path
stringlengths 7
265
| concatenated_notebook
stringlengths 46
17M
|
---|---|
Hecho/Results_StructLab15_5/.ipynb_checkpoints/Importing and exporting gocad property into python-checkpoint.ipynb | ###Markdown
Importing
###Code
def read_voxet(voxetname,propertyfile):
"""
Read a gocad property file and the geometry information from the .vo file
voxetname - is the path to the voxet file
propertyfile is the path to the binary file
Returns
origin numpy array
voxet_extent - is the length of each axis of the voxet
N is the number of steps in the voxet
array is the property values
steps is the size of the step vector for the voxet
"""
array = np.fromfile(propertyfile,dtype='float32')
array = array.newbyteorder()
with open(voxetname,'r') as file:
for l in file:
if 'AXIS_O ' in l:
origin = np.array(re.findall(r'[\d\.\d]+',l)).astype(float)
if 'AXIS_U ' in l:
U = float(re.findall(r'[\d\.\d]+',l)[0])
if 'AXIS_V ' in l:
V = float(re.findall(r'[\d\.\d]+',l)[1])
if 'AXIS_W ' in l:
W = float(re.findall(r'[\d\.\d]+',l)[2])
if 'AXIS_N ' in l:
N = np.array(re.findall(r'[\d\.\d]+',l)).astype(int)
voxet_extent = np.array([U,V,W])
steps = (maximum ) / (N-1)
return origin, voxet_extent, N, array, steps
origin, voxet_extent, nsteps, array = read_voxet("Hecho_Sparse.vo","Hecho_SparseResultBox_StructLab@@")
def write_property_to_gocad_voxet(propertyfilename, propertyvalues):
"""
This function writes a numpy array into the right format for a gocad
voxet property file. This assumet there is a property already added to the .vo file,
and is just updating the file.
propertyfile - string giving the path to the file to write
propertyvalues - numpy array nz,ny,nx ordering and in float format
"""
array = propertyvalues.newbyteorder()
array.tofile(propertyfilename)
array
write_property_to_gocad_voxet('../Results_/ResultVoxetResultBox_Strati@@',array)
###Output
_____no_output_____
###Markdown
Visualising using pyvista
###Code
# Create the spatial reference
grid = pv.UniformGrid()
# Set the grid dimensions: shape because we want to inject our values on the
# POINT data
grid.dimensions = N#slicedIm.shape
# Edit the spatial reference
grid.origin = origin#(0, 0, 0) # The bottom left corner of the data set
grid.spacing = steps # These are the cell sizes along each axis
# Add the data values to the cell data
# grid.point_arrays['Z'] =
grid.point_arrays["values"] = array.astype(float)
# Now plot the grid!
grid.plot(show_edges=False)
###Output
_____no_output_____
###Markdown
Visualise using matplotlib
###Code
array2 = array.reshape(N[2],N[1],N[0]) # [z,y,x]
plt.imshow(array2[:,0,:])
###Output
_____no_output_____ |
notebooks/Tuning/Bloom_Timing_202007F-allyears.ipynb | ###Markdown
202007F bloom timing
###Code
import datetime as dt
from erddapy import ERDDAP
import glob
import matplotlib.pyplot as plt
import netCDF4 as nc
import numpy as np
import pandas as pd
from pathlib import Path
import xarray as xr
from salishsea_tools import places
%matplotlib inline
###Output
_____no_output_____
###Markdown
Set up Model Results Getting
###Code
directory = '202007F'
dir_path = Path(f"/data/sallen/results/MEOPAR/202007/{directory}")
drop_vars = [
"time_centered",
"time_centered_bounds",
"time_counter_bounds",
"nav_lat",
"bounds_lat",
"nav_lon",
"bounds_lon",
"area",
"deptht_bounds",
]
chunks = {
"time_counter": 1,
"deptht": 1,
}
def prep_timeseries(dir_path, file_pattern, n_days):
ds_files = sorted(dir_path.glob(file_pattern))
ds = xr.open_mfdataset(
ds_files[:n_days],
chunks=chunks,
drop_variables=drop_vars,
)
S3y, S3x = places.PLACES['S3']['NEMO grid ji'][0], places.PLACES['S3']['NEMO grid ji'][1]
fourkm = 8
timeseries = ds.isel(deptht = 1, y = slice(S3y-fourkm, S3y+fourkm+1),
x = slice(S3x-fourkm, S3x+fourkm+1))
return timeseries
###Output
_____no_output_____
###Markdown
Set up Observations Getting
###Code
server = "https://salishsea.eos.ubc.ca/erddap"
protocol = "tabledap"
dataset_id = "ubcONCTWDP1mV18-01"
response = "nc"
variables = [
"latitude",
"longitude",
"chlorophyll",
"time",
]
fourkmlat = 4/110.574
fourkmlon = 4/(111.320*np.cos(50*np.pi/180.))
lon, lat = places.PLACES['S3']['lon lat']
###Output
_____no_output_____
###Markdown
Plotting Functions
###Code
def model_plot(timeseries2015):
fig, axs = plt.subplots(1, 2, figsize=(15, 4))
for i in range(timeseries2015.x.shape[0]):
for j in range(timeseries2015.y.shape[0]):
timeseries2015.diatoms[:, j, i].plot(ax=axs[0], color='blue', marker='o')
timeseries2015.diatoms[:, j, i].plot(ax=axs[1], color='blue', marker='o')
ax2 = axs[0].twinx()
for i in range(timeseries2015.x.shape[0]):
for j in range(timeseries2015.y.shape[0]):
timeseries2015.nitrate[:, j, i].plot(ax=ax2, color='lightblue', marker='o')
axs[0].grid()
for i in range(timeseries2015.x.shape[0]):
for j in range(timeseries2015.y.shape[0]):
(timeseries2015.diatoms+timeseries2015.flagellates+timeseries2015.ciliates)[:, j, i].plot(ax=axs[1], color='purple', marker='o', alpha=0.5);
def model_obs_comparison(timeseries2015, obs_pd, N2Chl=2.5):
fig, ax = plt.subplots(1, 1, figsize=(7, 4))
obs_pd['chlorophyll (ug/l)'].plot(ax=ax, marker='o', color='green', linewidth=0);
for i in range(timeseries2015.x.shape[0]):
for j in range(timeseries2015.y.shape[0]):
((timeseries2015.diatoms+timeseries2015.flagellates+timeseries2015.ciliates)[:, j, i]*N2Chl).plot(ax=ax, color='purple',
marker='.', alpha=0.5, linewidth=0);
###Output
_____no_output_____
###Markdown
2015 Model Results
###Code
year = 2015
months_regex = '0[23]'
file_pattern = f"SalishSea_1d_{year}{months_regex}*ptrc_T*.nc"
timeseries = prep_timeseries(dir_path, file_pattern, -1)
timeseries.load(scheduler='processes', num_workers=4);
model_plot(timeseries)
timeseries.diatoms[28-1:28-1+10].mean(axis=1).mean(axis=1).plot();
plt.grid()
###Output
_____no_output_____
###Markdown
Bloom is March 6. This bloom is three days early. Observations
###Code
constraints = {
"time>=": "2015-02-01T00:00:00Z",
"time<=": "2015-04-01T00:00:00Z",
"latitude>=": lat - fourkmlat,
"latitude<=": lat + fourkmlat,
"longitude>=": lon - fourkmlon,
"longitude<=": lon + fourkmlon,
}
obs = ERDDAP(server=server, protocol=protocol,)
obs.dataset_id = dataset_id
obs.variables = variables
obs.constraints = constraints
obs_pd = obs.to_pandas(index_col="time (UTC)", parse_dates=True,).dropna()
model_obs_comparison(timeseries, obs_pd, N2Chl=3)
obs_pd['chlorophyll (ug/l)'].groupby(pd.Grouper(freq='1D')).mean()
###Output
_____no_output_____
###Markdown
Bloom is March 9. 2016 Model Results
###Code
year = 2016
months_regex = '0[34]'
file_pattern = f"SalishSea_1d_{year}{months_regex}*ptrc_T*.nc"
timeseries = prep_timeseries(dir_path, file_pattern, -1)
timeseries.load(scheduler='processes', num_workers=4);
model_plot(timeseries)
timeseries.diatoms[29-1:32-1].mean(axis=1).mean(axis=1)
###Output
_____no_output_____
###Markdown
Bloom date is March 30. This value is 6 days late. Observations
###Code
constraints = {
"time>=": "2016-03-01T00:00:00Z",
"time<=": "2016-05-01T00:00:00Z",
"latitude>=": lat - fourkmlat,
"latitude<=": lat + fourkmlat,
"longitude>=": lon - fourkmlon,
"longitude<=": lon + fourkmlon,
}
obs = ERDDAP(server=server, protocol=protocol,)
obs.dataset_id = dataset_id
obs.variables = variables
obs.constraints = constraints
obs_pd = obs.to_pandas(index_col="time (UTC)", parse_dates=True,).dropna()
model_obs_comparison(timeseries, obs_pd, N2Chl=1.4)
obs_pd['chlorophyll (ug/l)'].groupby(pd.Grouper(freq='1D')).mean()[20:29]
###Output
_____no_output_____
###Markdown
Bloom date is March 24. 2017 Model Results
###Code
year = 2017
months_regex = '0[34]'
file_pattern = f"SalishSea_1d_{year}{months_regex}*ptrc_T*.nc"
timeseries = prep_timeseries(dir_path, file_pattern, -1)
timeseries.load(scheduler='processes', num_workers=4);
model_plot(timeseries)
timeseries.diatoms[32-1:42-1].mean(axis=1).mean(axis=1)
###Output
_____no_output_____
###Markdown
Bloom date is March 23. This date is 12 days late. Observations
###Code
constraints = {
"time>=": "2017-03-01T00:00:00Z",
"time<=": "2017-05-01T00:00:00Z",
"latitude>=": lat - fourkmlat,
"latitude<=": lat + fourkmlat,
"longitude>=": lon - fourkmlon,
"longitude<=": lon + fourkmlon,
}
obs = ERDDAP(server=server, protocol=protocol,)
obs.dataset_id = dataset_id
obs.variables = variables
obs.constraints = constraints
obs_pd = obs.to_pandas(index_col="time (UTC)", parse_dates=True,).dropna()
model_obs_comparison(timeseries, obs_pd, N2Chl=3)
###Output
_____no_output_____ |
2-Spit_Dataset.ipynb | ###Markdown
Split polyps / non-polyps datasetUse `polyps` and `non_polyps` from `cropped` to copy files into `train` and `validation` from `data_polyps`. This folder should already exists and have both subfolders (or ajust the script to create them). The current **data_polyps** folder will be the dataset folder for the next deep learning classifications.Therefore, we shall use a dataset split percentage such as **75% train** and **25% test**.We need `os` and `shutil` to manage the files, `random` to randomly split the dataset in train and validation subsets. You should have a folder structure such as:* `./data_polyps/train/polyps`* `./data_polyps/train/non_polyps`* `./data_polyps/validation/polyps`* `./data_polyps/validation/non_polyps`
###Code
import os
import random
import shutil
###Output
_____no_output_____
###Markdown
These lines are the locations for the source files (the entire dataset) and the future locations of the splitted dataset in train and validation subsets:
###Code
# Source dataset: from where to copy the files
sourceFolderClass1 = 'cropped/polyps'
sourceFolderClass2 = 'cropped/non_polyps'
# Destination folders: splitted dataset in train and validation for polyps and non-polyps
destFolderClass1_tr = 'data_polyps/train/polyps'
destFolderClass2_tr = 'data_polyps/train/non_polyps'
destFolderClass1_val = 'data_polyps/validation/polyps'
destFolderClass2_val = 'data_polyps/validation/non_polyps'
###Output
_____no_output_____
###Markdown
Get the list with all the files in the source folder:
###Code
sourceFiles1 = os.listdir(sourceFolderClass1)
sourceFiles2 = os.listdir(sourceFolderClass2)
print("Class 1 - polyps:", len(sourceFiles1))
print("Class 2 - non-polyps:", len(sourceFiles2))
###Output
Class 1 - polyps: 606
Class 2 - non-polyps: 606
###Markdown
Let's suffle the listw with the source files using a random seed:
###Code
random.seed(1)
random.shuffle(sourceFiles1)
random.shuffle(sourceFiles2)
###Output
_____no_output_____
###Markdown
We shall define a number of files to copy in the `validation` subfolder for each class. If you want a different split, you should modify `val_files`.
###Code
# No of file to copy in VALIDATION folder for each class
val_files = 151
# Copy the first 151 files for polyps and non-polyps into validation folders
print('--> Validation split ...')
for i in range(val_files):
# copy validation polyps
File1 = os.path.join(sourceFolderClass1,sourceFiles1[i])
File2 = os.path.join(destFolderClass1_val, sourceFiles1[i])
shutil.copy(File1,File2)
# copy validation non-polyps
File1 = os.path.join(sourceFolderClass2, sourceFiles2[i])
File2 = os.path.join(destFolderClass2_val, sourceFiles2[i])
shutil.copy(File1, File2)
print('--> Done!')
# Copy polyps to train
print('--> Train split ...')
for i in range(val_files,len(sourceFiles1)):
File1 = os.path.join(sourceFolderClass1, sourceFiles1[i])
File2 = os.path.join(destFolderClass1_tr, sourceFiles1[i])
shutil.copy(File1,File2)
# copy non-polyps to train
for i in range(val_files,len(sourceFiles2)):
File1 = os.path.join(sourceFolderClass2, sourceFiles2[i])
File2 = os.path.join(destFolderClass2_tr, sourceFiles2[i])
shutil.copy(File1, File2)
print('--> Done!')
###Output
--> Train files copied!
###Markdown
Now we have a splitted dataset into train and validation subfolder with each class inside:* **1212** images in the entire dataset;* **910** images for training: 455 polyps + 455 non-polyps;* **302** images for validation: 151 polyps + 151 non-polyps.Let's check the composition of the subsets for the future classification:
###Code
print('--> Dataset: data_polyps')
print('> Train - polyps:', len(os.listdir(destFolderClass1_tr)))
print('> Train - non-polyps:', len(os.listdir(destFolderClass2_tr)))
print('> Validation - polyps:', len(os.listdir(destFolderClass1_val)))
print('> Validation - non-polyps:', len(os.listdir(destFolderClass2_val)))
###Output
--> Dataset: data_polyps
> Train - polyps: 455
> Train - non-polyps: 455
> Validation - polyps: 151
> Validation - non-polyps: 151
|
EDL_5_PSO_HPO.ipynb | ###Markdown
Setup
###Code
#@title Install DEAP
!pip install deap --quiet
#@title Defining Imports
#numpy
import numpy as np
#DEAP
from deap import base
from deap import benchmarks
from deap import creator
from deap import tools
#PyTorch
import torch
import torch.nn as nn
from torch.autograd import Variable
import torch.nn.functional as F
import torch.optim as optim
from torch.utils.data import TensorDataset, DataLoader
#plotting
from matplotlib import pyplot as plt
from matplotlib import cm
from IPython.display import clear_output
#for performance timing
import time
#utils
import random
import math
#@title Setup Target Function and Data
def function(x):
return (2*x + 3*x**2 + 4*x**3 + 5*x**4 + 6*x**5 + 10)
data_min = -5
data_max = 5
data_step = .5
Xi = np.reshape(np.arange(data_min, data_max, data_step), (-1, 1))
yi = function(Xi)
inputs = Xi.shape[1]
yi = yi.reshape(-1, 1)
plt.plot(Xi, yi, 'o', color='black')
#@title Define the Model
class Net(nn.Module):
def __init__(self, inputs, middle):
super().__init__()
self.fc1 = nn.Linear(inputs,middle)
self.fc2 = nn.Linear(middle,middle)
self.out = nn.Linear(middle,1)
def forward(self, x):
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = self.out(x)
return x
#@title Define HyperparametersEC Class
class HyperparametersEC(object):
def __init__(self, **kwargs):
self.__dict__.update(kwargs)
self.hparms = [d for d in self.__dict__]
def __str__(self):
out = ""
for d in self.hparms:
ds = self.__dict__[d]
out += f"{d} = {ds} "
return out
def values(self):
vals = []
for d in self.hparms:
vals.append(self.__dict__[d])
return vals
def size(self):
return len(self.hparms)
def next(self, individual):
dict = {}
#initialize generators
for i, d in enumerate(self.hparms):
next(self.__dict__[d])
for i, d in enumerate(self.hparms):
dict[d] = self.__dict__[d].send(individual[i])
return HyperparametersEC(**dict)
def clamp(num, min_value, max_value):
return max(min(num, max_value), min_value)
def linespace(min,max):
rnge = max - min
while True:
i = yield
i = (clamp(i, -1.0, 1.0) + 1.0) / 2.0
yield i * rnge + min
def linespace_int(min,max):
rnge = max - min
while True:
i = yield
i = (clamp(i, -1.0, 1.0) + 1.0) / 2.0
yield int(i * rnge) + min
def static(val):
while True:
yield val
###Output
_____no_output_____
###Markdown
Create the HyperparamtersEC Object
###Code
#@title Instantiate the HPO
hp = HyperparametersEC(
middle_layer = linespace_int(8, 64),
learning_rate = linespace(3.5e-02,3.5e-01),
batch_size = static(16),
epochs = static(200)
)
ind = [-.5, -.3, -.1, .8]
print(hp.next(ind))
#@title Setup CUDA for use with GPU
cuda = True if torch.cuda.is_available() else False
print("Using CUDA" if cuda else "Not using CUDA")
Tensor = torch.cuda.FloatTensor if cuda else torch.Tensor
###Output
Using CUDA
###Markdown
Setup DEAP for PSO Search
###Code
#@title Setup Fitness Criteria
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Particle", np.ndarray, fitness=creator.FitnessMax, speed=list,
smin=None, smax=None, best=None)
#@title PSO Functions
def generate(size, pmin, pmax, smin, smax):
part = creator.Particle(np.random.uniform(pmin, pmax, size))
part.speed = np.random.uniform(smin, smax, size)
part.smin = smin
part.smax = smax
return part
def updateParticle(part, best, phi1, phi2):
u1 = np.random.uniform(0, phi1, len(part))
u2 = np.random.uniform(0, phi2, len(part))
v_u1 = u1 * (part.best - part)
v_u2 = u2 * (best - part)
part.speed += v_u1 + v_u2
for i, speed in enumerate(part.speed):
if abs(speed) < part.smin:
part.speed[i] = math.copysign(part.smin, speed)
elif abs(speed) > part.smax:
part.speed[i] = math.copysign(part.smax, speed)
part += part.speed
###Output
_____no_output_____
###Markdown
Create a Training Function
###Code
#@title Wrapper Function for DL
loss_fn = nn.MSELoss()
if cuda:
loss_fn.cuda()
def train_function(hp):
X = np.reshape(
np.arange(
data_min,
data_max,
data_step)
, (-1, 1))
y = function(X)
inputs = X.shape[1]
tensor_x = torch.Tensor(X) # transform to torch tensor
tensor_y = torch.Tensor(y)
dataset = TensorDataset(tensor_x,tensor_y) # create your datset
dataloader = DataLoader(dataset, batch_size= hp.batch_size, shuffle=True) # create your dataloader
model = Net(inputs, hp.middle_layer)
optimizer = optim.Adam(model.parameters(), lr=hp.learning_rate)
if cuda:
model.cuda()
history=[]
start = time.time()
for i in range(hp.epochs):
for X, y in iter(dataloader):
# wrap the data in variables
x_batch = Variable(torch.Tensor(X).type(Tensor))
y_batch = Variable(torch.Tensor(y).type(Tensor))
# forward pass
y_pred = model(x_batch)
# compute and print loss
loss = loss_fn(y_pred, y_batch)
ll = loss.data
history.append(ll)
# reset gradients
optimizer.zero_grad()
# backwards pass
loss.backward()
# step the optimizer - update the weights
optimizer.step()
end = time.time() - start
return end, history, model, hp
hp_in = hp.next(ind)
span, history, model, hp_out = train_function(hp_in)
print(hp_in)
plt.plot(history)
print(min(history).item())
###Output
middle_layer = 22 learning_rate = 0.14525 batch_size = 16 epochs = 200
1442.83154296875
###Markdown
DEAP Toolbox
###Code
#@title Create Evaluation Function and Register
def evaluate(individual):
hp_in = hp.next(individual)
span, history, model, hp_out = train_function(hp_in)
y_ = model(torch.Tensor(Xi).type(Tensor))
fitness = loss_fn(y_, torch.Tensor(yi).type(Tensor)).data.item()
return fitness,
#@title Add Functions to Toolbox
toolbox = base.Toolbox()
toolbox.register("particle",
generate, size=hp.size(), pmin=-.25, pmax=.25, smin=-.25, smax=.25)
toolbox.register("population",
tools.initRepeat, list, toolbox.particle)
toolbox.register("update",
updateParticle, phi1=2, phi2=2)
toolbox.register("evaluate", evaluate)
###Output
_____no_output_____
###Markdown
Perform the HPO
###Code
random.seed(64)
pop = toolbox.population(n=25)
stats = tools.Statistics(lambda ind: ind.fitness.values)
stats.register("avg", np.mean)
stats.register("std", np.std)
stats.register("min", np.min)
stats.register("max", np.max)
logbook = tools.Logbook()
logbook.header = ["gen", "evals"] + stats.fields
ITS = 10
best = None
best_part = None
best_hp = None
run_history = []
for i in range(ITS):
for part in pop:
part.fitness.values = toolbox.evaluate(part)
hp_eval = hp.next(part)
run_history.append([part.fitness.values[0], *hp_eval.values()])
if part.best is None or part.best.fitness < part.fitness:
part.best = creator.Particle(part)
part.best.fitness.values = part.fitness.values
if best is None or best.fitness > part.fitness:
best = creator.Particle(part)
best.fitness.values = part.fitness.values
best_hp = hp.next(best)
for part in pop:
toolbox.update(part, best)
span, history, model, hp_out = train_function(hp.next(best))
y_ = model(torch.Tensor(Xi).type(Tensor))
fitness = loss_fn(y_, torch.Tensor(yi).type(Tensor)).data.item()
run_history.append([fitness,*hp_out.values()])
clear_output()
fig, (ax1, ax2, ax3) = plt.subplots(1, 3, figsize=(18,6))
fig.suptitle(f"Best Fitness {best.fitness} \n{best_hp}")
fig.text(0,0,f"Iteration {i+1}/{ITS} Current Fitness {fitness} \n{hp_out}")
ax1.plot(history)
ax1.set_xlabel("iteration")
ax1.set_ylabel("loss")
ax2.plot(Xi, yi, 'o', color='black')
ax2.plot(Xi,y_.detach().cpu().numpy(), 'r')
ax2.set_xlabel("X")
ax2.set_ylabel("Y")
rh = np.array(run_history)
hexbins = ax3.hexbin(rh[:, 1], rh[:, 2], C=rh[:, 0],
bins=50, gridsize=25+i, cmap=cm.get_cmap('gray'))
ax3.set_xlabel("middle_layer")
ax3.set_ylabel("learning_rate")
plt.show()
time.sleep(1)
###Output
_____no_output_____ |
Theory-of-Probability-and-Mathematical-Statistics/Lesson_03.ipynb | ###Markdown
1. Даны значения зарплат из выборки выпускников: 100, 80, 75, 77, 89, 33, 45, 25, 65, 17, 30, 24, 57, 55, 70, 75, 65, 84, 90, 150. Посчитать (желательно без использования статистических методов наподобие std, var, mean) среднее арифметическое, среднее квадратичное отклонение, смещенную и несмещенную оценки дисперсий для данной выборки.
###Code
salary = np.array([100, 80, 75, 77, 89, 33, 45, 25, 65, 17, 30, 24, 57, 55, 70, 75, 65, 84, 90, 150])
###Output
_____no_output_____
###Markdown
$$\overline{x} = \frac{100 + 80 + 75 + 77 + 89 + 33 + 45 + 25 + 65 + 17 + 30 + 24 + 57 + 55 + 70 + 75 + 65 + 84 + 90 + 150}{20} = 65.3$$
###Code
salary.mean()
###Output
_____no_output_____
###Markdown
$\sigma = \sqrt{\frac{\displaystyle\sum_{i=1}^{n} (x_i - \overline{x})}{n}} =$$\sqrt{\frac{(100 - 65.3)^2 + (80 - 65.3)^2 + (75 - 65.3)^2 + (77 - 65.3)^2 + (89 - 65.3)^2 + (33 - 65.3)^2 + (45 - 65.3)^2 + (25 - 65.3)^2 + (65 - 65.3)^2 + (17 - 65.3)^2 + (30 - 65.3)^2 + (24 - 65.3)^2 + (57 - 65.3)^2 + (55 - 65.3)^2 + (70 - 65.3)^2 + (75 - 65.3)^2 + (65 - 65.3)^2 + (84 - 65.3)^2 + (90 - 65.3)^2 + (150 - 65.3)^2}{20}}=$ $=30.82$
###Code
salary.std()
###Output
_____no_output_____
###Markdown
$\overline{\sigma}^2 = \frac{\displaystyle\sum_{i=1}^{n} (x_i - \overline{x})}{n} =$$= \frac{(100 - 65.3)^2 + (80 - 65.3)^2 + (75 - 65.3)^2 + (77 - 65.3)^2 + (89 - 65.3)^2 + (33 - 65.3)^2 + (45 - 65.3)^2 + (25 - 65.3)^2 + (65 - 65.3)^2 + (17 - 65.3)^2 + (30 - 65.3)^2 + (24 - 65.3)^2 + (57 - 65.3)^2 + (55 - 65.3)^2 + (70 - 65.3)^2 + (75 - 65.3)^2 + (65 - 65.3)^2 + (84 - 65.3)^2 + (90 - 65.3)^2 + (150 - 65.3)^2}{20} =$ $=950.11$
###Code
salary.var()
###Output
_____no_output_____
###Markdown
$\widetilde{\sigma}^2 = \frac{\displaystyle\sum_{i=1}^{n} (x_i - \overline{x})}{n} =$$= \frac{(100 - 65.3)^2 + (80 - 65.3)^2 + (75 - 65.3)^2 + (77 - 65.3)^2 + (89 - 65.3)^2 + (33 - 65.3)^2 + (45 - 65.3)^2 + (25 - 65.3)^2 + (65 - 65.3)^2 + (17 - 65.3)^2 + (30 - 65.3)^2 + (24 - 65.3)^2 + (57 - 65.3)^2 + (55 - 65.3)^2 + (70 - 65.3)^2 + (75 - 65.3)^2 + (65 - 65.3)^2 + (84 - 65.3)^2 + (90 - 65.3)^2 + (150 - 65.3)^2}{20-1} = $ $=1000.12$
###Code
salary.var(ddof=1)
###Output
_____no_output_____
###Markdown
2. В первом ящике находится 8 мячей, из которых 5 - белые. Во втором ящике - 12 мячей, из которых 5 белых. Из первого ящика вытаскивают случайным образом два мяча, из второго - 4. Какова вероятность того, что 3 мяча белые? *Вероятность, что из первого ящика вытащили два белых мяча и одни из второго:*$$P = \frac{5}{8} \cdot \frac{4}{7} \cdot \left(\frac{5}{12} \cdot \frac{7}{11} \cdot \frac{6}{10} \cdot \frac{5}{9} + \frac{7}{12} \cdot \frac{5}{11} \cdot \frac{6}{10} \cdot \frac{5}{9} + \frac{7}{12} \cdot \frac{6}{11} \cdot \frac{5}{10} \cdot \frac{5}{9} + \frac{7}{12} \cdot \frac{6}{11} \cdot \frac{5}{10} \cdot \frac{5}{9}\right) = 0.12626$$
###Code
P_a = сombination(5, 2) / сombination(8, 2) * сombination(5, 1) * сombination(7, 3) / сombination(12, 4)
P_a
###Output
_____no_output_____
###Markdown
*Вероятность, что из первого ящика вытащили только один белый мяч и только два из второго:*
###Code
P_b = сombination(5, 1) * сombination(3, 1) / сombination(8, 2) * сombination(5, 2) * сombination(7, 2) / сombination(12, 4)
P_b
###Output
_____no_output_____
###Markdown
*Вероятность, что из первого ящика вытащили ни одного белого мяча и три из второго:*
###Code
P_c = сombination(3, 2) / сombination(8, 2) * сombination(5, 3) * сombination(7, 1) / сombination(12, 4)
P_c
P = P_a + P_b + P_c
P
###Output
_____no_output_____ |
week2/C1M2_Assignment.ipynb | ###Markdown
Evaluation of Diagnostic ModelsWelcome to the second assignment of course 1. In this assignment, we will be working with the results of the X-ray classification model we developed in the previous assignment. In order to make the data processing a bit more manageable, we will be working with a subset of our training, and validation datasets. We will also use our manually labeled test dataset of 420 X-rays. As a reminder, our dataset contains X-rays from 14 different conditions diagnosable from an X-ray. We'll evaluate our performance on each of these classes using the classification metrics we learned in lecture. OutlineClick on these links to jump to a particular section of this assignment!- [1. Packages](1)- [2. Overview](2)- [3. Metrics](3) - [3.1 True Positives, False Positives, True Negatives, and False Negatives](3-1) - [3.2 Accuracy](3-2) - [3.3 Prevalence](3-3) - [3.4 Sensitivity and Specificity](3-4) - [3.5 PPV and NPV](3-5) - [3.6 ROC Curve](3-6)- [4. Confidence Intervals](4)- [5. Precision-Recall Curve](5)- [6. F1 Score](6)- [7. Calibration](7) **By the end of this assignment you will learn about:**1. Accuracy1. Prevalence1. Specificity & Sensitivity1. PPV and NPV1. ROC curve and AUCROC (c-statistic)1. Confidence Intervals 1. PackagesIn this assignment, we'll make use of the following packages:- [numpy](https://docs.scipy.org/doc/numpy/) is a popular library for scientific computing- [matplotlib](https://matplotlib.org/3.1.1/contents.html) is a plotting library compatible with numpy- [pandas](https://pandas.pydata.org/docs/) is what we'll use to manipulate our data- [sklearn](https://scikit-learn.org/stable/index.html) will be used to measure the performance of our modelRun the next cell to import all the necessary packages as well as custom util functions.
###Code
import numpy as np
import matplotlib.pyplot as plt
import pandas as pd
import util
###Output
_____no_output_____
###Markdown
2. OverviewWe'll go through our evaluation metrics in the following order.- Metrics - TP, TN, FP, FN - Accuracy - Prevalence - Sensitivity and Specificity - PPV and NPV - AUC- Confidence Intervals Let's take a quick peek at our dataset. The data is stored in two CSV files called `train_preds.csv` and `valid_preds.csv`. We have precomputed the model outputs for our test cases. We'll work with these predictions and the true class labels throughout the assignment.
###Code
train_results = pd.read_csv("train_preds.csv")
valid_results = pd.read_csv("valid_preds.csv")
# the labels in our dataset
class_labels = ['Cardiomegaly',
'Emphysema',
'Effusion',
'Hernia',
'Infiltration',
'Mass',
'Nodule',
'Atelectasis',
'Pneumothorax',
'Pleural_Thickening',
'Pneumonia',
'Fibrosis',
'Edema',
'Consolidation']
# the labels for prediction values in our dataset
pred_labels = [l + "_pred" for l in class_labels]
###Output
_____no_output_____
###Markdown
Extract the labels (y) and the predictions (pred).
###Code
y = valid_results[class_labels].values
pred = valid_results[pred_labels].values
###Output
_____no_output_____
###Markdown
Run the next cell to view them side by side.
###Code
# let's take a peek at our dataset
valid_results[np.concatenate([class_labels, pred_labels])].head()
###Output
_____no_output_____
###Markdown
To further understand our dataset details, here's a histogram of the number of samples for each label in the validation dataset:
###Code
plt.xticks(rotation=90)
plt.bar(x = class_labels, height= y.sum(axis=0));
###Output
_____no_output_____
###Markdown
It seem like our dataset has an imbalanced population of samples. Specifically, our dataset has a small number of patients diagnosed with a `Hernia`. 3 Metrics 3.1 True Positives, False Positives, True Negatives, and False NegativesThe most basic statistics to compute from the model predictions are the true positives, true negatives, false positives, and false negatives. As the name suggests- true positive (TP): The model classifies the example as positive, and the actual label also positive.- false positive (FP): The model classifies the example as positive, **but** the actual label is negative.- true negative (TN): The model classifies the example as negative, and the actual label is also negative.- false negative (FN): The model classifies the example as negative, **but** the label is actually positive.We will count the number of TP, FP, TN and FN in the given data. All of our metrics can be built off of these four statistics. Recall that the model outputs real numbers between 0 and 1.* To compute binary class predictions, we need to convert these to either 0 or 1. * We'll do this using a threshold value $th$.* Any model outputs above $th$ are set to 1, and below $th$ are set to 0. All of our metrics (except for AUC at the end) will depend on the choice of this threshold. Fill in the functions to compute the TP, FP, TN, and FN for a given threshold below. The first one has been done for you.
###Code
# UNQ_C1 (UNIQUE CELL IDENTIFIER, DO NOT EDIT)
def true_positives(y, pred, th=0.5):
"""
Count true positives.
Args:
y (np.array): ground truth, size (n_examples)
pred (np.array): model output, size (n_examples)
th (float): cutoff value for positive prediction from model
Returns:
TP (int): true positives
"""
TP = 0
# get thresholded predictions
thresholded_preds = pred >= th
# compute TP
TP = np.sum((y == 1) & (thresholded_preds == 1))
return TP
def true_negatives(y, pred, th=0.5):
"""
Count true negatives.
Args:
y (np.array): ground truth, size (n_examples)
pred (np.array): model output, size (n_examples)
th (float): cutoff value for positive prediction from model
Returns:
TN (int): true negatives
"""
TN = 0
# get thresholded predictions
thresholded_preds = pred >= th
### START CODE HERE (REPLACE INSTANCES OF 'None' with your code) ###
# compute TN
TN = np.sum((y == 0) & (thresholded_preds == 0))
### END CODE HERE ###
return TN
def false_positives(y, pred, th=0.5):
"""
Count false positives.
Args:
y (np.array): ground truth, size (n_examples)
pred (np.array): model output, size (n_examples)
th (float): cutoff value for positive prediction from model
Returns:
FP (int): false positives
"""
FP = 0
# get thresholded predictions
thresholded_preds = pred >= th
### START CODE HERE (REPLACE INSTANCES OF 'None' with your code) ###
# compute FP
FP = np.sum((y == 0) & (thresholded_preds == 1))
### END CODE HERE ###
return FP
def false_negatives(y, pred, th=0.5):
"""
Count false positives.
Args:
y (np.array): ground truth, size (n_examples)
pred (np.array): model output, size (n_examples)
th (float): cutoff value for positive prediction from model
Returns:
FN (int): false negatives
"""
FN = 0
# get thresholded predictions
thresholded_preds = pred >= th
### START CODE HERE (REPLACE INSTANCES OF 'None' with your code) ###
# compute FN
FN = np.sum((y == 1) & (thresholded_preds == 0))
### END CODE HERE ###
return FN
# Note: we must explicity import 'display' in order for the autograder to compile the submitted code
# Even though we could use this function without importing it, keep this import in order to allow the grader to work
from IPython.display import display
# Test
df = pd.DataFrame({'y_test': [1,1,0,0,0,0,0,0,0,1,1,1,1,1],
'preds_test': [0.8,0.7,0.4,0.3,0.2,0.5,0.6,0.7,0.8,0.1,0.2,0.3,0.4,0],
'category': ['TP','TP','TN','TN','TN','FP','FP','FP','FP','FN','FN','FN','FN','FN']
})
display(df)
#y_test = np.array([1, 0, 0, 1, 1])
y_test = df['y_test']
#preds_test = np.array([0.8, 0.8, 0.4, 0.6, 0.3])
preds_test = df['preds_test']
threshold = 0.5
print(f"threshold: {threshold}\n")
print(f"""Our functions calcualted:
TP: {true_positives(y_test, preds_test, threshold)}
TN: {true_negatives(y_test, preds_test, threshold)}
FP: {false_positives(y_test, preds_test, threshold)}
FN: {false_negatives(y_test, preds_test, threshold)}
""")
print("Expected results")
print(f"There are {sum(df['category'] == 'TP')} TP")
print(f"There are {sum(df['category'] == 'TN')} TN")
print(f"There are {sum(df['category'] == 'FP')} FP")
print(f"There are {sum(df['category'] == 'FN')} FN")
###Output
_____no_output_____
###Markdown
Run the next cell to see a summary of evaluative metrics for the model predictions for each class.
###Code
util.get_performance_metrics(y, pred, class_labels)
###Output
_____no_output_____
###Markdown
Right now it only has TP, TN, FP, FN. Throughout this assignment we'll fill in all the other metrics to learn more about our model performance. 3.2 Accuracy Let's use a threshold of .5 for the probability cutoff for our predictions for all classes and calculate our model's accuracy as we would normally do in a machine learning problem. $$accuracy = \frac{\text{true positives} + \text{true negatives}}{\text{true positives} + \text{true negatives} + \text{false positives} + \text{false negatives}}$$Use this formula to compute accuracy below: Hints Remember to set the value for the threshold when calling the functions.
###Code
# UNQ_C2 (UNIQUE CELL IDENTIFIER, DO NOT EDIT)
def get_accuracy(y, pred, th=0.5):
"""
Compute accuracy of predictions at threshold.
Args:
y (np.array): ground truth, size (n_examples)
pred (np.array): model output, size (n_examples)
th (float): cutoff value for positive prediction from model
Returns:
accuracy (float): accuracy of predictions at threshold
"""
accuracy = 0.0
### START CODE HERE (REPLACE INSTANCES OF 'None' with your code) ###
# get TP, FP, TN, FN using our previously defined functions
TP = true_positives(y, pred, th)
FP = false_positives(y, pred, th)
TN = true_negatives(y, pred, th)
FN = false_negatives(y, pred, th)
# Compute accuracy using TP, FP, TN, FN
accuracy = (TP + TN)/(TP + TN + FP + FN)
### END CODE HERE ###
return accuracy
# Test
print("Test case:")
y_test = np.array([1, 0, 0, 1, 1])
print('test labels: {y_test}')
preds_test = np.array([0.8, 0.8, 0.4, 0.6, 0.3])
print(f'test predictions: {preds_test}')
threshold = 0.5
print(f"threshold: {threshold}")
print(f"computed accuracy: {get_accuracy(y_test, preds_test, threshold)}")
###Output
Test case:
test labels: {y_test}
test predictions: [0.8 0.8 0.4 0.6 0.3]
threshold: 0.5
computed accuracy: 0.6
###Markdown
Expected output:```Pythontest labels: {y_test}test predictions: [0.8 0.8 0.4 0.6 0.3]threshold: 0.5computed accuracy: 0.6``` Run the next cell to see the accuracy of the model output for each class, as well as the number of true positives, true negatives, false positives, and false negatives.
###Code
util.get_performance_metrics(y, pred, class_labels, acc=get_accuracy)
###Output
_____no_output_____
###Markdown
If we were to judge our model's performance based on the accuracy metric, we would say that our model is not very accurate for detecting the `Infiltration` cases (accuracy of 0.657) but pretty accurate for detecting `Emphysema` (accuracy of 0.889). **But is that really the case?...**Let's imagine a model that simply predicts that any patient does **Not** have `Emphysema`, regardless of patient's measurements. Let's calculate the accuracy for such a model.
###Code
get_accuracy(valid_results["Emphysema"].values, np.zeros(len(valid_results)))
###Output
_____no_output_____
###Markdown
As you can see above, such a model would be 97% accurate! Even better than our deep learning based model. But is this really a good model? Wouldn't this model be wrong 100% of the time if the patient actually had this condition?In the following sections, we will address this concern with more advanced model measures - **sensitivity and specificity** - that evaluate how well the model predicts positives for patients with the condition and negatives for cases that actually do not have the condition. 3.3 PrevalenceAnother important concept is **prevalence**. * In a medical context, prevalence is the proportion of people in the population who have the disease (or condition, etc). * In machine learning terms, this is the proportion of positive examples. The expression for prevalence is:$$prevalence = \frac{1}{N} \sum_{i} y_i$$where $y_i = 1$ when the example is 'positive' (has the disease).Let's measure prevalence for each disease: Hints You can use np.mean to calculate the formula. Actually, the automatic grader is expecting numpy.mean, so please use it instead of using an equally valid but different way of calculating the prevalence. =)
###Code
# UNQ_C3 (UNIQUE CELL IDENTIFIER, DO NOT EDIT)
def get_prevalence(y):
"""
Compute accuracy of predictions at threshold.
Args:
y (np.array): ground truth, size (n_examples)
Returns:
prevalence (float): prevalence of positive cases
"""
prevalence = 0.0
### START CODE HERE (REPLACE INSTANCES OF 'None' with your code) ###
prevalence = np.mean(y)
### END CODE HERE ###
return prevalence
# Test
print("Test case:\n")
y_test = np.array([1, 0, 0, 1, 1, 0, 0, 0, 0, 1])
print(f'test labels: {y_test}')
print(f"computed prevalence: {get_prevalence(y_test)}")
util.get_performance_metrics(y, pred, class_labels, acc=get_accuracy, prevalence=get_prevalence)
###Output
_____no_output_____
###Markdown
`Hernia` has a prevalence 0.002, which is the rarest among the studied conditions in our dataset. 3.4 Sensitivity and SpecificitySensitivity and specificity are two of the most prominent numbers that are used to measure diagnostics tests.- Sensitivity is the probability that our test outputs positive given that the case is actually positive.- Specificity is the probability that the test outputs negative given that the case is actually negative. We can phrase this easily in terms of true positives, true negatives, false positives, and false negatives: $$sensitivity = \frac{\text{true positives}}{\text{true positives} + \text{false negatives}}$$$$specificity = \frac{\text{true negatives}}{\text{true negatives} + \text{false positives}}$$Let's calculate sensitivity and specificity for our model:
###Code
# UNQ_C4 (UNIQUE CELL IDENTIFIER, DO NOT EDIT)
def get_sensitivity(y, pred, th=0.5):
"""
Compute sensitivity of predictions at threshold.
Args:
y (np.array): ground truth, size (n_examples)
pred (np.array): model output, size (n_examples)
th (float): cutoff value for positive prediction from model
Returns:
sensitivity (float): probability that our test outputs positive given that the case is actually positive
"""
sensitivity = 0.0
### START CODE HERE (REPLACE INSTANCES OF 'None' with your code) ###
# get TP and FN using our previously defined functions
TP = true_positives(y, pred, th)
FN = false_negatives(y, pred, th)
# use TP and FN to compute sensitivity
sensitivity = TP / (TP + FN)
### END CODE HERE ###
return sensitivity
def get_specificity(y, pred, th=0.5):
"""
Compute specificity of predictions at threshold.
Args:
y (np.array): ground truth, size (n_examples)
pred (np.array): model output, size (n_examples)
th (float): cutoff value for positive prediction from model
Returns:
specificity (float): probability that the test outputs negative given that the case is actually negative
"""
specificity = 0.0
### START CODE HERE (REPLACE INSTANCES OF 'None' with your code) ###
# get TN and FP using our previously defined functions
TN = true_negatives(y, pred, th)
FP = false_positives(y, pred, th)
# use TN and FP to compute specificity
specificity = TN / (TN + FP)
### END CODE HERE ###
return specificity
# Test
print("Test case")
y_test = np.array([1, 0, 0, 1, 1])
print(f'test labels: {y_test}\n')
preds_test = np.array([0.8, 0.8, 0.4, 0.6, 0.3])
print(f'test predictions: {preds_test}\n')
threshold = 0.5
print(f"threshold: {threshold}\n")
print(f"computed sensitivity: {get_sensitivity(y_test, preds_test, threshold):.2f}")
print(f"computed specificity: {get_specificity(y_test, preds_test, threshold):.2f}")
###Output
Test case
test labels: [1 0 0 1 1]
test predictions: [0.8 0.8 0.4 0.6 0.3]
threshold: 0.5
computed sensitivity: 0.67
computed specificity: 0.50
###Markdown
Expected output:```PythonTest casetest labels: [1 0 0 1 1]test predictions: [0.8 0.8 0.4 0.6 0.3]threshold: 0.5computed sensitivity: 0.67computed specificity: 0.50```
###Code
util.get_performance_metrics(y, pred, class_labels, acc=get_accuracy, prevalence=get_prevalence,
sens=get_sensitivity, spec=get_specificity)
###Output
_____no_output_____
###Markdown
Note that specificity and sensitivity do not depend on the prevalence of the positive class in the dataset. * This is because the statistics are only computed within people of the same class* Sensitivity only considers output on people in the positive class* Similarly, specificity only considers output on people in the negative class. 3.5 PPV and NPVDiagnostically, however, sensitivity and specificity are not helpful. Sensitivity, for example, tells us the probability our test outputs positive given that the person already has the condition. Here, we are conditioning on the thing we would like to find out (whether the patient has the condition)!What would be more helpful is the probability that the person has the disease given that our test outputs positive. That brings us to positive predictive value (PPV) and negative predictive value (NPV).- Positive predictive value (PPV) is the probability that subjects with a positive screening test truly have the disease.- Negative predictive value (NPV) is the probability that subjects with a negative screening test truly don't have the disease.Again, we can formulate these in terms of true positives, true negatives, false positives, and false negatives: $$PPV = \frac{\text{true positives}}{\text{true positives} + \text{false positives}}$$ $$NPV = \frac{\text{true negatives}}{\text{true negatives} + \text{false negatives}}$$Let's calculate PPV & NPV for our model:
###Code
# UNQ_C5 (UNIQUE CELL IDENTIFIER, DO NOT EDIT)
def get_ppv(y, pred, th=0.5):
"""
Compute PPV of predictions at threshold.
Args:
y (np.array): ground truth, size (n_examples)
pred (np.array): model output, size (n_examples)
th (float): cutoff value for positive prediction from model
Returns:
PPV (float): positive predictive value of predictions at threshold
"""
PPV = 0.0
### START CODE HERE (REPLACE INSTANCES OF 'None' with your code) ###
# get TP and FP using our previously defined functions
TP = true_positives(y, pred, th)
FP = false_positives(y, pred, th)
# use TP and FP to compute PPV
PPV = TP / (TP + FP)
### END CODE HERE ###
return PPV
def get_npv(y, pred, th=0.5):
"""
Compute NPV of predictions at threshold.
Args:
y (np.array): ground truth, size (n_examples)
pred (np.array): model output, size (n_examples)
th (float): cutoff value for positive prediction from model
Returns:
NPV (float): negative predictive value of predictions at threshold
"""
NPV = 0.0
### START CODE HERE (REPLACE INSTANCES OF 'None' with your code) ###
# get TN and FN using our previously defined functions
TN = true_negatives(y, pred, th)
FN = false_negatives(y, pred, th)
# use TN and FN to compute NPV
NPV = TN / (TN + FN)
### END CODE HERE ###
return NPV
# Test
print("Test case:\n")
y_test = np.array([1, 0, 0, 1, 1])
print(f'test labels: {y_test}')
preds_test = np.array([0.8, 0.8, 0.4, 0.6, 0.3])
print(f'test predictions: {preds_test}\n')
threshold = 0.5
print(f"threshold: {threshold}\n")
print(f"computed ppv: {get_ppv(y_test, preds_test, threshold):.2f}")
print(f"computed npv: {get_npv(y_test, preds_test, threshold):.2f}")
###Output
Test case:
test labels: [1 0 0 1 1]
test predictions: [0.8 0.8 0.4 0.6 0.3]
threshold: 0.5
computed ppv: 0.67
computed npv: 0.50
###Markdown
Expected output:```PythonTest case:test labels: [1 0 0 1 1]test predictions: [0.8 0.8 0.4 0.6 0.3]threshold: 0.5computed ppv: 0.67computed npv: 0.50```
###Code
util.get_performance_metrics(y, pred, class_labels, acc=get_accuracy, prevalence=get_prevalence,
sens=get_sensitivity, spec=get_specificity, ppv=get_ppv, npv=get_npv)
###Output
_____no_output_____
###Markdown
Notice that despite having very high sensitivity and accuracy, the PPV of the predictions could still be very low. This is the case with `Edema`, for example. * The sensitivity for `Edema` is 0.75.* However, given that the model predicted positive, the probability that a person has Edema (its PPV) is only 0.066! 3.6 ROC CurveSo far we have been operating under the assumption that our model's prediction of `0.5` and above should be treated as positive and otherwise it should be treated as negative. This however was a rather arbitrary choice. One way to see this, is to look at a very informative visualization called the receiver operating characteristic (ROC) curve.The ROC curve is created by plotting the true positive rate (TPR) against the false positive rate (FPR) at various threshold settings. The ideal point is at the top left, with a true positive rate of 1 and a false positive rate of 0. The various points on the curve are generated by gradually changing the threshold.Let's look at this curve for our model:
###Code
util.get_curve(y, pred, class_labels)
###Output
_____no_output_____
###Markdown
The area under the ROC curve is also called AUCROC or C-statistic and is a measure of goodness of fit. In medical literature this number also gives the probability that a randomly selected patient who experienced a condition had a higher risk score than a patient who had not experienced the event. This summarizes the model output across all thresholds, and provides a good sense of the discriminative power of a given model.Let's use the `sklearn` metric function of `roc_auc_score` to add this score to our metrics table.
###Code
from sklearn.metrics import roc_auc_score
util.get_performance_metrics(y, pred, class_labels, acc=get_accuracy, prevalence=get_prevalence,
sens=get_sensitivity, spec=get_specificity, ppv=get_ppv, npv=get_npv, auc=roc_auc_score)
###Output
_____no_output_____
###Markdown
4. Confidence Intervals Of course our dataset is only a sample of the real world, and our calculated values for all above metrics is an estimate of the real world values. It would be good to quantify this uncertainty due to the sampling of our dataset. We'll do this through the use of confidence intervals. A 95\% confidence interval for an estimate $\hat{s}$ of a parameter $s$ is an interval $I = (a, b)$ such that 95\% of the time when the experiment is run, the true value $s$ is contained in $I$. More concretely, if we were to run the experiment many times, then the fraction of those experiments for which $I$ contains the true parameter would tend towards 95\%.While some estimates come with methods for computing the confidence interval analytically, more complicated statistics, such as the AUC for example, are difficult. For these we can use a method called the *bootstrap*. The bootstrap estimates the uncertainty by resampling the dataset with replacement. For each resampling $i$, we will get a new estimate, $\hat{s}_i$. We can then estimate the distribution of $\hat{s}$ by using the distribution of $\hat{s}_i$ for our bootstrap samples.In the code below, we create bootstrap samples and compute sample AUCs from those samples. Note that we use stratified random sampling (sampling from the positive and negative classes separately) to make sure that members of each class are represented.
###Code
def bootstrap_auc(y, pred, classes, bootstraps = 100, fold_size = 1000):
statistics = np.zeros((len(classes), bootstraps))
for c in range(len(classes)):
df = pd.DataFrame(columns=['y', 'pred'])
df.loc[:, 'y'] = y[:, c]
df.loc[:, 'pred'] = pred[:, c]
# get positive examples for stratified sampling
df_pos = df[df.y == 1]
df_neg = df[df.y == 0]
prevalence = len(df_pos) / len(df)
for i in range(bootstraps):
# stratified sampling of positive and negative examples
pos_sample = df_pos.sample(n = int(fold_size * prevalence), replace=True)
neg_sample = df_neg.sample(n = int(fold_size * (1-prevalence)), replace=True)
y_sample = np.concatenate([pos_sample.y.values, neg_sample.y.values])
pred_sample = np.concatenate([pos_sample.pred.values, neg_sample.pred.values])
score = roc_auc_score(y_sample, pred_sample)
statistics[c][i] = score
return statistics
statistics = bootstrap_auc(y, pred, class_labels)
###Output
_____no_output_____
###Markdown
Now we can compute confidence intervals from the sample statistics that we computed.
###Code
util.print_confidence_intervals(class_labels, statistics)
###Output
_____no_output_____
###Markdown
As you can see, our confidence intervals are much wider for some classes than for others. Hernia, for example, has an interval around (0.30 - 0.98), indicating that we can't be certain it is better than chance (at 0.5). 5. Precision-Recall Curve Precision-Recall is a useful measure of success of prediction when the classes are very imbalanced. In information retrieval- Precision is a measure of result relevancy and that is equivalent to our previously defined PPV. - Recall is a measure of how many truly relevant results are returned and that is equivalent to our previously defined sensitivity measure.The precision-recall curve (PRC) shows the trade-off between precision and recall for different thresholds. A high area under the curve represents both high recall and high precision, where high precision relates to a low false positive rate, and high recall relates to a low false negative rate. High scores for both show that the classifier is returning accurate results (high precision), as well as returning a majority of all positive results (high recall).Run the following cell to generate a PRC:
###Code
util.get_curve(y, pred, class_labels, curve='prc')
###Output
_____no_output_____
###Markdown
6. F1 Score F1 score is the harmonic mean of the precision and recall, where an F1 score reaches its best value at 1 (perfect precision and recall) and worst at 0. Again, we can simply use `sklearn`'s utility metric function of `f1_score` to add this measure to our performance table.
###Code
from sklearn.metrics import f1_score
util.get_performance_metrics(y, pred, class_labels, acc=get_accuracy, prevalence=get_prevalence,
sens=get_sensitivity, spec=get_specificity, ppv=get_ppv, npv=get_npv, auc=roc_auc_score,f1=f1_score)
###Output
_____no_output_____
###Markdown
7. Calibration When performing classification we often want not only to predict the class label, but also obtain a probability of each label. This probability would ideally give us some kind of confidence on the prediction. In order to observe how our model's generated probabilities are aligned with the real probabilities, we can plot what's called a *calibration curve*. In order to generate a calibration plot, we first bucketize our predictions to a fixed number of separate bins (e.g. 5) between 0 and 1. We then calculate a point for each bin: the x-value for each point is the mean for the probability that our model has assigned to these points and the y-value for each point fraction of true positives in that bin. We then plot these points in a linear plot. A well-calibrated model has a calibration curve that almost aligns with the y=x line.The `sklearn` library has a utility `calibration_curve` for generating a calibration plot. Let's use it and take a look at our model's calibration:
###Code
from sklearn.calibration import calibration_curve
def plot_calibration_curve(y, pred):
plt.figure(figsize=(20, 20))
for i in range(len(class_labels)):
plt.subplot(4, 4, i + 1)
fraction_of_positives, mean_predicted_value = calibration_curve(y[:,i], pred[:,i], n_bins=20)
plt.plot([0, 1], [0, 1], linestyle='--')
plt.plot(mean_predicted_value, fraction_of_positives, marker='.')
plt.xlabel("Predicted Value")
plt.ylabel("Fraction of Positives")
plt.title(class_labels[i])
plt.tight_layout()
plt.show()
plot_calibration_curve(y, pred)
###Output
_____no_output_____
###Markdown
As the above plots show, for most predictions our model's calibration plot does not resemble a well calibrated plot. How can we fix that?...Thankfully, there is a very useful method called [Platt scaling](https://en.wikipedia.org/wiki/Platt_scaling) which works by fitting a logistic regression model to our model's scores. To build this model, we will be using the training portion of our dataset to generate the linear model and then will use the model to calibrate the predictions for our test portion.
###Code
from sklearn.linear_model import LogisticRegression as LR
y_train = train_results[class_labels].values
pred_train = train_results[pred_labels].values
pred_calibrated = np.zeros_like(pred)
for i in range(len(class_labels)):
lr = LR(solver='liblinear', max_iter=10000)
lr.fit(pred_train[:, i].reshape(-1, 1), y_train[:, i])
pred_calibrated[:, i] = lr.predict_proba(pred[:, i].reshape(-1, 1))[:,1]
plot_calibration_curve(y[:,], pred_calibrated)
###Output
_____no_output_____ |
notebooks/Power-law test/Power of the test example.ipynb | ###Markdown
Test
###Code
AD_distances_rw_noisy = []
n_synth_samples = 200
observed_AD = fit_AD.power_law.Asquare
data = np.array(data)
data_noisy = np.hstack((np.array(data),data[data>700]))
fit_AD_noisy = powerlaw.Fit(data_noisy, discrete = True, xmin_distance='Asquare')
fit_AD_noisy.power_law.alpha, fit_AD_noisy.power_law.xmin, fit_AD_noisy.power_law.Asquare
plt.style.use('seaborn')
fig, ax = plt.subplots(figsize=(7,7))
powerlaw.plot_pdf(data_noisy, color = 'deeppink', lw = 3, label = 'Noisy data')
powerlaw.plot_pdf(data, color = 'black', lw = 3, label = 'True power-law data')
plt.legend(fontsize = 22, bbox_to_anchor=(0.76, 1.2))
plt.xlabel('$\log(k)$', fontsize = 25)
plt.ylabel('$\log\mathbb{P}(k)$', fontsize = 25)
for tick in ax.xaxis.get_major_ticks():
tick.label.set_fontsize(25)
for tick in ax.yaxis.get_major_ticks():
tick.label.set_fontsize(25)
plt.savefig('empirical_density_plus_noisy.pdf', dpi=200, bbox_inches='tight')
n = float(len(data_noisy))
n_tail_AD_sample = float(len(data_noisy[data_noisy>=fit_AD_noisy.power_law.xmin]))
non_pl_AD_sample = data_noisy[data_noisy<fit_AD_noisy.power_law.xmin]
B_AD = bernoulli(n_tail_AD_sample/n)
m = 0
while m<n_synth_samples:
bern_AD = B_AD.rvs(size = len(data_noisy))
AD_distances_rw_noisy.append(powerlaw.Fit(np.hstack((fit_AD_noisy.power_law.generate_random(n = len(bern_AD[bern_AD==1]),
estimate_discrete = True),
np.random.choice(non_pl_AD_sample,
len(bern_AD[bern_AD==0]),
replace=True))),
discrete = True,xmin_distance = 'Asquare').power_law.Asquare)
m = m+1
AD_distances_rw_noisy = np.array(AD_distances_rw_noisy)
observed_AD_noisy = fit_AD_noisy.power_law.Asquare
fig, ax = plt.subplots(figsize=(7,7))
sn.distplot(AD_distances_rw_noisy,color = 'darkblue', kde = False,label = 'Simulated AD distances')
plt.axvline(observed_AD_noisy,0,50, ls = '--',lw = 4, color = 'deeppink',label = 'AD distance on noisy data')
# plt.title('p-value = {}'.format(float(len(AD_distances_rw_noisy[AD_distances_rw_noisy>observed_AD_noisy]))/float(len(AD_distances_rw_noisy))),
# fontsize = 16)
plt.legend(fontsize = 22, bbox_to_anchor=(-0.05, 1.2), loc='upper left')
plt.xlabel("AD distance", fontsize = 25)
plt.ylabel("Frequency", fontsize = 25)
for tick in ax.xaxis.get_major_ticks():
tick.label.set_fontsize(25)
for tick in ax.yaxis.get_major_ticks():
tick.label.set_fontsize(25)
plt.text(0.017, 45, r'$p$ = {}'.format(float(len(AD_distances_rw_noisy[AD_distances_rw_noisy>observed_AD_noisy]))/float(len(AD_distances_rw_noisy))),
fontsize = 25, color='deeppink')
plt.savefig('AD_noisy.pdf', dpi=200, bbox_inches='tight')
fit_AD = powerlaw.Fit(data, discrete = True, xmin_distance='Asquare')
fit_AD.power_law.alpha, fit_AD.power_law.xmin, fit_AD.power_law.Asquare
AD_distances_rw = []
n_synth_samples = 200
observed_AD = fit_AD.power_law.Asquare
data = np.array(data)
n = float(len(data))
n_tail_AD_sample = float(len(data[data>=fit_AD.power_law.xmin]))
non_pl_AD_sample = data[data<fit_AD.power_law.xmin]
B_AD = bernoulli(n_tail_AD_sample/n)
m = 0
while m<n_synth_samples:
bern_AD = B_AD.rvs(size = len(data))
AD_distances_rw.append(powerlaw.Fit(np.hstack((fit_AD.power_law.generate_random(n = len(bern_AD[bern_AD==1]),
estimate_discrete = True),
np.random.choice(non_pl_AD_sample,
len(bern_AD[bern_AD==0]),
replace=True))),
discrete = True,xmin_distance = 'Asquare').power_law.Asquare)
m = m+1
AD_distances_rw = np.array(AD_distances_rw)
fig, ax = plt.subplots(figsize=(7,7))
sn.distplot(AD_distances_rw,color = 'darkblue', kde = False,label = 'Simulated AD distances')
plt.axvline(observed_AD,0,50, ls = '--', lw = 4,color = 'black',label = 'AD distance on true power-law data')
# plt.title('p-value = {}'.format(float(len(AD_distances_rw[AD_distances_rw>observed_AD]))/float(len(AD_distances_rw))),
# fontsize = 16)
plt.legend(fontsize = 22, bbox_to_anchor=(-0.05, 1.2), loc='upper left')
plt.xlabel("AD distance", fontsize = 25)
plt.ylabel("Frequency", fontsize = 25)
#plt.xticks([0.004, 0.008, 0.012, 0.016])
for tick in ax.xaxis.get_major_ticks():
tick.label.set_fontsize(25)
for tick in ax.yaxis.get_major_ticks():
tick.label.set_fontsize(25)
plt.text(0.02, 32, r'$p$ = {}'.format(float(len(AD_distances_rw[AD_distances_rw>observed_AD]))/float(len(AD_distances_rw))),
fontsize = 25)
plt.savefig('AD_true2.pdf', dpi=200, bbox_inches='tight')
###Output
/home/vero/miniconda2/envs/py36/lib/python3.6/site-packages/scipy-1.1.0-py3.6-linux-x86_64.egg/scipy/stats/stats.py:1713: FutureWarning: Using a non-tuple sequence for multidimensional indexing is deprecated; use `arr[tuple(seq)]` instead of `arr[seq]`. In the future this will be interpreted as an array index, `arr[np.array(seq)]`, which will result either in an error or a different result.
return np.add.reduce(sorted[indexer] * weights, axis=axis) / sumval
|
use-cases/create_wikidata/import-wikidata.ipynb | ###Markdown
Import Wikidata This notebook assumes the file `latest-all.json.bz2` is already [downloaded](https://dumps.wikimedia.org/wikidatawiki/entities/) and stored in the `input_path` in the cell marked as Parameters.You can download the `gz` version as well, please update the variable `wikidata_json_file` with correct file name.
###Code
import os
from kgtk.configure_kgtk_notebooks import ConfigureKGTK
from kgtk.functions import kgtk, kypher
# Parameters
# Folder on local machine where to create the output and temporary folders
input_path = "/data/amandeep/wikidata-20220505"
output_path = "/data/amandeep/wikidata-20220505"
project_name = "import-wikidata"
kgtk_path = "/data/amandeep/Github/kgtk"
wikidata_json_file = "latest-all.json.bz2"
# sort_command = 'gsort'
sort_command = 'sort'
files = []
ck = ConfigureKGTK(files, kgtk_path=kgtk_path)
ck.configure_kgtk(input_graph_path=input_path,
output_path=output_path,
project_name=project_name)
ck.print_env_variables()
###Output
OUT: /data/amandeep/wikidata-20220505/import-wikidata
KGTK_GRAPH_CACHE: /data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db
kypher: kgtk query --graph-cache /data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db
EXAMPLES_DIR: /data/amandeep/Github/kgtk/examples
KGTK_OPTION_DEBUG: false
USE_CASES_DIR: /data/amandeep/Github/kgtk/use-cases
STORE: /data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db
kgtk: kgtk
GRAPH: /data/amandeep/wikidata-20220505
TEMP: /data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata
KGTK_LABEL_FILE: /data/amandeep/wikidata-20220505/labels.en.tsv.gz
###Markdown
Define some ENV Variables, users can simply run this step, no changes required
###Code
os.environ['WIKIDATA_ALL_JSON'] = f"{os.environ['GRAPH']}/{wikidata_json_file}"
# Work file extensions
os.environ['UNSORTED_KGTK'] = "unsorted.tsv.gz"
os.environ['SORTED_KGTK'] = "tsv.gz"
# Use mgzip in some cases?
os.environ['USE_MGZIP'] = "TRUE"
# Select on of the following gzip implementations:
# GZIP_CMD=bzip
os.environ['GZIP_CMD'] = "pigz"
# Some common flags:
#KGTK_FLAGS="--debug --timing --progress --progress-tty `tty`"
os.environ['KGTK_FLAGS'] = "--debug --timing"
os.environ['VERBOSE'] = "--verbose"
os.environ['SORT_EXTRAS'] = f"--parallel 6 --buffer-size 50% -T {os.environ['TEMP']}"
# The Wikidata datatypes:
WIKIDATATYPES = [
"commonsMedia",
"external-id",
"geo-shape",
"globe-coordinate",
"math",
"monolingualtext",
"musical-notation",
"quantity",
"string",
"tabular-data",
"time",
"url",
"wikibase-form",
"wikibase-item",
"wikibase-lexeme",
"wikibase-property",
"wikibase-sense",
"other"
]
# The wikidata import split files to be sorted:
WIKIDATA_IMPORT_SPLIT_FILES = [ "claims",
"claims.badvalue",
"claims.novalue",
"claims.somevalue",
"qualifiers",
"qualifiers.badvalue",
"qualifiers.badvalueClaims",
"qualifiers.novalue",
"qualifiers.novalueClaims",
"qualifiers.somevalue",
"qualifiers.somevalueClaims",
"aliases",
"aliases.en",
"descriptions",
"descriptions.en",
"labels",
"labels.en",
"sitelinks",
"sitelinks.en",
"sitelinks.en.qualifiers",
"sitelinks.qualifiers",
"metadata.node",
"metadata.property.datatypes",
"metadata.types"]
os.environ['SORT_COMMAND'] = sort_command
###Output
_____no_output_____
###Markdown
Run the `import-wikidata` command **NOTE**:This command is set to import only english labels/aliases/descriptions, controlled by parameters `--all-languages False` and `--lang en`.If you wish to import all languages, simple set `--all-languages True`.
###Code
!kgtk ${KGTK_FLAGS} \
import-wikidata \
-i ${WIKIDATA_ALL_JSON} \
--node-file ${TEMP}/metadata.node.${UNSORTED_KGTK} \
--minimal-edge-file ${TEMP}/claims.raw.${UNSORTED_KGTK} \
--minimal-qual-file ${TEMP}/qualifiers.raw.${UNSORTED_KGTK} \
--invalid-edge-file ${TEMP}/claims.badvalue.${UNSORTED_KGTK} \
--invalid-qual-file ${TEMP}/qualifiers.badvalue.${UNSORTED_KGTK} \
--node-file-id-only \
--explode-values False \
--all-languages True \
--lang en \
--alias-edges True \
--split-alias-file ${TEMP}/aliases.${UNSORTED_KGTK} \
--split-en-alias-file ${TEMP}/aliases.en.${UNSORTED_KGTK} \
--description-edges True \
--split-description-file ${TEMP}/descriptions.${UNSORTED_KGTK} \
--split-en-description-file ${TEMP}/descriptions.en.${UNSORTED_KGTK} \
--label-edges True \
--split-label-file ${TEMP}/labels.${UNSORTED_KGTK} \
--split-en-label-file ${TEMP}/labels.en.${UNSORTED_KGTK} \
--datatype-edges True \
--split-datatype-file ${TEMP}/metadata.property.datatypes.${UNSORTED_KGTK} \
--entry-type-edges True \
--split-type-file ${TEMP}/metadata.types.${UNSORTED_KGTK} \
--sitelink-edges True \
--sitelink-verbose-edges True \
--split-sitelink-file ${TEMP}/sitelinks.raw.${UNSORTED_KGTK} \
--split-en-sitelink-file ${TEMP}/sitelinks.en.raw.${UNSORTED_KGTK} \
--value-hash-width 6 \
--claim-id-hash-width 8 \
--use-kgtkwriter True \
--use-mgzip-for-input False \
--use-mgzip-for-output False \
--use-shm True \
--procs 12 \
--mapper-batch-size 5 \
--max-size-per-mapper-queue 3 \
--single-mapper-queue True \
--collect-results True \
--collect-seperately True\
--collector-batch-size 5 \
--collector-queue-per-proc-size 3 \
--progress-interval 500000 \
--clean \
--allow-end-of-day False \
--repair-month-or-day-zero \
--minimum-valid-year 1 \
--maximum-valid-year 9999 \
--validate-fromisoformat \
--repair-lax-coordinates \
--allow-language-suffixes \
--allow-wikidata-lq-strings \
| tee ${TEMP}/import-split-wikidata.log
###Output
kgtk import-wikidata version: 2021-11-17T01:38:17.437678+00:00#9z/aARcXhiV2hPdyVXjAREcpZwh2MawWFp6numz8GZBCtAg2WypLYAFpHjP43k97Zj8VHVaoel0oEit9KHXH0w==
Starting main process (pid 118098).
Processing.
Processing wikidata file /data/amandeep/wikidata-20220505/latest-all.json.bz2
Decompressing (bz2)
Creating the collector queue.
The collector node queue has been created (maxsize=36).
Creating the node_collector.
Creating the node collector process.
Starting the node collector process.
Started the node collector process.
The node collector is starting (pid 118140).
The collector edge queue has been created (maxsize=36).
Creating the edge_collector.
Creating the edge collector process.
Starting the edge collector process.
Started the edge collector process.
The edge collector is starting (pid 118141).
The collector qual queue has been created (maxsize=36).
Creating the qual_collector.
Creating the qual collector process.
Starting the qual collector process.
Started the qual collector process.
The qual collector is starting (pid 118142).
The collector invalid edge queue has been created (maxsize=36).
Creating the invalid_edge_collector.
Creating the invalid edge collector process.
Starting the invalid edge collector process.
Started the invalid edge collector process.
The invalid edge collector is starting (pid 118143).
The collector invalid qual queue has been created (maxsize=36).
Creating the invalid_qual_collector.
Creating the invalid qual collector process.
Starting the invalid qual collector process.
Started the invalid qual collector process.
The invalid qual collector is starting (pid 118144).
The collector description queue has been created (maxsize=36).
Creating the description collector.
Creating the description collector process.
Starting the description collector process.
Started the description collector process.
The description collector is starting (pid 118145).
The collector sitelink queue has been created (maxsize=36).
Creating the sitelink collector.
Creating the sitelink collector process.
Starting the sitelink collector process.
Started the sitelink collector process.
Sending the node header to the collector.
The sitelink collector is starting (pid 118146).
Sent the node header to the collector.
Sending the minimal edge file header to the collector.
Sent the minimal edge file header to the collector.
Sending the alias file header to the collector.
Sent the alias file header to the collector.
Sending the English alias file header to the collector.
Sent the English alias file header to the collector.
Sending the datatype file header to the collector.
Opening the node file in the node collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.node.unsorted.tsv.gz
Sent the datatype file header to the collector.
Sending the description file header to the collector.
Sent the description file header to the collector.
Sending the English description file header to the collector.
Sent the English description file header to the collector.
Sending the label file header to the collector.
Opening the minimal edge file in the edge collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.raw.unsorted.tsv.gz
Sent the label file header to the collector.
Sending the English label file header to the collector.
Sent the English label file header to the collector.
Sending the sitelink file header to the collector.
Sent the sitelink file header to the collector.
Sending the English sitelink file header to the collector.
Sent the English sitelink file header to the collector.
Sending the entry type file header to the collector.
Sent the entry type file header to the collector.
Sending the minimal invalid edge header to the collector.
Opening the description file in the description collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.unsorted.tsv.gz
Sent the minimal invalid edge header to the collector.
Sending the minimal qual file header to the collector.
Sent the minimal qual file header to the collector.
Sending the minimal invalid qual header to the collector.
Sent the minimal invalid qual header to the collector.
Opening the wikipedia_sitelink file in the sitelink collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.raw.unsorted.tsv.gz
Creating parallel processor for /data/amandeep/wikidata-20220505/latest-all.json.bz2
Opening the invalid edge file in the invalid edge collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.badvalue.unsorted.tsv.gz
Opening the minimal qual file in the qual collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.raw.unsorted.tsv.gz
Opening the qual file in the invalid qual collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.badvalue.unsorted.tsv.gz
Opening the alias file in the edge collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.unsorted.tsv.gz
Opening the English description file in the description collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.en.unsorted.tsv.gz
Opening the English wikipedia_sitelink file in the sitelink collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.raw.unsorted.tsv.gz
Opening the English alias file in the edge collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.en.unsorted.tsv.gz
Opening the datatype file in the edge collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.property.datatypes.unsorted.tsv.gz
Opening the label file in the edge collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/labels.unsorted.tsv.gz
Start parallel processing
Opening the English label file in the edge collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/labels.en.unsorted.tsv.gz
Opening the type file in the edge collector with KgtkWriter: /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.types.unsorted.tsv.gz
Starting worker process 0 (pid 118147).
Starting worker process 1 (pid 118148).
Starting worker process 2 (pid 118149).
Starting worker process 3 (pid 118150).
Starting worker process 4 (pid 118151).
Starting worker process 5 (pid 118152).
Starting worker process 6 (pid 118153).
Starting worker process 7 (pid 118154).
Starting worker process 8 (pid 118155).
Starting worker process 9 (pid 118156).
Starting worker process 10 (pid 118157).
Starting worker process 11 (pid 118158).
*** Sitelink collision #1 detected for Q7580-wikipedia_sitelink-dcda22 (http://lv.wikipedia.org/wiki/1743._gads)
*** Qualifier collision #1 detected for Q37062-P26-Q2028843-b2e6740f-0-P580-6f4356 (^1411-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q61814-P26-Q66516-1fa99291-0-P580-d435a1 (^1502-04-10T00:00:00Z/11)
*** Qualifier collision #1 detected for Q62481-P26-Q2086776-87b8910e-0-P580-360391 (^1561-10-12T00:00:00Z/11)
*** Qualifier collision #1 detected for Q70789-P26-Q935411-28987fd8-0-P580-941716 (^1463-05-10T00:00:00Z/11)
*** Qualifier collision #1 detected for Q89405-P26-Q101877-d20a377b-0-P580-2b9eed (^1560-07-01T00:00:00Z/11)
*** Qualifier collision #1 detected for Q150611-P26-Q233335-575116d2-0-P580-29c809 (^1521-05-25T00:00:00Z/11)
*** Qualifier collision #1 detected for Q165284-P26-Q353-84a8ff47-0-P580-776c43 (^1200-05-23T00:00:00Z/11)
*** Qualifier collision #1 detected for Q169992-P26-Q235487-0e315055-0-P580-7a47d9 (^1332-07-28T00:00:00Z/11)
*** Qualifier collision #1 detected for Q183698-P26-Q256222-4322595e-0-P580-1fecee (^1684-01-09T00:00:00Z/11)
*** Qualifier collision #1 detected for Q235447-P26-Q161958-0d89305f-0-P580-52c362 (^1406-10-26T00:00:00Z/11)
*** Qualifier collision #1 detected for Q256222-P26-Q183698-415fc5b0-0-P580-1fecee (^1684-01-09T00:00:00Z/11)
*** Qualifier collision #1 detected for Q380373-P26-Q1141121-48bebee4-0-P580-2e184a (^1294-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q380868-P26-Q384941-46f6240f-0-P580-4b742f (^1533-08-25T00:00:00Z/11)
*** Qualifier collision #1 detected for Q453771-P26-Q443876-84acba5b-0-P580-84a26a (^1446-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q477343-P26-Q3374718-c7014aa0-0-P580-a95d2d (^1573-10-27T00:00:00Z/11)
*** Sitelink collision #1 detected for Q702835-wikipedia_sitelink-6ce2fd (http://uk.wikipedia.org/wiki/Бессі_Купер)
*** Qualifier collision #1 detected for Q1834423-P26-Q322841-6c85598c-0-P580-876067 (^1559-06-16T00:00:00Z/11)
*** Alias collision #1 detected for Q2336516-alias-es-f24d14 ('Elecciones al Parlamento Europeo de 1989 en Dinamarca'@es)
*** Qualifier collision #1 detected for Q3007367-P26-Q430782-f64d3af2-0-P580-5b468d (^1555-02-07T00:00:00Z/11)
*** Sitelink collision #1 detected for Q4299475-wikipedia_sitelink-39fa76 (http://.wikipedia.org/wiki/Template:Bot)
*** Sitelink collision #1 detected for Q4847311-wikipedia_sitelink-c4b491 (http://.wikipedia.org/wiki/Template:Delete)
*** Sitelink collision #1 detected for Q5406510-wikipedia_sitelink-c7418e (http://.wikipedia.org/wiki/Template:=)
*** Sitelink collision #1 detected for Q5412328-wikipedia_sitelink-6bc2e1 (http://.wikipedia.org/wiki/Template:Trim)
*** Sitelink collision #1 detected for Q5607945-wikipedia_sitelink-6795ff (http://mr.wikipedia.org/wiki/वर्ग:मार्गक्रमण_साचे)
*** Sitelink collision #1 detected for Q5621274-wikipedia_sitelink-126246 (http://.wikipedia.org/wiki/Template:Column-count)
*** Sitelink collision #1 detected for Q5882248-wikipedia_sitelink-1cc4bd (http://.wikipedia.org/wiki/Template:Documentation_subpage)
*** Qualifier collision #1 detected for Q7529231-P26-Q6792225-896048a6-0-P580-5a896b (^1508-00-00T00:00:00Z/9)
*** Sitelink collision #1 detected for Q9737782-wikipedia_sitelink-0b4a66 (http://.wikipedia.org/wiki/Category:User_templates)
*** Sitelink collision #1 detected for Q10560270-wikipedia_sitelink-2fe24f (http://.wikipedia.org/wiki/Template:Under_construction)
*** Qualifier collision #1 detected for Q13058108-P159-Q1354-267a1462-0-P625-cb2660 (@23.728063/90.419591)
*** Sitelink collision #1 detected for Q13156670-wikipedia_sitelink-c35c37 (http://.wikipedia.org/wiki/Template:Interwiki_redirect)
*** Sitelink collision #1 detected for Q14511701-wikipedia_sitelink-5b1836 (http://.wikipedia.org/wiki/Template:TemplateData_header)
*** Sitelink collision #1 detected for Q14635514-wikipedia_sitelink-156619 (http://.wikipedia.org/wiki/Template:Reply_to)
*** Sitelink collision #1 detected for Q7253814-wikipedia_sitelink-9e2840 (http://.wikipedia.org/wiki/Module:String)
*** Sitelink collision #1 detected for Q7348344-wikipedia_sitelink-d451bf (http://.wikipedia.org/wiki/Module:Coordinates)
*** Sitelink collision #1 detected for Q15818920-wikipedia_sitelink-fa275b (http://.wikipedia.org/wiki/Template:Autoarchive_resolved_section)
The node collector called 500000 times: 2500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 500000 times: 0 nrows, 60662629 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 500000 times: 0 nrows, 81273275 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The sitelink collector called 500000 times: 0 nrows, 33927067 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q29053400-wikipedia_sitelink-b11f2e (http://.wikipedia.org/wiki/Category:Pages_with_template_loops)
The qual collector called 500000 times: 0 nrows, 0 erows, 7571086 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 1000000 times: 5000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 1000000 times: 0 nrows, 133466230 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 1000000 times: 0 nrows, 200314003 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
500000 lines processed by processor 5
500000 lines processed by processor 1
500000 lines processed by processor 9
500000 lines processed by processor 4
500000 lines processed by processor 10
500000 lines processed by processor 6
500000 lines processed by processor 7
500000 lines processed by processor 2
500000 lines processed by processor 11
500000 lines processed by processor 8
500000 lines processed by processor 3
*** Qualifier collision #1 detected for Q55579391-P26-Q121846-1952d1ff-0-P580-cae35d (^1284-00-00T00:00:00Z/9)
500000 lines processed by processor 0
*** Sitelink collision #1 detected for Q58832772-wikipedia_sitelink-ea0ae7 (http://.wikipedia.org/wiki/Module:LangSwitch)
The qual collector called 1000000 times: 0 nrows, 0 erows, 18531429 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 1500000 times: 7500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 1500000 times: 0 nrows, 183700332 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 1500000 times: 0 nrows, 268671270 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The qual collector called 1500000 times: 0 nrows, 0 erows, 28002684 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 2000000 times: 10000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 2000000 times: 0 nrows, 243125009 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 2000000 times: 0 nrows, 300937648 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q90722487-wikipedia_sitelink-24b73c (http://.wikipedia.org/wiki/Category:Pages_using_deprecated_source_tags)
*** Sitelink collision #1 detected for Q99735928-wikipedia_sitelink-a62902 (http://.wikipedia.org/wiki/Template:BCP47)
The sitelink collector called 1000000 times: 0 nrows, 40653120 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
1000000 lines processed by processor 5
*** Sitelink collision #1 detected for Q109249671-wikipedia_sitelink-f0183d (http://.wikipedia.org/wiki/Template:None)
1000000 lines processed by processor 8
1000000 lines processed by processor 10
1000000 lines processed by processor 7
1000000 lines processed by processor 0
1000000 lines processed by processor 6
1000000 lines processed by processor 11
1000000 lines processed by processor 9
1000000 lines processed by processor 4
1000000 lines processed by processor 1
1000000 lines processed by processor 2
1000000 lines processed by processor 3
The qual collector called 2000000 times: 0 nrows, 0 erows, 38508407 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q8058-P26-Q254085-4eab60ab-0-P580-8df26d (^1436-06-24T00:00:00Z/11)
*** Qualifier collision #1 detected for Q8384-P26-Q70590-6edd7354-0-P580-e23c66 (^1305-09-23T00:00:00Z/11)
*** Qualifier collision #1 detected for Q13167-P348-99b09e-08cc7a6d-0-P577-07f6e3 (^2016-07-12T00:00:00Z/11)
*** Qualifier collision #1 detected for Q57161-P26-Q441394-f0d02358-0-P580-77780b (^1308-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q57161-P26-Q467019-4ee33344-0-P580-9268e9 (^1324-02-25T00:00:00Z/11)
*** Qualifier collision #1 detected for Q74019-P26-Q540767-c098df36-0-P580-5774e5 (^1422-07-25T00:00:00Z/11)
*** Qualifier collision #1 detected for Q121130-P26-Q119431-af2d7776-0-P580-10c067 (^1197-05-25T00:00:00Z/11)
*** Qualifier collision #1 detected for Q151587-P26-Q7996-4448a491-0-P580-62d46c (^1572-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q153319-P26-Q57852-a80af489-0-P580-56d3ba (^1725-06-01T00:00:00Z/11)
*** Qualifier collision #1 detected for Q154998-P26-Q234549-77a9d927-0-P580-45ce34 (^1525-10-29T00:00:00Z/11)
*** Qualifier collision #1 detected for Q184868-P26-Q390071-b34d3d54-0-P580-90dfde (^1680-07-18T00:00:00Z/11)
*** Qualifier collision #1 detected for Q203647-P26-Q2284422-aed54bb0-0-P580-246002 (^1045-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q232137-P26-Q41847-0dcc4fd6-0-P580-fe3abc (^0956-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q263474-P26-Q3044-90d4ea9f-0-P580-9b0b8a (^0770-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q333359-P26-Q3052486-9cbd9d9e-0-P580-355ae9 (^0960-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q454810-P26-Q702209-74f88753-0-P580-e60df9 (^1476-08-25T00:00:00Z/11)
*** Qualifier collision #1 detected for Q598906-P26-Q1635933-0d160adf-0-P580-ec2def (^1236-11-01T00:00:00Z/11)
*** Qualifier collision #1 detected for Q672446-P26-Q2912335-f19e5091-0-P580-93d3bd (^1447-12-14T00:00:00Z/11)
*** Qualifier collision #1 detected for Q674931-P26-Q19601994-f7d507fb-0-P580-9b41a5 (^1222-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q694351-P26-Q329555-c88da6e5-0-P580-15a1f0 (^1381-09-02T00:00:00Z/11)
*** Qualifier collision #1 detected for Q702602-P26-Q79176-0f28ed9a-0-P580-676c21 (^1431-06-03T00:00:00Z/11)
*** Qualifier collision #1 detected for Q719501-P26-Q69462-4f695a08-0-P580-79dbc8 (^1512-07-06T00:00:00Z/11)
*** Sitelink collision #1 detected for Q921009-wikipedia_sitelink-53a877 (http://ceb.wikipedia.org/wiki/Balod_(lungsod_sa_Indiya))
*** Sitelink collision #1 detected for Q1007634-wikipedia_sitelink-ed9b51 (http://ko.wikipedia.org/wiki/에스텔리)
*** Sitelink collision #1 detected for Q1071820-wikipedia_sitelink-aab9e2 (http://br.wikipedia.org/wiki/Lagostomus)
*** Qualifier collision #1 detected for Q1106184-P26-Q4331742-bc45332b-0-P580-e7880a (^1555-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q1381324-P26-Q535528-9a0e7ede-0-P580-c9b00e (^1221-06-19T00:00:00Z/11)
*** Qualifier collision #1 detected for Q3139317-P159-Q9799-e865820c-0-P625-be8120 (@50.8802/5.9595)
The node collector called 2500000 times: 12500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 2500000 times: 0 nrows, 314121442 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q4115450-P159-Q191204-8fdad044-0-P625-a98823 (@35.569778/45.352163)
The description collector called 2500000 times: 0 nrows, 345747666 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q5411705-wikipedia_sitelink-7111a4 (http://.wikipedia.org/wiki/Template:Clear)
*** Sitelink collision #1 detected for Q5459259-wikipedia_sitelink-044e48 (http://.wikipedia.org/wiki/Template:Center)
*** Sitelink collision #1 detected for Q5622198-wikipedia_sitelink-a3c8ed (http://.wikipedia.org/wiki/Template:Done)
*** Sitelink collision #1 detected for Q5646673-wikipedia_sitelink-162b1a (http://.wikipedia.org/wiki/Template:Pp-template)
*** Sitelink collision #1 detected for Q6063221-wikipedia_sitelink-4fe51c (http://.wikipedia.org/wiki/Template:Mbox)
*** Sitelink collision #1 detected for Q6133158-wikipedia_sitelink-62de50 (http://.wikipedia.org/wiki/Template:@)
*** Qualifier collision #1 detected for Q6867218-P159-Q9268849-32a831ad-0-P625-51c420 (@52.223817/21.005108)
*** Qualifier collision #1 detected for Q9061646-P39-Q84701409-5a714518-0-P580-3e1e37 (^1116-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q9061646-P39-Q84701409-5a714518-0-P582-ac0fb1 (^1154-00-00T00:00:00Z/9)
*** Sitelink collision #1 detected for Q16051502-wikipedia_sitelink-1ba00f (http://arz.wikipedia.org/wiki/صوت_الصمت_2013)
*** Sitelink collision #1 detected for Q16748603-wikipedia_sitelink-1c7ff7 (http://.wikipedia.org/wiki/Module:No_globals)
*** Sitelink collision #1 detected for Q17347205-wikipedia_sitelink-9e0e56 (http://.wikipedia.org/wiki/Module:Category_handler/config)
The sitelink collector called 1500000 times: 0 nrows, 73918436 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q26878179-P26-Q55169081-de1c53f2-0-P580-01b412 (^1571-09-08T00:00:00Z/11)
The node collector called 3000000 times: 15000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 3000000 times: 0 nrows, 363934028 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q28941166-wikipedia_sitelink-d4f303 (http://fr.wikipedia.org/wiki/Tempête_de_neige_de_la_mi-mars_2017_dans_l'est_de_l'Amérique_du_Nord)
The description collector called 3000000 times: 0 nrows, 435008293 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The qual collector called 2500000 times: 0 nrows, 0 erows, 45967305 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 3500000 times: 17500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 3500000 times: 0 nrows, 439374610 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 3500000 times: 0 nrows, 558743824 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
1500000 lines processed by processor 5
1500000 lines processed by processor 7
1500000 lines processed by processor 4
1500000 lines processed by processor 9
1500000 lines processed by processor 11
1500000 lines processed by processor 8
1500000 lines processed by processor 0
1500000 lines processed by processor 1
1500000 lines processed by processor 10
1500000 lines processed by processor 6
1500000 lines processed by processor 2
*** Qualifier collision #1 detected for Q54902946-P26-Q31191593-fb18c102-0-P580-d109bb (^1560-12-15T00:00:00Z/11)
1500000 lines processed by processor 3
*** Sitelink collision #1 detected for Q56528384-wikipedia_sitelink-563962 (http://.wikipedia.org/wiki/Module:I18n/date)
*** Qualifier collision #1 detected for Q56582849-P26-Q72922-06e7a6cd-0-P580-c16f56 (^1499-01-21T00:00:00Z/11)
The qual collector called 3000000 times: 0 nrows, 0 erows, 56981081 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 4000000 times: 20000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 4000000 times: 0 nrows, 486362243 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 4000000 times: 0 nrows, 604767527 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q75458516-P26-Q7324457-79a267cb-0-P580-221dc5 (^1568-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q75552262-P26-Q75552257-6fa3779f-0-P580-04284b (^1556-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q75552257-P26-Q75552262-2af17717-0-P580-04284b (^1556-00-00T00:00:00Z/9)
The qual collector called 3500000 times: 0 nrows, 0 erows, 66478883 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 4500000 times: 22500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 4500000 times: 0 nrows, 548812363 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 4500000 times: 0 nrows, 643030994 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The sitelink collector called 2000000 times: 0 nrows, 81240831 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
2000000 lines processed by processor 5
2000000 lines processed by processor 7
2000000 lines processed by processor 4
2000000 lines processed by processor 11
2000000 lines processed by processor 8
2000000 lines processed by processor 2
2000000 lines processed by processor 9
2000000 lines processed by processor 10
2000000 lines processed by processor 0
2000000 lines processed by processor 1
2000000 lines processed by processor 6
2000000 lines processed by processor 3
The qual collector called 4000000 times: 0 nrows, 0 erows, 77003327 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q2323-wikipedia_sitelink-8e4ee7 (http://yo.wikipedia.org/wiki/8_February)
*** Sitelink collision #1 detected for Q8877-wikipedia_sitelink-dcbe09 (http://scn.wikipedia.org/wiki/Steven_Spielberg)
*** Sitelink collision #1 detected for Q9696-wikipedia_sitelink-c8fdf8 (http://haw.wikipedia.org/wiki/John_Fitzgerald_Kennedy)
*** Qualifier collision #1 detected for Q40433-P26-Q463669-cd43ed58-0-P580-480b99 (^1550-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q57654-P26-Q154041-8d52292f-0-P580-3b3df4 (^1572-07-20T00:00:00Z/11)
*** Qualifier collision #1 detected for Q66270-P26-Q325505-28bc872e-0-P580-08d4a0 (^1478-05-29T00:00:00Z/11)
*** Qualifier collision #1 detected for Q66516-P26-Q61814-43ebfd75-0-P580-d435a1 (^1502-04-10T00:00:00Z/11)
*** Qualifier collision #1 detected for Q101877-P26-Q89405-6e0cba4d-0-P580-2b9eed (^1560-07-01T00:00:00Z/11)
*** Qualifier collision #1 detected for Q155167-P26-Q269586-cc56bab6-0-P580-c54274 (^1334-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q168669-P26-Q193658-6bff08d2-0-P580-e8a3ec (^0939-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q241797-P26-Q7731-b7834ae7-0-P580-a01064 (^1671-02-01T00:00:00Z/11)
*** Qualifier collision #1 detected for Q262059-P26-Q187312-c501aba2-0-P580-7e48ad (^1302-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q267483-P26-Q57920-80635ac2-0-P580-c0fc4c (^1570-01-08T00:00:00Z/11)
*** Qualifier collision #1 detected for Q271799-P26-Q169319-a97c2304-0-P580-0d082c (^1523-12-11T00:00:00Z/11)
*** Qualifier collision #1 detected for Q326738-P26-Q684224-2df6ee20-0-P580-ff2137 (^1524-01-17T00:00:00Z/11)
*** Qualifier collision #1 detected for Q327572-P26-Q68952-ae5f6316-0-P580-5906e2 (^1563-05-10T00:00:00Z/11)
*** Qualifier collision #1 detected for Q374210-P26-Q4768218-c9e0eacd-0-P580-ef8382 (^1571-12-19T00:00:00Z/11)
*** Qualifier collision #1 detected for Q384941-P26-Q380868-4ca9581a-0-P580-4b742f (^1533-08-25T00:00:00Z/11)
*** Qualifier collision #1 detected for Q536174-P26-Q551752-c9a99a5e-0-P580-16c9b2 (^1229-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q574718-P26-Q21153658-ffa49040-0-P580-03dd18 (^1319-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q623188-P26-Q553289-7323bb58-0-P580-d8d288 (^1090-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q947423-P26-Q5358431-95b068e2-0-P580-6b2ce5 (^1152-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q2039358-P26-Q13474657-3f305fc3-0-P580-593f4e (^1558-00-00T00:00:00Z/9)
*** Sitelink collision #1 detected for Q6428609-wikipedia_sitelink-660dd5 (http://nah.wikipedia.org/wiki/Neneuhcāyōtl:Tlatequitiltilīlli_pt-1)
*** Sitelink collision #1 detected for Q6705618-wikipedia_sitelink-4b5e22 (http://.wikipedia.org/wiki/Template:Autotranslate)
The node collector called 5000000 times: 25000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 5000000 times: 0 nrows, 619728420 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q7221363-wikipedia_sitelink-78630c (http://.wikipedia.org/wiki/Category:Lua-based_templates)
*** Qualifier collision #1 detected for Q7324457-P26-Q75567328-84b7c804-0-P580-4e6c67 (^1553-11-24T00:00:00Z/11)
*** Qualifier collision #1 detected for Q7324457-P26-Q75458516-fee1a551-0-P580-221dc5 (^1568-00-00T00:00:00Z/9)
*** Sitelink collision #1 detected for Q7453435-wikipedia_sitelink-c016e9 (http://ku.wikipedia.org/wiki/Kategorî:Ewrasya)
The description collector called 5000000 times: 0 nrows, 710063872 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q10350561-wikipedia_sitelink-5f2907 (http://.wikipedia.org/wiki/Template:Lua)
*** Sitelink collision #1 detected for Q15116966-wikipedia_sitelink-74d712 (http://.wikipedia.org/wiki/Module:Message_box)
*** Sitelink collision #1 detected for Q15212145-wikipedia_sitelink-907e39 (http://.wikipedia.org/wiki/Template:LangSwitch)
*** Sitelink collision #1 detected for Q17121869-wikipedia_sitelink-582caf (http://.wikipedia.org/wiki/Module:Lua_banner)
The sitelink collector called 2500000 times: 0 nrows, 113956702 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q21153658-P26-Q574718-39f28f24-0-P580-03dd18 (^1319-00-00T00:00:00Z/9)
*** Sitelink collision #1 detected for Q26905108-wikipedia_sitelink-51f8f2 (http://.wikipedia.org/wiki/Module:I18n/complex_date)
The node collector called 5500000 times: 27500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 5500000 times: 0 nrows, 669593652 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 5500000 times: 0 nrows, 797514432 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The qual collector called 4500000 times: 0 nrows, 0 erows, 84435753 qrows, 0 invalid erows, 0 invalid qrows
2500000 lines processed by processor 5
2500000 lines processed by processor 7
2500000 lines processed by processor 4
2500000 lines processed by processor 11
2500000 lines processed by processor 0
2500000 lines processed by processor 8
2500000 lines processed by processor 9
The node collector called 6000000 times: 30000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 6000000 times: 0 nrows, 742817342 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
2500000 lines processed by processor 2
2500000 lines processed by processor 10
2500000 lines processed by processor 6
2500000 lines processed by processor 1
2500000 lines processed by processor 3
The description collector called 6000000 times: 0 nrows, 918682552 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The qual collector called 5000000 times: 0 nrows, 0 erows, 95556082 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 6500000 times: 0 nrows, 788430809 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 6500000 times: 32500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 6500000 times: 0 nrows, 944911355 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The qual collector called 5500000 times: 0 nrows, 0 erows, 104971336 qrows, 0 invalid erows, 0 invalid qrows
The sitelink collector called 3000000 times: 0 nrows, 121890140 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 7000000 times: 35000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 7000000 times: 0 nrows, 852807659 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 7000000 times: 0 nrows, 985723776 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
3000000 lines processed by processor 5
3000000 lines processed by processor 7
3000000 lines processed by processor 2
3000000 lines processed by processor 11
3000000 lines processed by processor 10
3000000 lines processed by processor 0
3000000 lines processed by processor 8
3000000 lines processed by processor 9
3000000 lines processed by processor 4
3000000 lines processed by processor 6
3000000 lines processed by processor 3
3000000 lines processed by processor 1
The qual collector called 6000000 times: 0 nrows, 0 erows, 115587156 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q3044-P26-Q263474-631d88d0-0-P580-9b0b8a (^0770-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q3044-P26-Q261866-27b1ed09-0-P580-3fbd66 (^0794-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q27932-P26-Q287503-29306074-0-P580-11c3a9 (^1237-04-25T00:00:00Z/11)
*** Qualifier collision #1 detected for Q38370-P26-Q80823-ae3ce4e4-0-P580-c9d352 (^1533-01-25T00:00:00Z/11)
*** Qualifier collision #1 detected for Q38370-P26-Q182637-8103e2ff-0-P580-7524c3 (^1536-05-30T00:00:00Z/11)
*** Qualifier collision #1 detected for Q38370-P26-Q57126-cb76b09d-0-P580-c55b0a (^1540-01-06T00:00:00Z/11)
*** Qualifier collision #1 detected for Q38370-P26-Q188926-259757b1-0-P580-3301d6 (^1540-07-28T00:00:00Z/11)
*** Qualifier collision #1 detected for Q38370-P26-Q192943-4b53adeb-0-P580-1ea2b6 (^1543-07-12T00:00:00Z/11)
*** Sitelink collision #1 detected for Q43682-wikipedia_sitelink-7b59de (http://ms.wikipedia.org/wiki/Philipp_Lahm)
*** Qualifier collision #1 detected for Q60563-P26-Q2915743-f5fdee07-0-P580-cade68 (^1169-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q65946-P26-Q462536-89b54878-0-P580-48f754 (^1407-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q66888-P26-Q3721846-b7243730-0-P580-4e0bc1 (^1571-01-09T00:00:00Z/11)
*** Qualifier collision #1 detected for Q66888-P26-Q23771111-34bc78ba-0-P580-713f01 (^1560-03-03T00:00:00Z/11)
*** Qualifier collision #1 detected for Q68304-P26-Q539111-dfcad6f4-0-P580-d0edbb (^1545-05-17T00:00:00Z/11)
*** Qualifier collision #1 detected for Q95627-P26-Q354945-873a167d-0-P580-3b86a9 (^1276-11-24T00:00:00Z/11)
*** Qualifier collision #1 detected for Q121846-P26-Q55579391-afdbc2b3-0-P580-cae35d (^1284-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q122794-P26-Q430950-e085ea2d-0-P580-94ae3a (^1577-10-20T00:00:00Z/11)
*** Qualifier collision #1 detected for Q124682-P26-Q337057-4fb67536-0-P580-db5ec5 (^1389-08-17T00:00:00Z/11)
*** Qualifier collision #1 detected for Q130005-P26-Q259564-c738415f-0-P580-a4a595 (^1045-01-23T00:00:00Z/11)
*** Qualifier collision #1 detected for Q132545-P26-Q131552-2fbc7eb5-0-P580-e56690 (^1533-10-28T00:00:00Z/11)
*** Qualifier collision #1 detected for Q134452-P26-Q201143-a2079e30-0-P580-7c0e43 (^1491-12-06T00:00:00Z/11)
*** Qualifier collision #1 detected for Q160349-P26-Q154064-ec5ff971-0-P580-7a7cba (^1385-07-17T00:00:00Z/11)
*** Qualifier collision #1 detected for Q220845-P26-Q936976-0f99833d-0-P580-5eeb19 (^1572-08-18T00:00:00Z/11)
*** Qualifier collision #1 detected for Q234257-P26-Q170398-56a0eb9a-0-P580-850b4d (^1816-01-24T00:00:00Z/11)
*** Qualifier collision #1 detected for Q259564-P26-Q130005-bd5ab415-0-P580-a4a595 (^1045-01-23T00:00:00Z/11)
*** Qualifier collision #1 detected for Q325583-P26-Q527486-704144b1-0-P580-f981af (^1577-05-19T00:00:00Z/11)
*** Qualifier collision #1 detected for Q326449-P26-Q23682783-85a9914e-0-P580-13178a (^1736-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q440132-P26-Q506527-db15118a-0-P580-2bef25 (^1524-11-06T00:00:00Z/11)
*** Qualifier collision #1 detected for Q469389-P26-Q1924994-36c61689-0-P580-017942 (^1377-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q658714-P26-Q20498980-28fdf4a6-0-P580-0672b7 (^1409-01-30T00:00:00Z/11)
*** Qualifier collision #1 detected for Q682736-P26-Q68285-f3f03090-0-P580-eae385 (^1460-11-19T00:00:00Z/11)
*** Qualifier collision #1 detected for Q684276-P26-Q61576937-87fbff2c-0-P580-2b5632 (^1217-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q684224-P26-Q326738-18c31ccf-0-P580-ff2137 (^1524-01-17T00:00:00Z/11)
*** Qualifier collision #1 detected for Q703249-P26-Q1309296-09047836-0-P580-a97c74 (^1228-00-00T00:00:00Z/9)
*** Sitelink collision #1 detected for Q1581723-wikipedia_sitelink-ff37b1 (http://eu.wikipedia.org/wiki/The_Love_Parade)
*** Qualifier collision #1 detected for Q2028843-P26-Q37062-259ae253-0-P580-6f4356 (^1411-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q6244699-P26-Q76366716-754c9057-0-P580-d7261a (^1579-04-27T00:00:00Z/11)
*** Sitelink collision #1 detected for Q7020051-wikipedia_sitelink-033b05 (http://fi.wikipedia.org/wiki/Luokka:Palkitut)
The node collector called 7500000 times: 37500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 7500000 times: 0 nrows, 922083165 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q15117391-wikipedia_sitelink-00ef81 (http://.wikipedia.org/wiki/Module:Message_box/configuration)
The description collector called 7500000 times: 0 nrows, 1062631793 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The sitelink collector called 3500000 times: 0 nrows, 153793624 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q25713407-wikipedia_sitelink-ff4697 (http://.wikipedia.org/wiki/Template:CURRENTCONTENTLANGUAGE)
The node collector called 8000000 times: 40000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 8000000 times: 0 nrows, 974216636 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The qual collector called 6500000 times: 0 nrows, 0 erows, 122984270 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 8000000 times: 0 nrows, 1155675148 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
3500000 lines processed by processor 0
3500000 lines processed by processor 5
3500000 lines processed by processor 7
3500000 lines processed by processor 11
3500000 lines processed by processor 2
3500000 lines processed by processor 10
3500000 lines processed by processor 8
3500000 lines processed by processor 9
3500000 lines processed by processor 4
3500000 lines processed by processor 3
3500000 lines processed by processor 6
3500000 lines processed by processor 1
The node collector called 8500000 times: 42500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 8500000 times: 0 nrows, 1047004832 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The qual collector called 7000000 times: 0 nrows, 0 erows, 134121504 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 8500000 times: 0 nrows, 1254745369 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q64944842-wikipedia_sitelink-8258da (http://.wikipedia.org/wiki/Module:Portal_navigation)
*** Qualifier collision #1 detected for Q65617406-P26-Q265478-6faeca05-0-P580-dc9c16 (^1884-05-30T00:00:00Z/11)
*** Qualifier collision #1 detected for Q76366716-P26-Q6244699-b5d45f0b-0-P580-d7261a (^1579-04-27T00:00:00Z/11)
The edge collector called 9000000 times: 0 nrows, 1092386056 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 9000000 times: 45000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The qual collector called 7500000 times: 0 nrows, 0 erows, 143533696 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 9000000 times: 0 nrows, 1286483409 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The sitelink collector called 4000000 times: 0 nrows, 162571004 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 9500000 times: 47500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 9500000 times: 0 nrows, 1155205313 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q102226589-wikipedia_sitelink-c65fff (http://.wikipedia.org/wiki/Template:User_mnw)
4000000 lines processed by processor 7
4000000 lines processed by processor 0
4000000 lines processed by processor 11
4000000 lines processed by processor 5
4000000 lines processed by processor 10
4000000 lines processed by processor 8
4000000 lines processed by processor 2
The description collector called 9500000 times: 0 nrows, 1331240615 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
4000000 lines processed by processor 9
4000000 lines processed by processor 4
4000000 lines processed by processor 3
4000000 lines processed by processor 1
4000000 lines processed by processor 6
The qual collector called 8000000 times: 0 nrows, 0 erows, 154200276 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q3740-wikipedia_sitelink-7aaed8 (http://.wikipedia.org/wiki/Category:Templates)
*** Sitelink collision #1 detected for Q8079-wikipedia_sitelink-ade6e5 (http://ga.wikipedia.org/wiki/Nintendo_Wii)
*** Qualifier collision #1 detected for Q41847-P26-Q232137-573ea212-0-P580-fe3abc (^0956-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q60386-P26-Q157776-b68a50b9-0-P580-e55fcf (^1478-09-06T00:00:00Z/11)
*** Qualifier collision #1 detected for Q60211-P26-Q264709-a5d5e20b-0-P580-7f1413 (^1564-12-17T00:00:00Z/11)
*** Qualifier collision #1 detected for Q70828-P26-Q110845-78948fbb-0-P580-189c4f (^1282-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q79176-P26-Q702602-bcda292d-0-P580-676c21 (^1431-06-03T00:00:00Z/11)
*** Sitelink collision #1 detected for Q112783-wikipedia_sitelink-d7b2c3 (http://uk.wikipedia.org/wiki/Вілкокс_(округ))
*** Qualifier collision #1 detected for Q119050-P26-Q26882160-c09f6014-0-P580-78cd0b (^1567-01-13T00:00:00Z/11)
*** Qualifier collision #1 detected for Q120365-P26-Q69620-c54a3667-0-P580-05429a (^1116-07-13T00:00:00Z/11)
*** Qualifier collision #1 detected for Q167782-P26-Q231794-aef59aa3-0-P580-7e2e98 (^1350-04-08T00:00:00Z/11)
*** Qualifier collision #1 detected for Q170586-P26-Q231742-11295529-0-P580-90c66e (^1313-07-00T00:00:00Z/10)
*** Qualifier collision #1 detected for Q172203-P26-Q229419-b442326a-0-P580-a50c51 (^1262-05-28T00:00:00Z/11)
*** Qualifier collision #1 detected for Q174964-P26-Q231798-bd2d3d6b-0-P580-dc0f7a (^1322-09-21T00:00:00Z/11)
*** Qualifier collision #1 detected for Q202566-P26-Q688471-440b6399-0-P580-283d12 (^1531-09-20T00:00:00Z/11)
*** Qualifier collision #1 detected for Q232801-P26-Q721680-fa26b14e-0-P580-70598b (^1473-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q270234-P26-Q210569-6b693078-0-P580-f6928a (^1446-06-20T00:00:00Z/11)
*** Qualifier collision #1 detected for Q325824-P26-Q547225-762b0607-0-P580-df29d7 (^1467-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q441394-P26-Q57161-47bffbac-0-P580-77780b (^1308-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q454769-P26-Q76956-91d862f6-0-P580-981a99 (^1245-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q455201-P26-Q152148-9eb66558-0-P580-e1de94 (^1389-05-02T00:00:00Z/11)
*** Qualifier collision #1 detected for Q479538-P26-Q98010-40ca7cda-0-P580-31ff5b (^1582-11-10T00:00:00Z/11)
*** Qualifier collision #1 detected for Q513315-P26-Q87066-cd6b2f7c-0-P580-3f638b (^1551-03-10T00:00:00Z/11)
*** Qualifier collision #1 detected for Q513315-P26-Q70019-5c7fa382-0-P580-f9548c (^1558-08-01T00:00:00Z/11)
*** Qualifier collision #1 detected for Q547225-P26-Q325824-31db3890-0-P580-df29d7 (^1467-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q567378-P26-Q434771-205319b2-0-P580-4d06ab (^1509-11-20T00:00:00Z/11)
*** Qualifier collision #1 detected for Q571597-P26-Q327750-b0a44162-0-P580-f16789 (^1555-09-10T00:00:00Z/11)
*** Qualifier collision #1 detected for Q687028-P26-Q2334373-525f829d-0-P580-a6af64 (^1556-02-16T00:00:00Z/11)
*** Qualifier collision #1 detected for Q1070853-P26-Q2467970-c7d5f6fa-0-P580-d71f7b (^1358-09-04T00:00:00Z/11)
*** Qualifier collision #1 detected for Q1141121-P26-Q380373-eeba5d95-0-P580-2e184a (^1294-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q1916706-P26-Q80714-35bbccc5-0-P580-d3fce7 (^1109-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q2049198-P26-Q63291-be046904-0-P580-f3b88a (^1372-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q2334373-P26-Q687028-b6b9f398-0-P580-a6af64 (^1556-02-16T00:00:00Z/11)
*** Qualifier collision #1 detected for Q2334373-P26-Q328693-3f939052-0-P580-0d15c6 (^1543-08-26T00:00:00Z/11)
*** Sitelink collision #1 detected for Q3926051-wikipedia_sitelink-a027cd (http://.wikipedia.org/wiki/Template:Tl)
*** Sitelink collision #1 detected for Q4481730-wikipedia_sitelink-1d5954 (http://.wikipedia.org/wiki/Template:Tracked)
*** Sitelink collision #1 detected for Q4989282-wikipedia_sitelink-8a2fcc (http://.wikipedia.org/wiki/Category:Pages_with_broken_file_links)
*** Sitelink collision #1 detected for Q5070586-wikipedia_sitelink-b1e4d1 (http://.wikipedia.org/wiki/Template:Shortcut)
*** Sitelink collision #1 detected for Q6027565-wikipedia_sitelink-91a43e (http://.wikipedia.org/wiki/Template:Tag)
*** Qualifier collision #1 detected for Q6940461-P159-Q61302-c45d5aa7-0-P625-dc88d7 (@28.6386/-106.0756)
*** Sitelink collision #1 detected for Q7643575-wikipedia_sitelink-d4d012 (http://.wikipedia.org/wiki/Template:Colon)
*** Qualifier collision #1 detected for Q9150575-P26-Q679083-79dd46a6-0-P580-5d5db4 (^1320-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q13972091-P26-Q75389849-5b19ecc3-0-P1319-532ed8 (^1509-07-04T00:00:00Z/11)
*** Qualifier collision #1 detected for Q13972091-P26-Q6469914-b9869239-0-P1319-839147 (^1520-00-00T00:00:00Z/9)
The node collector called 10000000 times: 50000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 10000000 times: 0 nrows, 1225496761 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q15281133-P26-Q75240211-8a7057f8-0-P580-97ad08 (^1526-07-20T00:00:00Z/11)
*** Sitelink collision #1 detected for Q15605797-wikipedia_sitelink-d3c200 (http://.wikipedia.org/wiki/Module:List)
The sitelink collector called 4500000 times: 0 nrows, 193397096 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q17347230-wikipedia_sitelink-be73cc (http://.wikipedia.org/wiki/Module:Category_handler/blacklist)
*** Qualifier collision #1 detected for Q20202663-P26-Q299612-893fda0a-0-P580-b50376 (^1080-00-00T00:00:00Z/9)
The description collector called 10000000 times: 0 nrows, 1410220232 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q20819962-wikipedia_sitelink-377adb (http://.wikipedia.org/wiki/Module:Fallback)
*** Qualifier collision #1 detected for Q26877297-P26-Q542751-a70d423c-0-P580-d584ea (^1488-02-17T00:00:00Z/11)
*** Qualifier collision #1 detected for Q27031232-P26-Q55217321-0fe60a4f-0-P580-7606e7 (^1280-00-00T00:00:00Z/9)
The qual collector called 8500000 times: 0 nrows, 0 erows, 161593444 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 10500000 times: 52500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 10500000 times: 0 nrows, 1291455380 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 10500000 times: 0 nrows, 1518697994 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q44191792-P26-Q54862322-4c83e8e6-0-P580-a27cd1 (^1567-08-21T00:00:00Z/11)
4500000 lines processed by processor 7
4500000 lines processed by processor 0
4500000 lines processed by processor 11
4500000 lines processed by processor 5
4500000 lines processed by processor 10
4500000 lines processed by processor 8
4500000 lines processed by processor 9
4500000 lines processed by processor 2
4500000 lines processed by processor 4
4500000 lines processed by processor 3
4500000 lines processed by processor 1
4500000 lines processed by processor 6
The qual collector called 9000000 times: 0 nrows, 0 erows, 172525914 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 11000000 times: 55000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 11000000 times: 0 nrows, 1351553522 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q64506586-P26-Q262726-b670dee9-0-P580-4a9d3d (^1298-00-00T00:00:00Z/9)
The description collector called 11000000 times: 0 nrows, 1600991120 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The sitelink collector called 5000000 times: 0 nrows, 203335940 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q75395291-P26-Q76157640-e3d697ee-0-P580-54254d (^1578-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q75420332-P26-Q208922-3b5559ee-0-P580-447dca (^1559-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q76157640-P26-Q75395291-d75eee5e-0-P580-54254d (^1578-00-00T00:00:00Z/9)
The edge collector called 11500000 times: 0 nrows, 1398071827 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 11500000 times: 57500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The qual collector called 9500000 times: 0 nrows, 0 erows, 181961851 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 11500000 times: 0 nrows, 1631823417 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
5000000 lines processed by processor 0
5000000 lines processed by processor 11
5000000 lines processed by processor 7
5000000 lines processed by processor 8
5000000 lines processed by processor 10
5000000 lines processed by processor 5
The node collector called 12000000 times: 60000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 12000000 times: 0 nrows, 1457551225 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
5000000 lines processed by processor 9
5000000 lines processed by processor 4
5000000 lines processed by processor 2
5000000 lines processed by processor 3
5000000 lines processed by processor 6
5000000 lines processed by processor 1
The qual collector called 10000000 times: 0 nrows, 0 erows, 192683479 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q7731-P26-Q259907-7f7cc241-0-P580-8d5052 (^1648-01-26T00:00:00Z/11)
*** Qualifier collision #1 detected for Q7731-P26-Q241797-ff9269a2-0-P580-a01064 (^1671-02-01T00:00:00Z/11)
*** Qualifier collision #1 detected for Q57920-P26-Q267483-a2460de3-0-P580-c0fc4c (^1570-01-08T00:00:00Z/11)
*** Qualifier collision #1 detected for Q62483-P26-Q229286-18b62769-0-P580-bebb21 (^1541-06-14T00:00:00Z/11)
*** Qualifier collision #1 detected for Q62483-P26-Q261905-fc01d066-0-P580-7aecc7 (^1546-07-18T00:00:00Z/11)
*** Qualifier collision #1 detected for Q69334-P26-Q2419674-1dc5e587-0-P580-e1ff18 (^1183-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q72922-P26-Q56582849-77ca7313-0-P580-c16f56 (^1499-01-21T00:00:00Z/11)
*** Qualifier collision #1 detected for Q76956-P26-Q454769-cf7fc40d-0-P580-981a99 (^1245-00-00T00:00:00Z/9)
*** Sitelink collision #1 detected for Q80235-wikipedia_sitelink-03e5ac (http://is.wikipedia.org/wiki/Tamarind)
*** Qualifier collision #1 detected for Q86055-P26-Q24661944-f75c4596-0-P580-54820b (^1472-10-19T00:00:00Z/11)
*** Sitelink collision #1 detected for Q151327-wikipedia_sitelink-9044c0 (http://oc.wikipedia.org/wiki/(333)_Badenia)
*** Qualifier collision #1 detected for Q168664-P26-Q15193-1b533b05-0-P580-a310ca (^1793-10-09T00:00:00Z/11)
*** Qualifier collision #1 detected for Q276526-P26-Q10855916-3e70b907-0-P580-f18c2a (^1392-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q287503-P26-Q316828-d4637da7-0-P580-9879f5 (^1261-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q316831-P26-Q238609-208f7dcc-0-P580-92ae06 (^1153-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q316831-P26-Q450971-656d5797-0-P580-5ed4f3 (^1177-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q336754-P26-Q2084307-30a93eb5-0-P580-b520a9 (^1318-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q370902-P26-Q75289133-2d7df0e9-0-P580-83a193 (^1275-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q430782-P26-Q3007367-9502d33f-0-P580-5b468d (^1555-02-07T00:00:00Z/11)
*** Qualifier collision #1 detected for Q443876-P26-Q453771-bbc80f51-0-P580-84a26a (^1446-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q563792-P26-Q4958342-a85e5b57-0-P580-acfb1b (^1391-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q588852-P26-Q58514-55e81240-0-P580-ae0480 (^1514-10-09T00:00:00Z/11)
*** Qualifier collision #1 detected for Q651948-P26-Q9165680-bf5d7e43-0-P580-a08da9 (^1396-03-06T00:00:00Z/11)
*** Qualifier collision #1 detected for Q679083-P26-Q9150575-c56910ae-0-P580-5d5db4 (^1320-00-00T00:00:00Z/9)
*** Sitelink collision #1 detected for Q929200-wikipedia_sitelink-7842a9 (http://vi.wikipedia.org/wiki/Ilicura_militaris)
*** Qualifier collision #1 detected for Q936976-P26-Q220845-281a5972-0-P580-5eeb19 (^1572-08-18T00:00:00Z/11)
*** Qualifier collision #1 detected for Q1166728-P26-Q1494018-db61e006-0-P580-3550f9 (^1285-00-00T00:00:00Z/9)
The description collector called 12000000 times: 0 nrows, 1675439151 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q1494018-P26-Q1166728-5c17988d-0-P580-3550f9 (^1285-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q1524640-P26-Q166853-40fa3891-0-P580-515f76 (^1375-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q2465095-P26-Q1167368-4ffb7291-0-P580-e64863 (^1257-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q2834394-P39-Q84701409-f487718d-0-P580-ac0fb1 (^1154-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q2834394-P39-Q84701409-f487718d-0-P582-35fc60 (^1173-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q2844574-P793-Q2238935-db1dea90-0-P585-ab2ece (^1350-00-00T00:00:00Z/7)
*** Alias collision #1 detected for Q4493910-alias-ru-70f749 ('Фёдоров А. В.'@ru)
*** Sitelink collision #1 detected for Q4608595-wikipedia_sitelink-4a0154 (http://.wikipedia.org/wiki/Template:Documentation)
*** Sitelink collision #1 detected for Q5611978-wikipedia_sitelink-3b808e (http://.wikipedia.org/wiki/Template:Welcome)
*** Qualifier collision #1 detected for Q6129540-P106-Q25393460-4c72cbac-0-P580-9eefc6 (^1552-07-17T00:00:00Z/11)
*** Sitelink collision #1 detected for Q6426831-wikipedia_sitelink-0d77ef (http://.wikipedia.org/wiki/Template:Edit_filter_warning)
*** Sitelink collision #1 detected for Q7605021-wikipedia_sitelink-3a136d (http://.wikipedia.org/wiki/Template:Comment)
The sitelink collector called 5500000 times: 0 nrows, 232340004 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q15098140-wikipedia_sitelink-bfa017 (http://.wikipedia.org/wiki/Module:Yesno)
*** Sitelink collision #1 detected for Q15117218-wikipedia_sitelink-4b5db5 (http://.wikipedia.org/wiki/Module:Category_handler)
*** Sitelink collision #1 detected for Q15506579-wikipedia_sitelink-c363ea (http://.wikipedia.org/wiki/Module:Documentation/config)
*** Sitelink collision #1 detected for Q8244473-wikipedia_sitelink-0e32ac (http://.wikipedia.org/wiki/Module:InfoboxImage)
The node collector called 12500000 times: 62500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 12500000 times: 0 nrows, 1530628094 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q20962109-wikipedia_sitelink-0f5e03 (http://.wikipedia.org/wiki/Module:ISOdate)
*** Sitelink collision #1 detected for Q22910717-wikipedia_sitelink-4da401 (http://.wikipedia.org/wiki/Template:Sandbox_other)
The description collector called 12500000 times: 0 nrows, 1757625754 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q25714577-wikipedia_sitelink-5c85f4 (http://.wikipedia.org/wiki/Module:WikidataIB)
*** Qualifier collision #1 detected for Q26877285-P26-Q828710-08b99587-0-P580-c30f0a (^1566-02-16T00:00:00Z/11)
The qual collector called 10500000 times: 0 nrows, 0 erows, 200088433 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 13000000 times: 65000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 13000000 times: 0 nrows, 1599577883 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 13000000 times: 0 nrows, 1879410082 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
5500000 lines processed by processor 0
5500000 lines processed by processor 11
5500000 lines processed by processor 7
5500000 lines processed by processor 8
5500000 lines processed by processor 10
5500000 lines processed by processor 5
5500000 lines processed by processor 9
5500000 lines processed by processor 2
5500000 lines processed by processor 4
5500000 lines processed by processor 3
5500000 lines processed by processor 6
5500000 lines processed by processor 1
The qual collector called 11000000 times: 0 nrows, 0 erows, 210987903 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q61140016-P26-Q44192051-99f17a00-0-P580-7a76d2 (^1575-01-30T00:00:00Z/11)
The sitelink collector called 6000000 times: 0 nrows, 243922942 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 13500000 times: 67500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 13500000 times: 0 nrows, 1654081431 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 13500000 times: 0 nrows, 1939040832 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q75390802-P26-Q6135465-d640c900-0-P580-91ce5e (^1422-10-20T00:00:00Z/11)
The qual collector called 11500000 times: 0 nrows, 0 erows, 220410486 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 14000000 times: 70000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 14000000 times: 0 nrows, 1703202908 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 14000000 times: 0 nrows, 1972258264 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
6000000 lines processed by processor 11
6000000 lines processed by processor 0
6000000 lines processed by processor 7
6000000 lines processed by processor 8
6000000 lines processed by processor 10
6000000 lines processed by processor 5
6000000 lines processed by processor 9
6000000 lines processed by processor 4
6000000 lines processed by processor 2
6000000 lines processed by processor 3
6000000 lines processed by processor 6
6000000 lines processed by processor 1
The node collector called 14500000 times: 72500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 14500000 times: 0 nrows, 1766161278 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The qual collector called 12000000 times: 0 nrows, 0 erows, 231223257 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q15193-P26-Q168664-80106bb4-0-P580-a310ca (^1793-10-09T00:00:00Z/11)
*** Qualifier collision #1 detected for Q53459-P26-Q93408-6fe4810b-0-P580-df7c8b (^1454-02-10T00:00:00Z/11)
*** Sitelink collision #1 detected for Q56226-wikipedia_sitelink-ff2d61 (http://sl.wikipedia.org/wiki/Kim_Džong-un)
*** Qualifier collision #1 detected for Q58514-P26-Q236220-01ae7b47-0-P580-766cef (^1476-09-08T00:00:00Z/11)
*** Qualifier collision #1 detected for Q58514-P26-Q201143-bc6f20e4-0-P580-5a4f65 (^1499-01-08T00:00:00Z/11)
*** Qualifier collision #1 detected for Q58514-P26-Q588852-cdd0895d-0-P580-ae0480 (^1514-10-09T00:00:00Z/11)
*** Qualifier collision #1 detected for Q69620-P26-Q120365-10ec8d2b-0-P580-05429a (^1116-07-13T00:00:00Z/11)
*** Qualifier collision #1 detected for Q91003-P26-Q72789-6b43d0ab-0-P580-4be813 (^1150-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q130969-P26-Q229189-a7f573de-0-P580-aacd85 (^1284-08-16T00:00:00Z/11)
*** Qualifier collision #1 detected for Q131412-P26-Q132548-dcd19f44-0-P580-3d6c00 (^1558-04-24T00:00:00Z/11)
*** Qualifier collision #1 detected for Q157789-P26-Q260926-aa804d7f-0-P580-d435a1 (^1502-04-10T00:00:00Z/11)
*** Qualifier collision #1 detected for Q157789-P26-Q233790-625e10c2-0-P580-4cdfa7 (^1518-10-09T00:00:00Z/11)
*** Qualifier collision #1 detected for Q160165-P26-Q63494-d339ca47-0-P580-b1f503 (^1710-11-11T00:00:00Z/11)
*** Qualifier collision #1 detected for Q178525-P26-Q134259-aaf86e95-0-P580-d2c4ca (^1137-07-25T00:00:00Z/11)
*** Qualifier collision #1 detected for Q178525-P26-Q102140-ab1e10d9-0-P580-8b694f (^1152-05-18T00:00:00Z/11)
*** Sitelink collision #1 detected for Q208420-wikipedia_sitelink-87d729 (http://pl.wikipedia.org/wiki/Triera)
*** Qualifier collision #1 detected for Q231476-P26-Q161866-fa54b200-0-P580-3b4b8f (^1403-02-07T00:00:00Z/11)
*** Qualifier collision #1 detected for Q231476-P26-Q449008-63ea5e99-0-P580-6fd386 (^1386-09-11T00:00:00Z/11)
*** Qualifier collision #1 detected for Q254927-P26-Q367001-9bafa4c7-0-P580-a91848 (^1112-02-03T00:00:00Z/11)
*** Qualifier collision #1 detected for Q259907-P26-Q7731-5a5cc2ce-0-P580-8d5052 (^1648-01-26T00:00:00Z/11)
*** Qualifier collision #1 detected for Q261847-P26-Q767582-bc9963e7-0-P580-5c7f0d (^1456-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q266309-P26-Q510987-645ea879-0-P580-5513d1 (^1272-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q283548-P26-Q450768-eb0b2e92-0-P580-26c3cf (^1572-08-17T00:00:00Z/11)
*** Sitelink collision #1 detected for Q397733-wikipedia_sitelink-d5cb47 (http://ar.wikipedia.org/wiki/سلوني)
*** Qualifier collision #1 detected for Q505918-P26-Q274025-fb8c2108-0-P580-e7b518 (^1545-02-15T00:00:00Z/11)
*** Qualifier collision #1 detected for Q540767-P26-Q74019-da034a0b-0-P580-5774e5 (^1422-07-25T00:00:00Z/11)
*** Qualifier collision #1 detected for Q702209-P26-Q454810-36c82016-0-P580-e60df9 (^1476-08-25T00:00:00Z/11)
*** Qualifier collision #1 detected for Q935411-P26-Q70789-de6fac79-0-P580-941716 (^1463-05-10T00:00:00Z/11)
*** Qualifier collision #1 detected for Q1360375-P26-Q4726173-be500fdb-0-P580-98b695 (^1253-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q2318556-P26-Q317621-b5da07f6-0-P580-a8e531 (^1575-06-14T00:00:00Z/11)
*** Qualifier collision #1 detected for Q3721525-P26-Q571632-481d6ba3-0-P580-10eca4 (^1436-02-12T00:00:00Z/11)
*** Qualifier collision #1 detected for Q3904375-P26-Q57231616-7c1080b1-0-P580-df5c65 (^1281-00-00T00:00:00Z/9)
*** Sitelink collision #1 detected for Q4048908-wikipedia_sitelink-0836ac (http://.wikipedia.org/wiki/Category:Hidden_categories)
*** Sitelink collision #1 detected for Q5324375-wikipedia_sitelink-91df00 (http://.wikipedia.org/wiki/Category:Maintenance)
*** Sitelink collision #1 detected for Q5626735-wikipedia_sitelink-7430af (http://.wikipedia.org/wiki/Template:Infobox)
*** Qualifier collision #1 detected for Q6135465-P26-Q75390802-f0852539-0-P580-91ce5e (^1422-10-20T00:00:00Z/11)
The description collector called 14500000 times: 0 nrows, 2017690517 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q6469914-P26-Q4497270-0dd2e8d0-0-P580-bd95e9 (^1511-08-28T00:00:00Z/11)
*** Sitelink collision #1 detected for Q8213590-wikipedia_sitelink-5ee1a4 (http://.wikipedia.org/wiki/Template:Sister_project)
*** Qualifier collision #1 detected for Q9264442-P159-Q270-adc8754c-0-P625-bdfc28 (@52.228472/21.013139)
The sitelink collector called 6500000 times: 0 nrows, 271891952 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q12118776-wikipedia_sitelink-2380bc (http://tt.wikipedia.org/wiki/Луминица_(Констанца))
*** Sitelink collision #1 detected for Q15408619-wikipedia_sitelink-e2b771 (http://.wikipedia.org/wiki/Module:TableTools)
*** Sitelink collision #1 detected for Q13107716-wikipedia_sitelink-595446 (http://.wikipedia.org/wiki/Module:Infobox)
*** Sitelink collision #1 detected for Q15818852-wikipedia_sitelink-659cea (http://.wikipedia.org/wiki/Template:Section_resolved)
*** Qualifier collision #1 detected for Q16566720-P26-Q319870-602b0c96-0-P580-ee5a8a (^1572-09-08T00:00:00Z/11)
*** Sitelink collision #1 detected for Q16830095-wikipedia_sitelink-064884 (http://.wikipedia.org/wiki/Module:Check_for_unknown_parameters)
*** Sitelink collision #1 detected for Q17347215-wikipedia_sitelink-aada88 (http://.wikipedia.org/wiki/Module:Category_handler/data)
*** Sitelink collision #1 detected for Q18123834-wikipedia_sitelink-e4e0f9 (http://.wikipedia.org/wiki/Template:Mono)
*** Sitelink collision #1 detected for Q18338361-wikipedia_sitelink-a89d12 (http://.wikipedia.org/wiki/Category:Pages_using_duplicate_arguments_in_template_calls)
The node collector called 15000000 times: 75000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 15000000 times: 0 nrows, 1833984215 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q23769960-P1416-Q26222380-85afc868-0-P580-896ff2 (^1999-12-01T00:00:00Z/11)
The description collector called 15000000 times: 0 nrows, 2108547178 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The qual collector called 12500000 times: 0 nrows, 0 erows, 238549976 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 15500000 times: 77500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 15500000 times: 0 nrows, 1905410846 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
6500000 lines processed by processor 11
6500000 lines processed by processor 7
6500000 lines processed by processor 0
6500000 lines processed by processor 8
6500000 lines processed by processor 5
6500000 lines processed by processor 10
6500000 lines processed by processor 9
6500000 lines processed by processor 4
6500000 lines processed by processor 2
6500000 lines processed by processor 3
6500000 lines processed by processor 6
6500000 lines processed by processor 1
The description collector called 15500000 times: 0 nrows, 2236189283 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The sitelink collector called 7000000 times: 0 nrows, 284558497 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q56528363-wikipedia_sitelink-12db12 (http://.wikipedia.org/wiki/Module:DateI18n)
The qual collector called 13000000 times: 0 nrows, 0 erows, 249491175 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q64944768-wikipedia_sitelink-f8a890 (http://.wikipedia.org/wiki/Template:Portal_navigation)
The node collector called 16000000 times: 80000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 16000000 times: 0 nrows, 1956689637 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 16000000 times: 0 nrows, 2275342115 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q75273844-P26-Q75273846-b5720745-0-P580-3f9e86 (^1468-07-08T00:00:00Z/11)
*** Qualifier collision #1 detected for Q75389849-P26-Q13972091-b1b4193f-0-P1319-532ed8 (^1509-07-04T00:00:00Z/11)
The qual collector called 13500000 times: 0 nrows, 0 erows, 258909784 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 16500000 times: 82500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 16500000 times: 0 nrows, 2011778907 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 16500000 times: 0 nrows, 2312084116 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
7000000 lines processed by processor 11
7000000 lines processed by processor 0
7000000 lines processed by processor 7
7000000 lines processed by processor 8
7000000 lines processed by processor 5
7000000 lines processed by processor 10
7000000 lines processed by processor 4
7000000 lines processed by processor 9
7000000 lines processed by processor 2
7000000 lines processed by processor 3
*** Sitelink collision #1 detected for Q105429923-wikipedia_sitelink-61eaae (http://.wikipedia.org/wiki/Special:RecentChanges)
7000000 lines processed by processor 6
7000000 lines processed by processor 1
The qual collector called 14000000 times: 0 nrows, 0 erows, 269782940 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q42305-P26-Q229807-78d461dc-0-P580-34f596 (^1191-05-12T00:00:00Z/11)
*** Qualifier collision #1 detected for Q51089-P26-Q378756-91878f66-0-P580-78aa9d (^1350-09-27T00:00:00Z/11)
*** Qualifier collision #1 detected for Q64222-P26-Q969770-fd31fc8c-0-P580-684a59 (^1433-11-12T00:00:00Z/11)
*** Qualifier collision #1 detected for Q68567-P26-Q53441-de9c32df-0-P580-4d0846 (^1115-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q68952-P26-Q327572-72aafaf0-0-P580-5906e2 (^1563-05-10T00:00:00Z/11)
*** Qualifier collision #1 detected for Q132548-P26-Q131412-dba1192f-0-P580-3d6c00 (^1558-04-24T00:00:00Z/11)
*** Qualifier collision #1 detected for Q155581-P26-Q61261-80392b57-0-P580-3550f9 (^1285-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q166853-P26-Q1524640-013a0a25-0-P580-515f76 (^1375-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q234549-P26-Q154998-45a333f4-0-P580-45ce34 (^1525-10-29T00:00:00Z/11)
*** Qualifier collision #1 detected for Q235484-P26-Q105378-affa9a7c-0-P580-e8c2d5 (^1168-02-01T00:00:00Z/11)
*** Qualifier collision #1 detected for Q238609-P26-Q316831-e3e16df6-0-P580-92ae06 (^1153-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q242636-P26-Q129308-cfe073cc-0-P580-c6fd17 (^1189-08-29T00:00:00Z/11)
*** Qualifier collision #1 detected for Q242636-P26-Q1502979-64af4f58-0-P580-366f19 (^1214-01-20T00:00:00Z/11)
*** Qualifier collision #1 detected for Q242636-P26-Q1381324-c86a9785-0-P580-762370 (^1217-09-00T00:00:00Z/10)
*** Qualifier collision #1 detected for Q260926-P26-Q157789-f6d156ff-0-P580-d435a1 (^1502-04-10T00:00:00Z/11)
*** Qualifier collision #1 detected for Q264709-P26-Q60211-e9a2f9fc-0-P580-7f1413 (^1564-12-17T00:00:00Z/11)
*** Qualifier collision #1 detected for Q266025-P26-Q312110-2dbf2ebf-0-P580-189c4f (^1282-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q273307-P26-Q1772833-b61045de-0-P580-a25d98 (^1271-00-00T00:00:00Z/9)
*** Sitelink collision #1 detected for Q282380-wikipedia_sitelink-bf04f9 (http://et.wikipedia.org/wiki/Jedwabne)
*** Qualifier collision #1 detected for Q325041-P26-Q2309561-25e0a31e-0-P580-f872f9 (^1515-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q435726-P26-Q719626-f865d7a4-0-P580-5a49c5 (^1496-11-21T00:00:00Z/11)
*** Qualifier collision #1 detected for Q450971-P26-Q316831-e5799852-0-P580-5ed4f3 (^1177-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q463669-P26-Q40433-6767e474-0-P580-480b99 (^1550-00-00T00:00:00Z/9)
*** Qualifier collision #1 detected for Q506527-P26-Q440132-e6b92d24-0-P580-2bef25 (^1524-11-06T00:00:00Z/11)
*** Qualifier collision #1 detected for Q553550-P26-Q465382-752c3e78-0-P580-6fd04d (^1540-02-08T00:00:00Z/11)
*** Qualifier collision #1 detected for Q926335-P26-Q2419674-95719c4f-0-P580-d5e047 (^1190-00-00T00:00:00Z/9)
*** Sitelink collision #1 detected for Q956852-wikipedia_sitelink-44d82a (http://zh-min-nan.wikipedia.org/wiki/Buffalo_(Missouri))
The node collector called 17000000 times: 85000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 17000000 times: 0 nrows, 2082839182 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q2299423-P159-Q1715-d2db66d9-0-P625-ceaec4 (@52.3704/9.7734)
*** Qualifier collision #1 detected for Q2467970-P26-Q1070853-48f1aeab-0-P580-d71f7b (^1358-09-04T00:00:00Z/11)
*** Qualifier collision #1 detected for Q2517901-P106-Q25393460-25aee660-0-P580-e5e8e2 (^1558-03-05T00:00:00Z/11)
*** Qualifier collision #1 detected for Q3997398-P159-Q101500-ee2c35e9-0-P625-79ba3e (@45.718139/9.715862)
*** Sitelink collision #1 detected for Q5640659-wikipedia_sitelink-53102e (http://.wikipedia.org/wiki/Template:Ombox)
*** Sitelink collision #1 detected for Q5843835-wikipedia_sitelink-6be4da (http://.wikipedia.org/wiki/Template:Fmbox)
*** Sitelink collision #1 detected for Q7009036-wikipedia_sitelink-904fb9 (http://lij.wikipedia.org/wiki/Categorîa:Bahrain)
The sitelink collector called 7500000 times: 0 nrows, 311160195 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 17000000 times: 0 nrows, 2383647839 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q13889180-wikipedia_sitelink-61fce0 (http://ceb.wikipedia.org/wiki/Neotanais_minimus)
*** Sitelink collision #1 detected for Q14357839-wikipedia_sitelink-379650 (http://.wikipedia.org/wiki/Module:Documentation)
*** Sitelink collision #1 detected for Q15379728-wikipedia_sitelink-f395bc (http://.wikipedia.org/wiki/Module:Arguments)
*** Sitelink collision #1 detected for Q16746551-wikipedia_sitelink-3b6af8 (http://.wikipedia.org/wiki/Template:Bulleted_list)
*** Sitelink collision #1 detected for Q17347224-wikipedia_sitelink-98d4f5 (http://.wikipedia.org/wiki/Module:Category_handler/shared)
*** Sitelink collision #1 detected for Q18577165-wikipedia_sitelink-79293a (http://.wikipedia.org/wiki/Template:Translatable_template)
*** Sitelink collision #1 detected for Q18577187-wikipedia_sitelink-8b452d (http://.wikipedia.org/wiki/Template:Translatable_template_name)
The node collector called 17500000 times: 87500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 17500000 times: 0 nrows, 2136259786 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Sitelink collision #1 detected for Q26905045-wikipedia_sitelink-969685 (http://.wikipedia.org/wiki/Module:Complex_date)
*** Sitelink collision #1 detected for Q28132212-wikipedia_sitelink-62565d (http://.wikipedia.org/wiki/Module:TNT)
*** Qualifier collision #1 detected for Q31191558-P26-Q61139836-6de8c7ca-0-P580-70d445 (^1577-02-18T00:00:00Z/11)
The qual collector called 14500000 times: 0 nrows, 0 erows, 277058427 qrows, 0 invalid erows, 0 invalid qrows
The description collector called 17500000 times: 0 nrows, 2471569069 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
7500000 lines processed by processor 11
7500000 lines processed by processor 0
7500000 lines processed by processor 8
7500000 lines processed by processor 7
7500000 lines processed by processor 10
7500000 lines processed by processor 5
The node collector called 18000000 times: 90000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 18000000 times: 0 nrows, 2210319612 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
7500000 lines processed by processor 9
7500000 lines processed by processor 4
7500000 lines processed by processor 2
7500000 lines processed by processor 6
7500000 lines processed by processor 3
The sitelink collector called 8000000 times: 0 nrows, 324984687 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
7500000 lines processed by processor 1
*** Qualifier collision #1 detected for Q54882974-P26-Q26205746-cdf8483f-0-P580-d027aa (^1549-02-16T00:00:00Z/11)
The description collector called 18000000 times: 0 nrows, 2585990048 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The qual collector called 15000000 times: 0 nrows, 0 erows, 287910963 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 18500000 times: 92500000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 18500000 times: 0 nrows, 2259350521 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
*** Qualifier collision #1 detected for Q75273846-P26-Q75273844-b3554203-0-P580-3f9e86 (^1468-07-08T00:00:00Z/11)
The description collector called 18500000 times: 0 nrows, 2615091276 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The qual collector called 15500000 times: 0 nrows, 0 erows, 297353277 qrows, 0 invalid erows, 0 invalid qrows
The node collector called 19000000 times: 95000000 nrows, 0 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
The edge collector called 19000000 times: 0 nrows, 2320200322 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
8000000 lines processed by processor 11
8000000 lines processed by processor 0
8000000 lines processed by processor 10
8000000 lines processed by processor 5
8000000 lines processed by processor 7
8000000 lines processed by processor 8
8000000 lines processed by processor 9
8000000 lines processed by processor 4
8000000 lines processed by processor 2
The description collector called 19000000 times: 0 nrows, 2654687085 erows, 0 qrows, 0 invalid erows, 0 invalid qrows
8000000 lines processed by processor 6
8000000 lines processed by processor 3
8000000 lines processed by processor 1
The qual collector called 16000000 times: 0 nrows, 0 erows, 308264519 qrows, 0 invalid erows, 0 invalid qrows
Done processing /data/amandeep/wikidata-20220505/latest-all.json.bz2
Telling the workers to shut down.
Exiting worker process 4 (pid 118151).
Exiting worker process 0 (pid 118147).
Exiting worker process 10 (pid 118157).
Exiting worker process 8 (pid 118155).
Waiting for the workers to shut down.
Exiting worker process 6 (pid 118153).
Exiting worker process 1 (pid 118148).
Exiting worker process 5 (pid 118152).
Exiting worker process 9 (pid 118156).
Exiting worker process 11 (pid 118158).
Exiting worker process 3 (pid 118150).
Exiting worker process 7 (pid 118154).
Exiting worker process 2 (pid 118149).
Worker shut down is complete.
Telling the node collector to shut down.
Waiting for the node collector to shut down.
Exiting the node collector (pid 118140).
The node collector has closed its output files.
Node collector shut down is complete.
Telling the edge collector to shut down.
Waiting for the edge collector to shut down.
Exiting the edge collector (pid 118141).
The edge collector has closed its output files.
Edge collector shut down is complete.
Telling the qual collector to shut down.
Waiting for the qual collector to shut down.
Exiting the qual collector (pid 118142).
The qual collector has closed its output files.
Qual collector shut down is complete.
Telling the invalid edge collector to shut down.
Waiting for the invalid edge collector to shut down.
Exiting the invalid edge collector (pid 118143).
The invalid edge collector has closed its output files.
Invalid edge collector shut down is complete.
Telling the invalid qual collector to shut down.
Waiting for the invalid qual collector to shut down.
Exiting the invalid qual collector (pid 118144).
The invalid qual collector has closed its output files.
Invalid qual collector shut down is complete.
Telling the description collector to shut down.
Waiting for the description collector to shut down.
Exiting the description collector (pid 118145).
The description collector has closed its output files.
Description collector shut down is complete.
Telling the sitelink collector to shut down.
Waiting for the sitelink collector to shut down.
Exiting the sitelink collector (pid 118146).
The sitelink collector has closed its output files.
Sitelink collector shut down is complete.
import complete
time taken : 45465.164197444916s
Timing: elapsed=12:37:48.283348 CPU=10:19:31.139152 ( 81.8%): import-wikidata -i /data/amandeep/wikidata-20220505/latest-all.json.bz2 --node-file /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.node.unsorted.tsv.gz --minimal-edge-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.raw.unsorted.tsv.gz --minimal-qual-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.raw.unsorted.tsv.gz --invalid-edge-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.badvalue.unsorted.tsv.gz --invalid-qual-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.badvalue.unsorted.tsv.gz --node-file-id-only --explode-values False --all-languages True --lang en --alias-edges True --split-alias-file /data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.unsorted.tsv.gz --split-en-alias-file /data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.en.unsorted.tsv.gz --description-edges True --split-description-file /data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.unsorted.tsv.gz --split-en-description-file /data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.en.unsorted.tsv.gz --label-edges True --split-label-file /data/amandeep/wikidata-20220505/import-wikidata/temp/labels.unsorted.tsv.gz --split-en-label-file /data/amandeep/wikidata-20220505/import-wikidata/temp/labels.en.unsorted.tsv.gz --datatype-edges True --split-datatype-file /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.property.datatypes.unsorted.tsv.gz --entry-type-edges True --split-type-file /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.types.unsorted.tsv.gz --sitelink-edges True --sitelink-verbose-edges True --split-sitelink-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.raw.unsorted.tsv.gz --split-en-sitelink-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.raw.unsorted.tsv.gz --value-hash-width 6 --claim-id-hash-width 8 --use-kgtkwriter True --use-mgzip-for-input False --use-mgzip-for-output False --use-shm True --procs 12 --mapper-batch-size 5 --max-size-per-mapper-queue 3 --single-mapper-queue True --collect-results True --collect-seperately True --collector-batch-size 5 --collector-queue-per-proc-size 3 --progress-interval 500000 --clean --allow-end-of-day False --repair-month-or-day-zero --minimum-valid-year 1 --maximum-valid-year 9999 --validate-fromisoformat --repair-lax-coordinates --allow-language-suffixes --allow-wikidata-lq-strings
###Markdown
Split `somevalue` and `novalue` from `claims.raw.unsorted.tsv.gz`
###Code
!kgtk ${KGTK_FLAGS} \
filter ${VERBOSE} --use-mgzip ${USE_MGZIP} \
--input-file ${TEMP}/claims.raw.${UNSORTED_KGTK} \
--first-match-only \
--pattern ";; novalue" -o ${TEMP}/claims.novalue.${UNSORTED_KGTK} \
--pattern ";; somevalue" -o ${TEMP}/claims.somevalue.${UNSORTED_KGTK} \
--reject-file ${TEMP}/claims.${UNSORTED_KGTK} \
| tee ${TEMP}/split-claims-missing-values.log
###Output
kgtk filter version: 2021-09-24T02:35:27.840163+00:00#gysblgql6Q7482L14Zozt/ne8Owd497FJa7MVp92+UbmixJKElkfg/GY5UmGBsog86NPtmYy+dXWa6PRMIyuIw==
Opening the input file: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.raw.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/claims.raw.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.raw.unsorted.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.novalue.unsorted.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.novalue.unsorted.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.somevalue.unsorted.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.somevalue.unsorted.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the reject file: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.unsorted.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.unsorted.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Applying a dispatched multiple-output object filter
Read 1362524112 rows, rejected 1361968102 rows, wrote 556010 rows.
Closing output files.
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
All output files have been closed.
Timing: elapsed=1:21:07.331541 CPU=4:34:39.248156 (338.6%): filter --verbose --use-mgzip TRUE --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.raw.unsorted.tsv.gz --first-match-only --pattern ;; novalue -o /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.novalue.unsorted.tsv.gz --pattern ;; somevalue -o /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.somevalue.unsorted.tsv.gz --reject-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.unsorted.tsv.gz
###Markdown
Split `somevalue` and `novalue` from `qualifiers.raw.tsv.gz`
###Code
!kgtk ${KGTK_FLAGS} \
filter ${VERBOSE} --use-mgzip ${USE_MGZIP} \
--input-file ${TEMP}/qualifiers.raw.${UNSORTED_KGTK} \
--first-match-only \
--pattern ";; novalue" -o ${TEMP}/qualifiers.novalue.${UNSORTED_KGTK} \
--pattern ";; somevalue" -o ${TEMP}/qualifiers.somevalue.${UNSORTED_KGTK} \
--reject-file - \
/ ifexists ${VERBOSE} \
--input-keys node1 \
--filter-file ${TEMP}/claims.novalue.${UNSORTED_KGTK} \
--filter-keys id \
--output-file ${TEMP}/qualifiers.novalueClaims.${UNSORTED_KGTK} \
--reject-file - \
/ ifexists ${VERBOSE} \
--input-keys node1 \
--filter-file ${TEMP}/claims.somevalue.${UNSORTED_KGTK} \
--filter-keys id \
--output-file ${TEMP}/qualifiers.somevalueClaims.${UNSORTED_KGTK} \
--reject-file - \
/ ifexists ${VERBOSE} \
--input-keys node1 \
--filter-file ${TEMP}/claims.badvalue.${UNSORTED_KGTK} \
--filter-keys id \
--output-file ${TEMP}/qualifiers.badvalueClaims.${UNSORTED_KGTK} \
--reject-file ${TEMP}/qualifiers.${UNSORTED_KGTK} \
| tee ${TEMP}/split-qualifiers-missing-values.log
###Output
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: -
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
kgtk filter version: 2021-09-24T02:35:27.840163+00:00#gysblgql6Q7482L14Zozt/ne8Owd497FJa7MVp92+UbmixJKElkfg/GY5UmGBsog86NPtmYy+dXWa6PRMIyuIw==
Opening the input file: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.raw.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: -
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '-' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading stdin
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.raw.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.raw.unsorted.tsv.gz
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '-' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading stdin
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: -
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '-' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading stdin
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.novalue.unsorted.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.novalue.unsorted.tsv.gz
header: id node1 label node2 node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.somevalue.unsorted.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.somevalue.unsorted.tsv.gz
header: id node1 label node2 node2;wikidatatype
Opening the reject file: -
KgtkWriter: writing stdout
header: id node1 label node2 node2;wikidatatype
Applying a dispatched multiple-output object filter
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.novalue.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/claims.novalue.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading gzip /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.novalue.unsorted.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.novalueClaims.unsorted.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing gzip /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.novalueClaims.unsorted.tsv.gz
header: id node1 label node2 node2;wikidatatype
Opening the reject file: -
KgtkWriter: writing stdout
header: id node1 label node2 node2;wikidatatype
Processing by cacheing the filter file's key set.
Building the filter key set from /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.novalue.unsorted.tsv.gz
There are 124282 entries in the filter key set.
Filtering records from -
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.somevalue.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/claims.somevalue.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading gzip /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.somevalue.unsorted.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.somevalueClaims.unsorted.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing gzip /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.somevalueClaims.unsorted.tsv.gz
header: id node1 label node2 node2;wikidatatype
Opening the reject file: -
KgtkWriter: writing stdout
header: id node1 label node2 node2;wikidatatype
Processing by cacheing the filter file's key set.
Building the filter key set from /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.somevalue.unsorted.tsv.gz
There are 431728 entries in the filter key set.
Filtering records from -
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.badvalue.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/claims.badvalue.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading gzip /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.badvalue.unsorted.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.badvalueClaims.unsorted.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing gzip /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.badvalueClaims.unsorted.tsv.gz
header: id node1 label node2 node2;wikidatatype
Opening the reject file: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing gzip /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing by cacheing the filter file's key set.
Building the filter key set from /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.badvalue.unsorted.tsv.gz
There are 50133 entries in the filter key set.
Filtering records from -
Read 308638139 rows, rejected 308457119 rows, wrote 181020 rows.
Closing output files.
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: not closing standard output
All output files have been closed.
Timing: elapsed=1:10:16.268229 CPU=0:19:02.118320 ( 27.1%): filter --verbose --use-mgzip TRUE --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.raw.unsorted.tsv.gz --first-match-only --pattern ;; novalue -o /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.novalue.unsorted.tsv.gz --pattern ;; somevalue -o /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.somevalue.unsorted.tsv.gz --reject-file -
Read 308457119 input records, accepted 39972 records, rejected 308417147 records.
KgtkWriter: closing the output file
KgtkWriter: not closing standard output
Timing: elapsed=1:10:16.404495 CPU=0:16:26.828742 ( 23.4%): ifexists --verbose --input-keys node1 --filter-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.novalue.unsorted.tsv.gz --filter-keys id --output-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.novalueClaims.unsorted.tsv.gz --reject-file -
Read 308417147 input records, accepted 368548 records, rejected 308048599 records.
KgtkWriter: closing the output file
KgtkWriter: not closing standard output
Timing: elapsed=1:10:16.610469 CPU=0:16:06.036942 ( 22.9%): ifexists --verbose --input-keys node1 --filter-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.somevalue.unsorted.tsv.gz --filter-keys id --output-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.somevalueClaims.unsorted.tsv.gz --reject-file -
Read 308048599 input records, accepted 14219 records, rejected 308034380 records.
KgtkWriter: closing the output file
KgtkWriter: closing the output file
Timing: elapsed=1:10:16.726708 CPU=1:09:22.133053 ( 98.7%): ifexists --verbose --input-keys node1 --filter-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.badvalue.unsorted.tsv.gz --filter-keys id --output-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.badvalueClaims.unsorted.tsv.gz --reject-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz
Timing: elapsed=1:10:29.856671 CPU=0:00:07.875724 ( 0.2%): filter --verbose --use-mgzip TRUE --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.raw.unsorted.tsv.gz --first-match-only --pattern ;; novalue -o /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.novalue.unsorted.tsv.gz --pattern ;; somevalue -o /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.somevalue.unsorted.tsv.gz --reject-file - / ifexists --verbose --input-keys node1 --filter-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.novalue.unsorted.tsv.gz --filter-keys id --output-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.novalueClaims.unsorted.tsv.gz --reject-file - / ifexists --verbose --input-keys node1 --filter-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.somevalue.unsorted.tsv.gz --filter-keys id --output-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.somevalueClaims.unsorted.tsv.gz --reject-file - / ifexists --verbose --input-keys node1 --filter-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.badvalue.unsorted.tsv.gz --filter-keys id --output-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.badvalueClaims.unsorted.tsv.gz --reject-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz
###Markdown
Split `sitelinks.raw.unsorted.tsv.gz`
###Code
!kgtk ${KGTK_FLAGS} \
filter ${VERBOSE} --use-mgzip=${USE_MGZIP} \
--input-file ${TEMP}/sitelinks.raw.${UNSORTED_KGTK} \
--pattern "; sitelink-badge,sitelink-language,sitelink-site,sitelink-title ;" \
--output-file ${TEMP}/sitelinks.qualifiers.${UNSORTED_KGTK} \
--reject-file ${TEMP}/sitelinks.${UNSORTED_KGTK} \
| tee ${TEMP}/split-sitelink-qualifiers.log
###Output
kgtk filter version: 2021-09-24T02:35:27.840163+00:00#gysblgql6Q7482L14Zozt/ne8Owd497FJa7MVp92+UbmixJKElkfg/GY5UmGBsog86NPtmYy+dXWa6PRMIyuIw==
Opening the input file: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.raw.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.raw.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.raw.unsorted.tsv.gz
header: id node1 label node2 lang
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.qualifiers.unsorted.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.qualifiers.unsorted.tsv.gz
header: id node1 label node2 lang
Opening the reject file: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.unsorted.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.unsorted.tsv.gz
header: id node1 label node2 lang
Applying a single general filter
Read 328865880 rows, rejected 82326179 rows, wrote 246539701 rows.
Keep counts: subject=0, predicate=246539701, object=0.
Reject counts: subject=0, predicate=82326179, object=0.
Closing output files.
KgtkWriter: closing the output file
KgtkWriter: closing the output file
All output files have been closed.
Timing: elapsed=0:23:09.677401 CPU=1:32:45.933341 (400.5%): filter --verbose --use-mgzip=TRUE --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.raw.unsorted.tsv.gz --pattern ; sitelink-badge,sitelink-language,sitelink-site,sitelink-title ; --output-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.qualifiers.unsorted.tsv.gz --reject-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.unsorted.tsv.gz
###Markdown
Split `sitelinks.en.raw.unsorted.tsv.gz`
###Code
!kgtk ${KGTK_FLAGS} \
filter ${VERBOSE} --use-mgzip=${USE_MGZIP} \
--input-file ${TEMP}/sitelinks.en.raw.${UNSORTED_KGTK} \
--pattern "; sitelink-badge,sitelink-language,sitelink-site,sitelink-title ;" \
--output-file ${TEMP}/sitelinks.en.qualifiers.${UNSORTED_KGTK} \
--reject-file ${TEMP}/sitelinks.en.${UNSORTED_KGTK} \
| tee ${TEMP}/split-sitelink-en-qualifiers.log
###Output
kgtk filter version: 2021-09-24T02:35:27.840163+00:00#gysblgql6Q7482L14Zozt/ne8Owd497FJa7MVp92+UbmixJKElkfg/GY5UmGBsog86NPtmYy+dXWa6PRMIyuIw==
Opening the input file: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.raw.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.raw.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.raw.unsorted.tsv.gz
header: id node1 label node2
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.qualifiers.unsorted.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.qualifiers.unsorted.tsv.gz
header: id node1 label node2
Opening the reject file: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.unsorted.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.unsorted.tsv.gz
header: id node1 label node2
Applying a single general filter
Read 55048589 rows, rejected 13745591 rows, wrote 41302998 rows.
Keep counts: subject=0, predicate=41302998, object=0.
Reject counts: subject=0, predicate=13745591, object=0.
Closing output files.
KgtkWriter: closing the output file
KgtkWriter: closing the output file
All output files have been closed.
Timing: elapsed=0:04:06.637752 CPU=0:16:28.598107 (400.8%): filter --verbose --use-mgzip=TRUE --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.raw.unsorted.tsv.gz --pattern ; sitelink-badge,sitelink-language,sitelink-site,sitelink-title ; --output-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.qualifiers.unsorted.tsv.gz --reject-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.unsorted.tsv.gz
###Markdown
Sort the files from `TEMP` to `OUT` folder
###Code
for TARGET in WIKIDATA_IMPORT_SPLIT_FILES:
print(f"Sort the {TARGET} file.")
input_file = f"{os.environ['TEMP']}/{TARGET}.{os.environ['UNSORTED_KGTK']}"
output_file = f"{os.environ['OUT']}/{TARGET}.{os.environ['SORTED_KGTK']}"
logfile = f"{os.environ['TEMP']}/{TARGET}-sorted.log"
sort_command = f"""kgtk {os.environ['KGTK_FLAGS']} \
sort {os.environ['VERBOSE']} \
--input-file {input_file} \
--output-file {output_file} \
--gzip-command {os.environ['GZIP_CMD']} \
--sort-command {os.environ['SORT_COMMAND']} \
--extra '{os.environ['SORT_EXTRAS']}' | tee {logfile}"""
!$sort_command
###Output
Sort the claims file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/claims.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/claims.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/claims.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/claims.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/claims.tsv.gz'
Monitoring the cat command (pid=175774).
Running the sort script (pid=175778).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 rank node2;wikidatatype
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Monitoring the sort command (pid=175786)
Cleanup.
Timing: elapsed=0:38:27.177785 CPU=0:00:06.266422 ( 0.3%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the claims.badvalue file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/claims.badvalue.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/claims.badvalue.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/claims.badvalue.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/claims.badvalue.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/claims.badvalue.tsv.gz'
Monitoring the cat command (pid=180475).
Running the sort script (pid=180479).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 rank node2;wikidatatype
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Exception looking for sort command:
RAN: /usr/bin/pgrep -g 180479 --newest sort
STDOUT:
STDERR:
Cleanup.
Timing: elapsed=0:00:06.927161 CPU=0:00:06.170400 ( 89.1%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.badvalue.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.badvalue.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the claims.novalue file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/claims.novalue.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/claims.novalue.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/claims.novalue.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/claims.novalue.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/claims.novalue.tsv.gz'
Monitoring the cat command (pid=180571).
Running the sort script (pid=180575).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 rank node2;wikidatatype
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Exception looking for sort command:
RAN: /usr/bin/pgrep -g 180575 --newest sort
STDOUT:
STDERR:
Cleanup.
Timing: elapsed=0:00:02.236354 CPU=0:00:05.542310 (247.8%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.novalue.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.novalue.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the claims.somevalue file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/claims.somevalue.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/claims.somevalue.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/claims.somevalue.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/claims.somevalue.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/claims.somevalue.tsv.gz'
Monitoring the cat command (pid=180673).
Running the sort script (pid=180677).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 rank node2;wikidatatype
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Exception looking for sort command:
RAN: /usr/bin/pgrep -g 180677 --newest sort
STDOUT:
STDERR:
Cleanup.
Timing: elapsed=0:00:02.156390 CPU=0:00:05.279872 (244.8%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.somevalue.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.somevalue.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the qualifiers file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.tsv.gz'
Monitoring the cat command (pid=180779).
Running the sort script (pid=180783).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 node2;wikidatatype
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Monitoring the sort command (pid=180791)
Cleanup.
Timing: elapsed=0:04:15.085880 CPU=0:00:05.685372 ( 2.2%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the qualifiers.badvalue file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.badvalue.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.badvalue.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.badvalue.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.badvalue.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.badvalue.tsv.gz'
Monitoring the cat command (pid=181176).
Running the sort script (pid=181180).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 node2;wikidatatype
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Exception looking for sort command:
RAN: /usr/bin/pgrep -g 181180 --newest sort
STDOUT:
STDERR:
Cleanup.
Timing: elapsed=0:00:03.547380 CPU=0:00:06.041493 (170.3%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.badvalue.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.badvalue.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the qualifiers.badvalueClaims file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.badvalueClaims.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.badvalueClaims.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.badvalueClaims.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.badvalueClaims.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.badvalueClaims.tsv.gz'
Monitoring the cat command (pid=181268).
Running the sort script (pid=181272).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 node2;wikidatatype
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Exception looking for sort command:
RAN: /usr/bin/pgrep -g 181272 --newest sort
STDOUT:
STDERR:
Cleanup.
Timing: elapsed=0:00:01.955742 CPU=0:00:05.388535 (275.5%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.badvalueClaims.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.badvalueClaims.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the qualifiers.novalue file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.novalue.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.novalue.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.novalue.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.novalue.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.novalue.tsv.gz'
Monitoring the cat command (pid=181345).
Running the sort script (pid=181349).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 node2;wikidatatype
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Exception looking for sort command:
RAN: /usr/bin/pgrep -g 181349 --newest sort
STDOUT:
STDERR:
Cleanup.
Timing: elapsed=0:00:02.107548 CPU=0:00:05.496728 (260.8%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.novalue.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.novalue.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the qualifiers.novalueClaims file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.novalueClaims.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.novalueClaims.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.novalueClaims.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.novalueClaims.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.novalueClaims.tsv.gz'
Monitoring the cat command (pid=181447).
Running the sort script (pid=181453).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 node2;wikidatatype
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Exception looking for sort command:
RAN: /usr/bin/pgrep -g 181453 --newest sort
STDOUT:
STDERR:
Cleanup.
Timing: elapsed=0:00:02.208194 CPU=0:00:05.602475 (253.7%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.novalueClaims.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.novalueClaims.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the qualifiers.somevalue file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.somevalue.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.somevalue.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.somevalue.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.somevalue.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.somevalue.tsv.gz'
Monitoring the cat command (pid=181543).
Running the sort script (pid=181547).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 node2;wikidatatype
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Exception looking for sort command:
RAN: /usr/bin/pgrep -g 181547 --newest sort
STDOUT:
STDERR:
Cleanup.
Timing: elapsed=0:00:02.095882 CPU=0:00:05.336625 (254.6%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.somevalue.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.somevalue.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the qualifiers.somevalueClaims file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.somevalueClaims.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.somevalueClaims.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.somevalueClaims.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.somevalueClaims.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.somevalueClaims.tsv.gz'
Monitoring the cat command (pid=181645).
Running the sort script (pid=181651).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 node2;wikidatatype
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Exception looking for sort command:
RAN: /usr/bin/pgrep -g 181651 --newest sort
STDOUT:
STDERR:
Cleanup.
Timing: elapsed=0:00:02.544968 CPU=0:00:05.689815 (223.6%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.somevalueClaims.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.somevalueClaims.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the aliases file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/aliases.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/aliases.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/aliases.tsv.gz'
Monitoring the cat command (pid=181750).
Running the sort script (pid=181754).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 lang
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 lang
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Monitoring the sort command (pid=181762)
Cleanup.
Timing: elapsed=0:01:36.071250 CPU=0:00:05.613290 ( 5.8%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/aliases.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the aliases.en file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/aliases.en.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/aliases.en.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.en.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.en.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/aliases.en.tsv.gz'
Monitoring the cat command (pid=181952).
Running the sort script (pid=181956).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Monitoring the sort command (pid=181964)
Cleanup.
Timing: elapsed=0:00:13.705462 CPU=0:00:05.731224 ( 41.8%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.en.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/aliases.en.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the descriptions file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/descriptions.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/descriptions.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/descriptions.tsv.gz'
Monitoring the cat command (pid=182059).
Running the sort script (pid=182063).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 lang
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 lang
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Monitoring the sort command (pid=182071)
Cleanup.
Timing: elapsed=1:06:40.955939 CPU=0:00:06.018089 ( 0.2%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/descriptions.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the descriptions.en file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/descriptions.en.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/descriptions.en.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.en.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.en.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/descriptions.en.tsv.gz'
Monitoring the cat command (pid=186189).
Running the sort script (pid=186193).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Monitoring the sort command (pid=186201)
Cleanup.
Timing: elapsed=0:00:46.518554 CPU=0:00:04.968905 ( 10.7%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.en.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/descriptions.en.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the labels file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/labels.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/labels.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/labels.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/labels.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/labels.tsv.gz'
Monitoring the cat command (pid=186298).
Running the sort script (pid=186302).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 lang
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 lang
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Monitoring the sort command (pid=186310)
Cleanup.
Timing: elapsed=0:12:25.459447 CPU=0:00:05.751043 ( 0.8%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/labels.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/labels.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the labels.en file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/labels.en.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/labels.en.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/labels.en.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/labels.en.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/labels.en.tsv.gz'
Monitoring the cat command (pid=187128).
Running the sort script (pid=187132).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Monitoring the sort command (pid=187140)
Cleanup.
Timing: elapsed=0:01:16.965917 CPU=0:00:06.148691 ( 8.0%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/labels.en.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/labels.en.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the sitelinks file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.tsv.gz'
Monitoring the cat command (pid=187343).
Running the sort script (pid=187347).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 lang
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 lang
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Monitoring the sort command (pid=187355)
Cleanup.
Timing: elapsed=0:01:27.116634 CPU=0:00:06.156457 ( 7.1%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the sitelinks.en file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.en.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.en.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.en.tsv.gz'
Monitoring the cat command (pid=187715).
Running the sort script (pid=187719).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Monitoring the sort command (pid=187735)
Cleanup.
Timing: elapsed=0:00:17.925203 CPU=0:00:05.605642 ( 31.3%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.en.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the sitelinks.en.qualifiers file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.en.qualifiers.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.en.qualifiers.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.qualifiers.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.qualifiers.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.en.qualifiers.tsv.gz'
Monitoring the cat command (pid=187877).
Running the sort script (pid=187881).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Monitoring the sort command (pid=187889)
Cleanup.
Timing: elapsed=0:00:33.135455 CPU=0:00:05.715740 ( 17.2%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.en.qualifiers.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.en.qualifiers.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the sitelinks.qualifiers file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.qualifiers.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.qualifiers.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.qualifiers.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.qualifiers.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.qualifiers.tsv.gz'
Monitoring the cat command (pid=188131).
Running the sort script (pid=188135).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 lang
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 lang
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Monitoring the sort command (pid=188144)
Cleanup.
Timing: elapsed=0:03:19.657764 CPU=0:00:05.317021 ( 2.7%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.qualifiers.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/sitelinks.qualifiers.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the metadata.node file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/metadata.node.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/metadata.node.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.node.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.node.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/metadata.node.tsv.gz'
Monitoring the cat command (pid=189086).
Running the sort script (pid=189090).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id
node1 column not found, assuming this is a KGTK node file
KgtkReader: is_edge_file=False is_node_file=True
KgtkReader: Special columns: node1=-1 label=-1 node2=-1 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1
Waiting for the sort command to complete.
Done reading the input file
Monitoring the sort command (pid=189098)
Cleanup.
Timing: elapsed=0:00:28.235616 CPU=0:00:05.945252 ( 21.1%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.node.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/metadata.node.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the metadata.property.datatypes file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/metadata.property.datatypes.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/metadata.property.datatypes.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.property.datatypes.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.property.datatypes.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/metadata.property.datatypes.tsv.gz'
Monitoring the cat command (pid=189196).
Running the sort script (pid=189200).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Exception looking for sort command:
RAN: /usr/bin/pgrep -g 189200 --newest sort
STDOUT:
STDERR:
Cleanup.
Timing: elapsed=0:00:02.100170 CPU=0:00:05.558517 (264.7%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.property.datatypes.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/metadata.property.datatypes.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
Sort the metadata.types file.
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/metadata.types.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/metadata.types.tsv.gz'
gunzip input file: '/data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.types.unsorted.tsv.gz'
full command: pigz -dc '/data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.types.unsorted.tsv.gz' | { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/metadata.types.tsv.gz'
Monitoring the cat command (pid=189262).
Running the sort script (pid=189268).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Done reading the input file
Monitoring the sort command (pid=189274)
Cleanup.
Timing: elapsed=0:00:36.869484 CPU=0:00:05.592611 ( 15.2%): sort --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.types.unsorted.tsv.gz --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/metadata.types.tsv.gz --gzip-command pigz --sort-command sort --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp
###Markdown
Build the `all.tsv.gz file`
###Code
!kgtk ${KGTK_FLAGS} \
cat ${VERBOSE} --use-mgzip=${USE_MGZIP} \
--input-file ${TEMP}/claims.${UNSORTED_KGTK} \
--input-file ${TEMP}/qualifiers.${UNSORTED_KGTK} \
--input-file ${TEMP}/aliases.${UNSORTED_KGTK} \
--input-file ${TEMP}/descriptions.${UNSORTED_KGTK} \
--input-file ${TEMP}/labels.${UNSORTED_KGTK} \
--input-file ${TEMP}/sitelinks.${UNSORTED_KGTK} \
--input-file ${TEMP}/sitelinks.qualifiers.${UNSORTED_KGTK} \
--input-file ${TEMP}/metadata.types.${UNSORTED_KGTK} \
--input-file ${TEMP}/metadata.property.datatypes.${UNSORTED_KGTK} \
/ sort ${VERBOSE} \
--gzip-command ${GZIP_CMD} \
--extra "${SORT_EXTRAS}" \
--output-file ${OUT}/all.${SORTED_KGTK} \
| tee ${TEMP}/build-all-edges.log
###Output
Using the sort command 'sort'
header pipe: read_fd=4 write_fd=5
sort options pipe: read_fd=6 write_fd=7
gzip output file: '/data/amandeep/wikidata-20220505/import-wikidata/data/all.tsv.gz'
sort command: { IFS= read -r header ; { printf "%s\n" "$header" >&5 ; } ; printf "%s\n" "$header" ; IFS= read -u 6 -r options ; LC_ALL=C sort -t ' ' $options ; } | pigz - > '/data/amandeep/wikidata-20220505/import-wikidata/data/all.tsv.gz'
Running the sort script (pid=158825).
Reading the KGTK input file header line with KgtkReader
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Starting kgtkcat pid=158741
Opening the 9 input files.
Opening file 1: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/claims.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.unsorted.tsv.gz
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '<4' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: reading file descriptor 4
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
The output file will be an edge file.
Mapping the 6 column names in /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.unsorted.tsv.gz.
Opening file 2: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Mapping the 5 column names in /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz.
Opening file 3: /data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.unsorted.tsv.gz
header: id node1 label node2 lang
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Mapping the 5 column names in /data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.unsorted.tsv.gz.
Opening file 4: /data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.unsorted.tsv.gz
header: id node1 label node2 lang
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Mapping the 5 column names in /data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.unsorted.tsv.gz.
Opening file 5: /data/amandeep/wikidata-20220505/import-wikidata/temp/labels.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/labels.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/labels.unsorted.tsv.gz
header: id node1 label node2 lang
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Mapping the 5 column names in /data/amandeep/wikidata-20220505/import-wikidata/temp/labels.unsorted.tsv.gz.
Opening file 6: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.unsorted.tsv.gz
header: id node1 label node2 lang
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Mapping the 5 column names in /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.unsorted.tsv.gz.
Opening file 7: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.qualifiers.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.qualifiers.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.qualifiers.unsorted.tsv.gz
header: id node1 label node2 lang
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Mapping the 5 column names in /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.qualifiers.unsorted.tsv.gz.
Opening file 8: /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.types.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.types.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.types.unsorted.tsv.gz
header: id node1 label node2
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Mapping the 4 column names in /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.types.unsorted.tsv.gz.
Opening file 9: /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.property.datatypes.unsorted.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.property.datatypes.unsorted.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.property.datatypes.unsorted.tsv.gz
header: id node1 label node2
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Mapping the 4 column names in /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.property.datatypes.unsorted.tsv.gz.
There are 7 merged columns.
Opening the output edge file: -
KgtkWriter: writing stdout
header: id node1 label node2 rank node2;wikidatatype lang
Copying data from file 1: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.unsorted.tsv.gz
Shortcut not possible: len(kr.column_names)=6 != len(kw.column_names)=7
Row by row file copy
header: id node1 label node2 rank node2;wikidatatype lang
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
KGTK header: id node1 label node2 rank node2;wikidatatype lang
sort options: --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp -k 1,1 -k 2,2 -k 3,3 -k 4,4
Waiting for the sort command to complete.
Read 1361968102 data lines from file 1: /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.unsorted.tsv.gz
Copying data from file 2: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz
Shortcut not possible: len(kr.column_names)=5 != len(kw.column_names)=7
Row by row file copy with a shuffle list: 0 1 2 3 5
Read 308034380 data lines from file 2: /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz
Copying data from file 3: /data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.unsorted.tsv.gz
Shortcut not possible: len(kr.column_names)=5 != len(kw.column_names)=7
Row by row file copy with a shuffle list: 0 1 2 3 6
Read 170178120 data lines from file 3: /data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.unsorted.tsv.gz
Copying data from file 4: /data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.unsorted.tsv.gz
Shortcut not possible: len(kr.column_names)=5 != len(kw.column_names)=7
Row by row file copy with a shuffle list: 0 1 2 3 6
Read 2670247344 data lines from file 4: /data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.unsorted.tsv.gz
Copying data from file 5: /data/amandeep/wikidata-20220505/import-wikidata/temp/labels.unsorted.tsv.gz
Shortcut not possible: len(kr.column_names)=5 != len(kw.column_names)=7
Row by row file copy with a shuffle list: 0 1 2 3 6
Read 739125735 data lines from file 5: /data/amandeep/wikidata-20220505/import-wikidata/temp/labels.unsorted.tsv.gz
Copying data from file 6: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.unsorted.tsv.gz
Shortcut not possible: len(kr.column_names)=5 != len(kw.column_names)=7
Row by row file copy with a shuffle list: 0 1 2 3 6
Read 82326179 data lines from file 6: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.unsorted.tsv.gz
Copying data from file 7: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.qualifiers.unsorted.tsv.gz
Shortcut not possible: len(kr.column_names)=5 != len(kw.column_names)=7
Row by row file copy with a shuffle list: 0 1 2 3 6
Read 246539701 data lines from file 7: /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.qualifiers.unsorted.tsv.gz
Copying data from file 8: /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.types.unsorted.tsv.gz
Shortcut not possible: len(kr.column_names)=4 != len(kw.column_names)=7
Row by row file copy
Read 96951235 data lines from file 8: /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.types.unsorted.tsv.gz
Copying data from file 9: /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.property.datatypes.unsorted.tsv.gz
Shortcut not possible: len(kr.column_names)=4 != len(kw.column_names)=7
Row by row file copy
Read 9984 data lines from file 9: /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.property.datatypes.unsorted.tsv.gz
Wrote 5675380780 lines total from 9 files
KgtkWriter: not closing standard output
Timing: elapsed=7:05:38.707032 CPU=6:22:33.602783 ( 89.9%): cat --verbose --use-mgzip=TRUE --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/labels.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.qualifiers.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.types.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.property.datatypes.unsorted.tsv.gz
Cleanup.
Timing: elapsed=8:15:48.511512 CPU=0:00:06.981474 ( 0.0%): sort --verbose --gzip-command pigz --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/all.tsv.gz
Timing: elapsed=8:15:54.400740 CPU=0:00:10.688431 ( 0.0%): cat --verbose --use-mgzip=TRUE --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/claims.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/qualifiers.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/aliases.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/descriptions.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/labels.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/sitelinks.qualifiers.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.types.unsorted.tsv.gz --input-file /data/amandeep/wikidata-20220505/import-wikidata/temp/metadata.property.datatypes.unsorted.tsv.gz / sort --verbose --gzip-command pigz --extra --parallel 6 --buffer-size 50% -T /data/amandeep/wikidata-20220505/import-wikidata/temp --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/all.tsv.gz
###Markdown
Check for unclaimed qualifiers
###Code
!kgtk ${KGTK_FLAGS} \
ifnotexists $VERBOSE --use-mgzip=$USE_MGZIP --presorted \
--input-file ${OUT}/qualifiers.${SORTED_KGTK} \
--input-keys node1 \
--filter-file ${OUT}/claims.${SORTED_KGTK} \
--filter-keys id \
--output-file ${OUT}/qualifiers.unclaimed.${SORTED_KGTK} \
| tee ${TEMP}/qualifiers.unclaimed.log
###Output
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/data/claims.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.unclaimed.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.unclaimed.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 308034380 input records, accepted 0 records, rejected 308034380 records.
Read 1361968102 filter records, 271770825 found matching input records, 1090197277 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=1:04:24.282472 CPU=1:01:51.930236 ( 96.1%): ifnotexists --verbose --use-mgzip=TRUE --presorted --input-file /data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.tsv.gz --input-keys node1 --filter-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.tsv.gz --filter-keys id --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/qualifiers.unclaimed.tsv.gz
###Markdown
Split edges by datatype
###Code
!kgtk ${KGTK_FLAGS} \
filter ${VERBOSE} \
--input-file ${OUT}/claims.${SORTED_KGTK} \
--obj "node2;wikidatatype" \
--first-match-only \
--pattern ";;commonsMedia" \
--output-file ${OUT}/claims.commonsMedia.${SORTED_KGTK} \
--pattern ";;external-id" \
--output-file ${OUT}/claims.external-id.${SORTED_KGTK} \
--pattern ";;geo-shape" \
--output-file ${OUT}/claims.geo-shape.${SORTED_KGTK} \
--pattern ";;globe-coordinate" \
--output-file ${OUT}/claims.globe-coordinate.${SORTED_KGTK} \
--pattern ";;math" \
--output-file ${OUT}/claims.math.${SORTED_KGTK} \
--pattern ";;monolingualtext" \
--output-file ${OUT}/claims.monolingualtext.${SORTED_KGTK} \
--pattern ";;musical-notation" \
--output-file ${OUT}/claims.musical-notation.${SORTED_KGTK} \
--pattern ";;quantity" \
--output-file ${OUT}/claims.quantity.${SORTED_KGTK} \
--pattern ";;string" \
--output-file ${OUT}/claims.string.${SORTED_KGTK} \
--pattern ";;tabular-data" \
--output-file ${OUT}/claims.tabular-data.${SORTED_KGTK} \
--pattern ";;time" \
--output-file ${OUT}/claims.time.${SORTED_KGTK} \
--pattern ";;url" \
--output-file ${OUT}/claims.url.${SORTED_KGTK} \
--pattern ";;wikibase-form" \
--output-file ${OUT}/claims.wikibase-form.${SORTED_KGTK} \
--pattern ";;wikibase-item" \
--output-file ${OUT}/claims.wikibase-item.${SORTED_KGTK} \
--pattern ";;wikibase-lexeme" \
--output-file ${OUT}/claims.wikibase-lexeme.${SORTED_KGTK} \
--pattern ";;wikibase-property" \
--output-file ${OUT}/claims.wikibase-property.${SORTED_KGTK} \
--pattern ";;wikibase-sense" \
--output-file ${OUT}/claims.wikibase-sense.${SORTED_KGTK} \
--reject-file ${OUT}/claims.other.${SORTED_KGTK} \
--use-mgzip ${USE_MGZIP} \
| tee ${TEMP}/edge-datatype-split.log
###Output
kgtk filter version: 2021-09-24T02:35:27.840163+00:00#gysblgql6Q7482L14Zozt/ne8Owd497FJa7MVp92+UbmixJKElkfg/GY5UmGBsog86NPtmYy+dXWa6PRMIyuIw==
Opening the input file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220505/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220505/import-wikidata/data/claims.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.commonsMedia.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.commonsMedia.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.external-id.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.external-id.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.geo-shape.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.geo-shape.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.globe-coordinate.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.globe-coordinate.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.math.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.math.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.monolingualtext.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.monolingualtext.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.musical-notation.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.musical-notation.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.quantity.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.quantity.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.string.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.string.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.tabular-data.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.tabular-data.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.time.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.time.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.url.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.url.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-form.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-form.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-item.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-item.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-lexeme.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-lexeme.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-property.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-property.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the output file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-sense.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-sense.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Opening the reject file: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.other.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220505/import-wikidata/data/claims.other.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Applying a dispatched multiple-output object filter
Read 1361968102 rows, rejected 0 rows, wrote 1361968102 rows.
Closing output files.
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
KgtkWriter: closing the output file
All output files have been closed.
Timing: elapsed=1:28:16.121467 CPU=4:51:32.610135 (330.3%): filter --verbose --input-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.tsv.gz --obj node2;wikidatatype --first-match-only --pattern ;;commonsMedia --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.commonsMedia.tsv.gz --pattern ;;external-id --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.external-id.tsv.gz --pattern ;;geo-shape --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.geo-shape.tsv.gz --pattern ;;globe-coordinate --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.globe-coordinate.tsv.gz --pattern ;;math --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.math.tsv.gz --pattern ;;monolingualtext --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.monolingualtext.tsv.gz --pattern ;;musical-notation --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.musical-notation.tsv.gz --pattern ;;quantity --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.quantity.tsv.gz --pattern ;;string --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.string.tsv.gz --pattern ;;tabular-data --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.tabular-data.tsv.gz --pattern ;;time --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.time.tsv.gz --pattern ;;url --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.url.tsv.gz --pattern ;;wikibase-form --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-form.tsv.gz --pattern ;;wikibase-item --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-item.tsv.gz --pattern ;;wikibase-lexeme --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-lexeme.tsv.gz --pattern ;;wikibase-property --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-property.tsv.gz --pattern ;;wikibase-sense --output-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.wikibase-sense.tsv.gz --reject-file /data/amandeep/wikidata-20220505/import-wikidata/data/claims.other.tsv.gz --use-mgzip TRUE
###Markdown
Extract qualifiers for edge datatype splits
###Code
for TARGET in WIKIDATATYPES:
print(f"Extract any qualifiers for the properties in claims.{TARGET}")
os.environ['TARGET'] = TARGET
!kgtk ${KGTK_FLAGS} \
ifexists ${VERBOSE} \
--input-file ${OUT}/qualifiers.${SORTED_KGTK} \
--filter-on ${OUT}/claims.${TARGET}.${SORTED_KGTK} \
--output-file ${OUT}/qualifiers.${TARGET}.${SORTED_KGTK} \
--input-keys node1 \
--filter-keys id \
--presorted \
--use-mgzip ${USE_MGZIP} \
| tee ${TEMP}/qualifiers.${TARGET}.log
###Output
Extract any qualifiers for the properties in claims.commonsMedia
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.commonsMedia.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.commonsMedia.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.commonsMedia.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.commonsMedia.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.commonsMedia.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 464056 records, rejected 305315947 records.
Read 5426154 filter records, 376326 found matching input records, 5049828 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:10:47.085673 CPU=0:10:50.451530 (100.5%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.commonsMedia.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.commonsMedia.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.external-id
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.external-id.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.external-id.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.external-id.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.external-id.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.external-id.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 6309903 records, rejected 299470100 records.
Read 188875219 filter records, 3510610 found matching input records, 185364608 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:16:52.937288 CPU=0:19:32.692672 (115.8%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.external-id.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.external-id.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.geo-shape
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.geo-shape.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.geo-shape.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.geo-shape.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.geo-shape.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.geo-shape.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 1754 records, rejected 305778249 records.
Read 28215 filter records, 1396 found matching input records, 26819 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:10:18.439706 CPU=0:10:17.922182 ( 99.9%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.geo-shape.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.geo-shape.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.globe-coordinate
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.globe-coordinate.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.globe-coordinate.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.globe-coordinate.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.globe-coordinate.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.globe-coordinate.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 162832 records, rejected 305617171 records.
Read 9156940 filter records, 155142 found matching input records, 9001798 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:10:32.063434 CPU=0:10:39.611307 (101.2%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.globe-coordinate.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.globe-coordinate.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.math
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.math.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.math.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.math.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.math.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.math.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 3807 records, rejected 305776196 records.
Read 24996 filter records, 3726 found matching input records, 21270 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:10:43.502118 CPU=0:10:46.397159 (100.4%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.math.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.math.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.monolingualtext
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.monolingualtext.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.monolingualtext.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.monolingualtext.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.monolingualtext.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.monolingualtext.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 317870 records, rejected 305462133 records.
Read 47753791 filter records, 231442 found matching input records, 47522349 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:12:29.399306 CPU=0:13:21.059256 (106.9%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.monolingualtext.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.monolingualtext.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.musical-notation
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.musical-notation.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.musical-notation.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.musical-notation.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.musical-notation.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.musical-notation.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 62 records, rejected 305779941 records.
Read 942 filter records, 38 found matching input records, 904 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:10:41.608581 CPU=0:10:44.486667 (100.4%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.musical-notation.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.musical-notation.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.quantity
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.quantity.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.quantity.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.quantity.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.quantity.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.quantity.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 54751054 records, rejected 251028949 records.
Read 86267605 filter records, 49747714 found matching input records, 36519891 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:15:38.545722 CPU=0:28:59.081318 (185.3%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.quantity.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.quantity.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.string
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.string.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.string.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.string.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.string.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.string.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 166537462 records, rejected 139242541 records.
Read 286774252 filter records, 163568733 found matching input records, 123205519 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:26:46.648710 CPU=1:07:50.078828 (253.3%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.string.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.string.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.tabular-data
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.tabular-data.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.tabular-data.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.tabular-data.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tabular-data.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tabular-data.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 12355 records, rejected 305767648 records.
Read 22880 filter records, 12334 found matching input records, 10546 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:10:29.056178 CPU=0:10:29.203481 (100.0%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.tabular-data.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tabular-data.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.time
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.time.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.time.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.time.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.time.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.time.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 1048220 records, rejected 304731783 records.
Read 54361593 filter records, 751395 found matching input records, 53610197 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:12:35.679876 CPU=0:13:09.415386 (104.5%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.time.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.time.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.url
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.url.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.url.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.url.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.url.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.url.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 2382690 records, rejected 303397313 records.
Read 8328249 filter records, 1750479 found matching input records, 6577770 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:11:04.391601 CPU=0:11:27.257304 (103.4%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.url.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.url.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.wikibase-form
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-form.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-form.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-form.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-form.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-form.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 28 records, rejected 305779975 records.
Read 8241 filter records, 25 found matching input records, 8216 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:10:17.631748 CPU=0:10:14.786283 ( 99.5%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-form.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-form.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.wikibase-item
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-item.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-item.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-item.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-item.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-item.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 73786828 records, rejected 231993175 records.
Read 670635690 filter records, 50007257 found matching input records, 620628432 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:34:14.591949 CPU=0:52:31.248502 (153.4%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-item.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-item.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.wikibase-lexeme
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-lexeme.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-lexeme.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-lexeme.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-lexeme.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-lexeme.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 338 records, rejected 305779665 records.
Read 4524 filter records, 279 found matching input records, 4245 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:10:08.115616 CPU=0:10:05.726132 ( 99.6%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-lexeme.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-lexeme.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.wikibase-property
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-property.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-property.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-property.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-property.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-property.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 683 records, rejected 305779320 records.
Read 39288 filter records, 552 found matching input records, 38736 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:10:21.397888 CPU=0:10:20.181629 ( 99.8%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-property.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-property.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.wikibase-sense
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-sense.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-sense.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-sense.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-sense.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-sense.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 61 records, rejected 305779942 records.
Read 47 filter records, 46 found matching input records, 1 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:09:44.827663 CPU=0:09:42.992229 ( 99.7%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.wikibase-sense.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.wikibase-sense.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
Extract any qualifiers for the properties in claims.other
KgtkIfEfexists version: 2020-12-03T17:23:24.872146+00:00#U5P2iPrj3w+Az10+UMbGGMcK/SHBl0wuwe3R1sFky9gXILt9e5oSjHFhPMQEWYVnQtoPd7FUqsZZqR3PfFWaAg==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the filter input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.other.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.other.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.other.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Input key columns: node1
Filter key columns: id
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.other.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.other.tsv.gz
header: id node1 label node2 node2;wikidatatype
Processing presorted files.
Read 305780003 input records, accepted 0 records, rejected 305780003 records.
Read 0 filter records, 0 found matching input records, 0 did not find matches.
KgtkWriter: closing the output file
Timing: elapsed=0:10:16.915168 CPU=0:10:14.315237 ( 99.6%): ifexists --verbose --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz --filter-on /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.other.tsv.gz --output-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.other.tsv.gz --input-keys node1 --filter-keys id --presorted --use-mgzip TRUE
###Markdown
Extract claims with a property in the node1 column
###Code
!kgtk $KGTK_FLAGS filter $VERBOSE --use-mgzip=$USE_MGZIP --regex\
--input-file $OUT/claims.$SORTED_KGTK \
-p '^P ;;' -o $OUT/claims.properties.$SORTED_KGTK \
| tee ${TEMP}/claims.properties.log
###Output
kgtk filter version: 2021-09-24T02:35:27.840163+00:00#gysblgql6Q7482L14Zozt/ne8Owd497FJa7MVp92+UbmixJKElkfg/GY5UmGBsog86NPtmYy+dXWa6PRMIyuIw==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.properties.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.properties.tsv.gz
header: id node1 label node2 rank node2;wikidatatype
Applying a multiple-output general regex match filter
Read 1357708626 rows, rejected 1357510220 rows, wrote 198406 rows.
Keep counts: subject=198406, predicate=0, object=0.
Reject counts: subject=1357510220, predicate=0, object=0.
Closing output files.
KgtkWriter: closing the output file
All output files have been closed.
Timing: elapsed=0:51:41.926642 CPU=0:51:39.721178 ( 99.9%): filter --verbose --use-mgzip=TRUE --regex --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.tsv.gz -p ^P ;; -o /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/claims.properties.tsv.gz
###Markdown
Extract qualifiers for claims with a property in node1 column
###Code
!kgtk $KGTK_FLAGS filter $VERBOSE --use-mgzip=$USE_MGZIP --regex \
--input-file $OUT/qualifiers.$SORTED_KGTK \
-p '^P ;;' -o $OUT/qualifiers.properties.$SORTED_KGTK \
| tee ${TEMP}/qualifiers.properties.log
###Output
kgtk filter version: 2021-09-24T02:35:27.840163+00:00#gysblgql6Q7482L14Zozt/ne8Owd497FJa7MVp92+UbmixJKElkfg/GY5UmGBsog86NPtmYy+dXWa6PRMIyuIw==
Opening the input file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
input format: kgtk
Using KGTK_GRAPH_CACHE='/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db'
Graph cache '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/temp.import-wikidata/wikidata.sqlite3.db': file '/data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz' not found in the cache.
KgtkReader: OK to use the fast read path.
KgtkReader: File_path.suffix: .gz
KgtkReader: reading mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz
header: id node1 label node2 node2;wikidatatype
node1 column found, this is a KGTK edge file
KgtkReader: is_edge_file=True is_node_file=False
KgtkReader: Special columns: node1=1 label=2 node2=3 id=0
KgtkReader: Reading a kgtk file using the fast path.
Opening the output file: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.properties.tsv.gz
File_path.suffix: .gz
KgtkWriter: writing mgzip with 3 threads: /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.properties.tsv.gz
header: id node1 label node2 node2;wikidatatype
Applying a multiple-output general regex match filter
Read 305780003 rows, rejected 305620319 rows, wrote 159684 rows.
Keep counts: subject=159684, predicate=0, object=0.
Reject counts: subject=305620319, predicate=0, object=0.
Closing output files.
KgtkWriter: closing the output file
All output files have been closed.
Timing: elapsed=0:11:44.249832 CPU=0:11:37.552965 ( 99.0%): filter --verbose --use-mgzip=TRUE --regex --input-file /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.tsv.gz -p ^P ;; -o /data/amandeep/wikidata-20220409/wikidata-dwd-v4/import-wikidata/data/qualifiers.properties.tsv.gz
###Markdown
Files in the output data Folder
###Code
!ls -lrth $OUT/data
###Output
total 115G
-rw-r--r-- 1 amandeep isdstaff 28G Apr 15 11:15 claims.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 994K Apr 15 11:16 claims.badvalue.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 1.7M Apr 15 11:16 claims.novalue.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 5.4M Apr 15 11:16 claims.somevalue.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 5.4G Apr 15 11:20 qualifiers.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 592K Apr 15 11:20 qualifiers.badvalue.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 242K Apr 15 11:20 qualifiers.badvalueClaims.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 862K Apr 15 11:20 qualifiers.novalue.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 573K Apr 15 11:20 qualifiers.novalueClaims.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 2.1M Apr 15 11:20 qualifiers.somevalue.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 6.7M Apr 15 11:20 qualifiers.somevalueClaims.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 184M Apr 15 11:20 aliases.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 181M Apr 15 11:20 aliases.en.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 694M Apr 15 11:21 descriptions.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 692M Apr 15 11:22 descriptions.en.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 2.2G Apr 15 11:23 labels.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 2.2G Apr 15 11:25 labels.en.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 1.8G Apr 15 11:26 sitelinks.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 287M Apr 15 11:27 sitelinks.en.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 507M Apr 15 11:27 sitelinks.en.qualifiers.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 3.3G Apr 15 11:30 sitelinks.qualifiers.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 204M Apr 15 11:31 metadata.node.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 54K Apr 15 11:31 metadata.property.datatypes.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 455M Apr 15 11:31 metadata.types.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 40G Apr 15 14:41 all.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 87 Apr 15 15:51 qualifiers.unclaimed.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 183M Apr 15 17:20 claims.commonsMedia.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 4.1G Apr 15 17:20 claims.external-id.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 778K Apr 15 17:20 claims.geo-shape.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 226M Apr 15 17:20 claims.globe-coordinate.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 686K Apr 15 17:20 claims.math.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 2.3G Apr 15 17:20 claims.monolingualtext.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 28K Apr 15 17:20 claims.musical-notation.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 2.0G Apr 15 17:21 claims.quantity.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 5.7G Apr 15 17:21 claims.string.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 420K Apr 15 17:21 claims.tabular-data.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 850M Apr 15 17:21 claims.time.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 205M Apr 15 17:21 claims.url.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 115K Apr 15 17:21 claims.wikibase-form.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 9.6G Apr 15 17:21 claims.wikibase-item.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 74K Apr 15 17:21 claims.wikibase-lexeme.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 634K Apr 15 17:21 claims.wikibase-property.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 960 Apr 15 17:21 claims.wikibase-sense.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 84 Apr 15 17:21 claims.other.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 15M Apr 15 17:32 qualifiers.commonsMedia.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 150M Apr 15 17:49 qualifiers.external-id.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 29K Apr 15 17:59 qualifiers.geo-shape.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 2.8M Apr 15 18:09 qualifiers.globe-coordinate.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 85K Apr 15 18:20 qualifiers.math.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 6.9M Apr 15 18:33 qualifiers.monolingualtext.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 1.8K Apr 15 18:43 qualifiers.musical-notation.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 891M Apr 15 18:59 qualifiers.quantity.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 2.7G Apr 15 19:26 qualifiers.string.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 201K Apr 15 19:36 qualifiers.tabular-data.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 16M Apr 15 19:49 qualifiers.time.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 39M Apr 15 20:00 qualifiers.url.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 1.1K Apr 15 20:10 qualifiers.wikibase-form.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 1.3G Apr 15 20:45 qualifiers.wikibase-item.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 9.2K Apr 15 20:55 qualifiers.wikibase-lexeme.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 18K Apr 15 21:05 qualifiers.wikibase-property.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 1.6K Apr 15 21:15 qualifiers.wikibase-sense.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 83 Apr 15 21:25 qualifiers.other.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 3.8M Apr 15 22:17 claims.properties.tsv.gz
-rw-r--r-- 1 amandeep isdstaff 2.8M Apr 15 22:29 qualifiers.properties.tsv.gz
|
src/test/datascience/notebook/withOutputForTrust.ipynb | ###Markdown
HELLO WORLD
###Code
with Error
import matplotlib
import matplotlib.pyplot as plt
import numpy as np
t = np.arange(0.0, 2.0, 0.01)
s = 1 + np.sin(2 * np.pi * t)
fig, ax = plt.subplots()
ax.plot(t, s)
ax.set(xlabel='time (s)', ylabel='voltage (mV)',
title='About as simple as it gets, folks')
ax.grid()
fig.savefig('test.png')
plt.show()
###Output
_____no_output_____
###Markdown
HELLO WORLD
###Code
with Error
import matplotlib
import matplotlib.pyplot as plt
import numpy as np
t = np.arange(0.0, 2.0, 0.01)
s = 1 + np.sin(2 * np.pi * t)
fig, ax = plt.subplots()
ax.plot(t, s)
ax.set(xlabel='time (s)', ylabel='voltage (mV)',
title='About as simple as it gets, folks')
ax.grid()
fig.savefig('test.png')
plt.show()
###Output
_____no_output_____ |
numba/numba-cpu/Numba_Cpu.ipynb | ###Markdown
Just-in-time Compilation with [Numba](http://numba.pydata.org/)
###Code
import math
import numpy as np
import matplotlib.pyplot as plt
import numba
###Output
Matplotlib is building the font cache using fc-list. This may take a moment.
###Markdown
Using `numba.jit`Numba offers `jit` which can used to decorate Python functions.
###Code
def is_prime(n):
if n <= 1:
raise ArithmeticError('"%s" <= 1' % n)
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
n = np.random.randint(2, 10000000, dtype=np.int64) # Get a random integer between 2 and 10000000
print(n, is_prime(n))
is_prime(1)
@numba.jit
def is_prime_jitted(n):
if n <= 1:
raise ArithmeticError('"%s" <= 1' % n)
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 100000, dtype=np.int64, size=10000)
%timeit p1 = [is_prime(n) for n in numbers]
%timeit p2 = [is_prime_jitted(n) for n in numbers]
###Output
116 ms ± 612 µs per loop (mean ± std. dev. of 7 runs, 10 loops each)
###Markdown
Using `numba.jit` with `nopython=True`
###Code
@numba.jit(nopython=True)
def is_prime_njitted(n):
if n <= 1:
raise ArithmeticError('n <= 1 doesn\'t work!')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 100000, dtype=np.int64, size=1000)
%timeit p1 = [is_prime_jitted(n) for n in numbers]
%timeit p2 = [is_prime_njitted(n) for n in numbers]
###Output
12.3 ms ± 67.6 µs per loop (mean ± std. dev. of 7 runs, 100 loops each)
433 µs ± 1.92 µs per loop (mean ± std. dev. of 7 runs, 1000 loops each)
###Markdown
Using ` @numba.jit(nopython=True)` is equivalent to using ` @numba.njit`
###Code
@numba.njit
def is_prime_njitted(n):
if n <= 1:
raise ArithmeticError('n <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 100000, dtype=np.int64, size=1000)
%time p = [is_prime_njitted(n) for n in numbers]
%time p = [is_prime_njitted(n) for n in numbers]
###Output
_____no_output_____
###Markdown
Use `cache=True` to cache the compiled function
###Code
import math
from numba import njit
@njit(cache=True)
def is_prime_njitted_cached(n):
if n <= 1:
raise ArithmeticError('n <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 100000, dtype=np.int64, size=1000)
%time p = [is_prime_njitted_cached(n) for n in numbers]
%time p = [is_prime_njitted_cached(n) for n in numbers]
###Output
_____no_output_____
###Markdown
Eager compilation using function signatures
###Code
import math
from numba import njit
@njit(['boolean(int64)', 'boolean(int32)'])
def is_prime_njitted_eager(n):
if n <= 1:
raise ArithmeticError('n <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 1000000, dtype=np.int64, size=1000)
%timeit p1 = [is_prime_njitted(n) for n in numbers]
%timeit p2 = [is_prime_njitted_eager(n) for n in numbers]
p1 = [is_prime_njitted_eager(n) for n in numbers.astype(np.int32)]
#p2 = [is_prime_njitted_eager(n) for n in numbers.astype(np.float64)]
###Output
_____no_output_____
###Markdown
Calculating and plotting the [Mandelbrot set](https://en.wikipedia.org/wiki/Mandelbrot_set)
###Code
X, Y = np.meshgrid(np.linspace(-2.0, 1, 1000), np.linspace(-1.0, 1.0, 1000))
def mandelbrot(X, Y, itermax):
mandel = np.empty(shape=X.shape, dtype=np.int32)
for i in range(X.shape[0]):
for j in range(X.shape[1]):
it = 0
cx = X[i, j]
cy = Y[i, j]
x = 0.0
y = 0.0
while x * x + y * y < 4.0 and it < itermax:
x, y = x * x - y * y + cx, 2.0 * x * y + cy
it += 1
mandel[i, j] = it
return mandel
fig = plt.figure(figsize=(15, 10))
ax = fig.add_subplot(111)
%time m = mandelbrot(X, Y, 100)
ax.imshow(np.log(1 + m), extent=[-2.0, 1, -1.0, 1.0]);
ax.set_aspect('equal')
ax.set_ylabel('Im[c]')
ax.set_xlabel('Re[c]');
@numba.njit(parallel=True)
def mandelbrot_jitted(X, Y, radius2, itermax):
mandel = np.empty(shape=X.shape, dtype=np.int32)
for i in numba.prange(X.shape[0]):
for j in numba.prange(X.shape[1]):
it = 0
cx = X[i, j]
cy = Y[i, j]
x = cx
y = cy
while x * x + y * y < 4.0 and it < itermax:
x, y = x * x - y * y + cx, 2.0 * x * y + cy
it += 1
mandel[i, j] = it
return mandel
fig = plt.figure(figsize=(15, 10))
ax = fig.add_subplot(111)
%timeit -n 1000 m = mandelbrot_jitted(X, Y, 4.0, 100)
ax.imshow(np.log(1 + m), extent=[-2.0, 1, -1.0, 1.0]);
ax.set_aspect('equal')
ax.set_ylabel('Im[c]')
ax.set_xlabel('Re[c]');
###Output
10.6 ms ± 15.2 µs per loop (mean ± std. dev. of 7 runs, 1000 loops each)
###Markdown
Getting parallelization information
###Code
mandelbrot_jitted.parallel_diagnostics(level=4)
###Output
================================================================================
Parallel Accelerator Optimizing: Function mandelbrot_jitted, <ipython-
input-27-3f338cd37bfc> (1)
================================================================================
Parallel loop listing for Function mandelbrot_jitted, <ipython-input-27-3f338cd37bfc> (1)
---------------------------------------------------------------|loop #ID
@numba.njit(parallel=True) |
def mandelbrot_jitted(X, Y, radius2, itermax): |
mandel = np.empty(shape=X.shape, dtype=np.int32) |
for i in numba.prange(X.shape[0]):-------------------------| #2
for j in numba.prange(X.shape[1]):---------------------| #1
it = 0 |
cx = X[i, j] |
cy = Y[i, j] |
x = cx |
y = cy |
while x * x + y * y < 4.0 and it < itermax: |
x, y = x * x - y * y + cx, 2.0 * x * y + cy |
it += 1 |
mandel[i, j] = it |
|
return mandel |
--------------------------------- Fusing loops ---------------------------------
Attempting fusion of parallel loops (combines loops with similar properties)...
----------------------------- Before Optimisation ------------------------------
Parallel region 0:
+--2 (parallel)
+--1 (parallel)
--------------------------------------------------------------------------------
------------------------------ After Optimisation ------------------------------
Parallel region 0:
+--2 (parallel)
+--1 (serial)
Parallel region 0 (loop #2) had 0 loop(s) fused and 1 loop(s) serialized as part
of the larger parallel loop (#2).
--------------------------------------------------------------------------------
--------------------------------------------------------------------------------
---------------------------Loop invariant code motion---------------------------
Allocation hoisting:
No allocation hoisting found
Instruction hoisting:
loop #2:
Has the following hoisted:
$42.4 = getattr(value=X, attr=shape)
$const42.5 = const(int, 1)
$42.6 = static_getitem(value=$42.4, index=1, index_var=$const42.5)
--------------------------------------------------------------------------------
###Markdown
Creating `ufuncs` using `numba.vectorize`
###Code
from math import sin
from numba import float64, int64
def my_numpy_sin(a, b): # NumPy doesn't have a ufunc version of sin(x)
return np.sin(a) + np.sin(b)
@np.vectorize
def my_sin(a, b):
return sin(a) + sin(b)
@numba.vectorize([float64(float64, float64), int64(int64, int64)], target='parallel')
def my_sin_numba(a, b):
return np.sin(a) + np.sin(b)
x = np.random.randint(0, 100, size=9000000)
y = np.random.randint(0, 100, size=9000000)
%time _ = my_numpy_sin(x, y)
%time _ = my_sin(x, y)
%time _ = my_sin_numba(x, y)
###Output
_____no_output_____
###Markdown
Vectorize the testing of prime numbers
###Code
@numba.vectorize('boolean(int64)')
def is_prime_v(n):
if n <= 1:
raise ArithmeticError(f'"0" <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 10000000000, dtype=np.int64, size=100000)
%timeit p = is_prime_v(numbers)
###Output
2.83 s ± 3.79 ms per loop (mean ± std. dev. of 7 runs, 1 loop each)
###Markdown
Parallelize the vectorized function
###Code
@numba.vectorize(['boolean(int64)', 'boolean(int32)'],
target='parallel')
def is_prime_vp(n):
if n <= 1:
raise ArithmeticError('n <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 10000000000, dtype=np.int64, size=100000)
%time p1 = is_prime_v(numbers)
%time p2 = is_prime_vp(numbers)
# Print the largest primes from to 1 and 10 millions
numbers = np.arange(1000000, 10000001, dtype=np.int32)
%time p1 = is_prime_vp(numbers)
primes = numbers[p1]
for n in primes[-10:]:
print(n)
###Output
_____no_output_____
###Markdown
Just-in-time Compilation with [Numba](http://numba.pydata.org/) Numba is a JIT compiler which translates Python code in native machine language* Using special decorators on Python functions Numba compiles them on the fly to machine code using LLVM* Numba is compatible with Numpy arrays which are the basis of many scientific packages in Python* It enables parallelization of machine code so that all the CPU cores are used
###Code
import math
import numpy as np
import matplotlib.pyplot as plt
import numba
###Output
_____no_output_____
###Markdown
Using `numba.jit`Numba offers `jit` which can used to decorate Python functions.
###Code
def is_prime(n):
if n <= 1:
raise ArithmeticError('"%s" <= 1' % n)
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
n = np.random.randint(2, 10000000, dtype=np.int64) # Get a random integer between 2 and 10000000
print(n, is_prime(n))
#is_prime(1)
@numba.jit
def is_prime_jitted(n):
if n <= 1:
raise ArithmeticError('"%s" <= 1' % n)
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 100000, dtype=np.int64, size=10000)
%time p1 = [is_prime(n) for n in numbers]
%time p2 = [is_prime_jitted(n) for n in numbers]
###Output
_____no_output_____
###Markdown
Using `numba.jit` with `nopython=True`
###Code
@numba.jit(nopython=True)
def is_prime_njitted(n):
if n <= 1:
raise ArithmeticError('"%s" <= 1' % n)
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 100000, dtype=np.int64, size=1000)
%time p1 = [is_prime_jitted(n) for n in numbers]
%time p2 = [is_prime_njitted(n) for n in numbers]
###Output
_____no_output_____
###Markdown
Using ` @numba.jit(nopython=True)` is equivalent to using ` @numba.njit`
###Code
@numba.njit
def is_prime_njitted(n):
if n <= 1:
raise ArithmeticError('n <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 100000, dtype=np.int64, size=1000)
%time p = [is_prime_njitted(n) for n in numbers]
%time p = [is_prime_njitted(n) for n in numbers]
###Output
_____no_output_____
###Markdown
Use `cache=True` to cache the compiled function
###Code
import math
from numba import njit
@njit(cache=True)
def is_prime_njitted_cached(n):
if n <= 1:
raise ArithmeticError('n <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 100000, dtype=np.int64, size=1000)
%time p = [is_prime_njitted_cached(n) for n in numbers]
%time p = [is_prime_njitted_cached(n) for n in numbers]
###Output
_____no_output_____
###Markdown
Vector Triad Benchmark Python vs Numpy vs Numba
###Code
from timeit import default_timer as timer
def vecTriad(a, b, c, d):
for j in range(a.shape[0]):
a[j] = b[j] + c[j] * d[j]
def vecTriadNumpy(a, b, c, d):
a[:] = b + c * d
@numba.njit()
def vecTriadNumba(a, b, c, d):
for j in range(a.shape[0]):
a[j] = b[j] + c[j] * d[j]
# Initialize Vectors
n = 10000 # Vector size
r = 100 # Iterations
a = np.zeros(n, dtype=np.float64)
b = np.empty_like(a)
b[:] = 1.0
c = np.empty_like(a)
c[:] = 1.0
d = np.empty_like(a)
d[:] = 1.0
# Python version
start = timer()
for i in range(r):
vecTriad(a, b, c, d)
end = timer()
mflops = 2.0 * r * n / ((end - start) * 1.0e6)
print(f'Python: Mflops/sec: {mflops}')
# Numpy version
start = timer()
for i in range(r):
vecTriadNumpy(a, b, c, d)
end = timer()
mflops = 2.0 * r * n / ((end - start) * 1.0e6)
print(f'Numpy: Mflops/sec: {mflops}')
# Numba version
vecTriadNumba(a, b, c, d) # Run once to avoid measuring the compilation overhead
start = timer()
for i in range(r):
vecTriadNumba(a, b, c, d)
end = timer()
mflops = 2.0 * r * n / ((end - start) * 1.0e6)
print(f'Numba: Mflops/sec: {mflops}')
###Output
_____no_output_____
###Markdown
Eager compilation using function signatures
###Code
import math
from numba import njit
@njit(['boolean(int64)', 'boolean(int32)'])
def is_prime_njitted_eager(n):
if n <= 1:
raise ArithmeticError('n <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 1000000, dtype=np.int64, size=1000)
%time p1 = [is_prime_njitted_eager(n) for n in numbers]
%time p2 = [is_prime_njitted_eager(n) for n in numbers]
p1 = [is_prime_njitted_eager(n) for n in numbers.astype(np.int32)]
#p2 = [is_prime_njitted_eager(n) for n in numbers.astype(np.float64)]
###Output
_____no_output_____
###Markdown
Calculating and plotting the [Mandelbrot set](https://en.wikipedia.org/wiki/Mandelbrot_set)
###Code
X, Y = np.meshgrid(np.linspace(-2.0, 1, 1000), np.linspace(-1.0, 1.0, 1000))
def mandelbrot(X, Y, itermax):
mandel = np.empty(shape=X.shape, dtype=np.int32)
for i in range(X.shape[0]):
for j in range(X.shape[1]):
it = 0
cx = X[i, j]
cy = Y[i, j]
x = 0.0
y = 0.0
while x * x + y * y < 4.0 and it < itermax:
x, y = x * x - y * y + cx, 2.0 * x * y + cy
it += 1
mandel[i, j] = it
return mandel
fig = plt.figure(figsize=(15, 10))
ax = fig.add_subplot(111)
%time m = mandelbrot(X, Y, 100)
ax.imshow(np.log(1 + m), extent=[-2.0, 1, -1.0, 1.0]);
ax.set_aspect('equal')
ax.set_ylabel('Im[c]')
ax.set_xlabel('Re[c]');
@numba.njit(parallel=True)
def mandelbrot_jitted(X, Y, radius2, itermax):
mandel = np.empty(shape=X.shape, dtype=np.int32)
for i in numba.prange(X.shape[0]):
for j in range(X.shape[1]):
it = 0
cx = X[i, j]
cy = Y[i, j]
x = cx
y = cy
while x * x + y * y < 4.0 and it < itermax:
x, y = x * x - y * y + cx, 2.0 * x * y + cy
it += 1
mandel[i, j] = it
return mandel
fig = plt.figure(figsize=(15, 10))
ax = fig.add_subplot(111)
%time m = mandelbrot_jitted(X, Y, 4.0, 100)
ax.imshow(np.log(1 + m), extent=[-2.0, 1, -1.0, 1.0]);
ax.set_aspect('equal')
ax.set_ylabel('Im[c]')
ax.set_xlabel('Re[c]');
###Output
_____no_output_____
###Markdown
Getting parallelization information
###Code
mandelbrot_jitted.parallel_diagnostics(level=3)
###Output
_____no_output_____
###Markdown
Creating `ufuncs` using `numba.vectorize`
###Code
from math import sin
from numba import float64, int64
def my_numpy_sin(a, b):
return np.sin(a) + np.sin(b)
@np.vectorize
def my_sin(a, b):
return sin(a) + sin(b)
@numba.vectorize([float64(float64, float64), int64(int64, int64)], target='parallel')
def my_sin_numba(a, b):
return np.sin(a) + np.sin(b)
x = np.random.randint(0, 100, size=9000000)
y = np.random.randint(0, 100, size=9000000)
%time _ = my_numpy_sin(x, y)
%time _ = my_sin(x, y)
%time _ = my_sin_numba(x, y)
###Output
_____no_output_____
###Markdown
Vectorize the testing of prime numbers
###Code
@numba.vectorize('boolean(int64)')
def is_prime_v(n):
if n <= 1:
raise ArithmeticError(f'"0" <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 10000000000, dtype=np.int64, size=100000)
%time p = is_prime_v(numbers)
###Output
_____no_output_____
###Markdown
Parallelize the vectorized function
###Code
@numba.vectorize(['boolean(int64)', 'boolean(int32)'],
target='parallel')
def is_prime_vp(n):
if n <= 1:
raise ArithmeticError('n <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 10000000000, dtype=np.int64, size=100000)
%time p1 = is_prime_v(numbers)
%time p2 = is_prime_vp(numbers)
# Print the largest primes from to 1 and 10 millions
numbers = np.arange(1000000, 10000001, dtype=np.int32)
%time p1 = is_prime_vp(numbers)
primes = numbers[p1]
for n in primes[-10:]:
print(n)
###Output
_____no_output_____
###Markdown
Just-in-time Compilation with [Numba](http://numba.pydata.org/) Numba is a JIT compiler which translates Python code in native machine language* Using special decorators on Python functions Numba compiles them on the fly to machine code using LLVM* Numba is compatible with Numpy arrays which are the basis of many scientific packages in Python* It enables parallelization of machine code so that all the CPU cores are used
###Code
import math
import numpy as np
import matplotlib.pyplot as plt
import numba
###Output
_____no_output_____
###Markdown
Using `numba.jit`Numba offers `jit` which can used to decorate Python functions.
###Code
def is_prime(n):
if n <= 1:
raise ArithmeticError('"%s" <= 1' % n)
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
n = np.random.randint(2, 10000000, dtype=np.int64) # Get a random integer between 2 and 10000000
print(n, is_prime(n))
#is_prime(1)
@numba.jit
def is_prime_jitted(n):
if n <= 1:
raise ArithmeticError('"%s" <= 1' % n)
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 100000, dtype=np.int64, size=10000)
%time p1 = [is_prime(n) for n in numbers]
%time p2 = [is_prime_jitted(n) for n in numbers]
###Output
CPU times: user 99.8 ms, sys: 58 µs, total: 99.9 ms
Wall time: 99.4 ms
###Markdown
Using `numba.jit` with `nopython=True`
###Code
@numba.jit(nopython=True)
def is_prime_njitted(n):
if n <= 1:
raise ArithmeticError('"%s" <= 1' % n)
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 100000, dtype=np.int64, size=1000)
%time p1 = [is_prime_jitted(n) for n in numbers]
%time p2 = [is_prime_njitted(n) for n in numbers]
###Output
CPU times: user 9.06 ms, sys: 4.55 ms, total: 13.6 ms
Wall time: 13.5 ms
###Markdown
Using ` @numba.jit(nopython=True)` is equivalent to using ` @numba.njit`
###Code
@numba.njit
def is_prime_njitted(n):
if n <= 1:
raise ArithmeticError('n <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 100000, dtype=np.int64, size=1000)
%time p = [is_prime_jitted(n) for n in numbers]
%time p = [is_prime_njitted(n) for n in numbers]
###Output
CPU times: user 11.9 ms, sys: 0 ns, total: 11.9 ms
Wall time: 11.9 ms
CPU times: user 74.7 ms, sys: 5.06 ms, total: 79.7 ms
Wall time: 88.7 ms
###Markdown
Use `cache=True` to cache the compiled function
###Code
import math
from numba import njit
@njit(cache=True)
def is_prime_njitted_cached(n):
if n <= 1:
raise ArithmeticError('n <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 100000, dtype=np.int64, size=1000)
%time p = [is_prime_njitted(n) for n in numbers]
%time p = [is_prime_njitted_cached(n) for n in numbers]
###Output
CPU times: user 916 µs, sys: 0 ns, total: 916 µs
Wall time: 922 µs
CPU times: user 80.8 ms, sys: 8.15 ms, total: 89 ms
Wall time: 90.3 ms
###Markdown
Vector Triad Benchmark Python vs Numpy vs Numba
###Code
from timeit import default_timer as timer
def vecTriad(a, b, c, d):
for j in range(a.shape[0]):
a[j] = b[j] + c[j] * d[j]
def vecTriadNumpy(a, b, c, d):
a[:] = b + c * d
@numba.njit()
def vecTriadNumba(a, b, c, d):
for j in range(a.shape[0]):
a[j] = b[j] + c[j] * d[j]
# Initialize Vectors
n = 10000 # Vector size
r = 100 # Iterations
a = np.zeros(n, dtype=np.float64)
b = np.empty_like(a)
b[:] = 1.0
c = np.empty_like(a)
c[:] = 1.0
d = np.empty_like(a)
d[:] = 1.0
# Python version
start = timer()
for i in range(r):
vecTriad(a, b, c, d)
end = timer()
mflops = 2.0 * r * n / ((end - start) * 1.0e6)
print(f'Python: Mflops/sec: {mflops}')
# Numpy version
start = timer()
for i in range(r):
vecTriadNumpy(a, b, c, d)
end = timer()
mflops = 2.0 * r * n / ((end - start) * 1.0e6)
print(f'Numpy: Mflops/sec: {mflops}')
# Numba version
vecTriadNumba(a, b, c, d) # Run once to avoid measuring the compilation overhead
start = timer()
for i in range(r):
vecTriadNumba(a, b, c, d)
end = timer()
mflops = 2.0 * r * n / ((end - start) * 1.0e6)
print(f'Numba: Mflops/sec: {mflops}')
###Output
Python: Mflops/sec: 4.054391116396938
Numpy: Mflops/sec: 1440.7789426876857
Numba: Mflops/sec: 3981.287948188027
###Markdown
Eager compilation using function signatures
###Code
import math
from numba import njit
@njit(['boolean(int64)', 'boolean(int32)'])
def is_prime_njitted_eager(n):
if n <= 1:
raise ArithmeticError('n <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 1000000, dtype=np.int64, size=1000)
# Run twice aft
%time p1 = [is_prime_njitted_eager(n) for n in numbers]
%time p2 = [is_prime_njitted_eager(n) for n in numbers]
p1 = [is_prime_njitted_eager(n) for n in numbers.astype(np.int32)]
#p2 = [is_prime_njitted_eager(n) for n in numbers.astype(np.float64)]
###Output
_____no_output_____
###Markdown
Calculating and plotting the [Mandelbrot set](https://en.wikipedia.org/wiki/Mandelbrot_set)
###Code
X, Y = np.meshgrid(np.linspace(-2.0, 1, 1000), np.linspace(-1.0, 1.0, 1000))
def mandelbrot(X, Y, itermax):
mandel = np.empty(shape=X.shape, dtype=np.int32)
for i in range(X.shape[0]):
for j in range(X.shape[1]):
it = 0
cx = X[i, j]
cy = Y[i, j]
x = 0.0
y = 0.0
while x * x + y * y < 4.0 and it < itermax:
x, y = x * x - y * y + cx, 2.0 * x * y + cy
it += 1
mandel[i, j] = it
return mandel
fig = plt.figure(figsize=(15, 10))
ax = fig.add_subplot(111)
%time m = mandelbrot(X, Y, 100)
ax.imshow(np.log(1 + m), extent=[-2.0, 1, -1.0, 1.0]);
ax.set_aspect('equal')
ax.set_ylabel('Im[c]')
ax.set_xlabel('Re[c]');
@numba.njit(parallel=True)
def mandelbrot_jitted(X, Y, radius2, itermax):
mandel = np.empty(shape=X.shape, dtype=np.int32)
for i in numba.prange(X.shape[0]):
for j in range(X.shape[1]):
it = 0
cx = X[i, j]
cy = Y[i, j]
x = cx
y = cy
while x * x + y * y < 4.0 and it < itermax:
x, y = x * x - y * y + cx, 2.0 * x * y + cy
it += 1
mandel[i, j] = it
return mandel
fig = plt.figure(figsize=(15, 10))
ax = fig.add_subplot(111)
%time m = mandelbrot_jitted(X, Y, 4.0, 100)
ax.imshow(np.log(1 + m), extent=[-2.0, 1, -1.0, 1.0]);
ax.set_aspect('equal')
ax.set_ylabel('Im[c]')
ax.set_xlabel('Re[c]');
###Output
CPU times: user 517 ms, sys: 0 ns, total: 517 ms
Wall time: 367 ms
###Markdown
Getting parallelization information
###Code
mandelbrot_jitted.parallel_diagnostics(level=3)
###Output
================================================================================
Parallel Accelerator Optimizing: Function mandelbrot_jitted, <ipython-
input-19-238a75aae604> (1)
================================================================================
Parallel loop listing for Function mandelbrot_jitted, <ipython-input-19-238a75aae604> (1)
---------------------------------------------------------------|loop #ID
@numba.njit(parallel=True) |
def mandelbrot_jitted(X, Y, radius2, itermax): |
mandel = np.empty(shape=X.shape, dtype=np.int32) |
for i in numba.prange(X.shape[0]):-------------------------| #0
for j in range(X.shape[1]): |
it = 0 |
cx = X[i, j] |
cy = Y[i, j] |
x = cx |
y = cy |
while x * x + y * y < 4.0 and it < itermax: |
x, y = x * x - y * y + cx, 2.0 * x * y + cy |
it += 1 |
mandel[i, j] = it |
|
return mandel |
--------------------------------- Fusing loops ---------------------------------
Attempting fusion of parallel loops (combines loops with similar properties)...
Following the attempted fusion of parallel for-loops there are 1 parallel for-
loop(s) (originating from loops labelled: #0).
--------------------------------------------------------------------------------
----------------------------- Before Optimisation ------------------------------
--------------------------------------------------------------------------------
------------------------------ After Optimisation ------------------------------
Parallel structure is already optimal.
--------------------------------------------------------------------------------
--------------------------------------------------------------------------------
---------------------------Loop invariant code motion---------------------------
Allocation hoisting:
No allocation hoisting found
###Markdown
Creating `ufuncs` using `numba.vectorize`
###Code
from math import sin
from numba import float64, int64
def my_numpy_sin(a, b):
return np.sin(a) + np.sin(b)
@np.vectorize
def my_sin(a, b):
return sin(a) + sin(b)
@numba.vectorize([float64(float64, float64), int64(int64, int64)], target='parallel')
def my_sin_numba(a, b):
return np.sin(a) + np.sin(b)
x = np.random.randint(0, 100, size=9000000)
y = np.random.randint(0, 100, size=9000000)
%time _ = my_numpy_sin(x, y)
%time _ = my_sin(x, y)
%time _ = my_sin_numba(x, y)
###Output
CPU times: user 293 ms, sys: 296 ms, total: 589 ms
Wall time: 591 ms
CPU times: user 2.14 s, sys: 229 ms, total: 2.37 s
Wall time: 2.37 s
CPU times: user 2.69 s, sys: 1.12 s, total: 3.82 s
Wall time: 377 ms
###Markdown
Vectorize the testing of prime numbers
###Code
@numba.vectorize('boolean(int64)')
def is_prime_v(n):
if n <= 1:
raise ArithmeticError(f'"0" <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 10000000000, dtype=np.int64, size=100000)
%time p = is_prime_v(numbers)
###Output
CPU times: user 2.19 s, sys: 0 ns, total: 2.19 s
Wall time: 2.19 s
###Markdown
Parallelize the vectorized function
###Code
@numba.vectorize(['boolean(int64)', 'boolean(int32)'],
target='parallel')
def is_prime_vp(n):
if n <= 1:
raise ArithmeticError('n <= 1')
if n == 2 or n == 3:
return True
elif n % 2 == 0:
return False
else:
n_sqrt = math.ceil(math.sqrt(n))
for i in range(3, n_sqrt):
if n % i == 0:
return False
return True
numbers = np.random.randint(2, 10000000000, dtype=np.int64, size=100000)
%time p1 = is_prime_v(numbers)
%time p2 = is_prime_vp(numbers)
# Print the largest primes from to 1 and 10 millions
numbers = np.arange(1000000, 10000001, dtype=np.int32)
%time p1 = is_prime_vp(numbers)
primes = numbers[p1]
for n in primes[-10:]:
print(n)
###Output
CPU times: user 9.85 s, sys: 1.73 ms, total: 9.85 s
Wall time: 836 ms
9999889
9999901
9999907
9999929
9999931
9999937
9999943
9999971
9999973
9999991
|
notebooks/10.00-Working-With-Symmetry.ipynb | ###Markdown
*This notebook contains material from [PyRosetta](https://RosettaCommons.github.io/PyRosetta.notebooks);content is available [on Github](https://github.com/RosettaCommons/PyRosetta.notebooks.git).* Working With SymmetryKeywords: symmetry, asymmetric, SetupForSymmetryMover, virtual OverviewSymmetry is an important concept to learn when working with biomolecules. When a protein is crystalized, it is in the precense of its symmetrical neighbors - which can be important if testing particular protocols or using crystal density for refinement or full structure building.Symmetry can also be useful for designing symmetrical structures or large repeating meta-proteins like protein cages. Symmetry In RosettaSo why do we care if our protein is symmetrical or not when it comes to Rosetta? Each residue and atom that is loaded into Rosetta takes time to both load, and time to score. Since scoring can happen thousands of times - even in a short protocol, anything we can do to speed this up becomes important. The most expensive operation in Rosetta is minimization, and by using symmetry - we can reduce the minimization time exponentially by minimizing a single copy instead of ALL copies. We will get into the details about how this works below.When we use symmetry in Rosetta - we are basically telling rosetta that the symmetrical partners are 'special', however, the total number of residues is now ALL residues, including symmetrical partners. Upon setting up symmety in Rosetta, Rosetta will replace the `Conformation` within the pose with a **Symmetrical** version, called the `SymmetricConformation`. If you know anything about classes, this `SymmetricConformation` is derived from the actual `Conformation` object, but contains extra information about the pose and some functions are replaced. Symmetric Scoring and MovingOk, so now lets assume that we have our symmetric pose. Now what? Well, the symmetric copies are all tied to their real counterparts. Once you move a chain, residue, or atom by packing or minimization, the symmetric copies of that residue are all moved in the same way. Cool. But what about scoring? Scoring works very similarly - instead of scoring each and every residue in our pose, Rosetta will score just our assymetric unit, and multiply that out to the number of symmetric copies we have. Intelligently, Rosetta will also figure out the symmetric interfaces that arise from the interactions of our assymetric unit to the symmetric copies and score them appropriately. Symmetry-aware moversMost of our common movers are symmetry-aware. At one point there were different symmetric and non-symmetric versions of particular code, such as MinMover and PackRotamersMover. Now though, Rosetta will automatically use the pose to figure out what needs to be done. You should seek original documentation (and contact the author if not explicit) to make sure that an uncommon protocol you are using is symmetry-aware. DocumentationMore information on RosettaSymmetry can be found in the following places:- https://www.rosettacommons.org/docs/latest/rosetta_basics/structural_concepts/symmetry- https://www.rosettacommons.org/demos/latest/tutorials/Symmetry/Symmetry- https://www.rosettacommons.org/docs/latest/application_documentation/utilities/make-symmdef-file- https://www.rosettacommons.org/docs/latest/scripting_documentation/RosettaScripts/Movers/movers_pages/SetupForSymmetryMover- https://www.rosettacommons.org/docs/latest/scripting_documentation/RosettaScripts/Movers/movers_pages/ExtractAsymmetricUnitMover
###Code
# Notebook setup
import sys
if 'google.colab' in sys.modules:
!pip install pyrosettacolabsetup
import pyrosettacolabsetup
pyrosettacolabsetup.setup()
print ("Notebook is set for PyRosetta use in Colab. Have fun!")
###Output
_____no_output_____
###Markdown
Here, we will use a few specific options. The first three options make Rosetta a bit more robust to input structures. The `-load_PDB_components` cannot be used with glycans, unfortunately, and our structure has a few very important glycans. Finally, we load a bunch of glycan-specific options, which we will cover in the next tutorial.
###Code
from pyrosetta import *
from pyrosetta.rosetta import *
from pyrosetta.teaching import *
import os
init('-ignore_unrecognized_res -load_PDB_components false -ignore_zero_occupancy false @inputs/glycan_flags')
###Output
PyRosetta-4 2019 [Rosetta PyRosetta4.Release.python36.mac 2019.39+release.93456a567a8125cafdf7f8cb44400bc20b570d81 2019-09-26T14:24:44] retrieved from: http://www.pyrosetta.org
(C) Copyright Rosetta Commons Member Institutions. Created in JHU by Sergey Lyskov and PyRosetta Team.
[0mcore.init: [0mChecking for fconfig files in pwd and ./rosetta/flags
[0mcore.init: [0mReading fconfig.../Users/jadolfbr/.rosetta/flags/common
[0mcore.init: [0m
[0mcore.init: [0m
[0mcore.init: [0mRosetta version: PyRosetta4.Release.python36.mac r233 2019.39+release.93456a567a8 93456a567a8125cafdf7f8cb44400bc20b570d81 http://www.pyrosetta.org 2019-09-26T14:24:44
[0mcore.init: [0mcommand: PyRosetta -ignore_unrecognized_res -load_PDB_components false -ignore_zero_occupancy false @inputs/glycan_flags -database /Users/jadolfbr/Library/Python/3.6/lib/python/site-packages/pyrosetta-2019.39+release.93456a567a8-py3.6-macosx-10.6-intel.egg/pyrosetta/database
[0mbasic.random.init_random_generator: [0m'RNG device' seed mode, using '/dev/urandom', seed=1544006277 seed_offset=0 real_seed=1544006277
[0mbasic.random.init_random_generator: [0mRandomGenerator:init: Normal mode, seed=1544006277 RG_type=mt19937
###Markdown
Creating a SymDef fileHere, we will start with how to create a basic symdef file for cyrstal symmetry. Note that there are ways to do this without a symdef file, but these do not currently work for glycan structures, which we will be using here. The `make_symdef_file.pl` file is within Rosetta3. To use it, you will need to download and licence Rosetta3. The code is in the `Rosetta/main/src/apps/public` directory. In the interest of reducing code drift, this file is NOT included in the tutorial directory as we may then have version drift. If you have done this, we can use the following command to create the symdef file. Here, the radius of symmetrical partners is 12A, which is certainly fairly large, but produces a very well represented crystal.
###Code
pdb = "inputs/1jnd.pdb"
base_cmd = f'cd inputs && make_symmdef_file.pl -r 12 -m CRYST -p {pdb}.pdb > {pdb}_crys.symm && cd -'
print(base_cmd)
###Output
cd inputs && make_symmdef_file.pl -r 12 -m CRYST -p inputs/1jnd.pdb.pdb > inputs/1jnd.pdb_crys.symm && cd -
###Markdown
Use this base command and the `os.system(cmd)` function to run the code or use the provided symdef file.
###Code
os.system('cp inputs/1jnd_crys.symm .')
###Output
_____no_output_____
###Markdown
Take a look at the symmetrized structure in pymol (`inputs/1jnd_symm.pdb`). What would happen if we increased the radius to 24 instead of 12? Setup a Symmetrized PoseHere, we will run a basic Rosetta protocol with symmetry. There are much more complicated things you can do with symmetry, but for now, we just want to symmetrically pack the protein. Please see the docs for more on symmetry. The full Rosetta C++ tutorial for symmetry is a great place to go from here: - https://www.rosettacommons.org/demos/latest/tutorials/Symmetry/Symmetry Lets first create a pose, and then use the `SetupForSymmetryMover` on the pose. Note this is an unrefined input structure. This is so that minmover will actually do something. A pareto-optimal refined structure can be found in the inputs as `1jnd_refined.pdb.gz`
###Code
p = pose_from_pdb('inputs/1jnd.pdb')
original = p.clone()
p.total_residue()
type(p.conformation())
symmetrize = rosetta.protocols.symmetry.SetupForSymmetryMover("1jnd_crys.symm")
symmetrize.apply(p)
print(p.total_residue())
print(type(p.conformation()))
###Output
3654
<class 'pyrosetta.rosetta.core.conformation.symmetry.SymmetricConformation'>
###Markdown
How many symmetric copies do we have in our pose?How do the scores compare for our original pose and our symmetrized version? Now lets use some of the functionality to understand how this all works. We can use the `SymetricInfo` object that is part of the `SymmetricConformation` to get at some info. Lets take a look at all residues and find the assymetric unit residues and equivalent residues for the rest.
###Code
print("AssymUnit? equivalent_res")
sym_info = p.conformation().Symmetry_Info()
for i in range(1, p.size()+1):
print(i, sym_info.bb_is_independent(i), sym_info.bb_follows(i))
###Output
AssymUnit? equivalent_res
1 True 0
2 True 0
3 True 0
4 True 0
5 True 0
6 True 0
7 True 0
8 True 0
9 True 0
10 True 0
11 True 0
12 True 0
13 True 0
14 True 0
15 True 0
16 True 0
17 True 0
18 True 0
19 True 0
20 True 0
21 True 0
22 True 0
23 True 0
24 True 0
25 True 0
26 True 0
27 True 0
28 True 0
29 True 0
30 True 0
31 True 0
32 True 0
33 True 0
34 True 0
35 True 0
36 True 0
37 True 0
38 True 0
39 True 0
40 True 0
41 True 0
42 True 0
43 True 0
44 True 0
45 True 0
46 True 0
47 True 0
48 True 0
49 True 0
50 True 0
51 True 0
52 True 0
53 True 0
54 True 0
55 True 0
56 True 0
57 True 0
58 True 0
59 True 0
60 True 0
61 True 0
62 True 0
63 True 0
64 True 0
65 True 0
66 True 0
67 True 0
68 True 0
69 True 0
70 True 0
71 True 0
72 True 0
73 True 0
74 True 0
75 True 0
76 True 0
77 True 0
78 True 0
79 True 0
80 True 0
81 True 0
82 True 0
83 True 0
84 True 0
85 True 0
86 True 0
87 True 0
88 True 0
89 True 0
90 True 0
91 True 0
92 True 0
93 True 0
94 True 0
95 True 0
96 True 0
97 True 0
98 True 0
99 True 0
100 True 0
101 True 0
102 True 0
103 True 0
104 True 0
105 True 0
106 True 0
107 True 0
108 True 0
109 True 0
110 True 0
111 True 0
112 True 0
113 True 0
114 True 0
115 True 0
116 True 0
117 True 0
118 True 0
119 True 0
120 True 0
121 True 0
122 True 0
123 True 0
124 True 0
125 True 0
126 True 0
127 True 0
128 True 0
129 True 0
130 True 0
131 True 0
132 True 0
133 True 0
134 True 0
135 True 0
136 True 0
137 True 0
138 True 0
139 True 0
140 True 0
141 True 0
142 True 0
143 True 0
144 True 0
145 True 0
146 True 0
147 True 0
148 True 0
149 True 0
150 True 0
151 True 0
152 True 0
153 True 0
154 True 0
155 True 0
156 True 0
157 True 0
158 True 0
159 True 0
160 True 0
161 True 0
162 True 0
163 True 0
164 True 0
165 True 0
166 True 0
167 True 0
168 True 0
169 True 0
170 True 0
171 True 0
172 True 0
173 True 0
174 True 0
175 True 0
176 True 0
177 True 0
178 True 0
179 True 0
180 True 0
181 True 0
182 True 0
183 True 0
184 True 0
185 True 0
186 True 0
187 True 0
188 True 0
189 True 0
190 True 0
191 True 0
192 True 0
193 True 0
194 True 0
195 True 0
196 True 0
197 True 0
198 True 0
199 True 0
200 True 0
201 True 0
202 True 0
203 True 0
204 True 0
205 True 0
206 True 0
207 True 0
208 True 0
209 True 0
210 True 0
211 True 0
212 True 0
213 True 0
214 True 0
215 True 0
216 True 0
217 True 0
218 True 0
219 True 0
220 True 0
221 True 0
222 True 0
223 True 0
224 True 0
225 True 0
226 True 0
227 True 0
228 True 0
229 True 0
230 True 0
231 True 0
232 True 0
233 True 0
234 True 0
235 True 0
236 True 0
237 True 0
238 True 0
239 True 0
240 True 0
241 True 0
242 True 0
243 True 0
244 True 0
245 True 0
246 True 0
247 True 0
248 True 0
249 True 0
250 True 0
251 True 0
252 True 0
253 True 0
254 True 0
255 True 0
256 True 0
257 True 0
258 True 0
259 True 0
260 True 0
261 True 0
262 True 0
263 True 0
264 True 0
265 True 0
266 True 0
267 True 0
268 True 0
269 True 0
270 True 0
271 True 0
272 True 0
273 True 0
274 True 0
275 True 0
276 True 0
277 True 0
278 True 0
279 True 0
280 True 0
281 True 0
282 True 0
283 True 0
284 True 0
285 True 0
286 True 0
287 True 0
288 True 0
289 True 0
290 True 0
291 True 0
292 True 0
293 True 0
294 True 0
295 True 0
296 True 0
297 True 0
298 True 0
299 True 0
300 True 0
301 True 0
302 True 0
303 True 0
304 True 0
305 True 0
306 True 0
307 True 0
308 True 0
309 True 0
310 True 0
311 True 0
312 True 0
313 True 0
314 True 0
315 True 0
316 True 0
317 True 0
318 True 0
319 True 0
320 True 0
321 True 0
322 True 0
323 True 0
324 True 0
325 True 0
326 True 0
327 True 0
328 True 0
329 True 0
330 True 0
331 True 0
332 True 0
333 True 0
334 True 0
335 True 0
336 True 0
337 True 0
338 True 0
339 True 0
340 True 0
341 True 0
342 True 0
343 True 0
344 True 0
345 True 0
346 True 0
347 True 0
348 True 0
349 True 0
350 True 0
351 True 0
352 True 0
353 True 0
354 True 0
355 True 0
356 True 0
357 True 0
358 True 0
359 True 0
360 True 0
361 True 0
362 True 0
363 True 0
364 True 0
365 True 0
366 True 0
367 True 0
368 True 0
369 True 0
370 True 0
371 True 0
372 True 0
373 True 0
374 True 0
375 True 0
376 True 0
377 True 0
378 True 0
379 True 0
380 True 0
381 True 0
382 True 0
383 True 0
384 True 0
385 True 0
386 True 0
387 True 0
388 True 0
389 True 0
390 True 0
391 True 0
392 True 0
393 True 0
394 True 0
395 True 0
396 True 0
397 True 0
398 True 0
399 True 0
400 True 0
401 True 0
402 True 0
403 True 0
404 True 0
405 False 1
406 False 2
407 False 3
408 False 4
409 False 5
410 False 6
411 False 7
412 False 8
413 False 9
414 False 10
415 False 11
416 False 12
417 False 13
418 False 14
419 False 15
420 False 16
421 False 17
422 False 18
423 False 19
424 False 20
425 False 21
426 False 22
427 False 23
428 False 24
429 False 25
430 False 26
431 False 27
432 False 28
433 False 29
434 False 30
435 False 31
436 False 32
437 False 33
438 False 34
439 False 35
440 False 36
441 False 37
442 False 38
443 False 39
444 False 40
445 False 41
446 False 42
447 False 43
448 False 44
449 False 45
450 False 46
451 False 47
452 False 48
453 False 49
454 False 50
455 False 51
456 False 52
457 False 53
458 False 54
459 False 55
460 False 56
461 False 57
462 False 58
463 False 59
464 False 60
465 False 61
466 False 62
467 False 63
468 False 64
469 False 65
470 False 66
471 False 67
472 False 68
473 False 69
474 False 70
475 False 71
476 False 72
477 False 73
478 False 74
479 False 75
480 False 76
481 False 77
482 False 78
483 False 79
484 False 80
485 False 81
486 False 82
487 False 83
488 False 84
489 False 85
490 False 86
491 False 87
492 False 88
493 False 89
494 False 90
495 False 91
496 False 92
497 False 93
498 False 94
499 False 95
500 False 96
501 False 97
502 False 98
503 False 99
504 False 100
505 False 101
506 False 102
507 False 103
508 False 104
509 False 105
510 False 106
511 False 107
512 False 108
513 False 109
514 False 110
515 False 111
516 False 112
517 False 113
518 False 114
519 False 115
520 False 116
521 False 117
522 False 118
523 False 119
524 False 120
525 False 121
526 False 122
527 False 123
528 False 124
529 False 125
530 False 126
531 False 127
532 False 128
533 False 129
534 False 130
535 False 131
536 False 132
537 False 133
538 False 134
539 False 135
540 False 136
541 False 137
542 False 138
543 False 139
544 False 140
545 False 141
546 False 142
547 False 143
548 False 144
549 False 145
550 False 146
551 False 147
552 False 148
553 False 149
554 False 150
555 False 151
556 False 152
557 False 153
558 False 154
559 False 155
560 False 156
561 False 157
562 False 158
563 False 159
564 False 160
565 False 161
566 False 162
567 False 163
568 False 164
569 False 165
570 False 166
571 False 167
572 False 168
573 False 169
574 False 170
575 False 171
576 False 172
577 False 173
578 False 174
579 False 175
580 False 176
581 False 177
582 False 178
583 False 179
584 False 180
585 False 181
586 False 182
587 False 183
588 False 184
589 False 185
590 False 186
591 False 187
592 False 188
593 False 189
594 False 190
595 False 191
596 False 192
597 False 193
598 False 194
599 False 195
600 False 196
601 False 197
602 False 198
603 False 199
604 False 200
605 False 201
606 False 202
607 False 203
608 False 204
609 False 205
610 False 206
611 False 207
612 False 208
613 False 209
614 False 210
615 False 211
616 False 212
617 False 213
618 False 214
619 False 215
620 False 216
621 False 217
622 False 218
623 False 219
624 False 220
625 False 221
626 False 222
627 False 223
628 False 224
629 False 225
630 False 226
631 False 227
632 False 228
633 False 229
634 False 230
635 False 231
636 False 232
637 False 233
638 False 234
639 False 235
640 False 236
641 False 237
642 False 238
643 False 239
644 False 240
645 False 241
646 False 242
647 False 243
648 False 244
649 False 245
650 False 246
651 False 247
652 False 248
653 False 249
654 False 250
655 False 251
656 False 252
657 False 253
658 False 254
659 False 255
660 False 256
661 False 257
662 False 258
663 False 259
664 False 260
665 False 261
666 False 262
667 False 263
668 False 264
669 False 265
670 False 266
671 False 267
672 False 268
673 False 269
674 False 270
675 False 271
676 False 272
677 False 273
678 False 274
679 False 275
680 False 276
681 False 277
682 False 278
683 False 279
684 False 280
685 False 281
686 False 282
687 False 283
688 False 284
689 False 285
690 False 286
691 False 287
692 False 288
693 False 289
694 False 290
695 False 291
696 False 292
697 False 293
698 False 294
699 False 295
700 False 296
701 False 297
702 False 298
703 False 299
704 False 300
705 False 301
706 False 302
707 False 303
708 False 304
709 False 305
710 False 306
711 False 307
712 False 308
713 False 309
714 False 310
715 False 311
716 False 312
717 False 313
718 False 314
719 False 315
720 False 316
721 False 317
722 False 318
723 False 319
724 False 320
725 False 321
726 False 322
727 False 323
728 False 324
729 False 325
730 False 326
731 False 327
732 False 328
733 False 329
734 False 330
735 False 331
736 False 332
737 False 333
738 False 334
739 False 335
740 False 336
741 False 337
742 False 338
743 False 339
744 False 340
745 False 341
746 False 342
747 False 343
748 False 344
749 False 345
750 False 346
751 False 347
752 False 348
753 False 349
754 False 350
755 False 351
756 False 352
757 False 353
758 False 354
759 False 355
760 False 356
761 False 357
762 False 358
763 False 359
764 False 360
765 False 361
766 False 362
767 False 363
768 False 364
769 False 365
770 False 366
771 False 367
772 False 368
773 False 369
774 False 370
775 False 371
776 False 372
777 False 373
778 False 374
779 False 375
780 False 376
781 False 377
782 False 378
783 False 379
784 False 380
785 False 381
786 False 382
787 False 383
788 False 384
789 False 385
790 False 386
791 False 387
792 False 388
793 False 389
794 False 390
795 False 391
796 False 392
797 False 393
798 False 394
799 False 395
800 False 396
801 False 397
802 False 398
803 False 399
804 False 400
805 False 401
806 False 402
807 False 403
808 False 404
809 False 1
810 False 2
811 False 3
812 False 4
813 False 5
814 False 6
815 False 7
816 False 8
817 False 9
818 False 10
819 False 11
820 False 12
821 False 13
822 False 14
823 False 15
824 False 16
825 False 17
826 False 18
827 False 19
828 False 20
829 False 21
830 False 22
831 False 23
832 False 24
833 False 25
834 False 26
835 False 27
836 False 28
837 False 29
838 False 30
839 False 31
840 False 32
841 False 33
842 False 34
843 False 35
844 False 36
845 False 37
846 False 38
847 False 39
848 False 40
849 False 41
850 False 42
851 False 43
852 False 44
853 False 45
854 False 46
855 False 47
856 False 48
857 False 49
858 False 50
859 False 51
860 False 52
861 False 53
862 False 54
863 False 55
864 False 56
865 False 57
866 False 58
867 False 59
868 False 60
869 False 61
870 False 62
871 False 63
872 False 64
873 False 65
874 False 66
875 False 67
876 False 68
877 False 69
878 False 70
879 False 71
880 False 72
881 False 73
882 False 74
883 False 75
884 False 76
885 False 77
886 False 78
887 False 79
888 False 80
889 False 81
890 False 82
891 False 83
###Markdown
Which residues are our original pose residues? Note that the final residues are called `Virtual` residues. Virtual residues are not scored. They have coordinates, and can move, but simply result in a score of zero. They are useful in some contexts to hide a part of the pose from the scoring machinery, and there are movers that can change residues to and from virtual. In this case, they are used for the FoldTree - in order to allow refinement of the full crystal environment. They allow relative movement of each subunit relative to each other. There are two virtual residues for each subunit
###Code
print(p.residue(3654))
print("Total Subunits:", (3654-18)/404)
print("Total Subunits:", sym_info.subunits())
score = get_score_function()
print(score(original))
print(score(p))
###Output
[0mcore.scoring.ScoreFunctionFactory: [0mSCOREFUNCTION: [32mref2015[0m
[0mcore.scoring.ScoreFunctionFactory: [0mThe -include_sugars flag was used with no sugar_bb weight set in the weights file. Setting sugar_bb weight to 1.0 by default.
-531.2534669946483
-1132.7274634504747
###Markdown
Running Protocols with SymmetryNow, lets try running a minimization with symmetry on.
###Code
mm = MoveMap()
mm.set_bb(True)
mm.set_chi(True)
minmover = rosetta.protocols.minimization_packing.MinMover()
minmover.score_function(score)
minmover.set_movemap(mm)
minmover.apply(p)
score(p)
###Output
_____no_output_____
###Markdown
How does our pose look? For being such a large pose, how was the speed of minimization? How does this compare to our refined pose? Try to copy a subunit to a new object in PyMol. Then use the align command to align it to our assymetric unit. What is the RMSD? Now lets pack with our symmetric structure.
###Code
from rosetta.core.pack.task import *
from rosetta.core.pack.task.operation import *
packer = PackRotamersMover()
tf = rosetta.core.pack.task.TaskFactory()
tf.push_back(RestrictToRepacking())
tf.push_back(IncludeCurrent())
packer.task_factory(tf)
p = original.clone()
symmetrize.apply(p)
packer.apply(p)
print("packed", score(p))
###Output
packed -1960.9189300248413
###Markdown
*This notebook contains material from [PyRosetta](https://RosettaCommons.github.io/PyRosetta.notebooks);content is available [on Github](https://github.com/RosettaCommons/PyRosetta.notebooks.git).* Working With SymmetryKeywords: symmetry, asymmetric, SetupForSymmetryMover, virtual OverviewSymmetry is an important concept to learn when working with biomolecules. When a protein is crystalized, it is in the precense of its symmetrical neighbors - which can be important if testing particular protocols or using crystal density for refinement or full structure building.Symmetry can also be useful for designing symmetrical structures or large repeating meta-proteins like protein cages. Symmetry In RosettaSo why do we care if our protein is symmetrical or not when it comes to Rosetta? Each residue and atom that is loaded into Rosetta takes time to both load, and time to score. Since scoring can happen thousands of times - even in a short protocol, anything we can do to speed this up becomes important. The most expensive operation in Rosetta is minimization, and by using symmetry - we can reduce the minimization time exponentially by minimizing a single copy instead of ALL copies. We will get into the details about how this works below.When we use symmetry in Rosetta - we are basically telling rosetta that the symmetrical partners are 'special', however, the total number of residues is now ALL residues, including symmetrical partners. Upon setting up symmety in Rosetta, Rosetta will replace the `Conformation` within the pose with a **Symmetrical** version, called the `SymmetricConformation`. If you know anything about classes, this `SymmetricConformation` is derived from the actual `Conformation` object, but contains extra information about the pose and some functions are replaced. Symmetric Scoring and MovingOk, so now lets assume that we have our symmetric pose. Now what? Well, the symmetric copies are all tied to their real counterparts. Once you move a chain, residue, or atom by packing or minimization, the symmetric copies of that residue are all moved in the same way. Cool. But what about scoring? Scoring works very similarly - instead of scoring each and every residue in our pose, Rosetta will score just our assymetric unit, and multiply that out to the number of symmetric copies we have. Intelligently, Rosetta will also figure out the symmetric interfaces that arise from the interactions of our assymetric unit to the symmetric copies and score them appropriately. Symmetry-aware moversMost of our common movers are symmetry-aware. At one point there were different symmetric and non-symmetric versions of particular code, such as MinMover and PackRotamersMover. Now though, Rosetta will automatically use the pose to figure out what needs to be done. You should seek original documentation (and contact the author if not explicit) to make sure that an uncommon protocol you are using is symmetry-aware. DocumentationMore information on RosettaSymmetry can be found in the following places:- https://www.rosettacommons.org/docs/latest/rosetta_basics/structural_concepts/symmetry- https://www.rosettacommons.org/demos/latest/tutorials/Symmetry/Symmetry- https://www.rosettacommons.org/docs/latest/application_documentation/utilities/make-symmdef-file- https://www.rosettacommons.org/docs/latest/scripting_documentation/RosettaScripts/Movers/movers_pages/SetupForSymmetryMover- https://www.rosettacommons.org/docs/latest/scripting_documentation/RosettaScripts/Movers/movers_pages/ExtractAsymmetricUnitMover
###Code
# Notebook setup
import sys
if 'google.colab' in sys.modules:
!pip install pyrosettacolabsetup
import pyrosettacolabsetup
pyrosettacolabsetup.mount_pyrosetta_install()
print ("Notebook is set for PyRosetta use in Colab. Have fun!")
###Output
_____no_output_____
###Markdown
Here, we will use a few specific options. The first three options make Rosetta a bit more robust to input structures. The `-load_PDB_components` cannot be used with glycans, unfortunately, and our structure has a few very important glycans. Finally, we load a bunch of glycan-specific options, which we will cover in the next tutorial.
###Code
from pyrosetta import *
from pyrosetta.rosetta import *
from pyrosetta.teaching import *
import os
init('-ignore_unrecognized_res -load_PDB_components false -ignore_zero_occupancy false @inputs/glycan_flags')
###Output
PyRosetta-4 2019 [Rosetta PyRosetta4.Release.python36.mac 2019.39+release.93456a567a8125cafdf7f8cb44400bc20b570d81 2019-09-26T14:24:44] retrieved from: http://www.pyrosetta.org
(C) Copyright Rosetta Commons Member Institutions. Created in JHU by Sergey Lyskov and PyRosetta Team.
[0mcore.init: [0mChecking for fconfig files in pwd and ./rosetta/flags
[0mcore.init: [0mReading fconfig.../Users/jadolfbr/.rosetta/flags/common
[0mcore.init: [0m
[0mcore.init: [0m
[0mcore.init: [0mRosetta version: PyRosetta4.Release.python36.mac r233 2019.39+release.93456a567a8 93456a567a8125cafdf7f8cb44400bc20b570d81 http://www.pyrosetta.org 2019-09-26T14:24:44
[0mcore.init: [0mcommand: PyRosetta -ignore_unrecognized_res -load_PDB_components false -ignore_zero_occupancy false @inputs/glycan_flags -database /Users/jadolfbr/Library/Python/3.6/lib/python/site-packages/pyrosetta-2019.39+release.93456a567a8-py3.6-macosx-10.6-intel.egg/pyrosetta/database
[0mbasic.random.init_random_generator: [0m'RNG device' seed mode, using '/dev/urandom', seed=1544006277 seed_offset=0 real_seed=1544006277
[0mbasic.random.init_random_generator: [0mRandomGenerator:init: Normal mode, seed=1544006277 RG_type=mt19937
###Markdown
Creating a SymDef fileHere, we will start with how to create a basic symdef file for cyrstal symmetry. Note that there are ways to do this without a symdef file, but these do not currently work for glycan structures, which we will be using here. The `make_symdef_file.pl` file is within Rosetta3. To use it, you will need to download and licence Rosetta3. The code is in the `Rosetta/main/src/apps/public` directory. In the interest of reducing code drift, this file is NOT included in the tutorial directory as we may then have version drift. If you have done this, we can use the following command to create the symdef file. Here, the radius of symmetrical partners is 12A, which is certainly fairly large, but produces a very well represented crystal.
###Code
pdb = "inputs/1jnd.pdb"
base_cmd = f'cd inputs && make_symmdef_file.pl -r 12 -m CRYST -p {pdb}.pdb > {pdb}_crys.symm && cd -'
print(base_cmd)
###Output
cd inputs && make_symmdef_file.pl -r 12 -m CRYST -p inputs/1jnd.pdb.pdb > inputs/1jnd.pdb_crys.symm && cd -
###Markdown
Use this base command and the `os.system(cmd)` function to run the code or use the provided symdef file.
###Code
os.system('cp inputs/1jnd_crys.symm .')
###Output
_____no_output_____
###Markdown
Take a look at the symmetrized structure in pymol (`inputs/1jnd_symm.pdb`). What would happen if we increased the radius to 24 instead of 12? Setup a Symmetrized PoseHere, we will run a basic Rosetta protocol with symmetry. There are much more complicated things you can do with symmetry, but for now, we just want to symmetrically pack the protein. Please see the docs for more on symmetry. The full Rosetta C++ tutorial for symmetry is a great place to go from here: - https://www.rosettacommons.org/demos/latest/tutorials/Symmetry/Symmetry Lets first create a pose, and then use the `SetupForSymmetryMover` on the pose. Note this is an unrefined input structure. This is so that minmover will actually do something. A pareto-optimal refined structure can be found in the inputs as `1jnd_refined.pdb.gz`
###Code
p = pose_from_pdb('inputs/1jnd.pdb')
original = p.clone()
p.total_residue()
type(p.conformation())
symmetrize = rosetta.protocols.symmetry.SetupForSymmetryMover("1jnd_crys.symm")
symmetrize.apply(p)
print(p.total_residue())
print(type(p.conformation()))
###Output
3654
<class 'pyrosetta.rosetta.core.conformation.symmetry.SymmetricConformation'>
###Markdown
How many symmetric copies do we have in our pose?How do the scores compare for our original pose and our symmetrized version? Now lets use some of the functionality to understand how this all works. We can use the `SymetricInfo` object that is part of the `SymmetricConformation` to get at some info. Lets take a look at all residues and find the assymetric unit residues and equivalent residues for the rest.
###Code
print("AssymUnit? equivalent_res")
sym_info = p.conformation().Symmetry_Info()
for i in range(1, p.size()+1):
print(i, sym_info.bb_is_independent(i), sym_info.bb_follows(i))
###Output
AssymUnit? equivalent_res
1 True 0
2 True 0
3 True 0
4 True 0
5 True 0
6 True 0
7 True 0
8 True 0
9 True 0
10 True 0
11 True 0
12 True 0
13 True 0
14 True 0
15 True 0
16 True 0
17 True 0
18 True 0
19 True 0
20 True 0
21 True 0
22 True 0
23 True 0
24 True 0
25 True 0
26 True 0
27 True 0
28 True 0
29 True 0
30 True 0
31 True 0
32 True 0
33 True 0
34 True 0
35 True 0
36 True 0
37 True 0
38 True 0
39 True 0
40 True 0
41 True 0
42 True 0
43 True 0
44 True 0
45 True 0
46 True 0
47 True 0
48 True 0
49 True 0
50 True 0
51 True 0
52 True 0
53 True 0
54 True 0
55 True 0
56 True 0
57 True 0
58 True 0
59 True 0
60 True 0
61 True 0
62 True 0
63 True 0
64 True 0
65 True 0
66 True 0
67 True 0
68 True 0
69 True 0
70 True 0
71 True 0
72 True 0
73 True 0
74 True 0
75 True 0
76 True 0
77 True 0
78 True 0
79 True 0
80 True 0
81 True 0
82 True 0
83 True 0
84 True 0
85 True 0
86 True 0
87 True 0
88 True 0
89 True 0
90 True 0
91 True 0
92 True 0
93 True 0
94 True 0
95 True 0
96 True 0
97 True 0
98 True 0
99 True 0
100 True 0
101 True 0
102 True 0
103 True 0
104 True 0
105 True 0
106 True 0
107 True 0
108 True 0
109 True 0
110 True 0
111 True 0
112 True 0
113 True 0
114 True 0
115 True 0
116 True 0
117 True 0
118 True 0
119 True 0
120 True 0
121 True 0
122 True 0
123 True 0
124 True 0
125 True 0
126 True 0
127 True 0
128 True 0
129 True 0
130 True 0
131 True 0
132 True 0
133 True 0
134 True 0
135 True 0
136 True 0
137 True 0
138 True 0
139 True 0
140 True 0
141 True 0
142 True 0
143 True 0
144 True 0
145 True 0
146 True 0
147 True 0
148 True 0
149 True 0
150 True 0
151 True 0
152 True 0
153 True 0
154 True 0
155 True 0
156 True 0
157 True 0
158 True 0
159 True 0
160 True 0
161 True 0
162 True 0
163 True 0
164 True 0
165 True 0
166 True 0
167 True 0
168 True 0
169 True 0
170 True 0
171 True 0
172 True 0
173 True 0
174 True 0
175 True 0
176 True 0
177 True 0
178 True 0
179 True 0
180 True 0
181 True 0
182 True 0
183 True 0
184 True 0
185 True 0
186 True 0
187 True 0
188 True 0
189 True 0
190 True 0
191 True 0
192 True 0
193 True 0
194 True 0
195 True 0
196 True 0
197 True 0
198 True 0
199 True 0
200 True 0
201 True 0
202 True 0
203 True 0
204 True 0
205 True 0
206 True 0
207 True 0
208 True 0
209 True 0
210 True 0
211 True 0
212 True 0
213 True 0
214 True 0
215 True 0
216 True 0
217 True 0
218 True 0
219 True 0
220 True 0
221 True 0
222 True 0
223 True 0
224 True 0
225 True 0
226 True 0
227 True 0
228 True 0
229 True 0
230 True 0
231 True 0
232 True 0
233 True 0
234 True 0
235 True 0
236 True 0
237 True 0
238 True 0
239 True 0
240 True 0
241 True 0
242 True 0
243 True 0
244 True 0
245 True 0
246 True 0
247 True 0
248 True 0
249 True 0
250 True 0
251 True 0
252 True 0
253 True 0
254 True 0
255 True 0
256 True 0
257 True 0
258 True 0
259 True 0
260 True 0
261 True 0
262 True 0
263 True 0
264 True 0
265 True 0
266 True 0
267 True 0
268 True 0
269 True 0
270 True 0
271 True 0
272 True 0
273 True 0
274 True 0
275 True 0
276 True 0
277 True 0
278 True 0
279 True 0
280 True 0
281 True 0
282 True 0
283 True 0
284 True 0
285 True 0
286 True 0
287 True 0
288 True 0
289 True 0
290 True 0
291 True 0
292 True 0
293 True 0
294 True 0
295 True 0
296 True 0
297 True 0
298 True 0
299 True 0
300 True 0
301 True 0
302 True 0
303 True 0
304 True 0
305 True 0
306 True 0
307 True 0
308 True 0
309 True 0
310 True 0
311 True 0
312 True 0
313 True 0
314 True 0
315 True 0
316 True 0
317 True 0
318 True 0
319 True 0
320 True 0
321 True 0
322 True 0
323 True 0
324 True 0
325 True 0
326 True 0
327 True 0
328 True 0
329 True 0
330 True 0
331 True 0
332 True 0
333 True 0
334 True 0
335 True 0
336 True 0
337 True 0
338 True 0
339 True 0
340 True 0
341 True 0
342 True 0
343 True 0
344 True 0
345 True 0
346 True 0
347 True 0
348 True 0
349 True 0
350 True 0
351 True 0
352 True 0
353 True 0
354 True 0
355 True 0
356 True 0
357 True 0
358 True 0
359 True 0
360 True 0
361 True 0
362 True 0
363 True 0
364 True 0
365 True 0
366 True 0
367 True 0
368 True 0
369 True 0
370 True 0
371 True 0
372 True 0
373 True 0
374 True 0
375 True 0
376 True 0
377 True 0
378 True 0
379 True 0
380 True 0
381 True 0
382 True 0
383 True 0
384 True 0
385 True 0
386 True 0
387 True 0
388 True 0
389 True 0
390 True 0
391 True 0
392 True 0
393 True 0
394 True 0
395 True 0
396 True 0
397 True 0
398 True 0
399 True 0
400 True 0
401 True 0
402 True 0
403 True 0
404 True 0
405 False 1
406 False 2
407 False 3
408 False 4
409 False 5
410 False 6
411 False 7
412 False 8
413 False 9
414 False 10
415 False 11
416 False 12
417 False 13
418 False 14
419 False 15
420 False 16
421 False 17
422 False 18
423 False 19
424 False 20
425 False 21
426 False 22
427 False 23
428 False 24
429 False 25
430 False 26
431 False 27
432 False 28
433 False 29
434 False 30
435 False 31
436 False 32
437 False 33
438 False 34
439 False 35
440 False 36
441 False 37
442 False 38
443 False 39
444 False 40
445 False 41
446 False 42
447 False 43
448 False 44
449 False 45
450 False 46
451 False 47
452 False 48
453 False 49
454 False 50
455 False 51
456 False 52
457 False 53
458 False 54
459 False 55
460 False 56
461 False 57
462 False 58
463 False 59
464 False 60
465 False 61
466 False 62
467 False 63
468 False 64
469 False 65
470 False 66
471 False 67
472 False 68
473 False 69
474 False 70
475 False 71
476 False 72
477 False 73
478 False 74
479 False 75
480 False 76
481 False 77
482 False 78
483 False 79
484 False 80
485 False 81
486 False 82
487 False 83
488 False 84
489 False 85
490 False 86
491 False 87
492 False 88
493 False 89
494 False 90
495 False 91
496 False 92
497 False 93
498 False 94
499 False 95
500 False 96
501 False 97
502 False 98
503 False 99
504 False 100
505 False 101
506 False 102
507 False 103
508 False 104
509 False 105
510 False 106
511 False 107
512 False 108
513 False 109
514 False 110
515 False 111
516 False 112
517 False 113
518 False 114
519 False 115
520 False 116
521 False 117
522 False 118
523 False 119
524 False 120
525 False 121
526 False 122
527 False 123
528 False 124
529 False 125
530 False 126
531 False 127
532 False 128
533 False 129
534 False 130
535 False 131
536 False 132
537 False 133
538 False 134
539 False 135
540 False 136
541 False 137
542 False 138
543 False 139
544 False 140
545 False 141
546 False 142
547 False 143
548 False 144
549 False 145
550 False 146
551 False 147
552 False 148
553 False 149
554 False 150
555 False 151
556 False 152
557 False 153
558 False 154
559 False 155
560 False 156
561 False 157
562 False 158
563 False 159
564 False 160
565 False 161
566 False 162
567 False 163
568 False 164
569 False 165
570 False 166
571 False 167
572 False 168
573 False 169
574 False 170
575 False 171
576 False 172
577 False 173
578 False 174
579 False 175
580 False 176
581 False 177
582 False 178
583 False 179
584 False 180
585 False 181
586 False 182
587 False 183
588 False 184
589 False 185
590 False 186
591 False 187
592 False 188
593 False 189
594 False 190
595 False 191
596 False 192
597 False 193
598 False 194
599 False 195
600 False 196
601 False 197
602 False 198
603 False 199
604 False 200
605 False 201
606 False 202
607 False 203
608 False 204
609 False 205
610 False 206
611 False 207
612 False 208
613 False 209
614 False 210
615 False 211
616 False 212
617 False 213
618 False 214
619 False 215
620 False 216
621 False 217
622 False 218
623 False 219
624 False 220
625 False 221
626 False 222
627 False 223
628 False 224
629 False 225
630 False 226
631 False 227
632 False 228
633 False 229
634 False 230
635 False 231
636 False 232
637 False 233
638 False 234
639 False 235
640 False 236
641 False 237
642 False 238
643 False 239
644 False 240
645 False 241
646 False 242
647 False 243
648 False 244
649 False 245
650 False 246
651 False 247
652 False 248
653 False 249
654 False 250
655 False 251
656 False 252
657 False 253
658 False 254
659 False 255
660 False 256
661 False 257
662 False 258
663 False 259
664 False 260
665 False 261
666 False 262
667 False 263
668 False 264
669 False 265
670 False 266
671 False 267
672 False 268
673 False 269
674 False 270
675 False 271
676 False 272
677 False 273
678 False 274
679 False 275
680 False 276
681 False 277
682 False 278
683 False 279
684 False 280
685 False 281
686 False 282
687 False 283
688 False 284
689 False 285
690 False 286
691 False 287
692 False 288
693 False 289
694 False 290
695 False 291
696 False 292
697 False 293
698 False 294
699 False 295
700 False 296
701 False 297
702 False 298
703 False 299
704 False 300
705 False 301
706 False 302
707 False 303
708 False 304
709 False 305
710 False 306
711 False 307
712 False 308
713 False 309
714 False 310
715 False 311
716 False 312
717 False 313
718 False 314
719 False 315
720 False 316
721 False 317
722 False 318
723 False 319
724 False 320
725 False 321
726 False 322
727 False 323
728 False 324
729 False 325
730 False 326
731 False 327
732 False 328
733 False 329
734 False 330
735 False 331
736 False 332
737 False 333
738 False 334
739 False 335
740 False 336
741 False 337
742 False 338
743 False 339
744 False 340
745 False 341
746 False 342
747 False 343
748 False 344
749 False 345
750 False 346
751 False 347
752 False 348
753 False 349
754 False 350
755 False 351
756 False 352
757 False 353
758 False 354
759 False 355
760 False 356
761 False 357
762 False 358
763 False 359
764 False 360
765 False 361
766 False 362
767 False 363
768 False 364
769 False 365
770 False 366
771 False 367
772 False 368
773 False 369
774 False 370
775 False 371
776 False 372
777 False 373
778 False 374
779 False 375
780 False 376
781 False 377
782 False 378
783 False 379
784 False 380
785 False 381
786 False 382
787 False 383
788 False 384
789 False 385
790 False 386
791 False 387
792 False 388
793 False 389
794 False 390
795 False 391
796 False 392
797 False 393
798 False 394
799 False 395
800 False 396
801 False 397
802 False 398
803 False 399
804 False 400
805 False 401
806 False 402
807 False 403
808 False 404
809 False 1
810 False 2
811 False 3
812 False 4
813 False 5
814 False 6
815 False 7
816 False 8
817 False 9
818 False 10
819 False 11
820 False 12
821 False 13
822 False 14
823 False 15
824 False 16
825 False 17
826 False 18
827 False 19
828 False 20
829 False 21
830 False 22
831 False 23
832 False 24
833 False 25
834 False 26
835 False 27
836 False 28
837 False 29
838 False 30
839 False 31
840 False 32
841 False 33
842 False 34
843 False 35
844 False 36
845 False 37
846 False 38
847 False 39
848 False 40
849 False 41
850 False 42
851 False 43
852 False 44
853 False 45
854 False 46
855 False 47
856 False 48
857 False 49
858 False 50
859 False 51
860 False 52
861 False 53
862 False 54
863 False 55
864 False 56
865 False 57
866 False 58
867 False 59
868 False 60
869 False 61
870 False 62
871 False 63
872 False 64
873 False 65
874 False 66
875 False 67
876 False 68
877 False 69
878 False 70
879 False 71
880 False 72
881 False 73
882 False 74
883 False 75
884 False 76
885 False 77
886 False 78
887 False 79
888 False 80
889 False 81
890 False 82
891 False 83
###Markdown
Which residues are our original pose residues? Note that the final residues are called `Virtual` residues. Virtual residues are not scored. They have coordinates, and can move, but simply result in a score of zero. They are useful in some contexts to hide a part of the pose from the scoring machinery, and there are movers that can change residues to and from virtual. In this case, they are used for the FoldTree - in order to allow refinement of the full crystal environment. They allow relative movement of each subunit relative to each other. There are two virtual residues for each subunit
###Code
print(p.residue(3654))
print("Total Subunits:", (3654-18)/404)
print("Total Subunits:", sym_info.subunits())
score = get_score_function()
print(score(original))
print(score(p))
###Output
[0mcore.scoring.ScoreFunctionFactory: [0mSCOREFUNCTION: [32mref2015[0m
[0mcore.scoring.ScoreFunctionFactory: [0mThe -include_sugars flag was used with no sugar_bb weight set in the weights file. Setting sugar_bb weight to 1.0 by default.
-531.2534669946483
-1132.7274634504747
###Markdown
Running Protocols with SymmetryNow, lets try running a minimization with symmetry on.
###Code
mm = MoveMap()
mm.set_bb(True)
mm.set_chi(True)
minmover = rosetta.protocols.minimization_packing.MinMover()
minmover.score_function(score)
minmover.set_movemap(mm)
if not os.getenv("DEBUG"):
minmover.apply(p)
score(p)
###Output
_____no_output_____
###Markdown
How does our pose look? For being such a large pose, how was the speed of minimization? How does this compare to our refined pose? Try to copy a subunit to a new object in PyMol. Then use the align command to align it to our assymetric unit. What is the RMSD? Now lets pack with our symmetric structure.
###Code
from rosetta.core.pack.task import *
from rosetta.core.pack.task.operation import *
packer = PackRotamersMover()
tf = rosetta.core.pack.task.TaskFactory()
tf.push_back(RestrictToRepacking())
tf.push_back(IncludeCurrent())
packer.task_factory(tf)
p = original.clone()
symmetrize.apply(p)
if not os.getenv("DEBUG"):
packer.apply(p)
print("packed", score(p))
###Output
packed -1960.9189300248413
###Markdown
*This notebook contains material from [PyRosetta](https://RosettaCommons.github.io/PyRosetta.notebooks);content is available [on Github](https://github.com/RosettaCommons/PyRosetta.notebooks.git).* Working With SymmetryKeywords: symmetry, asymmetric, SetupForSymmetryMover, virtual OverviewSymmetry is an important concept to learn when working with biomolecules. When a protein is crystalized, it is in the precense of its symmetrical neighbors - which can be important if testing particular protocols or using crystal density for refinement or full structure building.Symmetry can also be useful for designing symmetrical structures or large repeating meta-proteins like protein cages. Symmetry In RosettaSo why do we care if our protein is symmetrical or not when it comes to Rosetta? Each residue and atom that is loaded into Rosetta takes time to both load, and time to score. Since scoring can happen thousands of times - even in a short protocol, anything we can do to speed this up becomes important. The most expensive operation in Rosetta is minimization, and by using symmetry - we can reduce the minimization time exponentially by minimizing a single copy instead of ALL copies. We will get into the details about how this works below.When we use symmetry in Rosetta - we are basically telling rosetta that the symmetrical partners are 'special', however, the total number of residues is now ALL residues, including symmetrical partners. Upon setting up symmety in Rosetta, Rosetta will replace the `Conformation` within the pose with a **Symmetrical** version, called the `SymmetricConformation`. If you know anything about classes, this `SymmetricConformation` is derived from the actual `Conformation` object, but contains extra information about the pose and some functions are replaced. Symmetric Scoring and MovingOk, so now lets assume that we have our symmetric pose. Now what? Well, the symmetric copies are all tied to their real counterparts. Once you move a chain, residue, or atom by packing or minimization, the symmetric copies of that residue are all moved in the same way. Cool. But what about scoring? Scoring works very similarly - instead of scoring each and every residue in our pose, Rosetta will score just our assymetric unit, and multiply that out to the number of symmetric copies we have. Intelligently, Rosetta will also figure out the symmetric interfaces that arise from the interactions of our assymetric unit to the symmetric copies and score them appropriately. Symmetry-aware moversMost of our common movers are symmetry-aware. At one point there were different symmetric and non-symmetric versions of particular code, such as MinMover and PackRotamersMover. Now though, Rosetta will automatically use the pose to figure out what needs to be done. You should seek original documentation (and contact the author if not explicit) to make sure that an uncommon protocol you are using is symmetry-aware. DocumentationMore information on RosettaSymmetry can be found in the following places:- https://www.rosettacommons.org/docs/latest/rosetta_basics/structural_concepts/symmetry- https://www.rosettacommons.org/demos/latest/tutorials/Symmetry/Symmetry- https://www.rosettacommons.org/docs/latest/application_documentation/utilities/make-symmdef-file- https://www.rosettacommons.org/docs/latest/scripting_documentation/RosettaScripts/Movers/movers_pages/SetupForSymmetryMover- https://www.rosettacommons.org/docs/latest/scripting_documentation/RosettaScripts/Movers/movers_pages/ExtractAsymmetricUnitMover
###Code
# Notebook setup
import sys
if 'google.colab' in sys.modules:
!pip install pyrosettacolabsetup
import pyrosettacolabsetup
pyrosettacolabsetup.setup()
print ("Notebook is set for PyRosetta use in Colab. Have fun!")
###Output
_____no_output_____
###Markdown
Here, we will use a few specific options. The first three options make Rosetta a bit more robust to input structures. The `-load_PDB_components` cannot be used with glycans, unfortunately, and our structure has a few very important glycans. Finally, we load a bunch of glycan-specific options, which we will cover in the next tutorial.
###Code
from pyrosetta import *
from pyrosetta.rosetta import *
from pyrosetta.teaching import *
import os
init('-ignore_unrecognized_res -load_PDB_components false -ignore_zero_occupancy false @inputs/glycan_flags')
###Output
PyRosetta-4 2019 [Rosetta PyRosetta4.Release.python36.mac 2019.39+release.93456a567a8125cafdf7f8cb44400bc20b570d81 2019-09-26T14:24:44] retrieved from: http://www.pyrosetta.org
(C) Copyright Rosetta Commons Member Institutions. Created in JHU by Sergey Lyskov and PyRosetta Team.
[0mcore.init: [0mChecking for fconfig files in pwd and ./rosetta/flags
[0mcore.init: [0mReading fconfig.../Users/jadolfbr/.rosetta/flags/common
[0mcore.init: [0m
[0mcore.init: [0m
[0mcore.init: [0mRosetta version: PyRosetta4.Release.python36.mac r233 2019.39+release.93456a567a8 93456a567a8125cafdf7f8cb44400bc20b570d81 http://www.pyrosetta.org 2019-09-26T14:24:44
[0mcore.init: [0mcommand: PyRosetta -ignore_unrecognized_res -load_PDB_components false -ignore_zero_occupancy false @inputs/glycan_flags -database /Users/jadolfbr/Library/Python/3.6/lib/python/site-packages/pyrosetta-2019.39+release.93456a567a8-py3.6-macosx-10.6-intel.egg/pyrosetta/database
[0mbasic.random.init_random_generator: [0m'RNG device' seed mode, using '/dev/urandom', seed=1544006277 seed_offset=0 real_seed=1544006277
[0mbasic.random.init_random_generator: [0mRandomGenerator:init: Normal mode, seed=1544006277 RG_type=mt19937
###Markdown
Creating a SymDef fileHere, we will start with how to create a basic symdef file for cyrstal symmetry. Note that there are ways to do this without a symdef file, but these do not currently work for glycan structures, which we will be using here. The `make_symdef_file.pl` file is within Rosetta3. To use it, you will need to download and licence Rosetta3. The code is in the `Rosetta/main/src/apps/public` directory. In the interest of reducing code drift, this file is NOT included in the tutorial directory as we may then have version drift. If you have done this, we can use the following command to create the symdef file. Here, the radius of symmetrical partners is 12A, which is certainly fairly large, but produces a very well represented crystal.
###Code
pdb = "inputs/1jnd.pdb"
base_cmd = f'cd inputs && make_symmdef_file.pl -r 12 -m CRYST -p {pdb}.pdb > {pdb}_crys.symm && cd -'
print(base_cmd)
###Output
cd inputs && make_symmdef_file.pl -r 12 -m CRYST -p inputs/1jnd.pdb.pdb > inputs/1jnd.pdb_crys.symm && cd -
###Markdown
Use this base command and the `os.system(cmd)` function to run the code or use the provided symdef file.
###Code
os.system('cp inputs/1jnd_crys.symm .')
###Output
_____no_output_____
###Markdown
Take a look at the symmetrized structure in pymol (`inputs/1jnd_symm.pdb`). What would happen if we increased the radius to 24 instead of 12? Setup a Symmetrized PoseHere, we will run a basic Rosetta protocol with symmetry. There are much more complicated things you can do with symmetry, but for now, we just want to symmetrically pack the protein. Please see the docs for more on symmetry. The full Rosetta C++ tutorial for symmetry is a great place to go from here: - https://www.rosettacommons.org/demos/latest/tutorials/Symmetry/Symmetry Lets first create a pose, and then use the `SetupForSymmetryMover` on the pose. Note this is an unrefined input structure. This is so that minmover will actually do something. A pareto-optimal refined structure can be found in the inputs as `1jnd_refined.pdb.gz`
###Code
p = pose_from_pdb('inputs/1jnd.pdb')
original = p.clone()
p.total_residue()
type(p.conformation())
symmetrize = rosetta.protocols.symmetry.SetupForSymmetryMover("1jnd_crys.symm")
symmetrize.apply(p)
print(p.total_residue())
print(type(p.conformation()))
###Output
3654
<class 'pyrosetta.rosetta.core.conformation.symmetry.SymmetricConformation'>
###Markdown
How many symmetric copies do we have in our pose?How do the scores compare for our original pose and our symmetrized version? Now lets use some of the functionality to understand how this all works. We can use the `SymetricInfo` object that is part of the `SymmetricConformation` to get at some info. Lets take a look at all residues and find the assymetric unit residues and equivalent residues for the rest.
###Code
print("AssymUnit? equivalent_res")
sym_info = p.conformation().Symmetry_Info()
for i in range(1, p.size()+1):
print(i, sym_info.bb_is_independent(i), sym_info.bb_follows(i))
###Output
AssymUnit? equivalent_res
1 True 0
2 True 0
3 True 0
4 True 0
5 True 0
6 True 0
7 True 0
8 True 0
9 True 0
10 True 0
11 True 0
12 True 0
13 True 0
14 True 0
15 True 0
16 True 0
17 True 0
18 True 0
19 True 0
20 True 0
21 True 0
22 True 0
23 True 0
24 True 0
25 True 0
26 True 0
27 True 0
28 True 0
29 True 0
30 True 0
31 True 0
32 True 0
33 True 0
34 True 0
35 True 0
36 True 0
37 True 0
38 True 0
39 True 0
40 True 0
41 True 0
42 True 0
43 True 0
44 True 0
45 True 0
46 True 0
47 True 0
48 True 0
49 True 0
50 True 0
51 True 0
52 True 0
53 True 0
54 True 0
55 True 0
56 True 0
57 True 0
58 True 0
59 True 0
60 True 0
61 True 0
62 True 0
63 True 0
64 True 0
65 True 0
66 True 0
67 True 0
68 True 0
69 True 0
70 True 0
71 True 0
72 True 0
73 True 0
74 True 0
75 True 0
76 True 0
77 True 0
78 True 0
79 True 0
80 True 0
81 True 0
82 True 0
83 True 0
84 True 0
85 True 0
86 True 0
87 True 0
88 True 0
89 True 0
90 True 0
91 True 0
92 True 0
93 True 0
94 True 0
95 True 0
96 True 0
97 True 0
98 True 0
99 True 0
100 True 0
101 True 0
102 True 0
103 True 0
104 True 0
105 True 0
106 True 0
107 True 0
108 True 0
109 True 0
110 True 0
111 True 0
112 True 0
113 True 0
114 True 0
115 True 0
116 True 0
117 True 0
118 True 0
119 True 0
120 True 0
121 True 0
122 True 0
123 True 0
124 True 0
125 True 0
126 True 0
127 True 0
128 True 0
129 True 0
130 True 0
131 True 0
132 True 0
133 True 0
134 True 0
135 True 0
136 True 0
137 True 0
138 True 0
139 True 0
140 True 0
141 True 0
142 True 0
143 True 0
144 True 0
145 True 0
146 True 0
147 True 0
148 True 0
149 True 0
150 True 0
151 True 0
152 True 0
153 True 0
154 True 0
155 True 0
156 True 0
157 True 0
158 True 0
159 True 0
160 True 0
161 True 0
162 True 0
163 True 0
164 True 0
165 True 0
166 True 0
167 True 0
168 True 0
169 True 0
170 True 0
171 True 0
172 True 0
173 True 0
174 True 0
175 True 0
176 True 0
177 True 0
178 True 0
179 True 0
180 True 0
181 True 0
182 True 0
183 True 0
184 True 0
185 True 0
186 True 0
187 True 0
188 True 0
189 True 0
190 True 0
191 True 0
192 True 0
193 True 0
194 True 0
195 True 0
196 True 0
197 True 0
198 True 0
199 True 0
200 True 0
201 True 0
202 True 0
203 True 0
204 True 0
205 True 0
206 True 0
207 True 0
208 True 0
209 True 0
210 True 0
211 True 0
212 True 0
213 True 0
214 True 0
215 True 0
216 True 0
217 True 0
218 True 0
219 True 0
220 True 0
221 True 0
222 True 0
223 True 0
224 True 0
225 True 0
226 True 0
227 True 0
228 True 0
229 True 0
230 True 0
231 True 0
232 True 0
233 True 0
234 True 0
235 True 0
236 True 0
237 True 0
238 True 0
239 True 0
240 True 0
241 True 0
242 True 0
243 True 0
244 True 0
245 True 0
246 True 0
247 True 0
248 True 0
249 True 0
250 True 0
251 True 0
252 True 0
253 True 0
254 True 0
255 True 0
256 True 0
257 True 0
258 True 0
259 True 0
260 True 0
261 True 0
262 True 0
263 True 0
264 True 0
265 True 0
266 True 0
267 True 0
268 True 0
269 True 0
270 True 0
271 True 0
272 True 0
273 True 0
274 True 0
275 True 0
276 True 0
277 True 0
278 True 0
279 True 0
280 True 0
281 True 0
282 True 0
283 True 0
284 True 0
285 True 0
286 True 0
287 True 0
288 True 0
289 True 0
290 True 0
291 True 0
292 True 0
293 True 0
294 True 0
295 True 0
296 True 0
297 True 0
298 True 0
299 True 0
300 True 0
301 True 0
302 True 0
303 True 0
304 True 0
305 True 0
306 True 0
307 True 0
308 True 0
309 True 0
310 True 0
311 True 0
312 True 0
313 True 0
314 True 0
315 True 0
316 True 0
317 True 0
318 True 0
319 True 0
320 True 0
321 True 0
322 True 0
323 True 0
324 True 0
325 True 0
326 True 0
327 True 0
328 True 0
329 True 0
330 True 0
331 True 0
332 True 0
333 True 0
334 True 0
335 True 0
336 True 0
337 True 0
338 True 0
339 True 0
340 True 0
341 True 0
342 True 0
343 True 0
344 True 0
345 True 0
346 True 0
347 True 0
348 True 0
349 True 0
350 True 0
351 True 0
352 True 0
353 True 0
354 True 0
355 True 0
356 True 0
357 True 0
358 True 0
359 True 0
360 True 0
361 True 0
362 True 0
363 True 0
364 True 0
365 True 0
366 True 0
367 True 0
368 True 0
369 True 0
370 True 0
371 True 0
372 True 0
373 True 0
374 True 0
375 True 0
376 True 0
377 True 0
378 True 0
379 True 0
380 True 0
381 True 0
382 True 0
383 True 0
384 True 0
385 True 0
386 True 0
387 True 0
388 True 0
389 True 0
390 True 0
391 True 0
392 True 0
393 True 0
394 True 0
395 True 0
396 True 0
397 True 0
398 True 0
399 True 0
400 True 0
401 True 0
402 True 0
403 True 0
404 True 0
405 False 1
406 False 2
407 False 3
408 False 4
409 False 5
410 False 6
411 False 7
412 False 8
413 False 9
414 False 10
415 False 11
416 False 12
417 False 13
418 False 14
419 False 15
420 False 16
421 False 17
422 False 18
423 False 19
424 False 20
425 False 21
426 False 22
427 False 23
428 False 24
429 False 25
430 False 26
431 False 27
432 False 28
433 False 29
434 False 30
435 False 31
436 False 32
437 False 33
438 False 34
439 False 35
440 False 36
441 False 37
442 False 38
443 False 39
444 False 40
445 False 41
446 False 42
447 False 43
448 False 44
449 False 45
450 False 46
451 False 47
452 False 48
453 False 49
454 False 50
455 False 51
456 False 52
457 False 53
458 False 54
459 False 55
460 False 56
461 False 57
462 False 58
463 False 59
464 False 60
465 False 61
466 False 62
467 False 63
468 False 64
469 False 65
470 False 66
471 False 67
472 False 68
473 False 69
474 False 70
475 False 71
476 False 72
477 False 73
478 False 74
479 False 75
480 False 76
481 False 77
482 False 78
483 False 79
484 False 80
485 False 81
486 False 82
487 False 83
488 False 84
489 False 85
490 False 86
491 False 87
492 False 88
493 False 89
494 False 90
495 False 91
496 False 92
497 False 93
498 False 94
499 False 95
500 False 96
501 False 97
502 False 98
503 False 99
504 False 100
505 False 101
506 False 102
507 False 103
508 False 104
509 False 105
510 False 106
511 False 107
512 False 108
513 False 109
514 False 110
515 False 111
516 False 112
517 False 113
518 False 114
519 False 115
520 False 116
521 False 117
522 False 118
523 False 119
524 False 120
525 False 121
526 False 122
527 False 123
528 False 124
529 False 125
530 False 126
531 False 127
532 False 128
533 False 129
534 False 130
535 False 131
536 False 132
537 False 133
538 False 134
539 False 135
540 False 136
541 False 137
542 False 138
543 False 139
544 False 140
545 False 141
546 False 142
547 False 143
548 False 144
549 False 145
550 False 146
551 False 147
552 False 148
553 False 149
554 False 150
555 False 151
556 False 152
557 False 153
558 False 154
559 False 155
560 False 156
561 False 157
562 False 158
563 False 159
564 False 160
565 False 161
566 False 162
567 False 163
568 False 164
569 False 165
570 False 166
571 False 167
572 False 168
573 False 169
574 False 170
575 False 171
576 False 172
577 False 173
578 False 174
579 False 175
580 False 176
581 False 177
582 False 178
583 False 179
584 False 180
585 False 181
586 False 182
587 False 183
588 False 184
589 False 185
590 False 186
591 False 187
592 False 188
593 False 189
594 False 190
595 False 191
596 False 192
597 False 193
598 False 194
599 False 195
600 False 196
601 False 197
602 False 198
603 False 199
604 False 200
605 False 201
606 False 202
607 False 203
608 False 204
609 False 205
610 False 206
611 False 207
612 False 208
613 False 209
614 False 210
615 False 211
616 False 212
617 False 213
618 False 214
619 False 215
620 False 216
621 False 217
622 False 218
623 False 219
624 False 220
625 False 221
626 False 222
627 False 223
628 False 224
629 False 225
630 False 226
631 False 227
632 False 228
633 False 229
634 False 230
635 False 231
636 False 232
637 False 233
638 False 234
639 False 235
640 False 236
641 False 237
642 False 238
643 False 239
644 False 240
645 False 241
646 False 242
647 False 243
648 False 244
649 False 245
650 False 246
651 False 247
652 False 248
653 False 249
654 False 250
655 False 251
656 False 252
657 False 253
658 False 254
659 False 255
660 False 256
661 False 257
662 False 258
663 False 259
664 False 260
665 False 261
666 False 262
667 False 263
668 False 264
669 False 265
670 False 266
671 False 267
672 False 268
673 False 269
674 False 270
675 False 271
676 False 272
677 False 273
678 False 274
679 False 275
680 False 276
681 False 277
682 False 278
683 False 279
684 False 280
685 False 281
686 False 282
687 False 283
688 False 284
689 False 285
690 False 286
691 False 287
692 False 288
693 False 289
694 False 290
695 False 291
696 False 292
697 False 293
698 False 294
699 False 295
700 False 296
701 False 297
702 False 298
703 False 299
704 False 300
705 False 301
706 False 302
707 False 303
708 False 304
709 False 305
710 False 306
711 False 307
712 False 308
713 False 309
714 False 310
715 False 311
716 False 312
717 False 313
718 False 314
719 False 315
720 False 316
721 False 317
722 False 318
723 False 319
724 False 320
725 False 321
726 False 322
727 False 323
728 False 324
729 False 325
730 False 326
731 False 327
732 False 328
733 False 329
734 False 330
735 False 331
736 False 332
737 False 333
738 False 334
739 False 335
740 False 336
741 False 337
742 False 338
743 False 339
744 False 340
745 False 341
746 False 342
747 False 343
748 False 344
749 False 345
750 False 346
751 False 347
752 False 348
753 False 349
754 False 350
755 False 351
756 False 352
757 False 353
758 False 354
759 False 355
760 False 356
761 False 357
762 False 358
763 False 359
764 False 360
765 False 361
766 False 362
767 False 363
768 False 364
769 False 365
770 False 366
771 False 367
772 False 368
773 False 369
774 False 370
775 False 371
776 False 372
777 False 373
778 False 374
779 False 375
780 False 376
781 False 377
782 False 378
783 False 379
784 False 380
785 False 381
786 False 382
787 False 383
788 False 384
789 False 385
790 False 386
791 False 387
792 False 388
793 False 389
794 False 390
795 False 391
796 False 392
797 False 393
798 False 394
799 False 395
800 False 396
801 False 397
802 False 398
803 False 399
804 False 400
805 False 401
806 False 402
807 False 403
808 False 404
809 False 1
810 False 2
811 False 3
812 False 4
813 False 5
814 False 6
815 False 7
816 False 8
817 False 9
818 False 10
819 False 11
820 False 12
821 False 13
822 False 14
823 False 15
824 False 16
825 False 17
826 False 18
827 False 19
828 False 20
829 False 21
830 False 22
831 False 23
832 False 24
833 False 25
834 False 26
835 False 27
836 False 28
837 False 29
838 False 30
839 False 31
840 False 32
841 False 33
842 False 34
843 False 35
844 False 36
845 False 37
846 False 38
847 False 39
848 False 40
849 False 41
850 False 42
851 False 43
852 False 44
853 False 45
854 False 46
855 False 47
856 False 48
857 False 49
858 False 50
859 False 51
860 False 52
861 False 53
862 False 54
863 False 55
864 False 56
865 False 57
866 False 58
867 False 59
868 False 60
869 False 61
870 False 62
871 False 63
872 False 64
873 False 65
874 False 66
875 False 67
876 False 68
877 False 69
878 False 70
879 False 71
880 False 72
881 False 73
882 False 74
883 False 75
884 False 76
885 False 77
886 False 78
887 False 79
888 False 80
889 False 81
890 False 82
891 False 83
###Markdown
Which residues are our original pose residues? Note that the final residues are called `Virtual` residues. Virtual residues are not scored. They have coordinates, and can move, but simply result in a score of zero. They are useful in some contexts to hide a part of the pose from the scoring machinery, and there are movers that can change residues to and from virtual. In this case, they are used for the FoldTree - in order to allow refinement of the full crystal environment. They allow relative movement of each subunit relative to each other. There are two virtual residues for each subunit
###Code
print(p.residue(3654))
print("Total Subunits:", (3654-18)/404)
print("Total Subunits:", sym_info.subunits())
score = get_score_function()
print(score(original))
print(score(p))
###Output
[0mcore.scoring.ScoreFunctionFactory: [0mSCOREFUNCTION: [32mref2015[0m
[0mcore.scoring.ScoreFunctionFactory: [0mThe -include_sugars flag was used with no sugar_bb weight set in the weights file. Setting sugar_bb weight to 1.0 by default.
-531.2534669946483
-1132.7274634504747
###Markdown
Running Protocols with SymmetryNow, lets try running a minimization with symmetry on.
###Code
mm = MoveMap()
mm.set_bb(True)
mm.set_chi(True)
minmover = rosetta.protocols.minimization_packing.MinMover()
minmover.score_function(score)
minmover.set_movemap(mm)
if not os.getenv("DEBUG"):
minmover.apply(p)
score(p)
###Output
_____no_output_____
###Markdown
How does our pose look? For being such a large pose, how was the speed of minimization? How does this compare to our refined pose? Try to copy a subunit to a new object in PyMol. Then use the align command to align it to our assymetric unit. What is the RMSD? Now lets pack with our symmetric structure.
###Code
from rosetta.core.pack.task import *
from rosetta.core.pack.task.operation import *
packer = PackRotamersMover()
tf = rosetta.core.pack.task.TaskFactory()
tf.push_back(RestrictToRepacking())
tf.push_back(IncludeCurrent())
packer.task_factory(tf)
p = original.clone()
symmetrize.apply(p)
if not os.getenv("DEBUG"):
packer.apply(p)
print("packed", score(p))
###Output
packed -1960.9189300248413
###Markdown
*This notebook contains material from [PyRosetta](https://RosettaCommons.github.io/PyRosetta.notebooks);content is available [on Github](https://github.com/RosettaCommons/PyRosetta.notebooks.git).* Working With SymmetryKeywords: symmetry, asymmetric, SetupForSymmetryMover, virtual OverviewSymmetry is an important concept to learn when working with biomolecules. When a protein is crystalized, it is in the precense of its symmetrical neighbors - which can be important if testing particular protocols or using crystal density for refinement or full structure building.Symmetry can also be useful for designing symmetrical structures or large repeating meta-proteins like protein cages. Symmetry In RosettaSo why do we care if our protein is symmetrical or not when it comes to Rosetta? Each residue and atom that is loaded into Rosetta takes time to both load, and time to score. Since scoring can happen thousands of times - even in a short protocol, anything we can do to speed this up becomes important. The most expensive operation in Rosetta is minimization, and by using symmetry - we can reduce the minimization time exponentially by minimizing a single copy instead of ALL copies. We will get into the details about how this works below.When we use symmetry in Rosetta - we are basically telling rosetta that the symmetrical partners are 'special', however, the total number of residues is now ALL residues, including symmetrical partners. Upon setting up symmety in Rosetta, Rosetta will replace the `Conformation` within the pose with a **Symmetrical** version, called the `SymmetricConformation`. If you know anything about classes, this `SymmetricConformation` is derived from the actual `Conformation` object, but contains extra information about the pose and some functions are replaced. Symmetric Scoring and MovingOk, so now lets assume that we have our symmetric pose. Now what? Well, the symmetric copies are all tied to their real counterparts. Once you move a chain, residue, or atom by packing or minimization, the symmetric copies of that residue are all moved in the same way. Cool. But what about scoring? Scoring works very similarly - instead of scoring each and every residue in our pose, Rosetta will score just our assymetric unit, and multiply that out to the number of symmetric copies we have. Intelligently, Rosetta will also figure out the symmetric interfaces that arise from the interactions of our assymetric unit to the symmetric copies and score them appropriately. Symmetry-aware moversMost of our common movers are symmetry-aware. At one point there were different symmetric and non-symmetric versions of particular code, such as MinMover and PackRotamersMover. Now though, Rosetta will automatically use the pose to figure out what needs to be done. You should seek original documentation (and contact the author if not explicit) to make sure that an uncommon protocol you are using is symmetry-aware. DocumentationMore information on RosettaSymmetry can be found in the following places:- https://www.rosettacommons.org/docs/latest/rosetta_basics/structural_concepts/symmetry- https://www.rosettacommons.org/demos/latest/tutorials/Symmetry/Symmetry- https://www.rosettacommons.org/docs/latest/application_documentation/utilities/make-symmdef-file- https://www.rosettacommons.org/docs/latest/scripting_documentation/RosettaScripts/Movers/movers_pages/SetupForSymmetryMover- https://www.rosettacommons.org/docs/latest/scripting_documentation/RosettaScripts/Movers/movers_pages/ExtractAsymmetricUnitMover
###Code
# Notebook setup
import sys
if 'google.colab' in sys.modules:
!pip install pyrosettacolabsetup
import pyrosettacolabsetup
pyrosettacolabsetup.setup()
print ("Notebook is set for PyRosetta use in Colab. Have fun!")
###Output
_____no_output_____
###Markdown
Here, we will use a few specific options. The first three options make Rosetta a bit more robust to input structures. The `-load_PDB_components` cannot be used with glycans, unfortunately, and our structure has a few very important glycans. Finally, we load a bunch of glycan-specific options, which we will cover in the next tutorial.
###Code
from pyrosetta import *
from pyrosetta.rosetta import *
from pyrosetta.teaching import *
import os
init('-ignore_unrecognized_res -load_PDB_components false -ignore_zero_occupancy false @inputs/glycan_flags')
###Output
PyRosetta-4 2019 [Rosetta PyRosetta4.Release.python36.mac 2019.39+release.93456a567a8125cafdf7f8cb44400bc20b570d81 2019-09-26T14:24:44] retrieved from: http://www.pyrosetta.org
(C) Copyright Rosetta Commons Member Institutions. Created in JHU by Sergey Lyskov and PyRosetta Team.
[0mcore.init: [0mChecking for fconfig files in pwd and ./rosetta/flags
[0mcore.init: [0mReading fconfig.../Users/jadolfbr/.rosetta/flags/common
[0mcore.init: [0m
[0mcore.init: [0m
[0mcore.init: [0mRosetta version: PyRosetta4.Release.python36.mac r233 2019.39+release.93456a567a8 93456a567a8125cafdf7f8cb44400bc20b570d81 http://www.pyrosetta.org 2019-09-26T14:24:44
[0mcore.init: [0mcommand: PyRosetta -ignore_unrecognized_res -load_PDB_components false -ignore_zero_occupancy false @inputs/glycan_flags -database /Users/jadolfbr/Library/Python/3.6/lib/python/site-packages/pyrosetta-2019.39+release.93456a567a8-py3.6-macosx-10.6-intel.egg/pyrosetta/database
[0mbasic.random.init_random_generator: [0m'RNG device' seed mode, using '/dev/urandom', seed=1544006277 seed_offset=0 real_seed=1544006277
[0mbasic.random.init_random_generator: [0mRandomGenerator:init: Normal mode, seed=1544006277 RG_type=mt19937
###Markdown
Creating a SymDef fileHere, we will start with how to create a basic symdef file for cyrstal symmetry. Note that there are ways to do this without a symdef file, but these do not currently work for glycan structures, which we will be using here. The `make_symdef_file.pl` file is within Rosetta3. To use it, you will need to download and licence Rosetta3. The code is in the `Rosetta/main/src/apps/public` directory. In the interest of reducing code drift, this file is NOT included in the tutorial directory as we may then have version drift. If you have done this, we can use the following command to create the symdef file. Here, the radius of symmetrical partners is 12A, which is certainly fairly large, but produces a very well represented crystal.
###Code
pdb = "inputs/1jnd.pdb"
base_cmd = f'cd inputs && make_symmdef_file.pl -r 12 -m CRYST -p {pdb}.pdb > {pdb}_crys.symm && cd -'
print(base_cmd)
###Output
cd inputs && make_symmdef_file.pl -r 12 -m CRYST -p inputs/1jnd.pdb.pdb > inputs/1jnd.pdb_crys.symm && cd -
###Markdown
Use this base command and the `os.system(cmd)` function to run the code or use the provided symdef file.
###Code
os.system('cp inputs/1jnd_crys.symm .')
###Output
_____no_output_____
###Markdown
Take a look at the symmetrized structure in pymol (`inputs/1jnd_symm.pdb`). What would happen if we increased the radius to 24 instead of 12? Setup a Symmetrized PoseHere, we will run a basic Rosetta protocol with symmetry. There are much more complicated things you can do with symmetry, but for now, we just want to symmetrically pack the protein. Please see the docs for more on symmetry. The full Rosetta C++ tutorial for symmetry is a great place to go from here: - https://www.rosettacommons.org/demos/latest/tutorials/Symmetry/Symmetry Lets first create a pose, and then use the `SetupForSymmetryMover` on the pose. Note this is an unrefined input structure. This is so that minmover will actually do something. A pareto-optimal refined structure can be found in the inputs as `1jnd_refined.pdb.gz`
###Code
p = pose_from_pdb('inputs/1jnd.pdb')
original = p.clone()
p.total_residue()
type(p.conformation())
symmetrize = rosetta.protocols.symmetry.SetupForSymmetryMover("1jnd_crys.symm")
symmetrize.apply(p)
print(p.total_residue())
print(type(p.conformation()))
###Output
3654
<class 'pyrosetta.rosetta.core.conformation.symmetry.SymmetricConformation'>
###Markdown
How many symmetric copies do we have in our pose?How do the scores compare for our original pose and our symmetrized version? Now lets use some of the functionality to understand how this all works. We can use the `SymetricInfo` object that is part of the `SymmetricConformation` to get at some info. Lets take a look at all residues and find the assymetric unit residues and equivalent residues for the rest.
###Code
print("AssymUnit? equivalent_res")
sym_info = p.conformation().Symmetry_Info()
for i in range(1, p.size()+1):
print(i, sym_info.bb_is_independent(i), sym_info.bb_follows(i))
###Output
AssymUnit? equivalent_res
1 True 0
2 True 0
3 True 0
4 True 0
5 True 0
6 True 0
7 True 0
8 True 0
9 True 0
10 True 0
11 True 0
12 True 0
13 True 0
14 True 0
15 True 0
16 True 0
17 True 0
18 True 0
19 True 0
20 True 0
21 True 0
22 True 0
23 True 0
24 True 0
25 True 0
26 True 0
27 True 0
28 True 0
29 True 0
30 True 0
31 True 0
32 True 0
33 True 0
34 True 0
35 True 0
36 True 0
37 True 0
38 True 0
39 True 0
40 True 0
41 True 0
42 True 0
43 True 0
44 True 0
45 True 0
46 True 0
47 True 0
48 True 0
49 True 0
50 True 0
51 True 0
52 True 0
53 True 0
54 True 0
55 True 0
56 True 0
57 True 0
58 True 0
59 True 0
60 True 0
61 True 0
62 True 0
63 True 0
64 True 0
65 True 0
66 True 0
67 True 0
68 True 0
69 True 0
70 True 0
71 True 0
72 True 0
73 True 0
74 True 0
75 True 0
76 True 0
77 True 0
78 True 0
79 True 0
80 True 0
81 True 0
82 True 0
83 True 0
84 True 0
85 True 0
86 True 0
87 True 0
88 True 0
89 True 0
90 True 0
91 True 0
92 True 0
93 True 0
94 True 0
95 True 0
96 True 0
97 True 0
98 True 0
99 True 0
100 True 0
101 True 0
102 True 0
103 True 0
104 True 0
105 True 0
106 True 0
107 True 0
108 True 0
109 True 0
110 True 0
111 True 0
112 True 0
113 True 0
114 True 0
115 True 0
116 True 0
117 True 0
118 True 0
119 True 0
120 True 0
121 True 0
122 True 0
123 True 0
124 True 0
125 True 0
126 True 0
127 True 0
128 True 0
129 True 0
130 True 0
131 True 0
132 True 0
133 True 0
134 True 0
135 True 0
136 True 0
137 True 0
138 True 0
139 True 0
140 True 0
141 True 0
142 True 0
143 True 0
144 True 0
145 True 0
146 True 0
147 True 0
148 True 0
149 True 0
150 True 0
151 True 0
152 True 0
153 True 0
154 True 0
155 True 0
156 True 0
157 True 0
158 True 0
159 True 0
160 True 0
161 True 0
162 True 0
163 True 0
164 True 0
165 True 0
166 True 0
167 True 0
168 True 0
169 True 0
170 True 0
171 True 0
172 True 0
173 True 0
174 True 0
175 True 0
176 True 0
177 True 0
178 True 0
179 True 0
180 True 0
181 True 0
182 True 0
183 True 0
184 True 0
185 True 0
186 True 0
187 True 0
188 True 0
189 True 0
190 True 0
191 True 0
192 True 0
193 True 0
194 True 0
195 True 0
196 True 0
197 True 0
198 True 0
199 True 0
200 True 0
201 True 0
202 True 0
203 True 0
204 True 0
205 True 0
206 True 0
207 True 0
208 True 0
209 True 0
210 True 0
211 True 0
212 True 0
213 True 0
214 True 0
215 True 0
216 True 0
217 True 0
218 True 0
219 True 0
220 True 0
221 True 0
222 True 0
223 True 0
224 True 0
225 True 0
226 True 0
227 True 0
228 True 0
229 True 0
230 True 0
231 True 0
232 True 0
233 True 0
234 True 0
235 True 0
236 True 0
237 True 0
238 True 0
239 True 0
240 True 0
241 True 0
242 True 0
243 True 0
244 True 0
245 True 0
246 True 0
247 True 0
248 True 0
249 True 0
250 True 0
251 True 0
252 True 0
253 True 0
254 True 0
255 True 0
256 True 0
257 True 0
258 True 0
259 True 0
260 True 0
261 True 0
262 True 0
263 True 0
264 True 0
265 True 0
266 True 0
267 True 0
268 True 0
269 True 0
270 True 0
271 True 0
272 True 0
273 True 0
274 True 0
275 True 0
276 True 0
277 True 0
278 True 0
279 True 0
280 True 0
281 True 0
282 True 0
283 True 0
284 True 0
285 True 0
286 True 0
287 True 0
288 True 0
289 True 0
290 True 0
291 True 0
292 True 0
293 True 0
294 True 0
295 True 0
296 True 0
297 True 0
298 True 0
299 True 0
300 True 0
301 True 0
302 True 0
303 True 0
304 True 0
305 True 0
306 True 0
307 True 0
308 True 0
309 True 0
310 True 0
311 True 0
312 True 0
313 True 0
314 True 0
315 True 0
316 True 0
317 True 0
318 True 0
319 True 0
320 True 0
321 True 0
322 True 0
323 True 0
324 True 0
325 True 0
326 True 0
327 True 0
328 True 0
329 True 0
330 True 0
331 True 0
332 True 0
333 True 0
334 True 0
335 True 0
336 True 0
337 True 0
338 True 0
339 True 0
340 True 0
341 True 0
342 True 0
343 True 0
344 True 0
345 True 0
346 True 0
347 True 0
348 True 0
349 True 0
350 True 0
351 True 0
352 True 0
353 True 0
354 True 0
355 True 0
356 True 0
357 True 0
358 True 0
359 True 0
360 True 0
361 True 0
362 True 0
363 True 0
364 True 0
365 True 0
366 True 0
367 True 0
368 True 0
369 True 0
370 True 0
371 True 0
372 True 0
373 True 0
374 True 0
375 True 0
376 True 0
377 True 0
378 True 0
379 True 0
380 True 0
381 True 0
382 True 0
383 True 0
384 True 0
385 True 0
386 True 0
387 True 0
388 True 0
389 True 0
390 True 0
391 True 0
392 True 0
393 True 0
394 True 0
395 True 0
396 True 0
397 True 0
398 True 0
399 True 0
400 True 0
401 True 0
402 True 0
403 True 0
404 True 0
405 False 1
406 False 2
407 False 3
408 False 4
409 False 5
410 False 6
411 False 7
412 False 8
413 False 9
414 False 10
415 False 11
416 False 12
417 False 13
418 False 14
419 False 15
420 False 16
421 False 17
422 False 18
423 False 19
424 False 20
425 False 21
426 False 22
427 False 23
428 False 24
429 False 25
430 False 26
431 False 27
432 False 28
433 False 29
434 False 30
435 False 31
436 False 32
437 False 33
438 False 34
439 False 35
440 False 36
441 False 37
442 False 38
443 False 39
444 False 40
445 False 41
446 False 42
447 False 43
448 False 44
449 False 45
450 False 46
451 False 47
452 False 48
453 False 49
454 False 50
455 False 51
456 False 52
457 False 53
458 False 54
459 False 55
460 False 56
461 False 57
462 False 58
463 False 59
464 False 60
465 False 61
466 False 62
467 False 63
468 False 64
469 False 65
470 False 66
471 False 67
472 False 68
473 False 69
474 False 70
475 False 71
476 False 72
477 False 73
478 False 74
479 False 75
480 False 76
481 False 77
482 False 78
483 False 79
484 False 80
485 False 81
486 False 82
487 False 83
488 False 84
489 False 85
490 False 86
491 False 87
492 False 88
493 False 89
494 False 90
495 False 91
496 False 92
497 False 93
498 False 94
499 False 95
500 False 96
501 False 97
502 False 98
503 False 99
504 False 100
505 False 101
506 False 102
507 False 103
508 False 104
509 False 105
510 False 106
511 False 107
512 False 108
513 False 109
514 False 110
515 False 111
516 False 112
517 False 113
518 False 114
519 False 115
520 False 116
521 False 117
522 False 118
523 False 119
524 False 120
525 False 121
526 False 122
527 False 123
528 False 124
529 False 125
530 False 126
531 False 127
532 False 128
533 False 129
534 False 130
535 False 131
536 False 132
537 False 133
538 False 134
539 False 135
540 False 136
541 False 137
542 False 138
543 False 139
544 False 140
545 False 141
546 False 142
547 False 143
548 False 144
549 False 145
550 False 146
551 False 147
552 False 148
553 False 149
554 False 150
555 False 151
556 False 152
557 False 153
558 False 154
559 False 155
560 False 156
561 False 157
562 False 158
563 False 159
564 False 160
565 False 161
566 False 162
567 False 163
568 False 164
569 False 165
570 False 166
571 False 167
572 False 168
573 False 169
574 False 170
575 False 171
576 False 172
577 False 173
578 False 174
579 False 175
580 False 176
581 False 177
582 False 178
583 False 179
584 False 180
585 False 181
586 False 182
587 False 183
588 False 184
589 False 185
590 False 186
591 False 187
592 False 188
593 False 189
594 False 190
595 False 191
596 False 192
597 False 193
598 False 194
599 False 195
600 False 196
601 False 197
602 False 198
603 False 199
604 False 200
605 False 201
606 False 202
607 False 203
608 False 204
609 False 205
610 False 206
611 False 207
612 False 208
613 False 209
614 False 210
615 False 211
616 False 212
617 False 213
618 False 214
619 False 215
620 False 216
621 False 217
622 False 218
623 False 219
624 False 220
625 False 221
626 False 222
627 False 223
628 False 224
629 False 225
630 False 226
631 False 227
632 False 228
633 False 229
634 False 230
635 False 231
636 False 232
637 False 233
638 False 234
639 False 235
640 False 236
641 False 237
642 False 238
643 False 239
644 False 240
645 False 241
646 False 242
647 False 243
648 False 244
649 False 245
650 False 246
651 False 247
652 False 248
653 False 249
654 False 250
655 False 251
656 False 252
657 False 253
658 False 254
659 False 255
660 False 256
661 False 257
662 False 258
663 False 259
664 False 260
665 False 261
666 False 262
667 False 263
668 False 264
669 False 265
670 False 266
671 False 267
672 False 268
673 False 269
674 False 270
675 False 271
676 False 272
677 False 273
678 False 274
679 False 275
680 False 276
681 False 277
682 False 278
683 False 279
684 False 280
685 False 281
686 False 282
687 False 283
688 False 284
689 False 285
690 False 286
691 False 287
692 False 288
693 False 289
694 False 290
695 False 291
696 False 292
697 False 293
698 False 294
699 False 295
700 False 296
701 False 297
702 False 298
703 False 299
704 False 300
705 False 301
706 False 302
707 False 303
708 False 304
709 False 305
710 False 306
711 False 307
712 False 308
713 False 309
714 False 310
715 False 311
716 False 312
717 False 313
718 False 314
719 False 315
720 False 316
721 False 317
722 False 318
723 False 319
724 False 320
725 False 321
726 False 322
727 False 323
728 False 324
729 False 325
730 False 326
731 False 327
732 False 328
733 False 329
734 False 330
735 False 331
736 False 332
737 False 333
738 False 334
739 False 335
740 False 336
741 False 337
742 False 338
743 False 339
744 False 340
745 False 341
746 False 342
747 False 343
748 False 344
749 False 345
750 False 346
751 False 347
752 False 348
753 False 349
754 False 350
755 False 351
756 False 352
757 False 353
758 False 354
759 False 355
760 False 356
761 False 357
762 False 358
763 False 359
764 False 360
765 False 361
766 False 362
767 False 363
768 False 364
769 False 365
770 False 366
771 False 367
772 False 368
773 False 369
774 False 370
775 False 371
776 False 372
777 False 373
778 False 374
779 False 375
780 False 376
781 False 377
782 False 378
783 False 379
784 False 380
785 False 381
786 False 382
787 False 383
788 False 384
789 False 385
790 False 386
791 False 387
792 False 388
793 False 389
794 False 390
795 False 391
796 False 392
797 False 393
798 False 394
799 False 395
800 False 396
801 False 397
802 False 398
803 False 399
804 False 400
805 False 401
806 False 402
807 False 403
808 False 404
809 False 1
810 False 2
811 False 3
812 False 4
813 False 5
814 False 6
815 False 7
816 False 8
817 False 9
818 False 10
819 False 11
820 False 12
821 False 13
822 False 14
823 False 15
824 False 16
825 False 17
826 False 18
827 False 19
828 False 20
829 False 21
830 False 22
831 False 23
832 False 24
833 False 25
834 False 26
835 False 27
836 False 28
837 False 29
838 False 30
839 False 31
840 False 32
841 False 33
842 False 34
843 False 35
844 False 36
845 False 37
846 False 38
847 False 39
848 False 40
849 False 41
850 False 42
851 False 43
852 False 44
853 False 45
854 False 46
855 False 47
856 False 48
857 False 49
858 False 50
859 False 51
860 False 52
861 False 53
862 False 54
863 False 55
864 False 56
865 False 57
866 False 58
867 False 59
868 False 60
869 False 61
870 False 62
871 False 63
872 False 64
873 False 65
874 False 66
875 False 67
876 False 68
877 False 69
878 False 70
879 False 71
880 False 72
881 False 73
882 False 74
883 False 75
884 False 76
885 False 77
886 False 78
887 False 79
888 False 80
889 False 81
890 False 82
891 False 83
###Markdown
Which residues are our original pose residues? Note that the final residues are called `Virtual` residues. Virtual residues are not scored. They have coordinates, and can move, but simply result in a score of zero. They are useful in some contexts to hide a part of the pose from the scoring machinery, and there are movers that can change residues to and from virtual. In this case, they are used for the FoldTree - in order to allow refinement of the full crystal environment. They allow relative movement of each subunit relative to each other. There are two virtual residues for each subunit
###Code
print(p.residue(3654))
print("Total Subunits:", (3654-18)/404)
print("Total Subunits:", sym_info.subunits())
score = get_score_function()
print(score(original))
print(score(p))
###Output
[0mcore.scoring.ScoreFunctionFactory: [0mSCOREFUNCTION: [32mref2015[0m
[0mcore.scoring.ScoreFunctionFactory: [0mThe -include_sugars flag was used with no sugar_bb weight set in the weights file. Setting sugar_bb weight to 1.0 by default.
-531.2534669946483
-1132.7274634504747
###Markdown
Running Protocols with SymmetryNow, lets try running a minimization with symmetry on.
###Code
mm = MoveMap()
mm.set_bb(True)
mm.set_chi(True)
minmover = rosetta.protocols.minimization_packing.MinMover()
minmover.score_function(score)
minmover.set_movemap(mm)
if if not os.getenv("DEBUG"):
minmover.apply(p)
score(p)
###Output
_____no_output_____
###Markdown
How does our pose look? For being such a large pose, how was the speed of minimization? How does this compare to our refined pose? Try to copy a subunit to a new object in PyMol. Then use the align command to align it to our assymetric unit. What is the RMSD? Now lets pack with our symmetric structure.
###Code
from rosetta.core.pack.task import *
from rosetta.core.pack.task.operation import *
packer = PackRotamersMover()
tf = rosetta.core.pack.task.TaskFactory()
tf.push_back(RestrictToRepacking())
tf.push_back(IncludeCurrent())
packer.task_factory(tf)
p = original.clone()
symmetrize.apply(p)
if not os.getenv("DEBUG"):
packer.apply(p)
print("packed", score(p))
###Output
packed -1960.9189300248413
|
mind_models/MIND all architectures.ipynb | ###Markdown
1. Preprocessing
###Code
# remove users which have fewer than 5 interacations
print("Len before removal: ",len(merged))
_keys = merged["user"].value_counts()[merged["user"].value_counts() > 5].keys()
merged = merged[merged["user"].isin(_keys)]
print("Len after removal: ",len(merged))
user_enc = LabelEncoder()
article_enc = LabelEncoder()
merged["user_id"] = user_enc.fit_transform(merged["user"].values)
merged["article_id"] = article_enc.fit_transform(merged["news_id"].values)
import nltk
from nltk.corpus import stopwords
# Helper functions
def _removeNonAscii(s):
return "".join(i for i in s if ord(i)<128)
def make_lower_case(text):
return text.lower()
def remove_stop_words(text):
text = text.split()
stops = set(stopwords.words("english"))
text = [w for w in text if not w in stops]
text = " ".join(text)
return text
def remove_html(text):
html_pattern = re.compile('<.*?>')
return html_pattern.sub(r'', text)
def remove_punctuation(text):
text = re.sub(r'[^\w\s]', '', text)
return text
def text_to_list(text):
text = text.split(" ")
return text
def clean_title(df):
df["title_cleaned"] = df.title.apply(func = make_lower_case)
df["title_cleaned"] = df.title_cleaned.apply(func = remove_stop_words)
df["title_cleaned"] = df.title_cleaned.apply(func = remove_punctuation)
return df
def hyphen_to_underline(category):
"""
Convert hyphen to underline for the subcategories. So that Tfidf works correctly
"""
return category.replace("-","_")
merged = clean_title(merged)
merged["subcategory_cleaned"] = merged["sub_category"].apply(func = hyphen_to_underline)
###Output
_____no_output_____
###Markdown
Alternative to tf-idf End alternative to tfidf
###Code
vectorizer = TfidfVectorizer(analyzer="word", tokenizer=str.split)
item_ids = merged["article_id"].unique().tolist()
tfidf_matrix = vectorizer.fit_transform(merged["subcategory_cleaned"])
tfidf_feature_names = vectorizer.get_feature_names()
tfidf_matrix
item_ids = merged["article_id"].tolist()
def get_item_profile(item_id):
"""
item_id: the news article id
Return: an array of each n-gram in the item article.
with their n-gram id in tfidf_feature_names and weight.
"""
idx = item_ids.index(item_id) # returns the index to the item id
item_profile = tfidf_matrix[idx:idx+1]
return item_profile
def get_item_profiles(ids):
#print(ids)
item_profiles_list = [get_item_profile(x) for x in ids]
item_profiles = scipy.sparse.vstack(item_profiles_list)
return item_profiles
def build_user_profile(person_id):
interactions = merged[merged["user_id"] == person_id]["article_id"].values # gets all articles
user_item_profiles = get_item_profiles(interactions)
user_item_profiles = np.sum(user_item_profiles, axis=0)
user_profile_norm = sklearn.preprocessing.normalize(user_item_profiles)
return user_item_profiles
#t = build_user_profile(1)
def calculate_user_profiles(unique_user_ids):
user_profiles = {}
for idx in tqdm(unique_user_ids):
token_relevance = build_user_profile(idx).tolist()[0]
zipped = zip(tfidf_feature_names, token_relevance)
s = sorted(zipped, key=lambda x: -x[-1])[:6]
user_profiles[idx] = s
return user_profiles
user_profiles = calculate_user_profiles(merged["user_id"].unique())
subcategory_to_id = {name: idx+1 for idx, name in enumerate(tfidf_feature_names)}
id_to_subcategory = {idx: name for name, idx in subcategory_to_id.items()}
id_to_subcategory[0] = "Null"
subcategory_to_id["Null"] = 0
# add all id-category to the userprofile in df
profile_array = []
for index, row in tqdm(merged.iterrows()):
user_idx = row["user_id"]
profile = user_profiles[user_idx]
temp = []
for keyword_tuple in profile:
temp.append(subcategory_to_id[keyword_tuple[0]])
profile_array.append(temp)
merged["profile"] = profile_array
# add the id-category to the news articles
merged["subcategory_to_int"] = [subcategory_to_id[cat] for cat in merged["subcategory_cleaned"].values]
user_unique = merged.drop_duplicates("user_id")
userid_to_profile = user_unique[["user_id", "profile"]].set_index("user_id").to_dict()["profile"]
category_enc = LabelEncoder()
merged["main_category_int"] = category_enc.fit_transform(merged["category"].values)
article_id_to_category_int = merged[["article_id", "main_category_int"]].set_index("article_id").to_dict()
article_id_to_category_int = article_id_to_category_int["main_category_int"]
article_id_to_subcategory_int = merged[["article_id", "subcategory_to_int"]].set_index("article_id").to_dict()
article_id_to_subcategory_int = article_id_to_subcategory_int["subcategory_to_int"]
def train_test_split(df, user_id, article_id, have_timestamp, timestamp):
"""
params:
col_1: user_id
col_2: article_id
"""
df_test = df
if have_timestamp: # if df have timestamp; take last interacted article into test set
df_test = df_test.sort_values(timestamp).groupby(user_id).tail(1)
else:
df_test = df_test.sort_values(user_id).groupby(user_id).tail(1)
df_train = df.drop(index=df_test.index)
assert df_test.shape[0] + df_train.shape[0] == df.shape[0]
return df_train, df_test
df_train_true, df_test_true = train_test_split(merged, "user_id", "article_id", False, 0)
df_train_true.head(1)
def get_userid_to_article_history(df):
userid_to_article_history = {}
for user_id in tqdm(df["user_id"].unique()):
click_history = df[df["user_id"] == user_id]["article_id"].values
if len(click_history) < 30:
while len(click_history) < 30:
click_history = np.append(click_history, 0)
if len(click_history) > 30:
click_history = click_history[:30]
userid_to_article_history[user_id] = click_history
return userid_to_article_history
userid_to_article_history = get_userid_to_article_history(df_train_true)
all_article_ids = merged["article_id"].unique()
def negative_sampling(train_df, all_article_ids, user_id, article_id):
"""
Negative sample training instance; for each positive instance, add 4 negative articles
Return user_ids, news_ids, category_1, category_2, authors_onehotencoded, titles
"""
user_ids, user_click_history, articles, article_category, article_sub_category, labels = [], [], [], [], [], []
p0, p1, p2, p3, p4, p5, p6, p7, p8, p9 = [], [], [], [], [], [], [], [], [], []
user_item_set = set(zip(train_df[user_id],
train_df[article_id]))
num_negatives = 4
for (u, i) in tqdm(user_item_set):
user_ids.append(u)
user_click_history.append(userid_to_article_history[u])
profile = np.array(userid_to_profile[u])
p0.append(profile[0])
p1.append(profile[1])
p2.append(profile[2])
p3.append(profile[3])
p4.append(profile[4])
p5.append(profile[5])
article_category.append(article_id_to_category_int[i])
article_sub_category.append(article_id_to_subcategory_int[i])
articles.append(i)
labels.append(1)
for _ in range(num_negatives):
negative_item = np.random.choice(all_article_ids)
while (u, negative_item) in user_item_set:
negative_item = np.random.choice(all_article_ids)
user_ids.append(u)
user_click_history.append(userid_to_article_history[u])
p0.append(profile[0])
p1.append(profile[1])
p2.append(profile[2])
p3.append(profile[3])
p4.append(profile[4])
p5.append(profile[5])
article_category.append(article_id_to_category_int[negative_item])
article_sub_category.append(article_id_to_subcategory_int[negative_item])
articles.append(negative_item)
labels.append(0)
user_ids, user_click_history, p0, p1, p2, p3, p4, p5, articles,article_category,article_sub_category, labels = shuffle(user_ids,user_click_history, p0, p1, p2, p3, p4, p5, articles,article_category,article_sub_category, labels, random_state=0)
return pd.DataFrame(list(zip(user_ids,user_click_history,p0, p1, p2, p3, p4, p5, articles,article_category,article_sub_category, labels)), columns=["user_id","user_history","p0", "p1", "p2", "p3", "p4", "p5", "article_id","article_category","article_sub_category", "labels"])
df_train = negative_sampling(df_train_true, all_article_ids, "user_id", "article_id")
def fix_dftrain(df, column, max_len, padding):
i = 0
for i in tqdm(range(max_len)):
df[column + "_" + str(i)] = df[column].apply(lambda x: x[i] if i < len(x) else padding)
#df.drop(column, axis=1, inplace=True)
return df
df_train = fix_dftrain(df_train, "user_history", 30, 0)
df_train.drop(columns=["user_history"], inplace=True)
df_train.head()
# For each user; for each item the user has interacted with in the test set;
# Sample 99 items the user has not interacted with in the past and add the one test item
def negative_sample_testset(ordiginal_df, df_test, all_article_ids, user_id, article_id):
test_user_item_set = set(zip(df_test[user_id], df_test[article_id]))
user_interacted_items = ordiginal_df.groupby(user_id)[article_id].apply(list).to_dict()
users = []
p0, p1, p2, p3, p4, p5, p6, p7, p8, p9 = [], [], [], [], [], [], [], [], [], []
res_arr = []
article_category, article_sub_category = [], []
userid_to_true_item = {} # keep track of the real items
for (u,i) in tqdm(test_user_item_set):
interacted_items = user_interacted_items[u]
not_interacted_items = set(all_article_ids) - set(interacted_items)
selected_not_interacted = list(np.random.choice(list(not_interacted_items), 99))
test_items = selected_not_interacted + [i]
temp = []
profile = userid_to_profile[u]
for j in range(len(test_items)):
temp.append([u,
userid_to_article_history[u],
profile[0],
profile[1],
profile[2],
profile[3],
profile[4],
profile[5],
test_items[j], article_id_to_category_int[test_items[j]],
article_id_to_subcategory_int[test_items[j]]])
# user_click_history.append(userid_to_article_history[u])
res_arr.append(temp)
userid_to_true_item[u] = i
X_test = np.array(res_arr)
X_test = X_test.reshape(-1, X_test.shape[-1])
df_test = pd.DataFrame(X_test, columns=["user_id",
"click_history",
"p0",
"p1",
"p2",
"p3",
"p4",
"p5",
"article_id",
"category",
"sub_category"])
return X_test, df_test, userid_to_true_item
X_test, df_test, userid_to_true_item = negative_sample_testset(merged, df_test_true, merged["article_id"].unique(), "user_id", "article_id")
def fix_dftest(df, column, max_len, padding):
i = 0
for i in tqdm(range(max_len)):
df[column + "_" + str(i)] = df[column].apply(lambda x: x[i] if i < len(x) else padding)
#df.drop(column, axis=1, inplace=True)
return df
df_test = fix_dftest(df_test, "click_history", 30, 0)
df_test.drop(columns=["click_history"], inplace=True)
def getHitRatio(ranklist, gtItem):
for item in ranklist:
if item == gtItem:
return 1
return 0
def getNDCG(ranklist, gtItem):
for i in range(len(ranklist)):
item = ranklist[i]
if item == gtItem:
return math.log(2) / math.log(i+2)
return 0
def getNumHits(ranklist, gtItem):
h = 0
for item in ranklist:
for p in gtItem:
if
def evaluate_one_rating(model, user_id, user_profiles, all_articles,user_clicks, true_item, categories, sub_categories):
### Reshaping to make it on the right shape ###
#expanded_user_id = np.array([user_id]*100).reshape((100,1))
all_articles = np.array(all_articles).reshape(-1,1)
# predictions
#user_history, profile_input, item_input,category_input, subcategory_input
predictions = model.predict([user_clicks, user_profiles, all_articles, categories, sub_categories]) #TODO: add categories, sub_cat
predicted_labels = np.squeeze(predictions)
top_ten_items = [all_articles[i] for i in np.argsort(predicted_labels)[::-1][0:10].tolist()]
hr = getHitRatio(top_ten_items, true_item)
ndcg = getNDCG(top_ten_items, true_item)
hr_five = getHitRatio(top_ten_items[:5], true_item)
ndcg_five = getNDCG(top_ten_items[:5], true_item)
return hr, ndcg,hr_five,ndcg_five
def evalaute_model(model, df_test, userid_to_true_item):
print("Evaluate model")
hits = []
ndcgs = []
hits_five = []
ndcgs_five = []
users = df_test["user_id"].unique()
for user_id in tqdm(users):
user_df = df_test[df_test["user_id"] == user_id] # get the 100 samples for this user
true_item = userid_to_true_item[user_id] # get the actual true item in the test set
all_articles = user_df["article_id"].values.astype("int64") # get all possible articles
user_profiles = user_df.iloc[:, 1:7].values.astype("int64")# get the user_profile
user_clicks = user_df.iloc[:, 10:].values.astype("int64")
categories = user_df.iloc[:, 8].values.astype("int64")
sub_categories = user_df.iloc[:, 9].values.astype("int64")
ht, ndcg, ht_five, ndcg_five = evaluate_one_rating(model,
user_id,
user_profiles,
all_articles,user_clicks,
true_item,
categories,
sub_categories)
hits.append(ht)
ndcgs.append(ndcg)
hits_five.append(ht_five)
ndcgs_five.append(ndcg_five)
return hits, ndcgs,hits_five,ndcgs_five
def write_accuracy_results(model_name, hit_ten, ndcg_ten, hit_five, ndcg_five):
try:
file = open("performance.txt", "a")
s = model_name +": Hit@10 : "+ str(hit_ten)+", NDCG@10: "+ str(ndcg_ten)+", Hit@5:" + str(hit_five)+", ndcg@5 "+ str(ndcg_five) + "\n"
file.write(s)
file.close()
except:
print("error file wriite")
def write_category_results(model_name, hit_ten, ndcg_ten, hit_five, ndcg_five):
try:
file = open("category_performance.txt", "a")
s = model_name +": Hit@10 : "+ str(hit_ten)+", NDCG@10: "+ str(ndcg_ten)+", Hit@5:" + str(hit_five)+", ndcg@5 "+ str(ndcg_five) + "\n"
file.write(s)
file.close()
except:
print("error file wriite")
###Output
_____no_output_____
###Markdown
4. Models 4.1 Final model
###Code
# Params
num_unique_categories = len(subcategory_to_id)
num_users = len(merged["user_id"].unique()) +1
num_items = len(merged["article_id"].unique()) + 1
dims = 20
num_sub_categories = len(merged["subcategory_to_int"].unique()) +1
num_categories = len(merged["main_category_int"].unique()) +1
#@tf.autograph.experimental.do_not_convert
def get_model(num_users, num_items, dims,num_categories,num_sub_categories, dense_layers=[128, 64, 32, 8]):
#User features
user_history = Input(shape=(30,), name="user")
user_profile_input = Input(shape=(6,), name="profile")
#item features
item_input = Input(shape=(1,), name="item")
item_category = Input(shape=(1,), name="category")
item_subcategory = Input(shape=(1,), name="subcategory")
# User emb
click_history_emb = Embedding(output_dim=dims, input_dim=num_items+1, input_length=30, name="mf_user_emb")(user_history)
profile_emb = Embedding(output_dim=dims, input_dim=num_unique_categories, input_length=6, name="mf_profile_emb")(user_profile_input)
# Item emb
item_emb = Embedding(output_dim=dims, input_dim=num_items+1, input_length=1, name="mf_item_emb")(item_input)
category_emb = Embedding(output_dim=dims, input_dim=num_categories, input_length=1, name="cat_emb")(item_category)
subcategory_emb = Embedding(output_dim=dims, input_dim=num_sub_categories, input_length=1, name="subcat_emb")(item_subcategory)
### Wide
#wide_history = Flatten()(click_history_emb)
#wide_item = Flatten()(item_input)
wide = Concatenate(axis=1)([click_history_emb, item_emb])
wide = Flatten()(wide)
y_wide = Dense(2)(wide)
### Deep
deep_features = Concatenate(axis=1)([category_emb,subcategory_emb, profile_emb])
x_deep = LSTM(40)(deep_features)
print(x_deep.shape)
print(y_wide.shape)
final = Concatenate()([x_deep, y_wide])
final = BatchNormalization(axis=1)(final)
y = Dense(1, activation="sigmoid")(final)
model = Model(inputs=[user_history, user_profile_input, item_input, item_category, item_subcategory], outputs=y)
model.compile(
optimizer=Adam(0.01),
loss="binary_crossentropy",
metrics=["accuracy"],
)
return model
model = get_model(num_users, num_items, dims, num_categories,num_sub_categories)
###### Training ########
user_history = df_train.iloc[:, 11:].values.astype("int64")
profile_input = df_train.iloc[:, 1:7].values.astype("int64")
item_input = df_train.iloc[:, 7].values.reshape((-1,1)).astype("int64")
labels = df_train.iloc[:, 10].values.reshape((-1,1)).astype("int64")
category_input = df_train.iloc[:, 8].values.reshape((-1,1)).astype("int64")
subcategory_input = df_train.iloc[:, 9].values.reshape((-1,1)).astype("int64")
print(user_history.shape,profile_input.shape, item_input.shape, labels.shape )
all_user_ids = merged["user_id"].unique()
#user_input = df_train.iloc[:, 0].values.reshape((-1,1))
#profile_input = df_train.iloc[:, 1:6].values
#item_input = df_train.iloc[:, 7].values.reshape((-1,1))
#labels = df_train.iloc[:, 8].values.reshape((-1,1))
train_loss = []
val_loss = []
train_acc = []
val_acc = []
hits_list = []
ndcg_list = []
best_hits = 0
best_ndcgs = 0
best_hits_five = 0
best_ndcgs_five = 0
epochs=2
for epoch in range(epochs):
hist = model.fit([user_history, profile_input, item_input,category_input, subcategory_input ], labels, epochs=1,validation_split=0.1, shuffle=True, verbose=1)
train_loss.append(hist.history["loss"])
train_acc.append(hist.history["accuracy"])
val_loss.append(hist.history["val_loss"])
val_acc.append(hist.history["val_accuracy"])
hits, ndcgs, hits_five, ndcgs_five = evalaute_model( model, df_test, userid_to_true_item)
hits_list.append(np.average(hits))
ndcg_list.append(np.average(ndcgs))
temp_hits = np.average(hits)
temp_ndcgs = np.average(ndcgs)
if (temp_hits > best_hits):
best_hits = temp_hits
best_ndcgs = temp_ndcgs
best_hits_five = np.average(hits_five)
best_ndcgs_five = np.average(ndcgs_five)
print("Hit @ 10: {:.2f}".format(best_hits))
print("ncdgs @ 10: {:.2f}".format(best_ndcgs))
print("Hit @ 10: {:.2f}".format(best_hits_five))
print("ncdgs @ 10: {:.2f}".format(best_ndcgs_five))
write_accuracy_results("main", best_hits, best_ndcgs, best_hits_five, best_ndcgs_five)
import matplotlib.pyplot as plt
sns.set_style("darkgrid")
plt.plot(train_acc)
plt.plot(val_acc)
plt.title('model accuracy')
plt.ylabel('accuracy')
plt.xlabel('epoch')
plt.legend(['Train', 'Val'], loc='upper left')
plt.savefig("final_accuracy.pdf")
plt.show()
sns.set_style("darkgrid")
plt.plot(train_loss)
plt.plot(val_loss)
plt.title('Loss')
plt.ylabel('loss')
plt.xlabel('epoch')
plt.legend(['Train', 'Val'], loc='upper left')
plt.savefig("final_loss.pdf")
plt.show()
sns.set_style("darkgrid")
plt.plot(hits_list)
plt.plot(train_loss)
plt.title('Hit ratio vs Loss')
plt.xlabel('epoch')
plt.legend(['Hit@10', 'Train loss'], loc='upper left')
plt.savefig("final_hit_loss.pdf")
plt.show()
def get_article_category(article_id):
return merged[merged["article_id"] == article_id]["subcategory_cleaned"].values[0]
def get_userprofile_to_name(user_id, id_to_subcategory):
"""
Return array of strings with category names
"""
arr_profile = get_user_profile(df_train,user_id )
return [id_to_subcategory[elem] for elem in arr_profile]
def get_user_profile(df, user_id):
"""
Return the user profile given user_id
"""
return df[df["user_id"] == user_id].iloc[0, 1:7].values
def get_article_content(article_id):
article = merged[merged["article_id"] == article_id].head(1)
title = article["title"].values[0]
sub_category = article["sub_category"].values[0]
return title, sub_category
def get_item_features(user_id):
d = df_test[df_test["user_id"] == user_id]
return d["category"].values.reshape(-1,1), d["sub_category"].values.reshape(-1,1)
def get_item_features_one_item(article_id):
d = df_test[df_test["article_id"] == article_id]
return np.array(d["category"].values[0]), np.array(d["sub_category"].values[0])
def get_article_category(article_id, df):
"""
Return the article's category
type: int
"""
return df[df["article_id"] == article_id]["category"].values[0]
def get_article_subcategory(article_id, df):
"""
Return the article's category
type: int
"""
return df[df["article_id"] == article_id]["sub_category"].values[0]
def get_category_hit_ratio(user_profile, top_ten_categories):
for profile in user_profile:
for category in top_ten_categories:
if profile == category:
return 1
return 0
def get_ndcgs_category(user_profile, top_ten_categories):
for i in range(len(top_ten_categories)):
item = top_ten_categories[i]
for profile in user_profile:
if item == profile:
return math.log(2) / math.log(i+2)
return 0
def get_recommendations(user_id, df):
## Setup ###
user_profile = get_user_profile(df, user_id)
click_history = userid_to_article_history[user_id]
display_items = df[df["user_id"] == user_id]["article_id"].values.reshape(-1, 1).astype("int64")
user_profile = np.tile(user_profile, display_items.shape[0]).reshape(-1, 6).astype("int64")
category, sub_category = get_item_features(user_id)
user_ids = np.tile(np.array(user_id), display_items.shape[0]).reshape(-1,1).astype("int64")
category = np.asarray(category).astype("int64")
sub_category = np.asarray(sub_category).astype("int64")
click_history = np.tile(click_history, display_items.shape[0]).reshape(-1, 30).astype("int64")
## Preds ###
predictions = model.predict([click_history, user_profile, display_items, category, sub_category])
predicted_labels = np.squeeze(predictions)
top_ten_items = [display_items[i][0] for i in np.argsort(predicted_labels)[::-1][0:10].tolist()]
return top_ten_items
def predict_all_users(df):
hits_ten,ndcgs_ten = [], []
hits_five, ndcgs_five = [], []
counter = 0
for user_id in tqdm(df["user_id"].unique()):
top_ten_articles = get_recommendations(user_id, df)
top_ten_subcategories = [get_article_subcategory(_id, df) for _id in top_ten_articles]
user_profile = get_user_profile(df_test, user_id)
hit_ten = get_category_hit_ratio(user_profile, top_ten_subcategories)
ndcg_ten = get_ndcgs_category(user_profile, top_ten_subcategories)
hit_five = get_category_hit_ratio(user_profile, top_ten_subcategories[:5])
ndcg_five = get_ndcgs_category(user_profile, top_ten_subcategories[:5])
hits_ten.append(hit_ten)
ndcgs_ten.append(ndcg_ten)
hits_five.append(hit_five)
ndcgs_five.append(ndcg_five)
counter += 1
return np.average(hits_ten), np.average(ndcgs_ten), np.average(hits_five), np.average(ndcgs_five)
category_hits_ten, category_ndcg_ten,category_hits_five,category_ndcg_five = predict_all_users(df_test)
print(category_hits_ten)
print(category_ndcg_ten)
print(category_hits_five)
print(category_ndcg_five)
write_category_results("main", category_hits_ten, category_ndcg_ten, category_hits_five, category_ndcg_five)
###Output
_____no_output_____
###Markdown
4.1 Architecture 1
###Code
def evaluate_one_rating_arc1(model, user_id, all_articles, true_item):
### Reshaping to make it on the right shape ###
expanded_user_id = np.array([user_id]*100).reshape((100,1))
all_articles = np.array(all_articles).reshape(-1,1)
# predictions
predictions = model.predict([expanded_user_id, all_articles])
predicted_labels = np.squeeze(predictions)
top_ten_items = [all_articles[i] for i in np.argsort(predicted_labels)[::-1][0:10].tolist()]
hr = getHitRatio(top_ten_items, true_item)
ndcg = getNDCG(top_ten_items, true_item)
hr_five = getHitRatio(top_ten_items[:5], true_item)
ndcg_five = getNDCG(top_ten_items[:5], true_item)
return hr, ndcg, hr_five, ndcg_five
def evalaute_model_arc1(model, df_test, userid_to_true_item):
print("Evaluate model")
hits = []
ndcgs = []
hits_five, ndcgs_five = [], []
users = df_test["user_id"].unique()
for user_id in tqdm(users):
user_df = df_test[df_test["user_id"] == user_id] # get the 100 samples for this user
true_item = userid_to_true_item[user_id] # get the actual true item in the test set
all_articles = user_df["article_id"].values.astype("int64") # get all possible articles
ht, ndcg, hr_five, ndcg_five = evaluate_one_rating_arc1(model, user_id, all_articles, true_item)
hits.append(ht)
ndcgs.append(ndcg)
hits_five.append(hr_five)
ndcgs_five.append(ndcg_five)
return hits, ndcgs, hits_five, ndcgs_five
# Params
num_users = len(merged["user_id"].unique())
num_items = len(merged["article_id"].unique())
dims = 20
#@tf.autograph.experimental.do_not_convert
def get_model(num_users, num_items, dims, dense_layers=[128, 64, 32, 8]):
user_input = Input(shape=(1,), name="user")
item_input = Input(shape=(1,), name="item")
user_emb = Embedding(output_dim=dims, input_dim=num_users, input_length=1, name="mf_user_emb")(user_input)
item_emb = Embedding(output_dim=dims, input_dim=num_items, input_length=1, name="mf_item_emb")(item_input)
user_vecs = Reshape([dims])(user_emb)
item_vecs = Reshape([dims])(item_emb)
y = Dot(1, normalize=False)([user_vecs, item_vecs])
y = Dense(1, activation="sigmoid")(y)
model = Model(inputs=[user_input, item_input], outputs=y)
model.compile(
optimizer=Adam(0.01),
loss="binary_crossentropy",
metrics=["accuracy"],
)
return model
model_arc1 = get_model(num_users, num_items, dims)
df_train
###### Training ########
user_input = df_train.iloc[:, 0].values.reshape((-1,1))
item_input = df_train.iloc[:, 7].values.reshape((-1,1))
labels = df_train.iloc[:, 10].values.reshape((-1,1))
print(user_input.shape, item_input.shape, labels.shape )
all_user_ids = df_train["user_id"].unique()
#user_input = df_train.iloc[:, 0].values.reshape((-1,1))
#profile_input = df_train.iloc[:, 1:6].values
#item_input = df_train.iloc[:, 7].values.reshape((-1,1))
#labels = df_train.iloc[:, 8].values.reshape((-1,1))
train_loss = []
val_loss = []
train_acc = []
val_acc = []
hits_list = []
ndcg_list = []
best_hits = 0
best_ndcgs = 0
epochs=2
for epoch in range(epochs):
hist = model_arc1.fit([user_input, item_input], labels, epochs=1, shuffle=True, verbose=1, validation_split=0.1)
train_loss.append(hist.history["loss"])
train_acc.append(hist.history["accuracy"])
val_loss.append(hist.history["val_loss"])
val_acc.append(hist.history["val_accuracy"])
hits, ndcgs, hits_five, ndcgs_five = evalaute_model_arc1( model_arc1, df_test, userid_to_true_item)
hits_list.append(np.average(hits))
ndcg_list.append(np.average(ndcgs))
temp_hits = np.average(hits)
temp_ndcgs = np.average(ndcgs)
if (temp_hits > best_hits):
best_hits = temp_hits
best_ndcgs = temp_ndcgs
best_hits_five = np.average(hits_five)
best_ndcgs_five = np.average(ndcgs_five)
print(best_hits)
print(best_ndcgs)
print(best_hits_five)
print(best_ndcgs_five)
write_accuracy_results("arc1", best_hits, best_ndcgs, best_hits_five, best_ndcgs_five)
import matplotlib.pyplot as plt
sns.set_style("darkgrid")
plt.plot(train_acc)
plt.plot(val_acc)
plt.title('model accuracy')
plt.ylabel('accuracy')
plt.xlabel('epoch')
plt.legend(['Train', 'Val'], loc='upper left')
plt.savefig("arc1_accuracy.pdf")
plt.show()
sns.set_style("darkgrid")
plt.plot(train_loss)
plt.plot(val_loss)
plt.title('Loss')
plt.ylabel('loss')
plt.xlabel('epoch')
plt.legend(['Train', 'Val'], loc='upper left')
plt.savefig("arc1_loss.pdf")
plt.show()
sns.set_style("darkgrid")
plt.plot(hits_list)
plt.plot(train_loss)
plt.title('Hit ratio vs Loss')
plt.xlabel('epoch')
plt.legend(['Hit@10', 'Train loss'], loc='upper left')
plt.savefig("arc1_hit_loss.pdf")
plt.show()
def get_recommendations_arc1(user_id, df, model):
## Setup ###
display_items = df[df["user_id"] == user_id]["article_id"].values.reshape(-1, 1).astype("int64")
user_ids = np.tile(np.array(user_id), display_items.shape[0]).reshape(-1,1).astype("int64")
## Preds ###
predictions = model.predict([user_ids, display_items])
predicted_labels = np.squeeze(predictions)
top_ten_items = [display_items[i][0] for i in np.argsort(predicted_labels)[::-1][0:10].tolist()]
return top_ten_items
def get_category_hits_ndcg_arc1(df, model):
hits_ten,ndcgs_ten = [], []
hits_five, ndcgs_five = [], []
for user_id in tqdm(df["user_id"].unique()):
top_ten_articles = get_recommendations_arc1(user_id, df, model)
top_ten_subcategories = [get_article_subcategory(_id, df) for _id in top_ten_articles]
user_profile = userid_to_profile[user_id]
hit_ten = get_category_hit_ratio(user_profile, top_ten_subcategories)
ndcg_ten = get_ndcgs_category(user_profile, top_ten_subcategories)
hit_five = get_category_hit_ratio(user_profile, top_ten_subcategories[:5])
ndcg_five = get_ndcgs_category(user_profile, top_ten_subcategories[:5])
hits_ten.append(hit_ten)
ndcgs_ten.append(ndcg_ten)
hits_five.append(hit_five)
ndcgs_five.append(ndcg_five)
return np.average(hits_ten), np.average(ndcgs_ten), np.average(hits_five), np.average(ndcgs_five)
category_hits_ten, category_ndcg_ten, category_hits_five, category_ndcg_five = get_category_hits_ndcg_arc1(df_test,model_arc1)
print(category_hits_ten)
print(category_ndcg_ten)
print(category_hits_five)
print(category_ndcg_five)
write_category_results("arc1", category_hits_ten, category_ndcg_ten, category_hits_five, category_ndcg_five)
###Output
_____no_output_____
###Markdown
4.3 Architecture 2
###Code
def evaluate_one_rating_arc2(model, user_id, user_profiles, all_articles,user_clicks, true_item):
### Reshaping to make it on the right shape ###
#expanded_user_id = np.array([user_id]*100).reshape((100,1))
all_articles = np.array(all_articles).reshape(-1,1)
# predictions
predictions = model.predict([user_clicks, user_profiles, all_articles])
predicted_labels = np.squeeze(predictions)
top_ten_items = [all_articles[i] for i in np.argsort(predicted_labels)[::-1][0:10].tolist()]
hr = getHitRatio(top_ten_items, true_item)
ndcg = getNDCG(top_ten_items, true_item)
hr_five = getHitRatio(top_ten_items[:5], true_item)
ndcg_five = getNDCG(top_ten_items[:5], true_item)
return hr, ndcg, hr_five,ndcg_five
def evalaute_model_arc2(model, df_test, userid_to_true_item):
print("Evaluate model")
hits = []
ndcgs = []
hits_five, ndcgs_five = [], []
users = df_test["user_id"].unique()
for user_id in tqdm(users):
user_df = df_test[df_test["user_id"] == user_id] # get the 100 samples for this user
true_item = userid_to_true_item[user_id] # get the actual true item in the test set
all_articles = user_df["article_id"].values.astype("int64") # get all possible articles
user_profiles = user_df.iloc[:, 1:7].values.astype("int64")# get the user_profile
user_clicks = user_df.iloc[:, 10:].values.astype("int64")
ht, ndcg,hr_five,ndcg_five = evaluate_one_rating_arc2(model, user_id, user_profiles, all_articles,user_clicks, true_item)
hits.append(ht)
ndcgs.append(ndcg)
hits_five.append(hr_five)
ndcgs_five.append(ndcg_five)
return hits, ndcgs,hits_five,ndcgs_five
#@tf.autograph.experimental.do_not_convert
def get_model_arc2(num_users, num_items, dims, dense_layers=[128, 64, 32, 8]):
user_history = Input(shape=(30,), name="user")
user_profile_input = Input(shape=(6,), name="profile")
item_input = Input(shape=(1,), name="item")
mf_user_emb = Embedding(output_dim=dims, input_dim=num_items+1, input_length=30, name="mf_user_emb")(user_history)
mf_profile_emb = Embedding(output_dim=dims, input_dim=num_unique_categories, input_length=6, name="mf_profile_emb")(user_profile_input)
mf_item_emb = Embedding(output_dim=dims, input_dim=num_items+1, input_length=1, name="mf_item_emb")(item_input)
#profile_emb = GlobalAveragePooling1D()(mf_profile_emb)
profile_vecs = Flatten()(mf_user_emb)
user_vecs = Flatten()(mf_profile_emb)
item_vecs = Reshape([dims])(mf_item_emb)
user_vecs_complete = Concatenate(axis=1)([user_vecs, profile_vecs])
input_vecs = Concatenate()([user_vecs_complete, item_vecs])
x = Dense(128, activation="relu", name="dense_0")(input_vecs)
x = Dropout(0.5)(x)
y = Dense(1, activation="sigmoid", name="prediction")(x)
model = Model(inputs=[user_history, user_profile_input, item_input], outputs=y)
model.compile(
optimizer=Adam(0.01),
loss="binary_crossentropy",
metrics=["accuracy"],
)
return model
model_arc2 = get_model_arc2(num_users, num_items, dims)
###### Training ########
user_history = df_train.iloc[:, 11:].values.astype("int64")
profile_input = df_train.iloc[:, 1:7].values.astype("int64")
item_input = df_train.iloc[:, 7].values.reshape((-1,1)).astype("int64")
labels = df_train.iloc[:, 10].values.reshape((-1,1)).astype("int64")
print(user_history.shape,profile_input.shape, item_input.shape, labels.shape )
all_user_ids = merged["user_id"].unique()
epochs=2
train_loss = []
val_loss = []
train_acc = []
val_acc = []
hits_list = []
ndcg_list = []
best_hits = 0
best_ndcgs = 0
best_hits_five = 0
best_ndcgs_five = 0
for epoch in range(epochs):
hist = model_arc2.fit([user_history, profile_input, item_input], labels, epochs=1, shuffle=True, verbose=1, validation_split=0.1)
train_loss.append(hist.history["loss"])
train_acc.append(hist.history["accuracy"])
val_loss.append(hist.history["val_loss"])
val_acc.append(hist.history["val_accuracy"])
hits, ndcgs, hits_five, ndcgs_five = evalaute_model_arc2( model_arc2, df_test, userid_to_true_item)
hits_list.append(np.average(hits))
ndcg_list.append(np.average(ndcgs))
temp_hits = np.average(hits)
temp_ndcgs = np.average(ndcgs)
if (temp_hits > best_hits):
best_hits = temp_hits
best_hits_five = np.average(hits_five)
best_ndcgs_five = np.average(ndcgs_five)
best_ndcgs = temp_ndcgs
print("Hit @ 10: {:.2f}".format(best_hits))
print("ncdgs @ 10: {:.2f}".format(best_ndcgs))
print("Hit @ 5: {:.2f}".format(best_hits_five))
print("ncdgs @ 5: {:.2f}".format(best_ndcgs_five))
write_accuracy_results("arc2", best_hits, best_ndcgs, best_hits_five, best_ndcgs_five)
sns.set_style("darkgrid")
plt.plot(train_acc)
plt.plot(val_acc)
plt.title('model accuracy')
plt.ylabel('accuracy')
plt.xlabel('epoch')
plt.legend(['Train', 'Val'], loc='upper left')
plt.savefig("arc2_accuracy.pdf")
plt.show()
sns.set_style("darkgrid")
plt.plot(train_loss)
plt.plot(val_loss)
plt.title('Loss')
plt.ylabel('loss')
plt.xlabel('epoch')
plt.legend(['Train', 'Val'], loc='upper left')
plt.savefig("arc2_loss.pdf")
plt.show()
sns.set_style("darkgrid")
plt.plot(hits_list)
plt.plot(train_loss)
plt.title('Hit ratio vs Loss')
plt.xlabel('epoch')
plt.legend(['Hit@10', 'Train loss'], loc='upper left')
plt.savefig("arc2_hit_loss.pdf")
plt.show()
def get_recommendations_arc2(user_id, df, model):
#user_history, profile_input, item_input
## Setup ###
click_history = userid_to_article_history[user_id]
user_profile = get_user_profile(df, user_id)
display_items = df[df["user_id"] == user_id]["article_id"].values.reshape(-1, 1).astype("int64")
user_profile = np.tile(user_profile, display_items.shape[0]).reshape(-1, 6).astype("int64")
click_history = np.tile(np.array(click_history), display_items.shape[0]).reshape(-1,30).astype("int64")
## Preds ###
predictions = model.predict([click_history, user_profile,display_items])
predicted_labels = np.squeeze(predictions)
top_ten_items = [display_items[i][0] for i in np.argsort(predicted_labels)[::-1][0:10].tolist()]
return top_ten_items
def get_category_hits_ndcg_arc2(df, model):
hits_ten,ndcgs_ten = [], []
hits_five, ndcgs_five = [], []
for user_id in tqdm(df["user_id"].unique()):
top_ten_articles = get_recommendations_arc2(user_id, df, model)
top_ten_subcategories = [get_article_subcategory(_id, df) for _id in top_ten_articles]
user_profile = userid_to_profile[user_id]
hit_ten = get_category_hit_ratio(user_profile, top_ten_subcategories)
ndcg_ten = get_ndcgs_category(user_profile, top_ten_subcategories)
hit_five = get_category_hit_ratio(user_profile, top_ten_subcategories[:5])
ndcg_five = get_ndcgs_category(user_profile, top_ten_subcategories[:5])
hits_ten.append(hit_ten)
ndcgs_ten.append(ndcg_ten)
hits_five.append(hit_five)
ndcgs_five.append(ndcg_five)
return np.average(hits_ten), np.average(ndcgs_ten), np.average(hits_five), np.average(ndcgs_five)
category_hits_ten, category_ndcg_ten, category_hits_five, category_ndcg_five = get_category_hits_ndcg_arc2(df_test, model_arc2)
print(category_hits_ten)
print(category_ndcg_ten)
print(category_hits_five)
print(category_ndcg_five)
write_category_results("arc2", category_hits_ten, category_ndcg_ten, category_hits_five, category_ndcg_five)
###Output
_____no_output_____
###Markdown
4.4 Architecture 3
###Code
def evaluate_one_rating_arc3(model, user_id, user_profiles, all_articles,user_clicks, true_item, categories, sub_categories):
### Reshaping to make it on the right shape ###
#expanded_user_id = np.array([user_id]*100).reshape((100,1))
all_articles = np.array(all_articles).reshape(-1,1)
# predictions
#user_history, profile_input, item_input,category_input, subcategory_input
predictions = model.predict([user_clicks, user_profiles, all_articles, categories, sub_categories]) #TODO: add categories, sub_cat
predicted_labels = np.squeeze(predictions)
top_ten_items = [all_articles[i] for i in np.argsort(predicted_labels)[::-1][0:10].tolist()]
hr = getHitRatio(top_ten_items, true_item)
ndcg = getNDCG(top_ten_items, true_item)
hr_five = getHitRatio(top_ten_items[:5], true_item)
ndcg_five = getNDCG(top_ten_items[:5], true_item)
return hr, ndcg, hr_five,ndcg_five
def evalaute_model_arc3(model, df_test, userid_to_true_item):
print("Evaluate model")
hits = []
ndcgs = []
hits_five, ndcgs_five = [], []
users = df_test["user_id"].unique()
for user_id in tqdm(users):
user_df = df_test[df_test["user_id"] == user_id] # get the 100 samples for this user
true_item = userid_to_true_item[user_id] # get the actual true item in the test set
all_articles = user_df["article_id"].values.astype("int64") # get all possible articles
user_profiles = user_df.iloc[:, 1:7].values.astype("int64")# get the user_profile
user_clicks = user_df.iloc[:, 10:].values.astype("int64")
categories = user_df.iloc[:, 8].values.astype("int64")
sub_categories = user_df.iloc[:, 9].values.astype("int64")
ht, ndcg, hr_five,ndcg_five = evaluate_one_rating_arc3(model,
user_id,
user_profiles,
all_articles,user_clicks,
true_item,
categories,
sub_categories)
hits.append(ht)
ndcgs.append(ndcg)
hits_five.append(hr_five)
ndcgs_five.append(ndcg_five)
return hits, ndcgs, hits_five,ndcgs_five
#@tf.autograph.experimental.do_not_convert
def get_model_arc3(num_users, num_items, dims,num_categories,num_sub_categories, dense_layers=[128, 64, 32, 8]):
#User features
user_history = Input(shape=(30,), name="user")
user_profile_input = Input(shape=(6,), name="profile")
#item features
item_input = Input(shape=(1,), name="item")
item_category = Input(shape=(1,), name="category")
item_subcategory = Input(shape=(1,), name="subcategory")
# User emb
click_history_emb = Embedding(output_dim=dims, input_dim=num_items+1, input_length=30, name="mf_user_emb")(user_history)
profile_emb = Embedding(output_dim=dims, input_dim=num_unique_categories, input_length=6, name="mf_profile_emb")(user_profile_input)
#user_features = Concatenate(axis=1)([click_history_emb,profile_emb])
# Item emb
item_emb = Embedding(output_dim=dims, input_dim=num_items+1, input_length=1, name="mf_item_emb")(item_input)
category_emb = Embedding(output_dim=dims, input_dim=num_categories, input_length=1, name="cat_emb")(item_category)
subcategory_emb = Embedding(output_dim=dims, input_dim=num_sub_categories, input_length=1, name="subcat_emb")(item_subcategory)
item_features = Concatenate(axis=1)([item_emb,category_emb, subcategory_emb, profile_emb])
# User-tower
user_lstm = LSTM(40)(click_history_emb)
user_lstm = Dropout(0.5)(user_lstm)
user_lstm = BatchNormalization(axis=1)(user_lstm)
# Item tower
item_dense = Flatten()(item_features)
item_dense = Dense(128)(item_dense)
item_dense = Dropout(0.5)(item_dense)
item_dense = BatchNormalization(axis=1)(item_dense)
# Click predictor
final = Concatenate()([user_lstm,item_dense ])
y = Dense(1, activation="sigmoid")(final)
model = Model(inputs=[user_history, user_profile_input, item_input, item_category, item_subcategory], outputs=y)
model.compile(
optimizer=Adam(0.01),
loss="binary_crossentropy",
metrics=["accuracy"],
)
return model
model_arc3 = get_model_arc3(num_users, num_items, dims, num_categories,num_sub_categories)
###### Training ########
user_history = df_train.iloc[:, 11:].values.astype("int64")
profile_input = df_train.iloc[:, 1:7].values.astype("int64")
item_input = df_train.iloc[:, 7].values.reshape((-1,1)).astype("int64")
labels = df_train.iloc[:, 10].values.reshape((-1,1)).astype("int64")
category_input = df_train.iloc[:, 8].values.reshape((-1,1)).astype("int64")
subcategory_input = df_train.iloc[:, 9].values.reshape((-1,1)).astype("int64")
print(user_history.shape,profile_input.shape, item_input.shape, labels.shape )
all_user_ids = merged["user_id"].unique()
#user_input = df_train.iloc[:, 0].values.reshape((-1,1))
#profile_input = df_train.iloc[:, 1:6].values
#item_input = df_train.iloc[:, 7].values.reshape((-1,1))
#labels = df_train.iloc[:, 8].values.reshape((-1,1))
train_loss = []
val_loss = []
train_acc = []
val_acc = []
hits_list = []
ndcg_list = []
best_hits = 0
best_ndcgs = 0
best_hits_five = 0
best_ndcgs_five = 0
epochs=2
for epoch in range(epochs):
hist = model_arc3.fit([user_history, profile_input, item_input,category_input, subcategory_input ], labels, validation_split=0.1, epochs=1, shuffle=True, verbose=1)
train_loss.append(hist.history["loss"])
train_acc.append(hist.history["accuracy"])
val_loss.append(hist.history["val_loss"])
val_acc.append(hist.history["val_accuracy"])
hits, ndcgs, hits_five, ndcgs_five = evalaute_model_arc3( model_arc3, df_test, userid_to_true_item)
hits_list.append(np.average(hits))
ndcg_list.append(np.average(ndcgs))
temp_hits = np.average(hits)
temp_ndcgs = np.average(ndcgs)
if (temp_hits > best_hits):
best_hits = temp_hits
best_hits_five = np.average(hits_five)
best_ndcgs_five = np.average(ndcgs_five)
best_ndcgs = temp_ndcgs
print("Hit @ 10: {:.2f}".format(best_hits))
print("ncdgs @ 10: {:.2f}".format(best_ndcgs))
print("Hit @ 5: {:.2f}".format(best_hits_five))
print("ncdgs @ 5: {:.2f}".format(best_ndcgs_five))
write_accuracy_results("arc3", best_hits, best_ndcgs, best_hits_five, best_ndcgs_five)
sns.set_style("darkgrid")
plt.plot(train_acc)
plt.plot(val_acc)
plt.title('model accuracy')
plt.ylabel('accuracy')
plt.xlabel('epoch')
plt.legend(['Train', 'Val'], loc='upper left')
plt.savefig("arc3_accuracy.pdf")
plt.show()
sns.set_style("darkgrid")
plt.plot(train_loss)
plt.plot(val_loss)
plt.title('Loss')
plt.ylabel('loss')
plt.xlabel('epoch')
plt.legend(['Train', 'Val'], loc='upper left')
plt.savefig("arc3_loss.pdf")
plt.show()
sns.set_style("darkgrid")
plt.plot(hits_list)
plt.plot(train_loss)
plt.title('Hit ratio vs Loss')
plt.xlabel('epoch')
plt.legend(['Hit@10', 'Train loss'], loc='upper left')
plt.savefig("arc3_hit_loss.pdf")
plt.show()
def get_recommendations_arc3(user_id, df,model):
#user_history, profile_input, item_input,category_input, subcategory_input
## Setup ###
click_history = userid_to_article_history[user_id]
user_profile = get_user_profile(df, user_id)
display_items = df[df["user_id"] == user_id]["article_id"].values.reshape(-1, 1).astype("int64")
user_profile = np.tile(user_profile, display_items.shape[0]).reshape(-1, 6).astype("int64")
category, sub_category = get_item_features(user_id)
click_history = np.tile(np.array(click_history), display_items.shape[0]).reshape(-1,30).astype("int64")
category = np.asarray(category).astype("int64")
sub_category = np.asarray(sub_category).astype("int64")
#category = np.tile(category, display_items.shape[0]).reshape(-1,1).astype("int64")
#sub_category = np.tile(sub_category, display_items.shape[0]).reshape(-1,1).astype("int64")
## Preds ###
predictions = model.predict([click_history, user_profile, display_items, category, sub_category])
predicted_labels = np.squeeze(predictions)
top_ten_items = [display_items[i][0] for i in np.argsort(predicted_labels)[::-1][0:10].tolist()]
return top_ten_items
def predict_all_users_arc3(df, model):
hits_ten,ndcgs_ten = [], []
hits_five, ndcgs_five = [], []
counter = 0
for user_id in tqdm(df["user_id"].unique()):
top_ten_articles = get_recommendations_arc3(user_id, df, model)
assert len(top_ten_articles) == 10
top_ten_subcategories = [get_article_subcategory(_id, df) for _id in top_ten_articles]
user_profile = get_user_profile(df_test, user_id)
hit_ten = get_category_hit_ratio(user_profile, top_ten_subcategories)
ndcg_ten = get_ndcgs_category(user_profile, top_ten_subcategories)
hit_five = get_category_hit_ratio(user_profile, top_ten_subcategories[:5])
ndcg_five = get_ndcgs_category(user_profile, top_ten_subcategories[:5])
hits_ten.append(hit_ten)
ndcgs_ten.append(ndcg_ten)
hits_five.append(hit_five)
ndcgs_five.append(ndcg_five)
counter += 1
return np.average(hits_ten), np.average(ndcgs_ten), np.average(hits_five), np.average(ndcgs_five)
category_hits_ten, category_ndcg_ten,category_hits_five,category_ndcg_five = predict_all_users_arc3(df_test, model_arc3)
print(category_hits_ten)
print(category_ndcg_ten)
print(category_hits_five)
print(category_ndcg_five)
write_category_results("arc3", category_hits_ten, category_ndcg_ten, category_hits_five, category_ndcg_five)
###Output
_____no_output_____
###Markdown
4.5 Architecture 4
###Code
def evaluate_one_rating_arc4(model, user_id, user_profiles, all_articles,user_clicks, true_item, categories, sub_categories):
### Reshaping to make it on the right shape ###
#expanded_user_id = np.array([user_id]*100).reshape((100,1))
all_articles = np.array(all_articles).reshape(-1,1)
# predictions
#user_history, profile_input, item_input,category_input, subcategory_input
predictions = model.predict([user_clicks, user_profiles, all_articles, categories, sub_categories]) #TODO: add categories, sub_cat
predicted_labels = np.squeeze(predictions)
top_ten_items = [all_articles[i] for i in np.argsort(predicted_labels)[::-1][0:10].tolist()]
hr = getHitRatio(top_ten_items, true_item)
ndcg = getNDCG(top_ten_items, true_item)
hr_five = getHitRatio(top_ten_items[:5], true_item)
ndcg_five = getNDCG(top_ten_items[:5], true_item)
return hr, ndcg,hr_five,ndcg_five
def evalaute_model_arc4(model, df_test, userid_to_true_item):
print("Evaluate model")
hits = []
ndcgs = []
hits_five = []
ndcgs_five = []
users = df_test["user_id"].unique()
for user_id in tqdm(users):
user_df = df_test[df_test["user_id"] == user_id] # get the 100 samples for this user
true_item = userid_to_true_item[user_id] # get the actual true item in the test set
all_articles = user_df["article_id"].values.astype("int64") # get all possible articles
user_profiles = user_df.iloc[:, 1:7].values.astype("int64")# get the user_profile
user_clicks = user_df.iloc[:, 10:].values.astype("int64")
categories = user_df.iloc[:, 8].values.astype("int64")
sub_categories = user_df.iloc[:, 9].values.astype("int64")
ht, ndcg, hr_five, ndcg_five = evaluate_one_rating_arc4(model,
user_id,
user_profiles,
all_articles,user_clicks,
true_item,
categories,
sub_categories)
hits.append(ht)
ndcgs.append(ndcg)
hits_five.append(hr_five)
ndcgs_five.append(ndcg_five)
return hits, ndcgs, hits_five,ndcgs_five
#@tf.autograph.experimental.do_not_convert
def get_model_arc4(num_users, num_items, dims,num_categories,num_sub_categories, dense_layers=[128, 64, 32, 8]):
#User features
user_history = Input(shape=(30,), name="user")
user_profile_input = Input(shape=(6,), name="profile")
#item features
item_input = Input(shape=(1,), name="item")
item_category = Input(shape=(1,), name="category")
item_subcategory = Input(shape=(1,), name="subcategory")
# User emb
click_history_emb = Embedding(output_dim=dims, input_dim=num_items+1, input_length=30, name="mf_user_emb")(user_history)
profile_emb = Embedding(output_dim=dims, input_dim=num_unique_categories, input_length=6, name="mf_profile_emb")(user_profile_input)
# Item emb
item_emb = Embedding(output_dim=dims, input_dim=num_items+1, input_length=1, name="mf_item_emb")(item_input)
category_emb = Embedding(output_dim=dims, input_dim=num_categories, input_length=1, name="cat_emb")(item_category)
subcategory_emb = Embedding(output_dim=dims, input_dim=num_sub_categories, input_length=1, name="subcat_emb")(item_subcategory)
lstm_tower = Concatenate(axis=1)([click_history_emb,item_emb])
mlp_tower = Concatenate(axis=1)([profile_emb,category_emb, subcategory_emb])
mlp_tower = Flatten()(mlp_tower)
# Lstm-tower
lstm_tower = LSTM(40)(lstm_tower)
lstm_tower = Dropout(0.8)(lstm_tower)
lstm_tower = BatchNormalization(axis=1)(lstm_tower)
# MLP tower
mlp_tower = Dense(2)(mlp_tower)
mlp_tower = Dropout(0.2)(mlp_tower)
mlp_tower = BatchNormalization(axis=1)(mlp_tower)
# Click predictor
final = Concatenate()([lstm_tower,mlp_tower ])
final = BatchNormalization(axis=1)(final)
y = Dense(1, activation="sigmoid")(final)
model = Model(inputs=[user_history, user_profile_input, item_input, item_category, item_subcategory], outputs=y)
model.compile(
optimizer=Adam(0.01),
loss="binary_crossentropy",
metrics=["accuracy"],
)
return model
model_arc4 = get_model_arc4(num_users, num_items, dims, num_categories,num_sub_categories)
###### Training ########
user_history = df_train.iloc[:, 11:].values.astype("int64")
profile_input = df_train.iloc[:, 1:7].values.astype("int64")
item_input = df_train.iloc[:, 7].values.reshape((-1,1)).astype("int64")
labels = df_train.iloc[:, 10].values.reshape((-1,1)).astype("int64")
category_input = df_train.iloc[:, 8].values.reshape((-1,1)).astype("int64")
subcategory_input = df_train.iloc[:, 9].values.reshape((-1,1)).astype("int64")
print(user_history.shape,profile_input.shape, item_input.shape, labels.shape )
all_user_ids = merged["user_id"].unique()
#user_input = df_train.iloc[:, 0].values.reshape((-1,1))
#profile_input = df_train.iloc[:, 1:6].values
#item_input = df_train.iloc[:, 7].values.reshape((-1,1))
#labels = df_train.iloc[:, 8].values.reshape((-1,1))
train_loss = []
val_loss = []
train_acc = []
val_acc = []
hits_list = []
ndcg_list = []
best_hits = 0
best_ndcgs = 0
best_hits_five = 0
best_ndcgs_five = 0
epochs=2
for epoch in range(epochs):
hist = model_arc4.fit([user_history, profile_input, item_input,category_input, subcategory_input ], labels, epochs=1,validation_split=0.1, shuffle=True, verbose=1)
train_loss.append(hist.history["loss"])
train_acc.append(hist.history["accuracy"])
val_loss.append(hist.history["val_loss"])
val_acc.append(hist.history["val_accuracy"])
hits, ndcgs, hits_five, ndcgs_five = evalaute_model_arc4( model_arc4, df_test, userid_to_true_item)
hits_list.append(np.average(hits))
ndcg_list.append(np.average(ndcgs))
temp_hits = np.average(hits)
temp_ndcgs = np.average(ndcgs)
if (temp_hits > best_hits):
best_hits = temp_hits
best_ndcgs = temp_ndcgs
best_hits_five = np.average(hits_five)
best_ndcgs_five = np.average(ndcgs_five)
print("Hit @ 10: {:.2f}".format(best_hits))
print("ncdgs @ 10: {:.2f}".format(best_ndcgs))
print("Hit @ 5: {:.2f}".format(best_hits_five))
print("ncdgs @ 5: {:.2f}".format(best_ndcgs_five))
write_accuracy_results("arc4", best_hits, best_ndcgs, best_hits_five, best_ndcgs_five)
sns.set_style("darkgrid")
plt.plot(train_acc)
plt.plot(val_acc)
plt.title('model accuracy')
plt.ylabel('accuracy')
plt.xlabel('epoch')
plt.legend(['Train', 'Val'], loc='upper left')
plt.savefig("arc4_accuracy.pdf")
plt.show()
sns.set_style("darkgrid")
plt.plot(train_loss)
plt.plot(val_loss)
plt.title('Loss')
plt.ylabel('loss')
plt.xlabel('epoch')
plt.legend(['Train', 'Val'], loc='upper left')
plt.savefig("arc4_loss.pdf")
plt.show()
sns.set_style("darkgrid")
plt.plot(hits_list)
plt.plot(train_loss)
plt.title('Hit ratio vs Loss')
plt.xlabel('epoch')
plt.legend(['Hit@10', 'Train loss'], loc='upper left')
plt.savefig("arc4_hit_loss.pdf")
plt.show()
def get_recommendations_arc4(user_id, df, model):
## Setup ###
user_profile = get_user_profile(df, user_id)
click_history = userid_to_article_history[user_id]
display_items = df[df["user_id"] == user_id]["article_id"].values.reshape(-1, 1).astype("int64")
user_profile = np.tile(user_profile, display_items.shape[0]).reshape(-1, 6).astype("int64")
category, sub_category = get_item_features(user_id)
user_ids = np.tile(np.array(user_id), display_items.shape[0]).reshape(-1,1).astype("int64")
category = np.asarray(category).astype("int64")
sub_category = np.asarray(sub_category).astype("int64")
click_history = np.tile(click_history, display_items.shape[0]).reshape(-1, 30).astype("int64")
## Preds ###
predictions = model.predict([click_history, user_profile, display_items, category, sub_category])
predicted_labels = np.squeeze(predictions)
top_ten_items = [display_items[i][0] for i in np.argsort(predicted_labels)[::-1][0:10].tolist()]
return top_ten_items
def predict_all_users_arc4(df, model):
hits_ten,ndcgs_ten = [], []
hits_five, ndcgs_five = [], []
counter = 0
for user_id in tqdm(df["user_id"].unique()):
top_ten_articles = get_recommendations(user_id, df)
top_ten_subcategories = [get_article_subcategory(_id, df) for _id in top_ten_articles]
user_profile = get_user_profile(df_test, user_id)
hit_ten = get_category_hit_ratio(user_profile, top_ten_subcategories)
ndcg_ten = get_ndcgs_category(user_profile, top_ten_subcategories)
hit_five = get_category_hit_ratio(user_profile, top_ten_subcategories[:5])
ndcg_five = get_ndcgs_category(user_profile, top_ten_subcategories[:5])
hits_ten.append(hit_ten)
ndcgs_ten.append(ndcg_ten)
hits_five.append(hit_five)
ndcgs_five.append(ndcg_five)
counter += 1
return np.average(hits_ten), np.average(ndcgs_ten), np.average(hits_five), np.average(ndcgs_five)
category_hits_ten, category_ndcg_ten,category_hits_five,category_ndcg_five = predict_all_users_arc4(df_test, model_arc4)
print(category_hits_ten)
print(category_ndcg_ten)
print(category_hits_five)
print(category_ndcg_five)
write_category_results("arc4", category_hits_ten, category_ndcg_ten, category_hits_five, category_ndcg_five)
###Output
_____no_output_____ |
jupyter_notebook/preprocessing.ipynb | ###Markdown
1. Count labels in DB
###Code
import os
import torch
from tqdm import tqdm
db_type = 'NoKGenc'
db_name = 'px'
size = 1000
SPLIT = ['train','valid','test']
labels = list()
for split in SPLIT:
db_new = torch.load(f'/home/ssbae/bae/workspace/kgtxt/kg_txt_multimodal/gtx/data/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
for label in tqdm(db_new['label']):
labels+=label
label_count = dict()
for label in tqdm(labels):
if label not in label_count:
label_count[label]=0
label_count[label]+=1
label_count.pop(-100)
print(len(label_count))
# Prepare essential files
NUM_SPECIAL_TOKENS = 3
id2label = torch.load(f'/home/ssbae/bae/workspace/kgtxt/kg_txt_multimodal/gtx/data/{db_name}_{size}/{db_name}_{db_type}/id2label')
label2id = {v:k for k,v in id2label.items()}
# id2entity = {int(line.split('\t')[1])+NUM_SPECIAL_TOKENS:line.split('\t')[0].split('^^')[0] for line in open(os.path.join(f'/home/ssbae/bae/workspace/kgtxt/kg_txt_multimodal/gtx/data/{db_name}','entity2id.txt')).read().splitlines()[1:]}
# label2entity = {k:id2entity[v] for k,v in label2id.items()}
ori_label_count = label_count.copy()
a = torch.tensor([1,2,3,4,5])
b = torch.tensor([1,2,3,4,5])
a/b
import os
import torch
from tqdm import tqdm
db_type = 'UnifiedUniKGenc'
db_name = 'dx,prx'
size = 2000
id2label = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/node2uninode')
id2label
###Output
_____no_output_____
###Markdown
Visualize histogram
###Code
import matplotlib.pyplot as plt
import numpy as np
lower = 1000
upper = 1e10
NUM_SPECIAL_TOKENS = 3
db_name = 'px'
# Filter out labels
if db_name == 'px':
triples = [x.split() for x in open(os.path.join(f'../gtx/data/{db_name}','train2id.txt')).read().splitlines()[1:]]
drugname_nodes = set([int(t)+NUM_SPECIAL_TOKENS for h,t,r in triples if int(r) in [3,5,6]])
label_count = {k:v for k,v in ori_label_count.items() if label2id[k] in drugname_nodes}
filtered_label_counts = {k:v for k,v in label_count.items() if (v>lower) and (v<upper)}
num_labels = np.array([count for count in filtered_label_counts.values()])
print(len(num_labels))
# Plot histogram of label distribution
plt.hist(num_labels, bins=np.arange(num_labels.min(), num_labels.max() + 1000, 1000), rwidth=0.7,
density=True, cumulative=True,
range=(num_labels.min(), num_labels.max()))
plt.title(f'l:{lower},u:{upper},#labels:{len(num_labels)}')
# plt.savefig(f'data/{db_name}/edlabel_distribution.png')
plt.show()
# Generate dictionary for label --> AdmPred labels
# ap_label = {v:k for k,v in enumerate(label_count)}
# torch.save(ap_label,f'data/{db_name}/label2edlabel')
# print([label2entity[k] for k in torch.load(f'data/{db_name}/label2edlabel').keys()])
a = torch.eye(3)
b = torch.rand(3,4)
for _a,_b in zip(a,b):
print(len(_a))
print(_b)
###Output
_____no_output_____
###Markdown
2. Convert DB for AdmPred task
###Code
import os
import torch
import numpy as np
from numpy.random import choice
from tqdm import tqdm
db_name = 'dx,prx'
size = 2000
DB_type = ['NoKGenc','UniKGenc','UnifiedNoKGenc','UnifiedUniKGenc']
SPLIT = ['train','valid','test']
# # Prepare essential files
# NUM_SPECIAL_TOKENS = 3
# id2label = torch.load(f'data/{db_name}_{size}/{db_name}_{db_type}/id2label')
# label2id = {v:k for k,v in id2label.items()}
# id2entity = {int(line.split('\t')[1])+NUM_SPECIAL_TOKENS:line.split('\t')[0].split('^^')[0] for line in open(os.path.join(f'data/{db_name}','entity2id.txt')).read().splitlines()[1:]}
# label2entity = {k:id2entity[v] for k,v in label2id.items()}
adm2label = torch.load(f"../gtx/data/{'px' if db_name=='dx,prx' else 'dx,prx'}/adm2label")
label2aplabel = torch.load(f"../gtx/data/{'px' if db_name=='dx,prx' else 'dx,prx'}/label2aplabel")
for db_type in DB_type:
# Prepare essential files
if "Unified" not in db_type:
NUM_SPECIAL_TOKENS = 3
id2label = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/id2label')
label2id = {v:k for k,v in id2label.items()}
id2entity = {int(line.split('\t')[1])+NUM_SPECIAL_TOKENS:line.split('\t')[0].split('^^')[0] for line in open(os.path.join(f'../gtx/data/{db_name}','entity2id.txt')).read().splitlines()[1:]}
label2entity = {k:id2entity[v] for k,v in label2id.items()}
else:
id2entity = {v:k.split('\t')[0].split('^^')[0] for k,v in torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/unified_node').items()}
if db_type == 'NoKGenc':
global_label = list()
idx = 0
for split in SPLIT:
db = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
db_new = dict()
for k in db:
if k in ['label_mask','rc_index']:
continue
db_new[k] = list()
for in_db_idx, _input in tqdm(enumerate(db['input'])):
if db_type == 'NoKGenc':
if id2entity[_input[1]] in adm2label:
if any([True if label in label2aplabel else False for label in adm2label[id2entity[_input[1]]]]):
for k in db_new:
if k not in ['label','label_mask','rc_index']:
db_new[k].append(db[k][in_db_idx])
aplabel = [label2aplabel[k] for k in adm2label[id2entity[_input[1]]] if k in label2aplabel]
db_new['label'].append(aplabel)
global_label.append(aplabel)
else:
global_label.append(None)
else:
global_label.append(None)
else:
if global_label[idx] is not None:
for k in db_new:
if k not in ['label','label_mask','rc_index']:
db_new[k].append(db[k][in_db_idx])
db_new['label'].append(global_label[idx])
idx += 1
print(f'{db_name},{size},{split}',len(db_new['label']))
os.makedirs(f'../gtx/data/adm/{db_name}_{size}/{db_name}_{db_type}/{split}')
torch.save(db_new,f'../gtx/data/adm/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
# Open Adm Pred DB
labels = list()
db_new = torch.load(f'data/adm/{db_name}_{size}/{db_name}_NoKGenc/train/db')
num_samples = len(db_new['label'])
for label in tqdm(db_new['label']):
labels+=label
# Get sample frequency based class weights
from sklearn.utils.class_weight import compute_class_weight
import numpy as np
aplabel2weight = compute_class_weight(class_weight = "balanced" ,
classes=np.unique(labels),
y = labels)
torch.save(aplabel2weight,f'data/{db_name}/adm_class_weight')
###Output
_____no_output_____
###Markdown
3. Convert DB for ErrorDetection for Px,Dx
###Code
# Load D_ICD files
import torch
import pandas as pd
d_proc = pd.read_csv("../gtx/data/mimic_data/D_ICD_PROCEDURES.csv")
d_diag = pd.read_csv("../gtx/data/mimic_data/D_ICD_DIAGNOSES.csv")
def reformat(code, is_diag):
"""
Put a period in the right place because the MIMIC-3 data files exclude them.
Generally, procedure codes have dots after the first two digits,
while diagnosis codes have dots after the first three digits.
"""
code = ''.join(code.split('.'))
if is_diag:
if code.startswith('E'):
if len(code) > 4:
code = code[:4] + '.' + code[4:]
else:
if len(code) > 3:
code = code[:3] + '.' + code[3:]
else:
code = code[:2] + '.' + code[2:]
return code
d_diag['absolute_code'] = d_diag.apply(lambda row: str(reformat(str(row[1]), True)), axis=1)
d_proc['absolute_code'] = d_proc.apply(lambda row: str(reformat(str(row[1]), False)), axis=1)
d_diag['code_name'] = d_diag.apply(lambda row: str(row[3]).lower().strip(), axis=1)
d_proc['code_name'] = d_proc.apply(lambda row: str(row[3]).lower().strip(), axis=1)
d_diag_dict = d_diag[['absolute_code','code_name']].to_dict()
d_code2name = {d_diag_dict['absolute_code'][idx]:d_diag_dict['code_name'][idx] for idx in range(len(d_diag))}
d_name2codecat = {d_diag_dict['code_name'][idx]:dict([('large',d_diag_dict['absolute_code'][idx].split(".")[0]),('small',d_diag_dict['absolute_code'][idx].split(".")[-1] if len(d_diag_dict['absolute_code'][idx].split("."))>1 else "")]) for idx in range(len(d_diag))}
d_proc_dict = d_proc[['absolute_code','code_name']].to_dict()
p_code2name = {d_proc_dict['absolute_code'][idx]:d_proc_dict['code_name'][idx] for idx in range(len(d_proc))}
p_name2codecat = {d_proc_dict['code_name'][idx]:dict([('large',d_proc_dict['absolute_code'][idx].split(".")[0]),('small',d_proc_dict['absolute_code'][idx].split(".")[-1] if len(d_diag_dict['absolute_code'][idx].split("."))>1 else "")]) for idx in range(len(d_proc))}
code2name = dict()
for d in [d_code2name, p_code2name]:
for k,v in d.items():
code2name[k.lower()] = f'{v}'
name2codecat = dict()
for d in [d_name2codecat, p_name2codecat]:
for k,v in d.items():
v['large'] = v['large'].lower()
name2codecat[f'{k}'] = v
code2name_abs = dict()
for d in [d_code2name, p_code2name]:
for k,v in d.items():
code2name_abs[k.lower()] = f'{v}'
codebook = dict()
for k in code2name_abs:
try:
large, small = k.split(".")
except:
large, small = k, ""
if large not in codebook:
codebook[large] = list()
codebook[large].append(small)
import os
import torch
from copy import deepcopy
import numpy as np
from numpy.random import choice
from tqdm import tqdm
db_name = 'dx,prx'
size = 2000
DB_type = ['NoKGenc','UniKGenc','UnifiedNoKGenc','UnifiedUniKGenc']#,'UnifiedNoKGenc','UnifiedUniKGenc']
SPLIT = ['train','valid','test']
db_type = 'UnifiedNoKGenc'
uniid2entity = {v:k.split('\t')[0].split('^^')[0].strip('"').replace('\\"','"') for k,v in torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_UnifiedNoKGenc/unified_node').items()}
tot_amt = dict()
for split in SPLIT:
db = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
for idx in range(len(db['input'])):
ids = [x for x in db['input'][idx] if x>7]
for _id in ids:
if _id not in tot_amt:
tot_amt[_id] = 0
tot_amt[_id]+=1
code_frequency = {'.'.join([name2codecat[uniid2entity[k]]['large'],name2codecat[uniid2entity[k]]['small']]):1/v for k,v in tot_amt.items()}
uniid2entity
def reformat(code, is_diag):
"""
Put a period in the right place because the MIMIC-3 data files exclude them.
Generally, procedure codes have dots after the first two digits,
while diagnosis codes have dots after the first three digits.
"""
code = ''.join(code.split('.'))
if is_diag:
if code.startswith('e'):
if len(code) > 4:
code = code[:4] + '.' + code[4:]
else:
if len(code) > 3:
code = code[:3] + '.' + code[3:]
else:
code = code[:2] + '.' + code[2:]
return code
def corrupt_input_and_generate_label(inputs, mode, id2entity, entity2id, code_frequency=None):
if code_frequency is None:
raise ValueError("Must turn on non-uniform sampling")
try:
inputs=np.array(inputs)
inputs_ori = inputs.copy()
input_entities = np.array([id2entity[x] if x in id2entity else x for x in inputs])
if mode == 's':
codes = list()
f = list()
for x in input_entities:
if ("icd9_code" in x) and (x not in codes):
is_diag = True if 'diag' in x.split('/')[1] else False
code = name2codecat[code2name[reformat(x.split('/')[-1].strip(">"),is_diag = is_diag)]]
small_cat_length = len(codebook[code['large']])
if small_cat_length>=2:
codes.append(x)
f.append(code_frequency[".".join([code['large'],code['small']])])
p = [x/sum(f) for x in f]
corruption_target_codes = choice(codes,size=max(int(len(codes)*0.25),1), replace=False, p=p)
corruption_targets_idx = np.array([np.where(input_entities==code)[0][0] for code in corruption_target_codes])
for corruption_target in corruption_targets_idx:
code_entity = input_entities[corruption_target].split("/")
header = '/'.join(code_entity[:-1])
is_diag = True if 'diag' in header else False
code = reformat(code_entity[-1].strip(">"),is_diag=is_diag)
target_literal_idx = np.where(entity2id[code2name[code]] == inputs)[0][0]
icd_code = code2name[code]
codecat = name2codecat[icd_code]
large_cat, small_cat = codecat['large'], codecat['small']
small_cat_lists = codebook[large_cat].copy()
small_cat_lists.remove(small_cat)
for _ in range(50):
corrupted_small_cat = choice(small_cat_lists)
if code2name[".".join([large_cat,corrupted_small_cat])] in entity2id:
ERROR_FLAG=False
break
else:
ERROR_FLAG = True
if ERROR_FLAG:
raise ValueError()
inputs[target_literal_idx] = entity2id[code2name[".".join([large_cat,corrupted_small_cat])]]
inputs[corruption_target] = entity2id['/'.join([header,"".join([large_cat,corrupted_small_cat])])+">"]
labels = ~(inputs_ori==inputs)
except:
inputs = None
labels = None
return inputs, labels
for db_type in DB_type:
print(db_type)
if db_type == 'NoKGenc':
global_sample = list()
idx = 0
for split in SPLIT:
# Prepare essential files
NUM_SPECIAL_TOKENS = 3
id2entity = {int(line.split('\t')[1])+NUM_SPECIAL_TOKENS:line.split('\t')[0].split('^^')[0].strip('"').replace('\\"','"') for line in open(os.path.join(f'../gtx/data/{db_name}','entity2id.txt')).read().splitlines()[1:]}
id2entity[0]='PAD'
id2entity[1]='MASK'
id2entity[2]='CLS'
entity2id = {v:k for k,v in id2entity.items()}
uniid2entity = {v:k.split('\t')[0].split('^^')[0].strip('"').replace('\\"','"') for k,v in torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_UnifiedNoKGenc/unified_node').items()}
entity2uniid = {v:k for k,v in uniid2entity.items()}
id2uniid = {k:entity2uniid[v] for k,v in id2entity.items() if v in entity2uniid}
db = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
db_new = dict()
for k in db:
if k in ['rc_index', 'label_mask']:
continue
db_new[k] = list()
for in_db_idx, _input in tqdm(enumerate(db['input'])):
if db_type == 'NoKGenc':
x, y = corrupt_input_and_generate_label(inputs=db['input'][in_db_idx],mode='s',id2entity=id2entity, entity2id=entity2id, code_frequency=code_frequency)
sample = {
'input': x,
'label': y,
} if x is not None else None
if sample is not None:
for k in db_new:
if k in sample:
db_new[k].append(sample[k].tolist())
else:
db_new[k].append(db[k][in_db_idx])
global_sample.append(sample)
else:
if global_sample[idx] is not None:
sample = {k:global_sample[idx][k].copy() for k in global_sample[idx]}
actual_input = np.array(db['input'][in_db_idx].copy())
# Convert Non-unified sample to unifieid sample
if 'Unified' in db_type:
living_ids = sample['input'][sample['label']]
convertable_ids = np.array([True if living_id in id2uniid else False for living_id in living_ids])
sample['label'][sample['label']==True] = convertable_ids
actual_input[sample['label']] = np.array([id2uniid[x] for x in living_ids[convertable_ids]])
for k in db_new:
if k not in sample:
db_new[k].append(db[k][in_db_idx])
db_new['label'].append(sample['label'].tolist())
if 'Unified' in db_type:
db_new['input'].append(actual_input.tolist())
else:
db_new['input'].append(sample['input'].tolist())
idx += 1
print('*'*50)
print([uniid2entity[x] if 'Unified' in db_type else id2entity[x] for x in np.array(db['input'][0])[np.where(np.array(db_new['label'][0])==True)]])
print([uniid2entity[x] if 'Unified' in db_type else id2entity[x] for x in np.array(db_new['input'][0])[np.where(np.array(db_new['label'][0])==True)]])
print(np.where(np.array(db_new['label'][0])==True))
print('-'*50)
print(f'{db_name},{size},{split}',len(db_new['label']))
print(list(db_new.keys()))
print('*'*50)
os.makedirs(f'../gtx/data/ed/{db_name}_{size}/{db_name}_{db_type}/{split}', exist_ok=True)
torch.save(db_new,f'../gtx/data/ed/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
###Output
_____no_output_____
###Markdown
3-1. Convert DB for ErrorDetection for Rx
###Code
import os
import torch
import pandas as pd
from copy import deepcopy
import numpy as np
from numpy.random import choice
from tqdm import tqdm
db_name = 'px'
size = 1000
DB_type = ['UnifiedNoKGenc','UnifiedUniKGenc']
SPLIT = ['train','valid','test']
# Prepare essential files
NUM_SPECIAL_TOKENS = 3
id2entity = {int(line.split('\t')[1])+NUM_SPECIAL_TOKENS:line.split('\t')[0].split('^^')[0] for line in open(os.path.join(f'../gtx/data/{db_name}','entity2id.txt')).read().splitlines()[1:]}
id2entity[0]='PAD'
id2entity[1]='MASK'
id2entity[2]='CLS'
entity2id = {v:k for k,v in id2entity.items()}
uniid2entity = {v:k.split('\t')[0].split('^^')[0] for k,v in torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_UnifiedNoKGenc/unified_node').items()}
entity2uniid = {v:k for k,v in uniid2entity.items()}
uniid2id = {k:entity2id[v] for k,v in uniid2entity.items() if v in entity2id}
px = pd.read_csv('../gtx/data/mimic_data/PRESCRIPTIONS.csv')
px['DOSE'] = px.apply(lambda row: str(row[14])+str(row[15]), axis=1)
px_dict = px.to_dict()
CATEGORIES = ['DRUG_TYPE', 'DRUG', 'ROUTE', 'FORMULARY_DRUG_CD', 'DOSE']
pxcat2ids = {k:[f'"{str(x).lower()}"' for x in list(set(px_dict[k].values()))] for k in CATEGORIES}
id2pxcat = dict()
for k in pxcat2ids:
pxcat2ids[k] = [entity2uniid[x] for x in pxcat2ids[k] if x in entity2uniid]
for v in pxcat2ids[k]:
id2pxcat[v]=k
db_type = 'UnifiedNoKGenc'
uniid2entity = {v:k.split('\t')[0].split('^^')[0].strip('"').replace('\\"','"') for k,v in torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_UnifiedNoKGenc/unified_node').items()}
px2freq = {k:{v:0 for v in vl} for k,vl in pxcat2ids.items()}
# for k,vl in pxcat2ids.items()
for split in SPLIT:
db = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
for idx in range(len(db['input'])):
ids = [x for x in db['input'][idx] if x>7]
for _id in ids:
if _id in id2pxcat:
px2freq[id2pxcat[_id]][_id]+=1
import torch
label = torch.tensor([[1,1,0,0],[0,0,0,1],[1,1,1,1]])
torch.where(label==1, dim=1)
def corrupt_input_and_generate_label(inputs, literal_pos, pxcat2ids, id2pxcat):
# try:
corruptable_idx = np.array([i for i,(x,p) in enumerate(zip(inputs, literal_pos)) if (p==1) and (x in id2pxcat)])
inputs=np.array(inputs)
inputs_ori = inputs.copy()
corruption_targets_idx = choice(corruptable_idx,max(int(len(corruptable_idx)*0.2),1))
for corruption_target_idx in corruption_targets_idx:
corruption_target_id = inputs[corruption_target_idx]
pxcat = id2pxcat[corruption_target_id]
candidate = np.array(pxcat2ids[pxcat])
candidate[candidate!=corruption_target_id]
corrupted_target_id = choice(candidate)
inputs[corruption_target_idx] = corrupted_target_id
labels = ~(inputs_ori==inputs)
# except:
# inputs = None
# labels = None
return inputs, labels
for db_type in DB_type:
if db_type == 'UnifiedNoKGenc':
global_sample = list()
print(db_type)
idx = 0
for split in SPLIT:
db = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
db_new = dict()
for k in db:
if k in ['rc_index', 'label_mask']:
continue
db_new[k] = list()
for in_db_idx, _input in tqdm(enumerate(db['input'])):
if db_type == 'UnifiedNoKGenc':
x, y = corrupt_input_and_generate_label(inputs=db['input'][in_db_idx],literal_pos=db['label_mask'][in_db_idx],pxcat2ids=pxcat2ids,id2pxcat=id2pxcat)
sample = {
'input': x,
'label': y,
} if x is not None else None
if sample is not None:
for k in db_new:
if k in sample:
db_new[k].append(sample[k].tolist())
else:
db_new[k].append(db[k][in_db_idx])
global_sample.append(sample)
else:
if global_sample[idx] is not None:
sample = {k:global_sample[idx][k].copy() for k in global_sample[idx]}
actual_input = np.array(db['input'][in_db_idx].copy())
# Convert Non-unified sample to unifieid sample
if 'Unified' not in db_type:
living_ids = sample['input'][sample['label']]
actual_input[sample['label']] = np.array([uniid2id[x] for x in living_ids])
for k in db_new:
if k not in sample:
db_new[k].append(db[k][in_db_idx])
db_new['label'].append(sample['label'].tolist())
if 'Unified' not in db_type:
db_new['input'].append(actual_input.tolist())
else:
db_new['input'].append(sample['input'].tolist())
idx += 1
print('*'*50)
print([uniid2entity[x] if 'Unified' in db_type else id2entity[x] for x in np.array(db['input'][0])[np.where(np.array(db_new['label'][0])==True)]])
print([uniid2entity[x] if 'Unified' in db_type else id2entity[x] for x in np.array(db_new['input'][0])[np.where(np.array(db_new['label'][0])==True)]])
print(np.where(np.array(db_new['label'][0])==True))
print('-'*50)
print(f'{db_name},{size},{split}',len(db_new['label']))
print(list(db_new.keys()))
print('*'*50)
os.makedirs(f'../gtx/data/ed/{db_name}_{size}/{db_name}_{db_type}/{split}', exist_ok=True)
torch.save(db_new,f'../gtx/data/ed/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
###Output
UnifiedNoKGenc
###Markdown
4. Modify Pretraining Input for KnowMix strategy
###Code
import os
import torch
from tqdm import tqdm, trange
db_name = 'px'
size = 1000
DB_type = ['UnifiedNoKGenc','UnifiedUniKGenc']
SPLIT = ['train','valid','test']
know_list = list()
for db_type in DB_type:
global_idx=0
# Prepare essential files
if "Unified" not in db_type:
NUM_SPECIAL_TOKENS = 3
id2label = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/id2label')
label2id = {v:k for k,v in id2label.items()}
id2entity = {int(line.split('\t')[1])+NUM_SPECIAL_TOKENS:line.split('\t')[0].split('^^')[0] for line in open(os.path.join(f'../gtx/data/{db_name}','entity2id.txt')).read().splitlines()[1:]}
label2entity = {k:id2entity[v] for k,v in label2id.items()}
else:
id2entity = {v:k.split('\t')[0].split('^^')[0] for k,v in torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/unified_node').items()}
for split in SPLIT:
db = torch.load(f'../gtx/data/ed/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
db_new = {k:list() for k in db}
db_new['knowledge'] = list()
for idx in trange(len(db['input'])):
for k in db:
db_new[k].append(db[k][idx])
if db_type == 'UnifiedNoKGenc':
desc = [id2entity[x].replace('"','') if x>5 else "" for x in db_new['input'][idx]]
know_list.append(desc)
db_new['knowledge'].append(desc)
else:
db_new['knowledge'].append(know_list[global_idx])
global_idx += 1
os.makedirs(f'../gtx/data/ed/knowmix/{db_name}_{size}/{db_name}_{db_type}/{split}', exist_ok=True)
torch.save(db_new,f'../gtx/data/ed/knowmix/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
id2desc = {k:v.split('^^')[0].replace('"','') if '^^' in v else "" for k,v in id2entity.items()}
print(len(id2desc))
torch.save(id2desc,f'../gtx/data/ed/knowmix/{db_name}_{size}/{db_name}_{db_type}/id2desc')
# for k in db:
# print(k,db[k][-1])
# print("-"*30)
# for k in db_new:
# print(db_new[k][-1])
# print("-"*30)
!pip install transformers
###Output
_____no_output_____
###Markdown
5. Convert DB for ReAdmPred task
###Code
import os
import torch
import numpy as np
import pickle as pkl
from numpy.random import choice
from tqdm import tqdm
db_name = 'px'
size = 1000
DB_type = ['NoKGenc','UniKGenc','UnifiedNoKGenc','UnifiedUniKGenc']
SPLIT = ['train','valid','test']
for db_type in DB_type:
# Prepare essential files
if "Unified" not in db_type:
NUM_SPECIAL_TOKENS = 3
id2label = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/id2label')
label2id = {v:k for k,v in id2label.items()}
id2entity = {int(line.split('\t')[1])+NUM_SPECIAL_TOKENS:line.split('\t')[0].split('^^')[0] for line in open(os.path.join(f'../gtx/data/{db_name}','entity2id.txt')).read().splitlines()[1:]}
label2entity = {k:id2entity[v] for k,v in label2id.items()}
else:
id2entity = {v:k.split('\t')[0].split('^^')[0] for k,v in torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/unified_node').items()}
if db_type == 'NoKGenc':
global_label = list()
idx = 0
for split in SPLIT:
db = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
readmDB_path = f"../gtx/data/temporal/readm_{'dxpx' if db_name=='dx,prx' else 'rx'}_{split}.pkl"
with open(readmDB_path, 'rb') as f:
print(readmDB_path)
raw_readm_db = pkl.load(f)
readm_db = dict()
for sample in raw_readm_db:
hadm_id, label = sample
readm_db[str(hadm_id)] = label
print("# samples: ",len(readm_db))
db_new = dict()
for k in db:
if k in ['label_mask','rc_index']:
continue
db_new[k] = list()
for in_db_idx, _input in tqdm(enumerate(db['input'])):
if db_type == 'NoKGenc':
hadm_id = id2entity[_input[1]].split('/')[-1].replace('>','')
if hadm_id in readm_db:
for k in db_new:
if k not in ['label','label_mask','rc_index']:
db_new[k].append(db[k][in_db_idx])
db_new['label'].append(readm_db[hadm_id])
global_label.append(readm_db[hadm_id])
else:
global_label.append(None)
else:
if global_label[idx] is not None:
for k in db_new:
if k not in ['label','label_mask','rc_index']:
db_new[k].append(db[k][in_db_idx])
db_new['label'].append(global_label[idx])
idx += 1
print(f'{db_name},{size},{split}',len(db_new['label']))
if "Unified" in db_type:
os.makedirs(f'../gtx/data/temporal/readm/{db_name}_{size}/{db_name}_{db_type}/{split}', exist_ok=True)
torch.save(db_new,f'../gtx/data/temporal/readm/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
print("*** Saved! ***")
print(f"100th sample", [id2entity[x] for x in db_new['input'][99][1:30]])
print(f"100th label", db_new['label'][99])
###Output
_____no_output_____
###Markdown
6. Convert DB for NextDxPxPred task
###Code
import os
import torch
import numpy as np
import pickle as pkl
from numpy.random import choice
from tqdm import tqdm
db_name = 'px'
size = 1000
DB_type = ['NoKGenc','UniKGenc','UnifiedNoKGenc','UnifiedUniKGenc']
SPLIT = ['train','valid','test']
for db_type in DB_type:
# Prepare essential files
if "Unified" not in db_type:
NUM_SPECIAL_TOKENS = 3
id2label = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/id2label')
label2id = {v:k for k,v in id2label.items()}
id2entity = {int(line.split('\t')[1])+NUM_SPECIAL_TOKENS:line.split('\t')[0].split('^^')[0] for line in open(os.path.join(f'../gtx/data/{db_name}','entity2id.txt')).read().splitlines()[1:]}
label2entity = {k:id2entity[v] for k,v in label2id.items()}
else:
id2entity = {v:k.split('\t')[0].split('^^')[0] for k,v in torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/unified_node').items()}
if db_type == 'NoKGenc':
global_label = list()
idx = 0
for split in SPLIT:
db = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
nextdxDB_path = f"../gtx/data/temporal/nextdx_{'dxpx' if db_name=='dx,prx' else 'rx'}_{split}.pkl"
with open(nextdxDB_path, 'rb') as f:
print(nextdxDB_path)
raw_nextdx_db = pkl.load(f)
nextdx_db = dict()
for sample in raw_nextdx_db:
hadm_id, label = str(int(sample[0])), sample[1:]
nextdx_db[hadm_id] = label
print("# samples: ",len(nextdx_db))
db_new = dict()
for k in db:
if k in ['label_mask','rc_index']:
continue
db_new[k] = list()
for in_db_idx, _input in tqdm(enumerate(db['input'])):
if db_type == 'NoKGenc':
hadm_id = id2entity[_input[1]].split('/')[-1].replace('>','')
if hadm_id in nextdx_db:
for k in db_new:
if k not in ['label','label_mask','rc_index']:
db_new[k].append(db[k][in_db_idx])
db_new['label'].append(nextdx_db[hadm_id])
global_label.append(nextdx_db[hadm_id])
else:
global_label.append(None)
else:
if global_label[idx] is not None:
for k in db_new:
if k not in ['label','label_mask','rc_index']:
db_new[k].append(db[k][in_db_idx])
db_new['label'].append(global_label[idx])
idx += 1
print(f'{db_name},{size},{split}',len(db_new['label']))
if "Unified" in db_type:
os.makedirs(f'../gtx/data/temporal/nextdx/{db_name}_{size}/{db_name}_{db_type}/{split}', exist_ok=True)
torch.save(db_new,f'../gtx/data/temporal/nextdx/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
print("*** Saved! ***")
print(f"100th sample", [id2entity[x] for x in db_new['input'][99][100:105]])
print(f"100th label", db_new['label'][99][:5])
###Output
_____no_output_____
###Markdown
7. Convert DB for Mortaility Pred task
###Code
import os
import torch
import numpy as np
import pickle as pkl
from numpy.random import choice
from tqdm import tqdm
db_name = 'px'
size = 1000
# db_name = 'dx,prx'
# size = 2000
DB_type = ['NoKGenc','UniKGenc','UnifiedNoKGenc','UnifiedUniKGenc']
SPLIT = ['train','valid','test']
for db_type in DB_type:
# Prepare essential files
if "Unified" not in db_type:
NUM_SPECIAL_TOKENS = 3
id2label = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/id2label')
label2id = {v:k for k,v in id2label.items()}
id2entity = {int(line.split('\t')[1])+NUM_SPECIAL_TOKENS:line.split('\t')[0].split('^^')[0] for line in open(os.path.join(f'../gtx/data/{db_name}','entity2id.txt')).read().splitlines()[1:]}
label2entity = {k:id2entity[v] for k,v in label2id.items()}
else:
id2entity = {v:k.split('\t')[0].split('^^')[0] for k,v in torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/unified_node').items()}
if db_type == 'NoKGenc':
global_label = list()
idx = 0
for split in SPLIT:
db = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
death180DB_path = f"../gtx/data/temporal/death180_{'dxpx' if db_name=='dx,prx' else 'rx'}_{split}.pkl"
with open(death180DB_path, 'rb') as f:
print(death180DB_path)
raw_death180_db = pkl.load(f)
death180_db = dict()
for sample in raw_death180_db:
hadm_id, label = sample
death180_db[str(hadm_id)] = label
print("# samples: ",len(death180_db))
db_new = dict()
for k in db:
if k in ['label_mask','rc_index']:
continue
db_new[k] = list()
for in_db_idx, _input in tqdm(enumerate(db['input'])):
if db_type == 'NoKGenc':
hadm_id = id2entity[_input[1]].split('/')[-1].replace('>','')
if hadm_id in death180_db:
for k in db_new:
if k not in ['label','label_mask','rc_index']:
db_new[k].append(db[k][in_db_idx])
db_new['label'].append(death180_db[hadm_id])
global_label.append(death180_db[hadm_id])
else:
global_label.append(None)
else:
if global_label[idx] is not None:
for k in db_new:
if k not in ['label','label_mask','rc_index']:
db_new[k].append(db[k][in_db_idx])
db_new['label'].append(global_label[idx])
idx += 1
print(f'{db_name},{size},{split}',len(db_new['label']))
if "Unified" in db_type:
os.makedirs(f'../gtx/data/temporal/death180/{db_name}_{size}/{db_name}_{db_type}/{split}', exist_ok=True)
torch.save(db_new,f'../gtx/data/temporal/death180/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
print("*** Saved! ***")
print(f"100th sample", [id2entity[x] for x in db_new['input'][99][1:5]])
print(f"100th label", db_new['label'][99])
###Output
_____no_output_____
###Markdown
8. Prepare literal to word dictionary for word initialization
###Code
import os
import torch
from tqdm import tqdm, trange
db_name = 'px'
size = 1000
DB_type = ['UnifiedNoKGenc','UnifiedUniKGenc']
for db_type in DB_type:
global_idx=0
# Prepare essential files
id2entity = {v:k.split('\t')[0].split('^^')[0] for k,v in torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/unified_node').items()}
desc = [id2entity[x].replace('"','') if x>5 else "" for x in id2entity]
print(len(id2entity))
print(id2entity)
torch.save(desc,f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/lit2word')
torch.save(desc,f'../gtx/data/knowmix/{db_name}_{size}/{db_name}_{db_type}/lit2word')
###Output
_____no_output_____
###Markdown
IMPORTANT. FIX CLS ATTN MASK
###Code
import os
import torch
import gc
from tqdm import tqdm, trange
db_name = 'dx,prx'
size = 2000
TASK = ["","adm/","ed/","readm/","Death30/"]
DB_type = ['UnifiedUniKGenc']
SPLIT = ['train','valid','test']
for fusion in [True,False]:
for task in TASK:
if fusion:
task = task+'knowmix/'
for db_type in DB_type:
for split in SPLIT:
try:
db = torch.load(f'../gtx/data/{task}{db_name}_{size}/{db_name}_{db_type}/{split}/db')
except:
continue
db_length = len(db['input'])
for idx in trange(db_length):
_input, _mask = db['input'][idx], db['mask'][idx]
new_cls_mask = [1 if x!=0 else 0 for x in _input]
_mask[0] = torch.tensor(new_cls_mask)
db['mask'][idx] = _mask
if sum(db['mask'][idx][0])!= sum([x!=0 for x in _input]):
raise ValueError()
os.makedirs(f'../gtx/fixed_data/{task}/{db_name}_{size}/{db_name}_{db_type}/{split}', exist_ok=True)
torch.save(db,f'../gtx/fixed_data/{task}/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
os.remove(f'../gtx/data/{task}/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
del db
gc.collect()
for fusion in [True,False]:
for task in TASK:
if fusion:
task = task+'knowmix/'
for db_type in DB_type:
for split in SPLIT:
path = f'../gtx/data/{task}{db_name}_{size}/{db_name}_{db_type}/{split}/db'
print(path)
try:
db = torch.load(path)
except:
# print("fail")
continue
db_length = len(db['input'])
print(db['mask'][0][0])
import shutil
for fusion in [True,False]:
for task in TASK:
if fusion:
task = task+'knowmix/'
for db_type in DB_type:
count = 0
try:
shutil.copy(f'../gtx/data/{task}{db_name}_{size}/{db_name}_{db_type}/lit2word',
f'../gtx/fixed_data/{task}{db_name}_{size}/{db_name}_{db_type}/lit2word')
count += 1
except:
pass
try:
shutil.copy(f'../gtx/data/{task}{db_name}_{size}/{db_name}_{db_type}/id2label',
f'../gtx/fixed_data/{task}{db_name}_{size}/{db_name}_{db_type}/id2label')
count += 1
except:
pass
try:
shutil.copy(f'../gtx/data/{task}{db_name}_{size}/{db_name}_{db_type}/node2uninode',
f'../gtx/fixed_data/{task}{db_name}_{size}/{db_name}_{db_type}/node2uninode')
count += 1
except:
pass
try:
shutil.copy(f'../gtx/data/{task}{db_name}_{size}/{db_name}_{db_type}/unified_node',
f'../gtx/fixed_data/{task}{db_name}_{size}/{db_name}_{db_type}/unified_node')
count += 1
except:
pass
try:
shutil.copy(f'../gtx/data/{task}{db_name}_{size}/{db_name}_{db_type}/id2desc',
f'../gtx/fixed_data/{task}{db_name}_{size}/{db_name}_{db_type}/id2desc')
count += 1
except:
pass
print(task, db_type, count)
###Output
knowmix/ UnifiedUniKGenc 2
adm/knowmix/ UnifiedUniKGenc 1
ed/knowmix/ UnifiedUniKGenc 1
readm/knowmix/ UnifiedUniKGenc 1
Death30/knowmix/ UnifiedUniKGenc 1
UnifiedUniKGenc 4
adm/ UnifiedUniKGenc 0
ed/ UnifiedUniKGenc 0
readm/ UnifiedUniKGenc 0
Death30/ UnifiedUniKGenc 0
###Markdown
DB check
###Code
import torch
import os
db_name = 'px'
size = 1000
db_type = 'UnifiedUniKGenc'
split = 'valid'
db = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
if "Unified" not in db_type:
NUM_SPECIAL_TOKENS = 3
id2label = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/id2label')
label2id = {v:k for k,v in id2label.items()}
id2entity = {int(line.split('\t')[1])+NUM_SPECIAL_TOKENS:line.split('\t')[0].split('^^')[0] for line in open(os.path.join(f'../gtx/data/{db_name}','entity2id.txt')).read().splitlines()[1:]}
label2entity = {k:id2entity[v] for k,v in label2id.items()}
entity2id = {v:k for k,v in id2entity.items()}
else:
id2entity = {v:k.split('\t')[0].split('^^')[0] for k,v in torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/unified_node').items()}
entity2id = {v:k for k,v in id2entity.items()}
print(id2entity)
###Output
_____no_output_____
###Markdown
Extract HADM_ID
###Code
import torch
import os
db_name = 'dx,prx'
size = 2000
db_type = 'NoKGenc'
if "Unified" not in db_type:
NUM_SPECIAL_TOKENS = 3
id2label = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/id2label')
label2id = {v:k for k,v in id2label.items()}
id2entity = {int(line.split('\t')[1])+NUM_SPECIAL_TOKENS:line.split('\t')[0].split('^^')[0] for line in open(os.path.join(f'../gtx/data/{db_name}','entity2id.txt')).read().splitlines()[1:]}
label2entity = {k:id2entity[v] for k,v in label2id.items()}
entity2id = {v:k for k,v in id2entity.items()}
else:
id2entity = {v:k.split('\t')[0].split('^^')[0] for k,v in torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/unified_node').items()}
entity2id = {v:k for k,v in id2entity.items()}
import gc
seen_ids = list()
for split in ['train','valid','test']:
db = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
count = 0
with open(f'{db_name}_{split}.txt','w') as f:
for sample in db['input']:
aid = id2entity[sample[1]].split('/')[-1].replace('>','')
if aid in seen_ids and split != "train":
print("oh my god!")
print(aid)
count +=1
seen_ids.append(aid)
f.write(aid+'\n')
print(count)
del db
gc.collect()
print(df_discharge_smmary[df_discharge_smmary['HADM_ID']==167118].values[0,1])
###Output
_____no_output_____
###Markdown
Playground!
###Code
import pickle as pkl
with open('../gtx/data/temporal/icd2int.pkl', 'rb') as f:
icd2int = pkl.load(f)
with open('../gtx/data/temporal/nextdx_dxpx_test.pkl', 'rb') as f:
nextdx_dxpx = pkl.load(f)
with open('../gtx/data/temporal/nextdx_rx_test.pkl', 'rb') as f:
nextdx_rx = pkl.load(f)
with open('../gtx/data/temporal/readm_rx_test.pkl', 'rb') as f:
readm_rx = pkl.load(f)
with open('../gtx/data/temporal/readm_dxpx_test.pkl', 'rb') as f:
readm_dxpx = pkl.load(f)
for sample in nextdx_dxpx:
hadm_id, label = sample[0], sample[1:]
print(str(int(hadm_id)))
print(label)
import torch
db = torch.load(f'../gtx/data/dx,prx_2000/dx,prx_NoKGenc/train/db')
import pandas
df_diag = pandas.read_csv("../gtx/data/DIAGNOSES_ICD.csv")
df_note = pandas.read_csv("../gtx/data/DIAGNOSES_ICD.csv")
import torch
db_name = 'dx,prx'
size = 2000
db_type = 'UnifiedNoKGenc'
split="test"
db = torch.load(f'../gtx/data/{db_name}_{size}/{db_name}_{db_type}/{split}/db')
_input, _mask = db['input'][0], db['mask'][0]
new_cls_mask = [1 if x!=0 else 0 for x in _input]
_mask[0] = torch.tensor(new_cls_mask)
db['mask'][0] = _mask
db['mask'][0][0].sum()
sum([x!=0 for x in db['input'][0]])
###Output
_____no_output_____ |
08/assignment.ipynb | ###Markdown
PHYS 105A: Introduction to Scientific Computing Numerical IntegratorIn this assignment, we will see some of the limitations on numerical integrators, and find ways to improve them.
###Code
# Step 1. Import Libraries
import numpy as np
from matplotlib import pyplot as plt
# Step 2. Define standard numerical integrals
#
# There is nothing complicated that you need to do here.
# Simply copy and paste the functions in the lecture note from last week.
def trapezoidal(f, N=8, a=0, b=1):
______
def Simpson(f, N=8, a=0, b=1):
______
def Bode(f, N=8, a=0, b=1):
______
# Step 3. Define a function that breaks our integrators...
#
# Since we have worked on integrating pi for many times, let's define a quarter circle again!
def h(x):
return np.sqrt(1 - x * x)
X = np.linspace(0, 1, 5)
Y = h(X)
x = np.linspace(0, 1, 129) # define a fine grid for plotting
plt.plot(x, h(x))
plt.scatter(X, Y, color='r')
plt.fill_between(X, h(X), color='r', alpha=0.33)
plt.gca().set_aspect('equal')
# Step 4. Apply the integrators
Ns = [8, 16, 32, 64, 128, 256, 512, 1024]
err_t = [abs(trapezoidal(h, N) - np.pi/4) for N in Ns]
err_S = [abs(Simpson(h, N) - np.pi/4) for N in Ns]
err_B = [abs(Bode(h, N) - np.pi/4) for N in Ns]
plt.loglog(Ns, err_t, '+--', color='r', label='trapezoidal')
plt.loglog(Ns, err_S, 'o-.', color='g', label='Simpson')
plt.loglog(Ns, err_B, 'x:', color='b', label='Bode')
plt.xlabel('Number of sampling points')
plt.ylabel('Absolute errors')
plt.legend()
###Output
_____no_output_____
###Markdown
What is the convergent rate? Is this expected? Please write down your answers below.________
###Code
# Step 5. Idea: use smaller steps as we apporach one.
def trapezoidal_adaptive(h, N):
________
def Simpson_adaptive(h, N):
N = N//2 # Why do we need to divid by 2?
X = np.sin(np.pi/2 * np.arange(N+1)/N) # what is the distribution of grid points look like?
S = 0
for i in range(N):
S += Simpson(h, N=2, a=X[i], b=X[i+1])
return S
def Bode_adaptive(h, N):
________
# Step 6. Test the result
Ns = [8, 16, 32, 64, 128, 256, 512, 1024]
err_ta = [abs(trapezoidal_adaptive(h, N) - np.pi/4) for N in Ns]
err_Sa = [abs(Simpson_adaptive(h, N) - np.pi/4) for N in Ns]
err_Ba = [abs(Bode_adaptive(h, N) - np.pi/4) for N in Ns]
plt.loglog(Ns, err_t, '+--', color='r', label='trapezoidal')
plt.loglog(Ns, err_S, 'o-.', color='g', label='Simpson')
plt.loglog(Ns, err_B, 'x:', color='b', label='Bode')
plt.loglog(Ns, err_ta, '+-', color='r', label='adaptive trapezoidal')
plt.loglog(Ns, err_Sa, 'o-', color='g', label='adaptive Simpson')
plt.loglog(Ns, err_Ba, 'x-', color='b', label='adaptive Bode')
plt.xlabel('Number of sampling points')
plt.ylabel('Absolute errors')
plt.legend()
###Output
_____no_output_____ |
docs/tutorials/02_vibrational_structure.ipynb | ###Markdown
Vibrational structure Introduction The molecular Hamiltonian is $$\mathcal{H} = - \sum_I \frac{\nabla_{R_I}^2}{M_I} - \sum_i \frac{\nabla_{r_i}^2}{m_e} - \sum_I\sum_i \frac{Z_I e^2}{|R_I-r_i|} + \sum_i \sum_{j>i} \frac{e^2}{|r_i-r_j|} + \sum_I\sum_{J>I} \frac{Z_I Z_J e^2}{|R_I-R_J|}$$Because the nuclei are much heavier than the electrons they do not move on the same time scale and therefore, the behavior of nuclei and electrons can be decoupled. This is the Born-Oppenheimer approximation.Within the Born-Oppenheimer approximation, a molecular wave function is factorized as a product of an electronic part, which is the solution of the electronic Schroedinger equation, and a vibro-rotational one, which is the solution of the nuclear Schroedinger equation in the potential energy surface (PES) generated by sampling the eigenvalues of the electronic Schroedinger equation for different geometries.The nuclear Schroedinger equation is usually solved in two steps, in analogy with its electronic counterpart. A single-particle basis (the basis functions are called, in this case, modals) is obtained either by the harmonic approximation applied to the PES or from a vibrational self-consistent field (VSCF) calculation. Vibrational anharmonic correlations are added a-posteriori with perturbative or variational approaches.The latter include Vibrational Configuration Interaction (VCI) and Vibrational Coupled Cluster (VCC) for highly-accurate anharmonic energies. The main advantage of VCI and VCC over alternative approaches (such as perturbation theories) is that their accuracy can be systematically improved towards the complete basis set limit for a given PES. However, their applicability is limited to small molecules with up to about 10 atoms due to their unfavorable scaling with system size.To tackle the scaling problem we would like to use quantum algorithms.The nuclear Schroedinger equation is$$\mathcal{H}_{\text{vib}} |\Psi_{n}\rangle = E_{n} |\Psi_{n}\rangle$$The so-called Watson Hamiltonian (neglecting vibro-rotational coupling terms) is$$ \mathcal{H}_\text{vib}(Q_1, \ldots, Q_L) = - \frac{1}{2} \sum_{l=1}^{L} \frac{\partial^2}{\partial Q_l^2} + V(Q_1, \ldots, Q_L)$$where $Q_l$ are the harmonic mass-weighted normal coordinates.$\mathcal{H}_\text{vib}$ must be mapped to an operator that acts on the states of a given set of $N_q$ qubits in order to calculate its eigenfunctions on quantum hardware.In electronic structure calculations, the mapping is achieved by expressing the non-relativistic electronic Hamiltonian in second quantization, \textit{i.e.} by projecting it onto the complete set of antisymmetrized occupation number vectors (ONV) generated by a given (finite) set of orbitals.To encode the vibrational Hamiltonian in an analogous second quantization operators, we expand the potential $V(Q_1, \ldots, Q_L)$ with the $n$-body expansion as follows:$$ V(Q_1, \ldots, Q_L) = V_0 + \sum_{l=1}^L V^{[l]}(Q_l) + \sum_{l<m}^L V^{[l,m]}(Q_l, Q_m) + \sum_{l<m<n}^L V^{[l,m,n]}(Q_l, Q_m, Q_n) + \ldots$$where $V_0$ is the electronic energy of the reference geometry, the one-mode term $V^{[l]}(Q_l)$ represents the variation of the PES upon change of the $l$-th normal coordinate from the equilibrium position.Similarly, the two-body potential $V^{[l,m]}(Q_l, Q_m)$ represents the change in the exact PES upon a simultaneous displacement along the $l$-th and $m$-th coordinates. Often, including terms up to three-body in the $L$-body expansion is sufficient to obtain an accuracy of about 1~cm$^{-1}$. We highlight that the many-body expansion of the potential operator defining the Watson Hamiltonian contains arbitrarily high coupling terms. This is a crucial difference compared to the non-relativistic electronic-structure Hamiltonian that contains only pairwise interactions.A flexible second quantization form of the Watson Hamiltonian is obtained within the so-called n-mode representation. Let us assume that each mode $l$ is described by a $N_l$-dimensional basis set $S_l$ defined as follows:$$ \mathcal{S}_l = \{ \phi_1^{(l)} (Q_l) , \ldots , \phi_{N_l}^{(l)} (Q_l) \} \, .$$The $n$-mode wave function can be expanded in the product basis $\mathcal{S} = \otimes_{i=1}^L \mathcal{S}_i$ as the following CI-like expansion:$$ |\Psi\rangle = \sum_{k_1=1}^{N_1} \cdots \sum_{k_L=1}^{N_L} C_{k_1,\ldots,k_L} \phi_{k_1}^{(1)}(Q_1) \cdots \phi_{k_L}^{(L)}(Q_L) \, ,$$The many-body basis function $\phi_{k_1}^{(1)}(Q_1) \cdots \phi_{k_L}^{(L)}(Q_L)$ are encoded within the so-called $n$-mode second quantization as occupation-number vectors (ONVs) as follows:$$ \phi_{k_1}(Q_1) \cdots \phi_{k_L}(Q_L) \equiv |0_1 \cdots 1_{k_1} \cdots 0_{N_1}, 0_1 \cdots 1_{k_2} \cdots 0_{N_2}, \cdots , 0_1 \cdots 1_{k_L} \cdots 0_{N_L}\rangle \, .$$The ONV defined above is, therefore, the product of $L$ mode-specific ONVs, each one describing an individual mode. Since each mode is described by one and only one basis function, the occupation of each mode-specific ONV is one.From a theoretical perspective, each mode can be interpreted as a distinguishable quasi-particle (defined as phonons in solid-state physics). Distinguishability arises from the fact that the PES is not invariant by permutation of two modes, also in this case unlike the Coulomb interaction between two equal particles. From this perspective, a molecule can be interpreted as a collection of $L$ indistinguishable particles that interact through the PES operator.Based on this second-quantization representation we introduce a pair of creation and annihilation operators per mode $l$ \textit{and} per basis function $k_l$ defined as:$$ \begin{aligned} a_{k_l}^\dagger |\cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle &= | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle \\ a_{k_l}^\dagger | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle &= 0 \\ a_{k_l} | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle &= | \cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle \\ a_{k_l} | \cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle &= 0 \\ \end{aligned}$$with$$ \begin{aligned} \left[ a_{k_l}^\dagger, a_{h_m}^\dagger \right] &= 0 \\ \left[ a_{k_l}, a_{h_m} \right] &= 0 \\ \left[ a_{k_l}^\dagger, a_{h_m} \right] &= \delta_{l,m} \, , \delta_{k_l,h_m} \end{aligned}$$The second quantization form is obtained by expressing the potential as $$ \begin{aligned} \mathcal{H}_\text{vib}^{SQ} =& \sum_{l=1}^L \sum_{k_l,h_l}^{N_l} \langle \phi_{k_l} | T(Q_l) + V^{[l]}(Q_l) | \phi_{h_l} \rangle a_{k_l}^+ a_{h_l} \\ +& \sum_{l<m}^L \sum_{k_l,h_l}^{N_l} \sum_{k_m,h_m}^{N_m} \langle \phi_{k_l} \phi_{k_m} | V^{[l,m]}(Q_l, Q_m) | \phi_{h_l} \phi_{h_m} \rangle a_{k_l}^+ a_{k_m}^+ a_{h_l} a_{h_m} + \cdots \end{aligned}$$We highlight here the difference between the operators defined here above and the electronic structure one. First, as we already mentioned, the potential contains (in principle) three- and higher-body coupling terms that lead to strings with six (or more) second-quantization operators. Moreover, the Hamiltonian conserves the number of particles for each mode, as can be seen from the fact that the number of creation and annihilation operators for a given mode is the same in each term. Nevertheless, different modes are coupled by two- (and higher) body terms containing SQ operators belonging to different modes $l$ and $m$.Reference: Ollitrault, Pauline J., et al., arXiv:2003.12578 (2020). Compute the electronic potentialSolving the ESE for different nuclear configurations to obtain the PES function $V(Q_1, \ldots, Q_L)$. So far Qiskit gives the possibility to approximate the PES with a quartic force field. $$V(Q_1, \ldots, Q_L) = \frac{1}{2} \sum_{ij} k_{ij} Q_i Q_j + \frac{1}{6} \sum_{ijk} k_{ijk} Q_i Q_j Q_k + \frac{1}{16} \sum_{ijkl} k_{ijkl} Q_i Q_j Q_k Q_l$$The advantage of such form for the PES is that the anharmonic force fields ($k_{ij}$, $k_{ijk}$, $k_{ijkl}$) can be calculated by finite-difference approaches. For methods for which the nuclear energy Hessian can be calculated analytically with response theory-based methods (such as HF and DFT), the quartic force field can be calculated by semi-numerical differentiation as:$$k_{ijk} = \frac{H_{ij}(+\delta Q_k) - H_{ij}(-\delta Q_k)}{2\delta Q_k}$$and$$k_{ijkl} = \frac{H_{ij}(+\delta Q_k+\delta Q_l) - H_{ij}(+\delta Q_k-\delta Q_l) -H_{ij}(-\delta Q_k+\delta Q_l) + H_{ij}(-\delta Q_k+\delta Q_l)} {4\delta Q_k \delta Q_l}$$Such numerical procedure is implemented, for instance, in the Gaussian suite of programs.In practice this can be done with Qiskit using the GaussianForceDriver.
###Code
from qiskit_nature.drivers.second_quantization import GaussianForcesDriver
# if you ran Gaussian elsewhere and already have the output file
driver = GaussianForcesDriver(logfile='aux_files/CO2_freq_B3LYP_ccpVDZ.log')
# if you want to run the Gaussian job from Qiskit
# driver = GaussianForcesDriver(
# ['#p B3LYP/6-31g Freq=(Anharm) Int=Ultrafine SCF=VeryTight',
# '',
# 'CO2 geometry optimization B3LYP/6-31g',
# '',
# '0 1',
# 'C -0.848629 2.067624 0.160992',
# 'O 0.098816 2.655801 -0.159738',
# 'O -1.796073 1.479446 0.481721',
# '',
# ''
###Output
_____no_output_____
###Markdown
Map to a qubit HamiltonianNow that we have an approximation for the potential, we need to write the Hamiltonian in second quantization. To this end we need to select a modal basis to calculate the one-body integrals $\langle\phi_{k_i}| V(Q_i) | \phi_{h_i} \rangle$, two-body integrals $\langle\phi_{k_i} \phi_{k_j}| V(Q_i,Q_j) | \phi_{h_i} \phi_{h_j} \rangle$... In the simplest case, the $\phi$ functions are the harmonic-oscillator eigenfunctions for each mode. The main advantage of this choice is that the integrals of a PES expressed as a Taylor expansion are easy to calculate with such basis. A routine for computing these integrals is implemented in Qiskit. The bosonic operator, $\mathcal{H}_\text{vib}^{SQ}$, is then created and must be mapped to a qubit operator. The direct mapping introduced in the first section of this tutorial can be used is Qiskit as follows:
###Code
from qiskit_nature.problems.second_quantization import VibrationalStructureProblem
from qiskit_nature.converters.second_quantization import QubitConverter
from qiskit_nature.mappers.second_quantization import DirectMapper
vibrational_problem = VibrationalStructureProblem(driver, num_modals=2, truncation_order=2)
second_q_ops = vibrational_problem.second_q_ops()
###Output
_____no_output_____
###Markdown
The Vibrational operator for the problem now reads as
###Code
print(second_q_ops[0])
###Output
NIIIIIII * (1215.682529375+0j)
+ INIIIIII * (3656.9551768750007+0j)
+ IINIIIII * (682.5053337500001+0j)
+ II+-IIII * (-46.77167173323271+0j)
+ II-+IIII * (-46.77167173323271+0j)
+ IIINIIII * (2050.1464387500005+0j)
+ IIIINIII * (329.41209562500006+0j)
+ IIIIINII * (992.0224281250003+0j)
+ IIIIIINI * (328.12046812500006+0j)
+ IIIIIIIN * (985.5642906250002+0j)
+ NINIIIII * (5.039653750000002+0j)
+ INNIIIII * (15.118961250000009+0j)
+ NI+-IIII * (-89.0908653064951+0j)
+ IN+-IIII * (-267.27259591948535+0j)
+ NI-+IIII * (-89.0908653064951+0j)
+ IN-+IIII * (-267.27259591948535+0j)
+ NIINIIII * (15.118961250000009+0j)
+ ININIIII * (45.35688375000003+0j)
+ NIIINIII * (-6.3850425000000035+0j)
+ INIINIII * (-19.15512750000001+0j)
+ IININIII * (-2.5657231250000008+0j)
+ II+-NIII * (21.644966371722845+0j)
+ II-+NIII * (21.644966371722845+0j)
+ IIINNIII * (-7.697169375000003+0j)
+ +-II+-II * (-2.0085637500000004+0j)
+ -+II+-II * (-2.0085637500000004+0j)
+ +-II-+II * (-2.0085637500000004+0j)
+ -+II-+II * (-2.0085637500000004+0j)
+ NIIIINII * (-19.15512750000001+0j)
+ INIIINII * (-57.46538250000003+0j)
+ IINIINII * (-7.697169375000004+0j)
+ II+-INII * (64.93489911516855+0j)
+ II-+INII * (64.93489911516855+0j)
+ IIININII * (-23.091508125000015+0j)
+ NIIIIINI * (-4.595841875000001+0j)
+ INIIIINI * (-13.787525625000006+0j)
+ IINIIINI * (-1.683979375000001+0j)
+ II+-IINI * (6.412754934114709+0j)
+ II-+IINI * (6.412754934114709+0j)
+ IIINIINI * (-5.051938125000003+0j)
+ IIIININI * (-0.5510218750000002+0j)
+ IIIIINNI * (-1.6530656250000009+0j)
+ +-IIII+- * (3.5921675000000004+0j)
+ -+IIII+- * (3.5921675000000004+0j)
+ IIII+-+- * (7.946551250000004+0j)
+ IIII-++- * (7.946551250000004+0j)
+ +-IIII-+ * (3.5921675000000004+0j)
+ -+IIII-+ * (3.5921675000000004+0j)
+ IIII+--+ * (7.946551250000004+0j)
+ IIII-+-+ * (7.946551250000004+0j)
+ NIIIIIIN * (-13.787525625000006+0j)
+ INIIIIIN * (-41.362576875000016+0j)
+ IINIIIIN * (-5.051938125000002+0j)
+ II+-IIIN * (19.238264802344126+0j)
+ II-+IIIN * (19.238264802344126+0j)
+ IIINIIIN * (-15.15581437500001+0j)
+ IIIINIIN * (-1.6530656250000009+0j)
+ IIIIININ * (-4.959196875000003+0j)
###Markdown
In the previous cell we defined a bosonic transformation to express the Hamiltonian in the harmonic modal basis, with 2 modals per mode with the potential truncated at order 2 and the 'direct' boson to qubit mapping. The calculation is then ran as:
###Code
qubit_converter = QubitConverter(mapper=DirectMapper())
qubit_op = qubit_converter.convert(second_q_ops[0])
print(qubit_op)
###Output
5077.236560625012 * IIIIIIII
- 472.28961593750034 * ZIIIIIII
- 157.22939093749991 * IZIIIIII
- 467.5058515625001 * IIZIIIII
- 1.2397992187500007 * ZIZIIIII
- 0.4132664062500002 * IZZIIIII
- 155.20426031249983 * IIIZIIII
- 0.4132664062500002 * ZIIZIIII
- 0.13775546875000005 * IZIZIIII
- 1027.4430731249997 * IIIIZIII
- 3.7889535937500023 * ZIIIZIII
- 1.2629845312500008 * IZIIZIII
- 5.772877031250004 * IIZIZIII
- 1.9242923437500008 * IIIZZIII
- 342.04261812500033 * IIIIIZII
- 1.2629845312500005 * ZIIIIZII
- 0.42099484375000024 * IZIIIZII
- 1.924292343750001 * IIZIIZII
- 0.6414307812500002 * IIIZIZII
- 1810.6538965625004 * IIIIIIZI
- 10.340644218750004 * ZIIIIIZI
- 3.4468814062500015 * IZIIIIZI
- 14.366345625000008 * IIZIIIZI
- 4.788781875000002 * IIIZIIZI
+ 11.339220937500007 * IIIIZIZI
+ 3.779740312500002 * IIIIIZZI
- 601.9000340624999 * IIIIIIIZ
- 3.4468814062500015 * ZIIIIIIZ
- 1.1489604687500004 * IZIIIIIZ
- 4.788781875000002 * IIZIIIIZ
- 1.5962606250000009 * IIIZIIIZ
+ 3.779740312500002 * IIIIZIIZ
+ 1.2599134375000005 * IIIIIZIZ
+ 1.986637812500001 * XXXXIIII
+ 1.986637812500001 * YYXXIIII
+ 1.986637812500001 * XXYYIIII
+ 1.986637812500001 * YYYYIIII
- 84.41897986727398 * IIIIXXII
- 4.809566200586032 * ZIIIXXII
- 1.6031887335286772 * IZIIXXII
- 16.233724778792137 * IIZIXXII
- 5.411241592930711 * IIIZXXII
- 84.41897986727398 * IIIIYYII
- 4.809566200586032 * ZIIIYYII
- 1.6031887335286772 * IZIIYYII
- 16.233724778792137 * IIZIYYII
- 5.411241592930711 * IIIZYYII
+ 66.81814897987134 * IIIIXXZI
+ 66.81814897987134 * IIIIYYZI
+ 22.272716326623776 * IIIIXXIZ
+ 22.272716326623776 * IIIIYYIZ
+ 0.8980418750000001 * XXIIIIXX
+ 0.8980418750000001 * YYIIIIXX
+ 0.8980418750000001 * XXIIIIYY
+ 0.8980418750000001 * YYIIIIYY
- 0.5021409375000001 * IIXXIIXX
- 0.5021409375000001 * IIYYIIXX
- 0.5021409375000001 * IIXXIIYY
- 0.5021409375000001 * IIYYIIYY
###Markdown
To have a different number of modals per mode:
###Code
vibrational_problem = VibrationalStructureProblem(driver, num_modals=3, truncation_order=2)
second_q_ops = vibrational_problem.second_q_ops()
qubit_converter = QubitConverter(mapper = DirectMapper())
qubit_op = qubit_converter.convert(second_q_ops[0])
print(qubit_op)
###Output
11327.034966562516 * IIIIIIIIIIII
- 745.8599576562498 * ZIIIIIIIIIII
- 446.6739542187503 * IZIIIIIIIIII
- 148.69083703124986 * IIZIIIIIIIII
- 724.2074121874995 * IIIZIIIIIIII
- 3.4438867187500013 * ZIIZIIIIIIII
- 2.066332031250001 * IZIZIIIIIIII
- 0.6887773437500002 * IIZZIIIIIIII
- 431.8741484375 * IIIIZIIIIIII
- 2.066332031250001 * ZIIIZIIIIIII
- 1.2397992187500007 * IZIIZIIIIIII
- 0.4132664062500002 * IIZIZIIIIIII
- 143.32702593749988 * IIIIIZIIIIII
- 0.6887773437500002 * ZIIIIZIIIIII
- 0.4132664062500002 * IZIIIZIIIIII
- 0.13775546875000005 * IIZIIZIIIIII
- 1720.4111609375004 * IIIIIIZIIIII
- 10.524871093750003 * ZIIIIIZIIIII
- 6.314922656250003 * IZIIIIZIIIII
- 2.104974218750001 * IIZIIIZIIIII
- 16.035769531250004 * IIIZIIZIIIII
- 9.621461718750005 * IIIIZIZIIIII
- 3.207153906250001 * IIIIIZZIIIII
- 1030.4053903124998 * IIIIIIIZIIII
- 6.314922656250003 * ZIIIIIIZIIII
- 3.7889535937500023 * IZIIIIIZIIII
- 1.2629845312500008 * IIZIIIIZIIII
- 9.621461718750004 * IIIZIIIZIIII
- 5.772877031250004 * IIIIZIIZIIII
- 1.9242923437500008 * IIIIIZIZIIII
- 343.0300571875006 * IIIIIIIIZIII
- 2.1049742187500007 * ZIIIIIIIZIII
- 1.2629845312500005 * IZIIIIIIZIII
- 0.42099484375000024 * IIZIIIIIZIII
- 3.207153906250001 * IIIZIIIIZIII
- 1.924292343750001 * IIIIZIIIZIII
- 0.6414307812500002 * IIIIIZIIZIII
- 2992.18265640625 * IIIIIIIIIZII
- 28.72401171875001 * ZIIIIIIIIZII
- 17.234407031250004 * IZIIIIIIIZII
- 5.744802343750002 * IIZIIIIIIZII
- 39.90651562500001 * IIIZIIIIIZII
- 23.94390937500001 * IIIIZIIIIZII
- 7.981303125000004 * IIIIIZIIIZII
+ 31.497835937500014 * IIIIIIZIIZII
+ 18.89870156250001 * IIIIIIIZIZII
+ 6.299567187500003 * IIIIIIIIZZII
- 1788.3742817187504 * IIIIIIIIIIZI
- 17.234407031250008 * ZIIIIIIIIIZI
- 10.340644218750004 * IZIIIIIIIIZI
- 3.4468814062500015 * IIZIIIIIIIZI
- 23.94390937500001 * IIIZIIIIIIZI
- 14.366345625000008 * IIIIZIIIIIZI
- 4.788781875000002 * IIIIIZIIIIZI
+ 18.898701562500012 * IIIIIIZIIIZI
+ 11.339220937500007 * IIIIIIIZIIZI
+ 3.779740312500002 * IIIIIIIIZIZI
- 594.4734957812499 * IIIIIIIIIIIZ
- 5.744802343750002 * ZIIIIIIIIIIZ
- 3.4468814062500015 * IZIIIIIIIIIZ
- 1.1489604687500004 * IIZIIIIIIIIZ
- 7.981303125000003 * IIIZIIIIIIIZ
- 4.788781875000002 * IIIIZIIIIIIZ
- 1.5962606250000009 * IIIIIZIIIIIZ
+ 6.299567187500004 * IIIIIIZIIIIZ
+ 3.779740312500002 * IIIIIIIZIIIZ
+ 1.2599134375000005 * IIIIIIIIZIIZ
- 20.885040702719472 * XIXIIIIIIIII
- 20.885040702719472 * YIYIIIIIIIII
+ 0.9740782609865658 * XIXZIIIIIIII
+ 0.9740782609865658 * YIYZIIIIIIII
+ 0.5844469565919396 * XIXIZIIIIIII
+ 0.5844469565919396 * YIYIZIIIIIII
+ 0.19481565219731317 * XIXIIZIIIIII
+ 0.19481565219731317 * YIYIIZIIIIII
+ 2.9768830886019613 * XIXIIIZIIIII
+ 2.9768830886019613 * YIYIIIZIIIII
+ 1.7861298531611771 * XIXIIIIZIIII
+ 1.7861298531611771 * YIYIIIIZIIII
+ 0.5953766177203923 * XIXIIIIIZIII
+ 0.5953766177203923 * YIYIIIIIZIII
+ 8.124377387683996 * XIXIIIIIIZII
+ 8.124377387683996 * YIYIIIIIIZII
+ 4.874626432610398 * XIXIIIIIIIZI
+ 4.874626432610398 * YIYIIIIIIIZI
+ 1.6248754775367993 * XIXIIIIIIIIZ
+ 1.6248754775367993 * YIYIIIIIIIIZ
+ 7.946551250000002 * XXIXXIIIIIII
+ 7.946551250000002 * YYIXXIIIIIII
+ 7.946551250000002 * XXIYYIIIIIII
+ 7.946551250000002 * YYIYYIIIIIII
+ 4.245123515804727 * IXXXXIIIIIII
+ 4.245123515804727 * IYYXXIIIIIII
+ 4.245123515804727 * IXXYYIIIIIII
+ 4.245123515804727 * IYYYYIIIIIII
- 27.557296533490963 * IIIXIXIIIIII
+ 0.9740782609865657 * ZIIXIXIIIIII
+ 0.5844469565919395 * IZIXIXIIIIII
+ 0.19481565219731314 * IIZXIXIIIIII
- 27.557296533490963 * IIIYIYIIIIII
+ 0.9740782609865657 * ZIIYIYIIIIII
+ 0.5844469565919395 * IZIYIYIIIIII
+ 0.19481565219731314 * IIZYIYIIIIII
+ 4.535600550836601 * IIIXIXZIIIII
+ 4.535600550836601 * IIIYIYZIIIII
+ 2.721360330501961 * IIIXIXIZIIII
+ 2.721360330501961 * IIIYIYIZIIII
+ 0.9071201101673203 * IIIXIXIIZIII
+ 0.9071201101673203 * IIIYIYIIZIII
+ 11.287267124785771 * IIIXIXIIIZII
+ 11.287267124785771 * IIIYIYIIIZII
+ 6.772360274871463 * IIIXIXIIIIZI
+ 6.772360274871463 * IIIYIYIIIIZI
+ 2.2574534249571543 * IIIXIXIIIIIZ
+ 2.2574534249571543 * IIIYIYIIIIIZ
- 0.2755109375000001 * XIXXIXIIIIII
- 0.2755109375000001 * YIYXIXIIIIII
- 0.2755109375000001 * XIXYIYIIIIII
- 0.2755109375000001 * YIYYIYIIIIII
+ 4.1834668980774286 * XXIIXXIIIIII
+ 4.1834668980774286 * YYIIXXIIIIII
+ 4.1834668980774286 * XXIIYYIIIIII
+ 4.1834668980774286 * YYIIYYIIIIII
+ 1.986637812500001 * IXXIXXIIIIII
+ 1.986637812500001 * IYYIXXIIIIII
+ 1.986637812500001 * IXXIYYIIIIII
+ 1.986637812500001 * IYYIYYIIIIII
- 260.3514075 * IIIIIIXXIIII
- 11.336256250000002 * ZIIIIIXXIIII
- 6.801753750000003 * IZIIIIXXIIII
- 2.2672512500000006 * IIZIIIXXIIII
- 38.26325625000001 * IIIZIIXXIIII
- 22.95795375000001 * IIIIZIXXIIII
- 7.652651250000002 * IIIIIZXXIIII
- 260.3514075 * IIIIIIYYIIII
- 11.336256250000002 * ZIIIIIYYIIII
- 6.801753750000003 * IZIIIIYYIIII
- 2.2672512500000006 * IIZIIIYYIIII
- 38.26325625000001 * IIIZIIYYIIII
- 22.95795375000001 * IIIIZIYYIIII
- 7.652651250000002 * IIIIIZYYIIII
+ 157.49188750000002 * IIIIIIXXIZII
+ 157.49188750000002 * IIIIIIYYIZII
+ 94.49513250000001 * IIIIIIXXIIZI
+ 94.49513250000001 * IIIIIIYYIIZI
+ 31.498377500000004 * IIIIIIXXIIIZ
+ 31.498377500000004 * IIIIIIYYIIIZ
+ 3.206377467057354 * XIXIIIXXIIII
+ 3.206377467057354 * YIYIIIXXIIII
+ 3.206377467057354 * XIXIIIYYIIII
+ 3.206377467057354 * YIYIIIYYIIII
+ 10.822483185861422 * IIIXIXXXIIII
+ 10.822483185861422 * IIIYIYXXIIII
+ 10.822483185861422 * IIIXIXYYIIII
+ 10.822483185861422 * IIIYIYYYIIII
+ 4.373609679305444 * IIIIIIXIXIII
+ 2.9768830886019613 * ZIIIIIXIXIII
+ 1.786129853161177 * IZIIIIXIXIII
+ 0.5953766177203923 * IIZIIIXIXIII
+ 4.535600550836601 * IIIZIIXIXIII
+ 2.721360330501961 * IIIIZIXIXIII
+ 0.9071201101673203 * IIIIIZXIXIII
+ 4.373609679305444 * IIIIIIYIYIII
+ 2.9768830886019613 * ZIIIIIYIYIII
+ 1.786129853161177 * IZIIIIYIYIII
+ 0.5953766177203923 * IIZIIIYIYIII
+ 4.535600550836601 * IIIZIIYIYIII
+ 2.721360330501961 * IIIIZIYIYIII
+ 0.9071201101673203 * IIIIIZYIYIII
- 8.90893335364304 * IIIIIIXIXZII
- 8.90893335364304 * IIIIIIYIYZII
- 5.345360012185824 * IIIIIIXIXIZI
- 5.345360012185824 * IIIIIIYIYIZI
- 1.7817866707286079 * IIIIIIXIXIIZ
- 1.7817866707286079 * IIIIIIYIYIIZ
- 0.8419896875000004 * XIXIIIXIXIII
- 0.8419896875000004 * YIYIIIXIXIII
- 0.8419896875000004 * XIXIIIYIYIII
- 0.8419896875000004 * YIYIIIYIYIII
- 1.2828615625000004 * IIIXIXXIXIII
- 1.2828615625000004 * IIIYIYXIXIII
- 1.2828615625000004 * IIIXIXYIYIII
- 1.2828615625000004 * IIIYIYYIYIII
- 160.71040986809584 * IIIIIIIXXIII
- 8.015943667643384 * ZIIIIIIXXIII
- 4.809566200586032 * IZIIIIIXXIII
- 1.6031887335286772 * IIZIIIIXXIII
- 27.05620796465356 * IIIZIIIXXIII
- 16.233724778792137 * IIIIZIIXXIII
- 5.411241592930711 * IIIIIZIXXIII
- 160.71040986809584 * IIIIIIIYYIII
- 8.015943667643384 * ZIIIIIIYYIII
- 4.809566200586032 * IZIIIIIYYIII
- 1.6031887335286772 * IIZIIIIYYIII
- 27.05620796465356 * IIIZIIIYYIII
- 16.233724778792137 * IIIIZIIYYIII
- 5.411241592930711 * IIIIIZIYYIII
+ 111.36358163311888 * IIIIIIIXXZII
+ 111.36358163311888 * IIIIIIIYYZII
+ 66.81814897987134 * IIIIIIIXXIZI
+ 66.81814897987134 * IIIIIIIYYIZI
+ 22.272716326623776 * IIIIIIIXXIIZ
+ 22.272716326623776 * IIIIIIIYYIIZ
+ 2.267251250000001 * XIXIIIIXXIII
+ 2.267251250000001 * YIYIIIIXXIII
+ 2.267251250000001 * XIXIIIIYYIII
+ 2.267251250000001 * YIYIIIIYYIII
+ 7.6526512500000035 * IIIXIXIXXIII
+ 7.6526512500000035 * IIIYIYIXXIII
+ 7.6526512500000035 * IIIXIXIYYIII
+ 7.6526512500000035 * IIIYIYIYYIII
+ 3.5921675000000004 * XXIIIIIIIXXI
+ 3.5921675000000004 * YYIIIIIIIXXI
+ 3.5921675000000004 * XXIIIIIIIYYI
+ 3.5921675000000004 * YYIIIIIIIYYI
+ 5.912498983512137 * IXXIIIIIIXXI
+ 5.912498983512137 * IYYIIIIIIXXI
+ 5.912498983512137 * IXXIIIIIIYYI
+ 5.912498983512137 * IYYIIIIIIYYI
- 2.0085637500000013 * IIIXXIIIIXXI
- 2.0085637500000013 * IIIYYIIIIXXI
- 2.0085637500000013 * IIIXXIIIIYYI
- 2.0085637500000013 * IIIYYIIIIYYI
- 3.305978760488338 * IIIIXXIIIXXI
- 3.305978760488338 * IIIIYYIIIXXI
- 3.305978760488338 * IIIIXXIIIYYI
- 3.305978760488338 * IIIIYYIIIYYI
- 11.899156895555553 * IIIIIIIIIXIX
+ 8.124377387683996 * ZIIIIIIIIXIX
+ 4.874626432610398 * IZIIIIIIIXIX
+ 1.6248754775367993 * IIZIIIIIIXIX
+ 11.28726712478577 * IIIZIIIIIXIX
+ 6.772360274871463 * IIIIZIIIIXIX
+ 2.2574534249571543 * IIIIIZIIIXIX
- 8.90893335364304 * IIIIIIZIIXIX
- 5.345360012185823 * IIIIIIIZIXIX
- 1.7817866707286076 * IIIIIIIIZXIX
- 11.899156895555553 * IIIIIIIIIYIY
+ 8.124377387683996 * ZIIIIIIIIYIY
+ 4.874626432610398 * IZIIIIIIIYIY
+ 1.6248754775367993 * IIZIIIIIIYIY
+ 11.28726712478577 * IIIZIIIIIYIY
+ 6.772360274871463 * IIIIZIIIIYIY
+ 2.2574534249571543 * IIIIIZIIIYIY
- 8.90893335364304 * IIIIIIZIIYIY
- 5.345360012185823 * IIIIIIIZIYIY
- 1.7817866707286076 * IIIIIIIIZYIY
- 2.2979209375000007 * XIXIIIIIIXIX
- 2.2979209375000007 * YIYIIIIIIXIX
- 2.2979209375000007 * XIXIIIIIIYIY
- 2.2979209375000007 * YIYIIIIIIYIY
- 3.1925212500000018 * IIIXIXIIIXIX
- 3.1925212500000018 * IIIYIYIIIXIX
- 3.1925212500000018 * IIIXIXIIIYIY
- 3.1925212500000018 * IIIYIYIIIYIY
- 44.54543265324755 * IIIIIIXXIXIX
- 44.54543265324755 * IIIIIIYYIXIX
- 44.54543265324755 * IIIIIIXXIYIY
- 44.54543265324755 * IIIIIIYYIYIY
+ 2.5198268750000015 * IIIIIIXIXXIX
+ 2.5198268750000015 * IIIIIIYIYXIX
+ 2.5198268750000015 * IIIIIIXIXYIY
+ 2.5198268750000015 * IIIIIIYIYYIY
- 31.498377500000007 * IIIIIIIXXXIX
- 31.498377500000007 * IIIIIIIYYXIX
- 31.498377500000007 * IIIIIIIXXYIY
- 31.498377500000007 * IIIIIIIYYYIY
- 2.102429985900245 * XXIIIIIIIIXX
- 2.102429985900245 * YYIIIIIIIIXX
- 2.102429985900245 * XXIIIIIIIIYY
- 2.102429985900245 * YYIIIIIIIIYY
+ 0.8980418750000001 * IXXIIIIIIIXX
+ 0.8980418750000001 * IYYIIIIIIIXX
+ 0.8980418750000001 * IXXIIIIIIIYY
+ 0.8980418750000001 * IYYIIIIIIIYY
+ 1.175575188382615 * IIIXXIIIIIXX
+ 1.175575188382615 * IIIYYIIIIIXX
+ 1.175575188382615 * IIIXXIIIIIYY
+ 1.175575188382615 * IIIYYIIIIIYY
- 0.5021409375000001 * IIIIXXIIIIXX
- 0.5021409375000001 * IIIIYYIIIIXX
- 0.5021409375000001 * IIIIXXIIIIYY
- 0.5021409375000001 * IIIIYYIIIIYY
###Markdown
Now that the Hamiltonian is ready, it can be used in a quantum algorithm to find information about the vibrational structure of the corresponding molecule. Check out our tutorials on Ground State Calculation and Excited States Calculation to learn more about how to do that in Qiskit Nature!
###Code
import qiskit.tools.jupyter
%qiskit_version_table
%qiskit_copyright
###Output
_____no_output_____
###Markdown
Vibrational structure Introduction The molecular Hamiltonian is $$\mathcal{H} = - \sum_I \frac{\nabla_{R_I}^2}{M_I} - \sum_i \frac{\nabla_{r_i}^2}{m_e} - \sum_I\sum_i \frac{Z_I e^2}{|R_I-r_i|} + \sum_i \sum_{j>i} \frac{e^2}{|r_i-r_j|} + \sum_I\sum_{J>I} \frac{Z_I Z_J e^2}{|R_I-R_J|}$$Because the nuclei are much heavier than the electrons they do not move on the same time scale and therefore, the behavior of nuclei and electrons can be decoupled. This is the Born-Oppenheimer approximation.Within the Born-Oppenheimer approximation, a molecular wave function is factorized as a product of an electronic part, which is the solution of the electronic Schroedinger equation, and a vibro-rotational one, which is the solution of the nuclear Schroedinger equation in the potential energy surface (PES) generated by sampling the eigenvalues of the electronic Schroedinger equation for different geometries.The nuclear Schroedinger equation is usually solved in two steps, in analogy with its electronic counterpart. A single-particle basis (the basis functions are called, in this case, modals) is obtained either by the harmonic approximation applied to the PES or from a vibrational self-consistent field (VSCF) calculation. Vibrational anharmonic correlations are added a-posteriori with perturbative or variational approaches.The latter include Vibrational Configuration Interaction (VCI) and Vibrational Coupled Cluster (VCC) for highly-accurate anharmonic energies. The main advantage of VCI and VCC over alternative approaches (such as perturbation theories) is that their accuracy can be systematically improved towards the complete basis set limit for a given PES. However, their applicability is limited to small molecules with up to about 10 atoms due to their unfavorable scaling with system size.To tackle the scaling problem we would like to use quantum algorithms.The nuclear Schroedinger equation is$$\mathcal{H}_{\text{vib}} |\Psi_{n}\rangle = E_{n} |\Psi_{n}\rangle$$The so-called Watson Hamiltonian (neglecting vibro-rotational coupling terms) is$$ \mathcal{H}_\text{vib}(Q_1, \ldots, Q_L) = - \frac{1}{2} \sum_{l=1}^{L} \frac{\partial^2}{\partial Q_l^2} + V(Q_1, \ldots, Q_L)$$where $Q_l$ are the harmonic mass-weighted normal coordinates.$\mathcal{H}_\text{vib}$ must be mapped to an operator that acts on the states of a given set of $N_q$ qubits in order to calculate its eigenfunctions on quantum hardware.In electronic structure calculations, the mapping is achieved by expressing the non-relativistic electronic Hamiltonian in second quantization, \textit{i.e.} by projecting it onto the complete set of antisymmetrized occupation number vectors (ONV) generated by a given (finite) set of orbitals.To encode the vibrational Hamiltonian in an analogous second quantization operators, we expand the potential $V(Q_1, \ldots, Q_L)$ with the $n$-body expansion as follows:$$ V(Q_1, \ldots, Q_L) = V_0 + \sum_{l=1}^L V^{[l]}(Q_l) + \sum_{l<m}^L V^{[l,m]}(Q_l, Q_m) + \sum_{l<m<n}^L V^{[l,m,n]}(Q_l, Q_m, Q_n) + \ldots$$where $V_0$ is the electronic energy of the reference geometry, the one-mode term $V^{[l]}(Q_l)$ represents the variation of the PES upon change of the $l$-th normal coordinate from the equilibrium position.Similarly, the two-body potential $V^{[l,m]}(Q_l, Q_m)$ represents the change in the exact PES upon a simultaneous displacement along the $l$-th and $m$-th coordinates. Often, including terms up to three-body in the $L$-body expansion is sufficient to obtain an accuracy of about 1~cm$^{-1}$. We highlight that the many-body expansion of the potential operator defining the Watson Hamiltonian contains arbitrarily high coupling terms. This is a crucial difference compared to the non-relativistic electronic-structure Hamiltonian that contains only pairwise interactions.A flexible second quantization form of the Watson Hamiltonian is obtained within the so-called n-mode representation. Let us assume that each mode $l$ is described by a $N_l$-dimensional basis set $S_l$ defined as follows:$$ \mathcal{S}_l = \{ \phi_1^{(l)} (Q_l) , \ldots , \phi_{N_l}^{(l)} (Q_l) \} \, .$$The $n$-mode wave function can be expanded in the product basis $\mathcal{S} = \otimes_{i=1}^L \mathcal{S}_i$ as the following CI-like expansion:$$ |\Psi\rangle = \sum_{k_1=1}^{N_1} \cdots \sum_{k_L=1}^{N_L} C_{k_1,\ldots,k_L} \phi_{k_1}^{(1)}(Q_1) \cdots \phi_{k_L}^{(L)}(Q_L) \, ,$$The many-body basis function $\phi_{k_1}^{(1)}(Q_1) \cdots \phi_{k_L}^{(L)}(Q_L)$ are encoded within the so-called $n$-mode second quantization as occupation-number vectors (ONVs) as follows:$$ \phi_{k_1}(Q_1) \cdots \phi_{k_L}(Q_L) \equiv |0_1 \cdots 1_{k_1} \cdots 0_{N_1}, 0_1 \cdots 1_{k_2} \cdots 0_{N_2}, \cdots , 0_1 \cdots 1_{k_L} \cdots 0_{N_L}\rangle \, .$$The ONV defined above is, therefore, the product of $L$ mode-specific ONVs, each one describing an individual mode. Since each mode is described by one and only one basis function, the occupation of each mode-specific ONV is one.From a theoretical perspective, each mode can be interpreted as a distinguishable quasi-particle (defined as phonons in solid-state physics). Distinguishability arises from the fact that the PES is not invariant by permutation of two modes, also in this case unlike the Coulomb interaction between two equal particles. From this perspective, a molecule can be interpreted as a collection of $L$ indistinguishable particles that interact through the PES operator.Based on this second-quantization representation we introduce a pair of creation and annihilation operators per mode $l$ \textit{and} per basis function $k_l$ defined as:$$ \begin{aligned} a_{k_l}^\dagger |\cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle &= | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle \\ a_{k_l}^\dagger | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle &= 0 \\ a_{k_l} | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle &= | \cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle \\ a_{k_l} | \cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle &= 0 \\ \end{aligned}$$with$$ \begin{aligned} \left[ a_{k_l}^\dagger, a_{h_m}^\dagger \right] &= 0 \\ \left[ a_{k_l}, a_{h_m} \right] &= 0 \\ \left[ a_{k_l}^\dagger, a_{h_m} \right] &= \delta_{l,m} \, , \delta_{k_l,h_m} \end{aligned}$$The second quantization form is obtained by expressing the potential as $$ \begin{aligned} \mathcal{H}_\text{vib}^{SQ} =& \sum_{l=1}^L \sum_{k_l,h_l}^{N_l} \langle \phi_{k_l} | T(Q_l) + V^{[l]}(Q_l) | \phi_{h_l} \rangle a_{k_l}^+ a_{h_l} \\ +& \sum_{l<m}^L \sum_{k_l,h_l}^{N_l} \sum_{k_m,h_m}^{N_m} \langle \phi_{k_l} \phi_{k_m} | V^{[l,m]}(Q_l, Q_m) | \phi_{h_l} \phi_{h_m} \rangle a_{k_l}^+ a_{k_m}^+ a_{h_l} a_{h_m} + \cdots \end{aligned}$$We highlight here the difference between the operators defined here above and the electronic structure one. First, as we already mentioned, the potential contains (in principle) three- and higher-body coupling terms that lead to strings with six (or more) second-quantization operators. Moreover, the Hamiltonian conserves the number of particles for each mode, as can be seen from the fact that the number of creation and annihilation operators for a given mode is the same in each term. Nevertheless, different modes are coupled by two- (and higher) body terms containing SQ operators belonging to different modes $l$ and $m$.Reference: Ollitrault, Pauline J., et al., arXiv:2003.12578 (2020). Compute the electronic potentialSolving the ESE for different nuclear configurations to obtain the PES function $V(Q_1, \ldots, Q_L)$. So far Qiskit gives the possibility to approximate the PES with a quartic force field. $$V(Q_1, \ldots, Q_L) = \frac{1}{2} \sum_{ij} k_{ij} Q_i Q_j + \frac{1}{6} \sum_{ijk} k_{ijk} Q_i Q_j Q_k + \frac{1}{16} \sum_{ijkl} k_{ijkl} Q_i Q_j Q_k Q_l$$The advantage of such form for the PES is that the anharmonic force fields ($k_{ij}$, $k_{ijk}$, $k_{ijkl}$) can be calculated by finite-difference approaches. For methods for which the nuclear energy Hessian can be calculated analytically with response theory-based methods (such as HF and DFT), the quartic force field can be calculated by semi-numerical differentiation as:$$k_{ijk} = \frac{H_{ij}(+\delta Q_k) - H_{ij}(-\delta Q_k)}{2\delta Q_k}$$and$$k_{ijkl} = \frac{H_{ij}(+\delta Q_k+\delta Q_l) - H_{ij}(+\delta Q_k-\delta Q_l) -H_{ij}(-\delta Q_k+\delta Q_l) + H_{ij}(-\delta Q_k+\delta Q_l)} {4\delta Q_k \delta Q_l}$$Such numerical procedure is implemented, for instance, in the Gaussian suite of programs.In practice this can be done with Qiskit using the GaussianForceDriver.
###Code
from qiskit_nature.drivers.second_quantization import GaussianForcesDriver
# if you ran Gaussian elsewhere and already have the output file
driver = GaussianForcesDriver(logfile="aux_files/CO2_freq_B3LYP_ccpVDZ.log")
# if you want to run the Gaussian job from Qiskit
# driver = GaussianForcesDriver(
# ['#p B3LYP/6-31g Freq=(Anharm) Int=Ultrafine SCF=VeryTight',
# '',
# 'CO2 geometry optimization B3LYP/6-31g',
# '',
# '0 1',
# 'C -0.848629 2.067624 0.160992',
# 'O 0.098816 2.655801 -0.159738',
# 'O -1.796073 1.479446 0.481721',
# '',
# ''
###Output
_____no_output_____
###Markdown
Map to a qubit HamiltonianNow that we have an approximation for the potential, we need to write the Hamiltonian in second quantization. To this end we need to select a modal basis to calculate the one-body integrals $\langle\phi_{k_i}| V(Q_i) | \phi_{h_i} \rangle$, two-body integrals $\langle\phi_{k_i} \phi_{k_j}| V(Q_i,Q_j) | \phi_{h_i} \phi_{h_j} \rangle$... In the simplest case, the $\phi$ functions are the harmonic-oscillator eigenfunctions for each mode. The main advantage of this choice is that the integrals of a PES expressed as a Taylor expansion are easy to calculate with such basis. A routine for computing these integrals is implemented in Qiskit. The bosonic operator, $\mathcal{H}_\text{vib}^{SQ}$, is then created and must be mapped to a qubit operator. The direct mapping introduced in the first section of this tutorial can be used is Qiskit as follows:
###Code
from qiskit_nature.problems.second_quantization import VibrationalStructureProblem
from qiskit_nature.converters.second_quantization import QubitConverter
from qiskit_nature.mappers.second_quantization import DirectMapper
vibrational_problem = VibrationalStructureProblem(driver, num_modals=2, truncation_order=2)
second_q_ops = vibrational_problem.second_q_ops()
###Output
_____no_output_____
###Markdown
The Vibrational operator for the problem now reads as
###Code
print(second_q_ops[0])
###Output
NIIIIIII * (1215.682529375+0j)
+ INIIIIII * (3656.9551768750007+0j)
+ IINIIIII * (682.5053337500001+0j)
+ II+-IIII * (-46.77167173323271+0j)
+ II-+IIII * (-46.77167173323271+0j)
+ IIINIIII * (2050.1464387500005+0j)
+ IIIINIII * (329.41209562500006+0j)
+ IIIIINII * (992.0224281250003+0j)
+ IIIIIINI * (328.12046812500006+0j)
+ IIIIIIIN * (985.5642906250002+0j)
+ NINIIIII * (5.039653750000002+0j)
+ INNIIIII * (15.118961250000009+0j)
+ NI+-IIII * (-89.0908653064951+0j)
+ IN+-IIII * (-267.27259591948535+0j)
+ NI-+IIII * (-89.0908653064951+0j)
+ IN-+IIII * (-267.27259591948535+0j)
+ NIINIIII * (15.118961250000009+0j)
+ ININIIII * (45.35688375000003+0j)
+ NIIINIII * (-6.3850425000000035+0j)
+ INIINIII * (-19.15512750000001+0j)
+ IININIII * (-2.5657231250000008+0j)
+ II+-NIII * (21.644966371722845+0j)
+ II-+NIII * (21.644966371722845+0j)
+ IIINNIII * (-7.697169375000003+0j)
+ +-II+-II * (-2.0085637500000004+0j)
+ -+II+-II * (-2.0085637500000004+0j)
+ +-II-+II * (-2.0085637500000004+0j)
+ -+II-+II * (-2.0085637500000004+0j)
+ NIIIINII * (-19.15512750000001+0j)
+ INIIINII * (-57.46538250000003+0j)
+ IINIINII * (-7.697169375000004+0j)
+ II+-INII * (64.93489911516855+0j)
+ II-+INII * (64.93489911516855+0j)
+ IIININII * (-23.091508125000015+0j)
+ NIIIIINI * (-4.595841875000001+0j)
+ INIIIINI * (-13.787525625000006+0j)
+ IINIIINI * (-1.683979375000001+0j)
+ II+-IINI * (6.412754934114709+0j)
+ II-+IINI * (6.412754934114709+0j)
+ IIINIINI * (-5.051938125000003+0j)
+ IIIININI * (-0.5510218750000002+0j)
+ IIIIINNI * (-1.6530656250000009+0j)
+ +-IIII+- * (3.5921675000000004+0j)
+ -+IIII+- * (3.5921675000000004+0j)
+ IIII+-+- * (7.946551250000004+0j)
+ IIII-++- * (7.946551250000004+0j)
+ +-IIII-+ * (3.5921675000000004+0j)
+ -+IIII-+ * (3.5921675000000004+0j)
+ IIII+--+ * (7.946551250000004+0j)
+ IIII-+-+ * (7.946551250000004+0j)
+ NIIIIIIN * (-13.787525625000006+0j)
+ INIIIIIN * (-41.362576875000016+0j)
+ IINIIIIN * (-5.051938125000002+0j)
+ II+-IIIN * (19.238264802344126+0j)
+ II-+IIIN * (19.238264802344126+0j)
+ IIINIIIN * (-15.15581437500001+0j)
+ IIIINIIN * (-1.6530656250000009+0j)
+ IIIIININ * (-4.959196875000003+0j)
###Markdown
In the previous cell we defined a bosonic transformation to express the Hamiltonian in the harmonic modal basis, with 2 modals per mode with the potential truncated at order 2 and the 'direct' boson to qubit mapping. The calculation is then ran as:
###Code
qubit_converter = QubitConverter(mapper=DirectMapper())
qubit_op = qubit_converter.convert(second_q_ops[0])
print(qubit_op)
###Output
5077.236560625012 * IIIIIIII
- 472.28961593750034 * ZIIIIIII
- 157.22939093749991 * IZIIIIII
- 467.5058515625001 * IIZIIIII
- 1.2397992187500007 * ZIZIIIII
- 0.4132664062500002 * IZZIIIII
- 155.20426031249983 * IIIZIIII
- 0.4132664062500002 * ZIIZIIII
- 0.13775546875000005 * IZIZIIII
- 1027.4430731249997 * IIIIZIII
- 3.7889535937500023 * ZIIIZIII
- 1.2629845312500008 * IZIIZIII
- 5.772877031250004 * IIZIZIII
- 1.9242923437500008 * IIIZZIII
- 342.04261812500033 * IIIIIZII
- 1.2629845312500005 * ZIIIIZII
- 0.42099484375000024 * IZIIIZII
- 1.924292343750001 * IIZIIZII
- 0.6414307812500002 * IIIZIZII
- 1810.6538965625004 * IIIIIIZI
- 10.340644218750004 * ZIIIIIZI
- 3.4468814062500015 * IZIIIIZI
- 14.366345625000008 * IIZIIIZI
- 4.788781875000002 * IIIZIIZI
+ 11.339220937500007 * IIIIZIZI
+ 3.779740312500002 * IIIIIZZI
- 601.9000340624999 * IIIIIIIZ
- 3.4468814062500015 * ZIIIIIIZ
- 1.1489604687500004 * IZIIIIIZ
- 4.788781875000002 * IIZIIIIZ
- 1.5962606250000009 * IIIZIIIZ
+ 3.779740312500002 * IIIIZIIZ
+ 1.2599134375000005 * IIIIIZIZ
+ 1.986637812500001 * XXXXIIII
+ 1.986637812500001 * YYXXIIII
+ 1.986637812500001 * XXYYIIII
+ 1.986637812500001 * YYYYIIII
- 84.41897986727398 * IIIIXXII
- 4.809566200586032 * ZIIIXXII
- 1.6031887335286772 * IZIIXXII
- 16.233724778792137 * IIZIXXII
- 5.411241592930711 * IIIZXXII
- 84.41897986727398 * IIIIYYII
- 4.809566200586032 * ZIIIYYII
- 1.6031887335286772 * IZIIYYII
- 16.233724778792137 * IIZIYYII
- 5.411241592930711 * IIIZYYII
+ 66.81814897987134 * IIIIXXZI
+ 66.81814897987134 * IIIIYYZI
+ 22.272716326623776 * IIIIXXIZ
+ 22.272716326623776 * IIIIYYIZ
+ 0.8980418750000001 * XXIIIIXX
+ 0.8980418750000001 * YYIIIIXX
+ 0.8980418750000001 * XXIIIIYY
+ 0.8980418750000001 * YYIIIIYY
- 0.5021409375000001 * IIXXIIXX
- 0.5021409375000001 * IIYYIIXX
- 0.5021409375000001 * IIXXIIYY
- 0.5021409375000001 * IIYYIIYY
###Markdown
To have a different number of modals per mode:
###Code
vibrational_problem = VibrationalStructureProblem(driver, num_modals=3, truncation_order=2)
second_q_ops = vibrational_problem.second_q_ops()
qubit_converter = QubitConverter(mapper=DirectMapper())
qubit_op = qubit_converter.convert(second_q_ops[0])
print(qubit_op)
###Output
11327.034966562516 * IIIIIIIIIIII
- 745.8599576562498 * ZIIIIIIIIIII
- 446.6739542187503 * IZIIIIIIIIII
- 148.69083703124986 * IIZIIIIIIIII
- 724.2074121874995 * IIIZIIIIIIII
- 3.4438867187500013 * ZIIZIIIIIIII
- 2.066332031250001 * IZIZIIIIIIII
- 0.6887773437500002 * IIZZIIIIIIII
- 431.8741484375 * IIIIZIIIIIII
- 2.066332031250001 * ZIIIZIIIIIII
- 1.2397992187500007 * IZIIZIIIIIII
- 0.4132664062500002 * IIZIZIIIIIII
- 143.32702593749988 * IIIIIZIIIIII
- 0.6887773437500002 * ZIIIIZIIIIII
- 0.4132664062500002 * IZIIIZIIIIII
- 0.13775546875000005 * IIZIIZIIIIII
- 1720.4111609375004 * IIIIIIZIIIII
- 10.524871093750003 * ZIIIIIZIIIII
- 6.314922656250003 * IZIIIIZIIIII
- 2.104974218750001 * IIZIIIZIIIII
- 16.035769531250004 * IIIZIIZIIIII
- 9.621461718750005 * IIIIZIZIIIII
- 3.207153906250001 * IIIIIZZIIIII
- 1030.4053903124998 * IIIIIIIZIIII
- 6.314922656250003 * ZIIIIIIZIIII
- 3.7889535937500023 * IZIIIIIZIIII
- 1.2629845312500008 * IIZIIIIZIIII
- 9.621461718750004 * IIIZIIIZIIII
- 5.772877031250004 * IIIIZIIZIIII
- 1.9242923437500008 * IIIIIZIZIIII
- 343.0300571875006 * IIIIIIIIZIII
- 2.1049742187500007 * ZIIIIIIIZIII
- 1.2629845312500005 * IZIIIIIIZIII
- 0.42099484375000024 * IIZIIIIIZIII
- 3.207153906250001 * IIIZIIIIZIII
- 1.924292343750001 * IIIIZIIIZIII
- 0.6414307812500002 * IIIIIZIIZIII
- 2992.18265640625 * IIIIIIIIIZII
- 28.72401171875001 * ZIIIIIIIIZII
- 17.234407031250004 * IZIIIIIIIZII
- 5.744802343750002 * IIZIIIIIIZII
- 39.90651562500001 * IIIZIIIIIZII
- 23.94390937500001 * IIIIZIIIIZII
- 7.981303125000004 * IIIIIZIIIZII
+ 31.497835937500014 * IIIIIIZIIZII
+ 18.89870156250001 * IIIIIIIZIZII
+ 6.299567187500003 * IIIIIIIIZZII
- 1788.3742817187504 * IIIIIIIIIIZI
- 17.234407031250008 * ZIIIIIIIIIZI
- 10.340644218750004 * IZIIIIIIIIZI
- 3.4468814062500015 * IIZIIIIIIIZI
- 23.94390937500001 * IIIZIIIIIIZI
- 14.366345625000008 * IIIIZIIIIIZI
- 4.788781875000002 * IIIIIZIIIIZI
+ 18.898701562500012 * IIIIIIZIIIZI
+ 11.339220937500007 * IIIIIIIZIIZI
+ 3.779740312500002 * IIIIIIIIZIZI
- 594.4734957812499 * IIIIIIIIIIIZ
- 5.744802343750002 * ZIIIIIIIIIIZ
- 3.4468814062500015 * IZIIIIIIIIIZ
- 1.1489604687500004 * IIZIIIIIIIIZ
- 7.981303125000003 * IIIZIIIIIIIZ
- 4.788781875000002 * IIIIZIIIIIIZ
- 1.5962606250000009 * IIIIIZIIIIIZ
+ 6.299567187500004 * IIIIIIZIIIIZ
+ 3.779740312500002 * IIIIIIIZIIIZ
+ 1.2599134375000005 * IIIIIIIIZIIZ
- 20.885040702719472 * XIXIIIIIIIII
- 20.885040702719472 * YIYIIIIIIIII
+ 0.9740782609865658 * XIXZIIIIIIII
+ 0.9740782609865658 * YIYZIIIIIIII
+ 0.5844469565919396 * XIXIZIIIIIII
+ 0.5844469565919396 * YIYIZIIIIIII
+ 0.19481565219731317 * XIXIIZIIIIII
+ 0.19481565219731317 * YIYIIZIIIIII
+ 2.9768830886019613 * XIXIIIZIIIII
+ 2.9768830886019613 * YIYIIIZIIIII
+ 1.7861298531611771 * XIXIIIIZIIII
+ 1.7861298531611771 * YIYIIIIZIIII
+ 0.5953766177203923 * XIXIIIIIZIII
+ 0.5953766177203923 * YIYIIIIIZIII
+ 8.124377387683996 * XIXIIIIIIZII
+ 8.124377387683996 * YIYIIIIIIZII
+ 4.874626432610398 * XIXIIIIIIIZI
+ 4.874626432610398 * YIYIIIIIIIZI
+ 1.6248754775367993 * XIXIIIIIIIIZ
+ 1.6248754775367993 * YIYIIIIIIIIZ
+ 7.946551250000002 * XXIXXIIIIIII
+ 7.946551250000002 * YYIXXIIIIIII
+ 7.946551250000002 * XXIYYIIIIIII
+ 7.946551250000002 * YYIYYIIIIIII
+ 4.245123515804727 * IXXXXIIIIIII
+ 4.245123515804727 * IYYXXIIIIIII
+ 4.245123515804727 * IXXYYIIIIIII
+ 4.245123515804727 * IYYYYIIIIIII
- 27.557296533490963 * IIIXIXIIIIII
+ 0.9740782609865657 * ZIIXIXIIIIII
+ 0.5844469565919395 * IZIXIXIIIIII
+ 0.19481565219731314 * IIZXIXIIIIII
- 27.557296533490963 * IIIYIYIIIIII
+ 0.9740782609865657 * ZIIYIYIIIIII
+ 0.5844469565919395 * IZIYIYIIIIII
+ 0.19481565219731314 * IIZYIYIIIIII
+ 4.535600550836601 * IIIXIXZIIIII
+ 4.535600550836601 * IIIYIYZIIIII
+ 2.721360330501961 * IIIXIXIZIIII
+ 2.721360330501961 * IIIYIYIZIIII
+ 0.9071201101673203 * IIIXIXIIZIII
+ 0.9071201101673203 * IIIYIYIIZIII
+ 11.287267124785771 * IIIXIXIIIZII
+ 11.287267124785771 * IIIYIYIIIZII
+ 6.772360274871463 * IIIXIXIIIIZI
+ 6.772360274871463 * IIIYIYIIIIZI
+ 2.2574534249571543 * IIIXIXIIIIIZ
+ 2.2574534249571543 * IIIYIYIIIIIZ
- 0.2755109375000001 * XIXXIXIIIIII
- 0.2755109375000001 * YIYXIXIIIIII
- 0.2755109375000001 * XIXYIYIIIIII
- 0.2755109375000001 * YIYYIYIIIIII
+ 4.1834668980774286 * XXIIXXIIIIII
+ 4.1834668980774286 * YYIIXXIIIIII
+ 4.1834668980774286 * XXIIYYIIIIII
+ 4.1834668980774286 * YYIIYYIIIIII
+ 1.986637812500001 * IXXIXXIIIIII
+ 1.986637812500001 * IYYIXXIIIIII
+ 1.986637812500001 * IXXIYYIIIIII
+ 1.986637812500001 * IYYIYYIIIIII
- 260.3514075 * IIIIIIXXIIII
- 11.336256250000002 * ZIIIIIXXIIII
- 6.801753750000003 * IZIIIIXXIIII
- 2.2672512500000006 * IIZIIIXXIIII
- 38.26325625000001 * IIIZIIXXIIII
- 22.95795375000001 * IIIIZIXXIIII
- 7.652651250000002 * IIIIIZXXIIII
- 260.3514075 * IIIIIIYYIIII
- 11.336256250000002 * ZIIIIIYYIIII
- 6.801753750000003 * IZIIIIYYIIII
- 2.2672512500000006 * IIZIIIYYIIII
- 38.26325625000001 * IIIZIIYYIIII
- 22.95795375000001 * IIIIZIYYIIII
- 7.652651250000002 * IIIIIZYYIIII
+ 157.49188750000002 * IIIIIIXXIZII
+ 157.49188750000002 * IIIIIIYYIZII
+ 94.49513250000001 * IIIIIIXXIIZI
+ 94.49513250000001 * IIIIIIYYIIZI
+ 31.498377500000004 * IIIIIIXXIIIZ
+ 31.498377500000004 * IIIIIIYYIIIZ
+ 3.206377467057354 * XIXIIIXXIIII
+ 3.206377467057354 * YIYIIIXXIIII
+ 3.206377467057354 * XIXIIIYYIIII
+ 3.206377467057354 * YIYIIIYYIIII
+ 10.822483185861422 * IIIXIXXXIIII
+ 10.822483185861422 * IIIYIYXXIIII
+ 10.822483185861422 * IIIXIXYYIIII
+ 10.822483185861422 * IIIYIYYYIIII
+ 4.373609679305444 * IIIIIIXIXIII
+ 2.9768830886019613 * ZIIIIIXIXIII
+ 1.786129853161177 * IZIIIIXIXIII
+ 0.5953766177203923 * IIZIIIXIXIII
+ 4.535600550836601 * IIIZIIXIXIII
+ 2.721360330501961 * IIIIZIXIXIII
+ 0.9071201101673203 * IIIIIZXIXIII
+ 4.373609679305444 * IIIIIIYIYIII
+ 2.9768830886019613 * ZIIIIIYIYIII
+ 1.786129853161177 * IZIIIIYIYIII
+ 0.5953766177203923 * IIZIIIYIYIII
+ 4.535600550836601 * IIIZIIYIYIII
+ 2.721360330501961 * IIIIZIYIYIII
+ 0.9071201101673203 * IIIIIZYIYIII
- 8.90893335364304 * IIIIIIXIXZII
- 8.90893335364304 * IIIIIIYIYZII
- 5.345360012185824 * IIIIIIXIXIZI
- 5.345360012185824 * IIIIIIYIYIZI
- 1.7817866707286079 * IIIIIIXIXIIZ
- 1.7817866707286079 * IIIIIIYIYIIZ
- 0.8419896875000004 * XIXIIIXIXIII
- 0.8419896875000004 * YIYIIIXIXIII
- 0.8419896875000004 * XIXIIIYIYIII
- 0.8419896875000004 * YIYIIIYIYIII
- 1.2828615625000004 * IIIXIXXIXIII
- 1.2828615625000004 * IIIYIYXIXIII
- 1.2828615625000004 * IIIXIXYIYIII
- 1.2828615625000004 * IIIYIYYIYIII
- 160.71040986809584 * IIIIIIIXXIII
- 8.015943667643384 * ZIIIIIIXXIII
- 4.809566200586032 * IZIIIIIXXIII
- 1.6031887335286772 * IIZIIIIXXIII
- 27.05620796465356 * IIIZIIIXXIII
- 16.233724778792137 * IIIIZIIXXIII
- 5.411241592930711 * IIIIIZIXXIII
- 160.71040986809584 * IIIIIIIYYIII
- 8.015943667643384 * ZIIIIIIYYIII
- 4.809566200586032 * IZIIIIIYYIII
- 1.6031887335286772 * IIZIIIIYYIII
- 27.05620796465356 * IIIZIIIYYIII
- 16.233724778792137 * IIIIZIIYYIII
- 5.411241592930711 * IIIIIZIYYIII
+ 111.36358163311888 * IIIIIIIXXZII
+ 111.36358163311888 * IIIIIIIYYZII
+ 66.81814897987134 * IIIIIIIXXIZI
+ 66.81814897987134 * IIIIIIIYYIZI
+ 22.272716326623776 * IIIIIIIXXIIZ
+ 22.272716326623776 * IIIIIIIYYIIZ
+ 2.267251250000001 * XIXIIIIXXIII
+ 2.267251250000001 * YIYIIIIXXIII
+ 2.267251250000001 * XIXIIIIYYIII
+ 2.267251250000001 * YIYIIIIYYIII
+ 7.6526512500000035 * IIIXIXIXXIII
+ 7.6526512500000035 * IIIYIYIXXIII
+ 7.6526512500000035 * IIIXIXIYYIII
+ 7.6526512500000035 * IIIYIYIYYIII
+ 3.5921675000000004 * XXIIIIIIIXXI
+ 3.5921675000000004 * YYIIIIIIIXXI
+ 3.5921675000000004 * XXIIIIIIIYYI
+ 3.5921675000000004 * YYIIIIIIIYYI
+ 5.912498983512137 * IXXIIIIIIXXI
+ 5.912498983512137 * IYYIIIIIIXXI
+ 5.912498983512137 * IXXIIIIIIYYI
+ 5.912498983512137 * IYYIIIIIIYYI
- 2.0085637500000013 * IIIXXIIIIXXI
- 2.0085637500000013 * IIIYYIIIIXXI
- 2.0085637500000013 * IIIXXIIIIYYI
- 2.0085637500000013 * IIIYYIIIIYYI
- 3.305978760488338 * IIIIXXIIIXXI
- 3.305978760488338 * IIIIYYIIIXXI
- 3.305978760488338 * IIIIXXIIIYYI
- 3.305978760488338 * IIIIYYIIIYYI
- 11.899156895555553 * IIIIIIIIIXIX
+ 8.124377387683996 * ZIIIIIIIIXIX
+ 4.874626432610398 * IZIIIIIIIXIX
+ 1.6248754775367993 * IIZIIIIIIXIX
+ 11.28726712478577 * IIIZIIIIIXIX
+ 6.772360274871463 * IIIIZIIIIXIX
+ 2.2574534249571543 * IIIIIZIIIXIX
- 8.90893335364304 * IIIIIIZIIXIX
- 5.345360012185823 * IIIIIIIZIXIX
- 1.7817866707286076 * IIIIIIIIZXIX
- 11.899156895555553 * IIIIIIIIIYIY
+ 8.124377387683996 * ZIIIIIIIIYIY
+ 4.874626432610398 * IZIIIIIIIYIY
+ 1.6248754775367993 * IIZIIIIIIYIY
+ 11.28726712478577 * IIIZIIIIIYIY
+ 6.772360274871463 * IIIIZIIIIYIY
+ 2.2574534249571543 * IIIIIZIIIYIY
- 8.90893335364304 * IIIIIIZIIYIY
- 5.345360012185823 * IIIIIIIZIYIY
- 1.7817866707286076 * IIIIIIIIZYIY
- 2.2979209375000007 * XIXIIIIIIXIX
- 2.2979209375000007 * YIYIIIIIIXIX
- 2.2979209375000007 * XIXIIIIIIYIY
- 2.2979209375000007 * YIYIIIIIIYIY
- 3.1925212500000018 * IIIXIXIIIXIX
- 3.1925212500000018 * IIIYIYIIIXIX
- 3.1925212500000018 * IIIXIXIIIYIY
- 3.1925212500000018 * IIIYIYIIIYIY
- 44.54543265324755 * IIIIIIXXIXIX
- 44.54543265324755 * IIIIIIYYIXIX
- 44.54543265324755 * IIIIIIXXIYIY
- 44.54543265324755 * IIIIIIYYIYIY
+ 2.5198268750000015 * IIIIIIXIXXIX
+ 2.5198268750000015 * IIIIIIYIYXIX
+ 2.5198268750000015 * IIIIIIXIXYIY
+ 2.5198268750000015 * IIIIIIYIYYIY
- 31.498377500000007 * IIIIIIIXXXIX
- 31.498377500000007 * IIIIIIIYYXIX
- 31.498377500000007 * IIIIIIIXXYIY
- 31.498377500000007 * IIIIIIIYYYIY
- 2.102429985900245 * XXIIIIIIIIXX
- 2.102429985900245 * YYIIIIIIIIXX
- 2.102429985900245 * XXIIIIIIIIYY
- 2.102429985900245 * YYIIIIIIIIYY
+ 0.8980418750000001 * IXXIIIIIIIXX
+ 0.8980418750000001 * IYYIIIIIIIXX
+ 0.8980418750000001 * IXXIIIIIIIYY
+ 0.8980418750000001 * IYYIIIIIIIYY
+ 1.175575188382615 * IIIXXIIIIIXX
+ 1.175575188382615 * IIIYYIIIIIXX
+ 1.175575188382615 * IIIXXIIIIIYY
+ 1.175575188382615 * IIIYYIIIIIYY
- 0.5021409375000001 * IIIIXXIIIIXX
- 0.5021409375000001 * IIIIYYIIIIXX
- 0.5021409375000001 * IIIIXXIIIIYY
- 0.5021409375000001 * IIIIYYIIIIYY
###Markdown
Now that the Hamiltonian is ready, it can be used in a quantum algorithm to find information about the vibrational structure of the corresponding molecule. Check out our tutorials on Ground State Calculation and Excited States Calculation to learn more about how to do that in Qiskit Nature!
###Code
import qiskit.tools.jupyter
%qiskit_version_table
%qiskit_copyright
###Output
_____no_output_____
###Markdown
Vibrational structure Introduction The molecular Hamiltonian is $$\mathcal{H} = - \sum_I \frac{\nabla_{R_I}^2}{M_I} - \sum_i \frac{\nabla_{r_i}^2}{m_e} - \sum_I\sum_i \frac{Z_I e^2}{|R_I-r_i|} + \sum_i \sum_{j>i} \frac{e^2}{|r_i-r_j|} + \sum_I\sum_{J>I} \frac{Z_I Z_J e^2}{|R_I-R_J|}$$Because the nuclei are much heavier than the electrons they do not move on the same time scale and therefore, the behavior of nuclei and electrons can be decoupled. This is the Born-Oppenheimer approximation.Within the Born-Oppenheimer approximation, a molecular wave function is factorized as a product of an electronic part, which is the solution of the electronic Schroedinger equation, and a vibro-rotational one, which is the solution of the nuclear Schroedinger equation in the potential energy surface (PES) generated by sampling the eigenvalues of the electronic Schroedinger equation for different geometries.The nuclear Schroedinger equation is usually solved in two steps, in analogy with its electronic counterpart. A single-particle basis (the basis functions are called, in this case, modals) is obtained either by the harmonic approximation applied to the PES or from a vibrational self-consistent field (VSCF) calculation. Vibrational anharmonic correlations are added a-posteriori with perturbative or variational approaches.The latter include Vibrational Configuration Interaction (VCI) and Vibrational Coupled Cluster (VCC) for highly-accurate anharmonic energies. The main advantage of VCI and VCC over alternative approaches (such as perturbation theories) is that their accuracy can be systematically improved towards the complete basis set limit for a given PES. However, their applicability is limited to small molecules with up to about 10 atoms due to their unfavorable scaling with system size.To tackle the scaling problem we would like to use quantum algorithms.The nuclear Schroedinger equation is$$\mathcal{H}_{\text{vib}} |\Psi_{n}\rangle = E_{n} |\Psi_{n}\rangle$$The so-called Watson Hamiltonian (neglecting vibro-rotational coupling terms) is$$ \mathcal{H}_\text{vib}(Q_1, \ldots, Q_L) = - \frac{1}{2} \sum_{l=1}^{L} \frac{\partial^2}{\partial Q_l^2} + V(Q_1, \ldots, Q_L)$$where $Q_l$ are the harmonic mass-weighted normal coordinates.$\mathcal{H}_\text{vib}$ must be mapped to an operator that acts on the states of a given set of $N_q$ qubits in order to calculate its eigenfunctions on quantum hardware.In electronic structure calculations, the mapping is achieved by expressing the non-relativistic electronic Hamiltonian in second quantization, \textit{i.e.} by projecting it onto the complete set of antisymmetrized occupation number vectors (ONV) generated by a given (finite) set of orbitals.To encode the vibrational Hamiltonian in an analogous second quantization operators, we expand the potential $V(Q_1, \ldots, Q_L)$ with the $n$-body expansion as follows:$$ V(Q_1, \ldots, Q_L) = V_0 + \sum_{l=1}^L V^{[l]}(Q_l) + \sum_{l<m}^L V^{[l,m]}(Q_l, Q_m) + \sum_{l<m<n}^L V^{[l,m,n]}(Q_l, Q_m, Q_n) + \ldots$$where $V_0$ is the electronic energy of the reference geometry, the one-mode term $V^{[l]}(Q_l)$ represents the variation of the PES upon change of the $l$-th normal coordinate from the equilibrium position.Similarly, the two-body potential $V^{[l,m]}(Q_l, Q_m)$ represents the change in the exact PES upon a simultaneous displacement along the $l$-th and $m$-th coordinates. Often, including terms up to three-body in the $L$-body expansion is sufficient to obtain an accuracy of about 1~cm$^{-1}$. We highlight that the many-body expansion of the potential operator defining the Watson Hamiltonian contains arbitrarily high coupling terms. This is a crucial difference compared to the non-relativistic electronic-structure Hamiltonian that contains only pairwise interactions.A flexible second quantization form of the Watson Hamiltonian is obtained within the so-called n-mode representation. Let us assume that each mode $l$ is described by a $N_l$-dimensional basis set $S_l$ defined as follows:$$ \mathcal{S}_l = \{ \phi_1^{(l)} (Q_l) , \ldots , \phi_{N_l}^{(l)} (Q_l) \} \, .$$The $n$-mode wave function can be expanded in the product basis $\mathcal{S} = \otimes_{i=1}^L \mathcal{S}_i$ as the following CI-like expansion:$$ |\Psi\rangle = \sum_{k_1=1}^{N_1} \cdots \sum_{k_L=1}^{N_L} C_{k_1,\ldots,k_L} \phi_{k_1}^{(1)}(Q_1) \cdots \phi_{k_L}^{(L)}(Q_L) \, ,$$The many-body basis function $\phi_{k_1}^{(1)}(Q_1) \cdots \phi_{k_L}^{(L)}(Q_L)$ are encoded within the so-called $n$-mode second quantization as occupation-number vectors (ONVs) as follows:$$ \phi_{k_1}(Q_1) \cdots \phi_{k_L}(Q_L) \equiv |0_1 \cdots 1_{k_1} \cdots 0_{N_1}, 0_1 \cdots 1_{k_2} \cdots 0_{N_2}, \cdots , 0_1 \cdots 1_{k_L} \cdots 0_{N_L}\rangle \, .$$The ONV defined above is, therefore, the product of $L$ mode-specific ONVs, each one describing an individual mode. Since each mode is described by one and only one basis function, the occupation of each mode-specific ONV is one.From a theoretical perspective, each mode can be interpreted as a distinguishable quasi-particle (defined as phonons in solid-state physics). Distinguishability arises from the fact that the PES is not invariant by permutation of two modes, also in this case unlike the Coulomb interaction between two equal particles. From this perspective, a molecule can be interpreted as a collection of $L$ indistinguishable particles that interact through the PES operator.Based on this second-quantization representation we introduce a pair of creation and annihilation operators per mode $l$ \textit{and} per basis function $k_l$ defined as:$$ \begin{aligned} a_{k_l}^\dagger |\cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle &= | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle \\ a_{k_l}^\dagger | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle &= 0 \\ a_{k_l} | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle &= | \cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle \\ a_{k_l} | \cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle &= 0 \\ \end{aligned}$$with$$ \begin{aligned} \left[ a_{k_l}^\dagger, a_{h_m}^\dagger \right] &= 0 \\ \left[ a_{k_l}, a_{h_m} \right] &= 0 \\ \left[ a_{k_l}^\dagger, a_{h_m} \right] &= \delta_{l,m} \, , \delta_{k_l,h_m} \end{aligned}$$The second quantization form is obtained by expressing the potential as $$ \begin{aligned} \mathcal{H}_\text{vib}^{SQ} =& \sum_{l=1}^L \sum_{k_l,h_l}^{N_l} \langle \phi_{k_l} | T(Q_l) + V^{[l]}(Q_l) | \phi_{h_l} \rangle a_{k_l}^+ a_{h_l} \\ +& \sum_{l<m}^L \sum_{k_l,h_l}^{N_l} \sum_{k_m,h_m}^{N_m} \langle \phi_{k_l} \phi_{k_m} | V^{[l,m]}(Q_l, Q_m) | \phi_{h_l} \phi_{h_m} \rangle a_{k_l}^+ a_{k_m}^+ a_{h_l} a_{h_m} + \cdots \end{aligned}$$We highlight here the difference between the operators defined here above and the electronic structure one. First, as we already mentioned, the potential contains (in principle) three- and higher-body coupling terms that lead to strings with six (or more) second-quantization operators. Moreover, the Hamiltonian conserves the number of particles for each mode, as can be seen from the fact that the number of creation and annihilation operators for a given mode is the same in each term. Nevertheless, different modes are coupled by two- (and higher) body terms containing SQ operators belonging to different modes $l$ and $m$.Reference: Ollitrault, Pauline J., et al., arXiv:2003.12578 (2020). Compute the electronic potentialSolving the ESE for different nuclear configurations to obtain the PES function $V(Q_1, \ldots, Q_L)$. So far Qiskit gives the possibility to approximate the PES with a quartic force field. $$V(Q_1, \ldots, Q_L) = \frac{1}{2} \sum_{ij} k_{ij} Q_i Q_j + \frac{1}{6} \sum_{ijk} k_{ijk} Q_i Q_j Q_k + \frac{1}{16} \sum_{ijkl} k_{ijkl} Q_i Q_j Q_k Q_l$$The advantage of such form for the PES is that the anharmonic force fields ($k_{ij}$, $k_{ijk}$, $k_{ijkl}$) can be calculated by finite-difference approaches. For methods for which the nuclear energy Hessian can be calculated analytically with response theory-based methods (such as HF and DFT), the quartic force field can be calculated by semi-numerical differentiation as:$$k_{ijk} = \frac{H_{ij}(+\delta Q_k) - H_{ij}(-\delta Q_k)}{2\delta Q_k}$$and$$k_{ijkl} = \frac{H_{ij}(+\delta Q_k+\delta Q_l) - H_{ij}(+\delta Q_k-\delta Q_l) -H_{ij}(-\delta Q_k+\delta Q_l) + H_{ij}(-\delta Q_k+\delta Q_l)} {4\delta Q_k \delta Q_l}$$Such numerical procedure is implemented, for instance, in the Gaussian suite of programs.In practice this can be done with Qiskit using the GaussianForceDriver.
###Code
from qiskit_nature.drivers.second_quantization import GaussianForcesDriver
# if you ran Gaussian elsewhere and already have the output file
driver = GaussianForcesDriver(logfile='aux_files/CO2_freq_B3LYP_ccpVDZ.log')
# if you want to run the Gaussian job from Qiskit
# driver = GaussianForcesDriver(
# ['#p B3LYP/6-31g Freq=(Anharm) Int=Ultrafine SCF=VeryTight',
# '',
# 'CO2 geometry optimization B3LYP/6-31g',
# '',
# '0 1',
# 'C -0.848629 2.067624 0.160992',
# 'O 0.098816 2.655801 -0.159738',
# 'O -1.796073 1.479446 0.481721',
# '',
# ''
###Output
_____no_output_____
###Markdown
Map to a qubit HamiltonianNow that we have an approximation for the potential, we need to write the Hamiltonian in second quantization. To this end we need to select a modal basis to calculate the one-body integrals $\langle\phi_{k_i}| V(Q_i) | \phi_{h_i} \rangle$, two-body integrals $\langle\phi_{k_i} \phi_{k_j}| V(Q_i,Q_j) | \phi_{h_i} \phi_{h_j} \rangle$... In the simplest case, the $\phi$ functions are the harmonic-oscillator eigenfunctions for each mode. The main advantage of this choice is that the integrals of a PES expressed as a Taylor expansion are easy to calculate with such basis. A routine for computing these integrals is implemented in Qiskit. The bosonic operator, $\mathcal{H}_\text{vib}^{SQ}$, is then created and must be mapped to a qubit operator. The direct mapping introduced in the first section of this tutorial can be used is Qiskit as follows:
###Code
from qiskit_nature.problems.second_quantization import VibrationalStructureProblem
from qiskit_nature.converters.second_quantization import QubitConverter
from qiskit_nature.mappers.second_quantization import DirectMapper
vibrational_problem = VibrationalStructureProblem(driver, num_modals=2, truncation_order=2)
second_q_ops = vibrational_problem.second_q_ops()
###Output
_____no_output_____
###Markdown
The Vibrational operator for the problem now reads as
###Code
print(second_q_ops[0])
###Output
NIIIIIII * (1215.682529375+0j)
+ INIIIIII * (3656.9551768750007+0j)
+ IINIIIII * (682.5053337500001+0j)
+ II+-IIII * (-46.77167173323271+0j)
+ II-+IIII * (-46.77167173323271+0j)
+ IIINIIII * (2050.1464387500005+0j)
+ IIIINIII * (329.41209562500006+0j)
+ IIIIINII * (992.0224281250003+0j)
+ IIIIIINI * (328.12046812500006+0j)
+ IIIIIIIN * (985.5642906250002+0j)
+ NINIIIII * (5.039653750000002+0j)
+ INNIIIII * (15.118961250000009+0j)
+ NI+-IIII * (-89.0908653064951+0j)
+ IN+-IIII * (-267.27259591948535+0j)
+ NI-+IIII * (-89.0908653064951+0j)
+ IN-+IIII * (-267.27259591948535+0j)
+ NIINIIII * (15.118961250000009+0j)
+ ININIIII * (45.35688375000003+0j)
+ NIIINIII * (-6.3850425000000035+0j)
+ INIINIII * (-19.15512750000001+0j)
+ IININIII * (-2.5657231250000008+0j)
+ II+-NIII * (21.644966371722845+0j)
+ II-+NIII * (21.644966371722845+0j)
+ IIINNIII * (-7.697169375000003+0j)
+ +-II+-II * (-2.0085637500000004+0j)
+ -+II+-II * (-2.0085637500000004+0j)
+ +-II-+II * (-2.0085637500000004+0j)
+ -+II-+II * (-2.0085637500000004+0j)
+ NIIIINII * (-19.15512750000001+0j)
+ INIIINII * (-57.46538250000003+0j)
+ IINIINII * (-7.697169375000004+0j)
+ II+-INII * (64.93489911516855+0j)
+ II-+INII * (64.93489911516855+0j)
+ IIININII * (-23.091508125000015+0j)
+ NIIIIINI * (-4.595841875000001+0j)
+ INIIIINI * (-13.787525625000006+0j)
+ IINIIINI * (-1.683979375000001+0j)
+ II+-IINI * (6.412754934114709+0j)
+ II-+IINI * (6.412754934114709+0j)
+ IIINIINI * (-5.051938125000003+0j)
+ IIIININI * (-0.5510218750000002+0j)
+ IIIIINNI * (-1.6530656250000009+0j)
+ +-IIII+- * (3.5921675000000004+0j)
+ -+IIII+- * (3.5921675000000004+0j)
+ IIII+-+- * (7.946551250000004+0j)
+ IIII-++- * (7.946551250000004+0j)
+ +-IIII-+ * (3.5921675000000004+0j)
+ -+IIII-+ * (3.5921675000000004+0j)
+ IIII+--+ * (7.946551250000004+0j)
+ IIII-+-+ * (7.946551250000004+0j)
+ NIIIIIIN * (-13.787525625000006+0j)
+ INIIIIIN * (-41.362576875000016+0j)
+ IINIIIIN * (-5.051938125000002+0j)
+ II+-IIIN * (19.238264802344126+0j)
+ II-+IIIN * (19.238264802344126+0j)
+ IIINIIIN * (-15.15581437500001+0j)
+ IIIINIIN * (-1.6530656250000009+0j)
+ IIIIININ * (-4.959196875000003+0j)
###Markdown
In the previous cell we defined a bosonic transformation to express the Hamiltonian in the harmonic modal basis, with 2 modals per mode with the potential truncated at order 2 and the 'direct' boson to qubit mapping. The calculation is then ran as:
###Code
qubit_converter = QubitConverter(mapper=DirectMapper())
qubit_op = qubit_converter.convert(second_q_ops[0])
print(qubit_op)
###Output
5077.236560625012 * IIIIIIII
- 472.28961593750034 * ZIIIIIII
- 157.22939093749991 * IZIIIIII
- 467.5058515625001 * IIZIIIII
- 1.2397992187500007 * ZIZIIIII
- 0.4132664062500002 * IZZIIIII
- 155.20426031249983 * IIIZIIII
- 0.4132664062500002 * ZIIZIIII
- 0.13775546875000005 * IZIZIIII
- 1027.4430731249997 * IIIIZIII
- 3.7889535937500023 * ZIIIZIII
- 1.2629845312500008 * IZIIZIII
- 5.772877031250004 * IIZIZIII
- 1.9242923437500008 * IIIZZIII
- 342.04261812500033 * IIIIIZII
- 1.2629845312500005 * ZIIIIZII
- 0.42099484375000024 * IZIIIZII
- 1.924292343750001 * IIZIIZII
- 0.6414307812500002 * IIIZIZII
- 1810.6538965625004 * IIIIIIZI
- 10.340644218750004 * ZIIIIIZI
- 3.4468814062500015 * IZIIIIZI
- 14.366345625000008 * IIZIIIZI
- 4.788781875000002 * IIIZIIZI
+ 11.339220937500007 * IIIIZIZI
+ 3.779740312500002 * IIIIIZZI
- 601.9000340624999 * IIIIIIIZ
- 3.4468814062500015 * ZIIIIIIZ
- 1.1489604687500004 * IZIIIIIZ
- 4.788781875000002 * IIZIIIIZ
- 1.5962606250000009 * IIIZIIIZ
+ 3.779740312500002 * IIIIZIIZ
+ 1.2599134375000005 * IIIIIZIZ
+ 1.986637812500001 * XXXXIIII
+ 1.986637812500001 * YYXXIIII
+ 1.986637812500001 * XXYYIIII
+ 1.986637812500001 * YYYYIIII
- 84.41897986727398 * IIIIXXII
- 4.809566200586032 * ZIIIXXII
- 1.6031887335286772 * IZIIXXII
- 16.233724778792137 * IIZIXXII
- 5.411241592930711 * IIIZXXII
- 84.41897986727398 * IIIIYYII
- 4.809566200586032 * ZIIIYYII
- 1.6031887335286772 * IZIIYYII
- 16.233724778792137 * IIZIYYII
- 5.411241592930711 * IIIZYYII
+ 66.81814897987134 * IIIIXXZI
+ 66.81814897987134 * IIIIYYZI
+ 22.272716326623776 * IIIIXXIZ
+ 22.272716326623776 * IIIIYYIZ
+ 0.8980418750000001 * XXIIIIXX
+ 0.8980418750000001 * YYIIIIXX
+ 0.8980418750000001 * XXIIIIYY
+ 0.8980418750000001 * YYIIIIYY
- 0.5021409375000001 * IIXXIIXX
- 0.5021409375000001 * IIYYIIXX
- 0.5021409375000001 * IIXXIIYY
- 0.5021409375000001 * IIYYIIYY
###Markdown
To have a different number of modals per mode:
###Code
vibrational_problem = VibrationalStructureProblem(driver, num_modals=3, truncation_order=2)
second_q_ops = vibrational_problem.second_q_ops()
qubit_converter = QubitConverter(mapper = DirectMapper())
qubit_op = qubit_converter.convert(second_q_ops[0])
print(qubit_op)
###Output
11327.034966562516 * IIIIIIIIIIII
- 745.8599576562498 * ZIIIIIIIIIII
- 446.6739542187503 * IZIIIIIIIIII
- 148.69083703124986 * IIZIIIIIIIII
- 724.2074121874995 * IIIZIIIIIIII
- 3.4438867187500013 * ZIIZIIIIIIII
- 2.066332031250001 * IZIZIIIIIIII
- 0.6887773437500002 * IIZZIIIIIIII
- 431.8741484375 * IIIIZIIIIIII
- 2.066332031250001 * ZIIIZIIIIIII
- 1.2397992187500007 * IZIIZIIIIIII
- 0.4132664062500002 * IIZIZIIIIIII
- 143.32702593749988 * IIIIIZIIIIII
- 0.6887773437500002 * ZIIIIZIIIIII
- 0.4132664062500002 * IZIIIZIIIIII
- 0.13775546875000005 * IIZIIZIIIIII
- 1720.4111609375004 * IIIIIIZIIIII
- 10.524871093750003 * ZIIIIIZIIIII
- 6.314922656250003 * IZIIIIZIIIII
- 2.104974218750001 * IIZIIIZIIIII
- 16.035769531250004 * IIIZIIZIIIII
- 9.621461718750005 * IIIIZIZIIIII
- 3.207153906250001 * IIIIIZZIIIII
- 1030.4053903124998 * IIIIIIIZIIII
- 6.314922656250003 * ZIIIIIIZIIII
- 3.7889535937500023 * IZIIIIIZIIII
- 1.2629845312500008 * IIZIIIIZIIII
- 9.621461718750004 * IIIZIIIZIIII
- 5.772877031250004 * IIIIZIIZIIII
- 1.9242923437500008 * IIIIIZIZIIII
- 343.0300571875006 * IIIIIIIIZIII
- 2.1049742187500007 * ZIIIIIIIZIII
- 1.2629845312500005 * IZIIIIIIZIII
- 0.42099484375000024 * IIZIIIIIZIII
- 3.207153906250001 * IIIZIIIIZIII
- 1.924292343750001 * IIIIZIIIZIII
- 0.6414307812500002 * IIIIIZIIZIII
- 2992.18265640625 * IIIIIIIIIZII
- 28.72401171875001 * ZIIIIIIIIZII
- 17.234407031250004 * IZIIIIIIIZII
- 5.744802343750002 * IIZIIIIIIZII
- 39.90651562500001 * IIIZIIIIIZII
- 23.94390937500001 * IIIIZIIIIZII
- 7.981303125000004 * IIIIIZIIIZII
+ 31.497835937500014 * IIIIIIZIIZII
+ 18.89870156250001 * IIIIIIIZIZII
+ 6.299567187500003 * IIIIIIIIZZII
- 1788.3742817187504 * IIIIIIIIIIZI
- 17.234407031250008 * ZIIIIIIIIIZI
- 10.340644218750004 * IZIIIIIIIIZI
- 3.4468814062500015 * IIZIIIIIIIZI
- 23.94390937500001 * IIIZIIIIIIZI
- 14.366345625000008 * IIIIZIIIIIZI
- 4.788781875000002 * IIIIIZIIIIZI
+ 18.898701562500012 * IIIIIIZIIIZI
+ 11.339220937500007 * IIIIIIIZIIZI
+ 3.779740312500002 * IIIIIIIIZIZI
- 594.4734957812499 * IIIIIIIIIIIZ
- 5.744802343750002 * ZIIIIIIIIIIZ
- 3.4468814062500015 * IZIIIIIIIIIZ
- 1.1489604687500004 * IIZIIIIIIIIZ
- 7.981303125000003 * IIIZIIIIIIIZ
- 4.788781875000002 * IIIIZIIIIIIZ
- 1.5962606250000009 * IIIIIZIIIIIZ
+ 6.299567187500004 * IIIIIIZIIIIZ
+ 3.779740312500002 * IIIIIIIZIIIZ
+ 1.2599134375000005 * IIIIIIIIZIIZ
- 20.885040702719472 * XIXIIIIIIIII
- 20.885040702719472 * YIYIIIIIIIII
+ 0.9740782609865658 * XIXZIIIIIIII
+ 0.9740782609865658 * YIYZIIIIIIII
+ 0.5844469565919396 * XIXIZIIIIIII
+ 0.5844469565919396 * YIYIZIIIIIII
+ 0.19481565219731317 * XIXIIZIIIIII
+ 0.19481565219731317 * YIYIIZIIIIII
+ 2.9768830886019613 * XIXIIIZIIIII
+ 2.9768830886019613 * YIYIIIZIIIII
+ 1.7861298531611771 * XIXIIIIZIIII
+ 1.7861298531611771 * YIYIIIIZIIII
+ 0.5953766177203923 * XIXIIIIIZIII
+ 0.5953766177203923 * YIYIIIIIZIII
+ 8.124377387683996 * XIXIIIIIIZII
+ 8.124377387683996 * YIYIIIIIIZII
+ 4.874626432610398 * XIXIIIIIIIZI
+ 4.874626432610398 * YIYIIIIIIIZI
+ 1.6248754775367993 * XIXIIIIIIIIZ
+ 1.6248754775367993 * YIYIIIIIIIIZ
+ 7.946551250000002 * XXIXXIIIIIII
+ 7.946551250000002 * YYIXXIIIIIII
+ 7.946551250000002 * XXIYYIIIIIII
+ 7.946551250000002 * YYIYYIIIIIII
+ 4.245123515804727 * IXXXXIIIIIII
+ 4.245123515804727 * IYYXXIIIIIII
+ 4.245123515804727 * IXXYYIIIIIII
+ 4.245123515804727 * IYYYYIIIIIII
- 27.557296533490963 * IIIXIXIIIIII
+ 0.9740782609865657 * ZIIXIXIIIIII
+ 0.5844469565919395 * IZIXIXIIIIII
+ 0.19481565219731314 * IIZXIXIIIIII
- 27.557296533490963 * IIIYIYIIIIII
+ 0.9740782609865657 * ZIIYIYIIIIII
+ 0.5844469565919395 * IZIYIYIIIIII
+ 0.19481565219731314 * IIZYIYIIIIII
+ 4.535600550836601 * IIIXIXZIIIII
+ 4.535600550836601 * IIIYIYZIIIII
+ 2.721360330501961 * IIIXIXIZIIII
+ 2.721360330501961 * IIIYIYIZIIII
+ 0.9071201101673203 * IIIXIXIIZIII
+ 0.9071201101673203 * IIIYIYIIZIII
+ 11.287267124785771 * IIIXIXIIIZII
+ 11.287267124785771 * IIIYIYIIIZII
+ 6.772360274871463 * IIIXIXIIIIZI
+ 6.772360274871463 * IIIYIYIIIIZI
+ 2.2574534249571543 * IIIXIXIIIIIZ
+ 2.2574534249571543 * IIIYIYIIIIIZ
- 0.2755109375000001 * XIXXIXIIIIII
- 0.2755109375000001 * YIYXIXIIIIII
- 0.2755109375000001 * XIXYIYIIIIII
- 0.2755109375000001 * YIYYIYIIIIII
+ 4.1834668980774286 * XXIIXXIIIIII
+ 4.1834668980774286 * YYIIXXIIIIII
+ 4.1834668980774286 * XXIIYYIIIIII
+ 4.1834668980774286 * YYIIYYIIIIII
+ 1.986637812500001 * IXXIXXIIIIII
+ 1.986637812500001 * IYYIXXIIIIII
+ 1.986637812500001 * IXXIYYIIIIII
+ 1.986637812500001 * IYYIYYIIIIII
- 260.3514075 * IIIIIIXXIIII
- 11.336256250000002 * ZIIIIIXXIIII
- 6.801753750000003 * IZIIIIXXIIII
- 2.2672512500000006 * IIZIIIXXIIII
- 38.26325625000001 * IIIZIIXXIIII
- 22.95795375000001 * IIIIZIXXIIII
- 7.652651250000002 * IIIIIZXXIIII
- 260.3514075 * IIIIIIYYIIII
- 11.336256250000002 * ZIIIIIYYIIII
- 6.801753750000003 * IZIIIIYYIIII
- 2.2672512500000006 * IIZIIIYYIIII
- 38.26325625000001 * IIIZIIYYIIII
- 22.95795375000001 * IIIIZIYYIIII
- 7.652651250000002 * IIIIIZYYIIII
+ 157.49188750000002 * IIIIIIXXIZII
+ 157.49188750000002 * IIIIIIYYIZII
+ 94.49513250000001 * IIIIIIXXIIZI
+ 94.49513250000001 * IIIIIIYYIIZI
+ 31.498377500000004 * IIIIIIXXIIIZ
+ 31.498377500000004 * IIIIIIYYIIIZ
+ 3.206377467057354 * XIXIIIXXIIII
+ 3.206377467057354 * YIYIIIXXIIII
+ 3.206377467057354 * XIXIIIYYIIII
+ 3.206377467057354 * YIYIIIYYIIII
+ 10.822483185861422 * IIIXIXXXIIII
+ 10.822483185861422 * IIIYIYXXIIII
+ 10.822483185861422 * IIIXIXYYIIII
+ 10.822483185861422 * IIIYIYYYIIII
+ 4.373609679305444 * IIIIIIXIXIII
+ 2.9768830886019613 * ZIIIIIXIXIII
+ 1.786129853161177 * IZIIIIXIXIII
+ 0.5953766177203923 * IIZIIIXIXIII
+ 4.535600550836601 * IIIZIIXIXIII
+ 2.721360330501961 * IIIIZIXIXIII
+ 0.9071201101673203 * IIIIIZXIXIII
+ 4.373609679305444 * IIIIIIYIYIII
+ 2.9768830886019613 * ZIIIIIYIYIII
+ 1.786129853161177 * IZIIIIYIYIII
+ 0.5953766177203923 * IIZIIIYIYIII
+ 4.535600550836601 * IIIZIIYIYIII
+ 2.721360330501961 * IIIIZIYIYIII
+ 0.9071201101673203 * IIIIIZYIYIII
- 8.90893335364304 * IIIIIIXIXZII
- 8.90893335364304 * IIIIIIYIYZII
- 5.345360012185824 * IIIIIIXIXIZI
- 5.345360012185824 * IIIIIIYIYIZI
- 1.7817866707286079 * IIIIIIXIXIIZ
- 1.7817866707286079 * IIIIIIYIYIIZ
- 0.8419896875000004 * XIXIIIXIXIII
- 0.8419896875000004 * YIYIIIXIXIII
- 0.8419896875000004 * XIXIIIYIYIII
- 0.8419896875000004 * YIYIIIYIYIII
- 1.2828615625000004 * IIIXIXXIXIII
- 1.2828615625000004 * IIIYIYXIXIII
- 1.2828615625000004 * IIIXIXYIYIII
- 1.2828615625000004 * IIIYIYYIYIII
- 160.71040986809584 * IIIIIIIXXIII
- 8.015943667643384 * ZIIIIIIXXIII
- 4.809566200586032 * IZIIIIIXXIII
- 1.6031887335286772 * IIZIIIIXXIII
- 27.05620796465356 * IIIZIIIXXIII
- 16.233724778792137 * IIIIZIIXXIII
- 5.411241592930711 * IIIIIZIXXIII
- 160.71040986809584 * IIIIIIIYYIII
- 8.015943667643384 * ZIIIIIIYYIII
- 4.809566200586032 * IZIIIIIYYIII
- 1.6031887335286772 * IIZIIIIYYIII
- 27.05620796465356 * IIIZIIIYYIII
- 16.233724778792137 * IIIIZIIYYIII
- 5.411241592930711 * IIIIIZIYYIII
+ 111.36358163311888 * IIIIIIIXXZII
+ 111.36358163311888 * IIIIIIIYYZII
+ 66.81814897987134 * IIIIIIIXXIZI
+ 66.81814897987134 * IIIIIIIYYIZI
+ 22.272716326623776 * IIIIIIIXXIIZ
+ 22.272716326623776 * IIIIIIIYYIIZ
+ 2.267251250000001 * XIXIIIIXXIII
+ 2.267251250000001 * YIYIIIIXXIII
+ 2.267251250000001 * XIXIIIIYYIII
+ 2.267251250000001 * YIYIIIIYYIII
+ 7.6526512500000035 * IIIXIXIXXIII
+ 7.6526512500000035 * IIIYIYIXXIII
+ 7.6526512500000035 * IIIXIXIYYIII
+ 7.6526512500000035 * IIIYIYIYYIII
+ 3.5921675000000004 * XXIIIIIIIXXI
+ 3.5921675000000004 * YYIIIIIIIXXI
+ 3.5921675000000004 * XXIIIIIIIYYI
+ 3.5921675000000004 * YYIIIIIIIYYI
+ 5.912498983512137 * IXXIIIIIIXXI
+ 5.912498983512137 * IYYIIIIIIXXI
+ 5.912498983512137 * IXXIIIIIIYYI
+ 5.912498983512137 * IYYIIIIIIYYI
- 2.0085637500000013 * IIIXXIIIIXXI
- 2.0085637500000013 * IIIYYIIIIXXI
- 2.0085637500000013 * IIIXXIIIIYYI
- 2.0085637500000013 * IIIYYIIIIYYI
- 3.305978760488338 * IIIIXXIIIXXI
- 3.305978760488338 * IIIIYYIIIXXI
- 3.305978760488338 * IIIIXXIIIYYI
- 3.305978760488338 * IIIIYYIIIYYI
- 11.899156895555553 * IIIIIIIIIXIX
+ 8.124377387683996 * ZIIIIIIIIXIX
+ 4.874626432610398 * IZIIIIIIIXIX
+ 1.6248754775367993 * IIZIIIIIIXIX
+ 11.28726712478577 * IIIZIIIIIXIX
+ 6.772360274871463 * IIIIZIIIIXIX
+ 2.2574534249571543 * IIIIIZIIIXIX
- 8.90893335364304 * IIIIIIZIIXIX
- 5.345360012185823 * IIIIIIIZIXIX
- 1.7817866707286076 * IIIIIIIIZXIX
- 11.899156895555553 * IIIIIIIIIYIY
+ 8.124377387683996 * ZIIIIIIIIYIY
+ 4.874626432610398 * IZIIIIIIIYIY
+ 1.6248754775367993 * IIZIIIIIIYIY
+ 11.28726712478577 * IIIZIIIIIYIY
+ 6.772360274871463 * IIIIZIIIIYIY
+ 2.2574534249571543 * IIIIIZIIIYIY
- 8.90893335364304 * IIIIIIZIIYIY
- 5.345360012185823 * IIIIIIIZIYIY
- 1.7817866707286076 * IIIIIIIIZYIY
- 2.2979209375000007 * XIXIIIIIIXIX
- 2.2979209375000007 * YIYIIIIIIXIX
- 2.2979209375000007 * XIXIIIIIIYIY
- 2.2979209375000007 * YIYIIIIIIYIY
- 3.1925212500000018 * IIIXIXIIIXIX
- 3.1925212500000018 * IIIYIYIIIXIX
- 3.1925212500000018 * IIIXIXIIIYIY
- 3.1925212500000018 * IIIYIYIIIYIY
- 44.54543265324755 * IIIIIIXXIXIX
- 44.54543265324755 * IIIIIIYYIXIX
- 44.54543265324755 * IIIIIIXXIYIY
- 44.54543265324755 * IIIIIIYYIYIY
+ 2.5198268750000015 * IIIIIIXIXXIX
+ 2.5198268750000015 * IIIIIIYIYXIX
+ 2.5198268750000015 * IIIIIIXIXYIY
+ 2.5198268750000015 * IIIIIIYIYYIY
- 31.498377500000007 * IIIIIIIXXXIX
- 31.498377500000007 * IIIIIIIYYXIX
- 31.498377500000007 * IIIIIIIXXYIY
- 31.498377500000007 * IIIIIIIYYYIY
- 2.102429985900245 * XXIIIIIIIIXX
- 2.102429985900245 * YYIIIIIIIIXX
- 2.102429985900245 * XXIIIIIIIIYY
- 2.102429985900245 * YYIIIIIIIIYY
+ 0.8980418750000001 * IXXIIIIIIIXX
+ 0.8980418750000001 * IYYIIIIIIIXX
+ 0.8980418750000001 * IXXIIIIIIIYY
+ 0.8980418750000001 * IYYIIIIIIIYY
+ 1.175575188382615 * IIIXXIIIIIXX
+ 1.175575188382615 * IIIYYIIIIIXX
+ 1.175575188382615 * IIIXXIIIIIYY
+ 1.175575188382615 * IIIYYIIIIIYY
- 0.5021409375000001 * IIIIXXIIIIXX
- 0.5021409375000001 * IIIIYYIIIIXX
- 0.5021409375000001 * IIIIXXIIIIYY
- 0.5021409375000001 * IIIIYYIIIIYY
###Markdown
Now that the Hamiltonian is ready, it can be used in a quantum algorithm to find information about the vibrational structure of the corresponding molecule. Check out our tutorials on Ground State Calculation and Excited States Calculation to learn more about how to do that in Qiskit Nature!
###Code
import qiskit.tools.jupyter
%qiskit_version_table
%qiskit_copyright
###Output
_____no_output_____
###Markdown
Vibrational structure Introduction The molecular Hamiltonian is $$\mathcal{H} = - \sum_I \frac{\nabla_{R_I}^2}{M_I} - \sum_i \frac{\nabla_{r_i}^2}{m_e} - \sum_I\sum_i \frac{Z_I e^2}{|R_I-r_i|} + \sum_i \sum_{j>i} \frac{e^2}{|r_i-r_j|} + \sum_I\sum_{J>I} \frac{Z_I Z_J e^2}{|R_I-R_J|}$$Because the nuclei are much heavier than the electrons they do not move on the same time scale and therefore, the behavior of nuclei and electrons can be decoupled. This is the Born-Oppenheimer approximation.Within the Born-Oppenheimer approximation, a molecular wave function is factorized as a product of an electronic part, which is the solution of the electronic Schroedinger equation, and a vibro-rotational one, which is the solution of the nuclear Schroedinger equation in the potential energy surface (PES) generated by sampling the eigenvalues of the electronic Schroedinger equation for different geometries.The nuclear Schroedinger equation is usually solved in two steps, in analogy with its electronic counterpart. A single-particle basis (the basis functions are called, in this case, modals) is obtained either by the harmonic approximation applied to the PES or from a vibrational self-consistent field (VSCF) calculation. Vibrational anharmonic correlations are added a-posteriori with perturbative or variational approaches.The latter include Vibrational Configuration Interaction (VCI) and Vibrational Coupled Cluster (VCC) for highly-accurate anharmonic energies. The main advantage of VCI and VCC over alternative approaches (such as perturbation theories) is that their accuracy can be systematically improved towards the complete basis set limit for a given PES. However, their applicability is limited to small molecules with up to about 10 atoms due to their unfavorable scaling with system size.To tackle the scaling problem we would like to use quantum algorithms.The nuclear Schroedinger equation is$$\mathcal{H}_{\text{vib}} |\Psi_{n}\rangle = E_{n} |\Psi_{n}\rangle$$The so-called Watson Hamiltonian (neglecting vibro-rotational coupling terms) is$$ \mathcal{H}_\text{vib}(Q_1, \ldots, Q_L) = - \frac{1}{2} \sum_{l=1}^{L} \frac{\partial^2}{\partial Q_l^2} + V(Q_1, \ldots, Q_L)$$where $Q_l$ are the harmonic mass-weighted normal coordinates.$\mathcal{H}_\text{vib}$ must be mapped to an operator that acts on the states of a given set of $N_q$ qubits in order to calculate its eigenfunctions on quantum hardware.In electronic structure calculations, the mapping is achieved by expressing the non-relativistic electronic Hamiltonian in second quantization, \textit{i.e.} by projecting it onto the complete set of antisymmetrized occupation number vectors (ONV) generated by a given (finite) set of orbitals.To encode the vibrational Hamiltonian in an analogous second quantization operators, we expand the potential $V(Q_1, \ldots, Q_L)$ with the $n$-body expansion as follows:$$ V(Q_1, \ldots, Q_L) = V_0 + \sum_{l=1}^L V^{[l]}(Q_l) + \sum_{l<m}^L V^{[l,m]}(Q_l, Q_m) + \sum_{l<m<n}^L V^{[l,m,n]}(Q_l, Q_m, Q_n) + \ldots$$where $V_0$ is the electronic energy of the reference geometry, the one-mode term $V^{[l]}(Q_l)$ represents the variation of the PES upon change of the $l$-th normal coordinate from the equilibrium position.Similarly, the two-body potential $V^{[l,m]}(Q_l, Q_m)$ represents the change in the exact PES upon a simultaneous displacement along the $l$-th and $m$-th coordinates. Often, including terms up to three-body in the $L$-body expansion is sufficient to obtain an accuracy of about 1~cm$^{-1}$. We highlight that the many-body expansion of the potential operator defining the Watson Hamiltonian contains arbitrarily high coupling terms. This is a crucial difference compared to the non-relativistic electronic-structure Hamiltonian that contains only pairwise interactions.A flexible second quantization form of the Watson Hamiltonian is obtained within the so-called n-mode representation. Let us assume that each mode $l$ is described by a $N_l$-dimensional basis set $S_l$ defined as follows:$$ \mathcal{S}_l = \{ \phi_1^{(l)} (Q_l) , \ldots , \phi_{N_l}^{(l)} (Q_l) \} \, .$$The $n$-mode wave function can be expanded in the product basis $\mathcal{S} = \otimes_{i=1}^L \mathcal{S}_i$ as the following CI-like expansion:$$ |\Psi\rangle = \sum_{k_1=1}^{N_1} \cdots \sum_{k_L=1}^{N_L} C_{k_1,\ldots,k_L} \phi_{k_1}^{(1)}(Q_1) \cdots \phi_{k_L}^{(L)}(Q_L) \, ,$$The many-body basis function $\phi_{k_1}^{(1)}(Q_1) \cdots \phi_{k_L}^{(L)}(Q_L)$ are encoded within the so-called $n$-mode second quantization as occupation-number vectors (ONVs) as follows:$$ \phi_{k_1}(Q_1) \cdots \phi_{k_L}(Q_L) \equiv |0_1 \cdots 1_{k_1} \cdots 0_{N_1}, 0_1 \cdots 1_{k_2} \cdots 0_{N_2}, \cdots , 0_1 \cdots 1_{k_L} \cdots 0_{N_L}\rangle \, .$$The ONV defined above is, therefore, the product of $L$ mode-specific ONVs, each one describing an individual mode. Since each mode is described by one and only one basis function, the occupation of each mode-specific ONV is one.From a theoretical perspective, each mode can be interpreted as a distinguishable quasi-particle (defined as phonons in solid-state physics). Distinguishability arises from the fact that the PES is not invariant by permutation of two modes, also in this case unlike the Coulomb interaction between two equal particles. From this perspective, a molecule can be interpreted as a collection of $L$ indistinguishable particles that interact through the PES operator.Based on this second-quantization representation we introduce a pair of creation and annihilation operators per mode $l$ \textit{and} per basis function $k_l$ defined as:$$ \begin{aligned} a_{k_l}^\dagger |\cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle &= | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle \\ a_{k_l}^\dagger | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle &= 0 \\ a_{k_l} | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle &= | \cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle \\ a_{k_l} | \cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle &= 0 \\ \end{aligned}$$with$$ \begin{aligned} \left[ a_{k_l}^\dagger, a_{h_m}^\dagger \right] &= 0 \\ \left[ a_{k_l}, a_{h_m} \right] &= 0 \\ \left[ a_{k_l}^\dagger, a_{h_m} \right] &= \delta_{l,m} \, , \delta_{k_l,h_m} \end{aligned}$$The second quantization form is obtained by expressing the potential as $$ \begin{aligned} \mathcal{H}_\text{vib}^{SQ} =& \sum_{l=1}^L \sum_{k_l,h_l}^{N_l} \langle \phi_{k_l} | T(Q_l) + V^{[l]}(Q_l) | \phi_{h_l} \rangle a_{k_l}^+ a_{h_l} \\ +& \sum_{l<m}^L \sum_{k_l,h_l}^{N_l} \sum_{k_m,h_m}^{N_m} \langle \phi_{k_l} \phi_{k_m} | V^{[l,m]}(Q_l, Q_m) | \phi_{h_l} \phi_{h_m} \rangle a_{k_l}^+ a_{k_m}^+ a_{h_l} a_{h_m} + \cdots \end{aligned}$$We highlight here the difference between the operators defined here above and the electronic structure one. First, as we already mentioned, the potential contains (in principle) three- and higher-body coupling terms that lead to strings with six (or more) second-quantization operators. Moreover, the Hamiltonian conserves the number of particles for each mode, as can be seen from the fact that the number of creation and annihilation operators for a given mode is the same in each term. Nevertheless, different modes are coupled by two- (and higher) body terms containing SQ operators belonging to different modes $l$ and $m$.Reference: Ollitrault, Pauline J., et al., arXiv:2003.12578 (2020). Compute the electronic potentialSolving the ESE for different nuclear configurations to obtain the PES function $V(Q_1, \ldots, Q_L)$. So far Qiskit gives the possibility to approximate the PES with a quartic force field. $$V(Q_1, \ldots, Q_L) = \frac{1}{2} \sum_{ij} k_{ij} Q_i Q_j + \frac{1}{6} \sum_{ijk} k_{ijk} Q_i Q_j Q_k + \frac{1}{16} \sum_{ijkl} k_{ijkl} Q_i Q_j Q_k Q_l$$The advantage of such form for the PES is that the anharmonic force fields ($k_{ij}$, $k_{ijk}$, $k_{ijkl}$) can be calculated by finite-difference approaches. For methods for which the nuclear energy Hessian can be calculated analytically with response theory-based methods (such as HF and DFT), the quartic force field can be calculated by semi-numerical differentiation as:$$k_{ijk} = \frac{H_{ij}(+\delta Q_k) - H_{ij}(-\delta Q_k)}{2\delta Q_k}$$and$$k_{ijkl} = \frac{H_{ij}(+\delta Q_k+\delta Q_l) - H_{ij}(+\delta Q_k-\delta Q_l) -H_{ij}(-\delta Q_k+\delta Q_l) + H_{ij}(-\delta Q_k+\delta Q_l)} {4\delta Q_k \delta Q_l}$$Such numerical procedure is implemented, for instance, in the Gaussian suite of programs.In practice this can be done with Qiskit using the GaussianForceDriver.
###Code
from qiskit_nature.drivers import GaussianForcesDriver
# if you ran Gaussian elsewhere and already have the output file
driver = GaussianForcesDriver(logfile='aux_files/CO2_freq_B3LYP_ccpVDZ.log')
# if you want to run the Gaussian job from Qiskit
# driver = GaussianForcesDriver(
# ['#p B3LYP/6-31g Freq=(Anharm) Int=Ultrafine SCF=VeryTight',
# '',
# 'CO2 geometry optimization B3LYP/6-31g',
# '',
# '0 1',
# 'C -0.848629 2.067624 0.160992',
# 'O 0.098816 2.655801 -0.159738',
# 'O -1.796073 1.479446 0.481721',
# '',
# ''
###Output
/Users/bpa/opt/anaconda3/envs/qiskit-nature/lib/python3.7/site-packages/pyscf/lib/misc.py:46: H5pyDeprecationWarning: Using default_file_mode other than 'r' is deprecated. Pass the mode to h5py.File() instead.
h5py.get_config().default_file_mode = 'a'
###Markdown
Map to a qubit HamiltonianNow that we have an approximation for the potential, we need to write the Hamiltonian in second quantization. To this end we need to select a modal basis to calculate the one-body integrals $\langle\phi_{k_i}| V(Q_i) | \phi_{h_i} \rangle$, two-body integrals $\langle\phi_{k_i} \phi_{k_j}| V(Q_i,Q_j) | \phi_{h_i} \phi_{h_j} \rangle$... In the simplest case, the $\phi$ functions are the harmonic-oscillator eigenfunctions for each mode. The main advantage of this choice is that the integrals of a PES expressed as a Taylor expansion are easy to calculate with such basis. A routine for computing these integrals is implemented in Qiskit. The bosonic operator, $\mathcal{H}_\text{vib}^{SQ}$, is then created and must be mapped to a qubit operator. The direct mapping introduced in the first section of this tutorial can be used is Qiskit as follows:
###Code
from qiskit_nature.problems.second_quantization.vibrational.vibrational_structure_problem import VibrationalStructureProblem
from qiskit_nature.operators.second_quantization.qubit_converter import QubitConverter
from qiskit_nature.mappers.second_quantization import DirectMapper
vibrational_problem = VibrationalStructureProblem(driver, num_modals=2, truncation_order=2)
second_q_ops = vibrational_problem.second_q_ops()
###Output
_____no_output_____
###Markdown
The Vibrational operator for the problem now reads as
###Code
print(second_q_ops[0])
###Output
NIIIIIII * (1215.682529375+0j)
+ INIIIIII * (3656.9551768750007+0j)
+ IINIIIII * (682.5053337500001+0j)
+ II+-IIII * (-46.77167173323271+0j)
+ II-+IIII * (-46.77167173323271+0j)
+ IIINIIII * (2050.1464387500005+0j)
+ IIIINIII * (329.41209562500006+0j)
+ IIIIINII * (992.0224281250003+0j)
+ IIIIIINI * (328.12046812500006+0j)
+ IIIIIIIN * (985.5642906250002+0j)
+ NINIIIII * (5.039653750000002+0j)
+ INNIIIII * (15.118961250000009+0j)
+ NI+-IIII * (-89.0908653064951+0j)
+ IN+-IIII * (-267.27259591948535+0j)
+ NI-+IIII * (-89.0908653064951+0j)
+ IN-+IIII * (-267.27259591948535+0j)
+ NIINIIII * (15.118961250000009+0j)
+ ININIIII * (45.35688375000003+0j)
+ NIIINIII * (-6.3850425000000035+0j)
+ INIINIII * (-19.15512750000001+0j)
+ IININIII * (-2.5657231250000008+0j)
+ II+-NIII * (21.644966371722845+0j)
+ II-+NIII * (21.644966371722845+0j)
+ IIINNIII * (-7.697169375000003+0j)
+ +-II+-II * (-2.0085637500000004+0j)
+ -+II+-II * (-2.0085637500000004+0j)
+ +-II-+II * (-2.0085637500000004+0j)
+ -+II-+II * (-2.0085637500000004+0j)
+ NIIIINII * (-19.15512750000001+0j)
+ INIIINII * (-57.46538250000003+0j)
+ IINIINII * (-7.697169375000004+0j)
+ II+-INII * (64.93489911516855+0j)
+ II-+INII * (64.93489911516855+0j)
+ IIININII * (-23.091508125000015+0j)
+ NIIIIINI * (-4.595841875000001+0j)
+ INIIIINI * (-13.787525625000006+0j)
+ IINIIINI * (-1.683979375000001+0j)
+ II+-IINI * (6.412754934114709+0j)
+ II-+IINI * (6.412754934114709+0j)
+ IIINIINI * (-5.051938125000003+0j)
+ IIIININI * (-0.5510218750000002+0j)
+ IIIIINNI * (-1.6530656250000009+0j)
+ +-IIII+- * (3.5921675000000004+0j)
+ -+IIII+- * (3.5921675000000004+0j)
+ IIII+-+- * (7.946551250000004+0j)
+ IIII-++- * (7.946551250000004+0j)
+ +-IIII-+ * (3.5921675000000004+0j)
+ -+IIII-+ * (3.5921675000000004+0j)
+ IIII+--+ * (7.946551250000004+0j)
+ IIII-+-+ * (7.946551250000004+0j)
+ NIIIIIIN * (-13.787525625000006+0j)
+ INIIIIIN * (-41.362576875000016+0j)
+ IINIIIIN * (-5.051938125000002+0j)
+ II+-IIIN * (19.238264802344126+0j)
+ II-+IIIN * (19.238264802344126+0j)
+ IIINIIIN * (-15.15581437500001+0j)
+ IIIINIIN * (-1.6530656250000009+0j)
+ IIIIININ * (-4.959196875000003+0j)
###Markdown
In the previous cell we defined a bosonic transformation to express the Hamiltonian in the harmonic modal basis, with 2 modals per mode with the potential truncated at order 2 and the 'direct' boson to qubit mapping. The calculation is then ran as:
###Code
qubit_converter = QubitConverter(mapper=DirectMapper())
qubit_op = qubit_converter.convert(second_q_ops[0])
print(qubit_op)
###Output
5077.236560625012 * IIIIIIII
- 472.28961593750034 * ZIIIIIII
- 157.22939093749991 * IZIIIIII
- 467.5058515625001 * IIZIIIII
- 1.2397992187500007 * ZIZIIIII
- 0.4132664062500002 * IZZIIIII
- 155.20426031249983 * IIIZIIII
- 0.4132664062500002 * ZIIZIIII
- 0.13775546875000005 * IZIZIIII
- 1027.4430731249997 * IIIIZIII
- 3.7889535937500023 * ZIIIZIII
- 1.2629845312500008 * IZIIZIII
- 5.772877031250004 * IIZIZIII
- 1.9242923437500008 * IIIZZIII
- 342.04261812500033 * IIIIIZII
- 1.2629845312500005 * ZIIIIZII
- 0.42099484375000024 * IZIIIZII
- 1.924292343750001 * IIZIIZII
- 0.6414307812500002 * IIIZIZII
- 1810.6538965625004 * IIIIIIZI
- 10.340644218750004 * ZIIIIIZI
- 3.4468814062500015 * IZIIIIZI
- 14.366345625000008 * IIZIIIZI
- 4.788781875000002 * IIIZIIZI
+ 11.339220937500007 * IIIIZIZI
+ 3.779740312500002 * IIIIIZZI
- 601.9000340624999 * IIIIIIIZ
- 3.4468814062500015 * ZIIIIIIZ
- 1.1489604687500004 * IZIIIIIZ
- 4.788781875000002 * IIZIIIIZ
- 1.5962606250000009 * IIIZIIIZ
+ 3.779740312500002 * IIIIZIIZ
+ 1.2599134375000005 * IIIIIZIZ
+ 1.986637812500001 * XXXXIIII
+ 1.986637812500001 * YYXXIIII
+ 1.986637812500001 * XXYYIIII
+ 1.986637812500001 * YYYYIIII
- 84.41897986727398 * IIIIXXII
- 4.809566200586032 * ZIIIXXII
- 1.6031887335286772 * IZIIXXII
- 16.233724778792137 * IIZIXXII
- 5.411241592930711 * IIIZXXII
- 84.41897986727398 * IIIIYYII
- 4.809566200586032 * ZIIIYYII
- 1.6031887335286772 * IZIIYYII
- 16.233724778792137 * IIZIYYII
- 5.411241592930711 * IIIZYYII
+ 66.81814897987134 * IIIIXXZI
+ 66.81814897987134 * IIIIYYZI
+ 22.272716326623776 * IIIIXXIZ
+ 22.272716326623776 * IIIIYYIZ
+ 0.8980418750000001 * XXIIIIXX
+ 0.8980418750000001 * YYIIIIXX
+ 0.8980418750000001 * XXIIIIYY
+ 0.8980418750000001 * YYIIIIYY
- 0.5021409375000001 * IIXXIIXX
- 0.5021409375000001 * IIYYIIXX
- 0.5021409375000001 * IIXXIIYY
- 0.5021409375000001 * IIYYIIYY
###Markdown
To have a different number of modals per mode:
###Code
vibrational_problem = VibrationalStructureProblem(driver, num_modals=3, truncation_order=2)
second_q_ops = vibrational_problem.second_q_ops()
qubit_converter = QubitConverter(mapper = DirectMapper())
qubit_op = qubit_converter.convert(second_q_ops[0])
print(qubit_op)
###Output
11327.034966562516 * IIIIIIIIIIII
- 745.8599576562498 * ZIIIIIIIIIII
- 446.6739542187503 * IZIIIIIIIIII
- 148.69083703124986 * IIZIIIIIIIII
- 724.2074121874995 * IIIZIIIIIIII
- 3.4438867187500013 * ZIIZIIIIIIII
- 2.066332031250001 * IZIZIIIIIIII
- 0.6887773437500002 * IIZZIIIIIIII
- 431.8741484375 * IIIIZIIIIIII
- 2.066332031250001 * ZIIIZIIIIIII
- 1.2397992187500007 * IZIIZIIIIIII
- 0.4132664062500002 * IIZIZIIIIIII
- 143.32702593749988 * IIIIIZIIIIII
- 0.6887773437500002 * ZIIIIZIIIIII
- 0.4132664062500002 * IZIIIZIIIIII
- 0.13775546875000005 * IIZIIZIIIIII
- 1720.4111609375004 * IIIIIIZIIIII
- 10.524871093750003 * ZIIIIIZIIIII
- 6.314922656250003 * IZIIIIZIIIII
- 2.104974218750001 * IIZIIIZIIIII
- 16.035769531250004 * IIIZIIZIIIII
- 9.621461718750005 * IIIIZIZIIIII
- 3.207153906250001 * IIIIIZZIIIII
- 1030.4053903124998 * IIIIIIIZIIII
- 6.314922656250003 * ZIIIIIIZIIII
- 3.7889535937500023 * IZIIIIIZIIII
- 1.2629845312500008 * IIZIIIIZIIII
- 9.621461718750004 * IIIZIIIZIIII
- 5.772877031250004 * IIIIZIIZIIII
- 1.9242923437500008 * IIIIIZIZIIII
- 343.0300571875006 * IIIIIIIIZIII
- 2.1049742187500007 * ZIIIIIIIZIII
- 1.2629845312500005 * IZIIIIIIZIII
- 0.42099484375000024 * IIZIIIIIZIII
- 3.207153906250001 * IIIZIIIIZIII
- 1.924292343750001 * IIIIZIIIZIII
- 0.6414307812500002 * IIIIIZIIZIII
- 2992.18265640625 * IIIIIIIIIZII
- 28.72401171875001 * ZIIIIIIIIZII
- 17.234407031250004 * IZIIIIIIIZII
- 5.744802343750002 * IIZIIIIIIZII
- 39.90651562500001 * IIIZIIIIIZII
- 23.94390937500001 * IIIIZIIIIZII
- 7.981303125000004 * IIIIIZIIIZII
+ 31.497835937500014 * IIIIIIZIIZII
+ 18.89870156250001 * IIIIIIIZIZII
+ 6.299567187500003 * IIIIIIIIZZII
- 1788.3742817187504 * IIIIIIIIIIZI
- 17.234407031250008 * ZIIIIIIIIIZI
- 10.340644218750004 * IZIIIIIIIIZI
- 3.4468814062500015 * IIZIIIIIIIZI
- 23.94390937500001 * IIIZIIIIIIZI
- 14.366345625000008 * IIIIZIIIIIZI
- 4.788781875000002 * IIIIIZIIIIZI
+ 18.898701562500012 * IIIIIIZIIIZI
+ 11.339220937500007 * IIIIIIIZIIZI
+ 3.779740312500002 * IIIIIIIIZIZI
- 594.4734957812499 * IIIIIIIIIIIZ
- 5.744802343750002 * ZIIIIIIIIIIZ
- 3.4468814062500015 * IZIIIIIIIIIZ
- 1.1489604687500004 * IIZIIIIIIIIZ
- 7.981303125000003 * IIIZIIIIIIIZ
- 4.788781875000002 * IIIIZIIIIIIZ
- 1.5962606250000009 * IIIIIZIIIIIZ
+ 6.299567187500004 * IIIIIIZIIIIZ
+ 3.779740312500002 * IIIIIIIZIIIZ
+ 1.2599134375000005 * IIIIIIIIZIIZ
- 20.885040702719472 * XIXIIIIIIIII
- 20.885040702719472 * YIYIIIIIIIII
+ 0.9740782609865658 * XIXZIIIIIIII
+ 0.9740782609865658 * YIYZIIIIIIII
+ 0.5844469565919396 * XIXIZIIIIIII
+ 0.5844469565919396 * YIYIZIIIIIII
+ 0.19481565219731317 * XIXIIZIIIIII
+ 0.19481565219731317 * YIYIIZIIIIII
+ 2.9768830886019613 * XIXIIIZIIIII
+ 2.9768830886019613 * YIYIIIZIIIII
+ 1.7861298531611771 * XIXIIIIZIIII
+ 1.7861298531611771 * YIYIIIIZIIII
+ 0.5953766177203923 * XIXIIIIIZIII
+ 0.5953766177203923 * YIYIIIIIZIII
+ 8.124377387683996 * XIXIIIIIIZII
+ 8.124377387683996 * YIYIIIIIIZII
+ 4.874626432610398 * XIXIIIIIIIZI
+ 4.874626432610398 * YIYIIIIIIIZI
+ 1.6248754775367993 * XIXIIIIIIIIZ
+ 1.6248754775367993 * YIYIIIIIIIIZ
+ 7.946551250000002 * XXIXXIIIIIII
+ 7.946551250000002 * YYIXXIIIIIII
+ 7.946551250000002 * XXIYYIIIIIII
+ 7.946551250000002 * YYIYYIIIIIII
+ 4.245123515804727 * IXXXXIIIIIII
+ 4.245123515804727 * IYYXXIIIIIII
+ 4.245123515804727 * IXXYYIIIIIII
+ 4.245123515804727 * IYYYYIIIIIII
- 27.557296533490963 * IIIXIXIIIIII
+ 0.9740782609865657 * ZIIXIXIIIIII
+ 0.5844469565919395 * IZIXIXIIIIII
+ 0.19481565219731314 * IIZXIXIIIIII
- 27.557296533490963 * IIIYIYIIIIII
+ 0.9740782609865657 * ZIIYIYIIIIII
+ 0.5844469565919395 * IZIYIYIIIIII
+ 0.19481565219731314 * IIZYIYIIIIII
+ 4.535600550836601 * IIIXIXZIIIII
+ 4.535600550836601 * IIIYIYZIIIII
+ 2.721360330501961 * IIIXIXIZIIII
+ 2.721360330501961 * IIIYIYIZIIII
+ 0.9071201101673203 * IIIXIXIIZIII
+ 0.9071201101673203 * IIIYIYIIZIII
+ 11.287267124785771 * IIIXIXIIIZII
+ 11.287267124785771 * IIIYIYIIIZII
+ 6.772360274871463 * IIIXIXIIIIZI
+ 6.772360274871463 * IIIYIYIIIIZI
+ 2.2574534249571543 * IIIXIXIIIIIZ
+ 2.2574534249571543 * IIIYIYIIIIIZ
- 0.2755109375000001 * XIXXIXIIIIII
- 0.2755109375000001 * YIYXIXIIIIII
- 0.2755109375000001 * XIXYIYIIIIII
- 0.2755109375000001 * YIYYIYIIIIII
+ 4.1834668980774286 * XXIIXXIIIIII
+ 4.1834668980774286 * YYIIXXIIIIII
+ 4.1834668980774286 * XXIIYYIIIIII
+ 4.1834668980774286 * YYIIYYIIIIII
+ 1.986637812500001 * IXXIXXIIIIII
+ 1.986637812500001 * IYYIXXIIIIII
+ 1.986637812500001 * IXXIYYIIIIII
+ 1.986637812500001 * IYYIYYIIIIII
- 260.3514075 * IIIIIIXXIIII
- 11.336256250000002 * ZIIIIIXXIIII
- 6.801753750000003 * IZIIIIXXIIII
- 2.2672512500000006 * IIZIIIXXIIII
- 38.26325625000001 * IIIZIIXXIIII
- 22.95795375000001 * IIIIZIXXIIII
- 7.652651250000002 * IIIIIZXXIIII
- 260.3514075 * IIIIIIYYIIII
- 11.336256250000002 * ZIIIIIYYIIII
- 6.801753750000003 * IZIIIIYYIIII
- 2.2672512500000006 * IIZIIIYYIIII
- 38.26325625000001 * IIIZIIYYIIII
- 22.95795375000001 * IIIIZIYYIIII
- 7.652651250000002 * IIIIIZYYIIII
+ 157.49188750000002 * IIIIIIXXIZII
+ 157.49188750000002 * IIIIIIYYIZII
+ 94.49513250000001 * IIIIIIXXIIZI
+ 94.49513250000001 * IIIIIIYYIIZI
+ 31.498377500000004 * IIIIIIXXIIIZ
+ 31.498377500000004 * IIIIIIYYIIIZ
+ 3.206377467057354 * XIXIIIXXIIII
+ 3.206377467057354 * YIYIIIXXIIII
+ 3.206377467057354 * XIXIIIYYIIII
+ 3.206377467057354 * YIYIIIYYIIII
+ 10.822483185861422 * IIIXIXXXIIII
+ 10.822483185861422 * IIIYIYXXIIII
+ 10.822483185861422 * IIIXIXYYIIII
+ 10.822483185861422 * IIIYIYYYIIII
+ 4.373609679305444 * IIIIIIXIXIII
+ 2.9768830886019613 * ZIIIIIXIXIII
+ 1.786129853161177 * IZIIIIXIXIII
+ 0.5953766177203923 * IIZIIIXIXIII
+ 4.535600550836601 * IIIZIIXIXIII
+ 2.721360330501961 * IIIIZIXIXIII
+ 0.9071201101673203 * IIIIIZXIXIII
+ 4.373609679305444 * IIIIIIYIYIII
+ 2.9768830886019613 * ZIIIIIYIYIII
+ 1.786129853161177 * IZIIIIYIYIII
+ 0.5953766177203923 * IIZIIIYIYIII
+ 4.535600550836601 * IIIZIIYIYIII
+ 2.721360330501961 * IIIIZIYIYIII
+ 0.9071201101673203 * IIIIIZYIYIII
- 8.90893335364304 * IIIIIIXIXZII
- 8.90893335364304 * IIIIIIYIYZII
- 5.345360012185824 * IIIIIIXIXIZI
- 5.345360012185824 * IIIIIIYIYIZI
- 1.7817866707286079 * IIIIIIXIXIIZ
- 1.7817866707286079 * IIIIIIYIYIIZ
- 0.8419896875000004 * XIXIIIXIXIII
- 0.8419896875000004 * YIYIIIXIXIII
- 0.8419896875000004 * XIXIIIYIYIII
- 0.8419896875000004 * YIYIIIYIYIII
- 1.2828615625000004 * IIIXIXXIXIII
- 1.2828615625000004 * IIIYIYXIXIII
- 1.2828615625000004 * IIIXIXYIYIII
- 1.2828615625000004 * IIIYIYYIYIII
- 160.71040986809584 * IIIIIIIXXIII
- 8.015943667643384 * ZIIIIIIXXIII
- 4.809566200586032 * IZIIIIIXXIII
- 1.6031887335286772 * IIZIIIIXXIII
- 27.05620796465356 * IIIZIIIXXIII
- 16.233724778792137 * IIIIZIIXXIII
- 5.411241592930711 * IIIIIZIXXIII
- 160.71040986809584 * IIIIIIIYYIII
- 8.015943667643384 * ZIIIIIIYYIII
- 4.809566200586032 * IZIIIIIYYIII
- 1.6031887335286772 * IIZIIIIYYIII
- 27.05620796465356 * IIIZIIIYYIII
- 16.233724778792137 * IIIIZIIYYIII
- 5.411241592930711 * IIIIIZIYYIII
+ 111.36358163311888 * IIIIIIIXXZII
+ 111.36358163311888 * IIIIIIIYYZII
+ 66.81814897987134 * IIIIIIIXXIZI
+ 66.81814897987134 * IIIIIIIYYIZI
+ 22.272716326623776 * IIIIIIIXXIIZ
+ 22.272716326623776 * IIIIIIIYYIIZ
+ 2.267251250000001 * XIXIIIIXXIII
+ 2.267251250000001 * YIYIIIIXXIII
+ 2.267251250000001 * XIXIIIIYYIII
+ 2.267251250000001 * YIYIIIIYYIII
+ 7.6526512500000035 * IIIXIXIXXIII
+ 7.6526512500000035 * IIIYIYIXXIII
+ 7.6526512500000035 * IIIXIXIYYIII
+ 7.6526512500000035 * IIIYIYIYYIII
+ 3.5921675000000004 * XXIIIIIIIXXI
+ 3.5921675000000004 * YYIIIIIIIXXI
+ 3.5921675000000004 * XXIIIIIIIYYI
+ 3.5921675000000004 * YYIIIIIIIYYI
+ 5.912498983512137 * IXXIIIIIIXXI
+ 5.912498983512137 * IYYIIIIIIXXI
+ 5.912498983512137 * IXXIIIIIIYYI
+ 5.912498983512137 * IYYIIIIIIYYI
- 2.0085637500000013 * IIIXXIIIIXXI
- 2.0085637500000013 * IIIYYIIIIXXI
- 2.0085637500000013 * IIIXXIIIIYYI
- 2.0085637500000013 * IIIYYIIIIYYI
- 3.305978760488338 * IIIIXXIIIXXI
- 3.305978760488338 * IIIIYYIIIXXI
- 3.305978760488338 * IIIIXXIIIYYI
- 3.305978760488338 * IIIIYYIIIYYI
- 11.899156895555553 * IIIIIIIIIXIX
+ 8.124377387683996 * ZIIIIIIIIXIX
+ 4.874626432610398 * IZIIIIIIIXIX
+ 1.6248754775367993 * IIZIIIIIIXIX
+ 11.28726712478577 * IIIZIIIIIXIX
+ 6.772360274871463 * IIIIZIIIIXIX
+ 2.2574534249571543 * IIIIIZIIIXIX
- 8.90893335364304 * IIIIIIZIIXIX
- 5.345360012185823 * IIIIIIIZIXIX
- 1.7817866707286076 * IIIIIIIIZXIX
- 11.899156895555553 * IIIIIIIIIYIY
+ 8.124377387683996 * ZIIIIIIIIYIY
+ 4.874626432610398 * IZIIIIIIIYIY
+ 1.6248754775367993 * IIZIIIIIIYIY
+ 11.28726712478577 * IIIZIIIIIYIY
+ 6.772360274871463 * IIIIZIIIIYIY
+ 2.2574534249571543 * IIIIIZIIIYIY
- 8.90893335364304 * IIIIIIZIIYIY
- 5.345360012185823 * IIIIIIIZIYIY
- 1.7817866707286076 * IIIIIIIIZYIY
- 2.2979209375000007 * XIXIIIIIIXIX
- 2.2979209375000007 * YIYIIIIIIXIX
- 2.2979209375000007 * XIXIIIIIIYIY
- 2.2979209375000007 * YIYIIIIIIYIY
- 3.1925212500000018 * IIIXIXIIIXIX
- 3.1925212500000018 * IIIYIYIIIXIX
- 3.1925212500000018 * IIIXIXIIIYIY
- 3.1925212500000018 * IIIYIYIIIYIY
- 44.54543265324755 * IIIIIIXXIXIX
- 44.54543265324755 * IIIIIIYYIXIX
- 44.54543265324755 * IIIIIIXXIYIY
- 44.54543265324755 * IIIIIIYYIYIY
+ 2.5198268750000015 * IIIIIIXIXXIX
+ 2.5198268750000015 * IIIIIIYIYXIX
+ 2.5198268750000015 * IIIIIIXIXYIY
+ 2.5198268750000015 * IIIIIIYIYYIY
- 31.498377500000007 * IIIIIIIXXXIX
- 31.498377500000007 * IIIIIIIYYXIX
- 31.498377500000007 * IIIIIIIXXYIY
- 31.498377500000007 * IIIIIIIYYYIY
- 2.102429985900245 * XXIIIIIIIIXX
- 2.102429985900245 * YYIIIIIIIIXX
- 2.102429985900245 * XXIIIIIIIIYY
- 2.102429985900245 * YYIIIIIIIIYY
+ 0.8980418750000001 * IXXIIIIIIIXX
+ 0.8980418750000001 * IYYIIIIIIIXX
+ 0.8980418750000001 * IXXIIIIIIIYY
+ 0.8980418750000001 * IYYIIIIIIIYY
+ 1.175575188382615 * IIIXXIIIIIXX
+ 1.175575188382615 * IIIYYIIIIIXX
+ 1.175575188382615 * IIIXXIIIIIYY
+ 1.175575188382615 * IIIYYIIIIIYY
- 0.5021409375000001 * IIIIXXIIIIXX
- 0.5021409375000001 * IIIIYYIIIIXX
- 0.5021409375000001 * IIIIXXIIIIYY
- 0.5021409375000001 * IIIIYYIIIIYY
###Markdown
Now that the Hamiltonian is ready, it can be used in a quantum algorithm to find information about the vibrational structure of the corresponding molecule. Check out our tutorials on Ground State Calculation and Excited States Calculation to learn more about how to do that in Qiskit Nature!
###Code
import qiskit.tools.jupyter
%qiskit_version_table
%qiskit_copyright
###Output
_____no_output_____
###Markdown
Vibrational structure Introduction The molecular Hamiltonian is $$\mathcal{H} = - \sum_I \frac{\nabla_{R_I}^2}{M_I} - \sum_i \frac{\nabla_{r_i}^2}{m_e} - \sum_I\sum_i \frac{Z_I e^2}{|R_I-r_i|} + \sum_i \sum_{j>i} \frac{e^2}{|r_i-r_j|} + \sum_I\sum_{J>I} \frac{Z_I Z_J e^2}{|R_I-R_J|}$$Because the nuclei are much heavier than the electrons they do not move on the same time scale and therefore, the behavior of nuclei and electrons can be decoupled. This is the Born-Oppenheimer approximation.Within the Born-Oppenheimer approximation, a molecular wave function is factorized as a product of an electronic part, which is the solution of the electronic Schroedinger equation, and a vibro-rotational one, which is the solution of the nuclear Schroedinger equation in the potential energy surface (PES) generated by sampling the eigenvalues of the electronic Schroedinger equation for different geometries.The nuclear Schroedinger equation is usually solved in two steps, in analogy with its electronic counterpart. A single-particle basis (the basis functions are called, in this case, modals) is obtained either by the harmonic approximation applied to the PES or from a vibrational self-consistent field (VSCF) calculation. Vibrational anharmonic correlations are added a-posteriori with perturbative or variational approaches.The latter include Vibrational Configuration Interaction (VCI) and Vibrational Coupled Cluster (VCC) for highly-accurate anharmonic energies. The main advantage of VCI and VCC over alternative approaches (such as perturbation theories) is that their accuracy can be systematically improved towards the complete basis set limit for a given PES. However, their applicability is limited to small molecules with up to about 10 atoms due to their unfavorable scaling with system size.To tackle the scaling problem we would like to use quantum algorithms.The nuclear Schroedinger equation is$$\mathcal{H}_{\text{vib}} |\Psi_{n}\rangle = E_{n} |\Psi_{n}\rangle$$The so-called Watson Hamiltonian (neglecting vibro-rotational coupling terms) is$$ \mathcal{H}_\text{vib}(Q_1, \ldots, Q_L) = - \frac{1}{2} \sum_{l=1}^{L} \frac{\partial^2}{\partial Q_l^2} + V(Q_1, \ldots, Q_L)$$where $Q_l$ are the harmonic mass-weighted normal coordinates.$\mathcal{H}_\text{vib}$ must be mapped to an operator that acts on the states of a given set of $N_q$ qubits in order to calculate its eigenfunctions on quantum hardware.In electronic structure calculations, the mapping is achieved by expressing the non-relativistic electronic Hamiltonian in second quantization, \textit{i.e.} by projecting it onto the complete set of antisymmetrized occupation number vectors (ONV) generated by a given (finite) set of orbitals.To encode the vibrational Hamiltonian in an analogous second quantization operators, we expand the potential $V(Q_1, \ldots, Q_L)$ with the $n$-body expansion as follows:$$ V(Q_1, \ldots, Q_L) = V_0 + \sum_{l=1}^L V^{[l]}(Q_l) + \sum_{l<m}^L V^{[l,m]}(Q_l, Q_m) + \sum_{l<m<n}^L V^{[l,m,n]}(Q_l, Q_m, Q_n) + \ldots$$where $V_0$ is the electronic energy of the reference geometry, the one-mode term $V^{[l]}(Q_l)$ represents the variation of the PES upon change of the $l$-th normal coordinate from the equilibrium position.Similarly, the two-body potential $V^{[l,m]}(Q_l, Q_m)$ represents the change in the exact PES upon a simultaneous displacement along the $l$-th and $m$-th coordinates. Often, including terms up to three-body in the $L$-body expansion is sufficient to obtain an accuracy of about 1~cm$^{-1}$. We highlight that the many-body expansion of the potential operator defining the Watson Hamiltonian contains arbitrarily high coupling terms. This is a crucial difference compared to the non-relativistic electronic-structure Hamiltonian that contains only pairwise interactions.A flexible second quantization form of the Watson Hamiltonian is obtained within the so-called n-mode representation. Let us assume that each mode $l$ is described by a $N_l$-dimensional basis set $S_l$ defined as follows:$$ \mathcal{S}_l = \{ \phi_1^{(l)} (Q_l) , \ldots , \phi_{N_l}^{(l)} (Q_l) \} \, .$$The $n$-mode wave function can be expanded in the product basis $\mathcal{S} = \otimes_{i=1}^L \mathcal{S}_i$ as the following CI-like expansion:$$ |\Psi\rangle = \sum_{k_1=1}^{N_1} \cdots \sum_{k_L=1}^{N_L} C_{k_1,\ldots,k_L} \phi_{k_1}^{(1)}(Q_1) \cdots \phi_{k_L}^{(L)}(Q_L) \, ,$$The many-body basis function $\phi_{k_1}^{(1)}(Q_1) \cdots \phi_{k_L}^{(L)}(Q_L)$ are encoded within the so-called $n$-mode second quantization as occupation-number vectors (ONVs) as follows:$$ \phi_{k_1}(Q_1) \cdots \phi_{k_L}(Q_L) \equiv |0_1 \cdots 1_{k_1} \cdots 0_{N_1}, 0_1 \cdots 1_{k_2} \cdots 0_{N_2}, \cdots , 0_1 \cdots 1_{k_L} \cdots 0_{N_L}\rangle \, .$$The ONV defined above is, therefore, the product of $L$ mode-specific ONVs, each one describing an individual mode. Since each mode is described by one and only one basis function, the occupation of each mode-specific ONV is one.From a theoretical perspective, each mode can be interpreted as a distinguishable quasi-particle (defined as phonons in solid-state physics). Distinguishability arises from the fact that the PES is not invariant by permutation of two modes, also in this case unlike the Coulomb interaction between two equal particles. From this perspective, a molecule can be interpreted as a collection of $L$ indistinguishable particles that interact through the PES operator.Based on this second-quantization representation we introduce a pair of creation and annihilation operators per mode $l$ \textit{and} per basis function $k_l$ defined as:$$ \begin{aligned} a_{k_l}^\dagger |\cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle &= | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle \\ a_{k_l}^\dagger | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle &= 0 \\ a_{k_l} | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle &= | \cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle \\ a_{k_l} | \cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle &= 0 \\ \end{aligned}$$with$$ \begin{aligned} \left[ a_{k_l}^\dagger, a_{h_m}^\dagger \right] &= 0 \\ \left[ a_{k_l}, a_{h_m} \right] &= 0 \\ \left[ a_{k_l}^\dagger, a_{h_m} \right] &= \delta_{l,m} \, , \delta_{k_l,h_m} \end{aligned}$$The second quantization form is obtained by expressing the potential as $$ \begin{aligned} \mathcal{H}_\text{vib}^{SQ} =& \sum_{l=1}^L \sum_{k_l,h_l}^{N_l} \langle \phi_{k_l} | T(Q_l) + V^{[l]}(Q_l) | \phi_{h_l} \rangle a_{k_l}^+ a_{h_l} \\ +& \sum_{l<m}^L \sum_{k_l,h_l}^{N_l} \sum_{k_m,h_m}^{N_m} \langle \phi_{k_l} \phi_{k_m} | V^{[l,m]}(Q_l, Q_m) | \phi_{h_l} \phi_{h_m} \rangle a_{k_l}^+ a_{k_m}^+ a_{h_l} a_{h_m} + \cdots \end{aligned}$$We highlight here the difference between the operators defined here above and the electronic structure one. First, as we already mentioned, the potential contains (in principle) three- and higher-body coupling terms that lead to strings with six (or more) second-quantization operators. Moreover, the Hamiltonian conserves the number of particles for each mode, as can be seen from the fact that the number of creation and annihilation operators for a given mode is the same in each term. Nevertheless, different modes are coupled by two- (and higher) body terms containing SQ operators belonging to different modes $l$ and $m$.Reference: Ollitrault, Pauline J., et al., arXiv:2003.12578 (2020). Compute the electronic potentialSolving the ESE for different nuclear configurations to obtain the PES function $V(Q_1, \ldots, Q_L)$. So far Qiskit gives the possibility to approximate the PES with a quartic force field. $$V(Q_1, \ldots, Q_L) = \frac{1}{2} \sum_{ij} k_{ij} Q_i Q_j + \frac{1}{6} \sum_{ijk} k_{ijk} Q_i Q_j Q_k + \frac{1}{16} \sum_{ijkl} k_{ijkl} Q_i Q_j Q_k Q_l$$The advantage of such form for the PES is that the anharmonic force fields ($k_{ij}$, $k_{ijk}$, $k_{ijkl}$) can be calculated by finite-difference approaches. For methods for which the nuclear energy Hessian can be calculated analytically with response theory-based methods (such as HF and DFT), the quartic force field can be calculated by semi-numerical differentiation as:$$k_{ijk} = \frac{H_{ij}(+\delta Q_k) - H_{ij}(-\delta Q_k)}{2\delta Q_k}$$and$$k_{ijkl} = \frac{H_{ij}(+\delta Q_k+\delta Q_l) - H_{ij}(+\delta Q_k-\delta Q_l) -H_{ij}(-\delta Q_k+\delta Q_l) + H_{ij}(-\delta Q_k+\delta Q_l)} {4\delta Q_k \delta Q_l}$$Such numerical procedure is implemented, for instance, in the Gaussian suite of programs.In practice this can be done with Qiskit using the GaussianForceDriver.
###Code
from qiskit_nature.drivers import GaussianForcesDriver
# if you ran Gaussian elsewhere and already have the output file
driver = GaussianForcesDriver(logfile='aux_files/CO2_freq_B3LYP_ccpVDZ.log')
# if you want to run the Gaussian job from Qiskit
# driver = GaussianForcesDriver(
# ['#p B3LYP/6-31g Freq=(Anharm) Int=Ultrafine SCF=VeryTight',
# '',
# 'CO2 geometry optimization B3LYP/6-31g',
# '',
# '0 1',
# 'C -0.848629 2.067624 0.160992',
# 'O 0.098816 2.655801 -0.159738',
# 'O -1.796073 1.479446 0.481721',
# '',
# ''
###Output
_____no_output_____
###Markdown
Map to a qubit HamiltonianNow that we have an approximation for the potential, we need to write the Hamiltonian in second quantization. To this end we need to select a modal basis to calculate the one-body integrals $\langle\phi_{k_i}| V(Q_i) | \phi_{h_i} \rangle$, two-body integrals $\langle\phi_{k_i} \phi_{k_j}| V(Q_i,Q_j) | \phi_{h_i} \phi_{h_j} \rangle$... In the simplest case, the $\phi$ functions are the harmonic-oscillator eigenfunctions for each mode. The main advantage of this choice is that the integrals of a PES expressed as a Taylor expansion are easy to calculate with such basis. A routine for computing these integrals is implemented in Qiskit. The bosonic operator, $\mathcal{H}_\text{vib}^{SQ}$, is then created and must be mapped to a qubit operator. The direct mapping introduced in the first section of this tutorial can be used is Qiskit as follows:
###Code
from qiskit_nature.problems.second_quantization import VibrationalStructureProblem
from qiskit_nature.converters.second_quantization import QubitConverter
from qiskit_nature.mappers.second_quantization import DirectMapper
vibrational_problem = VibrationalStructureProblem(driver, num_modals=2, truncation_order=2)
second_q_ops = vibrational_problem.second_q_ops()
###Output
_____no_output_____
###Markdown
The Vibrational operator for the problem now reads as
###Code
print(second_q_ops[0])
###Output
NIIIIIII * (1215.682529375+0j)
+ INIIIIII * (3656.9551768750007+0j)
+ IINIIIII * (682.5053337500001+0j)
+ II+-IIII * (-46.77167173323271+0j)
+ II-+IIII * (-46.77167173323271+0j)
+ IIINIIII * (2050.1464387500005+0j)
+ IIIINIII * (329.41209562500006+0j)
+ IIIIINII * (992.0224281250003+0j)
+ IIIIIINI * (328.12046812500006+0j)
+ IIIIIIIN * (985.5642906250002+0j)
+ NINIIIII * (5.039653750000002+0j)
+ INNIIIII * (15.118961250000009+0j)
+ NI+-IIII * (-89.0908653064951+0j)
+ IN+-IIII * (-267.27259591948535+0j)
+ NI-+IIII * (-89.0908653064951+0j)
+ IN-+IIII * (-267.27259591948535+0j)
+ NIINIIII * (15.118961250000009+0j)
+ ININIIII * (45.35688375000003+0j)
+ NIIINIII * (-6.3850425000000035+0j)
+ INIINIII * (-19.15512750000001+0j)
+ IININIII * (-2.5657231250000008+0j)
+ II+-NIII * (21.644966371722845+0j)
+ II-+NIII * (21.644966371722845+0j)
+ IIINNIII * (-7.697169375000003+0j)
+ +-II+-II * (-2.0085637500000004+0j)
+ -+II+-II * (-2.0085637500000004+0j)
+ +-II-+II * (-2.0085637500000004+0j)
+ -+II-+II * (-2.0085637500000004+0j)
+ NIIIINII * (-19.15512750000001+0j)
+ INIIINII * (-57.46538250000003+0j)
+ IINIINII * (-7.697169375000004+0j)
+ II+-INII * (64.93489911516855+0j)
+ II-+INII * (64.93489911516855+0j)
+ IIININII * (-23.091508125000015+0j)
+ NIIIIINI * (-4.595841875000001+0j)
+ INIIIINI * (-13.787525625000006+0j)
+ IINIIINI * (-1.683979375000001+0j)
+ II+-IINI * (6.412754934114709+0j)
+ II-+IINI * (6.412754934114709+0j)
+ IIINIINI * (-5.051938125000003+0j)
+ IIIININI * (-0.5510218750000002+0j)
+ IIIIINNI * (-1.6530656250000009+0j)
+ +-IIII+- * (3.5921675000000004+0j)
+ -+IIII+- * (3.5921675000000004+0j)
+ IIII+-+- * (7.946551250000004+0j)
+ IIII-++- * (7.946551250000004+0j)
+ +-IIII-+ * (3.5921675000000004+0j)
+ -+IIII-+ * (3.5921675000000004+0j)
+ IIII+--+ * (7.946551250000004+0j)
+ IIII-+-+ * (7.946551250000004+0j)
+ NIIIIIIN * (-13.787525625000006+0j)
+ INIIIIIN * (-41.362576875000016+0j)
+ IINIIIIN * (-5.051938125000002+0j)
+ II+-IIIN * (19.238264802344126+0j)
+ II-+IIIN * (19.238264802344126+0j)
+ IIINIIIN * (-15.15581437500001+0j)
+ IIIINIIN * (-1.6530656250000009+0j)
+ IIIIININ * (-4.959196875000003+0j)
###Markdown
In the previous cell we defined a bosonic transformation to express the Hamiltonian in the harmonic modal basis, with 2 modals per mode with the potential truncated at order 2 and the 'direct' boson to qubit mapping. The calculation is then ran as:
###Code
qubit_converter = QubitConverter(mapper=DirectMapper())
qubit_op = qubit_converter.convert(second_q_ops[0])
print(qubit_op)
###Output
5077.236560625012 * IIIIIIII
- 472.28961593750034 * ZIIIIIII
- 157.22939093749991 * IZIIIIII
- 467.5058515625001 * IIZIIIII
- 1.2397992187500007 * ZIZIIIII
- 0.4132664062500002 * IZZIIIII
- 155.20426031249983 * IIIZIIII
- 0.4132664062500002 * ZIIZIIII
- 0.13775546875000005 * IZIZIIII
- 1027.4430731249997 * IIIIZIII
- 3.7889535937500023 * ZIIIZIII
- 1.2629845312500008 * IZIIZIII
- 5.772877031250004 * IIZIZIII
- 1.9242923437500008 * IIIZZIII
- 342.04261812500033 * IIIIIZII
- 1.2629845312500005 * ZIIIIZII
- 0.42099484375000024 * IZIIIZII
- 1.924292343750001 * IIZIIZII
- 0.6414307812500002 * IIIZIZII
- 1810.6538965625004 * IIIIIIZI
- 10.340644218750004 * ZIIIIIZI
- 3.4468814062500015 * IZIIIIZI
- 14.366345625000008 * IIZIIIZI
- 4.788781875000002 * IIIZIIZI
+ 11.339220937500007 * IIIIZIZI
+ 3.779740312500002 * IIIIIZZI
- 601.9000340624999 * IIIIIIIZ
- 3.4468814062500015 * ZIIIIIIZ
- 1.1489604687500004 * IZIIIIIZ
- 4.788781875000002 * IIZIIIIZ
- 1.5962606250000009 * IIIZIIIZ
+ 3.779740312500002 * IIIIZIIZ
+ 1.2599134375000005 * IIIIIZIZ
+ 1.986637812500001 * XXXXIIII
+ 1.986637812500001 * YYXXIIII
+ 1.986637812500001 * XXYYIIII
+ 1.986637812500001 * YYYYIIII
- 84.41897986727398 * IIIIXXII
- 4.809566200586032 * ZIIIXXII
- 1.6031887335286772 * IZIIXXII
- 16.233724778792137 * IIZIXXII
- 5.411241592930711 * IIIZXXII
- 84.41897986727398 * IIIIYYII
- 4.809566200586032 * ZIIIYYII
- 1.6031887335286772 * IZIIYYII
- 16.233724778792137 * IIZIYYII
- 5.411241592930711 * IIIZYYII
+ 66.81814897987134 * IIIIXXZI
+ 66.81814897987134 * IIIIYYZI
+ 22.272716326623776 * IIIIXXIZ
+ 22.272716326623776 * IIIIYYIZ
+ 0.8980418750000001 * XXIIIIXX
+ 0.8980418750000001 * YYIIIIXX
+ 0.8980418750000001 * XXIIIIYY
+ 0.8980418750000001 * YYIIIIYY
- 0.5021409375000001 * IIXXIIXX
- 0.5021409375000001 * IIYYIIXX
- 0.5021409375000001 * IIXXIIYY
- 0.5021409375000001 * IIYYIIYY
###Markdown
To have a different number of modals per mode:
###Code
vibrational_problem = VibrationalStructureProblem(driver, num_modals=3, truncation_order=2)
second_q_ops = vibrational_problem.second_q_ops()
qubit_converter = QubitConverter(mapper = DirectMapper())
qubit_op = qubit_converter.convert(second_q_ops[0])
print(qubit_op)
###Output
11327.034966562516 * IIIIIIIIIIII
- 745.8599576562498 * ZIIIIIIIIIII
- 446.6739542187503 * IZIIIIIIIIII
- 148.69083703124986 * IIZIIIIIIIII
- 724.2074121874995 * IIIZIIIIIIII
- 3.4438867187500013 * ZIIZIIIIIIII
- 2.066332031250001 * IZIZIIIIIIII
- 0.6887773437500002 * IIZZIIIIIIII
- 431.8741484375 * IIIIZIIIIIII
- 2.066332031250001 * ZIIIZIIIIIII
- 1.2397992187500007 * IZIIZIIIIIII
- 0.4132664062500002 * IIZIZIIIIIII
- 143.32702593749988 * IIIIIZIIIIII
- 0.6887773437500002 * ZIIIIZIIIIII
- 0.4132664062500002 * IZIIIZIIIIII
- 0.13775546875000005 * IIZIIZIIIIII
- 1720.4111609375004 * IIIIIIZIIIII
- 10.524871093750003 * ZIIIIIZIIIII
- 6.314922656250003 * IZIIIIZIIIII
- 2.104974218750001 * IIZIIIZIIIII
- 16.035769531250004 * IIIZIIZIIIII
- 9.621461718750005 * IIIIZIZIIIII
- 3.207153906250001 * IIIIIZZIIIII
- 1030.4053903124998 * IIIIIIIZIIII
- 6.314922656250003 * ZIIIIIIZIIII
- 3.7889535937500023 * IZIIIIIZIIII
- 1.2629845312500008 * IIZIIIIZIIII
- 9.621461718750004 * IIIZIIIZIIII
- 5.772877031250004 * IIIIZIIZIIII
- 1.9242923437500008 * IIIIIZIZIIII
- 343.0300571875006 * IIIIIIIIZIII
- 2.1049742187500007 * ZIIIIIIIZIII
- 1.2629845312500005 * IZIIIIIIZIII
- 0.42099484375000024 * IIZIIIIIZIII
- 3.207153906250001 * IIIZIIIIZIII
- 1.924292343750001 * IIIIZIIIZIII
- 0.6414307812500002 * IIIIIZIIZIII
- 2992.18265640625 * IIIIIIIIIZII
- 28.72401171875001 * ZIIIIIIIIZII
- 17.234407031250004 * IZIIIIIIIZII
- 5.744802343750002 * IIZIIIIIIZII
- 39.90651562500001 * IIIZIIIIIZII
- 23.94390937500001 * IIIIZIIIIZII
- 7.981303125000004 * IIIIIZIIIZII
+ 31.497835937500014 * IIIIIIZIIZII
+ 18.89870156250001 * IIIIIIIZIZII
+ 6.299567187500003 * IIIIIIIIZZII
- 1788.3742817187504 * IIIIIIIIIIZI
- 17.234407031250008 * ZIIIIIIIIIZI
- 10.340644218750004 * IZIIIIIIIIZI
- 3.4468814062500015 * IIZIIIIIIIZI
- 23.94390937500001 * IIIZIIIIIIZI
- 14.366345625000008 * IIIIZIIIIIZI
- 4.788781875000002 * IIIIIZIIIIZI
+ 18.898701562500012 * IIIIIIZIIIZI
+ 11.339220937500007 * IIIIIIIZIIZI
+ 3.779740312500002 * IIIIIIIIZIZI
- 594.4734957812499 * IIIIIIIIIIIZ
- 5.744802343750002 * ZIIIIIIIIIIZ
- 3.4468814062500015 * IZIIIIIIIIIZ
- 1.1489604687500004 * IIZIIIIIIIIZ
- 7.981303125000003 * IIIZIIIIIIIZ
- 4.788781875000002 * IIIIZIIIIIIZ
- 1.5962606250000009 * IIIIIZIIIIIZ
+ 6.299567187500004 * IIIIIIZIIIIZ
+ 3.779740312500002 * IIIIIIIZIIIZ
+ 1.2599134375000005 * IIIIIIIIZIIZ
- 20.885040702719472 * XIXIIIIIIIII
- 20.885040702719472 * YIYIIIIIIIII
+ 0.9740782609865658 * XIXZIIIIIIII
+ 0.9740782609865658 * YIYZIIIIIIII
+ 0.5844469565919396 * XIXIZIIIIIII
+ 0.5844469565919396 * YIYIZIIIIIII
+ 0.19481565219731317 * XIXIIZIIIIII
+ 0.19481565219731317 * YIYIIZIIIIII
+ 2.9768830886019613 * XIXIIIZIIIII
+ 2.9768830886019613 * YIYIIIZIIIII
+ 1.7861298531611771 * XIXIIIIZIIII
+ 1.7861298531611771 * YIYIIIIZIIII
+ 0.5953766177203923 * XIXIIIIIZIII
+ 0.5953766177203923 * YIYIIIIIZIII
+ 8.124377387683996 * XIXIIIIIIZII
+ 8.124377387683996 * YIYIIIIIIZII
+ 4.874626432610398 * XIXIIIIIIIZI
+ 4.874626432610398 * YIYIIIIIIIZI
+ 1.6248754775367993 * XIXIIIIIIIIZ
+ 1.6248754775367993 * YIYIIIIIIIIZ
+ 7.946551250000002 * XXIXXIIIIIII
+ 7.946551250000002 * YYIXXIIIIIII
+ 7.946551250000002 * XXIYYIIIIIII
+ 7.946551250000002 * YYIYYIIIIIII
+ 4.245123515804727 * IXXXXIIIIIII
+ 4.245123515804727 * IYYXXIIIIIII
+ 4.245123515804727 * IXXYYIIIIIII
+ 4.245123515804727 * IYYYYIIIIIII
- 27.557296533490963 * IIIXIXIIIIII
+ 0.9740782609865657 * ZIIXIXIIIIII
+ 0.5844469565919395 * IZIXIXIIIIII
+ 0.19481565219731314 * IIZXIXIIIIII
- 27.557296533490963 * IIIYIYIIIIII
+ 0.9740782609865657 * ZIIYIYIIIIII
+ 0.5844469565919395 * IZIYIYIIIIII
+ 0.19481565219731314 * IIZYIYIIIIII
+ 4.535600550836601 * IIIXIXZIIIII
+ 4.535600550836601 * IIIYIYZIIIII
+ 2.721360330501961 * IIIXIXIZIIII
+ 2.721360330501961 * IIIYIYIZIIII
+ 0.9071201101673203 * IIIXIXIIZIII
+ 0.9071201101673203 * IIIYIYIIZIII
+ 11.287267124785771 * IIIXIXIIIZII
+ 11.287267124785771 * IIIYIYIIIZII
+ 6.772360274871463 * IIIXIXIIIIZI
+ 6.772360274871463 * IIIYIYIIIIZI
+ 2.2574534249571543 * IIIXIXIIIIIZ
+ 2.2574534249571543 * IIIYIYIIIIIZ
- 0.2755109375000001 * XIXXIXIIIIII
- 0.2755109375000001 * YIYXIXIIIIII
- 0.2755109375000001 * XIXYIYIIIIII
- 0.2755109375000001 * YIYYIYIIIIII
+ 4.1834668980774286 * XXIIXXIIIIII
+ 4.1834668980774286 * YYIIXXIIIIII
+ 4.1834668980774286 * XXIIYYIIIIII
+ 4.1834668980774286 * YYIIYYIIIIII
+ 1.986637812500001 * IXXIXXIIIIII
+ 1.986637812500001 * IYYIXXIIIIII
+ 1.986637812500001 * IXXIYYIIIIII
+ 1.986637812500001 * IYYIYYIIIIII
- 260.3514075 * IIIIIIXXIIII
- 11.336256250000002 * ZIIIIIXXIIII
- 6.801753750000003 * IZIIIIXXIIII
- 2.2672512500000006 * IIZIIIXXIIII
- 38.26325625000001 * IIIZIIXXIIII
- 22.95795375000001 * IIIIZIXXIIII
- 7.652651250000002 * IIIIIZXXIIII
- 260.3514075 * IIIIIIYYIIII
- 11.336256250000002 * ZIIIIIYYIIII
- 6.801753750000003 * IZIIIIYYIIII
- 2.2672512500000006 * IIZIIIYYIIII
- 38.26325625000001 * IIIZIIYYIIII
- 22.95795375000001 * IIIIZIYYIIII
- 7.652651250000002 * IIIIIZYYIIII
+ 157.49188750000002 * IIIIIIXXIZII
+ 157.49188750000002 * IIIIIIYYIZII
+ 94.49513250000001 * IIIIIIXXIIZI
+ 94.49513250000001 * IIIIIIYYIIZI
+ 31.498377500000004 * IIIIIIXXIIIZ
+ 31.498377500000004 * IIIIIIYYIIIZ
+ 3.206377467057354 * XIXIIIXXIIII
+ 3.206377467057354 * YIYIIIXXIIII
+ 3.206377467057354 * XIXIIIYYIIII
+ 3.206377467057354 * YIYIIIYYIIII
+ 10.822483185861422 * IIIXIXXXIIII
+ 10.822483185861422 * IIIYIYXXIIII
+ 10.822483185861422 * IIIXIXYYIIII
+ 10.822483185861422 * IIIYIYYYIIII
+ 4.373609679305444 * IIIIIIXIXIII
+ 2.9768830886019613 * ZIIIIIXIXIII
+ 1.786129853161177 * IZIIIIXIXIII
+ 0.5953766177203923 * IIZIIIXIXIII
+ 4.535600550836601 * IIIZIIXIXIII
+ 2.721360330501961 * IIIIZIXIXIII
+ 0.9071201101673203 * IIIIIZXIXIII
+ 4.373609679305444 * IIIIIIYIYIII
+ 2.9768830886019613 * ZIIIIIYIYIII
+ 1.786129853161177 * IZIIIIYIYIII
+ 0.5953766177203923 * IIZIIIYIYIII
+ 4.535600550836601 * IIIZIIYIYIII
+ 2.721360330501961 * IIIIZIYIYIII
+ 0.9071201101673203 * IIIIIZYIYIII
- 8.90893335364304 * IIIIIIXIXZII
- 8.90893335364304 * IIIIIIYIYZII
- 5.345360012185824 * IIIIIIXIXIZI
- 5.345360012185824 * IIIIIIYIYIZI
- 1.7817866707286079 * IIIIIIXIXIIZ
- 1.7817866707286079 * IIIIIIYIYIIZ
- 0.8419896875000004 * XIXIIIXIXIII
- 0.8419896875000004 * YIYIIIXIXIII
- 0.8419896875000004 * XIXIIIYIYIII
- 0.8419896875000004 * YIYIIIYIYIII
- 1.2828615625000004 * IIIXIXXIXIII
- 1.2828615625000004 * IIIYIYXIXIII
- 1.2828615625000004 * IIIXIXYIYIII
- 1.2828615625000004 * IIIYIYYIYIII
- 160.71040986809584 * IIIIIIIXXIII
- 8.015943667643384 * ZIIIIIIXXIII
- 4.809566200586032 * IZIIIIIXXIII
- 1.6031887335286772 * IIZIIIIXXIII
- 27.05620796465356 * IIIZIIIXXIII
- 16.233724778792137 * IIIIZIIXXIII
- 5.411241592930711 * IIIIIZIXXIII
- 160.71040986809584 * IIIIIIIYYIII
- 8.015943667643384 * ZIIIIIIYYIII
- 4.809566200586032 * IZIIIIIYYIII
- 1.6031887335286772 * IIZIIIIYYIII
- 27.05620796465356 * IIIZIIIYYIII
- 16.233724778792137 * IIIIZIIYYIII
- 5.411241592930711 * IIIIIZIYYIII
+ 111.36358163311888 * IIIIIIIXXZII
+ 111.36358163311888 * IIIIIIIYYZII
+ 66.81814897987134 * IIIIIIIXXIZI
+ 66.81814897987134 * IIIIIIIYYIZI
+ 22.272716326623776 * IIIIIIIXXIIZ
+ 22.272716326623776 * IIIIIIIYYIIZ
+ 2.267251250000001 * XIXIIIIXXIII
+ 2.267251250000001 * YIYIIIIXXIII
+ 2.267251250000001 * XIXIIIIYYIII
+ 2.267251250000001 * YIYIIIIYYIII
+ 7.6526512500000035 * IIIXIXIXXIII
+ 7.6526512500000035 * IIIYIYIXXIII
+ 7.6526512500000035 * IIIXIXIYYIII
+ 7.6526512500000035 * IIIYIYIYYIII
+ 3.5921675000000004 * XXIIIIIIIXXI
+ 3.5921675000000004 * YYIIIIIIIXXI
+ 3.5921675000000004 * XXIIIIIIIYYI
+ 3.5921675000000004 * YYIIIIIIIYYI
+ 5.912498983512137 * IXXIIIIIIXXI
+ 5.912498983512137 * IYYIIIIIIXXI
+ 5.912498983512137 * IXXIIIIIIYYI
+ 5.912498983512137 * IYYIIIIIIYYI
- 2.0085637500000013 * IIIXXIIIIXXI
- 2.0085637500000013 * IIIYYIIIIXXI
- 2.0085637500000013 * IIIXXIIIIYYI
- 2.0085637500000013 * IIIYYIIIIYYI
- 3.305978760488338 * IIIIXXIIIXXI
- 3.305978760488338 * IIIIYYIIIXXI
- 3.305978760488338 * IIIIXXIIIYYI
- 3.305978760488338 * IIIIYYIIIYYI
- 11.899156895555553 * IIIIIIIIIXIX
+ 8.124377387683996 * ZIIIIIIIIXIX
+ 4.874626432610398 * IZIIIIIIIXIX
+ 1.6248754775367993 * IIZIIIIIIXIX
+ 11.28726712478577 * IIIZIIIIIXIX
+ 6.772360274871463 * IIIIZIIIIXIX
+ 2.2574534249571543 * IIIIIZIIIXIX
- 8.90893335364304 * IIIIIIZIIXIX
- 5.345360012185823 * IIIIIIIZIXIX
- 1.7817866707286076 * IIIIIIIIZXIX
- 11.899156895555553 * IIIIIIIIIYIY
+ 8.124377387683996 * ZIIIIIIIIYIY
+ 4.874626432610398 * IZIIIIIIIYIY
+ 1.6248754775367993 * IIZIIIIIIYIY
+ 11.28726712478577 * IIIZIIIIIYIY
+ 6.772360274871463 * IIIIZIIIIYIY
+ 2.2574534249571543 * IIIIIZIIIYIY
- 8.90893335364304 * IIIIIIZIIYIY
- 5.345360012185823 * IIIIIIIZIYIY
- 1.7817866707286076 * IIIIIIIIZYIY
- 2.2979209375000007 * XIXIIIIIIXIX
- 2.2979209375000007 * YIYIIIIIIXIX
- 2.2979209375000007 * XIXIIIIIIYIY
- 2.2979209375000007 * YIYIIIIIIYIY
- 3.1925212500000018 * IIIXIXIIIXIX
- 3.1925212500000018 * IIIYIYIIIXIX
- 3.1925212500000018 * IIIXIXIIIYIY
- 3.1925212500000018 * IIIYIYIIIYIY
- 44.54543265324755 * IIIIIIXXIXIX
- 44.54543265324755 * IIIIIIYYIXIX
- 44.54543265324755 * IIIIIIXXIYIY
- 44.54543265324755 * IIIIIIYYIYIY
+ 2.5198268750000015 * IIIIIIXIXXIX
+ 2.5198268750000015 * IIIIIIYIYXIX
+ 2.5198268750000015 * IIIIIIXIXYIY
+ 2.5198268750000015 * IIIIIIYIYYIY
- 31.498377500000007 * IIIIIIIXXXIX
- 31.498377500000007 * IIIIIIIYYXIX
- 31.498377500000007 * IIIIIIIXXYIY
- 31.498377500000007 * IIIIIIIYYYIY
- 2.102429985900245 * XXIIIIIIIIXX
- 2.102429985900245 * YYIIIIIIIIXX
- 2.102429985900245 * XXIIIIIIIIYY
- 2.102429985900245 * YYIIIIIIIIYY
+ 0.8980418750000001 * IXXIIIIIIIXX
+ 0.8980418750000001 * IYYIIIIIIIXX
+ 0.8980418750000001 * IXXIIIIIIIYY
+ 0.8980418750000001 * IYYIIIIIIIYY
+ 1.175575188382615 * IIIXXIIIIIXX
+ 1.175575188382615 * IIIYYIIIIIXX
+ 1.175575188382615 * IIIXXIIIIIYY
+ 1.175575188382615 * IIIYYIIIIIYY
- 0.5021409375000001 * IIIIXXIIIIXX
- 0.5021409375000001 * IIIIYYIIIIXX
- 0.5021409375000001 * IIIIXXIIIIYY
- 0.5021409375000001 * IIIIYYIIIIYY
###Markdown
Now that the Hamiltonian is ready, it can be used in a quantum algorithm to find information about the vibrational structure of the corresponding molecule. Check out our tutorials on Ground State Calculation and Excited States Calculation to learn more about how to do that in Qiskit Nature!
###Code
import qiskit.tools.jupyter
%qiskit_version_table
%qiskit_copyright
###Output
_____no_output_____
###Markdown
Vibrational structure Introduction The molecular Hamiltonian is $$\mathcal{H} = - \sum_I \frac{\nabla_{R_I}^2}{M_I} - \sum_i \frac{\nabla_{r_i}^2}{m_e} - \sum_I\sum_i \frac{Z_I e^2}{|R_I-r_i|} + \sum_i \sum_{j>i} \frac{e^2}{|r_i-r_j|} + \sum_I\sum_{J>I} \frac{Z_I Z_J e^2}{|R_I-R_J|}$$Because the nuclei are much heavier than the electrons they do not move on the same time scale and therefore, the behavior of nuclei and electrons can be decoupled. This is the Born-Oppenheimer approximation.Within the Born-Oppenheimer approximation, a molecular wave function is factorized as a product of an electronic part, which is the solution of the electronic Schroedinger equation, and a vibro-rotational one, which is the solution of the nuclear Schroedinger equation in the potential energy surface (PES) generated by sampling the eigenvalues of the electronic Schroedinger equation for different geometries.The nuclear Schroedinger equation is usually solved in two steps, in analogy with its electronic counterpart. A single-particle basis (the basis functions are called, in this case, modals) is obtained either by the harmonic approximation applied to the PES or from a vibrational self-consistent field (VSCF) calculation. Vibrational anharmonic correlations are added a-posteriori with perturbative or variational approaches.The latter include Vibrational Configuration Interaction (VCI) and Vibrational Coupled Cluster (VCC) for highly-accurate anharmonic energies. The main advantage of VCI and VCC over alternative approaches (such as perturbation theories) is that their accuracy can be systematically improved towards the complete basis set limit for a given PES. However, their applicability is limited to small molecules with up to about 10 atoms due to their unfavorable scaling with system size.To tackle the scaling problem we would like to use quantum algorithms.The nuclear Schroedinger equation is$$\mathcal{H}_{\text{vib}} |\Psi_{n}\rangle = E_{n} |\Psi_{n}\rangle$$The so-called Watson Hamiltonian (neglecting vibro-rotational coupling terms) is$$ \mathcal{H}_\text{vib}(Q_1, \ldots, Q_L) = - \frac{1}{2} \sum_{l=1}^{L} \frac{\partial^2}{\partial Q_l^2} + V(Q_1, \ldots, Q_L)$$where $Q_l$ are the harmonic mass-weighted normal coordinates.$\mathcal{H}_\text{vib}$ must be mapped to an operator that acts on the states of a given set of $N_q$ qubits in order to calculate its eigenfunctions on quantum hardware.In electronic structure calculations, the mapping is achieved by expressing the non-relativistic electronic Hamiltonian in second quantization, \textit{i.e.} by projecting it onto the complete set of antisymmetrized occupation number vectors (ONV) generated by a given (finite) set of orbitals.To encode the vibrational Hamiltonian in an analogous second quantization operators, we expand the potential $V(Q_1, \ldots, Q_L)$ with the $n$-body expansion as follows:$$ V(Q_1, \ldots, Q_L) = V_0 + \sum_{l=1}^L V^{[l]}(Q_l) + \sum_{l<m}^L V^{[l,m]}(Q_l, Q_m) + \sum_{l<m<n}^L V^{[l,m,n]}(Q_l, Q_m, Q_n) + \ldots$$where $V_0$ is the electronic energy of the reference geometry, the one-mode term $V^{[l]}(Q_l)$ represents the variation of the PES upon change of the $l$-th normal coordinate from the equilibrium position.Similarly, the two-body potential $V^{[l,m]}(Q_l, Q_m)$ represents the change in the exact PES upon a simultaneous displacement along the $l$-th and $m$-th coordinates. Often, including terms up to three-body in the $L$-body expansion is sufficient to obtain an accuracy of about 1~cm$^{-1}$. We highlight that the many-body expansion of the potential operator defining the Watson Hamiltonian contains arbitrarily high coupling terms. This is a crucial difference compared to the non-relativistic electronic-structure Hamiltonian that contains only pairwise interactions.A flexible second quantization form of the Watson Hamiltonian is obtained within the so-called n-mode representation. Let us assume that each mode $l$ is described by a $N_l$-dimensional basis set $S_l$ defined as follows:$$ \mathcal{S}_l = \{ \phi_1^{(l)} (Q_l) , \ldots , \phi_{N_l}^{(l)} (Q_l) \} \, .$$The $n$-mode wave function can be expanded in the product basis $\mathcal{S} = \otimes_{i=1}^L \mathcal{S}_i$ as the following CI-like expansion:$$ |\Psi\rangle = \sum_{k_1=1}^{N_1} \cdots \sum_{k_L=1}^{N_L} C_{k_1,\ldots,k_L} \phi_{k_1}^{(1)}(Q_1) \cdots \phi_{k_L}^{(L)}(Q_L) \, ,$$The many-body basis function $\phi_{k_1}^{(1)}(Q_1) \cdots \phi_{k_L}^{(L)}(Q_L)$ are encoded within the so-called $n$-mode second quantization as occupation-number vectors (ONVs) as follows:$$ \phi_{k_1}(Q_1) \cdots \phi_{k_L}(Q_L) \equiv |0_1 \cdots 1_{k_1} \cdots 0_{N_1}, 0_1 \cdots 1_{k_2} \cdots 0_{N_2}, \cdots , 0_1 \cdots 1_{k_L} \cdots 0_{N_L}\rangle \, .$$The ONV defined above is, therefore, the product of $L$ mode-specific ONVs, each one describing an individual mode. Since each mode is described by one and only one basis function, the occupation of each mode-specific ONV is one.From a theoretical perspective, each mode can be interpreted as a distinguishable quasi-particle (defined as phonons in solid-state physics). Distinguishability arises from the fact that the PES is not invariant by permutation of two modes, also in this case unlike the Coulomb interaction between two equal particles. From this perspective, a molecule can be interpreted as a collection of $L$ indistinguishable particles that interact through the PES operator.Based on this second-quantization representation we introduce a pair of creation and annihilation operators per mode $l$ \textit{and} per basis function $k_l$ defined as:$$ \begin{aligned} a_{k_l}^\dagger |\cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle &= | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle \\ a_{k_l}^\dagger | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle &= 0 \\ a_{k_l} | \cdots, 0_1 \cdots 1_{k_l} \cdots 0_{N_l}, \cdots\rangle &= | \cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle \\ a_{k_l} | \cdots, 0_1 \cdots 0_{k_l} \cdots 0_{N_l}, \cdots\rangle &= 0 \\ \end{aligned}$$with$$ \begin{aligned} \left[ a_{k_l}^\dagger, a_{h_m}^\dagger \right] &= 0 \\ \left[ a_{k_l}, a_{h_m} \right] &= 0 \\ \left[ a_{k_l}^\dagger, a_{h_m} \right] &= \delta_{l,m} \, , \delta_{k_l,h_m} \end{aligned}$$The second quantization form is obtained by expressing the potential as $$ \begin{aligned} \mathcal{H}_\text{vib}^{SQ} =& \sum_{l=1}^L \sum_{k_l,h_l}^{N_l} \langle \phi_{k_l} | T(Q_l) + V^{[l]}(Q_l) | \phi_{h_l} \rangle a_{k_l}^+ a_{h_l} \\ +& \sum_{l<m}^L \sum_{k_l,h_l}^{N_l} \sum_{k_m,h_m}^{N_m} \langle \phi_{k_l} \phi_{k_m} | V^{[l,m]}(Q_l, Q_m) | \phi_{h_l} \phi_{h_m} \rangle a_{k_l}^+ a_{k_m}^+ a_{h_l} a_{h_m} + \cdots \end{aligned}$$We highlight here the difference between the operators defined here above and the electronic structure one. First, as we already mentioned, the potential contains (in principle) three- and higher-body coupling terms that lead to strings with six (or more) second-quantization operators. Moreover, the Hamiltonian conserves the number of particles for each mode, as can be seen from the fact that the number of creation and annihilation operators for a given mode is the same in each term. Nevertheless, different modes are coupled by two- (and higher) body terms containing SQ operators belonging to different modes $l$ and $m$.Reference: Ollitrault, Pauline J., et al., arXiv:2003.12578 (2020). Compute the electronic potentialSolving the ESE for different nuclear configurations to obtain the PES function $V(Q_1, \ldots, Q_L)$. So far Qiskit gives the possibility to approximate the PES with a quartic force field. $$V(Q_1, \ldots, Q_L) = \frac{1}{2} \sum_{ij} k_{ij} Q_i Q_j + \frac{1}{6} \sum_{ijk} k_{ijk} Q_i Q_j Q_k + \frac{1}{16} \sum_{ijkl} k_{ijkl} Q_i Q_j Q_k Q_l$$The advantage of such form for the PES is that the anharmonic force fields ($k_{ij}$, $k_{ijk}$, $k_{ijkl}$) can be calculated by finite-difference approaches. For methods for which the nuclear energy Hessian can be calculated analytically with response theory-based methods (such as HF and DFT), the quartic force field can be calculated by semi-numerical differentiation as:$$k_{ijk} = \frac{H_{ij}(+\delta Q_k) - H_{ij}(-\delta Q_k)}{2\delta Q_k}$$and$$k_{ijkl} = \frac{H_{ij}(+\delta Q_k+\delta Q_l) - H_{ij}(+\delta Q_k-\delta Q_l) -H_{ij}(-\delta Q_k+\delta Q_l) + H_{ij}(-\delta Q_k+\delta Q_l)} {4\delta Q_k \delta Q_l}$$Such numerical procedure is implemented, for instance, in the Gaussian suite of programs.In practice this can be done with Qiskit using the GaussianForceDriver.
###Code
from qiskit_nature.drivers.second_quantization import GaussianForcesDriver
# if you ran Gaussian elsewhere and already have the output file
driver = GaussianForcesDriver(logfile="aux_files/CO2_freq_B3LYP_631g.log")
# if you want to run the Gaussian job from Qiskit
# driver = GaussianForcesDriver(
# ['#p B3LYP/6-31g Freq=(Anharm) Int=Ultrafine SCF=VeryTight',
# '',
# 'CO2 geometry optimization B3LYP/6-31g',
# '',
# '0 1',
# 'C -0.848629 2.067624 0.160992',
# 'O 0.098816 2.655801 -0.159738',
# 'O -1.796073 1.479446 0.481721',
# '',
# ''
###Output
_____no_output_____
###Markdown
Map to a qubit HamiltonianNow that we have an approximation for the potential, we need to write the Hamiltonian in second quantization. To this end we need to select a modal basis to calculate the one-body integrals $\langle\phi_{k_i}| V(Q_i) | \phi_{h_i} \rangle$, two-body integrals $\langle\phi_{k_i} \phi_{k_j}| V(Q_i,Q_j) | \phi_{h_i} \phi_{h_j} \rangle$... In the simplest case, the $\phi$ functions are the harmonic-oscillator eigenfunctions for each mode. The main advantage of this choice is that the integrals of a PES expressed as a Taylor expansion are easy to calculate with such basis. A routine for computing these integrals is implemented in Qiskit. The bosonic operator, $\mathcal{H}_\text{vib}^{SQ}$, is then created and must be mapped to a qubit operator. The direct mapping introduced in the first section of this tutorial can be used is Qiskit as follows:
###Code
from qiskit_nature.problems.second_quantization import VibrationalStructureProblem
from qiskit_nature.converters.second_quantization import QubitConverter
from qiskit_nature.mappers.second_quantization import DirectMapper
vibrational_problem = VibrationalStructureProblem(driver, num_modals=2, truncation_order=2)
second_q_ops = vibrational_problem.second_q_ops()
###Output
_____no_output_____
###Markdown
The Vibrational operator for the problem now reads as
###Code
print(second_q_ops[0])
###Output
NIIIIIII * (1268.0676746875001+0j)
+ INIIIIII * (3813.8767834375008+0j)
+ IINIIIII * (705.8633818750001+0j)
+ II+-IIII * (-46.025705898886045+0j)
+ II-+IIII * (-46.025705898886045+0j)
+ IIINIIII * (2120.1145593750007+0j)
+ IIIINIII * (238.31540750000005+0j)
+ IIIIINII * (728.9613775000003+0j)
+ IIIIIINI * (238.31540750000005+0j)
+ IIIIIIIN * (728.9613775000003+0j)
+ NINIIIII * (4.942542500000002+0j)
+ NI+-IIII * (-88.20174216876333+0j)
+ NI-+IIII * (-88.20174216876333+0j)
+ NIINIIII * (14.827627500000007+0j)
+ INNIIIII * (14.827627500000007+0j)
+ IN+-IIII * (-264.60522650629+0j)
+ IN-+IIII * (-264.60522650629+0j)
+ ININIIII * (44.482882500000024+0j)
+ NIIINIII * (-10.205891250000004+0j)
+ INIINIII * (-30.617673750000016+0j)
+ IININIII * (-4.194299375000002+0j)
+ II+-NIII * (42.67527310283147+0j)
+ II-+NIII * (42.67527310283147+0j)
+ IIINNIII * (-12.582898125000007+0j)
+ NIIIINII * (-30.61767375000002+0j)
+ INIIINII * (-91.85302125000007+0j)
+ IINIINII * (-12.582898125000007+0j)
+ II+-INII * (128.02581930849442+0j)
+ II-+INII * (128.02581930849442+0j)
+ IIININII * (-37.74869437500002+0j)
+ NIIIIINI * (-10.205891250000004+0j)
+ INIIIINI * (-30.617673750000016+0j)
+ IINIIINI * (-4.194299375000002+0j)
+ II+-IINI * (42.67527310283147+0j)
+ II-+IINI * (42.67527310283147+0j)
+ IIINIINI * (-12.582898125000007+0j)
+ IIIININI * (7.0983500000000035+0j)
+ IIIIINNI * (21.29505000000001+0j)
+ NIIIIIIN * (-30.61767375000002+0j)
+ INIIIIIN * (-91.85302125000007+0j)
+ IINIIIIN * (-12.582898125000007+0j)
+ II+-IIIN * (128.02581930849442+0j)
+ II-+IIIN * (128.02581930849442+0j)
+ IIINIIIN * (-37.74869437500002+0j)
+ IIIINIIN * (21.29505000000001+0j)
+ IIIIININ * (63.88515000000004+0j)
###Markdown
In the previous cell we defined a bosonic transformation to express the Hamiltonian in the harmonic modal basis, with 2 modals per mode with the potential truncated at order 2 and the 'direct' boson to qubit mapping. The calculation is then ran as:
###Code
qubit_converter = QubitConverter(mapper=DirectMapper())
qubit_op = qubit_converter.convert(second_q_ops[0])
print(qubit_op)
###Output
4854.200029687493 * IIIIIIII
- 342.57516687500015 * ZIIIIIII
- 111.85586312500007 * IZIIIIII
- 342.57516687500015 * IIZIIIII
+ 15.97128750000001 * ZIZIIIII
+ 5.323762500000003 * IZZIIIII
- 111.85586312500007 * IIIZIIII
+ 5.323762500000003 * ZIIZIIII
+ 1.7745875000000009 * IZIZIIII
- 1049.719110937499 * IIIIZIII
- 9.437173593750005 * ZIIIZIII
- 3.1457245312500017 * IZIIZIII
- 9.437173593750005 * IIZIZIII
- 3.1457245312500017 * IIIZZIII
- 349.4856346875002 * IIIIIZII
- 3.1457245312500017 * ZIIIIZII
- 1.0485748437500004 * IZIIIZII
- 3.1457245312500017 * IIZIIZII
- 1.0485748437500004 * IIIZIZII
- 1860.5306717187502 * IIIIIIZI
- 22.963255312500017 * ZIIIIIZI
- 7.654418437500004 * IZIIIIZI
- 22.963255312500017 * IIZIIIZI
- 7.654418437500004 * IIIZIIZI
+ 11.120720625000006 * IIIIZIZI
+ 3.706906875000002 * IIIIIZZI
- 618.5645973437502 * IIIIIIIZ
- 7.654418437500005 * ZIIIIIIZ
- 2.551472812500001 * IZIIIIIZ
- 7.654418437500005 * IIZIIIIZ
- 2.551472812500001 * IIIZIIIZ
+ 3.706906875000002 * IIIIZIIZ
+ 1.2356356250000005 * IIIIIZIZ
- 25.864048912543417 * IIIIXXII
- 32.006454827123605 * ZIIIXXII
- 10.668818275707867 * IZIIXXII
- 32.006454827123605 * IIZIXXII
- 10.668818275707867 * IIIZXXII
- 25.864048912543417 * IIIIYYII
- 32.006454827123605 * ZIIIYYII
- 10.668818275707867 * IZIIYYII
- 32.006454827123605 * IIZIYYII
- 10.668818275707867 * IIIZYYII
+ 66.1513066265725 * IIIIXXZI
+ 66.1513066265725 * IIIIYYZI
+ 22.050435542190833 * IIIIXXIZ
+ 22.050435542190833 * IIIIYYIZ
###Markdown
To have a different number of modals per mode:
###Code
vibrational_problem = VibrationalStructureProblem(driver, num_modals=3, truncation_order=2)
second_q_ops = vibrational_problem.second_q_ops()
qubit_converter = QubitConverter(mapper=DirectMapper())
qubit_op = qubit_converter.convert(second_q_ops[0])
print(qubit_op)
###Output
10788.719982656237 * IIIIIIIIIIII
- 541.6731217187498 * ZIIIIIIIIIII
- 315.1932645312502 * IZIIIIIIIIII
- 102.72856234375004 * IIZIIIIIIIII
- 541.6731217187498 * IIIZIIIIIIII
+ 44.36468750000001 * ZIIZIIIIIIII
+ 26.618812500000015 * IZIZIIIIIIII
+ 8.872937500000004 * IIZZIIIIIIII
- 13.32410345498359 * XIXIIIIIIIII
- 13.32410345498359 * YIYIIIIIIIII
- 12.54822855058883 * XIXZIIIIIIII
- 12.54822855058883 * YIYZIIIIIIII
- 315.1932645312502 * IIIIZIIIIIII
+ 26.61881250000001 * ZIIIZIIIIIII
+ 15.97128750000001 * IZIIZIIIIIII
+ 5.323762500000003 * IIZIZIIIIIII
- 7.528937130353299 * XIXIZIIIIIII
- 7.528937130353299 * YIYIZIIIIIII
- 102.72856234375004 * IIIIIZIIIIII
+ 8.872937500000003 * ZIIIIZIIIIII
+ 5.323762500000003 * IZIIIZIIIIII
+ 1.7745875000000009 * IIZIIZIIIIII
- 2.509645710117766 * XIXIIZIIIIII
- 2.509645710117766 * YIYIIZIIIIII
- 1730.9391493749995 * IIIIIIZIIIII
- 26.21437109375001 * ZIIIIIZIIIII
- 15.728622656250007 * IZIIIIZIIIII
- 5.242874218750002 * IIZIIIZIIIII
- 26.21437109375001 * IIIZIIZIIIII
+ 7.4145438259724985 * XIXIIIZIIIII
+ 7.4145438259724985 * YIYIIIZIIIII
- 15.728622656250007 * IIIIZIZIIIII
- 5.242874218750002 * IIIIIZZIIIII
- 1036.7963999999984 * IIIIIIIZIIII
- 15.728622656250007 * ZIIIIIIZIIII
- 9.437173593750005 * IZIIIIIZIIII
- 3.1457245312500017 * IIZIIIIZIIII
- 15.728622656250007 * IIIZIIIZIIII
+ 4.4487262955835 * XIXIIIIZIIII
+ 4.4487262955835 * YIYIIIIZIIII
- 9.437173593750005 * IIIIZIIZIIII
- 3.1457245312500017 * IIIIIZIZIIII
- 345.1780643749999 * IIIIIIIIZIII
- 5.242874218750002 * ZIIIIIIIZIII
- 3.1457245312500017 * IZIIIIIIZIII
- 1.0485748437500004 * IIZIIIIIZIII
- 5.242874218750002 * IIIZIIIIZIII
+ 1.4829087651944997 * XIXIIIIIZIII
+ 1.4829087651944997 * YIYIIIIIZIII
- 3.1457245312500017 * IIIIZIIIZIII
- 1.0485748437500004 * IIIIIZIIZIII
- 3015.4877554687487 * IIIIIIIIIZII
- 63.786820312500026 * ZIIIIIIIIZII
- 38.27209218750002 * IZIIIIIIIZII
- 12.757364062500006 * IIZIIIIIIZII
- 63.786820312500026 * IIIZIIIIIZII
+ 18.04163727731863 * XIXIIIIIIZII
+ 18.04163727731863 * YIYIIIIIIZII
- 38.27209218750002 * IIIIZIIIIZII
- 12.757364062500006 * IIIIIZIIIZII
+ 30.89089062500001 * IIIIIIZIIZII
+ 18.53453437500001 * IIIIIIIZIZII
+ 6.178178125000003 * IIIIIIIIZZII
- 1802.5210217187503 * IIIIIIIIIIZI
- 38.27209218750002 * ZIIIIIIIIIZI
- 22.963255312500017 * IZIIIIIIIIZI
- 7.654418437500004 * IIZIIIIIIIZI
- 38.27209218750002 * IIIZIIIIIIZI
+ 10.82498236639118 * XIXIIIIIIIZI
+ 10.82498236639118 * YIYIIIIIIIZI
- 22.963255312500017 * IIIIZIIIIIZI
- 7.654418437500004 * IIIIIZIIIIZI
+ 18.534534375000007 * IIIIIIZIIIZI
+ 11.120720625000006 * IIIIIIIZIIZI
+ 3.706906875000002 * IIIIIIIIZIZI
- 599.2280473437502 * IIIIIIIIIIIZ
- 12.757364062500006 * ZIIIIIIIIIIZ
- 7.654418437500005 * IZIIIIIIIIIZ
- 2.551472812500001 * IIZIIIIIIIIZ
- 12.757364062500006 * IIIZIIIIIIIZ
+ 3.608327455463727 * XIXIIIIIIIIZ
+ 3.608327455463727 * YIYIIIIIIIIZ
- 7.654418437500005 * IIIIZIIIIIIZ
- 2.551472812500001 * IIIIIZIIIIIZ
+ 6.178178125000002 * IIIIIIZIIIIZ
+ 3.706906875000002 * IIIIIIIZIIIZ
+ 1.2356356250000005 * IIIIIIIIZIIZ
- 2.8170754092577175 * IXXXXIIIIIII
- 2.8170754092577175 * IYYXXIIIIIII
- 2.8170754092577175 * IXXYYIIIIIII
- 2.8170754092577175 * IYYYYIIIIIII
- 13.3241034549836 * IIIXIXIIIIII
- 12.548228550588828 * ZIIXIXIIIIII
- 7.528937130353299 * IZIXIXIIIIII
- 2.509645710117766 * IIZXIXIIIIII
+ 3.5491750000000017 * XIXXIXIIIIII
+ 3.5491750000000017 * YIYXIXIIIIII
- 13.3241034549836 * IIIYIYIIIIII
- 12.548228550588828 * ZIIYIYIIIIII
- 7.528937130353299 * IZIYIYIIIIII
- 2.509645710117766 * IIZYIYIIIIII
+ 3.5491750000000017 * XIXYIYIIIIII
+ 3.5491750000000017 * YIYYIYIIIIII
+ 7.4145438259724985 * IIIXIXZIIIII
+ 7.4145438259724985 * IIIYIYZIIIII
+ 4.4487262955835 * IIIXIXIZIIII
+ 4.4487262955835 * IIIYIYIZIIII
+ 1.4829087651944997 * IIIXIXIIZIII
+ 1.4829087651944997 * IIIYIYIIZIII
+ 18.04163727731863 * IIIXIXIIIZII
+ 18.04163727731863 * IIIYIYIIIZII
+ 10.82498236639118 * IIIXIXIIIIZI
+ 10.82498236639118 * IIIYIYIIIIZI
+ 3.608327455463727 * IIIXIXIIIIIZ
+ 3.608327455463727 * IIIYIYIIIIIZ
+ 2.8170754092577175 * XXIIXXIIIIII
+ 2.8170754092577175 * YYIIXXIIIIII
+ 2.8170754092577175 * XXIIYYIIIIII
+ 2.8170754092577175 * YYIIYYIIIIII
- 74.16262749999993 * IIIIIIXXIIII
- 75.43993750000001 * ZIIIIIXXIIII
- 45.26396250000001 * IZIIIIXXIIII
- 15.087987500000002 * IIZIIIXXIIII
- 75.43993750000001 * IIIZIIXXIIII
+ 21.337636551415734 * XIXIIIXXIIII
+ 21.337636551415734 * YIYIIIXXIIII
- 45.26396250000001 * IIIIZIXXIIII
- 15.087987500000002 * IIIIIZXXIIII
- 74.16262749999993 * IIIIIIYYIIII
- 75.43993750000001 * ZIIIIIYYIIII
- 45.26396250000001 * IZIIIIYYIIII
- 15.087987500000002 * IIZIIIYYIIII
- 75.43993750000001 * IIIZIIYYIIII
+ 21.337636551415734 * XIXIIIYYIIII
+ 21.337636551415734 * YIYIIIYYIIII
- 45.26396250000001 * IIIIZIYYIIII
- 15.087987500000002 * IIIIIZYYIIII
+ 155.920125 * IIIIIIXXIZII
+ 155.920125 * IIIIIIYYIZII
+ 93.55207500000002 * IIIIIIXXIIZI
+ 93.55207500000002 * IIIIIIYYIIZI
+ 31.184025000000005 * IIIIIIXXIIIZ
+ 31.184025000000005 * IIIIIIYYIIIZ
+ 21.337636551415734 * IIIXIXXXIIII
+ 21.337636551415734 * IIIYIYXXIIII
+ 21.337636551415734 * IIIXIXYYIIII
+ 21.337636551415734 * IIIYIYYYIIII
- 9.180253761118216 * IIIIIIXIXIII
+ 7.414543825972498 * ZIIIIIXIXIII
+ 4.4487262955835 * IZIIIIXIXIII
+ 1.4829087651944997 * IIZIIIXIXIII
+ 7.414543825972498 * IIIZIIXIXIII
- 2.097149687500001 * XIXIIIXIXIII
- 2.097149687500001 * YIYIIIXIXIII
+ 4.4487262955835 * IIIIZIXIXIII
+ 1.4829087651944997 * IIIIIZXIXIII
- 9.180253761118216 * IIIIIIYIYIII
+ 7.414543825972498 * ZIIIIIYIYIII
+ 4.4487262955835 * IZIIIIYIYIII
+ 1.4829087651944997 * IIZIIIYIYIII
+ 7.414543825972498 * IIIZIIYIYIII
- 2.097149687500001 * XIXIIIYIYIII
- 2.097149687500001 * YIYIIIYIYIII
+ 4.4487262955835 * IIIIZIYIYIII
+ 1.4829087651944997 * IIIIIZYIYIII
- 8.737263295131783 * IIIIIIXIXZII
- 8.737263295131783 * IIIIIIYIYZII
- 5.24235797707907 * IIIIIIXIXIZI
- 5.24235797707907 * IIIIIIYIYIZI
- 1.7474526590263566 * IIIIIIXIXIIZ
- 1.7474526590263566 * IIIIIIYIYIIZ
- 2.097149687500001 * IIIXIXXIXIII
- 2.097149687500001 * IIIYIYXIXIII
- 2.097149687500001 * IIIXIXYIYIII
- 2.097149687500001 * IIIYIYYIYIII
- 29.42804386641884 * IIIIIIIXXIII
- 53.34409137853934 * ZIIIIIIXXIII
- 32.006454827123605 * IZIIIIIXXIII
- 10.668818275707867 * IIZIIIIXXIII
- 53.34409137853934 * IIIZIIIXXIII
+ 15.087987500000006 * XIXIIIIXXIII
+ 15.087987500000006 * YIYIIIIXXIII
- 32.006454827123605 * IIIIZIIXXIII
- 10.668818275707867 * IIIIIZIXXIII
- 29.42804386641884 * IIIIIIIYYIII
- 53.34409137853934 * ZIIIIIIYYIII
- 32.006454827123605 * IZIIIIIYYIII
- 10.668818275707867 * IIZIIIIYYIII
- 53.34409137853934 * IIIZIIIYYIII
+ 15.087987500000006 * XIXIIIIYYIII
+ 15.087987500000006 * YIYIIIIYYIII
- 32.006454827123605 * IIIIZIIYYIII
- 10.668818275707867 * IIIIIZIYYIII
+ 110.25217771095417 * IIIIIIIXXZII
+ 110.25217771095417 * IIIIIIIYYZII
+ 66.1513066265725 * IIIIIIIXXIZI
+ 66.1513066265725 * IIIIIIIYYIZI
+ 22.050435542190833 * IIIIIIIXXIIZ
+ 22.050435542190833 * IIIIIIIYYIIZ
+ 15.087987500000006 * IIIXIXIXXIII
+ 15.087987500000006 * IIIYIYIXXIII
+ 15.087987500000006 * IIIXIXIYYIII
+ 15.087987500000006 * IIIYIYIYYIII
- 42.38243941348044 * IIIIIIIIIXIX
+ 18.04163727731863 * ZIIIIIIIIXIX
+ 10.824982366391183 * IZIIIIIIIXIX
+ 3.608327455463727 * IIZIIIIIIXIX
+ 18.04163727731863 * IIIZIIIIIXIX
- 5.102945625000002 * XIXIIIIIIXIX
- 5.102945625000002 * YIYIIIIIIXIX
+ 10.824982366391183 * IIIIZIIIIXIX
+ 3.608327455463727 * IIIIIZIIIXIX
- 8.737263295131783 * IIIIIIZIIXIX
- 5.24235797707907 * IIIIIIIZIXIX
- 1.7474526590263566 * IIIIIIIIZXIX
- 42.38243941348044 * IIIIIIIIIYIY
+ 18.04163727731863 * ZIIIIIIIIYIY
+ 10.824982366391183 * IZIIIIIIIYIY
+ 3.608327455463727 * IIZIIIIIIYIY
+ 18.04163727731863 * IIIZIIIIIYIY
- 5.102945625000002 * XIXIIIIIIYIY
- 5.102945625000002 * YIYIIIIIIYIY
+ 10.824982366391183 * IIIIZIIIIYIY
+ 3.608327455463727 * IIIIIZIIIYIY
- 8.737263295131783 * IIIIIIZIIYIY
- 5.24235797707907 * IIIIIIIZIYIY
- 1.7474526590263566 * IIIIIIIIZYIY
- 5.102945625000002 * IIIXIXIIIXIX
- 5.102945625000002 * IIIYIYIIIXIX
- 5.102945625000002 * IIIXIXIIIYIY
- 5.102945625000002 * IIIYIYIIIYIY
- 44.100871084381666 * IIIIIIXXIXIX
- 44.100871084381666 * IIIIIIYYIXIX
- 44.100871084381666 * IIIIIIXXIYIY
- 44.100871084381666 * IIIIIIYYIYIY
+ 2.471271250000001 * IIIIIIXIXXIX
+ 2.471271250000001 * IIIIIIYIYXIX
+ 2.471271250000001 * IIIIIIXIXYIY
+ 2.471271250000001 * IIIIIIYIYYIY
- 31.184025000000013 * IIIIIIIXXXIX
- 31.184025000000013 * IIIIIIIYYXIX
- 31.184025000000013 * IIIIIIIXXYIY
- 31.184025000000013 * IIIIIIIYYYIY
###Markdown
Now that the Hamiltonian is ready, it can be used in a quantum algorithm to find information about the vibrational structure of the corresponding molecule. Check out our tutorials on Ground State Calculation and Excited States Calculation to learn more about how to do that in Qiskit Nature!
###Code
import qiskit.tools.jupyter
%qiskit_version_table
%qiskit_copyright
###Output
/home/oss/Files/Qiskit/src/qiskit-terra/main/qiskit/tools/jupyter/__init__.py:133: RuntimeWarning: matplotlib can't be found, ensure you have matplotlib and other visualization dependencies installed. You can run '!pip install qiskit-terra[visualization]' to install it from jupyter
warnings.warn(
|
notebooks/kervy/first_model-Copy1.ipynb | ###Markdown
Validation
###Code
X_train = proc_train.drop('sid', axis=1).reset_index(drop=True)
y_train = trn_session.target.reset_index(drop=True)
X_val = proc_val.drop('sid', axis=1).reset_index(drop=True)
y_val = val_session.target.reset_index(drop=True)
X_test = proc_test.drop('sid', axis=1).reset_index(drop=True)
temp_columns = X_train.columns
X_val = X_val[temp_columns]
X_test = X_test[temp_columns]
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
scaler.fit(X_train[X_train.columns[:32]])
X_train_sc = scaler.transform(X_train[X_train.columns[:32]])
X_val_sc = scaler.transform(X_val[X_val.columns[:32]])
from sklearn.decomposition import PCA
pca = PCA(n_components = 11).fit(X_train_sc)
X_train_pca = (pca.transform(X_train_sc))
X_val_pca = (pca.transform(X_val_sc))
X_train = pd.concat([pd.DataFrame(X_train), pd.DataFrame(X_train_pca)], axis=1)
X_val = pd.concat([pd.DataFrame(X_val), pd.DataFrame(X_val_pca)], axis=1)
# parameters for LightGBMClassifier
params = {
'objective' :'binary',
'max_depth' : 6,
'boosting_type' : 'dart',
'xgboost_dart_mode': True,
'learning_rate': 0.01,
'metric': 'binary_logloss',
'verbose': 1
#'bagging_fraction': 0.5,
}
d_train = lgbm.Dataset(X_train, label=trn_session.target)
d_valid = lgbm.Dataset(X_val, label=val_session.target)
clf = lgbm.train(params, d_train, valid_sets=[d_valid],
num_boost_round=700, verbose_eval=100)
# parameters for LightGBMClassifier
params = {
'objective' :'binary',
'max_depth' : 7,
'boosting_type' : 'dart',
'xgboost_dart_mode': True,
'learning_rate': 0.01,
'metric': 'binary_logloss',
'verbose': 1
#'bagging_fraction': 0.5,
}
d_train = lgbm.Dataset(X_train, label=trn_session.target)
d_valid = lgbm.Dataset(X_val, label=val_session.target)
clf = lgbm.train(params, d_train, valid_sets=[d_valid],
num_boost_round=700, verbose_eval=100)
# parameters for LightGBMClassifier
params = {
'objective' :'binary',
'max_depth' : 7,
'boosting_type' : 'dart',
'xgboost_dart_mode': True,
'learning_rate': 0.1,
'metric': 'binary_logloss',
'verbose': 1,
'bagging_fraction': 0.5,
}
d_train = lgbm.Dataset(X_train, label=trn_session.target)
d_valid = lgbm.Dataset(X_val, label=val_session.target)
clf = lgbm.train(params, d_train, valid_sets=[d_valid],
num_boost_round=70)
###Output
[1] valid_0's binary_logloss: 0.304912
[2] valid_0's binary_logloss: 0.298446
[3] valid_0's binary_logloss: 0.293581
[4] valid_0's binary_logloss: 0.28988
[5] valid_0's binary_logloss: 0.287032
[6] valid_0's binary_logloss: 0.284648
[7] valid_0's binary_logloss: 0.282814
[8] valid_0's binary_logloss: 0.282046
[9] valid_0's binary_logloss: 0.280683
[10] valid_0's binary_logloss: 0.279476
[11] valid_0's binary_logloss: 0.278594
[12] valid_0's binary_logloss: 0.278364
[13] valid_0's binary_logloss: 0.277627
[14] valid_0's binary_logloss: 0.277114
[15] valid_0's binary_logloss: 0.276616
[16] valid_0's binary_logloss: 0.276194
[17] valid_0's binary_logloss: 0.275836
[18] valid_0's binary_logloss: 0.275516
[19] valid_0's binary_logloss: 0.27526
[20] valid_0's binary_logloss: 0.275095
[21] valid_0's binary_logloss: 0.275047
[22] valid_0's binary_logloss: 0.274875
[23] valid_0's binary_logloss: 0.274775
[24] valid_0's binary_logloss: 0.27463
[25] valid_0's binary_logloss: 0.274525
[26] valid_0's binary_logloss: 0.274407
[27] valid_0's binary_logloss: 0.274323
[28] valid_0's binary_logloss: 0.274316
[29] valid_0's binary_logloss: 0.274214
[30] valid_0's binary_logloss: 0.274213
[31] valid_0's binary_logloss: 0.274194
[32] valid_0's binary_logloss: 0.274139
[33] valid_0's binary_logloss: 0.274112
[34] valid_0's binary_logloss: 0.2741
[35] valid_0's binary_logloss: 0.274092
[36] valid_0's binary_logloss: 0.274092
[37] valid_0's binary_logloss: 0.274083
[38] valid_0's binary_logloss: 0.274067
[39] valid_0's binary_logloss: 0.274
[40] valid_0's binary_logloss: 0.274003
[41] valid_0's binary_logloss: 0.274002
[42] valid_0's binary_logloss: 0.27401
[43] valid_0's binary_logloss: 0.274011
[44] valid_0's binary_logloss: 0.27402
[45] valid_0's binary_logloss: 0.274031
[46] valid_0's binary_logloss: 0.273999
[47] valid_0's binary_logloss: 0.273989
[48] valid_0's binary_logloss: 0.273984
[49] valid_0's binary_logloss: 0.273979
[50] valid_0's binary_logloss: 0.273986
[51] valid_0's binary_logloss: 0.27401
[52] valid_0's binary_logloss: 0.274033
[53] valid_0's binary_logloss: 0.274031
[54] valid_0's binary_logloss: 0.274061
[55] valid_0's binary_logloss: 0.274092
[56] valid_0's binary_logloss: 0.274089
[57] valid_0's binary_logloss: 0.274072
[58] valid_0's binary_logloss: 0.274033
[59] valid_0's binary_logloss: 0.274031
[60] valid_0's binary_logloss: 0.274013
[61] valid_0's binary_logloss: 0.273988
[62] valid_0's binary_logloss: 0.274007
[63] valid_0's binary_logloss: 0.274011
[64] valid_0's binary_logloss: 0.27401
[65] valid_0's binary_logloss: 0.274008
[66] valid_0's binary_logloss: 0.274017
[67] valid_0's binary_logloss: 0.274016
[68] valid_0's binary_logloss: 0.274006
[69] valid_0's binary_logloss: 0.274007
[70] valid_0's binary_logloss: 0.274006
###Markdown
Model
###Code
X_all = proc_all.drop('sid', axis=1).reset_index(drop=True)
y_all = all_session.target.reset_index(drop=True)
X_test = proc_test.drop('sid', axis=1).reset_index(drop=True)
temp_columns = X_all.columns
X_test = X_test[temp_columns]
scaler = StandardScaler()
scaler.fit(X_all[X_all.columns[:32]])
X_all_sc = scaler.transform(X_all[X_all.columns[:32]])
X_test_sc = scaler.transform(X_test[X_test.columns[:32]])
pca = PCA(n_components = 11).fit(X_all_sc)
X_all_pca = pd.DataFrame(pca.transform(X_all_sc))
X_test_pca = pd.DataFrame(pca.transform(X_test_sc))
X_all = pd.concat([pd.DataFrame(X_all), pd.DataFrame(X_all_pca)], axis=1)
X_test = pd.concat([pd.DataFrame(X_test), pd.DataFrame(X_test_pca)], axis=1)
import lightgbm as lgbm
# parameters for LightGBMClassifier
params = {
'objective' :'binary',
'max_depth' : 6,
'boosting_type' : 'dart',
'xgboost_dart_mode': True,
'learning_rate': 0.01,
#'metric': 'binary_logloss',
#'bagging_fraction': 0.5,
}
d_train = lgbm.Dataset(X_all, label=y_all)
#d_valid = lgbm.Dataset(X_val, label=y_val)
clf = lgbm.train(params, d_train,# valid_sets=[d_valid],
num_boost_round=700)
from matplotlib import rcParams
%matplotlib inline
rcParams['figure.figsize'] = 10, 10
lgbm.plot_importance(clf)
count += 1
preds=clf.predict(X_test)
test_session['target'] = preds
test_session.to_csv(f'data/submissions/kervy_{count}_submit.csv', index=False)
count = 14
###Output
_____no_output_____ |
notebooks/DBLP.citations_and_regressions.ipynb | ###Markdown
**Filter**- Keep only English language records.- Remove records that contain exactly 50 citations (I felt after plotting the histograms of the citation count that there was some discrepancy with this particular value -- better just remove it)**Transform**- Add an extra column for age.- Add an extra column for not containing any semantic words.
###Code
print (len(df_dblp[(df_dblp["lang"] == "en") & (df_dblp["indeg"] != 50)]))
df_dblp = df_dblp[(df_dblp["lang"] == "en") & (df_dblp["indeg"] != 50)]
df_dblp = df_dblp[df_dblp["year"] >= 1955] # just so that the z-scores can be calculated (we don't miss out on much)
df_dblp["age"] = 2019 - df_dblp["year"]
df_dblp["isUNK"] = (df_dblp["max_prog_word"] == "UNK").astype(int)
# for simplicity of descriptive analysis, let's remove docs which don't hit the lexicon
# In the regression we will have to add a dummy variable to account for this.
drop_misses = True
if drop_misses:
df_dblp = df_dblp[df_dblp["isUNK"] == 0]
print (len(df_dblp))
###Output
1159345
###Markdown
**Univariate analysis**
###Code
# quartile-ish bins of the innovation feature
df_dblp['max_prog_q'] = pd.qcut(df_dblp['max_prog'], 4, labels=["<25", "<50", "<75", "<100"], duplicates='drop')
## Used to do this for ACL' 19
#df_dblp['nprog_q'] = pd.qcut(df_dblp['nprog'].rank(method="first"), 4, labels=["<25", "<50", "<75", "<100"], duplicates='drop')
#df_dblp['nprog25_q'] = pd.qcut(df_dblp['nprog25'].rank(method="first"), 4, labels=["<25", "<50", "<75", "<100"], duplicates="drop")
#df_dblp['nprog50_q'] = pd.qcut(df_dblp['nprog50'].rank(method="first"), 4, labels=["<25", "<50", "<75", "<100"], duplicates="drop")
#df_dblp['nprog75_q'] = pd.qcut(df_dblp['nprog75'].rank(method="first"), 4, labels=["<25", "<50", "<75", "<100"], duplicates="drop")
#df_dblp['nprog90_q'] = pd.qcut(df_dblp['nprog90'].rank(method="first"), 4, labels=["<25", "<50", "<75", "<100"], duplicates="drop")
#df_dblp['nprog95_q'] = pd.qcut(df_dblp['nprog95'].rank(method="first"), 4, labels=["<25", "<50", "<75", "<100"], duplicates="drop")
#df_dblp['nprog99_q'] = pd.qcut(df_dblp['nprog99'].rank(method="first"), 4, labels=["<25", "<50", "<75", "<100"], duplicates="drop")
def give_me_zscores_per_year (frame, key):
years = frame.groupby("year").size().reset_index(name="counts")
years = list (years[years["counts"] > 1].year.values)
zscore_lookup = dict ()
for year in years:
docids = frame[frame["year"] == year]["docid"].values
zscores = zscore (frame[frame["year"] == year][key].values)
for i in range (len (docids)):
zscore_lookup[docids[i]] = zscores[i]
docids = frame["docid"].values
zscores = [zscore_lookup[docid] for docid in docids]
return zscores
df_dblp['max_progz'] = give_me_zscores_per_year (df_dblp, 'max_prog')
df_dblp['max_progz_q'] = pd.qcut(df_dblp['max_progz'], 4, labels=["<25", "<50", "<75", "<100"], duplicates='drop')
df_dblp_since_1980s = df_dblp[(df_dblp["year"] > 1980) & (df_dblp["year"] <= 2010)]
print (len(df_dblp_since_1980s))
df_dblp_since_1980s.to_csv ("../data/frames/emnlp/dblp.univariate.csv", sep=",", index=False)
sns.set_context("paper")
fig,ax = plt.subplots(1,1,figsize=[6,3.5])
#sns.barplot(y='indeg',x='max_prog_q',data=df_dblp_since_1980s,ax=ax);
sns.barplot(y='indeg',x='max_prog_q',data=df_dblp_since_1980s,ax=ax);
ax.set_title('Scientific articles');
sns.set_context("paper")
fig,ax = plt.subplots(1,1,figsize=[6,3.5])
#sns.barplot(y='indeg',x='max_prog_q',data=df_dblp_since_1980s,ax=ax);
sns.barplot(y='indeg',x='max_progz_q',data=df_dblp_since_1980s,ax=ax);
ax.set_title('Scientific articles');
###Output
_____no_output_____
###Markdown
```python Old (for ACL'19)sns.set_context("paper")fig,ax = plt.subplots(1,1,figsize=[6,3.5])sns.barplot(y='indeg',x='max_prog_q',data=df_dblp_since_1980s,ax=ax);ax.set_title('Scientific articles');``` ```python Old (for ACL'19)sns.set_context("paper")fig,ax = plt.subplots(1,1,figsize=[6,3.5],sharey=True)sns.barplot(y='indeg',x='nprog50_q',data=df_dblp_since_1980s,ax=ax);ax.set_title('>50 percentile')ax.set_xlabel('Intervals')ax.set_ylabel('Citations')``` **Multivariate regressions**
###Code
formulas_dblp = ["indeg ~ age + outdeg + nauthors + ntokens + bows + ninnovs"]
formulas_dblp.append(formulas_dblp[0]+" + max_prog")
formulas_dblp.append(formulas_dblp[0]+" + max_prog_q")
formulas_dblp.append(formulas_dblp[0]+" + max_progz")
formulas_dblp.append(formulas_dblp[0]+" + max_progz_q")
#formulas_dblp.append(formulas_dblp[0]+" + nprog50")
#formulas_dblp.append(formulas_dblp[0]+" + nprog50_q")
#formulas_dblp.append(formulas_dblp[0]+" + nprog25")
#formulas_dblp.append(formulas_dblp[0]+" + nprog25_q")
#formulas_dblp.append(formulas_dblp[0]+" + nprog75")
#formulas_dblp.append(formulas_dblp[0]+" + nprog75_q")
#formulas_dblp.append(formulas_dblp[1]+" + nprog99")
#formulas_dblp.append(formulas_dblp[1]+" + nprog99_q")
df_dblp_sample = df_dblp.sample (n=250000, axis=0)
print(len(df_dblp_sample[df_dblp_sample["max_progz_q"] == "<25"]))
print(len(df_dblp_sample[df_dblp_sample["max_progz_q"] == "<50"]))
print(len(df_dblp_sample[df_dblp_sample["max_progz_q"] == "<75"]))
print(len(df_dblp_sample[df_dblp_sample["max_progz_q"] == "<100"]))
print(len(df_dblp_sample))
print(Counter(df_dblp_sample[df_dblp_sample["max_progz_q"] == "<25"].year.values).most_common(25))
print(Counter(df_dblp_sample[df_dblp_sample["max_progz_q"] == "<50"].year.values).most_common(25))
print(Counter(df_dblp_sample[df_dblp_sample["max_progz_q"] == "<75"].year.values).most_common(25))
print(Counter(df_dblp_sample[df_dblp_sample["max_progz_q"] == "<100"].year.values).most_common(25))
from scipy.stats import ks_2samp
years = range (1990, 2016)
x = [Counter(df_dblp_sample[df_dblp_sample["max_progz_q"] == "<25"].year.values)[y] for y in years]
y = [Counter(df_dblp_sample[df_dblp_sample["max_progz_q"] == "<100"].year.values)[y] for y in years]
#y = [item[1] for item in Counter(df_dblp_sample[df_dblp_sample["max_progz_q"] == "<50"].year.values).most_common(10)]
ks_2samp(x, y)
results_dblp = []
for formula in formulas_dblp:
Y,X = patsy.dmatrices(formula,data=df_dblp_sample)
results_dblp.append(sm.GLM(Y, X, family=sm.families.Poisson()).fit())
lls = [result.llf for result in results_dblp]
print(lls)
print (max(lls))
for i,ll_0 in enumerate(lls):
for j,ll_1 in enumerate(lls[:i]):
chi2_score = ll_0-ll_1
dof = len(formulas_dblp[i].split("+")) - len(formulas_dblp[j].split('+'))
if i == 3: dof += 2 #quartiles
print(i,j,f'\\chi^2_{dof} = {chi2_score:.2f}, p < {chi2.sf(chi2_score, dof):.3e}')
for i in range (len (results_dblp)):
print(results_dblp[i].summary())
print ()
df_dblp_sample.to_csv ("../data/frames/emnlp/dblp.final", sep=",", index=False)
###Output
_____no_output_____ |
notebooks/03-Packages-Modules-Function.ipynb | ###Markdown
Packages, Modules, Methods, and Functions > The Python source distribution has long maintained the philosophy of "batteries included" -- having a rich and versatile standard library which is immediately available, without making the user download separate packages. This gives the Python language a head start in many projects.>> \- PEP 206 Applied Review Python and Jupyter Overview - We're working with Python through Jupyter, the most common IDE for data science. Fundamentals - Python's common *atomic*, or basic, data types are: - Integers - Floats (decimals) - Strings - Booleans - These simple types can be combined to form more complex types, including: - Lists: Ordered collections - Dictionaries: Key-value pairs - DataFrames: Tabular datasets Packages (aka *Modules*) So far we've seen several data types that Python offers out-of-the-box.However, to keep things organized, some Python functionality is stored in standalone *packages*, or libraries of code.The word "module" is generally synonymous with package; you will hear both in discussions of Python. For example, functionality related to the operating system -- such as creating files and folders -- is stored in a package called `os`.To use the tools in `os`, we *import* the package.
###Code
import os
###Output
_____no_output_____
###Markdown
Once we import it, we gain access to everything inside.With Jupyter's autocomplete, we can view what's available.
###Code
# Move your cursor the end of the below line and press tab.
os.
###Output
_____no_output_____
###Markdown
Some packages, like `os`, are bundled with every Python install; downloading Python guarantees you'll have these packages.Collectively, this group of packages is known as the *standard library*. Other packages must be downloaded separately, either because- they aren't sufficiently popular to merit inclusion in the standard library- *or* they change too quickly for the maintainers of Python to keep up The DataFrame type that we saw earlier is part of the `pandas` package (short for *Panel Data*), one such package.Since pandas is specific to data science and is still rapidly evolving, it is not part of the standard library. We can download packages like pandas from the internet using a website called PyPI, the *Python Package Index*.Fortunately, since we are using Binder today, that has been handled for us and pandas is already installed. It's possible to import packages under an *alias*, or a nickname.The community has adopted certain conventions for aliases for common packages;while following them isn't mandatory, it's highly recommended, as it makes your code easier for others to understand. pandas is conventionally imported under the alias `pd`.
###Code
import pandas as pd
# Importing pandas has given us access to the DataFrame, accessible as pd.DataFrame
pd.DataFrame
###Output
_____no_output_____
###Markdown
Question:What is the type of `pd`? Guess before you run the code below.
###Code
type(pd)
###Output
_____no_output_____
###Markdown
Third-party packages unlock a huge range of functionality that isn't available in native Python; much of Python's data science capabilities come from a handful of packages outside the standard library: - pandas- numpy (numerical computing)- scikit-learn (modeling)- scipy (scientific computing)- matplotlib (graphing) We won't have time to touch on most of these in this training, but if you're interested in one, google it! Your Turn1. Import the `numpy` library, listed above. Give it the alias "np".2. Using autocomplete, determine what variable or function inside the numpy library starts with "asco". *Hint: remember you'll need to preface the variable name with the package alias, e.g. `np.asco`* Dot Notation with Packages We've seen it a few times already, but now it's time to discuss it explicitly:things inside packages can be accessed with *dot-notation*. Dot notation looks like this:```pythonpd.Series``` or```pythonimport numpy as npnp.array``` You can read this is "the `array` variable, within the Numpy library". Packages can contain pretty much anything that's legal in Python;if it's code, it can be in a package.This flexibility is part of the reason that Python's package ecosystem is so expansive and powerful. Functions As you may have noticed already, occasionally we run code using parentheses.The feature that permits this in Python is **functions** -- code snippets wrapped up into a single name. For example, take the `type` function we saw above.```pythontype(x)``` `type` does some complex things under the hood -- it looks at the variable inside the parentheses, determines what type of thing it is, and then returns that type to the user.
###Code
x = 7
type(x)
###Output
_____no_output_____
###Markdown
But the beauty of `type`, and of all functions, is that you (as the user) don't need to know all the complex code that's necessary to figure out that x is an `int` -- you just need to remember that there's a `type` function to do that for you. Functions make you much more powerful, as they unlock lots of functionality within a simple interface. ```python Get the first few rows of the planes data.planes.head()``` ```python Read in the planes.csv file.pd.read_csv('../data/planes.csv')``` The variables within the parens are called function arguments, or simply **arguments**.Above, the string `'../data/planes.csv'` is the argument to the `pd.read_csv` function. Functions are integral to using Python, because it's much more efficient to use pre-written code than to always write your own. If you ever do want to write your own function -- perhaps to share with others, or to make it easier to reuse your work -- it's fairly simple to do so, but beyond the scope of this training. Objects and Dot Notation Dot-notation, which we discussed in relation to packages, has another use -- accessing things inside of *objects*.What's an object? Basically, a variable that contains other data or functionality inside of it that is exposed to users. For example, DataFrames are objects.
###Code
df = pd.DataFrame({'first_name': ['Ethan', 'Brad'], 'last_name': ['Swan', 'Boehmke']})
df
df.shape
df.describe()
###Output
_____no_output_____
###Markdown
You can see that DataFrames have a `shape` variable and a `describe` function inside of them, both accessible through dot notation.Variables inside an object are often called *attributes* and functions inside objects are called *methods*. On Consistency and Language Design One of the great things about Python is that its creators really cared about internal consistency. What that means to us, as users, is that syntax is consistent and predictable -- even across different uses that would appear to be different at first. Dot notation reveals something kind of cool about Python: packages are just like other objects, and the variables inside them are just attributes and methods!This standardization across packages and objects helps us remember a single, intuitive syntax that works for many different things. Functions, Objects, and Methods in the Context of DataFrames As we saw above, DataFrames are a type of Python object, so let's use them to explore the new Python features we've learned. Using the `read_csv` function from the Pandas package to read in a DataFrame
###Code
df = pd.read_csv('../data/airlines.csv')
###Output
_____no_output_____
###Markdown
Using the `type` function to determine the type of `df`
###Code
type(df)
###Output
_____no_output_____
###Markdown
Using the `head` method of the DataFrame to view some of its rows
###Code
df.head()
###Output
_____no_output_____
###Markdown
Examining the `columns` attribute of the DataFrame to see the names of its columns.
###Code
df.columns
###Output
_____no_output_____
###Markdown
Inspecting the `shape` attribute to find the *dimensions* (rows and columns) of the DataFrame.
###Code
df.shape
###Output
_____no_output_____
###Markdown
Calling the `describe` method to get a summary of the data in the DataFrame.
###Code
df.describe()
###Output
_____no_output_____
###Markdown
Now let's combine them: using the `type` function to determine what `df.describe` holds.
###Code
type(df.describe)
###Output
_____no_output_____
###Markdown
Question:Does this result make sense? What would happen if you added parens? i.e. type(df.describe()) Your TurnSpend some time using autocomplete to explore the methods and attributes of the `df` object we used above.Remember from the Jupyter lesson that you can use a question mark to see the documentation for a function or method (e.g. `df.describe?`). Deeper Dive on DataFrames Now that we understand objects and functions better, let's look more at DataFrames. What Are DataFrames Made of? Accessing an individual column of a DataFrame can be done by passing the column name as a string, in brackets.
###Code
carrier_column = df['carrier']
carrier_column
###Output
_____no_output_____
###Markdown
Individual columns are pandas `Series` objects.
###Code
type(carrier_column)
###Output
_____no_output_____
###Markdown
How are Series different from DataFrames? - They're always 1-dimensional - They have different attributes than DataFrames - For example, Series have a `to_list` method -- which doesn't make sense to have on DataFrames - They don't print in the pretty format of DataFrames, but in plain text (see above)
###Code
carrier_column.shape
df.shape
carrier_column.to_list()
df.to_list()
###Output
_____no_output_____
###Markdown
It's important to be familiar with Series because they are fundamentally the core of DataFrames.Not only are columns represented as Series, but so are rows!
###Code
# Fetch the first row of the DataFrame
first_row = df.loc[0]
first_row
type(first_row)
###Output
_____no_output_____
###Markdown
Whenever you select individual columns or rows, you'll get Series objects. What Can You Do with a Series? First, let's create our own Series object from scratch -- they don't need to come from a DataFrame.
###Code
# Pass a list in as an argument and it will be converted to a Series.
s = pd.Series([10, 20, 30, 40, 50])
s
# Pass a list in as an argument and it will be converted to a Series.
s = pd.Series([10, 20, 30, 40, 50])
s
###Output
_____no_output_____
###Markdown
There are 3 things to notice about this Series: - The values (10, 20, 30...) - The *dtype*, short for data type. - The *index* (0, 1, 2...) ValuesValues are fairly self-explanatory; we chose them in our input list. dtypeData types are also straightforward. Series are always homogeneous, holding only integers, floats, or generic Python objects (called just `object`). Because a Python object is general enough to contain any other type, any Series holding strings or other non-numeric data will typically default to be of type `object`. For example, going back to our carriers DataFrame, note that the carrier column is of type `object`.
###Code
df['carrier']
###Output
_____no_output_____
###Markdown
IndexIndexes are more interesting.Every Series has an index, a way to reference each element.The index of a Series is a lot like the keys of a dictionary: each index element corresponds to a value in the Series, and can be used to look up that element.
###Code
# Our index is a range from 0 (inclusive) to 5 (exclusive).
s.index
s
s[3]
###Output
_____no_output_____
###Markdown
In our example, the index is just the integers 0-4, so right now it looks no different that referencing elements of a regular Python list.*But* indexes can be changed to something different -- like the letters a-e, for example.
###Code
s.index = ['a', 'b', 'c', 'd', 'e']
s
###Output
_____no_output_____
###Markdown
Now to look up the value 40, we reference `'d'`.
###Code
s['d']
###Output
_____no_output_____
###Markdown
We saw earlier that rows of a DataFrame are Series.In such cases, the flexibility of Series indexes comes in handy;the index is set to the DataFrame column names.
###Code
df.head()
# Note that the index is ['carrier', 'name']
first_row = df.loc[0]
first_row
###Output
_____no_output_____
###Markdown
This is particularly handy because it means you can extract individual elements based on a column name.
###Code
first_row['carrier']
###Output
_____no_output_____
###Markdown
DataFrame Indexes It's not just Series that have indexes!DataFrames have them too.Take a look at the carrier DataFrame again and note the bold numbers on the left.
###Code
df.head()
###Output
_____no_output_____
###Markdown
These numbers are an index, just like the one we saw on our example Series.And DataFrame indexes support similar functionality.
###Code
# Our index is a range from 0 (inclusive) to 16 (exclusive).
df.index
###Output
_____no_output_____
###Markdown
When loading in a DataFrame, the default index will always be 0 to N-1, where N is the number of rows in your DataFrame.This is called a `RangeIndex`. Selecting individual rows by their index is done with the `.loc` accessor.An *accessor* is an attribute designed specifically to help users reference something else (like rows within a DataFrame).
###Code
# Get the row at index 4 (the fifth row).
df.loc[4]
###Output
_____no_output_____
###Markdown
As with Series, DataFrames support reassigning their index. However, with DataFrames it often makes sense to change one of your columns into the index. This is analogous to a primary key in relational databases: a way to rapidly look up rows within a table. In our case, maybe we will often use the carrier code (`carrier`) to look up the full name of the airline.In that case, it would make sense set the carrier column as our index.
###Code
df = df.set_index('carrier')
df.head()
###Output
_____no_output_____
###Markdown
Now the RangeIndex has been replaced with a more meaningful index, and it's possible to look up rows of the table by passing carrier code to the `.loc` accessor.
###Code
df.loc['UA']
###Output
_____no_output_____
###Markdown
Caution:Pandas does not require that indexes have unique values (that is, no duplicates) although many relational databases do have that requirement of a primary key. This means that it is *possible* to create a non-unique index, but highly inadvisable. Having duplicate values in your index can cause unexpected results when you refer to rows by index -- but multiple rows have that index. Don't do it if you can help it! When starting to work with a DataFrame, it's often a good idea to determine what column makes sense as your index and to set it immediately. This will make your code nicer -- by letting you directly look up values with the index -- and also make your selections and filters faster, because Pandas is optimized for operations by index. If you want to change the index of your DataFrame later, you can always `reset_index` (and then assign a new one).
###Code
df.head()
df = df.reset_index()
df.head()
###Output
_____no_output_____
###Markdown
Your TurnThe below cell has code to load in the first 100 rows of the airports data as `airports`.The data contains the airport code, airport name, and some basic facts about the airport location.1. What kind of index is the current index of `airports`? 2. Is this a good choice for the DataFrame's index? If not, what column or columns would be a better candidate?3. If you chose a different column to be the index, make it your index using `airports.set_index()`.4. Using your new index, look up "Pittsburgh-Monroeville Airport", code 4G0. What is its altitude?5. Reset your index in case you want to make a different column your index in the future.
###Code
airports = pd.read_csv('../data/airports.csv')
airports = airports.loc[0:100]
airports.head()
###Output
_____no_output_____
###Markdown
Packages, Modules, Methods, and Functions > The Python source distribution has long maintained the philosophy of "batteries included" -- having a rich and versatile standard library which is immediately available, without making the user download separate packages. This gives the Python language a head start in many projects.>> \- PEP 206 Applied Review Python and Jupyter Overview - We're working with Python through Jupyter, the most common IDE for data science. Fundamentals - Python's common *atomic*, or basic, data types are: - Integers - Floats (decimals) - Strings - Booleans - These simple types can be combined to form more complex types, including: - Lists: Ordered collections - Dictionaries: Key-value pairs - DataFrames: Tabular datasets Packages (aka *Modules*) So far we've seen several data types that Python offers out-of-the-box.However, to keep things organized, some Python functionality is stored in standalone *packages*, or libraries of code.The word "module" is generally synonymous with package; you will hear both in discussions of Python. For example, functionality related to the operating system -- such as creating files and folders -- is stored in a package called `os`.To use the tools in `os`, we *import* the package.
###Code
import os
###Output
_____no_output_____
###Markdown
Once we import it, we gain access to everything inside.With Jupyter's autocomplete, we can view what's available.
###Code
# Move your cursor the end of the below line and press tab.
os.
###Output
_____no_output_____
###Markdown
Some packages, like `os`, are bundled with every Python install; downloading Python guarantees you'll have these packages.Collectively, this group of packages is known as the *standard library*. Other packages must be downloaded separately, either because- they aren't sufficiently popular to merit inclusion in the standard library- *or* they change too quickly for the maintainers of Python to keep up The DataFrame type that we saw earlier is part of the `pandas` package (short for *Panel Data*), one such package.Since pandas is specific to data science and is still rapidly evolving, it is not part of the standard library. We can download packages like pandas from the internet using a website called PyPI, the *Python Package Index*.Fortunately, since we are using Binder today, that has been handled for us and pandas is already installed. It's possible to import packages under an *alias*, or a nickname.The community has adopted certain conventions for aliases for common packages;while following them isn't mandatory, it's highly recommended, as it makes your code easier for others to understand. pandas is conventionally imported under the alias `pd`.
###Code
import pandas as pd
# Importing pandas has given us access to the DataFrame, accessible as pd.DataFrame
pd.DataFrame
###Output
_____no_output_____
###Markdown
Question:What is the type of `pd`? Guess before you run the code below.
###Code
type(pd)
###Output
_____no_output_____
###Markdown
Third-party packages unlock a huge range of functionality that isn't available in native Python; much of Python's data science capabilities come from a handful of packages outside the standard library: - pandas- numpy (numerical computing)- scikit-learn (modeling)- scipy (scientific computing)- matplotlib (graphing) We won't have time to touch on most of these in this training, but if you're interested in one, google it! Your Turn1. Import the `numpy` library, listed above. Give it the alias "np".2. Using autocomplete, determine what variable or function inside the numpy library starts with "asco". *Hint: remember you'll need to preface the variable name with the package alias, e.g. `np.asco`* Dot Notation with Packages We've seen it a few times already, but now it's time to discuss it explicitly:things inside packages can be accessed with *dot-notation*. Dot notation looks like this:```pythonpd.Series``` or```pythonimport numpy as npnp.array``` You can read this is "the `array` variable, within the Numpy library". Packages can contain pretty much anything that's legal in Python;if it's code, it can be in a package.This flexibility is part of the reason that Python's package ecosystem is so expansive and powerful. Functions As you may have noticed already, occasionally we run code using parentheses.The feature that permits this in Python is **functions** -- code snippets wrapped up into a single name. For example, take the `type` function we saw above.```pythontype(x)``` `type` does some complex things under the hood -- it looks at the variable inside the parentheses, determines what type of thing it is, and then returns that type to the user.
###Code
x = 7
type(x)
###Output
_____no_output_____
###Markdown
But the beauty of `type`, and of all functions, is that you (as the user) don't need to know all the complex code that's necessary to figure out that x is an `int` -- you just need to remember that there's a `type` function to do that for you. Functions make you much more powerful, as they unlock lots of functionality within a simple interface. ```python Get the first few rows of the planes data.planes.head()``` ```python Read in the planes.csv file.pd.read_csv('../data/planes.csv')``` The variables within the parens are called function arguments, or simply **arguments**.Above, the string `'../data/planes.csv'` is the argument to the `pd.read_csv` function. Functions are integral to using Python, because it's much more efficient to use pre-written code than to always write your own. If you ever do want to write your own function -- perhaps to share with others, or to make it easier to reuse your work -- it's fairly simple to do so, but beyond the scope of this training. Objects and Dot Notation Dot-notation, which we discussed in relation to packages, has another use -- accessing things inside of *objects*.What's an object? Basically, a variable that contains other data or functionality inside of it that is exposed to users. For example, DataFrames are objects.
###Code
df = pd.DataFrame({'first_name': ['Ethan', 'Brad'], 'last_name': ['Swan', 'Boehmke']})
df
df.shape
df.describe()
###Output
_____no_output_____
###Markdown
You can see that DataFrames have a `shape` variable and a `describe` function inside of them, both accessible through dot notation.Variables inside an object are often called *attributes* and functions inside objects are called *methods*. On Consistency and Language Design One of the great things about Python is that its creators really cared about internal consistency. What that means to us, as users, is that syntax is consistent and predictable -- even across different uses that would appear to be different at first. Dot notation reveals something kind of cool about Python: packages are just like other objects, and the variables inside them are just attributes and methods!This standardization across packages and objects helps us remember a single, intuitive syntax that works for many different things. Functions, Objects, and Methods in the Context of DataFrames As we saw above, DataFrames are a type of Python object, so let's use them to explore the new Python features we've learned. Using the `read_csv` function from the Pandas package to read in a DataFrame
###Code
df = pd.read_csv('../data/airlines.csv')
###Output
_____no_output_____
###Markdown
Using the `type` function to determine the type of `df`
###Code
type(df)
###Output
_____no_output_____
###Markdown
Using the `head` method of the DataFrame to view some of its rows
###Code
df.head()
###Output
_____no_output_____
###Markdown
Examining the `columns` attribute of the DataFrame to see the names of its columns.
###Code
df.columns
###Output
_____no_output_____
###Markdown
Inspecting the `shape` attribute to find the *dimensions* (rows and columns) of the DataFrame.
###Code
df.shape
###Output
_____no_output_____
###Markdown
Calling the `describe` method to get a summary of the data in the DataFrame.
###Code
df.describe()
###Output
_____no_output_____
###Markdown
Now let's combine them: using the `type` function to determine what `df.describe` holds.
###Code
type(df.describe)
###Output
_____no_output_____
###Markdown
Question:Does this result make sense? What would happen if you added parens? i.e. type(df.describe()) Your TurnSpend some time using autocomplete to explore the methods and attributes of the `df` object we used above.Remember from the Jupyter lesson that you can use a question mark to see the documentation for a function or method (e.g. `df.describe?`). Deeper Dive on DataFrames Now that we understand objects and functions better, let's look more at DataFrames. What Are DataFrames Made of? Accessing an individual column of a DataFrame can be done by passing the column name as a string, in brackets.
###Code
carrier_column = df['carrier']
carrier_column
###Output
_____no_output_____
###Markdown
Individual columns are pandas `Series` objects.
###Code
type(carrier_column)
###Output
_____no_output_____
###Markdown
How are Series different from DataFrames? - They're always 1-dimensional - They have different attributes than DataFrames - For example, Series have a `to_list` method -- which doesn't make sense to have on DataFrames - They don't print in the pretty format of DataFrames, but in plain text (see above)
###Code
carrier_column.shape
df.shape
carrier_column.to_list()
df.to_list()
###Output
_____no_output_____
###Markdown
It's important to be familiar with Series because they are fundamentally the core of DataFrames.Not only are columns represented as Series, but so are rows!
###Code
# Fetch the first row of the DataFrame
first_row = df.loc[0]
first_row
type(first_row)
###Output
_____no_output_____
###Markdown
Whenever you select individual columns or rows, you'll get Series objects. What Can You Do with a Series? First, let's create our own Series object from scratch -- they don't need to come from a DataFrame.
###Code
# Pass a list in as an argument and it will be converted to a Series.
s = pd.Series([10, 20, 30, 40, 50])
s
# Pass a list in as an argument and it will be converted to a Series.
s = pd.Series([10, 20, 30, 40, 50])
s
###Output
_____no_output_____
###Markdown
There are 3 things to notice about this Series: - The values (10, 20, 30...) - The *dtype*, short for data type. - The *index* (0, 1, 2...) ValuesValues are fairly self-explanatory; we chose them in our input list. dtypeData types are also straightforward. Series are always homogeneous, holding only integers, floats, or generic Python objects (called just `object`). Because a Python object is general enough to contain any other type, any Series holding strings or other non-numeric data will typically default to be of type `object`. For example, going back to our carriers DataFrame, note that the carrier column is of type `object`.
###Code
df['carrier']
###Output
_____no_output_____
###Markdown
IndexIndexes are more interesting.Every Series has an index, a way to reference each element.The index of a Series is a lot like the keys of a dictionary: each index element corresponds to a value in the Series, and can be used to look up that element.
###Code
# Our index is a range from 0 (inclusive) to 5 (exclusive).
s.index
s
s[3]
###Output
_____no_output_____
###Markdown
In our example, the index is just the integers 0-4, so right now it looks no different that referencing elements of a regular Python list.*But* indexes can be changed to something different -- like the letters a-e, for example.
###Code
s.index = ['a', 'b', 'c', 'd', 'e']
s
###Output
_____no_output_____
###Markdown
Now to look up the value 40, we reference `'d'`.
###Code
s['d']
###Output
_____no_output_____
###Markdown
We saw earlier that rows of a DataFrame are Series.In such cases, the flexibility of Series indexes comes in handy;the index is set to the DataFrame column names.
###Code
df.head()
# Note that the index is ['carrier', 'name']
first_row = df.loc[0]
first_row
###Output
_____no_output_____
###Markdown
This is particularly handy because it means you can extract individual elements based on a column name.
###Code
first_row['carrier']
###Output
_____no_output_____
###Markdown
DataFrame Indexes It's not just Series that have indexes!DataFrames have them too.Take a look at the carrier DataFrame again and note the bold numbers on the left.
###Code
df.head()
###Output
_____no_output_____
###Markdown
These numbers are an index, just like the one we saw on our example Series.And DataFrame indexes support similar functionality.
###Code
# Our index is a range from 0 (inclusive) to 16 (exclusive).
df.index
###Output
_____no_output_____
###Markdown
When loading in a DataFrame, the default index will always be 0 to N-1, where N is the number of rows in your DataFrame.This is called a `RangeIndex`. Selecting individual rows by their index is done with the `.loc` accessor.An *accessor* is an attribute designed specifically to help users reference something else (like rows within a DataFrame).
###Code
# Get the row at index 4 (the fifth row).
df.loc[4]
###Output
_____no_output_____
###Markdown
As with Series, DataFrames support reassigning their index. However, with DataFrames it often makes sense to change one of your columns into the index. This is analogous to a primary key in relational databases: a way to rapidly look up rows within a table. In our case, maybe we will often use the carrier code (`carrier`) to look up the full name of the airline.In that case, it would make sense set the carrier column as our index.
###Code
df = df.set_index('carrier')
df.head()
###Output
_____no_output_____
###Markdown
Now the RangeIndex has been replaced with a more meaningful index, and it's possible to look up rows of the table by passing carrier code to the `.loc` accessor.
###Code
df.loc['UA']
###Output
_____no_output_____
###Markdown
Caution:Pandas does not require that indexes have unique values (that is, no duplicates) although many relational databases do have that requirement of a primary key. This means that it is *possible* to create a non-unique index, but highly inadvisable. Having duplicate values in your index can cause unexpected results when you refer to rows by index -- but multiple rows have that index. Don't do it if you can help it! When starting to work with a DataFrame, it's often a good idea to determine what column makes sense as your index and to set it immediately. This will make your code nicer -- by letting you directly look up values with the index -- and also make your selections and filters faster, because Pandas is optimized for operations by index. If you want to change the index of your DataFrame later, you can always `reset_index` (and then assign a new one).
###Code
df.head()
df = df.reset_index()
df.head()
###Output
_____no_output_____
###Markdown
Your TurnThe below cell has code to load in the first 100 rows of the airports data as `airports`.The data contains the airport code, airport name, and some basic facts about the airport location.1. What kind of index is the current index of `airports`? 2. Is this a good choice for the DataFrame's index? If not, what column or columns would be a better candidate?3. If you chose a different column to be the index, make it your index using `airports.set_index()`.4. Using your new index, look up "Pittsburgh-Monroeville Airport", code 4G0. What is its altitude?5. Reset your index in case you want to make a different column your index in the future.
###Code
airports = pd.read_csv('../data/airports.csv')
airports = airports.loc[0:100]
airports.head()
###Output
_____no_output_____
###Markdown
Packages, Modules, Methods, and Functions > The Python source distribution has long maintained the philosophy of "batteries included" -- having a rich and versatile standard library which is immediately available, without making the user download separate packages. This gives the Python language a head start in many projects.>> \- PEP 206 Applied Review Python and Jupyter Overview - We're working with Python through Jupyter, the most common IDE for data science. Fundamentals - Python's common *atomic*, or basic, data types are: - Integers - Floats (decimals) - Strings - Booleans - These simple types can be combined to form more complex types, including: - Lists: Ordered collections - Dictionaries: Key-value pairs - DataFrames: Tabular datasets Packages (aka *Modules*) So far we've seen several data types that Python offers out-of-the-box.However, to keep things organized, some Python functionality is stored in standalone *packages*, or libraries of code.The word "module" is generally synonymous with package; you will hear both in discussions of Python. For example, functionality related to the operating system -- such as creating files and folders -- is stored in a package called `os`.To use the tools in `os`, we *import* the package.
###Code
import os
###Output
_____no_output_____
###Markdown
Once we import it, we gain access to everything inside.With Jupyter's autocomplete, we can view what's available.
###Code
# Move your cursor the end of the below line and press tab.
os.
###Output
_____no_output_____
###Markdown
Some packages, like `os`, are bundled with every Python install; downloading Python guarantees you'll have these packages.Collectively, this group of packages is known as the *standard library*. Other packages must be downloaded separately, either because- they aren't sufficiently popular to merit inclusion in the standard library- *or* they change too quickly for the maintainers of Python to keep up The DataFrame type that we saw earlier is part of the `pandas` package (short for *Panel Data*), one such package.Since pandas is specific to data science and is still rapidly evolving, it is not part of the standard library. We can download packages like pandas from the internet using a website called PyPI, the *Python Package Index*.Fortunately, since we are using Binder today, that has been handled for us and pandas is already installed. It's possible to import packages under an *alias*, or a nickname.The community has adopted certain conventions for aliases for common packages;while following them isn't mandatory, it's highly recommended, as it makes your code easier for others to understand. pandas is conventionally imported under the alias `pd`.
###Code
import pandas as pd
# Importing pandas has given us access to the DataFrame, accessible as pd.DataFrame
pd.DataFrame
###Output
_____no_output_____
###Markdown
Question:What is the type of `pd`? Guess before you run the code below.
###Code
type(pd)
###Output
_____no_output_____
###Markdown
Third-party packages unlock a huge range of functionality that isn't available in native Python; much of Python's data science capabilities come from a handful of packages outside the standard library: - pandas- numpy (numerical computing)- scikit-learn (modeling)- scipy (scientific computing)- matplotlib (graphing) We won't have time to touch on most of these in this training, but if you're interested in one, google it! Your Turn1. Import the `numpy` library, listed above. Give it the alias "np".2. Using autocomplete, determine what variable inside the numpy library starts with "eig". *Hint: remember you'll need to preface the variable name with the package alias, e.g. `np.eig`* Dot Notation with Packages We've seen it a few times already, but now it's time to discuss it explicitly:things inside packages can be accessed with *dot-notation*. Dot notation looks like this:```pythonpd.Series``` or```pythonimport numpy as npnp.array``` You can read this is "the `array` variable, within the Numpy library". Packages can contain pretty much anything that's legal in Python;if it's code, it can be in a package.This flexibility is part of the reason that Python's package ecosystem is so expansive and powerful. Functions As you may have noticed already, occasionally we run code using parentheses.The feature that permits this in Python is **functions** -- code snippets wrapped up into a single name. For example, take the `type` function we saw above.```pythontype(x)``` `type` does some complex things under the hood -- it looks at the variable inside the parentheses, determines what type of thing it is, and then returns that type to the user.
###Code
x = 7
type(x)
###Output
_____no_output_____
###Markdown
But the beauty of `type`, and of all functions, is that you (as the user) don't need to know all the complex code that's necessary to figure out that x is an `int` -- you just need to remember that there's a `type` function to do that for you. Functions make you much more powerful, as they unlock lots of functionality within a simple interface. ```python Get the first few rows of the planes data.planes.head()``` ```python Read in the planes.csv file.pd.read_csv('../data/planes.csv')``` The variables within the parens are called function arguments, or simply **arguments**.Above, the string `'../data/planes.csv'` is the argument to the `pd.read_csv` function. Functions are integral to using Python, because it's much more efficient to use pre-written code than to always write your own. If you ever do want to write your own function -- perhaps to share with others, or to make it easier to reuse your work -- it's fairly simple to do so, but beyond the scope of this training. Objects and Dot Notation Dot-notation, which we discussed in relation to packages, has another use -- accessing things inside of *objects*.What's an object? Basically, a variable that contains other data or functionality inside of it that is exposed to users. For example, DataFrames are objects.
###Code
df = pd.DataFrame({'first_name': ['Ethan', 'Mark'], 'last_name': ['Swan', 'Roepke']})
df
df.shape
df.describe()
###Output
_____no_output_____
###Markdown
You can see that DataFrames have a `shape` variable and a `describe` function inside of them, both accessible through dot notation.Variables inside an object are often called *attributes* and functions inside objects are called *methods*. On Consistency and Language Design One of the great things about Python is that its creators really cared about internal consistency. What that means to us, as users, is that syntax is consistent and predictable -- even across different uses that would appear to be different at first. Dot notation reveals something kind of cool about Python: packages are just like other objects, and the variables inside them are just attributes and methods!This standardization across packages and objects helps us remember a single, intuitive syntax that works for many different things. Functions, Objects, and Methods in the Context of DataFrames As we saw above, DataFrames are a type of Python object, so let's use them to explore the new Python features we've learned. Using the `read_csv` function from the Pandas package to read in a DataFrame
###Code
df = pd.read_csv('../data/airlines.csv')
###Output
_____no_output_____
###Markdown
Using the `type` function to determine the type of `df`
###Code
type(df)
###Output
_____no_output_____
###Markdown
Using the `head` method of the DataFrame to view some of its rows
###Code
df.head()
###Output
_____no_output_____
###Markdown
Examining the `columns` attribute of the DataFrame to see the names of its columns.
###Code
df.columns
###Output
_____no_output_____
###Markdown
Inspecting the `shape` attribute to find the *dimensions* (rows and columns) of the DataFrame.
###Code
df.shape
###Output
_____no_output_____
###Markdown
Calling the `describe` method to get a summary of the data in the DataFrame.
###Code
df.describe()
###Output
_____no_output_____
###Markdown
Now let's combine them: using the `type` function to determine what `df.describe` holds.
###Code
type(df.describe)
###Output
_____no_output_____
###Markdown
Question:Does this result make sense? What would happen if you added parens? i.e. type(df.describe()) Your TurnSpend some time using autocomplete to explore the methods and attributes of the `df` object we used above.Remember from the Jupyter lesson that you can use a question mark to see the documentation for a function or method (e.g. `df.describe?`). Deeper Dive on DataFrames Now that we understand objects and functions better, let's look more at DataFrames. What Are DataFrames Made of? Accessing an individual column of a DataFrame can be done by passing the column name as a string, in brackets.
###Code
carrier_column = df['carrier']
carrier_column
###Output
_____no_output_____
###Markdown
Individual columns are pandas `Series` objects.
###Code
type(carrier_column)
###Output
_____no_output_____
###Markdown
How are Series different from DataFrames? - They're always 1-dimensional - They have different attributes than DataFrames - For example, Series have a `to_list` method -- which doesn't make sense to have on DataFrames - They don't print in the pretty format of DataFrames, but in plain text (see above)
###Code
carrier_column.shape
df.shape
carrier_column.to_list()
df.to_list()
###Output
_____no_output_____
###Markdown
It's important to be familiar with Series because they are fundamentally the core of DataFrames.Not only are columns represented as Series, but so are rows!
###Code
# Fetch the first row of the DataFrame
first_row = df.loc[0]
first_row
type(first_row)
###Output
_____no_output_____
###Markdown
Whenever you select individual columns or rows, you'll get Series objects. What Can You Do with a Series? First, let's create our own Series object from scratch -- they don't need to come from a DataFrame.
###Code
# Pass a list in as an argument and it will be converted to a Series.
s = pd.Series([10, 20, 30, 40, 50])
s
# Pass a list in as an argument and it will be converted to a Series.
s = pd.Series([10, 20, 30, 40, 50])
s
###Output
_____no_output_____
###Markdown
There are 3 things to notice about this Series: - The values (10, 20, 30...) - The *dtype*, short for data type. - The *index* (0, 1, 2...) ValuesValues are fairly self-explanatory; we chose them in our input list. dtypeData types are also straightforward. Series are always homogeneous, holding only integers, floats, or generic Python objects (called just `object`). Because a Python object is general enough to contain any other type, any Series holding strings or other non-numeric data will typically default to be of type `object`. For example, going back to our carriers DataFrame, note that the carrier column is of type `object`.
###Code
df['carrier']
###Output
_____no_output_____
###Markdown
IndexIndexes are more interesting.Every Series has an index, a way to reference each element.The index of a Series is a lot like the keys of a dictionary: each index element corresponds to a value in the Series, and can be used to look up that element.
###Code
# Our index is a range from 0 (inclusive) to 5 (exclusive).
s.index
s
s[3]
###Output
_____no_output_____
###Markdown
In our example, the index is just the integers 0-4, so right now it looks no different that referencing elements of a regular Python list.*But* indexes can be changed to something different -- like the letters a-e, for example.
###Code
s.index = ['a', 'b', 'c', 'd', 'e']
s
###Output
_____no_output_____
###Markdown
Now to look up the value 40, we reference `'d'`.
###Code
s['d']
###Output
_____no_output_____
###Markdown
We saw earlier that rows of a DataFrame are Series.In such cases, the flexibility of Series indexes comes in handy;the index is set to the DataFrame column names.
###Code
df.head()
# Note that the index is ['carrier', 'name']
first_row = df.loc[0]
first_row
###Output
_____no_output_____
###Markdown
This is particularly handy because it means you can extract individual elements based on a column name.
###Code
first_row['carrier']
###Output
_____no_output_____
###Markdown
DataFrame Indexes It's not just Series that have indexes!DataFrames have them too.Take a look at the carrier DataFrame again and note the bold numbers on the left.
###Code
df.head()
###Output
_____no_output_____
###Markdown
These numbers are an index, just like the one we saw on our example Series.And DataFrame indexes support similar functionality.
###Code
# Our index is a range from 0 (inclusive) to 16 (exclusive).
df.index
###Output
_____no_output_____
###Markdown
When loading in a DataFrame, the default index will always be 0 to N-1, where N is the number of rows in your DataFrame.This is called a `RangeIndex`. Selecting individual rows by their index is done with the `.loc` accessor.An *accessor* is an attribute designed specifically to help users reference something else (like rows within a DataFrame).
###Code
# Get the row at index 4 (the fifth row).
df.loc[4]
###Output
_____no_output_____
###Markdown
As with Series, DataFrames support reassigning their index. However, with DataFrames it often makes sense to change one of your columns into the index. This is analogous to a primary key in relational databases: a way to rapidly look up rows within a table. In our case, maybe we will often use the carrier code (`carrier`) to look up the full name of the airline.In that case, it would make sense set the carrier column as our index.
###Code
df = df.set_index('carrier')
df.head()
###Output
_____no_output_____
###Markdown
Now the RangeIndex has been replaced with a more meaningful index, and it's possible to look up rows of the table by passing carrier code to the `.loc` accessor.
###Code
df.loc['UA']
###Output
_____no_output_____
###Markdown
Caution:Pandas does not require that indexes have unique values (that is, no duplicates) although many relational databases do have that requirement of a primary key. This means that it is *possible* to create a non-unique index, but highly inadvisable. Having duplicate values in your index can cause unexpected results when you refer to rows by index -- but multiple rows have that index. Don't do it if you can help it! When starting to work with a DataFrame, it's often a good idea to determine what column makes sense as your index and to set it immediately. This will make your code nicer -- by letting you directly look up values with the index -- and also make your selections and filters faster, because Pandas is optimized for operations by index. If you want to change the index of your DataFrame later, you can always `reset_index` (and then assign a new one).
###Code
df.head()
df = df.reset_index()
df.head()
###Output
_____no_output_____
###Markdown
Your TurnThe below cell has code to load in the first 100 rows of the airports data as `airports`.The data contains the airport code, airport name, and some basic facts about the airport location.1. What kind of index is the current index of `airports`? 2. Is this a good choice for the DataFrame's index? If not, what column or columns would be a better candidate?3. If you chose a different column to be the index, make it your index using `airports.set_index()`.4. Using your new index, look up "Pittsburgh-Monroeville Airport", code 4G0. What is its altitude?5. Reset your index in case you want to make a different column your index in the future.
###Code
airports = pd.read_csv('../data/airports.csv')
airports = airports.loc[0:100]
airports.head()
###Output
_____no_output_____
###Markdown
Packages, Modules, Methods, and Functions > The Python source distribution has long maintained the philosophy of "batteries included" -- having a rich and versatile standard library which is immediately available, without making the user download separate packages. This gives the Python language a head start in many projects.>> \- PEP 206 Applied Review Python and Jupyter Overview - We're working with Python through Jupyter, the most common IDE for data science. Fundamentals - Python's common *atomic*, or basic, data types are: - Integers - Floats (decimals) - Strings - Booleans - These simple types can be combined to form more complex types, including: - Lists: Ordered collections - Dictionaries: Key-value pairs - DataFrames: Tabular datasets Packages (aka *Modules*) So far we've seen several data types that Python offers out-of-the-box.However, to keep things organized, some Python functionality is stored in standalone *packages*, or libraries of code.The word "module" is generally synonymous with package; you will hear both in discussions of Python. For example, functionality related to the operating system -- such as creating files and folders -- is stored in a package called `os`.To use the tools in `os`, we *import* the package.
###Code
import os
###Output
_____no_output_____
###Markdown
Once we import it, we gain access to everything inside.With Jupyter's autocomplete, we can view what's available.
###Code
# Move your cursor the end of the below line and press tab.
os.
###Output
_____no_output_____
###Markdown
Some packages, like `os`, are bundled with every Python install; downloading Python guarantees you'll have these packages.Collectively, this group of packages is known as the *standard library*. Other packages must be downloaded separately, either because- they aren't sufficiently popular to merit inclusion in the standard library- *or* they change too quickly for the maintainers of Python to keep up The DataFrame type that we saw earlier is part of the `pandas` package (short for *Panel Data*), one such package.Since pandas is specific to data science and is still rapidly evolving, it is not part of the standard library. We can download packages like pandas from the internet using a website called PyPI, the *Python Package Index*.Fortunately, since we are using Binder today, that has been handled for us and pandas is already installed. It's possible to import packages under an *alias*, or a nickname.The community has adopted certain conventions for aliases for common packages;while following them isn't mandatory, it's highly recommended, as it makes your code easier for others to understand. pandas is conventionally imported under the alias `pd`.
###Code
import pandas as pd
# Importing pandas has given us access to the DataFrame, accessible as pd.DataFrame
pd.DataFrame
###Output
_____no_output_____
###Markdown
Question:What is the type of `pd`? Guess before you run the code below.
###Code
type(pd)
###Output
_____no_output_____
###Markdown
Third-party packages unlock a huge range of functionality that isn't available in native Python; much of Python's data science capabilities come from a handful of packages outside the standard library: - pandas- numpy (numerical computing)- scikit-learn (modeling)- scipy (scientific computing)- matplotlib (graphing) We won't have time to touch on most of these in this training, but if you're interested in one, google it! Your Turn1. Import the `numpy` library, listed above. Give it the alias "np".2. Using autocomplete, determine what variable inside the numpy library starts with "asco". *Hint: remember you'll need to preface the variable name with the package alias, e.g. `np.asco`* Dot Notation with Packages We've seen it a few times already, but now it's time to discuss it explicitly:things inside packages can be accessed with *dot-notation*. Dot notation looks like this:```pythonpd.Series``` or```pythonimport numpy as npnp.array``` You can read this is "the `array` variable, within the Numpy library". Packages can contain pretty much anything that's legal in Python;if it's code, it can be in a package.This flexibility is part of the reason that Python's package ecosystem is so expansive and powerful. Functions As you may have noticed already, occasionally we run code using parentheses.The feature that permits this in Python is **functions** -- code snippets wrapped up into a single name. For example, take the `type` function we saw above.```pythontype(x)``` `type` does some complex things under the hood -- it looks at the variable inside the parentheses, determines what type of thing it is, and then returns that type to the user.
###Code
x = 7
type(x)
###Output
_____no_output_____
###Markdown
But the beauty of `type`, and of all functions, is that you (as the user) don't need to know all the complex code that's necessary to figure out that x is an `int` -- you just need to remember that there's a `type` function to do that for you. Functions make you much more powerful, as they unlock lots of functionality within a simple interface. ```python Get the first few rows of the planes data.planes.head()``` ```python Read in the planes.csv file.pd.read_csv('../data/planes.csv')``` The variables within the parens are called function arguments, or simply **arguments**.Above, the string `'../data/planes.csv'` is the argument to the `pd.read_csv` function. Functions are integral to using Python, because it's much more efficient to use pre-written code than to always write your own. If you ever do want to write your own function -- perhaps to share with others, or to make it easier to reuse your work -- it's fairly simple to do so, but beyond the scope of this training. Objects and Dot Notation Dot-notation, which we discussed in relation to packages, has another use -- accessing things inside of *objects*.What's an object? Basically, a variable that contains other data or functionality inside of it that is exposed to users. For example, DataFrames are objects.
###Code
df = pd.DataFrame({'first_name': ['Ethan', 'Mark'], 'last_name': ['Swan', 'Roepke']})
df
df.shape
df.describe()
###Output
_____no_output_____
###Markdown
You can see that DataFrames have a `shape` variable and a `describe` function inside of them, both accessible through dot notation.Variables inside an object are often called *attributes* and functions inside objects are called *methods*. On Consistency and Language Design One of the great things about Python is that its creators really cared about internal consistency. What that means to us, as users, is that syntax is consistent and predictable -- even across different uses that would appear to be different at first. Dot notation reveals something kind of cool about Python: packages are just like other objects, and the variables inside them are just attributes and methods!This standardization across packages and objects helps us remember a single, intuitive syntax that works for many different things. Functions, Objects, and Methods in the Context of DataFrames As we saw above, DataFrames are a type of Python object, so let's use them to explore the new Python features we've learned. Using the `read_csv` function from the Pandas package to read in a DataFrame
###Code
df = pd.read_csv('../data/airlines.csv')
###Output
_____no_output_____
###Markdown
Using the `type` function to determine the type of `df`
###Code
type(df)
###Output
_____no_output_____
###Markdown
Using the `head` method of the DataFrame to view some of its rows
###Code
df.head()
###Output
_____no_output_____
###Markdown
Examining the `columns` attribute of the DataFrame to see the names of its columns.
###Code
df.columns
###Output
_____no_output_____
###Markdown
Inspecting the `shape` attribute to find the *dimensions* (rows and columns) of the DataFrame.
###Code
df.shape
###Output
_____no_output_____
###Markdown
Calling the `describe` method to get a summary of the data in the DataFrame.
###Code
df.describe()
###Output
_____no_output_____
###Markdown
Now let's combine them: using the `type` function to determine what `df.describe` holds.
###Code
type(df.describe)
###Output
_____no_output_____
###Markdown
Question:Does this result make sense? What would happen if you added parens? i.e. type(df.describe()) Your TurnSpend some time using autocomplete to explore the methods and attributes of the `df` object we used above.Remember from the Jupyter lesson that you can use a question mark to see the documentation for a function or method (e.g. `df.describe?`). Deeper Dive on DataFrames Now that we understand objects and functions better, let's look more at DataFrames. What Are DataFrames Made of? Accessing an individual column of a DataFrame can be done by passing the column name as a string, in brackets.
###Code
carrier_column = df['carrier']
carrier_column
###Output
_____no_output_____
###Markdown
Individual columns are pandas `Series` objects.
###Code
type(carrier_column)
###Output
_____no_output_____
###Markdown
How are Series different from DataFrames? - They're always 1-dimensional - They have different attributes than DataFrames - For example, Series have a `to_list` method -- which doesn't make sense to have on DataFrames - They don't print in the pretty format of DataFrames, but in plain text (see above)
###Code
carrier_column.shape
df.shape
carrier_column.to_list()
df.to_list()
###Output
_____no_output_____
###Markdown
It's important to be familiar with Series because they are fundamentally the core of DataFrames.Not only are columns represented as Series, but so are rows!
###Code
# Fetch the first row of the DataFrame
first_row = df.loc[0]
first_row
type(first_row)
###Output
_____no_output_____
###Markdown
Whenever you select individual columns or rows, you'll get Series objects. What Can You Do with a Series? First, let's create our own Series object from scratch -- they don't need to come from a DataFrame.
###Code
# Pass a list in as an argument and it will be converted to a Series.
s = pd.Series([10, 20, 30, 40, 50])
s
# Pass a list in as an argument and it will be converted to a Series.
s = pd.Series([10, 20, 30, 40, 50])
s
###Output
_____no_output_____
###Markdown
There are 3 things to notice about this Series: - The values (10, 20, 30...) - The *dtype*, short for data type. - The *index* (0, 1, 2...) ValuesValues are fairly self-explanatory; we chose them in our input list. dtypeData types are also straightforward. Series are always homogeneous, holding only integers, floats, or generic Python objects (called just `object`). Because a Python object is general enough to contain any other type, any Series holding strings or other non-numeric data will typically default to be of type `object`. For example, going back to our carriers DataFrame, note that the carrier column is of type `object`.
###Code
df['carrier']
###Output
_____no_output_____
###Markdown
IndexIndexes are more interesting.Every Series has an index, a way to reference each element.The index of a Series is a lot like the keys of a dictionary: each index element corresponds to a value in the Series, and can be used to look up that element.
###Code
# Our index is a range from 0 (inclusive) to 5 (exclusive).
s.index
s
s[3]
###Output
_____no_output_____
###Markdown
In our example, the index is just the integers 0-4, so right now it looks no different that referencing elements of a regular Python list.*But* indexes can be changed to something different -- like the letters a-e, for example.
###Code
s.index = ['a', 'b', 'c', 'd', 'e']
s
###Output
_____no_output_____
###Markdown
Now to look up the value 40, we reference `'d'`.
###Code
s['d']
###Output
_____no_output_____
###Markdown
We saw earlier that rows of a DataFrame are Series.In such cases, the flexibility of Series indexes comes in handy;the index is set to the DataFrame column names.
###Code
df.head()
# Note that the index is ['carrier', 'name']
first_row = df.loc[0]
first_row
###Output
_____no_output_____
###Markdown
This is particularly handy because it means you can extract individual elements based on a column name.
###Code
first_row['carrier']
###Output
_____no_output_____
###Markdown
DataFrame Indexes It's not just Series that have indexes!DataFrames have them too.Take a look at the carrier DataFrame again and note the bold numbers on the left.
###Code
df.head()
###Output
_____no_output_____
###Markdown
These numbers are an index, just like the one we saw on our example Series.And DataFrame indexes support similar functionality.
###Code
# Our index is a range from 0 (inclusive) to 16 (exclusive).
df.index
###Output
_____no_output_____
###Markdown
When loading in a DataFrame, the default index will always be 0 to N-1, where N is the number of rows in your DataFrame.This is called a `RangeIndex`. Selecting individual rows by their index is done with the `.loc` accessor.An *accessor* is an attribute designed specifically to help users reference something else (like rows within a DataFrame).
###Code
# Get the row at index 4 (the fifth row).
df.loc[4]
###Output
_____no_output_____
###Markdown
As with Series, DataFrames support reassigning their index. However, with DataFrames it often makes sense to change one of your columns into the index. This is analogous to a primary key in relational databases: a way to rapidly look up rows within a table. In our case, maybe we will often use the carrier code (`carrier`) to look up the full name of the airline.In that case, it would make sense set the carrier column as our index.
###Code
df = df.set_index('carrier')
df.head()
###Output
_____no_output_____
###Markdown
Now the RangeIndex has been replaced with a more meaningful index, and it's possible to look up rows of the table by passing carrier code to the `.loc` accessor.
###Code
df.loc['UA']
###Output
_____no_output_____
###Markdown
Caution:Pandas does not require that indexes have unique values (that is, no duplicates) although many relational databases do have that requirement of a primary key. This means that it is *possible* to create a non-unique index, but highly inadvisable. Having duplicate values in your index can cause unexpected results when you refer to rows by index -- but multiple rows have that index. Don't do it if you can help it! When starting to work with a DataFrame, it's often a good idea to determine what column makes sense as your index and to set it immediately. This will make your code nicer -- by letting you directly look up values with the index -- and also make your selections and filters faster, because Pandas is optimized for operations by index. If you want to change the index of your DataFrame later, you can always `reset_index` (and then assign a new one).
###Code
df.head()
df = df.reset_index()
df.head()
###Output
_____no_output_____
###Markdown
Your TurnThe below cell has code to load in the first 100 rows of the airports data as `airports`.The data contains the airport code, airport name, and some basic facts about the airport location.1. What kind of index is the current index of `airports`? 2. Is this a good choice for the DataFrame's index? If not, what column or columns would be a better candidate?3. If you chose a different column to be the index, make it your index using `airports.set_index()`.4. Using your new index, look up "Pittsburgh-Monroeville Airport", code 4G0. What is its altitude?5. Reset your index in case you want to make a different column your index in the future.
###Code
airports = pd.read_csv('../data/airports.csv')
airports = airports.loc[0:100]
airports.head()
###Output
_____no_output_____ |
get_related_categories_online.ipynb | ###Markdown
Get all categories from various online websites. This data can be used for categorization in machine learning
###Code
import requests
from time import sleep
import random
import json
import pprint as pp
url = "http://developer.shopclues.com/api/v1/category"
headers = {
'authorization': "Bearer 11111112172617652167452716",
'content-type': "application/json",
'cache-control': "no-cache"
}
visited = {}
unvisited = {}
while True:
response = requests.request("GET", url, headers=headers)
if response.status_code == 200 and not isinstance(json.loads(response.text)['data'], basestring):
data = json.loads(response.text)
break
for cat in data['data']:
if cat['is_leaf_category'] == 'N':
unvisited.update({
cat["category_id"]: {
"name":cat['category'],
"parent":0
}
})
else:
visited.update({
cat["category_id"]: {
"name":cat['category'],
"parent":0
}
})
print(len(visited))
print(len(unvisited))
while unvisited:
unv = unvisited.keys()[0]
print(unv)
while True:
sleep_time = random.randint(1, 2000)
querystring = {"category_id":unv}
response = requests.request("GET", url, headers=headers, params=querystring)
sleep(sleep_time/1000)
if response.status_code == 200 and not isinstance(json.loads(response.text)['data'], basestring):
break
print("status " + str(response.status_code))
data = json.loads(response.text)
for cat in data['data']:
if cat['is_leaf_category'] == 'N':
unvisited.update({
cat["category_id"]: {
"name":cat['category'],
"parent":int(unv)
}
})
else:
visited.update({
cat["category_id"]: {
"name":cat['category'],
"parent":int(unv)
}
})
visited.update({unv:unvisited.get(unv)})
del unvisited[unv]
print(str(len(visited)) + " " + str(len(unvisited)))
#pp.pprint(visited)
#pp.pprint(unvisited)
list_categories = []
for x in visited:
parent_id = str(visited[x]['parent'])
parent_cat = ""
while parent_id != '0' and parent_id in visited:
parent_cat = str(visited[parent_id]['name']) + " > " + parent_cat
parent_id = str(visited[parent_id]['parent'])
list_categories.append([
x,
visited[x]['name'],
parent_cat,
visited[x]['parent']
])
import csv
myfile = open("sclues_categories_"+str(random.randint(1, 2000))+".csv", 'wb')
wr = csv.writer(myfile, quoting=csv.QUOTE_ALL)
wr.writerows(list_categories)
###Output
_____no_output_____ |
Pfizer_FamaFrench3_Model.ipynb | ###Markdown
Pzifer Stock Analysis Fama French 3 Factor Model The Fama and French model has three factors: size of firms, book-to-market values and excess return on the market. In other words, the three factors used are SMB (small minus big), HML (high minus low) and the portfolio's return less the risk free rate of return.
###Code
#Importing Packages
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
import scipy.stats as ss
from sklearn.metrics import mean_squared_error
from sklearn.metrics import r2_score
df_pfe = pd.read_csv ('pfe_fama3_new.csv')
df_pfe
df_pfe.shape
df_pfe['stock_return'] = (df_pfe['Adj Close']-df_pfe['Yest_Close'])/(df_pfe['Yest_Close'])
T = df_pfe.shape[0]
Y = df_pfe['stock_return'].values
columns = ['MktRF','SMB','HML']
X=df_pfe[columns]
X = np.column_stack([np.ones((len(X),1)),X])
N = X.shape
Y=np.asarray(Y)
Y = Y.reshape(T,1)
'REGRESSION STARTS:'
'Linear Regression of Y: T x 1 on'
'Regressors X: T x N'
invXX = np.linalg.inv(X.transpose()@X)
'OLS estimator beta: N x 1'
beta_hat = [email protected]()@Y
'Predictive value of Y_t using OLS'
y_hat = X@beta_hat;
'Residuals from OLS: Y - X*beta'
residuals = Y - y_hat;
'variance of Y_t or residuals'
sigma2 = (1/T)*(residuals.transpose()@residuals)
'standard deviation of Y_t or residuals'
sig = np.sqrt(sigma2)
'variance-covariance matrix of beta_hat'
'N x N: on-diagnal variance(beta_j)'
'N x N: off-diagnal cov(beta_i, beta_j)'
varcov_beta_hat = (sigma2)*invXX
var_beta_hat = np.sqrt(T*np.diag(varcov_beta_hat))
'Calculate R-square'
R_square = 1 - residuals.transpose()@residuals/(T*np.var(Y))
adj_R_square = 1-(1-R_square)*(T-1)/(T-N[1])
'Test Each Coefficient: beta_i'
't-test stat: N x 1'
t_stat = beta_hat.transpose()/var_beta_hat
' t-test significance level: N x 1'
p_val_t = 1-ss.norm.cdf(t_stat)
'Test of Joint Significance of Model'
F_stat = beta_hat.transpose()@varcov_beta_hat@beta_hat/\
(residuals.transpose()@residuals)
'size: (1 x N)*(N x N)*(N x 1)/((1 x T) * (T x 1)) = 1 x 1'
p_val_F = 1-ss.f.cdf(F_stat,N[1]-1,T-N[1])
print(' REGRESSION STATISTICS \n')
print('------------------------\n')
print('\n Joint significance of all coefficients\n',[F_stat,p_val_F])
print('Beta Values \n',beta_hat)
print('P values \n',p_val_t)
print('R-Square is \n',R_square)
print('Adjusted R Square \n',adj_R_square)
print('Standard Error \n',sig)
print('Observations \n',T)
print('-------------------------\n')
plt.plot(y_hat, color='blue')
plt.plot(Y, color = 'red')
plt.show()
pred = pd.DataFrame(y_hat)
act = pd.DataFrame(Y)
plot_df = pd.DataFrame({"actual": act[0], "predictions": pred[0]})
plot_df.plot(figsize=(20, 6), title='Predictions using FF3 using Linear Regression')
mse = mean_squared_error(Y,y_hat)
rmse = np.sqrt(mse)
print('RMSE-------',rmse)
print('R-Squared--',r2_score(Y,y_hat))
###Output
RMSE------- 0.008998722544327587
R-Squared-- 0.3501952868736381
|
examples/notebooks/consumption_savings_misperceived_rho.ipynb | ###Markdown
Over-persistent Bias in Income Expectation and Consumption - Tao Wang and Julien Acalin - Topics of Advanced Macroeconomics by Professor Chris Carroll What does this notebook do?- We replicate this [notebook](https://github.com/econ-ark/DemARK/blob/master/notebooks/IncExpectationExample.ipynb) using dolo. The original notebook used HARK to explore the implications for a consumption saving problem with AR1 income process when the agents perceive the permanent income more persistent than actual income process.
###Code
from dolo import *
import matplotlib.pyplot as plt
import seaborn
from matplotlib import pyplot as plt
model = yaml_import("../models/consumption_savings_persistent_income.yaml")
###Output
_____no_output_____
###Markdown
To undertake the thought experiment, we first solve the decision rule of an agent the perceived persistence parameter. Then we simulate the model using the income process according to the actual persistence parameter. To make the comparison, we also compare the decision rules with different beliefs.
###Code
CorrAct = 0.92 # actual rho
CorrPcvd =0.94 # perceived rho
CorrPcvd1 =0.90 # underperceived rho
#### We first solve the decision rule using perceived $\rho$ greater than actual
model.exogenous.rho[0][0]=CorrPcvd # set rho to be corrPcvd
drPcvd = time_iteration(model) # solve the decision rule based on perceived rho
tabPcvd = tabulate(model, drPcvd,'w') ## tabulate the decision rules in two scenarios
#### We also solve the decision rule using perceived $\rho$ smaller than actual
model.exogenous.rho[0][0]=CorrPcvd1 # set rho to be corrPcvd
drPcvd1 = time_iteration(model) # solve the decision rule based on perceived rho
tabPcvd1 = tabulate(model, drPcvd1,'w') ## tabulate the decision rules in two scenarios
#### then we also solve the decision rule using the actual $\rho$
model.exogenous.rho[0][0]=CorrAct # set rho to be the actual
drRE = time_iteration(model) # solve the decision rule under rational expectation
tabRE = tabulate(model, drRE,'w') ## tabulate the decision rules in two scenarios
###Output
Solving WITH complementarities.
------------------------------------------------
| N | Error | Gain | Time | nit |
------------------------------------------------
| 1 | 7.000e-01 | nan | 0.073 | 5 |
###Markdown
Comparing the Decision Rules under Misperception and Rational Expectation
###Code
## plot the decision rule
#stable_wealth = model.eval_formula('1/r+(1-1/r)*w(0)', tab)
#plt.plot(tab['w'], tab['w'],'k--')
#plt.plot(tab['w'], stable_wealth,'k--)
plt.plot(tabPcvd['w'], tabPcvd['c'],label=r'$\rho={}$'.format(CorrPcvd))
plt.plot(tabPcvd1['w'], tabPcvd1['c'],label=r'$\rho={}$'.format(CorrPcvd1))
plt.plot(tabRE['w'], tabRE['c'],label=r'$\rho={}$'.format(CorrAct))
plt.xlabel(r"$w_t$")
plt.ylabel(r"$c_t$")
plt.legend(loc=0)
plt.grid()
###Output
_____no_output_____
###Markdown
Why does higehr perceived persistence lead to lower consumption?This is due to precautionary saving motive. We know for any AR1 process $y_t=\rho y_{t-1} +\epsilon_t$, the unconditional variance$$Var(y) = \frac{\sigma^2_\epsilon}{1-\rho^2}$$Higher $\rho$, higher serial correlation of income leads to higher variance of income. This increases precautionary saving for given wealth. Simulation We now simulate the model using the decision rule under perceived income process, but with the actual income process.
###Code
## We have set the model back to actual rho, let's check
model.exogenous.rho[0][0]
## parameters for simulation
n_sim = 10
T_sim=100
## simulation
dp = model.exogenous.discretize() ## discretize is to generate income shocks by the exogeous process
sim_shock = dp.simulate(n_sim, T_sim, i0=1)
# Plot the simulated ture income shocks
for i in range(n_sim):
plt.plot(sim_shock[:,i,0], color='red', alpha=0.5)
plt.title('Simulated Income Process under Actual Parameter')
## now simulate with different decision rules
sim = simulate(model, drPcvd, i0=1, N=100) # simulate with the actual
# process but using the decision rule under misperception
sim_long = simulate(model, drPcvd, i0=1, N=1000, T=200) # simulate with the actual
# process but using the decision rule under misperception
sim_long1 = simulate(model, drPcvd1, i0=1, N=1000, T=200) # simulate with the actual
# process but using the decision rule under misperception
sim_long_RE = simulate(model, drRE, i0=1, N=1000, T=200) # simulate with the actual process
# and rational expectation rule
###Output
_____no_output_____
###Markdown
Simulated wealth distribution We care about the implication of the misperception on the wealth distribution.
###Code
seaborn.distplot(sim_long.sel(T=199, V='w'),color='b',label=r'Perceived $\rho$>actual')
seaborn.distplot(sim_long1.sel(T=199, V='w'),color='r',label=r'Perceived $\rho$<actual')
seaborn.distplot(sim_long_RE.sel(T=199, V='w'), color='y',label='Rational Expectation')
plt.xlabel(r"$w$")
plt.legend(loc=0)
###Output
[33mFutureWarning[0m:/Users/Myworld/anaconda3/lib/python3.7/site-packages/scipy/stats/stats.py:1713
Using a non-tuple sequence for multidimensional indexing is deprecated; use `arr[tuple(seq)]` instead of `arr[seq]`. In the future this will be interpreted as an array index, `arr[np.array(seq)]`, which will result either in an error or a different result.
|
src/notebooks/46-add-text-annotation-on-scatterplot.ipynb | ###Markdown
Welcome in the introductory template of the python graph gallery. Here is how to proceed to add a new `.ipynb` file that will be converted to a blogpost in the gallery! Notebook Metadata It is very important to add the following fields to your notebook. It helps building the page later on:- **slug**: the URL of the blogPost. It should be exactly the same as the file title. Example: `70-basic-density-plot-with-seaborn`- **chartType**: the chart type like density or heatmap. For a complete list see [here](https://github.com/holtzy/The-Python-Graph-Gallery/blob/master/src/util/sectionDescriptions.js), it must be one of the `id` options.- **title**: what will be written in big on top of the blogpost! use html syntax there.- **description**: what will be written just below the title, centered text.- **keyword**: list of keywords related with the blogpost- **seoDescription**: a description for the bloppost meta. Should be a bit shorter than the description and must not contain any html syntax. Add a chart description A chart example always come with some explanation. It must:contain keywordslink to related pages like the parent page (graph section)give explanations. In depth for complicated charts. High level for beginner level charts Add a chart
###Code
import seaborn as sns, numpy as np
np.random.seed(0)
x = np.random.randn(100)
ax = sns.distplot(x)
###Output
_____no_output_____
###Markdown
Basic scatterplot You can create a [basic scatterplot](http://python-graph-gallery.com/40-basic-scatterplot-seaborn/) using `regplot()` function of [seaborn](http://python-graph-gallery.com/seaborn/) library. The following parameters should be provided:* `data` : dataset* `x` : positions of points on the X axis* `y` : positions of points on the Y axis* `fit_reg` : if True, show the linear regression fit line* `marker` : marker shape* `color` : the color of markers
###Code
import pandas as pd
import numpy as np
import matplotlib.pylab as plt
import seaborn as sns
# Create dataframe
df = pd.DataFrame({
'x': [1, 1.5, 3, 4, 5],
'y': [5, 15, 5, 10, 2],
'group': ['A','other group','B','C','D']
})
sns.regplot(data=df, x="x", y="y", fit_reg=False, marker="+", color="skyblue")
plt.show()
###Output
_____no_output_____
###Markdown
Add one annotation Once you have created the dataset and plotted the scatterplot with the previous code, you can use `text()` function of matplotlib to add annotation. The following parameters should be provided:* `x` : the position to place the text in x axis* `y` : the position to place the text in y axis* `s`: the textYou can also specify the additional parameters such as `horizontalalignment`, `size`, `color`, `weight` to design your text.
###Code
# basic plot
sns.regplot(data=df, x="x", y="y", fit_reg=False, marker="o", color="skyblue", scatter_kws={'s':400})
# add text annotation
plt.text(3+0.2, 4.5, "An annotation", horizontalalignment='left', size='medium', color='black', weight='semibold')
plt.show()
###Output
_____no_output_____
###Markdown
Use a loop to annotate each marker If you want to annotate every markers, it is practical to use a loop as follow:
###Code
# basic plot
sns.regplot(data=df, x="x", y="y", fit_reg=False, marker="o", color="skyblue", scatter_kws={'s':400})
# add annotations one by one with a loop
for line in range(0,df.shape[0]):
plt.text(df.x[line]+0.2, df.y[line], df.group[line], horizontalalignment='left', size='medium', color='black', weight='semibold')
plt.show()
###Output
_____no_output_____ |
teaching_material/session_14/Introduction_to_Social_Data_Science_Text_as_Data.ipynb | ###Markdown
Session 14: Text data Introduced by Terne Sasha Thorn JakobsenWe introduce the concept of **Text as Data**, and apply our newly acquired knowledge of supervised learning to a text classification problem. Lecture videosView the videos and follow along in this notebook, which I go through in the videos!Part 1, Intro and preprocessing: https://youtu.be/Gv7IU18hbXwPart 2, Lexicons for sentiment analysis: https://youtu.be/B_5k_QkCHLI Part 3, Feature extraction: https://youtu.be/HV3Vl-P3kqs Part 4, Text classification: https://www.youtube.com/watch?v=0RYmNQwnhOE&feature=youtu.be Required readings - PML: following sections from chapter 8: - Preparing the IMDb movie review data for text processing - Introducing the bag-of-words model - Training a logistic regression model for document classification Gentzkow, M., Kelly, B.T. and Taddy, M., 2017. ["Text as data"](http://www.nber.org/papers/w23276.pdf) (No. w23276). *National Bureau of Economic Research*.Jurafsky, D., & Martin, J. H. (2019). Vector Semantics and Embeddings. Speech and Language Processing, 3rd ed. draft. https://web.stanford.edu/~jurafsky/slp3/6.pdf*(PML) Raschka, Sebastian, and Mirjalili, Vahid. Python Machine Learning - Second Edition. Birmingham: Packt Publishing, Limited, 2017. Chapter 8* Inspirational readingsGorrell, Genevieve et al. “Twits, Twats and Twaddle: Trends in Online Abuse towards UK Politicians.” ICWSM (2018). https://gate-socmedia.group.shef.ac.uk/wp-content/uploads/2019/07/Gorrell-Greenwood.pdfPang, Bo et al. “Thumbs up? Sentiment Classification using Machine Learning Techniques.” EMNLP (2002). https://www.aclweb.org/anthology/W02-1011.pdf Course page: https://abjer.github.io/isds2020 MotivationWhy work with text data... Huge amount of data! May teach us about:- how we communicate with eachother- public opinions (e.g. towards politicians, reflecting who we are going to vote for)- general knowledge: we can extract information and knowledge from documents, and we can summarize information from large corpuses to just a few lines of text if needed. **Agenda**- Preprocessing text- Lexicons- Feature extraction- Text classification Import packages
###Code
!pip install pyprind
!pip install afinn
!pip install vaderSentiment
# from the textbook, for printing a process bar.
import pyprind
# basic packages
import pandas as pd
import os
import numpy as np
import re # python regular expressions
import string # for efficient operations with strings
import matplotlib.pyplot as plt
%matplotlib inline
# NLTK: A basic, popular NLP package. Find many examples of applications at https://www.nltk.org/book/
# Install guide: https://www.nltk.org/install.html
import nltk
nltk.download('punkt') # you will probably need to do this
nltk.download('wordnet') # and this
nltk.download('stopwords') # aand this
nltk.download('sentiwordnet')
# for vectorization
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.feature_extraction.text import TfidfTransformer
#Vader Lexicon for sentiment analysis
from vaderSentiment.vaderSentiment import SentimentIntensityAnalyzer
# similarity/distance measures
from scipy.spatial import distance
from sklearn.metrics.pairwise import linear_kernel
# for classification
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import confusion_matrix
from sklearn.model_selection import GridSearchCV
from sklearn.pipeline import Pipeline
# Lexicons for sentiment analysis
from vaderSentiment import vaderSentiment
from afinn import Afinn
# to display images in notebook
from IPython.display import Image
###Output
_____no_output_____
###Markdown
Preparing data (following PML chapter 8) Data source:Andrew L. Maas, Raymond E. Daly, Peter T. Pham, Dan Huang, Andrew Y. Ng, and Christopher Potts. (2011). Learning Word Vectors for Sentiment Analysis. The 49th Annual Meeting of the Association for Computational Linguistics (ACL 2011).Download from http://ai.stanford.edu/~amaas/data/sentiment/. (just click the link and put the files in the same folder as the notebook).If you are a mac user you can also just:
###Code
# download the data with wget (for mac users. Windows users just click the link above)
!wget http://ai.stanford.edu/~amaas/data/sentiment/aclImdb_v1.tar.gz
# unpack
import tarfile
with tarfile.open("aclImdb_v1.tar.gz", "r:gz") as tar:
tar.extractall()
# load data into a pandas DataFrame
basepath = "aclImdb"
labels = {"pos":1, "neg":0}
pbar = pyprind.ProgBar(50000)
df = pd.DataFrame()
for s in ("test", "train"):
for l in ("pos", "neg"):
path = os.path.join(basepath,s,l)
for file in sorted(os.listdir(path)):
with open(os.path.join(path,file), "r", encoding="utf-8") as infile:
txt = infile.read()
# Here I also append s ("train" or "test") to later use the predefined
# split of the data. They didn't do this in the textbook.
df = df.append([[txt, labels[l], s]], ignore_index=True)
pbar.update()
df.columns = ["review", "sentiment", "set"]
df # look at the dataframe
# dividing into train and test set again.
df_train = df[df.set=="train"]
df_test = df[df.set=="test"]
# Permute data such that pos and neg samples are mixed
np.random.seed(0)
df_train = df.reindex(np.random.permutation(df_train.index))
df_test = df.reindex(np.random.permutation(df_test.index))
# save dataframes:
df_train.to_csv("train.csv", index=False)
df_test.to_csv("test.csv", index=False)
# load saved dataframes:
df_train = pd.read_csv("train.csv")
df_test = pd.read_csv("test.csv")
df_train.head()
###Output
_____no_output_____
###Markdown
Preprocessing herein text normalization (i.e. standardizing the text format) Tokenization (segmentation)
###Code
sentence = df_train.review.values[0]
print(sentence)
# word tokenization (segmentation)
# by blank space (crude)
sent = sentence.split(" ")
print(sent)
# by using a package such as NLTK.
sent = nltk.tokenize.word_tokenize(sentence)
print()
print(" NLTK word tokenization: ", sent)
# nltk.tokenize.TweetTokenizer() is another option, and good for social media text.
# Sentence segmentation (sentence splitting)
sents = nltk.tokenize.sent_tokenize(sentence)
print()
print("Sent tokenization", sents)
# There are many other more complex versions used for specific cases/tasks/models and sometime you may want to costumize the segmentation.
# Learn more: https://web.stanford.edu/~jurafsky/slp3/2.pdf
###Output
_____no_output_____
###Markdown
Stemming or lemmatising **Stemming**: Stripping the word down to it's central meaning (it's stem) by removing affixes. Stemming usually only removes suffixes (i.e. affixes at the end of the words).E.g.: sings --> singlaughing --> laughwonderful --> wonder**Lemmatizing**: "the task of determining that two words have the same root, despite their surface differences. For example, the words sang, sung, and sings are forms of the verb sing. The word sing is the common lemma of these words, and a lemmatizer maps from all of these to sing." (Jurafsky, D., & Martin, J. H., 2019. Chap. 2, p. 2)
###Code
def stem(word):
# find suffixes and return stems
# (.*?) matches any character in front of a word (non greedy version)
regexp = r'^(.*?)(ing|ly|ed|ious|ies|ive|es|s|ment)?$'
stem, suffix = re.findall(regexp, word)[0]
return stem
# Tokenize the text first
tokens = nltk.word_tokenize(sentence)
#print(tokens)
print([stem(t) for t in tokens]) # hmm not so good
# using NLTK's Porter stemmer
porter = nltk.PorterStemmer()
#print(sentence)
print([porter.stem(t) for t in tokens])
# using the WordNet lemmatizer through NLTK
# WordNet is a large lexical database of English (think "lexicon")
wnl = nltk.WordNetLemmatizer()
#print(sentence)
print([wnl.lemmatize(t) for t in tokens])
# note the differences.. this lemmatizer knows that the word "based" does not have the same meaning as "base" here.
# Seems more comprehensible. Or lot less has actually changed. (examples: "goes" became "go" and "villagers" became "villager")
###Output
_____no_output_____
###Markdown
Casing, stopwords, punctuation etc.
###Code
# Casing (lower casing is normal procedure such that the word "That", after a punctuation, and "that" are not treated as two seperate features.
sent = sentence.lower()
print(sent)
# Note: you may want some exceptions, e.g. distinction between US and us can be important for some tasks.
# lowercase and then tokenize all texts in the training set – in one list comprehension:
train_sents = [nltk.word_tokenize(i.lower()) for i in df_train.review.values]
print(train_sents[0])
# And do the same with the test set
test_sents = [nltk.word_tokenize(i.lower()) for i in df_test.review.values]
# Removing certain words (stopwords)
stop_words_list = nltk.corpus.stopwords.words("english")
print(stop_words_list)
print()
sent_sw_removed = [i for i in nltk.word_tokenize(sentence.lower()) if i not in stop_words_list]
print(sent_sw_removed)
#train_sents_sw_removed = [[i for i in sent if i not in stop_words_list] for sent in train_sents] # nested list comprehension; lists in list.
#test_sents_sw_removed = [[i for i in sent if i not in stop_words_list] for sent in test_sents]
#print(train_sents_sw_removed[0])
# unfortunately the tokenization does not totally align with the stopwords – the simple split at whitespace may be more appropiate before stopword removal.
# Removing punctuation, two ways:
punct_removed_1 = sentence.translate(str.maketrans('', '', string.punctuation))
print(punct_removed_1)
#def removePunctuation (word):
# return re.sub("[^\w\s\-\']", "", word)
punct_removed_2 = re.sub(r'[^\w\s]','',sentence) # learn more about regex at https://www.datacamp.com/community/tutorials/python-regular-expression-tutorial or find a cheat sheet.
print(punct_removed_2)
###Output
_____no_output_____
###Markdown
Exercise 1: preprocessingMake a preprocessing function that takes a single string and 1) Lowercase the words, 2) split the text into words (tokenize), 3) either stem or lemmatize words.Feel free to add more steps of preprocessing. For example stopword removal or removal of what seems to be HTML elements (such as "") in the text, and removal of punctuation, and handling of emoticons as in the textbook.
###Code
def preprocess(text):
# YOUR CODE HERE
return lemmas # return a list of stems/lemmas
print(preprocess(sentence))
# Have a look at the size of the vocabulary of the train data that
# has only been lower-cased and tokenized (a few cells above).
all_words = []
for i in train_sents:
all_words.extend(i)
V = set(all_words)
print(len(all_words))
print(len(V))
# Now do it yourself with your train data after preprocessing:
# YOUR CODE HERE
sorted(V)
###Output
_____no_output_____
###Markdown
Notes: without preprocessing we see a lot of "words" in the vocabulary that are not words. This is a lesson in how important it is to look at your (training!) data.Consider altering your preprocessing if you notice many mistakes in the vocab. Lexicons for sentiment analysis Vader
###Code
# Vader lexicon
analyser = vaderSentiment.SentimentIntensityAnalyzer()
snt = analyser.polarity_scores(sentence)
#print(sentence)
print(snt)
compound = snt["compound"] # how to scoring works: https://github.com/cjhutto/vaderSentiment#about-the-scoring
###Output
_____no_output_____
###Markdown
Afinn
###Code
# Afinn lexicon
afn = Afinn(emoticons=True)
print(afn.score(sentence))
example_neg = "congrats on making an all-together predictable movie"
example_pos = "OMG I literally died!"
print(afn.score(example_neg))
print(afn.score(example_pos))
###Output
_____no_output_____
###Markdown
SentiWordNet
###Code
# lexicon can be accessed with NLTK:
good = nltk.corpus.sentiwordnet.senti_synsets('good', 'a') # a=adjective,n=noun
for i in good:
print(i)
print()
print("good.a.01 Definition: ", nltk.corpus.wordnet.synset('good.a.01').definition())
print("good.s.20 Definition: ", nltk.corpus.wordnet.synset('good.s.20').definition())
print("good.a.03 Definition: ", nltk.corpus.wordnet.synset('good.a.03').definition())
###Output
_____no_output_____
###Markdown
Feature representation/extraction BoW (Bag of Words)- Represent the texts with a **Term-Document frequency matrix** where each text is represented by a vector of word counts. n_samples x n_features matrix. n_features=size of vocabulary.
###Code
Image("term_document.png") # (Jurafsky, D., & Martin, J. H., 2019. Chap. 6, p. 7)
# CountVectorizer has a build-in tokenizer and lowercases by default. Also has an option to remove stopwords.
vectorizer = CountVectorizer()
# However, you can override the default tokenization with your own defined function, like so:
#vectorizer = CountVectorizer(tokenizer=preprocess)
# fit and transform train
X_train_bow = vectorizer.fit_transform(df_train.review.values)
# Only tranform test: never fitting your vectorizer on the test set (it is cheating). OOV words are handled automatically be sklearn's vectorizer.
X_test_bow = vectorizer.transform(df_test.review.values)
print(X_train_bow.shape)
print(len(vectorizer.vocabulary_))
X_train_bow[0]
###Output
_____no_output_____
###Markdown
Only 238 nonzero elements in the vector of the first text, i.e. 238 unique features/words out of 74,849.
###Code
X_train_bow[0].toarray()
###Output
_____no_output_____
###Markdown
N-grams - Collection of 1 or more tokens.- Bag of words lacks word order and context (semantics). n-grams to the rescue!
###Code
example = "The cat in the hat"
def make_ngrams(sentence,n):
tokens = sentence.split(" ")
ngrams = zip(*[tokens[i:] for i in range(n)])
return [" ".join(ngram) for ngram in ngrams]
print("Unigrams:",make_ngrams(example,1))
print("Bigrams:",make_ngrams(example,2))
print("Trigrams:",make_ngrams(example,3))
print("4-grams:",make_ngrams(example,4))
print("5-grams:",make_ngrams(example,5))
# n=1-5
vectorizer = CountVectorizer(ngram_range=(1,5)) #tokenizer=preprocess)
X_train_5gram = vectorizer.fit_transform(df_train.review.values)
print(X_train_5gram.shape)
X_train_5gram[0]
###Output
_____no_output_____
###Markdown
- But now we have problems with high dimensionalty and uniqeness of features!- N-grams are used for many applications but are especially known from **Language Models**: In short, probalistic models that learn to predict the next word in a sequence of words, given the "history" (the previous words), simply by storing the probability of this event occuring in the given text, e.g. P(hat|The cat in the). But instead of using ALL previous word (which would be a combination of words unique to the given text), the history is approximated by a few previous words (n-grams), e.g. P(hat|the). This is the n-gram language model. Term Frequency–Inverse Document Frequency (TF-IDF)The tf-idf value of term t in document d is:TF-IDF(t,d) = tf(t,d) x idf(t)where,tf(t,d) = count of term t in document dN = total number of documentsdf(t) = number of documents that term t occurs inidf(t) = N/df(t)A "term" is a token in our case.
###Code
Image("tf_idf.png") # (Jurafsky, D., & Martin, J. H., 2019. Chap. 6, p. 14)
tfidf = TfidfVectorizer()
X_train_tfidf = tfidf.fit_transform(df_train.review.values)
###Output
_____no_output_____
###Markdown
Document similarity with tf-idf vectors
###Code
#1-distance.cosine(X_train_tfidf[0].toarray(), X_train_tfidf[1].toarray())
# https://scikit-learn.org/stable/modules/metrics.html#cosine-similarity
cosine_similarities = linear_kernel(X_train_tfidf[0], X_train_tfidf).flatten()
indices = cosine_similarities.argsort()[::-1] # in descending order
print("most similar:",indices[:10])
print("least similar", indices[-9:])
#print(df_train.review.values[0])
print()
print("most similar: ", df_train.review.values[23558])
print()
print("least similar: ", df_train.review.values[23603])
###Output
most similar: Okay, so I'm not a big video game buff, but was the game House of the Dead really famous enough to make a movie from? Sure, they went as far as to actually put in quick video game clips throughout the movie, as though justifying any particular scene of violence, but there are dozens and dozens of games that look exactly the same, with the hand in the bottom on the screen, supposedly your own, holding whatever weapon and goo-ing all kinds of aliens or walking dead or snipers or whatever the case may be.<br /><br />It's an interesting premise in House of the Dead, with a lot of college kids (LOADED college kids, as it were, kids who are able to pay some fisherman something like $1,500 just for a ride after they miss their boat) trying to get out to this island for what is supposed to be the rave of the year. The first thing that comes to mind about House of the Dead after watching it is that it has become increasingly clear that modern horror movies have become nothing more than an exercise in coming up with creative ways to get a lot of scantily clad teenagers into exactly the same situations. At least in this case, the fact that they were on their way to a rave excuses the way the girls are dressed. They look badly out of place running around the woods in cute little halter-tops, but at least they THOUGHT they were dressed for the occasion.<br /><br />Clint Howard, tellingly the most interesting character in the film by far, delivers an absolutely awful performance, the greatness of which overshadows every other actor in the movie. I can't stand it when well-known actors change their accents in movies, it is so rarely effective, and Howard here shows that it is equally flat to have an well-known actor pretend that he's this hardened fisherman with a raspy voice from years of breathing salty air. He didn't even rasp well. It sounded like he was eating a cinnamon roll before shooting and accidentally inhaled some powdered sugar or something. Real tough there, Clint! I expected more from him, but then again, he did agree to a part in this mess.<br /><br />Once we get to the island, the movie temporarily turns into any one of the Friday the 13th movies that took place at Camp Crystal Lake. Lots of teenagers played by actors who were way too old for their parts getting naked and then killed. The nudity was impressive, I guess, but let's consider something for a minute. These kids pay almost two grand to get out to this island to go to the Rave Of The Year, find NO ONE, and say, well, who wants a beer! Even the guy who pulled that stack of hundreds out of his wallet to get them all over there didn't think anything of it that they found a full bar and not a single solitary person in sight. Here you have the input from director Uwe Boll - There's alcohol! They won't notice that the party they came for consists of no one but themselves!<br /><br />So not only do they start drinking, not minding the fact that the whole party seems to have vacated the island, but when one of the girls goes off into the dark woods to find out where everyone is (dragging one other girl and one of the guys reluctantly along), the guy and the girl who stay behind to get smashed decide that it would be a great idea to strip down for a quickie now that they're alone. It's like they expected to find the island empty, and now that they rest of the people that they came over with were gone for a little while, they would have some privacy since there's no one else around. Brilliant!<br /><br />Now for the things that everyone hated, judging by the reviews that I've read about the movie. Yes, intersplicing shots from the video game into the movie, mostly in order to show that, yes, the movie was being faithful to/directly copying the video game. Sure, it was a stupid idea. I can't imagine who thought up that little nugget, but worse than that is the Matrix-style bullet time scenes that were thrown in over and over and over and over. After the first time (at which point I found it pretentious and cheesy for a movie like this to have a shot like that as though it was something original) it is noticeable more for the technique of the shot itself rather than any dramatic meaning or creation of any kind of tension for the film.<br /><br />One of the things that makes a zombie film scary and gets you on the edge of your seat is to have them slowly but relentlessly coming after the living humans, who are much faster but getting tired, running out of places to run, and with a terrifying shortage of things with which to fight the zombies off with. The first two are done right in the movie, the kids are terrified and don't have a lot of places to run since they're on an island, but since they caught a ride over with a smuggler, they find themselves heavily armed. And I mean that very strongly. I mean, these people have everything from machine guns to hand grenades, which removes most of the tension of the impending walking dead.<br /><br />Then you have what I call the techno-slasher scene. Since the rave never happened, and I guess since Uwe Boll thought people were going to be disappointed at not hearing any techno music in the movie, there's one scene right in the middle where all the humans are fighting off the living dead, and amazingly enough it turns into something of a music video. There's techno music blasting as the shots are edited together faster and faster until it's nothing but a blur of gory shot, mostly only about 5 frames long (which is about 1/6 of a second) flashing across the screen in time with the speed techno music. Clever, I guess, but it has no place in a horror movie because it completely removes any sense of scariness or tension of even the gross-out effect because you can't see any one thing for long enough to react to it. You're just watching these shots fly across the screen and wondering what the hell the director was thinking when he decided that it would be a good idea to put something like this in the movie.<br /><br />I've seen a lot of people compare this movie to Resident Evil, mostly claiming that it copies the premise of it, and they're exactly right. I appreciate that at least here, as was not the case in Resident Evil, it wasn't some man-made virus that turned people into walking dead that were able to infect other people, changing them the way vampires turn others into vampires. 28 Days Later was also clearly an inspiration for this movie, it's just too bad that House of the Dead didn't do a single original thing, except for the somewhat moronic idea of putting in quick shots of the video game on which it is based, just in case you forget. I really think that this should have been a much better movie. While obviously I can't say that I know much about the game it's based on, just the title and the movie poster deserve a much better movie, but unfortunately I think that's more often the case than not with horror movies. It's really kind of sad when a movie comes out that is so obviously advertised as a no-holds-barred horror film, and the scariest thing in the entire movie is the closing shot, which suggests the possibility of a sequel.
least similar: Cool idea... botched writing, botched directing, botched editing, botched acting. Sorta makes me wish I could play God and strike everyone involved in making this film with several bolts of lightning.
###Markdown
Vector semantics / Vector Space Models / (word) embeddingsSemantics: The *meaning* or catagory of a word.Context is important to understand a word and give it a descriptive representation (encoding it's meaning). Assumption/intution: Two words are similar if they appear in similar contexts.Word2Vec architectures:- CBOW: Learn word embeddings by predicting a focus word given a context- Skip-gram: Learn word embeddings by predicting context words given focus word(https://arxiv.org/pdf/1301.3781.pdf ,https://papers.nips.cc/paper/5021-distributed-representations-of-words-and-phrases-and-their-compositionality.pdf, https://radimrehurek.com/gensim/models/word2vec.html)
###Code
from gensim.models import Word2Vec
# using the train_sents from earlier (the lowercased and tokenized sentences)
model = Word2Vec(train_sents, size=50)
print(model.wv['good'])
# Reducing the 50-dimensional vectors to 2 dimensions in order to visualise selected words.
from sklearn.decomposition import PCA
words = ["drama","comedy", "good", "great", "bad", "horrible", "cat", "dog"]
X = [model.wv['drama'], model.wv['comedy'],
model.wv['good'], model.wv['great'],
model.wv['bad'], model.wv['horrible'],
model.wv['cat'], model.wv['dog']]
pca = PCA(n_components=2)
X_r = pca.fit(X).transform(X)
plt.figure(figsize=(6,6))
plt.scatter(X_r[:,0], X_r[:,1], edgecolors='k', c='r')
for word, (x,y) in zip(words, X_r):
plt.text(x+0.2, y+0.1, word)
###Output
_____no_output_____
###Markdown
Text classification Exercise 2: Lexicon-based sentiment classification (no Machine Learning)Use the Vader lexicon to classify each review in the test set. Use the "compound" score where compound0=positive review (1). Report the classification accuracy.
###Code
#Your code here
###Output
_____no_output_____
###Markdown
Text classification with logistic regression
###Code
# Get feature vectors
tfidf = TfidfVectorizer()
# use your own preprocessing function in the vectorizer when you've finished that exercise:
#tfidf = TfidfVectorizer(tokenizer=preprocess)
X_train_tfidf = tfidf.fit_transform(df_train.review.values)
X_test_tfidf = tfidf.transform(df_test.review.values)
# labels
y_train = df_train.sentiment.values
y_test = df_test.sentiment.values
# classifier
lr = LogisticRegression(random_state=0)
#training
lr.fit(X_train_tfidf,y_train)
#testing
train_preds = lr.predict(X_train_tfidf)
test_preds = lr.predict(X_test_tfidf)
print("training accuracy:", np.mean([(train_preds==y_train)]))
print("testing accuracy:", np.mean([(test_preds==y_test)]))
###Output
training accuracy: 0.93328
testing accuracy: 0.88316
###Markdown
Let's look at the features' coeffiecients
###Code
features = ['_'.join(s.split()) for s in tfidf.get_feature_names()]
coefficients = lr.coef_
coefs_df = pd.DataFrame.from_records(coefficients, columns=features)
coefs_df
print(coefs_df.T.sort_values(by=[0], ascending=False).head(20))
print()
print(coefs_df.T.sort_values(by=[0], ascending=True).head(20))
###Output
0
great 7.597993
excellent 6.185474
best 5.127967
perfect 4.818600
wonderful 4.676444
amazing 4.164898
well 4.061736
loved 3.835260
fun 3.805573
today 3.782701
love 3.782314
favorite 3.743822
enjoyed 3.513010
highly 3.390157
brilliant 3.353821
it 3.330147
superb 3.321679
and 3.176546
definitely 3.031838
still 2.983629
0
worst -9.242321
bad -7.956060
awful -6.493183
waste -6.278238
boring -6.020011
poor -5.448303
terrible -4.848693
nothing -4.761642
worse -4.577197
no -4.394958
horrible -4.208934
poorly -4.167156
dull -4.155190
unfortunately -3.984436
script -3.871557
annoying -3.827654
stupid -3.818522
ridiculous -3.668310
minutes -3.600162
instead -3.526372
###Markdown
Exercise 3: Experiment with different features (counts and tf-idf) and n_gram ranges ((1,1), (1,2),(1,3) and (1,4)). Do 3-fold cross-validation using sklearn's GridSearchCV – as in the PML textbook (from page 268) – to see which combinations are best. Then, using this best combination, train a model with the full train set and test it on the test set. Report results. (Hint: You can use the parameter "use_idf" in the TfidfTransformer() – not vectorizer – to easliy test the usage of either just term frequencies or tf-idf frequencies).
###Code
# YOUR CODE HERE
###Output
_____no_output_____
###Markdown
Final exercise: Cross-domain evaluation1. Download the Airline sentiment dataset here (you may need to login to download it): https://www.kaggle.com/crowdflower/twitter-airline-sentimentGeneral note on tweets: Tweets are messy (generally require a lot a of preproccesing if used to train a ML model).2. Load the data into a dataframe named tweet_df. 3. As you can see, the samples have three labels (neg, pos, neutral). You have to either relabel neutral tweets as positive or remove neutral tweets from the dataframe entirely.4. Extract features from the tweets using the tf-idf vectorizer previously fitted on the Imdb review training data.5. Use your previously trained logistic regression classifier and make predictions over your vectorized tweets. Report testing accuracy.6. Finally, answer these questions: A) How well does your model perform in this other domain? B) Can you identify the types of errors it makes? (hint: you can make a confusion matrix and you look at the words, and their respective coefficients, of relevant examples of misclassification). C) Now, fit a new vectorizer and logistic regression classifier on the airline tweets (remove mentions, @user, as the very least amount of preprocessing. there are plenty of examples of how to do this with python regular expressions if you google it). What are the difference between most important features (high and low coeffiecients) between this model and your original model trained on the Imdb reviews? And what does this mean for the models' ability to generalize to new data?
###Code
# YOUR CODE HERE
###Output
_____no_output_____ |
docs/source/cookbook_services.ipynb | ###Markdown
Cookbook SMRT Link Webservices using pbcommand
###Code
# https://github.com/PacificBiosciences/pbcommand
# install via
# pip install -e git://github.com/PacificBiosciences/pbcommand.git#egg=pbcommand`
import pbcommand
from pbcommand.services import ServiceAccessLayer
print pbcommand.get_version()
###Output
0.6.0
###Markdown
Configure and Get Status
###Code
host = "smrtlink-beta"
port = 8081
sal = ServiceAccessLayer(host, port)
sal.get_status()
###Output
_____no_output_____
###Markdown
Get SubreadSet from SMRT Link Services Get SubreadSet details by UUID
###Code
# Example SubreadSet
subreadset_uuid = "4463c7d8-4b6e-43a3-93ff-325628066a0b"
ds = sal.get_subreadset_by_id(subreadset_uuid)
ds['metadataContextId'], ds['name'], ds['id'], ds['jobId'], ds['wellName'], ds['path'], ds['uuid']
# or get by Int id
dx = sal.get_subreadset_by_id(ds['id'])
dx['uuid'], ds['uuid']
# Get the Import Job associated with the SubreadSet. This Job computes the metrics at import time
import_job_id = ds['jobId']
###Output
_____no_output_____
###Markdown
Get SubreadSet Import Job and Import Report Metrics
###Code
import_job = sal.get_import_job_by_id(import_job_id)
import_job
import_job.state
import_job.name
import_report_attributes = sal.get_import_job_report_attrs(import_job.id)
import_report_attributes # This are what are displayed in SMRT Link for Import Job Types
###Output
_____no_output_____
###Markdown
Get Analysis (pbsmrtpipe) Job
###Code
analysis_job_id = 12524 # Random Example Job
analysis_job = sal.get_analysis_job_by_id(analysis_job_id)
analysis_job
analysis_job.settings['pipelineId']
###Output
_____no_output_____
###Markdown
Get the Entry Points to the Pipeline
###Code
# Get Entry Points Use
eps = sal.get_analysis_job_entry_points(analysis_job_id)
for e in eps:
print e
###Output
JobEntryPoint(job_id=12524, dataset_uuid=u'3adaa998-8ba9-4f7c-97c4-2ef343b419bb', dataset_metatype=u'PacBio.DataSet.SubreadSet')
JobEntryPoint(job_id=12524, dataset_uuid=u'c2f73d21-b3bf-4b69-a3a3-6beeb7b22e26', dataset_metatype=u'PacBio.DataSet.ReferenceSet')
###Markdown
Get Report Metrics for Analysis Job
###Code
analysis_report_attributes = sal.get_analysis_job_report_attrs(analysis_job_id)
analysis_report_attributes
###Output
_____no_output_____
###Markdown
Example of Getting Mulitple Jobs and extracting Metrics to build a Table
###Code
# Grab some example jobs
job_ids = [12357, 12359, 12360, 12361, 12362, 12367]
def get_attrs_from_job_id(sal_, job_id_):
d = sal_.get_analysis_job_report_attrs(job_id_)
d['job_id'] = job_id_
d['host'] = host
d['port'] = port
return d
datum = [get_attrs_from_job_id(sal, x) for x in job_ids]
# Plot some data
import matplotlib.pyplot as plt
import matplotlib
import pandas as pd
import seaborn as sns
sns.set_style("whitegrid")
%matplotlib inline
df = pd.DataFrame(datum)
df
sns.barplot(x="job_id", y="mapping_stats.mapped_readlength_mean", data=df)
###Output
_____no_output_____ |
LS_DS_123_Make_explanatory_visualizations.ipynb | ###Markdown
_Lambda School Data Science_ Choose appropriate visualizationsRecreate this [example by FiveThirtyEight:](https://fivethirtyeight.com/features/al-gores-new-movie-exposes-the-big-flaw-in-online-movie-ratings/)Using this data:https://github.com/fivethirtyeight/data/tree/master/inconvenient-sequel Stretch goalsRecreate more examples from [FiveThityEight's shared data repository](https://data.fivethirtyeight.com/).For example:- [thanksgiving-2015](https://fivethirtyeight.com/features/heres-what-your-part-of-america-eats-on-thanksgiving/) ([`altair`](https://altair-viz.github.io/gallery/index.htmlmaps))- [candy-power-ranking](https://fivethirtyeight.com/features/the-ultimate-halloween-candy-power-ranking/) ([`statsmodels`](https://www.statsmodels.org/stable/index.html))
###Code
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
df = pd.read_csv('https://raw.githubusercontent.com/fivethirtyeight/data/master/inconvenient-sequel/ratings.csv')
df.timestamp = pd.to_datetime(df.timestamp)
df.set_index('timestamp', inplace=True)
pct_respondents = df[df['category'] == 'IMDb users']['respondents'] / df[df['category'] == 'IMDb users']['respondents'].max()
import matplotlib.ticker as ticker
plt.style.use('fivethirtyeight')
ax = pct_respondents.plot(color = 'skyblue', ylim = (0,1.03), legend=False)
vals = ax.get_yticks()
ax.set_yticklabels(['{:,.0%}'.format(x) for x in vals])
ax.set(xlabel='')
ax.set_xticklabels(['','JULY 23, 2017', 'JULY 30', 'AUG. 6', 'AUG. 13', 'AUG. 20', 'AUG. 27'])
ax.set_yticklabels(['0 ', '20 ', '40 ', '60 ', '80 ', '100%'])
ax.tick_params(axis='x',labelrotation=0)
long_title = "'An Inconvenient Sequel' was doomed before its release"
long_title += '\nShare of tickets sold and IMDb reviews for "An Inconvenient Sequel"'
ax.set_title(y=1.3, label = long_title);
# ax.text(x=0, y=0, s = 'Share of tickets sold and IMDb reviews for "An Inconvenient Sequel"')
# ax.text(x=0, y=0, s = 'Posted through Aug.27, by day')
###Output
_____no_output_____
###Markdown
_Lambda School Data Science_ Choose appropriate visualizationsRecreate this [example by FiveThirtyEight:](https://fivethirtyeight.com/features/al-gores-new-movie-exposes-the-big-flaw-in-online-movie-ratings/)Using this data:https://github.com/fivethirtyeight/data/tree/master/inconvenient-sequel Stretch goalsRecreate more examples from [FiveThityEight's shared data repository](https://data.fivethirtyeight.com/).For example:- [thanksgiving-2015](https://fivethirtyeight.com/features/heres-what-your-part-of-america-eats-on-thanksgiving/) ([`altair`](https://altair-viz.github.io/gallery/index.htmlmaps))- [candy-power-ranking](https://fivethirtyeight.com/features/the-ultimate-halloween-candy-power-ranking/) ([`statsmodels`](https://www.statsmodels.org/stable/index.html)) Data Wrangling and Exploration
###Code
import pandas as pd
import matplotlib.pyplot as plt
url = 'https://raw.githubusercontent.com/fivethirtyeight/data/master/inconvenient-sequel/ratings.csv'
df = pd.read_csv(url)
# df.head()
df.timestamp = pd.to_datetime(df.timestamp)
df.timestamp.describe()
df.set_index('timestamp', inplace = True)
df.head()
length, width = df.shape
print(df.shape)
print(length*width)
# Use Value Counts rather than Unique
df.category.value_counts()
df.columns
###Output
_____no_output_____
###Markdown
Suggested Correct Version of this problem
###Code
cols2 = ['1_pct','2_pct', '3_pct', '4_pct', '5_pct',
'6_pct', '7_pct', '8_pct', '9_pct', '10_pct']
pct_votes = df[cols2].tail(1)
pct_votes
# Why inplace = True fails is beyond me, better to make a copy
pct_votes_new = pct_votes.reset_index(drop=True)
pct_votes_new
pct_votes_new.rename(columns={"1_pct": "1",
"2_pct": "2",
"3_pct": "3",
"4_pct": "4",
"5_pct": "5",
"6_pct": "6",
"7_pct": "7",
"8_pct": "8",
"9_pct": "9",
"10_pct": "10",},
inplace = True)
pct_votes_new = pct_votes_new.T
pct_votes_new
# fig, ax = plt.subplots()
plt.style.use("fivethirtyeight")
# fig = plt.figure();
# fig.patch.set_alpha(0.0)
ax = pct_votes_new.plot.bar(color = '#EC713B', width = 0.9) # All bars are the same color and width
ax.tick_params(labelrotation = 0, colors = 'gray')
y_ticks = [0,10,20,30,40]
ax.set_yticks(y_ticks)
ax.legend().set_visible(False)
ax.set_facecolor("white")
ax.set_ylabel("Percent of total votes", fontweight='bold')
ax.set_xlabel("Rating", fontweight='bold')
ax.text(-1.5,45,s="'An Inconvenient Sequel: Truth to Power' is divisive", fontsize=18, weight='bold')
ax.text(-1.5,42,s="IMDb Ratings for the film as of Aug. 29", fontsize=16)
# ax.set_title("'An Inconvenient Sequel: Truth to Power' is divisive \n IMDb Ratings for the film as of Aug. 29", loc = 'left')
# ax.spines['bottom'].set_color('white')
# ax.spines['top'].set_color('white')
# ax.spines['right'].set_color('white')
# ax.spines['left'].set_color('white')
# ax.tick_params(axis = 'x', color = 'lightgray')
# ax.tick_params(axis = 'y', color = 'blue')
# ax.yaxis.label.set_color('black')
# ax.xaxis.label.set_color('black')
# ax.patch.set_facecolor('white')
plt.show()
# plt.savefig('538.png', transparent=True)
###Output
_____no_output_____
###Markdown
Alternate Attempt at this problem
###Code
# Slicing out relevant columns
cols = ['1_votes', '2_votes', '3_votes', '4_votes', '5_votes',
'6_votes', '7_votes', '8_votes', '9_votes', '10_votes']
df_new = df[cols]
sum_votes = pd.Series(df_new.sum())
sum_votes = 100 * sum_votes / sum_votes.sum()
sum_votes.rename(index={"1_votes": "1",
"2_votes": "2",
"3_votes": "3",
"4_votes": "4",
"5_votes": "5",
"6_votes": "6",
"7_votes": "7",
"8_votes": "8",
"9_votes": "9",
"10_votes": "10",},
inplace = True)
sum_votes
plt.style.use("fivethirtyeight")
ax = sum_votes.plot.bar(color = '#EC713B', width = 0.9)
ax.tick_params(labelrotation = 0, colors = 'gray')
y_ticks = [0,10,20,30,40]
ax.set_yticks(y_ticks)
ax.legend().set_visible(False)
ax.set_facecolor("white")
ax.set_ylabel("Percent of total votes", fontweight='bold')
ax.set_xlabel("Rating", fontweight='bold')
ax.text(-1.5,45,s="'An Inconvenient Sequel: Truth to Power' is divisive", fontsize=18, weight='bold')
ax.text(-1.5,42,s="IMDb Ratings for the film as of Aug. 29", fontsize=18)
# ax.set_title("'An Inconvenient Sequel: Truth to Power' is divisive \n IMDb Ratings for the film as of Aug. 29", loc = 'left')
# ax.spines['bottom'].set_color('white')
# ax.spines['top'].set_color('white')
# ax.spines['right'].set_color('white')
# ax.spines['left'].set_color('white')
# ax.tick_params(axis = 'x', color = 'lightgray')
# ax.tick_params(axis = 'y', color = 'blue')
# ax.yaxis.label.set_color('black')
# ax.xaxis.label.set_color('black')
plt.show()
###Output
_____no_output_____
###Markdown
STRETCH GOAL
###Code
# Bad Drivers - https://github.com/fivethirtyeight/data/tree/master/bad-drivers
url2 = 'https://raw.githubusercontent.com/fivethirtyeight/data/master/bad-drivers/bad-drivers.csv'
driver_df = pd.read_csv(url2)
driver_df.head()
driver_df.describe()
driver_df.columns
driver_df.rename(columns = {'Number of drivers involved in fatal collisions per billion miles' : 'no_fatal',
'Percentage Of Drivers Involved In Fatal Collisions Who Were Speeding' : 'pct_fatal',
'Percentage Of Drivers Involved In Fatal Collisions Who Were Alcohol-Impaired': 'pct_fatal_alcohol',
'Percentage Of Drivers Involved In Fatal Collisions Who Were Not Distracted': 'pct_fatal_nodistraction',
'Percentage Of Drivers Involved In Fatal Collisions Who Had Not Been Involved In Any Previous Accidents' : 'pct_fatal_noprev',
'Car Insurance Premiums ($)' : 'ins_premiums',
'Losses incurred by insurance companies for collisions per insured driver ($)' : 'ins_losses'}, inplace = True)
driver_df.head()
# import altair as alt
# from vega_datasets import data
no_fatal = driver_df.no_fatal.sort_values()
no_fatal
plt.style.use("fivethirtyeight")
# fig = plt.figure();
# fig.patch.set_alpha(0.0)
ax = no_fatal.plot.bar(width = 0.9) # All bars are the same color and width (color = '#EC713B')
ax.tick_params(labelrotation = 90, colors = 'gray')
y_ticks = [0,5,10,15,20,25]
ax.set_yticks(y_ticks)
ax.legend().set_visible(False)
ax.set_facecolor("white")
ax.set_ylabel("Drivers involved in fatal collisions", fontweight='bold')
ax.set_xlabel("State", fontweight='bold')
ax.text(-1.5,28,s="'Drivers involved in fatal collisions per billion miles by State", fontsize=18, weight='bold')
# ax.text(-1.5,42,s="IMDb Ratings for the film as of Aug. 29", fontsize=16)
# ax.set_title("'An Inconvenient Sequel: Truth to Power' is divisive \n IMDb Ratings for the film as of Aug. 29", loc = 'left')
# ax.spines['bottom'].set_color('white')
# ax.spines['top'].set_color('white')
# ax.spines['right'].set_color('white')
# ax.spines['left'].set_color('white')
# ax.tick_params(axis = 'x', color = 'lightgray')
# ax.tick_params(axis = 'y', color = 'blue')
# ax.yaxis.label.set_color('black')
# ax.xaxis.label.set_color('black')
# ax.patch.set_facecolor('white')
plt.show()
###Output
_____no_output_____
###Markdown
_Lambda School Data Science_ Choose appropriate visualizationsRecreate this [example by FiveThirtyEight:](https://fivethirtyeight.com/features/al-gores-new-movie-exposes-the-big-flaw-in-online-movie-ratings/) create data by hand and plot it
###Code
from IPython.display import display, Image
url = 'https://fivethirtyeight.com/wp-content/uploads/2017/09/mehtahickey-inconvenient-0830-1.png'
example = Image(url=url, width=400)
display(example)
%matplotlib inline
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
# set up some fake data similar to above chart
fake = pd.Series( [38,3,2,1,2,4,6,5,5,33] ,index=range(1,11) )
plt.style.available
plt.style.use('fivethirtyeight')
#plt.style.use('seaborn-white')
fake.plot.bar();
# create an object handle for the chart
# this will allow setting of Chart Obj parameters
ax = fake.plot.bar(color='#EC713B', width=0.92)
ax.tick_params(labelrotation=0) # rotate the x labels
# define the title
#ax.set_title('Xxx')
ax.set(title='"An Inconvenient Sequel: Truth to Power" is divisive',
xlabel='Rating',
ylabel='Percent of total votes');
display(example)
###Output
_____no_output_____
###Markdown
Read thru the actual data and plot it
###Code
df = pd.read_csv('https://raw.githubusercontent.com/fivethirtyeight/data/master/inconvenient-sequel/ratings.csv')
df.head()
# don't skip any columns!!!
pd.options.display.max_columns=None
df.head()
# Vertically show all columns/features of 1 row/instance
df.sample(1).T
df.timestamp.describe()
# timestamp not being treated as a datetime
# convert timestamp data to datetime format
df.timestamp=pd.to_datetime(df.timestamp)
# set the index to timestamp instead of count_integers
df.set_index('timestamp', inplace=True)
df
df.info()
df.category.value_counts()
# like a where clause in sql
# booleaN INDEXING
# filter out the falses; accept the trues
df[df.category=='IMDb users']
lastday=df['2017-08-29']
lastday[lastday.category=='IMDb users'].respondents.plot()
# the last timestamp/ row/
df.tail(1)
final =df.tail(1)
columns=['1_pct', '2_pct','3_pct','4_pct','5_pct','6_pct','7_pct','8_pct','9_pct','10_pct',]
data = final[columns].T
data=fake
#data.plot.bar()
colors=['C1','C3','C3','C3','C3','C3','C3','C3','C3','C1']
ax = data.plot.bar(color=colors, width=0.92, legend=False)
ax.tick_params(labelrotation=0) # rotate the x labels
# define the title
#ax.set_title('Xxx')
ax.set(title='"An Inconvenient Sequel: Truth to Power" is divisive',
xlabel='Rating',
ylabel='Percent of total votes');
#Aprint(fake)
fake.head()
data.head()
###Output
_____no_output_____
###Markdown
Explanatory Visualization : my assignment Try out Altair and Reproduce this Chart: Altair’s main purpose is to convert plot specifications to a JSON string that conforms to the Vega-Lite schema- [thanksgiving-2015](https://fivethirtyeight.com/features/heres-what-your-part-of-america-eats-on-thanksgiving/) ([`altair`](https://altair-viz.github.io/gallery/index.htmlmaps))
###Code
from IPython.display import display, Image
url = 'https://fivethirtyeight.com/wp-content/uploads/2015/11/hickey-side-dish-1.png?w=575'
example = Image(url=url, width=400)
display(example)
###Output
_____no_output_____
###Markdown
Get the data into pandasfrom https://github.com/fivethirtyeight/data/blob/master/thanksgiving-2015/thanksgiving-2015-poll-data.csv
###Code
%matplotlib inline
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
data_url='https://raw.githubusercontent.com/fivethirtyeight/data/master/thanksgiving-2015/thanksgiving-2015-poll-data.csv'
df=pd.read_csv(data_url)
pd.options.display.max_columns=None
df.head()
df.sample(1).T
###Output
_____no_output_____
###Markdown
Plot a Chloropleth Map (maps where the color of each shape is based on the value of an associated variable)
###Code
# Altair’s main purpose is to convert plot specifications to a JSON string
# that conforms to the Vega-Lite schema
import altair as alt
# vega lite sample datasets lib
from vega_datasets import data
states = alt.topo_feature(data.us_10m.url, 'states')
#df = pd.read_json(data.us_10m.url)
print(states)
print(data.us_10m.url)
# print a chloropleth
alt.Chart(states).mark_geoshape().encode(
).project(
type='albersUsa'
).properties(
width=800,
height=600)
counties = alt.topo_feature(data.us_10m.url, 'counties')
unemp_data = data.unemployment.url
alt.Chart(counties).mark_geoshape().encode(
color='rate:Q'
).transform_lookup(
lookup='id',
from_=alt.LookupData(unemp_data, 'id', ['rate'])
).project(
type='albersUsa'
).properties(
width=500,
height=300
)
states = alt.topo_feature(data.us_10m.url, 'states')
pop_eng_hur_url = data.population_engineers_hurricanes.url
pop_eng_hur_df= pd.read_csv(pop_eng_hur_url)
pop_eng_hur_df
variable_list = ['state']
print(states)
alt.Chart(states).mark_geoshape().encode(
alt.Color(alt.repeat('row'), type='nominal')
).transform_lookup(
lookup='id',
from_=alt.LookupData(pop_eng_hur_df, 'id', variable_list)
).properties(
width=500,
height=300
).project(
type='albersUsa'
).repeat(
row=variable_list
).resolve_scale(
color='independent'
)
variable_list = ['population']
alt.Chart(states).mark_geoshape().encode(
alt.Color(alt.repeat('row'), type='quantitative')
).transform_lookup(
lookup='id',
from_=alt.LookupData(pop_eng_hur_df, 'id', variable_list)
).properties(
width=500,
height=300
).project(
type='albersUsa'
).repeat(
row=variable_list
).resolve_scale(
color='independent'
)
###Output
_____no_output_____
###Markdown
GeoPandas playground- ([`GeoPandas`](http://geopandas.org/mapping.html))
###Code
# install geopandas
!pip install geopandas
!pip install descartes
# create maps by using the plot() method on a GeoSeries or GeoDataFrame.
import geopandas
# create GeoDataFrames
world = geopandas.read_file(geopandas.datasets.get_path('naturalearth_lowres'))
cities = geopandas.read_file(geopandas.datasets.get_path('naturalearth_cities'))
# Examine country GeoDataFrame
world.head(5)
# basic plot
world.plot(column='iso_a3');
# Check original projection
# (it's Platte Carre! x-y are long and lat)
world.crs
# Visualize
ax = world.plot()
ax.set_title("WGS84 (lat/lon)");
# Reproject to Mercator (after dropping Antartica)
world = world[(world.name != "Antarctica") & (world.name != "Fr. S. Antarctic Lands")]
world = world.to_crs({'init': 'epsg:3395'}) # world.to_crs(epsg=3395) would also work
ax = world.plot(column='continent')
ax.set_title("Mercator");
# Plot by GDP per capta
world = world[(world.pop_est>0) & (world.name!="Antarctica")]
world['gdp_per_cap'] = world.gdp_md_est / world.pop_est
world.plot(column='gdp_per_cap');
df = geopandas.read_file(geopandas.datasets.get_path('nybb'))
#Web map tiles are typically provided in Web Mercator (EPSG 3857), so we need to make sure to convert our data first to the same CRS to combine our polygons and background tiles in the same map:
ax = df.plot(figsize=(10, 10), alpha=0.5, edgecolor='k')
# Web map tiles are typically provided in Web Mercator (EPSG 3857),
# so we need to make sure to convert our data first to the same CRS
# to combine our polygons and background tiles in the same map:
df = df.to_crs(epsg=3857)
!pip install geos
!pip install cython
!pip install contextily
import contextily as ctx
def add_basemap(ax, zoom, url='http://tile.stamen.com/terrain/tileZ/tileX/tileY.png'):
xmin, xmax, ymin, ymax = ax.axis()
basemap, extent = ctx.bounds2img(xmin, ymin, xmax, ymax, zoom=zoom, url=url)
ax.imshow(basemap, extent=extent, interpolation='bilinear')
# restore original x/y limits
ax.axis((xmin, xmax, ymin, ymax))
###Output
_____no_output_____ |
Generative Adversarial Networks (GANs) Specialization/deep_convolutional_GANs/C1_W2_Assignment.ipynb | ###Markdown
Deep Convolutional GAN (DCGAN) GoalIn this notebook, you're going to create another GAN using the MNIST dataset. You will implement a Deep Convolutional GAN (DCGAN), a very successful and influential GAN model developed in 2015.*Note: [here](https://arxiv.org/pdf/1511.06434v1.pdf) is the paper if you are interested! It might look dense now, but soon you'll be able to understand many parts of it :)* Learning Objectives1. Get hands-on experience making a widely used GAN: Deep Convolutional GAN (DCGAN).2. Train a powerful generative model.Figure: Architectural drawing of a generator from DCGAN from [Radford et al (2016)](https://arxiv.org/pdf/1511.06434v1.pdf). Getting Started DCGANHere are the main features of DCGAN (don't worry about memorizing these, you will be guided through the implementation!): <!-- ```Architecture guidelines for stable Deep Convolutional GANs• Replace any pooling layers with strided convolutions (discriminator) and fractional-stridedconvolutions (generator).• Use BatchNorm in both the generator and the discriminator.• Remove fully connected hidden layers for deeper architectures.• Use ReLU activation in generator for all layers except for the output, which uses Tanh.• Use LeakyReLU activation in the discriminator for all layers.``` -->* Use convolutions without any pooling layers* Use batchnorm in both the generator and the discriminator* Don't use fully connected hidden layers* Use ReLU activation in the generator for all layers except for the output, which uses a Tanh activation.* Use LeakyReLU activation in the discriminator for all layers except for the output, which does not use an activationYou will begin by importing some useful packages and data that will help you create your GAN. You are also provided a visualizer function to help see the images your GAN will create.
###Code
import torch
from torch import nn
from tqdm.auto import tqdm
from torchvision import transforms
from torchvision.datasets import MNIST
from torchvision.utils import make_grid
from torch.utils.data import DataLoader
import matplotlib.pyplot as plt
torch.manual_seed(0) # Set for testing purposes, please do not change!
def show_tensor_images(image_tensor, num_images=25, size=(1, 28, 28)):
'''
Function for visualizing images: Given a tensor of images, number of images, and
size per image, plots and prints the images in an uniform grid.
'''
image_tensor = (image_tensor + 1) / 2
image_unflat = image_tensor.detach().cpu()
image_grid = make_grid(image_unflat[:num_images], nrow=5)
plt.imshow(image_grid.permute(1, 2, 0).squeeze())
plt.show()
###Output
_____no_output_____
###Markdown
GeneratorThe first component you will make is the generator. You may notice that instead of passing in the image dimension, you will pass the number of image channels to the generator. This is because with DCGAN, you use convolutions which don’t depend on the number of pixels on an image. However, the number of channels is important to determine the size of the filters.You will build a generator using 4 layers (3 hidden layers + 1 output layer). As before, you will need to write a function to create a single block for the generator's neural network. Since in DCGAN the activation function will be different for the output layer, you will need to check what layer is being created. You are supplied with some tests following the code cell so you can see if you're on the right track!At the end of the generator class, you are given a forward pass function that takes in a noise vector and generates an image of the output dimension using your neural network. You are also given a function to create a noise vector. These functions are the same as the ones from the last assignment.Optional hint for make_gen_block1. You'll find [nn.ConvTranspose2d](https://pytorch.org/docs/master/generated/torch.nn.ConvTranspose2d.html) and [nn.BatchNorm2d](https://pytorch.org/docs/master/generated/torch.nn.BatchNorm2d.html) useful!
###Code
# UNQ_C1 (UNIQUE CELL IDENTIFIER, DO NOT EDIT)
# GRADED FUNCTION: Generator
class Generator(nn.Module):
'''
Generator Class
Values:
z_dim: the dimension of the noise vector, a scalar
im_chan: the number of channels in the images, fitted for the dataset used, a scalar
(MNIST is black-and-white, so 1 channel is your default)
hidden_dim: the inner dimension, a scalar
'''
def __init__(self, z_dim=10, im_chan=1, hidden_dim=64):
super(Generator, self).__init__()
self.z_dim = z_dim
# Build the neural network
self.gen = nn.Sequential(
self.make_gen_block(z_dim, hidden_dim * 4),
self.make_gen_block(hidden_dim * 4, hidden_dim * 2, kernel_size=4, stride=1),
self.make_gen_block(hidden_dim * 2, hidden_dim),
self.make_gen_block(hidden_dim, im_chan, kernel_size=4, final_layer=True),
)
def make_gen_block(self, input_channels, output_channels, kernel_size=3, stride=2, final_layer=False):
'''
Function to return a sequence of operations corresponding to a generator block of DCGAN,
corresponding to a transposed convolution, a batchnorm (except for in the last layer), and an activation.
Parameters:
input_channels: how many channels the input feature representation has
output_channels: how many channels the output feature representation should have
kernel_size: the size of each convolutional filter, equivalent to (kernel_size, kernel_size)
stride: the stride of the convolution
final_layer: a boolean, true if it is the final layer and false otherwise
(affects activation and batchnorm)
'''
# Steps:
# 1) Do a transposed convolution using the given parameters.
# 2) Do a batchnorm, except for the last layer.
# 3) Follow each batchnorm with a ReLU activation.
# 4) If its the final layer, use a Tanh activation after the deconvolution.
# Build the neural block
if not final_layer:
return nn.Sequential(
#### START CODE HERE ####
nn.ConvTranspose2d(in_channels=input_channels, out_channels=output_channels, stride=stride, kernel_size=kernel_size),
nn.BatchNorm2d(num_features=output_channels),
nn.ReLU(),
#### END CODE HERE ####
)
else: # Final Layer
return nn.Sequential(
#### START CODE HERE ####
nn.ConvTranspose2d(in_channels=input_channels, out_channels=output_channels, stride=stride, kernel_size=kernel_size),
nn.Tanh(),
#### END CODE HERE ####
)
def unsqueeze_noise(self, noise):
'''
Function for completing a forward pass of the generator: Given a noise tensor,
returns a copy of that noise with width and height = 1 and channels = z_dim.
Parameters:
noise: a noise tensor with dimensions (n_samples, z_dim)
'''
return noise.view(len(noise), self.z_dim, 1, 1)
def forward(self, noise):
'''
Function for completing a forward pass of the generator: Given a noise tensor,
returns generated images.
Parameters:
noise: a noise tensor with dimensions (n_samples, z_dim)
'''
x = self.unsqueeze_noise(noise)
return self.gen(x)
def get_noise(n_samples, z_dim, device='cpu'):
'''
Function for creating noise vectors: Given the dimensions (n_samples, z_dim)
creates a tensor of that shape filled with random numbers from the normal distribution.
Parameters:
n_samples: the number of samples to generate, a scalar
z_dim: the dimension of the noise vector, a scalar
device: the device type
'''
return torch.randn(n_samples, z_dim, device=device)
# UNQ_C2 (UNIQUE CELL IDENTIFIER, DO NOT EDIT)
'''
Test your make_gen_block() function
'''
gen = Generator()
num_test = 100
# Test the hidden block
test_hidden_noise = get_noise(num_test, gen.z_dim)
test_hidden_block = gen.make_gen_block(10, 20, kernel_size=4, stride=1)
test_uns_noise = gen.unsqueeze_noise(test_hidden_noise)
hidden_output = test_hidden_block(test_uns_noise)
# Check that it works with other strides
test_hidden_block_stride = gen.make_gen_block(20, 20, kernel_size=4, stride=2)
test_final_noise = get_noise(num_test, gen.z_dim) * 20
test_final_block = gen.make_gen_block(10, 20, final_layer=True)
test_final_uns_noise = gen.unsqueeze_noise(test_final_noise)
final_output = test_final_block(test_final_uns_noise)
# Test the whole thing:
test_gen_noise = get_noise(num_test, gen.z_dim)
test_uns_gen_noise = gen.unsqueeze_noise(test_gen_noise)
gen_output = gen(test_uns_gen_noise)
###Output
_____no_output_____
###Markdown
Here's the test for your generator block:
###Code
# UNIT TESTS
assert tuple(hidden_output.shape) == (num_test, 20, 4, 4)
assert hidden_output.max() > 1
assert hidden_output.min() == 0
assert hidden_output.std() > 0.2
assert hidden_output.std() < 1
assert hidden_output.std() > 0.5
assert tuple(test_hidden_block_stride(hidden_output).shape) == (num_test, 20, 10, 10)
assert final_output.max().item() == 1
assert final_output.min().item() == -1
assert tuple(gen_output.shape) == (num_test, 1, 28, 28)
assert gen_output.std() > 0.5
assert gen_output.std() < 0.8
print("Success!")
###Output
Success!
###Markdown
DiscriminatorThe second component you need to create is the discriminator.You will use 3 layers in your discriminator's neural network. Like with the generator, you will need create the function to create a single neural network block for the discriminator.There are also tests at the end for you to use.Optional hint for make_disc_block1. You'll find [nn.Conv2d](https://pytorch.org/docs/master/generated/torch.nn.Conv2d.html), [nn.BatchNorm2d](https://pytorch.org/docs/master/generated/torch.nn.BatchNorm2d.html), and [nn.LeakyReLU](https://pytorch.org/docs/master/generated/torch.nn.LeakyReLU.html) useful!
###Code
# UNQ_C3 (UNIQUE CELL IDENTIFIER, DO NOT EDIT)
# GRADED FUNCTION: Discriminator
class Discriminator(nn.Module):
'''
Discriminator Class
Values:
im_chan: the number of channels in the images, fitted for the dataset used, a scalar
(MNIST is black-and-white, so 1 channel is your default)
hidden_dim: the inner dimension, a scalar
'''
def __init__(self, im_chan=1, hidden_dim=16):
super(Discriminator, self).__init__()
self.disc = nn.Sequential(
self.make_disc_block(im_chan, hidden_dim),
self.make_disc_block(hidden_dim, hidden_dim * 2),
self.make_disc_block(hidden_dim * 2, 1, final_layer=True),
)
def make_disc_block(self, input_channels, output_channels, kernel_size=4, stride=2, final_layer=False):
'''
Function to return a sequence of operations corresponding to a discriminator block of DCGAN,
corresponding to a convolution, a batchnorm (except for in the last layer), and an activation.
Parameters:
input_channels: how many channels the input feature representation has
output_channels: how many channels the output feature representation should have
kernel_size: the size of each convolutional filter, equivalent to (kernel_size, kernel_size)
stride: the stride of the convolution
final_layer: a boolean, true if it is the final layer and false otherwise
(affects activation and batchnorm)
'''
# Steps:
# 1) Add a convolutional layer using the given parameters.
# 2) Do a batchnorm, except for the last layer.
# 3) Follow each batchnorm with a LeakyReLU activation with slope 0.2.
# Build the neural block
if not final_layer:
return nn.Sequential(
#### START CODE HERE #### #
nn.Conv2d(in_channels=input_channels, out_channels=output_channels, stride=stride, kernel_size=kernel_size),
nn.BatchNorm2d(num_features=output_channels),
nn.LeakyReLU(0.2)
#### END CODE HERE ####
)
else: # Final Layer
return nn.Sequential(
#### START CODE HERE #### #
nn.Conv2d(in_channels=input_channels, out_channels=output_channels, stride=stride, kernel_size=kernel_size),
#### END CODE HERE ####
)
def forward(self, image):
'''
Function for completing a forward pass of the discriminator: Given an image tensor,
returns a 1-dimension tensor representing fake/real.
Parameters:
image: a flattened image tensor with dimension (im_dim)
'''
disc_pred = self.disc(image)
return disc_pred.view(len(disc_pred), -1)
# UNQ_C4 (UNIQUE CELL IDENTIFIER, DO NOT EDIT)
'''
Test your make_disc_block() function
'''
num_test = 100
gen = Generator()
disc = Discriminator()
test_images = gen(get_noise(num_test, gen.z_dim))
# Test the hidden block
test_hidden_block = disc.make_disc_block(1, 5, kernel_size=6, stride=3)
hidden_output = test_hidden_block(test_images)
# Test the final block
test_final_block = disc.make_disc_block(1, 10, kernel_size=2, stride=5, final_layer=True)
final_output = test_final_block(test_images)
# Test the whole thing:
disc_output = disc(test_images)
###Output
_____no_output_____
###Markdown
Here's a test for your discriminator block:
###Code
# Test the hidden block
assert tuple(hidden_output.shape) == (num_test, 5, 8, 8)
# Because of the LeakyReLU slope
assert -hidden_output.min() / hidden_output.max() > 0.15
assert -hidden_output.min() / hidden_output.max() < 0.25
assert hidden_output.std() > 0.5
assert hidden_output.std() < 1
# Test the final block
assert tuple(final_output.shape) == (num_test, 10, 6, 6)
assert final_output.max() > 1.0
assert final_output.min() < -1.0
assert final_output.std() > 0.3
assert final_output.std() < 0.6
# Test the whole thing:
assert tuple(disc_output.shape) == (num_test, 1)
assert disc_output.std() > 0.25
assert disc_output.std() < 0.5
print("Success!")
###Output
Success!
###Markdown
TrainingNow you can put it all together!Remember that these are your parameters: * criterion: the loss function * n_epochs: the number of times you iterate through the entire dataset when training * z_dim: the dimension of the noise vector * display_step: how often to display/visualize the images * batch_size: the number of images per forward/backward pass * lr: the learning rate * beta_1, beta_2: the momentum term * device: the device type Change runtime type` and set hardware accelerator to GPU and replace`device = "cpu"`with`device = "cuda"`. The code should then run without any more changes, over 1,000 times faster. -->
###Code
criterion = nn.BCEWithLogitsLoss()
z_dim = 64
display_step = 500
batch_size = 128
# A learning rate of 0.0002 works well on DCGAN
lr = 0.0002
# These parameters control the optimizer's momentum, which you can read more about here:
# https://distill.pub/2017/momentum/ but you don’t need to worry about it for this course!
beta_1 = 0.5
beta_2 = 0.999
device = 'cuda'
# You can tranform the image values to be between -1 and 1 (the range of the tanh activation)
transform = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize((0.5,), (0.5,)),
])
dataloader = DataLoader(
MNIST('.', download=False, transform=transform),
batch_size=batch_size,
shuffle=True)
###Output
_____no_output_____
###Markdown
Then, you can initialize your generator, discriminator, and optimizers.
###Code
gen = Generator(z_dim).to(device)
gen_opt = torch.optim.Adam(gen.parameters(), lr=lr, betas=(beta_1, beta_2))
disc = Discriminator().to(device)
disc_opt = torch.optim.Adam(disc.parameters(), lr=lr, betas=(beta_1, beta_2))
# You initialize the weights to the normal distribution
# with mean 0 and standard deviation 0.02
def weights_init(m):
if isinstance(m, nn.Conv2d) or isinstance(m, nn.ConvTranspose2d):
torch.nn.init.normal_(m.weight, 0.0, 0.02)
if isinstance(m, nn.BatchNorm2d):
torch.nn.init.normal_(m.weight, 0.0, 0.02)
torch.nn.init.constant_(m.bias, 0)
gen = gen.apply(weights_init)
disc = disc.apply(weights_init)
###Output
_____no_output_____
###Markdown
Finally, you can train your GAN!For each epoch, you will process the entire dataset in batches. For every batch, you will update the discriminator and generator. Then, you can see DCGAN's results! Here's roughly the progression you should be expecting. On GPU this takes about 30 seconds per thousand steps. On CPU, this can take about 8 hours per thousand steps. You might notice that in the image of Step 5000, the generator is disproprotionately producing things that look like ones. If the discriminator didn't learn to detect this imbalance quickly enough, then the generator could just produce more ones. As a result, it may have ended up tricking the discriminator so well that there would be no more improvement, known as mode collapse: 
###Code
n_epochs = 50
cur_step = 0
mean_generator_loss = 0
mean_discriminator_loss = 0
for epoch in range(n_epochs):
# Dataloader returns the batches
for real, _ in tqdm(dataloader):
cur_batch_size = len(real)
real = real.to(device)
## Update discriminator ##
disc_opt.zero_grad()
fake_noise = get_noise(cur_batch_size, z_dim, device=device)
fake = gen(fake_noise)
disc_fake_pred = disc(fake.detach())
disc_fake_loss = criterion(disc_fake_pred, torch.zeros_like(disc_fake_pred))
disc_real_pred = disc(real)
disc_real_loss = criterion(disc_real_pred, torch.ones_like(disc_real_pred))
disc_loss = (disc_fake_loss + disc_real_loss) / 2
# Keep track of the average discriminator loss
mean_discriminator_loss += disc_loss.item() / display_step
# Update gradients
disc_loss.backward(retain_graph=True)
# Update optimizer
disc_opt.step()
## Update generator ##
gen_opt.zero_grad()
fake_noise_2 = get_noise(cur_batch_size, z_dim, device=device)
fake_2 = gen(fake_noise_2)
disc_fake_pred = disc(fake_2)
gen_loss = criterion(disc_fake_pred, torch.ones_like(disc_fake_pred))
gen_loss.backward()
gen_opt.step()
# Keep track of the average generator loss
mean_generator_loss += gen_loss.item() / display_step
## Visualization code ##
if cur_step % display_step == 0 and cur_step > 0:
print(f"Step {cur_step}: Generator loss: {mean_generator_loss}, discriminator loss: {mean_discriminator_loss}")
show_tensor_images(fake)
show_tensor_images(real)
mean_generator_loss = 0
mean_discriminator_loss = 0
cur_step += 1
###Output
_____no_output_____ |
lectures/lecture_10.ipynb | ###Markdown
Lecture 10 - Basics of Curve Fitting: Bayesian Linear Regression
###Code
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
sns.set_context('talk')
sns.set_style('white')
%matplotlib inline
###Output
_____no_output_____
###Markdown
Objectives+ Maximum Posterior Estimate+ Bayesian Linear Regression+ The evidence approximation+ Automatic relevance determination. Readings+ These notes.+ [Ch. 3 of Bishop, 2006](http://www.amazon.com/Pattern-Recognition-Learning-Information-Statistics/dp/0387310738)+ [Mathematicalmonk, Bayesian Linear Regression](https://www.youtube.com/watch?v=dtkGq9tdYcI) Plese see the lecturee 9 before you start on this one.We just repeat some of the code that we developed there.In particular, we load the essential modules and we redefine the basis function classes.
###Code
# Linear Basis
class LinearBasis(object):
"""
Represents a 1D linear basis.
"""
def __init__(self):
self.num_basis = 2 # The number of basis functions
def __call__(self, x):
"""
``x`` should be a 1D array.
"""
return [1., x[0]]
# We need a generic function that computes the design matrix
def compute_design_matrix(X, phi):
"""
Arguments:
X - The observed inputs (1D array)
phi - The basis functions.
"""
num_observations = X.shape[0]
num_basis = phi.num_basis
Phi = np.ndarray((num_observations, num_basis))
for i in range(num_observations):
Phi[i, :] = phi(X[i, :])
return Phi
# Here is a class for the polynomials:
class PolynomialBasis(object):
"""
A set of linear basis functions.
Arguments:
degree - The degree of the polynomial.
"""
def __init__(self, degree):
self.degree = degree
self.num_basis = degree + 1
def __call__(self, x):
return np.array([x[0] ** i for i in range(self.degree + 1)])
class FourierBasis(object):
"""
A set of linear basis functions.
Arguments:
num_terms - The number of Fourier terms.
L - The period of the function.
"""
def __init__(self, num_terms, L):
self.num_terms = num_terms
self.L = L
self.num_basis = 2 * num_terms
def __call__(self, x):
res = np.ndarray((self.num_basis,))
for i in range(num_terms):
res[2 * i] = np.cos(2 * i * np.pi / self.L * x[0])
res[2 * i + 1] = np.sin(2 * (i+1) * np.pi / self.L * x[0])
return res
class RadialBasisFunctions(object):
"""
A set of linear basis functions.
Arguments:
X - The centers of the radial basis functions.
ell - The assumed lengthscale.
"""
def __init__(self, X, ell):
self.X = X
self.ell = ell
self.num_basis = X.shape[0]
def __call__(self, x):
return np.exp(-.5 * (x - self.X) ** 2 / self.ell ** 2).flatten()
class StepFunctionBasis(object):
"""
A set of step functions.
Arguments:
X - The centers of the step functions.
"""
def __init__(self, X):
self.X = X
self.num_basis = X.shape[0]
def __call__(self, x):
res = np.ones((self.num_basis, ))
res[x < self.X.flatten()] = 0.
return res
###Output
_____no_output_____
###Markdown
We will use the motorcycle data set of lecture 7.
###Code
data = np.loadtxt('motor.dat')
X = data[:, 0][:, None]
Y = data[:, 1]
###Output
_____no_output_____
###Markdown
Probabilistic Regression - Version 2+ We wish to model the data using some **fixed** basis/features:$$y(\mathbf{x};\mathbf{w}) = \sum_{j=1}^{m} w_{j}\phi_{j}(\mathbf{x}) = \mathbf{w^{T}\boldsymbol{\phi}(\mathbf{x})}$$+ We *model the measurement process* using a **likelihood** function:$$\mathbf{y}_{1:n} | \mathbf{x}_{1:n}, \mathbf{w}, \sigma \sim N(\mathbf{w^{T}\boldsymbol{\phi}(\mathbf{x})}, \sigma^2).$$+ We *model the uncertainty in the model parameters* using a **prior**:$$\mathbf{w} \sim p(\mathbf{w}).$$ Gaussian Prior on the Weights+ Consider the following **prior** on $\mathbf{w}$:$$p(\mathbf{w}|\alpha) = \mathcal{N}\left(\mathbf{w}|\mathbf{0},\alpha^{-1}\mathbf{I}\right) = \left(\frac{\alpha}{2\pi}\right)^{\frac{m}{2}}\exp\left\{-\frac{\alpha}{2}\lVert\mathbf{w}\rVert^2\right\}.$$+ We say:> Before we see the data, we beleive that $\mathbf{w}$ must be around zero with a precision of $\alpha$. Graphical Representation
###Code
from graphviz import Digraph
g = Digraph('bayes_regression')
g.node('alpha', label='<α>', style='filled')
g.node('w', label='<<b>w</b>>')
g.node('sigma', label='<σ>', style='filled')
with g.subgraph(name='cluster_0') as sg:
sg.node('xj', label='<<b>x</b><sub>j</sub>>', style='filled')
sg.node('yj', label='<y<sub>j</sub>>', style='filled')
sg.attr(label='j=1,...,n')
sg.attr(labelloc='b')
g.edge('alpha', 'w')
g.edge('sigma', 'yj')
g.edge('w', 'yj')
g.edge('xj', 'yj')
g.render('bayes_regression', format='png')
g
###Output
_____no_output_____
###Markdown
The Posterior of the Weights+ Combining the likelihood and the prior, we get using Bayes rule:$$p(\mathbf{w}|\mathbf{x}_{1:n},\mathbf{y}_{1:n}, \sigma,\alpha) = \frac{p(\mathbf{y}_{1:n}|\mathbf{x}_{1:n}, \mathbf{w}, \sigma)p(\mathbf{w}|\alpha)}{\int p(\mathbf{y}_{1:n}|\mathbf{x}_{1:n}, \mathbf{w}', \sigma)p(\mathbf{w}'|\alpha)d\mathbf{w}'}.$$+ We say> The posterior summarizes our state of knowledge about $\mathbf{w}$ after we see the data,if we know $\alpha$ and $\sigma$. Maximum Posterior Estimate+ We can find a point estimate of $\mathbf{w}$ by solving:$$\mathbf{w}_{\mbox{MPE}} = \arg\max_{\mathbf{w}} p(\mathbf{y}_{1:n}|\mathbf{x}_{1:n}, \mathbf{w}, \sigma)p(\mathbf{w}|\alpha).$$+ For Gaussian likelihood and weights:$$\log p(\mathbf{w}|\mathbf{x}_{1:n},\mathbf{y}_{1:n}, \sigma,\alpha) = - \frac{1}{2\sigma^2}\lVert\mathbf{\Phi}\mathbf{w}-\mathbf{y}_{1:n}\rVert^2 -\frac{\alpha}{2}\lVert\mathbf{w}\rVert^2.$$+ With maximum:$$\mathbf{w}_{\mbox{MPE}} = \left(\sigma^{-2}\mathbf{\Phi}^T\mathbf{\Phi}+\alpha\mathbf{I}\right)^{-1}\mathbf{\Phi}^T\mathbf{y}_{1:n}.$$+ But, no analytic formula for $\sigma$... The Stable Way to Compute the MAP Estimate+ Construct the positive-definite matrix:$$\mathbf{A} = \left(\sigma^{-2}\mathbf{\Phi}^T\mathbf{\Phi}+\alpha\mathbf{I}\right)$$+ Compute the [Cholesky decomposition](https://en.wikipedia.org/wiki/Cholesky_decomposition) of $\mathbf{A}$:$$\mathbf{A} = \mathbf{L}\mathbf{L}^T,$$where $\mathbf{L}$ is lower triangular.+ Then, solve the system:$$\mathbf{L}\mathbf{L}^T\mathbf{w} = \mathbf{\Phi}^T\mathbf{y}_{1:n},$$doing a forward and a backward substitution.+ [scipy.linalg.cho_factor](http://docs.scipy.org/doc/scipy-0.14.0/reference/generated/scipy.linalg.cho_factor.htmlscipy.linalg.cho_factor) and [scipy.linalg.cho_solve](http://docs.scipy.org/doc/scipy-0.14.0/reference/generated/scipy.linalg.cho_solve.html) can be used for this. Radial Basis Functions
###Code
import scipy.linalg
ell = 2.
alpha = 5
sigma = 20.28
Xc = np.linspace(0, 60, 20)
phi = RadialBasisFunctions(Xc, ell)
Phi = compute_design_matrix(X, phi)
A = np.dot(Phi.T, Phi) / sigma ** 2. + alpha * np.eye(Phi.shape[1])
L = scipy.linalg.cho_factor(A)
w_MPE = scipy.linalg.cho_solve(L, np.dot(Phi.T, Y))
print('w_MPE:')
print(w_MPE)
# Let's predict on these points:
X_p = np.linspace(0, 60, 100)[:, None]
Phi_p = compute_design_matrix(X_p, phi)
Y_p = np.dot(Phi_p, w_MPE)
Y_l = Y_p - 2. * sigma # Lower predictive bound
Y_u = Y_p + 2. * sigma # Upper predictive bound
fig, ax = plt.subplots()
ax.plot(X, Y, 'x', markeredgewidth=2, label='Observations')
ax.plot(X_p, Y_p, label='MPE Prediction (Radial Basis Functions, alpha=%1.2f)' % alpha)
ax.fill_between(X_p.flatten(), Y_l, Y_u, color=sns.color_palette()[1], alpha=0.25)
ax.set_xlabel('$x$')
ax.set_ylabel('$y$')
plt.legend(loc='best');
###Output
_____no_output_____
###Markdown
Questions+ Experiment with different alphas.+ When are we underfitting?+ When are we overfitting?+ Which one (if any) gives you the best fit? Issues with Maximum Posterior Estimate+ How many basis functions should I use?+ Which basis functions should I use?+ How do I pick the parameters of the basis functions, e.g., the lengthscale $\ell$ of the RBFs, $\alpha$, etc.? Probabilistic Regression - Version 3 - Bayesian Linear Regression+ For Gaussian likelihood and weights, the posterior is Gaussian:$$p(\mathbf{w}|\mathbf{x}_{1:n},\mathbf{y}_{1:n}, \sigma, \alpha) = \mathcal{N}\left(\mathbf{w}|\mathbf{m}, \mathbf{S}\right),$$where$$\mathbf{S} = \left(\sigma^{-2}\mathbf{\Phi}^T\mathbf{\Phi}+\alpha\mathbf{I}\right)^{-1},$$and$$\mathbf{m} = \sigma^{-2}\mathbf{S}\Phi^T\mathbf{y}_{1:n}.$$+ In general: [Markov Chain Monte Carlo](https://en.wikipedia.org/wiki/Markov_chain_Monte_Carlo). Posterior Predictive Distribution+ Using probability theory, we ask: What do we know about $y$ at a new $\mathbf{x}$ after seeing the data.+ We have using the sum rule:$$p(y|\mathbf{x}, \mathbf{x}_{1:n}, \mathbf{y}_{1:n}, \sigma, \alpha) = \int p(y | \mathbf{x}, \mathbf{w}, \sigma) p(\mathbf{w}|\mathbf{x}_{1:n}, \mathbf{y}_{1:n},\sigma,\alpha)d\mathbf{w}.$$+ For Gaussian likelihood and prior:$$p(y|\mathbf{x}, \mathbf{x}_{1:n}, \mathbf{y}_{1:n}, \sigma, \alpha) = \mathcal{N}\left(y|m(\mathbf{x}), s^2(\mathbf{x})\right),$$where$$m(\mathbf{x}) = \mathbf{m}^T\boldsymbol{\phi}(\mathbf{x})\;\mbox{and}\;s(\mathbf{x}) = \boldsymbol{\phi}(\mathbf{x})^T\mathbf{S}\boldsymbol{\phi}(\mathbf{x}) + \sigma^2.$$ Predictive Uncertainty+ The **predictive uncertainty** is:$$s^2(\mathbf{x}) = \boldsymbol{\phi}(\mathbf{x})^T\mathbf{S}\boldsymbol{\phi}(\mathbf{x}) + \sigma^2.$$+ $\sigma^2$ corresponds to the measurement noise.+ $\boldsymbol{\phi}(\mathbf{x})^T\mathbf{S}\boldsymbol{\phi}(\mathbf{x})$ is the epistemic uncertainty induced by limited data.
###Code
import scipy.linalg
ell = 2.
alpha = 0.001
sigma = 20.28
Xc = np.linspace(0, 60, 20)
phi = RadialBasisFunctions(Xc, ell)
Phi = compute_design_matrix(X, phi)
A = np.dot(Phi.T, Phi) / sigma ** 2. + alpha * np.eye(Phi.shape[1])
L = scipy.linalg.cho_factor(A)
m = scipy.linalg.cho_solve(L, np.dot(Phi.T, Y) / sigma ** 2) # The posterior mean of w
S = scipy.linalg.cho_solve(L, np.eye(Phi.shape[1])) # The posterior covariance of w
Phi_p = compute_design_matrix(X_p, phi)
Y_p = np.dot(Phi_p, m) # The mean prediction
V_p_ep = np.einsum('ij,jk,ik->i', Phi_p, S, Phi_p) # The epistemic uncertainty
S_p_ep = np.sqrt(V_p_ep)
V_p = V_p_ep + sigma ** 2 # Full uncertainty
S_p = np.sqrt(V_p)
Y_l_ep = Y_p - 2. * S_p_ep # Lower epistemic predictive bound
Y_u_ep = Y_p + 2. * S_p_ep # Upper epistemic predictive bound
Y_l = Y_p - 2. * S_p # Lower predictive bound
Y_u = Y_p + 2. * S_p # Upper predictive bound
fig, ax = plt.subplots()
ax.plot(X, Y, 'x', markeredgewidth=2, label='Observations')
ax.plot(X_p, Y_p, label='Bayesian Prediction (Radial Basis Functions, alpha=%1.2f)' % alpha)
ax.fill_between(X_p.flatten(), Y_l_ep, Y_u_ep, color=sns.color_palette()[2], alpha=0.25)
ax.fill_between(X_p.flatten(), Y_l, Y_l_ep, color=sns.color_palette()[1], alpha=0.25)
ax.fill_between(X_p.flatten(), Y_u_ep, Y_u, color=sns.color_palette()[1], alpha=0.25)
ax.set_xlabel('$x$')
ax.set_ylabel('$y$')
plt.legend(loc='best');
###Output
_____no_output_____
###Markdown
Sampling Posterior Models+ We can actually sample models (functions) from the posterior. Here is how: + Sample a $\mathbf{w}$ from $p(\mathbf{w}|\mathbf{x}_{1:n},\mathbf{y}_{1:n}, \sigma, \alpha)$. + Look at the sampled model: $$ y(\mathbf{x};\mathbf{w}) = \mathbf{w}^T\boldsymbol{\phi}(\mathbf{x}). $$
###Code
# We have m, S, X_p, and Phi_p from before
fig, ax = plt.subplots()
ax.plot(X, Y, 'x', markeredgewidth=2, label='Observations')
for i in range(10):
w = np.random.multivariate_normal(m, S)
Y_p_s = np.dot(Phi_p, w)
ax.plot(X_p, Y_p_s, color=sns.color_palette()[2], linewidth=0.5);
ax.set_xlabel('$x$')
ax.set_ylabel('$y$')
###Output
_____no_output_____
###Markdown
Issues with Bayesian Linear Regression+ How many basis functions should I use?+ Which basis functions should I use?+ How do I pick the parameters of the basis functions, e.g., the lengthscale $\ell$ of the RBFs, $\alpha$, etc.?+ Questions+ Experiment with different alphas, ells, and sigmas.+ When are we underfitting?+ When are we overfitting?+ Which one (if any) gives you the best fit?+ In the figure, right above: Increase the number of posterior $\mathbf{w}$ samples to get a sense of the epistemic uncertainty induced by the limited data. Probabilistic Regression - Version 4 - Hierarchical Priors+ So, how do we find all the parameters like $\sigma$, $\alpha$, $\ell$, etc?+ These are all called **hyper-parameters** of the model.+ Call all of them$$\boldsymbol{\theta} = \{\sigma, \alpha, \ell,\dots\}.$$ Hierarchical Priors+ Model:$$y(\mathbf{x};\mathbf{w}) = \sum_{j=1}^{m} w_{j}\phi_{j}(\mathbf{x}) = \mathbf{w^{T}\boldsymbol{\phi}(\mathbf{x})}$$+ Likelihood:$$\mathbf{y}_{1:n} | \mathbf{x}_{1:n}, \mathbf{w}, \boldsymbol{\theta} \sim p(\mathbf{y}_{1:n}|\mathbf{x}_{1:n}, \mathbf{w}, \boldsymbol{\theta}).$$+ Weight prior:$$\mathbf{w} | \boldsymbol{\theta} \sim p(\mathbf{w}| \boldsymbol{\theta}).$$+ Hyper-prior:$$\boldsymbol{\theta} \sim p(\boldsymbol{\theta}).$$Graphically:
###Code
g2 = Digraph('full_bayes_regression_2')
g2.node('alpha', label='<α>')
g2.node('w', label='<<b>w</b>>')
g2.node('sigma', label='<σ>')
with g2.subgraph(name='cluster_0') as sg:
sg.node('xj', label='<<b>x</b><sub>j</sub>>', style='filled')
sg.node('yj', label='<y<sub>j</sub>>', style='filled')
sg.attr(label='j=1,...,n')
sg.attr(labelloc='b')
g2.edge('alpha', 'w')
g2.edge('sigma', 'yj')
g2.edge('w', 'yj')
g2.edge('xj', 'yj')
g2.render('full_bayes_regression_2', format='png')
g2
###Output
_____no_output_____
###Markdown
Fully Bayesian Solution+ Just write down the posterior of everything:$$p(\mathbf{w}, \boldsymbol{\theta}|\mathbf{x}_{1:n}, \mathbf{y}_{1:n}) \propto p(\mathbf{y}_{1:n}|\mathbf{x}_{1:n}|\mathbf{w},\boldsymbol{\theta})p(\mathbf{w}|\boldsymbol{\theta})p(\boldsymbol{\theta}).$$+ and, somehow, sample from it... The Evidence Approximation+ Look at the marginal posterior of $\boldsymbol{\theta}$:$$p(\boldsymbol{\theta}|\mathbf{x}_{1:n}, \mathbf{y}_{1:n}) \propto \int p(\mathbf{y}_{1:n}|\mathbf{x}_{1:n}|\mathbf{w},\boldsymbol{\theta})p(\mathbf{w}|\boldsymbol{\theta})p(\boldsymbol{\theta})d\mathbf{w}.$$+ Assume that the hyper-prior is relatively flat:$$p(\boldsymbol{\theta}) \propto 1,$$+ Use a MAP estimate for $\boldsymbol{\theta}$:$$\boldsymbol{\theta}_{\mbox{EV}} = \arg\max_{\boldsymbol{\theta}}\int p(\mathbf{y}_{1:n}|\mathbf{x}_{1:n}|\mathbf{w},\boldsymbol{\theta})p(\mathbf{w}|\boldsymbol{\theta})d\mathbf{w}.$$+ Analytical for Gaussian likelihood and prior. Implementation Evidence Approximation+ There is a fast algorithm for the evidence approximation for Bayesian linear regression.+ It would take about an hour to go over it. See Ch. 3 of (Bishop, 2006).+ We will use the implementation found in [scikit-learn](http://scikit-learn.org).+ If you don't have it:```conda install scikit-learn``` Radial Basis Functions
###Code
from sklearn.linear_model import BayesianRidge
ell = 2.
Xc = np.linspace(0, 60, 50)
phi = RadialBasisFunctions(Xc, ell)
Phi = compute_design_matrix(X, phi)
regressor = BayesianRidge()
regressor.fit(Phi, Y)
# They are using different names:
sigma = np.sqrt(1. / regressor.alpha_)
print('best sigma:', sigma)
alpha = regressor.lambda_
print('best alpha:', alpha)
A = np.dot(Phi.T, Phi) / sigma ** 2. + alpha * np.eye(Phi.shape[1])
L = scipy.linalg.cho_factor(A)
m = scipy.linalg.cho_solve(L, np.dot(Phi.T, Y) / sigma ** 2) # The posterior mean of w
S = scipy.linalg.cho_solve(L, np.eye(Phi.shape[1])) # The posterior covariance of w
Phi_p = compute_design_matrix(X_p, phi)
Y_p = np.dot(Phi_p, m) # The mean prediction
V_p_ep = np.einsum('ij,jk,ik->i', Phi_p, S, Phi_p) # The epistemic uncertainty
S_p_ep = np.sqrt(V_p_ep)
V_p = V_p_ep + sigma ** 2 # Full uncertainty
S_p = np.sqrt(V_p)
Y_l_ep = Y_p - 2. * S_p_ep # Lower epistemic predictive bound
Y_u_ep = Y_p + 2. * S_p_ep # Upper epistemic predictive bound
Y_l = Y_p - 2. * S_p # Lower predictive bound
Y_u = Y_p + 2. * S_p # Upper predictive bound
fig, ax = plt.subplots()
ax.plot(X, Y, 'x', markeredgewidth=2, label='Observations')
ax.plot(X_p, Y_p, label='Bayesian Prediction (Radial Basis Functions, alpha=%1.2f)' % alpha)
ax.fill_between(X_p.flatten(), Y_l_ep, Y_u_ep, color=sns.color_palette()[2], alpha=0.25)
ax.fill_between(X_p.flatten(), Y_l, Y_l_ep, color=sns.color_palette()[1], alpha=0.25)
ax.fill_between(X_p.flatten(), Y_u_ep, Y_u, color=sns.color_palette()[1], alpha=0.25)
ax.set_xlabel('$x$')
ax.set_ylabel('$y$')
plt.legend(loc='best');
###Output
_____no_output_____
###Markdown
Issues with Bayesian Linear Regression+ How many basis functions should I use?+ Which basis functions should I use? Questions+ Try the evidence approximation with the Fourier basis.+ Try the evidence approximation with the Step function basis. Probabilistic Regression - Version 5 - Automatic Relevance Determination+ Use a different precision $\alpha_i$ for each weight:$$p(w_j | \alpha_j) \propto \exp\left\{-\alpha_jw_j^2\right\},$$+ so that:$$p(\mathbf{w}|\boldsymbol{\alpha}) = \prod_{j=1}^mp(w_j|\alpha_j).$$Graphically:
###Code
g3 = Digraph('full_bayes_regression_3')
g3.node('sigma', label='<σ>')
with g3.subgraph(name='cluster_0') as sg:
sg.node('xj', label='<<b>x</b><sub>j</sub>>', style='filled')
sg.node('yj', label='<y<sub>j</sub>>', style='filled')
sg.attr(label='j=1,...,n')
sg.attr(labelloc='b')
with g3.subgraph(name='cluster_1') as sg:
sg.node('alphai', label='<α<sub>i</sub>>')
sg.node('wi', label='<w<sub>i</sub>>')
sg.attr(label='i=1,...,m')
sg.attr(labelloc='b')
g3.edge('alphai', 'wi')
g3.edge('sigma', 'yj')
g3.edge('wi', 'yj')
g3.edge('xj', 'yj')
g3.render('full_bayes_regression_3', format='png')
g3
###Output
_____no_output_____
###Markdown
Again, we need advanced techniques to carry out full Bayesian inference.A quick way out is to maximize the **evidence** with respect to all the $\alpha_j$'s. Radial Basis Functions
###Code
from sklearn.linear_model import ARDRegression
ell = 2.
Xc = np.linspace(0, 60, 50)
phi = RadialBasisFunctions(Xc, ell)
Phi = compute_design_matrix(X, phi)
regressor = ARDRegression()
regressor.fit(Phi, Y)
# They are using different names:
sigma = np.sqrt(1. / regressor.alpha_)
print('best sigma:', sigma)
alpha = regressor.lambda_
print('best alpha:', alpha)
A = np.dot(Phi.T, Phi) / sigma ** 2. + alpha * np.eye(Phi.shape[1])
L = scipy.linalg.cho_factor(A)
m = scipy.linalg.cho_solve(L, np.dot(Phi.T, Y) / sigma ** 2) # The posterior mean of w
S = scipy.linalg.cho_solve(L, np.eye(Phi.shape[1])) # The posterior covariance of w
Phi_p = compute_design_matrix(X_p, phi)
Y_p = np.dot(Phi_p, m) # The mean prediction
V_p_ep = np.einsum('ij,jk,ik->i', Phi_p, S, Phi_p) # The epistemic uncertainty
S_p_ep = np.sqrt(V_p_ep)
V_p = V_p_ep + sigma ** 2 # Full uncertainty
S_p = np.sqrt(V_p)
Y_l_ep = Y_p - 2. * S_p_ep # Lower epistemic predictive bound
Y_u_ep = Y_p + 2. * S_p_ep # Upper epistemic predictive bound
Y_l = Y_p - 2. * S_p # Lower predictive bound
Y_u = Y_p + 2. * S_p # Upper predictive bound
fig, ax = plt.subplots()
ax.plot(X, Y, 'x', markeredgewidth=2, label='Observations')
ax.plot(X_p, Y_p, label='Bayesian Prediction (Radial Basis Functions, ARD)')
ax.fill_between(X_p.flatten(), Y_l_ep, Y_u_ep, color=sns.color_palette()[2], alpha=0.25)
ax.fill_between(X_p.flatten(), Y_l, Y_l_ep, color=sns.color_palette()[1], alpha=0.25)
ax.fill_between(X_p.flatten(), Y_u_ep, Y_u, color=sns.color_palette()[1], alpha=0.25)
ax.set_xlabel('$x$')
ax.set_ylabel('$y$')
plt.legend(loc='best');
###Output
_____no_output_____
###Markdown
Lecture 10 - Uncertainty Propagation: Perturbative Methods Objectives+ To remember the Taylor expansion of multivaritae real functions+ To use the Laplace approximation to approximate arbitrary probability densities as Gaussians+ To use the Taylor expansion and the Laplace approximation to (approximately) propagate uncertainties through models+ To use the method of adjoints to calculate derivatives of dynamical systems or partial differential equations with respect to uncertain parameters Readings+ None
###Code
import numpy as np
import math
import scipy.stats as st
import scipy
import matplotlib as mpl
import matplotlib.pyplot as plt
%matplotlib inline
import seaborn as sns
sns.set_style('white')
sns.set_context('talk')
import warnings
warnings.filterwarnings('ignore')
###Output
_____no_output_____
###Markdown
The Taylor Expansion in 1DLet $g:\mathbb{R}\rightarrow\mathbb{R}$ be a smooth real function and $x_0\in\mathbb{R}$.The [Taylor series expansion](https://en.wikipedia.org/wiki/Taylor_series) of $g(x)$ abount $x_0$ is:$$g(x) = g(x_0) + \frac{dg(x_0)}{dx}(x-x_0) + \frac{1}{2}\frac{d^2g(x_0)}{dx^2}(x-x_0)^2+\dots + \frac{1}{n!}\frac{d^ng(x_0)}{dx^n}(x-x_0)^n+\dots$$You can use the Taylor expansion to approximate any function as a polynomial. Example: 1D Taylor Series ExpansionTake $g(x) = \sin(x)$ and $x_0=0$.We have:$$\frac{dg(0)}{dx} = \cos(x)|_{x=0} = 1,$$$$\frac{d^2g(0)}{dx^2} = -\sin(x)_{x=0} = 0,$$$$\frac{d^3g(0)}{dx^3} = -\cos(x)_{x=0} = -1,$$and in general:$$\sin(x) = \sum_{k=0}^{\infty} \frac{(-1)^k}{(2k+1)!}x^{2k+1}.$$
###Code
max_taylor_order = 3
fig, ax = plt.subplots()
x = np.linspace(-np.pi, np.pi, 100)
y_true = np.sin(x)
y_taylor = np.zeros(x.shape)
for k in range(max_taylor_order):
y_taylor += (-1.) ** k * 1. / math.factorial(2 * k + 1) * x ** (2 * k + 1)
ax.plot(x, y_true, label='$\sin(x)$')
ax.plot(x, y_taylor, '--', label='Truncated Taylor series')
plt.legend(loc='best')
ax.set_ylim(-1.5, 1.5)
ax.set_title('Maximum Taylor order: %d' % max_taylor_order)
ax.set_xlabel('$x$')
ax.set_ylabel('$g(x)$');
###Output
_____no_output_____
###Markdown
Questions+ Start increasing the maximum order of the series expansion until you get a satisfactory approximation. The Taylor Expansion in Higher DimensionsLet $g:\mathbb{R}^d\rightarrow \mathbb{R}$ and $x_0\in\mathbb{R}^d$.The Taylor series expansion is:$$g(x) = g(x_0) + \sum_{i=1}^d\frac{\partial g(x_0)}{\partial x_i}(x_i-x_{0i})+\frac{1}{2}\sum_{i,j=1}^d\frac{\partial^2g(x_0)}{\partial x_i\partial x_j}(x_i-x_{0i})(x-x_{0j}) + \dots$$Another way of writing this is:$$g(x) = g(x_0) + \nabla g(x_0)^T(x-x_0) + \frac{1}{2}(x-x_0)^T\nabla^2 g(x_0) (x-x_0),$$where the *Jacobian* is defined as:$$\nabla g(x_0) = \left(\frac{\partial g(x_0)}{\partial x_1},\dots,\frac{\partial g(x_0)}{\partial x_1}\right),$$and the *Hessian* is:$$\nabla^2 g(x_0) = \left(\frac{\partial^2g(x_0)}{\partial x_i\partial x_j}\right)_{i,j=1}^d.$$ The Laplace Approximation in 1DIf you are not interested in probabilities of rare events, you may approximate any probability density as a Gaussian (assuming that it is sufficiently narrow).Let $X$ be a random variable with probability density $p(x)$.Because $p(x)$ is positive, it is better to work with its logarithm.First, we find the maximum of $\log p(x)$ which is called the *nominal value* (or just maximum):$$x_0 = \arg\max_x \log p(x).$$Then, we take the Taylor expansion of $\log p(x)$ about $x=x_0$:$$\log p(x) = \log p(x_0) + \frac{d\log p(x_0)}{dx} (x-x_0) + \frac{1}{2}\frac{d^2\log p(x_0)}{dx^2} (x-x_0)^2 + \dots.$$Since $x_0$ is a critical point of $\log p(x)$, we must have that:$$\frac{d\log p(x_0)}{dx} = 0.$$So, the expansion becomes:$$\log p(x) = \frac{1}{2}\frac{d^2\log p(x_0)}{dx^2} (x-x_0)^2 + \text{const}.$$Therefore,$$p(x) \propto \exp\left\{ \frac{1}{2}\frac{d^2\log p(x_0)}{dx^2} (x-x_0)^2 \right\}.$$Since $x_0$ is a maximum of $\log p(x)$, the matrix $\frac{d^2\log p(x_0)}{dx^2}$ must be a negative number.Therefore, the number:$$c^2 = -\left[\frac{d^2\log p(x_0)}{dx^2}\right]^{-1},$$is positive.By inspection then, we see that:$$p(x) \propto \exp\left\{-\frac{(x-x_0)^2}{2c^2}\right\}.$$Ignoring all higher order terms, we conclude:$$p(x) \approx \mathcal{N}\left(x|x_0, -\left[\frac{d^2\log p(x_0)}{dx^2}\right]^{-1}\right).$$This is the Laplace approximation in one dimension. ExampleLet's just approximate a Gamma distribution with a Gaussian.We will do a case that works and a case that does not work.
###Code
# Construct the ``true random variable``
alpha = 10
X = st.gamma(a=alpha)
# Find the maximum using an optimization method (the minus is because we will use a minimization method)
minus_log_pdf_true = lambda x: -np.log(X.pdf(x))
res = scipy.optimize.minimize_scalar(minus_log_pdf_true, bounds=[1., 8])
# This is the maximum of the pdf
x_0 = res.x
# This is the value of the pdf at the maximum:
p_0 = np.exp(-res.fun)
# The derivative of the pdf at the maximum should be exaclty zero.
# Let's verify it using numerical integration
grad_m_log_p_0 = scipy.misc.derivative(minus_log_pdf_true, x_0, dx=1e-6, n=1)
print('grad minus log p at x0 is: ', grad_m_log_p_0)
# We need the second derivative.
# We will get it using numerical integration as well:
grad_2_m_log_p_0 = scipy.misc.derivative(minus_log_pdf_true, x_0, dx=1e-6, n=2)
# The standard deviation of the Gaussian approximation to p(x) is:
c = np.sqrt(1. / grad_2_m_log_p_0)
# Using scipy code, the random variable that approximates X is:
Z = st.norm(loc=x_0, scale=c)
# Let's plot everything
fig, ax = plt.subplots()
x = np.linspace(x_0 - 6 * c, x_0 + 6 * c, 200)
pdf_true = X.pdf(x)
# Plot the truth:
ax.plot(x, pdf_true)
# Mark the location of the maximum:
# Plot the approximation
ax.plot(x, Z.pdf(x), '--')
ax.plot([x_0] * 10, np.linspace(0, p_0, 10), ':')
plt.legend(['PDF of Gamma(%.2f)' % alpha, 'Laplace approximation'], loc='best')
ax.set_xlabel('$x$')
ax.set_ylabel('PDF');
###Output
grad minus log p at x0 is: 1.7763568394002505e-09
###Markdown
The Laplace Approximation in Many DimensionsThen, we take the Taylor expansion of $\log p(x)$ about $x=x_0$:$$\log p(x) = \log p(x_0) + \nabla \log p(x_0) (x-x_0) + \frac{1}{2}(x-x_0)^T\nabla^2 \log p(x_0) (x-x_0) + \dots.$$Since $x_0$ is a critical point of $\log p(x)$, we must have that:$$\nabla \log p(x_0) = 0.$$So, the expansion becomes:$$\log p(x) = \frac{1}{2}(x-x_0)^T\nabla^2 \log p(x_0) (x-x_0) + \text{const}.$$Therefore,$$p(x) \propto \exp\left\{ \frac{1}{2}(x-x_0)^T\nabla^2 \log p(x_0) (x-x_0) \right\}.$$Since $x_0$ is a maximum of $\log p(x)$, the matrix $\nabla^2 \log p(x_0)$ must be negative definite.Therefore, the matrix:$$C = -(\nabla^2\log p(x_0))^{-1},$$is positive definite.By inspection then, we see that:$$p(x) \propto \exp\left\{-\frac{1}{2}(x-x_0)^TC^{-1}(x-x_0)\right\}.$$Ignoring all higher order terms, we conclude:$$p(x) \approx \mathcal{N}\left(x|x_0, -(\nabla^2\log p(x_0))^{-1}\right).$$This is the Laplace approximation in many dimensions. Questions+ Experiment with various values of alpha for the true Gamma distribution. What happens when alpha is smaller than one. Why doesn't the method work at for that parameter? Perturbation Approach in 1DLet $X\sim p$ be a random vector and $Y=f(X)$ a scalar quantity of interest that depends on $X$.Our goal is to estimate the first order statistics of $Y$.In particular, the mean:$$\mathbb{E}[f(X)] = \int f(x) p(x)dx,$$and the variance:$$\mathbb{V}[f(X)] = \int \left(f(x)-\mathbb{E}[f(X)]\right)^2p(x)dx.$$First, we take the laplace approximation for the random vector and approximate its density as a Gaussian.That is, we write:$$p(x) \approx \mathcal{N}\left(x|\mu, \sigma^2\right),$$with $\mu$ and $\Sigma$ as given by the Laplace approximation.Now, we take the Taylor expansion of $f(x)$ around $x=\mu$:$$f(x) = f(\mu) + \frac{df(\mu)}{dx} (x-\mu)+\dots$$Now, let's take the expecation over the Laplace approximation of $p(x)$.$$\begin{array}{ccc}\mathbb{E}[f(X)] &=& \mathbb{E}\left[f(\mu) + \frac{df(\mu)}{dx} (X-\mu) + \dots\right]\\&=& f(\mu) + \frac{df(\mu)}{dx}\mathbb{E}[X-\mu] + \dots\\&=& f(\mu) + \dots\end{array}$$So, to *second order* we have:$$\mathbb{E}[f(X)] \approx f(\mu).$$You can, of course, construct higher order approximations in this way.Let's now do the variance.We will use the formula:$$\mathbb{V}[f(X)] = \mathbb{E}[f^2(X)] - \left[\mathbb{E}[f(X)]\right]^2.$$Keeping up to second order terms, we get:$$\begin{array}{ccc}\mathbb{E}[f^2(X)] &=& \mathbb{E}\left[\left(f(\mu) + \frac{df(\mu)}{dx} (X-\mu) + \dots\right)^2\right]\\&=& f^2(\mu) + \left(\frac{df(\mu)}{dx}\right)^2\mathbb{E}[(X-\mu)^2] + 2f(\mu)\frac{df(\mu)}{dx}\mathbb{E}[X-\mu] + \dots\\&=& f^2(\mu) + \left(\frac{df(\mu)}{dx}\right)^2\sigma^2 + \dots\end{array}$$Therefore, for the variance we get:$$\begin{array}{ccc}\mathbb{V}[f(X)] &=& f^2(\mu) + \left(\frac{df(\mu)}{dx}\right)^2\sigma^2 + \dots - \left(f(\mu) + \dots\right)^2\\&=& f^2(\mu) + \left(\frac{df(\mu)}{dx}\right)^2\sigma^2 - f^2(\mu) + \dots\\&=& \left(\frac{df(\mu)}{dx}\right)^2\sigma^2 + \dots\end{array}$$So, to *first order* we have:$$\mathbb{V}[f(X)] \approx \left(\frac{df(\mu)}{dx}\right)^2\sigma^2$$And, of course, we can go to higher order approximations.Now, having approximations for the mean and the variance, we may approximate the full density of the random variable $Y=f(X)$ by a Gaussian:$$p(y) \approx \mathcal{N}\left(y|f(\mu), \left(\frac{df(\mu)}{dx}\right)^2\sigma^2\right).$$ Example: 1D Propagation of UncertaintyLet's solve a (very simple) uncertainty propagation problem using these techniques.Consider:$$X\sim \mathcal{N}(\pi/2, 0.1^2),$$and$$f(x) = \sin(x) e^{-0.5x}.$$We have:
###Code
# Defining the densit of X:
mu = 1.1
sigma = 0.1
X = st.norm(loc=mu, scale=sigma)
# The function through which we want to propagate uncertainties
f = lambda x: np.sin(x) * np.exp(-0.5 * x)
fig1, ax1 = plt.subplots()
x = np.linspace(0., np.pi, 200)
ax1.plot(x, f(x))
ax1.set_xlabel('$x$')
ax1.set_ylabel('$f(x)$', color=sns.color_palette()[0])
ax1.tick_params('y', colors=sns.color_palette()[0])
ax2 = ax1.twinx()
ax2.plot(x, X.pdf(x), color=sns.color_palette()[1])
ax2.set_ylabel('$p(x)$', color=sns.color_palette()[1])
ax2.tick_params('y', colors=sns.color_palette()[1])
# The function at mu
f_at_mu = f(mu)
# The first derivative of the function at mu
df_at_mu = scipy.misc.derivative(f, mu, dx=1e-8, n=1)
# We can now construct a Gaussian approximation to the distribution of f(X)
exp_f_X = f_at_mu
var_f_X = f_at_mu ** 2 * sigma ** 2
f_X = st.norm(loc=exp_f_X, scale=np.sqrt(var_f_X))
# We will compare to the histogram of 10,000 samples
y_samples = [f(X.rvs()) for _ in range(10000)]
fig, ax = plt.subplots()
y = np.linspace(exp_f_X - 6 * np.sqrt(var_f_X), exp_f_X + 6 * np.sqrt(var_f_X), 100)
ax.plot(y, f_X.pdf(y))
ax.set_xlim(0., 0.6)
ax.hist(y_samples, bins=10, normed=True, alpha=0.5);
ax.set_xlabel('$y$')
ax.set_ylabel('$p(y)$');
###Output
_____no_output_____
###Markdown
Perturbation Approach in Many DimensionsLet $X\sim\mathcal{N}(\mu,\Sigma)$ be a d-dimensional Gaussian random vector.If $X$ was not Gaussian, we could use the Laplace approximation.Let $f(x)$ be a m-dimensional function of $x$:$$f:\mathbb{R}^d\rightarrow \mathbb{R}^m.$$To get the Taylor series expansion of $f(x)$, you need to work out the Taylor series expansion of each output component and put everything back together.You will get:$$f(x) = f(\mu) + \nabla f(\mu) (x - \mu) + \dots,$$where $\nabla f(\mu)\in\mathbb{R}^{m\times d}$ is the Jacobian matrix defined by:$$\nabla f(\mu) = \left(\begin{array}{ccc}\frac{\partial f_1(\mu)}{\partial x_1} & \dots & \frac{\partial f_1(\mu)}{\partial x_d}\\\vdots & \ddots & \vdots\\\frac{\partial f_m(\mu)}{\partial x_1} & \dots & \frac{\partial f_m(\mu)}{\partial x_d}\end{array}\right)$$To first order, the expectation of $Y = f(X)$ is:$$\mathbb{E}[f(X)] \approx f(\mu),$$while the covariance matrix is:$$\mathbb{C}[f(X)] \approx \nabla f(\mu)\Sigma \left(\nabla f(\mu)\right)^T,$$Putting these two things together, we may approximate the density of $Y=f(X)$ with a multivariate Gaussian:$$p(y) \approx \mathcal{N}\left(y|f(\mu), \nabla f(\mu)\Sigma \left(\nabla f(\mu)\right)^T\right).$$ Perturbation Approach for Stochastic Dynamical Systems with Low Dimensional, Small UncertaintiesPotentially using the Laplace approximation, assume that we have a $d$-dimensional random vector:$$X\sim\mathcal{N}(\mu, \Sigma).$$Consider the $m$-dimensional dynamical system:$$\frac{dy}{dt} = g(y;X),$$with initial conditions$$y(0) = y_0(X).$$Since $X$ is a random vector, the response of the dynamical system $Y(t)$ is a stochastic process:$$Y(t) = y(t; X),$$where $y(t;X)$ is the solution of the initial value problem for a specific realization of the random vector $X$. We will approximate this stochastic process with a Gaussian process:$$Y(\cdot) \sim \mbox{GP}\left(m(\cdot), k(\cdot, \cdot)\right).$$This can easily be done, using the results of the previous sections.The mean is:$$m(t) = \mathbb{E}[Y(t)] = \mathbb{E}[y(t;X)] = y(t;\mu).$$The covariance between two times is:$$k(t,t') = \mathbb{C}[Y(t), Y(t')] = \mathbb{C}[y(t;X), y(t';X)] = \nabla_x y(t;\mu) \Sigma \left(\nabla_x y(t';\mu)\right)^T.$$The only complicating factor, is that we need a way to get $\nabla_x y(t;\mu)$.For this, we need the method of adjoints. Calculating Sensitivities in Dynamical Systems using the Method of AjdointsWe will show how we can compute $\nabla_x y(t;\mu)$ using the method of adjoints.The idea is simple.We will derive the differential equation that $\nabla_x y(t;\mu)$ satisfies and we will be solving it as we solve for $y(t;\mu)$.Let $i\in\{1,\dots,m\}$ and $j\in\{1,\dots,d\}$.We start with the initial conditions.We have:$$\frac{\partial y_i(0;\mu)}{\partial x_j} = \frac{\partial y_{0i}(\mu)}{\partial x_j}.$$Now, let's do the derivatives:$$\begin{array}{ccc}\frac{d}{dt}\frac{\partial y_i(t;\mu)}{\partial x_j} &=& \frac{\partial }{\partial x_j}\frac{dy_i(t,\mu)}{dt} \\&=& \frac{\partial }{\partial x_j} g_i(y(t;\mu); \mu)\\&=& \sum_{r=1}^m\frac{\partial g_i(y(t;\mu); \mu)}{\partial y_r}\frac{\partial y_r(t;\mu)}{\partial x_j} + \frac{\partial g_i(y(t;\mu); \mu)}{\partial x_j}.\end{array}$$So, we see that the dynamical system that $\nabla_x y(t;\mu)$ satisfies is:$$\frac{d}{dt}\nabla_x y(t;\mu) = \left((\nabla_y g(y;\mu)\right)^T \nabla_x y(t;\mu) + \nabla_x g(y;\mu),$$with initial conditions$$\nabla_x y(0;\mu) = \nabla_x y_0(\mu).$$This is known as the *adjoint dynamical* system. Example: Dynamical System with Uncertain ParamtersTake the random vector:$$X = (X_1, X_2),$$and assume that the components are independent Gaussian:$$X_i \sim \mathcal{N}(\mu_i, \sigma_i^2).$$So, for the full random vector we have a mean:$$\mu = (\mu_1, \mu_2),$$and a covariance matrix:$$\Sigma = \operatorname{diag}(\sigma_1^2,\sigma_2^2).$$Consider the ODE: \begin{align*} &\dot{y} = \frac{d y(t)}{dt} =-X_1y(t) \equiv g(y,X),\\ &\qquad y(0) = X_2 \equiv y_0(X). \end{align*}The adjoint system describes the evolution of the derivative:$$\nabla_x y(t;\mu) = \left(\frac{\partial y(t;\mu)}{\partial x_1}, \frac{\partial y(t;\mu)}{\partial x_2}\right).$$According to the formulas, we wrote before we need:$$\nabla_x y_0(\mu) = (0, 1),$$$$\frac{\partial g(y;\mu)}{\partial y} = -\mu_1,$$and$$\nabla_x g(y;\mu) = (-y, 0).$$Putting everything together, the adjoint dynamical system is:$$\begin{array}{ccc}\frac{d}{dt}\frac{\partial y}{\partial x_1} &=& -x_1 \frac{\partial y}{\partial x_1} - y,\\\frac{d}{dt}\frac{\partial y}{\partial x_2} &=& -x_1 \frac{\partial y}{\partial x_2},\\\end{array}$$with initial conditions:$$\begin{array}{ccc}\frac{d}{dt}\frac{\partial y(0)}{\partial x_1} &=& 0,\\\frac{d}{dt}\frac{\partial y(0)}{\partial x_2} &=& 1.\end{array}$$
###Code
# Let's extend the solver object we had before so that it also gives the adjoint solution
import scipy.integrate
class Ex1Solver(object):
"""
An object that can solver the afforementioned ODE problem.
It will work just like a multivariate function.
"""
def __init__(self, nt=100, T=5):
"""
This is the initializer of the class.
Arguments:
nt - The number of timesteps.
T - The final time.
"""
self.nt = nt
self.T = T
self.t = np.linspace(0, T, nt) # The timesteps on which we will get the solution
# The following are not essential, but they are convenient
self.num_input = 2 # The number of inputs the class accepts
self.num_output = nt # The number of outputs the class returns
def __call__(self, x):
"""
This special class method emulates a function call.
Arguments:
x - A 1D numpy array with 2 elements. This represents the stochastic input x = (x1, x2).
"""
# The dynamics of the adjoint z = y, dy/dx1, dy/dx2
def g(z, t, x):
return -x[0] * z[0], -x[0] * z[1] - z[0], -x[0] * z[2]
# The initial condition
y0 = (x[1], 0, 1)
# We are ready to solve the ODE
y = scipy.integrate.odeint(g, y0, self.t, args=(x,))
return y
mu = np.array([0.05, 8.])
Sigma = np.diag([0.01 ** 2, 0.01 ** 2])
solver = Ex1Solver(nt=500, T=100)
z_at_mu = solver(mu)
y_at_mu = z_at_mu[:, 0]
grad_x_y_at_mu = z_at_mu[:, 1:]
C = np.dot(grad_x_y_at_mu, np.dot(Sigma, grad_x_y_at_mu.T))
# For comparison, let's compute the mean and the variance with 1,000 LHS samples
import pyDOE
num_lhs = 1000
X_lhs = pyDOE.lhs(2, num_lhs) # These are uniformly distributed - Turn them to standard normal
X_samples = mu + np.dot(st.norm.ppf(X_lhs), np.sqrt(Sigma))
s = 0.
s2 = 0.
for x in X_samples:
y = solver(x)[:, 0]
s += y
s2 += y ** 2
y_mu_lhs = s / num_lhs
y_var_lhs = s2 / num_lhs - y_mu_lhs ** 2
# Make the figure
fig1, ax1 = plt.subplots()
# Plot the mean and compare to LHS
ax1.plot(solver.t, y_mu_lhs, color=sns.color_palette()[0], label='LHS mean ($n=%d$)' % num_lhs)
ax1.plot(solver.t, y_at_mu, '--', color=sns.color_palette()[0], label='Perturbation mean')
ax1.set_xlabel('$t$')
ax1.set_ylabel('$\mu(t)$', color=sns.color_palette()[0])
ax1.tick_params('y', colors=sns.color_palette()[0])
plt.legend(loc='upper right')
# Plot variance and compare to LHS
ax2 = ax1.twinx()
ax2.plot(solver.t, y_var_lhs, color=sns.color_palette()[1], label='LHS variance ($n=%d$)' % num_lhs)
ax2.plot(solver.t, np.diag(C), '--', color=sns.color_palette()[1], label='Perturbation variance')
ax2.set_ylabel('$\sigma^2(t) = k(t, t)$', color=sns.color_palette()[1])
ax2.tick_params('y', colors=sns.color_palette()[1])
plt.legend(loc='center right');
# Let's do 95% intervals
s = np.sqrt(np.diag(C))
l = y_at_mu - 2 * s
u = y_at_mu + 2 * s
fig, ax = plt.subplots()
ax.plot(solver.t, y_at_mu)
ax.fill_between(solver.t, l, u, alpha=0.25)
ax.set_xlabel('$t$')
ax.set_ylabel('$y(t)$')
# Let's take some sample paths
L = np.linalg.cholesky(C + 1e-10 * np.eye(C.shape[0])) # add something to the diagonal for numerical stability
fig, ax = plt.subplots()
ax.set_xlabel('$t$')
ax.set_ylabel('$y(t)$')
for _ in range(5):
xi = np.random.randn(L.shape[0])
y = y_at_mu + np.dot(L, xi)
ax.plot(solver.t, y, 'r', lw=1)
###Output
_____no_output_____
###Markdown
Lecture 10 - Uncertainty Propagation: Perturbative Methods Objectives+ To remember the Taylor expansion of multivaritae real functions+ To use the Laplace approximation to approximate arbitrary probability densities as Gaussians+ To use the Taylor expansion and the Laplace approximation to (approximately) propagate uncertainties through models+ To use the method of adjoints to calculate derivatives of dynamical systems or partial differential equations with respect to uncertain parameters Readings+ None
###Code
import numpy as np
import math
import scipy.stats as st
import scipy
import matplotlib as mpl
import matplotlib.pyplot as plt
%matplotlib inline
import seaborn as sns
sns.set_style('white')
sns.set_context('talk')
import warnings
warnings.filterwarnings('ignore')
###Output
_____no_output_____
###Markdown
The Taylor Expansion in 1DLet $g:\mathbb{R}\rightarrow\mathbb{R}$ be a smooth real function and $x_0\in\mathbb{R}$.The [Taylor series expansion](https://en.wikipedia.org/wiki/Taylor_series) of $g(x)$ abount $x_0$ is:$$g(x) = g(x_0) + \frac{dg(x_0)}{dx}(x-x_0) + \frac{1}{2}\frac{d^2g(x_0)}{dx^2}(x-x_0)^2+\dots + \frac{1}{n!}\frac{d^ng(x_0)}{dx^n}(x-x_0)^n+\dots$$You can use the Taylor expansion to approximate any function as a polynomial. Example: 1D Taylor Series ExpansionTake $g(x) = \sin(x)$ and $x_0=0$.We have:$$\frac{dg(0)}{dx} = \cos(x)|_{x=0} = 1,$$$$\frac{d^2g(0)}{dx^2} = -\sin(x)_{x=0} = 0,$$$$\frac{d^3g(0)}{dx^3} = -\cos(x)_{x=0} = -1,$$and in general:$$\sin(x) = \sum_{k=0}^{\infty} \frac{(-1)^k}{(2k+1)!}x^{2k+1}.$$
###Code
max_taylor_order = 3
fig, ax = plt.subplots()
x = np.linspace(-np.pi, np.pi, 100)
y_true = np.sin(x)
y_taylor = np.zeros(x.shape)
for k in range(max_taylor_order):
y_taylor += (-1.) ** k * 1. / math.factorial(2 * k + 1) * x ** (2 * k + 1)
ax.plot(x, y_true, label='$\sin(x)$')
ax.plot(x, y_taylor, '--', label='Truncated Taylor series')
plt.legend(loc='best')
ax.set_ylim(-1.5, 1.5)
ax.set_title('Maximum Taylor order: %d' % max_taylor_order)
ax.set_xlabel('$x$')
ax.set_ylabel('$g(x)$');
###Output
_____no_output_____
###Markdown
Questions+ Start increasing the maximum order of the series expansion until you get a satisfactory approximation. The Taylor Expansion in Higher DimensionsLet $g:\mathbb{R}^d\rightarrow \mathbb{R}$ and $x_0\in\mathbb{R}^d$.The Taylor series expansion is:$$g(x) = g(x_0) + \sum_{i=1}^d\frac{\partial g(x_0)}{\partial x_i}(x_i-x_{0i})+\frac{1}{2}\sum_{i,j=1}^d\frac{\partial^2g(x_0)}{\partial x_i\partial x_j}(x_i-x_{0i})(x-x_{0j}) + \dots$$Another way of writing this is:$$g(x) = g(x_0) + \nabla g(x_0)^T(x-x_0) + \frac{1}{2}(x-x_0)^T\nabla^2 g(x_0) (x-x_0),$$where the *Jacobian* is defined as:$$\nabla g(x_0) = \left(\frac{\partial g(x_0)}{\partial x_1},\dots,\frac{\partial g(x_0)}{\partial x_1}\right),$$and the *Hessian* is:$$\nabla^2 g(x_0) = \left(\frac{\partial^2g(x_0)}{\partial x_i\partial x_j}\right)_{i,j=1}^d.$$ The Laplace Approximation in 1DIf you are not interested in probabilities of rare events, you may approximate any probability density as a Gaussian (assuming that it is sufficiently narrow).Let $X$ be a random variable with probability density $p(x)$.Because $p(x)$ is positive, it is better to work with its logarithm.First, we find the maximum of $\log p(x)$ which is called the *nominal value* (or just maximum):$$x_0 = \arg\max_x \log p(x).$$Then, we take the Taylor expansion of $\log p(x)$ about $x=x_0$:$$\log p(x) = \log p(x_0) + \frac{d\log p(x_0)}{dx} (x-x_0) + \frac{1}{2}\frac{d^2\log p(x_0)}{dx^2} (x-x_0)^2 + \dots.$$Since $x_0$ is a critical point of $\log p(x)$, we must have that:$$\frac{d\log p(x_0)}{dx} = 0.$$So, the expansion becomes:$$\log p(x) = \frac{1}{2}\frac{d^2\log p(x_0)}{dx^2} (x-x_0)^2 + \text{const}.$$Therefore,$$p(x) \propto \exp\left\{ \frac{1}{2}\frac{d^2\log p(x_0)}{dx^2} (x-x_0)^2 \right\}.$$Since $x_0$ is a maximum of $\log p(x)$, the matrix $\frac{d^2\log p(x_0)}{dx^2}$ must be a negative number.Therefore, the number:$$c^2 = -\left[\frac{d^2\log p(x_0)}{dx^2}\right]^{-1},$$is positive.By inspection then, we see that:$$p(x) \propto \exp\left\{-\frac{(x-x_0)^2}{2c^2}\right\}.$$Ignoring all higher order terms, we conclude:$$p(x) \approx \mathcal{N}\left(x|x_0, -\left[\frac{d^2\log p(x_0)}{dx^2}\right]^{-1}\right).$$This is the Laplace approximation in one dimension. ExampleLet's just approximate a Gamma distribution with a Gaussian.We will do a case that works and a case that does not work.
###Code
# Construct the ``true random variable``
alpha = 10
X = st.gamma(a=alpha)
# Find the maximum using an optimization method (the minus is because we will use a minimization method)
minus_log_pdf_true = lambda x: -np.log(X.pdf(x))
res = scipy.optimize.minimize_scalar(minus_log_pdf_true, bounds=[1., 8])
# This is the maximum of the pdf
x_0 = res.x
# This is the value of the pdf at the maximum:
p_0 = np.exp(-res.fun)
# The derivative of the pdf at the maximum should be exaclty zero.
# Let's verify it using numerical integration
grad_m_log_p_0 = scipy.misc.derivative(minus_log_pdf_true, x_0, dx=1e-6, n=1)
print('grad minus log p at x0 is: ', grad_m_log_p_0)
# We need the second derivative.
# We will get it using numerical integration as well:
grad_2_m_log_p_0 = scipy.misc.derivative(minus_log_pdf_true, x_0, dx=1e-6, n=2)
# The standard deviation of the Gaussian approximation to p(x) is:
c = np.sqrt(1. / grad_2_m_log_p_0)
# Using scipy code, the random variable that approximates X is:
Z = st.norm(loc=x_0, scale=c)
# Let's plot everything
fig, ax = plt.subplots()
x = np.linspace(x_0 - 6 * c, x_0 + 6 * c, 200)
pdf_true = X.pdf(x)
# Plot the truth:
ax.plot(x, pdf_true)
# Mark the location of the maximum:
# Plot the approximation
ax.plot(x, Z.pdf(x), '--')
ax.plot([x_0] * 10, np.linspace(0, p_0, 10), ':')
plt.legend(['PDF of Gamma(%.2f)' % alpha, 'Laplace approximation'], loc='best')
ax.set_xlabel('$x$')
ax.set_ylabel('PDF');
###Output
grad minus log p at x0 is: 1.7763568394002505e-09
###Markdown
The Laplace Approximation in Many DimensionsThen, we take the Taylor expansion of $\log p(x)$ about $x=x_0$:$$\log p(x) = \log p(x_0) + \nabla \log p(x_0) (x-x_0) + \frac{1}{2}(x-x_0)^T\nabla^2 \log p(x_0) (x-x_0) + \dots.$$Since $x_0$ is a critical point of $\log p(x)$, we must have that:$$\nabla \log p(x_0) = 0.$$So, the expansion becomes:$$\log p(x) = \frac{1}{2}(x-x_0)^T\nabla^2 \log p(x_0) (x-x_0) + \text{const}.$$Therefore,$$p(x) \propto \exp\left\{ \frac{1}{2}(x-x_0)^T\nabla^2 \log p(x_0) (x-x_0) \right\}.$$Since $x_0$ is a maximum of $\log p(x)$, the matrix $\nabla^2 \log p(x_0)$ must be negative definite.Therefore, the matrix:$$C = -(\nabla^2\log p(x_0))^{-1},$$is positive definite.By inspection then, we see that:$$p(x) \propto \exp\left\{-\frac{1}{2}(x-x_0)^TC^{-1}(x-x_0)\right\}.$$Ignoring all higher order terms, we conclude:$$p(x) \approx \mathcal{N}\left(x|x_0, -(\nabla^2\log p(x_0))^{-1}\right).$$This is the Laplace approximation in many dimensions. Questions+ Experiment with various values of alpha for the true Gamma distribution. What happens when alpha is smaller than one. Why doesn't the method work at for that parameter? Perturbation Approach in 1DLet $X\sim p$ be a random vector and $Y=f(X)$ a scalar quantity of interest that depends on $X$.Our goal is to estimate the first order statistics of $Y$.In particular, the mean:$$\mathbb{E}[f(X)] = \int f(x) p(x)dx,$$and the variance:$$\mathbb{V}[f(X)] = \int \left(f(x)-\mathbb{E}[f(X)]\right)^2p(x)dx.$$First, we take the laplace approximation for the random vector and approximate its density as a Gaussian.That is, we write:$$p(x) \approx \mathcal{N}\left(x|\mu, \sigma^2\right),$$with $\mu$ and $\Sigma$ as given by the Laplace approximation.Now, we take the Taylor expansion of $f(x)$ around $x=\mu$:$$f(x) = f(\mu) + \frac{df(\mu)}{dx} (x-\mu)+\dots$$Now, let's take the expecation over the Laplace approximation of $p(x)$.$$\begin{array}{ccc}\mathbb{E}[f(X)] &=& \mathbb{E}\left[f(\mu) + \frac{df(\mu)}{dx} (X-\mu) + \dots\right]\\&=& f(\mu) + \frac{df(\mu)}{dx}\mathbb{E}[X-\mu] + \dots\\&=& f(\mu) + \dots\end{array}$$So, to *second order* we have:$$\mathbb{E}[f(X)] \approx f(\mu).$$You can, of course, construct higher order approximations in this way.Let's now do the variance.We will use the formula:$$\mathbb{V}[f(X)] = \mathbb{E}[f^2(X)] - \left[\mathbb{E}[f(X)]\right]^2.$$Keeping up to second order terms, we get:$$\begin{array}{ccc}\mathbb{E}[f^2(X)] &=& \mathbb{E}\left[\left(f(\mu) + \frac{df(\mu)}{dx} (X-\mu) + \dots\right)^2\right]\\&=& f^2(\mu) + \left(\frac{df(\mu)}{dx}\right)^2\mathbb{E}[(X-\mu)^2] + 2f(\mu)\frac{df(\mu)}{dx}\mathbb{E}[X-\mu] + \dots\\&=& f^2(\mu) + \left(\frac{df(\mu)}{dx}\right)^2\sigma^2 + \dots\end{array}$$Therefore, for the variance we get:$$\begin{array}{ccc}\mathbb{V}[f(X)] &=& f^2(\mu) + \left(\frac{df(\mu)}{dx}\right)^2\sigma^2 + \dots - \left(f(\mu) + \dots\right)^2\\&=& f^2(\mu) + \left(\frac{df(\mu)}{dx}\right)^2\sigma^2 - f^2(\mu) + \dots\\&=& \left(\frac{df(\mu)}{dx}\right)^2\sigma^2 + \dots\end{array}$$So, to *first order* we have:$$\mathbb{V}[f(X)] \approx \left(\frac{df(\mu)}{dx}\right)^2\sigma^2$$And, of course, we can go to higher order approximations.Now, having approximations for the mean and the variance, we may approximate the full density of the random variable $Y=f(X)$ by a Gaussian:$$p(y) \approx \mathcal{N}\left(y|f(\mu), \left(\frac{df(\mu)}{dx}\right)^2\sigma^2\right).$$ Example: 1D Propagation of UncertaintyLet's solve a (very simple) uncertainty propagation problem using these techniques.Consider:$$X\sim \mathcal{N}(\pi/2, 0.1^2),$$and$$f(x) = \sin(x) e^{-0.5x}.$$We have:
###Code
# Defining the densit of X:
mu = 1.1
sigma = 0.1
X = st.norm(loc=mu, scale=sigma)
# The function through which we want to propagate uncertainties
f = lambda x: np.sin(x) * np.exp(-0.5 * x)
fig1, ax1 = plt.subplots()
x = np.linspace(0., np.pi, 200)
ax1.plot(x, f(x))
ax1.set_xlabel('$x$')
ax1.set_ylabel('$f(x)$', color=sns.color_palette()[0])
ax1.tick_params('y', colors=sns.color_palette()[0])
ax2 = ax1.twinx()
ax2.plot(x, X.pdf(x), color=sns.color_palette()[1])
ax2.set_ylabel('$p(x)$', color=sns.color_palette()[1])
ax2.tick_params('y', colors=sns.color_palette()[1])
# The function at mu
f_at_mu = f(mu)
# The first derivative of the function at mu
df_at_mu = scipy.misc.derivative(f, mu, dx=1e-8, n=1)
# We can now construct a Gaussian approximation to the distribution of f(X)
exp_f_X = f_at_mu
var_f_X = f_at_mu ** 2 * sigma ** 2
f_X = st.norm(loc=exp_f_X, scale=np.sqrt(var_f_X))
# We will compare to the histogram of 10,000 samples
y_samples = [f(X.rvs()) for _ in range(10000)]
fig, ax = plt.subplots()
y = np.linspace(exp_f_X - 6 * np.sqrt(var_f_X), exp_f_X + 6 * np.sqrt(var_f_X), 100)
ax.plot(y, f_X.pdf(y))
ax.set_xlim(0., 0.6)
ax.hist(y_samples, bins=10, density=True, alpha=0.5);
ax.set_xlabel('$y$')
ax.set_ylabel('$p(y)$');
###Output
_____no_output_____
###Markdown
Perturbation Approach in Many DimensionsLet $X\sim\mathcal{N}(\mu,\Sigma)$ be a d-dimensional Gaussian random vector.If $X$ was not Gaussian, we could use the Laplace approximation.Let $f(x)$ be a m-dimensional function of $x$:$$f:\mathbb{R}^d\rightarrow \mathbb{R}^m.$$To get the Taylor series expansion of $f(x)$, you need to work out the Taylor series expansion of each output component and put everything back together.You will get:$$f(x) = f(\mu) + \nabla f(\mu) (x - \mu) + \dots,$$where $\nabla f(\mu)\in\mathbb{R}^{m\times d}$ is the Jacobian matrix defined by:$$\nabla f(\mu) = \left(\begin{array}{ccc}\frac{\partial f_1(\mu)}{\partial x_1} & \dots & \frac{\partial f_1(\mu)}{\partial x_d}\\\vdots & \ddots & \vdots\\\frac{\partial f_m(\mu)}{\partial x_1} & \dots & \frac{\partial f_m(\mu)}{\partial x_d}\end{array}\right)$$To first order, the expectation of $Y = f(X)$ is:$$\mathbb{E}[f(X)] \approx f(\mu),$$while the covariance matrix is:$$\mathbb{C}[f(X)] \approx \nabla f(\mu)\Sigma \left(\nabla f(\mu)\right)^T,$$Putting these two things together, we may approximate the density of $Y=f(X)$ with a multivariate Gaussian:$$p(y) \approx \mathcal{N}\left(y|f(\mu), \nabla f(\mu)\Sigma \left(\nabla f(\mu)\right)^T\right).$$ Perturbation Approach for Stochastic Dynamical Systems with Low Dimensional, Small UncertaintiesPotentially using the Laplace approximation, assume that we have a $d$-dimensional random vector:$$X\sim\mathcal{N}(\mu, \Sigma).$$Consider the $m$-dimensional dynamical system:$$\frac{dy}{dt} = g(y;X),$$with initial conditions$$y(0) = y_0(X).$$Since $X$ is a random vector, the response of the dynamical system $Y(t)$ is a stochastic process:$$Y(t) = y(t; X),$$where $y(t;X)$ is the solution of the initial value problem for a specific realization of the random vector $X$. We will approximate this stochastic process with a Gaussian process:$$Y(\cdot) \sim \text{GP}\left(m(\cdot), k(\cdot, \cdot)\right).$$This can easily be done, using the results of the previous sections.The mean is:$$m(t) = \mathbb{E}[Y(t)] = \mathbb{E}[y(t;X)] = y(t;\mu).$$The covariance between two times is:$$k(t,t') = \mathbb{C}[Y(t), Y(t')] = \mathbb{C}[y(t;X), y(t';X)] = \nabla_x y(t;\mu) \Sigma \left(\nabla_x y(t';\mu)\right)^T.$$The only complicating factor, is that we need a way to get $\nabla_x y(t;\mu)$.For this, we need the method of adjoints. Calculating Sensitivities in Dynamical Systems using the Method of AjdointsWe will show how we can compute $\nabla_x y(t;\mu)$ using the method of adjoints.The idea is simple.We will derive the differential equation that $\nabla_x y(t;\mu)$ satisfies and we will be solving it as we solve for $y(t;\mu)$.Let $i\in\{1,\dots,m\}$ and $j\in\{1,\dots,d\}$.We start with the initial conditions.We have:$$\frac{\partial y_i(0;\mu)}{\partial x_j} = \frac{\partial y_{0i}(\mu)}{\partial x_j}.$$Now, let's do the derivatives:$$\begin{array}{ccc}\frac{d}{dt}\frac{\partial y_i(t;\mu)}{\partial x_j} &=& \frac{\partial }{\partial x_j}\frac{dy_i(t,\mu)}{dt} \\&=& \frac{\partial }{\partial x_j} g_i(y(t;\mu); \mu)\\&=& \sum_{r=1}^m\frac{\partial g_i(y(t;\mu); \mu)}{\partial y_r}\frac{\partial y_r(t;\mu)}{\partial x_j} + \frac{\partial g_i(y(t;\mu); \mu)}{\partial x_j}.\end{array}$$So, we see that the dynamical system that $\nabla_x y(t;\mu)$ satisfies is:$$\frac{d}{dt}\nabla_x y(t;\mu) = \left((\nabla_y g(y;\mu)\right)^T \nabla_x y(t;\mu) + \nabla_x g(y;\mu),$$with initial conditions$$\nabla_x y(0;\mu) = \nabla_x y_0(\mu).$$This is known as the *adjoint dynamical* system. Example: Dynamical System with Uncertain ParamtersTake the random vector:$$X = (X_1, X_2),$$and assume that the components are independent Gaussian:$$X_i \sim \mathcal{N}(\mu_i, \sigma_i^2).$$So, for the full random vector we have a mean:$$\mu = (\mu_1, \mu_2),$$and a covariance matrix:$$\Sigma = \operatorname{diag}(\sigma_1^2,\sigma_2^2).$$Consider the ODE: \begin{align*} &\dot{y} = \frac{d y(t)}{dt} =-X_1y(t) \equiv g(y,X),\\ &\qquad y(0) = X_2 \equiv y_0(X). \end{align*}The adjoint system describes the evolution of the derivative:$$\nabla_x y(t;\mu) = \left(\frac{\partial y(t;\mu)}{\partial x_1}, \frac{\partial y(t;\mu)}{\partial x_2}\right).$$According to the formulas, we wrote before we need:$$\nabla_x y_0(\mu) = (0, 1),$$$$\frac{\partial g(y;\mu)}{\partial y} = -\mu_1,$$and$$\nabla_x g(y;\mu) = (-y, 0).$$Putting everything together, the adjoint dynamical system is:$$\begin{array}{ccc}\frac{d}{dt}\frac{\partial y}{\partial x_1} &=& -x_1 \frac{\partial y}{\partial x_1} - y,\\\frac{d}{dt}\frac{\partial y}{\partial x_2} &=& -x_1 \frac{\partial y}{\partial x_2},\\\end{array}$$with initial conditions:$$\begin{array}{ccc}\frac{d}{dt}\frac{\partial y(0)}{\partial x_1} &=& 0,\\\frac{d}{dt}\frac{\partial y(0)}{\partial x_2} &=& 1.\end{array}$$
###Code
# Let's extend the solver object we had before so that it also gives the adjoint solution
import scipy.integrate
class Ex1Solver(object):
"""
An object that can solver the afforementioned ODE problem.
It will work just like a multivariate function.
"""
def __init__(self, nt=100, T=5):
"""
This is the initializer of the class.
Arguments:
nt - The number of timesteps.
T - The final time.
"""
self.nt = nt
self.T = T
self.t = np.linspace(0, T, nt) # The timesteps on which we will get the solution
# The following are not essential, but they are convenient
self.num_input = 2 # The number of inputs the class accepts
self.num_output = nt # The number of outputs the class returns
def __call__(self, x):
"""
This special class method emulates a function call.
Arguments:
x - A 1D numpy array with 2 elements. This represents the stochastic input x = (x1, x2).
"""
# The dynamics of the adjoint z = y, dy/dx1, dy/dx2
def g(z, t, x):
return -x[0] * z[0], -x[0] * z[1] - z[0], -x[0] * z[2]
# The initial condition
y0 = (x[1], 0, 1)
# We are ready to solve the ODE
y = scipy.integrate.odeint(g, y0, self.t, args=(x,))
return y
mu = np.array([0.05, 8.])
Sigma = np.diag([0.01 ** 2, 0.01 ** 2])
solver = Ex1Solver(nt=500, T=100)
z_at_mu = solver(mu)
y_at_mu = z_at_mu[:, 0]
grad_x_y_at_mu = z_at_mu[:, 1:]
C = np.dot(grad_x_y_at_mu, np.dot(Sigma, grad_x_y_at_mu.T))
# For comparison, let's compute the mean and the variance with 1,000 LHS samples
import pyDOE
num_lhs = 1000
X_lhs = pyDOE.lhs(2, num_lhs) # These are uniformly distributed - Turn them to standard normal
X_samples = mu + np.dot(st.norm.ppf(X_lhs), np.sqrt(Sigma))
s = 0.
s2 = 0.
for x in X_samples:
y = solver(x)[:, 0]
s += y
s2 += y ** 2
y_mu_lhs = s / num_lhs
y_var_lhs = s2 / num_lhs - y_mu_lhs ** 2
# Make the figure
fig1, ax1 = plt.subplots()
# Plot the mean and compare to LHS
ax1.plot(solver.t, y_mu_lhs, color=sns.color_palette()[0], label='LHS mean ($n=%d$)' % num_lhs)
ax1.plot(solver.t, y_at_mu, '--', color=sns.color_palette()[0], label='Perturbation mean')
ax1.set_xlabel('$t$')
ax1.set_ylabel('$\mu(t)$', color=sns.color_palette()[0])
ax1.tick_params('y', colors=sns.color_palette()[0])
plt.legend(loc='upper right')
# Plot variance and compare to LHS
ax2 = ax1.twinx()
ax2.plot(solver.t, y_var_lhs, color=sns.color_palette()[1], label='LHS variance ($n=%d$)' % num_lhs)
ax2.plot(solver.t, np.diag(C), '--', color=sns.color_palette()[1], label='Perturbation variance')
ax2.set_ylabel('$\sigma^2(t) = k(t, t)$', color=sns.color_palette()[1])
ax2.tick_params('y', colors=sns.color_palette()[1])
plt.legend(loc='center right');
# Let's do 95% intervals
s = np.sqrt(np.diag(C))
l = y_at_mu - 2 * s
u = y_at_mu + 2 * s
fig, ax = plt.subplots()
ax.plot(solver.t, y_at_mu)
ax.fill_between(solver.t, l, u, alpha=0.25)
ax.set_xlabel('$t$')
ax.set_ylabel('$y(t)$')
# Let's take some sample paths
L = np.linalg.cholesky(C + 1e-10 * np.eye(C.shape[0])) # add something to the diagonal for numerical stability
fig, ax = plt.subplots()
ax.set_xlabel('$t$')
ax.set_ylabel('$y(t)$')
for _ in range(5):
xi = np.random.randn(L.shape[0])
y = y_at_mu + np.dot(L, xi)
ax.plot(solver.t, y, 'r', lw=1)
###Output
_____no_output_____ |
python36/F_DAWP_Chs_12_13.ipynb | ###Markdown
Derivatives Analytics with Python **_Chapters 12 & 13_****Wiley Finance (2015)** Dr. Yves J. HilpischThe Python Quants [email protected] | http://tpq.ioPython online training | http://training.tpq.ioDX Analytics library | http://dx-analytics.com
###Code
from pylab import plt
plt.style.use('seaborn')
%matplotlib inline
###Output
_____no_output_____
###Markdown
Chapter 12: Valuation
###Code
%run 12_val/BCC97_simulation.py
np.array((S0, r0, kappa_r, theta_r, sigma_r))
opt
###Output
_____no_output_____
###Markdown
Simulation
###Code
plot_rate_paths(r)
plt.savefig('../images/12_val/rate_paths.pdf')
plot_volatility_paths(v)
plt.savefig('../images/12_val/volatility_paths.pdf')
plot_index_paths(S)
plt.savefig('../images/12_val/ES50_paths.pdf')
plot_index_histogram(S)
plt.savefig('../images/12_val/ES50_histogram.pdf')
###Output
_____no_output_____
###Markdown
European Options Valuation
###Code
%run 12_val/BCC97_valuation_comparison.py
%time compare_values(M0=50, I=50000)
%time compare_values(M0=50, I=200000)
%time compare_values(M0=200, I=50000)
###Output
T | K | C0 | MCS | DIFF
0.083 | 3050 | 193.692 | 193.241 | -0.450
0.083 | 3225 | 62.147 | 61.595 | -0.552
0.083 | 3400 | 1.967 | 2.581 | 0.614
0.500 | 3050 | 259.126 | 259.140 | 0.014
0.500 | 3225 | 146.891 | 149.090 | 2.199
0.500 | 3400 | 67.142 | 71.122 | 3.980
1.000 | 3050 | 321.419 | 322.117 | 0.697
1.000 | 3225 | 216.227 | 219.528 | 3.301
1.000 | 3400 | 133.950 | 139.285 | 5.335
1.500 | 3050 | 378.978 | 377.499 | -1.479
1.500 | 3225 | 276.942 | 278.276 | 1.334
1.500 | 3400 | 193.333 | 197.137 | 3.804
2.000 | 3050 | 435.337 | 435.067 | -0.270
2.000 | 3225 | 335.010 | 337.597 | 2.587
2.000 | 3400 | 250.314 | 255.541 | 5.227
3.000 | 3050 | 549.127 | 552.842 | 3.715
3.000 | 3225 | 450.522 | 457.774 | 7.252
3.000 | 3400 | 364.049 | 374.340 | 10.291
CPU times: user 21.7 s, sys: 5.14 s, total: 26.9 s
Wall time: 25.5 s
###Markdown
American Options Valuation
###Code
%run 12_val/BCC97_american_valuation.py
%time lsm_compare_values(M0=150, I=50000)
###Output
T | K | P0 | LSM | DIFF
0.083 | 3050 | 17.681 | 17.431 | -0.250
0.083 | 3225 | 61.131 | 61.001 | -0.130
0.083 | 3400 | 175.947 | 181.570 | 5.623
0.500 | 3050 | 77.963 | 84.277 | 6.314
0.500 | 3225 | 140.428 | 149.270 | 8.842
0.500 | 3400 | 235.379 | 246.150 | 10.771
1.000 | 3050 | 124.220 | 136.155 | 11.934
1.000 | 3225 | 192.808 | 207.958 | 15.149
1.000 | 3400 | 284.311 | 302.051 | 17.740
1.500 | 3050 | 155.970 | 174.854 | 18.884
1.500 | 3225 | 226.234 | 250.278 | 24.044
1.500 | 3400 | 314.923 | 344.215 | 29.292
2.000 | 3050 | 177.841 | 206.460 | 28.619
2.000 | 3225 | 247.834 | 282.910 | 35.077
2.000 | 3400 | 333.458 | 376.640 | 43.182
3.000 | 3050 | 201.032 | 249.858 | 48.826
3.000 | 3225 | 267.549 | 328.487 | 60.937
3.000 | 3400 | 346.197 | 421.464 | 75.267
CPU times: user 39.8 s, sys: 7.42 s, total: 47.2 s
Wall time: 43.5 s
###Markdown
Chapter 13: Dynamic Hedging BSM Model
###Code
%run 13_dyh/BSM_lsm_hedging_algorithm.py
S, po, vt, errs, t = BSM_hedge_run(p=25)
plot_hedge_path(S, po, vt, errs, t)
# plt.savefig('../images/13_dyh/BSM_hedge_run_1.pdf')
S, po, vt, errs, t = BSM_hedge_run(p=5)
plot_hedge_path(S, po, vt, errs, t)
plt.savefig('../images/13_dyh/BSM_hedge_run_2.pdf')
%run 13_dyh/BSM_lsm_hedging_histogram.py
%time pl_list = BSM_dynamic_hedge_mcs()
plot_hedge_histogram(pl_list)
plt.savefig('../images/13_dyh/BSM_hedge_histo.pdf')
%time pl_list = BSM_dynamic_hedge_mcs(M=200, I=150000)
plot_hedge_histogram(pl_list)
plt.savefig('../images/13_dyh/BSM_hedge_histo_more.pdf')
###Output
_____no_output_____
###Markdown
BCC97 Model
###Code
%run 13_dyh/BCC97_lsm_hedging_algorithm.py
S, po, vt, errs, t = BCC97_hedge_run(2)
plot_hedge_path(S, po, vt, errs, t)
plt.savefig('../images/13_dyh/BCC_hedge_run_1.pdf')
S, po, vt, errs, t = BCC97_hedge_run(8)
plot_hedge_path(S, po, vt, errs, t + 1)
plt.savefig('../images/13_dyh/BCC_hedge_run_2.pdf')
S, po, vt, errs, t = BCC97_hedge_run(4)
plot_hedge_path(S, po, vt, errs, t + 1)
plt.savefig('../images/13_dyh/BCC_hedge_run_3.pdf')
%run 13_dyh/BCC97_lsm_hedging_histogram.py
%time pl_list = BCC97_hedge_simulation(M=150, I=150000)
plot_hedge_histogram(pl_list)
plt.savefig('../images/13_dyh/BCC_hedge_histo.pdf')
###Output
_____no_output_____ |
Python and Numpy/Python_Numpy_Tutorial.ipynb | ###Markdown
Introduction Python is a great general-purpose programming language on its own, but with the help of a few popular libraries (numpy, scipy, matplotlib) it becomes a powerful environment for scientific computing.We expect that many of you will have some experience with Python and numpy; for the rest of you, this section will serve as a quick crash course both on the Python programming language and on the use of Python for scientific computing.Some of you may have previous knowledge in Matlab, in which case we also recommend the numpy for Matlab users page (https://docs.scipy.org/doc/numpy-dev/user/numpy-for-matlab-users.html). In this tutorial, we will cover:* Basic Python: Basic data types (Containers, Lists, Dictionaries, Sets, Tuples), Functions, Classes* Numpy: Arrays, Array indexing, Datatypes, Array math, Broadcasting* Matplotlib: Plotting, Subplots, Images* IPython: Creating notebooks, Typical workflows Basics of Python Python is a high-level, dynamically typed multiparadigm programming language. Python code is often said to be almost like pseudocode, since it allows you to express very powerful ideas in very few lines of code while being very readable. As an example, here is an implementation of the classic quicksort algorithm in Python:
###Code
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
print quicksort([3,6,8,10,1,2,1])
###Output
[1, 1, 2, 3, 6, 8, 10]
###Markdown
Python versions There are currently two different supported versions of Python, 2.7 and 3.4. Somewhat confusingly, Python 3.0 introduced many backwards-incompatible changes to the language, so code written for 2.7 may not work under 3.4 and vice versa. For this class all code will use Python 2.7.You can check your Python version at the command line by running `python --version`. Basic data types Numbers Integers and floats work as you would expect from other languages:
###Code
x = 3
print x, type(x)
print x + 1 # Addition;
print x - 1 # Subtraction;
print x * 2 # Multiplication;
print x ** 2 # Exponentiation;
x += 1
print x # Prints "4"
x *= 2
print x # Prints "8"
y = 2.5
print type(y) # Prints "<type 'float'>"
print y, y + 1, y * 2, y ** 2 # Prints "2.5 3.5 5.0 6.25"
###Output
<type 'float'>
2.5 3.5 5.0 6.25
###Markdown
Note that unlike many languages, Python does not have unary increment (x++) or decrement (x--) operators.Python also has built-in types for long integers and complex numbers; you can find all of the details in the [documentation](https://docs.python.org/2/library/stdtypes.htmlnumeric-types-int-float-long-complex). Booleans Python implements all of the usual operators for Boolean logic, but uses English words rather than symbols (`&&`, `||`, etc.):
###Code
t, f = True, False
print type(t) # Prints "<type 'bool'>"
###Output
<type 'bool'>
###Markdown
Now we let's look at the operations:
###Code
print t and f # Logical AND;
print t or f # Logical OR;
print not t # Logical NOT;
print t != f # Logical XOR;
###Output
False
True
False
True
###Markdown
Strings
###Code
hello = 'hello' # String literals can use single quotes
world = "world" # or double quotes; it does not matter.
print hello, len(hello)
hw = hello + ' ' + world # String concatenation
print hw # prints "hello world"
hw12 = '%s %s %d' % (hello, world, 12) # sprintf style string formatting
print hw12 # prints "hello world 12"
###Output
hello world 12
###Markdown
String objects have a bunch of useful methods; for example:
###Code
s = "hello"
print s.capitalize() # Capitalize a string; prints "Hello"
print s.upper() # Convert a string to uppercase; prints "HELLO"
print s.rjust(7) # Right-justify a string, padding with spaces; prints " hello"
print s.center(7) # Center a string, padding with spaces; prints " hello "
print s.replace('l', '(ell)') # Replace all instances of one substring with another;
# prints "he(ell)(ell)o"
print ' world '.strip() # Strip leading and trailing whitespace; prints "world"
###Output
Hello
HELLO
hello
hello
he(ell)(ell)o
world
###Markdown
You can find a list of all string methods in the [documentation](https://docs.python.org/2/library/stdtypes.htmlstring-methods). Containers Python includes several built-in container types: lists, dictionaries, sets, and tuples. Lists A list is the Python equivalent of an array, but is resizeable and can contain elements of different types:
###Code
xs = [3, 1, 2] # Create a list
print xs, xs[2]
print xs[-1] # Negative indices count from the end of the list; prints "2"
xs[2] = 'foo' # Lists can contain elements of different types
print xs
xs.append('bar') # Add a new element to the end of the list
print xs
x = xs.pop() # Remove and return the last element of the list
print x, xs
###Output
bar [3, 1, 'foo']
###Markdown
As usual, you can find all the gory details about lists in the [documentation](https://docs.python.org/2/tutorial/datastructures.htmlmore-on-lists). Slicing In addition to accessing list elements one at a time, Python provides concise syntax to access sublists; this is known as slicing:
###Code
nums = range(5) # range is a built-in function that creates a list of integers
print nums # Prints "[0, 1, 2, 3, 4]"
print nums[2:4] # Get a slice from index 2 to 4 (exclusive); prints "[2, 3]"
print nums[2:] # Get a slice from index 2 to the end; prints "[2, 3, 4]"
print nums[:2] # Get a slice from the start to index 2 (exclusive); prints "[0, 1]"
print nums[:] # Get a slice of the whole list; prints ["0, 1, 2, 3, 4]"
print nums[:-1] # Slice indices can be negative; prints ["0, 1, 2, 3]"
nums[2:4] = [8, 9] # Assign a new sublist to a slice
print nums # Prints "[0, 1, 8, 9, 4]"
###Output
[0, 1, 2, 3, 4]
[2, 3]
[2, 3, 4]
[0, 1]
[0, 1, 2, 3, 4]
[0, 1, 2, 3]
[0, 1, 8, 9, 4]
###Markdown
Loops You can loop over the elements of a list like this:
###Code
animals = ['cat', 'dog', 'monkey']
for animal in animals:
print animal
###Output
cat
dog
monkey
###Markdown
If you want access to the index of each element within the body of a loop, use the built-in `enumerate` function:
###Code
animals = ['cat', 'dog', 'monkey']
for idx, animal in enumerate(animals):
print '#%d: %s' % (idx + 1, animal)
###Output
#1: cat
#2: dog
#3: monkey
###Markdown
List comprehensions: When programming, frequently we want to transform one type of data into another. As a simple example, consider the following code that computes square numbers:
###Code
nums = [0, 1, 2, 3, 4]
squares = []
for x in nums:
squares.append(x ** 2)
print squares
###Output
[0, 1, 4, 9, 16]
###Markdown
You can make this code simpler using a list comprehension:
###Code
nums = [0, 1, 2, 3, 4]
squares = [x ** 2 for x in nums]
print squares
###Output
[0, 1, 4, 9, 16]
###Markdown
List comprehensions can also contain conditions:
###Code
nums = [0, 1, 2, 3, 4]
even_squares = [x ** 2 for x in nums if x % 2 == 0]
print even_squares
###Output
[0, 4, 16]
###Markdown
Dictionaries A dictionary stores (key, value) pairs, similar to a `Map` in Java or an object in Javascript. You can use it like this:
###Code
d = {'cat': 'cute', 'dog': 'furry'} # Create a new dictionary with some data
print d['cat'] # Get an entry from a dictionary; prints "cute"
print 'cat' in d # Check if a dictionary has a given key; prints "True"
d['fish'] = 'wet' # Set an entry in a dictionary
print d['fish'] # Prints "wet"
print d['monkey'] # KeyError: 'monkey' not a key of d
print d.get('monkey', 'N/A') # Get an element with a default; prints "N/A"
print d.get('fish', 'N/A') # Get an element with a default; prints "wet"
del d['fish'] # Remove an element from a dictionary
print d.get('fish', 'N/A') # "fish" is no longer a key; prints "N/A"
###Output
N/A
###Markdown
You can find all you need to know about dictionaries in the [documentation](https://docs.python.org/2/library/stdtypes.htmldict). It is easy to iterate over the keys in a dictionary:
###Code
d = {'person': 2, 'cat': 4, 'spider': 8}
for animal in d:
legs = d[animal]
print 'A %s has %d legs' % (animal, legs)
###Output
A person has 2 legs
A spider has 8 legs
A cat has 4 legs
###Markdown
If you want access to keys and their corresponding values, use the iteritems method:
###Code
d = {'person': 2, 'cat': 4, 'spider': 8}
for animal, legs in d.iteritems():
print 'A %s has %d legs' % (animal, legs)
###Output
A person has 2 legs
A spider has 8 legs
A cat has 4 legs
###Markdown
Dictionary comprehensions: These are similar to list comprehensions, but allow you to easily construct dictionaries. For example:
###Code
nums = [0, 1, 2, 3, 4]
even_num_to_square = {x: x ** 2 for x in nums if x % 2 == 0}
print even_num_to_square
###Output
{0: 0, 2: 4, 4: 16}
###Markdown
Sets A set is an unordered collection of distinct elements. As a simple example, consider the following:
###Code
animals = {'cat', 'dog'}
print 'cat' in animals # Check if an element is in a set; prints "True"
print 'fish' in animals # prints "False"
animals.add('fish') # Add an element to a set
print 'fish' in animals
print len(animals) # Number of elements in a set;
animals.add('cat') # Adding an element that is already in the set does nothing
print len(animals)
animals.remove('cat') # Remove an element from a set
print len(animals)
###Output
3
2
###Markdown
_Loops_: Iterating over a set has the same syntax as iterating over a list; however since sets are unordered, you cannot make assumptions about the order in which you visit the elements of the set:
###Code
animals = {'cat', 'dog', 'fish'}
for idx, animal in enumerate(animals):
print '#%d: %s' % (idx + 1, animal)
# Prints "#1: fish", "#2: dog", "#3: cat"
###Output
#1: fish
#2: dog
#3: cat
###Markdown
Set comprehensions: Like lists and dictionaries, we can easily construct sets using set comprehensions:
###Code
from math import sqrt
print {int(sqrt(x)) for x in range(30)}
###Output
set([0, 1, 2, 3, 4, 5])
###Markdown
Tuples A tuple is an (immutable) ordered list of values. A tuple is in many ways similar to a list; one of the most important differences is that tuples can be used as keys in dictionaries and as elements of sets, while lists cannot. Here is a trivial example:
###Code
d = {(x, x + 1): x for x in range(10)} # Create a dictionary with tuple keys
t = (5, 6) # Create a tuple
print type(t)
print d[t]
print d[(1, 2)]
t[0] = 1
###Output
_____no_output_____
###Markdown
Functions Python functions are defined using the `def` keyword. For example:
###Code
def sign(x):
if x > 0:
return 'positive'
elif x < 0:
return 'negative'
else:
return 'zero'
for x in [-1, 0, 1]:
print sign(x)
###Output
negative
zero
positive
###Markdown
We will often define functions to take optional keyword arguments, like this:
###Code
def hello(name, loud=False):
if loud:
print 'HELLO, %s' % name.upper()
else:
print 'Hello, %s!' % name
hello('Bob')
hello('Fred', loud=True)
###Output
Hello, Bob!
HELLO, FRED
###Markdown
Classes The syntax for defining classes in Python is straightforward:
###Code
class Greeter:
# Constructor
def __init__(self, name):
self.name = name # Create an instance variable
# Instance method
def greet(self, loud=False):
if loud:
print 'HELLO, %s!' % self.name.upper()
else:
print 'Hello, %s' % self.name
g = Greeter('Fred') # Construct an instance of the Greeter class
g.greet() # Call an instance method; prints "Hello, Fred"
g.greet(loud=True) # Call an instance method; prints "HELLO, FRED!"
###Output
Hello, Fred
HELLO, FRED!
###Markdown
Numpy Numpy is the core library for scientific computing in Python. It provides a high-performance multidimensional array object, and tools for working with these arrays. If you are already familiar with MATLAB, you might find this [tutorial](http://wiki.scipy.org/NumPy_for_Matlab_Users) useful to get started with Numpy. To use Numpy, we first need to import the `numpy` package:
###Code
import numpy as np
###Output
_____no_output_____
###Markdown
Arrays A numpy array is a grid of values, all of the same type, and is indexed by a tuple of nonnegative integers. The number of dimensions is the rank of the array; the shape of an array is a tuple of integers giving the size of the array along each dimension. We can initialize numpy arrays from nested Python lists, and access elements using square brackets:
###Code
a = np.array([1, 2, 3]) # Create a rank 1 array
print type(a), a.shape, a[0], a[1], a[2]
a[0] = 5 # Change an element of the array
print a
b = np.array([[1,2,3],[4,5,6]]) # Create a rank 2 array
print b
print b.shape
print b[0, 0], b[0, 1], b[1, 0]
###Output
(2, 3)
1 2 4
###Markdown
Numpy also provides many functions to create arrays:
###Code
a = np.zeros((2,2)) # Create an array of all zeros
print a
b = np.ones((1,2)) # Create an array of all ones
print b
c = np.full((2,2), 7) # Create a constant array
print c
d = np.eye(2) # Create a 2x2 identity matrix
print d
e = np.random.random((2,2)) # Create an array filled with random values
print e
###Output
[[ 0.09477679 0.79267634]
[ 0.78291274 0.38962829]]
###Markdown
Array indexing Numpy offers several ways to index into arrays. Slicing: Similar to Python lists, numpy arrays can be sliced. Since arrays may be multidimensional, you must specify a slice for each dimension of the array:
###Code
import numpy as np
# Create the following rank 2 array with shape (3, 4)
# [[ 1 2 3 4]
# [ 5 6 7 8]
# [ 9 10 11 12]]
a = np.array([[1,2,3,4], [5,6,7,8], [9,10,11,12]])
# Use slicing to pull out the subarray consisting of the first 2 rows
# and columns 1 and 2; b is the following array of shape (2, 2):
# [[2 3]
# [6 7]]
b = a[:2, 1:3]
print b
###Output
[[2 3]
[6 7]]
###Markdown
A slice of an array is a view into the same data, so modifying it will modify the original array.
###Code
print a[0, 1]
b[0, 0] = 77 # b[0, 0] is the same piece of data as a[0, 1]
print a[0, 1]
###Output
2
77
###Markdown
You can also mix integer indexing with slice indexing. However, doing so will yield an array of lower rank than the original array. Note that this is quite different from the way that MATLAB handles array slicing:
###Code
# Create the following rank 2 array with shape (3, 4)
a = np.array([[1,2,3,4], [5,6,7,8], [9,10,11,12]])
print a
###Output
[[ 1 2 3 4]
[ 5 6 7 8]
[ 9 10 11 12]]
###Markdown
Two ways of accessing the data in the middle row of the array.Mixing integer indexing with slices yields an array of lower rank,while using only slices yields an array of the same rank as theoriginal array:
###Code
row_r1 = a[1, :] # Rank 1 view of the second row of a
row_r2 = a[1:2, :] # Rank 2 view of the second row of a
row_r3 = a[[1], :] # Rank 2 view of the second row of a
print row_r1, row_r1.shape
print row_r2, row_r2.shape
print row_r3, row_r3.shape
# We can make the same distinction when accessing columns of an array:
col_r1 = a[:, 1]
col_r2 = a[:, 1:2]
print col_r1, col_r1.shape
print
print col_r2, col_r2.shape
###Output
[ 2 6 10] (3,)
[[ 2]
[ 6]
[10]] (3, 1)
###Markdown
Integer array indexing: When you index into numpy arrays using slicing, the resulting array view will always be a subarray of the original array. In contrast, integer array indexing allows you to construct arbitrary arrays using the data from another array. Here is an example:
###Code
a = np.array([[1,2], [3, 4], [5, 6]])
# An example of integer array indexing.
# The returned array will have shape (3,) and
print a[[0, 1, 2], [0, 1, 0]]
# The above example of integer array indexing is equivalent to this:
print np.array([a[0, 0], a[1, 1], a[2, 0]])
# When using integer array indexing, you can reuse the same
# element from the source array:
print a[[0, 0], [1, 1]]
# Equivalent to the previous integer array indexing example
print np.array([a[0, 1], a[0, 1]])
###Output
[2 2]
[2 2]
###Markdown
One useful trick with integer array indexing is selecting or mutating one element from each row of a matrix:
###Code
# Create a new array from which we will select elements
a = np.array([[1,2,3], [4,5,6], [7,8,9], [10, 11, 12]])
print a
# Create an array of indices
b = np.array([0, 2, 0, 1])
# Select one element from each row of a using the indices in b
print a[np.arange(4), b] # Prints "[ 1 6 7 11]"
# Mutate one element from each row of a using the indices in b
a[np.arange(4), b] += 10
print a
###Output
[[11 2 3]
[ 4 5 16]
[17 8 9]
[10 21 12]]
###Markdown
Boolean array indexing: Boolean array indexing lets you pick out arbitrary elements of an array. Frequently this type of indexing is used to select the elements of an array that satisfy some condition. Here is an example:
###Code
import numpy as np
a = np.array([[1,2], [3, 4], [5, 6]])
bool_idx = (a > 2) # Find the elements of a that are bigger than 2;
# this returns a numpy array of Booleans of the same
# shape as a, where each slot of bool_idx tells
# whether that element of a is > 2.
print bool_idx
# We use boolean array indexing to construct a rank 1 array
# consisting of the elements of a corresponding to the True values
# of bool_idx
print a[bool_idx]
# We can do all of the above in a single concise statement:
print a[a > 2]
###Output
[3 4 5 6]
[3 4 5 6]
###Markdown
For brevity we have left out a lot of details about numpy array indexing; if you want to know more you should read the documentation. Datatypes Every numpy array is a grid of elements of the same type. Numpy provides a large set of numeric datatypes that you can use to construct arrays. Numpy tries to guess a datatype when you create an array, but functions that construct arrays usually also include an optional argument to explicitly specify the datatype. Here is an example:
###Code
x = np.array([1, 2]) # Let numpy choose the datatype
y = np.array([1.0, 2.0]) # Let numpy choose the datatype
z = np.array([1, 2], dtype=np.int64) # Force a particular datatype
print x.dtype, y.dtype, z.dtype
###Output
int64 float64 int64
###Markdown
You can read all about numpy datatypes in the [documentation](http://docs.scipy.org/doc/numpy/reference/arrays.dtypes.html). Array math Basic mathematical functions operate elementwise on arrays, and are available both as operator overloads and as functions in the numpy module:
###Code
x = np.array([[1,2],[3,4]], dtype=np.float64)
y = np.array([[5,6],[7,8]], dtype=np.float64)
# Elementwise sum; both produce the array
print x + y
print np.add(x, y)
# Elementwise difference; both produce the array
print x - y
print np.subtract(x, y)
# Elementwise product; both produce the array
print x * y
print np.multiply(x, y)
# Elementwise division; both produce the array
# [[ 0.2 0.33333333]
# [ 0.42857143 0.5 ]]
print x / y
print np.divide(x, y)
# Elementwise square root; produces the array
# [[ 1. 1.41421356]
# [ 1.73205081 2. ]]
print np.sqrt(x)
###Output
[[ 1. 1.41421356]
[ 1.73205081 2. ]]
###Markdown
Note that unlike MATLAB, `*` is elementwise multiplication, not matrix multiplication. We instead use the dot function to compute inner products of vectors, to multiply a vector by a matrix, and to multiply matrices. dot is available both as a function in the numpy module and as an instance method of array objects:
###Code
x = np.array([[1,2],[3,4]])
y = np.array([[5,6],[7,8]])
v = np.array([9,10])
w = np.array([11, 12])
# Inner product of vectors; both produce 219
print v.dot(w)
print np.dot(v, w)
# Matrix / vector product; both produce the rank 1 array [29 67]
print x.dot(v)
print np.dot(x, v)
# Matrix / matrix product; both produce the rank 2 array
# [[19 22]
# [43 50]]
print x.dot(y)
print np.dot(x, y)
###Output
[[19 22]
[43 50]]
[[19 22]
[43 50]]
###Markdown
Numpy provides many useful functions for performing computations on arrays; one of the most useful is `sum`:
###Code
x = np.array([[1,2],[3,4]])
print np.sum(x) # Compute sum of all elements; prints "10"
print np.sum(x, axis=0) # Compute sum of each column; prints "[4 6]"
print np.sum(x, axis=1) # Compute sum of each row; prints "[3 7]"
###Output
10
[4 6]
[3 7]
###Markdown
You can find the full list of mathematical functions provided by numpy in the [documentation](http://docs.scipy.org/doc/numpy/reference/routines.math.html).Apart from computing mathematical functions using arrays, we frequently need to reshape or otherwise manipulate data in arrays. The simplest example of this type of operation is transposing a matrix; to transpose a matrix, simply use the T attribute of an array object:
###Code
print x
print x.T
v = np.array([[1,2,3]])
print v
print v.T
###Output
[1 2 3]
[1 2 3]
###Markdown
Broadcasting Broadcasting is a powerful mechanism that allows numpy to work with arrays of different shapes when performing arithmetic operations. Frequently we have a smaller array and a larger array, and we want to use the smaller array multiple times to perform some operation on the larger array.For example, suppose that we want to add a constant vector to each row of a matrix. We could do it like this:
###Code
# We will add the vector v to each row of the matrix x,
# storing the result in the matrix y
x = np.array([[1,2,3], [4,5,6], [7,8,9], [10, 11, 12]])
v = np.array([1, 0, 1])
y = np.empty_like(x) # Create an empty matrix with the same shape as x
# Add the vector v to each row of the matrix x with an explicit loop
for i in range(4):
y[i, :] = x[i, :] + v
print y
###Output
[[ 2 2 4]
[ 5 5 7]
[ 8 8 10]
[11 11 13]]
###Markdown
This works; however when the matrix `x` is very large, computing an explicit loop in Python could be slow. Note that adding the vector v to each row of the matrix `x` is equivalent to forming a matrix `vv` by stacking multiple copies of `v` vertically, then performing elementwise summation of `x` and `vv`. We could implement this approach like this:
###Code
vv = np.tile(v, (4, 1)) # Stack 4 copies of v on top of each other
print vv # Prints "[[1 0 1]
# [1 0 1]
# [1 0 1]
# [1 0 1]]"
y = x + vv # Add x and vv elementwise
print y
###Output
[[ 2 2 4]
[ 5 5 7]
[ 8 8 10]
[11 11 13]]
###Markdown
Numpy broadcasting allows us to perform this computation without actually creating multiple copies of v. Consider this version, using broadcasting:
###Code
import numpy as np
# We will add the vector v to each row of the matrix x,
# storing the result in the matrix y
x = np.array([[1,2,3], [4,5,6], [7,8,9], [10, 11, 12]])
v = np.array([1, 0, 1])
y = x + v # Add v to each row of x using broadcasting
print y
###Output
[[ 2 2 4]
[ 5 5 7]
[ 8 8 10]
[11 11 13]]
###Markdown
The line `y = x + v` works even though `x` has shape `(4, 3)` and `v` has shape `(3,)` due to broadcasting; this line works as if v actually had shape `(4, 3)`, where each row was a copy of `v`, and the sum was performed elementwise.Broadcasting two arrays together follows these rules:1. If the arrays do not have the same rank, prepend the shape of the lower rank array with 1s until both shapes have the same length.2. The two arrays are said to be compatible in a dimension if they have the same size in the dimension, or if one of the arrays has size 1 in that dimension.3. The arrays can be broadcast together if they are compatible in all dimensions.4. After broadcasting, each array behaves as if it had shape equal to the elementwise maximum of shapes of the two input arrays.5. In any dimension where one array had size 1 and the other array had size greater than 1, the first array behaves as if it were copied along that dimensionIf this explanation does not make sense, try reading the explanation from the [documentation](http://docs.scipy.org/doc/numpy/user/basics.broadcasting.html) or this [explanation](http://wiki.scipy.org/EricsBroadcastingDoc).Functions that support broadcasting are known as universal functions. You can find the list of all universal functions in the [documentation](http://docs.scipy.org/doc/numpy/reference/ufuncs.htmlavailable-ufuncs).Here are some applications of broadcasting:
###Code
# Compute outer product of vectors
v = np.array([1,2,3]) # v has shape (3,)
w = np.array([4,5]) # w has shape (2,)
# To compute an outer product, we first reshape v to be a column
# vector of shape (3, 1); we can then broadcast it against w to yield
# an output of shape (3, 2), which is the outer product of v and w:
print np.reshape(v, (3, 1)) * w
# Add a vector to each row of a matrix
x = np.array([[1,2,3], [4,5,6]])
# x has shape (2, 3) and v has shape (3,) so they broadcast to (2, 3),
# giving the following matrix:
print x + v
# Add a vector to each column of a matrix
# x has shape (2, 3) and w has shape (2,).
# If we transpose x then it has shape (3, 2) and can be broadcast
# against w to yield a result of shape (3, 2); transposing this result
# yields the final result of shape (2, 3) which is the matrix x with
# the vector w added to each column. Gives the following matrix:
print (x.T + w).T
# Another solution is to reshape w to be a row vector of shape (2, 1);
# we can then broadcast it directly against x to produce the same
# output.
print x + np.reshape(w, (2, 1))
# Multiply a matrix by a constant:
# x has shape (2, 3). Numpy treats scalars as arrays of shape ();
# these can be broadcast together to shape (2, 3), producing the
# following array:
print x * 2
###Output
[[ 2 4 6]
[ 8 10 12]]
###Markdown
Broadcasting typically makes your code more concise and faster, so you should strive to use it where possible. This brief overview has touched on many of the important things that you need to know about numpy, but is far from complete. Check out the [numpy reference](http://docs.scipy.org/doc/numpy/reference/) to find out much more about numpy. Matplotlib Matplotlib is a plotting library. In this section give a brief introduction to the `matplotlib.pyplot` module, which provides a plotting system similar to that of MATLAB.
###Code
import matplotlib.pyplot as plt
###Output
_____no_output_____
###Markdown
By running this special iPython command, we will be displaying plots inline:
###Code
%matplotlib inline
###Output
_____no_output_____
###Markdown
Plotting The most important function in `matplotlib` is plot, which allows you to plot 2D data. Here is a simple example:
###Code
# Compute the x and y coordinates for points on a sine curve
x = np.arange(0, 3 * np.pi, 0.1)
y = np.sin(x)
# Plot the points using matplotlib
plt.plot(x, y)
###Output
_____no_output_____
###Markdown
With just a little bit of extra work we can easily plot multiple lines at once, and add a title, legend, and axis labels:
###Code
y_sin = np.sin(x)
y_cos = np.cos(x)
# Plot the points using matplotlib
plt.plot(x, y_sin)
plt.plot(x, y_cos)
plt.xlabel('x axis label')
plt.ylabel('y axis label')
plt.title('Sine and Cosine')
plt.legend(['Sine', 'Cosine'])
###Output
_____no_output_____
###Markdown
Subplots You can plot different things in the same figure using the subplot function. Here is an example:
###Code
# Compute the x and y coordinates for points on sine and cosine curves
x = np.arange(0, 3 * np.pi, 0.1)
y_sin = np.sin(x)
y_cos = np.cos(x)
# Set up a subplot grid that has height 2 and width 1,
# and set the first such subplot as active.
plt.subplot(2, 1, 1)
# Make the first plot
plt.plot(x, y_sin)
plt.title('Sine')
# Set the second subplot as active, and make the second plot.
plt.subplot(2, 1, 2)
plt.plot(x, y_cos)
plt.title('Cosine')
# Show the figure.
plt.show()
###Output
_____no_output_____ |
hw2test.ipynb | ###Markdown
Module 2.4: Sequential Logic**Prev: [Control Flow](2.3_control_flow.ipynb)****Next: [FIR Filter](2.5_exercise.ipynb)** MotivationYou can't write any meaningful digital logic without state. You can't write any meaningful digital logic without state. You can't write any meaningful digital logic....Get it? Because without storing intermediate results, you can't get anywhere.Ok, that bad joke aside, this module will describe how to express common sequential patterns in Chisel. By the end of the module, you should be able to implement and test a shift register in Chisel.It's important to emphasize that this section will probably not dramatically impress you. Chisel's power is not in new sequential logic patterns, but in the parameterization of a design. Before we demonstrate that capability, we have to learn what these sequential patterns are. Thus, this section will show you that Chisel can do pretty much what Verilog can do - you just need to learn the Chisel syntax. Setup
###Code
val path = System.getProperty("user.dir") + "/source/load-ivy.sc"
interp.load.module(ammonite.ops.Path(java.nio.file.FileSystems.getDefault().getPath(path)))
import chisel3._
import chisel3.util._
import chisel3.iotesters.{ChiselFlatSpec, Driver, PeekPokeTester}
###Output
_____no_output_____
###Markdown
--- RegistersThe basic stateful element in Chisel is the register, denoted `Reg`.A `Reg` holds its output value until the rising edge of its clock, at which time it takes on the value of its input.By default, every Chisel `Module` has an implicit clock that is used by every register in the design.This saves you from always specifying the same clock all over your code.**Example: Using a Register**The following code block implements a module that takes the input, adds 1 to it, and connects it as the input of a register.*Note: The implicit clock can be overridden for multi-clock designs. See the appendix for an example.*
###Code
class RegisterModule extends Module {
val io = IO(new Bundle {
val a = Input (UInt(4.W))
val b = Input (UInt(2.W))
val c = Input (UInt(3.W))
val out = Output(UInt())
})
val sum = Reg(UInt())
val prod = Reg(UInt())
sum := io.a + io.b
prod := sum * io.c
io.out := prod - sum
}
println(getFirrtl(new RegisterModule))
println(getVerilog(new RegisterModule))
###Output
[[35minfo[0m] [0.000] Elaborating design...
[[35minfo[0m] [0.070] Done elaborating.
circuit cmd7WrapperHelperRegisterModule :
module cmd7WrapperHelperRegisterModule :
input clock : Clock
input reset : UInt<1>
output io : {flip a : UInt<4>, flip b : UInt<2>, flip c : UInt<3>, out : UInt}
reg sum : UInt, clock @[cmd7.sc 9:16]
reg prod : UInt, clock @[cmd7.sc 10:17]
node _T_8 = add(io.a, io.b) @[cmd7.sc 11:15]
node _T_9 = tail(_T_8, 1) @[cmd7.sc 11:15]
sum <= _T_9 @[cmd7.sc 11:7]
node _T_10 = mul(sum, io.c) @[cmd7.sc 12:15]
prod <= _T_10 @[cmd7.sc 12:8]
node _T_11 = sub(prod, sum) @[cmd7.sc 13:18]
node _T_12 = asUInt(_T_11) @[cmd7.sc 13:18]
node _T_13 = tail(_T_12, 1) @[cmd7.sc 13:18]
io.out <= _T_13 @[cmd7.sc 13:10]
[[35minfo[0m] [0.000] Elaborating design...
[[35minfo[0m] [0.002] Done elaborating.
Total FIRRTL Compile Time: 1529.4 ms
module cmd7WrapperHelperRegisterModule( // @[:@3.2]
input clock, // @[:@4.4]
input reset, // @[:@5.4]
input [3:0] io_a, // @[:@6.4]
input [1:0] io_b, // @[:@6.4]
input [2:0] io_c, // @[:@6.4]
output [6:0] io_out // @[:@6.4]
);
reg [3:0] sum; // @[cmd7.sc 9:16:@8.4]
reg [31:0] _RAND_0;
reg [6:0] prod; // @[cmd7.sc 10:17:@9.4]
reg [31:0] _RAND_1;
wire [3:0] _GEN_0; // @[cmd7.sc 11:15:@10.4]
wire [4:0] _T_8; // @[cmd7.sc 11:15:@10.4]
wire [3:0] _T_9; // @[cmd7.sc 11:15:@11.4]
wire [3:0] _GEN_1; // @[cmd7.sc 12:15:@13.4]
wire [6:0] _T_10; // @[cmd7.sc 12:15:@13.4]
wire [6:0] _GEN_2; // @[cmd7.sc 13:18:@15.4]
wire [7:0] _T_11; // @[cmd7.sc 13:18:@15.4]
wire [7:0] _T_12; // @[cmd7.sc 13:18:@16.4]
wire [6:0] _T_13; // @[cmd7.sc 13:18:@17.4]
assign _GEN_0 = {{2'd0}, io_b}; // @[cmd7.sc 11:15:@10.4]
assign _T_8 = io_a + _GEN_0; // @[cmd7.sc 11:15:@10.4]
assign _T_9 = _T_8[3:0]; // @[cmd7.sc 11:15:@11.4]
assign _GEN_1 = {{1'd0}, io_c}; // @[cmd7.sc 12:15:@13.4]
assign _T_10 = sum * _GEN_1; // @[cmd7.sc 12:15:@13.4]
assign _GEN_2 = {{3'd0}, sum}; // @[cmd7.sc 13:18:@15.4]
assign _T_11 = prod - _GEN_2; // @[cmd7.sc 13:18:@15.4]
assign _T_12 = $unsigned(_T_11); // @[cmd7.sc 13:18:@16.4]
assign _T_13 = _T_12[6:0]; // @[cmd7.sc 13:18:@17.4]
assign io_out = _T_13;
`ifdef RANDOMIZE
integer initvar;
initial begin
`ifndef verilator
#0.002 begin end
`endif
`ifdef RANDOMIZE_REG_INIT
_RAND_0 = {1{$random}};
sum = _RAND_0[3:0];
`endif // RANDOMIZE_REG_INIT
`ifdef RANDOMIZE_REG_INIT
_RAND_1 = {1{$random}};
prod = _RAND_1[6:0];
`endif // RANDOMIZE_REG_INIT
end
`endif // RANDOMIZE
always @(posedge clock) begin
sum <= _T_9;
prod <= _T_10;
end
endmodule
###Markdown
The register is created by calling `Reg(tpe)`, where `tpe` is a variable that encodes the type of register we want.In this example, `tpe` is a 12-bit `UInt`.Look at what the tester above is doing.Between calls to `poke()` and `expect`, there is a call to `step(1)`.This tells the test harness to tick the clock once, which will cause the register to pass its input to its output.Calling `step(n)` will tick the clock `n` times.The astute observer will notice that previous testers testing combinational logic did not call `step()`. This is because calling `poke()` on an input immediately propagates the updated values through combinational logic. Calling `step()` is only needed to update state elements in sequential logic. The code block below will show the verilog generated by `RegisterModule`.Note:* The module has an input for clock (and reset) that you didn't add- this is the implicit clock* The variable `register` shows up as `reg [11:0]`, as expected* There is a block sectioned off by ` `ifdef Randomize` that initialized the register to some random variable before simulation starts* `register` is updated on `posedge clock`
###Code
println(getVerilog(new RegisterModule))
###Output
[[35minfo[0m] [0.001] Elaborating design...
[[35minfo[0m] [0.004] Done elaborating.
Total FIRRTL Compile Time: 157.5 ms
module cmd2WrapperHelperRegisterModule( // @[:@3.2]
input clock, // @[:@4.4]
input reset, // @[:@5.4]
input [11:0] io_in, // @[:@6.4]
output [11:0] io_out // @[:@6.4]
);
reg [11:0] register; // @[cmd2.sc 7:21:@8.4]
reg [31:0] _RAND_0;
wire [12:0] _T_6; // @[cmd2.sc 8:21:@9.4]
wire [11:0] _T_7; // @[cmd2.sc 8:21:@10.4]
assign _T_6 = io_in + 12'h1; // @[cmd2.sc 8:21:@9.4]
assign _T_7 = _T_6[11:0]; // @[cmd2.sc 8:21:@10.4]
assign io_out = register;
`ifdef RANDOMIZE
integer initvar;
initial begin
`ifndef verilator
#0.002 begin end
`endif
`ifdef RANDOMIZE_REG_INIT
_RAND_0 = {1{$random}};
register = _RAND_0[11:0];
`endif // RANDOMIZE_REG_INIT
end
`endif // RANDOMIZE
always @(posedge clock) begin
register <= _T_7;
end
endmodule
###Markdown
One important note is that Chisel distinguishes between types (like `UInt`) and hardware nodes (like the literal `2.U`, or the output of `myReg`). While```scalaval myReg = Reg(UInt(2.W))```is legal because a Reg needs a data type as a model,```scalaval myReg = Reg(2.U)```is an error because `2.U` is already a hardware node and can't be used as a model. **Example: RegNext**Chisel has a convenience register object for registers with simple input connections. The previous `Module` can be shortened to the following `Module`. Notice how we didn't need to specify the register bitwidth this time. It gets inferred from the register's output connection, in this case `io.out`.
###Code
class RegNextModule extends Module {
val io = IO(new Bundle {
val in = Input (UInt(12.W))
val out = Output(UInt(12.W))
})
// register bitwidth is inferred from io.out
io.out := RegNext(io.in + 1.U)
}
class RegNextModuleTester(c: RegNextModule) extends PeekPokeTester(c) {
for (i <- 0 until 100) {
poke(c.io.in, i)
step(1)
expect(c.io.out, i+1)
}
}
assert(chisel3.iotesters.Driver(() => new RegNextModule) { c => new RegNextModuleTester(c) })
println("SUCCESS!!")
###Output
[[35minfo[0m] [0.000] Elaborating design...
[[35minfo[0m] [0.004] Done elaborating.
Total FIRRTL Compile Time: 41.4 ms
Total FIRRTL Compile Time: 15.9 ms
End of dependency graph
Circuit state created
[[35minfo[0m] [0.000] SEED 1536081558494
test cmd4WrapperHelperRegNextModule Success: 100 tests passed in 105 cycles taking 0.149247 seconds
[[35minfo[0m] [0.147] RAN 100 CYCLES PASSED
SUCCESS!!
###Markdown
The Verilog looks almost the same as before, though the register name is generated instead of explicity defined.
###Code
println(getVerilog(new RegNextModule))
###Output
[[35minfo[0m] [0.000] Elaborating design...
[[35minfo[0m] [0.003] Done elaborating.
Total FIRRTL Compile Time: 22.6 ms
module cmd4WrapperHelperRegNextModule( // @[:@3.2]
input clock, // @[:@4.4]
input reset, // @[:@5.4]
input [11:0] io_in, // @[:@6.4]
output [11:0] io_out // @[:@6.4]
);
wire [12:0] _T_5; // @[cmd4.sc 8:27:@8.4]
wire [11:0] _T_6; // @[cmd4.sc 8:27:@9.4]
reg [11:0] _T_8; // @[cmd4.sc 8:20:@10.4]
reg [31:0] _RAND_0;
assign _T_5 = io_in + 12'h1; // @[cmd4.sc 8:27:@8.4]
assign _T_6 = _T_5[11:0]; // @[cmd4.sc 8:27:@9.4]
assign io_out = _T_8;
`ifdef RANDOMIZE
integer initvar;
initial begin
`ifndef verilator
#0.002 begin end
`endif
`ifdef RANDOMIZE_REG_INIT
_RAND_0 = {1{$random}};
_T_8 = _RAND_0[11:0];
`endif // RANDOMIZE_REG_INIT
end
`endif // RANDOMIZE
always @(posedge clock) begin
_T_8 <= _T_6;
end
endmodule
###Markdown
--- `RegInit`The register in `RegisterModule` was initialized to random data for simulation.Unless otherwised specified, registers do not have a reset value (or a reset).The way to create a register that resets to a given value is with `RegInit`.For instance, a 12-bit register initialized to zero can be created with the following.Both versions below are valid and do the same thing:```scalaval myReg = RegInit(UInt(12.W), 0.U)val myReg = RegInit(0.U(12.W))```The first version has two arguments.The first argument is a type node that specified the datatype and its width.The second argument is a hardware node that specified the reset value, in this case 0.The second version has one argument.It is a hardware node that specifies the reset value, but normally `0.U`.**Example: Initialized Register** The following demonstrates using `RegInit()`, initialized to zero.
###Code
class RegInitModule extends Module {
val io = IO(new Bundle {
val in = Input (UInt(12.W))
val out = Output(UInt(12.W))
})
val register = RegInit(0.U(12.W))
register := io.in + 1.U
io.out := register
}
println(getVerilog(new RegInitModule))
###Output
[[35minfo[0m] [0.000] Elaborating design...
[[35minfo[0m] [0.006] Done elaborating.
Total FIRRTL Compile Time: 20.3 ms
module cmd6WrapperHelperRegInitModule( // @[:@3.2]
input clock, // @[:@4.4]
input reset, // @[:@5.4]
input [11:0] io_in, // @[:@6.4]
output [11:0] io_out // @[:@6.4]
);
reg [11:0] register; // @[cmd6.sc 7:25:@8.4]
reg [31:0] _RAND_0;
wire [12:0] _T_7; // @[cmd6.sc 8:21:@9.4]
wire [11:0] _T_8; // @[cmd6.sc 8:21:@10.4]
assign _T_7 = io_in + 12'h1; // @[cmd6.sc 8:21:@9.4]
assign _T_8 = _T_7[11:0]; // @[cmd6.sc 8:21:@10.4]
assign io_out = register;
`ifdef RANDOMIZE
integer initvar;
initial begin
`ifndef verilator
#0.002 begin end
`endif
`ifdef RANDOMIZE_REG_INIT
_RAND_0 = {1{$random}};
register = _RAND_0[11:0];
`endif // RANDOMIZE_REG_INIT
end
`endif // RANDOMIZE
always @(posedge clock) begin
if (reset) begin
register <= 12'h0;
end else begin
register <= _T_8;
end
end
endmodule
###Markdown
Note that the generated verilog now has a block that checks `if (reset)` to reset the register to 0.Also note that this is inside the `always @(posedge clock)` block.Chisel's implicit reset is active high and synchronous.The register is still initialized to random junk before reset is called.The `PeekPokeTesters` always call reset before running your test, but you can manually call reset as well using the `reset(n)` function, where reset is high for `n` cycles. --- Control FlowRegisters are very similar to wires in terms of control flow.They have last connect semantics and can be assigned to conditionally with `when`, `elsewhen`, and `otherwise`.**Example: Register Control Flow**The following example finds the maximum value in a sequence of inputs using conditional register assignments.
###Code
class FindMax extends Module {
val io = IO(new Bundle {
val in = Input(UInt(10.W))
val max = Output(UInt(10.W))
})
val max = RegInit(0.U(10.W))
when (io.in > max) {
max := io.in
}
io.max := max
}
assert(chisel3.iotesters.Driver(() => new FindMax) {
c => new PeekPokeTester(c) {
expect(c.io.max, 0)
poke(c.io.in, 1)
step(1)
expect(c.io.max, 1)
poke(c.io.in, 3)
step(1)
expect(c.io.max, 3)
poke(c.io.in, 2)
step(1)
expect(c.io.max, 3)
poke(c.io.in, 24)
step(1)
expect(c.io.max, 24)
}
})
println("SUCCESS!!")
###Output
[[35minfo[0m] [0.000] Elaborating design...
[[35minfo[0m] [0.010] Done elaborating.
Total FIRRTL Compile Time: 21.9 ms
Total FIRRTL Compile Time: 19.0 ms
End of dependency graph
Circuit state created
[[35minfo[0m] [0.001] SEED 1536081843926
test cmd7WrapperHelperFindMax Success: 5 tests passed in 9 cycles taking 0.008140 seconds
[[35minfo[0m] [0.006] RAN 4 CYCLES PASSED
SUCCESS!!
###Markdown
--- Other Register ExamplesOperations called on a register are performed on the **output** of the register, and the kind of operations depend on the register's type.That means that you can write```scalaval reg: UInt = Reg(UInt(4.W))```which means the value `reg` is of type `UInt` and you can do things you can normally do with `UInt`s, like `+`, `-`, etc.You aren't restricted to using `UInt`s with registers, you can use any subclass of the base type `chisel3.Data`. This includes `SInt` for signed integers and a lot of other things.**Example: Comb Filter**The following example shows a comb filter.
###Code
class Comb extends Module {
val io = IO(new Bundle {
val in = Input(SInt(12.W))
val out = Output(SInt(12.W))
})
val delay: SInt = Reg(SInt(12.W))
delay := io.in
io.out := io.in - delay
}
println(getVerilog(new Comb))
###Output
[[35minfo[0m] [0.000] Elaborating design...
[[35minfo[0m] [0.009] Done elaborating.
Total FIRRTL Compile Time: 27.1 ms
module cmd8WrapperHelperComb( // @[:@3.2]
input clock, // @[:@4.4]
input reset, // @[:@5.4]
input [11:0] io_in, // @[:@6.4]
output [11:0] io_out // @[:@6.4]
);
reg [11:0] delay; // @[cmd8.sc 7:24:@8.4]
reg [31:0] _RAND_0;
wire [12:0] _T_5; // @[cmd8.sc 9:19:@10.4]
wire [11:0] _T_6; // @[cmd8.sc 9:19:@11.4]
wire [11:0] _T_7; // @[cmd8.sc 9:19:@12.4]
assign _T_5 = $signed(io_in) - $signed(delay); // @[cmd8.sc 9:19:@10.4]
assign _T_6 = _T_5[11:0]; // @[cmd8.sc 9:19:@11.4]
assign _T_7 = $signed(_T_6); // @[cmd8.sc 9:19:@12.4]
assign io_out = _T_7;
`ifdef RANDOMIZE
integer initvar;
initial begin
`ifndef verilator
#0.002 begin end
`endif
`ifdef RANDOMIZE_REG_INIT
_RAND_0 = {1{$random}};
delay = _RAND_0[11:0];
`endif // RANDOMIZE_REG_INIT
end
`endif // RANDOMIZE
always @(posedge clock) begin
delay <= io_in;
end
endmodule
###Markdown
--- Exercises**Exercise: Shift Register**Given your new-found registering knowledge, build a module that implements a shift register for a LFSR. Specifically:- Each element is a single bit wide.- Has a 4-bit output signal.- Takes a single input bit, which is the next value into the shift register.- Outputs the parallel output of the shift register, with the most significant bit being the last element of the shift register and the least significant bit being the first element of the shift register. `Cat` may come in handy.- **The output initializes at `b0001`.**- Shifts each clock cycle (no enable signal).- **Note in Chisel, subword assignment IS ILLEGAL**; something like `out(0) := in` will not work.A basic Module skeleton, testvector, and Driver invocation is provided below. The first register has been provided for you.
###Code
class MyShiftRegister(val init: Int = 1) extends Module {
val io = IO(new Bundle {
val in = Input(Bool())
val out = Output(UInt(4.W))
})
val state = RegInit(UInt(4.W), init.U)
val next_state = Wire(UInt(4.W))
next_state := Cat(state(2), state(1), state(0), io.in)
state := next_state
io.out := state
}
class MyShiftRegisterTester(c: MyShiftRegister) extends PeekPokeTester(c) {
var state = c.init
for (i <- 0 until 10) {
// poke in LSB of i (i % 2)
poke(c.io.in, i % 2)
// update expected state
state = ((state * 2) + (i % 2)) & 0xf
step(1)
expect(c.io.out, state)
}
}
assert(chisel3.iotesters.Driver(() => new MyShiftRegister()) {
c => new MyShiftRegisterTester(c)
})
println("SUCCESS!!")
###Output
[[35minfo[0m] [0.000] Elaborating design...
[[35minfo[0m] [0.007] Done elaborating.
Total FIRRTL Compile Time: 18.0 ms
Total FIRRTL Compile Time: 16.0 ms
End of dependency graph
Circuit state created
[[35minfo[0m] [0.000] SEED 1536083837322
test cmd19WrapperHelperMyShiftRegister Success: 10 tests passed in 15 cycles taking 0.007899 seconds
[[35minfo[0m] [0.007] RAN 10 CYCLES PASSED
SUCCESS!!
###Markdown
Solution val nextState = (state << 1) | io.in state := nextState io.out := state **Exercise: Parameterized Shift Register (Optional)**Write a shift register that is parameterized by its delay (`n`), its initial value (`init`), and also has an enable input signal (`en`).
###Code
// n is the output width (number of delays - 1)
// init state to init
class MyOptionalShiftRegister(val n: Int, val init: BigInt = 1) extends Module {
val io = IO(new Bundle {
val en = Input(Bool())
val in = Input(Bool())
val out = Output(UInt(n.W))
})
val state = RegInit(init.U(n.W))
val next_state = Wire(UInt(n.W))
// Can just use bit shifting and bitwise OR
next_state := ((state << 1) | io.in)
when (io.en) {state := next_state}
// I think io.out should be assigned to state, as in the previous exercise.
io.out := next_state
}
class MyOptionalShiftRegisterTester(c: MyOptionalShiftRegister) extends PeekPokeTester(c) {
val inSeq = Seq(0, 1, 1, 1, 0, 1, 1, 0, 0, 1)
var state = c.init
var i = 0
poke(c.io.en, 1)
while (i < 10 * c.n) {
// poke in repeated inSeq
val toPoke = inSeq(i % inSeq.length)
poke(c.io.in, toPoke)
// update expected state
state = ((state * 2) + toPoke) & BigInt("1"*c.n, 2)
expect(c.io.out, state)
step(1)
i += 1
}
}
// test different depths
for (i <- Seq(3, 4, 8, 24, 65)) {
println(s"Testing n=$i")
assert(chisel3.iotesters.Driver(() => new MyOptionalShiftRegister(n = i)) {
c => new MyOptionalShiftRegisterTester(c)
})
}
println("SUCCESS!!")
###Output
Testing n=3
[[35minfo[0m] [0.000] Elaborating design...
[[35minfo[0m] [0.005] Done elaborating.
Total FIRRTL Compile Time: 14.2 ms
Total FIRRTL Compile Time: 15.0 ms
End of dependency graph
Circuit state created
[[35minfo[0m] [0.001] SEED 1536110690413
test cmd8WrapperHelperMyOptionalShiftRegister Success: 30 tests passed in 35 cycles taking 0.010246 seconds
[[35minfo[0m] [0.007] RAN 30 CYCLES PASSED
Testing n=4
[[35minfo[0m] [0.000] Elaborating design...
[[35minfo[0m] [0.003] Done elaborating.
Total FIRRTL Compile Time: 15.4 ms
Total FIRRTL Compile Time: 11.4 ms
End of dependency graph
Circuit state created
[[35minfo[0m] [0.000] SEED 1536110690483
test cmd8WrapperHelperMyOptionalShiftRegister Success: 40 tests passed in 45 cycles taking 0.005827 seconds
[[35minfo[0m] [0.006] RAN 40 CYCLES PASSED
Testing n=8
[[35minfo[0m] [0.000] Elaborating design...
[[35minfo[0m] [0.002] Done elaborating.
Total FIRRTL Compile Time: 15.6 ms
Total FIRRTL Compile Time: 18.6 ms
End of dependency graph
Circuit state created
[[35minfo[0m] [0.000] SEED 1536110690540
test cmd8WrapperHelperMyOptionalShiftRegister Success: 80 tests passed in 85 cycles taking 0.010338 seconds
[[35minfo[0m] [0.010] RAN 80 CYCLES PASSED
Testing n=24
[[35minfo[0m] [0.000] Elaborating design...
[[35minfo[0m] [0.002] Done elaborating.
Total FIRRTL Compile Time: 11.8 ms
Total FIRRTL Compile Time: 14.4 ms
End of dependency graph
Circuit state created
[[35minfo[0m] [0.000] SEED 1536110690619
test cmd8WrapperHelperMyOptionalShiftRegister Success: 240 tests passed in 245 cycles taking 0.030431 seconds
[[35minfo[0m] [0.030] RAN 240 CYCLES PASSED
Testing n=65
[[35minfo[0m] [0.000] Elaborating design...
[[35minfo[0m] [0.002] Done elaborating.
Total FIRRTL Compile Time: 15.5 ms
Total FIRRTL Compile Time: 9.0 ms
End of dependency graph
Circuit state created
[[35minfo[0m] [0.001] SEED 1536110690697
test cmd8WrapperHelperMyOptionalShiftRegister Success: 650 tests passed in 655 cycles taking 0.067013 seconds
[[35minfo[0m] [0.067] RAN 650 CYCLES PASSED
SUCCESS!!
###Markdown
Solution val nextState = (state << 1) | io.in when (io.en) { state := nextState } io.out := nextState --- Appendix: Explicit clock and resetChisel modules have a default clock and reset that are implicitly used by every register created inside them.There are times where you want to be able to override this default behavior; perhaps you have a black box that generates a clock or reset signal, or you have a multi-clock design.Chisel provides constructs for dealing with these cases.Clocks and resets can be overridden separately or together with `withClock() {}`, `withReset() {}`, and `withClockAndReset() {}`.The following code blocks will give examples of using these functions.One thing to be aware of is that `reset` (as of this tutorial's writing) is always synchronous and of type `Bool`.Clocks have their own type in Chisel (`Clock`) and should be declared as such.*`Bool`s can be converted to `Clock`s by calling `asClock()` on them, but you should be careful that you aren't doing something silly.*Also note that `chisel-testers` do not currently have complete support for multi-clock designs.**Example: Multi-Clock Module**A module with multiple clocks and reset signals.
###Code
// we need to import multi-clock features
import chisel3.experimental.{withClock, withReset, withClockAndReset}
class ClockExamples extends Module {
val io = IO(new Bundle {
val in = Input(UInt(10.W))
val alternateReset = Input(Bool())
val alternateClock = Input(Clock())
val outImplicit = Output(UInt())
val outAlternateReset = Output(UInt())
val outAlternateClock = Output(UInt())
val outAlternateBoth = Output(UInt())
})
val imp = RegInit(0.U(10.W))
imp := io.in
io.outImplicit := imp
withReset(io.alternateReset) {
// everything in this scope with have alternateReset as the reset
val altRst = RegInit(0.U(10.W))
altRst := io.in
io.outAlternateReset := altRst
}
withClock(io.alternateClock) {
val altClk = RegInit(0.U(10.W))
altClk := io.in
io.outAlternateClock := altClk
}
withClockAndReset(io.alternateClock, io.alternateReset) {
val alt = RegInit(0.U(10.W))
alt := io.in
io.outAlternateBoth := alt
}
}
println(getVerilog(new ClockExamples))
###Output
[[35minfo[0m] [0.000] Elaborating design...
[[35minfo[0m] [0.007] Done elaborating.
Total FIRRTL Compile Time: 19.3 ms
module cmd10WrapperHelperClockExamples( // @[:@3.2]
input clock, // @[:@4.4]
input reset, // @[:@5.4]
input [9:0] io_in, // @[:@6.4]
input io_alternateReset, // @[:@6.4]
input io_alternateClock, // @[:@6.4]
output [9:0] io_outImplicit, // @[:@6.4]
output [9:0] io_outAlternateReset, // @[:@6.4]
output [9:0] io_outAlternateClock, // @[:@6.4]
output [9:0] io_outAlternateBoth // @[:@6.4]
);
reg [9:0] imp; // @[cmd10.sc 14:20:@8.4]
reg [31:0] _RAND_0;
reg [9:0] _T_13; // @[cmd10.sc 20:25:@11.4]
reg [31:0] _RAND_1;
reg [9:0] _T_16; // @[cmd10.sc 26:25:@14.4]
reg [31:0] _RAND_2;
reg [9:0] _T_19; // @[cmd10.sc 32:22:@17.4]
reg [31:0] _RAND_3;
assign io_outImplicit = imp;
assign io_outAlternateReset = _T_13;
assign io_outAlternateClock = _T_16;
assign io_outAlternateBoth = _T_19;
`ifdef RANDOMIZE
integer initvar;
initial begin
`ifndef verilator
#0.002 begin end
`endif
`ifdef RANDOMIZE_REG_INIT
_RAND_0 = {1{$random}};
imp = _RAND_0[9:0];
`endif // RANDOMIZE_REG_INIT
`ifdef RANDOMIZE_REG_INIT
_RAND_1 = {1{$random}};
_T_13 = _RAND_1[9:0];
`endif // RANDOMIZE_REG_INIT
`ifdef RANDOMIZE_REG_INIT
_RAND_2 = {1{$random}};
_T_16 = _RAND_2[9:0];
`endif // RANDOMIZE_REG_INIT
`ifdef RANDOMIZE_REG_INIT
_RAND_3 = {1{$random}};
_T_19 = _RAND_3[9:0];
`endif // RANDOMIZE_REG_INIT
end
`endif // RANDOMIZE
always @(posedge clock) begin
if (reset) begin
imp <= 10'h0;
end else begin
imp <= io_in;
end
if (io_alternateReset) begin
_T_13 <= 10'h0;
end else begin
_T_13 <= io_in;
end
end
always @(posedge io_alternateClock) begin
if (reset) begin
_T_16 <= 10'h0;
end else begin
_T_16 <= io_in;
end
if (io_alternateReset) begin
_T_19 <= 10'h0;
end else begin
_T_19 <= io_in;
end
end
endmodule
|
site/en/r2/tutorials/keras/save_and_restore_models.ipynb | ###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject-based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install h5py pyyaml
###Output
_____no_output_____
###Markdown
Get an example datasetWe'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/) to train our model to demonstrate saving weights. To speed up these demonstration runs, only use the first 1000 examples:
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
import os
!pip install tensorflow==2.0.0-beta1
import tensorflow as tf
from tensorflow import keras
tf.__version__
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Let's build a simple model we'll use to demonstrate saving and loading weights.
###Code
# Returns a short sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training The primary use case is to automatically save checkpoints *during* and at *the end* of training. This way you can use a trained model without having to retrain it, or pick-up training where you left of—in case the training process was interrupted.`tf.keras.callbacks.ModelCheckpoint` is a callback that performs this task. The callback takes a couple of arguments to configure checkpointing. Checkpoint callback usageTrain the model and pass it the `ModelCheckpoint` callback:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create checkpoint callback
cp_callback = tf.keras.callbacks.ModelCheckpoint(checkpoint_path,
save_weights_only=True,
verbose=1)
model = create_model()
model.fit(train_images, train_labels, epochs = 10,
validation_data = (test_images,test_labels),
callbacks = [cp_callback]) # pass callback to training
# This may generate warnings related to saving the state of the optimizer.
# These warnings (and similar warnings throughout this notebook)
# are in place to discourage outdated usage, and can be ignored.
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from only weights, you must have a model with the same architecture as the original model. Since it's the same model architecture, we can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
model = create_model()
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint, and re-evaluate:
###Code
model.load_weights(checkpoint_path)
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to give the resulting checkpoints unique names, and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every 5-epochs:
###Code
# include the epoch in the file name. (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(
checkpoint_path, verbose=1, save_weights_only=True,
# Save weights, every 5-epochs.
period=5)
model = create_model()
model.save_weights(checkpoint_path.format(epoch=0))
model.fit(train_images, train_labels,
epochs = 50, callbacks = [cp_callback],
validation_data = (test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
model = create_model()
model.load_weights(latest)
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights.* An index file that indicates which weights are stored in a which shard.If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsAbove you saw how to load the weights into a model.Manually saving the weights is just as simple, use the `Model.save_weights` method.
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Restore the weights
model = create_model()
model.load_weights('./checkpoints/my_checkpoint')
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables), and the model configuration. This allows you to export a model so it can be used without access to the original python code. Since the optimizer-state is recovered you can even resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) As an HDF5 fileKeras provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob.
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
# Save entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now recreate the model from that file:
###Code
# Recreate the exact same model, including weights and optimizer.
new_model = keras.models.load_model('my_model.h5')
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configurationKeras saves models by inspecting the architecture. Currently, it is not able to save TensorFlow optimizers (from `tf.train`). When using those you will need to re-compile the model after loading, and you will lose the state of the optimizer. As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions. Build a fresh model:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`, and place it in a time-stamped directory:
###Code
import time
saved_model_path = "./saved_models/{}".format(int(time.time()))
tf.keras.experimental.export_saved_model(model, saved_model_path)
saved_model_path
###Output
_____no_output_____
###Markdown
List your saved models:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh keras model from the saved model.
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
new_model.summary()
###Output
_____no_output_____
###Markdown
Run the restored model.
###Code
model.predict(test_images).shape
# The model has to be compiled before evaluating.
# This step is not required if the saved model is only being deployed.
new_model.compile(optimizer=model.optimizer, # keep the optimizer that was loaded
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Evaluate the restored model.
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject_based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install h5py pyyaml
###Output
_____no_output_____
###Markdown
Get an example datasetWe'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/) to train our model to demonstrate saving weights. To speed up these demonstration runs, only use the first 1000 examples:
###Code
from __future__ import absolute_import, division, print_function
import os
!pip install tf-nightly-2.0-preview
import tensorflow as tf
keras = tf.keras
tf.__version__
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Let's build a simple model we'll use to demonstrate saving and loading weights.
###Code
# Returns a short sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation=tf.keras.activations.relu, input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation=tf.keras.activations.softmax)
])
model.compile(optimizer='adam',
loss=tf.keras.losses.sparse_categorical_crossentropy,
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training The primary use case is to automatically save checkpoints *during* and at *the end* of training. This way you can use a trained model without having to retrain it, or pick-up training where you left of—in case the training process was interrupted.`tf.keras.callbacks.ModelCheckpoint` is a callback that performs this task. The callback takes a couple of arguments to configure checkpointing. Checkpoint callback usageTrain the model and pass it the `ModelCheckpoint` callback:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create checkpoint callback
cp_callback = tf.keras.callbacks.ModelCheckpoint(checkpoint_path,
save_weights_only=True,
verbose=1)
model = create_model()
model.fit(train_images, train_labels, epochs = 10,
validation_data = (test_images,test_labels),
callbacks = [cp_callback]) # pass callback to training
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from only weights, you must have a model with the same architecture as the original model. Since it's the same model architecture, we can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
model = create_model()
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint, and re-evaluate:
###Code
model.load_weights(checkpoint_path)
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to give the resulting checkpoints unique names, and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every 5-epochs:
###Code
# include the epoch in the file name. (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(
checkpoint_path, verbose=1, save_weights_only=True,
# Save weights, every 5-epochs.
period=5)
model = create_model()
model.save_weights(checkpoint_path.format(epoch=0))
model.fit(train_images, train_labels,
epochs = 50, callbacks = [cp_callback],
validation_data = (test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
model = create_model()
model.load_weights(latest)
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights. * An index file that indicates which weights are stored in a which shard. If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsAbove you saw how to load the weights into a model.Manually saving the weights is just as simple, use the `Model.save_weights` method.
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Restore the weights
model = create_model()
model.load_weights('./checkpoints/my_checkpoint')
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe entire model can be saved to a file that contains the weight values, the model's configuration, and even the optimizer's configuration (depends on set up). This allows you to checkpoint a model and resume training later—from the exact same state—without access to the original code.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) As an HDF5 fileKeras provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob.
###Code
model = create_model()
# You need to use a keras.optimizer to restore the optimizer state from an HDF5 file.
model.compile(optimizer='adam',
loss=tf.keras.losses.sparse_categorical_crossentropy,
metrics=['accuracy'])
model.fit(train_images, train_labels, epochs=5)
# Save entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now recreate the model from that file:
###Code
# Recreate the exact same model, including weights and optimizer.
new_model = keras.models.load_model('my_model.h5')
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject_based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install h5py pyyaml
###Output
_____no_output_____
###Markdown
Get an example datasetWe'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/) to train our model to demonstrate saving weights. To speed up these demonstration runs, only use the first 1000 examples:
###Code
from __future__ import absolute_import, division, print_function
import os
!pip install tf-nightly-2.0-preview
import tensorflow as tf
keras = tf.keras
tf.__version__
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Let's build a simple model we'll use to demonstrate saving and loading weights.
###Code
# Returns a short sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation=tf.keras.activations.relu, input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation=tf.keras.activations.softmax)
])
model.compile(optimizer='adam',
loss=tf.keras.losses.sparse_categorical_crossentropy,
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training The primary use case is to automatically save checkpoints *during* and at *the end* of training. This way you can use a trained model without having to retrain it, or pick-up training where you left of—in case the training process was interrupted.`tf.keras.callbacks.ModelCheckpoint` is a callback that performs this task. The callback takes a couple of arguments to configure checkpointing. Checkpoint callback usageTrain the model and pass it the `ModelCheckpoint` callback:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create checkpoint callback
cp_callback = tf.keras.callbacks.ModelCheckpoint(checkpoint_path,
save_weights_only=True,
verbose=1)
model = create_model()
model.fit(train_images, train_labels, epochs = 10,
validation_data = (test_images,test_labels),
callbacks = [cp_callback]) # pass callback to training
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from only weights, you must have a model with the same architecture as the original model. Since it's the same model architecture, we can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
model = create_model()
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint, and re-evaluate:
###Code
model.load_weights(checkpoint_path)
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to give the resulting checkpoints unique names, and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every 5-epochs:
###Code
# include the epoch in the file name. (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(
checkpoint_path, verbose=1, save_weights_only=True,
# Save weights, every 5-epochs.
period=5)
model = create_model()
model.save_weights(checkpoint_path.format(epoch=0))
model.fit(train_images, train_labels,
epochs = 50, callbacks = [cp_callback],
validation_data = (test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
model = create_model()
model.load_weights(latest)
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights. * An index file that indicates which weights are stored in a which shard. If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsAbove you saw how to load the weights into a model.Manually saving the weights is just as simple, use the `Model.save_weights` method.
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Restore the weights
model = create_model()
model.load_weights('./checkpoints/my_checkpoint')
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables), and the model configuration. This allows you to export a model so it can be used without access to the original python code. Since the optimizer-state is recovered you can even resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) As an HDF5 fileKeras provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob.
###Code
model = create_model()
model.compile(optimizer='adam',
loss=tf.keras.losses.sparse_categorical_crossentropy,
metrics=['accuracy'])
model.fit(train_images, train_labels, epochs=5)
# Save entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now recreate the model from that file:
###Code
# Recreate the exact same model, including weights and optimizer.
new_model = keras.models.load_model('my_model.h5')
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configuration As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions. Build a fresh model:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`:
###Code
saved_model_path = tf.keras.experimental.export(model, "./saved_models")
###Output
_____no_output_____
###Markdown
Saved models are placed in a time-stamped directory:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh keras model from the saved model.
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
new_model.summary()
###Output
_____no_output_____
###Markdown
Run the restored model.
###Code
model.predict(test_images).shape
# The re-loaded model needs to be re-compiled.
new_model.compile(optimizer=model.optimizer, # keep the optimizer that was loaded
loss=tf.keras.losses.sparse_categorical_crossentropy,
metrics=['accuracy'])
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject-based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install h5py pyyaml
###Output
_____no_output_____
###Markdown
Get an example datasetWe'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/) to train our model to demonstrate saving weights. To speed up these demonstration runs, only use the first 1000 examples:
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
import os
!pip install tensorflow==2.0.0-alpha0
import tensorflow as tf
from tensorflow import keras
tf.__version__
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Let's build a simple model we'll use to demonstrate saving and loading weights.
###Code
# Returns a short sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training The primary use case is to automatically save checkpoints *during* and at *the end* of training. This way you can use a trained model without having to retrain it, or pick-up training where you left of—in case the training process was interrupted.`tf.keras.callbacks.ModelCheckpoint` is a callback that performs this task. The callback takes a couple of arguments to configure checkpointing. Checkpoint callback usageTrain the model and pass it the `ModelCheckpoint` callback:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create checkpoint callback
cp_callback = tf.keras.callbacks.ModelCheckpoint(checkpoint_path,
save_weights_only=True,
verbose=1)
model = create_model()
model.fit(train_images, train_labels, epochs = 10,
validation_data = (test_images,test_labels),
callbacks = [cp_callback]) # pass callback to training
# This may generate warnings related to saving the state of the optimizer.
# These warnings (and similar warnings throughout this notebook)
# are in place to discourage outdated usage, and can be ignored.
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from only weights, you must have a model with the same architecture as the original model. Since it's the same model architecture, we can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
model = create_model()
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint, and re-evaluate:
###Code
model.load_weights(checkpoint_path)
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to give the resulting checkpoints unique names, and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every 5-epochs:
###Code
# include the epoch in the file name. (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(
checkpoint_path, verbose=1, save_weights_only=True,
# Save weights, every 5-epochs.
period=5)
model = create_model()
model.save_weights(checkpoint_path.format(epoch=0))
model.fit(train_images, train_labels,
epochs = 50, callbacks = [cp_callback],
validation_data = (test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
model = create_model()
model.load_weights(latest)
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights.* An index file that indicates which weights are stored in a which shard.If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsAbove you saw how to load the weights into a model.Manually saving the weights is just as simple, use the `Model.save_weights` method.
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Restore the weights
model = create_model()
model.load_weights('./checkpoints/my_checkpoint')
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables), and the model configuration. This allows you to export a model so it can be used without access to the original python code. Since the optimizer-state is recovered you can even resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) As an HDF5 fileKeras provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob.
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
# Save entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now recreate the model from that file:
###Code
# Recreate the exact same model, including weights and optimizer.
new_model = keras.models.load_model('my_model.h5')
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configurationKeras saves models by inspecting the architecture. Currently, it is not able to save TensorFlow optimizers (from `tf.train`). When using those you will need to re-compile the model after loading, and you will lose the state of the optimizer. As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions. Build a fresh model:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`, and place it in a time-stamped directory:
###Code
import time
saved_model_path = "./saved_models/{}".format(int(time.time()))
tf.keras.experimental.export_saved_model(model, saved_model_path)
saved_model_path
###Output
_____no_output_____
###Markdown
List your saved models:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh keras model from the saved model.
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
new_model.summary()
###Output
_____no_output_____
###Markdown
Run the restored model.
###Code
model.predict(test_images).shape
# The model has to be compiled before evaluating.
# This step is not required if the saved model is only being deployed.
new_model.compile(optimizer=model.optimizer, # keep the optimizer that was loaded
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Evaluate the restored model.
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject-based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
try:
# %tensorflow_version only exists in Colab.
%tensorflow_version 2.x
except Exception:
pass
!pip install pyyaml h5py # Required to save models in HDF5 format
from __future__ import absolute_import, division, print_function, unicode_literals
import os
import tensorflow as tf
from tensorflow import keras
print(tf.version.VERSION)
###Output
_____no_output_____
###Markdown
Get an example datasetTo demonstrate how to save and load weights, you'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/). To speed up these runs, use the first 1000 examples:
###Code
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Start by building a simple sequential model:
###Code
# Define a simple sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
# Display the model's architecture
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training You can use a trained model without having to retrain it, or pick-up training where you left off—in case the training process was interrupted. The `tf.keras.callbacks.ModelCheckpoint` callback allows to continually save the model both *during* and at *the end* of training. Checkpoint callback usageCreate a `tf.keras.callbacks.ModelCheckpoint` callback that saves weights only during training:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create a callback that saves the model's weights
cp_callback = tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_path,
save_weights_only=True,
verbose=1)
# Train the model with the new callback
model.fit(train_images,
train_labels,
epochs=10,
validation_data=(test_images,test_labels),
callbacks=[cp_callback]) # Pass callback to training
# This may generate warnings related to saving the state of the optimizer.
# These warnings (and similar warnings throughout this notebook)
# are in place to discourage outdated usage, and can be ignored.
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from weights-only, you must have a model with the same architecture as the original model. Since it's the same model architecture, you can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
# Create a basic model instance
model = create_model()
# Evaluate the model
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint and re-evaluate:
###Code
# Loads the weights
model.load_weights(checkpoint_path)
# Re-evaluate the model
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to provide unique names for checkpoints and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every five epochs:
###Code
# Include the epoch in the file name (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create a callback that saves the model's weights every 5 epochs
cp_callback = tf.keras.callbacks.ModelCheckpoint(
filepath=checkpoint_path,
verbose=1,
save_weights_only=True,
period=5)
# Create a new model instance
model = create_model()
# Save the weights using the `checkpoint_path` format
model.save_weights(checkpoint_path.format(epoch=0))
# Train the model with the new callback
model.fit(train_images,
train_labels,
epochs=50,
callbacks=[cp_callback],
validation_data=(test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
# Create a new model instance
model = create_model()
# Load the previously saved weights
model.load_weights(latest)
# Re-evaluate the model
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights.* An index file that indicates which weights are stored in a which shard.If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsYou saw how to load the weights into a model. Manually saving them is just as simple with the `Model.save_weights` method. By default, `tf.keras`—and `save_weights` in particular—uses the TensorFlow [checkpoints](../../guide/keras/checkpoints) format with a `.ckpt` extension (saving in [HDF5](https://js.tensorflow.org/tutorials/import-keras.html) with a `.h5` extension is covered in the [Save and serialize models](../../guide/keras/saving_and_serializingweights-only_saving_in_savedmodel_format) guide):
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Create a new model instance
model = create_model()
# Restore the weights
model.load_weights('./checkpoints/my_checkpoint')
# Evaluate the model
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables) and the model configuration. This allows you to export a model so it can be used without access to the original Python code. Since the optimizer-state is recovered, you can resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) Save model as an HDF5 fileKeras also provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob:
###Code
# Create a new model instance
model = create_model()
# Train the model
model.fit(train_images, train_labels, epochs=5)
# Save the entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now, recreate the model from that file:
###Code
# Recreate the exact same model, including its weights and the optimizer
new_model = keras.models.load_model('my_model.h5')
# Show the model architecture
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configurationKeras saves models by inspecting the architecture. Currently, it is not able to save TensorFlow optimizers (from `tf.train`). When using those you will need to re-compile the model after loading, and you will lose the state of the optimizer. As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions.Build a new model, then train it:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`, and place it in a time-stamped directory with `tf.keras.experimental.export_saved_model`:
###Code
import time
saved_model_path = "./saved_models/{}".format(int(time.time()))
tf.keras.experimental.export_saved_model(model, saved_model_path)
saved_model_path
###Output
_____no_output_____
###Markdown
List your saved models:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh Keras model from the saved model:
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
# Check its architecture
new_model.summary()
###Output
_____no_output_____
###Markdown
Run a prediction with the restored model:
###Code
model.predict(test_images).shape
# The model has to be compiled before evaluating.
# This step is not required if the saved model is only being deployed.
new_model.compile(optimizer=model.optimizer, # Keep the optimizer that was loaded
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Evaluate the restored model
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject-based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
try:
%tensorflow_version 2.x # Colab only.
except Exception:
pass
!pip install pyyaml h5py # Required to save models in HDF5 format
from __future__ import absolute_import, division, print_function, unicode_literals
import os
import tensorflow as tf
from tensorflow import keras
print(tf.version.VERSION)
###Output
_____no_output_____
###Markdown
Get an example datasetTo demonstrate how to save and load weights, you'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/). To speed up these runs, use the first 1000 examples:
###Code
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Start by building a simple sequential model:
###Code
# Define a simple sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
# Display the model's architecture
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training You can use a trained model without having to retrain it, or pick-up training where you left off—in case the training process was interrupted. The `tf.keras.callbacks.ModelCheckpoint` callback allows to continually save the model both *during* and at *the end* of training. Checkpoint callback usageCreate a `tf.keras.callbacks.ModelCheckpoint` callback that saves weights only during training:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create a callback that saves the model's weights
cp_callback = tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_path,
save_weights_only=True,
verbose=1)
# Train the model with the new callback
model.fit(train_images,
train_labels,
epochs=10,
validation_data=(test_images,test_labels),
callbacks=[cp_callback]) # Pass callback to training
# This may generate warnings related to saving the state of the optimizer.
# These warnings (and similar warnings throughout this notebook)
# are in place to discourage outdated usage, and can be ignored.
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from weights-only, you must have a model with the same architecture as the original model. Since it's the same model architecture, you can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
# Create a basic model instance
model = create_model()
# Evaluate the model
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint and re-evaluate:
###Code
# Loads the weights
model.load_weights(checkpoint_path)
# Re-evaluate the model
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to provide unique names for checkpoints and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every five epochs:
###Code
# Include the epoch in the file name (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create a callback that saves the model's weights every 5 epochs
cp_callback = tf.keras.callbacks.ModelCheckpoint(
filepath=checkpoint_path,
verbose=1,
save_weights_only=True,
period=5)
# Create a new model instance
model = create_model()
# Save the weights using the `checkpoint_path` format
model.save_weights(checkpoint_path.format(epoch=0))
# Train the model with the new callback
model.fit(train_images,
train_labels,
epochs=50,
callbacks=[cp_callback],
validation_data=(test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
# Create a new model instance
model = create_model()
# Load the previously saved weights
model.load_weights(latest)
# Re-evaluate the model
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights.* An index file that indicates which weights are stored in a which shard.If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsYou saw how to load the weights into a model. Manually saving them is just as simple with the `Model.save_weights` method. By default, `tf.keras`—and `save_weights` in particular—uses the TensorFlow [checkpoints](../../guide/keras/checkpoints) format with a `.ckpt` extension (saving in [HDF5](https://js.tensorflow.org/tutorials/import-keras.html) with a `.h5` extension is covered in the [Save and serialize models](../../guide/keras/saving_and_serializingweights-only_saving_in_savedmodel_format) guide):
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Create a new model instance
model = create_model()
# Restore the weights
model.load_weights('./checkpoints/my_checkpoint')
# Evaluate the model
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables) and the model configuration. This allows you to export a model so it can be used without access to the original Python code. Since the optimizer-state is recovered, you can resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) Save model as an HDF5 fileKeras also provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob:
###Code
# Create a new model instance
model = create_model()
# Train the model
model.fit(train_images, train_labels, epochs=5)
# Save the entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now, recreate the model from that file:
###Code
# Recreate the exact same model, including its weights and the optimizer
new_model = keras.models.load_model('my_model.h5')
# Show the model architecture
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configurationKeras saves models by inspecting the architecture. Currently, it is not able to save TensorFlow optimizers (from `tf.train`). When using those you will need to re-compile the model after loading, and you will lose the state of the optimizer. As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions.Build a new model, then train it:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`, and place it in a time-stamped directory with `tf.keras.experimental.export_saved_model`:
###Code
import time
saved_model_path = "./saved_models/{}".format(int(time.time()))
tf.keras.experimental.export_saved_model(model, saved_model_path)
saved_model_path
###Output
_____no_output_____
###Markdown
List your saved models:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh Keras model from the saved model:
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
# Check its architecture
new_model.summary()
###Output
_____no_output_____
###Markdown
Run a prediction with the restored model:
###Code
model.predict(test_images).shape
# The model has to be compiled before evaluating.
# This step is not required if the saved model is only being deployed.
new_model.compile(optimizer=model.optimizer, # Keep the optimizer that was loaded
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Evaluate the restored model
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject-based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install h5py pyyaml
###Output
_____no_output_____
###Markdown
Get an example datasetWe'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/) to train our model to demonstrate saving weights. To speed up these demonstration runs, only use the first 1000 examples:
###Code
from __future__ import absolute_import, division, print_function
import os
!pip install tensorflow==2.0.0-alpha0
import tensorflow as tf
from tensorflow import keras
tf.__version__
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Let's build a simple model we'll use to demonstrate saving and loading weights.
###Code
# Returns a short sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training The primary use case is to automatically save checkpoints *during* and at *the end* of training. This way you can use a trained model without having to retrain it, or pick-up training where you left of—in case the training process was interrupted.`tf.keras.callbacks.ModelCheckpoint` is a callback that performs this task. The callback takes a couple of arguments to configure checkpointing. Checkpoint callback usageTrain the model and pass it the `ModelCheckpoint` callback:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create checkpoint callback
cp_callback = tf.keras.callbacks.ModelCheckpoint(checkpoint_path,
save_weights_only=True,
verbose=1)
model = create_model()
model.fit(train_images, train_labels, epochs = 10,
validation_data = (test_images,test_labels),
callbacks = [cp_callback]) # pass callback to training
# This may generate warnings related to saving the state of the optimizer.
# These warnings (and similar warnings throughout this notebook)
# are in place to discourage outdated usage, and can be ignored.
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from only weights, you must have a model with the same architecture as the original model. Since it's the same model architecture, we can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
model = create_model()
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint, and re-evaluate:
###Code
model.load_weights(checkpoint_path)
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to give the resulting checkpoints unique names, and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every 5-epochs:
###Code
# include the epoch in the file name. (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(
checkpoint_path, verbose=1, save_weights_only=True,
# Save weights, every 5-epochs.
period=5)
model = create_model()
model.save_weights(checkpoint_path.format(epoch=0))
model.fit(train_images, train_labels,
epochs = 50, callbacks = [cp_callback],
validation_data = (test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
model = create_model()
model.load_weights(latest)
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights. * An index file that indicates which weights are stored in a which shard. If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsAbove you saw how to load the weights into a model.Manually saving the weights is just as simple, use the `Model.save_weights` method.
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Restore the weights
model = create_model()
model.load_weights('./checkpoints/my_checkpoint')
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables), and the model configuration. This allows you to export a model so it can be used without access to the original python code. Since the optimizer-state is recovered you can even resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) As an HDF5 fileKeras provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob.
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
# Save entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now recreate the model from that file:
###Code
# Recreate the exact same model, including weights and optimizer.
new_model = keras.models.load_model('my_model.h5')
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configurationKeras saves models by inspecting the architecture. Currently, it is not able to save TensorFlow optimizers (from `tf.train`). When using those you will need to re-compile the model after loading, and you will lose the state of the optimizer. As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions. Build a fresh model:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`, and place it in a time-stamped directory:
###Code
import time
saved_model_path = "./saved_models/{}".format(int(time.time()))
tf.keras.experimental.export_saved_model(model, saved_model_path)
saved_model_path
###Output
_____no_output_____
###Markdown
List your saved models:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh keras model from the saved model.
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
new_model.summary()
###Output
_____no_output_____
###Markdown
Run the restored model.
###Code
model.predict(test_images).shape
# The model has to be compiled before evaluating.
# This step is not required if the saved model is only being deployed.
new_model.compile(optimizer=model.optimizer, # keep the optimizer that was loaded
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Evaluate the restored model.
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject-based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install h5py pyyaml
###Output
_____no_output_____
###Markdown
Get an example datasetWe'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/) to train our model to demonstrate saving weights. To speed up these demonstration runs, only use the first 1000 examples:
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
import os
!pip install tensorflow==2.0.0-beta0
import tensorflow as tf
from tensorflow import keras
tf.__version__
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Let's build a simple model we'll use to demonstrate saving and loading weights.
###Code
# Returns a short sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training The primary use case is to automatically save checkpoints *during* and at *the end* of training. This way you can use a trained model without having to retrain it, or pick-up training where you left of—in case the training process was interrupted.`tf.keras.callbacks.ModelCheckpoint` is a callback that performs this task. The callback takes a couple of arguments to configure checkpointing. Checkpoint callback usageTrain the model and pass it the `ModelCheckpoint` callback:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create checkpoint callback
cp_callback = tf.keras.callbacks.ModelCheckpoint(checkpoint_path,
save_weights_only=True,
verbose=1)
model = create_model()
model.fit(train_images, train_labels, epochs = 10,
validation_data = (test_images,test_labels),
callbacks = [cp_callback]) # pass callback to training
# This may generate warnings related to saving the state of the optimizer.
# These warnings (and similar warnings throughout this notebook)
# are in place to discourage outdated usage, and can be ignored.
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from only weights, you must have a model with the same architecture as the original model. Since it's the same model architecture, we can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
model = create_model()
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint, and re-evaluate:
###Code
model.load_weights(checkpoint_path)
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to give the resulting checkpoints unique names, and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every 5-epochs:
###Code
# include the epoch in the file name. (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(
checkpoint_path, verbose=1, save_weights_only=True,
# Save weights, every 5-epochs.
period=5)
model = create_model()
model.save_weights(checkpoint_path.format(epoch=0))
model.fit(train_images, train_labels,
epochs = 50, callbacks = [cp_callback],
validation_data = (test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
model = create_model()
model.load_weights(latest)
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights.* An index file that indicates which weights are stored in a which shard.If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsAbove you saw how to load the weights into a model.Manually saving the weights is just as simple, use the `Model.save_weights` method.
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Restore the weights
model = create_model()
model.load_weights('./checkpoints/my_checkpoint')
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables), and the model configuration. This allows you to export a model so it can be used without access to the original python code. Since the optimizer-state is recovered you can even resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) As an HDF5 fileKeras provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob.
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
# Save entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now recreate the model from that file:
###Code
# Recreate the exact same model, including weights and optimizer.
new_model = keras.models.load_model('my_model.h5')
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configurationKeras saves models by inspecting the architecture. Currently, it is not able to save TensorFlow optimizers (from `tf.train`). When using those you will need to re-compile the model after loading, and you will lose the state of the optimizer. As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions. Build a fresh model:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`, and place it in a time-stamped directory:
###Code
import time
saved_model_path = "./saved_models/{}".format(int(time.time()))
tf.keras.experimental.export_saved_model(model, saved_model_path)
saved_model_path
###Output
_____no_output_____
###Markdown
List your saved models:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh keras model from the saved model.
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
new_model.summary()
###Output
_____no_output_____
###Markdown
Run the restored model.
###Code
model.predict(test_images).shape
# The model has to be compiled before evaluating.
# This step is not required if the saved model is only being deployed.
new_model.compile(optimizer=model.optimizer, # keep the optimizer that was loaded
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Evaluate the restored model.
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject-based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install h5py pyyaml
###Output
_____no_output_____
###Markdown
Get an example datasetWe'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/) to train our model to demonstrate saving weights. To speed up these demonstration runs, only use the first 1000 examples:
###Code
from __future__ import absolute_import, division, print_function
import os
!pip install tf-nightly-2.0-preview
import tensorflow as tf
from tensorflow import keras
tf.__version__
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Let's build a simple model we'll use to demonstrate saving and loading weights.
###Code
# Returns a short sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training The primary use case is to automatically save checkpoints *during* and at *the end* of training. This way you can use a trained model without having to retrain it, or pick-up training where you left of—in case the training process was interrupted.`tf.keras.callbacks.ModelCheckpoint` is a callback that performs this task. The callback takes a couple of arguments to configure checkpointing. Checkpoint callback usageTrain the model and pass it the `ModelCheckpoint` callback:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create checkpoint callback
cp_callback = tf.keras.callbacks.ModelCheckpoint(checkpoint_path,
save_weights_only=True,
verbose=1)
model = create_model()
model.fit(train_images, train_labels, epochs = 10,
validation_data = (test_images,test_labels),
callbacks = [cp_callback]) # pass callback to training
# This may generate warnings related to saving the state of the optimizer.
# These warnings (and similar warnings throughout this notebook)
# are in place to discourage outdated usage, and can be ignored.
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from only weights, you must have a model with the same architecture as the original model. Since it's the same model architecture, we can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
model = create_model()
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint, and re-evaluate:
###Code
model.load_weights(checkpoint_path)
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to give the resulting checkpoints unique names, and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every 5-epochs:
###Code
# include the epoch in the file name. (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(
checkpoint_path, verbose=1, save_weights_only=True,
# Save weights, every 5-epochs.
period=5)
model = create_model()
model.save_weights(checkpoint_path.format(epoch=0))
model.fit(train_images, train_labels,
epochs = 50, callbacks = [cp_callback],
validation_data = (test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
model = create_model()
model.load_weights(latest)
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights. * An index file that indicates which weights are stored in a which shard. If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsAbove you saw how to load the weights into a model.Manually saving the weights is just as simple, use the `Model.save_weights` method.
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Restore the weights
model = create_model()
model.load_weights('./checkpoints/my_checkpoint')
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables), and the model configuration. This allows you to export a model so it can be used without access to the original python code. Since the optimizer-state is recovered you can even resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) As an HDF5 fileKeras provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob.
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
# Save entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now recreate the model from that file:
###Code
# Recreate the exact same model, including weights and optimizer.
new_model = keras.models.load_model('my_model.h5')
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configurationKeras saves models by inspecting the architecture. Currently, it is not able to save TensorFlow optimizers (from `tf.train`). When using those you will need to re-compile the model after loading, and you will lose the state of the optimizer. As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions. Build a fresh model:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`, and place it in a time-stamped directory:
###Code
import time
saved_model_path = "./saved_models/{}".format(int(time.time()))
tf.keras.experimental.export_saved_model(model, saved_model_path)
saved_model_path
###Output
_____no_output_____
###Markdown
List your saved models:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh keras model from the saved model.
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
new_model.summary()
###Output
_____no_output_____
###Markdown
Run the restored model.
###Code
model.predict(test_images).shape
# The model has to be compiled before evaluating.
# This step is not required if the saved model is only being deployed.
new_model.compile(optimizer=model.optimizer, # keep the optimizer that was loaded
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Evaluate the restored model.
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject-based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install h5py pyyaml
###Output
_____no_output_____
###Markdown
Get an example datasetWe'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/) to train our model to demonstrate saving weights. To speed up these demonstration runs, only use the first 1000 examples:
###Code
from __future__ import absolute_import, division, print_function
import os
!pip install tf-nightly-2.0-preview
import tensorflow as tf
from tensorflow import keras
tf.__version__
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Let's build a simple model we'll use to demonstrate saving and loading weights.
###Code
# Returns a short sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training The primary use case is to automatically save checkpoints *during* and at *the end* of training. This way you can use a trained model without having to retrain it, or pick-up training where you left of—in case the training process was interrupted.`tf.keras.callbacks.ModelCheckpoint` is a callback that performs this task. The callback takes a couple of arguments to configure checkpointing. Checkpoint callback usageTrain the model and pass it the `ModelCheckpoint` callback:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create checkpoint callback
cp_callback = tf.keras.callbacks.ModelCheckpoint(checkpoint_path,
save_weights_only=True,
verbose=1)
model = create_model()
model.fit(train_images, train_labels, epochs = 10,
validation_data = (test_images,test_labels),
callbacks = [cp_callback]) # pass callback to training
# This may generate warnings related to saving the state of the optimizer.
# These warnings (and similar warnings throughout this notebook)
# are in place to discourage outdated usage, and can be ignored.
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from only weights, you must have a model with the same architecture as the original model. Since it's the same model architecture, we can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
model = create_model()
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint, and re-evaluate:
###Code
model.load_weights(checkpoint_path)
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to give the resulting checkpoints unique names, and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every 5-epochs:
###Code
# include the epoch in the file name. (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(
checkpoint_path, verbose=1, save_weights_only=True,
# Save weights, every 5-epochs.
period=5)
model = create_model()
model.save_weights(checkpoint_path.format(epoch=0))
model.fit(train_images, train_labels,
epochs = 50, callbacks = [cp_callback],
validation_data = (test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
model = create_model()
model.load_weights(latest)
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights. * An index file that indicates which weights are stored in a which shard. If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsAbove you saw how to load the weights into a model.Manually saving the weights is just as simple, use the `Model.save_weights` method.
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Restore the weights
model = create_model()
model.load_weights('./checkpoints/my_checkpoint')
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables), and the model configuration. This allows you to export a model so it can be used without access to the original python code. Since the optimizer-state is recovered you can even resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) As an HDF5 fileKeras provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob.
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
# Save entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now recreate the model from that file:
###Code
# Recreate the exact same model, including weights and optimizer.
new_model = keras.models.load_model('my_model.h5')
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configurationKeras saves models by inspecting the architecture. Currently, it is not able to save TensorFlow optimizers (from `tf.train`). When using those you will need to re-compile the model after loading, and you will lose the state of the optimizer. As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions. Build a fresh model:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`:
###Code
saved_model_path = tf.keras.experimental.export(model, "./saved_models")
###Output
_____no_output_____
###Markdown
Saved models are placed in a time-stamped directory:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh keras model from the saved model.
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
new_model.summary()
###Output
_____no_output_____
###Markdown
Run the restored model.
###Code
model.predict(test_images).shape
# The model has to be compiled before evaluating.
# This step is not required if the saved model is only being deployed.
new_model.compile(optimizer=model.optimizer, # keep the optimizer that was loaded
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Evaluate the restored model.
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject-based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install tensorflow==2.0.0-beta1
!pip install pyyaml h5py # Required to save models in HDF5 format
from __future__ import absolute_import, division, print_function, unicode_literals
import os
import tensorflow as tf
from tensorflow import keras
print(tf.version.VERSION)
###Output
_____no_output_____
###Markdown
Get an example datasetTo demonstrate how to save and load weights, you'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/). To speed up these runs, use the first 1000 examples:
###Code
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Start by building a simple sequential model:
###Code
# Define a simple sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
# Display the model's architecture
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training You can use a trained model without having to retrain it, or pick-up training where you left off—in case the training process was interrupted. The `tf.keras.callbacks.ModelCheckpoint` callback allows to continually save the model both *during* and at *the end* of training. Checkpoint callback usageCreate a `tf.keras.callbacks.ModelCheckpoint` callback that saves weights only during training:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create a callback that saves the model's weights
cp_callback = tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_path,
save_weights_only=True,
verbose=1)
# Train the model with the new callback
model.fit(train_images,
train_labels,
epochs=10,
validation_data=(test_images,test_labels),
callbacks=[cp_callback]) # Pass callback to training
# This may generate warnings related to saving the state of the optimizer.
# These warnings (and similar warnings throughout this notebook)
# are in place to discourage outdated usage, and can be ignored.
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from weights-only, you must have a model with the same architecture as the original model. Since it's the same model architecture, you can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
# Create a basic model instance
model = create_model()
# Evaluate the model
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint and re-evaluate:
###Code
# Loads the weights
model.load_weights(checkpoint_path)
# Re-evaluate the model
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to provide unique names for checkpoints and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every five epochs:
###Code
# Include the epoch in the file name (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create a callback that saves the model's weights every 5 epochs
cp_callback = tf.keras.callbacks.ModelCheckpoint(
filepath=checkpoint_path,
verbose=1,
save_weights_only=True,
period=5)
# Create a new model instance
model = create_model()
# Save the weights using the `checkpoint_path` format
model.save_weights(checkpoint_path.format(epoch=0))
# Train the model with the new callback
model.fit(train_images,
train_labels,
epochs=50,
callbacks=[cp_callback],
validation_data=(test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
# Create a new model instance
model = create_model()
# Load the previously saved weights
model.load_weights(latest)
# Re-evaluate the model
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights.* An index file that indicates which weights are stored in a which shard.If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsYou saw how to load the weights into a model. Manually saving them is just as simple with the `Model.save_weights` method. By default, `tf.keras`—and `save_weights` in particular—uses the TensorFlow [checkpoints](../../guide/keras/checkpoints) format with a `.ckpt` extension (saving in [HDF5](https://js.tensorflow.org/tutorials/import-keras.html) with a `.h5` extension is covered in the [Save and serialize models](../../guide/keras/saving_and_serializingweights-only_saving_in_savedmodel_format) guide):
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Create a new model instance
model = create_model()
# Restore the weights
model.load_weights('./checkpoints/my_checkpoint')
# Evaluate the model
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables) and the model configuration. This allows you to export a model so it can be used without access to the original Python code. Since the optimizer-state is recovered, you can resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) Save model as an HDF5 fileKeras also provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob:
###Code
# Create a new model instance
model = create_model()
# Train the model
model.fit(train_images, train_labels, epochs=5)
# Save the entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now, recreate the model from that file:
###Code
# Recreate the exact same model, including its weights and the optimizer
new_model = keras.models.load_model('my_model.h5')
# Show the model architecture
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configurationKeras saves models by inspecting the architecture. Currently, it is not able to save TensorFlow optimizers (from `tf.train`). When using those you will need to re-compile the model after loading, and you will lose the state of the optimizer. As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions.Build a new model, then train it:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`, and place it in a time-stamped directory with `tf.keras.experimental.export_saved_model`:
###Code
import time
saved_model_path = "./saved_models/{}".format(int(time.time()))
tf.keras.experimental.export_saved_model(model, saved_model_path)
saved_model_path
###Output
_____no_output_____
###Markdown
List your saved models:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh Keras model from the saved model:
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
# Check its architecture
new_model.summary()
###Output
_____no_output_____
###Markdown
Run a prediction with the restored model:
###Code
model.predict(test_images).shape
# The model has to be compiled before evaluating.
# This step is not required if the saved model is only being deployed.
new_model.compile(optimizer=model.optimizer, # Keep the optimizer that was loaded
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Evaluate the restored model
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject-based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install h5py pyyaml
###Output
_____no_output_____
###Markdown
Get an example datasetWe'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/) to train our model to demonstrate saving weights. To speed up these demonstration runs, only use the first 1000 examples:
###Code
from __future__ import absolute_import, division, print_function
import os
!pip install tf-nightly-2.0-preview
import tensorflow as tf
from tensorflow import keras
tf.__version__
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Let's build a simple model we'll use to demonstrate saving and loading weights.
###Code
# Returns a short sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training The primary use case is to automatically save checkpoints *during* and at *the end* of training. This way you can use a trained model without having to retrain it, or pick-up training where you left of—in case the training process was interrupted.`tf.keras.callbacks.ModelCheckpoint` is a callback that performs this task. The callback takes a couple of arguments to configure checkpointing. Checkpoint callback usageTrain the model and pass it the `ModelCheckpoint` callback:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create checkpoint callback
cp_callback = tf.keras.callbacks.ModelCheckpoint(checkpoint_path,
save_weights_only=True,
verbose=1)
model = create_model()
model.fit(train_images, train_labels, epochs = 10,
validation_data = (test_images,test_labels),
callbacks = [cp_callback]) # pass callback to training
# This may generate warnings related to saving the state of the optimizer.
# These warnings (and similar warnings throughout this notebook)
# are in place to discourage outdated usage, and can be ignored.
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from only weights, you must have a model with the same architecture as the original model. Since it's the same model architecture, we can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
model = create_model()
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint, and re-evaluate:
###Code
model.load_weights(checkpoint_path)
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to give the resulting checkpoints unique names, and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every 5-epochs:
###Code
# include the epoch in the file name. (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(
checkpoint_path, verbose=1, save_weights_only=True,
# Save weights, every 5-epochs.
period=5)
model = create_model()
model.save_weights(checkpoint_path.format(epoch=0))
model.fit(train_images, train_labels,
epochs = 50, callbacks = [cp_callback],
validation_data = (test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
model = create_model()
model.load_weights(latest)
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights. * An index file that indicates which weights are stored in a which shard. If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsAbove you saw how to load the weights into a model.Manually saving the weights is just as simple, use the `Model.save_weights` method.
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Restore the weights
model = create_model()
model.load_weights('./checkpoints/my_checkpoint')
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables), and the model configuration. This allows you to export a model so it can be used without access to the original python code. Since the optimizer-state is recovered you can even resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) As an HDF5 fileKeras provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob.
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
# Save entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now recreate the model from that file:
###Code
# Recreate the exact same model, including weights and optimizer.
new_model = keras.models.load_model('my_model.h5')
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configurationKeras saves models by inspecting the architecture. Currently, it is not able to save TensorFlow optimizers (from `tf.train`). When using those you will need to re-compile the model after loading, and you will lose the state of the optimizer. As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions. Build a fresh model:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`, and place it in a time-stamped directory:
###Code
import time
saved_model_path = "./saved_models/{}".format(int(time.time()))
tf.keras.experimental.export_saved_model(model, saved_model_path)
saved_model_path
###Output
_____no_output_____
###Markdown
List your saved models:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh keras model from the saved model.
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
new_model.summary()
###Output
_____no_output_____
###Markdown
Run the restored model.
###Code
model.predict(test_images).shape
# The model has to be compiled before evaluating.
# This step is not required if the saved model is only being deployed.
new_model.compile(optimizer=model.optimizer, # keep the optimizer that was loaded
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Evaluate the restored model.
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject-based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install h5py pyyaml
###Output
_____no_output_____
###Markdown
Get an example datasetWe'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/) to train our model to demonstrate saving weights. To speed up these demonstration runs, only use the first 1000 examples:
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
import os
!pip install tensorflow==2.0.0-beta1
import tensorflow as tf
from tensorflow import keras
tf.__version__
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Let's build a simple model we'll use to demonstrate saving and loading weights.
###Code
# Returns a short sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training The primary use case is to automatically save checkpoints *during* and at *the end* of training. This way you can use a trained model without having to retrain it, or pick-up training where you left of—in case the training process was interrupted.`tf.keras.callbacks.ModelCheckpoint` is a callback that performs this task. The callback takes a couple of arguments to configure checkpointing. Checkpoint callback usageTrain the model and pass it the `ModelCheckpoint` callback:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create checkpoint callback
cp_callback = tf.keras.callbacks.ModelCheckpoint(checkpoint_path,
save_weights_only=True,
verbose=1)
model = create_model()
model.fit(train_images, train_labels, epochs = 10,
validation_data = (test_images,test_labels),
callbacks = [cp_callback]) # pass callback to training
# This may generate warnings related to saving the state of the optimizer.
# These warnings (and similar warnings throughout this notebook)
# are in place to discourage outdated usage, and can be ignored.
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from only weights, you must have a model with the same architecture as the original model. Since it's the same model architecture, we can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
model = create_model()
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint, and re-evaluate:
###Code
model.load_weights(checkpoint_path)
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to give the resulting checkpoints unique names, and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every 5-epochs:
###Code
# include the epoch in the file name. (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(
checkpoint_path, verbose=1, save_weights_only=True,
# Save weights, every 5-epochs.
period=5)
model = create_model()
model.save_weights(checkpoint_path.format(epoch=0))
model.fit(train_images, train_labels,
epochs = 50, callbacks = [cp_callback],
validation_data = (test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
model = create_model()
model.load_weights(latest)
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights.* An index file that indicates which weights are stored in a which shard.If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsAbove you saw how to load the weights into a model.Manually saving the weights is just as simple, use the `Model.save_weights` method.
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Restore the weights
model = create_model()
model.load_weights('./checkpoints/my_checkpoint')
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables), and the model configuration. This allows you to export a model so it can be used without access to the original python code. Since the optimizer-state is recovered you can even resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) As an HDF5 fileKeras provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob.
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
# Save entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now recreate the model from that file:
###Code
# Recreate the exact same model, including weights and optimizer.
new_model = keras.models.load_model('my_model.h5')
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configurationKeras saves models by inspecting the architecture. Currently, it is not able to save TensorFlow optimizers (from `tf.train`). When using those you will need to re-compile the model after loading, and you will lose the state of the optimizer. As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions. Build a fresh model:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`, and place it in a time-stamped directory:
###Code
import time
saved_model_path = "./saved_models/{}".format(int(time.time()))
tf.keras.experimental.export_saved_model(model, saved_model_path)
saved_model_path
###Output
_____no_output_____
###Markdown
List your saved models:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh keras model from the saved model.
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
new_model.summary()
###Output
_____no_output_____
###Markdown
Run the restored model.
###Code
model.predict(test_images).shape
# The model has to be compiled before evaluating.
# This step is not required if the saved model is only being deployed.
new_model.compile(optimizer=model.optimizer, # keep the optimizer that was loaded
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Evaluate the restored model.
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject_based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install h5py pyyaml
###Output
_____no_output_____
###Markdown
Get an example datasetWe'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/) to train our model to demonstrate saving weights. To speed up these demonstration runs, only use the first 1000 examples:
###Code
from __future__ import absolute_import, division, print_function
import os
!pip install tf-nightly-2.0-preview
import tensorflow as tf
keras = tf.keras
tf.__version__
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Let's build a simple model we'll use to demonstrate saving and loading weights.
###Code
# Returns a short sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training The primary use case is to automatically save checkpoints *during* and at *the end* of training. This way you can use a trained model without having to retrain it, or pick-up training where you left of—in case the training process was interrupted.`tf.keras.callbacks.ModelCheckpoint` is a callback that performs this task. The callback takes a couple of arguments to configure checkpointing. Checkpoint callback usageTrain the model and pass it the `ModelCheckpoint` callback:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create checkpoint callback
cp_callback = tf.keras.callbacks.ModelCheckpoint(checkpoint_path,
save_weights_only=True,
verbose=1)
model = create_model()
model.fit(train_images, train_labels, epochs = 10,
validation_data = (test_images,test_labels),
callbacks = [cp_callback]) # pass callback to training
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from only weights, you must have a model with the same architecture as the original model. Since it's the same model architecture, we can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
model = create_model()
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint, and re-evaluate:
###Code
model.load_weights(checkpoint_path)
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to give the resulting checkpoints unique names, and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every 5-epochs:
###Code
# include the epoch in the file name. (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(
checkpoint_path, verbose=1, save_weights_only=True,
# Save weights, every 5-epochs.
period=5)
model = create_model()
model.save_weights(checkpoint_path.format(epoch=0))
model.fit(train_images, train_labels,
epochs = 50, callbacks = [cp_callback],
validation_data = (test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
model = create_model()
model.load_weights(latest)
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights. * An index file that indicates which weights are stored in a which shard. If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsAbove you saw how to load the weights into a model.Manually saving the weights is just as simple, use the `Model.save_weights` method.
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Restore the weights
model = create_model()
model.load_weights('./checkpoints/my_checkpoint')
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables), and the model configuration. This allows you to export a model so it can be used without access to the original python code. Since the optimizer-state is recovered you can even resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) As an HDF5 fileKeras provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob.
###Code
model = create_model()
model.compile(optimizer='adam',
loss=tf.keras.losses.sparse_categorical_crossentropy,
metrics=['accuracy'])
model.fit(train_images, train_labels, epochs=5)
# Save entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now recreate the model from that file:
###Code
# Recreate the exact same model, including weights and optimizer.
new_model = keras.models.load_model('my_model.h5')
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configuration As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions. Build a fresh model:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`:
###Code
saved_model_path = tf.keras.experimental.export(model, "./saved_models")
###Output
_____no_output_____
###Markdown
Saved models are placed in a time-stamped directory:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh keras model from the saved model.
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
new_model.summary()
###Output
_____no_output_____
###Markdown
Run the restored model.
###Code
model.predict(test_images).shape
# The re-loaded model needs to be re-compiled.
new_model.compile(optimizer=model.optimizer, # keep the optimizer that was loaded
loss=tf.keras.losses.sparse_categorical_crossentropy,
metrics=['accuracy'])
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject-based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install h5py pyyaml
###Output
_____no_output_____
###Markdown
Get an example datasetWe'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/) to train our model to demonstrate saving weights. To speed up these demonstration runs, only use the first 1000 examples:
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
import os
!pip install tensorflow==2.0.0-beta0
import tensorflow as tf
from tensorflow import keras
tf.__version__
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Let's build a simple model we'll use to demonstrate saving and loading weights.
###Code
# Returns a short sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training The primary use case is to automatically save checkpoints *during* and at *the end* of training. This way you can use a trained model without having to retrain it, or pick-up training where you left of—in case the training process was interrupted.`tf.keras.callbacks.ModelCheckpoint` is a callback that performs this task. The callback takes a couple of arguments to configure checkpointing. Checkpoint callback usageTrain the model and pass it the `ModelCheckpoint` callback:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create checkpoint callback
cp_callback = tf.keras.callbacks.ModelCheckpoint(checkpoint_path,
save_weights_only=True,
verbose=1)
model = create_model()
model.fit(train_images, train_labels, epochs = 10,
validation_data = (test_images,test_labels),
callbacks = [cp_callback]) # pass callback to training
# This may generate warnings related to saving the state of the optimizer.
# These warnings (and similar warnings throughout this notebook)
# are in place to discourage outdated usage, and can be ignored.
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from only weights, you must have a model with the same architecture as the original model. Since it's the same model architecture, we can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
model = create_model()
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint, and re-evaluate:
###Code
model.load_weights(checkpoint_path)
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to give the resulting checkpoints unique names, and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every 5-epochs:
###Code
# include the epoch in the file name. (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(
checkpoint_path, verbose=1, save_weights_only=True,
# Save weights, every 5-epochs.
period=5)
model = create_model()
model.save_weights(checkpoint_path.format(epoch=0))
model.fit(train_images, train_labels,
epochs = 50, callbacks = [cp_callback],
validation_data = (test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
model = create_model()
model.load_weights(latest)
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights.* An index file that indicates which weights are stored in a which shard.If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsAbove you saw how to load the weights into a model.Manually saving the weights is just as simple, use the `Model.save_weights` method.
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Restore the weights
model = create_model()
model.load_weights('./checkpoints/my_checkpoint')
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables), and the model configuration. This allows you to export a model so it can be used without access to the original python code. Since the optimizer-state is recovered you can even resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) As an HDF5 fileKeras provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob.
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
# Save entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now recreate the model from that file:
###Code
# Recreate the exact same model, including weights and optimizer.
new_model = keras.models.load_model('my_model.h5')
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configurationKeras saves models by inspecting the architecture. Currently, it is not able to save TensorFlow optimizers (from `tf.train`). When using those you will need to re-compile the model after loading, and you will lose the state of the optimizer. As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions. Build a fresh model:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`, and place it in a time-stamped directory:
###Code
import time
saved_model_path = "./saved_models/{}".format(int(time.time()))
tf.keras.experimental.export_saved_model(model, saved_model_path)
saved_model_path
###Output
_____no_output_____
###Markdown
List your saved models:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh keras model from the saved model.
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
new_model.summary()
###Output
_____no_output_____
###Markdown
Run the restored model.
###Code
model.predict(test_images).shape
# The model has to be compiled before evaluating.
# This step is not required if the saved model is only being deployed.
new_model.compile(optimizer=model.optimizer, # keep the optimizer that was loaded
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Evaluate the restored model.
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject_based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install h5py pyyaml
###Output
_____no_output_____
###Markdown
Get an example datasetWe'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/) to train our model to demonstrate saving weights. To speed up these demonstration runs, only use the first 1000 examples:
###Code
from __future__ import absolute_import, division, print_function
import os
!pip install tf-nightly-2.0-preview
import tensorflow as tf
keras = tf.keras
tf.__version__
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Let's build a simple model we'll use to demonstrate saving and loading weights.
###Code
# Returns a short sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training The primary use case is to automatically save checkpoints *during* and at *the end* of training. This way you can use a trained model without having to retrain it, or pick-up training where you left of—in case the training process was interrupted.`tf.keras.callbacks.ModelCheckpoint` is a callback that performs this task. The callback takes a couple of arguments to configure checkpointing. Checkpoint callback usageTrain the model and pass it the `ModelCheckpoint` callback:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create checkpoint callback
cp_callback = tf.keras.callbacks.ModelCheckpoint(checkpoint_path,
save_weights_only=True,
verbose=1)
model = create_model()
model.fit(train_images, train_labels, epochs = 10,
validation_data = (test_images,test_labels),
callbacks = [cp_callback]) # pass callback to training
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from only weights, you must have a model with the same architecture as the original model. Since it's the same model architecture, we can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
model = create_model()
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint, and re-evaluate:
###Code
model.load_weights(checkpoint_path)
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to give the resulting checkpoints unique names, and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every 5-epochs:
###Code
# include the epoch in the file name. (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(
checkpoint_path, verbose=1, save_weights_only=True,
# Save weights, every 5-epochs.
period=5)
model = create_model()
model.save_weights(checkpoint_path.format(epoch=0))
model.fit(train_images, train_labels,
epochs = 50, callbacks = [cp_callback],
validation_data = (test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
model = create_model()
model.load_weights(latest)
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights. * An index file that indicates which weights are stored in a which shard. If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsAbove you saw how to load the weights into a model.Manually saving the weights is just as simple, use the `Model.save_weights` method.
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Restore the weights
model = create_model()
model.load_weights('./checkpoints/my_checkpoint')
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables), and the model configuration. This allows you to export a model so it can be used without access to the original python code. Since the optimizer-state is recovered you can even resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) As an HDF5 fileKeras provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob.
###Code
model = create_model()
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
model.fit(train_images, train_labels, epochs=5)
# Save entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now recreate the model from that file:
###Code
# Recreate the exact same model, including weights and optimizer.
new_model = keras.models.load_model('my_model.h5')
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configuration As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions. Build a fresh model:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`:
###Code
saved_model_path = tf.keras.experimental.export(model, "./saved_models")
###Output
_____no_output_____
###Markdown
Saved models are placed in a time-stamped directory:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh keras model from the saved model.
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
new_model.summary()
###Output
_____no_output_____
###Markdown
Run the restored model.
###Code
model.predict(test_images).shape
# The re-loaded model needs to be re-compiled.
new_model.compile(optimizer=model.optimizer, # keep the optimizer that was loaded
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What's NextThat was a quick guide to saving and loading in with `tf.keras`.* The [tf.keras guide](https://www.tensorflow.org/guide/keras) shows more about saving and loading models with `tf.keras`.* See [Saving in eager](https://www.tensorflow.org/guide/eagerobject_based_saving) for saving during eager execution.* The [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide has low-level details about TensorFlow saving.
###Code
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#@title MIT License
#
# Copyright (c) 2017 François Chollet
#
# Permission is hereby granted, free of charge, to any person obtaining a
# copy of this software and associated documentation files (the "Software"),
# to deal in the Software without restriction, including without limitation
# the rights to use, copy, modify, merge, publish, distribute, sublicense,
# and/or sell copies of the Software, and to permit persons to whom the
# Software is furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in
# all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
# THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
# DEALINGS IN THE SOFTWARE.
###Output
_____no_output_____
###Markdown
Save and restore models View on TensorFlow.org Run in Google Colab View source on GitHub Model progress can be saved during—and after—training. This means a model can resume where it left off and avoid long training times. Saving also means you can share your model and others can recreate your work. When publishing research models and techniques, most machine learning practitioners share:* code to create the model, and* the trained weights, or parameters, for the modelSharing this data helps others understand how the model works and try it themselves with new data.Caution: Be careful with untrusted code—TensorFlow models are code. See [Using TensorFlow Securely](https://github.com/tensorflow/tensorflow/blob/master/SECURITY.md) for details. OptionsThere are different ways to save TensorFlow models—depending on the API you're using. This guide uses [tf.keras](https://www.tensorflow.org/guide/keras), a high-level API to build and train models in TensorFlow. For other approaches, see the TensorFlow [Save and Restore](https://www.tensorflow.org/guide/saved_model) guide or [Saving in eager](https://www.tensorflow.org/guide/eagerobject-based_saving). Setup Installs and imports Install and import TensorFlow and dependencies:
###Code
!pip install h5py pyyaml
###Output
_____no_output_____
###Markdown
Get an example datasetWe'll use the [MNIST dataset](http://yann.lecun.com/exdb/mnist/) to train our model to demonstrate saving weights. To speed up these demonstration runs, only use the first 1000 examples:
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
import os
!pip install tensorflow==2.0.0-alpha0
import tensorflow as tf
from tensorflow import keras
tf.__version__
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
train_labels = train_labels[:1000]
test_labels = test_labels[:1000]
train_images = train_images[:1000].reshape(-1, 28 * 28) / 255.0
test_images = test_images[:1000].reshape(-1, 28 * 28) / 255.0
###Output
_____no_output_____
###Markdown
Define a model Let's build a simple model we'll use to demonstrate saving and loading weights.
###Code
# Returns a short sequential model
def create_model():
model = tf.keras.models.Sequential([
keras.layers.Dense(512, activation='relu', input_shape=(784,)),
keras.layers.Dropout(0.2),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
return model
# Create a basic model instance
model = create_model()
model.summary()
###Output
_____no_output_____
###Markdown
Save checkpoints during training The primary use case is to automatically save checkpoints *during* and at *the end* of training. This way you can use a trained model without having to retrain it, or pick-up training where you left of—in case the training process was interrupted.`tf.keras.callbacks.ModelCheckpoint` is a callback that performs this task. The callback takes a couple of arguments to configure checkpointing. Checkpoint callback usageTrain the model and pass it the `ModelCheckpoint` callback:
###Code
checkpoint_path = "training_1/cp.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
# Create checkpoint callback
cp_callback = tf.keras.callbacks.ModelCheckpoint(checkpoint_path,
save_weights_only=True,
verbose=1)
model = create_model()
model.fit(train_images, train_labels, epochs = 10,
validation_data = (test_images,test_labels),
callbacks = [cp_callback]) # pass callback to training
# This may generate warnings related to saving the state of the optimizer.
# These warnings (and similar warnings throughout this notebook)
# are in place to discourage outdated usage, and can be ignored.
###Output
_____no_output_____
###Markdown
This creates a single collection of TensorFlow checkpoint files that are updated at the end of each epoch:
###Code
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Create a new, untrained model. When restoring a model from only weights, you must have a model with the same architecture as the original model. Since it's the same model architecture, we can share weights despite that it's a different *instance* of the model.Now rebuild a fresh, untrained model, and evaluate it on the test set. An untrained model will perform at chance levels (~10% accuracy):
###Code
model = create_model()
loss, acc = model.evaluate(test_images, test_labels)
print("Untrained model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Then load the weights from the checkpoint, and re-evaluate:
###Code
model.load_weights(checkpoint_path)
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Checkpoint callback optionsThe callback provides several options to give the resulting checkpoints unique names, and adjust the checkpointing frequency.Train a new model, and save uniquely named checkpoints once every 5-epochs:
###Code
# include the epoch in the file name. (uses `str.format`)
checkpoint_path = "training_2/cp-{epoch:04d}.ckpt"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(
checkpoint_path, verbose=1, save_weights_only=True,
# Save weights, every 5-epochs.
period=5)
model = create_model()
model.save_weights(checkpoint_path.format(epoch=0))
model.fit(train_images, train_labels,
epochs = 50, callbacks = [cp_callback],
validation_data = (test_images,test_labels),
verbose=0)
###Output
_____no_output_____
###Markdown
Now, look at the resulting checkpoints and choose the latest one:
###Code
! ls {checkpoint_dir}
latest = tf.train.latest_checkpoint(checkpoint_dir)
latest
###Output
_____no_output_____
###Markdown
Note: the default tensorflow format only saves the 5 most recent checkpoints.To test, reset the model and load the latest checkpoint:
###Code
model = create_model()
model.load_weights(latest)
loss, acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
What are these files? The above code stores the weights to a collection of [checkpoint](https://www.tensorflow.org/guide/saved_modelsave_and_restore_variables)-formatted files that contain only the trained weights in a binary format. Checkpoints contain:* One or more shards that contain your model's weights. * An index file that indicates which weights are stored in a which shard. If you are only training a model on a single machine, you'll have one shard with the suffix: `.data-00000-of-00001` Manually save weightsAbove you saw how to load the weights into a model.Manually saving the weights is just as simple, use the `Model.save_weights` method.
###Code
# Save the weights
model.save_weights('./checkpoints/my_checkpoint')
# Restore the weights
model = create_model()
model.load_weights('./checkpoints/my_checkpoint')
loss,acc = model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
Save the entire modelThe model and optimizer can be saved to a file that contains both their state (weights and variables), and the model configuration. This allows you to export a model so it can be used without access to the original python code. Since the optimizer-state is recovered you can even resume training from exactly where you left off.Saving a fully-functional model is very useful—you can load them in TensorFlow.js ([HDF5](https://js.tensorflow.org/tutorials/import-keras.html), [Saved Model](https://js.tensorflow.org/tutorials/import-saved-model.html)) and then train and run them in web browsers, or convert them to run on mobile devices using TensorFlow Lite ([HDF5](https://www.tensorflow.org/lite/convert/python_apiexporting_a_tfkeras_file_), [Saved Model](https://www.tensorflow.org/lite/convert/python_apiexporting_a_savedmodel_)) As an HDF5 fileKeras provides a basic save format using the [HDF5](https://en.wikipedia.org/wiki/Hierarchical_Data_Format) standard. For our purposes, the saved model can be treated as a single binary blob.
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
# Save entire model to a HDF5 file
model.save('my_model.h5')
###Output
_____no_output_____
###Markdown
Now recreate the model from that file:
###Code
# Recreate the exact same model, including weights and optimizer.
new_model = keras.models.load_model('my_model.h5')
new_model.summary()
###Output
_____no_output_____
###Markdown
Check its accuracy:
###Code
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____
###Markdown
This technique saves everything:* The weight values* The model's configuration(architecture)* The optimizer configurationKeras saves models by inspecting the architecture. Currently, it is not able to save TensorFlow optimizers (from `tf.train`). When using those you will need to re-compile the model after loading, and you will lose the state of the optimizer. As a `saved_model` Caution: This method of saving a `tf.keras` model is experimental and may change in future versions. Build a fresh model:
###Code
model = create_model()
model.fit(train_images, train_labels, epochs=5)
###Output
_____no_output_____
###Markdown
Create a `saved_model`, and place it in a time-stamped directory:
###Code
import time
saved_model_path = "./saved_models/{}".format(int(time.time()))
tf.keras.experimental.export_saved_model(model, saved_model_path)
saved_model_path
###Output
_____no_output_____
###Markdown
List your saved models:
###Code
!ls saved_models/
###Output
_____no_output_____
###Markdown
Reload a fresh keras model from the saved model.
###Code
new_model = tf.keras.experimental.load_from_saved_model(saved_model_path)
new_model.summary()
###Output
_____no_output_____
###Markdown
Run the restored model.
###Code
model.predict(test_images).shape
# The model has to be compiled before evaluating.
# This step is not required if the saved model is only being deployed.
new_model.compile(optimizer=model.optimizer, # keep the optimizer that was loaded
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Evaluate the restored model.
loss, acc = new_model.evaluate(test_images, test_labels)
print("Restored model, accuracy: {:5.2f}%".format(100*acc))
###Output
_____no_output_____ |
examples/mp/jupyter/green_truck.ipynb | ###Markdown
There must be enough volume for a truck before it leaves a hub. In another words, the shipments for a truck must arrive at the hub from all spokes before the truck leaves. The constraint can be expressed as the following: For each route s-h and leaving truck of type t: Cumulated inbound volume arrived before the loading time of the truck >= Cumulated outbound volume upto the loading time of the truck(including the shipments being loaded).
###Code
for (s,h,dist) in routes:
for t in truckTypeIds:
model.add_constraint(
# The expression below defines the indices of the trucks unloaded before truck t starts loading.
model.sum(inVolumeThroughHubOnTruck[(o, h, s), t1]
for (o,h0,s0) in triples if h0 == h and s0 == s
for t1 in truckTypeIds
for (o2,h2,dist1) in routes if h2 == h0 and o2 == o
if earliestUnloadingTime[(o, h, dist1), t1] <= latestLoadingTime[(s, h, dist), t])
>=
# The expression below defines the indices of the trucks left before truck t starts loading.
model.sum(outVolumeThroughHubOnTruck[(o, h, s), t2]
for (o,h0,s0) in triples if h0 == h and s0 == s
for t2 in truckTypeIds
for (o2,h2,dist2) in routes if h2 == h0 and o2 == o
if latestLoadingTime[(o, h, dist2), t2] <= latestLoadingTime[(s, h, dist), t])
)
###Output
_____no_output_____
###Markdown
Express the objective
###Code
totalCost = model.sum(2 * r.distance * truckTypeInfos[t].costPerMile * truckOnRoute[r, t] for r in routes for t in truckTypeIds)
model.minimize(totalCost)
###Output
_____no_output_____
###Markdown
Solve with Decision Optimization You will get the best solution found after n seconds, due to a time limit parameter.
###Code
model.print_information()
assert model.solve(), "!!! Solve of the model fails"
model.report()
###Output
_____no_output_____
###Markdown
Step 5: Investigate the solution and then run an example analysis
###Code
#solution object model
_result = namedtuple('result', ['totalCost'])
_nbTrucksOnRouteRes = namedtuple('nbTrucksOnRouteRes', ['spoke', 'hub', 'truckType', 'nbTruck'])
_volumeThroughHubOnTruckRes = namedtuple('volumeThroughHubOnTruckRes', ['origin', 'hub', 'destination', 'truckType', 'quantity'])
_aggregatedReport = namedtuple('aggregatedReport', ['spoke', 'hub', 'truckType', 'quantity'])
# Post processing: result data structures are exported as post-processed tuple or list of tuples
# Solve objective value
import pandas as pd
result = _result(totalCost.solution_value)
nbTrucksOnRouteRes = pd.DataFrame([_nbTrucksOnRouteRes(r.spoke, r.hub, t, int(truckOnRoute[r, t]))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
# Volume shipped into each hub by each type of trucks and each pair (origin, destination)
inVolumeThroughHubOnTruckRes = pd.DataFrame([_volumeThroughHubOnTruckRes(tr.origin, tr.hub, tr.destination, t, int(inVolumeThroughHubOnTruck[tr, t]))
for tr in triples
for t in truckTypeIds
if int(inVolumeThroughHubOnTruck[tr, t]) > 0])
# Volume shipped from each hub by each type of trucks and each pair (origin, destination)
outVolumeThroughHubOnTruckRes = pd.DataFrame([_volumeThroughHubOnTruckRes(tr.origin, tr.hub, tr.destination, t, int(outVolumeThroughHubOnTruck[tr, t]))
for tr in triples
for t in truckTypeIds
if int(outVolumeThroughHubOnTruck[tr, t]) > 0])
inBoundAggregated = pd.DataFrame([_aggregatedReport(r.spoke, r.hub, t, sum(int(inVolumeThroughHubOnTruck[tr, t])
for tr in triples if tr.origin == r.spoke and tr.hub == r.hub))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
outBoundAggregated = pd.DataFrame([_aggregatedReport(r.spoke, r.hub, t, sum(int(outVolumeThroughHubOnTruck[tr, t])
for tr in triples if tr.destination == r.spoke and tr.hub == r.hub))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
from IPython.display import display
display(nbTrucksOnRouteRes)
display(inVolumeThroughHubOnTruckRes)
display(outVolumeThroughHubOnTruckRes)
display(inBoundAggregated)
display(outBoundAggregated)
###Output
_____no_output_____
###Markdown
Use decision optimization to help a trucking company manage its shipments.This tutorial includes everything you need to set up decision optimization engines, build mathematical programming models, and arrive at managing a truck fleet.When you finish this tutorial, you'll have a foundational knowledge of _Prescriptive Analytics_.>This notebook is part of the [Prescriptive Analytics for Python](http://ibmdecisionoptimization.github.io/docplex-doc/)>It requires an [installation of CPLEX Optimizers](http://ibmdecisionoptimization.github.io/docplex-doc/getting_started.html)Discover us [here](https://developer.ibm.com/docloud)Table of contents:- [The business problem](The-business-problem:--Games-Scheduling-in-the-National-Football-League)* [How decision optimization (prescriptive analytics) can help](How--decision-optimization-can-help)* [Use decision optimization](Use-decision-optimization) * [Step 1: Import the library](Step-1:-Import-the-library) - [Step 2: Model the Data](Step-2:-Model-the-data) * [Step 3: Prepare the data](Step-3:-Prepare-the-data) - [Step 4: Set up the prescriptive model](Step-4:-Set-up-the-prescriptive-model) * [Define the decision variables](Define-the-decision-variables) * [Express the business constraints](Express-the-business-constraints) * [Express the objective](Express-the-objective) * [Solve with Decision Optimization](Solve-with-Decision-Optimization) * [Step 5: Investigate the solution and run an example analysis](Step-5:-Investigate-the-solution-and-then-run-an-example-analysis)* [Summary](Summary) The business problem: Transportation Optimization Problem * A trucking company has a hub and spoke system. The shipments to be delivered are specified by an originating spoke, a destination spoke, and a shipment volume. The trucks have different types defined by a maximum capacity, a speed, and a cost per mile. The model assigns the correct number of trucks to each route in order to minimize the cost of transshipment and meet the volume requirements. There is a minimum departure time and a maximum return time for trucks at a spoke, and a load and unload time at the hub. Trucks of different types travel at different speeds. Therefore, shipments are available at each hub in a timely manner. Volume availability constraints are taken into account, meaning that the shipments that will be carried back from a hub to a spoke by a truck must be available for loading before the truck leaves.* The assumptions are: * Exactly the same number of trucks that go from spoke to hub return from hub to spoke. * Each truck arrives at a hub as early as possible and leaves as late as possible. * The shipments can be broken arbitrarily into smaller packages and shipped through different paths. How decision optimization can help* Prescriptive analytics (decision optimization) technology recommends actions that are based on desired outcomes. It takes into account specific scenarios, resources, and knowledge of past and current events. With this insight, your organization can make better decisions and have greater control of business outcomes. * Prescriptive analytics is the next step on the path to insight-based actions. It creates value through synergy with predictive analytics, which analyzes data to predict future outcomes. * Prescriptive analytics takes that insight to the next level by suggesting the optimal way to handle that future situation. Organizations that can act fast in dynamic conditions and make superior decisions in uncertain environments gain a strong competitive advantage. With prescriptive analytics, you can: * Automate the complex decisions and trade-offs to better manage your limited resources.* Take advantage of a future opportunity or mitigate a future risk.* Proactively update recommendations based on changing events.* Meet operational goals, increase customer loyalty, prevent threats and fraud, and optimize business processes. Use decision optimization Step 1: Import the libraryRun the following code to import the Decision Optimization CPLEX Modeling library. The *DOcplex* library contains the two modeling packages, Mathematical Programming (docplex.mp) and Constraint Programming (docplex.cp).
###Code
import sys
try:
import docplex.mp
except:
raise Exception('Please install docplex. See https://pypi.org/project/docplex/')
###Output
_____no_output_____
###Markdown
If *CPLEX* is not installed, install CPLEX Community edition.
###Code
try:
import cplex
except:
raise Exception('Please install CPLEX. See https://pypi.org/project/cplex/')
###Output
_____no_output_____
###Markdown
Step 2: Model the dataIn this scenario, the data is simple and is delivered in the json format under the Optimization github.
###Code
from collections import namedtuple
_parameters = namedtuple('parameters', ['maxTrucks', 'maxVolume'])
_location = namedtuple('location', ['name'])
_spoke = namedtuple('spoke', ['name', 'minDepTime', 'maxArrTime'])
_truckType = namedtuple('truckType', ['truckType', 'capacity', 'costPerMile', 'milesPerHour'])
_loadTimeInfo = namedtuple('loadTimeInfo', ['hub', 'truckType', 'loadTime'])
_routeInfo = namedtuple('routeInfo', ['spoke', 'hub', 'distance'])
_triple = namedtuple('triple', ['origin', 'hub', 'destination'])
_shipment = namedtuple('shipment', ['origin', 'destination', 'totalVolume'])
import requests
import json
import decimal
r = requests.get("https://github.com/vberaudi/utwt/blob/master/trucking.json?raw=true")
json_data = json.loads(r.text, parse_float=decimal.Decimal )
def read_json_tuples(name, my_namedtuple):
json_fragment = json_data[name]
length = len(my_namedtuple._fields)
ret = []
for t in json_fragment:
#print t
ret2 = [0 for i in range(length)]
for i in range(length):
field = my_namedtuple._fields[i]
ret2[i] = t[field]
ret.append(my_namedtuple(*tuple(ret2)))
return ret
def read_json_tuple(name, my_namedtuple):
json_fragment = json_data[name]
length = len(my_namedtuple._fields)
ret = [0 for i in range(length)]
for i in range(length):
field = my_namedtuple._fields[i]
ret[i] = json_fragment[field]
return my_namedtuple(*tuple(ret))
###Output
_____no_output_____
###Markdown
Use basic HTML and a stylesheet to format the data.
###Code
CSS = """
body {
margin: 0;
font-family: Helvetica;
}
table.dataframe {
border-collapse: collapse;
border: none;
}
table.dataframe tr {
border: none;
}
table.dataframe td, table.dataframe th {
margin: 0;
border: 1px solid white;
padding-left: 0.25em;
padding-right: 0.25em;
}
table.dataframe th:not(:empty) {
background-color: #fec;
text-align: left;
font-weight: normal;
}
table.dataframe tr:nth-child(2) th:empty {
border-left: none;
border-right: 1px dashed #888;
}
table.dataframe td {
border: 2px solid #ccf;
background-color: #f4f4ff;
}
table.dataframe thead th:first-child {
display: none;
}
table.dataframe tbody th {
display: none;
}
"""
from IPython.core.display import HTML
HTML('<style>{}</style>'.format(CSS))
parameters = read_json_tuple(name='Parameters', my_namedtuple=_parameters)
hubs = read_json_tuples(name='Hubs', my_namedtuple=_location)
truckTypes = read_json_tuples(name='TruckTypes', my_namedtuple=_truckType)
spokes = read_json_tuples(name='Spokes', my_namedtuple=_spoke)
loadTimes = read_json_tuples(name='LoadTimes', my_namedtuple=_loadTimeInfo)
routes = read_json_tuples(name='Routes', my_namedtuple=_routeInfo)
shipments = read_json_tuples(name='Shipments', my_namedtuple=_shipment)
###Output
_____no_output_____
###Markdown
Step 3: Prepare the dataGiven the number of teams in each division and the number of intradivisional and interdivisional games to be played, you can calculate the total number of teams and the number of weeks in the schedule, assuming every team plays exactly one game per week. The season is split into halves, and the number of the intradivisional games that each team must play in the first half of the season is calculated.
###Code
maxTrucks = parameters.maxTrucks;
maxVolume = parameters.maxVolume;
hubIds = {h.name for h in hubs}
spokeIds = {s.name for s in spokes}
spoke = {s.name : s for s in spokes}
truckTypeIds = {ttis.truckType for ttis in truckTypes}
truckTypeInfos = {tti.truckType : tti for tti in truckTypes}
loadTime = {(lt.hub , lt.truckType) : lt.loadTime for lt in loadTimes}
# feasible pathes from spokes to spokes via one hub
triples = {_triple(r1.spoke, r1.hub, r2.spoke) for r1 in routes for r2 in routes if (r1 != r2 and r1.hub == r2.hub)}
###Output
_____no_output_____
###Markdown
Some asserts to check the data follows the guidelines.
###Code
# Make sure the data is consistent: latest arrive time >= earliest departure time
for s in spokeIds:
assert spoke[s].maxArrTime > spoke[s].minDepTime, "inconsistent data"
# The following assertion is to make sure that the spoke
# in each route is indeed in the set of Spokes.
for r in routes:
assert r.spoke in spokeIds, "some route is not in the spokes"
# The following assertion is to make sure that the hub
# in each route are indeed in the set of Hubs.
for r in routes:
assert r.hub in hubIds, "some route is not in the hubs"
# The following assertion is to make sure that the origin
# of each shipment is indeed in the set of Spokes.
for s in shipments:
assert s.origin in spokeIds, "origin is not in the set of Spokes"
# The following assertion is to make sure that the destination
# of each shipment is indeed in the set of Spokes.
for s in shipments:
assert s.destination in spokeIds, "shipment is not in the set of Spokes"
from math import ceil, floor
# the earliest unloading time at a hub for each type of trucks
earliestUnloadingTime = {(r, t) : int(ceil(loadTime[r.hub, t] + spoke[r.spoke].minDepTime + 60 * r.distance / truckTypeInfos[t].milesPerHour)) for t in truckTypeIds for r in routes}
# the latest loading time at a hub for each type of trucks
latestLoadingTime = {(r, t) : int(floor(spoke[r.spoke].maxArrTime - loadTime[r.hub, t] - 60 * r.distance / truckTypeInfos[t].milesPerHour)) for t in truckTypeIds for r in routes}
# Compute possible truck types that can be assigned on a route
# A type of truck can be assigned on a route only if it can make it to the hub and back
# before the max arrival time at the spoke.
possibleTruckOnRoute = {(r, t) : 1 if earliestUnloadingTime[r, t] < latestLoadingTime[r, t] else 0 for t in truckTypeIds for r in routes}
###Output
_____no_output_____
###Markdown
Step 4: Set up the prescriptive model
###Code
from docplex.mp.environment import Environment
env = Environment()
env.print_information()
###Output
_____no_output_____
###Markdown
Create the DOcplex modelThe model contains all the business constraints and defines the objective.
###Code
from docplex.mp.model import Model
model = Model("truck")
###Output
_____no_output_____
###Markdown
Define the decision variables
###Code
truckOnRoute = model.integer_var_matrix(keys1=routes, keys2=truckTypeIds, lb=0, ub=maxTrucks, name="TruckOnRoute")
# This represents the volumes shipped out from each hub
# by each type of trucks on each triple
# The volumes are distinguished by trucktypes because trucks of different types
# arrive at a hub at different times and the timing is used in defining
# the constraints for volume availability for the trucks leaving the hub.
outVolumeThroughHubOnTruck = model.integer_var_matrix(keys1=triples, keys2=truckTypeIds, lb=0, ub=maxVolume, name="OutVolumeThroughHubOnTruck")
# This represents the volume shipped into each hub by each type of trucks on each triple
# It is used in defining timing constraints.
inVolumeThroughHubOnTruck = model.integer_var_matrix(keys1=triples, keys2=truckTypeIds, lb=0, ub=maxVolume, name="InVolumeThroughHubOnTruck")
###Output
_____no_output_____
###Markdown
Express the business constraints The number of trucks of each type should be less than "maxTrucks", and if a type of truck is impossible for a route, its number should be zero
###Code
for r in routes:
for t in truckTypeIds:
model.add_constraint(truckOnRoute[r, t] <= possibleTruckOnRoute[r, t] * maxTrucks)
###Output
_____no_output_____
###Markdown
On each route s-h, the total inbound volume carried by trucks of each type should be less than the total capacity of the trucks of this type.
###Code
for (s,h,dist) in routes:
for t in truckTypeIds:
model.add_constraint(
model.sum(inVolumeThroughHubOnTruck[(s1, h1, dest), t] for (s1, h1, dest) in triples if s == s1 and h1 == h)
<= truckOnRoute[(s, h, dist), t] * truckTypeInfos[t].capacity
)
###Output
_____no_output_____
###Markdown
On any triple, the total flows in the hub = the total flows out the hub
###Code
for tr in triples:
model.add_constraint(
model.sum(inVolumeThroughHubOnTruck[tr, t] for t in truckTypeIds)
== model.sum(outVolumeThroughHubOnTruck[tr, t] for t in truckTypeIds)
)
###Output
_____no_output_____
###Markdown
The sum of flows between any origin-destination pair via all hubs is equal to the shipment between the o-d pair.
###Code
for (o,d,v) in shipments:
model.add_constraint(model.sum(inVolumeThroughHubOnTruck[(o1, h, d1), t] for t in truckTypeIds for (o1, h, d1) in triples if o1 == o and d1 == d) == v)
###Output
_____no_output_____
###Markdown
There must be enough volume for a truck before it leaves a hub. In another words, the shipments for a truck must arrive at the hub from all spokes before the truck leaves. The constraint can be expressed as the following: For each route s-h and leaving truck of type t: Cumulated inbound volume arrived before the loading time of the truck >= Cumulated outbound volume upto the loading time of the truck(including the shipments being loaded).
###Code
for (s,h,dist) in routes:
for t in truckTypeIds:
model.add_constraint(
# The expression below defines the indices of the trucks unloaded before truck t starts loading.
model.sum(inVolumeThroughHubOnTruck[(o, h, s), t1]
for (o,h0,s0) in triples if h0 == h and s0 == s
for t1 in truckTypeIds
for (o2,h2,dist1) in routes if h2 == h0 and o2 == o
if earliestUnloadingTime[(o, h, dist1), t1] <= latestLoadingTime[(s, h, dist), t])
>=
# The expression below defines the indices of the trucks left before truck t starts loading.
model.sum(outVolumeThroughHubOnTruck[(o, h, s), t2]
for (o,h0,s0) in triples if h0 == h and s0 == s
for t2 in truckTypeIds
for (o2,h2,dist2) in routes if h2 == h0 and o2 == o
if latestLoadingTime[(o, h, dist2), t2] <= latestLoadingTime[(s, h, dist), t])
)
###Output
_____no_output_____
###Markdown
Express the objective
###Code
totalCost = model.sum(2 * r.distance * truckTypeInfos[t].costPerMile * truckOnRoute[r, t] for r in routes for t in truckTypeIds)
model.minimize(totalCost)
###Output
_____no_output_____
###Markdown
Solve with Decision Optimization You will get the best solution found after n seconds, due to a time limit parameter.
###Code
model.print_information()
assert model.solve(), "!!! Solve of the model fails"
model.report()
###Output
_____no_output_____
###Markdown
Step 5: Investigate the solution and then run an example analysis
###Code
#solution object model
_result = namedtuple('result', ['totalCost'])
_nbTrucksOnRouteRes = namedtuple('nbTrucksOnRouteRes', ['spoke', 'hub', 'truckType', 'nbTruck'])
_volumeThroughHubOnTruckRes = namedtuple('volumeThroughHubOnTruckRes', ['origin', 'hub', 'destination', 'truckType', 'quantity'])
_aggregatedReport = namedtuple('aggregatedReport', ['spoke', 'hub', 'truckType', 'quantity'])
# Post processing: result data structures are exported as post-processed tuple or list of tuples
# Solve objective value
import pandas as pd
result = _result(totalCost.solution_value)
nbTrucksOnRouteRes = pd.DataFrame([_nbTrucksOnRouteRes(r.spoke, r.hub, t, int(truckOnRoute[r, t]))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
# Volume shipped into each hub by each type of trucks and each pair (origin, destination)
inVolumeThroughHubOnTruckRes = pd.DataFrame([_volumeThroughHubOnTruckRes(tr.origin, tr.hub, tr.destination, t, int(inVolumeThroughHubOnTruck[tr, t]))
for tr in triples
for t in truckTypeIds
if int(inVolumeThroughHubOnTruck[tr, t]) > 0])
# Volume shipped from each hub by each type of trucks and each pair (origin, destination)
outVolumeThroughHubOnTruckRes = pd.DataFrame([_volumeThroughHubOnTruckRes(tr.origin, tr.hub, tr.destination, t, int(outVolumeThroughHubOnTruck[tr, t]))
for tr in triples
for t in truckTypeIds
if int(outVolumeThroughHubOnTruck[tr, t]) > 0])
inBoundAggregated = pd.DataFrame([_aggregatedReport(r.spoke, r.hub, t, sum(int(inVolumeThroughHubOnTruck[tr, t])
for tr in triples if tr.origin == r.spoke and tr.hub == r.hub))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
outBoundAggregated = pd.DataFrame([_aggregatedReport(r.spoke, r.hub, t, sum(int(outVolumeThroughHubOnTruck[tr, t])
for tr in triples if tr.destination == r.spoke and tr.hub == r.hub))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
from IPython.display import display
display(nbTrucksOnRouteRes)
display(inVolumeThroughHubOnTruckRes)
display(outVolumeThroughHubOnTruckRes)
display(inBoundAggregated)
display(outBoundAggregated)
###Output
_____no_output_____
###Markdown
Use decision optimization to help a trucking company manage its shipments.This tutorial includes everything you need to set up decision optimization engines, build mathematical programming models, and arrive at managing a truck fleet.When you finish this tutorial, you'll have a foundational knowledge of _Prescriptive Analytics_.>This notebook is part of **[Prescriptive Analytics for Python](http://ibmdecisionoptimization.github.io/docplex-doc/)**>>It requires either an [installation of CPLEX Optimizers](http://ibmdecisionoptimization.github.io/docplex-doc/getting_started.html) or it can be run on [IBM Cloud Pak for Data as a Service](https://www.ibm.com/products/cloud-pak-for-data/as-a-service/) (Sign up for a [free IBM Cloud account](https://dataplatform.cloud.ibm.com/registration/stepone?context=wdp&apps=all>)and you can start using `IBM Cloud Pak for Data as a Service` right away).>> CPLEX is available on IBM Cloud Pack for Data and IBM Cloud Pak for Data as a Service:> - IBM Cloud Pak for Data as a Service: Depends on the runtime used:> - Python 3.x runtime: Community edition> - Python 3.x + DO runtime: full edition> - Cloud Pack for Data: Community edition is installed by default. Please install `DO` addon in `Watson Studio Premium` for the full editionTable of contents:- [The business problem](The-business-problem:--Games-Scheduling-in-the-National-Football-League)* [How decision optimization (prescriptive analytics) can help](How--decision-optimization-can-help)* [Use decision optimization](Use-decision-optimization) * [Step 1: Import the library](Step-1:-Import-the-library) - [Step 2: Model the Data](Step-2:-Model-the-data) * [Step 3: Prepare the data](Step-3:-Prepare-the-data) - [Step 4: Set up the prescriptive model](Step-4:-Set-up-the-prescriptive-model) * [Define the decision variables](Define-the-decision-variables) * [Express the business constraints](Express-the-business-constraints) * [Express the objective](Express-the-objective) * [Solve with Decision Optimization](Solve-with-Decision-Optimization) * [Step 5: Investigate the solution and run an example analysis](Step-5:-Investigate-the-solution-and-then-run-an-example-analysis)* [Summary](Summary) The business problem: Transportation Optimization Problem * A trucking company has a hub and spoke system. The shipments to be delivered are specified by an originating spoke, a destination spoke, and a shipment volume. The trucks have different types defined by a maximum capacity, a speed, and a cost per mile. The model assigns the correct number of trucks to each route in order to minimize the cost of transshipment and meet the volume requirements. There is a minimum departure time and a maximum return time for trucks at a spoke, and a load and unload time at the hub. Trucks of different types travel at different speeds. Therefore, shipments are available at each hub in a timely manner. Volume availability constraints are taken into account, meaning that the shipments that will be carried back from a hub to a spoke by a truck must be available for loading before the truck leaves.* The assumptions are: * Exactly the same number of trucks that go from spoke to hub return from hub to spoke. * Each truck arrives at a hub as early as possible and leaves as late as possible. * The shipments can be broken arbitrarily into smaller packages and shipped through different paths. How decision optimization can help* Prescriptive analytics (decision optimization) technology recommends actions that are based on desired outcomes. It takes into account specific scenarios, resources, and knowledge of past and current events. With this insight, your organization can make better decisions and have greater control of business outcomes. * Prescriptive analytics is the next step on the path to insight-based actions. It creates value through synergy with predictive analytics, which analyzes data to predict future outcomes. * Prescriptive analytics takes that insight to the next level by suggesting the optimal way to handle that future situation. Organizations that can act fast in dynamic conditions and make superior decisions in uncertain environments gain a strong competitive advantage. With prescriptive analytics, you can: * Automate the complex decisions and trade-offs to better manage your limited resources.* Take advantage of a future opportunity or mitigate a future risk.* Proactively update recommendations based on changing events.* Meet operational goals, increase customer loyalty, prevent threats and fraud, and optimize business processes. Use decision optimization Step 1: Import the libraryRun the following code to import the Decision Optimization CPLEX Modeling library. The *DOcplex* library contains the two modeling packages, Mathematical Programming (docplex.mp) and Constraint Programming (docplex.cp).
###Code
import sys
try:
import docplex.mp
except:
raise Exception('Please install docplex. See https://pypi.org/project/docplex/')
###Output
_____no_output_____
###Markdown
If *CPLEX* is not installed, you can install CPLEX Community edition.
###Code
try:
import cplex
except:
raise Exception('Please install CPLEX. See https://pypi.org/project/cplex/')
###Output
_____no_output_____
###Markdown
Step 2: Model the dataIn this scenario, the data is simple and is delivered in the json format under the Optimization github.
###Code
from collections import namedtuple
_parameters = namedtuple('parameters', ['maxTrucks', 'maxVolume'])
_location = namedtuple('location', ['name'])
_spoke = namedtuple('spoke', ['name', 'minDepTime', 'maxArrTime'])
_truckType = namedtuple('truckType', ['truckType', 'capacity', 'costPerMile', 'milesPerHour'])
_loadTimeInfo = namedtuple('loadTimeInfo', ['hub', 'truckType', 'loadTime'])
_routeInfo = namedtuple('routeInfo', ['spoke', 'hub', 'distance'])
_triple = namedtuple('triple', ['origin', 'hub', 'destination'])
_shipment = namedtuple('shipment', ['origin', 'destination', 'totalVolume'])
import requests
import json
import decimal
r = requests.get("https://github.com/vberaudi/utwt/blob/master/trucking.json?raw=true")
json_data = json.loads(r.text, parse_float=decimal.Decimal )
def read_json_tuples(name, my_namedtuple):
json_fragment = json_data[name]
length = len(my_namedtuple._fields)
ret = []
for t in json_fragment:
#print t
ret2 = [0 for i in range(length)]
for i in range(length):
field = my_namedtuple._fields[i]
ret2[i] = t[field]
ret.append(my_namedtuple(*tuple(ret2)))
return ret
def read_json_tuple(name, my_namedtuple):
json_fragment = json_data[name]
length = len(my_namedtuple._fields)
ret = [0 for i in range(length)]
for i in range(length):
field = my_namedtuple._fields[i]
ret[i] = json_fragment[field]
return my_namedtuple(*tuple(ret))
###Output
_____no_output_____
###Markdown
Use basic HTML and a stylesheet to format the data.
###Code
CSS = """
body {
margin: 0;
font-family: Helvetica;
}
table.dataframe {
border-collapse: collapse;
border: none;
}
table.dataframe tr {
border: none;
}
table.dataframe td, table.dataframe th {
margin: 0;
border: 1px solid white;
padding-left: 0.25em;
padding-right: 0.25em;
}
table.dataframe th:not(:empty) {
background-color: #fec;
text-align: left;
font-weight: normal;
}
table.dataframe tr:nth-child(2) th:empty {
border-left: none;
border-right: 1px dashed #888;
}
table.dataframe td {
border: 2px solid #ccf;
background-color: #f4f4ff;
}
table.dataframe thead th:first-child {
display: none;
}
table.dataframe tbody th {
display: none;
}
"""
from IPython.core.display import HTML
HTML('<style>{}</style>'.format(CSS))
parameters = read_json_tuple(name='Parameters', my_namedtuple=_parameters)
hubs = read_json_tuples(name='Hubs', my_namedtuple=_location)
truckTypes = read_json_tuples(name='TruckTypes', my_namedtuple=_truckType)
spokes = read_json_tuples(name='Spokes', my_namedtuple=_spoke)
loadTimes = read_json_tuples(name='LoadTimes', my_namedtuple=_loadTimeInfo)
routes = read_json_tuples(name='Routes', my_namedtuple=_routeInfo)
shipments = read_json_tuples(name='Shipments', my_namedtuple=_shipment)
###Output
_____no_output_____
###Markdown
Step 3: Prepare the dataGiven the number of teams in each division and the number of intradivisional and interdivisional games to be played, you can calculate the total number of teams and the number of weeks in the schedule, assuming every team plays exactly one game per week. The season is split into halves, and the number of the intradivisional games that each team must play in the first half of the season is calculated.
###Code
maxTrucks = parameters.maxTrucks;
maxVolume = parameters.maxVolume;
hubIds = {h.name for h in hubs}
spokeIds = {s.name for s in spokes}
spoke = {s.name : s for s in spokes}
truckTypeIds = {ttis.truckType for ttis in truckTypes}
truckTypeInfos = {tti.truckType : tti for tti in truckTypes}
loadTime = {(lt.hub , lt.truckType) : lt.loadTime for lt in loadTimes}
# feasible pathes from spokes to spokes via one hub
triples = {_triple(r1.spoke, r1.hub, r2.spoke) for r1 in routes for r2 in routes if (r1 != r2 and r1.hub == r2.hub)}
###Output
_____no_output_____
###Markdown
Some asserts to check the data follows the guidelines.
###Code
# Make sure the data is consistent: latest arrive time >= earliest departure time
for s in spokeIds:
assert spoke[s].maxArrTime > spoke[s].minDepTime, "inconsistent data"
# The following assertion is to make sure that the spoke
# in each route is indeed in the set of Spokes.
for r in routes:
assert r.spoke in spokeIds, "some route is not in the spokes"
# The following assertion is to make sure that the hub
# in each route are indeed in the set of Hubs.
for r in routes:
assert r.hub in hubIds, "some route is not in the hubs"
# The following assertion is to make sure that the origin
# of each shipment is indeed in the set of Spokes.
for s in shipments:
assert s.origin in spokeIds, "origin is not in the set of Spokes"
# The following assertion is to make sure that the destination
# of each shipment is indeed in the set of Spokes.
for s in shipments:
assert s.destination in spokeIds, "shipment is not in the set of Spokes"
from math import ceil, floor
# the earliest unloading time at a hub for each type of trucks
earliestUnloadingTime = {(r, t) : int(ceil(loadTime[r.hub, t] + spoke[r.spoke].minDepTime + 60 * r.distance / truckTypeInfos[t].milesPerHour)) for t in truckTypeIds for r in routes}
# the latest loading time at a hub for each type of trucks
latestLoadingTime = {(r, t) : int(floor(spoke[r.spoke].maxArrTime - loadTime[r.hub, t] - 60 * r.distance / truckTypeInfos[t].milesPerHour)) for t in truckTypeIds for r in routes}
# Compute possible truck types that can be assigned on a route
# A type of truck can be assigned on a route only if it can make it to the hub and back
# before the max arrival time at the spoke.
possibleTruckOnRoute = {(r, t) : 1 if earliestUnloadingTime[r, t] < latestLoadingTime[r, t] else 0 for t in truckTypeIds for r in routes}
###Output
_____no_output_____
###Markdown
Step 4: Set up the prescriptive model
###Code
from docplex.mp.environment import Environment
env = Environment()
env.print_information()
###Output
_____no_output_____
###Markdown
Create the DOcplex modelThe model contains all the business constraints and defines the objective.
###Code
from docplex.mp.model import Model
model = Model("truck")
###Output
_____no_output_____
###Markdown
Define the decision variables
###Code
truckOnRoute = model.integer_var_matrix(keys1=routes, keys2=truckTypeIds, lb=0, ub=maxTrucks, name="TruckOnRoute")
# This represents the volumes shipped out from each hub
# by each type of trucks on each triple
# The volumes are distinguished by trucktypes because trucks of different types
# arrive at a hub at different times and the timing is used in defining
# the constraints for volume availability for the trucks leaving the hub.
outVolumeThroughHubOnTruck = model.integer_var_matrix(keys1=triples, keys2=truckTypeIds, lb=0, ub=maxVolume, name="OutVolumeThroughHubOnTruck")
# This represents the volume shipped into each hub by each type of trucks on each triple
# It is used in defining timing constraints.
inVolumeThroughHubOnTruck = model.integer_var_matrix(keys1=triples, keys2=truckTypeIds, lb=0, ub=maxVolume, name="InVolumeThroughHubOnTruck")
###Output
_____no_output_____
###Markdown
Express the business constraints The number of trucks of each type should be less than "maxTrucks", and if a type of truck is impossible for a route, its number should be zero
###Code
for r in routes:
for t in truckTypeIds:
model.add_constraint(truckOnRoute[r, t] <= possibleTruckOnRoute[r, t] * maxTrucks)
###Output
_____no_output_____
###Markdown
On each route s-h, the total inbound volume carried by trucks of each type should be less than the total capacity of the trucks of this type.
###Code
for (s,h,dist) in routes:
for t in truckTypeIds:
model.add_constraint(
model.sum(inVolumeThroughHubOnTruck[(s1, h1, dest), t] for (s1, h1, dest) in triples if s == s1 and h1 == h)
<= truckOnRoute[(s, h, dist), t] * truckTypeInfos[t].capacity
)
###Output
_____no_output_____
###Markdown
On any triple, the total flows in the hub = the total flows out the hub
###Code
for tr in triples:
model.add_constraint(
model.sum(inVolumeThroughHubOnTruck[tr, t] for t in truckTypeIds)
== model.sum(outVolumeThroughHubOnTruck[tr, t] for t in truckTypeIds)
)
###Output
_____no_output_____
###Markdown
The sum of flows between any origin-destination pair via all hubs is equal to the shipment between the o-d pair.
###Code
for (o,d,v) in shipments:
model.add_constraint(model.sum(inVolumeThroughHubOnTruck[(o1, h, d1), t] for t in truckTypeIds for (o1, h, d1) in triples if o1 == o and d1 == d) == v)
###Output
_____no_output_____
###Markdown
There must be enough volume for a truck before it leaves a hub. In another words, the shipments for a truck must arrive at the hub from all spokes before the truck leaves. The constraint can be expressed as the following: For each route s-h and leaving truck of type t: Cumulated inbound volume arrived before the loading time of the truck >= Cumulated outbound volume upto the loading time of the truck(including the shipments being loaded).
###Code
for (s,h,dist) in routes:
for t in truckTypeIds:
model.add_constraint(
# The expression below defines the indices of the trucks unloaded before truck t starts loading.
model.sum(inVolumeThroughHubOnTruck[(o, h, s), t1]
for (o,h0,s0) in triples if h0 == h and s0 == s
for t1 in truckTypeIds
for (o2,h2,dist1) in routes if h2 == h0 and o2 == o
if earliestUnloadingTime[(o, h, dist1), t1] <= latestLoadingTime[(s, h, dist), t])
>=
# The expression below defines the indices of the trucks left before truck t starts loading.
model.sum(outVolumeThroughHubOnTruck[(o, h, s), t2]
for (o,h0,s0) in triples if h0 == h and s0 == s
for t2 in truckTypeIds
for (o2,h2,dist2) in routes if h2 == h0 and o2 == o
if latestLoadingTime[(o, h, dist2), t2] <= latestLoadingTime[(s, h, dist), t])
)
###Output
_____no_output_____
###Markdown
Express the objective
###Code
totalCost = model.sum(2 * r.distance * truckTypeInfos[t].costPerMile * truckOnRoute[r, t] for r in routes for t in truckTypeIds)
model.minimize(totalCost)
###Output
_____no_output_____
###Markdown
Solve with Decision Optimization You will get the best solution found after n seconds, due to a time limit parameter.
###Code
model.print_information()
assert model.solve(), "!!! Solve of the model fails"
model.report()
###Output
_____no_output_____
###Markdown
Step 5: Investigate the solution and then run an example analysis
###Code
#solution object model
_result = namedtuple('result', ['totalCost'])
_nbTrucksOnRouteRes = namedtuple('nbTrucksOnRouteRes', ['spoke', 'hub', 'truckType', 'nbTruck'])
_volumeThroughHubOnTruckRes = namedtuple('volumeThroughHubOnTruckRes', ['origin', 'hub', 'destination', 'truckType', 'quantity'])
_aggregatedReport = namedtuple('aggregatedReport', ['spoke', 'hub', 'truckType', 'quantity'])
# Post processing: result data structures are exported as post-processed tuple or list of tuples
# Solve objective value
import pandas as pd
result = _result(totalCost.solution_value)
nbTrucksOnRouteRes = pd.DataFrame([_nbTrucksOnRouteRes(r.spoke, r.hub, t, int(truckOnRoute[r, t]))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
# Volume shipped into each hub by each type of trucks and each pair (origin, destination)
inVolumeThroughHubOnTruckRes = pd.DataFrame([_volumeThroughHubOnTruckRes(tr.origin, tr.hub, tr.destination, t, int(inVolumeThroughHubOnTruck[tr, t]))
for tr in triples
for t in truckTypeIds
if int(inVolumeThroughHubOnTruck[tr, t]) > 0])
# Volume shipped from each hub by each type of trucks and each pair (origin, destination)
outVolumeThroughHubOnTruckRes = pd.DataFrame([_volumeThroughHubOnTruckRes(tr.origin, tr.hub, tr.destination, t, int(outVolumeThroughHubOnTruck[tr, t]))
for tr in triples
for t in truckTypeIds
if int(outVolumeThroughHubOnTruck[tr, t]) > 0])
inBoundAggregated = pd.DataFrame([_aggregatedReport(r.spoke, r.hub, t, sum(int(inVolumeThroughHubOnTruck[tr, t])
for tr in triples if tr.origin == r.spoke and tr.hub == r.hub))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
outBoundAggregated = pd.DataFrame([_aggregatedReport(r.spoke, r.hub, t, sum(int(outVolumeThroughHubOnTruck[tr, t])
for tr in triples if tr.destination == r.spoke and tr.hub == r.hub))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
from IPython.display import display
display(nbTrucksOnRouteRes)
display(inVolumeThroughHubOnTruckRes)
display(outVolumeThroughHubOnTruckRes)
display(inBoundAggregated)
display(outBoundAggregated)
###Output
_____no_output_____
###Markdown
Use basic HTML and a stylesheet to format the data.
###Code
CSS = """
body {
margin: 0;
font-family: Helvetica;
}
table.dataframe {
border-collapse: collapse;
border: none;
}
table.dataframe tr {
border: none;
}
table.dataframe td, table.dataframe th {
margin: 0;
border: 1px solid white;
padding-left: 0.25em;
padding-right: 0.25em;
}
table.dataframe th:not(:empty) {
background-color: #fec;
text-align: left;
font-weight: normal;
}
table.dataframe tr:nth-child(2) th:empty {
border-left: none;
border-right: 1px dashed #888;
}
table.dataframe td {
border: 2px solid #ccf;
background-color: #f4f4ff;
}
table.dataframe thead th:first-child {
display: none;
}
table.dataframe tbody th {
display: none;
}
"""
from IPython.core.display import HTML
HTML('<style>{}</style>'.format(CSS))
parameters = read_json_tuple(name='Parameters', my_namedtuple=_parameters)
hubs = read_json_tuples(name='Hubs', my_namedtuple=_location)
truckTypes = read_json_tuples(name='TruckTypes', my_namedtuple=_truckType)
spokes = read_json_tuples(name='Spokes', my_namedtuple=_spoke)
loadTimes = read_json_tuples(name='LoadTimes', my_namedtuple=_loadTimeInfo)
routes = read_json_tuples(name='Routes', my_namedtuple=_routeInfo)
shipments = read_json_tuples(name='Shipments', my_namedtuple=_shipment)
###Output
_____no_output_____
###Markdown
Step 3: Prepare the dataGiven the number of teams in each division and the number of intradivisional and interdivisional games to be played, you can calculate the total number of teams and the number of weeks in the schedule, assuming every team plays exactly one game per week. The season is split into halves, and the number of the intradivisional games that each team must play in the first half of the season is calculated.
###Code
maxTrucks = parameters.maxTrucks;
maxVolume = parameters.maxVolume;
hubIds = {h.name for h in hubs}
spokeIds = {s.name for s in spokes}
spoke = {s.name : s for s in spokes}
truckTypeIds = {ttis.truckType for ttis in truckTypes}
truckTypeInfos = {tti.truckType : tti for tti in truckTypes}
loadTime = {(lt.hub , lt.truckType) : lt.loadTime for lt in loadTimes}
# feasible pathes from spokes to spokes via one hub
triples = {_triple(r1.spoke, r1.hub, r2.spoke) for r1 in routes for r2 in routes if (r1 != r2 and r1.hub == r2.hub)}
###Output
_____no_output_____
###Markdown
Some asserts to check the data follows the guidelines.
###Code
# Make sure the data is consistent: latest arrive time >= earliest departure time
for s in spokeIds:
assert spoke[s].maxArrTime > spoke[s].minDepTime, "inconsistent data"
# The following assertion is to make sure that the spoke
# in each route is indeed in the set of Spokes.
for r in routes:
assert r.spoke in spokeIds, "some route is not in the spokes"
# The following assertion is to make sure that the hub
# in each route are indeed in the set of Hubs.
for r in routes:
assert r.hub in hubIds, "some route is not in the hubs"
# The following assertion is to make sure that the origin
# of each shipment is indeed in the set of Spokes.
for s in shipments:
assert s.origin in spokeIds, "origin is not in the set of Spokes"
# The following assertion is to make sure that the destination
# of each shipment is indeed in the set of Spokes.
for s in shipments:
assert s.destination in spokeIds, "shipment is not in the set of Spokes"
from math import ceil, floor
# the earliest unloading time at a hub for each type of trucks
earliestUnloadingTime = {(r, t) : int(ceil(loadTime[r.hub, t] + spoke[r.spoke].minDepTime + 60 * r.distance / truckTypeInfos[t].milesPerHour)) for t in truckTypeIds for r in routes}
# the latest loading time at a hub for each type of trucks
latestLoadingTime = {(r, t) : int(floor(spoke[r.spoke].maxArrTime - loadTime[r.hub, t] - 60 * r.distance / truckTypeInfos[t].milesPerHour)) for t in truckTypeIds for r in routes}
# Compute possible truck types that can be assigned on a route
# A type of truck can be assigned on a route only if it can make it to the hub and back
# before the max arrival time at the spoke.
possibleTruckOnRoute = {(r, t) : 1 if earliestUnloadingTime[r, t] < latestLoadingTime[r, t] else 0 for t in truckTypeIds for r in routes}
###Output
_____no_output_____
###Markdown
Step 4: Set up the prescriptive model
###Code
from docplex.mp.environment import Environment
env = Environment()
env.print_information()
###Output
_____no_output_____
###Markdown
Create the DOcplex modelThe model contains all the business constraints and defines the objective.
###Code
from docplex.mp.model import Model
model = Model("truck")
###Output
_____no_output_____
###Markdown
Define the decision variables
###Code
truckOnRoute = model.integer_var_matrix(keys1=routes, keys2=truckTypeIds, lb=0, ub=maxTrucks, name="TruckOnRoute")
# This represents the volumes shipped out from each hub
# by each type of trucks on each triple
# The volumes are distinguished by trucktypes because trucks of different types
# arrive at a hub at different times and the timing is used in defining
# the constraints for volume availability for the trucks leaving the hub.
outVolumeThroughHubOnTruck = model.integer_var_matrix(keys1=triples, keys2=truckTypeIds, lb=0, ub=maxVolume, name="OutVolumeThroughHubOnTruck")
# This represents the volume shipped into each hub by each type of trucks on each triple
# It is used in defining timing constraints.
inVolumeThroughHubOnTruck = model.integer_var_matrix(keys1=triples, keys2=truckTypeIds, lb=0, ub=maxVolume, name="InVolumeThroughHubOnTruck")
###Output
_____no_output_____
###Markdown
Express the business constraints The number of trucks of each type should be less than "maxTrucks", and if a type of truck is impossible for a route, its number should be zero
###Code
for r in routes:
for t in truckTypeIds:
model.add_constraint(truckOnRoute[r, t] <= possibleTruckOnRoute[r, t] * maxTrucks)
###Output
_____no_output_____
###Markdown
On each route s-h, the total inbound volume carried by trucks of each type should be less than the total capacity of the trucks of this type.
###Code
for (s,h,dist) in routes:
for t in truckTypeIds:
model.add_constraint(
model.sum(inVolumeThroughHubOnTruck[(s1, h1, dest), t] for (s1, h1, dest) in triples if s == s1 and h1 == h)
<= truckOnRoute[(s, h, dist), t] * truckTypeInfos[t].capacity
)
###Output
_____no_output_____
###Markdown
On any triple, the total flows in the hub = the total flows out the hub
###Code
for tr in triples:
model.add_constraint(
model.sum(inVolumeThroughHubOnTruck[tr, t] for t in truckTypeIds)
== model.sum(outVolumeThroughHubOnTruck[tr, t] for t in truckTypeIds)
)
###Output
_____no_output_____
###Markdown
The sum of flows between any origin-destination pair via all hubs is equal to the shipment between the o-d pair.
###Code
for (o,d,v) in shipments:
model.add_constraint(model.sum(inVolumeThroughHubOnTruck[(o1, h, d1), t] for t in truckTypeIds for (o1, h, d1) in triples if o1 == o and d1 == d) == v)
###Output
_____no_output_____
###Markdown
There must be enough volume for a truck before it leaves a hub. In another words, the shipments for a truck must arrive at the hub from all spokes before the truck leaves. The constraint can be expressed as the following: For each route s-h and leaving truck of type t: Cumulated inbound volume arrived before the loading time of the truck >= Cumulated outbound volume upto the loading time of the truck(including the shipments being loaded).
###Code
for (s,h,dist) in routes:
for t in truckTypeIds:
model.add_constraint(
# The expression below defines the indices of the trucks unloaded before truck t starts loading.
model.sum(inVolumeThroughHubOnTruck[(o, h, s), t1]
for (o,h0,s0) in triples if h0 == h and s0 == s
for t1 in truckTypeIds
for (o2,h2,dist1) in routes if h2 == h0 and o2 == o
if earliestUnloadingTime[(o, h, dist1), t1] <= latestLoadingTime[(s, h, dist), t])
>=
# The expression below defines the indices of the trucks left before truck t starts loading.
model.sum(outVolumeThroughHubOnTruck[(o, h, s), t2]
for (o,h0,s0) in triples if h0 == h and s0 == s
for t2 in truckTypeIds
for (o2,h2,dist2) in routes if h2 == h0 and o2 == o
if latestLoadingTime[(o, h, dist2), t2] <= latestLoadingTime[(s, h, dist), t])
)
###Output
_____no_output_____
###Markdown
Express the objective
###Code
totalCost = model.sum(2 * r.distance * truckTypeInfos[t].costPerMile * truckOnRoute[r, t] for r in routes for t in truckTypeIds)
model.minimize(totalCost)
###Output
_____no_output_____
###Markdown
Solve with Decision Optimization You will get the best solution found after n seconds, due to a time limit parameter.
###Code
model.print_information()
assert model.solve(), "!!! Solve of the model fails"
model.report()
###Output
_____no_output_____
###Markdown
Step 5: Investigate the solution and then run an example analysis
###Code
#solution object model
_result = namedtuple('result', ['totalCost'])
_nbTrucksOnRouteRes = namedtuple('nbTrucksOnRouteRes', ['spoke', 'hub', 'truckType', 'nbTruck'])
_volumeThroughHubOnTruckRes = namedtuple('volumeThroughHubOnTruckRes', ['origin', 'hub', 'destination', 'truckType', 'quantity'])
_aggregatedReport = namedtuple('aggregatedReport', ['spoke', 'hub', 'truckType', 'quantity'])
# Post processing: result data structures are exported as post-processed tuple or list of tuples
# Solve objective value
import pandas as pd
result = _result(totalCost.solution_value)
nbTrucksOnRouteRes = pd.DataFrame([_nbTrucksOnRouteRes(r.spoke, r.hub, t, int(truckOnRoute[r, t]))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
# Volume shipped into each hub by each type of trucks and each pair (origin, destination)
inVolumeThroughHubOnTruckRes = pd.DataFrame([_volumeThroughHubOnTruckRes(tr.origin, tr.hub, tr.destination, t, int(inVolumeThroughHubOnTruck[tr, t]))
for tr in triples
for t in truckTypeIds
if int(inVolumeThroughHubOnTruck[tr, t]) > 0])
# Volume shipped from each hub by each type of trucks and each pair (origin, destination)
outVolumeThroughHubOnTruckRes = pd.DataFrame([_volumeThroughHubOnTruckRes(tr.origin, tr.hub, tr.destination, t, int(outVolumeThroughHubOnTruck[tr, t]))
for tr in triples
for t in truckTypeIds
if int(outVolumeThroughHubOnTruck[tr, t]) > 0])
inBoundAggregated = pd.DataFrame([_aggregatedReport(r.spoke, r.hub, t, sum(int(inVolumeThroughHubOnTruck[tr, t])
for tr in triples if tr.origin == r.spoke and tr.hub == r.hub))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
outBoundAggregated = pd.DataFrame([_aggregatedReport(r.spoke, r.hub, t, sum(int(outVolumeThroughHubOnTruck[tr, t])
for tr in triples if tr.destination == r.spoke and tr.hub == r.hub))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
from IPython.display import display
display(nbTrucksOnRouteRes)
display(inVolumeThroughHubOnTruckRes)
display(outVolumeThroughHubOnTruckRes)
display(inBoundAggregated)
display(outBoundAggregated)
###Output
_____no_output_____
###Markdown
Use decision optimization to help a trucking company manage its shipments.This tutorial includes everything you need to set up decision optimization engines, build mathematical programming models, and arrive at managing a truck fleet.When you finish this tutorial, you'll have a foundational knowledge of _Prescriptive Analytics_.>This notebook is part of **[Prescriptive Analytics for Python](http://ibmdecisionoptimization.github.io/docplex-doc/)**>>It requires either an [installation of CPLEX Optimizers](http://ibmdecisionoptimization.github.io/docplex-doc/getting_started.html) or it can be run on [IBM Watson Studio Cloud](https://www.ibm.com/cloud/watson-studio/) (Sign up for a [free IBM Cloud account](https://dataplatform.cloud.ibm.com/registration/stepone?context=wdp&apps=all>)and you can start using Watson Studio Cloud right away).Table of contents:- [The business problem](The-business-problem:--Games-Scheduling-in-the-National-Football-League)* [How decision optimization (prescriptive analytics) can help](How--decision-optimization-can-help)* [Use decision optimization](Use-decision-optimization) * [Step 1: Import the library](Step-1:-Import-the-library) - [Step 2: Model the Data](Step-2:-Model-the-data) * [Step 3: Prepare the data](Step-3:-Prepare-the-data) - [Step 4: Set up the prescriptive model](Step-4:-Set-up-the-prescriptive-model) * [Define the decision variables](Define-the-decision-variables) * [Express the business constraints](Express-the-business-constraints) * [Express the objective](Express-the-objective) * [Solve with Decision Optimization](Solve-with-Decision-Optimization) * [Step 5: Investigate the solution and run an example analysis](Step-5:-Investigate-the-solution-and-then-run-an-example-analysis)* [Summary](Summary) The business problem: Transportation Optimization Problem * A trucking company has a hub and spoke system. The shipments to be delivered are specified by an originating spoke, a destination spoke, and a shipment volume. The trucks have different types defined by a maximum capacity, a speed, and a cost per mile. The model assigns the correct number of trucks to each route in order to minimize the cost of transshipment and meet the volume requirements. There is a minimum departure time and a maximum return time for trucks at a spoke, and a load and unload time at the hub. Trucks of different types travel at different speeds. Therefore, shipments are available at each hub in a timely manner. Volume availability constraints are taken into account, meaning that the shipments that will be carried back from a hub to a spoke by a truck must be available for loading before the truck leaves.* The assumptions are: * Exactly the same number of trucks that go from spoke to hub return from hub to spoke. * Each truck arrives at a hub as early as possible and leaves as late as possible. * The shipments can be broken arbitrarily into smaller packages and shipped through different paths. How decision optimization can help* Prescriptive analytics (decision optimization) technology recommends actions that are based on desired outcomes. It takes into account specific scenarios, resources, and knowledge of past and current events. With this insight, your organization can make better decisions and have greater control of business outcomes. * Prescriptive analytics is the next step on the path to insight-based actions. It creates value through synergy with predictive analytics, which analyzes data to predict future outcomes. * Prescriptive analytics takes that insight to the next level by suggesting the optimal way to handle that future situation. Organizations that can act fast in dynamic conditions and make superior decisions in uncertain environments gain a strong competitive advantage. With prescriptive analytics, you can: * Automate the complex decisions and trade-offs to better manage your limited resources.* Take advantage of a future opportunity or mitigate a future risk.* Proactively update recommendations based on changing events.* Meet operational goals, increase customer loyalty, prevent threats and fraud, and optimize business processes. Use decision optimization Step 1: Import the libraryRun the following code to import the Decision Optimization CPLEX Modeling library. The *DOcplex* library contains the two modeling packages, Mathematical Programming (docplex.mp) and Constraint Programming (docplex.cp).
###Code
import sys
try:
import docplex.mp
except:
raise Exception('Please install docplex. See https://pypi.org/project/docplex/')
###Output
_____no_output_____
###Markdown
If *CPLEX* is not installed, install CPLEX Community edition.
###Code
try:
import cplex
except:
raise Exception('Please install CPLEX. See https://pypi.org/project/cplex/')
###Output
_____no_output_____
###Markdown
Step 2: Model the dataIn this scenario, the data is simple and is delivered in the json format under the Optimization github.
###Code
from collections import namedtuple
_parameters = namedtuple('parameters', ['maxTrucks', 'maxVolume'])
_location = namedtuple('location', ['name'])
_spoke = namedtuple('spoke', ['name', 'minDepTime', 'maxArrTime'])
_truckType = namedtuple('truckType', ['truckType', 'capacity', 'costPerMile', 'milesPerHour'])
_loadTimeInfo = namedtuple('loadTimeInfo', ['hub', 'truckType', 'loadTime'])
_routeInfo = namedtuple('routeInfo', ['spoke', 'hub', 'distance'])
_triple = namedtuple('triple', ['origin', 'hub', 'destination'])
_shipment = namedtuple('shipment', ['origin', 'destination', 'totalVolume'])
import requests
import json
import decimal
r = requests.get("https://github.com/vberaudi/utwt/blob/master/trucking.json?raw=true")
json_data = json.loads(r.text, parse_float=decimal.Decimal )
def read_json_tuples(name, my_namedtuple):
json_fragment = json_data[name]
length = len(my_namedtuple._fields)
ret = []
for t in json_fragment:
#print t
ret2 = [0 for i in range(length)]
for i in range(length):
field = my_namedtuple._fields[i]
ret2[i] = t[field]
ret.append(my_namedtuple(*tuple(ret2)))
return ret
def read_json_tuple(name, my_namedtuple):
json_fragment = json_data[name]
length = len(my_namedtuple._fields)
ret = [0 for i in range(length)]
for i in range(length):
field = my_namedtuple._fields[i]
ret[i] = json_fragment[field]
return my_namedtuple(*tuple(ret))
###Output
_____no_output_____
###Markdown
Use basic HTML and a stylesheet to format the data.
###Code
CSS = """
body {
margin: 0;
font-family: Helvetica;
}
table.dataframe {
border-collapse: collapse;
border: none;
}
table.dataframe tr {
border: none;
}
table.dataframe td, table.dataframe th {
margin: 0;
border: 1px solid white;
padding-left: 0.25em;
padding-right: 0.25em;
}
table.dataframe th:not(:empty) {
background-color: #fec;
text-align: left;
font-weight: normal;
}
table.dataframe tr:nth-child(2) th:empty {
border-left: none;
border-right: 1px dashed #888;
}
table.dataframe td {
border: 2px solid #ccf;
background-color: #f4f4ff;
}
table.dataframe thead th:first-child {
display: none;
}
table.dataframe tbody th {
display: none;
}
"""
from IPython.core.display import HTML
HTML('<style>{}</style>'.format(CSS))
parameters = read_json_tuple(name='Parameters', my_namedtuple=_parameters)
hubs = read_json_tuples(name='Hubs', my_namedtuple=_location)
truckTypes = read_json_tuples(name='TruckTypes', my_namedtuple=_truckType)
spokes = read_json_tuples(name='Spokes', my_namedtuple=_spoke)
loadTimes = read_json_tuples(name='LoadTimes', my_namedtuple=_loadTimeInfo)
routes = read_json_tuples(name='Routes', my_namedtuple=_routeInfo)
shipments = read_json_tuples(name='Shipments', my_namedtuple=_shipment)
###Output
_____no_output_____
###Markdown
Step 3: Prepare the dataGiven the number of teams in each division and the number of intradivisional and interdivisional games to be played, you can calculate the total number of teams and the number of weeks in the schedule, assuming every team plays exactly one game per week. The season is split into halves, and the number of the intradivisional games that each team must play in the first half of the season is calculated.
###Code
maxTrucks = parameters.maxTrucks;
maxVolume = parameters.maxVolume;
hubIds = {h.name for h in hubs}
spokeIds = {s.name for s in spokes}
spoke = {s.name : s for s in spokes}
truckTypeIds = {ttis.truckType for ttis in truckTypes}
truckTypeInfos = {tti.truckType : tti for tti in truckTypes}
loadTime = {(lt.hub , lt.truckType) : lt.loadTime for lt in loadTimes}
# feasible pathes from spokes to spokes via one hub
triples = {_triple(r1.spoke, r1.hub, r2.spoke) for r1 in routes for r2 in routes if (r1 != r2 and r1.hub == r2.hub)}
###Output
_____no_output_____
###Markdown
Some asserts to check the data follows the guidelines.
###Code
# Make sure the data is consistent: latest arrive time >= earliest departure time
for s in spokeIds:
assert spoke[s].maxArrTime > spoke[s].minDepTime, "inconsistent data"
# The following assertion is to make sure that the spoke
# in each route is indeed in the set of Spokes.
for r in routes:
assert r.spoke in spokeIds, "some route is not in the spokes"
# The following assertion is to make sure that the hub
# in each route are indeed in the set of Hubs.
for r in routes:
assert r.hub in hubIds, "some route is not in the hubs"
# The following assertion is to make sure that the origin
# of each shipment is indeed in the set of Spokes.
for s in shipments:
assert s.origin in spokeIds, "origin is not in the set of Spokes"
# The following assertion is to make sure that the destination
# of each shipment is indeed in the set of Spokes.
for s in shipments:
assert s.destination in spokeIds, "shipment is not in the set of Spokes"
from math import ceil, floor
# the earliest unloading time at a hub for each type of trucks
earliestUnloadingTime = {(r, t) : int(ceil(loadTime[r.hub, t] + spoke[r.spoke].minDepTime + 60 * r.distance / truckTypeInfos[t].milesPerHour)) for t in truckTypeIds for r in routes}
# the latest loading time at a hub for each type of trucks
latestLoadingTime = {(r, t) : int(floor(spoke[r.spoke].maxArrTime - loadTime[r.hub, t] - 60 * r.distance / truckTypeInfos[t].milesPerHour)) for t in truckTypeIds for r in routes}
# Compute possible truck types that can be assigned on a route
# A type of truck can be assigned on a route only if it can make it to the hub and back
# before the max arrival time at the spoke.
possibleTruckOnRoute = {(r, t) : 1 if earliestUnloadingTime[r, t] < latestLoadingTime[r, t] else 0 for t in truckTypeIds for r in routes}
###Output
_____no_output_____
###Markdown
Step 4: Set up the prescriptive model
###Code
from docplex.mp.environment import Environment
env = Environment()
env.print_information()
###Output
_____no_output_____
###Markdown
Create the DOcplex modelThe model contains all the business constraints and defines the objective.
###Code
from docplex.mp.model import Model
model = Model("truck")
###Output
_____no_output_____
###Markdown
Define the decision variables
###Code
truckOnRoute = model.integer_var_matrix(keys1=routes, keys2=truckTypeIds, lb=0, ub=maxTrucks, name="TruckOnRoute")
# This represents the volumes shipped out from each hub
# by each type of trucks on each triple
# The volumes are distinguished by trucktypes because trucks of different types
# arrive at a hub at different times and the timing is used in defining
# the constraints for volume availability for the trucks leaving the hub.
outVolumeThroughHubOnTruck = model.integer_var_matrix(keys1=triples, keys2=truckTypeIds, lb=0, ub=maxVolume, name="OutVolumeThroughHubOnTruck")
# This represents the volume shipped into each hub by each type of trucks on each triple
# It is used in defining timing constraints.
inVolumeThroughHubOnTruck = model.integer_var_matrix(keys1=triples, keys2=truckTypeIds, lb=0, ub=maxVolume, name="InVolumeThroughHubOnTruck")
###Output
_____no_output_____
###Markdown
Express the business constraints The number of trucks of each type should be less than "maxTrucks", and if a type of truck is impossible for a route, its number should be zero
###Code
for r in routes:
for t in truckTypeIds:
model.add_constraint(truckOnRoute[r, t] <= possibleTruckOnRoute[r, t] * maxTrucks)
###Output
_____no_output_____
###Markdown
On each route s-h, the total inbound volume carried by trucks of each type should be less than the total capacity of the trucks of this type.
###Code
for (s,h,dist) in routes:
for t in truckTypeIds:
model.add_constraint(
model.sum(inVolumeThroughHubOnTruck[(s1, h1, dest), t] for (s1, h1, dest) in triples if s == s1 and h1 == h)
<= truckOnRoute[(s, h, dist), t] * truckTypeInfos[t].capacity
)
###Output
_____no_output_____
###Markdown
On any triple, the total flows in the hub = the total flows out the hub
###Code
for tr in triples:
model.add_constraint(
model.sum(inVolumeThroughHubOnTruck[tr, t] for t in truckTypeIds)
== model.sum(outVolumeThroughHubOnTruck[tr, t] for t in truckTypeIds)
)
###Output
_____no_output_____
###Markdown
The sum of flows between any origin-destination pair via all hubs is equal to the shipment between the o-d pair.
###Code
for (o,d,v) in shipments:
model.add_constraint(model.sum(inVolumeThroughHubOnTruck[(o1, h, d1), t] for t in truckTypeIds for (o1, h, d1) in triples if o1 == o and d1 == d) == v)
###Output
_____no_output_____
###Markdown
There must be enough volume for a truck before it leaves a hub. In another words, the shipments for a truck must arrive at the hub from all spokes before the truck leaves. The constraint can be expressed as the following: For each route s-h and leaving truck of type t: Cumulated inbound volume arrived before the loading time of the truck >= Cumulated outbound volume upto the loading time of the truck(including the shipments being loaded).
###Code
for (s,h,dist) in routes:
for t in truckTypeIds:
model.add_constraint(
# The expression below defines the indices of the trucks unloaded before truck t starts loading.
model.sum(inVolumeThroughHubOnTruck[(o, h, s), t1]
for (o,h0,s0) in triples if h0 == h and s0 == s
for t1 in truckTypeIds
for (o2,h2,dist1) in routes if h2 == h0 and o2 == o
if earliestUnloadingTime[(o, h, dist1), t1] <= latestLoadingTime[(s, h, dist), t])
>=
# The expression below defines the indices of the trucks left before truck t starts loading.
model.sum(outVolumeThroughHubOnTruck[(o, h, s), t2]
for (o,h0,s0) in triples if h0 == h and s0 == s
for t2 in truckTypeIds
for (o2,h2,dist2) in routes if h2 == h0 and o2 == o
if latestLoadingTime[(o, h, dist2), t2] <= latestLoadingTime[(s, h, dist), t])
)
###Output
_____no_output_____
###Markdown
Express the objective
###Code
totalCost = model.sum(2 * r.distance * truckTypeInfos[t].costPerMile * truckOnRoute[r, t] for r in routes for t in truckTypeIds)
model.minimize(totalCost)
###Output
_____no_output_____
###Markdown
Solve with Decision Optimization You will get the best solution found after n seconds, due to a time limit parameter.
###Code
model.print_information()
assert model.solve(), "!!! Solve of the model fails"
model.report()
###Output
_____no_output_____
###Markdown
Step 5: Investigate the solution and then run an example analysis
###Code
#solution object model
_result = namedtuple('result', ['totalCost'])
_nbTrucksOnRouteRes = namedtuple('nbTrucksOnRouteRes', ['spoke', 'hub', 'truckType', 'nbTruck'])
_volumeThroughHubOnTruckRes = namedtuple('volumeThroughHubOnTruckRes', ['origin', 'hub', 'destination', 'truckType', 'quantity'])
_aggregatedReport = namedtuple('aggregatedReport', ['spoke', 'hub', 'truckType', 'quantity'])
# Post processing: result data structures are exported as post-processed tuple or list of tuples
# Solve objective value
import pandas as pd
result = _result(totalCost.solution_value)
nbTrucksOnRouteRes = pd.DataFrame([_nbTrucksOnRouteRes(r.spoke, r.hub, t, int(truckOnRoute[r, t]))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
# Volume shipped into each hub by each type of trucks and each pair (origin, destination)
inVolumeThroughHubOnTruckRes = pd.DataFrame([_volumeThroughHubOnTruckRes(tr.origin, tr.hub, tr.destination, t, int(inVolumeThroughHubOnTruck[tr, t]))
for tr in triples
for t in truckTypeIds
if int(inVolumeThroughHubOnTruck[tr, t]) > 0])
# Volume shipped from each hub by each type of trucks and each pair (origin, destination)
outVolumeThroughHubOnTruckRes = pd.DataFrame([_volumeThroughHubOnTruckRes(tr.origin, tr.hub, tr.destination, t, int(outVolumeThroughHubOnTruck[tr, t]))
for tr in triples
for t in truckTypeIds
if int(outVolumeThroughHubOnTruck[tr, t]) > 0])
inBoundAggregated = pd.DataFrame([_aggregatedReport(r.spoke, r.hub, t, sum(int(inVolumeThroughHubOnTruck[tr, t])
for tr in triples if tr.origin == r.spoke and tr.hub == r.hub))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
outBoundAggregated = pd.DataFrame([_aggregatedReport(r.spoke, r.hub, t, sum(int(outVolumeThroughHubOnTruck[tr, t])
for tr in triples if tr.destination == r.spoke and tr.hub == r.hub))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
from IPython.display import display
display(nbTrucksOnRouteRes)
display(inVolumeThroughHubOnTruckRes)
display(outVolumeThroughHubOnTruckRes)
display(inBoundAggregated)
display(outBoundAggregated)
###Output
_____no_output_____
###Markdown
Use decision optimization to help a trucking company manage its shipments.This tutorial includes everything you need to set up decision optimization engines, build mathematical programming models, and arrive at managing a truck fleet.When you finish this tutorial, you'll have a foundational knowledge of _Prescriptive Analytics_.>This notebook is part of the [Prescriptive Analytics for Python](https://rawgit.com/IBMDecisionOptimization/docplex-doc/master/docs/index.html)>It requires an [installation of CPLEX Optimizers](http://ibmdecisionoptimization.github.io/docplex-doc/getting_started.html)Discover us [here](https://developer.ibm.com/docloud)Table of contents:- [The business problem](The-business-problem:--Games-Scheduling-in-the-National-Football-League)* [How decision optimization (prescriptive analytics) can help](How--decision-optimization-can-help)* [Use decision optimization](Use-decision-optimization) * [Step 1: Import the library](Step-1:-Import-the-library) - [Step 2: Model the Data](Step-2:-Model-the-data) * [Step 3: Prepare the data](Step-3:-Prepare-the-data) - [Step 4: Set up the prescriptive model](Step-4:-Set-up-the-prescriptive-model) * [Define the decision variables](Define-the-decision-variables) * [Express the business constraints](Express-the-business-constraints) * [Express the objective](Express-the-objective) * [Solve with Decision Optimization](Solve-with-Decision-Optimization) * [Step 5: Investigate the solution and run an example analysis](Step-5:-Investigate-the-solution-and-then-run-an-example-analysis)* [Summary](Summary) The business problem: Transportation Optimization Problem * A trucking company has a hub and spoke system. The shipments to be delivered are specified by an originating spoke, a destination spoke, and a shipment volume. The trucks have different types defined by a maximum capacity, a speed, and a cost per mile. The model assigns the correct number of trucks to each route in order to minimize the cost of transshipment and meet the volume requirements. There is a minimum departure time and a maximum return time for trucks at a spoke, and a load and unload time at the hub. Trucks of different types travel at different speeds. Therefore, shipments are available at each hub in a timely manner. Volume availability constraints are taken into account, meaning that the shipments that will be carried back from a hub to a spoke by a truck must be available for loading before the truck leaves.* The assumptions are: * Exactly the same number of trucks that go from spoke to hub return from hub to spoke. * Each truck arrives at a hub as early as possible and leaves as late as possible. * The shipments can be broken arbitrarily into smaller packages and shipped through different paths. How decision optimization can help* Prescriptive analytics (decision optimization) technology recommends actions that are based on desired outcomes. It takes into account specific scenarios, resources, and knowledge of past and current events. With this insight, your organization can make better decisions and have greater control of business outcomes. * Prescriptive analytics is the next step on the path to insight-based actions. It creates value through synergy with predictive analytics, which analyzes data to predict future outcomes. * Prescriptive analytics takes that insight to the next level by suggesting the optimal way to handle that future situation. Organizations that can act fast in dynamic conditions and make superior decisions in uncertain environments gain a strong competitive advantage. With prescriptive analytics, you can: * Automate the complex decisions and trade-offs to better manage your limited resources.* Take advantage of a future opportunity or mitigate a future risk.* Proactively update recommendations based on changing events.* Meet operational goals, increase customer loyalty, prevent threats and fraud, and optimize business processes. Use decision optimization Step 1: Import the libraryRun the following code to import the Decision Optimization CPLEX Modeling library. The *DOcplex* library contains the two modeling packages, Mathematical Programming (docplex.mp) and Constraint Programming (docplex.cp).
###Code
import sys
try:
import docplex.mp
except:
raise Exception('Please install docplex. See https://pypi.org/project/docplex/')
###Output
_____no_output_____
###Markdown
If *CPLEX* is not installed, install CPLEX Community edition.
###Code
try:
import cplex
except:
raise Exception('Please install CPLEX. See https://pypi.org/project/cplex/')
###Output
_____no_output_____
###Markdown
Step 2: Model the dataIn this scenario, the data is simple and is delivered in the json format under the Optimization github.
###Code
from collections import namedtuple
_parameters = namedtuple('parameters', ['maxTrucks', 'maxVolume'])
_location = namedtuple('location', ['name'])
_spoke = namedtuple('spoke', ['name', 'minDepTime', 'maxArrTime'])
_truckType = namedtuple('truckType', ['truckType', 'capacity', 'costPerMile', 'milesPerHour'])
_loadTimeInfo = namedtuple('loadTimeInfo', ['hub', 'truckType', 'loadTime'])
_routeInfo = namedtuple('routeInfo', ['spoke', 'hub', 'distance'])
_triple = namedtuple('triple', ['origin', 'hub', 'destination'])
_shipment = namedtuple('shipment', ['origin', 'destination', 'totalVolume'])
import requests
import json
import decimal
r = requests.get("https://github.com/vberaudi/utwt/blob/master/trucking.json?raw=true")
json_data = json.loads(r.text, parse_float=decimal.Decimal )
def read_json_tuples(name, my_namedtuple):
json_fragment = json_data[name]
length = len(my_namedtuple._fields)
ret = []
for t in json_fragment:
#print t
ret2 = [0 for i in range(length)]
for i in range(length):
field = my_namedtuple._fields[i]
ret2[i] = t[field]
ret.append(my_namedtuple(*tuple(ret2)))
return ret
def read_json_tuple(name, my_namedtuple):
json_fragment = json_data[name]
length = len(my_namedtuple._fields)
ret = [0 for i in range(length)]
for i in range(length):
field = my_namedtuple._fields[i]
ret[i] = json_fragment[field]
return my_namedtuple(*tuple(ret))
###Output
_____no_output_____
###Markdown
Use decision optimization to help a trucking company manage its shipments.This tutorial includes everything you need to set up decision optimization engines, build mathematical programming models, and arrive at managing a truck fleet.When you finish this tutorial, you'll have a foundational knowledge of _Prescriptive Analytics_.>This notebook is part of **[Prescriptive Analytics for Python](http://ibmdecisionoptimization.github.io/docplex-doc/)**>>It requires either an [installation of CPLEX Optimizers](http://ibmdecisionoptimization.github.io/docplex-doc/getting_started.html) or it can be run on [IBM Cloud Pak for Data as a Service](https://www.ibm.com/products/cloud-pak-for-data/as-a-service/) (Sign up for a [free IBM Cloud account](https://dataplatform.cloud.ibm.com/registration/stepone?context=wdp&apps=all>)and you can start using `IBM Cloud Pak for Data as a Service` right away).>> CPLEX is available on IBM Cloud Pack for Data and IBM Cloud Pak for Data as a Service:> - IBM Cloud Pak for Data as a Service: Depends on the runtime used:> - Python 3.x runtime: Community edition> - Python 3.x + DO runtime: full edition> - Cloud Pack for Data: Community edition is installed by default. Please install `DO` addon in `Watson Studio Premium` for the full editionTable of contents:- [The business problem](The-business-problem:--Games-Scheduling-in-the-National-Football-League)* [How decision optimization (prescriptive analytics) can help](How--decision-optimization-can-help)* [Use decision optimization](Use-decision-optimization) * [Step 1: Import the library](Step-1:-Import-the-library) - [Step 2: Model the Data](Step-2:-Model-the-data) * [Step 3: Prepare the data](Step-3:-Prepare-the-data) - [Step 4: Set up the prescriptive model](Step-4:-Set-up-the-prescriptive-model) * [Define the decision variables](Define-the-decision-variables) * [Express the business constraints](Express-the-business-constraints) * [Express the objective](Express-the-objective) * [Solve with Decision Optimization](Solve-with-Decision-Optimization) * [Step 5: Investigate the solution and run an example analysis](Step-5:-Investigate-the-solution-and-then-run-an-example-analysis)* [Summary](Summary) The business problem: Transportation Optimization Problem * A trucking company has a hub and spoke system. The shipments to be delivered are specified by an originating spoke, a destination spoke, and a shipment volume. The trucks have different types defined by a maximum capacity, a speed, and a cost per mile. The model assigns the correct number of trucks to each route in order to minimize the cost of transshipment and meet the volume requirements. There is a minimum departure time and a maximum return time for trucks at a spoke, and a load and unload time at the hub. Trucks of different types travel at different speeds. Therefore, shipments are available at each hub in a timely manner. Volume availability constraints are taken into account, meaning that the shipments that will be carried back from a hub to a spoke by a truck must be available for loading before the truck leaves.* The assumptions are: * Exactly the same number of trucks that go from spoke to hub return from hub to spoke. * Each truck arrives at a hub as early as possible and leaves as late as possible. * The shipments can be broken arbitrarily into smaller packages and shipped through different paths. How decision optimization can help* Prescriptive analytics (decision optimization) technology recommends actions that are based on desired outcomes. It takes into account specific scenarios, resources, and knowledge of past and current events. With this insight, your organization can make better decisions and have greater control of business outcomes. * Prescriptive analytics is the next step on the path to insight-based actions. It creates value through synergy with predictive analytics, which analyzes data to predict future outcomes. * Prescriptive analytics takes that insight to the next level by suggesting the optimal way to handle that future situation. Organizations that can act fast in dynamic conditions and make superior decisions in uncertain environments gain a strong competitive advantage. With prescriptive analytics, you can: * Automate the complex decisions and trade-offs to better manage your limited resources.* Take advantage of a future opportunity or mitigate a future risk.* Proactively update recommendations based on changing events.* Meet operational goals, increase customer loyalty, prevent threats and fraud, and optimize business processes. Use decision optimization Step 1: Import the libraryRun the following code to import the Decision Optimization CPLEX Modeling library. The *DOcplex* library contains the two modeling packages, Mathematical Programming (docplex.mp) and Constraint Programming (docplex.cp).
###Code
import sys
try:
import docplex.mp
except:
raise Exception('Please install docplex. See https://pypi.org/project/docplex/')
###Output
_____no_output_____
###Markdown
If *CPLEX* is not installed, you can install CPLEX Community edition.
###Code
try:
import cplex
except:
raise Exception('Please install CPLEX. See https://pypi.org/project/cplex/')
###Output
_____no_output_____
###Markdown
Step 2: Model the dataIn this scenario, the data is simple and is delivered in the json format under the Optimization github.
###Code
from collections import namedtuple
_parameters = namedtuple('parameters', ['maxTrucks', 'maxVolume'])
_location = namedtuple('location', ['name'])
_spoke = namedtuple('spoke', ['name', 'minDepTime', 'maxArrTime'])
_truckType = namedtuple('truckType', ['truckType', 'capacity', 'costPerMile', 'milesPerHour'])
_loadTimeInfo = namedtuple('loadTimeInfo', ['hub', 'truckType', 'loadTime'])
_routeInfo = namedtuple('routeInfo', ['spoke', 'hub', 'distance'])
_triple = namedtuple('triple', ['origin', 'hub', 'destination'])
_shipment = namedtuple('shipment', ['origin', 'destination', 'totalVolume'])
import requests
import json
import decimal
r = requests.get("https://github.com/vberaudi/utwt/blob/master/trucking.json?raw=true")
json_data = json.loads(r.text, parse_float=decimal.Decimal )
def read_json_tuples(name, my_namedtuple):
json_fragment = json_data[name]
length = len(my_namedtuple._fields)
ret = []
for t in json_fragment:
#print t
ret2 = [0 for i in range(length)]
for i in range(length):
field = my_namedtuple._fields[i]
ret2[i] = t[field]
ret.append(my_namedtuple(*tuple(ret2)))
return ret
def read_json_tuple(name, my_namedtuple):
json_fragment = json_data[name]
length = len(my_namedtuple._fields)
ret = [0 for i in range(length)]
for i in range(length):
field = my_namedtuple._fields[i]
ret[i] = json_fragment[field]
return my_namedtuple(*tuple(ret))
###Output
_____no_output_____
###Markdown
Use basic HTML and a stylesheet to format the data.
###Code
CSS = """
body {
margin: 0;
font-family: Helvetica;
}
table.dataframe {
border-collapse: collapse;
border: none;
}
table.dataframe tr {
border: none;
}
table.dataframe td, table.dataframe th {
margin: 0;
border: 1px solid white;
padding-left: 0.25em;
padding-right: 0.25em;
}
table.dataframe th:not(:empty) {
background-color: #fec;
text-align: left;
font-weight: normal;
}
table.dataframe tr:nth-child(2) th:empty {
border-left: none;
border-right: 1px dashed #888;
}
table.dataframe td {
border: 2px solid #ccf;
background-color: #f4f4ff;
}
table.dataframe thead th:first-child {
display: none;
}
table.dataframe tbody th {
display: none;
}
"""
from IPython.core.display import HTML
HTML('<style>{}</style>'.format(CSS))
parameters = read_json_tuple(name='Parameters', my_namedtuple=_parameters)
hubs = read_json_tuples(name='Hubs', my_namedtuple=_location)
truckTypes = read_json_tuples(name='TruckTypes', my_namedtuple=_truckType)
spokes = read_json_tuples(name='Spokes', my_namedtuple=_spoke)
loadTimes = read_json_tuples(name='LoadTimes', my_namedtuple=_loadTimeInfo)
routes = read_json_tuples(name='Routes', my_namedtuple=_routeInfo)
shipments = read_json_tuples(name='Shipments', my_namedtuple=_shipment)
###Output
_____no_output_____
###Markdown
Step 3: Prepare the dataGiven the number of teams in each division and the number of intradivisional and interdivisional games to be played, you can calculate the total number of teams and the number of weeks in the schedule, assuming every team plays exactly one game per week. The season is split into halves, and the number of the intradivisional games that each team must play in the first half of the season is calculated.
###Code
maxTrucks = parameters.maxTrucks;
maxVolume = parameters.maxVolume;
hubIds = {h.name for h in hubs}
spokeIds = {s.name for s in spokes}
spoke = {s.name : s for s in spokes}
truckTypeIds = {ttis.truckType for ttis in truckTypes}
truckTypeInfos = {tti.truckType : tti for tti in truckTypes}
loadTime = {(lt.hub , lt.truckType) : lt.loadTime for lt in loadTimes}
# feasible pathes from spokes to spokes via one hub
triples = {_triple(r1.spoke, r1.hub, r2.spoke) for r1 in routes for r2 in routes if (r1 != r2 and r1.hub == r2.hub)}
###Output
_____no_output_____
###Markdown
Some asserts to check the data follows the guidelines.
###Code
# Make sure the data is consistent: latest arrive time >= earliest departure time
for s in spokeIds:
assert spoke[s].maxArrTime > spoke[s].minDepTime, "inconsistent data"
# The following assertion is to make sure that the spoke
# in each route is indeed in the set of Spokes.
for r in routes:
assert r.spoke in spokeIds, "some route is not in the spokes"
# The following assertion is to make sure that the hub
# in each route are indeed in the set of Hubs.
for r in routes:
assert r.hub in hubIds, "some route is not in the hubs"
# The following assertion is to make sure that the origin
# of each shipment is indeed in the set of Spokes.
for s in shipments:
assert s.origin in spokeIds, "origin is not in the set of Spokes"
# The following assertion is to make sure that the destination
# of each shipment is indeed in the set of Spokes.
for s in shipments:
assert s.destination in spokeIds, "shipment is not in the set of Spokes"
from math import ceil, floor
# the earliest unloading time at a hub for each type of trucks
earliestUnloadingTime = {(r, t) : int(ceil(loadTime[r.hub, t] + spoke[r.spoke].minDepTime + 60 * r.distance / truckTypeInfos[t].milesPerHour)) for t in truckTypeIds for r in routes}
# the latest loading time at a hub for each type of trucks
latestLoadingTime = {(r, t) : int(floor(spoke[r.spoke].maxArrTime - loadTime[r.hub, t] - 60 * r.distance / truckTypeInfos[t].milesPerHour)) for t in truckTypeIds for r in routes}
# Compute possible truck types that can be assigned on a route
# A type of truck can be assigned on a route only if it can make it to the hub and back
# before the max arrival time at the spoke.
possibleTruckOnRoute = {(r, t) : 1 if earliestUnloadingTime[r, t] < latestLoadingTime[r, t] else 0 for t in truckTypeIds for r in routes}
###Output
_____no_output_____
###Markdown
Step 4: Set up the prescriptive model
###Code
from docplex.mp.environment import Environment
env = Environment()
env.print_information()
###Output
_____no_output_____
###Markdown
Create the DOcplex modelThe model contains all the business constraints and defines the objective.
###Code
from docplex.mp.model import Model
model = Model("truck")
###Output
_____no_output_____
###Markdown
Define the decision variables
###Code
truckOnRoute = model.integer_var_matrix(keys1=routes, keys2=truckTypeIds, lb=0, ub=maxTrucks, name="TruckOnRoute")
# This represents the volumes shipped out from each hub
# by each type of trucks on each triple
# The volumes are distinguished by trucktypes because trucks of different types
# arrive at a hub at different times and the timing is used in defining
# the constraints for volume availability for the trucks leaving the hub.
outVolumeThroughHubOnTruck = model.integer_var_matrix(keys1=triples, keys2=truckTypeIds, lb=0, ub=maxVolume, name="OutVolumeThroughHubOnTruck")
# This represents the volume shipped into each hub by each type of trucks on each triple
# It is used in defining timing constraints.
inVolumeThroughHubOnTruck = model.integer_var_matrix(keys1=triples, keys2=truckTypeIds, lb=0, ub=maxVolume, name="InVolumeThroughHubOnTruck")
###Output
_____no_output_____
###Markdown
Express the business constraints The number of trucks of each type should be less than "maxTrucks", and if a type of truck is impossible for a route, its number should be zero
###Code
for r in routes:
for t in truckTypeIds:
model.add_constraint(truckOnRoute[r, t] <= possibleTruckOnRoute[r, t] * maxTrucks)
###Output
_____no_output_____
###Markdown
On each route s-h, the total inbound volume carried by trucks of each type should be less than the total capacity of the trucks of this type.
###Code
for (s,h,dist) in routes:
for t in truckTypeIds:
model.add_constraint(
model.sum(inVolumeThroughHubOnTruck[(s1, h1, dest), t] for (s1, h1, dest) in triples if s == s1 and h1 == h)
<= truckOnRoute[(s, h, dist), t] * truckTypeInfos[t].capacity
)
###Output
_____no_output_____
###Markdown
On any triple, the total flows in the hub = the total flows out the hub
###Code
for tr in triples:
model.add_constraint(
model.sum(inVolumeThroughHubOnTruck[tr, t] for t in truckTypeIds)
== model.sum(outVolumeThroughHubOnTruck[tr, t] for t in truckTypeIds)
)
###Output
_____no_output_____
###Markdown
The sum of flows between any origin-destination pair via all hubs is equal to the shipment between the o-d pair.
###Code
for (o,d,v) in shipments:
model.add_constraint(model.sum(inVolumeThroughHubOnTruck[(o1, h, d1), t] for t in truckTypeIds for (o1, h, d1) in triples if o1 == o and d1 == d) == v)
###Output
_____no_output_____
###Markdown
There must be enough volume for a truck before it leaves a hub. In another words, the shipments for a truck must arrive at the hub from all spokes before the truck leaves. The constraint can be expressed as the following: For each route s-h and leaving truck of type t: Cumulated inbound volume arrived before the loading time of the truck >= Cumulated outbound volume upto the loading time of the truck(including the shipments being loaded).
###Code
for (s,h,dist) in routes:
for t in truckTypeIds:
model.add_constraint(
# The expression below defines the indices of the trucks unloaded before truck t starts loading.
model.sum(inVolumeThroughHubOnTruck[(o, h, s), t1]
for (o,h0,s0) in triples if h0 == h and s0 == s
for t1 in truckTypeIds
for (o2,h2,dist1) in routes if h2 == h0 and o2 == o
if earliestUnloadingTime[(o, h, dist1), t1] <= latestLoadingTime[(s, h, dist), t])
>=
# The expression below defines the indices of the trucks left before truck t starts loading.
model.sum(outVolumeThroughHubOnTruck[(o, h, s), t2]
for (o,h0,s0) in triples if h0 == h and s0 == s
for t2 in truckTypeIds
for (o2,h2,dist2) in routes if h2 == h0 and o2 == o
if latestLoadingTime[(o, h, dist2), t2] <= latestLoadingTime[(s, h, dist), t])
)
###Output
_____no_output_____
###Markdown
Express the objective
###Code
totalCost = model.sum(2 * r.distance * truckTypeInfos[t].costPerMile * truckOnRoute[r, t] for r in routes for t in truckTypeIds)
model.minimize(totalCost)
###Output
_____no_output_____
###Markdown
Solve with Decision Optimization You will get the best solution found after n seconds, due to a time limit parameter.
###Code
model.print_information()
assert model.solve(), "!!! Solve of the model fails"
model.report()
###Output
_____no_output_____
###Markdown
Step 5: Investigate the solution and then run an example analysis
###Code
#solution object model
_result = namedtuple('result', ['totalCost'])
_nbTrucksOnRouteRes = namedtuple('nbTrucksOnRouteRes', ['spoke', 'hub', 'truckType', 'nbTruck'])
_volumeThroughHubOnTruckRes = namedtuple('volumeThroughHubOnTruckRes', ['origin', 'hub', 'destination', 'truckType', 'quantity'])
_aggregatedReport = namedtuple('aggregatedReport', ['spoke', 'hub', 'truckType', 'quantity'])
# Post processing: result data structures are exported as post-processed tuple or list of tuples
# Solve objective value
import pandas as pd
result = _result(totalCost.solution_value)
nbTrucksOnRouteRes = pd.DataFrame([_nbTrucksOnRouteRes(r.spoke, r.hub, t, int(truckOnRoute[r, t]))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
# Volume shipped into each hub by each type of trucks and each pair (origin, destination)
inVolumeThroughHubOnTruckRes = pd.DataFrame([_volumeThroughHubOnTruckRes(tr.origin, tr.hub, tr.destination, t, int(inVolumeThroughHubOnTruck[tr, t]))
for tr in triples
for t in truckTypeIds
if int(inVolumeThroughHubOnTruck[tr, t]) > 0])
# Volume shipped from each hub by each type of trucks and each pair (origin, destination)
outVolumeThroughHubOnTruckRes = pd.DataFrame([_volumeThroughHubOnTruckRes(tr.origin, tr.hub, tr.destination, t, int(outVolumeThroughHubOnTruck[tr, t]))
for tr in triples
for t in truckTypeIds
if int(outVolumeThroughHubOnTruck[tr, t]) > 0])
inBoundAggregated = pd.DataFrame([_aggregatedReport(r.spoke, r.hub, t, sum(int(inVolumeThroughHubOnTruck[tr, t])
for tr in triples if tr.origin == r.spoke and tr.hub == r.hub))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
outBoundAggregated = pd.DataFrame([_aggregatedReport(r.spoke, r.hub, t, sum(int(outVolumeThroughHubOnTruck[tr, t])
for tr in triples if tr.destination == r.spoke and tr.hub == r.hub))
for r in routes
for t in truckTypeIds
if int(truckOnRoute[r, t]) > 0])
from IPython.display import display
display(nbTrucksOnRouteRes)
display(inVolumeThroughHubOnTruckRes)
display(outVolumeThroughHubOnTruckRes)
display(inBoundAggregated)
display(outBoundAggregated)
###Output
_____no_output_____
###Markdown
Use decision optimization to help a trucking company manage its shipments.This tutorial includes everything you need to set up decision optimization engines, build mathematical programming models, and arrive at managing a truck fleet.When you finish this tutorial, you'll have a foundational knowledge of _Prescriptive Analytics_.>This notebook is part of **[Prescriptive Analytics for Python](http://ibmdecisionoptimization.github.io/docplex-doc/)**>>It requires either an [installation of CPLEX Optimizers](http://ibmdecisionoptimization.github.io/docplex-doc/getting_started.html) or it can be run on [IBM Watson Studio Cloud](https://www.ibm.com/cloud/watson-studio/>) (Sign up for a [free IBM Cloud account](https://dataplatform.cloud.ibm.com/registration/stepone?context=wdp&apps=all>)and you can start using Watson Studio Cloud right away).Table of contents:- [The business problem](The-business-problem:--Games-Scheduling-in-the-National-Football-League)* [How decision optimization (prescriptive analytics) can help](How--decision-optimization-can-help)* [Use decision optimization](Use-decision-optimization) * [Step 1: Import the library](Step-1:-Import-the-library) - [Step 2: Model the Data](Step-2:-Model-the-data) * [Step 3: Prepare the data](Step-3:-Prepare-the-data) - [Step 4: Set up the prescriptive model](Step-4:-Set-up-the-prescriptive-model) * [Define the decision variables](Define-the-decision-variables) * [Express the business constraints](Express-the-business-constraints) * [Express the objective](Express-the-objective) * [Solve with Decision Optimization](Solve-with-Decision-Optimization) * [Step 5: Investigate the solution and run an example analysis](Step-5:-Investigate-the-solution-and-then-run-an-example-analysis)* [Summary](Summary) The business problem: Transportation Optimization Problem * A trucking company has a hub and spoke system. The shipments to be delivered are specified by an originating spoke, a destination spoke, and a shipment volume. The trucks have different types defined by a maximum capacity, a speed, and a cost per mile. The model assigns the correct number of trucks to each route in order to minimize the cost of transshipment and meet the volume requirements. There is a minimum departure time and a maximum return time for trucks at a spoke, and a load and unload time at the hub. Trucks of different types travel at different speeds. Therefore, shipments are available at each hub in a timely manner. Volume availability constraints are taken into account, meaning that the shipments that will be carried back from a hub to a spoke by a truck must be available for loading before the truck leaves.* The assumptions are: * Exactly the same number of trucks that go from spoke to hub return from hub to spoke. * Each truck arrives at a hub as early as possible and leaves as late as possible. * The shipments can be broken arbitrarily into smaller packages and shipped through different paths. How decision optimization can help* Prescriptive analytics (decision optimization) technology recommends actions that are based on desired outcomes. It takes into account specific scenarios, resources, and knowledge of past and current events. With this insight, your organization can make better decisions and have greater control of business outcomes. * Prescriptive analytics is the next step on the path to insight-based actions. It creates value through synergy with predictive analytics, which analyzes data to predict future outcomes. * Prescriptive analytics takes that insight to the next level by suggesting the optimal way to handle that future situation. Organizations that can act fast in dynamic conditions and make superior decisions in uncertain environments gain a strong competitive advantage. With prescriptive analytics, you can: * Automate the complex decisions and trade-offs to better manage your limited resources.* Take advantage of a future opportunity or mitigate a future risk.* Proactively update recommendations based on changing events.* Meet operational goals, increase customer loyalty, prevent threats and fraud, and optimize business processes. Use decision optimization Step 1: Import the libraryRun the following code to import the Decision Optimization CPLEX Modeling library. The *DOcplex* library contains the two modeling packages, Mathematical Programming (docplex.mp) and Constraint Programming (docplex.cp).
###Code
import sys
try:
import docplex.mp
except:
raise Exception('Please install docplex. See https://pypi.org/project/docplex/')
###Output
_____no_output_____
###Markdown
If *CPLEX* is not installed, install CPLEX Community edition.
###Code
try:
import cplex
except:
raise Exception('Please install CPLEX. See https://pypi.org/project/cplex/')
###Output
_____no_output_____
###Markdown
Step 2: Model the dataIn this scenario, the data is simple and is delivered in the json format under the Optimization github.
###Code
from collections import namedtuple
_parameters = namedtuple('parameters', ['maxTrucks', 'maxVolume'])
_location = namedtuple('location', ['name'])
_spoke = namedtuple('spoke', ['name', 'minDepTime', 'maxArrTime'])
_truckType = namedtuple('truckType', ['truckType', 'capacity', 'costPerMile', 'milesPerHour'])
_loadTimeInfo = namedtuple('loadTimeInfo', ['hub', 'truckType', 'loadTime'])
_routeInfo = namedtuple('routeInfo', ['spoke', 'hub', 'distance'])
_triple = namedtuple('triple', ['origin', 'hub', 'destination'])
_shipment = namedtuple('shipment', ['origin', 'destination', 'totalVolume'])
import requests
import json
import decimal
r = requests.get("https://github.com/vberaudi/utwt/blob/master/trucking.json?raw=true")
json_data = json.loads(r.text, parse_float=decimal.Decimal )
def read_json_tuples(name, my_namedtuple):
json_fragment = json_data[name]
length = len(my_namedtuple._fields)
ret = []
for t in json_fragment:
#print t
ret2 = [0 for i in range(length)]
for i in range(length):
field = my_namedtuple._fields[i]
ret2[i] = t[field]
ret.append(my_namedtuple(*tuple(ret2)))
return ret
def read_json_tuple(name, my_namedtuple):
json_fragment = json_data[name]
length = len(my_namedtuple._fields)
ret = [0 for i in range(length)]
for i in range(length):
field = my_namedtuple._fields[i]
ret[i] = json_fragment[field]
return my_namedtuple(*tuple(ret))
###Output
_____no_output_____
###Markdown
Use basic HTML and a stylesheet to format the data.
###Code
CSS = """
body {
margin: 0;
font-family: Helvetica;
}
table.dataframe {
border-collapse: collapse;
border: none;
}
table.dataframe tr {
border: none;
}
table.dataframe td, table.dataframe th {
margin: 0;
border: 1px solid white;
padding-left: 0.25em;
padding-right: 0.25em;
}
table.dataframe th:not(:empty) {
background-color: #fec;
text-align: left;
font-weight: normal;
}
table.dataframe tr:nth-child(2) th:empty {
border-left: none;
border-right: 1px dashed #888;
}
table.dataframe td {
border: 2px solid #ccf;
background-color: #f4f4ff;
}
table.dataframe thead th:first-child {
display: none;
}
table.dataframe tbody th {
display: none;
}
"""
from IPython.core.display import HTML
HTML('<style>{}</style>'.format(CSS))
parameters = read_json_tuple(name='Parameters', my_namedtuple=_parameters)
hubs = read_json_tuples(name='Hubs', my_namedtuple=_location)
truckTypes = read_json_tuples(name='TruckTypes', my_namedtuple=_truckType)
spokes = read_json_tuples(name='Spokes', my_namedtuple=_spoke)
loadTimes = read_json_tuples(name='LoadTimes', my_namedtuple=_loadTimeInfo)
routes = read_json_tuples(name='Routes', my_namedtuple=_routeInfo)
shipments = read_json_tuples(name='Shipments', my_namedtuple=_shipment)
###Output
_____no_output_____
###Markdown
Step 3: Prepare the dataGiven the number of teams in each division and the number of intradivisional and interdivisional games to be played, you can calculate the total number of teams and the number of weeks in the schedule, assuming every team plays exactly one game per week. The season is split into halves, and the number of the intradivisional games that each team must play in the first half of the season is calculated.
###Code
maxTrucks = parameters.maxTrucks;
maxVolume = parameters.maxVolume;
hubIds = {h.name for h in hubs}
spokeIds = {s.name for s in spokes}
spoke = {s.name : s for s in spokes}
truckTypeIds = {ttis.truckType for ttis in truckTypes}
truckTypeInfos = {tti.truckType : tti for tti in truckTypes}
loadTime = {(lt.hub , lt.truckType) : lt.loadTime for lt in loadTimes}
# feasible pathes from spokes to spokes via one hub
triples = {_triple(r1.spoke, r1.hub, r2.spoke) for r1 in routes for r2 in routes if (r1 != r2 and r1.hub == r2.hub)}
###Output
_____no_output_____
###Markdown
Some asserts to check the data follows the guidelines.
###Code
# Make sure the data is consistent: latest arrive time >= earliest departure time
for s in spokeIds:
assert spoke[s].maxArrTime > spoke[s].minDepTime, "inconsistent data"
# The following assertion is to make sure that the spoke
# in each route is indeed in the set of Spokes.
for r in routes:
assert r.spoke in spokeIds, "some route is not in the spokes"
# The following assertion is to make sure that the hub
# in each route are indeed in the set of Hubs.
for r in routes:
assert r.hub in hubIds, "some route is not in the hubs"
# The following assertion is to make sure that the origin
# of each shipment is indeed in the set of Spokes.
for s in shipments:
assert s.origin in spokeIds, "origin is not in the set of Spokes"
# The following assertion is to make sure that the destination
# of each shipment is indeed in the set of Spokes.
for s in shipments:
assert s.destination in spokeIds, "shipment is not in the set of Spokes"
from math import ceil, floor
# the earliest unloading time at a hub for each type of trucks
earliestUnloadingTime = {(r, t) : int(ceil(loadTime[r.hub, t] + spoke[r.spoke].minDepTime + 60 * r.distance / truckTypeInfos[t].milesPerHour)) for t in truckTypeIds for r in routes}
# the latest loading time at a hub for each type of trucks
latestLoadingTime = {(r, t) : int(floor(spoke[r.spoke].maxArrTime - loadTime[r.hub, t] - 60 * r.distance / truckTypeInfos[t].milesPerHour)) for t in truckTypeIds for r in routes}
# Compute possible truck types that can be assigned on a route
# A type of truck can be assigned on a route only if it can make it to the hub and back
# before the max arrival time at the spoke.
possibleTruckOnRoute = {(r, t) : 1 if earliestUnloadingTime[r, t] < latestLoadingTime[r, t] else 0 for t in truckTypeIds for r in routes}
###Output
_____no_output_____
###Markdown
Step 4: Set up the prescriptive model
###Code
from docplex.mp.environment import Environment
env = Environment()
env.print_information()
###Output
_____no_output_____
###Markdown
Create the DOcplex modelThe model contains all the business constraints and defines the objective.
###Code
from docplex.mp.model import Model
model = Model("truck")
###Output
_____no_output_____
###Markdown
Define the decision variables
###Code
truckOnRoute = model.integer_var_matrix(keys1=routes, keys2=truckTypeIds, lb=0, ub=maxTrucks, name="TruckOnRoute")
# This represents the volumes shipped out from each hub
# by each type of trucks on each triple
# The volumes are distinguished by trucktypes because trucks of different types
# arrive at a hub at different times and the timing is used in defining
# the constraints for volume availability for the trucks leaving the hub.
outVolumeThroughHubOnTruck = model.integer_var_matrix(keys1=triples, keys2=truckTypeIds, lb=0, ub=maxVolume, name="OutVolumeThroughHubOnTruck")
# This represents the volume shipped into each hub by each type of trucks on each triple
# It is used in defining timing constraints.
inVolumeThroughHubOnTruck = model.integer_var_matrix(keys1=triples, keys2=truckTypeIds, lb=0, ub=maxVolume, name="InVolumeThroughHubOnTruck")
###Output
_____no_output_____
###Markdown
Express the business constraints The number of trucks of each type should be less than "maxTrucks", and if a type of truck is impossible for a route, its number should be zero
###Code
for r in routes:
for t in truckTypeIds:
model.add_constraint(truckOnRoute[r, t] <= possibleTruckOnRoute[r, t] * maxTrucks)
###Output
_____no_output_____
###Markdown
On each route s-h, the total inbound volume carried by trucks of each type should be less than the total capacity of the trucks of this type.
###Code
for (s,h,dist) in routes:
for t in truckTypeIds:
model.add_constraint(
model.sum(inVolumeThroughHubOnTruck[(s1, h1, dest), t] for (s1, h1, dest) in triples if s == s1 and h1 == h)
<= truckOnRoute[(s, h, dist), t] * truckTypeInfos[t].capacity
)
###Output
_____no_output_____
###Markdown
On any triple, the total flows in the hub = the total flows out the hub
###Code
for tr in triples:
model.add_constraint(
model.sum(inVolumeThroughHubOnTruck[tr, t] for t in truckTypeIds)
== model.sum(outVolumeThroughHubOnTruck[tr, t] for t in truckTypeIds)
)
###Output
_____no_output_____
###Markdown
The sum of flows between any origin-destination pair via all hubs is equal to the shipment between the o-d pair.
###Code
for (o,d,v) in shipments:
model.add_constraint(model.sum(inVolumeThroughHubOnTruck[(o1, h, d1), t] for t in truckTypeIds for (o1, h, d1) in triples if o1 == o and d1 == d) == v)
###Output
_____no_output_____ |
jupyterhub/notebooks/zz_under_construction/zz_old/talks/StartupML/Jan-20-2017/SparkMLTensorflowAI-HybridCloud-ContinuousDeployment.ipynb | ###Markdown
Where Am I? Startup.ML Conference - San Francisco - Jan 20, 2017 Who Am I? Chris Fregly Research Scientist @ **[PipelineIO](http://pipeline.io)**  Video Series Author "High Performance Tensorflow in Production" @ [OReilly](http://oreilly.com) (Coming Soon) Founder @ **[Advanced Spark and Tensorflow Meetup](http://http://www.meetup.com/Advanced-Spark-and-TensorFlow-Meetup/)** **[Github Repo](https://github.com/fluxcapacitor/pipeline)**  **[DockerHub Repo](https://hub.docker.com/u/fluxcapacitor/)** **[Slideshare](http://www.slideshare.net/cfregly)** **[YouTube](https://www.youtube.com/playlist?list=PL7pBcJ870QHeNRBXdKirc4fdtbtbB5Xy-)** Who Was I? Software Engineer @ **Netflix**, **Databricks**, **IBM Spark Tech Center**   1. Infrastructure and Tools DockerImages, ContainersUseful Docker Image: **[AWS + GPU + Docker + Tensorflow + Spark](https://github.com/fluxcapacitor/pipeline/wiki/AWS-GPU-TensorFlow-Docker)** KubernetesContainer Orchestration Across Clusters WeavescopeKubernetes Cluster Visualization Jupyter NotebooksWhat We're Using Here for Everything! AirflowInvoke Any Type of Workflow on Any Type of Schedule  GithubCommit New Model to Github, Airflow Workflow Triggered for Continuous Deployment  DockerHubMaintains Docker Images  Continuous DeploymentNot Just for Code, Also for ML/AI Models! Canary ReleaseDeploy and Compare New Model Alongside Existing Metrics and DashboardsNot Just System Metrics, ML/AI Model Prediction MetricsNetflixOSS-basedPrometheusGrafanaElasticsearch Separate Cluster ConcernsTraining/Admin ClusterPrediction Cluster Hybrid Cloud Deployment for eXtreme High Availability (XHA)AWS and Google Cloud Apache Spark Tensorflow + Tensorflow Serving 2. Model Deployment Bundles KeyValueie. RecommendationsIn-memory: Redis, MemcacheOn-disk: Cassandra, RocksDBFirst-class Servable in [Tensorflow Serving](https://github.com/tensorflow/serving/tree/master/tensorflow_serving/servables) PMMLIt's Useful and [Well-Supported](https://github.com/jpmml)Apple, Cisco, [Airbnb](http://nerds.airbnb.com/architecting-machine-learning-system-risk/), HomeAway, etcPlease Don't Re-build It - Reduce Your Technical Debt! Native CodeHand-coded (Python + Pickling)Generate Java Code from PMML? Tensorflow Model Exports[freeze_graph.py](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/python/tools/freeze_graph.py): Combine Tensorflow Graph (Static) with Trained Weights (Checkpoints) into Single Deployable Model 3. Model Deployments and Rollbacks MutableEach New Model is Deployed to Live, Running Container ImmutableEach New Model is a New Docker Image 4. Optimizing Tensorflow Models for Serving Python Scripts[optimize_graph_for_inference.py](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/python/tools/optimize_for_inference.py)[Pete Warden's Blog](https://petewarden.com/2016/12/30/rewriting-tensorflow-graphs-with-the-gtt/)[Graph Transform Tool](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/tools/graph_transforms/README.md) Compile (Tensorflow 1.0+) [XLA](https://www.tensorflow.org/versions/master/experimental/xla/) CompilerCompiles 3 graph operations (input, operation, output) into 1 operationRemoves need for Tensorflow Runtime (20 MB is significant on tiny devices)Allows new backends for hardware-specific optimizations (better portability) [tfcompile](https://github.com/tensorflow/tensorflow/tree/master/tensorflow/compiler/aot)Convert Graph into executable code Compress/Distill Ensemble ModelsConvert ensembles or other complex models into smaller modelsRe-score training data with output of model being distilled Train smaller model to produce same outputOutput of smaller model learns more information than original label 5. Optimizing Serving Runtime Environment Throughput**Option 1**: Add more Tensorflow Serving servers behind **load balancer****Option 2**: Enable **request batching** in each Tensorflow Serving**Option Trade-offs**: Higher Latency (bad) for Higher Throughput (good)```$TENSORFLOW_SERVING_HOME/bazel-bin/tensorflow_serving/model_servers/tensorflow_model_server --port=9000 --model_name=tensorflow_minimal --model_base_path=/root/models/tensorflow_minimal/export--enable_batching=true--max_batch_size=1000000--batch_timeout_micros=10000--max_enqueued_batches=1000000``` LatencyThe deeper the model, the longer the latencyStart inference in parallel where possible (ie. user inference in parallel with item inference)Pre-load common inputs from database (ie. user attributes, item attributes) Pre-compute/partial-compute common inputs (ie. popular word embeddings) MemoryWord embeddings are **huge**!Use **hashId** for each wordOff-load embedding matrices to **parameter server** and share between serving servers 6. Demos!! Train and Deploy Tensorflow AI Model (Simple Model, Immutable Deploy) Train Tensorflow AI Model
###Code
import numpy as np
import os
import tensorflow as tf
from tensorflow.contrib.session_bundle import exporter
import time
# make things wide
from IPython.core.display import display, HTML
display(HTML("<style>.container { width:100% !important; }</style>"))
from IPython.display import clear_output, Image, display, HTML
def strip_consts(graph_def, max_const_size=32):
"""Strip large constant values from graph_def."""
strip_def = tf.GraphDef()
for n0 in graph_def.node:
n = strip_def.node.add()
n.MergeFrom(n0)
if n.op == 'Const':
tensor = n.attr['value'].tensor
size = len(tensor.tensor_content)
if size > max_const_size:
tensor.tensor_content = "<stripped %d bytes>"%size
return strip_def
def show_graph(graph_def=None, width=1200, height=800, max_const_size=32, ungroup_gradients=False):
if not graph_def:
graph_def = tf.get_default_graph().as_graph_def()
"""Visualize TensorFlow graph."""
if hasattr(graph_def, 'as_graph_def'):
graph_def = graph_def.as_graph_def()
strip_def = strip_consts(graph_def, max_const_size=max_const_size)
data = str(strip_def)
if ungroup_gradients:
data = data.replace('"gradients/', '"b_')
#print(data)
code = """
<script>
function load() {{
document.getElementById("{id}").pbtxt = {data};
}}
</script>
<link rel="import" href="https://tensorboard.appspot.com/tf-graph-basic.build.html" onload=load()>
<div style="height:600px">
<tf-graph-basic id="{id}"></tf-graph-basic>
</div>
""".format(data=repr(data), id='graph'+str(np.random.rand()))
iframe = """
<iframe seamless style="width:{}px;height:{}px;border:0" srcdoc="{}"></iframe>
""".format(width, height, code.replace('"', '"'))
display(HTML(iframe))
# If this errors out, increment the `export_version` variable, restart the Kernel, and re-run
flags = tf.app.flags
FLAGS = flags.FLAGS
flags.DEFINE_integer("batch_size", 10, "The batch size to train")
flags.DEFINE_integer("epoch_number", 10, "Number of epochs to run trainer")
flags.DEFINE_integer("steps_to_validate", 1,
"Steps to validate and print loss")
flags.DEFINE_string("checkpoint_dir", "./checkpoint/",
"indicates the checkpoint dirctory")
#flags.DEFINE_string("model_path", "./model/", "The export path of the model")
flags.DEFINE_string("model_path", "/root/pipeline/prediction.ml/tensorflow/models/tensorflow_minimal/export/", "The export path of the model")
#flags.DEFINE_integer("export_version", 27, "The version number of the model")
from datetime import datetime
seconds_since_epoch = int(datetime.now().strftime("%s"))
export_version = seconds_since_epoch
# If this errors out, increment the `export_version` variable, restart the Kernel, and re-run
def main():
# Define training data
x = np.ones(FLAGS.batch_size)
y = np.ones(FLAGS.batch_size)
# Define the model
X = tf.placeholder(tf.float32, shape=[None], name="X")
Y = tf.placeholder(tf.float32, shape=[None], name="yhat")
w = tf.Variable([1.0], name="weight")
b = tf.Variable([1.0], name="bias")
loss = tf.square(Y - tf.matmul(X, w) - b)
train_op = tf.train.GradientDescentOptimizer(0.01).minimize(loss)
predict_op = tf.mul(X, w) + b
saver = tf.train.Saver()
checkpoint_dir = FLAGS.checkpoint_dir
checkpoint_file = checkpoint_dir + "/checkpoint.ckpt"
if not os.path.exists(checkpoint_dir):
os.makedirs(checkpoint_dir)
# Start the session
with tf.Session() as sess:
sess.run(tf.initialize_all_variables())
ckpt = tf.train.get_checkpoint_state(checkpoint_dir)
if ckpt and ckpt.model_checkpoint_path:
print("Continue training from the model {}".format(ckpt.model_checkpoint_path))
saver.restore(sess, ckpt.model_checkpoint_path)
saver_def = saver.as_saver_def()
print(saver_def.filename_tensor_name)
print(saver_def.restore_op_name)
# Start training
start_time = time.time()
for epoch in range(FLAGS.epoch_number):
sess.run(train_op, feed_dict={X: x, Y: y})
# Start validating
if epoch % FLAGS.steps_to_validate == 0:
end_time = time.time()
print("[{}] Epoch: {}".format(end_time - start_time, epoch))
saver.save(sess, checkpoint_file)
tf.train.write_graph(sess.graph_def, checkpoint_dir, 'trained_model.pb', as_text=False)
tf.train.write_graph(sess.graph_def, checkpoint_dir, 'trained_model.txt', as_text=True)
start_time = end_time
# Print model variables
w_value, b_value = sess.run([w, b])
print("The model of w: {}, b: {}".format(w_value, b_value))
# Export the model
print("Exporting trained model to {}".format(FLAGS.model_path))
model_exporter = exporter.Exporter(saver)
model_exporter.init(
sess.graph.as_graph_def(),
named_graph_signatures={
'inputs': exporter.generic_signature({"features": X}),
'outputs': exporter.generic_signature({"prediction": predict_op})
})
model_exporter.export(FLAGS.model_path, tf.constant(export_version), sess)
print('Done exporting!')
if __name__ == "__main__":
main()
show_graph()
###Output
_____no_output_____
###Markdown
Commit and Deploy New Tensorflow AI Model Commit Model to Github
###Code
!ls -l /root/pipeline/prediction.ml/tensorflow/models/tensorflow_minimal/export
!ls -l /root/pipeline/prediction.ml/tensorflow/models/tensorflow_minimal/export/00000027
!git status
!git add --all /root/pipeline/prediction.ml/tensorflow/models/tensorflow_minimal/export/00000027/
!git status
!git commit -m "updated tensorflow model"
!git status
# If this fails with "Permission denied", use terminal within jupyter to manually `git push`
!git push
###Output
warning: push.default is unset; its implicit value is changing in
Git 2.0 from 'matching' to 'simple'. To squelch this message
and maintain the current behavior after the default changes, use:
git config --global push.default matching
To squelch this message and adopt the new behavior now, use:
git config --global push.default simple
When push.default is set to 'matching', git will push local branches
to the remote branches that already exist with the same name.
In Git 2.0, Git will default to the more conservative 'simple'
behavior, which only pushes the current branch to the corresponding
remote branch that 'git pull' uses to update the current branch.
See 'git help config' and search for 'push.default' for further information.
(the 'simple' mode was introduced in Git 1.7.11. Use the similar mode
'current' instead of 'simple' if you sometimes use older versions of Git)
Permission denied (publickey).
fatal: Could not read from remote repository.
Please make sure you have the correct access rights
and the repository exists.
###Markdown
[Airflow Workflow](http://demo.pipeline.io:8080/admin/) Deploys New Model through Github Post-Commit [Webhook](https://github.com/fluxcapacitor/pipeline/blob/master/scheduler.ml/airflow/github_webhook) to Triggers
###Code
from IPython.core.display import display, HTML
display(HTML("<style>.container { width:100% !important; }</style>"))
from IPython.display import clear_output, Image, display, HTML
html = '<iframe width=100% height=500px src="http://demo.pipeline.io:8080/admin">'
display(HTML(html))
###Output
_____no_output_____
###Markdown
Train and Deploy Spark ML Model (Airbnb Model, Mutable Deploy) Scale Out Spark Training Cluster Kubernetes [CLI](https://github.com/fluxcapacitor/pipeline/wiki/Kubernetes-Commands)
###Code
!kubectl scale --context=awsdemo --replicas=2 rc spark-worker-2-0-1
!kubectl get pod --context=awsdemo
###Output
NAME READY STATUS RESTARTS AGE
airflow-z2txg 1/1 Running 0 51m
hdfs-gd1pf 1/1 Running 0 11d
hystrix-rdx5g 1/1 Running 0 9d
jupyterhub-master-sqqjf 1/1 Running 0 11d
metastore-1-2-1-jw8kx 1/1 Running 0 11d
mysql-master-586zp 1/1 Running 0 11d
prediction-codegen-b8c5b3016dc1eb4e655146be94cd2d56-p5m8f 1/1 Running 0 9d
prediction-pmml-7db0fe5cbac0a14d38069985acd5b119-jv3ln 1/1 Running 0 9d
prediction-tensorflow-60175981688eb64d9663a29c3ced8f45-4zndp 1/1 Running 0 9d
spark-master-2-0-1-28vrg 1/1 Running 0 11d
spark-worker-2-0-1-49qqg 1/1 Running 0 11d
spark-worker-2-0-1-56hqq 1/1 Running 0 5d
turbine-2gvb2 1/1 Running 0 9d
weavescope-app-c9khj 1/1 Running 0 11d
weavescope-probe-0mtn7 1/1 Running 0 11d
weavescope-probe-7qtz4 1/1 Running 0 11d
weavescope-probe-kqt3z 1/1 Running 0 11d
web-home-0c9jw 1/1 Running 0 11d
###Markdown
Weavescope Kubernetes [AWS Cluster](http://kubernetes-aws.demo.pipeline.io) Visualization
###Code
from IPython.core.display import display, HTML
display(HTML("<style>.container { width:100% !important; }</style>"))
from IPython.display import clear_output, Image, display, HTML
html = '<iframe width=100% height=500px src="http://kubernetes-aws.demo.pipeline.io">'
display(HTML(html))
###Output
_____no_output_____
###Markdown
Generate PMML from Spark ML Model
###Code
from pyspark.ml.linalg import Vectors
from pyspark.ml.feature import VectorAssembler, StandardScaler
from pyspark.ml.feature import OneHotEncoder, StringIndexer
from pyspark.ml import Pipeline, PipelineModel
from pyspark.ml.regression import LinearRegression
# You may need to Reconnect (more than Restart) the Kernel to pick up changes to these sett
import os
master = '--master spark://spark-master-2-1-0:7077'
conf = '--conf spark.cores.max=1 --conf spark.executor.memory=512m'
packages = '--packages com.amazonaws:aws-java-sdk:1.7.4,org.apache.hadoop:hadoop-aws:2.7.1'
jars = '--jars /root/lib/jpmml-sparkml-package-1.0-SNAPSHOT.jar'
py_files = '--py-files /root/lib/jpmml.py'
os.environ['PYSPARK_SUBMIT_ARGS'] = master \
+ ' ' + conf \
+ ' ' + packages \
+ ' ' + jars \
+ ' ' + py_files \
+ ' ' + 'pyspark-shell'
print(os.environ['PYSPARK_SUBMIT_ARGS'])
from pyspark.sql import SparkSession
spark = SparkSession.builder.getOrCreate()
###Output
_____no_output_____
###Markdown
**Step 0**: Load Libraries and Data
###Code
df = spark.read.format("csv") \
.option("inferSchema", "true").option("header", "true") \
.load("s3a://datapalooza/airbnb/airbnb.csv.bz2")
df.registerTempTable("df")
print(df.head())
print(df.count())
###Output
198576
###Markdown
**Step 1**: Clean, Filter, and Summarize the Data
###Code
df_filtered = df.filter("price >= 50 AND price <= 750 AND bathrooms > 0.0 AND bedrooms is not null")
df_filtered.registerTempTable("df_filtered")
df_final = spark.sql("""
select
id,
city,
case when state in('NY', 'CA', 'London', 'Berlin', 'TX' ,'IL', 'OR', 'DC', 'WA')
then state
else 'Other'
end as state,
space,
cast(price as double) as price,
cast(bathrooms as double) as bathrooms,
cast(bedrooms as double) as bedrooms,
room_type,
host_is_super_host,
cancellation_policy,
cast(case when security_deposit is null
then 0.0
else security_deposit
end as double) as security_deposit,
price_per_bedroom,
cast(case when number_of_reviews is null
then 0.0
else number_of_reviews
end as double) as number_of_reviews,
cast(case when extra_people is null
then 0.0
else extra_people
end as double) as extra_people,
instant_bookable,
cast(case when cleaning_fee is null
then 0.0
else cleaning_fee
end as double) as cleaning_fee,
cast(case when review_scores_rating is null
then 80.0
else review_scores_rating
end as double) as review_scores_rating,
cast(case when square_feet is not null and square_feet > 100
then square_feet
when (square_feet is null or square_feet <=100) and (bedrooms is null or bedrooms = 0)
then 350.0
else 380 * bedrooms
end as double) as square_feet
from df_filtered
""").persist()
df_final.registerTempTable("df_final")
df_final.select("square_feet", "price", "bedrooms", "bathrooms", "cleaning_fee").describe().show()
print(df_final.count())
print(df_final.schema)
# Most popular cities
spark.sql("""
select
state,
count(*) as ct,
avg(price) as avg_price,
max(price) as max_price
from df_final
group by state
order by count(*) desc
""").show()
# Most expensive popular cities
spark.sql("""
select
city,
count(*) as ct,
avg(price) as avg_price,
max(price) as max_price
from df_final
group by city
order by avg(price) desc
""").filter("ct > 25").show()
###Output
+-------------------+---+------------------+---------+
| city| ct| avg_price|max_price|
+-------------------+---+------------------+---------+
| Palm Beach| 26| 348.7692307692308| 701.0|
| Watsonville| 38| 313.3157894736842| 670.0|
| Malibu|136| 280.9852941176471| 750.0|
| Avalon| 38|262.42105263157896| 701.0|
| Capitola| 35| 246.4| 650.0|
| Tamarama| 72| 238.5| 750.0|
| Manhattan Beach|109|232.10091743119267| 700.0|
|Rancho Palos Verdes| 39|230.02564102564102| 750.0|
| Avalon Beach| 38|229.60526315789474| 620.0|
| Newport| 52| 223.8653846153846| 750.0|
| Darling Point| 29|221.51724137931035| 623.0|
| Middle Park| 34| 212.7941176470588| 671.0|
| Balmain| 55|212.56363636363636| 712.0|
| North Bondi|180|206.68333333333334| 750.0|
| Bronte|144|203.70833333333334| 750.0|
| Queenscliff| 40| 201.925| 650.0|
| Lilyfield| 26|198.92307692307693| 701.0|
| Freshwater| 54| 198.5185185185185| 650.0|
| La Jolla| 52|197.82692307692307| 649.0|
| Marina del Rey|205| 196.6390243902439| 550.0|
+-------------------+---+------------------+---------+
only showing top 20 rows
###Markdown
**Step 2**: Define Continous and Categorical Features
###Code
continuous_features = ["bathrooms", \
"bedrooms", \
"security_deposit", \
"cleaning_fee", \
"extra_people", \
"number_of_reviews", \
"square_feet", \
"review_scores_rating"]
categorical_features = ["room_type", \
"host_is_super_host", \
"cancellation_policy", \
"instant_bookable", \
"state"]
###Output
_____no_output_____
###Markdown
**Step 3**: Split Data into Training and Validation
###Code
[training_dataset, validation_dataset] = df_final.randomSplit([0.8, 0.2])
###Output
_____no_output_____
###Markdown
**Step 4**: Continous Feature Pipeline
###Code
continuous_feature_assembler = VectorAssembler(inputCols=continuous_features, outputCol="unscaled_continuous_features")
continuous_feature_scaler = StandardScaler(inputCol="unscaled_continuous_features", outputCol="scaled_continuous_features", \
withStd=True, withMean=False)
###Output
_____no_output_____
###Markdown
**Step 5**: Categorical Feature Pipeline
###Code
categorical_feature_indexers = [StringIndexer(inputCol=x, \
outputCol="{}_index".format(x)) \
for x in categorical_features]
categorical_feature_one_hot_encoders = [OneHotEncoder(inputCol=x.getOutputCol(), \
outputCol="oh_encoder_{}".format(x.getOutputCol() )) \
for x in categorical_feature_indexers]
###Output
_____no_output_____
###Markdown
**Step 6**: Assemble our Features and Feature Pipeline
###Code
feature_cols_lr = [x.getOutputCol() \
for x in categorical_feature_one_hot_encoders]
feature_cols_lr.append("scaled_continuous_features")
feature_assembler_lr = VectorAssembler(inputCols=feature_cols_lr, \
outputCol="features_lr")
###Output
_____no_output_____
###Markdown
**Step 7**: Train a Linear Regression Model
###Code
linear_regression = LinearRegression(featuresCol="features_lr", \
labelCol="price", \
predictionCol="price_prediction", \
maxIter=10, \
regParam=0.3, \
elasticNetParam=0.8)
estimators_lr = \
[continuous_feature_assembler, continuous_feature_scaler] \
+ categorical_feature_indexers + categorical_feature_one_hot_encoders \
+ [feature_assembler_lr] + [linear_regression]
pipeline = Pipeline(stages=estimators_lr)
pipeline_model = pipeline.fit(training_dataset)
print(pipeline_model)
###Output
PipelineModel_4bea9edae088d627db1d
###Markdown
**Step 8**: Convert PipelineModel to PMML
###Code
from jpmml import toPMMLBytes
model_bytes = toPMMLBytes(spark, training_dataset, pipeline_model)
print(model_bytes.decode("utf-8"))
###Output
<?xml version="1.0" encoding="UTF-8" standalone="yes"?>
<PMML xmlns="http://www.dmg.org/PMML-4_3" version="4.3">
<Header>
<Application/>
<Timestamp>2017-03-27T17:08:12Z</Timestamp>
</Header>
<DataDictionary>
<DataField name="bathrooms" optype="continuous" dataType="double"/>
<DataField name="bedrooms" optype="continuous" dataType="double"/>
<DataField name="security_deposit" optype="continuous" dataType="double"/>
<DataField name="cleaning_fee" optype="continuous" dataType="double"/>
<DataField name="extra_people" optype="continuous" dataType="double"/>
<DataField name="number_of_reviews" optype="continuous" dataType="double"/>
<DataField name="square_feet" optype="continuous" dataType="double"/>
<DataField name="review_scores_rating" optype="continuous" dataType="double"/>
<DataField name="room_type" optype="categorical" dataType="string">
<Value value="Entire home/apt"/>
<Value value="Private room"/>
<Value value="Shared room"/>
</DataField>
<DataField name="host_is_super_host" optype="categorical" dataType="string">
<Value value="0.0"/>
<Value value="1.0"/>
</DataField>
<DataField name="cancellation_policy" optype="categorical" dataType="string">
<Value value="strict"/>
<Value value="moderate"/>
<Value value="flexible"/>
<Value value="super_strict_30"/>
<Value value="no_refunds"/>
<Value value="super_strict_60"/>
<Value value="long_term"/>
</DataField>
<DataField name="instant_bookable" optype="categorical" dataType="string">
<Value value="0.0"/>
<Value value="1.0"/>
</DataField>
<DataField name="state" optype="categorical" dataType="string">
<Value value="Other"/>
<Value value="NY"/>
<Value value="CA"/>
<Value value="Berlin"/>
<Value value="IL"/>
<Value value="TX"/>
<Value value="WA"/>
<Value value="DC"/>
<Value value="OR"/>
<Value value="London"/>
</DataField>
<DataField name="price" optype="continuous" dataType="double"/>
</DataDictionary>
<TransformationDictionary>
<DerivedField name="scaled_continuous_features[0]" optype="continuous" dataType="double">
<Apply function="*">
<FieldRef field="bathrooms"/>
<Constant dataType="double">2.0694178044699</Constant>
</Apply>
</DerivedField>
<DerivedField name="scaled_continuous_features[1]" optype="continuous" dataType="double">
<Apply function="*">
<FieldRef field="bedrooms"/>
<Constant dataType="double">1.1841578139669175</Constant>
</Apply>
</DerivedField>
<DerivedField name="scaled_continuous_features[2]" optype="continuous" dataType="double">
<Apply function="*">
<FieldRef field="security_deposit"/>
<Constant dataType="double">0.005528293625471707</Constant>
</Apply>
</DerivedField>
<DerivedField name="scaled_continuous_features[3]" optype="continuous" dataType="double">
<Apply function="*">
<FieldRef field="cleaning_fee"/>
<Constant dataType="double">0.023503907888529584</Constant>
</Apply>
</DerivedField>
<DerivedField name="scaled_continuous_features[4]" optype="continuous" dataType="double">
<Apply function="*">
<FieldRef field="extra_people"/>
<Constant dataType="double">0.053941703607268326</Constant>
</Apply>
</DerivedField>
<DerivedField name="scaled_continuous_features[5]" optype="continuous" dataType="double">
<Apply function="*">
<FieldRef field="number_of_reviews"/>
<Constant dataType="double">0.037033530093691217</Constant>
</Apply>
</DerivedField>
<DerivedField name="scaled_continuous_features[6]" optype="continuous" dataType="double">
<Apply function="*">
<FieldRef field="square_feet"/>
<Constant dataType="double">0.002693576320427388</Constant>
</Apply>
</DerivedField>
<DerivedField name="scaled_continuous_features[7]" optype="continuous" dataType="double">
<Apply function="*">
<FieldRef field="review_scores_rating"/>
<Constant dataType="double">0.11363684571655443</Constant>
</Apply>
</DerivedField>
</TransformationDictionary>
<RegressionModel functionName="regression">
<MiningSchema>
<MiningField name="price" usageType="target"/>
<MiningField name="bathrooms"/>
<MiningField name="bedrooms"/>
<MiningField name="security_deposit"/>
<MiningField name="cleaning_fee"/>
<MiningField name="extra_people"/>
<MiningField name="number_of_reviews"/>
<MiningField name="square_feet"/>
<MiningField name="review_scores_rating"/>
<MiningField name="room_type"/>
<MiningField name="host_is_super_host"/>
<MiningField name="cancellation_policy"/>
<MiningField name="instant_bookable"/>
<MiningField name="state"/>
</MiningSchema>
<RegressionTable intercept="-34.92863985637842">
<NumericPredictor name="scaled_continuous_features[0]" coefficient="17.086657246509073"/>
<NumericPredictor name="scaled_continuous_features[1]" coefficient="22.136272333729234"/>
<NumericPredictor name="scaled_continuous_features[2]" coefficient="0.9746404215648721"/>
<NumericPredictor name="scaled_continuous_features[3]" coefficient="23.743529537063555"/>
<NumericPredictor name="scaled_continuous_features[4]" coefficient="2.1885813420248064"/>
<NumericPredictor name="scaled_continuous_features[5]" coefficient="-2.702884377424204"/>
<NumericPredictor name="scaled_continuous_features[6]" coefficient="3.0590826753812905"/>
<NumericPredictor name="scaled_continuous_features[7]" coefficient="4.51103964412197"/>
<CategoricalPredictor name="room_type" value="Entire home/apt" coefficient="27.953591583546153"/>
<CategoricalPredictor name="room_type" value="Private room" coefficient="-11.84412411692917"/>
<CategoricalPredictor name="host_is_super_host" value="0.0" coefficient="-5.518313707404658"/>
<CategoricalPredictor name="cancellation_policy" value="strict" coefficient="2.690406709014169"/>
<CategoricalPredictor name="cancellation_policy" value="moderate" coefficient="-4.820333309812547"/>
<CategoricalPredictor name="cancellation_policy" value="flexible" coefficient="0.0"/>
<CategoricalPredictor name="cancellation_policy" value="super_strict_30" coefficient="66.07552484327911"/>
<CategoricalPredictor name="cancellation_policy" value="no_refunds" coefficient="0.0"/>
<CategoricalPredictor name="cancellation_policy" value="super_strict_60" coefficient="62.65987622321582"/>
<CategoricalPredictor name="instant_bookable" value="0.0" coefficient="7.019590939009696"/>
<CategoricalPredictor name="state" value="Other" coefficient="-11.107524809481783"/>
<CategoricalPredictor name="state" value="NY" coefficient="21.4058345812419"/>
<CategoricalPredictor name="state" value="CA" coefficient="12.697922398918772"/>
<CategoricalPredictor name="state" value="Berlin" coefficient="-49.63938639709832"/>
<CategoricalPredictor name="state" value="IL" coefficient="15.983445099508659"/>
<CategoricalPredictor name="state" value="TX" coefficient="32.22381075322937"/>
<CategoricalPredictor name="state" value="WA" coefficient="-8.022834073105122"/>
<CategoricalPredictor name="state" value="DC" coefficient="5.913735195331112"/>
<CategoricalPredictor name="state" value="OR" coefficient="-16.436721245351528"/>
</RegressionTable>
</RegressionModel>
</PMML>
###Markdown
Push PMML to Live, Running Spark ML Model Server (Mutable)
###Code
import urllib.request
namespace = 'default'
model_name = 'airbnb'
version = '1'
update_url = 'http://prediction-pmml-aws.demo.pipeline.io/update-pmml-model/%s/%s/%s' % (namespace, model_name, version)
update_headers = {}
update_headers['Content-type'] = 'application/xml'
req = urllib.request.Request(update_url, \
headers=update_headers, \
data=model_bytes)
resp = urllib.request.urlopen(req)
print(resp.status) # Should return Http Status 200
import urllib.request
update_url = 'http://prediction-pmml-gcp.demo.pipeline.io/update-pmml/pmml_airbnb'
update_headers = {}
update_headers['Content-type'] = 'application/xml'
req = urllib.request.Request(update_url, \
headers=update_headers, \
data=pmmlBytes)
resp = urllib.request.urlopen(req)
print(resp.status) # Should return Http Status 200
import urllib.parse
import json
namespace = 'default'
model_name = 'airbnb'
version = '1'
evaluate_url = 'http://prediction-pmml-aws.demo.pipeline.io/evaluate-pmml-model/%s/%s/%s' % (namespace, model_name, version)
evaluate_headers = {}
evaluate_headers['Content-type'] = 'application/json'
input_params = '{"bathrooms":5.0, \
"bedrooms":4.0, \
"security_deposit":175.00, \
"cleaning_fee":25.0, \
"extra_people":1.0, \
"number_of_reviews": 2.0, \
"square_feet": 250.0, \
"review_scores_rating": 2.0, \
"room_type": "Entire home/apt", \
"host_is_super_host": "0.0", \
"cancellation_policy": "flexible", \
"instant_bookable": "1.0", \
"state": "CA"}'
encoded_input_params = input_params.encode('utf-8')
req = urllib.request.Request(evaluate_url, \
headers=evaluate_headers, \
data=encoded_input_params)
resp = urllib.request.urlopen(req)
print(resp.read())
###Output
_____no_output_____
###Markdown
Deploy Java-based Model (Simple Model, Mutable Deploy)
###Code
from urllib import request
sourceBytes = ' \n\
private String str; \n\
\n\
public void initialize(Map<String, Object> args) { \n\
} \n\
\n\
public Object predict(Map<String, Object> inputs) { \n\
String id = (String)inputs.get("id"); \n\
\n\
return id.equals("21619"); \n\
} \n\
'.encode('utf-8')
from urllib import request
namespace = 'default'
model_name = 'java_equals'
version = '1'
update_url = 'http://prediction-java-aws.demo.pipeline.io/update-java/%s/%s/%s' % (namespace, model_name, version)
update_headers = {}
update_headers['Content-type'] = 'text/plain'
req = request.Request("%s" % update_url, headers=update_headers, data=sourceBytes)
resp = request.urlopen(req)
generated_code = resp.read()
print(generated_code.decode('utf-8'))
from urllib import request
namespace = 'default'
model_name = 'java_equals'
version = '1'
evaluate_url = 'http://prediction-java-aws.demo.pipeline.io/evaluate-java/%s/%s/%s' % (namespace, model_name, version)
evaluate_headers = {}
evaluate_headers['Content-type'] = 'application/json'
input_params = '{"id":"21618"}'
encoded_input_params = input_params.encode('utf-8')
req = request.Request(evaluate_url, headers=evaluate_headers, data=encoded_input_params)
resp = request.urlopen(req)
print(resp.read()) # Should return false
from urllib import request
namespace = 'default'
model_name = 'java_equals'
version = '1'
evaluate_url = 'http://prediction-java-aws.demo.pipeline.io/evaluate-java/%s/%s/%s' % (namespace, model_name, version)
evaluate_headers = {}
evaluate_headers['Content-type'] = 'application/json'
input_params = '{"id":"21619"}'
encoded_input_params = input_params.encode('utf-8')
req = request.Request(evaluate_url, headers=evaluate_headers, data=encoded_input_params)
resp = request.urlopen(req)
print(resp.read()) # Should return true
###Output
b'true'
###Markdown
Deploy Java Model (HttpClient Model, Mutable Deploy)
###Code
from urllib import request
sourceBytes = ' \n\
public Map<String, Object> data = new HashMap<String, Object>(); \n\
\n\
public void initialize(Map<String, Object> args) { \n\
data.put("url", "http://demo.pipeline.io:9040/prediction/"); \n\
} \n\
\n\
public Object predict(Map<String, Object> inputs) { \n\
try { \n\
String userId = (String)inputs.get("userId"); \n\
String itemId = (String)inputs.get("itemId"); \n\
String url = data.get("url") + "/" + userId + "/" + itemId; \n\
\n\
return org.apache.http.client.fluent.Request \n\
.Get(url) \n\
.execute() \n\
.returnContent(); \n\
\n\
} catch(Exception exc) { \n\
System.out.println(exc); \n\
throw exc; \n\
} \n\
} \n\
'.encode('utf-8')
from urllib import request
name = 'codegen_httpclient'
# Note: Must have trailing '/'
update_url = 'http://prediction-codegen-aws.demo.pipeline.io/update-codegen/%s/' % name
update_headers = {}
update_headers['Content-type'] = 'text/plain'
req = request.Request("%s" % update_url, headers=update_headers, data=sourceBytes)
resp = request.urlopen(req)
generated_code = resp.read()
print(generated_code.decode('utf-8'))
from urllib import request
name = 'codegen_httpclient'
# Note: Must have trailing '/'
update_url = 'http://prediction-codegen-gcp.demo.pipeline.io/update-codegen/%s/' % name
update_headers = {}
update_headers['Content-type'] = 'text/plain'
req = request.Request("%s" % update_url, headers=update_headers, data=sourceBytes)
resp = request.urlopen(req)
generated_code = resp.read()
print(generated_code.decode('utf-8'))
from urllib import request
name = 'codegen_httpclient'
evaluate_url = 'http://prediction-codegen-aws.demo.pipeline.io/evaluate-codegen/%s' % name
evaluate_headers = {}
evaluate_headers['Content-type'] = 'application/json'
input_params = '{"userId":"21619", "itemId":"10006"}'
encoded_input_params = input_params.encode('utf-8')
req = request.Request(evaluate_url, headers=evaluate_headers, data=encoded_input_params)
resp = request.urlopen(req)
print(resp.read()) # Should return float
from urllib import request
name = 'codegen_httpclient'
evaluate_url = 'http://prediction-codegen-gcp.demo.pipeline.io/evaluate-codegen/%s' % name
evaluate_headers = {}
evaluate_headers['Content-type'] = 'application/json'
input_params = '{"userId":"21619", "itemId":"10006"}'
encoded_input_params = input_params.encode('utf-8')
req = request.Request(evaluate_url, headers=evaluate_headers, data=encoded_input_params)
resp = request.urlopen(req)
print(resp.read()) # Should return float
###Output
_____no_output_____
###Markdown
Load Test and Compare Cloud Providers (AWS and Google) Monitor Performance Across Cloud Providers NetflixOSS Services [Dashboard](http://hystrix.demo.pipeline.io/hystrix-dashboard/monitor/monitor.html?streams=%5B%7B%22name%22%3A%22Predictions%20-%20AWS%22%2C%22stream%22%3A%22http%3A%2F%2Fturbine-aws.demo.pipeline.io%2Fturbine.stream%22%2C%22auth%22%3A%22%22%2C%22delay%22%3A%22%22%7D%2C%7B%22name%22%3A%22Predictions%20-%20GCP%22%2C%22stream%22%3A%22http%3A%2F%2Fturbine-gcp.demo.pipeline.io%2Fturbine.stream%22%2C%22auth%22%3A%22%22%2C%22delay%22%3A%22%22%7D%5D) (Hystrix)
###Code
from IPython.core.display import display, HTML
display(HTML("<style>.container { width:100% !important; }</style>"))
from IPython.display import clear_output, Image, display, HTML
html = '<iframe width=100% height=500px src="http://hystrix.demo.pipeline.io/hystrix-dashboard/monitor/monitor.html?streams=%5B%7B%22name%22%3A%22Predictions%20-%20AWS%22%2C%22stream%22%3A%22http%3A%2F%2Fturbine-aws.demo.pipeline.io%2Fturbine.stream%22%2C%22auth%22%3A%22%22%2C%22delay%22%3A%22%22%7D%2C%7B%22name%22%3A%22Predictions%20-%20GCP%22%2C%22stream%22%3A%22http%3A%2F%2Fturbine-gcp.demo.pipeline.io%2Fturbine.stream%22%2C%22auth%22%3A%22%22%2C%22delay%22%3A%22%22%7D%5D">'
display(HTML(html))
###Output
_____no_output_____
###Markdown
Start Load Tests Run JMeter Tests from Local Laptop (Limited by Laptop) Run Headless JMeter Tests from Training Clusters in Cloud
###Code
# Spark ML - PMML - Airbnb
!kubectl create --context=awsdemo -f /root/pipeline/loadtest.ml/loadtest-aws-airbnb-rc.yaml
!kubectl create --context=gcpdemo -f /root/pipeline/loadtest.ml/loadtest-aws-airbnb-rc.yaml
# Codegen - Java - Simple
!kubectl create --context=awsdemo -f /root/pipeline/loadtest.ml/loadtest-aws-equals-rc.yaml
!kubectl create --context=gcpdemo -f /root/pipeline/loadtest.ml/loadtest-aws-equals-rc.yaml
# Tensorflow AI - Tensorflow Serving - Simple
!kubectl create --context=awsdemo -f /root/pipeline/loadtest.ml/loadtest-aws-minimal-rc.yaml
!kubectl create --context=gcpdemo -f /root/pipeline/loadtest.ml/loadtest-aws-minimal-rc.yaml
###Output
_____no_output_____
###Markdown
End Load Tests
###Code
!kubectl delete --context=awsdemo rc loadtest-aws-airbnb
!kubectl delete --context=gcpdemo rc loadtest-aws-airbnb
!kubectl delete --context=awsdemo rc loadtest-aws-equals
!kubectl delete --context=gcpdemo rc loadtest-aws-equals
!kubectl delete --context=awsdemo rc loadtest-aws-minimal
!kubectl delete --context=gcpdemo rc loadtest-aws-minimal
###Output
_____no_output_____
###Markdown
Rolling Deploy Tensorflow AI (Simple Model, Immutable Deploy) Kubernetes [CLI](https://github.com/fluxcapacitor/pipeline/wiki/Kubernetes-Commands)
###Code
!kubectl rolling-update prediction-tensorflow --context=awsdemo --image-pull-policy=Always --image=fluxcapacitor/prediction-tensorflow
!kubectl get pod --context=awsdemo
!kubectl rolling-update prediction-tensorflow --context=gcpdemo --image-pull-policy=Always --image=fluxcapacitor/prediction-tensorflow
!kubectl get pod --context=gcpdemo
###Output
_____no_output_____ |
temperatures-dijon.ipynb | ###Markdown
Quelle température fera à Dijon en 2050 ? Et en 2100?En **2010** la température moyenne à Dijon a été de **9.07°C**. Le modèle créé dans ce notebook, prédit **10.91°C** en **2050** et **12.02°C** en **2100**. *Licence: Creative Commons Attribution-Share Alike 3.0 [Source](https://commons.wikimedia.org/wiki/File:Dijon_Panorama_01.jpg)* Mais comment fait-on ces calculs ?On a besoin des données historiques des températures à Dijon, notamment à partir de 1950. On va décrire toutes les étapes pour créer le modèle qui va nous permettre de prédire la température moyenne qui fera à Dijon dans le futur. Aperçu du modèleLes *points bleus* réprésentent les températures moyennes annuelles au fil des années. La *ligne rouge*, indique les prédictions du modèle pour les mêmes dates.Voici les étapes : 1. Obtenir les données**Source des données** : Berkeley Earth (http://berkeleyearth.org) ** Licence des données **: CC BY-NC-SA 4.0 2. Réunir les outilsAu lieu de coder tout à la main, on va prendre appui sur les librairies les plus populaires de Python pour la science des données :
###Code
import numpy as np # Bibliotheque de fonctions mathématiques optimisées
import pandas as pd # Structures de données pour analyse de données
%matplotlib inline
import matplotlib.pyplot as plt # Bibliothèque de visualisation 2D
import seaborn; seaborn.set() # Evolution de matplotlib
###Output
_____no_output_____
###Markdown
3. Charger et nettoyer les données
###Code
temp = pd.read_csv('data/dijon-temp.csv')
temp = temp.drop(['City', 'Country', 'Latitude', 'Longitude', 'AverageTemperatureUncertainty', 'Unnamed: 0'], axis=1)
temp = temp.rename(columns={'dt': 'date', 'AverageTemperature': 'avg'})
temp['an'] = temp['date'].str[:4].astype(int)
temp['mois'] = temp['date'].str[5:7].astype(int)
temp = temp.dropna(axis = 0 , how = 'any')
temp = temp.drop(['date'], axis=1)
temp.head()
###Output
_____no_output_____
###Markdown
4. Explorer les températures moyennes mensuelles
###Code
avg = temp['avg']
print('Nombre de mois: ', len(temp))
print('Mois temp < -5° : ', np.sum(avg < -5))
print('Mois temp < 0° : ', np.sum(avg < 0))
print('Mois temp >0°<5° : ', np.sum((avg > 0) & (avg < 5)))
print('Mois temp >5°<10° : ', np.sum((avg > 5) & (avg < 10)))
print('Mois temp >10°<15° : ', np.sum((avg > 10) & (avg < 15)))
print('Mois temp >15°<20° : ', np.sum((avg > 15) & (avg < 20)))
print('Mois temp >20°<25° : ', np.sum((avg > 20) & (avg < 25)))
print('Mois temp >25°<30° : ', np.sum((avg > 25) & (avg < 30)))
print('Mois temp >30°<35° : ', np.sum((avg > 30) & (avg < 35)))
max = np.max(temp)
print('Mois le plus chaud: en ', max['an'], ' le mois ', max['mois'], 'avec ', max['avg'])
min = np.min(temp)
print('Mois le plus froid:en ', min['an'], ' le mois ', max['mois'], ' avec ', min['avg'])
###Output
Nombre de mois: 3166
Mois temp < -5° : 10
Mois temp < 0° : 274
Mois temp >0°<5° : 840
Mois temp >5°<10° : 644
Mois temp >10°<15° : 606
Mois temp >15°<20° : 765
Mois temp >20°<25° : 36
Mois temp >25°<30° : 0
Mois temp >30°<35° : 0
Mois le plus chaud: en 2013.0 le mois 12.0 avec 23.224
Mois le plus froid:en 1743.0 le mois 12.0 avec -7.075
###Markdown
Fréquence des températures moyennes mensuellesQuelles températures moyennes sont les plus fréquentes ? :
###Code
a=plt.hist(temp['avg'], 40)
###Output
_____no_output_____
###Markdown
5. Créer le modèle sur les températures moyennes annuellesOn va d'abord charger l'algorithme de regression linéaire à partir de la librairie de machine learning :
###Code
from sklearn import linear_model
###Output
_____no_output_____
###Markdown
Puis calculer les températures moyennes annuelles (les données d'origine donnent les températures moyennes mensuelles) :
###Code
temp_an = temp.groupby(['an'], as_index=False).mean()
temp_an = temp_an.drop(['mois'], axis=1)
temp_an.head()
###Output
_____no_output_____
###Markdown
On va afficher les données pour vérifier que tout semble correct. On peut constater qu'autour de 1750 il y a eu des mesures qui ne semblent pas exactes :
###Code
temp_an.plot(kind='scatter', x='an', y='avg', figsize=(12,8))
###Output
_____no_output_____
###Markdown
Maintenant, il faut préparer les données pour appliquer la régression linéaire :
###Code
temp_an.insert(0, 'ones', 1)
temp_an.head()
X = temp_an[['ones','an']]
X = np.matrix(X.values)
y = temp_an['avg']
y = y.values.reshape(267,1)
y = np.matrix(y)
theta = np.matrix( np.array([0, 0]) )
X.shape, y.shape, theta.shape
model = linear_model.LinearRegression()
model.fit(X, y)
years = np.array(X[:, 1].A1)
predicted_temp = model.predict(X).flatten()
fig, ax = plt.subplots(figsize=(12, 8))
ax.plot(years, predicted_temp, 'r', label='Prediction')
ax.scatter(temp_an.an, temp_an.avg, label='Training data')
###Output
_____no_output_____
###Markdown
La hausse de température est évidente. Mais on a l'impression qu'elle est plus importante à partir de 1950. Evolution à partir de 1950Pour vérifier cette hypothèse, on va créer un modèle seulement avec les données à partir de 1950 :
###Code
temp_1950 = temp[temp['an'] > 1950]
temp_1950.head()
temp_1950_an = temp_1950.groupby(['an'], as_index=False).mean()
temp_1950_an = temp_1950_an.drop(['mois'], axis=1)
temp_1950_an.head()
temp_1950_an.insert(0, 'ones', 1)
X = temp_1950_an[['ones', 'an']]
X = np.matrix( X.values )
y = temp_1950_an[['avg']]
y = y.values.reshape(63, 1)
y = np.matrix( y )
theta = np.matrix( np.array( [0, 0]))
X.shape, y.shape, theta.shape
model = linear_model.LinearRegression()
model.fit(X, y)
years = np.array(X[:, 1].A1)
predicted_temp = model.predict(X).flatten()
fig, ax = plt.subplots(figsize=(12, 8))
ax.plot(years, predicted_temp, 'r', label='Prediction')
ax.scatter(temp_1950_an.an, temp_1950_an.avg, label='Training data')
###Output
_____no_output_____
###Markdown
La hausse semble bien plus nette avec le nouveau modèle ! Predictions pour 2050 et 2100Avec le modèle calculé, il suffit d'une petite manipulation pour faire nos prédictions
###Code
years = np.array([2010,2050,2100])
df = pd.DataFrame({'an':years})
df.insert(0,'ones',1)
Z = np.matrix(df)
predicted_temp = model.predict(Z).flatten()
print('Température moyenne en 2010 : ', predicted_temp[0], '°C')
print('Température moyenne en 2050 : ', predicted_temp[1], '°C')
print('Température moyenne en 2100 : ', predicted_temp[2], '°C')
###Output
Température moyenne en 2010 : 10.0233472942 °C
Température moyenne en 2050 : 10.9135571477 °C
Température moyenne en 2100 : 12.0263194644 °C
|
both machine learning models/exercise-model-validation.ipynb | ###Markdown
**This notebook is an exercise in the [Introduction to Machine Learning](https://www.kaggle.com/learn/intro-to-machine-learning) course. You can reference the tutorial at [this link](https://www.kaggle.com/dansbecker/model-validation).**--- RecapYou've built a model. In this exercise you will test how good your model is.Run the cell below to set up your coding environment where the previous exercise left off.
###Code
# Code you have previously used to load data
import pandas as pd
from sklearn.tree import DecisionTreeRegressor
# Path of the file to read
iowa_file_path = '../input/home-data-for-ml-course/train.csv'
home_data = pd.read_csv(iowa_file_path)
y = home_data.SalePrice
feature_columns = ['LotArea', 'YearBuilt', '1stFlrSF', '2ndFlrSF', 'FullBath', 'BedroomAbvGr', 'TotRmsAbvGrd']
X = home_data[feature_columns]
# Specify Model
iowa_model = DecisionTreeRegressor()
# Fit Model
iowa_model.fit(X, y)
print("First in-sample predictions:", iowa_model.predict(X.head()))
print("Actual target values for those homes:", y.head().tolist())
# Set up code checking
from learntools.core import binder
binder.bind(globals())
from learntools.machine_learning.ex4 import *
print("Setup Complete")
###Output
First in-sample predictions: [208500. 181500. 223500. 140000. 250000.]
Actual target values for those homes: [208500, 181500, 223500, 140000, 250000]
Setup Complete
###Markdown
Exercises Step 1: Split Your DataUse the `train_test_split` function to split up your data.Give it the argument `random_state=1` so the `check` functions know what to expect when verifying your code.Recall, your features are loaded in the DataFrame **X** and your target is loaded in **y**.
###Code
# Import the train_test_split function and uncomment
from sklearn.model_selection import train_test_split
train_X, val_X, train_y, val_y = train_test_split(X, y, random_state = 1)
step_1.check()
# Check your answer
step_1.check()
# The lines below will show you a hint or the solution.
# step_1.hint()
# step_1.solution()
###Output
_____no_output_____
###Markdown
Step 2: Specify and Fit the ModelCreate a `DecisionTreeRegressor` model and fit it to the relevant data.Set `random_state` to 1 again when creating the model.
###Code
# You imported DecisionTreeRegressor in your last exercise
# and that code has been copied to the setup code above. So, no need to
# import it again
# Specify the model
iowa_model = DecisionTreeRegressor(random_state=1)
# Fit iowa_model with the training data.
iowa_model.fit(train_X, train_y)
# Check your answer
step_2.check()
# step_2.hint()
# step_2.solution()
###Output
_____no_output_____
###Markdown
Step 3: Make Predictions with Validation data
###Code
# Predict with all validation observations
val_predictions = iowa_model.predict(val_X)
# Check your answer
step_3.check()
# step_3.hint()
# step_3.solution()
###Output
_____no_output_____
###Markdown
Inspect your predictions and actual values from validation data.
###Code
# print the top few validation predictions
printval_predictions = iowa_model.predict(val_X)
# print the top few actual prices from validation data
print(____)
###Output
<learntools.core.constants.PlaceholderValue object at 0x7fd99a323190>
###Markdown
What do you notice that is different from what you saw with in-sample predictions (which are printed after the top code cell in this page).Do you remember why validation predictions differ from in-sample (or training) predictions? This is an important idea from the last lesson. Step 4: Calculate the Mean Absolute Error in Validation Data
###Code
from sklearn.metrics import mean_absolute_error
val_mae = mean_absolute_error(val_y, val_predictions)
# uncomment following line to see the validation_mae
#print(val_mae)
# Check your answer
step_4.check()
# step_4.hint()
# step_4.solution()
###Output
_____no_output_____ |
.ipynb_checkpoints/Advanced_lane_finding-checkpoint.ipynb | ###Markdown
Self-Driving Car Engineer Nanodegree Advanced Lane Finding ProjectThe goals / steps of this project are the following:Compute the camera calibration matrix and distortion coefficients given a set of chessboard images.Apply a distortion correction to raw images.Use color transforms, gradients, etc., to create a thresholded binary image.Apply a perspective transform to rectify binary image ("birds-eye view").Detect lane pixels and fit to find the lane boundary.Determine the curvature of the lane and vehicle position with respect to center.Warp the detected lane boundaries back onto the original image.Output visual display of the lane boundaries and numerical estimation of lane curvature and vehicle position.--- Import Packages
###Code
#importing some useful packages
#!pip install opencv-python
#!conda install -c conda-forge opencv
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
import numpy as np
import cv2
import glob
import os
%matplotlib inline
###Output
_____no_output_____
###Markdown
Compute camera calibration
###Code
# Add code for camera calibration here
def calibrate_camera(images_path):
images = glob.glob(images_path)
#define the number of inner corner in both x and y
nx = 9
ny = 6
# Arrays to store object ponts and image ponts from all the images
object_points = [] # 3D points in real world space
image_points = [] # 2D points in image plane space
objp = np.zeros((nx * ny, 3), np.float32)
objp[:, :2] = np.mgrid[0:nx, 0:ny].T.reshape(-1, 2)
for image in images:
#read image into memory
img = mpimg.imread(image)
#convert to gray scale before applying camera calibration
# using RGB2GRAY as I used mpimg to read the image
gray = cv2.cvtColor(img, cv2.COLOR_RGB2GRAY)
# find corners of chessboard as used in the lessons
ret, corners = cv2.findChessboardCorners(gray, (nx, ny), None)
#add result to object_points and image_points if corners were found with sucess
if ret == True:
image_points.append(corners)
object_points.append(objp)
cv2.drawChessboardCorners(img, (nx, ny), corners, ret)
cv2.imwrite('images_after_calibration/' + os.path.basename(image), img)
#use open cv calibrate camera function
# the gray.shape[::-1] means the height and width of the image, as explained in te lesson 6 from camera calibration
ret, mtx, dist, rvecs, tvecs = cv2.calibrateCamera(object_points, image_points, gray.shape[::-1], None, None)
#return mtx and dist only because we only need these parameters to undistort the actual road image
return mtx, dist
###Output
_____no_output_____
###Markdown
Distortion correction
###Code
# Add code for distortion correction here
def undistort_image_computed(image, mtx, dist):
#apply cv2 function undistort with mtx and dist collected from the camera calibration step
dst = cv2.undistort(image, mtx, dist, None, mtx)
#cv2.imwrite('images_after_undistortion/' + os.path.basename(image_path), dst)
return dst
def undistort_image(image_path, mtx, dist):
image = cv2.imread(image_path)
#apply cv2 function undistort with mtx and dist collected from the camera calibration step
dst = cv2.undistort(image, mtx, dist, None, mtx)
cv2.imwrite('images_after_undistortion/' + os.path.basename(image_path), dst)
return dst
def undistort_images(images_directory, images_names, mtx, dist):
undistorted_images = []
for img in images_names:
dst = undistort_image(str(images_directory + img), mtx, dist)
undistorted_images.append(dst)
return undistorted_images
###Output
_____no_output_____
###Markdown
Color and gradient transformation
###Code
# Add code for color and gradient transformation here
def get_images_with_hls_channel(images_directory, images_names):
images_with_hls_channel = []
for img in images_names:
image_channels = []
image = cv2.imread(str(images_directory + img))
hls_image = cv2.cvtColor(image, cv2.COLOR_BGR2HLS)
h = hls_image[:,:,0]
l = hls_image[:,:,1]
s = hls_image[:,:,2]
image_channels.append(h)
image_channels.append(l)
image_channels.append(s)
cv2.imwrite('images_gradient_h_channel/' + os.path.basename(img), h)
cv2.imwrite('images_gradient_l_channel/' + os.path.basename(img), l)
cv2.imwrite('images_gradient_s_channel/' + os.path.basename(img), s)
images_with_hls_channel.append(image_channels)
return images_with_hls_channel
def extract_s_channel_from_image(image):
hls_image = cv2.cvtColor(image, cv2.COLOR_BGR2HLS)
s = hls_image[:,:,2]
return s
def extract_sobel_x_from_image(gray):
# Sobel x as taken from lesson
sobelx = cv2.Sobel(gray, cv2.CV_64F, 1, 0) # Take the derivative in x
abs_sobelx = np.absolute(sobelx) # Absolute x derivative to accentuate lines away from horizontal
scaled_sobel = np.uint8(255*abs_sobelx/np.max(abs_sobelx))
# Threshold x gradient
thresh_min = 20
thresh_max = 100
sxbinary = np.zeros_like(scaled_sobel)
sxbinary[(scaled_sobel >= thresh_min) & (scaled_sobel <= thresh_max)] = 1
return sxbinary
def apply_combined_sobelx_and_color_channel_gradient(images_directory, images_names):
channel_processed_images = []
for img in images_names:
image = cv2.imread(str(images_directory + img))
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
sxbinary = extract_sobel_x_from_image(gray)
s_channel = extract_s_channel_from_image(image)
# Threshold color channel
s_thresh_min = 170
s_thresh_max = 255
s_binary = np.zeros_like(s_channel)
s_binary[(s_channel >= s_thresh_min) & (s_channel <= s_thresh_max)] = 1
# Stack each channel to view their individual contributions in green and blue respectively
# This returns a stack of the two binary images, whose components you can see as different colors
color_binary = np.dstack((np.zeros_like(sxbinary), sxbinary, s_binary)) * 255
# Combine the two binary thresholds
combined_binary = np.zeros_like(sxbinary)
combined_binary[(s_binary == 1) | (sxbinary == 1)] = 1
height, width = gray.shape
final_region_of_interest_mask = np.zeros_like(combined_binary)
region_of_interest_vertices = np.array([[0,height-1], [width/2, int(0.5*height)], [width-1, height-1]], dtype=np.int32)
cv2.fillPoly(final_region_of_interest_mask, [region_of_interest_vertices], 1)
region_image = cv2.bitwise_and(combined_binary, final_region_of_interest_mask)
cv2.imwrite('images_with_gradients_applied_and_region_of_interest/' + os.path.basename(img), region_image * 255)
channel_processed_images.append(region_image)
return channel_processed_images
def apply_combined_gradient_to_image(image):
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
sxbinary = extract_sobel_x_from_image(gray)
s_channel = extract_s_channel_from_image(image)
# Threshold color channel
s_thresh_min = 170
s_thresh_max = 255
s_binary = np.zeros_like(s_channel)
s_binary[(s_channel >= s_thresh_min) & (s_channel <= s_thresh_max)] = 1
# Stack each channel to view their individual contributions in green and blue respectively
# This returns a stack of the two binary images, whose components you can see as different colors
color_binary = np.dstack((np.zeros_like(sxbinary), sxbinary, s_binary)) * 255
# Combine the two binary thresholds
combined_binary = np.zeros_like(sxbinary)
combined_binary[(s_binary == 1) | (sxbinary == 1)] = 1
height, width = gray.shape
final_region_of_interest_mask = np.zeros_like(combined_binary)
region_of_interest_vertices = np.array([[0,height-1], [width/2, int(0.5*height)], [width-1, height-1]], dtype=np.int32)
cv2.fillPoly(final_region_of_interest_mask, [region_of_interest_vertices], 1)
region_image = cv2.bitwise_and(combined_binary, final_region_of_interest_mask)
return region_image
###Output
_____no_output_____
###Markdown
Perspective transformation
###Code
# Add code for perspective transformation here
def warp_images(images, output_folder_path):
warped_images = []
minvs = []
for img in images:
#image = cv2.imread(str(images_directory + img))
#image_rgb = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
warp_result = warp(img)
warped_image = warp_result[0]
Minv = warp_result[2]
#output_name = str(output_folder_path + img)
#plt.imshow(warped_image)
#cv2.imwrite(output_name, warped_image)
warped_images.append(warped_image)
minvs.append(Minv)
return warped_images, minvs
def warp(img, src=None, dst=None):
# Define image size to work with
img_size = (img.shape[1], img.shape[0])
if src is None:
src = np.float32(
[[290, 700], # Bottom left corner
[600, 460], # Top left corner
[730, 460], # Top right corner
[1120, 700]]) # Bottom right corner
if dst is None:
dst = np.float32(
[[250, 720], # Bottom left corner
[250, 0], # Top left corner
[1000, 0], # Top right corner
[1000, 720]]) # Bottom right corner
# Compute the perspective transform, M
M = cv2.getPerspectiveTransform(src, dst)
# Compute the opposite matrix
Minv = cv2.getPerspectiveTransform(dst, src)
# Create warped image - uses linear interpolation as defined in opencv and in the lesson from camera calibration
warped_image = cv2.warpPerspective(img, M, img_size, flags=cv2.INTER_LINEAR)
return warped_image, M, Minv
###Output
_____no_output_____
###Markdown
Detect lane pixels and fit to find the lane boundary
###Code
# Add code for detect lane pixels and fit to find the lane boundary here
def find_all_histograms(images_directory, images_names):
histograms = []
for img in images_names:
image = mpimg.imread(str(images_directory + img))
histogram = hist(image)
#cv2.imwrite('histograms/' + os.path.basename(img), histogram)
#plt.plot(histogram)
histograms.append(histogram)
return histograms
#as in the class
def hist(img):
# Grab only the bottom half of the image
# Lane lines are likely to be mostly vertical nearest to the car
bottom_half = img[img.shape[0]//2:,:]
# Sum across image pixels vertically - make sure to set an `axis`
# i.e. the highest areas of vertical lines should be larger values
histogram = np.sum(bottom_half, axis=0)
return histogram
def find_lane_pixels(binary_warped, nwindows = 10, margin = 100, minpix = 50):
histogram = hist(binary_warped)
# Create an output image to draw on and visualize the result
out_img = np.dstack((binary_warped, binary_warped, binary_warped))
# Find the peak of the left and right halves of the histogram
# These will be the starting point for the left and right lines
midpoint = np.int(histogram.shape[0]//2)
leftx_base = np.argmax(histogram[:midpoint])
rightx_base = np.argmax(histogram[midpoint:]) + midpoint
# Set height of windows - based on nwindows above and image shape
window_height = np.int(binary_warped.shape[0]//nwindows)
# Identify the x and y positions of all nonzero pixels in the image
nonzero = binary_warped.nonzero()
nonzeroy = np.array(nonzero[0])
nonzerox = np.array(nonzero[1])
# Current positions to be updated later for each window in nwindows
leftx_current = leftx_base
rightx_current = rightx_base
# Create empty lists to receive left and right lane pixel indices
left_lane_inds = []
right_lane_inds = []
# Step through the windows one by one
for window in range(nwindows):
# Identify window boundaries in x and y (and right and left)
win_y_low = binary_warped.shape[0] - (window+1)*window_height
win_y_high = binary_warped.shape[0] - window*window_height
win_xleft_low = leftx_current - margin
win_xleft_high = leftx_current + margin
win_xright_low = rightx_current - margin
win_xright_high = rightx_current + margin
# Draw the windows on the visualization image
cv2.rectangle(out_img,(win_xleft_low,win_y_low),
(win_xleft_high,win_y_high),(0,255,0), 2)
cv2.rectangle(out_img,(win_xright_low,win_y_low),
(win_xright_high,win_y_high),(0,255,0), 2)
# Identify the nonzero pixels in x and y within the window #
good_left_inds = ((nonzeroy >= win_y_low) & (nonzeroy < win_y_high) &
(nonzerox >= win_xleft_low) & (nonzerox < win_xleft_high)).nonzero()[0]
good_right_inds = ((nonzeroy >= win_y_low) & (nonzeroy < win_y_high) &
(nonzerox >= win_xright_low) & (nonzerox < win_xright_high)).nonzero()[0]
# Append these indices to the lists
left_lane_inds.append(good_left_inds)
right_lane_inds.append(good_right_inds)
# If you found > minpix pixels, recenter next window on their mean position
if len(good_left_inds) > minpix:
leftx_current = np.int(np.mean(nonzerox[good_left_inds]))
if len(good_right_inds) > minpix:
rightx_current = np.int(np.mean(nonzerox[good_right_inds]))
# Concatenate the arrays of indices (previously was a list of lists of pixels)
try:
left_lane_inds = np.concatenate(left_lane_inds)
right_lane_inds = np.concatenate(right_lane_inds)
except ValueError:
# Avoids an error if the above is not implemented fully
pass
# Extract left and right line pixel positions
leftx = nonzerox[left_lane_inds]
lefty = nonzeroy[left_lane_inds]
rightx = nonzerox[right_lane_inds]
righty = nonzeroy[right_lane_inds]
#print(binary_warped)
#cv2.imwrite('sliding_windows_search/' + str(os.path.basename(binary_warped)), out_img)
return leftx, lefty, rightx, righty, out_img
def fit_polynomial(binary_warped):
# Find our lane pixels first
leftx, lefty, rightx, righty, out_img = find_lane_pixels(binary_warped)
# Fit a second order polynomial to each using `np.polyfit`
left_fit = np.polyfit(lefty, leftx, 2)
right_fit = np.polyfit(righty, rightx, 2)
# Generate x and y values for plotting
ploty = np.linspace(0, binary_warped.shape[0]-1, binary_warped.shape[0] )
try:
left_fitx = left_fit[0]*ploty**2 + left_fit[1]*ploty + left_fit[2]
right_fitx = right_fit[0]*ploty**2 + right_fit[1]*ploty + right_fit[2]
except TypeError:
# Avoids an error if `left` and `right_fit` are still none or incorrect
print('The function failed to fit a line!')
left_fitx = 1*ploty**2 + 1*ploty
right_fitx = 1*ploty**2 + 1*ploty
## Visualization ##
# Colors in the left and right lane regions
out_img[lefty, leftx] = [255, 0, 0]
out_img[righty, rightx] = [0, 0, 255]
# Plots the left and right polynomials on the lane lines
#plt.plot(left_fitx, ploty, color='yellow')
#plt.plot(right_fitx, ploty, color='yellow')
return (left_fit, right_fit), (left_fitx, ploty), (right_fitx, ploty), out_img
def fit_poly(img_shape, leftx, lefty, rightx, righty):
left_fit = np.polyfit(lefty, leftx, 2)
right_fit = np.polyfit(righty, rightx, 2)
# Generate x and y values for plotting
ploty = np.linspace(0, img_shape[0]-1, img_shape[0])
### TO-DO: Calc both polynomials using ploty, left_fit and right_fit ###
left_fitx = left_fit[0]*ploty**2 + left_fit[1]*ploty + left_fit[2]
right_fitx = right_fit[0]*ploty**2 + right_fit[1]*ploty + right_fit[2]
return left_fit, right_fit, left_fitx, right_fitx, ploty
def search_around_poly(binary_warped, left_fit, right_fit, margin = 100):
# Grab activated pixels
nonzero = binary_warped.nonzero()
nonzeroy = np.array(nonzero[0])
nonzerox = np.array(nonzero[1])
left_lane_inds = ((nonzerox > (left_fit[0]*(nonzeroy**2) + left_fit[1]*nonzeroy +
left_fit[2] - margin)) & (nonzerox < (left_fit[0]*(nonzeroy**2) +
left_fit[1]*nonzeroy + left_fit[2] + margin)))
right_lane_inds = ((nonzerox > (right_fit[0]*(nonzeroy**2) + right_fit[1]*nonzeroy +
right_fit[2] - margin)) & (nonzerox < (right_fit[0]*(nonzeroy**2) +
right_fit[1]*nonzeroy + right_fit[2] + margin)))
# Again, extract left and right line pixel positions
leftx = nonzerox[left_lane_inds]
lefty = nonzeroy[left_lane_inds]
rightx = nonzerox[right_lane_inds]
righty = nonzeroy[right_lane_inds]
# Fit new polynomials
left_fit, right_fit, left_fitx, right_fitx, ploty = fit_poly(binary_warped.shape, leftx, lefty, rightx, righty)
## Visualization ##
# Create an image to draw on and an image to show the selection window
out_img = np.dstack((binary_warped, binary_warped, binary_warped))*255
window_img = np.zeros_like(out_img)
# Color in left and right line pixels
out_img[nonzeroy[left_lane_inds], nonzerox[left_lane_inds]] = [255, 0, 0]
out_img[nonzeroy[right_lane_inds], nonzerox[right_lane_inds]] = [0, 0, 255]
# Generate a polygon to illustrate the search window area
# And recast the x and y points into usable format for cv2.fillPoly()
left_line_window1 = np.array([np.transpose(np.vstack([left_fitx-margin, ploty]))])
left_line_window2 = np.array([np.flipud(np.transpose(np.vstack([left_fitx+margin,
ploty])))])
left_line_pts = np.hstack((left_line_window1, left_line_window2))
right_line_window1 = np.array([np.transpose(np.vstack([right_fitx-margin, ploty]))])
right_line_window2 = np.array([np.flipud(np.transpose(np.vstack([right_fitx+margin,
ploty])))])
right_line_pts = np.hstack((right_line_window1, right_line_window2))
# Draw the lane onto the warped blank image
cv2.fillPoly(window_img, np.int_([left_line_pts]), (0,255, 0))
cv2.fillPoly(window_img, np.int_([right_line_pts]), (0,255, 0))
result = cv2.addWeighted(out_img, 1, window_img, 0.3, 0)
# Plot the polynomial lines onto the image
# plt.plot(left_fitx, ploty, color='yellow')
#plt.plot(right_fitx, ploty, color='yellow')
## End visualization steps ##
return (left_fit, right_fit), (left_fitx, ploty), (right_fitx, ploty), result
###Output
_____no_output_____
###Markdown
Determine the curvature of the lane and vehicle position with respect to center
###Code
# Add code for determine the curvature of the lane and vehicle position with respect to center here
#this code was taken from the lessons, with some slight changes
def measure_curvature_real(ploty, leftx, rightx, image_shape):
'''
Calculates the curvature of polynomial functions in meters.
'''
# ploty = np.linspace(0, image_shape[0] - 1, image_shape[0])
leftx = leftx[::-1] # Reverse to match top-to-bottom in y
rightx = rightx[::-1] # Reverse to match top-to-bottom in y
# Define conversions in x and y from pixels space to meters
ym_per_pix = 30/720 # meters per pixel in y dimension
xm_per_pix = 3.7/700 # meters per pixel in x dimension
print(ploty)
print(leftx)
left_fit_cr = np.polyfit(ploty*ym_per_pix, leftx*xm_per_pix, 2)
right_fit_cr = np.polyfit(ploty*ym_per_pix, rightx*xm_per_pix, 2)
# Define y-value where we want radius of curvature
# We'll choose the maximum y-value, corresponding to the bottom of the image
y_eval = np.max(ploty)
# Calculation of R_curve (radius of curvature)
left_curverad = ((1 + (2*left_fit_cr[0]*y_eval*ym_per_pix + left_fit_cr[1])**2)**1.5) / np.absolute(2*left_fit_cr[0])
right_curverad = ((1 + (2*right_fit_cr[0]*y_eval*ym_per_pix + right_fit_cr[1])**2)**1.5) / np.absolute(2*right_fit_cr[0])
return left_curverad, right_curverad
def measure_curvature_pixels(ploty, left_fit, right_fit):
'''
Calculates the curvature of polynomial functions in pixels.
'''
# Define y-value where we want radius of curvature
# We'll choose the maximum y-value, corresponding to the bottom of the image
y_eval = np.max(ploty)
# Calculation of R_curve (radius of curvature)
left_curverad = ((1 + (2*left_fit[0]*y_eval + left_fit[1])**2)**1.5) / np.absolute(2*left_fit[0])
right_curverad = ((1 + (2*right_fit[0]*y_eval + right_fit[1])**2)**1.5) / np.absolute(2*right_fit[0])
return left_curverad, right_curverad
def car_offset(leftx, rightx, img_shape, xm_per_pix=3.7/800):
## Image mid horizontal position
mid_imgx = img_shape[1]//2
## Car position with respect to the lane
car_pos = (leftx[-1] + rightx[-1])/2
## Horizontal car offset
offsetx = (mid_imgx - car_pos) * xm_per_pix
return offsetx
###Output
_____no_output_____
###Markdown
Warp the detected lane boundaries back onto the original image
###Code
# Add code for warp the detected lane boundaries back onto the original image here
def compose_image_with_lines(img, warped_img, left_fitx, right_fitx, ploty, Minv):
# Create an image to draw the lines on
#print("Image = " + str(img.shape))
#print("Warped image = " + str(warped_img.shape))
#print("Left fit X = " + str(left_fit[0]))
#print("Right fit X = " + str(right_fit[0]))
#print("Plot y = " + str(ploty))
#print("Minv = " + str(Minv))
#plt.imshow(warped_img)
warp_zero = np.zeros_like(warped_img).astype(np.uint8)
#one for each color channel
color_warp = np.dstack((warp_zero, warp_zero, warp_zero))
# Recast the x and y points into usable format for cv2.fillPoly()
#left_fitx = left_fit[0]
#right_fitx = right_fit[0]
points_left = np.array([np.transpose(np.vstack([left_fitx, ploty]))])
points_right = np.array([np.flipud(np.transpose(np.vstack([right_fitx, ploty])))])
points = np.hstack((points_left, points_right))
# Draw the lane onto the warped blank image
cv2.fillPoly(color_warp, np.int_([points]), (0,255, 0))
# Warp the blank back to original image space using inverse perspective matrix (Minv)
composed_warp = cv2.warpPerspective(color_warp, Minv, (img.shape[1], img.shape[0]))
#plt.imshow(composed_warp)
weighted_composed_warp = cv2.addWeighted(img, 1, composed_warp, 0.3, 0)
#plt.imshow(weighted_composed_warp)
return weighted_composed_warp
###Output
_____no_output_____
###Markdown
Output visual display of the lane boundaries and numerical estimation of lane curvature and vehicle position
###Code
# Add code for output visual display of the lane boundaries and numerical estimation of lane curvature and vehicle position here
def add_image_real_time_info(img, leftx, rightx, ploty, xm_per_pix=3.7/800, ym_per_pix = 25/720):
curvature_rads = measure_curvature_real(leftx, rightx, img.shape)
offsetx = car_offset(leftx, rightx, img.shape)
image_with_info = img.copy()
cv2.putText(image_with_info, 'Left curvature: {:.2f} m'.format(curvature_rads[0]),
(60, 60), cv2.FONT_HERSHEY_SIMPLEX, 1.5, (255,255,255), 5)
cv2.putText(image_with_info, 'Right curvature: {:.2f} m'.format(curvature_rads[1]),
(60, 110), cv2.FONT_HERSHEY_SIMPLEX, 1.5, (255,255,255), 5)
cv2.putText(image_with_info, 'Horizontal car offset: {:.2f} m'.format(offsetx),
(60, 160), cv2.FONT_HERSHEY_SIMPLEX, 1.5, (255,255,255), 5)
return image_with_info
###Output
_____no_output_____
###Markdown
Project 2 Pipeline
###Code
# Add new pipeline here
imagesDir = os.listdir("test_images/") # get the test images
original_images = []
for img in imagesDir:
image = image = cv2.imread(str("test_images/" + img))
image_rgb = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
original_images.append(image_rgb)
mtx, dist = calibrate_camera('./camera_cal/calibration*.jpg')
undistorted_images = undistort_images("test_images/", imagesDir, mtx, dist)
images_with_hls_channel = get_images_with_hls_channel("images_after_undistortion/", imagesDir)
channel_processed_images = apply_combined_sobelx_and_color_channel_gradient("images_after_undistortion/", imagesDir)
#original_warped_images = warp_images("test_images/", imagesDir, 'original_warped_images/')
#thresholded_warped_images = warp_images("images_with_gradients_applied_and_region_of_interest/", imagesDir, 'thresholded_warped_images/')
result = warp_images(channel_processed_images, 'thresholded_warped_images/')
thresholded_warped_images = result[0]
minvs = result[1]
histograms = find_all_histograms("thresholded_warped_images/", imagesDir)
sliding_windows_images = []
right_fits = []
left_fits = []
lines_fits_array = []
for i in range(len(histograms)):
lines_fit, left_points, right_points, out_img = fit_polynomial(thresholded_warped_images[i])
left_fits.append(left_points)
right_fits.append(right_points)
sliding_windows_images.append(out_img)
lines_fits_array.append(lines_fit)
#plt.imshow(sliding_windows_images[2])
optimized_lane_finding = []
left_fits_optimized = []
right_fits_optimized = []
plot_ys = []
for i in range(len(histograms)):
lines_fit, left_points, right_points, out_img = search_around_poly(thresholded_warped_images[i], lines_fits_array[i][0], lines_fits_array[i][1])
#result = search_around_poly(thresholded_warped_images[i], left_fits[i], right_fits[i])
optimized_lane_finding.append(out_img)
left_fits_optimized.append(left_points)
right_fits_optimized.append(right_points)
plot_ys.append(left_points)
#plt.imshow(optimized_lane_finding[2])
composed_images = []
for i in range(len(original_images)):
composed = compose_image_with_lines(original_images[i], thresholded_warped_images[i], left_fits_optimized[i][0], right_fits_optimized[i][0], left_fits_optimized[i][1], minvs[i])
composed_images.append(composed)
#plt.imshow(composed_images[7])
plt.imshow(composed_images[1])
plt.imshow(sliding_windows_images[3])
plt.imshow(optimized_lane_finding[3])
histograms = find_all_histograms("thresholded_warped_images/", imagesDir)
plt.plot(histograms[0])
histograms = find_all_histograms("thresholded_warped_images/", imagesDir)
for histogram in histograms:
plt.plot(histogram)
#printing curvatures for testing purposes
for i in range(len(optimized_lane_finding)):
#curvatures = measure_curvature_real(plot_ys[i], left_fits[i], right_fits[i], optimized_lane_finding[i].shape)
curvatures = measure_curvature_real(plot_ys[i], left_fits[i], right_fits[i], optimized_lane_finding[i].shape)
# Print the results
print('Left line curvature:', curvatures[0], 'm')
print('Right line curvature:', curvatures[1], 'm')
class Pipeline:
def __init__(self, cal_path):
# Make a list of calibration images
# Calibrate camera
self.mtx, self.dist = calibrate_camera(cal_path)
self.lines_fit = None
def __call__(self, img):
# STEP 1: UNDISTORT (using camera calibration step matrix and dist)
undistorted = undistort_image_computed(img, self.mtx, self.dist)
#plt.imshow(undistorted)
# STEP 3: APPLY COLOR SPACE TRANSFORM AND SOBEL THRESHOLDING
thresholded = apply_combined_gradient_to_image(undistorted)
#plt.imshow(thresholded)
# STEP 4: WARP BINARY IMAGE INTO TOP-DOWN VIEW
warped, M, Minv = warp(thresholded)
#plt.imshow(wad)
lines_fit, left_points, right_points, out_img = fit_polynomial(warped)
lines_fit1, left_points1, right_points1, out_img1 = search_around_poly(warped, lines_fit[0], lines_fit[1])
composed = compose_image_with_lines(img, warped, left_points1[0], right_points1[0], left_points1[1], Minv)
composed_with_info = add_image_real_time_info(composed, left_points1[0], right_points1[0], left_points1[1])
return composed_with_info
#example of the pipeline applied to 1 single image
img = mpimg.imread('test_images/test6.jpg')
# Process video frames with our 'process_image' function
process_image = Pipeline('./camera_cal/calibration*.jpg')
# Apply pipeline
processed = process_image(img)
# Plot the 2 images
f, (ax1, ax2) = plt.subplots(1, 2, figsize=(24, 9))
f.tight_layout()
ax1.imshow(img)
ax1.set_title('Original Image', fontsize=50)
ax2.imshow(processed, cmap='gray')
ax2.set_title('Processed Image', fontsize=50)
plt.subplots_adjust(left=0., right=1, top=0.9, bottom=0.)
# Import everything needed to edit/save/watch video clips
#!pip install moviepy
from moviepy.editor import VideoFileClip
from IPython.display import HTML
previous_image = None
def process_image(image):
result = process_image_with_drawing_lines(image)
if(result == None):
return previous_image
else:
previous_image = result
return result
def apply_video(input_video, output_video):
# Process video frames with our 'process_image' function
process_image = Pipeline('./camera_cal/calibration*.jpg')
## You may uncomment the following line for a subclip of the first 5 seconds
#clip1 = VideoFileClip(input_video).subclip(0,5)
clip1 = VideoFileClip(input_video)
white_clip = clip1.fl_image(process_image)
%time white_clip.write_videofile(output_video, audio=False)
###Output
_____no_output_____
###Markdown
Let's try the one with the solid white lane on the right first ...
###Code
input_video = './harder_challenge_video.mp4'
output_video = './harder_challenge_video_output.mp4'
apply_video(input_video, output_video)
###Output
Moviepy - Building video ./harder_challenge_video_output.mp4.
Moviepy - Writing video ./harder_challenge_video_output.mp4
###Markdown
Play the video inline, or if you prefer find the video in your filesystem (should be in the same directory) and play it in your video player of choice. Now for the one with the solid yellow lane on the left. This one's more tricky!
###Code
input_video = './project_video.mp4'
output_video = './project_video_output.mp4'
apply_video(input_video, output_video)
###Output
[MoviePy] >>>> Building video test_videos_output/solidYellowLeft.mp4
[MoviePy] Writing video test_videos_output/solidYellowLeft.mp4
|
notebooks/ivis_cnn_backbone_fashion_mnist.ipynb | ###Markdown
How to use Ivis to reduce dimensionality of image data A common objective of image analysis is dimensionality reduction. Over the last couple of months we made some adjustments to our [`Ivis`](https://github.com/beringresearch/ivis) library with the goal to make it easy to create and apply custom Neural Network backbones for dimensionality reduction of complex datasets such as images.In this notebook we will demo how to create a custom neural network and then use it to reduce dimensionality of an imaging dataset using Ivis.
###Code
!pip install ivis
import tensorflow as tf
gpus = tf.config.experimental.list_physical_devices('GPU')
if gpus:
try:
# Currently, memory growth needs to be the same across GPUs
for gpu in gpus:
tf.config.experimental.set_memory_growth(gpu, True)
logical_gpus = tf.config.experimental.list_logical_devices('GPU')
print(len(gpus), "Physical GPUs,", len(logical_gpus), "Logical GPUs")
except RuntimeError as e:
# Memory growth must be set before GPUs have been initialized
print(e)
# Ivis uses several stochastic processes.
# To make sure that results are consistent from one run to another,
# we fix the random seed
import os
os.environ["PYTHONHASHSEED"]="1234"
import random
import numpy as np
np.random.seed(1234)
random.seed(1234)
tf.random.set_seed(1234)
import matplotlib.pyplot as plt
from ivis import Ivis
###Output
_____no_output_____
###Markdown
1. Data import To start, we will use the Fashion MNIST dataset. Each image in this dataset is 28x28 pixels.
###Code
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.fashion_mnist.load_data()
x_train = x_train.astype('float32') / 255.
x_test = x_test.astype('float32') / 255.
x_train = x_train[..., tf.newaxis]
x_test = x_test[..., tf.newaxis]
###Output
_____no_output_____
###Markdown
2. Nearest neighbour retrieval Image representation Ivis uses a nearest neighbour matrix to identify positive and negative observations that are then fed into the triplet loss function. For tabular data, nearest neighbours are computed directly from the input matrix using the Annoy library. However, since images are represented as multidimensional arrays, we will use a convolutional Autoencoder to first extract latent image features, which will then be fed into our nearest neighbour algorithm. > Note: By default, to extract nearest eighbours from a multidimensional array, Ivis will simply flattent the data prior to feeding it into the Annoy algorithm. However, Ivis will still be trained on the original multidimensional dataset.
###Code
class Autoencoder(tf.keras.Model):
def __init__(self):
super(Autoencoder, self).__init__()
self.latent_dim = 128
self.encoder = tf.keras.Sequential([
tf.keras.layers.Conv2D(16, (3, 3), activation='relu', padding='same'),
tf.keras.layers.MaxPooling2D((2, 2), padding='same'),
tf.keras.layers.Conv2D(8, (3, 3), activation='relu', padding='same'),
tf.keras.layers.MaxPooling2D((2, 2), padding='same'),
tf.keras.layers.Conv2D(8, (3, 3), activation='relu', padding='same'),
tf.keras.layers.MaxPooling2D((2, 2), padding='same'),
])
self.decoder = tf.keras.Sequential([
tf.keras.layers.Conv2D(8, (3, 3), activation='relu', padding='same'),
tf.keras.layers.UpSampling2D((2, 2)),
tf.keras.layers.Conv2D(8, (3, 3), activation='relu', padding='same'),
tf.keras.layers.UpSampling2D((2, 2)),
tf.keras.layers.Conv2D(16, (3, 3), activation='relu'),
tf.keras.layers.UpSampling2D((2, 2)),
])
def call(self, x):
encoded = self.encoder(x)
decoded = self.decoder(encoded)
return decoded
autoencoder = Autoencoder()
autoencoder.compile(optimizer='adam', loss=tf.keras.losses.MeanSquaredError())
autoencoder.fit(x_train, x_train,
# In the interest of time, epochs are set to 10
epochs=10,
shuffle=True,
validation_data=(x_test, x_test))
###Output
_____no_output_____
###Markdown
The final result is a matrix of latent image representations with shape 60,000 x 128.
###Code
encoder = tf.keras.Model(inputs=autoencoder.encoder.input, outputs=autoencoder.encoder.output)
x_train_encoded = encoder.predict(x_train)
x_train_encoded = x_train_encoded.reshape((60000, 128))
###Output
_____no_output_____
###Markdown
Nearest neighbour retrieval We are now ready to extract the nearest neighbour matrix. In this example, we're using the built-in Annoy algorithm, but it can be substituted for your favourite approach.
###Code
from ivis.data.neighbour_retrieval import AnnoyKnnMatrix
annoy = AnnoyKnnMatrix.build(x_train_encoded, path='annoy.index')
nearest_neighbours = annoy.get_neighbour_indices()
###Output
1%| | 390/60000 [00:00<00:15, 3893.01it/s]
###Markdown
3. Dimensionality reduction Finally, we're ready to train Ivis. We define a simple custom convolutional network that will be used as a Siamese Network backbone.
###Code
def create_model():
model = tf.keras.models.Sequential()
model.add(tf.keras.layers.BatchNormalization(input_shape=x_train.shape[1:]))
model.add(tf.keras.layers.Conv2D(64, (5, 5), padding='same', activation='elu'))
model.add(tf.keras.layers.MaxPooling2D(pool_size=(2, 2), strides=(2,2)))
model.add(tf.keras.layers.Dropout(0.25))
model.add(tf.keras.layers.BatchNormalization(input_shape=x_train.shape[1:]))
model.add(tf.keras.layers.Conv2D(128, (5, 5), padding='same', activation='elu'))
model.add(tf.keras.layers.MaxPooling2D(pool_size=(2, 2)))
model.add(tf.keras.layers.Dropout(0.25))
model.add(tf.keras.layers.BatchNormalization(input_shape=x_train.shape[1:]))
model.add(tf.keras.layers.Conv2D(256, (5, 5), padding='same', activation='elu'))
model.add(tf.keras.layers.MaxPooling2D(pool_size=(2, 2), strides=(2,2)))
model.add(tf.keras.layers.Flatten())
return model
base_model = create_model()
###Output
_____no_output_____
###Markdown
> NOTE: It's recommended to train Ivis on a GPU, but if that isn't accessible, you can reduce the `epochs` hyperparameter to save some time.
###Code
# The base_model and the pre-computed nearest neighbour matrix is passed directly into ivis
ivis = Ivis(model=base_model, epochs=5,
neighbour_matrix=nearest_neighbours)
ivis.fit(x_train)
###Output
Training neural network
Epoch 1/5
469/469 [==============================] - 108s 224ms/step - loss: 0.1462
Epoch 2/5
469/469 [==============================] - 106s 226ms/step - loss: 0.0988
Epoch 3/5
469/469 [==============================] - 116s 247ms/step - loss: 0.0685
Epoch 4/5
469/469 [==============================] - 109s 232ms/step - loss: 0.0589
Epoch 5/5
469/469 [==============================] - 151s 321ms/step - loss: 0.0537
###Markdown
4. Visualise Embeddings
###Code
from IPython.display import set_matplotlib_formats
set_matplotlib_formats('retina')
###Output
_____no_output_____
###Markdown
Final embeddins can be obtained using the `transform` method. Here we examine trainig and testing set embeddings.
###Code
embeddings_train = ivis.transform(x_train)
embeddings_test = ivis.transform(x_test)
fig, axs = plt.subplots(1, 2, figsize=(9, 3))
axs[0].scatter(embeddings_train[:, 0], embeddings_train[:, 1], c=y_train, s=0.1)
axs[0].set_xlabel('ivis 1')
axs[0].set_ylabel('ivis 1')
axs[0].set_title('Training set')
axs[1].scatter(embeddings_test[:, 0], embeddings_test[:, 1], c=y_test, s=0.1)
axs[1].set_xlabel('ivis 1')
axs[1].set_ylabel('ivis 1')
axs[1].set_title('Testing set')
###Output
_____no_output_____
###Markdown
How to use Ivis to reduce dimensionality of image data A common objective of image analysis is dimensionality reduction. Over the last couple of months we made some adjustments to our [`Ivis`](https://github.com/beringresearch/ivis) library with the goal to make it easy to create and apply custom Neural Network backbones for dimensionality reduction of complex datasets such as images.In this notebook we will demo how to create a custom neural network and then use it to reduce dimensionality of an imaging dataset using Ivis.
###Code
!pip install ivis
# Ivis uses several stochastic processes.
# To make sure that results are consistent from one run to another,
# we fix the random seed
import os
os.environ["PYTHONHASHSEED"]="1234"
import random
import numpy as np
import tensorflow as tf
np.random.seed(1234)
random.seed(1234)
tf.random.set_seed(1234)
import matplotlib.pyplot as plt
from ivis import Ivis
###Output
_____no_output_____
###Markdown
1. Data import To start, we will use the Fashion MNIST dataset. Each image in this dataset is 28x28 pixels.
###Code
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.fashion_mnist.load_data()
x_train = x_train.astype('float32') / 255.
x_test = x_test.astype('float32') / 255.
x_train = x_train[..., tf.newaxis]
x_test = x_test[..., tf.newaxis]
###Output
_____no_output_____
###Markdown
2. Nearest neighbour retrieval Image representation Ivis uses a nearest neighbour matrix to identify positive and negative observations that are then fed into the triplet loss function. For tabular data, nearest neighbours are computed directly from the input matrix using the Annoy library. However, since images are represented as multidimensional arrays, we will use a convolutional Autoencoder to first extract latent image features, which will then be fed into our nearest neighbour algorithm. > Note: By default, to extract nearest eighbours from a multidimensional array, Ivis will simply flattent the data prior to feeding it into the Annoy algorithm. However, Ivis will still be trained on the original multidimensional dataset.
###Code
class Autoencoder(tf.keras.Model):
def __init__(self):
super(Autoencoder, self).__init__()
self.latent_dim = latent_dim
self.encoder = tf.keras.Sequential([
tf.keras.layers.Conv2D(16, (3, 3), activation='relu', padding='same'),
tf.keras.layers.MaxPooling2D((2, 2), padding='same'),
tf.keras.layers.Conv2D(8, (3, 3), activation='relu', padding='same'),
tf.keras.layers.MaxPooling2D((2, 2), padding='same'),
tf.keras.layers.Conv2D(8, (3, 3), activation='relu', padding='same'),
tf.keras.layers.MaxPooling2D((2, 2), padding='same'),
])
self.decoder = tf.keras.Sequential([
tf.keras.layers.Conv2D(8, (3, 3), activation='relu', padding='same'),
tf.keras.layers.UpSampling2D((2, 2)),
tf.keras.layers.Conv2D(8, (3, 3), activation='relu', padding='same'),
tf.keras.layers.UpSampling2D((2, 2)),
tf.keras.layers.Conv2D(16, (3, 3), activation='relu'),
tf.keras.layers.UpSampling2D((2, 2)),
])
def call(self, x):
encoded = self.encoder(x)
decoded = self.decoder(encoded)
return decoded
autoencoder = Autoencoder()
autoencoder.compile(optimizer='adam', loss=tf.keras.losses.MeanSquaredError())
autoencoder.fit(x_train, x_train,
# In the interest of time, epochs are set to 10
epochs=10,
shuffle=True,
validation_data=(x_test, x_test))
###Output
Epoch 1/10
1875/1875 [==============================] - 9s 5ms/step - loss: 0.0419 - val_loss: 0.0246
Epoch 2/10
1875/1875 [==============================] - 10s 5ms/step - loss: 0.0242 - val_loss: 0.0234
Epoch 3/10
1875/1875 [==============================] - 9s 5ms/step - loss: 0.0232 - val_loss: 0.0227
Epoch 4/10
1875/1875 [==============================] - 9s 5ms/step - loss: 0.0226 - val_loss: 0.0222
Epoch 5/10
1875/1875 [==============================] - 10s 5ms/step - loss: 0.0221 - val_loss: 0.0220
Epoch 6/10
1875/1875 [==============================] - 9s 5ms/step - loss: 0.0217 - val_loss: 0.0217
Epoch 7/10
1875/1875 [==============================] - 10s 5ms/step - loss: 0.0215 - val_loss: 0.0214
Epoch 8/10
1875/1875 [==============================] - 9s 5ms/step - loss: 0.0213 - val_loss: 0.0212
Epoch 9/10
1875/1875 [==============================] - 9s 5ms/step - loss: 0.0211 - val_loss: 0.0211
Epoch 10/10
1875/1875 [==============================] - 9s 5ms/step - loss: 0.0210 - val_loss: 0.0210
###Markdown
The final result is a matrix of latent image representations with shape 60,000 x 128.
###Code
encoder = tf.keras.Model(inputs=autoencoder.encoder.input, outputs=autoencoder.encoder.output)
x_train_encoded = encoder.predict(x_train)
x_train_encoded = x_train_encoded.reshape((60000, 128))
###Output
_____no_output_____
###Markdown
Nearest neighbour retrieval We are now ready to extract the nearest neighbour matrix. In this example, we're using the built-in Annoy algorithm, but it can be substituted for your favourite approach.
###Code
from ivis.data.neighbour_retrieval import AnnoyKnnMatrix
annoy = AnnoyKnnMatrix.build(x_train_encoded, path='annoy.index')
nearest_neighbours = annoy.get_neighbour_indices()
###Output
Extracting KNN neighbours
###Markdown
3. Dimensionality reduction Finally, we're ready to train Ivis. We define a simple custom convolutional network that will be used to as a Siamese Network backbone.
###Code
def create_model():
model = tf.keras.models.Sequential()
model.add(tf.keras.layers.BatchNormalization(input_shape=x_train.shape[1:]))
model.add(tf.keras.layers.Conv2D(64, (5, 5), padding='same', activation='elu'))
model.add(tf.keras.layers.MaxPooling2D(pool_size=(2, 2), strides=(2,2)))
model.add(tf.keras.layers.Dropout(0.25))
model.add(tf.keras.layers.BatchNormalization(input_shape=x_train.shape[1:]))
model.add(tf.keras.layers.Conv2D(128, (5, 5), padding='same', activation='elu'))
model.add(tf.keras.layers.MaxPooling2D(pool_size=(2, 2)))
model.add(tf.keras.layers.Dropout(0.25))
model.add(tf.keras.layers.BatchNormalization(input_shape=x_train.shape[1:]))
model.add(tf.keras.layers.Conv2D(256, (5, 5), padding='same', activation='elu'))
model.add(tf.keras.layers.MaxPooling2D(pool_size=(2, 2), strides=(2,2)))
model.add(tf.keras.layers.Flatten())
return model
base_model = create_model()
###Output
_____no_output_____
###Markdown
> NOTE: It's recommended to train Ivis on a GPU, but if that isn't accessible, you can reduce the `epochs` hyperparameter to save some time.
###Code
# The base_model and the pre-computed nearest neighbour matrix is passed directly into ivis
ivis = Ivis(model=base_model, epochs=5,
neighbour_matrix=nearest_neighbours)
ivis.fit(x_train)
###Output
Training neural network
Epoch 1/5
469/469 [==============================] - 106s 223ms/step - loss: 0.0523
Epoch 2/5
469/469 [==============================] - 102s 218ms/step - loss: 0.0445
Epoch 3/5
469/469 [==============================] - 104s 221ms/step - loss: 0.0435
Epoch 4/5
469/469 [==============================] - 103s 220ms/step - loss: 0.0441
Epoch 5/5
469/469 [==============================] - 104s 221ms/step - loss: 0.0420
###Markdown
4. Visualise Embeddings
###Code
from IPython.display import set_matplotlib_formats
set_matplotlib_formats('retina')
###Output
_____no_output_____
###Markdown
Final embeddins can be obtained using the `transform` method. Here we examine trainig and testing set embeddings.
###Code
embeddings_train = ivis.transform(x_train)
embeddings_test = ivis.transform(x_test)
fig, axs = plt.subplots(1, 2, figsize=(9, 3))
axs[0].scatter(embeddings_train[:, 0], embeddings_train[:, 1], c=y_train, s=0.1)
axs[0].set_xlabel('ivis 1')
axs[0].set_ylabel('ivis 1')
axs[0].set_title('Training set')
axs[1].scatter(embeddings_test[:, 0], embeddings_test[:, 1], c=y_test, s=0.1)
axs[1].set_xlabel('ivis 1')
axs[1].set_ylabel('ivis 1')
axs[1].set_title('Testing set')
###Output
_____no_output_____ |
tutorial013Exercise.ipynb | ###Markdown
In class exercise for Tutorial 13: Looping back and forward a little Set up your GitHub Repo for the exerciseFor this exercise we will ask you to go back to the beginning of the semester and use GitHub. We will also practice with loops and if statements.To get started follow these instructions: (1) Create a new GitHub repository under your account on GitHub.com called 'FDS-2022-Exercises' (2) Clone the repository locally on your computer. Say under your local 'git' folder (3) Initialize it as your like it (add a readme file, a license, etc) (4) Create a new file inside the repository locally: tutorial13Exercise_.ipynb (5) Add the file to the Gitreposiory (6) Commit the to the repository and push it to the cloud. (7) Use the above jupyter notebook and the repository you set up to complete the exercises below.The PDF submitted on canvas of tutorial13Exercise_YourInitials.ipynb will need to report the URL of the jupyter notebook from your repository on GitHub.com. You can add the URL in the following cell. URL of your tutorial13Exercise on GitHub: Simulating dataCreate 5 distributions of 1,000 normally distributed random data with the following characteristics: - Dist 1: mean = 5 SD = 2 - Dist 2: mean = 7 SD = 4 - Dist 3: mean = 9 SD = 6 - Dist 4: mean = 11 SD = 8 - Dist 5: mean = 13 SD = 10 Use for loops to generate the distributions
###Code
# import what you need for numerical arrays and pretty plots
# create arrays for your means and SDs values
# create the distributions and store them
# into a single numpy array 'dist'
# Plot the KDEs of the distributions in a single figure
# Plot the KDEs of the distributions in multiple subplots
#
# hint: you might need to use zip() to return two counters as a tuple
# zip(my_index_vals, my_axis_vals)
# https://docs.python.org/3/library/functions.html#zip
###Output
_____no_output_____ |
notebooks/users/john-dileo/session-data-mappings/first-view-of-csv-data.ipynb | ###Markdown
this is a first view of the CSV export From the GSheet document created by John: https://docs.google.com/spreadsheets/d/1KjYydaCgeMm-Xwh3PQEeY82MQLDlJGZ-/
###Code
import osbot_jupyter
%%HTML
<iframe src="https://docs.google.com/spreadsheets/d/1KjYydaCgeMm-Xwh3PQEeY82MQLDlJGZ-/" width="100%" , height="500">
import pandas as pd
csv_file = './Session Data and Tags--20190601.xlsx - Sheet1.csv'
df_sessions = pd.read_csv(csv_file)
df_sessions
df_sessions.columns
import qgrid
columns = ['Title', 'Track', 'Type', 'Organiser(s)', 'Participants',
'Day', 'Time', 'Synopsis?', 'Locked?',
# 'OWASP Type', 'CSF Category', 'SAMM 2.0 Practice & Stream', 'Tags',
# 'Notes','Remote Participants'
]
qgrid.show_grid(df_sessions[columns])
df_sessions['CSF Category'].group_by('CSF Category')
###Output
_____no_output_____ |
notebook/ASL_MobileNetV2.ipynb | ###Markdown
Determine the dimension of the images
###Code
dim1 = []
dim2 = []
for image_filename in os.listdir(train_dir+'A'):
img = mpimg.imread(train_dir+'A'+'/'+image_filename)
d1,d2,colors = img.shape
dim1.append(d1)
dim2.append(d2)
sns.jointplot(dim1,dim2)
print(np.mean(dim1))
print(np.mean(dim2))
###Output
200.0
200.0
###Markdown
`Height` is 200 and `Width` is 200
###Code
image_paths = list(paths.list_images("train"))
np.random.shuffle(image_paths)
image_paths[:5]
###Output
_____no_output_____
###Markdown
Counting number of images per class
###Code
labels = []
for image_path in image_paths:
label = image_path.split("/")[1]
labels.append(label)
class_count = Counter(labels)
pprint(class_count)
###Output
Counter({'Q': 3000,
'G': 3000,
'H': 3000,
'space': 3000,
'K': 3000,
'N': 3000,
'F': 3000,
'V': 3000,
'R': 3000,
'A': 3000,
'U': 3000,
'nothing': 3000,
'W': 3000,
'L': 3000,
'S': 3000,
'I': 3000,
'del': 3000,
'Y': 3000,
'O': 3000,
'D': 3000,
'T': 3000,
'C': 3000,
'E': 3000,
'P': 3000,
'J': 3000,
'M': 3000,
'Z': 3000,
'B': 3000,
'X': 3000})
###Markdown
Wow! Balanced Dataset Define the Hyperparamteres
###Code
TRAIN_SPLIT = 0.9
BATCH_SIZE = 256
AUTO = tf.data.AUTOTUNE
EPOCHS = 100
IMG_SIZE = 224
NUM_CLASSES=29
###Output
_____no_output_____
###Markdown
Splitting the dataset
###Code
i = int(len(image_paths) * TRAIN_SPLIT)
train_paths = image_paths[:i]
train_labels = labels[:i]
validation_paths = image_paths[i:]
validation_labels = labels[i:]
print(len(train_paths), len(validation_paths))
###Output
78300 8700
###Markdown
Labelling the dataset
###Code
le = LabelEncoder()
train_labels_le = le.fit_transform(train_labels)
validation_labels_le = le.transform(validation_labels)
print(train_labels_le[:5])
###Output
[16 6 7 28 10]
###Markdown
Preprocessing the data
###Code
@tf.function
def load_images(image_path, label):
image = tf.io.read_file(image_path)
image = tf.image.decode_jpeg(image, channels=3)
image = tf.cast(image, tf.float32) / 255.
image = tf.image.resize(image, (IMG_SIZE, IMG_SIZE))
return (image, label)
data_augmentation = tf.keras.Sequential(
[
tf.keras.layers.experimental.preprocessing.RandomFlip("horizontal"),
tf.keras.layers.experimental.preprocessing.RandomRotation(factor=0.02),
tf.keras.layers.experimental.preprocessing.RandomZoom(
height_factor=0.2, width_factor=0.2
),
],
name="data_augmentation",
)
###Output
_____no_output_____
###Markdown
Creating the `Data` Pipeline
###Code
train_ds = tf.data.Dataset.from_tensor_slices((train_paths, train_labels_le))
train_ds = (
train_ds
.shuffle(1024)
.map(load_images, num_parallel_calls=AUTO)
.batch(BATCH_SIZE)
.map(lambda x, y: (data_augmentation(x), y), num_parallel_calls=AUTO)
.prefetch(AUTO)
)
val_ds = tf.data.Dataset.from_tensor_slices((validation_paths, validation_labels_le))
val_ds = (
val_ds
.map(load_images, num_parallel_calls=AUTO)
.batch(BATCH_SIZE)
.prefetch(AUTO)
)
###Output
_____no_output_____
###Markdown
Define the Model
###Code
def get_training_model(trainable=False):
# Load the MobileNetV2 model but exclude the classification layers
EXTRACTOR = MobileNetV2(weights="imagenet", include_top=False,
input_shape=(224, 224, 3))
# We will set it to both True and False
EXTRACTOR.trainable = trainable
# Construct the head of the model that will be placed on top of the
# the base model
class_head = EXTRACTOR.output
class_head = GlobalAveragePooling2D()(class_head)
class_head = Dense(512, activation="relu")(class_head)
class_head = Dropout(0.5)(class_head)
class_head = Dense(NUM_CLASSES, activation="softmax")(class_head)
# Create the new model
classifier = tf.keras.Model(inputs=EXTRACTOR.input, outputs=class_head)
# Compile and return the model
classifier.compile(loss="sparse_categorical_crossentropy",
optimizer="adam",
metrics=["accuracy"])
return classifier
###Output
_____no_output_____
###Markdown
Plot
###Code
def plot_hist(hist):
plt.plot(hist.history["accuracy"])
plt.plot(hist.history["val_accuracy"])
plt.plot(hist.history["loss"])
plt.plot(hist.history["val_loss"])
plt.title("Training Progress")
plt.ylabel("Accuracy/Loss")
plt.xlabel("Epochs")
plt.legend(["train_accuracy", "val_accuracy", "train_loss", "val_loss"], loc="upper left")
plt.show()
###Output
_____no_output_____
###Markdown
Define the Callback
###Code
train_callbacks = [
tf.keras.callbacks.EarlyStopping(
monitor='val_accuracy', patience=2, restore_best_weights=True)
]
###Output
_____no_output_____
###Markdown
Train the Model
###Code
classifier = get_training_model()
h = classifier.fit(train_ds,
validation_data=val_ds,
epochs=EPOCHS,
batch_size=BATCH_SIZE,
callbacks=train_callbacks)
accuracy = classifier.evaluate(val_ds)[1] * 100
print("Accuracy: {:.2f}%".format(accuracy))
plot_hist(h)
###Output
Downloading data from https://storage.googleapis.com/tensorflow/keras-applications/mobilenet_v2/mobilenet_v2_weights_tf_dim_ordering_tf_kernels_1.0_224_no_top.h5
9412608/9406464 [==============================] - 0s 0us/step
Epoch 1/100
306/306 [==============================] - 670s 2s/step - loss: 1.2481 - accuracy: 0.6500 - val_loss: 0.1612 - val_accuracy: 0.9552
Epoch 2/100
306/306 [==============================] - 757s 2s/step - loss: 0.2466 - accuracy: 0.9238 - val_loss: 0.0905 - val_accuracy: 0.9755
Epoch 3/100
306/306 [==============================] - 757s 2s/step - loss: 0.1761 - accuracy: 0.9439 - val_loss: 0.0652 - val_accuracy: 0.9824
Epoch 4/100
306/306 [==============================] - 759s 2s/step - loss: 0.1444 - accuracy: 0.9543 - val_loss: 0.0504 - val_accuracy: 0.9851
Epoch 5/100
306/306 [==============================] - 757s 2s/step - loss: 0.1253 - accuracy: 0.9584 - val_loss: 0.0447 - val_accuracy: 0.9872
Epoch 6/100
306/306 [==============================] - 756s 2s/step - loss: 0.1111 - accuracy: 0.9641 - val_loss: 0.0385 - val_accuracy: 0.9886
Epoch 7/100
306/306 [==============================] - 760s 2s/step - loss: 0.0989 - accuracy: 0.9666 - val_loss: 0.0368 - val_accuracy: 0.9900
Epoch 8/100
306/306 [==============================] - 762s 2s/step - loss: 0.0954 - accuracy: 0.9679 - val_loss: 0.0362 - val_accuracy: 0.9897
Epoch 9/100
306/306 [==============================] - 758s 2s/step - loss: 0.0914 - accuracy: 0.9694 - val_loss: 0.0342 - val_accuracy: 0.9891
34/34 [==============================] - 10s 277ms/step - loss: 0.0368 - accuracy: 0.9900
Accuracy: 99.00%
###Markdown
Saving our model
###Code
classifier.save('asl_model')
!du -lh asl_model
###Output
4.0K asl_model/assets
17M asl_model/variables
20M asl_model
###Markdown
Saving the h5 file
###Code
KERAS_ASL_FILE = 'asl.h5'
classifier.save(KERAS_ASL_FILE)
###Output
_____no_output_____
###Markdown
Helper Function - To determine the file size of our model
###Code
import os
from sys import getsizeof
def get_file_size(file_path):
size = os.path.getsize(file_path)
return size
def convert_bytes(size, unit=None):
if unit == "KB":
return print('File Size: ' + str(round(size/1024, 3)) + 'Kilobytes')
elif unit == 'MB':
return print('File Size: ' + str(round(size/(1024*1024), 3)) + 'Megabytes')
else:
return print('File Size: ' + str(size) + 'bytes')
convert_bytes(get_file_size(KERAS_ASL_FILE), "MB")
###Output
File Size: 16.75Megabytes
###Markdown
Wow! 16MB
###Code
converter = tf.lite.TFLiteConverter.from_saved_model("asl_model")
converter.optimizations = [tf.lite.Optimize.DEFAULT]
tflite_model = converter.convert()
open("asl_optimise.tflite", 'wb').write(tflite_model)
print('Model size is %f MBs.' % (len(tflite_model) / 1024 / 1024.0))
###Output
Model size is 3.146927 MBs.
###Markdown
Zipping our model together
###Code
!tar cvf asl_model.tar.gz asl_model asl.h5 asl.tflite asl_optimise.tflite
###Output
asl_model/
asl_model/assets/
asl_model/variables/
asl_model/variables/variables.data-00000-of-00001
asl_model/variables/variables.index
asl_model/saved_model.pb
asl.h5
asl.tflite
asl_optimise.tflite
###Markdown
Testing Pipeline
###Code
def preprocess_image(image_path):
image = tf.io.read_file(image_path)
image = tf.image.decode_jpeg(image, channels=3)
image = tf.cast(image, tf.float32) / 255.
image = tf.image.resize(image, (IMG_SIZE, IMG_SIZE))
return image
test_image_paths = (list(paths.list_images("test")))
print(f"Total test images: {len(test_image_paths)}")
test_image_paths[:28]
test_ds = tf.data.Dataset.from_tensor_slices(test_image_paths)
test_ds = (
test_ds
.map(preprocess_image)
.batch(BATCH_SIZE)
)
test_predictions = np.argmax(classifier.predict(test_ds), 1)
test_predictions.shape
test_predictions[:28]
test_predictions_le = le.inverse_transform(test_predictions)
test_predictions_le[:28]
interpreter = tf.lite.Interpreter(model_path = 'asl.tflite')
input_details = interpreter.get_input_details()
output_details = interpreter.get_output_details()
print("Input Shape:", input_details[0]['shape'])
print("Input Type:", input_details[0]['dtype'])
print("Output Shape:", output_details[0]['shape'])
print("Output Type:", output_details[0]['dtype'])
interpreter.resize_tensor_input(input_details[0]['index'], (28, 224, 224, 3))
interpreter.resize_tensor_input(output_details[0]['index'], (28, 29))
interpreter.allocate_tensors()
input_details = interpreter.get_input_details()
output_details = interpreter.get_output_details()
print("Input Shape:", input_details[0]['shape'])
print("Input Type:", input_details[0]['dtype'])
print("Output Shape:", output_details[0]['shape'])
print("Output Type:", output_details[0]['dtype'])
test_imgs = next(iter(test_ds))
interpreter.set_tensor(input_details[0]['index'], test_imgs)
interpreter.invoke()
tflite_model_predictions = interpreter.get_tensor(output_details[0]['index'])
print("Prediction results shape:", tflite_model_predictions.shape)
prediction_classes = np.argmax(tflite_model_predictions, axis=1)
prediction_classes[:28]
prediction_classes_le = le.inverse_transform(prediction_classes)
prediction_classes_le[:28]
###Output
_____no_output_____
###Markdown
American Sign Language - MobileNetV2Author: [Sayan Nath](https://github.com/sayannath)Dataset Link: [Kaggle ASL](https://www.kaggle.com/grassknoted/asl-alphabet) Initial Setup
###Code
!nvidia-smi
!pip install -q kaggle
!pip install -qq tensorflow-addons
from google.colab import files
files.upload()
###Output
_____no_output_____
###Markdown
Data Gathering
###Code
!mkdir ~p ~/.kaggle
!cp kaggle.json ~/.kaggle/
#Change the permission
!chmod 600 ~/.kaggle/kaggle.json
!kaggle datasets download -d grassknoted/asl-alphabet
!unzip -q asl-alphabet.zip
mkdir train
import shutil
shutil.make_archive('dataset', 'zip', '/content/asl_alphabet_train/asl_alphabet_train')
!pip install patool
import patoolib
patoolib.extract_archive("dataset.zip", outdir="train/")
rm -rf asl_alphabet_train
###Output
_____no_output_____
###Markdown
Setting up Path
###Code
train_dir = 'train/'
###Output
_____no_output_____
###Markdown
Import the modules
###Code
import tensorflow as tf
tf.random.set_seed(42)
print(tf.__version__)
from imutils import paths
from pprint import pprint
from collections import Counter
import tensorflow_hub as hub
from sklearn.preprocessing import LabelEncoder
from tensorflow.keras.utils import to_categorical
from tensorflow.keras.models import load_model, Sequential
from tensorflow.keras.applications import MobileNetV2
from tensorflow.keras.layers import *
import matplotlib.pyplot as plt
import re
import os
import pandas as pd
import matplotlib.image as mpimg
import seaborn as sns
import numpy as np
np.random.seed(42)
# from tensorflow.keras import mixed_precision
# mixed_precision.set_global_policy('mixed_float16')
###Output
_____no_output_____ |
bronze/B84_Phase_Kickback.ipynb | ###Markdown
prepared by Abuzer Yakaryilmaz (QLatvia) updated by Özlem Salehi | October 26, 2020 This cell contains some macros. If there is a problem with displaying mathematical formulas, please run this cell to load these macros. $ \newcommand{\bra}[1]{\langle 1|} $$ \newcommand{\ket}[1]{|1\rangle} $$ \newcommand{\braket}[2]{\langle 1|2\rangle} $$ \newcommand{\dot}[2]{ 1 \cdot 2} $$ \newcommand{\biginner}[2]{\left\langle 1,2\right\rangle} $$ \newcommand{\mymatrix}[2]{\left( \begin{array}{1} 2\end{array} \right)} $$ \newcommand{\myvector}[1]{\mymatrix{c}{1}} $$ \newcommand{\myrvector}[1]{\mymatrix{r}{1}} $$ \newcommand{\mypar}[1]{\left( 1 \right)} $$ \newcommand{\mybigpar}[1]{ \Big( 1 \Big)} $$ \newcommand{\sqrttwo}{\frac{1}{\sqrt{2}}} $$ \newcommand{\dsqrttwo}{\dfrac{1}{\sqrt{2}}} $$ \newcommand{\onehalf}{\frac{1}{2}} $$ \newcommand{\donehalf}{\dfrac{1}{2}} $$ \newcommand{\hadamard}{ \mymatrix{rr}{ \sqrttwo & \sqrttwo \\ \sqrttwo & -\sqrttwo }} $$ \newcommand{\vzero}{\myvector{1\\0}} $$ \newcommand{\vone}{\myvector{0\\1}} $$ \newcommand{\vhadamardzero}{\myvector{ \sqrttwo \\ \sqrttwo } } $$ \newcommand{\vhadamardone}{ \myrvector{ \sqrttwo \\ -\sqrttwo } } $$ \newcommand{\myarray}[2]{ \begin{array}{1}2\end{array}} $$ \newcommand{\X}{ \mymatrix{cc}{0 & 1 \\ 1 & 0} } $$ \newcommand{\Z}{ \mymatrix{rr}{1 & 0 \\ 0 & -1} } $$ \newcommand{\Htwo}{ \mymatrix{rrrr}{ \frac{1}{2} & \frac{1}{2} & \frac{1}{2} & \frac{1}{2} \\ \frac{1}{2} & -\frac{1}{2} & \frac{1}{2} & -\frac{1}{2} \\ \frac{1}{2} & \frac{1}{2} & -\frac{1}{2} & -\frac{1}{2} \\ \frac{1}{2} & -\frac{1}{2} & -\frac{1}{2} & \frac{1}{2} } } $$ \newcommand{\CNOT}{ \mymatrix{cccc}{1 & 0 & 0 & 0 \\ 0 & 1 & 0 & 0 \\ 0 & 0 & 0 & 1 \\ 0 & 0 & 1 & 0} } $$ \newcommand{\norm}[1]{ \left\lVert 1 \right\rVert } $ Phase Kickback Consider the quantum state $\ket{-}=\frac{1}{\sqrt{2}}\ket{0}-\frac{1}{\sqrt{2}}\ket{1}$. Let's check the effect of applying NOT operator to the quantum state $\ket{-}$. \begin{align*}\ket{-} \xrightarrow{NOT} &= \frac{1}{\sqrt{2}} \ket{1} - \frac{1}{\sqrt{2}} \ket{0}= \text{-} \left(\frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1}\right)= \text{-}\ket{-}\end{align*} So we see that $NOT\ket{-} = \text{-}\ket{-}$. This is called phase kickback._The eigenvalue is kicked back to the control register._ _Note: $\ket{-}$ is an eigenstate of the NOT operator with eigenvalue -1._ Now we will observe another interesting quantum effect here. We will apply a Controlled-NOT operator, but the controller qubit will be affected(!) Suppose that the second qubit is in state $\ket{-}$. When the first qubit is in state $\ket{0}$, then CNOT does not have any effect.$\ket{0}\ket{-} \xrightarrow{CNOT} \ket{0}\ket{-}$ When the first qubit is in state $\ket{1}$, then we have the following state.$\ket{1}\ket{-} \xrightarrow{CNOT} \text{-}\ket{1}\ket{-}$ Now suppose that first qubit is in state $\alpha \ket{0} + \beta \ket{1}$ and the second qubit is in state $\ket{-}$, that is we have the following quantum state $\alpha \ket{0}\ket{-} + \beta \ket{1}\ket{-}$.$\alpha \ket{0}\ket{-} + \beta \ket{1}\ket{-} \xrightarrow{CNOT} \alpha \ket{0}\ket{-} ~-~ \beta \ket{1}\ket{-} = (\alpha \ket{0} - \beta \ket{1})\ket{-}$ The state of the first qubit after the CNOT is $\alpha \ket{0} - \beta \ket{1}$The sign of $ \ket{1} $ in the first qubit changes sign after the CNOT operator. Task 1Create a quantum circuit with two qubits.Set the state of the first qubit to $ \ket{0} $.Set the state of the second qubit to $ \ket{1} $.Apply Hadamard to both qubits.Apply CNOT operator, where the controller qubit is the first qubit and the target qubit is the second qubit.Apply Hadamard to both qubits.Measure the outcomes.We start in quantum state $ \ket{01} $. What is the outcome?
###Code
# import all necessary objects and methods for quantum circuits
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
#
# your code is here
#
###Output
_____no_output_____
###Markdown
click for our solution Analysis The quantum state of the first qubit before CNOT:$$ \ket{0} \xrightarrow{H} \frac{1}{\sqrt{2}} \ket{0} + \frac{1}{\sqrt{2}} \ket{1}.$$The quantum state of the second qubit before CNOT:$$ \ket{1} \xrightarrow{H} \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1}.$$ The quantum state of the composite system:$$ \mypar{ \frac{1}{\sqrt{2}} \ket{0} + \frac{1}{\sqrt{2}} \ket{1} } \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$ CNOT affects when the first qubit has the value 1.Let's rewrite the composite state as below to explicitly represent the effect of CNOT.$$ \frac{1}{\sqrt{2}} \ket{0} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } + \frac{1}{\sqrt{2}} \ket{1} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$ CNOT flips the state of the second qubit.After CNOT, we have:$$ \frac{1}{\sqrt{2}} \ket{0} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } + \frac{1}{\sqrt{2}} \ket{1} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{1} - \frac{1}{\sqrt{2}} \ket{0} }$$Remark that $\ket{0}$ and $ \ket{1} $ are swapped in the second qubit.Thus the last equation can be equivalently written as follows:$$ \frac{1}{\sqrt{2}} \ket{0} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } - \frac{1}{\sqrt{2}} \ket{1} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$It is easy to see from the last expression, that the quantum states of the qubits are separable (no correlation):$$ \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$Note that the sign of $ \ket{1} $ in the first qubit is flipped. If we apply Hadamard to each qubit, both qubits evolve to state $ \ket{1} $.Hence, the final state is $ \ket{11} $. Task 2 Create a curcuit with 7 qubits.Set the states of the first six qubits to $ \ket{0} $.Set the state of the last qubit to $ \ket{1} $.Apply Hadamard operators to all qubits.Apply CNOT operator (first-qubit,last-qubit) Apply CNOT operator (fourth-qubit,last-qubit)Apply CNOT operator (fifth-qubit,last-qubit)Apply Hadamard operators to all qubits.Measure all qubits. For each CNOT operator, do we have a phase-kickback effect?
###Code
# import all necessary objects and methods for quantum circuits
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
#
# your code is here
#
###Output
_____no_output_____
###Markdown
Abuzer Yakaryilmaz | May 1, 2019 (updated) This cell contains some macros. If there is a problem with displaying mathematical formulas, please run this cell to load these macros. $ \newcommand{\bra}[1]{\langle 1|} $$ \newcommand{\ket}[1]{|1\rangle} $$ \newcommand{\braket}[2]{\langle 1|2\rangle} $$ \newcommand{\dot}[2]{ 1 \cdot 2} $$ \newcommand{\biginner}[2]{\left\langle 1,2\right\rangle} $$ \newcommand{\mymatrix}[2]{\left( \begin{array}{1} 2\end{array} \right)} $$ \newcommand{\myvector}[1]{\mymatrix{c}{1}} $$ \newcommand{\myrvector}[1]{\mymatrix{r}{1}} $$ \newcommand{\mypar}[1]{\left( 1 \right)} $$ \newcommand{\mybigpar}[1]{ \Big( 1 \Big)} $$ \newcommand{\sqrttwo}{\frac{1}{\sqrt{2}}} $$ \newcommand{\dsqrttwo}{\dfrac{1}{\sqrt{2}}} $$ \newcommand{\onehalf}{\frac{1}{2}} $$ \newcommand{\donehalf}{\dfrac{1}{2}} $$ \newcommand{\hadamard}{ \mymatrix{rr}{ \sqrttwo & \sqrttwo \\ \sqrttwo & -\sqrttwo }} $$ \newcommand{\vzero}{\myvector{1\\0}} $$ \newcommand{\vone}{\myvector{0\\1}} $$ \newcommand{\vhadamardzero}{\myvector{ \sqrttwo \\ \sqrttwo } } $$ \newcommand{\vhadamardone}{ \myrvector{ \sqrttwo \\ -\sqrttwo } } $$ \newcommand{\myarray}[2]{ \begin{array}{1}2\end{array}} $$ \newcommand{\X}{ \mymatrix{cc}{0 & 1 \\ 1 & 0} } $$ \newcommand{\Z}{ \mymatrix{rr}{1 & 0 \\ 0 & -1} } $$ \newcommand{\Htwo}{ \mymatrix{rrrr}{ \frac{1}{2} & \frac{1}{2} & \frac{1}{2} & \frac{1}{2} \\ \frac{1}{2} & -\frac{1}{2} & \frac{1}{2} & -\frac{1}{2} \\ \frac{1}{2} & \frac{1}{2} & -\frac{1}{2} & -\frac{1}{2} \\ \frac{1}{2} & -\frac{1}{2} & -\frac{1}{2} & \frac{1}{2} } } $$ \newcommand{\CNOT}{ \mymatrix{cccc}{1 & 0 & 0 & 0 \\ 0 & 1 & 0 & 0 \\ 0 & 0 & 0 & 1 \\ 0 & 0 & 1 & 0} } $$ \newcommand{\norm}[1]{ \left\lVert 1 \right\rVert } $ Phase KickbackWe observe another interesting quantum effect here.We apply a Controlled-NOT operator, but the controller qubit will be affected(!) Task 1Create a quantum circuit with two qubits.Set the state of the first qubit to $ \ket{0} $.Set the state of the second qubit to $ \ket{1} $.Apply Hadamard to both qubits.Apply CNOT operator, where the controller qubit is the first qubit and the target qubit is the second qubit.Apply Hadamard to both qubits.Measure the outcomes.We start in quantum state $ \ket{01} $. What is the outcome?
###Code
# import all necessary objects and methods for quantum circuits
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
#
# your code is here
qreg1 = QuantumRegister(2) # quantum register with 2 qubits
creg1 = ClassicalRegister(2) # classical register with 2 bits
mycircuit1 = QuantumCircuit(qreg1,creg1) # quantum circuit with quantum and classical registers
# the first qubit is in |0>
# set the second qubit to |1>
mycircuit1.x(qreg1[1]) # apply x-gate (NOT operator)
# apply Hadamard to both qubits.
mycircuit1.h(qreg1[0])
mycircuit1.h(qreg1[1])
# apply CNOT operator, where the controller qubit is the first qubit and the target qubit is the second qubit.
mycircuit1.cx(qreg1[0],qreg1[1])
# apply Hadamard to both qubits.
mycircuit1.h(qreg1[0])
mycircuit1.h(qreg1[1])
# measure both qubits
mycircuit1.measure(qreg1,creg1)
# execute the circuit 100 times in the local simulator
job = execute(mycircuit1,Aer.get_backend('qasm_simulator'),shots=100)
counts = job.result().get_counts(mycircuit1)
# print the reverse of the outcome
for outcome in counts:
reverse_outcome = ''
for i in outcome:
reverse_outcome = i + reverse_outcome
print("Start state: 01, Result state: ",reverse_outcome," Observed",counts[outcome],"times")
###Output
Start state: 01, Result state: 11 Observed 100 times
###Markdown
click for our solution The effect of CNOT The quantum state of the first qubit before CNOT:$$ \ket{0} \xrightarrow{H} \frac{1}{\sqrt{2}} \ket{0} + \frac{1}{\sqrt{2}} \ket{1}.$$The quantum state of the second qubit before CNOT:$$ \ket{1} \xrightarrow{H} \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1}.$$ The quantum state of the composite system:$$ \mypar{ \frac{1}{\sqrt{2}} \ket{0} + \frac{1}{\sqrt{2}} \ket{1} } \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$ CNOT affects when the first qubit has the value 1.Let's rewrite the composite state as below to explicitly represent the effect of CNOT.$$ \frac{1}{\sqrt{2}} \ket{0} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } + \frac{1}{\sqrt{2}} \ket{1} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$ CNOT flips the state of the second qubit.After CNOT, we have:$$ \frac{1}{\sqrt{2}} \ket{0} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } + \frac{1}{\sqrt{2}} \ket{1} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{1} - \frac{1}{\sqrt{2}} \ket{0} }$$Remark that $\ket{0}$ and $ \ket{1} $ are swapped in the second qubit.If we write the quantum state of the second qubit as before, the sign of $ \ket{1} $ in the first qubit should be flipped.Thus the last equation can be equivalently written as follows:$$ \frac{1}{\sqrt{2}} \ket{0} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } - \frac{1}{\sqrt{2}} \ket{1} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$ Before CNOT operator, the sign of $ \ket{1} $ in the first qubit is positive. After CNOT operator, its sign changes to negative.This is called phase kickback. After CNOT It is easy to see from the last expression, that the quantum states of the qubits are separable (no correlation):$$ \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$If we apply Hadamard to each qubit, both qubits evolve to state $ \ket{1} $.The final state is $ \ket{11} $. Task 2 Create a curcuit with 7 qubits.Set the states of the first six qubits to $ \ket{0} $.Set the state of the last qubit to $ \ket{1} $.Apply Hadamard operators to all qubits.Apply CNOT operator (first-qubit,last-qubit) Apply CNOT operator (fourth-qubit,last-qubit)Apply CNOT operator (fifth-qubit,last-qubit)Apply Hadamard operators to all qubits.Measure all qubits. For each CNOT operator, do we have a phase-kickback effect? - Anwer: YES
###Code
# import all necessary objects and methods for quantum circuits
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
#
# your code is here
#
# import all necessary objects and methods for quantum circuits
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
# Create a circuit with 7 qubits.
n = 7
qreg2 = QuantumRegister(n) # quantum register with 7 qubits
creg2 = ClassicalRegister(n) # classical register with 7 bits
mycircuit2 = QuantumCircuit(qreg2,creg2) # quantum circuit with quantum and classical registers
# the first six qubits are already in |0>
# set the last qubit to |1>
mycircuit2.x(qreg2[n-1]) # apply x-gate (NOT operator)
# apply Hadamard to all qubits.
for i in range(n):
mycircuit2.h(qreg2[i])
# apply CNOT operator (first-qubit,last-qubit)
# apply CNOT operator (fourth-qubit,last-qubit)
# apply CNOT operator (fifth-qubit,last-qubit)
mycircuit2.cx(qreg2[0],qreg2[n-1])
mycircuit2.cx(qreg2[3],qreg2[n-1])
mycircuit2.cx(qreg2[4],qreg2[n-1])
# apply Hadamard to all qubits.
for i in range(n):
mycircuit2.h(qreg2[i])
# measure all qubits
mycircuit2.measure(qreg2,creg2)
# execute the circuit 100 times in the local simulator
job = execute(mycircuit2,Aer.get_backend('qasm_simulator'),shots=100)
counts = job.result().get_counts(mycircuit2)
# print the reverse of the outcome
for outcome in counts:
reverse_outcome = ''
for i in outcome:
reverse_outcome = i + reverse_outcome
print(reverse_outcome,"is observed",counts[outcome],"times")
for i in range(len(reverse_outcome)):
print("Final q-bit value for nth q-bit",(i+1),"is",reverse_outcome[i])
###Output
1001101 is observed 100 times
Final q-bit value for nth q-bit 1 is 1
Final q-bit value for nth q-bit 2 is 0
Final q-bit value for nth q-bit 3 is 0
Final q-bit value for nth q-bit 4 is 1
Final q-bit value for nth q-bit 5 is 1
Final q-bit value for nth q-bit 6 is 0
Final q-bit value for nth q-bit 7 is 1
###Markdown
prepared by Abuzer Yakaryilmaz (QLatvia) updated by Özlem Salehi | October 26, 2020 This cell contains some macros. If there is a problem with displaying mathematical formulas, please run this cell to load these macros. $ \newcommand{\bra}[1]{\langle 1|} $$ \newcommand{\ket}[1]{|1\rangle} $$ \newcommand{\braket}[2]{\langle 1|2\rangle} $$ \newcommand{\dot}[2]{ 1 \cdot 2} $$ \newcommand{\biginner}[2]{\left\langle 1,2\right\rangle} $$ \newcommand{\mymatrix}[2]{\left( \begin{array}{1} 2\end{array} \right)} $$ \newcommand{\myvector}[1]{\mymatrix{c}{1}} $$ \newcommand{\myrvector}[1]{\mymatrix{r}{1}} $$ \newcommand{\mypar}[1]{\left( 1 \right)} $$ \newcommand{\mybigpar}[1]{ \Big( 1 \Big)} $$ \newcommand{\sqrttwo}{\frac{1}{\sqrt{2}}} $$ \newcommand{\dsqrttwo}{\dfrac{1}{\sqrt{2}}} $$ \newcommand{\onehalf}{\frac{1}{2}} $$ \newcommand{\donehalf}{\dfrac{1}{2}} $$ \newcommand{\hadamard}{ \mymatrix{rr}{ \sqrttwo & \sqrttwo \\ \sqrttwo & -\sqrttwo }} $$ \newcommand{\vzero}{\myvector{1\\0}} $$ \newcommand{\vone}{\myvector{0\\1}} $$ \newcommand{\vhadamardzero}{\myvector{ \sqrttwo \\ \sqrttwo } } $$ \newcommand{\vhadamardone}{ \myrvector{ \sqrttwo \\ -\sqrttwo } } $$ \newcommand{\myarray}[2]{ \begin{array}{1}2\end{array}} $$ \newcommand{\X}{ \mymatrix{cc}{0 & 1 \\ 1 & 0} } $$ \newcommand{\Z}{ \mymatrix{rr}{1 & 0 \\ 0 & -1} } $$ \newcommand{\Htwo}{ \mymatrix{rrrr}{ \frac{1}{2} & \frac{1}{2} & \frac{1}{2} & \frac{1}{2} \\ \frac{1}{2} & -\frac{1}{2} & \frac{1}{2} & -\frac{1}{2} \\ \frac{1}{2} & \frac{1}{2} & -\frac{1}{2} & -\frac{1}{2} \\ \frac{1}{2} & -\frac{1}{2} & -\frac{1}{2} & \frac{1}{2} } } $$ \newcommand{\CNOT}{ \mymatrix{cccc}{1 & 0 & 0 & 0 \\ 0 & 1 & 0 & 0 \\ 0 & 0 & 0 & 1 \\ 0 & 0 & 1 & 0} } $$ \newcommand{\norm}[1]{ \left\lVert 1 \right\rVert } $ Phase Kickback Consider the quantum state $\ket{-}=\frac{1}{\sqrt{2}}\ket{0}-\frac{1}{\sqrt{2}}\ket{1}$. Let's check the effect of applying NOT operator to the quantum state $\ket{-}$. \begin{align*}\ket{-} \xrightarrow{NOT} &= \frac{1}{\sqrt{2}} \ket{1} - \frac{1}{\sqrt{2}} \ket{0}= \text{-} \left(\frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1}\right)= \text{-}\ket{-}\end{align*} So we see that $NOT\ket{-} = \text{-}\ket{-}$. This is called phase kickback._The eigenvalue is kicked back to the control register._ _Note: $\ket{-}$ is an eigenstate of the NOT operator with eigenvalue -1._ Now we will observe another interesting quantum effect here. We will apply a Controlled-NOT operator, but the controller qubit will be affected(!) Suppose that the second qubit is in state $\ket{-}$. When the first qubit is in state $\ket{0}$, then CNOT does not have any effect.$\ket{0}\ket{-} \xrightarrow{CNOT} \ket{0}\ket{-}$ When the first qubit is in state $\ket{1}$, then we have the following state.$\ket{1}\ket{-} \xrightarrow{CNOT} \text{-}\ket{1}\ket{-}$ Now suppose that first qubit is in state $\alpha \ket{0} + \beta \ket{1}$ and the second qubit is in state $\ket{-}$, that is we have the following quantum state $\alpha \ket{0}\ket{-} + \beta \ket{1}\ket{-}$.$\alpha \ket{0}\ket{-} + \beta \ket{1}\ket{-} \xrightarrow{CNOT} \alpha \ket{0}\ket{-} ~-~ \beta \ket{1}\ket{-} = (\alpha \ket{0} - \beta \ket{1})\ket{-}$ The state of the first qubit after the CNOT is $\alpha \ket{0} - \beta \ket{1}$The sign of $ \ket{1} $ in the first qubit changes sign after the CNOT operator. Task 1Create a quantum circuit with two qubits.Set the state of the first qubit to $ \ket{0} $.Set the state of the second qubit to $ \ket{1} $.Apply Hadamard to both qubits.Apply CNOT operator, where the controller qubit is the first qubit and the target qubit is the second qubit.Apply Hadamard to both qubits.Measure the outcomes.We start in quantum state $ \ket{01} $. What is the outcome?
###Code
# import all necessary objects and methods for quantum circuits
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
#
# your code is here
#
###Output
_____no_output_____
###Markdown
click for our solution Analysis The quantum state of the first qubit before CNOT:$$ \ket{0} \xrightarrow{H} \frac{1}{\sqrt{2}} \ket{0} + \frac{1}{\sqrt{2}} \ket{1}.$$The quantum state of the second qubit before CNOT:$$ \ket{1} \xrightarrow{H} \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1}.$$ The quantum state of the composite system:$$ \mypar{ \frac{1}{\sqrt{2}} \ket{0} + \frac{1}{\sqrt{2}} \ket{1} } \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$ CNOT affects when the first qubit has the value 1.Let's rewrite the composite state as below to explicitly represent the effect of CNOT.$$ \frac{1}{\sqrt{2}} \ket{0} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } + \frac{1}{\sqrt{2}} \ket{1} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$ CNOT flips the state of the second qubit.After CNOT, we have:$$ \frac{1}{\sqrt{2}} \ket{0} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } + \frac{1}{\sqrt{2}} \ket{1} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{1} - \frac{1}{\sqrt{2}} \ket{0} }$$Remark that $\ket{0}$ and $ \ket{1} $ are swapped in the second qubit.Thus the last equation can be equivalently written as follows:$$ \frac{1}{\sqrt{2}} \ket{0} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } - \frac{1}{\sqrt{2}} \ket{1} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$It is easy to see from the last expression, that the quantum states of the qubits are separable (no correlation):$$ \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$Note that the sign of $ \ket{1} $ in the first qubit is flipped. If we apply Hadamard to each qubit, both qubits evolve to state $ \ket{1} $.Hence, the final state is $ \ket{11} $. Task 2 Create a curcuit with 7 qubits.Set the states of the first six qubits to $ \ket{0} $.Set the state of the last qubit to $ \ket{1} $.Apply Hadamard operators to all qubits.Apply CNOT operator (first-qubit,last-qubit) Apply CNOT operator (fourth-qubit,last-qubit)Apply CNOT operator (fifth-qubit,last-qubit)Apply Hadamard operators to all qubits.Measure all qubits. For each CNOT operator, do we have a phase-kickback effect?
###Code
# import all necessary objects and methods for quantum circuits
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
#
# your code is here
#
###Output
_____no_output_____
###Markdown
Prepared by Abuzer Yakaryilmaz This cell contains some macros. If there is a problem with displaying mathematical formulas, please run this cell to load these macros. $ \newcommand{\bra}[1]{\langle 1|} $$ \newcommand{\ket}[1]{|1\rangle} $$ \newcommand{\braket}[2]{\langle 1|2\rangle} $$ \newcommand{\dot}[2]{ 1 \cdot 2} $$ \newcommand{\biginner}[2]{\left\langle 1,2\right\rangle} $$ \newcommand{\mymatrix}[2]{\left( \begin{array}{1} 2\end{array} \right)} $$ \newcommand{\myvector}[1]{\mymatrix{c}{1}} $$ \newcommand{\myrvector}[1]{\mymatrix{r}{1}} $$ \newcommand{\mypar}[1]{\left( 1 \right)} $$ \newcommand{\mybigpar}[1]{ \Big( 1 \Big)} $$ \newcommand{\sqrttwo}{\frac{1}{\sqrt{2}}} $$ \newcommand{\dsqrttwo}{\dfrac{1}{\sqrt{2}}} $$ \newcommand{\onehalf}{\frac{1}{2}} $$ \newcommand{\donehalf}{\dfrac{1}{2}} $$ \newcommand{\hadamard}{ \mymatrix{rr}{ \sqrttwo & \sqrttwo \\ \sqrttwo & -\sqrttwo }} $$ \newcommand{\vzero}{\myvector{1\\0}} $$ \newcommand{\vone}{\myvector{0\\1}} $$ \newcommand{\vhadamardzero}{\myvector{ \sqrttwo \\ \sqrttwo } } $$ \newcommand{\vhadamardone}{ \myrvector{ \sqrttwo \\ -\sqrttwo } } $$ \newcommand{\myarray}[2]{ \begin{array}{1}2\end{array}} $$ \newcommand{\X}{ \mymatrix{cc}{0 & 1 \\ 1 & 0} } $$ \newcommand{\Z}{ \mymatrix{rr}{1 & 0 \\ 0 & -1} } $$ \newcommand{\Htwo}{ \mymatrix{rrrr}{ \frac{1}{2} & \frac{1}{2} & \frac{1}{2} & \frac{1}{2} \\ \frac{1}{2} & -\frac{1}{2} & \frac{1}{2} & -\frac{1}{2} \\ \frac{1}{2} & \frac{1}{2} & -\frac{1}{2} & -\frac{1}{2} \\ \frac{1}{2} & -\frac{1}{2} & -\frac{1}{2} & \frac{1}{2} } } $$ \newcommand{\CNOT}{ \mymatrix{cccc}{1 & 0 & 0 & 0 \\ 0 & 1 & 0 & 0 \\ 0 & 0 & 0 & 1 \\ 0 & 0 & 1 & 0} } $$ \newcommand{\norm}[1]{ \left\lVert 1 \right\rVert } $ Phase KickbackWe observe another interesting quantum effect here.We will apply a Controlled-NOT operator, but the controller qubit will be affected(!) Suppose that the state of the first qubit is $\alpha\ket{0}+ \beta \ket{1} = \myvector{\alpha \\ \beta}$ and the state of the second qubit is $\ket{-}=\frac{1}{\sqrt{2}}\ket{0}-\frac{1}{\sqrt{2}}\ket{1} = \myvector{\frac{1}{\sqrt{2}} \\ -\frac{1}{\sqrt{2}} }$The state of the overall system is represented by the vector $\frac{1}{\sqrt{2}}\myvector{\alpha\\-\alpha\\ \beta\\ -\beta}$After applying CNOT where the first qubit is the controler and the second is the target, we have $$\CNOT \frac{1}{\sqrt{2}}\myvector{\alpha\\-\alpha\\ \beta\\ -\beta}= \frac{1}{\sqrt{2}}\myvector{\alpha\\-\alpha\\ -\beta\\ \beta} =\myvector{\alpha \\ -\beta} \otimes \myvector{\frac{1}{\sqrt{2}} \\ -\frac{1}{\sqrt{2}} }$$ The state of the first qubit after the CNOT is $\alpha \ket{0} - \beta \ket{1}$The sign of $ \ket{1} $ in the first qubit changes sign after the CNOT operator.This is called phase kickback. Task 1Create a quantum circuit with two qubits.Set the state of the first qubit to $ \ket{0} $.Set the state of the second qubit to $ \ket{1} $.Apply Hadamard to both qubits.Apply CNOT operator, where the controller qubit is the first qubit and the target qubit is the second qubit.Apply Hadamard to both qubits.Measure the outcomes.We start in quantum state $ \ket{01} $. What is the outcome?
###Code
# import all necessary objects and methods for quantum circuits
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
#
# your code is here
#
###Output
_____no_output_____
###Markdown
click for our solution Analysis The quantum state of the first qubit before CNOT:$$ \ket{0} \xrightarrow{H} \frac{1}{\sqrt{2}} \ket{0} + \frac{1}{\sqrt{2}} \ket{1}.$$The quantum state of the second qubit before CNOT:$$ \ket{1} \xrightarrow{H} \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1}.$$ The quantum state of the composite system:$$ \mypar{ \frac{1}{\sqrt{2}} \ket{0} + \frac{1}{\sqrt{2}} \ket{1} } \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$ CNOT affects when the first qubit has the value 1.Let's rewrite the composite state as below to explicitly represent the effect of CNOT.$$ \frac{1}{\sqrt{2}} \ket{0} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } + \frac{1}{\sqrt{2}} \ket{1} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$ CNOT flips the state of the second qubit.After CNOT, we have:$$ \frac{1}{\sqrt{2}} \ket{0} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } + \frac{1}{\sqrt{2}} \ket{1} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{1} - \frac{1}{\sqrt{2}} \ket{0} }$$Remark that $\ket{0}$ and $ \ket{1} $ are swapped in the second qubit.Thus the last equation can be equivalently written as follows:$$ \frac{1}{\sqrt{2}} \ket{0} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } - \frac{1}{\sqrt{2}} \ket{1} \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$It is easy to see from the last expression, that the quantum states of the qubits are separable (no correlation):$$ \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} } \otimes \mypar{ \frac{1}{\sqrt{2}} \ket{0} - \frac{1}{\sqrt{2}} \ket{1} }$$Note that the sign of $ \ket{1} $ in the first qubit is flipped. If we apply Hadamard to each qubit, both qubits evolve to state $ \ket{1} $.Hence, the final state is $ \ket{11} $. Task 2 Create a curcuit with 7 qubits.Set the states of the first six qubits to $ \ket{0} $.Set the state of the last qubit to $ \ket{1} $.Apply Hadamard operators to all qubits.Apply CNOT operator (first-qubit,last-qubit) Apply CNOT operator (fourth-qubit,last-qubit)Apply CNOT operator (fifth-qubit,last-qubit)Apply Hadamard operators to all qubits.Measure all qubits. For each CNOT operator, do we have a phase-kickback effect?
###Code
# import all necessary objects and methods for quantum circuits
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, execute, Aer
#
# your code is here
#
###Output
_____no_output_____ |
Dia_1/Dia_1_grupo1/Dia_1.ipynb | ###Markdown
Ipython NotebookFernando Perez, el Colombiano que creo Ipython una de las interfaces Python mas importantes en el mundo en los ultimos 10 años.https://en.wikipedia.org/wiki/Fernando_P%C3%A9rez_(software_developer)https://pybonacci.es/2013/05/16/entrevista-a-fernando-perez-creador-de-ipython/http://fperez.org/personal.html Ejemplo de lo que puedes lograrhttp://nbviewer.jupyter.org/github/glue-viz/example_data_viewers/blob/master/mario/mario.ipynb Opreaciones Matematicas **Suma** : $2+3$
###Code
2+38
###Output
_____no_output_____
###Markdown
**Multiplicación**: $2x3$
###Code
2*30
###Output
_____no_output_____
###Markdown
**División**: $\frac{2}{3}$
###Code
2/
###Output
_____no_output_____
###Markdown
**Potencia**: $ 2^{3}$
###Code
2**3
###Output
_____no_output_____
###Markdown
Funciones TrigonometricasEn las siguientes celdas vamos a calcular los valores de funciones comunes en nuestras clases de matemáticas.Para esto, necesitamos importar la libreria numpy.
###Code
# Importar una libreria en Python
import numpy as np # el comando "as nb" sirve para asignarle un codigo mas corto a la libreria y ser mas rapido.
np.sin(3)
(np.sin(3))*(np.sin(2))
###Output
_____no_output_____
###Markdown
Logaritmo y Exponencial: $ln(3), e^{3}$
###Code
np.log(3)
np.exp(3)
###Output
_____no_output_____
###Markdown
Reto de Programación- Resolver la ecuación $$x^2 - x - 12 = 0$$- Encuentre la hipotenusa y los ángulos del triángulo rectangulo de lados a = 4 y b = 5 Variables Una variable es un espacio para guardar valores modificables o constantes.----```pythonnombre_de_la_variable = valor_de_la_variable```---**Los distintos tipos de variables son:****Enteros (**`int`**): 1, 2, 3, -10, -103**** Números continuos (**`float`**): 0.666, -10.678**** Cadena de texto (**`str`**): 'clubes', 'clubes de ciencia', 'Roberto'****Booleano (verdadero / Falso): `True`, `False` **
###Code
# Ejemplo
a = 5
print (a) # Imprimir mi variable
###Output
5
###Markdown
Variable tipo `int`
###Code
b = -15
print (b)
###Output
-15
###Markdown
Variable tipo `float`
###Code
c = 3.1416
print (c)
###Output
3.1416
###Markdown
Variable tipo `str`
###Code
d = 'clubes de ciencia'
print (d)
###Output
clubes de ciencia
###Markdown
Variable tipo `bool`
###Code
e = False
print (e)
###Output
False
###Markdown
Como averiguo el tipo de una variable ??Utilizando la función `type`:```pythontype(nombre_de_la_variable)```
###Code
print (type(a))
print (type(b))
print (type(c))
print (type(d))
print (type(e))
###Output
<class 'int'>
<class 'int'>
<class 'float'>
<class 'str'>
<class 'bool'>
###Markdown
Reto de Programación Variables para guardar colecciones de datos>** Python tiene otro 3 tipos de variables mas complejas que pueden almacenar colecciones de datos>como los visotos anteriormente**- Listas- Tuplas- Diccionarios ListasLas listas permiten guardar colecciones de datos con diferentes tipos:```pythonint; str; float; bool```Una lista se crea de la siguiente forma:```pythonnombre_de_la_lista = [valor_1, valor_2, valor_3]```Los valores de la lista pueden ser modificados.
###Code
# Ejemplo
mi_lista = [1,2,3,5,6,-3.1416]
mi_lista_diversa = [1,2,'clubes', 'de', 'ciencia', 3.1416, False]
print (mi_lista)
print (mi_lista_diversa)
###Output
[1, 2, 3, 5, 6, -3.1416]
[1, 2, 'clubes', 'de', 'ciencia', 3.1416, False]
###Markdown
Como puedo mirar un elemento o elementos de mi lista??Para leer el elemento de la posición `n`, se usa:```pythonmi_lista[n]```
###Code
# Ejemplo
print (mi_lista[0]) # Leer el primer elemento que se encuentra en la posición n=0
print (mi_lista_diversa[0])
print (type(mi_lista[5])) # Leer el tipo de variable en la posición n=5
###Output
1
1
<class 'float'>
###Markdown
** Como leer los elementos entre la posición n y m??**```pythonmi_lista[n:m+1]```
###Code
#Ejemplo
print (mi_lista[0:3]) # Leer entre n=0 y m=2
###Output
[1, 2, 3]
###Markdown
Reto de Programación TuplasLas tuplas permiten guardar colecciones de datos de diferentes tipos:```pythonint; str; float; bool```Una tupla se crea de la siguiente forma:```pythonmi_tupla = ('cadena de texto', 15, 2.8, 'otro dato', 25)```Los valores de una tupla no pueden ser modificados. Sus elementos se leen como en las listas
###Code
#Ejemplo
mi_lista = ('cadena de texto', 15, 2.8, 'otro dato', 25)
print (mi_lista)
print (mi_lista[2]) # leer el tercer elemento de la tupla
print (mi_lista[2:4]) # leer los dos ultimos elementos de la tupla
###Output
('cadena de texto', 15, 2.8, 'otro dato', 25)
2.8
(2.8, 'otro dato')
###Markdown
Reto de Programación DiccionariosMientras que en las listas y tuplas se accede a los elementos por un número de indice, en los diccionarios se utilizan claves(numericas ó de texto) para acceder a los elementos. Los elementos guardados en cada clave son de diferentes tipos, incluso listas u otros diccionarios.```pythonint; str; float; bool, list, dict```Una diccionario se crea de la siguiente forma:```pythonmi_diccionario = {'grupo_1':4, 'grupo_2':6, 'grupo_3':7, 'grupo_4':3}```Acceder al valor de la clave `grupo_2`:```pythonprint (mi_diccionario['grupo_2'])```
###Code
# Ejemplo 1
mi_diccionario = {'grupo_1':4, 'grupo_2':6, 'grupo_3':7, 'grupo_4':3}
print (mi_diccionario['grupo_2'])
# Ejemplo 2 con diferentes tipos de elementos
informacion_persona = {'nombres':'Elon', 'apellidos':'Musk', 'edad':45, 'nacionalidad':'Sudafricano',
'educacion':['Administracion de empresas','Física'],'empresas':['Zip2','PyPal','SpaceX','SolarCity']}
print (informacion_persona['educacion'])
print (informacion_persona['empresas'])
###Output
['Administracion de empresas', 'Física']
['Zip2', 'PyPal', 'SpaceX', 'SolarCity']
###Markdown
Reto de Programación Estructuras de control condicionalesLas estructuras de control condicionales nos permiten evaluar si una o mas condiciones se cumplen, y respecto a estoejecutar la siguiente accion.Primero usamos:```pythonif```Despues algun operador relacional para comparar```python== igual que!= diferente de < menor que> mayor que<= menor igual que>= mayor igual que```Cuando se evalua mas de una conición:```pythonand, & (y)or, | (ó)```
###Code
# Ejemplo
color_semaforo = 'amarillo'
if color_semaforo == 'verde':
print ("Cruzar la calle")
else:
print ("Esperar")
# ejemplo
dia_semana = 'lunes'
if dia_semana == 'sabado' or dia_semana == 'domingo':
print ('Me levanto a las 10 de la mañana')
else:
print ('Me levanto antes de las 7am')
# Ejemplo
costo_compra = 90
if costo_compra <= 100:
print ("Pago en efectivo")
elif costo_compra > 100 and costo_compra < 300:
print ("Pago con tarjeta de débito")
else:
print ("Pago con tarjeta de crédito")
###Output
_____no_output_____
###Markdown
Reto de Programación Estructuras de control iterativas(cíclicas o bucles)Estas estructuras nos permiten ejecutar un mismo codigo, de manera repetida, mientras se cumpla una condición. Bucle WhileEste bucle ejecuta una misma acción mientras determinada condición se cumpla:```pythonanio = 2001while anio <= 2012: print ("Informes del Año", str(anio)) anio = anio + 1 aumentamos anio en 1```En este ejemplo la condición es menor que 2012
###Code
# ejemplo
anio = 2001
while anio <= 2012:
print ("Informes del Año", str(anio))
anio = anio + 1 # aumentamos anio en 1
# ejemplo
cuenta = 10
while cuenta >= 0:
print ('faltan '+str(cuenta)+' minutos')
cuenta += -1
###Output
faltan 10 minutos
faltan 9 minutos
faltan 8 minutos
faltan 7 minutos
faltan 6 minutos
faltan 5 minutos
faltan 4 minutos
faltan 3 minutos
faltan 2 minutos
faltan 1 minutos
faltan 0 minutos
###Markdown
Reto de Programación Bucle forEn Python el bucle for nos permite iterar sobre variables que guardan colecciones de datos, como : tuplas y listas.```pythonmi_lista = ['Juan', 'Antonio', 'Pedro', 'Herminio']for nombre in mi_lista: print (nombre)```En el codigo vemos que la orden es ir por cada uno de los elementos de la lista para imprimirlos.
###Code
# Ejemplo
mi_tupla = ('rosa', 'verde', 'celeste', 'amarillo')
for color in mi_tupla:
print (color)
# Ejemplo
dias_semana = ['lunes','martes','miercoles','jueves','viernes','sabado','domingo']
for i in dias_semana:
if (i == dias_semana[-1]) or (i == dias_semana[-2]):
print ('Hoy seguire aprendiendo de programación')
else:
print ('Hoy tengo que ir al colegio')
###Output
Hoy tengo que ir al colegio
Hoy tengo que ir al colegio
Hoy tengo que ir al colegio
Hoy tengo que ir al colegio
Hoy tengo que ir al colegio
Hoy seguire aprendiendo de programación
Hoy seguire aprendiendo de programación
|
Hugging Face Transformers Generating Blog Posts.ipynb | ###Markdown
0. Install and Import Dependencies
###Code
!pip install transformers
import tensorflow as tf
from transformers import GPT2LMHeadModel, GPT2Tokenizer
tokenizer = GPT2Tokenizer.from_pretrained("gpt2-large")
model = GPT2LMHeadModel.from_pretrained("gpt2-large", pad_token_id=tokenizer.eos_token_id)
sentence = 'Once Nasreddin was invited to deliver a sermon. When he got on the pulpit, he asked, Do you know what I am going to say? The audience replied "no", so he announced, I have no desire to speak to people who dont even know what I will be talking about! and left. The people felt embarrassed and called him back again the next day. This time, when he asked the same question, the people replied yes. So Nasreddin said, Well, since you already know what I am going to say, I wont waste any more of your time! and left.\
Now the people were really perplexed. They decided to try one more time and once again invited the Mulla to speak the following week. Once again he asked the same question – Do you know what I am going to say? Now the people were prepared and so half of them answered "yes" while the other half replied "no". So Nasreddin said '
input_ids = tokenizer.encode(sentence, return_tensors='pt')
input_ids
# generate text until the output length (which includes the context length) reaches 50
output = model.generate(input_ids, max_length=2000, num_beams=5, no_repeat_ngram_size=2, early_stopping=True)
output
tokenizer.decode(output[0],skip_special_tokens=True)
print(tokenizer.decode(output[0], skip_special_tokens=True))
###Output
_____no_output_____ |
notebook/Composer/DeepFashion/Composer_DeepFashion.ipynb | ###Markdown
DeepFashion
###Code
%matplotlib inline
import os,sys
pwd = os.getcwd()
parent = '/'.join(pwd.split('/')[:-3])
sys.path.insert(0,parent)
os.chdir(parent)
#%%
print('-'*30)
print(os.getcwd())
print('-'*30)
#%%
import torch
import torch.optim as optim
import torch.nn as nn
import torch.nn.functional as F
import pandas as pd
from core.DAZLE import DAZLE
from core.DeepFashionDataLoader import DeepFashionDataLoader
from core.helper_func import eval_zs_gzsl,visualize_attention,eval_zs_gzsl_k#,get_attribute_attention_stats
from global_setting import NFS_path_AoA,save_NFS
#from core.Scheduler import Scheduler
import importlib
import pdb
import numpy as np
import argparse
import time
from importlib import reload
import matplotlib.pyplot as plt
import core.helper_func
reload(core.helper_func)
from core.helper_func import next_unseen_batch,compute_mean_real_unseen,compute_mean_real_seen,compute_generated_quality,evaluate_quality,evaluate_quality_omni,selective_sample,eval_zs_gzsl,visualize_attention,eval_zs_gzsl_k,get_attr_entropy
###Output
_____no_output_____
###Markdown
Load data
###Code
parser = argparse.ArgumentParser()
parser.add_argument('--critic_iter', type=int, default=5, help='critic iteration, following WGAN-GP')
parser.add_argument('--lambda1', type=float, default=10, help='gradient penalty regularizer, following WGAN-GP')
parser.add_argument('--nz', type=int, default=312, help='size of the latent z vector')
parser.add_argument('--normalize_V', type=bool, default=False, help='normalize_V')
parser.add_argument('--gen_hidden', type=int, default=1024, help='gen_hidden')
opt = parser.parse_known_args()[0] #omit unknown arguments
print(opt)
save_path = save_NFS+'results/Relase_DeepFashion_Composer_timing/'
idx_GPU = 7
device = torch.device("cuda:{}".format(idx_GPU) if torch.cuda.is_available() else "cpu")
torch.backends.cudnn.benchmark = True
dataloader = DeepFashionDataLoader(NFS_path_AoA,device,is_balance = True)
full_att = dataloader.att.clone().detach()
full_init_w2v_att = dataloader.w2v_att.clone().detach()
n_attr = 300
attr_entropy = get_attr_entropy(full_att.cpu().numpy())
idx_attr_dis = np.argsort(attr_entropy)[:n_attr]
init_w2v_att = full_init_w2v_att[idx_attr_dis]
att = full_att[:,idx_attr_dis]
att = F.normalize(att,dim=1)
dataloader.att = att
dataloader.w2v_att = init_w2v_att
###Output
_____no_output_____
###Markdown
Pretrain DAZLE
###Code
#%%
seed = 214
torch.manual_seed(seed)
torch.cuda.manual_seed_all(seed)
print('Randomize seed {}'.format(seed))
#%%
batch_size = 50
nepoches = 20
niters = dataloader.ntrain * nepoches//batch_size
dim_f = 2048
dim_v = 300
init_w2v_att = dataloader.w2v_att
att = dataloader.att#dataloader.normalize_att#
normalize_att = None#dataloader.normalize_att
#assert (att.min().item() == 0 and att.max().item() == 1)
trainable_w2v = True
lambda_1 = 0.0
lambda_2 = 0.0
lambda_3 = 0.0
bias = 0
prob_prune = 0
uniform_att_1 = False
uniform_att_2 = True
dataloader.seeker[:] = 0
print('seeker ',dataloader.seeker)
seenclass = dataloader.seenclasses
unseenclass = dataloader.unseenclasses
desired_mass = 1#unseenclass.size(0)/(seenclass.size(0)+unseenclass.size(0))
report_interval = niters//nepoches#10000//batch_size#
model = DAZLE(dim_f,dim_v,init_w2v_att,att,normalize_att,
seenclass,unseenclass,
lambda_1,lambda_2,lambda_3,
device,
trainable_w2v,normalize_V=opt.normalize_V,normalize_F=True,is_conservative=False,
uniform_att_1=uniform_att_1,uniform_att_2=uniform_att_2,
prob_prune=prob_prune,desired_mass=desired_mass, is_conv=False,
is_bias=False)
#%%
lr = 0.0001
weight_decay = 0.0001#0.000#0.#
momentum = 0.9#0.#
optimizer_m = optim.RMSprop( model.parameters() ,lr=lr,weight_decay=weight_decay, momentum=momentum)
#%%
print('-'*30)
print('learing rate {}'.format(lr))
print('trainable V {}'.format(trainable_w2v))
print('lambda_1 {}'.format(lambda_1))
print('lambda_2 {}'.format(lambda_2))
print('lambda_3 {}'.format(lambda_3))
print('optimized seen only')
print('optimizer: RMSProp with momentum = {} and weight_decay = {}'.format(momentum,weight_decay))
print('-'*30)
save_dir_phase_1 = save_path+'phase_1_checkpoint/'
run_phase_1 = False
if not os.path.isdir(save_dir_phase_1):
run_phase_1 = True
os.mkdir(save_path)
if run_phase_1:
#%% phase 1 convergence of attention model
for i in range(0,2000):
tic = time.time()
model.train()
optimizer_m.zero_grad()
batch_label, batch_feature, batch_att = dataloader.next_batch(batch_size)
out_package = model(batch_feature)
in_package = out_package
in_package['batch_label'] = batch_label
out_package=model.compute_loss(in_package)
loss,loss_CE,loss_w2v,loss_prune,loss_pmp = out_package['loss'],out_package['loss_CE'],out_package['loss_w2v'],out_package['loss_prune'],out_package['loss_pmp']
entropy = out_package['entropy']
entropy_A_p = out_package['entropy_A_p']
loss.backward()
optimizer_m.step()
#print("Elapse time training {}".format(time.time()-tic))
if i%100==0:
print('-'*30)
acc_seen, acc_novel, H, acc_zs = eval_zs_gzsl(dataloader,model,device,bias_seen=-bias,bias_unseen=bias)
print('iter {} loss {} loss_CE {} loss_w2v {} loss_prune: {} loss_pmp: {}'.format(i,loss.item(),loss_CE.item(),loss_w2v.item(),loss_prune.item(),loss_pmp.item()))
print('entropy {} entropy_A_p {}'.format(entropy,entropy_A_p))
print('acc_seen {} acc_novel {} H {}'.format(acc_seen, acc_novel, H))
print('acc_zs {}'.format(acc_zs))
os.mkdir(save_dir_phase_1)
torch.save(model.state_dict(), save_dir_phase_1+'model_phase_1')
else:
print('-'*30)
model.load_state_dict(torch.load(save_dir_phase_1+'model_phase_1'))
print("Load checkpoint")
acc_seen, acc_novel, H, acc_zs = eval_zs_gzsl(dataloader,model,device,bias_seen=-bias,bias_unseen=bias)
print('acc_seen {} acc_novel {} H {}'.format(acc_seen, acc_novel, H))
print("Add bias")
test_bias = 2
acc_seen, acc_novel, H, acc_zs = eval_zs_gzsl(dataloader,model,device,bias_seen=-test_bias,bias_unseen=test_bias)
print('acc_seen {} acc_novel {} H {}'.format(acc_seen, acc_novel, H))
###Output
Add bias
bias_seen -2 bias_unseen 2
acc_seen 0.34127628803253174 acc_novel 0.22246943414211273 H 0.269353858302795
###Markdown
Dense Feature Composition
###Code
del model
del optimizer_m
model = DAZLE(dim_f,dim_v,init_w2v_att,att,normalize_att,
seenclass,unseenclass,
lambda_1,lambda_2,lambda_3,
device,
trainable_w2v,normalize_V=opt.normalize_V,normalize_F=True,is_conservative=True,
uniform_att_1=uniform_att_1,uniform_att_2=uniform_att_2,
prob_prune=prob_prune,desired_mass=desired_mass, is_conv=False,
is_bias=False,margin=1)
print('-'*30)
model.load_state_dict(torch.load(save_dir_phase_1+'model_phase_1'))
print("Load checkpoint")
acc_seen, acc_novel, H, acc_zs = eval_zs_gzsl(dataloader,model,device,bias_seen=-bias,bias_unseen=bias)
print('acc_seen {} acc_novel {} H {}'.format(acc_seen, acc_novel, H))
lr = 0.0001
weight_decay = 0.0001#0.000#0.#
momentum = 0.#0.#
optimizer_m = optim.RMSprop( model.parameters() ,lr=lr,weight_decay=weight_decay, momentum=momentum)
from core.Composer_AttEmb import Composer_AttEmb
pGenerator = Composer_AttEmb(device=device,base_model = model, k=5,n_comp=1,T=5)
###Output
Attribute Embed
Inherit V
Repurpose W_1
Inherit W_1
Using OMP
nearest neighbour k 5
T 5
n_comp 1
###Markdown
RemarkTo prevent seen class bias, we add a fix margin of size 1 to unseen class scores following the DAZLE work.Learning this margin as a function of input could further improve performances.
###Code
import core.Margin
reload(core.Margin)
from core.Margin import Margin
margin_model = Margin(base_model=model,second_order=True,activate=True)
print("No hidden layer")
lr_p = 0.001
weight_decay_p = 0.01#0.000#0.#
momentum_p = 0.0#0.#
optimizer_p_m = optim.RMSprop( margin_model.parameters() ,lr=lr_p,weight_decay=weight_decay_p, momentum=momentum_p)
margin_model.linear1.weight.data *= 0
margin_model.linear1.bias.data *= 0
margin_model.linear1.bias.data += 1
print(margin_model.linear1.weight)
print(margin_model.linear1.bias)
margin_model.linear1.weight.requires_grad = False
margin_model.linear1.bias.requires_grad = False
model.W_1.requires_grad = True
model.V.requires_grad = True
model.W_2.requires_grad = False
model.W_3.requires_grad = False
seed = 113
torch.manual_seed(seed)
torch.cuda.manual_seed_all(seed)
np.random.seed(seed)
best_performance = [0,0,0,0]
lamb = 1#0.7
for i in range(0,4000):
tic = time.time()
batch_label, batch_feature, batch_att = dataloader.next_batch(batch_size)
Hs_seen = model.extract_attention(batch_feature)['Hs']
### random mini-batch from generator ###
unseen_labels = model.unseenclass[np.random.randint(model.unseenclass.size(0),size=batch_label.size(0))]
att_unseen = model.att[unseen_labels]
Hs_unseen = pGenerator.compose(Hs=Hs_seen,labels_s=batch_label,labels_t=unseen_labels)
### random mini-batch from generator ###
if Hs_unseen.size(0) == 0:
continue
## seen reinforcement
optimizer_m.zero_grad()
optimizer_p_m.zero_grad()
out_package_seen = margin_model.compute_attribute_embed(Hs_seen)
in_package_seen = out_package_seen
in_package_seen['batch_label'] = batch_label
loss_CE_seen = margin_model.compute_aug_cross_entropy(in_package_seen,is_conservative=True)
out_package_unseen = margin_model.compute_attribute_embed(Hs_unseen)
in_package_unseen = out_package_unseen
in_package_unseen['batch_label'] = unseen_labels
loss_pmp = model.compute_loss_Laplace(in_package_unseen)
loss_CE_unseen = margin_model.compute_aug_cross_entropy(in_package_unseen)
if lamb == -1:
lamb = loss_CE_seen.item()/loss_CE_unseen.item()
print("lamb:",lamb)
loss_s_u = loss_CE_seen + lamb*loss_CE_unseen#0.4*loss_pmp #
loss_s_u.backward()
optimizer_p_m.step()
optimizer_m.step()
if i%100==0:
print('-'*30)
print(i)
bias = 0
acc_seen, acc_novel, H, acc_zs = eval_zs_gzsl(dataloader,margin_model,device,bias_seen=-bias,bias_unseen=bias)
if H > best_performance[2]:
best_performance = [acc_seen, acc_novel, H, acc_zs]
stats_package = {'iter':i, 'loss_s_u':loss_s_u.item(),
'acc_seen':best_performance[0], 'acc_novel':best_performance[1], 'H':best_performance[2], 'acc_zs':best_performance[3]}
print(stats_package)
tic = time.time()
###Output
load data from hdf
1..10..11..13..14..16..17..18..20..21..22..23..24..25..27..28..3..31..32..33..34..35..36..37..39..4..40..41..43..46..47..5..6..7..8..9..
Elapsed time 35.505596999999966
|
.ipynb_checkpoints/Conv3D-checkpoint.ipynb | ###Markdown
Training
###Code
#Loss
criterion = nn.MSELoss()
#Optimizer
optimizer = torch.optim.Adam(model.parameters(), lr=0.005)
# y = torch.from_numpy(simple_mean(angle=0,cluster=4)).to(device,dtype=torch.float)
#Epochs
n_epochs = 7
for epoch in range(1, n_epochs+1):
# monitor training loss
train_loss = 0.0
#Training
for images,y in dl:
images,y = images.cuda(), y.cuda()
optimizer.zero_grad()
out = model(images)
temp_out = torch.reshape(out, (out.shape[0],out.shape[3],out.shape[4]))
loss = criterion(temp_out, y)
loss.backward()
optimizer.step()
train_loss += loss.item()
train_loss = train_loss/len(dl)
print('Epoch: {} \tTraining Loss: {:.6f}'.format(epoch, train_loss))
torch.cuda.empty_cache()
###Output
_____no_output_____
###Markdown
CHECK
###Code
def ims(img):
plt.imshow(np.transpose(img, (1, 2, 0)),cmap='gray',vmax=1)
###Output
_____no_output_____
###Markdown
10 exps (i.e, 53*3 = 159 images)
###Code
len(data)
dataiter = iter(dl)
images,_ = dataiter.next()
# images = images.to('cpu',dtype=torch.float)
# print(type(images),images.shape)
#Sample outputs
output = model(images.to(device=device))
images = images.cpu().numpy().reshape(3,160,120)
output = output.view(160, 120)
output = output.cpu().detach().numpy()
out = np.mean([image for image in images],axis=0)
print(images.shape)
#Reconstructed Images
fig, axes = plt.subplots(nrows=1, ncols=2, sharex=True, sharey=True, figsize=(12,4))
axes[0].imshow(output,cmap='gray',vmin=0,vmax=1)
axes[0].set_title('Model')
axes[1].imshow(out,cmap='gray',vmin=0,vmax=1)
axes[1].set_title('Average')
plt.show()
# plt.show()
###Output
(3, 160, 120)
|
03 - Lakehouse Devday - Unified Data Lake.ipynb | ###Markdown
Part 2.2: Unified Data Lake  MERGE INTO Support INSERT or UPDATE parquet: 7-step processWith a legacy data pipeline, to insert or update a table, you must:1. Identify the new rows to be inserted2. Identify the rows that will be replaced (i.e. updated)3. Identify all of the rows that are not impacted by the insert or update4. Create a new temp based on all three insert statements5. Delete the original table (and all of those associated files)6. "Rename" the temp table back to the original table name7. Drop the temp table INSERT or UPDATE with Delta Lake2-step process: 1. Identify rows to insert or update2. Use `MERGE`
###Code
%sql
USE aws_lakehouse_devday;
CREATE TABLE IF NOT EXISTS IOTDevicesSilver (device_name STRING, latest_CO2 INT)
USING Delta;
MERGE INTO IOTDevicesSilver
USING IOTEventsBronze
ON IOTEventsBronze.device_name = IOTDevicesSilver.device_name
WHEN MATCHED
THEN UPDATE SET latest_CO2 = c02_level
WHEN NOT MATCHED
THEN INSERT (device_name, latest_CO2) VALUES (device_name, c02_level);
SELECT * FROM IOTDevicesSilver;
%sql
OPTIMIZE IOTDevicesSilver ZORDER BY device_name
%sql
DESCRIBE HISTORY IOTDevicesSilver
%sql
SELECT * FROM IOTDevicesSilver VERSION AS OF 1
%sql
Describe detail IOTDevicesSilver
###Output
_____no_output_____
###Markdown
This is so cool!
###Code
%sql
SELECT * FROM IOTDevicesSilver TIMESTAMP AS OF '2021-11-17T12:26:12.000+0000'
spark.readStream.format('delta').table('IOTEventsBronze') \
.writeStream.format('delta') \
.option('checkpointLocation', '/aws-lakehouse-devday/lakehouse/IOTEvents/check-point') \
.trigger(once=True) \
.table('IOTEventsSilverRealtime')
%sql
SELECT * FROM IOTEventsSilverRealtime ORDER BY battery_level DESC
--select count(*) from IOTEventsSilverRealtime; -> 160K
###Output
_____no_output_____ |
Machine Learning/17_Air_Passengers_Time_Series_Modeling.ipynb | ###Markdown
Problem Statement:The Air Passenger dataset provides monthly total of US airline passengers, from 1949 to 1960.This dataset is of a time series class. Objective:• Check for the stationarity of your data using Rolling Statistics and Dickey fuller test• If stationarity is present, remove it using differencing in Python• Perform ARIMA modeling in Python after obtaining ACF and PACF plots
###Code
import numpy as np
import pandas as pd
from datetime import datetime as dt
from statsmodels.tsa.stattools import adfuller, acf, pacf
from statsmodels.tsa.arima_model import ARIMA
import math
import matplotlib.pyplot as plt
%matplotlib inline
from matplotlib.pylab import rcParams
rcParams['figure.figsize'] = 15,6
import warnings
warnings.filterwarnings('ignore')
data = pd.read_csv('C:/data/AirPassengers.csv')
data.info()
data.head()
data.describe()
data['Month'].head()
#display total monthly passenger count
data['Month'] = data['Month'].apply(lambda x: dt(int(x[:4]),int(x[5:]),15))
data = data.set_index('Month')
data.head()
#assigning passenger data time series variable
ts = data['#Passengers']
plt.plot(ts)
#transformig series using log
ts_log = np.log(ts)
def test_stationarity(timeseries):
rolmean = timeseries.rolling(window=52,center=False).mean()
rolstd = timeseries.rolling(window=52,center=False).std()
orig = plt.plot(timeseries,color='blue',label='Original')
mean = plt.plot(rolmean,color='red',label='Rolling Mean')
std = plt.plot(rolstd,color='black',label='Rolling Std')
plt.legend(loc='best')
plt.title('Rolling Mean & Standard Deviation')
plt.show(block=False)
print('Result of Dickey-Fluller Test :')
dftest = adfuller(timeseries,autolag='AIC')
dfoutput = pd.Series(dftest[0:4],index=['Test Statistic','p-value','#Lags Used',
'Number of Obervations Used'])
for key,value in dftest[4].items():
dfoutput['Cretical value(%s)'%key] = value
print(dfoutput)
test_stationarity(data['#Passengers'])
plt.plot(ts_log)
movingAverage = ts_log.rolling(window=12).mean()
movingSTD = ts_log.rolling(window=12).std()
plt.plot(ts_log)
plt.plot(movingAverage, color='red')
ts_log_mv_diff = ts_log - movingAverage
ts_log_mv_diff.head(12)
ts_log_mv_diff.dropna(inplace= True)
ts_log_mv_diff.head(10)
test_stationarity(ts_log_mv_diff)
#we can reject null hypothesis
###Output
_____no_output_____
###Markdown
Fit a model using an ARIMA algorithm Calculate forecasts to find out the p and q values for the model,we will use ACF and PACF plots
###Code
plt.plot(np.arange(0,11),acf(ts_log_mv_diff,nlags=10))
plt.axhline(y=0,linestyle='--',color='gray')
plt.axhline(y=-7.96/np.sqrt(len(ts_log_mv_diff)),linestyle='--',color='gray')
plt.axhline(y=7.96/np.sqrt(len(ts_log_mv_diff)),linestyle='--',color='gray')
plt.title('Autocorerelation Function')
plt.show()
plt.plot(np.arange(0,11),pacf(ts_log_mv_diff,nlags=10))
plt.axhline(y=0,linestyle='--',color='gray')
plt.axhline(y=-7.96/np.sqrt(len(ts_log_mv_diff)),linestyle='--',color='gray')
plt.axhline(y=7.96/np.sqrt(len(ts_log_mv_diff)),linestyle='--',color='gray')
plt.title('Partial Autocorerelation Function')
plt.show()
model = ARIMA(ts_log,order=(1,1,0))
result_ARIMA = model.fit(disp=-1)
plt.plot(ts_log_mv_diff)
plt.plot(result_ARIMA.fittedvalues,color='red')
plt.title('RSS: %.4f'%sum((result_ARIMA.fittedvalues[1:]) -ts_log_mv_diff)**2)
predictions_Arima_diff = pd.Series(result_ARIMA.fittedvalues,copy=True)
predictions_Arima_diff.head()
predictions_Arima_diff_cumsum = predictions_Arima_diff.cumsum()
predictions_Arima_diff_cumsum.head()
predictions_Arima_log = pd.Series(ts_log.iloc[0],index=ts_log.index)
predictions_Arima_log = predictions_Arima_log.add(predictions_Arima_diff_cumsum,fill_value=0)
predictions_Arima_log.head()
predictions_Arima = np.exp(predictions_Arima_log)
plt.plot(ts)
plt.plot(predictions_Arima)
plt.title('RMSE: %.4f'%np.sqrt(sum((predictions_Arima-ts)**2)/len(ts)))
###Output
_____no_output_____ |
notebooks/Python Loops.ipynb | ###Markdown
Looping in PythonNow that we know about basic collection types in Python, let's see how to iterate over them Python range functionBefore we take a look at the loops, it is a good time to learn about the python built in function: ```range``````range()``` as its name implies, returns a list of numbers in the given range. Let's see a few examples:
###Code
print range(10)
###Output
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
###Markdown
By default ```range``` starts at the value 0 (as seen above) and goes till one value before the value specified to it.We can give it an explicit starting value as well
###Code
print range(2, 10)
###Output
[2, 3, 4, 5, 6, 7, 8, 9]
###Markdown
One more possible variation is to specify the step size between successive values
###Code
print range(0, 101, 10) #Step size of 10
###Output
[0, 10, 20, 30, 40, 50, 60, 70, 80, 90, 100]
###Markdown
For loopLet's see how to print each element of a list
###Code
for i in range(10):
print i
###Output
0
1
2
3
4
5
6
7
8
9
###Markdown
If we wanted to print the square of each number in the list?
###Code
list1 = range(10)
for i in list1:
print i ** 2
###Output
0
1
4
9
16
25
36
49
64
81
###Markdown
For loop over a stringYou can loop through the characters of a String too
###Code
name = 'Alice'
for c in name:
print c
###Output
A
l
i
c
e
###Markdown
Looping over a tupleLooping through a tuple is similar
###Code
tup = (1, 2, 3, 4)
for i in tup:
print i
###Output
1
2
3
4
###Markdown
Looping over a dictionaryUnlike lists, dictionaries contain key, value pairs instead of a single object. Looping through dicts is a bit different as we will see __Printing all the keys of a dictionary__
###Code
d = {}
d['foo'] = 1
d['bar'] = 2
d['baz'] = 3
for k in d:
print k
###Output
baz
foo
bar
###Markdown
*Notice the keys are not printed in the same order in which they were added to the dict. Dictionaries do not maintain order* __Printing the values of the dictionary__
###Code
for v in d.values():
print v
###Output
3
1
2
###Markdown
__Printing both key and values in a dictionary__
###Code
for k, v in d.items():
print k, v
###Output
baz 3
foo 1
bar 2
###Markdown
While loops in PythonWhile loops in Python are similar to other languages
###Code
i = 10
while i > 0:
print i
i = i -1
###Output
10
9
8
7
6
5
4
3
2
1
|
courses/machine_learning/deepdive2/tensorflow_extended/labs/Simple_TFX_Pipeline_for_Vertex_Pipelines.ipynb | ###Markdown
Creating Simple TFX Pipeline for Vertex Pipelines Learning objectives1. Prepare example data.2. Create a pipeline.3. Run the pipeline on Vertex Pipelines. IntroductionIn this notebook, you will create a simple TFX pipeline and run it usingGoogle Cloud Vertex Pipelines. This notebook is based on the TFX pipelineyou built in[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).If you are not familiar with TFX and you have not read that tutorial yet, youshould read it before proceeding with this notebook.Google Cloud Vertex Pipelines helps you to automate, monitor, and governyour ML systems by orchestrating your ML workflow in a serverless manner. Youcan define your ML pipelines using Python with TFX, and then execute yourpipelines on Google Cloud. See[Vertex Pipelines introduction](https://cloud.google.com/vertex-ai/docs/pipelines/introduction)to learn more about Vertex Pipelines. Install python packages You will install required Python packages including TFX and KFP to author MLpipelines and submit jobs to Vertex Pipelines.
###Code
# Use the latest version of pip.
!pip install --upgrade pip
!pip install --upgrade "tfx[kfp]<2"
###Output
Requirement already satisfied: pip in /opt/conda/lib/python3.7/site-packages (22.0.4)
Collecting tfx[kfp]<2
Downloading tfx-1.7.1-py3-none-any.whl (2.5 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m2.5/2.5 MB[0m [31m27.6 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hRequirement already satisfied: tfx-bsl<1.8.0,>=1.7.0 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.7.0)
Collecting packaging<21,>=20
Downloading packaging-20.9-py2.py3-none-any.whl (40 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m40.9/40.9 KB[0m [31m6.3 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: google-apitools<1,>=0.5 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (0.5.31)
Requirement already satisfied: tensorflow-serving-api!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<3,>=1.15 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (2.8.0)
Requirement already satisfied: keras-tuner<2,>=1.0.4 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.1.0)
Requirement already satisfied: apache-beam[gcp]<3,>=2.36 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (2.37.0)
Collecting google-api-python-client<2,>=1.8
Downloading google_api_python_client-1.12.11-py2.py3-none-any.whl (62 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m62.1/62.1 KB[0m [31m9.5 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: google-cloud-aiplatform<2,>=1.6.2 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.11.0)
Requirement already satisfied: numpy<2,>=1.16 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.19.5)
Collecting tensorflow-model-analysis<0.39,>=0.38.0
Downloading tensorflow_model_analysis-0.38.0-py3-none-any.whl (1.8 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.8/1.8 MB[0m [31m58.0 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting pyarrow<6,>=1
Downloading pyarrow-5.0.0-cp37-cp37m-manylinux2014_x86_64.whl (23.6 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m23.6/23.6 MB[0m [31m45.6 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hCollecting click<8,>=7
Downloading click-7.1.2-py2.py3-none-any.whl (82 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m82.8/82.8 KB[0m [31m13.5 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting tensorflow-data-validation<1.8.0,>=1.7.0
Downloading tensorflow_data_validation-1.7.0-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (1.4 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.4/1.4 MB[0m [31m68.5 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: grpcio<2,>=1.28.1 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.44.0)
Collecting ml-pipelines-sdk==1.7.1
Downloading ml_pipelines_sdk-1.7.1-py3-none-any.whl (1.3 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.3/1.3 MB[0m [31m55.5 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: protobuf<4,>=3.13 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (3.19.4)
Requirement already satisfied: google-cloud-bigquery<3,>=2.26.0 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (2.34.2)
Collecting docker<5,>=4.1
Downloading docker-4.4.4-py2.py3-none-any.whl (147 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m147.0/147.0 KB[0m [31m21.7 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: tensorflow-transform<1.8.0,>=1.7.0 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.7.0)
Requirement already satisfied: jinja2<4,>=2.7.3 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (2.11.3)
Collecting pyyaml<6,>=3.12
Downloading PyYAML-5.4.1-cp37-cp37m-manylinux1_x86_64.whl (636 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m636.6/636.6 KB[0m [31m49.5 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: absl-py<2.0.0,>=0.9 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (0.15.0)
Collecting portpicker<2,>=1.3.1
Downloading portpicker-1.5.0-py3-none-any.whl (14 kB)
Collecting attrs<21,>=19.3.0
Downloading attrs-20.3.0-py2.py3-none-any.whl (49 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m49.3/49.3 KB[0m [31m6.9 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: tensorflow-hub<0.13,>=0.9.0 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (0.12.0)
Collecting ml-metadata<1.8.0,>=1.7.0
Downloading ml_metadata-1.7.0-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (6.6 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m6.6/6.6 MB[0m [31m60.8 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0mm
[?25hCollecting kubernetes<13,>=10.0.1
Downloading kubernetes-12.0.1-py2.py3-none-any.whl (1.7 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.7/1.7 MB[0m [31m79.5 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5
Downloading tensorflow-2.8.0-cp37-cp37m-manylinux2010_x86_64.whl (497.5 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m497.5/497.5 MB[0m [31m1.6 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hCollecting kfp-pipeline-spec<0.2,>=0.1.10
Downloading kfp_pipeline_spec-0.1.14-py3-none-any.whl (18 kB)
Collecting kfp<2,>=1.8.5
Downloading kfp-1.8.12.tar.gz (301 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m301.2/301.2 KB[0m [31m32.4 MB/s[0m eta [36m0:00:00[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hRequirement already satisfied: six in /opt/conda/lib/python3.7/site-packages (from absl-py<2.0.0,>=0.9->tfx[kfp]<2) (1.15.0)
Collecting httplib2<0.20.0,>=0.8
Downloading httplib2-0.19.1-py3-none-any.whl (95 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m95.4/95.4 KB[0m [31m12.9 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: pytz>=2018.3 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2021.3)
Requirement already satisfied: proto-plus<2,>=1.7.1 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.20.3)
Requirement already satisfied: fastavro<2,>=0.23.6 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.4.10)
Requirement already satisfied: pydot<2,>=1.2.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.4.2)
Requirement already satisfied: hdfs<3.0.0,>=2.1.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.6.0)
Requirement already satisfied: pymongo<4.0.0,>=3.8.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.12.3)
Requirement already satisfied: typing-extensions>=3.7.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.10.0.2)
Requirement already satisfied: crcmod<2.0,>=1.7 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.7)
Requirement already satisfied: requests<3.0.0,>=2.24.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.27.1)
Requirement already satisfied: python-dateutil<3,>=2.8.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.8.2)
Collecting dill<0.3.2,>=0.3.1.1
Downloading dill-0.3.1.1.tar.gz (151 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m152.0/152.0 KB[0m [31m24.3 MB/s[0m eta [36m0:00:00[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hRequirement already satisfied: cloudpickle<3,>=2.0.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.0.0)
Requirement already satisfied: orjson<4.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.6.7)
Requirement already satisfied: oauth2client<5,>=2.0.1 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (4.1.3)
Collecting google-cloud-videointelligence<2,>=1.8.0
Downloading google_cloud_videointelligence-1.16.2-py2.py3-none-any.whl (183 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m183.9/183.9 KB[0m [31m26.5 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting google-cloud-spanner<2,>=1.13.0
Downloading google_cloud_spanner-1.19.2-py2.py3-none-any.whl (255 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m255.5/255.5 KB[0m [31m32.3 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: cachetools<5,>=3.1.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (4.2.4)
Requirement already satisfied: google-auth<3,>=1.18.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.35.0)
Requirement already satisfied: google-cloud-dlp<4,>=3.0.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.6.2)
Collecting google-cloud-datastore<2,>=1.8.0
Downloading google_cloud_datastore-1.15.4-py2.py3-none-any.whl (134 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m134.2/134.2 KB[0m [31m20.8 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting google-cloud-language<2,>=1.3.0
Downloading google_cloud_language-1.3.1-py2.py3-none-any.whl (83 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m83.6/83.6 KB[0m [31m11.2 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: grpcio-gcp<1,>=0.2.2 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.2.2)
Requirement already satisfied: google-cloud-pubsub<3,>=2.1.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.11.0)
Collecting google-cloud-bigtable<2,>=0.31.1
Downloading google_cloud_bigtable-1.7.1-py2.py3-none-any.whl (267 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m267.7/267.7 KB[0m [31m28.3 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: google-cloud-bigquery-storage>=2.6.3 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.13.0)
Requirement already satisfied: google-cloud-pubsublite<2,>=1.2.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.4.1)
Collecting google-cloud-vision<2,>=0.38.0
Downloading google_cloud_vision-1.0.1-py2.py3-none-any.whl (435 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m435.1/435.1 KB[0m [31m47.1 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting google-cloud-core<2,>=0.28.1
Downloading google_cloud_core-1.7.2-py2.py3-none-any.whl (28 kB)
Requirement already satisfied: google-cloud-recommendations-ai<=0.2.0,>=0.1.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.2.0)
Requirement already satisfied: websocket-client>=0.32.0 in /opt/conda/lib/python3.7/site-packages (from docker<5,>=4.1->tfx[kfp]<2) (1.3.1)
Collecting uritemplate<4dev,>=3.0.0
Downloading uritemplate-3.0.1-py2.py3-none-any.whl (15 kB)
Requirement already satisfied: google-api-core<3dev,>=1.21.0 in /opt/conda/lib/python3.7/site-packages (from google-api-python-client<2,>=1.8->tfx[kfp]<2) (2.5.0)
Requirement already satisfied: google-auth-httplib2>=0.0.3 in /opt/conda/lib/python3.7/site-packages (from google-api-python-client<2,>=1.8->tfx[kfp]<2) (0.1.0)
Requirement already satisfied: fasteners>=0.14 in /opt/conda/lib/python3.7/site-packages (from google-apitools<1,>=0.5->tfx[kfp]<2) (0.17.3)
Requirement already satisfied: google-cloud-storage<3.0.0dev,>=1.32.0 in /opt/conda/lib/python3.7/site-packages (from google-cloud-aiplatform<2,>=1.6.2->tfx[kfp]<2) (2.2.1)
Requirement already satisfied: google-resumable-media<3.0dev,>=0.6.0 in /opt/conda/lib/python3.7/site-packages (from google-cloud-bigquery<3,>=2.26.0->tfx[kfp]<2) (2.3.2)
Requirement already satisfied: MarkupSafe>=0.23 in /opt/conda/lib/python3.7/site-packages (from jinja2<4,>=2.7.3->tfx[kfp]<2) (2.0.1)
Requirement already satisfied: scipy in /opt/conda/lib/python3.7/site-packages (from keras-tuner<2,>=1.0.4->tfx[kfp]<2) (1.7.3)
Requirement already satisfied: tensorboard in /opt/conda/lib/python3.7/site-packages (from keras-tuner<2,>=1.0.4->tfx[kfp]<2) (2.6.0)
Requirement already satisfied: ipython in /opt/conda/lib/python3.7/site-packages (from keras-tuner<2,>=1.0.4->tfx[kfp]<2) (7.32.0)
Requirement already satisfied: kt-legacy in /opt/conda/lib/python3.7/site-packages (from keras-tuner<2,>=1.0.4->tfx[kfp]<2) (1.0.4)
Collecting google-cloud-storage<3.0.0dev,>=1.32.0
Downloading google_cloud_storage-1.44.0-py2.py3-none-any.whl (106 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m106.8/106.8 KB[0m [31m15.5 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting requests-toolbelt<1,>=0.8.0
Downloading requests_toolbelt-0.9.1-py2.py3-none-any.whl (54 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m54.3/54.3 KB[0m [31m8.2 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting kfp-server-api<2.0.0,>=1.1.2
Downloading kfp-server-api-1.8.1.tar.gz (54 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m54.2/54.2 KB[0m [31m9.2 MB/s[0m eta [36m0:00:00[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hCollecting jsonschema<4,>=3.0.1
Downloading jsonschema-3.2.0-py2.py3-none-any.whl (56 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m56.3/56.3 KB[0m [31m10.4 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting tabulate<1,>=0.8.6
Downloading tabulate-0.8.9-py3-none-any.whl (25 kB)
Collecting Deprecated<2,>=1.2.7
Downloading Deprecated-1.2.13-py2.py3-none-any.whl (9.6 kB)
Collecting strip-hints<1,>=0.1.8
Downloading strip-hints-0.1.10.tar.gz (29 kB)
Preparing metadata (setup.py) ... [?25ldone
[?25hCollecting docstring-parser<1,>=0.7.3
Downloading docstring_parser-0.14.1-py3-none-any.whl (33 kB)
Collecting fire<1,>=0.3.1
Downloading fire-0.4.0.tar.gz (87 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m87.7/87.7 KB[0m [31m415.4 kB/s[0m eta [36m0:00:00[0ma [36m0:00:01[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hRequirement already satisfied: pydantic<2,>=1.8.2 in /opt/conda/lib/python3.7/site-packages (from kfp<2,>=1.8.5->tfx[kfp]<2) (1.9.0)
Collecting typer<1.0,>=0.3.2
Downloading typer-0.4.1-py3-none-any.whl (27 kB)
Requirement already satisfied: certifi>=14.05.14 in /opt/conda/lib/python3.7/site-packages (from kubernetes<13,>=10.0.1->tfx[kfp]<2) (2021.10.8)
Requirement already satisfied: setuptools>=21.0.0 in /opt/conda/lib/python3.7/site-packages (from kubernetes<13,>=10.0.1->tfx[kfp]<2) (59.8.0)
Requirement already satisfied: urllib3>=1.24.2 in /opt/conda/lib/python3.7/site-packages (from kubernetes<13,>=10.0.1->tfx[kfp]<2) (1.26.8)
Requirement already satisfied: requests-oauthlib in /opt/conda/lib/python3.7/site-packages (from kubernetes<13,>=10.0.1->tfx[kfp]<2) (1.3.1)
Requirement already satisfied: pyparsing>=2.0.2 in /opt/conda/lib/python3.7/site-packages (from packaging<21,>=20->tfx[kfp]<2) (3.0.7)
Requirement already satisfied: psutil in /opt/conda/lib/python3.7/site-packages (from portpicker<2,>=1.3.1->tfx[kfp]<2) (5.9.0)
Collecting libclang>=9.0.1
Downloading libclang-14.0.1-py2.py3-none-manylinux1_x86_64.whl (14.5 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m14.5/14.5 MB[0m [31m71.9 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hRequirement already satisfied: keras-preprocessing>=1.1.1 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.1.2)
Collecting tf-estimator-nightly==2.8.0.dev2021122109
Downloading tf_estimator_nightly-2.8.0.dev2021122109-py2.py3-none-any.whl (462 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m462.5/462.5 KB[0m [31m41.4 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting numpy<2,>=1.16
Downloading numpy-1.21.6-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (15.7 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m15.7/15.7 MB[0m [31m70.3 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hCollecting tensorboard
Downloading tensorboard-2.8.0-py3-none-any.whl (5.8 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m5.8/5.8 MB[0m [31m96.3 MB/s[0m eta [36m0:00:00[0mta [36m0:00:01[0m
[?25hRequirement already satisfied: h5py>=2.9.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (3.1.0)
Requirement already satisfied: astunparse>=1.6.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.6.3)
Requirement already satisfied: termcolor>=1.1.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.1.0)
Collecting keras<2.9,>=2.8.0rc0
Downloading keras-2.8.0-py2.py3-none-any.whl (1.4 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.4/1.4 MB[0m [31m62.4 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting tensorflow-io-gcs-filesystem>=0.23.1
Downloading tensorflow_io_gcs_filesystem-0.25.0-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (2.1 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m2.1/2.1 MB[0m [31m82.0 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: gast>=0.2.1 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (0.4.0)
Requirement already satisfied: flatbuffers>=1.12 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.12)
Requirement already satisfied: opt-einsum>=2.3.2 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (3.3.0)
Requirement already satisfied: wrapt>=1.11.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.12.1)
Requirement already satisfied: google-pasta>=0.1.1 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (0.2.0)
Collecting joblib<0.15,>=0.12
Downloading joblib-0.14.1-py2.py3-none-any.whl (294 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m294.9/294.9 KB[0m [31m34.9 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting pyfarmhash<0.4,>=0.2
Downloading pyfarmhash-0.3.2.tar.gz (99 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m99.9/99.9 KB[0m [31m15.7 MB/s[0m eta [36m0:00:00[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hRequirement already satisfied: pandas<2,>=1.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow-data-validation<1.8.0,>=1.7.0->tfx[kfp]<2) (1.3.5)
Requirement already satisfied: tensorflow-metadata<1.8,>=1.7.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow-data-validation<1.8.0,>=1.7.0->tfx[kfp]<2) (1.7.0)
Requirement already satisfied: ipywidgets<8,>=7 in /opt/conda/lib/python3.7/site-packages (from tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (7.6.5)
Requirement already satisfied: wheel<1.0,>=0.23.0 in /opt/conda/lib/python3.7/site-packages (from astunparse>=1.6.0->tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (0.37.1)
Requirement already satisfied: googleapis-common-protos<2.0dev,>=1.52.0 in /opt/conda/lib/python3.7/site-packages (from google-api-core<3dev,>=1.21.0->google-api-python-client<2,>=1.8->tfx[kfp]<2) (1.54.0)
Requirement already satisfied: grpcio-status<2.0dev,>=1.33.2 in /opt/conda/lib/python3.7/site-packages (from google-api-core<3dev,>=1.21.0->google-api-python-client<2,>=1.8->tfx[kfp]<2) (1.44.0)
Requirement already satisfied: rsa<5,>=3.1.4 in /opt/conda/lib/python3.7/site-packages (from google-auth<3,>=1.18.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (4.8)
Requirement already satisfied: pyasn1-modules>=0.2.1 in /opt/conda/lib/python3.7/site-packages (from google-auth<3,>=1.18.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.2.7)
Requirement already satisfied: grpc-google-iam-v1<0.13dev,>=0.12.3 in /opt/conda/lib/python3.7/site-packages (from google-cloud-bigtable<2,>=0.31.1->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.12.3)
Collecting google-cloud-core<2,>=0.28.1
Downloading google_cloud_core-1.7.1-py2.py3-none-any.whl (28 kB)
Downloading google_cloud_core-1.7.0-py2.py3-none-any.whl (28 kB)
Downloading google_cloud_core-1.6.0-py2.py3-none-any.whl (28 kB)
Downloading google_cloud_core-1.5.0-py2.py3-none-any.whl (27 kB)
Downloading google_cloud_core-1.4.4-py2.py3-none-any.whl (27 kB)
Downloading google_cloud_core-1.4.3-py2.py3-none-any.whl (27 kB)
Downloading google_cloud_core-1.4.2-py2.py3-none-any.whl (26 kB)
Downloading google_cloud_core-1.4.1-py2.py3-none-any.whl (26 kB)
INFO: pip is looking at multiple versions of google-cloud-bigtable to determine which version is compatible with other requirements. This could take a while.
Collecting google-cloud-bigtable<2,>=0.31.1
Downloading google_cloud_bigtable-1.7.0-py2.py3-none-any.whl (267 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m267.7/267.7 KB[0m [31m35.5 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-cloud-bigquery-storage to determine which version is compatible with other requirements. This could take a while.
Collecting google-cloud-bigquery-storage>=2.6.3
Downloading google_cloud_bigquery_storage-2.13.1-py2.py3-none-any.whl (180 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m180.1/180.1 KB[0m [31m27.5 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-auth-httplib2 to determine which version is compatible with other requirements. This could take a while.
Collecting google-auth-httplib2>=0.0.3
Downloading google_auth_httplib2-0.1.0-py2.py3-none-any.whl (9.3 kB)
INFO: pip is looking at multiple versions of google-auth to determine which version is compatible with other requirements. This could take a while.
Collecting google-auth<3,>=1.18.0
Downloading google_auth-1.35.0-py2.py3-none-any.whl (152 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m152.9/152.9 KB[0m [31m21.4 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-api-core[grpc] to determine which version is compatible with other requirements. This could take a while.
Collecting google-api-core[grpc]!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.0,<3.0.0dev,>=1.31.5
Downloading google_api_core-2.7.3-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.6/114.6 KB[0m [31m19.6 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-api-core to determine which version is compatible with other requirements. This could take a while.
Downloading google_api_core-2.7.2-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.6/114.6 KB[0m [31m19.7 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.7.1-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.7/114.7 KB[0m [31m17.4 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.7.0-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.7/114.7 KB[0m [31m17.4 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.6.1-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.6/114.6 KB[0m [31m17.4 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.6.0-py2.py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.6/114.6 KB[0m [31m18.7 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.5.0-py2.py3-none-any.whl (111 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m111.8/111.8 KB[0m [31m11.0 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-api-core[grpc] to determine which version is compatible with other requirements. This could take a while.
Downloading google_api_core-2.4.0-py2.py3-none-any.whl (111 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m111.7/111.7 KB[0m [31m15.5 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-api-core to determine which version is compatible with other requirements. This could take a while.
Downloading google_api_core-2.3.2-py2.py3-none-any.whl (109 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m109.8/109.8 KB[0m [31m15.1 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-1.31.5-py2.py3-none-any.whl (93 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m93.3/93.3 KB[0m [31m14.2 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: overrides<7.0.0,>=6.0.1 in /opt/conda/lib/python3.7/site-packages (from google-cloud-pubsublite<2,>=1.2.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (6.1.0)
Requirement already satisfied: google-crc32c<2.0dev,>=1.0 in /opt/conda/lib/python3.7/site-packages (from google-resumable-media<3.0dev,>=0.6.0->google-cloud-bigquery<3,>=2.26.0->tfx[kfp]<2) (1.1.2)
Requirement already satisfied: cached-property in /opt/conda/lib/python3.7/site-packages (from h5py>=2.9.0->tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.5.2)
Requirement already satisfied: docopt in /opt/conda/lib/python3.7/site-packages (from hdfs<3.0.0,>=2.1.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.6.2)
Collecting pyparsing>=2.0.2
Downloading pyparsing-2.4.7-py2.py3-none-any.whl (67 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m67.8/67.8 KB[0m [31m10.8 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: backcall in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.2.0)
Requirement already satisfied: decorator in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (5.1.1)
Requirement already satisfied: prompt-toolkit!=3.0.0,!=3.0.1,<3.1.0,>=2.0.0 in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (3.0.27)
Requirement already satisfied: pygments in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (2.11.2)
Requirement already satisfied: jedi>=0.16 in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.18.1)
Requirement already satisfied: matplotlib-inline in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.1.3)
Requirement already satisfied: pexpect>4.3 in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (4.8.0)
Requirement already satisfied: traitlets>=4.2 in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (5.1.1)
Requirement already satisfied: pickleshare in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.7.5)
Requirement already satisfied: jupyterlab-widgets>=1.0.0 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.0.2)
Requirement already satisfied: nbformat>=4.2.0 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (5.2.0)
Requirement already satisfied: ipython-genutils~=0.2.0 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.2.0)
Requirement already satisfied: widgetsnbextension~=3.5.0 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (3.5.2)
Requirement already satisfied: ipykernel>=4.5.1 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (6.9.2)
Requirement already satisfied: importlib-metadata in /opt/conda/lib/python3.7/site-packages (from jsonschema<4,>=3.0.1->kfp<2,>=1.8.5->tfx[kfp]<2) (4.11.3)
Requirement already satisfied: pyrsistent>=0.14.0 in /opt/conda/lib/python3.7/site-packages (from jsonschema<4,>=3.0.1->kfp<2,>=1.8.5->tfx[kfp]<2) (0.18.1)
Requirement already satisfied: pyasn1>=0.1.7 in /opt/conda/lib/python3.7/site-packages (from oauth2client<5,>=2.0.1->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.4.8)
Requirement already satisfied: idna<4,>=2.5 in /opt/conda/lib/python3.7/site-packages (from requests<3.0.0,>=2.24.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.3)
Requirement already satisfied: charset-normalizer~=2.0.0 in /opt/conda/lib/python3.7/site-packages (from requests<3.0.0,>=2.24.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.0.12)
Requirement already satisfied: google-auth-oauthlib<0.5,>=0.4.1 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.4.6)
Requirement already satisfied: markdown>=2.6.8 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (3.3.6)
Requirement already satisfied: tensorboard-plugin-wit>=1.6.0 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (1.8.1)
Requirement already satisfied: werkzeug>=0.11.15 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (2.0.3)
Requirement already satisfied: tensorboard-data-server<0.7.0,>=0.6.0 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.6.1)
Requirement already satisfied: oauthlib>=3.0.0 in /opt/conda/lib/python3.7/site-packages (from requests-oauthlib->kubernetes<13,>=10.0.1->tfx[kfp]<2) (3.2.0)
Requirement already satisfied: cffi>=1.0.0 in /opt/conda/lib/python3.7/site-packages (from google-crc32c<2.0dev,>=1.0->google-resumable-media<3.0dev,>=0.6.0->google-cloud-bigquery<3,>=2.26.0->tfx[kfp]<2) (1.15.0)
Requirement already satisfied: debugpy<2.0,>=1.0.0 in /opt/conda/lib/python3.7/site-packages (from ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.5.1)
Requirement already satisfied: tornado<7.0,>=4.2 in /opt/conda/lib/python3.7/site-packages (from ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (6.1)
Requirement already satisfied: jupyter-client<8.0 in /opt/conda/lib/python3.7/site-packages (from ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (7.1.2)
Requirement already satisfied: nest-asyncio in /opt/conda/lib/python3.7/site-packages (from ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.5.4)
Requirement already satisfied: parso<0.9.0,>=0.8.0 in /opt/conda/lib/python3.7/site-packages (from jedi>=0.16->ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.8.3)
Requirement already satisfied: zipp>=0.5 in /opt/conda/lib/python3.7/site-packages (from importlib-metadata->jsonschema<4,>=3.0.1->kfp<2,>=1.8.5->tfx[kfp]<2) (3.7.0)
Requirement already satisfied: jupyter-core in /opt/conda/lib/python3.7/site-packages (from nbformat>=4.2.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (4.9.2)
Requirement already satisfied: typing-utils>=0.0.3 in /opt/conda/lib/python3.7/site-packages (from overrides<7.0.0,>=6.0.1->google-cloud-pubsublite<2,>=1.2.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.1.0)
Requirement already satisfied: ptyprocess>=0.5 in /opt/conda/lib/python3.7/site-packages (from pexpect>4.3->ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.7.0)
Requirement already satisfied: wcwidth in /opt/conda/lib/python3.7/site-packages (from prompt-toolkit!=3.0.0,!=3.0.1,<3.1.0,>=2.0.0->ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.2.5)
Requirement already satisfied: notebook>=4.4.1 in /opt/conda/lib/python3.7/site-packages (from widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (6.4.10)
Requirement already satisfied: pycparser in /opt/conda/lib/python3.7/site-packages (from cffi>=1.0.0->google-crc32c<2.0dev,>=1.0->google-resumable-media<3.0dev,>=0.6.0->google-cloud-bigquery<3,>=2.26.0->tfx[kfp]<2) (2.21)
Requirement already satisfied: entrypoints in /opt/conda/lib/python3.7/site-packages (from jupyter-client<8.0->ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.4)
Requirement already satisfied: pyzmq>=13 in /opt/conda/lib/python3.7/site-packages (from jupyter-client<8.0->ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (22.3.0)
Requirement already satisfied: nbconvert>=5 in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (6.4.4)
Requirement already satisfied: argon2-cffi in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (21.3.0)
Requirement already satisfied: terminado>=0.8.3 in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.13.3)
Requirement already satisfied: prometheus-client in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.13.1)
Requirement already satisfied: Send2Trash>=1.8.0 in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.8.0)
Requirement already satisfied: nbclient<0.6.0,>=0.5.0 in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.5.13)
Requirement already satisfied: mistune<2,>=0.8.1 in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.8.4)
Requirement already satisfied: beautifulsoup4 in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (4.10.0)
Requirement already satisfied: defusedxml in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.7.1)
Requirement already satisfied: bleach in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (4.1.0)
Requirement already satisfied: testpath in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.6.0)
Requirement already satisfied: jupyterlab-pygments in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.1.2)
Requirement already satisfied: pandocfilters>=1.4.1 in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.5.0)
Requirement already satisfied: argon2-cffi-bindings in /opt/conda/lib/python3.7/site-packages (from argon2-cffi->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (21.2.0)
Requirement already satisfied: soupsieve>1.2 in /opt/conda/lib/python3.7/site-packages (from beautifulsoup4->nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (2.3.1)
Requirement already satisfied: webencodings in /opt/conda/lib/python3.7/site-packages (from bleach->nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.5.1)
Building wheels for collected packages: kfp, dill, fire, kfp-server-api, pyfarmhash, strip-hints
Building wheel for kfp (setup.py) ... [?25ldone
[?25h Created wheel for kfp: filename=kfp-1.8.12-py3-none-any.whl size=419048 sha256=fc5fc7f3a9b5f676cd53e5cd69e492ed3e995211cc4b6b8cfc5a06b54796f1d4
Stored in directory: /home/jupyter/.cache/pip/wheels/54/0c/4a/3fc55077bc88cc17eacaae34c5fd3f6178c1d16d2ee3b0afdf
Building wheel for dill (setup.py) ... [?25ldone
[?25h Created wheel for dill: filename=dill-0.3.1.1-py3-none-any.whl size=78544 sha256=6d34132d6cad3e94afaaf4057513f0bcf6eec4626ab3183f05c6bb5e4d8f93bf
Stored in directory: /home/jupyter/.cache/pip/wheels/a4/61/fd/c57e374e580aa78a45ed78d5859b3a44436af17e22ca53284f
Building wheel for fire (setup.py) ... [?25ldone
[?25h Created wheel for fire: filename=fire-0.4.0-py2.py3-none-any.whl size=115942 sha256=a0e96813de3269a653f0bbe1d106b94a930df65b64cfc8c8afd4091e2af841b1
Stored in directory: /home/jupyter/.cache/pip/wheels/8a/67/fb/2e8a12fa16661b9d5af1f654bd199366799740a85c64981226
Building wheel for kfp-server-api (setup.py) ... [?25ldone
[?25h Created wheel for kfp-server-api: filename=kfp_server_api-1.8.1-py3-none-any.whl size=95549 sha256=1d5907bdf9958cf5fd1529a0fa69ee8a91fefca39b8c3942766a2365d59478f3
Stored in directory: /home/jupyter/.cache/pip/wheels/f5/4e/2e/6795bd3ed456a43652e7de100aca275ec179c9a8dfbcc65626
Building wheel for pyfarmhash (setup.py) ... [?25ldone
[?25h Created wheel for pyfarmhash: filename=pyfarmhash-0.3.2-cp37-cp37m-linux_x86_64.whl size=108625 sha256=1ba31109887fb167782b9678b72ce85552e486cea10a26e776ef9b5d238c512d
Stored in directory: /home/jupyter/.cache/pip/wheels/53/58/7a/3b040f3a2ee31908e3be916e32660db6db53621ce6eba838dc
Building wheel for strip-hints (setup.py) ... [?25ldone
[?25h Created wheel for strip-hints: filename=strip_hints-0.1.10-py2.py3-none-any.whl size=22302 sha256=1fa632e6899f84956f31c089308aec5d16a45626c95b97bbb9dd78e5851779bb
Stored in directory: /home/jupyter/.cache/pip/wheels/5e/14/c3/6e44e9b2545f2d570b03f5b6d38c00b7534aa8abb376978363
Successfully built kfp dill fire kfp-server-api pyfarmhash strip-hints
Installing collected packages: tf-estimator-nightly, tabulate, pyfarmhash, libclang, keras, joblib, uritemplate, tensorflow-io-gcs-filesystem, strip-hints, pyyaml, pyparsing, portpicker, numpy, kfp-pipeline-spec, fire, docstring-parser, dill, Deprecated, click, attrs, typer, requests-toolbelt, pyarrow, packaging, ml-metadata, kfp-server-api, jsonschema, httplib2, docker, kubernetes, google-api-core, tensorboard, google-cloud-core, google-api-python-client, tensorflow, ml-pipelines-sdk, google-cloud-vision, google-cloud-videointelligence, google-cloud-storage, google-cloud-spanner, google-cloud-language, google-cloud-datastore, google-cloud-bigtable, kfp, tensorflow-model-analysis, tensorflow-data-validation, tfx
Attempting uninstall: keras
Found existing installation: keras 2.6.0
Uninstalling keras-2.6.0:
Successfully uninstalled keras-2.6.0
Attempting uninstall: joblib
Found existing installation: joblib 1.0.1
Uninstalling joblib-1.0.1:
Successfully uninstalled joblib-1.0.1
Attempting uninstall: uritemplate
Found existing installation: uritemplate 4.1.1
Uninstalling uritemplate-4.1.1:
Successfully uninstalled uritemplate-4.1.1
Attempting uninstall: pyyaml
Found existing installation: PyYAML 6.0
Uninstalling PyYAML-6.0:
Successfully uninstalled PyYAML-6.0
Attempting uninstall: pyparsing
Found existing installation: pyparsing 3.0.7
Uninstalling pyparsing-3.0.7:
Successfully uninstalled pyparsing-3.0.7
Attempting uninstall: numpy
Found existing installation: numpy 1.19.5
Uninstalling numpy-1.19.5:
Successfully uninstalled numpy-1.19.5
Attempting uninstall: dill
Found existing installation: dill 0.3.4
Uninstalling dill-0.3.4:
Successfully uninstalled dill-0.3.4
Attempting uninstall: click
Found existing installation: click 8.0.4
Uninstalling click-8.0.4:
Successfully uninstalled click-8.0.4
Attempting uninstall: attrs
Found existing installation: attrs 21.4.0
Uninstalling attrs-21.4.0:
Successfully uninstalled attrs-21.4.0
Attempting uninstall: pyarrow
Found existing installation: pyarrow 7.0.0
Uninstalling pyarrow-7.0.0:
Successfully uninstalled pyarrow-7.0.0
Attempting uninstall: packaging
Found existing installation: packaging 21.3
Uninstalling packaging-21.3:
Successfully uninstalled packaging-21.3
Attempting uninstall: jsonschema
Found existing installation: jsonschema 4.4.0
Uninstalling jsonschema-4.4.0:
Successfully uninstalled jsonschema-4.4.0
Attempting uninstall: httplib2
Found existing installation: httplib2 0.20.4
Uninstalling httplib2-0.20.4:
Successfully uninstalled httplib2-0.20.4
Attempting uninstall: docker
Found existing installation: docker 5.0.3
Uninstalling docker-5.0.3:
Successfully uninstalled docker-5.0.3
Attempting uninstall: kubernetes
Found existing installation: kubernetes 23.3.0
Uninstalling kubernetes-23.3.0:
Successfully uninstalled kubernetes-23.3.0
Attempting uninstall: google-api-core
Found existing installation: google-api-core 2.5.0
Uninstalling google-api-core-2.5.0:
Successfully uninstalled google-api-core-2.5.0
Attempting uninstall: tensorboard
Found existing installation: tensorboard 2.6.0
Uninstalling tensorboard-2.6.0:
Successfully uninstalled tensorboard-2.6.0
Attempting uninstall: google-cloud-core
Found existing installation: google-cloud-core 2.2.3
Uninstalling google-cloud-core-2.2.3:
Successfully uninstalled google-cloud-core-2.2.3
Attempting uninstall: google-api-python-client
Found existing installation: google-api-python-client 2.41.0
Uninstalling google-api-python-client-2.41.0:
Successfully uninstalled google-api-python-client-2.41.0
Attempting uninstall: tensorflow
Found existing installation: tensorflow 2.6.3
Uninstalling tensorflow-2.6.3:
Successfully uninstalled tensorflow-2.6.3
Attempting uninstall: google-cloud-vision
Found existing installation: google-cloud-vision 2.7.1
Uninstalling google-cloud-vision-2.7.1:
Successfully uninstalled google-cloud-vision-2.7.1
Attempting uninstall: google-cloud-videointelligence
Found existing installation: google-cloud-videointelligence 2.6.1
Uninstalling google-cloud-videointelligence-2.6.1:
Successfully uninstalled google-cloud-videointelligence-2.6.1
Attempting uninstall: google-cloud-storage
Found existing installation: google-cloud-storage 2.2.1
Uninstalling google-cloud-storage-2.2.1:
Successfully uninstalled google-cloud-storage-2.2.1
Attempting uninstall: google-cloud-spanner
Found existing installation: google-cloud-spanner 3.13.0
Uninstalling google-cloud-spanner-3.13.0:
Successfully uninstalled google-cloud-spanner-3.13.0
Attempting uninstall: google-cloud-language
Found existing installation: google-cloud-language 2.4.1
Uninstalling google-cloud-language-2.4.1:
Successfully uninstalled google-cloud-language-2.4.1
Attempting uninstall: google-cloud-datastore
Found existing installation: google-cloud-datastore 2.5.1
Uninstalling google-cloud-datastore-2.5.1:
Successfully uninstalled google-cloud-datastore-2.5.1
Attempting uninstall: google-cloud-bigtable
Found existing installation: google-cloud-bigtable 2.7.0
Uninstalling google-cloud-bigtable-2.7.0:
Successfully uninstalled google-cloud-bigtable-2.7.0
[31mERROR: pip's dependency resolver does not currently take into account all the packages that are installed. This behaviour is the source of the following dependency conflicts.
tensorflow-io 0.21.0 requires tensorflow<2.7.0,>=2.6.0, but you have tensorflow 2.8.0 which is incompatible.
tensorflow-io 0.21.0 requires tensorflow-io-gcs-filesystem==0.21.0, but you have tensorflow-io-gcs-filesystem 0.25.0 which is incompatible.
statsmodels 0.13.2 requires packaging>=21.3, but you have packaging 20.9 which is incompatible.
pandas-profiling 3.1.0 requires joblib~=1.0.1, but you have joblib 0.14.1 which is incompatible.
cloud-tpu-client 0.10 requires google-api-python-client==1.8.0, but you have google-api-python-client 1.12.11 which is incompatible.
black 22.1.0 requires click>=8.0.0, but you have click 7.1.2 which is incompatible.[0m[31m
[0mSuccessfully installed Deprecated-1.2.13 attrs-20.3.0 click-7.1.2 dill-0.3.1.1 docker-4.4.4 docstring-parser-0.14.1 fire-0.4.0 google-api-core-1.31.5 google-api-python-client-1.12.11 google-cloud-bigtable-1.7.1 google-cloud-core-2.2.2 google-cloud-datastore-1.15.4 google-cloud-language-1.3.1 google-cloud-spanner-1.19.2 google-cloud-storage-2.1.0 google-cloud-videointelligence-1.16.2 google-cloud-vision-1.0.1 httplib2-0.19.1 joblib-0.14.1 jsonschema-3.2.0 keras-2.8.0 kfp-1.8.12 kfp-pipeline-spec-0.1.14 kfp-server-api-1.8.1 kubernetes-12.0.1 libclang-14.0.1 ml-metadata-1.7.0 ml-pipelines-sdk-1.7.1 numpy-1.21.6 packaging-20.9 portpicker-1.5.0 pyarrow-5.0.0 pyfarmhash-0.3.2 pyparsing-2.4.7 pyyaml-5.4.1 requests-toolbelt-0.9.1 strip-hints-0.1.10 tabulate-0.8.9 tensorboard-2.8.0 tensorflow-2.8.0 tensorflow-data-validation-1.7.0 tensorflow-io-gcs-filesystem-0.25.0 tensorflow-model-analysis-0.38.0 tf-estimator-nightly-2.8.0.dev2021122109 tfx-1.7.1 typer-0.4.1 uritemplate-3.0.1
###Markdown
Did you restart the runtime? You can restart runtime with following cell.
###Code
# docs_infra: no_execute
import sys
if not 'google.colab' in sys.modules:
# Automatically restart kernel after installs
import IPython
app = IPython.Application.instance()
app.kernel.do_shutdown(True)
###Output
_____no_output_____
###Markdown
Check the package versions
###Code
import tensorflow as tf
print('TensorFlow version: {}'.format(tf.__version__))
from tfx import v1 as tfx
print('TFX version: {}'.format(tfx.__version__))
import kfp
print('KFP version: {}'.format(kfp.__version__))
###Output
TensorFlow version: 2.8.0
TFX version: 1.7.1
KFP version: 1.8.12
###Markdown
Set up variablesYou will set up some variables used to customize the pipelines below. Followinginformation is required:* GCP Project id. See[Identifying your project id](https://cloud.google.com/resource-manager/docs/creating-managing-projectsidentifying_projects).* GCP Region to run pipelines. For more information about the regions thatVertex Pipelines is available in, see the[Vertex AI locations guide](https://cloud.google.com/vertex-ai/docs/general/locationsfeature-availability).* Google Cloud Storage Bucket to store pipeline outputs.**Enter required values in the cell below before running it**.
###Code
GOOGLE_CLOUD_PROJECT = 'qwiklabs-gcp-01-2e305ff9c72b' # <--- ENTER THIS
GOOGLE_CLOUD_REGION = 'us-central1' # <--- ENTER THIS
GCS_BUCKET_NAME = 'qwiklabs-gcp-01-2e305ff9c72b' # <--- ENTER THIS
if not (GOOGLE_CLOUD_PROJECT and GOOGLE_CLOUD_REGION and GCS_BUCKET_NAME):
from absl import logging
logging.error('Please set all required parameters.')
###Output
_____no_output_____
###Markdown
Set `gcloud` to use your project.
###Code
!gcloud config set project {GOOGLE_CLOUD_PROJECT}
PIPELINE_NAME = 'penguin-vertex-pipelines'
# Path to various pipeline artifact.
PIPELINE_ROOT = 'gs://{}/pipeline_root/{}'.format(
GCS_BUCKET_NAME, PIPELINE_NAME)
# Paths for users' Python module.
MODULE_ROOT = 'gs://{}/pipeline_module/{}'.format(
GCS_BUCKET_NAME, PIPELINE_NAME)
# Paths for input data.
DATA_ROOT = 'gs://{}/data/{}'.format(GCS_BUCKET_NAME, PIPELINE_NAME)
# This is the path where your model will be pushed for serving.
SERVING_MODEL_DIR = 'gs://{}/serving_model/{}'.format(
GCS_BUCKET_NAME, PIPELINE_NAME)
print('PIPELINE_ROOT: {}'.format(PIPELINE_ROOT))
###Output
PIPELINE_ROOT: gs://qwiklabs-gcp-01-2e305ff9c72b/pipeline_root/penguin-vertex-pipelines
###Markdown
Prepare example dataYou will use the same[Palmer Penguins dataset](https://allisonhorst.github.io/palmerpenguins/articles/intro.html)as[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).There are four numeric features in this dataset which were already normalizedto have range [0,1]. You will build a classification model which predicts the`species` of penguins. You need to make your own copy of the dataset. Because TFX ExampleGen readsinputs from a directory, You need to create a directory and copy dataset to iton GCS.
###Code
!gsutil cp gs://download.tensorflow.org/data/palmer_penguins/penguins_processed.csv {DATA_ROOT}/
###Output
Copying gs://download.tensorflow.org/data/palmer_penguins/penguins_processed.csv [Content-Type=application/octet-stream]...
/ [1 files][ 25.0 KiB/ 25.0 KiB]
Operation completed over 1 objects/25.0 KiB.
###Markdown
Take a quick look at the CSV file.
###Code
!gsutil cat {DATA_ROOT}/penguins_processed.csv | head
###Output
species,culmen_length_mm,culmen_depth_mm,flipper_length_mm,body_mass_g
0,0.2545454545454545,0.6666666666666666,0.15254237288135594,0.2916666666666667
0,0.26909090909090905,0.5119047619047618,0.23728813559322035,0.3055555555555556
0,0.29818181818181805,0.5833333333333334,0.3898305084745763,0.1527777777777778
0,0.16727272727272732,0.7380952380952381,0.3559322033898305,0.20833333333333334
0,0.26181818181818167,0.892857142857143,0.3050847457627119,0.2638888888888889
0,0.24727272727272717,0.5595238095238096,0.15254237288135594,0.2569444444444444
0,0.25818181818181823,0.773809523809524,0.3898305084745763,0.5486111111111112
0,0.32727272727272727,0.5357142857142859,0.1694915254237288,0.1388888888888889
0,0.23636363636363636,0.9642857142857142,0.3220338983050847,0.3055555555555556
###Markdown
Create a pipelineTFX pipelines are defined using Python APIs. You will define a pipeline whichconsists of three components, CsvExampleGen, Trainer and Pusher. The pipelineand model definition is almost the same as[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).The only difference is that you don't need to set `metadata_connection_config`which is used to locate[ML Metadata](https://www.tensorflow.org/tfx/guide/mlmd) database. BecauseVertex Pipelines uses a managed metadata service, users don't need to careof it, and you don't need to specify the parameter.Before actually define the pipeline, you need to write a model code for theTrainer component first. Write model code.You will use the same model code as in the[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).
###Code
_trainer_module_file = 'penguin_trainer.py'
%%writefile {_trainer_module_file}
# Copied from https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple
from typing import List
from absl import logging
import tensorflow as tf
from tensorflow import keras
from tensorflow_transform.tf_metadata import schema_utils
from tfx import v1 as tfx
from tfx_bsl.public import tfxio
from tensorflow_metadata.proto.v0 import schema_pb2
_FEATURE_KEYS = [
'culmen_length_mm', 'culmen_depth_mm', 'flipper_length_mm', 'body_mass_g'
]
_LABEL_KEY = 'species'
_TRAIN_BATCH_SIZE = 20
_EVAL_BATCH_SIZE = 10
# Since you're not generating or creating a schema, you will instead create
# a feature spec. Since there are a fairly small number of features this is
# manageable for this dataset.
_FEATURE_SPEC = {
**{
feature: tf.io.FixedLenFeature(shape=[1], dtype=tf.float32)
for feature in _FEATURE_KEYS
},
_LABEL_KEY: tf.io.FixedLenFeature(shape=[1], dtype=tf.int64)
}
def _input_fn(file_pattern: List[str],
data_accessor: tfx.components.DataAccessor,
schema: schema_pb2.Schema,
batch_size: int) -> tf.data.Dataset:
"""Generates features and label for training.
Args:
file_pattern: List of paths or patterns of input tfrecord files.
data_accessor: DataAccessor for converting input to RecordBatch.
schema: schema of the input data.
batch_size: representing the number of consecutive elements of returned
dataset to combine in a single batch
Returns:
A dataset that contains (features, indices) tuple where features is a
dictionary of Tensors, and indices is a single Tensor of label indices.
"""
return data_accessor.tf_dataset_factory(
file_pattern,
tfxio.TensorFlowDatasetOptions(
batch_size=batch_size, label_key=_LABEL_KEY),
schema=schema).repeat()
def _make_keras_model() -> tf.keras.Model:
"""Creates a DNN Keras model for classifying penguin data.
Returns:
A Keras Model.
"""
# The model below is built with Functional API, please refer to
# https://www.tensorflow.org/guide/keras/overview for all API options.
inputs = [keras.layers.Input(shape=(1,), name=f) for f in _FEATURE_KEYS]
d = keras.layers.concatenate(inputs)
for _ in range(2):
d = keras.layers.Dense(8, activation='relu')(d)
outputs = keras.layers.Dense(3)(d)
model = keras.Model(inputs=inputs, outputs=outputs)
model.compile(
optimizer=keras.optimizers.Adam(1e-2),
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=[keras.metrics.SparseCategoricalAccuracy()])
model.summary(print_fn=logging.info)
return model
# TFX Trainer will call this function.
def run_fn(fn_args: tfx.components.FnArgs):
"""Train the model based on given args.
Args:
fn_args: Holds args used to train the model as name/value pairs.
"""
# This schema is usually either an output of SchemaGen or a manually-curated
# version provided by pipeline author. A schema can also derived from TFT
# graph if a Transform component is used. In the case when either is missing,
# `schema_from_feature_spec` could be used to generate schema from very simple
# feature_spec, but the schema returned would be very primitive.
schema = schema_utils.schema_from_feature_spec(_FEATURE_SPEC)
train_dataset = _input_fn(
fn_args.train_files,
fn_args.data_accessor,
schema,
batch_size=_TRAIN_BATCH_SIZE)
eval_dataset = _input_fn(
fn_args.eval_files,
fn_args.data_accessor,
schema,
batch_size=_EVAL_BATCH_SIZE)
model = _make_keras_model()
model.fit(
train_dataset,
steps_per_epoch=fn_args.train_steps,
validation_data=eval_dataset,
validation_steps=fn_args.eval_steps)
# The result of the training should be saved in `fn_args.serving_model_dir`
# directory.
model.save(fn_args.serving_model_dir, save_format='tf')
###Output
Writing penguin_trainer.py
###Markdown
Copy the module file to GCS which can be accessed from the pipeline components.Because model training happens on GCP, you need to upload this model definition. Otherwise, you might want to build a container image including the module fileand use the image to run the pipeline.
###Code
!gsutil cp {_trainer_module_file} {MODULE_ROOT}/
###Output
Copying file://penguin_trainer.py [Content-Type=text/x-python]...
/ [1 files][ 3.8 KiB/ 3.8 KiB]
Operation completed over 1 objects/3.8 KiB.
###Markdown
Write a pipeline definitionYou will define a function to create a TFX pipeline.
###Code
# Copied from https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple and
# slightly modified because you don't need `metadata_path` argument.
def _create_pipeline(pipeline_name: str, pipeline_root: str, data_root: str,
module_file: str, serving_model_dir: str,
) -> tfx.dsl.Pipeline:
"""Creates a three component penguin pipeline with TFX."""
# Brings data into the pipeline.
example_gen = tfx.components.CsvExampleGen(input_base=data_root)
# Uses user-provided Python function that trains a model.
trainer = tfx.components.Trainer(
module_file=module_file,
examples=example_gen.outputs['examples'],
train_args=tfx.proto.TrainArgs(num_steps=100),
eval_args=tfx.proto.EvalArgs(num_steps=5))
# Pushes the model to a filesystem destination.
pusher = tfx.components.Pusher(
model=trainer.outputs['model'],
push_destination=tfx.proto.PushDestination(
filesystem=tfx.proto.PushDestination.Filesystem(
base_directory=serving_model_dir)))
# Following three components will be included in the pipeline.
components = [
example_gen,
trainer,
pusher,
]
return tfx.dsl.Pipeline(
pipeline_name=pipeline_name,
pipeline_root=pipeline_root,
components=components)
###Output
_____no_output_____
###Markdown
Run the pipeline on Vertex Pipelines.You used `LocalDagRunner` which runs on local environment in[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).TFX provides multiple orchestrators to run your pipeline. In this tutorial youwill use the Vertex Pipelines together with the Kubeflow V2 dag runner. You need to define a runner to actually run the pipeline. You will compileyour pipeline into our pipeline definition format using TFX APIs.
###Code
import os
PIPELINE_DEFINITION_FILE = PIPELINE_NAME + '_pipeline.json'
runner = tfx.orchestration.experimental.KubeflowV2DagRunner(
config=tfx.orchestration.experimental.KubeflowV2DagRunnerConfig(),
output_filename=PIPELINE_DEFINITION_FILE)
# Following function will write the pipeline definition to PIPELINE_DEFINITION_FILE.
_ = runner.run(
_create_pipeline(
pipeline_name=PIPELINE_NAME,
pipeline_root=PIPELINE_ROOT,
data_root=DATA_ROOT,
module_file=os.path.join(MODULE_ROOT, _trainer_module_file),
serving_model_dir=SERVING_MODEL_DIR))
###Output
_____no_output_____
###Markdown
The generated definition file can be submitted using kfp client.
###Code
# docs_infra: no_execute
from google.cloud import aiplatform
from google.cloud.aiplatform import pipeline_jobs
import logging
logging.getLogger().setLevel(logging.INFO)
aiplatform.init(project=GOOGLE_CLOUD_PROJECT, location=GOOGLE_CLOUD_REGION)
job = pipeline_jobs.PipelineJob(template_path=PIPELINE_DEFINITION_FILE,
display_name=PIPELINE_NAME)
job.submit()
###Output
INFO:google.cloud.aiplatform.pipeline_jobs:Creating PipelineJob
INFO:google.cloud.aiplatform.pipeline_jobs:PipelineJob created. Resource name: projects/202132981718/locations/us-central1/pipelineJobs/penguin-vertex-pipelines-20220510100554
INFO:google.cloud.aiplatform.pipeline_jobs:To use this PipelineJob in another session:
INFO:google.cloud.aiplatform.pipeline_jobs:pipeline_job = aiplatform.PipelineJob.get('projects/202132981718/locations/us-central1/pipelineJobs/penguin-vertex-pipelines-20220510100554')
INFO:google.cloud.aiplatform.pipeline_jobs:View Pipeline Job:
https://console.cloud.google.com/vertex-ai/locations/us-central1/pipelines/runs/penguin-vertex-pipelines-20220510100554?project=202132981718
###Markdown
Creating a Simple TFX Pipeline for Vertex AI Pipelines Learning objectives1. Prepare example data.2. Create a pipeline.3. Run the pipeline on Vertex AI Pipelines. IntroductionIn this notebook, you will create a simple TFX pipeline and run it usingGoogle Cloud Vertex Pipelines. This notebook is based on the TFX pipelineyou built in[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).If you are not familiar with TFX and you have not read that tutorial yet, youshould read it before proceeding with this notebook.Google Cloud Vertex Pipelines helps you to automate, monitor, and governyour ML systems by orchestrating your ML workflow in a serverless manner. Youcan define your ML pipelines using Python with TFX, and then execute yourpipelines on Google Cloud. See[Vertex Pipelines introduction](https://cloud.google.com/vertex-ai/docs/pipelines/introduction)to learn more about Vertex Pipelines.Each learning objective will correspond to a _TODO_ in this student lab notebook -- try to complete this notebook first and then review the [solution notebook](../solutions/Simple_TFX_Pipeline_for_Vertex_Pipelines.ipynb) Install python packages You will install required Python packages including TFX and KFP to author MLpipelines and submit jobs to Vertex Pipelines.
###Code
# Use the latest version of pip.
!pip install --upgrade pip
!pip install --upgrade "tfx[kfp]<2"
###Output
Requirement already satisfied: pip in /opt/conda/lib/python3.7/site-packages (22.0.4)
Collecting tfx[kfp]<2
Downloading tfx-1.7.1-py3-none-any.whl (2.5 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m2.5/2.5 MB[0m [31m27.6 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hRequirement already satisfied: tfx-bsl<1.8.0,>=1.7.0 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.7.0)
Collecting packaging<21,>=20
Downloading packaging-20.9-py2.py3-none-any.whl (40 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m40.9/40.9 KB[0m [31m6.3 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: google-apitools<1,>=0.5 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (0.5.31)
Requirement already satisfied: tensorflow-serving-api!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<3,>=1.15 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (2.8.0)
Requirement already satisfied: keras-tuner<2,>=1.0.4 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.1.0)
Requirement already satisfied: apache-beam[gcp]<3,>=2.36 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (2.37.0)
Collecting google-api-python-client<2,>=1.8
Downloading google_api_python_client-1.12.11-py2.py3-none-any.whl (62 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m62.1/62.1 KB[0m [31m9.5 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: google-cloud-aiplatform<2,>=1.6.2 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.11.0)
Requirement already satisfied: numpy<2,>=1.16 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.19.5)
Collecting tensorflow-model-analysis<0.39,>=0.38.0
Downloading tensorflow_model_analysis-0.38.0-py3-none-any.whl (1.8 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.8/1.8 MB[0m [31m58.0 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting pyarrow<6,>=1
Downloading pyarrow-5.0.0-cp37-cp37m-manylinux2014_x86_64.whl (23.6 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m23.6/23.6 MB[0m [31m45.6 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hCollecting click<8,>=7
Downloading click-7.1.2-py2.py3-none-any.whl (82 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m82.8/82.8 KB[0m [31m13.5 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting tensorflow-data-validation<1.8.0,>=1.7.0
Downloading tensorflow_data_validation-1.7.0-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (1.4 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.4/1.4 MB[0m [31m68.5 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: grpcio<2,>=1.28.1 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.44.0)
Collecting ml-pipelines-sdk==1.7.1
Downloading ml_pipelines_sdk-1.7.1-py3-none-any.whl (1.3 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.3/1.3 MB[0m [31m55.5 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: protobuf<4,>=3.13 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (3.19.4)
Requirement already satisfied: google-cloud-bigquery<3,>=2.26.0 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (2.34.2)
Collecting docker<5,>=4.1
Downloading docker-4.4.4-py2.py3-none-any.whl (147 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m147.0/147.0 KB[0m [31m21.7 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: tensorflow-transform<1.8.0,>=1.7.0 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.7.0)
Requirement already satisfied: jinja2<4,>=2.7.3 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (2.11.3)
Collecting pyyaml<6,>=3.12
Downloading PyYAML-5.4.1-cp37-cp37m-manylinux1_x86_64.whl (636 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m636.6/636.6 KB[0m [31m49.5 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: absl-py<2.0.0,>=0.9 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (0.15.0)
Collecting portpicker<2,>=1.3.1
Downloading portpicker-1.5.0-py3-none-any.whl (14 kB)
Collecting attrs<21,>=19.3.0
Downloading attrs-20.3.0-py2.py3-none-any.whl (49 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m49.3/49.3 KB[0m [31m6.9 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: tensorflow-hub<0.13,>=0.9.0 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (0.12.0)
Collecting ml-metadata<1.8.0,>=1.7.0
Downloading ml_metadata-1.7.0-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (6.6 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m6.6/6.6 MB[0m [31m60.8 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0mm
[?25hCollecting kubernetes<13,>=10.0.1
Downloading kubernetes-12.0.1-py2.py3-none-any.whl (1.7 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.7/1.7 MB[0m [31m79.5 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5
Downloading tensorflow-2.8.0-cp37-cp37m-manylinux2010_x86_64.whl (497.5 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m497.5/497.5 MB[0m [31m1.6 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hCollecting kfp-pipeline-spec<0.2,>=0.1.10
Downloading kfp_pipeline_spec-0.1.14-py3-none-any.whl (18 kB)
Collecting kfp<2,>=1.8.5
Downloading kfp-1.8.12.tar.gz (301 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m301.2/301.2 KB[0m [31m32.4 MB/s[0m eta [36m0:00:00[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hRequirement already satisfied: six in /opt/conda/lib/python3.7/site-packages (from absl-py<2.0.0,>=0.9->tfx[kfp]<2) (1.15.0)
Collecting httplib2<0.20.0,>=0.8
Downloading httplib2-0.19.1-py3-none-any.whl (95 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m95.4/95.4 KB[0m [31m12.9 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: pytz>=2018.3 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2021.3)
Requirement already satisfied: proto-plus<2,>=1.7.1 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.20.3)
Requirement already satisfied: fastavro<2,>=0.23.6 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.4.10)
Requirement already satisfied: pydot<2,>=1.2.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.4.2)
Requirement already satisfied: hdfs<3.0.0,>=2.1.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.6.0)
Requirement already satisfied: pymongo<4.0.0,>=3.8.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.12.3)
Requirement already satisfied: typing-extensions>=3.7.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.10.0.2)
Requirement already satisfied: crcmod<2.0,>=1.7 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.7)
Requirement already satisfied: requests<3.0.0,>=2.24.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.27.1)
Requirement already satisfied: python-dateutil<3,>=2.8.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.8.2)
Collecting dill<0.3.2,>=0.3.1.1
Downloading dill-0.3.1.1.tar.gz (151 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m152.0/152.0 KB[0m [31m24.3 MB/s[0m eta [36m0:00:00[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hRequirement already satisfied: cloudpickle<3,>=2.0.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.0.0)
Requirement already satisfied: orjson<4.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.6.7)
Requirement already satisfied: oauth2client<5,>=2.0.1 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (4.1.3)
Collecting google-cloud-videointelligence<2,>=1.8.0
Downloading google_cloud_videointelligence-1.16.2-py2.py3-none-any.whl (183 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m183.9/183.9 KB[0m [31m26.5 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting google-cloud-spanner<2,>=1.13.0
Downloading google_cloud_spanner-1.19.2-py2.py3-none-any.whl (255 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m255.5/255.5 KB[0m [31m32.3 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: cachetools<5,>=3.1.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (4.2.4)
Requirement already satisfied: google-auth<3,>=1.18.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.35.0)
Requirement already satisfied: google-cloud-dlp<4,>=3.0.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.6.2)
Collecting google-cloud-datastore<2,>=1.8.0
Downloading google_cloud_datastore-1.15.4-py2.py3-none-any.whl (134 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m134.2/134.2 KB[0m [31m20.8 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting google-cloud-language<2,>=1.3.0
Downloading google_cloud_language-1.3.1-py2.py3-none-any.whl (83 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m83.6/83.6 KB[0m [31m11.2 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: grpcio-gcp<1,>=0.2.2 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.2.2)
Requirement already satisfied: google-cloud-pubsub<3,>=2.1.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.11.0)
Collecting google-cloud-bigtable<2,>=0.31.1
Downloading google_cloud_bigtable-1.7.1-py2.py3-none-any.whl (267 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m267.7/267.7 KB[0m [31m28.3 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: google-cloud-bigquery-storage>=2.6.3 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.13.0)
Requirement already satisfied: google-cloud-pubsublite<2,>=1.2.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.4.1)
Collecting google-cloud-vision<2,>=0.38.0
Downloading google_cloud_vision-1.0.1-py2.py3-none-any.whl (435 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m435.1/435.1 KB[0m [31m47.1 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting google-cloud-core<2,>=0.28.1
Downloading google_cloud_core-1.7.2-py2.py3-none-any.whl (28 kB)
Requirement already satisfied: google-cloud-recommendations-ai<=0.2.0,>=0.1.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.2.0)
Requirement already satisfied: websocket-client>=0.32.0 in /opt/conda/lib/python3.7/site-packages (from docker<5,>=4.1->tfx[kfp]<2) (1.3.1)
Collecting uritemplate<4dev,>=3.0.0
Downloading uritemplate-3.0.1-py2.py3-none-any.whl (15 kB)
Requirement already satisfied: google-api-core<3dev,>=1.21.0 in /opt/conda/lib/python3.7/site-packages (from google-api-python-client<2,>=1.8->tfx[kfp]<2) (2.5.0)
Requirement already satisfied: google-auth-httplib2>=0.0.3 in /opt/conda/lib/python3.7/site-packages (from google-api-python-client<2,>=1.8->tfx[kfp]<2) (0.1.0)
Requirement already satisfied: fasteners>=0.14 in /opt/conda/lib/python3.7/site-packages (from google-apitools<1,>=0.5->tfx[kfp]<2) (0.17.3)
Requirement already satisfied: google-cloud-storage<3.0.0dev,>=1.32.0 in /opt/conda/lib/python3.7/site-packages (from google-cloud-aiplatform<2,>=1.6.2->tfx[kfp]<2) (2.2.1)
Requirement already satisfied: google-resumable-media<3.0dev,>=0.6.0 in /opt/conda/lib/python3.7/site-packages (from google-cloud-bigquery<3,>=2.26.0->tfx[kfp]<2) (2.3.2)
Requirement already satisfied: MarkupSafe>=0.23 in /opt/conda/lib/python3.7/site-packages (from jinja2<4,>=2.7.3->tfx[kfp]<2) (2.0.1)
Requirement already satisfied: scipy in /opt/conda/lib/python3.7/site-packages (from keras-tuner<2,>=1.0.4->tfx[kfp]<2) (1.7.3)
Requirement already satisfied: tensorboard in /opt/conda/lib/python3.7/site-packages (from keras-tuner<2,>=1.0.4->tfx[kfp]<2) (2.6.0)
Requirement already satisfied: ipython in /opt/conda/lib/python3.7/site-packages (from keras-tuner<2,>=1.0.4->tfx[kfp]<2) (7.32.0)
Requirement already satisfied: kt-legacy in /opt/conda/lib/python3.7/site-packages (from keras-tuner<2,>=1.0.4->tfx[kfp]<2) (1.0.4)
Collecting google-cloud-storage<3.0.0dev,>=1.32.0
Downloading google_cloud_storage-1.44.0-py2.py3-none-any.whl (106 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m106.8/106.8 KB[0m [31m15.5 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting requests-toolbelt<1,>=0.8.0
Downloading requests_toolbelt-0.9.1-py2.py3-none-any.whl (54 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m54.3/54.3 KB[0m [31m8.2 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting kfp-server-api<2.0.0,>=1.1.2
Downloading kfp-server-api-1.8.1.tar.gz (54 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m54.2/54.2 KB[0m [31m9.2 MB/s[0m eta [36m0:00:00[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hCollecting jsonschema<4,>=3.0.1
Downloading jsonschema-3.2.0-py2.py3-none-any.whl (56 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m56.3/56.3 KB[0m [31m10.4 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting tabulate<1,>=0.8.6
Downloading tabulate-0.8.9-py3-none-any.whl (25 kB)
Collecting Deprecated<2,>=1.2.7
Downloading Deprecated-1.2.13-py2.py3-none-any.whl (9.6 kB)
Collecting strip-hints<1,>=0.1.8
Downloading strip-hints-0.1.10.tar.gz (29 kB)
Preparing metadata (setup.py) ... [?25ldone
[?25hCollecting docstring-parser<1,>=0.7.3
Downloading docstring_parser-0.14.1-py3-none-any.whl (33 kB)
Collecting fire<1,>=0.3.1
Downloading fire-0.4.0.tar.gz (87 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m87.7/87.7 KB[0m [31m415.4 kB/s[0m eta [36m0:00:00[0ma [36m0:00:01[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hRequirement already satisfied: pydantic<2,>=1.8.2 in /opt/conda/lib/python3.7/site-packages (from kfp<2,>=1.8.5->tfx[kfp]<2) (1.9.0)
Collecting typer<1.0,>=0.3.2
Downloading typer-0.4.1-py3-none-any.whl (27 kB)
Requirement already satisfied: certifi>=14.05.14 in /opt/conda/lib/python3.7/site-packages (from kubernetes<13,>=10.0.1->tfx[kfp]<2) (2021.10.8)
Requirement already satisfied: setuptools>=21.0.0 in /opt/conda/lib/python3.7/site-packages (from kubernetes<13,>=10.0.1->tfx[kfp]<2) (59.8.0)
Requirement already satisfied: urllib3>=1.24.2 in /opt/conda/lib/python3.7/site-packages (from kubernetes<13,>=10.0.1->tfx[kfp]<2) (1.26.8)
Requirement already satisfied: requests-oauthlib in /opt/conda/lib/python3.7/site-packages (from kubernetes<13,>=10.0.1->tfx[kfp]<2) (1.3.1)
Requirement already satisfied: pyparsing>=2.0.2 in /opt/conda/lib/python3.7/site-packages (from packaging<21,>=20->tfx[kfp]<2) (3.0.7)
Requirement already satisfied: psutil in /opt/conda/lib/python3.7/site-packages (from portpicker<2,>=1.3.1->tfx[kfp]<2) (5.9.0)
Collecting libclang>=9.0.1
Downloading libclang-14.0.1-py2.py3-none-manylinux1_x86_64.whl (14.5 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m14.5/14.5 MB[0m [31m71.9 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hRequirement already satisfied: keras-preprocessing>=1.1.1 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.1.2)
Collecting tf-estimator-nightly==2.8.0.dev2021122109
Downloading tf_estimator_nightly-2.8.0.dev2021122109-py2.py3-none-any.whl (462 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m462.5/462.5 KB[0m [31m41.4 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting numpy<2,>=1.16
Downloading numpy-1.21.6-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (15.7 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m15.7/15.7 MB[0m [31m70.3 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hCollecting tensorboard
Downloading tensorboard-2.8.0-py3-none-any.whl (5.8 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m5.8/5.8 MB[0m [31m96.3 MB/s[0m eta [36m0:00:00[0mta [36m0:00:01[0m
[?25hRequirement already satisfied: h5py>=2.9.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (3.1.0)
Requirement already satisfied: astunparse>=1.6.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.6.3)
Requirement already satisfied: termcolor>=1.1.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.1.0)
Collecting keras<2.9,>=2.8.0rc0
Downloading keras-2.8.0-py2.py3-none-any.whl (1.4 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.4/1.4 MB[0m [31m62.4 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting tensorflow-io-gcs-filesystem>=0.23.1
Downloading tensorflow_io_gcs_filesystem-0.25.0-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (2.1 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m2.1/2.1 MB[0m [31m82.0 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: gast>=0.2.1 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (0.4.0)
Requirement already satisfied: flatbuffers>=1.12 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.12)
Requirement already satisfied: opt-einsum>=2.3.2 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (3.3.0)
Requirement already satisfied: wrapt>=1.11.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.12.1)
Requirement already satisfied: google-pasta>=0.1.1 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (0.2.0)
Collecting joblib<0.15,>=0.12
Downloading joblib-0.14.1-py2.py3-none-any.whl (294 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m294.9/294.9 KB[0m [31m34.9 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting pyfarmhash<0.4,>=0.2
Downloading pyfarmhash-0.3.2.tar.gz (99 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m99.9/99.9 KB[0m [31m15.7 MB/s[0m eta [36m0:00:00[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hRequirement already satisfied: pandas<2,>=1.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow-data-validation<1.8.0,>=1.7.0->tfx[kfp]<2) (1.3.5)
Requirement already satisfied: tensorflow-metadata<1.8,>=1.7.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow-data-validation<1.8.0,>=1.7.0->tfx[kfp]<2) (1.7.0)
Requirement already satisfied: ipywidgets<8,>=7 in /opt/conda/lib/python3.7/site-packages (from tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (7.6.5)
Requirement already satisfied: wheel<1.0,>=0.23.0 in /opt/conda/lib/python3.7/site-packages (from astunparse>=1.6.0->tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (0.37.1)
Requirement already satisfied: googleapis-common-protos<2.0dev,>=1.52.0 in /opt/conda/lib/python3.7/site-packages (from google-api-core<3dev,>=1.21.0->google-api-python-client<2,>=1.8->tfx[kfp]<2) (1.54.0)
Requirement already satisfied: grpcio-status<2.0dev,>=1.33.2 in /opt/conda/lib/python3.7/site-packages (from google-api-core<3dev,>=1.21.0->google-api-python-client<2,>=1.8->tfx[kfp]<2) (1.44.0)
Requirement already satisfied: rsa<5,>=3.1.4 in /opt/conda/lib/python3.7/site-packages (from google-auth<3,>=1.18.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (4.8)
Requirement already satisfied: pyasn1-modules>=0.2.1 in /opt/conda/lib/python3.7/site-packages (from google-auth<3,>=1.18.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.2.7)
Requirement already satisfied: grpc-google-iam-v1<0.13dev,>=0.12.3 in /opt/conda/lib/python3.7/site-packages (from google-cloud-bigtable<2,>=0.31.1->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.12.3)
Collecting google-cloud-core<2,>=0.28.1
Downloading google_cloud_core-1.7.1-py2.py3-none-any.whl (28 kB)
Downloading google_cloud_core-1.7.0-py2.py3-none-any.whl (28 kB)
Downloading google_cloud_core-1.6.0-py2.py3-none-any.whl (28 kB)
Downloading google_cloud_core-1.5.0-py2.py3-none-any.whl (27 kB)
Downloading google_cloud_core-1.4.4-py2.py3-none-any.whl (27 kB)
Downloading google_cloud_core-1.4.3-py2.py3-none-any.whl (27 kB)
Downloading google_cloud_core-1.4.2-py2.py3-none-any.whl (26 kB)
Downloading google_cloud_core-1.4.1-py2.py3-none-any.whl (26 kB)
INFO: pip is looking at multiple versions of google-cloud-bigtable to determine which version is compatible with other requirements. This could take a while.
Collecting google-cloud-bigtable<2,>=0.31.1
Downloading google_cloud_bigtable-1.7.0-py2.py3-none-any.whl (267 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m267.7/267.7 KB[0m [31m35.5 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-cloud-bigquery-storage to determine which version is compatible with other requirements. This could take a while.
Collecting google-cloud-bigquery-storage>=2.6.3
Downloading google_cloud_bigquery_storage-2.13.1-py2.py3-none-any.whl (180 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m180.1/180.1 KB[0m [31m27.5 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-auth-httplib2 to determine which version is compatible with other requirements. This could take a while.
Collecting google-auth-httplib2>=0.0.3
Downloading google_auth_httplib2-0.1.0-py2.py3-none-any.whl (9.3 kB)
INFO: pip is looking at multiple versions of google-auth to determine which version is compatible with other requirements. This could take a while.
Collecting google-auth<3,>=1.18.0
Downloading google_auth-1.35.0-py2.py3-none-any.whl (152 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m152.9/152.9 KB[0m [31m21.4 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-api-core[grpc] to determine which version is compatible with other requirements. This could take a while.
Collecting google-api-core[grpc]!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.0,<3.0.0dev,>=1.31.5
Downloading google_api_core-2.7.3-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.6/114.6 KB[0m [31m19.6 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-api-core to determine which version is compatible with other requirements. This could take a while.
Downloading google_api_core-2.7.2-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.6/114.6 KB[0m [31m19.7 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.7.1-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.7/114.7 KB[0m [31m17.4 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.7.0-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.7/114.7 KB[0m [31m17.4 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.6.1-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.6/114.6 KB[0m [31m17.4 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.6.0-py2.py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.6/114.6 KB[0m [31m18.7 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.5.0-py2.py3-none-any.whl (111 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m111.8/111.8 KB[0m [31m11.0 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-api-core[grpc] to determine which version is compatible with other requirements. This could take a while.
Downloading google_api_core-2.4.0-py2.py3-none-any.whl (111 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m111.7/111.7 KB[0m [31m15.5 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-api-core to determine which version is compatible with other requirements. This could take a while.
Downloading google_api_core-2.3.2-py2.py3-none-any.whl (109 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m109.8/109.8 KB[0m [31m15.1 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-1.31.5-py2.py3-none-any.whl (93 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m93.3/93.3 KB[0m [31m14.2 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: overrides<7.0.0,>=6.0.1 in /opt/conda/lib/python3.7/site-packages (from google-cloud-pubsublite<2,>=1.2.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (6.1.0)
Requirement already satisfied: google-crc32c<2.0dev,>=1.0 in /opt/conda/lib/python3.7/site-packages (from google-resumable-media<3.0dev,>=0.6.0->google-cloud-bigquery<3,>=2.26.0->tfx[kfp]<2) (1.1.2)
Requirement already satisfied: cached-property in /opt/conda/lib/python3.7/site-packages (from h5py>=2.9.0->tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.5.2)
Requirement already satisfied: docopt in /opt/conda/lib/python3.7/site-packages (from hdfs<3.0.0,>=2.1.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.6.2)
Collecting pyparsing>=2.0.2
Downloading pyparsing-2.4.7-py2.py3-none-any.whl (67 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m67.8/67.8 KB[0m [31m10.8 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: backcall in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.2.0)
Requirement already satisfied: decorator in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (5.1.1)
Requirement already satisfied: prompt-toolkit!=3.0.0,!=3.0.1,<3.1.0,>=2.0.0 in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (3.0.27)
Requirement already satisfied: pygments in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (2.11.2)
Requirement already satisfied: jedi>=0.16 in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.18.1)
Requirement already satisfied: matplotlib-inline in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.1.3)
Requirement already satisfied: pexpect>4.3 in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (4.8.0)
Requirement already satisfied: traitlets>=4.2 in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (5.1.1)
Requirement already satisfied: pickleshare in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.7.5)
Requirement already satisfied: jupyterlab-widgets>=1.0.0 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.0.2)
Requirement already satisfied: nbformat>=4.2.0 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (5.2.0)
Requirement already satisfied: ipython-genutils~=0.2.0 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.2.0)
Requirement already satisfied: widgetsnbextension~=3.5.0 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (3.5.2)
Requirement already satisfied: ipykernel>=4.5.1 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (6.9.2)
Requirement already satisfied: importlib-metadata in /opt/conda/lib/python3.7/site-packages (from jsonschema<4,>=3.0.1->kfp<2,>=1.8.5->tfx[kfp]<2) (4.11.3)
Requirement already satisfied: pyrsistent>=0.14.0 in /opt/conda/lib/python3.7/site-packages (from jsonschema<4,>=3.0.1->kfp<2,>=1.8.5->tfx[kfp]<2) (0.18.1)
Requirement already satisfied: pyasn1>=0.1.7 in /opt/conda/lib/python3.7/site-packages (from oauth2client<5,>=2.0.1->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.4.8)
Requirement already satisfied: idna<4,>=2.5 in /opt/conda/lib/python3.7/site-packages (from requests<3.0.0,>=2.24.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.3)
Requirement already satisfied: charset-normalizer~=2.0.0 in /opt/conda/lib/python3.7/site-packages (from requests<3.0.0,>=2.24.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.0.12)
Requirement already satisfied: google-auth-oauthlib<0.5,>=0.4.1 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.4.6)
Requirement already satisfied: markdown>=2.6.8 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (3.3.6)
Requirement already satisfied: tensorboard-plugin-wit>=1.6.0 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (1.8.1)
Requirement already satisfied: werkzeug>=0.11.15 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (2.0.3)
Requirement already satisfied: tensorboard-data-server<0.7.0,>=0.6.0 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.6.1)
Requirement already satisfied: oauthlib>=3.0.0 in /opt/conda/lib/python3.7/site-packages (from requests-oauthlib->kubernetes<13,>=10.0.1->tfx[kfp]<2) (3.2.0)
Requirement already satisfied: cffi>=1.0.0 in /opt/conda/lib/python3.7/site-packages (from google-crc32c<2.0dev,>=1.0->google-resumable-media<3.0dev,>=0.6.0->google-cloud-bigquery<3,>=2.26.0->tfx[kfp]<2) (1.15.0)
Requirement already satisfied: debugpy<2.0,>=1.0.0 in /opt/conda/lib/python3.7/site-packages (from ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.5.1)
Requirement already satisfied: tornado<7.0,>=4.2 in /opt/conda/lib/python3.7/site-packages (from ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (6.1)
Requirement already satisfied: jupyter-client<8.0 in /opt/conda/lib/python3.7/site-packages (from ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (7.1.2)
Requirement already satisfied: nest-asyncio in /opt/conda/lib/python3.7/site-packages (from ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.5.4)
Requirement already satisfied: parso<0.9.0,>=0.8.0 in /opt/conda/lib/python3.7/site-packages (from jedi>=0.16->ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.8.3)
Requirement already satisfied: zipp>=0.5 in /opt/conda/lib/python3.7/site-packages (from importlib-metadata->jsonschema<4,>=3.0.1->kfp<2,>=1.8.5->tfx[kfp]<2) (3.7.0)
Requirement already satisfied: jupyter-core in /opt/conda/lib/python3.7/site-packages (from nbformat>=4.2.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (4.9.2)
Requirement already satisfied: typing-utils>=0.0.3 in /opt/conda/lib/python3.7/site-packages (from overrides<7.0.0,>=6.0.1->google-cloud-pubsublite<2,>=1.2.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.1.0)
Requirement already satisfied: ptyprocess>=0.5 in /opt/conda/lib/python3.7/site-packages (from pexpect>4.3->ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.7.0)
Requirement already satisfied: wcwidth in /opt/conda/lib/python3.7/site-packages (from prompt-toolkit!=3.0.0,!=3.0.1,<3.1.0,>=2.0.0->ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.2.5)
Requirement already satisfied: notebook>=4.4.1 in /opt/conda/lib/python3.7/site-packages (from widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (6.4.10)
Requirement already satisfied: pycparser in /opt/conda/lib/python3.7/site-packages (from cffi>=1.0.0->google-crc32c<2.0dev,>=1.0->google-resumable-media<3.0dev,>=0.6.0->google-cloud-bigquery<3,>=2.26.0->tfx[kfp]<2) (2.21)
Requirement already satisfied: entrypoints in /opt/conda/lib/python3.7/site-packages (from jupyter-client<8.0->ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.4)
Requirement already satisfied: pyzmq>=13 in /opt/conda/lib/python3.7/site-packages (from jupyter-client<8.0->ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (22.3.0)
Requirement already satisfied: nbconvert>=5 in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (6.4.4)
Requirement already satisfied: argon2-cffi in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (21.3.0)
Requirement already satisfied: terminado>=0.8.3 in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.13.3)
Requirement already satisfied: prometheus-client in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.13.1)
Requirement already satisfied: Send2Trash>=1.8.0 in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.8.0)
Requirement already satisfied: nbclient<0.6.0,>=0.5.0 in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.5.13)
Requirement already satisfied: mistune<2,>=0.8.1 in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.8.4)
Requirement already satisfied: beautifulsoup4 in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (4.10.0)
Requirement already satisfied: defusedxml in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.7.1)
Requirement already satisfied: bleach in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (4.1.0)
Requirement already satisfied: testpath in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.6.0)
Requirement already satisfied: jupyterlab-pygments in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.1.2)
Requirement already satisfied: pandocfilters>=1.4.1 in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.5.0)
Requirement already satisfied: argon2-cffi-bindings in /opt/conda/lib/python3.7/site-packages (from argon2-cffi->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (21.2.0)
Requirement already satisfied: soupsieve>1.2 in /opt/conda/lib/python3.7/site-packages (from beautifulsoup4->nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (2.3.1)
Requirement already satisfied: webencodings in /opt/conda/lib/python3.7/site-packages (from bleach->nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.5.1)
Building wheels for collected packages: kfp, dill, fire, kfp-server-api, pyfarmhash, strip-hints
Building wheel for kfp (setup.py) ... [?25ldone
[?25h Created wheel for kfp: filename=kfp-1.8.12-py3-none-any.whl size=419048 sha256=fc5fc7f3a9b5f676cd53e5cd69e492ed3e995211cc4b6b8cfc5a06b54796f1d4
Stored in directory: /home/jupyter/.cache/pip/wheels/54/0c/4a/3fc55077bc88cc17eacaae34c5fd3f6178c1d16d2ee3b0afdf
Building wheel for dill (setup.py) ... [?25ldone
[?25h Created wheel for dill: filename=dill-0.3.1.1-py3-none-any.whl size=78544 sha256=6d34132d6cad3e94afaaf4057513f0bcf6eec4626ab3183f05c6bb5e4d8f93bf
Stored in directory: /home/jupyter/.cache/pip/wheels/a4/61/fd/c57e374e580aa78a45ed78d5859b3a44436af17e22ca53284f
Building wheel for fire (setup.py) ... [?25ldone
[?25h Created wheel for fire: filename=fire-0.4.0-py2.py3-none-any.whl size=115942 sha256=a0e96813de3269a653f0bbe1d106b94a930df65b64cfc8c8afd4091e2af841b1
Stored in directory: /home/jupyter/.cache/pip/wheels/8a/67/fb/2e8a12fa16661b9d5af1f654bd199366799740a85c64981226
Building wheel for kfp-server-api (setup.py) ... [?25ldone
[?25h Created wheel for kfp-server-api: filename=kfp_server_api-1.8.1-py3-none-any.whl size=95549 sha256=1d5907bdf9958cf5fd1529a0fa69ee8a91fefca39b8c3942766a2365d59478f3
Stored in directory: /home/jupyter/.cache/pip/wheels/f5/4e/2e/6795bd3ed456a43652e7de100aca275ec179c9a8dfbcc65626
Building wheel for pyfarmhash (setup.py) ... [?25ldone
[?25h Created wheel for pyfarmhash: filename=pyfarmhash-0.3.2-cp37-cp37m-linux_x86_64.whl size=108625 sha256=1ba31109887fb167782b9678b72ce85552e486cea10a26e776ef9b5d238c512d
Stored in directory: /home/jupyter/.cache/pip/wheels/53/58/7a/3b040f3a2ee31908e3be916e32660db6db53621ce6eba838dc
Building wheel for strip-hints (setup.py) ... [?25ldone
[?25h Created wheel for strip-hints: filename=strip_hints-0.1.10-py2.py3-none-any.whl size=22302 sha256=1fa632e6899f84956f31c089308aec5d16a45626c95b97bbb9dd78e5851779bb
Stored in directory: /home/jupyter/.cache/pip/wheels/5e/14/c3/6e44e9b2545f2d570b03f5b6d38c00b7534aa8abb376978363
Successfully built kfp dill fire kfp-server-api pyfarmhash strip-hints
Installing collected packages: tf-estimator-nightly, tabulate, pyfarmhash, libclang, keras, joblib, uritemplate, tensorflow-io-gcs-filesystem, strip-hints, pyyaml, pyparsing, portpicker, numpy, kfp-pipeline-spec, fire, docstring-parser, dill, Deprecated, click, attrs, typer, requests-toolbelt, pyarrow, packaging, ml-metadata, kfp-server-api, jsonschema, httplib2, docker, kubernetes, google-api-core, tensorboard, google-cloud-core, google-api-python-client, tensorflow, ml-pipelines-sdk, google-cloud-vision, google-cloud-videointelligence, google-cloud-storage, google-cloud-spanner, google-cloud-language, google-cloud-datastore, google-cloud-bigtable, kfp, tensorflow-model-analysis, tensorflow-data-validation, tfx
Attempting uninstall: keras
Found existing installation: keras 2.6.0
Uninstalling keras-2.6.0:
Successfully uninstalled keras-2.6.0
Attempting uninstall: joblib
Found existing installation: joblib 1.0.1
Uninstalling joblib-1.0.1:
Successfully uninstalled joblib-1.0.1
Attempting uninstall: uritemplate
Found existing installation: uritemplate 4.1.1
Uninstalling uritemplate-4.1.1:
Successfully uninstalled uritemplate-4.1.1
Attempting uninstall: pyyaml
Found existing installation: PyYAML 6.0
Uninstalling PyYAML-6.0:
Successfully uninstalled PyYAML-6.0
Attempting uninstall: pyparsing
Found existing installation: pyparsing 3.0.7
Uninstalling pyparsing-3.0.7:
Successfully uninstalled pyparsing-3.0.7
Attempting uninstall: numpy
Found existing installation: numpy 1.19.5
Uninstalling numpy-1.19.5:
Successfully uninstalled numpy-1.19.5
Attempting uninstall: dill
Found existing installation: dill 0.3.4
Uninstalling dill-0.3.4:
Successfully uninstalled dill-0.3.4
Attempting uninstall: click
Found existing installation: click 8.0.4
Uninstalling click-8.0.4:
Successfully uninstalled click-8.0.4
Attempting uninstall: attrs
Found existing installation: attrs 21.4.0
Uninstalling attrs-21.4.0:
Successfully uninstalled attrs-21.4.0
Attempting uninstall: pyarrow
Found existing installation: pyarrow 7.0.0
Uninstalling pyarrow-7.0.0:
Successfully uninstalled pyarrow-7.0.0
Attempting uninstall: packaging
Found existing installation: packaging 21.3
Uninstalling packaging-21.3:
Successfully uninstalled packaging-21.3
Attempting uninstall: jsonschema
Found existing installation: jsonschema 4.4.0
Uninstalling jsonschema-4.4.0:
Successfully uninstalled jsonschema-4.4.0
Attempting uninstall: httplib2
Found existing installation: httplib2 0.20.4
Uninstalling httplib2-0.20.4:
Successfully uninstalled httplib2-0.20.4
Attempting uninstall: docker
Found existing installation: docker 5.0.3
Uninstalling docker-5.0.3:
Successfully uninstalled docker-5.0.3
Attempting uninstall: kubernetes
Found existing installation: kubernetes 23.3.0
Uninstalling kubernetes-23.3.0:
Successfully uninstalled kubernetes-23.3.0
Attempting uninstall: google-api-core
Found existing installation: google-api-core 2.5.0
Uninstalling google-api-core-2.5.0:
Successfully uninstalled google-api-core-2.5.0
Attempting uninstall: tensorboard
Found existing installation: tensorboard 2.6.0
Uninstalling tensorboard-2.6.0:
Successfully uninstalled tensorboard-2.6.0
Attempting uninstall: google-cloud-core
Found existing installation: google-cloud-core 2.2.3
Uninstalling google-cloud-core-2.2.3:
Successfully uninstalled google-cloud-core-2.2.3
Attempting uninstall: google-api-python-client
Found existing installation: google-api-python-client 2.41.0
Uninstalling google-api-python-client-2.41.0:
Successfully uninstalled google-api-python-client-2.41.0
Attempting uninstall: tensorflow
Found existing installation: tensorflow 2.6.3
Uninstalling tensorflow-2.6.3:
Successfully uninstalled tensorflow-2.6.3
Attempting uninstall: google-cloud-vision
Found existing installation: google-cloud-vision 2.7.1
Uninstalling google-cloud-vision-2.7.1:
Successfully uninstalled google-cloud-vision-2.7.1
Attempting uninstall: google-cloud-videointelligence
Found existing installation: google-cloud-videointelligence 2.6.1
Uninstalling google-cloud-videointelligence-2.6.1:
Successfully uninstalled google-cloud-videointelligence-2.6.1
Attempting uninstall: google-cloud-storage
Found existing installation: google-cloud-storage 2.2.1
Uninstalling google-cloud-storage-2.2.1:
Successfully uninstalled google-cloud-storage-2.2.1
Attempting uninstall: google-cloud-spanner
Found existing installation: google-cloud-spanner 3.13.0
Uninstalling google-cloud-spanner-3.13.0:
Successfully uninstalled google-cloud-spanner-3.13.0
Attempting uninstall: google-cloud-language
Found existing installation: google-cloud-language 2.4.1
Uninstalling google-cloud-language-2.4.1:
Successfully uninstalled google-cloud-language-2.4.1
Attempting uninstall: google-cloud-datastore
Found existing installation: google-cloud-datastore 2.5.1
Uninstalling google-cloud-datastore-2.5.1:
Successfully uninstalled google-cloud-datastore-2.5.1
Attempting uninstall: google-cloud-bigtable
Found existing installation: google-cloud-bigtable 2.7.0
Uninstalling google-cloud-bigtable-2.7.0:
Successfully uninstalled google-cloud-bigtable-2.7.0
[31mERROR: pip's dependency resolver does not currently take into account all the packages that are installed. This behaviour is the source of the following dependency conflicts.
tensorflow-io 0.21.0 requires tensorflow<2.7.0,>=2.6.0, but you have tensorflow 2.8.0 which is incompatible.
tensorflow-io 0.21.0 requires tensorflow-io-gcs-filesystem==0.21.0, but you have tensorflow-io-gcs-filesystem 0.25.0 which is incompatible.
statsmodels 0.13.2 requires packaging>=21.3, but you have packaging 20.9 which is incompatible.
pandas-profiling 3.1.0 requires joblib~=1.0.1, but you have joblib 0.14.1 which is incompatible.
cloud-tpu-client 0.10 requires google-api-python-client==1.8.0, but you have google-api-python-client 1.12.11 which is incompatible.
black 22.1.0 requires click>=8.0.0, but you have click 7.1.2 which is incompatible.[0m[31m
[0mSuccessfully installed Deprecated-1.2.13 attrs-20.3.0 click-7.1.2 dill-0.3.1.1 docker-4.4.4 docstring-parser-0.14.1 fire-0.4.0 google-api-core-1.31.5 google-api-python-client-1.12.11 google-cloud-bigtable-1.7.1 google-cloud-core-2.2.2 google-cloud-datastore-1.15.4 google-cloud-language-1.3.1 google-cloud-spanner-1.19.2 google-cloud-storage-2.1.0 google-cloud-videointelligence-1.16.2 google-cloud-vision-1.0.1 httplib2-0.19.1 joblib-0.14.1 jsonschema-3.2.0 keras-2.8.0 kfp-1.8.12 kfp-pipeline-spec-0.1.14 kfp-server-api-1.8.1 kubernetes-12.0.1 libclang-14.0.1 ml-metadata-1.7.0 ml-pipelines-sdk-1.7.1 numpy-1.21.6 packaging-20.9 portpicker-1.5.0 pyarrow-5.0.0 pyfarmhash-0.3.2 pyparsing-2.4.7 pyyaml-5.4.1 requests-toolbelt-0.9.1 strip-hints-0.1.10 tabulate-0.8.9 tensorboard-2.8.0 tensorflow-2.8.0 tensorflow-data-validation-1.7.0 tensorflow-io-gcs-filesystem-0.25.0 tensorflow-model-analysis-0.38.0 tf-estimator-nightly-2.8.0.dev2021122109 tfx-1.7.1 typer-0.4.1 uritemplate-3.0.1
###Markdown
Did you restart the runtime? You can restart runtime with following cell.
###Code
# docs_infra: no_execute
import sys
if not 'google.colab' in sys.modules:
# Automatically restart kernel after installs
import IPython
app = IPython.Application.instance()
app.kernel.do_shutdown(True)
###Output
_____no_output_____
###Markdown
Check the package versions
###Code
import tensorflow as tf
print('TensorFlow version: {}'.format(tf.__version__))
from tfx import v1 as tfx
print('TFX version: {}'.format(tfx.__version__))
import kfp
print('KFP version: {}'.format(kfp.__version__))
###Output
TensorFlow version: 2.8.0
TFX version: 1.7.1
KFP version: 1.8.12
###Markdown
Set up variablesYou will set up some variables used to customize the pipelines below. Followinginformation is required:* GCP Project id. See[Identifying your project id](https://cloud.google.com/resource-manager/docs/creating-managing-projectsidentifying_projects).* GCP Region to run pipelines. For more information about the regions thatVertex Pipelines is available in, see the[Vertex AI locations guide](https://cloud.google.com/vertex-ai/docs/general/locationsfeature-availability).* Google Cloud Storage Bucket to store pipeline outputs.**Enter required values in the cell below before running it**.
###Code
GOOGLE_CLOUD_PROJECT = 'qwiklabs-gcp-01-2e305ff9c72b' # Replace this with your Project-ID
GOOGLE_CLOUD_REGION = 'us-central1' # Replace this with your region
GCS_BUCKET_NAME = 'qwiklabs-gcp-01-2e305ff9c72b' # Replace this with your Cloud Storage bucket
if not (GOOGLE_CLOUD_PROJECT and GOOGLE_CLOUD_REGION and GCS_BUCKET_NAME):
from absl import logging
logging.error('Please set all required parameters.')
###Output
_____no_output_____
###Markdown
Set `gcloud` to use your project.
###Code
!gcloud config set project {GOOGLE_CLOUD_PROJECT}
PIPELINE_NAME = 'penguin-vertex-pipelines'
# Path to various pipeline artifact.
PIPELINE_ROOT = 'gs://{}/pipeline_root/{}'.format(
GCS_BUCKET_NAME, PIPELINE_NAME)
# Paths for users' Python module.
MODULE_ROOT = 'gs://{}/pipeline_module/{}'.format(
GCS_BUCKET_NAME, PIPELINE_NAME)
# Paths for input data.
DATA_ROOT = 'gs://{}/data/{}'.format(GCS_BUCKET_NAME, PIPELINE_NAME)
# This is the path where your model will be pushed for serving.
SERVING_MODEL_DIR = 'gs://{}/serving_model/{}'.format(
GCS_BUCKET_NAME, PIPELINE_NAME)
print('PIPELINE_ROOT: {}'.format(PIPELINE_ROOT))
###Output
PIPELINE_ROOT: gs://qwiklabs-gcp-01-2e305ff9c72b/pipeline_root/penguin-vertex-pipelines
###Markdown
Prepare example dataYou will use the same[Palmer Penguins dataset](https://allisonhorst.github.io/palmerpenguins/articles/intro.html)as[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).There are four numeric features in this dataset which were already normalizedto have range [0,1]. You will build a classification model which predicts the`species` of penguins. You need to make your own copy of the dataset. Because TFX ExampleGen readsinputs from a directory, You need to create a directory and copy dataset to iton GCS.
###Code
!gsutil cp gs://download.tensorflow.org/data/palmer_penguins/penguins_processed.csv {DATA_ROOT}/
###Output
Copying gs://download.tensorflow.org/data/palmer_penguins/penguins_processed.csv [Content-Type=application/octet-stream]...
/ [1 files][ 25.0 KiB/ 25.0 KiB]
Operation completed over 1 objects/25.0 KiB.
###Markdown
Take a quick look at the CSV file.
###Code
# Review the contents of the CSV file
# TODO 1: Your code goes here
###Output
species,culmen_length_mm,culmen_depth_mm,flipper_length_mm,body_mass_g
0,0.2545454545454545,0.6666666666666666,0.15254237288135594,0.2916666666666667
0,0.26909090909090905,0.5119047619047618,0.23728813559322035,0.3055555555555556
0,0.29818181818181805,0.5833333333333334,0.3898305084745763,0.1527777777777778
0,0.16727272727272732,0.7380952380952381,0.3559322033898305,0.20833333333333334
0,0.26181818181818167,0.892857142857143,0.3050847457627119,0.2638888888888889
0,0.24727272727272717,0.5595238095238096,0.15254237288135594,0.2569444444444444
0,0.25818181818181823,0.773809523809524,0.3898305084745763,0.5486111111111112
0,0.32727272727272727,0.5357142857142859,0.1694915254237288,0.1388888888888889
0,0.23636363636363636,0.9642857142857142,0.3220338983050847,0.3055555555555556
###Markdown
Create a pipelineTFX pipelines are defined using Python APIs. You will define a pipeline whichconsists of three components, CsvExampleGen, Trainer and Pusher. The pipelineand model definition is almost the same as[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).The only difference is that you don't need to set `metadata_connection_config`which is used to locate[ML Metadata](https://www.tensorflow.org/tfx/guide/mlmd) database. BecauseVertex Pipelines uses a managed metadata service, users don't need to careof it, and you don't need to specify the parameter.Before actually define the pipeline, you need to write a model code for theTrainer component first. Write model code.You will use the same model code as in the[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).
###Code
_trainer_module_file = 'penguin_trainer.py'
%%writefile {_trainer_module_file}
# Copied from https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple
from typing import List
from absl import logging
import tensorflow as tf
from tensorflow import keras
from tensorflow_transform.tf_metadata import schema_utils
from tfx import v1 as tfx
from tfx_bsl.public import tfxio
from tensorflow_metadata.proto.v0 import schema_pb2
_FEATURE_KEYS = [
'culmen_length_mm', 'culmen_depth_mm', 'flipper_length_mm', 'body_mass_g'
]
_LABEL_KEY = 'species'
_TRAIN_BATCH_SIZE = 20
_EVAL_BATCH_SIZE = 10
# Since you're not generating or creating a schema, you will instead create
# a feature spec. Since there are a fairly small number of features this is
# manageable for this dataset.
_FEATURE_SPEC = {
**{
feature: tf.io.FixedLenFeature(shape=[1], dtype=tf.float32)
for feature in _FEATURE_KEYS
},
_LABEL_KEY: tf.io.FixedLenFeature(shape=[1], dtype=tf.int64)
}
def _input_fn(file_pattern: List[str],
data_accessor: tfx.components.DataAccessor,
schema: schema_pb2.Schema,
batch_size: int) -> tf.data.Dataset:
"""Generates features and label for training.
Args:
file_pattern: List of paths or patterns of input tfrecord files.
data_accessor: DataAccessor for converting input to RecordBatch.
schema: schema of the input data.
batch_size: representing the number of consecutive elements of returned
dataset to combine in a single batch
Returns:
A dataset that contains (features, indices) tuple where features is a
dictionary of Tensors, and indices is a single Tensor of label indices.
"""
return data_accessor.tf_dataset_factory(
file_pattern,
tfxio.TensorFlowDatasetOptions(
batch_size=batch_size, label_key=_LABEL_KEY),
schema=schema).repeat()
def _make_keras_model() -> tf.keras.Model:
"""Creates a DNN Keras model for classifying penguin data.
Returns:
A Keras Model.
"""
# The model below is built with Functional API, please refer to
# https://www.tensorflow.org/guide/keras/overview for all API options.
inputs = [keras.layers.Input(shape=(1,), name=f) for f in _FEATURE_KEYS]
d = keras.layers.concatenate(inputs)
for _ in range(2):
d = keras.layers.Dense(8, activation='relu')(d)
outputs = keras.layers.Dense(3)(d)
model = keras.Model(inputs=inputs, outputs=outputs)
model.compile(
optimizer=keras.optimizers.Adam(1e-2),
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=[keras.metrics.SparseCategoricalAccuracy()])
model.summary(print_fn=logging.info)
return model
# TFX Trainer will call this function.
def run_fn(fn_args: tfx.components.FnArgs):
"""Train the model based on given args.
Args:
fn_args: Holds args used to train the model as name/value pairs.
"""
# This schema is usually either an output of SchemaGen or a manually-curated
# version provided by pipeline author. A schema can also derived from TFT
# graph if a Transform component is used. In the case when either is missing,
# `schema_from_feature_spec` could be used to generate schema from very simple
# feature_spec, but the schema returned would be very primitive.
schema = schema_utils.schema_from_feature_spec(_FEATURE_SPEC)
train_dataset = _input_fn(
fn_args.train_files,
fn_args.data_accessor,
schema,
batch_size=_TRAIN_BATCH_SIZE)
eval_dataset = _input_fn(
fn_args.eval_files,
fn_args.data_accessor,
schema,
batch_size=_EVAL_BATCH_SIZE)
model = _make_keras_model()
model.fit(
train_dataset,
steps_per_epoch=fn_args.train_steps,
validation_data=eval_dataset,
validation_steps=fn_args.eval_steps)
# The result of the training should be saved in `fn_args.serving_model_dir`
# directory.
model.save(fn_args.serving_model_dir, save_format='tf')
###Output
Writing penguin_trainer.py
###Markdown
Copy the module file to GCS which can be accessed from the pipeline components.Because model training happens on GCP, you need to upload this model definition. Otherwise, you might want to build a container image including the module fileand use the image to run the pipeline.
###Code
!gsutil cp {_trainer_module_file} {MODULE_ROOT}/
###Output
Copying file://penguin_trainer.py [Content-Type=text/x-python]...
/ [1 files][ 3.8 KiB/ 3.8 KiB]
Operation completed over 1 objects/3.8 KiB.
###Markdown
Write a pipeline definitionYou will define a function to create a TFX pipeline.
###Code
# Copied from https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple and
# slightly modified because you don't need `metadata_path` argument.
def _create_pipeline(pipeline_name: str, pipeline_root: str, data_root: str,
module_file: str, serving_model_dir: str,
) -> tfx.dsl.Pipeline:
"""Creates a three component penguin pipeline with TFX."""
# Brings data into the pipeline.
example_gen = # TODO 2: Your code goes here
# Uses user-provided Python function that trains a model.
trainer = tfx.components.Trainer(
module_file=module_file,
examples=example_gen.outputs['examples'],
train_args=tfx.proto.TrainArgs(num_steps=100),
eval_args=tfx.proto.EvalArgs(num_steps=5))
# Pushes the model to a filesystem destination.
pusher = tfx.components.Pusher(
model=trainer.outputs['model'],
push_destination=tfx.proto.PushDestination(
filesystem=tfx.proto.PushDestination.Filesystem(
base_directory=serving_model_dir)))
# Following three components will be included in the pipeline.
components = [
example_gen,
trainer,
pusher,
]
return tfx.dsl.Pipeline(
pipeline_name=pipeline_name,
pipeline_root=pipeline_root,
components=components)
###Output
_____no_output_____
###Markdown
Run the pipeline on Vertex Pipelines.You used `LocalDagRunner` which runs on local environment in[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).TFX provides multiple orchestrators to run your pipeline. In this tutorial youwill use the Vertex Pipelines together with the Kubeflow V2 dag runner. You need to define a runner to actually run the pipeline. You will compileyour pipeline into our pipeline definition format using TFX APIs.
###Code
import os
PIPELINE_DEFINITION_FILE = PIPELINE_NAME + '_pipeline.json'
runner = tfx.orchestration.experimental.KubeflowV2DagRunner(
config=tfx.orchestration.experimental.KubeflowV2DagRunnerConfig(),
output_filename=PIPELINE_DEFINITION_FILE)
# Following function will write the pipeline definition to PIPELINE_DEFINITION_FILE.
_ = runner.run(
_create_pipeline(
pipeline_name=PIPELINE_NAME,
pipeline_root=PIPELINE_ROOT,
data_root=DATA_ROOT,
module_file=os.path.join(MODULE_ROOT, _trainer_module_file),
serving_model_dir=SERVING_MODEL_DIR))
###Output
_____no_output_____
###Markdown
The generated definition file can be submitted using kfp client.
###Code
# docs_infra: no_execute
from google.cloud import aiplatform
from google.cloud.aiplatform import pipeline_jobs
import logging
logging.getLogger().setLevel(logging.INFO)
aiplatform.init(project=GOOGLE_CLOUD_PROJECT, location=GOOGLE_CLOUD_REGION)
# Create a job to submit the pipeline
job = # TODO 3: Your code goes here
job.submit()
###Output
INFO:google.cloud.aiplatform.pipeline_jobs:Creating PipelineJob
INFO:google.cloud.aiplatform.pipeline_jobs:PipelineJob created. Resource name: projects/202132981718/locations/us-central1/pipelineJobs/penguin-vertex-pipelines-20220510100554
INFO:google.cloud.aiplatform.pipeline_jobs:To use this PipelineJob in another session:
INFO:google.cloud.aiplatform.pipeline_jobs:pipeline_job = aiplatform.PipelineJob.get('projects/202132981718/locations/us-central1/pipelineJobs/penguin-vertex-pipelines-20220510100554')
INFO:google.cloud.aiplatform.pipeline_jobs:View Pipeline Job:
https://console.cloud.google.com/vertex-ai/locations/us-central1/pipelines/runs/penguin-vertex-pipelines-20220510100554?project=202132981718
###Markdown
Creating Simple TFX Pipeline for Vertex Pipelines Learning objectives1. Prepare example data.2. Create a pipeline.3. Run the pipeline on Vertex Pipelines. IntroductionIn this notebook, you will create a simple TFX pipeline and run it usingGoogle Cloud Vertex Pipelines. This notebook is based on the TFX pipelineyou built in[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).If you are not familiar with TFX and you have not read that tutorial yet, youshould read it before proceeding with this notebook.Google Cloud Vertex Pipelines helps you to automate, monitor, and governyour ML systems by orchestrating your ML workflow in a serverless manner. Youcan define your ML pipelines using Python with TFX, and then execute yourpipelines on Google Cloud. See[Vertex Pipelines introduction](https://cloud.google.com/vertex-ai/docs/pipelines/introduction)to learn more about Vertex Pipelines.Each learning objective will correspond to a _TODO_ in this student lab notebook -- try to complete this notebook first and then review the [solution notebook](../solutions/Simple_TFX_Pipeline_for_Vertex_Pipelines.ipynb) Install python packages You will install required Python packages including TFX and KFP to author MLpipelines and submit jobs to Vertex Pipelines.
###Code
# Use the latest version of pip.
!pip install --upgrade pip
!pip install --upgrade "tfx[kfp]<2"
###Output
Requirement already satisfied: pip in /opt/conda/lib/python3.7/site-packages (22.0.4)
Collecting tfx[kfp]<2
Downloading tfx-1.7.1-py3-none-any.whl (2.5 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m2.5/2.5 MB[0m [31m27.6 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hRequirement already satisfied: tfx-bsl<1.8.0,>=1.7.0 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.7.0)
Collecting packaging<21,>=20
Downloading packaging-20.9-py2.py3-none-any.whl (40 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m40.9/40.9 KB[0m [31m6.3 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: google-apitools<1,>=0.5 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (0.5.31)
Requirement already satisfied: tensorflow-serving-api!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<3,>=1.15 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (2.8.0)
Requirement already satisfied: keras-tuner<2,>=1.0.4 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.1.0)
Requirement already satisfied: apache-beam[gcp]<3,>=2.36 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (2.37.0)
Collecting google-api-python-client<2,>=1.8
Downloading google_api_python_client-1.12.11-py2.py3-none-any.whl (62 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m62.1/62.1 KB[0m [31m9.5 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: google-cloud-aiplatform<2,>=1.6.2 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.11.0)
Requirement already satisfied: numpy<2,>=1.16 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.19.5)
Collecting tensorflow-model-analysis<0.39,>=0.38.0
Downloading tensorflow_model_analysis-0.38.0-py3-none-any.whl (1.8 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.8/1.8 MB[0m [31m58.0 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting pyarrow<6,>=1
Downloading pyarrow-5.0.0-cp37-cp37m-manylinux2014_x86_64.whl (23.6 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m23.6/23.6 MB[0m [31m45.6 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hCollecting click<8,>=7
Downloading click-7.1.2-py2.py3-none-any.whl (82 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m82.8/82.8 KB[0m [31m13.5 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting tensorflow-data-validation<1.8.0,>=1.7.0
Downloading tensorflow_data_validation-1.7.0-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (1.4 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.4/1.4 MB[0m [31m68.5 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: grpcio<2,>=1.28.1 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.44.0)
Collecting ml-pipelines-sdk==1.7.1
Downloading ml_pipelines_sdk-1.7.1-py3-none-any.whl (1.3 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.3/1.3 MB[0m [31m55.5 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: protobuf<4,>=3.13 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (3.19.4)
Requirement already satisfied: google-cloud-bigquery<3,>=2.26.0 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (2.34.2)
Collecting docker<5,>=4.1
Downloading docker-4.4.4-py2.py3-none-any.whl (147 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m147.0/147.0 KB[0m [31m21.7 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: tensorflow-transform<1.8.0,>=1.7.0 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (1.7.0)
Requirement already satisfied: jinja2<4,>=2.7.3 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (2.11.3)
Collecting pyyaml<6,>=3.12
Downloading PyYAML-5.4.1-cp37-cp37m-manylinux1_x86_64.whl (636 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m636.6/636.6 KB[0m [31m49.5 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: absl-py<2.0.0,>=0.9 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (0.15.0)
Collecting portpicker<2,>=1.3.1
Downloading portpicker-1.5.0-py3-none-any.whl (14 kB)
Collecting attrs<21,>=19.3.0
Downloading attrs-20.3.0-py2.py3-none-any.whl (49 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m49.3/49.3 KB[0m [31m6.9 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: tensorflow-hub<0.13,>=0.9.0 in /opt/conda/lib/python3.7/site-packages (from tfx[kfp]<2) (0.12.0)
Collecting ml-metadata<1.8.0,>=1.7.0
Downloading ml_metadata-1.7.0-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (6.6 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m6.6/6.6 MB[0m [31m60.8 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0mm
[?25hCollecting kubernetes<13,>=10.0.1
Downloading kubernetes-12.0.1-py2.py3-none-any.whl (1.7 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.7/1.7 MB[0m [31m79.5 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5
Downloading tensorflow-2.8.0-cp37-cp37m-manylinux2010_x86_64.whl (497.5 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m497.5/497.5 MB[0m [31m1.6 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hCollecting kfp-pipeline-spec<0.2,>=0.1.10
Downloading kfp_pipeline_spec-0.1.14-py3-none-any.whl (18 kB)
Collecting kfp<2,>=1.8.5
Downloading kfp-1.8.12.tar.gz (301 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m301.2/301.2 KB[0m [31m32.4 MB/s[0m eta [36m0:00:00[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hRequirement already satisfied: six in /opt/conda/lib/python3.7/site-packages (from absl-py<2.0.0,>=0.9->tfx[kfp]<2) (1.15.0)
Collecting httplib2<0.20.0,>=0.8
Downloading httplib2-0.19.1-py3-none-any.whl (95 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m95.4/95.4 KB[0m [31m12.9 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: pytz>=2018.3 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2021.3)
Requirement already satisfied: proto-plus<2,>=1.7.1 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.20.3)
Requirement already satisfied: fastavro<2,>=0.23.6 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.4.10)
Requirement already satisfied: pydot<2,>=1.2.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.4.2)
Requirement already satisfied: hdfs<3.0.0,>=2.1.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.6.0)
Requirement already satisfied: pymongo<4.0.0,>=3.8.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.12.3)
Requirement already satisfied: typing-extensions>=3.7.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.10.0.2)
Requirement already satisfied: crcmod<2.0,>=1.7 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.7)
Requirement already satisfied: requests<3.0.0,>=2.24.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.27.1)
Requirement already satisfied: python-dateutil<3,>=2.8.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.8.2)
Collecting dill<0.3.2,>=0.3.1.1
Downloading dill-0.3.1.1.tar.gz (151 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m152.0/152.0 KB[0m [31m24.3 MB/s[0m eta [36m0:00:00[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hRequirement already satisfied: cloudpickle<3,>=2.0.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.0.0)
Requirement already satisfied: orjson<4.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.6.7)
Requirement already satisfied: oauth2client<5,>=2.0.1 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (4.1.3)
Collecting google-cloud-videointelligence<2,>=1.8.0
Downloading google_cloud_videointelligence-1.16.2-py2.py3-none-any.whl (183 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m183.9/183.9 KB[0m [31m26.5 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting google-cloud-spanner<2,>=1.13.0
Downloading google_cloud_spanner-1.19.2-py2.py3-none-any.whl (255 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m255.5/255.5 KB[0m [31m32.3 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: cachetools<5,>=3.1.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (4.2.4)
Requirement already satisfied: google-auth<3,>=1.18.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.35.0)
Requirement already satisfied: google-cloud-dlp<4,>=3.0.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.6.2)
Collecting google-cloud-datastore<2,>=1.8.0
Downloading google_cloud_datastore-1.15.4-py2.py3-none-any.whl (134 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m134.2/134.2 KB[0m [31m20.8 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting google-cloud-language<2,>=1.3.0
Downloading google_cloud_language-1.3.1-py2.py3-none-any.whl (83 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m83.6/83.6 KB[0m [31m11.2 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: grpcio-gcp<1,>=0.2.2 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.2.2)
Requirement already satisfied: google-cloud-pubsub<3,>=2.1.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.11.0)
Collecting google-cloud-bigtable<2,>=0.31.1
Downloading google_cloud_bigtable-1.7.1-py2.py3-none-any.whl (267 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m267.7/267.7 KB[0m [31m28.3 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: google-cloud-bigquery-storage>=2.6.3 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.13.0)
Requirement already satisfied: google-cloud-pubsublite<2,>=1.2.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (1.4.1)
Collecting google-cloud-vision<2,>=0.38.0
Downloading google_cloud_vision-1.0.1-py2.py3-none-any.whl (435 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m435.1/435.1 KB[0m [31m47.1 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting google-cloud-core<2,>=0.28.1
Downloading google_cloud_core-1.7.2-py2.py3-none-any.whl (28 kB)
Requirement already satisfied: google-cloud-recommendations-ai<=0.2.0,>=0.1.0 in /opt/conda/lib/python3.7/site-packages (from apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.2.0)
Requirement already satisfied: websocket-client>=0.32.0 in /opt/conda/lib/python3.7/site-packages (from docker<5,>=4.1->tfx[kfp]<2) (1.3.1)
Collecting uritemplate<4dev,>=3.0.0
Downloading uritemplate-3.0.1-py2.py3-none-any.whl (15 kB)
Requirement already satisfied: google-api-core<3dev,>=1.21.0 in /opt/conda/lib/python3.7/site-packages (from google-api-python-client<2,>=1.8->tfx[kfp]<2) (2.5.0)
Requirement already satisfied: google-auth-httplib2>=0.0.3 in /opt/conda/lib/python3.7/site-packages (from google-api-python-client<2,>=1.8->tfx[kfp]<2) (0.1.0)
Requirement already satisfied: fasteners>=0.14 in /opt/conda/lib/python3.7/site-packages (from google-apitools<1,>=0.5->tfx[kfp]<2) (0.17.3)
Requirement already satisfied: google-cloud-storage<3.0.0dev,>=1.32.0 in /opt/conda/lib/python3.7/site-packages (from google-cloud-aiplatform<2,>=1.6.2->tfx[kfp]<2) (2.2.1)
Requirement already satisfied: google-resumable-media<3.0dev,>=0.6.0 in /opt/conda/lib/python3.7/site-packages (from google-cloud-bigquery<3,>=2.26.0->tfx[kfp]<2) (2.3.2)
Requirement already satisfied: MarkupSafe>=0.23 in /opt/conda/lib/python3.7/site-packages (from jinja2<4,>=2.7.3->tfx[kfp]<2) (2.0.1)
Requirement already satisfied: scipy in /opt/conda/lib/python3.7/site-packages (from keras-tuner<2,>=1.0.4->tfx[kfp]<2) (1.7.3)
Requirement already satisfied: tensorboard in /opt/conda/lib/python3.7/site-packages (from keras-tuner<2,>=1.0.4->tfx[kfp]<2) (2.6.0)
Requirement already satisfied: ipython in /opt/conda/lib/python3.7/site-packages (from keras-tuner<2,>=1.0.4->tfx[kfp]<2) (7.32.0)
Requirement already satisfied: kt-legacy in /opt/conda/lib/python3.7/site-packages (from keras-tuner<2,>=1.0.4->tfx[kfp]<2) (1.0.4)
Collecting google-cloud-storage<3.0.0dev,>=1.32.0
Downloading google_cloud_storage-1.44.0-py2.py3-none-any.whl (106 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m106.8/106.8 KB[0m [31m15.5 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting requests-toolbelt<1,>=0.8.0
Downloading requests_toolbelt-0.9.1-py2.py3-none-any.whl (54 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m54.3/54.3 KB[0m [31m8.2 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting kfp-server-api<2.0.0,>=1.1.2
Downloading kfp-server-api-1.8.1.tar.gz (54 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m54.2/54.2 KB[0m [31m9.2 MB/s[0m eta [36m0:00:00[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hCollecting jsonschema<4,>=3.0.1
Downloading jsonschema-3.2.0-py2.py3-none-any.whl (56 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m56.3/56.3 KB[0m [31m10.4 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting tabulate<1,>=0.8.6
Downloading tabulate-0.8.9-py3-none-any.whl (25 kB)
Collecting Deprecated<2,>=1.2.7
Downloading Deprecated-1.2.13-py2.py3-none-any.whl (9.6 kB)
Collecting strip-hints<1,>=0.1.8
Downloading strip-hints-0.1.10.tar.gz (29 kB)
Preparing metadata (setup.py) ... [?25ldone
[?25hCollecting docstring-parser<1,>=0.7.3
Downloading docstring_parser-0.14.1-py3-none-any.whl (33 kB)
Collecting fire<1,>=0.3.1
Downloading fire-0.4.0.tar.gz (87 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m87.7/87.7 KB[0m [31m415.4 kB/s[0m eta [36m0:00:00[0ma [36m0:00:01[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hRequirement already satisfied: pydantic<2,>=1.8.2 in /opt/conda/lib/python3.7/site-packages (from kfp<2,>=1.8.5->tfx[kfp]<2) (1.9.0)
Collecting typer<1.0,>=0.3.2
Downloading typer-0.4.1-py3-none-any.whl (27 kB)
Requirement already satisfied: certifi>=14.05.14 in /opt/conda/lib/python3.7/site-packages (from kubernetes<13,>=10.0.1->tfx[kfp]<2) (2021.10.8)
Requirement already satisfied: setuptools>=21.0.0 in /opt/conda/lib/python3.7/site-packages (from kubernetes<13,>=10.0.1->tfx[kfp]<2) (59.8.0)
Requirement already satisfied: urllib3>=1.24.2 in /opt/conda/lib/python3.7/site-packages (from kubernetes<13,>=10.0.1->tfx[kfp]<2) (1.26.8)
Requirement already satisfied: requests-oauthlib in /opt/conda/lib/python3.7/site-packages (from kubernetes<13,>=10.0.1->tfx[kfp]<2) (1.3.1)
Requirement already satisfied: pyparsing>=2.0.2 in /opt/conda/lib/python3.7/site-packages (from packaging<21,>=20->tfx[kfp]<2) (3.0.7)
Requirement already satisfied: psutil in /opt/conda/lib/python3.7/site-packages (from portpicker<2,>=1.3.1->tfx[kfp]<2) (5.9.0)
Collecting libclang>=9.0.1
Downloading libclang-14.0.1-py2.py3-none-manylinux1_x86_64.whl (14.5 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m14.5/14.5 MB[0m [31m71.9 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hRequirement already satisfied: keras-preprocessing>=1.1.1 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.1.2)
Collecting tf-estimator-nightly==2.8.0.dev2021122109
Downloading tf_estimator_nightly-2.8.0.dev2021122109-py2.py3-none-any.whl (462 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m462.5/462.5 KB[0m [31m41.4 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting numpy<2,>=1.16
Downloading numpy-1.21.6-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (15.7 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m15.7/15.7 MB[0m [31m70.3 MB/s[0m eta [36m0:00:00[0m00:01[0m00:01[0m
[?25hCollecting tensorboard
Downloading tensorboard-2.8.0-py3-none-any.whl (5.8 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m5.8/5.8 MB[0m [31m96.3 MB/s[0m eta [36m0:00:00[0mta [36m0:00:01[0m
[?25hRequirement already satisfied: h5py>=2.9.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (3.1.0)
Requirement already satisfied: astunparse>=1.6.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.6.3)
Requirement already satisfied: termcolor>=1.1.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.1.0)
Collecting keras<2.9,>=2.8.0rc0
Downloading keras-2.8.0-py2.py3-none-any.whl (1.4 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m1.4/1.4 MB[0m [31m62.4 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting tensorflow-io-gcs-filesystem>=0.23.1
Downloading tensorflow_io_gcs_filesystem-0.25.0-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (2.1 MB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m2.1/2.1 MB[0m [31m82.0 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: gast>=0.2.1 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (0.4.0)
Requirement already satisfied: flatbuffers>=1.12 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.12)
Requirement already satisfied: opt-einsum>=2.3.2 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (3.3.0)
Requirement already satisfied: wrapt>=1.11.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.12.1)
Requirement already satisfied: google-pasta>=0.1.1 in /opt/conda/lib/python3.7/site-packages (from tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (0.2.0)
Collecting joblib<0.15,>=0.12
Downloading joblib-0.14.1-py2.py3-none-any.whl (294 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m294.9/294.9 KB[0m [31m34.9 MB/s[0m eta [36m0:00:00[0m
[?25hCollecting pyfarmhash<0.4,>=0.2
Downloading pyfarmhash-0.3.2.tar.gz (99 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m99.9/99.9 KB[0m [31m15.7 MB/s[0m eta [36m0:00:00[0m
[?25h Preparing metadata (setup.py) ... [?25ldone
[?25hRequirement already satisfied: pandas<2,>=1.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow-data-validation<1.8.0,>=1.7.0->tfx[kfp]<2) (1.3.5)
Requirement already satisfied: tensorflow-metadata<1.8,>=1.7.0 in /opt/conda/lib/python3.7/site-packages (from tensorflow-data-validation<1.8.0,>=1.7.0->tfx[kfp]<2) (1.7.0)
Requirement already satisfied: ipywidgets<8,>=7 in /opt/conda/lib/python3.7/site-packages (from tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (7.6.5)
Requirement already satisfied: wheel<1.0,>=0.23.0 in /opt/conda/lib/python3.7/site-packages (from astunparse>=1.6.0->tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (0.37.1)
Requirement already satisfied: googleapis-common-protos<2.0dev,>=1.52.0 in /opt/conda/lib/python3.7/site-packages (from google-api-core<3dev,>=1.21.0->google-api-python-client<2,>=1.8->tfx[kfp]<2) (1.54.0)
Requirement already satisfied: grpcio-status<2.0dev,>=1.33.2 in /opt/conda/lib/python3.7/site-packages (from google-api-core<3dev,>=1.21.0->google-api-python-client<2,>=1.8->tfx[kfp]<2) (1.44.0)
Requirement already satisfied: rsa<5,>=3.1.4 in /opt/conda/lib/python3.7/site-packages (from google-auth<3,>=1.18.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (4.8)
Requirement already satisfied: pyasn1-modules>=0.2.1 in /opt/conda/lib/python3.7/site-packages (from google-auth<3,>=1.18.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.2.7)
Requirement already satisfied: grpc-google-iam-v1<0.13dev,>=0.12.3 in /opt/conda/lib/python3.7/site-packages (from google-cloud-bigtable<2,>=0.31.1->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.12.3)
Collecting google-cloud-core<2,>=0.28.1
Downloading google_cloud_core-1.7.1-py2.py3-none-any.whl (28 kB)
Downloading google_cloud_core-1.7.0-py2.py3-none-any.whl (28 kB)
Downloading google_cloud_core-1.6.0-py2.py3-none-any.whl (28 kB)
Downloading google_cloud_core-1.5.0-py2.py3-none-any.whl (27 kB)
Downloading google_cloud_core-1.4.4-py2.py3-none-any.whl (27 kB)
Downloading google_cloud_core-1.4.3-py2.py3-none-any.whl (27 kB)
Downloading google_cloud_core-1.4.2-py2.py3-none-any.whl (26 kB)
Downloading google_cloud_core-1.4.1-py2.py3-none-any.whl (26 kB)
INFO: pip is looking at multiple versions of google-cloud-bigtable to determine which version is compatible with other requirements. This could take a while.
Collecting google-cloud-bigtable<2,>=0.31.1
Downloading google_cloud_bigtable-1.7.0-py2.py3-none-any.whl (267 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m267.7/267.7 KB[0m [31m35.5 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-cloud-bigquery-storage to determine which version is compatible with other requirements. This could take a while.
Collecting google-cloud-bigquery-storage>=2.6.3
Downloading google_cloud_bigquery_storage-2.13.1-py2.py3-none-any.whl (180 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m180.1/180.1 KB[0m [31m27.5 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-auth-httplib2 to determine which version is compatible with other requirements. This could take a while.
Collecting google-auth-httplib2>=0.0.3
Downloading google_auth_httplib2-0.1.0-py2.py3-none-any.whl (9.3 kB)
INFO: pip is looking at multiple versions of google-auth to determine which version is compatible with other requirements. This could take a while.
Collecting google-auth<3,>=1.18.0
Downloading google_auth-1.35.0-py2.py3-none-any.whl (152 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m152.9/152.9 KB[0m [31m21.4 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-api-core[grpc] to determine which version is compatible with other requirements. This could take a while.
Collecting google-api-core[grpc]!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.0,<3.0.0dev,>=1.31.5
Downloading google_api_core-2.7.3-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.6/114.6 KB[0m [31m19.6 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-api-core to determine which version is compatible with other requirements. This could take a while.
Downloading google_api_core-2.7.2-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.6/114.6 KB[0m [31m19.7 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.7.1-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.7/114.7 KB[0m [31m17.4 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.7.0-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.7/114.7 KB[0m [31m17.4 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.6.1-py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.6/114.6 KB[0m [31m17.4 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.6.0-py2.py3-none-any.whl (114 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m114.6/114.6 KB[0m [31m18.7 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-2.5.0-py2.py3-none-any.whl (111 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m111.8/111.8 KB[0m [31m11.0 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-api-core[grpc] to determine which version is compatible with other requirements. This could take a while.
Downloading google_api_core-2.4.0-py2.py3-none-any.whl (111 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m111.7/111.7 KB[0m [31m15.5 MB/s[0m eta [36m0:00:00[0m
[?25hINFO: pip is looking at multiple versions of google-api-core to determine which version is compatible with other requirements. This could take a while.
Downloading google_api_core-2.3.2-py2.py3-none-any.whl (109 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m109.8/109.8 KB[0m [31m15.1 MB/s[0m eta [36m0:00:00[0m
[?25h Downloading google_api_core-1.31.5-py2.py3-none-any.whl (93 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m93.3/93.3 KB[0m [31m14.2 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: overrides<7.0.0,>=6.0.1 in /opt/conda/lib/python3.7/site-packages (from google-cloud-pubsublite<2,>=1.2.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (6.1.0)
Requirement already satisfied: google-crc32c<2.0dev,>=1.0 in /opt/conda/lib/python3.7/site-packages (from google-resumable-media<3.0dev,>=0.6.0->google-cloud-bigquery<3,>=2.26.0->tfx[kfp]<2) (1.1.2)
Requirement already satisfied: cached-property in /opt/conda/lib/python3.7/site-packages (from h5py>=2.9.0->tensorflow!=2.0.*,!=2.1.*,!=2.2.*,!=2.3.*,!=2.4.*,!=2.5.*,!=2.6.*,!=2.7.*,<2.9,>=1.15.5->tfx[kfp]<2) (1.5.2)
Requirement already satisfied: docopt in /opt/conda/lib/python3.7/site-packages (from hdfs<3.0.0,>=2.1.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.6.2)
Collecting pyparsing>=2.0.2
Downloading pyparsing-2.4.7-py2.py3-none-any.whl (67 kB)
[2K [90m━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━[0m [32m67.8/67.8 KB[0m [31m10.8 MB/s[0m eta [36m0:00:00[0m
[?25hRequirement already satisfied: backcall in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.2.0)
Requirement already satisfied: decorator in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (5.1.1)
Requirement already satisfied: prompt-toolkit!=3.0.0,!=3.0.1,<3.1.0,>=2.0.0 in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (3.0.27)
Requirement already satisfied: pygments in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (2.11.2)
Requirement already satisfied: jedi>=0.16 in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.18.1)
Requirement already satisfied: matplotlib-inline in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.1.3)
Requirement already satisfied: pexpect>4.3 in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (4.8.0)
Requirement already satisfied: traitlets>=4.2 in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (5.1.1)
Requirement already satisfied: pickleshare in /opt/conda/lib/python3.7/site-packages (from ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.7.5)
Requirement already satisfied: jupyterlab-widgets>=1.0.0 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.0.2)
Requirement already satisfied: nbformat>=4.2.0 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (5.2.0)
Requirement already satisfied: ipython-genutils~=0.2.0 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.2.0)
Requirement already satisfied: widgetsnbextension~=3.5.0 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (3.5.2)
Requirement already satisfied: ipykernel>=4.5.1 in /opt/conda/lib/python3.7/site-packages (from ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (6.9.2)
Requirement already satisfied: importlib-metadata in /opt/conda/lib/python3.7/site-packages (from jsonschema<4,>=3.0.1->kfp<2,>=1.8.5->tfx[kfp]<2) (4.11.3)
Requirement already satisfied: pyrsistent>=0.14.0 in /opt/conda/lib/python3.7/site-packages (from jsonschema<4,>=3.0.1->kfp<2,>=1.8.5->tfx[kfp]<2) (0.18.1)
Requirement already satisfied: pyasn1>=0.1.7 in /opt/conda/lib/python3.7/site-packages (from oauth2client<5,>=2.0.1->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.4.8)
Requirement already satisfied: idna<4,>=2.5 in /opt/conda/lib/python3.7/site-packages (from requests<3.0.0,>=2.24.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (3.3)
Requirement already satisfied: charset-normalizer~=2.0.0 in /opt/conda/lib/python3.7/site-packages (from requests<3.0.0,>=2.24.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (2.0.12)
Requirement already satisfied: google-auth-oauthlib<0.5,>=0.4.1 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.4.6)
Requirement already satisfied: markdown>=2.6.8 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (3.3.6)
Requirement already satisfied: tensorboard-plugin-wit>=1.6.0 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (1.8.1)
Requirement already satisfied: werkzeug>=0.11.15 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (2.0.3)
Requirement already satisfied: tensorboard-data-server<0.7.0,>=0.6.0 in /opt/conda/lib/python3.7/site-packages (from tensorboard->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.6.1)
Requirement already satisfied: oauthlib>=3.0.0 in /opt/conda/lib/python3.7/site-packages (from requests-oauthlib->kubernetes<13,>=10.0.1->tfx[kfp]<2) (3.2.0)
Requirement already satisfied: cffi>=1.0.0 in /opt/conda/lib/python3.7/site-packages (from google-crc32c<2.0dev,>=1.0->google-resumable-media<3.0dev,>=0.6.0->google-cloud-bigquery<3,>=2.26.0->tfx[kfp]<2) (1.15.0)
Requirement already satisfied: debugpy<2.0,>=1.0.0 in /opt/conda/lib/python3.7/site-packages (from ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.5.1)
Requirement already satisfied: tornado<7.0,>=4.2 in /opt/conda/lib/python3.7/site-packages (from ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (6.1)
Requirement already satisfied: jupyter-client<8.0 in /opt/conda/lib/python3.7/site-packages (from ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (7.1.2)
Requirement already satisfied: nest-asyncio in /opt/conda/lib/python3.7/site-packages (from ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.5.4)
Requirement already satisfied: parso<0.9.0,>=0.8.0 in /opt/conda/lib/python3.7/site-packages (from jedi>=0.16->ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.8.3)
Requirement already satisfied: zipp>=0.5 in /opt/conda/lib/python3.7/site-packages (from importlib-metadata->jsonschema<4,>=3.0.1->kfp<2,>=1.8.5->tfx[kfp]<2) (3.7.0)
Requirement already satisfied: jupyter-core in /opt/conda/lib/python3.7/site-packages (from nbformat>=4.2.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (4.9.2)
Requirement already satisfied: typing-utils>=0.0.3 in /opt/conda/lib/python3.7/site-packages (from overrides<7.0.0,>=6.0.1->google-cloud-pubsublite<2,>=1.2.0->apache-beam[gcp]<3,>=2.36->tfx[kfp]<2) (0.1.0)
Requirement already satisfied: ptyprocess>=0.5 in /opt/conda/lib/python3.7/site-packages (from pexpect>4.3->ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.7.0)
Requirement already satisfied: wcwidth in /opt/conda/lib/python3.7/site-packages (from prompt-toolkit!=3.0.0,!=3.0.1,<3.1.0,>=2.0.0->ipython->keras-tuner<2,>=1.0.4->tfx[kfp]<2) (0.2.5)
Requirement already satisfied: notebook>=4.4.1 in /opt/conda/lib/python3.7/site-packages (from widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (6.4.10)
Requirement already satisfied: pycparser in /opt/conda/lib/python3.7/site-packages (from cffi>=1.0.0->google-crc32c<2.0dev,>=1.0->google-resumable-media<3.0dev,>=0.6.0->google-cloud-bigquery<3,>=2.26.0->tfx[kfp]<2) (2.21)
Requirement already satisfied: entrypoints in /opt/conda/lib/python3.7/site-packages (from jupyter-client<8.0->ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.4)
Requirement already satisfied: pyzmq>=13 in /opt/conda/lib/python3.7/site-packages (from jupyter-client<8.0->ipykernel>=4.5.1->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (22.3.0)
Requirement already satisfied: nbconvert>=5 in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (6.4.4)
Requirement already satisfied: argon2-cffi in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (21.3.0)
Requirement already satisfied: terminado>=0.8.3 in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.13.3)
Requirement already satisfied: prometheus-client in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.13.1)
Requirement already satisfied: Send2Trash>=1.8.0 in /opt/conda/lib/python3.7/site-packages (from notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.8.0)
Requirement already satisfied: nbclient<0.6.0,>=0.5.0 in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.5.13)
Requirement already satisfied: mistune<2,>=0.8.1 in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.8.4)
Requirement already satisfied: beautifulsoup4 in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (4.10.0)
Requirement already satisfied: defusedxml in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.7.1)
Requirement already satisfied: bleach in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (4.1.0)
Requirement already satisfied: testpath in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.6.0)
Requirement already satisfied: jupyterlab-pygments in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.1.2)
Requirement already satisfied: pandocfilters>=1.4.1 in /opt/conda/lib/python3.7/site-packages (from nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (1.5.0)
Requirement already satisfied: argon2-cffi-bindings in /opt/conda/lib/python3.7/site-packages (from argon2-cffi->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (21.2.0)
Requirement already satisfied: soupsieve>1.2 in /opt/conda/lib/python3.7/site-packages (from beautifulsoup4->nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (2.3.1)
Requirement already satisfied: webencodings in /opt/conda/lib/python3.7/site-packages (from bleach->nbconvert>=5->notebook>=4.4.1->widgetsnbextension~=3.5.0->ipywidgets<8,>=7->tensorflow-model-analysis<0.39,>=0.38.0->tfx[kfp]<2) (0.5.1)
Building wheels for collected packages: kfp, dill, fire, kfp-server-api, pyfarmhash, strip-hints
Building wheel for kfp (setup.py) ... [?25ldone
[?25h Created wheel for kfp: filename=kfp-1.8.12-py3-none-any.whl size=419048 sha256=fc5fc7f3a9b5f676cd53e5cd69e492ed3e995211cc4b6b8cfc5a06b54796f1d4
Stored in directory: /home/jupyter/.cache/pip/wheels/54/0c/4a/3fc55077bc88cc17eacaae34c5fd3f6178c1d16d2ee3b0afdf
Building wheel for dill (setup.py) ... [?25ldone
[?25h Created wheel for dill: filename=dill-0.3.1.1-py3-none-any.whl size=78544 sha256=6d34132d6cad3e94afaaf4057513f0bcf6eec4626ab3183f05c6bb5e4d8f93bf
Stored in directory: /home/jupyter/.cache/pip/wheels/a4/61/fd/c57e374e580aa78a45ed78d5859b3a44436af17e22ca53284f
Building wheel for fire (setup.py) ... [?25ldone
[?25h Created wheel for fire: filename=fire-0.4.0-py2.py3-none-any.whl size=115942 sha256=a0e96813de3269a653f0bbe1d106b94a930df65b64cfc8c8afd4091e2af841b1
Stored in directory: /home/jupyter/.cache/pip/wheels/8a/67/fb/2e8a12fa16661b9d5af1f654bd199366799740a85c64981226
Building wheel for kfp-server-api (setup.py) ... [?25ldone
[?25h Created wheel for kfp-server-api: filename=kfp_server_api-1.8.1-py3-none-any.whl size=95549 sha256=1d5907bdf9958cf5fd1529a0fa69ee8a91fefca39b8c3942766a2365d59478f3
Stored in directory: /home/jupyter/.cache/pip/wheels/f5/4e/2e/6795bd3ed456a43652e7de100aca275ec179c9a8dfbcc65626
Building wheel for pyfarmhash (setup.py) ... [?25ldone
[?25h Created wheel for pyfarmhash: filename=pyfarmhash-0.3.2-cp37-cp37m-linux_x86_64.whl size=108625 sha256=1ba31109887fb167782b9678b72ce85552e486cea10a26e776ef9b5d238c512d
Stored in directory: /home/jupyter/.cache/pip/wheels/53/58/7a/3b040f3a2ee31908e3be916e32660db6db53621ce6eba838dc
Building wheel for strip-hints (setup.py) ... [?25ldone
[?25h Created wheel for strip-hints: filename=strip_hints-0.1.10-py2.py3-none-any.whl size=22302 sha256=1fa632e6899f84956f31c089308aec5d16a45626c95b97bbb9dd78e5851779bb
Stored in directory: /home/jupyter/.cache/pip/wheels/5e/14/c3/6e44e9b2545f2d570b03f5b6d38c00b7534aa8abb376978363
Successfully built kfp dill fire kfp-server-api pyfarmhash strip-hints
Installing collected packages: tf-estimator-nightly, tabulate, pyfarmhash, libclang, keras, joblib, uritemplate, tensorflow-io-gcs-filesystem, strip-hints, pyyaml, pyparsing, portpicker, numpy, kfp-pipeline-spec, fire, docstring-parser, dill, Deprecated, click, attrs, typer, requests-toolbelt, pyarrow, packaging, ml-metadata, kfp-server-api, jsonschema, httplib2, docker, kubernetes, google-api-core, tensorboard, google-cloud-core, google-api-python-client, tensorflow, ml-pipelines-sdk, google-cloud-vision, google-cloud-videointelligence, google-cloud-storage, google-cloud-spanner, google-cloud-language, google-cloud-datastore, google-cloud-bigtable, kfp, tensorflow-model-analysis, tensorflow-data-validation, tfx
Attempting uninstall: keras
Found existing installation: keras 2.6.0
Uninstalling keras-2.6.0:
Successfully uninstalled keras-2.6.0
Attempting uninstall: joblib
Found existing installation: joblib 1.0.1
Uninstalling joblib-1.0.1:
Successfully uninstalled joblib-1.0.1
Attempting uninstall: uritemplate
Found existing installation: uritemplate 4.1.1
Uninstalling uritemplate-4.1.1:
Successfully uninstalled uritemplate-4.1.1
Attempting uninstall: pyyaml
Found existing installation: PyYAML 6.0
Uninstalling PyYAML-6.0:
Successfully uninstalled PyYAML-6.0
Attempting uninstall: pyparsing
Found existing installation: pyparsing 3.0.7
Uninstalling pyparsing-3.0.7:
Successfully uninstalled pyparsing-3.0.7
Attempting uninstall: numpy
Found existing installation: numpy 1.19.5
Uninstalling numpy-1.19.5:
Successfully uninstalled numpy-1.19.5
Attempting uninstall: dill
Found existing installation: dill 0.3.4
Uninstalling dill-0.3.4:
Successfully uninstalled dill-0.3.4
Attempting uninstall: click
Found existing installation: click 8.0.4
Uninstalling click-8.0.4:
Successfully uninstalled click-8.0.4
Attempting uninstall: attrs
Found existing installation: attrs 21.4.0
Uninstalling attrs-21.4.0:
Successfully uninstalled attrs-21.4.0
Attempting uninstall: pyarrow
Found existing installation: pyarrow 7.0.0
Uninstalling pyarrow-7.0.0:
Successfully uninstalled pyarrow-7.0.0
Attempting uninstall: packaging
Found existing installation: packaging 21.3
Uninstalling packaging-21.3:
Successfully uninstalled packaging-21.3
Attempting uninstall: jsonschema
Found existing installation: jsonschema 4.4.0
Uninstalling jsonschema-4.4.0:
Successfully uninstalled jsonschema-4.4.0
Attempting uninstall: httplib2
Found existing installation: httplib2 0.20.4
Uninstalling httplib2-0.20.4:
Successfully uninstalled httplib2-0.20.4
Attempting uninstall: docker
Found existing installation: docker 5.0.3
Uninstalling docker-5.0.3:
Successfully uninstalled docker-5.0.3
Attempting uninstall: kubernetes
Found existing installation: kubernetes 23.3.0
Uninstalling kubernetes-23.3.0:
Successfully uninstalled kubernetes-23.3.0
Attempting uninstall: google-api-core
Found existing installation: google-api-core 2.5.0
Uninstalling google-api-core-2.5.0:
Successfully uninstalled google-api-core-2.5.0
Attempting uninstall: tensorboard
Found existing installation: tensorboard 2.6.0
Uninstalling tensorboard-2.6.0:
Successfully uninstalled tensorboard-2.6.0
Attempting uninstall: google-cloud-core
Found existing installation: google-cloud-core 2.2.3
Uninstalling google-cloud-core-2.2.3:
Successfully uninstalled google-cloud-core-2.2.3
Attempting uninstall: google-api-python-client
Found existing installation: google-api-python-client 2.41.0
Uninstalling google-api-python-client-2.41.0:
Successfully uninstalled google-api-python-client-2.41.0
Attempting uninstall: tensorflow
Found existing installation: tensorflow 2.6.3
Uninstalling tensorflow-2.6.3:
Successfully uninstalled tensorflow-2.6.3
Attempting uninstall: google-cloud-vision
Found existing installation: google-cloud-vision 2.7.1
Uninstalling google-cloud-vision-2.7.1:
Successfully uninstalled google-cloud-vision-2.7.1
Attempting uninstall: google-cloud-videointelligence
Found existing installation: google-cloud-videointelligence 2.6.1
Uninstalling google-cloud-videointelligence-2.6.1:
Successfully uninstalled google-cloud-videointelligence-2.6.1
Attempting uninstall: google-cloud-storage
Found existing installation: google-cloud-storage 2.2.1
Uninstalling google-cloud-storage-2.2.1:
Successfully uninstalled google-cloud-storage-2.2.1
Attempting uninstall: google-cloud-spanner
Found existing installation: google-cloud-spanner 3.13.0
Uninstalling google-cloud-spanner-3.13.0:
Successfully uninstalled google-cloud-spanner-3.13.0
Attempting uninstall: google-cloud-language
Found existing installation: google-cloud-language 2.4.1
Uninstalling google-cloud-language-2.4.1:
Successfully uninstalled google-cloud-language-2.4.1
Attempting uninstall: google-cloud-datastore
Found existing installation: google-cloud-datastore 2.5.1
Uninstalling google-cloud-datastore-2.5.1:
Successfully uninstalled google-cloud-datastore-2.5.1
Attempting uninstall: google-cloud-bigtable
Found existing installation: google-cloud-bigtable 2.7.0
Uninstalling google-cloud-bigtable-2.7.0:
Successfully uninstalled google-cloud-bigtable-2.7.0
[31mERROR: pip's dependency resolver does not currently take into account all the packages that are installed. This behaviour is the source of the following dependency conflicts.
tensorflow-io 0.21.0 requires tensorflow<2.7.0,>=2.6.0, but you have tensorflow 2.8.0 which is incompatible.
tensorflow-io 0.21.0 requires tensorflow-io-gcs-filesystem==0.21.0, but you have tensorflow-io-gcs-filesystem 0.25.0 which is incompatible.
statsmodels 0.13.2 requires packaging>=21.3, but you have packaging 20.9 which is incompatible.
pandas-profiling 3.1.0 requires joblib~=1.0.1, but you have joblib 0.14.1 which is incompatible.
cloud-tpu-client 0.10 requires google-api-python-client==1.8.0, but you have google-api-python-client 1.12.11 which is incompatible.
black 22.1.0 requires click>=8.0.0, but you have click 7.1.2 which is incompatible.[0m[31m
[0mSuccessfully installed Deprecated-1.2.13 attrs-20.3.0 click-7.1.2 dill-0.3.1.1 docker-4.4.4 docstring-parser-0.14.1 fire-0.4.0 google-api-core-1.31.5 google-api-python-client-1.12.11 google-cloud-bigtable-1.7.1 google-cloud-core-2.2.2 google-cloud-datastore-1.15.4 google-cloud-language-1.3.1 google-cloud-spanner-1.19.2 google-cloud-storage-2.1.0 google-cloud-videointelligence-1.16.2 google-cloud-vision-1.0.1 httplib2-0.19.1 joblib-0.14.1 jsonschema-3.2.0 keras-2.8.0 kfp-1.8.12 kfp-pipeline-spec-0.1.14 kfp-server-api-1.8.1 kubernetes-12.0.1 libclang-14.0.1 ml-metadata-1.7.0 ml-pipelines-sdk-1.7.1 numpy-1.21.6 packaging-20.9 portpicker-1.5.0 pyarrow-5.0.0 pyfarmhash-0.3.2 pyparsing-2.4.7 pyyaml-5.4.1 requests-toolbelt-0.9.1 strip-hints-0.1.10 tabulate-0.8.9 tensorboard-2.8.0 tensorflow-2.8.0 tensorflow-data-validation-1.7.0 tensorflow-io-gcs-filesystem-0.25.0 tensorflow-model-analysis-0.38.0 tf-estimator-nightly-2.8.0.dev2021122109 tfx-1.7.1 typer-0.4.1 uritemplate-3.0.1
###Markdown
Did you restart the runtime? You can restart runtime with following cell.
###Code
# docs_infra: no_execute
import sys
if not 'google.colab' in sys.modules:
# Automatically restart kernel after installs
import IPython
app = IPython.Application.instance()
app.kernel.do_shutdown(True)
###Output
_____no_output_____
###Markdown
Check the package versions
###Code
import tensorflow as tf
print('TensorFlow version: {}'.format(tf.__version__))
from tfx import v1 as tfx
print('TFX version: {}'.format(tfx.__version__))
import kfp
print('KFP version: {}'.format(kfp.__version__))
###Output
TensorFlow version: 2.8.0
TFX version: 1.7.1
KFP version: 1.8.12
###Markdown
Set up variablesYou will set up some variables used to customize the pipelines below. Followinginformation is required:* GCP Project id. See[Identifying your project id](https://cloud.google.com/resource-manager/docs/creating-managing-projectsidentifying_projects).* GCP Region to run pipelines. For more information about the regions thatVertex Pipelines is available in, see the[Vertex AI locations guide](https://cloud.google.com/vertex-ai/docs/general/locationsfeature-availability).* Google Cloud Storage Bucket to store pipeline outputs.**Enter required values in the cell below before running it**.
###Code
GOOGLE_CLOUD_PROJECT = 'qwiklabs-gcp-01-2e305ff9c72b' # Replace this with your Project-ID
GOOGLE_CLOUD_REGION = 'us-central1' # Replace this with your region
GCS_BUCKET_NAME = 'qwiklabs-gcp-01-2e305ff9c72b' # Replace this with your Cloud Storage bucket
if not (GOOGLE_CLOUD_PROJECT and GOOGLE_CLOUD_REGION and GCS_BUCKET_NAME):
from absl import logging
logging.error('Please set all required parameters.')
###Output
_____no_output_____
###Markdown
Set `gcloud` to use your project.
###Code
!gcloud config set project {GOOGLE_CLOUD_PROJECT}
PIPELINE_NAME = 'penguin-vertex-pipelines'
# Path to various pipeline artifact.
PIPELINE_ROOT = 'gs://{}/pipeline_root/{}'.format(
GCS_BUCKET_NAME, PIPELINE_NAME)
# Paths for users' Python module.
MODULE_ROOT = 'gs://{}/pipeline_module/{}'.format(
GCS_BUCKET_NAME, PIPELINE_NAME)
# Paths for input data.
DATA_ROOT = 'gs://{}/data/{}'.format(GCS_BUCKET_NAME, PIPELINE_NAME)
# This is the path where your model will be pushed for serving.
SERVING_MODEL_DIR = 'gs://{}/serving_model/{}'.format(
GCS_BUCKET_NAME, PIPELINE_NAME)
print('PIPELINE_ROOT: {}'.format(PIPELINE_ROOT))
###Output
PIPELINE_ROOT: gs://qwiklabs-gcp-01-2e305ff9c72b/pipeline_root/penguin-vertex-pipelines
###Markdown
Prepare example dataYou will use the same[Palmer Penguins dataset](https://allisonhorst.github.io/palmerpenguins/articles/intro.html)as[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).There are four numeric features in this dataset which were already normalizedto have range [0,1]. You will build a classification model which predicts the`species` of penguins. You need to make your own copy of the dataset. Because TFX ExampleGen readsinputs from a directory, You need to create a directory and copy dataset to iton GCS.
###Code
!gsutil cp gs://download.tensorflow.org/data/palmer_penguins/penguins_processed.csv {DATA_ROOT}/
###Output
Copying gs://download.tensorflow.org/data/palmer_penguins/penguins_processed.csv [Content-Type=application/octet-stream]...
/ [1 files][ 25.0 KiB/ 25.0 KiB]
Operation completed over 1 objects/25.0 KiB.
###Markdown
Take a quick look at the CSV file.
###Code
# Review the contents of the CSV file
# TODO 1: Your code goes here
###Output
species,culmen_length_mm,culmen_depth_mm,flipper_length_mm,body_mass_g
0,0.2545454545454545,0.6666666666666666,0.15254237288135594,0.2916666666666667
0,0.26909090909090905,0.5119047619047618,0.23728813559322035,0.3055555555555556
0,0.29818181818181805,0.5833333333333334,0.3898305084745763,0.1527777777777778
0,0.16727272727272732,0.7380952380952381,0.3559322033898305,0.20833333333333334
0,0.26181818181818167,0.892857142857143,0.3050847457627119,0.2638888888888889
0,0.24727272727272717,0.5595238095238096,0.15254237288135594,0.2569444444444444
0,0.25818181818181823,0.773809523809524,0.3898305084745763,0.5486111111111112
0,0.32727272727272727,0.5357142857142859,0.1694915254237288,0.1388888888888889
0,0.23636363636363636,0.9642857142857142,0.3220338983050847,0.3055555555555556
###Markdown
Create a pipelineTFX pipelines are defined using Python APIs. You will define a pipeline whichconsists of three components, CsvExampleGen, Trainer and Pusher. The pipelineand model definition is almost the same as[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).The only difference is that you don't need to set `metadata_connection_config`which is used to locate[ML Metadata](https://www.tensorflow.org/tfx/guide/mlmd) database. BecauseVertex Pipelines uses a managed metadata service, users don't need to careof it, and you don't need to specify the parameter.Before actually define the pipeline, you need to write a model code for theTrainer component first. Write model code.You will use the same model code as in the[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).
###Code
_trainer_module_file = 'penguin_trainer.py'
%%writefile {_trainer_module_file}
# Copied from https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple
from typing import List
from absl import logging
import tensorflow as tf
from tensorflow import keras
from tensorflow_transform.tf_metadata import schema_utils
from tfx import v1 as tfx
from tfx_bsl.public import tfxio
from tensorflow_metadata.proto.v0 import schema_pb2
_FEATURE_KEYS = [
'culmen_length_mm', 'culmen_depth_mm', 'flipper_length_mm', 'body_mass_g'
]
_LABEL_KEY = 'species'
_TRAIN_BATCH_SIZE = 20
_EVAL_BATCH_SIZE = 10
# Since you're not generating or creating a schema, you will instead create
# a feature spec. Since there are a fairly small number of features this is
# manageable for this dataset.
_FEATURE_SPEC = {
**{
feature: tf.io.FixedLenFeature(shape=[1], dtype=tf.float32)
for feature in _FEATURE_KEYS
},
_LABEL_KEY: tf.io.FixedLenFeature(shape=[1], dtype=tf.int64)
}
def _input_fn(file_pattern: List[str],
data_accessor: tfx.components.DataAccessor,
schema: schema_pb2.Schema,
batch_size: int) -> tf.data.Dataset:
"""Generates features and label for training.
Args:
file_pattern: List of paths or patterns of input tfrecord files.
data_accessor: DataAccessor for converting input to RecordBatch.
schema: schema of the input data.
batch_size: representing the number of consecutive elements of returned
dataset to combine in a single batch
Returns:
A dataset that contains (features, indices) tuple where features is a
dictionary of Tensors, and indices is a single Tensor of label indices.
"""
return data_accessor.tf_dataset_factory(
file_pattern,
tfxio.TensorFlowDatasetOptions(
batch_size=batch_size, label_key=_LABEL_KEY),
schema=schema).repeat()
def _make_keras_model() -> tf.keras.Model:
"""Creates a DNN Keras model for classifying penguin data.
Returns:
A Keras Model.
"""
# The model below is built with Functional API, please refer to
# https://www.tensorflow.org/guide/keras/overview for all API options.
inputs = [keras.layers.Input(shape=(1,), name=f) for f in _FEATURE_KEYS]
d = keras.layers.concatenate(inputs)
for _ in range(2):
d = keras.layers.Dense(8, activation='relu')(d)
outputs = keras.layers.Dense(3)(d)
model = keras.Model(inputs=inputs, outputs=outputs)
model.compile(
optimizer=keras.optimizers.Adam(1e-2),
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=[keras.metrics.SparseCategoricalAccuracy()])
model.summary(print_fn=logging.info)
return model
# TFX Trainer will call this function.
def run_fn(fn_args: tfx.components.FnArgs):
"""Train the model based on given args.
Args:
fn_args: Holds args used to train the model as name/value pairs.
"""
# This schema is usually either an output of SchemaGen or a manually-curated
# version provided by pipeline author. A schema can also derived from TFT
# graph if a Transform component is used. In the case when either is missing,
# `schema_from_feature_spec` could be used to generate schema from very simple
# feature_spec, but the schema returned would be very primitive.
schema = schema_utils.schema_from_feature_spec(_FEATURE_SPEC)
train_dataset = _input_fn(
fn_args.train_files,
fn_args.data_accessor,
schema,
batch_size=_TRAIN_BATCH_SIZE)
eval_dataset = _input_fn(
fn_args.eval_files,
fn_args.data_accessor,
schema,
batch_size=_EVAL_BATCH_SIZE)
model = _make_keras_model()
model.fit(
train_dataset,
steps_per_epoch=fn_args.train_steps,
validation_data=eval_dataset,
validation_steps=fn_args.eval_steps)
# The result of the training should be saved in `fn_args.serving_model_dir`
# directory.
model.save(fn_args.serving_model_dir, save_format='tf')
###Output
Writing penguin_trainer.py
###Markdown
Copy the module file to GCS which can be accessed from the pipeline components.Because model training happens on GCP, you need to upload this model definition. Otherwise, you might want to build a container image including the module fileand use the image to run the pipeline.
###Code
!gsutil cp {_trainer_module_file} {MODULE_ROOT}/
###Output
Copying file://penguin_trainer.py [Content-Type=text/x-python]...
/ [1 files][ 3.8 KiB/ 3.8 KiB]
Operation completed over 1 objects/3.8 KiB.
###Markdown
Write a pipeline definitionYou will define a function to create a TFX pipeline.
###Code
# Copied from https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple and
# slightly modified because you don't need `metadata_path` argument.
def _create_pipeline(pipeline_name: str, pipeline_root: str, data_root: str,
module_file: str, serving_model_dir: str,
) -> tfx.dsl.Pipeline:
"""Creates a three component penguin pipeline with TFX."""
# Brings data into the pipeline.
example_gen = # TODO 2: Your code goes here
# Uses user-provided Python function that trains a model.
trainer = tfx.components.Trainer(
module_file=module_file,
examples=example_gen.outputs['examples'],
train_args=tfx.proto.TrainArgs(num_steps=100),
eval_args=tfx.proto.EvalArgs(num_steps=5))
# Pushes the model to a filesystem destination.
pusher = tfx.components.Pusher(
model=trainer.outputs['model'],
push_destination=tfx.proto.PushDestination(
filesystem=tfx.proto.PushDestination.Filesystem(
base_directory=serving_model_dir)))
# Following three components will be included in the pipeline.
components = [
example_gen,
trainer,
pusher,
]
return tfx.dsl.Pipeline(
pipeline_name=pipeline_name,
pipeline_root=pipeline_root,
components=components)
###Output
_____no_output_____
###Markdown
Run the pipeline on Vertex Pipelines.You used `LocalDagRunner` which runs on local environment in[Simple TFX Pipeline Tutorial](https://www.tensorflow.org/tfx/tutorials/tfx/penguin_simple).TFX provides multiple orchestrators to run your pipeline. In this tutorial youwill use the Vertex Pipelines together with the Kubeflow V2 dag runner. You need to define a runner to actually run the pipeline. You will compileyour pipeline into our pipeline definition format using TFX APIs.
###Code
import os
PIPELINE_DEFINITION_FILE = PIPELINE_NAME + '_pipeline.json'
runner = tfx.orchestration.experimental.KubeflowV2DagRunner(
config=tfx.orchestration.experimental.KubeflowV2DagRunnerConfig(),
output_filename=PIPELINE_DEFINITION_FILE)
# Following function will write the pipeline definition to PIPELINE_DEFINITION_FILE.
_ = runner.run(
_create_pipeline(
pipeline_name=PIPELINE_NAME,
pipeline_root=PIPELINE_ROOT,
data_root=DATA_ROOT,
module_file=os.path.join(MODULE_ROOT, _trainer_module_file),
serving_model_dir=SERVING_MODEL_DIR))
###Output
_____no_output_____
###Markdown
The generated definition file can be submitted using kfp client.
###Code
# docs_infra: no_execute
from google.cloud import aiplatform
from google.cloud.aiplatform import pipeline_jobs
import logging
logging.getLogger().setLevel(logging.INFO)
aiplatform.init(project=GOOGLE_CLOUD_PROJECT, location=GOOGLE_CLOUD_REGION)
# Create a job to submit the pipeline
job = # TODO 3: Your code goes here
job.submit()
###Output
INFO:google.cloud.aiplatform.pipeline_jobs:Creating PipelineJob
INFO:google.cloud.aiplatform.pipeline_jobs:PipelineJob created. Resource name: projects/202132981718/locations/us-central1/pipelineJobs/penguin-vertex-pipelines-20220510100554
INFO:google.cloud.aiplatform.pipeline_jobs:To use this PipelineJob in another session:
INFO:google.cloud.aiplatform.pipeline_jobs:pipeline_job = aiplatform.PipelineJob.get('projects/202132981718/locations/us-central1/pipelineJobs/penguin-vertex-pipelines-20220510100554')
INFO:google.cloud.aiplatform.pipeline_jobs:View Pipeline Job:
https://console.cloud.google.com/vertex-ai/locations/us-central1/pipelines/runs/penguin-vertex-pipelines-20220510100554?project=202132981718
|
ICON_data_demo_colab_v02.ipynb | ###Markdown
Ionospheric Connection Explorer (ICON) on Jupyter Notebook v2.0 - Access all data products and data documents on the website: - ICON Rules of the Road - Please review the RotR for the ICON products, and consult with instrument scientists to support your analyses.- ICON Public Data - All ICON Data Products are available in NetCDF formats at this FTP site.LEVEL.2 Data products: - L2.1 MIGHTI -- Line-of-Sight Winds - L2.2 MIGHTI -- Neutral Vector Winds -- Zonal Wind, Meridional Wind, Field-aligned Wind - L2.3 MIGHTI-A/B* -- Neutral Temperature- L2.4 FUV--Column O/$N_{2}$- L2.5 FUV--Nighttime $O^{+}$ Density, NmF2, HmF2- L2.6 EUV--Daytime $O^{+}$ Density, NmF2, HmF2- L2.7 IVM-A/B* -- Ion Drift, Ion Densities, Ion Temperature - A/B* are two identical instruments pointing to different directions. Note: - IDL version:> ICON_IDL_EUVIVM_Tutorial.pro> ICON_IDL_MIGHTIIVM_Tutorial.pro> ICON_IDL_MIGHTI_Tutorial.pro- ICON demo on InGeo: https://ingeo.datatransport.org/v3/workspace Go To examples > tutorials- Please read variable notes and quality flags for caveats, limitations, and best practices.- Please check official data sources for the latest version of the data.- Page Maintanence: Yen-Jung Wu yjwu@dn {dn=ssl.berkeley.edu}- Code Contributor: Dr. Colin Triplett triplett@dn and Dr. Brian Harding bharding@dn Why Colab? Where is Colab running? How to run the script locally?1. According to https://colab.research.google.com/notebooks/intro.ipynb, Colaboratory, or "Colab" for short, allows you to write and execute Python in your browser, with > - Zero configuration required> - Free access to GPUs> - Easy sharing2. Google Colab provides free but limited GPUs, runtimes, and memory. Each runtime will be terminated after 12 hours.3. Because of the runtime limit, running the script on Colab (free version) directly is only good for demonstration and quick view purposes, but not effective for any scientific analysis.> - Download the notebook and run on your local machine.> - Same notebook for running locally: https://github.com/YJWu-SSL/ICON_Data_Demo/blob/master/ICON_data_demo_Local_v02.ipynb 1. Get Ready Install necessary Python packages that are not mounted on Google Colab by default. The netCDF file reader/writer (netCDF4) is one of them
###Code
!pip install netCDF4
###Output
Collecting netCDF4
[?25l Downloading https://files.pythonhosted.org/packages/1a/71/a321abeee439caf94850b4f68ecef68d2ad584a5a9566816c051654cff94/netCDF4-1.5.5.1-cp36-cp36m-manylinux2014_x86_64.whl (4.7MB)
[K |████████████████████████████████| 4.7MB 8.5MB/s
[?25hCollecting cftime
[?25l Downloading https://files.pythonhosted.org/packages/21/65/b5a4965862b5d4a9347050f31f1a13c4ec873ebec92d52c958b9d57753f2/cftime-1.4.1-cp36-cp36m-manylinux2014_x86_64.whl (316kB)
[K |████████████████████████████████| 317kB 40.8MB/s
[?25hRequirement already satisfied: numpy>=1.9 in /usr/local/lib/python3.6/dist-packages (from netCDF4) (1.19.5)
Installing collected packages: cftime, netCDF4
Successfully installed cftime-1.4.1 netCDF4-1.5.5.1
###Markdown
Import Python packages
###Code
import numpy as np
import matplotlib
import matplotlib.pyplot as plt
from matplotlib.dates import DateFormatter, MinuteLocator
import matplotlib.gridspec as gridspec
from matplotlib.ticker import MaxNLocator
from datetime import datetime, timedelta
import bisect
import netCDF4
import pandas as pd
import seaborn as sns
import pylab
import os
import glob
from ftplib import FTP
import fnmatch
import matplotlib.dates as mdates
'''Optional: Specify the plotting style '''
plt.style.use('seaborn')
plt.rcParams.update({'font.size': 15,\
'xtick.labelsize' : 15,\
'ytick.labelsize' : 15,\
'axes.titlesize' : 16,\
'axes.labelsize' : 16,\
'date.autoformatter.minute': '%H:%M' })
###Output
_____no_output_____
###Markdown
Additional functions
###Code
def download_ICON_ftp(ftp, target_YYYY, target_doy, ICON_product, ICON_instrument, ICON_level='LEVEL.2',MTAB=None):
'''
INPUTS
------
ftp class : ftp class that has logged in to the ftp site ftp=libftp.ftp('address')
target_YYYY str : the year of the target day
target_doy str : the day of year of the target day
ICON_product str : i.e. 'L2.1','L2.2','L2.3','L2.4','L2.5','L2.6','L2.7'
ICON_instrument str : has to be one of the following ['MIGHTI','IVM-A','FUV','EUV']
Optional:
ICON_level str : 'LEVEL.2','LEVEL.1'
MTAB str : A required input when retrieving L2.3 MIGHTI Temperature, MTAB='A' for MIGHTI-A, MTAB='B' for MIGHTI-B
RESULT
-------
Download (retrieve) the latest version of ICON data from the FTP site hosting at UC Berkeley (https://icon.ssl.berkeley.edu/Data)
'''
target_dir='/pub/%s/%s/%s/%s' % (ICON_level, ICON_instrument,target_YYYY,target_doy)
if target_dir in ftp.nlst('/pub/%s/%s/%s/' % (ICON_level, ICON_instrument,target_YYYY)):
ftp.cwd(target_dir+'/ZIP/')
filenames=ftp.nlst()
if not (ICON_product == 'L2-3'):
if ftp.nlst("ICON_%s*" % (ICON_product)):
select_fn=sorted(ftp.nlst("ICON_%s*" % (ICON_product)), key=lambda x: ftp.voidcmd(f"MDTM {x}"))[-1]
else:
raise Exception('File does not exist!')
else:
if (MTAB == None):
raise Exception('Please specify MIGHTI-A or -B in keyword MTAB, MTAB="A" for example ')
else:
if ftp.nlst('ICON_%s_%s-%s*' % (ICON_product, ICON_instrument,MTAB)):
select_fn=sorted(ftp.nlst('ICON_%s_%s-%s*' % (ICON_product, ICON_instrument,MTAB)), key=lambda x: ftp.voidcmd(f"MDTM {x}"))[-1]
else:
raise Exception('File does not exist!')
download_file = open(select_fn, 'wb')
ftp.retrbinary('RETR '+ select_fn, download_file.write)
download_file.close()
print('%s has been retrieved' % select_fn)
else:
raise Exception('/pub/%s/%s/%s/%s/ZIP/ does not exist!' % (ICON_level, ICON_instrument,target_YYYY,target_doy))
return
class OOMFormatter(matplotlib.ticker.ScalarFormatter):
'''
Math text formatter on colorbars
source:
https://stackoverflow.com/questions/42656139/set-scientific-notation-with-fixed-exponent-and-significant-digits-for-multiple
'''
def __init__(self, order=0, fformat="%1.1f", offset=True, mathText=True):
self.oom = order
self.fformat = fformat
matplotlib.ticker.ScalarFormatter.__init__(self,useOffset=offset,useMathText=mathText)
def _set_order_of_magnitude(self):
self.orderOfMagnitude = self.oom
def _set_format(self, vmin=None, vmax=None):
self.format = self.fformat
if self._useMathText:
self.format = r'$\mathdefault{%s}$' % self.format
###Output
_____no_output_____
###Markdown
2. Download ICON data from the FTP site
###Code
'''Insert a target day'''
target_datetime=datetime(2020,3,24) # (year, month, day)
target_YYYY='%4i' % target_datetime.year
target_mm='%02i' % target_datetime.month
target_dd='%02i' % target_datetime.day
target_doy='%03i' % ((target_datetime-datetime(np.int(target_YYYY),1,1)).days+1)
target_date_str='%s-%s-%s' % (target_YYYY,target_mm,target_dd)
if not glob.glob('ICON*%s*' % target_date_str):
ftp=FTP('icon-science.ssl.berkeley.edu')
ftp.login()
download_ICON_ftp(ftp, target_YYYY, target_doy, ICON_product='L2-2', ICON_instrument='MIGHTI')
download_ICON_ftp(ftp, target_YYYY, target_doy, ICON_product='L2-3', ICON_instrument='MIGHTI',MTAB='A')
download_ICON_ftp(ftp, target_YYYY, target_doy, ICON_product='L2-4', ICON_instrument='FUV')
download_ICON_ftp(ftp, target_YYYY, target_doy, ICON_product='L2-5', ICON_instrument='FUV')
download_ICON_ftp(ftp, target_YYYY, target_doy, ICON_product='L2-6', ICON_instrument='EUV')
download_ICON_ftp(ftp, target_YYYY, target_doy, ICON_product='L2-7', ICON_instrument='IVM-A')
#
ftp.quit()
'''Unzip the file'''
!unzip '*.ZIP'
###Output
Archive: ICON_L2-7_IVM-A_2020-03-24_v02r002.ZIP
ICON archive of file set ICON_L2-7_IVM-A_2020-03-24.
inflating: 084/ICON_L2-7_IVM-A_2020-03-24_v02r002.NC
Archive: ICON_L2-5_FUV_Night_2020-03-24_v03r002.ZIP
ICON archive of file set ICON_L2-5_FUV_Night_2020-03-24.
inflating: 084/ICON_L2-5_FUV_Night_2020-03-24_v03r002.NC
Archive: ICON_L2-2_MIGHTI_2020-03-24_v04r001.ZIP
ICON archive of file set ICON_L2-2_MIGHTI_2020-03-24.
inflating: 084/ICON_L2-2_MIGHTI_Vector-Wind-Green_2020-03-24_v04r001.NC
inflating: 084/ICON_L2-2_MIGHTI_Vector-Wind-Red_2020-03-24_v04r001.NC
Archive: ICON_L2-6_EUV_2020-03-24_v02r002.ZIP
ICON archive of file set ICON_L2-6_EUV_2020-03-24.
inflating: 084/ICON_L2-6_EUV_2020-03-24_v02r002.NC
Archive: ICON_L2-4_FUV_Day_2020-03-24_v03r001.ZIP
ICON archive of file set ICON_L2-4_FUV_Day_2020-03-24.
inflating: 084/ICON_L2-4_FUV_Day_2020-03-24_v03r001.NC
Archive: ICON_L2-3_MIGHTI-A_Temperature_2020-03-24_v04r000.ZIP
ICON archive of file set ICON_L2-3_MIGHTI-A_Temperature_2020-03-24.
inflating: 084/ICON_L2-3_MIGHTI-A_Temperature_2020-03-24_v04r000.NC
6 archives were successfully processed.
###Markdown
3. Read ICON netCDF files
###Code
'''
Alternative way to deal with netCDF file other than dict is xarray
http://xarray.pydata.org/en/stable/io.html
'''
# import xarray as xr
# with xr.open_dataset(fn_L27) as ds:
# print(ds.keys())
'''MIGHTI L2.2 Vector Wind'''
MT_color= 'Green'
fn_L22=glob.glob('/content/%s/ICON_L2-2_*%s*.NC' % (target_doy,MT_color))[0]
## Load winds
dm_g = {}
with netCDF4.Dataset(fn_L22) as d:
time_msec = np.ma.filled(d.variables['Epoch'][...], np.nan) # Time in msec since 1970-01-01
dm_g['time_ms'] = time_msec
dm_g['time'] = np.array([datetime(1970,1,1) + timedelta(seconds = 1e-3*s) for s in time_msec])
dm_g['orb_num'] = np.ma.filled(d.variables['ICON_L22_Orbit_Number'][...], np.nan)
dm_g['uzon'] = np.ma.filled(d.variables['ICON_L22_Zonal_Wind'][...], np.nan) #>0: Eastward, <0: Westward
dm_g['umer'] = np.ma.filled(d.variables['ICON_L22_Meridional_Wind'][...], np.nan) #>0: northward, <0: southward
dm_g['tang_alt'] = np.ma.filled(d.variables['ICON_L22_Altitude'][...], np.nan)
dm_g['tang_lon'] = np.ma.filled(d.variables['ICON_L22_Longitude'][...], np.nan)
dm_g['tang_lat'] = np.ma.filled(d.variables['ICON_L22_Latitude'][...], np.nan)
dm_g['tang_slt'] = np.ma.filled(d.variables['ICON_L22_Local_Solar_Time'][...], np.nan)
# Simple quality control
wind_quality = np.ma.filled(d.variables['ICON_L22_Wind_Quality'][...], np.nan)
good_data = (wind_quality == 1)| (wind_quality == 0.5)
dm_g['uzon'][~good_data] = np.nan
dm_g['umer'][~good_data] = np.nan
print('Orbit numbers: %04i to %04i' % (np.nanmin(dm_g['orb_num']),np.nanmax(dm_g['orb_num'])))
'''MIGHTI L2.2 Vector Wind'''
MT_color= 'Red'
fn_L22=glob.glob('/content/%s/ICON_L2-2_*%s*.NC' % (target_doy,MT_color))[0]
### Load winds
dm_r = {}
with netCDF4.Dataset(fn_L22) as d:
time_msec = np.ma.filled(d.variables['Epoch'][...], np.nan) # Time in msec since 1970-01-01
dm_r['time_ms'] = time_msec
dm_r['time'] = np.array([datetime(1970,1,1) + timedelta(seconds = 1e-3*s) for s in time_msec])
dm_r['orb_num'] = np.ma.filled(d.variables['ICON_L22_Orbit_Number'][...], np.nan)
dm_r['uzon'] = np.ma.filled(d.variables['ICON_L22_Zonal_Wind'][...], np.nan) #>0: Eastward, <0: Westward
dm_r['umer'] = np.ma.filled(d.variables['ICON_L22_Meridional_Wind'][...], np.nan) #>0: northward, <0: southward
dm_r['tang_alt'] = np.ma.filled(d.variables['ICON_L22_Altitude'][...], np.nan)
dm_r['tang_lon'] = np.ma.filled(d.variables['ICON_L22_Longitude'][...], np.nan)
dm_r['tang_lat'] = np.ma.filled(d.variables['ICON_L22_Latitude'][...], np.nan)
dm_r['tang_slt'] = np.ma.filled(d.variables['ICON_L22_Local_Solar_Time'][...], np.nan)
# Simple quality control
wind_quality = np.ma.filled(d.variables['ICON_L22_Wind_Quality'][...], np.nan)
good_data = (wind_quality == 1)| (wind_quality == 0.5)
dm_r['uzon'][~good_data] = np.nan
dm_r['umer'][~good_data] = np.nan
print('Orbit numbers: %04i to %04i' % (np.nanmin(dm_r['orb_num']),np.nanmax(dm_r['orb_num'])))
'''MIGHTI L2.3 Temperature'''
MTAB='A'
fn_L23=glob.glob('/content/%s/ICON_L2-3_MIGHTI-%s*.NC' % (target_doy, MTAB))[0]
#### Load Temperature ###
dTN = {}
with netCDF4.Dataset(fn_L23) as d:
time_msec = np.ma.filled(d.variables['Epoch'][...], np.nan) # Time in msec since 1970-01-01
dTN['time'] = np.array([datetime(1970,1,1) + timedelta(seconds = 1e-3*s) for s in time_msec])
dTN['TN'] = np.ma.filled(d.variables['ICON_L23_MIGHTI_%s_Temperature' % (MTAB)][...], np.nan)
dTN['mlat'] = np.ma.filled(d.variables['ICON_L23_MIGHTI_%s_Tangent_Magnetic_Longitude' % (MTAB)][...], np.nan)
dTN['tang_slt'] = np.ma.filled(d.variables['ICON_L23_MIGHTI_%s_Tangent_Local_Solar_Time' % (MTAB)][...], np.nan)
dTN['tang_alt'] = np.ma.filled(d.variables['ICON_L23_MIGHTI_%s_Tangent_Altitude' % (MTAB)][...], np.nan)
dTN['tang_lat'] = np.ma.filled(d.variables['ICON_L23_MIGHTI_%s_Tangent_Latitude' % (MTAB)][...], np.nan)
dTN['tang_lon'] = np.ma.filled(d.variables['ICON_L23_MIGHTI_%s_Tangent_Longitude' % (MTAB)][...], np.nan)
dTN['SC_lat'] = np.ma.filled(d.variables['ICON_L23_Observatory_Latitude'][...], np.nan)
dTN['SC_lon'] = np.ma.filled(d.variables['ICON_L23_Observatory_Longitude'][...], np.nan)
dTN['orb_num'] = np.ma.filled(d.variables['ICON_L23_Orbit_Number'][...], np.nan)
# Simple quality control
saa_flag = np.ma.filled(d.variables['ICON_L1_MIGHTI_%s_Quality_Flag_South_Atlantic_Anomaly' % (MTAB)][...], np.nan) # SAA flag
cali_flag = np.ma.filled(d.variables['ICON_L1_MIGHTI_%s_Quality_Flag_Bad_Calibration' % (MTAB)][...], np.nan) # Calibration flag
good_data = (dTN['TN'] > 0)
dTN['TN'][~good_data] = np.nan
good_data = (saa_flag == 0) & (cali_flag == 0)#
dTN['TN'][:,~good_data] = np.nan # only good data are shown
print('Orbit number: %04i to %04i' % (np.nanmin(dTN['orb_num']),np.nanmax(dTN['orb_num'])))
'''FUV L2.4 Disk O/N2'''
fn_L24 =glob.glob('/content/%s/ICON_L2-4_FUV*.NC' % (target_doy))[0]
# Load L2.4 disk O/N2 #
dON2 = {}
with netCDF4.Dataset(fn_L24) as d:
time_msec = np.ma.filled(d.variables['Epoch'][...], np.nan) # Time in msec since 1970-01-01
dON2['time'] = np.array([datetime(1970,1,1) + timedelta(seconds = 1e-3*s) for s in time_msec])
dON2['disk_ON2'] = np.ma.filled(d.variables['ICON_L24_Disk_ON2'][...], np.nan)
dON2['disk_slt'] = np.ma.filled(d.variables['ICON_L24_Local_Solar_Time_Disk'][...], np.nan)
dON2['disk_lon'] = np.ma.filled(d.variables['ICON_L24_Disk_Longitude'][...], np.nan)
dON2['disk_lat'] = np.ma.filled(d.variables['ICON_L24_Disk_Latitude'][...], np.nan)
dON2['disk_mlon'] = np.ma.filled(d.variables['ICON_L24_Disk_Magnetic_Longitude'][...], np.nan)
dON2['disk_mlat'] = np.ma.filled(d.variables['ICON_L24_Disk_Magnetic_Latitude'][...], np.nan)
dON2['SC_lon'] = np.ma.filled(d.variables['ICON_L24_Observatory_Longitude'][...], np.nan)
dON2['SC_lat'] = np.ma.filled(d.variables['ICON_L24_Observatory_Latitude'][...], np.nan)
# dON2['orb_num'] = np.ma.filled(d.variables['ICON_L24_Orbit_Number'][...], np.nan)
# Simple quality control
model_flag = np.ma.filled(d.variables['ICON_L24_Disk_Retrieval_Flags'][...], np.nan)
good_data = ( model_flag == 0)
dON2['disk_ON2'][~good_data] = np.nan
print( (np.nanmin(dON2['time']),np.nanmax(dON2['time'])))
'''FUV L2.5 Nighttime Ionosphere'''
fn_L25 =glob.glob('/content/%s/ICON_L2-5_FUV*.NC' % (target_doy))[0]
# Load L2.6 Daytime plasma observations #
dL25 = {}
with netCDF4.Dataset(fn_L25) as d:
time_msec = np.ma.filled(d.variables['Epoch'][...], np.nan) # Time in msec since 1970-01-01
dL25['time'] = np.array([datetime(1970,1,1) + timedelta(seconds = 1e-3*s) for s in time_msec])
dL25['HmF2'] = np.ma.filled(d.variables['ICON_L25_HMF2'][...], np.nan)
dL25['NmF2'] = np.ma.filled(d.variables['ICON_L25_NMF2'][...], np.nan)
dL25['O_plus'] = np.ma.filled(d.variables['ICON_L25_O_Plus_Density'][...], np.nan)
dL25['orb_num'] = np.ma.filled(d.variables['ICON_L25_Orbit_Number'][...], np.nan)
dL25['tang_slt'] = np.ma.filled(d.variables['ICON_L25_Local_Solar_Time'][...], np.nan)
dL25['HmF2_lon'] = np.ma.filled(d.variables['ICON_L25_Longitude'][...], np.nan)
dL25['HmF2_lat'] = np.ma.filled(d.variables['ICON_L25_Latitude'][...], np.nan)
dL25['HmF2_mlon'] = np.ma.filled(d.variables['ICON_L25_Magnetic_Longitude'][...], np.nan)
dL25['HmF2_mlat'] = np.ma.filled(d.variables['ICON_L25_Magnetic_Latitude'][...], np.nan)
# Simple quality control
L25_flag = np.ma.filled(d.variables['ICON_L25_Quality'][...], np.nan)
good_data = ( L25_flag == 1)# Allow L26_flag=0 and 1. 0 =No issues reported, 1 = Moderate issue(s) identified, use results with caution, 2 = Severe issue(s) identified.,
dL25['HmF2'][~good_data] = np.nan
dL25['NmF2'][~good_data] = np.nan
dL25['HmF2_mlon'][~good_data] = np.nan
dL25['HmF2_mlat'][~good_data] = np.nan
# dL25['Oplus'][~good_data,:] = np.nan
print('Orbit number: %04i to %04i' % (np.nanmin(dL25['orb_num']),np.nanmax(dL25['orb_num'])))
'''EUV L2.6 Daytime Ionosphere'''
fn_L26 =glob.glob('/content/%s/ICON_L2-6_EUV*.NC' % (target_doy))[0]
# Load L2.6 Daytime plasma observations #
dEUV = {}
with netCDF4.Dataset(fn_L26) as d:
time_msec = np.ma.filled(d.variables['Epoch'][...], np.nan) # Time in msec since 1970-01-01
dEUV['time'] = np.array([datetime(1970,1,1) + timedelta(seconds = 1e-3*s) for s in time_msec])
dEUV['HmF2'] = np.ma.filled(d.variables['ICON_L26_HmF2'][...], np.nan)
dEUV['NmF2'] = np.ma.filled(d.variables['ICON_L26_NmF2'][...], np.nan)
dEUV['Oplus'] = np.ma.filled(d.variables['ICON_L26_Oplus'][...], np.nan)
dEUV['orb_num'] = np.ma.filled(d.variables['ICON_L26_Orbit_Number'][...], np.nan)
dEUV['tang_slt'] = np.ma.filled(d.variables['ICON_L26_Local_Solar_Time'][...], np.nan)
dEUV['tang_lon'] = np.ma.filled(d.variables['ICON_L26_Longitude'][...], np.nan)
dEUV['tang_lat'] = np.ma.filled(d.variables['ICON_L26_Latitude'][...], np.nan)
dEUV['tang_alt'] = np.ma.filled(d.variables['ICON_L26_Altitude'][...], np.nan)
dEUV['tang_mlon'] = np.ma.filled(d.variables['ICON_L26_Magnetic_Longitude'][...], np.nan)
dEUV['tang_mlat'] = np.ma.filled(d.variables['ICON_L26_Magnetic_Latitude'][...], np.nan)
# Simple quality control
L26_flag = np.ma.filled(d.variables['ICON_L26_Flag'][...], np.nan)
good_data = ( L26_flag == 0)|( L26_flag == 1 ) # Allow L26_flag=0 and 1. 0 =No issues reported, 1 = Moderate issue(s) identified, use results with caution, 2 = Severe issue(s) identified.,
dEUV['HmF2'][~good_data] = np.nan
dEUV['NmF2'][~good_data] = np.nan
dEUV['Oplus'][~good_data,:] = np.nan
print('Orbit number: %04i to %04i' % (np.nanmin(dEUV['orb_num']),np.nanmax(dEUV['orb_num'])))
'''IVM L2.7 In-situ Plasma Measurement'''
fn_L27 =glob.glob('/content/%s/ICON_L2-7_IVM-A*.NC' % (target_doy))[0]
#### Load ion velocity
di = {}
with netCDF4.Dataset(fn_L27) as d:
time_msec = np.ma.filled(d.variables['Epoch'][...], np.nan) # Time in msec since 1970-01-01
di['time'] = np.array([datetime(1970,1,1) + timedelta(seconds = 1e-3*s) for s in time_msec])
di['vmer'] = np.ma.filled(d.variables['ICON_L27_Ion_Velocity_Meridional'][...], np.nan) # Mag Meridional (Upward/poleward) ion velocity
di['Ni'] = np.ma.filled(d.variables['ICON_L27_Ion_Density'][...], np.nan)
di['mlat'] = np.ma.filled(d.variables['ICON_L27_Magnetic_Latitude'][...], np.nan)
di['mlon'] = np.ma.filled(d.variables['ICON_L27_Magnetic_Longitude'][...], np.nan)
di['slt'] = np.ma.filled(d.variables['ICON_L27_Solar_Local_Time'][...], np.nan)
di['alt'] = np.ma.filled(d.variables['ICON_L27_Altitude'][...], np.nan)
di['lon'] = np.ma.filled(d.variables['ICON_L27_Longitude'][...], np.nan)
di['lat'] = np.ma.filled(d.variables['ICON_L27_Latitude'][...], np.nan)
di['orb_num'] = np.ma.filled(d.variables['ICON_L27_Orbit_Number'][...], np.nan) # Local solar time
# In latest released version v02r002, use the flags below
DM_flag = np.ma.filled(d.variables['ICON_L27_DM_Flag'][...], np.nan) # Quality control variable for Drift Meter
RPA_flag = np.ma.filled(d.variables['ICON_L27_RPA_Flag'][...], np.nan) # Quality control variable for RPA
good_data = (DM_flag == 0) & (RPA_flag < 2)
di['Ni'][~good_data] = np.nan
di['mlat'][~good_data] = np.nan
print('Orbit number: %04i to %04i' % (np.nanmin(di['orb_num']),np.nanmax(di['orb_num'])))
###Output
Orbit number: 2466 to 2481
###Markdown
Example 1: Visualizing data in a single orbit* This practice will demostrate the how to use *orbit number* and how to compare different ICON product.* Use L2.2 Vector Wind and L2.3 Temperature for demonstration.
###Code
# Select a particular orbit
target_orbit_num = 2470
dTN_select_orb_num_idx = np.argwhere(dTN['orb_num']== target_orbit_num)
dTN_select_idx = dTN_select_orb_num_idx[:,0]
select_dTN = {}
select_dTN['orb_num'] = dTN['orb_num'][dTN_select_idx]
select_dTN['time'] = dTN['time'][dTN_select_idx]
select_dTN['TN'] = dTN['TN'][:,dTN_select_idx]
select_dTN['tang_slt'] = dTN['tang_slt'][:,dTN_select_idx]
select_dTN['tang_lon'] = dTN['tang_lon'][:,dTN_select_idx]
select_dTN['tang_lat'] = dTN['tang_lat'][:,dTN_select_idx]
select_dTN['tang_alt'] = dTN['tang_alt'][:,dTN_select_idx]
dm_select_idx=np.where((dm_g['time']>=select_dTN['time'][0]) & (dm_g['time'] < select_dTN['time'][-1]))
select_dm_g = {}
select_dm_g['orb_num'] = dm_g['orb_num'][dm_select_idx]
select_dm_g['time'] = dm_g['time'][dm_select_idx]
select_dm_g['uzon'] = dm_g['uzon'][dm_select_idx,:][0,:,:].transpose()
select_dm_g['umer'] = dm_g['umer'][dm_select_idx,:][0,:,:].transpose()
select_dm_g['tang_slt'] = dm_g['tang_slt'][dm_select_idx,:][0,:,:].transpose()
select_dm_g['tang_lon'] = dm_g['tang_lon'][dm_select_idx,:][0,:,:].transpose()
select_dm_g['tang_lat'] = dm_g['tang_lat'][dm_select_idx,:][0,:,:].transpose()
select_dm_g['tang_alt'] = dm_g['tang_alt'][:]
dm_select_idx=np.where((dm_r['time']>=select_dTN['time'][0]) & (dm_r['time'] < select_dTN['time'][-1]))
select_dm_r = {}
select_dm_r['orb_num'] = dm_r['orb_num'][dm_select_idx]
select_dm_r['time'] = dm_r['time'][dm_select_idx]
select_dm_r['uzon'] = dm_r['uzon'][dm_select_idx,:][0,:,:].transpose()
select_dm_r['umer'] = dm_r['umer'][dm_select_idx,:][0,:,:].transpose()
select_dm_r['tang_slt'] = dm_r['tang_slt'][dm_select_idx,:][0,:,:].transpose()
select_dm_r['tang_lon'] = dm_r['tang_lon'][dm_select_idx,:][0,:,:].transpose()
select_dm_r['tang_lat'] = dm_r['tang_lat'][dm_select_idx,:][0,:,:].transpose()
select_dm_r['tang_alt'] = dm_r['tang_alt'][:]
print('Selected time: %s to %s' % (select_dTN['time'][0].strftime("%m-%d-%y %H:%M:%S"),select_dTN['time'][-1].strftime("%m-%d-%y %H:%M:%S")))
###Output
Selected time: 03-24-20 06:10:28 to 03-24-20 07:46:00
###Markdown
Quick view of a single orbit L2.2 Vector Wind* Daytime observations of the red line (630.0 nm) emission span the altitude range of ~180 to 300 km while observations of the green line (557.7 nm) emission span the altitude range of 90 to ~190 km. * Nighttime observations of the red line emission span the altitude range of ~210 to 300 km while those of the green line span 90 to ~109 km.* Daytime observations are provided at a 30-s cadence while nighttime observations are provided at a 60-s cadence.* How to deal with the overlapped altitudes?
###Code
X_g, Y_g = np.meshgrid(select_dm_g['time'],select_dm_g['tang_alt'])
X_r, Y_r = np.meshgrid(select_dm_r['time'],select_dm_r['tang_alt'])
show_time=select_dm_g['time']
# ## Wind ###
cmap='bwr'
vmax=200
vmin=-200
variable_name='MIGHTI L2.2 Meridional Wind'
cb_label='Meridional Wind (m/s)'
xlabel='UTC'
ylabel='Altitude (km)'
plt.close()
fig,ax=plt.subplots(figsize=(16,4))
fig.suptitle('%s %s' % (variable_name, show_time[0].strftime("%m-%d-%Y")),size=20,x=0.5)
gs = gridspec.GridSpec(1,2)
ax0=plt.subplot(gs[0,0])
im = ax0.pcolor(X_g,Y_g,select_dm_g['umer'] ,cmap=cmap,vmin=vmin,vmax=vmax)
ax0.set_ylim([90,300])
ax0.set_title('Green')
ax0.set_xlabel(xlabel)
ax0.set_ylabel(ylabel)
color_bar=fig.colorbar(im)
color_bar.set_label(cb_label,size=16)
ax0=plt.subplot(gs[0,1])
im = ax0.pcolor(X_r,Y_r,select_dm_r['umer'] ,cmap=cmap,vmin=vmin,vmax=vmax)
ax0.set_ylim([90,300])
ax0.set_title('Red')
ax0.set_xlabel(xlabel)
ax0.set_ylabel(ylabel)
color_bar=fig.colorbar(im)
color_bar.set_label(cb_label,size=16)
fig.autofmt_xdate(rotation=45)
left = 0.1 # the left side of the subplots of the figure
right = 0.9 # the right side of the subplots of the figure
bottom = 0.05 # the bottom of the subplots of the figure
top = 0.8 # the top of the subplots of the figure
wspace = 0.2#0.2 # the amount of width reserved for blank space between subplots
hspace = 0.3#0.2 # the amount of height reserved for white space between subplots
plt.subplots_adjust(left, bottom, right, top, wspace, hspace)
###Output
_____no_output_____
###Markdown
Extension: Visualizing the neutral wind and temperature at mesosphere and the lower thermosphere at a time.
###Code
## Temperature ###
target_1_array=select_dTN['TN']
Y_1_array=select_dTN['tang_alt']
X_1_array, _ = np.meshgrid(select_dTN['time'],select_dTN['tang_alt'][:,0])
## Green Line Wind ###
target_2_array=select_dm_g['uzon']
X_2_array, Y_2_array = np.meshgrid(select_dm_g['time'],select_dm_g['tang_alt'])
# ## Temperature ###
cmap_1='plasma'
vmax_1=300
vmin_1=150
variable_name_1='ICON L2.3-%s Temperature' % (MTAB)
xlabel_1='UTC'
ylabel_1='Altitude (km)'
cb_label_1='Temperature (K)'
tang_alt_idx=5
# ## Wind ###
cmap_2='bwr'
vmax_2=200
vmin_2=-200
variable_name_2='ICON L2.2 Zonal Wind'
xlabel_2='UTC'
ylabel_2='Altitude (km)'
cb_label_2='Zonal Wind (m/s)'
######################## PLOT #############################
show_time=select_dTN['time']
fig,ax=plt.subplots(figsize=(8,8))#fig=pp.figure(figsize=(12,10)
fig.suptitle('UTC %s to %s' % (show_time[0].strftime("%H:%M:%S"),show_time[-1].strftime("%H:%M:%S %m-%d-%y")),size=20,x=0.43)
gs = gridspec.GridSpec(3, 1, height_ratios=[1,1,1])
ax0=plt.subplot(gs[0,0])
im = ax0.pcolor(X_1_array,Y_1_array,target_1_array ,cmap=cmap_1,vmin=vmin_1,vmax=vmax_1)#,norm=colors.Normalize(vmin=0, vmax=150))
ax0.set_ylim([90,115])
ax0.set_title(variable_name_1)
ax0.set_xlabel(xlabel_1)
ax0.set_ylabel(ylabel_1)
color_bar=fig.colorbar(im)
color_bar.set_label(cb_label_1)
ax0=plt.subplot(gs[1,0])
im = ax0.pcolor(X_2_array,Y_2_array,target_2_array ,cmap=cmap_2,vmin=vmin_2,vmax=vmax_2)#,norm=colors.Normalize(vmin=0, vmax=150))
ax0.set_ylim([90,115])
ax0.set_title(variable_name_2)
ax0.set_xlabel(xlabel_2)
ax0.set_ylabel(ylabel_2)
color_bar=fig.colorbar(im)
color_bar.set_label(cb_label_2)
ax0=plt.subplot(gs[2,0])
im=ax0.scatter(select_dTN['tang_lon'][tang_alt_idx,:],select_dTN['tang_lat'][tang_alt_idx,:],c=select_dTN['tang_slt'][tang_alt_idx,:],cmap='twilight_shifted')
ax0.set_title('Tangent point at ~%03i km' % select_dTN['tang_alt'][tang_alt_idx,1])
ax0.set_xlabel('Longitude')
ax0.set_ylabel('Latitude')
noon_idx=np.argmin(np.abs(select_dTN['tang_slt'][tang_alt_idx,:]-12))
noon_lon=select_dTN['tang_lon'][tang_alt_idx,noon_idx]
noon_lat=select_dTN['tang_lat'][tang_alt_idx,noon_idx]
ax0.plot(noon_lon,noon_lat,marker='o',color='red',label='Noon time')
color_bar=fig.colorbar(im)
color_bar.set_label('Solar Local Time (hr)')
plt.tight_layout(rect=[0, 0.0, 1.05, 0.95])
fig.autofmt_xdate(rotation=45)
plt.legend()
left = 0.1 # the left side of the subplots of the figure
right = 0.9 # the right side of the subplots of the figure
bottom = 0.05 # the bottom of the subplots of the figure
top = 0.9 # the top of the subplots of the figure
wspace = 0.1#0.2 # the amount of width reserved for blank space between subplots
hspace = 0.8#0.2 # the amount of height reserved for white space between subplots
plt.subplots_adjust(left, bottom, right, top, wspace, hspace)
###Output
_____no_output_____
###Markdown
Example 2: Ionospheric measurements by EUV, FUV and IVM- L2.5 FUV: Remotely sensed ***nighttime*** O+ profile, HmF2 and NmF2 on the FUV's limb.- L2.6 EUV: Remotely sensed ***daytime*** O+ profile, HmF2 and NmF2 on the EUV's limb.- L2.7 IVM: ***In-situ*** ion density, temperature, and composition at S/C altitude (~600 km)
###Code
di_select_orb_num_idx = np.argwhere(di['orb_num']== target_orbit_num)
di_select_idx = di_select_orb_num_idx[:,0]
select_di = {}
select_di['orb_num'] = di['orb_num'][di_select_idx]
select_di['time'] = di['time'][di_select_idx]
select_di['Ni'] = di['Ni'][di_select_idx]
select_di['vmer'] = di['vmer'][di_select_idx]
select_di['slt'] = di['slt'][di_select_idx]
select_di['lon'] = di['lon'][di_select_idx]
select_di['lat'] = di['lat'][di_select_idx]
select_di['mlon'] = di['mlon'][di_select_idx]
select_di['mlat'] = di['mlat'][di_select_idx]
dEUV_select_idx=np.where((dEUV['time']>=select_di['time'][0]) & (dEUV['time'] < select_di['time'][-1]))
select_dEUV = {}
select_dEUV['orb_num'] = dEUV['orb_num'][dEUV_select_idx]
select_dEUV['time'] = dEUV['time'][dEUV_select_idx]
select_dEUV['Oplus'] = dEUV['Oplus'][dEUV_select_idx,:][0,:,:].transpose()
select_dEUV['HmF2'] = dEUV['HmF2'][dEUV_select_idx]
select_dEUV['NmF2'] = dEUV['NmF2'][dEUV_select_idx]
select_dEUV['tang_slt'] = dEUV['tang_slt'][dEUV_select_idx]
select_dEUV['tang_lon'] = dEUV['tang_lon'][dEUV_select_idx]
select_dEUV['tang_lat'] = dEUV['tang_lat'][dEUV_select_idx]
select_dEUV['tang_alt'] = dEUV['tang_alt'][dEUV_select_idx,:][0,:,:].transpose()
select_dEUV['tang_mlon'] = dEUV['tang_mlon'][dEUV_select_idx]
select_dEUV['tang_mlat'] = dEUV['tang_mlat'][dEUV_select_idx]
# dON2_select_idx=np.where((dON2['time']>=select_di['time'][0]) & (dON2['time'] < select_di['time'][-1]))
# select_dON2 = {}
# select_dON2['time'] = dON2['time'][dON2_select_idx]
# select_dON2['disk_ON2'] = dON2['disk_ON2'][dON2_select_idx]
# select_dON2['disk_slt'] = dON2['disk_slt'][dON2_select_idx]
# select_dON2['disk_lon'] = dON2['disk_lon'][dON2_select_idx]
# select_dON2['disk_lat'] = dON2['disk_lat'][dON2_select_idx]
# select_dON2['disk_mlon'] = dON2['disk_mlon'][dON2_select_idx]
# select_dON2['disk_mlat'] = dON2['disk_mlat'][dON2_select_idx]
dL25_select_idx=np.where((dL25['time']>=select_di['time'][0]) & (dL25['time'] < select_di['time'][-1]))
select_dL25 = {}
select_dL25['time'] = dL25['time'][dL25_select_idx]
select_dL25['HmF2'] = dL25['HmF2'][dL25_select_idx]
select_dL25['NmF2'] = dL25['NmF2'][dL25_select_idx]
select_dL25['HmF2_lon'] = dL25['HmF2_lon'][dL25_select_idx]
select_dL25['HmF2_lat'] = dL25['HmF2_lat'][dL25_select_idx]
select_dL25['HmF2_mlon'] = dL25['HmF2_mlon'][dL25_select_idx]
select_dL25['HmF2_mlat'] = dL25['HmF2_mlat'][dL25_select_idx]
select_dL25['tang_slt'] = dL25['tang_slt'][dL25_select_idx]
print('Selected time: %s to %s' % (select_di['time'][0].strftime("%m-%d-%y %H:%M:%S"),select_di['time'][-1].strftime("%m-%d-%y %H:%M:%S")))
###Output
Selected time: 03-24-20 06:10:19 to 03-24-20 07:46:40
###Markdown
Visualizing HmF2 from EUV and FUV
###Code
plt.close()
fig,ax=plt.subplots(figsize=(12,4))
fig.suptitle('ICON HmF2 %s' % ( show_time[0].strftime("%m-%d-%Y")),size=20,x=0.5)
gs = gridspec.GridSpec(1,2)
ax0=plt.subplot(gs[0])
ax0.plot(select_dEUV['time'],select_dEUV['HmF2'],'ro',label='Daytime (EUV)')
ax0.plot(select_dL25['time'],np.nanmean(select_dL25['HmF2'],axis=1),'bo',label='Nighttime (FUV)')
ax0.set_xlabel('UTC')
ax0.set_ylabel('HmF2 (km)')
ax0.legend(fontsize=15)
ax0=plt.subplot(gs[1])
im=ax0.scatter(select_dEUV['time'],select_dEUV['tang_mlat'],c=select_dEUV['tang_slt'],vmax=24,vmin=0,cmap='twilight_shifted')
im=ax0.scatter(select_dL25['time'],select_dL25['HmF2_mlat'][:,3],c=select_dL25['tang_slt'][:,3],vmax=24,vmin=0,cmap='twilight_shifted')
ax0.set_ylabel('Mag. Latitude')
ax0.set_xlabel('UTC')
color_bar=fig.colorbar(im)
color_bar.set_label('Solar Local Time (hr)')
fig.autofmt_xdate(rotation=45)
plt.tight_layout(rect=[0, 0.0, 1.0, 0.95])
###Output
/usr/local/lib/python3.6/dist-packages/ipykernel_launcher.py:9: RuntimeWarning: Mean of empty slice
if __name__ == '__main__':
###Markdown
Extension: Visualizing the in-situ IVM ion density and EUV O+ density profilt at tangent points
###Code
show_time=select_di['time']
### IVM Ion Density ####
target_2_array=select_di['Ni']
X_2_array=select_di['time']
## EUV Oplus ###
target_1_array=select_dEUV['Oplus']
X_1_array, Y_1_array = np.meshgrid(select_dEUV['time'],select_dEUV['tang_alt'][:,0])
cmap_1='plasma'
vmax_1=8*10**5
vmin_1=1*10**5
variable_name_1='ICON L2.6 EUV Oplus %s' % show_time[0].strftime("%m-%d-%y")
xlabel_1='UTC'
ylabel_1='Altitude (km)'
cb_label_1='O+ Density (#/cm^3)'
######################## PLOT #############################
fig,ax=plt.subplots(figsize=(10,5))#fig=pp.figure(figsize=(12,10)
gs = gridspec.GridSpec(1, 1)
ax0=plt.subplot(gs[0,0])
im = ax0.pcolor(X_1_array,Y_1_array,target_1_array ,cmap=cmap_1,vmin=vmin_1,vmax=vmax_1)#,norm=colors.Normalize(vmin=0, vmax=150))
ax0.set_ylim([200,600])
ax0.set_xlim([show_time[0],show_time[-1]])
ax0.set_title(variable_name_1)
ax0.set_xlabel(xlabel_1)
ax0.set_ylabel(ylabel_1)
color_bar=fig.colorbar(im,format=OOMFormatter(5, mathText=True))
color_bar.set_label(cb_label_1)
ax2 = ax0.twinx()
ax2.plot(X_2_array,target_2_array/100000.,'g.',label='IVM Ion Density')
ax2.grid(None)
leg=plt.legend(loc='best')
for text in leg.get_texts():
plt.setp(text, color = 'g',size=20)
ax2.tick_params(axis='y', colors="g")
ax0.set_xlim([show_time[0],show_time[-1]])
myFmt = mdates.DateFormatter('%H:%M')
plt.gca().xaxis.set_major_formatter(myFmt)
plt.tight_layout(rect=[0, 0.0, 1.05, 0.95])
###Output
_____no_output_____
###Markdown
Example 3: IVM-MIGHTI - Neutral wind driven electrodynamic interactions- Once per orbit, ICON crosses the equator in the daytime.- IVM measures the vertical drift at the equator- MIGHTI measures the wind profile at the northern footpoint of the magnetic field line.
###Code
'''The IVM-MIGHTI pair of equator crossing with minimum time difference during'''
icon_near_equator = (abs(di['mlat']) < 1.) # ICON is within 1 degree of equator
daytime = abs(di['slt'] - 12) < 6 # ICON is in daytime hours
ii = np.where(icon_near_equator & daytime)
t0 = di['time'][ii]
print('ICON near equator starting at %s' % t0[0])
dm=dm_g
# Find MIGHTI index that matches this time
# Note: in practice, the true MIGHTI-IVM conjunction may happen a couple minutes before or after this time.
# Magnetic field line tracing is recommended to find true conjunctions.
# find_min is an array for the minimum time difference between each t0 and MIGHTI.
find_min=[(np.nanmin(abs((dm['time']-i)))) if not np.all(np.isnan(dm['uzon'][np.argmin(abs((dm['time']-i))),:])) else (np.nan) for i in t0]
# find_argmin is an array for the argument (index) of the minimum time difference between each t0 and MIGHTI.
find_argmin=[(np.argmin(abs((dm['time']-i)))) if not np.all(np.isnan(dm['uzon'][np.argmin(abs((dm['time']-i))),:])) else (np.nan) for i in t0]
# Transfer find_min (datetime.timedelta object) to a float array time_diff.
time_diff=[(i.total_seconds()) if not pd.isnull(i) else (np.nan) for i in find_min]
m_idx=find_argmin[np.nanargmin(time_diff)]
i_idx=np.nanargmin(time_diff)
#### Plot the profiles ###
plt.close()
plt.figure(figsize=(6,8))
plt.plot(dm['uzon'][m_idx,:], dm['tang_alt'], 'C0-', label='Zonal Neutral Wind')
plt.plot(dm['umer'][m_idx,:], dm['tang_alt'], 'C1-', label='Meridional Neutral Wind')
plt.plot(di['vmer'][ii][i_idx], di['alt'][ii][i_idx], 'ks', label='Vertical Ion Velocity')
plt.ylabel('Altitude [km]')
plt.xlabel('Velocity [m/s]')
plt.title('Observations of neutral wind and ion velocity\non nearly identical magnetic field lines')
# plt.ylim([90,250])
plt.legend(loc='upper left')
plt.tight_layout(rect=[0, 0.0, 1.05, 0.95])
###Output
_____no_output_____ |
embeddings.ipynb | ###Markdown
Texata finals 2015Álvaro Barbero Jiménez Semantic embeddings In this notebook I will compute the semantic embedding models required for some of the tasks. I will use the data I previously loaded into Elasticsearch, by making use of the convenient PostsCorpus class I created. This way I don't need to keep all the data in memory, instead I can efficiently iterate through the text stored in ElasticSearch. CISCO data embedding First I will create a semantic embedding by using all the data provided by CISCO:
###Code
from texata import text
corpus = text.PostsCorpus(["support-forums"])
###Output
_____no_output_____
###Markdown
Now let's compute a word2vec embedding using all the texts in the data:
###Code
from texata import semantic
model = semantic.computewordembedding(corpus)
model.save('models/word2vec-cisco.pkl')
###Output
_____no_output_____
###Markdown
Stackexchange embedding I will also compute and embedding using data from some relevant communities of Stackexchange. I hope that with this I will capture a significant vocabulary of tech-words:
###Code
from texata import text
corpus = text.PostsCorpus([
"dsp.stackexchange.com",
"networkengineering.stackexchange.com",
"reverseengineering.stackexchange.com",
"robotics.stackexchange.com",
"security.stackexchange.com",
"serverfault.com"
])
from texata import semantic
model = semantic.computewordembedding(corpus)
model.save('models/word2vec-stackexchange.pkl')
###Output
_____no_output_____
###Markdown
Fun with embeddings! Import necessary libraries
###Code
import pandas as pd
import numpy as np
from sklearn.metrics.pairwise import cosine_similarity, euclidean_distances
from sklearn.manifold import TSNE
import matplotlib.pyplot as plt
import matplotlib.cm as cm
from sklearn.preprocessing import normalize
###Output
_____no_output_____
###Markdown
Load the embeddings from disk
###Code
#Use the output from the word2vec notebook
#embeddings = pd.DataFrame.from_csv('embeddings.csv')
#reverse_dictionary = np.array(list(embeddings.index))
# Use embeddings from polyglot
# https://sites.google.com/site/rmyeid/projects/polyglot
reverse_dictionary, embeddings = pd.read_pickle('polyglot-en.pkl')
embeddings = normalize(embeddings)
reverse_dictionary = np.array(reverse_dictionary)
dictionary = dict(zip(reverse_dictionary, range(embeddings.shape[0])))
print(embeddings.shape)
###Output
(100004, 64)
###Markdown
Find the most similar words
###Code
def most_similar(word, embeddings, reverse_dictionary, top_k=8):
valid_word = dictionary[word]
similarity = cosine_similarity(embeddings, embeddings[valid_word, :].reshape(1, -1))
nearest = (-similarity).argsort(axis=0)[1:top_k + 1].flatten()
return reverse_dictionary[nearest]
most_similar("king", embeddings, reverse_dictionary)
###Output
_____no_output_____
###Markdown
Analogies Question task set downloaded from: http://download.tensorflow.org/data/questions-words.txt
###Code
questions = pd.read_table('questions-words.txt', comment=':', sep=' ', header=None)
print(questions.shape)
def evaluate_analogy(question):
try:
word1, word2, word3, word4 = question
key1 = dictionary[word1.lower()]
key2 = dictionary[word2.lower()]
key3 = dictionary[word3.lower()]
key4 = dictionary[word4.lower()]
vec1 = embeddings[key1, :]
vec2 = embeddings[key2, :]
vec3 = embeddings[key3, :]
vec4 = embeddings[key4, :]
predict = vec2-vec1+vec3
sim = np.matmul(predict, embeddings.T)
nearest = np.argsort(-sim)[:4]
return word4.lower() in reverse_dictionary[nearest]
except:
pass
results = [evaluate_analogy(questions.iloc[i]) for i in range(questions.shape[0])]
clean_results = [res for res in results if res is not None]
accuracy = np.mean(clean_results)
print(accuracy)
###Output
0.34814977009
###Markdown
Visualization Embedding matrix
###Code
plt.figure(figsize=(15, 5))
plt.imshow(embeddings.T, aspect=300, cmap=cm.jet)
plt.xlabel("vocabulary")
plt.ylabel("embeddings dimensions")
plt.show()
###Output
_____no_output_____
###Markdown
tSNE
###Code
tsne = TSNE(perplexity=30, n_components=2, init='pca', n_iter=5000, method='exact')
plot_only = 500 # Plot only 500 words
low_dim_embs = tsne.fit_transform(np.array(embeddings)[:plot_only, :])
labels = [reverse_dictionary[i] for i in range(plot_only)]
plt.figure(figsize=(18, 18)) # in inches
for i, label in enumerate(labels):
x, y = low_dim_embs[i, :]
plt.scatter(x, y)
plt.annotate(label,
xy=(x, y),
xytext=(5, 2),
textcoords='offset points',
ha='right',
va='bottom')
#plt.savefig("tSNE.png")
plt.show()
###Output
_____no_output_____
###Markdown
**Custom Embeddings**This notebook should be ideally run on google colab. For Google colab, 1. Make sure you have added the shared folder "digital-forest". 2. Mount the google drive onto the colab environment. 1. Go to the folder icon on the left 2. Click on th folder icon with google drive icon. 3. This should mount the drive. 4. Now all files in your drive are directly accessible in your colab environment.For running on local environment, 1. Make sure to change the root path to the local directory.2. If any errors make sure to double check the file directory. 1. Load the data **Note:-** Here the directory should match the directory from your google colab drive. To get this1. Explore the folders in the files section2. Right Click on the folder whose path you woukld like to import.3. Click on Copy Path from the dropdown
###Code
mdpi_dir = '/content/drive/MyDrive/digital-forest/mdpi'
# Get a list of all files in given directory
from os import walk
filenames = next(walk(mdpi_dir), (None, None, []))[2] # [] if no file
filenames[:3]
###Output
_____no_output_____
###Markdown
2. Get text data from all files
###Code
import imp
from bs4 import BeautifulSoup
import re
def extract_text_from_html(mdpi_dir, mdpi_file_name):
with open(mdpi_dir + '/' + mdpi_file_name, "r", encoding='utf-8') as f:
html_file = f.read()
soup = BeautifulSoup(html_file, 'html.parser')
article = soup.find('article')
text_list = article.find_all(text=True)
article_text = " ".join(text_list)
# Remove \n characters
clean_text = article_text.replace('\n', ' ')
# Remove special characters and numbers
clean_text = re.sub('[^.,A-Za-z]+', ' ', clean_text)
# Convert all text to lower
clean_text = clean_text.lower()
return clean_text
# Get all the text data from the articles
mdpi_corpus = []
failed_files = []
for file_name in filenames:
# There might be possible exceptions from extracting text.
# This will catch the exceptions and we can analyze why it failed for some files
try:
extracted_text = extract_text_from_html(mdpi_dir, file_name)
mdpi_corpus.append(extracted_text)
except Exception as e:
failed_files.append(file_name)
print("Error while extracting text for {}".format(file_name), e)
print("Successfully processed {} records".format(len(mdpi_corpus)))
###Output
Successfully processed 402 records
###Markdown
3. Setup glove embeddings **This is required on google colab as data is not stored permenantly.**
###Code
!wget https://nlp.stanford.edu/data/glove.6B.zip
!unzip "glove.6B.zip"
###Output
Archive: glove.6B.zip
inflating: glove.6B.50d.txt
inflating: glove.6B.100d.txt
inflating: glove.6B.200d.txt
inflating: glove.6B.300d.txt
###Markdown
4. Setup pipeline to make custom embeddings
###Code
import gensim
from gensim.test.utils import get_tmpfile, datapath
from gensim.models import KeyedVectors
from gensim.scripts.glove2word2vec import glove2word2vec
from gensim.models import Word2Vec
glove_file = datapath('/content/glove.6B.50d.txt')
tmp_file = get_tmpfile("test_word2vec.txt")
_ = glove2word2vec(glove_file, tmp_file)
glove_vectors = KeyedVectors.load_word2vec_format(tmp_file)
###Output
_____no_output_____
###Markdown
4.1 Process the corpus to the input format required by Word2Vec algorithm
###Code
import nltk
from nltk.tokenize import word_tokenize, sent_tokenize
nltk.download('punkt')
# First we combine all the records into one single string
full_text = " ".join(mdpi_corpus)
sentences = []
for document in mdpi_corpus:
# Break down each document in the corpus to list of sentences
sent_list = sent_tokenize(document)
# For each sentence break it into list of words
for sent in sent_list:
word_list = word_tokenize(sent)
sentences.append(word_tokenize(sent))
print("We have {} sentences in the corpus".format(len(sentences)))
###Output
We have 377179 sentences in the corpus
###Markdown
4.2 Setup Word2Vec model
###Code
# build a word2vec model on your dataset
base_model = Word2Vec(size=50, window=5, min_count=3, workers=4)
base_model.build_vocab(sentences)
total_examples = base_model.corpus_count
# Unique words in the vocabulary
len(base_model.wv.vocab)
# Statistics of our vocabulary
unique_words = set(base_model.wv.vocab.keys()) - set(glove_vectors.vocab.keys())
common_words = set(base_model.wv.vocab.keys()).intersection(set(glove_vectors.vocab.keys()))
print("Unique words to our corpus {}".format(len(unique_words)))
print("Common words between corpus and glove {}".format(len(common_words)))
###Output
Unique words to our corpus 6268
Common words between corpus and glove 20260
###Markdown
4.3 Train Word2Vec model
###Code
# update our model with GloVe's vocabulary & weights
base_model.build_vocab([list(glove_vectors.vocab.keys())], update=True)
# train on your data
base_model.train(sentences, total_examples=total_examples, epochs=100)
base_model_wv = base_model.wv
###Output
_____no_output_____
###Markdown
4.4 Analyze our embeddings
###Code
list(unique_words)[:10]
'geoinform' in common_words
base_model_wv.most_similar('geoinform')
###Output
_____no_output_____
###Markdown
1. Data Import and Cleaning
###Code
whitepapers = read_csv('whitepapers.csv')
# merge description and document text
whitepapers['text'] = whitepapers.description + ' ' + whitepapers.document_text
# filter down to relevant entries
df = whitepapers.drop(columns=['description', 'document_text', 'document_tokens'])
del whitepapers
# tokenize (aka .split()++, thank you nltk)
train_txt = ''
for _, row in df.iterrows():
train_txt += row['text'].lower()
tokens = word_tokenize(train_txt)
del df
# word2idx and idx2word setup
unique_tokens = set(tokens)
w2x = {word: idx for (idx, word) in enumerate(unique_tokens)}
x2w = {idx: word for (idx, word) in enumerate(unique_tokens)}
indices = [w2x[w] for w in tokens]
# generate training data
window = 2
train_data = []
for idx in range(len(indices)):
for r in range(-window, window + 1):
cxt = idx + r
if not ((cxt < 0) or (cxt >= len(indices)) or (idx == cxt)):
train_data.append([indices[idx], indices[cxt]])
train_data = np.array(train_data)
train_data = torch.LongTensor(train_data)
# record vocab_size
vocab_size = len(unique_tokens)
# sanity check
for [x,y] in train_data[200100:200105]:
print(x2w[int(x)], x2w[int(y)])
# clean memory
del indices
del tokens
###Output
_____no_output_____
###Markdown
2. Continuous Bag-of-Words Model
###Code
class CBOW(nn.Module):
def __init__(self, vocab_size, embedding_dim, hidden_dim,
context_size, batch_size):
super(CBOW, self).__init__()
self.batch_size = batch_size
self.embed = nn.Embedding(vocab_size, embedding_dim)
self.fc1 = nn.Linear(embedding_dim, hidden_dim)
self.fc2 = nn.Linear(hidden_dim, vocab_size)
self.out = nn.Softmax(dim=2)
def forward(self, x):
x = self.embed(x).view(self.batch_size, 1, -1)
x = F.relu(self.fc1(x))
x = self.fc2(x)
return self.out(x).squeeze()
model = CBOW(vocab_size=vocab_size, embedding_dim=100, hidden_dim=128, context_size=2, batch_size=256).cuda()
def one_hot(idx_batch):
one_hot_mat = torch.zeros((len(idx_batch), vocab_size)).float()
indices = torch.LongTensor(idx_batch).view(-1, 1)
one_hot_mat.scatter_(1, indices, 1.0)
return one_hot_mat
def mat_loss(pred, gt):
delta = pred.float() - gt.float()
norm = torch.norm(delta, p=2, dim=1)
return (torch.sum(norm) / gt.shape[1])
def batchify(data, batch_size, use_cuda=False):
rm_size = len(data) % batch_size
x, y = data[:-rm_size, 0], data[:-rm_size, 1]
if use_cuda:
x = x.view(-1, batch_size).cuda()
else:
x = x.view(-1, batch_size)
y = y.view(-1, batch_size)
return x, y
x, y = batchify(train_data, batch_size=256, use_cuda=True)
def train(x_train, y_train, num_epochs, use_cuda=False):
loss_fn = mat_loss
optimizer = optim.SGD(model.parameters(), lr=1e-2)
scheduler = optim.lr_scheduler.StepLR(optimizer,
step_size=10,
gamma=0.5)
for epoch in tnrange(num_epochs, desc='epoch'):
total_loss = 0
for batch_idx in tqdm_notebook(range(x_train.shape[0]),
desc='batch', leave=False):
x = x_train[batch_idx, :]
y = y_train[batch_idx, :]
model.zero_grad()
log_prob = model(x)
gt = one_hot(y)
if use_cuda:
gt = gt.cuda()
loss = loss_fn(log_prob, gt)
loss.backward()
scheduler.step()
total_loss += loss.data
print(total_loss)
torch.save(model, 'models/model_{}'.format(total_loss))
print("Successfully Saved model_{}!".format(total_loss))
train(x, y, num_epochs=100, use_cuda=True)
###Output
_____no_output_____ |
nlp/v1_scripts/doc2vec_UCI_save_embedding.ipynb | ###Markdown
###Code
!pip install PyDrive
from google.colab import drive
drive.mount('/content/gdrive')
import numpy as np
import pandas as pd
import matplotlib
import matplotlib.pyplot as plt
from itertools import product
import re
import time
import tensorflow as tf
from tensorflow import keras
###Output
_____no_output_____
###Markdown
Read Data
###Code
def convert_label(row):
if row["Classes"] == 'EI':
return 0
if row["Classes"] == 'IE':
return 1
if row["Classes"] == 'N':
return 2
data_path = '/content/gdrive/My Drive/Colab Notebooks/UCI/'
splice_df = pd.read_csv(data_path+'splice.data', header=None)
splice_df.columns = ['Classes', 'Name', 'Seq']
splice_df.Seq = splice_df.Seq.str.replace(' ', '').replace('D', 'N').replace('S', 'N').replace('R', 'N')
splice_df["Label"] = splice_df.apply(lambda row: convert_label(row), axis=1)
splice_df.head()
def n_gram(x, word_size=3):
arr_x = [c for c in x]
words = tf.strings.ngrams(arr_x, ngram_width=word_size, separator='').numpy()
words = list(pd.Series(words).apply(lambda b: b.decode('utf-8')))
return words
splice_df["ngram"] = splice_df.Seq.apply(n_gram)
splice_df.head()
from sklearn.model_selection import train_test_split
from sklearn.manifold import TSNE
xtrain_full, xtest, ytrain_full, ytest = train_test_split(splice_df, splice_df.Label, test_size=0.2, random_state=100, stratify=splice_df.Label)
xtrain, xval, ytrain, yval = train_test_split(xtrain_full, ytrain_full, test_size=0.2, random_state=100, stratify=ytrain_full)
print("shape of training, validation, test set\n", xtrain.shape, xval.shape, xtest.shape, ytrain.shape, yval.shape, ytest.shape)
word_size = 3
vocab = [''.join(c) for c in product('ATCGN', repeat=word_size)]
from gensim.models import Doc2Vec
from gensim.models.doc2vec import TaggedDocument
xtrain_tagged = xtrain.apply(
lambda r: TaggedDocument(words=r["ngram"], tags=[r["Label"]]), axis=1
)
xval_tagged = xval.apply(
lambda r: TaggedDocument(words=r["ngram"], tags=[r["Label"]]), axis=1
)
xtest_tagged = xtest.apply(
lambda r: TaggedDocument(words=r["ngram"], tags=[r["Label"]]), axis=1
)
xtrain_tagged.values[0]
import multiprocessing
from tqdm import tqdm
import time
tqdm.pandas(desc="progress-bar")
cores = multiprocessing.cpu_count()
cores
# model_dm = Doc2Vec(dm=1, vector_size=10, negative=5, hs=0, \
# min_count=2, sample=0, workers=cores)
# model_dm.build_vocab(xtrain_tagged.values)
# for epoch in range(10):
# model_dm.train(xtrain_tagged.values, \
# total_examples=len(xtrain_tagged.values), epochs=1)
# model_dm.alpha -= 0.002
# model_dm.min_alpha = model_dm.alpha
# xtrain_vec = getVec(model_dm, xtrain_tagged)
def getVec(model, tagged_docs, epochs=20):
sents = tagged_docs.values
regressors = [model.infer_vector(doc.words, epochs=epochs) for doc in sents]
return np.array(regressors)
def plotVec(ax, x, y, title="title"):
scatter = ax.scatter(x[:, 0], x[:, 1], c=y,
cmap=matplotlib.colors.ListedColormap(["red", "blue", "yellow"]))
ax.set_title(title)
ax.legend(*scatter.legend_elements(), loc=0, title="Classes")
def doc2vec_training(embed_size_list=[30,50,80,100], figsize=(10,50), verbose=0):
num_model = len(embed_size_list)
fig, axes = plt.subplots(num_model, 2, figsize=figsize)
counter = 0
model_list = []
hist_list = []
es_cb = keras.callbacks.EarlyStopping(patience=50, restore_best_weights=True)
for embed_size in embed_size_list:
start = time.time()
print("training doc2vec for embedding size =", embed_size)
model_dm = Doc2Vec(dm=1, vector_size=embed_size, negative=5, hs=0, \
min_count=2, sample=0, workers=cores)
if verbose == 1:
model_dm.build_vocab([x for x in tqdm(xtrain_tagged.values)])
else:
model_dm.build_vocab(xtrain_tagged.values)
for epoch in range(80):
if verbose == 1:
model_dm.train([x for x in tqdm(xtrain_tagged.values)], \
total_examples=len(xtrain_tagged.values), epochs=1)
else:
model_dm.train(xtrain_tagged.values, \
total_examples=len(xtrain_tagged.values), epochs=1)
model_dm.alpha -= 0.002
model_dm.min_alpha = model_dm.alpha
xtrain_vec = getVec(model_dm, xtrain_tagged)
xval_vec = getVec(model_dm, xval_tagged)
xtest_vec = getVec(model_dm, xtest_tagged)
np.savetxt(data_path + 'doc2vec_uci_size30_train.csv', xtrain_vec, delimiter=",")
np.savetxt(data_path + 'doc2vec_uci_size30_val.csv', xval_vec, delimiter=",")
np.savetxt(data_path + 'doc2vec_uci_size30_test.csv', xtest_vec, delimiter=",")
print("the shape for training vector is", xtrain_vec.shape, \
"the shape for test vector is", xtest_vec.shape)
# xtrain_tsne = TSNE(n_components=2, metric="cosine").fit_transform(xtrain_vec)
# xtest_tsne = TSNE(n_components=2, metric="cosine").fit_transform(xtest_vec)
# plotVec(axes[counter, 0], xtrain_tsne, ytrain, title="TSNE, training, embedding="+str(embed_size))
# plotVec(axes[counter, 1], xtest_tsne, ytest, title="TSNE, test, embedding="+str(embed_size))
counter += 1
print("training NN for embedding size =", embed_size)
model = keras.Sequential([
keras.layers.Dense(128, activation="relu", input_shape=[embed_size]),
keras.layers.Dropout(0.2),
keras.layers.Dense(64, activation="relu"),
keras.layers.Dropout(0.2),
keras.layers.Dense(32, activation="relu"),
keras.layers.Dropout(0.2),
keras.layers.Dense(16, activation="relu"),
keras.layers.Dropout(0.2),
keras.layers.Dense(3, activation="softmax")
])
model.compile(keras.optimizers.SGD(momentum=0.9), \
keras.losses.SparseCategoricalCrossentropy(), metrics=["accuracy"])
hist = model.fit(xtrain_vec, ytrain, \
epochs=1000, callbacks=[es_cb], validation_data=(xval_vec, yval))
train_loss, train_acc = model.evaluate(xtrain_vec, ytrain)
val_loss, val_acc = model.evaluate(xval_vec, yval)
test_loss, test_acc = model.evaluate(xtest_vec, ytest)
print("Evaluation on training set: loss", np.round(train_loss, 3), \
"accuracy", np.round(train_acc, 4))
print("Evaluation on validation set: loss", np.round(val_loss, 3), \
"accuracy", np.round(val_acc, 4))
print("Evaluation on test set: loss", np.round(test_loss, 3), \
"accuracy", np.round(test_acc, 4))
model_list.append(model)
hist_list.append(hist)
end = time.time()
print("running time in ", end - start, "seconds")
print("\n\n")
return model_list, hist_list
embed_size_list = [30]
num_model = len(embed_size_list)
model_list, hist_list = doc2vec_training(embed_size_list=embed_size_list, figsize=(10,20))
for i in range(len(embed_size_list)):
s = embed_size_list[i]
filename = data_path + "doc2vec_uci_size30_history.csv"
hist_df = pd.DataFrame(hist_list[i].history)
with open(filename, mode='w') as f:
hist_df.to_csv(f)
fig, axes = plt.subplots(num_model, 2, figsize=(10, 5))
for i in range(num_model):
hist = hist_list[i]
ax1 = axes[0]
ax2 = axes[1]
ax1.plot(hist.history['loss'], label='training')
ax1.plot(hist.history['val_loss'], label='validation')
ax1.set_title('model loss, embedding = '+str(embed_size_list[i]))
ax1.set_xlabel('epoch')
ax1.set_ylabel('loss')
ax1.legend(['train', 'test'], loc='upper left')
ax2.plot(hist.history['accuracy'], label='training')
ax2.plot(hist.history['val_accuracy'], label='validation')
ax2.set_title('model accuracy, embedding = '+str(embed_size_list[i]))
ax2.set_xlabel('epoch')
ax2.set_ylabel('accuracy')
ax2.legend(['train', 'validation'], loc='upper left')
fig.tight_layout()
###Output
_____no_output_____ |
Algo3 - Random Forest/Random Forest.ipynb | ###Markdown
Below are some script cells
###Code
# Original Testing Random Forest
# ===========================================================
forest_acc = []
for tree_i in random_forest:
accuracy = []
for row in training_data:
classification = tree_i.classify(row, tree_i.root)
if len(classification) == 1:
# print("======", row, tree.classify(row, tree.root).get(row[-1], 0))
accuracy.append(int(classification.get(row[-1], 0) > 0))
else:
# print("======", row, tree.classify(row, tree.root).get(row[-1], 0))
tot = sum(classification.values())
accuracy.append(classification.get(row[-1], 0) / tot)
forest_acc.append(sum(accuracy) / len(accuracy))
print(np.average(forest_acc))
end = time.time()
print("time = %.03fs" % (end - start))
# sklearn lib version
# ===========================================================
from sklearn.ensemble import RandomForestClassifier
from sklearn.datasets import make_classification
df = pd.read_csv('clinvar_conflicting_mapped.csv', low_memory=False)
df.head()
df = df.sample(n = df.shape[0])
all_rows = df.values.tolist()
row_num = len(all_rows)
training_percentage = 0.01 # percent of partition of training dataset
training_size = int(row_num * training_percentage)
testing_size = row_num - training_size
training_attribute = list(df.columns)
training_data = all_rows[: training_size] # training data should include header row
testing_data = all_rows[training_size: ] # testing data don't need to include header row
# X, y = make_classification(n_samples=1000, n_features=4,
# n_informative=2, n_redundant=0,
# random_state=0, shuffle=False)
X = [row[: -1] for row in training_data]
Y = [row[-1] for row in training_data]
clf = RandomForestClassifier(max_depth=2, random_state=0)
clf.fit(X, Y)
test = [row[: -1] for row in testing_data]
actual_label = [row[-1] for row in testing_data]
result = clf.predict(test)
accuracy = 0
for i in range(len(result)):
accuracy += int(result[i] == actual_label[i])
accuracy /= len(result)
accuracy
def test(p):
return 2 * p * (1 - p)
test(0.999)
# training_attribute - acc
attribute_acc = [0.6742062692718905, 0.6754536157553033, 0.6850566341788431, 0.7187853479399414, 0.704456358368068, 0.7360932488340022, 0.6692362520724545, 0.7198041433596231, 0.6726528967878891, 0.6803306630305095, 0.6753761408184452, 0.7310651254319227, 0.7217642592621287, 0.7095270929854193, 0.7073268047786541, 0.6674039698157647, 0.7182546446224646, 0.7231936718471574, 0.7169724344174659, 0.6964764398717016, 0.7249174891922463, 0.7072841935633822, 0.6843128747850071, 0.7285239475029828, 0.6901080000619799, 0.714163967956366, 0.7057928010288672, 0.6890775834017695, 0.7248981204580318, 0.6749965136278414, 0.7111385716720642, 0.7120217859522444, 0.6904372685436262, 0.7136371383857323, 0.7124672668391775, 0.7131412987898414]
importance = Table().with_columns('ATT', training_attribute[: -1], 'ACC', attribute_acc)
importance.sort('ACC', descending=True).show(5)
importance.bar('ATT')
a, b = ROC.column('FPR'), ROC.column('SENSITIVITY')
a
b
###Output
_____no_output_____ |
files/_archive/subset_active.ipynb | ###Markdown
This notebook explores how we can subset the active sentences data to just inmates who have served at least 75 percent of their sentence.
###Code
importlib.reload(build)
importlib.reload(config)
pd.set_option('display.max_columns', None)
pd.set_option('display.max_rows', None)
dataset_3 = pd.read_csv('../data/dataset_main_active3.csv')
dataset_3.head()
grouping_target = config.grouping_target
print(grouping_target)
train_data, test_data, validate_data, active_sentences, active_almost_cmomplete = build.split_and_process(dataset_3, config, grouping_target)
active_almost_cmomplete.head()
active_almost_cmomplete.columns
active_almost_cmomplete.shape
###Output
_____no_output_____
###Markdown
IGNORE EVERYTHING BELOW - already in the build_dataset.py file
###Code
# Create a variable that stores today's date
today = pd.to_datetime('today')
print(today)
# Check that EARLIEST_SENTENCE_EFFECTIVE_DT, END_DATE is datetime
#active_sentences['EARLIEST_SENTENCE_EFFECTIVE_DT'].dtype
active_sentences['END_DATE'].dtype
# Convert END_DATE to datetime
active_sentences['END_DATE'] = pd.to_datetime(active_sentences['END_DATE'], yearfirst=True)
active_sentences['END_DATE'].dtype
active_sentences['sent_total'] = (active_sentences['END_DATE'] - active_sentences['EARLIEST_SENTENCE_EFFECTIVE_DT']).dt.days
active_sentences['sent_complete'] = (today - active_sentences['EARLIEST_SENTENCE_EFFECTIVE_DT']).dt.days
active_sentences['pct_sent_complete'] = active_sentences['sent_complete'] / active_sentences['sent_total']
sentence_subset = active_sentences[['EARLIEST_SENTENCE_EFFECTIVE_DT', 'END_DATE', 'sent_total', 'sent_complete', 'pct_sent_complete']]
sentence_subset.head()
potential_release = sentence_subset.loc[sentence_subset['pct_sent_complete'] >= 0.75, :]
potential_release.shape
10762 / 32711
test_subset = build.get_sentence_almost_complete(active_sentences)
###Output
_____no_output_____ |
examples/pendulum/Analysis_Pendulum.ipynb | ###Markdown
Setup
###Code
# Figure parameters
plot_save_path = './analysis_results/'
font = {'family': 'DejaVu Sans', 'size': 18}
matplotlib.rc('font', **font)
fontsize = 18
figsize = (15, 10)
dpisave = 300
# Initialize the compute device
DEVICE = '/GPU:0'
GPUS = tf.config.experimental.list_physical_devices('GPU')
if GPUS:
try:
for gpu in GPUS:
tf.config.experimental.set_memory_growth(gpu, True)
except RuntimeError as e:
print(e)
else:
DEVICE = '/CPU:0'
tf.keras.backend.set_floatx('float64') # !! Set precision for the entire model here
print("TensorFlow version: {}".format(tf.__version__))
print("Eager execution: {}".format(tf.executing_eagerly()))
print("Num GPUs available: {}".format(len(GPUS)))
print("Training at precision: {}".format(tf.keras.backend.floatx()))
print("Training on device: {}".format(DEVICE))
###Output
_____no_output_____
###Markdown
Load model and data
###Code
# SET THIS PATH (w/o file extension). Both '.pkl' and '.h5' files should have same name
model_path = './trained_models/pendulum_2021-10-05-0004/epoch_10_loss_-1.5'
hyp_params_path = model_path + '.pkl'
weight_path = model_path + '.h5'
# Load the hyper parameters
hyp_params = pickle.load(open(hyp_params_path, 'rb'))
# Set Tensorflow backend precision
tf.keras.backend.set_floatx(hyp_params['precision'])
print("Using precision: {}\n".format(tf.keras.backend.floatx()))
# Load evenly spaced rings for test trajectories
test_data = pickle.load(open('evenly_spaced_trajs.pkl', 'rb'))
test_data = tf.cast(test_data, dtype=hyp_params['precision'])
print("Test data shape: {}".format(test_data.shape))
# Fix hyper parameters for running the model on test data
hyp_params['pretrain'] = False
hyp_params['batch_size'] = test_data.shape[0]
hyp_params['num_time_steps'] = test_data.shape[1]
hyp_params['latent_dim'] = test_data.shape[2]
hyp_params['phys_dim'] = test_data.shape[2]
# Load the trained DLDMD model weights
model = dl.DLDMD(hyp_params)
model.num_pred_steps = model.num_time_steps
model.time_final = int(model.num_time_steps*model.delta_t)
model(test_data)
model.load_weights(weight_path)
# Initialize the loss function
loss = lf.LossDLDMD(hyp_params)
print("Number of prediction steps: ", model.num_pred_steps)
###Output
_____no_output_____
###Markdown
Run the DLDMD model
###Code
with tf.device(DEVICE):
[y, x_ae, x_adv, y_adv, weights, evals, evecs, phi] = model(test_data, training=False)
losses = loss([y, x_ae, x_adv, y_adv, weights, evals, evecs, phi], test_data)
print("Loss: {loss:2.7f}".format(loss=losses.numpy()))
print("Log10 Loss: {loss:2.7f}".format(loss=np.log10(losses.numpy())))
###Output
_____no_output_____
###Markdown
Run standard DMD
###Code
# EDMD on the unencoded data
[phim, evals, evecs, x_pred] = edmd(test_data, num_pred=test_data.shape[1])
x_pred = np.real(tf.transpose(x_pred, perm=[0, 2, 1]))
###Output
_____no_output_____
###Markdown
Visualize results
###Code
fs = 20
ts = 20
lw = 2.0
ms = 20.0
figsize = (12, 12)
skip = 1
# DLDMD reconstruction
fig = plt.figure(1, figsize=figsize)
for ii in range(0, test_data.shape[0], skip):
plt.plot(test_data[ii, :, 0], test_data[ii, :, 1], 'k', linestyle='solid', lw=lw)
plt.plot(x_adv[ii, :, 0], x_adv[ii, :, 1], 'k', linestyle='dotted', ms=ms)
plt.plot(test_data[ii, :, 0], test_data[ii, :, 1], 'k', linestyle='solid', lw=lw, label='Test data')
plt.plot(x_adv[ii, 0, 0], x_adv[ii, 0, 1], 'k', linestyle='dotted', ms=20*ms, label='DLDMD')
plt.xlabel(r'$x$', fontsize=fs)
plt.ylabel(r'$\dot{x}$', fontsize=fs)
plt.legend(fontsize=fs, loc='upper right')
plt.axis('equal')
ax = plt.gca()
ax.tick_params(axis='both', which='major', labelsize=ts)
ax.tick_params(axis='both', which='minor', labelsize=ts)
# DMD reconstruction
fig = plt.figure(2, figsize=figsize)
for ii in range(0, test_data.shape[0], skip):
plt.plot(test_data[ii, :, 0], test_data[ii, :, 1], 'k', linestyle='solid', lw=lw)
plt.plot(x_pred[ii, :, 0], x_pred[ii, :, 1], 'k', linestyle='dotted', ms=ms)
plt.plot(test_data[ii, :, 0], test_data[ii, :, 1], 'k', linestyle='solid', lw=lw, label='Test data')
plt.plot(x_pred[ii, 0, 0], x_pred[ii, 0, 1], 'k', linestyle='dotted', ms=20*ms, label='DMD')
plt.xlabel(r'$x$', fontsize=fs)
plt.ylabel(r'$\dot{x}$', fontsize=fs)
plt.legend(fontsize=fs)
plt.axis('equal')
ax = plt.gca()
ax.tick_params(axis='both', which='major', labelsize=ts)
ax.tick_params(axis='both', which='minor', labelsize=ts)
# Plot the trajectories in phase space and latent space
fig = plt.figure(3, figsize=figsize)
for ii in range(0, test_data.shape[0], skip):
plt.plot(test_data[ii, :, 0], test_data[ii, :, 1], 'k', linestyle='solid', lw=lw)
plt.xlabel(r'$x$', fontsize=fs)
plt.ylabel(r'$\dot{x}$', fontsize=fs)
plt.axis('equal')
ax = plt.gca()
ax.tick_params(axis='both', which='major', labelsize=ts)
ax.tick_params(axis='both', which='minor', labelsize=ts)
# Plot the trajectories in latent space
fig = plt.figure(4, figsize=figsize)
for ii in range(y_adv.shape[0]):
plt.plot(y[ii, :, 0], y[ii, :, 1], 'k', linestyle='solid', ms=ms)
plt.xlabel(r'$\tilde{x}_{1}$', fontsize=fs)
plt.ylabel(r'$\tilde{x}_{2}$', fontsize=fs)
plt.axis('equal')
ax = plt.gca()
ax.tick_params(axis='both', which='major', labelsize=ts)
ax.tick_params(axis='both', which='minor', labelsize=ts)
plt.show()
###Output
_____no_output_____
###Markdown
Setup
###Code
# Figure parameters
plot_save_path = './analysis_results/'
font = {'family': 'DejaVu Sans', 'size': 18}
matplotlib.rc('font', **font)
fontsize = 18
figsize = (15, 10)
dpisave = 300
# Initialize the compute device
DEVICE = '/GPU:0'
GPUS = tf.config.experimental.list_physical_devices('GPU')
if GPUS:
try:
for gpu in GPUS:
tf.config.experimental.set_memory_growth(gpu, True)
except RuntimeError as e:
print(e)
else:
DEVICE = '/CPU:0'
tf.keras.backend.set_floatx('float64') # !! Set precision for the entire model here
print("TensorFlow version: {}".format(tf.__version__))
print("Eager execution: {}".format(tf.executing_eagerly()))
print("Num GPUs available: {}".format(len(GPUS)))
print("Training at precision: {}".format(tf.keras.backend.floatx()))
print("Training on device: {}".format(DEVICE))
###Output
TensorFlow version: 2.5.0
Eager execution: True
Num GPUs available: 2
Training at precision: float64
Training on device: /GPU:0
###Markdown
Load model and data
###Code
# SET THIS PATH (w/o file extension). Both '.pkl' and '.h5' files should have same name
model_path = './trained_models/pendulum_2021-06-08-2132/epoch_100_loss_-2.68'
hyp_params_path = model_path + '.pkl'
weight_path = model_path + '.h5'
# Load the hyper parameters
hyp_params = pickle.load(open(hyp_params_path, 'rb'))
# Set Tensorflow backend precision
tf.keras.backend.set_floatx(hyp_params['precision'])
print("Using precision: {}\n".format(tf.keras.backend.floatx()))
# Load evenly spaced rings for test trajectories (from Lusch et al 2018)
rings = np.loadtxt('PendulumRings.csv', delimiter=',')
test_data = rings.reshape((rings.shape[0], int(rings.shape[1]/2), 2))
test_data = tf.cast(test_data, dtype=hyp_params['precision'])
print("Test data shape: {}".format(test_data.shape))
# Fix hyper parameters for running the model on test data
hyp_params['pretrain'] = False
hyp_params['batch_size'] = test_data.shape[0]
hyp_params['num_time_steps'] = test_data.shape[1]
hyp_params['latent_dim'] = test_data.shape[2]
hyp_params['phys_dim'] = test_data.shape[2]
# Load the trained DLDMD model weights
model = dl.DLDMD(hyp_params)
model.num_pred_steps = model.num_time_steps
model.time_final = int(model.num_time_steps*model.delta_t)
model(test_data)
model.load_weights(weight_path)
# Initialize the loss function
loss = lf.LossDLDMD(hyp_params)
print("Number of prediction steps: ", model.num_pred_steps)
###Output
Number of prediction steps: 1500
###Markdown
Run the DLDMD model
###Code
with tf.device(DEVICE):
[y, x_ae, x_adv, y_adv_real, y_adv_imag, weights, Lam, Phi, b] = model(test_data, training=False)
losses = loss([y, x_ae, x_adv, y_adv_real, y_adv_imag, weights, Lam, Phi, b], test_data)
y_adv = y_adv_real
print("Loss: {loss:2.7f}".format(loss=losses.numpy()))
print("Log10 Loss: {loss:2.7f}".format(loss=np.log10(losses.numpy())))
###Output
Loss: 2.5822053
Log10 Loss: 0.4119908
###Markdown
Run standard DMD
###Code
# Standard DMD on the unencoded data
[x_dmd_r, x_dmd_i, Lam_dmd, Phi_dmd, b_dmd] = dmd(test_data, num_pred=test_data.shape[1], t_final=30, delta_t=0.02)
x_dmd = x_dmd_r
###Output
_____no_output_____
###Markdown
Visualize results
###Code
fig = plt.figure(3141, figsize=(25,10))
ax1 = plt.subplot(1, 2, 1)
ax2 = plt.subplot(1, 2, 2)
for ii in range(test_data.shape[0]):
ax1.plot(test_data[ii, :, 0], test_data[ii, :, 1], 'r-', lw=0.5)
ax1.plot(x_dmd[ii, :, 0], x_dmd[ii, :, 1], 'b.', ms=0.5)
ax2.plot(test_data[ii, :, 0], test_data[ii, :, 1], 'r-', lw=0.5)
ax2.plot(x_adv[ii, :, 0], x_adv[ii, :, 1], 'b.', ms=0.5)
ax1.plot(x_dmd[:, 0, 0], x_dmd[:, 0, 1], 'go', label='initial condition')
ax2.plot(x_adv[:, 0, 0], x_adv[:, 0, 1], 'go', label='initial condition')
ax1.plot(x_dmd[0, 0, 0], x_dmd[0, 0, 1], 'b.', label='dmd')
ax2.plot(x_adv[0, 0, 0], x_adv[0, 0, 1], 'b.', label='dldmd')
ax1.plot(test_data[0, :, 0], test_data[0, :, 1], 'r-', lw=0.5, label='test data')
ax2.plot(test_data[0, :, 0], test_data[0, :, 1], 'r-', lw=0.5, label='test data')
ax1.legend()
ax2.legend()
ax1.axis('equal')
ax2.axis('equal')
ax1.set_title('standard DMD')
ax2.set_title('DLDMD')
plt.suptitle("DLDMD vs DMD predictions of nonlinear pendulum phase orbits")
plt.show()
fig = plt.figure(1, figsize=(20,20))
ax1 = plt.subplot(2, 2, 1)
ax2 = plt.subplot(2, 2, 2)
ax3 = plt.subplot(2, 2, 3)
ax4 = plt.subplot(2, 2, 4)
for ii in range(test_data.shape[0]):
ax1.plot(test_data[ii, :, 0], test_data[ii, :, 1], '-')
ax2.plot(x_adv[ii, :, 0], x_adv[ii, :, 1], '-')
ax3.plot(y_adv[ii, :, 0], y_adv[ii, :, 1], '-')
ax4.plot(x_ae[ii, :, 0], x_ae[ii, :, 1], '-')
ax1.plot(test_data[:, 0, 0], test_data[:, 0, 1], '.')
ax2.plot(x_adv[:, 0, 0], x_adv[:, 0, 1], '.')
ax3.plot(y_adv[:, 0, 0], y_adv[:, 0, 1], '.')
ax4.plot(x_ae[:, 0, 0], x_ae[:, 0, 1], '.')
ax1.axis('equal')
ax1.set_xlim([-3.1, 3.1])
ax1.set_ylim([-3.0, 3.0])
ax1.set_xlabel("$x_1$", fontsize=fontsize)
ax1.set_ylabel("$x_2$", fontsize=fontsize)
ax1.set_title("Test Data (x)", fontsize=fontsize)
ax2.axis('equal')
ax2.set_xlim([-3.1, 3.1])
ax2.set_ylim([-3.0, 3.0])
ax2.set_xlabel("$x_1$", fontsize=fontsize)
ax2.set_ylabel("$x_2$", fontsize=fontsize)
ax2.set_title("Encoded-Advanced-Decoded (x_adv))", fontsize=fontsize)
ax3.axis('equal')
ax3.set_xlabel("$y_1$", fontsize=fontsize)
ax3.set_ylabel("$y_2$", fontsize=fontsize)
ax3.set_title("Encoded-Advanced (y_adv))", fontsize=fontsize)
ax4.axis('equal')
ax4.set_xlim([-3.1, 3.1])
ax4.set_ylim([-3.0, 3.0])
ax4.set_xlabel("$x_1$", fontsize=fontsize)
ax4.set_ylabel("$x_2$", fontsize=fontsize)
ax4.set_title("Encoded-Decoded (x_ae)", fontsize=fontsize)
plt.show()
# Eigenvalues
angle = np.linspace(0, 2*np.pi, 150)
radius = 1.0
x = radius * np.cos(angle)
y = radius * np.sin(angle)
fig = plt.figure(123, figsize=figsize)
ax1 = plt.subplot(1, 1, 1)
s1 = ax1.scatter(np.real(Lam[:]), np.imag(Lam[:]))
ax1.plot(x, y, 'r--')
ax1.axis('equal')
ax1.set_xlabel("$Re(\lambda)$", fontsize=fontsize)
ax1.set_ylabel("$Imag(\lambda)$", fontsize=fontsize)
ax1.set_title("$\lambda$", fontsize=fontsize)
plt.tight_layout()
plt.show()
###Output
_____no_output_____ |
Chapter04/Exercise4.01/Exercise4.01.ipynb | ###Markdown
Exercise 4.01: Comparing IQ Scores for Different Test Groups by Using a Box PlotIn this exercise, we will compare IQ scores among different test groups using a box plot of the Seaborn library to demonstrate how easy and efficient it is to create plots with Seaborn provided that we have a proper DataFrame. This exercise also shows how to quickly change the style and context of a Figure using the preconfigurations supplied by Seaborn.Let's compare IQ scores among different test groups using the Seaborn library: Import the necessary modules and enable plotting within a Jupyter Notebook.
###Code
%matplotlib inline
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
###Output
_____no_output_____
###Markdown
Use the pandas read_csv() function to read the data located in the data folder:
###Code
mydata = pd.read_csv("../../Datasets/iq_scores.csv")
mydata
###Output
_____no_output_____
###Markdown
Access the data of each test group in the column. Convert them into a list using the tolist() method. Once the data of each test group has been converted into a list, assign this list to variables of each respective test group:
###Code
group_a = mydata[mydata.columns[0]].tolist()
group_b = mydata[mydata.columns[1]].tolist()
group_c = mydata[mydata.columns[2]].tolist()
group_d = mydata[mydata.columns[3]].tolist()
###Output
_____no_output_____
###Markdown
Print the values of each group to check whether the data inside it is converted into a list. This can be done with the help of the print() function:
###Code
print(group_a)
print(group_b)
print(group_c)
print(group_d)
###Output
[93, 99, 91, 110, 80, 113, 111, 115, 98, 74, 96, 80, 83, 102, 60, 91, 82, 90, 97, 101, 89, 89, 117, 91, 104, 104, 102, 128, 106, 111, 79, 92, 97, 101, 106, 110, 93, 93, 106, 108, 85, 83, 108, 94, 79, 87, 113, 112, 111, 111, 79, 116, 104, 84, 116, 111, 103, 103, 112, 68, 54, 80, 86, 119, 81, 84, 91, 96, 116, 125, 99, 58, 102, 77, 98, 100, 90, 106, 109, 114, 102, 102, 112, 103, 98, 96, 85, 97, 110, 131, 92, 79, 115, 122, 95, 105, 74, 85, 85, 95]
###Markdown
Once we have the data for each test group, we need to construct a DataFrame from this given data. This can be done with the help of the pd.DataFrame() function, which is provided by pandas.
###Code
data = pd.DataFrame({'Groups': ['Group A'] * len(group_a) + ['Group B'] * len(group_b) + ['Group C'] * len(group_c) + ['Group D'] * len(group_d),
'IQ score': group_a + group_b + group_c + group_d})
data
###Output
_____no_output_____
###Markdown
If you don’t create your own DataFrame, it is often helpful to print the column names, which is done by calling print(data.columns). The output is as follows:
###Code
print(data.columns)
###Output
Index(['Groups', 'IQ score'], dtype='object')
###Markdown
You can see that our DataFrame has two variables with the labels Groups and IQ score. This is especially interesting since we can use them to specify which variable to plot on the x-axis and which one on the y-axis. Now, since we have the DataFrame, we need to create a box plot using the boxplot() function provided by Seaborn. Within this function, specify the variables for both the axes along with the DataFrame. Make Groups the variable to plot on the x-axis and IQ score the variable for the y-axis. Pass data as a parameter. Here, data is the DataFrame that we obtained from the previous step. Moreover, use the whitegrid style, set the context to talk, and remove all axes splines, except the one on the bottom.
###Code
plt.figure(dpi=300)
# Set style to 'whitegrid'
sns.set_style('whitegrid')
# Set context to 'talk'
sns.set_context('talk')
# Create boxplot
sns.boxplot('Groups', 'IQ score', data=data)
# Despine
sns.despine(left=True, right=True, top=True)
# Add title
plt.title('IQ scores for different test groups')
# Show plot
plt.show()
###Output
_____no_output_____
###Markdown
Exercise 4.01: Comparing IQ Scores for Different Test Groups by Using a Box PlotIn this exercise, we will compare IQ scores among different test groups using a box plot of the Seaborn library to demonstrate how easy and efficient it is to create plots with Seaborn provided that we have a proper DataFrame. This exercise also shows how to quickly change the style and context of a Figure using the preconfigurations supplied by Seaborn.Let's compare IQ scores among different test groups using the Seaborn library: Import the necessary modules and enable plotting within a Jupyter Notebook.
###Code
%matplotlib inline
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
###Output
_____no_output_____
###Markdown
Use the pandas read_csv() function to read the data located in the data folder:
###Code
mydata = pd.read_csv("../../Datasets/iq_scores.csv")
###Output
_____no_output_____
###Markdown
Access the data of each test group in the column. Convert them into a list using the tolist() method. Once the data of each test group has been converted into a list, assign this list to variables of each respective test group:
###Code
group_a = mydata[mydata.columns[0]].tolist()
group_b = mydata[mydata.columns[1]].tolist()
group_c = mydata[mydata.columns[2]].tolist()
group_d = mydata[mydata.columns[3]].tolist()
###Output
_____no_output_____
###Markdown
Print the values of each group to check whether the data inside it is converted into a list. This can be done with the help of the print() function:
###Code
print(group_a)
print(group_b)
print(group_c)
print(group_d)
###Output
[93, 99, 91, 110, 80, 113, 111, 115, 98, 74, 96, 80, 83, 102, 60, 91, 82, 90, 97, 101, 89, 89, 117, 91, 104, 104, 102, 128, 106, 111, 79, 92, 97, 101, 106, 110, 93, 93, 106, 108, 85, 83, 108, 94, 79, 87, 113, 112, 111, 111, 79, 116, 104, 84, 116, 111, 103, 103, 112, 68, 54, 80, 86, 119, 81, 84, 91, 96, 116, 125, 99, 58, 102, 77, 98, 100, 90, 106, 109, 114, 102, 102, 112, 103, 98, 96, 85, 97, 110, 131, 92, 79, 115, 122, 95, 105, 74, 85, 85, 95]
###Markdown
Once we have the data for each test group, we need to construct a DataFrame from this given data. This can be done with the help of the pd.DataFrame() function, which is provided by pandas.
###Code
data = pd.DataFrame({'Groups': ['Group A'] * len(group_a) + ['Group B'] * len(group_b) + ['Group C'] * len(group_c) + ['Group D'] * len(group_d),
'IQ score': group_a + group_b + group_c + group_d})
###Output
_____no_output_____
###Markdown
If you don’t create your own DataFrame, it is often helpful to print the column names, which is done by calling print(data.columns). The output is as follows:
###Code
print(data.columns)
###Output
Index(['Groups', 'IQ score'], dtype='object')
###Markdown
You can see that our DataFrame has two variables with the labels Groups and IQ score. This is especially interesting since we can use them to specify which variable to plot on the x-axis and which one on the y-axis. Now, since we have the DataFrame, we need to create a box plot using the boxplot() function provided by Seaborn. Within this function, specify the variables for both the axes along with the DataFrame. Make Groups the variable to plot on the x-axis and IQ score the variable for the y-axis. Pass data as a parameter. Here, data is the DataFrame that we obtained from the previous step. Moreover, use the whitegrid style, set the context to talk, and remove all axes splines, except the one on the bottom.
###Code
plt.figure(dpi=300)
# Set style to 'whitegrid'
sns.set_style('whitegrid')
# Set context to 'talk'
sns.set_context('talk')
# Create boxplot
sns.boxplot('Groups', 'IQ score', data=data)
# Despine
sns.despine(left=True, right=True, top=True)
# Add title
plt.title('IQ scores for different test groups')
# Show plot
plt.show()
###Output
_____no_output_____
###Markdown
Comparing IQ Scores for Different Test Groups by Using a Box PlotIn this exercise, we will compare IQ scores among different test groups using a box plot of the Seaborn library to demonstrate how easy and efficient it is to create plots with Seaborn provided that we have a proper DataFrame. This exercise also shows how to quickly change the style and context of a Figure using the preconfigurations supplied by Seaborn.Let's compare IQ scores among different test groups using the Seaborn library: Import the necessary modules and enable plotting within a Jupyter Notebook.
###Code
%matplotlib inline
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
###Output
_____no_output_____
###Markdown
Use the pandas read_csv() function to read the data located in the data folder:
###Code
mydata = pd.read_csv("../../Datasets/iq_scores.csv")
###Output
_____no_output_____
###Markdown
Access the data of each test group in the column. Convert them into a list using the tolist() method. Once the data of each test group has been converted into a list, assign this list to variables of each respective test group:
###Code
group_a = mydata[mydata.columns[0]].tolist()
group_b = mydata[mydata.columns[1]].tolist()
group_c = mydata[mydata.columns[2]].tolist()
group_d = mydata[mydata.columns[3]].tolist()
###Output
_____no_output_____
###Markdown
Print the values of each group to check whether the data inside it is converted into a list. This can be done with the help of the print() function:
###Code
print(group_a)
print(group_b)
print(group_c)
print(group_d)
###Output
[93, 99, 91, 110, 80, 113, 111, 115, 98, 74, 96, 80, 83, 102, 60, 91, 82, 90, 97, 101, 89, 89, 117, 91, 104, 104, 102, 128, 106, 111, 79, 92, 97, 101, 106, 110, 93, 93, 106, 108, 85, 83, 108, 94, 79, 87, 113, 112, 111, 111, 79, 116, 104, 84, 116, 111, 103, 103, 112, 68, 54, 80, 86, 119, 81, 84, 91, 96, 116, 125, 99, 58, 102, 77, 98, 100, 90, 106, 109, 114, 102, 102, 112, 103, 98, 96, 85, 97, 110, 131, 92, 79, 115, 122, 95, 105, 74, 85, 85, 95]
###Markdown
Once we have the data for each test group, we need to construct a DataFrame from this given data. This can be done with the help of the pd.DataFrame() function, which is provided by pandas.
###Code
data = pd.DataFrame({'Groups': ['Group A'] * len(group_a) + ['Group B'] * len(group_b) + ['Group C'] * len(group_c) + ['Group D'] * len(group_d),
'IQ score': group_a + group_b + group_c + group_d})
###Output
_____no_output_____
###Markdown
If you don’t create your own DataFrame, it is often helpful to print the column names, which is done by calling print(data.columns). The output is as follows:
###Code
print(data.columns)
###Output
Index(['Groups', 'IQ score'], dtype='object')
###Markdown
You can see that our DataFrame has two variables with the labels Groups and IQ score. This is especially interesting since we can use them to specify which variable to plot on the x-axis and which one on the y-axis. Now, since we have the DataFrame, we need to create a box plot using the boxplot() function provided by Seaborn. Within this function, specify the variables for both the axes along with the DataFrame. Make Groups the variable to plot on the x-axis and IQ score the variable for the y-axis. Pass data as a parameter. Here, data is the DataFrame that we obtained from the previous step. Moreover, use the whitegrid style, set the context to talk, and remove all axes splines, except the one on the bottom.
###Code
plt.figure(dpi=300)
# Set style to 'whitegrid'
sns.set_style('whitegrid')
# Set context to 'talk'
sns.set_context('talk')
# Create boxplot
sns.boxplot('Groups', 'IQ score', data=data)
# Despine
sns.despine(left=True, right=True, top=True)
# Add title
plt.title('IQ scores for different test groups')
# Show plot
plt.show()
###Output
_____no_output_____ |
week6_outro/practice_mcts.ipynb | ###Markdown
Seminar: Monte-carlo tree searchIn this seminar, we'll implement a vanilla MCTS planning and use it to solve some Gym envs.But before we do that, we first need to modify gym env to allow saving and loading game states to facilitate backtracking.
###Code
from gym.core import Wrapper
from pickle import dumps,loads
from collections import namedtuple
#a container for get_result function below. Works just like tuple, but prettier
ActionResult = namedtuple("action_result",("snapshot","observation","reward","is_done","info"))
class WithSnapshots(Wrapper):
"""
Creates a wrapper that supports saving and loading environemnt states.
Required for planning algorithms.
This class will have access to the core environment as self.env, e.g.:
- self.env.reset() #reset original env
- self.env.ale.cloneState() #make snapshot for atari. load with .restoreState()
- ...
You can also use reset, step and render directly for convenience.
- s, r, done, _ = self.step(action) #step, same as self.env.step(action)
- self.render(close=True) #close window, same as self.env.render(close=True)
"""
def get_snapshot(self):
"""
:returns: environment state that can be loaded with load_snapshot
Snapshots guarantee same env behaviour each time they are loaded.
Warning! Snapshots can be arbitrary things (strings, integers, json, tuples)
Don't count on them being pickle strings when implementing MCTS.
Developer Note: Make sure the object you return will not be affected by
anything that happens to the environment after it's saved.
You shouldn't, for example, return self.env.
In case of doubt, use pickle.dumps or deepcopy.
"""
#self.render() #close popup windows since we can't pickle them
if self.unwrapped.viewer is not None:
self.unwrapped.viewer.close()
self.unwrapped.viewer = None
return dumps(self.env)
def load_snapshot(self,snapshot):
"""
Loads snapshot as current env state.
Should not change snapshot inplace (in case of doubt, deepcopy).
"""
assert not hasattr(self,"_monitor") or hasattr(self.env,"_monitor"), "can't backtrack while recording"
#self.render()#close=True #close popup windows since we can't load into them
self.env = loads(snapshot)
def get_result(self,snapshot,action):
"""
A convenience function that
- loads snapshot,
- commits action via self.step,
- and takes snapshot again :)
:returns: next snapshot, next_observation, reward, is_done, info
Basically it returns next snapshot and everything that env.step would have returned.
"""
#<your code here load,commit,take snapshot>
self.load_snapshot(snapshot)
o,r,is_done,info = self.step(action)
next_snapshot = self.get_snapshot()
return ActionResult(next_snapshot,o,r,is_done,info)
#return ActionResult(<next_snapshot>, #fill in the variables
# <next_observation>,
# <reward>, <is_done>, <info>)
###Output
_____no_output_____
###Markdown
try out snapshots:
###Code
#make env
env = WithSnapshots(gym.make("CartPole-v0"))
env.reset()
n_actions = env.action_space.n
print("initial_state:")
plt.imshow(env.render('rgb_array'))
#create first snapshot
snap0 = env.get_snapshot()
#play without making snapshots (faster)
while True:
is_done = env.step(env.action_space.sample())[2]
if is_done:
print("Whoops! We died!")
break
print("final state:")
plt.imshow(env.render('rgb_array'))
plt.show()
#reload initial state
env.load_snapshot(snap0)
print("\n\nAfter loading snapshot")
plt.imshow(env.render('rgb_array'))
plt.show()
#get outcome (snapshot, observation, reward, is_done, info)
res = env.get_result(snap0,env.action_space.sample())
snap1, observation, reward = res[:3]
#second step
res2 = env.get_result(snap1,env.action_space.sample())
###Output
_____no_output_____
###Markdown
MCTS: Monte-Carlo tree searchIn this section, we'll implement the vanilla MCTS algorithm with UCB1-based node selection.We will start by implementing the `Node` class - a simple class that acts like MCTS node and supports some of the MCTS algorithm steps.This MCTS implementation makes some assumptions about the environment, you can find those _in the notes section at the end of the notebook_.
###Code
assert isinstance(env,WithSnapshots)
class Node:
""" a tree node for MCTS """
#metadata:
parent = None #parent Node
value_sum = 0. #sum of state values from all visits (numerator)
times_visited = 0 #counter of visits (denominator)
def __init__(self,parent,action,):
"""
Creates and empty node with no children.
Does so by commiting an action and recording outcome.
:param parent: parent Node
:param action: action to commit from parent Node
"""
self.parent = parent
self.action = action
self.children = set() #set of child nodes
#get action outcome and save it
res = env.get_result(parent.snapshot,action)
self.snapshot,self.observation,self.immediate_reward,self.is_done,_ = res
def is_leaf(self):
return len(self.children)==0
def is_root(self):
return self.parent is None
def get_mean_value(self):
return self.value_sum / self.times_visited if self.times_visited !=0 else 0
def ucb_score(self,scale=10,max_value=1e100):
"""
Computes ucb1 upper bound using current value and visit counts for node and it's parent.
:param scale: Multiplies upper bound by that. From hoeffding inequality, assumes reward range to be [0,scale].
:param max_value: a value that represents infinity (for unvisited nodes)
"""
if self.times_visited == 0:
return max_value
#compute ucb-1 additive component (to be added to mean value)
#hint: you can use self.parent.times_visited for N times node was considered,
# and self.times_visited for n times it was visited
#if self.parent is None:
# return max_value
U = np.sqrt(2*np.log(self.parent.times_visited)/self.times_visited)#<your code here>
return self.get_mean_value() + scale*U
#MCTS steps
def select_best_leaf(self):
"""
Picks the leaf with highest priority to expand
Does so by recursively picking nodes with best UCB-1 score until it reaches the leaf.
"""
if self.is_leaf():
return self
children = list(self.children)
children_ucb = [node.ucb_score() for node in children]
best_child = children[np.argmax(children_ucb)]#<select best child node in terms of node.ucb_score()>
return best_child.select_best_leaf()
def expand(self):
"""
Expands the current node by creating all possible child nodes.
Then returns one of those children.
"""
assert not self.is_done, "can't expand from terminal state"
for action in range(n_actions):
self.children.add(Node(self,action))
return self.select_best_leaf()
def rollout(self,t_max=10**4):
"""
Play the game from this state to the end (done) or for t_max steps.
On each step, pick action at random (hint: env.action_space.sample()).
Compute sum of rewards from current state till
Note 1: use env.action_space.sample() for random action
Note 2: if node is terminal (self.is_done is True), just return 0
"""
#set env into the appropriate state
env.load_snapshot(self.snapshot)
obs = self.observation
is_done = self.is_done
#<your code here - rollout and compute reward>
rollout_reward = 0
t = 0
gamma = 1
cof = 1
while not is_done and t < t_max:
o,r,is_done,_ = env.step(env.action_space.sample())
rollout_reward += ( cof* r)
t += 1
cof *= gamma
return rollout_reward
def propagate(self,child_value):
"""
Uses child value (sum of rewards) to update parents recursively.
"""
#compute node value
gamma = 1
my_value = self.immediate_reward + gamma * child_value
#update value_sum and times_visited
self.value_sum+=my_value
self.times_visited+=1
#propagate upwards
if not self.is_root():
self.parent.propagate(my_value)
def safe_delete(self):
"""safe delete to prevent memory leak in some python versions"""
del self.parent
for child in self.children:
child.safe_delete()
del child
class Root(Node):
def __init__(self,snapshot,observation):
"""
creates special node that acts like tree root
:snapshot: snapshot (from env.get_snapshot) to start planning from
:observation: last environment observation
"""
self.parent = self.action = None
self.children = set() #set of child nodes
#root: load snapshot and observation
self.snapshot = snapshot
self.observation = observation
self.immediate_reward = 0
self.is_done=False
@staticmethod
def from_node(node):
"""initializes node as root"""
root = Root(node.snapshot,node.observation)
#copy data
copied_fields = ["value_sum","times_visited","children","is_done"]
for field in copied_fields:
setattr(root,field,getattr(node,field))
return root
###Output
_____no_output_____
###Markdown
Main MCTS loopWith all we implemented, MCTS boils down to a trivial piece of code.
###Code
def plan_mcts(root,n_iters=10):
"""
builds tree with monte-carlo tree search for n_iters iterations
:param root: tree node to plan from
:param n_iters: how many select-expand-simulate-propagete loops to make
"""
for _ in range(n_iters):
node = root.select_best_leaf()#<select best leaf>
if node.is_done:
node.propagate(0)
else: #node is not terminal
#<expand-simulate-propagate loop>
node_child =node.expand()
r = node_child.rollout()#10**4
node.propagate(r)
###Output
_____no_output_____
###Markdown
Plan and executeIn this section, we use the MCTS implementation to find optimal policy.
###Code
root_observation = env.reset()
root_snapshot = env.get_snapshot()
root = Root(root_snapshot,root_observation)
#plan from root:
plan_mcts(root,n_iters=1000)
from IPython.display import clear_output
from itertools import count
from gym.wrappers import Monitor
total_reward = 0 #sum of rewards
test_env = loads(root_snapshot) #env used to show progress
for i in count():
if i % 5 == 0:
print(i)
#get best child
children = list(root.children)
best_child = children[np.argmax([child.get_mean_value() for child in children])]
#<select child with highest mean reward>
#take action
s,r,done,_ = test_env.step(best_child.action)
#show image
clear_output(True)
plt.title("step %i"%i)
plt.imshow(test_env.render('rgb_array'))
plt.show()
total_reward += r
if done:
print("Finished with reward = ",total_reward)
break
#discard unrealized part of the tree [because not every child matters :(]
for child in root.children:
if child != best_child:
child.safe_delete()
#declare best child a new root
root = Root.from_node(best_child)
if root.is_leaf():
plan_mcts(root,10)
assert not root.is_leaf(), "We ran out of tree! Need more planning! Try growing tree right inside the loop."
#you may want to expand tree here
#<your code here>
#root.expand()
###Output
_____no_output_____
###Markdown
Submit to Coursera
###Code
from submit import submit_mcts
submit_mcts(total_reward,<EMAIL>, <TOKEN>)
###Output
_____no_output_____
###Markdown
Seminar: Monte-carlo tree search (5 pts)Monte Carlo tree search (MCTS) is a heuristic search algorithm, which shows cool results in challenging domains such as Go and chess. The algorithm builds a search tree, iteratively traverses it, and evaluates its nodes using a Monte-Carlo simulation.In this seminar, we'll implement a MCTS([[1]](1), [[2]](2)) planning and use it to solve some Gym envs.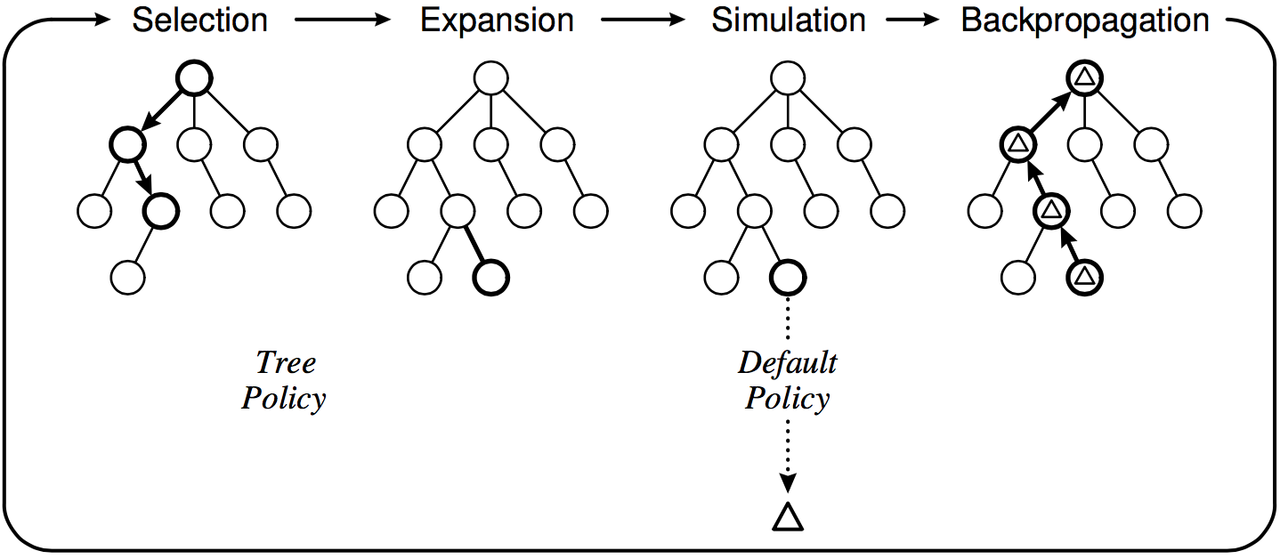 __How it works?__We just start with an empty tree and expand it. There are several common procedures.__1) Selection__Starting from the root, recursively select the node that corresponds to the tree policy. There are several options for tree policies, which we saw earlier as exploration strategies: epsilon-greedy, Thomson sampling, UCB-1. It was shown that in MCTS, UCB-1 achieves a good result. Further, we will consider the one, but you can try to use others.Following the UCB-1 tree policy, we will choose an action that, on one hand, we expect to have the highest return, and on the other hand, we haven't explored much.$$\DeclareMathOperator*{\argmax}{arg\,max}$$$$\dot{a} = \argmax_{a} \dot{Q}(s, a)$$$$\dot{Q}(s, a) = Q(s, a) + C_p \sqrt{\frac{2 \log {N}}{n_a}}$$where: - $N$ - number of times we have visited state $s$,- $n_a$ - number of times we have taken action $a$,- $C_p$ - exploration balance parameter, which is performed between exploration and exploitation. Using Hoeffding inequality for rewards $R \in [0,1]$ it can be shown [[3]](3) that optimal $C_p = 1/\sqrt{2}$. For rewards outside this range, the parameter should be tuned. We'll be using 10, but you can experiment with other values.__2) Expansion__After the selection procedure, we can achieve a leaf node or node in which we don't complete actions. In this case, we expand the tree by feasible actions and get new state nodes. __3) Simulation__How we can estimate node Q-values? The idea is to estimate action values for a given _rollout policy_ by averaging the return of many simulated trajectories from the current node. Simply, we can play with random or some special policy or use some model that can estimate it.__4) Backpropagation__The reward of the last simulation is backed up through the traversed nodes and propagates Q-value estimations, upwards to the root.$$Q({\text{parent}}, a) = r + \gamma \cdot Q({\text{child}}, a)$$There are a lot modifications of MCTS, more details about it you can find in this paper [[4]](4)
###Code
import sys, os
if 'google.colab' in sys.modules and not os.path.exists('.setup_complete'):
!wget -q https://raw.githubusercontent.com/yandexdataschool/Practical_RL/master/setup_colab.sh -O- | bash
!wget -q https://raw.githubusercontent.com/yandexdataschool/Practical_RL/coursera/grading.py -O ../grading.py
!wget -q https://raw.githubusercontent.com/yandexdataschool/Practical_RL/coursera/week6_outro/submit.py
!touch .setup_complete
# This code creates a virtual display to draw game images on.
# It will have no effect if your machine has a monitor.
if type(os.environ.get("DISPLAY")) is not str or len(os.environ.get("DISPLAY")) == 0:
!bash ../xvfb start
os.environ['DISPLAY'] = ':1'
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
###Output
_____no_output_____
###Markdown
---But before we do that, we first need to make a wrapper for Gym environments to allow saving and loading game states to facilitate backtracking.
###Code
import gym
from gym.core import Wrapper
from pickle import dumps, loads
from collections import namedtuple
# a container for get_result function below. Works just like tuple, but prettier
ActionResult = namedtuple(
"action_result", ("snapshot", "observation", "reward", "is_done", "info"))
class WithSnapshots(Wrapper):
"""
Creates a wrapper that supports saving and loading environemnt states.
Required for planning algorithms.
This class will have access to the core environment as self.env, e.g.:
- self.env.reset() #reset original env
- self.env.ale.cloneState() #make snapshot for atari. load with .restoreState()
- ...
You can also use reset() and step() directly for convenience.
- s = self.reset() # same as self.env.reset()
- s, r, done, _ = self.step(action) # same as self.env.step(action)
Note that while you may use self.render(), it will spawn a window that cannot be pickled.
Thus, you will need to call self.close() before pickling will work again.
"""
def get_snapshot(self, render=False):
"""
:returns: environment state that can be loaded with load_snapshot
Snapshots guarantee same env behaviour each time they are loaded.
Warning! Snapshots can be arbitrary things (strings, integers, json, tuples)
Don't count on them being pickle strings when implementing MCTS.
Developer Note: Make sure the object you return will not be affected by
anything that happens to the environment after it's saved.
You shouldn't, for example, return self.env.
In case of doubt, use pickle.dumps or deepcopy.
"""
if render:
self.render() # close popup windows since we can't pickle them
self.close()
if self.unwrapped.viewer is not None:
self.unwrapped.viewer.close()
self.unwrapped.viewer = None
return dumps(self.env)
def load_snapshot(self, snapshot, render=False):
"""
Loads snapshot as current env state.
Should not change snapshot inplace (in case of doubt, deepcopy).
"""
assert not hasattr(self, "_monitor") or hasattr(
self.env, "_monitor"), "can't backtrack while recording"
if render:
self.render() # close popup windows since we can't load into them
self.close()
self.env = loads(snapshot)
def get_result(self, snapshot, action):
"""
A convenience function that
- loads snapshot,
- commits action via self.step,
- and takes snapshot again :)
:returns: next snapshot, next_observation, reward, is_done, info
Basically it returns next snapshot and everything that env.step would have returned.
"""
self.load_snapshot(snapshot)
s,r,is_done,info = self.env.step(action)
snap = self.get_snapshot()
return ActionResult(
snap,
s,
r,
is_done,
info
)
###Output
_____no_output_____
###Markdown
Try out snapshots:Let`s check our wrapper. At first, reset environment and save it, further randomly play some actions and restore our environment from the snapshot. It should be the same as our previous initial state.
###Code
# make env
env = WithSnapshots(gym.make("CartPole-v0"))
env.reset()
n_actions = env.action_space.n
print("initial_state:")
plt.imshow(env.render('rgb_array'))
env.close()
# create first snapshot
snap0 = env.get_snapshot()
# play without making snapshots (faster)
while True:
is_done = env.step(env.action_space.sample())[2]
if is_done:
print("Whoops! We died!")
break
print("final state:")
plt.imshow(env.render('rgb_array'))
env.close()
# reload initial state
env.load_snapshot(snap0)
print("\n\nAfter loading snapshot")
plt.imshow(env.render('rgb_array'))
env.close()
# get outcome (snapshot, observation, reward, is_done, info)
res = env.get_result(snap0, env.action_space.sample())
snap1, observation, reward = res[:3]
# second step
res2 = env.get_result(snap1, env.action_space.sample())
###Output
_____no_output_____
###Markdown
MCTS: Monte-Carlo tree searchWe will start by implementing the `Node` class - a simple class that acts like MCTS node and supports some of the MCTS algorithm steps.This MCTS implementation makes some assumptions about the environment, you can find those _in the notes section at the end of the notebook_.
###Code
assert isinstance(env, WithSnapshots)
id_ = 0
from numpy.core.numeric import roll
class Node:
"""A tree node for MCTS.
Each Node corresponds to the result of performing a particular action (self.action)
in a particular state (self.parent), and is essentially one arm in the multi-armed bandit that
we model in that state."""
# metadata:
parent = None # parent Node
qvalue_sum = 0. # sum of Q-values from all visits (numerator)
times_visited = 0 # counter of visits (denominator)
id='*'
def __init__(self, parent, action,id=0):
"""
Creates and empty node with no children.
Does so by commiting an action and recording outcome.
:param parent: parent Node
:param action: action to commit from parent Node
"""
self.parent = parent
self.action = action
self.children = set() # set of child nodes
# get action outcome and save it
res = env.get_result(parent.snapshot, action)
self.snapshot, self.observation, self.immediate_reward, self.is_done, _ = res
self.id = self.parent.id+f"{action}"
def is_leaf(self):
return len(self.children) == 0
def is_root(self):
return self.parent is None
def get_qvalue_estimate(self):
return self.qvalue_sum / self.times_visited if self.times_visited != 0 else 0
def ucb_score(self, scale=10, max_value=1e100):
"""
Computes ucb1 upper bound using current value and visit counts for node and it's parent.
:param scale: Multiplies upper bound by that. From Hoeffding inequality,
assumes reward range to be [0, scale].
:param max_value: a value that represents infinity (for unvisited nodes).
"""
if self.times_visited == 0:
return max_value
# compute ucb-1 additive component (to be added to mean value)
# hint: you can use self.parent.times_visited for N times node was considered,
# and self.times_visited for n times it was visited
U = np.sqrt(2 * np.log(self.parent.times_visited) / self.times_visited)
return self.get_qvalue_estimate() + scale * U
# MCTS steps
def select_best_leaf(self):
"""
Picks the leaf with the highest priority to expand.
Does so by recursively picking nodes with the best UCB-1 score until it reaches a leaf.
"""
if self.is_leaf():
# print(f'==> bestleaf: {self.id}')
return self
children = self.children
# Select the child node with the highest UCB score. You might want to implement some heuristics
# to break ties in a smart way, although CartPole should work just fine without them.
max_sco = 0.0
s = []
for i in children:
s.append(f'{i.ucb_score()}')
if i.ucb_score() > max_sco:
max_sco = i.ucb_score()
best_child = i
# print(f'[{self.id}] {s}')
# best_child = <YOUR CODE>
return best_child.select_best_leaf()
def expand(self):
"""
Expands the current node by creating all possible child nodes.
Then returns one of those children.
"""
assert not self.is_done, "can't expand from terminal state"
for action in range(n_actions):
self.children.add(Node(self, action))
# If you have implemented any heuristics in select_best_leaf(), they will be used here.
# Otherwise, this is equivalent to picking some undefined newly created child node.
return self.select_best_leaf()
def rollout(self, t_max=10**4):
"""
Play the game from this state to the end (done) or for t_max steps.
On each step, pick action at random (hint: env.action_space.sample()).
Compute sum of rewards from the current state until the end of the episode.
Note 1: use env.action_space.sample() for picking a random action.
Note 2: if the node is terminal (self.is_done is True), just return self.immediate_reward.
"""
# set env into the appropriate state
env.load_snapshot(self.snapshot)
obs = self.observation
is_done = self.is_done
# <YOUR CODE: perform rollout and compute reward>
if is_done:
return self.immediate_reward
rollout_reward = 0
for _ in range(t_max):
s_next, r, do,_ = env.step(env.action_space.sample())
rollout_reward += r
if do: break
return rollout_reward
def propagate(self, child_qvalue):
"""
Uses child Q-value (sum of rewards) to update parents recursively.
"""
# compute node Q-value
my_qvalue = self.immediate_reward + child_qvalue
# update qvalue_sum and times_visited
self.qvalue_sum += my_qvalue
self.times_visited += 1
# propagate upwards
if not self.is_root():
self.parent.propagate(my_qvalue)
def safe_delete(self):
"""safe delete to prevent memory leak in some python versions"""
if self.parent == None : print(f'del:[{self.id}\t{self.children}]')
del self.parent
for child in self.children:
child.safe_delete()
del child
class Root(Node):
def __init__(self, snapshot, observation):
"""
creates special node that acts like tree root
:snapshot: snapshot (from env.get_snapshot) to start planning from
:observation: last environment observation
"""
self.parent = self.action = None
self.children = set() # set of child nodes
# root: load snapshot and observation
self.snapshot = snapshot
self.observation = observation
self.immediate_reward = 0
self.is_done = False
@staticmethod
def from_node(node):
"""initializes node as root"""
root = Root(node.snapshot, node.observation)
# copy data
copied_fields = ["qvalue_sum", "times_visited", "children", "is_done"]
for field in copied_fields:
setattr(root, field, getattr(node, field))
return root
###Output
_____no_output_____
###Markdown
Main MCTS loopWith all we implemented, MCTS boils down to a trivial piece of code.
###Code
def plan_mcts(root, n_iters=10):
"""
builds tree with monte-carlo tree search for n_iters iterations
:param root: tree node to plan from
:param n_iters: how many select-expand-simulate-propagete loops to make
"""
for _ in range(n_iters):
# print('--'*10)
node = root.select_best_leaf()
if node.is_done:
# All rollouts from a terminal node are empty, and thus have 0 reward.
node.propagate(0)
else:
# Expand the best leaf. Perform a rollout from it. Propagate the results upwards.
# Note that here you have some leeway in choosing where to propagate from.
# Any reasonable choice should work.
# <YOUR CODE>
if node.is_root():
new_node = node.expand()
else:
# for cl in node.parent.children:
# cl.expand()
node.expand()
new_node = node
rollout_reward = new_node.rollout()
# print(f'+++> newnode: [{new_node.id}\t{new_node.immediate_reward}\t{new_node.qvalue_sum}\t{new_node.times_visited}] [{rollout_reward}] ')
new_node.propagate(rollout_reward)
###Output
_____no_output_____
###Markdown
Plan and executeLet's use our MCTS implementation to find the optimal policy.
###Code
env = WithSnapshots(gym.make("CartPole-v0"))
root_observation = env.reset()
root_snapshot = env.get_snapshot()
root = Root(root_snapshot, root_observation)
# plan from root:
root = Root(root_snapshot, root_observation)
plan_mcts(root, n_iters=1000)
# print(f"{1321651:.3g}")
# import copy
# saved_root = copy.deepcopy(root)
# root = saved_root
# count()
# root = Root(root_snapshot, root_observation)
# plan_mcts(root, n_iters=10)
# def show_tree(node ,level = 0):
# print(f'[{node.id}]:\t{"*"*level}{node.action}=>{node.get_qvalue_estimate()}')
# if node.is_leaf(): return print('-'*level)
# for cl in node.children:
# show_tree(cl,level+1)
# return 0
# show_tree(root)
root.children.copy().pop().is_leaf()
from IPython.display import clear_output
from itertools import count
from gym.wrappers import Monitor
total_reward = 0 # sum of rewards
test_env = loads(root_snapshot) # env used to show progress
for i in count():
# get best child
# best_child = <YOUR CODE: select child with the highest mean reward>
# best_child = None
max_sco = 0.0
best_child = None
for j in root.children:
if j.get_qvalue_estimate() >= max_sco:
max_sco = j.get_qvalue_estimate()
best_child = j
# take action
s, r, done, _ = test_env.step(best_child.action)
# show image
clear_output(True)
plt.title("step %i %f" % (i,total_reward))
plt.imshow(test_env.render('rgb_array'))
plt.show()
total_reward += r
if done:
print("Finished with reward = ", total_reward)
break
# discard unrealized part of the tree [because not every child matters :(]
for child in root.children:
if child != best_child:
child.safe_delete()
# declare best child a new root
root = Root.from_node(best_child)
assert not root.is_leaf(), \
"We ran out of tree! Need more planning! Try growing the tree right inside the loop."
# You may want to run more planning here
if root.children.copy().pop().is_leaf():
print('growing the tree')
plan_mcts(root, n_iters=1000)
from submit import submit_mcts
submit_mcts(total_reward, '[email protected]', 'qv3PCtdXdGJYYAJ1')
###Output
Submitted to Coursera platform. See results on assignment page!
###Markdown
Bonus assignments (10+pts each)There's a few things you might want to try if you want to dig deeper: Node selection and expansion"Analyze this" assignmentUCB-1 is a weak bound as it relies on a very general bounds (Hoeffding Inequality, to be exact). * Try playing with the exploration parameter $C_p$. The theoretically optimal $C_p$ you can get from a max reward of the environment (max reward for CartPole is 200).* Use using a different exploration strategy (bayesian UCB, for example)* Expand not all but several random actions per `expand` call. See __the notes below__ for details.The goal is to find out what gives the optimal performance for `CartPole-v0` for different time budgets (i.e. different n_iter in plan_mcts.)Evaluate your results on `Acrobot-v1` - do the results change and if so, how can you explain it? Atari-RAM"Build this" assignmentApply MCTS to play Atari games. In particular, let's start with ```gym.make("MsPacman-ramDeterministic-v0")```.This requires two things:* Slightly modify WithSnapshots wrapper to work with atari. * Atari has a special interface for snapshots: ``` snapshot = self.env.ale.cloneState() ... self.env.ale.restoreState(snapshot) ``` * Try it on the env above to make sure it does what you told it to. * Run MCTS on the game above. * Start with small tree size to speed-up computations * You will probably want to rollout for 10-100 steps (t_max) for starters * Consider using discounted rewards (see __notes at the end__) * Try a better rollout policy Integrate learning into planningPlanning on each iteration is a costly thing to do. You can speed things up drastically if you train a classifier to predict which action will turn out to be best according to MCTS.To do so, just record which action did the MCTS agent take on each step and fit something to [state, mcts_optimal_action]* You can also use optimal actions from discarded states to get more (dirty) samples. Just don't forget to fine-tune without them.* It's also worth a try to use P(best_action|state) from your model to select best nodes in addition to UCB* If your model is lightweight enough, try using it as a rollout policy.While CartPole is glorious enough, try expanding this to ```gym.make("MsPacmanDeterministic-v0")```* See previous section on how to wrap atari* Also consider what [AlphaGo Zero](https://deepmind.com/blog/alphago-zero-learning-scratch/) did in this area. Integrate planning into learning _(this will likely take long time, better consider this as side project when all other deadlines are met)_Incorporate planning into the agent architecture. The goal is to implement [Value Iteration Networks](https://arxiv.org/abs/1602.02867).Remember [week5 assignment](https://github.com/yandexdataschool/Practical_RL/blob/coursera/week5_policy_based/practice_a3c.ipynb)? You will need to switch it into a maze-like game, like MsPacman, and implement a special layer that performs value iteration-like update to a recurrent memory. This can be implemented the same way you did in the POMDP assignment. Notes AssumptionsThe full list of assumptions is:* __Finite number of actions__: we enumerate all actions in `expand`.* __Episodic (finite) MDP__: while technically it works for infinite MDPs, we perform a rollout for $10^4$ steps. If you are knowingly infinite, please adjust `t_max` to something more reasonable.* __Deterministic MDP__: `Node` represents the single outcome of taking `self.action` in `self.parent`, and does not support the situation where taking an action in a state may lead to different rewards and next states.* __No discounted rewards__: we assume $\gamma=1$. If that isn't the case, you only need to change two lines in `rollout()` and use `my_qvalue = self.immediate_reward + gamma * child_qvalue` for `propagate()`.* __pickleable env__: won't work if e.g. your env is connected to a web-browser surfing the internet. For custom envs, you may need to modify get_snapshot/load_snapshot from `WithSnapshots`. On `get_best_leaf` and `expand` functionsThis MCTS implementation only selects leaf nodes for expansion.This doesn't break things down because `expand` adds all possible actions. Hence, all non-leaf nodes are by design fully expanded and shouldn't be selected.If you want to only add a few random action on each expand, you will also have to modify `get_best_leaf` to consider returning non-leafs. Rollout policyWe use a simple uniform policy for rollouts. This introduces a negative bias to good situations that can be messed up completely with random bad action. As a simple example, if you tend to rollout with uniform policy, you better don't use sharp knives and walk near cliffs.You can improve that by integrating a reinforcement _learning_ algorithm with a computationally light agent. You can even train this agent on optimal policy found by the tree search. Contributions* Reusing some code from 5vision [solution for deephack.RL](https://github.com/5vision/uct_atari), code by Mikhail Pavlov* Using some code from [this gist](https://gist.github.com/blole/dfebbec182e6b72ec16b66cc7e331110) References* [1] _Coulom R. (2007) Efficient Selectivity and Backup Operators in Monte-Carlo Tree Search. In: van den Herik H.J., Ciancarini P., Donkers H.H.L.M.. (eds) Computers and Games. CG 2006. Lecture Notes in Computer Science, vol 4630. Springer, Berlin, Heidelberg_* [2] _Kocsis L., Szepesvári C. (2006) Bandit Based Monte-Carlo Planning. In: Fürnkranz J., Scheffer T., Spiliopoulou M. (eds) Machine Learning: ECML 2006. ECML 2006. Lecture Notes in Computer Science, vol 4212. Springer, Berlin, Heidelberg_* [3] _Kocsis, Levente, Csaba Szepesvári, and Jan Willemson. "Improved monte-carlo search." Univ. Tartu, Estonia, Tech. Rep 1 (2006)._* [4] _C. B. Browne et al., "A Survey of Monte Carlo Tree Search Methods," in IEEE Transactions on Computational Intelligence and AI in Games, vol. 4, no. 1, pp. 1-43, March 2012, doi: 10.1109/TCIAIG.2012.2186810._
###Code
###Output
_____no_output_____
###Markdown
Seminar: Monte-carlo tree searchIn this seminar, we'll implement a vanilla MCTS planning and use it to solve some Gym envs.But before we do that, we first need to modify gym env to allow saving and loading game states to facilitate backtracking.
###Code
from gym.core import Wrapper
from pickle import dumps,loads
from collections import namedtuple
#a container for get_result function below. Works just like tuple, but prettier
ActionResult = namedtuple("action_result",("snapshot","observation","reward","is_done","info"))
class WithSnapshots(Wrapper):
"""
Creates a wrapper that supports saving and loading environemnt states.
Required for planning algorithms.
This class will have access to the core environment as self.env, e.g.:
- self.env.reset() #reset original env
- self.env.ale.cloneState() #make snapshot for atari. load with .restoreState()
- ...
You can also use reset, step and render directly for convenience.
- s, r, done, _ = self.step(action) #step, same as self.env.step(action)
- self.render(close=True) #close window, same as self.env.render(close=True)
"""
def get_snapshot(self):
"""
:returns: environment state that can be loaded with load_snapshot
Snapshots guarantee same env behaviour each time they are loaded.
Warning! Snapshots can be arbitrary things (strings, integers, json, tuples)
Don't count on them being pickle strings when implementing MCTS.
Developer Note: Make sure the object you return will not be affected by
anything that happens to the environment after it's saved.
You shouldn't, for example, return self.env.
In case of doubt, use pickle.dumps or deepcopy.
"""
self.render() #close popup windows since we can't pickle them
if self.unwrapped.viewer is not None:
self.unwrapped.viewer.close()
self.unwrapped.viewer = None
return dumps(self.env)
def load_snapshot(self,snapshot):
"""
Loads snapshot as current env state.
Should not change snapshot inplace (in case of doubt, deepcopy).
"""
assert not hasattr(self,"_monitor") or hasattr(self.env,"_monitor"), "can't backtrack while recording"
self.render(close=True) #close popup windows since we can't load into them
self.env = loads(snapshot)
def get_result(self,snapshot,action):
"""
A convenience function that
- loads snapshot,
- commits action via self.step,
- and takes snapshot again :)
:returns: next snapshot, next_observation, reward, is_done, info
Basically it returns next snapshot and everything that env.step would have returned.
"""
<your code here load,commit,take snapshot>
return ActionResult(<next_snapshot>, #fill in the variables
<next_observation>,
<reward>, <is_done>, <info>)
###Output
_____no_output_____
###Markdown
try out snapshots:
###Code
#make env
env = WithSnapshots(gym.make("CartPole-v0"))
env.reset()
n_actions = env.action_space.n
print("initial_state:")
plt.imshow(env.render('rgb_array'))
#create first snapshot
snap0 = env.get_snapshot()
#play without making snapshots (faster)
while True:
is_done = env.step(env.action_space.sample())[2]
if is_done:
print("Whoops! We died!")
break
print("final state:")
plt.imshow(env.render('rgb_array'))
plt.show()
#reload initial state
env.load_snapshot(snap0)
print("\n\nAfter loading snapshot")
plt.imshow(env.render('rgb_array'))
plt.show()
#get outcome (snapshot, observation, reward, is_done, info)
res = env.get_result(snap0,env.action_space.sample())
snap1, observation, reward = res[:3]
#second step
res2 = env.get_result(snap1,env.action_space.sample())
###Output
_____no_output_____
###Markdown
MCTS: Monte-Carlo tree searchIn this section, we'll implement the vanilla MCTS algorithm with UCB1-based node selection.We will start by implementing the `Node` class - a simple class that acts like MCTS node and supports some of the MCTS algorithm steps.This MCTS implementation makes some assumptions about the environment, you can find those _in the notes section at the end of the notebook_.
###Code
assert isinstance(env,WithSnapshots)
class Node:
""" a tree node for MCTS """
#metadata:
parent = None #parent Node
value_sum = 0. #sum of state values from all visits (numerator)
times_visited = 0 #counter of visits (denominator)
def __init__(self,parent,action,):
"""
Creates and empty node with no children.
Does so by commiting an action and recording outcome.
:param parent: parent Node
:param action: action to commit from parent Node
"""
self.parent = parent
self.action = action
self.children = set() #set of child nodes
#get action outcome and save it
res = env.get_result(parent.snapshot,action)
self.snapshot,self.observation,self.immediate_reward,self.is_done,_ = res
def is_leaf(self):
return len(self.children)==0
def is_root(self):
return self.parent is None
def get_mean_value(self):
return self.value_sum / self.times_visited if self.times_visited !=0 else 0
def ucb_score(self,scale=10,max_value=1e100):
"""
Computes ucb1 upper bound using current value and visit counts for node and it's parent.
:param scale: Multiplies upper bound by that. From hoeffding inequality, assumes reward range to be [0,scale].
:param max_value: a value that represents infinity (for unvisited nodes)
"""
if self.times_visited == 0:
return max_value
#compute ucb-1 additive component (to be added to mean value)
#hint: you can use self.parent.times_visited for N times node was considered,
# and self.times_visited for n times it was visited
U = <your code here>
return self.get_mean_value() + scale*U
#MCTS steps
def select_best_leaf(self):
"""
Picks the leaf with highest priority to expand
Does so by recursively picking nodes with best UCB-1 score until it reaches the leaf.
"""
if self.is_leaf():
return self
children = self.children
best_child = <select best child node in terms of node.ucb_score()>
return best_child.select_best_leaf()
def expand(self):
"""
Expands the current node by creating all possible child nodes.
Then returns one of those children.
"""
assert not self.is_done, "can't expand from terminal state"
for action in range(n_actions):
self.children.add(Node(self,action))
return self.select_best_leaf()
def rollout(self,t_max=10**4):
"""
Play the game from this state to the end (done) or for t_max steps.
On each step, pick action at random (hint: env.action_space.sample()).
Compute sum of rewards from current state till
Note 1: use env.action_space.sample() for random action
Note 2: if node is terminal (self.is_done is True), just return 0
"""
#set env into the appropriate state
env.load_snapshot(self.snapshot)
obs = self.observation
is_done = self.is_done
<your code here - rollout and compute reward>
return rollout_reward
def propagate(self,child_value):
"""
Uses child value (sum of rewards) to update parents recursively.
"""
#compute node value
my_value = self.immediate_reward + child_value
#update value_sum and times_visited
self.value_sum+=my_value
self.times_visited+=1
#propagate upwards
if not self.is_root():
self.parent.propagate(my_value)
def safe_delete(self):
"""safe delete to prevent memory leak in some python versions"""
del self.parent
for child in self.children:
child.safe_delete()
del child
class Root(Node):
def __init__(self,snapshot,observation):
"""
creates special node that acts like tree root
:snapshot: snapshot (from env.get_snapshot) to start planning from
:observation: last environment observation
"""
self.parent = self.action = None
self.children = set() #set of child nodes
#root: load snapshot and observation
self.snapshot = snapshot
self.observation = observation
self.immediate_reward = 0
self.is_done=False
@staticmethod
def from_node(node):
"""initializes node as root"""
root = Root(node.snapshot,node.observation)
#copy data
copied_fields = ["value_sum","times_visited","children","is_done"]
for field in copied_fields:
setattr(root,field,getattr(node,field))
return root
###Output
_____no_output_____
###Markdown
Main MCTS loopWith all we implemented, MCTS boils down to a trivial piece of code.
###Code
def plan_mcts(root,n_iters=10):
"""
builds tree with monte-carlo tree search for n_iters iterations
:param root: tree node to plan from
:param n_iters: how many select-expand-simulate-propagete loops to make
"""
for _ in range(n_iters):
node = <select best leaf>
if node.is_done:
node.propagate(0)
else: #node is not terminal
<expand-simulate-propagate loop>
###Output
_____no_output_____
###Markdown
Plan and executeIn this section, we use the MCTS implementation to find optimal policy.
###Code
root_observation = env.reset()
root_snapshot = env.get_snapshot()
root = Root(root_snapshot,root_observation)
#plan from root:
plan_mcts(root,n_iters=1000)
from IPython.display import clear_output
from itertools import count
from gym.wrappers import Monitor
total_reward = 0 #sum of rewards
test_env = loads(root_snapshot) #env used to show progress
for i in count():
#get best child
best_child = <select child with highest mean reward>
#take action
s,r,done,_ = test_env.step(best_child.action)
#show image
clear_output(True)
plt.title("step %i"%i)
plt.imshow(test_env.render('rgb_array'))
plt.show()
total_reward += r
if done:
print("Finished with reward = ",total_reward)
break
#discard unrealized part of the tree [because not every child matters :(]
for child in root.children:
if child != best_child:
child.safe_delete()
#declare best child a new root
root = Root.from_node(best_child)
assert not root.is_leaf(), "We ran out of tree! Need more planning! Try growing tree right inside the loop."
#you may want to expand tree here
#<your code here>
###Output
_____no_output_____
###Markdown
Submit to Coursera
###Code
from submit import submit_mcts
submit_mcts(total_reward, <EMAIL>, <TOKEN>)
###Output
_____no_output_____
###Markdown
Seminar: Monte-carlo tree searchIn this seminar, we'll implement a vanilla MCTS planning and use it to solve some Gym envs.But before we do that, we first need to modify gym env to allow saving and loading game states to facilitate backtracking.
###Code
from collections import namedtuple
from pickle import dumps, loads
from gym.core import Wrapper
#a container for get_result function below. Works just like tuple, but prettier
ActionResult = namedtuple("action_result", ("snapshot", "observation", "reward", "is_done", "info"))
class WithSnapshots(Wrapper):
"""
Creates a wrapper that supports saving and loading environemnt states.
Required for planning algorithms.
This class will have access to the core environment as self.env, e.g.:
- self.env.reset() #reset original env
- self.env.ale.cloneState() #make snapshot for atari. load with .restoreState()
- ...
You can also use reset, step and render directly for convenience.
- s, r, done, _ = self.step(action) #step, same as self.env.step(action)
- self.render(close=True) #close window, same as self.env.render(close=True)
"""
def get_snapshot(self):
"""
:returns: environment state that can be loaded with load_snapshot
Snapshots guarantee same env behaviour each time they are loaded.
Warning! Snapshots can be arbitrary things (strings, integers, json, tuples)
Don't count on them being pickle strings when implementing MCTS.
Developer Note: Make sure the object you return will not be affected by
anything that happens to the environment after it's saved.
You shouldn't, for example, return self.env.
In case of doubt, use pickle.dumps or deepcopy.
"""
self.render() #close popup windows since we can't pickle them
if self.unwrapped.viewer is not None:
self.unwrapped.viewer.close()
self.unwrapped.viewer = None
return dumps(self.env)
def load_snapshot(self, snapshot):
"""
Loads snapshot as current env state.
Should not change snapshot inplace (in case of doubt, deepcopy).
"""
assert not hasattr(self, "_monitor") or hasattr(self.env, "_monitor"), "can't backtrack while recording"
self.close() # close popup windows since we can't load into them
self.env = loads(snapshot)
def get_result(self, snapshot, action):
"""
A convenience function that
- loads snapshot,
- commits action via self.step,
- and takes snapshot again :)
:returns: next snapshot, next_observation, reward, is_done, info
Basically it returns next snapshot and everything that env.step would have returned.
"""
<your code here load,commit,take snapshot>
return ActionResult(<next_snapshot>, #fill in the variables
<next_observation>,
<reward>, <is_done>, <info>)
###Output
_____no_output_____
###Markdown
try out snapshots:
###Code
#make env
env = WithSnapshots(gym.make("CartPole-v0"))
env.reset()
n_actions = env.action_space.n
print("initial_state:")
plt.imshow(env.render('rgb_array'))
#create first snapshot
snap0 = env.get_snapshot()
#play without making snapshots (faster)
while True:
is_done = env.step(env.action_space.sample())[2]
if is_done:
print("Whoops! We died!")
break
print("final state:")
plt.imshow(env.render('rgb_array'))
plt.show()
#reload initial state
env.load_snapshot(snap0)
print("\n\nAfter loading snapshot")
plt.imshow(env.render('rgb_array'))
plt.show()
#get outcome (snapshot, observation, reward, is_done, info)
res = env.get_result(snap0,env.action_space.sample())
snap1, observation, reward = res[:3]
#second step
res2 = env.get_result(snap1,env.action_space.sample())
###Output
_____no_output_____
###Markdown
MCTS: Monte-Carlo tree searchIn this section, we'll implement the vanilla MCTS algorithm with UCB1-based node selection.We will start by implementing the `Node` class - a simple class that acts like MCTS node and supports some of the MCTS algorithm steps.This MCTS implementation makes some assumptions about the environment, you can find those _in the notes section at the end of the notebook_.
###Code
assert isinstance(env,WithSnapshots)
class Node:
""" a tree node for MCTS """
#metadata:
parent = None #parent Node
value_sum = 0. #sum of state values from all visits (numerator)
times_visited = 0 #counter of visits (denominator)
def __init__(self,parent,action,):
"""
Creates and empty node with no children.
Does so by commiting an action and recording outcome.
:param parent: parent Node
:param action: action to commit from parent Node
"""
self.parent = parent
self.action = action
self.children = set() #set of child nodes
#get action outcome and save it
res = env.get_result(parent.snapshot,action)
self.snapshot,self.observation,self.immediate_reward,self.is_done,_ = res
def is_leaf(self):
return len(self.children)==0
def is_root(self):
return self.parent is None
def get_mean_value(self):
return self.value_sum / self.times_visited if self.times_visited !=0 else 0
def ucb_score(self,scale=10,max_value=1e100):
"""
Computes ucb1 upper bound using current value and visit counts for node and it's parent.
:param scale: Multiplies upper bound by that. From hoeffding inequality, assumes reward range to be [0,scale].
:param max_value: a value that represents infinity (for unvisited nodes)
"""
if self.times_visited == 0:
return max_value
#compute ucb-1 additive component (to be added to mean value)
#hint: you can use self.parent.times_visited for N times node was considered,
# and self.times_visited for n times it was visited
U = <your code here>
return self.get_mean_value() + scale*U
#MCTS steps
def select_best_leaf(self):
"""
Picks the leaf with highest priority to expand
Does so by recursively picking nodes with best UCB-1 score until it reaches the leaf.
"""
if self.is_leaf():
return self
children = self.children
best_child = <select best child node in terms of node.ucb_score()>
return best_child.select_best_leaf()
def expand(self):
"""
Expands the current node by creating all possible child nodes.
Then returns one of those children.
"""
assert not self.is_done, "can't expand from terminal state"
for action in range(n_actions):
self.children.add(Node(self,action))
return self.select_best_leaf()
def rollout(self,t_max=10**4):
"""
Play the game from this state to the end (done) or for t_max steps.
On each step, pick action at random (hint: env.action_space.sample()).
Compute sum of rewards from current state till
Note 1: use env.action_space.sample() for random action
Note 2: if node is terminal (self.is_done is True), just return 0
"""
#set env into the appropriate state
env.load_snapshot(self.snapshot)
obs = self.observation
is_done = self.is_done
<your code here - rollout and compute reward>
return rollout_reward
def propagate(self,child_value):
"""
Uses child value (sum of rewards) to update parents recursively.
"""
#compute node value
my_value = self.immediate_reward + child_value
#update value_sum and times_visited
self.value_sum+=my_value
self.times_visited+=1
#propagate upwards
if not self.is_root():
self.parent.propagate(my_value)
def safe_delete(self):
"""safe delete to prevent memory leak in some python versions"""
del self.parent
for child in self.children:
child.safe_delete()
del child
class Root(Node):
def __init__(self,snapshot,observation):
"""
creates special node that acts like tree root
:snapshot: snapshot (from env.get_snapshot) to start planning from
:observation: last environment observation
"""
self.parent = self.action = None
self.children = set() #set of child nodes
#root: load snapshot and observation
self.snapshot = snapshot
self.observation = observation
self.immediate_reward = 0
self.is_done=False
@staticmethod
def from_node(node):
"""initializes node as root"""
root = Root(node.snapshot,node.observation)
#copy data
copied_fields = ["value_sum","times_visited","children","is_done"]
for field in copied_fields:
setattr(root,field,getattr(node,field))
return root
###Output
_____no_output_____
###Markdown
Main MCTS loopWith all we implemented, MCTS boils down to a trivial piece of code.
###Code
def plan_mcts(root,n_iters=10):
"""
builds tree with monte-carlo tree search for n_iters iterations
:param root: tree node to plan from
:param n_iters: how many select-expand-simulate-propagete loops to make
"""
for _ in range(n_iters):
node = <select best leaf>
if node.is_done:
node.propagate(0)
else: #node is not terminal
<expand-simulate-propagate loop>
###Output
_____no_output_____
###Markdown
Plan and executeIn this section, we use the MCTS implementation to find optimal policy.
###Code
root_observation = env.reset()
root_snapshot = env.get_snapshot()
root = Root(root_snapshot,root_observation)
#plan from root:
plan_mcts(root,n_iters=1000)
from IPython.display import clear_output
from itertools import count
from gym.wrappers import Monitor
total_reward = 0 #sum of rewards
test_env = loads(root_snapshot) #env used to show progress
for i in count():
#get best child
best_child = <select child with highest mean reward>
#take action
s,r,done,_ = test_env.step(best_child.action)
#show image
clear_output(True)
plt.title("step %i"%i)
plt.imshow(test_env.render('rgb_array'))
plt.show()
total_reward += r
if done:
print("Finished with reward = ",total_reward)
break
#discard unrealized part of the tree [because not every child matters :(]
for child in root.children:
if child != best_child:
child.safe_delete()
#declare best child a new root
root = Root.from_node(best_child)
assert not root.is_leaf(), "We ran out of tree! Need more planning! Try growing tree right inside the loop."
#you may want to expand tree here
#<your code here>
###Output
_____no_output_____
###Markdown
Submit to Coursera
###Code
from submit import submit_mcts
submit_mcts(total_reward, <EMAIL>, <TOKEN>)
###Output
_____no_output_____
###Markdown
Seminar: Monte-carlo tree searchIn this seminar, we'll implement a vanilla MCTS planning and use it to solve some Gym envs.But before we do that, we first need to modify gym env to allow saving and loading game states to facilitate backtracking.
###Code
from collections import namedtuple
from pickle import dumps, loads
from gym.core import Wrapper
# a container for get_result function below. Works just like tuple, but prettier
ActionResult = namedtuple(
"action_result", ("snapshot", "observation", "reward", "is_done", "info"))
class WithSnapshots(Wrapper):
"""
Creates a wrapper that supports saving and loading environemnt states.
Required for planning algorithms.
This class will have access to the core environment as self.env, e.g.:
- self.env.reset() #reset original env
- self.env.ale.cloneState() #make snapshot for atari. load with .restoreState()
- ...
You can also use reset() and step() directly for convenience.
- s = self.reset() # same as self.env.reset()
- s, r, done, _ = self.step(action) # same as self.env.step(action)
Note that while you may use self.render(), it will spawn a window that cannot be pickled.
Thus, you will need to call self.close() before pickling will work again.
"""
def get_snapshot(self, render=False):
"""
:returns: environment state that can be loaded with load_snapshot
Snapshots guarantee same env behaviour each time they are loaded.
Warning! Snapshots can be arbitrary things (strings, integers, json, tuples)
Don't count on them being pickle strings when implementing MCTS.
Developer Note: Make sure the object you return will not be affected by
anything that happens to the environment after it's saved.
You shouldn't, for example, return self.env.
In case of doubt, use pickle.dumps or deepcopy.
"""
if render:
self.render() # close popup windows since we can't pickle them
self.close()
if self.unwrapped.viewer is not None:
self.unwrapped.viewer.close()
self.unwrapped.viewer = None
return dumps(self.env)
def load_snapshot(self, snapshot, render=False):
"""
Loads snapshot as current env state.
Should not change snapshot inplace (in case of doubt, deepcopy).
"""
assert not hasattr(self, "_monitor") or hasattr(
self.env, "_monitor"), "can't backtrack while recording"
if render:
self.render() # close popup windows since we can't load into them
self.close()
self.env = loads(snapshot)
def get_result(self, snapshot, action):
"""
A convenience function that
- loads snapshot,
- commits action via self.step,
- and takes snapshot again :)
:returns: next snapshot, next_observation, reward, is_done, info
Basically it returns next snapshot and everything that env.step would have returned.
"""
<your code here load, commit, take snapshot >
return ActionResult(< next_snapshot > , #fill in the variables
< next_observation > ,
< reward > , < is_done > , < info > )
###Output
_____no_output_____
###Markdown
try out snapshots:
###Code
# make env
env = WithSnapshots(gym.make("CartPole-v0"))
env.reset()
n_actions = env.action_space.n
print("initial_state:")
plt.imshow(env.render('rgb_array'))
env.close()
# create first snapshot
snap0 = env.get_snapshot()
# play without making snapshots (faster)
while True:
is_done = env.step(env.action_space.sample())[2]
if is_done:
print("Whoops! We died!")
break
print("final state:")
plt.imshow(env.render('rgb_array'))
env.close()
# reload initial state
env.load_snapshot(snap0)
print("\n\nAfter loading snapshot")
plt.imshow(env.render('rgb_array'))
env.close()
# get outcome (snapshot, observation, reward, is_done, info)
res = env.get_result(snap0, env.action_space.sample())
snap1, observation, reward = res[:3]
# second step
res2 = env.get_result(snap1, env.action_space.sample())
###Output
_____no_output_____
###Markdown
MCTS: Monte-Carlo tree searchIn this section, we'll implement the vanilla MCTS algorithm with UCB1-based node selection.We will start by implementing the `Node` class - a simple class that acts like MCTS node and supports some of the MCTS algorithm steps.This MCTS implementation makes some assumptions about the environment, you can find those _in the notes section at the end of the notebook_.
###Code
assert isinstance(env,WithSnapshots)
class Node:
""" a tree node for MCTS """
#metadata:
parent = None #parent Node
value_sum = 0. #sum of state values from all visits (numerator)
times_visited = 0 #counter of visits (denominator)
def __init__(self,parent,action,):
"""
Creates and empty node with no children.
Does so by commiting an action and recording outcome.
:param parent: parent Node
:param action: action to commit from parent Node
"""
self.parent = parent
self.action = action
self.children = set() #set of child nodes
#get action outcome and save it
res = env.get_result(parent.snapshot,action)
self.snapshot,self.observation,self.immediate_reward,self.is_done,_ = res
def is_leaf(self):
return len(self.children)==0
def is_root(self):
return self.parent is None
def get_mean_value(self):
return self.value_sum / self.times_visited if self.times_visited !=0 else 0
def ucb_score(self,scale=10,max_value=1e100):
"""
Computes ucb1 upper bound using current value and visit counts for node and it's parent.
:param scale: Multiplies upper bound by that. From hoeffding inequality, assumes reward range to be [0,scale].
:param max_value: a value that represents infinity (for unvisited nodes)
"""
if self.times_visited == 0:
return max_value
#compute ucb-1 additive component (to be added to mean value)
#hint: you can use self.parent.times_visited for N times node was considered,
# and self.times_visited for n times it was visited
U = <your code here>
return self.get_mean_value() + scale*U
#MCTS steps
def select_best_leaf(self):
"""
Picks the leaf with highest priority to expand
Does so by recursively picking nodes with best UCB-1 score until it reaches the leaf.
"""
if self.is_leaf():
return self
children = self.children
best_child = <select best child node in terms of node.ucb_score()>
return best_child.select_best_leaf()
def expand(self):
"""
Expands the current node by creating all possible child nodes.
Then returns one of those children.
"""
assert not self.is_done, "can't expand from terminal state"
for action in range(n_actions):
self.children.add(Node(self,action))
return self.select_best_leaf()
def rollout(self,t_max=10**4):
"""
Play the game from this state to the end (done) or for t_max steps.
On each step, pick action at random (hint: env.action_space.sample()).
Compute sum of rewards from current state till
Note 1: use env.action_space.sample() for random action
Note 2: if node is terminal (self.is_done is True), just return 0
"""
#set env into the appropriate state
env.load_snapshot(self.snapshot)
obs = self.observation
is_done = self.is_done
<your code here - rollout and compute reward>
return rollout_reward
def propagate(self,child_value):
"""
Uses child value (sum of rewards) to update parents recursively.
"""
#compute node value
my_value = self.immediate_reward + child_value
#update value_sum and times_visited
self.value_sum+=my_value
self.times_visited+=1
#propagate upwards
if not self.is_root():
self.parent.propagate(my_value)
def safe_delete(self):
"""safe delete to prevent memory leak in some python versions"""
del self.parent
for child in self.children:
child.safe_delete()
del child
class Root(Node):
def __init__(self,snapshot,observation):
"""
creates special node that acts like tree root
:snapshot: snapshot (from env.get_snapshot) to start planning from
:observation: last environment observation
"""
self.parent = self.action = None
self.children = set() #set of child nodes
#root: load snapshot and observation
self.snapshot = snapshot
self.observation = observation
self.immediate_reward = 0
self.is_done=False
@staticmethod
def from_node(node):
"""initializes node as root"""
root = Root(node.snapshot,node.observation)
#copy data
copied_fields = ["value_sum","times_visited","children","is_done"]
for field in copied_fields:
setattr(root,field,getattr(node,field))
return root
###Output
_____no_output_____
###Markdown
Main MCTS loopWith all we implemented, MCTS boils down to a trivial piece of code.
###Code
def plan_mcts(root,n_iters=10):
"""
builds tree with monte-carlo tree search for n_iters iterations
:param root: tree node to plan from
:param n_iters: how many select-expand-simulate-propagete loops to make
"""
for _ in range(n_iters):
node = <select best leaf>
if node.is_done:
node.propagate(0)
else: #node is not terminal
<expand-simulate-propagate loop>
###Output
_____no_output_____
###Markdown
Plan and executeIn this section, we use the MCTS implementation to find optimal policy.
###Code
env = WithSnapshots(gym.make("CartPole-v0"))
root_observation = env.reset()
root_snapshot = env.get_snapshot()
root = Root(root_snapshot, root_observation)
#plan from root:
plan_mcts(root,n_iters=1000)
from IPython.display import clear_output
from itertools import count
from gym.wrappers import Monitor
total_reward = 0 #sum of rewards
test_env = loads(root_snapshot) #env used to show progress
for i in count():
#get best child
best_child = <select child with highest mean reward>
#take action
s,r,done,_ = test_env.step(best_child.action)
#show image
clear_output(True)
plt.title("step %i"%i)
plt.imshow(test_env.render('rgb_array'))
plt.show()
total_reward += r
if done:
print("Finished with reward = ",total_reward)
break
#discard unrealized part of the tree [because not every child matters :(]
for child in root.children:
if child != best_child:
child.safe_delete()
#declare best child a new root
root = Root.from_node(best_child)
assert not root.is_leaf(), "We ran out of tree! Need more planning! Try growing tree right inside the loop."
#you may want to expand tree here
#<your code here>
###Output
_____no_output_____
###Markdown
Submit to Coursera
###Code
from submit import submit_mcts
submit_mcts(total_reward, <EMAIL>, <TOKEN>)
###Output
_____no_output_____
###Markdown
Seminar: Monte-carlo tree search (5 pts)Monte Carlo tree search (MCTS) is a heuristic search algorithm, which shows cool results in challenging domains such as Go and chess. The algorithm builds a search tree, iteratively traverses it, and evaluates its nodes using a Monte-Carlo simulation.In this seminar, we'll implement a MCTS([[1]](1), [[2]](2)) planning and use it to solve some Gym envs.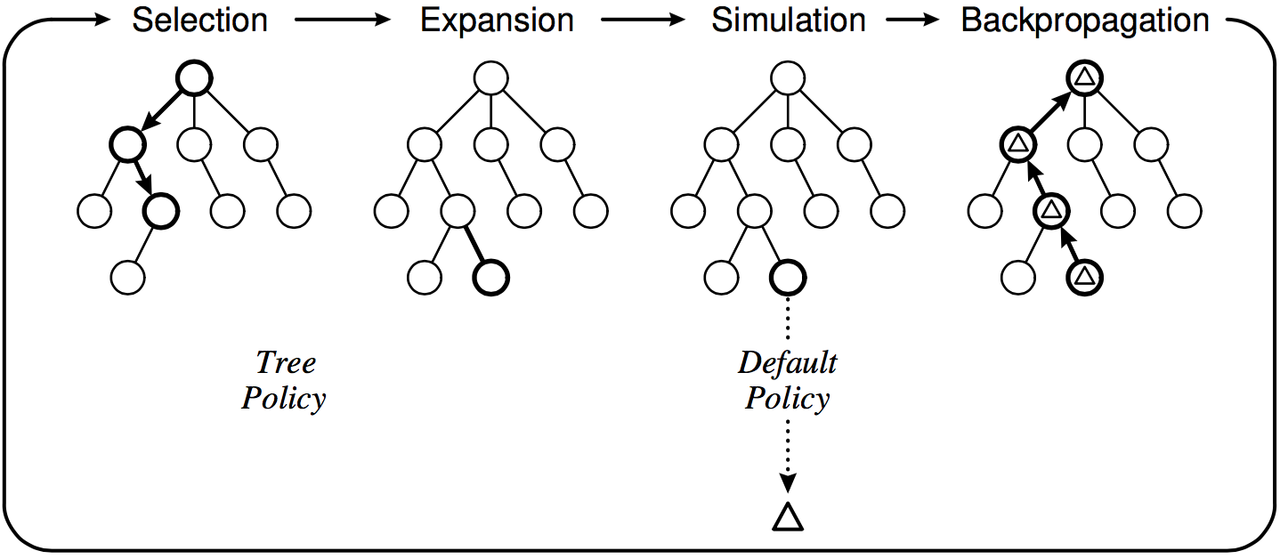 __How it works?__We just start with an empty tree and expand it. There are several common procedures.__1) Selection__Starting from the root, recursively select the node that corresponds to the tree policy. There are several options for tree policies, which we saw earlier as exploration strategies: epsilon-greedy, Thomson sampling, UCB-1. It was shown that in MCTS, UCB-1 achieves a good result. Further, we will consider the one, but you can try to use others.Following the UCB-1 tree policy, we will choose an action that, on one hand, we expect to have the highest return, and on the other hand, we haven't explored much.$$\DeclareMathOperator*{\argmax}{arg\,max}$$$$\dot{a} = \argmax_{a} \dot{Q}(s, a)$$$$\dot{Q}(s, a) = Q(s, a) + C_p \sqrt{\frac{2 \log {N}}{n_a}}$$where: - $N$ - number of times we have visited state $s$,- $n_a$ - number of times we have taken action $a$,- $C_p$ - exploration balance parameter, which is performed between exploration and exploitation. Using Hoeffding inequality for rewards $R \in [0,1]$ it can be shown [[3]](3) that optimal $C_p = 1/\sqrt{2}$. For rewards outside this range, the parameter should be tuned. We'll be using 10, but you can experiment with other values.__2) Expansion__After the selection procedure, we can achieve a leaf node or node in which we don't complete actions. In this case, we expand the tree by feasible actions and get new state nodes. __3) Simulation__How we can estimate node Q-values? The idea is to estimate action values for a given _rollout policy_ by averaging the return of many simulated trajectories from the current node. Simply, we can play with random or some special policy or use some model that can estimate it.__4) Backpropagation__The reward of the last simulation is backed up through the traversed nodes and propagates Q-value estimations, upwards to the root.$$Q({\text{parent}}, a) = r + \gamma \cdot Q({\text{child}}, a)$$There are a lot modifications of MCTS, more details about it you can find in this paper [[4]](4)
###Code
import sys, os
if 'google.colab' in sys.modules and not os.path.exists('.setup_complete'):
!wget -q https://raw.githubusercontent.com/yandexdataschool/Practical_RL/master/setup_colab.sh -O- | bash
!wget -q https://raw.githubusercontent.com/yandexdataschool/Practical_RL/coursera/grading.py -O ../grading.py
!wget -q https://raw.githubusercontent.com/yandexdataschool/Practical_RL/coursera/week6_outro/submit.py
!touch .setup_complete
# This code creates a virtual display to draw game images on.
# It will have no effect if your machine has a monitor.
if type(os.environ.get("DISPLAY")) is not str or len(os.environ.get("DISPLAY")) == 0:
!bash ../xvfb start
os.environ['DISPLAY'] = ':1'
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
###Output
_____no_output_____
###Markdown
---But before we do that, we first need to make a wrapper for Gym environments to allow saving and loading game states to facilitate backtracking.
###Code
import gym
from gym.core import Wrapper
from pickle import dumps, loads
from collections import namedtuple
# a container for get_result function below. Works just like tuple, but prettier
ActionResult = namedtuple(
"action_result", ("snapshot", "observation", "reward", "is_done", "info"))
class WithSnapshots(Wrapper):
"""
Creates a wrapper that supports saving and loading environemnt states.
Required for planning algorithms.
This class will have access to the core environment as self.env, e.g.:
- self.env.reset() #reset original env
- self.env.ale.cloneState() #make snapshot for atari. load with .restoreState()
- ...
You can also use reset() and step() directly for convenience.
- s = self.reset() # same as self.env.reset()
- s, r, done, _ = self.step(action) # same as self.env.step(action)
Note that while you may use self.render(), it will spawn a window that cannot be pickled.
Thus, you will need to call self.close() before pickling will work again.
"""
def get_snapshot(self, render=False):
"""
:returns: environment state that can be loaded with load_snapshot
Snapshots guarantee same env behaviour each time they are loaded.
Warning! Snapshots can be arbitrary things (strings, integers, json, tuples)
Don't count on them being pickle strings when implementing MCTS.
Developer Note: Make sure the object you return will not be affected by
anything that happens to the environment after it's saved.
You shouldn't, for example, return self.env.
In case of doubt, use pickle.dumps or deepcopy.
"""
if render:
self.render() # close popup windows since we can't pickle them
self.close()
if self.unwrapped.viewer is not None:
self.unwrapped.viewer.close()
self.unwrapped.viewer = None
return dumps(self.env)
def load_snapshot(self, snapshot, render=False):
"""
Loads snapshot as current env state.
Should not change snapshot inplace (in case of doubt, deepcopy).
"""
assert not hasattr(self, "_monitor") or hasattr(
self.env, "_monitor"), "can't backtrack while recording"
if render:
self.render() # close popup windows since we can't load into them
self.close()
self.env = loads(snapshot)
def get_result(self, snapshot, action):
"""
A convenience function that
- loads snapshot,
- commits action via self.step,
- and takes snapshot again :)
:returns: next snapshot, next_observation, reward, is_done, info
Basically it returns next snapshot and everything that env.step would have returned.
"""
<YOUR CODE: load, commit, take snapshot>
return ActionResult(
<YOUR CODE: next_snapshot>, # fill in the variables
<YOUR CODE: next_observation>,
<YOUR CODE: reward>,
<YOUR CODE: is_done>,
<YOUR CODE: info>,
)
###Output
_____no_output_____
###Markdown
Try out snapshots:Let`s check our wrapper. At first, reset environment and save it, further randomly play some actions and restore our environment from the snapshot. It should be the same as our previous initial state.
###Code
# make env
env = WithSnapshots(gym.make("CartPole-v0"))
env.reset()
n_actions = env.action_space.n
print("initial_state:")
plt.imshow(env.render('rgb_array'))
env.close()
# create first snapshot
snap0 = env.get_snapshot()
# play without making snapshots (faster)
while True:
is_done = env.step(env.action_space.sample())[2]
if is_done:
print("Whoops! We died!")
break
print("final state:")
plt.imshow(env.render('rgb_array'))
env.close()
# reload initial state
env.load_snapshot(snap0)
print("\n\nAfter loading snapshot")
plt.imshow(env.render('rgb_array'))
env.close()
# get outcome (snapshot, observation, reward, is_done, info)
res = env.get_result(snap0, env.action_space.sample())
snap1, observation, reward = res[:3]
# second step
res2 = env.get_result(snap1, env.action_space.sample())
###Output
_____no_output_____
###Markdown
MCTS: Monte-Carlo tree searchWe will start by implementing the `Node` class - a simple class that acts like MCTS node and supports some of the MCTS algorithm steps.This MCTS implementation makes some assumptions about the environment, you can find those _in the notes section at the end of the notebook_.
###Code
assert isinstance(env, WithSnapshots)
class Node:
"""A tree node for MCTS.
Each Node corresponds to the result of performing a particular action (self.action)
in a particular state (self.parent), and is essentially one arm in the multi-armed bandit that
we model in that state."""
# metadata:
parent = None # parent Node
qvalue_sum = 0. # sum of Q-values from all visits (numerator)
times_visited = 0 # counter of visits (denominator)
def __init__(self, parent, action):
"""
Creates and empty node with no children.
Does so by commiting an action and recording outcome.
:param parent: parent Node
:param action: action to commit from parent Node
"""
self.parent = parent
self.action = action
self.children = set() # set of child nodes
# get action outcome and save it
res = env.get_result(parent.snapshot, action)
self.snapshot, self.observation, self.immediate_reward, self.is_done, _ = res
def is_leaf(self):
return len(self.children) == 0
def is_root(self):
return self.parent is None
def get_qvalue_estimate(self):
return self.qvalue_sum / self.times_visited if self.times_visited != 0 else 0
def ucb_score(self, scale=10, max_value=1e100):
"""
Computes ucb1 upper bound using current value and visit counts for node and it's parent.
:param scale: Multiplies upper bound by that. From Hoeffding inequality,
assumes reward range to be [0, scale].
:param max_value: a value that represents infinity (for unvisited nodes).
"""
if self.times_visited == 0:
return max_value
# compute ucb-1 additive component (to be added to mean value)
# hint: you can use self.parent.times_visited for N times node was considered,
# and self.times_visited for n times it was visited
U = <YOUR CODE>
return self.get_qvalue_estimate() + scale * U
# MCTS steps
def select_best_leaf(self):
"""
Picks the leaf with the highest priority to expand.
Does so by recursively picking nodes with the best UCB-1 score until it reaches a leaf.
"""
if self.is_leaf():
return self
children = self.children
# Select the child node with the highest UCB score. You might want to implement some heuristics
# to break ties in a smart way, although CartPole should work just fine without them.
best_child = <YOUR CODE>
return best_child.select_best_leaf()
def expand(self):
"""
Expands the current node by creating all possible child nodes.
Then returns one of those children.
"""
assert not self.is_done, "can't expand from terminal state"
for action in range(n_actions):
self.children.add(Node(self, action))
# If you have implemented any heuristics in select_best_leaf(), they will be used here.
# Otherwise, this is equivalent to picking some undefined newly created child node.
return self.select_best_leaf()
def rollout(self, t_max=10**4):
"""
Play the game from this state to the end (done) or for t_max steps.
On each step, pick action at random (hint: env.action_space.sample()).
Compute sum of rewards from the current state until the end of the episode.
Note 1: use env.action_space.sample() for picking a random action.
Note 2: if the node is terminal (self.is_done is True), just return self.immediate_reward.
"""
# set env into the appropriate state
env.load_snapshot(self.snapshot)
obs = self.observation
is_done = self.is_done
<YOUR CODE: perform rollout and compute reward>
return rollout_reward
def propagate(self, child_qvalue):
"""
Uses child Q-value (sum of rewards) to update parents recursively.
"""
# compute node Q-value
my_qvalue = self.immediate_reward + child_qvalue
# update qvalue_sum and times_visited
self.qvalue_sum += my_qvalue
self.times_visited += 1
# propagate upwards
if not self.is_root():
self.parent.propagate(my_qvalue)
def safe_delete(self):
"""safe delete to prevent memory leak in some python versions"""
del self.parent
for child in self.children:
child.safe_delete()
del child
class Root(Node):
def __init__(self, snapshot, observation):
"""
creates special node that acts like tree root
:snapshot: snapshot (from env.get_snapshot) to start planning from
:observation: last environment observation
"""
self.parent = self.action = None
self.children = set() # set of child nodes
# root: load snapshot and observation
self.snapshot = snapshot
self.observation = observation
self.immediate_reward = 0
self.is_done = False
@staticmethod
def from_node(node):
"""initializes node as root"""
root = Root(node.snapshot, node.observation)
# copy data
copied_fields = ["qvalue_sum", "times_visited", "children", "is_done"]
for field in copied_fields:
setattr(root, field, getattr(node, field))
return root
###Output
_____no_output_____
###Markdown
Main MCTS loopWith all we implemented, MCTS boils down to a trivial piece of code.
###Code
def plan_mcts(root, n_iters=10):
"""
builds tree with monte-carlo tree search for n_iters iterations
:param root: tree node to plan from
:param n_iters: how many select-expand-simulate-propagete loops to make
"""
for _ in range(n_iters):
node = <YOUR CODE: select best leaf>
if node.is_done:
# All rollouts from a terminal node are empty, and thus have 0 reward.
node.propagate(0)
else:
# Expand the best leaf. Perform a rollout from it. Propagate the results upwards.
# Note that here you have some leeway in choosing where to propagate from.
# Any reasonable choice should work.
<YOUR CODE>
###Output
_____no_output_____
###Markdown
Plan and executeLet's use our MCTS implementation to find the optimal policy.
###Code
env = WithSnapshots(gym.make("CartPole-v0"))
root_observation = env.reset()
root_snapshot = env.get_snapshot()
root = Root(root_snapshot, root_observation)
# plan from root:
plan_mcts(root, n_iters=1000)
# import copy
# saved_root = copy.deepcopy(root)
# root = saved_root
from IPython.display import clear_output
from itertools import count
from gym.wrappers import Monitor
total_reward = 0 # sum of rewards
test_env = loads(root_snapshot) # env used to show progress
for i in count():
# get best child
best_child = <YOUR CODE: select child with the highest mean reward>
# take action
s, r, done, _ = test_env.step(best_child.action)
# show image
clear_output(True)
plt.title("step %i" % i)
plt.imshow(test_env.render('rgb_array'))
plt.show()
total_reward += r
if done:
print("Finished with reward = ", total_reward)
break
# discard unrealized part of the tree [because not every child matters :(]
for child in root.children:
if child != best_child:
child.safe_delete()
# declare best child a new root
root = Root.from_node(best_child)
assert not root.is_leaf(), \
"We ran out of tree! Need more planning! Try growing the tree right inside the loop."
# You may want to run more planning here
# <YOUR CODE>
from submit import submit_mcts
submit_mcts(total_reward, '[email protected]', 'YourAssignmentToken')
###Output
_____no_output_____
###Markdown
Seminar: Monte-carlo tree searchIn this seminar, we'll implement a vanilla MCTS planning and use it to solve some Gym envs.But before we do that, we first need to modify gym env to allow saving and loading game states to facilitate backtracking.
###Code
from collections import namedtuple
from pickle import dumps, loads
from gym.core import Wrapper
#a container for get_result function below. Works just like tuple, but prettier
ActionResult = namedtuple("action_result", ("snapshot", "observation", "reward", "is_done", "info"))
class WithSnapshots(Wrapper):
"""
Creates a wrapper that supports saving and loading environemnt states.
Required for planning algorithms.
This class will have access to the core environment as self.env, e.g.:
- self.env.reset() #reset original env
- self.env.ale.cloneState() #make snapshot for atari. load with .restoreState()
- ...
You can also use reset, step and render directly for convenience.
- s, r, done, _ = self.step(action) #step, same as self.env.step(action)
- self.render(close=True) #close window, same as self.env.render(close=True)
"""
def get_snapshot(self):
"""
:returns: environment state that can be loaded with load_snapshot
Snapshots guarantee same env behaviour each time they are loaded.
Warning! Snapshots can be arbitrary things (strings, integers, json, tuples)
Don't count on them being pickle strings when implementing MCTS.
Developer Note: Make sure the object you return will not be affected by
anything that happens to the environment after it's saved.
You shouldn't, for example, return self.env.
In case of doubt, use pickle.dumps or deepcopy.
"""
self.render() #close popup windows since we can't pickle them
if self.unwrapped.viewer is not None:
self.unwrapped.viewer.close()
self.unwrapped.viewer = None
return dumps(self.env)
def load_snapshot(self, snapshot):
"""
Loads snapshot as current env state.
Should not change snapshot inplace (in case of doubt, deepcopy).
"""
assert not hasattr(self, "_monitor") or hasattr(self.env, "_monitor"), "can't backtrack while recording"
self.close() # close popup windows since we can't load into them
self.env = loads(snapshot)
def get_result(self, snapshot, action):
"""
A convenience function that
- loads snapshot,
- commits action via self.step,
- and takes snapshot again :)
:returns: next snapshot, next_observation, reward, is_done, info
Basically it returns next snapshot and everything that env.step would have returned.
"""
<your code here load,commit,take snapshot>
return ActionResult(<next_snapshot>, #fill in the variables
<next_observation>,
<reward>, <is_done>, <info>)
###Output
_____no_output_____
###Markdown
try out snapshots:
###Code
#make env
env = WithSnapshots(gym.make("CartPole-v0"))
env.reset()
n_actions = env.action_space.n
print("initial_state:")
plt.imshow(env.render('rgb_array'))
#create first snapshot
snap0 = env.get_snapshot()
#play without making snapshots (faster)
while True:
is_done = env.step(env.action_space.sample())[2]
if is_done:
print("Whoops! We died!")
break
print("final state:")
plt.imshow(env.render('rgb_array'))
plt.show()
#reload initial state
env.load_snapshot(snap0)
print("\n\nAfter loading snapshot")
plt.imshow(env.render('rgb_array'))
plt.show()
#get outcome (snapshot, observation, reward, is_done, info)
res = env.get_result(snap0,env.action_space.sample())
snap1, observation, reward = res[:3]
#second step
res2 = env.get_result(snap1,env.action_space.sample())
###Output
_____no_output_____
###Markdown
MCTS: Monte-Carlo tree searchIn this section, we'll implement the vanilla MCTS algorithm with UCB1-based node selection.We will start by implementing the `Node` class - a simple class that acts like MCTS node and supports some of the MCTS algorithm steps.This MCTS implementation makes some assumptions about the environment, you can find those _in the notes section at the end of the notebook_.
###Code
assert isinstance(env,WithSnapshots)
class Node:
""" a tree node for MCTS """
#metadata:
parent = None #parent Node
value_sum = 0. #sum of state values from all visits (numerator)
times_visited = 0 #counter of visits (denominator)
def __init__(self,parent,action,):
"""
Creates and empty node with no children.
Does so by commiting an action and recording outcome.
:param parent: parent Node
:param action: action to commit from parent Node
"""
self.parent = parent
self.action = action
self.children = set() #set of child nodes
#get action outcome and save it
res = env.get_result(parent.snapshot,action)
self.snapshot,self.observation,self.immediate_reward,self.is_done,_ = res
def is_leaf(self):
return len(self.children)==0
def is_root(self):
return self.parent is None
def get_mean_value(self):
return self.value_sum / self.times_visited if self.times_visited !=0 else 0
def ucb_score(self,scale=10,max_value=1e100):
"""
Computes ucb1 upper bound using current value and visit counts for node and it's parent.
:param scale: Multiplies upper bound by that. From hoeffding inequality, assumes reward range to be [0,scale].
:param max_value: a value that represents infinity (for unvisited nodes)
"""
if self.times_visited == 0:
return max_value
#compute ucb-1 additive component (to be added to mean value)
#hint: you can use self.parent.times_visited for N times node was considered,
# and self.times_visited for n times it was visited
U = <your code here>
return self.get_mean_value() + scale*U
#MCTS steps
def select_best_leaf(self):
"""
Picks the leaf with highest priority to expand
Does so by recursively picking nodes with best UCB-1 score until it reaches the leaf.
"""
if self.is_leaf():
return self
children = self.children
best_child = <select best child node in terms of node.ucb_score()>
return best_child.select_best_leaf()
def expand(self):
"""
Expands the current node by creating all possible child nodes.
Then returns one of those children.
"""
assert not self.is_done, "can't expand from terminal state"
for action in range(n_actions):
self.children.add(Node(self,action))
return self.select_best_leaf()
def rollout(self,t_max=10**4):
"""
Play the game from this state to the end (done) or for t_max steps.
On each step, pick action at random (hint: env.action_space.sample()).
Compute sum of rewards from current state till
Note 1: use env.action_space.sample() for random action
Note 2: if node is terminal (self.is_done is True), just return 0
"""
#set env into the appropriate state
env.load_snapshot(self.snapshot)
obs = self.observation
is_done = self.is_done
<your code here - rollout and compute reward>
return rollout_reward
def propagate(self,child_value):
"""
Uses child value (sum of rewards) to update parents recursively.
"""
#compute node value
my_value = self.immediate_reward + child_value
#update value_sum and times_visited
self.value_sum+=my_value
self.times_visited+=1
#propagate upwards
if not self.is_root():
self.parent.propagate(my_value)
def safe_delete(self):
"""safe delete to prevent memory leak in some python versions"""
del self.parent
for child in self.children:
child.safe_delete()
del child
class Root(Node):
def __init__(self,snapshot,observation):
"""
creates special node that acts like tree root
:snapshot: snapshot (from env.get_snapshot) to start planning from
:observation: last environment observation
"""
self.parent = self.action = None
self.children = set() #set of child nodes
#root: load snapshot and observation
self.snapshot = snapshot
self.observation = observation
self.immediate_reward = 0
self.is_done=False
@staticmethod
def from_node(node):
"""initializes node as root"""
root = Root(node.snapshot,node.observation)
#copy data
copied_fields = ["value_sum","times_visited","children","is_done"]
for field in copied_fields:
setattr(root,field,getattr(node,field))
return root
###Output
_____no_output_____
###Markdown
Main MCTS loopWith all we implemented, MCTS boils down to a trivial piece of code.
###Code
def plan_mcts(root,n_iters=10):
"""
builds tree with monte-carlo tree search for n_iters iterations
:param root: tree node to plan from
:param n_iters: how many select-expand-simulate-propagete loops to make
"""
for _ in range(n_iters):
node = <select best leaf>
if node.is_done:
node.propagate(0)
else: #node is not terminal
<expand-simulate-propagate loop>
###Output
_____no_output_____
###Markdown
Plan and executeIn this section, we use the MCTS implementation to find optimal policy.
###Code
root_observation = env.reset()
root_snapshot = env.get_snapshot()
root = Root(root_snapshot,root_observation)
#plan from root:
plan_mcts(root,n_iters=1000)
from IPython.display import clear_output
from itertools import count
from gym.wrappers import Monitor
total_reward = 0 #sum of rewards
test_env = loads(root_snapshot) #env used to show progress
for i in count():
#get best child
best_child = <select child with highest mean reward>
#take action
s,r,done,_ = test_env.step(best_child.action)
#show image
clear_output(True)
plt.title("step %i"%i)
plt.imshow(test_env.render('rgb_array'))
plt.show()
total_reward += r
if done:
print("Finished with reward = ",total_reward)
break
#discard unrealized part of the tree [because not every child matters :(]
for child in root.children:
if child != best_child:
child.safe_delete()
#declare best child a new root
root = Root.from_node(best_child)
assert not root.is_leaf(), "We ran out of tree! Need more planning! Try growing tree right inside the loop."
#you may want to expand tree here
#<your code here>
###Output
_____no_output_____
###Markdown
Submit to Coursera
###Code
from submit import submit_mcts
submit_mcts(total_reward, <EMAIL>, <TOKEN>)
###Output
_____no_output_____
###Markdown
Seminar: Monte-carlo tree searchIn this seminar, we'll implement a vanilla MCTS planning and use it to solve some Gym envs.But before we do that, we first need to modify gym env to allow saving and loading game states to facilitate backtracking.
###Code
from gym.core import Wrapper
from pickle import dumps,loads
from collections import namedtuple
#a container for get_result function below. Works just like tuple, but prettier
ActionResult = namedtuple("action_result",("snapshot","observation","reward","is_done","info"))
class WithSnapshots(Wrapper):
"""
Creates a wrapper that supports saving and loading environemnt states.
Required for planning algorithms.
This class will have access to the core environment as self.env, e.g.:
- self.env.reset() #reset original env
- self.env.ale.cloneState() #make snapshot for atari. load with .restoreState()
- ...
You can also use reset, step and render directly for convenience.
- s, r, done, _ = self.step(action) #step, same as self.env.step(action)
- self.render(close=True) #close window, same as self.env.render(close=True)
"""
def get_snapshot(self):
"""
:returns: environment state that can be loaded with load_snapshot
Snapshots guarantee same env behaviour each time they are loaded.
Warning! Snapshots can be arbitrary things (strings, integers, json, tuples)
Don't count on them being pickle strings when implementing MCTS.
Developer Note: Make sure the object you return will not be affected by
anything that happens to the environment after it's saved.
You shouldn't, for example, return self.env.
In case of doubt, use pickle.dumps or deepcopy.
"""
self.render() #close popup windows since we can't pickle them
if self.unwrapped.viewer is not None:
self.unwrapped.viewer.close()
self.unwrapped.viewer = None
return dumps(self.env)
def load_snapshot(self,snapshot):
"""
Loads snapshot as current env state.
Should not change snapshot inplace (in case of doubt, deepcopy).
"""
assert not hasattr(self,"_monitor") or hasattr(self.env,"_monitor"), "can't backtrack while recording"
# self.render(close=True) #close popup windows since we can't load into them
self.env = loads(snapshot)
def get_result(self,snapshot,action):
"""
A convenience function that
- loads snapshot,
- commits action via self.step,
- and takes snapshot again :)
:returns: next snapshot, next_observation, reward, is_done, info
Basically it returns next snapshot and everything that env.step would have returned.
"""
self.load_snapshot(snapshot)
s, r, done, info = self.step(action)
next_snapshot = self.get_snapshot()
return ActionResult(next_snapshot, s, r, done, info)
###Output
_____no_output_____
###Markdown
try out snapshots:
###Code
#make env
env = WithSnapshots(gym.make("CartPole-v0"))
env.reset()
n_actions = env.action_space.n
print("initial_state:")
plt.imshow(env.render('rgb_array'))
#create first snapshot
snap0 = env.get_snapshot()
#play without making snapshots (faster)
while True:
is_done = env.step(env.action_space.sample())[2]
if is_done:
print("Whoops! We died!")
break
print("final state:")
plt.imshow(env.render('rgb_array'))
plt.show()
#reload initial state
env.load_snapshot(snap0)
print("\n\nAfter loading snapshot")
plt.imshow(env.render('rgb_array'))
plt.show()
#get outcome (snapshot, observation, reward, is_done, info)
res = env.get_result(snap0,env.action_space.sample())
snap1, observation, reward = res[:3]
#second step
res2 = env.get_result(snap1,env.action_space.sample())
###Output
_____no_output_____
###Markdown
MCTS: Monte-Carlo tree searchIn this section, we'll implement the vanilla MCTS algorithm with UCB1-based node selection.We will start by implementing the `Node` class - a simple class that acts like MCTS node and supports some of the MCTS algorithm steps.This MCTS implementation makes some assumptions about the environment, you can find those _in the notes section at the end of the notebook_.
###Code
assert isinstance(env,WithSnapshots)
class Node:
""" a tree node for MCTS """
#metadata:
parent = None #parent Node
value_sum = 0. #sum of state values from all visits (numerator)
times_visited = 0 #counter of visits (denominator)
def __init__(self,parent,action,):
"""
Creates and empty node with no children.
Does so by commiting an action and recording outcome.
:param parent: parent Node
:param action: action to commit from parent Node
"""
self.parent = parent
self.action = action
self.children = set() #set of child nodes
#get action outcome and save it
# res contains snapshot, obs, reward, done, info
res = env.get_result(parent.snapshot,action)
self.snapshot,self.observation,self.immediate_reward,self.is_done,_ = res
def is_leaf(self):
return len(self.children)==0
def is_root(self):
return self.parent is None
def get_mean_value(self):
return self.value_sum / self.times_visited if self.times_visited !=0 else 0
def ucb_score(self,scale=10,max_value=1e100):
"""
Computes ucb1 upper bound using current value and visit counts for node and it's parent.
:param scale: Multiplies upper bound by that. From hoeffding inequality, assumes reward range to be [0,scale].
:param max_value: a value that represents infinity (for unvisited nodes)
"""
if self.times_visited == 0:
return max_value
#compute ucb-1 additive component (to be added to mean value)
#hint: you can use self.parent.times_visited for N times node was considered,
# and self.times_visited for n times it was visited
U = np.sqrt(2*np.log(self.parent.times_visited)/self.times_visited)
return self.get_mean_value() + scale*U
#MCTS steps
def select_best_leaf(self):
"""
Picks the leaf with highest priority to expand
Does so by recursively picking nodes with best UCB-1 score until it reaches the leaf.
"""
if self.is_leaf():
return self
children = self.children
best_child = max([(child.ucb_score(), child) for child in children],
key=lambda x: x[0])[1]
return best_child.select_best_leaf()
def expand(self):
"""
Expands the current node by creating all possible child nodes.
Then returns one of those children.
"""
assert not self.is_done, "can't expand from terminal state"
for action in range(n_actions):
self.children.add(Node(self,action))
return self.select_best_leaf()
def rollout(self,t_max=10**4):
"""
Play the game from this state to the end (done) or for t_max steps.
On each step, pick action at random (hint: env.action_space.sample()).
Compute sum of rewards from current state till
Note 1: use env.action_space.sample() for random action
Note 2: if node is terminal (self.is_done is True), just return 0
"""
#set env into the appropriate state
env.load_snapshot(self.snapshot)
obs = self.observation
is_done = self.is_done
rollout_reward = 0
while not is_done and t_max>0:
t_max -= 1
_, r, is_done, _ = env.step(env.action_space.sample())
rollout_reward += r
return rollout_reward
def propagate(self,child_value):
"""
Uses child value (sum of rewards) to update parents recursively.
"""
#compute node value
my_value = self.immediate_reward + child_value
#update value_sum and times_visited
self.value_sum+=my_value
self.times_visited+=1
#propagate upwards
if not self.is_root():
self.parent.propagate(my_value)
def safe_delete(self):
"""safe delete to prevent memory leak in some python versions"""
del self.parent
for child in self.children:
child.safe_delete()
del child
class Root(Node):
def __init__(self,snapshot,observation):
"""
creates special node that acts like tree root
:snapshot: snapshot (from env.get_snapshot) to start planning from
:observation: last environment observation
"""
self.parent = self.action = None
self.children = set() #set of child nodes
#root: load snapshot and observation
self.snapshot = snapshot
self.observation = observation
self.immediate_reward = 0
self.is_done=False
@staticmethod
def from_node(node):
"""initializes node as root"""
root = Root(node.snapshot,node.observation)
#copy data
copied_fields = ["value_sum","times_visited","children","is_done"]
for field in copied_fields:
setattr(root,field,getattr(node,field))
return root
###Output
_____no_output_____
###Markdown
Main MCTS loopWith all we implemented, MCTS boils down to a trivial piece of code.
###Code
def plan_mcts(root,n_iters=10):
"""
builds tree with monte-carlo tree search for n_iters iterations
:param root: tree node to plan from
:param n_iters: how many select-expand-simulate-propagete loops to make
"""
for _ in range(n_iters):
node = root.select_best_leaf()
if node.is_done:
node.propagate(0)
else: #node is not terminal
node_child = node.expand()
child_reward = node_child.rollout()
node.propagate(child_reward)
###Output
_____no_output_____
###Markdown
Plan and executeIn this section, we use the MCTS implementation to find optimal policy.
###Code
root_observation = env.reset()
root_snapshot = env.get_snapshot()
root = Root(root_snapshot,root_observation)
#plan from root:
plan_mcts(root,n_iters=1000)
from IPython.display import clear_output
from itertools import count
from gym.wrappers import Monitor
total_reward = 0 #sum of rewards
test_env = loads(root_snapshot) #env used to show progress
for i in count():
#get best child
best_child = <select child with highest mean reward>
#take action
s,r,done,_ = test_env.step(best_child.action)
#show image
clear_output(True)
plt.title("step %i"%i)
plt.imshow(test_env.render('rgb_array'))
plt.show()
total_reward += r
if done:
print("Finished with reward = ",total_reward)
break
#discard unrealized part of the tree [because not every child matters :(]
for child in root.children:
if child != best_child:
child.safe_delete()
#declare best child a new root
root = Root.from_node(best_child)
assert not root.is_leaf(), "We ran out of tree! Need more planning! Try growing tree right inside the loop."
#you may want to expand tree here
#<your code here>
###Output
_____no_output_____
###Markdown
Submit to Coursera
###Code
from submit import submit_mcts
submit_mcts(200.0, "[email protected]", "npnUphFB1r7YBgf6")
#submit_mcts(total_reward, <EMAIL>, <TOKEN>)
###Output
Submitted to Coursera platform. See results on assignment page!
|
tf_cifar_10.ipynb | ###Markdown
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
(train_images,train_labels),(test_images,test_labels) = tf.keras.datasets.cifar10.load_data()
print("Size of training data set ",train_images.size)
print("\nFirst Image of Training Set")
plt.figure()
plt.imshow(train_images[0])
plt.show()
print("\nTraining data set Image shape",train_images.shape)
print("\nTraining data set Lable shape",train_labels.shape)
class_names = [
"airplane",
"automobile",
"bird",
"cat",
"deer",
"dog",
"frog",
"horse",
"ship",
"truck"]
train_labels = train_labels.flatten()
plt.figure(figsize=(10,10))
for i in range(36):
plt.subplot(6,6,i+1)
plt.xticks([])
plt.yticks([])
plt.grid(False)
plt.imshow(train_images[i])
plt.xlabel(class_names[train_labels[i]])
plt.show()
train_images = train_images/255.0
test_images = test_images/255.0
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3,3), padding='same', activation=tf.nn.relu,input_shape=(32, 32, 3)),
tf.keras.layers.MaxPooling2D((2, 2), strides=2),
tf.keras.layers.Dropout(rate=0.3),
tf.keras.layers.Conv2D(64, (3,3), padding='same', activation=tf.nn.relu),
tf.keras.layers.MaxPooling2D((2, 2), strides=2),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(256, activation=tf.nn.relu),
tf.keras.layers.Dense(10, activation=tf.nn.softmax)
])
model.compile(optimizer='adam',
loss="sparse_categorical_crossentropy",
metrics=['accuracy'])
model.fit(train_images, train_labels, epochs=50)
test_loss, test_acc = model.evaluate(test_images, test_labels)
predictions = model.predict(test_images)
class_names[np.argmax(predictions[1904])]
plt.figure()
plt.imshow(test_images[1904])
plt.show()
###Output
_____no_output_____ |
tutorials/Step_5d_Build_CNN_Tf_DSX.ipynb | ###Markdown
SETI CNN using TF and Binary DS
###Code
import requests
import json
#import ibmseti
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
import tensorflow as tf
import pickle
import time
#!sudo pip install sklearn
import os
from sklearn.metrics import confusion_matrix
from sklearn import metrics
###Output
_____no_output_____
###Markdown
Import dataset readerThe following cell will load a python code to read the SETI dataset.
###Code
!wget --output-document SETI.zip https://ibm.box.com/shared/static/jhqdhcblhua5dx2t7ixwm88okitjrl6l.zip
!unzip -o SETI.zip
import SETI
###Output
_____no_output_____
###Markdown
Download data
###Code
ds_directory = 'SETI/SETI_ds_64x128/'
ds_name = 'SETI/SETI64x128.tar.gz'
###Output
_____no_output_____
###Markdown
!rm -r SETI/*!mkdir SETIos.system('wget --output-document '+ ds_name +' xxxxx.gz')os.system('tar -xvf '+ ds_name) @hidden_cell!rm -r SETI/*!mkdir SETIos.system('wget --output-document '+ ds_name +' https://ibm.box.com/shared/static/zl3e3y7780h3bsqwt1g5elg57jvw7a3z.gz')os.system('tar -xvf '+ ds_name)
###Code
print os.popen("ls -lrt "+ ds_directory).read() # to verify
###Output
total 7152
-rw-r----- 1 sd22-2e55b7df66e8c3-b01c69100280 users 1665328 May 22 21:11 train-images-idx3-ubyte.gz
-rw-r----- 1 sd22-2e55b7df66e8c3-b01c69100280 users 306 May 22 21:11 train-labels-idx1-ubyte.gz
-rw-r----- 1 sd22-2e55b7df66e8c3-b01c69100280 users 765379 May 22 21:11 test-images-idx3-ubyte.gz
-rw-r----- 1 sd22-2e55b7df66e8c3-b01c69100280 users 172 May 22 21:11 test-labels-idx1-ubyte.gz
###Markdown
Load data SETI
###Code
#from tensorflow.examples.tutorials.mnist import input_data
#dataset = input_data.read_data_sets("MNIST_data/", one_hot=True)
dataset = SETI.read_data_sets(ds_directory, one_hot=True, validation_size=0)
dataset.train.images.shape
###Output
Extracting SETI/SETI_ds_64x128/train-images-idx3-ubyte.gz
Extracting SETI/SETI_ds_64x128/train-labels-idx1-ubyte.gz
Extracting SETI/SETI_ds_64x128/test-images-idx3-ubyte.gz
Extracting SETI/SETI_ds_64x128/test-labels-idx1-ubyte.gz
###Markdown
Network Parameters
###Code
# Parameters
decay_rate=0.98
decay_steps=1000
learning_rate = 0.05
training_epochs = 20
batch_size = 50
display_step = 100
#check point directory
chk_directory = 'SETI/save/'
checkpoint_path = chk_directory+'model.ckpt'
n_classes = 4 # number of possible classifications for the problem
dropout = 0.50 # Dropout, probability to keep units
height = 64 # height of the image in pixels
width = 128 # width of the image in pixels
n_input = width * height # number of pixels in one image
###Output
_____no_output_____
###Markdown
Inputs
###Code
x = tf.placeholder(tf.float32, shape=[None, n_input])
y_ = tf.placeholder(tf.float32, shape=[None, n_classes])
x_image = tf.reshape(x, [-1,height,width,1])
x_image
###Output
_____no_output_____
###Markdown
Convolutional Layer 1
###Code
W_conv1 = tf.Variable(tf.truncated_normal([5, 5, 1, 32], stddev=0.1))
b_conv1 = tf.Variable(tf.constant(0.1, shape=[32])) # need 32 biases for 32 outputs
convolve1 = tf.nn.conv2d(x_image, W_conv1, strides=[1, 1, 1, 1], padding='SAME') + b_conv1
h_conv1 = tf.nn.relu(convolve1)
conv1 = tf.nn.max_pool(h_conv1, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME') #max_pool_2x2
conv1
###Output
_____no_output_____
###Markdown
Convolutional Layer 2
###Code
W_conv2 = tf.Variable(tf.truncated_normal([5, 5, 32, 64], stddev=0.1))
b_conv2 = tf.Variable(tf.constant(0.1, shape=[64])) #need 64 biases for 64 outputs
convolve2= tf.nn.conv2d(conv1, W_conv2, strides=[1, 1, 1, 1], padding='SAME')+ b_conv2
h_conv2 = tf.nn.relu(convolve2)
conv2 = tf.nn.max_pool(h_conv2, ksize=[1, 2, 2, 1], strides=[1, 4, 4, 1], padding='SAME') #max_pool_2x2
conv2
###Output
_____no_output_____
###Markdown
Convolutional Layer 3
###Code
W_conv3 = tf.Variable(tf.truncated_normal([5, 5, 64, 128], stddev=0.1))
b_conv3 = tf.Variable(tf.constant(0.1, shape=[128])) #need 64 biases for 64 outputs
convolve3= tf.nn.conv2d(conv2, W_conv3, strides=[1, 1, 1, 1], padding='SAME')+ b_conv3
h_conv3 = tf.nn.relu(convolve3)
conv3 = tf.nn.max_pool(h_conv3, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME') #max_pool_2x2
conv3
###Output
_____no_output_____
###Markdown
Convolutional Layer 4
###Code
W_conv4 = tf.Variable(tf.truncated_normal([5, 5, 128, 256], stddev=0.1))
b_conv4 = tf.Variable(tf.constant(0.1, shape=[256])) #need 64 biases for 64 outputs
convolve4= tf.nn.conv2d(conv3, W_conv4, strides=[1, 1, 1, 1], padding='SAME')+ b_conv4
h_conv4 = tf.nn.relu(convolve4)
conv4 = tf.nn.max_pool(h_conv4, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME') #max_pool_2x2
###Output
_____no_output_____
###Markdown
Fully Connected Layer 1
###Code
input_layer = conv2
dim = input_layer.get_shape().as_list()
dim
dims= dim[1]*dim[2]*dim[3]
nodes1 = 1024
prv_layer_matrix = tf.reshape(input_layer, [-1, dims])
W_fc1 = tf.Variable(tf.truncated_normal([dims, nodes1], stddev=0.1))
b_fc1 = tf.Variable(tf.constant(0.1, shape=[nodes1])) # need 1024 biases for 1024 outputs
h_fcl1 = tf.matmul(prv_layer_matrix, W_fc1) + b_fc1
fc_layer1 = tf.nn.relu(h_fcl1) # ???
fc_layer1
###Output
_____no_output_____
###Markdown
Droupout 1
###Code
keep_prob = tf.placeholder(tf.float32)
layer_drop1 = tf.nn.dropout(fc_layer1, keep_prob)
###Output
_____no_output_____
###Markdown
Fully Connected Layer 2
###Code
nodes2 = 256
W_fc2 = tf.Variable(tf.truncated_normal([layer_drop1.get_shape().as_list()[1], nodes2], stddev=0.1))
b_fc2 = tf.Variable(tf.constant(0.1, shape=[nodes2]))
h_fcl2 = tf.matmul(layer_drop1, W_fc2) + b_fc2
fc_layer2 = tf.nn.relu(h_fcl2) # ???
fc_layer2
###Output
_____no_output_____
###Markdown
Droupout 2
###Code
layer_drop2 = tf.nn.dropout(fc_layer2, keep_prob)
###Output
_____no_output_____
###Markdown
Readout Layer
###Code
W_fc = tf.Variable(tf.truncated_normal([nodes1, n_classes], stddev=0.1)) #1024 neurons
b_fc = tf.Variable(tf.constant(0.1, shape=[n_classes])) # 10 possibilities for classes [0,1,2,3]
fc = tf.matmul(layer_drop1, W_fc) + b_fc
y_CNN= tf.nn.softmax(fc)
###Output
_____no_output_____
###Markdown
Loss function
###Code
#cross_entropy = tf.reduce_mean(-tf.reduce_sum(y_ * tf.log(y_l4_conv), reduction_indices=[1]))
cross_entropy = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=y_CNN, labels=y_))
###Output
_____no_output_____
###Markdown
Training
###Code
# Create a variable to track the global step.
global_step = tf.Variable(0, trainable=False)
# create learning_decay
lr = tf.train.exponential_decay( learning_rate,
global_step,
decay_steps,
decay_rate, staircase=True )
# Use the optimizer to apply the gradients that minimize the loss
# (and also increment the global step counter) as a single training step.
optimizer = tf.train.GradientDescentOptimizer(lr)
train_op = optimizer.minimize(cross_entropy, global_step=global_step)
#train_op = tf.train.AdamOptimizer(learning_rate=learning_rate).minimize(cross_entropy)
###Output
_____no_output_____
###Markdown
Evaluation
###Code
correct_prediction = tf.equal(tf.argmax(y_CNN,1), tf.argmax(y_,1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
###Output
_____no_output_____
###Markdown
Create checkpoint directory
###Code
directory = os.path.dirname(chk_directory)
try:
os.stat(directory)
ckpt = tf.train.get_checkpoint_state(chk_directory)
print ckpt
except:
os.mkdir(directory)
###Output
model_checkpoint_path: "SETI/save/model.ckpt-240"
all_model_checkpoint_paths: "SETI/save/model.ckpt-240"
###Markdown
Training
###Code
# Initializing the variables
init = tf.initialize_all_variables()
loss_values = []
with tf.Session() as sess:
X_test = dataset.test.images
y_test = dataset.test.labels
sess.run(init)
saver = tf.train.Saver(tf.all_variables())
# load previously trained model if appilcable
ckpt = tf.train.get_checkpoint_state(chk_directory)
if ckpt:
print "loading model: ",ckpt.model_checkpoint_path
saver.restore(sess, ckpt.model_checkpoint_path)
#step = 0
num_examples = dataset.train.num_examples
# Training cycle
for epoch in range(training_epochs):
avg_loss = 0.
avg_accuracy = 0.
#dataset.shuffle_data()
total_batch = int(num_examples / batch_size)
# Loop over all batches
for step in range(total_batch):
start = time.time()
x_batch, y_batch = dataset.train.next_batch(batch_size,shuffle=True)
train_op.run(feed_dict={x: x_batch, y_: y_batch, keep_prob: dropout})
loss, acc = sess.run([cross_entropy, accuracy], feed_dict={x: x_batch,y_: y_batch,keep_prob: 1.})
end = time.time()
avg_loss += loss / total_batch
avg_accuracy += acc / total_batch
if step % display_step == 1000:
# Calculate batch loss and accuracy
loss, acc = sess.run([cross_entropy, accuracy], feed_dict={x: x_batch,y_: y_batch,keep_prob: 1.})
#train_accuracy = accuracy.eval(feed_dict={x:x_batch, y_: y_batch, keep_prob: 0.5})
test_accuracy = sess.run(accuracy, feed_dict={x: X_test[0:100], y_: y_test[0:100], keep_prob: 1.})
print("Iter " + str(step) + \
", Training time= " + "{:.5f}".format(end - start) + \
", Minibatch Loss= " + "{:.6f}".format(loss) + \
", Training Accuracy= " + "{:.5f}".format(acc) + \
", Test Accuracy= " + "{:.5f}".format(test_accuracy) )
# save model every 1 epochs
if epoch >= 0 and epoch % 1 == 0:
# Save model
#print ("model saved to {}".format(checkpoint_path))
#saver.save(sess, checkpoint_path, global_step = epoch)
plr = sess.run(lr)
loss_values.append(avg_loss)
#print(sess.run(tf.train.global_step()))
print "Epoch:", '%04d' % (epoch+1), "lr=", "{:.9f}".format(plr), "cost=", "{:.9f}".format(avg_loss) ,"Acc=", "{:.9f}".format(avg_accuracy)
print("Optimization Finished!")
print ("model saved to {}".format(checkpoint_path))
saver.save(sess, checkpoint_path, global_step = (epoch+1)*step)
# Calculate accuracy for test images
#print("Testing Accuracy:", sess.run(accuracy, feed_dict={x: X_test[0:30], y_: y_test[0:30], keep_prob: 1.}))
# Find the labels of test set
y_pred_lb = sess.run(tf.argmax(y_CNN,1), feed_dict={x: X_test[0:100], y_: y_test[0:100], keep_prob: 1.})
y_pred = sess.run(y_CNN, feed_dict={x: X_test[0:100], y_: y_test[0:100], keep_prob: 1.})
# lets save kernels
kernels_l1 = sess.run(tf.reshape(tf.transpose(W_conv1, perm=[2, 3, 0, 1]),[32,-1]))
kernels_l2 = sess.run(tf.reshape(tf.transpose(W_conv2, perm=[2, 3, 0, 1]),[32*64,-1]))
%matplotlib inline
import numpy as np
import matplotlib.pyplot as plt
plt.plot([np.mean(loss_values[i:i+5]) for i in range(len(loss_values))])
plt.show()
###Output
_____no_output_____
###Markdown
Evaluation
###Code
y_ = np.argmax(y_test[0:100],1) # ground truth
print metrics.classification_report(y_true= y_, y_pred= y_pred_lb)
print metrics.confusion_matrix(y_true= y_, y_pred= y_pred_lb)
print("Classification accuracy: %0.6f" % metrics.accuracy_score(y_true= y_, y_pred= y_pred_lb) )
print("Log Loss: %0.6f" % metrics.log_loss(y_true= y_, y_pred= y_pred, labels=range(4)) )
###Output
precision recall f1-score support
0 0.00 0.00 0.00 25
1 0.00 0.00 0.00 28
2 0.30 1.00 0.46 23
3 1.00 1.00 1.00 24
avg / total 0.31 0.47 0.35 100
[[ 0 0 25 0]
[ 0 0 28 0]
[ 0 0 23 0]
[ 0 0 0 24]]
Classification accuracy: 0.470000
Log Loss: 0.972129
###Markdown
Viz
###Code
!wget --output-document utils1.py http://deeplearning.net/tutorial/code/utils.py
import utils1
from utils1 import tile_raster_images
#from utils import tile_raster_images
import matplotlib.pyplot as plt
from PIL import Image
%matplotlib inline
image = Image.fromarray(tile_raster_images(kernels_l1, img_shape=(5, 5) ,tile_shape=(4, 8), tile_spacing=(1, 1)))
### Plot image
plt.rcParams['figure.figsize'] = (18.0, 18.0)
imgplot = plt.imshow(image)
imgplot.set_cmap('gray')
image = Image.fromarray(tile_raster_images(kernels_l2, img_shape=(5, 5) ,tile_shape=(4, 12), tile_spacing=(1, 1)))
### Plot image
plt.rcParams['figure.figsize'] = (18.0, 18.0)
imgplot = plt.imshow(image)
imgplot.set_cmap('gray')
import numpy as np
plt.rcParams['figure.figsize'] = (5.0, 5.0)
sampleimage1 = X_test[3]
plt.imshow(np.reshape(sampleimage1,[64,128]), cmap="gray")
# Launch the graph
with tf.Session() as sess:
sess.run(init)
saver = tf.train.Saver(tf.all_variables())
# load previously trained model if appilcable
ckpt = tf.train.get_checkpoint_state(chk_directory)
if ckpt:
print "loading model: ",ckpt.model_checkpoint_path
saver.restore(sess, ckpt.model_checkpoint_path)
ActivatedUnits1 = sess.run(convolve1,feed_dict={x:np.reshape(sampleimage1,[1,64*128],order='F'),keep_prob:1.0})
plt.figure(1, figsize=(20,20))
n_columns = 3
n_rows = 3
for i in range(9):
plt.subplot(n_rows, n_columns, i+1)
plt.title('Filter ' + str(i))
plt.imshow(ActivatedUnits1[0,:,:,i], interpolation="nearest", cmap="gray")
###Output
_____no_output_____
###Markdown
-------------------- SETI CNN using TF and Binary DS
###Code
import requests
import json
#import ibmseti
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
import tensorflow as tf
import pickle
import time
#!sudo pip install sklearn
import os
from sklearn.metrics import confusion_matrix
from sklearn import metrics
###Output
_____no_output_____
###Markdown
Import dataset readerThe following cell will load a python code to read the SETI dataset.
###Code
!wget --output-document SETI.zip https://ibm.box.com/shared/static/jhqdhcblhua5dx2t7ixwm88okitjrl6l.zip
!unzip -o SETI.zip
import SETI
###Output
_____no_output_____
###Markdown
Download data
###Code
ds_directory = 'SETI/SETI_ds_64x128/'
ds_name = 'SETI/SETI64x128.tar.gz'
###Output
_____no_output_____
###Markdown
!rm -r SETI/*!mkdir SETIos.system('wget --output-document '+ ds_name +' xxxxx.gz')os.system('tar -xvf '+ ds_name) @hidden_cell!rm -r SETI/*!mkdir SETIos.system('wget --output-document '+ ds_name +' https://ibm.box.com/shared/static/zl3e3y7780h3bsqwt1g5elg57jvw7a3z.gz')os.system('tar -xvf '+ ds_name)
###Code
print os.popen("ls -lrt "+ ds_directory).read() # to verify
###Output
total 7152
-rw-r----- 1 sd22-2e55b7df66e8c3-b01c69100280 users 1665328 May 22 21:11 train-images-idx3-ubyte.gz
-rw-r----- 1 sd22-2e55b7df66e8c3-b01c69100280 users 306 May 22 21:11 train-labels-idx1-ubyte.gz
-rw-r----- 1 sd22-2e55b7df66e8c3-b01c69100280 users 765379 May 22 21:11 test-images-idx3-ubyte.gz
-rw-r----- 1 sd22-2e55b7df66e8c3-b01c69100280 users 172 May 22 21:11 test-labels-idx1-ubyte.gz
###Markdown
Load data SETI
###Code
#from tensorflow.examples.tutorials.mnist import input_data
#dataset = input_data.read_data_sets("MNIST_data/", one_hot=True)
dataset = SETI.read_data_sets(ds_directory, one_hot=True, validation_size=0)
dataset.train.images.shape
###Output
Extracting SETI/SETI_ds_64x128/train-images-idx3-ubyte.gz
Extracting SETI/SETI_ds_64x128/train-labels-idx1-ubyte.gz
Extracting SETI/SETI_ds_64x128/test-images-idx3-ubyte.gz
Extracting SETI/SETI_ds_64x128/test-labels-idx1-ubyte.gz
###Markdown
Network Parameters
###Code
# Parameters
decay_rate=0.98
decay_steps=1000
learning_rate = 0.05
training_epochs = 20
batch_size = 50
display_step = 100
#check point directory
chk_directory = 'SETI/save/'
checkpoint_path = chk_directory+'model.ckpt'
n_classes = 4 # number of possible classifications for the problem
dropout = 0.50 # Dropout, probability to keep units
height = 64 # height of the image in pixels
width = 128 # width of the image in pixels
n_input = width * height # number of pixels in one image
###Output
_____no_output_____
###Markdown
Inputs
###Code
x = tf.placeholder(tf.float32, shape=[None, n_input])
y_ = tf.placeholder(tf.float32, shape=[None, n_classes])
x_image = tf.reshape(x, [-1,height,width,1])
x_image
###Output
_____no_output_____
###Markdown
Convolutional Layer 1
###Code
W_conv1 = tf.Variable(tf.truncated_normal([5, 5, 1, 32], stddev=0.1))
b_conv1 = tf.Variable(tf.constant(0.1, shape=[32])) # need 32 biases for 32 outputs
convolve1 = tf.nn.conv2d(x_image, W_conv1, strides=[1, 1, 1, 1], padding='SAME') + b_conv1
h_conv1 = tf.nn.relu(convolve1)
conv1 = tf.nn.max_pool(h_conv1, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME') #max_pool_2x2
conv1
###Output
_____no_output_____
###Markdown
Convolutional Layer 2
###Code
W_conv2 = tf.Variable(tf.truncated_normal([5, 5, 32, 64], stddev=0.1))
b_conv2 = tf.Variable(tf.constant(0.1, shape=[64])) #need 64 biases for 64 outputs
convolve2= tf.nn.conv2d(conv1, W_conv2, strides=[1, 1, 1, 1], padding='SAME')+ b_conv2
h_conv2 = tf.nn.relu(convolve2)
conv2 = tf.nn.max_pool(h_conv2, ksize=[1, 2, 2, 1], strides=[1, 4, 4, 1], padding='SAME') #max_pool_2x2
conv2
###Output
_____no_output_____
###Markdown
Convolutional Layer 3
###Code
W_conv3 = tf.Variable(tf.truncated_normal([5, 5, 64, 128], stddev=0.1))
b_conv3 = tf.Variable(tf.constant(0.1, shape=[128])) #need 64 biases for 64 outputs
convolve3= tf.nn.conv2d(conv2, W_conv3, strides=[1, 1, 1, 1], padding='SAME')+ b_conv3
h_conv3 = tf.nn.relu(convolve3)
conv3 = tf.nn.max_pool(h_conv3, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME') #max_pool_2x2
conv3
###Output
_____no_output_____
###Markdown
Convolutional Layer 4
###Code
W_conv4 = tf.Variable(tf.truncated_normal([5, 5, 128, 256], stddev=0.1))
b_conv4 = tf.Variable(tf.constant(0.1, shape=[256])) #need 64 biases for 64 outputs
convolve4= tf.nn.conv2d(conv3, W_conv4, strides=[1, 1, 1, 1], padding='SAME')+ b_conv4
h_conv4 = tf.nn.relu(convolve4)
conv4 = tf.nn.max_pool(h_conv4, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME') #max_pool_2x2
###Output
_____no_output_____
###Markdown
Fully Connected Layer 1
###Code
input_layer = conv2
dim = input_layer.get_shape().as_list()
dim
dims= dim[1]*dim[2]*dim[3]
nodes1 = 1024
prv_layer_matrix = tf.reshape(input_layer, [-1, dims])
W_fc1 = tf.Variable(tf.truncated_normal([dims, nodes1], stddev=0.1))
b_fc1 = tf.Variable(tf.constant(0.1, shape=[nodes1])) # need 1024 biases for 1024 outputs
h_fcl1 = tf.matmul(prv_layer_matrix, W_fc1) + b_fc1
fc_layer1 = tf.nn.relu(h_fcl1) # ???
fc_layer1
###Output
_____no_output_____
###Markdown
Droupout 1
###Code
keep_prob = tf.placeholder(tf.float32)
layer_drop1 = tf.nn.dropout(fc_layer1, keep_prob)
###Output
_____no_output_____
###Markdown
Fully Connected Layer 2
###Code
nodes2 = 256
W_fc2 = tf.Variable(tf.truncated_normal([layer_drop1.get_shape().as_list()[1], nodes2], stddev=0.1))
b_fc2 = tf.Variable(tf.constant(0.1, shape=[nodes2]))
h_fcl2 = tf.matmul(layer_drop1, W_fc2) + b_fc2
fc_layer2 = tf.nn.relu(h_fcl2) # ???
fc_layer2
###Output
_____no_output_____
###Markdown
Droupout 2
###Code
layer_drop2 = tf.nn.dropout(fc_layer2, keep_prob)
###Output
_____no_output_____
###Markdown
Readout Layer
###Code
W_fc = tf.Variable(tf.truncated_normal([nodes1, n_classes], stddev=0.1)) #1024 neurons
b_fc = tf.Variable(tf.constant(0.1, shape=[n_classes])) # 10 possibilities for classes [0,1,2,3]
fc = tf.matmul(layer_drop1, W_fc) + b_fc
y_CNN= tf.nn.softmax(fc)
###Output
_____no_output_____
###Markdown
Loss function
###Code
#cross_entropy = tf.reduce_mean(-tf.reduce_sum(y_ * tf.log(y_l4_conv), reduction_indices=[1]))
cross_entropy = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=y_CNN, labels=y_))
###Output
_____no_output_____
###Markdown
Training
###Code
# Create a variable to track the global step.
global_step = tf.Variable(0, trainable=False)
# create learning_decay
lr = tf.train.exponential_decay( learning_rate,
global_step,
decay_steps,
decay_rate, staircase=True )
# Use the optimizer to apply the gradients that minimize the loss
# (and also increment the global step counter) as a single training step.
optimizer = tf.train.GradientDescentOptimizer(lr)
train_op = optimizer.minimize(cross_entropy, global_step=global_step)
#train_op = tf.train.AdamOptimizer(learning_rate=learning_rate).minimize(cross_entropy)
###Output
_____no_output_____
###Markdown
Evaluation
###Code
correct_prediction = tf.equal(tf.argmax(y_CNN,1), tf.argmax(y_,1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
###Output
_____no_output_____
###Markdown
Create checkpoint directory
###Code
directory = os.path.dirname(chk_directory)
try:
os.stat(directory)
ckpt = tf.train.get_checkpoint_state(chk_directory)
print ckpt
except:
os.mkdir(directory)
###Output
model_checkpoint_path: "SETI/save/model.ckpt-240"
all_model_checkpoint_paths: "SETI/save/model.ckpt-240"
###Markdown
Training
###Code
# Initializing the variables
init = tf.initialize_all_variables()
loss_values = []
with tf.Session() as sess:
X_test = dataset.test.images
y_test = dataset.test.labels
sess.run(init)
saver = tf.train.Saver(tf.all_variables())
# load previously trained model if appilcable
ckpt = tf.train.get_checkpoint_state(chk_directory)
if ckpt:
print "loading model: ",ckpt.model_checkpoint_path
saver.restore(sess, ckpt.model_checkpoint_path)
#step = 0
num_examples = dataset.train.num_examples
# Training cycle
for epoch in range(training_epochs):
avg_loss = 0.
avg_accuracy = 0.
#dataset.shuffle_data()
total_batch = int(num_examples / batch_size)
# Loop over all batches
for step in range(total_batch):
start = time.time()
x_batch, y_batch = dataset.train.next_batch(batch_size,shuffle=True)
train_op.run(feed_dict={x: x_batch, y_: y_batch, keep_prob: dropout})
loss, acc = sess.run([cross_entropy, accuracy], feed_dict={x: x_batch,y_: y_batch,keep_prob: 1.})
end = time.time()
avg_loss += loss / total_batch
avg_accuracy += acc / total_batch
if step % display_step == 1000:
# Calculate batch loss and accuracy
loss, acc = sess.run([cross_entropy, accuracy], feed_dict={x: x_batch,y_: y_batch,keep_prob: 1.})
#train_accuracy = accuracy.eval(feed_dict={x:x_batch, y_: y_batch, keep_prob: 0.5})
test_accuracy = sess.run(accuracy, feed_dict={x: X_test[0:100], y_: y_test[0:100], keep_prob: 1.})
print("Iter " + str(step) + \
", Training time= " + "{:.5f}".format(end - start) + \
", Minibatch Loss= " + "{:.6f}".format(loss) + \
", Training Accuracy= " + "{:.5f}".format(acc) + \
", Test Accuracy= " + "{:.5f}".format(test_accuracy) )
# save model every 1 epochs
if epoch >= 0 and epoch % 1 == 0:
# Save model
#print ("model saved to {}".format(checkpoint_path))
#saver.save(sess, checkpoint_path, global_step = epoch)
plr = sess.run(lr)
loss_values.append(avg_loss)
#print(sess.run(tf.train.global_step()))
print "Epoch:", '%04d' % (epoch+1), "lr=", "{:.9f}".format(plr), "cost=", "{:.9f}".format(avg_loss) ,"Acc=", "{:.9f}".format(avg_accuracy)
print("Optimization Finished!")
print ("model saved to {}".format(checkpoint_path))
saver.save(sess, checkpoint_path, global_step = (epoch+1)*step)
# Calculate accuracy for test images
#print("Testing Accuracy:", sess.run(accuracy, feed_dict={x: X_test[0:30], y_: y_test[0:30], keep_prob: 1.}))
# Find the labels of test set
y_pred_lb = sess.run(tf.argmax(y_CNN,1), feed_dict={x: X_test[0:100], y_: y_test[0:100], keep_prob: 1.})
y_pred = sess.run(y_CNN, feed_dict={x: X_test[0:100], y_: y_test[0:100], keep_prob: 1.})
# lets save kernels
kernels_l1 = sess.run(tf.reshape(tf.transpose(W_conv1, perm=[2, 3, 0, 1]),[32,-1]))
kernels_l2 = sess.run(tf.reshape(tf.transpose(W_conv2, perm=[2, 3, 0, 1]),[32*64,-1]))
%matplotlib inline
import numpy as np
import matplotlib.pyplot as plt
plt.plot([np.mean(loss_values[i:i+5]) for i in range(len(loss_values))])
plt.show()
###Output
_____no_output_____
###Markdown
Evaluation
###Code
y_ = np.argmax(y_test[0:100],1) # ground truth
print metrics.classification_report(y_true= y_, y_pred= y_pred_lb)
print metrics.confusion_matrix(y_true= y_, y_pred= y_pred_lb)
print("Classification accuracy: %0.6f" % metrics.accuracy_score(y_true= y_, y_pred= y_pred_lb) )
print("Log Loss: %0.6f" % metrics.log_loss(y_true= y_, y_pred= y_pred, labels=range(4)) )
###Output
precision recall f1-score support
0 0.00 0.00 0.00 25
1 0.00 0.00 0.00 28
2 0.30 1.00 0.46 23
3 1.00 1.00 1.00 24
avg / total 0.31 0.47 0.35 100
[[ 0 0 25 0]
[ 0 0 28 0]
[ 0 0 23 0]
[ 0 0 0 24]]
Classification accuracy: 0.470000
Log Loss: 0.972129
###Markdown
Viz
###Code
!wget --output-document utils1.py http://deeplearning.net/tutorial/code/utils.py
import utils1
from utils1 import tile_raster_images
#from utils import tile_raster_images
import matplotlib.pyplot as plt
from PIL import Image
%matplotlib inline
image = Image.fromarray(tile_raster_images(kernels_l1, img_shape=(5, 5) ,tile_shape=(4, 8), tile_spacing=(1, 1)))
### Plot image
plt.rcParams['figure.figsize'] = (18.0, 18.0)
imgplot = plt.imshow(image)
imgplot.set_cmap('gray')
image = Image.fromarray(tile_raster_images(kernels_l2, img_shape=(5, 5) ,tile_shape=(4, 12), tile_spacing=(1, 1)))
### Plot image
plt.rcParams['figure.figsize'] = (18.0, 18.0)
imgplot = plt.imshow(image)
imgplot.set_cmap('gray')
import numpy as np
plt.rcParams['figure.figsize'] = (5.0, 5.0)
sampleimage1 = X_test[3]
plt.imshow(np.reshape(sampleimage1,[64,128]), cmap="gray")
# Launch the graph
with tf.Session() as sess:
sess.run(init)
saver = tf.train.Saver(tf.all_variables())
# load previously trained model if appilcable
ckpt = tf.train.get_checkpoint_state(chk_directory)
if ckpt:
print "loading model: ",ckpt.model_checkpoint_path
saver.restore(sess, ckpt.model_checkpoint_path)
ActivatedUnits1 = sess.run(convolve1,feed_dict={x:np.reshape(sampleimage1,[1,64*128],order='F'),keep_prob:1.0})
plt.figure(1, figsize=(20,20))
n_columns = 3
n_rows = 3
for i in range(9):
plt.subplot(n_rows, n_columns, i+1)
plt.title('Filter ' + str(i))
plt.imshow(ActivatedUnits1[0,:,:,i], interpolation="nearest", cmap="gray")
###Output
_____no_output_____
###Markdown
-------------------- SETI CNN using TF and Binary DS
###Code
import sys
sys.path.insert(0, "/opt/DL/tensorflow/lib/python2.7/site-packages/")
import requests
import json
#import ibmseti
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
import tensorflow as tf
import pickle
import time
#!sudo pip install sklearn
import os
from sklearn.metrics import confusion_matrix
from sklearn import metrics
###Output
_____no_output_____
###Markdown
Import dataset readerThe following cell will load a python code to read the SETI dataset.
###Code
!wget --output-document SETI.zip https://ibm.box.com/shared/static/jhqdhcblhua5dx2t7ixwm88okitjrl6l.zip
!unzip -o SETI.zip
import SETI
###Output
--2017-07-31 20:12:10-- https://ibm.box.com/shared/static/jhqdhcblhua5dx2t7ixwm88okitjrl6l.zip
Resolving ibm.box.com (ibm.box.com)... 107.152.27.197, 107.152.26.197
Connecting to ibm.box.com (ibm.box.com)|107.152.27.197|:443... connected.
HTTP request sent, awaiting response... 301 Moved Permanently
Location: https://ibm.ent.box.com/shared/static/jhqdhcblhua5dx2t7ixwm88okitjrl6l.zip [following]
--2017-07-31 20:12:11-- https://ibm.ent.box.com/shared/static/jhqdhcblhua5dx2t7ixwm88okitjrl6l.zip
Resolving ibm.ent.box.com (ibm.ent.box.com)... 107.152.26.211, 107.152.27.211
Connecting to ibm.ent.box.com (ibm.ent.box.com)|107.152.26.211|:443... connected.
HTTP request sent, awaiting response... 302 Found
Location: https://public.boxcloud.com/d/1/0vLBgnHguBZThithxqVJjl-22BB1thiBHMXPqx5XoUWmJZ8VEfnF4kVOxduoURdq6HNp32zo4RLszlhfYfaS3mZFBHCccf0VliQKTgwhrwF1OMqhpWqZa1Xh7OSfaRyjrS2LIfgRfAwMhiynbZezu0C_fy9tvm6QD1VDgIH_b9C2-S4kSUuupSuT9qqWHjefhAfBRmbe7fhOIukEiPpRZb6z83s2sbNT3Kfky5AbL8xlZGseIaH1gVb2C5HMgws0ssZyipnsKMnSDjEoAaXreG3zryFxs4OrWYIt0x1KSgIDp6_Fv3g2FnJhFH4pg-nUbWlNfsPdtTz0roCblpzIqRk0MUfTC_zZnPLNmDLFnbIlg16YiWG1CZj-V1oIkGjgDYlB_bE5Ty1x6yhJNN3caC9dQULiAVKV2o3166dU5IqtUibuy9lE675X02CzkEWSuQuL4zLtaFNk1nLv1uuvu_Lp6V-Wp9_AvWtmYw2PMpCMA47VWtBHmS5qCT_lQRCn3x3cWYCXHaUWWG-IG5SESzittFDCRyZuTM0YJMjeLlK7ZDlGrslqWJ1jysaewfXBnNf-JrjnF_aVxpJDCq4U5FqnpZjIWJTVQdROe4Euv55mfBayCUW6NVJaqaj1f7ed5B5wByUM9lSupR-fnVjzQGTA50PIhvRYgrSbzh-bwX6ppz0MCeFUICfLxn7r7m80s11s6cP7mPdMhL65lSqbxuvwHmPmeCz_aQS1WJV1BxjCO6GergorBkH8PffQxHxwH7DQRF2KXXp6lqsuHLPqqvSNrqFVLoYGbgU89UwZMyTECdODoBTWxbpCaJ2GtBujUsodaAeyLAR6sMCJbh_M7VXEN4Hre9Y_QvWEJ_us7A_CQkyUdw_7jaG_HVsMUeGHemEC8yMDJjkhzrDdjqEYDlqt0YVHMxDhHW_-aaCV5P987ipuCayqIjR3IiLA6h-zVWhdn8-sTGy1fyTspE2lcFIJJHIGqQQtVCZKTbTDY_vx0Mz8K8rHQiRU1MUdbqOyXryx3aR-mU_oCzi_Jsz8Av297Cz-d5cas2JyDbM90kZx5fNjIxDxqKqNKddSUr4ozpHupulMCI37Aq6AuSQptSZopXiOaoIeTEpaVN2QGS0ykxTRyeLWzNghH8PRv-uyBTcFNBvStDJ4FJ61xSHL7aA4/download [following]
--2017-07-31 20:12:11-- https://public.boxcloud.com/d/1/0vLBgnHguBZThithxqVJjl-22BB1thiBHMXPqx5XoUWmJZ8VEfnF4kVOxduoURdq6HNp32zo4RLszlhfYfaS3mZFBHCccf0VliQKTgwhrwF1OMqhpWqZa1Xh7OSfaRyjrS2LIfgRfAwMhiynbZezu0C_fy9tvm6QD1VDgIH_b9C2-S4kSUuupSuT9qqWHjefhAfBRmbe7fhOIukEiPpRZb6z83s2sbNT3Kfky5AbL8xlZGseIaH1gVb2C5HMgws0ssZyipnsKMnSDjEoAaXreG3zryFxs4OrWYIt0x1KSgIDp6_Fv3g2FnJhFH4pg-nUbWlNfsPdtTz0roCblpzIqRk0MUfTC_zZnPLNmDLFnbIlg16YiWG1CZj-V1oIkGjgDYlB_bE5Ty1x6yhJNN3caC9dQULiAVKV2o3166dU5IqtUibuy9lE675X02CzkEWSuQuL4zLtaFNk1nLv1uuvu_Lp6V-Wp9_AvWtmYw2PMpCMA47VWtBHmS5qCT_lQRCn3x3cWYCXHaUWWG-IG5SESzittFDCRyZuTM0YJMjeLlK7ZDlGrslqWJ1jysaewfXBnNf-JrjnF_aVxpJDCq4U5FqnpZjIWJTVQdROe4Euv55mfBayCUW6NVJaqaj1f7ed5B5wByUM9lSupR-fnVjzQGTA50PIhvRYgrSbzh-bwX6ppz0MCeFUICfLxn7r7m80s11s6cP7mPdMhL65lSqbxuvwHmPmeCz_aQS1WJV1BxjCO6GergorBkH8PffQxHxwH7DQRF2KXXp6lqsuHLPqqvSNrqFVLoYGbgU89UwZMyTECdODoBTWxbpCaJ2GtBujUsodaAeyLAR6sMCJbh_M7VXEN4Hre9Y_QvWEJ_us7A_CQkyUdw_7jaG_HVsMUeGHemEC8yMDJjkhzrDdjqEYDlqt0YVHMxDhHW_-aaCV5P987ipuCayqIjR3IiLA6h-zVWhdn8-sTGy1fyTspE2lcFIJJHIGqQQtVCZKTbTDY_vx0Mz8K8rHQiRU1MUdbqOyXryx3aR-mU_oCzi_Jsz8Av297Cz-d5cas2JyDbM90kZx5fNjIxDxqKqNKddSUr4ozpHupulMCI37Aq6AuSQptSZopXiOaoIeTEpaVN2QGS0ykxTRyeLWzNghH8PRv-uyBTcFNBvStDJ4FJ61xSHL7aA4/download
Resolving public.boxcloud.com (public.boxcloud.com)... 107.152.27.200, 107.152.26.200
Connecting to public.boxcloud.com (public.boxcloud.com)|107.152.27.200|:443... connected.
HTTP request sent, awaiting response... 200 OK
Length: 3288 (3.2K) [application/zip]
Saving to: ‘SETI.zip’
SETI.zip 100%[===================>] 3.21K --.-KB/s in 0s
2017-07-31 20:12:11 (221 MB/s) - ‘SETI.zip’ saved [3288/3288]
Archive: SETI.zip
inflating: SETI.py
inflating: __MACOSX/._SETI.py
###Markdown
Download data
###Code
ds_directory = 'SETI/SETI_ds_64x128/'
ds_name = 'SETI/SETI64x128.tar.gz'
###Output
_____no_output_____
###Markdown
!rm -r SETI/*!mkdir SETIos.system('wget --output-document '+ ds_name +' xxxxx.gz')os.system('tar -xvf '+ ds_name) @hidden_cell!rm -r SETI/*!mkdir SETIos.system('wget --output-document '+ ds_name +' https://ibm.box.com/shared/static/zl3e3y7780h3bsqwt1g5elg57jvw7a3z.gz')os.system('tar -xvf '+ ds_name)
###Code
print os.popen("ls -lrt "+ ds_directory).read() # to verify
###Output
###Markdown
Load data SETI
###Code
#from tensorflow.examples.tutorials.mnist import input_data
#dataset = input_data.read_data_sets("MNIST_data/", one_hot=True)
dataset = SETI.read_data_sets(ds_directory, one_hot=True, validation_size=0)
dataset.train.images.shape
###Output
_____no_output_____
###Markdown
Network Parameters
###Code
# Parameters
decay_rate=0.98
decay_steps=1000
learning_rate = 0.05
training_epochs = 20
batch_size = 50
display_step = 100
#check point directory
chk_directory = 'SETI/save/'
checkpoint_path = chk_directory+'model.ckpt'
n_classes = 4 # number of possible classifications for the problem
dropout = 0.50 # Dropout, probability to keep units
height = 64 # height of the image in pixels
width = 128 # width of the image in pixels
n_input = width * height # number of pixels in one image
###Output
_____no_output_____
###Markdown
Inputs
###Code
x = tf.placeholder(tf.float32, shape=[None, n_input])
y_ = tf.placeholder(tf.float32, shape=[None, n_classes])
x_image = tf.reshape(x, [-1,height,width,1])
x_image
###Output
_____no_output_____
###Markdown
Convolutional Layer 1
###Code
W_conv1 = tf.Variable(tf.truncated_normal([5, 5, 1, 32], stddev=0.1))
b_conv1 = tf.Variable(tf.constant(0.1, shape=[32])) # need 32 biases for 32 outputs
convolve1 = tf.nn.conv2d(x_image, W_conv1, strides=[1, 1, 1, 1], padding='SAME') + b_conv1
h_conv1 = tf.nn.relu(convolve1)
conv1 = tf.nn.max_pool(h_conv1, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME') #max_pool_2x2
conv1
###Output
_____no_output_____
###Markdown
Convolutional Layer 2
###Code
W_conv2 = tf.Variable(tf.truncated_normal([5, 5, 32, 64], stddev=0.1))
b_conv2 = tf.Variable(tf.constant(0.1, shape=[64])) #need 64 biases for 64 outputs
convolve2= tf.nn.conv2d(conv1, W_conv2, strides=[1, 1, 1, 1], padding='SAME')+ b_conv2
h_conv2 = tf.nn.relu(convolve2)
conv2 = tf.nn.max_pool(h_conv2, ksize=[1, 2, 2, 1], strides=[1, 4, 4, 1], padding='SAME') #max_pool_2x2
conv2
###Output
_____no_output_____
###Markdown
Convolutional Layer 3
###Code
W_conv3 = tf.Variable(tf.truncated_normal([5, 5, 64, 128], stddev=0.1))
b_conv3 = tf.Variable(tf.constant(0.1, shape=[128])) #need 64 biases for 64 outputs
convolve3= tf.nn.conv2d(conv2, W_conv3, strides=[1, 1, 1, 1], padding='SAME')+ b_conv3
h_conv3 = tf.nn.relu(convolve3)
conv3 = tf.nn.max_pool(h_conv3, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME') #max_pool_2x2
conv3
###Output
_____no_output_____
###Markdown
Convolutional Layer 4
###Code
W_conv4 = tf.Variable(tf.truncated_normal([5, 5, 128, 256], stddev=0.1))
b_conv4 = tf.Variable(tf.constant(0.1, shape=[256])) #need 64 biases for 64 outputs
convolve4= tf.nn.conv2d(conv3, W_conv4, strides=[1, 1, 1, 1], padding='SAME')+ b_conv4
h_conv4 = tf.nn.relu(convolve4)
conv4 = tf.nn.max_pool(h_conv4, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME') #max_pool_2x2
###Output
_____no_output_____
###Markdown
Fully Connected Layer 1
###Code
input_layer = conv2
dim = input_layer.get_shape().as_list()
dim
dims= dim[1]*dim[2]*dim[3]
nodes1 = 1024
prv_layer_matrix = tf.reshape(input_layer, [-1, dims])
W_fc1 = tf.Variable(tf.truncated_normal([dims, nodes1], stddev=0.1))
b_fc1 = tf.Variable(tf.constant(0.1, shape=[nodes1])) # need 1024 biases for 1024 outputs
h_fcl1 = tf.matmul(prv_layer_matrix, W_fc1) + b_fc1
fc_layer1 = tf.nn.relu(h_fcl1) # ???
fc_layer1
###Output
_____no_output_____
###Markdown
Droupout 1
###Code
keep_prob = tf.placeholder(tf.float32)
layer_drop1 = tf.nn.dropout(fc_layer1, keep_prob)
###Output
_____no_output_____
###Markdown
Fully Connected Layer 2
###Code
nodes2 = 256
W_fc2 = tf.Variable(tf.truncated_normal([layer_drop1.get_shape().as_list()[1], nodes2], stddev=0.1))
b_fc2 = tf.Variable(tf.constant(0.1, shape=[nodes2]))
h_fcl2 = tf.matmul(layer_drop1, W_fc2) + b_fc2
fc_layer2 = tf.nn.relu(h_fcl2) # ???
fc_layer2
###Output
_____no_output_____
###Markdown
Droupout 2
###Code
layer_drop2 = tf.nn.dropout(fc_layer2, keep_prob)
###Output
_____no_output_____
###Markdown
Readout Layer
###Code
W_fc = tf.Variable(tf.truncated_normal([nodes1, n_classes], stddev=0.1)) #1024 neurons
b_fc = tf.Variable(tf.constant(0.1, shape=[n_classes])) # 10 possibilities for classes [0,1,2,3]
fc = tf.matmul(layer_drop1, W_fc) + b_fc
y_CNN= tf.nn.softmax(fc)
###Output
_____no_output_____
###Markdown
Loss function
###Code
#cross_entropy = tf.reduce_mean(-tf.reduce_sum(y_ * tf.log(y_l4_conv), reduction_indices=[1]))
cross_entropy = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=y_CNN, labels=y_))
###Output
_____no_output_____
###Markdown
Training
###Code
# Create a variable to track the global step.
global_step = tf.Variable(0, trainable=False)
# create learning_decay
lr = tf.train.exponential_decay( learning_rate,
global_step,
decay_steps,
decay_rate, staircase=True )
# Use the optimizer to apply the gradients that minimize the loss
# (and also increment the global step counter) as a single training step.
optimizer = tf.train.GradientDescentOptimizer(lr)
train_op = optimizer.minimize(cross_entropy, global_step=global_step)
#train_op = tf.train.AdamOptimizer(learning_rate=learning_rate).minimize(cross_entropy)
###Output
_____no_output_____
###Markdown
Evaluation
###Code
correct_prediction = tf.equal(tf.argmax(y_CNN,1), tf.argmax(y_,1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
###Output
_____no_output_____
###Markdown
Create checkpoint directory
###Code
directory = os.path.dirname(chk_directory)
try:
os.stat(directory)
ckpt = tf.train.get_checkpoint_state(chk_directory)
print ckpt
except:
os.mkdir(directory)
###Output
model_checkpoint_path: "SETI/save/model.ckpt-240"
all_model_checkpoint_paths: "SETI/save/model.ckpt-240"
###Markdown
Training
###Code
# Initializing the variables
init = tf.initialize_all_variables()
loss_values = []
with tf.Session() as sess:
X_test = dataset.test.images
y_test = dataset.test.labels
sess.run(init)
saver = tf.train.Saver(tf.all_variables())
# load previously trained model if appilcable
ckpt = tf.train.get_checkpoint_state(chk_directory)
if ckpt:
print "loading model: ",ckpt.model_checkpoint_path
saver.restore(sess, ckpt.model_checkpoint_path)
#step = 0
num_examples = dataset.train.num_examples
# Training cycle
for epoch in range(training_epochs):
avg_loss = 0.
avg_accuracy = 0.
#dataset.shuffle_data()
total_batch = int(num_examples / batch_size)
# Loop over all batches
for step in range(total_batch):
start = time.time()
x_batch, y_batch = dataset.train.next_batch(batch_size,shuffle=True)
train_op.run(feed_dict={x: x_batch, y_: y_batch, keep_prob: dropout})
loss, acc = sess.run([cross_entropy, accuracy], feed_dict={x: x_batch,y_: y_batch,keep_prob: 1.})
end = time.time()
avg_loss += loss / total_batch
avg_accuracy += acc / total_batch
if step % display_step == 1000:
# Calculate batch loss and accuracy
loss, acc = sess.run([cross_entropy, accuracy], feed_dict={x: x_batch,y_: y_batch,keep_prob: 1.})
#train_accuracy = accuracy.eval(feed_dict={x:x_batch, y_: y_batch, keep_prob: 0.5})
test_accuracy = sess.run(accuracy, feed_dict={x: X_test[0:100], y_: y_test[0:100], keep_prob: 1.})
print("Iter " + str(step) + \
", Training time= " + "{:.5f}".format(end - start) + \
", Minibatch Loss= " + "{:.6f}".format(loss) + \
", Training Accuracy= " + "{:.5f}".format(acc) + \
", Test Accuracy= " + "{:.5f}".format(test_accuracy) )
# save model every 1 epochs
if epoch >= 0 and epoch % 1 == 0:
# Save model
#print ("model saved to {}".format(checkpoint_path))
#saver.save(sess, checkpoint_path, global_step = epoch)
plr = sess.run(lr)
loss_values.append(avg_loss)
#print(sess.run(tf.train.global_step()))
print "Epoch:", '%04d' % (epoch+1), "lr=", "{:.9f}".format(plr), "cost=", "{:.9f}".format(avg_loss) ,"Acc=", "{:.9f}".format(avg_accuracy)
print("Optimization Finished!")
print ("model saved to {}".format(checkpoint_path))
saver.save(sess, checkpoint_path, global_step = (epoch+1)*step)
# Calculate accuracy for test images
#print("Testing Accuracy:", sess.run(accuracy, feed_dict={x: X_test[0:30], y_: y_test[0:30], keep_prob: 1.}))
# Find the labels of test set
y_pred_lb = sess.run(tf.argmax(y_CNN,1), feed_dict={x: X_test[0:100], y_: y_test[0:100], keep_prob: 1.})
y_pred = sess.run(y_CNN, feed_dict={x: X_test[0:100], y_: y_test[0:100], keep_prob: 1.})
# lets save kernels
kernels_l1 = sess.run(tf.reshape(tf.transpose(W_conv1, perm=[2, 3, 0, 1]),[32,-1]))
kernels_l2 = sess.run(tf.reshape(tf.transpose(W_conv2, perm=[2, 3, 0, 1]),[32*64,-1]))
%matplotlib inline
import numpy as np
import matplotlib.pyplot as plt
plt.plot([np.mean(loss_values[i:i+5]) for i in range(len(loss_values))])
plt.show()
###Output
_____no_output_____
###Markdown
Evaluation
###Code
y_ = np.argmax(y_test[0:100],1) # ground truth
print metrics.classification_report(y_true= y_, y_pred= y_pred_lb)
print metrics.confusion_matrix(y_true= y_, y_pred= y_pred_lb)
print("Classification accuracy: %0.6f" % metrics.accuracy_score(y_true= y_, y_pred= y_pred_lb) )
print("Log Loss: %0.6f" % metrics.log_loss(y_true= y_, y_pred= y_pred, labels=range(4)) )
###Output
precision recall f1-score support
0 0.00 0.00 0.00 25
1 0.00 0.00 0.00 28
2 0.30 1.00 0.46 23
3 1.00 1.00 1.00 24
avg / total 0.31 0.47 0.35 100
[[ 0 0 25 0]
[ 0 0 28 0]
[ 0 0 23 0]
[ 0 0 0 24]]
Classification accuracy: 0.470000
Log Loss: 0.972129
###Markdown
Viz
###Code
!wget --output-document utils1.py http://deeplearning.net/tutorial/code/utils.py
import utils1
from utils1 import tile_raster_images
#from utils import tile_raster_images
import matplotlib.pyplot as plt
from PIL import Image
%matplotlib inline
image = Image.fromarray(tile_raster_images(kernels_l1, img_shape=(5, 5) ,tile_shape=(4, 8), tile_spacing=(1, 1)))
### Plot image
plt.rcParams['figure.figsize'] = (18.0, 18.0)
imgplot = plt.imshow(image)
imgplot.set_cmap('gray')
image = Image.fromarray(tile_raster_images(kernels_l2, img_shape=(5, 5) ,tile_shape=(4, 12), tile_spacing=(1, 1)))
### Plot image
plt.rcParams['figure.figsize'] = (18.0, 18.0)
imgplot = plt.imshow(image)
imgplot.set_cmap('gray')
import numpy as np
plt.rcParams['figure.figsize'] = (5.0, 5.0)
sampleimage1 = X_test[3]
plt.imshow(np.reshape(sampleimage1,[64,128]), cmap="gray")
# Launch the graph
with tf.Session() as sess:
sess.run(init)
saver = tf.train.Saver(tf.all_variables())
# load previously trained model if appilcable
ckpt = tf.train.get_checkpoint_state(chk_directory)
if ckpt:
print "loading model: ",ckpt.model_checkpoint_path
saver.restore(sess, ckpt.model_checkpoint_path)
ActivatedUnits1 = sess.run(convolve1,feed_dict={x:np.reshape(sampleimage1,[1,64*128],order='F'),keep_prob:1.0})
plt.figure(1, figsize=(20,20))
n_columns = 3
n_rows = 3
for i in range(9):
plt.subplot(n_rows, n_columns, i+1)
plt.title('Filter ' + str(i))
plt.imshow(ActivatedUnits1[0,:,:,i], interpolation="nearest", cmap="gray")
###Output
_____no_output_____ |
Section9_Assessment3/section9_assessment3.ipynb | ###Markdown
Table of Contents1 Load the database2 Assessment 33 Create database4 Connect to the database5 Create table students6 INSERT some data into the tables Load the database
###Code
import numpy as np
import pandas as pd
import sys
sys.path.append('../utils')
import psycopg2
import util_database
from util_database import *
display(show_method_attributes(util_database))
dbname, dbuser, dbpass, dbport = get_postgres_configs('school')
%load_ext sql
%sql postgres://postgres:$dbpass@localhost:$dbport/$dbname
###Output
_____no_output_____
###Markdown
Assessment 3Complete the following task:Create a new database called "School" this database should have two tables: teachers and students.The students table should have columns for student_id, first_name,last_name, homeroom_number, phone,email, and graduation year.The teachers table should have columns for teacher_id, first_name, last_name,homeroom_number, department, email, and phone.The constraints are mostly up to you, but your table constraints do have to consider the following: We must have a phone number to contact students in case of an emergency. We must have ids as the primary key of the tablesPhone numbers and emails must be unique to the individual.Once you've made the tables, insert a student named Mark Watney (student_id=1) who has a phone number of 777-555-1234 and doesn't have an email. He graduates in 2035 and has 5 as a homeroom number.Then insert a teacher names Jonas Salk (teacher_id = 1) who as a homeroom number of 5 and is from the Biology department. His contact info is: [email protected] and a phone number of 777-555-4321. Create database- Go to pgadmin and create the database. Connect to the database
###Code
print(get_postgres_configs.__doc__)
dbname, dbuser, dbpass, dbport = get_postgres_configs('school')
%load_ext sql
%sql postgres://postgres:$dbpass@localhost:$dbport/$dbname
###Output
_____no_output_____
###Markdown
Create table students
###Code
%sql drop table if exists students
%sql drop table if exists teachers
%%sql
create table students(
student_id serial primary key,
first_name varchar(50) not null,
last_name varchar(50) not null,
homeroom_number int unique,
phone varchar(20) not null unique,
email varchar(512) unique,
graduation_year int
);
%%sql
create table teachers(
teacher_id serial primary key,
first_name varchar(50) not null,
last_name varchar(50) not null,
homeroom_number int unique,
phone varchar(20) not null unique,
email varchar(512) unique,
department varchar(512)
);
###Output
* postgres://postgres:***@localhost:5432/school
Done.
###Markdown
INSERT some data into the tables
###Code
%%sql
insert into students(first_name, last_name, phone,graduation_year,homeroom_number)
values
('Mark', 'Watney','777-555-1234',2035,5);
%sql select * from students;
%%sql
insert into teachers(first_name, last_name, phone,department,homeroom_number,email)
values
('Jonas', 'Salk','777-555-4321','Biology',5,'[email protected]');
%sql select * from teachers;
###Output
* postgres://postgres:***@localhost:5432/school
1 rows affected.
|
archived-datasets/omop-drugbank/process-omop-drugbank.ipynb | ###Markdown
Process OMOP to DrugBank mappings DataJupyter Notebook to download and preprocess files to transform to BioLink RDF. Download filesThe download can be defined:* in this Jupyter Notebook using Python* as a Bash script in the `download/download.sh` file, and executed using `d2s download omop-drugbank`
###Code
import os
# Variables and path for the dataset
dataset_id = 'omop-drugbank'
input_folder = '/notebooks/workspace/input/' + dataset_id
mapping_folder = '/notebooks/datasets/' + dataset_id + '/mapping'
os.makedirs(input_folder, exist_ok=True)
# Use input folder as working folder
os.chdir(input_folder)
# Download OMOP to DrugBank mappings
os.system('wget -N https://raw.githubusercontent.com/OHDSI/KnowledgeBase/master/LAERTES/terminology-mappings/RxNORM-to-UNII-PreferredName-To-DrugBank/rxnorm-drugbank-omop-mapping-CLEANED.tsv')
###Output
_____no_output_____ |
demos/20200509.ipynb | ###Markdown
Scikit-Learn Estimators
###Code
from yellowbrick.datasets import load_concrete
ds = load_concrete(return_dataset=True)
print(ds.README)
X, y = load_concrete()
X.head()
y.head()
X.shape
y.shape
import seaborn as sns
sns.pairplot(X)
from sklearn.linear_model import LinearRegression
model = LinearRegression(fit_intercept=False)
model.fit(X,y)
model.score(X,y)
model.predict([[150.3, 32, 0.0, 75, 0.25, 750.0, 342.1, 152]])
from sklearn.ensemble import RandomForestRegressor
model = RandomForestRegressor(n_estimators=50)
model.fit(X, y)
model.score(X, y)
model.predict([[150.3, 32, 0.0, 75, 0.25, 750.0, 342.1, 152]])
for attr in dir(model):
if attr.endswith("_") and not attr.startswith("_"):
print(attr)
from sklearn.neural_network import MLPRegressor
model = MLPRegressor(hidden_layer_sizes=(100, 100, 100), activation="relu")
model.fit(X, y)
model.score(X, y)
from sklearn.preprocessing import StandardScaler, RobustScaler
scale = StandardScaler().fit(X)
Xp = scale.transform(X)
import pandas as pd
pd.DataFrame(Xp).head()
for estimator in (
LinearRegression(fit_intercept=False),
RandomForestRegressor(n_estimators=50),
MLPRegressor(hidden_layer_sizes=(100,100,100), activation="relu")):
estimator.fit(Xp, y)
print(estimator.score(Xp, y))
Xp = RobustScaler().fit_transform(X)
for estimator in (
LinearRegression(fit_intercept=False),
RandomForestRegressor(n_estimators=50),
MLPRegressor(hidden_layer_sizes=(100,100,100), activation="relu")):
estimator.fit(Xp, y)
print(estimator.score(Xp, y))
from sklearn.pipeline import Pipeline
from sklearn.model_selection import train_test_split as tts
models = [
Pipeline([
("std", StandardScaler()),
("reg", LinearRegression(fit_intercept=False)),
]),
Pipeline([
("std", StandardScaler()),
("reg", RandomForestRegressor(n_estimators=50)),
]),
Pipeline([
("std", StandardScaler()),
("reg", MLPRegressor(hidden_layer_sizes=(100,100,100))),
]),
Pipeline([
("std", RobustScaler()),
("reg", LinearRegression(fit_intercept=False)),
]),
Pipeline([
("std", RobustScaler()),
("reg", RandomForestRegressor(n_estimators=50)),
]),
Pipeline([
("std", RobustScaler()),
("reg", MLPRegressor(hidden_layer_sizes=(100,100,100))),
]),
]
X_train, X_test, y_train, y_test = tts(X, y, test_size=0.2)
for model in models:
model.fit(X_train, y_train)
score = model.score(X_test, y_test)
print(model, score)
print("\n\n")
from sklearn.compose import ColumnTransformer
from sklearn.preprocessing import Binarizer
model = Pipeline([
("cols", ColumnTransformer([
("bin", Binarizer(), ["slag", "ash", "splast", "age"]),
("std", RobustScaler(), ["cement", "water", "coarse", "fine"]),
], remainder="drop")),
("reg", RandomForestRegressor(n_estimators=50))
])
model.fit(X_train, y_train)
model.score(X_test, y_test)
model = Pipeline([
("cols", ColumnTransformer([
("bin", Binarizer(), ["slag", "ash", "splast"]),
("std", RobustScaler(), ["cement", "water", "coarse", "fine"]),
], remainder="drop")),
("reg", MLPRegressor(hidden_layer_sizes=(100,100,100)))
])
model.fit(X_train, y_train)
model.score(X_test, y_test)
from sklearn.model_selection import cross_val_score
model = Pipeline([
("std", RobustScaler()),
("reg", MLPRegressor(hidden_layer_sizes=(100,100,100))),
])
cross_val_score(model, X, y, cv=12)
###Output
/Users/benjamin/.pyenv/versions/3.7.3/Python.framework/Versions/3.7/lib/python3.7/site-packages/sklearn/neural_network/_multilayer_perceptron.py:571: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (200) reached and the optimization hasn't converged yet.
% self.max_iter, ConvergenceWarning)
/Users/benjamin/.pyenv/versions/3.7.3/Python.framework/Versions/3.7/lib/python3.7/site-packages/sklearn/neural_network/_multilayer_perceptron.py:571: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (200) reached and the optimization hasn't converged yet.
% self.max_iter, ConvergenceWarning)
/Users/benjamin/.pyenv/versions/3.7.3/Python.framework/Versions/3.7/lib/python3.7/site-packages/sklearn/neural_network/_multilayer_perceptron.py:571: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (200) reached and the optimization hasn't converged yet.
% self.max_iter, ConvergenceWarning)
/Users/benjamin/.pyenv/versions/3.7.3/Python.framework/Versions/3.7/lib/python3.7/site-packages/sklearn/neural_network/_multilayer_perceptron.py:571: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (200) reached and the optimization hasn't converged yet.
% self.max_iter, ConvergenceWarning)
/Users/benjamin/.pyenv/versions/3.7.3/Python.framework/Versions/3.7/lib/python3.7/site-packages/sklearn/neural_network/_multilayer_perceptron.py:571: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (200) reached and the optimization hasn't converged yet.
% self.max_iter, ConvergenceWarning)
/Users/benjamin/.pyenv/versions/3.7.3/Python.framework/Versions/3.7/lib/python3.7/site-packages/sklearn/neural_network/_multilayer_perceptron.py:571: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (200) reached and the optimization hasn't converged yet.
% self.max_iter, ConvergenceWarning)
/Users/benjamin/.pyenv/versions/3.7.3/Python.framework/Versions/3.7/lib/python3.7/site-packages/sklearn/neural_network/_multilayer_perceptron.py:571: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (200) reached and the optimization hasn't converged yet.
% self.max_iter, ConvergenceWarning)
/Users/benjamin/.pyenv/versions/3.7.3/Python.framework/Versions/3.7/lib/python3.7/site-packages/sklearn/neural_network/_multilayer_perceptron.py:571: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (200) reached and the optimization hasn't converged yet.
% self.max_iter, ConvergenceWarning)
/Users/benjamin/.pyenv/versions/3.7.3/Python.framework/Versions/3.7/lib/python3.7/site-packages/sklearn/neural_network/_multilayer_perceptron.py:571: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (200) reached and the optimization hasn't converged yet.
% self.max_iter, ConvergenceWarning)
/Users/benjamin/.pyenv/versions/3.7.3/Python.framework/Versions/3.7/lib/python3.7/site-packages/sklearn/neural_network/_multilayer_perceptron.py:571: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (200) reached and the optimization hasn't converged yet.
% self.max_iter, ConvergenceWarning)
/Users/benjamin/.pyenv/versions/3.7.3/Python.framework/Versions/3.7/lib/python3.7/site-packages/sklearn/neural_network/_multilayer_perceptron.py:571: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (200) reached and the optimization hasn't converged yet.
% self.max_iter, ConvergenceWarning)
/Users/benjamin/.pyenv/versions/3.7.3/Python.framework/Versions/3.7/lib/python3.7/site-packages/sklearn/neural_network/_multilayer_perceptron.py:571: ConvergenceWarning: Stochastic Optimizer: Maximum iterations (200) reached and the optimization hasn't converged yet.
% self.max_iter, ConvergenceWarning)
|
demonstration of TEB to IQU.ipynb | ###Markdown
Load data
###Code
data_IQU = hp.read_map('./map_mock_des128.fits', field=(0,1,2))
data_IQU[0] *= 1e-5
###Output
NSIDE = 128
ORDERING = RING in fits file
INDXSCHM = IMPLICIT
###Markdown
Convert to TEB (remembering mask problems)
###Code
data_TEB = qu2teb(data_IQU, 128, 128*3-1)
plt.figure(figsize=(14,5))
hp.mollview(data_IQU[0], min=-0.01,max=0.01, sub=(2,3,1), title='I')
hp.mollview(data_IQU[1], min=-0.01,max=0.01, sub=(2,3,2), title='Q')
hp.mollview(data_IQU[2], min=-0.01,max=0.01, sub=(2,3,3), title='U')
hp.mollview(data_TEB[0], min=-0.01,max=0.01, sub=(2,3,4), title='T')
hp.mollview(data_TEB[1], min=-0.01,max=0.01, sub=(2,3,5), title='E')
hp.mollview(data_TEB[2], min=-0.01,max=0.01, sub=(2,3,6), title='B')
###Output
_____no_output_____
###Markdown
Recover IQU - ud_grade to higher nside
###Code
recovered_IQU = teb2iqu(data_TEB, 128, 128*3-1)
hp.gnomview(data_IQU[1], reso = 13, rot=(40,-38), min = -0.01,max=0.01, sub = (1,2,1) ,
title = str('Residual var = ' + str(np.var(data_IQU[1]-recovered_IQU[1]))))
hp.gnomview(recovered_IQU[1], reso = 13, rot=(40,-38), min = -0.01,max=0.01, sub = (1,2,2), title=None)
data_TEB_high_nside = iqu2teb(hp.ud_grade(data_IQU,512), 512, 512*3-1)
recovered_IQU_high_nside = hp.ud_grade(teb2iqu(data_TEB_high_nside, 512, 512*3-1),128)
hp.gnomview(data_IQU[1], reso = 13, rot=(40,-38), min = -0.01,max=0.01, sub = (1,2,1) ,
title = str('Residual var = ' + str(np.var(data_IQU[1]-recovered_IQU_high_nside[1]))))
hp.gnomview(recovered_IQU_high_nside[1], reso = 13, rot=(40,-38), min = -0.01,max=0.01, sub = (1,2,2), title=None)
###Output
_____no_output_____ |
array_strings/ipynb/one_edit_distance.ipynb | ###Markdown
Given two strings s and t, determine if they are both one edit distance apart.Note: There are 3 possiblities to satisify one edit distance apart:Insert a character into s to get tDelete a character from s to get tReplace a character of s to get tExample 1:Input: s = "ab", t = "acb"Output: trueExplanation: We can insert 'c' into s to get t.Example 2:Input: s = "cab", t = "ad"Output: falseExplanation: We cannot get t from s by only one step.Example 3:Input: s = "1203", t = "1213"Output: trueExplanation: We can replace '0' with '1' to get t. Approach 1: One pass algorithmIntuitionFirst of all, let's ensure that the string lengths are not too far from each other. If the length difference is 2 or more characters, then s and t couldn't be one edit away strings.For the next let's assume that s is always shorter or the same length as t. If not, one could always call isOneEditDistance(t, s) to inverse the string order.Now it's time to pass along the strings and to look for the first different character.If there is no differences between the first len(s) characters, only two situations are possible :1)The strings are equal.2)The strings are one edit away distance.Now what if there is a different character so that s[i] != t[i].If the strings are of the same length, all next characters should be equal to keep one edit away distance. To verify it, one has to compare the substrings of s and t both starting from the i + 1th character.If t is one character longer than s, the additional character t[i] should be the only difference between both strings. To verify it, one has to compare a substring of s starting from the ith character and a substring of t starting from the i + 1th character.
###Code
def one_edit_distance(ls,t):
ns, nt = len(ls), len(t)
if ns > nt:
return False
if nt - ns > 1:
return False
for i in range(ns):
if ls[i] != t[i]:
if ns == nt:
return ls[i + 1:] == t[i + 1:]
else:
return ls[i:] == t[i + 1:]
return ns + 1 == nt
print(one_edit_distance('abc','abhh'))
print(one_edit_distance('ab','acb'))
print(one_edit_distance('ba','abc'))
print(one_edit_distance('cab','ad'))
print(one_edit_distance('1203','1213'))
#olution with print and commit
def one_edit_distance(ls,t):
ns, nt = len(ls), len(t)
print('ns',ns)
print('nt',nt)
# Ensure that s is shorter than t.
if ns > nt:
return False
# The strings are NOT one edit away distance
# if the length diff is more than 1.
if nt - ns > 1:
return False
for i in range(ns):
print('\nls[i]',ls[i])
print('t[i]',t[i])
print('ls[i:]',ls[i:] )
if ls[i] != t[i]:
# if strings have the same length
if ns == nt:
return ls[i + 1:] == t[i + 1:]
# if strings have different lengths
else:
return ls[i:] == t[i + 1:]
print('ls[i + 1:]',ls[i + 1:])
print('t[i + 1:]',t[i + 1:])
# If there is no diffs on ns distance
# the strings are one edit away only if
# t has one more character.
return ns + 1 == nt
print(one_edit_distance('abc','abhh'))
###Output
ns 3
nt 4
ls[i] a
t[i] a
ls[i:] abc
ls[i + 1:] bc
t[i + 1:] bhh
ls[i] b
t[i] b
ls[i:] bc
ls[i + 1:] c
t[i + 1:] hh
ls[i] c
t[i] h
ls[i:] c
False
|
.ipynb_checkpoints/classify task-checkpoint.ipynb | ###Markdown
Training
###Code
import torch
def get_device():
if torch.cuda.is_available():
if torch.cuda.get_device_name(0) == 'GeForce GT 730':
device = 'cpu'
else:
device = 'cuda'
else:
device = 'cpu'
return torch.device(device)
device = get_device()
import random
import time
import numpy as np
from torch.utils.data import DataLoader
import os
import sys
import pandas as pd
from gensim.models.word2vec import Word2Vec
root = 'data/'
train_data = pd.read_pickle(root+'train/blocks.pkl')
val_data = pd.read_pickle(root + 'dev/blocks.pkl')
test_data = pd.read_pickle(root+'test/blocks.pkl')
word2vec = Word2Vec.load(root+"train/embedding/node_w2v_128").wv
embeddings = np.zeros((word2vec.vectors.shape[0] + 1, word2vec.vectors.shape[1]), dtype="float32")
embeddings[:word2vec.vectors.shape[0]] = word2vec.vectors
USE_GPU = False
HIDDEN_DIM = 100
ENCODE_DIM = 128
LABELS = 104
EPOCHS = 15
BATCH_SIZE = 128
MAX_TOKENS = word2vec.vectors.shape[0]
EMBEDDING_DIM = word2vec.vectors.shape[1]
model = BatchProgramClassifier(EMBEDDING_DIM,HIDDEN_DIM,MAX_TOKENS+1,ENCODE_DIM,LABELS,BATCH_SIZE,
USE_GPU, embeddings)
if USE_GPU:
model.cuda()
parameters = model.parameters()
optimizer = torch.optim.Adamax(parameters)
loss_function = torch.nn.CrossEntropyLoss()
train_loss_ = []
val_loss_ = []
train_acc_ = []
val_acc_ = []
best_acc = 0.0
print('Start training...')
# training procedure
best_model = model
for epoch in range(EPOCHS):
start_time = time.time()
total_acc = 0.0
total_loss = 0.0
total = 0.0
i = 0
model.train()
while i < len(train_data):
train_inputs, train_labels = get_batch(train_data, i, BATCH_SIZE)
i += BATCH_SIZE
if USE_GPU:
train_inputs, train_labels = train_inputs.cuda(), train_labels.cuda()
model.zero_grad()
model.batch_size = len(train_labels)
model.hidden = model.init_hidden()
output = model(train_inputs)
loss = loss_function(output, Variable(train_labels))
loss.backward()
optimizer.step()
print('[Epoch: %3d/%3d] [data: %d/%d] Training Loss: %.4f'
% (epoch + 1, EPOCHS, i, len(train_data), loss))
# calc training acc
_, predicted = torch.max(output.data, 1)
total_acc += (predicted == train_labels).sum()
total += len(train_labels)
total_loss += loss.item()*len(train_inputs)
train_loss_.append(total_loss / total)
train_acc_.append(total_acc.item() / total)
# validation epoch
total_acc = 0.0
total_loss = 0.0
total = 0.0
i = 0
model.eval()
with torch.no_grad():
while i < len(val_data):
val_inputs, val_labels = get_batch(val_data, i, BATCH_SIZE)
i += BATCH_SIZE
if USE_GPU:
val_inputs, val_labels = val_inputs.cuda(), val_labels.cuda()
model.batch_size = len(val_labels)
model.hidden = model.init_hidden()
output = model(val_inputs)
loss = loss_function(output, Variable(val_labels))
# calc valing acc
_, predicted = torch.max(output.data, 1)
total_acc += (predicted == val_labels).sum()
total += len(val_labels)
total_loss += loss.item()*len(val_inputs)
val_loss_.append(total_loss / total)
val_acc_.append(total_acc.item() / total)
end_time = time.time()
if total_acc/total > best_acc:
best_model = model
print('[Epoch: %3d/%3d] Training Loss: %.4f, Validation Loss: %.4f,'
' Training Acc: %.3f, Validation Acc: %.3f, Time Cost: %.3f s'
% (epoch + 1, EPOCHS, train_loss_[epoch], val_loss_[epoch],
train_acc_[epoch], val_acc_[epoch], end_time - start_time))
total_acc = 0.0
total_loss = 0.0
total = 0.0
i = 0
model = best_model
model.eval()
with torch.no_grad():
while i < len(test_data):
test_inputs, test_labels = get_batch(test_data, i, BATCH_SIZE)
i += BATCH_SIZE
if USE_GPU:
test_inputs, test_labels = test_inputs.cuda(), test_labels.cuda()
model.batch_size = len(test_labels)
model.hidden = model.init_hidden()
output = model(test_inputs)
loss = loss_function(output, Variable(test_labels))
_, predicted = torch.max(output.data, 1)
total_acc += (predicted == test_labels).sum()
total += len(test_labels)
total_loss += loss.item() * len(test_inputs)
print("Testing results(Acc):", total_acc.item() / total)
# train.py
import pandas as pd
import random
import torch
import time
import numpy as np
from gensim.models.word2vec import Word2Vec
from model import BatchProgramClassifier
from torch.autograd import Variable
from torch.utils.data import DataLoader
import os
import sys
def get_batch(dataset, idx, bs):
tmp = dataset.iloc[idx: idx+bs]
data, labels = [], []
for _, item in tmp.iterrows():
data.append(item[1])
labels.append(item[2]-1)
return data, torch.LongTensor(labels)
root = 'data/'
train_data = pd.read_pickle(root+'train/blocks.pkl')
val_data = pd.read_pickle(root + 'dev/blocks.pkl')
test_data = pd.read_pickle(root+'test/blocks.pkl')
word2vec = Word2Vec.load(root+"train/embedding/node_w2v_128").wv
embeddings = np.zeros((word2vec.syn0.shape[0] + 1, word2vec.syn0.shape[1]), dtype="float32")
embeddings[:word2vec.syn0.shape[0]] = word2vec.syn0
HIDDEN_DIM = 100
ENCODE_DIM = 128
LABELS = 104
EPOCHS = 15
BATCH_SIZE = 64
USE_GPU = True
MAX_TOKENS = word2vec.syn0.shape[0]
EMBEDDING_DIM = word2vec.syn0.shape[1]
model = BatchProgramClassifier(EMBEDDING_DIM,HIDDEN_DIM,MAX_TOKENS+1,ENCODE_DIM,LABELS,BATCH_SIZE,
USE_GPU, embeddings)
if USE_GPU:
model.cuda()
parameters = model.parameters()
optimizer = torch.optim.Adamax(parameters)
loss_function = torch.nn.CrossEntropyLoss()
train_loss_ = []
val_loss_ = []
train_acc_ = []
val_acc_ = []
best_acc = 0.0
print('Start training...')
# training procedure
best_model = model
for epoch in range(EPOCHS):
start_time = time.time()
total_acc = 0.0
total_loss = 0.0
total = 0.0
i = 0
while i < len(train_data):
batch = get_batch(train_data, i, BATCH_SIZE)
i += BATCH_SIZE
train_inputs, train_labels = batch
if USE_GPU:
train_inputs, train_labels = train_inputs, train_labels.cuda()
model.zero_grad()
model.batch_size = len(train_labels)
model.hidden = model.init_hidden()
output = model(train_inputs)
loss = loss_function(output, Variable(train_labels))
loss.backward()
optimizer.step()
# calc training acc
_, predicted = torch.max(output.data, 1)
total_acc += (predicted == train_labels).sum()
total += len(train_labels)
total_loss += loss.item()*len(train_inputs)
train_loss_.append(total_loss / total)
train_acc_.append(total_acc.item() / total)
# validation epoch
total_acc = 0.0
total_loss = 0.0
total = 0.0
i = 0
while i < len(val_data):
batch = get_batch(val_data, i, BATCH_SIZE)
i += BATCH_SIZE
val_inputs, val_labels = batch
if USE_GPU:
val_inputs, val_labels = val_inputs, val_labels.cuda()
model.batch_size = len(val_labels)
model.hidden = model.init_hidden()
output = model(val_inputs)
loss = loss_function(output, Variable(val_labels))
# calc valing acc
_, predicted = torch.max(output.data, 1)
total_acc += (predicted == val_labels).sum()
total += len(val_labels)
total_loss += loss.item()*len(val_inputs)
val_loss_.append(total_loss / total)
val_acc_.append(total_acc.item() / total)
end_time = time.time()
if total_acc/total > best_acc:
best_model = model
print('[Epoch: %3d/%3d] Training Loss: %.4f, Validation Loss: %.4f,'
' Training Acc: %.3f, Validation Acc: %.3f, Time Cost: %.3f s'
% (epoch + 1, EPOCHS, train_loss_[epoch], val_loss_[epoch],
train_acc_[epoch], val_acc_[epoch], end_time - start_time))
total_acc = 0.0
total_loss = 0.0
total = 0.0
i = 0
model = best_model
while i < len(test_data):
batch = get_batch(test_data, i, BATCH_SIZE)
i += BATCH_SIZE
test_inputs, test_labels = batch
if USE_GPU:
test_inputs, test_labels = test_inputs, test_labels.cuda()
model.batch_size = len(test_labels)
model.hidden = model.init_hidden()
output = model(test_inputs)
loss = loss_function(output, Variable(test_labels))
_, predicted = torch.max(output.data, 1)
total_acc += (predicted == test_labels).sum()
total += len(test_labels)
total_loss += loss.item() * len(test_inputs)
print("Testing results(Acc):", total_acc.item() / total)
###Output
_____no_output_____
###Markdown
generate preprocessed data
###Code
import pandas as pd
import os
def parse_source(root, output_file, option):
path = root+output_file
if os.path.exists(path) and option is 'existing':
source = pd.read_pickle(path)
else:
from pycparser import c_parser
parser = c_parser.CParser()
source = pd.read_pickle(root+'programs.pkl')
source.columns = ['id', 'code', 'label']
source['code'] = source['code'].apply(parser.parse)
source.to_pickle(path)
return source
#s = parse_source(root='data/', output_file='ast.pkl',option='existing')
def split_data(root,ratio):
data = pd.read_pickle(root+'ast.pkl')
data_num = len(data)
ratios = [int(r) for r in ratio.split(':')]
train_split = int(ratios[0]/sum(ratios)*data_num)
val_split = train_split + int(ratios[1]/sum(ratios)*data_num)
data = data.sample(frac=1, random_state=666)
train = data.iloc[:train_split]
dev = data.iloc[train_split:val_split]
test = data.iloc[val_split:]
def check_or_create(path):
if not os.path.exists(path):
os.mkdir(path)
train_path = root+'train/'
check_or_create(train_path)
train_file_path = train_path+'train_.pkl'
train.to_pickle(train_file_path)
dev_path = root+'dev/'
check_or_create(dev_path)
dev_file_path = dev_path+'dev_.pkl'
dev.to_pickle(dev_file_path)
test_path = root+'test/'
check_or_create(test_path)
test_file_path = test_path+'test_.pkl'
test.to_pickle(test_file_path)
def dictionary_and_embedding(root, input_file, size):
if not input_file:
input_file = 'data/train/train_.pkl'
trees = pd.read_pickle(input_file)
if not os.path.exists(root+'train/embedding'):
os.mkdir(root+'train/embedding')
from prepare_data import get_sequences
def trans_to_sequences(ast):
sequence = []
get_sequences(ast, sequence)
return sequence
corpus = trees['code'].apply(trans_to_sequences) # every row of corpus is list of str
str_corpus = [' '.join(c) for c in corpus] # str_corpus is list of str
trees['code'] = pd.Series(str_corpus)
# the first saveral ones are alway the same in every row, can we delete them ?
trees.to_csv(root+'train/programs_ns.tsv') # tsv?
from gensim.models.word2vec import Word2Vec
w2v = Word2Vec(corpus, size=size, workers=16, sg=1, min_count=3)
w2v.save(root+'train/embedding/node_w2v_' + str(size))
def generate_block_seqs(root,data_path,part,size=128):
from prepare_data import get_blocks as func
from gensim.models.word2vec import Word2Vec
word2vec = Word2Vec.load(root+'train/embedding/node_w2v_' + str(size)).wv
vocab = word2vec.vocab
max_token = word2vec.vectors.shape[0]
# Attribute `syn0` will be removed in 4.0.0, use self.vectors instead
def tree_to_index(node):
token = node.token
result = [vocab[token].index if token in vocab else max_token]
children = node.children
for child in children:
result.append(tree_to_index(child))
return result
def trans2seq(r):
blocks = []
func(r, blocks)
tree = []
for b in blocks:
btree = tree_to_index(b)
tree.append(btree)
return tree
trees = pd.read_pickle(data_path)
trees['code'] = trees['code'].apply(trans2seq)
trees.to_pickle(root+part+'/blocks.pkl')
generate_block_seqs(root='data/', data_path='data/train/train_.pkl', part='train')
generate_block_seqs(root='data/', data_path='data/dev/dev_.pkl', part='dev')
generate_block_seqs(root='data/', data_path='data/test/test_.pkl', part='test')
# source code
import pandas as pd
import os
class Pipeline:
def __init__(self, ratio, root):
self.ratio = ratio
self.root = root
self.sources = None
self.train_file_path = None
self.dev_file_path = None
self.test_file_path = None
self.size = None
# parse source code
def parse_source(self, output_file, option):
path = self.root+output_file
if os.path.exists(path) and option is 'existing':
source = pd.read_pickle(path)
else:
from pycparser import c_parser
parser = c_parser.CParser()
source = pd.read_pickle(self.root+'programs.pkl')
source.columns = ['id', 'code', 'label']
source['code'] = source['code'].apply(parser.parse)
source.to_pickle(path)
self.sources = source
return source
# split data for training, developing and testing
def split_data(self):
data = self.sources
data_num = len(data)
ratios = [int(r) for r in self.ratio.split(':')]
train_split = int(ratios[0]/sum(ratios)*data_num)
val_split = train_split + int(ratios[1]/sum(ratios)*data_num)
data = data.sample(frac=1, random_state=666)
train = data.iloc[:train_split]
dev = data.iloc[train_split:val_split]
test = data.iloc[val_split:]
def check_or_create(path):
if not os.path.exists(path):
os.mkdir(path)
train_path = self.root+'train/'
check_or_create(train_path)
self.train_file_path = train_path+'train_.pkl'
train.to_pickle(self.train_file_path)
dev_path = self.root+'dev/'
check_or_create(dev_path)
self.dev_file_path = dev_path+'dev_.pkl'
dev.to_pickle(self.dev_file_path)
test_path = self.root+'test/'
check_or_create(test_path)
self.test_file_path = test_path+'test_.pkl'
test.to_pickle(self.test_file_path)
# construct dictionary and train word embedding
def dictionary_and_embedding(self, input_file, size):
self.size = size
if not input_file:
input_file = self.train_file_path
trees = pd.read_pickle(input_file)
if not os.path.exists(self.root+'train/embedding'):
os.mkdir(self.root+'train/embedding')
from prepare_data import get_sequences
def trans_to_sequences(ast):
sequence = []
get_sequences(ast, sequence)
return sequence
corpus = trees['code'].apply(trans_to_sequences) # every row of corpus is list of str
str_corpus = [' '.join(c) for c in corpus] # str_corpus is list of str
trees['code'] = pd.Series(str_corpus)
# the first saveral ones are alway the same in every row, can we delete them ?
trees.to_csv(self.root+'train/programs_ns.tsv')
from gensim.models.word2vec import Word2Vec
w2v = Word2Vec(corpus, size=size, workers=16, sg=1, min_count=3)
w2v.save(self.root+'train/embedding/node_w2v_' + str(size))
# generate block sequences with index representations
def generate_block_seqs(self,data_path,part):
from prepare_data import get_blocks as func
from gensim.models.word2vec import Word2Vec
word2vec = Word2Vec.load(self.root+'train/embedding/node_w2v_' + str(self.size)).wv
vocab = word2vec.vocab
max_token = word2vec.vectors.shape[0]
# Attribute `syn0` will be removed in 4.0.0, use self.vectors instead
def tree_to_index(node):
token = node.token
result = [vocab[token].index if token in vocab else max_token]
children = node.children
for child in children:
result.append(tree_to_index(child))
return result
def trans2seq(r):
blocks = []
func(r, blocks)
tree = []
for b in blocks:
btree = tree_to_index(b)
tree.append(btree)
return tree
trees = pd.read_pickle(data_path)
trees['code'] = trees['code'].apply(trans2seq)
trees.to_pickle(self.root+part+'/blocks.pkl')
# run for processing data to train
def run(self):
print('parse source code...')
self.parse_source(output_file='ast.pkl',option='existing')
print('split data...')
self.split_data()
print('train word embedding...')
self.dictionary_and_embedding(None,128)
print('generate block sequences...')
self.generate_block_seqs(self.train_file_path, 'train')
self.generate_block_seqs(self.dev_file_path, 'dev')
self.generate_block_seqs(self.test_file_path, 'test')
ppl = Pipeline('3:1:1', 'data/')
ppl.run()
###Output
_____no_output_____ |
workspace/modeling_edited.ipynb | ###Markdown
Modeling ExerciseThis exercise is designed to both help you to learn `git` and `github` as well as some concepts from modeling that will be important. If you need some ideas take a look at the [course notes](http://github.com/mandli/intro-numerical-methods). Reading DataTake the data you read in from `get_hydro_data.ipynb` and write a function that read in the stream flow data and precip and returns the data
###Code
def read_streamflow_data(path):
return pd.read_csv(path, header=0,
usecols=['dateTime', 'X_00060_00000'],
parse_dates=['dateTime'],
dtype = {'X_00060_00000': float}, )
data = read_streamflow_data("temp_data/streamflow_data.csv")
print(data)
###Output
dateTime X_00060_00000
0 2018-08-01 05:00:00+00:00 155.0
1 2018-08-01 05:05:00+00:00 152.0
2 2018-08-01 05:10:00+00:00 164.0
3 2018-08-01 05:15:00+00:00 161.0
4 2018-08-01 05:20:00+00:00 160.0
5 2018-08-01 05:25:00+00:00 151.0
6 2018-08-01 05:30:00+00:00 153.0
7 2018-08-01 05:35:00+00:00 157.0
8 2018-08-01 05:40:00+00:00 150.0
9 2018-08-01 05:45:00+00:00 156.0
10 2018-08-01 05:50:00+00:00 170.0
11 2018-08-01 05:55:00+00:00 159.0
12 2018-08-01 06:00:00+00:00 160.0
13 2018-08-01 06:05:00+00:00 158.0
14 2018-08-01 06:10:00+00:00 155.0
15 2018-08-01 06:15:00+00:00 152.0
16 2018-08-01 06:20:00+00:00 157.0
17 2018-08-01 06:25:00+00:00 155.0
18 2018-08-01 06:30:00+00:00 163.0
19 2018-08-01 06:35:00+00:00 165.0
20 2018-08-01 06:40:00+00:00 155.0
21 2018-08-01 06:45:00+00:00 158.0
22 2018-08-01 06:50:00+00:00 158.0
23 2018-08-01 06:55:00+00:00 152.0
24 2018-08-01 07:00:00+00:00 166.0
25 2018-08-01 07:05:00+00:00 160.0
26 2018-08-01 07:10:00+00:00 159.0
27 2018-08-01 07:15:00+00:00 152.0
28 2018-08-01 07:20:00+00:00 154.0
29 2018-08-01 07:25:00+00:00 143.0
... ... ...
33148 2018-12-02 03:30:00+00:00 356.0
33149 2018-12-02 03:35:00+00:00 371.0
33150 2018-12-02 03:40:00+00:00 365.0
33151 2018-12-02 03:45:00+00:00 365.0
33152 2018-12-02 03:50:00+00:00 384.0
33153 2018-12-02 03:55:00+00:00 378.0
33154 2018-12-02 04:00:00+00:00 381.0
33155 2018-12-02 04:05:00+00:00 381.0
33156 2018-12-02 04:10:00+00:00 377.0
33157 2018-12-02 04:15:00+00:00 371.0
33158 2018-12-02 04:20:00+00:00 376.0
33159 2018-12-02 04:25:00+00:00 374.0
33160 2018-12-02 04:30:00+00:00 374.0
33161 2018-12-02 04:35:00+00:00 371.0
33162 2018-12-02 04:40:00+00:00 367.0
33163 2018-12-02 04:45:00+00:00 368.0
33164 2018-12-02 04:50:00+00:00 363.0
33165 2018-12-02 04:55:00+00:00 368.0
33166 2018-12-02 05:00:00+00:00 361.0
33167 2018-12-02 05:05:00+00:00 366.0
33168 2018-12-02 05:10:00+00:00 371.0
33169 2018-12-02 05:15:00+00:00 364.0
33170 2018-12-02 05:20:00+00:00 366.0
33171 2018-12-02 05:25:00+00:00 366.0
33172 2018-12-02 05:30:00+00:00 365.0
33173 2018-12-02 05:35:00+00:00 361.0
33174 2018-12-02 05:40:00+00:00 361.0
33175 2018-12-02 05:45:00+00:00 360.0
33176 2018-12-02 05:50:00+00:00 361.0
33177 2018-12-02 05:55:00+00:00 359.0
[33178 rows x 2 columns]
###Markdown
Plot the DataNow write a function that plots the data you read in from above.
###Code
def plot_streamflow_data(x, y):
plt.plot(x, y, '.')
plot_streamflow_data(data.values[:, 0], data.values[:, 1])
###Output
/opt/conda/lib/python3.7/site-packages/pandas/plotting/_converter.py:129: FutureWarning: Using an implicitly registered datetime converter for a matplotlib plotting method. The converter was registered by pandas on import. Future versions of pandas will require you to explicitly register matplotlib converters.
To register the converters:
>>> from pandas.plotting import register_matplotlib_converters
>>> register_matplotlib_converters()
warnings.warn(msg, FutureWarning)
###Markdown
Make a ModelWrite a function that takes the data from above and fits a curve to it. Justify your approach to this problem as well.
###Code
def fit_streamflow_data(x, y):
""""""
pass
###Output
_____no_output_____
###Markdown
Pull It All TogetherUse all three functions above and make a prediction where there is no data. Think about where your prediction may be valid or invalid. Make sure to mention this in your `doc-string`.
###Code
def streamflow_prediction(x, y):
""""""
pass
###Output
_____no_output_____ |
notebooks/00_moving_avg.ipynb | ###Markdown
Moving Average Forecast> Practical Assignment 1
###Code
#hide
from nbdev.showdoc import *
###Output
_____no_output_____
###Markdown
1. Calculate MAD, MSE, RMSE, and MAPE error for the 6 mo Moving Average forecast and compare them to relevant accuracy measures for the 3mo Moving Average Forecast. Which seems to be a more accurate model? Why? Does your conclusion depend on which measure you use?2. Generate the cumulative error graph and turning point graph for the 6mo Moving Average forecast and compare them to the results for 3mo Moving Average Forecast. Which models seems to perform better?3. Repeat the exercises for the Seasonally Adjusted Data, i.e. generate 3mo and 6mo Moving Average forecasts, calculate accuracy measures, generate cumulative error and turning point graphs and compare the two models.4. In general, how do measures of accuracy and graphs compare between the NSA and SA data? In other words, are errors larger/smaller, is there difference in turning point predictions, etc.
###Code
#export
import pandas as pd
import numpy as np
import math
import matplotlib.pyplot as plt
###Output
_____no_output_____
###Markdown
Question 1 Read data from excel
###Code
df = pd.read_excel("../data/unemployment_rate.xls")
print(df.head())
###Output
observation_date UNRATE_SA UNRATENSA
0 1948-01-01 3.4 4.0
1 1948-02-01 3.8 4.7
2 1948-03-01 4.0 4.5
3 1948-04-01 3.9 4.0
4 1948-05-01 3.5 3.4
###Markdown
Find Moving Average forecast
###Code
#export
def moving_avg(n_month, df, df_column):
"""
Get an array of n-month moving averages.
Arguments:
n_month: the number of continuous months for moving average
df: dataframe
df_column: the column containing data
"""
# get number of rows
num_dates = df.shape[0]
ma_forecasts = np.zeros(num_dates)
for idx in range(n_month, num_dates):
ma = np.mean(df_column[idx-n_month:idx])
ma_forecasts[idx] = ma
return ma_forecasts
# 3 month moving average
ma_3mo = moving_avg(3, df, df['UNRATENSA'])
df['3mo-ma-forecast'] = ma_3mo
# 6 month moving average
ma_6mo = moving_avg(6, df, df['UNRATENSA'])
df['6mo-ma-forecast'] = ma_6mo
print(df[0:7])
###Output
observation_date UNRATE_SA UNRATENSA 3mo-ma-forecast 6mo-ma-forecast
0 1948-01-01 3.4 4.0 0.000000 0.000000
1 1948-02-01 3.8 4.7 0.000000 0.000000
2 1948-03-01 4.0 4.5 0.000000 0.000000
3 1948-04-01 3.9 4.0 4.400000 0.000000
4 1948-05-01 3.5 3.4 4.400000 0.000000
5 1948-06-01 3.6 3.9 3.966667 0.000000
6 1948-07-01 3.6 3.9 3.766667 4.083333
###Markdown
MAD, MSE, RMSE, MAPE (Q1)
###Code
#export
def mad(n_month, predicted, target):
"""Mean absolute deviation"""
abs_deviations = [abs(predicted[i] - target[i]) for i in range(n_month, len(predicted))]
return np.mean(abs_deviations)
def mse(n_month, predicted, target):
"""Mean squared error"""
squared_errors = [(predicted[i] - target[i])**2 for i in range(n_month, len(predicted))]
return np.mean(squared_errors)
def rmse(n_month, predicted, target):
"""Root mean squared error"""
return math.sqrt(mse(n_month, predicted, target))
def mape(n_month, predicted, target):
"""Mean abosolute percentage error (in %)"""
abs_percent = [abs(predicted[i] - target[i]) / target[i] * 100 for i in range(n_month, len(predicted))]
return np.mean(abs_percent)
print("3 month")
print("MAD: ", mad(3, df['3mo-ma-forecast'], df['UNRATENSA']))
print("MSE: ", mse(3, df['3mo-ma-forecast'], df['UNRATENSA']))
print("RMSE: ", rmse(3, df['3mo-ma-forecast'], df['UNRATENSA']))
print("MAPE: ", mape(3, df['3mo-ma-forecast'], df['UNRATENSA']))
print("6 month")
print("MAD: ", mad(6, df['6mo-ma-forecast'], df['UNRATENSA']))
print("MSE: ", mse(6, df['6mo-ma-forecast'], df['UNRATENSA']))
print("RMSE: ", rmse(6, df['6mo-ma-forecast'], df['UNRATENSA']))
print("MAPE: ", mape(6, df['6mo-ma-forecast'], df['UNRATENSA']))
###Output
6 month
MAD: 0.4855672105672106
MSE: 0.4253978891478891
RMSE: 0.6522253361744613
MAPE: 8.743678060315847
###Markdown
Q1 Text AnswerAccording to the MAD, MSE, RMSE, and MAPE error measures calculated above, the 3-month moving average model is more accurate. All error measures for the 3-month model are lower than the 6-month model. My conclusion depends on all 4 error measures. Question 2 Cumulative error graph (Q2)
###Code
#export
def cumulative_error(n_month, predicted, target):
errors = [target[i] - predicted[i] for i in range(n_month, len(predicted))]
return np.cumsum(errors)
cum_error_3mo = cumulative_error(3, df['3mo-ma-forecast'], df['UNRATENSA'])
cum_error_6mo = cumulative_error(6, df['6mo-ma-forecast'], df['UNRATENSA'])
%matplotlib inline
plt.plot(range(3, len(cum_error_3mo)+3), cum_error_3mo, label='3-month')
plt.plot(range(6, len(cum_error_6mo)+6), cum_error_6mo, label='6-month')
plt.title("cumulative error graph")
plt.legend()
plt.show()
###Output
_____no_output_____
###Markdown
Turning point graph (Q2)
###Code
#export
def change_in_variable(n_month, target):
return [target[i] - target[i-1] for i in range(n_month+1, len(target))]
def change_in_forecast(n_month, predicted):
return [predicted[i] - predicted[i-1] for i in range(n_month+1, len(predicted))]
change_variable_3mo = change_in_variable(3, df['UNRATENSA'])
change_forecast_3mo = change_in_forecast(3, df['3mo-ma-forecast'])
change_variable_6mo = change_in_variable(6, df['UNRATENSA'])
change_forecast_6mo = change_in_forecast(6, df['6mo-ma-forecast'])
#export
def turning_point_graph(n_month, change_in_variable, change_in_forecast):
fig, ax = plt.subplots()
ax.axhline(y=0, color='k', lw=1)
ax.axvline(x=0, color='k', lw=1)
ax.axline([0, 0], [1, 1], color='r', lw=1.5)
ax.scatter(change_in_forecast, change_in_variable, s=1)
plt.xlabel("Forecast Change")
plt.ylabel("Actual Change")
plt.title(f"turning point graph - {n_month} month")
plt.show()
turning_point_graph(3, change_variable_3mo, change_forecast_3mo)
turning_point_graph(6, change_variable_6mo, change_forecast_6mo)
fig, ax = plt.subplots()
ax.axhline(y=0, color='k', lw=1)
ax.axvline(x=0, color='k', lw=1)
ax.axline([0, 0], [1, 1], color='r', lw=1.5)
ax.scatter(change_forecast_3mo, change_variable_3mo, s=1, label='3-month')
ax.scatter(change_forecast_6mo, change_variable_6mo, s=1, label='6-month')
plt.xlabel("Forecast Change")
plt.ylabel("Actual Change")
plt.title(f"turning point graph")
plt.legend()
plt.show()
###Output
_____no_output_____
###Markdown
Q2 Text AnswerAccording to the cumulative error graph, the 3-month moving average model performs better because its cumulative errors are lower. Based on the turning point graph, it is hard to tell which model performs better. Either model lands close to the perfect prediction line. Question 3 Repeat for Seasonally Adjusted Data (Q3) Get forecasts
###Code
# 3 month moving average
ma_3mo = moving_avg(3, df, df['UNRATE_SA'])
df['sa-3mo-ma-forecast'] = ma_3mo
# 6 month moving average
ma_6mo = moving_avg(6, df, df['UNRATE_SA'])
df['sa-6mo-ma-forecast'] = ma_6mo
print(df[0:7])
###Output
observation_date UNRATE_SA UNRATENSA 3mo-ma-forecast 6mo-ma-forecast \
0 1948-01-01 3.4 4.0 0.000000 0.000000
1 1948-02-01 3.8 4.7 0.000000 0.000000
2 1948-03-01 4.0 4.5 0.000000 0.000000
3 1948-04-01 3.9 4.0 4.400000 0.000000
4 1948-05-01 3.5 3.4 4.400000 0.000000
5 1948-06-01 3.6 3.9 3.966667 0.000000
6 1948-07-01 3.6 3.9 3.766667 4.083333
sa-3mo-ma-forecast sa-6mo-ma-forecast
0 0.000000 0.0
1 0.000000 0.0
2 0.000000 0.0
3 3.733333 0.0
4 3.900000 0.0
5 3.800000 0.0
6 3.666667 3.7
###Markdown
Get error measures
###Code
print("3 month")
print("MAD: ", mad(3, df['sa-3mo-ma-forecast'], df['UNRATE_SA']))
print("MSE: ", mse(3, df['sa-3mo-ma-forecast'], df['UNRATE_SA']))
print("RMSE: ", rmse(3, df['sa-3mo-ma-forecast'], df['UNRATE_SA']))
print("MAPE: ", mape(3, df['sa-3mo-ma-forecast'], df['UNRATE_SA']))
print("6 month")
print("MAD: ", mad(6, df['sa-6mo-ma-forecast'], df['UNRATE_SA']))
print("MSE: ", mse(6, df['sa-6mo-ma-forecast'], df['UNRATE_SA']))
print("RMSE: ", rmse(6, df['sa-6mo-ma-forecast'], df['UNRATE_SA']))
print("MAPE: ", mape(6, df['sa-6mo-ma-forecast'], df['UNRATE_SA']))
###Output
6 month
MAD: 0.3013597513597514
MSE: 0.18971315721315724
RMSE: 0.4355607388334688
MAPE: 5.243036319115769
###Markdown
Graph
###Code
cum_error_3mo = cumulative_error(3, df['sa-3mo-ma-forecast'], df['UNRATE_SA'])
cum_error_6mo = cumulative_error(6, df['sa-6mo-ma-forecast'], df['UNRATE_SA'])
plt.plot(range(3, len(cum_error_3mo)+3), cum_error_3mo, label='3-month')
plt.plot(range(6, len(cum_error_6mo)+6), cum_error_6mo, label='6-month')
plt.title("cumulative error graph - seasonally adjusted")
plt.legend()
plt.show()
change_variable_3mo = change_in_variable(3, df['UNRATE_SA'])
change_forecast_3mo = change_in_forecast(3, df['sa-3mo-ma-forecast'])
change_variable_6mo = change_in_variable(6, df['UNRATE_SA'])
change_forecast_6mo = change_in_forecast(6, df['sa-6mo-ma-forecast'])
turning_point_graph(3, change_variable_3mo, change_forecast_3mo)
turning_point_graph(6, change_variable_6mo, change_forecast_6mo)
fig, ax = plt.subplots()
ax.axhline(y=0, color='k', lw=1)
ax.axvline(x=0, color='k', lw=1)
ax.axline([0, 0], [1, 1], color='r', lw=1.5)
ax.scatter(change_forecast_3mo, change_variable_3mo, s=1, label='3-month')
ax.scatter(change_forecast_6mo, change_variable_6mo, s=1, label='6-month')
plt.xlabel("Forecast Change")
plt.ylabel("Actual Change")
plt.title(f"turning point graph - seasonally adjusted")
plt.legend()
plt.show()
###Output
_____no_output_____ |
tutorials/learn_bivariate_normal.ipynb | ###Markdown
Your First Flow The TaskLet's begin our Normalizing Flow journey with a simple example! We'll train a flow to transform standard normal noise to samples from an arbitrary bivariate normal distribution.That is, the base distribution is,$$\begin{aligned} X &\sim \mathcal{N}\left(\mu=\begin{bmatrix} 0 \\ 0\end{bmatrix}, \Sigma=\begin{bmatrix} 1 & 0 \\ 0 & 1\end{bmatrix} \right)\end{aligned},$$the target distribution to learn is,$$\begin{aligned} Y' &\sim \mathcal{N}\left(\mu=\begin{bmatrix} 5 \\ 5\end{bmatrix}, \Sigma=\begin{bmatrix} 0.5 & 0 \\ 0 & 0.5\end{bmatrix} \right)\end{aligned},$$and the task is to learn some bijection $g_\theta$ so that$$\begin{aligned} Y &\triangleq g_\theta(X) \\ &\sim Y'\end{aligned}$$approximately holds.We will define our Normalizing Flow, $g_\theta$, by a single affine transformation,$$\begin{aligned} g_\theta(\mathbf{x}) &\triangleq \begin{bmatrix} \mu_1 \\ \mu_2(x_1)\end{bmatrix} + \begin{bmatrix} \sigma_1 \\ \sigma_2(x_1)\end{bmatrix}\otimes\begin{bmatrix} x_1 \\ x_2\end{bmatrix}.\end{aligned}$$In this notation, $\mathbf{x}=(x_1,x_2)^T$, $\otimes$ denotes element-wise multiplication, and the parameters are the scalars $\mu_1,\sigma_1$ and the parameters of the neural networks $\mu_2(\cdot)$ and $\sigma_2(\cdot)$. (Think of the NNs as very simple shallow feedforward nets in this example.) This is an example of [Inverse Autoregressive Flow](/dev/bibliographykingma2016improving).There are several metrics we could use to train $Y$ to be close in distribution to $Y'$. Denote the target distribution of $Y'$ by $p(\cdot)$ and the learnable distribution of the normalizing flow, $Y$, as $q_\theta(\cdot)$ (in the following sections, we will explain how to calculate $q_\theta$ from $g_\theta$). Let's use the forward [KL-divergence](https://en.wikipedia.org/wiki/Kullback%E2%80%93Leibler_divergence),$$\begin{aligned}\text{KL}\{p\ ||\ q_\theta\} &\triangleq \mathbb{E}_{p(\cdot)}\left[\log\frac{p(Y')}{q_\theta(Y')}\right] \\&= -\mathbb{E}_{p(\cdot)}\left[\log q_\theta(Y')\right] + C,\end{aligned}$$where C is a constant that does not depend on $\theta$. In practice, we draw a finite sample, $\{y_1,\ldots,y_M\}$, from $p$ and optimize a [Monte Carlo estimate](https://en.wikipedia.org/wiki/Monte_Carlo_integration) of the KL-divergence with stochastic gradient descent so that the loss is,$$\begin{aligned} \mathcal{L}(\theta) &= -\frac{1}{M}\sum^M_{m=1}\log(q_\theta(y_m))\end{aligned}$$*So, to summarize, the task at hand is to learn how to transform standard bivariate normal noise into another bivariate normal distribution using an affine transformation, and we will do so by matching distributions with the KL-divergence metric.* ImplementationFirst, we import the relevant libraries:
###Code
import torch
import flowtorch.bijectors as B
import flowtorch.distributions as D
import flowtorch.parameters as P
###Output
_____no_output_____
###Markdown
The base and target distributions are defined using standard PyTorch:
###Code
base_dist = torch.distributions.Independent(
torch.distributions.Normal(torch.zeros(2), torch.ones(2)),
1
)
target_dist = torch.distributions.Independent(
torch.distributions.Normal(torch.zeros(2)+5, torch.ones(2)*0.5),
1
)
###Output
_____no_output_____
###Markdown
Note the use of [`torch.distributions.Independent`](https://pytorch.org/docs/stable/distributions.htmlindependent) so that our base and target distributions are *vector valued*. A Normalizing Flow is created in two steps. First, we create a "plan" for the flow as a [`flowtorch.bijectors.Bijector`](https://flowtorch.ai/api/flowtorch.bijectors.bijector) object,
###Code
# Lazily instantiated flow
bijector = B.AffineAutoregressive()
###Output
_____no_output_____
###Markdown
This plan is then made concrete by combining it with the base distributions, which provides the input shape, and constructing a [`flowtorch.distributions.Flow`](https://flowtorch.ai/api/flowtorch.distributions.flow) object, an extension of [`torch.distributions.Distribution`](https://pytorch.org/docs/stable/distributions.htmldistribution):
###Code
# Instantiate transformed distribution and parameters
flow = D.Flow(base_dist, bijector)
###Output
_____no_output_____
###Markdown
At this point, we have an object, `flow`, for the distribution, $q_\theta(\cdot)$, that follows the standard PyTorch interface. We can visualize samples from the base and target distributions as well as the flow before training:
###Code
import matplotlib.pyplot as plt
plt.axis([-4., 8., -4., 8.])
base_samples = base_dist.sample((1000,))
target_samples = target_dist.sample((1000,))
flow_samples = flow.sample((1000,))
plt.scatter(base_samples[:,0], base_samples[:,1], label='base', c='grey')
plt.scatter(target_samples[:,0], target_samples[:,1], label='target', c='blue')
plt.scatter(flow_samples[:,0], flow_samples[:,1], label='flow', c='red')
plt.legend()
plt.show()
###Output
_____no_output_____
###Markdown
Since `flow` follows the standard inference for [`torch.distributions`](https://pytorch.org/docs/stable/distributions.html), it can be trained with the following code, which will be familiar to PyTorch users:
###Code
# Training loop
opt = torch.optim.Adam(flow.parameters(), lr=5e-3)
for idx in range(3001):
opt.zero_grad()
# Minimize KL(p || q)
y = target_dist.sample((1000,))
loss = -flow.log_prob(y).mean()
if idx % 500 == 0:
print('epoch', idx, 'loss', loss.item())
loss.backward()
opt.step()
###Output
epoch 0 loss 20.42441177368164
epoch 500 loss 3.5910611152648926
epoch 1000 loss 3.3313257694244385
epoch 1500 loss 3.024606704711914
epoch 2000 loss 2.4530959129333496
epoch 2500 loss 1.5909523963928223
epoch 3000 loss 1.478494644165039
###Markdown
Note how we obtain the learnable parameters of the normalizing flow from the `flow` object, which is a [`torch.nn.Module`](https://pytorch.org/docs/stable/generated/torch.nn.Module.htmltorch.nn.Module). Visualizing samples after learning, we see that we have been successful in matching the target distribution:
###Code
fig = plt.figure()
plt.axis([-4., 8., -4., 8.])
flow_samples = flow.sample((1000,))
plt.scatter(base_samples[:,0], base_samples[:,1], label='base', c='grey')
plt.scatter(target_samples[:,0], target_samples[:,1], label='target', c='blue')
plt.scatter(flow_samples[:,0], flow_samples[:,1], label='flow', c='red')
plt.legend()
plt.show()
###Output
_____no_output_____ |
Classification/Support Vector Machine/Example Usage - SVM.ipynb | ###Markdown
Support Vector Machinehttps://scikit-learn.org/stable/modules/svm.html Dummy Data Generation
###Code
import numpy as np
X = np.array([[-1, -1], [-2, -1], [-3, -2], [1, 1], [2, 1], [3, 2]])
Y = np.array([1, 1, 1, 2, 2, 2])
###Output
_____no_output_____
###Markdown
Training
###Code
from sklearn import svm
clf = svm.SVC(kernel= 'linear', gamma='scale')
clf.fit(X, Y)
###Output
_____no_output_____
###Markdown
Prediction
###Code
print(clf.predict([[-0.8, -1]]))
print(clf.predict([[-0.8, -1],[1, 2]]))
###Output
[1]
[1 2]
###Markdown
Accuracy
###Code
from sklearn.metrics import accuracy_score
predictions = clf.predict(X)
accuracy = accuracy_score(Y,predictions)
print (accuracy)
###Output
1.0
|
Bank_Fantasy/Golden_Bridge/forecast_experiemnts.ipynb | ###Markdown
Forecasting Experiments - Finxed Window Solution* Try different forecasting methods on 2 representative sample data, try to see whether we can find a method works for both time series in order to forecast well.* Fixed Window solution means, it's using all the historical data as the training data.
###Code
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from sklearn.metrics import mean_squared_error
from math import sqrt
import statsmodels.api as sm
import itertools
from sklearn.model_selection import TimeSeriesSplit
from statsmodels.tsa.statespace.sarimax import SARIMAX
from statsmodels.tsa.api import ExponentialSmoothing, SimpleExpSmoothing, Holt
from statsmodels.tsa.statespace.sarimax import SARIMAX
from statsmodels.graphics.tsaplots import plot_pacf
import warnings
warnings.filterwarnings("ignore")
sample_train_df1 = pd.read_pickle('sample_train_df1.pkl')
sample_test_df1 = pd.read_pickle('sample_test_df1.pkl')
print(sample_train_df1.shape, sample_test_df1.shape)
sample_train_df2 = pd.read_pickle('sample_train_df2.pkl')
sample_test_df2 = pd.read_pickle('sample_test_df2.pkl')
print(sample_train_df2.shape, sample_test_df2.shape)
sample_train_df1.head()
train1_col = sample_train_df1['client_count']
train2_col = sample_train_df2['client_count']
test1_col = sample_test_df1['client_count']
test2_col = sample_test_df2['client_count']
# Generate logged moving average for both time series sequences
ts_log_train1 = np.log(train1_col)
ts_moving_avg_train1 = ts_log_train1.rolling(window=4,center=False).mean().dropna()
ts_log_test1 = np.log(test1_col)
ts_moving_avg_test1 = ts_log_test1.rolling(window=4,center=False).mean().dropna()
ts_log_train2 = np.log(train2_col)
ts_moving_avg_train2 = ts_log_train2.rolling(window=4,center=False).mean().dropna()
ts_log_test2 = np.log(test2_col)
ts_moving_avg_test2 = ts_log_test2.rolling(window=4,center=False).mean().dropna()
print(ts_moving_avg_train1.shape, ts_moving_avg_train2.shape, ts_moving_avg_test1.shape, ts_moving_avg_test2.shape)
ts_moving_avg_test1.head()
plt.figure(figsize=(9,7))
plt.plot(ts_log_train1.index, ts_log_train1, label='Logged Train', color='green')
plt.plot(ts_log_test1.index, ts_log_test1, label='Logged Test', color='green')
plt.plot(ts_moving_avg_train1.index, ts_moving_avg_train1, label='Logged Moving Average Train', color='purple')
plt.plot(ts_moving_avg_test1.index, ts_moving_avg_test1, label='Logged Moving Average Test', color='purple')
plt.legend(loc='best')
plt.title("Naive Forecast - Sample 1 - Original Time Series")
plt.show()
###Output
_____no_output_____
###Markdown
Logged Time Series* Without using logged moving average but just use the logged original time series. * Because logged time series has no null value and keeps the original time series pattern. * It's in the same scale as logged moving average, which makes the comparison between RMSE values easier.* Compare between different forecasting methods, to see which one works better for the original time series. Approach 1 - Weighted Naive Forecast* As we can see logged ts is in dropping trend. So we can assume testing data is w*latest_window of training data. * Latest window of training data has the same size as the testing data, and it's the closest to the testing data. * w is the weight. We can assume there is a linear regression relationshio between testing data and the latest window of the training data. * The reason I chose w=2/3 here is because the latest window of training data is between [4, 6], while the testing data seems between [2, 4]. * Also tried a few other weights and this one works better.
###Code
train = ts_log_train1.copy()
test = ts_log_test1.copy()
w = 2/3
y_pred = train.iloc[-len(test):]*w
y_pred.index = test.index
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(y_pred.index, y_pred, label='Weighted Naive Forecast')
plt.legend(loc='best')
plt.title("Weighted Naive Forecast - Sample 1 - Logged Time Series")
plt.show()
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
###Output
_____no_output_____
###Markdown
Approach 2 - Exponetial Smoothing* The idea is, the weights will decrease from the latest to the oldest data* `Yt+1 = α*Yt + α(1-α)*Yt-1 + α(1-α)(1-α)*Yt-2 + ... + α*pow(1-α, n)*Yt-n` * α is in [0,1] range
###Code
title = 'Exponential Smoothing'
train = ts_log_train1.copy()
test = ts_log_test1.copy()
model_fit = SimpleExpSmoothing(np.array(train)).fit(smoothing_level=0.99,optimized=False)
y_pred = model_fit.forecast(len(test))
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(test.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 1 - Logged Time Series")
plt.show()
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
###Output
_____no_output_____
###Markdown
Note* The exponential smoothing appears like a horizontal line might be because it puts too much weights on the last time point. * Larger `α` (smoothing level), smaller RMSE, but also means higher weights on latest time points. So overall looks like constant over the time. Approach 3 - Holt's Linear TrendThis method helps to decompose the time series into trend, seasonality and residual. It applies exponential smoothing to both Level data and Trend data.* Level data - the average value in the series* Each equation here is exponential smoothing* Additive method - Forecast result = Trent Equation + Level Equation* Multiplication method - Forecast result = Trent Equation*Level Equation* This approach is neither additive nor multiplication, but linear
###Code
title = "Holt's Linear Trend"
train = ts_log_train1.copy()
test = ts_log_test1.copy()
model_fit = Holt(np.asarray(train)).fit(smoothing_level = 0.8,smoothing_slope = 0.6)
y_pred = model_fit.forecast(len(test))
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(test.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 1 - Logged Time Series")
plt.show()
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
###Output
_____no_output_____
###Markdown
Approach 4 - Holt-Winters* It considers both trend and seasonality.* Each equation here is exponential smoothing.* Additive method - Forecast result = Trent Equation + Level Equation + Seasonality Equation* Multiplication method - Forecast result = Trent Equation*Level Equation*Seasonality Equation
###Code
title = "Holt Winters"
train = ts_log_train1.copy()
test = ts_log_test1.copy()
model_fit = ExponentialSmoothing(np.asarray(train) ,seasonal_periods=6, trend='mul',
seasonal='add', damped=False).fit(smoothing_level = 0.8,smoothing_slope = 0.6)
y_pred = model_fit.forecast(len(test))
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(test.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 1 - Logged Time Series")
plt.show()
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
###Output
_____no_output_____
###Markdown
Approach 5 - Seasonal ARIMA (SARIMA)* ARIMA is trying to tell the correlations between the data. Seasonal ARIMA takes into account the seasonality* p: The number of lag observations included in the model, also called the lag order. It is The lag value where the PACF chart crosses the upper confidence interval for the first time* d: The number of times that the raw observations are differenced, also called the degree of differencing.* q: The size of the moving average window, also called the order of moving average.
###Code
title = "SARIMA"
train = ts_log_train1.copy()
test = ts_log_test1.copy()
plot_pacf(train, lags=20);
# check time series cross validation
tscv = TimeSeriesSplit(n_splits=20)
ct = 0
for cv_train_index, cv_test_index in tscv.split(train.values):
print("TRAIN:", cv_train_index, "TEST:", cv_test_index)
ct += 1
if ct ==4:
break
p = d = q = range(0, 3)
pdq = list(itertools.product(p, d, q))
bestAIC = np.inf
best_cvAIC = np.inf
bestParam = None
best_cvParam = None
X = train.values
# grid search with cross validation
for param in pdq:
for cv_train_index, cv_test_index in tscv.split(train.values):
X_train, X_test = X[cv_train_index], X[cv_test_index]
try:
model = sm.tsa.statespace.SARIMAX(X_train,
order=param,
seasonal_order=(0,1,1,7))
results = model.fit()
#if current run of AIC is better than the best one so far, overwrite it
if results.aic < bestAIC:
bestAIC = results.aic
bestParam = param
# apply the best order on testig data
best_model = sm.tsa.statespace.SARIMAX(X_train,
order=param,
seasonal_order=(0,1,1,7))
results = best_model.fit()
if results.aic < best_cvAIC:
best_cvAIC = results.aic
best_cvParam = bestParam
except:
pass
print('the best AIC, the best CV AIC and order are:',bestAIC,best_cvAIC,bestParam)
p = d = q = range(0, 3)
pdq = list(itertools.product(p, d, q))
bestAIC = np.inf
bestParam = None
best_itrAIC = np.inf
best_itrParam = None
X_train = train.values[:-len(test)]
X_test = train.values[-len(test):]
print(len(X_train), len(X_test))
# grid search without cross validation
for param in pdq:
try:
model = sm.tsa.statespace.SARIMAX(X_train,
order=param,
seasonal_order=(0,1,1,7))
results = model.fit()
#if current run of AIC is better than the best one so far, overwrite it
if results.aic < bestAIC:
bestAIC = results.aic
bestParam = param
# apply the best order on testig data
best_model = sm.tsa.statespace.SARIMAX(X_train,
order=param,
seasonal_order=(0,1,1,7))
results = best_model.fit()
if results.aic < best_itrAIC:
best_itrAIC = results.aic
best_itrParam = bestParam
except:
pass
print('the best AIC, the best iteration AIC and order are:',bestAIC,best_itrAIC,bestParam)
model_fit = SARIMAX(train, order=(1, 1, 1),seasonal_order=(0,1,1,7)).fit() # order means (p,d,q) for ARIMA
y_pred = model_fit.predict(start="2019-05-22", end="2019-06-04", dynamic=True)
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(test.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 1 - Logged Time Series")
plt.show()
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
model_fit = SARIMAX(train, order=(2,1,2),seasonal_order=(0,1,1,7)).fit() # order means (p,d,q) for ARIMA
y_pred = model_fit.predict(start="2019-05-22", end="2019-06-04", dynamic=True)
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(test.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 1 - Logged Time Series")
plt.show()
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
###Output
_____no_output_____
###Markdown
Note:* For SARIMA, this time series works better when there is seasonal order.* Looking at the pattern of the whole time series, the latest values are decreasing. Grid search with corss validation (CV) doesn't work better than grid search without CV. * This is because earlier data as training could help less to the testing data.* However optimized order from Grid Search doesn't give better result as order (1,1,1) for this time series. Try Some winning methods on Sample 2
###Code
title = "Holt's Linear Trend"
train = ts_log_train2.copy()
test = ts_log_test2.copy()
model_fit = Holt(np.asarray(train)).fit()
y_pred = model_fit.forecast(len(test))
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(test.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 2 - Logged Time Series")
plt.show()
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
title = "Holt Winters"
train = ts_log_train2.copy()
test = ts_log_test2.copy()
model_fit = ExponentialSmoothing(np.asarray(train) ,seasonal_periods=9, trend='add',
seasonal='add', damped=False).fit()
y_pred = model_fit.forecast(len(test))
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(test.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 2 - Logged Time Series")
plt.show()
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
title = "SARIMA"
train = ts_log_train2.copy()
test = ts_log_test2.copy()
plot_pacf(train, lags=20);
p = d = q = range(0, 3)
pdq = list(itertools.product(p, d, q))
bestAIC = np.inf
bestParam = None
best_itrAIC = np.inf
best_itrParam = None
X_train = train.values[:-len(test)]
X_test = train.values[-len(test):]
print(len(X_train), len(X_test))
# grid search without cross validation
for param in pdq:
try:
model = sm.tsa.statespace.SARIMAX(X_train,
order=param,
seasonal_order=(0,1,1,7))
results = model.fit()
#if current run of AIC is better than the best one so far, overwrite it
if results.aic < bestAIC:
bestAIC = results.aic
bestParam = param
# apply the best order on testig data
best_model = sm.tsa.statespace.SARIMAX(X_train,
order=param,
seasonal_order=(0,1,1,7))
results = best_model.fit()
if results.aic < best_itrAIC:
best_itrAIC = results.aic
best_itrParam = bestParam
except:
pass
print('the best AIC, the best iteration AIC and order are:',bestAIC,best_itrAIC,bestParam)
model_fit = SARIMAX(train, order=(0,2,2),seasonal_order=(0,1,1,7)).fit() # order means (p,d,q) for ARIMA
y_pred = model_fit.predict(start="2019-05-22", end="2019-06-02", dynamic=True)
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(y_pred.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 2 - Logged Time Series")
plt.show()
# Need to make sure test and y_pred have matched dates
del y_pred['2019-05-28']
del y_pred['2019-06-01']
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
model_fit = SARIMAX(train, order=(2,1,1),seasonal_order=(0,1,1,7)).fit() # order means (p,d,q) for ARIMA
y_pred = model_fit.predict(start="2019-05-22", end="2019-06-02", dynamic=True)
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(y_pred.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 2 - Logged Time Series")
plt.show()
# Need to make sure test and y_pred have matched dates
del y_pred['2019-05-28']
del y_pred['2019-06-01']
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
###Output
_____no_output_____
###Markdown
Note* One of the drawback of SARIMA here is, it defines start and end time, and if testing data has some date is missing, you need to remove some records from y_pred to make sure they have matched dates, in order to calculate RMSE.* In Sample 2, the testing data is no longer as linear as Sample 1, models such as Holt Winters which can capture both trend and seasonality might be better. Logged Moving Average Approach 1 - Weighted Naive Forecast
###Code
title = 'Weighted Naive Forecast'
train = ts_moving_avg_train1.copy()
test = ts_moving_avg_test1.copy()
w = 2/3
y_pred = train.iloc[-len(test):]*w
y_pred.index = test.index
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(y_pred.index, y_pred, label='Weighted Naive Forecast')
plt.legend(loc='best')
plt.title(title + " - Sample 1 - Logged Moving Average Time Series")
plt.show()
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
###Output
_____no_output_____
###Markdown
Approach 2 - Exponential Smoothing
###Code
title = 'Exponential Smoothing'
train = ts_moving_avg_train1.copy()
test = ts_moving_avg_test1.copy()
model_fit = SimpleExpSmoothing(np.array(train)).fit(smoothing_level=0.99,optimized=False)
y_pred = model_fit.forecast(len(test))
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(test.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 1 - Logged Moving Average Time Series")
plt.show()
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
###Output
_____no_output_____
###Markdown
Approach 3 - Holt's Linear Trend
###Code
title = "Holt's Linear Trend"
train = ts_moving_avg_train1.copy()
test = ts_moving_avg_test1.copy()
model_fit = Holt(np.asarray(train)).fit(smoothing_level = 0.8,smoothing_slope = 0.6)
y_pred = model_fit.forecast(len(test))-0.5
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(test.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 1 - Logged Moving Average Time Series")
plt.show()
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
###Output
_____no_output_____
###Markdown
Approach 4 - Holt Winters
###Code
title = "Holt Winters"
train = ts_moving_avg_train1.copy()
test = ts_moving_avg_test1.copy()
model_fit = ExponentialSmoothing(np.asarray(train) ,seasonal_periods=6, trend='add',
seasonal='add', damped=False).fit(smoothing_level = 0.8,smoothing_slope = 0.6)
y_pred = model_fit.forecast(len(test))-0.5
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(test.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 1 - Logged Moving Average Time Series")
plt.show()
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
###Output
_____no_output_____
###Markdown
Approach 5 - SARIMA
###Code
title = "SARIMA"
train = ts_moving_avg_train1.copy()
test = ts_moving_avg_test1.copy()
plot_pacf(train, lags=20);
p = d = q = range(0, 3)
pdq = list(itertools.product(p, d, q))
bestAIC = np.inf
bestParam = None
best_itrAIC = np.inf
best_itrParam = None
X_train = train.values[:-len(test)]
X_test = train.values[-len(test):]
print(len(X_train), len(X_test))
# grid search without cross validation
for param in pdq:
try:
model = sm.tsa.statespace.SARIMAX(X_train,
order=param,
seasonal_order=(0,1,1,7))
results = model.fit()
#if current run of AIC is better than the best one so far, overwrite it
if results.aic < bestAIC:
bestAIC = results.aic
bestParam = param
# apply the best order on testig data
best_model = sm.tsa.statespace.SARIMAX(X_train,
order=param,
seasonal_order=(0,1,1,7))
results = best_model.fit()
if results.aic < best_itrAIC:
best_itrAIC = results.aic
best_itrParam = bestParam
except:
pass
print('the best AIC, the best iteration AIC and order are:',bestAIC,best_itrAIC,bestParam)
model_fit = SARIMAX(train, order=(1, 1, 2),seasonal_order=(0,1,1,7)).fit() # order means (p,d,q) for ARIMA
y_pred = model_fit.predict(start="2019-05-25", end="2019-06-04", dynamic=True)
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(test.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 1 - Logged Moving Average Time Series")
plt.show()
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
model_fit = SARIMAX(train, order=(1, 1, 1),seasonal_order=(1,1,1,2)).fit() # order means (p,d,q) for ARIMA
y_pred = model_fit.predict(start="2019-05-25", end="2019-06-04", dynamic=True)
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(test.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 1 - Logged Moving Average Time Series")
plt.show()
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
###Output
_____no_output_____
###Markdown
Sample 2
###Code
title = "SARIMA"
train = ts_moving_avg_train2.copy()
test = ts_moving_avg_test2.copy()
plot_pacf(train, lags=20);
p = d = q = range(0, 3)
pdq = list(itertools.product(p, d, q))
bestAIC = np.inf
bestParam = None
best_itrAIC = np.inf
best_itrParam = None
X_train = train.values[:-len(test)]
X_test = train.values[-len(test):]
print(len(X_train), len(X_test))
# grid search without cross validation
for param in pdq:
try:
model = sm.tsa.statespace.SARIMAX(X_train,
order=param,
seasonal_order=(0,1,1,2))
results = model.fit()
#if current run of AIC is better than the best one so far, overwrite it
if results.aic < bestAIC:
bestAIC = results.aic
bestParam = param
# apply the best order on testig data
best_model = sm.tsa.statespace.SARIMAX(X_train,
order=param,
seasonal_order=(0,1,1,2))
results = best_model.fit()
if results.aic < best_itrAIC:
best_itrAIC = results.aic
best_itrParam = bestParam
except:
pass
print('the best AIC, the best iteration AIC and order are:',bestAIC,best_itrAIC,bestParam)
model_fit = SARIMAX(train, order=(2, 0, 1),seasonal_order=(0,1,1,2)).fit() # order means (p,d,q) for ARIMA
y_pred = model_fit.predict(start="2019-05-25", end="2019-06-02", dynamic=True)
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(y_pred.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 2 - Logged Moving Average Time Series")
plt.show()
del y_pred['2019-05-28']
del y_pred['2019-06-01']
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
model_fit = SARIMAX(train, order=(1, 1, 1),seasonal_order=(0,1,1,2)).fit() # order means (p,d,q) for ARIMA
y_pred = model_fit.predict(start="2019-05-25", end="2019-06-02", dynamic=True)
plt.figure(figsize=(9,7))
plt.plot(train.index, train, label='Train')
plt.plot(test.index, test, label='Test')
plt.plot(y_pred.index, y_pred, label=title)
plt.legend(loc='best')
plt.title(title + " - Sample 2 - Logged Moving Average Time Series")
plt.show()
del y_pred['2019-05-28']
del y_pred['2019-06-01']
rmse = sqrt(mean_squared_error(test, y_pred))
print('RMSE:', rmse)
###Output
_____no_output_____ |
notebooks/Lab 4 - Believer-Skeptic.ipynb | ###Markdown
Believer-Skeptic meets Actor-Critic
###Code
from __future__ import division
import ADMCode
from ADMCode import visualize as vis
from ADMCode import believer_skeptic
import numpy as np
from numpy.random import sample as rs
import pandas as pd
import sys
import os
# from ipywidgets import interactive
import matplotlib.pyplot as plt
import seaborn as sns
import warnings
# Temporary for now until push changes to PIP
#sys.path.insert(0,'../ADMCode')
#import believer_skeptic
warnings.simplefilter('ignore', np.RankWarning)
warnings.filterwarnings("ignore", module="matplotlib")
warnings.filterwarnings("ignore")
sns.set(style='white', font_scale=1.3)
%matplotlib inline
###Output
_____no_output_____
###Markdown
Believer-Skeptic model Recall from the previous lecture that the Believer Skeptic model posits that decisions arise from the competitive architecture of the cortico-basal ganglia thalamus (CBGT) pathways. The critical assumptions of the model are:* CBGT pathways are organized as action channels.* Within-channel competition between the direct (Believers) and indirect (Skeptic) pathways map to the accumulation rate of the decision for an action channel.* Greater within-channel competition slows the accumulation of evidence for the specific decision.* Hyper-direct pathways act as a global emergency break.* For multi-choice decisions, the first accumulation process to hit its decision boundary wins.
###Code
from IPython.display import Image
from IPython.core.display import HTML
Image(url='https://github.com/CoAxLab/AdaptiveDecisionMaking_2018/blob/master/notebooks/images/believer-skeptic.png?raw=true', width=500)
Image(url='https://github.com/CoAxLab/AdaptiveDecisionMaking_2018/blob/master/notebooks/images/believer-skeptic_to_accumulation.png?raw=true', width=500)
###Output
_____no_output_____
###Markdown
Iowa Gambling Task (IGT) The IGT is a popular neuropsychology task that looks at strategic decision-making. Get the most points possible after a specific number of draws from four decks. Participants can only pull from one deck at a time. Two decks (C & D) are advantageous, meaning that they'll pay off in the long run. Two decks are disadvantageous (A & B) meaning that you will lose in the long run if you select from these decks.
###Code
Image(url='https://github.com/CoAxLab/AdaptiveDecisionMaking_2018/blob/master/notebooks/images/IGT.png?raw=true', width=500)
###Output
_____no_output_____
###Markdown
Parameter definitions**$v_d$** : drift rate of the direct (Go) pathway**$v_i$** : drift rate of the indirect (Go) pathway**$a$** : boundary height (of the $v_d-v_i$ process)**$tr$** : non-decision time**$\gamma$ or $xb$** : exponential term on the $v_d-v_i$ process**$\alpha^G$ or $aGo$** : learning rate on the direct (Go) pathway**$\alpha^N$ or $aNo$** : learning rate on the indirect (NoGo) pathway Diffusion & update equations The Go (G; direct pathway) and NoGo (N; indirect pathway) processes on each trial $t$ are simulated for every $j$ action channel at each timestep $\tau$ $(\Delta \tau = 1ms)$ as:$G_{j,t}(\tau) = G_{j,t}(\tau - \Delta \tau) + \upsilon ^G _{j,t} \Delta \tau + \epsilon^G_j (\tau)$$N_{j,t}(\tau) = N_{j,t}(\tau - \Delta \tau) + \upsilon ^N _{j,t} \Delta \tau + \epsilon^N_j (\tau)$where $G_{j,t}(\tau - \Delta \tau)$ and $N_{j,t}(\tau - \Delta \tau)$ are the state of the processes at the previous update. $\epsilon^{G/N}_j (\tau)$ represents the diffusion noise at each update as a normal distribution (i.e., $\epsilon^{G/N}_j (\tau) ~ N(0,\sigma^2)$).Finally the action value for each channel and each pathway is reflected by the $\upsilon^{G/N}_{j,t}$ terms for the $j^{th}$ action on trial $t$. The execution processThe execution process $\theta$ for each action channel $j$ is then computed in the network output layer as teh difference between the $G$ and $N$ processes.$\theta_{j,t}(\tau) = [G_{j,t}(\tau) - N_{j,t}(\tau)] \cdot cosh(\gamma \cdot \tau)$Where $cosh(\gamma \cdot \tau)$ is an exponential term where the drift process accelerates to the decision boundary as time progresses. The learning processAt the end of the simulated trial a reward signal $r(t)$ is given, reflecting the value of the card selected. We then update the value of each target deck $q_j$ as$q_j(t+1) = q_j(t) + \alpha \cdot [r(t) - q_j(t)]$.To implement this as a learning rule, we $q_j$ to estimate softmax probability of selecting each deck $j$.$p_j(t) = \frac{\exp{\beta \cdot q_j(t)}}{\Sigma^n_i \exp{\beta \cdot q_i(t)}}$As usual, $\beta$ is the inverse temperature paremeter. We will assume that it is held constant, but will use the probability of selecting each deck (i.e., the probability of each action $j$) to update the action values which, in turn, impact the selection "policy" by the drift process. Specifically, we can now estimate the reward prediction error ($\delta$) on each trial as the difference in selection procbability from the previous trial (which is updated by $r(t)$).$\delta_j(t) = p_j(t) - p_j(t-1)$Using $\delta_j(t)$ we can then update the value functions for the $G$ and $N$ processes as$\upsilon^G_{j,t+1} = \upsilon^G_{j,t} + \alpha^G \cdot \delta_j(t)$$\upsilon^N_{j,t+1} = \upsilon^N_{j,t} + \alpha^N \cdot \delta_j(t)$Here $\alpha^G$ and $\alpha^N$ are the learning rates for the Go (G) and NoGo (N) procecesses. For the purposes here, we want to simulate four action channels that race to be the first to make a decision
###Code
Image(url='https://github.com/CoAxLab/AdaptiveDecisionMaking_2018/blob/master/notebooks/images/multichannel_selection.png?raw=true', width=700)
###Output
_____no_output_____
###Markdown
Performing the IGT with a 4-choice believer-skeptic model
###Code
# Define the DDM parameters as an object to pass
p={'vd':np.asarray([.7]*4), 'vi':np.asarray([.25]*4), 'a':.25, 'tr':.3, 'xb':.00005}
p
###Output
_____no_output_____
###Markdown
Implementing learning in the Believer-Skeptic network (insert learning equations here)
###Code
# Learning rates on the Go (direct) and NoGo (indirect) pathways
aGo=.1
aNo=.1
# Now run one simulation of the IGT with the believer-skeptic model
# With singleProcess==0, we are approximating the direct-indirect competition
# on each run by simulating a single process based on the difference in the
# two drift rates
igtData = pd.read_csv("https://github.com/CoAxLab/AdaptiveDecisionMaking_2018/blob/master/data/IGTCards.csv?raw=true")
outdf, agentdf = believer_skeptic.play_IGT(p, feedback=igtData, beta=.09, nblocks=2,
alphaGo=aGo, alphaNo=aNo, singleProcess=0)
# Look at the selection rate of our simulated agent
# 0 = Deck A
# 1 = Deck B
# 2 = Deck C
# 3 = Deck D
print(agentdf.rt.mean())
agentdf.iloc[:, :].choice.value_counts().sort_index()
###Output
0.63724
###Markdown
Let's look at the within-channel competition (direct-indirect) $V_{\Delta}$ for each action channel.
###Code
_=[agentdf['vDelta{}'.format(i)].plot(label=str(i)) for i in range(4)]
_=plt.legend()
sns.despine()
###Output
_____no_output_____
###Markdown
We can see how this competition emerges by looking at the separate drift rates for the direct ($v_d$) & indirect ($v_i$) pathways.
###Code
_=[agentdf['vd{}'.format(i)].plot(label=str(i)) for i in range(4)]
_=plt.legend()
sns.despine()
_=[agentdf['vi{}'.format(i)].plot(label=str(i)) for i in range(4)]
_=plt.legend()
sns.despine()
###Output
_____no_output_____
###Markdown
Next let's look at the q-value for each action.
###Code
_=[outdf['q{}'.format(i)].plot(label=str(i)) for i in range(4)]
_=plt.legend()
sns.despine()
###Output
_____no_output_____
###Markdown
Next let's look at the action policy (i.e. $\beta$).
###Code
_=[outdf['p{}'.format(i)].plot(label=str(i)) for i in range(4)]
_=plt.legend()
sns.despine()
# Take another look at our parameters
p
###Output
_____no_output_____
###Markdown
For simplicity sake we modeled the competitive process as a dual process, but you can also run the full competition model (it's just slower). Here's how that foramlly works.
###Code
# This is a snippet from believer_skeptic.py, specifically the
# simulate_multirace function.
single_process=0
si=.1
tb=1.0
dt=.001
nresp = p['vd'].size
dx = si * np.sqrt(dt)
nTime = np.ceil((tb-p['tr'])/dt).astype(int)
xtb = believer_skeptic.temporal_dynamics(p, np.cumsum([dt]*nTime))
# Run the process model
Pd = .5 * (1 + (p['vd'] * np.sqrt(dt))/si)
Pi = .5 * (1 + (p['vi'] * np.sqrt(dt))/si)
direct = xtb * np.where((rs((nresp, nTime)).T < Pd),dx,-dx).T
indirect = np.where((rs((nresp, nTime)).T < Pi),dx,-dx).T
execution = np.cumsum(direct-indirect, axis=1)
act_ix, rt, rt_ix = believer_skeptic.analyze_multiresponse(execution, p)
nsteps_to_rt = np.argmax((execution.T>=p['a']).T, axis=1)
rts = p['tr'] + nsteps_to_rt*dt
# set non responses to 999
rts[rts==p['tr']]=999
# get accumulator with fastest RT (winner) in each cond
act_ix = np.argmin(rts)
winner, rt=act_ix, rts[act_ix]
rt_ix = np.ceil((rt-p['tr'])/dt).astype(int)
actions = np.arange(nresp)
losers = actions[actions!=act_ix]
print(act_ix)
plt.plot(execution[act_ix][:rt_ix], color='b')
for l in losers:
plt.plot(execution[l][:rt_ix], color='r', alpha=.3)
sns.despine()
###Output
3
|
Ch12_Optimization_Algorithms/Gradient_Descent.ipynb | ###Markdown
Gradient Descent In this section we are going to introduce the basic concepts underlying gradient descent. This is brief by necessity. See e.g. :cite:Boyd.Vandenberghe.2004 for an in-depth introduction to convex optimization. Although the latter is rarely used directly in deep learning, an understanding of gradient descent is key to understanding stochastic gradient descent algorithms. For instance, the optimization problem might diverge due to an overly large learning rate. This phenomenon can already be seen in gradient descent. Likewise, preconditioning is a common technique in gradient descent and carries over to more advanced algorithms. Let's start with a simple special case. Gradient Descent in One Dimension Gradient descent in one dimension is an excellent example to explain why the gradient descent algorithm may reduce the value of the objective function. Consider some continously differentiable real-valued function $f: \mathbb{R} \rightarrow \mathbb{R}$. Using a Taylor expansion (:numref:chapter_math) we obtain that$$f(x + \epsilon) = f(x) + \epsilon f'(x) + O(\epsilon^2).$$ :eqlabel:gd-taylorThat is, in first approximation $f(x+\epsilon)$ is given by the function value $f(x)$ and the first derivative $f'(x)$ at $x$. It is not unreasonable to assume that for small $\epsilon$ moving in the direction of the negative gradient will decrease $f$. To keep things simple we pick a fixed step size $\eta > 0$ and choose $\epsilon = -\eta f'(x)$. Plugging this into the Taylor expansion above we get$$f(x - \eta f'(x)) = f(x) - \eta f'^2(x) + O(\eta^2 f'^2(x)).$$If the derivative $f'(x) \neq 0$ does not vanish we make progress since $\eta f'^2(x)>0$. Moreover, we can always choose $\eta$ small enough for the higher order terms to become irrelevant. Hence we arrive at$$f(x - \eta f'(x)) \lessapprox f(x).$$This means that, if we use$$x \leftarrow x - \eta f'(x)$$to iterate $x$, the value of function $f(x)$ might decline. Therefore, in gradient descent we first choose an initial value $x$ and a constant $\eta > 0$ and then use them to continuously iterate $x$ until the stop condition is reached, for example, when the magnitude of the gradient $|f'(x)|$ is small enough or the number of iterations has reached a certain value.For simplicity we choose the objective function $f(x)=x^2$ to illustrate how to implement gradient descent. Although we know that $x=0$ is the solution to minimize $f(x)$, we still use this simple function to observe how $x$ changes. As always, we begin by importing all required modules.
###Code
import sys
sys.path.insert(0, '..')
%matplotlib inline
import d2l
import numpy as np
import math
def f(x): return x**2 # objective function
def gradf(x): return 2 * x # its derivative
###Output
_____no_output_____
###Markdown
Next, we use $x=10$ as the initial value and assume $\eta=0.2$. Using gradient descent to iterate $x$ for 10 times we can see that, eventually, the value of $x$ approaches the optimal solution.
###Code
def gd(eta):
x = 10
results = [x]
for i in range(10):
x -= eta * gradf(x)
results.append(x)
print('epoch 10, x:', x)
return results
res = gd(0.2)
###Output
epoch 10, x: 0.06046617599999997
###Markdown
The progress of optimizing over $x$ can be plotted as follows.
###Code
def show_trace(res):
n = max(abs(min(res)), abs(max(res)))
f_line = np.arange(-n, n, 0.01)
d2l.set_figsize((3.5, 2.5))
d2l.plot([f_line, res], [[f(x) for x in f_line], [f(x) for x in res]],'x', 'f(x)', fmts=['-', '-o'])
show_trace(res)
###Output
_____no_output_____
###Markdown
Learning Rate The learning rate $\eta$ can be set by the algorithm designer. If we use a learning rate that is too small, it will cause $x$ to update very slowly, requiring more iterations to get a better solution. To show what happens in such a case, consider the progress in the same optimization problem for $\eta = 0.05$. As we can see, even after 10 steps we are still very far from the optimal solution.
###Code
show_trace(gd(0.05))
###Output
epoch 10, x: 3.4867844009999995
###Markdown
Conversely, if we use an excessively high learning rate, $\left|\eta f'(x)\right|$ might be too large for the first-order Taylor expansion formula. That is, the term $O(\eta^2 f'^2(x))$ in :eqref:gd-taylor might become significant. In this case, we cannot guarantee that the iteration of $x$ will be able to lower the value of $f(x)$. For example, when we set the learning rate to $\eta=1.1$, $x$ overshoots the optimal solution $x=0$ and gradually diverges.
###Code
show_trace(gd(1.1))
###Output
epoch 10, x: 61.917364224000096
###Markdown
Local Minima To illustrate what happens for nonconvex functions consider the case of $f(x) = x \cdot \cos c x$. This function has infinitely many local minima. Depending on our choice of learning rate and depending on how well conditioned the problem is, we may end up with one of many solutions. The example below illustrates how an (unrealistically) high learning rate will lead to a poor local minimum.
###Code
c = 0.15 * math.pi
def f(x): return x*math.cos(c * x)
def gradf(x): return math.cos(c * x) - c * x * math.sin(c * x)
show_trace(gd(2))
###Output
epoch 10, x: -1.528165927635083
###Markdown
Multivariate Gradient Descent Now that have a better intuition of the univariate case, let us consider the situation where $\mathbf{x} \in \mathbb{R}^d$. That is, the objective function $f: \mathbb{R}^d \to \mathbb{R}$ maps vectors into scalars. Correspondingly its gradient is multivariate, too. It is a vector consisting of $d$ partial derivatives:$$\nabla f(\mathbf{x}) = \bigg[\frac{\partial f(\mathbf{x})}{\partial x_1}, \frac{\partial f(\mathbf{x})}{\partial x_2}, \ldots, \frac{\partial f(\mathbf{x})}{\partial x_d}\bigg]^\top.$$Each partial derivative element $\partial f(\mathbf{x})/\partial x_i$ in the gradient indicates the rate of change of $f$ at $\mathbf{x}$ with respect to the input $x_i$. As before in the univariate case we can use the corresponding Taylor approximation for multivariate functions to get some idea of what we should do. In particular, we have that$$f(\mathbf{x} + \mathbf{\epsilon}) = f(\mathbf{x}) + \mathbf{\epsilon}^\top \nabla f(\mathbf{x}) + O(|\mathbf{\epsilon}|^2).$$ :eqlabel:gd-multi-taylorIn other words, up to second order terms in $\mathbf{epsilon}$ the direction of steepest descent is given by the negative gradient $-\nabla f(\mathbf{x})$. Choosing a suitable learning rate $\eta > 0$ yields the prototypical gradient descent algorithm:$\mathbf{x} \leftarrow \mathbf{x} - \eta \nabla f(\mathbf{x}).$To see how the algorithm behaves in practice let's construct an objective function $f(\mathbf{x})=x_1^2+2x_2^2$ with a two-dimensional vector $\mathbf{x} = [x_1, x_2]^\top$ as input and a scalar as output. The gradient is given by $\nabla f(\mathbf{x}) = [2x_1, 4x_2]^\top$. We will observe the trajectory of $\mathbf{x}$ by gradient descent from the initial position $[-5,-2]$. We need two more helper functions. The first uses an update function and applies it $20$ times to the initial value. The second helper visualizes the trajectory of $\mathbf{x}$. Next, we observe the trajectory of the optimization variable $\mathbf{x}$ for learning rate $\eta = 0.1$. We can see that after 20 steps the value of $\mathbf{x}$ approaches its minimum at $[0, 0]$. Progress is fairly well-behaved albeit rather slow.
###Code
def f(x1, x2): return x1 ** 2 + 2 * x2 ** 2 # objective
def gradf(x1, x2): return (2 * x1, 4 * x2) # gradient
def gd(x1, x2, s1, s2):
(g1, g2) = gradf(x1, x2) # compute gradient
return (x1 -eta * g1, x2 -eta * g2, 0, 0) # update variables
eta = 0.1
d2l.show_trace_2d(f, d2l.train_2d(gd))
###Output
epoch 20, x1 -0.057646, x2 -0.000073
###Markdown
Adaptive Methods As we could see in :numref:section_gd-learningrate, getting the learning rate $\eta$ 'just right' is tricky. If we pick it too small, we make no progress. If we pick it too large, the solution oscillates and in the worst case it might even diverge. What if we could determine $\eta$ automatically or get rid of having to select a step size at all? Second order methods that look not only at the value and gradient of the objective but also at its curvature can help in this case. While these methods cannot be applied to deep learning directly due to the computational cost, they provide useful intuition into how to design advanced optimization algorithms that mimic many of the desirable properties of the algorithms outlined below. Newton's Method Reviewing the Taylor expansion of $f$ there's no need to stop after the first term. In fact, we can write it as$$f(\mathbf{x} + \mathbf{\epsilon}) = f(\mathbf{x}) + \mathbf{\epsilon}^\top \nabla f(\mathbf{x}) + \frac{1}{2} \mathbf{\epsilon}^\top \nabla \nabla^\top f(\mathbf{x}) \mathbf{\epsilon} + O(|\mathbf{\epsilon}|^3)$$ :eqlabel:gd-hot-taylorTo avoid cumbersome notation we define $H_f := \nabla \nabla^\top f(\mathbf{x})$ to be the Hessian of $f$. This is a $d \times d$ matrix. For small $d$ and simple problems $H_f$ is easy to compute. For deep networks, on the other hand, $H_f$ may be prohibitively large, due to the cost of storing $O(d^2)$ entries. Furthermore it may be too expensive to compute via backprop as we would need to apply backprop to the backpropagation call graph. For now let us ignore such considerations and look at what algorithm we'd get.After all, the minimum of $f$ satisfies $\nabla f(\mathbf{x}) = 0$. Taking derivatives of :eqref:gd-hot-taylor with regard to $\mathbf{\epsilon}$ and ignoring higher order terms we arrive at$$\nabla f(\mathbf{x}) + H_f \mathbf{\epsilon} = 0 \text{ and hence } \mathbf{\epsilon} = -H_f^{-1} \nabla f(\mathbf{x}).$$That is, we need to invert the Hessian $H_f$ as part of the optimization problem.For $f(x) = \frac{1}{2} x^2$ we have $\nabla f(x) = x$ and $H_f = 1$. Hence for any $x$ we obtain $\epsilon = -x$. In other words, a single step is sufficient to converge perfectly without the need for any adjustment! Alas, we got a bit lucky here since the Taylor expansion was exact. Let's see what happens in other problems
###Code
c = 0.5
def f(x): return math.cosh(c * x) # objective
def gradf(x): return c * math.sinh(c * x) # derivative
def hessf(x): return c**2 * math.cosh(c * x) # hessian
# hide learning rate for now
def newton(eta = 1):
x = 10
results = [x]
for i in range(10):
x -= eta * gradf(x) / hessf(x)
results.append(x)
print('epoch 10, x:', x)
return results
show_trace(newton())
###Output
epoch 10, x: 0.0
###Markdown
Now let's see what happens when we have a nonconvex function, such as $f(x) = x \cos(c x)$. After all, note that in Newton's method we end up dividing by the Hessian. This means that if the second derivative is negative we would walk into the direction of increasing $f$. That is a fatal flaw of the algorithm. Let's see what happens in practice.
###Code
c = 0.15 * math.pi
def f(x): return x*math.cos(c * x)
def gradf(x): return math.cos(c * x) - c * x * math.sin(c * x)
def hessf(x): return - 2 * c * math.sin(c * x) - x * c**2 * math.cos(c * x)
show_trace(newton())
###Output
epoch 10, x: 26.83413291324767
###Markdown
This went spectacularly wrong. How can we fix it? One way would be to 'fix' the Hessian by taking its absolute value instead. Another strategy is to bring back the learning rate. This seems to defeat the purpose, but not quite. Having second order information allows us to be cautious whenever the curvature is large and to take longer steps whenever the objective is flat. Let's see how this works with a slightly smaller learning rate, say $\eta = 0.5$. As we can see, we have quite an efficient algorithm.
###Code
show_trace(newton(0.5))
###Output
epoch 10, x: 7.269860168684531
###Markdown
Gradient Descent In this section we are going to introduce the basic concepts underlying gradient descent. This is brief by necessity. See e.g. :cite:Boyd.Vandenberghe.2004 for an in-depth introduction to convex optimization. Although the latter is rarely used directly in deep learning, an understanding of gradient descent is key to understanding stochastic gradient descent algorithms. For instance, the optimization problem might diverge due to an overly large learning rate. This phenomenon can already be seen in gradient descent. Likewise, preconditioning is a common technique in gradient descent and carries over to more advanced algorithms. Let's start with a simple special case. Gradient Descent in One Dimension Gradient descent in one dimension is an excellent example to explain why the gradient descent algorithm may reduce the value of the objective function. Consider some continously differentiable real-valued function $f: \mathbb{R} \rightarrow \mathbb{R}$. Using a Taylor expansion (:numref:chapter_math) we obtain that$$f(x + \epsilon) = f(x) + \epsilon f'(x) + O(\epsilon^2).$$ :eqlabel:gd-taylorThat is, in first approximation $f(x+\epsilon)$ is given by the function value $f(x)$ and the first derivative $f'(x)$ at $x$. It is not unreasonable to assume that for small $\epsilon$ moving in the direction of the negative gradient will decrease $f$. To keep things simple we pick a fixed step size $\eta > 0$ and choose $\epsilon = -\eta f'(x)$. Plugging this into the Taylor expansion above we get$$f(x - \eta f'(x)) = f(x) - \eta f'^2(x) + O(\eta^2 f'^2(x)).$$If the derivative $f'(x) \neq 0$ does not vanish we make progress since $\eta f'^2(x)>0$. Moreover, we can always choose $\eta$ small enough for the higher order terms to become irrelevant. Hence we arrive at$$f(x - \eta f'(x)) \lessapprox f(x).$$This means that, if we use$$x \leftarrow x - \eta f'(x)$$to iterate $x$, the value of function $f(x)$ might decline. Therefore, in gradient descent we first choose an initial value $x$ and a constant $\eta > 0$ and then use them to continuously iterate $x$ until the stop condition is reached, for example, when the magnitude of the gradient $|f'(x)|$ is small enough or the number of iterations has reached a certain value.For simplicity we choose the objective function $f(x)=x^2$ to illustrate how to implement gradient descent. Although we know that $x=0$ is the solution to minimize $f(x)$, we still use this simple function to observe how $x$ changes. As always, we begin by importing all required modules.
###Code
import sys
sys.path.insert(0, '..')
%matplotlib inline
import d2l
import numpy as np
import math
def f(x): return x**2 # objective function
def gradf(x): return 2 * x # its derivative
###Output
_____no_output_____
###Markdown
Next, we use $x=10$ as the initial value and assume $\eta=0.2$. Using gradient descent to iterate $x$ for 10 times we can see that, eventually, the value of $x$ approaches the optimal solution.
###Code
def gd(eta):
x = 10
results = [x]
for i in range(10):
x -= eta * gradf(x)
results.append(x)
print('epoch 10, x:', x)
return results
res = gd(0.2)
###Output
epoch 10, x: 0.06046617599999997
###Markdown
The progress of optimizing over $x$ can be plotted as follows.
###Code
def show_trace(res):
n = max(abs(min(res)), abs(max(res)))
f_line = np.arange(-n, n, 0.01)
d2l.set_figsize((3.5, 2.5))
d2l.plot([f_line, res], [[f(x) for x in f_line], [f(x) for x in res]],'x', 'f(x)', fmts=['-', '-o'])
show_trace(res)
###Output
_____no_output_____
###Markdown
Learning Rate The learning rate $\eta$ can be set by the algorithm designer. If we use a learning rate that is too small, it will cause $x$ to update very slowly, requiring more iterations to get a better solution. To show what happens in such a case, consider the progress in the same optimization problem for $\eta = 0.05$. As we can see, even after 10 steps we are still very far from the optimal solution.
###Code
show_trace(gd(0.05))
###Output
epoch 10, x: 3.4867844009999995
###Markdown
Conversely, if we use an excessively high learning rate, $\left|\eta f'(x)\right|$ might be too large for the first-order Taylor expansion formula. That is, the term $O(\eta^2 f'^2(x))$ in :eqref:gd-taylor might become significant. In this case, we cannot guarantee that the iteration of $x$ will be able to lower the value of $f(x)$. For example, when we set the learning rate to $\eta=1.1$, $x$ overshoots the optimal solution $x=0$ and gradually diverges.
###Code
show_trace(gd(1.1))
###Output
epoch 10, x: 61.917364224000096
###Markdown
Local Minima To illustrate what happens for nonconvex functions consider the case of $f(x) = x \cdot \cos c x$. This function has infinitely many local minima. Depending on our choice of learning rate and depending on how well conditioned the problem is, we may end up with one of many solutions. The example below illustrates how an (unrealistically) high learning rate will lead to a poor local minimum.
###Code
c = 0.15 * math.pi
def f(x): return x*math.cos(c * x)
def gradf(x): return math.cos(c * x) - c * x * math.sin(c * x)
show_trace(gd(2))
###Output
epoch 10, x: -1.528165927635083
###Markdown
Multivariate Gradient Descent Now that have a better intuition of the univariate case, let us consider the situation where $\mathbf{x} \in \mathbb{R}^d$. That is, the objective function $f: \mathbb{R}^d \to \mathbb{R}$ maps vectors into scalars. Correspondingly its gradient is multivariate, too. It is a vector consisting of $d$ partial derivatives:$$\nabla f(\mathbf{x}) = \bigg[\frac{\partial f(\mathbf{x})}{\partial x_1}, \frac{\partial f(\mathbf{x})}{\partial x_2}, \ldots, \frac{\partial f(\mathbf{x})}{\partial x_d}\bigg]^\top.$$Each partial derivative element $\partial f(\mathbf{x})/\partial x_i$ in the gradient indicates the rate of change of $f$ at $\mathbf{x}$ with respect to the input $x_i$. As before in the univariate case we can use the corresponding Taylor approximation for multivariate functions to get some idea of what we should do. In particular, we have that$$f(\mathbf{x} + \mathbf{\epsilon}) = f(\mathbf{x}) + \mathbf{\epsilon}^\top \nabla f(\mathbf{x}) + O(|\mathbf{\epsilon}|^2).$$ :eqlabel:gd-multi-taylorIn other words, up to second order terms in $\mathbf{epsilon}$ the direction of steepest descent is given by the negative gradient $-\nabla f(\mathbf{x})$. Choosing a suitable learning rate $\eta > 0$ yields the prototypical gradient descent algorithm:$\mathbf{x} \leftarrow \mathbf{x} - \eta \nabla f(\mathbf{x}).$To see how the algorithm behaves in practice let's construct an objective function $f(\mathbf{x})=x_1^2+2x_2^2$ with a two-dimensional vector $\mathbf{x} = [x_1, x_2]^\top$ as input and a scalar as output. The gradient is given by $\nabla f(\mathbf{x}) = [2x_1, 4x_2]^\top$. We will observe the trajectory of $\mathbf{x}$ by gradient descent from the initial position $[-5,-2]$. We need two more helper functions. The first uses an update function and applies it $20$ times to the initial value. The second helper visualizes the trajectory of $\mathbf{x}$. Next, we observe the trajectory of the optimization variable $\mathbf{x}$ for learning rate $\eta = 0.1$. We can see that after 20 steps the value of $\mathbf{x}$ approaches its minimum at $[0, 0]$. Progress is fairly well-behaved albeit rather slow.
###Code
def f(x1, x2): return x1 ** 2 + 2 * x2 ** 2 # objective
def gradf(x1, x2): return (2 * x1, 4 * x2) # gradient
def gd(x1, x2, s1, s2):
(g1, g2) = gradf(x1, x2) # compute gradient
return (x1 -eta * g1, x2 -eta * g2, 0, 0) # update variables
eta = 0.1
d2l.show_trace_2d(f, d2l.train_2d(gd))
###Output
epoch 20, x1 -0.057646, x2 -0.000073
###Markdown
Adaptive Methods As we could see in :numref:section_gd-learningrate, getting the learning rate $\eta$ 'just right' is tricky. If we pick it too small, we make no progress. If we pick it too large, the solution oscillates and in the worst case it might even diverge. What if we could determine $\eta$ automatically or get rid of having to select a step size at all? Second order methods that look not only at the value and gradient of the objective but also at its curvature can help in this case. While these methods cannot be applied to deep learning directly due to the computational cost, they provide useful intuition into how to design advanced optimization algorithms that mimic many of the desirable properties of the algorithms outlined below. Newton's Method Reviewing the Taylor expansion of $f$ there's no need to stop after the first term. In fact, we can write it as$$f(\mathbf{x} + \mathbf{\epsilon}) = f(\mathbf{x}) + \mathbf{\epsilon}^\top \nabla f(\mathbf{x}) + \frac{1}{2} \mathbf{\epsilon}^\top \nabla \nabla^\top f(\mathbf{x}) \mathbf{\epsilon} + O(|\mathbf{\epsilon}|^3)$$ :eqlabel:gd-hot-taylorTo avoid cumbersome notation we define $H_f := \nabla \nabla^\top f(\mathbf{x})$ to be the Hessian of $f$. This is a $d \times d$ matrix. For small $d$ and simple problems $H_f$ is easy to compute. For deep networks, on the other hand, $H_f$ may be prohibitively large, due to the cost of storing $O(d^2)$ entries. Furthermore it may be too expensive to compute via backprop as we would need to apply backprop to the backpropagation call graph. For now let us ignore such considerations and look at what algorithm we'd get.After all, the minimum of $f$ satisfies $\nabla f(\mathbf{x}) = 0$. Taking derivatives of :eqref:gd-hot-taylor with regard to $\mathbf{\epsilon}$ and ignoring higher order terms we arrive at$$\nabla f(\mathbf{x}) + H_f \mathbf{\epsilon} = 0 \text{ and hence } \mathbf{\epsilon} = -H_f^{-1} \nabla f(\mathbf{x}).$$That is, we need to invert the Hessian $H_f$ as part of the optimization problem.For $f(x) = \frac{1}{2} x^2$ we have $\nabla f(x) = x$ and $H_f = 1$. Hence for any $x$ we obtain $\epsilon = -x$. In other words, a single step is sufficient to converge perfectly without the need for any adjustment! Alas, we got a bit lucky here since the Taylor expansion was exact. Let's see what happens in other problems
###Code
c = 0.5
def f(x): return math.cosh(c * x) # objective
def gradf(x): return c * math.sinh(c * x) # derivative
def hessf(x): return c**2 * math.cosh(c * x) # hessian
# hide learning rate for now
def newton(eta = 1):
x = 10
results = [x]
for i in range(10):
x -= eta * gradf(x) / hessf(x)
results.append(x)
print('epoch 10, x:', x)
return results
show_trace(newton())
###Output
epoch 10, x: 0.0
###Markdown
Now let's see what happens when we have a nonconvex function, such as $f(x) = x \cos(c x)$. After all, note that in Newton's method we end up dividing by the Hessian. This means that if the second derivative is negative we would walk into the direction of increasing $f$. That is a fatal flaw of the algorithm. Let's see what happens in practice.
###Code
c = 0.15 * math.pi
def f(x): return x*math.cos(c * x)
def gradf(x): return math.cos(c * x) - c * x * math.sin(c * x)
def hessf(x): return - 2 * c * math.sin(c * x) - x * c**2 * math.cos(c * x)
show_trace(newton())
###Output
epoch 10, x: 26.83413291324767
###Markdown
This went spectacularly wrong. How can we fix it? One way would be to 'fix' the Hessian by taking its absolute value instead. Another strategy is to bring back the learning rate. This seems to defeat the purpose, but not quite. Having second order information allows us to be cautious whenever the curvature is large and to take longer steps whenever the objective is flat. Let's see how this works with a slightly smaller learning rate, say $\eta = 0.5$. As we can see, we have quite an efficient algorithm.
###Code
show_trace(newton(0.5))
###Output
epoch 10, x: 7.269860168684531
###Markdown
Gradient Descent In this section we are going to introduce the basic concepts underlying gradient descent. This is brief by necessity. See e.g. :cite:Boyd.Vandenberghe.2004 for an in-depth introduction to convex optimization. Although the latter is rarely used directly in deep learning, an understanding of gradient descent is key to understanding stochastic gradient descent algorithms. For instance, the optimization problem might diverge due to an overly large learning rate. This phenomenon can already be seen in gradient descent. Likewise, preconditioning is a common technique in gradient descent and carries over to more advanced algorithms. Let's start with a simple special case. Gradient Descent in One Dimension Gradient descent in one dimension is an excellent example to explain why the gradient descent algorithm may reduce the value of the objective function. Consider some continously differentiable real-valued function $f: \mathbb{R} \rightarrow \mathbb{R}$. Using a Taylor expansion (:numref:chapter_math) we obtain that$$f(x + \epsilon) = f(x) + \epsilon f'(x) + O(\epsilon^2).$$ :eqlabel:gd-taylorThat is, in first approximation $f(x+\epsilon)$ is given by the function value $f(x)$ and the first derivative $f'(x)$ at $x$. It is not unreasonable to assume that for small $\epsilon$ moving in the direction of the negative gradient will decrease $f$. To keep things simple we pick a fixed step size $\eta > 0$ and choose $\epsilon = -\eta f'(x)$. Plugging this into the Taylor expansion above we get$$f(x - \eta f'(x)) = f(x) - \eta f'^2(x) + O(\eta^2 f'^2(x)).$$If the derivative $f'(x) \neq 0$ does not vanish we make progress since $\eta f'^2(x)>0$. Moreover, we can always choose $\eta$ small enough for the higher order terms to become irrelevant. Hence we arrive at$$f(x - \eta f'(x)) \lessapprox f(x).$$This means that, if we use$$x \leftarrow x - \eta f'(x)$$to iterate $x$, the value of function $f(x)$ might decline. Therefore, in gradient descent we first choose an initial value $x$ and a constant $\eta > 0$ and then use them to continuously iterate $x$ until the stop condition is reached, for example, when the magnitude of the gradient $|f'(x)|$ is small enough or the number of iterations has reached a certain value.For simplicity we choose the objective function $f(x)=x^2$ to illustrate how to implement gradient descent. Although we know that $x=0$ is the solution to minimize $f(x)$, we still use this simple function to observe how $x$ changes. As always, we begin by importing all required modules.
###Code
import sys
sys.path.insert(0, '..')
%matplotlib inline
import d2l
import numpy as np
import math
def f(x): return x**2 # objective function
def gradf(x): return 2 * x # its derivative
###Output
_____no_output_____
###Markdown
Next, we use $x=10$ as the initial value and assume $\eta=0.2$. Using gradient descent to iterate $x$ for 10 times we can see that, eventually, the value of $x$ approaches the optimal solution.
###Code
def gd(eta):
x = 10
results = [x]
for i in range(10):
x -= eta * gradf(x)
results.append(x)
print('epoch 10, x:', x)
return results
res = gd(0.2)
###Output
epoch 10, x: 0.06046617599999997
###Markdown
The progress of optimizing over $x$ can be plotted as follows.
###Code
def show_trace(res):
n = max(abs(min(res)), abs(max(res)))
f_line = np.arange(-n, n, 0.01)
d2l.set_figsize((3.5, 2.5))
d2l.plot([f_line, res], [[f(x) for x in f_line], [f(x) for x in res]],'x', 'f(x)', fmts=['-', '-o'])
show_trace(res)
###Output
_____no_output_____
###Markdown
Learning Rate The learning rate $\eta$ can be set by the algorithm designer. If we use a learning rate that is too small, it will cause $x$ to update very slowly, requiring more iterations to get a better solution. To show what happens in such a case, consider the progress in the same optimization problem for $\eta = 0.05$. As we can see, even after 10 steps we are still very far from the optimal solution.
###Code
show_trace(gd(0.05))
###Output
epoch 10, x: 3.4867844009999995
###Markdown
Conversely, if we use an excessively high learning rate, $\left|\eta f'(x)\right|$ might be too large for the first-order Taylor expansion formula. That is, the term $O(\eta^2 f'^2(x))$ in :eqref:gd-taylor might become significant. In this case, we cannot guarantee that the iteration of $x$ will be able to lower the value of $f(x)$. For example, when we set the learning rate to $\eta=1.1$, $x$ overshoots the optimal solution $x=0$ and gradually diverges.
###Code
show_trace(gd(1.1))
###Output
epoch 10, x: 61.917364224000096
###Markdown
Local Minima To illustrate what happens for nonconvex functions consider the case of $f(x) = x \cdot \cos c x$. This function has infinitely many local minima. Depending on our choice of learning rate and depending on how well conditioned the problem is, we may end up with one of many solutions. The example below illustrates how an (unrealistically) high learning rate will lead to a poor local minimum.
###Code
c = 0.15 * math.pi
def f(x): return x*math.cos(c * x)
def gradf(x): return math.cos(c * x) - c * x * math.sin(c * x)
show_trace(gd(2))
###Output
epoch 10, x: -1.528165927635083
###Markdown
Multivariate Gradient Descent Now that have a better intuition of the univariate case, let us consider the situation where $\mathbf{x} \in \mathbb{R}^d$. That is, the objective function $f: \mathbb{R}^d \to \mathbb{R}$ maps vectors into scalars. Correspondingly its gradient is multivariate, too. It is a vector consisting of $d$ partial derivatives:$$\nabla f(\mathbf{x}) = \bigg[\frac{\partial f(\mathbf{x})}{\partial x_1}, \frac{\partial f(\mathbf{x})}{\partial x_2}, \ldots, \frac{\partial f(\mathbf{x})}{\partial x_d}\bigg]^\top.$$Each partial derivative element $\partial f(\mathbf{x})/\partial x_i$ in the gradient indicates the rate of change of $f$ at $\mathbf{x}$ with respect to the input $x_i$. As before in the univariate case we can use the corresponding Taylor approximation for multivariate functions to get some idea of what we should do. In particular, we have that$$f(\mathbf{x} + \mathbf{\epsilon}) = f(\mathbf{x}) + \mathbf{\epsilon}^\top \nabla f(\mathbf{x}) + O(|\mathbf{\epsilon}|^2).$$ :eqlabel:gd-multi-taylorIn other words, up to second order terms in $\mathbf{epsilon}$ the direction of steepest descent is given by the negative gradient $-\nabla f(\mathbf{x})$. Choosing a suitable learning rate $\eta > 0$ yields the prototypical gradient descent algorithm:$\mathbf{x} \leftarrow \mathbf{x} - \eta \nabla f(\mathbf{x}).$To see how the algorithm behaves in practice let's construct an objective function $f(\mathbf{x})=x_1^2+2x_2^2$ with a two-dimensional vector $\mathbf{x} = [x_1, x_2]^\top$ as input and a scalar as output. The gradient is given by $\nabla f(\mathbf{x}) = [2x_1, 4x_2]^\top$. We will observe the trajectory of $\mathbf{x}$ by gradient descent from the initial position $[-5,-2]$. We need two more helper functions. The first uses an update function and applies it $20$ times to the initial value. The second helper visualizes the trajectory of $\mathbf{x}$. Next, we observe the trajectory of the optimization variable $\mathbf{x}$ for learning rate $\eta = 0.1$. We can see that after 20 steps the value of $\mathbf{x}$ approaches its minimum at $[0, 0]$. Progress is fairly well-behaved albeit rather slow.
###Code
def f(x1, x2): return x1 ** 2 + 2 * x2 ** 2 # objective
def gradf(x1, x2): return (2 * x1, 4 * x2) # gradient
def gd(x1, x2, s1, s2):
(g1, g2) = gradf(x1, x2) # compute gradient
return (x1 -eta * g1, x2 -eta * g2, 0, 0) # update variables
eta = 0.1
d2l.show_trace_2d(f, d2l.train_2d(gd))
###Output
epoch 20, x1 -0.057646, x2 -0.000073
###Markdown
Adaptive Methods As we could see in :numref:section_gd-learningrate, getting the learning rate $\eta$ 'just right' is tricky. If we pick it too small, we make no progress. If we pick it too large, the solution oscillates and in the worst case it might even diverge. What if we could determine $\eta$ automatically or get rid of having to select a step size at all? Second order methods that look not only at the value and gradient of the objective but also at its curvature can help in this case. While these methods cannot be applied to deep learning directly due to the computational cost, they provide useful intuition into how to design advanced optimization algorithms that mimic many of the desirable properties of the algorithms outlined below. Newton's Method Reviewing the Taylor expansion of $f$ there's no need to stop after the first term. In fact, we can write it as$$f(\mathbf{x} + \mathbf{\epsilon}) = f(\mathbf{x}) + \mathbf{\epsilon}^\top \nabla f(\mathbf{x}) + \frac{1}{2} \mathbf{\epsilon}^\top \nabla \nabla^\top f(\mathbf{x}) \mathbf{\epsilon} + O(|\mathbf{\epsilon}|^3)$$ :eqlabel:gd-hot-taylorTo avoid cumbersome notation we define $H_f := \nabla \nabla^\top f(\mathbf{x})$ to be the Hessian of $f$. This is a $d \times d$ matrix. For small $d$ and simple problems $H_f$ is easy to compute. For deep networks, on the other hand, $H_f$ may be prohibitively large, due to the cost of storing $O(d^2)$ entries. Furthermore it may be too expensive to compute via backprop as we would need to apply backprop to the backpropagation call graph. For now let us ignore such considerations and look at what algorithm we'd get.After all, the minimum of $f$ satisfies $\nabla f(\mathbf{x}) = 0$. Taking derivatives of :eqref:gd-hot-taylor with regard to $\mathbf{\epsilon}$ and ignoring higher order terms we arrive at$$\nabla f(\mathbf{x}) + H_f \mathbf{\epsilon} = 0 \text{ and hence } \mathbf{\epsilon} = -H_f^{-1} \nabla f(\mathbf{x}).$$That is, we need to invert the Hessian $H_f$ as part of the optimization problem.For $f(x) = \frac{1}{2} x^2$ we have $\nabla f(x) = x$ and $H_f = 1$. Hence for any $x$ we obtain $\epsilon = -x$. In other words, a single step is sufficient to converge perfectly without the need for any adjustment! Alas, we got a bit lucky here since the Taylor expansion was exact. Let's see what happens in other problems
###Code
c = 0.5
def f(x): return math.cosh(c * x) # objective
def gradf(x): return c * math.sinh(c * x) # derivative
def hessf(x): return c**2 * math.cosh(c * x) # hessian
# hide learning rate for now
def newton(eta = 1):
x = 10
results = [x]
for i in range(10):
x -= eta * gradf(x) / hessf(x)
results.append(x)
print('epoch 10, x:', x)
return results
show_trace(newton())
###Output
epoch 10, x: 0.0
###Markdown
Now let's see what happens when we have a nonconvex function, such as $f(x) = x \cos(c x)$. After all, note that in Newton's method we end up dividing by the Hessian. This means that if the second derivative is negative we would walk into the direction of increasing $f$. That is a fatal flaw of the algorithm. Let's see what happens in practice.
###Code
c = 0.15 * math.pi
def f(x): return x*math.cos(c * x)
def gradf(x): return math.cos(c * x) - c * x * math.sin(c * x)
def hessf(x): return - 2 * c * math.sin(c * x) - x * c**2 * math.cos(c * x)
show_trace(newton())
###Output
epoch 10, x: 26.83413291324767
###Markdown
This went spectacularly wrong. How can we fix it? One way would be to 'fix' the Hessian by taking its absolute value instead. Another strategy is to bring back the learning rate. This seems to defeat the purpose, but not quite. Having second order information allows us to be cautious whenever the curvature is large and to take longer steps whenever the objective is flat. Let's see how this works with a slightly smaller learning rate, say $\eta = 0.5$. As we can see, we have quite an efficient algorithm.
###Code
show_trace(newton(0.5))
###Output
epoch 10, x: 7.269860168684531
###Markdown
Gradient Descent In this section we are going to introduce the basic concepts underlying gradient descent. This is brief by necessity. See e.g. :cite:Boyd.Vandenberghe.2004 for an in-depth introduction to convex optimization. Although the latter is rarely used directly in deep learning, an understanding of gradient descent is key to understanding stochastic gradient descent algorithms. For instance, the optimization problem might diverge due to an overly large learning rate. This phenomenon can already be seen in gradient descent. Likewise, preconditioning is a common technique in gradient descent and carries over to more advanced algorithms. Let's start with a simple special case. Gradient Descent in One Dimension Gradient descent in one dimension is an excellent example to explain why the gradient descent algorithm may reduce the value of the objective function. Consider some continously differentiable real-valued function $f: \mathbb{R} \rightarrow \mathbb{R}$. Using a Taylor expansion (:numref:chapter_math) we obtain that$$f(x + \epsilon) = f(x) + \epsilon f'(x) + O(\epsilon^2).$$ :eqlabel:gd-taylorThat is, in first approximation $f(x+\epsilon)$ is given by the function value $f(x)$ and the first derivative $f'(x)$ at $x$. It is not unreasonable to assume that for small $\epsilon$ moving in the direction of the negative gradient will decrease $f$. To keep things simple we pick a fixed step size $\eta > 0$ and choose $\epsilon = -\eta f'(x)$. Plugging this into the Taylor expansion above we get$$f(x - \eta f'(x)) = f(x) - \eta f'^2(x) + O(\eta^2 f'^2(x)).$$If the derivative $f'(x) \neq 0$ does not vanish we make progress since $\eta f'^2(x)>0$. Moreover, we can always choose $\eta$ small enough for the higher order terms to become irrelevant. Hence we arrive at$$f(x - \eta f'(x)) \lessapprox f(x).$$This means that, if we use$$x \leftarrow x - \eta f'(x)$$to iterate $x$, the value of function $f(x)$ might decline. Therefore, in gradient descent we first choose an initial value $x$ and a constant $\eta > 0$ and then use them to continuously iterate $x$ until the stop condition is reached, for example, when the magnitude of the gradient $|f'(x)|$ is small enough or the number of iterations has reached a certain value.For simplicity we choose the objective function $f(x)=x^2$ to illustrate how to implement gradient descent. Although we know that $x=0$ is the solution to minimize $f(x)$, we still use this simple function to observe how $x$ changes. As always, we begin by importing all required modules.
###Code
import sys
sys.path.insert(0, '..')
%matplotlib inline
import d2l
import numpy as np
import math
def f(x): return x**2 # objective function
def gradf(x): return 2 * x # its derivative
###Output
_____no_output_____
###Markdown
Next, we use $x=10$ as the initial value and assume $\eta=0.2$. Using gradient descent to iterate $x$ for 10 times we can see that, eventually, the value of $x$ approaches the optimal solution.
###Code
def gd(eta):
x = 10
results = [x]
for i in range(10):
x -= eta * gradf(x)
results.append(x)
print('epoch 10, x:', x)
return results
res = gd(0.2)
###Output
epoch 10, x: 0.06046617599999997
###Markdown
The progress of optimizing over $x$ can be plotted as follows.
###Code
def show_trace(res):
n = max(abs(min(res)), abs(max(res)))
f_line = np.arange(-n, n, 0.01)
d2l.set_figsize((3.5, 2.5))
d2l.plot([f_line, res], [[f(x) for x in f_line], [f(x) for x in res]],'x', 'f(x)', fmts=['-', '-o'])
show_trace(res)
###Output
_____no_output_____
###Markdown
Learning Rate The learning rate $\eta$ can be set by the algorithm designer. If we use a learning rate that is too small, it will cause $x$ to update very slowly, requiring more iterations to get a better solution. To show what happens in such a case, consider the progress in the same optimization problem for $\eta = 0.05$. As we can see, even after 10 steps we are still very far from the optimal solution.
###Code
show_trace(gd(0.05))
###Output
epoch 10, x: 3.4867844009999995
###Markdown
Conversely, if we use an excessively high learning rate, $\left|\eta f'(x)\right|$ might be too large for the first-order Taylor expansion formula. That is, the term $O(\eta^2 f'^2(x))$ in :eqref:gd-taylor might become significant. In this case, we cannot guarantee that the iteration of $x$ will be able to lower the value of $f(x)$. For example, when we set the learning rate to $\eta=1.1$, $x$ overshoots the optimal solution $x=0$ and gradually diverges.
###Code
show_trace(gd(1.1))
###Output
epoch 10, x: 61.917364224000096
###Markdown
Local Minima To illustrate what happens for nonconvex functions consider the case of $f(x) = x \cdot \cos c x$. This function has infinitely many local minima. Depending on our choice of learning rate and depending on how well conditioned the problem is, we may end up with one of many solutions. The example below illustrates how an (unrealistically) high learning rate will lead to a poor local minimum.
###Code
c = 0.15 * math.pi
def f(x): return x*math.cos(c * x)
def gradf(x): return math.cos(c * x) - c * x * math.sin(c * x)
show_trace(gd(2))
###Output
epoch 10, x: -1.528165927635083
###Markdown
Multivariate Gradient Descent Now that have a better intuition of the univariate case, let us consider the situation where $\mathbf{x} \in \mathbb{R}^d$. That is, the objective function $f: \mathbb{R}^d \to \mathbb{R}$ maps vectors into scalars. Correspondingly its gradient is multivariate, too. It is a vector consisting of $d$ partial derivatives:$$\nabla f(\mathbf{x}) = \bigg[\frac{\partial f(\mathbf{x})}{\partial x_1}, \frac{\partial f(\mathbf{x})}{\partial x_2}, \ldots, \frac{\partial f(\mathbf{x})}{\partial x_d}\bigg]^\top.$$Each partial derivative element $\partial f(\mathbf{x})/\partial x_i$ in the gradient indicates the rate of change of $f$ at $\mathbf{x}$ with respect to the input $x_i$. As before in the univariate case we can use the corresponding Taylor approximation for multivariate functions to get some idea of what we should do. In particular, we have that$$f(\mathbf{x} + \mathbf{\epsilon}) = f(\mathbf{x}) + \mathbf{\epsilon}^\top \nabla f(\mathbf{x}) + O(|\mathbf{\epsilon}|^2).$$ :eqlabel:gd-multi-taylorIn other words, up to second order terms in $\mathbf{epsilon}$ the direction of steepest descent is given by the negative gradient $-\nabla f(\mathbf{x})$. Choosing a suitable learning rate $\eta > 0$ yields the prototypical gradient descent algorithm:$\mathbf{x} \leftarrow \mathbf{x} - \eta \nabla f(\mathbf{x}).$To see how the algorithm behaves in practice let's construct an objective function $f(\mathbf{x})=x_1^2+2x_2^2$ with a two-dimensional vector $\mathbf{x} = [x_1, x_2]^\top$ as input and a scalar as output. The gradient is given by $\nabla f(\mathbf{x}) = [2x_1, 4x_2]^\top$. We will observe the trajectory of $\mathbf{x}$ by gradient descent from the initial position $[-5,-2]$. We need two more helper functions. The first uses an update function and applies it $20$ times to the initial value. The second helper visualizes the trajectory of $\mathbf{x}$. Next, we observe the trajectory of the optimization variable $\mathbf{x}$ for learning rate $\eta = 0.1$. We can see that after 20 steps the value of $\mathbf{x}$ approaches its minimum at $[0, 0]$. Progress is fairly well-behaved albeit rather slow.
###Code
def f(x1, x2): return x1 ** 2 + 2 * x2 ** 2 # objective
def gradf(x1, x2): return (2 * x1, 4 * x2) # gradient
def gd(x1, x2, s1, s2):
(g1, g2) = gradf(x1, x2) # compute gradient
return (x1 -eta * g1, x2 -eta * g2, 0, 0) # update variables
eta = 0.1
d2l.show_trace_2d(f, d2l.train_2d(gd))
###Output
epoch 20, x1 -0.057646, x2 -0.000073
###Markdown
Adaptive Methods As we could see in :numref:section_gd-learningrate, getting the learning rate $\eta$ 'just right' is tricky. If we pick it too small, we make no progress. If we pick it too large, the solution oscillates and in the worst case it might even diverge. What if we could determine $\eta$ automatically or get rid of having to select a step size at all? Second order methods that look not only at the value and gradient of the objective but also at its curvature can help in this case. While these methods cannot be applied to deep learning directly due to the computational cost, they provide useful intuition into how to design advanced optimization algorithms that mimic many of the desirable properties of the algorithms outlined below. Newton's Method Reviewing the Taylor expansion of $f$ there's no need to stop after the first term. In fact, we can write it as$$f(\mathbf{x} + \mathbf{\epsilon}) = f(\mathbf{x}) + \mathbf{\epsilon}^\top \nabla f(\mathbf{x}) + \frac{1}{2} \mathbf{\epsilon}^\top \nabla \nabla^\top f(\mathbf{x}) \mathbf{\epsilon} + O(|\mathbf{\epsilon}|^3)$$ :eqlabel:gd-hot-taylorTo avoid cumbersome notation we define $H_f := \nabla \nabla^\top f(\mathbf{x})$ to be the Hessian of $f$. This is a $d \times d$ matrix. For small $d$ and simple problems $H_f$ is easy to compute. For deep networks, on the other hand, $H_f$ may be prohibitively large, due to the cost of storing $O(d^2)$ entries. Furthermore it may be too expensive to compute via backprop as we would need to apply backprop to the backpropagation call graph. For now let us ignore such considerations and look at what algorithm we'd get.After all, the minimum of $f$ satisfies $\nabla f(\mathbf{x}) = 0$. Taking derivatives of :eqref:gd-hot-taylor with regard to $\mathbf{\epsilon}$ and ignoring higher order terms we arrive at$$\nabla f(\mathbf{x}) + H_f \mathbf{\epsilon} = 0 \text{ and hence } \mathbf{\epsilon} = -H_f^{-1} \nabla f(\mathbf{x}).$$That is, we need to invert the Hessian $H_f$ as part of the optimization problem.For $f(x) = \frac{1}{2} x^2$ we have $\nabla f(x) = x$ and $H_f = 1$. Hence for any $x$ we obtain $\epsilon = -x$. In other words, a single step is sufficient to converge perfectly without the need for any adjustment! Alas, we got a bit lucky here since the Taylor expansion was exact. Let's see what happens in other problems
###Code
c = 0.5
def f(x): return math.cosh(c * x) # objective
def gradf(x): return c * math.sinh(c * x) # derivative
def hessf(x): return c**2 * math.cosh(c * x) # hessian
# hide learning rate for now
def newton(eta = 1):
x = 10
results = [x]
for i in range(10):
x -= eta * gradf(x) / hessf(x)
results.append(x)
print('epoch 10, x:', x)
return results
show_trace(newton())
###Output
epoch 10, x: 0.0
###Markdown
Now let's see what happens when we have a nonconvex function, such as $f(x) = x \cos(c x)$. After all, note that in Newton's method we end up dividing by the Hessian. This means that if the second derivative is negative we would walk into the direction of increasing $f$. That is a fatal flaw of the algorithm. Let's see what happens in practice.
###Code
c = 0.15 * math.pi
def f(x): return x*math.cos(c * x)
def gradf(x): return math.cos(c * x) - c * x * math.sin(c * x)
def hessf(x): return - 2 * c * math.sin(c * x) - x * c**2 * math.cos(c * x)
show_trace(newton())
###Output
epoch 10, x: 26.83413291324767
###Markdown
This went spectacularly wrong. How can we fix it? One way would be to 'fix' the Hessian by taking its absolute value instead. Another strategy is to bring back the learning rate. This seems to defeat the purpose, but not quite. Having second order information allows us to be cautious whenever the curvature is large and to take longer steps whenever the objective is flat. Let's see how this works with a slightly smaller learning rate, say $\eta = 0.5$. As we can see, we have quite an efficient algorithm.
###Code
show_trace(newton(0.5))
###Output
epoch 10, x: 7.269860168684531
|
Tournments/.ipynb_checkpoints/LiarsGame-checkpoint.ipynb | ###Markdown
Game1. In Liars Game, a community of $n$ players start with the same amount of money each.2. Every round each individual selects how much they want to contribute to the central pot.3. After each round the central point is distributed between the entire community.4. The community does not like selfish people, so they kick out the person who gives the least amount of money each round.5. This also means that if you give too much in the beginning, you will not have enough in the end to survive.6. What strategy increases the odds of you coming out victorious? Links- GitRepo: https://github.com/migueltorrescosta/nash_equilibria/tree/main/tournments/liars_game Remark- The Uniformly Random function might not perform very well, but for now it is the only one introducing a significant amount of randomness. Without it the other strategies become deterministic and provide for a very boring analysis- In a game where all opponents always put everything, then you are limited to do the same. However as soon as someone else has a different strategy, you can beat it by putting slightly more and then always putting almost everything. Current Best Strategies:1. Slightly More2. Exponential Decay3. Everything Except on Initial4. Everything 5. Ninety Percentile6. Uniformly Random7. Half8. Tenth Percentile9. Two Over N Players(descriptions below) All imports
###Code
import random
import pandas as pd
from abc import abstractmethod
import seaborn as sns
from tqdm import tqdm
import itertools
cm = sns.light_palette("green", as_cmap=True)
###Output
_____no_output_____
###Markdown
Game Class
###Code
class LiarsGame:
def __init__(self, initial_money, strategies, verbose=False):
assert len(strategies) > 2, "You need at least 3 players to start a game"
assert initial_money > 0
# Secret attributes
self.__players = [strategy(name=strategy.__name__) for strategy in strategies]
self.__initial_n_players = len(self.__players)
self.__money = {player: initial_money for player in self.__players}
self.__game_history = pd.DataFrame(
columns=[player.name for player in self.__players],
index=[],
data=0
)
self.__verbose = verbose
self.__eliminations = []
self.__run_game()
def __repr__(self):
return f"LiarsGame: {self.n_players} with {self.total_money}¥"
def __weight(self, coin_list):
return sum([self.__coin_weights[i] for i in coin_list])
# Accessible attributes
@property
def money(self):
return self.__money
@property
def total_money(self):
return sum(self.__money.values())
@property
def players(self):
return self.__players
@property
def n_players(self):
return len(self.__players)
@property
def eliminations(self):
return self.__eliminations
@property
def game_history(self):
return self.__game_history
def show_game_history_heatmap(self):
return self.__game_history.T.style.background_gradient(cmap=cm).set_precision(2).highlight_null('red')
def show_game_history_bar_plot(self):
return self.__game_history.plot.bar(
stacked=True,
figsize=(20,10),
width=.95
).legend(
loc="center right",
bbox_to_anchor=(0, 0.5),
prop={'size': 18}
)
def my_money(self, player):
return self.__money[player]
# Key Methods
def __run_round(self):
self.__game_history.loc[self.__initial_n_players - self.n_players] = {
player.name: self.money[player] for player in self.players}
current_move = {
player: max([min([player.move(self), 1]), 0])*self.money[player]
for player in self.players
}
if self.__verbose:
for player in self.players:
print(
f"{player.name}: {current_move[player]:.2f} / {self.money[player]:.2f}")
print("\n" + "="*50 + "\n")
lowest_contribution = min(current_move.values())
smallest_contributor = random.choice([
player
for player in self.__players
if current_move[player]==lowest_contribution
])
self.__eliminations.append(smallest_contributor)
current_move[smallest_contributor] = self.__money[smallest_contributor]
pot = sum(current_move.values())
self.__players = [
player for player in self.__players if player != smallest_contributor]
self.__money = {
player: self.__money[player] -
current_move[player] + pot/self.n_players
for player in self.players
}
def __run_game(self):
while self.n_players > 1:
self.__run_round()
winner = self.players[0]
self.__game_history.loc[self.__initial_n_players - self.n_players] = {winner.name: self.money[winner]}
self.__eliminations.append(winner)
if self.__verbose:
print(f"Winner: {winner.name}")
return winner.name
###Output
_____no_output_____
###Markdown
Player Parent Class
###Code
class Player:
def __init__(self, name):
self.name = name
def __repr__(self):
return self.name
@abstractmethod
def move(self):
pass
###Output
_____no_output_____
###Markdown
Submitted Strategies
###Code
# Always contributes half of their wealth
class half(Player):
def move(self, status):
return .5
# Always contributes all their wealth
class everything(Player):
def move(self, status):
return 1
# Always contributes 90% of their wealth
class ninety_percentile(Player):
def move(self, status):
return .9
# Always contributes 10% of their wealth
class tenth_percentile(Player):
def move(self, status):
return .1
# Contributes an uniformly random amount of their wealth
class uniformly_random(Player):
def move(self, status):
return random.random()
# Contributes a weird amount
class two_over_n_players(Player):
def __init__(self, name):
self.name = name
self.initial_number_of_players = None
def move(self, status):
if not self.initial_number_of_players:
self.initial_number_of_players = status.n_players
return 2*(1 - status.n_players/self.initial_number_of_players)
# In the first random it contributes a random amount, otherwise it contributes everything
class everything_except_on_initial(Player):
def __init__(self, name):
self.name = name
self.is_first_move = True
def move(self, status):
if self.is_first_move:
self.is_first_move = False
return random.random()
else:
return 1
# Contributes an amount that converges to 1 exponentially
class exponential_decay(Player):
def __init__(self, name):
self.name = name
self.initial_number_of_players = None
def move(self, status):
if not self.initial_number_of_players:
self.initial_number_of_players = status.n_players
return 1 - 0.3**(1 + self.initial_number_of_players - status.n_players)
# First round it contributes an uniformly random amount, after that it contributes the minimum needed to ensure survival.
class slightly_more(Player):
def __init__(self, name):
self.name = name
self.is_first_move = True
def move(self, status):
if self.is_first_move:
self.is_first_move = False
return random.random()
else:
least_money = min(status.money.values())
return least_money/status.my_money(self) + 10e-9
###Output
_____no_output_____
###Markdown
Your playgroundEdit the code below with your own ideas :)
###Code
class your_custom_player():
def __init__(self, name):
self.name = name
self.initial_number_of_players = None
def move(self, status):
if not self.initial_number_of_players:
self.initial_number_of_players = status.n_players
return 2*(1 - status.n_players/self.initial_number_of_players)
###Output
_____no_output_____
###Markdown
Sample Run
###Code
initial_money = 100
best_strategies = [
exponential_decay,
half,
everything,
tenth_percentile,
ninety_percentile,
two_over_n_players,
uniformly_random,
everything_except_on_initial,
slightly_more
]
x = LiarsGame(
initial_money= initial_money,
strategies=best_strategies
)
x.show_game_history_heatmap()
x.show_game_history_bar_plot()
###Output
_____no_output_____
###Markdown
Distribution of Rounds survivedThe more rounds one survives, the better the strategy is
###Code
runs = 100000
strategy_names = [strategy.__name__ for strategy in best_strategies]
rounds_survived = pd.DataFrame(
columns=range(len(strategy_names)),
index=strategy_names,
data=0
)
for _ in tqdm(range(runs)):
eliminations = LiarsGame(initial_money=initial_money, strategies=best_strategies).eliminations
for (i, player) in enumerate(eliminations):
rounds_survived[i][player.name] += 1
rounds_survived.T.plot.bar(
stacked=True,
figsize=(20,5),
width=.5
).legend(
loc="center right",
bbox_to_anchor=(0, 0.5),
prop={'size': 18}
)
rounds_survived.style.background_gradient(cmap=cm)
###Output
_____no_output_____ |
examples/notebooks/PoC_EODC.ipynb | ###Markdown
OpenEO Connection to EODC Backend
###Code
import logging
import openeo
from openeo.auth.auth_bearer import BearerAuth
logging.basicConfig(level=logging.INFO)
# Define constants
# Connection
EODC_DRIVER_URL = "http://openeo.eodc.eu"
OUTPUT_FILE = "/tmp/openeo_eodc_output.tiff"
EODC_USER = "user1"
EODC_PWD = "Test123#"
# Data
PRODUCT_ID = "s2a_prd_msil1c"
DATE_START = "2017-01-01"
DATE_END = "2017-01-08"
IMAGE_LEFT = 652000
IMAGE_RIGHT = 672000
IMAGE_TOP = 5161000
IMAGE_BOTTOM = 5181000
IMAGE_SRS = "EPSG:32632"
# Processes
NDVI_RED = "B04"
NDVI_NIR = "B08"
# Connect with EODC backend
session = openeo.session(EODC_USER, endpoint=EODC_DRIVER_URL)
# Login
token = session.auth(EODC_USER, EODC_PWD, BearerAuth)
session
# Retrieve the list of available collections
collections = session.imagecollections()
list(collections)[:5]
# Get detailed information about a collection
collection = session.get_collection(PRODUCT_ID)
collection
# Select collection product
s2a_prd_msil1c = session.image(PRODUCT_ID)
s2a_prd_msil1c
# Get list of available processes
processes = session.get_all_processes()
processes
# Specifying the date range and the bounding box
timeseries = s2a_prd_msil1c.filter_bbox(west=IMAGE_LEFT, east=IMAGE_RIGHT, north=IMAGE_TOP,
south=IMAGE_BOTTOM, crs=IMAGE_SRS)
timeseries = timeseries.filter_temporal(DATE_START, DATE_END)
timeseries
# Applying some operations on the data
timeseries = timeseries.ndvi(NDVI_RED, NDVI_NIR)
timeseries = timeseries.min_time()
timeseries
# Sending the job to the backend
job = timeseries.create_job()
job
# Queue job to start the processing
status = job.queue()
# Get Status of Job
status = job.status()
status
# Download job result
from openeo.rest.job import ClientJob
job = ClientJob(107, session)
job.download(OUTPUT_FILE)
job
# Showing the result
!gdalinfo -hist "/tmp/openeo_eodc_output.tiff"
###Output
_____no_output_____
###Markdown
OpenEO Connection to EODC Backend
###Code
import logging
import openeo
from openeo.auth.auth_bearer import BearerAuth
logging.basicConfig(level=logging.INFO)
# Define constants
# Connection
EODC_DRIVER_URL = "http://openeo.eodc.eu"
OUTPUT_FILE = "/tmp/openeo_eodc_output.tiff"
EODC_USER = "user1"
EODC_PWD = "Test123#"
# Data
PRODUCT_ID = "s2a_prd_msil1c"
DATE_START = "2017-01-01"
DATE_END = "2017-01-08"
IMAGE_LEFT = 652000
IMAGE_RIGHT = 672000
IMAGE_TOP = 5161000
IMAGE_BOTTOM = 5181000
IMAGE_SRS = "EPSG:32632"
# Processes
NDVI_RED = "B04"
NDVI_NIR = "B08"
# Connect with EODC backend
session = openeo.session(EODC_USER, endpoint=EODC_DRIVER_URL)
# Login
token = session.auth(EODC_USER, EODC_PWD, BearerAuth)
session
# Retrieve the list of available collections
collections = session.imagecollections()
list(collections)[:5]
# Get detailed information about a collection
collection = session.get_collection(PRODUCT_ID)
collection
# Select collection product
s2a_prd_msil1c = session.image(PRODUCT_ID)
s2a_prd_msil1c
# Get list of available processes
processes = session.get_all_processes()
processes
# Specifying the date range and the bounding box
timeseries = s2a_prd_msil1c.filter_bbox(west=IMAGE_LEFT, east=IMAGE_RIGHT, north=IMAGE_TOP,
south=IMAGE_BOTTOM, crs=IMAGE_SRS)
timeseries = timeseries.filter_temporal(DATE_START, DATE_END)
timeseries
# Applying some operations on the data
timeseries = timeseries.ndvi(NDVI_RED, NDVI_NIR)
timeseries = timeseries.min_time()
timeseries
# Sending the job to the backend
job = timeseries.send_job()
job
# Queue job to start the processing
status = job.queue()
# Get Status of Job
status = job.status()
status
# Download job result
from openeo.rest.job import ClientJob
job = ClientJob(107, session)
job.download(OUTPUT_FILE)
job
# Showing the result
!gdalinfo -hist "/tmp/openeo_eodc_output.tiff"
###Output
_____no_output_____ |
Recipes/CGC/projects_detailOne.ipynb | ###Markdown
What are the details of one of my _projects_? OverviewThere are a number of API calls related to projects. Here we focus on _getting the details_ of a single project. As with any **detail**-type call, we will get extensive information about one project but must first know that project's id. Prerequisites 1. You need to be a member (or owner) of _at least one_ project. 2. You need your _authentication token_ and the API needs to know about it. See **Setup_API_environment.ipynb** for details. 3. You understand how to list projects you are a member of (we will just use that call directly here). ImportsWe import the _Api_ class from the official sevenbridges-python bindings below.
###Code
import sevenbridges as sbg
###Output
_____no_output_____
###Markdown
Initialize the objectThe _Api_ object needs to know your **auth\_token** and the correct path. Here we assume you are using the .sbgrc file in your home directory. For other options see Setup_API_environment.ipynb
###Code
# [USER INPUT] specify platform {cgc, sbg}
prof = 'cgc'
config_config_file = sbg.Config(profile=prof)
api = sbg.Api(config=config_config_file)
###Output
_____no_output_____
###Markdown
List some projects & get details of one of themWe start by listing all of your projects, then get more information on the first one. A **detail**-call for projects returns the following *attributes*:* **description** The user specified project description* **id** _Unique_ identifier for the project, generated based on Project Name* **name** Name of project specified by the user, maybe _non-unique_* **href** Address1 of the project.* **tags** List of tags, currently tags[0] = 'tcga' if protected data is used on the **CGC**. This does not apply to **SBPLAT*** **type** (unimportant) this is _always_ equal to 'v2'1 This is the address where, by using API you can get this resource
###Code
# [USER INPUT] project index
p_index = 0
existing_projects = [p for p in api.projects.query().all()]
single_project = api.projects.get(id=existing_projects[p_index].id)
print('You have selected project (%s).' % (single_project.name))
if hasattr(single_project, 'description'):
# Need to check if description has been entered, GUI created project have default text,
# but it is not in the metadata.
print('Project description: %s \n' % (single_project.description))
if len(single_project.tags) > 0:
if single_project.tags[0] == 'tcga':
print('This is a CONTROLLED data project.')
else:
print('This is an OPEN data project.')
###Output
_____no_output_____ |
Exercises01-06 and Activities/Exercises01-06 and Activities01-02.ipynb | ###Markdown
Tokenization
###Code
import nltk
nltk.download('punkt')
from nltk import tokenize
tokenize.sent_tokenize(raw_txt)
txt_sents = tokenize.sent_tokenize(raw_txt)
type(txt_sents), len(txt_sents)
txt_words = [tokenize.word_tokenize(sent) for sent in txt_sents]
type(txt_words), type(txt_words[0])
print(txt_words[:2])
###Output
[['Welcome', 'to', 'the', 'world', 'of', 'Deep', 'Learning', 'for', 'NLP', '!'], ['We', "'re", 'in', 'this', 'together', ',', 'and', 'we', "'ll", 'learn', 'together', '.']]
###Markdown
Normalizing case
###Code
#You needn't run this
raw_txt = raw_txt.lower()
txt_sents = [sent.lower() for sent in txt_sents]
txt_sents
txt_words = [tokenize.word_tokenize(sent) for sent in txt_sents]
print(txt_words[:2])
###Output
[['welcome', 'to', 'the', 'world', 'of', 'deep', 'learning', 'for', 'nlp', '!'], ['we', "'re", 'in', 'this', 'together', ',', 'and', 'we', "'ll", 'learn', 'together', '.']]
###Markdown
Removing punctuation
###Code
from string import punctuation
list_punct = list(punctuation)
print(list_punct)
def drop_punct(input_tokens):
return [token for token in input_tokens if token not in list_punct]
drop_punct(["let",".","us",".","go","!"])
txt_words_nopunct = [drop_punct(sent) for sent in txt_words]
print(txt_words_nopunct)
###Output
[['welcome', 'to', 'the', 'world', 'of', 'deep', 'learning', 'for', 'nlp'], ['we', "'re", 'in', 'this', 'together', 'and', 'we', "'ll", 'learn', 'together'], ['nlp', 'is', 'amazing', 'and', 'deep', 'learning', 'makes', 'it', 'even', 'more', 'fun'], ['let', "'s", 'learn']]
###Markdown
Removing stop words
###Code
import nltk
nltk.download("stopwords")
from nltk.corpus import stopwords
list_stop = stopwords.words("english")
len(list_stop)
print(list_stop[:50])
###Output
['i', 'me', 'my', 'myself', 'we', 'our', 'ours', 'ourselves', 'you', "you're", "you've", "you'll", "you'd", 'your', 'yours', 'yourself', 'yourselves', 'he', 'him', 'his', 'himself', 'she', "she's", 'her', 'hers', 'herself', 'it', "it's", 'its', 'itself', 'they', 'them', 'their', 'theirs', 'themselves', 'what', 'which', 'who', 'whom', 'this', 'that', "that'll", 'these', 'those', 'am', 'is', 'are', 'was', 'were', 'be']
###Markdown
Exercise 01: Tokenizing, Case Normalization, Punctuation and Stop Word Removal
###Code
import nltk
from nltk import tokenize
raw_txt = """Welcome to the world of Deep Learning for NLP! We're in this together, and we'll learn together. NLP is amazing, and Deep Learning makes it even more fun. Let's learn!"""
txt_sents = tokenize.sent_tokenize(raw_txt.lower())
txt_words = [tokenize.word_tokenize(sent) for sent in txt_sents]
from string import punctuation
stop_punct = list(punctuation)
from nltk.corpus import stopwords
stop_nltk = stopwords.words("english")
stop_final = stop_punct + stop_nltk
def drop_stop(input_tokens):
return [token for token in input_tokens if token not in stop_final]
txt_words_nostop = [drop_stop(sent) for sent in txt_words]
print(txt_words_nostop[0])
###Output
['welcome', 'world', 'deep', 'learning', 'nlp']
###Markdown
Stemming
###Code
from nltk.stem import PorterStemmer
stemmer_p = PorterStemmer()
print(stemmer_p.stem("driving"))
txt = "I mustered all my drive, drove to the driving school!"
tokens = tokenize.word_tokenize(txt)
print([stemmer_p.stem(word) for word in tokens])
###Output
['I', 'muster', 'all', 'my', 'drive', ',', 'drove', 'to', 'the', 'drive', 'school', '!']
###Markdown
Lemmatization
###Code
nltk.download('wordnet')
from nltk.stem import WordNetLemmatizer
lemmatizer = WordNetLemmatizer()
lemmatizer.lemmatize("ponies")
###Output
_____no_output_____
###Markdown
Exercise 02: Stemming Our Data
###Code
from nltk.stem import PorterStemmer
stemmer_p = PorterStemmer()
print([stemmer_p.stem(token) for token in txt_words_nostop[0]])
###Output
['welcom', 'world', 'deep', 'learn', 'nlp']
###Markdown
Applying stemmer to all the sentences
###Code
txt_words_stem = [[stemmer_p.stem(token) for token in sent] for sent in txt_words_nostop]
txt_words_stem
###Output
_____no_output_____
###Markdown
Downloading Text Corpora using NLTK
###Code
import nltk
nltk.download()
alice_raw = nltk.corpus.gutenberg.raw('carroll-alice.txt')
alice_raw[:800]
###Output
_____no_output_____
###Markdown
Representation 1. One hot encoding
###Code
txt_words_nostop
###Output
_____no_output_____
###Markdown
Exercise 03: Creating One-Hot Encoding for Our Data
###Code
print(txt_words_nostop)
target_terms = ["nlp","deep","learn"]
def get_onehot(sent):
return [1 if term in sent else 0 for term in target_terms]
one_hot_mat = [get_onehot(sent) for sent in txt_words_nostop]
import numpy as np
np.array(one_hot_mat)
###Output
_____no_output_____
###Markdown
Term Frequencies
###Code
from sklearn.feature_extraction.text import CountVectorizer
vectorizer = CountVectorizer(max_features = 5)
vectorizer.fit(txt_sents)
vectorizer.vocabulary_
txt_dtm = vectorizer.fit_transform(txt_sents)
txt_dtm.toarray()
txt_sents
def do_nothing(doc):
return doc
vectorizer = CountVectorizer(max_features=5,
preprocessor=do_nothing,
tokenizer=do_nothing)
txt_dtm = vectorizer.fit_transform(txt_words_stem)
txt_dtm.toarray()
vectorizer.vocabulary_
txt_words_stem
###Output
_____no_output_____
###Markdown
Exercise 04: Document Term Matrix with TF-IDF
###Code
from sklearn.feature_extraction.text import TfidfVectorizer
vectorizer_tfidf = TfidfVectorizer(max_features=5)
vectorizer_tfidf.fit(txt_sents)
vectorizer_tfidf.vocabulary_
txt_tfidf = vectorizer_tfidf.transform(txt_sents)
txt_tfidf.toarray()
vectorizer_tfidf.idf_
###Output
_____no_output_____
###Markdown
Training Our Own Embeddings
###Code
import gensim.downloader as api
from gensim.models import word2vec
#Another way of loading the data. if this doesn't work, you could use the text8 corpus local file
dataset = api.load("text8")
###Output
[==================================================] 100.0% 31.6/31.6MB downloaded
###Markdown
To ensure reproducible results, set random seed as 1
###Code
np.random.seed(1)
dataset = word2vec.Text8Corpus("text8")
model = word2vec.Word2Vec(dataset)
print(model.wv["animal"])
len(model.wv["animal"])
model.wv.most_similar("animal")
model.wv.most_similar("happiness")
###Output
_____no_output_____
###Markdown
Semantic Regularities in Word Embeddings
###Code
model.wv.most_similar(positive=['woman', 'king'], negative=['man'], topn=5)
model.wv.most_similar(positive=['uncle', 'woman'], negative=['man'], topn=5)
###Output
_____no_output_____
###Markdown
Exercise 05: Vectors for Phrases
###Code
v1 = model.wv['get']
v2 = model.wv['happy']
res1 = (v1+v2)/2
v1 = model.wv['make']
v2 = model.wv['merry']
res2 = (v1+v2)/2
model.wv.cosine_similarities(res1, [res2])
###Output
_____no_output_____
###Markdown
Effect of Parameters - 'size' of the Vector
###Code
model = word2vec.Word2Vec(dataset, size=30)
model.wv.most_similar(positive=['woman', 'king'], negative=['man'], topn=5)
###Output
_____no_output_____
###Markdown
Effect of parameters - skipgram vs. CBOW Rare terms - oeuvre
###Code
model = word2vec.Word2Vec(dataset)
model.wv.most_similar("oeuvre", topn=5)
model_sg = word2vec.Word2Vec(dataset, sg=1)
model_sg.wv.most_similar("oeuvre", topn=5)
###Output
_____no_output_____
###Markdown
Exercise 06: Training Word Vectors on Different Datasets
###Code
nltk.download('brown')
nltk.download('movie_reviews')
from nltk.corpus import brown, movie_reviews
model_brown = word2vec.Word2Vec(brown.sents(), sg=1)
model_movie = word2vec.Word2Vec(movie_reviews.sents(), sg=1)
model_brown.wv.most_similar('money', topn=5)
model_movie.wv.most_similar('money', topn=5)
###Output
_____no_output_____
###Markdown
Using Pre-Trained Word Vectors
###Code
from gensim.scripts.glove2word2vec import glove2word2vec
glove_input_file = 'glove.6B.100d.txt'
word2vec_output_file = 'glove.6B.100d.w2vformat.txt'
glove2word2vec(glove_input_file, word2vec_output_file)
from gensim.models.keyedvectors import KeyedVectors
glove_model = KeyedVectors.load_word2vec_format("glove.6B.100d.w2vformat.txt", binary=False)
glove_model.most_similar("money", topn=5)
glove_model.most_similar(positive=['woman', 'king'], negative=['man'], topn=5)
###Output
_____no_output_____
###Markdown
Bias in Embeddings – A Word of Caution
###Code
model.wv.most_similar(positive=['woman', 'doctor'], negative=['man'], topn=5)
model.wv.most_similar(positive=['woman', 'smart'], negative=['man'], topn=5)
###Output
_____no_output_____
###Markdown
Activity 01: Text Preprocessing of the 'Alice in Wonderland' Text
###Code
alice_raw[:800]
###Output
_____no_output_____
###Markdown
Solution
###Code
txt_sents = tokenize.sent_tokenize(alice_raw.lower())
txt_words = [tokenize.word_tokenize(sent) for sent in txt_sents]
from string import punctuation
stop_punct = list(punctuation)
from nltk.corpus import stopwords
stop_nltk = stopwords.words("english")
stop_context = ["--", "said"]
stop_final = stop_punct + stop_nltk + stop_context
def drop_stop(input_tokens):
return [token for token in input_tokens if token not in stop_final]
alice_words_nostop = [drop_stop(sent) for sent in txt_words]
print(alice_words_nostop[:2])
from nltk.stem import PorterStemmer
stemmer_p = PorterStemmer()
alice_words_stem = [[stemmer_p.stem(token) for token in sent] for sent in alice_words_nostop]
print(alice_words_stem[:5])
###Output
[['alic', "'s", 'adventur', 'wonderland', 'lewi', 'carrol', '1865', 'chapter', 'i.', 'rabbit-hol', 'alic', 'begin', 'get', 'tire', 'sit', 'sister', 'bank', 'noth', 'twice', 'peep', 'book', 'sister', 'read', 'pictur', 'convers', "'and", 'use', 'book', 'thought', 'alic', "'without", 'pictur', 'convers'], ['consid', 'mind', 'well', 'could', 'hot', 'day', 'made', 'feel', 'sleepi', 'stupid', 'whether', 'pleasur', 'make', 'daisy-chain', 'would', 'worth', 'troubl', 'get', 'pick', 'daisi', 'suddenli', 'white', 'rabbit', 'pink', 'eye', 'ran', 'close'], ['noth', 'remark', 'alic', 'think', 'much', 'way', 'hear', 'rabbit', 'say', "'oh", 'dear'], ['oh', 'dear'], ['shall', 'late']]
###Markdown
Activity 02: Text Representation for Alice in Wonderland Solution
###Code
#From activity 01, print the first 3 sentences from the result after stop word removal. This is the data you will work with.
print(alice_words_nostop[:3])
from gensim.models import word2vec
model = word2vec.Word2Vec(alice_words_nostop)
model.wv.most_similar("rabbit", topn=5)
model = word2vec.Word2Vec(alice_words_nostop, window=2)
model.wv.most_similar("rabbit", topn=5)
model = word2vec.Word2Vec(alice_words_nostop, window=5, sg=1)
model.wv.most_similar("rabbit", topn=5)
v1 = model.wv['white']
v2 = model.wv['rabbit']
res1 = (v1+v2)/2
v1 = model.wv['mad']
v2 = model.wv['hatter']
res2 = (v1+v2)/2
model.wv.cosine_similarities(res1, [res2])
from gensim.models.keyedvectors import KeyedVectors
glove_model = KeyedVectors.load_word2vec_format("glove.6B.100d.w2vformat.txt", binary=False)
v1 = glove_model['white']
v2 = glove_model['rabbit']
res1 = (v1+v2)/2
v1 = glove_model['mad']
v2 = glove_model['hatter']
res2 = (v1+v2)/2
glove_model.cosine_similarities(res1, [res2])
###Output
_____no_output_____ |
examples/model_displacement.ipynb | ###Markdown
Generates 10 random 3D coordinates, rotate and translate them, and show howthe displacement can be fitted and predicted with `ModelDisplacement`.
###Code
%matplotlib notebook
import numpy as np
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
from ecoggui import ModelDisplacement
# Make a random flat grid. Note that it works for any solid objects.
n_samples = 6 ** 2
X = np.meshgrid(np.linspace(0, 1., np.sqrt(n_samples)), # x axis
np.linspace(0, 1., np.sqrt(n_samples))) # y axis
X = np.mat(np.transpose([ii.ravel() for ii in X]))
X = np.hstack((X, np.zeros((len(X), 1)))) # add z axis
plt.scatter(X[:, 0], X[:, 1])
plt.show()
# Random rotation and translation
R = np.mat(np.random.rand(3, 3))
t = np.mat(np.random.rand(3, 1))
# make R a proper rotation matrix, force orthonormal
U, S, Vt = np.linalg.svd(R)
R = U * Vt
# remove reflection
if np.linalg.det(R) < 0:
Vt[2, :] *= -1
R = U * Vt
# Displace X points
Y = R * X.T + np.tile(t, (1, n_samples))
Y = Y.T
# To avoid confusion, we'll now treat these matrices as array:
X, Y = np.array(X), np.array(Y)
# Plot
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.scatter(X[:, 0], X[:, 1], X[:, 2], s=100, c='k')
ax.scatter(Y[:, 0], Y[:, 1], Y[:, 2], s=100, c='b')
plt.show()
# recover the transformation
displacer = ModelDisplacement()
displacer.fit(X, Y)
Xt = displacer.transform(X)
# Compute the error between the true Y position and the predicted X position
# from the rotation + translation fit
err = np.sqrt(np.sum((Xt - Y) ** 2))
print(err)
# Plot
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.scatter(X[:, 0], X[:, 1], X[:, 2], s=100, c='k')
ax.scatter(Y[:, 0], Y[:, 1], Y[:, 2], s=100, c='b')
ax.scatter(Xt[:, 0], Xt[:, 1], Xt[:, 2], s=40, c='r')
ax.legend(['X', 'Y', 'predicted X'])
plt.show()
###Output
2.30755522366e-15
|
notebooks/1.0-xs-scraper.ipynb | ###Markdown
get current price
###Code
soup.find_all('div',{'class':'element element--intraday'})[0].find_all('h3',{'class':'intraday__price'})
data = soup.find_all('div',{'class':'element element--intraday'})[0].find('h3',{'class':'intraday__price'}).text
data
data.strip('$\n')
def get_current_price(ticker):
""" input: ticker, output:price """
r = requests.get('https://www.marketwatch.com/investing/stock/%s/charts?mod=mw_quote_tab' %ticker)
soup = bs4.BeautifulSoup(r.content, 'html.parser')
soup.find_all('div',{'class':'element element--intraday'})[0].find_all('h3',{'class':'intraday__price'})
price = soup.find_all('div',{'class':'element element--intraday'})[0].find('h3',{'class':'intraday__price'}).text.strip('$\n')
return price
get_current_price("spce")
###Output
_____no_output_____
###Markdown
get historical price data
###Code
from datetime import datetime
a = str(datetime.now().month)
b = str(datetime.now().day -1)
c = str(datetime.now().year -1)
d = str(datetime.now().year)
last_year = a + '/' + b + '/' + c
this_year = a + '/' + b + '/' + d
last_year, this_year
r = requests.get('https://www.marketwatch.com/investing/stock/spce/download-data?startDate=07/14/2020&endDate=07/14/2021')
soup = bs4.BeautifulSoup(r.content,'html.parser')
soup
soup.find_all('a',{'class':'link link--csv m100'})
link = soup.find_all('a',{'class':'link link--csv m100'},href=True)[0]['href'].replace(' ','%')
link
import pandas as pd
pd.read_csv(link)
# need to fix the start year and the end year time
link
link_parts = link.split('startdate=')
link_parts
link_parts[1][13:]
from datetime import datetime
a = str(datetime.now().month)
b = str(datetime.now().day -1)
c = str(datetime.now().year -1)
d = str(datetime.now().year)
last_year = a + '/' + b + '/' + c
this_year = a + '/' + b + '/' + d
last_year, this_year
go
link_parts
link = link_parts[0] + 'startdate=' + last_year + '%2000' + link_parts[1][13:]
link
#fixing enddate time
link_parts = link.split('&daterange')
link_parts[0]
link_parts[0][:-8]+'2000:00:00&daterange' + link_parts[1]
hist_link = link_parts[0][:-8]+'2000:00:00&daterange' + link_parts[1]
hist_link
year_example_link = 'https://www.marketwatch.com/investing/stock/spce/downloaddatapartial?startdate=7/14/2020%2000:00:00&enddate=07/14/2021%2000:00:00&daterange=d30&frequency=p1d&csvdownload=true&downloadpartial=false&newdates=false'
year_example_link
class MarketScraper:
def __init__(self):
a = str(datetime.now().month)
b = str(datetime.now().day -1)
c = str(datetime.now().year -2)
d = str(datetime.now().year)
yesterday = str(a + '/' + b + '/' + d)
last_year = str(a + '/' + b + '/' + c)
self.yesterday = yesterday
self.last_year = last_year
def get_current_price(ticker):
""" input: ticker, output:price """
r = requests.get('https://www.marketwatch.com/investing/stock/%s/charts?mod=mw_quote_tab' %ticker)
soup = bs4.BeautifulSoup(r.content, 'html.parser')
soup.find_all('div',{'class':'element element--intraday'})[0].find_all('h3',{'class':'intraday__price'})
price = soup.find_all('div',{'class':'element element--intraday'})[0].find('h3',{'class':'intraday__price'}).text.strip('$\n')
return price
def get_price_hist(self,ticker):
hist_link = 'https://www.marketwatch.com/investing/stock/{}/downloaddatapartial?startdate={}%2000:00:00&enddate={}%2000:00:00&daterange=d30&frequency=p1d&csvdownload=true&downloadpartial=false&newdates=false'.format(ticker,self.last_year,self.yesterday)
return pd.read_csv(hist_link)
import pandas as pd
ms = MarketScraper()
goog_data = ms.get_price_hist('goog')
goog_data
cd
goog_data.to_csv('~/Documents/advantage-investing/data/raw/Goog.csv')
###Output
_____no_output_____
###Markdown
getting marketcap, eps, ect
###Code
r=requests.get('https://www.marketwatch.com/investing/stock/SPCE?mod=mw_quote_tab')
soup = bs4.BeautifulSoup(r.content, 'html.parser')
soup_text = soup.find_all('ul',{'class':'list list--kv list--col50'})[0].text.split('\n')
soup_text
scrap_date = soup_metrics.index('Short Interest')+1
soup_metrics = list(filter(None, soup_text))
scrap_date = soup_metrics.index('Short Interest')+2
soup_metrics.pop(scrap_date)
soup_metrics
metrics = soup_metrics[0:][::2] # even
values = soup_metrics[1:][::2] # odd
pd.DataFrame([values],columns=metrics)
###Output
_____no_output_____ |
01_basic-python/01_semantics.ipynb | ###Markdown
[Table of contents](../toc.ipynb) Python semanticsRemember that semantics is the meaning of the language. Variables are actually pointersThe next slides of topic (variables are actually pointers) are a condensed version of notebook 03 from *A Whirlwind Tour of Python* [[VanderPlas2016]](../references.bib), which is under CC0 license.Assigning a variable in Python is very easy, just use the equal sign:```pythonmy_var = 7``` Comparision with CHowever, in contrast to other languages like C, the above line of code should be read like:**`my_var` points to a memory bucket which contains currently an integer of seven.**This is very different from C where a similar line of code would be```Cint my_var = 7;```This C code line could be read as:**A container called `my_var` is defined to store integers and it contains currently seven.** ConsequencesBecause Python variables just point to some object in the memory: * there is no need to declare a variable type * the variable type may change * and this is the reason why Python is called dynamically typed language Hence you can do things like this in Python which won't work in statically typed languages like C.
###Code
my_var = 7 # integer
my_var = 7.1 # float
my_var = "Some string" # string
my_var = [0, 1, "a list with a string"] # list with intergers and a string
print (my_var)
###Output
[0, 1, 'a list with a string']
###Markdown
Variables as pointers in practiceBecause variables are actually pointers, you might wonder how this code is interpreted.
###Code
x = [0, 1, 2, 3]
y = x
print(y)
x[3] = 99
print(y)
###Output
[0, 1, 2, 99]
###Markdown
Here, the last entry of x was changed and because y point to x, the print y command showed the changed values.However, if we change the bucket where x points to something different, y still points to the "old" bucket.
###Code
x = 77.7
print(y)
###Output
[0, 1, 2, 99]
###Markdown
Is this safe?You might wonder if this will cause trouble in equations. But it is safe because:* Numbers, strings and other basic types are immutable* This means you can not change their value. You can only change what values the variable points to.
###Code
x = 10
y = x
# Here actually the variable is changed so that it points to another integer which is 15. Hence, y does not change.
x += 5
print("x =", x)
print("y =", y)
###Output
x = 15
y = 10
###Markdown
Python loves objects!Because there is no need to define the type of a variable it is often said that Python is type-free. **This is wrong!**If no type is given, the Python interpreter selects a type and we can read the type.
###Code
x = 7
type(x)
x = [0, 1, 2, "string"]
type(x)
x = {"a": 3, "b": 4.4}
type(x)
###Output
_____no_output_____
###Markdown
Hence, Python has types and the type is not linked to the variable but to the object. You have seen here some of the basic types like `int` (integer), `list`, and `dict` (dictionary). Everything is an objectYou can access different properties of objects with the period `.`The last line of code set x as pointer to a dictionary and the keys of a dictionary can be accessed with `.keys()` method.
###Code
x = {"a": 3, "b": 4.4}
x.keys()
###Output
_____no_output_____
###Markdown
Also very basic objects like integers have attributes and methods.
###Code
x = 9
print(x.real) # real attribute of x
print(x.bit_length()) # compute bitlength of x with .bitlenght() method
###Output
9
4
###Markdown
OperatorsPython's operators can be categorized into:* Arithmetic Operators* Comparison (Relational) Operators* Assignment Operators* Logical Operators* Bitwise Operators* Membership Operators* Identity Operators Arithmetic operatorsThe arithmetic operators in Python are:Operator | Description | Code example--- | --- | ---Addition | Sum of two variables | `a + b`Subtraction | Difference of two variables | `a - b`Multiplication | Product of two variables | `a * b` Exponentiation | - | `a ** b`Division | Quotient of two variables | `a / b`Floor division | Quotient without fractional part | `a // b`Modulus | Returns integer which remains after division | `a % b`Negation | Negative of variable | `-a`Matrix product | Introduced in Python 3.5, requires numpy | `a @ b`
###Code
# here some operators in combination
((5 + 3) / 4) ** 2
# true division
21 / 2
# floor division
21 // 2
# modulus
21 % 2
###Output
_____no_output_____
###Markdown
Comparison operatorsThe comparison operators in Python return a bool (`True` or `False`) and these operators are:Code example | Description --- | --- `a == b` | a equal b`a != b` | a not equal b`a < b` | a less than b`a <= b` | a less or equal b`a > b` | a greater than b`a >= b` | a greater or equal b
###Code
a = 1
b = 5
a < b
a != b
a > b
# check floor division
25 // 2 == 12
# 30 is between 24 and 50
24 < 30 < 50
###Output
_____no_output_____
###Markdown
Assignment operatorsBeside the simple assignment `=`, there are:Code example | Description --- | --- `a += 5` | Add And`a -= 5` | Subtract And`a *= 5` | Multiply And`a **= 5` | Exponent And`a /= 5` | Division And`a %= 5` | Modulus And`a //= 5` | Floor division And
###Code
a = 5
a += 5
print(a)
a -= 10
print(a)
###Output
0
###Markdown
Logical operatorsThese operators are designed for boolean variables.There are logical `and`, `or`, `not`. The operator `xor` is missing but can be constructed.
###Code
a = True
b = False
a and b
a or b
# The return value will be True if the statement(s) are not True
not(a,b)
###Output
_____no_output_____
###Markdown
Bitwise operators* These operators are rather advanced and barely used for standard tasks. * They compare the binary representation of numbers, which can be accessed with `bin()` function.
###Code
bin(10)
###Output
_____no_output_____
###Markdown
Which is read as: `0b` binary format, $1 \cdot 2^3 + 0 \cdot 2^2 + 1 \cdot 2^1 + 0 \cdot 2^0 = 10$Fore sake of completeness the operators are:* Bitwise AND `a & b`* Bitwise OR `a | b`* Bitwise XOR `a ^ b`* Bitshift left `a << b`* Bitshift right `a >> b`* Binary complement (flipping the bit) `~a` Membership operatorsThe membership operators are designed to find values in lists, tuples or strings.The two operators are `in` and `not in` and they return `True` or `False`.
###Code
a = 2
b = [0, 1, 2, 3, 4]
a in b
c = 8
c not in b
strg = "hi here is a text"
"text" in strg
###Output
_____no_output_____
###Markdown
Identity operatorsThey compare memory location of objects and are called `is` and `is not`.
###Code
a = 5
b = 2
a is b
a = 10
b = a
a is b
###Output
_____no_output_____
###Markdown
[Table of contents](../toc.ipynb) Python semanticsRemember that semantics is the meaning of the language. Variables are actually pointersThe next slides of topic (variables are actually pointers) are a condensed version of notebook 03 from *A Whirlwind Tour of Python* [[VanderPlas2016]](../references.bib), which is under CC0 license.Assigning a variable in Python is very easy, just use the equal sign:```pythonmy_var = 7``` Comparision with CHowever, in contrast to other languages like C, the above line of code should be read like:**`my_var` points to a memory bucket which contains currently an integer of seven.**This is very different from C where a similar line of code would be```Cint my_var = 7;```This C code line could be read as:**A container called `my_var` is defined to store integers and it contains currently seven.** ConsequencesBecause Python variables just point to some object in the memory: * there is no need to declare a variable type * the variable type may change * and this is the reason why Python is called dynamically typed language Hence you can do things like this in Python which won't work in statically typed languages like C.
###Code
my_var = 7 # integer
my_var = 7.1 # float
my_var = "Some string" # string
my_var = [0, 1, "a list with a string"] # list with intergers and a string
###Output
_____no_output_____
###Markdown
Variables as pointers in practiceBecause variables are actually pointers, you might wonder how this code is interpreted.
###Code
x = [0, 1, 2, 3]
y = x
print(y)
x[3] = 99
print(y)
###Output
[0, 1, 2, 99]
###Markdown
Here, the last entry of x was changed and because y point to x, the print y command showed the changed values.However, if we change the bucket where x points to something different, y still points to the "old" bucket.
###Code
x = 77.7
print(y)
###Output
[0, 1, 2, 99]
###Markdown
Is this safe?You might wonder if this will cause trouble in equations. But it is safe because:* Numbers, strings and other basic types are immutable* This means you can not change their value. You can only change what values the variable points to.
###Code
x = 10
y = x
# Here actually the variable is changed so that it points to another integer which is 15. Hence, y does not change.
x += 5
print("x =", x)
print("y =", y)
###Output
x = 15
y = 10
###Markdown
Python loves objects!Because there is no need to define the type of a variable it is often said that Python is type-free. **This is wrong!**If no type is given, the Python interpreter selects a type and we can read the type.
###Code
x = 7
type(x)
x = [0, 1, 2, "string"]
type(x)
x = {"a": 3, "b": 4.4}
type(x)
###Output
_____no_output_____
###Markdown
Hence, Python has types and the type is not linked to the variable but to the object. You have seen here some of the basic types like `int` (integer), `list`, and `dict` (dictionary). Everything is an objectYou can access different properties of objects with the period `.`The last line of code set x as pointer to a dictionary and the keys of a dictionary can be accessed with `.keys()` method.
###Code
x = {"a": 3, "b": 4.4}
x.keys()
###Output
_____no_output_____
###Markdown
Also very basic objects like integers have attributes and methods.
###Code
x = 9
print(x.real) # real attribute of x
print(x.bit_length()) # compute bitlength of x with .bitlenght() method
###Output
9
4
###Markdown
OperatorsPython's operators can be categorized into:* Arithmetic Operators* Comparison (Relational) Operators* Assignment Operators* Logical Operators* Bitwise Operators* Membership Operators* Identity Operators Arithmetic operatorsThe arithmetic operators in Python are:Operator | Description | Code example--- | --- | ---Addition | Sum of two variables | `a + b`Subtraction | Difference of two variables | `a - b`Multiplication | Product of two variables | `a * b` Exponentiation | - | `a ** b`Division | Quotient of two variables | `a / b`Floor division | Quotient without fractional part | `a // b`Modulus | Returns integer which remains after division | `a % b`Negation | Negative of variable | `-a`Matrix product | Introduced in Python 3.5, requires numpy | `a @ b`
###Code
# here some operators in combination
((5 + 3) / 4) ** 2
# true division
21 / 2
# floor division
21 // 2
# modulus
21 % 2
###Output
_____no_output_____
###Markdown
Comparison operatorsThe comparison operators in Python return a bool (`True` or `False`) and these operators are:Code example | Description --- | --- `a == b` | a equal b`a != b` | a not equal b`a < b` | a less than b`a <= b` | a less or equal b`a > b` | a greater than b`a >= b` | a greater or equal b
###Code
a = 1
b = 5
a < b
a != b
a > b
# check floor division
25 // 2 == 12
# 30 is between 24 and 50
24 < 30 < 50
###Output
_____no_output_____
###Markdown
Assignment operatorsBeside the simple assignment `=`, there are:Code example | Description --- | --- `a += 5` | Add And`a -= 5` | Subtract And`a *= 5` | Multiply And`a **= 5` | Exponent And`a /= 5` | Division And`a %= 5` | Modulus And`a //= 5` | Floor division And
###Code
a = 5
a += 5
print(a)
a -= 10
print(a)
###Output
0
###Markdown
Logical operatorsThese operators are designed for boolean variables.There are logical `and`, `or`, `not`. The operator `xor` is missing but can be constructed.
###Code
a = True
b = False
a and b
a or b
not(a, b)
###Output
_____no_output_____
###Markdown
Bitwise operators* These operators are rather advanced and barely used for standard tasks. * They compare the binary representation of numbers, which can be accessed with `bin()` function.
###Code
bin(10)
###Output
_____no_output_____
###Markdown
Which is read as: `0b` binary format, $1 \cdot 2^3 + 0 \cdot 2^2 + 1 \cdot 2^1 + 0 \cdot 2^0 = 10$Fore sake of completeness the operators are:* Bitwise AND `a & b`* Bitwise OR `a | b`* Bitwise XOR `a ^ b`* Bitshift left `a << b`* Bitshift right `a >> b`* Binary complement (flipping the bit) `~a` Membership operatorsThe membership operators are designed to find values in lists, tuples or strings.The two operators are `in` and `not in` and they return `True` or `False`.
###Code
a = 2
b = [0, 1, 2, 3, 4]
a in b
c = 8
c not in b
strg = "hi here is a text"
"text" in strg
###Output
_____no_output_____
###Markdown
Identity operatorsThey compare memory location of objects and are called `is` and `is not`.
###Code
a = 5
b = 2
a is b
a = 10
b = a
a is b
###Output
_____no_output_____
###Markdown
[Table of contents](../toc.ipynb) Python semanticsRemember that semantics is the meaning of the language. Variables are actually pointersThe next slides of topic (variables are actually pointers) are a condensed version of notebook 03 from *A Whirlwind Tour of Python* [[VanderPlas2016]](../references.bib), which is under CC0 license.Assigning a variable in Python is very easy, just use the equal sign:```pythonmy_var = 7``` Comparision with CHowever, in contrast to other languages like C, the above line of code should be read like:**`my_var` points to a memory bucket which contains currently an integer of seven.**This is very different from C where a similar line of code would be```Cint my_var = 7;```This C code line could be read as:**A container called `my_var` is defined to store integers and it contains currently seven.** ConsequencesBecause Python variables just point to some object in the memory: * there is no need to declare a variable type * the variable type may change * and this is the reason why Python is called dynamically typed language Hence you can do things like this in Python which won't work in statically typed languages like C.
###Code
my_var = 7 # integer
my_var = 7.1 # float
my_var = "Some string" # string
my_var = [0, 1, "a list with a string"] # list with intergers and a string
###Output
_____no_output_____
###Markdown
Variables as pointers in practiceBecause variables are actually pointers, you might wonder how this code is interpreted.
###Code
x = [0, 1, 2, 3]
y = x
print(y)
x[3] = 99
print(y)
###Output
[0, 1, 2, 99]
###Markdown
Here, the last entry of x was changed and because y point to x, the print y command showed the changed values.However, if we change the bucket where x points to something different, y still points to the "old" bucket.
###Code
x = 77.7
print(y)
###Output
[0, 1, 2, 99]
###Markdown
Is this safe?You might wonder if this will cause trouble in equations. But it is safe because:* Numbers, strings and other basic types are immutable* This means you can not change their value. You can only change what values the variable points to.
###Code
x = 10
y = x
# Here actually the variable is changed so that it points to another integer which is 15. Hence, y does not change.
x += 5
print("x =", x)
print("y =", y)
###Output
x = 15
y = 10
###Markdown
Python loves objects!Because there is no need to define the type of a variable it is often said that Python is type-free. **This is wrong!**If no type is given, the Python interpreter selects a type and we can read the type.
###Code
x = 7
type(x)
x = [0, 1, 2, "string"]
type(x)
x = {"a": 3, "b": 4.4}
type(x)
###Output
_____no_output_____
###Markdown
Hence, Python has types and the type is not linked to the variable but to the object. You have seen here some of the basic types like `int` (integer), `list`, and `dict` (dictionary). Everything is an objectYou can access different properties of objects with the period `.`The last line of code set x as pointer to a dictionary and the keys of a dictionary can be accessed with `.keys()` method.
###Code
x = {"a": 3, "b": 4.4}
x.keys()
###Output
_____no_output_____
###Markdown
Also very basic objects like integers have attributes and methods.
###Code
x = 9
print(x.real) # real attribute of x
print(x.bit_length()) # compute bitlength of x with .bitlenght() method
###Output
9
4
###Markdown
OperatorsPython's operators can be categorized into:* Arithmetic Operators* Comparison (Relational) Operators* Assignment Operators* Logical Operators* Bitwise Operators* Membership Operators* Identity Operators Arithmetic operatorsThe arithmetic operators in Python are:Operator | Description | Code example--- | --- | ---Addition | Sum of two variables | `a + b`Subtraction | Difference of two variables | `a - b`Multiplication | Product of two variables | `a * b` Exponentiation | - | `a ** b`Division | Quotient of two variables | `a / b`Floor division | Quotient without fractional part | `a // b`Modulus | Returns integer which remains after division | `a % b`Negation | Negative of variable | `-a`Matrix product | Introduced in Python 3.5, requires numpy | `a @ b`
###Code
# here some operators in combination
((5 + 3) / 4) ** 2
# true division
21 / 2
# floor division
21 // 2
# modulus
21 % 2
###Output
_____no_output_____
###Markdown
Comparison operatorsThe comparison operators in Python return a bool (`True` or `False`) and these operators are:Code example | Description --- | --- `a == b` | a equal b`a != b` | a not equal b`a < b` | a less than b`a <= b` | a less or equal b`a > b` | a greater than b`a >= b` | a greater or equal b
###Code
a = 1
b = 5
a < b
a != b
a > b
# check floor division
25 // 2 == 12
# 30 is between 24 and 50
24 < 30 < 50
###Output
_____no_output_____
###Markdown
Assignment operatorsBeside the simple assignment `=`, there are:Code example | Description --- | --- `a += 5` | Add And`a -= 5` | Subtract And`a *= 5` | Multiply And`a **= 5` | Exponent And`a /= 5` | Division And`a %= 5` | Modulus And`a //= 5` | Floor division And
###Code
a = 5
a += 5
print(a)
a -= 10
print(a)
###Output
0
###Markdown
Logical operatorsThese operators are designed for boolean variables.There are logical `and`, `or`, `not`. The operator `xor` is missing but can be constructed.
###Code
a = True
b = False
a and b
a or b
not(a and b) # a and b gives False and hence not(a and b) returns True
###Output
_____no_output_____
###Markdown
Bitwise operators* These operators are rather advanced and barely used for standard tasks. * They compare the binary representation of numbers, which can be accessed with `bin()` function.
###Code
bin(10)
###Output
_____no_output_____
###Markdown
Which is read as: `0b` binary format, $1 \cdot 2^3 + 0 \cdot 2^2 + 1 \cdot 2^1 + 0 \cdot 2^0 = 10$Fore sake of completeness the operators are:* Bitwise AND `a & b`* Bitwise OR `a | b`* Bitwise XOR `a ^ b`* Bitshift left `a << b`* Bitshift right `a >> b`* Binary complement (flipping the bit) `~a` Membership operatorsThe membership operators are designed to find values in lists, tuples or strings.The two operators are `in` and `not in` and they return `True` or `False`.
###Code
a = 2
b = [0, 1, 2, 3, 4]
a in b
c = 8
c not in b
strg = "hi here is a text"
"text" in strg
###Output
_____no_output_____
###Markdown
Identity operatorsThey compare memory location of objects and are called `is` and `is not`.
###Code
a = 5
b = 2
a is b
a = 10
b = a
a is b
###Output
_____no_output_____
###Markdown
[Table of contents](../toc.ipynb) Python semanticsRemember that semantics is the meaning of the language. Variables are actually pointersThe next slides of topic (variables are actually pointers) are a condensed version of notebook 03 from *A Whirlwind Tour of Python* [[VanderPlas2016]](./references.bib), which is under CC0 license.Assigning a variable in Python is very easy, just use the equal sign
###Code
my_var = 7
###Output
_____no_output_____
###Markdown
Comparision with CHowever, in contrast to other languages like C, the above line of code should be read like:**`my_var` points to a memory bucket which contains currently an integer of seven.**This is very different from C where a similar line of code would be```Cint my_var = 7;```This C code line could be read as:**A container called `my_var` is defined to store integers and it contains currently seven.** ConsequencesBecause Python variables just point to some object in the memory: * there is no need to declare a variable type * the variable type may change * and this is the reason why Python is called dynamically typed language Hence you can do things like this in Python which won't work in statically typed languages like C
###Code
my_var = 7 # integer
my_var = 7.1 # float
my_var = "Some string" # string
my_var = [0, 1, "a list with a string"] # list with intergers and a string
###Output
_____no_output_____
###Markdown
Variables as pointers in practiceBecause variables are actually pointers, you might wonder how this code is interpreted.
###Code
x = [0, 1, 2, 3]
y = x
print(y)
x[3] = 99
print(y)
###Output
[0, 1, 2, 99]
###Markdown
Here, the last entry of x was changed and because y point to x, the print y command showed the changed values.However, if we change the bucket where x points to something different, y still points to the "old" bucket.
###Code
x = 77.7
print(y)
###Output
[0, 1, 2, 99]
###Markdown
Is this safe?You might wonder if this will cause trouble in equations. But it is safe because:* Numbers, strings and other basic types are immutable* This means you can not their value. You can only change what values the variable points to.
###Code
x = 10
y = x
x += 5 # Here actually the variable is changed so that it points to another integer which is 15. Hence, y does not change.
print("x =", x)
print("y =", y)
###Output
x = 15
y = 10
###Markdown
Python loves objects!Because there is no need to define the type of a variable it is often said that Python is type-free. **This is wrong!**If no type is given, the Python interpreter selects a type and we can read the type.
###Code
x = 7
type(x)
x = [0, 1, 2, "string"]
type(x)
x = {"a": 3, "b": 4.4}
type(x)
###Output
_____no_output_____
###Markdown
Hence, Python has types and the type are not linked to the variable but to the object.You have seen here some of the basic types like `int` (integer), `list`, and `dict` (dictionary). Everything is an objectYou can access different properties of objects with the period `.`The last line of code set x as pointer to a dictionary and the keys of a dictionary can be accessed with `.keys()` method.
###Code
x = {"a": 3, "b": 4.4}
x.keys()
###Output
_____no_output_____
###Markdown
Also very basic objects like integers have attributes and methods.
###Code
x = 9
print(x.real) # real attribute of x
print(x.bit_length()) # compute bitlength of x with .bitlenght() method
###Output
9
4
###Markdown
OperatorsPython's operators can be categorized into:* Arithmetic Operators* Comparison (Relational) Operators* Assignment Operators* Logical Operators* Bitwise Operators* Membership Operators* Identity Operators Arithmetic operatorsThe arithmetic operators in Python areOperator | Description | Code example--- | --- | ---Addition | Sum of two variables | `a + b`Subtraction | Difference of two variables | `a - b`Multiplication | Product of two variables | `a * b` Exponentiation | - | `a ** b`Division | Quotient of two variables | `a / b`Floor division | Quotient without fractional part | `a // b`Modulus | Returns integer which remains after division | `a % b`Negation | Negative of variable | `-a`Matrix product | Introduced in Python 3.5, requires numpy | `a @ b`
###Code
# here some operators in combination
((5 + 3) / 4) ** 2
# true division
21 / 2
# floor division
21 // 2
# modulus
21 % 2
###Output
_____no_output_____
###Markdown
Comparison operatorsThe comparison operators in Python return a bool (`True` or `False`) and these operators areCode example | Description --- | --- `a == b` | a equal b`a != b` | a not equal b`a < b` | a less than b`a <= b` | a less or equal b`a > b` | a greater than b`a >= b` | a greater or equal b
###Code
a = 1; b = 5
a < b
a != b
a > b
# check floor division
25 // 2 == 12
# 30 is between 24 and 50
24 < 30 < 50
###Output
_____no_output_____
###Markdown
Assignment operatorsBeside the simple assignment `=`, there are Code example | Description --- | --- `a += 5` | Add And`a -= 5` | Subtract And`a *= 5` | Multiply And`a **= 5` | Exponent And`a /= 5` | Division And`a %= 5` | Modulus And`a //= 5` | Floor division And
###Code
a = 5
a += 5
print(a)
a -= 10
print(a)
###Output
0
###Markdown
Logical operatorsThese operators are designed for boolean variables.There are logical `and`, `or`, `not`. The operator `xor` is missing but can be constructed.
###Code
a = True; b = False
a and b
a or b
not(a, b)
###Output
_____no_output_____
###Markdown
Bitwise operators* These operators are rather advanced and barely used for standard tasks. * They compare the binary representation of numbers, which can be accessed with `bin()` function.
###Code
bin(10)
###Output
_____no_output_____
###Markdown
Which is read as: `0b` binary format, $1 \cdot 2^3 + 0 \cdot 2^2 + 1 \cdot 2^1 + 0 \cdot 2^0 = 10$Fore sake of completeness the operators are:* Bitwise AND `a & b`* Bitwise OR `a | b`* Bitwise XOR `a ^ b`* Bitshift left `a << b`* Bitshift right `a >> b`* Binary complement (flipping the bit) `~a` Membership operatorsThe membership operators are designed to find values in lists, tuples or strings.The two operators are `in` and `not in` and they return `True` or `False`.
###Code
a = 2
b = [0, 1, 2, 3, 4]
a in b
c = 8
c not in b
strg = "hi here is a text"
"text" in strg
###Output
_____no_output_____
###Markdown
Identity operatorsThey compare memory location of objects and are called `is` and `is not`.
###Code
a = 5; b = 2
a is b
a = 10; b = a
a is b
###Output
_____no_output_____
###Markdown
[Table of contents](../toc.ipynb) Python semanticsRemember that semantics is the meaning of the language. Variables are actually pointersThe next slides of topic (variables are actually pointers) are a condensed version of notebook 03 from *A Whirlwind Tour of Python* [[VanderPlas2016]](./references.bib), which is under CC0 license.Assigning a variable in Python is very easy, just use the equal sign:```pythonmy_var = 7``` Comparision with CHowever, in contrast to other languages like C, the above line of code should be read like:**`my_var` points to a memory bucket which contains currently an integer of seven.**This is very different from C where a similar line of code would be```Cint my_var = 7;```This C code line could be read as:**A container called `my_var` is defined to store integers and it contains currently seven.** ConsequencesBecause Python variables just point to some object in the memory: * there is no need to declare a variable type * the variable type may change * and this is the reason why Python is called dynamically typed language Hence you can do things like this in Python which won't work in statically typed languages like C.
###Code
my_var = 7 # integer
my_var = 7.1 # float
my_var = "Some string" # string
my_var = [0, 1, "a list with a string"] # list with intergers and a string
###Output
_____no_output_____
###Markdown
Variables as pointers in practiceBecause variables are actually pointers, you might wonder how this code is interpreted.
###Code
x = [0, 1, 2, 3]
y = x
print(y)
x[3] = 99
print(y)
###Output
[0, 1, 2, 99]
###Markdown
Here, the last entry of x was changed and because y point to x, the print y command showed the changed values.However, if we change the bucket where x points to something different, y still points to the "old" bucket.
###Code
x = 77.7
print(y)
###Output
[0, 1, 2, 99]
###Markdown
Is this safe?You might wonder if this will cause trouble in equations. But it is safe because:* Numbers, strings and other basic types are immutable* This means you can not their value. You can only change what values the variable points to.
###Code
x = 10
y = x
x += 5 # Here actually the variable is changed so that it points to another integer which is 15. Hence, y does not change.
print("x =", x)
print("y =", y)
###Output
x = 15
y = 10
###Markdown
Python loves objects!Because there is no need to define the type of a variable it is often said that Python is type-free. **This is wrong!**If no type is given, the Python interpreter selects a type and we can read the type.
###Code
x = 7
type(x)
x = [0, 1, 2, "string"]
type(x)
x = {"a": 3, "b": 4.4}
type(x)
###Output
_____no_output_____
###Markdown
Hence, Python has types and the type are not linked to the variable but to the object.You have seen here some of the basic types like `int` (integer), `list`, and `dict` (dictionary). Everything is an objectYou can access different properties of objects with the period `.`The last line of code set x as pointer to a dictionary and the keys of a dictionary can be accessed with `.keys()` method.
###Code
x = {"a": 3, "b": 4.4}
x.keys()
###Output
_____no_output_____
###Markdown
Also very basic objects like integers have attributes and methods.
###Code
x = 9
print(x.real) # real attribute of x
print(x.bit_length()) # compute bitlength of x with .bitlenght() method
###Output
9
4
###Markdown
OperatorsPython's operators can be categorized into:* Arithmetic Operators* Comparison (Relational) Operators* Assignment Operators* Logical Operators* Bitwise Operators* Membership Operators* Identity Operators Arithmetic operatorsThe arithmetic operators in Python are:Operator | Description | Code example--- | --- | ---Addition | Sum of two variables | `a + b`Subtraction | Difference of two variables | `a - b`Multiplication | Product of two variables | `a * b` Exponentiation | - | `a ** b`Division | Quotient of two variables | `a / b`Floor division | Quotient without fractional part | `a // b`Modulus | Returns integer which remains after division | `a % b`Negation | Negative of variable | `-a`Matrix product | Introduced in Python 3.5, requires numpy | `a @ b`
###Code
# here some operators in combination
((5 + 3) / 4) ** 2
# true division
21 / 2
# floor division
21 // 2
# modulus
21 % 2
###Output
_____no_output_____
###Markdown
Comparison operatorsThe comparison operators in Python return a bool (`True` or `False`) and these operators are:Code example | Description --- | --- `a == b` | a equal b`a != b` | a not equal b`a < b` | a less than b`a <= b` | a less or equal b`a > b` | a greater than b`a >= b` | a greater or equal b
###Code
a = 1; b = 5
a < b
a != b
a > b
# check floor division
25 // 2 == 12
# 30 is between 24 and 50
24 < 30 < 50
###Output
_____no_output_____
###Markdown
Assignment operatorsBeside the simple assignment `=`, there are:Code example | Description --- | --- `a += 5` | Add And`a -= 5` | Subtract And`a *= 5` | Multiply And`a **= 5` | Exponent And`a /= 5` | Division And`a %= 5` | Modulus And`a //= 5` | Floor division And
###Code
a = 5
a += 5
print(a)
a -= 10
print(a)
###Output
0
###Markdown
Logical operatorsThese operators are designed for boolean variables.There are logical `and`, `or`, `not`. The operator `xor` is missing but can be constructed.
###Code
a = True; b = False
a and b
a or b
not(a, b)
###Output
_____no_output_____
###Markdown
Bitwise operators* These operators are rather advanced and barely used for standard tasks. * They compare the binary representation of numbers, which can be accessed with `bin()` function.
###Code
bin(10)
###Output
_____no_output_____
###Markdown
Which is read as: `0b` binary format, $1 \cdot 2^3 + 0 \cdot 2^2 + 1 \cdot 2^1 + 0 \cdot 2^0 = 10$Fore sake of completeness the operators are:* Bitwise AND `a & b`* Bitwise OR `a | b`* Bitwise XOR `a ^ b`* Bitshift left `a << b`* Bitshift right `a >> b`* Binary complement (flipping the bit) `~a` Membership operatorsThe membership operators are designed to find values in lists, tuples or strings.The two operators are `in` and `not in` and they return `True` or `False`.
###Code
a = 2
b = [0, 1, 2, 3, 4]
a in b
c = 8
c not in b
strg = "hi here is a text"
"text" in strg
###Output
_____no_output_____
###Markdown
Identity operatorsThey compare memory location of objects and are called `is` and `is not`.
###Code
a = 5; b = 2
a is b
a = 10; b = a
a is b
###Output
_____no_output_____ |
multiple_models/multiple_models_plots.ipynb | ###Markdown
K = 4
###Code
gen_data_ex = lambda : gen_data(nobs=1000, a=0.0, num_params=4)
llr_stats = plot_true2(gen_data_ex,setup_test)
gen_data_ex = lambda : gen_data(nobs=1000, a=0.25, num_params=4)
llr_stats = plot_true2(gen_data_ex,setup_test)
gen_data_ex = lambda : gen_data(nobs=1000, a=0.5, num_params=4)
llr_stats = plot_true2(gen_data_ex,setup_test)
###Output
_____no_output_____
###Markdown
K = 9
###Code
gen_data_ex = lambda : gen_data(nobs=1000, a=0.0, num_params=9)
llr_stats = plot_true2(gen_data_ex,setup_test)
gen_data_ex = lambda : gen_data(nobs=1000, a=0.25, num_params=9)
llr_stats = plot_true2(gen_data_ex,setup_test)
gen_data_ex = lambda : gen_data(nobs=1000, a=0.5, num_params=9)
llr_stats = plot_true2(gen_data_ex,setup_test)
###Output
_____no_output_____
###Markdown
K = 19
###Code
gen_data_ex = lambda : gen_data(nobs=1000, a=0.0, num_params=19)
llr_stats = plot_true2(gen_data_ex,setup_test)
gen_data_ex = lambda : gen_data(nobs=1000, a=0.25, num_params=19)
llr_stats = plot_true2(gen_data_ex,setup_test)
gen_data_ex = lambda : gen_data(nobs=1000, a=0.5, num_params=19)
llr_stats = plot_true2(gen_data_ex,setup_test)
###Output
_____no_output_____
###Markdown
K = 4
###Code
gen_data_ex = lambda : gen_data(nobs=1000, a=0.0, num_params=4)
llr_stats = plot_true2(gen_data_ex,setup_test)
gen_data_ex = lambda : gen_data(nobs=1000, a=0.25, num_params=4)
llr_stats = plot_true2(gen_data_ex,setup_test)
gen_data_ex = lambda : gen_data(nobs=1000, a=0.5, num_params=4)
llr_stats = plot_true2(gen_data_ex,setup_test)
###Output
_____no_output_____
###Markdown
K = 9
###Code
gen_data_ex = lambda : gen_data(nobs=1000, a=0.0, num_params=9)
llr_stats = plot_true2(gen_data_ex,setup_test)
gen_data_ex = lambda : gen_data(nobs=1000, a=0.25, num_params=9)
llr_stats = plot_true2(gen_data_ex,setup_test)
gen_data_ex = lambda : gen_data(nobs=1000, a=0.5, num_params=9)
llr_stats = plot_true2(gen_data_ex,setup_test)
###Output
_____no_output_____
###Markdown
K = 19
###Code
gen_data_ex = lambda : gen_data(nobs=1000, a=0.0, num_params=19)
llr_stats = plot_true2(gen_data_ex,setup_test)
gen_data_ex = lambda : gen_data(nobs=1000, a=0.25, num_params=19)
llr_stats = plot_true2(gen_data_ex,setup_test)
gen_data_ex = lambda : gen_data(nobs=1000, a=0.5, num_params=19)
llr_stats = plot_true2(gen_data_ex,setup_test)
###Output
_____no_output_____ |
OpenID Connect inspect setup.ipynb | ###Markdown
Set up OpenID APIsInspect an OpenID connect well-known JSON for the variables you need elsewhere
###Code
# Enter the .well-known URL for the auth service you want to use
WELL_KNOWN_URL = input('Enter .well-known/openid-configuration URL: ') or 'https://accounts.google.com/.well-known/openid-configuration'
import json
import requests
from urllib.parse import urlparse
def get_json(url):
resp = requests.get(url)
try:
resp.raise_for_status()
except Exception as ex:
print(resp.content)
raise ex
return resp.json()
url_info = urlparse(WELL_KNOWN_URL)
DOMAIN, _, _ = url_info.netloc.partition(':') # removes the port
print(f"Looking up details for {DOMAIN}")
SSL_KEY_PATH = "/Users/nick/Devel/cloud_storage/BankingCerts/Fractal2/obseal-obwac/transport-WIM6jzA_tjOdB5nqga0dAAjrdp4.pem" #"/Users/nick/Devel/cloud_storage/BankingCerts/Fractal/eidas/QWAC.key" #"./local/TPP_OB_Transport.key"
SSL_CERT_PATH = "/Users/nick/Devel/cloud_storage/BankingCerts/Fractal2/obseal-obwac/transport.key" #"/Users/nick/Devel/cloud_storage/BankingCerts/Fractal/eidas/QWAC.2m-5W3Zk1oWnTKzaKEFSNQAt8_U.pem" #"./local/TTP_OB_Transport.pem"
try:
WELL_KNOWN = get_json(WELL_KNOWN_URL)
except:
WELL_KNOWN = json.loads(input("Enter your .well-known JSON here: "))
print(json.dumps(WELL_KNOWN, indent=2))
AUTH_METHODS = WELL_KNOWN.get('token_endpoint_auth_methods_supported', [])
AUTHORIZATION_URL = WELL_KNOWN.get('authorization_endpoint')
TOKEN_URL = WELL_KNOWN.get('token_endpoint')
REGISTER_URL = WELL_KNOWN.get('registration_endpoint')
ISSUER = WELL_KNOWN.get('issuer')
print(f"Issuer: {ISSUER}")
print(f"Token endpoint auth methods: {', '.join(AUTH_METHODS)}")
print(f"AUTHORIZATION_URL: {AUTHORIZATION_URL}")
print(f"TOKEN_URL: {TOKEN_URL}")
print(f"REGISTER_URL: {REGISTER_URL}")
#assert "code id_token" in WELL_KNOWN.get("response_types_supported", [])
assert "authorization_code" in WELL_KNOWN.get("grant_types_supported", [])
assert "client_credentials" in WELL_KNOWN.get("grant_types_supported", [])
#assert "refresh_token" in WELL_KNOWN.get("grant_types_supported", [])
#assert "openid" in WELL_KNOWN.get("scopes_supported", [])
assert "accounts" in WELL_KNOWN.get("scopes_supported", [])
#assert "payments" in WELL_KNOWN.get("scopes_supported", [])
if "private_key_jwt" in AUTH_METHODS:
assert "PS256" in WELL_KNOWN.get('token_endpoint_auth_signing_alg_values_supported', [])
assert "PS256" in WELL_KNOWN.get('request_object_signing_alg_values_supported', [])
#assert "PS256" in WELL_KNOWN.get('id_token_signing_alg_values_supported', [])
###Output
_____no_output_____
###Markdown
Create APIs for Dynamic Registration
###Code
# Enter intended project's APImetrics API key here:
API_KEY = input("Enter your APImetrics API key for the intended project: ")
SOFTWARE_STATEMENT = input("Enter the Software statement you wish to use: ") or "{{software_statement}}"
if len(AUTH_METHODS) == 1:
AUTH_METHOD = AUTH_METHODS[0]
else:
AUTH_METHOD = input('Auth method (tls_client_auth): ') or 'tls_client_auth'
# Helper functions
import requests
import json
import urllib
from apimetrics_api import APImetricsAPI
# An instance of the class that calls the APImetrics API
CLIENT = APImetricsAPI(API_KEY)
# First, create APImetrics API Auth Setting
tag = 'auth:apimetrics_api'
if tag not in CLIENT.auths_by_tag:
setup = {
"meta": {
"domain": "client.apimetrics.io",
"documentation": {
"keys": "https://client.apimetrics.io/settings/api-key",
"docs": "https://apimetrics.readme.io/v2/reference",
"apps": "",
"provider": "https://client.apimetrics.io/",
},
"name": "APImetrics API",
"tags": [tag],
"description": "API that allows you to call APImetrics' API.",
},
"settings": {"auth_type": "MANUAL"}
}
auth = CLIENT.create_auth(setup)
print(f"Created Auth Setting {auth['meta']['name']} with id {auth['id']}")
else:
print(f"Found Auth Setting {CLIENT.auths_by_tag[tag]}")
# Next, Create Token
if CLIENT.auths_by_tag[tag] not in CLIENT.tokens_by_auth:
setup = {
'meta': {
'name': 'Project Access Token',
'domain': 'client.apimetrics.io',
'auth_id': CLIENT.auths_by_tag[tag]
},
'token': {
'headers': [
{
'p_key': 'Authorization',
'p_val': f'Bearer {API_KEY}',
},
],
}
}
token = CLIENT.create_token(setup)
print(f"Created Auth Token {token['meta']['name']} with id {token['id']}")
# Create Auth Setting for Bank API
tag = 'auth:bank_matls'
if tag not in CLIENT.auths_by_tag:
ssl_key = None
ssl_cert = None
if SSL_KEY_PATH:
with open(SSL_KEY_PATH) as stream:
ssl_key = stream.read()
if SSL_CERT_PATH:
with open(SSL_CERT_PATH) as stream:
ssl_cert = stream.read()
setup = {
"access": {
"keys": False,
"org_keys": False,
"org_settings": True,
"settings": False,
},
"keys": {},
"meta": {
"domain": DOMAIN,
"documentation": {"keys": "", "docs": "", "apps": "", "provider": ""},
"name": "Transport MATLS",
"tags": [tag],
"description": "Mutual Authenticated TLS for calls to bank APIs",
},
"settings": {
"auth_type": "MANUAL",
"ssl_key": ssl_key,
"ssl_cert": ssl_cert,
},
}
# if OAUTH_METHOD == "client_secret_post" or OAUTH_METHOD == "client_secret_basic":
# setup["keys"]["client_id"] = CLIENT_ID
# setup["keys"]["client_secret"] = CLIENT_SECRET
auth = CLIENT.create_auth(setup)
print(f"Created Auth Setting {auth['meta']['name']} with id {auth['id']}")
# For user authentication
tag = 'jwt:sign:dynamic_registration'
if tag not in CLIENT.calls_by_tag:
auth_tag = 'auth:apimetrics_api'
auth_id = CLIENT.auths_by_tag[auth_tag]
token_id = CLIENT.tokens_by_auth[auth_id]
if "tls_client_auth" == AUTH_METHOD:
payload = {
"redirect_uris": [
"https://google.api.expert/get",
"https://client.apimetrics.io/tokens/callback/"
],
"token_endpoint_auth_method": "tls_client_auth",
"tls_client_auth_dn": "C = GB, O = OpenBanking, OU = 00158000016i44aAAA, CN = ccS2Kwyg38PrOmCQO5WOXU",
"grant_types": [
"authorization_code",
"client_credentials"
],
"response_types": [
"code id_token"
],
"software_id": "{{ssa_id}}",
"software_statement": SOFTWARE_STATEMENT,
"application_type": "web",
"scope": "openid accounts payments" if "payments" in WELL_KNOWN.get("scopes_supported", []) else "openid accounts",
"id_token_signed_response_alg": "PS256",
"request_object_signing_alg": "PS256"
}
elif "private_key_jwt" == AUTH_METHOD:
payload = {
"redirect_uris": [
"https://google.api.expert/get",
"https://client.apimetrics.io/tokens/callback/"
],
"token_endpoint_auth_method": "private_key_jwt",
"token_endpoint_auth_signing_alg": "PS256",
"grant_types": [
"authorization_code",
"client_credentials"
],
"response_types": [
"code id_token"
],
"software_id": "{{ssa_id}}",
"software_statement": SOFTWARE_STATEMENT,
"application_type": "web",
"scope": "openid accounts payments" if "payments" in WELL_KNOWN.get("scopes_supported", []) else "openid accounts",
"id_token_signed_response_alg": "PS256",
"request_object_signing_alg": "PS256"
}
else:
payload = {
"redirect_uris": [
"https://google.api.expert/get",
"https://client.apimetrics.io/tokens/callback/"
],
"token_endpoint_auth_method": AUTH_METHOD,
"grant_types": [
"authorization_code",
"client_credentials"
],
"response_types": [
"code id_token"
],
"software_id": "{{ssa_id}}",
"software_statement": SOFTWARE_STATEMENT,
"application_type": "web",
"scope": "openid accounts payments" if "payments" in WELL_KNOWN.get("scopes_supported", []) else "openid accounts",
"id_token_signed_response_alg": "PS256",
"request_object_signing_alg": "PS256"
}
if "refresh_token" in WELL_KNOWN.get('grant_types_supported', []):
payload['grant_types'].append('refresh_token')
body = {
"header": {},
"payload": payload,
"private_key": {
"versionId": '{{kid_cert_version}}'
}
}
body_str = json.dumps(body, indent=2)
setup = {
"meta": {
"tags": ["api_type:create", "sector:devtools", tag],
"name": "Sign Dynamic Client Registration JWT with KMS Cert",
"workspace": "global",
},
"request": {
"body": body_str,
"parameters": [
{"value": "{{kid}}", "key": "kid"},
{"value": "PS256", "key": "alg"},
{"value": "{{ssa_id}}", "key": "iss"},
{"value": "{{aud}}", "key": "aud"},
{"value": "10m", "key": "expiresIn"},
{"value": "%%GUID%%", "key": "jti"},
],
"url": "https://us-central1-viatests.cloudfunctions.net/jwt-kms-signer",
"auth_id": auth_id,
"headers": [
{"key": "Accept", "value": "application/json"},
{"key": "Content-Type", "value": "application/json"},
],
"token_id": token_id,
"method": "POST",
},
}
call = CLIENT.create_call(setup)
print(f"Created Call {call['meta']['name']} with id {call['id']}")
conditions = {
"conditions": [
{
"source": "RESPONSE_BODY",
"test_result_on_true": None,
"val": "",
"variable_path": "token",
"variable_name": "JWT_TOKEN",
"test_result_on_false": "CONTENT_ERROR",
"condition": "EXISTS"
},
]
}
CLIENT.set_call_conditions(call['id'], conditions)
tag = 'banks:3.1:dynamic_registration:create'
if tag not in CLIENT.calls_by_tag:
setup = {
"meta": {
"description": None,
"tags": [
"api_type:create",
"sector:financial",
tag,
],
"name": "Dynamic Client Registration: Create",
"workspace": "global"
},
"request": {
"body": "__JWT_TOKEN__",
"parameters": [],
"url": REGISTER_URL,
"auth_id": CLIENT.auths_by_tag['auth:bank_matls'],
"headers": [
{
"value": "application/jose",
"key": "Content-Type"
}
],
"token_id": None,
"method": "POST"
}
}
call = CLIENT.create_call(setup)
print(f"Created Call {call['meta']['name']} with id {call['id']}")
tag = 'banks:3.1:dynamic_registration'
if tag not in CLIENT.workflows_by_tag:
call_tags = [
'jwt:sign:dynamic_registration',
'banks:3.1:dynamic_registration:create',
]
for t in call_tags:
assert t in CLIENT.calls_by_tag, f"API {t} does not exist"
setup = {
"meta": {
"name": "Dynamic Client Registration",
"workspace": "global",
"tags": [tag],
},
"workflow": {
"handle_cookies": False,
"stop_on_failure": True,
"call_ids": [CLIENT.calls_by_tag[t] for t in call_tags]
}
}
workflow = CLIENT.create_workflow(setup)
print(f"Created Workflow {workflow['meta']['name']} with id {workflow['id']}")
###Output
_____no_output_____
###Markdown
Set Environment Variables
###Code
CLIENT.set_env_variable("global", "ssa_id", input("Software Statement ID: ") or "ccS2Kwyg38PrOmCQO5WOXU")
#CLIENT.set_env_variable("global", "software_statement", input("Software Statement: ") )
CLIENT.set_env_variable("global", "kid", input("Key ID: ") or "Lu1iJaKRqUvGS25yv-040yYGT2o" )
CLIENT.set_env_variable("global", "kid_cert_version", input("KMS cert version: ") or "1")
CLIENT.set_env_variable("global", "aud", ISSUER)
CLIENT.set_env_variable("global", "redirect_uri", "https://client.apimetrics.io/tokens/callback/")
###Output
_____no_output_____ |
model-selection/model_selection_auc_01.ipynb | ###Markdown
ROC / AUC Table of Contents
###Code
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from collections import defaultdict
from sklearn.model_selection import train_test_split
from sklearn.base import BaseEstimator
from sklearn.base import TransformerMixin
from sklearn.ensemble import RandomForestClassifier
from sklearn.preprocessing import LabelEncoder
from sklearn.preprocessing import OneHotEncoder
from sklearn.preprocessing import StandardScaler
from sklearn.model_selection import cross_val_score
from sklearn.pipeline import make_pipeline
from sklearn.compose import make_column_transformer
print(f'numpy: {np.__version__}')
print(f'pandas: {pd.__version__}')
###Output
numpy: 1.17.5
pandas: 0.25.3
###Markdown
ROC/AUC for Binary Calssification We will analyze a employee retention. Our goal is to find the employees that are likely to leave in the future.
###Code
hr_retention_url = 'https://raw.githubusercontent.com/martin-fabbri/colab-notebooks/master/data/model-selection/hr_retention.csv'
df = pd.read_csv(hr_retention_url)
df.info()
###Output
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 12000 entries, 0 to 11999
Data columns (total 7 columns):
s 12000 non-null float64
lpr 12000 non-null float64
np 12000 non-null int64
anh 12000 non-null int64
tic 12000 non-null int64
newborn 12000 non-null int64
left 12000 non-null int64
dtypes: float64(2), int64(5)
memory usage: 656.4 KB
###Markdown
Variables definition:- **s**: The satisfaction level on a scale of 0 to 1- **lpe**: Last project evaluation by a client on a scale of 0 to 1- **np**: Represents the number of projects worked on by employee in the last 12 month- **anh**: Average number of hours worked in the last 12 month for that employee- **tic**: Amount of time the employee spent in the company, measured in years- **newborn**: This variable will take the value 1 if the employee had a newborn within the last 12 month and 0 otherwise- **left**: 1 if the employee left the company, 0 if they're still working here. This is our response variable
###Code
df.head()
X = df.drop(['left'], axis=1)
y = df['left']
print('labels distribution:', np.bincount(y) / y.size)
test_size = 0.2
random_state = 43
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=test_size,
random_state=random_state,
stratify=y)
###Output
labels distribution: [0.83333333 0.16666667]
###Markdown
PreprocessingWe will perform generic preprocessing task including:- Normalize numeric columns- One-hot-encode categorical columns. The ***newborn*** variable is treated as a categorical variable.
###Code
column_trans = make_column_transformer(
(StandardScaler(), ['s', 'lpr', 'np', 'anh', 'tic']),
(OneHotEncoder(), ['newborn']),
remainder='passthrough'
)
###Output
_____no_output_____
###Markdown
Model Building
###Code
rf = RandomForestClassifier(max_depth = 4, random_state=43)
pipe = make_pipeline(column_trans, rf)
cross_val_score(pipe, X, y, cv=3, scoring='accuracy').mean()
###Output
_____no_output_____
###Markdown
After training our model, we need to evaluate whether its any good or not and the most straightforward and intuitive metric for a supervised classifier's performance is accuracy. Unfortunately, there are circumstances where simple accuracy does not work well. For example, with a disease that only affects 1 in a million people, a completely bogus screening test that always reports "negative" will be 99.9999% accurate. Unlike accuracy, ROC curves are less sensitive to class imbalance; the bogus screening test would have an AUC of 0.5, which is like not having a test at all. >First column name | Second column name>--- | --->Row 1, Col 1 | Row 1, Col 2>Row 2, Col 1 | Row 2, Col 2--- **ROC curve (Receiver Operating Characteristic)** is a commonly used way to visualize the performance of a binary classifier and AUC (Area Under the ROC Curve) is used to summarize its performance in a single number. Most machine learning algorithms have the ability to produce probability scores that tells us the strength in which it thinks a given observation is positive. Turning these probability scores into yes or no predictions requires setting a threshold; cases with scores above the threshold are classified as positive, and vice versa. Different threshold values can lead to different result:- A higher threshold is more conservative about labeling a case as positive; this makes it less likely to produce false positive (an observation that has a negative label but gets classified as positive by the model) results but more likely to miss cases that are in fact positive (lower true positive rate)- A lower threshold produces positive labels more liberally, so it creates more false positives but also generate more true positivesA quick refresher on terminology:\begin{align}[\text{true positive rate}]&= \frac{[\text{ positive data points with positive predictions}]}{\text{[ all positive data points]}} \\&= \frac{[\text{ true positives}]}{[\text{ true positives}] + [\text{ false negatives}]}\end{align}true positive rate is also known as **recall** or **sensitivity**\begin{align}[\text{false positive rate}]&= \frac{[\text{ positive data points with positive predictions}]}{\text{[ all negative data points]}} \\&= \frac{[\text{ false positives}]}{[\text{ false positives}] + [\text{ true negatives}]}\end{align}The ROC curve is created by plotting the true positive rate (when it's actually a yes, how often does it predict yes?) on the y axis against the false positive rate (when it's actually a no, how often does it predict yes?) on the x axis at various cutoff settings, giving us a picture of the whole spectrum of the trade-off we're making between the two measures.If all these true/false positive terminology is confusing to you, consider reading the material at the following link. [Blog: Simple guide to confusion matrix terminology](http://www.dataschool.io/simple-guide-to-confusion-matrix-terminology/)
###Code
###Output
_____no_output_____ |
compas.ipynb | ###Markdown
Testing DEMV on Compas dataset
###Code
import pandas as pd
from sklearn.linear_model import LogisticRegression
from sklearn.pipeline import Pipeline
from sklearn.preprocessing import StandardScaler
from fairlearn.reductions import ExponentiatedGradient, BoundedGroupLoss, ZeroOneLoss, DemographicParity, GridSearch
from utils import *
from demv import DEMV
import warnings
warnings.filterwarnings('ignore')
sns.set_style('whitegrid')
data = pd.read_csv('data2/compas.csv', index_col=0)
label = 'two_year_recid'
sensitive_vars = ['sex','race']
protected_group = {'sex':0, 'race': 0}
pipeline = Pipeline([
('scaler', StandardScaler()),
('classifier', LogisticRegression(class_weight='balanced', solver='liblinear'))
])
###Output
_____no_output_____
###Markdown
Biased Data
###Code
model, biasmetrics = cross_val(pipeline, data, label, protected_group, sensitive_vars, positive_label=0)
print_metrics(biasmetrics)
###Output
Statistical parity: -0.265 +- 0.041
Disparate impact: 0.614 +- 0.055
Zero one loss: 0.136 +- 0.051
F1 score: 0.667 +- 0.018
Accuracy score: 0.667 +- 0.018
###Markdown
DEMV Data
###Code
demv = DEMV(round_level=1)
demv_data = data.copy()
model, demv_metrics = cross_val(pipeline, demv_data, label, protected_group, sensitive_vars, debiaser=demv, positive_label=0)
print_metrics(demv_metrics)
###Output
Statistical parity: -0.105 +- 0.045
Disparate impact: 0.824 +- 0.068
Zero one loss: 0.102 +- 0.043
F1 score: 0.664 +- 0.017
Accuracy score: 0.664 +- 0.017
###Markdown
DEMV Evaluation
###Code
metrics = eval_demv(29, 116, data.copy(), pipeline, label, protected_group, sensitive_vars, positive_label=0)
%load_ext autoreload
%autoreload 2
%reload_ext autoreload
###Output
_____no_output_____
###Markdown
Blackbox Postprocessing Plot
###Code
df = prepareplots(metrics,'compas')
points = preparepoints(blackboxmetrics,demv.get_iters())
plot_metrics_curves(df, points, 'Compas binary dataset')
unprivpergentage(data,protected_group, demv.get_iters())
save_metrics('blackbox', 'compas', blackboxmetrics)
###Output
_____no_output_____
###Markdown
COMPAS Case Study: Fairness of a Machine Learning This notebook explores the difficulties in making algorithms fair to all groups. In this example, we explore the performance of COMPAS - an algorithm used by parole boards in prisons in the US to assess risk of re-offending ('recidivism').The notebook is reconstructed largely from Farhan Rahman's work at https://towardsdatascience.com/compas-case-study-fairness-of-a-machine-learning-model-f0f804108751The above analysis was based on orginal wotk by ProPublica: https://www.propublica.org/article/machine-bias-risk-assessments-in-criminal-sentencingAdditional material included is from *The Alignment Problem* by Brian Christian. The use of evidence in parole decisions*"Our law punishes people for what they do, not who they are. Dispensing punishment on the basis of an immutable characteristic flatly contravenes this guiding principle"*, US Supreme Court Chief Justice John Roberts.In 1927, the new chairman of the Parole Board of Illinois, Hinton Clabaugh, commissioned a study on the workings of the parole system in the state. He was particularly interested in whether the right people for parole could be selected - those who would not commit further crime. On reviewing evidence, he concluded that certain characteristics, such as having served less than a year, or coming from a farming background, were associated with a lower probability of re-offending. Meanwhile other characteristics, such as those "living in the criminal underworld" were associated with a higher probability of re-offending. He recommended that the Parole Board be given a summary sheet for each man to be paroled which would list key attributes of the person and their association with re-offense rates. By 1951 a *'Manual of Parole Prediction'* had been written for Illinois, outlining the evidence gathered. At the end of the book is a present section on *'Scoring by Machine Methods'*, which considers use of punch-card machines to automate processing of data and outputting individualized prediction of likelihood to re-offend. Update of such methods was, however, slow. By 1970 only two states in the US used predictive modeling in parole decisions.But that was to change with the development, in 1998, of *'Correctional Offender Management Profiling for Alternative Sanctions'*, or *'COMPAS'*. About COMPASCOMPAS, an acronym for *Correctional Offender Management Profiling for Alternative Sanctions*, is an assistive software and support tool used to predict recidivism risk, that is the risk that a criminal defendant will re-offend. * COMPAS is helpful in ways that it provides scores from 1 (being lowest risk) to 10 (being highest risk).* It also provides a category based evaluation labeled as high risk of recidivism, medium risk of recidivism, or low risk of recidivism. For simplifying things we can convert this multi-class classification problem into binary classification combining the medium risk and high risk of recidivism vs. low risk of recidivism.* The input used for prediction of recidivism is wide-scale and uses 137 factors including age, gender, and criminal history of the defendant as the input. COMPAS **does not** use race as an input.* Race is not an explicit feature considered by the model. Use of COMPAS, and the bias uncovered by ProPublicaCOMPAS has been used by the U.S. states of New York, Wisconsin, California, Florida’s Broward County, and other jurisdictions. Depending on the scores generated by this software, the judge can decide upon whether to detain the defendant prior to trial and/or when sentencing. It has been observed that the Defendants who are classified medium or high risk (scores of 5–10), are more likely to be held in prison while awaiting trial than those classified as low risk (scores of 1–4). Although this software might seem to be assistive and helpful but it suffers from machine bias. According to an investigative journal ProPublica:* Though the accuracy is similar for white and black people, the prediction fails differently for the black defendants:| | White | African-American ||-------------------------------------------|-------|------------------|| Labelled high risk, but did not re-offend | 24% | 45% || Labelled lower risk, but did re-offend | 48% | 28% |* Overall, COMPAS correctly predicts recidivism 61 percent of the time. But black people are almost twice as likely as white people to be labeled a higher risk but not actually re-offend. It makes the opposite mistake among white people: They are much more likely than black people to be labeled lower risk but go on to commit other crimes. This a major risk of machine learning models might possess and when it comes to someone’s freedom it’s a flaw that shouldn’t go unnoticed.To get what ProPublica claimed let’s try to make our own model which replicates their analysis. Formulating ProPublica analysisBefore starting any analysis, we need to have the proper tools for that — importing the required libraries.
###Code
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.metrics import confusion_matrix
###Output
_____no_output_____
###Markdown
Read in the dataAfter importing the libraries we need to import the data and to know how the data looks like use the following code to visualize it.Data may be read from local file, or from the internet.
###Code
read_local = False
if read_local:
df = pd.read_csv('compas_data.csv')
else:
url = 'https://raw.githubusercontent.com/propublica/compas-analysis/master/compas-scores-two-years.csv'
df = pd.read_csv(url)
df.to_csv('compas_data.csv')
df.head()
###Output
_____no_output_____
###Markdown
As stated earlier to make this into a binary classification problem we carry out the following section. Transform into a binary classification problemFirst, let’s make this a binary classification problem. We will add a new column that translates the risk score (decile_score) into a binary label.Any score 5 or higher (Medium or High risk) means that a defendant is treated as a likely recividist, and a score of 4 or lower (Low risk) means that a defendant is treated as unlikely to re-offend. As we know that creating a new feature with the help of the existing one might help us to envision data that might go unexplored. The code to do the same is given below:
###Code
# turn into a binary classification problem
# create feature is_med_or_high_risk
df['is_med_or_high_risk'] = (df['decile_score']>=5).astype(int)
df.head()
###Output
_____no_output_____
###Markdown
Evaluate model performanceTo evaluate the performance of the model, we will compare the model’s predictions to the “truth”:* The risk score prediction of the COMPAS system is in the `decile_score` column,* The classification of COMPAS as medium/high risk or low risk is in the `is_med_or_high_risk` column* The “true” recidivism value (whether or not the defendant committed another crime in the next two years) is in the `two_year_recid` column. AccuracyLet’s start by computing the accuracy, and the overall recidivism rate.
###Code
# classification accuracy
accuracy = np.mean(df['is_med_or_high_risk']==df['two_year_recid'])
print (f'Accuracy: {accuracy:0.3f}')
# Two year recidivism rate
recidivism = np.mean(df['two_year_recid'])
print (f'Recidivism: {recidivism:0.3f}')
###Output
Recidivism: 0.451
###Markdown
This, itself, might already be considered problematic…The accuracy score includes both kinds of errors:* false positives (defendant is predicted as medium/high risk but does not re-offend)* false negatives (defendant is predicted as low risk, but does re-offend)but these errors have different costs. It can be useful to pull them out separately, to see the rate of different types of errors. Confusion matrices, false positives, and false negativesIf we create a confusion matrix, we can use it to derive a whole set of classifier metrics:* True Positive Rate (TPR) also called recall or sensitivity* True Negative Rate (TNR) also called specificity* Positive Predictive Value (PPV) also called precision* Negative Predictive Value (NPV)* False Positive Rate (FPR)* False Discovery Rate (FDR)* False Negative Rate (FNR)* False Omission Rate (FOR) Lets plot a confusion matrix of predicted vs. actual:
###Code
cm = pd.crosstab(df['is_med_or_high_risk'], df['two_year_recid'],
rownames=['Predicted'], colnames=['Actual'])
p = plt.figure(figsize=(5,5));
p = sns.heatmap(cm, annot=True, fmt="d", cbar=False)
###Output
_____no_output_____
###Markdown
We can also use `sklearn`'s `confusion_matrix` to pull out these values and compute any metrics of interest:
###Code
[[tn , fp],[fn , tp]] = confusion_matrix(df['two_year_recid'], df['is_med_or_high_risk'])
print("True negatives: ", tn)
print("False positives: ", fp)
print("False negatives: ", fn)
print("True positives: ", tp)
###Output
True negatives: 2681
False positives: 1282
False negatives: 1216
True positives: 2035
###Markdown
We can replot our confusion matrices, normalising by row or column.
###Code
# Normlaise by row
cm = pd.crosstab(df['is_med_or_high_risk'], df['two_year_recid'],
rownames=['Predicted'], colnames=['Actual'], normalize='index')
p = plt.figure(figsize=(5,5));
p = sns.heatmap(cm, annot=True, fmt=".2f", cbar=False)
###Output
_____no_output_____
###Markdown
When we normalise by row we can more readily see what happened to our 'predicted' groups. We can see that for those predicted not to re-offend, 69% did not re-offend, and 31% did. For those predicted to re-offend, 61% did re-offend, and 39% did not.
###Code
# Normalise by column
cm = pd.crosstab(df['is_med_or_high_risk'], df['two_year_recid'],
rownames=['Predicted'], colnames=['Actual'], normalize='columns')
p = plt.figure(figsize=(5,5));
p = sns.heatmap(cm, annot=True, fmt=".2f", cbar=False)
###Output
_____no_output_____
###Markdown
When we normalise by column we can more readily see what predictions were made for the groups that did or did not re-offend. We can see that of those that did not re-offend, 68% were predicted not to re-offend, whereas 32% were predicted to offend. Of those who went on to re-offend, 63% were predicted to re-offend, whereas 37% were predicted not to re-offend. If we show false positive rate and false negative rate, we see that a defendant has a similar likelihood of being wrongly labeled a likely recidivist and of being wrongly labeled as unlikely to re-offend.
###Code
fpr = fp/(fp+tn)
fnr = fn/(fn+tp)
print(f'False positive rate (overall): {fpr:0.3f}')
print(f'False negative rate (overall): {fnr:0.3f}')
###Output
False positive rate (overall): 0.323
False negative rate (overall): 0.374
###Markdown
Analyse risk scoreWe can also directly evaluate the risk score, instead of just the labels. The risk score is meant to indicate the probability that a defendant will re-offend.
###Code
d = df.groupby('decile_score').agg({'two_year_recid': 'mean'})
# plot
sns.lineplot(data=d);
plt.ylim(0,1);
plt.ylabel('Recidivism rate');
###Output
_____no_output_____
###Markdown
Defendants with a higher COMPAS score indeed had higher rates of recidivism. Receiver-Operator Characteristic curveWe can plot a receiver-operator characteristic curve, and calculate area under curve.
###Code
from sklearn.metrics import roc_curve, roc_auc_score
fpr, tpr, thresholds = roc_curve(df['two_year_recid'], df['decile_score'])
sns.scatterplot(x=fpr, y=tpr, );
sns.lineplot(x=fpr, y=tpr);
plt.ylabel("TPR");
plt.xlabel("FPR");
auc = roc_auc_score(df['two_year_recid'], df['decile_score'])
print (f'ROC AUC: {auc:0.3}')
###Output
ROC AUC: 0.702
###Markdown
FairnessCOMPAS has been under scrutiny for issues related for fairness with respect to race of the defendant.Race is not an explicit input to COMPAS, but some of the questions that are used as input may have strong correlations with race. When we use inputs that correlate with race, race becomes a *redundant variable* - removing it will change little.First, we will find out how frequently each race is represented in the data (this is recorded, but not used in the COMPAS algorithm):
###Code
df['race'].value_counts()
###Output
_____no_output_____
###Markdown
We will focus specifically on African-American or Caucasian defendants, since they are the subject of the ProPublica claim. Accuracy by race
###Code
# Restrict data to black and white pwoplw
df = df[df.race.isin(["African-American","Caucasian"])]
# compare accuracy
results = (df['two_year_recid']==df['is_med_or_high_risk']).astype(int).groupby(
df['race']).mean()
results.round(3)
###Output
_____no_output_____
###Markdown
It isn’t exactly the same, but it’s similar — within a few points. This is a type of fairness known as **overall accuracy equality**. Confusion matrices, and false positives/negatives by race Calculate for black people
###Code
# Black
mask = df['race']=='African-American'
df_masked = df[mask]
[[tn , fp],[fn , tp]] = confusion_matrix(
df_masked['two_year_recid'], df_masked['is_med_or_high_risk'])
false_pos_as_frac_of_all = fp / (fp + tp + fn + tn)
false_neg_as_frac_of_all = fn / (fp + tp + fn + tn)
prop_errors_false_positive = fp / (fp + fn)
prop_no_crime_identified_as_high_risk = fp / (fp + tn)
prop_crime_identified_as_low_risk = fn / (fn + tp)
print("True negatives: ", tn)
print("False positives: ", fp)
print("False negatives: ", fn)
print("True positives: ", tp)
print(f"False positives as fraction of all: {false_pos_as_frac_of_all:0.3f}")
print(f"False negatives as fraction of all: {false_neg_as_frac_of_all:0.3f}")
print(f"Proportion of errors that are false positive: {prop_errors_false_positive:0.3f}")
print(f"Proportion of non-offenders who were identified as high risk: {prop_no_crime_identified_as_high_risk:0.3f}")
print(f"Proportion of offenders who were identified as low risk: {prop_crime_identified_as_low_risk:0.3f}")
###Output
True negatives: 990
False positives: 805
False negatives: 532
True positives: 1369
False positives as fraction of all: 0.218
False negatives as fraction of all: 0.144
Proportion of errors that are false positive: 0.602
Proportion of non-offenders who were identified as high risk: 0.448
Proportion of offenders who were identified as low risk: 0.280
###Markdown
Calculate for white people
###Code
# White
mask = df['race']=='Caucasian'
df_masked = df[mask]
[[tn , fp],[fn , tp]] = confusion_matrix(
df_masked['two_year_recid'], df_masked['is_med_or_high_risk'])
false_pos_as_frac_of_all = fp / (fp + tp + fn + tn)
false_neg_as_frac_of_all = fn / (fp + tp + fn + tn)
prop_errors_false_positive = fp / (fp + fn)
prop_no_crime_identified_as_high_risk = fp / (fp + tn)
prop_crime_identified_as_low_risk = fn / (fn + tp)
print("True negatives: ", tn)
print("False positives: ", fp)
print("False negatives: ", fn)
print("True positives: ", tp)
print(f"False positives as fraction of all: {false_pos_as_frac_of_all:0.3f}")
print(f"False negatives as fraction of all: {false_neg_as_frac_of_all:0.3f}")
print(f"Proportion of errors that are false positive: {prop_errors_false_positive:0.3f}")
print(f"Proportion of non-offenders who were identified as high risk: {prop_no_crime_identified_as_high_risk:0.3f}")
print(f"Proportion of offenders who were identified as low risk: {prop_crime_identified_as_low_risk:0.3f}")
###Output
True negatives: 1139
False positives: 349
False negatives: 461
True positives: 505
False positives as fraction of all: 0.142
False negatives as fraction of all: 0.188
Proportion of errors that are false positive: 0.431
Proportion of non-offenders who were identified as high risk: 0.235
Proportion of offenders who were identified as low risk: 0.477
###Markdown
Here we see a clear disparity by race:* 45% of black people who did not go on to re-offend were classed as high risk of offending, whereas the same figure for white people was 24%.* 48% of white people who went on to re-offend were classed as low risk, whereas the same figure for black people was 28%* 22% of all black people are incorrectly assessed as high risk, compared to 14% of white people.* 19% of white people are incorrectly assessed as low risk, compared to 14% of black people.* For black people, the algorithm incorrectly classifies more people as high risk than low risk. 60% of errors are false positives.* For white people, the algorithm incorrectly classifies more people as low risk than high risk. 43% of errors are false positives. Positive predictive value by raceNext, let’s see whether a defendant who is classified as medium/high risk has the same probability of recidivism for the two groups.In other words, we will compute the PPV for each group:
###Code
# compute PPV
results = df[df['is_med_or_high_risk']==1]['two_year_recid'].groupby(
df['race']).mean()
results.round(3)
###Output
_____no_output_____
###Markdown
Again, similar (within a few points). This is a type of fairness known as **predictive parity**. Assessment of risk score by race
###Code
# calibration plot
d = pd.DataFrame(
df.groupby(['decile_score','race']).agg({'two_year_recid': 'mean'}))
d = d.reset_index()
im = sns.lineplot(
data=d, x='decile_score', y='two_year_recid', hue='race');
im.set(ylim=(0,1));
###Output
_____no_output_____
###Markdown
We can see that for both African-American and Caucasian defendants, for any given COMPAS score, recidivism rates are similar. This is a type of fairness known as **calibration** Frequency of risk score by raceNext, we will look at the frequency with which defendants of each race are assigned each COMPAS score:
###Code
# frequency plot
g = sns.FacetGrid(df, col="race", margin_titles=True);
g.map(plt.hist, "decile_score", bins=10);
###Output
_____no_output_____
###Markdown
We observe that Caucasian defendants in this sample are more likely to be assigned a low risk score.However, to evaluate whether this is *unfair*, we need to know the true prevalence — whether the rates of recidivism are the same in both populations, according to the data:
###Code
# base rates
results = df.groupby('race').agg({'two_year_recid': 'mean',
'is_med_or_high_risk': 'mean',
'decile_score': 'mean'})
results.round(3)
###Output
_____no_output_____ |
Projects/BigMartSales-ExtraTrees_IsolationForest_GradientBoosting.ipynb | ###Markdown
Exploring the datasets
###Code
print(data.shape)
data.describe()
# we see that these are the numeric columns, we will verify this in a moment and make changes if needed.
# we will see if the numerical columns have some relationship with eachother
# before that we will check if pandas infered all the columns correctly, make changes if not.
data.info()
# we see that all of them to be correct, except year column. we will check the values first.
# before everything else we will check if there are any missing data in the dataset
data.isna().sum()
# we see missing data in Two variables, the third ids due to appending the test set.
data['Item_Weight'].interpolate(inplace= True)
# Let's check the values in there.
print(data['Item_Weight'].isna().sum())
data['Item_Weight'].sample(10)
# we see that the value has been filled
# We need to impute the values on this variable
print(data['Outlet_Size'].isna().sum())
data['Outlet_Size'].fillna(data['Outlet_Size'].mode()[0], inplace= True)
print(data['Outlet_Size'].isna().sum())
# we imputed the missing values, now we will proceed
print(f'Number of uniqure values in "Estd.Year" is: {data.Outlet_Establishment_Year.nunique()}')
print(data.Outlet_Establishment_Year.value_counts(dropna= False))
# we will convert this in to an object as there aren't many values
data['Outlet_Establishment_Year']= data.Outlet_Establishment_Year.astype('object')
print(data.Outlet_Establishment_Year.dtype)
# we see that data type is now an object
# we will now see a pairpolot of the numerical columns
num_cols= [*data.select_dtypes(['int64', 'float64']).columns]
sns.pairplot(data[num_cols])
# we don't see a lot of serious relationships betweem the variables and the target variables,
# we will plot a more meaningful plot using seaborn
num_cols.remove('Item_Outlet_Sales')
num_cols
plt.figure(figsize= (24, 9))
count= 1
for col in num_cols:
plt.subplot(3, 2, count)
sns.regplot(x= col, y= 'Item_Outlet_Sales', data= data)
plt.xlabel(col)
count+=1
plt.subplot(3, 2, count)
sns.distplot(data.loc[data[col].notnull(), col])
count+= 1
# We can't see no clear relationship in the data
data.head()
# We'll check the values of categorical columns ('objects')
obj_cols= [*data.select_dtypes('object').columns]
obj_cols
for col in obj_cols:
if data[col].nunique() > 10:
print(f'Number of unique values in {col} is {data[col].nunique()} so not printing values.')
print(" ")
else:
print(f'Values in {col} are: \n {data[col].value_counts()}')
print(" ")
# we see that there are duplicate values in the Item_Fat_Content. We'll work on it
data['Item_Fat_Content'].value_counts(dropna= False)
data['Item_Fat_Content'].replace({'LF': 'Low Fat', 'low fat': 'Low Fat', 'reg': 'Regular'}, inplace= True)
# We will check the values again
data['Item_Fat_Content'].value_counts(dropna= False)
# seems we have replace correctly
# We also saw that there are 1559 unique values in Item_Identifier column and the total number of observations are close to 8k
# let's check this out
print(data['Item_Identifier'].sample(10)) # Let's try extracting the first three letters from the variable and check if there's a pattern
print(data['Item_Identifier'].str[:3].value_counts(dropna= False)) # looks like there are 71 values
print(data['Item_Identifier'].str[:2].value_counts(dropna= False)) # looks like there are only 3 values if we extract 2 letters
data['Item_Identifier']= data['Item_Identifier'].str[:2]
# We see that there is a value called NC, non-consumable, we will have to change fat content
data['Item_Fat_Content']= np.where(data['Item_Identifier']== 'NC', 'Non-durable', data['Item_Fat_Content'])
data.sample(10)
plt.figure(figsize= (24, 12))
for idx, col in enumerate(obj_cols):
plt.subplot(3, 3, idx+1)
sns.boxplot(col, 'Item_Outlet_Sales', data= data)
data.boxplot(column= 'Item_Outlet_Sales', by= ['Item_Fat_Content', 'Item_Identifier'], figsize= (12, 4), rot= 45)
data.boxplot(column= 'Item_Outlet_Sales', by= ['Outlet_Location_Type', 'Outlet_Size'], figsize= (12, 4), rot= 45)
# we'll create a dummy dataframe from the preprocessed dataFrame
df= pd.get_dummies(data, drop_first= True)
for col in num_cols:
print(f'Minimum value in {col} is: {data[col].min()}')
print(" ")
print(f'Minimum value in {col} is: {data[col].max()}')
print(" ")
# seems like there
df['Non-Visible']= np.where(df['Item_Visibility']==0, 1, 0)
df['Non-Visible'].value_counts(dropna= False)
df.head()
df.isna().sum()
X, y= df.drop('Item_Outlet_Sales', axis= 1), df.Item_Outlet_Sales
X.shape, y.shape
y.head()
X_train, X_test, y_train, y_test= train_test_split(X, y, test_size= 0.2, random_state= 123)
lr = LinearRegression()
lr.fit(X_train, y_train)
lr_pred= lr.predict(X_test)
print(np.sqrt(mean_squared_error(y_test, lr_pred)))
rf= RandomForestRegressor(max_depth= 5)
rf.fit(X_train, y_train)
rf_pred= rf.predict(X_test)
print(np.sqrt(mean_squared_error(y_test, rf_pred)))
# We get a better score from a RandomForest Model
gbm= GradientBoostingRegressor(max_depth= 2)
gbm.fit(X_train, y_train)
gbm_pred= gbm.predict(X_test)
print(np.sqrt(mean_squared_error(y_test, gbm_pred)))
# we get a slightly better score.
from sklearn.metrics import make_scorer
# creating a custom scoring function for cross validation
def RMSE(y_true, y_pred):
RMSE = np.sqrt(np.mean((y_true - y_pred) ** 2))
return RMSE
rmse= make_scorer(RMSE, greater_is_better= False)
score= cross_val_score(estimator= gbm, X= X_train, y= y_train, scoring= rmse, cv= 5,\
n_jobs= -1, verbose= 1)
score.mean(), score.std()
# we get negative score as the scorer function returns negative score in cross validation
et= ExtraTreesRegressor()
et.fit(X_train, y_train)
et_pred= et.predict(X_test)
print(np.sqrt(mean_squared_error(y_test, et_pred)))
# we get a slightly better score.
iso_forest= IsolationForest(contamination= 'auto', behaviour= 'New')
outliers= iso_forest.fit_predict(X, y)
pd.Series(outliers).value_counts(dropna= False)
# -1 indicate that the values are outliers
# we will remove the outliers from the original predictor(X) and traget(y) variables
out_bool= outliers == 1
X_new, y_new= X[out_bool], y[out_bool]
X_new.shape, y_new.shape
# we'll now create new train and test values
X_train, X_test, y_train, y_test= train_test_split(X_new, y_new, random_state= 123, test_size= 0.2)
# splitting data 80/20
lr= LinearRegression()
lr.fit(X_train, y_train)
lr_pred= lr.predict(X_test)
print(np.sqrt(mean_squared_error(y_test, lr_pred)))
rf= RandomForestRegressor(max_depth= 5)
rf.fit(X_train, y_train)
rf_pred= rf.predict(X_test)
print(np.sqrt(mean_squared_error(y_test, rf_pred)))
# the score improves a little.
gbm= GradientBoostingRegressor(max_depth= 2)
gbm.fit(X_train, y_train)
gbm_pred= gbm.predict(X_test)
print(np.sqrt(mean_squared_error(y_test, gbm_pred)))
# We see a slight improvement in the socre after removing the outliers
# we can perform a GridSearch to see if we can improve the score further
gbm_params= {'max_depth': np.arange(1, 10, 2), "max_features": [.7, .8, .9],
'max_leaf_nodes': np.arange(2, 10, 2), "min_samples_leaf": np.arange(1, 10, 2),
'min_samples_split': np.arange(2, 10, 2)}
gbm_grid= GridSearchCV(gbm, gbm_params, scoring= rmse, n_jobs= -1, cv= 3, verbose= 1)
gbm_grid.fit(X_train, y_train)
gbm_grid_pred= gbm_grid.predict(X_test)
print(np.sqrt(mean_squared_error(y_test, gbm_grid_pred)))
# We see almost the same score
###Output
1099.34614041462
|
spark_ecosystem/spark/voc_to_delta.ipynb | ###Markdown
Land image data into Delta Table with Spark
###Code
import pyspark
spark = pyspark.sql.SparkSession.builder.appName("MyApp") \
.config("spark.jars.packages", "io.delta:delta-core_2.11:0.6.0") \
.getOrCreate()
sc = spark.sparkContext
sc.addPyFile("/usr/lib/spark/jars/delta-core_2.11-0.6.0.jar")
from delta.tables import *
RAW_DATA_BUCKET = ""
import boto3
import os
def load_voc_image_names(bucket_name, prefix):
s3 = boto3.resource('s3')
bucket = s3.Bucket(bucket_name)
files = []
for obj in bucket.objects.filter(Prefix=os.path.join(prefix, "JPEGImages")):
if obj.key.endswith('.jpg'):
files.append(obj.key)
return(files)
V1_DATA_FOLDER = "v1"
keys = load_voc_image_names(RAW_DATA_BUCKET, V1_DATA_FOLDER)
import io
import random
random.seed(42)
def load_image_list(bucket_name, prefix, list_name):
s3 = boto3.resource('s3')
bucket = s3.Bucket(bucket_name)
path = os.path.join(prefix, "ImageSets", "Main", f"{list_name}.txt")
response = bucket.Object(path)
data = response.get()['Body'].read()
return data.decode('utf8').split('\n')
train_list = load_image_list(RAW_DATA_BUCKET, V1_DATA_FOLDER, 'train')
val_list = load_image_list(RAW_DATA_BUCKET, V1_DATA_FOLDER, 'val')
random.shuffle(val_list)
val_list = val_list[:1000]
train_files = [k for k in keys if os.path.basename(k).split('.')[0] in train_list]
val_files = [k for k in keys if os.path.basename(k).split('.')[0] in val_list]
print(f"{len(train_files)} files in training dataset")
print(f"{len(val_files)} files in val dataset")
def download_from_s3(row):
s3 = boto3.client('s3')
bucket = RAW_DATA_BUCKET
key = row.image_key
filename = os.path.basename(str(key))
basename = filename.split('.')[0]
response = s3.get_object(Bucket=bucket, Key=key)
body = response["Body"]
contents = bytearray(body.read())
body.close()
annotation_key = key.replace("JPEGImages", "Annotations").replace('.jpg', '.xml')
response = s3.get_object(Bucket=bucket, Key=annotation_key)
data = response['Body'].read()
annotations = data.decode('utf8')
if len(contents):
return (key, contents, annotations)
from pyspark.sql.types import StructType, StructField, IntegerType, BinaryType, StringType
from pyspark.sql import Row
def write_to_delta(files, bucket, table, append=False):
rdd1 = sc.parallelize(files)
row_rdd = rdd1.map(lambda x: Row(x))
rows_df = sqlContext.createDataFrame(row_rdd,['image_key'])
images_rdd = (
rows_df
.rdd
.map(download_from_s3)
)
schema = StructType([StructField("key", StringType(), False),
StructField("image", BinaryType(), False),
StructField("annotations", StringType(), False)]
)
image_df = (
images_rdd
.toDF(schema)
)
if append:
mode = "append"
else:
mode = "overwrite"
(
image_df
.write
.format("delta")
.mode(mode)
.option("compression", "gzip")
.save(f"s3://{bucket}/{table}")
)
DELTA_BUCKET = ""
TRAIN_TABLE_NAME = "train"
VAL_TABLE_NAME = "val"
write_to_delta(train_files, DELTA_BUCKET, TRAIN_TABLE_NAME)
write_to_delta(val_files, DELTA_BUCKET, VAL_TABLE_NAME)
train_df = spark.read.format("delta").option("versionAsOf", 0).load(f"s3://{DELTA_BUCKET}/{TRAIN_TABLE_NAME}/")
train_df.show(10)
###Output
_____no_output_____
###Markdown
Update our Delta Table with new images
###Code
updated_keys = load_voc_image_names(RAW_DATA_BUCKET, 'v2')
train_list2 = load_image_list(RAW_DATA_BUCKET, 'v2', 'train')
train_files2 = [k for k in updated_keys if os.path.basename(k).split('.')[0] in train_list2]
print(f"{len(train_files2)} files in the new training dataset")
write_to_delta(train_files2, DELTA_BUCKET, 'train', append=True)
###Output
_____no_output_____ |
module3-cross-validation/Jen_Banks__LS_DS_223_assignment.ipynb | ###Markdown
Lambda School Data Science*Unit 2, Sprint 2, Module 3*--- Cross-Validation Assignment- [ ] [Review requirements for your portfolio project](https://lambdaschool.github.io/ds/unit2), then submit your dataset.- [ ] Continue to participate in our Kaggle challenge. - [ ] Use scikit-learn for hyperparameter optimization with RandomizedSearchCV.- [ ] Submit your predictions to our Kaggle competition. (Go to our Kaggle InClass competition webpage. Use the blue **Submit Predictions** button to upload your CSV file. Or you can use the Kaggle API to submit your predictions.)- [ ] Commit your notebook to your fork of the GitHub repo.You won't be able to just copy from the lesson notebook to this assignment.- Because the lesson was ***regression***, but the assignment is ***classification.***- Because the lesson used [TargetEncoder](https://contrib.scikit-learn.org/categorical-encoding/targetencoder.html), which doesn't work as-is for _multi-class_ classification.So you will have to adapt the example, which is good real-world practice.1. Use a model for classification, such as [RandomForestClassifier](https://scikit-learn.org/stable/modules/generated/sklearn.ensemble.RandomForestClassifier.html)2. Use hyperparameters that match the classifier, such as `randomforestclassifier__ ...`3. Use a metric for classification, such as [`scoring='accuracy'`](https://scikit-learn.org/stable/modules/model_evaluation.htmlcommon-cases-predefined-values)4. If you’re doing a multi-class classification problem — such as whether a waterpump is functional, functional needs repair, or nonfunctional — then use a categorical encoding that works for multi-class classification, such as [OrdinalEncoder](https://contrib.scikit-learn.org/categorical-encoding/ordinal.html) (not [TargetEncoder](https://contrib.scikit-learn.org/categorical-encoding/targetencoder.html)) Stretch Goals Reading- Jake VanderPlas, [Python Data Science Handbook, Chapter 5.3](https://jakevdp.github.io/PythonDataScienceHandbook/05.03-hyperparameters-and-model-validation.html), Hyperparameters and Model Validation- Jake VanderPlas, [Statistics for Hackers](https://speakerdeck.com/jakevdp/statistics-for-hackers?slide=107)- Ron Zacharski, [A Programmer's Guide to Data Mining, Chapter 5](http://guidetodatamining.com/chapter5/), 10-fold cross validation- Sebastian Raschka, [A Basic Pipeline and Grid Search Setup](https://github.com/rasbt/python-machine-learning-book/blob/master/code/bonus/svm_iris_pipeline_and_gridsearch.ipynb)- Peter Worcester, [A Comparison of Grid Search and Randomized Search Using Scikit Learn](https://blog.usejournal.com/a-comparison-of-grid-search-and-randomized-search-using-scikit-learn-29823179bc85) Doing- Add your own stretch goals!- Try other [categorical encodings](https://contrib.scikit-learn.org/categorical-encoding/). See the previous assignment notebook for details.- In additon to `RandomizedSearchCV`, scikit-learn has [`GridSearchCV`](https://scikit-learn.org/stable/modules/generated/sklearn.model_selection.GridSearchCV.html). Another library called scikit-optimize has [`BayesSearchCV`](https://scikit-optimize.github.io/notebooks/sklearn-gridsearchcv-replacement.html). Experiment with these alternatives.- _[Introduction to Machine Learning with Python](http://shop.oreilly.com/product/0636920030515.do)_ discusses options for "Grid-Searching Which Model To Use" in Chapter 6:> You can even go further in combining GridSearchCV and Pipeline: it is also possible to search over the actual steps being performed in the pipeline (say whether to use StandardScaler or MinMaxScaler). This leads to an even bigger search space and should be considered carefully. Trying all possible solutions is usually not a viable machine learning strategy. However, here is an example comparing a RandomForestClassifier and an SVC ...The example is shown in [the accompanying notebook](https://github.com/amueller/introduction_to_ml_with_python/blob/master/06-algorithm-chains-and-pipelines.ipynb), code cells 35-37. Could you apply this concept to your own pipelines? BONUS: Stacking!Here's some code you can use to "stack" multiple submissions, which is another form of ensembling:```pythonimport pandas as pd Filenames of your submissions you want to ensemblefiles = ['submission-01.csv', 'submission-02.csv', 'submission-03.csv']target = 'status_group'submissions = (pd.read_csv(file)[[target]] for file in files)ensemble = pd.concat(submissions, axis='columns')majority_vote = ensemble.mode(axis='columns')[0]sample_submission = pd.read_csv('sample_submission.csv')submission = sample_submission.copy()submission[target] = majority_votesubmission.to_csv('my-ultimate-ensemble-submission.csv', index=False)```
###Code
%%capture
import sys
# If you're on Colab:
if 'google.colab' in sys.modules:
DATA_PATH = 'https://raw.githubusercontent.com/LambdaSchool/DS-Unit-2-Kaggle-Challenge/master/data/'
!pip install category_encoders==2.*
# If you're working locally:
else:
DATA_PATH = '../data/'
import pandas as pd
# Merge train_features.csv & train_labels.csv
train_orig = pd.merge(pd.read_csv(DATA_PATH+'waterpumps/train_features.csv'),
pd.read_csv(DATA_PATH+'waterpumps/train_labels.csv'))
# Read test_features.csv & sample_submission.csv
test_orig = pd.read_csv(DATA_PATH+'waterpumps/test_features.csv')
sample_submission = pd.read_csv(DATA_PATH+'waterpumps/sample_submission.csv')
# imports
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
import category_encoders as ce
from sklearn.impute import SimpleImputer
from sklearn.ensemble import RandomForestClassifier, BaggingClassifier
from sklearn.ensemble import AdaBoostClassifier, VotingClassifier
from sklearn.ensemble import GradientBoostingClassifier
from sklearn.pipeline import make_pipeline
from sklearn.preprocessing import StandardScaler
# Split train data set
train=train_orig.copy()
test=test_orig.copy()
train, val = train_test_split(train, test_size=0.2)
# Data Wranggling
def wrangle(X):
"""Wrangle train, validate, and test sets in the same way"""
# Prevent SettingWithCopyWarning
X = X.copy()
# About 6.1% of 'funder' cell are missing, fill with mode
X['funder']=X['funder'].fillna(X.funder.mode()[0], inplace=False)
# About 6.2% of 'installer' cell are missing, fill with mode
X['installer']=X['installer'].fillna(X['installer'].mode()[0], inplace=False)
# About 3% of the time, latitude has small values near zero,
# outside Tanzania, so we'll treat these values like zero.
X['latitude'] = X['latitude'].replace(-2e-08, 0)
# About 5.7% of 'public_meeting' cell are missing, fill with mode
X['public_meeting']=X['public_meeting'].fillna(X['public_meeting'].mode()[0],
inplace=False)
# About 6.5% of 'public_meeting' cell are missing, fill with mode
X['scheme_management']=X['scheme_management'].fillna(X['scheme_management'].mode()[0],
inplace=False)
# About 5.2% of 'public_meeting' cell are missing, fill with mode
X['permit']=X['permit'].fillna(X['permit'].mode()[0], inplace=False)
# When columns have zeros and shouldn't, they are like null values.
# So we will replace the zeros with nulls, and impute missing values later.
# Also create a "missing indicator" column, because the fact that
# values are missing may be a predictive signal.
cols_with_zeros = ['longitude', 'latitude', 'construction_year',
'gps_height', 'population']
for col in cols_with_zeros:
X[col] = X[col].replace(0, np.nan)
X[col+'_MISSING'] = X[col].isnull()
# Drop duplicate columns
duplicates = ['quantity_group', 'payment_type']
X = X.drop(columns=duplicates)
# Drop recorded_by (never varies) and id (always varies, random)
unusable_variance = ['recorded_by', 'id']
X = X.drop(columns=unusable_variance)
# Convert date_recorded to datetime
X['date_recorded'] = pd.to_datetime(X['date_recorded'], infer_datetime_format=True)
# Extract components from date_recorded, then drop the original column
X['year_recorded'] = X['date_recorded'].dt.year
X['month_recorded'] = X['date_recorded'].dt.month
X['day_recorded'] = X['date_recorded'].dt.day
X = X.drop(columns='date_recorded')
# Engineer feature: how many years from construction_year to date_recorded
X['years'] = X['year_recorded'] - X['construction_year']
X['years_MISSING'] = X['years'].isnull()
# return the wrangled dataframe
return X
train = wrangle(train)
val = wrangle(val)
test = wrangle(test)
# The status_group column is the target
target = 'status_group'
# Get a dataframe with all train columns except the target
train_features = train.drop(columns=[target])
# Get a list of the numeric features
numeric_features = train_features.select_dtypes(include='number').columns.tolist()
# Get a series with the cardinality of the nonnumeric features
cardinality = train_features.select_dtypes(exclude='number').nunique()
# Get a list of all categorical features with cardinality <= 50
categorical_features = cardinality[cardinality <= 50].index.tolist()
# Combine the lists
features = numeric_features + categorical_features
# Arrange data into X features matrix and y target vector
X_train = train[features]
y_train = train[target]
X_val = val[features]
y_val = val[target]
X_test = test[features]
#using RandomForest, ordinal encoding
%matplotlib inline
import matplotlib.pyplot as plt
from sklearn.model_selection import validation_curve
pipeline_rf_ord=make_pipeline(
ce.OrdinalEncoder(),
SimpleImputer(),
RandomForestClassifier()
)
depth =range( 1, 30, 3)
train_scores, val_scores=validation_curve(
pipeline_rf_ord, X_train,y_train,
param_name='randomforestclassifier__max_depth',
param_range=depth, scoring='accuracy',
cv= 3,
n_jobs=-1
)
pipeline_rf_ord.fit(X_train, y_train)
print(pipeline_rf_ord.score(X_train, y_train))
print(pipeline_rf_ord.score(X_val, y_val))
# Plotting Validation Curve with RandomForest, max depth Hyperparameter
plt.figure(dpi=100)
plt.plot(depth, np.mean(train_scores, axis=1), color='blue', label='training accuracy')
plt.plot(depth, np.mean(val_scores, axis= 1), color='red', label= 'validation accuracy')
plt.title('Validation Curve')
plt.xlabel('Most Complexity: RandomForestClassifier_Max_depth')
plt.ylabel('Model Score: Accuracy')
plt.legend();
#Zooming In
plt.figure(dpi=100)
plt.plot(depth, np.mean(train_scores, axis=1), color='blue', label='training accuracy')
plt.plot(depth, np.mean(val_scores, axis= 1), color='red', label= 'validation accuracy')
plt.title('Validation Curve, Zoomed In')
plt.xlabel('Most Complexity: RandomForestClassifier_Max_depth')
plt.ylabel('Model Score: Accuracy')
plt.ylim(0.78, 0.83)
plt.legend();
# Creating a prediction file
y_pred_rf_ord=pipeline_rf_ord.predict(X_test)
submission_rf_ord=sample_submission.copy()
submission_rf_ord['status_group']=y_pred_rf_ord
submission_rf_ord.to_csv('submission_rf_ord.csv', index=False)
# Using Bagging ensemble, no replacement
from sklearn.tree import DecisionTreeClassifier
pipeline_bag1=make_pipeline(
ce.OrdinalEncoder(),
SimpleImputer(),
StandardScaler(),
BaggingClassifier(DecisionTreeClassifier(),
n_estimators=100,
max_features=0.6,
max_samples=0.4,
bootstrap=False,
bootstrap_features= False)
)
feat =[ 0.3, .5, 0.8]
train_scores, val_scores=validation_curve(
pipeline_bag1, X_train,y_train,
param_name='baggingclassifier__max_features',
param_range=feat, scoring='accuracy',
cv= 3,
n_jobs=-1
)
pipeline_bag1.fit(X_train, y_train)
print(pipeline_bag1.score(X_train, y_train))
print(pipeline_bag1.score(X_val, y_val))
# Creating a prediction file
y_pred_bag1=pipeline_bag1.predict(X_test)
submission_bag1=sample_submission.copy()
submission_bag1['status_group']=y_pred_bag1
submission_bag1.to_csv('submission_bag811.csv', index=False)
# Plotting Validation Curve with DecisioonTreeClassifier, max_features Hyperparameter
plt.figure(dpi=100)
plt.plot(feat, np.mean(train_scores, axis=1), color='blue', label='training accuracy')
plt.plot(feat, np.mean(val_scores, axis= 1), color='red', label= 'validation accuracy')
plt.title('Validation Curve')
plt.xlabel('Most Complexity: DecisionTreeClassifier_Max_depth')
plt.ylabel('Model Score: Accuracy')
plt.legend();
###Output
_____no_output_____
###Markdown
Using RandomizedSearchCV
###Code
#With RandomizedSearchCV on RandomforestClassifier
pipeline_rf_rand=make_pipeline(
ce.OrdinalEncoder(),
SimpleImputer(),
RandomForestClassifier()
)
param_distribution={
'simpleimputer__strategy':['mean','most_frequent', 'median'],
'randomforestclassifier__max_depth':[3,5,10],
'randomforestclassifier__n_estimators':[10,20,30],
'randomforestclassifier__criterion':['gini', 'entropy']
}
search=RandomizedSearchCV(
pipeline_rf_rand,
param_distributions=param_distribution,
n_iter=20,
cv=3,
scoring='accuracy',
return_train_score=True,
verbose=10,
n_jobs=-1
)
search.fit(X_train, y_train)
print(search.score(X_train, y_train))
print(search.score(X_val, y_val))
print("Best Parameters", search.best_params_)
print("Best Accuracy score", search.best_score_)
pipeline_rf_rand_sample=make_pipeline(
ce.OrdinalEncoder(),
SimpleImputer(strategy='median'),
RandomForestClassifier(n_estimators= 30,
max_depth=10,
criterion='gini')
)
pipeline_rf_rand_sample.fit(X_train, y_train)
print(pipeline_rf_rand_sample.score(X_train, y_train))
print(pipeline_rf_rand_sample.score(X_val, y_val))
# Creating a prediction file
y_pred_rf_rand=search.predict(X_test)
submission_rf_rand=sample_submission.copy()
submission_rf_rand['status_group']=y_pred_rf_rand
submission_rf_rand.to_csv('submission_rf_rand.csv', index=False)
#With RandomizedSearchCV on BaggingClassifier
pipeline_bag_rand=make_pipeline(
ce.OrdinalEncoder(),
SimpleImputer(),
StandardScaler(),
BaggingClassifier()
)
param_distribution={
'simpleimputer__strategy':['mean','most_frequent', 'median'],
'baggingclassifier__max_features':[.3,.5,1.0],
'baggingclassifier__n_estimators':[30,50,1000],
'baggingclassifier__bootstrap':[False, True]
}
search1=RandomizedSearchCV(
pipeline_bag_rand,
param_distributions=param_distribution,
n_iter=20,
cv=3,
scoring='accuracy',
return_train_score=True,
verbose=10,
n_jobs=-1
)
search1.fit(X_train, y_train)
print(search1.score(X_train, y_train))
print(search1.score(X_val, y_val))
print("Best Parameters", search1.best_params_)
print("Best Accuracy score", search1.best_score_)
# Creating a prediction file
y_pred_bag_rand=search1.predict(X_test)
submission_bag_rand=sample_submission.copy()
submission_bag_rand['status_group']=y_pred_bag_rand
submission_bag_rand.to_csv('submission_bag_rand.csv', index=False)
# Checking for detailed report
import pandas as pd
pd.DataFrame(search1.cv_results_).sort_values(by='rank_test_score').T
#With RandomizedSearchCV on RandomForestClassifier with function
from xgboost.sklearn import XGBClassifier
classifier=make_pipeline(
ce.OrdinalEncoder(),
SimpleImputer(),
RandomForestClassifier()
)
params={
'simpleimputer__strategy':['mean','most_frequent', 'median'],
'randomforestclassifier__min_samples_leaf':[3,5,10],
'randomforestclassifier__n_estimators':[50,100],
'randomforestclassifier__criterion':['gini', 'entropy']
}
def hypertuning(classifier, param_dist, n_iters, X,y ):
search2=RandomizedSearchCV(
classifier,
param_distributions=param_dist,
n_jobs=-1,
n_iter=n_iters,
verbose=10,
cv=3)
search2.fit(X_train, y_train)
hyper_params=search2.best_params_
hyper_score=search2.best_score_
return hyper_params, hyper_score
hyper_params, hyper_score=hypertuning(classifier, params, 5, X_train,y_train)
hyper_params, hyper_score
# Applying the given hyperparameters
classifier2=make_pipeline(
ce.OrdinalEncoder(),
SimpleImputer(strategy= 'median'),
RandomForestClassifier(criterion='entropy',
min_samples_leaf= 3,
n_estimators=50)
)
classifier2.fit(X_train, y_train)
print(classifier2.score(X_train, y_train))
print(classifier2.score(X_val, y_val))
# Creating a prediction file
y_pred_rf_func=classifier2.predict(X_test)
submission_rf_func=sample_submission.copy()
submission_rf_func['status_group']=y_pred_rf_func
submission_rf_func.to_csv('submission_rf_rand.csv', index=False)
#With RandomizedSearchCV on XGBoost with function
pipeline_xgb=make_pipeline(
ce.OrdinalEncoder(),
SimpleImputer(),
StandardScaler(),
XGBClassifier(n_estimators=50)
)
params_xgb={
'simpleimputer__strategy':['mean','most_frequent', 'median'],
'xgbclassifier__min_child_weight':[1,5,10],
'xgbclassifier__max_depth':[5,10, 15],
'xgbclassifier__learning_rate':[0.3, 0.5, 1.0],
'xgbclassifier__scale_pos_weight':[0.5,1],
}
hyper_params, hyper_score=hypertuning(pipeline_xgb, params_xgb, 5, X_train,y_train)
hyper_params, hyper_score
#Applying hyperparameters
pipeline_xgb_sample=make_pipeline(
ce.OrdinalEncoder(),
SimpleImputer(strategy='most_frequent'),
StandardScaler(),
XGBClassifier(n_estimators=50, learning_rate=.3, max_depth= 10, min_child_weight=1, scale_pos_weight= 0.5)
)
pipeline_xgb_sample.fit(X_train, y_train)
print(pipeline_xgb_sample.score(X_train, y_train))
print(pipeline_xgb_sample.score(X_val, y_val))
# Creating a prediction file
y_pred_xgb_func=pipeline_xgb_sample.predict(X_test)
submission_xgb_func=sample_submission.copy()
submission_xgb_func['status_group']=y_pred_xgb_func
submission_xgb_func.to_csv('submission_xgb_rand.csv', index=False)
#Creating a csv file for sample submission
sample_submission.to_csv('sample_submission.csv', index=False)
# Stacking
import pandas as pd
# Filenames of your submissions you want to ensemble
files = ['submission_rf_ord.csv', 'submission_bag811.csv', 'submission_bag_rand.csv', 'submission_rf_rand.csv', 'submission_xgb_rand.csv']
target = 'status_group'
submissions = (pd.read_csv(file)[[target]] for file in files)
ensemble = pd.concat(submissions, axis='columns')
majority_vote = ensemble.mode(axis='columns')[0]
sample_submission = pd.read_csv('sample_submission.csv')
submission = sample_submission.copy()
submission[target] = majority_vote
submission.to_csv('my-ultimate-ensemble-submission.csv', index=False)
# Stacking data, the mode/observation will be final prediction
ensemble
###Output
_____no_output_____
###Markdown
Applying the highest score to the whole TRAIN dataset
###Code
#Copying and wrangling data
train_whole=train_orig.copy()
test_whole=test_orig.copy()
train_whole = wrangle(train_whole)
test_whole = wrangle(test_whole)
# The status_group column is the target
target = 'status_group'
# Get a dataframe with all train columns except the target
train_features2 = train_whole.drop(columns=[target])
# Get a list of the numeric features
numeric_features2 = train_features2.select_dtypes(include='number').columns.tolist()
# Get a series with the cardinality of the nonnumeric features
cardinality2 = train_features2.select_dtypes(exclude='number').nunique()
# Get a list of all categorical features with cardinality <= 50
categorical_features2 = cardinality2[cardinality2 <= 50].index.tolist()
# Combine the lists
features2 = numeric_features2 + categorical_features2
# Arrange data into X features matrix and y target vector
X_train_whole = train_whole[features2]
y_train_whole = train_whole[target]
X_test_whole = test_whole[features2]
# Applying the given hyperparameters
classifier_whole=make_pipeline(
ce.OrdinalEncoder(),
SimpleImputer(strategy= 'median'),
RandomForestClassifier(criterion='entropy',
min_samples_leaf= 3,
n_estimators=50)
)
classifier_whole.fit(X_train_whole, y_train_whole)
print(classifier_whole.score(X_train_whole, y_train_whole))
y_pred_whole=classifier_whole.predict(X_test_whole)
submission_whole=sample_submission.copy()
submission_whole['status_group']=y_pred_whole
submission_whole.to_csv('submission_whole.csv', index=False)
###Output
_____no_output_____ |
_build/jupyter_execute/02_linear_regression.ipynb | ###Markdown
Linear Regression Modelgiven a dataset $\left \{ (x^{(1)},y^{(1)}),...,(x^{(n)},y^{(n)}) \right \} $, where $x^{(i)} \in \mathbb{R}^{d},\ y^{(i)} \in \mathbb{R}$.linear regression is trying to model $y^{(i)}$ from $x^{(i)}$ by a linear model:$$h_{\theta}(x^{(i)}) = \theta_{0} + \theta_{1}x_{1}^{(i)} + \theta_{2}x_{2}^{(i)} + ... + \theta_{d}x_{d}^{(i)}\approx y^{(i)}$$for the sake of simplicity, we set $x_{0}^{(i)}=1,\ x^{(i)} = (x_{0}^{(i)},...,x_{d}^{(i)}),\ \theta=(\theta_{0},...,\theta_{d})$, then:$$h_{\theta}(x^{(i)}) = \sum_{j=0}^{d}\theta_{j}x_{j}^{(i)} = \theta^{T}x^{(i)}$$ Loss Functiongiven $\hat{y}=(h_{\theta}^{(1)},...,h_{\theta}^{(n)}),\ y=(y^{(1)},...,y^{(n)})$.we want to approximate $y$ by $\hat{y}$, or equivalent to say, we want to minimize the distance between $\hat{y}$ and $y$.using euclidean distance, we derive the loss function for linear regression:$$J(\theta) = \frac{1}{2}\sum_{i=1}^{n}(h_{\theta}x^{(i)} - y^{(i)})^2$$ Gradient Descentafter defining model and loss function, our goal now is to find the model that minimize the loss function:$$\hat{\theta} = \underset{\theta}{argmin}\ J(\theta)$$to minimize the loss function, we can set $$\nabla J(\theta)=0$$but to find the analytic solution of this equation is usually impossible.alternatively, we can init $\theta$ randomly, then iteratively move $\theta$ towards the direction that makes $J(\theta)$ smaller.remmenber the oposite direction of gradient is the fastest direction that makes functions smaller, we derive gradient descent:$$\theta := \theta - \alpha\nabla{J(\theta )}$$$\alpha > 0$ is called the learning rateappendix: proof of opposite gradient as fastest descentfor all $l\in \mathbb{R}^{d}, \left \| l \right \| =1$$$\lim_{x \to 0} \frac{J(\theta + x l) - J(\theta ) }{x}=l \cdot \nabla{J(\theta )} >= -\left \| l \right \|\left \|J(\theta) \right \| = -\left \| J(\theta )\right \| $$equality obtained only if $l$ is in the opposite direction of $\nabla{J(\theta )}$
###Code
import numpy as np
X = 2 * np.random.rand(100, 1)
y = 4 + 3 * X + np.random.randn(100, 1)
X_new = np.array([[0], [2]])
"""LinearRegression actually uses SVD to get pseudoinverse, based on scipy.linalg.lstsq()"""
from sklearn.linear_model import LinearRegression
lin_reg = LinearRegression()
lin_reg.fit(X, y)
lin_reg.intercept_, lin_reg.coef_
y_predict = lin_reg.predict(X_new)
y_predict
import matplotlib.pyplot as plt
plt.plot(X_new, y_predict, "r-", linewidth=2, label="Predictions")
plt.plot(X, y, "b.")
plt.xlabel("$x_1$", fontsize=18)
plt.ylabel("$y$", rotation=0, fontsize=18)
plt.legend(loc="upper left", fontsize=14)
plt.axis([0, 2, 0, 15])
plt.show()
###Output
_____no_output_____
###Markdown
stochastic gradient descentin practice, to update $\theta$ by gradient descent(concretely batch gradient descent), at each step, we need to calculate all sample's gradient, too slow.to fix this problem, we can only use one sample's gradient at a time, choose that sample randomly:$$\theta := \theta - \alpha{l(h_{\theta}(x^{(i)}), y^{(i)})}$$
###Code
from sklearn.linear_model import SGDRegressor
sgd_reg = SGDRegressor(max_iter=1000, tol=1e-3, penalty=None, eta0=0.1)
sgd_reg.fit(X, y.ravel())
sgd_reg.intercept_, sgd_reg.coef_
###Output
_____no_output_____
###Markdown
mini-batch gradient descentsgd too random, batch gradient descent too slow, we can use some(not one, not all) samples at a time:$$\theta := \theta - \alpha\sum_{i \in batch}{l(h_{\theta}(x^{(i)}), y^{(i)})}$$ update rule of linear regressionfor linear regression, we have:$$\begin{equation}\begin{split} \frac{\partial }{\partial \theta_{j}}J(\theta ) &= \frac{\partial }{\partial \theta_{j}}\frac{1}{2}\sum_{i=1}^{n}(h_{\theta }(x^{(i)}) - y^{(i)})^2 \\ &=\sum_{i=1}^{n}(h_{\theta }(x^{(i)}) - y^{(i)})\cdot{}\frac{\partial }{\partial \theta_{j}}(h_{\theta }(x^{(i)}) - y^{(i)})\\ & =\sum_{i=1}^{n}(h_{\theta }(x^{(i)}) - y^{(i)})x_{j}^{(i)}\end{split}\end{equation}$$so we have the update rule for linear regression:$$\theta_{j}: =\theta_{j} - \alpha\sum_{i=1}^{n} (h_{\theta }(x^{(i)}) - y^{(i)})x_{j}^{(i)} $$combine all dimensions, we have:$$\theta: =\theta - \alpha\sum_{i=1}^{n} (h_{\theta }(x^{(i)}) - y^{(i)})\cdot x^{(i)} $$ matrix formdefine $X = [(x^{(1)})^{T},...,(x^{(n)})^{T}]$, then we can write $J(\theta)$ in matrix form:$$\begin{equation}\begin{split}J(\theta) &= \frac{1}{2}\sum_{i=1}^{n}(h_{\theta}x^{(i)} - y^{(i)})^2 \\&= \frac{1}{2}\sum_{i=1}^{n}(\theta^{T}x^{(i)} - y^{(i)})^2 \\&= \frac{1}{2}(X\theta - y)^{T}(X\theta - y)\end{split}\end{equation}$$we then have:$$\begin{equation}\begin{split}\nabla{J(\theta )} &= \nabla\frac{1}{2}(X\theta - y)^{T}(X\theta - y) \\&= \frac{1}{2}\nabla(\theta^{T}X^{T}X\theta - y^{T}(X\theta) - (X\theta)^{T}y) \\&= \frac{1}{2}\nabla(\theta^{T}X^{T}X\theta - 2(X^{T}y)^{T}\theta) \\&= \frac{1}{2}(2X^{T}X\theta - 2(X^{T}y)) \\&= X^{T}X\theta - X^{T}y\end{split}\end{equation}$$here we use1.$a^{T}b=b^{T}a$, obvious.2.$\nabla{a^{T}x}=a$, obvious.3.$\nabla{x^{T}Ax} = (A + A^{T})x$, proof:$$\begin{equation}\begin{split}\frac{\partial}{\partial x_{i}}x^{T}Ax &= \frac{\partial}{\partial x_{i}}{\sum_{j=1}^{n}}{\sum_{k=1}^{n}}a_{jk}x_{j}x_{k} \\&= \frac{\partial}{\partial x_{i}}(\sum_{j\ne{i}}a_{ji}x_{j}x_{i} + \sum_{k\ne{i}}a_{ik}x_{i}x_{k} + a_{ii}x_{i}^{2}) \\&= \sum_{j\ne{i}}a_{ji}x_{j} + \sum_{k\ne{i}}a_{ik}x_{k} + 2a_{ii}x_{ii} \\&= \sum_{j=1}^{n}a_{ji}x_{j} + \sum_{k=1}^{n}a_{ik}x_{k} \\&= (A^{T}x)_{i} + (Ax)_{i}\end{split}\end{equation}$$finally, we get the matrix form of the update rule:$$\theta: =\theta - \alpha X^{T}(X\theta-\mathbf{y} ) $$ analytic solutionfrom above, we have:$$\nabla{J(\theta )} = X^{T}X\theta - X^{T}y$$so the equation of zero gradient change to:$$X^{T}X\theta - X^{T}y = 0$$if $X^{T}X$ is invertible:$$\theta = (X^{T}X)^{-1}X^{T}y$$if $X^{T}X$ is not invertible, the equation also have solution. proof:on one hand $X\theta = 0 \Rightarrow X^{T}X\theta=0$on the other hand $X^{T}X\theta=0 \Rightarrow \theta^{T}X^{T}X\theta=0 \Rightarrow (X\theta)^{T}X\theta=0 \Rightarrow X\theta=0$so $X\theta=0 \Leftrightarrow X^{T}X\theta=0$, that is to say $null(X^{T}X)=null(X)$we easily derive from the above that $rank(X^{T}X) = rank(X) = rank(X^{T})$but $range(X^{T}X) \subseteq range(X^{T})$, so we must have $range(X^{T}X)=range(X^{T})$
###Code
X_b = np.c_[np.ones((100, 1)), X]
theta_best = np.linalg.inv(X_b.T.dot(X_b)).dot(X_b.T).dot(y)
theta_best
###Output
_____no_output_____
###Markdown
geometric interpretation of the linear regressionconsider the linear space $S = span\left \{ columns\ of\ X \right \}$, linear combination of $S$ could be write as $X\theta$, then:$X\theta$ is the projection of $y$ on $S \Leftrightarrow$ $X\theta - y$ orthogonal with $S \Leftrightarrow$ orthogonal with $columns\ of\ X \Leftrightarrow X^{T}(X\theta - y)=0$so linear regression could be interpret as finding the projection of $y$ on $S$. probabilistic interpretationassume targets and inputs are related via:$$y^{(i)} = \theta^{T}x^{(i)} + \epsilon^{(i)}$$where $\epsilon^{(i)}$ is the error term, assume that $\epsilon^{(i)}$ are distributed IID according to Gaussian with mean 0 and variance $\sigma^{2}$, i.e the density of $\epsilon^{(i)}$ is given by:$$p(\epsilon^{(i)}) = \frac{1}{\sqrt{2\pi}\sigma}exp\left (-\frac{(\epsilon^{(i)})^{2}}{2\sigma^{2}}\right )$$this implies:$$p(y^{(i)}|x^{(i)}; \theta) = \frac{1}{\sqrt{2\pi}\sigma}exp\left ( -\frac{(y^{(i)} - \theta^{T}x^{(i)})^{2}}{2\sigma^{2}}\right)$$we should denote that $\theta$ is not a random variable.given $X$ and $\theta$, what is the probability of $y$? we call it the likelihood function:$$L(\theta) = L(\theta; X,y) = p(y|X; \theta)$$for the above assumptions:$$\begin{equation}\begin{split}L(\theta) &= \prod_{i=1}^{n}p(y^{(i)}|x^{(i)}; \theta) \\&= \prod_{i=1}^{n}\frac{1}{\sqrt{2\pi}\sigma}exp\left ( -\frac{(y^{(i)} - \theta^{T}x^{(i)})^{2}}{2\sigma^{2}}\right)\end{split}\end{equation}$$we should choose $\theta$ so as to make the data as high probability as possible, i.e we should choose $\theta$ to maxmize $L(\theta)$, this is called maximum likelihood. one step further:$$maximize\ L(\theta) \Leftrightarrow maxmize\ log(L(\theta))$$so we maximize the log likelihood, this is simpler.$$\begin{equation}\begin{split}l(\theta) &= log(L(\theta)) \\&= log\prod_{i=1}^{n}\frac{1}{\sqrt{2\pi}\sigma}exp\left ( -\frac{(y^{(i)} - \theta^{T}x^{(i)})^{2}}{2\sigma^{2}}\right) \\&= \sum_{i=1}^{n}log\frac{1}{\sqrt{2\pi}\sigma}exp\left ( -\frac{(y^{(i)} - \theta^{T}x^{(i)})^{2}}{2\sigma^{2}}\right) \\&= n\ log\frac{1}{\sqrt{2\pi}\sigma} - \frac{1}{2\sigma^{2}}\sum_{i=1}^{n}(y^{(i)} - \theta^{T}x^{(i)})^{2}\end{split}\end{equation}$$hence, maximizing $l(\theta)$ gives the same answer as minimizing$$\frac{1}{2}\sum_{i=1}^{n}(y^{(i)} - \theta^{T}x^{(i)})^{2} = J(\theta)$$so linear regression $\Leftrightarrow $ maximum likelihood given Gaussian error regularizationto lower variance $\Rightarrow $ to limit model's complexity $\Rightarrow $ to prevent the absolute value of parameters to be too large $\Rightarrow $ we add punishment term concerning the absolute value of parameters on $J(\theta)$choose $\lambda{\left \| \theta \right \|}_{2}^{2}$ as the punishment term:$$J(\theta) := J(\theta) + \lambda{\left \| \theta \right \|}_{2}^{2}$$$\lambda$ is the regularization hyperparameter, linear regression with $l_{2}$ loss is ridge regression.otherwise, if we choose $\lambda{\left \| \theta \right \|}_{1}$ as the punishment term:$$J(\theta) := J(\theta) + \lambda{\left \| \theta \right \|}_{1}$$that is called lasso regression. probabilistic interpretation of regularizationas before, we assume:$$p(y^{(i)}|x^{(i)}; \theta) = \frac{1}{\sqrt{2\pi}\sigma}exp\left ( -\frac{(y^{(i)} - \theta^{T}x^{(i)})^{2}}{2\sigma^{2}}\right)$$in bayes perspective, we can also assume $\theta \sim N(0, \sigma_{0})$, that is:$$p(\theta) = \frac{1}{\sqrt{2\pi}\sigma_{0}}exp\left ( -\frac{\left \| \theta \right \|_{2}^{2}}{2\sigma_{0}^{2}}\right)$$by bayes rule:$$P(\theta|y)=\frac{P(y|\theta)P(\theta)}{P(y)}$$thus we have maximum a posteriori estimation(MAP):$$\begin{equation}\begin{split}\hat{\theta} =&\underset{\theta}{argmax}P(\theta|y)\\=&\underset{\theta}{argmax}P(y|\theta)P(\theta) \\=&\underset{\theta}{argmax}\ logP(y|\theta)P(\theta) \\=&\underset{\theta}{argmax}\ log\ exp\left ( \sum_{i=1}^{n}-\frac{(y^{(i)} - \theta^{T}x^{(i)})^{2}}{2\sigma^{2}}\right)exp\left ( -\frac{\left \| \theta \right \|_{2}^{2}}{2\sigma_{0}^{2}}\right)\\=&\underset{\theta}{argmin}\left(\sum_{i=1}^{n}\frac{(y^{(i)} - \theta^{T}x^{(i)})^{2}}{2\sigma^{2}} + \frac{\left \| \theta \right \|_{2}^{2}}{2\sigma_{0}^{2}}\right)\\=&\underset{\theta}{argmin}\left(\sum_{i=1}^{n}(y^{(i)} - \theta^{T}x^{(i)})^{2} + \frac{\sigma^{2}}{\sigma_{0}^{2}}\left \| \theta \right \|_{2}^{2}\right)\end{split}\end{equation}$$that is exactly minimize:$$J(\theta) = \frac{1}{2}\sum_{i=1}^{n}(y^{(i)} - \theta^{T}x^{(i)})^2 + \lambda{\left \| \theta \right \|}^{2}$$with $\lambda=\frac{\sigma^{2}}{2\sigma_{0}^{2}}$in one word:1. linear regression $\Leftrightarrow $ maximum likelihood estimation(MLE) where noise is guassian.2. ridge regression $\Leftrightarrow $ maximum a posteriori estimation(MAP) where noise and prior are guassian.
###Code
from sklearn.linear_model import Ridge
ridge_reg = Ridge(alpha=1, solver="cholesky", random_state=42)
ridge_reg.fit(X, y)
ridge_reg.predict([[1.5]])
"""set SGDRegressor penalty=l2"""
sgd_reg = SGDRegressor(penalty="l2", max_iter=1000, tol=1e-3, random_state=42)
sgd_reg.fit(X, y.ravel())
sgd_reg.predict([[1.5]])
###Output
_____no_output_____
###Markdown
Lasso
###Code
"""use Lasso or set SGDRegressor penalty=l1"""
from sklearn.linear_model import Lasso
lasso_reg = Lasso(alpha=0.1)
lasso_reg.fit(X, y)
lasso_reg.predict([[1.5]])
"""combination of ridge and lasso"""
from sklearn.linear_model import ElasticNet
elastic_net = ElasticNet(alpha=0.1, l1_ratio=0.5)
elastic_net.fit(X, y)
elastic_net.predict([[1.5]])
###Output
_____no_output_____
###Markdown
Polynomial Regression
###Code
m = 100
X = 6 * np.random.rand(m, 1) - 3
y = 0.5 * X**2 + X + 2 + np.random.randn(m, 1)
plt.plot(X, y, "b.")
plt.xlabel("$x_1$", fontsize=18)
plt.ylabel("$y$", rotation=0, fontsize=18)
plt.axis([-3, 3, 0, 10])
plt.show()
from sklearn.preprocessing import PolynomialFeatures
poly_features = PolynomialFeatures(degree=2, include_bias=False)
X_poly = poly_features.fit_transform(X)
lin_reg = LinearRegression()
lin_reg.fit(X_poly, y)
lin_reg.intercept_, lin_reg.coef_
X_new=np.linspace(-3, 3, 100).reshape(100, 1)
X_new_poly = poly_features.transform(X_new)
y_new = lin_reg.predict(X_new_poly)
plt.plot(X, y, "b.")
plt.plot(X_new, y_new, "r-", linewidth=2, label="Predictions")
plt.xlabel("$x_1$", fontsize=18)
plt.ylabel("$y$", rotation=0, fontsize=18)
plt.legend(loc="upper left", fontsize=14)
plt.axis([-3, 3, 0, 10])
plt.show()
"""using pipeline"""
from sklearn.preprocessing import StandardScaler
from sklearn.pipeline import Pipeline
def polynomial_regression(degree, include_bias=True):
return Pipeline([
("poly_features", PolynomialFeatures(degree=degree, include_bias=False)),
("std_scaler", StandardScaler()),
("lin_reg", LinearRegression()),
])
poly_reg = polynomial_regression(degree=2)
poly_reg.fit(X, y)
X_new = np.linspace(-3, 3, 100).reshape(100, 1)
y_new = poly_reg.predict(X_new)
plt.plot(X, y, "b.")
plt.plot(X_new, y_new, "r-", linewidth=2, label="Predictions")
plt.xlabel("$x_1$", fontsize=18)
plt.ylabel("$y$", rotation=0, fontsize=18)
plt.legend(loc="upper left", fontsize=14)
plt.axis([-3, 3, 0, 10])
plt.show()
###Output
_____no_output_____ |
ELECTRAbase/addUNKtokens_lowercase/addUNKtokens_lowercase.ipynb | ###Markdown
Get data
###Code
df = pd.read_csv('./../../../labeledTweets/allLabeledTweets.csv')
df = df[['id', 'message', 'label']]
df = df.drop_duplicates()
print(df.shape[0])
df.head()
df['label'].value_counts()
newLine ="\\n|\\r"
urls = '(https?:\/\/(?:www\.|(?!www))[a-zA-Z0-9][a-zA-Z0-9-]+[a-zA-Z0-9]\.[^\s]{2,}|www\.[a-zA-Z0-9][a-zA-Z0-9-]+[a-zA-Z0-9]\.[^\s]{2,}|https?:\/\/(?:www\.|(?!www))[a-zA-Z0-9]+\.[^\s]{2,}|www\.[a-zA-Z0-9]+\.[^\s]{2,})'
numbers = '\d+((\.|\-)\d+)?'
mentions = '\B\@([\w\-]+)'
hashtag = '#'
whitespaces = '\s+'
leadTrailWhitespace = '^\s+|\s+?$'
df['clean_message'] = df['message']
df['clean_message'] = df['clean_message'].str.replace(newLine,' ',regex=True)
df['clean_message'] = df['clean_message'].str.replace(urls,' URL ',regex=True)
df['clean_message'] = df['clean_message'].str.replace(mentions,' MENTION ',regex=True)
df['clean_message'] = df['clean_message'].str.replace(numbers,' NMBR ',regex=True)
df['clean_message'] = df['clean_message'].str.replace(hashtag,' ',regex=True)
df['clean_message'] = df['clean_message'].str.replace(whitespaces,' ',regex=True)
df['clean_message'] = df['clean_message'].str.replace(leadTrailWhitespace,'',regex=True)
df.head()
###Output
_____no_output_____
###Markdown
Train, validate split (balanced)
###Code
df_0 = df[df['label']==0]
df_1 = df[df['label']==1]
df_2 = df[df['label']==2]
trainLabelSize = round(df_1.shape[0]*0.85)
trainLabelSize
df_0 = df_0.sample(trainLabelSize, random_state=42)
df_1 = df_1.sample(trainLabelSize, random_state=42)
df_2 = df_2.sample(trainLabelSize, random_state=42)
df_train = pd.concat([df_0, df_1, df_2])
# Shuffle rows
df_train = sklearn.utils.shuffle(df_train, random_state=42)
df_train['label'].value_counts()
df_val = df.merge(df_train, on=['id', 'message', 'label', 'clean_message'], how='left', indicator=True)
df_val = df_val[df_val['_merge']=='left_only']
df_val = df_val[['id', 'message', 'label', 'clean_message']]
df_val['label'].value_counts()
###Output
_____no_output_____
###Markdown
Tokenizer "google/electra-base-discriminator"
###Code
tokenizer = ElectraTokenizerFast.from_pretrained('google/electra-base-discriminator', do_lower_case=True)
###Output
_____no_output_____
###Markdown
Find popular UNK tokens
###Code
unk_tokens = []
for message in df.clean_message.values:
list_of_space_separated_pieces = message.strip().split()
ids = [tokenizer(piece, add_special_tokens=False)["input_ids"] for piece in list_of_space_separated_pieces]
unk_indices = [i for i, encoded in enumerate(ids) if tokenizer.unk_token_id in encoded]
unknown_strings = [piece for i, piece in enumerate(list_of_space_separated_pieces) if i in unk_indices]
for unk_str in unknown_strings:
unk_tokens.append(unk_str)
import collections
counter=collections.Counter(unk_tokens)
print(counter.most_common(100))
most_common_values= [word for word, word_count in counter.most_common(100)]
tokenizer.add_tokens(most_common_values, special_tokens=True)
###Output
_____no_output_____
###Markdown
Find max length for tokenizer
###Code
token_lens = []
for txt in list(df.clean_message.values):
tokens = tokenizer.encode(txt, max_length=512, truncation=True)
token_lens.append(len(tokens))
max_length = max(token_lens)
max_length
###Output
_____no_output_____
###Markdown
Encode messages
###Code
encoded_data_train = tokenizer.batch_encode_plus(
df_train["clean_message"].values.tolist(),
add_special_tokens=True,
return_attention_mask=True,
padding='max_length',
truncation=True,
max_length=max_length,
return_tensors='pt'
)
encoded_data_val = tokenizer.batch_encode_plus(
df_val["clean_message"].values.tolist(),
add_special_tokens=True,
return_attention_mask=True,
padding='max_length',
truncation=True,
max_length=max_length,
return_tensors='pt'
)
input_ids_train = encoded_data_train['input_ids']
attention_masks_train = encoded_data_train['attention_mask']
labels_train = torch.tensor(df_train.label.values)
input_ids_val = encoded_data_val['input_ids']
attention_masks_val = encoded_data_val['attention_mask']
labels_val = torch.tensor(df_val.label.values)
dataset_train = TensorDataset(input_ids_train, attention_masks_train, labels_train)
dataset_val = TensorDataset(input_ids_val, attention_masks_val, labels_val)
len(dataset_train), len(dataset_val)
###Output
_____no_output_____
###Markdown
Model "google/electra-base-discriminator"
###Code
model = ElectraForSequenceClassification.from_pretrained("google/electra-base-discriminator",
num_labels=3,
output_attentions=False,
output_hidden_states=False)
model.resize_token_embeddings(len(tokenizer))
from torch.utils.data import DataLoader, RandomSampler, SequentialSampler
batch_size = 32
dataloader_train = DataLoader(dataset_train, sampler=RandomSampler(dataset_train), batch_size=batch_size)
dataloader_validation = DataLoader(dataset_val, sampler=SequentialSampler(dataset_val), batch_size=batch_size)
from transformers import get_linear_schedule_with_warmup
optimizer = torch.optim.AdamW(model.parameters(), lr=1e-5, eps=1e-8)
# optimizer = torch.optim.SGD(model.parameters(), lr=0.0001)
epochs = 5
scheduler = get_linear_schedule_with_warmup(optimizer, num_warmup_steps=0, num_training_steps=len(dataloader_train)*epochs)
# Function to measure weighted F1
from sklearn.metrics import f1_score
def f1_score_func(preds, labels):
preds_flat = np.argmax(preds, axis=1).flatten()
labels_flat = labels.flatten()
return f1_score(labels_flat, preds_flat, average='weighted')
import random
seed_val = 17
random.seed(seed_val)
np.random.seed(seed_val)
torch.manual_seed(seed_val)
torch.cuda.manual_seed_all(seed_val)
# device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
device = torch.device('cpu')
model.to(device)
print(device)
# Function to evaluate model. Returns average validation loss, predictions, true values
def evaluate(dataloader_val):
model.eval()
loss_val_total = 0
predictions, true_vals = [], []
progress_bar = tqdm(dataloader_val, desc='Validating:', leave=False, disable=False)
for batch in progress_bar:
batch = tuple(b.to(device) for b in batch)
inputs = {'input_ids': batch[0], 'attention_mask': batch[1], 'labels': batch[2]}
with torch.no_grad():
outputs = model(**inputs)
loss = outputs[0]
logits = outputs[1]
loss_val_total += loss.item()
logits = logits.detach().cpu().numpy()
label_ids = inputs['labels'].cpu().numpy()
predictions.append(logits)
true_vals.append(label_ids)
loss_val_avg = loss_val_total/len(dataloader_val)
predictions = np.concatenate(predictions, axis=0)
true_vals = np.concatenate(true_vals, axis=0)
return loss_val_avg, predictions, true_vals
###Output
_____no_output_____
###Markdown
Evaluate untrained model
###Code
_, predictions, true_vals = evaluate(dataloader_validation)
from sklearn.metrics import classification_report, confusion_matrix
preds_flat = np.argmax(predictions, axis=1).flatten()
print(classification_report(true_vals, preds_flat))
print(f1_score_func(predictions, true_vals))
pd.DataFrame(confusion_matrix(true_vals, preds_flat),
index = [['actual', 'actual', 'actual'], ['neutral', 'positive', 'negative']],
columns = [['predicted', 'predicted', 'predicted'], ['neutral', 'positive', 'negative']])
###Output
_____no_output_____
###Markdown
Train
###Code
for epoch in tqdm(range(1, epochs+1)):
model.train()
loss_train_total = 0
progress_bar = tqdm(dataloader_train, desc='Epoch {:1d}'.format(epoch), leave=False, disable=False)
for batch in progress_bar:
model.zero_grad()
batch = tuple(b.to(device) for b in batch)
inputs = {'input_ids': batch[0], 'attention_mask': batch[1], 'labels': batch[2]}
outputs = model(**inputs)
loss = outputs[0]
loss_train_total += loss.item()
loss.backward()
torch.nn.utils.clip_grad_norm_(model.parameters(), 1.0)
optimizer.step()
scheduler.step()
progress_bar.set_postfix({'training_loss': '{:.3f}'.format(loss.item()/len(batch))})
torch.save(model.state_dict(), f'modelsUNK/finetuned_ELECTRAbase_epoch_{epoch}.model')
tqdm.write(f'\nEpoch {epoch}')
loss_train_avg = loss_train_total/len(dataloader_train)
tqdm.write(f'Training loss: {loss_train_avg}')
val_loss, predictions, true_vals = evaluate(dataloader_validation)
val_f1 = f1_score_func(predictions, true_vals)
tqdm.write(f'Validation loss: {val_loss}')
tqdm.write(f'F1 Score (Weighted): {val_f1}')
preds_flat = np.argmax(predictions, axis=1).flatten()
print('Classification report:')
print(classification_report(true_vals, preds_flat))
print('Confusion matrix:')
print(pd.DataFrame(confusion_matrix(true_vals, preds_flat),
index = [['actual', 'actual', 'actual'], ['neutral', 'positive', 'negative']],
columns = [['predicted', 'predicted', 'predicted'], ['neutral', 'positive', 'negative']]))
###Output
_____no_output_____
###Markdown
Evaluate best model
###Code
model.load_state_dict(torch.load('modelsBase/finetuned_ELECTRAbase_epoch_X.model', map_location=torch.device('cpu')))
_, predictions, true_vals = evaluate(dataloader_validation)
preds_flat = np.argmax(predictions, axis=1).flatten()
print(f1_score_func(predictions, true_vals))
print(classification_report(true_vals, preds_flat))
pd.DataFrame(confusion_matrix(true_vals, preds_flat),
index = [['actual', 'actual', 'actual'], ['neutral', 'positive', 'negative']],
columns = [['predicted', 'predicted', 'predicted'], ['neutral', 'positive', 'negative']])
###Output
_____no_output_____ |
src/automation/kallisto_bash_script_generator.ipynb | ###Markdown
Table of Contents
###Code
import os
import pandas as pd
# params
directory = '../input/rawseq'
length = 180
sigma = 60
btstrp = 200
thrds = 6
# sequences:
seqs = next(os.walk(directory))[1]
# params
directory = '../input/rawseq'
length = 180
sigma = 60
btstrp = 200
thrds = 6
# sequences:
seqs = next(os.walk(directory))[1]
def explicit_kallisto(directory, files, res_dir):
"""
TODO: Make a function that allows you to systematically
set up each parameter for each sequencing run individually.
"""
if type(directory) is not str:
raise ValueError('directory must be a str')
if type(files) is not list:
raise ValueError('files must be a list')
print('This sequence file contains a Kallisto_Info file\
and cannot be processed at the moment.')
return '# {0} could not be processed'.format(res_dir), ''
def implicit_kallisto(directory, files, res_dir):
"""
A function to write a Kallisto command with standard parameter
setup
"""
if type(directory) is not str:
raise ValueError('directory must be a str')
if type(files) is not list:
raise ValueError('files must be a list')
# parts of each kallisto statement
# information
info = '# kallisto command for {0}'.format(directory)
# transcript file location:
k_head = 'kallisto quant -i input/transcripts.idx -o '
# output file location
k_output = 'input/kallisto_all/' + res_dir + '/kallisto '
# parameter info:
k_params = '--single -s {0} -l {1} -b {2} -t {3} --bias --fusion'.format(sigma, length, btstrp, thrds)
# what files to use:
k_files = ''
# go through each file and add it to the command
# unless it's a SampleSheet.csv file, in which
# case you should ignore it.
for y in files:
if y != 'SampleSheet.csv':
if directory[:3] == '../':
d = directory[3:]
else:
d = directory[:]
k_files += ' '+ d + '/' + y
# all together now:
kallisto = k_head + k_output + k_params + k_files +';'
return info, kallisto
def walk_seq_directories(directory):
"""
Given a directory, walk through it,
find all the rna-seq repository folders
and generate kallisto commands
"""
kallisto = ''
#directory contains all the projects, walk through it:
for x in os.walk(directory):
# first directory is always parent
# if it's not the parent, move forward:
if x[0] != directory:
# cut the head off and get the project name:
res_dir = x[0][len(directory)+1:]
# if this project has attributes explicitly written in
# use those parameter specs:
if 'Kallisto_Info.csv' in x[2]:
info, command = explicit_kallisto(x[0], x[2], res_dir)
continue
# otherwise, best guesses:
info, command = implicit_kallisto(x[0], x[2], res_dir)
kallisto += info + '\n' + command + '\n'
if not os.path.exists('../input/kallisto_all/' + res_dir):
os.makedirs('../input/kallisto_all/' + res_dir)
return kallisto
with open('../kallisto_commands.sh', 'w') as f:
f.write('#!/bin/bash\n')
f.write('# make transcript index\n')
f.write('kallisto index -i input/transcripts.idx input/c_elegans_WBcel235.rel79.cdna.all.fa;\n')
kallisto = walk_seq_directories(directory)
f.write(kallisto)
###Output
_____no_output_____ |
archive/4-cliques-triangles-structures-student.ipynb | ###Markdown
Load DataAs usual, let's start by loading some network data. This time round, we have a [physician trust](http://konect.cc/networks/moreno_innovation) network, but slightly modified such that it is undirected rather than directed.> This directed network captures innovation spread among 246 physicians in for towns in Illinois, Peoria, Bloomington, Quincy and Galesburg. The data was collected in 1966. A node represents a physician and an edge between two physicians shows that the left physician told that the righ physician is his friend or that he turns to the right physician if he needs advice or is interested in a discussion. There always only exists one edge between two nodes even if more than one of the listed conditions are true.
###Code
# Load the network.
G = cf.load_physicians_network()
# Make a Circos plot of the graph
from nxviz import CircosPlot
c = CircosPlot(G)
c.draw()
###Output
_____no_output_____
###Markdown
QuestionWhat can you infer about the structure of the graph from the Circos plot? Structures in a GraphWe can leverage what we have learned in the previous notebook to identify special structures in a graph. In a network, cliques are one of these special structures. CliquesIn a social network, cliques are groups of people in which everybody knows everybody. **Questions:**1. What is the simplest clique?1. What is the simplest complex clique?Let's try implementing a simple algorithm that finds out whether a node is present in a simple complex clique.
###Code
# Example code.
def in_triangle(G, node):
"""
Returns whether a given node is present in a triangle relationship or not.
"""
# We first assume that the node is not present in a triangle.
is_in_triangle = False
# Then, iterate over every pair of the node's neighbors.
for nbr1, nbr2 in combinations(G.neighbors(node), 2):
# Check to see if there is an edge between the node's neighbors.
# If there is an edge, then the given node is present in a triangle.
if G.has_edge(nbr1, nbr2):
is_in_triangle = True
# We break because any triangle that is present automatically
# satisfies the problem requirements.
break
return is_in_triangle
in_triangle(G, 3)
###Output
_____no_output_____
###Markdown
In reality, NetworkX already has a function that *counts* the number of triangles that any given node is involved in. This is probably more useful than knowing whether a node is present in a triangle or not, but the above code was simply for practice.
###Code
nx.triangles(G, 3)
###Output
_____no_output_____
###Markdown
ExerciseCan you write a function that takes in one node and its associated graph as an input, and returns a list or set of itself + all other nodes that it is in a triangle relationship with? Do not return the triplets, but the `set`/`list` of nodes. (5 min.)**Possible Implementation:** If I check every pair of my neighbors, any pair that are also connected in the graph are in a triangle relationship with me.Hint: Python's [`itertools`](https://docs.python.org/3/library/itertools.html) module has a `combinations` function that may be useful.Hint: NetworkX graphs have a `.has_edge(node1, node2)` function that checks whether an edge exists between two nodes.Verify your answer by drawing out the subgraph composed of those nodes.
###Code
# Possible answer
def get_triangles(G, node):
neighbors = set(G.neighbors(node))
triangle_nodes = set()
"""
Fill in the rest of the code below.
"""
return triangle_nodes
# Verify your answer with the following funciton call. Should return something of the form:
# {3, 9, 11, 41, 42, 67}
get_triangles(G, 3)
# Then, draw out those nodes.
nx.draw(G.subgraph(get_triangles(G, 3)), with_labels=True)
# Compare for yourself that those are the only triangles that node 3 is involved in.
neighbors3 = G.neighbors(3)
neighbors3.append(3)
nx.draw(G.subgraph(neighbors3), with_labels=True)
###Output
_____no_output_____
###Markdown
Friend Recommendation: Open TrianglesNow that we have some code that identifies closed triangles, we might want to see if we can do some friend recommendations by looking for open triangles.Open triangles are like those that we described earlier on - A knows B and B knows C, but C's relationship with A isn't captured in the graph. What are the two general scenarios for finding open triangles that a given node is involved in?1. The given node is the centre node.1. The given node is one of the termini nodes. ExerciseCan you write a function that identifies, for a given node, the other two nodes that it is involved with in an open triangle, if there is one? (5 min.)Note: For this exercise, only consider the case when the node of interest is the centre node.**Possible Implementation:** Check every pair of my neighbors, and if they are not connected to one another, then we are in an open triangle relationship.
###Code
# Fill in your code here.
def get_open_triangles(G, node):
"""
There are many ways to represent this. One may choose to represent only the nodes involved
in an open triangle; this is not the approach taken here.
Rather, we have a code that explicitly enumrates every open triangle present.
"""
open_triangle_nodes = []
neighbors = set(G.neighbors(node))
for n1, n2 in combinations(G.neighbors(node), 2):
...
return open_triangle_nodes
# # Uncomment the following code if you want to draw out each of the triplets.
# nodes = get_open_triangles(G, 2)
# for i, triplet in enumerate(nodes):
# fig = plt.figure(i)
# nx.draw(G.subgraph(triplet), with_labels=True)
print(get_open_triangles(G, 3))
len(get_open_triangles(G, 3))
###Output
_____no_output_____
###Markdown
Triangle closure is also the core idea behind social networks' friend recommendation systems; of course, it's definitely more complicated than what we've implemented here. CliquesWe have figured out how to find triangles. Now, let's find out what **cliques** are present in the network. Recall: what is the definition of a clique?- NetworkX has a [clique-finding](https://networkx.github.io/documentation/networkx-1.10/reference/generated/networkx.algorithms.clique.find_cliques.html) algorithm implemented.- This algorithm finds all maximally-sized cliques for a given node.- Note that maximal cliques of size `n` include all cliques of `size < n`
###Code
list(nx.find_cliques(G))
###Output
_____no_output_____
###Markdown
ExerciseTry writing a function `maximal_cliques_of_size(size, G)` that implements a search for all maximal cliques of a given size. (3 min.)
###Code
def maximal_cliqes_of_size(size, G):
return ______________________
maximal_cliqes_of_size(2, G)
###Output
_____no_output_____
###Markdown
Connected ComponentsFrom [Wikipedia](https://en.wikipedia.org/wiki/Connected_component_%28graph_theory%29):> In graph theory, a connected component (or just component) of an undirected graph is a subgraph in which any two vertices are connected to each other by paths, and which is connected to no additional vertices in the supergraph.NetworkX also implements a [function](https://networkx.github.io/documentation/networkx-1.9.1/reference/generated/networkx.algorithms.components.connected.connected_component_subgraphs.html) that identifies connected component subgraphs.Remember how based on the Circos plot above, we had this hypothesis that the physician trust network may be divided into subgraphs. Let's check that, and see if we can redraw the Circos visualization.
###Code
ccsubgraph_nodes = list(nx.connected_components(G))
ccsubgraph_nodes
###Output
_____no_output_____
###Markdown
ExerciseDraw a circos plot of the graph, but now colour and order the nodes by their connected component subgraph. (5 min.)Recall Circos API:```pythonc = CircosPlot(G, node_order='...', node_color='...')c.draw()plt.show() or plt.savefig(...)```
###Code
# Start by labelling each node in the master graph G by some number
# that represents the subgraph that contains the node.
for i, g in enumerate(_____________):
# Fill in code below.
c = CircosPlot(G, _________)
c.draw()
plt.savefig('images/physicians.png', dpi=300)
###Output
_____no_output_____
###Markdown
Load DataAs usual, let's start by loading some network data. This time round, we have a [physician trust](http://konect.uni-koblenz.de/networks/moreno_innovation) network, but slightly modified such that it is undirected rather than directed.> This directed network captures innovation spread among 246 physicians in for towns in Illinois, Peoria, Bloomington, Quincy and Galesburg. The data was collected in 1966. A node represents a physician and an edge between two physicians shows that the left physician told that the righ physician is his friend or that he turns to the right physician if he needs advice or is interested in a discussion. There always only exists one edge between two nodes even if more than one of the listed conditions are true.
###Code
# Load the network.
G = cf.load_physicians_network()
# Make a Circos plot of the graph
from nxviz import CircosPlot
c = CircosPlot(G)
c.draw()
###Output
_____no_output_____
###Markdown
QuestionWhat can you infer about the structure of the graph from the Circos plot? Structures in a GraphWe can leverage what we have learned in the previous notebook to identify special structures in a graph. In a network, cliques are one of these special structures. CliquesIn a social network, cliques are groups of people in which everybody knows everybody. **Questions:**1. What is the simplest clique?1. What is the simplest complex clique?Let's try implementing a simple algorithm that finds out whether a node is present in a simple complex clique.
###Code
# Example code.
def in_triangle(G, node):
"""
Returns whether a given node is present in a triangle relationship or not.
"""
# We first assume that the node is not present in a triangle.
is_in_triangle = False
# Then, iterate over every pair of the node's neighbors.
for nbr1, nbr2 in combinations(G.neighbors(node), 2):
# Check to see if there is an edge between the node's neighbors.
# If there is an edge, then the given node is present in a triangle.
if G.has_edge(nbr1, nbr2):
is_in_triangle = True
# We break because any triangle that is present automatically
# satisfies the problem requirements.
break
return is_in_triangle
in_triangle(G, 3)
###Output
_____no_output_____
###Markdown
In reality, NetworkX already has a function that *counts* the number of triangles that any given node is involved in. This is probably more useful than knowing whether a node is present in a triangle or not, but the above code was simply for practice.
###Code
nx.triangles(G, 3)
###Output
_____no_output_____
###Markdown
ExerciseCan you write a function that takes in one node and its associated graph as an input, and returns a list or set of itself + all other nodes that it is in a triangle relationship with? Do not return the triplets, but the `set`/`list` of nodes. (5 min.)**Possible Implementation:** If I check every pair of my neighbors, any pair that are also connected in the graph are in a triangle relationship with me.Hint: Python's [`itertools`](https://docs.python.org/3/library/itertools.html) module has a `combinations` function that may be useful.Hint: NetworkX graphs have a `.has_edge(node1, node2)` function that checks whether an edge exists between two nodes.Verify your answer by drawing out the subgraph composed of those nodes.
###Code
# Possible answer
def get_triangles(G, node):
neighbors = set(G.neighbors(node))
triangle_nodes = set()
"""
Fill in the rest of the code below.
"""
return triangle_nodes
# Verify your answer with the following funciton call. Should return something of the form:
# {3, 9, 11, 41, 42, 67}
get_triangles(G, 3)
# Then, draw out those nodes.
nx.draw(G.subgraph(get_triangles(G, 3)), with_labels=True)
# Compare for yourself that those are the only triangles that node 3 is involved in.
neighbors3 = G.neighbors(3)
neighbors3.append(3)
nx.draw(G.subgraph(neighbors3), with_labels=True)
###Output
_____no_output_____
###Markdown
Friend Recommendation: Open TrianglesNow that we have some code that identifies closed triangles, we might want to see if we can do some friend recommendations by looking for open triangles.Open triangles are like those that we described earlier on - A knows B and B knows C, but C's relationship with A isn't captured in the graph. What are the two general scenarios for finding open triangles that a given node is involved in?1. The given node is the centre node.1. The given node is one of the termini nodes. ExerciseCan you write a function that identifies, for a given node, the other two nodes that it is involved with in an open triangle, if there is one? (5 min.)Note: For this exercise, only consider the case when the node of interest is the centre node.**Possible Implementation:** Check every pair of my neighbors, and if they are not connected to one another, then we are in an open triangle relationship.
###Code
# Fill in your code here.
def get_open_triangles(G, node):
"""
There are many ways to represent this. One may choose to represent only the nodes involved
in an open triangle; this is not the approach taken here.
Rather, we have a code that explicitly enumrates every open triangle present.
"""
open_triangle_nodes = []
neighbors = set(G.neighbors(node))
for n1, n2 in combinations(G.neighbors(node), 2):
...
return open_triangle_nodes
# # Uncomment the following code if you want to draw out each of the triplets.
# nodes = get_open_triangles(G, 2)
# for i, triplet in enumerate(nodes):
# fig = plt.figure(i)
# nx.draw(G.subgraph(triplet), with_labels=True)
print(get_open_triangles(G, 3))
len(get_open_triangles(G, 3))
###Output
_____no_output_____
###Markdown
Triangle closure is also the core idea behind social networks' friend recommendation systems; of course, it's definitely more complicated than what we've implemented here. CliquesWe have figured out how to find triangles. Now, let's find out what **cliques** are present in the network. Recall: what is the definition of a clique?- NetworkX has a [clique-finding](https://networkx.github.io/documentation/networkx-1.10/reference/generated/networkx.algorithms.clique.find_cliques.html) algorithm implemented.- This algorithm finds all maximally-sized cliques for a given node.- Note that maximal cliques of size `n` include all cliques of `size < n`
###Code
list(nx.find_cliques(G))
###Output
_____no_output_____
###Markdown
ExerciseTry writing a function `maximal_cliques_of_size(size, G)` that implements a search for all maximal cliques of a given size. (3 min.)
###Code
def maximal_cliqes_of_size(size, G):
return ______________________
maximal_cliqes_of_size(2, G)
###Output
_____no_output_____
###Markdown
Connected ComponentsFrom [Wikipedia](https://en.wikipedia.org/wiki/Connected_component_%28graph_theory%29):> In graph theory, a connected component (or just component) of an undirected graph is a subgraph in which any two vertices are connected to each other by paths, and which is connected to no additional vertices in the supergraph.NetworkX also implements a [function](https://networkx.github.io/documentation/networkx-1.9.1/reference/generated/networkx.algorithms.components.connected.connected_component_subgraphs.html) that identifies connected component subgraphs.Remember how based on the Circos plot above, we had this hypothesis that the physician trust network may be divided into subgraphs. Let's check that, and see if we can redraw the Circos visualization.
###Code
ccsubgraph_nodes = list(nx.connected_components(G))
ccsubgraph_nodes
###Output
_____no_output_____
###Markdown
ExerciseDraw a circos plot of the graph, but now colour and order the nodes by their connected component subgraph. (5 min.)Recall Circos API:```pythonc = CircosPlot(G, node_order='...', node_color='...')c.draw()plt.show() or plt.savefig(...)```
###Code
# Start by labelling each node in the master graph G by some number
# that represents the subgraph that contains the node.
for i, g in enumerate(_____________):
# Fill in code below.
c = CircosPlot(G, _________)
c.draw()
plt.savefig('images/physicians.png', dpi=300)
###Output
_____no_output_____ |
playground/keras_backend_functions.ipynb | ###Markdown
1. Setup
###Code
import keras.backend as K
import numpy as np
import tensorflow as tf
sess = tf.Session()
###Output
_____no_output_____
###Markdown
2. K.abs()
###Code
a = [-1, 4, -2, 5, 0]
print(K.abs(a).eval(session=sess))
###Output
[1 4 2 5 0]
###Markdown
3. K.mean()
###Code
a = [-1., 4., -2., 5., 0.]
a = tf.convert_to_tensor(a)
print(K.mean(a).eval(session=sess))
print(K.mean(K.abs(a)).eval(session=sess))
a = [
[-1., 4., -2., 5., 0.],
[-1., 4., -2., 5., 0.],
]
a = tf.convert_to_tensor(a)
print(K.mean(a).eval(session=sess))
print(K.mean(a, axis=None).eval(session=sess))
print(K.mean(a, axis=-1).eval(session=sess))
###Output
1.2
1.2
[1.2 1.2]
|
HW4/HW4_plotting.ipynb | ###Markdown
Data-X Spring 2019: Homework 04 Name: Shun Lin SID: 26636176In this homework, you will do some exercises with plotting. REMEMBER TO DISPLAY ALL OUTPUTS. If the question asks you to do something, make sure to print your results. 1. Data:__Data Source__:Data file is uploaded to bCourses and is named: __Energy.csv__The dataset was created by Angeliki Xifara ( Civil/Structural Engineer) and was processed by Athanasios Tsanas, Oxford Centre for Industrial and Applied Mathematics, University of Oxford, UK).__Data Description__:The dataset contains eight attributes of a building (or features, denoted by X1...X8) and response being the heating load on the building, y1. * X1 Relative Compactness * X2 Surface Area * X3 Wall Area * X4 Roof Area * X5 Overall Height * X6 Orientation * X7 Glazing Area * X8 Glazing Area Distribution * y1 Heating Load Q1.1Read the data file in python. Check if there are any NaN values, and print the results.
###Code
# your code
# Load required modules
import pandas as pd
import numpy as np
import seaborn as sns
import matplotlib.pyplot as plt
df=pd.read_csv('Energy.csv')
sum_of_nans = sum(len(df) - df.count())
print("There are " + str(sum_of_nans) + " Nan values in the dataframe")
df.head()
###Output
There are 0 Nan values in the dataframe
###Markdown
Q 1.2 Describe (using python function) data features in terms of type, distribution range (max and min), and mean values.
###Code
# your code
df.describe(include='all')
###Output
_____no_output_____
###Markdown
Q 1.3Plot feature distributions for all the attributes in the dataset (Hint - Histograms are one way to plot data distributions). This step should give you clues about data sufficiency.
###Code
# your code
plot1_3 = df.hist(figsize=(10, 10))
###Output
_____no_output_____
###Markdown
Q1.4Create a combined line and scatter plot for attributes 'X1' and 'X2' with a marker (*). You can choose either of the attributes as x & y. Label your axes and give a title to your plot.
###Code
# your code
plot1_4 = df.plot(x='X1', y='X2', style='*-', title="X1 vs X2 (Relative Compactness VS Surface Area)", legend=False)
plot1_4.set_xlabel("X1 (Relative Compactness)")
plot1_4.set_ylabel("X2 (Surface Area)")
###Output
_____no_output_____
###Markdown
Q1.5Create a scatter plot for how 'Wall Area' changes with 'Relative Compactness'. Give different colors for different 'Overall Height' and different bubble sizes by 'Roof Area'. Label the axes and give a title. Add a legend to your plot.
###Code
# your code
wall_area = df["X3"]
relative_compactness = df["X1"]
overall_height = df["X5"]
max_overall_height = max(overall_height)
roof_area = df["X4"]
# create plots
f, ax = plt.subplots(figsize=(10, 10))
colors_list = ["green", "blue"]
lines_map = ()
heights_map = ()
for i, height in enumerate(overall_height.unique()):
color = colors_list[i]
x = df[overall_height == height]["X1"]
y = df[overall_height == height]["X3"]
sizes = df[overall_height == height]["X4"]
lines_map = lines_map + (ax.scatter(x, y, c=color, s=sizes),)
heights_map = heights_map + (height,)
ax.set_ylabel("wall area")
ax.set_xlabel("relative compactness")
ax.set_title("Wall Area vs Relative Compactness", fontsize=20, fontweight="bold")
ax.legend(lines_map, heights_map, loc="best")
###Output
_____no_output_____
###Markdown
2. Q 2.1a. Create a dataframe called `icecream` that has column `Flavor` with entries `Strawberry`, `Vanilla`, and `Chocolate` and another column with `Price` with entries `3.50`, `3.00`, and `4.25`. Print the dataframe.
###Code
# your code
icecream = pd.DataFrame([["Strawberry", 3.5], ["Vanilla", 3.00],["Chocolate", 4.25]], columns=['Flavor', 'Price'])
print(icecream)
###Output
Flavor Price
0 Strawberry 3.50
1 Vanilla 3.00
2 Chocolate 4.25
###Markdown
Q 2.1b Create a bar chart representing the three flavors and their associated prices. Label the axes and give a title.
###Code
# your code
plot2_1b = icecream.plot.bar(x="Flavor", y="Price", title="Flavor vs price")
plot2_1b.set_ylabel("Price")
###Output
_____no_output_____
###Markdown
Q 2.2 Create 9 random plots in a figure (Hint: There is a numpy function for generating random data). The top three should be scatter plots (one with green dots, one with purple crosses, and one with blue triangles. The middle three graphs should be a line graph, a horizontal bar chart, and a histogram. The bottom three graphs should be trignometric functions (one sin, one cosine, one tangent). Keep in mind the range and conditions for the trignometric functions. All these plots should be on the same figure and not 9 independent figures.
###Code
# your code
# generate different random data for each plot
random_data = {}
for i in range(1, 10):
random_x = 10 * np.random.normal(size=400)
if i >= 7:
random_x = np.random.uniform(-np.pi, np.pi, 1000)
if i == 7:
random_y = np.sin(random_x)
elif i == 8:
random_y = np.cos(random_x)
elif i == 9:
eps = 0.05
random_x = np.random.uniform(-np.pi/2+eps, np.pi/2 - eps, 1000)
random_y = np.tan(random_x)
else:
random_y = 10 * np.random.normal(size=400)
random_data[i] = {}
random_data[i]["x"] = random_x
random_data[i]["y"] = random_y
# create plots
f, ax = plt.subplots(nrows=3,ncols=3, figsize=(20, 20))
# top three plots
ax[0,0].scatter(random_data[1]["x"], random_data[1]["y"], marker=".", c="green")
ax[0,1].scatter(random_data[2]["x"], random_data[2]["y"], marker="+", c="purple")
ax[0,2].scatter(random_data[3]["x"], random_data[3]["y"], marker="^", c="blue")
# middle three plots
ax[1,0].plot(random_data[4]["x"], random_data[4]["y"], color="green")
ax[1,1].barh(random_data[5]["x"], random_data[5]["y"], color="purple")
ax[1,2].hist(random_data[6]["x"], color="blue")
# bottom three plots
# sin plot
ax[2,0].scatter(random_data[7]["x"], random_data[7]["y"], c="green")
# cos plot
ax[2,1].scatter(random_data[8]["x"], random_data[8]["y"], c = "purple")
# tangent plot
ax[2,2].scatter(random_data[9]["x"], random_data[9]["y"], c="blue")
###Output
_____no_output_____
###Markdown
3. Q 3.1Load the 'Iris' dataset using seaborn. Create a box plot for the attributes 'sepal_length', sepal_width', 'petal_length' and 'petal_width' in the Iris dataset.
###Code
# your code
iris = sns.load_dataset("iris")
iris.boxplot(column=["sepal_length", "sepal_width", "petal_length", "petal_width"])
###Output
_____no_output_____
###Markdown
Q 3.2In a few sentences explain what can you interpret from the above box plot.
###Code
solution_text = "The box plot above shows that there are some outliers from the sepal_width column, that the mean for sepal_length is the highest while the mean for petal_width is the smallest. We can also see that sepal_width has less spread (values are closer to one another), while petal_length has the most spread and values varies from one another. From those box plots we can also see that sepal_length has the highest value while petal_width has the lowest value."
print(solution_text)
###Output
The box plot above shows that there are some outliers from the sepal_width column, that the mean for sepal_length is the highest while the mean for petal_width is the smallest. We can also see that sepal_width has less spread (values are closer to one another), while petal_length has the most spread and values varies from one another. From those box plots we can also see that sepal_length has the highest value while petal_width has the lowest value.
###Markdown
Q 4.The data files needed: `google_data.txt, ny_temps.txt & yahoo_data.txt`Use your knowledge with `Python, NumPy, pandas and matplotlib` to reproduce the plot below:
###Code
# your code
# import data
google_data = pd.read_csv('google_data.txt',sep="\t")
yahoo_data = pd.read_csv('yahoo_data.txt',sep="\t")
ny_temps = pd.read_csv('ny_temps.txt',sep='\t')
f3, ax3 = plt.subplots(figsize=(12, 12))
# plot yahoo and google
l1, = ax3.plot(yahoo_data["Modified Julian Date"], yahoo_data["Stock Value"], color="purple", label='Yahoo! Stock Value')
l2, = ax3.plot(google_data["Modified Julian Date"], google_data["Stock Value"], color="green", label="Google Stock Value")
# set left y label
ax3.set_ylabel("Value (Dollars)",color="purple", labelpad=20, fontsize=15)
# set x label and size
ax3.set_xlabel("Date (MJD)", labelpad=20, fontsize=15)
# set title
ax3.set_title("New York Temperature, Google, and Yahoo!", pad=25, fontsize=20, fontweight="bold")
# turn on left minor tick
plt.minorticks_on()
# create right axis for ny temperature
ax4 = ax3.twinx()
# plot ny_temperature
l3, = ax4.plot(ny_temps["Modified Julian Date"], ny_temps["Max Temperature"], "--", color="blue", label="NY Mon. High Temp")
# set right y label
ax4.set_ylabel("Temperature (°F)",color="blue", labelpad=20, fontsize=15)
# set left and right ticks color
ax3.tick_params("y", colors="green")
ax4.tick_params("y", colors="blue")
# set lengends
plt.legend((l1,l2, l3), ('Yahoo! Stock Value', "Google Stock Value", "NY Mon. High Temp"), loc=[0.01,0.5], frameon=False, prop={'size': 12})
# set right y axis tick range
plt.yticks(np.arange(-150, 150, 50))
# turn on right minor ticks
plt.minorticks_on()
###Output
_____no_output_____ |
notebook/1_big_picture.ipynb | ###Markdown
AI Based Investment Process  จากภาพข้างต้นรวบรวมข้อมูลแบบเดียวกัน แต่เป็นของ Crypto Currency - Market Place - Social Trading - Steaming Pro (Robinhood.com) Crypto จากเงินต้น 10k เดือนถัดไปเก็บเพิ่มเดือนละ 10k ได้เดือนละ 50% ผ่านไป 1 ปี จะมีเงินเก็บดังนี้
###Code
x0 = 10*1000
y = 10*1000
n = 1*12
x = x0
for i in range(n):
x = x + 0.3*x + y
print('เงินต้น :',x0+n*y)
print('ผลลัพธ์ :',x)
print('ส่วนต่าง :',x-x0-n*y)
###Output
เงินต้น : 130000
ผลลัพธ์ : 976250.35530751
ส่วนต่าง : 846250.35530751
###Markdown
ถ้าถอนมา 2.75M เหลือเงินต้น 2M และถอนมาใช้เดือนละ 50k จะได้
###Code
x20 = 2000000
y2 = -50000
time = 24
x2 = x20
for i in range(24):
x2 = x2 + 0.2*x2 + y2
print('เงินต้น :',x20)
print('ผลลัพธ์ :',x2)
print('ส่วนต่าง :',x2-x20)
###Output
เงินต้น : 2000000
ผลลัพธ์ : 139369482.60593396
ส่วนต่าง : 137369482.60593396
###Markdown
จากเงินต้น 10k วันละ 3%
###Code
x0 = 10*1000
n = 365
x = 10*1000
for i in range(n):
x = x + 0.03*x
print('เงินต้น :',x0)
print('ผลลัพธ์ :', round(x/(10**6),2), "M")
print('ส่วนต่าง :',round((x-x0)/(10**6),2), "M")
###Output
เงินต้น : 10000
ผลลัพธ์ : 484.83 M
ส่วนต่าง : 484.82 M
###Markdown
Goal - เงินต้น 15k - +1%/day- trigger every 15min
###Code
initial_money = 15000
interest = 0.01
number_of_day = 365
x = initial_money
for i in range(number_of_day):
x = x + interest*x
print('เงินต้น :',initial_money)
print('ผลลัพธ์ :',x)
print('ส่วนต่าง :',x - initial_money)
###Output
เงินต้น : 15000
ผลลัพธ์ : 566751.5149933076
ส่วนต่าง : 551751.5149933076
###Markdown
หุ้น เก็บปีแรก 100k ปีถัดไปเก็บเพิ่มปีละ 100k ผ่านไป 25 ปี (อายุ 50) จะมีเงินเก็บดังนี้
###Code
principal0 = 100*1000
annual_add = 100*1000
year = 50-24
all_principal = principal0
all_money = principal0
interest = 0.12
for i in range(year):
all_principal = all_principal + i*annual_add
all_money = (all_money + i*annual_add)*(1+interest)
print('เงินต้น :', round(all_principal/(10**6), 2), 'M')
print('ผลลัพธ์ :', round(all_money/(10**6), 2), 'M')
print('ส่วนต่าง :', round((all_money-all_principal)/(10**6), 2),'M')
###Output
เงินต้น : 32.6 M
ผลลัพธ์ : 117.95 M
ส่วนต่าง : 85.35 M
|
02_Dimensional_analysis.ipynb | ###Markdown
Universidade Federal do Rio Grande do Sul (UFRGS) Programa de Pós-Graduação em Engenharia Civil (PPGEC) PEC00144: Experimental Methods in Civil Engineering Part I: Analysis[2. Dimensional analysis and similarity](section_2) [2.1. Quantities, units, dimensions](section_21) [2.2. Dataframe for scales computation](section_22) [2.3. The Theorem of Pi's by Vaschy-Buckinghan](section_23) [2.4. Change of base units](section_24) [2.5. Change of units is like change of scale](section_25) [2.6. Famous Pi-Numbers](section_26) [2.7. Assignment](section_27) ---_Prof. Marcelo M. Rocha, Dr.techn._ [(ORCID)](https://orcid.org/0000-0001-5640-1020) _Porto Alegre, RS, Brazil_
###Code
# Importing Python modules required for this notebook
# (this cell must be executed with "shift+enter" before any other Python cell)
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
###Output
_____no_output_____
###Markdown
2. Dimensional analysis and similarity 2.1. Quantities, units, dimensions Prof. G.I. Barenblatt has written in his book _Dimensional Analysis_ (1987): >_In fact, the idea on which dimensional analysis is based is very simple, and can be understood by all: physical laws do not depend on arbitrariness in the choice of basic units of measurement. An important conclusion can be drawn from this simple idea using simple arguments: the functions that express physical laws must possess a certain fundamental property, which in mathematics is called generalized homogeneity or symmetry. This property allows the number of arguments in these functions to be reduced, thereby making it simpler to obtain them (by calculating them or determining them experimentally). This is, in fact, the entire content of dimensional analysis - there is nothing more to it._In other words, the units resulting from both sides of an equation must be the same.Although simple, this idea has allowed great scientists like Newton, Rayleigh, Maxwell, Reynolds, Kolmogorov, Cauchy, and many others, to proposed many fundamental physical principles.To illustrate how the law of _dimensional homogeneity_ can be applied, let us investigate the free vibration frequency, $f_{\rm n}$, of an undamped mass-spring system with one degree of freedom.The frequency $f_{\rm n}$ could be derived from mechanical principles like dynamicequilibrium equations, but we take the alternative dimensional path instead.The system above _may_ involve several physical quantities like: * the instantaneous position, $u$, * the oscillating mass, $m$, * the time, $t$, * the displacement amplitude, $u_0$, * the spring linear stiffness, $k$, and also * the free vibration frequency, $f_{\rm n}$. The completeness of this set of quantities is essential for the success of ourdimensional analysis. The analysis process requires an investigation of possiblerelationships among the _candidate_ quantities above and the frequency $f_{\rm n}$. Let us take a look at their _units of measurement_: \begin{align*}[ u ] &= [L]^{1}[M]^{0}[T]^{0} \\[ m ] &= [L]^{0}[M]^{1}[T]^{0} \\[ t ] &= [L]^{0}[M]^{0}[T]^{1} \\[ u_0 ] &= [L]^{1}[M]^{0}[T]^{0} \\[ k ] &= [L]^{0}[M]^{1}[T]^{-2} \\[ f_{\rm n}] &= [L]^{0}[M]^{0}[T]^{-1}\end{align*}where the brackets mean "units of". One can observe that all measurement units have beenwritten in terms of only three _fundamental units_: length, $[L]$, mass, $[M]$, and time, $[T]$. Most principles in solid mechanics and structural dynamics may be expressed with these three units only. Eventually the temperature, $\Theta$, may be also required.For the sake of simplicity, hereafter brackets will be ommited.The quantities directly subordinated to the fundamental units are called _fundamentalquantities_, which are the length, the mass and the time, while other units and quantitiesare called _derived_ units and quantities, like stiffness, frequency, acceleration, etc. The dependency of derived units on the fundamental units are expressed through _dimensional formulae_, as shown above, which are always _power-law monomials_. The exponents applyed to the fundamental units in these formulae are called _dimensions_ or _dimension exponents_.In the case of using the _International System_ (SI), the three fundamental units are themeter (m), the kilogram (kg), and the second (s). In the example above this means that:\begin{align*}[ u ] &= {\rm m}^{1}{\rm kg}^{0}{\rm s}^{0} = {\rm m} \\[ m ] &= {\rm m}^{0}{\rm kg}^{1}{\rm s}^{0} = {\rm kg} \\[ t ] &= {\rm m}^{0}{\rm kg}^{0}{\rm s}^{1} = {\rm s} \\[ u_0 ] &= {\rm m}^{1}{\rm kg}^{0}{\rm s}^{0} = {\rm m} \\[ k ] &= {\rm m}^{0}{\rm kg}^{1}{\rm s}^{-2} = {\rm kg}/{\rm s}^{2} = {\rm N/m} \\[ f_{\rm n}] &= {\rm m}^{0}{\rm kg}^{0}{\rm s}^{-1} = 1/{\rm s} = {\rm Hz}\end{align*} 2.2. Dataframe for scales computation To facilitate the scales calculations as presented in this _notebook_, let us now import a ``pandas`` dataframe with physical quantities commonly used in engineering mechanics andstructural dynamics, aerodynamics, and fluid and soil mechanics:
###Code
DimData = pd.read_excel('resources/DimData.xlsx',
index_col = 0,
sheet_name = 'DimData')
DimData
###Output
_____no_output_____
###Markdown
Other quantities may be easily included in this dataframe by editing the Excel file``DimData.xlsx``. Each entry in the table corresponds to a dimensional quantity, being the lastthree columns the respective dimension exponents in the dimensional formulae.Quantities like angles, strains, Poison's coefficient and ratio of critical damping areby definition non-dimensional, so there is no need of including them in this table.Relations between length measures, the so-called _form factors_, must also not be included.Observe that, for the sake of clearness, we are using Unicodevariable names, like greek letters, which is a remarkable Python's feature.To access the $LMT$ exponents of a set of quantities, let us say the ones selected forthe oscillator problem above, the dataframe ```loc``` view may be used:
###Code
par = ['L', 'm', 't', 'L', 'k', 'f'] # selected dimensional quantities
LMT = ['L', 'M', 'T'] # three last columns of dataframe
DimMat = DimData.loc[par, LMT] # the dimensional matrix
DimMat
###Output
_____no_output_____
###Markdown
According to ``pandas`` module, a subset of a dataframe is itself also a dataframe.If only the dimension exponents are to be retrieved, the attribute ``values`` must be called,returning a ``numpy`` 2D array.This array will be required in the following sections, where we show how the information provided in the dataframe ``DimData`` can be used to carry out units and scales changes for designing reduced scale models.
###Code
print(DimMat.values)
###Output
[[ 1 0 0]
[ 0 1 0]
[ 0 0 1]
[ 1 0 0]
[ 0 1 -2]
[ 0 0 -1]]
###Markdown
Observe that the dataframe indices are the codes to access a specific quantity, while the following first column, which header is ``descriptor``, corresponds to a description to help identification. The dataframe also brings a column named ``latex`` with the $\LaTeX$ code for eventual automaticplot labelling. 2.3. The Vaschy-Buckingham's $\Pi$-Theorem The Vaschy-Buckingham's $\Pi$-Theorem may be expressed as follows:>Let us assume that a given physical phenomenon involves $n$ _dimensional quantities_, denoted as $p_1, p_2, \dots, p_n$. For this phenomenon a mathematical model may be expressed as a general relation:\begin{equation} f\left ( p_1, p_2, \dots, p_n \right ) = 0 \end{equation}>This relation may be replaced by another one with $r = n - k$ _non-dimensional quantities_:\begin{equation} F\left ( \Pi_1, \Pi_2, \dots, \Pi_r \right ) = 0\end{equation}where $k$ is the number of fundamental units required to compose the units of all dimensional quantities involved. The $\Pi$-numbers have the general form:\begin{equation}\Pi = p_1^{\alpha_1} \; p_2^{\alpha_2} \; \dots \; p_n^{\alpha_n}\end{equation}where the exponents $\vec{\alpha} = \left [ \alpha_1 \; \alpha_2 \; \dots \; \alpha_n \right ]$ are such that the condition of non-dimensionality must always be fulfilled.Recalling that in the present context the only required fundamental units are $L$, $M$, and $T$, then $k = 3$. A demonstration of this theorem can be found in the literatura and will not bereplicated here.To apply this theorem, let us assemble a _dimensional matrix_, $\bf D$, with one column foreach fundamental unit and one row for each quantity involved in the analysis. Thedataframe ``DimMat`` defined in the previous section is an computational example of a dimensional matrix. To build up $\Pi$-numbers we propose now some vector, $\vec{\alpha}$, such that (for $k = 3$):\begin{equation}\vec{\alpha}\,{\bf D} = \left[0 \hspace{5mm} 0 \hspace{5mm} 0 \right]\end{equation}There is no general rule for specifying $\vec{\alpha}$.The practical principle that guides any proposition is some possible physical meaning.If the dimensional analysis subject is not well known, relevant $\Pi$-numbers willalways result from some educated guess.Even the choice of a relevant set of quantities is not always obvious, for it mayboth exceed or lack completeness. At this point, one can clearly see that _dimensional analysis is not a panacea for model proposition_ and a good measure of experience, or even luck, may be required.Going back to the oscillator problem, possible $\Pi$-numbers for the involved quantities are:\begin{align*} \Pi_1 &= u^1 u_0^{-1} \\ \Pi_2 &= t^1 \; f_{\rm n}^{1} \\ \Pi_3 &= m^{-1/2} \; k^{1/2} \; f_{\rm n}^{-1} \end{align*}where we can se that $\Pi_3$ is clearly the most interesting, with $$\vec{\alpha}_3 = \left [ 0 \;\; {-1/2} \;\;\; 0 \;\;\; 0 \;\; 1/2 \;\; {-1} \right]$$The product $\vec{\alpha}_3{\bf D}$ evidentiates its non-dimensionality:
###Code
α3 = np.array([0, -1/2, 0, 0, 1/2, -1])
print(np.matmul(α3, DimMat.values))
###Output
[0. 0. 0.]
###Markdown
Representing physical phenomena as relationships among non-dimensional quantities certainly implies independence of the chosen units system, but it does not ensure independence of length scale, which is the holy grail of any mathematical model.On the other hand, models may eventually include the length scale dependence itself whenever enough data is available. For the oscillator example above, it can be shown both experimentally or from physical principles, that $\Pi_3 \approx 2\pi$ for small $u_0$. This constant results is, within some limits, also (length) scale independent. The whole point here is that this result _can be experimentally obtained through dimensionalanalysis of experimental outcomes even if the applicable principles of physics werenot known in advance_.This justifies the importance of dimensional analsys, for it has allowed great cientiststo propose models with $\Pi$-numbers governing many important phenomena. Some remarkableexamples will be shown in section 2.6. 2.4. Change of base units Dimensional formulae allow us to calculate derived units after a change of the base units.One can, for instance, change from S.I. to British unit system and use the dimensionalformulae to calculate the unit equivalence of any required physical quantity. In thissection it is shown that the same calculation for changing the system of units can alsobe used for changing scales and designing experimental reduced models.We recall that the choice of $L$, $M$, and $T$ as fundamental base units, although now internationally acknowledged, is in some way arbitrary. However, it is always possibleto replace these three quantities and calculate a new dimensional matrix for otherthree quantities according to our convenience.Assuming that the base units $L$, $M$, and $T$ ($LMT$ for short) are to be replaced by a new set of units $A$, $B$ e $C$ ($ABC$ for short). The dimensional matrix forthis new base can always be written as:\begin{align*} A &= L^{a_{L}} M^{a_{M}} T^{a_{T}} \\ B &= L^{b_{L}} M^{b_{M}} T^{b_{T}} \\ C &= L^{c_{L}} M^{c_{M}} T^{c_{T}}\end{align*}As an example, let us propose a new base by selecting three quantities from the dataframe```DimData```. For instance, let us take $A = L$ (length), $B = \rho$ (density), and $C = a$ (acceleration):
###Code
ABC = ['L', 'ρ', 'a']
base = DimData.loc[ABC, LMT]
base
###Output
_____no_output_____
###Markdown
The new base _must include quantities with linearly independent dimensions_, only! It is not possible, for instance, to choose length, area and volume, for their dimensions are multiples one of each other.Now we take logarithms from both sides of the equation above:\begin{align*}\log A &= \log \left ( L^{a_{L}} M^{a_{M}} T^{a_{T}} \right ) = a_{L}\log L + a_{M}\log M + a_{T}\log T \\\log B &= \log \left ( L^{b_{L}} M^{b_{M}} T^{b_{T}} \right ) = b_{L}\log L + b_{M}\log M + b_{T}\log T \\\log C &= \log \left ( L^{c_{L}} M^{c_{M}} T^{c_{T}} \right ) = c_{L}\log L + c_{M}\log M + c_{T}\log T\end{align*}In matrix notation:$$\left [ \begin{array}{ccc} a_{L}& a_{M}& a_{T} \\ b_{L}& b_{M}& b_{T} \\ c_{L}& c_{M}& c_{T} \end{array} \right ] \left [ \begin{array}{ccc} \log L \\ \log M \\ \log T \end{array} \right ] = \left [ \begin{array}{ccc} \log A \\ \log B \\ \log C \end{array} \right ] $$By isolating the vector with the fundamental base it gives: $$\left [ \begin{array}{ccc} \log L \\ \log M \\ \log T \end{array} \right ] = \left [ \begin{array}{ccc} a_{A}& a_{B}& a_{C} \\ b_{A}& b_{B}& b_{C} \\ c_{A}& c_{B}& c_{C} \end{array} \right ] \left [ \begin{array}{ccc} \log A \\ \log B \\ \log C \end{array} \right ] $$where:$$\left [ \begin{array}{ccc} a_{A}& a_{B}& a_{C} \\ b_{A}& b_{B}& b_{C} \\ c_{A}& c_{B}& c_{C} \end{array} \right ] = \left [ \begin{array}{ccc} a_{L}& a_{M}& a_{T} \\ b_{L}& b_{M}& b_{T} \\ c_{L}& c_{M}& c_{T} \end{array} \right ] ^{-1} $$This is the dimension matrix of base $LMT$ according to the new base $ABC$.Now it becomes clear why the quantities in the new base must have linearly independentdimensions, otherwise the matrix inversion is not possible.Going back to the monomial form we get:\begin{align*} L &= A^{a_{A}} B^{a_{B}} C^{a_{C}} \\ M &= A^{b_{A}} B^{b_{B}} C^{b_{C}} \\ T &= A^{c_{A}} B^{c_{B}} C^{c_{C}}\end{align*}The inversion of the dimension matrix is carried out in Python with the ```numpy``` module as follows:
###Code
i_base = np.linalg.inv(base)
i_base
###Output
_____no_output_____
###Markdown
Now we take a look in what happens with the dimensions of any derived quantity, $X$,after the base change. The dimensional formula using the fundamental base is$$ X = L^{\alpha_{L}} M^{\beta_{M}} T^{\gamma_{T}} $$Replacing the previous result yields:$$ X = \left ( A^{a_{A}} B^{a_{B}} C^{a_{C}} \right )^{\alpha_{L}} \left ( A^{b_{A}} B^{b_{B}} C^{b_{C}} \right )^{\beta_{M}} \left ( A^{c_{A}} B^{c_{B}} C^{c_{C}} \right )^{\gamma_{T}} $$Rearranging terms:$$ X = A^{(a_{A}\alpha_{L} + b_{A}\beta_{M} + c_{A}\gamma_{T})} B^{(a_{B}\alpha_{L} + b_{B}\beta_{M} + c_{B}\gamma_{T})} C^{(a_{C}\alpha_{L} + b_{C}\beta_{M} + c_{C}\gamma_{T})} = A^{\alpha_{A}} B^{\beta_{B}} C^{\gamma_{C}} $$Finally, the dimensions of $X$ in the new base $ABC$ can be calculated as:$$\left [ \begin{array}{ccc} \alpha_{A} \\ \beta_{B} \\ \gamma_{C} \end{array} \right ] = \left [ \begin{array}{ccc} a_{A}& a_{B}& a_{C} \\ b_{A}& b_{B}& b_{C} \\ c_{A}& c_{B}& c_{C} \end{array} \right ] ^{T} \left [ \begin{array}{ccc} \alpha_{L} \\ \beta_{M} \\ \gamma_{T} \end{array} \right ] $$ This matrix multiplication can be carried out with ``numpy``. We keep the computationalstrategy previously adopted and use the result to create a new ``pandas`` dataframe from scratch:
###Code
NewMat = pd.DataFrame(data = np.matmul(DimMat.values, i_base),
index = DimMat.index,
columns = ABC)
NewMat
###Output
_____no_output_____
###Markdown
This new dimension matrix contains the dimensions of the selected quantities in the new base.These dimensions can be used for changing the unit system in the much same way it can be usedfor changing scales. 2.5. Changing units is like changing scales Como já mencionado, uma das aplicações da mudança de base é a mudança de unidades das grandezas fundamentais. A nova matriz dimensional nos permite calcular o efeito desta mudança nas grandezas derivadas. Por exemplo, vamos admitir que desejamos utilizar a milha nautica ao invés do metro como unidade de comprimento, e a hora ao invés do segundo como unidade do tempo. Vejamos como isso repercute na unidade de velocidade, sendo que a fórmula de cálculo é a mesma que foi anteriormente utilizada para a dimensão:\begin{equation} X = A^{\alpha_{A}} B^{\beta_{B}} C^{\gamma_{C}}\end{equation}For instance, let us take a change from meters per second (m/s) to knots (nautical miles per hour).The units for the fundamental quantities are:
###Code
λ_L = 1852/1 # 1852 meters is 1 nautical mile
λ_m = 1/1 # mass does not matter for velocity
λ_t = 3600/1 # 3600 seconds is 1 hour
scales = np.tile([λ_L, λ_m, λ_t],(1,1))
###Output
_____no_output_____
###Markdown
Now we get the dimensions of velocity and apply the equation:
###Code
Dim_v = DimData.loc[['v'], LMT] # LMT dimensions of velocity must be as list
λ_v = np.prod(scales**Dim_v, axis=1); # applies formula e returns also a vector
print('Velocity of 1 knot is equal to {0:5.3f}m/s.'.format(λ_v[0]))
###Output
Velocity of 1 knot is equal to 0.514m/s.
###Markdown
what means that 1m/s corresponds to approximately 1.94knots. This is not a very excitingresult, for the same number could have been obtained simply dividing 3600 by 1852. However, the main goal here is to demonstrate a computational procedure that is as much concise as general.Furthermore, the same procedure can be used to calculate scale changes of any dimensionalquantities, with scales being specified for quantities other then the fundamental ones.This calculation is required for designing reduced scale models, as will be shown innext class. As an example, let us calculate the scale for velocity with scale changes being specifiedfor length, $L$, density, $\rho$, and acceleration, $a$. We assume that only the lengthscale is changed, with $\lambda_L = 1:160$. This is a typical situation of a reduced scale model tested in a wind tunnel, where fluid density (air) and gravitycannot be changed. In Python, the new base and the scales definition is done as follows:
###Code
ABC = ['L', 'ρ', 'a'] # define quantities for the new base
base = DimData.loc[ABC, LMT] # get dimensional matrix
i_base = np.linalg.inv(base) # get LMT dimensions in the new base
λ_L = 1/160 # length scale for the reduced model
λ_ρ = 1/1 # density remains unchanged (same fluid)
λ_a = 1/1 # acceleration remains unchanged (same gravity)
scales = np.tile([λ_L, λ_ρ, λ_a],(1,1))
###Output
_____no_output_____
###Markdown
Now we change base, calculate new dimensions and apply scales:
###Code
DimMat = DimData.loc[['F','σ','v'], LMT] # LMT dimensions of velocity must be as list
NewMat = pd.DataFrame(data = np.matmul(DimMat.values, i_base),
index = DimMat.index,
columns = ABC)
λ_vec = np.prod(scales**NewMat, axis=1);
print('Velocity in model scale is {0:6.4f} times the prototype velocity.'.format(λ_vec[2]))
1/λ_vec
###Output
Velocity in model scale is 0.0791 times the prototype velocity.
###Markdown
Universidade Federal do Rio Grande do Sul (UFRGS) Programa de Pós-Graduação em Engenharia Civil (PPGEC) PEC00144: Experimental Methods in Civil Engineering Part I: Analysis[2. Dimensional analysis and similarity](section_2) [2.1. Quantities, units, dimensions](section_21) [2.2. Dataframe for scales computation](section_22) [2.3. The Theorem of Pi's by Vaschy-Buckinghan](section_23) [2.4. Change of base units](section_24) [2.5. Change of units is like change of scale](section_25) [2.6. Famous Pi-Numbers](section_26) [2.7. Assignment](section_27) ---_Prof. Marcelo M. Rocha, Dr.techn._ [(ORCID)](https://orcid.org/0000-0001-5640-1020) _Porto Alegre, RS, Brazil_
###Code
# Importing Python modules required for this notebook
# (this cell must be executed with "shift+enter" before any other Python cell)
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
###Output
_____no_output_____
###Markdown
2. Dimensional analysis and similarity 2.1. Quantities, units, dimensions Prof. G.I. Barenblatt has written in his book _Dimensional Analysis_ (1987): >_In fact, the idea on which dimensional analysis is based is very simple, and can be understood by all: physical laws do not depend on arbitrariness in the choice of basic units of measurement. An important conclusion can be drawn from this simple idea using simple arguments: the functions that express physical laws must possess a certain fundamental property, which in mathematics is called generalized homogeneity or symmetry. This property allows the number of arguments in these functions to be reduced, thereby making it simpler to obtain them (by calculating them or determining them experimentally). This is, in fact, the entire content of dimensional analysis - there is nothing more to it._In other words, the units resulting from both sides of an equation must be the same.Although simple, this idea has allowed great scientists like Newton, Rayleigh, Maxwell, Reynolds, Kolmogorov, Cauchy, and many others, to proposed many fundamental physical principles.To illustrate how the law of _dimensional homogeneity_ can be applied, let us investigate the free vibration frequency, $f_{\rm n}$, of an undamped mass-spring system with one degree of freedom.The frequency $f_{\rm n}$ could be derived from mechanical principles like dynamicequilibrium equations, but we take the alternative dimensional path instead.The system above _may_ involve several physical quantities like: * the instantaneous position, $u$, * the oscillating mass, $m$, * the time, $t$, * the displacement amplitude, $u_0$, * the spring linear stiffness, $k$, and also * the free vibration frequency, $f_{\rm n}$. The completeness of this set of quantities is essential for the success of ourdimensional analysis. The analysis process requires an investigation of possiblerelationships among the _candidate_ quantities above and the frequency $f_{\rm n}$. Let us take a look at their _units of measurement_: \begin{align*}[ u ] &= [L]^{1}[M]^{0}[T]^{0} \\[ m ] &= [L]^{0}[M]^{1}[T]^{0} \\[ t ] &= [L]^{0}[M]^{0}[T]^{1} \\[ u_0 ] &= [L]^{1}[M]^{0}[T]^{0} \\[ k ] &= [L]^{0}[M]^{1}[T]^{-2} \\[ f_{\rm n}] &= [L]^{0}[M]^{0}[T]^{-1}\end{align*}where the brackets mean "units of". One can observe that all measurement units have beenwritten in terms of only three _fundamental units_: length, $[L]$, mass, $[M]$, and time, $[T]$. Most principles in solid mechanics and structural dynamics may be expressed with these three units only. Eventually the temperature, $\Theta$, may be also required.For the sake of simplicity, hereafter brackets will be ommited.The quantities directly subordinated to the fundamental units are called _fundamentalquantities_, which are the length, the mass and the time, while other units and quantitiesare called _derived_ units and quantities, like stiffness, frequency, acceleration, etc. The dependency of derived units on the fundamental units are expressed through _dimensional formulae_, as shown above, which are always _power-law monomials_. The exponents applyed to the fundamental units in these formulae are called _dimensions_ or _dimension exponents_.In the case of using the _International System_ (SI), the three fundamental units are themeter (m), the kilogram (kg), and the second (s). In the example above this means that:\begin{align*}[ u ] &= {\rm m}^{1}{\rm kg}^{0}{\rm s}^{0} = {\rm m} \\[ m ] &= {\rm m}^{0}{\rm kg}^{1}{\rm s}^{0} = {\rm kg} \\[ t ] &= {\rm m}^{0}{\rm kg}^{0}{\rm s}^{1} = {\rm s} \\[ u_0 ] &= {\rm m}^{1}{\rm kg}^{0}{\rm s}^{0} = {\rm m} \\[ k ] &= {\rm m}^{0}{\rm kg}^{1}{\rm s}^{-2} = {\rm kg}/{\rm s}^{2} = {\rm N/m} \\[ f_{\rm n}] &= {\rm m}^{0}{\rm kg}^{0}{\rm s}^{-1} = 1/{\rm s} = {\rm Hz}\end{align*} 2.2. Dataframe for scales computation To facilitate the scales calculations as presented in this _notebook_, let us now import a ``pandas`` dataframe with physical quantities commonly used in engineering mechanics andstructural dynamics, aerodynamics, and fluid and soil mechanics:
###Code
# Please Google "pandas read_excel()"
DimData = pd.read_excel('resources/DimData.xlsx',
header = 0,
index_col = 0,
sheet_name = 'DimData')
print(DimData)
###Output
descriptor latex L M T
a Acceleration a 1 0 -2
α Angular acceleration \alpha 0 0 -2
ω Angular frequency \omega 0 0 -1
A Area A 2 0 0
EA Axial stiffness EA 1 1 -2
EI Beam bending stiffness EI 3 1 -2
GAs Beam shear stiffness GA_s 1 1 -2
ρ Density \rho -3 1 0
μ Dynamic viscosity \mu -1 1 -1
F Force F 1 1 -2
q Force per unit length q 0 1 -2
f frequency f 0 0 -1
υ Kinematic viscosity \nu 2 0 -1
L Length L 1 0 0
m Mass m 0 1 0
im Mass inertia per unit length i_m 1 1 0
μA Mass per unit area \mu_A -2 1 0
μL Mass per unit length \mu_L -1 1 0
M Moment M 2 1 -2
I Moment of inertia I 4 0 0
W Resistent modulus W 3 0 0
Im Rotational mass inertia I_m 2 1 0
k Spring stiffness k 0 1 -2
σ Stress \sigma -1 1 -2
t Time t 0 0 1
v Velocity v 1 0 -1
c Viscous damping c 0 1 -1
V Volume V 3 0 0
###Markdown
Other quantities may be easily included in this dataframe by editing the Excel file``DimData.xlsx``. Each entry in the table corresponds to a dimensional quantity, being the lastthree columns the respective dimension exponents in the dimensional formulae.Quantities like angles, strains, Poison's coefficient and ratio of critical damping areby definition non-dimensional, so there is no need of including them in this table.Relations between length measures, the so-called _form factors_, must also not be included.Observe that, for the sake of clearness, we are using Unicodevariable names, like greek letters, which is a remarkable Python's feature.To access the $LMT$ exponents of a set of quantities, let us say the ones selected forthe oscillator problem above, the dataframe ```loc``` view may be used:
###Code
par = ['L', 'm', 't', 'L', 'k', 'f'] # selected dimensional quantities
LMT = ['L', 'M', 'T'] # three last columns of dataframe
DimMat = DimData.loc[par, LMT] # the dimensional matrix
print(DimMat)
###Output
L M T
L 1 0 0
m 0 1 0
t 0 0 1
L 1 0 0
k 0 1 -2
f 0 0 -1
###Markdown
According to ``pandas`` module, a subset of a dataframe is itself also a dataframe.If only the dimension exponents are to be retrieved, the attribute ``values`` must be called,returning a ``numpy`` 2D array.This array will be required in the following sections, where we show how the information provided in the dataframe ``DimData`` can be used to carry out units and scales changes for designing reduced scale models.
###Code
print(DimMat.values)
###Output
[[ 1 0 0]
[ 0 1 0]
[ 0 0 1]
[ 1 0 0]
[ 0 1 -2]
[ 0 0 -1]]
###Markdown
Observe that the dataframe indices are the codes to access a specific quantity, while the following first column, which header is ``descriptor``, corresponds to a description to help identification. The dataframe also brings a column named ``latex`` with the $\LaTeX$ code for eventual automaticplot labelling. 2.3. The Vaschy-Buckingham's $\Pi$-Theorem The Vaschy-Buckingham's $\Pi$-Theorem may be expressed as follows:>Let us assume that a given physical phenomenon involves $n$ _dimensional quantities_, denoted as $p_1, p_2, \dots, p_n$. For this phenomenon a mathematical model may be expressed as a general relation:\begin{equation} f\left ( p_1, p_2, \dots, p_n \right ) = 0 \end{equation}>This relation may be replaced by another one with $r = n - k$ _non-dimensional quantities_:\begin{equation} F\left ( \Pi_1, \Pi_2, \dots, \Pi_r \right ) = 0\end{equation}where $k$ is the number of fundamental units required to compose the units of all dimensional quantities involved. The $\Pi$-numbers have the general form:\begin{equation}\Pi = p_1^{\alpha_1} \; p_2^{\alpha_2} \; \dots \; p_n^{\alpha_n}\end{equation}where the exponents $\vec{\alpha} = \left [ \alpha_1 \; \alpha_2 \; \dots \; \alpha_n \right ]$ are such that the condition of non-dimensionality must always be fulfilled.Recalling that in the present context the only required fundamental units are $L$, $M$, and $T$, then $k = 3$. A demonstration of this theorem can be found in the literatura and will not bereplicated here.To apply this theorem, let us assemble a _dimensional matrix_, $\bf D$, with one column foreach fundamental unit and one row for each quantity involved in the analysis. Thedataframe ``DimMat`` defined in the previous section is an computational example of a dimensional matrix. To build up $\Pi$-numbers we propose now some vector, $\vec{\alpha}$, such that (for $k = 3$):\begin{equation}\vec{\alpha}\,{\bf D} = \left[0 \hspace{5mm} 0 \hspace{5mm} 0 \right]\end{equation}There is no general rule for specifying $\vec{\alpha}$.The practical principle that guides any proposition is some possible physical meaning.If the dimensional analysis subject is not well known, relevant $\Pi$-numbers willalways result from some educated guess.Even the choice of a relevant set of quantities is not always obvious, for it mayboth exceed or lack completeness. At this point, one can clearly see that _dimensional analysis is not a panacea for model proposition_ and a good measure of experience, or even luck, may be required.Going back to the oscillator problem, possible $\Pi$-numbers for the involved quantities are:\begin{align*} \Pi_1 &= u^1 u_0^{-1} \\ \Pi_2 &= t^1 \; f_{\rm n}^{1} \\ \Pi_3 &= m^{-1/2} \; k^{1/2} \; f_{\rm n}^{-1} = \frac{\sqrt{k/m}}{f_{\rm n}}\end{align*}where we can se that $\Pi_3$ is clearly the most interesting, with $$\vec{\alpha}_3 = \left [ 0 \;\; {-1/2} \;\;\; 0 \;\;\; 0 \;\; 1/2 \;\; {-1} \right]$$The product $\vec{\alpha}_3{\bf D}$ evidentiates its non-dimensionality:
###Code
par = ['L', 'm', 't', 'L', 'k', 'f'] # selected dimensional quantities
α3 = np.array([ 0, -1/2, 0, 0, 1/2, -1 ])
print(np.matmul(α3, DimMat.values))
###Output
[0. 0. 0.]
###Markdown
Representing physical phenomena as relationships among non-dimensional quantities certainly implies independence of the chosen units system, but it does not ensure independence of length scale, which is the holy grail of any mathematical model.On the other hand, models may eventually include the length scale dependence itself whenever enough data is available. For the oscillator example above, it can be shown both experimentally or from physical principles, that $\Pi_3 \approx 2\pi$ for small $u_0$. This constant results is, within some limits, also (length) scale independent. The whole point here is that this result _can be experimentally obtained through dimensionalanalysis of experimental outcomes even if the applicable principles of physics werenot known in advance_.This justifies the importance of dimensional analsys, for it has allowed great cientiststo propose models with $\Pi$-numbers governing many important phenomena. Some remarkableexamples will be shown in section 2.6. 2.4. Change of base units Dimensional formulae allow us to calculate derived units after a change of the base units.One can, for instance, change from S.I. to British unit system and use the dimensionalformulae to calculate the unit equivalence of any required physical quantity. In thissection it is shown that the same calculation for changing the system of units can alsobe used for changing scales and designing experimental reduced models.We recall that the choice of $L$, $M$, and $T$ as fundamental base units, although now internationally acknowledged, is in some way arbitrary. However, it is always possibleto replace these three quantities and calculate a new dimensional matrix for otherthree quantities according to our convenience.Assuming that the base units $L$, $M$, and $T$ ($LMT$ for short) are to be replaced by a new set of units $A$, $B$ e $C$ ($ABC$ for short). The dimensional matrix forthis new base can always be written as:\begin{align*} A &= L^{a_{L}} M^{a_{M}} T^{a_{T}} \\ B &= L^{b_{L}} M^{b_{M}} T^{b_{T}} \\ C &= L^{c_{L}} M^{c_{M}} T^{c_{T}}\end{align*}As an example, let us propose a new base by selecting three quantities from the dataframe```DimData```. For instance, let us take $A = L$ (length), $B = \rho$ (density), and $C = a$ (acceleration):
###Code
ABC = ['L', 'ρ', 'a']
base = DimData.loc[ABC, LMT]
print(base)
###Output
L M T
L 1 0 0
ρ -3 1 0
a 1 0 -2
###Markdown
The new base _must include quantities with linearly independent dimensions_, only! It is not possible, for instance, to choose length, area and volume, for their dimensions are multiples one of each other.Now we take logarithms from both sides of the equation above:\begin{align*}\log A &= \log \left ( L^{a_{L}} M^{a_{M}} T^{a_{T}} \right ) = a_{L}\log L + a_{M}\log M + a_{T}\log T \\\log B &= \log \left ( L^{b_{L}} M^{b_{M}} T^{b_{T}} \right ) = b_{L}\log L + b_{M}\log M + b_{T}\log T \\\log C &= \log \left ( L^{c_{L}} M^{c_{M}} T^{c_{T}} \right ) = c_{L}\log L + c_{M}\log M + c_{T}\log T\end{align*}In matrix notation:$$\left [ \begin{array}{ccc} a_{L}& a_{M}& a_{T} \\ b_{L}& b_{M}& b_{T} \\ c_{L}& c_{M}& c_{T} \end{array} \right ] \left [ \begin{array}{ccc} \log L \\ \log M \\ \log T \end{array} \right ] = \left [ \begin{array}{ccc} \log A \\ \log B \\ \log C \end{array} \right ] $$By isolating the vector with the fundamental base it gives: $$\left [ \begin{array}{ccc} \log L \\ \log M \\ \log T \end{array} \right ] = \left [ \begin{array}{ccc} a_{A}& a_{B}& a_{C} \\ b_{A}& b_{B}& b_{C} \\ c_{A}& c_{B}& c_{C} \end{array} \right ] \left [ \begin{array}{ccc} \log A \\ \log B \\ \log C \end{array} \right ] $$where:$$\left [ \begin{array}{ccc} a_{A}& a_{B}& a_{C} \\ b_{A}& b_{B}& b_{C} \\ c_{A}& c_{B}& c_{C} \end{array} \right ] = \left [ \begin{array}{ccc} a_{L}& a_{M}& a_{T} \\ b_{L}& b_{M}& b_{T} \\ c_{L}& c_{M}& c_{T} \end{array} \right ] ^{-1} $$This is the dimension matrix of base $LMT$ according to the new base $ABC$.Now it becomes clear why the quantities in the new base must have linearly independentdimensions, otherwise the matrix inversion is not possible.Going back to the monomial form we get:\begin{align*} L &= A^{a_{A}} B^{a_{B}} C^{a_{C}} \\ M &= A^{b_{A}} B^{b_{B}} C^{b_{C}} \\ T &= A^{c_{A}} B^{c_{B}} C^{c_{C}}\end{align*}The inversion of the dimension matrix is carried out in Python with the ```numpy``` module as follows:
###Code
i_base = np.linalg.inv(base)
i_base
###Output
_____no_output_____
###Markdown
Now we take a look in what happens with the dimensions of any derived quantity, $X$,after the base change. The dimensional formula using the fundamental base is$$ X = L^{\alpha_{L}} M^{\beta_{M}} T^{\gamma_{T}} $$Replacing the previous result yields:$$ X = \left ( A^{a_{A}} B^{a_{B}} C^{a_{C}} \right )^{\alpha_{L}} \left ( A^{b_{A}} B^{b_{B}} C^{b_{C}} \right )^{\beta_{M}} \left ( A^{c_{A}} B^{c_{B}} C^{c_{C}} \right )^{\gamma_{T}} $$Rearranging terms:$$ X = A^{(a_{A}\alpha_{L} + b_{A}\beta_{M} + c_{A}\gamma_{T})} B^{(a_{B}\alpha_{L} + b_{B}\beta_{M} + c_{B}\gamma_{T})} C^{(a_{C}\alpha_{L} + b_{C}\beta_{M} + c_{C}\gamma_{T})} = A^{\alpha_{A}} B^{\beta_{B}} C^{\gamma_{C}} $$Finally, the dimensions of $X$ in the new base $ABC$ can be calculated as:$$\left [ \begin{array}{ccc} \alpha_{A} \\ \beta_{B} \\ \gamma_{C} \end{array} \right ] = \left [ \begin{array}{ccc} a_{A}& a_{B}& a_{C} \\ b_{A}& b_{B}& b_{C} \\ c_{A}& c_{B}& c_{C} \end{array} \right ] ^{T} \left [ \begin{array}{ccc} \alpha_{L} \\ \beta_{M} \\ \gamma_{T} \end{array} \right ] $$ This matrix multiplication can be carried out with ``numpy``. We keep the computationalstrategy previously adopted and use the result to create a new ``pandas`` dataframe from scratch:
###Code
NewMat = pd.DataFrame(data = np.matmul(DimMat.values, i_base),
index = DimMat.index,
columns = ABC)
print(NewMat)
###Output
L ρ a
L 1.0 0.0 0.0
m 3.0 1.0 0.0
t 0.5 0.0 -0.5
L 1.0 0.0 0.0
k 2.0 1.0 1.0
f -0.5 0.0 0.5
###Markdown
This new dimension matrix contains the dimensions of the selected quantities in the new base.These dimensions can be used for changing the unit system in the much same way it can be usedfor changing scales. 2.5. Changing units is like changing scales Como já mencionado, uma das aplicações da mudança de base é a mudança de unidades das grandezas fundamentais. A nova matriz dimensional nos permite calcular o efeito desta mudança nas grandezas derivadas. Por exemplo, vamos admitir que desejamos utilizar a milha nautica ao invés do metro como unidade de comprimento, e a hora ao invés do segundo como unidade do tempo. Vejamos como isso repercute na unidade de velocidade, sendo que a fórmula de cálculo é a mesma que foi anteriormente utilizada para a dimensão:\begin{equation} X = A^{\alpha_{A}} B^{\beta_{B}} C^{\gamma_{C}}\end{equation}For instance, let us take a change from meters per second (m/s) to knots (nautical miles per hour).The units for the fundamental quantities are:
###Code
λ_L = 1852/1 # 1852 meters is 1 nautical mile
λ_m = 1/1 # mass does not matter for velocity
λ_t = 3600/1 # 3600 seconds is 1 hour
scales = np.tile([λ_L, λ_m, λ_t],(1,1))
print(scales)
###Output
[[1.852e+03 1.000e+00 3.600e+03]]
###Markdown
Now we get the dimensions of velocity and apply the equation:
###Code
Dim_v = DimData.loc[['v'], LMT] # LMT dimensions of velocity must be as list
λ_v = np.prod(scales**Dim_v, axis=1); # applies formula e returns also a vector
print('Velocity of 1 knot is equal to {0:5.3f}m/s.'.format(λ_v[0]))
###Output
Velocity of 1 knot is equal to 0.514m/s.
###Markdown
what means that 1m/s corresponds to approximately 1.94knots. This is not a very excitingresult, for the same number could have been obtained simply dividing 3600 by 1852. However, the main goal here is to demonstrate a computational procedure that is as much concise as general.Furthermore, the same procedure can be used to calculate scale changes of any dimensionalquantities, with scales being specified for quantities other then the fundamental ones.This calculation is required for designing reduced scale models, as will be shown innext class. As an example, let us calculate the scale for velocity with scale changes being specifiedfor length, $L$, density, $\rho$, and acceleration, $a$. We assume that only the lengthscale is changed, with $\lambda_L = 1:160$. This is a typical situation of a reduced scale model tested in a wind tunnel, where fluid density (air) and gravitycannot be changed. In Python, the new base and the scales definition is done as follows:
###Code
ABC = ['L', 'ρ', 'a'] # define quantities for the new base
base = DimData.loc[ABC, LMT] # get dimensional matrix
i_base = np.linalg.inv(base) # get LMT dimensions in the new base
λ_L = 1/160 # length scale for the reduced model
λ_ρ = 1/1 # density remains unchanged (same fluid)
λ_a = 1/1 # acceleration remains unchanged (same gravity)
scales = np.tile([λ_L, λ_ρ, λ_a],(4,1))
print(scales)
###Output
[[0.00625 1. 1. ]
[0.00625 1. 1. ]
[0.00625 1. 1. ]
[0.00625 1. 1. ]]
###Markdown
Now we change base, calculate new dimensions and apply scales:
###Code
DimMat = DimData.loc[['t','F','σ','v'], LMT] # LMT dimensions of velocity must be as list
NewMat = pd.DataFrame(data = np.matmul(DimMat.values, i_base),
index = DimMat.index,
columns = ABC)
λ_vec = np.prod(scales**NewMat, axis=1);
print('Velocity in model scale is {0:6.4f} times the prototype velocity.'.format(λ_vec[3]))
1/λ_vec
###Output
Velocity in model scale is 0.0791 times the prototype velocity.
|
code/Project2-v2.ipynb | ###Markdown
PROJECT 2 : TEAM 11 Members: Talia Tandler, SeungU Lyu
###Code
# Configure Jupyter so figures appear in the notebook
%matplotlib inline
# Configure Jupyter to display the assigned value after an assignment
%config InteractiveShell.ast_node_interactivity='last_expr_or_assign'
# import functions from the modsim.py module
from modsim import *
import math
###Output
_____no_output_____
###Markdown
http://www.worldometers.info/world-population/us-population/US pop in 2017 = 324,459,463https://www.cdc.gov/vaccines/imz-managers/coverage/childvaxview/data-reports/mmr/trend/index.htmlMMR immunization rate in 2017 = 90.7%https://www.acha.org/documents/Programs_Services/ConEd/Measles_Update_2015_Implications_for_the_College_Setting.pdfMMR Measles importatoin number = 33/yearhttps://wwwnc.cdc.gov/travel/yellowbook/2018/infectious-diseases-related-to-travel/measles-rubeolaMeasles incubation period 11 days average, infectious period 2-4 days before rash to after rash.https://www.researchgate.net/figure/Daily-average-number-of-contacts-per-person-in-age-group-j-The-average-number-of_fig2_228649013Daily average number of contacts per person. https://academic.oup.com/jid/article/189/Supplement_1/S91/8250771999 overall immunity for measles were expected to be 93% for US population.https://www.statista.com/statistics/195943/birth-rate-in-the-united-states-since-1990/Birth rate = 12.2/1000 per year = 0.0122 Death rate = 8.4/1000 per year = 0.0084
###Code
pop = 1000 #population
init_im = 0.5 #initial immunity of the US population
beta = 0.9
gamma = 1/7 #US recovery rate from measles
sigma = 0.091 #US rate from exposure period of 11 days to infected
contact_num = beta/gamma
###Output
_____no_output_____
###Markdown
What would be the impact of getting rid of the MMR vaccination in newborn babies over the course of 10 years?Background/why we thought this was interesting-Explanation of the disease: symptoms, contagious- Reference the fake study where the guy said vaccinations are bad for your baby --WHAT IF PEOPLE BELIEVED HIM???- MMR is very super infections and has high herd immunity so when you drop the vaccination rate, it drops the infection rate. MethodologyIn order to creat this model, we:1. Did background research on MMR vaccination and the measles diseases and found a set of constants we would implement in our model.2. Put the variables into a state function.3. Ran the simulation based on measles infections every day.4. Expanded simuluation for a course of 10 years.5. Implemented the idea of a measles outbreak to make the simulation more realistic.6. Created graphs to visually represent our results.
###Code
def make_system (pop, init_im, beta, gamma, sigma):
"""Make a system object for the SCIR model
pop: Total US population
init_im: Initial Population Immunity
gamm: recovery rate for infected people
sigma: rate of incubation group moving to infectious group
return: System object"""
init = State(S = int(pop*(1 - init_im)), E = 0, I = 1, R = int(pop*init_im))
init /= np.sum(init)
#S: susceptible, E: exposed period, I: infected, R: recovered(immune to disease)
t0 = 0
t_end = 365 #number of days in 1 year
return System(init = init,
beta = beta,
gamma = gamma,
sigma = sigma,
t0 = t0,
t_end = t_end,
init_im = init_im)
def update_func(state, time, system):
"""Update the SEIR model
state: starting variables of SEIR
t: time step
system: includes alpha,beta,gamma,omega rates
contact: current contact number for the state
"""
unpack(system)
s,e,i,r = state
#current population
total_pop = s+e+i+r
#change rate for each status
ds = (-beta*s*i)/total_pop #change in number of people susceptible
de = ((beta*s*i)/total_pop) - sigma*e #change in number of people moving to exposed period
di = sigma*e - gamma*i #change in people moving to infectious period
dr = gamma*i #change in people recovered
s += ds #number of people susceptible
e += de #number of people exposed
i += di #number of people infected
r += dr #number of people recovered
return State(S=s, E=e, I=i, R=r)
def run_simulation(system, update_func):
"""Runs a simulation of the system.
system: System object
update_func: function that updates state
returns: TimeFrame
"""
unpack(system)
#creates timeframe to save daily states
frame = TimeFrame(columns=init.index)
frame.row[t0] = init
for time in linrange(t0, t_end):
frame.row[time+1] = update_func(frame.row[time], time, system)
return frame
def plot_results (S,E,I,R):
plot(S, '--', label = 'Susceptible')
plot(E, '-', label = 'Exposded')
plot(I, '.', label = 'Infected')
plot(R, ':', label = 'Recovered')
decorate(xlabel='Time (days)',
ylabel = 'Fraction of population')
system = make_system(pop, init_im, beta, gamma, sigma)
results = run_simulation(system, update_func)
results
plot_results(results.S, results.E, results.I, results.R)
imax = max(results.I)
def calc_highest_infected(results):
"""Fraction of population infected during the simulation.
results: DataFrame with columns S, I, R
returns: fraction of population
"""
return max(results.I)
def sweep_init_im(imun_rate_array):
"""Sweep a range of values for beta.
beta_array: array of beta values
gamma: recovery rate
returns: SweepSeries that maps from beta to total infected
"""
sweep = SweepSeries()
for init_im in imun_rate_array:
system = make_system(pop, init_im, beta, gamma, sigma)
results = run_simulation(system, update_func)
sweep[system.init_im] = calc_highest_infected(results)*pop
return sweep
imun_rate_array = linspace(0, 1, 11)
sweep = sweep_init_im(imun_rate_array)
sweep
plot(sweep)
decorate(xlabel='Immunity Rate',
ylabel = 'Highest number of people infected during 1 outbreak')
###Output
_____no_output_____
###Markdown
PROJECT 2 : TEAM 11 Members: Talia Tandler, SeungU Lyu
###Code
# Configure Jupyter so figures appear in the notebook
%matplotlib inline
# Configure Jupyter to display the assigned value after an assignment
%config InteractiveShell.ast_node_interactivity='last_expr_or_assign'
# import functions from the modsim.py module
from modsim import *
import math
###Output
_____no_output_____
###Markdown
http://www.worldometers.info/world-population/us-population/US pop in 2017 = 324,459,463https://wwwnc.cdc.gov/travel/yellowbook/2018/infectious-diseases-related-to-travel/measles-rubeolaMeasles incubation period 11 days average, infectious period 2-4 days before rash to after rash.
###Code
pop = 999 #population
init_im = 0.85 #initial immunity of the US population
beta = 0.9
gamma = 1/7 #US recovery rate from measles
sigma = 0.091 #US rate from exposure period of 11 days to infected
###Output
_____no_output_____
###Markdown
What will be the result of lowering immunity rate for measles in a small community during a outbreak?Measles is a highly infectious disease that can infect about 90% of people come to contact with the patient. However, the disease is not treated dangerous these days because of the MMR vaccination, which can effectively prevent people to get the disease. Due to the high vaccination rate, THe United States declared free of circulating measles in 2000 with 911 cases from 2001 to 2011. The only occurrences of measles nowadays in the U.S is due to importation from other countries. Because of its high infectious rate upon contact, herd immunity is considered really important for measles. In 2015, Disney Measles Outbreak happened, causing more than 159 people to be infected during a single outbreak countrywide. Only 50~86% people exposed to this outbreak were vaccinated, causing a bigger outbreak. Recent Anti-Vaccination Movement in the U.S was pointed out to be the major reason, lowering the population immunity rate, causing the herd immunity to not function as expected. Starter of this movement, Andrew Wakefield, stated that MMR vaccination can cause Autism to newborn children with the mercury content inside the specific vaccine. Due to the fear of getting an autistic child, a number of parents refused to vaccinate their children.This simulation looks to find the effect of changing the immunity rate of a community toward measles to check out the effectiveness of vaccination toward the disease. MethodologyIn order to creat this model, we:1. Did background research on MMR vaccination and the measles diseases and found a set of constants we would implement in our model.2. Put the variables into a state function.3. Set the initial population to 1000, and one person infected with measles.4. Ran the simulation based on measles infections every day.5. Set a condition where the outbreak ends when the infected people is less than one person. 6. Created graphs to visually represent our results.
###Code
def make_system (pop, init_im, beta, gamma, sigma):
"""Make a system object for the SCIR model
pop: Total US population
init_im: Initial Population Immunity
gamm: recovery rate for infected people
sigma: rate of incubation group moving to infectious group
return: System object"""
init = State(S = int(pop*(1 - init_im)), E = 0, I = 1, R = int(pop*init_im))
init /= np.sum(init)
#S: susceptible, E: exposed period, I: infected, R: recovered(immune to disease)
t0 = 0
t_end = 365 #number of days in 1 year
return System(init = init,
beta = beta,
gamma = gamma,
sigma = sigma,
t0 = t0,
t_end = t_end,
init_im = init_im)
def update_func(state, time, system):
"""Update the SEIR model
state: starting variables of SEIR
t: time step
system: includes alpha,beta,gamma,omega rates
contact: current contact number for the state
"""
unpack(system)
s,e,i,r = state
# if time>30 and i<0.001:
# e = 0
# i = 0
#current population
total_pop = s+e+i+r
#change rate for each status
ds = (-beta*s*i)/total_pop #change in number of people susceptible
de = ((beta*s*i)/total_pop) - sigma*e #change in number of people moving to exposed period
di = sigma*e - gamma*i #change in people moving to infectious period
dr = gamma*i #change in people recovered
s += ds #number of people susceptible
e += de #number of people exposed
i += di #number of people infected
r += dr #number of people recovered
return State(S=s, E=e, I=i, R=r)
def run_simulation(system, update_func):
"""Runs a simulation of the system.
system: System object
update_func: function that updates state
returns: TimeFrame
"""
unpack(system)
#creates timeframe to save daily states
frame = TimeFrame(columns=init.index)
frame.row[t0] = init
for time in linrange(t0, t_end):
frame.row[time+1] = update_func(frame.row[time], time, system)
return frame
def plot_results (S,E,I,R):
plot(S, '--', label = 'Susceptible')
plot(E, '-', label = 'Exposded')
plot(I, '.', label = 'Infected')
plot(R, ':', label = 'Recovered')
decorate(xlabel='Time (days)',
ylabel = 'Fraction of population')
init_im = 0.8
system = make_system(pop, init_im, beta, gamma, sigma)
results = run_simulation(system, update_func)
results
#plot(results.I)
plot_results(results.S, results.E, results.I, results.R)
plot(results.I)
def calc_highest_infected(results):
"""Fraction of population infected during the simulation.
results: DataFrame with columns S, I, R
returns: fraction of population
"""
return max(results.I)
def calc_fraction_infected(results):
return (get_first_value(results.S) - get_last_value(results.S))/get_first_value(results.S)
def sweep_init_im(imun_rate_array):
"""Sweep a range of values for beta.
beta_array: array of beta values
gamma: recovery rate
returns: SweepSeries that maps from beta to total infected
"""
sweep = SweepSeries()
for init_im in imun_rate_array:
system = make_system(pop, init_im, beta, gamma, sigma)
results = run_simulation(system, update_func)
sweep[system.init_im] = calc_highest_infected(results)*pop
return sweep
def sweep_init_im2(imun_rate_array):
"""Sweep a range of values for beta.
beta_array: array of beta values
gamma: recovery rate
returns: SweepSeries that maps from beta to total infected
"""
sweep = SweepSeries()
for init_im in imun_rate_array:
system = make_system(pop, init_im, beta, gamma, sigma)
results = run_simulation(system, update_func)
sweep[system.init_im] = calc_fraction_infected(results)*100
return sweep
imun_rate_array = linspace(0, 1, 50)
sweep = sweep_init_im2(imun_rate_array)
sweep
plot(sweep)
decorate(xlabel='Immunity Rate',
ylabel = 'Highest number of people infected during 1 outbreak')
###Output
_____no_output_____ |
Transfer Learning via InceptionV3.ipynb | ###Markdown
Transfer Learning via InceptionV3 **Below is a demo and a brief introduction of how to work with transfer learning . I have used imagenet model "INCEPTIONV3" as my base model for better diagnosis purpose than the self model that I have laid architecture of and trained in the other notebook .**
###Code
import pandas as pd
import cv2
import numpy as np
import os
from random import shuffle
from tqdm import tqdm
import scipy
import skimage
from skimage.transform import resize
print(os.listdir("../chest_xray/"))
print(os.listdir("../chest_xray/train/"))
TRAIN_DIR = "../chest_xray/train/"
TEST_DIR = "../chest_xray/test/"
def get_label(Dir):
for nextdir in os.listdir(Dir):
if not nextdir.startswith('.'):
if nextdir in ['NORMAL']:
label = 0
elif nextdir in ['PNEUMONIA']:
label = 1
else:
label = 2
return nextdir, label
###Output
_____no_output_____
###Markdown
**Pre-processing**
###Code
def preprocessing_data(Dir):
X = []
y = []
for nextdir in os.listdir(Dir):
nextdir, label = get_label(Dir)
temp = Dir + nextdir
for image_filename in tqdm(os.listdir(temp)):
path = os.path.join(temp + '/' , image_filename)
img = cv2.imread(path,cv2.IMREAD_GRAYSCALE)
if img is not None:
img = skimage.transform.resize(img, (150, 150, 3))
img = np.asarray(img)
X.append(img)
y.append(label)
X = np.asarray(X)
y = np.asarray(y)
return X,y
def get_data(Dir):
X = []
y = []
for nextDir in os.listdir(Dir):
if not nextDir.startswith('.'):
if nextDir in ['NORMAL']:
label = 0
elif nextDir in ['PNEUMONIA']:
label = 1
else:
label = 2
temp = Dir + nextDir
for file in tqdm(os.listdir(temp)):
img = cv2.imread(temp + '/' + file)
if img is not None:
img = skimage.transform.resize(img, (150, 150, 3))
#img_file = scipy.misc.imresize(arr=img_file, size=(299, 299, 3))
img = np.asarray(img)
X.append(img)
y.append(label)
X = np.asarray(X)
y = np.asarray(y)
return X,y
#X_train, y_train = get_data(TRAIN_DIR)
import h5py
from keras.utils.np_utils import to_categorical
#------- Read training images---------------------------------------------------
NUM_CLASSES = 2 # Binary output
IMAGE_DIMS = (150,150,3)
file_name = 'X_train.h5' # File name for saving your training images
try:
with h5py.File(file_name) as hf:
X_train, y_train = hf['imgs'][:], hf['labels'][:]
print("Loaded images from {}".format(file_name))
except (IOError,OSError, KeyError):
print("Error in reading {}. Processing all images...".format(file_name))
#root_dir = "../chest_xray/train/" # changed to your needs
X_train, y_train = get_data(TRAIN_DIR)
y_train = to_categorical(y_train, NUM_CLASSES)
# Save the training dictionary
with h5py.File(file_name,'w') as hf:
hf.create_dataset('imgs', data=X_train)
hf.create_dataset('labels', data=y_train)
#X_test , y_test = get_data(TEST_DIR)
NUM_CLASSES = 2 # Binary output
IMAGE_DIMS = (150,150,3)
file_name = 'X_test.h5' # File name for saving your training images
try:
with h5py.File(file_name) as hf:
X_test, y_test = hf['imgs'][:], hf['labels'][:]
print("Loaded images from {}".format(file_name))
except (IOError,OSError, KeyError):
print("Error in reading {}. Processing all images...".format(file_name))
#root_dir = "../chest_xray/test/" # changed to your needs
X_test, y_test = get_data(TEST/_DIR)
y_test = to_categorical(y_test, NUM_CLASSES)
# Save the training dictionary
with h5py.File(file_name,'w') as hf:
hf.create_dataset('imgs', data=X_test)
hf.create_dataset('labels', data=y_test)
#X_train, y_train = get_data(TRAIN_DIR)
#X_test , y_test = get_data(TEST_DIR)
print(X_train.shape,'\n',X_test.shape)
print(y_train.shape,'\n',y_test.shape)
###Output
(5216, 2)
(624, 2)
###Markdown
We already tranform the data to categorical
###Code
#from keras.utils.np_utils import to_categorical
#y_train = to_categorical(y_train, 2)
#y_test = to_categorical(y_test, 2)
Pimages = os.listdir(TRAIN_DIR + "PNEUMONIA")
Nimages = os.listdir(TRAIN_DIR + "NORMAL")
###Output
_____no_output_____
###Markdown
Plotting the XRays of No Pneumonia and Pneumonia patients.
###Code
import matplotlib.pyplot as plt
def plotter(i):
imagep1 = cv2.imread(TRAIN_DIR+"PNEUMONIA/"+Pimages[i])
imagep1 = skimage.transform.resize(imagep1, (150, 150, 3) , mode = 'reflect')
imagen1 = cv2.imread(TRAIN_DIR+"NORMAL/"+Nimages[i])
imagen1 = skimage.transform.resize(imagen1, (150, 150, 3))
pair = np.concatenate((imagen1, imagep1), axis=1)
print("(Left) - No Pneumonia Vs (Right) - Pneumonia")
print("-----------------------------------------------------------------------------------------------------------------------------------")
plt.figure(figsize=(10,5))
plt.imshow(pair)
plt.show()
for i in range(5,10):
plotter(i)
from keras.callbacks import ReduceLROnPlateau , ModelCheckpoint , LearningRateScheduler
lr_reduce = ReduceLROnPlateau(monitor='val_acc', factor=0.1, epsilon=0.0001, patience=1, verbose=1)
###Output
/home/martin/anaconda3/envs/tfgpu/lib/python3.6/site-packages/keras/callbacks.py:928: UserWarning: `epsilon` argument is deprecated and will be removed, use `min_delta` insted.
warnings.warn('`epsilon` argument is deprecated and '
###Markdown
**Reducing the learning rate timely using callbacks in Keras and also checkpointing the model when achieved the best so far quantity that is monitored , in this dataset I am monitoring validation accuracy.** **Saving the weights of the best model after checkpointing in transferlearning_weights.hdf5 .**
###Code
filepath="transferlearning_weights.hdf5"
checkpoint = ModelCheckpoint(filepath, monitor='val_acc', verbose=1, save_best_only=True, mode='max')
from keras.models import Sequential , Model
from keras.layers import Dense , Activation
from keras.layers import Dropout , GlobalAveragePooling2D
from keras.layers import Flatten
from keras.constraints import maxnorm
from keras.optimizers import SGD , RMSprop , Adadelta , Adam
from keras.layers import Conv2D , BatchNormalization
from keras.layers import MaxPooling2D
from keras.utils import np_utils
from keras import backend as K
K.set_image_dim_ordering('th')
from sklearn.model_selection import GridSearchCV
from keras.wrappers.scikit_learn import KerasClassifier
X_train=X_train.reshape(5216,3,150,150)
X_test=X_test.reshape(624,3,150,150)
###Output
_____no_output_____
###Markdown
**Importing InceptionV3 from Keras but with no weights. Also define the necessary input shape of the resized images which were resized initially. The default image size is 299 X 299 for InceptionV3.**
###Code
from keras.applications.inception_v3 import InceptionV3
# create the base pre-trained model
base_model = InceptionV3(weights=None, include_top=False, input_shape=(3, 150, 150))
#base_model = InceptionV3(weights=None, include_top=False, input_shape=(150, 150, 3))
#base_model = InceptionV3()
###Output
_____no_output_____
###Markdown
**The output of the above model was being fed into the after layers that we are laying down. There is no rule of thumb for laying down the after layers after InceptionV3 but practice and intuition. ** **The layers were checked multiple times for the output and finally the second last layer of BatchNormalization() did some good for the model. **
###Code
x = base_model.output
x = Dropout(0.5)(x)
x = GlobalAveragePooling2D()(x)
x = Dense(128, activation='relu')(x)
x = BatchNormalization()(x)
predictions = Dense(2, activation='sigmoid')(x)
###Output
_____no_output_____
###Markdown
**Here the I have not debarred any of the InceptionV3 layers to train themselves and rather training only the added layers. I have trained the whole network from start for the different hyperparameters. This approach gave me one of the best results so far. **
###Code
base_model.load_weights("./inceptionweights/inception_v3_weights.h5")
###Output
_____no_output_____
###Markdown
**Loading the best set of weights on the model which were attained by checkpointing and saving the weights in between the epochs and also prior to overfitting of the model. **
###Code
model = Model(inputs=base_model.input, outputs=predictions)
model.compile(loss='categorical_crossentropy',
optimizer='adam',
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
**Architecture of Layers. **
###Code
print(model.summary())
batch_size = 64
epochs = 10
history = model.fit(X_train, y_train, validation_data = (X_test , y_test) ,callbacks=[lr_reduce,checkpoint] ,
epochs=epochs)
model.load_weights("transferlearning_weights.hdf5")
###Output
_____no_output_____
###Markdown
**The best validation accuracy achieved is 86.86 % which is better than before. The model will tend to overfit because the model architecture is very much complex and also we are short of data . So better save the weights before 4th epoch prior to overfitting and also not deteriorating the performance of the model. **
###Code
import matplotlib.pyplot as plt
plt.plot(history.history['acc'])
plt.plot(history.history['val_acc'])
plt.title('model accuracy')
plt.ylabel('accuracy')
plt.xlabel('epoch')
plt.legend(['train', 'test'], loc='upper left')
plt.show()
# summarize history for loss
plt.plot(history.history['loss'])
plt.plot(history.history['val_loss'])
plt.title('model loss')
plt.ylabel('loss')
plt.xlabel('epoch')
plt.legend(['train', 'test'], loc='upper left')
plt.show()
from sklearn.metrics import confusion_matrix
pred = model.predict(X_test)
pred = np.argmax(pred,axis = 1)
y_true = np.argmax(y_test,axis = 1)
###Output
_____no_output_____
###Markdown
**Confusion matrix is very much necessary for the above model because we are having unequal number of people with pneumonia and no pneumonia. In the above dataset we are having more people suffering from pneumonia than normal people. So, as I told in other notebook , accuracy won't be the soul criteria for determining model performance.**
###Code
CM = confusion_matrix(y_true, pred)
from mlxtend.plotting import plot_confusion_matrix
fig, ax = plot_confusion_matrix(conf_mat=CM , figsize=(5, 5))
plt.show()
###Output
_____no_output_____
###Markdown
**Notice how the confusion matrix changed from other notebook and became even better than before. **
###Code
341 / (341 + 49)
###Output
_____no_output_____
###Markdown
This model even has greater precision than before . Earlier the precision was 78.4 % but now it is having 85.64 % as its precision . Therefore I have minimized false positive in the denominator of the formula . This will even make more sure than before that a person not suffering from pneumonia shouldn't be diagnosed as being suffering from pneumonia. This is what precision is .
###Code
341 / (4341 + 53)
###Output
_____no_output_____
###Markdown
The recall for the above trained model is approx. 95 % which is also quite impressive . Also as I told in other notebook more priority is to given to recall when compare to precision for this dataset.
###Code
#2*0.9487*0.8564 / (0.9487 + 0.8564)
###Output
_____no_output_____
###Markdown
Transfer Learning via InceptionV3 **Below is a demo and a brief introduction of how to work with transfer learning . I have used imagenet model "INCEPTIONV3" as my base model for better diagnosis purpose than the self model that I have laid architecture of and trained in the other notebook .**
###Code
import pandas as pd
import cv2
import numpy as np
import os
from random import shuffle
from tqdm import tqdm
import scipy
import skimage
from skimage.transform import resize
print(os.listdir("../input/chest-xray-pneumonia/chest_xray/chest_xray/"))
print(os.listdir("../input/chest-xray-pneumonia/chest_xray/chest_xray/train/"))
TRAIN_DIR = "../input/chest-xray-pneumonia/chest_xray/chest_xray/train/"
TEST_DIR = "../input/chest-xray-pneumonia/chest_xray/chest_xray/test/"
def get_label(Dir):
for nextdir in os.listdir(Dir):
if not nextdir.startswith('.'):
if nextdir in ['NORMAL']:
label = 0
elif nextdir in ['PNEUMONIA']:
label = 1
else:
label = 2
return nextdir, label
###Output
_____no_output_____
###Markdown
**Pre-processing**
###Code
def preprocessing_data(Dir):
X = []
y = []
for nextdir in os.listdir(Dir):
nextdir, label = get_label(Dir)
temp = Dir + nextdir
for image_filename in tqdm(os.listdir(temp)):
path = os.path.join(temp + '/' , image_filename)
img = cv2.imread(path,cv2.IMREAD_GRAYSCALE)
if img is not None:
img = skimage.transform.resize(img, (150, 150, 3))
img = np.asarray(img)
X.append(img)
y.append(label)
X = np.asarray(X)
y = np.asarray(y)
return X,y
def get_data(Dir):
X = []
y = []
for nextDir in os.listdir(Dir):
if not nextDir.startswith('.'):
if nextDir in ['NORMAL']:
label = 0
elif nextDir in ['PNEUMONIA']:
label = 1
else:
label = 2
temp = Dir + nextDir
for file in tqdm(os.listdir(temp)):
img = cv2.imread(temp + '/' + file)
if img is not None:
img = skimage.transform.resize(img, (150, 150, 3))
#img_file = scipy.misc.imresize(arr=img_file, size=(299, 299, 3))
img = np.asarray(img)
X.append(img)
y.append(label)
X = np.asarray(X)
y = np.asarray(y)
return X,y
X_train, y_train = get_data(TRAIN_DIR)
X_test , y_test = get_data(TEST_DIR)
print(X_train.shape,'\n',X_test.shape)
print(y_train.shape,'\n',y_test.shape)
from keras.utils.np_utils import to_categorical
y_train = to_categorical(y_train, 2)
y_test = to_categorical(y_test, 2)
Pimages = os.listdir(TRAIN_DIR + "PNEUMONIA")
Nimages = os.listdir(TRAIN_DIR + "NORMAL")
###Output
_____no_output_____
###Markdown
Plotting the XRays of No Pneumonia and Pneumonia patients.
###Code
import matplotlib.pyplot as plt
def plotter(i):
imagep1 = cv2.imread(TRAIN_DIR+"PNEUMONIA/"+Pimages[i])
imagep1 = skimage.transform.resize(imagep1, (150, 150, 3) , mode = 'reflect')
imagen1 = cv2.imread(TRAIN_DIR+"NORMAL/"+Nimages[i])
imagen1 = skimage.transform.resize(imagen1, (150, 150, 3))
pair = np.concatenate((imagen1, imagep1), axis=1)
print("(Left) - No Pneumonia Vs (Right) - Pneumonia")
print("-----------------------------------------------------------------------------------------------------------------------------------")
plt.figure(figsize=(10,5))
plt.imshow(pair)
plt.show()
for i in range(5,10):
plotter(i)
from keras.callbacks import ReduceLROnPlateau , ModelCheckpoint , LearningRateScheduler
lr_reduce = ReduceLROnPlateau(monitor='val_acc', factor=0.1, epsilon=0.0001, patience=1, verbose=1)
###Output
/opt/conda/lib/python3.6/site-packages/Keras-2.1.5-py3.6.egg/keras/callbacks.py:919: UserWarning: `epsilon` argument is deprecated and will be removed, use `min_delta` insted.
###Markdown
**Reducing the learning rate timely using callbacks in Keras and also checkpointing the model when achieved the best so far quantity that is monitored , in this dataset I am monitoring validation accuracy.** **Saving the weights of the best model after checkpointing in transferlearning_weights.hdf5 .**
###Code
filepath="transferlearning_weights.hdf5"
checkpoint = ModelCheckpoint(filepath, monitor='val_acc', verbose=1, save_best_only=True, mode='max')
from keras.models import Sequential , Model
from keras.layers import Dense , Activation
from keras.layers import Dropout , GlobalAveragePooling2D
from keras.layers import Flatten
from keras.constraints import maxnorm
from keras.optimizers import SGD , RMSprop , Adadelta , Adam
from keras.layers import Conv2D , BatchNormalization
from keras.layers import MaxPooling2D
from keras.utils import np_utils
from keras import backend as K
K.set_image_dim_ordering('th')
from sklearn.model_selection import GridSearchCV
from keras.wrappers.scikit_learn import KerasClassifier
X_train=X_train.reshape(5216,3,150,150)
X_test=X_test.reshape(624,3,150,150)
###Output
_____no_output_____
###Markdown
**Importing InceptionV3 from Keras but with no weights. Also define the necessary input shape of the resized images which were resized initially. The default image size is 299 X 299 for InceptionV3.**
###Code
from keras.applications.inception_v3 import InceptionV3
# create the base pre-trained model
base_model = InceptionV3(weights=None, include_top=False , input_shape=(3, 150, 150))
###Output
_____no_output_____
###Markdown
**The output of the above model was being fed into the after layers that we are laying down. There is no rule of thumb for laying down the after layers after InceptionV3 but practice and intuition. ** **The layers were checked multiple times for the output and finally the second last layer of BatchNormalization() did some good for the model. **
###Code
x = base_model.output
x = Dropout(0.5)(x)
x = GlobalAveragePooling2D()(x)
x = Dense(128, activation='relu')(x)
x = BatchNormalization()(x)
predictions = Dense(2, activation='sigmoid')(x)
###Output
_____no_output_____
###Markdown
**Here the I have not debarred any of the InceptionV3 layers to train themselves and rather training only the added layers. I have trained the whole network from start for the different hyperparameters. This approach gave me one of the best results so far. **
###Code
base_model.load_weights("../input/inceptionweights/inception_v3_weights.h5")
###Output
_____no_output_____
###Markdown
**Loading the best set of weights on the model which were attained by checkpointing and saving the weights in between the epochs and also prior to overfitting of the model. **
###Code
model = Model(inputs=base_model.input, outputs=predictions)
model.compile(loss='categorical_crossentropy',
optimizer='adam',
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
**Architecture of Layers. **
###Code
print(model.summary())
batch_size = 64
epochs = 10
history = model.fit(X_train, y_train, validation_data = (X_test , y_test) ,callbacks=[lr_reduce,checkpoint] ,
epochs=epochs)
model.load_weights("transferlearning_weights.hdf5")
###Output
_____no_output_____
###Markdown
**The best validation accuracy achieved is 86.86 % which is better than before. The model will tend to overfit because the model architecture is very much complex and also we are short of data . So better save the weights before 4th epoch prior to overfitting and also not deteriorating the performance of the model. **
###Code
import matplotlib.pyplot as plt
plt.plot(history.history['acc'])
plt.plot(history.history['val_acc'])
plt.title('model accuracy')
plt.ylabel('accuracy')
plt.xlabel('epoch')
plt.legend(['train', 'test'], loc='upper left')
plt.show()
# summarize history for loss
plt.plot(history.history['loss'])
plt.plot(history.history['val_loss'])
plt.title('model loss')
plt.ylabel('loss')
plt.xlabel('epoch')
plt.legend(['train', 'test'], loc='upper left')
plt.show()
from sklearn.metrics import confusion_matrix
pred = model.predict(X_test)
pred = np.argmax(pred,axis = 1)
y_true = np.argmax(y_test,axis = 1)
###Output
_____no_output_____
###Markdown
**Confusion matrix is very much necessary for the above model because we are having unequal number of people with pneumonia and no pneumonia. In the above dataset we are having more people suffering from pneumonia than normal people. So, as I told in other notebook , accuracy won't be the soul criteria for determining model performance.**
###Code
CM = confusion_matrix(y_true, pred)
from mlxtend.plotting import plot_confusion_matrix
fig, ax = plot_confusion_matrix(conf_mat=CM , figsize=(5, 5))
plt.show()
###Output
_____no_output_____
###Markdown
**Notice how the confusion matrix changed from other notebook and became even better than before. **
###Code
370 / (370 + 62)
###Output
_____no_output_____
###Markdown
This model even has greater precision than before . Earlier the precision was 78.4 % but now it is having 85.64 % as its precision . Therefore I have minimized false positive in the denominator of the formula . This will even make more sure than before that a person not suffering from pneumonia shouldn't be diagnosed as being suffering from pneumonia. This is what precision is .
###Code
370 / (370 + 20)
###Output
_____no_output_____
###Markdown
The recall for the above trained model is approx. 95 % which is also quite impressive . Also as I told in other notebook more priority is to given to recall when compare to precision for this dataset.
###Code
2*0.9487*0.8564 / (0.9487 + 0.8564)
###Output
_____no_output_____
###Markdown
Transfer Learning via InceptionV3 **Below is a demo and a brief introduction of how to work with transfer learning . I have used imagenet model "INCEPTIONV3" as my base model for better diagnosis purpose than the self model that I have laid architecture of and trained in the other notebook .**
###Code
import pandas as pd
import cv2
import numpy as np
import os
from random import shuffle
from tqdm import tqdm
import scipy
import skimage
from skimage.transform import resize
print(os.listdir("../input/chest-xray-pneumonia/chest_xray/chest_xray/"))
print(os.listdir("../input/chest-xray-pneumonia/chest_xray/chest_xray/train/"))
TRAIN_DIR = "../input/chest-xray-pneumonia/chest_xray/chest_xray/train/"
TEST_DIR = "../input/chest-xray-pneumonia/chest_xray/chest_xray/test/"
def get_label(Dir):
for nextdir in os.listdir(Dir):
if not nextdir.startswith('.'):
if nextdir in ['NORMAL']:
label = 0
elif nextdir in ['PNEUMONIA']:
label = 1
else:
label = 2
return nextdir, label
###Output
_____no_output_____
###Markdown
**Pre-processing**
###Code
def preprocessing_data(Dir):
X = []
y = []
for nextdir in os.listdir(Dir):
nextdir, label = get_label(Dir)
temp = Dir + nextdir
for image_filename in tqdm(os.listdir(temp)):
path = os.path.join(temp + '/' , image_filename)
img = cv2.imread(path,cv2.IMREAD_GRAYSCALE)
if img is not None:
img = skimage.transform.resize(img, (150, 150, 3))
img = np.asarray(img)
X.append(img)
y.append(label)
X = np.asarray(X)
y = np.asarray(y)
return X,y
def get_data(Dir):
X = []
y = []
for nextDir in os.listdir(Dir):
if not nextDir.startswith('.'):
if nextDir in ['NORMAL']:
label = 0
elif nextDir in ['PNEUMONIA']:
label = 1
else:
label = 2
temp = Dir + nextDir
for file in tqdm(os.listdir(temp)):
img = cv2.imread(temp + '/' + file)
if img is not None:
img = skimage.transform.resize(img, (150, 150, 3))
#img_file = scipy.misc.imresize(arr=img_file, size=(299, 299, 3))
img = np.asarray(img)
X.append(img)
y.append(label)
X = np.asarray(X)
y = np.asarray(y)
return X,y
X_train, y_train = get_data(TRAIN_DIR)
X_test , y_test = get_data(TEST_DIR)
print(X_train.shape,'\n',X_test.shape)
print(y_train.shape,'\n',y_test.shape)
from keras.utils.np_utils import to_categorical
y_train = to_categorical(y_train, 2)
y_test = to_categorical(y_test, 2)
Pimages = os.listdir(TRAIN_DIR + "PNEUMONIA")
Nimages = os.listdir(TRAIN_DIR + "NORMAL")
###Output
_____no_output_____
###Markdown
Plotting the XRays of No Pneumonia and Pneumonia patients.
###Code
import matplotlib.pyplot as plt
def plotter(i):
imagep1 = cv2.imread(TRAIN_DIR+"PNEUMONIA/"+Pimages[i])
imagep1 = skimage.transform.resize(imagep1, (150, 150, 3) , mode = 'reflect')
imagen1 = cv2.imread(TRAIN_DIR+"NORMAL/"+Nimages[i])
imagen1 = skimage.transform.resize(imagen1, (150, 150, 3))
pair = np.concatenate((imagen1, imagep1), axis=1)
print("(Left) - No Pneumonia Vs (Right) - Pneumonia")
print("-----------------------------------------------------------------------------------------------------------------------------------")
plt.figure(figsize=(10,5))
plt.imshow(pair)
plt.show()
for i in range(5,10):
plotter(i)
from keras.callbacks import ReduceLROnPlateau , ModelCheckpoint , LearningRateScheduler
lr_reduce = ReduceLROnPlateau(monitor='val_acc', factor=0.1, epsilon=0.0001, patience=1, verbose=1)
###Output
/opt/conda/lib/python3.6/site-packages/Keras-2.1.5-py3.6.egg/keras/callbacks.py:919: UserWarning: `epsilon` argument is deprecated and will be removed, use `min_delta` insted.
###Markdown
**Reducing the learning rate timely using callbacks in Keras and also checkpointing the model when achieved the best so far quantity that is monitored , in this dataset I am monitoring validation accuracy.** **Saving the weights of the best model after checkpointing in transferlearning_weights.hdf5 .**
###Code
filepath="transferlearning_weights.hdf5"
checkpoint = ModelCheckpoint(filepath, monitor='val_acc', verbose=1, save_best_only=True, mode='max')
from keras.models import Sequential , Model
from keras.layers import Dense , Activation
from keras.layers import Dropout , GlobalAveragePooling2D
from keras.layers import Flatten
from keras.constraints import maxnorm
from keras.optimizers import SGD , RMSprop , Adadelta , Adam
from keras.layers import Conv2D , BatchNormalization
from keras.layers import MaxPooling2D
from keras.utils import np_utils
from keras import backend as K
K.set_image_dim_ordering('th')
from sklearn.model_selection import GridSearchCV
from keras.wrappers.scikit_learn import KerasClassifier
X_train=X_train.reshape(5216,3,150,150)
X_test=X_test.reshape(624,3,150,150)
###Output
_____no_output_____
###Markdown
**Importing InceptionV3 from Keras but with no weights. Also define the necessary input shape of the resized images which were resized initially. The default image size is 299 X 299 for InceptionV3.**
###Code
from keras.applications.inception_v3 import InceptionV3
# create the base pre-trained model
base_model = InceptionV3(weights=None, include_top=False , input_shape=(3, 150, 150))
###Output
_____no_output_____
###Markdown
**The output of the above model was being fed into the after layers that we are laying down. There is no rule of thumb for laying down the after layers after InceptionV3 but practice and intuition. ** **The layers were checked multiple times for the output and finally the second last layer of BatchNormalization() did some good for the model. **
###Code
x = base_model.output
x = Dropout(0.5)(x)
x = GlobalAveragePooling2D()(x)
x = Dense(128, activation='relu')(x)
x = BatchNormalization()(x)
predictions = Dense(2, activation='sigmoid')(x)
###Output
_____no_output_____
###Markdown
**Here the I have not debarred any of the InceptionV3 layers to train themselves and rather training only the added layers. I have trained the whole network from start for the different hyperparameters. This approach gave me one of the best results so far. **
###Code
base_model.load_weights("../input/inceptionweights/inception_v3_weights.h5")
###Output
_____no_output_____
###Markdown
**Loading the best set of weights on the model which were attained by checkpointing and saving the weights in between the epochs and also prior to overfitting of the model. **
###Code
model = Model(inputs=base_model.input, outputs=predictions)
model.compile(loss='categorical_crossentropy',
optimizer='adam',
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
**Architecture of Layers. **
###Code
print(model.summary())
batch_size = 64
epochs = 10
history = model.fit(X_train, y_train, validation_data = (X_test , y_test) ,callbacks=[lr_reduce,checkpoint] ,
epochs=epochs)
model.load_weights("transferlearning_weights.hdf5")
###Output
_____no_output_____
###Markdown
**The model will tend to overfit because the model architecture is very much complex and also we are short of data . So better save the weights before 4th epoch prior to overfitting and also not deteriorating the performance of the model. **
###Code
import matplotlib.pyplot as plt
plt.plot(history.history['acc'])
plt.plot(history.history['val_acc'])
plt.title('model accuracy')
plt.ylabel('accuracy')
plt.xlabel('epoch')
plt.legend(['train', 'test'], loc='upper left')
plt.show()
# summarize history for loss
plt.plot(history.history['loss'])
plt.plot(history.history['val_loss'])
plt.title('model loss')
plt.ylabel('loss')
plt.xlabel('epoch')
plt.legend(['train', 'test'], loc='upper left')
plt.show()
from sklearn.metrics import confusion_matrix
pred = model.predict(X_test)
pred = np.argmax(pred,axis = 1)
y_true = np.argmax(y_test,axis = 1)
###Output
_____no_output_____
###Markdown
**Confusion matrix is very much necessary for the above model because we are having unequal number of people with pneumonia and no pneumonia. In the above dataset we are having more people suffering from pneumonia than normal people. So, as I told in other notebook , accuracy won't be the soul criteria for determining model performance.**
###Code
CM = confusion_matrix(y_true, pred)
from mlxtend.plotting import plot_confusion_matrix
fig, ax = plot_confusion_matrix(conf_mat=CM , figsize=(5, 5))
plt.show()
###Output
_____no_output_____
###Markdown
**Notice how the confusion matrix changed from other notebook and became even better than before. **
###Code
370 / (370 + 62)
###Output
_____no_output_____
###Markdown
This model even has greater precision than before . Earlier the precision was 78.4 % but now it is having 85.64 % as its precision . Therefore I have minimized false positive in the denominator of the formula . This will even make more sure than before that a person not suffering from pneumonia shouldn't be diagnosed as being suffering from pneumonia. This is what precision is .
###Code
370 / (370 + 20)
###Output
_____no_output_____
###Markdown
The recall for the above trained model is approx. 95 % which is also quite impressive . Also as I told in other notebook more priority is to given to recall when compare to precision for this dataset.
###Code
2*0.9487*0.8564 / (0.9487 + 0.8564)
###Output
_____no_output_____ |
PIFuHD_Demo.ipynb | ###Markdown
PIFuHD Demo: https://shunsukesaito.github.io/PIFuHD/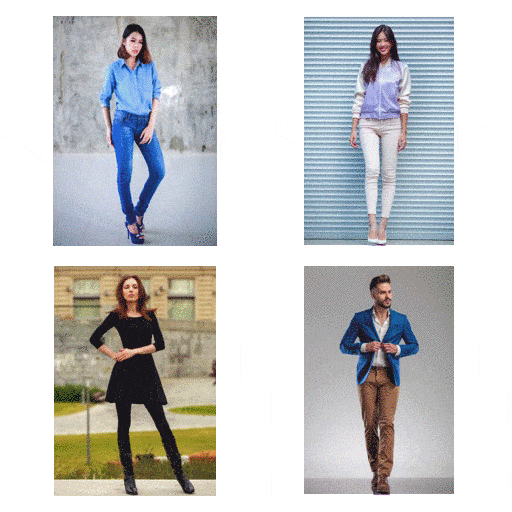Made by [](https://twitter.com/psyth91)To see how the model works, visit the project repository.[](https://github.com/facebookresearch/pifuhd) NoteMake sure that your runtime type is 'Python 3 with GPU acceleration'. To do so, go to Edit > Notebook settings > Hardware Accelerator > Select "GPU". More Info- Paper: https://arxiv.org/pdf/2004.00452.pdf- Repo: https://github.com/facebookresearch/pifuhd- Project Page: https://shunsukesaito.github.io/PIFuHD/- 1-minute/5-minute Presentation (see below)
###Code
import IPython
IPython.display.HTML('<h2>1-Minute Presentation</h2><iframe width="720" height="405" src="https://www.youtube.com/embed/-1XYTmm8HhE" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe><br><h2>5-Minute Presentation</h2><iframe width="720" height="405" src="https://www.youtube.com/embed/uEDqCxvF5yc" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>')
###Output
_____no_output_____
###Markdown
Requirements- Python 3- PyTorch tested on 1.4.0- json- PIL- skimage- tqdm- numpy- cv2 Help! I'm new to Google ColabYou can check out the following youtube video on how to upload your own picture and run PIFuHD. **Note that with new update, you can upload your own picture more easily with GUI down below.**
###Code
import IPython
IPython.display.HTML('<iframe width="720" height="405" src="https://www.youtube.com/embed/LWDGR5v3-3o" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>')
###Output
_____no_output_____
###Markdown
Clone PIFuHD repository
###Code
!git clone https://github.com/facebookresearch/pifuhd
###Output
Cloning into 'pifuhd'...
remote: Enumerating objects: 213, done.[K
remote: Total 213 (delta 0), reused 0 (delta 0), pack-reused 213[K
Receiving objects: 100% (213/213), 402.72 KiB | 2.22 MiB/s, done.
Resolving deltas: 100% (104/104), done.
###Markdown
Configure input data
###Code
cd /content/pifuhd/sample_images
###Output
/content/pifuhd/sample_images
###Markdown
**If you want to upload your own picture, run the next cell**. Otherwise, go to the next next cell. Currently PNG, JPEG files are supported.
###Code
from google.colab import files
filename = list(files.upload().keys())[0]
import os
try:
image_path = '/content/pifuhd/sample_images/%s' % filename
except:
image_path = '/content/pifuhd/sample_images/godel_stand.jpg' # example image
image_dir = os.path.dirname(image_path)
file_name = os.path.splitext(os.path.basename(image_path))[0]
# output pathes
obj_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.obj' % file_name
out_img_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.png' % file_name
video_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.mp4' % file_name
video_display_path = '/content/pifuhd/results/pifuhd_final/result_%s_256_display.mp4' % file_name
cd /content
###Output
/content
###Markdown
Preprocess (for cropping image)
###Code
!git clone https://github.com/Daniil-Osokin/lightweight-human-pose-estimation.pytorch.git
cd /content/lightweight-human-pose-estimation.pytorch/
!wget https://download.01.org/opencv/openvino_training_extensions/models/human_pose_estimation/checkpoint_iter_370000.pth
import torch
import cv2
import numpy as np
from models.with_mobilenet import PoseEstimationWithMobileNet
from modules.keypoints import extract_keypoints, group_keypoints
from modules.load_state import load_state
from modules.pose import Pose, track_poses
import demo
def get_rect(net, images, height_size):
net = net.eval()
stride = 8
upsample_ratio = 4
num_keypoints = Pose.num_kpts
previous_poses = []
delay = 33
for image in images:
rect_path = image.replace('.%s' % (image.split('.')[-1]), '_rect.txt')
img = cv2.imread(image, cv2.IMREAD_COLOR)
orig_img = img.copy()
orig_img = img.copy()
heatmaps, pafs, scale, pad = demo.infer_fast(net, img, height_size, stride, upsample_ratio, cpu=False)
total_keypoints_num = 0
all_keypoints_by_type = []
for kpt_idx in range(num_keypoints): # 19th for bg
total_keypoints_num += extract_keypoints(heatmaps[:, :, kpt_idx], all_keypoints_by_type, total_keypoints_num)
pose_entries, all_keypoints = group_keypoints(all_keypoints_by_type, pafs)
for kpt_id in range(all_keypoints.shape[0]):
all_keypoints[kpt_id, 0] = (all_keypoints[kpt_id, 0] * stride / upsample_ratio - pad[1]) / scale
all_keypoints[kpt_id, 1] = (all_keypoints[kpt_id, 1] * stride / upsample_ratio - pad[0]) / scale
current_poses = []
rects = []
for n in range(len(pose_entries)):
if len(pose_entries[n]) == 0:
continue
pose_keypoints = np.ones((num_keypoints, 2), dtype=np.int32) * -1
valid_keypoints = []
for kpt_id in range(num_keypoints):
if pose_entries[n][kpt_id] != -1.0: # keypoint was found
pose_keypoints[kpt_id, 0] = int(all_keypoints[int(pose_entries[n][kpt_id]), 0])
pose_keypoints[kpt_id, 1] = int(all_keypoints[int(pose_entries[n][kpt_id]), 1])
valid_keypoints.append([pose_keypoints[kpt_id, 0], pose_keypoints[kpt_id, 1]])
valid_keypoints = np.array(valid_keypoints)
if pose_entries[n][10] != -1.0 or pose_entries[n][13] != -1.0:
pmin = valid_keypoints.min(0)
pmax = valid_keypoints.max(0)
center = (0.5 * (pmax[:2] + pmin[:2])).astype(np.int)
radius = int(0.65 * max(pmax[0]-pmin[0], pmax[1]-pmin[1]))
elif pose_entries[n][10] == -1.0 and pose_entries[n][13] == -1.0 and pose_entries[n][8] != -1.0 and pose_entries[n][11] != -1.0:
# if leg is missing, use pelvis to get cropping
center = (0.5 * (pose_keypoints[8] + pose_keypoints[11])).astype(np.int)
radius = int(1.45*np.sqrt(((center[None,:] - valid_keypoints)**2).sum(1)).max(0))
center[1] += int(0.05*radius)
else:
center = np.array([img.shape[1]//2,img.shape[0]//2])
radius = max(img.shape[1]//2,img.shape[0]//2)
x1 = center[0] - radius
y1 = center[1] - radius
rects.append([x1, y1, 2*radius, 2*radius])
np.savetxt(rect_path, np.array(rects), fmt='%d')
net = PoseEstimationWithMobileNet()
checkpoint = torch.load('checkpoint_iter_370000.pth', map_location='cpu')
load_state(net, checkpoint)
get_rect(net.cuda(), [image_path], 512)
###Output
_____no_output_____
###Markdown
Download the Pretrained Model
###Code
cd /content/pifuhd/
!sh ./scripts/download_trained_model.sh
!ls checkpoints/
###Output
pifuhd.pt
###Markdown
Run PIFuHD!
###Code
# Warning: all images with the corresponding rectangle files under -i will be processed.
!python -m apps.simple_test -r 256 --use_rect -i $image_dir
# seems that 256 is the maximum resolution that can fit into Google Colab.
# If you want to reconstruct a higher-resolution mesh, please try with your own machine.
###Output
Resuming from ./checkpoints/pifuhd.pt
Warning: opt is overwritten.
test data size: 2
initialize network with normal
initialize network with normal
generate mesh (test) ...
0% 0/2 [00:00<?, ?it/s]./results/pifuhd_final/recon/result_godel_stand_256.obj
50% 1/2 [00:08<00:08, 8.70s/it]./results/pifuhd_final/recon/result_test_256.obj
100% 2/2 [00:16<00:00, 8.46s/it]
###Markdown
Render the result
###Code
#!pip uninstall pytorch3d
# If you get an error in the next cell, you can instead try the following command (don't forget to comment out the one above!).
# Note that this error is caused by inconsistent cuda version for the pytorch3d package and pytorch in Colab environment.
# Thus, this issue may persist unless pytorch3d in the pip package is updated with the cuda version consistent with pytorch in Colab.
# Also please be aware that the following command is much slower as it builds pytorch3d from scratch.
# !pip install 'git+https://github.com/facebookresearch/pytorch3d.git@stable'
# You can try another solution below as well. This is also slow and requires you to restart the runtime.
# !pip install 'torch==1.6.0+cu101' -f https://download.pytorch.org/whl/torch_stable.html
# !pip install 'torchvision==0.7.0+cu101' -f https://download.pytorch.org/whl/torch_stable.html
# !pip install 'pytorch3d==0.2.5'
#!conda install -c pytorch pytorch=1.7.0 torchvision cudatoolkit=10.1
!pip install 'torch==1.6.0+cu101' -f https://download.pytorch.org/whl/torch_stable.html
!pip install 'torchvision==0.7.0+cu101' -f https://download.pytorch.org/whl/torch_stable.html
!pip install 'pytorch3d==0.2.5'
!pwd
#from lib.colab_util import *
from lib.colab_util import set_renderer
from lib.colab_util import video
from lib.colab_util import generate_video_from_obj
renderer = set_renderer()
generate_video_from_obj(obj_path, out_img_path, video_path, renderer)
# we cannot play a mp4 video generated by cv2
!ffmpeg -i $video_path -vcodec libx264 $video_display_path -y -loglevel quiet
video(video_display_path)
###Output
_____no_output_____
###Markdown
Tips for Inputs: My results are broken!(Kudos to those who share results on twitter with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag!!!!)Due to the limited variation in the training data, your results might be broken sometimes. Here I share some useful tips to get resonable results. * Use high-res image. The model is trained with 1024x1024 images. Use at least 512x512 with fine-details. Low-res images and JPEG artifacts may result in unsatisfactory results. * Use an image with a single person. If the image contain multiple people, reconstruction quality is likely degraded.* Front facing with standing works best (or with fashion pose)* The entire body is covered within the image. (Note: now missing legs is partially supported)* Make sure the input image is well lit. Exteremy dark or bright image and strong shadow often create artifacts.* I recommend nearly parallel camera angle to the ground. High camera height may result in distorted legs or high heels. * If the background is cluttered, use less complex background or try removing it using https://www.remove.bg/ before processing.* It's trained with human only. Anime characters may not work well (To my surprise, indeed many people tried it!!).* Search on twitter with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag to get a better sense of what succeeds and what fails. Share your result! Please share your results with[ pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag on Twitter. Sharing your good/bad results helps and encourages the authors to further push towards producition-quality human digitization at home.**As the tweet buttom below doesn't add the result video automatically, please download the result video above and manually add it to the tweet.**
###Code
import IPython
IPython.display.HTML('<a href="https://twitter.com/intent/tweet?button_hashtag=pifuhd&ref_src=twsrc%5Etfw" class="twitter-hashtag-button" data-size="large" data-text="Google Colab Link: " data-url="https://bit.ly/37sfogZ" data-show-count="false">Tweet #pifuhd</a><script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script> (Don\'t forget to add your result to the tweet!)')
###Output
_____no_output_____
###Markdown
Cool ApplicationsSpecial thanks to those who play with PIFuHD and came up with many creative applications!! If you made any cool applications, please tweet your demo with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live). I'm constantly checking results there.If you need complete texture on the mesh, please try my previous work [PIFu](https://github.com/shunsukesaito/PIFu) as well! It supports 3D reconstruction + texturing from a single image although the geometry quality may not be as good as PIFuHD.
###Code
IPython.display.HTML('<h2>Rigging (Mixamo) + Photoreal Rendering (Blender)</h2><blockquote class="twitter-tweet"><p lang="pt" dir="ltr">vcs ainda tem a PACHORRA de me dizer que eu não sei dançar<a href="https://twitter.com/hashtag/b3d?src=hash&ref_src=twsrc%5Etfw">#b3d</a> <a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> <a href="https://t.co/kHCnLh6zxH">pic.twitter.com/kHCnLh6zxH</a></p>— lukas arendero (@lukazvd) <a href="https://twitter.com/lukazvd/status/1274810484798128131?ref_src=twsrc%5Etfw">June 21, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>FaceApp + Rigging (Mixamo)</h2><blockquote class="twitter-tweet"><p lang="ja" dir="ltr">カツラかぶってる自分に見える <a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> <a href="https://t.co/V8o7VduTiG">pic.twitter.com/V8o7VduTiG</a></p>— Shuhei Tsuchida (@shuhei2306) <a href="https://twitter.com/shuhei2306/status/1274507242910314498?ref_src=twsrc%5Etfw">June 21, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>Rigging (Mixamo) + AR (Adobe Aero)</AR><blockquote class="twitter-tweet"><p lang="ja" dir="ltr">写真→PIFuHD→Mixamo→AdobeAeroでサウンド付きARを作成。Zip化してLINEでARコンテンツを共有。<br>写真が1枚あれば簡単にARの3Dアニメーションが作れる時代…凄い。<a href="https://twitter.com/hashtag/PIFuHD?src=hash&ref_src=twsrc%5Etfw">#PIFuHD</a> <a href="https://twitter.com/hashtag/AdobeAero?src=hash&ref_src=twsrc%5Etfw">#AdobeAero</a> <a href="https://twitter.com/hashtag/Mixamo?src=hash&ref_src=twsrc%5Etfw">#Mixamo</a> <a href="https://t.co/CbiMi4gZ0K">pic.twitter.com/CbiMi4gZ0K</a></p>— モジョン (@mojon1) <a href="https://twitter.com/mojon1/status/1273217947872317441?ref_src=twsrc%5Etfw">June 17, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>3D Printing</h2><blockquote class="twitter-tweet"><p lang="ja" dir="ltr"><a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> 楽しい〜<br>小さい自分プリントした <a href="https://t.co/4qyWuij0Hs">pic.twitter.com/4qyWuij0Hs</a></p>— isb (@vxzxzxzxv) <a href="https://twitter.com/vxzxzxzxv/status/1273136266406694913?ref_src=twsrc%5Etfw">June 17, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script>')
###Output
_____no_output_____
###Markdown
PIFuHD Demo: https://shunsukesaito.github.io/PIFuHD/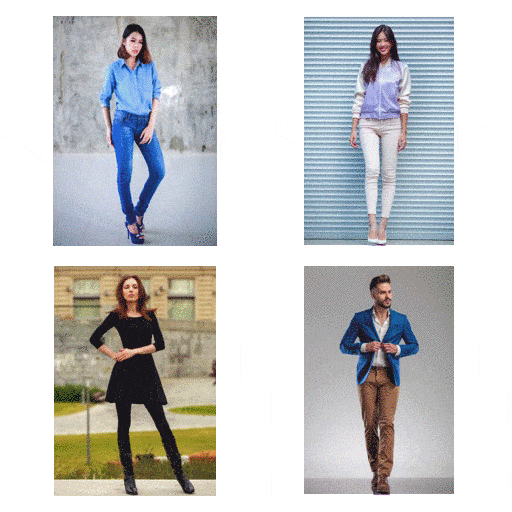Made by [](https://twitter.com/psyth91)To see how the model works, visit the project repository.[](https://github.com/facebookresearch/pifuhd) NoteMake sure that your runtime type is 'Python 3 with GPU acceleration'. To do so, go to Edit > Notebook settings > Hardware Accelerator > Select "GPU". More Info- Paper: https://arxiv.org/pdf/2004.00452.pdf- Repo: https://github.com/facebookresearch/pifuhd- Project Page: https://shunsukesaito.github.io/PIFuHD/- 1-minute/5-minute Presentation (see below)
###Code
import IPython
IPython.display.HTML('<h2>1-Minute Presentation</h2><iframe width="720" height="405" src="https://www.youtube.com/embed/-1XYTmm8HhE" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe><br><h2>5-Minute Presentation</h2><iframe width="720" height="405" src="https://www.youtube.com/embed/uEDqCxvF5yc" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>')
###Output
_____no_output_____
###Markdown
Requirements- Python 3- PyTorch tested on 1.4.0- json- PIL- skimage- tqdm- numpy- cv2 Help! I'm new to Google ColabYou can check out the following youtube video on how to upload your own picture and run PIFuHD. **Note that with new update, you can upload your own picture more easily with GUI down below.**
###Code
import IPython
IPython.display.HTML('<iframe width="720" height="405" src="https://www.youtube.com/embed/LWDGR5v3-3o" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>')
###Output
_____no_output_____
###Markdown
Clone PIFuHD repository
###Code
!git clone https://github.com/facebookresearch/pifuhd
###Output
Cloning into 'pifuhd'...
remote: Enumerating objects: 4, done.[K
remote: Counting objects: 100% (4/4), done.[K
remote: Compressing objects: 100% (4/4), done.[K
remote: Total 195 (delta 0), reused 1 (delta 0), pack-reused 191[K
Receiving objects: 100% (195/195), 399.23 KiB | 14.26 MiB/s, done.
Resolving deltas: 100% (93/93), done.
###Markdown
Configure input data
###Code
cd /content/pifuhd/sample_images
###Output
/content/pifuhd/sample_images
###Markdown
**If you want to upload your own picture, run the next cell**. Otherwise, go to the next next cell. Currently PNG, JPEG files are supported.
###Code
from google.colab import files
filename = list(files.upload().keys())[0]
import os
try:
image_path = '/content/pifuhd/sample_images/%s' % filename
except:
image_path = '/content/pifuhd/sample_images/test.png' # example image
image_dir = os.path.dirname(image_path)
file_name = os.path.splitext(os.path.basename(image_path))[0]
# output pathes
obj_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.obj' % file_name
out_img_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.png' % file_name
video_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.mp4' % file_name
video_display_path = '/content/pifuhd/results/pifuhd_final/result_%s_256_display.mp4' % file_name
cd /content
###Output
/content
###Markdown
Preprocess (for cropping image)
###Code
!git clone https://github.com/Daniil-Osokin/lightweight-human-pose-estimation.pytorch.git
cd /content/lightweight-human-pose-estimation.pytorch/
!wget https://download.01.org/opencv/openvino_training_extensions/models/human_pose_estimation/checkpoint_iter_370000.pth
import torch
import cv2
import numpy as np
from models.with_mobilenet import PoseEstimationWithMobileNet
from modules.keypoints import extract_keypoints, group_keypoints
from modules.load_state import load_state
from modules.pose import Pose, track_poses
import demo
def get_rect(net, images, height_size):
net = net.eval()
stride = 8
upsample_ratio = 4
num_keypoints = Pose.num_kpts
previous_poses = []
delay = 33
for image in images:
rect_path = image.replace('.%s' % (image.split('.')[-1]), '_rect.txt')
img = cv2.imread(image, cv2.IMREAD_COLOR)
orig_img = img.copy()
orig_img = img.copy()
heatmaps, pafs, scale, pad = demo.infer_fast(net, img, height_size, stride, upsample_ratio, cpu=False)
total_keypoints_num = 0
all_keypoints_by_type = []
for kpt_idx in range(num_keypoints): # 19th for bg
total_keypoints_num += extract_keypoints(heatmaps[:, :, kpt_idx], all_keypoints_by_type, total_keypoints_num)
pose_entries, all_keypoints = group_keypoints(all_keypoints_by_type, pafs, demo=True)
for kpt_id in range(all_keypoints.shape[0]):
all_keypoints[kpt_id, 0] = (all_keypoints[kpt_id, 0] * stride / upsample_ratio - pad[1]) / scale
all_keypoints[kpt_id, 1] = (all_keypoints[kpt_id, 1] * stride / upsample_ratio - pad[0]) / scale
current_poses = []
rects = []
for n in range(len(pose_entries)):
if len(pose_entries[n]) == 0:
continue
pose_keypoints = np.ones((num_keypoints, 2), dtype=np.int32) * -1
valid_keypoints = []
for kpt_id in range(num_keypoints):
if pose_entries[n][kpt_id] != -1.0: # keypoint was found
pose_keypoints[kpt_id, 0] = int(all_keypoints[int(pose_entries[n][kpt_id]), 0])
pose_keypoints[kpt_id, 1] = int(all_keypoints[int(pose_entries[n][kpt_id]), 1])
valid_keypoints.append([pose_keypoints[kpt_id, 0], pose_keypoints[kpt_id, 1]])
valid_keypoints = np.array(valid_keypoints)
if pose_entries[n][10] != -1.0 or pose_entries[n][13] != -1.0:
pmin = valid_keypoints.min(0)
pmax = valid_keypoints.max(0)
center = (0.5 * (pmax[:2] + pmin[:2])).astype(np.int)
radius = int(0.65 * max(pmax[0]-pmin[0], pmax[1]-pmin[1]))
elif pose_entries[n][10] == -1.0 and pose_entries[n][13] == -1.0 and pose_entries[n][8] != -1.0 and pose_entries[n][11] != -1.0:
# if leg is missing, use pelvis to get cropping
center = (0.5 * (pose_keypoints[8] + pose_keypoints[11])).astype(np.int)
radius = int(1.45*np.sqrt(((center[None,:] - valid_keypoints)**2).sum(1)).max(0))
center[1] += int(0.05*radius)
else:
center = np.array([img.shape[1]//2,img.shape[0]//2])
radius = max(img.shape[1]//2,img.shape[0]//2)
x1 = center[0] - radius
y1 = center[1] - radius
rects.append([x1, y1, 2*radius, 2*radius])
np.savetxt(rect_path, np.array(rects), fmt='%d')
net = PoseEstimationWithMobileNet()
checkpoint = torch.load('checkpoint_iter_370000.pth', map_location='cpu')
load_state(net, checkpoint)
get_rect(net.cuda(), [image_path], 512)
###Output
_____no_output_____
###Markdown
Download the Pretrained Model
###Code
cd /content/pifuhd/
!sh ./scripts/download_trained_model.sh
###Output
+ mkdir -p checkpoints
+ cd checkpoints
+ wget https://dl.fbaipublicfiles.com/pifuhd/checkpoints/pifuhd.pt pifuhd.pt
--2020-09-19 01:18:11-- https://dl.fbaipublicfiles.com/pifuhd/checkpoints/pifuhd.pt
Resolving dl.fbaipublicfiles.com (dl.fbaipublicfiles.com)... 172.67.9.4, 104.22.75.142, 104.22.74.142, ...
Connecting to dl.fbaipublicfiles.com (dl.fbaipublicfiles.com)|172.67.9.4|:443... connected.
HTTP request sent, awaiting response... 200 OK
Length: 1548375177 (1.4G) [application/octet-stream]
Saving to: ‘pifuhd.pt’
pifuhd.pt 100%[===================>] 1.44G 81.3MB/s in 21s
2020-09-19 01:18:33 (71.7 MB/s) - ‘pifuhd.pt’ saved [1548375177/1548375177]
--2020-09-19 01:18:33-- http://pifuhd.pt/
Resolving pifuhd.pt (pifuhd.pt)... failed: Name or service not known.
wget: unable to resolve host address ‘pifuhd.pt’
FINISHED --2020-09-19 01:18:33--
Total wall clock time: 21s
Downloaded: 1 files, 1.4G in 21s (71.7 MB/s)
###Markdown
Run PIFuHD!
###Code
# Warning: all images with the corresponding rectangle files under -i will be processed.
!python -m apps.simple_test -r 256 --use_rect -i $image_dir
# seems that 256 is the maximum resolution that can fit into Google Colab.
# If you want to reconstruct a higher-resolution mesh, please try with your own machine.
###Output
Resuming from ./checkpoints/pifuhd.pt
Warning: opt is overwritten.
test data size: 1
initialize network with normal
initialize network with normal
generate mesh (test) ...
0% 0/1 [00:00<?, ?it/s]./results/pifuhd_final/recon/result_test_256.obj
100% 1/1 [00:05<00:00, 5.90s/it]
###Markdown
Render the result
###Code
# This command takes a few minutes
!pip install pytorch3d
from lib.colab_util import generate_video_from_obj, set_renderer, video
renderer = set_renderer()
generate_video_from_obj(obj_path, out_img_path, video_path, renderer)
# we cannot play a mp4 video generated by cv2
!ffmpeg -i $video_path -vcodec libx264 $video_display_path -y -loglevel quiet
video(video_display_path)
###Output
_____no_output_____
###Markdown
Tips for Inputs: My results are broken!(Kudos to those who share results on twitter with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag!!!!)Due to the limited variation in the training data, your results might be broken sometimes. Here I share some useful tips to get resonable results. * Use high-res image. The model is trained with 1024x1024 images. Use at least 512x512 with fine-details. Low-res images and JPEG artifacts may result in unsatisfactory results. * Use an image with a single person. If the image contain multiple people, reconstruction quality is likely degraded.* Front facing with standing works best (or with fashion pose)* The entire body is covered within the image. (Note: now missing legs is partially supported)* Make sure the input image is well lit. Exteremy dark or bright image and strong shadow often create artifacts.* I recommend nearly parallel camera angle to the ground. High camera height may result in distorted legs or high heels. * If the background is cluttered, use less complex background or try removing it using https://www.remove.bg/ before processing.* It's trained with human only. Anime characters may not work well (To my surprise, indeed many people tried it!!).* Search on twitter with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag to get a better sense of what succeeds and what fails. Share your result! Please share your results with[ pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag on Twitter. Sharing your good/bad results helps and encourages the authors to further push towards producition-quality human digitization at home.**As the tweet buttom below doesn't add the result video automatically, please download the result video above and manually add it to the tweet.**
###Code
import IPython
IPython.display.HTML('<a href="https://twitter.com/intent/tweet?button_hashtag=pifuhd&ref_src=twsrc%5Etfw" class="twitter-hashtag-button" data-size="large" data-text="Google Colab Link: " data-url="https://bit.ly/37sfogZ" data-show-count="false">Tweet #pifuhd</a><script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script> (Don\'t forget to add your result to the tweet!)')
###Output
_____no_output_____
###Markdown
Cool ApplicationsSpecial thanks to those who play with PIFuHD and came up with many creative applications!! If you made any cool applications, please tweet your demo with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live). I'm constantly checking results there.If you need complete texture on the mesh, please try my previous work [PIFu](https://github.com/shunsukesaito/PIFu) as well! It supports 3D reconstruction + texturing from a single image although the geometry quality may not be as good as PIFuHD.
###Code
IPython.display.HTML('<h2>Rigging (Mixamo) + Photoreal Rendering (Blender)</h2><blockquote class="twitter-tweet"><p lang="pt" dir="ltr">vcs ainda tem a PACHORRA de me dizer que eu não sei dançar<a href="https://twitter.com/hashtag/b3d?src=hash&ref_src=twsrc%5Etfw">#b3d</a> <a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> <a href="https://t.co/kHCnLh6zxH">pic.twitter.com/kHCnLh6zxH</a></p>— lukas arendero (@lukazvd) <a href="https://twitter.com/lukazvd/status/1274810484798128131?ref_src=twsrc%5Etfw">June 21, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>FaceApp + Rigging (Mixamo)</h2><blockquote class="twitter-tweet"><p lang="ja" dir="ltr">カツラかぶってる自分に見える <a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> <a href="https://t.co/V8o7VduTiG">pic.twitter.com/V8o7VduTiG</a></p>— Shuhei Tsuchida (@shuhei2306) <a href="https://twitter.com/shuhei2306/status/1274507242910314498?ref_src=twsrc%5Etfw">June 21, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>Rigging (Mixamo) + AR (Adobe Aero)</AR><blockquote class="twitter-tweet"><p lang="ja" dir="ltr">写真→PIFuHD→Mixamo→AdobeAeroでサウンド付きARを作成。Zip化してLINEでARコンテンツを共有。<br>写真が1枚あれば簡単にARの3Dアニメーションが作れる時代…凄い。<a href="https://twitter.com/hashtag/PIFuHD?src=hash&ref_src=twsrc%5Etfw">#PIFuHD</a> <a href="https://twitter.com/hashtag/AdobeAero?src=hash&ref_src=twsrc%5Etfw">#AdobeAero</a> <a href="https://twitter.com/hashtag/Mixamo?src=hash&ref_src=twsrc%5Etfw">#Mixamo</a> <a href="https://t.co/CbiMi4gZ0K">pic.twitter.com/CbiMi4gZ0K</a></p>— モジョン (@mojon1) <a href="https://twitter.com/mojon1/status/1273217947872317441?ref_src=twsrc%5Etfw">June 17, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>3D Printing</h2><blockquote class="twitter-tweet"><p lang="ja" dir="ltr"><a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> 楽しい〜<br>小さい自分プリントした <a href="https://t.co/4qyWuij0Hs">pic.twitter.com/4qyWuij0Hs</a></p>— isb (@vxzxzxzxv) <a href="https://twitter.com/vxzxzxzxv/status/1273136266406694913?ref_src=twsrc%5Etfw">June 17, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script>')
###Output
_____no_output_____
###Markdown
PIFuHD Demo: https://shunsukesaito.github.io/PIFuHD/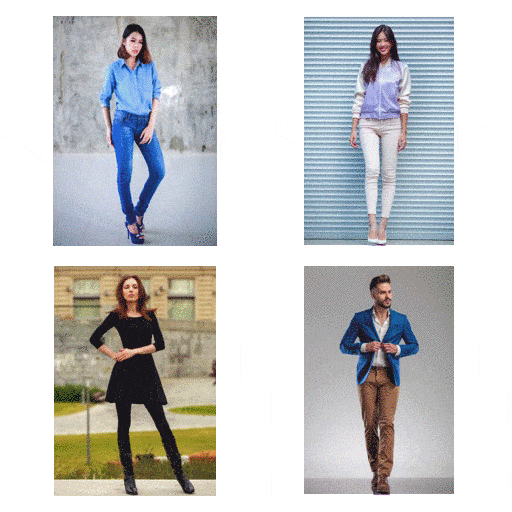Made by [](https://twitter.com/psyth91)To see how the model works, visit the project repository.[](https://github.com/facebookresearch/pifuhd) NoteMake sure that your runtime type is 'Python 3 with GPU acceleration'. To do so, go to Edit > Notebook settings > Hardware Accelerator > Select "GPU". More Info- Paper: https://arxiv.org/pdf/2004.00452.pdf- Repo: https://github.com/facebookresearch/pifuhd- Project Page: https://shunsukesaito.github.io/PIFuHD/- 1-minute/5-minute Presentation (see below)
###Code
import IPython
IPython.display.HTML('<h2>1-Minute Presentation</h2><iframe width="720" height="405" src="https://www.youtube.com/embed/-1XYTmm8HhE" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe><br><h2>5-Minute Presentation</h2><iframe width="720" height="405" src="https://www.youtube.com/embed/uEDqCxvF5yc" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>')
###Output
_____no_output_____
###Markdown
Requirements- Python 3- PyTorch tested on 1.4.0- json- PIL- skimage- tqdm- numpy- cv2 Help! I'm new to Google ColabYou can check out the following youtube video on how to upload your own picture and run PIFuHD. **Note that with new update, you can upload your own picture more easily with GUI down below.**
###Code
import IPython
IPython.display.HTML('<iframe width="720" height="405" src="https://www.youtube.com/embed/LWDGR5v3-3o" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>')
###Output
_____no_output_____
###Markdown
Clone PIFuHD repository
###Code
!git clone https://github.com/facebookresearch/pifuhd
###Output
Cloning into 'pifuhd'...
remote: Enumerating objects: 6, done.[K
remote: Counting objects: 100% (6/6), done.[K
remote: Compressing objects: 100% (6/6), done.[K
remote: Total 173 (delta 0), reused 2 (delta 0), pack-reused 167[K
Receiving objects: 100% (173/173), 395.96 KiB | 1.32 MiB/s, done.
Resolving deltas: 100% (79/79), done.
###Markdown
Configure input data
###Code
cd /content/pifuhd/sample_images
###Output
/content/pifuhd/sample_images
###Markdown
**If you want to upload your own picture, run the next cell**. Otherwise, go to the next next cell. Currently PNG, JPEG files are supported.
###Code
from google.colab import files
filename = list(files.upload().keys())[0]
import os
try:
image_path = '/content/pifuhd/sample_images/%s' % filename
except:
image_path = '/content/pifuhd/sample_images/test.png' # example image
image_dir = os.path.dirname(image_path)
file_name = os.path.splitext(os.path.basename(image_path))[0]
# output pathes
obj_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.obj' % file_name
out_img_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.png' % file_name
video_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.mp4' % file_name
video_display_path = '/content/pifuhd/results/pifuhd_final/result_%s_256_display.mp4' % file_name
cd /content
###Output
/content
###Markdown
Preprocess (for cropping image)
###Code
!git clone https://github.com/Daniil-Osokin/lightweight-human-pose-estimation.pytorch.git
cd /content/lightweight-human-pose-estimation.pytorch/
!wget https://download.01.org/opencv/openvino_training_extensions/models/human_pose_estimation/checkpoint_iter_370000.pth
import torch
import cv2
import numpy as np
from models.with_mobilenet import PoseEstimationWithMobileNet
from modules.keypoints import extract_keypoints, group_keypoints
from modules.load_state import load_state
from modules.pose import Pose, track_poses
import demo
def get_rect(net, images, height_size):
net = net.eval()
stride = 8
upsample_ratio = 4
num_keypoints = Pose.num_kpts
previous_poses = []
delay = 33
for image in images:
rect_path = image.replace('.%s' % (image.split('.')[-1]), '_rect.txt')
img = cv2.imread(image, cv2.IMREAD_COLOR)
orig_img = img.copy()
orig_img = img.copy()
heatmaps, pafs, scale, pad = demo.infer_fast(net, img, height_size, stride, upsample_ratio, cpu=False)
total_keypoints_num = 0
all_keypoints_by_type = []
for kpt_idx in range(num_keypoints): # 19th for bg
total_keypoints_num += extract_keypoints(heatmaps[:, :, kpt_idx], all_keypoints_by_type, total_keypoints_num)
pose_entries, all_keypoints = group_keypoints(all_keypoints_by_type, pafs, demo=True)
for kpt_id in range(all_keypoints.shape[0]):
all_keypoints[kpt_id, 0] = (all_keypoints[kpt_id, 0] * stride / upsample_ratio - pad[1]) / scale
all_keypoints[kpt_id, 1] = (all_keypoints[kpt_id, 1] * stride / upsample_ratio - pad[0]) / scale
current_poses = []
rects = []
for n in range(len(pose_entries)):
if len(pose_entries[n]) == 0:
continue
pose_keypoints = np.ones((num_keypoints, 2), dtype=np.int32) * -1
valid_keypoints = []
for kpt_id in range(num_keypoints):
if pose_entries[n][kpt_id] != -1.0: # keypoint was found
pose_keypoints[kpt_id, 0] = int(all_keypoints[int(pose_entries[n][kpt_id]), 0])
pose_keypoints[kpt_id, 1] = int(all_keypoints[int(pose_entries[n][kpt_id]), 1])
valid_keypoints.append([pose_keypoints[kpt_id, 0], pose_keypoints[kpt_id, 1]])
valid_keypoints = np.array(valid_keypoints)
if pose_entries[n][10] != -1.0 or pose_entries[n][13] != -1.0:
pmin = valid_keypoints.min(0)
pmax = valid_keypoints.max(0)
center = (0.5 * (pmax[:2] + pmin[:2])).astype(np.int)
radius = int(0.65 * max(pmax[0]-pmin[0], pmax[1]-pmin[1]))
elif pose_entries[n][10] == -1.0 and pose_entries[n][13] == -1.0 and pose_entries[n][8] != -1.0 and pose_entries[n][11] != -1.0:
# if leg is missing, use pelvis to get cropping
center = (0.5 * (pose_keypoints[8] + pose_keypoints[11])).astype(np.int)
radius = int(1.45*np.sqrt(((center[None,:] - valid_keypoints)**2).sum(1)).max(0))
center[1] += int(0.05*radius)
else:
center = np.array([img.shape[1]//2,img.shape[0]//2])
radius = max(img.shape[1]//2,img.shape[0]//2)
x1 = center[0] - radius
y1 = center[1] - radius
rects.append([x1, y1, 2*radius, 2*radius])
np.savetxt(rect_path, np.array(rects), fmt='%d')
net = PoseEstimationWithMobileNet()
checkpoint = torch.load('checkpoint_iter_370000.pth', map_location='cpu')
load_state(net, checkpoint)
get_rect(net.cuda(), [image_path], 512)
###Output
_____no_output_____
###Markdown
Download the Pretrained Model
###Code
cd /content/pifuhd/
!sh ./scripts/download_trained_model.sh
###Output
+ mkdir -p checkpoints
+ cd checkpoints
+ wget https://dl.fbaipublicfiles.com/pifuhd/checkpoints/pifuhd.pt pifuhd.pt
--2020-07-05 20:22:21-- https://dl.fbaipublicfiles.com/pifuhd/checkpoints/pifuhd.pt
Resolving dl.fbaipublicfiles.com (dl.fbaipublicfiles.com)... 104.22.74.142, 172.67.9.4, 104.22.75.142, ...
Connecting to dl.fbaipublicfiles.com (dl.fbaipublicfiles.com)|104.22.74.142|:443... connected.
HTTP request sent, awaiting response... 200 OK
Length: 1548375177 (1.4G) [application/octet-stream]
Saving to: ‘pifuhd.pt’
pifuhd.pt 100%[===================>] 1.44G 9.95MB/s in 2m 24s
2020-07-05 20:24:46 (10.2 MB/s) - ‘pifuhd.pt’ saved [1548375177/1548375177]
--2020-07-05 20:24:46-- http://pifuhd.pt/
Resolving pifuhd.pt (pifuhd.pt)... failed: Name or service not known.
wget: unable to resolve host address ‘pifuhd.pt’
FINISHED --2020-07-05 20:24:46--
Total wall clock time: 2m 25s
Downloaded: 1 files, 1.4G in 2m 24s (10.2 MB/s)
###Markdown
Run PIFuHD!
###Code
# Warning: all images with the corresponding rectangle files under -i will be processed.
!python -m apps.simple_test -r 256 --use_rect -i $image_dir
# seems that 256 is the maximum resolution that can fit into Google Colab.
# If you want to reconstruct a higher-resolution mesh, please try with your own machine.
###Output
/usr/bin/python3: Error while finding module specification for 'apps.simple_test' (ModuleNotFoundError: No module named 'apps')
###Markdown
Render the result
###Code
# This command takes a few minutes
!pip install 'git+https://github.com/facebookresearch/pytorch3d.git@stable'
from lib.colab_util import generate_video_from_obj, set_renderer, video
renderer = set_renderer()
generate_video_from_obj(obj_path, out_img_path, video_path, renderer)
# we cannot play a mp4 video generated by cv2
!ffmpeg -i $video_path -vcodec libx264 $video_display_path -y -loglevel quiet
video(video_display_path)
###Output
/usr/local/lib/python3.6/dist-packages/pytorch3d/io/obj_io.py:70: UserWarning: Faces have invalid indices
warnings.warn("Faces have invalid indices")
###Markdown
Tips for Inputs: My results are broken!(Kudos to those who share results on twitter with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag!!!!)Due to the limited variation in the training data, your results might be broken sometimes. Here I share some useful tips to get resonable results. * Use high-res image. The model is trained with 1024x1024 images. Use at least 512x512 with fine-details. Low-res images and JPEG artifacts may result in unsatisfactory results. * Use an image with a single person. If the image contain multiple people, reconstruction quality is likely degraded.* Front facing with standing works best (or with fashion pose)* The entire body is covered within the image. (Note: now missing legs is partially supported)* Make sure the input image is well lit. Exteremy dark or bright image and strong shadow often create artifacts.* I recommend nearly parallel camera angle to the ground. High camera height may result in distorted legs or high heels. * If the background is cluttered, use less complex background or try removing it using https://www.remove.bg/ before processing.* It's trained with human only. Anime characters may not work well (To my surprise, indeed many people tried it!!).* Search on twitter with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag to get a better sense of what succeeds and what fails. Share your result! Please share your results with[ pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag on Twitter. Sharing your good/bad results helps and encourages the authors to further push towards producition-quality human digitization at home.**As the tweet buttom below doesn't add the result video automatically, please download the result video above and manually add it to the tweet.**
###Code
import IPython
IPython.display.HTML('<a href="https://twitter.com/intent/tweet?button_hashtag=pifuhd&ref_src=twsrc%5Etfw" class="twitter-hashtag-button" data-size="large" data-text="Google Colab Link: " data-url="https://bit.ly/37sfogZ" data-show-count="false">Tweet #pifuhd</a><script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script> (Don\'t forget to add your result to the tweet!)')
###Output
_____no_output_____
###Markdown
Cool ApplicationsSpecial thanks to those who play with PIFuHD and came up with many creative applications!! If you made any cool applications, please tweet your demo with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live). I'm constantly checking results there.If you need complete texture on the mesh, please try my previous work [PIFu](https://github.com/shunsukesaito/PIFu) as well! It supports 3D reconstruction + texturing from a single image although the geometry quality may not be as good as PIFuHD.
###Code
IPython.display.HTML('<h2>Rigging (Mixamo) + Photoreal Rendering (Blender)</h2><blockquote class="twitter-tweet"><p lang="pt" dir="ltr">vcs ainda tem a PACHORRA de me dizer que eu não sei dançar<a href="https://twitter.com/hashtag/b3d?src=hash&ref_src=twsrc%5Etfw">#b3d</a> <a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> <a href="https://t.co/kHCnLh6zxH">pic.twitter.com/kHCnLh6zxH</a></p>— lukas arendero (@lukazvd) <a href="https://twitter.com/lukazvd/status/1274810484798128131?ref_src=twsrc%5Etfw">June 21, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>FaceApp + Rigging (Mixamo)</h2><blockquote class="twitter-tweet"><p lang="ja" dir="ltr">カツラかぶってる自分に見える <a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> <a href="https://t.co/V8o7VduTiG">pic.twitter.com/V8o7VduTiG</a></p>— Shuhei Tsuchida (@shuhei2306) <a href="https://twitter.com/shuhei2306/status/1274507242910314498?ref_src=twsrc%5Etfw">June 21, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>Rigging (Mixamo) + AR (Adobe Aero)</AR><blockquote class="twitter-tweet"><p lang="ja" dir="ltr">写真→PIFuHD→Mixamo→AdobeAeroでサウンド付きARを作成。Zip化してLINEでARコンテンツを共有。<br>写真が1枚あれば簡単にARの3Dアニメーションが作れる時代…凄い。<a href="https://twitter.com/hashtag/PIFuHD?src=hash&ref_src=twsrc%5Etfw">#PIFuHD</a> <a href="https://twitter.com/hashtag/AdobeAero?src=hash&ref_src=twsrc%5Etfw">#AdobeAero</a> <a href="https://twitter.com/hashtag/Mixamo?src=hash&ref_src=twsrc%5Etfw">#Mixamo</a> <a href="https://t.co/CbiMi4gZ0K">pic.twitter.com/CbiMi4gZ0K</a></p>— モジョン (@mojon1) <a href="https://twitter.com/mojon1/status/1273217947872317441?ref_src=twsrc%5Etfw">June 17, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>3D Printing</h2><blockquote class="twitter-tweet"><p lang="ja" dir="ltr"><a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> 楽しい〜<br>小さい自分プリントした <a href="https://t.co/4qyWuij0Hs">pic.twitter.com/4qyWuij0Hs</a></p>— isb (@vxzxzxzxv) <a href="https://twitter.com/vxzxzxzxv/status/1273136266406694913?ref_src=twsrc%5Etfw">June 17, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script>')
###Output
_____no_output_____
###Markdown
PIFuHD Demo: https://shunsukesaito.github.io/PIFuHD/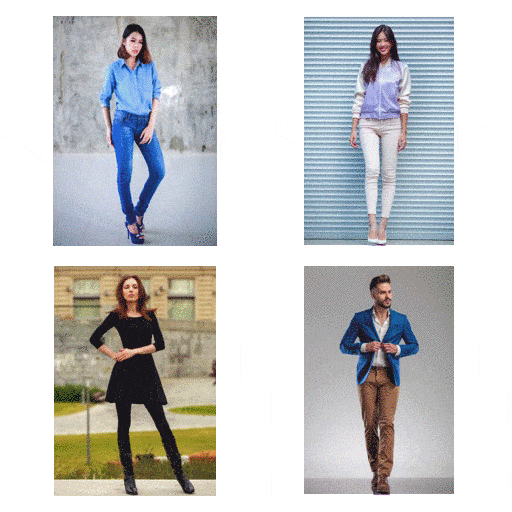Made by [](https://twitter.com/psyth91)To see how the model works, visit the project repository.[](https://github.com/facebookresearch/pifuhd) NoteMake sure that your runtime type is 'Python 3 with GPU acceleration'. To do so, go to Edit > Notebook settings > Hardware Accelerator > Select "GPU". More Info- Paper: https://arxiv.org/pdf/2004.00452.pdf- Repo: https://github.com/facebookresearch/pifuhd- Project Page: https://shunsukesaito.github.io/PIFuHD/- 1-minute/5-minute Presentation (see below)
###Code
import IPython
IPython.display.HTML('<h2>1-Minute Presentation</h2><iframe width="720" height="405" src="https://www.youtube.com/embed/-1XYTmm8HhE" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe><br><h2>5-Minute Presentation</h2><iframe width="720" height="405" src="https://www.youtube.com/embed/uEDqCxvF5yc" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>')
###Output
_____no_output_____
###Markdown
Requirements- Python 3- PyTorch tested on 1.4.0- json- PIL- skimage- tqdm- numpy- cv2 Help! I'm new to Google ColabYou can check out the following youtube video on how to upload your own picture and run PIFuHD. **Note that with new update, you can upload your own picture more easily with GUI down below.**
###Code
import IPython
IPython.display.HTML('<iframe width="720" height="405" src="https://www.youtube.com/embed/LWDGR5v3-3o" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>')
###Output
_____no_output_____
###Markdown
Clone PIFuHD repository
###Code
!git clone https://github.com/facebookresearch/pifuhd
###Output
Cloning into 'pifuhd'...
remote: Enumerating objects: 4, done.[K
remote: Counting objects: 100% (4/4), done.[K
remote: Compressing objects: 100% (4/4), done.[K
remote: Total 195 (delta 0), reused 1 (delta 0), pack-reused 191[K
Receiving objects: 100% (195/195), 399.23 KiB | 14.26 MiB/s, done.
Resolving deltas: 100% (93/93), done.
###Markdown
Configure input data
###Code
cd /content/pifuhd/sample_images
###Output
/content/pifuhd/sample_images
###Markdown
**If you want to upload your own picture, run the next cell**. Otherwise, go to the next next cell. Currently PNG, JPEG files are supported.
###Code
from google.colab import files
filename = list(files.upload().keys())[0]
import os
try:
image_path = '/content/pifuhd/sample_images/%s' % filename
except:
image_path = '/content/pifuhd/sample_images/test.png' # example image
image_dir = os.path.dirname(image_path)
file_name = os.path.splitext(os.path.basename(image_path))[0]
# output pathes
obj_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.obj' % file_name
out_img_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.png' % file_name
video_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.mp4' % file_name
video_display_path = '/content/pifuhd/results/pifuhd_final/result_%s_256_display.mp4' % file_name
cd /content
###Output
/content
###Markdown
Preprocess (for cropping image)
###Code
!git clone https://github.com/Daniil-Osokin/lightweight-human-pose-estimation.pytorch.git
cd /content/lightweight-human-pose-estimation.pytorch/
!wget https://download.01.org/opencv/openvino_training_extensions/models/human_pose_estimation/checkpoint_iter_370000.pth
import torch
import cv2
import numpy as np
from models.with_mobilenet import PoseEstimationWithMobileNet
from modules.keypoints import extract_keypoints, group_keypoints
from modules.load_state import load_state
from modules.pose import Pose, track_poses
import demo
def get_rect(net, images, height_size):
net = net.eval()
stride = 8
upsample_ratio = 4
num_keypoints = Pose.num_kpts
previous_poses = []
delay = 33
for image in images:
rect_path = image.replace('.%s' % (image.split('.')[-1]), '_rect.txt')
img = cv2.imread(image, cv2.IMREAD_COLOR)
orig_img = img.copy()
orig_img = img.copy()
heatmaps, pafs, scale, pad = demo.infer_fast(net, img, height_size, stride, upsample_ratio, cpu=False)
total_keypoints_num = 0
all_keypoints_by_type = []
for kpt_idx in range(num_keypoints): # 19th for bg
total_keypoints_num += extract_keypoints(heatmaps[:, :, kpt_idx], all_keypoints_by_type, total_keypoints_num)
pose_entries, all_keypoints = group_keypoints(all_keypoints_by_type, pafs, demo=True)
for kpt_id in range(all_keypoints.shape[0]):
all_keypoints[kpt_id, 0] = (all_keypoints[kpt_id, 0] * stride / upsample_ratio - pad[1]) / scale
all_keypoints[kpt_id, 1] = (all_keypoints[kpt_id, 1] * stride / upsample_ratio - pad[0]) / scale
current_poses = []
rects = []
for n in range(len(pose_entries)):
if len(pose_entries[n]) == 0:
continue
pose_keypoints = np.ones((num_keypoints, 2), dtype=np.int32) * -1
valid_keypoints = []
for kpt_id in range(num_keypoints):
if pose_entries[n][kpt_id] != -1.0: # keypoint was found
pose_keypoints[kpt_id, 0] = int(all_keypoints[int(pose_entries[n][kpt_id]), 0])
pose_keypoints[kpt_id, 1] = int(all_keypoints[int(pose_entries[n][kpt_id]), 1])
valid_keypoints.append([pose_keypoints[kpt_id, 0], pose_keypoints[kpt_id, 1]])
valid_keypoints = np.array(valid_keypoints)
if pose_entries[n][10] != -1.0 or pose_entries[n][13] != -1.0:
pmin = valid_keypoints.min(0)
pmax = valid_keypoints.max(0)
center = (0.5 * (pmax[:2] + pmin[:2])).astype(np.int)
radius = int(0.65 * max(pmax[0]-pmin[0], pmax[1]-pmin[1]))
elif pose_entries[n][10] == -1.0 and pose_entries[n][13] == -1.0 and pose_entries[n][8] != -1.0 and pose_entries[n][11] != -1.0:
# if leg is missing, use pelvis to get cropping
center = (0.5 * (pose_keypoints[8] + pose_keypoints[11])).astype(np.int)
radius = int(1.45*np.sqrt(((center[None,:] - valid_keypoints)**2).sum(1)).max(0))
center[1] += int(0.05*radius)
else:
center = np.array([img.shape[1]//2,img.shape[0]//2])
radius = max(img.shape[1]//2,img.shape[0]//2)
x1 = center[0] - radius
y1 = center[1] - radius
rects.append([x1, y1, 2*radius, 2*radius])
np.savetxt(rect_path, np.array(rects), fmt='%d')
net = PoseEstimationWithMobileNet()
checkpoint = torch.load('checkpoint_iter_370000.pth', map_location='cpu')
load_state(net, checkpoint)
get_rect(net.cuda(), [image_path], 512)
###Output
_____no_output_____
###Markdown
Download the Pretrained Model
###Code
cd /content/pifuhd/
!sh ./scripts/download_trained_model.sh
###Output
+ mkdir -p checkpoints
+ cd checkpoints
+ wget https://dl.fbaipublicfiles.com/pifuhd/checkpoints/pifuhd.pt pifuhd.pt
--2020-09-19 01:18:11-- https://dl.fbaipublicfiles.com/pifuhd/checkpoints/pifuhd.pt
Resolving dl.fbaipublicfiles.com (dl.fbaipublicfiles.com)... 172.67.9.4, 104.22.75.142, 104.22.74.142, ...
Connecting to dl.fbaipublicfiles.com (dl.fbaipublicfiles.com)|172.67.9.4|:443... connected.
HTTP request sent, awaiting response... 200 OK
Length: 1548375177 (1.4G) [application/octet-stream]
Saving to: ‘pifuhd.pt’
pifuhd.pt 100%[===================>] 1.44G 81.3MB/s in 21s
2020-09-19 01:18:33 (71.7 MB/s) - ‘pifuhd.pt’ saved [1548375177/1548375177]
--2020-09-19 01:18:33-- http://pifuhd.pt/
Resolving pifuhd.pt (pifuhd.pt)... failed: Name or service not known.
wget: unable to resolve host address ‘pifuhd.pt’
FINISHED --2020-09-19 01:18:33--
Total wall clock time: 21s
Downloaded: 1 files, 1.4G in 21s (71.7 MB/s)
###Markdown
Run PIFuHD!
###Code
# Warning: all images with the corresponding rectangle files under -i will be processed.
!python -m apps.simple_test -r 256 --use_rect -i $image_dir
# seems that 256 is the maximum resolution that can fit into Google Colab.
# If you want to reconstruct a higher-resolution mesh, please try with your own machine.
###Output
Resuming from ./checkpoints/pifuhd.pt
Warning: opt is overwritten.
test data size: 1
initialize network with normal
initialize network with normal
generate mesh (test) ...
0% 0/1 [00:00<?, ?it/s]./results/pifuhd_final/recon/result_test_256.obj
100% 1/1 [00:05<00:00, 5.90s/it]
###Markdown
Render the result
###Code
# This command takes a few minutes
!pip install pytorch3d
from lib.colab_util import generate_video_from_obj, set_renderer, video
renderer = set_renderer()
generate_video_from_obj(obj_path, out_img_path, video_path, renderer)
# we cannot play a mp4 video generated by cv2
!ffmpeg -i $video_path -vcodec libx264 $video_display_path -y -loglevel quiet
video(video_display_path)
###Output
_____no_output_____
###Markdown
Tips for Inputs: My results are broken!(Kudos to those who share results on twitter with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag!!!!)Due to the limited variation in the training data, your results might be broken sometimes. Here I share some useful tips to get resonable results. * Use high-res image. The model is trained with 1024x1024 images. Use at least 512x512 with fine-details. Low-res images and JPEG artifacts may result in unsatisfactory results. * Use an image with a single person. If the image contain multiple people, reconstruction quality is likely degraded.* Front facing with standing works best (or with fashion pose)* The entire body is covered within the image. (Note: now missing legs is partially supported)* Make sure the input image is well lit. Exteremy dark or bright image and strong shadow often create artifacts.* I recommend nearly parallel camera angle to the ground. High camera height may result in distorted legs or high heels. * If the background is cluttered, use less complex background or try removing it using https://www.remove.bg/ before processing.* It's trained with human only. Anime characters may not work well (To my surprise, indeed many people tried it!!).* Search on twitter with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag to get a better sense of what succeeds and what fails. Share your result! Please share your results with[ pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag on Twitter. Sharing your good/bad results helps and encourages the authors to further push towards producition-quality human digitization at home.**As the tweet buttom below doesn't add the result video automatically, please download the result video above and manually add it to the tweet.**
###Code
import IPython
IPython.display.HTML('<a href="https://twitter.com/intent/tweet?button_hashtag=pifuhd&ref_src=twsrc%5Etfw" class="twitter-hashtag-button" data-size="large" data-text="Google Colab Link: " data-url="https://bit.ly/37sfogZ" data-show-count="false">Tweet #pifuhd</a><script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script> (Don\'t forget to add your result to the tweet!)')
###Output
_____no_output_____
###Markdown
Cool ApplicationsSpecial thanks to those who play with PIFuHD and came up with many creative applications!! If you made any cool applications, please tweet your demo with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live). I'm constantly checking results there.If you need complete texture on the mesh, please try my previous work [PIFu](https://github.com/shunsukesaito/PIFu) as well! It supports 3D reconstruction + texturing from a single image although the geometry quality may not be as good as PIFuHD.
###Code
IPython.display.HTML('<h2>Rigging (Mixamo) + Photoreal Rendering (Blender)</h2><blockquote class="twitter-tweet"><p lang="pt" dir="ltr">vcs ainda tem a PACHORRA de me dizer que eu não sei dançar<a href="https://twitter.com/hashtag/b3d?src=hash&ref_src=twsrc%5Etfw">#b3d</a> <a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> <a href="https://t.co/kHCnLh6zxH">pic.twitter.com/kHCnLh6zxH</a></p>— lukas arendero (@lukazvd) <a href="https://twitter.com/lukazvd/status/1274810484798128131?ref_src=twsrc%5Etfw">June 21, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>FaceApp + Rigging (Mixamo)</h2><blockquote class="twitter-tweet"><p lang="ja" dir="ltr">カツラかぶってる自分に見える <a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> <a href="https://t.co/V8o7VduTiG">pic.twitter.com/V8o7VduTiG</a></p>— Shuhei Tsuchida (@shuhei2306) <a href="https://twitter.com/shuhei2306/status/1274507242910314498?ref_src=twsrc%5Etfw">June 21, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>Rigging (Mixamo) + AR (Adobe Aero)</AR><blockquote class="twitter-tweet"><p lang="ja" dir="ltr">写真→PIFuHD→Mixamo→AdobeAeroでサウンド付きARを作成。Zip化してLINEでARコンテンツを共有。<br>写真が1枚あれば簡単にARの3Dアニメーションが作れる時代…凄い。<a href="https://twitter.com/hashtag/PIFuHD?src=hash&ref_src=twsrc%5Etfw">#PIFuHD</a> <a href="https://twitter.com/hashtag/AdobeAero?src=hash&ref_src=twsrc%5Etfw">#AdobeAero</a> <a href="https://twitter.com/hashtag/Mixamo?src=hash&ref_src=twsrc%5Etfw">#Mixamo</a> <a href="https://t.co/CbiMi4gZ0K">pic.twitter.com/CbiMi4gZ0K</a></p>— モジョン (@mojon1) <a href="https://twitter.com/mojon1/status/1273217947872317441?ref_src=twsrc%5Etfw">June 17, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>3D Printing</h2><blockquote class="twitter-tweet"><p lang="ja" dir="ltr"><a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> 楽しい〜<br>小さい自分プリントした <a href="https://t.co/4qyWuij0Hs">pic.twitter.com/4qyWuij0Hs</a></p>— isb (@vxzxzxzxv) <a href="https://twitter.com/vxzxzxzxv/status/1273136266406694913?ref_src=twsrc%5Etfw">June 17, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script>')
###Output
_____no_output_____
###Markdown
PIFuHD Demo: https://shunsukesaito.github.io/PIFuHD/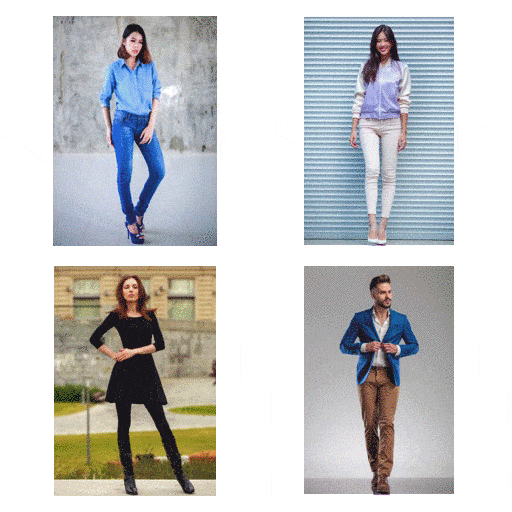Made by [](https://twitter.com/psyth91)To see how the model works, visit the project repository.[](https://github.com/facebookresearch/pifuhd) NoteMake sure that your runtime type is 'Python 3 with GPU acceleration'. To do so, go to Edit > Notebook settings > Hardware Accelerator > Select "GPU". More Info- Paper: https://arxiv.org/pdf/2004.00452.pdf- Repo: https://github.com/facebookresearch/pifuhd- Project Page: https://shunsukesaito.github.io/PIFuHD/- 1-minute/5-minute Presentation (see below)
###Code
import IPython
IPython.display.HTML('<h2>1-Minute Presentation</h2><iframe width="720" height="405" src="https://www.youtube.com/embed/-1XYTmm8HhE" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe><br><h2>5-Minute Presentation</h2><iframe width="720" height="405" src="https://www.youtube.com/embed/uEDqCxvF5yc" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>')
###Output
_____no_output_____
###Markdown
Requirements- Python 3- PyTorch tested on 1.4.0- json- PIL- skimage- tqdm- numpy- cv2 Install pytorch3d for Rendering
###Code
!pip install 'torch==1.6.0+cu101' -f https://download.pytorch.org/whl/torch_stable.html
!pip install 'torchvision==0.7.0+cu101' -f https://download.pytorch.org/whl/torch_stable.html
!pip install 'pytorch3d==0.2.5'
###Output
Requirement already satisfied: pytorch3d==0.2.5 in /usr/local/lib/python3.7/dist-packages (0.2.5)
Requirement already satisfied: torchvision>=0.4 in /usr/local/lib/python3.7/dist-packages (from pytorch3d==0.2.5) (0.7.0+cu101)
Requirement already satisfied: fvcore in /usr/local/lib/python3.7/dist-packages (from pytorch3d==0.2.5) (0.1.3.post20210227)
Requirement already satisfied: torch==1.6.0 in /usr/local/lib/python3.7/dist-packages (from torchvision>=0.4->pytorch3d==0.2.5) (1.6.0+cu101)
Requirement already satisfied: numpy in /usr/local/lib/python3.7/dist-packages (from torchvision>=0.4->pytorch3d==0.2.5) (1.19.5)
Requirement already satisfied: pillow>=4.1.1 in /usr/local/lib/python3.7/dist-packages (from torchvision>=0.4->pytorch3d==0.2.5) (7.0.0)
Requirement already satisfied: tqdm in /usr/local/lib/python3.7/dist-packages (from fvcore->pytorch3d==0.2.5) (4.41.1)
Requirement already satisfied: yacs>=0.1.6 in /usr/local/lib/python3.7/dist-packages (from fvcore->pytorch3d==0.2.5) (0.1.8)
Requirement already satisfied: iopath>=0.1.2 in /usr/local/lib/python3.7/dist-packages (from fvcore->pytorch3d==0.2.5) (0.1.4)
Requirement already satisfied: termcolor>=1.1 in /usr/local/lib/python3.7/dist-packages (from fvcore->pytorch3d==0.2.5) (1.1.0)
Requirement already satisfied: tabulate in /usr/local/lib/python3.7/dist-packages (from fvcore->pytorch3d==0.2.5) (0.8.9)
Requirement already satisfied: pyyaml>=5.1 in /usr/local/lib/python3.7/dist-packages (from fvcore->pytorch3d==0.2.5) (5.4.1)
Requirement already satisfied: future in /usr/local/lib/python3.7/dist-packages (from torch==1.6.0->torchvision>=0.4->pytorch3d==0.2.5) (0.16.0)
Requirement already satisfied: portalocker in /usr/local/lib/python3.7/dist-packages (from iopath>=0.1.2->fvcore->pytorch3d==0.2.5) (2.2.1)
###Markdown
IMPORTANT: RESTART RUNTIME Clone PIFuHD repository
###Code
!git clone https://github.com/facebookresearch/pifuhd
###Output
Cloning into 'pifuhd'...
remote: Enumerating objects: 213, done.[K
Receiving objects: 0% (1/213)
Receiving objects: 1% (3/213)
Receiving objects: 2% (5/213)
Receiving objects: 3% (7/213)
Receiving objects: 4% (9/213)
Receiving objects: 5% (11/213)
Receiving objects: 6% (13/213)
Receiving objects: 7% (15/213)
Receiving objects: 8% (18/213)
Receiving objects: 9% (20/213)
Receiving objects: 10% (22/213)
Receiving objects: 11% (24/213)
Receiving objects: 12% (26/213)
Receiving objects: 13% (28/213)
Receiving objects: 14% (30/213)
Receiving objects: 15% (32/213)
Receiving objects: 16% (35/213)
Receiving objects: 17% (37/213)
Receiving objects: 18% (39/213)
Receiving objects: 19% (41/213)
Receiving objects: 20% (43/213)
Receiving objects: 21% (45/213)
Receiving objects: 22% (47/213)
Receiving objects: 23% (49/213)
Receiving objects: 24% (52/213)
Receiving objects: 25% (54/213)
Receiving objects: 26% (56/213)
Receiving objects: 27% (58/213)
Receiving objects: 28% (60/213)
Receiving objects: 29% (62/213)
Receiving objects: 30% (64/213)
Receiving objects: 31% (67/213)
Receiving objects: 32% (69/213)
Receiving objects: 33% (71/213)
Receiving objects: 34% (73/213)
Receiving objects: 35% (75/213)
Receiving objects: 36% (77/213)
Receiving objects: 37% (79/213)
Receiving objects: 38% (81/213)
Receiving objects: 39% (84/213)
Receiving objects: 40% (86/213)
Receiving objects: 41% (88/213)
Receiving objects: 42% (90/213)
Receiving objects: 43% (92/213)
Receiving objects: 44% (94/213)
Receiving objects: 45% (96/213)
Receiving objects: 46% (98/213)
Receiving objects: 47% (101/213)
Receiving objects: 48% (103/213)
Receiving objects: 49% (105/213)
Receiving objects: 50% (107/213)
Receiving objects: 51% (109/213)
Receiving objects: 52% (111/213)
Receiving objects: 53% (113/213)
Receiving objects: 54% (116/213)
Receiving objects: 55% (118/213)
Receiving objects: 56% (120/213)
Receiving objects: 57% (122/213)
Receiving objects: 58% (124/213)
Receiving objects: 59% (126/213)
Receiving objects: 60% (128/213)
Receiving objects: 61% (130/213)
Receiving objects: 62% (133/213)
Receiving objects: 63% (135/213)
remote: Total 213 (delta 0), reused 0 (delta 0), pack-reused 213[K
Receiving objects: 64% (137/213)
Receiving objects: 65% (139/213)
Receiving objects: 66% (141/213)
Receiving objects: 67% (143/213)
Receiving objects: 68% (145/213)
Receiving objects: 69% (147/213)
Receiving objects: 70% (150/213)
Receiving objects: 71% (152/213)
Receiving objects: 72% (154/213)
Receiving objects: 73% (156/213)
Receiving objects: 74% (158/213)
Receiving objects: 75% (160/213)
Receiving objects: 76% (162/213)
Receiving objects: 77% (165/213)
Receiving objects: 78% (167/213)
Receiving objects: 79% (169/213)
Receiving objects: 80% (171/213)
Receiving objects: 81% (173/213)
Receiving objects: 82% (175/213)
Receiving objects: 83% (177/213)
Receiving objects: 84% (179/213)
Receiving objects: 85% (182/213)
Receiving objects: 86% (184/213)
Receiving objects: 87% (186/213)
Receiving objects: 88% (188/213)
Receiving objects: 89% (190/213)
Receiving objects: 90% (192/213)
Receiving objects: 91% (194/213)
Receiving objects: 92% (196/213)
Receiving objects: 93% (199/213)
Receiving objects: 94% (201/213)
Receiving objects: 95% (203/213)
Receiving objects: 96% (205/213)
Receiving objects: 97% (207/213)
Receiving objects: 98% (209/213)
Receiving objects: 99% (211/213)
Receiving objects: 100% (213/213)
Receiving objects: 100% (213/213), 402.72 KiB | 23.69 MiB/s, done.
Resolving deltas: 0% (0/104)
Resolving deltas: 2% (3/104)
Resolving deltas: 3% (4/104)
Resolving deltas: 5% (6/104)
Resolving deltas: 11% (12/104)
Resolving deltas: 13% (14/104)
Resolving deltas: 17% (18/104)
Resolving deltas: 19% (20/104)
Resolving deltas: 20% (21/104)
Resolving deltas: 21% (22/104)
Resolving deltas: 22% (23/104)
Resolving deltas: 23% (24/104)
Resolving deltas: 25% (26/104)
Resolving deltas: 28% (30/104)
Resolving deltas: 30% (32/104)
Resolving deltas: 32% (34/104)
Resolving deltas: 33% (35/104)
Resolving deltas: 34% (36/104)
Resolving deltas: 35% (37/104)
Resolving deltas: 36% (38/104)
Resolving deltas: 37% (39/104)
Resolving deltas: 38% (40/104)
Resolving deltas: 39% (41/104)
Resolving deltas: 40% (42/104)
Resolving deltas: 41% (43/104)
Resolving deltas: 42% (44/104)
Resolving deltas: 45% (47/104)
Resolving deltas: 46% (48/104)
Resolving deltas: 51% (54/104)
Resolving deltas: 56% (59/104)
Resolving deltas: 62% (65/104)
Resolving deltas: 64% (67/104)
Resolving deltas: 65% (68/104)
Resolving deltas: 83% (87/104)
Resolving deltas: 92% (96/104)
Resolving deltas: 94% (98/104)
Resolving deltas: 95% (99/104)
Resolving deltas: 97% (101/104)
Resolving deltas: 100% (104/104)
Resolving deltas: 100% (104/104), done.
###Markdown
Configure input data
###Code
cd /content/pifuhd/sample_images
###Output
/content/pifuhd/sample_images
###Markdown
**If you want to upload your own picture, run the next cell**. Otherwise, go to the next next cell. Currently PNG, JPEG files are supported.**YOUR IMAGE SHOULD BE AROUND 60KB IN SIZE, OTHERWISE YOU WILL GET AN OUT OF MEMORY ERROR**
###Code
from google.colab import files
filename = list(files.upload().keys())[0]
import os
try:
image_path = '/content/pifuhd/sample_images/%s' % filename
except:
image_path = '/content/pifuhd/sample_images/test.png' # example image
image_dir = os.path.dirname(image_path)
file_name = os.path.splitext(os.path.basename(image_path))[0]
# output pathes
obj_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.obj' % file_name
out_img_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.png' % file_name
video_path = '/content/pifuhd/results/pifuhd_final/recon/result_%s_256.mp4' % file_name
video_display_path = '/content/pifuhd/results/pifuhd_final/result_%s_256_display.mp4' % file_name
cd /content
###Output
/content
###Markdown
Preprocess (for cropping image)
###Code
!git clone https://github.com/Daniil-Osokin/lightweight-human-pose-estimation.pytorch.git
cd /content/lightweight-human-pose-estimation.pytorch/
!wget https://download.01.org/opencv/openvino_training_extensions/models/human_pose_estimation/checkpoint_iter_370000.pth
import torch
import cv2
import numpy as np
from models.with_mobilenet import PoseEstimationWithMobileNet
from modules.keypoints import extract_keypoints, group_keypoints
from modules.load_state import load_state
from modules.pose import Pose, track_poses
import demo
def get_rect(net, images, height_size):
net = net.eval()
stride = 8
upsample_ratio = 4
num_keypoints = Pose.num_kpts
previous_poses = []
delay = 33
for image in images:
rect_path = image.replace('.%s' % (image.split('.')[-1]), '_rect.txt')
img = cv2.imread(image, cv2.IMREAD_COLOR)
orig_img = img.copy()
orig_img = img.copy()
heatmaps, pafs, scale, pad = demo.infer_fast(net, img, height_size, stride, upsample_ratio, cpu=False)
total_keypoints_num = 0
all_keypoints_by_type = []
for kpt_idx in range(num_keypoints): # 19th for bg
total_keypoints_num += extract_keypoints(heatmaps[:, :, kpt_idx], all_keypoints_by_type, total_keypoints_num)
pose_entries, all_keypoints = group_keypoints(all_keypoints_by_type, pafs)
for kpt_id in range(all_keypoints.shape[0]):
all_keypoints[kpt_id, 0] = (all_keypoints[kpt_id, 0] * stride / upsample_ratio - pad[1]) / scale
all_keypoints[kpt_id, 1] = (all_keypoints[kpt_id, 1] * stride / upsample_ratio - pad[0]) / scale
current_poses = []
rects = []
for n in range(len(pose_entries)):
if len(pose_entries[n]) == 0:
continue
pose_keypoints = np.ones((num_keypoints, 2), dtype=np.int32) * -1
valid_keypoints = []
for kpt_id in range(num_keypoints):
if pose_entries[n][kpt_id] != -1.0: # keypoint was found
pose_keypoints[kpt_id, 0] = int(all_keypoints[int(pose_entries[n][kpt_id]), 0])
pose_keypoints[kpt_id, 1] = int(all_keypoints[int(pose_entries[n][kpt_id]), 1])
valid_keypoints.append([pose_keypoints[kpt_id, 0], pose_keypoints[kpt_id, 1]])
valid_keypoints = np.array(valid_keypoints)
if pose_entries[n][10] != -1.0 or pose_entries[n][13] != -1.0:
pmin = valid_keypoints.min(0)
pmax = valid_keypoints.max(0)
center = (0.5 * (pmax[:2] + pmin[:2])).astype(np.int)
radius = int(0.65 * max(pmax[0]-pmin[0], pmax[1]-pmin[1]))
elif pose_entries[n][10] == -1.0 and pose_entries[n][13] == -1.0 and pose_entries[n][8] != -1.0 and pose_entries[n][11] != -1.0:
# if leg is missing, use pelvis to get cropping
center = (0.5 * (pose_keypoints[8] + pose_keypoints[11])).astype(np.int)
radius = int(1.45*np.sqrt(((center[None,:] - valid_keypoints)**2).sum(1)).max(0))
center[1] += int(0.05*radius)
else:
center = np.array([img.shape[1]//2,img.shape[0]//2])
radius = max(img.shape[1]//2,img.shape[0]//2)
x1 = center[0] - radius
y1 = center[1] - radius
rects.append([x1, y1, 2*radius, 2*radius])
np.savetxt(rect_path, np.array(rects), fmt='%d')
net = PoseEstimationWithMobileNet()
checkpoint = torch.load('checkpoint_iter_370000.pth', map_location='cpu')
load_state(net, checkpoint)
get_rect(net.cuda(), [image_path], 512)
###Output
_____no_output_____
###Markdown
Download the Pretrained Model
###Code
cd /content/pifuhd/
!sh ./scripts/download_trained_model.sh
###Output
+ mkdir -p checkpoints
+ cd checkpoints
+ wget https://dl.fbaipublicfiles.com/pifuhd/checkpoints/pifuhd.pt pifuhd.pt
--2020-09-19 01:18:11-- https://dl.fbaipublicfiles.com/pifuhd/checkpoints/pifuhd.pt
Resolving dl.fbaipublicfiles.com (dl.fbaipublicfiles.com)... 172.67.9.4, 104.22.75.142, 104.22.74.142, ...
Connecting to dl.fbaipublicfiles.com (dl.fbaipublicfiles.com)|172.67.9.4|:443... connected.
HTTP request sent, awaiting response... 200 OK
Length: 1548375177 (1.4G) [application/octet-stream]
Saving to: ‘pifuhd.pt’
pifuhd.pt 100%[===================>] 1.44G 81.3MB/s in 21s
2020-09-19 01:18:33 (71.7 MB/s) - ‘pifuhd.pt’ saved [1548375177/1548375177]
--2020-09-19 01:18:33-- http://pifuhd.pt/
Resolving pifuhd.pt (pifuhd.pt)... failed: Name or service not known.
wget: unable to resolve host address ‘pifuhd.pt’
FINISHED --2020-09-19 01:18:33--
Total wall clock time: 21s
Downloaded: 1 files, 1.4G in 21s (71.7 MB/s)
###Markdown
Run PIFuHD!
###Code
# Warning: all images with the corresponding rectangle files under -i will be processed.
!python -m apps.simple_test -r 256 --use_rect -i $image_dir
# seems that 256 is the maximum resolution that can fit into Google Colab.
# If you want to reconstruct a higher-resolution mesh, please try with your own machine.
###Output
Resuming from ./checkpoints/pifuhd.pt
Warning: opt is overwritten.
test data size: 1
initialize network with normal
initialize network with normal
generate mesh (test) ...
0% 0/1 [00:00<?, ?it/s]./results/pifuhd_final/recon/result_test_256.obj
100% 1/1 [00:05<00:00, 5.90s/it]
###Markdown
Render the result
###Code
from lib.colab_util import generate_video_from_obj, set_renderer, video
renderer = set_renderer()
generate_video_from_obj(obj_path, out_img_path, video_path, renderer)
# we cannot play a mp4 video generated by cv2
!ffmpeg -i $video_path -vcodec libx264 $video_display_path -y -loglevel quiet
video(video_display_path)
###Output
_____no_output_____
###Markdown
Tips for Inputs: My results are broken!(Kudos to those who share results on twitter with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag!!!!)Due to the limited variation in the training data, your results might be broken sometimes. Here I share some useful tips to get resonable results. * Use high-res image. The model is trained with 1024x1024 images. Use at least 512x512 with fine-details. Low-res images and JPEG artifacts may result in unsatisfactory results. * Use an image with a single person. If the image contain multiple people, reconstruction quality is likely degraded.* Front facing with standing works best (or with fashion pose)* The entire body is covered within the image. (Note: now missing legs is partially supported)* Make sure the input image is well lit. Exteremy dark or bright image and strong shadow often create artifacts.* I recommend nearly parallel camera angle to the ground. High camera height may result in distorted legs or high heels. * If the background is cluttered, use less complex background or try removing it using https://www.remove.bg/ before processing.* It's trained with human only. Anime characters may not work well (To my surprise, indeed many people tried it!!).* Search on twitter with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag to get a better sense of what succeeds and what fails. Share your result! Please share your results with[ pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live) tag on Twitter. Sharing your good/bad results helps and encourages the authors to further push towards producition-quality human digitization at home.**As the tweet buttom below doesn't add the result video automatically, please download the result video above and manually add it to the tweet.**
###Code
import IPython
IPython.display.HTML('<a href="https://twitter.com/intent/tweet?button_hashtag=pifuhd&ref_src=twsrc%5Etfw" class="twitter-hashtag-button" data-size="large" data-text="Google Colab Link: " data-url="https://bit.ly/37sfogZ" data-show-count="false">Tweet #pifuhd</a><script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script> (Don\'t forget to add your result to the tweet!)')
###Output
_____no_output_____
###Markdown
Cool ApplicationsSpecial thanks to those who play with PIFuHD and came up with many creative applications!! If you made any cool applications, please tweet your demo with [pifuhd](https://twitter.com/search?q=%23pifuhd&src=recent_search_click&f=live). I'm constantly checking results there.If you need complete texture on the mesh, please try my previous work [PIFu](https://github.com/shunsukesaito/PIFu) as well! It supports 3D reconstruction + texturing from a single image although the geometry quality may not be as good as PIFuHD.
###Code
IPython.display.HTML('<h2>Rigging (Mixamo) + Photoreal Rendering (Blender)</h2><blockquote class="twitter-tweet"><p lang="pt" dir="ltr">vcs ainda tem a PACHORRA de me dizer que eu não sei dançar<a href="https://twitter.com/hashtag/b3d?src=hash&ref_src=twsrc%5Etfw">#b3d</a> <a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> <a href="https://t.co/kHCnLh6zxH">pic.twitter.com/kHCnLh6zxH</a></p>— lukas arendero (@lukazvd) <a href="https://twitter.com/lukazvd/status/1274810484798128131?ref_src=twsrc%5Etfw">June 21, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>FaceApp + Rigging (Mixamo)</h2><blockquote class="twitter-tweet"><p lang="ja" dir="ltr">カツラかぶってる自分に見える <a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> <a href="https://t.co/V8o7VduTiG">pic.twitter.com/V8o7VduTiG</a></p>— Shuhei Tsuchida (@shuhei2306) <a href="https://twitter.com/shuhei2306/status/1274507242910314498?ref_src=twsrc%5Etfw">June 21, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>Rigging (Mixamo) + AR (Adobe Aero)</AR><blockquote class="twitter-tweet"><p lang="ja" dir="ltr">写真→PIFuHD→Mixamo→AdobeAeroでサウンド付きARを作成。Zip化してLINEでARコンテンツを共有。<br>写真が1枚あれば簡単にARの3Dアニメーションが作れる時代…凄い。<a href="https://twitter.com/hashtag/PIFuHD?src=hash&ref_src=twsrc%5Etfw">#PIFuHD</a> <a href="https://twitter.com/hashtag/AdobeAero?src=hash&ref_src=twsrc%5Etfw">#AdobeAero</a> <a href="https://twitter.com/hashtag/Mixamo?src=hash&ref_src=twsrc%5Etfw">#Mixamo</a> <a href="https://t.co/CbiMi4gZ0K">pic.twitter.com/CbiMi4gZ0K</a></p>— モジョン (@mojon1) <a href="https://twitter.com/mojon1/status/1273217947872317441?ref_src=twsrc%5Etfw">June 17, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script><h2>3D Printing</h2><blockquote class="twitter-tweet"><p lang="ja" dir="ltr"><a href="https://twitter.com/hashtag/pifuhd?src=hash&ref_src=twsrc%5Etfw">#pifuhd</a> 楽しい〜<br>小さい自分プリントした <a href="https://t.co/4qyWuij0Hs">pic.twitter.com/4qyWuij0Hs</a></p>— isb (@vxzxzxzxv) <a href="https://twitter.com/vxzxzxzxv/status/1273136266406694913?ref_src=twsrc%5Etfw">June 17, 2020</a></blockquote> <script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script>')
###Output
_____no_output_____ |
Query Optimization/algorithms/ikkbz_hist/histogram.ipynb | ###Markdown
HistogramsAllows you to approximate the selectivity using a histogram for following selections:- $A = c$- $A > c$- $A_1 = A_2$ Example for $A=c$
###Code
hist = Histogram()
hist.add_interval(0, 20, 1)\
.add_interval(20, 40, 3)\
.add_interval(40, 60, 4)\
.add_interval(60, 80, 2)\
.add_interval(80, 100, 0)
tex, _ = approx_equals_constant_verbose(hist, 50)
display(Math(tex))
###Output
_____no_output_____
###Markdown
Exercise 12.2:
###Code
hist = Histogram()
hist.add_interval(0, 20, 1)\
.add_interval(20, 40, 3)\
.add_interval(40, 60, 4)\
.add_interval(60, 80, 2)\
.add_interval(80, 100, 0)
tex, _ = approx_greater_constant_verbose(hist, 54)
display(Math(tex))
###Output
_____no_output_____
###Markdown
so we get a selecitivy of approx. 0.32 having 10 elements this leads to $0.32 \cdot 10 = 3.2$ elements. Exercise 12.3
###Code
hist_r = Histogram()
hist_r.add_interval(0, 20, 1)\
.add_interval(20, 40, 3)\
.add_interval(40, 60, 4)\
.add_interval(60, 80, 2)\
.add_interval(80, 100, 0)
hist_s = Histogram()
hist_s.add_interval(0, 10, 2)\
.add_interval(10, 20, 4)\
.add_interval(20, 40, 1)\
.add_interval(40, 50, 6)\
.add_interval(50, 100, 4)
tex, _ = approx_join_verbose(hist_r, hist_s)
display(Math(tex))
###Output
_____no_output_____
###Markdown
HistogramsAllows you to approximate the selectivity using a histogram for following selections:- $A = c$- $A > c$- $A_1 = A_2$ Example for $A=c$
###Code
hist = Histogram()
hist.add_interval(0, 20, 1)\
.add_interval(20, 40, 3)\
.add_interval(40, 60, 4)\
.add_interval(60, 80, 2)\
.add_interval(80, 100, 0)
tex, _ = approx_equals_constant_verbose(hist, 50)
display(Math(tex))
###Output
_____no_output_____
###Markdown
Exercise 12.2:
###Code
hist = Histogram()
hist.add_interval(0, 20, 1)\
.add_interval(20, 40, 3)\
.add_interval(40, 60, 4)\
.add_interval(60, 80, 2)\
.add_interval(80, 100, 0)
tex, _ = approx_greater_constant_verbose(hist, 54)
display(Math(tex))
###Output
_____no_output_____
###Markdown
so we get a selecitivy of approx. 0.32 having 10 elements this leads to $0.32 \cdot 10 = 3.2$ elements. Exercise 12.3
###Code
hist_r = Histogram()
hist_r.add_interval(0, 20, 1)\
.add_interval(20, 40, 3)\
.add_interval(40, 60, 4)\
.add_interval(60, 80, 2)\
.add_interval(80, 100, 0)
hist_s = Histogram()
hist_s.add_interval(0, 10, 2)\
.add_interval(10, 20, 4)\
.add_interval(20, 40, 1)\
.add_interval(40, 50, 6)\
.add_interval(50, 100, 4)
tex, _ = approx_join_verbose(hist_r, hist_s)
display(Math(tex))
###Output
_____no_output_____
###Markdown
HistogramsAllows you to approximate the selectivity using a histogram for following selections:- $A = c$- $A > c$- $A_1 = A_2$ Example for $A=c$
###Code
hist = Histogram()
hist.add_interval(0, 20, 1)\
.add_interval(20, 40, 3)\
.add_interval(40, 60, 4)\
.add_interval(60, 80, 2)\
.add_interval(80, 100, 0)
tex, _ = approx_equals_constant_verbose(hist, 50)
display(Math(tex))
###Output
_____no_output_____
###Markdown
Exercise 12.2:
###Code
hist = Histogram()
hist.add_interval(0, 20, 1)\
.add_interval(20, 40, 3)\
.add_interval(40, 60, 4)\
.add_interval(60, 80, 2)\
.add_interval(80, 100, 0)
tex, _ = approx_greater_constant_verbose(hist, 54)
display(Math(tex))
###Output
_____no_output_____
###Markdown
so we get a selecitivy of approx. 0.32 having 10 elements this leads to $0.32 \cdot 10 = 3.2$ elements. Exercise 12.3
###Code
hist_r = Histogram()
hist_r.add_interval(0, 20, 1)\
.add_interval(20, 40, 3)\
.add_interval(40, 60, 4)\
.add_interval(60, 80, 2)\
.add_interval(80, 100, 0)
hist_s = Histogram()
hist_s.add_interval(0, 10, 2)\
.add_interval(10, 20, 4)\
.add_interval(20, 40, 1)\
.add_interval(40, 50, 6)\
.add_interval(50, 100, 4)
tex, _ = approx_join_verbose(hist_r, hist_s)
display(Math(tex))
###Output
_____no_output_____ |
paper/08_Robustness/02_attentivefp_retrieve.ipynb | ###Markdown
1) classification-scaffold split
###Code
task_names = ["BACE" , "BBBP", "HIV" , ] # "Tox21","SIDER", "ToxCast",
task_types = ["classification", "classification", "classification", "classification", "classification"]
res1 = []
for task_name,task_type in zip(task_names, task_types):
for seed in random_seeds: #
file_path = "/raid/shenwanxiang/08_Robustness/dataset_induces/split"
vl_path = os.path.join(file_path, task_name,"%s" % seed,"attfp_saved_val.csv")
vl_pred_path = os.path.join(file_path, task_name,"%s" % seed, "attfp_pred_val.csv")
ts_path = os.path.join(file_path, task_name,"%s" % seed, "attfp_saved_test.csv")
ts_pred_path = os.path.join(file_path, task_name,"%s" % seed, "attfp_pred_test.csv")
df_true = pd.read_csv(ts_path)
df_pred = pd.read_csv(ts_pred_path)
df_true_val = pd.read_csv(vl_path)
df_pred_val = pd.read_csv(vl_pred_path)
# if len(df_true.columns[1:]) > 1:
test_rocs = []
valid_rocs = []
for i in df_true.columns[1:]:
dfi = df_true[i].to_frame(name = 'true').join(df_pred[i].to_frame(name = 'pred'))
dfi = dfi.dropna()
try:
test_ = roc_auc(dfi.true.tolist(), dfi.pred.tolist())
except:
test_ = np.nan
dfi = df_true_val[i].to_frame(name = 'true').join(df_pred_val[i].to_frame(name = 'pred'))
dfi = dfi.dropna()
try:
val_ = roc_auc(dfi.true.tolist(), dfi.pred.tolist())
except:
val_ = np.nan
test_rocs.append(test_)
valid_rocs.append(val_)
test_roc = np.nanmean(test_rocs)
valid_roc = np.nanmean(valid_rocs)
final_res = {"task_name":task_name, "seed":seed, "valid_roc": valid_roc, "test_roc": test_roc}
res1.append(final_res)
df1 = pd.DataFrame(res1)
df1
###Output
_____no_output_____
###Markdown
2) classification-random split
###Code
task_names = ["Tox21","SIDER", "ToxCast"]
task_types = ["classification", "classification", "classification", "classification", "classification"]
res2 = []
for task_name,task_type in zip(task_names, task_types):
for seed in random_seeds: #
file_path = "/raid/shenwanxiang/08_Robustness/dataset_induces/split"
vl_path = os.path.join(file_path, task_name,"%s" % seed,"attfp_saved_val.csv")
vl_pred_path = os.path.join(file_path, task_name,"%s" % seed, "attfp_pred_val.csv")
ts_path = os.path.join(file_path, task_name,"%s" % seed, "attfp_saved_test.csv")
ts_pred_path = os.path.join(file_path, task_name,"%s" % seed, "attfp_pred_test.csv")
df_true = pd.read_csv(ts_path)
df_pred = pd.read_csv(ts_pred_path)
df_true_val = pd.read_csv(vl_path)
df_pred_val = pd.read_csv(vl_pred_path)
# if len(df_true.columns[1:]) > 1:
test_rocs = []
valid_rocs = []
for i in df_true.columns[1:]:
dfi = df_true[i].to_frame(name = 'true').join(df_pred[i].to_frame(name = 'pred'))
dfi = dfi.dropna()
try:
test_ = roc_auc(dfi.true.tolist(), dfi.pred.tolist())
except:
test_ = np.nan
dfi = df_true_val[i].to_frame(name = 'true').join(df_pred_val[i].to_frame(name = 'pred'))
dfi = dfi.dropna()
try:
val_ = roc_auc(dfi.true.tolist(), dfi.pred.tolist())
except:
val_ = np.nan
test_rocs.append(test_)
valid_rocs.append(val_)
test_roc = np.nanmean(test_rocs)
valid_roc = np.nanmean(valid_rocs)
final_res = {"task_name":task_name, "seed":seed, "valid_roc": valid_roc, "test_roc": test_roc}
res2.append(final_res)
df2 = pd.DataFrame(res2)
df2
###Output
_____no_output_____
###Markdown
03) regression
###Code
task_names = [ "FreeSolv", "ESOL" , "Malaria"]
task_types = ["regression", "regression", "regression"]
res3 = []
for task_name,task_type in zip(task_names, task_types):
for seed in random_seeds: #
file_path = "/raid/shenwanxiang/08_Robustness/dataset_induces/split"
vl_path = os.path.join(file_path, task_name,"%s" % seed,"attfp_saved_val.csv")
vl_pred_path = os.path.join(file_path, task_name,"%s" % seed, "attfp_pred_val.csv")
ts_path = os.path.join(file_path, task_name,"%s" % seed, "attfp_saved_test.csv")
ts_pred_path = os.path.join(file_path, task_name,"%s" % seed, "attfp_pred_test.csv")
df_true = pd.read_csv(ts_path)
df_pred = pd.read_csv(ts_pred_path)
df_true_val = pd.read_csv(vl_path)
df_pred_val = pd.read_csv(vl_pred_path)
test_rmses = []
valid_rmses = []
for i in df_true.columns[1:]:
dfi = df_true[i].to_frame(name = 'true').join(df_pred[i].to_frame(name = 'pred'))
dfi = dfi.dropna()
try:
test_ = rmse(dfi.true.tolist(), dfi.pred.tolist())
except:
test_ = np.nan
dfi = df_true_val[i].to_frame(name = 'true').join(df_pred_val[i].to_frame(name = 'pred'))
dfi = dfi.dropna()
try:
val_ = rmse(dfi.true.tolist(), dfi.pred.tolist())
except:
val_ = np.nan
test_rmses.append(test_)
valid_rmses.append(val_)
test_rmse = np.nanmean(test_rmses)
valid_rmse = np.nanmean(valid_rmses)
final_res = {"task_name":task_name, "seed":seed, "valid_rmse": valid_rmse, "test_rmse": test_rmse}
res3.append(final_res)
df3 = pd.DataFrame(res3)
df3
model_name = 'AttentiveFP'
df1['test_metric'] = 'ROC_AUC'
df1['test_performance'] = df1.test_roc
df1['model'] = model_name
df1['split'] = 'scaffold'
df1 = df1[["task_name","seed", "split", "test_metric","test_performance","model"]]
df2['test_metric'] = 'ROC_AUC'
df2['test_performance'] = df2.test_roc
df2['model'] = model_name
df2['split'] = 'random'
df2 = df2[["task_name","seed", "split", "test_metric","test_performance","model"]]
df3['test_metric'] = 'RMSE'
df3['test_performance'] = df3.test_rmse
df3['model'] = model_name
df3['split'] = 'random'
df3 = df3[["task_name","seed", "split", "test_metric","test_performance","model"]]
df1.append(df2).append(df3).round(3).to_csv('./results_attentivefp.csv')
###Output
_____no_output_____ |
.ipynb_checkpoints/Main-checkpoint.ipynb | ###Markdown
Determining the best neighborhood in Pittsburgh Our Metric We determined the best neighborhood in Pittsburgh by seeing which has the lowest rate of crime, estimated percent of population below the poverty line, and housing conditions. These three pieces of data can best be surmised as the total quality of life within a neighborhood. Lowest rate of crimeThis section is focused on calculating the neighborhood that has the lowest crime per 100 persons.
###Code
#Imports
import pandas as pd
import numpy as np
import math
%matplotlib inline
import matplotlib.pyplot as plt
#Intializing the pandas dataframe
crime = pd.read_csv("public-safety.csv", index_col="Neighborhood")
#dropping the unnecessary columns from the dataset
crime = crime.drop(crime.columns[[7,8,9,10,11,12,13]], axis=1)
#creating the new column by which I will be using as the main metric
crime["Total Crime per 100 persons"] = crime['Part 1 Crime per 100 Persons (2010)'] + crime['Part 2 Crime per 100 Persons (2010)']
crime.head(5)
#Sorting the values just to get an idea of what neighborhoods have high crime rates
crime.sort_values(by=['Part 1 Crime per 100 Persons (2010)'], ascending=False).head(5)
#Sorting to see what datasets have low crime
crime.sort_values(by=['Part 2 Crime per 100 Persons (2010)'], ascending=True).head(5)
#Again sorting to see what neighborhoods have low crime
crime.sort_values(by=['Part 1 Crime per 100 Persons (2010)'], ascending=True).head(5)
#Creating a new sorted data set to graph the lowest crime neighborhoods
crime_data = crime.sort_values(by=['Total Crime per 100 persons'], ascending=True)
crime_data['Total Crime per 100 persons'][:10].plot(kind="bar", ylabel='Crime per 100 Persons (2010)', title='Crime per 100 Persons vs. Neighborhood')
#Listing the top 10 data sets and their total crimes per 100 persons
crime_data['Total Crime per 100 persons'][:10]
###Output
_____no_output_____
###Markdown
So from the end results we see Fairywood seems to be the lowest crime rate neighborhood. So our current scores are looking like1. Fairywood 10pts2. New Homestead 7pts3. Squirrel Hill North 3 pts4. Neighborhoods 4-10 1pt Population under poverty line This section was focused on taking data about education and income and determining the neighborhood with the least amount of population below the poverty line.
###Code
#Loading in Education - Income
inc = pd.read_csv("education-income.csv", index_col="Neighborhood")
inc.dropna()
#Just Checking the Raw data
inc.plot.bar()
#Dropping unnessisary columns
newpov = inc.drop(inc.columns[[0,1,2,3,4,5,6,7,8,9,10,11,12,13]], axis=1)
#Checking if everything is correct
newpov.head(5)
#Cleaning the Data, Some columns had 0% and 100% for poverty, which seems inaccurate
newpov = newpov.drop(['Chateau'])
newpov = newpov.drop(['North Shore'])
newpov = newpov.drop(['South Shore'])
newpov = newpov.drop(['West End'])
#Opening the data again
newpov.head(5)
#Converting the Est Percent Under Poverty (2010) to floats instead of strings
count = 0
#Instance Variable for a loop that goes through all values of newpov
while count < len(newpov):
#Casting the strings inside newpov as a float by chopping off the % value from the end
newpov['Est. Percent Under Poverty (2010)'][count] = float(newpov['Est. Percent Under Poverty (2010)'][count][:3])
count = count + 1
#Openning the data up to check work
newpov.head(5)
#Now that the data is numerical, we can now order it
newpov = newpov.sort_values(by=["Est. Percent Under Poverty (2010)"], ascending=True)
newpov.head(5)
#Finally we are able to plot the data, only plotting the first ten to be readable.
newpov['Est. Percent Under Poverty (2010)'][:10].plot(kind="bar", ylabel="Est. Percent Under Poverty (2010) in Percent", title="Est. Percent Under Poverty plotted by Neighborhood for the ten lowest poverty rated neighborhoods in Pittsburgh")
#Dropping the head so it is easier to read
newpov.head(10)
#Just to get an overall picture of the city, here is the plot with all the values
newpov['Est. Percent Under Poverty (2010)'].plot(kind="bar", ylabel="Est. Percent Under Poverty (2010)",title="Est. Percent Under Poverty plotted by Neighborhood for every neighborhood in Pittsburgh")
###Output
_____no_output_____
###Markdown
After analyzing the data and adding up the scores the scores from before and now:1. Fairywood & Regent Square 10 pts2. Swisshelm Park 8 pts3. Stript District 7 pts4. Lincoln Place & Stanton Heights 4 pts Housing Conditions This final section is focused on the quality of houses compared to the population.
###Code
import pandas as pd
file = pd.read_csv("built-enviornment-conditions.csv")
file
df = pd.read_csv("built-enviornment-conditions.csv")
df[u'% Good / Excellent Condition Buildings (2009)'] = df[u'% Good / Excellent Condition Buildings (2009)'].str.strip('%').astype(float)/100
df[u'% Average Condition Buildings (2009)'] = df[u'% Average Condition Buildings (2009)'].str.strip('%').astype(float)/100
df['%Average on Good&Average Condition Buildings'] = df[['% Good / Excellent Condition Buildings (2009)', '% Average Condition Buildings (2009)']].mean(axis=1)
df
newdata = df.sort_values("% Good / Excellent Condition Buildings (2009)", ascending=False).head(20)
df1 = newdata.loc[:,['Neighborhood','% Good / Excellent Condition Buildings (2009)','% Average Condition Buildings (2009)','%Average on Good&Average Condition Buildings']]
df1
condition = df1.sort_values("%Average on Good&Average Condition Buildings", ascending=False).head(15)
condition
df = pd.read_csv("built-enviornment-conditions.csv")
def con(column):
column = column.replace(',', '')
column = int(column)
return column
df['Population (2010)'] = df['Population (2010)'].map(con)
df
SquareM = df['Land Area (acres)'].str.replace(',', '').astype(float)*0.0015625
df['Population Density'] = df['Population (2010)']/SquareM
df2 = df.sort_values("Population Density", ascending=True).head(45)
density = df2.loc[:,['Neighborhood','Population (2010)','Land Area (acres)','Population Density','Sector #']]
density
import matplotlib.pyplot as plt
plt.figure(figsize = (8,40))
plt.barh(density['Neighborhood'], density['Population Density'])
plt.title('Differences in Population Density')
plt.show()
df3 = pd.merge(condition,density,on='Neighborhood',how='inner')
df3
df3.loc[:,['Neighborhood','% Good / Excellent Condition Buildings (2009)','% Average Condition Buildings (2009)','%Average on Good&Average Condition Buildings','Population Density','Sector #']]
###Output
_____no_output_____
###Markdown
Urban Diversity Within Seattle’s Neighborhoods Contents1. Data Collection2. Data Cleaning3. Exploration4. Modeling5. Interpretation Data Collection Web scraping neighborhood list from https://seattle.findwell.com/seattle-neighborhoods/
###Code
from bs4 import BeautifulSoup
import requests #Get the webpage
import pandas as pd
def getSoup(url):
webpage = requests.get(url)
return webpage, BeautifulSoup(webpage.content, 'html.parser')
#obtain the website and display html content
page, soup = getSoup('https://seattle.findwell.com/seattle-neighborhoods/')
soup
seaList = soup.findAll(class_="col-sm-2")
len(seaList)
a = seaList[0]
temp = a.findAll('a')
central = [name.get_text() for name in temp]
central
central_df = pd.DataFrame(central)
central_df['Zone'] = 'Central'
central_df
b = seaList[1]
temp = b.findAll('a')
north_east = [name.get_text() for name in temp]
north_east
ne_df = pd.DataFrame(north_east)
ne_df['Zone'] = 'North East'
ne_df
c = seaList[2]
temp = c.findAll('a')
north_west = [name.get_text() for name in temp]
north_west
nw_df = pd.DataFrame(north_west)
nw_df['Zone'] = 'North West'
nw_df
d = seaList[3]
temp = d.findAll('a')
south = [name.get_text() for name in temp]
south
s_df = pd.DataFrame(south)
s_df['Zone'] = 'South'
s_df
e = seaList[4]
temp = e.findAll('a')
west = [name.get_text() for name in temp]
west
# Delete 'West Seattle'
del west[0]
west
west_df = pd.DataFrame(west)
west_df['Zone'] = 'West'
west_df
frames = [central_df, ne_df, nw_df, s_df, west_df]
neigh_df = pd.concat(frames, ignore_index=True)
neigh_df
# rename first column
neigh_df.rename(columns={0:"Neighborhood"}, inplace=True)
neigh_df.head()
# save to csv
sea_neigh_list.to_csv('Data/sea_neigh_list.csv', index=False)
###Output
_____no_output_____
###Markdown
Latitud and Longitude for each neighborhood
###Code
#read neighborhoods df
neighborhoods = pd.read_csv('Data/sea_neigh_list.csv')
neighborhoods.head()
#read coordinates df
coordinates = pd.read_csv('Data/coordinates_shaped.csv')
coordinates.head()
# @hidden_cell
# check how to Load data from local files IBM https://dataplatform.cloud.ibm.com/docs/content/wsj/analyze-data/load-and-access-data.html
body = client_11766bbc34154972b7ed0fd38ff1d66a.get_object(Bucket='ourserafinal-donotdelete-pr-vjm5icmxxrql9r',Key='data_asset/coordinates.csv_shaped_f635055.csv')['Body']
# add missing __iter__ method, so pandas accepts body as file-like object
if not hasattr(body, "__iter__"): body.__iter__ = types.MethodType( __iter__, body )
coordinates = pd.read_csv(body)
import types
from botocore.client import Config
import ibm_boto3
def __iter__(self): return 0
# @hidden_cell
# The following code accesses a file in your IBM Cloud Object Storage. It includes your credentials.
# You might want to remove those credentials before you share the notebook.
client_11766bbc34154972b7ed0fd38ff1d66a = ibm_boto3.client(service_name='s3',
ibm_api_key_id='yT4fd-K-ufE94VcOehEdAHG-Yxg5NIWisZ0veFxZ6938',
ibm_auth_endpoint="https://iam.ng.bluemix.net/oidc/token",
config=Config(signature_version='oauth'),
endpoint_url='https://s3-api.us-geo.objectstorage.service.networklayer.com')
body = client_11766bbc34154972b7ed0fd38ff1d66a.get_object(Bucket='ourserafinal-donotdelete-pr-vjm5icmxxrql9r',Key='sea_neigh_list.csv')['Body']
# add missing __iter__ method, so pandas accepts body as file-like object
if not hasattr(body, "__iter__"): body.__iter__ = types.MethodType( __iter__, body )
neighborhoods = pd.read_csv(body)
###Output
_____no_output_____
###Markdown
Merge both data frames
###Code
data = pd.merge(neighborhoods, coordinates, on='Neighborhood', how='inner')
data.head()
data.shape
# save to csv
data.to_csv('Data/data.csv', index=False)
###Output
_____no_output_____
###Markdown
Exploration and Clustering
###Code
data = pd.read_csv('Data/data.csv')
data.head()
#!pip install folium
import folium
latitude = 47.60621
longitude = -122.33207
seattle_map = folium.Map(location=[latitude, longitude], zoom_start=11)
# add markers for B
for lat, lng, label in zip(data.Latitude, data.Longitude, data.Neighborhood):
label = folium.Popup(label, parse_html=True)
folium.CircleMarker([lat, lng], radius=5, popup=label, color='purple', fill=True, parse_html=False).add_to(seattle_map)
seattle_map
###Output
_____no_output_____
###Markdown
Explore Neighborhoods with Foursquare API
###Code
import numpy as np
import requests
# @hidden_cell
CLIENT_ID = 'SRJ3OGDDEJTQEZBPDGROOVSOUF1ROULP0G4NRGTRJBLE5TS2' # your Foursquare ID
CLIENT_SECRET = '2JDU24ZYQRACKICJBENQKFJBAFAVZJL3TMROIP13M20ZO4IJ' # your Foursquare Secret
VERSION = '20200104' # Foursquare API version
LIMIT = 100
def getVenues(zones, names, latitudes, longitudes, radius=500): #radius -> meters
venues_list=[]
for zone, name, lat, lng in zip (zones, names, latitudes, longitudes):
print(name)
# create the API request URL
url = 'https://api.foursquare.com/v2/venues/explore?&client_id={}&client_secret={}&v={}&ll={},{}&radius={}&limit={}'.format(
CLIENT_ID,
CLIENT_SECRET,
VERSION,
lat,
lng,
radius,
LIMIT)
# make the GET request
results = requests.get(url).json()["response"]['groups'][0]['items']
# return only relevant information for each nearby venue
venues_list.append([(
zone,
name,
v['venue']['name'],
v['venue']['categories'][0]['name']) for v in results])
nearby_venues = pd.DataFrame([item for venue_list in venues_list for item in venue_list])
nearby_venues.columns = ['Zone', 'Neighborhood', 'Venue', 'Venue Category']
return(nearby_venues)
seattle_venues = getVenues(zones = data['Zone'],
names = data['Neighborhood'],
latitudes = data['Latitude'],
longitudes = data['Longitude']
)
print(seattle_venues.shape)
seattle_venues.head(10)
#Unique categories
print(len(seattle_venues['Venue Category'].unique()), 'unique venues')
print(seattle_venues['Venue Category'].unique())
seattle_venues.describe()
###Output
_____no_output_____
###Markdown
10 most popular venues in each neighborhood
###Code
# one hot encoding
seattle_onehot = pd.get_dummies(seattle_venues[['Venue Category']], prefix="", prefix_sep="")
# add neighborhood column back to dataframe
seattle_onehot['Neighborhood'] = seattle_venues['Neighborhood']
# move neighborhood column to the first column
fixed_columns = [seattle_onehot.columns[-1]] + list(seattle_onehot.columns[:-1])
seattle_onehot = seattle_onehot[fixed_columns]
#seattle_onehot.head()
seattle_onehot.shape
# group rows by neighborhood and by taking the mean of the frequency of occurrence of each category
sea_grouped = seattle_onehot.groupby('Neighborhood').mean().reset_index()
sea_grouped.shape
top_venues = 10
for hood in sea_grouped['Neighborhood']:
print("----" + hood + "----")
temp = sea_grouped[sea_grouped['Neighborhood'] == hood].T.reset_index()
temp.columns = ['Venue','Freq.']
temp = temp.iloc[1:]
temp['Freq.'] = temp['Freq.'].astype(float)
temp = temp.round({'Freq.': 2})
print(temp.sort_values('Freq.', ascending=False).reset_index(drop=True).head(top_venues))
print('\n')
###Output
----Admiral----
Venue Freq.
0 Coffee Shop 0.12
1 Pub 0.05
2 Grocery Store 0.05
3 Thai Restaurant 0.02
4 Salon / Barbershop 0.02
5 Convenience Store 0.02
6 Market 0.02
7 Gym / Fitness Center 0.02
8 Falafel Restaurant 0.02
9 Cocktail Bar 0.02
----Alki----
Venue Freq.
0 Coffee Shop 0.08
1 Beach 0.06
2 Park 0.06
3 Restaurant 0.06
4 Italian Restaurant 0.06
5 Art Gallery 0.06
6 Sports Bar 0.03
7 Fish & Chips Shop 0.03
8 Beach Bar 0.03
9 Market 0.03
----Ballard----
Venue Freq.
0 Hobby Shop 0.17
1 French Restaurant 0.17
2 Jewelry Store 0.17
3 Park 0.17
4 Gift Shop 0.17
5 Coffee Shop 0.17
6 Movie Theater 0.00
7 Museum 0.00
8 Motel 0.00
9 Music Store 0.00
----Beacon Hill----
Venue Freq.
0 Bus Station 0.5
1 Light Rail Station 0.5
2 Yoga Studio 0.0
3 Monument / Landmark 0.0
4 Motel 0.0
5 Movie Theater 0.0
6 Museum 0.0
7 Music Store 0.0
8 Music Venue 0.0
9 Nail Salon 0.0
----Belltown----
Venue Freq.
0 Bar 0.07
1 Sushi Restaurant 0.05
2 Cocktail Bar 0.05
3 Coffee Shop 0.05
4 New American Restaurant 0.04
5 Gym 0.03
6 Lounge 0.03
7 Breakfast Spot 0.03
8 Bakery 0.03
9 Pizza Place 0.03
----Blue Ridge/North Beach----
Venue Freq.
0 Home Service 0.33
1 Construction & Landscaping 0.33
2 Park 0.33
3 Music Venue 0.00
4 Office 0.00
5 Noodle House 0.00
6 Nightclub 0.00
7 New American Restaurant 0.00
8 Nail Salon 0.00
9 Music Store 0.00
----Broadmoor----
Venue Freq.
0 Golf Course 0.25
1 Playground 0.25
2 Garden 0.25
3 Soccer Field 0.25
4 Yoga Studio 0.00
5 Music Venue 0.00
6 Noodle House 0.00
7 Nightclub 0.00
8 New American Restaurant 0.00
9 Nail Salon 0.00
----Broadview----
Venue Freq.
0 Concert Hall 0.67
1 Trail 0.33
2 Music Venue 0.00
3 Office 0.00
4 Noodle House 0.00
5 Nightclub 0.00
6 New American Restaurant 0.00
7 Nail Salon 0.00
8 Music Store 0.00
9 Organic Grocery 0.00
----Bryant----
Venue Freq.
0 Bus Station 0.1
1 Trail 0.1
2 Café 0.1
3 Mexican Restaurant 0.1
4 Coffee Shop 0.1
5 Grocery Store 0.1
6 Italian Restaurant 0.1
7 Seafood Restaurant 0.1
8 Martial Arts Dojo 0.1
9 Chinese Restaurant 0.1
----Capitol Hill----
Venue Freq.
0 Cocktail Bar 0.11
1 American Restaurant 0.08
2 Coffee Shop 0.08
3 Bar 0.08
4 Italian Restaurant 0.05
5 Café 0.05
6 Restaurant 0.05
7 Thai Restaurant 0.05
8 Noodle House 0.03
9 Frozen Yogurt Shop 0.03
----Central District----
Venue Freq.
0 Ethiopian Restaurant 0.27
1 Fried Chicken Joint 0.07
2 Farmers Market 0.07
3 Bakery 0.07
4 Thai Restaurant 0.07
5 Grocery Store 0.07
6 Gym Pool 0.07
7 Asian Restaurant 0.07
8 Bar 0.07
9 Baseball Field 0.07
----Columbia City----
Venue Freq.
0 Pizza Place 0.08
1 Yoga Studio 0.05
2 Gym 0.05
3 Coffee Shop 0.05
4 Vietnamese Restaurant 0.05
5 Park 0.02
6 Sports Bar 0.02
7 Soccer Field 0.02
8 Caribbean Restaurant 0.02
9 Sandwich Place 0.02
----Crown Hill----
Venue Freq.
0 Burger Joint 0.1
1 Taco Place 0.1
2 Pizza Place 0.1
3 Sports Bar 0.1
4 Coffee Shop 0.1
5 Mexican Restaurant 0.1
6 Pet Store 0.1
7 Fast Food Restaurant 0.1
8 Sandwich Place 0.1
9 Grocery Store 0.1
----Denny-Blaine----
Venue Freq.
0 Park 0.67
1 Monument / Landmark 0.17
2 Beach 0.17
3 Yoga Studio 0.00
4 Nail Salon 0.00
5 Office 0.00
6 Noodle House 0.00
7 Nightclub 0.00
8 New American Restaurant 0.00
9 Music Store 0.00
----Downtown----
Venue Freq.
0 Hotel 0.09
1 Coffee Shop 0.09
2 Seafood Restaurant 0.05
3 Cocktail Bar 0.04
4 Café 0.03
5 American Restaurant 0.03
6 Steakhouse 0.02
7 Sushi Restaurant 0.02
8 Scenic Lookout 0.02
9 Breakfast Spot 0.02
----Eastlake----
Venue Freq.
0 Sandwich Place 0.13
1 Italian Restaurant 0.10
2 Coffee Shop 0.10
3 Playground 0.06
4 Mexican Restaurant 0.06
5 Pizza Place 0.06
6 Bus Stop 0.06
7 Harbor / Marina 0.06
8 Grocery Store 0.03
9 Steakhouse 0.03
----Fauntleroy----
Venue Freq.
0 Construction & Landscaping 0.11
1 Botanical Garden 0.11
2 Cupcake Shop 0.11
3 Coffee Shop 0.11
4 Deli / Bodega 0.11
5 Park 0.11
6 Other Repair Shop 0.11
7 Fish & Chips Shop 0.11
8 Cosmetics Shop 0.11
9 Japanese Restaurant 0.00
----First Hill----
Venue Freq.
0 Sandwich Place 0.11
1 Coffee Shop 0.09
2 Hotel 0.07
3 Pizza Place 0.05
4 Pharmacy 0.05
5 Bakery 0.05
6 Asian Restaurant 0.05
7 Food & Drink Shop 0.02
8 Gift Shop 0.02
9 Taco Place 0.02
----Fremont----
Venue Freq.
0 Coffee Shop 0.06
1 Thai Restaurant 0.05
2 Bar 0.05
3 Pub 0.04
4 Music Venue 0.02
5 Pizza Place 0.02
6 Spa 0.02
7 Park 0.02
8 Dessert Shop 0.02
9 Eye Doctor 0.01
----Georgetown----
Venue Freq.
0 Pizza Place 0.05
1 Bar 0.05
2 Café 0.05
3 Park 0.05
4 Dive Bar 0.04
5 Lounge 0.04
6 Mexican Restaurant 0.04
7 Dessert Shop 0.04
8 Furniture / Home Store 0.04
9 Brewery 0.04
----Green Lake----
Venue Freq.
0 Frozen Yogurt Shop 0.08
1 Coffee Shop 0.08
2 Spa 0.08
3 Gym 0.08
4 Yoga Studio 0.04
5 Brazilian Restaurant 0.04
6 Thai Restaurant 0.04
7 Mexican Restaurant 0.04
8 Boat Rental 0.04
9 Burger Joint 0.04
----Greenwood----
Venue Freq.
0 Coffee Shop 0.10
1 Spa 0.06
2 Bar 0.06
3 Mexican Restaurant 0.06
4 Bookstore 0.04
5 Pizza Place 0.04
6 Playground 0.02
7 French Restaurant 0.02
8 Sandwich Place 0.02
9 Comic Shop 0.02
----Haller Lake----
Venue Freq.
0 Lake 0.2
1 Coffee Shop 0.2
2 Playground 0.2
3 Park 0.2
4 Dance Studio 0.2
5 Yoga Studio 0.0
6 New American Restaurant 0.0
7 Optical Shop 0.0
8 Office 0.0
9 Noodle House 0.0
----Hawthorne Hills----
Venue Freq.
0 Construction & Landscaping 0.25
1 Café 0.25
2 Bank 0.25
3 Auto Workshop 0.25
4 Poke Place 0.00
5 Other Repair Shop 0.00
6 Motel 0.00
7 Movie Theater 0.00
8 Museum 0.00
9 Music Store 0.00
----International District----
Venue Freq.
0 Chinese Restaurant 0.16
1 Vietnamese Restaurant 0.09
2 Café 0.05
3 Hotpot Restaurant 0.05
4 Bakery 0.05
5 Japanese Restaurant 0.04
6 Dessert Shop 0.04
7 Bubble Tea Shop 0.04
8 Cantonese Restaurant 0.04
9 Thai Restaurant 0.04
----Junction----
Venue Freq.
0 Pizza Place 0.10
1 Coffee Shop 0.08
2 American Restaurant 0.04
3 Yoga Studio 0.02
4 Salon / Barbershop 0.02
5 Design Studio 0.02
6 Deli / Bodega 0.02
7 Ramen Restaurant 0.02
8 Cupcake Shop 0.02
9 Italian Restaurant 0.02
----Lake City----
Venue Freq.
0 Mexican Restaurant 0.06
1 Pizza Place 0.06
2 Thai Restaurant 0.06
3 Bar 0.06
4 Bank 0.06
5 Burger Joint 0.03
6 Beer Bar 0.03
7 Beer Store 0.03
8 Sushi Restaurant 0.03
9 Gastropub 0.03
----Laurelhurst----
Venue Freq.
0 Salon / Barbershop 0.08
1 Café 0.08
2 Thai Restaurant 0.08
3 Gift Shop 0.08
4 Coffee Shop 0.08
5 Pharmacy 0.08
6 Food Truck 0.08
7 Lawyer 0.08
8 Bus Stop 0.08
9 Juice Bar 0.08
----Leschi----
Venue Freq.
0 Park 0.12
1 Playground 0.12
2 Coffee Shop 0.12
3 Pizza Place 0.12
4 Vietnamese Restaurant 0.12
5 Pet Store 0.12
6 Grocery Store 0.12
7 Gym 0.12
8 Yoga Studio 0.00
9 Nightclub 0.00
----Loyal Heights----
Venue Freq.
0 Pizza Place 0.29
1 Park 0.29
2 Hobby Shop 0.14
3 Soccer Field 0.14
4 Coffee Shop 0.14
5 Noodle House 0.00
6 Nightclub 0.00
7 New American Restaurant 0.00
8 Nail Salon 0.00
9 Music Venue 0.00
----Madison Park----
Venue Freq.
0 Bar 0.12
1 Bank 0.08
2 Park 0.08
3 Mexican Restaurant 0.04
4 Café 0.04
5 Beach 0.04
6 Bath House 0.04
7 Food & Drink Shop 0.04
8 Bakery 0.04
###Markdown
Making Clusters
###Code
#Create a DF with the common venues
def return_most_common_venues(row, num_top_venues):
row_categories = row.iloc[1:]
row_categories_sorted = row_categories.sort_values(ascending=False)
return row_categories_sorted.index.values[0:num_top_venues]
top_venues = 10
indicators = ['st', 'nd', 'rd']
# create columns according to number of top venues
columns = ['Neighborhood']
for ind in np.arange(top_venues):
try:
columns.append('{}{} Most Common Venue'.format(ind+1, indicators[ind]))
except:
columns.append('{}th Most Common Venue'.format(ind+1))
venues_sorted = pd.DataFrame(columns=columns)
venues_sorted['Neighborhood'] = sea_grouped['Neighborhood']
for ind in np.arange(sea_grouped.shape[0]):
venues_sorted.iloc[ind, 1:] = return_most_common_venues(sea_grouped.iloc[ind, :], top_venues)
venues_sorted.head()
from sklearn.cluster import KMeans
import matplotlib.cm as cm
import matplotlib.colors as colors
# set number of clusters
kclusters = 5
sea_clusters = sea_grouped.drop('Neighborhood', 1)
# run k-means clustering
kmeans = KMeans(n_clusters=kclusters, random_state=0).fit(sea_clusters)
# check cluster labels generated for each row in the dataframe
kmeans.labels_[0:10]
# add clustering labels
venues_sorted.insert(0, 'Cluster Labels', kmeans.labels_)
sea_merged = data
# merge toronto_grouped with toronto_data to add latitude/longitude for each neighborhood
sea_merged = sea_merged.join(venues_sorted.set_index('Neighborhood'), on='Neighborhood')
sea_merged.head()
# create map
map_clusters = folium.Map(location=[latitude, longitude], zoom_start=11)
# set color scheme for the clusters
x = np.arange(kclusters)
ys = [i + x + (i*x)**2 for i in range(kclusters)]
colors_array = cm.rainbow(np.linspace(0, 1, len(ys)))
rainbow = [colors.rgb2hex(i) for i in colors_array]
# add markers to the map
markers_colors = []
for lat, lon, poi, cluster in zip(sea_merged['Latitude'], sea_merged['Longitude'], sea_merged['Neighborhood'], sea_merged['Cluster Labels']):
label = folium.Popup(str(poi) + ' Cluster ' + str(cluster), parse_html=True)
folium.CircleMarker(
[lat, lon],
radius=5,
popup=label,
color=rainbow[cluster-1],
fill=True,
fill_color=rainbow[cluster-1],
fill_opacity=0.7).add_to(map_clusters)
map_clusters
sea_merged
import matplotlib.pyplot as plt
sea_merged.groupby(['Zone', '1st Most Common Venue']).size().unstack().plot(kind='barh', figsize=(20,20), stacked=True)
###Output
_____no_output_____
###Markdown
Cluster Examination Cluster 1
###Code
#Cluster 0
sea_merged.loc[sea_merged['Cluster Labels'] == 0, sea_merged.columns[[0] + [1] + list(range(5, sea_merged.shape[1]))]]
###Output
_____no_output_____
###Markdown
Cluster 2
###Code
#Cluster 1
sea_merged.loc[sea_merged['Cluster Labels'] == 1, sea_merged.columns[[0] + [1] + list(range(5, sea_merged.shape[1]))]]
###Output
_____no_output_____
###Markdown
Cluster 3
###Code
sea_merged.loc[sea_merged['Cluster Labels'] == 2, sea_merged.columns[[0] + [1] + list(range(5, sea_merged.shape[1]))]]
###Output
_____no_output_____
###Markdown
Cluster 4
###Code
sea_merged.loc[sea_merged['Cluster Labels'] == 3, sea_merged.columns[[0] + [1] + list(range(5, sea_merged.shape[1]))]]
###Output
_____no_output_____
###Markdown
Cluster 5
###Code
sea_merged.loc[sea_merged['Cluster Labels'] == 4, sea_merged.columns[[0] + [1] + list(range(5, sea_merged.shape[1]))]]
###Output
_____no_output_____
###Markdown
Pre-processing
###Code
'''STEP 1: Pre-processing the dataframes'''
# NY live COVID data
url = 'https://raw.githubusercontent.com/nytimes/covid-19-data/master/us-states.csv'
df = pd.read_csv(url, parse_dates=[0]) # Cols: date, state, fips, cases, deaths
df.drop(['fips'], axis=1, inplace=True)
date_df = df.groupby('date')
date_df.sum()['cases']
df = df.merge(pop_df.iloc[:,:2], left_on='state', right_on='State')
df.drop('State', axis=1, inplace=True)
'''STEP 1: Pre-processing the dataframes'''
# NY live COVID data
url = 'https://raw.githubusercontent.com/nytimes/covid-19-data/master/us-states.csv'
df = pd.read_csv(url, parse_dates=[0]) # Cols: date, state, fips, cases, deaths
# State population
# Made redundant with plotly dataset
# url = 'https://en.wikipedia.org/wiki/List_of_states_and_territories_of_the_United_States_by_population'
# pop_df = pd.read_html(url)[0]
# pop_df = pop_df.iloc[:,2:4]
# pop_df = pop_df.droplevel(0, axis=1)
# pop_df = pop_df[:52]
# def pre_process(total_pop):
# '''Pre-process population values where in some cases the references might
# have '...[..]' pattern signifying reference
# '''
# try:
# num = int(total_pop)
# except:
# m = re.match(r'\d+[\d+]', total_pop)
# num = int(m.group(0))
# return num
# pop_df.rename(columns={pop_df.columns[1]: 'Recent_population'}, inplace=True)
# pop_df['Recent_population'] = pop_df['Recent_population'].apply(pre_process)
# pop_df.set_index('State').T.to_dict('list')
url = 'https://raw.githubusercontent.com/plotly/datasets/master/2014_usa_states.csv'
pop_df = pd.read_csv(url)
population_dict = pop_df.drop(['Postal'], axis=1).set_index('State').T.to_dict('list')
# If Postal code is needed, we can keep it as the first dict item
'''STEP 2: Merging the dataframes'''
df = df.merge(pop_df.iloc[:,1:3], left_on='state', right_on='State')
df.drop('State', axis=1, inplace=True)
# remove_states = []
# for state in df.state.unique():
# if state not in population_dict.keys():
# remove_states.append(state)
# df=df[~df.state.isin(remove_states)]
df
date_df =df.groupby
population_dict
df.set_index('date', inplace=True)
state_df = df.groupby('state')
rolling_daily = state_df.rolling(5).mean().diff()
rolling_daily.index #.iloc[0]
fig, ax = plt.subplots(figsize=(10,6))
for i, (state_name, state) in enumerate(rolling_daily.groupby(level=0)):
if i<20 or i>30:
continue
total_pop = population_dict[state_name]
dates = state.index.get_level_values(1)
ax.plot(dates, state.cases/total_pop, label=state_name)
plt.legend(bbox_to_anchor=(1.05, 1));
###Output
_____no_output_____
###Markdown
ARIMA
###Code
# for i, (state_name, state) in enumerate(rolling_daily.groupby(level=0)):
# rolling_daily.cases
def ARIMA_forecast(series, split=0.8, **kwargs):
L = len(series)
size = int(np.floor(L*split))
n_preds = L - size
# size = L - n_preds
train, test = list(series[:size]), list(series[size:])
mode = kwargs.get('mode', 'Single')
order = kwargs.get('order', (5,1,0))
history = list(train)#[x for x in train]
predictions = list()
# 1 by 1
if mode=='Single':
predictions, stderr, bounds = [],[],[]
# print('1 by 1')
for t in range(len(test)):
model = ARIMA(history, order=order)
model_fit = model.fit(disp=0)
output = model_fit.forecast()
ypred = round(output[0][0])
predictions.append(ypred)
stderr.append(output[1])
bounds.append(output[2])
obs = test[t]
history.append(obs)
# print(f'predicted={ypred}, expected={obs}')
results = (np.squeeze(predictions), np.squeeze(stderr), np.squeeze(bounds))
error = mean_squared_error(test, predictions)
print(error)
# All at once
elif mode=='All':
# print('All at once')
model = ARIMA(history, order=(5,1,0))
model_fit = model.fit(disp=0)
results = model_fit.forecast(steps=n_preds)
predictions, stderr, bounds = results
error = mean_squared_error(test, predictions)
# print(error)
# print(f'Test MSE: {error:0.3f}')
return {'error': error,
'train': train,
'test': test,
'results': results,
}
# we get daily cases by making the model with cases have d=1. So the result of daily_cases having d=0 is the same
output = ARIMA_forecast(state_df.cases, 0.9, **{'mode':'All'})
###Output
_____no_output_____
###Markdown
1. Load and Preprocess Dataset
###Code
X_DICT_TRAIN, Y_DICT_TRAIN, X_DICT_VALIDATION, Y_DICT_VALIDATION = load_data(path = './data/')
plot_train_data(X_DICT_TRAIN, Y_DICT_TRAIN, image_number = 1)
N_BANDS = 8
N_CLASSES = 1 # buildings, roads, trees, crops and water
N_EPOCHS = 10
PATCH_SZ = 160 # should divide by 16
BATCH_SIZE = 100
TRAIN_SZ = 5000 # train size
VAL_SZ = 500 # validation size
channel='all'# 0: Buildings , 1: Roads & Tracks, 2: Trees , 3: Crops, 4: Water or 'all'
x_train, y_train = get_patches(X_DICT_TRAIN, Y_DICT_TRAIN, n_patches=TRAIN_SZ, sz=PATCH_SZ, channel=channel)
x_val, y_val = get_patches(X_DICT_VALIDATION, Y_DICT_VALIDATION, n_patches=VAL_SZ, sz=PATCH_SZ, channel=channel)
y_train = y_train[:,:, :,2:3]
y_val = y_val[:,:, :,2:3]
print("Train shape is: {} and Train Ground truth shape is: {}".format(x_train.shape, y_train.shape))
print("Validation shape is: {} and Validation Ground truth shape is: {}".format(x_val.shape, y_val.shape))
###Output
Train shape is: (5000, 160, 160, 8) and Train Ground truth shape is: (5000, 160, 160, 1)
Validation shape is: (500, 160, 160, 8) and Validation Ground truth shape is: (500, 160, 160, 1)
###Markdown
2. Build and Train U-net Model
###Code
model = unet_model( n_classes= N_CLASSES,
im_sz= PATCH_SZ,
n_channels = N_BANDS,
n_filters_start = 32,
growth_factor = 2,
upconv=True)
model.summary()
callbacks_list = get_callbacks()
model.fit(x_train, y_train, batch_size=BATCH_SIZE, epochs=N_EPOCHS,
verbose=1, shuffle=True, validation_data=(x_val, y_val), callbacks=callbacks_list)
###Output
Train on 5000 samples, validate on 500 samples
Epoch 1/10
4900/5000 [============================>.] - ETA: 0s - loss: 0.1174 - accuracy: 0.9494 - precision: 0.9764 - recall: 0.7740
Epoch 00001: val_loss improved from inf to 0.13955, saving model to ./models/UNet_(11-26-2020 , 20:05:29)
WARNING:tensorflow:From /home/amir/anaconda3/envs/nlp/lib/python3.6/site-packages/tensorflow_core/python/ops/resource_variable_ops.py:1786: calling BaseResourceVariable.__init__ (from tensorflow.python.ops.resource_variable_ops) with constraint is deprecated and will be removed in a future version.
Instructions for updating:
If using Keras pass *_constraint arguments to layers.
INFO:tensorflow:Assets written to: ./models/UNet_(11-26-2020 , 20:05:29)/assets
5000/5000 [==============================] - 60s 12ms/sample - loss: 0.1174 - accuracy: 0.9494 - precision: 0.9764 - recall: 0.7744 - val_loss: 0.1396 - val_accuracy: 0.9439 - val_precision: 0.9737 - val_recall: 0.7424
Epoch 2/10
4900/5000 [============================>.] - ETA: 0s - loss: 0.1141 - accuracy: 0.9507 - precision: 0.9769 - recall: 0.7808
Epoch 00002: val_loss did not improve from 0.13955
5000/5000 [==============================] - 22s 4ms/sample - loss: 0.1143 - accuracy: 0.9506 - precision: 0.9769 - recall: 0.7808 - val_loss: 0.1400 - val_accuracy: 0.9432 - val_precision: 0.9731 - val_recall: 0.7411
Epoch 3/10
4900/5000 [============================>.] - ETA: 0s - loss: 0.1143 - accuracy: 0.9507 - precision: 0.9769 - recall: 0.7803
Epoch 00003: val_loss did not improve from 0.13955
5000/5000 [==============================] - 22s 4ms/sample - loss: 0.1146 - accuracy: 0.9506 - precision: 0.9769 - recall: 0.7796 - val_loss: 0.1436 - val_accuracy: 0.9434 - val_precision: 0.9754 - val_recall: 0.7266
Epoch 4/10
4900/5000 [============================>.] - ETA: 0s - loss: 0.1135 - accuracy: 0.9510 - precision: 0.9768 - recall: 0.7845
Epoch 00004: val_loss did not improve from 0.13955
5000/5000 [==============================] - 22s 4ms/sample - loss: 0.1133 - accuracy: 0.9511 - precision: 0.9768 - recall: 0.7845 - val_loss: 0.1555 - val_accuracy: 0.9417 - val_precision: 0.9748 - val_recall: 0.7192
Epoch 5/10
4900/5000 [============================>.] - ETA: 0s - loss: 0.1120 - accuracy: 0.9516 - precision: 0.9771 - recall: 0.7870
Epoch 00005: val_loss did not improve from 0.13955
5000/5000 [==============================] - 22s 4ms/sample - loss: 0.1119 - accuracy: 0.9516 - precision: 0.9772 - recall: 0.7867 - val_loss: 0.1470 - val_accuracy: 0.9423 - val_precision: 0.9771 - val_recall: 0.7074
Epoch 6/10
4900/5000 [============================>.] - ETA: 0s - loss: 0.1123 - accuracy: 0.9515 - precision: 0.9773 - recall: 0.7851
Epoch 00006: val_loss did not improve from 0.13955
5000/5000 [==============================] - 22s 4ms/sample - loss: 0.1121 - accuracy: 0.9516 - precision: 0.9773 - recall: 0.7852 - val_loss: 0.1483 - val_accuracy: 0.9421 - val_precision: 0.9744 - val_recall: 0.7245
Epoch 7/10
4900/5000 [============================>.] - ETA: 0s - loss: 0.1115 - accuracy: 0.9518 - precision: 0.9773 - recall: 0.7871
Epoch 00007: val_loss did not improve from 0.13955
5000/5000 [==============================] - 22s 4ms/sample - loss: 0.1114 - accuracy: 0.9519 - precision: 0.9773 - recall: 0.7877 - val_loss: 0.1416 - val_accuracy: 0.9426 - val_precision: 0.9708 - val_recall: 0.7518
Epoch 8/10
4900/5000 [============================>.] - ETA: 0s - loss: 0.1110 - accuracy: 0.9521 - precision: 0.9774 - recall: 0.7884
Epoch 00008: val_loss did not improve from 0.13955
5000/5000 [==============================] - 22s 4ms/sample - loss: 0.1109 - accuracy: 0.9521 - precision: 0.9775 - recall: 0.7875 - val_loss: 0.1462 - val_accuracy: 0.9436 - val_precision: 0.9763 - val_recall: 0.7232
Epoch 9/10
4900/5000 [============================>.] - ETA: 0s - loss: 0.1101 - accuracy: 0.9524 - precision: 0.9775 - recall: 0.7905
Epoch 00009: val_loss improved from 0.13955 to 0.13883, saving model to ./models/UNet_(11-26-2020 , 20:05:29)
INFO:tensorflow:Assets written to: ./models/UNet_(11-26-2020 , 20:05:29)/assets
5000/5000 [==============================] - 28s 6ms/sample - loss: 0.1102 - accuracy: 0.9524 - precision: 0.9775 - recall: 0.7907 - val_loss: 0.1388 - val_accuracy: 0.9447 - val_precision: 0.9757 - val_recall: 0.7351
Epoch 10/10
4900/5000 [============================>.] - ETA: 0s - loss: 0.1096 - accuracy: 0.9526 - precision: 0.9776 - recall: 0.7917
Epoch 00010: val_loss did not improve from 0.13883
5000/5000 [==============================] - 22s 4ms/sample - loss: 0.1096 - accuracy: 0.9527 - precision: 0.9776 - recall: 0.7915 - val_loss: 0.1480 - val_accuracy: 0.9432 - val_precision: 0.9790 - val_recall: 0.7018
###Markdown
3. Load trained Model
###Code
model_path = "/home/amir/Desktop/Tree Counting/Counting Trees/models/UNet_(11-26-2020 , 19:51:28)"
model = model_load(model_path)
###Output
_____no_output_____
###Markdown
4. Evaluate trained Model
###Code
y_pred = model.predict(x_val)
number = 123 #56 ,34
img = y_pred[number,:,:,:]
opened_img, number_of_objects, blob_labels = post_processing(img)
print("Number of Trees: {}".format(number_of_objects[-1]))
f, axs = plt.subplots(1,4, figsize=(23,23))
ep.plot_rgb(x_val[number,:,:,:].transpose([2,0,1]),
rgb=[0, 1, 2],
title="Satellite Image",
stretch=True,
ax=axs[0])
axs[1].imshow(y_val[number,:,:,:])
axs[1].set_title("Ground Truth")
axs[2].imshow(opened_img)
axs[2].set_title("Prediction")
axs[3].imshow(blob_labels, cmap='gist_ncar')
axs[3].set_title("Number of Trees: {}".format(number_of_objects[-1]))
f.show()
###Output
_____no_output_____
###Markdown
ChallengeIn this challenge, we will practice on dimensionality reduction with PCA and selection of variables with RFE. We will use the _data set_ [Fifa 2019](https://www.kaggle.com/karangadiya/fifa19), originally containing 89 variables from over 18 thousand players of _game_ FIFA 2019. _Setup_
###Code
pip install loguru
from math import sqrt
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
import scipy.stats as sct
import seaborn as sns
import statsmodels.api as sm
import statsmodels.stats as st
from sklearn.decomposition import PCA
from loguru import logger
# Algumas configurações para o matplotlib.
%matplotlib inline
from IPython.core.pylabtools import figsize
figsize(12, 8)
sns.set()
fifa = pd.read_csv("fifa.csv")
columns_to_drop = ["Unnamed: 0", "ID", "Name", "Photo", "Nationality", "Flag",
"Club", "Club Logo", "Value", "Wage", "Special", "Preferred Foot",
"International Reputation", "Weak Foot", "Skill Moves", "Work Rate",
"Body Type", "Real Face", "Position", "Jersey Number", "Joined",
"Loaned From", "Contract Valid Until", "Height", "Weight", "LS",
"ST", "RS", "LW", "LF", "CF", "RF", "RW", "LAM", "CAM", "RAM", "LM",
"LCM", "CM", "RCM", "RM", "LWB", "LDM", "CDM", "RDM", "RWB", "LB", "LCB",
"CB", "RCB", "RB", "Release Clause"
]
try:
fifa.drop(columns_to_drop, axis=1, inplace=True)
except KeyError:
logger.warning(f"Columns already dropped")
###Output
_____no_output_____
###Markdown
Starts analysis
###Code
fifa.head()
###Output
_____no_output_____
###Markdown
Questão 1Qual fração da variância consegue ser explicada pelo primeiro componente principal de `fifa`? Responda como um único float (entre 0 e 1) arredondado para três casas decimais.
###Code
def q1():
# Retorne aqui o resultado da questão 1.
pass
###Output
_____no_output_____
###Markdown
Questão 2Quantos componentes principais precisamos para explicar 95% da variância total? Responda como un único escalar inteiro.
###Code
def q2():
# Retorne aqui o resultado da questão 2.
pass
###Output
_____no_output_____
###Markdown
Questão 3Qual são as coordenadas (primeiro e segundo componentes principais) do ponto `x` abaixo? O vetor abaixo já está centralizado. Cuidado para __não__ centralizar o vetor novamente (por exemplo, invocando `PCA.transform()` nele). Responda como uma tupla de float arredondados para três casas decimais.
###Code
x = [0.87747123, -1.24990363, -1.3191255, -36.7341814,
-35.55091139, -37.29814417, -28.68671182, -30.90902583,
-42.37100061, -32.17082438, -28.86315326, -22.71193348,
-38.36945867, -20.61407566, -22.72696734, -25.50360703,
2.16339005, -27.96657305, -33.46004736, -5.08943224,
-30.21994603, 3.68803348, -36.10997302, -30.86899058,
-22.69827634, -37.95847789, -22.40090313, -30.54859849,
-26.64827358, -19.28162344, -34.69783578, -34.6614351,
48.38377664, 47.60840355, 45.76793876, 44.61110193,
49.28911284
]
def q3():
# Retorne aqui o resultado da questão 3.
pass
###Output
_____no_output_____
###Markdown
Questão 4Realiza RFE com estimador de regressão linear para selecionar cinco variáveis, eliminando uma a uma. Quais são as variáveis selecionadas? Responda como uma lista de nomes de variáveis.
###Code
def q4():
# Retorne aqui o resultado da questão 4.
pass
###Output
_____no_output_____ |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.