/installation/"
{...props}
/>
),
);
function Router(props: { children?: React.ReactNode }) {
const { children } = props;
if (typeof window === 'undefined') {
return <StaticRouter location="/">{children}</StaticRouter>;
}
return <MemoryRouter>{children}</MemoryRouter>;
}
export default function LinkRouter() {
return (
<Box sx={{ typography: 'body1' }}>
<Router>
<Link component={RouterLink} to="/">
With prop forwarding
</Link>
<br />
<Link component={LinkBehavior}>Without prop forwarding</Link>
</Router>
</Box>
);
}
| 3,641 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/LinkRouter.tsx.preview | <Router>
<Link component={RouterLink} to="/">
With prop forwarding
</Link>
<br />
<Link component={LinkBehavior}>Without prop forwarding</Link>
</Router> | 3,642 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/LinkRouterWithTheme.js | import * as React from 'react';
import PropTypes from 'prop-types';
import { Link as RouterLink, MemoryRouter } from 'react-router-dom';
import { StaticRouter } from 'react-router-dom/server';
import { ThemeProvider, createTheme } from '@mui/material/styles';
import Button from '@mui/material/Button';
import Stack from '@mui/material/Stack';
import Link from '@mui/material/Link';
const LinkBehavior = React.forwardRef((props, ref) => {
const { href, ...other } = props;
// Map href (MUI) -> to (react-router)
return <RouterLink data-testid="custom-link" ref={ref} to={href} {...other} />;
});
LinkBehavior.propTypes = {
href: PropTypes.oneOfType([
PropTypes.shape({
hash: PropTypes.string,
pathname: PropTypes.string,
search: PropTypes.string,
}),
PropTypes.string,
]).isRequired,
};
function Router(props) {
const { children } = props;
if (typeof window === 'undefined') {
return <StaticRouter location="/">{children}</StaticRouter>;
}
return <MemoryRouter>{children}</MemoryRouter>;
}
Router.propTypes = {
children: PropTypes.node,
};
const theme = createTheme({
components: {
MuiLink: {
defaultProps: {
component: LinkBehavior,
},
},
MuiButtonBase: {
defaultProps: {
LinkComponent: LinkBehavior,
},
},
},
});
export default function LinkRouterWithTheme() {
return (
<Stack sx={{ typography: 'body1' }} alignItems="center" spacing={1}>
<ThemeProvider theme={theme}>
<Router>
<Link href="/">Link</Link>
<Button href="/" variant="contained">
Link
</Button>
</Router>
</ThemeProvider>
</Stack>
);
}
| 3,643 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/LinkRouterWithTheme.tsx | import * as React from 'react';
import {
Link as RouterLink,
LinkProps as RouterLinkProps,
MemoryRouter,
} from 'react-router-dom';
import { StaticRouter } from 'react-router-dom/server';
import { ThemeProvider, createTheme } from '@mui/material/styles';
import Button from '@mui/material/Button';
import Stack from '@mui/material/Stack';
import Link, { LinkProps } from '@mui/material/Link';
const LinkBehavior = React.forwardRef<
HTMLAnchorElement,
Omit<RouterLinkProps, 'to'> & { href: RouterLinkProps['to'] }
>((props, ref) => {
const { href, ...other } = props;
// Map href (MUI) -> to (react-router)
return <RouterLink data-testid="custom-link" ref={ref} to={href} {...other} />;
});
function Router(props: { children?: React.ReactNode }) {
const { children } = props;
if (typeof window === 'undefined') {
return <StaticRouter location="/">{children}</StaticRouter>;
}
return <MemoryRouter>{children}</MemoryRouter>;
}
const theme = createTheme({
components: {
MuiLink: {
defaultProps: {
component: LinkBehavior,
} as LinkProps,
},
MuiButtonBase: {
defaultProps: {
LinkComponent: LinkBehavior,
},
},
},
});
export default function LinkRouterWithTheme() {
return (
<Stack sx={{ typography: 'body1' }} alignItems="center" spacing={1}>
<ThemeProvider theme={theme}>
<Router>
<Link href="/">Link</Link>
<Button href="/" variant="contained">
Link
</Button>
</Router>
</ThemeProvider>
</Stack>
);
}
| 3,644 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/LinkRouterWithTheme.tsx.preview | <ThemeProvider theme={theme}>
<Router>
<Link href="/">Link</Link>
<Button href="/" variant="contained">
Link
</Button>
</Router>
</ThemeProvider> | 3,645 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/ListRouter.js | import * as React from 'react';
import PropTypes from 'prop-types';
import List from '@mui/material/List';
import Box from '@mui/material/Box';
import ListItem from '@mui/material/ListItem';
import Paper from '@mui/material/Paper';
import ListItemIcon from '@mui/material/ListItemIcon';
import ListItemText from '@mui/material/ListItemText';
import Divider from '@mui/material/Divider';
import InboxIcon from '@mui/icons-material/Inbox';
import DraftsIcon from '@mui/icons-material/Drafts';
import Typography from '@mui/material/Typography';
import {
Link as RouterLink,
Route,
Routes,
MemoryRouter,
useLocation,
} from 'react-router-dom';
import { StaticRouter } from 'react-router-dom/server';
function Router(props) {
const { children } = props;
if (typeof window === 'undefined') {
return <StaticRouter location="/drafts">{children}</StaticRouter>;
}
return (
<MemoryRouter initialEntries={['/drafts']} initialIndex={0}>
{children}
</MemoryRouter>
);
}
Router.propTypes = {
children: PropTypes.node,
};
const Link = React.forwardRef(function Link(itemProps, ref) {
return <RouterLink ref={ref} {...itemProps} role={undefined} />;
});
function ListItemLink(props) {
const { icon, primary, to } = props;
return (
<li>
<ListItem button component={Link} to={to}>
{icon ? <ListItemIcon>{icon}</ListItemIcon> : null}
<ListItemText primary={primary} />
</ListItem>
</li>
);
}
ListItemLink.propTypes = {
icon: PropTypes.element,
primary: PropTypes.string.isRequired,
to: PropTypes.string.isRequired,
};
function Content() {
const location = useLocation();
return (
<Typography variant="body2" sx={{ pb: 2 }} color="text.secondary">
Current route: {location.pathname}
</Typography>
);
}
export default function ListRouter() {
return (
<Router>
<Box sx={{ width: 360 }}>
<Routes>
<Route path="*" element={<Content />} />
</Routes>
<Paper elevation={0}>
<List aria-label="main mailbox folders">
<ListItemLink to="/inbox" primary="Inbox" icon={<InboxIcon />} />
<ListItemLink to="/drafts" primary="Drafts" icon={<DraftsIcon />} />
</List>
<Divider />
<List aria-label="secondary mailbox folders">
<ListItemLink to="/trash" primary="Trash" />
<ListItemLink to="/spam" primary="Spam" />
</List>
</Paper>
</Box>
</Router>
);
}
| 3,646 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/ListRouter.tsx | import * as React from 'react';
import List from '@mui/material/List';
import Box from '@mui/material/Box';
import ListItem from '@mui/material/ListItem';
import Paper from '@mui/material/Paper';
import ListItemIcon from '@mui/material/ListItemIcon';
import ListItemText from '@mui/material/ListItemText';
import Divider from '@mui/material/Divider';
import InboxIcon from '@mui/icons-material/Inbox';
import DraftsIcon from '@mui/icons-material/Drafts';
import Typography from '@mui/material/Typography';
import {
Link as RouterLink,
LinkProps as RouterLinkProps,
Route,
Routes,
MemoryRouter,
useLocation,
} from 'react-router-dom';
import { StaticRouter } from 'react-router-dom/server';
function Router(props: { children?: React.ReactNode }) {
const { children } = props;
if (typeof window === 'undefined') {
return <StaticRouter location="/drafts">{children}</StaticRouter>;
}
return (
<MemoryRouter initialEntries={['/drafts']} initialIndex={0}>
{children}
</MemoryRouter>
);
}
interface ListItemLinkProps {
icon?: React.ReactElement;
primary: string;
to: string;
}
const Link = React.forwardRef<HTMLAnchorElement, RouterLinkProps>(function Link(
itemProps,
ref,
) {
return <RouterLink ref={ref} {...itemProps} role={undefined} />;
});
function ListItemLink(props: ListItemLinkProps) {
const { icon, primary, to } = props;
return (
<li>
<ListItem button component={Link} to={to}>
{icon ? <ListItemIcon>{icon}</ListItemIcon> : null}
<ListItemText primary={primary} />
</ListItem>
</li>
);
}
function Content() {
const location = useLocation();
return (
<Typography variant="body2" sx={{ pb: 2 }} color="text.secondary">
Current route: {location.pathname}
</Typography>
);
}
export default function ListRouter() {
return (
<Router>
<Box sx={{ width: 360 }}>
<Routes>
<Route path="*" element={<Content />} />
</Routes>
<Paper elevation={0}>
<List aria-label="main mailbox folders">
<ListItemLink to="/inbox" primary="Inbox" icon={<InboxIcon />} />
<ListItemLink to="/drafts" primary="Drafts" icon={<DraftsIcon />} />
</List>
<Divider />
<List aria-label="secondary mailbox folders">
<ListItemLink to="/trash" primary="Trash" />
<ListItemLink to="/spam" primary="Spam" />
</List>
</Paper>
</Box>
</Router>
);
}
| 3,647 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/TabsRouter.js | import * as React from 'react';
import PropTypes from 'prop-types';
import Box from '@mui/material/Box';
import Tabs from '@mui/material/Tabs';
import Tab from '@mui/material/Tab';
import Typography from '@mui/material/Typography';
import {
MemoryRouter,
Route,
Routes,
Link,
matchPath,
useLocation,
} from 'react-router-dom';
import { StaticRouter } from 'react-router-dom/server';
function Router(props) {
const { children } = props;
if (typeof window === 'undefined') {
return <StaticRouter location="/drafts">{children}</StaticRouter>;
}
return (
<MemoryRouter initialEntries={['/drafts']} initialIndex={0}>
{children}
</MemoryRouter>
);
}
Router.propTypes = {
children: PropTypes.node,
};
function useRouteMatch(patterns) {
const { pathname } = useLocation();
for (let i = 0; i < patterns.length; i += 1) {
const pattern = patterns[i];
const possibleMatch = matchPath(pattern, pathname);
if (possibleMatch !== null) {
return possibleMatch;
}
}
return null;
}
function MyTabs() {
// You need to provide the routes in descendant order.
// This means that if you have nested routes like:
// users, users/new, users/edit.
// Then the order should be ['users/add', 'users/edit', 'users'].
const routeMatch = useRouteMatch(['/inbox/:id', '/drafts', '/trash']);
const currentTab = routeMatch?.pattern?.path;
return (
<Tabs value={currentTab}>
<Tab label="Inbox" value="/inbox/:id" to="/inbox/1" component={Link} />
<Tab label="Drafts" value="/drafts" to="/drafts" component={Link} />
<Tab label="Trash" value="/trash" to="/trash" component={Link} />
</Tabs>
);
}
function CurrentRoute() {
const location = useLocation();
return (
<Typography variant="body2" sx={{ pb: 2 }} color="text.secondary">
Current route: {location.pathname}
</Typography>
);
}
export default function TabsRouter() {
return (
<Router>
<Box sx={{ width: '100%' }}>
<Routes>
<Route path="*" element={<CurrentRoute />} />
</Routes>
<MyTabs />
</Box>
</Router>
);
}
| 3,648 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/TabsRouter.tsx | import * as React from 'react';
import Box from '@mui/material/Box';
import Tabs from '@mui/material/Tabs';
import Tab from '@mui/material/Tab';
import Typography from '@mui/material/Typography';
import {
MemoryRouter,
Route,
Routes,
Link,
matchPath,
useLocation,
} from 'react-router-dom';
import { StaticRouter } from 'react-router-dom/server';
function Router(props: { children?: React.ReactNode }) {
const { children } = props;
if (typeof window === 'undefined') {
return <StaticRouter location="/drafts">{children}</StaticRouter>;
}
return (
<MemoryRouter initialEntries={['/drafts']} initialIndex={0}>
{children}
</MemoryRouter>
);
}
function useRouteMatch(patterns: readonly string[]) {
const { pathname } = useLocation();
for (let i = 0; i < patterns.length; i += 1) {
const pattern = patterns[i];
const possibleMatch = matchPath(pattern, pathname);
if (possibleMatch !== null) {
return possibleMatch;
}
}
return null;
}
function MyTabs() {
// You need to provide the routes in descendant order.
// This means that if you have nested routes like:
// users, users/new, users/edit.
// Then the order should be ['users/add', 'users/edit', 'users'].
const routeMatch = useRouteMatch(['/inbox/:id', '/drafts', '/trash']);
const currentTab = routeMatch?.pattern?.path;
return (
<Tabs value={currentTab}>
<Tab label="Inbox" value="/inbox/:id" to="/inbox/1" component={Link} />
<Tab label="Drafts" value="/drafts" to="/drafts" component={Link} />
<Tab label="Trash" value="/trash" to="/trash" component={Link} />
</Tabs>
);
}
function CurrentRoute() {
const location = useLocation();
return (
<Typography variant="body2" sx={{ pb: 2 }} color="text.secondary">
Current route: {location.pathname}
</Typography>
);
}
export default function TabsRouter() {
return (
<Router>
<Box sx={{ width: '100%' }}>
<Routes>
<Route path="*" element={<CurrentRoute />} />
</Routes>
<MyTabs />
</Box>
</Router>
);
}
| 3,649 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/TabsRouter.tsx.preview | <Router>
<Box sx={{ width: '100%' }}>
<Routes>
<Route path="*" element={<CurrentRoute />} />
</Routes>
<MyTabs />
</Box>
</Router> | 3,650 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/routing/routing.md | # Routing libraries
<p class="description">By default, the navigation is performed with a native <a> element. You can customize it, for instance, using Next.js's Link or react-router.</p>
## Navigation components
There are two main components available to perform navigations.
The most common one is the [`Link`](/material-ui/react-link/) as its name might suggest.
It renders a native `<a>` element and applies the `href` as an attribute.
{{"demo": "LinkDemo.js"}}
You can also make a button perform navigation actions.
If your component is extending [`ButtonBase`](/material-ui/api/button-base/), providing a `href` prop enables the link mode.
For instance, with a `Button` component:
{{"demo": "ButtonDemo.js"}}
## Global theme Link
In real-life applications, using a native `<a>` element is rarely enough.
You can improve the user experience by using an enhanced Link component systematically.
The Material UI theme lets you configure this component once.
For instance, with react-router:
```tsx
import { Link as RouterLink, LinkProps as RouterLinkProps } from 'react-router-dom';
import { LinkProps } from '@mui/material/Link';
const LinkBehavior = React.forwardRef<
HTMLAnchorElement,
Omit<RouterLinkProps, 'to'> & { href: RouterLinkProps['to'] }
>((props, ref) => {
const { href, ...other } = props;
// Map href (Material UI) -> to (react-router)
return <RouterLink ref={ref} to={href} {...other} />;
});
const theme = createTheme({
components: {
MuiLink: {
defaultProps: {
component: LinkBehavior,
} as LinkProps,
},
MuiButtonBase: {
defaultProps: {
LinkComponent: LinkBehavior,
},
},
},
});
```
{{"demo": "LinkRouterWithTheme.js", "defaultCodeOpen": false}}
:::warning
This approach has limitations with TypeScript.
The `href` prop only accepts a string.
In the event you need to provide a richer structure, see the next section.
:::
## `component` prop
You can achieve the integration with third-party routing libraries with the `component` prop.
You can learn more about this prop in the **[composition guide](/material-ui/guides/composition/#component-prop)**.
## react-router examples
Here are a few demos with [react-router](https://github.com/remix-run/react-router).
You can apply the same strategy with all the components: BottomNavigation, Card, etc.
### Link
{{"demo": "LinkRouter.js"}}
### Button
{{"demo": "ButtonRouter.js"}}
**Note**: The button base component adds the `role="button"` attribute when it identifies the intent to render a button without a native `<button>` element.
This can create issues when rendering a link.
If you are not using one of the `href`, `to`, or `component="a"` props, you need to override the `role` attribute.
The above demo achieves this by setting `role={undefined}` **after** the spread props.
```jsx
const LinkBehavior = React.forwardRef((props, ref) => (
<RouterLink ref={ref} to="/" {...props} role={undefined} />
));
```
### Tabs
{{"demo": "TabsRouter.js", "defaultCodeOpen": false}}
### List
{{"demo": "ListRouter.js"}}
## More examples
### Next.js Pages Router
The [example folder](https://github.com/mui/material-ui/tree/HEAD/examples/material-ui-nextjs-pages-router-ts) provides an adapter for the use of [Next.js's Link component](https://nextjs.org/docs/pages/api-reference/components/link) with Material UI.
- The first version of the adapter is the [`NextLinkComposed`](https://github.com/mui/material-ui/blob/-/examples/material-ui-nextjs-pages-router-ts/src/Link.tsx) component.
This component is unstyled and only responsible for handling the navigation.
The prop `href` was renamed `to` to avoid a naming conflict.
This is similar to react-router's Link component.
```tsx
import Button from '@mui/material/Button';
import { NextLinkComposed } from '../src/Link';
export default function Index() {
return (
<Button
component={NextLinkComposed}
to={{
pathname: '/about',
query: { name: 'test' },
}}
>
Button link
</Button>
);
}
```
- The second version of the adapter is the `Link` component.
This component is styled.
It uses the [Material UI Link component](/material-ui/react-link/) with `NextLinkComposed`.
```tsx
import Link from '../src/Link';
export default function Index() {
return (
<Link
href={{
pathname: '/about',
query: { name: 'test' },
}}
>
Link
</Link>
);
}
```
| 3,651 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/server-rendering/server-rendering.md | # Server rendering
<p class="description">The most common use case for server-side rendering is to handle the initial render when a user (or search engine crawler) first requests your app.</p>
When the server receives the request, it renders the required component(s) into an HTML string, and then sends it as a response to the client.
From that point on, the client takes over rendering duties.
## Material UI on the server
Material UI was designed from the ground-up with the constraint of rendering on the server, but it's up to you to make sure it's correctly integrated.
It's important to provide the page with the required CSS, otherwise the page will render with just the HTML then wait for the CSS to be injected by the client, causing it to flicker (FOUC).
To inject the style down to the client, we need to:
1. Create a fresh, new [`emotion cache`](https://emotion.sh/docs/@emotion/cache) instance on every request.
2. Render the React tree with the server-side collector.
3. Pull the CSS out.
4. Pass the CSS along to the client.
On the client-side, the CSS will be injected a second time before removing the server-side injected CSS.
## Setting up
In the following recipe, we are going to look at how to set up server-side rendering.
### The theme
Create a theme that will be shared between the client and the server:
`theme.js`
```js
import { createTheme } from '@mui/material/styles';
import { red } from '@mui/material/colors';
// Create a theme instance.
const theme = createTheme({
palette: {
primary: {
main: '#556cd6',
},
secondary: {
main: '#19857b',
},
error: {
main: red.A400,
},
},
});
export default theme;
```
### The server-side
The following is the outline for what the server-side is going to look like.
We are going to set up an [Express middleware](https://expressjs.com/en/guide/using-middleware.html) using [app.use](https://expressjs.com/en/api.html) to handle all requests that come into the server.
If you're unfamiliar with Express or middleware, know that the `handleRender` function will be called every time the server receives a request.
`server.js`
```js
import express from 'express';
// We are going to fill these out in the sections to follow.
function renderFullPage(html, css) {
/* ... */
}
function handleRender(req, res) {
/* ... */
}
const app = express();
// This is fired every time the server-side receives a request.
app.use(handleRender);
const port = 3000;
app.listen(port);
```
### Handling the request
The first thing that we need to do on every request is to create a new `emotion cache`.
When rendering, we will wrap `App`, the root component,
inside a [`CacheProvider`](https://emotion.sh/docs/cache-provider) and [`ThemeProvider`](/system/styles/api/#themeprovider) to make the style configuration and the `theme` available to all components in the component tree.
The key step in server-side rendering is to render the initial HTML of the component **before** we send it to the client-side. To do this, we use [ReactDOMServer.renderToString()](https://react.dev/reference/react-dom/server/renderToString).
Material UI uses Emotion as its default styled engine.
We need to extract the styles from the Emotion instance.
For this, we need to share the same cache configuration for both the client and server:
`createEmotionCache.js`
```js
import createCache from '@emotion/cache';
export default function createEmotionCache() {
return createCache({ key: 'css' });
}
```
With this we are creating a new Emotion cache instance and using this to extract the critical styles for the html as well.
We will see how this is passed along in the `renderFullPage` function.
```jsx
import express from 'express';
import * as React from 'react';
import * as ReactDOMServer from 'react-dom/server';
import CssBaseline from '@mui/material/CssBaseline';
import { ThemeProvider } from '@mui/material/styles';
import { CacheProvider } from '@emotion/react';
import createEmotionServer from '@emotion/server/create-instance';
import App from './App';
import theme from './theme';
import createEmotionCache from './createEmotionCache';
function handleRender(req, res) {
const cache = createEmotionCache();
const { extractCriticalToChunks, constructStyleTagsFromChunks } =
createEmotionServer(cache);
// Render the component to a string.
const html = ReactDOMServer.renderToString(
<CacheProvider value={cache}>
<ThemeProvider theme={theme}>
{/* CssBaseline kickstart an elegant, consistent, and simple baseline
to build upon. */}
<CssBaseline />
<App />
</ThemeProvider>
</CacheProvider>,
);
// Grab the CSS from emotion
const emotionChunks = extractCriticalToChunks(html);
const emotionCss = constructStyleTagsFromChunks(emotionChunks);
// Send the rendered page back to the client.
res.send(renderFullPage(html, emotionCss));
}
const app = express();
app.use('/build', express.static('build'));
// This is fired every time the server-side receives a request.
app.use(handleRender);
const port = 3000;
app.listen(port);
```
### Inject initial component HTML and CSS
The final step on the server-side is to inject the initial component HTML and CSS into a template to be rendered on the client-side.
```js
function renderFullPage(html, css) {
return `
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>My page</title>
${css}
<meta name="viewport" content="initial-scale=1, width=device-width" />
<link rel="preconnect" href="https://fonts.googleapis.com" />
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin />
<link
rel="stylesheet"
href="https://fonts.googleapis.com/css2?family=Roboto:wght@300;400;500;600;700&display=swap"
/>
</head>
<body>
<div id="root">${html}</div>
</body>
</html>
`;
}
```
### The client-side
The client-side is straightforward.
All we need to do is use the same cache configuration as the server-side.
Let's take a look at the client file:
`client.js`
```jsx
import * as React from 'react';
import * as ReactDOM from 'react-dom';
import CssBaseline from '@mui/material/CssBaseline';
import { ThemeProvider } from '@mui/material/styles';
import { CacheProvider } from '@emotion/react';
import App from './App';
import theme from './theme';
import createEmotionCache from './createEmotionCache';
const cache = createEmotionCache();
function Main() {
return (
<CacheProvider value={cache}>
<ThemeProvider theme={theme}>
{/* CssBaseline kickstart an elegant, consistent, and simple baseline
to build upon. */}
<CssBaseline />
<App />
</ThemeProvider>
</CacheProvider>
);
}
ReactDOM.hydrate(<Main />, document.querySelector('#root'));
```
## Reference implementations
Here is [the reference implementation of this tutorial](https://github.com/mui/material-ui/tree/HEAD/examples/material-ui-express-ssr).
You can more SSR implementations in the GitHub repository under the `/examples` folder, see [the other examples](/material-ui/getting-started/example-projects/).
| 3,652 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/shadow-dom/ShadowDOMDemo.js | import * as React from 'react';
export default function ShadowDOMDemo() {
return (
<iframe
title="codesandbox"
src="https://codesandbox.io/embed/rki9k5?hidenavigation=1&fontsize=14&view=preview"
style={{
width: '100%',
height: 350,
border: 0,
}}
sandbox="allow-modals allow-forms allow-popups allow-scripts allow-same-origin hidenavigation"
/>
);
}
| 3,653 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/shadow-dom/shadow-dom.md | # Shadow DOM
<p class="description">The shadow DOM lets you encapsulate parts of an app to keep them separate from global styles that target the regular DOM tree.</p>
## How to use the shadow DOM with Material UI
### 1. Styles
The shadow DOM is an API that provides a way to attach a hidden separated DOM to an element.
This is useful when you need to keep the structure, style, and behavior of different components separate from the rest of the code on the page, to prevent conflicts.
See [the MDN docs on the shadow DOM](https://developer.mozilla.org/en-US/docs/Web/API/Web_components/Using_shadow_DOM) for more information.
The following code snippet shows how to apply styles inside of the shadow DOM:
```tsx
const container = document.querySelector('#root');
const shadowContainer = container.attachShadow({ mode: 'open' });
const emotionRoot = document.createElement('style');
const shadowRootElement = document.createElement('div');
shadowContainer.appendChild(emotionRoot);
shadowContainer.appendChild(shadowRootElement);
const cache = createCache({
key: 'css',
prepend: true,
container: emotionRoot,
});
ReactDOM.createRoot(shadowRootElement).render(
<CacheProvider value={cache}>
<App />
</CacheProvider>,
);
```
### 2. Theme
Material UI components like `Menu`, `Dialog`, `Popover` and others use [`Portal`](/material-ui/react-portal/) to render a new "subtree" in a container outside of current DOM hierarchy.
By default, this container is `document.body`.
But since the styles are applied only inside of the Shadow DOM, we need to render portals inside the Shadow DOM container as well:
```tsx
const theme = createTheme({
components: {
MuiPopover: {
defaultProps: {
container: shadowRootElement,
},
},
MuiPopper: {
defaultProps: {
container: shadowRootElement,
},
},
MuiModal: {
defaultProps: {
container: shadowRootElement,
},
},
},
});
// ...
<ThemeProvider theme={theme}>
<App />
</ThemeProvider>;
```
## Demo
In the example below you can see that the component outside of the shadow DOM is affected by global styles, while the component inside of the shadow DOM is not:
{{"demo": "ShadowDOMDemo.js", "hideToolbar": true, "bg": true}}
| 3,654 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/styled-components/styled-components.md | # Using styled-components
<p class="description">Learn how to use styled-components instead of Emotion with Material UI.</p>
:::error
As of late 2021, [styled-components](https://github.com/styled-components/styled-components) is **not compatible** with server-rendered Material UI projects.
This is because `babel-plugin-styled-components` isn't able to work with the `styled()` utility inside `@mui` packages.
See [this GitHub issue](https://github.com/mui/material-ui/issues/29742) for more details.
We **strongly recommend** using Emotion for SSR projects.
:::
By default, Material UI uses [Emotion](https://github.com/emotion-js/emotion) to generate CSS styles.
All components rely on the `styled()` API to inject CSS into the page.
This API is supported by multiple popular styling libraries, which makes it possible to switch between them in Material UI.
MUI provides two different packages to wrap your chosen styling solution for compatibility with Material UI:
- `@mui/styled-engine`: a thin wrapper around Emotion's [`styled()`](https://emotion.sh/docs/styled) API that includes required utilities like the `<GlobalStyles />` component, the `css` and `keyframe` helpers, and more. This is the default, and you do not need to install it.
- `@mui/styled-engine-sc`: a similar wrapper, but specifically tailored for styled-components. You must install and implement this package to use styled-components with Material UI.
These two packages implement the same interface, making them interchangeable.
## Bundler configuration
By default, `@mui/material` has `@mui/styled-engine` as a dependency.
To use styled-components, you need to configure your bundler to replace it with `@mui/styled-engine-sc`.
### With yarn
If you're using yarn, you can configure it using a package resolution:
**package.json**
<!-- #default-branch-switch -->
```diff
{
"dependencies": {
- "@mui/styled-engine": "latest"
+ "@mui/styled-engine": "npm:@mui/styled-engine-sc@latest"
},
+ "resolutions": {
+ "@mui/styled-engine": "npm:@mui/styled-engine-sc@latest"
+ },
}
```
### With npm
Because package resolutions aren't available with npm, you must update your bundler's config to add this alias.
The example below shows how to do this with Webpack:
**webpack.config.js**
```diff
module.exports = {
//...
+ resolve: {
+ alias: {
+ '@mui/styled-engine': '@mui/styled-engine-sc'
+ },
+ },
};
```
For TypeScript, you must also update the `tsconfig.json` as shown here:
**tsconfig.json**
```diff
{
"compilerOptions": {
+ "paths": {
+ "@mui/styled-engine": ["./node_modules/@mui/styled-engine-sc"]
+ }
},
}
```
### Next.js
**next.config.js**
```diff
+const withTM = require('next-transpile-modules')([
+ '@mui/material',
+ '@mui/system',
+ '@mui/icons-material', // If @mui/icons-material is being used
+]);
+module.exports = withTM({
webpack: (config) => {
config.resolve.alias = {
...config.resolve.alias,
+ '@mui/styled-engine': '@mui/styled-engine-sc',
};
return config;
}
+});
```
:::info
**Versions compatibility**
To ensure compatibility, it's essential to align the major version of `@mui/styled-engine-sc` with that of the `styled-components` package you're using. For instance, if you opt for `styled-components` version 5, it's necessary to use `@mui/styled-engine-sc` version 5. Similarly, if your preference is `styled-components` version 6, you'll need to upgrade `@mui/styled-engine-sc` to its version 6, which is currently in an alpha state.
:::
## Ready-to-use examples
MUI provides boilerplate examples of Create React App with Material UI and styled-components in both JavaScript and TypeScript:
<!-- #default-branch-switch -->
- [Material UI + CRA + styled-components (JavaScript)](https://github.com/mui/material-ui/tree/master/examples/material-ui-cra-styled-components)
- [Material UI + CRA + styled-components (TypeScript)](https://github.com/mui/material-ui/tree/master/examples/material-ui-cra-styled-components-ts)
:::warning
`@emotion/react`, `@emotion/styled`, and `styled-components` are optional peer dependencies of `@mui/material`, so you need to install them yourself.
See the [Installation guide](/material-ui/getting-started/installation/) for more info.
:::
| 3,655 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/testing/testing.md | # Testing
<p class="description">Write tests to prevent regressions and write better code.</p>
## Userspace
It's generally recommended to test your application without tying the tests too closely to Material UI.
This is how Material UI components are tested internally.
A library that has a first-class API for this approach is [`@testing-library/react`](https://testing-library.com/docs/react-testing-library/intro/).
For example, when rendering a `TextField` your test should not need to query for the specific Material UI instance of the `TextField` but rather for the `input`, or `[role="textbox"]`.
By not relying on the React component tree you make your test more robust against internal changes in Material UI or, if you need snapshot testing, adding additional wrapper components such as context providers.
We don't recommend snapshot testing though.
["Effective snapshot testing" by Kent C. Dodds](https://kentcdodds.com/blog/effective-snapshot-testing) goes into more details why snapshot testing might be misleading for React component tests.
## Internal
MUI has **a wide range** of tests for Material UI so we can
iterate with confidence on the components, for instance, the visual regression tests provided by [Argos-CI](https://app.argos-ci.com/mui/material-ui/builds) have proven to be really helpful.
To learn more about the internal tests, you can have a look at the [README](https://github.com/mui/material-ui/blob/HEAD/test/README.md).
| 3,656 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/theme-scoping/theme-scoping.md | # Theme scoping
<p class="description">Learn how to use multiple styling solutions in a single Material UI app.</p>
:::warning
Having more than one styling library can introduce unnecessary complexity to your project.
You should have a very good reason to do this.
:::
Starting with [v5.12.0](https://github.com/mui/material-ui/releases/tag/v5.12.0), Material UI can coexist with other component libraries that depend on Emotion or styled-components—this is possible thanks to _theme scoping_.
To do this, you need to render Material UI's `ThemeProvider` as an inner provider and use the `THEME_ID` to store the theme, as shown below:
```js
import { ThemeProvider, THEME_ID, createTheme } from '@mui/material/styles';
import { AnotherThemeProvider } from 'another-ui-library';
const materialTheme = createTheme(/* your theme */);
function App() {
return (
<AnotherThemeProvider>
<ThemeProvider theme={{ [THEME_ID]: materialTheme }}>
{/* components from another library and Material UI */}
</ThemeProvider>
</AnotherThemeProvider>
);
}
```
The Material UI theme will be separated from the other library, so when you use APIs such as `styled`, the `sx` prop, and `useTheme`, you'll be able to access Material UI's theme like you normally would.
## Minimum version
[Theme scoping](https://github.com/mui/material-ui/pull/36664) was introduced in Material UI v5.12.0, so make sure you're running that version or higher.
### Using with Theme UI
Render Material UI's theme provider below Theme UI's provider and assign the Material theme to the `THEME_ID` property:
```js
import { ThemeUIProvider } from 'theme-ui';
import { createTheme as materialCreateTheme, THEME_ID } from '@mui/material/styles';
const themeUITheme = {
fonts: {
body: 'system-ui, sans-serif',
heading: '"Avenir Next", sans-serif',
monospace: 'Menlo, monospace',
},
colors: {
text: '#000',
background: '#fff',
primary: '#33e',
},
};
const materialTheme = materialCreateTheme();
function App() {
return (
<ThemeUIProvider theme={themeUITheme}>
<MaterialThemeProvider theme={{ [THEME_ID]: materialTheme }}>
Theme UI components and Material UI components
</MaterialThemeProvider>
</ThemeUIProvider>
);
}
```
### Using with Chakra UI
Render Material UI's theme provider below Chakra UI's provider and assign the material theme to the `THEME_ID` property:
```js
import { ChakraProvider, extendTheme as chakraExtendTheme } from '@chakra-ui/react';
import {
ThemeProvider as MaterialThemeProvider,
createTheme as muiCreateTheme,
THEME_ID,
} from '@mui/material/styles';
const chakraTheme = chakraExtendTheme();
const materialTheme = muiCreateTheme();
function App() {
return (
<ChakraProvider theme={chakraTheme} resetCSS>
<MaterialThemeProvider theme={{ [THEME_ID]: materialTheme }}>
Chakra UI components and Material UI components
</MaterialThemeProvider>
</ChakraProvider>
);
}
```
| 3,657 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/typescript/typescript.md | # TypeScript
<p class="description">You can add static typing to JavaScript to improve developer productivity and code quality thanks to TypeScript.</p>
## Minimum configuration
<!-- #default-branch-switch -->
Material UI requires a minimum version of TypeScript 3.5. Have a look at the [Create React App with TypeScript](https://github.com/mui/material-ui/tree/master/examples/material-ui-cra-ts) example.
For types to work, it's recommended that you have at least the following options enabled in your `tsconfig.json`:
```json
{
"compilerOptions": {
"lib": ["es6", "dom"],
"noImplicitAny": true,
"noImplicitThis": true,
"strictNullChecks": true
}
}
```
The strict mode options are the same that are required for every types package
published in the `@types/` namespace.
Using a less strict `tsconfig.json` or omitting some of the libraries might cause errors.
To get the best type experience with the types we recommend setting `"strict": true`.
## Handling `value` and event handlers
Many components concerned with user input offer a `value` prop or event handlers
which include the current `value`. In most situations that `value` is only handled
within React which allows it be of any type, such as objects or arrays.
However, that type cannot be verified at compile time in situations where it depends
on the component's children e.g. for `Select` or `RadioGroup`. This means that
the soundest option is to type it as `unknown` and let the developer decide
how they want to narrow that type down. We do not offer the possibility to use a generic
type in those cases for [the same reasons `event.target` is not generic in React](https://github.com/DefinitelyTyped/DefinitelyTyped/issues/11508#issuecomment-256045682).
The demos include typed variants that use type casting. It is an acceptable tradeoff
because the types are all located in a single file and are very basic. You have to decide for yourself
if the same tradeoff is acceptable for you. The library types are strict
by default and loose via opt-in.
## Customization of `Theme`
Moved to [/customization/theming/#custom-variables](/material-ui/customization/theming/#custom-variables).
## Complications with the `component` prop
Because of some TypeScript limitations, using the `component` prop can be problematic if you are creating your custom component based on the Material UI's components.
For the composition of the components, you will likely need to use one of these two options:
1. Wrap the Material UI component in order to enhance it
2. Use the `styled()` utility in order to customize the styles of the component
If you are using the first option, take a look at the [composition guide](/material-ui/guides/composition/#with-typescript) for more details.
If you are using the `styled()` utility (regardless of whether it comes from `@mui/material` or `@emotion/styled`), you will need to cast the resulting component as shown below:
```tsx
import Button from '@mui/material/Button';
import { styled } from '@mui/material/styles';
const CustomButton = styled(Button)({
// your custom styles go here
}) as typeof Button;
```
| 3,658 |
0 | petrpan-code/mui/material-ui/docs/data/material/guides | petrpan-code/mui/material-ui/docs/data/material/guides/understand-mui-packages/understand-mui-packages.md | # Understanding MUI packages
<p class="description">An overview of MUI's packages and the relationships between them.</p>
## Overview
- If you want to build a design system based on Material Design, use `@mui/material`.
- If you want to build with components that give you complete control over your app's CSS, use `@mui/base`.
- For CSS utilities to help in laying out custom designs with Material UI or Base UI, use `@mui/system`.
### Glossary
- **install** refers to running `yarn add $module` or `npm install $module`.
- **import** refers to making a module API available in your code by adding `import ... from '$module'`.
## MUI packages
The following is an up-to-date list of `@mui` public packages.
- `@mui/material`
- `@mui/base`
- `@mui/system`
- `@mui/styled-engine`
- `@mui/styled-engine-sc`
### Understanding MUI's products
As a company, MUI maintains a suite of open-source and open-core React UI projects.
These projects live within two product lines: MUI Core and MUI X.
The following chart illustrates how MUI's packages are related to one another:
<img src="/static/images/packages/mui-packages.png" style="width: 814px; margin-top: 4px; margin-bottom: 8px;" alt="The first half of the image shows @mui/material and @mui/base as component libraries, and @mui/system and styled engines as styling solutions, both under the MUI Core umbrella. The second half shows @mui/x-data-grid and @mui/x-date-pickers as components from MUI X." width="1628" height="400" />
In this article, we'll only cover the MUI Core packages.
Visit the [MUI X Overview](/x/introduction/) for more information about our collection of advanced components.
## Component libraries
### Material UI
Material UI is a comprehensive library of components that features our implementation of Google's Material Design.
`@mui/system` is included as dependency, meaning you don't need to install or import it separately—you can import its components and functions directly from `@mui/material`.
### Base UI
[Base UI](/base-ui/) is a library of unstyled React UI components and hooks.
With Base UI, you gain complete control over your app's CSS and accessibility features.
The Base package includes prebuilt components with production-ready functionality, along with low-level hooks for transferring that functionality to other components.
### Using them together
Imagine you're working on an application that uses `@mui/material` with a custom theme, and you need to develop a new switch component that looks very different from the one found in Material Design.
In this case, instead of overriding all the styles on the Material UI `Switch` component, you can use the `styled` API to customize the Base `SwitchUnstyled` component from scratch:
```js
import { styled } from '@mui/material/styles';
import { SwitchUnstyled, switchUnstyledClasses } from '@mui/base/SwitchUnstyled';
const Root = styled('span')(`
position: relative;
display: inline-block;
width: 32px;
height: 20px;
& .${switchUnstyledClasses.track} {
// ...css
}
& .${switchUnstyledClasses.thumb} {
// ...css
}
`);
export default function CustomSwitch() {
const label = { slotProps: { input: { 'aria-label': 'Demo switch' } } };
return <SwitchUnstyled component={Root} {...label} />;
}
```
## Styling
### Styled engines
Material UI relies on styling engines to inject CSS into your app.
These engines come in two packages:
- `@mui/styled-engine`
- `@mui/styled-engine-sc`
By default, Material UI uses [Emotion](https://emotion.sh/docs/styled) as its styling engine—it's included in the [installation](/material-ui/getting-started/installation/) process.
If you plan to stick with Emotion, then `@mui/styled-engine` is a dependency in your app, and you don't need to install it separately.
If you prefer to use [styled-components](https://styled-components.com/docs/basics#getting-started), then you need to install `@mui/styled-engine-sc` in place of the Emotion packages.
See the [styled-components guide](/material-ui/guides/styled-components/) for more details.
In either case, you won't interact much with either of these packages beyond installation—they're used internally in `@mui/system`.
:::warning
Prior to v5, Material UI used `@mui/styles` as a JSS wrapper.
This package is now deprecated and will be removed in the future.
Check out [the guide to migrating from v4 to v5](/material-ui/migration/migration-v4/) to learn how to upgrade to a newer solution.
:::
### MUI System
MUI System is a collection of CSS utilities to help you rapidly lay out custom designs.
It uses the Emotion adapter (`@mui/styled-engine`) as the default style engine to create the CSS utilities.
#### Advantages of MUI System
- You have full control of the `theme` object.
- You can use `sx` prop normally as the `styled` API supports it by default.
- You can have themeable components by using `styled` via slots and variants.
:::warning
To use MUI System, you must install either Emotion or styled-components, because the respective `styled-engine` package depends on it.
:::
<img src="/static/images/packages/mui-system.png" style="width: 814px; margin-top: 4px; margin-bottom: 8px;" alt="A diagram showing an arrow going from @mui/system to @mui/styled-engine, with a note that it is the default engine. Then, from @mui/styled-engine a solid arrow points to @emotion/react and @emotion/styled while a dashed arrow points to @mui/styled-engine-sc, which points to styled-components." width="1628" height="400" />
| 3,659 |
0 | petrpan-code/mui/material-ui/docs/data/material/migration | petrpan-code/mui/material-ui/docs/data/material/migration/migration-grid-v2/GridDisableEqualOverflow.js | import * as React from 'react';
import Box from '@mui/material/Box';
import Grid2 from '@mui/material/Unstable_Grid2';
import Typography from '@mui/material/Typography';
export default function GridDisableEqualOverflow() {
return (
<Box sx={{ pt: 3 }}>
<Box sx={{ border: '1px solid', borderColor: 'primary.main' }}>
<Grid2
container
spacing={2}
disableEqualOverflow
sx={{ bgcolor: 'rgba(255 255 255 / 0.6)' }}
>
<Grid2
height={100}
display="flex"
flexDirection="column"
alignItems="center"
justifyContent="center"
textAlign="center"
xs
>
ver.2 <br />
Top and left overflow
</Grid2>
</Grid2>
</Box>
<Typography variant="body2" sx={{ mt: 3, color: 'text.secondary' }}>
The overflow represents the negative margin of the grid.
</Typography>
</Box>
);
}
| 3,660 |
0 | petrpan-code/mui/material-ui/docs/data/material/migration | petrpan-code/mui/material-ui/docs/data/material/migration/migration-grid-v2/GridsDiff.js | import * as React from 'react';
import Box from '@mui/material/Box';
import Grid from '@mui/material/Grid';
import Grid2 from '@mui/material/Unstable_Grid2';
import Typography from '@mui/material/Typography';
export default function GridsDiff() {
return (
<div>
<Box
sx={{
pt: 3,
display: 'flex',
flexWrap: 'wrap',
gap: 4,
justifyContent: 'space-between',
}}
>
<Box sx={{ border: '1px solid', borderColor: 'primary.main' }}>
<Grid
container
spacing={2}
sx={{ bgcolor: 'rgba(255 255 255 / 0.72)', width: 160 }}
>
<Grid
item
height={100}
display="flex"
alignItems="center"
justifyContent="center"
textAlign="center"
xs
>
ver.1 <br />
Top and left
</Grid>
</Grid>
</Box>
<Box sx={{ border: '1px solid', borderColor: 'primary.main' }}>
<Grid2
container
spacing={2}
sx={{ bgcolor: 'rgba(255 255 255 / 0.6)', width: 160 }}
>
<Grid2
height={100}
display="flex"
alignItems="center"
justifyContent="center"
textAlign="center"
xs
>
ver.2 <br />
All sides
</Grid2>
</Grid2>
</Box>
</Box>
<Typography variant="body2" sx={{ mt: 3, color: 'text.secondary' }}>
The overflow represents the negative margin of the grid.
</Typography>
</div>
);
}
| 3,661 |
0 | petrpan-code/mui/material-ui/docs/data/material/migration | petrpan-code/mui/material-ui/docs/data/material/migration/migration-grid-v2/migration-grid-v2.md | # Migration to Grid v2
<p class="description">This guide explains how and why to migrate from Material UI Grid v1 to v2.</p>
## Why you should migrate
Grid v2 has several new feature and many improvements over the original:
- Grid v2 uses CSS variables which remove CSS specificity from class selectors.
Now you can use `sx` prop on the Grid to control any style you'd like.
- All grids are considered items without specifying the `item` prop.
- The long-awaited [offset feature](/material-ui/react-grid2/#offset) gives you more flexibility for positioning.
- [Nested grids](/material-ui/react-grid2/#nested-grid) now have no depth limitation.
- The `disableEqualOverflow` flag disables the horizontal scrollbar in smaller viewports.
:::info
Grid v2 is currently considered `Unstable_` as we give the community time to try it out and offer feedback.
We will make it stable and deprecate v1 in the next major release of Material UI.
:::
## With Material UI v4
The Grid v2 is introduced in Material UI v5, so you have to follow the [Material UI migration guide](/material-ui/migration/migration-v4/) first.
## With Material UI v5
The migration is expected to be smooth since most of the APIs remains the same. However, there is one breaking change that we want to clarify:
The default implementation of the negative margin in Grid v2 is spread equally on all sides (same as the Grid in Material UI v4).
{{"demo": "GridsDiff.js", "bg": true, "hideToolbar": true}}
### Import
```diff
-import Grid from '@mui/material/Grid';
+import Grid from '@mui/material/Unstable_Grid2';
```
### Remove props
The `item` and `zeroMinWidth` props have been removed in Grid v2:
```diff
-<Grid item zeroMinWidth xs={6}>
+<Grid xs={6}>
```
### Negative margins
If you want to apply the negative margins similar to the Grid v1, specify `disableEqualOverflow: true` on the grid container:
{{"demo": "GridDisableEqualOverflow.js", "bg": true, "hideToolbar": true}}
To apply to all grids, add the default props to the theme:
```js
import { createTheme, ThemeProvider } from '@mui/material/styles';
import Grid from '@mui/material/Unstable_Grid2';
const theme = createTheme({
components: {
MuiGrid2: {
defaultProps: {
// all grids under this theme will apply
// negative margin on the top and left sides.
disableEqualOverflow: true,
},
},
},
});
function Demo() {
return (
<ThemeProvider theme={theme}>
<Grid container>...grids</Grid>
</ThemeProvider>
);
}
```
## Documentation page
Check out [Grid v2 docs](/material-ui/react-grid2/#fluid-grids) for all the demos and code samples.
| 3,662 |
0 | petrpan-code/mui/material-ui/docs/data/material/migration | petrpan-code/mui/material-ui/docs/data/material/migration/migration-v0x/migration-v0x.md | # Migration from v0.x to v1
<p class="description">Yeah, v1 has been released! Take advantage of 2 years worth of effort.</p>
## FAQ
### Woah - the API is way different! Does that mean 1.0 is completely different, I'll have to learn the basics all over again, and migrating will be practically impossible?
I'm glad you asked! The answer is no. The core concepts haven't changed.
You will notice that the API provides more flexibility, but this has a cost –
lower-level components that abstract less complexity.
### What motivated such a large change?
Material UI was started [4 years ago](https://github.com/mui/material-ui/commit/28b768913b75752ecf9b6bb32766e27c241dbc46).
The ecosystem has evolved a lot since then, we have also learned a lot.
[@nathanmarks](https://github.com/nathanmarks/) started an ambitious task, rebuilding Material UI from the **ground-up**
taking advantage of this knowledge to address long-standing issues. To name some of the major changes:
- New styling solution using CSS-in-JS (better [customization](/material-ui/customization/how-to-customize/) power, better performance)
- New theme handling (nesting, self-supporting, etc.)
- Blazing fast documentation thanks to [Next.js](https://github.com/vercel/next.js)
- Way better [test coverage](/material-ui/guides/testing/) (99%+, run on all the major browsers, [visual regression tests](https://app.argos-ci.com/mui/material-ui/builds))
- Full [server-side rendering](/material-ui/guides/server-rendering/) support
- Wide range of [supported browsers](/material-ui/getting-started/supported-platforms/)
### Where should I start in a migration?
1. Start by installing the v1.x version of Material UI along side the v0.x version.
With yarn:
```bash
yarn add material-ui
yarn add @material-ui/core
```
Or with npm:
```bash
npm install material-ui
npm install @material-ui/core
```
then
```js
import FlatButton from 'material-ui/FlatButton'; // v0.x
import Button from '@material-ui/core/Button'; // v1.x
```
2. Run [the migration helper](https://github.com/mui/material-ui/tree/master/packages/mui-codemod) on your project.
3. `MuiThemeProvider` is optional for v1.x., but if you have a custom theme, you are free to use v0.x and v1.x versions of the component at the same time, like this:
```jsx
import * as React from 'react';
import { MuiThemeProvider, createMuiTheme } from '@material-ui/core/styles'; // v1.x
import { MuiThemeProvider as V0MuiThemeProvider } from 'material-ui';
import getMuiTheme from 'material-ui/styles/getMuiTheme';
const theme = createMuiTheme({
/* theme for v1.x */
});
const themeV0 = getMuiTheme({
/* theme for v0.x */
});
function App() {
return (
<MuiThemeProvider theme={theme}>
<V0MuiThemeProvider muiTheme={themeV0}>{/*Components*/}</V0MuiThemeProvider>
</MuiThemeProvider>
);
}
export default App;
```
4. After that, you are free to migrate one component instance at the time.
## Components
### Autocomplete
Material UI doesn't provide a high-level API for solving this problem.
You're encouraged you to explore [the solutions the React community has built](/material-ui/react-autocomplete/).
In the future, we will look into providing a simple component to solve the simple use cases: [#9997](https://github.com/mui/material-ui/issues/9997).
### Svg Icon
Run [the migration helper](https://github.com/mui/material-ui/tree/master/packages/mui-codemod) on your project.
This will apply a change such as the following:
```diff
-import AddIcon from 'material-ui/svg-icons/Add';
+import AddIcon from '@mui/icons-material/Add';
<AddIcon />
```
### Flat Button
```diff
-import FlatButton from 'material-ui/FlatButton';
+import Button from '@material-ui/core/Button';
-<FlatButton />
+<Button />
```
### Raised Button
RaisedButton upgrade path:
```diff
-import RaisedButton from 'material-ui/RaisedButton';
+import Button from '@material-ui/core/Button';
-<RaisedButton />
+<Button variant="contained" />
```
### Subheader
```diff
-import Subheader from 'material-ui/Subheader';
+import ListSubheader from '@material-ui/core/ListSubheader';
-<Subheader>Sub Heading</Subheader>
+<ListSubheader>Sub Heading</ListSubheader>
```
### Toggle
```diff
-import Toggle from 'material-ui/Toggle';
+import Switch from '@material-ui/core/Switch';
-<Toggle
- toggled={this.state.checkedA}
- onToggle={this.handleToggle}
-/>
+<Switch
+ checked={this.state.checkedA}
+ onChange={this.handleSwitch}
+/>
```
### Menu Item
```diff
-import MenuItem from 'material-ui/MenuItem';
+import MenuItem from '@material-ui/core/MenuItem';
-<MenuItem primaryText="Profile" />
+<MenuItem>Profile</MenuItem>
```
### Font Icon
```diff
-import FontIcon from 'material-ui/FontIcon';
+import Icon from '@material-ui/core/Icon';
-<FontIcon>home</FontIcon>
+<Icon>home</Icon>
```
### Circular Progress
```diff
-import CircularProgress from 'material-ui/CircularProgress';
+import CircularProgress from '@material-ui/core/CircularProgress';
-<CircularProgress mode="indeterminate" />
+<CircularProgress variant="indeterminate" />
```
### Drop Down Menu
```diff
-import DropDownMenu from 'material-ui/DropDownMenu';
+import Select from '@material-ui/core/Select';
-<DropDownMenu></DropDownMenu>
+<Select value={this.state.value}></Select>
```
### To be continued…
Have you successfully migrated your app, and wish to help the community?
There is an open issue in order to finish this migration guide [#7195](https://github.com/mui/material-ui/issues/7195). Any pull request is welcomed 😊.
| 3,663 |
0 | petrpan-code/mui/material-ui/docs/data/material/migration | petrpan-code/mui/material-ui/docs/data/material/migration/migration-v3/migration-v3.md | # Migration from v3 to v4
<p class="description">Yeah, v4 has been released!</p>
Looking for the v3 docs? You can [find the latest version here](https://mui.com/versions/).
:::info
This document is a work in progress.
Have you upgraded your site and run into something that's not covered here?
[Add your changes on GitHub](https://github.com/mui/material-ui/blob/HEAD/docs/data/material/migration/migration-v3/migration-v3.md).
:::
## Introduction
This is a reference for upgrading your site from Material UI v3 to v4.
While there's a lot covered here, you probably won't need to do everything for your site.
We'll do our best to keep things easy to follow, and as sequential as possible so you can quickly get rocking on v4!
## Why you should migrate
This documentation page covers the **how** of migrating from v3 to v4.
The **why** is covered in the [release blog post on Medium](https://mui.com/blog/material-ui-v4-is-out/).
## Updating your dependencies
The very first thing you will need to do is to update your dependencies.
### Update Material UI version
You need to update your `package.json` to use the latest version of Material UI.
```json
"dependencies": {
"@material-ui/core": "^4.0.0"
}
```
Or run
```bash
npm install @material-ui/core
or
yarn add @material-ui/core
```
### Update React version
The minimum required version of React was increased from `react@^16.3.0` to `react@^16.8.0`.
This allows us to rely on [Hooks](https://legacy.reactjs.org/docs/hooks-intro.html) (we no longer use the class API).
### Update Material UI Styles version
If you were previously using `@material-ui/styles` with v3 you need to update your `package.json` to use the latest version of Material UI Styles.
```json
"dependencies": {
"@material-ui/styles": "^4.0.0"
}
```
Or run
```bash
npm install @material-ui/styles
or
yarn add @material-ui/styles
```
## Handling breaking changes
### Core
- Every component forward their ref.
This is implemented by using `React.forwardRef()`.
This affects the internal component tree and display name and therefore might break shallow or snapshot tests.
`innerRef` will no longer return a ref to the instance (or nothing if the inner component is a function component) but a ref to its root component.
The corresponding API docs list the root component.
### Styles
- ⚠️ Material UI depends on JSS v10. JSS v10 is not backward compatible with v9.
Make sure JSS v9 is not installed in your environment.
(Removing `react-jss` from your `package.json` can help).
The StylesProvider component replaces the JssProvider one.
- Remove the first option argument of `withTheme()`.
(The first argument was a placeholder for a potential future option that never arose.)
It matches the [emotion API](https://emotion.sh/docs/introduction) and the [styled-components API](https://styled-components.com).
```diff
-const DeepChild = withTheme()(DeepChildRaw);
+const DeepChild = withTheme(DeepChildRaw);
```
- Rename `convertHexToRGB` to `hexToRgb`.
```diff
-import { convertHexToRgb } from '@material-ui/core/styles/colorManipulator';
+import { hexToRgb } from '@material-ui/core/styles';
```
- Scope the [keyframes API](https://cssinjs.org/jss-syntax/#keyframes-animation). You should apply the following changes in your codebase.
It helps isolating the animation logic:
```diff
rippleVisible: {
opacity: 0.3,
- animation: 'mui-ripple-enter 100ms cubic-bezier(0.4, 0, 0.2, 1)',
+ animation: '$mui-ripple-enter 100ms cubic-bezier(0.4, 0, 0.2, 1)',
},
'@keyframes mui-ripple-enter': {
'0%': {
opacity: 0.1,
},
'100%': {
opacity: 0.3,
},
},
```
### Theme
- The `theme.palette.augmentColor()` method no longer performs a side effect on its input color.
To use it correctly, you have to use the returned value.
```diff
-const background = { main: color };
-theme.palette.augmentColor(background);
+const background = theme.palette.augmentColor({ main: color });
console.log({ background });
```
- You can safely remove the next variant from the theme creation:
```diff
typography: {
- useNextVariants: true,
},
```
- `theme.spacing.unit` usage is deprecated, you can use the new API:
```diff
label: {
[theme.breakpoints.up('sm')]: {
- paddingTop: theme.spacing.unit * 12,
+ paddingTop: theme.spacing(12),
},
}
```
_Tip: you can provide more than 1 argument: `theme.spacing(1, 2) // = '8px 16px'`_.
You can use [the migration helper](https://github.com/mui/material-ui/tree/master/packages/mui-codemod/README.md#theme-spacing-api) on your project to make this smoother.
### Layout
- [Grid] In order to support arbitrary spacing values and to remove the need to mentally count by 8, we are changing the spacing API:
```diff
/**
* Defines the space between the type `item` component.
* It can only be used on a type `container` component.
*/
- spacing: PropTypes.oneOf([0, 8, 16, 24, 32, 40]),
+ spacing: PropTypes.oneOf([0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]),
```
Going forward, you can use the theme to implement [a custom Grid spacing transformation function](https://mui.com/system/spacing/#transformation).
- [Container] Moved from `@material-ui/lab` to `@material-ui/core`.
```diff
-import Container from '@material-ui/lab/Container';
+import Container from '@material-ui/core/Container';
```
### TypeScript
#### `value` type
Normalized `value` prop type for input components to use `unknown`. This affects
`InputBase`, `NativeSelect`, `OutlinedInput`, `Radio`, `RadioGroup`, `Select`, `SelectInput`, `Switch`, `TextArea`, and `TextField`.
```diff
function MySelect({ children }) {
- const handleChange = (event: any, value: string) => {
+ const handleChange = (event: any, value: unknown) => {
// handle value
};
return <Select onChange={handleChange}>{children}</Select>
}
```
This change is explained in more detail in the [TypeScript guide](/material-ui/guides/typescript/#handling-value-and-event-handlers)
### Button
- [Button] Remove the deprecated button variants (flat, raised and fab):
```diff
-<Button variant="raised" />
+<Button variant="contained" />
```
```diff
-<Button variant="flat" />
+<Button variant="text" />
```
```diff
-import Button from '@material-ui/core/Button';
-<Button variant="fab" />
+import Fab from '@material-ui/core/Fab';
+<Fab />
```
```diff
-import Button from '@material-ui/core/Button';
-<Button variant="extendedFab" />
+import Fab from '@material-ui/core/Fab';
+<Fab variant="extended" />
```
- [ButtonBase] The component passed to the `component` prop needs to be able to hold a ref.
The [composition guide](/material-ui/guides/composition/#caveat-with-refs) explains the migration strategy.
This also applies to `BottomNavigationAction`, `Button`, `CardActionArea`, `Checkbox`, `ExpansionPanelSummary`, `Fab`, `IconButton`, `MenuItem`, `Radio`, `StepButton`, `Tab`, `TableSortLabel` as well as `ListItem` if the `button` prop is true.
### Card
- [CardActions] Rename the `disableActionSpacing` prop to `disableSpacing`.
- [CardActions] Remove the `disableActionSpacing` CSS class.
- [CardActions] Rename the `action` CSS class to `spacing`.
### ClickAwayListener
- [ClickAwayListener] Hide react-event-listener props.
### Dialog
- [DialogActions] Rename the `disableActionSpacing` prop to `disableSpacing`.
- [DialogActions] Rename the `action` CSS class to `spacing`.
- [DialogContentText] Use typography variant `body1` instead of `subtitle1`.
- [Dialog] The child needs to be able to hold a ref. The [composition guide](/material-ui/guides/composition/#caveat-with-refs)
explains the migration strategy.
### Divider
- [Divider] Remove the deprecated `inset` prop.
```diff
-<Divider inset />
+<Divider variant="inset" />
```
### ExpansionPanel
- [ExpansionPanelActions] Rename the `action` CSS class to `spacing`.
- [ExpansionPanel] Increase the CSS specificity of the `disabled` and `expanded` style rules.
- [ExpansionPanel] Rename the `CollapseProps` prop to `TransitionProps`.
### List
- [List] Rework the list components to match the specification:
- The `ListItemAvatar` component is required when using an avatar.
- The `ListItemIcon` component is required when using a left checkbox.
- The `edge` property should be set on the icon buttons.
- [List] `dense` no longer reduces the top and bottom padding of the `List` element.
- [ListItem] Increase the CSS specificity of the `disabled` and `focusVisible` style rules.
### Menu
- [MenuItem] Remove the fixed height of the MenuItem.
The padding and line-height are used by the browser to compute the height.
### Modal
- [Modal] The child needs to be able to hold a ref. The [composition guide](/material-ui/guides/composition/#caveat-with-refs) explains
the migration strategy.
This also applies to `Dialog` and `Popover`.
- [Modal] Remove the classes customization API for the Modal component (-74% bundle size reduction when used standalone).
- [Modal] event.defaultPrevented is now ignored.
The new logic closes the Modal even if `event.preventDefault()` is called on the key down escape event.
`event.preventDefault()` is meant to stop default behaviors like clicking a checkbox to check it, hitting a button to submit a form, and hitting left arrow to move the cursor in a text input etc.
Only special HTML elements have these default behaviors.
You should use `event.stopPropagation()` if you don't want to trigger an `onClose` event on the modal.
### Paper
- [Paper] Reduce the default elevation.
Change the default Paper elevation to match the Card and the Expansion Panel:
```diff
-<Paper />
+<Paper elevation={2} />
```
This affects the `ExpansionPanel` as well.
### Portal
- [Portal] The child needs to be able to hold a ref when `disablePortal` is used. The [composition guide](/material-ui/guides/composition/#caveat-with-refs) explains
the migration strategy.
### Slide
- [Slide] The child needs to be able to hold a ref. The [composition guide](/material-ui/guides/composition/#caveat-with-refs) explains
the migration strategy.
### Slider
- [Slider] Move from `@material-ui/lab` to `@material-ui/core`.
```diff
-import Slider from '@material-ui/lab/Slider'
+import Slider from '@material-ui/core/Slider'
```
### Switch
- [Switch] Refactor the implementation to make it easier to override the styles.
Rename the class names to match the specification wording:
```diff
-icon
-bar
+thumb
+track
```
### Snackbar
- [Snackbar] Match the new specification.
- Change the dimensions
- Change the default transition from `Slide` to `Grow`.
### SvgIcon
- [SvgIcon] Rename nativeColor -> htmlColor.
React solved the same problem with the `for` HTML attribute, they have decided to call the prop `htmlFor`. This change follows the same reasoning.
```diff
-<AddIcon nativeColor="#fff" />
+<AddIcon htmlColor="#fff" />
```
### Tabs
- [Tab] Remove the `labelContainer`, `label` and `labelWrapped` class keys for simplicity.
This has allowed us to remove 2 intermediary DOM elements.
You should be able to move the custom styles to the `root` class key.
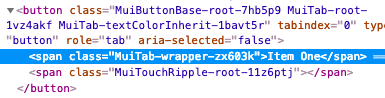
- [Tabs] Remove deprecated fullWidth and scrollable props:
```diff
-<Tabs fullWidth scrollable />
+<Tabs variant="scrollable" />
```
### Table
- [TableCell] Remove the deprecated `numeric` property:
```diff
-<TableCell numeric>{row.calories}</TableCell>
+<TableCell align="right">{row.calories}</TableCell>
```
- [TableRow] Remove the fixed height CSS property.
The cell height is computed by the browser using the padding and line-height.
- [TableCell] Move the `dense` mode to a different property:
```diff
-<TableCell padding="dense" />
+<TableCell size="small" />
```
- [TablePagination] The component no longer tries to fix invalid (`page`, `count`, `rowsPerPage`) property combinations. It raises a warning instead.
### TextField
- [InputLabel] You should be able to override all the styles of the FormLabel component using the CSS API of the InputLabel component.
The `FormLabelClasses` property has been removed.
```diff
<InputLabel
- FormLabelClasses={{ asterisk: 'bar' }}
+ classes={{ asterisk: 'bar' }}
>
Foo
</InputLabel>
```
- [InputBase] Change the default box sizing model.
It uses the following CSS now:
```css
box-sizing: border-box;
```
This solves issues with the `fullWidth` prop.
- [InputBase] Remove the `inputType` class from `InputBase`.
### Tooltip
- [Tooltip] The child needs to be able to hold a ref. The [composition guide](/material-ui/guides/composition/#caveat-with-refs) explains
the migration strategy.
- [Tooltip] Appears only after focus-visible focus instead of any focus.
### Typography
- [Typography] Remove the deprecated typography variants. You can upgrade by performing the following replacements:
- display4 => h1
- display3 => h2
- display2 => h3
- display1 => h4
- headline => h5
- title => h6
- subheading => subtitle1
- body2 => body1
- body1 (default) => body2 (default)
- [Typography] Remove the opinionated `display: block` default typography style.
You can use the new `display?: 'initial' | 'inline' | 'block';` property.
- [Typography] Rename the `headlineMapping` property to `variantMapping` to better align with its purpose.
```diff
-<Typography headlineMapping={headlineMapping}>
+<Typography variantMapping={variantMapping}>
```
- [Typography] Change the default variant from `body2` to `body1`.
A font size of 16px is a better default than 14px.
Bootstrap, material.io, and even the documentation use 16px as a default font size.
14px like Ant Design uses is understandable, as Chinese users have a different alphabet.
12px is recommended as the default font size for Japanese.
- [Typography] Remove the default color from the typography variants.
The color should inherit most of the time. It's the default behavior of the web.
- [Typography] Rename `color="default"` to `color="initial"` following the logic of [this thread](https://github.com/mui/material-ui/issues/13028).
The usage of _default_ should be avoided, it lacks semantic.
### Node
- [Drop node 6 support](https://github.com/nodejs/Release/blob/eb91c94681ea968a69bf4a4fe85c656ed44263b3/README.md#release-schedule), you should upgrade to node 8.
### UMD
- This change eases the use of Material UI with a CDN:
```diff
const {
Button,
TextField,
-} = window['material-ui'];
+} = MaterialUI;
```
It's consistent with other React projects:
- material-ui => MaterialUI
- react-dom => ReactDOM
- prop-types => PropTypes
| 3,664 |
0 | petrpan-code/mui/material-ui/docs/data/material/migration | petrpan-code/mui/material-ui/docs/data/material/migration/migration-v4/migrating-from-jss.md | # Migrating from JSS (optional)
<p class="description">This guide explains how to migrate from JSS to Emotion when updating from Material UI v4 to v5.</p>
## Material UI v5 migration
1. [Getting started](/material-ui/migration/migration-v4/)
2. [Breaking changes part one: style and theme](/material-ui/migration/v5-style-changes/)
3. [Breaking changes part two: components](/material-ui/migration/v5-component-changes/)
4. Migrating from JSS 👈 _you are here_
5. [Troubleshooting](/material-ui/migration/troubleshooting/)
## Migrating from JSS to Emotion
One of the biggest changes in v5 is the replacement of JSS for [Emotion](https://emotion.sh/docs/introduction) (or [styled-components](https://styled-components.com/) as an alternative) as a default styling solution .
Note that you may continue to use JSS for adding overrides for the components (e.g. `makeStyles`, `withStyles`) even after migrating to v5.
Then, if at any point you want to move over to the new styling engine, you can refactor your components progressively.
:::info
If you are using Next.js and you are not sure how to configure SSR to work with both Emotion & JSS, take a look a this [example project](https://github.com/mui/material-ui/tree/master/examples/material-ui-nextjs-ts-v4-v5-migration).
:::
This document reviews all the steps necessary to migrate away from JSS.
While you can use either of the following two options, the first is considered preferable:
### 1. Use styled or sx API
#### Codemod
We provide [a codemod](https://github.com/mui/material-ui/blob/master/packages/mui-codemod/README.md#jss-to-styled) to help migrate JSS styles to `styled` API, but this approach increases the CSS specificity.
:::info
Normally you wouldn't write styles like this.
But this is the best transformation that we could create with a codemod.
If you want to refine them later, you can refer to the examples shown in the sections below.
:::
```bash
npx @mui/codemod v5.0.0/jss-to-styled <path>
```
Example transformation:
```diff
import Typography from '@mui/material/Typography';
-import makeStyles from '@mui/styles/makeStyles';
+import { styled } from '@mui/material/styles';
-const useStyles = makeStyles((theme) => ({
- root: {
- display: 'flex',
- alignItems: 'center',
- backgroundColor: theme.palette.primary.main
- },
- cta: {
- borderRadius: theme.shape.radius
- },
- content: {
- color: theme.palette.common.white,
- fontSize: 16,
- lineHeight: 1.7
- },
-}))
+const PREFIX = 'MyCard';
+const classes = {
+ root: `${PREFIX}-root`,
+ cta: `${PREFIX}-cta`,
+ content: `${PREFIX}-content`,
+}
+const Root = styled('div')(({ theme }) => ({
+ [`&.${classes.root}`]: {
+ display: 'flex',
+ alignItems: 'center',
+ backgroundColor: theme.palette.primary.main
+ },
+ [`& .${classes.cta}`]: {
+ borderRadius: theme.shape.radius
+ },
+ [`& .${classes.content}`]: {
+ color: theme.palette.common.white,
+ fontSize: 16,
+ lineHeight: 1.7
+ },
+}))
export const MyCard = () => {
- const classes = useStyles();
return (
- <div className={classes.root}>
+ <Root className={classes.root}>
{/* The benefit of this approach is that the code inside Root stays the same. */}
<Typography className={classes.content}>...</Typography>
<Button className={classes.cta}>Go</Button>
- </div>
+ </Root>
)
}
```
:::success
You should run this codemod on a small chunk of files and then check the changes before continuing, because in some cases you might need to adjust the code after the transformation—this codemod won't cover all cases.
:::
#### Manual
We recommend `sx` API over `styled` for creating responsive styles or overriding minor CSS.
[Read more about `sx` here](/system/getting-started/the-sx-prop/).
```diff
import Chip from '@mui/material/Chip';
-import makeStyles from '@mui/styles/makeStyles';
+import Box from '@mui/material/Box';
-const useStyles = makeStyles((theme) => ({
- wrapper: {
- display: 'flex',
- },
- chip: {
- padding: theme.spacing(1, 1.5),
- boxShadow: theme.shadows[1],
- }
-}));
function App() {
- const classes = useStyles();
return (
- <div className={classes.wrapper}>
- <Chip className={classes.chip} label="Chip" />
- </div>
+ <Box sx={{ display: 'flex' }}>
+ <Chip label="Chip" sx={{ py: 1, px: 1.5, boxShadow: 1 }} />
+ </Box>
);
}
```
In some cases, you might want to create multiple styled components in a file instead of increasing CSS specificity.
For example:
```diff
-import makeStyles from '@mui/styles/makeStyles';
+import { styled } from '@mui/material/styles';
-const useStyles = makeStyles((theme) => ({
- root: {
- display: 'flex',
- alignItems: 'center',
- borderRadius: 20,
- background: theme.palette.grey[50],
- },
- label: {
- color: theme.palette.primary.main,
- }
-}))
+const Root = styled('div')(({ theme }) => ({
+ display: 'flex',
+ alignItems: 'center',
+ borderRadius: 20,
+ background: theme.palette.grey[50],
+}))
+const Label = styled('span')(({ theme }) => ({
+ color: theme.palette.primary.main,
+}))
function Status({ label }) {
- const classes = useStyles();
return (
- <div className={classes.root}>
- {icon}
- <span className={classes.label}>{label}</span>
- </div>
+ <Root>
+ {icon}
+ <Label>{label}</Label>
+ </Root>
)
}
```
:::success
[This jss-to-styled tool](https://siriwatk.dev/tool/jss-to-styled) helps convert JSS to multiple styled components without increasing CSS specificity.
This tool is _not_ maintained by MUI.
:::
### 2. Use [tss-react](https://github.com/garronej/tss-react)
:::error
This API will not work if you are [using `styled-components` as the underlying styling engine in place of `@emotion`](/material-ui/guides/interoperability/#styled-components).
:::
The API is similar to JSS `makeStyles`, but under the hood, it uses `@emotion/react`.
It also features much better TypeScript support than v4's `makeStyles`.
In order to use it, you'll need to add it to your project's dependencies:
With npm:
```bash
npm install tss-react
```
With yarn:
```bash
yarn add tss-react
```
#### Codemod
We provide [a codemod](https://github.com/mui/material-ui/blob/master/packages/mui-codemod/README.md#jss-to-tss-react) to help migrate JSS styles to the `tss-react` API.
```bash
npx @mui/codemod v5.0.0/jss-to-tss-react <path>
```
Example transformation:
```diff
import * as React from 'react';
-import makeStyles from '@material-ui/styles/makeStyles';
+import { makeStyles } from 'tss-react/mui';
import Button from '@mui/material/Button';
import Link from '@mui/material/Link';
-const useStyles = makeStyles((theme) => {
+const useStyles = makeStyles()((theme) => {
return {
root: {
color: theme.palette.primary.main,
},
apply: {
marginRight: theme.spacing(2),
},
};
});
function Apply() {
- const classes = useStyles();
+ const { classes } = useStyles();
return (
<div className={classes.root}>
<Button component={Link} to="https://support.mui.com" className={classes.apply}>
Apply now
</Button>
</div>
);
}
export default Apply;
```
If you were using the `$` syntax and `clsx` to combine multiple CSS classes,
the transformation would look like this:
```diff
import * as React from 'react';
-import { makeStyles } from '@material-ui/core/styles';
-import clsx from 'clsx';
+import { makeStyles } from 'tss-react/mui';
-const useStyles = makeStyles((theme) => ({
+const useStyles = makeStyles<void, 'child' | 'small'>()((theme, _params, classes) => ({
parent: {
padding: 30,
- '&:hover $child': {
+ [`&:hover .${classes.child}`]: {
backgroundColor: 'red',
},
},
small: {},
child: {
backgroundColor: 'blue',
height: 50,
- '&$small': {
+ [`&.${classes.small}`]: {
backgroundColor: 'lightblue',
height: 30
}
},
}));
function App() {
- const classes = useStyles();
+ const { classes, cx } = useStyles();
return (
<div className={classes.parent}>
<div className={classes.child}>
Background turns red when the mouse hovers over the parent.
</div>
- <div className={clsx(classes.child, classes.small)}>
+ <div className={cx(classes.child, classes.small)}>
Background turns red when the mouse hovers over the parent.
I am smaller than the other child.
</div>
</div>
);
}
export default App;
```
:::error
When using JavaScript (rather than TypeScript), remove `<void, 'child' | 'small'>`.
:::
The following is a comprehensive example using the `$` syntax, `useStyles()` parameters, merging in classes from a `classes` prop ([see doc](https://docs.tss-react.dev/your-own-classes-prop)) and [an explicit name for the stylesheet](https://docs.tss-react.dev/api/makestyles#naming-the-stylesheets-useful-for-debugging-and-theme-style-overrides).
```diff
-import clsx from 'clsx';
-import { makeStyles, createStyles } from '@material-ui/core/styles';
+import { makeStyles } from 'tss-react/mui';
-const useStyles = makeStyles((theme) => createStyles<
- 'root' | 'small' | 'child', {color: 'primary' | 'secondary', padding: number}
->
-({
- root: ({color, padding}) => ({
+const useStyles = makeStyles<{color: 'primary' | 'secondary', padding: number}, 'child' | 'small'>({name: 'App'})((theme, { color, padding }, classes) => ({
+ root: {
padding: padding,
- '&:hover $child': {
+ [`&:hover .${classes.child}`]: {
backgroundColor: theme.palette[color].main,
}
- }),
+ },
small: {},
child: {
border: '1px solid black',
height: 50,
- '&$small': {
+ [`&.${classes.small}`]: {
height: 30
}
}
-}), {name: 'App'});
+}));
function App({classes: classesProp}: {classes?: any}) {
- const classes = useStyles({color: 'primary', padding: 30, classes: classesProp});
+ const { classes, cx } = useStyles({
+ color: 'primary',
+ padding: 30
+ }, {
+ props: {
+ classes: classesProp
+ }
+ });
return (
<div className={classes.root}>
<div className={classes.child}>
The Background take the primary theme color when the mouse hovers the parent.
</div>
- <div className={clsx(classes.child, classes.small)}>
+ <div className={cx(classes.child, classes.small)}>
The Background take the primary theme color when the mouse hovers the parent.
I am smaller than the other child.
</div>
</div>
);
}
export default App;
```
After running the codemod, search your code for "TODO jss-to-tss-react codemod" to find cases that the codemod could not handle reliably.
There may be other cases beyond those with TODO comments that are not handled fully by the codemod—particularly if parts of the styles are returned by functions.
If the styles buried within a function use the `$` syntax or `useStyles` params, then those styles won't be migrated appropriately.
:::error
You should drop [`clsx`](https://www.npmjs.com/package/clsx) in favor of [`cx`](https://emotion.sh/docs/@emotion/css#cx).
The key advantage of `cx` is that it detects Emotion-generated class names to ensure that styles are overwritten in the correct order.
The default precedence of styles from multiple CSS classes is different between JSS and tss-react and some manual re-ordering of `cx` parameters
may be necessary—see [this issue comment](https://github.com/mui/material-ui/pull/31802#issuecomment-1093478971) for more details.
:::
To ensure that your class names always includes the actual name of your components, you can provide the `name` as an implicitly named key (`name: { App }`).
See [this tss-react doc](https://docs.tss-react.dev/api/makestyles#naming-the-stylesheets-useful-for-debugging-and-theme-style-overrides) for details.
You may end up with eslint warnings [like this one](https://user-images.githubusercontent.com/6702424/148657837-eae48942-fb86-4516-abe4-5dc10f44f0be.png) if you deconstruct more than one item.
Don't hesitate to disable `eslint(prefer-const)`, [like this](https://github.com/thieryw/gitlanding/blob/b2b0c71d95cfd353979c86dfcfa1646ef1665043/.eslintrc.js#L17) in a regular project, or [like this](https://github.com/InseeFrLab/onyxia/blob/a264ec6a6a7110cb1a17b2e22cc0605901db6793/package.json#L133) in a CRA.
#### withStyles()
`tss-react` also features a [type-safe implementation](https://docs.tss-react.dev/api/withstyles) of [v4's `withStyles()`](https://v4.mui.com/styles/api/#withstyles-styles-options-higher-order-component).
:::info
The equivalent of the `$` syntax is also supported in tss's `withStyles()`.
[See doc](https://docs.tss-react.dev/nested-selectors#withstyles).
:::
```diff
-import Button from '@material-ui/core/Button';
+import Button from '@mui/material/Button';
-import withStyles from '@material-ui/styles/withStyles';
+import { withStyles } from 'tss-react/mui';
const MyCustomButton = withStyles(
+ Button,
(theme) => ({
root: {
minHeight: '30px',
},
textPrimary: {
color: theme.palette.text.primary,
},
'@media (min-width: 960px)': {
textPrimary: {
fontWeight: 'bold',
},
},
}),
-)(Button);
+);
export default MyCustomButton;
```
#### Theme style overrides
[Global theme overrides](https://v4.mui.com/customization/components/#global-theme-override) are supported out of the box by TSS.
Follow the instructions in the relevant section of the [Breaking changes](/material-ui/migration/v5-style-changes/#restructure-component-definitions) doc, and [provide a `name` to `makeStyles`](https://docs.tss-react.dev/api/makestyles#naming-the-stylesheets-useful-for-debugging-and-theme-style-overrides).
In Material UI v5, [style overrides also accept callbacks](https://mui.com/material-ui/customization/theme-components/).
By default, TSS is only able to provide the theme.
If you want to provide the props and the `ownerState`, [please refer to this documentation](https://docs.tss-react.dev/mui-global-styleoverrides).
:::warning
tss-react is _not_ maintained by MUI.
If you have any question about how to setup SSR (Next.js), or if you are wondering
how to customize the `theme` object, please refer to the tss-react documentation—particularly the [MUI integration section](https://github.com/garronej/tss-react#mui-integration).
You can also [submit an issue](https://github.com/garronej/tss-react/issues/new) for any bug or feature request, and [start a discussion](https://github.com/garronej/tss-react/discussions) if you need help.
:::
## Complete the migration
Once you migrate all of the styling, remove unnecessary `@mui/styles` by uninstalling the package.
With npm:
```bash
npm uninstall @mui/styles
```
With yarn:
```bash
yarn remove @mui/styles
```
:::warning
`@emotion/styled` is a peer dependency of `@mui/material`.
You must keep it in your dependencies even if you never explicitly use it.
:::
| 3,665 |
0 | petrpan-code/mui/material-ui/docs/data/material/migration | petrpan-code/mui/material-ui/docs/data/material/migration/migration-v4/migration-v4.md | # Migrating to v5: getting started
<p class="description">This guide explains how and why to migrate from Material UI v4 to v5.</p>
## Material UI v5 migration
1. Getting started 👈 _you are here_
2. [Breaking changes part one: style and theme](/material-ui/migration/v5-style-changes/)
3. [Breaking changes part two: components](/material-ui/migration/v5-component-changes/)
4. [Migrating from JSS](/material-ui/migration/migrating-from-jss/)
5. [Troubleshooting](/material-ui/migration/troubleshooting/)
## Introduction
This is the first document in a multi-part series to walk you through upgrading your app from Material UI v4 to v5.
We highly recommend running our [codemods](#run-codemods) for efficiency—these will automatically address many of the [breaking changes](#address-breaking-changes) introduced in v5.
One of the biggest changes in v5 is the replacement of JSS for [Emotion](https://emotion.sh/docs/introduction) as a default styling solution.
Note that you may continue to use JSS for adding overrides to the components (e.g. `makeStyles`, `withStyles`) even after migrating to v5.
Once you've completed the rest of the v5 upgrade, we recommend progressively moving over to the new styling engine.
This process is covered in [Migrating from JSS](/material-ui/migration/migrating-from-jss/).
:::info
Need to refer back to an older version of the docs? Check out [the v4 documentation here](https://v4.mui.com/).
:::
:::info
If you are using Next.js and you are not sure how to configure SSR to work with both Emotion & JSS, take a look a this [example project](https://github.com/mui/material-ui/tree/master/examples/material-ui-nextjs-ts-v4-v5-migration).
:::
## Why you should migrate
Material UI v5 includes many bug fixes and improvements over v4.
Chief among these improvements is the new styling engine, which offers significant advancements in performance when it comes to dynamic styles, as well as a more enjoyable developer experience.
Additionally, v5 is the only version that fully supports React 18, so you will need to migrate to take advantage of the latest React features.
To learn more, check out [the blog post about the release of Material UI v5](https://mui.com/blog/mui-core-v5/).
:::success
Create small commits as you go to ensure a smooth migration.
If you encounter any issues along the way, check the [Troubleshooting](/material-ui/migration/troubleshooting/) doc.
For problems not addressed there, please [create an issue](https://github.com/mui/material-ui/issues/new?assignees=&labels=status%3A+needs+triage&template=1.bug.yml) with this title format: **[Migration] Summary of your issue**.
:::
## Supported browsers and Node versions
The targets of the default bundle have changed in v5.
The exact versions will be pinned on release from the browserslist query `"> 0.5%, last 2 versions, Firefox ESR, not dead, not IE 11, maintained node versions"`.
The default bundle supports the following minimum versions:
<!-- #stable-snapshot -->
- Node 12 (up from 8)
- Chrome 90 (up from 49)
- Edge 91 (up from 14)
- Firefox 78 (up from 52)
- Safari 14 (macOS) and 12.5 (iOS) (up from 10)
- and more (see [.browserslistrc (`stable` entry)](https://github.com/mui/material-ui/blob/v5.0.0/.browserslistrc#L11))
Material UI no longer supports IE 11.
If you need to support IE 11, check out our [legacy bundle](/material-ui/guides/minimizing-bundle-size/#legacy-bundle).
## Update React & TypeScript version
### Update React
The minimum supported version of React has been increased from v16.8.0 to v17.0.0.
If you are using a React version below 17.0.0, update your packages to at least v4.11.2 for Material UI and v17.0.0 for React.
<codeblock storageKey="package-manager">
```bash npm
npm install @material-ui/core@^4.11.2 react@^17.0.0
```
```bash yarn
yarn upgrade @material-ui/core@^4.11.2 react@^17.0.0
```
</codeblock>
### Update TypeScript
The minimum supported version of TypeScript has been increased from v3.2 to v3.5.
:::info
We try to align with types released by [DefinitelyTyped](https://github.com/DefinitelyTyped/DefinitelyTyped) (i.e. packages published on npm under the `@types` namespace).
We will not change the minimum supported version in a minor version of Material UI.
However, we generally recommend not to use a TypeScript version older than the lowest supported version of DefinitelyTyped.
:::
If your project includes these packages, you'll need to update them:
- `react-scripts`
- `@types/react`
- `@types/react-dom`
:::warning
Make sure that your application is still running without errors, and commit the changes before continuing to the next step.
:::
## Set up `ThemeProvider`
Before upgrading to v5, please make sure that `ThemeProvider` is defined at the root of your application and in tests—even if you are using the default theme—and `useStyles` is _not_ called before `ThemeProvider`.
Eventually you may want to [migrate from JSS to Emotion](/material-ui/migration/migrating-from-jss/), but in the meantime you can continue to use JSS with the `@mui/styles` package.
This package requires `ThemeProvider`.
The root of your application should look something like this:
```js
import { ThemeProvider, createMuiTheme, makeStyles } from '@material-ui/core/styles';
const theme = createMuiTheme();
const useStyles = makeStyles((theme) => {
root: {
// some CSS that accesses the theme
}
});
function App() {
const classes = useStyles(); // ❌ If you have this, consider moving it
// inside of a component wrapped with <ThemeProvider />
return <ThemeProvider theme={theme}>{children}</ThemeProvider>;
}
```
:::warning
Make sure that your application is still running without errors, and commit the changes before continuing to the next step.
:::
## Update MUI packages
### Material UI v5 and `@mui/styles`
Install the Material UI v5 packages.
<codeblock storageKey="package-manager">
```bash npm
npm install @mui/material @mui/styles
```
```bash yarn
yarn add @mui/material @mui/styles
```
</codeblock>
If you're using `@material-ui/lab` or `@material-ui/icons`, you will need to install the new packages.
### `@material-ui/lab`
<codeblock storageKey="package-manager">
```bash npm
npm install @mui/lab
```
```bash yarn
yarn add @mui/lab
```
</codeblock>
### `@material-ui/icons`
<codeblock storageKey="package-manager">
```bash npm
npm install @mui/icons-material
```
```bash yarn
yarn add @mui/icons-material
```
</codeblock>
### Date and time pickers
The date and time picker components have been moved to MUI X.
If you are using `@material-ui/date-pickers` or the pickers in the `@mui/lab` package, you will need to migrate to `@mui/x-date-pickers`.
See [Migration from the lab](https://mui.com/x/migration/migration-pickers-lab/) for details.
### Peer dependencies
Next, add the Emotion packages.
<codeblock storageKey="package-manager">
```bash npm
npm install @emotion/react @emotion/styled
```
```bash yarn
yarn add @emotion/react @emotion/styled
```
</codeblock>
#### styled-components (optional)
If you want to use Material UI v5 with styled-components instead of Emotion, check out [the Material UI installation guide](/material-ui/getting-started/installation/).
Note that if your app uses server-side rendering (SSR), there is a [known bug](https://github.com/mui/material-ui/issues/29742) with the Babel plugin for styled-components which prevents `@mui/styled-engine-sc` (the adapter for styled-components) from being used.
We strongly recommend using the default setup with Emotion instead.
:::warning
Make sure that your application is still running without errors, and commit the changes before continuing to the next step.
:::
### Replace all imports
With the release of v5, the names of all related packages were changed from `@material-ui/*` to `@mui/*` as part of our updated branding. See [this blog post](/blog/material-ui-is-now-mui/) for details.
<details>
<summary>Updated package names</summary>
```text
@material-ui/core -> @mui/material
@material-ui/unstyled -> @mui/base
@material-ui/icons -> @mui/icons-material
@material-ui/styles -> @mui/styles
@material-ui/system -> @mui/system
@material-ui/lab -> @mui/lab
@material-ui/types -> @mui/types
@material-ui/styled-engine -> @mui/styled-engine
@material-ui/styled-engine-sc ->@mui/styled-engine-sc
@material-ui/private-theming -> @mui/private-theming
@material-ui/codemod -> @mui/codemod
@material-ui/docs -> @mui/docs
@material-ui/envinfo -> @mui/envinfo
```
</details>
### Remove old packages
Once you've installed all the necessary packages and ensured that your app still runs, you can safely remove the old `@material-ui/*` packages by running `npm uninstall @material-ui/*` or `yarn remove @material-ui/*`.
:::success
The [preset-safe codemod](#preset-safe) (explained in more detail below) handles this automatically.
:::
## Fix CSS specificity (optional)
If you want to apply styles to components by importing a CSS file, you need to bump up the specificity to be able to target the correct components.
Consider the following example:
```js
import './style.css';
import Chip from '@mui/material/Chip';
const ChipWithGreenIcon = () => (
<Chip
classes={{ deleteIcon: 'green' }}
label="delete icon is green"
onDelete={() => {}}
/>
);
```
In this example, in order to correctly apply a particular style to the delete icon of `Chip`, one option is to increase the specificity of your CSS classes, as shown below:
```css
.MuiChip-root .green {
color: green;
}
```
By contrast, the following CSS snippet will not apply the style to the delete icon:
```css
.green {
color: green;
}
```
## Run codemods
The following codemods will automatically adjust the bulk of your code to account for breaking changes in v5.
Make sure that your application still runs without errors after running each codemod, and commit the changes before continuing to the next step.
### preset-safe
This codemod contains most of the transformers that are necessary for migration. It should be only applied **once per folder.**
```bash
npx @mui/codemod v5.0.0/preset-safe <path>
```
:::info
If you want to run the transformers one by one, check out the [preset-safe codemod](https://github.com/mui/material-ui/blob/master/packages/mui-codemod/README.md#-preset-safe) for more details.
:::
### variant-prop
This codemod transforms the `<TextField/>`, `<FormControl/>`, and `<Select/>` components by applying `variant="standard"` if no variant is defined—the default variant has changed from `"standard"` in v4 to `"outlined"` in v5.
:::error
You should _not_ use this codemod if you have already defined `variant: "outlined"` as the default in the theme.
:::
```js
// ❌ if you have a theme setup like this, don't run this codemod.
// these default props can be removed later because `outlined` is the default value in v5
createMuiTheme({
components: {
MuiTextField: {
defaultProps: {
variant: 'outlined',
},
},
},
});
```
If you want to keep `variant="standard"` in your components, run this codemod or else configure the corresponding default theme props.
```bash
npx @mui/codemod v5.0.0/variant-prop <path>
```
For more details, check out the [variant-prop codemod README](https://github.com/mui/material-ui/blob/master/packages/mui-codemod/README.md#variant-prop).
### link-underline-hover
This codemod transforms the `<Link />` component by applying `underline="hover"` if there is no `underline` prop defined—the default `underline` has changed from `"hover"` in v4 to `"always"` in v5.
:::error
You should _not_ use this codemod if you have already defined `underline: "always"` as the default in the theme.
:::
```js
// if you have theme setup like this, ❌ don't run this codemod.
// this default props can be removed later because `always` is the default value in v5
createMuiTheme({
components: {
MuiLink: {
defaultProps: {
underline: 'always',
},
},
},
});
```
If you want to keep `underline="hover"`, run this codemod or else configure the corresponding default theme props.
```bash
npx @mui/codemod v5.0.0/link-underline-hover <path>
```
For more details, check out the [link-underline-hover codemod README](https://github.com/mui/material-ui/blob/master/packages/mui-codemod/README.md#link-underline-hover).
## Address breaking changes
The codemods handle many of the breaking changes, but others must be addressed manually.
Whether or not you choose to use the codemods, you are now ready to move on to the first of two [breaking changes](/material-ui/migration/v5-style-changes/) documents.
| 3,666 |
0 | petrpan-code/mui/material-ui/docs/data/material/migration | petrpan-code/mui/material-ui/docs/data/material/migration/migration-v4/troubleshooting.md | # Troubleshooting
<p class="description">This document covers known issues and common problems encountered when migrating from Material UI v4 to v5.</p>
## Material UI v5 migration
1. [Getting started](/material-ui/migration/migration-v4/)
2. [Breaking changes part one: style and theme](/material-ui/migration/v5-style-changes/)
3. [Breaking changes part two: components](/material-ui/migration/v5-component-changes/)
4. [Migrating from JSS](/material-ui/migration/migrating-from-jss/)
5. Troubleshooting 👈 _you are here_
## Styles broken after migrating to v5
There are two reasons why component styles may be broken after you've completed all steps in the migration process.
First, check if you have configured the `StyledEngineProvider` correctly, as shown in the [Style library](/material-ui/migration/v5-style-changes/#style-library) section.
If the `StyledEngineProvider` is already used at the top of your application and the styles are still broken, it may be the case that you still have `@material-ui/core` in your application.
This could be caused by other dependencies in the app that still rely on Material UI v4.
To check this, run `npm ls @material-ui/core` (or `yarn why @material-ui/core`).
If your project contains such dependencies, you will see a list that looks something like this:
```bash
$ npm ls @material-ui/core
[email protected] /path/to/project
└─┬ @mui/[email protected]
└── @material-ui/[email protected]
```
The output above indicates that `@material-ui/core` is a dependency of `@mui/x-data-grid`.
In this specific example, you would need to bump the version of `@mui/x-data-grid` to [v5](https://www.npmjs.com/package/@mui/x-data-grid) so that it depends on `@mui/material` instead.
## Storybook and Emotion
If your project uses Storybook v6.x, you will need to update the `.storybook/main.js` webpack config to use the most recent version of Emotion:
```js
// .storybook/main.js
const path = require('path');
const toPath = (filePath) => path.join(process.cwd(), filePath);
module.exports = {
webpackFinal: async (config) => {
return {
...config,
resolve: {
...config.resolve,
alias: {
...config.resolve.alias,
'@emotion/core': toPath('node_modules/@emotion/react'),
'emotion-theming': toPath('node_modules/@emotion/react'),
},
},
};
},
};
```
Next, update `.storybook/preview.js` to prevent Storybook's Docs tab from displaying an empty page:
```js
// .storybook/preview.js
import { ThemeProvider, createTheme } from '@mui/material/styles';
import { ThemeProvider as Emotion10ThemeProvider } from 'emotion-theming';
const defaultTheme = createTheme(); // or your custom theme
const withThemeProvider = (Story, context) => {
return (
<Emotion10ThemeProvider theme={defaultTheme}>
<ThemeProvider theme={defaultTheme}>
<Story {...context} />
</ThemeProvider>
</Emotion10ThemeProvider>
);
};
export const decorators = [withThemeProvider];
// ...other storybook exports
```
:::info
This solution has been tested on the following versions:
```json
{
"@storybook/react": "6.3.8",
"@storybook/addon-docs": "6.3.8",
"@emotion/react": "11.4.1",
"@emotion/styled": "11.3.0",
"@mui/material": "5.0.2"
}
```
Note that this is a workaround that may not be suitable for your situation if you are using different versions.
For more details, checkout these GitHub issues:
- https://github.com/storybookjs/storybook/issues/16099
- https://github.com/mui/material-ui/issues/24282#issuecomment-796755133
:::
## Cannot read property scrollTop of null
This error comes from `Fade`, `Grow`, `Slide`, `Zoom` components due to a missing DOM node.
Make sure that the children forward the `ref` to the DOM for custom components:
```jsx
// Ex. 1-1 ❌ This will cause an error because the Fragment is not a DOM node:
<Fade in>
<React.Fragment>
<CustomComponent />
</React.Fragment>
</Fade>
```
```jsx
// Ex. 1-2 ✅ Add a DOM node such as this div:
<Fade in>
<div>
<CustomComponent />
</div>
</Fade>
```
```jsx
// Ex. 2-1 ❌ This will cause an error because `CustomComponent` does not forward `ref` to the DOM:
function CustomComponent() {
return <div>...</div>;
}
<Fade in>
<CustomComponent />
</Fade>;
```
```jsx
// Ex. 2-2 ✅ Add `React.forwardRef` to forward `ref` to the DOM:
const CustomComponent = React.forwardRef(function CustomComponent(props, ref) {
return (
<div ref={ref}>
...
</div>
)
})
<Fade in>
<CustomComponent />
</Fade>
```
For more details, checkout [this issue](https://github.com/mui/material-ui/issues/27154) on GitHub.
## [Types] Property "palette", "spacing" does not exist on type 'DefaultTheme'
This error arises because `makeStyles` is now exported from the `@mui/styles` package, which does not know about `Theme` in the core package.
To fix this, you need to augment the `DefaultTheme` (empty object) in `@mui/styles` with `Theme` from the core.
Read more about module augmentation in [the official TypeScript docs](https://www.typescriptlang.org/docs/handbook/declaration-merging.html#module-augmentation).
### TypeScript
Add this snippet to your theme file:
```ts
// it could be your App.tsx file or theme file that is included in your tsconfig.json
import { Theme } from '@mui/material/styles';
declare module '@mui/styles/defaultTheme' {
// eslint-disable-next-line @typescript-eslint/no-empty-interface (remove this line if you don't have the rule enabled)
interface DefaultTheme extends Theme {}
}
```
### JavaScript
If you are using an IDE like VSCode which is able to infer types from a `d.ts` file, create `index.d.ts` in your `src` folder and add the following lines of code:
```js
// index.d.ts
declare module '@mui/private-theming' {
import type { Theme } from '@mui/material/styles';
interface DefaultTheme extends Theme {}
}
```
## [Jest] SyntaxError: Unexpected token 'export'
`@mui/material/colors/red` is considered private since v1.0.0.
To fix this error, you must replace the import.
For more details, see [this GitHub issue](https://github.com/mui/material-ui/issues/27296).
We recommend using this codemod to fix all imports in your project:
```bash
npx @mui/codemod v5.0.0/optimal-imports <path>
```
You can fix it manually like this:
```diff
-import red from '@mui/material/colors/red';
+import { red } from '@mui/material/colors';
```
## makeStyles - TypeError: Cannot read property 'drawer' of undefined
This error occurs when calling `useStyles` or `withStyles` outside of the scope of `<ThemeProvider>`, as in the following example:
```js
import * as React from 'react';
import { ThemeProvider, createTheme } from '@mui/material/styles';
import makeStyles from '@mui/styles/makeStyles';
import Card from '@mui/material/Card';
import CssBaseline from '@mui/material/CssBaseline';
const useStyles = makeStyles((theme) => ({
root: {
display: 'flex',
backgroundColor: theme.palette.primary.main,
color: theme.palette.common.white,
},
}));
const theme = createTheme();
function App() {
const classes = useStyles(); // ❌ called outside of ThemeProvider
return (
<ThemeProvider theme={theme}>
<CssBaseline />
<Card className={classes.root}>...</Card>
</ThemeProvider>
);
}
export default App;
```
You can fix this by moving `useStyles` inside another component so that it is called under `<ThemeProvider>`:
```js
// ...imports
function AppContent(props) {
const classes = useStyles(); // ✅ This is safe because it is called inside ThemeProvider
return <Card className={classes.root}>...</Card>;
}
function App(props) {
return (
<ThemeProvider theme={theme}>
<CssBaseline />
<AppContent {...props} />
</ThemeProvider>
);
}
export default App;
```
## TypeError: Cannot read properties of undefined (reading 'pxToRem')
This error results from trying to access an empty theme.
Make sure that you have addressed the following issues:
1. `styled` should only be imported from `@mui/material/styles` (if you are not using the standalone `@mui/system`):
```js
import { styled } from '@mui/material/styles';
```
2. `useStyles` cannot be called outside of `<ThemeProvider>`.
To fix this problem, follow [the instructions in this section](#makestyles-typeerror-cannot-read-property-drawer-of-undefined).
For more details, see [this GitHub issue](https://github.com/mui/material-ui/issues/28496).
## Still having problems?
If you're encountering a problem not covered here, please [create a GitHub issue](https://github.com/mui/material-ui/issues/new?assignees=&labels=status%3A+needs+triage&template=1.bug.yml) with this title format: **[Migration] Summary of your issue**.
| 3,667 |
0 | petrpan-code/mui/material-ui/docs/data/material/migration | petrpan-code/mui/material-ui/docs/data/material/migration/migration-v4/v5-component-changes.md | # Breaking changes in v5, part two: core components
<p class="description">This is a reference guide to the breaking changes introduced in Material UI v5, and how to migrating from v4. This part covers changes to components.</p>
## Material UI v5 migration
1. [Getting started](/material-ui/migration/migration-v4/)
2. [Breaking changes part one: style and theme](/material-ui/migration/v5-style-changes/)
3. Breaking changes part two: components 👈 _you are here_
4. [Migrating from JSS](/material-ui/migration/migrating-from-jss/)
5. [Troubleshooting](/material-ui/migration/troubleshooting/)
## Breaking changes, part two
Material UI v5 introduces a number of breaking changes from v4.
Many of these changes can be resolved automatically using [the codemods](/material-ui/migration/migration-v4/#run-codemods) described in the [main migration guide](/material-ui/migration/migration-v4/).
The following document lists all breaking changes related to components in v5 and how to address them.
If you haven't already, please be sure to review [Breaking changes in v5 part one: styles and themes](/material-ui/migration/v5-style-changes/) to continue the migration process.
:::warning
Breaking changes that are handled by the codemods are denoted by a ✅ emoji in the table of contents on the right side of the screen.
If you have already followed the instructions in the main migration guide and run the codemods, then you should not need to take any further action on these items.
All other changes must be handled manually.
:::
As the core components use Emotion as their style engine, the props used by Emotion are not intercepted. The prop `as` in the following code snippet will not be propagated to `SomeOtherComponent`.
```jsx
<MuiComponent component={SomeOtherComponent} as="button" />
```
## AppBar
### Fix z-index issues
Remove z-index when position static and relative. This avoids the creation of a stacking context and rendering issues.
### Replace color prop for dark mode
The `color` prop has no longer any effect in dark mode. The app bar uses the background color required by the elevation to follow the [Material Design guidelines](https://m2.material.io/design/color/dark-theme.html). Use `enableColorOnDark` to restore the behavior of v4.
```jsx
<AppBar enableColorOnDark />
```
## Alert
### ✅ Update import
Move the component from the lab to the core. The component is now stable.
```diff
-import Alert from '@mui/lab/Alert';
-import AlertTitle from '@mui/lab/AlertTitle';
+import Alert from '@mui/material/Alert';
+import AlertTitle from '@mui/material/AlertTitle';
```
## Autocomplete
### ✅ Update import
Move the component from the lab to the core. The component is now stable.
```diff
-import Autocomplete from '@mui/lab/Autocomplete';
-import useAutocomplete from '@mui/lab/useAutocomplete';
+import Autocomplete from '@mui/material/Autocomplete';
+import useAutocomplete from '@mui/material/useAutocomplete';
```
### Remove debug prop
Remove `debug` prop. There are a couple of simpler alternatives: `open={true}`, Chrome devtools ["Emulate focused"](https://twitter.com/sulco/status/1305841873945272321), or React devtools prop setter.
### Update `renderOption`
`renderOption` should now return the full DOM structure of the option.
It makes customizations easier. You can recover from the change with:
```diff
<Autocomplete
- renderOption={(option, { selected }) => (
- <React.Fragment>
+ renderOption={(props, option, { selected }) => (
+ <li {...props}>
<Checkbox
icon={icon}
checkedIcon={checkedIcon}
style={{ marginRight: 8 }}
checked={selected}
/>
{option.title}
- </React.Fragment>
+ </li>
)}
/>
```
### ✅ Rename `closeIcon` to `clearIcon`
Rename `closeIcon` prop to `clearIcon` to avoid confusion.
```diff
-<Autocomplete closeIcon={defaultClearIcon} />
+<Autocomplete clearIcon={defaultClearIcon} />
```
### Rename reason arguments
The following values of the reason argument in `onChange` and `onClose` were renamed for consistency:
1. `create-option` to `createOption`
2. `select-option` to `selectOption`
3. `remove-option` to `removeOption`
Change the CSS rules that use `[data-focus="true"]` to use `.Mui-focused`. The `data-focus` attribute is not set on the focused option anymore; instead, global class names are used.
```diff
-'.MuiAutocomplete-option[data-focus="true"]': {
+'.MuiAutocomplete-option.Mui-focused': {
```
### ✅ Rename getOptionSelected
Rename `getOptionSelected` to `isOptionEqualToValue` to better describe its purpose.
```diff
<Autocomplete
- getOptionSelected={(option, value) => option.title === value.title}
+ isOptionEqualToValue={(option, value) => option.title === value.title}
```
## Avatar
### ✅ Rename circle
Rename `circle` to `circular` for consistency:
```diff
-<Avatar variant="circle">
-<Avatar classes={{ circle: 'className' }}>
+<Avatar variant="circular">
+<Avatar classes={{ circular: 'className' }}>
```
Since `circular` is the default value, the variant prop can be deleted:
```diff
-<Avatar variant="circle">
+<Avatar>
```
### ✅ Update AvatarGroup import
Move the AvatarGroup from the lab to the core.
```diff
-import AvatarGroup from '@mui/lab/AvatarGroup';
+import AvatarGroup from '@mui/material/AvatarGroup';
```
## Badge
### ✅ Rename circle and rectangle
Rename `circle` to `circular` and `rectangle` to `rectangular` for consistency.
```diff
-<Badge overlap="circle">
-<Badge overlap="rectangle">
+<Badge overlap="circular">
+<Badge overlap="rectangular">
```
```diff
<Badge classes={{
- anchorOriginTopRightRectangle: 'className',
- anchorOriginBottomRightRectangle: 'className',
- anchorOriginTopLeftRectangle: 'className',
- anchorOriginBottomLeftRectangle: 'className',
- anchorOriginTopRightCircle: 'className',
- anchorOriginBottomRightCircle: 'className',
- anchorOriginTopLeftCircle: 'className',
+ anchorOriginTopRightRectangular: 'className',
+ anchorOriginBottomRightRectangular: 'className',
+ anchorOriginTopLeftRectangular: 'className',
+ anchorOriginBottomLeftRectangular: 'className',
+ anchorOriginTopRightCircular: 'className',
+ anchorOriginBottomRightCircular: 'className',
+ anchorOriginTopLeftCircular: 'className',
}}>
```
## BottomNavigation
### Update event type (TypeScript)
The `event` in `onChange` is now typed as a `React.SyntheticEvent` instead of a `React.ChangeEvent`.
```diff
-<BottomNavigation onChange={(event: React.ChangeEvent<{}>) => {}} />
+<BottomNavigation onChange={(event: React.SyntheticEvent) => {}} />
```
## BottomNavigationAction
### Remove span and wrapper
Remove the `span` element that wraps the children.
Remove the `wrapper` classKey too.
You can find out more details about this change in [this GitHub pull request](https://github.com/mui/material-ui/pull/26923).
```diff
<button class="MuiBottomNavigationAction-root">
- <span class="MuiBottomNavigationAction-wrapper">
{icon}
<span class="MuiBottomNavigationAction-label">
{label}
</span>
- </span>
</button>
```
## Box
### ✅ Update borderRadius prop value
The `borderRadius` system prop value transformation has been changed.
If it receives a number, it multiplies this value with the `theme.shape.borderRadius` value.
Use a string to provide an explicit px value.
```diff
-<Box borderRadius="borderRadius">
+<Box borderRadius={1}>
```
```diff
-<Box borderRadius={16}>
+<Box borderRadius="16px">
```
### ✅ Apply sx API
The Box system props have an optional alternative API in v5, using the `sx` prop.
Check out the System docs to learn more about [the tradeoffs of this API](/system/getting-started/usage/#api-tradeoff).
```jsx
<Box border="1px dashed grey" p={[2, 3, 4]} m={2}>
<Box sx={{ border: "1px dashed grey", p: [2, 3, 4], m: 2 }}>
```
### ✅ Rename CSS properties
The following properties have been renamed because they are considered deprecated CSS properties by the CSS specification:
1. `gridGap` to `gap`
2. `gridColumnGap` to `columnGap`
3. `gridRowGap` to `rowGap`
```diff
-<Box gridGap={1}>
-<Box gridColumnGap={2}>
-<Box gridRowGap={3}>
+<Box gap={1}>
+<Box columnGap={2}>
+<Box rowGap={3}>
```
:::info
The system grid function was not documented in v4.
:::
### Remove clone prop
The `clone` prop was removed because its behavior can be obtained by applying the `sx` prop directly to the child if it is a Material UI component.
```diff
-<Box sx={{ border: '1px dashed grey' }} clone>
- <Button>Save</Button>
-</Box>
+<Button sx={{ border: '1px dashed grey' }}>Save</Button>
```
### Replace render prop with `sx`
The ability to pass a render prop was removed because its behavior can be obtained by applying the `sx` prop directly to the child if it is a Material UI component.
```diff
-<Box sx={{ border: '1px dashed grey' }}>
- {(props) => <Button {...props}>Save</Button>}
-</Box>
+<Button sx={{ border: '1px dashed grey' }}>Save</Button>
```
For non-Material UI components, use the `component` prop.
```diff
-<Box sx={{ border: '1px dashed grey' }}>
- {(props) => <button {...props}>Save</button>}
-</Box>
+<Box component="button" sx={{ border: '1px dashed grey' }}>Save</Box>
```
## Button
### ✅ Remove default color prop
The button `color` prop is now "primary" by default, and "default" has been removed.
This makes the button closer to the Material Design guidelines and simplifies the API.
```diff
-<Button color="default">
+<Button>
```
:::info
If you prefer to use the `default` color in v4, take a look at this [CodeSandbox demo](https://codesandbox.io/s/mimic-v4-button-default-color-bklx8?file=/src/Demo.tsx) to see how to make it work in v5.
:::
### Remove span and label
The `span` element that wraps children has been removed.
The `label` classKey is also removed.
You can find out more details about this change in [this GitHub pull request](https://github.com/mui/material-ui/pull/26666), it used to be necessary for iOS.
```diff
<button class="MuiButton-root">
- <span class="MuiButton-label">
children
- </span>
</button>
```
## Chip
### ✅ Rename default to filled
Rename `default` variant to `filled` for consistency.
Since `filled` is the default value, the variant prop can be deleted:
```diff
-<Chip variant="default">
+<Chip>
```
## Checkbox
### Set to "primary" by default
The checkbox color prop is now "primary" by default.
To continue using the "secondary" color, you must explicitly indicate `secondary`.
This brings the checkbox closer to the Material Design guidelines.
```diff
-<Checkbox />
+<Checkbox color="secondary" />
```
### Update CSS class names
The component doesn't have `.MuiIconButton-root` and `.MuiIconButton-label` class names anymore.
Target `.MuiButtonBase-root` instead.
```diff
-<span class="MuiIconButton-root MuiButtonBase-root MuiCheckbox-root PrivateSwitchBase-root">
- <span class="MuiIconButton-label">
- <input class="PrivateSwitchBase-input">
+<span class="MuiButtonBase-root MuiCheckbox-root PrivateSwitchBase-root">
+ <span class="PrivateSwitchBase-input">
```
## CircularProgress
### ✅ Rename static to determinate
The `static` variant has been renamed to `determinate`, and the previous appearance of `determinate` has been replaced by that of `static`.
This was an exception to Material Design, and was removed from the specification.
```diff
-<CircularProgress variant="static" classes={{ static: 'className' }} />
+<CircularProgress variant="determinate" classes={{ determinate: 'className' }} />
```
:::error
If you had previously customized `determinate`, then your customizations are most likely no longer valid.
Please remove them.
:::
## Collapse
### ✅ Rename collapsedHeight prop
The `collapsedHeight` prop was renamed `collapsedSize` to support the horizontal direction.
```diff
-<Collapse collapsedHeight={40}>
+<Collapse collapsedSize={40}>
```
The `classes.container` key was changed to match the convention of the other components.
```diff
-<Collapse classes={{ container: 'collapse' }}>
+<Collapse classes={{ root: 'collapse' }}>
```
## CssBaseline
### Update styled-engine
The component was migrated to use the `@mui/styled-engine` (`emotion` or `styled-components`) instead of `jss`.
You should remove the `@global` key when defining the style overrides for it.
You could also start using the CSS template syntax over the JavaScript object syntax.
```diff
const theme = createTheme({
components: {
MuiCssBaseline: {
- styleOverrides: {
- '@global': {
- html: {
- WebkitFontSmoothing: 'auto',
- },
- },
- },
+ styleOverrides: `
+ html {
+ -webkit-font-smoothing: auto;
+ }
+ `
},
},
});
```
### Update body font size
The `body` font size has changed from `theme.typography.body2` (`0.875rem`) to `theme.typography.body1` (`1rem`).
To return to the previous size, you can override it in the theme:
```js
const theme = createMuiTheme({
components: {
MuiCssBaseline: {
styleOverrides: {
body: {
fontSize: '0.875rem',
lineHeight: 1.43,
letterSpacing: '0.01071em',
},
},
},
},
});
```
## Dialog
### ✅ Update transition props
The `on*` transition props were removed.
Use `TransitionProps` instead.
```diff
<Dialog
- onEnter={onEnter}
- onEntered={onEntered}
- onEntering={onEntering}
- onExit={onExit}
- onExited={onExited}
- onExiting={onExiting}
+ TransitionProps={{
+ onEnter,
+ onEntered,
+ onEntering,
+ onExit,
+ onExited,
+ onExiting,
+ }}
>
```
### ✅ Remove disableBackdropClick prop
Remove the `disableBackdropClick` prop because it is redundant.
Ignore close events from `onClose` when `reason === 'backdropClick'` instead.
```diff
<Dialog
- disableBackdropClick
- onClose={handleClose}
+ onClose={(event, reason) => {
+ if (reason !== 'backdropClick') {
+ handleClose(event, reason);
+ }
+ }}
/>
```
### Remove withMobileDialog component
Remove the `withMobileDialog` higher-order component.
:::warning
This is handled in the [preset-safe codemod](#preset-safe) by applying hard-coded function to prevent application crash, but further fixes are required.
:::
The hook API allows a simpler and more flexible solution:
```diff
-import withMobileDialog from '@mui/material/withMobileDialog';
+import { useTheme, useMediaQuery } from '@mui/material';
function ResponsiveDialog(props) {
- const { fullScreen } = props;
+ const theme = useTheme();
+ const fullScreen = useMediaQuery(theme.breakpoints.down('sm'));
const [open, setOpen] = React.useState(false);
// ...
-export default withMobileDialog()(ResponsiveDialog);
+export default ResponsiveDialog;
```
### ✅ Remove disableTypography prop
Flatten DialogTitle DOM structure and remove the `disableTypography` prop.
```diff
-<DialogTitle disableTypography>
- <Typography variant="h4" component="h2">
+<DialogTitle>
+ <Typography variant="h4" component="span">
My header
</Typography>
```
## Divider
### Replace background-color with border-color
Use `border-color` instead of `background-color`.
This prevents inconsistent height on scaled screens.
If you have customized the color of the border, you will need to update the CSS property override:
```diff
.MuiDivider-root {
- background-color: #f00;
+ border-color: #f00;
}
```
### Support "middle" variant with "vertical" orientation
In v4, using `orientation="vertical"` and `variant="middle"` was adding a left and right margins of `16px` in the component.
In the v5, to avoid fixed spacing on the component, this margin was removed.
:::info
If you want to use the previous margin values, this change can be made in your theme with the following code. See the example on [CodeSandbox demo](https://codesandbox.io/s/v5-migration-vertical-alignment-middle-divider-45vepj?file=/src/index.tsx).
:::
```diff
const theme = createTheme({
components: {
MuiDivider: {
+ styleOverrides: {
+ root: ({ ownerState, theme }) => ({
+ ...(ownerState.orientation === 'vertical' && ownerState.variant === 'middle' && {
+ marginLeft: theme.spacing(2),
+ marginRight: theme.spacing(2),
+ }),
+ })
+ }
},
},
});
```
## ExpansionPanel
### ✅ Rename components
Rename the `ExpansionPanel` components to `Accordion` to use a more common naming convention:
```diff
-import ExpansionPanel from '@mui/material/ExpansionPanel';
-import ExpansionPanelSummary from '@mui/material/ExpansionPanelSummary';
-import ExpansionPanelDetails from '@mui/material/ExpansionPanelDetails';
-import ExpansionPanelActions from '@mui/material/ExpansionPanelActions';
+import Accordion from '@mui/material/Accordion';
+import AccordionSummary from '@mui/material/AccordionSummary';
+import AccordionDetails from '@mui/material/AccordionDetails';
+import AccordionActions from '@mui/material/AccordionActions';
-<ExpansionPanel>
+<Accordion>
- <ExpansionPanelSummary>
+ <AccordionSummary>
<Typography>Location</Typography>
<Typography>Select trip destination</Typography>
- </ExpansionPanelSummary>
+ </AccordionSummary>
- <ExpansionPanelDetails>
+ <AccordionDetails>
<Chip label="Barbados" onDelete={() => {}} />
<Typography variant="caption">Select your destination of choice</Typography>
- </ExpansionPanelDetails>
+ </AccordionDetails>
<Divider />
- <ExpansionPanelActions>
+ <AccordionActions>
<Button size="small">Cancel</Button>
<Button size="small">Save</Button>
- </ExpansionPanelActions>
+ </AccordionActions>
-</ExpansionPanel>
+</Accordion>
```
### Update event type (TypeScript)
The `event` in `onChange` is now typed as a `React.SyntheticEvent` instead of a `React.ChangeEvent`.
```diff
-<Accordion onChange={(event: React.ChangeEvent<{}>, expanded: boolean) => {}} />
+<Accordion onChange={(event: React.SyntheticEvent, expanded: boolean) => {}} />
```
## ExpansionPanelDetails
### Remove display: flex
Remove `display: flex` from `AccordionDetails` (formerly `ExpansionPanelDetails`) as it was too opinionated—most developers expect `display: block`.
## ExpansionPanelSummary
### Rename focused to focusVisible
Rename `focused` to `focusVisible` for consistency:
```diff
<AccordionSummary
classes={{
- focused: 'custom-focus-visible-classname',
+ focusVisible: 'custom-focus-visible-classname',
}}
/>
```
### Remove IconButtonProps prop
Remove `IconButtonProps` prop from `AccordionSummary` (formerly `ExpansionPanelSummary`).
The component renders a `<div>` element instead of an `IconButton`, so the prop is no longer necessary.
## Fab
### ✅ Rename round to circular
```diff
-<Fab variant="round">
+<Fab variant="circular">
```
### Remove span and label
The `span` element that wraps children has been removed.
The `label` classKey is also removed.
You can find out more details about this change in [this GitHub pull request](https://github.com/mui/material-ui/pull/27112), it used to be necessary for iOS.
```diff
<button class="MuiFab-root">
- <span class="MuiFab-label">
{children}
- </span>
</button>
```
## FormControl
### ✅ Update default variant
Change the default variant from `standard` to `outlined`.
`standard` has been removed from the Material Design guidelines.
:::success
This is handled in [variant-prop codemod](#variant-prop)—read the details before running this codemod.
:::
```diff
-<FormControl value="Standard" />
-<FormControl value="Outlined" variant="outlined" />
+<FormControl value="Standard" variant="standard" />
+<FormControl value="Outlined" />
```
## FormControlLabel
### Add required label prop
The `label` prop is now required.
If you were using a `FormControlLabel` without a `label`, you can replace it with just the value of the `control` prop.
```diff
-<FormControlLabel control={<Checkbox />} />
+<Checkbox />
```
## Grid
### ✅ Rename justify prop
Rename the `justify` prop to `justifyContent` to align with the CSS property name.
```diff
-<Grid justify="center">
+<Grid justifyContent="center">
```
### ✅ Remove align and justify props and classes
The props `alignItems`, `alignContent`, and `justifyContent`—along with their classes and style overrides keys—have been removed:
"align-items-xs-center", "align-items-xs-flex-start", "align-items-xs-flex-end", "align-items-xs-baseline", "align-content-xs-center", "align-content-xs-flex-start", "align-content-xs-flex-end", "align-content-xs-space-between", "align-content-xs-space-around", "justify-content-xs-center", "justify-content-xs-flex-end", "justify-content-xs-space-between", "justify-content-xs-space-around" and "justify-content-xs-space-evenly".
These props are now considered part of the System, not the `Grid` component itself.
If you still wish to add overrides for them, you can use the [callback as a value in `styleOverrides`](/material-ui/customization/theme-components/#overrides-based-on-props).
```diff
const theme = createTheme({
components: {
MuiGrid: {
- styleOverrides: {
- 'align-items-xs-flex-end': {
- marginTop: 20,
- },
- },
+ styleOverrides: ({ ownerState }) => ({
+ ...ownerState.alignItems === 'flex-end' && {
+ marginTop: 20,
+ },
+ }),
},
},
});
```
### Change negative margins
The negative margins apply only to the top and left sides of the grid container.
If you need negative margins on all sides, we recommend using the new Grid v2 instead:
```diff
- import Grid from '@mui/material/Grid';
+ import Grid from '@mui/material/Unstable_Grid2';
```
To learn more about the Grid v2, check out the [demos](/material-ui/react-grid2/#whats-changed) and the [Grid migration guide](/material-ui/migration/migration-grid-v2/).
:::info
Grid v2 was introduced in Material UI v5.9.1 and features negative margins on all sides by default.
:::
## GridList
### ✅ Rename GridList component
Rename the `GridList` components to `ImageList` to align with the current Material Design naming.
### Rename GridList props
- Rename the GridList `spacing` prop to `gap` to align with the CSS attribute.
- Rename the GridList `cellHeight` prop to `rowHeight`.
- Add the `variant` prop to GridList.
- Rename the GridListItemBar `actionPosition` prop to `position`. (Note also the related classname changes.)
### Use CSS object-fit
Use CSS `object-fit`. For IE11 support either use a polyfill such as
[this npm package](https://www.npmjs.com/package/object-fit-images), or else continue to use the v4 component.
```diff
-import GridList from '@mui/material/GridList';
-import GridListTile from '@mui/material/GridListTile';
-import GridListTileBar from '@mui/material/GridListTileBar';
+import ImageList from '@mui/material/ImageList';
+import ImageListItem from '@mui/material/ImageListItem';
+import ImageListItemBar from '@mui/material/ImageListItemBar';
-<GridList spacing={8} cellHeight={200}>
- <GridListTile>
+<ImageList gap={8} rowHeight={200}>
+ <ImageListItem>
<img src="file.jpg" alt="Image title" />
- <GridListTileBar
+ <ImageListItemBar
title="Title"
subtitle="Subtitle"
/>
- </GridListTile>
-</GridList>
+ </ImageListItem>
+</ImageList>
```
## Hidden
### Replace deprecated component
This component is deprecated because its functionality can be created with the [`sx`](/system/getting-started/the-sx-prop/) prop or the [`useMediaQuery`](/material-ui/react-use-media-query/) hook.
:::success
This is handled in the [preset-safe codemod](#preset-safe) by applying fake `Hidden` component to prevent application crash, but further fixes are required.
:::
Use the `sx` prop to replace `implementation="css"`:
```diff
-<Hidden implementation="css" xlUp><Paper /></Hidden>
-<Hidden implementation="css" xlUp><button /></Hidden>
+<Paper sx={{ display: { xl: 'none', xs: 'block' } }} />
+<Box component="button" sx={{ display: { xl: 'none', xs: 'block' } }} />
```
```diff
-<Hidden implementation="css" mdDown><Paper /></Hidden>
-<Hidden implementation="css" mdDown><button /></Hidden>
+<Paper sx={{ display: { xs: 'none', md: 'block' } }} />
+<Box component="button" sx={{ display: { xs: 'none', md: 'block' } }} />
```
Use the `useMediaQuery` hook to replace `implementation="js"`:
```diff
-<Hidden implementation="js" xlUp><Paper /></Hidden>
+const hidden = useMediaQuery(theme => theme.breakpoints.up('xl'));
+return hidden ? null : <Paper />;
```
## Icon
### Remove fontSize="default"
The default value of `fontSize` was changed from `default` to `medium` for consistency.
In the unlikely event that you were using the value `default`, the prop can be removed:
```diff
-<Icon fontSize="default">icon-name</Icon>
+<Icon>icon-name</Icon>
```
## IconButton
### ✅ Update size prop
Padding for the default size has been reduced to 8px, bringing it down to 40px.
For the old default size of 48px, use `size="large"`.
The change was made to better match Google's products when Material Design stopped documenting the icon button pattern.
```diff
- <IconButton>
+ <IconButton size="large">
```
### Remove span and label
The `span` element that wraps children has been removed.
The `label` classKey is also removed.
You can find out more details about this change in [this GitHub pull request](https://github.com/mui/material-ui/pull/26666), it used to be necessary for iOS.
```diff
<button class="MuiIconButton-root">
- <span class="MuiIconButton-label">
<svg />
- </span>
</button>
```
## Link
### ✅ Update default underline prop
The default `underline` prop is changed from `"hover"` to `"always"`.
To recreate the behavior from v4, apply `defaultProps` in the theme.
:::success
This is handled in [link-underline-hover codemod](#link-underline-hover)—read the details before running this codemod.
:::
```js
createTheme({
components: {
MuiLink: {
defaultProps: {
underline: 'hover',
},
},
},
});
```
## Menu
### ✅ Update transition props
The `on*` transition props were removed.
Use `TransitionProps` instead.
```diff
<Menu
- onEnter={onEnter}
- onEntered={onEntered}
- onEntering={onEntering}
- onExit={onExit}
- onExited={onExited}
- onExiting={onExiting}
+ TransitionProps={{
+ onEnter,
+ onEntered,
+ onEntering,
+ onExit,
+ onExited,
+ onExiting,
+ }}
>
```
:::info
The `selectedMenu` variant will no longer vertically align the selected item with the anchor.
:::
### Change default anchorOrigin.vertical value
Change the default value of `anchorOrigin.vertical` to follow the Material Design guidelines.
The menu is now displayed below the anchor instead of on top of it.
You can restore the previous behavior with:
```diff
<Menu
+ anchorOrigin={{
+ vertical: 'top',
+ horizontal: 'left',
+ }}
```
## MenuItem
### Update CSS class names
The `MenuItem` component inherits the `ButtonBase` component instead of `ListItem`.
The class names related to "MuiListItem-\*" have been removed, and theming `ListItem` no longer has an effect on `MenuItem`.
```diff
-<li className="MuiButtonBase-root MuiMenuItem-root MuiListItem-root">
+<li className="MuiButtonBase-root MuiMenuItem-root">
```
### Replace listItemClasses prop
prop `listItemClasses` is removed, use `classes` instead.
```diff
-<MenuItem listItemClasses={{...}}>
+<MenuItem classes={{...}}>
```
Read more about the [MenuItem CSS API](/material-ui/api/menu-item/#css).
## Modal
### ✅ Remove disableBackdropClick prop
Remove the `disableBackdropClick` prop because it is redundant.
Use `onClose` with `reason === 'backdropClick'` instead.
```diff
<Modal
- disableBackdropClick
- onClose={handleClose}
+ onClose={(event, reason) => {
+ if (reason !== 'backdropClick') {
+ handleClose(event, reason);
+ }
+ }}
/>
```
### ✅ Remove `onEscapeKeyDown` prop
Remove the `onEscapeKeyDown` prop because it is redundant.
Use `onClose` with `reason === "escapeKeyDown"` instead.
```diff
<Modal
- onEscapeKeyDown={handleEscapeKeyDown}
+ onClose={(event, reason) => {
+ if (reason === 'escapeKeyDown') {
+ handleEscapeKeyDown(event);
+ }
+ }}
/>
```
### Remove `onRendered` prop
Remove the `onRendered` prop.
Depending on your use case, you can either use a [callback ref](https://legacy.reactjs.org/docs/refs-and-the-dom.html#callback-refs) on the child element, or an effect hook in the child component.
## NativeSelect
### Remove selectMenu slot
Merge the `selectMenu` slot into `select`.
The `selectMenu` slot was redundant.
The `root` slot is no longer applied to the select, but to the root.
```diff
-<NativeSelect classes={{ root: 'class1', select: 'class2', selectMenu: 'class3' }} />
+<NativeSelect classes={{ select: 'class1 class2 class3' }} />
```
## OutlinedInput
### Replace labelWidth prop
Remove the `labelWidth` prop.
The `label` prop now fulfills the same purpose, using CSS layout instead of JavaScript measurement to render the gap in the outlined.
```diff
-<OutlinedInput labelWidth={20} />
+<OutlinedInput label="First Name" />
```
## Paper
### Change dark mode background opacity
Change the background opacity based on the elevation in dark mode.
This change was made to better conform to the Material Design guidelines.
You can revert it in the theme:
```diff
const theme = createTheme({
components: {
MuiPaper: {
+ styleOverrides: { root: { backgroundImage: 'unset' } },
},
},
});
```
## Pagination
### ✅ Update import
Move the component from the lab to the core.
The component is now stable.
```diff
-import Pagination from '@mui/lab/Pagination';
-import PaginationItem from '@mui/lab/PaginationItem';
-import { usePagination } from '@mui/lab/Pagination';
+import Pagination from '@mui/material/Pagination';
+import PaginationItem from '@mui/material/PaginationItem';
+import usePagination from '@mui/material/usePagination';
```
### ✅ Rename round to circular
```diff
-<Pagination shape="round">
-<PaginationItem shape="round">
+<Pagination shape="circular">
+<PaginationItem shape="circular">
```
## Popover
### ✅ Update transition props
The `on*` transition props were removed.
Use `TransitionProps` instead.
```diff
<Popover
- onEnter={onEnter}
- onEntered={onEntered}
- onEntering={onEntering}
- onExit={onExit}
- onExited={onExited}
- onExiting={onExiting}
+ TransitionProps={{
+ onEnter,
+ onEntered,
+ onEntering,
+ onExit,
+ onExited,
+ onExiting,
+ }}
>
```
### Remove getContentAnchorEl prop
The `getContentAnchorEl` prop was removed to simplify the positioning logic.
## Popper
### Upgrade from v1 to v2
Upgrade [Popper.js](https://popper.js.org/) from v1 to v2.
The CSS prefixes have changed:
```diff
popper: {
zIndex: 1,
- '&[x-placement*="bottom"] .arrow': {
+ '&[data-popper-placement*="bottom"] .arrow': {
```
Method names have changed:
```diff
-popperRef.current.scheduleUpdate()
+popperRef.current.update()
```
```diff
-popperRef.current.update()
+popperRef.current.forceUpdate()
```
The Modifiers API has been changed too significantly to fully cover here.
Read the [Popper.js migration guide](https://popper.js.org/docs/v2/migration-guide/) for complete details.
## Portal
### Remove onRendered prop
Remove the `onRendered` prop.
Depending on your use case, you can either use a [callback ref](https://legacy.reactjs.org/docs/refs-and-the-dom.html#callback-refs) on the child element, or an effect hook in the child component.
## Radio
### Update default color prop
The radio color prop is now "primary" by default.
To continue using the "secondary" color, you must explicitly indicate `secondary`.
This brings the radio closer to the Material Design guidelines.
```diff
-<Radio />
+<Radio color="secondary" />
```
### Update CSS classes
This component no longer has the class names `.MuiIconButton-root` or `.MuiIconButton-label`.
Instead, target `.MuiButtonBase-root`.
```diff
- <span class="MuiIconButton-root MuiButtonBase-root MuiRadio-root PrivateSwitchBase-root">
- <span class="MuiIconButton-label">
- <input class="PrivateSwitchBase-input">
+ <span class="MuiButtonBase-root MuiRadio-root PrivateSwitchBase-root">
+ <span class="PrivateSwitchBase-input">
```
## Rating
### ✅ Update imports
Move the component from the lab to the core.
The component is now stable.
```diff
-import Rating from '@mui/lab/Rating';
+import Rating from '@mui/material/Rating';
```
### Change default empty icon
Change the default empty icon to improve accessibility.
If you have a custom `icon` prop but no `emptyIcon` prop, you can restore the previous behavior with:
```diff
<Rating
icon={customIcon}
+ emptyIcon={null}
/>
```
### Rename visuallyhidden
Rename `visuallyhidden` to `visuallyHidden` for consistency:
```diff
<Rating
classes={{
- visuallyhidden: 'custom-visually-hidden-classname',
+ visuallyHidden: 'custom-visually-hidden-classname',
}}
/>
```
## RootRef
### Remove component
This component has been removed.
You can get a reference to the underlying DOM node of our components via `ref` prop.
The component relied on [`ReactDOM.findDOMNode`](https://react.dev/reference/react-dom/findDOMNode) which is [deprecated in `React.StrictMode`](https://react.dev/reference/react/StrictMode#warning-about-deprecated-finddomnode-usage).
:::success
This is handled in the [preset-safe codemod](#preset-safe) by applying fake `RootRef` component to prevent application crash, but further fixes are required.
:::
```diff
-<RootRef rootRef={ref}>
- <Button />
-</RootRef>
+<Button ref={ref} />
```
## Select
### ✅ Update default variant
Change the default variant from `standard` to `outlined`.
`standard` has been removed from the Material Design guidelines.
If you are composing the `Select` with a form control component, you only need to update `FormControl`—the select inherits the variant from its context.
:::success
This is handled in [variant-prop codemod](#variant-prop)—read the details before running this codemod.
:::
```diff
-<Select value="Standard" />
-<Select value="Outlined" variant="outlined" />
+<Select value="Standard" variant="standard" />
+<Select value="Outlined" />
```
### Replace labelWidth prop
Remove the `labelWidth` prop.
The `label` prop now fulfills the same purpose, using the CSS layout instead of JavaScript measurements to render the gap in the `outlined` variant.
The `TextField` already handles this by default.
```diff
-<Select variant="outlined" labelWidth={20} />
+<Select variant="outlined" label="Gender" />
```
### Remove selectMenu slot
Merge the `selectMenu` slot into `select`.
The `selectMenu` slot was redundant.
The `root` slot is no longer applied to the select, but to the root.
```diff
-<Select classes={{ root: 'class1', select: 'class2', selectMenu: 'class3' }} />
+<Select classes={{ select: 'class1 class2 class3' }} />
```
### Update event type (TypeScript)
The `event` in `onChange` is now typed as a `SelectChangeEvent<T>` instead of a `React.ChangeEvent`.
```diff
+ import Select, { SelectChangeEvent } from '@mui/material/Select';
-<Select onChange={(event: React.SyntheticEvent, value: unknown) => {}} />
+<Select onChange={(event: SelectChangeEvent<T>, child: React.ReactNode) => {}} />
```
This was necessary to prevent overriding the `event.target` of the events that caused the change.
## Skeleton
### ✅ Update import
Move the component from the lab to the core.
The component is now stable.
```diff
-import Skeleton from '@mui/lab/Skeleton';
+import Skeleton from '@mui/material/Skeleton';
```
### ✅ Rename circle and rect
Rename `circle` to `circular` and `rect` to `rectangular` for consistency:
```diff
-<Skeleton variant="circle" />
-<Skeleton variant="rect" />
-<Skeleton classes={{ circle: 'custom-circle-classname', rect: 'custom-rect-classname', }} />
+<Skeleton variant="circular" />
+<Skeleton variant="rectangular" />
+<Skeleton classes={{ circular: 'custom-circle-classname', rectangular: 'custom-rect-classname', }} />
```
## Slider
### Update event type (TypeScript)
The `event` in `onChange` is now typed as a `React.SyntheticEvent` instead of a `React.ChangeEvent`.
```diff
-<Slider onChange={(event: React.SyntheticEvent, value: unknown) => {}} />
+<Slider onChange={(event: Event, value: unknown) => {}} />
```
This was necessary to prevent overriding the `event.target` of the events that caused the change.
### Replace ValueLabelComponent and ThumbComponent props
The `ValueLabelComponent` and `ThumbComponent` props are now part of the `components` prop.
```diff
<Slider
- ValueLabelComponent={CustomValueLabel}
- ThumbComponent={CustomThumb}
+ components={{
+ ValueLabel: CustomValueLabel,
+ Thumb: CustomThumb,
+ }}
/>
```
### Refactor CSS
Rework the CSS to match the latest [Material Design guidelines](https://m2.material.io/components/sliders) and make custom styles more intuitive.
[See documentation](/material-ui/react-slider/).
<a href="/material-ui/react-slider/#continuous-sliders"><img width="247" alt="" src="https://user-images.githubusercontent.com/3165635/121884800-a8808600-cd13-11eb-8cdf-e25de8f1ba73.png" style="margin: auto"></a>
You can reduce the density of the slider, closer to v4 with the [`size="small"` prop](/material-ui/react-slider/#sizes).
## Snackbar
### Update default positioning
The notification now displays at the bottom left on large screens.
This better matches the behavior of Gmail, Google Keep, material.io, etc.
You can restore the v4 behavior with:
```diff
-<Snackbar />
+<Snackbar anchorOrigin={{ vertical: 'bottom', horizontal: 'center' }} />
```
### ✅ Update transition props
The `on*` transition props were removed.
Use `TransitionProps` instead.
```diff
<Snackbar
- onEnter={onEnter}
- onEntered={onEntered}
- onEntering={onEntering}
- onExit={onExit}
- onExited={onExited}
- onExiting={onExiting}
+ TransitionProps={{
+ onEnter,
+ onEntered,
+ onEntering,
+ onExit,
+ onExited,
+ onExiting,
+ }}
>
```
## SpeedDial
### ✅ Update import
Move the component from the lab to the core.
The component is now stable.
```diff
-import SpeedDial from '@mui/lab/SpeedDial';
-import SpeedDialAction from '@mui/lab/SpeedDialAction';
-import SpeedDialIcon from '@mui/lab/SpeedDialIcon';
+import SpeedDial from '@mui/material/SpeedDial';
+import SpeedDialAction from '@mui/material/SpeedDialAction';
+import SpeedDialIcon from '@mui/material/SpeedDialIcon';
```
## Stepper
### Update component structure
The root component `Paper` was replaced with a `<div>`.
`Stepper` no longer has elevation, and it does not inherit props from `Paper` anymore.
This change is meant to encourage composition.
```diff
+<Paper square elevation={2}>
- <Stepper elevation={2}>
+ <Stepper>
<Step>
<StepLabel>Hello world</StepLabel>
</Step>
</Stepper>
+<Paper>
```
### Remove built-in padding
The built-in 24px padding has been removed.
To keep it intact, add the following:
```diff
-<Stepper>
+<Stepper style={{ padding: 24 }}>
<Step>
<StepLabel>Hello world</StepLabel>
</Step>
</Stepper>
```
## SvgIcon
### Remove fontSize="default"
The default value of `fontSize` was changed from `default` to `medium` for consistency.
In the unlikely event that you were using the value `default`, the prop can be removed:
```diff
-<SvgIcon fontSize="default">
+<SvgIcon>
<path d="M10 20v-6h4v6h5v-8h3L12 3 2 12h3v8z" />
</SvgIcon>
```
## Switch
### Remove second onChange argument
The second argument from `onChange` has been deprecated.
You can pull out the checked state by accessing `event.target.checked`.
```diff
function MySwitch() {
- const handleChange = (event: React.ChangeEvent<HTMLInputElement>, checked: boolean) => {
+ const handleChange = (event: React.ChangeEvent<HTMLInputElement>) => {
+ const checked = event.target.checked;
};
return <Switch onChange={handleChange} />;
}
```
### Update default color prop
The `color` prop is now "primary" by default.
To continue using the "secondary" color, you must explicitly indicate `secondary`.
This brings `Switch` closer to the Material Design guidelines.
```diff
-<Switch />
+<Switch color="secondary" />
```
### Update CSS classes
This component no longer has the `.MuiIconButton-root` and `.MuiIconButton-label`.
Instead, target `.MuiButtonBase-root`.
```diff
<span class="MuiSwitch-root">
- <span class="MuiIconButton-root MuiButtonBase-root MuiSwitch-switchBase PrivateSwitchBase-root">
- <span class="MuiIconButton-label">
- <input class="MuiSwitch-input PrivateSwitchBase-input">
+ <span class="MuiButtonBase-root MuiSwitch-switchBase PrivateSwitchBase-root">
+ <span class="MuiSwitch-input PrivateSwitchBase-input">
```
## Table
### Rename default padding prop value
Rename the `default` value of the `padding` prop to `normal`.
```diff
-<Table padding="default" />
-<TableCell padding="default" />
+<Table padding="normal" />
+<TableCell padding="normal" />
```
## TablePagination
### Customize labels with getItemAriaLabel prop
The customization of the table pagination's actions labels must be done with the `getItemAriaLabel` prop.
This increases consistency with the `Pagination` component.
```diff
<TablePagination
- backIconButtonText="Back"
- nextIconButtonText="Next"
+ getItemAriaLabel={…}
```
### ✅ Rename onChangeRowsPerPage and onChangePage
Rename `onChangeRowsPerPage` to `onRowsPerPageChange` and `onChangePage` to `onPageChange` for API consistency.
```diff
<TablePagination
- onChangeRowsPerPage={()=>{}}
- onChangePage={()=>{}}
+ onRowsPerPageChange={()=>{}}
+ onPageChange={()=>{}}
```
### Separate label classes
Separate classes for different table pagination labels.
```diff
<TablePagination
- classes={{ caption: 'foo' }}
+ classes={{ selectLabel: 'foo', displayedRows: 'foo' }}
/>
```
### Move custom class on input to select
Move the custom class on `input` to `select`.
The `input` key is applied on another element.
```diff
<TablePagination
- classes={{ input: 'foo' }}
+ classes={{ select: 'foo' }}
/>
```
## Tabs
### Update default indicatorColor and textColor prop values
Change the default `indicatorColor` and `textColor` prop values to "primary".
This is done to match the most common use cases with Material Design.
If you'd prefer to keep the v4 color styles, use `"secondary"` and `"inherit"`, respectively, as shown below:
```diff
-<Tabs />
+<Tabs indicatorColor="secondary" textColor="inherit" />
```
### Update event type (TypeScript)
The `event` in `onChange` is now typed as a `React.SyntheticEvent` instead of a `React.ChangeEvent`.
```diff
-<Tabs onChange={(event: React.ChangeEvent<{}>, value: unknown) => {}} />
+<Tabs onChange={(event: React.SyntheticEvent, value: unknown) => {}} />
```
### ✅ Add new scroll button props
The API that controls the scroll buttons has been split into two props.
- The `scrollButtons` prop controls when the scroll buttons are displayed depending on the space available.
- The `allowScrollButtonsMobile` prop removes the CSS media query that systematically hides the scroll buttons on mobile.
```diff
-<Tabs scrollButtons="on" />
-<Tabs scrollButtons="desktop" />
-<Tabs scrollButtons="off" />
+<Tabs scrollButtons allowScrollButtonsMobile />
+<Tabs scrollButtons />
+<Tabs scrollButtons={false} />
```
## Tab
### Update default minWidth and maxWidth
Default minimum and maximum widths have been changed to match the [Material Design specifications](https://m2.material.io/components/tabs#specs):
- `minWidth` was changed from 72px to 90px.
- `maxWidth` was changed from 264px to 360px.
### Remove span and wrapper
The `span` element that wraps children has been removed.
The `wrapper` classKey is also removed.
You can find out more details about this change in [this GitHub pull request](https://github.com/mui/material-ui/pull/26926).
```diff
<button class="MuiTab-root">
- <span class="MuiTab-wrapper">
{icon}
{label}
- </span>
</button>
```
## TextField
### ✅ Update default variant
Change the default variant from `standard` to `outlined`.
`standard` has been removed from the Material Design guidelines.
:::success
This is handled in [variant-prop codemod](#variant-prop)—read the details before running this codemod.
:::
```diff
-<TextField value="Standard" />
-<TextField value="Outlined" variant="outlined" />
+<TextField value="Standard" variant="standard" />
+<TextField value="Outlined" />
```
### ✅ Rename rowsMax
Rename `rowsMax` prop to `maxRows` for consistency with HTML attributes.
```diff
-<TextField rowsMax={6}>
+<TextField maxRows={6}>
```
### ✅ Replace rows with minRows
Rename `rows` prop to `minRows` for dynamic resizing.
You need to use the `minRows` prop in the following case:
```diff
-<TextField rows={2} maxRows={5} />
+<TextField minRows={2} maxRows={5} />
```
### Forward ref instead of inputRef prop
Change ref forwarding expectations on custom `inputComponent`.
The component should forward the `ref` prop instead of the `inputRef` prop.
```diff
-function NumberFormatCustom(props) {
- const { inputRef, onChange, ...other } = props;
+const NumberFormatCustom = React.forwardRef(function NumberFormatCustom(
+ props,
+ ref,
+) {
const { onChange, ...other } = props;
return (
<NumberFormat
{...other}
- getInputRef={inputRef}
+ getInputRef={ref}
```
### Rename marginDense and inputMarginDense classes
Rename `marginDense` and `inputMarginDense` classes to `sizeSmall` and `inputSizeSmall` to match the prop.
```diff
-<Input margin="dense" />
+<Input size="small" />
```
### Update InputAdornment position prop
Set the InputAdornment `position` prop to `start` or `end`.
Use `start` if used as the value of the `startAdornment` prop.
Use `end` if used as the value of the `endAdornment` prop.
```diff
-<TextField startAdornment={<InputAdornment>kg</InputAdornment>} />
-<TextField endAdornment={<InputAdornment>kg</InputAdornment>} />
+<TextField startAdornment={<InputAdornment position="start">kg</InputAdornment>} />
+<TextField endAdornment={<InputAdornment position="end">kg</InputAdornment>} />
```
## TextareaAutosize
### ✅ Replace rows with minRows
Remove the `rows` prop, use the `minRows` prop instead.
This change aims to clarify the behavior of the prop.
```diff
-<TextareaAutosize rows={2} />
+<TextareaAutosize minRows={2} />
```
### ✅ Rename rowsMax
Rename `rowsMax` prop to `maxRows` for consistency with HTML attributes.
```diff
-<TextareaAutosize rowsMax={6}>
+<TextareaAutosize maxRows={6}>
```
### ✅ Rename rowsMin
Rename `rowsMin` prop with `minRows` for consistency with HTML attributes.
```diff
-<TextareaAutosize rowsMin={1}>
+<TextareaAutosize minRows={1}>
```
## ToggleButton
### ✅ Update import
Move the component from the lab to the core.
The component is now stable.
```diff
-import ToggleButton from '@mui/lab/ToggleButton';
-import ToggleButtonGroup from '@mui/lab/ToggleButtonGroup';
+import ToggleButton from '@mui/material/ToggleButton';
+import ToggleButtonGroup from '@mui/material/ToggleButtonGroup';
```
### Remove span and label
The `span` element that wraps children has been removed.
The `label` classKey is also removed.
You can find out more details about this change in [this GitHub pull request](https://github.com/mui/material-ui/pull/27111).
```diff
<button class="MuiToggleButton-root">
- <span class="MuiToggleButton-label">
{children}
- </span>
</button>
```
## Tooltip
### Interactive by default
Tooltips are now interactive by default.
The previous default behavior failed the [success criterion 1.4.3 ("hoverable") in WCAG 2.1](https://www.w3.org/TR/WCAG21/#content-on-hover-or-focus).
To reflect the new default value, the prop was renamed to `disableInteractive`.
If you want to restore the v4 behavior, you can apply the following diff:
```diff
-<Tooltip>
+<Tooltip disableInteractive>
# Interactive tooltips no longer need the `interactive` prop.
-<Tooltip interactive>
+<Tooltip>
```
## Typography
### Remove srOnly variant
Remove the `srOnly` variant.
You can use the `visuallyHidden` utility in conjunction with the `sx` prop instead.
```diff
+import { visuallyHidden } from '@mui/utils';
-<Typography variant="srOnly">Create a user</Typography>
+<span style={visuallyHidden}>Create a user</span>
```
### Remove color and style override keys
The following classes and style overrides keys were removed:
"colorInherit", "colorPrimary", "colorSecondary", "colorTextPrimary", "colorTextSecondary", "colorError", "displayInline", and "displayBlock".
These props are now considered part of the System rather than the `Typography` component itself.
If you still wish to add overrides for them, you can use the [callback as a value in `styleOverrides`](/material-ui/customization/theme-components/#overrides-based-on-props).
For example:
```diff
const theme = createTheme({
components: {
MuiTypography: {
- styleOverrides: {
- colorSecondary: {
- marginTop: '20px',
- },
- },
+ styleOverrides: ({ ownerState }) => ({
+ ...ownerState.color === 'secondary' && {
+ marginTop: '20px',
+ },
+ }),
},
},
});
```
## Theme
### Default background colors
The default background color is now `#fff` in light mode and `#121212` in dark mode.
This matches the Material Design guidelines.
### ✅ Breakpoint behavior
Breakpoints are now treated as values instead of [ranges](https://v4.mui.com/customization/breakpoints/#default-breakpoints).
The behavior of `down(key)` was changed to define a media query below the value defined by the corresponding breakpoint (exclusive), rather than the breakpoint above.
`between(start, end)` was also updated to define a media query for the values between the actual start (inclusive) and end (exclusive) values.
When using the `down()` breakpoints utility, you need to update the breakpoint key by one step up.
When using `between(start, end)`, the end breakpoint should also be updated by one step up.
Here are some examples of the changes required:
```diff
-theme.breakpoints.down('sm') // '@media (max-width:959.95px)' - [0, sm + 1) => [0, md)
+theme.breakpoints.down('md') // '@media (max-width:959.95px)' - [0, md)
```
```diff
-theme.breakpoints.between('sm', 'md') // '@media (min-width:600px) and (max-width:1279.95px)' - [sm, md + 1) => [0, lg)
+theme.breakpoints.between('sm', 'lg') // '@media (min-width:600px) and (max-width:1279.95px)' - [0, lg)
```
```diff
-theme.breakpoints.between('sm', 'xl') // '@media (min-width:600px)'
+theme.breakpoints.up('sm') // '@media (min-width:600px)'
```
The same should be done when using the `Hidden` component:
```diff
-<Hidden smDown>{...}</Hidden> // '@media (min-width:600px)'
+<Hidden mdDown>{...}</Hidden> // '@media (min-width:600px)'
```
### Breakpoint sizes
The default breakpoints were changed to better match common use cases as well as the Material Design guidelines.
You can find out more details about this change in [this GitHub issue](https://github.com/mui/material-ui/issues/21902)
```diff
{
xs: 0,
sm: 600,
- md: 960,
+ md: 900,
- lg: 1280,
+ lg: 1200,
- xl: 1920,
+ xl: 1536,
}
```
If you prefer the old breakpoint values, use the snippet below:
```js
import { createTheme } from '@mui/material/styles';
const theme = createTheme({
breakpoints: {
values: {
xs: 0,
sm: 600,
md: 960,
lg: 1280,
xl: 1920,
},
},
});
```
### ✅ Replace theme.breakpoints.width
The `theme.breakpoints.width` utility has been removed because it was redundant.
Use `theme.breakpoints.values` to get the same values.
```diff
-theme.breakpoints.width('md')
+theme.breakpoints.values.md
```
### Update theme.palette.augmentColor helper
The signature of `theme.palette.augmentColor` helper has changed:
```diff
-theme.palette.augmentColor(red);
+theme.palette.augmentColor({ color: red, name: 'brand' });
```
### Remove theme.typography.round helper
The `theme.typography.round` helper was removed because it was no longer used.
If you need it, use the function below:
```js
function round(value) {
return Math.round(value * 1e5) / 1e5;
}
```
## @mui/types
### Rename the exported Omit type
The module is now called `DistributiveOmit`.
This change removes the confusion with the built-in `Omit` helper introduced in TypeScript v3.5.
The built-in `Omit`, while similar, is non-distributive.
This leads to differences when applied to union types.
[See this Stack Overflow answer for further details](https://stackoverflow.com/a/57103940/1009797).
```diff
-import { Omit } from '@mui/types';
+import { DistributiveOmit } from '@mui/types';
```
| 3,668 |
0 | petrpan-code/mui/material-ui/docs/data/material/migration | petrpan-code/mui/material-ui/docs/data/material/migration/migration-v4/v5-style-changes.md | # Breaking changes in v5, part one: styles and themes
<p class="description">This is a reference guide to the breaking changes introduced in Material UI v5, and how to migrating from v4. This part covers changes to styling and theming.</p>
## Material UI v5 migration
1. [Getting started](/material-ui/migration/migration-v4/)
2. Breaking changes part one: style and theme 👈 _you are here_
3. [Breaking changes part two: components](/material-ui/migration/v5-component-changes/)
4. [Migrating from JSS](/material-ui/migration/migrating-from-jss/)
5. [Troubleshooting](/material-ui/migration/troubleshooting/)
## Breaking changes, part one
Material UI v5 introduces a number of breaking changes from v4.
Many of these changes can be resolved automatically using [the codemods](/material-ui/migration/migration-v4/#run-codemods) described in the [main migration guide](/material-ui/migration/migration-v4/).
The following document lists all breaking changes related to styles and themes in v5 and how to address them.
After you're finished here, please move on to [Breaking changes in v5 part two: components](/material-ui/migration/v5-component-changes/) to continue the migration process.
:::warning
Breaking changes that are handled by codemods are denoted by a ✅ emoji in the table of contents on the right side of the screen.
If you have already followed the instructions in the main migration guide and run the codemods, then you should not need to take any further action on these items.
All other changes must be handled manually.
:::
## Migrate theme styleOverrides to Emotion
Although your style overrides defined in the theme may partially work, there is an important difference regarding how the nested elements are styled.
The `$` syntax (local rule reference) used with JSS will not work with Emotion.
You need to replace those selectors with a valid class selector.
### Replace state class names
```diff
const theme = createTheme({
components: {
MuiOutlinedInput: {
styleOverrides: {
root: {
- '&$focused': {
+ '&.Mui-focused': {
borderWidth: 1,
}
}
}
}
}
});
```
### Replace nested classes selectors with global class names
```diff
const theme = createTheme({
components: {
MuiOutlinedInput: {
styleOverrides: {
root: {
- '& $notchedOutline': {
+ '& .MuiOutlinedInput-notchedOutline': {
borderWidth: 1,
}
}
}
}
}
});
```
:::info
For each component, we export a `[component]Classes` constant that contains all nested classes for that component.
You can rely on this instead of hardcoding the classes.
:::
```diff
+import { outlinedInputClasses } from '@mui/material/OutlinedInput';
const theme = createTheme({
components: {
MuiOutlinedInput: {
styleOverrides: {
root: {
- '& $notchedOutline': {
+ [`& .${outlinedInputClasses.notchedOutline}`]: {
borderWidth: 1,
}
}
}
}
}
});
```
Take a look at the complete [list of global state classnames](/material-ui/customization/how-to-customize/#state-classes) available.
## ref
### Refactor non-ref-forwarding class components
Support for non-ref-forwarding class components in the `component` prop or as immediate `children` has been dropped.
If you were using `unstable_createStrictModeTheme` or didn't see any warnings related to `findDOMNode` in `React.StrictMode` then you don't need to take any further action.
Otherwise check out the [Caveat with refs](/material-ui/guides/composition/#caveat-with-refs) section in the Composition guide to find out how to migrate.
This change affects almost all components where you're using the `component` prop or passing `children` to components that require `children` to be elements (e.g. `<MenuList><CustomMenuItem /></MenuList>`).
### Fix ref type specificity
For some components, you may get a type error when passing `ref`.
To avoid the error, you should use a specific element type.
For example, `Card` expects the type of `ref` to be `HTMLDivElement`, and `ListItem` expects its `ref` type to be `HTMLLIElement`.
Here is an example:
```diff
import * as React from 'react';
import Card from '@mui/material/Card';
import ListItem from '@mui/material/ListItem';
export default function SpecificRefType() {
- const cardRef = React.useRef<HTMLElement>(null);
+ const cardRef = React.useRef<HTMLDivElement>(null);
- const listItemRef = React.useRef<HTMLElement>(null);
+ const listItemRef = React.useRef<HTMLLIElement>(null);
return (
<div>
<Card ref={cardRef}></Card>
<ListItem ref={listItemRef}></ListItem>
</div>
);
}
```
Here are the specific element types that each component expects:
#### @mui/material
- [Accordion](/material-ui/api/accordion/) - `HTMLDivElement`
- [Alert](/material-ui/api/alert/) - `HTMLDivElement`
- [Avatar](/material-ui/api/avatar/) - `HTMLDivElement`
- [ButtonGroup](/material-ui/api/button-group/) - `HTMLDivElement`
- [Card](/material-ui/api/card/) - `HTMLDivElement`
- [Dialog](/material-ui/api/dialog/) - `HTMLDivElement`
- [ImageList](/material-ui/api/image-list/) - `HTMLUListElement`
- [List](/material-ui/api/list/) - `HTMLUListElement`
- [Tab](/material-ui/api/tab/) - `HTMLDivElement`
- [Tabs](/material-ui/api/tabs/) - `HTMLDivElement`
- [ToggleButton](/material-ui/api/toggle-button/) - `HTMLButtonElement`
#### @mui/lab
- [Timeline](/material-ui/api/timeline/) - `HTMLUListElement`
## Style library
### ✅ Adjust CSS injection order
The style library used by default in v5 is [Emotion](https://emotion.sh/docs/introduction).
If you were using JSS for the style overrides of Material UI components—for example, those created by `makeStyles`—you will need to take care of the CSS injection order.
JSS `<style`>' elements need to be injected in the `<head>` after Emotion `<style>`' elements.
To do so, you need to have the `StyledEngineProvider` with the `injectFirst` option at the top of your component tree, as shown here:
```jsx
import * as React from 'react';
import { StyledEngineProvider } from '@mui/material/styles';
export default function GlobalCssPriority() {
return (
{/* Inject Emotion before JSS */}
<StyledEngineProvider injectFirst>
{/* Your component tree. Now you can override Material UI's styles. */}
</StyledEngineProvider>
);
}
```
### ✅ Add prepend to createCache
If you have a custom cache and are using Emotion to style your app, it will override the cache provided by Material UI.
To correct the injection order, add the `prepend` option to `createCache`, as shown below:
```diff
import * as React from 'react';
import { CacheProvider } from '@emotion/react';
import createCache from '@emotion/cache';
const cache = createCache({
key: 'css',
+ prepend: true,
});
export default function PlainCssPriority() {
return (
<CacheProvider value={cache}>
{/* Your component tree. Now you can override Material UI's styles. */}
</CacheProvider>
);
}
```
:::warning
If you are using styled-components and have a `StyleSheetManager` with a custom `target`, make sure that the target is the first element in the HTML `<head>`.
To see how it can be done, take a look at the [`StyledEngineProvider` implementation](https://github.com/mui/material-ui/blob/-/packages/mui-styled-engine-sc/src/StyledEngineProvider/StyledEngineProvider.js) in the `@mui/styled-engine-sc` package.
:::
## Theme structure
### ✅ Add adaptV4Theme helper
The structure of the theme has changed in v5. You need to update its shape.
For a smoother transition, the `adaptV4Theme` helper allows you to iteratively upgrade some of the theme changes to the new theme structure.
```diff
-import { createMuiTheme } from '@mui/material/styles';
+import { createTheme, adaptV4Theme } from '@mui/material/styles';
-const theme = createMuiTheme({
+const theme = createTheme(adaptV4Theme({
// v4 theme
-});
+}));
```
:::warning
This adapter only handles the input arguments of `createTheme`.
If you modify the shape of the theme after its creation, you need to migrate the structure manually.
:::
The following changes are supported by the adapter:
### Remove gutters
The "gutters" abstraction hasn't proven to be used frequently enough to be valuable.
```diff
-theme.mixins.gutters(),
+paddingLeft: theme.spacing(2),
+paddingRight: theme.spacing(2),
+[theme.breakpoints.up('sm')]: {
+ paddingLeft: theme.spacing(3),
+ paddingRight: theme.spacing(3),
+},
```
### ✅ Remove px suffix
`theme.spacing` now returns single values with px units by default.
This change improves the integration with styled-components & Emotion.
Before:
```js
theme.spacing(2) => 16
```
After:
```js
theme.spacing(2) => '16px'
```
### ✅ Rename theme.palette.type
The `theme.palette.type` key was renamed to `theme.palette.mode`, to better follow the "dark mode" terminology that is usually used for describing this feature.
```diff
import { createTheme } from '@mui/material/styles';
-const theme = createTheme({ palette: { type: 'dark' } }),
+const theme = createTheme({ palette: { mode: 'dark' } }),
```
### Change default theme.palette.info colors
The default `theme.palette.info` colors were changed to pass the AA accessibility standard contrast ratio in both light and dark modes.
```diff
info = {
- main: cyan[500],
+ main: lightBlue[700], // lightBlue[400] in "dark" mode
- light: cyan[300],
+ light: lightBlue[500], // lightBlue[300] in "dark" mode
- dark: cyan[700],
+ dark: lightBlue[900], // lightBlue[700] in "dark" mode
}
```
### Change default theme.palette.success colors
The default `theme.palette.success` colors were changed to pass the AA accessibility standard contrast ratio in both light and dark modes.
```diff
success = {
- main: green[500],
+ main: green[800], // green[400] in "dark" mode
- light: green[300],
+ light: green[500], // green[300] in "dark" mode
- dark: green[700],
+ dark: green[900], // green[700] in "dark" mode
}
```
### Change default theme.palette.warning colors
The default `theme.palette.warning` colors were changed to pass the AA accessibility standard contrast ratio in both light and dark modes.
```diff
warning = {
- main: orange[500],
+ main: '#ED6C02', // orange[400] in "dark" mode
- light: orange[300],
+ light: orange[500], // orange[300] in "dark" mode
- dark: orange[700],
+ dark: orange[900], // orange[700] in "dark" mode
}
```
### Restore theme.palette.text.hint key (if needed)
The `theme.palette.text.hint` key was unused in Material UI components, and has been removed.
If you depend on it, you can add it back:
```diff
import { createTheme } from '@mui/material/styles';
-const theme = createTheme(),
+const theme = createTheme({
+ palette: { text: { hint: 'rgba(0, 0, 0, 0.38)' } },
+});
```
### Restructure component definitions
The component definitions in the theme were restructured under the `components` key to make them easier to find.
#### 1. props
```diff
import { createTheme } from '@mui/material/styles';
const theme = createTheme({
- props: {
- MuiButton: {
- disableRipple: true,
- },
- },
+ components: {
+ MuiButton: {
+ defaultProps: {
+ disableRipple: true,
+ },
+ },
+ },
});
```
#### 2. overrides
```diff
import { createTheme } from '@mui/material/styles';
const theme = createTheme({
- overrides: {
- MuiButton: {
- root: { padding: 0 },
- },
- },
+ components: {
+ MuiButton: {
+ styleOverrides: {
+ root: { padding: 0 },
+ },
+ },
+ },
});
```
## @mui/styles
### Update ThemeProvider import
If you are using the utilities from `@mui/styles` together with the `@mui/material`, you should replace the use of `ThemeProvider` from `@mui/styles` with the one exported from `@mui/material/styles`.
This way, the `theme` provided in the context will be available in both the styling utilities exported from `@mui/styles`, like `makeStyles`, `withStyles`, etc., along with the Material UI components.
```diff
-import { ThemeProvider } from '@mui/styles';
+import { ThemeProvider } from '@mui/material/styles';
```
Make sure to add a `ThemeProvider` at the root of your application, as the `defaultTheme` is no longer available in the utilities coming from `@mui/styles`.
### ✅ Add module augmentation for DefaultTheme (TypeScript)
The `@mui/styles` package is no longer part of `@mui/material/styles`.
If you are using `@mui/styles` together with `@mui/material` you need to add a module augmentation for the `DefaultTheme`.
```ts
// in the file where you are creating the theme (invoking the function `createTheme()`)
import { Theme } from '@mui/material/styles';
declare module '@mui/styles' {
interface DefaultTheme extends Theme {}
}
```
## @mui/material/colors
### ✅ Change color imports
Nested imports of more than one level are private. For example, you can no longer import `red` from `@mui/material/colors/red`.
```diff
-import red from '@mui/material/colors/red';
+import { red } from '@mui/material/colors';
```
## @mui/material/styles
### ✅ Rename fade to alpha
`fade` was renamed to `alpha` to better describe its functionality.
The previous name caused confusion when the input color already had an alpha value. The helper overrides the alpha value of the color.
```diff
-import { fade } from '@mui/material/styles';
+import { alpha } from '@mui/material/styles';
const classes = makeStyles(theme => ({
- backgroundColor: fade(theme.palette.primary.main, theme.palette.action.selectedOpacity),
+ backgroundColor: alpha(theme.palette.primary.main, theme.palette.action.selectedOpacity),
}));
```
### ✅ Update createStyles import
The `createStyles` function from `@mui/material/styles` was moved to the one exported from `@mui/styles`. It is necessary for removing the dependency on `@mui/styles` in the Material UI npm package.
```diff
-import { createStyles } from '@mui/material/styles';
+import { createStyles } from '@mui/styles';
```
### ✅ Update createGenerateClassName import
The `createGenerateClassName` function is no longer exported from `@mui/material/styles`. You should import it directly from `@mui/styles`.
```diff
-import { createGenerateClassName } from '@mui/material/styles';
+import { createGenerateClassName } from '@mui/styles';
```
To generate custom class names without using `@mui/styles`, check out [ClassName Generator](/material-ui/experimental-api/classname-generator/) for more details.
### ✅ Rename createMuiTheme
The function `createMuiTheme` was renamed to `createTheme` to make it more intuitive to use with `ThemeProvider`.
```diff
-import { createMuiTheme } from '@mui/material/styles';
+import { createTheme } from '@mui/material/styles';
-const theme = createMuiTheme({
+const theme = createTheme({
```
### ✅ Update MuiThemeProvider import
The `MuiThemeProvider` component is no longer exported from `@mui/material/styles`. Use `ThemeProvider` instead.
```diff
-import { MuiThemeProvider } from '@mui/material/styles';
+import { ThemeProvider } from '@mui/material/styles';
```
### ✅ Update jssPreset import
The `jssPreset` object is no longer exported from `@mui/material/styles`. You should import it directly from `@mui/styles`.
```diff
-import { jssPreset } from '@mui/material/styles';
+import { jssPreset } from '@mui/styles';
```
### ✅ Update `makeStyles` import
The `makeStyles` JSS utility is no longer exported from `@mui/material/styles`.
You can use `@mui/styles/makeStyles` instead.
Make sure to add a `ThemeProvider` at the root of your application, as the `defaultTheme` is no longer available.
If you are using this utility together with `@mui/material`, it's recommended that you use the `ThemeProvider` component from `@mui/material/styles` instead.
```diff
-import { makeStyles } from '@mui/material/styles';
+import { makeStyles } from '@mui/styles';
+import { createTheme, ThemeProvider } from '@mui/material/styles';
+const theme = createTheme();
const useStyles = makeStyles((theme) => ({
background: theme.palette.primary.main,
}));
function Component() {
const classes = useStyles();
return <div className={classes.root} />
}
// In the root of your app
function App(props) {
- return <Component />;
+ return <ThemeProvider theme={theme}><Component {...props} /></ThemeProvider>;
}
```
### ✅ Update ServerStyleSheets import
The `ServerStyleSheets` component is no longer exported from `@mui/material/styles`. You should import it directly from `@mui/styles`.
```diff
-import { ServerStyleSheets } from '@mui/material/styles';
+import { ServerStyleSheets } from '@mui/styles';
```
### styled
The `styled` JSS utility is no longer exported from `@mui/material/styles`. You can use the one exported from `@mui/styles` instead.
Make sure to add a `ThemeProvider` at the root of your application, as the `defaultTheme` is no longer available.
If you are using this utility together with `@mui/material`, it's recommended you use the `ThemeProvider` component from `@mui/material/styles` instead.
```diff
-import { styled } from '@mui/material/styles';
+import { styled } from '@mui/styles';
+import { createTheme, ThemeProvider } from '@mui/material/styles';
+const theme = createTheme();
const MyComponent = styled('div')(({ theme }) => ({ background: theme.palette.primary.main }));
function App(props) {
- return <MyComponent />;
+ return <ThemeProvider theme={theme}><MyComponent {...props} /></ThemeProvider>;
}
```
### ✅ Update StylesProvider import
The `StylesProvider` component is no longer exported from `@mui/material/styles`. You should import it directly from `@mui/styles`.
```diff
-import { StylesProvider } from '@mui/material/styles';
+import { StylesProvider } from '@mui/styles';
```
### ✅ Update useThemeVariants import
The `useThemeVariants` hook is no longer exported from `@mui/material/styles`.
You should import it directly from `@mui/styles`.
```diff
-import { useThemeVariants } from '@mui/material/styles';
+import { useThemeVariants } from '@mui/styles';
```
### ✅ Update withStyles import
The `withStyles` JSS utility is no longer exported from `@mui/material/styles`.
You can use `@mui/styles/withStyles` instead.
Make sure to add a `ThemeProvider` at the root of your application, as the `defaultTheme` is no longer available.
If you are using this utility together with `@mui/material`, you should use the `ThemeProvider` component from `@mui/material/styles` instead.
```diff
-import { withStyles } from '@mui/material/styles';
+import { withStyles } from '@mui/styles';
+import { createTheme, ThemeProvider } from '@mui/material/styles';
+const defaultTheme = createTheme();
const MyComponent = withStyles((props) => {
const { classes, className, ...other } = props;
return <div className={clsx(className, classes.root)} {...other} />
})(({ theme }) => ({ root: { background: theme.palette.primary.main }}));
function App() {
- return <MyComponent />;
+ return <ThemeProvider theme={defaultTheme}><MyComponent /></ThemeProvider>;
}
```
### ✅ Replace innerRef with ref
Replace the `innerRef` prop with the `ref` prop. Refs are now automatically forwarded to the inner component.
```diff
import * as React from 'react';
import { withStyles } from '@mui/styles';
const MyComponent = withStyles({
root: {
backgroundColor: 'red',
},
})(({ classes }) => <div className={classes.root} />);
function MyOtherComponent(props) {
const ref = React.useRef();
- return <MyComponent innerRef={ref} />;
+ return <MyComponent ref={ref} />;
}
```
### Update withTheme import
The `withTheme` HOC utility has been removed from the `@mui/material/styles` package. You can use `@mui/styles/withTheme` instead.
Make sure to add a `ThemeProvider` at the root of your application, as the `defaultTheme` is no longer available.
If you are using this utility together with `@mui/material`, it's recommended you use the `ThemeProvider` component from `@mui/material/styles` instead.
```diff
-import { withTheme } from '@mui/material/styles';
+import { withTheme } from '@mui/styles';
+import { createTheme, ThemeProvider } from '@mui/material/styles';
+const theme = createTheme();
const MyComponent = withTheme(({ theme }) => <div>{theme.direction}</div>);
function App(props) {
- return <MyComponent />;
+ return <ThemeProvider theme={theme}><MyComponent {...props} /></ThemeProvider>;
}
```
### ✅ Remove withWidth
This HOC was removed. If you need this feature, you can try [the alternative that uses the `useMediaQuery` hook](/material-ui/react-use-media-query/#migrating-from-withwidth).
## @mui/icons-material
### Reduce GitHub icon size
The GitHub icon was reduced in size from 24px to 22px wide to match the size of the other icons.
## @material-ui/pickers
We have a [dedicated page](/material-ui/guides/pickers-migration/) for migrating `@material-ui/pickers` to v5.
## System
### ✅ Rename gap props
The following system functions and properties were renamed because they are considered deprecated CSS:
- `gridGap` becomes `gap`
- `gridRowGap` becomes `rowGap`
- `gridColumnGap` becomes `columnGap`
### ✅ Add spacing units to gap props
Use a spacing unit in `gap`, `rowGap`, and `columnGap`. If you were using a number previously, you need to mention the px to bypass the new transformation with `theme.spacing`.
```diff
<Box
- gap={2}
+ gap="2px"
>
```
Replace `css` prop with `sx` to avoid collision with styled-components and Emotion's `css` prop.
```diff
-<Box css={{ color: 'primary.main' }} />
+<Box sx={{ color: 'primary.main' }} />
```
:::warning
The system grid function was not documented in v4.
:::
| 3,669 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/GlobalCss.js | import * as React from 'react';
import { makeStyles } from '@mui/styles';
const useStyles = makeStyles({
'@global': {
'.cssjss-advanced-global-root': {
height: 100,
width: 100,
backgroundColor: 'blue',
},
'.cssjss-advanced-global-child': {
height: 8,
backgroundColor: 'red',
},
},
});
export default function GlobalCss() {
useStyles();
return (
<div className="cssjss-advanced-global-root">
<div className="cssjss-advanced-global-child" />
</div>
);
}
| 3,670 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/GlobalCss.tsx | import * as React from 'react';
import { makeStyles } from '@mui/styles';
const useStyles = makeStyles({
'@global': {
'.cssjss-advanced-global-root': {
height: 100,
width: 100,
backgroundColor: 'blue',
},
'.cssjss-advanced-global-child': {
height: 8,
backgroundColor: 'red',
},
},
});
export default function GlobalCss() {
useStyles();
return (
<div className="cssjss-advanced-global-root">
<div className="cssjss-advanced-global-child" />
</div>
);
}
| 3,671 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/GlobalCss.tsx.preview | <div className="cssjss-advanced-global-child" /> | 3,672 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/HybridGlobalCss.js | import * as React from 'react';
import clsx from 'clsx';
import { makeStyles } from '@mui/styles';
const useStyles = makeStyles({
root: {
'&.root': {
height: 100,
width: 100,
backgroundColor: 'blue',
},
'& .child': {
height: 8,
backgroundColor: 'red',
},
},
});
export default function HybridGlobalCss() {
const classes = useStyles();
return (
<div className={clsx(classes.root, 'root')}>
<div className="child" />
</div>
);
}
| 3,673 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/HybridGlobalCss.tsx | import * as React from 'react';
import clsx from 'clsx';
import { makeStyles } from '@mui/styles';
const useStyles = makeStyles({
root: {
'&.root': {
height: 100,
width: 100,
backgroundColor: 'blue',
},
'& .child': {
height: 8,
backgroundColor: 'red',
},
},
});
export default function HybridGlobalCss() {
const classes = useStyles();
return (
<div className={clsx(classes.root, 'root')}>
<div className="child" />
</div>
);
}
| 3,674 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/HybridGlobalCss.tsx.preview | <div className="child" /> | 3,675 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/StringTemplates.js | import * as React from 'react';
import { jssPreset, StylesProvider, makeStyles } from '@mui/styles';
import { create } from 'jss';
import jssTemplate from 'jss-plugin-template';
const jss = create({
plugins: [jssTemplate(), ...jssPreset().plugins],
});
const useStyles = makeStyles({
root: `
background: linear-gradient(45deg, #fe6b8b 30%, #ff8e53 90%);
border-radius: 3px;
font-size: 16px;
border: 0;
color: white;
height: 48px;
padding: 0 30px;
box-shadow: 0 3px 5px 2px rgba(255, 105, 135, 0.3);
`,
});
function Child() {
const classes = useStyles();
return (
<button type="button" className={classes.root}>
String templates
</button>
);
}
function StringTemplates() {
return (
<StylesProvider jss={jss}>
<Child />
</StylesProvider>
);
}
export default StringTemplates;
| 3,676 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/ThemeNesting.js | import * as React from 'react';
import { ThemeProvider, makeStyles } from '@mui/styles';
const useStyles = makeStyles((theme) => ({
root: {
background: theme.background,
border: 0,
fontSize: 16,
borderRadius: 3,
boxShadow: theme.boxShadow,
color: 'white',
height: 48,
padding: '0 30px',
},
}));
function DeepChild() {
const classes = useStyles();
return (
<button type="button" className={classes.root}>
Theme nesting
</button>
);
}
export default function ThemeNesting() {
return (
<div>
<ThemeProvider
theme={{
background: 'linear-gradient(45deg, #FE6B8B 30%, #FF8E53 90%)',
boxShadow: '0 3px 5px 2px rgba(255, 105, 135, .3)',
}}
>
<DeepChild />
<br />
<br />
<ThemeProvider
theme={(outerTheme) => ({
...outerTheme,
background: 'linear-gradient(45deg, #2196F3 30%, #21CBF3 90%)',
boxShadow: '0 3px 5px 2px rgba(33, 203, 243, .3)',
})}
>
<DeepChild />
</ThemeProvider>
</ThemeProvider>
</div>
);
}
| 3,677 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/ThemeNesting.tsx | import * as React from 'react';
import { ThemeProvider, makeStyles } from '@mui/styles';
interface MyTheme {
background: string;
boxShadow: string;
}
const useStyles = makeStyles((theme: MyTheme) => ({
root: {
background: theme.background,
border: 0,
fontSize: 16,
borderRadius: 3,
boxShadow: theme.boxShadow,
color: 'white',
height: 48,
padding: '0 30px',
},
}));
function DeepChild() {
const classes = useStyles();
return (
<button type="button" className={classes.root}>
Theme nesting
</button>
);
}
export default function ThemeNesting() {
return (
<div>
<ThemeProvider<MyTheme>
theme={{
background: 'linear-gradient(45deg, #FE6B8B 30%, #FF8E53 90%)',
boxShadow: '0 3px 5px 2px rgba(255, 105, 135, .3)',
}}
>
<DeepChild />
<br />
<br />
<ThemeProvider<MyTheme>
theme={(outerTheme) => ({
...outerTheme,
background: 'linear-gradient(45deg, #2196F3 30%, #21CBF3 90%)',
boxShadow: '0 3px 5px 2px rgba(33, 203, 243, .3)',
})}
>
<DeepChild />
</ThemeProvider>
</ThemeProvider>
</div>
);
}
| 3,678 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/Theming.js | import * as React from 'react';
import { ThemeProvider, makeStyles } from '@mui/styles';
const themeInstance = {
background: 'linear-gradient(45deg, #FE6B8B 30%, #FF8E53 90%)',
};
const useStyles = makeStyles((theme) => ({
root: {
background: theme.background,
border: 0,
fontSize: 16,
borderRadius: 3,
boxShadow: '0 3px 5px 2px rgba(255, 105, 135, .3)',
color: 'white',
height: 48,
padding: '0 30px',
},
}));
function DeepChild() {
const classes = useStyles();
return (
<button type="button" className={classes.root}>
Theming
</button>
);
}
export default function Theming() {
return (
<ThemeProvider theme={themeInstance}>
<DeepChild />
</ThemeProvider>
);
}
| 3,679 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/Theming.tsx | import * as React from 'react';
import { ThemeProvider, makeStyles } from '@mui/styles';
const themeInstance = {
background: 'linear-gradient(45deg, #FE6B8B 30%, #FF8E53 90%)',
};
const useStyles = makeStyles((theme: typeof themeInstance) => ({
root: {
background: theme.background,
border: 0,
fontSize: 16,
borderRadius: 3,
boxShadow: '0 3px 5px 2px rgba(255, 105, 135, .3)',
color: 'white',
height: 48,
padding: '0 30px',
},
}));
function DeepChild() {
const classes = useStyles();
return (
<button type="button" className={classes.root}>
Theming
</button>
);
}
export default function Theming() {
return (
<ThemeProvider theme={themeInstance}>
<DeepChild />
</ThemeProvider>
);
}
| 3,680 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/Theming.tsx.preview | <ThemeProvider theme={themeInstance}>
<DeepChild />
</ThemeProvider> | 3,681 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/UseTheme.js | import * as React from 'react';
import { ThemeProvider, useTheme } from '@mui/styles';
function DeepChild() {
const theme = useTheme();
return <span>{`spacing ${theme.spacing}`}</span>;
}
export default function UseTheme() {
return (
<ThemeProvider
theme={{
spacing: '8px',
}}
>
<DeepChild />
</ThemeProvider>
);
}
| 3,682 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/UseTheme.tsx | import * as React from 'react';
import { ThemeProvider, useTheme } from '@mui/styles';
interface MyTheme {
spacing: string;
}
function DeepChild() {
const theme = useTheme<MyTheme>();
return <span>{`spacing ${theme.spacing}`}</span>;
}
export default function UseTheme() {
return (
<ThemeProvider<MyTheme>
theme={{
spacing: '8px',
}}
>
<DeepChild />
</ThemeProvider>
);
}
| 3,683 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/UseTheme.tsx.preview | <ThemeProvider<MyTheme>
theme={{
spacing: '8px',
}}
>
<DeepChild />
</ThemeProvider> | 3,684 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/WithTheme.js | import * as React from 'react';
import PropTypes from 'prop-types';
import { ThemeProvider, withTheme } from '@mui/styles';
function DeepChildRaw(props) {
return <span>{`spacing ${props.theme.spacing}`}</span>;
}
DeepChildRaw.propTypes = {
theme: PropTypes.shape({
spacing: PropTypes.string.isRequired,
}).isRequired,
};
const DeepChild = withTheme(DeepChildRaw);
function WithTheme() {
return (
<ThemeProvider
theme={{
spacing: '8px',
}}
>
<DeepChild />
</ThemeProvider>
);
}
export default WithTheme;
| 3,685 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/WithTheme.tsx | import * as React from 'react';
import { ThemeProvider, WithTheme as WithThemeProps, withTheme } from '@mui/styles';
interface Theme {
spacing: string;
}
interface Props extends WithThemeProps<Theme> {}
function DeepChildRaw(props: Props) {
return <span>{`spacing ${props.theme.spacing}`}</span>;
}
const DeepChild = withTheme<Theme, typeof DeepChildRaw>(DeepChildRaw);
function WithTheme() {
return (
<ThemeProvider
theme={{
spacing: '8px',
}}
>
<DeepChild />
</ThemeProvider>
);
}
export default WithTheme;
| 3,686 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/advanced/advanced.md | # Advanced (LEGACY)
<p class="description">This section covers more advanced usage of @mui/styles.</p>
:::error
`@mui/styles` was deprecated with the release of MUI Core v5 in late 2021.
It depended on [JSS](https://cssinjs.org/) as a styling solution, which is no longer used in `@mui/material`.
`@mui/styles` is not compatible with [React.StrictMode](https://react.dev/reference/react/StrictMode) or React 18, and it will not be updated.
This documentation remains here for those working on legacy projects, but we **strongly discourage** you from using `@mui/styles` when creating a new app with Material UI—you _will_ face unresolvable dependency issues.
Please use [`@mui/system`](/system/getting-started/) instead.
:::
## Theming
Add a `ThemeProvider` to the top level of your app to pass a theme down the React component tree. Then, you can access the theme object in style functions.
:::info
This example creates a theme object for custom-built components. If you intend to use some of Material UI's components you need to provide a richer theme structure using the `createTheme()` method. Head to the [theming section](/material-ui/customization/theming/) to learn how to build your custom Material UI theme.
:::
```jsx
import { ThemeProvider } from '@mui/styles';
import DeepChild from './my_components/DeepChild';
const theme = {
background: 'linear-gradient(45deg, #FE6B8B 30%, #FF8E53 90%)',
};
function Theming() {
return (
<ThemeProvider theme={theme}>
<DeepChild />
</ThemeProvider>
);
}
```
{{"demo": "Theming.js"}}
### Accessing the theme in a component
You might need to access the theme variables inside your React components.
#### `useTheme` hook
For use in function components:
```jsx
import { useTheme } from '@mui/styles';
function DeepChild() {
const theme = useTheme();
return <span>{`spacing ${theme.spacing}`}</span>;
}
```
{{"demo": "UseTheme.js"}}
#### `withTheme` HOC
For use in class or function components:
```jsx
import { withTheme } from '@mui/styles';
function DeepChildRaw(props) {
return <span>{`spacing ${props.theme.spacing}`}</span>;
}
const DeepChild = withTheme(DeepChildRaw);
```
{{"demo": "WithTheme.js"}}
### Theme nesting
You can nest multiple theme providers.
This can be really useful when dealing with different areas of your application that have distinct appearance from each other.
```jsx
<ThemeProvider theme={outerTheme}>
<Child1 />
<ThemeProvider theme={innerTheme}>
<Child2 />
</ThemeProvider>
</ThemeProvider>
```
{{"demo": "ThemeNesting.js"}}
The inner theme will **override** the outer theme.
You can extend the outer theme by providing a function:
```jsx
<ThemeProvider theme={…} >
<Child1 />
<ThemeProvider theme={outerTheme => ({ darkMode: true, ...outerTheme })}>
<Child2 />
</ThemeProvider>
</ThemeProvider>
```
## Overriding styles - `classes` prop
The `makeStyles` (hook generator) and `withStyles` (HOC) APIs allow the creation of multiple style rules per style sheet. Each style rule has its own class name.
The class names are provided to the component with the `classes` variable.
This is particularly useful when styling nested elements in a component.
```jsx
// A style sheet
const useStyles = makeStyles({
root: {}, // a style rule
label: {}, // a nested style rule
});
function Nested(props) {
const classes = useStyles();
return (
<button className={classes.root}>
{/* 'jss1' */}
<span className={classes.label}>{/* 'jss2' nested */}</span>
</button>
);
}
function Parent() {
return <Nested />;
}
```
However, the class names are often non-deterministic. How can a parent component override the style of a nested element?
### `withStyles`
This is the simplest case. The wrapped component accepts a `classes` prop,
it simply merges the class names provided with the style sheet.
```jsx
const Nested = withStyles({
root: {}, // a style rule
label: {}, // a nested style rule
})(({ classes }) => (
<button className={classes.root}>
<span className={classes.label}>{/* 'jss2 my-label' Nested*/}</span>
</button>
));
function Parent() {
return <Nested classes={{ label: 'my-label' }} />;
}
```
### `makeStyles`
The hook API requires a bit more work. You have to forward the parent props to the hook as a first argument.
```jsx
const useStyles = makeStyles({
root: {}, // a style rule
label: {}, // a nested style rule
});
function Nested(props) {
const classes = useStyles(props);
return (
<button className={classes.root}>
<span className={classes.label}>{/* 'jss2 my-label' nested */}</span>
</button>
);
}
function Parent() {
return <Nested classes={{ label: 'my-label' }} />;
}
```
## JSS plugins
JSS uses plugins to extend its core, allowing you to cherry-pick the features you need,
and only pay the performance overhead for what you are using.
Not all the plugins are available in Material UI by default. The following (which is a subset of
[jss-preset-default](https://cssinjs.org/jss-preset-default/)) are included:
- [jss-plugin-rule-value-function](https://cssinjs.org/jss-plugin-rule-value-function/)
- [jss-plugin-global](https://cssinjs.org/jss-plugin-global/)
- [jss-plugin-nested](https://cssinjs.org/jss-plugin-nested/)
- [jss-plugin-camel-case](https://cssinjs.org/jss-plugin-camel-case/)
- [jss-plugin-default-unit](https://cssinjs.org/jss-plugin-default-unit/)
- [jss-plugin-vendor-prefixer](https://cssinjs.org/jss-plugin-vendor-prefixer/)
- [jss-plugin-props-sort](https://cssinjs.org/jss-plugin-props-sort/)
Of course, you are free to use additional plugins. Here is an example with the [jss-rtl](https://github.com/alitaheri/jss-rtl) plugin.
```jsx
import { create } from 'jss';
import { StylesProvider, jssPreset } from '@mui/styles';
import rtl from 'jss-rtl';
const jss = create({
plugins: [...jssPreset().plugins, rtl()],
});
export default function App() {
return <StylesProvider jss={jss}>...</StylesProvider>;
}
```
## String templates
If you prefer CSS syntax over JSS, you can use the [jss-plugin-template](https://cssinjs.org/jss-plugin-template/) plugin.
```jsx
const useStyles = makeStyles({
root: `
background: linear-gradient(45deg, #fe6b8b 30%, #ff8e53 90%);
border-radius: 3px;
font-size: 16px;
border: 0;
color: white;
height: 48px;
padding: 0 30px;
box-shadow: 0 3px 5px 2px rgba(255, 105, 135, 0.3);
`,
});
```
Note that this doesn't support selectors, or nested rules.
{{"demo": "StringTemplates.js"}}
## CSS injection order
:::warning
It's **really important** to understand how the CSS specificity is calculated by the browser, as it's one of the key elements to know when overriding styles.
Read this section from the MDN docs for more information: [How is specificity calculated?](https://developer.mozilla.org/en-US/docs/Web/CSS/Specificity#How_is_specificity_calculated)
:::
By default, the style tags are injected **last** in the `<head>` element of the page.
They gain more specificity than any other style tags on your page e.g. CSS modules, styled components.
### injectFirst
The `StylesProvider` component has an `injectFirst` prop to inject the style tags **first** in the head (less priority):
```jsx
import { StylesProvider } from '@mui/styles';
<StylesProvider injectFirst>
{/* Your component tree.
Styled components can override Material UI's styles. */}
</StylesProvider>;
```
### `makeStyles` / `withStyles` / `styled`
The injection of style tags happens in the **same order** as the `makeStyles` / `withStyles` / `styled` invocations. For instance the color red wins in this case:
```jsx
import clsx from 'clsx';
import { makeStyles } from '@mui/styles';
const useStylesBase = makeStyles({
root: {
color: 'blue', // 🔵
},
});
const useStyles = makeStyles({
root: {
color: 'red', // 🔴
},
});
export default function MyComponent() {
// Order doesn't matter
const classes = useStyles();
const classesBase = useStylesBase();
// Order doesn't matter
const className = clsx(classes.root, classesBase.root);
// color: red 🔴 wins.
return <div className={className} />;
}
```
The hook call order and the class name concatenation order **don't matter**.
### insertionPoint
JSS [provides a mechanism](https://github.com/cssinjs/jss/blob/master/docs/setup.md#specify-the-dom-insertion-point) to control this situation.
By adding an `insertionPoint` within the HTML you can [control the order](https://cssinjs.org/jss-api/#attach-style-sheets-in-a-specific-order) that the CSS rules are applied to your components.
#### HTML comment
The simplest approach is to add an HTML comment to the `<head>` that determines where JSS will inject the styles:
```html
<head>
<!-- jss-insertion-point -->
<link href="..." />
</head>
```
```jsx
import { create } from 'jss';
import { StylesProvider, jssPreset } from '@mui/styles';
const jss = create({
...jssPreset(),
// Define a custom insertion point that JSS will look for when injecting the styles into the DOM.
insertionPoint: 'jss-insertion-point',
});
export default function App() {
return <StylesProvider jss={jss}>...</StylesProvider>;
}
```
#### Other HTML elements
[Create React App](https://github.com/facebook/create-react-app) strips HTML comments when creating the production build.
To get around this issue, you can provide a DOM element (other than a comment) as the JSS insertion point, for example, a `<noscript>` element:
```jsx
<head>
<noscript id="jss-insertion-point" />
<link href="..." />
</head>
```
```jsx
import { create } from 'jss';
import { StylesProvider, jssPreset } from '@mui/styles';
const jss = create({
...jssPreset(),
// Define a custom insertion point that JSS will look for when injecting the styles into the DOM.
insertionPoint: document.getElementById('jss-insertion-point'),
});
export default function App() {
return <StylesProvider jss={jss}>...</StylesProvider>;
}
```
#### JS createComment
codesandbox.io prevents access to the `<head>` element.
To get around this issue, you can use the JavaScript `document.createComment()` API:
```jsx
import { create } from 'jss';
import { StylesProvider, jssPreset } from '@mui/styles';
const styleNode = document.createComment('jss-insertion-point');
document.head.insertBefore(styleNode, document.head.firstChild);
const jss = create({
...jssPreset(),
// Define a custom insertion point that JSS will look for when injecting the styles into the DOM.
insertionPoint: 'jss-insertion-point',
});
export default function App() {
return <StylesProvider jss={jss}>...</StylesProvider>;
}
```
## Server-side rendering
This example returns a string of HTML and inlines the critical CSS required, right before it's used:
```jsx
import * as ReactDOMServer from 'react-dom/server';
import { ServerStyleSheets } from '@mui/styles';
function render() {
const sheets = new ServerStyleSheets();
const html = ReactDOMServer.renderToString(sheets.collect(<App />));
const css = sheets.toString();
return `
<!DOCTYPE html>
<html>
<head>
<style id="jss-server-side">${css}</style>
</head>
<body>
<div id="root">${html}</div>
</body>
</html>
`;
}
```
You can [follow the server side guide](/material-ui/guides/server-rendering/) for a more detailed example, or read the [`ServerStyleSheets` API documentation](/system/styles/api/#serverstylesheets).
### CSS prefixing
Be aware that some CSS features [require](https://github.com/cssinjs/jss/issues/279) an additional postprocessing step
that adds vendor-specific prefixes.
These prefixes are automatically added to the client thanks to [`jss-plugin-vendor-prefixer`](https://www.npmjs.com/package/jss-plugin-vendor-prefixer).
The CSS served on this documentation is processed with [`autoprefixer`](https://www.npmjs.com/package/autoprefixer).
You can use [the documentation implementation](https://github.com/mui/material-ui/blob/47aa5aeaec1d4ac2c08fd0e84277d6b91e497557/pages/_document.js#L123) as inspiration.
Be aware that it has an implication with the performance of the page.
It's a must-do for static pages, but it needs to be put in balance with not doing anything when rendering dynamic pages.
### Gatsby
There is [an official Gatsby plugin](https://github.com/hupe1980/gatsby-plugin-material-ui) that enables server-side rendering for `@mui/styles`.
Refer to the plugin's page for setup and usage instructions.
<!-- #default-branch-switch -->
Refer to [this example Gatsby project](https://github.com/mui/material-ui/tree/v4.x/examples/gatsby) for an usage example.
### Next.js Pages Router
You need to have a custom `pages/_document.js`, then copy [this logic](https://github.com/mui/material-ui/blob/v4.x/examples/nextjs/pages/_document.js#L52-L59) to inject the server-side rendered styles into the `<head>` element.
<!-- #default-branch-switch -->
Refer to [this example project](https://github.com/mui/material-ui/tree/v4.x/examples/nextjs) for an up-to-date usage example.
## Class names
The class names are generated by [the class name generator](/system/styles/api/#creategenerateclassname-options-class-name-generator).
### Default
By default, the class names generated by `@mui/styles` are **non-deterministic**; you can't rely on them to stay the same. Let's take the following style as an example:
```js
const useStyles = makeStyles({
root: {
opacity: 1,
},
});
```
This will generate a class name such as `makeStyles-root-123`.
You have to use the `classes` prop of a component to override the styles.
The non-deterministic nature of the class names enables style isolation.
- In **development**, the class name is: `.makeStyles-root-123`, following this logic:
```js
const sheetName = 'makeStyles';
const ruleName = 'root';
const identifier = 123;
const className = `${sheetName}-${ruleName}-${identifier}`;
```
- In **production**, the class name is: `.jss123`, following this logic:
```js
const productionPrefix = 'jss';
const identifier = 123;
const className = `${productionPrefix}${identifier}`;
```
However, when the following conditions are met, the class names are **deterministic**:
- Only one theme provider is used (**No theme nesting**)
- The style sheet has a name that starts with `Mui` (all Material UI components).
- The `disableGlobal` option of the [class name generator](/system/styles/api/#creategenerateclassname-options-class-name-generator) is `false` (the default).
## Global CSS
### `jss-plugin-global`
The [`jss-plugin-global`](#jss-plugins) plugin is installed in the default preset.
You can use it to define global class names.
{{"demo": "GlobalCss.js"}}
### Hybrid
You can also combine JSS generated class names with global ones.
{{"demo": "HybridGlobalCss.js"}}
## CSS prefixes
JSS uses feature detection to apply the correct prefixes.
[Don't be surprised](https://github.com/mui/material-ui/issues/9293) if you can't see a specific prefix in the latest version of Chrome. Your browser probably doesn't need it.
## TypeScript usage
Using `withStyles` in TypeScript can be a little tricky, but there are some utilities to make the experience as painless as possible.
### Using `createStyles` to defeat type widening
A frequent source of confusion is TypeScript's [type widening](https://mariusschulz.com/blog/literal-type-widening-in-typescript), which causes this example not to work as expected:
```ts
const styles = {
root: {
display: 'flex',
flexDirection: 'column',
},
};
withStyles(styles);
// ^^^^^^
// Types of property 'flexDirection' are incompatible.
// Type 'string' is not assignable to type '"-moz-initial" | "inherit" | "initial" | "revert" | "unset" | "column" | "column-reverse" | "row"...'.
```
The problem is that the type of the `flexDirection` prop is inferred as `string`, which is too wide. To fix this, you can pass the styles object directly to `withStyles`:
```ts
withStyles({
root: {
display: 'flex',
flexDirection: 'column',
},
});
```
However type widening rears its ugly head once more if you try to make the styles depend on the theme:
```ts
withStyles(({ palette, spacing }) => ({
root: {
display: 'flex',
flexDirection: 'column',
padding: spacing.unit,
backgroundColor: palette.background.default,
color: palette.primary.main,
},
}));
```
This is because TypeScript [widens the return types of function expressions](https://github.com/Microsoft/TypeScript/issues/241).
Because of this, using the `createStyles` helper function to construct your style rules object is recommended:
```ts
// Non-dependent styles
const styles = createStyles({
root: {
display: 'flex',
flexDirection: 'column',
},
});
// Theme-dependent styles
const styles = ({ palette, spacing }: Theme) =>
createStyles({
root: {
display: 'flex',
flexDirection: 'column',
padding: spacing.unit,
backgroundColor: palette.background.default,
color: palette.primary.main,
},
});
```
`createStyles` is just the identity function; it doesn't "do anything" at runtime, just helps guide type inference at compile time.
### Media queries
`withStyles` allows a styles object with top level media-queries like so:
```ts
const styles = createStyles({
root: {
minHeight: '100vh',
},
'@media (min-width: 960px)': {
root: {
display: 'flex',
},
},
});
```
To allow these styles to pass TypeScript however, the definitions have to be unambiguous concerning the names for CSS classes and actual CSS property names. Due to this, class names that are equal to CSS properties should be avoided.
```ts
// error because TypeScript thinks `@media (min-width: 960px)` is a class name
// and `content` is the CSS property
const ambiguousStyles = createStyles({
content: {
minHeight: '100vh',
},
'@media (min-width: 960px)': {
content: {
display: 'flex',
},
},
});
// works just fine
const ambiguousStyles = createStyles({
contentClass: {
minHeight: '100vh',
},
'@media (min-width: 960px)': {
contentClass: {
display: 'flex',
},
},
});
```
### Augmenting your props using `WithStyles`
Since a component decorated with `withStyles(styles)` gets a special `classes` prop injected, you will want to define its props accordingly:
```ts
const styles = (theme: Theme) =>
createStyles({
root: {
/* ... */
},
paper: {
/* ... */
},
button: {
/* ... */
},
});
interface Props {
// non-style props
foo: number;
bar: boolean;
// injected style props
classes: {
root: string;
paper: string;
button: string;
};
}
```
However this isn't very [DRY](https://en.wikipedia.org/wiki/Don%27t_repeat_yourself) because it requires you to maintain the class names (`'root'`, `'paper'`, `'button'`, ...) in two different places. We provide a type operator `WithStyles` to help with this, so that you can just write:
```ts
import { createStyles, WithStyles } from '@mui/styles';
const styles = (theme: Theme) =>
createStyles({
root: {
/* ... */
},
paper: {
/* ... */
},
button: {
/* ... */
},
});
interface Props extends WithStyles<typeof styles> {
foo: number;
bar: boolean;
}
```
### Decorating components
Applying `withStyles(styles)` as a function works as expected:
```tsx
const DecoratedSFC = withStyles(styles)(({ text, type, color, classes }: Props) => (
<Typography variant={type} color={color} classes={classes}>
{text}
</Typography>
));
const DecoratedClass = withStyles(styles)(
class extends React.Component<Props> {
render() {
const { text, type, color, classes } = this.props;
return (
<Typography variant={type} color={color} classes={classes}>
{text}
</Typography>
);
}
},
);
```
Unfortunately due to a [current limitation of TypeScript decorators](https://github.com/Microsoft/TypeScript/issues/4881), `withStyles(styles)` can't be used as a decorator in TypeScript.
| 3,687 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/api/api.md | ---
title: Styles API
---
# API (LEGACY)
<p class="description">The API reference for @mui/styles.</p>
:::error
`@mui/styles` was deprecated with the release of MUI Core v5 in late 2021.
It depended on [JSS](https://cssinjs.org/) as a styling solution, which is no longer used in `@mui/material`.
`@mui/styles` is not compatible with [React.StrictMode](https://react.dev/reference/react/StrictMode) or React 18, and it will not be updated.
This documentation remains here for those working on legacy projects, but we **strongly discourage** you from using `@mui/styles` when creating a new app with Material UI—you _will_ face unresolvable dependency issues.
Please use [`@mui/system`](/system/getting-started/) instead.
:::
## `createGenerateClassName([options]) => class name generator`
A function which returns [a class name generator function](https://cssinjs.org/jss-api/#generate-your-class-names).
### Arguments
1. `options` (_object_ [optional]):
- `options.disableGlobal` (_bool_ [optional]): Defaults to `false`. Disable the generation of deterministic class names.
- `options.productionPrefix` (_string_ [optional]): Defaults to `'jss'`. The string used to prefix the class names in production.
- `options.seed` (_string_ [optional]): Defaults to `''`. The string used to uniquely identify the generator. It can be used to avoid class name collisions when using multiple generators in the same document.
### Returns
`class name generator`: The generator should be provided to JSS.
### Examples
```jsx
import * as React from 'react';
import { StylesProvider, createGenerateClassName } from '@mui/styles';
const generateClassName = createGenerateClassName({
productionPrefix: 'c',
});
export default function App() {
return <StylesProvider generateClassName={generateClassName}>...</StylesProvider>;
}
```
## `createStyles(styles) => styles`
This function doesn't really "do anything" at runtime, it's just the identity
function. Its only purpose is to defeat **TypeScript**'s type widening when providing
style rules to `makeStyles`/`withStyles` which are a function of the `Theme`.
### Arguments
1. `styles` (_object_): A styles object.
### Returns
`styles`: A styles object.
### Examples
```jsx
import { createStyles, makeStyles } from '@mui/styles';
import { createTheme, ThemeProvider } from '@mui/material/styles';
const useStyles = makeStyles((theme: Theme) =>
createStyles({
root: {
backgroundColor: theme.palette.red,
},
}),
);
const theme = createTheme();
export default function MyComponent() {
const classes = useStyles();
return (
<ThemeProvider theme={theme}>
<div className={classes.root} />
</ThemeProvider>
);
}
```
## `makeStyles(styles, [options]) => hook`
Link a style sheet with a function component using the **hook** pattern.
### Arguments
1. `styles` (_Function | Object_): A function generating the styles or a styles object.
It will be linked to the component.
Use the function signature if you need to have access to the theme. It's provided as the first argument.
2. `options` (_object_ [optional]):
- `options.defaultTheme` (_object_ [optional]): The default theme to use if a theme isn't supplied through a Theme Provider.
- `options.name` (_string_ [optional]): The name of the style sheet. Useful for debugging.
- `options.flip` (_bool_ [optional]): When set to `false`, this sheet will opt-out the `rtl` transformation. When set to `true`, the styles are inversed. When set to `null`, it follows `theme.direction`.
- The other keys are forwarded to the options argument of [jss.createStyleSheet([styles], [options])](https://cssinjs.org/jss-api/#create-style-sheet).
### Returns
`hook`: A hook. This hook can be used in a function component. The documentation often calls this returned hook `useStyles`.
It accepts one argument: the props that will be used for "interpolation" in
the style sheet.
### Examples
```jsx
import * as React from 'react';
import { makeStyles } from '@mui/styles';
const useStyles = makeStyles({
root: {
backgroundColor: 'red',
color: (props) => props.color,
},
});
export default function MyComponent(props) {
const classes = useStyles(props);
return <div className={classes.root} />;
}
```
## `ServerStyleSheets`
This is a class helper to handle server-side rendering. [You can follow this guide for a practical approach](/material-ui/guides/server-rendering/).
```jsx
import * as ReactDOMServer from 'react-dom/server';
import { ServerStyleSheets } from '@mui/styles';
const sheets = new ServerStyleSheets();
const html = ReactDOMServer.renderToString(sheets.collect(<App />));
const cssString = sheets.toString();
const response = `
<!DOCTYPE html>
<html>
<head>
<style id="jss-server-side">${cssString}</style>
</head>
<body>${html}</body>
</html>
`;
```
### `new ServerStyleSheets([options])`
The instantiation accepts an options object as a first argument.
1. `options` (_object_ [optional]): The options are spread as props to the [`StylesProvider`](#stylesprovider) component.
### `sheets.collect(node) => React element`
The method wraps your React node in a provider element.
It collects the style sheets during the rendering so they can be later sent to the client.
### `sheets.toString() => CSS string`
The method returns the collected styles.
⚠️ You must call `.collect()` before using this method.
### `sheets.getStyleElement() => CSS React element`
The method is an alternative to `.toString()` when you are rendering the whole page with React.
⚠️ You must call `.collect()` before using this method.
## `styled(Component)(styles, [options]) => Component`
Link a style sheet with a function component using the **styled components** pattern.
### Arguments
1. `Component`: The component that will be wrapped.
2. `styles` (_Function | Object_): A function generating the styles or a styles object.
It will be linked to the component.
Use the function signature if you need to have access to the theme. It's provided as property of the first argument.
3. `options` (_object_ [optional]):
- `options.defaultTheme` (_object_ [optional]): The default theme to use if a theme isn't supplied through a Theme Provider.
- `options.withTheme` (_bool_ [optional]): Defaults to `false`. Provide the `theme` object to the component as a prop.
- `options.name` (_string_ [optional]): The name of the style sheet. Useful for debugging.
If the value isn't provided, it will try to fallback to the name of the component.
- `options.flip` (_bool_ [optional]): When set to `false`, this sheet will opt-out the `rtl` transformation. When set to `true`, the styles are inversed. When set to `null`, it follows `theme.direction`.
- The other keys are forwarded to the options argument of [jss.createStyleSheet([styles], [options])](https://cssinjs.org/jss-api/#create-style-sheet).
### Returns
`Component`: The new component created.
### Examples
```jsx
import * as React from 'react';
import { styled, ThemeProvider } from '@mui/styles';
import { createTheme } from '@mui/material/styles';
const MyComponent = styled('div')({
backgroundColor: 'red',
});
const MyThemeComponent = styled('div')(({ theme }) => ({
padding: theme.spacing(1),
}));
const theme = createTheme();
export default function StyledComponents() {
return (
<ThemeProvider theme={theme}>
<MyThemeComponent>
<MyComponent />
</MyThemeComponent>
<ThemeProvider>
);
}
```
## `StylesProvider`
This component allows you to change the behavior of the styling solution. It makes the options available down the React tree thanks to the context.
It should preferably be used at **the root of your component tree**.
### Props
| Name | Type | Default | Description |
| :---------------- | :----- | :------ | :------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| children \* | node | | Your component tree. |
| disableGeneration | bool | false | You can disable the generation of the styles with this option. It can be useful when traversing the React tree outside of the HTML rendering step on the server. Let's say you are using react-apollo to extract all the queries made by the interface server-side. You can significantly speed up the traversal with this prop. |
| generateClassName | func | | JSS's class name generator. |
| injectFirst | bool | false | By default, the styles are injected last in the `<head>` element of the page. As a result, they gain more specificity than any other style sheet. If you want to override Material UI's styles, set this prop. |
| jss | object | | JSS's instance. |
### Examples
```jsx
import * as React from 'react';
import { StylesProvider } from '@mui/styles';
function App() {
return <StylesProvider jss={jss}>...</StylesProvider>;
}
```
## `ThemeProvider`
This component takes a `theme` prop, and makes it available down the React tree thanks to the context.
It should preferably be used at **the root of your component tree**.
### Props
| Name | Type | Default | Description |
| :--------------- | :--------------------------------------- | :------ | :-------------------------------------------------------------------- |
| children \* | node | | Your component tree. |
| theme \* | union: object | func | | A theme object. You can provide a function to extend the outer theme. |
### Examples
```jsx
import * as React from 'react';
import { ThemeProvider } from '@mui/material/styles';
const theme = {};
function App() {
return <ThemeProvider theme={theme}>...</ThemeProvider>;
}
```
## `useTheme() => theme`
This hook returns the `theme` object so it can be used inside a function component.
### Returns
`theme`: The theme object previously injected in the context.
### Examples
```jsx
import * as React from 'react';
import { useTheme } from '@mui/material/styles';
export default function MyComponent() {
const theme = useTheme();
return <div>{`spacing ${theme.spacing}`}</div>;
}
```
## `withStyles(styles, [options]) => higher-order component`
Link a style sheet with a component using the **higher-order component** pattern.
It does not modify the component passed to it; instead, it returns a new component with a `classes` prop.
This `classes` object contains the name of the class names injected in the DOM.
Some implementation details that might be interesting to being aware of:
- It adds a `classes` prop so you can override the injected class names from the outside.
- It forwards refs to the inner component.
- It does **not** copy over statics.
For instance, it can be used to define a `getInitialProps()` static method (next.js).
### Arguments
1. `styles` (_Function | Object_): A function generating the styles or a styles object.
It will be linked to the component.
Use the function signature if you need to have access to the theme. It's provided as the first argument.
2. `options` (_object_ [optional]):
- `options.defaultTheme` (_object_ [optional]): The default theme to use if a theme isn't supplied through a Theme Provider.
- `options.withTheme` (_bool_ [optional]): Defaults to `false`. Provide the `theme` object to the component as a prop.
- `options.name` (_string_ [optional]): The name of the style sheet. Useful for debugging.
If the value isn't provided, it will try to fallback to the name of the component.
- `options.flip` (_bool_ [optional]): When set to `false`, this sheet will opt-out the `rtl` transformation. When set to `true`, the styles are inversed. When set to `null`, it follows `theme.direction`.
- The other keys are forwarded to the options argument of [jss.createStyleSheet([styles], [options])](https://cssinjs.org/jss-api/#create-style-sheet).
### Returns
`higher-order component`: Should be used to wrap a component.
### Examples
```jsx
import * as React from 'react';
import { withStyles } from '@mui/styles';
const styles = {
root: {
backgroundColor: 'red',
},
};
function MyComponent(props) {
return <div className={props.classes.root} />;
}
export default withStyles(styles)(MyComponent);
```
Also, you can use as [decorators](https://babeljs.io/docs/babel-plugin-proposal-decorators) like so:
```jsx
import * as React from 'react';
import { withStyles } from '@mui/styles';
const styles = {
root: {
backgroundColor: 'red',
},
};
@withStyles(styles)
class MyComponent extends React.Component {
render() {
return <div className={this.props.classes.root} />;
}
}
export default MyComponent;
```
## `withTheme(Component) => Component`
Provide the `theme` object as a prop of the input component so it can be used
in the render method.
### Arguments
1. `Component`: The component that will be wrapped.
### Returns
`Component`: The new component created. Does forward refs to the inner component.
### Examples
```jsx
import * as React from 'react';
import { withTheme } from '@mui/styles';
function MyComponent(props) {
return <div>{props.theme.direction}</div>;
}
export default withTheme(MyComponent);
```
| 3,688 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/basics/AdaptingHOC.js | import * as React from 'react';
import PropTypes from 'prop-types';
import { withStyles } from '@mui/styles';
import Button from '@mui/material/Button';
const styles = {
root: {
background: (props) =>
props.color === 'red'
? 'linear-gradient(45deg, #FE6B8B 30%, #FF8E53 90%)'
: 'linear-gradient(45deg, #2196F3 30%, #21CBF3 90%)',
border: 0,
borderRadius: 3,
boxShadow: (props) =>
props.color === 'red'
? '0 3px 5px 2px rgba(255, 105, 135, .3)'
: '0 3px 5px 2px rgba(33, 203, 243, .3)',
color: 'white',
height: 48,
padding: '0 30px',
margin: 8,
},
};
function MyButtonRaw(props) {
const { classes, color, ...other } = props;
return <Button className={classes.root} {...other} />;
}
MyButtonRaw.propTypes = {
classes: PropTypes.object.isRequired,
color: PropTypes.oneOf(['blue', 'red']).isRequired,
};
const MyButton = withStyles(styles)(MyButtonRaw);
export default function AdaptingHOC() {
return (
<React.Fragment>
<MyButton color="red">Red</MyButton>
<MyButton color="blue">Blue</MyButton>
</React.Fragment>
);
}
| 3,689 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/basics/AdaptingHOC.tsx | import * as React from 'react';
import { withStyles, WithStyles } from '@mui/styles';
import Button, { ButtonProps } from '@mui/material/Button';
const styles = {
root: {
background: (props: MyButtonRawProps) =>
props.color === 'red'
? 'linear-gradient(45deg, #FE6B8B 30%, #FF8E53 90%)'
: 'linear-gradient(45deg, #2196F3 30%, #21CBF3 90%)',
border: 0,
borderRadius: 3,
boxShadow: (props: MyButtonRawProps) =>
props.color === 'red'
? '0 3px 5px 2px rgba(255, 105, 135, .3)'
: '0 3px 5px 2px rgba(33, 203, 243, .3)',
color: 'white',
height: 48,
padding: '0 30px',
margin: 8,
},
};
interface MyButtonRawProps {
color: 'red' | 'blue';
}
// @babel-ignore-comment-in-output These are the props available inside MyButtonRaw
// @babel-ignore-comment-in-output They're different from MyButtonRawProps which are the props available for dynamic styling.
type MyButtonRawInnerProps = MyButtonRawProps & WithStyles<typeof styles>;
function MyButtonRaw(
props: MyButtonRawInnerProps & Omit<ButtonProps, keyof MyButtonRawInnerProps>,
) {
const { classes, color, ...other } = props;
return <Button className={classes.root} {...other} />;
}
const MyButton = withStyles(styles)(MyButtonRaw);
export default function AdaptingHOC() {
return (
<React.Fragment>
<MyButton color="red">Red</MyButton>
<MyButton color="blue">Blue</MyButton>
</React.Fragment>
);
}
| 3,690 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/basics/AdaptingHOC.tsx.preview | <React.Fragment>
<MyButton color="red">Red</MyButton>
<MyButton color="blue">Blue</MyButton>
</React.Fragment> | 3,691 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/basics/AdaptingHook.js | import * as React from 'react';
import PropTypes from 'prop-types';
import { makeStyles } from '@mui/styles';
import Button from '@mui/material/Button';
const useStyles = makeStyles({
root: {
background: (props) =>
props.color === 'red'
? 'linear-gradient(45deg, #FE6B8B 30%, #FF8E53 90%)'
: 'linear-gradient(45deg, #2196F3 30%, #21CBF3 90%)',
border: 0,
borderRadius: 3,
boxShadow: (props) =>
props.color === 'red'
? '0 3px 5px 2px rgba(255, 105, 135, .3)'
: '0 3px 5px 2px rgba(33, 203, 243, .3)',
color: 'white',
height: 48,
padding: '0 30px',
margin: 8,
},
});
function MyButton(props) {
const { color, ...other } = props;
const classes = useStyles(props);
return <Button className={classes.root} {...other} />;
}
MyButton.propTypes = {
color: PropTypes.oneOf(['blue', 'red']).isRequired,
};
export default function AdaptingHook() {
return (
<React.Fragment>
<MyButton color="red">Red</MyButton>
<MyButton color="blue">Blue</MyButton>
</React.Fragment>
);
}
| 3,692 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/basics/AdaptingHook.tsx | import * as React from 'react';
import { makeStyles } from '@mui/styles';
import Button, { ButtonProps as MuiButtonProps } from '@mui/material/Button';
interface Props {
color: 'red' | 'blue';
}
const useStyles = makeStyles({
root: {
background: (props: Props) =>
props.color === 'red'
? 'linear-gradient(45deg, #FE6B8B 30%, #FF8E53 90%)'
: 'linear-gradient(45deg, #2196F3 30%, #21CBF3 90%)',
border: 0,
borderRadius: 3,
boxShadow: (props: Props) =>
props.color === 'red'
? '0 3px 5px 2px rgba(255, 105, 135, .3)'
: '0 3px 5px 2px rgba(33, 203, 243, .3)',
color: 'white',
height: 48,
padding: '0 30px',
margin: 8,
},
});
function MyButton(props: Props & Omit<MuiButtonProps, keyof Props>) {
const { color, ...other } = props;
const classes = useStyles(props);
return <Button className={classes.root} {...other} />;
}
export default function AdaptingHook() {
return (
<React.Fragment>
<MyButton color="red">Red</MyButton>
<MyButton color="blue">Blue</MyButton>
</React.Fragment>
);
}
| 3,693 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/basics/AdaptingHook.tsx.preview | <React.Fragment>
<MyButton color="red">Red</MyButton>
<MyButton color="blue">Blue</MyButton>
</React.Fragment> | 3,694 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/basics/AdaptingStyledComponents.js | import * as React from 'react';
import { styled } from '@mui/styles';
import Button from '@mui/material/Button';
const MyButton = styled(({ color, ...other }) => <Button {...other} />)({
background: (props) =>
props.color === 'red'
? 'linear-gradient(45deg, #FE6B8B 30%, #FF8E53 90%)'
: 'linear-gradient(45deg, #2196F3 30%, #21CBF3 90%)',
border: 0,
borderRadius: 3,
boxShadow: (props) =>
props.color === 'red'
? '0 3px 5px 2px rgba(255, 105, 135, .3)'
: '0 3px 5px 2px rgba(33, 203, 243, .3)',
color: 'white',
height: 48,
padding: '0 30px',
margin: 8,
});
export default function AdaptingStyledComponents() {
return (
<React.Fragment>
<MyButton color="red">Red</MyButton>
<MyButton color="blue">Blue</MyButton>
</React.Fragment>
);
}
| 3,695 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/basics/AdaptingStyledComponents.tsx | import * as React from 'react';
import { styled } from '@mui/styles';
import Button, { ButtonProps } from '@mui/material/Button';
interface MyButtonProps {
color: 'red' | 'blue';
}
const MyButton = styled(
({ color, ...other }: MyButtonProps & Omit<ButtonProps, keyof MyButtonProps>) => (
<Button {...other} />
),
)({
background: (props: MyButtonProps) =>
props.color === 'red'
? 'linear-gradient(45deg, #FE6B8B 30%, #FF8E53 90%)'
: 'linear-gradient(45deg, #2196F3 30%, #21CBF3 90%)',
border: 0,
borderRadius: 3,
boxShadow: (props: MyButtonProps) =>
props.color === 'red'
? '0 3px 5px 2px rgba(255, 105, 135, .3)'
: '0 3px 5px 2px rgba(33, 203, 243, .3)',
color: 'white',
height: 48,
padding: '0 30px',
margin: 8,
});
export default function AdaptingStyledComponents() {
return (
<React.Fragment>
<MyButton color="red">Red</MyButton>
<MyButton color="blue">Blue</MyButton>
</React.Fragment>
);
}
| 3,696 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/basics/AdaptingStyledComponents.tsx.preview | <React.Fragment>
<MyButton color="red">Red</MyButton>
<MyButton color="blue">Blue</MyButton>
</React.Fragment> | 3,697 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/basics/HigherOrderComponent.js | import * as React from 'react';
import PropTypes from 'prop-types';
import { withStyles } from '@mui/styles';
import Button from '@mui/material/Button';
const styles = {
root: {
background: 'linear-gradient(45deg, #FE6B8B 30%, #FF8E53 90%)',
border: 0,
borderRadius: 3,
boxShadow: '0 3px 5px 2px rgba(255, 105, 135, .3)',
color: 'white',
height: 48,
padding: '0 30px',
},
};
function UnstyledComponent(props) {
const { classes } = props;
return <Button className={classes.root}>Styled with HOC API</Button>;
}
UnstyledComponent.propTypes = {
classes: PropTypes.object.isRequired,
};
export default withStyles(styles)(UnstyledComponent);
| 3,698 |
0 | petrpan-code/mui/material-ui/docs/data/styles | petrpan-code/mui/material-ui/docs/data/styles/basics/HigherOrderComponent.tsx | import * as React from 'react';
import { withStyles, WithStyles } from '@mui/styles';
import Button from '@mui/material/Button';
const styles = {
root: {
background: 'linear-gradient(45deg, #FE6B8B 30%, #FF8E53 90%)',
border: 0,
borderRadius: 3,
boxShadow: '0 3px 5px 2px rgba(255, 105, 135, .3)',
color: 'white',
height: 48,
padding: '0 30px',
},
};
function UnstyledComponent(props: WithStyles<typeof styles>) {
const { classes } = props;
return <Button className={classes.root}>Styled with HOC API</Button>;
}
export default withStyles(styles)(UnstyledComponent);
| 3,699 |
Subsets and Splits