path
stringlengths 7
265
| concatenated_notebook
stringlengths 46
17M
|
---|---|
15/code.ipynb | ###Markdown
15. Classifying Images with Deep Convolutional Neural Networks Contents- [15.01. The building blocks of CNNs][1501] - [15.01.01. Understanding CNNs and features hierachies][150101] - [15.01.02. Performing discrete convolutions][150102] - [15.01.02.01. Discrete convolutions in one dimension][15010201] - [15.01.02.02. Padding inputs to control the size of the output feature maps][15010202] - [15.01.02.03. Determining the size of the convolution output][15010203] - [15.01.02.04. Performing a discrete convoution in 2D][15010204] - [15.01.03. Subsampling layers][150103]- [15.02. Putting everything together - implementing a CNN][1502] - [15.02.01. Working with multiple input or color channels][150201] - [15.02.02. Regularizing an NN with dropout][150202] - [15.02.03. Loss functions for classification][150203]- [15.03. Implementing a deep CNN using TensorFlow][1503] - [15.03.01. The multilayer CNN architecture][150301] - [15.03.02. Loading and preprocessing the data][150302] - [15.03.03. Implemeting a CNN using the TensorFlow Keras API][150303] - [15.03.03.01. Configuration CNN layers in Keras][15030301] - [15.03.03.02. Constructing a CNN in Keras][15030302]- [15.04. Gender classigcation from face images using][1504] - [15.04.01. Loading the CelebA dataset][150401] - [15.04.02. Image transformation and data augmentation][150401] - [15.04.03. Training a CNN gender classifier][150403]- [15.05. Summary][1505]
###Code
%load_ext watermark
%watermark -u -d -t -v -p numpy,scipy,pandas,matplotlib,sklearn,tensorflow,tensorflow_datasets,nltk
###Output
Last updated: 2021-05-02 17:49:05
Python implementation: CPython
Python version : 3.7.10
IPython version : 7.22.0
numpy : 1.19.2
scipy : 1.6.2
pandas : 1.2.2
matplotlib : 3.3.2
sklearn : 0.24.1
tensorflow : 2.0.0
tensorflow_datasets: not installed
nltk : 3.5
###Markdown
15.01. The building blocks of CNNs 15.01.01. Understanding CNNs and features hierachies **salient (relevant) features****feature hierarchy****feature map****local receptive field**2 ideas:- **sparse connectivity**- **parameter-sharing**$w_{ij}$ input unit $i$, output unit $j$pooling layers 15.01.02. Performing discrete convolutions **(discrete) convolution** 15.01.02.01. Dscrete convolutions in one dimension vec $x$, $w$ $y = x \ast w$signal $x$, filter/kernel $w$$$y = x \ast w \rightarrow y[i] = \sum_{k=-\infty}^{+\infty}{x[i-k]w[k]}$$**(zero-)padding**$m ≤ n$ $x^p$$x$ have $n$ elements, $w$ have $m$ elementspadded vector $x^p$$$y = x \ast w \rightarrow y[i] = \sum_{k=0}^{k=m-1}{x^p[i+m-k] w[k]}$$$w^r$$x[i:i+m]$ 15.01.02.02. Padding inputs to control the size of the output feature maps 15.01.02.03. Determining the size of the convolution output
###Code
import numpy as np
def convld(x, w, p=0, s=1):
w_rot = np.array(w[::-1])
x_padded = np.array(x)
if p > 0:
zero_pad = np.zeros(shape=p)
x_padded = np.concatenate([zero_pad, x_padded, zero_pad])
res = []
for i in range(0, int(len(x)/s), s):
res.append(np.sum(x_padded[i:i+w_rot.shape[0]] * w_rot))
return np.array(res)
# Testing:
x = [1, 3, 2, 4, 5, 6, 1, 3]
w = [1, 0, 3, 1, 2]
print('Convid implementation:', convld(x, w, p=2, s=1))
print('Numpy Results:', np.convolve(x, w, mode='same'))
###Output
Numpy Results: [ 5 14 16 26 24 34 19 22]
###Markdown
15.01.02.04. Performing a discrete convoution in 2D
###Code
import numpy as np
import scipy.signal
def conv2d(X, W, p=(0,0), s=(1,1)):
W_rot = np.array(W)[::-1,::-1]
X_orig = np.array(X)
n1 = X_orig.shape[0] + 2*p[0]
n2 = X_orig.shape[1] + 2*p[1]
X_padded = np.zeros(shape=(n1, n2))
X_padded[p[0]:p[0]+X_orig.shape[0],
p[1]:p[1]+X_orig.shape[1]] = X_orig
res = []
for i in range(0, int((X_padded.shape[0] - W_rot.shape[0])/s[0])+1, s[0]):
res.append([])
for j in range(0, int((X_padded.shape[1] - W_rot.shape[1])/s[1])+1, s[1]):
X_sub = X_padded[i:i+W_rot.shape[0], j:j+W_rot.shape[1]]
res[-1].append(np.sum(X_sub * W_rot))
return(np.array(res))
X = [[1,3,2,4], [5,6,1,3], [1,2,0,2], [3,4,3,2]]
W = [[1,0,3], [1,2,1], [0,1,1]]
Y = conv2d(X,W, p=(1,1), s=(1,1))
print('Conv2d Impplementation:\n', Y)
fig, ax = plt.subplots(ncols=2, sharey=True)
ax[0].imshow(Y)
ax[1].imshow(X)
for i in range(4):
for j in range(4):
ax[0].text(j, i, Y[i, j], ha='center', va='center', color='w')
X = np.array(X)
for i in range(4):
for j in range(4):
ax[1].text(j, i, X[i, j], ha='center', va='center', color='w')
ax[0].set_yticks(np.arange(4))
ax[0].set_title('Y')
ax[1].set_title('X')
fig.tight_layout()
plt.show()
print('SciPy Results:\n', scipy.signal.convolve2d(X, W, mode='same'))
###Output
SciPy Results:
[[11 25 32 13]
[19 25 24 13]
[13 28 25 17]
[11 17 14 9]]
###Markdown
15.01.03. Subsampling layers 15.02. Putting everything together - implementing a CNN 15.02.01. Working with multiple input or color channels Reading an image file `unit8`: unsigned 8-bit integer
###Code
import tensorflow as tf
img_raw = tf.io.read_file('watchmen.png')
img = tf.image.decode_image(img_raw)
print('Image shape:', img.shape)
import imageio
img = imageio.imread('watchmen.png')
print('Image shape:', img.shape)
print('Number data type:', img.dtype)
print(img[100:102, 100:102, :])
###Output
Image shape: (332, 332, 4)
Number data type: uint8
[[[255 236 0 255]
[255 236 0 255]]
[[255 236 0 255]
[255 236 0 255]]]
###Markdown
15.02.02. Regularizing an NN with dropout
###Code
from tensorflow import keras
conv_layer = keras.layers.Conv2D(
filters=16,
kernel_size=(3,3), kernel_regularizer=keras.regularizers.l2(.001))
fc_layer = keras.layers.Dense(
units=16,
kernel_regularizer=keras.regularizers.l2(.001))
###Output
_____no_output_____
###Markdown
15.02.03. Loss functions for classification
###Code
import tensorflow_datasets as tfds
### Binary Crossentropy
bce_probas = tf.keras.losses.BinaryCrossentropy(from_logits=False)
bce_logits = tf.keras.losses.BinaryCrossentropy(from_logits=True)
logits = tf.constant([0.8])
probas = tf.keras.activations.sigmoid(logits)
tf.print(
'BCE \n(w Probas): {:.4f}'.format(
bce_probas(y_true=[1], y_pred=probas)),
'(w Logits): {:.4f}\n'.format(
bce_logits(y_true=[1], y_pred=logits)))
### Categorical Crossentropy
cce_probas = tf.keras.losses.CategoricalCrossentropy(from_logits=False)
cce_logits = tf.keras.losses.CategoricalCrossentropy(from_logits=True)
logits = tf.constant([[1.5, 0.8, 2.1]])
probas = tf.keras.activations.softmax(logits)
tf.print(
'CCE \n(w Probas): {:.4f}'.format(
cce_probas(y_true=[0, 0, 1], y_pred=probas)),
'(w Logits): {:.4f}\n'.format(
cce_logits(y_true=[0, 0, 1], y_pred=logits)))
### Sparcse Categorical Crossentropy
sp_cce_probas = tf.keras.losses.SparseCategoricalCrossentropy(from_logits=False)
sp_cce_logits = tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True)
tf.print(
'Sparse CCE \n(w Probas): {:.4f}'.format(
sp_cce_probas(y_true=[2], y_pred=probas)),
'(w Logits): {:.4f}'.format(
sp_cce_logits(y_true=[2], y_pred=logits)))
###Output
BCE
(w Probas): 0.3711 (w Logits): 0.3711
CCE
(w Probas): 0.5996 (w Logits): 0.5996
Sparse CCE
(w Probas): 0.5996 (w Logits): 0.5996
###Markdown
15.03. Implementing a deep CNN using TensorFlow 15.03.01. The multilayer CNN architecture 15.03.02. Loading and preprocessing the data
###Code
import tensorflow_datasets as tfds
## Loading the data
mnist_bldr = tfds.builder('mnist')
mnist_bldr.download_and_prepare()
datasets = mnist_bldr.as_dataset(shuffle_files=False)
mnist_train_orig = datasets['train']
mnist_test_orig = datasets['test']
BUFFER_SIZE = 10000
BATCH_SIZE = 64
NUM_EPOCHS = 20
mnist_train = mnist_train_orig.map(
lambda item: (tf.cast(item['image'], tf.float32)/255.0,
tf.cast(item['label'], tf.int32)))
mnist_test = mnist_test_orig.map(
lambda item: (tf.cast(item['image'], tf.float32)/255.0,
tf.cast(item['label'], tf.int32)))
tf.random.set_seed(1)
mnist_train = mnist_train.shuffle(buffer_size=BUFFER_SIZE,
reshuffle_each_iteration=False)
mnist_valid = mnist_train.take(10000).batch(BATCH_SIZE)
mnist_train = mnist_train.skip(10000).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
15.03.03. Implemeting a CNN using the TensorFlow Keras API 15.03.03.01. Configuration CNN layers in Keras 15.03.03.02. Constructing a CNN in Keras
###Code
model = tf.keras.Sequential()
model.add(tf.keras.layers.Conv2D(
filters=32,
kernel_size=(5,5),
strides=(1,1),
padding='same',
data_format='channels_last',
name='conv_1',
activation='relu'))
model.add(tf.keras.layers.MaxPool2D(
pool_size=(2,2),
name='pool_1'))
model.add(tf.keras.layers.Conv2D(
filters=64,
kernel_size=(5,5),
strides=(1,1),
padding='same',
name='conv_2',
activation='relu'))
model.add(tf.keras.layers.MaxPool2D(
pool_size=(2,2),
name='pool_2'))
model.compute_output_shape(input_shape=(16,28,28,1))
model.add(tf.keras.layers.Dense(
units=1024, name='fc_1', activation='relu'))
model.add(tf.keras.layers.Dropout(
rate=.5))
model.add(tf.keras.layers.Dense(
units=10, name='fc_2', activation='softmax'))
###Output
_____no_output_____ |
guides/ipynb/keras_tuner/getting_started.ipynb | ###Markdown
Getting started with KerasTuner**Authors:** Luca Invernizzi, James Long, Francois Chollet, Tom O'Malley, Haifeng Jin**Date created:** 2019/05/31**Last modified:** 2021/10/27**Description:** The basics of using KerasTuner to tune model hyperparameters.
###Code
!pip install keras-tuner -q
###Output
_____no_output_____
###Markdown
IntroductionKerasTuner is a general-purpose hyperparameter tuning library. It has strongintegration with Keras workflows, but it isn't limited to them: you could useit to tune scikit-learn models, or anything else. In this tutorial, you willsee how to tune model architecture, training process, and data preprocessingsteps with KerasTuner. Let's start from a simple example. Tune the model architectureThe first thing we need to do is writing a function, which returns a compiledKeras model. It takes an argument `hp` for defining the hyperparameters whilebuilding the model. Define the search spaceIn the following code example, we define a Keras model with two `Dense` layers.We want to tune the number of units in the first `Dense` layer. We just definean integer hyperparameter with `hp.Int('units', min_value=32, max_value=512, step=32)`,whose range is from 32 to 512 inclusive. When sampling from it, the minimumstep for walking through the interval is 32.
###Code
from tensorflow import keras
from tensorflow.keras import layers
def build_model(hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
# Define the hyperparameter.
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer="adam", loss="categorical_crossentropy", metrics=["accuracy"],
)
return model
###Output
_____no_output_____
###Markdown
You can quickly test if the model builds successfully.
###Code
import keras_tuner
build_model(keras_tuner.HyperParameters())
###Output
_____no_output_____
###Markdown
There are many other types of hyperparameters as well. We can define multiplehyperparameters in the function. In the following code, we tune the whether touse a `Dropout` layer with `hp.Boolean()`, tune which activation function touse with `hp.Choice()`, tune the learning rate of the optimizer with`hp.Float()`.
###Code
def build_model(hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
# Tune number of units.
units=hp.Int("units", min_value=32, max_value=512, step=32),
# Tune the activation function to use.
activation=hp.Choice("activation", ["relu", "tanh"]),
)
)
# Tune whether to use dropout.
if hp.Boolean("dropout"):
model.add(layers.Dropout(rate=0.25))
model.add(layers.Dense(10, activation="softmax"))
# Define the optimizer learning rate as a hyperparameter.
learning_rate = hp.Float("lr", min_value=1e-4, max_value=1e-2, sampling="log")
model.compile(
optimizer=keras.optimizers.Adam(learning_rate=learning_rate),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
build_model(keras_tuner.HyperParameters())
###Output
_____no_output_____
###Markdown
As shown below, the hyperparameters are actual values. In fact, they are justfunctions returning actual values. For example, `hp.Int()` returns an `int`value. Therefore, you can put them into variables, for loops, or ifconditions.
###Code
hp = keras_tuner.HyperParameters()
print(hp.Int("units", min_value=32, max_value=512, step=32))
###Output
_____no_output_____
###Markdown
You can also define the hyperparameters in advance and keep your Keras code ina separate function.
###Code
def call_existing_code(units, activation, dropout, lr):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(layers.Dense(units=units, activation=activation))
if dropout:
model.add(layers.Dropout(rate=0.25))
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer=keras.optimizers.Adam(learning_rate=lr),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
def build_model(hp):
units = hp.Int("units", min_value=32, max_value=512, step=32)
activation = hp.Choice("activation", ["relu", "tanh"])
dropout = hp.Boolean("dropout")
lr = hp.Float("lr", min_value=1e-4, max_value=1e-2, sampling="log")
# call existing model-building code with the hyperparameter values.
model = call_existing_code(
units=units, activation=activation, dropout=dropout, lr=lr
)
return model
build_model(keras_tuner.HyperParameters())
###Output
_____no_output_____
###Markdown
Each of the hyperparameters is uniquely identified by its name (the firstargument). To tune the number of units in different `Dense` layers separatelyas different hyperparameters, we give them different names as `f"units_{i}"`.Notably, this is also an example of creating conditional hyperparameters.There are many hyperparameters specifying the number of units in the `Dense`layers. The number of such hyperparameters is decided by the number of layers,which is also a hyperparameter. Therefore, the total number of hyperparametersused may be different from trial to trial. Some hyperparameter is only usedwhen a certain condition is satisfied. For example, `units_3` is only usedwhen `num_layers` is larger than 3. With KerasTuner, you can easily definesuch hyperparameters dynamically while creating the model.
###Code
def build_model(hp):
model = keras.Sequential()
model.add(layers.Flatten())
# Tune the number of layers.
for i in range(hp.Int("num_layers", 1, 3)):
model.add(
layers.Dense(
# Tune number of units separately.
units=hp.Int(f"units_{i}", min_value=32, max_value=512, step=32),
activation=hp.Choice("activation", ["relu", "tanh"]),
)
)
if hp.Boolean("dropout"):
model.add(layers.Dropout(rate=0.25))
model.add(layers.Dense(10, activation="softmax"))
learning_rate = hp.Float("lr", min_value=1e-4, max_value=1e-2, sampling="log")
model.compile(
optimizer=keras.optimizers.Adam(learning_rate=learning_rate),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
build_model(keras_tuner.HyperParameters())
###Output
_____no_output_____
###Markdown
Start the searchAfter defining the search space, we need to select a tuner class to run thesearch. You may choose from `RandomSearch`, `BayesianOptimization` and`Hyperband`, which correspond to different tuning algorithms. Here we use`RandomSearch` as an example.To initialize the tuner, we need to specify several arguments in the initializer.* `hypermodel`. The model-building function, which is `build_model` in our case.* `objective`. The name of the objective to optimize (whether to minimize ormaximize is automatically inferred for built-in metrics). We will introduce howto use custom metrics later in this tutorial.* `max_trials`. The total number of trials to run during the search.* `executions_per_trial`. The number of models that should be built and fit foreach trial. Different trials have different hyperparameter values. Theexecutions within the same trial have the same hyperparameter values. Thepurpose of having multiple executions per trial is to reduce results varianceand therefore be able to more accurately assess the performance of a model. Ifyou want to get results faster, you could set `executions_per_trial=1` (singleround of training for each model configuration).* `overwrite`. Control whether to overwrite the previous results in the samedirectory or resume the previous search instead. Here we set `overwrite=True`to start a new search and ignore any previous results.* `directory`. A path to a directory for storing the search results.* `project_name`. The name of the sub-directory in the `directory`.
###Code
tuner = keras_tuner.RandomSearch(
hypermodel=build_model,
objective="val_accuracy",
max_trials=3,
executions_per_trial=2,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
###Output
_____no_output_____
###Markdown
You can print a summary of the search space:
###Code
tuner.search_space_summary()
###Output
_____no_output_____
###Markdown
Before starting the search, let's prepare the MNIST dataset.
###Code
from tensorflow import keras
import numpy as np
(x, y), (x_test, y_test) = keras.datasets.mnist.load_data()
x_train = x[:-10000]
x_val = x[-10000:]
y_train = y[:-10000]
y_val = y[-10000:]
x_train = np.expand_dims(x_train, -1).astype("float32") / 255.0
x_val = np.expand_dims(x_val, -1).astype("float32") / 255.0
x_test = np.expand_dims(x_test, -1).astype("float32") / 255.0
num_classes = 10
y_train = keras.utils.to_categorical(y_train, num_classes)
y_val = keras.utils.to_categorical(y_val, num_classes)
y_test = keras.utils.to_categorical(y_test, num_classes)
###Output
_____no_output_____
###Markdown
Then, start the search for the best hyperparameter configuration.All the arguments passed to `search` is passed to `model.fit()` in eachexecution. Remember to pass `validation_data` to evaluate the model.
###Code
tuner.search(x_train, y_train, epochs=2, validation_data=(x_val, y_val))
###Output
_____no_output_____
###Markdown
During the `search`, the model-building function is called with differenthyperparameter values in different trial. In each trial, the tuner wouldgenerate a new set of hyperparameter values to build the model. The model isthen fit and evaluated. The metrics are recorded. The tuner progressivelyexplores the space and finally finds a good set of hyperparameter values. Query the resultsWhen search is over, you can retrieve the best model(s). The model is saved atits best performing epoch evaluated on the `validation_data`.
###Code
# Get the top 2 models.
models = tuner.get_best_models(num_models=2)
best_model = models[0]
# Build the model.
# Needed for `Sequential` without specified `input_shape`.
best_model.build(input_shape=(None, 28, 28))
best_model.summary()
###Output
_____no_output_____
###Markdown
You can also print a summary of the search results.
###Code
tuner.results_summary()
###Output
_____no_output_____
###Markdown
You will find detailed logs, checkpoints, etc, in the folder`my_dir/helloworld`, i.e. `directory/project_name`.You can also visualize the tuning results using TensorBoard and HParams plugin.For more information, please following[this link](https://keras.io/guides/keras_tuner/visualize_tuning/). Retrain the modelIf you want to train the model with the entire dataset, you may retrieve thebest hyperparameters and retrain the model by yourself.
###Code
# Get the top 2 hyperparameters.
best_hps = tuner.get_best_hyperparameters(5)
# Build the model with the best hp.
model = build_model(best_hps[0])
# Fit with the entire dataset.
x_all = np.concatenate((x_train, x_val))
y_all = np.concatenate((y_train, y_val))
model.fit(x=x_all, y=y_all, epochs=1)
###Output
_____no_output_____
###Markdown
Tune model trainingTo tune the model building process, we need to subclass the `HyperModel` class,which also makes it easy to share and reuse hypermodels.We need to override `HyperModel.build()` and `HyperModel.fit()` to tune themodel building and training process respectively. A `HyperModel.build()`method is the same as the model-building function, which creates a Keras modelusing the hyperparameters and returns it.In `HyperModel.fit()`, you can access the model returned by`HyperModel.build()`,`hp` and all the arguments passed to `search()`. You needto train the model and return the training history.In the following code, we will tune the `shuffle` argument in `model.fit()`.It is generally not needed to tune the number of epochs because a built-incallback is passed to `model.fit()` to save the model at its best epochevaluated by the `validation_data`.> **Note**: The `**kwargs` should always be passed to `model.fit()` because itcontains the callbacks for model saving and tensorboard plugins.
###Code
class MyHyperModel(keras_tuner.HyperModel):
def build(self, hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer="adam", loss="categorical_crossentropy", metrics=["accuracy"],
)
return model
def fit(self, hp, model, *args, **kwargs):
return model.fit(
*args,
# Tune whether to shuffle the data in each epoch.
shuffle=hp.Boolean("shuffle"),
**kwargs,
)
###Output
_____no_output_____
###Markdown
Again, we can do a quick check to see if the code works correctly.
###Code
hp = keras_tuner.HyperParameters()
hypermodel = MyHyperModel()
model = hypermodel.build(hp)
hypermodel.fit(hp, model, np.random.rand(100, 28, 28), np.random.rand(100, 10))
###Output
_____no_output_____
###Markdown
Tune data preprocessingTo tune data preprocessing, we just add an additional step in`HyperModel.fit()`, where we can access the dataset from the arguments. In thefollowing code, we tune whether to normalize the data before training themodel. This time we explicitly put `x` and `y` in the function signaturebecause we need to use them.
###Code
class MyHyperModel(keras_tuner.HyperModel):
def build(self, hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer="adam", loss="categorical_crossentropy", metrics=["accuracy"],
)
return model
def fit(self, hp, model, x, y, **kwargs):
if hp.Boolean("normalize"):
x = layers.Normalization()(x)
return model.fit(
x,
y,
# Tune whether to shuffle the data in each epoch.
shuffle=hp.Boolean("shuffle"),
**kwargs,
)
hp = keras_tuner.HyperParameters()
hypermodel = MyHyperModel()
model = hypermodel.build(hp)
hypermodel.fit(hp, model, np.random.rand(100, 28, 28), np.random.rand(100, 10))
###Output
_____no_output_____
###Markdown
If a hyperparameter is used both in `build()` and `fit()`, you can define it in`build()` and use `hp.get(hp_name)` to retrieve it in `fit()`. We use theimage size as an example. It is both used as the input shape in `build()`, andused by data prerprocessing step to crop the images in `fit()`.
###Code
class MyHyperModel(keras_tuner.HyperModel):
def build(self, hp):
image_size = hp.Int("image_size", 10, 28)
inputs = keras.Input(shape=(image_size, image_size))
outputs = layers.Flatten()(inputs)
outputs = layers.Dense(
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)(outputs)
outputs = layers.Dense(10, activation="softmax")(outputs)
model = keras.Model(inputs, outputs)
model.compile(
optimizer="adam", loss="categorical_crossentropy", metrics=["accuracy"],
)
return model
def fit(self, hp, model, x, y, validation_data=None, **kwargs):
if hp.Boolean("normalize"):
x = layers.Normalization()(x)
image_size = hp.get("image_size")
cropped_x = x[:, :image_size, :image_size, :]
if validation_data:
x_val, y_val = validation_data
cropped_x_val = x_val[:, :image_size, :image_size, :]
validation_data = (cropped_x_val, y_val)
return model.fit(
cropped_x,
y,
# Tune whether to shuffle the data in each epoch.
shuffle=hp.Boolean("shuffle"),
validation_data=validation_data,
**kwargs,
)
tuner = keras_tuner.RandomSearch(
MyHyperModel(),
objective="val_accuracy",
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="tune_hypermodel",
)
tuner.search(x_train, y_train, epochs=2, validation_data=(x_val, y_val))
###Output
_____no_output_____
###Markdown
Retrain the modelUsing `HyperModel` also allows you to retrain the best model by yourself.
###Code
hypermodel = MyHyperModel()
best_hp = tuner.get_best_hyperparameters()[0]
model = hypermodel.build(best_hp)
hypermodel.fit(best_hp, model, x_all, y_all, epochs=1)
###Output
_____no_output_____
###Markdown
Specify the tuning objectiveIn all previous examples, we all just used validation accuracy(`"val_accuracy"`) as the tuning objective to select the best model. Actually,you can use any metric as the objective. The most commonly used metric is`"val_loss"`, which is the validation loss. Built-in metric as the objectiveThere are many other built-in metrics in Keras you can use as the objective.Here is [a list of the built-in metrics](https://keras.io/api/metrics/).To use a built-in metric as the objective, you need to follow these steps:* Compile the model with the the built-in metric. For example, you want to use`MeanAbsoluteError()`. You need to compile the model with`metrics=[MeanAbsoluteError()]`. You may also use its name string instead:`metrics=["mean_absolute_error"]`. The name string of the metric is alwaysthe snake case of the class name.* Identify the objective name string. The name string of the objective isalways in the format of `f"val_{metric_name_string}"`. For example, theobjective name string of mean squared error evaluated on the validation datashould be `"val_mean_absolute_error"`.* Wrap it into `keras_tuner.Objective`. We usually need to wrap the objectiveinto a `keras_tuner.Objective` object to specify the direction to optimize theobjective. For example, we want to minimize the mean squared error, we can use`keras_tuner.Objective("val_mean_absolute_error", "min")`. The direction shouldbe either `"min"` or `"max"`.* Pass the wrapped objective to the tuner.You can see the following barebone code example.
###Code
def build_regressor(hp):
model = keras.Sequential(
[
layers.Dense(units=hp.Int("units", 32, 128, 32), activation="relu"),
layers.Dense(units=1),
]
)
model.compile(
optimizer="adam",
loss="mean_squared_error",
# Objective is one of the metrics.
metrics=[keras.metrics.MeanAbsoluteError()],
)
return model
tuner = keras_tuner.RandomSearch(
hypermodel=build_regressor,
# The objective name and direction.
# Name is the f"val_{snake_case_metric_class_name}".
objective=keras_tuner.Objective("val_mean_absolute_error", direction="min"),
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="built_in_metrics",
)
tuner.search(
x=np.random.rand(100, 10),
y=np.random.rand(100, 1),
validation_data=(np.random.rand(20, 10), np.random.rand(20, 1)),
)
tuner.results_summary()
###Output
_____no_output_____
###Markdown
Custom metric as the objectiveYou may implement your own metric and use it as the hyperparameter searchobjective. Here, we use mean squared error (MSE) as an example. First, weimplement the MSE metric by subclassing `keras.metrics.Metric`. Remember togive a name to your metric using the `name` argument of `super().__init__()`,which will be used later. Note: MSE is actully a build-in metric, which can beimported with `keras.metrics.MeanSquaredError`. This is just an example to showhow to use a custom metric as the hyperparameter search objective.For more information about implementing custom metrics, please see [thistutorial](https://keras.io/api/metrics/creating-custom-metrics). If you wouldlike a metric with a different function signature than `update_state(y_true,y_pred, sample_weight)`, you can override the `train_step()` method of yourmodel following [thistutorial](https://keras.io/guides/customizing_what_happens_in_fit/going-lowerlevel).
###Code
import tensorflow as tf
class CustomMetric(keras.metrics.Metric):
def __init__(self, **kwargs):
# Specify the name of the metric as "custom_metric".
super().__init__(name="custom_metric", **kwargs)
self.sum = self.add_weight(name="sum", initializer="zeros")
self.count = self.add_weight(name="count", dtype=tf.int32, initializer="zeros")
def update_state(self, y_true, y_pred, sample_weight=None):
values = tf.math.squared_difference(y_pred, y_true)
count = tf.shape(y_true)[0]
if sample_weight is not None:
sample_weight = tf.cast(sample_weight, self.dtype)
values *= sample_weight
count *= sample_weight
self.sum.assign_add(tf.reduce_sum(values))
self.count.assign_add(count)
def result(self):
return self.sum / tf.cast(self.count, tf.float32)
def reset_states(self):
self.sum.assign(0)
self.count.assign(0)
###Output
_____no_output_____
###Markdown
Run the search with the custom objective.
###Code
def build_regressor(hp):
model = keras.Sequential(
[
layers.Dense(units=hp.Int("units", 32, 128, 32), activation="relu"),
layers.Dense(units=1),
]
)
model.compile(
optimizer="adam",
loss="mean_squared_error",
# Put custom metric into the metrics.
metrics=[CustomMetric()],
)
return model
tuner = keras_tuner.RandomSearch(
hypermodel=build_regressor,
# Specify the name and direction of the objective.
objective=keras_tuner.Objective("val_custom_metric", direction="min"),
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="custom_metrics",
)
tuner.search(
x=np.random.rand(100, 10),
y=np.random.rand(100, 1),
validation_data=(np.random.rand(20, 10), np.random.rand(20, 1)),
)
tuner.results_summary()
###Output
_____no_output_____
###Markdown
If your custom objective is hard to put into a custom metric, you can alsoevaluate the model by yourself in `HyperModel.fit()` and return the objectivevalue. The objective value would be minimized by default. In this case, youdon't need to specify the `objective` when initializing the tuner. However, inthis case, the metric value will not be tracked in the Keras logs by onlyKerasTuner logs. Therefore, these values would not be displayed by anyTensorBoard view using the Keras metrics.
###Code
class HyperRegressor(keras_tuner.HyperModel):
def build(self, hp):
model = keras.Sequential(
[
layers.Dense(units=hp.Int("units", 32, 128, 32), activation="relu"),
layers.Dense(units=1),
]
)
model.compile(
optimizer="adam", loss="mean_squared_error",
)
return model
def fit(self, hp, model, x, y, validation_data, **kwargs):
model.fit(x, y, **kwargs)
x_val, y_val = validation_data
y_pred = model.predict(x_val)
# Return a single float to minimize.
return np.mean(np.abs(y_pred - y_val))
tuner = keras_tuner.RandomSearch(
hypermodel=HyperRegressor(),
# No objective to specify.
# Objective is the return value of `HyperModel.fit()`.
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="custom_eval",
)
tuner.search(
x=np.random.rand(100, 10),
y=np.random.rand(100, 1),
validation_data=(np.random.rand(20, 10), np.random.rand(20, 1)),
)
tuner.results_summary()
###Output
_____no_output_____
###Markdown
If you have multiple metrics to track in KerasTuner, but only use one of themas the objective, you can return a dictionary, whose keys are the metric namesand the values are the metrics values, for example, return `{"metric_a": 1.0,"metric_b", 2.0}`. Use one of the keys as the objective name, for example,`keras_tuner.Objective("metric_a", "min")`.
###Code
class HyperRegressor(keras_tuner.HyperModel):
def build(self, hp):
model = keras.Sequential(
[
layers.Dense(units=hp.Int("units", 32, 128, 32), activation="relu"),
layers.Dense(units=1),
]
)
model.compile(
optimizer="adam", loss="mean_squared_error",
)
return model
def fit(self, hp, model, x, y, validation_data, **kwargs):
model.fit(x, y, **kwargs)
x_val, y_val = validation_data
y_pred = model.predict(x_val)
# Return a dictionary of metrics for KerasTuner to track.
return {
"metric_a": -np.mean(np.abs(y_pred - y_val)),
"metric_b": np.mean(np.square(y_pred - y_val)),
}
tuner = keras_tuner.RandomSearch(
hypermodel=HyperRegressor(),
# Objective is one of the keys.
# Maximize the negative MAE, equivalent to minimize MAE.
objective=keras_tuner.Objective("metric_a", "max"),
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="custom_eval_dict",
)
tuner.search(
x=np.random.rand(100, 10),
y=np.random.rand(100, 1),
validation_data=(np.random.rand(20, 10), np.random.rand(20, 1)),
)
tuner.results_summary()
###Output
_____no_output_____
###Markdown
Tune end-to-end workflowsIn some cases, it is hard to align your code into build and fit functions. Youcan also keep your end-to-end workflow in one place by overriding`Tuner.run_trial()`, which gives you full control of a trial. You can see itas a black-box optimizer for anything. Tune any functionFor example, you can find a value of `x`, which minimizes `f(x)=x*x+1`. In thefollowing code, we just define `x` as a hyperparameter, and return `f(x)` asthe objective value. The `hypermodel` and `objective` argument for initializingthe tuner can be omitted.
###Code
class MyTuner(keras_tuner.RandomSearch):
def run_trial(self, trial, *args, **kwargs):
# Get the hp from trial.
hp = trial.hyperparameters
# Define "x" as a hyperparameter.
x = hp.Float("x", min_value=-1.0, max_value=1.0)
# Return the objective value to minimize.
return x * x + 1
tuner = MyTuner(
# No hypermodel or objective specified.
max_trials=20,
overwrite=True,
directory="my_dir",
project_name="tune_anything",
)
# No need to pass anything to search()
# unless you use them in run_trial().
tuner.search()
print(tuner.get_best_hyperparameters()[0].get("x"))
###Output
_____no_output_____
###Markdown
Keep Keras code separateYou can keep all your Keras code unchanged and use KerasTuner to tune it. Itis useful if you cannot modify the Keras code for some reason.It also gives you more flexibility. You don't have to separate the modelbuilding and training code apart. However, this workflow would not help yousave the model or connect with the TensorBoard plugins.To save the model, you can use `trial.trial_id`, which is a string to uniquelyidentify a trial, to construct different paths to save the models fromdifferent trials.
###Code
import os
def keras_code(units, optimizer, saving_path):
# Build model
model = keras.Sequential(
[layers.Dense(units=units, activation="relu"), layers.Dense(units=1),]
)
model.compile(
optimizer=optimizer, loss="mean_squared_error",
)
# Prepare data
x_train = np.random.rand(100, 10)
y_train = np.random.rand(100, 1)
x_val = np.random.rand(20, 10)
y_val = np.random.rand(20, 1)
# Train & eval model
model.fit(x_train, y_train)
# Save model
model.save(saving_path)
# Return a single float as the objective value.
# You may also return a dictionary
# of {metric_name: metric_value}.
y_pred = model.predict(x_val)
return np.mean(np.abs(y_pred - y_val))
class MyTuner(keras_tuner.RandomSearch):
def run_trial(self, trial, **kwargs):
hp = trial.hyperparameters
return keras_code(
units=hp.Int("units", 32, 128, 32),
optimizer=hp.Choice("optimizer", ["adam", "adadelta"]),
saving_path=os.path.join("/tmp", trial.trial_id),
)
tuner = MyTuner(
max_trials=3, overwrite=True, directory="my_dir", project_name="keep_code_separate",
)
tuner.search()
# Retraining the model
best_hp = tuner.get_best_hyperparameters()[0]
keras_code(**best_hp.values, saving_path="/tmp/best_model")
###Output
_____no_output_____
###Markdown
KerasTuner includes pre-made tunable applications: HyperResNet and HyperXceptionThese are ready-to-use hypermodels for computer vision.They come pre-compiled with `loss="categorical_crossentropy"` and`metrics=["accuracy"]`.
###Code
from keras_tuner.applications import HyperResNet
hypermodel = HyperResNet(input_shape=(28, 28, 1), classes=10)
tuner = keras_tuner.RandomSearch(
hypermodel,
objective="val_accuracy",
max_trials=2,
overwrite=True,
directory="my_dir",
project_name="built_in_hypermodel",
)
tuner.search(
x_train[:100], y_train[:100], epochs=1, validation_data=(x_val[:100], y_val[:100])
)
###Output
_____no_output_____
###Markdown
Getting started with KerasTuner**Authors:** Luca Invernizzi, James Long, Francois Chollet, Tom O'Malley, Haifeng Jin**Date created:** 2019/05/31**Last modified:** 2021/10/27**Description:** The basics of using KerasTuner to tune model hyperparameters. shellpip install keras-tuner -q IntroductionKerasTuner is a general-purpose hyperparameter tuning library. It has strongintegration with Keras workflows, but it isn't limited to them: you could useit to tune scikit-learn models, or anything else. In this tutorial, you willsee how to tune model architecture, training process, and data preprocessingsteps with KerasTuner. Let's start from a simple example. Tune the model architectureThe first thing we need to do is writing a function, which returns a compiledKeras model. It takes an argument `hp` for defining the hyperparameters whilebuilding the model. Define the search spaceIn the following code example, we define a Keras model with two `Dense` layers.We want to tune the number of units in the first `Dense` layer. We just definean integer hyperparameter with `hp.Int('units', min_value=32, max_value=512, step=32)`,whose range is from 32 to 512 inclusive. When sampling from it, the minimumstep for walking through the interval is 32.
###Code
from tensorflow import keras
from tensorflow.keras import layers
def build_model(hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
# Define the hyperparameter.
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer="adam", loss="categorical_crossentropy", metrics=["accuracy"],
)
return model
###Output
_____no_output_____
###Markdown
You can quickly test if the model builds successfully.
###Code
import keras_tuner as kt
build_model(kt.HyperParameters())
###Output
_____no_output_____
###Markdown
There are many other types of hyperparameters as well. We can define multiplehyperparameters in the function. In the following code, we tune the whether touse a `Dropout` layer with `hp.Boolean()`, tune which activation function touse with `hp.Choice()`, tune the learning rate of the optimizer with`hp.Float()`.
###Code
def build_model(hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
# Tune number of units.
units=hp.Int("units", min_value=32, max_value=512, step=32),
# Tune the activation function to use.
activation=hp.Choice("activation", ["relu", "tanh"]),
)
)
# Tune whether to use dropout.
if hp.Boolean("dropout"):
model.add(layers.Dropout(rate=0.25))
model.add(layers.Dense(10, activation="softmax"))
# Define the optimizer learning rate as a hyperparameter.
learning_rate = hp.Float("lr", min_value=1e-4, max_value=1e-2, sampling="log")
model.compile(
optimizer=keras.optimizers.Adam(learning_rate=learning_rate),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
build_model(kt.HyperParameters())
###Output
_____no_output_____
###Markdown
As shown below, the hyperparameters are actual values. In fact, they are justfunctions returning actual values. For example, `hp.Int()` returns an `int`value. Therefore, you can put them into variables, for loops, or ifconditions.
###Code
hp = kt.HyperParameters()
print(hp.Int("units", min_value=32, max_value=512, step=32))
###Output
_____no_output_____
###Markdown
You can also define the hyperparameters in advance and keep your Keras code ina separate function.
###Code
def call_existing_code(units, activation, dropout, lr):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(layers.Dense(units=units, activation=activation))
if dropout:
model.add(layers.Dropout(rate=0.25))
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer=keras.optimizers.Adam(learning_rate=lr),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
def build_model(hp):
units = hp.Int("units", min_value=32, max_value=512, step=32)
activation = hp.Choice("activation", ["relu", "tanh"])
dropout = hp.Boolean("dropout")
lr = hp.Float("lr", min_value=1e-4, max_value=1e-2, sampling="log")
# call existing model-building code with the hyperparameter values.
model = call_existing_code(
units=units, activation=activation, dropout=dropout, lr=lr
)
return model
build_model(kt.HyperParameters())
###Output
_____no_output_____
###Markdown
Each of the hyperparameters is uniquely identified by its name (the firstargument). To tune the number of units in different `Dense` layers separatelyas different hyperparameters, we give them different names as `f"units_{i}"`.Notably, this is also an example of creating conditional hyperparameters.There are many hyperparameters specifying the number of units in the `Dense`layers. The number of such hyperparameters is decided by the number of layers,which is also a hyperparameter. Therefore, the total number of hyperparametersused may be different from trial to trial. Some hyperparameter is only usedwhen a certain condition is satisfied. For example, `units_3` is only usedwhen `num_layers` is larger than 3. With KerasTuner, you can easily definesuch hyperparameters dynamically while creating the model.
###Code
def build_model(hp):
model = keras.Sequential()
model.add(layers.Flatten())
# Tune the number of layers.
for i in range(hp.Int("num_layers", 1, 3)):
model.add(
layers.Dense(
# Tune number of units separately.
units=hp.Int(f"units_{i}", min_value=32, max_value=512, step=32),
activation=hp.Choice("activation", ["relu", "tanh"]),
)
)
if hp.Boolean("dropout"):
model.add(layers.Dropout(rate=0.25))
model.add(layers.Dense(10, activation="softmax"))
learning_rate = hp.Float("lr", min_value=1e-4, max_value=1e-2, sampling="log")
model.compile(
optimizer=keras.optimizers.Adam(learning_rate=learning_rate),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
build_model(kt.HyperParameters())
###Output
_____no_output_____
###Markdown
Start the searchAfter defining the search space, we need to select a tuner class to run thesearch. You may choose from `RandomSearch`, `BayesianOptimization` and`Hyperband`, which correspond to different tuning algorithms. Here we use`RandomSearch` as an example.To initialize the tuner, we need to specify several arguments in the initializer.* `hypermodel`. The model-building function, which is `build_model` in our case.* `objective`. The name of the objective to optimize (whether to minimize ormaximize is automatically inferred for built-in metrics). We will introduce howto use custom metrics later in this tutorial.* `max_trials`. The total number of trials to run during the search.* `executions_per_trial`. The number of models that should be built and fit foreach trial. Different trials have different hyperparameter values. Theexecutions within the same trial have the same hyperparameter values. Thepurpose of having multiple executions per trial is to reduce results varianceand therefore be able to more accurately assess the performance of a model. Ifyou want to get results faster, you could set `executions_per_trial=1` (singleround of training for each model configuration).* `overwrite`. Control whether to overwrite the previous results in the samedirectory or resume the previous search instead. Here we set `overwrite=True`to start a new search and ignore any previous results.* `directory`. A path to a directory for storing the search results.* `project_name`. The name of the sub-directory in the `directory`.
###Code
tuner = kt.RandomSearch(
hypermodel=build_model,
objective="val_accuracy",
max_trials=3,
executions_per_trial=2,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
###Output
_____no_output_____
###Markdown
You can print a summary of the search space:
###Code
tuner.search_space_summary()
###Output
_____no_output_____
###Markdown
Before starting the search, let's prepare the MNIST dataset.
###Code
from tensorflow import keras
import numpy as np
(x, y), (x_test, y_test) = keras.datasets.mnist.load_data()
x_train = x[:-10000]
x_val = x[-10000:]
y_train = y[:-10000]
y_val = y[-10000:]
x_train = np.expand_dims(x_train, -1).astype("float32") / 255.0
x_val = np.expand_dims(x_val, -1).astype("float32") / 255.0
x_test = np.expand_dims(x_test, -1).astype("float32") / 255.0
num_classes = 10
y_train = keras.utils.to_categorical(y_train, num_classes)
y_val = keras.utils.to_categorical(y_val, num_classes)
y_test = keras.utils.to_categorical(y_test, num_classes)
###Output
_____no_output_____
###Markdown
Then, start the search for the best hyperparameter configuration.All the arguments passed to `search` is passed to `model.fit()` in eachexecution. Remember to pass `validation_data` to evaluate the model.
###Code
tuner.search(x_train, y_train, epochs=2, validation_data=(x_val, y_val))
###Output
_____no_output_____
###Markdown
During the `search`, the model-building function is called with differenthyperparameter values in different trial. In each trial, the tuner wouldgenerate a new set of hyperparameter values to build the model. The model isthen fit and evaluated. The metrics are recorded. The tuner progressivelyexplores the space and finally finds a good set of hyperparameter values. Query the resultsWhen search is over, you can retrieve the best model(s). The model is saved atits best performing epoch evaluated on the `validation_data`.
###Code
# Get the top 2 models.
models = tuner.get_best_models(num_models=2)
best_model = models[0]
# Build the model.
# Needed for `Sequential` without specified `input_shape`.
best_model.build(input_shape=(None, 28, 28))
best_model.summary()
###Output
_____no_output_____
###Markdown
You can also print a summary of the search results.
###Code
tuner.results_summary()
###Output
_____no_output_____
###Markdown
You will find detailed logs, checkpoints, etc, in the folder`my_dir/helloworld`, i.e. `directory/project_name`.You can also visualize the tuning results using TensorBoard and HParams plugin.For more information, please following[this link](https://keras.io/guides/keras_tuner/visualize_tuning/). Retrain the modelIf you want to train the model with the entire dataset, you may retrieve thebest hyperparameters and retrain the model by yourself.
###Code
# Get the top 2 hyperparameters.
best_hps = tuner.get_best_hyperparameters(5)
# Build the model with the best hp.
model = build_model(best_hps[0])
# Fit with the entire dataset.
x_all = np.concatenate((x_train, x_val))
y_all = np.concatenate((y_train, y_val))
model.fit(x=x_all, y=y_all, epochs=1)
###Output
_____no_output_____
###Markdown
Tune model trainingTo tune the model building process, we need to subclass the `HyperModel` class,which also makes it easy to share and reuse hypermodels.We need to override `HyperModel.build()` and `HyperModel.fit()` to tune themodel building and training process respectively. A `HyperModel.build()`method is the same as the model-building function, which creates a Keras modelusing the hyperparameters and returns it.In `HyperModel.fit()`, you can access the model returned by`HyperModel.build()`,`hp` and all the arguments passed to `search()`. You needto train the model and return the training history.In the following code, we will tune the `shuffle` argument in `model.fit()`.It is generally not needed to tune the number of epochs because a built-incallback is passed to `model.fit()` to save the model at its best epochevaluated by the `validation_data`.> **Note**: The `**kwargs` should always be passed to `model.fit()` because itcontains the callbacks for model saving and tensorboard plugins.
###Code
class MyHyperModel(kt.HyperModel):
def build(self, hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer="adam", loss="categorical_crossentropy", metrics=["accuracy"],
)
return model
def fit(self, hp, model, *args, **kwargs):
return model.fit(
*args,
# Tune whether to shuffle the data in each epoch.
shuffle=hp.Boolean("shuffle"),
**kwargs,
)
###Output
_____no_output_____
###Markdown
Again, we can do a quick check to see if the code works correctly.
###Code
hp = kt.HyperParameters()
hypermodel = MyHyperModel()
model = hypermodel.build(hp)
hypermodel.fit(hp, model, np.random.rand(100, 28, 28), np.random.rand(100, 10))
###Output
_____no_output_____
###Markdown
Tune data preprocessingTo tune data preprocessing, we just add an additional step in`HyperModel.fit()`, where we can access the dataset from the arguments. In thefollowing code, we tune whether to normalize the data before training themodel. This time we explicitly put `x` and `y` in the function signaturebecause we need to use them.
###Code
class MyHyperModel(kt.HyperModel):
def build(self, hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer="adam", loss="categorical_crossentropy", metrics=["accuracy"],
)
return model
def fit(self, hp, model, x, y, **kwargs):
if hp.Boolean("normalize"):
x = layers.Normalization()(x)
return model.fit(
x,
y,
# Tune whether to shuffle the data in each epoch.
shuffle=hp.Boolean("shuffle"),
**kwargs,
)
hp = kt.HyperParameters()
hypermodel = MyHyperModel()
model = hypermodel.build(hp)
hypermodel.fit(hp, model, np.random.rand(100, 28, 28), np.random.rand(100, 10))
###Output
_____no_output_____
###Markdown
If a hyperparameter is used both in `build()` and `fit()`, you can define it in`build()` and use `hp.get(hp_name)` to retrieve it in `fit()`. We use theimage size as an example. It is both used as the input shape in `build()`, andused by data prerprocessing step to crop the images in `fit()`.
###Code
class MyHyperModel(kt.HyperModel):
def build(self, hp):
image_size = hp.Int("image_size", 10, 28)
inputs = keras.Input(shape=(image_size, image_size))
outputs = layers.Flatten()(inputs)
outputs = layers.Dense(
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)(outputs)
outputs = layers.Dense(10, activation="softmax")(outputs)
model = keras.Model(inputs, outputs)
model.compile(
optimizer="adam", loss="categorical_crossentropy", metrics=["accuracy"],
)
return model
def fit(self, hp, model, x, y, validation_data=None, **kwargs):
if hp.Boolean("normalize"):
x = layers.Normalization()(x)
image_size = hp.get("image_size")
cropped_x = x[:, :image_size, :image_size, :]
if validation_data:
x_val, y_val = validation_data
cropped_x_val = x_val[:, :image_size, :image_size, :]
validation_data = (cropped_x_val, y_val)
return model.fit(
cropped_x,
y,
# Tune whether to shuffle the data in each epoch.
shuffle=hp.Boolean("shuffle"),
validation_data=validation_data,
**kwargs,
)
tuner = kt.RandomSearch(
MyHyperModel(),
objective="val_accuracy",
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="tune_hypermodel",
)
tuner.search(x_train, y_train, epochs=2, validation_data=(x_val, y_val))
###Output
_____no_output_____
###Markdown
Retrain the modelUsing `HyperModel` also allows you to retrain the best model by yourself.
###Code
hypermodel = MyHyperModel()
best_hp = tuner.get_best_hyperparameters()[0]
model = hypermodel.build(best_hp)
hypermodel.fit(best_hp, model, x_all, y_all, epochs=1)
###Output
_____no_output_____
###Markdown
Specify the tuning objectiveIn all previous examples, we all just used validation accuracy(`"val_accuracy"`) as the tuning objective to select the best model. Actually,you can use any metric as the objective. The most commonly used metric is`"val_loss"`, which is the validation loss. Built-in metric as the objectiveThere are many other built-in metrics in Keras you can use as the objective.Here is [a list of the built-in metrics](https://keras.io/api/metrics/).To use a built-in metric as the objective, you need to follow these steps:* Compile the model with the the built-in metric. For example, you want to use`MeanAbsoluteError()`. You need to compile the model with`metrics=[MeanAbsoluteError()]`. You may also use its name string instead:`metrics=["mean_absolute_error"]`. The name string of the metric is alwaysthe snake case of the class name.* Identify the objective name string. The name string of the objective isalways in the format of `f"val_{metric_name_string}"`. For example, theobjective name string of mean squared error evaluated on the validation datashould be `"val_mean_absolute_error"`.* Wrap it into `kt.Objective`. We usually need to wrap the objective into a`kt.Objective` object to specify the direction to optimize the objective. Forexample, we want to minimize the mean squared error, we can use`kt.Objective("val_mean_absolute_error", "min")`. The direction should beeither `"min"` or `"max"`.* Pass the wrapped objective to the tuner.You can see the following barebone code example.
###Code
def build_regressor(hp):
model = keras.Sequential(
[
layers.Dense(units=hp.Int("units", 32, 128, 32), activation="relu"),
layers.Dense(units=1),
]
)
model.compile(
optimizer="adam",
loss="mean_squared_error",
# Objective is one of the metrics.
metrics=[keras.metrics.MeanAbsoluteError()],
)
return model
tuner = kt.RandomSearch(
hypermodel=build_regressor,
# The objective name and direction.
# Name is the f"val_{snake_case_metric_class_name}".
objective=kt.Objective("val_mean_absolute_error", direction="min"),
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="built_in_metrics",
)
tuner.search(
x=np.random.rand(100, 10),
y=np.random.rand(100, 1),
validation_data=(np.random.rand(20, 10), np.random.rand(20, 1)),
)
tuner.results_summary()
###Output
_____no_output_____
###Markdown
Custom metric as the objectiveYou may implement your own metric and use it as the hyperparameter searchobjective. Here, we use mean squared error (MSE) as an example. First, weimplement the MSE metric by subclassing `keras.metrics.Metric`. Remember togive a name to your metric using the `name` argument of `super().__init__()`,which will be used later. Note: MSE is actully a build-in metric, which can beimported with `keras.metrics.MeanSquaredError`. This is just an example to showhow to use a custom metric as the hyperparameter search objective.For more information about implementing custom metrics, please see [thistutorial](https://keras.io/api/metrics/creating-custom-metrics). If you wouldlike a metric with a different function signature than `update_state(y_true,y_pred, sample_weight)`, you can override the `train_step()` method of yourmodel following [thistutorial](https://keras.io/guides/customizing_what_happens_in_fit/going-lowerlevel).
###Code
import tensorflow as tf
class CustomMetric(keras.metrics.Metric):
def __init__(self, **kwargs):
# Specify the name of the metric as "custom_metric".
super().__init__(name="custom_metric", **kwargs)
self.sum = self.add_weight(name="sum", initializer="zeros")
self.count = self.add_weight(name="count", dtype=tf.int32, initializer="zeros")
def update_state(self, y_true, y_pred, sample_weight=None):
values = tf.math.squared_difference(y_pred, y_true)
count = tf.shape(y_true)[0]
if sample_weight is not None:
sample_weight = tf.cast(sample_weight, self.dtype)
values *= sample_weight
count *= sample_weight
self.sum.assign_add(tf.reduce_sum(values))
self.count.assign_add(count)
def result(self):
return self.sum / tf.cast(self.count, tf.float32)
def reset_states(self):
self.sum.assign(0)
self.count.assign(0)
###Output
_____no_output_____
###Markdown
Run the search with the custom objective.
###Code
def build_regressor(hp):
model = keras.Sequential(
[
layers.Dense(units=hp.Int("units", 32, 128, 32), activation="relu"),
layers.Dense(units=1),
]
)
model.compile(
optimizer="adam",
loss="mean_squared_error",
# Put custom metric into the metrics.
metrics=[CustomMetric()],
)
return model
tuner = kt.RandomSearch(
hypermodel=build_regressor,
# Specify the name and direction of the objective.
objective=kt.Objective("val_custom_metric", direction="min"),
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="custom_metrics",
)
tuner.search(
x=np.random.rand(100, 10),
y=np.random.rand(100, 1),
validation_data=(np.random.rand(20, 10), np.random.rand(20, 1)),
)
tuner.results_summary()
###Output
_____no_output_____
###Markdown
If your custom objective is hard to put into a custom metric, you can alsoevaluate the model by yourself in `HyperModel.fit()` and return the objectivevalue. The objective value would be minimized by default. In this case, youdon't need to specify the `objective` when initializing the tuner. However, inthis case, the metric value will not be tracked in the Keras logs by onlyKerasTuner logs. Therefore, these values would not be displayed by anyTensorBoard view using the Keras metrics.
###Code
class HyperRegressor(kt.HyperModel):
def build(self, hp):
model = keras.Sequential(
[
layers.Dense(units=hp.Int("units", 32, 128, 32), activation="relu"),
layers.Dense(units=1),
]
)
model.compile(
optimizer="adam", loss="mean_squared_error",
)
return model
def fit(self, hp, model, x, y, validation_data, **kwargs):
model.fit(x, y, **kwargs)
x_val, y_val = validation_data
y_pred = model.predict(x_val)
# Return a single float to minimize.
return np.mean(np.abs(y_pred - y_val))
tuner = kt.RandomSearch(
hypermodel=HyperRegressor(),
# No objective to specify.
# Objective is the return value of `HyperModel.fit()`.
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="custom_eval",
)
tuner.search(
x=np.random.rand(100, 10),
y=np.random.rand(100, 1),
validation_data=(np.random.rand(20, 10), np.random.rand(20, 1)),
)
tuner.results_summary()
###Output
_____no_output_____
###Markdown
If you have multiple metrics to track in KerasTuner, but only use one of themas the objective, you can return a dictionary, whose keys are the metric namesand the values are the metrics values, for example, return `{"metric_a": 1.0,"metric_b", 2.0}`. Use one of the keys as the objective name, for example,`kt.Objective("metric_a", "min")`.
###Code
class HyperRegressor(kt.HyperModel):
def build(self, hp):
model = keras.Sequential(
[
layers.Dense(units=hp.Int("units", 32, 128, 32), activation="relu"),
layers.Dense(units=1),
]
)
model.compile(
optimizer="adam", loss="mean_squared_error",
)
return model
def fit(self, hp, model, x, y, validation_data, **kwargs):
model.fit(x, y, **kwargs)
x_val, y_val = validation_data
y_pred = model.predict(x_val)
# Return a dictionary of metrics for KerasTuner to track.
return {
"metric_a": -np.mean(np.abs(y_pred - y_val)),
"metric_b": np.mean(np.square(y_pred - y_val)),
}
tuner = kt.RandomSearch(
hypermodel=HyperRegressor(),
# Objective is one of the keys.
# Maximize the negative MAE, equivalent to minimize MAE.
objective=kt.Objective("metric_a", "max"),
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="custom_eval_dict",
)
tuner.search(
x=np.random.rand(100, 10),
y=np.random.rand(100, 1),
validation_data=(np.random.rand(20, 10), np.random.rand(20, 1)),
)
tuner.results_summary()
###Output
_____no_output_____
###Markdown
Tune end-to-end workflowsIn some cases, it is hard to align your code into build and fit functions. Youcan also keep your end-to-end workflow in one place by overriding`Tuner.run_trial()`, which gives you full control of a trial. You can see itas a black-box optimizer for anything. Tune any functionFor example, you can find a value of `x`, which minimizes `f(x)=x*x+1`. In thefollowing code, we just define `x` as a hyperparameter, and return `f(x)` asthe objective value. The `hypermodel` and `objective` argument for initializingthe tuner can be omitted.
###Code
class MyTuner(kt.RandomSearch):
def run_trial(self, trial, *args, **kwargs):
# Get the hp from trial.
hp = trial.hyperparameters
# Define "x" as a hyperparameter.
x = hp.Float("x", min_value=-1.0, max_value=1.0)
# Return the objective value to minimize.
return x * x + 1
tuner = MyTuner(
# No hypermodel or objective specified.
max_trials=20,
overwrite=True,
directory="my_dir",
project_name="tune_anything",
)
# No need to pass anything to search()
# unless you use them in run_trial().
tuner.search()
print(tuner.get_best_hyperparameters()[0].get("x"))
###Output
_____no_output_____
###Markdown
Keep Keras code separateYou can keep all your Keras code unchanged and use KerasTuner to tune it. Itis useful if you cannot modify the Keras code for some reason.It also gives you more flexibility. You don't have to separate the modelbuilding and training code apart. However, this workflow would not help yousave the model or connect with the TensorBoard plugins.To save the model, you can use `trial.trial_id`, which is a string to uniquelyidentify a trial, to construct different paths to save the models fromdifferent trials.
###Code
import os
def keras_code(units, optimizer, saving_path):
# Build model
model = keras.Sequential(
[layers.Dense(units=units, activation="relu"), layers.Dense(units=1),]
)
model.compile(
optimizer=optimizer, loss="mean_squared_error",
)
# Prepare data
x_train = np.random.rand(100, 10)
y_train = np.random.rand(100, 1)
x_val = np.random.rand(20, 10)
y_val = np.random.rand(20, 1)
# Train & eval model
model.fit(x_train, y_train)
# Save model
model.save(saving_path)
# Return a single float as the objective value.
# You may also return a dictionary
# of {metric_name: metric_value}.
y_pred = model.predict(x_val)
return np.mean(np.abs(y_pred - y_val))
class MyTuner(kt.RandomSearch):
def run_trial(self, trial, **kwargs):
hp = trial.hyperparameters
return keras_code(
units=hp.Int("units", 32, 128, 32),
optimizer=hp.Choice("optimizer", ["adam", "adadelta"]),
saving_path=os.path.join("/tmp", trial.trial_id),
)
tuner = MyTuner(
max_trials=3, overwrite=True, directory="my_dir", project_name="keep_code_separate",
)
tuner.search()
# Retraining the model
best_hp = tuner.get_best_hyperparameters()[0]
keras_code(**best_hp.values, saving_path="/tmp/best_model")
###Output
_____no_output_____
###Markdown
KerasTuner includes pre-made tunable applications: HyperResNet and HyperXceptionThese are ready-to-use hypermodels for computer vision.They come pre-compiled with `loss="categorical_crossentropy"` and`metrics=["accuracy"]`.
###Code
from keras_tuner.applications import HyperResNet
hypermodel = HyperResNet(input_shape=(28, 28, 1), classes=10)
tuner = kt.RandomSearch(
hypermodel,
objective="val_accuracy",
max_trials=2,
overwrite=True,
directory="my_dir",
project_name="built_in_hypermodel",
)
tuner.search(
x_train[:100], y_train[:100], epochs=1, validation_data=(x_val[:100], y_val[:100])
)
###Output
_____no_output_____
###Markdown
Getting started with KerasTuner**Authors:** Luca Invernizzi, James Long, Francois Chollet, Tom O'Malley, Haifeng Jin**Date created:** 2019/05/31**Last modified:** 2021/10/27**Description:** The basics of using KerasTuner to tune model hyperparameters.
###Code
!pip install keras-tuner -q
###Output
_____no_output_____
###Markdown
IntroductionKerasTuner is a general-purpose hyperparameter tuning library. It has strongintegration with Keras workflows, but it isn't limited to them: you could useit to tune scikit-learn models, or anything else. In this tutorial, you willsee how to tune model architecture, training process, and data preprocessingsteps with KerasTuner. Let's start from a simple example. Tune the model architectureThe first thing we need to do is writing a function, which returns a compiledKeras model. It takes an argument `hp` for defining the hyperparameters whilebuilding the model. Define the search spaceIn the following code example, we define a Keras model with two `Dense` layers.We want to tune the number of units in the first `Dense` layer. We just definean integer hyperparameter with `hp.Int('units', min_value=32, max_value=512, step=32)`,whose range is from 32 to 512 inclusive. When sampling from it, the minimumstep for walking through the interval is 32.
###Code
from tensorflow import keras
from tensorflow.keras import layers
def build_model(hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
# Define the hyperparameter.
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer="adam", loss="categorical_crossentropy", metrics=["accuracy"],
)
return model
###Output
_____no_output_____
###Markdown
You can quickly test if the model builds successfully.
###Code
import keras_tuner as kt
build_model(kt.HyperParameters())
###Output
_____no_output_____
###Markdown
There are many other types of hyperparameters as well. We can define multiplehyperparameters in the function. In the following code, we tune the whether touse a `Dropout` layer with `hp.Boolean()`, tune which activation function touse with `hp.Choice()`, tune the learning rate of the optimizer with`hp.Float()`.
###Code
def build_model(hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
# Tune number of units.
units=hp.Int("units", min_value=32, max_value=512, step=32),
# Tune the activation function to use.
activation=hp.Choice("activation", ["relu", "tanh"]),
)
)
# Tune whether to use dropout.
if hp.Boolean("dropout"):
model.add(layers.Dropout(rate=0.25))
model.add(layers.Dense(10, activation="softmax"))
# Define the optimizer learning rate as a hyperparameter.
learning_rate = hp.Float("lr", min_value=1e-4, max_value=1e-2, sampling="log")
model.compile(
optimizer=keras.optimizers.Adam(learning_rate=learning_rate),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
build_model(kt.HyperParameters())
###Output
_____no_output_____
###Markdown
As shown below, the hyperparameters are actual values. In fact, they are justfunctions returning actual values. For example, `hp.Int()` returns an `int`value. Therefore, you can put them into variables, for loops, or ifconditions.
###Code
hp = kt.HyperParameters()
print(hp.Int("units", min_value=32, max_value=512, step=32))
###Output
_____no_output_____
###Markdown
You can also define the hyperparameters in advance and keep your Keras code ina separate function.
###Code
def call_existing_code(units, activation, dropout, lr):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(layers.Dense(units=units, activation=activation))
if dropout:
model.add(layers.Dropout(rate=0.25))
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer=keras.optimizers.Adam(learning_rate=lr),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
def build_model(hp):
units = hp.Int("units", min_value=32, max_value=512, step=32)
activation = hp.Choice("activation", ["relu", "tanh"])
dropout = hp.Boolean("dropout")
lr = hp.Float("lr", min_value=1e-4, max_value=1e-2, sampling="log")
# call existing model-building code with the hyperparameter values.
model = call_existing_code(
units=units, activation=activation, dropout=dropout, lr=lr
)
return model
build_model(kt.HyperParameters())
###Output
_____no_output_____
###Markdown
Each of the hyperparameters is uniquely identified by its name (the firstargument). To tune the number of units in different `Dense` layers separatelyas different hyperparameters, we give them different names as `f"units_{i}"`.Notably, this is also an example of creating conditional hyperparameters.There are many hyperparameters specifying the number of units in the `Dense`layers. The number of such hyperparameters is decided by the number of layers,which is also a hyperparameter. Therefore, the total number of hyperparametersused may be different from trial to trial. Some hyperparameter is only usedwhen a certain condition is satisfied. For example, `units_3` is only usedwhen `num_layers` is larger than 3. With KerasTuner, you can easily definesuch hyperparameters dynamically while creating the model.
###Code
def build_model(hp):
model = keras.Sequential()
model.add(layers.Flatten())
# Tune the number of layers.
for i in range(hp.Int("num_layers", 1, 3)):
model.add(
layers.Dense(
# Tune number of units separately.
units=hp.Int(f"units_{i}", min_value=32, max_value=512, step=32),
activation=hp.Choice("activation", ["relu", "tanh"]),
)
)
if hp.Boolean("dropout"):
model.add(layers.Dropout(rate=0.25))
model.add(layers.Dense(10, activation="softmax"))
learning_rate = hp.Float("lr", min_value=1e-4, max_value=1e-2, sampling="log")
model.compile(
optimizer=keras.optimizers.Adam(learning_rate=learning_rate),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
build_model(kt.HyperParameters())
###Output
_____no_output_____
###Markdown
Start the searchAfter defining the search space, we need to select a tuner class to run thesearch. You may choose from `RandomSearch`, `BayesianOptimization` and`Hyperband`, which correspond to different tuning algorithms. Here we use`RandomSearch` as an example.To initialize the tuner, we need to specify several arguments in the initializer.* `hypermodel`. The model-building function, which is `build_model` in our case.* `objective`. The name of the objective to optimize (whether to minimize ormaximize is automatically inferred for built-in metrics). We will introduce howto use custom metrics later in this tutorial.* `max_trials`. The total number of trials to run during the search.* `executions_per_trial`. The number of models that should be built and fit foreach trial. Different trials have different hyperparameter values. Theexecutions within the same trial have the same hyperparameter values. Thepurpose of having multiple executions per trial is to reduce results varianceand therefore be able to more accurately assess the performance of a model. Ifyou want to get results faster, you could set `executions_per_trial=1` (singleround of training for each model configuration).* `overwrite`. Control whether to overwrite the previous results in the samedirectory or resume the previous search instead. Here we set `overwrite=True`to start a new search and ignore any previous results.* `directory`. A path to a directory for storing the search results.* `project_name`. The name of the sub-directory in the `directory`.
###Code
tuner = kt.RandomSearch(
hypermodel=build_model,
objective="val_accuracy",
max_trials=3,
executions_per_trial=2,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
###Output
_____no_output_____
###Markdown
You can print a summary of the search space:
###Code
tuner.search_space_summary()
###Output
_____no_output_____
###Markdown
Before starting the search, let's prepare the MNIST dataset.
###Code
from tensorflow import keras
import numpy as np
(x, y), (x_test, y_test) = keras.datasets.mnist.load_data()
x_train = x[:-10000]
x_val = x[-10000:]
y_train = y[:-10000]
y_val = y[-10000:]
x_train = np.expand_dims(x_train, -1).astype("float32") / 255.0
x_val = np.expand_dims(x_val, -1).astype("float32") / 255.0
x_test = np.expand_dims(x_test, -1).astype("float32") / 255.0
num_classes = 10
y_train = keras.utils.to_categorical(y_train, num_classes)
y_val = keras.utils.to_categorical(y_val, num_classes)
y_test = keras.utils.to_categorical(y_test, num_classes)
###Output
_____no_output_____
###Markdown
Then, start the search for the best hyperparameter configuration.All the arguments passed to `search` is passed to `model.fit()` in eachexecution. Remember to pass `validation_data` to evaluate the model.
###Code
tuner.search(x_train, y_train, epochs=2, validation_data=(x_val, y_val))
###Output
_____no_output_____
###Markdown
During the `search`, the model-building function is called with differenthyperparameter values in different trial. In each trial, the tuner wouldgenerate a new set of hyperparameter values to build the model. The model isthen fit and evaluated. The metrics are recorded. The tuner progressivelyexplores the space and finally finds a good set of hyperparameter values. Query the resultsWhen search is over, you can retrieve the best model(s). The model is saved atits best performing epoch evaluated on the `validation_data`.
###Code
# Get the top 2 models.
models = tuner.get_best_models(num_models=2)
best_model = models[0]
# Build the model.
# Needed for `Sequential` without specified `input_shape`.
best_model.build(input_shape=(None, 28, 28))
best_model.summary()
###Output
_____no_output_____
###Markdown
You can also print a summary of the search results.
###Code
tuner.results_summary()
###Output
_____no_output_____
###Markdown
You will find detailed logs, checkpoints, etc, in the folder`my_dir/helloworld`, i.e. `directory/project_name`.You can also visualize the tuning results using TensorBoard and HParams plugin.For more information, please following[this link](https://keras.io/guides/keras_tuner/visualize_tuning/). Retrain the modelIf you want to train the model with the entire dataset, you may retrieve thebest hyperparameters and retrain the model by yourself.
###Code
# Get the top 2 hyperparameters.
best_hps = tuner.get_best_hyperparameters(5)
# Build the model with the best hp.
model = build_model(best_hps[0])
# Fit with the entire dataset.
x_all = np.concatenate((x_train, x_val))
y_all = np.concatenate((y_train, y_val))
model.fit(x=x_all, y=y_all, epochs=1)
###Output
_____no_output_____
###Markdown
Tune model trainingTo tune the model building process, we need to subclass the `HyperModel` class,which also makes it easy to share and reuse hypermodels.We need to override `HyperModel.build()` and `HyperModel.fit()` to tune themodel building and training process respectively. A `HyperModel.build()`method is the same as the model-building function, which creates a Keras modelusing the hyperparameters and returns it.In `HyperModel.fit()`, you can access the model returned by`HyperModel.build()`,`hp` and all the arguments passed to `search()`. You needto train the model and return the training history.In the following code, we will tune the `shuffle` argument in `model.fit()`.It is generally not needed to tune the number of epochs because a built-incallback is passed to `model.fit()` to save the model at its best epochevaluated by the `validation_data`.> **Note**: The `**kwargs` should always be passed to `model.fit()` because itcontains the callbacks for model saving and tensorboard plugins.
###Code
class MyHyperModel(kt.HyperModel):
def build(self, hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer="adam", loss="categorical_crossentropy", metrics=["accuracy"],
)
return model
def fit(self, hp, model, *args, **kwargs):
return model.fit(
*args,
# Tune whether to shuffle the data in each epoch.
shuffle=hp.Boolean("shuffle"),
**kwargs,
)
###Output
_____no_output_____
###Markdown
Again, we can do a quick check to see if the code works correctly.
###Code
hp = kt.HyperParameters()
hypermodel = MyHyperModel()
model = hypermodel.build(hp)
hypermodel.fit(hp, model, np.random.rand(100, 28, 28), np.random.rand(100, 10))
###Output
_____no_output_____
###Markdown
Tune data preprocessingTo tune data preprocessing, we just add an additional step in`HyperModel.fit()`, where we can access the dataset from the arguments. In thefollowing code, we tune whether to normalize the data before training themodel. This time we explicitly put `x` and `y` in the function signaturebecause we need to use them.
###Code
class MyHyperModel(kt.HyperModel):
def build(self, hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer="adam", loss="categorical_crossentropy", metrics=["accuracy"],
)
return model
def fit(self, hp, model, x, y, **kwargs):
if hp.Boolean("normalize"):
x = layers.Normalization()(x)
return model.fit(
x,
y,
# Tune whether to shuffle the data in each epoch.
shuffle=hp.Boolean("shuffle"),
**kwargs,
)
hp = kt.HyperParameters()
hypermodel = MyHyperModel()
model = hypermodel.build(hp)
hypermodel.fit(hp, model, np.random.rand(100, 28, 28), np.random.rand(100, 10))
###Output
_____no_output_____
###Markdown
If a hyperparameter is used both in `build()` and `fit()`, you can define it in`build()` and use `hp.get(hp_name)` to retrieve it in `fit()`. We use theimage size as an example. It is both used as the input shape in `build()`, andused by data prerprocessing step to crop the images in `fit()`.
###Code
class MyHyperModel(kt.HyperModel):
def build(self, hp):
image_size = hp.Int("image_size", 10, 28)
inputs = keras.Input(shape=(image_size, image_size))
outputs = layers.Flatten()(inputs)
outputs = layers.Dense(
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)(outputs)
outputs = layers.Dense(10, activation="softmax")(outputs)
model = keras.Model(inputs, outputs)
model.compile(
optimizer="adam", loss="categorical_crossentropy", metrics=["accuracy"],
)
return model
def fit(self, hp, model, x, y, validation_data=None, **kwargs):
if hp.Boolean("normalize"):
x = layers.Normalization()(x)
image_size = hp.get("image_size")
cropped_x = x[:, :image_size, :image_size, :]
if validation_data:
x_val, y_val = validation_data
cropped_x_val = x_val[:, :image_size, :image_size, :]
validation_data = (cropped_x_val, y_val)
return model.fit(
cropped_x,
y,
# Tune whether to shuffle the data in each epoch.
shuffle=hp.Boolean("shuffle"),
validation_data=validation_data,
**kwargs,
)
tuner = kt.RandomSearch(
MyHyperModel(),
objective="val_accuracy",
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="tune_hypermodel",
)
tuner.search(x_train, y_train, epochs=2, validation_data=(x_val, y_val))
###Output
_____no_output_____
###Markdown
Retrain the modelUsing `HyperModel` also allows you to retrain the best model by yourself.
###Code
hypermodel = MyHyperModel()
best_hp = tuner.get_best_hyperparameters()[0]
model = hypermodel.build(best_hp)
hypermodel.fit(best_hp, model, x_all, y_all, epochs=1)
###Output
_____no_output_____
###Markdown
Specify the tuning objectiveIn all previous examples, we all just used validation accuracy(`"val_accuracy"`) as the tuning objective to select the best model. Actually,you can use any metric as the objective. The most commonly used metric is`"val_loss"`, which is the validation loss. Built-in metric as the objectiveThere are many other built-in metrics in Keras you can use as the objective.Here is [a list of the built-in metrics](https://keras.io/api/metrics/).To use a built-in metric as the objective, you need to follow these steps:* Compile the model with the the built-in metric. For example, you want to use`MeanAbsoluteError()`. You need to compile the model with`metrics=[MeanAbsoluteError()]`. You may also use its name string instead:`metrics=["mean_absolute_error"]`. The name string of the metric is alwaysthe snake case of the class name.* Identify the objective name string. The name string of the objective isalways in the format of `f"val_{metric_name_string}"`. For example, theobjective name string of mean squared error evaluated on the validation datashould be `"val_mean_absolute_error"`.* Wrap it into `kt.Objective`. We usually need to wrap the objective into a`kt.Objective` object to specify the direction to optimize the objective. Forexample, we want to minimize the mean squared error, we can use`kt.Objective("val_mean_absolute_error", "min")`. The direction should beeither `"min"` or `"max"`.* Pass the wrapped objective to the tuner.You can see the following barebone code example.
###Code
def build_regressor(hp):
model = keras.Sequential(
[
layers.Dense(units=hp.Int("units", 32, 128, 32), activation="relu"),
layers.Dense(units=1),
]
)
model.compile(
optimizer="adam",
loss="mean_squared_error",
# Objective is one of the metrics.
metrics=[keras.metrics.MeanAbsoluteError()],
)
return model
tuner = kt.RandomSearch(
hypermodel=build_regressor,
# The objective name and direction.
# Name is the f"val_{snake_case_metric_class_name}".
objective=kt.Objective("val_mean_absolute_error", direction="min"),
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="built_in_metrics",
)
tuner.search(
x=np.random.rand(100, 10),
y=np.random.rand(100, 1),
validation_data=(np.random.rand(20, 10), np.random.rand(20, 1)),
)
tuner.results_summary()
###Output
_____no_output_____
###Markdown
Custom metric as the objectiveYou may implement your own metric and use it as the hyperparameter searchobjective. Here, we use mean squared error (MSE) as an example. First, weimplement the MSE metric by subclassing `keras.metrics.Metric`. Remember togive a name to your metric using the `name` argument of `super().__init__()`,which will be used later. Note: MSE is actully a build-in metric, which can beimported with `keras.metrics.MeanSquaredError`. This is just an example to showhow to use a custom metric as the hyperparameter search objective.For more information about implementing custom metrics, please see [thistutorial](https://keras.io/api/metrics/creating-custom-metrics). If you wouldlike a metric with a different function signature than `update_state(y_true,y_pred, sample_weight)`, you can override the `train_step()` method of yourmodel following [thistutorial](https://keras.io/guides/customizing_what_happens_in_fit/going-lowerlevel).
###Code
import tensorflow as tf
class CustomMetric(keras.metrics.Metric):
def __init__(self, **kwargs):
# Specify the name of the metric as "custom_metric".
super().__init__(name="custom_metric", **kwargs)
self.sum = self.add_weight(name="sum", initializer="zeros")
self.count = self.add_weight(name="count", dtype=tf.int32, initializer="zeros")
def update_state(self, y_true, y_pred, sample_weight=None):
values = tf.math.squared_difference(y_pred, y_true)
count = tf.shape(y_true)[0]
if sample_weight is not None:
sample_weight = tf.cast(sample_weight, self.dtype)
values *= sample_weight
count *= sample_weight
self.sum.assign_add(tf.reduce_sum(values))
self.count.assign_add(count)
def result(self):
return self.sum / tf.cast(self.count, tf.float32)
def reset_states(self):
self.sum.assign(0)
self.count.assign(0)
###Output
_____no_output_____
###Markdown
Run the search with the custom objective.
###Code
def build_regressor(hp):
model = keras.Sequential(
[
layers.Dense(units=hp.Int("units", 32, 128, 32), activation="relu"),
layers.Dense(units=1),
]
)
model.compile(
optimizer="adam",
loss="mean_squared_error",
# Put custom metric into the metrics.
metrics=[CustomMetric()],
)
return model
tuner = kt.RandomSearch(
hypermodel=build_regressor,
# Specify the name and direction of the objective.
objective=kt.Objective("val_custom_metric", direction="min"),
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="custom_metrics",
)
tuner.search(
x=np.random.rand(100, 10),
y=np.random.rand(100, 1),
validation_data=(np.random.rand(20, 10), np.random.rand(20, 1)),
)
tuner.results_summary()
###Output
_____no_output_____
###Markdown
If your custom objective is hard to put into a custom metric, you can alsoevaluate the model by yourself in `HyperModel.fit()` and return the objectivevalue. The objective value would be minimized by default. In this case, youdon't need to specify the `objective` when initializing the tuner. However, inthis case, the metric value will not be tracked in the Keras logs by onlyKerasTuner logs. Therefore, these values would not be displayed by anyTensorBoard view using the Keras metrics.
###Code
class HyperRegressor(kt.HyperModel):
def build(self, hp):
model = keras.Sequential(
[
layers.Dense(units=hp.Int("units", 32, 128, 32), activation="relu"),
layers.Dense(units=1),
]
)
model.compile(
optimizer="adam", loss="mean_squared_error",
)
return model
def fit(self, hp, model, x, y, validation_data, **kwargs):
model.fit(x, y, **kwargs)
x_val, y_val = validation_data
y_pred = model.predict(x_val)
# Return a single float to minimize.
return np.mean(np.abs(y_pred - y_val))
tuner = kt.RandomSearch(
hypermodel=HyperRegressor(),
# No objective to specify.
# Objective is the return value of `HyperModel.fit()`.
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="custom_eval",
)
tuner.search(
x=np.random.rand(100, 10),
y=np.random.rand(100, 1),
validation_data=(np.random.rand(20, 10), np.random.rand(20, 1)),
)
tuner.results_summary()
###Output
_____no_output_____
###Markdown
If you have multiple metrics to track in KerasTuner, but only use one of themas the objective, you can return a dictionary, whose keys are the metric namesand the values are the metrics values, for example, return `{"metric_a": 1.0,"metric_b", 2.0}`. Use one of the keys as the objective name, for example,`kt.Objective("metric_a", "min")`.
###Code
class HyperRegressor(kt.HyperModel):
def build(self, hp):
model = keras.Sequential(
[
layers.Dense(units=hp.Int("units", 32, 128, 32), activation="relu"),
layers.Dense(units=1),
]
)
model.compile(
optimizer="adam", loss="mean_squared_error",
)
return model
def fit(self, hp, model, x, y, validation_data, **kwargs):
model.fit(x, y, **kwargs)
x_val, y_val = validation_data
y_pred = model.predict(x_val)
# Return a dictionary of metrics for KerasTuner to track.
return {
"metric_a": -np.mean(np.abs(y_pred - y_val)),
"metric_b": np.mean(np.square(y_pred - y_val)),
}
tuner = kt.RandomSearch(
hypermodel=HyperRegressor(),
# Objective is one of the keys.
# Maximize the negative MAE, equivalent to minimize MAE.
objective=kt.Objective("metric_a", "max"),
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="custom_eval_dict",
)
tuner.search(
x=np.random.rand(100, 10),
y=np.random.rand(100, 1),
validation_data=(np.random.rand(20, 10), np.random.rand(20, 1)),
)
tuner.results_summary()
###Output
_____no_output_____
###Markdown
Tune end-to-end workflowsIn some cases, it is hard to align your code into build and fit functions. Youcan also keep your end-to-end workflow in one place by overriding`Tuner.run_trial()`, which gives you full control of a trial. You can see itas a black-box optimizer for anything. Tune any functionFor example, you can find a value of `x`, which minimizes `f(x)=x*x+1`. In thefollowing code, we just define `x` as a hyperparameter, and return `f(x)` asthe objective value. The `hypermodel` and `objective` argument for initializingthe tuner can be omitted.
###Code
class MyTuner(kt.RandomSearch):
def run_trial(self, trial, *args, **kwargs):
# Get the hp from trial.
hp = trial.hyperparameters
# Define "x" as a hyperparameter.
x = hp.Float("x", min_value=-1.0, max_value=1.0)
# Return the objective value to minimize.
return x * x + 1
tuner = MyTuner(
# No hypermodel or objective specified.
max_trials=20,
overwrite=True,
directory="my_dir",
project_name="tune_anything",
)
# No need to pass anything to search()
# unless you use them in run_trial().
tuner.search()
print(tuner.get_best_hyperparameters()[0].get("x"))
###Output
_____no_output_____
###Markdown
Keep Keras code separateYou can keep all your Keras code unchanged and use KerasTuner to tune it. Itis useful if you cannot modify the Keras code for some reason.It also gives you more flexibility. You don't have to separate the modelbuilding and training code apart. However, this workflow would not help yousave the model or connect with the TensorBoard plugins.To save the model, you can use `trial.trial_id`, which is a string to uniquelyidentify a trial, to construct different paths to save the models fromdifferent trials.
###Code
import os
def keras_code(units, optimizer, saving_path):
# Build model
model = keras.Sequential(
[layers.Dense(units=units, activation="relu"), layers.Dense(units=1),]
)
model.compile(
optimizer=optimizer, loss="mean_squared_error",
)
# Prepare data
x_train = np.random.rand(100, 10)
y_train = np.random.rand(100, 1)
x_val = np.random.rand(20, 10)
y_val = np.random.rand(20, 1)
# Train & eval model
model.fit(x_train, y_train)
# Save model
model.save(saving_path)
# Return a single float as the objective value.
# You may also return a dictionary
# of {metric_name: metric_value}.
y_pred = model.predict(x_val)
return np.mean(np.abs(y_pred - y_val))
class MyTuner(kt.RandomSearch):
def run_trial(self, trial, **kwargs):
hp = trial.hyperparameters
return keras_code(
units=hp.Int("units", 32, 128, 32),
optimizer=hp.Choice("optimizer", ["adam", "adadelta"]),
saving_path=os.path.join("/tmp", trial.trial_id),
)
tuner = MyTuner(
max_trials=3, overwrite=True, directory="my_dir", project_name="keep_code_separate",
)
tuner.search()
# Retraining the model
best_hp = tuner.get_best_hyperparameters()[0]
keras_code(**best_hp.values, saving_path="/tmp/best_model")
###Output
_____no_output_____
###Markdown
KerasTuner includes pre-made tunable applications: HyperResNet and HyperXceptionThese are ready-to-use hypermodels for computer vision.They come pre-compiled with `loss="categorical_crossentropy"` and`metrics=["accuracy"]`.
###Code
from keras_tuner.applications import HyperResNet
hypermodel = HyperResNet(input_shape=(28, 28, 1), classes=10)
tuner = kt.RandomSearch(
hypermodel,
objective="val_accuracy",
max_trials=2,
overwrite=True,
directory="my_dir",
project_name="built_in_hypermodel",
)
tuner.search(
x_train[:100], y_train[:100], epochs=1, validation_data=(x_val[:100], y_val[:100])
)
###Output
_____no_output_____
###Markdown
Getting started with KerasTuner**Authors:** Luca Invernizzi, James Long, Francois Chollet, Tom O'Malley, Haifeng Jin**Date created:** 2019/05/31**Last modified:** 2021/06/07**Description:** The basics of using KerasTuner to tune Keras model's hyperparameters. Setup
###Code
!pip install keras-tuner -q
###Output
_____no_output_____
###Markdown
IntroductionHere's how to perform hyperparameter tuning for a single-layer dense neuralnetwork using random search.First, we need to prepare the dataset -- let's use MNIST dataset as an example.
###Code
from tensorflow import keras
import numpy as np
(x, y), (x_test, y_test) = keras.datasets.mnist.load_data()
x_train = x[:-10000]
x_val = x[-10000:]
y_train = y[:-10000]
y_val = y[-10000:]
x_train = np.expand_dims(x_train, -1).astype("float32") / 255.0
x_val = np.expand_dims(x_val, -1).astype("float32") / 255.0
x_test = np.expand_dims(x_test, -1).astype("float32") / 255.0
num_classes = 10
y_train = keras.utils.to_categorical(y_train, num_classes)
y_val = keras.utils.to_categorical(y_val, num_classes)
y_test = keras.utils.to_categorical(y_test, num_classes)
###Output
_____no_output_____
###Markdown
Prepare a model-building functionThen, we define a model-building function. It takes an argument `hp` fromwhich you can sample hyperparameters, such as`hp.Int('units', min_value=32, max_value=512, step=32)`(an integer from a certain range).This function returns a compiled model.
###Code
from tensorflow.keras import layers
from kerastuner import RandomSearch
def build_model(hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer=keras.optimizers.Adam(
hp.Choice("learning_rate", values=[1e-2, 1e-3, 1e-4])
),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
###Output
_____no_output_____
###Markdown
Start the searchNext, let's instantiate a tuner. You should specify the model-building function, thename of the objective to optimize (whether to minimize or maximize isautomatically inferred for built-in metrics), the total number of trials(`max_trials`) to test, and the number of models that should be built and fitfor each trial (`executions_per_trial`).We use the `overwrite` argument to control whether to overwrite the previousresults in the same directory or resume the previous search instead. Here weset `overwrite=True` to start a new search and ignore any previous results.Available tuners are `RandomSearch`, `BayesianOptimization` and `Hyperband`.**Note:** the purpose of having multiple executions per trial is to reduceresults variance and therefore be able to more accurately assess theperformance of a model. If you want to get results faster, you could set`executions_per_trial=1` (single round of training for each modelconfiguration).
###Code
tuner = RandomSearch(
build_model,
objective="val_accuracy",
max_trials=3,
executions_per_trial=2,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
###Output
_____no_output_____
###Markdown
You can print a summary of the search space:
###Code
tuner.search_space_summary()
###Output
_____no_output_____
###Markdown
Then, start the search for the best hyperparameter configuration.The call to `search` has the same signature as `model.fit()`.
###Code
tuner.search(x_train, y_train, epochs=2, validation_data=(x_val, y_val))
###Output
_____no_output_____
###Markdown
Here's what happens in `search`: models are built iteratively by calling themodel-building function, which populates the hyperparameter space (searchspace) tracked by the `hp` object. The tuner progressively explores the space,recording metrics for each configuration. Query the resultsWhen search is over, you can retrieve the best model(s):
###Code
models = tuner.get_best_models(num_models=2)
###Output
_____no_output_____
###Markdown
Or print a summary of the results:
###Code
tuner.results_summary()
###Output
_____no_output_____
###Markdown
You will also find detailed logs, checkpoints, etc, in the folder `my_dir/helloworld`, i.e. `directory/project_name`. The search space may contain conditional hyperparametersBelow, we have a `for` loop creating a tunable number of layers,which themselves involve a tunable `units` parameter.This can be pushed to any level of parameter interdependency, including recursion.Note that all parameter names should be unique (here, in the loop over `i`,we name the inner parameters `'units_' + str(i)`).
###Code
def build_model(hp):
model = keras.Sequential()
model.add(layers.Flatten())
for i in range(hp.Int("num_layers", 2, 20)):
model.add(
layers.Dense(
units=hp.Int("units_" + str(i), min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer=keras.optimizers.Adam(hp.Choice("learning_rate", [1e-2, 1e-3, 1e-4])),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
###Output
_____no_output_____
###Markdown
You can use a HyperModel subclass instead of a model-building functionThis makes it easy to share and reuse hypermodels.A `HyperModel` subclass only needs to implement a `build(self, hp)` method.
###Code
from kerastuner import HyperModel
class MyHyperModel(HyperModel):
def __init__(self, classes):
self.classes = classes
def build(self, hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(self.classes, activation="softmax"))
model.compile(
optimizer=keras.optimizers.Adam(
hp.Choice("learning_rate", values=[1e-2, 1e-3, 1e-4])
),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
hypermodel = MyHyperModel(classes=10)
tuner = RandomSearch(
hypermodel,
objective="val_accuracy",
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
tuner.search(x_train, y_train, epochs=2, validation_data=(x_val, y_val))
###Output
_____no_output_____
###Markdown
KerasTuner includes pre-made tunable applications: HyperResNet and HyperXceptionThese are ready-to-use hypermodels for computer vision.They come pre-compiled with `loss="categorical_crossentropy"` and `metrics=["accuracy"]`.
###Code
from kerastuner.applications import HyperResNet
hypermodel = HyperResNet(input_shape=(28, 28, 1), classes=10)
tuner = RandomSearch(
hypermodel,
objective="val_accuracy",
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
tuner.search(
x_train[:100], y_train[:100], epochs=1, validation_data=(x_val[:100], y_val[:100])
)
###Output
_____no_output_____
###Markdown
You can easily restrict the search space to just a few parametersIf you have an existing hypermodel, and you want to search over only a few parameters(such as the learning rate), you can do so by passing a `hyperparameters` argumentto the tuner constructor, as well as `tune_new_entries=False` to specify that parametersthat you didn't list in `hyperparameters` should not be tuned. For these parameters, the defaultvalue gets used.
###Code
from kerastuner import HyperParameters
from kerastuner.applications import HyperXception
hypermodel = HyperXception(input_shape=(28, 28, 1), classes=10)
hp = HyperParameters()
# This will override the `learning_rate` parameter with your
# own selection of choices
hp.Choice("learning_rate", values=[1e-2, 1e-3, 1e-4])
tuner = RandomSearch(
hypermodel,
hyperparameters=hp,
# `tune_new_entries=False` prevents unlisted parameters from being tuned
tune_new_entries=False,
objective="val_accuracy",
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
tuner.search(
x_train[:100], y_train[:100], epochs=1, validation_data=(x_val[:100], y_val[:100])
)
###Output
_____no_output_____
###Markdown
About parameter default valuesWhenever you register a hyperparameter inside a model-building function or the `build` method of a hypermodel,you can specify a default value:```pythonhp.Int("units", min_value=32, max_value=512, step=32, default=128)```If you don't, hyperparameters always have a default default (for `Int`, it is equal to `min_value`). Fixing values in a hypermodelWhat if you want to do the reverse -- tune all available parameters in a hypermodel, **except** one (the learning rate)?Pass a `hyperparameters` argument with a `Fixed` entry (or any number of `Fixed` entries), and specify `tune_new_entries=True`.
###Code
hypermodel = HyperXception(input_shape=(28, 28, 1), classes=10)
hp = HyperParameters()
hp.Fixed("learning_rate", value=1e-4)
tuner = RandomSearch(
hypermodel,
hyperparameters=hp,
tune_new_entries=True,
objective="val_accuracy",
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
tuner.search(
x_train[:100], y_train[:100], epochs=1, validation_data=(x_val[:100], y_val[:100])
)
###Output
_____no_output_____
###Markdown
Overriding compilation argumentsIf you have a hypermodel for which you want to change the existing optimizer,loss, or metrics, you can do so by passing these argumentsto the tuner constructor:
###Code
hypermodel = HyperXception(input_shape=(28, 28, 1), classes=10)
tuner = RandomSearch(
hypermodel,
optimizer=keras.optimizers.Adam(1e-3),
loss="mse",
metrics=[
keras.metrics.Precision(name="precision"),
keras.metrics.Recall(name="recall"),
],
objective="val_loss",
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
tuner.search(
x_train[:100], y_train[:100], epochs=1, validation_data=(x_val[:100], y_val[:100])
)
###Output
_____no_output_____
###Markdown
Getting started with KerasTuner**Authors:** Luca Invernizzi, James Long, Francois Chollet, Tom O'Malley, Haifeng Jin**Date created:** 2019/05/31**Last modified:** 2021/06/07**Description:** The basics of using KerasTuner to tune model hyperparameters. Setup
###Code
!pip install keras-tuner -q
###Output
_____no_output_____
###Markdown
IntroductionHere's how to perform hyperparameter tuning for a single-layer dense neuralnetwork using random search.First, we need to prepare the dataset -- let's use MNIST dataset as an example.
###Code
from tensorflow import keras
import numpy as np
(x, y), (x_test, y_test) = keras.datasets.mnist.load_data()
x_train = x[:-10000]
x_val = x[-10000:]
y_train = y[:-10000]
y_val = y[-10000:]
x_train = np.expand_dims(x_train, -1).astype("float32") / 255.0
x_val = np.expand_dims(x_val, -1).astype("float32") / 255.0
x_test = np.expand_dims(x_test, -1).astype("float32") / 255.0
num_classes = 10
y_train = keras.utils.to_categorical(y_train, num_classes)
y_val = keras.utils.to_categorical(y_val, num_classes)
y_test = keras.utils.to_categorical(y_test, num_classes)
###Output
_____no_output_____
###Markdown
Prepare a model-building functionThen, we define a model-building function. It takes an argument `hp` fromwhich you can sample hyperparameters, such as`hp.Int('units', min_value=32, max_value=512, step=32)`(an integer from a certain range).This function returns a compiled model.
###Code
from tensorflow.keras import layers
from keras_tuner import RandomSearch
def build_model(hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer=keras.optimizers.Adam(
hp.Choice("learning_rate", values=[1e-2, 1e-3, 1e-4])
),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
###Output
_____no_output_____
###Markdown
Start the searchNext, let's instantiate a tuner. You should specify the model-building function, thename of the objective to optimize (whether to minimize or maximize isautomatically inferred for built-in metrics), the total number of trials(`max_trials`) to test, and the number of models that should be built and fitfor each trial (`executions_per_trial`).We use the `overwrite` argument to control whether to overwrite the previousresults in the same directory or resume the previous search instead. Here weset `overwrite=True` to start a new search and ignore any previous results.Available tuners are `RandomSearch`, `BayesianOptimization` and `Hyperband`.**Note:** the purpose of having multiple executions per trial is to reduceresults variance and therefore be able to more accurately assess theperformance of a model. If you want to get results faster, you could set`executions_per_trial=1` (single round of training for each modelconfiguration).
###Code
tuner = RandomSearch(
build_model,
objective="val_accuracy",
max_trials=3,
executions_per_trial=2,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
###Output
_____no_output_____
###Markdown
You can print a summary of the search space:
###Code
tuner.search_space_summary()
###Output
_____no_output_____
###Markdown
Then, start the search for the best hyperparameter configuration.The call to `search` has the same signature as `model.fit()`.
###Code
tuner.search(x_train, y_train, epochs=2, validation_data=(x_val, y_val))
###Output
_____no_output_____
###Markdown
Here's what happens in `search`: models are built iteratively by calling themodel-building function, which populates the hyperparameter space (searchspace) tracked by the `hp` object. The tuner progressively explores the space,recording metrics for each configuration. Query the resultsWhen search is over, you can retrieve the best model(s):
###Code
models = tuner.get_best_models(num_models=2)
###Output
_____no_output_____
###Markdown
Or print a summary of the results:
###Code
tuner.results_summary()
###Output
_____no_output_____
###Markdown
You will also find detailed logs, checkpoints, etc, in the folder `my_dir/helloworld`, i.e. `directory/project_name`. The search space may contain conditional hyperparametersBelow, we have a `for` loop creating a tunable number of layers,which themselves involve a tunable `units` parameter.This can be pushed to any level of parameter interdependency, including recursion.Note that all parameter names should be unique (here, in the loop over `i`,we name the inner parameters `'units_' + str(i)`).
###Code
def build_model(hp):
model = keras.Sequential()
model.add(layers.Flatten())
for i in range(hp.Int("num_layers", 2, 20)):
model.add(
layers.Dense(
units=hp.Int("units_" + str(i), min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(10, activation="softmax"))
model.compile(
optimizer=keras.optimizers.Adam(hp.Choice("learning_rate", [1e-2, 1e-3, 1e-4])),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
###Output
_____no_output_____
###Markdown
You can use a HyperModel subclass instead of a model-building functionThis makes it easy to share and reuse hypermodels.A `HyperModel` subclass only needs to implement a `build(self, hp)` method.
###Code
from keras_tuner import HyperModel
class MyHyperModel(HyperModel):
def __init__(self, classes):
self.classes = classes
def build(self, hp):
model = keras.Sequential()
model.add(layers.Flatten())
model.add(
layers.Dense(
units=hp.Int("units", min_value=32, max_value=512, step=32),
activation="relu",
)
)
model.add(layers.Dense(self.classes, activation="softmax"))
model.compile(
optimizer=keras.optimizers.Adam(
hp.Choice("learning_rate", values=[1e-2, 1e-3, 1e-4])
),
loss="categorical_crossentropy",
metrics=["accuracy"],
)
return model
hypermodel = MyHyperModel(classes=10)
tuner = RandomSearch(
hypermodel,
objective="val_accuracy",
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
tuner.search(x_train, y_train, epochs=2, validation_data=(x_val, y_val))
###Output
_____no_output_____
###Markdown
KerasTuner includes pre-made tunable applications: HyperResNet and HyperXceptionThese are ready-to-use hypermodels for computer vision.They come pre-compiled with `loss="categorical_crossentropy"` and `metrics=["accuracy"]`.
###Code
from keras_tuner.applications import HyperResNet
hypermodel = HyperResNet(input_shape=(28, 28, 1), classes=10)
tuner = RandomSearch(
hypermodel,
objective="val_accuracy",
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
tuner.search(
x_train[:100], y_train[:100], epochs=1, validation_data=(x_val[:100], y_val[:100])
)
###Output
_____no_output_____
###Markdown
You can easily restrict the search space to just a few parametersIf you have an existing hypermodel, and you want to search over only a few parameters(such as the learning rate), you can do so by passing a `hyperparameters` argumentto the tuner constructor, as well as `tune_new_entries=False` to specify that parametersthat you didn't list in `hyperparameters` should not be tuned. For these parameters, the defaultvalue gets used.
###Code
from keras_tuner import HyperParameters
from keras_tuner.applications import HyperXception
hypermodel = HyperXception(input_shape=(28, 28, 1), classes=10)
hp = HyperParameters()
# This will override the `learning_rate` parameter with your
# own selection of choices
hp.Choice("learning_rate", values=[1e-2, 1e-3, 1e-4])
tuner = RandomSearch(
hypermodel,
hyperparameters=hp,
# `tune_new_entries=False` prevents unlisted parameters from being tuned
tune_new_entries=False,
objective="val_accuracy",
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
tuner.search(
x_train[:100], y_train[:100], epochs=1, validation_data=(x_val[:100], y_val[:100])
)
###Output
_____no_output_____
###Markdown
About parameter default valuesWhenever you register a hyperparameter inside a model-building function or the `build` method of a hypermodel,you can specify a default value:```pythonhp.Int("units", min_value=32, max_value=512, step=32, default=128)```If you don't, hyperparameters always have a default default (for `Int`, it is equal to `min_value`). Fixing values in a hypermodelWhat if you want to do the reverse -- tune all available parameters in a hypermodel, **except** one (the learning rate)?Pass a `hyperparameters` argument with a `Fixed` entry (or any number of `Fixed` entries), and specify `tune_new_entries=True`.
###Code
hypermodel = HyperXception(input_shape=(28, 28, 1), classes=10)
hp = HyperParameters()
hp.Fixed("learning_rate", value=1e-4)
tuner = RandomSearch(
hypermodel,
hyperparameters=hp,
tune_new_entries=True,
objective="val_accuracy",
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
tuner.search(
x_train[:100], y_train[:100], epochs=1, validation_data=(x_val[:100], y_val[:100])
)
###Output
_____no_output_____
###Markdown
Overriding compilation argumentsIf you have a hypermodel for which you want to change the existing optimizer,loss, or metrics, you can do so by passing these argumentsto the tuner constructor:
###Code
hypermodel = HyperXception(input_shape=(28, 28, 1), classes=10)
tuner = RandomSearch(
hypermodel,
optimizer=keras.optimizers.Adam(1e-3),
loss="mse",
metrics=[
keras.metrics.Precision(name="precision"),
keras.metrics.Recall(name="recall"),
],
objective="val_loss",
max_trials=3,
overwrite=True,
directory="my_dir",
project_name="helloworld",
)
tuner.search(
x_train[:100], y_train[:100], epochs=1, validation_data=(x_val[:100], y_val[:100])
)
###Output
_____no_output_____ |
tutorials/W3D4_ReinforcementLearning/W3D4_Tutorial2.ipynb | ###Markdown
Neuromatch Academy: Week 3, Day 4, Tutorial 2 Learning to Act: Multi-Armed Bandits__Content creators:__ Marcelo Mattar and Eric DeWitt with help from Byron Galbraith__Content reviewers:__ Matt Krause and Michael Waskom --- Tutorial Objectives In this tutorial you will use 'bandits' to understand the fundementals of how a policy interacts with the learning algorithm in reinforcement learning. * You will understand the fundemental tradeoff between exploration and exploitation in a policy.* You will understand how the learning rate interacts with exploration to find the best available action. --- Setup
###Code
# Imports
import numpy as np
import matplotlib.pyplot as plt
#@title Figure settings
import ipywidgets as widgets # interactive display
%config InlineBackend.figure_format = 'retina'
plt.style.use("https://raw.githubusercontent.com/NeuromatchAcademy/course-content/master/nma.mplstyle")
#@title Helper functions
np.set_printoptions(precision=3)
def plot_choices(q, epsilon, choice_fn, n_steps=1000, rng_seed=1):
np.random.seed(rng_seed)
counts = np.zeros_like(q)
for t in range(n_steps):
action = choice_fn(q, epsilon)
counts[action] += 1
fig, ax = plt.subplots()
ax.bar(range(len(q)), counts/n_steps)
ax.set(ylabel='% chosen', xlabel='action', ylim=(0,1), xticks=range(len(q)))
def plot_multi_armed_bandit_results(results):
fig, (ax1, ax2, ax3) = plt.subplots(ncols=3, figsize=(20, 4))
ax1.plot(results['rewards'])
ax1.set(title=f"Total Reward: {np.sum(results['rewards']):.2f}",
xlabel='step', ylabel='reward')
ax2.plot(results['qs'])
ax2.set(xlabel='step', ylabel='value')
ax2.legend(range(len(results['mu'])))
ax3.plot(results['mu'], label='latent')
ax3.plot(results['qs'][-1], label='learned')
ax3.set(xlabel='action', ylabel='value')
ax3.legend()
def plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal):
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(16, 6))
ax1.plot(np.mean(trial_rewards, axis=1).T)
ax1.set(title=f'Average Reward ({fixed})', xlabel='step', ylabel='reward')
ax1.legend(labels)
ax2.plot(np.mean(trial_optimal, axis=1).T)
ax2.set(title=f'Performance ({fixed})', xlabel='step', ylabel='% optimal')
ax2.legend(labels)
###Output
_____no_output_____
###Markdown
--- Section 1: Multi-Armed Bandits
###Code
#@title Video 1: Multi-Armed Bandits
# Insert the ID of the corresponding youtube video
from IPython.display import YouTubeVideo
video = YouTubeVideo(id="kdiXr1zsfo0", width=854, height=480, fs=1)
print("Video available at https://youtu.be/" + video.id)
video
###Output
_____no_output_____
###Markdown
Consider the following learning problem. You are faced repeatedly with a choice among $k$ different options, or actions. After each choice you receive a reward signal in the form of a numerical value, where the larger value is the better. Your objective is to maximize the expected total reward over some time period, for example, over 1000 action selections, or time steps.This is the original form of the k-armed bandit problem. This name derives from the colloquial name for a slot machine, the "one-armed bandit", because it has the one lever to pull, and it is often rigged to take more money than it pays out over time. The multi-armed bandit extension is to imagine, for instance, that you are faced with multiple slot machines that you can play, but only one at a time. Which machine should you play, i.e. which arm should you pull, which action should you take, at any given time to maximize your total payout.While there are many different levels of sophistication and assumptions in how the rewards are determined, for simplicity's sake we will assume that each action results in a reward drawn from a fixed Gaussian distribution with unknown mean and unit variance. This problem setting is referred to as the *environment*, and goal is to find the arm with the highest mean value.We will solve this *optimization problem* with an *agent*, in this case an algorithm that takes in rewards and returns actions. --- Section 2: Choosing an Action The first thing our agent needs to be able to do is choose which arm to pull. The strategy for choosing actions based on our expectations is called a *policy* (often denoted $\pi$). We could have a random policy -- just pick an arm at random each time -- though this doesn't seem likely to be capable of optimizing our reward. We want some intentionality, and to do that we need a way of describing our beliefs about the arms' reward potential. We do this with an action-value function\begin{align}q(a) = \mathbb{E} [r_{t} | a_{t} = a]\end{align}where the value $q$ for taking action $a \in A$ at time $t$ is equal to the expected value of the reward $r_t$ given that we took action $a$ at that time. In practice, this is often represented as an array of values, where each action's value is a different element in the array.Great, now that we have a way to describe our beliefs about the values each action should return, let's come up with a policy.An obvious choice would be to take the action with the highest expected value. This is referred to as the *greedy* policy\begin{align}a_{t} = \text{argmax}_{a} \; q_{t} (a)\end{align}where our choice action is the one that maximizes the current value function.So far so good, but it can't be this easy. And, in fact, the greedy policy does have a fatal flaw: it easily gets trapped in local maxima. It never explores to see what it hasn't seen before if one option is already better than the others. This leads us to a fundamental challenge in coming up with effective polices. Section 2.1: The Exploitation-Exploration DilemmaIf we never try anything new, if we always stick to the safe bet, we don't know what we are missing. Sometimes we aren't missing much of anything, and regret not sticking with our preferred choice, yet other times we stumble upon something new that was way better than we thought.This is the exploitation-exploration dilemma: do you go with you best choice now, or risk the less certain option with the hope of finding something better. Too much exploration, however, means you may end up with a sub-optimal reward once it's time to stop.In order to avoid getting stuck in local minima while also maximizing reward, effective policies need some way to balance between these two aims.A simple extension to our greedy policy is to add some randomness. For instance, a coin flip -- heads we take the best choice now, tails we pick one at random. This is referred to as the $\epsilon$-greedy policy:\begin{align}P (a_{t} = a) = \begin{cases} 1 - \epsilon + \epsilon/N & \quad \text{if } a_{t} = \text{argmax}_{a} \; q_{t} (a) \\ \epsilon/N & \quad \text{else} \end{cases} \end{align}which is to say that with probability 1 - $\epsilon$ for $\epsilon \in [0,1]$ we select the greedy choice, and otherwise we select an action at random (including the greedy option).Despite its relative simplicity, the epsilon-greedy policy is quite effective, which leads to its general popularity. Exercise 1: Implement Epsilon-GreedyIn this exercise you will implement the epsilon-greedy algorithm for deciding which action to take from a set of possible actions given their value function and a probability $\epsilon$ of simply chosing one at random. TIP: You may find [`np.random.random`](https://numpy.org/doc/stable/reference/random/generated/numpy.random.random.html), [`np.random.choice`](https://numpy.org/doc/stable/reference/random/generated/numpy.random.choice.html), and [`np.argmax`](https://numpy.org/doc/stable/reference/generated/numpy.argmax.html) useful here.
###Code
def epsilon_greedy(q, epsilon):
"""Epsilon-greedy policy: selects the maximum value action with probabilty
(1-epsilon) and selects randomly with epsilon probability.
Args:
q (ndarray): an array of action values
epsilon (float): probability of selecting an action randomly
Returns:
int: the chosen action
"""
#####################################################################
## TODO for students: implement the epsilon greedy decision algorithm
# Fill out function and remove
raise NotImplementedError("Student excercise: implement the epsilon greedy decision algorithm")
#####################################################################
# write a boolean expression that determines if we should take the best action
be_greedy = ...
if be_greedy:
# write an expression for selecting the best action from the action values
action = ...
else:
# write an expression for selecting a random action
action = ...
return action
# Uncomment once the epsilon_greedy function is complete
# q = [-2, 5, 0, 1]
# epsilon = 0.1
# plot_choices(q, epsilon, epsilon_greedy)
# to_remove solution
def epsilon_greedy(q, epsilon):
"""Epsilon-greedy policy: selects the maximum value action with probabilty
(1-epsilon) and selects randomly with epsilon probability.
Args:
q (ndarray): an array of action values
epsilon (float): probability of selecting an action randomly
Returns:
int: the chosen action
"""
# write a boolean expression that determines if we should take the best action
be_greedy = np.random.random() > epsilon
if be_greedy:
# write an expression for selecting the best action from the action values
action = np.argmax(q)
else:
# write an expression for selecting a random action
action = np.random.choice(len(q))
return action
q = [-2, 5, 0, 1]
epsilon = 0.1
with plt.xkcd():
plot_choices(q, epsilon, epsilon_greedy)
###Output
_____no_output_____
###Markdown
This is what we should expect, that the action with the largest value (action 1) is selected about (1-$\epsilon$) of the time, or 90% for $\epsilon = 0.1$, and the remaining 10% is split evenly amongst the other options. Use the demo below to explore how changing $\epsilon$ affects the distribution of selected actions. Interactive Demo: Changing EpsilonEpsilon is our one parameter for balancing exploitation and exploration. Given a set of values $q = [-2, 5, 0, 1]$, use the widget below to see how changing $\epsilon$ influences our selection of the max value 5 (action = 1) vs the others. At the extremes of its range (0 and 1), the $\epsilon$-greedy policy reproduces two other policies. What are they?
###Code
#@title
#@markdown Make sure you execute this cell to enable the widget!
@widgets.interact(epsilon=widgets.FloatSlider(0.1, min=0.0, max=1.0))
def explore_epilson_values(epsilon=0.1):
q = [-2, 5, 0, 1]
plot_choices(q, epsilon, epsilon_greedy, rng_seed=None)
#to_remove explanation
"""
When epsilon is zero, the agent always chooses the currently best option; it
becomes greedy. When epsilon is 1, the agent chooses randomly.
""";
###Output
_____no_output_____
###Markdown
--- Section 3: Learning from RewardsNow that we have a policy for deciding what to do, how do we learn from our actions?One way to do this is just keep a record of every result we ever got and use the averages for each action. If we have a potentially very long running episode, the computational cost of keeping all these values and recomputing the mean over and over again isn't ideal. Instead we can use a streaming mean calculation, which looks like this:\begin{align}q_{t+1}(a) \leftarrow q_{t}(a) + \frac{1}{n_t} (r_{t} - q_{t}(a))\end{align}where our action-value function $q_t(a)$ is the mean of the rewards seen so far, $n_t$ is the number of actions taken by time $t$, and $r_t$ is the reward just received for taking action $a$.This still requires us to remember how many actions we've taken, so let's generalize this a bit further and replace the action total with a general parameter $\alpha$, which we will call the learning rate\begin{align}q_{t+1}(a) \leftarrow q_{t}(a) + \alpha (r_{t} - q_{t}(a)).\end{align} Exercise 2: Updating Action ValuesIn this exercise you will implement the action-value update rule above. The function will take in the action-value function represented as an array `q`, the action taken, the reward received, and the learning rate, `alpha`. The function will return the updated value for the selection action.
###Code
def update_action_value(q, action, reward, alpha):
""" Compute the updated action value given the learning rate and observed
reward.
Args:
q (ndarray): an array of action values
action (int): the action taken
reward (float): the reward received for taking the action
alpha (float): the learning rate
Returns:
float: the updated value for the selected action
"""
#####################################################
## TODO for students: compute the action value update
# Fill out function and remove
raise NotImplementedError("Student excercise: compute the action value update")
#####################################################
# write an expression for the updated action value
value = ...
return value
# Uncomment once the update_action_value function is complete
# q = [-2, 5, 0, 1]
# action = 2
# print(f"Original q({action}) value = {q[action]}")
# q[action] = update_action_value(q, 2, 10, 0.01)
# print(f"Updated q({action}) value = {q[action]}")
# to_remove solution
def update_action_value(q, action, reward, alpha):
""" Compute the updated action value given the learning rate and observed
reward.
Args:
q (ndarray): an array of action values
action (int): the action taken
reward (float): the reward received for taking the action
alpha (float): the learning rate
Returns:
float: the updated value for the selected action
"""
# write an expression for the updated action value
value = q[action] + alpha * (reward - q[action])
return value
q = [-2, 5, 0, 1]
action = 2
print(f"Original q({action}) value = {q[action]}")
q[action] = update_action_value(q, 2, 10, 0.01)
print(f"Updated q({action}) value = {q[action]}")
###Output
_____no_output_____
###Markdown
--- Section 4: Solving Multi-Armed BanditsNow that we have both a policy and a learning rule, we can combine these to solve our original multi-armed bandit task. Recall that we have some number of arms that give rewards drawn from Gaussian distributions with unknown mean and unit variance, and our goal is to find the arm with the highest mean.First, let's see how we will simulate this environment by reading through the annotated code below.
###Code
def multi_armed_bandit(n_arms, epsilon, alpha, n_steps):
""" A Gaussian multi-armed bandit using an epsilon-greedy policy. For each
action, rewards are randomly sampled from normal distribution, with a mean
associated with that arm and unit variance.
Args:
n_arms (int): number of arms or actions
epsilon (float): probability of selecting an action randomly
alpha (float): the learning rate
n_steps (int): number of steps to evaluate
Returns:
dict: a dictionary containing the action values, actions, and rewards from
the evaluation along with the true arm parameters mu and the optimality of
the chosen actions.
"""
# Gaussian bandit parameters
mu = np.random.normal(size=n_arms)
# evaluation and reporting state
q = np.zeros(n_arms)
qs = np.zeros((n_steps, n_arms))
rewards = np.zeros(n_steps)
actions = np.zeros(n_steps)
optimal = np.zeros(n_steps)
# run the bandit
for t in range(n_steps):
# choose an action
action = epsilon_greedy(q, epsilon)
actions[t] = action
# copmute rewards for all actions
all_rewards = np.random.normal(mu)
# observe the reward for the chosen action
reward = all_rewards[action]
rewards[t] = reward
# was it the best possible choice?
optimal_action = np.argmax(all_rewards)
optimal[t] = action == optimal_action
# update the action value
q[action] = update_action_value(q, action, reward, alpha)
qs[t] = q
results = {
'qs': qs,
'actions': actions,
'rewards': rewards,
'mu': mu,
'optimal': optimal
}
return results
###Output
_____no_output_____
###Markdown
We can use our multi-armed bandit method to evaluate how our epsilon-greedy policy and learning rule perform at solving the task. First we will set our environment to have 10 arms and our agent parameters to $\epsilon=0.1$ and $\alpha=0.01$. In order to get a good sense of the agent's performance, we will run the episode for 1000 steps.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
n_arms = 10
epsilon = 0.1
alpha = 0.01
n_steps = 1000
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(16, 6))
ax1.plot(results['rewards'])
ax1.set(title=f'Observed Reward ($\epsilon$={epsilon}, $\\alpha$={alpha})',
xlabel='step', ylabel='reward')
ax2.plot(results['qs'])
ax2.set(title=f'Action Values ($\epsilon$={epsilon}, $\\alpha$={alpha})',
xlabel='step', ylabel='value')
ax2.legend(range(n_arms));
###Output
_____no_output_____
###Markdown
Alright, we got some rewards that are kind of all over the place, but the agent seemed to settle in on the first arm as the preferred choice of action relatively quickly. Let's see how well we did at recovering the true means of the Gaussian random variables behind the arms.
###Code
fig, ax = plt.subplots()
ax.plot(results['mu'], label='latent')
ax.plot(results['qs'][-1], label='learned')
ax.set(title=f'$\epsilon$={epsilon}, $\\alpha$={alpha}',
xlabel='action', ylabel='value')
ax.legend();
###Output
_____no_output_____
###Markdown
Well, we seem to have found a very good estimate for action 0, but most of the others are not great. In fact, we can see the effect of the local maxima trap at work -- the greedy part of our algorithm locked onto action 0, which is actually the 2nd best choice to action 6. Since these are the means of Gaussian random variables, we can see that the overlap between the two would be quite high, so even if we did explore action 6, we may draw a sample that is still lower than our estimate for action 0.However, this was just one choice of parameters. Perhaps there is a better combination? Interactive Demo: Changing Epsilon and AlphaUse the widget below to explore how varying the values of $\epsilon$ (exploitation-exploration tradeoff), $\alpha$ (learning rate), and even the number of actions $k$, changes the behavior of our agent.
###Code
#@title
#@markdown Make sure you execute this cell to enable the widget!
@widgets.interact_manual(k=widgets.IntSlider(10, min=2, max=15),
epsilon=widgets.FloatSlider(0.1, min=0.0, max=1.0),
alpha=widgets.FloatLogSlider(0.01, min=-3, max=0))
def explore_bandit_parameters(k=10, epsilon=0.1, alpha=0.001):
results = multi_armed_bandit(k, epsilon, alpha, 1000)
plot_multi_armed_bandit_results(results)
###Output
_____no_output_____
###Markdown
While we can see how changing the epsilon and alpha values impact the agent's behavior, this doesn't give as a great sense of which combination is optimal. Due to the stochastic nature of both our rewards and our policy, a single trial run isn't sufficient to give us this information. Let's run mulitple trials and compare the average performance.First we will look at differet values for $\epsilon \in [0.0, 0.1, 0.2]$ to a fixed $\alpha=0.1$. We will run 200 trials as a nice balance between speed and accuracy.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
epsilons = [0.0, 0.1, 0.2]
alpha = 0.1
n_trials = 200
trial_rewards = np.zeros((len(epsilons), n_trials, n_steps))
trial_optimal = np.zeros((len(epsilons), n_trials, n_steps))
for i, epsilon in enumerate(epsilons):
for n in range(n_trials):
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
trial_rewards[i, n] = results['rewards']
trial_optimal[i, n] = results['optimal']
labels = [f'$\epsilon$={e}' for e in epsilons]
fixed = f'$\\alpha$={alpha}'
plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal)
###Output
_____no_output_____
###Markdown
On the left we have plotted the average reward over time, and we see that while $\epsilon=0$ (the greedy policy) does well initially, $\epsilon=0.1$ starts to do slightly better in the long run, while $\epsilon=0.2$ does the worst. Looking on the right, we see the percentage of times the optimal action (the best possible choice at time $t$) was taken, and here again we see a similar pattern of $\epsilon=0.1$ starting out a bit slower but eventually having a slight edge in the longer run.We can also do the same for the learning rates. We will evaluate $\alpha \in [0.01, 0.1, 1.0]$ to a fixed $\epsilon=0.1$.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
epsilon = 0.1
alphas = [0.01, 0.1, 1.0]
n_trials = 200
trial_rewards = np.zeros((len(epsilons), n_trials, n_steps))
trial_optimal = np.zeros((len(epsilons), n_trials, n_steps))
for i, alpha in enumerate(alphas):
for n in range(n_trials):
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
trial_rewards[i, n] = results['rewards']
trial_optimal[i, n] = results['optimal']
labels = [f'$\\alpha$={a}' for a in alphas]
fixed = f'$\epsilon$={epsilon}'
plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal)
###Output
_____no_output_____
###Markdown
Tutorial 2: Learning to Act: Multi-Armed Bandits**Week 3, Day 4: Reinforcement Learning****By Neuromatch Academy**__Content creators:__ Marcelo Mattar and Eric DeWitt with help from Byron Galbraith__Content reviewers:__ Matt Krause and Michael Waskom --- Tutorial Objectives In this tutorial you will use 'bandits' to understand the fundementals of how a policy interacts with the learning algorithm in reinforcement learning. * You will understand the fundemental tradeoff between exploration and exploitation in a policy.* You will understand how the learning rate interacts with exploration to find the best available action. --- Setup
###Code
# Imports
import numpy as np
import matplotlib.pyplot as plt
#@title Figure settings
import ipywidgets as widgets # interactive display
%config InlineBackend.figure_format = 'retina'
plt.style.use("https://raw.githubusercontent.com/NeuromatchAcademy/course-content/master/nma.mplstyle")
#@title Helper functions
np.set_printoptions(precision=3)
def plot_choices(q, epsilon, choice_fn, n_steps=1000, rng_seed=1):
np.random.seed(rng_seed)
counts = np.zeros_like(q)
for t in range(n_steps):
action = choice_fn(q, epsilon)
counts[action] += 1
fig, ax = plt.subplots()
ax.bar(range(len(q)), counts/n_steps)
ax.set(ylabel='% chosen', xlabel='action', ylim=(0,1), xticks=range(len(q)))
def plot_multi_armed_bandit_results(results):
fig, (ax1, ax2, ax3) = plt.subplots(ncols=3, figsize=(20, 4))
ax1.plot(results['rewards'])
ax1.set(title=f"Total Reward: {np.sum(results['rewards']):.2f}",
xlabel='step', ylabel='reward')
ax2.plot(results['qs'])
ax2.set(xlabel='step', ylabel='value')
ax2.legend(range(len(results['mu'])))
ax3.plot(results['mu'], label='latent')
ax3.plot(results['qs'][-1], label='learned')
ax3.set(xlabel='action', ylabel='value')
ax3.legend()
def plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal):
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(16, 6))
ax1.plot(np.mean(trial_rewards, axis=1).T)
ax1.set(title=f'Average Reward ({fixed})', xlabel='step', ylabel='reward')
ax1.legend(labels)
ax2.plot(np.mean(trial_optimal, axis=1).T)
ax2.set(title=f'Performance ({fixed})', xlabel='step', ylabel='% optimal')
ax2.legend(labels)
###Output
_____no_output_____
###Markdown
--- Section 1: Multi-Armed Bandits
###Code
#@title Video 1: Multi-Armed Bandits
# Insert the ID of the corresponding youtube video
from IPython.display import YouTubeVideo
video = YouTubeVideo(id="kdiXr1zsfo0", width=854, height=480, fs=1)
print("Video available at https://youtu.be/" + video.id)
video
###Output
_____no_output_____
###Markdown
Consider the following learning problem. You are faced repeatedly with a choice among $k$ different options, or actions. After each choice you receive a reward signal in the form of a numerical value, where the larger value is the better. Your objective is to maximize the expected total reward over some time period, for example, over 1000 action selections, or time steps.This is the original form of the k-armed bandit problem. This name derives from the colloquial name for a slot machine, the "one-armed bandit", because it has the one lever to pull, and it is often rigged to take more money than it pays out over time. The multi-armed bandit extension is to imagine, for instance, that you are faced with multiple slot machines that you can play, but only one at a time. Which machine should you play, i.e. which arm should you pull, which action should you take, at any given time to maximize your total payout.While there are many different levels of sophistication and assumptions in how the rewards are determined, for simplicity's sake we will assume that each action results in a reward drawn from a fixed Gaussian distribution with unknown mean and unit variance. This problem setting is referred to as the *environment*, and goal is to find the arm with the highest mean value.We will solve this *optimization problem* with an *agent*, in this case an algorithm that takes in rewards and returns actions. --- Section 2: Choosing an Action The first thing our agent needs to be able to do is choose which arm to pull. The strategy for choosing actions based on our expectations is called a *policy* (often denoted $\pi$). We could have a random policy -- just pick an arm at random each time -- though this doesn't seem likely to be capable of optimizing our reward. We want some intentionality, and to do that we need a way of describing our beliefs about the arms' reward potential. We do this with an action-value function\begin{align}q(a) = \mathbb{E} [r_{t} | a_{t} = a]\end{align}where the value $q$ for taking action $a \in A$ at time $t$ is equal to the expected value of the reward $r_t$ given that we took action $a$ at that time. In practice, this is often represented as an array of values, where each action's value is a different element in the array.Great, now that we have a way to describe our beliefs about the values each action should return, let's come up with a policy.An obvious choice would be to take the action with the highest expected value. This is referred to as the *greedy* policy\begin{align}a_{t} = \text{argmax}_{a} \; q_{t} (a)\end{align}where our choice action is the one that maximizes the current value function.So far so good, but it can't be this easy. And, in fact, the greedy policy does have a fatal flaw: it easily gets trapped in local maxima. It never explores to see what it hasn't seen before if one option is already better than the others. This leads us to a fundamental challenge in coming up with effective polices. Section 2.1: The Exploitation-Exploration DilemmaIf we never try anything new, if we always stick to the safe bet, we don't know what we are missing. Sometimes we aren't missing much of anything, and regret not sticking with our preferred choice, yet other times we stumble upon something new that was way better than we thought.This is the exploitation-exploration dilemma: do you go with you best choice now, or risk the less certain option with the hope of finding something better. Too much exploration, however, means you may end up with a sub-optimal reward once it's time to stop.In order to avoid getting stuck in local minima while also maximizing reward, effective policies need some way to balance between these two aims.A simple extension to our greedy policy is to add some randomness. For instance, a coin flip -- heads we take the best choice now, tails we pick one at random. This is referred to as the $\epsilon$-greedy policy:\begin{align}P (a_{t} = a) = \begin{cases} 1 - \epsilon + \epsilon/N & \quad \text{if } a_{t} = \text{argmax}_{a} \; q_{t} (a) \\ \epsilon/N & \quad \text{else} \end{cases} \end{align}which is to say that with probability 1 - $\epsilon$ for $\epsilon \in [0,1]$ we select the greedy choice, and otherwise we select an action at random (including the greedy option).Despite its relative simplicity, the epsilon-greedy policy is quite effective, which leads to its general popularity. Exercise 1: Implement Epsilon-GreedyIn this exercise you will implement the epsilon-greedy algorithm for deciding which action to take from a set of possible actions given their value function and a probability $\epsilon$ of simply chosing one at random. TIP: You may find [`np.random.random`](https://numpy.org/doc/stable/reference/random/generated/numpy.random.random.html), [`np.random.choice`](https://numpy.org/doc/stable/reference/random/generated/numpy.random.choice.html), and [`np.argmax`](https://numpy.org/doc/stable/reference/generated/numpy.argmax.html) useful here.
###Code
def epsilon_greedy(q, epsilon):
"""Epsilon-greedy policy: selects the maximum value action with probabilty
(1-epsilon) and selects randomly with epsilon probability.
Args:
q (ndarray): an array of action values
epsilon (float): probability of selecting an action randomly
Returns:
int: the chosen action
"""
#####################################################################
## TODO for students: implement the epsilon greedy decision algorithm
# Fill out function and remove
raise NotImplementedError("Student excercise: implement the epsilon greedy decision algorithm")
#####################################################################
# write a boolean expression that determines if we should take the best action
be_greedy = ...
if be_greedy:
# write an expression for selecting the best action from the action values
action = ...
else:
# write an expression for selecting a random action
action = ...
return action
# Uncomment once the epsilon_greedy function is complete
# q = [-2, 5, 0, 1]
# epsilon = 0.1
# plot_choices(q, epsilon, epsilon_greedy)
# to_remove solution
def epsilon_greedy(q, epsilon):
"""Epsilon-greedy policy: selects the maximum value action with probabilty
(1-epsilon) and selects randomly with epsilon probability.
Args:
q (ndarray): an array of action values
epsilon (float): probability of selecting an action randomly
Returns:
int: the chosen action
"""
# write a boolean expression that determines if we should take the best action
be_greedy = np.random.random() > epsilon
if be_greedy:
# write an expression for selecting the best action from the action values
action = np.argmax(q)
else:
# write an expression for selecting a random action
action = np.random.choice(len(q))
return action
q = [-2, 5, 0, 1]
epsilon = 0.1
with plt.xkcd():
plot_choices(q, epsilon, epsilon_greedy)
###Output
_____no_output_____
###Markdown
This is what we should expect, that the action with the largest value (action 1) is selected about (1-$\epsilon$) of the time, or 90% for $\epsilon = 0.1$, and the remaining 10% is split evenly amongst the other options. Use the demo below to explore how changing $\epsilon$ affects the distribution of selected actions. Interactive Demo: Changing EpsilonEpsilon is our one parameter for balancing exploitation and exploration. Given a set of values $q = [-2, 5, 0, 1]$, use the widget below to see how changing $\epsilon$ influences our selection of the max value 5 (action = 1) vs the others. At the extremes of its range (0 and 1), the $\epsilon$-greedy policy reproduces two other policies. What are they?
###Code
#@title
#@markdown Make sure you execute this cell to enable the widget!
@widgets.interact(epsilon=widgets.FloatSlider(0.1, min=0.0, max=1.0))
def explore_epilson_values(epsilon=0.1):
q = [-2, 5, 0, 1]
plot_choices(q, epsilon, epsilon_greedy, rng_seed=None)
#to_remove explanation
"""
When epsilon is zero, the agent always chooses the currently best option; it
becomes greedy. When epsilon is 1, the agent chooses randomly.
""";
###Output
_____no_output_____
###Markdown
--- Section 3: Learning from RewardsNow that we have a policy for deciding what to do, how do we learn from our actions?One way to do this is just keep a record of every result we ever got and use the averages for each action. If we have a potentially very long running episode, the computational cost of keeping all these values and recomputing the mean over and over again isn't ideal. Instead we can use a streaming mean calculation, which looks like this:\begin{align}q_{t+1}(a) \leftarrow q_{t}(a) + \frac{1}{n_t} (r_{t} - q_{t}(a))\end{align}where our action-value function $q_t(a)$ is the mean of the rewards seen so far, $n_t$ is the number of actions taken by time $t$, and $r_t$ is the reward just received for taking action $a$.This still requires us to remember how many actions we've taken, so let's generalize this a bit further and replace the action total with a general parameter $\alpha$, which we will call the learning rate\begin{align}q_{t+1}(a) \leftarrow q_{t}(a) + \alpha (r_{t} - q_{t}(a)).\end{align} Exercise 2: Updating Action ValuesIn this exercise you will implement the action-value update rule above. The function will take in the action-value function represented as an array `q`, the action taken, the reward received, and the learning rate, `alpha`. The function will return the updated value for the selection action.
###Code
def update_action_value(q, action, reward, alpha):
""" Compute the updated action value given the learning rate and observed
reward.
Args:
q (ndarray): an array of action values
action (int): the action taken
reward (float): the reward received for taking the action
alpha (float): the learning rate
Returns:
float: the updated value for the selected action
"""
#####################################################
## TODO for students: compute the action value update
# Fill out function and remove
raise NotImplementedError("Student excercise: compute the action value update")
#####################################################
# write an expression for the updated action value
value = ...
return value
# Uncomment once the update_action_value function is complete
# q = [-2, 5, 0, 1]
# action = 2
# print(f"Original q({action}) value = {q[action]}")
# q[action] = update_action_value(q, 2, 10, 0.01)
# print(f"Updated q({action}) value = {q[action]}")
# to_remove solution
def update_action_value(q, action, reward, alpha):
""" Compute the updated action value given the learning rate and observed
reward.
Args:
q (ndarray): an array of action values
action (int): the action taken
reward (float): the reward received for taking the action
alpha (float): the learning rate
Returns:
float: the updated value for the selected action
"""
# write an expression for the updated action value
value = q[action] + alpha * (reward - q[action])
return value
q = [-2, 5, 0, 1]
action = 2
print(f"Original q({action}) value = {q[action]}")
q[action] = update_action_value(q, 2, 10, 0.01)
print(f"Updated q({action}) value = {q[action]}")
###Output
_____no_output_____
###Markdown
--- Section 4: Solving Multi-Armed BanditsNow that we have both a policy and a learning rule, we can combine these to solve our original multi-armed bandit task. Recall that we have some number of arms that give rewards drawn from Gaussian distributions with unknown mean and unit variance, and our goal is to find the arm with the highest mean.First, let's see how we will simulate this environment by reading through the annotated code below.
###Code
def multi_armed_bandit(n_arms, epsilon, alpha, n_steps):
""" A Gaussian multi-armed bandit using an epsilon-greedy policy. For each
action, rewards are randomly sampled from normal distribution, with a mean
associated with that arm and unit variance.
Args:
n_arms (int): number of arms or actions
epsilon (float): probability of selecting an action randomly
alpha (float): the learning rate
n_steps (int): number of steps to evaluate
Returns:
dict: a dictionary containing the action values, actions, and rewards from
the evaluation along with the true arm parameters mu and the optimality of
the chosen actions.
"""
# Gaussian bandit parameters
mu = np.random.normal(size=n_arms)
# evaluation and reporting state
q = np.zeros(n_arms)
qs = np.zeros((n_steps, n_arms))
rewards = np.zeros(n_steps)
actions = np.zeros(n_steps)
optimal = np.zeros(n_steps)
# run the bandit
for t in range(n_steps):
# choose an action
action = epsilon_greedy(q, epsilon)
actions[t] = action
# copmute rewards for all actions
all_rewards = np.random.normal(mu)
# observe the reward for the chosen action
reward = all_rewards[action]
rewards[t] = reward
# was it the best possible choice?
optimal_action = np.argmax(all_rewards)
optimal[t] = action == optimal_action
# update the action value
q[action] = update_action_value(q, action, reward, alpha)
qs[t] = q
results = {
'qs': qs,
'actions': actions,
'rewards': rewards,
'mu': mu,
'optimal': optimal
}
return results
###Output
_____no_output_____
###Markdown
We can use our multi-armed bandit method to evaluate how our epsilon-greedy policy and learning rule perform at solving the task. First we will set our environment to have 10 arms and our agent parameters to $\epsilon=0.1$ and $\alpha=0.01$. In order to get a good sense of the agent's performance, we will run the episode for 1000 steps.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
n_arms = 10
epsilon = 0.1
alpha = 0.01
n_steps = 1000
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(16, 6))
ax1.plot(results['rewards'])
ax1.set(title=f'Observed Reward ($\epsilon$={epsilon}, $\\alpha$={alpha})',
xlabel='step', ylabel='reward')
ax2.plot(results['qs'])
ax2.set(title=f'Action Values ($\epsilon$={epsilon}, $\\alpha$={alpha})',
xlabel='step', ylabel='value')
ax2.legend(range(n_arms));
###Output
_____no_output_____
###Markdown
Alright, we got some rewards that are kind of all over the place, but the agent seemed to settle in on the first arm as the preferred choice of action relatively quickly. Let's see how well we did at recovering the true means of the Gaussian random variables behind the arms.
###Code
fig, ax = plt.subplots()
ax.plot(results['mu'], label='latent')
ax.plot(results['qs'][-1], label='learned')
ax.set(title=f'$\epsilon$={epsilon}, $\\alpha$={alpha}',
xlabel='action', ylabel='value')
ax.legend();
###Output
_____no_output_____
###Markdown
Well, we seem to have found a very good estimate for action 0, but most of the others are not great. In fact, we can see the effect of the local maxima trap at work -- the greedy part of our algorithm locked onto action 0, which is actually the 2nd best choice to action 6. Since these are the means of Gaussian random variables, we can see that the overlap between the two would be quite high, so even if we did explore action 6, we may draw a sample that is still lower than our estimate for action 0.However, this was just one choice of parameters. Perhaps there is a better combination? Interactive Demo: Changing Epsilon and AlphaUse the widget below to explore how varying the values of $\epsilon$ (exploitation-exploration tradeoff), $\alpha$ (learning rate), and even the number of actions $k$, changes the behavior of our agent.
###Code
#@title
#@markdown Make sure you execute this cell to enable the widget!
@widgets.interact_manual(k=widgets.IntSlider(10, min=2, max=15),
epsilon=widgets.FloatSlider(0.1, min=0.0, max=1.0),
alpha=widgets.FloatLogSlider(0.01, min=-3, max=0))
def explore_bandit_parameters(k=10, epsilon=0.1, alpha=0.001):
results = multi_armed_bandit(k, epsilon, alpha, 1000)
plot_multi_armed_bandit_results(results)
###Output
_____no_output_____
###Markdown
While we can see how changing the epsilon and alpha values impact the agent's behavior, this doesn't give as a great sense of which combination is optimal. Due to the stochastic nature of both our rewards and our policy, a single trial run isn't sufficient to give us this information. Let's run mulitple trials and compare the average performance.First we will look at differet values for $\epsilon \in [0.0, 0.1, 0.2]$ to a fixed $\alpha=0.1$. We will run 200 trials as a nice balance between speed and accuracy.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
epsilons = [0.0, 0.1, 0.2]
alpha = 0.1
n_trials = 200
trial_rewards = np.zeros((len(epsilons), n_trials, n_steps))
trial_optimal = np.zeros((len(epsilons), n_trials, n_steps))
for i, epsilon in enumerate(epsilons):
for n in range(n_trials):
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
trial_rewards[i, n] = results['rewards']
trial_optimal[i, n] = results['optimal']
labels = [f'$\epsilon$={e}' for e in epsilons]
fixed = f'$\\alpha$={alpha}'
plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal)
###Output
_____no_output_____
###Markdown
On the left we have plotted the average reward over time, and we see that while $\epsilon=0$ (the greedy policy) does well initially, $\epsilon=0.1$ starts to do slightly better in the long run, while $\epsilon=0.2$ does the worst. Looking on the right, we see the percentage of times the optimal action (the best possible choice at time $t$) was taken, and here again we see a similar pattern of $\epsilon=0.1$ starting out a bit slower but eventually having a slight edge in the longer run.We can also do the same for the learning rates. We will evaluate $\alpha \in [0.01, 0.1, 1.0]$ to a fixed $\epsilon=0.1$.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
epsilon = 0.1
alphas = [0.01, 0.1, 1.0]
n_trials = 200
trial_rewards = np.zeros((len(epsilons), n_trials, n_steps))
trial_optimal = np.zeros((len(epsilons), n_trials, n_steps))
for i, alpha in enumerate(alphas):
for n in range(n_trials):
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
trial_rewards[i, n] = results['rewards']
trial_optimal[i, n] = results['optimal']
labels = [f'$\\alpha$={a}' for a in alphas]
fixed = f'$\epsilon$={epsilon}'
plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal)
###Output
_____no_output_____
###Markdown
Tutorial 2: Learning to Act: Multi-Armed Bandits**Week 3, Day 4: Reinforcement Learning****By Neuromatch Academy**__Content creators:__ Marcelo Mattar and Eric DeWitt with help from Byron Galbraith__Content reviewers:__ Matt Krause and Michael Waskom **Our 2021 Sponsors, including Presenting Sponsor Facebook Reality Labs** --- Tutorial Objectives In this tutorial you will use 'bandits' to understand the fundementals of how a policy interacts with the learning algorithm in reinforcement learning. * You will understand the fundemental tradeoff between exploration and exploitation in a policy.* You will understand how the learning rate interacts with exploration to find the best available action. --- Setup
###Code
# Imports
import numpy as np
import matplotlib.pyplot as plt
#@title Figure settings
import ipywidgets as widgets # interactive display
%config InlineBackend.figure_format = 'retina'
plt.style.use("https://raw.githubusercontent.com/NeuromatchAcademy/course-content/master/nma.mplstyle")
#@title Helper functions
np.set_printoptions(precision=3)
def plot_choices(q, epsilon, choice_fn, n_steps=1000, rng_seed=1):
np.random.seed(rng_seed)
counts = np.zeros_like(q)
for t in range(n_steps):
action = choice_fn(q, epsilon)
counts[action] += 1
fig, ax = plt.subplots()
ax.bar(range(len(q)), counts/n_steps)
ax.set(ylabel='% chosen', xlabel='action', ylim=(0,1), xticks=range(len(q)))
def plot_multi_armed_bandit_results(results):
fig, (ax1, ax2, ax3) = plt.subplots(ncols=3, figsize=(20, 4))
ax1.plot(results['rewards'])
ax1.set(title=f"Total Reward: {np.sum(results['rewards']):.2f}",
xlabel='step', ylabel='reward')
ax2.plot(results['qs'])
ax2.set(xlabel='step', ylabel='value')
ax2.legend(range(len(results['mu'])))
ax3.plot(results['mu'], label='latent')
ax3.plot(results['qs'][-1], label='learned')
ax3.set(xlabel='action', ylabel='value')
ax3.legend()
def plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal):
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(16, 6))
ax1.plot(np.mean(trial_rewards, axis=1).T)
ax1.set(title=f'Average Reward ({fixed})', xlabel='step', ylabel='reward')
ax1.legend(labels)
ax2.plot(np.mean(trial_optimal, axis=1).T)
ax2.set(title=f'Performance ({fixed})', xlabel='step', ylabel='% optimal')
ax2.legend(labels)
###Output
_____no_output_____
###Markdown
--- Section 1: Multi-Armed Bandits
###Code
# @title Video 1: Multi-Armed Bandits
from ipywidgets import widgets
out2 = widgets.Output()
with out2:
from IPython.display import IFrame
class BiliVideo(IFrame):
def __init__(self, id, page=1, width=400, height=300, **kwargs):
self.id=id
src = 'https://player.bilibili.com/player.html?bvid={0}&page={1}'.format(id, page)
super(BiliVideo, self).__init__(src, width, height, **kwargs)
video = BiliVideo(id="", width=854, height=480, fs=1)
print('Video available at https://www.bilibili.com/video/{0}'.format(video.id))
display(video)
out1 = widgets.Output()
with out1:
from IPython.display import YouTubeVideo
video = YouTubeVideo(id="kdiXr1zsfo0", width=854, height=480, fs=1, rel=0)
print('Video available at https://youtube.com/watch?v=' + video.id)
display(video)
out = widgets.Tab([out1, out2])
out.set_title(0, 'Youtube')
out.set_title(1, 'Bilibili')
display(out)
###Output
_____no_output_____
###Markdown
Consider the following learning problem. You are faced repeatedly with a choice among $k$ different options, or actions. After each choice you receive a reward signal in the form of a numerical value, where the larger value is the better. Your objective is to maximize the expected total reward over some time period, for example, over 1000 action selections, or time steps.This is the original form of the k-armed bandit problem. This name derives from the colloquial name for a slot machine, the "one-armed bandit", because it has the one lever to pull, and it is often rigged to take more money than it pays out over time. The multi-armed bandit extension is to imagine, for instance, that you are faced with multiple slot machines that you can play, but only one at a time. Which machine should you play, i.e. which arm should you pull, which action should you take, at any given time to maximize your total payout.While there are many different levels of sophistication and assumptions in how the rewards are determined, for simplicity's sake we will assume that each action results in a reward drawn from a fixed Gaussian distribution with unknown mean and unit variance. This problem setting is referred to as the *environment*, and goal is to find the arm with the highest mean value.We will solve this *optimization problem* with an *agent*, in this case an algorithm that takes in rewards and returns actions. --- Section 2: Choosing an Action The first thing our agent needs to be able to do is choose which arm to pull. The strategy for choosing actions based on our expectations is called a *policy* (often denoted $\pi$). We could have a random policy -- just pick an arm at random each time -- though this doesn't seem likely to be capable of optimizing our reward. We want some intentionality, and to do that we need a way of describing our beliefs about the arms' reward potential. We do this with an action-value function\begin{align}q(a) = \mathbb{E} [r_{t} | a_{t} = a]\end{align}where the value $q$ for taking action $a \in A$ at time $t$ is equal to the expected value of the reward $r_t$ given that we took action $a$ at that time. In practice, this is often represented as an array of values, where each action's value is a different element in the array.Great, now that we have a way to describe our beliefs about the values each action should return, let's come up with a policy.An obvious choice would be to take the action with the highest expected value. This is referred to as the *greedy* policy\begin{align}a_{t} = \text{argmax}_{a} \; q_{t} (a)\end{align}where our choice action is the one that maximizes the current value function.So far so good, but it can't be this easy. And, in fact, the greedy policy does have a fatal flaw: it easily gets trapped in local maxima. It never explores to see what it hasn't seen before if one option is already better than the others. This leads us to a fundamental challenge in coming up with effective polices. Section 2.1: The Exploitation-Exploration DilemmaIf we never try anything new, if we always stick to the safe bet, we don't know what we are missing. Sometimes we aren't missing much of anything, and regret not sticking with our preferred choice, yet other times we stumble upon something new that was way better than we thought.This is the exploitation-exploration dilemma: do you go with you best choice now, or risk the less certain option with the hope of finding something better. Too much exploration, however, means you may end up with a sub-optimal reward once it's time to stop.In order to avoid getting stuck in local minima while also maximizing reward, effective policies need some way to balance between these two aims.A simple extension to our greedy policy is to add some randomness. For instance, a coin flip -- heads we take the best choice now, tails we pick one at random. This is referred to as the $\epsilon$-greedy policy:\begin{align}P (a_{t} = a) = \begin{cases} 1 - \epsilon + \epsilon/N & \quad \text{if } a_{t} = \text{argmax}_{a} \; q_{t} (a) \\ \epsilon/N & \quad \text{else} \end{cases} \end{align}which is to say that with probability 1 - $\epsilon$ for $\epsilon \in [0,1]$ we select the greedy choice, and otherwise we select an action at random (including the greedy option).Despite its relative simplicity, the epsilon-greedy policy is quite effective, which leads to its general popularity. Exercise 1: Implement Epsilon-GreedyIn this exercise you will implement the epsilon-greedy algorithm for deciding which action to take from a set of possible actions given their value function and a probability $\epsilon$ of simply chosing one at random. TIP: You may find [`np.random.random`](https://numpy.org/doc/stable/reference/random/generated/numpy.random.random.html), [`np.random.choice`](https://numpy.org/doc/stable/reference/random/generated/numpy.random.choice.html), and [`np.argmax`](https://numpy.org/doc/stable/reference/generated/numpy.argmax.html) useful here.
###Code
def epsilon_greedy(q, epsilon):
"""Epsilon-greedy policy: selects the maximum value action with probabilty
(1-epsilon) and selects randomly with epsilon probability.
Args:
q (ndarray): an array of action values
epsilon (float): probability of selecting an action randomly
Returns:
int: the chosen action
"""
#####################################################################
## TODO for students: implement the epsilon greedy decision algorithm
# Fill out function and remove
raise NotImplementedError("Student excercise: implement the epsilon greedy decision algorithm")
#####################################################################
# write a boolean expression that determines if we should take the best action
be_greedy = ...
if be_greedy:
# write an expression for selecting the best action from the action values
action = ...
else:
# write an expression for selecting a random action
action = ...
return action
# Uncomment once the epsilon_greedy function is complete
# q = [-2, 5, 0, 1]
# epsilon = 0.1
# plot_choices(q, epsilon, epsilon_greedy)
# to_remove solution
def epsilon_greedy(q, epsilon):
"""Epsilon-greedy policy: selects the maximum value action with probabilty
(1-epsilon) and selects randomly with epsilon probability.
Args:
q (ndarray): an array of action values
epsilon (float): probability of selecting an action randomly
Returns:
int: the chosen action
"""
# write a boolean expression that determines if we should take the best action
be_greedy = np.random.random() > epsilon
if be_greedy:
# write an expression for selecting the best action from the action values
action = np.argmax(q)
else:
# write an expression for selecting a random action
action = np.random.choice(len(q))
return action
q = [-2, 5, 0, 1]
epsilon = 0.1
with plt.xkcd():
plot_choices(q, epsilon, epsilon_greedy)
###Output
_____no_output_____
###Markdown
This is what we should expect, that the action with the largest value (action 1) is selected about (1-$\epsilon$) of the time, or 90% for $\epsilon = 0.1$, and the remaining 10% is split evenly amongst the other options. Use the demo below to explore how changing $\epsilon$ affects the distribution of selected actions. Interactive Demo: Changing EpsilonEpsilon is our one parameter for balancing exploitation and exploration. Given a set of values $q = [-2, 5, 0, 1]$, use the widget below to see how changing $\epsilon$ influences our selection of the max value 5 (action = 1) vs the others. At the extremes of its range (0 and 1), the $\epsilon$-greedy policy reproduces two other policies. What are they?
###Code
#@title
#@markdown Make sure you execute this cell to enable the widget!
@widgets.interact(epsilon=widgets.FloatSlider(0.1, min=0.0, max=1.0))
def explore_epilson_values(epsilon=0.1):
q = [-2, 5, 0, 1]
plot_choices(q, epsilon, epsilon_greedy, rng_seed=None)
#to_remove explanation
"""
When epsilon is zero, the agent always chooses the currently best option; it
becomes greedy. When epsilon is 1, the agent chooses randomly.
""";
###Output
_____no_output_____
###Markdown
--- Section 3: Learning from RewardsNow that we have a policy for deciding what to do, how do we learn from our actions?One way to do this is just keep a record of every result we ever got and use the averages for each action. If we have a potentially very long running episode, the computational cost of keeping all these values and recomputing the mean over and over again isn't ideal. Instead we can use a streaming mean calculation, which looks like this:\begin{align}q_{t+1}(a) \leftarrow q_{t}(a) + \frac{1}{n_t} (r_{t} - q_{t}(a))\end{align}where our action-value function $q_t(a)$ is the mean of the rewards seen so far, $n_t$ is the number of actions taken by time $t$, and $r_t$ is the reward just received for taking action $a$.This still requires us to remember how many actions we've taken, so let's generalize this a bit further and replace the action total with a general parameter $\alpha$, which we will call the learning rate\begin{align}q_{t+1}(a) \leftarrow q_{t}(a) + \alpha (r_{t} - q_{t}(a)).\end{align} Exercise 2: Updating Action ValuesIn this exercise you will implement the action-value update rule above. The function will take in the action-value function represented as an array `q`, the action taken, the reward received, and the learning rate, `alpha`. The function will return the updated value for the selection action.
###Code
def update_action_value(q, action, reward, alpha):
""" Compute the updated action value given the learning rate and observed
reward.
Args:
q (ndarray): an array of action values
action (int): the action taken
reward (float): the reward received for taking the action
alpha (float): the learning rate
Returns:
float: the updated value for the selected action
"""
#####################################################
## TODO for students: compute the action value update
# Fill out function and remove
raise NotImplementedError("Student excercise: compute the action value update")
#####################################################
# write an expression for the updated action value
value = ...
return value
# Uncomment once the update_action_value function is complete
# q = [-2, 5, 0, 1]
# action = 2
# print(f"Original q({action}) value = {q[action]}")
# q[action] = update_action_value(q, 2, 10, 0.01)
# print(f"Updated q({action}) value = {q[action]}")
# to_remove solution
def update_action_value(q, action, reward, alpha):
""" Compute the updated action value given the learning rate and observed
reward.
Args:
q (ndarray): an array of action values
action (int): the action taken
reward (float): the reward received for taking the action
alpha (float): the learning rate
Returns:
float: the updated value for the selected action
"""
# write an expression for the updated action value
value = q[action] + alpha * (reward - q[action])
return value
q = [-2, 5, 0, 1]
action = 2
print(f"Original q({action}) value = {q[action]}")
q[action] = update_action_value(q, 2, 10, 0.01)
print(f"Updated q({action}) value = {q[action]}")
###Output
_____no_output_____
###Markdown
--- Section 4: Solving Multi-Armed BanditsNow that we have both a policy and a learning rule, we can combine these to solve our original multi-armed bandit task. Recall that we have some number of arms that give rewards drawn from Gaussian distributions with unknown mean and unit variance, and our goal is to find the arm with the highest mean.First, let's see how we will simulate this environment by reading through the annotated code below.
###Code
def multi_armed_bandit(n_arms, epsilon, alpha, n_steps):
""" A Gaussian multi-armed bandit using an epsilon-greedy policy. For each
action, rewards are randomly sampled from normal distribution, with a mean
associated with that arm and unit variance.
Args:
n_arms (int): number of arms or actions
epsilon (float): probability of selecting an action randomly
alpha (float): the learning rate
n_steps (int): number of steps to evaluate
Returns:
dict: a dictionary containing the action values, actions, and rewards from
the evaluation along with the true arm parameters mu and the optimality of
the chosen actions.
"""
# Gaussian bandit parameters
mu = np.random.normal(size=n_arms)
# evaluation and reporting state
q = np.zeros(n_arms)
qs = np.zeros((n_steps, n_arms))
rewards = np.zeros(n_steps)
actions = np.zeros(n_steps)
optimal = np.zeros(n_steps)
# run the bandit
for t in range(n_steps):
# choose an action
action = epsilon_greedy(q, epsilon)
actions[t] = action
# copmute rewards for all actions
all_rewards = np.random.normal(mu)
# observe the reward for the chosen action
reward = all_rewards[action]
rewards[t] = reward
# was it the best possible choice?
optimal_action = np.argmax(all_rewards)
optimal[t] = action == optimal_action
# update the action value
q[action] = update_action_value(q, action, reward, alpha)
qs[t] = q
results = {
'qs': qs,
'actions': actions,
'rewards': rewards,
'mu': mu,
'optimal': optimal
}
return results
###Output
_____no_output_____
###Markdown
We can use our multi-armed bandit method to evaluate how our epsilon-greedy policy and learning rule perform at solving the task. First we will set our environment to have 10 arms and our agent parameters to $\epsilon=0.1$ and $\alpha=0.01$. In order to get a good sense of the agent's performance, we will run the episode for 1000 steps.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
n_arms = 10
epsilon = 0.1
alpha = 0.01
n_steps = 1000
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(16, 6))
ax1.plot(results['rewards'])
ax1.set(title=f'Observed Reward ($\epsilon$={epsilon}, $\\alpha$={alpha})',
xlabel='step', ylabel='reward')
ax2.plot(results['qs'])
ax2.set(title=f'Action Values ($\epsilon$={epsilon}, $\\alpha$={alpha})',
xlabel='step', ylabel='value')
ax2.legend(range(n_arms));
###Output
_____no_output_____
###Markdown
Alright, we got some rewards that are kind of all over the place, but the agent seemed to settle in on the first arm as the preferred choice of action relatively quickly. Let's see how well we did at recovering the true means of the Gaussian random variables behind the arms.
###Code
fig, ax = plt.subplots()
ax.plot(results['mu'], label='latent')
ax.plot(results['qs'][-1], label='learned')
ax.set(title=f'$\epsilon$={epsilon}, $\\alpha$={alpha}',
xlabel='action', ylabel='value')
ax.legend();
###Output
_____no_output_____
###Markdown
Well, we seem to have found a very good estimate for action 0, but most of the others are not great. In fact, we can see the effect of the local maxima trap at work -- the greedy part of our algorithm locked onto action 0, which is actually the 2nd best choice to action 6. Since these are the means of Gaussian random variables, we can see that the overlap between the two would be quite high, so even if we did explore action 6, we may draw a sample that is still lower than our estimate for action 0.However, this was just one choice of parameters. Perhaps there is a better combination? Interactive Demo: Changing Epsilon and AlphaUse the widget below to explore how varying the values of $\epsilon$ (exploitation-exploration tradeoff), $\alpha$ (learning rate), and even the number of actions $k$, changes the behavior of our agent.
###Code
#@title
#@markdown Make sure you execute this cell to enable the widget!
@widgets.interact_manual(k=widgets.IntSlider(10, min=2, max=15),
epsilon=widgets.FloatSlider(0.1, min=0.0, max=1.0),
alpha=widgets.FloatLogSlider(0.01, min=-3, max=0))
def explore_bandit_parameters(k=10, epsilon=0.1, alpha=0.001):
results = multi_armed_bandit(k, epsilon, alpha, 1000)
plot_multi_armed_bandit_results(results)
###Output
_____no_output_____
###Markdown
While we can see how changing the epsilon and alpha values impact the agent's behavior, this doesn't give as a great sense of which combination is optimal. Due to the stochastic nature of both our rewards and our policy, a single trial run isn't sufficient to give us this information. Let's run mulitple trials and compare the average performance.First we will look at differet values for $\epsilon \in [0.0, 0.1, 0.2]$ to a fixed $\alpha=0.1$. We will run 200 trials as a nice balance between speed and accuracy.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
epsilons = [0.0, 0.1, 0.2]
alpha = 0.1
n_trials = 200
trial_rewards = np.zeros((len(epsilons), n_trials, n_steps))
trial_optimal = np.zeros((len(epsilons), n_trials, n_steps))
for i, epsilon in enumerate(epsilons):
for n in range(n_trials):
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
trial_rewards[i, n] = results['rewards']
trial_optimal[i, n] = results['optimal']
labels = [f'$\epsilon$={e}' for e in epsilons]
fixed = f'$\\alpha$={alpha}'
plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal)
###Output
_____no_output_____
###Markdown
On the left we have plotted the average reward over time, and we see that while $\epsilon=0$ (the greedy policy) does well initially, $\epsilon=0.1$ starts to do slightly better in the long run, while $\epsilon=0.2$ does the worst. Looking on the right, we see the percentage of times the optimal action (the best possible choice at time $t$) was taken, and here again we see a similar pattern of $\epsilon=0.1$ starting out a bit slower but eventually having a slight edge in the longer run.We can also do the same for the learning rates. We will evaluate $\alpha \in [0.01, 0.1, 1.0]$ to a fixed $\epsilon=0.1$.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
epsilon = 0.1
alphas = [0.01, 0.1, 1.0]
n_trials = 200
trial_rewards = np.zeros((len(epsilons), n_trials, n_steps))
trial_optimal = np.zeros((len(epsilons), n_trials, n_steps))
for i, alpha in enumerate(alphas):
for n in range(n_trials):
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
trial_rewards[i, n] = results['rewards']
trial_optimal[i, n] = results['optimal']
labels = [f'$\\alpha$={a}' for a in alphas]
fixed = f'$\epsilon$={epsilon}'
plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal)
###Output
_____no_output_____
###Markdown
Tutorial 2: Learning to Act: Multi-Armed Bandits**Week 3, Day 4: Reinforcement Learning****By Neuromatch Academy**__Content creators:__ Marcelo Mattar and Eric DeWitt with help from Byron Galbraith__Content reviewers:__ Ella Batty, Matt Krause and Michael Waskom **Our 2021 Sponsors, including Presenting Sponsor Facebook Reality Labs** --- Tutorial Objectives *Estimated timing of tutorial: 45 min*In this tutorial you will use 'bandits' to understand the fundementals of how a policy interacts with the learning algorithm in reinforcement learning. * You will understand the fundemental tradeoff between exploration and exploitation in a policy.* You will understand how the learning rate interacts with exploration to find the best available action.
###Code
# @title Tutorial slides
# @markdown These are the slides for all videos in this tutorial.
from IPython.display import IFrame
IFrame(src=f"https://mfr.ca-1.osf.io/render?url=https://osf.io/2jzdu/?direct%26mode=render%26action=download%26mode=render", width=854, height=480)
###Output
_____no_output_____
###Markdown
--- Setup
###Code
# Imports
import numpy as np
import matplotlib.pyplot as plt
#@title Figure Settings
import ipywidgets as widgets # interactive display
%config InlineBackend.figure_format = 'retina'
plt.style.use("https://raw.githubusercontent.com/NeuromatchAcademy/course-content/master/nma.mplstyle")
#@title Plotting Functions
np.set_printoptions(precision=3)
def plot_choices(q, epsilon, choice_fn, n_steps=1000, rng_seed=1):
np.random.seed(rng_seed)
counts = np.zeros_like(q)
for t in range(n_steps):
action = choice_fn(q, epsilon)
counts[action] += 1
fig, ax = plt.subplots()
ax.bar(range(len(q)), counts/n_steps)
ax.set(ylabel='% chosen', xlabel='action', ylim=(0,1), xticks=range(len(q)))
def plot_multi_armed_bandit_results(results):
fig, (ax1, ax2, ax3) = plt.subplots(ncols=3, figsize=(20, 4))
ax1.plot(results['rewards'])
ax1.set(title=f"Total Reward: {np.sum(results['rewards']):.2f}",
xlabel='step', ylabel='reward')
ax2.plot(results['qs'])
ax2.set(xlabel='step', ylabel='value')
ax2.legend(range(len(results['mu'])))
ax3.plot(results['mu'], label='latent')
ax3.plot(results['qs'][-1], label='learned')
ax3.set(xlabel='action', ylabel='value')
ax3.legend()
def plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal):
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(16, 6))
ax1.plot(np.mean(trial_rewards, axis=1).T)
ax1.set(title=f'Average Reward ({fixed})', xlabel='step', ylabel='reward')
ax1.legend(labels)
ax2.plot(np.mean(trial_optimal, axis=1).T)
ax2.set(title=f'Performance ({fixed})', xlabel='step', ylabel='% optimal')
ax2.legend(labels)
###Output
_____no_output_____
###Markdown
--- Section 1: Multi-Armed Bandits
###Code
# @title Video 1: Multi-Armed Bandits
from ipywidgets import widgets
out2 = widgets.Output()
with out2:
from IPython.display import IFrame
class BiliVideo(IFrame):
def __init__(self, id, page=1, width=400, height=300, **kwargs):
self.id=id
src = 'https://player.bilibili.com/player.html?bvid={0}&page={1}'.format(id, page)
super(BiliVideo, self).__init__(src, width, height, **kwargs)
video = BiliVideo(id="BV1M54y1B7S3", width=854, height=480, fs=1)
print('Video available at https://www.bilibili.com/video/{0}'.format(video.id))
display(video)
out1 = widgets.Output()
with out1:
from IPython.display import YouTubeVideo
video = YouTubeVideo(id="kdiXr1zsfo0", width=854, height=480, fs=1, rel=0)
print('Video available at https://youtube.com/watch?v=' + video.id)
display(video)
out = widgets.Tab([out1, out2])
out.set_title(0, 'Youtube')
out.set_title(1, 'Bilibili')
display(out)
###Output
_____no_output_____
###Markdown
Consider the following learning problem. You are faced repeatedly with a choice among $k$ different options, or actions. After each choice you receive a reward signal in the form of a numerical value, where the larger value is the better. Your objective is to maximize the expected total reward over some time period, for example, over 1000 action selections, or time steps.This is the original form of the k-armed bandit problem. This name derives from the colloquial name for a slot machine, the "one-armed bandit", because it has the one lever to pull, and it is often rigged to take more money than it pays out over time. The multi-armed bandit extension is to imagine, for instance, that you are faced with multiple slot machines that you can play, but only one at a time. Which machine should you play, i.e. which arm should you pull, which action should you take, at any given time to maximize your total payout.While there are many different levels of sophistication and assumptions in how the rewards are determined, for simplicity's sake we will assume that each action results in a reward drawn from a fixed Gaussian distribution with unknown mean and unit variance. This problem setting is referred to as the *environment*, and goal is to find the arm with the highest mean value.We will solve this *optimization problem* with an *agent*, in this case an algorithm that takes in rewards and returns actions. --- Section 2: Choosing an Action *Estimated timing to here from start of tutorial: 10 min*The first thing our agent needs to be able to do is choose which arm to pull. The strategy for choosing actions based on our expectations is called a *policy* (often denoted $\pi$). We could have a random policy -- just pick an arm at random each time -- though this doesn't seem likely to be capable of optimizing our reward. We want some intentionality, and to do that we need a way of describing our beliefs about the arms' reward potential. We do this with an action-value function\begin{align}q(a) = \mathbb{E} [r_{t} | a_{t} = a]\end{align}where the value $q$ for taking action $a \in A$ at time $t$ is equal to the expected value of the reward $r_t$ given that we took action $a$ at that time. In practice, this is often represented as an array of values, where each action's value is a different element in the array.Great, now that we have a way to describe our beliefs about the values each action should return, let's come up with a policy.An obvious choice would be to take the action with the highest expected value. This is referred to as the *greedy* policy\begin{align}a_{t} = \text{argmax}_{a} \; q_{t} (a)\end{align}where our choice action is the one that maximizes the current value function.So far so good, but it can't be this easy. And, in fact, the greedy policy does have a fatal flaw: it easily gets trapped in local maxima. It never explores to see what it hasn't seen before if one option is already better than the others. This leads us to a fundamental challenge in coming up with effective polices. **The Exploitation-Exploration Dilemma**If we never try anything new, if we always stick to the safe bet, we don't know what we are missing. Sometimes we aren't missing much of anything, and regret not sticking with our preferred choice, yet other times we stumble upon something new that was way better than we thought.This is the exploitation-exploration dilemma: do you go with you best choice now, or risk the less certain option with the hope of finding something better. Too much exploration, however, means you may end up with a sub-optimal reward once it's time to stop.In order to avoid getting stuck in local minima while also maximizing reward, effective policies need some way to balance between these two aims.A simple extension to our greedy policy is to add some randomness. For instance, a coin flip -- heads we take the best choice now, tails we pick one at random. This is referred to as the $\epsilon$-greedy policy:\begin{align}P (a_{t} = a) = \begin{cases} 1 - \epsilon + \epsilon/N & \quad \text{if } a_{t} = \text{argmax}_{a} \; q_{t} (a) \\ \epsilon/N & \quad \text{else} \end{cases} \end{align}which is to say that with probability 1 - $\epsilon$ for $\epsilon \in [0,1]$ we select the greedy choice, and otherwise we select an action at random (including the greedy option).Despite its relative simplicity, the epsilon-greedy policy is quite effective, which leads to its general popularity. Coding Exercise 2: Implement Epsilon-Greedy*Referred to in video as Exercise 1*In this exercise you will implement the epsilon-greedy algorithm for deciding which action to take from a set of possible actions given their value function and a probability $\epsilon$ of simply chosing one at random. TIP: You may find [`np.random.random`](https://numpy.org/doc/stable/reference/random/generated/numpy.random.random.html), [`np.random.choice`](https://numpy.org/doc/stable/reference/random/generated/numpy.random.choice.html), and [`np.argmax`](https://numpy.org/doc/stable/reference/generated/numpy.argmax.html) useful here.
###Code
def epsilon_greedy(q, epsilon):
"""Epsilon-greedy policy: selects the maximum value action with probabilty
(1-epsilon) and selects randomly with epsilon probability.
Args:
q (ndarray): an array of action values
epsilon (float): probability of selecting an action randomly
Returns:
int: the chosen action
"""
#####################################################################
## TODO for students: implement the epsilon greedy decision algorithm
# Fill out function and remove
raise NotImplementedError("Student excercise: implement the epsilon greedy decision algorithm")
#####################################################################
# write a boolean expression that determines if we should take the best action
be_greedy = ...
if be_greedy:
# write an expression for selecting the best action from the action values
action = ...
else:
# write an expression for selecting a random action
action = ...
return action
# Set parameters
q = [-2, 5, 0, 1]
epsilon = 0.1
# Visualize
plot_choices(q, epsilon, epsilon_greedy)
# to_remove solution
def epsilon_greedy(q, epsilon):
"""Epsilon-greedy policy: selects the maximum value action with probabilty
(1-epsilon) and selects randomly with epsilon probability.
Args:
q (ndarray): an array of action values
epsilon (float): probability of selecting an action randomly
Returns:
int: the chosen action
"""
# write a boolean expression that determines if we should take the best action
be_greedy = np.random.random() > epsilon
if be_greedy:
# write an expression for selecting the best action from the action values
action = np.argmax(q)
else:
# write an expression for selecting a random action
action = np.random.choice(len(q))
return action
# Set parameters
q = [-2, 5, 0, 1]
epsilon = 0.1
# Visualize
with plt.xkcd():
plot_choices(q, epsilon, epsilon_greedy)
###Output
_____no_output_____
###Markdown
This is what we should expect, that the action with the largest value (action 1) is selected about (1-$\epsilon$) of the time, or 90% for $\epsilon = 0.1$, and the remaining 10% is split evenly amongst the other options. Use the demo below to explore how changing $\epsilon$ affects the distribution of selected actions. Interactive Demo 2: Changing EpsilonEpsilon is our one parameter for balancing exploitation and exploration. Given a set of values $q = [-2, 5, 0, 1]$, use the widget below to see how changing $\epsilon$ influences our selection of the max value 5 (action = 1) vs the others. At the extremes of its range (0 and 1), the $\epsilon$-greedy policy reproduces two other policies. What are they?
###Code
#@title
#@markdown Make sure you execute this cell to enable the widget!
@widgets.interact(epsilon=widgets.FloatSlider(0.1, min=0.0, max=1.0))
def explore_epilson_values(epsilon=0.1):
q = [-2, 5, 0, 1]
plot_choices(q, epsilon, epsilon_greedy, rng_seed=None)
# to_remove explanation
"""
When epsilon is zero, the agent always chooses the currently best option; it
becomes greedy. When epsilon is 1, the agent chooses randomly.
""";
###Output
_____no_output_____
###Markdown
--- Section 3: Learning from Rewards*Estimated timing to here from start of tutorial: 25 min*Now that we have a policy for deciding what to do, how do we learn from our actions?One way to do this is just keep a record of every result we ever got and use the averages for each action. If we have a potentially very long running episode, the computational cost of keeping all these values and recomputing the mean over and over again isn't ideal. Instead we can use a streaming mean calculation, which looks like this:\begin{align}q_{t+1}(a) \leftarrow q_{t}(a) + \frac{1}{n_t} (r_{t} - q_{t}(a))\end{align}where our action-value function $q_t(a)$ is the mean of the rewards seen so far, $n_t$ is the number of actions taken by time $t$, and $r_t$ is the reward just received for taking action $a$.This still requires us to remember how many actions we've taken, so let's generalize this a bit further and replace the action total with a general parameter $\alpha$, which we will call the learning rate\begin{align}q_{t+1}(a) \leftarrow q_{t}(a) + \alpha (r_{t} - q_{t}(a)).\end{align} Coding Exercise 3: Updating Action Values*Referred to in video as Exercise 2*In this exercise you will implement the action-value update rule above. The function will take in the action-value function represented as an array `q`, the action taken, the reward received, and the learning rate, `alpha`. The function will return the updated value for the selection action.
###Code
def update_action_value(q, action, reward, alpha):
""" Compute the updated action value given the learning rate and observed
reward.
Args:
q (ndarray): an array of action values
action (int): the action taken
reward (float): the reward received for taking the action
alpha (float): the learning rate
Returns:
float: the updated value for the selected action
"""
#####################################################
## TODO for students: compute the action value update
# Fill out function and remove
raise NotImplementedError("Student excercise: compute the action value update")
#####################################################
# Write an expression for the updated action value
value = ...
return value
# Set parameters
q = [-2, 5, 0, 1]
action = 2
print(f"Original q({action}) value = {q[action]}")
# Update action
q[action] = update_action_value(q, 2, 10, 0.01)
print(f"Updated q({action}) value = {q[action]}")
# to_remove solution
def update_action_value(q, action, reward, alpha):
""" Compute the updated action value given the learning rate and observed
reward.
Args:
q (ndarray): an array of action values
action (int): the action taken
reward (float): the reward received for taking the action
alpha (float): the learning rate
Returns:
float: the updated value for the selected action
"""
# Write an expression for the updated action value
value = q[action] + alpha * (reward - q[action])
return value
# Set parameters
q = [-2, 5, 0, 1]
action = 2
print(f"Original q({action}) value = {q[action]}")
# Update action
q[action] = update_action_value(q, 2, 10, 0.01)
print(f"Updated q({action}) value = {q[action]}")
###Output
_____no_output_____
###Markdown
You should see```Original q(2) value = 0Updated q(2) value = 0.1``` --- Section 4: Solving Multi-Armed Bandits*Estimated timing to here from start of tutorial: 31 min*Now that we have both a policy and a learning rule, we can combine these to solve our original multi-armed bandit task. Recall that we have some number of arms that give rewards drawn from Gaussian distributions with unknown mean and unit variance, and our goal is to find the arm with the highest mean.First, let's see how we will simulate this environment by reading through the annotated code below.
###Code
def multi_armed_bandit(n_arms, epsilon, alpha, n_steps):
""" A Gaussian multi-armed bandit using an epsilon-greedy policy. For each
action, rewards are randomly sampled from normal distribution, with a mean
associated with that arm and unit variance.
Args:
n_arms (int): number of arms or actions
epsilon (float): probability of selecting an action randomly
alpha (float): the learning rate
n_steps (int): number of steps to evaluate
Returns:
dict: a dictionary containing the action values, actions, and rewards from
the evaluation along with the true arm parameters mu and the optimality of
the chosen actions.
"""
# Gaussian bandit parameters
mu = np.random.normal(size=n_arms)
# Evaluation and reporting state
q = np.zeros(n_arms)
qs = np.zeros((n_steps, n_arms))
rewards = np.zeros(n_steps)
actions = np.zeros(n_steps)
optimal = np.zeros(n_steps)
# Run the bandit
for t in range(n_steps):
# Choose an action
action = epsilon_greedy(q, epsilon)
actions[t] = action
# Compute rewards for all actions
all_rewards = np.random.normal(mu)
# Observe the reward for the chosen action
reward = all_rewards[action]
rewards[t] = reward
# Was it the best possible choice?
optimal_action = np.argmax(all_rewards)
optimal[t] = action == optimal_action
# Update the action value
q[action] = update_action_value(q, action, reward, alpha)
qs[t] = q
results = {
'qs': qs,
'actions': actions,
'rewards': rewards,
'mu': mu,
'optimal': optimal
}
return results
###Output
_____no_output_____
###Markdown
We can use our multi-armed bandit method to evaluate how our epsilon-greedy policy and learning rule perform at solving the task. First we will set our environment to have 10 arms and our agent parameters to $\epsilon=0.1$ and $\alpha=0.01$. In order to get a good sense of the agent's performance, we will run the episode for 1000 steps.
###Code
# @markdown Execute to see visualization
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
n_arms = 10
epsilon = 0.1
alpha = 0.01
n_steps = 1000
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(16, 6))
ax1.plot(results['rewards'])
ax1.set(title=f'Observed Reward ($\epsilon$={epsilon}, $\\alpha$={alpha})',
xlabel='step', ylabel='reward')
ax2.plot(results['qs'])
ax2.set(title=f'Action Values ($\epsilon$={epsilon}, $\\alpha$={alpha})',
xlabel='step', ylabel='value')
ax2.legend(range(n_arms));
###Output
_____no_output_____
###Markdown
Alright, we got some rewards that are kind of all over the place, but the agent seemed to settle in on the first arm as the preferred choice of action relatively quickly. Let's see how well we did at recovering the true means of the Gaussian random variables behind the arms.
###Code
# @markdown Execute to see visualization
fig, ax = plt.subplots()
ax.plot(results['mu'], label='latent')
ax.plot(results['qs'][-1], label='learned')
ax.set(title=f'$\epsilon$={epsilon}, $\\alpha$={alpha}',
xlabel='action', ylabel='value')
ax.legend();
###Output
_____no_output_____
###Markdown
Well, we seem to have found a very good estimate for action 0, but most of the others are not great. In fact, we can see the effect of the local maxima trap at work -- the greedy part of our algorithm locked onto action 0, which is actually the 2nd best choice to action 6. Since these are the means of Gaussian random variables, we can see that the overlap between the two would be quite high, so even if we did explore action 6, we may draw a sample that is still lower than our estimate for action 0.However, this was just one choice of parameters. Perhaps there is a better combination? Interactive Demo 4: Changing Epsilon and Alpha*Referred to in video as Exercise 3*Use the widget below to explore how varying the values of $\epsilon$ (exploitation-exploration tradeoff), $\alpha$ (learning rate), and even the number of actions $k$, changes the behavior of our agent.
###Code
#@title
#@markdown Make sure you execute this cell to enable the widget!
@widgets.interact_manual(k=widgets.IntSlider(10, min=2, max=15),
epsilon=widgets.FloatSlider(0.1, min=0.0, max=1.0),
alpha=widgets.FloatLogSlider(0.01, min=-3, max=0))
def explore_bandit_parameters(k=10, epsilon=0.1, alpha=0.001):
results = multi_armed_bandit(k, epsilon, alpha, 1000)
plot_multi_armed_bandit_results(results)
###Output
_____no_output_____
###Markdown
While we can see how changing the epsilon and alpha values impact the agent's behavior, this doesn't give as a great sense of which combination is optimal. Due to the stochastic nature of both our rewards and our policy, a single trial run isn't sufficient to give us this information. Let's run mulitple trials and compare the average performance.First we will look at different values for $\epsilon \in [0.0, 0.1, 0.2]$ to a fixed $\alpha=0.1$. We will run 200 trials as a nice balance between speed and accuracy.
###Code
# @markdown Execute this cell to see visualization
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
epsilons = [0.0, 0.1, 0.2]
alpha = 0.1
n_trials = 200
trial_rewards = np.zeros((len(epsilons), n_trials, n_steps))
trial_optimal = np.zeros((len(epsilons), n_trials, n_steps))
for i, epsilon in enumerate(epsilons):
for n in range(n_trials):
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
trial_rewards[i, n] = results['rewards']
trial_optimal[i, n] = results['optimal']
labels = [f'$\epsilon$={e}' for e in epsilons]
fixed = f'$\\alpha$={alpha}'
plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal)
###Output
_____no_output_____
###Markdown
On the left we have plotted the average reward over time, and we see that while $\epsilon=0$ (the greedy policy) does well initially, $\epsilon=0.1$ starts to do slightly better in the long run, while $\epsilon=0.2$ does the worst. Looking on the right, we see the percentage of times the optimal action (the best possible choice at time $t$) was taken, and here again we see a similar pattern of $\epsilon=0.1$ starting out a bit slower but eventually having a slight edge in the longer run.We can also do the same for the learning rates. We will evaluate $\alpha \in [0.01, 0.1, 1.0]$ to a fixed $\epsilon=0.1$.
###Code
# @markdown Execute this cell to see visualization
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
epsilon = 0.1
alphas = [0.01, 0.1, 1.0]
n_trials = 200
trial_rewards = np.zeros((len(epsilons), n_trials, n_steps))
trial_optimal = np.zeros((len(epsilons), n_trials, n_steps))
for i, alpha in enumerate(alphas):
for n in range(n_trials):
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
trial_rewards[i, n] = results['rewards']
trial_optimal[i, n] = results['optimal']
labels = [f'$\\alpha$={a}' for a in alphas]
fixed = f'$\epsilon$={epsilon}'
plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal)
###Output
_____no_output_____
###Markdown
Tutorial 2: Learning to Act: Multi-Armed Bandits**Week 3, Day 4: Reinforcement Learning****By Neuromatch Academy**__Content creators:__ Marcelo Mattar and Eric DeWitt with help from Byron Galbraith__Content reviewers:__ Matt Krause and Michael Waskom --- Tutorial Objectives In this tutorial you will use 'bandits' to understand the fundementals of how a policy interacts with the learning algorithm in reinforcement learning. * You will understand the fundemental tradeoff between exploration and exploitation in a policy.* You will understand how the learning rate interacts with exploration to find the best available action. --- Setup
###Code
# Imports
import numpy as np
import matplotlib.pyplot as plt
#@title Figure settings
import ipywidgets as widgets # interactive display
%config InlineBackend.figure_format = 'retina'
plt.style.use("https://raw.githubusercontent.com/NeuromatchAcademy/course-content/master/nma.mplstyle")
#@title Helper functions
np.set_printoptions(precision=3)
def plot_choices(q, epsilon, choice_fn, n_steps=1000, rng_seed=1):
np.random.seed(rng_seed)
counts = np.zeros_like(q)
for t in range(n_steps):
action = choice_fn(q, epsilon)
counts[action] += 1
fig, ax = plt.subplots()
ax.bar(range(len(q)), counts/n_steps)
ax.set(ylabel='% chosen', xlabel='action', ylim=(0,1), xticks=range(len(q)))
def plot_multi_armed_bandit_results(results):
fig, (ax1, ax2, ax3) = plt.subplots(ncols=3, figsize=(20, 4))
ax1.plot(results['rewards'])
ax1.set(title=f"Total Reward: {np.sum(results['rewards']):.2f}",
xlabel='step', ylabel='reward')
ax2.plot(results['qs'])
ax2.set(xlabel='step', ylabel='value')
ax2.legend(range(len(results['mu'])))
ax3.plot(results['mu'], label='latent')
ax3.plot(results['qs'][-1], label='learned')
ax3.set(xlabel='action', ylabel='value')
ax3.legend()
def plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal):
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(16, 6))
ax1.plot(np.mean(trial_rewards, axis=1).T)
ax1.set(title=f'Average Reward ({fixed})', xlabel='step', ylabel='reward')
ax1.legend(labels)
ax2.plot(np.mean(trial_optimal, axis=1).T)
ax2.set(title=f'Performance ({fixed})', xlabel='step', ylabel='% optimal')
ax2.legend(labels)
###Output
_____no_output_____
###Markdown
--- Section 1: Multi-Armed Bandits
###Code
#@title Video 1: Multi-Armed Bandits
# Insert the ID of the corresponding youtube video
from IPython.display import YouTubeVideo
video = YouTubeVideo(id="kdiXr1zsfo0", width=854, height=480, fs=1)
print("Video available at https://youtu.be/" + video.id)
video
###Output
_____no_output_____
###Markdown
Consider the following learning problem. You are faced repeatedly with a choice among $k$ different options, or actions. After each choice you receive a reward signal in the form of a numerical value, where the larger value is the better. Your objective is to maximize the expected total reward over some time period, for example, over 1000 action selections, or time steps.This is the original form of the k-armed bandit problem. This name derives from the colloquial name for a slot machine, the "one-armed bandit", because it has the one lever to pull, and it is often rigged to take more money than it pays out over time. The multi-armed bandit extension is to imagine, for instance, that you are faced with multiple slot machines that you can play, but only one at a time. Which machine should you play, i.e. which arm should you pull, which action should you take, at any given time to maximize your total payout.While there are many different levels of sophistication and assumptions in how the rewards are determined, for simplicity's sake we will assume that each action results in a reward drawn from a fixed Gaussian distribution with unknown mean and unit variance. This problem setting is referred to as the *environment*, and goal is to find the arm with the highest mean value.We will solve this *optimization problem* with an *agent*, in this case an algorithm that takes in rewards and returns actions. --- Section 2: Choosing an Action The first thing our agent needs to be able to do is choose which arm to pull. The strategy for choosing actions based on our expectations is called a *policy* (often denoted $\pi$). We could have a random policy -- just pick an arm at random each time -- though this doesn't seem likely to be capable of optimizing our reward. We want some intentionality, and to do that we need a way of describing our beliefs about the arms' reward potential. We do this with an action-value function\begin{align}q(a) = \mathbb{E} [r_{t} | a_{t} = a]\end{align}where the value $q$ for taking action $a \in A$ at time $t$ is equal to the expected value of the reward $r_t$ given that we took action $a$ at that time. In practice, this is often represented as an array of values, where each action's value is a different element in the array.Great, now that we have a way to describe our beliefs about the values each action should return, let's come up with a policy.An obvious choice would be to take the action with the highest expected value. This is referred to as the *greedy* policy\begin{align}a_{t} = \text{argmax}_{a} \; q_{t} (a)\end{align}where our choice action is the one that maximizes the current value function.So far so good, but it can't be this easy. And, in fact, the greedy policy does have a fatal flaw: it easily gets trapped in local maxima. It never explores to see what it hasn't seen before if one option is already better than the others. This leads us to a fundamental challenge in coming up with effective polices. Section 2.1: The Exploitation-Exploration DilemmaIf we never try anything new, if we always stick to the safe bet, we don't know what we are missing. Sometimes we aren't missing much of anything, and regret not sticking with our preferred choice, yet other times we stumble upon something new that was way better than we thought.This is the exploitation-exploration dilemma: do you go with you best choice now, or risk the less certain option with the hope of finding something better. Too much exploration, however, means you may end up with a sub-optimal reward once it's time to stop.In order to avoid getting stuck in local minima while also maximizing reward, effective policies need some way to balance between these two aims.A simple extension to our greedy policy is to add some randomness. For instance, a coin flip -- heads we take the best choice now, tails we pick one at random. This is referred to as the $\epsilon$-greedy policy:\begin{align}P (a_{t} = a) = \begin{cases} 1 - \epsilon + \epsilon/N & \quad \text{if } a_{t} = \text{argmax}_{a} \; q_{t} (a) \\ \epsilon/N & \quad \text{else} \end{cases} \end{align}which is to say that with probability 1 - $\epsilon$ for $\epsilon \in [0,1]$ we select the greedy choice, and otherwise we select an action at random (including the greedy option).Despite its relative simplicity, the epsilon-greedy policy is quite effective, which leads to its general popularity. Exercise 1: Implement Epsilon-GreedyIn this exercise you will implement the epsilon-greedy algorithm for deciding which action to take from a set of possible actions given their value function and a probability $\epsilon$ of simply chosing one at random. TIP: You may find [`np.random.random`](https://numpy.org/doc/stable/reference/random/generated/numpy.random.random.html), [`np.random.choice`](https://numpy.org/doc/stable/reference/random/generated/numpy.random.choice.html), and [`np.argmax`](https://numpy.org/doc/stable/reference/generated/numpy.argmax.html) useful here.
###Code
def epsilon_greedy(q, epsilon):
"""Epsilon-greedy policy: selects the maximum value action with probabilty
(1-epsilon) and selects randomly with epsilon probability.
Args:
q (ndarray): an array of action values
epsilon (float): probability of selecting an action randomly
Returns:
int: the chosen action
"""
#####################################################################
## TODO for students: implement the epsilon greedy decision algorithm
# Fill out function and remove
raise NotImplementedError("Student excercise: implement the epsilon greedy decision algorithm")
#####################################################################
# write a boolean expression that determines if we should take the best action
be_greedy = ...
if be_greedy:
# write an expression for selecting the best action from the action values
action = ...
else:
# write an expression for selecting a random action
action = ...
return action
# Uncomment once the epsilon_greedy function is complete
# q = [-2, 5, 0, 1]
# epsilon = 0.1
# plot_choices(q, epsilon, epsilon_greedy)
# to_remove solution
def epsilon_greedy(q, epsilon):
"""Epsilon-greedy policy: selects the maximum value action with probabilty
(1-epsilon) and selects randomly with epsilon probability.
Args:
q (ndarray): an array of action values
epsilon (float): probability of selecting an action randomly
Returns:
int: the chosen action
"""
# write a boolean expression that determines if we should take the best action
be_greedy = np.random.random() > epsilon
if be_greedy:
# write an expression for selecting the best action from the action values
action = np.argmax(q)
else:
# write an expression for selecting a random action
action = np.random.choice(len(q))
return action
q = [-2, 5, 0, 1]
epsilon = 0.1
with plt.xkcd():
plot_choices(q, epsilon, epsilon_greedy)
###Output
_____no_output_____
###Markdown
This is what we should expect, that the action with the largest value (action 1) is selected about (1-$\epsilon$) of the time, or 90% for $\epsilon = 0.1$, and the remaining 10% is split evenly amongst the other options. Use the demo below to explore how changing $\epsilon$ affects the distribution of selected actions. Interactive Demo: Changing EpsilonEpsilon is our one parameter for balancing exploitation and exploration. Given a set of values $q = [-2, 5, 0, 1]$, use the widget below to see how changing $\epsilon$ influences our selection of the max value 5 (action = 1) vs the others. At the extremes of its range (0 and 1), the $\epsilon$-greedy policy reproduces two other policies. What are they?
###Code
#@title
#@markdown Make sure you execute this cell to enable the widget!
@widgets.interact(epsilon=widgets.FloatSlider(0.1, min=0.0, max=1.0))
def explore_epilson_values(epsilon=0.1):
q = [-2, 5, 0, 1]
plot_choices(q, epsilon, epsilon_greedy, rng_seed=None)
#to_remove explanation
"""
When epsilon is zero, the agent always chooses the currently best option; it
becomes greedy. When epsilon is 1, the agent chooses randomly.
""";
###Output
_____no_output_____
###Markdown
--- Section 3: Learning from RewardsNow that we have a policy for deciding what to do, how do we learn from our actions?One way to do this is just keep a record of every result we ever got and use the averages for each action. If we have a potentially very long running episode, the computational cost of keeping all these values and recomputing the mean over and over again isn't ideal. Instead we can use a streaming mean calculation, which looks like this:\begin{align}q_{t+1}(a) \leftarrow q_{t}(a) + \frac{1}{n_t} (r_{t} - q_{t}(a))\end{align}where our action-value function $q_t(a)$ is the mean of the rewards seen so far, $n_t$ is the number of actions taken by time $t$, and $r_t$ is the reward just received for taking action $a$.This still requires us to remember how many actions we've taken, so let's generalize this a bit further and replace the action total with a general parameter $\alpha$, which we will call the learning rate\begin{align}q_{t+1}(a) \leftarrow q_{t}(a) + \alpha (r_{t} - q_{t}(a)).\end{align} Exercise 2: Updating Action ValuesIn this exercise you will implement the action-value update rule above. The function will take in the action-value function represented as an array `q`, the action taken, the reward received, and the learning rate, `alpha`. The function will return the updated value for the selection action.
###Code
def update_action_value(q, action, reward, alpha):
""" Compute the updated action value given the learning rate and observed
reward.
Args:
q (ndarray): an array of action values
action (int): the action taken
reward (float): the reward received for taking the action
alpha (float): the learning rate
Returns:
float: the updated value for the selected action
"""
#####################################################
## TODO for students: compute the action value update
# Fill out function and remove
raise NotImplementedError("Student excercise: compute the action value update")
#####################################################
# write an expression for the updated action value
value = ...
return value
# Uncomment once the update_action_value function is complete
# q = [-2, 5, 0, 1]
# action = 2
# print(f"Original q({action}) value = {q[action]}")
# q[action] = update_action_value(q, 2, 10, 0.01)
# print(f"Updated q({action}) value = {q[action]}")
# to_remove solution
def update_action_value(q, action, reward, alpha):
""" Compute the updated action value given the learning rate and observed
reward.
Args:
q (ndarray): an array of action values
action (int): the action taken
reward (float): the reward received for taking the action
alpha (float): the learning rate
Returns:
float: the updated value for the selected action
"""
# write an expression for the updated action value
value = q[action] + alpha * (reward - q[action])
return value
q = [-2, 5, 0, 1]
action = 2
print(f"Original q({action}) value = {q[action]}")
q[action] = update_action_value(q, 2, 10, 0.01)
print(f"Updated q({action}) value = {q[action]}")
###Output
_____no_output_____
###Markdown
--- Section 4: Solving Multi-Armed BanditsNow that we have both a policy and a learning rule, we can combine these to solve our original multi-armed bandit task. Recall that we have some number of arms that give rewards drawn from Gaussian distributions with unknown mean and unit variance, and our goal is to find the arm with the highest mean.First, let's see how we will simulate this environment by reading through the annotated code below.
###Code
def multi_armed_bandit(n_arms, epsilon, alpha, n_steps):
""" A Gaussian multi-armed bandit using an epsilon-greedy policy. For each
action, rewards are randomly sampled from normal distribution, with a mean
associated with that arm and unit variance.
Args:
n_arms (int): number of arms or actions
epsilon (float): probability of selecting an action randomly
alpha (float): the learning rate
n_steps (int): number of steps to evaluate
Returns:
dict: a dictionary containing the action values, actions, and rewards from
the evaluation along with the true arm parameters mu and the optimality of
the chosen actions.
"""
# Gaussian bandit parameters
mu = np.random.normal(size=n_arms)
# evaluation and reporting state
q = np.zeros(n_arms)
qs = np.zeros((n_steps, n_arms))
rewards = np.zeros(n_steps)
actions = np.zeros(n_steps)
optimal = np.zeros(n_steps)
# run the bandit
for t in range(n_steps):
# choose an action
action = epsilon_greedy(q, epsilon)
actions[t] = action
# copmute rewards for all actions
all_rewards = np.random.normal(mu)
# observe the reward for the chosen action
reward = all_rewards[action]
rewards[t] = reward
# was it the best possible choice?
optimal_action = np.argmax(all_rewards)
optimal[t] = action == optimal_action
# update the action value
q[action] = update_action_value(q, action, reward, alpha)
qs[t] = q
results = {
'qs': qs,
'actions': actions,
'rewards': rewards,
'mu': mu,
'optimal': optimal
}
return results
###Output
_____no_output_____
###Markdown
We can use our multi-armed bandit method to evaluate how our epsilon-greedy policy and learning rule perform at solving the task. First we will set our environment to have 10 arms and our agent parameters to $\epsilon=0.1$ and $\alpha=0.01$. In order to get a good sense of the agent's performance, we will run the episode for 1000 steps.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
n_arms = 10
epsilon = 0.1
alpha = 0.01
n_steps = 1000
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(16, 6))
ax1.plot(results['rewards'])
ax1.set(title=f'Observed Reward ($\epsilon$={epsilon}, $\\alpha$={alpha})',
xlabel='step', ylabel='reward')
ax2.plot(results['qs'])
ax2.set(title=f'Action Values ($\epsilon$={epsilon}, $\\alpha$={alpha})',
xlabel='step', ylabel='value')
ax2.legend(range(n_arms));
###Output
_____no_output_____
###Markdown
Alright, we got some rewards that are kind of all over the place, but the agent seemed to settle in on the first arm as the preferred choice of action relatively quickly. Let's see how well we did at recovering the true means of the Gaussian random variables behind the arms.
###Code
fig, ax = plt.subplots()
ax.plot(results['mu'], label='latent')
ax.plot(results['qs'][-1], label='learned')
ax.set(title=f'$\epsilon$={epsilon}, $\\alpha$={alpha}',
xlabel='action', ylabel='value')
ax.legend();
###Output
_____no_output_____
###Markdown
Well, we seem to have found a very good estimate for action 0, but most of the others are not great. In fact, we can see the effect of the local maxima trap at work -- the greedy part of our algorithm locked onto action 0, which is actually the 2nd best choice to action 6. Since these are the means of Gaussian random variables, we can see that the overlap between the two would be quite high, so even if we did explore action 6, we may draw a sample that is still lower than our estimate for action 0.However, this was just one choice of parameters. Perhaps there is a better combination? Interactive Demo: Changing Epsilon and AlphaUse the widget below to explore how varying the values of $\epsilon$ (exploitation-exploration tradeoff), $\alpha$ (learning rate), and even the number of actions $k$, changes the behavior of our agent.
###Code
#@title
#@markdown Make sure you execute this cell to enable the widget!
@widgets.interact_manual(k=widgets.IntSlider(10, min=2, max=15),
epsilon=widgets.FloatSlider(0.1, min=0.0, max=1.0),
alpha=widgets.FloatLogSlider(0.01, min=-3, max=0))
def explore_bandit_parameters(k=10, epsilon=0.1, alpha=0.001):
results = multi_armed_bandit(k, epsilon, alpha, 1000)
plot_multi_armed_bandit_results(results)
###Output
_____no_output_____
###Markdown
While we can see how changing the epsilon and alpha values impact the agent's behavior, this doesn't give as a great sense of which combination is optimal. Due to the stochastic nature of both our rewards and our policy, a single trial run isn't sufficient to give us this information. Let's run mulitple trials and compare the average performance.First we will look at differet values for $\epsilon \in [0.0, 0.1, 0.2]$ to a fixed $\alpha=0.1$. We will run 200 trials as a nice balance between speed and accuracy.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
epsilons = [0.0, 0.1, 0.2]
alpha = 0.1
n_trials = 200
trial_rewards = np.zeros((len(epsilons), n_trials, n_steps))
trial_optimal = np.zeros((len(epsilons), n_trials, n_steps))
for i, epsilon in enumerate(epsilons):
for n in range(n_trials):
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
trial_rewards[i, n] = results['rewards']
trial_optimal[i, n] = results['optimal']
labels = [f'$\epsilon$={e}' for e in epsilons]
fixed = f'$\\alpha$={alpha}'
plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal)
###Output
_____no_output_____
###Markdown
On the left we have plotted the average reward over time, and we see that while $\epsilon=0$ (the greedy policy) does well initially, $\epsilon=0.1$ starts to do slightly better in the long run, while $\epsilon=0.2$ does the worst. Looking on the right, we see the percentage of times the optimal action (the best possible choice at time $t$) was taken, and here again we see a similar pattern of $\epsilon=0.1$ starting out a bit slower but eventually having a slight edge in the longer run.We can also do the same for the learning rates. We will evaluate $\alpha \in [0.01, 0.1, 1.0]$ to a fixed $\epsilon=0.1$.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
epsilon = 0.1
alphas = [0.01, 0.1, 1.0]
n_trials = 200
trial_rewards = np.zeros((len(epsilons), n_trials, n_steps))
trial_optimal = np.zeros((len(epsilons), n_trials, n_steps))
for i, alpha in enumerate(alphas):
for n in range(n_trials):
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
trial_rewards[i, n] = results['rewards']
trial_optimal[i, n] = results['optimal']
labels = [f'$\\alpha$={a}' for a in alphas]
fixed = f'$\epsilon$={epsilon}'
plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal)
###Output
_____no_output_____
###Markdown
Tutorial 2: Learning to Act: Multi-Armed Bandits**Week 3, Day 4: Reinforcement Learning****By Neuromatch Academy**__Content creators:__ Marcelo Mattar and Eric DeWitt with help from Byron Galbraith__Content reviewers:__ Ella Batty, Matt Krause and Michael Waskom **Our 2021 Sponsors, including Presenting Sponsor Facebook Reality Labs** --- Tutorial Objectives *Estimated timing of tutorial: 45 min*In this tutorial you will use 'bandits' to understand the fundementals of how a policy interacts with the learning algorithm in reinforcement learning. * You will understand the fundemental tradeoff between exploration and exploitation in a policy.* You will understand how the learning rate interacts with exploration to find the best available action.
###Code
# @title Tutorial slides
# @markdown These are the slides for all videos in this tutorial.
from IPython.display import IFrame
IFrame(src=f"https://mfr.ca-1.osf.io/render?url=https://osf.io/2jzdu/?direct%26mode=render%26action=download%26mode=render", width=854, height=480)
###Output
_____no_output_____
###Markdown
--- Setup
###Code
# Imports
import numpy as np
import matplotlib.pyplot as plt
#@title Figure Settings
import ipywidgets as widgets # interactive display
%config InlineBackend.figure_format = 'retina'
plt.style.use("https://raw.githubusercontent.com/NeuromatchAcademy/course-content/master/nma.mplstyle")
#@title Plotting Functions
np.set_printoptions(precision=3)
def plot_choices(q, epsilon, choice_fn, n_steps=1000, rng_seed=1):
np.random.seed(rng_seed)
counts = np.zeros_like(q)
for t in range(n_steps):
action = choice_fn(q, epsilon)
counts[action] += 1
fig, ax = plt.subplots()
ax.bar(range(len(q)), counts/n_steps)
ax.set(ylabel='% chosen', xlabel='action', ylim=(0,1), xticks=range(len(q)))
def plot_multi_armed_bandit_results(results):
fig, (ax1, ax2, ax3) = plt.subplots(ncols=3, figsize=(20, 4))
ax1.plot(results['rewards'])
ax1.set(title=f"Total Reward: {np.sum(results['rewards']):.2f}",
xlabel='step', ylabel='reward')
ax2.plot(results['qs'])
ax2.set(xlabel='step', ylabel='value')
ax2.legend(range(len(results['mu'])))
ax3.plot(results['mu'], label='latent')
ax3.plot(results['qs'][-1], label='learned')
ax3.set(xlabel='action', ylabel='value')
ax3.legend()
def plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal):
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(16, 6))
ax1.plot(np.mean(trial_rewards, axis=1).T)
ax1.set(title=f'Average Reward ({fixed})', xlabel='step', ylabel='reward')
ax1.legend(labels)
ax2.plot(np.mean(trial_optimal, axis=1).T)
ax2.set(title=f'Performance ({fixed})', xlabel='step', ylabel='% optimal')
ax2.legend(labels)
###Output
_____no_output_____
###Markdown
--- Section 1: Multi-Armed Bandits
###Code
# @title Video 1: Multi-Armed Bandits
from ipywidgets import widgets
out2 = widgets.Output()
with out2:
from IPython.display import IFrame
class BiliVideo(IFrame):
def __init__(self, id, page=1, width=400, height=300, **kwargs):
self.id=id
src = 'https://player.bilibili.com/player.html?bvid={0}&page={1}'.format(id, page)
super(BiliVideo, self).__init__(src, width, height, **kwargs)
video = BiliVideo(id="BV1M54y1B7S3", width=854, height=480, fs=1)
print('Video available at https://www.bilibili.com/video/{0}'.format(video.id))
display(video)
out1 = widgets.Output()
with out1:
from IPython.display import YouTubeVideo
video = YouTubeVideo(id="kdiXr1zsfo0", width=854, height=480, fs=1, rel=0)
print('Video available at https://youtube.com/watch?v=' + video.id)
display(video)
out = widgets.Tab([out1, out2])
out.set_title(0, 'Youtube')
out.set_title(1, 'Bilibili')
display(out)
###Output
_____no_output_____
###Markdown
Consider the following learning problem. You are faced repeatedly with a choice among $k$ different options, or actions. After each choice you receive a reward signal in the form of a numerical value, where the larger value is the better. Your objective is to maximize the expected total reward over some time period, for example, over 1000 action selections, or time steps.This is the original form of the k-armed bandit problem. This name derives from the colloquial name for a slot machine, the "one-armed bandit", because it has the one lever to pull, and it is often rigged to take more money than it pays out over time. The multi-armed bandit extension is to imagine, for instance, that you are faced with multiple slot machines that you can play, but only one at a time. Which machine should you play, i.e., which arm should you pull, which action should you take, at any given time to maximize your total payout.While there are many different levels of sophistication and assumptions in how the rewards are determined, for simplicity's sake we will assume that each action results in a reward drawn from a fixed Gaussian distribution with unknown mean and unit variance. This problem setting is referred to as the *environment*, and goal is to find the arm with the highest mean value.We will solve this *optimization problem* with an *agent*, in this case an algorithm that takes in rewards and returns actions. --- Section 2: Choosing an Action *Estimated timing to here from start of tutorial: 10 min*The first thing our agent needs to be able to do is choose which arm to pull. The strategy for choosing actions based on our expectations is called a *policy* (often denoted $\pi$). We could have a random policy -- just pick an arm at random each time -- though this doesn't seem likely to be capable of optimizing our reward. We want some intentionality, and to do that we need a way of describing our beliefs about the arms' reward potential. We do this with an action-value function\begin{align}q(a) = \mathbb{E} [r_{t} | a_{t} = a]\end{align}where the value $q$ for taking action $a \in A$ at time $t$ is equal to the expected value of the reward $r_t$ given that we took action $a$ at that time. In practice, this is often represented as an array of values, where each action's value is a different element in the array.Great, now that we have a way to describe our beliefs about the values each action should return, let's come up with a policy.An obvious choice would be to take the action with the highest expected value. This is referred to as the *greedy* policy\begin{align}a_{t} = \text{argmax}_{a} \; q_{t} (a)\end{align}where our choice action is the one that maximizes the current value function.So far so good, but it can't be this easy. And, in fact, the greedy policy does have a fatal flaw: it easily gets trapped in local maxima. It never explores to see what it hasn't seen before if one option is already better than the others. This leads us to a fundamental challenge in coming up with effective polices. **The Exploitation-Exploration Dilemma**If we never try anything new, if we always stick to the safe bet, we don't know what we are missing. Sometimes we aren't missing much of anything, and regret not sticking with our preferred choice, yet other times we stumble upon something new that was way better than we thought.This is the exploitation-exploration dilemma: do you go with you best choice now, or risk the less certain option with the hope of finding something better. Too much exploration, however, means you may end up with a sub-optimal reward once it's time to stop.In order to avoid getting stuck in local minima while also maximizing reward, effective policies need some way to balance between these two aims.A simple extension to our greedy policy is to add some randomness. For instance, a coin flip -- heads we take the best choice now, tails we pick one at random. This is referred to as the $\epsilon$-greedy policy:\begin{align}P (a_{t} = a) = \begin{cases} 1 - \epsilon + \epsilon/N & \quad \text{if } a_{t} = \text{argmax}_{a} \; q_{t} (a) \\ \epsilon/N & \quad \text{else} \end{cases} \end{align}which is to say that with probability 1 - $\epsilon$ for $\epsilon \in [0,1]$ we select the greedy choice, and otherwise we select an action at random (including the greedy option).Despite its relative simplicity, the epsilon-greedy policy is quite effective, which leads to its general popularity. Coding Exercise 2: Implement Epsilon-Greedy*Referred to in video as Exercise 1*In this exercise you will implement the epsilon-greedy algorithm for deciding which action to take from a set of possible actions given their value function and a probability $\epsilon$ of simply chosing one at random. TIP: You may find [`np.random.random`](https://numpy.org/doc/stable/reference/random/generated/numpy.random.random.html), [`np.random.choice`](https://numpy.org/doc/stable/reference/random/generated/numpy.random.choice.html), and [`np.argmax`](https://numpy.org/doc/stable/reference/generated/numpy.argmax.html) useful here.
###Code
def epsilon_greedy(q, epsilon):
"""Epsilon-greedy policy: selects the maximum value action with probabilty
(1-epsilon) and selects randomly with epsilon probability.
Args:
q (ndarray): an array of action values
epsilon (float): probability of selecting an action randomly
Returns:
int: the chosen action
"""
#####################################################################
## TODO for students: implement the epsilon greedy decision algorithm
# Fill out function and remove
raise NotImplementedError("Student excercise: implement the epsilon greedy decision algorithm")
#####################################################################
# write a boolean expression that determines if we should take the best action
be_greedy = ...
if be_greedy:
# write an expression for selecting the best action from the action values
action = ...
else:
# write an expression for selecting a random action
action = ...
return action
# Set parameters
q = [-2, 5, 0, 1]
epsilon = 0.1
# Visualize
plot_choices(q, epsilon, epsilon_greedy)
# to_remove solution
def epsilon_greedy(q, epsilon):
"""Epsilon-greedy policy: selects the maximum value action with probabilty
(1-epsilon) and selects randomly with epsilon probability.
Args:
q (ndarray): an array of action values
epsilon (float): probability of selecting an action randomly
Returns:
int: the chosen action
"""
# write a boolean expression that determines if we should take the best action
be_greedy = np.random.random() > epsilon
if be_greedy:
# write an expression for selecting the best action from the action values
action = np.argmax(q)
else:
# write an expression for selecting a random action
action = np.random.choice(len(q))
return action
# Set parameters
q = [-2, 5, 0, 1]
epsilon = 0.1
# Visualize
with plt.xkcd():
plot_choices(q, epsilon, epsilon_greedy)
###Output
_____no_output_____
###Markdown
This is what we should expect, that the action with the largest value (action 1) is selected about (1-$\epsilon$) of the time, or 90% for $\epsilon = 0.1$, and the remaining 10% is split evenly amongst the other options. Use the demo below to explore how changing $\epsilon$ affects the distribution of selected actions. Interactive Demo 2: Changing EpsilonEpsilon is our one parameter for balancing exploitation and exploration. Given a set of values $q = [-2, 5, 0, 1]$, use the widget below to see how changing $\epsilon$ influences our selection of the max value 5 (action = 1) vs the others. At the extremes of its range (0 and 1), the $\epsilon$-greedy policy reproduces two other policies. What are they?
###Code
#@title
#@markdown Make sure you execute this cell to enable the widget!
@widgets.interact(epsilon=widgets.FloatSlider(0.1, min=0.0, max=1.0))
def explore_epilson_values(epsilon=0.1):
q = [-2, 5, 0, 1]
plot_choices(q, epsilon, epsilon_greedy, rng_seed=None)
# to_remove explanation
"""
When epsilon is zero, the agent always chooses the currently best option; it
becomes greedy. When epsilon is 1, the agent chooses randomly.
""";
###Output
_____no_output_____
###Markdown
--- Section 3: Learning from Rewards*Estimated timing to here from start of tutorial: 25 min*Now that we have a policy for deciding what to do, how do we learn from our actions?One way to do this is just keep a record of every result we ever got and use the averages for each action. If we have a potentially very long running episode, the computational cost of keeping all these values and recomputing the mean over and over again isn't ideal. Instead we can use a streaming mean calculation, which looks like this:\begin{align}q_{t+1}(a) \leftarrow q_{t}(a) + \frac{1}{n_t} (r_{t} - q_{t}(a))\end{align}where our action-value function $q_t(a)$ is the mean of the rewards seen so far, $n_t$ is the number of actions taken by time $t$, and $r_t$ is the reward just received for taking action $a$.This still requires us to remember how many actions we've taken, so let's generalize this a bit further and replace the action total with a general parameter $\alpha$, which we will call the learning rate\begin{align}q_{t+1}(a) \leftarrow q_{t}(a) + \alpha (r_{t} - q_{t}(a)).\end{align} Coding Exercise 3: Updating Action Values*Referred to in video as Exercise 2*In this exercise you will implement the action-value update rule above. The function will take in the action-value function represented as an array `q`, the action taken, the reward received, and the learning rate, `alpha`. The function will return the updated value for the selection action.
###Code
def update_action_value(q, action, reward, alpha):
""" Compute the updated action value given the learning rate and observed
reward.
Args:
q (ndarray): an array of action values
action (int): the action taken
reward (float): the reward received for taking the action
alpha (float): the learning rate
Returns:
float: the updated value for the selected action
"""
#####################################################
## TODO for students: compute the action value update
# Fill out function and remove
raise NotImplementedError("Student excercise: compute the action value update")
#####################################################
# Write an expression for the updated action value
value = ...
return value
# Set parameters
q = [-2, 5, 0, 1]
action = 2
print(f"Original q({action}) value = {q[action]}")
# Update action
q[action] = update_action_value(q, 2, 10, 0.01)
print(f"Updated q({action}) value = {q[action]}")
# to_remove solution
def update_action_value(q, action, reward, alpha):
""" Compute the updated action value given the learning rate and observed
reward.
Args:
q (ndarray): an array of action values
action (int): the action taken
reward (float): the reward received for taking the action
alpha (float): the learning rate
Returns:
float: the updated value for the selected action
"""
# Write an expression for the updated action value
value = q[action] + alpha * (reward - q[action])
return value
# Set parameters
q = [-2, 5, 0, 1]
action = 2
print(f"Original q({action}) value = {q[action]}")
# Update action
q[action] = update_action_value(q, 2, 10, 0.01)
print(f"Updated q({action}) value = {q[action]}")
###Output
_____no_output_____
###Markdown
You should see```Original q(2) value = 0Updated q(2) value = 0.1``` --- Section 4: Solving Multi-Armed Bandits*Estimated timing to here from start of tutorial: 31 min*Now that we have both a policy and a learning rule, we can combine these to solve our original multi-armed bandit task. Recall that we have some number of arms that give rewards drawn from Gaussian distributions with unknown mean and unit variance, and our goal is to find the arm with the highest mean.First, let's see how we will simulate this environment by reading through the annotated code below.
###Code
def multi_armed_bandit(n_arms, epsilon, alpha, n_steps):
""" A Gaussian multi-armed bandit using an epsilon-greedy policy. For each
action, rewards are randomly sampled from normal distribution, with a mean
associated with that arm and unit variance.
Args:
n_arms (int): number of arms or actions
epsilon (float): probability of selecting an action randomly
alpha (float): the learning rate
n_steps (int): number of steps to evaluate
Returns:
dict: a dictionary containing the action values, actions, and rewards from
the evaluation along with the true arm parameters mu and the optimality of
the chosen actions.
"""
# Gaussian bandit parameters
mu = np.random.normal(size=n_arms)
# Evaluation and reporting state
q = np.zeros(n_arms)
qs = np.zeros((n_steps, n_arms))
rewards = np.zeros(n_steps)
actions = np.zeros(n_steps)
optimal = np.zeros(n_steps)
# Run the bandit
for t in range(n_steps):
# Choose an action
action = epsilon_greedy(q, epsilon)
actions[t] = action
# Compute rewards for all actions
all_rewards = np.random.normal(mu)
# Observe the reward for the chosen action
reward = all_rewards[action]
rewards[t] = reward
# Was it the best possible choice?
optimal_action = np.argmax(all_rewards)
optimal[t] = action == optimal_action
# Update the action value
q[action] = update_action_value(q, action, reward, alpha)
qs[t] = q
results = {
'qs': qs,
'actions': actions,
'rewards': rewards,
'mu': mu,
'optimal': optimal
}
return results
###Output
_____no_output_____
###Markdown
We can use our multi-armed bandit method to evaluate how our epsilon-greedy policy and learning rule perform at solving the task. First we will set our environment to have 10 arms and our agent parameters to $\epsilon=0.1$ and $\alpha=0.01$. In order to get a good sense of the agent's performance, we will run the episode for 1000 steps.
###Code
# @markdown Execute to see visualization
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
n_arms = 10
epsilon = 0.1
alpha = 0.01
n_steps = 1000
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(16, 6))
ax1.plot(results['rewards'])
ax1.set(title=f'Observed Reward ($\epsilon$={epsilon}, $\\alpha$={alpha})',
xlabel='step', ylabel='reward')
ax2.plot(results['qs'])
ax2.set(title=f'Action Values ($\epsilon$={epsilon}, $\\alpha$={alpha})',
xlabel='step', ylabel='value')
ax2.legend(range(n_arms));
###Output
_____no_output_____
###Markdown
Alright, we got some rewards that are kind of all over the place, but the agent seemed to settle in on the first arm as the preferred choice of action relatively quickly. Let's see how well we did at recovering the true means of the Gaussian random variables behind the arms.
###Code
# @markdown Execute to see visualization
fig, ax = plt.subplots()
ax.plot(results['mu'], label='latent')
ax.plot(results['qs'][-1], label='learned')
ax.set(title=f'$\epsilon$={epsilon}, $\\alpha$={alpha}',
xlabel='action', ylabel='value')
ax.legend();
###Output
_____no_output_____
###Markdown
Well, we seem to have found a very good estimate for action 0, but most of the others are not great. In fact, we can see the effect of the local maxima trap at work -- the greedy part of our algorithm locked onto action 0, which is actually the 2nd best choice to action 6. Since these are the means of Gaussian random variables, we can see that the overlap between the two would be quite high, so even if we did explore action 6, we may draw a sample that is still lower than our estimate for action 0.However, this was just one choice of parameters. Perhaps there is a better combination? Interactive Demo 4: Changing Epsilon and Alpha*Referred to in video as Exercise 3*Use the widget below to explore how varying the values of $\epsilon$ (exploitation-exploration tradeoff), $\alpha$ (learning rate), and even the number of actions $k$, changes the behavior of our agent.
###Code
#@title
#@markdown Make sure you execute this cell to enable the widget!
@widgets.interact_manual(k=widgets.IntSlider(10, min=2, max=15),
epsilon=widgets.FloatSlider(0.1, min=0.0, max=1.0),
alpha=widgets.FloatLogSlider(0.01, min=-3, max=0))
def explore_bandit_parameters(k=10, epsilon=0.1, alpha=0.001):
results = multi_armed_bandit(k, epsilon, alpha, 1000)
plot_multi_armed_bandit_results(results)
###Output
_____no_output_____
###Markdown
While we can see how changing the epsilon and alpha values impact the agent's behavior, this doesn't give as a great sense of which combination is optimal. Due to the stochastic nature of both our rewards and our policy, a single trial run isn't sufficient to give us this information. Let's run mulitple trials and compare the average performance.First we will look at different values for $\epsilon \in [0.0, 0.1, 0.2]$ to a fixed $\alpha=0.1$. We will run 200 trials as a nice balance between speed and accuracy.
###Code
# @markdown Execute this cell to see visualization
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
epsilons = [0.0, 0.1, 0.2]
alpha = 0.1
n_trials = 200
trial_rewards = np.zeros((len(epsilons), n_trials, n_steps))
trial_optimal = np.zeros((len(epsilons), n_trials, n_steps))
for i, epsilon in enumerate(epsilons):
for n in range(n_trials):
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
trial_rewards[i, n] = results['rewards']
trial_optimal[i, n] = results['optimal']
labels = [f'$\epsilon$={e}' for e in epsilons]
fixed = f'$\\alpha$={alpha}'
plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal)
###Output
_____no_output_____
###Markdown
On the left we have plotted the average reward over time, and we see that while $\epsilon=0$ (the greedy policy) does well initially, $\epsilon=0.1$ starts to do slightly better in the long run, while $\epsilon=0.2$ does the worst. Looking on the right, we see the percentage of times the optimal action (the best possible choice at time $t$) was taken, and here again we see a similar pattern of $\epsilon=0.1$ starting out a bit slower but eventually having a slight edge in the longer run.We can also do the same for the learning rates. We will evaluate $\alpha \in [0.01, 0.1, 1.0]$ to a fixed $\epsilon=0.1$.
###Code
# @markdown Execute this cell to see visualization
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
epsilon = 0.1
alphas = [0.01, 0.1, 1.0]
n_trials = 200
trial_rewards = np.zeros((len(epsilons), n_trials, n_steps))
trial_optimal = np.zeros((len(epsilons), n_trials, n_steps))
for i, alpha in enumerate(alphas):
for n in range(n_trials):
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
trial_rewards[i, n] = results['rewards']
trial_optimal[i, n] = results['optimal']
labels = [f'$\\alpha$={a}' for a in alphas]
fixed = f'$\epsilon$={epsilon}'
plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal)
###Output
_____no_output_____
###Markdown
[](https://kaggle.com/kernels/welcome?src=https://raw.githubusercontent.com/NeuromatchAcademy/course-content/master/tutorials/W3D4_ReinforcementLearning/W3D4_Tutorial2.ipynb) Tutorial 2: Learning to Act: Multi-Armed Bandits**Week 3, Day 4: Reinforcement Learning****By Neuromatch Academy**__Content creators:__ Marcelo Mattar and Eric DeWitt with help from Byron Galbraith__Content reviewers:__ Matt Krause and Michael Waskom **Our 2021 Sponsors, including Presenting Sponsor Facebook Reality Labs** --- Tutorial Objectives In this tutorial you will use 'bandits' to understand the fundementals of how a policy interacts with the learning algorithm in reinforcement learning. * You will understand the fundemental tradeoff between exploration and exploitation in a policy.* You will understand how the learning rate interacts with exploration to find the best available action. --- Setup
###Code
# Imports
import numpy as np
import matplotlib.pyplot as plt
#@title Figure settings
import ipywidgets as widgets # interactive display
%config InlineBackend.figure_format = 'retina'
plt.style.use("https://raw.githubusercontent.com/NeuromatchAcademy/course-content/master/nma.mplstyle")
#@title Helper functions
np.set_printoptions(precision=3)
def plot_choices(q, epsilon, choice_fn, n_steps=1000, rng_seed=1):
np.random.seed(rng_seed)
counts = np.zeros_like(q)
for t in range(n_steps):
action = choice_fn(q, epsilon)
counts[action] += 1
fig, ax = plt.subplots()
ax.bar(range(len(q)), counts/n_steps)
ax.set(ylabel='% chosen', xlabel='action', ylim=(0,1), xticks=range(len(q)))
def plot_multi_armed_bandit_results(results):
fig, (ax1, ax2, ax3) = plt.subplots(ncols=3, figsize=(20, 4))
ax1.plot(results['rewards'])
ax1.set(title=f"Total Reward: {np.sum(results['rewards']):.2f}",
xlabel='step', ylabel='reward')
ax2.plot(results['qs'])
ax2.set(xlabel='step', ylabel='value')
ax2.legend(range(len(results['mu'])))
ax3.plot(results['mu'], label='latent')
ax3.plot(results['qs'][-1], label='learned')
ax3.set(xlabel='action', ylabel='value')
ax3.legend()
def plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal):
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(16, 6))
ax1.plot(np.mean(trial_rewards, axis=1).T)
ax1.set(title=f'Average Reward ({fixed})', xlabel='step', ylabel='reward')
ax1.legend(labels)
ax2.plot(np.mean(trial_optimal, axis=1).T)
ax2.set(title=f'Performance ({fixed})', xlabel='step', ylabel='% optimal')
ax2.legend(labels)
###Output
_____no_output_____
###Markdown
--- Section 1: Multi-Armed Bandits
###Code
# @title Video 1: Multi-Armed Bandits
from ipywidgets import widgets
out2 = widgets.Output()
with out2:
from IPython.display import IFrame
class BiliVideo(IFrame):
def __init__(self, id, page=1, width=400, height=300, **kwargs):
self.id=id
src = 'https://player.bilibili.com/player.html?bvid={0}&page={1}'.format(id, page)
super(BiliVideo, self).__init__(src, width, height, **kwargs)
video = BiliVideo(id="", width=854, height=480, fs=1)
print('Video available at https://www.bilibili.com/video/{0}'.format(video.id))
display(video)
out1 = widgets.Output()
with out1:
from IPython.display import YouTubeVideo
video = YouTubeVideo(id="kdiXr1zsfo0", width=854, height=480, fs=1, rel=0)
print('Video available at https://youtube.com/watch?v=' + video.id)
display(video)
out = widgets.Tab([out1, out2])
out.set_title(0, 'Youtube')
out.set_title(1, 'Bilibili')
display(out)
###Output
_____no_output_____
###Markdown
Consider the following learning problem. You are faced repeatedly with a choice among $k$ different options, or actions. After each choice you receive a reward signal in the form of a numerical value, where the larger value is the better. Your objective is to maximize the expected total reward over some time period, for example, over 1000 action selections, or time steps.This is the original form of the k-armed bandit problem. This name derives from the colloquial name for a slot machine, the "one-armed bandit", because it has the one lever to pull, and it is often rigged to take more money than it pays out over time. The multi-armed bandit extension is to imagine, for instance, that you are faced with multiple slot machines that you can play, but only one at a time. Which machine should you play, i.e. which arm should you pull, which action should you take, at any given time to maximize your total payout.While there are many different levels of sophistication and assumptions in how the rewards are determined, for simplicity's sake we will assume that each action results in a reward drawn from a fixed Gaussian distribution with unknown mean and unit variance. This problem setting is referred to as the *environment*, and goal is to find the arm with the highest mean value.We will solve this *optimization problem* with an *agent*, in this case an algorithm that takes in rewards and returns actions. --- Section 2: Choosing an Action The first thing our agent needs to be able to do is choose which arm to pull. The strategy for choosing actions based on our expectations is called a *policy* (often denoted $\pi$). We could have a random policy -- just pick an arm at random each time -- though this doesn't seem likely to be capable of optimizing our reward. We want some intentionality, and to do that we need a way of describing our beliefs about the arms' reward potential. We do this with an action-value function\begin{align}q(a) = \mathbb{E} [r_{t} | a_{t} = a]\end{align}where the value $q$ for taking action $a \in A$ at time $t$ is equal to the expected value of the reward $r_t$ given that we took action $a$ at that time. In practice, this is often represented as an array of values, where each action's value is a different element in the array.Great, now that we have a way to describe our beliefs about the values each action should return, let's come up with a policy.An obvious choice would be to take the action with the highest expected value. This is referred to as the *greedy* policy\begin{align}a_{t} = \text{argmax}_{a} \; q_{t} (a)\end{align}where our choice action is the one that maximizes the current value function.So far so good, but it can't be this easy. And, in fact, the greedy policy does have a fatal flaw: it easily gets trapped in local maxima. It never explores to see what it hasn't seen before if one option is already better than the others. This leads us to a fundamental challenge in coming up with effective polices. Section 2.1: The Exploitation-Exploration DilemmaIf we never try anything new, if we always stick to the safe bet, we don't know what we are missing. Sometimes we aren't missing much of anything, and regret not sticking with our preferred choice, yet other times we stumble upon something new that was way better than we thought.This is the exploitation-exploration dilemma: do you go with you best choice now, or risk the less certain option with the hope of finding something better. Too much exploration, however, means you may end up with a sub-optimal reward once it's time to stop.In order to avoid getting stuck in local minima while also maximizing reward, effective policies need some way to balance between these two aims.A simple extension to our greedy policy is to add some randomness. For instance, a coin flip -- heads we take the best choice now, tails we pick one at random. This is referred to as the $\epsilon$-greedy policy:\begin{align}P (a_{t} = a) = \begin{cases} 1 - \epsilon + \epsilon/N & \quad \text{if } a_{t} = \text{argmax}_{a} \; q_{t} (a) \\ \epsilon/N & \quad \text{else} \end{cases} \end{align}which is to say that with probability 1 - $\epsilon$ for $\epsilon \in [0,1]$ we select the greedy choice, and otherwise we select an action at random (including the greedy option).Despite its relative simplicity, the epsilon-greedy policy is quite effective, which leads to its general popularity. Exercise 1: Implement Epsilon-GreedyIn this exercise you will implement the epsilon-greedy algorithm for deciding which action to take from a set of possible actions given their value function and a probability $\epsilon$ of simply chosing one at random. TIP: You may find [`np.random.random`](https://numpy.org/doc/stable/reference/random/generated/numpy.random.random.html), [`np.random.choice`](https://numpy.org/doc/stable/reference/random/generated/numpy.random.choice.html), and [`np.argmax`](https://numpy.org/doc/stable/reference/generated/numpy.argmax.html) useful here.
###Code
def epsilon_greedy(q, epsilon):
"""Epsilon-greedy policy: selects the maximum value action with probabilty
(1-epsilon) and selects randomly with epsilon probability.
Args:
q (ndarray): an array of action values
epsilon (float): probability of selecting an action randomly
Returns:
int: the chosen action
"""
#####################################################################
## TODO for students: implement the epsilon greedy decision algorithm
# Fill out function and remove
raise NotImplementedError("Student excercise: implement the epsilon greedy decision algorithm")
#####################################################################
# write a boolean expression that determines if we should take the best action
be_greedy = ...
if be_greedy:
# write an expression for selecting the best action from the action values
action = ...
else:
# write an expression for selecting a random action
action = ...
return action
# Uncomment once the epsilon_greedy function is complete
# q = [-2, 5, 0, 1]
# epsilon = 0.1
# plot_choices(q, epsilon, epsilon_greedy)
# to_remove solution
def epsilon_greedy(q, epsilon):
"""Epsilon-greedy policy: selects the maximum value action with probabilty
(1-epsilon) and selects randomly with epsilon probability.
Args:
q (ndarray): an array of action values
epsilon (float): probability of selecting an action randomly
Returns:
int: the chosen action
"""
# write a boolean expression that determines if we should take the best action
be_greedy = np.random.random() > epsilon
if be_greedy:
# write an expression for selecting the best action from the action values
action = np.argmax(q)
else:
# write an expression for selecting a random action
action = np.random.choice(len(q))
return action
q = [-2, 5, 0, 1]
epsilon = 0.1
with plt.xkcd():
plot_choices(q, epsilon, epsilon_greedy)
###Output
_____no_output_____
###Markdown
This is what we should expect, that the action with the largest value (action 1) is selected about (1-$\epsilon$) of the time, or 90% for $\epsilon = 0.1$, and the remaining 10% is split evenly amongst the other options. Use the demo below to explore how changing $\epsilon$ affects the distribution of selected actions. Interactive Demo: Changing EpsilonEpsilon is our one parameter for balancing exploitation and exploration. Given a set of values $q = [-2, 5, 0, 1]$, use the widget below to see how changing $\epsilon$ influences our selection of the max value 5 (action = 1) vs the others. At the extremes of its range (0 and 1), the $\epsilon$-greedy policy reproduces two other policies. What are they?
###Code
#@title
#@markdown Make sure you execute this cell to enable the widget!
@widgets.interact(epsilon=widgets.FloatSlider(0.1, min=0.0, max=1.0))
def explore_epilson_values(epsilon=0.1):
q = [-2, 5, 0, 1]
plot_choices(q, epsilon, epsilon_greedy, rng_seed=None)
#to_remove explanation
"""
When epsilon is zero, the agent always chooses the currently best option; it
becomes greedy. When epsilon is 1, the agent chooses randomly.
""";
###Output
_____no_output_____
###Markdown
--- Section 3: Learning from RewardsNow that we have a policy for deciding what to do, how do we learn from our actions?One way to do this is just keep a record of every result we ever got and use the averages for each action. If we have a potentially very long running episode, the computational cost of keeping all these values and recomputing the mean over and over again isn't ideal. Instead we can use a streaming mean calculation, which looks like this:\begin{align}q_{t+1}(a) \leftarrow q_{t}(a) + \frac{1}{n_t} (r_{t} - q_{t}(a))\end{align}where our action-value function $q_t(a)$ is the mean of the rewards seen so far, $n_t$ is the number of actions taken by time $t$, and $r_t$ is the reward just received for taking action $a$.This still requires us to remember how many actions we've taken, so let's generalize this a bit further and replace the action total with a general parameter $\alpha$, which we will call the learning rate\begin{align}q_{t+1}(a) \leftarrow q_{t}(a) + \alpha (r_{t} - q_{t}(a)).\end{align} Exercise 2: Updating Action ValuesIn this exercise you will implement the action-value update rule above. The function will take in the action-value function represented as an array `q`, the action taken, the reward received, and the learning rate, `alpha`. The function will return the updated value for the selection action.
###Code
def update_action_value(q, action, reward, alpha):
""" Compute the updated action value given the learning rate and observed
reward.
Args:
q (ndarray): an array of action values
action (int): the action taken
reward (float): the reward received for taking the action
alpha (float): the learning rate
Returns:
float: the updated value for the selected action
"""
#####################################################
## TODO for students: compute the action value update
# Fill out function and remove
raise NotImplementedError("Student excercise: compute the action value update")
#####################################################
# write an expression for the updated action value
value = ...
return value
# Uncomment once the update_action_value function is complete
# q = [-2, 5, 0, 1]
# action = 2
# print(f"Original q({action}) value = {q[action]}")
# q[action] = update_action_value(q, 2, 10, 0.01)
# print(f"Updated q({action}) value = {q[action]}")
# to_remove solution
def update_action_value(q, action, reward, alpha):
""" Compute the updated action value given the learning rate and observed
reward.
Args:
q (ndarray): an array of action values
action (int): the action taken
reward (float): the reward received for taking the action
alpha (float): the learning rate
Returns:
float: the updated value for the selected action
"""
# write an expression for the updated action value
value = q[action] + alpha * (reward - q[action])
return value
q = [-2, 5, 0, 1]
action = 2
print(f"Original q({action}) value = {q[action]}")
q[action] = update_action_value(q, 2, 10, 0.01)
print(f"Updated q({action}) value = {q[action]}")
###Output
_____no_output_____
###Markdown
--- Section 4: Solving Multi-Armed BanditsNow that we have both a policy and a learning rule, we can combine these to solve our original multi-armed bandit task. Recall that we have some number of arms that give rewards drawn from Gaussian distributions with unknown mean and unit variance, and our goal is to find the arm with the highest mean.First, let's see how we will simulate this environment by reading through the annotated code below.
###Code
def multi_armed_bandit(n_arms, epsilon, alpha, n_steps):
""" A Gaussian multi-armed bandit using an epsilon-greedy policy. For each
action, rewards are randomly sampled from normal distribution, with a mean
associated with that arm and unit variance.
Args:
n_arms (int): number of arms or actions
epsilon (float): probability of selecting an action randomly
alpha (float): the learning rate
n_steps (int): number of steps to evaluate
Returns:
dict: a dictionary containing the action values, actions, and rewards from
the evaluation along with the true arm parameters mu and the optimality of
the chosen actions.
"""
# Gaussian bandit parameters
mu = np.random.normal(size=n_arms)
# evaluation and reporting state
q = np.zeros(n_arms)
qs = np.zeros((n_steps, n_arms))
rewards = np.zeros(n_steps)
actions = np.zeros(n_steps)
optimal = np.zeros(n_steps)
# run the bandit
for t in range(n_steps):
# choose an action
action = epsilon_greedy(q, epsilon)
actions[t] = action
# copmute rewards for all actions
all_rewards = np.random.normal(mu)
# observe the reward for the chosen action
reward = all_rewards[action]
rewards[t] = reward
# was it the best possible choice?
optimal_action = np.argmax(all_rewards)
optimal[t] = action == optimal_action
# update the action value
q[action] = update_action_value(q, action, reward, alpha)
qs[t] = q
results = {
'qs': qs,
'actions': actions,
'rewards': rewards,
'mu': mu,
'optimal': optimal
}
return results
###Output
_____no_output_____
###Markdown
We can use our multi-armed bandit method to evaluate how our epsilon-greedy policy and learning rule perform at solving the task. First we will set our environment to have 10 arms and our agent parameters to $\epsilon=0.1$ and $\alpha=0.01$. In order to get a good sense of the agent's performance, we will run the episode for 1000 steps.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
n_arms = 10
epsilon = 0.1
alpha = 0.01
n_steps = 1000
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(16, 6))
ax1.plot(results['rewards'])
ax1.set(title=f'Observed Reward ($\epsilon$={epsilon}, $\\alpha$={alpha})',
xlabel='step', ylabel='reward')
ax2.plot(results['qs'])
ax2.set(title=f'Action Values ($\epsilon$={epsilon}, $\\alpha$={alpha})',
xlabel='step', ylabel='value')
ax2.legend(range(n_arms));
###Output
_____no_output_____
###Markdown
Alright, we got some rewards that are kind of all over the place, but the agent seemed to settle in on the first arm as the preferred choice of action relatively quickly. Let's see how well we did at recovering the true means of the Gaussian random variables behind the arms.
###Code
fig, ax = plt.subplots()
ax.plot(results['mu'], label='latent')
ax.plot(results['qs'][-1], label='learned')
ax.set(title=f'$\epsilon$={epsilon}, $\\alpha$={alpha}',
xlabel='action', ylabel='value')
ax.legend();
###Output
_____no_output_____
###Markdown
Well, we seem to have found a very good estimate for action 0, but most of the others are not great. In fact, we can see the effect of the local maxima trap at work -- the greedy part of our algorithm locked onto action 0, which is actually the 2nd best choice to action 6. Since these are the means of Gaussian random variables, we can see that the overlap between the two would be quite high, so even if we did explore action 6, we may draw a sample that is still lower than our estimate for action 0.However, this was just one choice of parameters. Perhaps there is a better combination? Interactive Demo: Changing Epsilon and AlphaUse the widget below to explore how varying the values of $\epsilon$ (exploitation-exploration tradeoff), $\alpha$ (learning rate), and even the number of actions $k$, changes the behavior of our agent.
###Code
#@title
#@markdown Make sure you execute this cell to enable the widget!
@widgets.interact_manual(k=widgets.IntSlider(10, min=2, max=15),
epsilon=widgets.FloatSlider(0.1, min=0.0, max=1.0),
alpha=widgets.FloatLogSlider(0.01, min=-3, max=0))
def explore_bandit_parameters(k=10, epsilon=0.1, alpha=0.001):
results = multi_armed_bandit(k, epsilon, alpha, 1000)
plot_multi_armed_bandit_results(results)
###Output
_____no_output_____
###Markdown
While we can see how changing the epsilon and alpha values impact the agent's behavior, this doesn't give as a great sense of which combination is optimal. Due to the stochastic nature of both our rewards and our policy, a single trial run isn't sufficient to give us this information. Let's run mulitple trials and compare the average performance.First we will look at differet values for $\epsilon \in [0.0, 0.1, 0.2]$ to a fixed $\alpha=0.1$. We will run 200 trials as a nice balance between speed and accuracy.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
epsilons = [0.0, 0.1, 0.2]
alpha = 0.1
n_trials = 200
trial_rewards = np.zeros((len(epsilons), n_trials, n_steps))
trial_optimal = np.zeros((len(epsilons), n_trials, n_steps))
for i, epsilon in enumerate(epsilons):
for n in range(n_trials):
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
trial_rewards[i, n] = results['rewards']
trial_optimal[i, n] = results['optimal']
labels = [f'$\epsilon$={e}' for e in epsilons]
fixed = f'$\\alpha$={alpha}'
plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal)
###Output
_____no_output_____
###Markdown
On the left we have plotted the average reward over time, and we see that while $\epsilon=0$ (the greedy policy) does well initially, $\epsilon=0.1$ starts to do slightly better in the long run, while $\epsilon=0.2$ does the worst. Looking on the right, we see the percentage of times the optimal action (the best possible choice at time $t$) was taken, and here again we see a similar pattern of $\epsilon=0.1$ starting out a bit slower but eventually having a slight edge in the longer run.We can also do the same for the learning rates. We will evaluate $\alpha \in [0.01, 0.1, 1.0]$ to a fixed $\epsilon=0.1$.
###Code
# set for reproducibility, comment out / change seed value for different results
np.random.seed(1)
epsilon = 0.1
alphas = [0.01, 0.1, 1.0]
n_trials = 200
trial_rewards = np.zeros((len(epsilons), n_trials, n_steps))
trial_optimal = np.zeros((len(epsilons), n_trials, n_steps))
for i, alpha in enumerate(alphas):
for n in range(n_trials):
results = multi_armed_bandit(n_arms, epsilon, alpha, n_steps)
trial_rewards[i, n] = results['rewards']
trial_optimal[i, n] = results['optimal']
labels = [f'$\\alpha$={a}' for a in alphas]
fixed = f'$\epsilon$={epsilon}'
plot_parameter_performance(labels, fixed, trial_rewards, trial_optimal)
###Output
_____no_output_____ |
Sequoia Image Processing Tutorial.ipynb | ###Markdown
Parrot Sequoia Image Processing Tutorial OverviewMicasense has made an open python library with image processing tools and tutorials for their RedEdge sensor. These tools are also higly relevant for processing Parrot Sequoia images. I have added some functionality to the micasense library, which enables processing of Sequoia imagery. This tutorial is similar to the `MicaSense RedEdge Image Processing Tutorial 1`. It walks through how to convert Sequoia data from raw images to irradiance normalised with data from the sunshine sensor and then to reflectance. Vignette correction and removal of fish-eye distortion is also part of the workflow.The code is found in the file `sequoiautils.py`. Most of the code has originally been shared by other users in the [Parrot developer forum](https://forum.developer.parrot.com/c/sequoia) (see details in the relevant sections below and in the script).This tutorial assumes you have gone through the basic setup [here](./MicaSense Image Processing Setup.html) and your system is set up and ready to go. Opening an image with pyplotSequoia 16-bit images can be read with pyplot directly into numpy arrays using the pyplot `imread` function or the matplotlib `imread` function, and then we can display the image inline using the `imshow` function of `matplotlib`.
###Code
import cv2
import matplotlib.pyplot as plt
import numpy as np
import os,glob
import math
%matplotlib inline
imagePath = os.path.join('.','data','Sequoia','0077')
imageName = os.path.join(imagePath,'IMG_180413_080658_0000_NIR.TIF')
# Read raw image DN values
# reads 16 bit tif - this will likely not work for 12 bit images
imageRaw=plt.imread(imageName)
# Display the image
fig, ax = plt.subplots(figsize=(8,6))
ax.imshow(imageRaw, cmap='gray')
plt.show()
###Output
_____no_output_____
###Markdown
Adding a colorbarWe will use start by using a plotting function in `micasense.plotutils` that adds a colorbar to the display, so that we can more easily see changes in the values in the images and also see the range of the image values after various conversions. This function also colorizes the grayscale images, so that changes can more easily be seen. Depending on your viewing style, you may prefer a different color map and you can also select that colormap here or browsing the colormaps on the [matplotlib site](https://matplotlib.org/users/colormaps.html).
###Code
import micasense.plotutils as plotutils
# Optional: pick a color map that fits your viewing style
# one of 'gray, viridis, plasma, inferno, magma, nipy_spectral'
plotutils.colormap('viridis')
plotutils.plotwithcolorbar(imageRaw, title='Raw image values with colorbar');
###Output
_____no_output_____
###Markdown
Reading Sequoia MetadataIn order to perform various processing on the images, we need to read the metadata of each image. For this we use ExifTool. We can read standard image capture metadata such as location, UTC time, imager exposure and gain, but also Sequoia specific metadata which can make processing workflows easier.
###Code
import micasense.metadata as metadata
exiftoolPath = None
if os.name == 'nt':
exiftoolPath = 'C:/exiftool/exiftool.exe'
# get image metadata
meta = metadata.Metadata(imageName, exiftoolPath=exiftoolPath)
cameraMake = meta.get_item('EXIF:Make')
cameraModel = meta.get_item('EXIF:Model')
firmwareVersion = meta.get_item('EXIF:Software')
bandName = meta.get_item('XMP:BandName')
print('{0} {1} firmware version: {2}'.format(cameraMake,
cameraModel,
firmwareVersion))
print('Exposure Time: {0} seconds'.format(meta.get_item('EXIF:ExposureTime')))
print('Imager Gain: {0}'.format(meta.get_item('EXIF:ISO')/100.0))
print('Size: {0}x{1} pixels'.format(meta.get_item('EXIF:ImageWidth'),meta.get_item('EXIF:ImageHeight')))
print('Band Name: {0}'.format(bandName))
print('Center Wavelength: {0} nm'.format(meta.get_item('XMP:CentralWavelength')))
print('Bandwidth: {0} nm'.format(meta.get_item('XMP:WavelengthFWHM')))
print('Focal Length: {0}'.format(meta.get_item('EXIF:FocalLength')))
###Output
Parrot Sequoia firmware version: v1.4.1
Exposure Time: 0.003000000027 seconds
Imager Gain: 6.21
Size: 1280x960 pixels
Band Name: NIR
Center Wavelength: 790.0 nm
Bandwidth: 40.0 nm
Focal Length: 3.979999908
###Markdown
Converting raw Sequoia images to irradianceThis step includes vignette correction and conversion of raw image to irradiance using the sensor calibration model.First a vignette map is created following the procedure described in the [Application note: How to correct vignetting in images](https://forum.developer.parrot.com/uploads/default/original/2X/b/b9b5e49bc21baf8778659d8ed75feb4b2db5c45a.pdf). The implemented code is written by [seanmcleod](https://forum.developer.parrot.com/t/vignetting-correction-sample-code/5614). The vignette map will be multiplied by the raw image values to reverse the darkening seen at the image corners. See the `vignette_correction` function for the details of the vignette parameters and their use.```python V = vignette_correction(meta, xDim, yDim)```The conversion of raw image to irradiance is done following the procedure described in the [Application note: Pixel value to irradiance using the sensor calibration model](https://forum.developer.parrot.com/uploads/default/original/2X/3/383261d35e33f1f375ee49e9c7a9b10071d2bf9d.pdf). The procedure is described in more details in this [document](https://onedrive.live.com/?authkey=%21ACzNLk1ORe37aRQ&cid=C34147D823D8DFEF&id=C34147D823D8DFEF%2115414&parId=C34147D823D8DFEF%21106&o=OneUp).
###Code
import micasense.sequoiautils as msutils
SequoiaIrradiance, V = msutils.sequoia_irradiance(meta, imageRaw)
plotutils.plotwithcolorbar(V,'Vignette Factor');
plotutils.plotwithcolorbar(SequoiaIrradiance,'Sequoia irradiance image with vignette factor applied');
###Output
_____no_output_____
###Markdown
Sunshine calibration of Sequoia irradiance imageNext step is to calculate the sunshine irradiance. This is used to normalise the images in an image dataset according to variations in the incomming solar radiation. The implemented code for retrieving the sunshine sensor data is written by [Yu-Hsuan Tu](https://github.com/dobedobedo/Parrot_Sequoia_Image_Handler/tree/master/Modules/Dependency).
###Code
# Sunshine sensor Irradiance
SunIrradiance = msutils.GetSunIrradiance(meta)
print ('Sunshine sensor irradiance: ', SunIrradiance)
# Light calibrated sequoia irradiance
SequoiaIrradianceCalibrated = SequoiaIrradiance/SunIrradiance
plotutils.plotwithcolorbar(SequoiaIrradianceCalibrated,'Light calibrated Sequoia irradiance image');
###Output
280
Sunshine sensor irradiance: 0.14
###Markdown
Convert irradiance to reflectanceThe calibrated iradiance image can now be converted into reflectance. To do this, an image of a reflectance panel with known reflectance is required. The irradiance values of the panel image is then used to determine a scale factor between irradiance and reflectance.For now I do not have a Sequoia example. But the procedure is similar to the RedEdge procedure described in the `MicaSense RedEdge Image Processing Tutorial 1`. Undistorting imagesFinally, lens distortion effects can be removed from the images using the information in the [Application note: How to correct distortion in images](https://forum.developer.parrot.com/uploads/default/original/2X/e/ec302e9e4498cba5165711c2a52fa2c37be10431.pdf). The implemented code is originally written in Matlab by [muzammil360](https://github.com/muzammil360/SeqUDR) and has been modified and rewritten in Python.Generally for photogrammetry processes on raw (or irradiance/reflectance) images, this step is not required, as the photogrammetry process will optimize a lens distortion model as part of it's bulk bundle adjustment.
###Code
# correct for lens distortions to make straight lines straight
undistortedImage = msutils.correct_lens_distortion_sequoia(meta, SequoiaIrradianceCalibrated)
plotutils.plotwithcolorbar(undistortedImage, 'Undistorted image');
###Output
_____no_output_____
###Markdown
Parrot Sequoia Image Processing Tutorial OverviewMicasense has made an open python library with image processing tools and tutorials for their RedEdge sensor. These tools are also higly relevant for processing Parrot Sequoia images. I have added some functionality to the micasense library, which enables processing of Sequoia imagery. This tutorial is similar to the `MicaSense RedEdge Image Processing Tutorial 1`. It walks through how to convert Sequoia data from raw images to irradiance normalised with data from the sunshine sensor and then to reflectance. Vignette correction and removal of fish-eye distortion is also part of the workflow.The code is found in the file `sequoiautils.py`. Most of the code has originally been shared by other users in the [Parrot developer forum](https://forum.developer.parrot.com/c/sequoia) (see details in the relevant sections below and in the script).This tutorial assumes you have gone through the basic setup [here](./MicaSense Image Processing Setup.html) and your system is set up and ready to go. Opening an image with pyplotSequoia 16-bit images can be read with pyplot directly into numpy arrays using the pyplot `imread` function or the matplotlib `imread` function, and then we can display the image inline using the `imshow` function of `matplotlib`.
###Code
import cv2
import matplotlib.pyplot as plt
import numpy as np
import os,glob
import math
%matplotlib inline
imagePath = os.path.join('.','data','Sequoia','DCIM','IX-02-54613_0033')
imageName = os.path.join(imagePath,'IMG_200714_121144_0011_NIR.TIF')
# Read raw image DN values
# reads 16 bit tif - this will likely not work for 12 bit images
imageRaw=plt.imread(imageName)
# Display the image
fig, ax = plt.subplots(figsize=(8,6))
ax.imshow(imageRaw, cmap='gray')
plt.show()
###Output
_____no_output_____
###Markdown
Adding a colorbarWe will use start by using a plotting function in `micasense.plotutils` that adds a colorbar to the display, so that we can more easily see changes in the values in the images and also see the range of the image values after various conversions. This function also colorizes the grayscale images, so that changes can more easily be seen. Depending on your viewing style, you may prefer a different color map and you can also select that colormap here or browsing the colormaps on the [matplotlib site](https://matplotlib.org/users/colormaps.html).
###Code
import micasense.plotutils as plotutils
# Optional: pick a color map that fits your viewing style
# one of 'gray, viridis, plasma, inferno, magma, nipy_spectral'
plotutils.colormap('viridis')
plotutils.plotwithcolorbar(imageRaw, title='Raw image values with colorbar');
###Output
_____no_output_____
###Markdown
Reading Sequoia MetadataIn order to perform various processing on the images, we need to read the metadata of each image. For this we use ExifTool. We can read standard image capture metadata such as location, UTC time, imager exposure and gain, but also Sequoia specific metadata which can make processing workflows easier.
###Code
import micasense.metadata as metadata
exiftoolPath = None
if os.name == 'nt':
exiftoolPath = 'C:/exiftool/exiftool.exe'
# get image metadata
meta = metadata.Metadata(imageName, exiftoolPath=exiftoolPath)
cameraMake = meta.get_item('EXIF:Make')
cameraModel = meta.get_item('EXIF:Model')
firmwareVersion = meta.get_item('EXIF:Software')
bandName = meta.get_item('XMP:BandName')
print('{0} {1} firmware version: {2}'.format(cameraMake,
cameraModel,
firmwareVersion))
print('Exposure Time: {0} seconds'.format(meta.get_item('EXIF:ExposureTime')))
print('Imager Gain: {0}'.format(meta.get_item('EXIF:ISO')/100.0))
print('Size: {0}x{1} pixels'.format(meta.get_item('EXIF:ImageWidth'),meta.get_item('EXIF:ImageHeight')))
print('Band Name: {0}'.format(bandName))
print('Center Wavelength: {0} nm'.format(meta.get_item('XMP:CentralWavelength')))
print('Bandwidth: {0} nm'.format(meta.get_item('XMP:WavelengthFWHM')))
print('Focal Length: {0}'.format(meta.get_item('EXIF:FocalLength')))
###Output
Parrot Sequoia firmware version: v1.7.2
Exposure Time: 0.003000000027 seconds
Imager Gain: 1.0
Size: 1280x960 pixels
Band Name: NIR
Center Wavelength: 790.0 nm
Bandwidth: 40.0 nm
Focal Length: 3.979999908
###Markdown
Converting raw Sequoia images to irradianceThis step includes vignette correction and conversion of raw image to irradiance using the sensor calibration model.First a vignette map is created following the procedure described in the [Application note: How to correct vignetting in images](https://forum.developer.parrot.com/uploads/default/original/2X/b/b9b5e49bc21baf8778659d8ed75feb4b2db5c45a.pdf). The implemented code is written by [seanmcleod](https://forum.developer.parrot.com/t/vignetting-correction-sample-code/5614). The vignette map will be multiplied by the raw image values to reverse the darkening seen at the image corners. See the `vignette_correction` function for the details of the vignette parameters and their use.```python V = vignette_correction(meta, xDim, yDim)```The conversion of raw image to irradiance is done following the procedure described in the [Application note: Pixel value to irradiance using the sensor calibration model](https://forum.developer.parrot.com/uploads/default/original/2X/3/383261d35e33f1f375ee49e9c7a9b10071d2bf9d.pdf). The procedure is described in more details in this [document](https://onedrive.live.com/?authkey=%21ACzNLk1ORe37aRQ&cid=C34147D823D8DFEF&id=C34147D823D8DFEF%2115414&parId=C34147D823D8DFEF%21106&o=OneUp).
###Code
import micasense.sequoiautils as msutils
SequoiaIrradiance, V = msutils.sequoia_irradiance(meta, imageRaw)
plotutils.plotwithcolorbar(V,'Vignette Factor');
plotutils.plotwithcolorbar(SequoiaIrradiance,'Sequoia irradiance image with vignette factor applied');
###Output
_____no_output_____
###Markdown
Sunshine calibration of Sequoia irradiance imageNext step is to calculate the sunshine irradiance. This is used to normalise the images in an image dataset according to variations in the incomming solar radiation. The implemented code for retrieving the sunshine sensor data is written by [Yu-Hsuan Tu](https://github.com/dobedobedo/Parrot_Sequoia_Image_Handler/tree/master/Modules/Dependency).
###Code
# Sunshine sensor Irradiance
SunIrradiance = msutils.GetSunIrradiance(meta)
print ('Sunshine sensor irradiance: ', SunIrradiance)
# Light calibrated sequoia irradiance
SequoiaIrradianceCalibrated = SequoiaIrradiance/SunIrradiance
plotutils.plotwithcolorbar(SequoiaIrradianceCalibrated,'Light calibrated Sequoia irradiance image');
###Output
Sunshine sensor irradiance: 17.01
###Markdown
Convert irradiance to reflectanceThe calibrated iradiance image can now be converted into reflectance. To do this, an image of a reflectance panel with known reflectance is required. The irradiance values of the panel image is then used to determine a scale factor between irradiance and reflectance.For now I do not have a Sequoia example. But the procedure is similar to the RedEdge procedure described in the `MicaSense RedEdge Image Processing Tutorial 1`. Undistorting imagesFinally, lens distortion effects can be removed from the images using the information in the [Application note: How to correct distortion in images](https://forum.developer.parrot.com/uploads/default/original/2X/e/ec302e9e4498cba5165711c2a52fa2c37be10431.pdf). The implemented code is originally written in Matlab by [muzammil360](https://github.com/muzammil360/SeqUDR) and has been modified and rewritten in Python.Generally for photogrammetry processes on raw (or irradiance/reflectance) images, this step is not required, as the photogrammetry process will optimize a lens distortion model as part of it's bulk bundle adjustment.
###Code
# correct for lens distortions to make straight lines straight
undistortedImage = msutils.correct_lens_distortion_sequoia(meta, SequoiaIrradianceCalibrated)
plotutils.plotwithcolorbar(undistortedImage, 'Undistorted image');
###Output
_____no_output_____ |
book/python/04-algo_thinking.ipynb | ###Markdown
Algorithmic Thinking What is Algorithms? An algorithm is a **sequence** combination of finite steps to solve a particular problem. > “Algorithmic thinking is likely to cause the most disruptive paradigm shift in the sciences since quantum mechanics.” —Bernard ChazelleFor example: Multiple two numbers - **Step-1:** Take two inputs(a,b) - **Step-2:** Multiply `a` and `b` and store in `sum`- **Step-3:** Print `sum` Importance of algorithms - To improve the efficiency of a computer program - Proper utilization of resources Algorithmic Thinking: The Ultimate Steps - **Step-1:** Understabd the problem - **Step-2:** Formulate it - **Step-3:** Design an algorithm- **Step-4:** Implement it - **Step-5:** Run the code and solve the original problem Understanding the Problem- Understabd the description of the problem - What are the input/output? - Do a few examples by hand - Think about special cases Formulate the Problem - Think about the data and the best way to represent them(graph, strings, etc)- What mathematical criterion corresponds to the desired output? Design an Algorithm - Is the formulated problem amenable to certain algorithm design technique (greedy, divide-and-conquer etc.)- What data structures match Examples \begin{example}Write a Python Program to Add Two Integers \end{example} - Start - Inputs A, B(INT) - SUM = A + B - PRINT SUM - End
###Code
A = int(input())
B = int(input())
SUM = A+B
print(SUM)
###Output
10
36
46
###Markdown
\begin{example}Write a Python Program to Compute the Average of Two Integers \end{example} - Start - INPUT A, B(INT) - AVG = A+B/2- PRINT AVG - End
###Code
X = int(input())
Y = int(input())
AVG = (X+Y)/2
print(AVG)
###Output
10
36
23.0
|
Notebooks/Analisis_bivariado.ipynb | ###Markdown
**Análisis bivariado - LoL e-sports**
###Code
!pip install pandas_profiling --upgrade
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
import pandas_profiling
pd.set_option('display.max_rows',200)
pd.set_option('display.max_columns',200)
url_teams = 'https://raw.githubusercontent.com/cporras/lol-esports/main/Data/df_teams.csv'
df = pd.read_csv(url_teams)
df.drop(['position','player','champion','game','date','teamkills','teamdeaths','firstbloodkill','firstbloodassist','firstbloodvictim','turretplates','opp_turretplates','heralds','opp_heralds'],axis=1,inplace=True)
df['quadrakills'] = df['quadrakills'].fillna(0)
df['pentakills'] = df['pentakills'].fillna(0)
df.head()
df.shape
###Output
_____no_output_____
###Markdown
**Profiling del data frame**
###Code
features = ['result','gamelength','kills','deaths','assists','doublekills','triplekills','quadrakills','pentakills','firstblood','team kpm','ckpm','firstdragon','dragons','opp_dragons','firstherald','firstbaron','barons','opp_barons','firsttower','towers','opp_towers','firstmidtower','firsttothreetowers','inhibitors','opp_inhibitors','damagetochampions','dpm','damagetakenperminute','wardsplaced','wpm','wardskilled','wcpm','controlwardsbought','totalgold','earned gpm','goldspent','total cs','minionkills','monsterkills','monsterkillsownjungle','monsterkillsenemyjungle','cspm','goldat10','xpat10','csat10','golddiffat10','xpdiffat10','csdiffat10','killsat10','assistsat10','deathsat10','opp_killsat10','opp_assistsat10','opp_deathsat10','goldat15','xpat15','csat15','golddiffat15','xpdiffat15','csdiffat15','killsat15','assistsat15','deathsat15','opp_killsat15','opp_assistsat15','opp_deathsat15']
df_feat = df[features]
profile = pandas_profiling.ProfileReport(df_feat)
profile
###Output
_____no_output_____
###Markdown
El reporte generado es muy pesado y Colab no lo está cargando, genero el archivo en HTML, lo descargo y presento más abajo algunos datos interesante
###Code
profile.to_file('profile_report.html')
###Output
_____no_output_____
###Markdown
Es posible ver el reporte en GitHub: [profile_reporte.html](https://media.githubusercontent.com/media/cporras/lol-esports/main/Data/profile_report.html) (Pesa más de 250MB)
###Code
sns.scatterplot(data = df_feat, y= 'result', x= 'kills')
###Output
_____no_output_____
###Markdown
Media de resultado agrupado por asesinatos del equipo
###Code
df_feat.groupby('kills').mean()['result']
# lo podemos ver graficamente
pd.crosstab(df_feat['kills'],df['result']).plot(kind='barh', stacked=True)
df_feat.groupby('kills').mean()['result'].plot.line()
###Output
_____no_output_____
###Markdown
**Diferencia de Oro a los 15 minutos vs resultado de la partida**
###Code
# golddiffat15 vs result
plt.figure(figsize=(10, 7))
sns.boxplot(df_feat['result'], df_feat['golddiffat15'], palette = 'viridis')
plt.title('Relación entre golddiffat15 y result', fontsize = 20)
plt.show()
###Output
/usr/local/lib/python3.7/dist-packages/seaborn/_decorators.py:43: FutureWarning: Pass the following variables as keyword args: x, y. From version 0.12, the only valid positional argument will be `data`, and passing other arguments without an explicit keyword will result in an error or misinterpretation.
FutureWarning
|
code/model.ipynb | ###Markdown
load training dataset
###Code
# 合并后的数据集
import pandas as pd
from sklearn.model_selection import train_test_split
df = pd.read_csv("../data/dataset/training.csv")
features = ['Chr_', 'Start', 'SIFT_score', 'Polyphen2_HDIV_score', 'Polyphen2_HVAR_score','LRT_score', 'MutationTaster_score', 'MutationAssessor_score', 'FATHMM_score', 'RadialSVM_score', 'VEST3_score', 'CADD_phred', 'A_Ref_', 'A_Alt_']
x_train_all, x_valid_all, y_train, y_valid = train_test_split(df, df.iloc[:, 0], test_size=0.3, random_state=0)
# only need some features as input of classifier
x_train = x_train_all[features]
x_valid = x_valid_all[features]
###Output
_____no_output_____
###Markdown
train the predictor
###Code
# 调参后,分类器使用最优参数
from lightgbm.sklearn import LGBMClassifier
num_leaves = 48
n_estimators = 100
max_depth = 8
learning_rate = 0.05
early_stopping_rounds = 10
num_round = 700
optimized_classifier = LGBMClassifier(boosting="gbdt", num_leaves=100, max_depth=8, learning_rate=0.03, n_estimators=150, metrics='auc', min_child_samples=20, min_child_weight=0.001, bagging_fraction=0.6, feature_fraction=0.5, reg_alpha=0.08, reg_lambda=0.3, num_round=50, early_stopping_rounds=50)
optimized_classifier.fit(x_train, y_train, eval_set=[(x_valid, y_valid)], categorical_feature=["Chr_", "A_Ref_", "A_Alt_"], early_stopping_rounds=early_stopping_rounds)
optimized_classifier
###Output
_____no_output_____
###Markdown
save the model
###Code
optimized_classifier.booster_.save_model('ppsnv_final.model')
###Output
_____no_output_____
###Markdown
visualize the importances of features
###Code
from matplotlib import pyplot as plt
import numpy as np
fig = plt.figure(figsize=(12,4))
min_ = np.sum(optimized_classifier.feature_importances_)
max_ = np.max(optimized_classifier.feature_importances_)
importances = (optimized_classifier.feature_importances_) / (min_)
plt.title("Importance of features used in our model")
plt.xlabel("Features", fontsize=14)
plt.ylabel("Importance", fontsize=14)
feature_names = optimized_classifier.feature_name_
feature_names[1] = "Location"
df = pd.DataFrame({"feature_name":feature_names, "importance": importances})
df.sort_values("importance", ascending=False, inplace=True)
plt.bar(df["feature_name"], df["importance"])
import pandas as pd
plt.xticks(rotation=60)
plt.savefig("features_importances.png", dpi=300, bbox_inches = 'tight')
# 在swissvar上比较不同分类器性能
import pandas as pd
import numpy as np
features = ['Chr', 'Start', 'SIFT_score', 'Polyphen2_HDIV_score', 'Polyphen2_HVAR_score','LRT_score', 'MutationTaster_score', 'MutationAssessor_score', 'FATHMM_score', 'RadialSVM_score', 'VEST3_score', 'CADD_phred', 'A_Ref', 'A_Alt']
labels = ["SIFT_Label", "Polyphen2_HDIV_Label", "Polyphen2_HVAR_Label", "LRT_Label", "MutationTaster_Label", "MutationAssessor_Label", "FATHMM_Label", "RadialSVM_Label", "VEST3_score", "CADD_phred"]
swissvar_test_all = pd.read_csv("filter/swissvar_with_labels.csv")
comparators = ['MCAP', 'REVEL', 'MVP_score','DANN_score', 'GERP++_RS']
swissvar_compare = swissvar_test_all.dropna(subset=comparators)
swissvar_input = swissvar_compare[features]
# 对分数做归一化
comparator_to_normalized = ["MCAP", 'GERP++_RS']
max_min_scaler = lambda x: (x - np.min(x)) / (np.max(x) - np.min(x))
for c in comparators:
if c in comparator_to_normalized:
swissvar_compare[c + "_normalized"] = swissvar_compare[[c]].apply(max_min_scaler)
else:
swissvar_compare[c + "_normalized"] = swissvar_compare[c]
swissvar_output = optimized_classifier.predict_proba(swissvar_input)
swissvar_output = swissvar_output[:, 1]
swissvar_label = swissvar_compare["True Label"]
import sklearn.metrics as me
compare_classifiers = ["MCAP_normalized", "REVEL_normalized", "MVP_score_normalized", "DANN_score_normalized", "GERP++_RS_normalized"]
swissvar_probs = [swissvar_output, swissvar_compare["MCAP_normalized"], swissvar_compare["REVEL_normalized"], swissvar_compare["MVP_score_normalized"], swissvar_compare["DANN_score_normalized"]]
# 画roc曲线
import matplotlib.pyplot as plt
def draw_roc_curve_2(ax, label, pred_probs, classifiers):
fpr_tpr_aucs = []
for pred_prob in pred_probs:
assert(len(pred_prob)==len(label))
fpr, tpr, thres= me.roc_curve(label, pred_prob)
auc = me.auc(fpr, tpr)
fpr_tpr_aucs.append([fpr, tpr, auc])
for i in range(len(fpr_tpr_aucs)):
ax.plot(fpr_tpr_aucs[i][0], fpr_tpr_aucs[i][1], label='{} (AUC={})'.format(classifiers[i], str(round(fpr_tpr_aucs[i][2], 3))))
#绘制对角线
ax.plot([0,1],[0,1],linestyle='--',color='grey')
#调整字体大小
ax.legend(fontsize=12)
ax.set_title("SwissVarSelected")
classifers = ["proposed", "MCAP", "REVEL", "MVP", "DANN", "GERP++"]
draw_roc_curve(swissvar_label, swissvar_probs, classifers)
# 在exovar上比较不同分类器性能
import pandas as pd
import numpy as np
# features = ['Chr', 'Start', 'End', 'Ref', 'Alt', 'SIFT_score', 'Polyphen2_HDIV_score', 'Polyphen2_HVAR_score','LRT_score', 'MutationTaster_score', 'MutationAssessor_score', 'FATHMM_score', 'RadialSVM_score', 'VEST3_score', 'CADD_phred', 'A_Ref', 'A_Alt']
features = ['Chr', 'Start', 'SIFT_score', 'Polyphen2_HDIV_score', 'Polyphen2_HVAR_score','LRT_score', 'MutationTaster_score', 'MutationAssessor_score', 'FATHMM_score', 'RadialSVM_score', 'VEST3_score', 'CADD_phred', 'A_Ref', 'A_Alt']
labels = ["SIFT_Label", "Polyphen2_HDIV_Label", "Polyphen2_HVAR_Label", "LRT_Label", "MutationTaster_Label", "MutationAssessor_Label", "FATHMM_Label", "RadialSVM_Label", "VEST3_score", "CADD_phred"]
exovar_test_all = pd.read_csv("filter/ExoVarFiltered.hg19_multianno.csv")
comparators = ['MCAP', 'REVEL', 'MVP_score','DANN_score', 'GERP++_RS']
exovar_compare = exovar_test_all.dropna(subset=comparators)
exovar_input = exovar_compare[features]
# 对分数做归一化
comparator_to_normalized = ["MCAP", 'GERP++_RS']
max_min_scaler = lambda x: (x - np.min(x)) / (np.max(x) - np.min(x))
for c in comparators:
if c in comparator_to_normalized:
exovar_compare[c + "_normalized"] = exovar_compare[[c]].apply(max_min_scaler)
else:
exovar_compare[c + "_normalized"] = exovar_compare[c]
exovar_output = optimized_classifier.predict_proba(exovar_input)
exovar_output = exovar_output[:, 1]
exovar_label = exovar_compare["True Label"]
import sklearn.metrics as me
compare_classifiers = ["MCAP_normalized", "REVEL_normalized", "MVP_score_normalized", "DANN_score_normalized"]
exovar_probs = [exovar_output, exovar_compare["MCAP_normalized"], exovar_compare["REVEL_normalized"], exovar_compare["MVP_score_normalized"], exovar_compare["DANN_score_normalized"]]
# 画roc曲线
import matplotlib.pyplot as plt
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(20, 8))
def draw_roc_curve_1(ax, label, pred_probs, classifiers):
fpr_tpr_aucs = []
for pred_prob in pred_probs:
assert(len(pred_prob)==len(label))
fpr, tpr, thres= me.roc_curve(label, pred_prob)
auc = me.auc(fpr, tpr)
fpr_tpr_aucs.append([fpr, tpr, auc])
# ax = plt.subplot(121)
for i in range(len(fpr_tpr_aucs)):
ax.plot(fpr_tpr_aucs[i][0], fpr_tpr_aucs[i][1], label='{} (AUC={})'.format(classifiers[i], str(round(fpr_tpr_aucs[i][2], 3))))
#绘制对角线
ax.plot([0,1],[0,1],linestyle='--',color='grey')
ax.set_xlabel("False Positive Rate", fontsize=15)
ax.set_ylabel("True Positive Rate", fontsize=15)
ax.set_title("ExoVar")
ax.text(-0.2, 1, "a.", fontsize=20)
#调整字体大小
ax.legend(fontsize=12)
def draw_roc_curve_2(ax, label, pred_probs, classifiers):
fpr_tpr_aucs = []
for pred_prob in pred_probs:
assert(len(pred_prob)==len(label))
fpr, tpr, thres= me.roc_curve(label, pred_prob)
auc = me.auc(fpr, tpr)
fpr_tpr_aucs.append([fpr, tpr, auc])
# fig, ax = plt.subplots(figsize=(10,8))
# ax = plt.subplots(122)
for i in range(len(fpr_tpr_aucs)):
ax.plot(fpr_tpr_aucs[i][0], fpr_tpr_aucs[i][1], label='{} (AUC={})'.format(classifiers[i], str(round(fpr_tpr_aucs[i][2], 3))))
#绘制对角线
ax.plot([0,1],[0,1],linestyle='--',color='grey')
ax.set_xlabel("False Positive Rate", fontsize=15)
ax.set_ylabel("True Positive Rate", fontsize=15)
#调整字体大小
ax.legend(fontsize=12)
ax.set_title("SwissVarSelected", pad=12)
ax.text(-0.2, 1, "b.", fontsize=20)
# plt.savefig("result.png", dpi=1000, bbox_inches = 'tight')
classifers = ["proposed", "MCAP", "REVEL", "MVP", "DANN"]
draw_roc_curve_1(ax1, exovar_label, exovar_probs, classifers)
draw_roc_curve_2(ax2, swissvar_label, swissvar_probs, classifers)
plt.savefig("result.png", dpi=1000, bbox_inches = 'tight')
###Output
_____no_output_____
###Markdown
1. Import Packages
###Code
# in this section we import the packages used
import os
import cv2
import numpy as np
import pandas as pd
import tensorflow as tf
import tensorflow_probability as tfp
import matplotlib.pyplot as plt
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Conv2D, Flatten, MaxPool2D, MaxPooling2D, Dropout, Activation
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.preprocessing.image import ImageDataGenerator
from tensorflow.keras import backend as K
tfd = tfp.distributions
tfpl = tfp.layers
###Output
_____no_output_____
###Markdown
2. Load and Generate Image
###Code
labels = pd.read_csv('./trainLabels_cropped.csv')
root_dir=os.path.join('./levels')
batch_size=64
img_width=256
img_height=256
# use image generator to import and modulate the images
# Image generator also splits the data in the training dataset and the test dataset
def image_generator(root_dir):
datagen = ImageDataGenerator(rescale=1/255,
#shear_range=0.2,
#zoom_range=0.2,
horizontal_flip=True,
vertical_flip=True,
validation_split=0.2)
train_generator = datagen.flow_from_directory(root_dir,
target_size = (img_width, img_height),
batch_size = batch_size,
class_mode = 'categorical',
subset='training')
test_generator = datagen.flow_from_directory(root_dir,
target_size=(img_width, img_height),
batch_size = batch_size,
class_mode = 'categorical',
subset='validation')
return train_generator, test_generator
# KL divergence funcction below uses the lambda function to pass the an
# input to the Kernel_divergence of either the filpout layer or the reprametrization layer
train_generator, test_generator = image_generator(root_dir)
divergence_fn = lambda q,p,_:tfd.kl_divergence(q,p)/train_generator.samples
###Output
_____no_output_____
###Markdown
3. Bayesian by backprop Moedels In this section we introduced 3 bayesian models:+ Bayesian Model 1 is a Bayesian by back prop model using the reparameterization trick the reparameterization trick is an approximative way in solving Bayesian function+ Bayesian Model 2 is another Bayesian by backprop methods that replaces the reparameterization trick/ reparameterization layers with the flipout layers. The flipout layers use Mont carol approximation to solve the Bayesian function. One advantage of that method is its faster training time and more weights/ more degrees of freedom.+ Bayesian Model 3 is an improvement over Bayesian model 2 with the training process with different layer shapes and different filter sizes. Bayesian Model 1
###Code
# Model 1 below is the deepest modle it uses reprametrization layers to implement bayes by back prop
model_bayes = Sequential([
tfpl.Convolution2DReparameterization(input_shape=(img_width, img_height,3),padding="same",filters=8, kernel_size=16, activation='relu',
kernel_prior_fn = tfpl.default_multivariate_normal_fn,
kernel_posterior_fn=tfpl.default_mean_field_normal_fn(is_singular=False),
kernel_divergence_fn = divergence_fn,
bias_prior_fn = tfpl.default_multivariate_normal_fn,
bias_posterior_fn=tfpl.default_mean_field_normal_fn(is_singular=False),
bias_divergence_fn = divergence_fn),
Conv2D(64, (3,3), activation='relu'),
Conv2D(64, (3,3), activation='relu'),
MaxPooling2D(2,2),
Conv2D(128, (3,3), activation='relu'),
Conv2D(128, (3,3), activation='relu'),
MaxPooling2D(2,2),
Conv2D(256, (3,3), activation='relu'),
Conv2D(256, (3,3), activation='relu'),
Conv2D(256, (3,3), activation='relu'),
Conv2D(256, (3,3), activation='relu'),
Conv2D(256, (3,3), activation='relu'),
MaxPooling2D(2,2),
Flatten(),
Dense(512, activation='relu'),
Dropout(0.2),
tfpl.DenseReparameterization(units=tfpl.OneHotCategorical.params_size(5), activation=None,
kernel_prior_fn = tfpl.default_multivariate_normal_fn,
kernel_posterior_fn=tfpl.default_mean_field_normal_fn(is_singular=False),
kernel_divergence_fn = divergence_fn,
bias_prior_fn = tfpl.default_multivariate_normal_fn,
bias_posterior_fn=tfpl.default_mean_field_normal_fn(is_singular=False),
bias_divergence_fn = divergence_fn
),
tfpl.OneHotCategorical(5)
])
model_bayes.summary()
learning_rate = 0.005
def negative_log_likelihood(y_true, y_pred):
return -y_pred.log_prob(y_true)
###Output
_____no_output_____
###Markdown
Bayesian Model 2
###Code
# Model 2 is similar to modle 1 but replaces the repramaterization layers with filpout layers
# filpout layers use monte carol approximation as a method to control the variance
# flip out layers introduce more parameters (almost double) compared to the previously mentioned reprameterization layers
# flipout layers have been noteiced to train faster
model_bayes = tf.keras.Sequential([
tf.keras.Input(shape=(img_width, img_height, 3),name="basket"),
tfpl.Convolution2DFlipout(16, kernel_size=5, strides=(1,1), data_format="channels_last",
padding="same", activation=tf.nn.relu, name="conv_tfp_1a",
kernel_divergence_fn=divergence_fn),
MaxPool2D(strides=(4,4), pool_size=(4,4), padding="same"),
Conv2D(32, (3,3), activation='relu'),
tfpl.Convolution2DFlipout(32, kernel_size=3, strides=(1,1), data_format="channels_last",
padding="same", activation=tf.nn.relu, name="conv_tfp_1b",
kernel_divergence_fn=divergence_fn),
MaxPool2D(strides=(4,4), pool_size=(4,4), padding="same"),
Conv2D(64, (3,3), activation='relu'),
MaxPooling2D(2,2),
Flatten(),
Dense(1024, activation='relu'),
Dense(512, activation='relu'),
Dropout(0.2),
tfpl.DenseFlipout(units=5, kernel_divergence_fn=divergence_fn),
])
model_bayes.summary()
learning_rate = 1.0e-3
def negative_log_likelihood(y_true, y_pred):
y_pred = tfp.distributions.MultivariateNormalTriL(y_pred)
return -tf.reduce_mean(y_pred.log_prob(y_true))
###Output
_____no_output_____
###Markdown
Bayesian Model 3
###Code
# Model 3 is an imporovment upon Bayesain model 2
# where the layer sizes have been adjusted to provide the best results
model_bayes = tf.keras.Sequential([
tf.keras.Input(shape=(img_width, img_height, 3),name="basket"),
tfpl.Convolution2DFlipout(16, kernel_size=5, strides=(1,1), data_format="channels_last",
padding="same", activation=tf.nn.relu, name="conv_tfp_1a",
kernel_divergence_fn=divergence_fn),
MaxPool2D(strides=(2,2), pool_size=(2,2), padding="same"),
Conv2D(128, (3,3), activation='relu'),
MaxPool2D(strides=(2,2), pool_size=(2,2), padding="same"),
tfpl.Convolution2DFlipout(32, kernel_size=3, strides=(1,1), data_format="channels_last",
padding="same", activation=tf.nn.relu, name="conv_tfp_1b",
kernel_divergence_fn=divergence_fn),
MaxPool2D(strides=(2,2), pool_size=(2,2), padding="same"),
Conv2D(128, (3,3), activation='relu'),
MaxPooling2D(2,2),
Flatten(),
Dense(1024, activation='relu'),
Dense(512, activation='relu'),
Dropout(0.2),
tfpl.DenseFlipout(units=5,activation='softmax', kernel_divergence_fn=divergence_fn),
])
model_bayes.summary()
learning_rate = 1.0e-3
def negative_log_likelihood(y_true, y_pred):
y_pred = tfp.distributions.MultivariateNormalTriL(y_pred)
return -tf.reduce_mean(y_pred.log_prob(y_true))
###Output
_____no_output_____
###Markdown
4. Compile and Train the Model
###Code
# class0-5 is used to track accuracy of different classes (severity levels of the disease)
def class0(y_true, y_pred):
class_id_true = K.argmax(y_true, axis=-1)
class_id_preds = K.argmax(y_pred, axis=-1)
accuracy_mask = K.cast(K.equal(class_id_preds, 0), 'int32')
class_acc_tensor = K.cast(K.equal(class_id_true, class_id_preds), 'int32') * accuracy_mask
class_acc = K.sum(class_acc_tensor) / K.maximum(K.sum(accuracy_mask), 1)
return class_acc
def class1(y_true, y_pred):
class_id_true = K.argmax(y_true, axis=-1)
class_id_preds = K.argmax(y_pred, axis=-1)
accuracy_mask = K.cast(K.equal(class_id_preds, 1), 'int32')
class_acc_tensor = K.cast(K.equal(class_id_true, class_id_preds), 'int32') * accuracy_mask
class_acc = K.sum(class_acc_tensor) / K.maximum(K.sum(accuracy_mask), 1)
return class_acc
def class2(y_true, y_pred):
class_id_true = K.argmax(y_true, axis=-1)
class_id_preds = K.argmax(y_pred, axis=-1)
accuracy_mask = K.cast(K.equal(class_id_preds, 2), 'int32')
class_acc_tensor = K.cast(K.equal(class_id_true, class_id_preds), 'int32') * accuracy_mask
class_acc = K.sum(class_acc_tensor) / K.maximum(K.sum(accuracy_mask), 1)
return class_acc
def class3(y_true, y_pred):
class_id_true = K.argmax(y_true, axis=-1)
class_id_preds = K.argmax(y_pred, axis=-1)
accuracy_mask = K.cast(K.equal(class_id_preds, 3), 'int32')
class_acc_tensor = K.cast(K.equal(class_id_true, class_id_preds), 'int32') * accuracy_mask
class_acc = K.sum(class_acc_tensor) / K.maximum(K.sum(accuracy_mask), 1)
return class_acc
def class4(y_true, y_pred):
class_id_true = K.argmax(y_true, axis=-1)
class_id_preds = K.argmax(y_pred, axis=-1)
accuracy_mask = K.cast(K.equal(class_id_preds, 4), 'int32')
class_acc_tensor = K.cast(K.equal(class_id_true, class_id_preds), 'int32') * accuracy_mask
class_acc = K.sum(class_acc_tensor) / K.maximum(K.sum(accuracy_mask), 1)
return class_acc
model_bayes.compile(loss = negative_log_likelihood,
optimizer = Adam(learning_rate=learning_rate),
metrics=[tf.keras.metrics.CategoricalAccuracy(),class0,class1,class2,class3,class4],
experimental_run_tf_function = False)
history = model_bayes.fit(train_generator,
steps_per_epoch = train_generator.samples // batch_size,
validation_data = test_generator,
validation_steps = test_generator.samples // batch_size,
epochs = 100)
###Output
_____no_output_____
###Markdown
5. Data Analysis
###Code
# this section displays the plots of the model's accuracy and loss over the epochs
np.savez("history_plots.npz",history.history)
fig, (ax1,ax2) = plt.subplots(1,2,figsize=(12, 3))
fig.suptitle("Bayesian Model 2's accuracy and Loss",fontsize=12)
ax1.plot(history.history['categorical_accuracy'],label='train')
ax1.plot(history.history['val_categorical_accuracy'],label='test')
ax1.title.set_text('model accuracy')
ax1.set_ylabel('accuracy')
ax1.set_xlabel('epoch')
ax1.legend()
ax2.plot(history.history['loss'],label='train')
ax2.plot(history.history['val_loss'],label='test')
ax2.title.set_text('model loss')
ax2.set_ylabel('loss')
ax2.set_xlabel('epoch')
ax2.legend()
# the function import and predict calcualtes and dispalys the prediction accuacy
# Since our model provide the prediction in a distribution we take 300 samples form this distribution
# we display all the samples in the 95 percnetile and ignore the rest ( the outliers)
# the result is a bar, and the thickness of the bar represents the variance of prediction/ equivlent to the uncertainty
def import_and_predict_bayes(image, true_label):
#read image
img = cv2.imread(image)
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
#show the image
plt.imshow(img)
plt.axis('off')
img_resize = (cv2.resize(img, dsize=(256, 256), interpolation=cv2.INTER_CUBIC))/255.
predicted_probabilities = np.empty(shape=(300, 5))
predicted_probabilities2 = np.empty(shape=(300, 5))
predicted_probabilities3 = np.empty(shape=(300, 5))
for i in range(300):
predicted_probabilities2[i] =model_bayes.predict(img_resize[np.newaxis,...])
pct_2p5 = np.array([np.percentile(predicted_probabilities2[:, i], 2.5) for i in range(5)])
pct_97p5 = np.array([np.percentile(predicted_probabilities2[:, i], 97.5) for i in range(5)])
fig, ax = plt.subplots(figsize=(12, 6))
bar = ax.bar(np.arange(5), pct_97p5-0.02, color='red')
bar[true_label].set_color('green')
bar = ax.bar(np.arange(5), pct_2p5-0.02, color='white')
ax.set_xticklabels([''] + [x for x in label],fontsize=20)
ax.set_ylim([0, 1])
ax.set_ylabel('Probability',fontsize=16)
plt.show()
# the function import_and_predict2 similar to the previous function in calcuated the prediction accuracy
# yet it takes T=10 samples form the prediction accuracy
#it calcuates the variance
# and form the variance it drives the two types of uncertainty (aleatoric and epistemic)
def import_and_predict2(image_data, label, T):
#read image
img = cv2.imread(image_data)
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
# resize and reshape the image
img_resize = (cv2.resize(img, dsize=(256, 256), interpolation=cv2.INTER_CUBIC))/255.
img_reshape = img_resize[np.newaxis,...]
#predict the image
prediction = model_bayes.predict(img_reshape)
p_hat=[]
for t in range (T):
p_hat.append(model_bayes.predict(img_reshape)[0])
p_hat=np.array(p_hat)
prediction = np.mean(p_hat, axis=0)
label_prediction = label[np.argmax(prediction)]
aleatoric = np.mean(p_hat*(1-p_hat), axis=0)
epistemic = np.mean(p_hat**2, axis=0) - np.mean(p_hat, axis=0)**2
label_prediction = [np.argmax(prediction)]
return prediction, label_prediction, aleatoric, epistemic
# Testing and displaying the clcuated accurca
label = os.listdir(root_dir)
image0_dir = os.path.join(root_dir+'/0/20_right.jpeg')
prediction = import_and_predict_bayes(image0_dir2,label.index('0'))
# This loop passes over all images and calculates the accuracy and uncertinty
input_dir=root_dir
Class_Names=["0","1","2","3","4"]
total_prediction=np.empty(5)
total_label_prediction=np.empty(1)
total_aleatoric=np.empty(5)
total_epistemic=np.empty(5)
total_accuracy=np.empty(1)
total_tracker=np.empty(1)
tracker=np.empty(1)
for Class in Class_Names:
path = os.path.join(input_dir,Class)
files = os.listdir(path)
for file in files:
T=10 # T should be 2 or greater
image_dir=os.path.join(path,file)
prediction, label_prediction, aleatoric, epistemic=import_and_predict2(image_dir, label,T)
total_prediction=np.vstack((total_prediction,prediction))
total_label_prediction=np.vstack((total_label_prediction,label_prediction))
total_aleatoric=np.vstack((total_aleatoric,aleatoric))
total_epistemic=np.vstack((total_epistemic,epistemic))
tracker=Class_Names.index(Class)
total_tracker=np.vstack((total_tracker,tracker))
if(int("".join(map(str, label_prediction)))==Class_Names.index(Class)):
total_accuracy=np.vstack((total_accuracy,1))
else:
total_accuracy=np.vstack((total_accuracy,0))
total_prediction1=total_prediction[1:,:]
total_label_prediction1=total_label_prediction[1:,:]
total_aleatoric1=total_aleatoric[1:,:]
total_epistemic1=total_epistemic[1:,:]
total_accuracy1=total_accuracy[1:,:]
total_tracker1=total_tracker[1:,:]
np.savez("dataDR_bayes_700.npz",total_prediction1,total_label_prediction1,total_aleatoric1,total_epistemic1,total_accuracy1,total_tracker1)
###Output
_____no_output_____
###Markdown
Preprocess funnction
###Code
import re
def pre_process(text):
#Data Cleaning
# lowercase
text=text.lower()
#remove tags
text=re.sub("<!--?.*?-->","",text)
# remove special characters and digits
text=re.sub("(\\d|\\W)+"," ",text)
return text
# keyword extraction
def sort_coo(coo_matrix):
tuples = zip(coo_matrix.col, coo_matrix.data)
return sorted(tuples, key=lambda x: (x[1], x[0]), reverse=True)
def extract_topn_from_vector(feature_names, sorted_items, topn=10):
"""get the feature names and tf-idf score of top n items"""
sorted_items = sorted_items[:topn]
score_vals = []
feature_vals = []
# word index and corresponding tf-idf score
for idx, score in sorted_items:
#keep track of feature name and its corresponding score
score_vals.append(round(score, 3))
feature_vals.append(feature_names[idx])
#results = zip(feature_vals,score_vals)
results= {}
for idx in range(len(feature_vals)):
results[feature_vals[idx]]=score_vals[idx]
return results
#this is a mapping of index to
feature_names=tfidf.get_feature_names()
# get the document that we want to extract keywords from
doc=X['text']
#generate tf-idf for the given document
tf_idf_vector=tfidf.transform(doc)
#sort the tf-idf vectors by descending order of scores
sorted_items=sort_coo(tf_idf_vector.tocoo())
#extract only the top n
keywords=extract_topn_from_vector(feature_names,sorted_items,10)
print("\n===Keywords===")
x = []
values = []
for k in keywords:
x.append(k)
values.append(keywords[k])
print(k,keywords[k])
import matplotlib
font = {'family' : 'normal',
'weight' : 'bold',
'size' : 34}
matplotlib.rc('font', **font)
plt.figure(figsize=(70, 30))
plt.bar(x, values)
plt.savefig('keywords.png')
###Output
findfont: Font family ['normal'] not found. Falling back to DejaVu Sans.
###Markdown
Read and preprocess data
###Code
X = pd.read_csv('development.csv')
test_set = pd.read_csv('evaluation.csv')
y_train = X['class']
X['text'] = X['text'].apply(lambda x:pre_process(x))
test_set['text'] = test_set['text'].apply(lambda x:pre_process(x))
#show the second 'text' just for fun
X['text']
###Output
_____no_output_____
###Markdown
GridSearch and cross validation
###Code
from sklearn.feature_extraction.text import CountVectorizer, TfidfVectorizer
from stop_words import get_stop_words
from sklearn.model_selection import GridSearchCV
from sklearn.linear_model import SGDClassifier
from sklearn.pipeline import Pipeline
from sklearn.metrics import f1_score, make_scorer
text_clf = Pipeline([
('tfidf', TfidfVectorizer(ngram_range=(1,2), stop_words=stop_words, use_idf=True,
max_df=0.35)),
('clf', SGDClassifier(loss='hinge', penalty='l2', random_state=42, max_iter=500,
class_weight= {'pos':1, 'neg':3},tol=1e-5, n_jobs=-1, alpha=1e-5)),
])
stop_words = get_stop_words('it')
parameters = {}
def my_f1(y_true, y_pred):
return f1_score(y_true, y_pred, pos_label='pos')
f1 = make_scorer(my_f1)
gs_clf = GridSearchCV(text_clf, parameters, cv=5, n_jobs=-1, scoring=f1)
gs_clf = gs_clf.fit(X['text'], X['class'])
gs_clf.best_estimator_[0]
gs_clf.best_score_
df = pd.DataFrame()
y_test = gs_clf.predict(test_set['text'])
df['Id'] = [j for j in range(0, y_test.shape[0])]
df['Predicted'] =y_test
df.to_csv('76_fine_tuned_SGD.csv', index=False)
###Output
_____no_output_____
###Markdown
Setting Vectorizer
###Code
# in case u need the emojis use this pattern for any tokenizer: r'[^\s]+'
# vectorizer classes are defined here
# count_vec = CountVectorizer(token_pattern=r'[^\s]+')
tfidf = TfidfVectorizer(ngram_range=(1,2), stop_words=stop_words, use_idf=True,
max_df=0.35)
count_vec.fit(X['text'].values)
tfidf.fit(X['text'].values)
###Output
_____no_output_____
###Markdown
Vectorization
###Code
X_train1 = count_vec.transform(X['text'].values)
X_train2 = tfidf.transform(X['text'].values)
# tsvd = TruncatedSVD(n_components=50).fit(X_train2)
###Output
_____no_output_____
###Markdown
Classification
###Code
from sklearn.linear_model import LogisticRegression
from sklearn.ensemble import RandomForestClassifier, GradientBoostingClassifier
from sklearn.svm import LinearSVC
from sklearn.datasets import make_classification
###Output
_____no_output_____
###Markdown
logistic regression parameters (C)
###Code
c_vals = [2000,5000,7000,10000]
c_vals
models = []
# X_train=tsvd.transform(X_train2)
for c in tqdm(c_vals):
model= LogisticRegression(C=c, random_state=700,penalty='l1',solver='liblinear')
model.fit(X_train2, X['class'])
models.append(model)
# model=LinearSVC(random_state=0, tol=1e-5)
# model.fit(X_train1, y_train)
probs = pd.DataFrame()
X_test = tfidf.transform(test_set['text'].values)
# X_test=tsvd.transform(X_test)
for i in tqdm(range(len(models))):
probs['prob_'+str(i)] = models[i].predict_proba(X_test)[:,1]
###Output
100%|██████████| 4/4 [00:53<00:00, 13.42s/it]
100%|██████████| 4/4 [00:00<00:00, 76.24it/s]
###Markdown
undersampling and model averaging
###Code
n = 301
# fnumber = 74
transform = 0
name = str(fnumber)+'_'+str(n)+'_probs_c110_log_undrsmpl_tfidf_18k_rep_true_l1_liblinear.csv'
vectorizers = [tfidf, count_vec]
test_set = pd.read_csv('evaluation.csv')
sel_pos = (X['class']=='pos')
sel_neg = (X['class']=='neg')
models = []
for i in tqdm(range(n)):
X_pos = X[sel_pos].sample(9222, replace=True)
X_neg = X[sel_neg].sample(9222, replace=True)
X_balanced = pd.concat([X_neg, X_pos]).sample(frac=1)
X_train = tfidf.transform(X_balanced['text'].values)
model = SGDClassifier(loss='hinge', penalty='l2', random_state=42, max_iter=500,
tol=1e-5, n_jobs=-1, alpha=1e-5)
X_balanced['class'] = X_balanced['class'].replace('pos',1)
X_balanced['class'] = X_balanced['class'].replace('neg',0)
if transform == 1:
model.fit(X_train, X_balanced['class'])
else:
model.fit(X_train, X_balanced['class'])
models.append(model)
probs = pd.DataFrame()
X_test = tfidf.transform(test_set['text'].values)
loss = 'hing'
for i in tqdm(range(n)):
if loss=='hing':
probs['prob_'+str(i)] = models[i].predict(X_test)
else:
probs['prob_'+str(i)] = models[i].predict_proba(X_test)[:,1]
probs.to_csv(name, index=False)
fnumber+=1
# X_balanced
y_prob = probs.mean(axis=1)
y_test=y_prob>=0.5
df=pd.DataFrame()
a = []
# i=12
submission_name = 'aggr_'+name
for y in y_test:
if y:
a.append('pos')
else:
a.append('neg')
df['Id'] = [i for i in range(0, y_test.shape[0])]
df['Predicted'] = a
df.to_csv(submission_name, index=False)
###Output
_____no_output_____
###Markdown
Best Models Details
###Code
# score :96
SGDClassifier(alpha=1e-05, average=False, class_weight=None,
early_stopping=False, epsilon=0.1, eta0=0.0, fit_intercept=True,
l1_ratio=0.15, learning_rate='optimal', loss='hinge', max_iter=10,
n_iter_no_change=5, n_jobs=None, penalty='l2', power_t=0.5,
random_state=42, shuffle=True, tol=None, validation_fraction=0.1,
verbose=0, warm_start=False)
TfidfVectorizer(analyzer='word', binary=False, decode_error='strict',
dtype=<class 'numpy.float64'>, encoding='utf-8',
input='content', lowercase=True, max_df=0.85, max_features=None,
min_df=1, ngram_range=(1, 2), norm='l2', preprocessor=None,
smooth_idf=True,
stop_words=stop_words,
strip_accents=None, sublinear_tf=False,
token_pattern='(?u)\\b\\w\\w+\\b', tokenizer=None, use_idf=True,
vocabulary=None)
# score :96.3
SGDClassifier(alpha=1e-05, average=False, class_weight=None,
early_stopping=False, epsilon=0.1, eta0=0.0, fit_intercept=True,
l1_ratio=0.15, learning_rate='optimal', loss='hinge', max_iter=20,
n_iter_no_change=5, n_jobs=None, penalty='l2', power_t=0.5,
random_state=42, shuffle=True, tol=None, validation_fraction=0.1,
verbose=0, warm_start=False)
TfidfVectorizer(analyzer='word', binary=False, decode_error='strict',
dtype=<class 'numpy.float64'>, encoding='utf-8',
input='content', lowercase=True, max_df=0.75, max_features=None,
min_df=1, ngram_range=(1, 2), norm='l2', preprocessor=None,
smooth_idf=True,
stop_words=['a', 'abbia', 'abbiamo', 'abbiano', 'abbiate', 'ad',
'adesso', 'agl', 'agli', 'ai', 'al', 'all', 'alla',
'alle', 'allo', 'allora', 'altre', 'altri', 'altro',
'anche', 'ancora', 'avemmo', 'avendo', 'avere',
'avesse', 'avessero', 'avessi', 'avessimo',
'aveste', 'avesti', ...],
strip_accents=None, sublinear_tf=False,
token_pattern='(?u)\\b\\w\\w+\\b', tokenizer=None, use_idf=True,
vocabulary=None)
#score :97.41
SGDClassifier(alpha=1e-05, average=False, class_weight={'neg': 3, 'pos': 1},
early_stopping=False, epsilon=0.1, eta0=0.0, fit_intercept=True,
l1_ratio=0.15, learning_rate='optimal', loss='hinge',
max_iter=500, n_iter_no_change=5, n_jobs=-1, penalty='l2',
power_t=0.5, random_state=42, shuffle=True, tol=1e-05,
validation_fraction=0.1, verbose=0, warm_start=False)
TfidfVectorizer(analyzer='word', binary=False, decode_error='strict',
dtype=<class 'numpy.float64'>, encoding='utf-8',
input='content', lowercase=True, max_df=0.35, max_features=None,
min_df=1, ngram_range=(1, 2), norm='l2', preprocessor=None,
smooth_idf=True,
stop_words=['a', 'abbia', 'abbiamo', 'abbiano', 'abbiate', 'ad',
'adesso', 'agl', 'agli', 'ai', 'al', 'all', 'alla',
'alle', 'allo', 'allora', 'altre', 'altri', 'altro',
'anche', 'ancora', 'avemmo', 'avendo', 'avere',
'avesse', 'avessero', 'avessi', 'avessimo',
'aveste', 'avesti', ...],
strip_accents=None, sublinear_tf=False,
token_pattern='(?u)\\b\\w\\w+\\b', tokenizer=None, use_idf=True,
vocabulary=None)
###Output
_____no_output_____
###Markdown
###Code
import
###Output
_____no_output_____
###Markdown
Pre-processing TO-DO: - Impute missing values (dont use fillna) - Work with categorical variables (wind direction -> one hot) - Create date related features (day of week, month, etc) Temporary pre processing Drop columns with all NaNs or 1 unique value, drop categorical data
###Code
df = df[df.columns[df.nunique()>1]]
###Output
_____no_output_____
###Markdown
Wind: -direccion M_DD(cat), -v_media M_Ff, -> numerical columns, no missing values -v_maxima en 10 mins anteriores M_ff10, -> Fill missing values with 0 -maxima en periodo anterior M_ff3 -> Fill missing values with 0 Supossing that missing data in maximum wind gust columns corresponds to no wind, we set theses values to 0
###Code
df.fillna({'M_ff10':0,'M_ff3':0}, inplace = True)
###Output
D:\ProgramData\Anaconda3\lib\site-packages\pandas\core\generic.py:4355: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame
See the caveats in the documentation: http://pandas.pydata.org/pandas-docs/stable/indexing.html#indexing-view-versus-copy
self._update_inplace(new_data)
###Markdown
Categorical weather from station: -M_WW -> If no data, set to 0 (it will be treated as a new category). It seems that only data is present if there is rain, so 0 can be interpreted as no-rain -M_W1 -> drop, very sparse data (TO BE REVIEWED) -M_W2 -> drop, very sparse data (TO BE REVIEWED)
###Code
df.loc[df['M_WW']==' ', 'M_WW'] = 0
df.drop(columns= ['M_W1', 'M_W2'], inplace= True)
###Output
D:\ProgramData\Anaconda3\lib\site-packages\pandas\core\indexing.py:537: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: http://pandas.pydata.org/pandas-docs/stable/indexing.html#indexing-view-versus-copy
self.obj[item] = s
D:\ProgramData\Anaconda3\lib\site-packages\ipykernel_launcher.py:2: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame
See the caveats in the documentation: http://pandas.pydata.org/pandas-docs/stable/indexing.html#indexing-view-versus-copy
###Markdown
Tn, Tx, min y max temp en las 12 horas previasDrop them
###Code
df.drop(columns= ['M_Tn', 'M_Tx'], inplace= True)
###Output
D:\ProgramData\Anaconda3\lib\site-packages\ipykernel_launcher.py:1: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame
See the caveats in the documentation: http://pandas.pydata.org/pandas-docs/stable/indexing.html#indexing-view-versus-copy
"""Entry point for launching an IPython kernel.
###Markdown
PrecipitationsM_RRR, set no precipitation to 0
###Code
df.loc[df['M_RRR']=='No precipitation', 'M_RRR'] = 0
###Output
D:\ProgramData\Anaconda3\lib\site-packages\pandas\core\indexing.py:537: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: http://pandas.pydata.org/pandas-docs/stable/indexing.html#indexing-view-versus-copy
self.obj[item] = s
###Markdown
Categorical columns encodings, train test split, normalization
###Code
cat_columns = ['M_DD', 'M_WW']
for col in cat_columns:
df = pd.concat([df,pd.get_dummies(df[col], prefix=col)],axis=1).drop([col],axis=1)
Y = df['Y']
df.drop(columns= ['date', 'Y'], inplace= True)
train = df.iloc[0:100].copy()
test = df.iloc[8001:].copy()
Y_train = Y.iloc[0:100].copy()
Y_test = Y.iloc[8001:].copy()
train = df.iloc[0:8000].copy()
test = df.iloc[8001:].copy()
Y_train = Y.iloc[0:8000].copy()
Y_test = Y.iloc[8001:].copy()
cs = MinMaxScaler()
train = cs.fit_transform(train)
test = cs.transform(test)
import seaborn as sns
sns.pairplot(data = df)
###Output
_____no_output_____
###Markdown
Fake image data
###Code
images_train = np.random.randn(len(train), 10,10,1 )
images_test = np.random.randn(len(test), 10,10,1 )
images_train.shape
train.shape
###Output
_____no_output_____
###Markdown
Model
###Code
from keras import metrics
from keras.losses import mean_squared_error
from keras.callbacks import TensorBoard
from keras import backend as K
from keras.optimizers import Adam
from keras.layers import Dense, Flatten, Input, Conv2D, Conv2DTranspose, BatchNormalization, Activation, MaxPooling2D, concatenate, Dropout
from keras.models import Model
# Callbacks:
# tensorboard --logdir=D:\imageColorization\logs
tensorboarder = TensorBoard(log_dir='./logs', histogram_freq=0, batch_size=32, write_graph=True, write_grads=False, write_images=False, embeddings_freq=0, embeddings_layer_names=None, embeddings_metadata=None, embeddings_data=None, update_freq='epoch')
numeric_input = Input(shape=(train.shape[1],))
image_input = Input(shape=(10,10,1))
# Convolution
def convBlock(x, number_of_filters, kernel_size = (3,3)):
x = Conv2D(number_of_filters, kernel_size, strides = 1, padding="same", activation='relu')(x)
x = Dropout(0)(x) # No dropout for now
x = BatchNormalization()(x)
return x
x = convBlock(image_input, 16)
#x = convBlock(x, 4)
x = convBlock(x, 16, kernel_size = (2,2))
x = MaxPooling2D(pool_size=(2, 2))(x)
x = convBlock(x, 32, kernel_size = (2,2))
x = MaxPooling2D(pool_size=(2, 2))(x)
image_output = Flatten()(x)
merged = concatenate([image_output, numeric_input])
use_images=True
if use_images:
x = Dense(40, activation="relu")(merged)
else:
x = Dense(1000, activation="sigmoid")(numeric_input)
x = BatchNormalization()(x)
x = Dense(500, activation="relu")(x)
x = BatchNormalization()(x)
# x = Dense(500, activation="tanh")(x)
#x = Dropout(0.5)(x)
x = Dense(1, activation="linear")(x)
if use_images:
model = Model(inputs=[image_input, numeric_input], outputs=x)
else:
model = Model(inputs=numeric_input, outputs=x)
opt = Adam(lr=1e-4, beta_1=0.9, beta_2=0.999, decay=0)
model.compile(loss='mean_squared_error',#mean_absolute_error_none,#
optimizer=opt,
metrics=['mae'])
model.summary()
from keras.utils import plot_model
plot_model(model, show_shapes=True, show_layer_names=True, to_file='model.png')
from IPython.display import Image
Image(retina=True, filename='model.png')
if use_images:
hist = model.fit([images_train, train], Y_train.astype(float),
validation_data = ([images_test, test], Y_test.astype(float)),
epochs=1000)
else:
hist = model.fit(train, Y_train.astype(float),
validation_data = (test, Y_test.astype(float)),
epochs=20, callbacks = [tensorboarder], shuffle = True)
import matplotlib.pyplot as plt
plt.plot(hist.history['val_loss'][0:], label='val')
plt.plot(hist.history['loss'][0:], label='loss')
plt.legend()
import matplotlib.pyplot as plt
plt.plot(hist.history['loss'][0:], label='loss')
plt.legend()
import matplotlib.pyplot as plt
plt.plot(hist.history['val_loss'][:], label='val')
plt.legend()
test[3]
predictions = model.predict(test)
predictions = model.predict([images_test, test])
((Y_test.mean()-Y_test)**2).pow(1./2).mean()
((predictions.squeeze()-Y_test)**2).pow(1./2).mean()
predictions
import matplotlib.pyplot as plt
plt.scatter(predictions, Y_test)
plt.hist(Y_test, bins=100)
###Output
_____no_output_____ |
notebooks/11.02-Understanding-Cross-Validation-Bootstrapping-and-McNemar's-Test.ipynb | ###Markdown
*This notebook contains an excerpt from the book [Machine Learning for OpenCV](https://www.packtpub.com/big-data-and-business-intelligence/machine-learning-opencv) by Michael Beyeler.The code is released under the [MIT license](https://opensource.org/licenses/MIT),and is available on [GitHub](https://github.com/mbeyeler/opencv-machine-learning).**Note that this excerpt contains only the raw code - the book is rich with additional explanations and illustrations.If you find this content useful, please consider supporting the work by[buying the book](https://www.packtpub.com/big-data-and-business-intelligence/machine-learning-opencv)!* Understanding Cross-ValidationCross-validation is a method of evaluating the generalization performance of a model thatis generally more stable and thorough than splitting the dataset into training and test sets.The most commonly used version of cross-validation is $k$-fold cross-validation, where $k$ is anumber specified by the user (usually five or ten). Here, the dataset is partitioned into kparts of more or less equal size, called folds. For a dataset that contains $N$ data points, eachfold should thus have approximately $N / k$ samples. Then a series of models is trained onthe data, using $k - 1$ folds for training and one remaining fold for testing. The procedure isrepeated for $k$ iterations, each time choosing a different fold for testing, until every fold hasserved as a test set once.Refer to the book for an illustration of $k$-fold cross-validation for different values of $k$. Do you know what makes cross-validation different from just splitting the data into training and test sets? Manually implementing cross-validation in OpenCVThe easiest way to perform cross-validation in OpenCV is to do the data splits by hand.For example, in order to implement two-fold cross-validation, we would follow thefollowing procedure.Load the dataset:
###Code
from sklearn.datasets import load_iris
import numpy as np
iris = load_iris()
X = iris.data.astype(np.float32)
y = iris.target
###Output
_____no_output_____
###Markdown
Split the data into two equally sized parts:
###Code
from sklearn.model_selection import train_test_split
X_fold1, X_fold2, y_fold1, y_fold2 = train_test_split(
X, y, random_state=37, train_size=0.5
)
###Output
_____no_output_____
###Markdown
Instantiate the classifier:
###Code
import cv2
knn = cv2.ml.KNearest_create()
knn.setDefaultK(1)
###Output
_____no_output_____
###Markdown
Train the classifier on the first fold, then predict the labels of the second fold:
###Code
knn.train(X_fold1, cv2.ml.ROW_SAMPLE, y_fold1)
_, y_hat_fold2 = knn.predict(X_fold2)
###Output
_____no_output_____
###Markdown
Train the classifier on the second fold, then predict the labels of the first fold:
###Code
knn.train(X_fold2, cv2.ml.ROW_SAMPLE, y_fold2)
_, y_hat_fold1 = knn.predict(X_fold1)
###Output
_____no_output_____
###Markdown
Compute accuracy scores for both folds:
###Code
from sklearn.metrics import accuracy_score
accuracy_score(y_fold1, y_hat_fold1)
accuracy_score(y_fold2, y_hat_fold2)
###Output
_____no_output_____
###Markdown
This procedure will yield two accuracy scores, one for the first fold (92% accuracy), and onefor the second fold (88% accuracy). On average, our classifier thus achieved 90% accuracyon unseen data. Automating cross-validation using scikit-learnInstantiate the classifier:
###Code
from sklearn.neighbors import KNeighborsClassifier
model = KNeighborsClassifier(n_neighbors=1)
###Output
_____no_output_____
###Markdown
Perform cross-validation with the cross_val_score function. This functiontakes as input a model, the full dataset (`X`), the target labels (`y`) and an integervalue for the number of folds (`cv`). It is not necessary to split the data byhand—the function will do that automatically depending on the number of folds.After the cross-validation is completed, the function returns the test scores:
###Code
from sklearn.model_selection import cross_val_score
scores = cross_val_score(model, X, y, cv=5)
scores
###Output
_____no_output_____
###Markdown
In order to get a sense how the model did on average, we can look at the mean andstandard deviation of the five scores:
###Code
scores.mean(), scores.std()
###Output
_____no_output_____
###Markdown
With five folds, we have a much better idea about how robust the classifier is on average.We see that $k$-NN with $k=1$ achieves on average 96% accuracy, and this value fluctuatesfrom run to run with a standard deviation of roughly 2.5%. Implementing leave-one-out cross-validationAnother popular way to implement cross-validation is to choose the number of folds equalto the number of data points in the dataset. In other words, if there are $N$ data points, we set$k=N$. This means that we will end up having to do $N$ iterations of cross-validation, but inevery iteration, the training set will consist of only a single data point. The advantage of thisprocedure is that we get to use all-but-one data point for training. Hence, this procedure isalso known as leave-one-out cross-validation.In scikit-learn, this functionality is provided by the `LeaveOneOut` method from the`model_selection` module:
###Code
from sklearn.model_selection import LeaveOneOut
###Output
_____no_output_____
###Markdown
This object can be passed directly to the `cross_val_score` function in the following way:
###Code
scores = cross_val_score(model, X, y, cv=LeaveOneOut())
###Output
_____no_output_____
###Markdown
Because every test set now contains a single data point, we would expect the scorer toreturn 150 values—one for each data point in the dataset. Each of these points we could geteither right or wrong. Thus, we expect `scores` to be a list of ones (1) and zeros (0), whichcorresponds to correct and incorrect classifications, respectively:
###Code
scores
###Output
_____no_output_____
###Markdown
If we want to know the average performance of the classifier, we would still compute themean and standard deviation of the scores:
###Code
scores.mean(), scores.std()
###Output
_____no_output_____
###Markdown
We can see this scoring scheme returns very similar results to five-fold cross-validation. Estimating robustness using bootstrappingAn alternative procedure to $k$-fold cross-validation is **bootstrapping**.Instead of splitting the data into folds, bootstrapping builds a training set by drawingsamples randomly from the dataset. Typically, a bootstrap is formed by drawing sampleswith replacement. Imagine putting all of the data points into a bag and then drawingrandomly from the bag. After drawing a sample, we would put it back in the bag. Thisallows for some samples to show up multiple times in the training set, which is somethingcross-validation does not allow.The classifier is then tested on all samples that are not part of the bootstrap (the so-called**out-of-bag** examples), and the procedure is repeated a large number of times (say, 10,000times). Thus, we get a distribution of the model's score that allows us to estimate therobustness of the model. Bootstrapping can be implemented with the following procedure.Instantiate the classifier:
###Code
knn = cv2.ml.KNearest_create()
knn.setDefaultK(1)
###Output
_____no_output_____
###Markdown
From our dataset with $N$ samples, randomly choose $N$ samples with replacementto form a bootstrap. This can be done most easily with the choice function fromNumPy's random module. We tell the function to draw len(`X`) samples in therange `[0, len(X)-1]` with replacement (`replace=True`). The function thenreturns a list of indices, from which we form our bootstrap:
###Code
idx_boot = np.random.choice(len(X), size=len(X), replace=True)
X_boot = X[idx_boot, :]
y_boot = y[idx_boot]
###Output
_____no_output_____
###Markdown
Put all samples that do not show in the bootstrap in the out-of-bag set:
###Code
idx_oob = np.array([x not in idx_boot
for x in np.arange(len(X))], dtype=np.bool)
X_oob = X[idx_oob, :]
y_oob = y[idx_oob]
###Output
_____no_output_____
###Markdown
Train the classifier on the bootstrap samples:
###Code
knn.train(X_boot, cv2.ml.ROW_SAMPLE, y_boot)
###Output
_____no_output_____
###Markdown
Test the classifier on the out-of-bag samples:
###Code
_, y_hat = knn.predict(X_oob)
accuracy_score(y_oob, y_hat)
###Output
_____no_output_____
###Markdown
Then we want to repeat these steps up to 10,000 times to get 10,000accuracy scores, then average the scores to get an idea of the classifier's meanperformance.For our convenience, we can build a function so that it is easy to run theprocedure for some `n_iter` number of times. We also pass a model (our $k$-NN classifier,`model`), the feature matrix (`X`), and the vector with all class labels (`y`):
###Code
def yield_bootstrap(model, X, y, n_iter=10000):
for _ in range(n_iter):
# train the classifier on bootstrap
idx_boot = np.random.choice(len(X), size=len(X),
replace=True)
X_boot = X[idx_boot, :]
y_boot = y[idx_boot]
model.train(X_boot, cv2.ml.ROW_SAMPLE, y_boot)
# test classifier on out-of-bag examples
idx_oob = np.array([x not in idx_boot
for x in np.arange(len(X))],
dtype=np.bool)
X_oob = X[idx_oob, :]
y_oob = y[idx_oob]
_, y_hat = model.predict(X_oob)
# return accuracy
yield accuracy_score(y_oob, y_hat)
###Output
_____no_output_____
###Markdown
To make sure we all get the same result, let's fix the seed of the random number generator:
###Code
np.random.seed(42)
###Output
_____no_output_____
###Markdown
Now, let's run the procedure for `n_iter=10` times by converting the function output to alist:
###Code
list(yield_bootstrap(knn, X, y, n_iter=10))
###Output
_____no_output_____
###Markdown
As you can see, for this small sample we get accuracy scores anywhere between 92% and98%. To get a more reliable estimate of the model's performance, we repeat the procedure1,000 times and calculate both mean and standard deviation of the resulting scores:
###Code
acc = list(yield_bootstrap(knn, X, y, n_iter=1000))
np.mean(acc), np.std(acc)
###Output
_____no_output_____
###Markdown
You are always welcome to increase the number of repetitions. But once `n_iter` is largeenough, the procedure should be robust to the randomness of the sampling procedure. Inthis case, we do not expect to see any more changes to the distribution of score values as wekeep increasing `n_iter` to, for example, 10,000 iterations:
###Code
acc = list(yield_bootstrap(knn, X, y, n_iter=10000))
np.mean(acc), np.std(acc)
###Output
_____no_output_____
###Markdown
Typically, the scores obtained with bootstrapping would be used in a **statistical test** toassess the **significance** of our result. Let's have a look at how that is done. Implementing Student's t-testOne of the most famous statistical tests is **Student's $t$-test**. You might have heard of itbefore: it allows us to determine whether two sets of data are significantly different fromone another. This was a really important test for William Sealy Gosset, the inventor of thetest, who worked at the Guinness brewery and wanted to know whether two batches ofstout differed in quality.In practice, the $t$-test allows us to determine whether two data samples come fromunderlying distributions with the same mean or **expected value**.For our purposes, this means that we can use the $t$-test to determine whether the test scoresof two independent classifiers have the same mean value. We start by hypothesizing thatthe two sets of test scores are identical. We call this the **null hypothesis** because this is thehypothesis we want to nullify, that is, we are looking for evidence to **reject** the hypothesisbecause we want to ensure that one classifier is significantly better than the other.We accept or reject a null hypothesis based on a parameter known as the $p$-value that the $t$-testreturns. The $p$-value takes on values between 0 and 1. A $p$-value of 0.05 would meanthat the null hypothesis is right only 5 out of 100 times. A small $p$-value thus indicatesstrong evidence that the hypothesis can be safely rejected. It is customary to use $p=0.05$ as acut-off value below which we reject the null hypothesis.If this is all too confusing, think of it this way: when we run a $t$-test for the purpose ofcomparing classifier test scores, we are looking to obtain a small $p$-value because that meansthat the two classifiers give significantly different results.We can implement Student's $t$-test with SciPy's `ttest_ind` function from the `stats`module:
###Code
from scipy.stats import ttest_ind
###Output
_____no_output_____
###Markdown
Let's start with a simple example. Assume we ran five-fold cross-validation on twoclassifiers and obtained the following scores:
###Code
scores_a = [1, 1, 1, 1, 1]
scores_b = [0, 0, 0, 0, 0]
###Output
_____no_output_____
###Markdown
This means that Model A achieved 100% accuracy in all five folds, whereas Model B got 0%accuracy. In this case, it is clear that the two results are significantly different. If we run the$t$-test on this data, we should thus find a really small $p$-value:
###Code
ttest_ind(scores_a, scores_b)
###Output
_____no_output_____
###Markdown
And we do! We actually get the smallest possible $p$-value, $p=0.0$.On the other hand, what if the two classifiers got exactly the same numbers, except duringdifferent folds. In this case, we would expect the two classifiers to be equivalent, which isindicated by a really large $p$-value:
###Code
scores_a = [0.9, 0.9, 0.9, 0.8, 0.8]
scores_b = [0.8, 0.8, 0.9, 0.9, 0.9]
ttest_ind(scores_a, scores_b)
###Output
_____no_output_____
###Markdown
Analogous to the aforementioned, we get the largest possible $p$-value, $p=1.0$. To see what happens in a more realistic example, let's return to our $k$-NN classifier fromearlier example. Using the test scores obtained from the ten-fold cross-validation procedure,we can compare two different $k$-NN classifiers with the following procedure.Obtain a set of test scores for Model A. We choose Model A to be the $k$-NNclassifier from earlier ($k=1$):
###Code
k1 = KNeighborsClassifier(n_neighbors=1)
scores_k1 = cross_val_score(k1, X, y, cv=10)
np.mean(scores_k1), np.std(scores_k1)
###Output
_____no_output_____
###Markdown
Obtain a set of test scores for Model B. Let's choose Model B to be a $k$-NNclassifier with $k=3$:
###Code
k3 = KNeighborsClassifier(n_neighbors=3)
scores_k3 = cross_val_score(k3, X, y, cv=10)
np.mean(scores_k3), np.std(scores_k3)
###Output
_____no_output_____
###Markdown
Apply the $t$-test to both sets of scores:
###Code
ttest_ind(scores_k1, scores_k3)
###Output
_____no_output_____
###Markdown
As you can see, this is a good example of two classifiers giving different cross-validationscores (96.0% and 96.7%) that turn out to be not significantly different! Because we get alarge $p$-value ($p=0.777$), we expect the two classifiers to be equivalent 77 out of 100 times. Implementing McNemar's testA more advanced statistical technique is McNemar's test. This test can be used on paireddata to determine whether there are any differences between the two samples. As in thecase of the $t$-test, we can use McNemar's test to determine whether two models givesignificantly different classification results.McNemar's test operates on pairs of data points. This means that we need to know, for bothclassifiers, how they classified each data point. Based on the number of data points that thefirst classifier got right but the second got wrong and vice versa, we can determine whetherthe two classifiers are equivalent.
###Code
from scipy.stats import binom
def mcnemar_midp(b, c):
"""
Compute McNemar's test using the "mid-p" variant suggested by:
M.W. Fagerland, S. Lydersen, P. Laake. 2013. The McNemar test for
binary matched-pairs data: Mid-p and asymptotic are better than exact
conditional. BMC Medical Research Methodology 13: 91.
`b` is the number of observations correctly labeled by the first---but
not the second---system; `c` is the number of observations correctly
labeled by the second---but not the first---system.
"""
n = b + c
x = min(b, c)
dist = binom(n, .5)
p = 2. * dist.cdf(x)
midp = p - dist.pmf(x)
return midp
###Output
_____no_output_____
###Markdown
Let's assume the preceding Model A and Model B were applied to the same five data points.Whereas Model A classified every data point correctly (denoted with a 1), Model B got all ofthem wrong (denoted with a 0):
###Code
scores_a = np.array([1, 1, 1, 1, 1])
scores_b = np.array([0, 0, 0, 0, 0])
###Output
_____no_output_____
###Markdown
McNemar's test wants to know two things:- How many data points did Model A get right but Model B get wrong?- How many data points did Model A get wrong but Model B get right?We can check which data points Model A got right but Model B got wrong as follows:
###Code
a1_b0 = scores_a * (1 - scores_b)
a1_b0
###Output
_____no_output_____
###Markdown
Of course, this applies to all of the data points. The opposite is true for the data points thatModel B got right and Model A got wrong:
###Code
a0_b1 = (1 - scores_a) * scores_b
a0_b1
###Output
_____no_output_____
###Markdown
Feeding these numbers to McNemar's test should return a small $p$-value because the twoclassifiers are obviously different:
###Code
mcnemar_midp(a1_b0.sum(), a0_b1.sum())
###Output
_____no_output_____
###Markdown
And it does!We can apply McNemar's test to a more complicated example, but we cannot operate oncross-validation scores anymore. The reason is that we need to know the classification resultfor every data point, not just an average. Hence, it makes more sense to apply McNemar'stest to the leave-one-out cross-validation.Going back to $k$-NN with $k=1$ and $k=3$, we can calculate their scores as follows:
###Code
scores_k1 = cross_val_score(k1, X, y, cv=LeaveOneOut())
scores_k3 = cross_val_score(k3, X, y, cv=LeaveOneOut())
###Output
_____no_output_____
###Markdown
The number of data points that one of the classifiers got right but the other got wrong are asfollows:
###Code
np.sum(scores_k1 * (1 - scores_k3))
np.sum((1 - scores_k3) * scores_k3)
###Output
_____no_output_____
###Markdown
We got no differences whatsoever! Now it becomes clear why the $t$-test led us to believethat the two classifiers are identical. As a result, if we feed the two sums into McNemar'stest function, we get the largest possible $p$-value, $p=1.0$:
###Code
mcnemar_midp(np.sum(scores_k1 * (1 - scores_k3)),
np.sum((1 - scores_k1) * scores_k3))
###Output
_____no_output_____
###Markdown
*This notebook contains an excerpt from the book [Machine Learning for OpenCV](https://www.packtpub.com/big-data-and-business-intelligence/machine-learning-opencv) by Michael Beyeler.The code is released under the [MIT license](https://opensource.org/licenses/MIT),and is available on [GitHub](https://github.com/mbeyeler/opencv-machine-learning).**Note that this excerpt contains only the raw code - the book is rich with additional explanations and illustrations.If you find this content useful, please consider supporting the work by[buying the book](https://www.packtpub.com/big-data-and-business-intelligence/machine-learning-opencv)!* Understanding Cross-ValidationCross-validation is a method of evaluating the generalization performance of a model thatis generally more stable and thorough than splitting the dataset into training and test sets.The most commonly used version of cross-validation is $k$-fold cross-validation, where $k$ is anumber specified by the user (usually five or ten). Here, the dataset is partitioned into kparts of more or less equal size, called folds. For a dataset that contains $N$ data points, eachfold should thus have approximately $N / k$ samples. Then a series of models is trained onthe data, using $k - 1$ folds for training and one remaining fold for testing. The procedure isrepeated for $k$ iterations, each time choosing a different fold for testing, until every fold hasserved as a test set once.Refer to the book for an illustration of $k$-fold cross-validation for different values of $k$. Do you know what makes cross-validation different from just splitting the data into training and test sets? Manually implementing cross-validation in OpenCVThe easiest way to perform cross-validation in OpenCV is to do the data splits by hand.For example, in order to implement two-fold cross-validation, we would follow thefollowing procedure.Load the dataset:
###Code
from sklearn.datasets import load_iris
import numpy as np
iris = load_iris()
X = iris.data.astype(np.float32)
y = iris.target
###Output
_____no_output_____
###Markdown
Split the data into two equally sized parts:
###Code
from sklearn.model_selection import train_test_split
X_fold1, X_fold2, y_fold1, y_fold2 = train_test_split(
X, y, random_state=37, train_size=0.5
)
###Output
_____no_output_____
###Markdown
Instantiate the classifier:
###Code
import cv2
knn = cv2.ml.KNearest_create()
knn.setDefaultK(1)
###Output
_____no_output_____
###Markdown
Train the classifier on the first fold, then predict the labels of the second fold:
###Code
knn.train(X_fold1, cv2.ml.ROW_SAMPLE, y_fold1)
_, y_hat_fold2 = knn.predict(X_fold2)
###Output
_____no_output_____
###Markdown
Train the classifier on the second fold, then predict the labels of the first fold:
###Code
knn.train(X_fold2, cv2.ml.ROW_SAMPLE, y_fold2)
_, y_hat_fold1 = knn.predict(X_fold1)
###Output
_____no_output_____
###Markdown
Compute accuracy scores for both folds:
###Code
from sklearn.metrics import accuracy_score
accuracy_score(y_fold1, y_hat_fold1)
accuracy_score(y_fold2, y_hat_fold2)
###Output
_____no_output_____
###Markdown
This procedure will yield two accuracy scores, one for the first fold (92% accuracy), and onefor the second fold (88% accuracy). On average, our classifier thus achieved 90% accuracyon unseen data. Automating cross-validation using scikit-learnInstantiate the classifier:
###Code
from sklearn.neighbors import KNeighborsClassifier
model = KNeighborsClassifier(n_neighbors=1)
###Output
_____no_output_____
###Markdown
Perform cross-validation with the cross_val_score function. This functiontakes as input a model, the full dataset (`X`), the target labels (`y`) and an integervalue for the number of folds (`cv`). It is not necessary to split the data byhand—the function will do that automatically depending on the number of folds.After the cross-validation is completed, the function returns the test scores:
###Code
from sklearn.model_selection import cross_val_score
scores = cross_val_score(model, X, y, cv=5)
scores
###Output
_____no_output_____
###Markdown
In order to get a sense how the model did on average, we can look at the mean andstandard deviation of the five scores:
###Code
scores.mean(), scores.std()
###Output
_____no_output_____
###Markdown
With five folds, we have a much better idea about how robust the classifier is on average.We see that $k$-NN with $k=1$ achieves on average 96% accuracy, and this value fluctuatesfrom run to run with a standard deviation of roughly 2.5%. Implementing leave-one-out cross-validationAnother popular way to implement cross-validation is to choose the number of folds equalto the number of data points in the dataset. In other words, if there are $N$ data points, we set$k=N$. This means that we will end up having to do $N$ iterations of cross-validation, but inevery iteration, the training set will consist of only a single data point. The advantage of thisprocedure is that we get to use all-but-one data point for training. Hence, this procedure isalso known as leave-one-out cross-validation.In scikit-learn, this functionality is provided by the `LeaveOneOut` method from the`model_selection` module:
###Code
from sklearn.model_selection import LeaveOneOut
###Output
_____no_output_____
###Markdown
This object can be passed directly to the `cross_val_score` function in the following way:
###Code
scores = cross_val_score(model, X, y, cv=LeaveOneOut())
###Output
_____no_output_____
###Markdown
Because every test set now contains a single data point, we would expect the scorer toreturn 150 values—one for each data point in the dataset. Each of these points we could geteither right or wrong. Thus, we expect `scores` to be a list of ones (1) and zeros (0), whichcorresponds to correct and incorrect classifications, respectively:
###Code
scores
###Output
_____no_output_____
###Markdown
If we want to know the average performance of the classifier, we would still compute themean and standard deviation of the scores:
###Code
scores.mean(), scores.std()
###Output
_____no_output_____
###Markdown
We can see this scoring scheme returns very similar results to five-fold cross-validation. Estimating robustness using bootstrappingAn alternative procedure to $k$-fold cross-validation is **bootstrapping**.Instead of splitting the data into folds, bootstrapping builds a training set by drawingsamples randomly from the dataset. Typically, a bootstrap is formed by drawing sampleswith replacement. Imagine putting all of the data points into a bag and then drawingrandomly from the bag. After drawing a sample, we would put it back in the bag. Thisallows for some samples to show up multiple times in the training set, which is somethingcross-validation does not allow.The classifier is then tested on all samples that are not part of the bootstrap (the so-called**out-of-bag** examples), and the procedure is repeated a large number of times (say, 10,000times). Thus, we get a distribution of the model's score that allows us to estimate therobustness of the model. Bootstrapping can be implemented with the following procedure.Instantiate the classifier:
###Code
knn = cv2.ml.KNearest_create()
knn.setDefaultK(1)
###Output
_____no_output_____
###Markdown
From our dataset with $N$ samples, randomly choose $N$ samples with replacementto form a bootstrap. This can be done most easily with the choice function fromNumPy's random module. We tell the function to draw len(`X`) samples in therange `[0, len(X)-1]` with replacement (`replace=True`). The function thenreturns a list of indices, from which we form our bootstrap:
###Code
idx_boot = np.random.choice(len(X), size=len(X), replace=True)
X_boot = X[idx_boot, :]
y_boot = y[idx_boot]
###Output
_____no_output_____
###Markdown
Put all samples that do not show in the bootstrap in the out-of-bag set:
###Code
idx_oob = np.array([x not in idx_boot
for x in np.arange(len(X))], dtype=np.bool)
X_oob = X[idx_oob, :]
y_oob = y[idx_oob]
###Output
_____no_output_____
###Markdown
Train the classifier on the bootstrap samples:
###Code
knn.train(X_boot, cv2.ml.ROW_SAMPLE, y_boot)
###Output
_____no_output_____
###Markdown
Test the classifier on the out-of-bag samples:
###Code
_, y_hat = knn.predict(X_oob)
accuracy_score(y_oob, y_hat)
###Output
_____no_output_____
###Markdown
Then we want to repeat these steps up to 10,000 times to get 10,000accuracy scores, then average the scores to get an idea of the classifier's meanperformance.For our convenience, we can build a function so that it is easy to run theprocedure for some `n_iter` number of times. We also pass a model (our $k$-NN classifier,`model`), the feature matrix (`X`), and the vector with all class labels (`y`):
###Code
def yield_bootstrap(model, X, y, n_iter=10000):
for _ in range(n_iter):
# train the classifier on bootstrap
idx_boot = np.random.choice(len(X), size=len(X),
replace=True)
X_boot = X[idx_boot, :]
y_boot = y[idx_boot]
knn.train(X_boot, cv2.ml.ROW_SAMPLE, y_boot)
# test classifier on out-of-bag examples
idx_oob = np.array([x not in idx_boot
for x in np.arange(len(X))],
dtype=np.bool)
X_oob = X[idx_oob, :]
y_oob = y[idx_oob]
_, y_hat = knn.predict(X_oob)
# return accuracy
yield accuracy_score(y_oob, y_hat)
###Output
_____no_output_____
###Markdown
To make sure we all get the same result, let's fix the seed of the random number generator:
###Code
np.random.seed(42)
###Output
_____no_output_____
###Markdown
Now, let's run the procedure for `n_iter=10` times by converting the function output to alist:
###Code
list(yield_bootstrap(knn, X, y, n_iter=10))
###Output
_____no_output_____
###Markdown
As you can see, for this small sample we get accuracy scores anywhere between 92% and98%. To get a more reliable estimate of the model's performance, we repeat the procedure1,000 times and calculate both mean and standard deviation of the resulting scores:
###Code
acc = list(yield_bootstrap(knn, X, y, n_iter=1000))
np.mean(acc), np.std(acc)
###Output
_____no_output_____
###Markdown
You are always welcome to increase the number of repetitions. But once `n_iter` is largeenough, the procedure should be robust to the randomness of the sampling procedure. Inthis case, we do not expect to see any more changes to the distribution of score values as wekeep increasing `n_iter` to, for example, 10,000 iterations:
###Code
acc = list(yield_bootstrap(knn, X, y, n_iter=10000))
np.mean(acc), np.std(acc)
###Output
_____no_output_____
###Markdown
Typically, the scores obtained with bootstrapping would be used in a **statistical test** toassess the **significance** of our result. Let's have a look at how that is done. Implementing Student's t-testOne of the most famous statistical tests is **Student's $t$-test**. You might have heard of itbefore: it allows us to determine whether two sets of data are significantly different fromone another. This was a really important test for William Sealy Gosset, the inventor of thetest, who worked at the Guinness brewery and wanted to know whether two batches ofstout differed in quality.In practice, the $t$-test allows us to determine whether two data samples come fromunderlying distributions with the same mean or **expected value**.For our purposes, this means that we can use the $t$-test to determine whether the test scoresof two independent classifiers have the same mean value. We start by hypothesizing thatthe two sets of test scores are identical. We call this the **null hypothesis** because this is thehypothesis we want to nullify, that is, we are looking for evidence to **reject** the hypothesisbecause we want to ensure that one classifier is significantly better than the other.We accept or reject a null hypothesis based on a parameter known as the $p$-value that the $t$-testreturns. The $p$-value takes on values between 0 and 1. A $p$-value of 0.05 would meanthat the null hypothesis is right only 5 out of 100 times. A small $p$-value thus indicatesstrong evidence that the hypothesis can be safely rejected. It is customary to use $p=0.05$ as acut-off value below which we reject the null hypothesis.If this is all too confusing, think of it this way: when we run a $t$-test for the purpose ofcomparing classifier test scores, we are looking to obtain a small $p$-value because that meansthat the two classifiers give significantly different results.We can implement Student's $t$-test with SciPy's `ttest_ind` function from the `stats`module:
###Code
from scipy.stats import ttest_ind
###Output
_____no_output_____
###Markdown
Let's start with a simple example. Assume we ran five-fold cross-validation on twoclassifiers and obtained the following scores:
###Code
scores_a = [1, 1, 1, 1, 1]
scores_b = [0, 0, 0, 0, 0]
###Output
_____no_output_____
###Markdown
This means that Model A achieved 100% accuracy in all five folds, whereas Model B got 0%accuracy. In this case, it is clear that the two results are significantly different. If we run the$t$-test on this data, we should thus find a really small $p$-value:
###Code
ttest_ind(scores_a, scores_b)
###Output
_____no_output_____
###Markdown
And we do! We actually get the smallest possible $p$-value, $p=0.0$.On the other hand, what if the two classifiers got exactly the same numbers, except duringdifferent folds. In this case, we would expect the two classifiers to be equivalent, which isindicated by a really large $p$-value:
###Code
scores_a = [0.9, 0.9, 0.9, 0.8, 0.8]
scores_b = [0.8, 0.8, 0.9, 0.9, 0.9]
ttest_ind(scores_a, scores_b)
###Output
_____no_output_____
###Markdown
Analogous to the aforementioned, we get the largest possible $p$-value, $p=1.0$. To see what happens in a more realistic example, let's return to our $k$-NN classifier fromearlier example. Using the test scores obtained from the ten-fold cross-validation procedure,we can compare two different $k$-NN classifiers with the following procedure.Obtain a set of test scores for Model A. We choose Model A to be the $k$-NNclassifier from earlier ($k=1$):
###Code
k1 = KNeighborsClassifier(n_neighbors=1)
scores_k1 = cross_val_score(k1, X, y, cv=10)
np.mean(scores_k1), np.std(scores_k1)
###Output
_____no_output_____
###Markdown
Obtain a set of test scores for Model B. Let's choose Model B to be a $k$-NNclassifier with $k=3$:
###Code
k3 = KNeighborsClassifier(n_neighbors=3)
scores_k3 = cross_val_score(k3, X, y, cv=10)
np.mean(scores_k3), np.std(scores_k3)
###Output
_____no_output_____
###Markdown
Apply the $t$-test to both sets of scores:
###Code
ttest_ind(scores_k1, scores_k3)
###Output
_____no_output_____
###Markdown
As you can see, this is a good example of two classifiers giving different cross-validationscores (96.0% and 96.7%) that turn out to be not significantly different! Because we get alarge $p$-value ($p=0.777$), we expect the two classifiers to be equivalent 77 out of 100 times. Implementing McNemar's testA more advanced statistical technique is McNemar's test. This test can be used on paireddata to determine whether there are any differences between the two samples. As in thecase of the $t$-test, we can use McNemar's test to determine whether two models givesignificantly different classification results.McNemar's test operates on pairs of data points. This means that we need to know, for bothclassifiers, how they classified each data point. Based on the number of data points that thefirst classifier got right but the second got wrong and vice versa, we can determine whetherthe two classifiers are equivalent.
###Code
from scipy.stats import binom
def mcnemar_midp(b, c):
"""
Compute McNemar's test using the "mid-p" variant suggested by:
M.W. Fagerland, S. Lydersen, P. Laake. 2013. The McNemar test for
binary matched-pairs data: Mid-p and asymptotic are better than exact
conditional. BMC Medical Research Methodology 13: 91.
`b` is the number of observations correctly labeled by the first---but
not the second---system; `c` is the number of observations correctly
labeled by the second---but not the first---system.
"""
n = b + c
x = min(b, c)
dist = binom(n, .5)
p = 2. * dist.cdf(x)
midp = p - dist.pmf(x)
return midp
###Output
_____no_output_____
###Markdown
Let's assume the preceding Model A and Model B were applied to the same five data points.Whereas Model A classified every data point correctly (denoted with a 1), Model B got all ofthem wrong (denoted with a 0):
###Code
scores_a = np.array([1, 1, 1, 1, 1])
scores_b = np.array([0, 0, 0, 0, 0])
###Output
_____no_output_____
###Markdown
McNemar's test wants to know two things:- How many data points did Model A get right but Model B get wrong?- How many data points did Model A get wrong but Model B get right?We can check which data points Model A got right but Model B got wrong as follows:
###Code
a1_b0 = scores_a * (1 - scores_b)
a1_b0
###Output
_____no_output_____
###Markdown
Of course, this applies to all of the data points. The opposite is true for the data points thatModel B got right and Model A got wrong:
###Code
a0_b1 = (1 - scores_a) * scores_b
a0_b1
###Output
_____no_output_____
###Markdown
Feeding these numbers to McNemar's test should return a small $p$-value because the twoclassifiers are obviously different:
###Code
mcnemar_midp(a1_b0.sum(), a0_b1.sum())
###Output
_____no_output_____
###Markdown
And it does!We can apply McNemar's test to a more complicated example, but we cannot operate oncross-validation scores anymore. The reason is that we need to know the classification resultfor every data point, not just an average. Hence, it makes more sense to apply McNemar'stest to the leave-one-out cross-validation.Going back to $k$-NN with $k=1$ and $k=3$, we can calculate their scores as follows:
###Code
scores_k1 = cross_val_score(k1, X, y, cv=LeaveOneOut())
scores_k3 = cross_val_score(k3, X, y, cv=LeaveOneOut())
###Output
_____no_output_____
###Markdown
The number of data points that one of the classifiers got right but the other got wrong are asfollows:
###Code
np.sum(scores_k1 * (1 - scores_k3))
np.sum((1 - scores_k3) * scores_k3)
###Output
_____no_output_____
###Markdown
We got no differences whatsoever! Now it becomes clear why the $t$-test led us to believethat the two classifiers are identical. As a result, if we feed the two sums into McNemar'stest function, we get the largest possible $p$-value, $p=1.0$:
###Code
mcnemar_midp(np.sum(scores_k1 * (1 - scores_k3)),
np.sum((1 - scores_k1) * scores_k3))
###Output
_____no_output_____ |
scripts/getStats.ipynb | ###Markdown
/* * Copyright 2021 ConsenSys Software Inc. * * Licensed under the Apache License, Version 2.0 (the "License"); you may * not use this file except in compliance with the License. You may obtain * a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software dis- * tributed under the License is distributed on an "AS IS" BASIS, WITHOUT * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the * License for the specific language governing permissions and limitations * under the License. */ Notebook descriptionThis notebook contains some basic processing to automate the collection of statistics relating to the Dafny files.By creating functions to perform analysis of Dafny files, additional results can easily be added to the pandas dataframe.The use of a pandas dataframe provides many options for visualisation and the data can easily by stored in a csv.The data can also easily be supplemented with timestamps to faciliate time series analysis.This file is a working file and will be converted to a python script in due course. TODO: Reformat function documentation to standard style used within this repo Libraries
###Code
# import libraries
import os
import subprocess
import pandas as pd
import re
import numpy as np
import time
import shutil
###Output
_____no_output_____
###Markdown
File processing functions
###Code
# find *.dfy files, within a given local repo path
# this function will search all subfolders of dirName
# a sorted list of files is returned
def getListOfDafnyFiles(dirName,exclude_folders=[]):
listOfFile = os.listdir(dirName)
allFiles = list()
for entry in listOfFile:
fullPath = os.path.join(dirName, entry)
# if entry is a directory then append the list of files in this directory to allFiles
if os.path.isdir(fullPath):
if os.path.abspath(fullPath) not in exclude_folders:
allFiles = allFiles + getListOfDafnyFiles(fullPath, exclude_folders)
# else append file only if it is a Dafny file
else:
if entry.endswith(".dfy"):
allFiles.append(fullPath)
return sorted(allFiles)
# find folders within the repo that have *.dfy files
# a sorted list of folders is returned (i.e. full path of each folder)
def getListOfDafnyFolders(dafnyFiles):
listOfDirectories = list()
for file in dafnyFiles:
listOfDirectories.append(os.path.dirname(file))
return sorted(list(set(listOfDirectories)))
# get folder for an individual dafny file
# i.e. for the full path of a dafny file, the filename and repo path are striped
def getFolder(repo, dafny_file):
repo_path, folder = os.path.dirname(dafny_file).split(repo,1)
return folder
###Output
_____no_output_____
###Markdown
Test file processing functions
###Code
# test the getListOfDafnyFiles, getListOfDafnyFolders and getFolder functions
# local repo path needs to be set prior to running the tests and `if False`
# must be changed to `if True`
if False:
repo_directory = "/home/roberto/projects_offline/lavoro/consensys/content/eth2.0-dafny-for-stats"
exclude_folders_rel_path = ["src/dafny/libraries/integers"]
exclude_folders_full_path = [os.path.join(repo_directory,f) for f in exclude_folders]
print("Test getListOfDafnyFiles: ")
files = getListOfDafnyFiles(repo_directory, exclude_folders_full_path)
for i in files:
print(i)
print("Length of returned list: ", len(files))
print("Test getListOfDafnyFolders: ")
directories = getListOfDafnyFolders(files)
for i in directories:
print(i)
print("Length of returned list: ", len(directories))
print("Test getFolder for each file in files: ")
for file in files:
print(getFolder(repo_directory, file))
###Output
_____no_output_____
###Markdown
Functions to collect statistics
###Code
# count the number of "lemmas" in a given dafny file
# this function uses a subprocess call
# an alternative method would be to read and search the file directly
def getLemmas(dafny_file):
cmd = "cat " + dafny_file +"| grep lemma | wc -l"
result = subprocess.run(['/bin/bash', '-i', '-c', cmd], stdout=subprocess.PIPE)
return result.stdout.strip().decode('ascii')
# count the number of "function methods" in a given dafny file
# this function uses a subprocess call
# an alternative method would be to read and search the file directly
def getFunctions(dafny_file):
cmd = "cat " + dafny_file +"| grep function | grep method | wc -l"
result = subprocess.run(['/bin/bash', '-i', '-c', cmd], stdout=subprocess.PIPE)
return result.stdout.strip().decode('ascii')
# count the number of ghost (= function and lemmas) processes
# ignores function methods
# to be referred to as "Theorems" in the data display
def getGhost(dafny_file):
tmp_file = open(dafny_file, "r")
count = 0
for line in tmp_file.readlines():
if line.strip().startswith(("function", "lemma")):
if not line.strip().startswith("function method"):
count += 1
#print(line)
tmp_file.close()
return count
# count the number of non-ghost ()= function methods and methods and predicates) processes
# to be referred to as "Implementations" in the data display
def getNonGhost(dafny_file):
tmp_file = open(dafny_file, "r")
count = 0
for line in tmp_file.readlines():
if line.strip().startswith(("function method", "method", "predicate")):
count += 1
#print(line)
tmp_file.close()
return count
# count the number of lines of code
# the count occurs after the dafny file is printed used the compiler
# the count also occurs after this output has been cleaned
def getLoC(dafny_file):
show_ghost = True
executable = "dafny"
args = []
args += ['/rprint:-']
args += ["/noAutoReq"]
args += ["/noVerify"]
args += ["/env:0"]
if show_ghost:
args += ["/printMode:NoIncludes"]
else:
args += ["/printMode:NoGhost"]
args += [dafny_file]
cmd = ' '.join([executable] + args)
result = subprocess.run(['/bin/bash', '-i', '-c', cmd], stdout=subprocess.PIPE)
output = result.stdout.decode('ascii')
#print(type(result.stdout.decode('ascii')))
#print(result.stdout.decode('ascii'))
#remove this section once code has be tested OR comment out
#tmp_file = open("tmp.txt", "w")
#tmp_file.write(result.stdout.decode('ascii'))
#tmp_file.close()
######---------------------
count = 0
for line in output.splitlines():
# clean output i.e. remove comment at start and verifier status
if line.startswith(("Dafny program verifier did not attempt verification", "//")):
#print(i)
pass
else:
if line.strip():
count += 1
#print(line)
#print("#LoC: ", count)
return count
# count the number of lines included in the license comment
# assumes license comment is at the start of the file and is of format /* ... */
# assumes that it has been confirmed that the file has a license comment
def getLicenseLineCount(dafny_file):
tmp_file = open(dafny_file, "r")
count = 0
flag = 0
for line in tmp_file.readlines():
tmp_line = line.strip()
cleaned = ' '.join(i for i in tmp_line.split() if i not in ["//", "/*", "/**", "*", "*/"])
if (not flag) and (tmp_line.startswith("/*")):
if cleaned:
count += 1
flag = 1
elif flag:
if cleaned:
count += 1
if tmp_line.startswith("*/"):
tmp_file.close()
return count
# count the number of lines of documentation
# don't include license comment or empty comment lines
def getDocumentation(dafny_file):
tmp_file = open(dafny_file, "r")
count = 0
license_flag = 0
for line in tmp_file.readlines():
tmp_line = line.strip()
if tmp_line.startswith(("//", "/*", "/**", "*", "*/")):
cleaned = ' '.join(i for i in tmp_line.split() if i not in ["//", "/*", "/**", "*", "*/"])
if cleaned:
#print(cleaned)
count += 1
#print(line)
if tmp_line.startswith("* Copyright 2021 ConsenSys Software Inc."):
license_flag = 1
tmp_file.close()
if license_flag:
count -= getLicenseLineCount(dafny_file)
#print(getLicenseLineCount(dafny_file))
return count
# count the number of theorems (getGhost) and implementations (getNonGhost) proved
# i.e. check that the number of errors when verified is zero
# TODO: include arguments for getGhost and getNonGhost to reduce duplicate processing
def getProved(dafny_file):
cmd = "dafny /dafnyVerify:1 /compile:0 " + dafny_file
result = subprocess.run(['/bin/bash', '-i', '-c', cmd], stdout=subprocess.PIPE)
output = result.stdout.decode('ascii')
for line in output.splitlines():
if line.startswith("Dafny program verifier finished with "):
# check no errors
#print(line, re.findall(r'\d+', line)[1], type(re.findall(r'\d+', line)[1]))
if not int(re.findall(r'\d+', line)[1]):
return (getGhost(dafny_file) + getNonGhost(dafny_file))
else:
pass
# if the verifier doesn't finish, return -1
return 0
###Output
_____no_output_____
###Markdown
Test statistics functions
###Code
# s/False/True if need to run the tests
if False:
# test file options:
test_file = "/Users/joannefuller/Documents/vscode/eth2.0-dafny/src/dafny/ssz/BytesAndBits.dfy"
#test_file = "/Users/joannefuller/Documents/vscode/eth2.0-dafny/test/dafny/merkle/Merkleise.test.dfy"
#test_file = "/Users/joannefuller/Documents/vscode/eth2.0-dafny/test/dafny/ssz/BitListSeDes.tests.dfy"
#test_file = "/Users/joannefuller/Documents/vscode/eth2.0-dafny/src/dafny/ssz/BitListSeDes.dfy"
#test_file = "/Users/joannefuller/Documents/vscode/eth2.0-dafny/src/dafny/merkle/Merkleise.dfy"
#print("Lemmas ...")
#print(getLemmas(test_file))
#print("Function methods ...")
#print(getFunctions(test_file))
#print("LoC ...")
#print(getLoC(test_file))
#print("Documentation ...")
#print(getDocumentation(test_file))
print("Proved (verified from compile) ...")
print(getProved(test_file))
#print("Ghost ...")
#rint(getGhost(test_file))
#print("NonGhost ...")
#print(getNonGhost(test_file))
###Output
Proved (verified from compile) ...
CompletedProcess(args=['/bin/bash', '-i', '-c', 'dafny /dafnyVerify:1 /compile:0 /noCheating:1 /vcsMaxKeepGoingSplits:10 /vcsCores:12 /vcsMaxCost:1000 /vcsKeepGoingTimeout:30 /vcsFinalAssertTimeout:90 /verifySeparately /Users/joannefuller/Documents/vscode/eth2.0-dafny/src/dafny/ssz/BytesAndBits.dfy'], returncode=2, stdout=b'(0,-1): Error: Unable to open included file\nError opening file "/Users/joannefuller/Documents/vscode/eth2.0-dafny/src/dafny/ssz/BytesAndBits.dfy": Could not find a part of the path \'/Users/joannefuller/Documents/vscode/eth2.0-dafny/src/dafny/ssz/BytesAndBits.dfy\'.\n')
0
###Markdown
Collate results into a pandas dataframeOne row per Dafny file.
###Code
import tempfile
# create a pandas dataframe to store stats relating to the dafny files
column_list = ['Files', 'Folder', '#LoC', 'Theorems', 'Implementations', "Documentation", "#Doc/#LoC (%)", "Proved"]
# list here all the directory not to include in the stat collection with path relative to the root of the repo
exclude_folders_rel_path = ["src/dafny/libraries/integers"]
# performs a clean checkout from GitHub before collecting the stats
with tempfile.TemporaryDirectory() as repo_directory:
# subprocess.run(['/bin/bash','-c','git clone [email protected]:PegaSysEng/eth2.0-dafny.git ' + repo_directory], stdout=subprocess.PIPE)
repo_directory = "/Users/franck/development/eth2.0-dafny/src/dafny/"
exclude_folders_full_path = [os.path.join(repo_directory,f) for f in exclude_folders_rel_path]
files = getListOfDafnyFiles(repo_directory, exclude_folders_full_path)
df = pd.DataFrame(columns=column_list)
# collect data for each dafny file
for file in files:
loc = getLoC(file)
ghost = getGhost(file)
nonghost = getNonGhost(file)
doc = getDocumentation(file)
proved = getProved(file)
df2 = pd.DataFrame([[os.path.basename(file),
getFolder(repo_directory, file),
loc ,
ghost,
nonghost,
doc,
round(doc/loc * 100),
proved]],
columns=column_list)
df = df.append(df2, ignore_index=True)
# create and append totals for numeric columns
totals = pd.DataFrame([["",
"TOTAL",
df['#LoC'].sum(),
df['Theorems'].sum(),
df['Implementations'].sum(),
df['Documentation'].sum(),
round(df['Documentation'].sum()/df['#LoC'].sum() * 100),
df['Proved'].sum()]],
columns=column_list)
df = df.append(totals, ignore_index=True)
# convert numeric columns to int64
numCols = ['#LoC', 'Theorems', 'Implementations', "Documentation", "#Doc/#LoC (%)", "Proved"]
df[numCols] = df[numCols].astype("int64")
#display a sample of rows
df.head(len(df))
# create a pandas dataframe to store stats relating to the dafny files
column_list = ['Files', 'Folder', '#LoC', 'Theorems', 'Implementations', "Documentation", "#Doc/#LoC (%)", "Proved"]
# list here all the directory not to include in the stat collection with path relative to the root of the repo
exclude_folders_rel_path = ["src/dafny/libraries/integers"]
# performs a clean checkout from GitHub before collecting the stats
with tempfile.TemporaryDirectory() as repo_directory:
# subprocess.run(['/bin/bash','-c','git clone [email protected]:PegaSysEng/eth2.0-dafny.git ' + repo_directory], stdout=subprocess.PIPE)
repo_directory = "/Users/franck/development/eth2.0-dafny/src/dafny/"
exclude_folders_full_path = [os.path.join(repo_directory,f) for f in exclude_folders_rel_path]
files = getListOfDafnyFiles(repo_directory, exclude_folders_full_path)
df = pd.DataFrame(columns=column_list)
# collect data for each dafny file
for file in files:
loc = getLoC(file)
ghost = getGhost(file)
nonghost = getNonGhost(file)
doc = getDocumentation(file)
proved = getProved(file)
df2 = pd.DataFrame([[os.path.basename(file),
getFolder(repo_directory, file),
loc ,
ghost,
nonghost,
doc,
round(doc/loc * 100),
proved]],
columns=column_list)
df = df.append(df2, ignore_index=True)
# create and append totals for numeric columns
totals = pd.DataFrame([["",
"TOTAL",
df['#LoC'].sum(),
df['Theorems'].sum(),
df['Implementations'].sum(),
df['Documentation'].sum(),
round(df['Documentation'].sum()/df['#LoC'].sum() * 100),
df['Proved'].sum()]],
columns=column_list)
df = df.append(totals, ignore_index=True)
###Output
_____no_output_____
###Markdown
Alternative formatMay be useful for github
###Code
from tabulate import tabulate
output = tabulate(df, headers='keys', tablefmt='github')
with open('../wiki/stats.md', 'w') as f:
f.write(output)
timestr = time.strftime("%Y-%m-%d-%H:%M")
mdfile = 'data/md/data' + timestr + '.md'
with open(mdfile, 'w') as f:
f.write(output)
# sys.stdout = f # Change the standard output to the file we created.
# print('This message will be written to a file.')
# sys.stdout = original_stdout #
###Output
_____no_output_____
###Markdown
| | Files | Folder | LoC | Theorems | Implementations | Documentation | Doc/LoC (%) | Proved ||----|---------------------|----------|--------|------------|-------------------|-----------------|-----------------|----------|| 0 | ForkChoice.dfy | | 226 | 3 | 15 | 172 | 76 | 18 || 1 | ForkChoiceTypes.dfy | | 7 | 0 | 0 | 17 | 243 | 0 || 2 | | TOTAL | 233 | 3 | 15 | 189 | 81 | 18 | Group dataOne row per folder.
###Code
# create a pandas dataframe to store stats relating to the dafny files
# stats grouped by folder
column_list = ['Folder', '#Files', '#LoC', 'Theorems', 'Implementations', "Documentation", "#Doc/#LoC (%)", "Proved"]
df_grouped = pd.DataFrame(columns=column_list)
with tempfile.TemporaryDirectory() as repo_directory:
subprocess.run(['/bin/bash','-c','git clone [email protected]:PegaSysEng/eth2.0-dafny.git ' + repo_directory], stdout=subprocess.PIPE)
exclude_folders_full_path = [os.path.join(repo_directory,f) for f in exclude_folders_rel_path]
# TODO: We currently get the list of folders out of the list of files and then in the `for` loop
# we retrieve the list of files again for each folder. We may want to think of a more elegant
# implementation.
allFiles = getListOfDafnyFiles(repo_directory, exclude_folders_full_path)
folders = getListOfDafnyFolders(allFiles)
for folder in folders:
files = getListOfDafnyFiles(folder)
nFiles = 0
nLoc = 0
nGhost = 0
nNonGhost = 0
nDoc = 0
nProved = 0
for file in files:
nFiles += 1
nLoc += getLoC(file)
nGhost += getGhost(file)
nNonGhost += getNonGhost(file)
nDoc += getDocumentation(file)
nProved += getProved(file)
df2 = pd.DataFrame([[getFolder(repo_directory, files[0]),
nFiles,
nLoc ,
nGhost,
nNonGhost,
nDoc,
round(nDoc/nLoc * 100),
nProved]],
columns=column_list)
df_grouped = df_grouped.append(df2, ignore_index=True)
#display a sample of rows
df_grouped.head(len(df_grouped))
###Output
_____no_output_____
###Markdown
Print dataframe to .csv, .tex and .pdf
###Code
# create filenames that include the current data string
timestr = time.strftime("%Y-%m-%d-%H:%M")
rawfile = 'data' + timestr + '.csv'
grouped_rawfile = 'dataGrouped' + timestr + '.csv'
filename = 'data' + timestr + '.tex'
pdffile = 'data' + timestr + '.pdf'
# check if data directory already exists and create if necessary
if not os.path.exists('data'):
os.makedirs('data')
#print to csv file without an index
df.to_csv("data/csv/" + rawfile, index = False)
df_grouped.to_csv("data/csv/" + grouped_rawfile, index = False)
#print to pdf via latex
template = r'''\documentclass[a4paper, 12pt]{{article}}
\usepackage[landscape]{{geometry}}
\usepackage{{booktabs}}
\begin{{document}}
\section*{{https://github.com/ConsenSys/eth2.0-dafny}}
\subsection*{{Data collected: {}}}
\scriptsize
{}
\vspace{{2em}}
{}
\end{{document}}
'''
with open(filename, 'w') as f:
f.write(template.format(time.strftime("%Y-%m-%d-%H:%M"), df.to_latex(index=False), df_grouped.to_latex(index=False)))
subprocess.call(['pdflatex', filename])
# remove surplus files and move .csv, .tex and .pdf files to the data folder
os.remove('data' + timestr + '.log')
os.remove('data' + timestr + '.aux')
shutil.move(filename, "data/tex/" + filename)
shutil.move(pdffile, "data/pdf/" + pdffile)
###Output
_____no_output_____
###Markdown
/* * Copyright 2020 ConsenSys AG. * * Licensed under the Apache License, Version 2.0 (the "License"); you may * not use this file except in compliance with the License. You may obtain * a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software dis- * tributed under the License is distributed on an "AS IS" BASIS, WITHOUT * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the * License for the specific language governing permissions and limitations * under the License. */ Notebook descriptionThis notebook contains some basic processing to automate the collection of statistics relating to the Dafny files.By creating functions to perform analysis of Dafny files, additional results can easily be added to the pandas dataframe.The use of a pandas dataframe provides many options for visualisation and the data can easily by stored in a csv.The data can also easily be supplemented with timestamps to faciliate time series analysis.This file is a working file and will be converted to a python script in due course. TODO: Reformat function documentation to standard style used within this repo Libraries
###Code
# import libraries
import os
import subprocess
import pandas as pd
import re
import numpy as np
import time
import shutil
###Output
_____no_output_____
###Markdown
File processing functions
###Code
# find *.dfy files, within a given local repo path
# this function will search all subfolders of dirName
# a sorted list of files is returned
def getListOfDafnyFiles(dirName,exclude_folders=[]):
listOfFile = os.listdir(dirName)
allFiles = list()
for entry in listOfFile:
fullPath = os.path.join(dirName, entry)
# if entry is a directory then append the list of files in this directory to allFiles
if os.path.isdir(fullPath):
if os.path.abspath(fullPath) not in exclude_folders:
allFiles = allFiles + getListOfDafnyFiles(fullPath, exclude_folders)
# else append file only if it is a Dafny file
else:
if entry.endswith(".dfy"):
allFiles.append(fullPath)
return sorted(allFiles)
# find folders within the repo that have *.dfy files
# a sorted list of folders is returned (i.e. full path of each folder)
def getListOfDafnyFolders(dafnyFiles):
listOfDirectories = list()
for file in dafnyFiles:
listOfDirectories.append(os.path.dirname(file))
return sorted(list(set(listOfDirectories)))
# get folder for an individual dafny file
# i.e. for the full path of a dafny file, the filename and repo path are striped
def getFolder(repo, dafny_file):
repo_path, folder = os.path.dirname(dafny_file).split(repo,1)
return folder
###Output
_____no_output_____
###Markdown
Test file processing functions
###Code
# test the getListOfDafnyFiles, getListOfDafnyFolders and getFolder functions
# local repo path needs to be set prior to running the tests and `if False`
# must be changed to `if True`
if False:
repo_directory = "/home/roberto/projects_offline/lavoro/consensys/content/eth2.0-dafny-for-stats"
exclude_folders_rel_path = ["src/dafny/libraries/integers"]
exclude_folders_full_path = [os.path.join(repo_directory,f) for f in exclude_folders]
print("Test getListOfDafnyFiles: ")
files = getListOfDafnyFiles(repo_directory, exclude_folders_full_path)
for i in files:
print(i)
print("Length of returned list: ", len(files))
print("Test getListOfDafnyFolders: ")
directories = getListOfDafnyFolders(files)
for i in directories:
print(i)
print("Length of returned list: ", len(directories))
print("Test getFolder for each file in files: ")
for file in files:
print(getFolder(repo_directory, file))
###Output
_____no_output_____
###Markdown
Functions to collect statistics
###Code
# count the number of "lemmas" in a given dafny file
# this function uses a subprocess call
# an alternative method would be to read and search the file directly
def getLemmas(dafny_file):
cmd = "cat " + dafny_file +"| grep lemma | wc -l"
result = subprocess.run(['/bin/bash', '-i', '-c', cmd], stdout=subprocess.PIPE)
return result.stdout.strip().decode('ascii')
# count the number of "function methods" in a given dafny file
# this function uses a subprocess call
# an alternative method would be to read and search the file directly
def getFunctions(dafny_file):
cmd = "cat " + dafny_file +"| grep function | grep method | wc -l"
result = subprocess.run(['/bin/bash', '-i', '-c', cmd], stdout=subprocess.PIPE)
return result.stdout.strip().decode('ascii')
# count the number of ghost (= function and lemmas) processes
# ignores function methods
# to be referred to as "Theorems" in the data display
def getGhost(dafny_file):
tmp_file = open(dafny_file, "r")
count = 0
for line in tmp_file.readlines():
if line.strip().startswith(("function", "lemma")):
if not line.strip().startswith("function method"):
count += 1
#print(line)
tmp_file.close()
return count
# count the number of non-ghost ()= function methods and methods and predicates) processes
# to be referred to as "Implementations" in the data display
def getNonGhost(dafny_file):
tmp_file = open(dafny_file, "r")
count = 0
for line in tmp_file.readlines():
if line.strip().startswith(("function method", "method", "predicate")):
count += 1
#print(line)
tmp_file.close()
return count
# count the number of lines of code
# the count occurs after the dafny file is printed used the compiler
# the count also occurs after this output has been cleaned
def getLoC(dafny_file):
show_ghost = True
executable = "dafny"
args = []
args += ['/rprint:-']
args += ["/noAutoReq"]
args += ["/noVerify"]
args += ["/nologo"]
args += ["/env:0"]
if show_ghost:
args += ["/printMode:NoIncludes"]
else:
args += ["/printMode:NoGhost"]
args += [dafny_file]
cmd = ' '.join([executable] + args)
result = subprocess.run(['/bin/bash', '-i', '-c', cmd], stdout=subprocess.PIPE)
output = result.stdout.decode('ascii')
#print(type(result.stdout.decode('ascii')))
#print(result.stdout.decode('ascii'))
#remove this section once code has be tested OR comment out
#tmp_file = open("tmp.txt", "w")
#tmp_file.write(result.stdout.decode('ascii'))
#tmp_file.close()
######---------------------
count = 0
for line in output.splitlines():
# clean output i.e. remove comment at start and verifier status
if line.startswith(("Dafny program verifier finished", "//")):
#print(i)
pass
else:
if line.strip():
count += 1
#print(line)
#print("#LoC: ", count)
return count
# count the number of lines included in the license comment
# assumes license comment is at the start of the file and is of format /* ... */
# assumes that it has been confirmed that the file has a license comment
def getLicenseLineCount(dafny_file):
tmp_file = open(dafny_file, "r")
count = 0
flag = 0
for line in tmp_file.readlines():
tmp_line = line.strip()
cleaned = ' '.join(i for i in tmp_line.split() if i not in ["//", "/*", "/**", "*", "*/"])
if (not flag) and (tmp_line.startswith("/*")):
if cleaned:
count += 1
flag = 1
elif flag:
if cleaned:
count += 1
if tmp_line.startswith("*/"):
tmp_file.close()
return count
# count the number of lines of documentation
# don't include license comment or empty comment lines
def getDocumentation(dafny_file):
tmp_file = open(dafny_file, "r")
count = 0
license_flag = 0
for line in tmp_file.readlines():
tmp_line = line.strip()
if tmp_line.startswith(("//", "/*", "/**", "*", "*/")):
cleaned = ' '.join(i for i in tmp_line.split() if i not in ["//", "/*", "/**", "*", "*/"])
if cleaned:
#print(cleaned)
count += 1
#print(line)
if tmp_line.startswith("* Copyright 2020 ConsenSys AG."):
license_flag = 1
tmp_file.close()
if license_flag:
count -= getLicenseLineCount(dafny_file)
#print(getLicenseLineCount(dafny_file))
return count
# count the number of theorems (getGhost) and implementations (getNonGhost) proved
# i.e. check that the number of errors when verified is zero
# TODO: include arguments for getGhost and getNonGhost to reduce duplicate processing
def getProved(dafny_file):
cmd = "dafny /dafnyVerify:1 /compile:0 " + dafny_file
result = subprocess.run(['/bin/bash', '-i', '-c', cmd], stdout=subprocess.PIPE)
output = result.stdout.decode('ascii')
for line in output.splitlines():
if line.startswith("Dafny program verifier finished with "):
# check no errors
#print(line, re.findall(r'\d+', line)[1], type(re.findall(r'\d+', line)[1]))
if not int(re.findall(r'\d+', line)[1]):
return (getGhost(dafny_file) + getNonGhost(dafny_file))
else:
pass
# if the verifier doesn't finish, return -1
return 0
###Output
_____no_output_____
###Markdown
Test statistics functions
###Code
# s/False/True if need to run the tests
if False:
# test file options:
test_file = "/Users/joannefuller/Documents/vscode/eth2.0-dafny/src/dafny/ssz/BytesAndBits.dfy"
#test_file = "/Users/joannefuller/Documents/vscode/eth2.0-dafny/test/dafny/merkle/Merkleise.test.dfy"
#test_file = "/Users/joannefuller/Documents/vscode/eth2.0-dafny/test/dafny/ssz/BitListSeDes.tests.dfy"
#test_file = "/Users/joannefuller/Documents/vscode/eth2.0-dafny/src/dafny/ssz/BitListSeDes.dfy"
#test_file = "/Users/joannefuller/Documents/vscode/eth2.0-dafny/src/dafny/merkle/Merkleise.dfy"
#print("Lemmas ...")
#print(getLemmas(test_file))
#print("Function methods ...")
#print(getFunctions(test_file))
#print("LoC ...")
#print(getLoC(test_file))
#print("Documentation ...")
#print(getDocumentation(test_file))
print("Proved (verified from compile) ...")
print(getProved(test_file))
#print("Ghost ...")
#rint(getGhost(test_file))
#print("NonGhost ...")
#print(getNonGhost(test_file))
###Output
_____no_output_____
###Markdown
Collate results into a pandas dataframeOne row per Dafny file.
###Code
import tempfile
# create a pandas dataframe to store stats relating to the dafny files
column_list = ['Files', 'Folder', '#LoC', 'Theorems', 'Implementations', "Documentation", "#Doc/#LoC (%)", "Proved"]
# list here all the directory not to include in the stat collection with path relative to the root of the repo
exclude_folders_rel_path = ["src/dafny/libraries/integers"]
# performs a clean checkout from GitHub before collecting the stats
with tempfile.TemporaryDirectory() as repo_directory:
subprocess.run(['/bin/bash','-c','git clone [email protected]:PegaSysEng/eth2.0-dafny.git ' + repo_directory], stdout=subprocess.PIPE)
exclude_folders_full_path = [os.path.join(repo_directory,f) for f in exclude_folders_rel_path]
files = getListOfDafnyFiles(repo_directory, exclude_folders_full_path)
df = pd.DataFrame(columns=column_list)
# collect data for each dafny file
for file in files:
loc = getLoC(file)
ghost = getGhost(file)
nonghost = getNonGhost(file)
doc = getDocumentation(file)
proved = getProved(file)
df2 = pd.DataFrame([[os.path.basename(file),
getFolder(repo_directory, file),
loc ,
ghost,
nonghost,
doc,
round(doc/loc * 100),
proved]],
columns=column_list)
df = df.append(df2, ignore_index=True)
# create and append totals for numeric columns
totals = pd.DataFrame([["",
"TOTAL",
df['#LoC'].sum(),
df['Theorems'].sum(),
df['Implementations'].sum(),
df['Documentation'].sum(),
round(df['Documentation'].sum()/df['#LoC'].sum() * 100),
df['Proved'].sum()]],
columns=column_list)
df = df.append(totals, ignore_index=True)
# convert numeric columns to int64
numCols = ['#LoC', 'Theorems', 'Implementations', "Documentation", "#Doc/#LoC (%)", "Proved"]
df[numCols] = df[numCols].astype("int64")
#display a sample of rows
df.head(len(df))
###Output
_____no_output_____
###Markdown
Alternative formatMay be useful for github
###Code
from tabulate import tabulate
print(tabulate(df, headers='keys', tablefmt='github'))
###Output
_____no_output_____
###Markdown
Group dataOne row per folder.
###Code
# create a pandas dataframe to store stats relating to the dafny files
# stats grouped by folder
column_list = ['Folder', '#Files', '#LoC', 'Theorems', 'Implementations', "Documentation", "#Doc/#LoC (%)", "Proved"]
df_grouped = pd.DataFrame(columns=column_list)
with tempfile.TemporaryDirectory() as repo_directory:
subprocess.run(['/bin/bash','-c','git clone [email protected]:PegaSysEng/eth2.0-dafny.git ' + repo_directory], stdout=subprocess.PIPE)
exclude_folders_full_path = [os.path.join(repo_directory,f) for f in exclude_folders_rel_path]
# TODO: We currently get the list of folders out of the list of files and then in the `for` loop
# we retrieve the list of files again for each folder. We may want to think of a more elegant
# implementation.
allFiles = getListOfDafnyFiles(repo_directory, exclude_folders_full_path)
folders = getListOfDafnyFolders(allFiles)
for folder in folders:
files = getListOfDafnyFiles(folder)
nFiles = 0
nLoc = 0
nGhost = 0
nNonGhost = 0
nDoc = 0
nProved = 0
for file in files:
nFiles += 1
nLoc += getLoC(file)
nGhost += getGhost(file)
nNonGhost += getNonGhost(file)
nDoc += getDocumentation(file)
nProved += getProved(file)
df2 = pd.DataFrame([[getFolder(repo_directory, file),
nFiles,
nLoc ,
nGhost,
nNonGhost,
nDoc,
round(nDoc/nLoc * 100),
nProved]],
columns=column_list)
df_grouped = df_grouped.append(df2, ignore_index=True)
#display a sample of rows
df_grouped.head(len(df_grouped))
###Output
_____no_output_____
###Markdown
Print dataframe to .csv, .tex and .pdf
###Code
# create filenames that include the current data string
timestr = time.strftime("%Y%m%d")
rawfile = 'data' + timestr + '.csv'
grouped_rawfile = 'dataGrouped' + timestr + '.csv'
filename = 'data' + timestr + '.tex'
pdffile = 'data' + timestr + '.pdf'
# check if data directory already exists and create if necessary
if not os.path.exists('data'):
os.makedirs('data')
#print to csv file without an index
df.to_csv("data/" + rawfile, index = False)
df_grouped.to_csv("data/" + grouped_rawfile, index = False)
#print to pdf via latex
template = r'''\documentclass[a4paper, 12pt]{{article}}
\usepackage[landscape]{{geometry}}
\usepackage{{booktabs}}
\begin{{document}}
\section*{{https://github.com/PegaSysEng/eth2.0-dafny}}
\subsection*{{Data collected: {}}}
\scriptsize
{}
\vspace{{2em}}
{}
\end{{document}}
'''
with open(filename, 'w') as f:
f.write(template.format(time.strftime("%Y-%m-%d"), df.to_latex(index=False), df_grouped.to_latex(index=False)))
subprocess.call(['pdflatex', filename])
# remove surplus files and move .csv, .tex and .pdf files to the data folder
os.remove('data' + timestr + '.log')
os.remove('data' + timestr + '.aux')
shutil.move(filename, "data/" + filename)
shutil.move(pdffile, "data/" + pdffile)
###Output
_____no_output_____ |
nbs/61_tutorial.medical_imaging.ipynb | ###Markdown
To use `fastai.medical.imaging` you'll need to:```bashconda install pyarrowpip install pydicom kornia opencv-python scikit-image``` To run this tutorial on Google Colab, you'll need to uncomment the following two lines and run the cell:
###Code
#!conda install pyarrow
#!pip install pydicom kornia opencv-python scikit-image nbdev
from fastai.basics import *
from fastai.callback.all import *
from fastai.vision.all import *
from fastai.medical.imaging import *
import pydicom
import pandas as pd
#hide
from nbdev.showdoc import *
###Output
_____no_output_____
###Markdown
Tutorial - Binary classification of chest X-rays> In this tutorial we will build a classifier that distinguishes between chest X-rays with pneumothorax and chest X-rays without pneumothorax. The image data is loaded directly from the DICOM source files, so no prior DICOM data handling is needed. This tutorial also goes through what DICOM images are and review at a high level how to evaluate the results of the classifier. Download and import of X-ray DICOM files First, we will use the `untar_data` function to download the _siim_small_ folder containing a subset (250 DICOM files, \~30MB) of the [SIIM-ACR Pneumothorax Segmentation](https://doi.org/10.1007/s10278-019-00299-9) \[1\] dataset.The downloaded _siim_small_ folder will be stored in your _\~/.fastai/data/_ directory. The variable `pneumothorax-source` will store the absolute path to the _siim_small_ folder as soon as the download is complete.
###Code
pneumothorax_source = untar_data(URLs.SIIM_SMALL)
###Output
_____no_output_____
###Markdown
The _siim_small_ folder has the following directory/file structure:  What are DICOMs? **DICOM**(**D**igital **I**maging and **CO**mmunications in **M**edicine) is the de-facto standard that establishes rules that allow medical images(X-Ray, MRI, CT) and associated information to be exchanged between imaging equipment from different vendors, computers, and hospitals. The DICOM format provides a suitable means that meets health infomation exchange (HIE) standards for transmision of health related data among facilites and HL7 standards which is the messaging standard that enables clinical applications to exchange dataDICOM files typically have a `.dcm` extension and provides a means of storing data in separate ‘tags’ such as patient information as well as image/pixel data. A DICOM file consists of a header and image data sets packed into a single file. By extracting data from these tags one can access important information regarding the patient demographics, study parameters, etc.16 bit DICOM images have values ranging from `-32768` to `32768` while 8-bit greyscale images store values from `0` to `255`. The value ranges in DICOM images are useful as they correlate with the [Hounsfield Scale](https://en.wikipedia.org/wiki/Hounsfield_scale) which is a quantitative scale for describing radiodensity Plotting the DICOM data To analyze our dataset, we load the paths to the DICOM files with the `get_dicom_files` function. When calling the function, we append _train/_ to the `pneumothorax_source` path to choose the folder where the DICOM files are located. We store the path to each DICOM file in the `items` list.
###Code
items = get_dicom_files(pneumothorax_source/f"train/")
###Output
_____no_output_____
###Markdown
Next, we split the `items` list into a train `trn` and validation `val` list using the `RandomSplitter` function:
###Code
trn,val = RandomSplitter()(items)
###Output
_____no_output_____
###Markdown
Pydicom is a python package for parsing DICOM files, making it easier to access the `header` of the DICOM as well as coverting the raw `pixel_data` into pythonic structures for easier manipulation. `fastai.medical.imaging` uses `pydicom.dcmread` to load the DICOM file.To plot an X-ray, we can select an entry in the `items` list and load the DICOM file with `dcmread`.
###Code
patient = 7
xray_sample = items[patient].dcmread()
###Output
_____no_output_____
###Markdown
To view the `header`
###Code
xray_sample
###Output
_____no_output_____
###Markdown
Explanation of each element is beyond the scope of this tutorial but [this](http://dicom.nema.org/medical/dicom/current/output/chtml/part03/sect_C.7.6.3.htmlsect_C.7.6.3.1.4) site has some excellent information about each of the entries Some key pointers on the tag information above:- **Pixel Data** (7fe0 0010) - This is where the raw pixel data is stored. The order of pixels encoded for each image plane is left to right, top to bottom, i.e., the upper left pixel (labeled 1,1) is encoded first- **Photometric Interpretation** (0028, 0004) - also known as color space. In this case it is `MONOCHROME2` where pixel data is represented as a single monochrome image plane where low values=dark, high values=bright. If the colorspace was `MONOCHROME` then the low values=bright and high values=dark info.- **Samples per Pixel** (0028, 0002) - This should be 1 as this image is monochrome. This value would be 3 if the color space was RGB for example- **Bits Stored** (0028 0101) - Number of bits stored for each pixel sample. Typical 8 bit images have a pixel range between `0` and `255`- **Pixel Represenation**(0028 0103) - can either be unsigned(0) or signed(1)- **Lossy Image Compression** (0028 2110) - `00` image has not been subjected to lossy compression. `01` image has been subjected to lossy compression.- **Lossy Image Compression Method** (0028 2114) - states the type of lossy compression used (in this case `ISO_10918_1` represents JPEG Lossy Compression)- **Pixel Data** (7fe0, 0010) - Array of 161452 elements represents the image pixel data that pydicom uses to convert the pixel data into an image. What does `PixelData` look like?
###Code
xray_sample.PixelData[:200]
###Output
_____no_output_____
###Markdown
Because of the complexity in interpreting `PixelData`, pydicom provides an easy way to get it in a convenient form: `pixel_array` which returns a `numpy.ndarray` containing the pixel data:
###Code
xray_sample.pixel_array, xray_sample.pixel_array.shape
###Output
_____no_output_____
###Markdown
You can then use the `show` function to view the image
###Code
xray_sample.show()
###Output
_____no_output_____
###Markdown
You can also conveniently create a dataframe with all the `tag` information as columns for all the images in a dataset by using `from_dicoms`
###Code
dicom_dataframe = pd.DataFrame.from_dicoms(items)
dicom_dataframe[:5]
###Output
_____no_output_____
###Markdown
Next, we need to load the labels for the dataset. We import the _labels.csv_ file using pandas and print the first five entries. The **file** column shows the relative path to the _.dcm_ file and the **label** column indicates whether the chest x-ray has a pneumothorax or not.
###Code
df = pd.read_csv(pneumothorax_source/f"labels.csv")
df.head()
###Output
_____no_output_____
###Markdown
Now, we use the `DataBlock` class to prepare the DICOM data for training. As we are dealing with DICOM images, we need to use `PILDicom` as the `ImageBlock` category. This is so the `DataBlock` will know how to open the DICOM images. As this is a binary classification task we will use `CategoryBlock`
###Code
pneumothorax = DataBlock(blocks=(ImageBlock(cls=PILDicom), CategoryBlock),
get_x=lambda x:pneumothorax_source/f"{x[0]}",
get_y=lambda x:x[1],
batch_tfms=aug_transforms(size=224))
dls = pneumothorax.dataloaders(df.values, num_workers=0)
###Output
_____no_output_____
###Markdown
Additionally, we plot a first batch with the specified transformations:
###Code
dls = pneumothorax.dataloaders(df.values)
dls.show_batch(max_n=16)
###Output
_____no_output_____
###Markdown
Training We can then use the `cnn_learner` function and initiate the training.
###Code
learn = cnn_learner(dls, resnet34, metrics=accuracy)
###Output
_____no_output_____
###Markdown
Note that if you do not select a loss or optimizer function, fastai will try to choose the best selection for the task. You can check the loss function by calling `loss_func`
###Code
learn.loss_func
###Output
_____no_output_____
###Markdown
And you can do the same for the optimizer by calling `opt_func`
###Code
learn.opt_func
###Output
_____no_output_____
###Markdown
Use `lr_find` to try to find the best learning rate
###Code
learn.lr_find()
learn.fit_one_cycle(1)
learn.predict(pneumothorax_source/f"train/Pneumothorax/000004.dcm")
###Output
_____no_output_____
###Markdown
When predicting on an image `learn.predict` returns a tuple (class, class tensor and [probabilities of each class]).In this dataset there are only 2 classes `No Pneumothorax` and `Pneumothorax` hence the reason why each probability has 2 values, the first value is the probability whether the image belongs to `class 0` or `No Pneumothorax` and the second value is the probability whether the image belongs to `class 1` or `Pneumothorax`
###Code
tta = learn.tta(use_max=True)
learn.show_results(max_n=16)
interp = Interpretation.from_learner(learn)
interp.plot_top_losses(2)
###Output
_____no_output_____
###Markdown
Result Evaluation Medical models are predominantly high impact so it is important to know how good a model is at detecting a certain condition. This model has an accuracy of 56%. Accuracy can be defined as the number of correctly predicted data points out of all the data points. However in this context we can define accuracy as the probability that the model is correct and the patient has the condition **PLUS** the probability that the model is correct and the patient does not have the condition There are some other key terms that need to be used when evaluating medical models: **False Positive & False Negative** - **False Positive** is an error in which a test result improperly indicates presence of a condition, such as a disease (the result is positive), when in reality it is not present- **False Negative** is an error in which a test result improperly indicates no presence of a condition (the result is negative), when in reality it is present **Sensitivity & Specificity** - **Sensitivity or True Positive Rate** is where the model classifies a patient has the disease given the patient actually does have the disease. Sensitivity quantifies the avoidance of false negativesExample: A new test was tested on 10,000 patients, if the new test has a sensitivity of 90% the test will correctly detect 9,000 (True Positive) patients but will miss 1000 (False Negative) patients that have the condition but were tested as not having the condition- **Specificity or True Negative Rate** is where the model classifies a patient as not having the disease given the patient actually does not have the disease. Specificity quantifies the avoidance of false positives [Understanding and using sensitivity, specificity and predictive values](https://www.ncbi.nlm.nih.gov/pmc/articles/PMC2636062/) is a great paper if you are interested in learning more about understanding sensitivity, specificity and predictive values. **PPV and NPV** Most medical testing is evaluated via **PPV** (Positive Predictive Value) or **NPV** (Negative Predictive Value).**PPV** - if the model predicts a patient has a condition what is the probability that the patient actually has the condition**NPV** - if the model predicts a patient does not have a condition what is the probability that the patient actually does not have the conditionThe ideal value of the PPV, with a perfect test, is 1 (100%), and the worst possible value would be zeroThe ideal value of the NPV, with a perfect test, is 1 (100%), and the worst possible value would be zero **Confusion Matrix** The confusion matrix is plotted against the `valid` dataset
###Code
interp = ClassificationInterpretation.from_learner(learn)
losses,idxs = interp.top_losses()
len(dls.valid_ds)==len(losses)==len(idxs)
interp.plot_confusion_matrix(figsize=(7,7))
###Output
_____no_output_____
###Markdown
You can also reproduce the results interpreted from plot_confusion_matrix like so:
###Code
upp, low = interp.confusion_matrix()
tn, fp = upp[0], upp[1]
fn, tp = low[0], low[1]
print(tn, fp, fn, tp)
###Output
23 13 12 2
###Markdown
Note that **Sensitivity = True Positive/(True Positive + False Negative)**
###Code
sensitivity = tp/(tp + fn)
sensitivity
###Output
_____no_output_____
###Markdown
In this case the model has a sensitivity of 40% and hence is only capable of correctly detecting 40% True Positives (i.e. who have Pneumothorax) but will miss 60% of False Negatives (patients that actually have Pneumothorax but were told they did not! Not a good situation to be in).This is also know as a **Type II error** **Specificity = True Negative/(False Positive + True Negative)**
###Code
specificity = tn/(fp + tn)
specificity
###Output
_____no_output_____
###Markdown
The model has a specificity of 63% and hence can correctly detect 63% of the time that a patient does **not** have Pneumothorax but will incorrectly classify that 37% of the patients have Pneumothorax (False Postive) but actually do not.This is also known as a **Type I error** **Positive Predictive Value (PPV)**
###Code
ppv = tp/(tp+fp)
ppv
###Output
_____no_output_____
###Markdown
In this case the model performs poorly in correctly predicting patients with Pneumothorax **Negative Predictive Value (NPV)**
###Code
npv = tn/(tn+fn)
npv
###Output
_____no_output_____
###Markdown
This model is better at predicting patients with No Pneumothorax **Calculating Accuracy** The accuracy of this model as mentioned before was 56% but how was this calculated? We can consider accuracy as: **accuracy = sensitivity x prevalence + specificity * (1 - prevalence)** Where **prevalence** is a statistical concept referring to the number of cases of a disease that are present in a particular population at a given time. The prevalence in this case is how many patients in the valid dataset have the condition compared to the total number.To view the files in the valid dataset you call `dls.valid_ds.cat`
###Code
val = dls.valid_ds.cat
#val[0]
###Output
_____no_output_____
###Markdown
There are 15 Pneumothorax images in the valid set (which has a total of 50 images and can be checked by using `len(dls.valid_ds)`) so the prevalence here is 15/50 = 0.3
###Code
prevalence = 15/50
prevalence
accuracy = (sensitivity * prevalence) + (specificity * (1 - prevalence))
accuracy
###Output
_____no_output_____
###Markdown
To use `fastai.medical.imaging` you'll need to:```bashconda install pyarrowpip install pydicom kornia opencv-python scikit-image``` To run this tutorial on Google Colab, you'll need to uncomment the following two lines and run the cell:
###Code
#!conda install pyarrow
#!pip install pydicom kornia opencv-python scikit-image nbdev
from fastai.basics import *
from fastai.callback.all import *
from fastai.vision.all import *
from fastai.medical.imaging import *
import pydicom
import pandas as pd
#hide
from nbdev.showdoc import *
###Output
_____no_output_____
###Markdown
Tutorial - Binary classification of chest X-rays> In this tutorial we will build a classifier that distinguishes between chest X-rays with pneumothorax and chest X-rays without pneumothorax. The image data is loaded directly from the DICOM source files, so no prior DICOM data handling is needed. This tutorial also goes through what DICOM images are and review at a high level how to evaluate the results of the classifier. Download and import of X-ray DICOM files First, we will use the `untar_data` function to download the _siim_small_ folder containing a subset (250 DICOM files, \~30MB) of the [SIIM-ACR Pneumothorax Segmentation](https://doi.org/10.1007/s10278-019-00299-9) \[1\] dataset.The downloaded _siim_small_ folder will be stored in your _\~/.fastai/data/_ directory. The variable `pneumothorax-source` will store the absolute path to the _siim_small_ folder as soon as the download is complete.
###Code
pneumothorax_source = untar_data(URLs.SIIM_SMALL)
###Output
_____no_output_____
###Markdown
The _siim_small_ folder has the following directory/file structure:  What are DICOMs? **DICOM**(**D**igital **I**maging and **CO**mmunications in **M**edicine) is the de-facto standard that establishes rules that allow medical images(X-Ray, MRI, CT) and associated information to be exchanged between imaging equipment from different vendors, computers, and hospitals. The DICOM format provides a suitable means that meets health infomation exchange (HIE) standards for transmision of health related data among facilites and HL7 standards which is the messaging standard that enables clinical applications to exchange dataDICOM files typically have a `.dcm` extension and provides a means of storing data in separate ‘tags’ such as patient information as well as image/pixel data. A DICOM file consists of a header and image data sets packed into a single file. By extracting data from these tags one can access important information regarding the patient demographics, study parameters, etc.16 bit DICOM images have values ranging from `-32768` to `32768` while 8-bit greyscale images store values from `0` to `255`. The value ranges in DICOM images are useful as they correlate with the [Hounsfield Scale](https://en.wikipedia.org/wiki/Hounsfield_scale) which is a quantitative scale for describing radiodensity Plotting the DICOM data To analyze our dataset, we load the paths to the DICOM files with the `get_dicom_files` function. When calling the function, we append _train/_ to the `pneumothorax_source` path to choose the folder where the DICOM files are located. We store the path to each DICOM file in the `items` list.
###Code
items = get_dicom_files(pneumothorax_source/f"train/")
###Output
_____no_output_____
###Markdown
Next, we split the `items` list into a train `trn` and validation `val` list using the `RandomSplitter` function:
###Code
trn,val = RandomSplitter()(items)
###Output
_____no_output_____
###Markdown
Pydicom is a python package for parsing DICOM files, making it easier to access the `header` of the DICOM as well as coverting the raw `pixel_data` into pythonic structures for easier manipulation. `fastai.medical.imaging` uses `pydicom.dcmread` to load the DICOM file.To plot an X-ray, we can select an entry in the `items` list and load the DICOM file with `dcmread`.
###Code
patient = 7
xray_sample = items[patient].dcmread()
###Output
_____no_output_____
###Markdown
To view the `header`
###Code
xray_sample
###Output
_____no_output_____
###Markdown
Explanation of each element is beyond the scope of this tutorial but [this](http://dicom.nema.org/medical/dicom/current/output/chtml/part03/sect_C.7.6.3.htmlsect_C.7.6.3.1.4) site has some excellent information about each of the entries Some key pointers on the tag information above:- **Pixel Data** (7fe0 0010) - This is where the raw pixel data is stored. The order of pixels encoded for each image plane is left to right, top to bottom, i.e., the upper left pixel (labeled 1,1) is encoded first- **Photometric Interpretation** (0028, 0004) - also known as color space. In this case it is `MONOCHROME2` where pixel data is represented as a single monochrome image plane where low values=dark, high values=bright. If the colorspace was `MONOCHROME` then the low values=bright and high values=dark info.- **Samples per Pixel** (0028, 0002) - This should be 1 as this image is monochrome. This value would be 3 if the color space was RGB for example- **Bits Stored** (0028 0101) - Number of bits stored for each pixel sample. Typical 8 bit images have a pixel range between `0` and `255`- **Pixel Represenation**(0028 0103) - can either be unsigned(0) or signed(1)- **Lossy Image Compression** (0028 2110) - `00` image has not been subjected to lossy compression. `01` image has been subjected to lossy compression.- **Lossy Image Compression Method** (0028 2114) - states the type of lossy compression used (in this case `ISO_10918_1` represents JPEG Lossy Compression)- **Pixel Data** (7fe0, 0010) - Array of 161452 elements represents the image pixel data that pydicom uses to convert the pixel data into an image. What does `PixelData` look like?
###Code
xray_sample.PixelData[:200]
###Output
_____no_output_____
###Markdown
Because of the complexity in interpreting `PixelData`, pydicom provides an easy way to get it in a convenient form: `pixel_array` which returns a `numpy.ndarray` containing the pixel data:
###Code
xray_sample.pixel_array, xray_sample.pixel_array.shape
###Output
_____no_output_____
###Markdown
You can then use the `show` function to view the image
###Code
xray_sample.show()
###Output
_____no_output_____
###Markdown
You can also conveniently create a dataframe with all the `tag` information as columns for all the images in a dataset by using `from_dicoms`
###Code
dicom_dataframe = pd.DataFrame.from_dicoms(items)
dicom_dataframe[:5]
###Output
_____no_output_____
###Markdown
Next, we need to load the labels for the dataset. We import the _labels.csv_ file using pandas and print the first five entries. The **file** column shows the relative path to the _.dcm_ file and the **label** column indicates whether the chest x-ray has a pneumothorax or not.
###Code
df = pd.read_csv(pneumothorax_source/f"labels.csv")
df.head()
###Output
_____no_output_____
###Markdown
Now, we use the `DataBlock` class to prepare the DICOM data for training. As we are dealing with DICOM images, we need to use `PILDicom` as the `ImageBlock` category. This is so the `DataBlock` will know how to open the DICOM images. As this is a binary classification task we will use `CategoryBlock`
###Code
pneumothorax = DataBlock(blocks=(ImageBlock(cls=PILDicom), CategoryBlock),
get_x=lambda x:pneumothorax_source/f"{x[0]}",
get_y=lambda x:x[1],
batch_tfms=aug_transforms(size=224))
dls = pneumothorax.dataloaders(df.values, num_workers=0)
###Output
_____no_output_____
###Markdown
Additionally, we plot a first batch with the specified transformations:
###Code
dls = pneumothorax.dataloaders(df.values)
dls.show_batch(max_n=16)
###Output
_____no_output_____
###Markdown
Training We can then use the `cnn_learner` function and initiate the training.
###Code
learn = cnn_learner(dls, resnet34, metrics=accuracy)
###Output
_____no_output_____
###Markdown
Note that if you do not select a loss or optimizer function, fastai will try to choose the best selection for the task. You can check the loss function by calling `loss_func`
###Code
learn.loss_func
###Output
_____no_output_____
###Markdown
And you can do the same for the optimizer by calling `opt_func`
###Code
learn.opt_func
###Output
_____no_output_____
###Markdown
Use `lr_find` to try to find the best learning rate
###Code
learn.lr_find()
learn.fit_one_cycle(1)
learn.predict(pneumothorax_source/f"train/Pneumothorax/000004.dcm")
###Output
_____no_output_____
###Markdown
When predicting on an image `learn.predict` returns a tuple (class, class tensor and [probabilities of each class]).In this dataset there are only 2 classes `No Pneumothorax` and `Pneumothorax` hence the reason why each probability has 2 values, the first value is the probability whether the image belongs to `class 0` or `No Pneumothorax` and the second value is the probability whether the image belongs to `class 1` or `Pneumothorax`
###Code
tta = learn.tta(use_max=True)
learn.show_results(max_n=16)
interp = Interpretation.from_learner(learn)
interp.plot_top_losses(2)
###Output
_____no_output_____
###Markdown
Result Evaluation Medical models are predominantly high impact so it is important to know how good a model is at detecting a certain condition. This model has an accuracy of 56%. Accuracy can be defined as the number of correctly predicted data points out of all the data points. However in this context we can define accuracy as the probability that the model is correct and the patient has the condition **PLUS** the probability that the model is correct and the patient does not have the condition There are some other key terms that need to be used when evaluating medical models: **False Positive & False Negative** - **False Positive** is an error in which a test result improperly indicates presence of a condition, such as a disease (the result is positive), when in reality it is not present- **False Negative** is an error in which a test result improperly indicates no presence of a condition (the result is negative), when in reality it is present **Sensitivity & Specificity** - **Sensitivity or True Positive Rate** is where the model classifies a patient has the disease given the patient actually does have the disease. Sensitivity quantifies the avoidance of false negativesExample: A new test was tested on 10,000 patients, if the new test has a sensitivity of 90% the test will correctly detect 9,000 (True Positive) patients but will miss 1000 (False Negative) patients that have the condition but were tested as not having the condition- **Specificity or True Negative Rate** is where the model classifies a patient as not having the disease given the patient actually does not have the disease. Specificity quantifies the avoidance of false positives [Understanding and using sensitivity, specificity and predictive values](https://www.ncbi.nlm.nih.gov/pmc/articles/PMC2636062/) is a great paper if you are interested in learning more about understanding sensitivity, specificity and predictive values. **PPV and NPV** Most medical testing is evaluated via **PPV** (Positive Predictive Value) or **NPV** (Negative Predictive Value).**PPV** - if the model predicts a patient has a condition what is the probability that the patient actually has the condition**NPV** - if the model predicts a patient does not have a condition what is the probability that the patient actually does not have the conditionThe ideal value of the PPV, with a perfect test, is 1 (100%), and the worst possible value would be zeroThe ideal value of the NPV, with a perfect test, is 1 (100%), and the worst possible value would be zero **Confusion Matrix** The confusion matrix is plotted against the `valid` dataset
###Code
interp = ClassificationInterpretation.from_learner(learn)
losses,idxs = interp.top_losses()
len(dls.valid_ds)==len(losses)==len(idxs)
interp.plot_confusion_matrix(figsize=(7,7))
###Output
_____no_output_____
###Markdown
You can also reproduce the results interpreted from plot_confusion_matrix like so:
###Code
upp, low = interp.confusion_matrix()
tn, fp = upp[0], upp[1]
fn, tp = low[0], low[1]
print(tn, fp, fn, tp)
###Output
23 13 12 2
###Markdown
Note that **Sensitivity = True Positive/(True Positive + False Negative)**
###Code
sensitivity = tp/(tp + fn)
sensitivity
###Output
_____no_output_____
###Markdown
In this case the model has a sensitivity of 40% and hence is only capable of correctly detecting 40% True Positives (i.e. who have Pneumothorax) but will miss 60% of False Negatives (patients that actually have Pneumothorax but were told they did not! Not a good situation to be in).This is also know as a **Type II error** **Specificity = True Negative/(False Positive + True Negative)**
###Code
specificity = tn/(fp + tn)
specificity
###Output
_____no_output_____
###Markdown
The model has a specificity of 63% and hence can correctly detect 63% of the time that a patient does **not** have Pneumothorax but will incorrectly classify that 37% of the patients have Pneumothorax (False Postive) but actually do not.This is also known as a **Type I error** **Positive Predictive Value (PPV)**
###Code
ppv = tp/(tp+fp)
ppv
###Output
_____no_output_____
###Markdown
In this case the model performs poorly in correctly predicting patients with Pneumothorax **Negative Predictive Value (NPV)**
###Code
npv = tn/(tn+fn)
npv
###Output
_____no_output_____
###Markdown
This model is better at predicting patients with No Pneumothorax **Calculating Accuracy** The accuracy of this model as mentioned before was 56% but how was this calculated? We can consider accuracy as: **accuracy = sensitivity x prevalence + specificity * (1 - prevalence)** Where **prevalence** is a statistical concept referring to the number of cases of a disease that are present in a particular population at a given time. The prevalence in this case is how many patients in the valid dataset have the condition compared to the total number.To view the files in the valid dataset you call `dls.valid_ds.cat`
###Code
val = dls.valid_ds.cat
#val[0]
###Output
_____no_output_____
###Markdown
There are 15 Pneumothorax images in the valid set (which has a total of 50 images and can be checked by using `len(dls.valid_ds)`) so the prevalence here is 15/50 = 0.3
###Code
prevalence = 15/50
prevalence
accuracy = (sensitivity * prevalence) + (specificity * (1 - prevalence))
accuracy
###Output
_____no_output_____ |
Tutorial/Exercises-5.ipynb | ###Markdown
Make a bar plot of the months in which movies with "Christmas" in their title tend to be released in the USA.
###Code
stNick = release_dates[release_dates.title.str.contains('Christmas')]
stNick.date.dt.month.value_counts().sort_index().plot(kind='bar')
###Output
_____no_output_____
###Markdown
Make a bar plot of the months in which movies whose titles start with "The Hobbit" are released in the USA.
###Code
frodoBaggins = release_dates[release_dates.title.str.startswith('The Hobbit')]
frodoBaggins.date.dt.month.value_counts().sort_index().plot(kind='bar')
###Output
_____no_output_____
###Markdown
Make a bar plot of the day of the week on which movies with "Romance" in their title tend to be released in the USA.
###Code
ronJeremy = release_dates[release_dates.title.str.contains('Romance')]
ronJeremy.date.dt.dayofweek.value_counts().sort_index().plot(kind='bar')
###Output
_____no_output_____
###Markdown
Make a bar plot of the day of the week on which movies with "Action" in their title tend to be released in the USA.
###Code
jamesBond = release_dates[release_dates.title.str.contains('Action')]
jamesBond.date.dt.dayofweek.value_counts().sort_index().plot(kind='bar')
###Output
_____no_output_____
###Markdown
On which date was each Judi Dench movie from the 1990s released in the USA?
###Code
judiDench = pd.merge(cast[(cast.name=='Judi Dench')],release_dates[release_dates.country=='USA'])
judiDench[judiDench.year//10 == 199]
###Output
_____no_output_____
###Markdown
In which months do films with Judi Dench tend to be released in the USA?
###Code
judiDench.date.dt.month.value_counts().sort_index()
###Output
_____no_output_____
###Markdown
In which months do films with Tom Cruise tend to be released in the USA?
###Code
tommyC =pd.merge(cast[(cast.name=='Tom Cruise')],release_dates[release_dates.country=='USA'])
tommyC.date.dt.month.value_counts().sort_index()
###Output
_____no_output_____ |
module4-classification-metrics/Travis_Cain_LS_DS14_224_assignment.ipynb | ###Markdown
Lambda School Data Science*Unit 2, Sprint 2, Module 4*--- Classification Metrics Assignment- [ ] If you haven't yet, [review requirements for your portfolio project](https://lambdaschool.github.io/ds/unit2), then submit your dataset.- [ ] Plot a confusion matrix for your Tanzania Waterpumps model.- [ ] Continue to participate in our Kaggle challenge. Every student should have made at least one submission that scores at least 70% accuracy (well above the majority class baseline).- [ ] Submit your final predictions to our Kaggle competition. Optionally, go to **My Submissions**, and _"you may select up to 1 submission to be used to count towards your final leaderboard score."_- [ ] Commit your notebook to your fork of the GitHub repo.- [ ] Read [Maximizing Scarce Maintenance Resources with Data: Applying predictive modeling, precision at k, and clustering to optimize impact](https://towardsdatascience.com/maximizing-scarce-maintenance-resources-with-data-8f3491133050), by Lambda DS3 student Michael Brady. His blog post extends the Tanzania Waterpumps scenario, far beyond what's in the lecture notebook. Stretch Goals Reading- [Attacking discrimination with smarter machine learning](https://research.google.com/bigpicture/attacking-discrimination-in-ml/), by Google Research, with interactive visualizations. _"A threshold classifier essentially makes a yes/no decision, putting things in one category or another. We look at how these classifiers work, ways they can potentially be unfair, and how you might turn an unfair classifier into a fairer one. As an illustrative example, we focus on loan granting scenarios where a bank may grant or deny a loan based on a single, automatically computed number such as a credit score."_- [Notebook about how to calculate expected value from a confusion matrix by treating it as a cost-benefit matrix](https://github.com/podopie/DAT18NYC/blob/master/classes/13-expected_value_cost_benefit_analysis.ipynb)- [Visualizing Machine Learning Thresholds to Make Better Business Decisions](https://blog.insightdatascience.com/visualizing-machine-learning-thresholds-to-make-better-business-decisions-4ab07f823415) Doing- [ ] Share visualizations in our Slack channel!- [ ] RandomizedSearchCV / GridSearchCV, for model selection. (See module 3 assignment notebook)- [ ] Stacking Ensemble. (See module 3 assignment notebook)- [ ] More Categorical Encoding. (See module 2 assignment notebook)
###Code
%%capture
import sys
# If you're on Colab:
if 'google.colab' in sys.modules:
DATA_PATH = 'https://raw.githubusercontent.com/LambdaSchool/DS-Unit-2-Kaggle-Challenge/master/data/'
!pip install category_encoders==2.*
# If you're working locally:
else:
DATA_PATH = '../data/'
import pandas as pd
# Merge train_features.csv & train_labels.csv
train = pd.merge(pd.read_csv(DATA_PATH+'waterpumps/train_features.csv'),
pd.read_csv(DATA_PATH+'waterpumps/train_labels.csv'))
# Read test_features.csv & sample_submission.csv
test = pd.read_csv(DATA_PATH+'waterpumps/test_features.csv')
sample_submission = pd.read_csv(DATA_PATH+'waterpumps/sample_submission.csv')
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
import seaborn as sns
# Split train into train & val
train, val = train_test_split(train, train_size=0.80, test_size=0.20,
stratify=train['status_group'], random_state=42)
def wrangle(X):
"""Wrangle train, validate, and test sets in the same way"""
# Prevent SettingWithCopyWarning
X = X.copy()
# About 3% of the time, latitude has small values near zero,
# outside Tanzania, so we'll treat these values like zero.
X['latitude'] = X['latitude'].replace(-2e-08, 0)
# When columns have zeros and shouldn't, they are like null values.
# So we will replace the zeros with nulls, and impute missing values later.
# Also create a "missing indicator" column, because the fact that
# values are missing may be a predictive signal.
cols_with_zeros = ['longitude', 'latitude', 'construction_year',
'gps_height', 'population']
for col in cols_with_zeros:
X[col] = X[col].replace(0, np.nan)
X[col+'_MISSING'] = X[col].isnull()
# Drop duplicate columns
duplicates = ['quantity_group', 'payment_type']
X = X.drop(columns=duplicates)
# Drop recorded_by (never varies) and id (always varies, random)
unusable_variance = ['recorded_by', 'id']
X = X.drop(columns=unusable_variance)
# return the wrangled dataframe
return X
train = wrangle(train)
val = wrangle(val)
test = wrangle(test)
# The status_group column is the target
target = 'status_group'
# Get a dataframe with all train columns except the target
train_features = train.drop(columns=[target])
# Get a list of the numeric features
numeric_features = train_features.select_dtypes(include='number').columns.tolist()
# Get a series with the cardinality of the nonnumeric features
cardinality = train_features.select_dtypes(exclude='number').nunique()
# Get a list of all categorical features with cardinality <= 50
categorical_features = cardinality[cardinality <= 50].index.tolist()
# Combine the lists
features = numeric_features + categorical_features
cardinality
X_train = train[features]
y_train = train[target]
X_val = val[features]
y_val = val[target]
X_test = test[features]
X_train = train.drop(columns=target)
y_train = train[target]
X_val = val.drop(columns=target)
y_val = val[target]
X_test = test
import category_encoders as ce
from sklearn.impute import SimpleImputer
from sklearn.metrics import accuracy_score
from sklearn.pipeline import make_pipeline
from sklearn.ensemble import RandomForestClassifier
pipeline = make_pipeline(
ce.OrdinalEncoder(),
SimpleImputer(strategy='mean'),
RandomForestClassifier(n_estimators=100, random_state=42, n_jobs=-1)
)
# Fit on train, score on val
pipeline.fit(X_train, y_train)
y_pred = pipeline.predict(X_val)
print('Validation Accuracy', accuracy_score(y_val, y_pred))
from sklearn.metrics import confusion_matrix
confusion_matrix(y_val, y_pred)
import matplotlib.pyplot as plt
from sklearn.metrics import plot_confusion_matrix
plt.rcParams['figure.dpi'] = 200
plot_confusion_matrix(pipeline, X_val, y_val, values_format='.0f', xticks_rotation='vertical');
###Output
_____no_output_____ |
src/jupyter/visualize.ipynb | ###Markdown
Cifar 10 samples
###Code
num_images = 100
images = load_neurify_mnist("./data/mnist_neurify", range(num_images)).reshape(num_images, -1)
plt.subplot(2,5,1)
plt.imshow(images[3].reshape(28,28), cmap="gray")
plt.axis("off")
plt.subplot(2,5,2)
plt.imshow(images[2].reshape(28,28), cmap="gray")
plt.axis("off")
plt.subplot(2,5,3)
plt.imshow(images[1].reshape(28,28), cmap="gray")
plt.axis("off")
plt.subplot(2,5,4)
plt.imshow(images[18].reshape(28,28), cmap="gray")
plt.axis("off")
plt.subplot(2,5,5)
plt.imshow(images[4].reshape(28,28), cmap="gray")
plt.axis("off")
plt.subplot(2,5,6)
plt.imshow(images[8].reshape(28,28), cmap="gray")
plt.axis("off")
plt.subplot(2,5,7)
plt.imshow(images[-2].reshape(28,28), cmap="gray")
plt.axis("off")
plt.subplot(2,5,8)
plt.imshow(images[0].reshape(28,28), cmap="gray")
plt.axis("off")
plt.subplot(2,5,9)
plt.imshow(images[-16].reshape(28,28), cmap="gray")
plt.axis("off")
plt.subplot(2,5,10)
plt.imshow(images[-1].reshape(28,28), cmap="gray")
plt.axis("off")
###Output
_____no_output_____
###Markdown
Plot Relu linear relaxation
###Code
x = np.linspace(-2, 2, 1000)
x_to_zero = np.linspace(-2, 0, 1000)
x_zero_up = np.linspace(0, 2, 1000)
x_long = np.linspace(-4, 4, 1000)
relu = x_long.copy()
relu[relu < 0] = 0
relax_low_1 = np.zeros_like(x_to_zero)
relax_low_2 = x_zero_up
relax_up = 0.5 * x + 1
v_line = np.linspace(-0.1, 0.1, 10)
plt.axhline(y=0, color='k', alpha=0.25)
plt.axvline(x=0, color='k', alpha=0.25)
plt.xticks([])
plt.yticks([])
plt.plot(x_long, relu, "black", x_to_zero, relax_low_1, x_zero_up, relax_low_2, x, relax, np.zeros_like(v_line) - 2, v_line, "red", np.zeros_like(v_line) + 2, v_line, "red")
plt.axis((-3, 3, -1, 3))
plt.fill_between(x, np.concatenate((relax_low_1, relax_low_2))[::2], relax_up, alpha=0.5)
plt.grid(True, which='both')
plt.xlabel("z")
plt.ylabel("y")
###Output
_____no_output_____
###Markdown
Neurify Relu linear relaxation
###Code
x = np.linspace(-2, 2, 1000)
x_long = np.linspace(-4, 4, 1000)
relu = x_long.copy()
relu[relu < 0] = 0
relax_low = 0.5 * x
relax_up = 0.5 * x + 1
v_line = np.linspace(-0.1, 0.1, 10)
plt.xticks([])
plt.yticks([])
v_line = np.linspace(-0.1, 0.1, 10)
plt.plot(x_long, relu, "k", x, relax_low, "b", x, relax_up, "g", np.zeros_like(v_line) - 2, v_line, "r", np.zeros_like(v_line) + 2, v_line, "r")
plt.axhline(y=0, color='k', alpha=0.25)
plt.axvline(x=0, color='k', alpha=0.25)
plt.axis((-3, 3, -1.5, 2.5))
plt.fill_between(x, 0.5*x, 0.5*x + 1, alpha=0.5)
plt.grid(True, which='both')
plt.xlabel("z")
plt.ylabel("y")
###Output
_____no_output_____
###Markdown
Sigmoid naive linear relaxation
###Code
start, end = -4, 0.5
x_long = np.linspace(-6, 2, 1000)
x = np.linspace(start, end, 1000)
sigmoid = lambda x: 1/(1 + np.exp(-x))
d_sigmoid = lambda x: sigmoid(x)*(1 - sigmoid(x))
s = sigmoid(x)
min_d = d_sigmoid(x[0])
relax_low = min_d * x + (s[0] - min_d*start)
relax_up = min_d * x + (s[-1] - min_d*end)
v_line = np.linspace(-0.1, 0.1, 10)
relax_low_opt = d_tangent*x - d_tangent * tangent_point + sigmoid(tangent_point)
relax_up_opt = ((s[-1] - s[0])/(x[-1] - x[0]))*(x - x[-1]) + (s[-1])
plt.xticks([])
plt.yticks([])
plt.plot(x_long, sigmoid(x_long), "k", x, relax_low, "r", x, relax_up, "r", x, relax_low_opt, "b", x, relax_up_opt, "b")
plt.axhline(y=0, color='k', alpha=0.25)
plt.axvline(x=0, color='k', alpha=0.25)
plt.axis((-5, 1.5, -0.2, 0.75))
plt.fill_between(x, relax_low, relax_up, alpha=0.5, facecolor="red")
plt.fill_between(x, relax_low_opt, relax_up_opt, alpha=0.5)
plt.grid(True, which='both')
plt.xlabel("z")
plt.ylabel("y")
start, end = -4, 0.5
x_long = np.linspace(-6, 4, 1000)
x = np.linspace(start, end, 1000)
sigmoid = lambda x: 1/(1 + np.exp(-x))
d_sigmoid = lambda x: sigmoid(x)*(1 - sigmoid(x))
s = sigmoid(x)
min_d = d_sigmoid(x[0])
relax_low = min_d * x + (s[0] - min_d*start)
relax_up = min_d * x + (s[-1] - min_d*end)
tangent_point = (x[0]**2 - x[-1]**2)/(2*(x[0] - x[-1]))
d_tangent = d_sigmoid(tangent_point)
relax_low_opt = d_tangent*x - d_tangent * tangent_point + sigmoid(tangent_point)
relax_up_opt = ((s[-1] - s[0])/(x[-1] - x[0]))*(x - x[-1]) + (s[-1])
v_line = np.linspace(-0.1, 0.1, 10)
plt.xticks([])
plt.yticks([])
plt.plot(x_long, sigmoid(x_long), "k", x, relax_low, "b", x, relax_up, "g")
plt.axhline(y=0, color='k', alpha=0.25)
plt.axvline(x=0, color='k', alpha=0.25)
plt.axis((-6, 4, -0.2, 1))
plt.fill_between(x, relax_low, relax_up, alpha=0.5)
plt.grid(True, which='both')
plt.xlabel("z")
plt.ylabel("y")
###Output
_____no_output_____
###Markdown
Naive vs. symbolic interval propagation
###Code
x = np.linspace(-2, 2, 1000)
relu = x.copy()
relu[relu < 0] = 0
v_line = np.linspace(-0.1, 0.1, 10)
plt.plot(x, relu, "k")
plt.axhline(y=0, color='k', alpha=0.25)
plt.axvline(x=0, color='k', alpha=0.25)
plt.axis((-3, 3, -1, 3))
plt.fill_between(x, np.zeros_like(x), np.zeros_like(x) + 2, facecolor="red", alpha=0.4)
plt.fill_between(x, 0.5*x, 0.5*x + 1, facecolor="blue", alpha=0.4)
plt.grid(True, which='both')
plt.xlabel("z")
plt.ylabel("y")
plt.legend(["Relu", "_no_label_", "_no_label", "Symbolic relaxation", "Naive relaxation"])
###Output
/Users/patrick/.local/share/virtualenvs/src-mm8Q-jZ2/lib/python3.6/site-packages/ipykernel_launcher.py:18: UserWarning: The handle <matplotlib.lines.Line2D object at 0x132e90a58> has a label of '_no_label_' which cannot be automatically added to the legend.
/Users/patrick/.local/share/virtualenvs/src-mm8Q-jZ2/lib/python3.6/site-packages/ipykernel_launcher.py:18: UserWarning: The handle <matplotlib.lines.Line2D object at 0x133022e80> has a label of '_no_label' which cannot be automatically added to the legend.
###Markdown
Adversarial example
###Code
nnet = NNET("./data/models_nnet/neurify/mnist24.nnet")
model = nnet.from_nnet_to_verinet_nn()
solver = VeriNet(model, max_procs=20)
image = load_neurify_mnist("./data/mnist_neurify/test_images_100/", list(range(100))).reshape((100, -1))[2]
correct_class = int(model(torch.Tensor(image)).argmax(dim=1))
eps = 5
input_bounds = np.zeros((*image.shape, 2), dtype=np.float32)
input_bounds[:, 0] = image - eps
input_bounds[:, 1] = image + eps
input_bounds = nnet.normalize_input(input_bounds)
objective = ArbitraryAdversarialObjective(correct_class, input_bounds, output_size=10)
solver.verify(objective, timeout=3600, no_split=False, verbose=False)
counter_example = np.array(solver.counter_example)
diff = (abs(counter_example - nnet.normalize_input(image)) * 255).astype(np.int32) * 10
print(correct_class)
print(int(model(torch.Tensor(counter_example)).argmax(dim=1)))
plt.subplot(1,3,1)
plt.imshow(image.reshape((28,28)), cmap="gray")
plt.axis('off')
plt.subplot(1,3,2)
plt.imshow(diff.reshape((28, 28)), cmap="gray", vmin=0, vmax=255)
plt.axis('off')
plt.subplot(1,3,3)
plt.imshow(counter_example.reshape(28, 28), cmap="gray")
plt.axis('off')
#print(counter_example)
start, end = -4, 0.5
x_long = np.linspace(-6, 6, 1000)
x = np.linspace(start, end, 1000)
sigmoid = lambda x: 1/(1 + np.exp(-x))
d_sigmoid = lambda x: sigmoid(x)*(1 - sigmoid(x))
s = sigmoid(x)
min_d = d_sigmoid(x[0])
relax_up_opt = ((s[-1] - s[0])/(x[-1] - x[0]))*(x - x[-1]) + (s[-1])
v_line_start = np.linspace(0, s[0], 100)
v_line_end = np.linspace(0, s[-1], 100)
plt.xticks([])
plt.yticks([])
plt.plot(x_long, sigmoid(x_long), "k", x, relax_up_opt, "b", np.zeros_like(v_line_start)+start, v_line_start, "r--",
np.zeros_like(v_line_end)+end, v_line_end, "r--")
plt.axhline(y=0, color='k', alpha=0.25)
plt.axvline(x=0, color='k', alpha=0.25)
plt.axis((-5, 5, -0.2, 1.2))
plt.fill_between(x, s, relax_up_opt, alpha=0.5)
plt.grid(True, which='both')
plt.xlabel("z")
plt.ylabel("y")
start, end = -0.5, 4
x_long = np.linspace(-6, 6, 1000)
x = np.linspace(start, end, 1000)
sigmoid = lambda x: 1/(1 + np.exp(-x))
d_sigmoid = lambda x: sigmoid(x)*(1 - sigmoid(x))
s = sigmoid(x)
min_d = d_sigmoid(x[0])
v_line = np.linspace(-0.1, 0.1, 10)
tangent_point = (x[-1]**2 - x[0]**2)/(2*(x[-1] - x[0]))
d_tangent = d_sigmoid(tangent_point)
relax_up_opt = d_tangent*x - d_tangent * tangent_point + sigmoid(tangent_point)
v_line_start = np.linspace(0, relax_up_opt[0], 100)
v_line_end = np.linspace(0, relax_up_opt[-1], 100)
v_line_tan = np.linspace(0, sigmoid(tangent_point), 100)
plt.xticks([])
plt.yticks([])
plt.plot(x_long, sigmoid(x_long), "k", x, relax_up_opt, "b", np.zeros_like(v_line_start)+start, v_line_start, "r--",
np.zeros_like(v_line_end)+end, v_line_end, "r--", np.zeros_like(v_line_tan)+tangent_point, v_line_tan, "g--")
plt.axhline(y=0, color='k', alpha=0.25)
plt.axvline(x=0, color='k', alpha=0.25)
plt.axis((-5, 5, -0.2, 1.2))
plt.fill_between(x, s, relax_up_opt, alpha=0.5)
plt.grid(True, which='both')
plt.xlabel("z")
plt.ylabel("y")
start, end = -2, 3
x_long = np.linspace(-6, 6, 1000)
x = np.linspace(start, end, 1000)
sigmoid = lambda x: 1/(1 + np.exp(-x))
d_sigmoid = lambda x: sigmoid(x)*(1 - sigmoid(x))
s = sigmoid(x)
min_d = d_sigmoid(x[0])
v_line = np.linspace(-0.1, 0.1, 10)
tangent_point = (x[-1]**2 - x[0]**2)/(2*(x[-1] - x[0]))
d_tangent = d_sigmoid(tangent_point)
relax_up_opt = d_tangent*x - d_tangent * tangent_point + sigmoid(tangent_point)
v_line_start = np.linspace(0, relax_up_opt[0], 100)
v_line_end = np.linspace(0, relax_up_opt[-1], 100)
v_line_tan = np.linspace(0, sigmoid(tangent_point), 100)
plt.xticks([])
plt.yticks([])
plt.plot(x_long, sigmoid(x_long), "k", x, relax_up_opt, "b", np.zeros_like(v_line_start)+start, v_line_start, "r--",
np.zeros_like(v_line_end)+end, v_line_end, "r--", np.zeros_like(v_line_tan)+tangent_point, v_line_tan, "g--")
plt.axhline(y=0, color='k', alpha=0.25)
plt.axvline(x=0, color='k', alpha=0.25)
plt.axis((-5, 5, -0.2, 1.2))
#plt.fill_between(x, s, relax_up_opt, alpha=0.5)
plt.grid(True, which='both')
plt.xlabel("z")
plt.ylabel("y")
start, end = -2, 3
x_long = np.linspace(-6, 6, 1000)
x = np.linspace(start, end, 1000)
sigmoid = lambda x: 1/(1 + np.exp(-x))
d_sigmoid = lambda x: sigmoid(x)*(1 - sigmoid(x))
s = sigmoid(x)
min_d = d_sigmoid(x[0])
v_line = np.linspace(-0.1, 0.1, 10)
tangent_point = 0.9
tangent_point_opt = (x[-1]**2 - x[0]**2)/(2*(x[-1] - x[0]))
d_tangent = d_sigmoid(tangent_point)
relax_up_opt = d_tangent*x - d_tangent * tangent_point + sigmoid(tangent_point)
v_line_start = np.linspace(0, relax_up_opt[0], 100)
v_line_end = np.linspace(0, relax_up_opt[-1], 100)
v_line_tan = np.linspace(0, sigmoid(tangent_point), 100)
v_line_tan_opt = np.linspace(0, sigmoid(tangent_point_opt), 100)
plt.xticks([])
plt.yticks([])
plt.plot(x_long, sigmoid(x_long), "k", x, relax_up_opt, "b", np.zeros_like(v_line_start)+start, v_line_start, "r--",
np.zeros_like(v_line_end)+end, v_line_end, "r--", np.zeros_like(v_line_tan)+tangent_point, v_line_tan, "g--",
np.zeros_like(v_line_tan) + tangent_point_opt, v_line_tan_opt, "y--")
plt.axhline(y=0, color='k', alpha=0.25)
plt.axvline(x=0, color='k', alpha=0.25)
plt.axis((-5, 5, -0.2, 1.2))
plt.fill_between(x, s, relax_up_opt, alpha=0.5)
plt.grid(True, which='both')
plt.xlabel("z")
plt.ylabel("y")
start, end = -2, 3
x_long = np.linspace(-6, 6, 1000)
x = np.linspace(start, end, 1000)
sigmoid = lambda x: 1/(1 + np.exp(-x))
d_sigmoid = lambda x: sigmoid(x)*(1 - sigmoid(x))
s = sigmoid(x)
min_d = d_sigmoid(x[0])
v_line = np.linspace(-0.1, 0.1, 10)
d_tangent = (s[-1] - s[0]) / (x[-1] - x[0])
intercept = d_tangent*x - d_tangent * x[0] + s[0]
v_line_start = np.linspace(0, s[0], 100)
v_line_end = np.linspace(0, s[-1], 100)
plt.xticks([])
plt.yticks([])
plt.plot(x_long, sigmoid(x_long), "k", x, intercept, "b", np.zeros_like(v_line_start)+start, v_line_start, "r--",
np.zeros_like(v_line_end)+end, v_line_end, "r--")
plt.axhline(y=0, color='k', alpha=0.25)
plt.axvline(x=0, color='k', alpha=0.25)
plt.axis((-5, 5, -0.2, 1.2))
plt.grid(True, which='both')
plt.xlabel("z")
plt.ylabel("y")
start, end = -2, 3
x_long = np.linspace(-6, 6, 1000)
x = np.linspace(start, end, 1000)
sigmoid = lambda x: 1/(1 + np.exp(-x))
d_sigmoid = lambda x: sigmoid(x)*(1 - sigmoid(x))
s = sigmoid(x)
min_d = d_sigmoid(x[0])
v_line = np.linspace(-0.1, 0.1, 10)
d_tangent = (s[-1] - s[0]) / (x[-1] - x[0])
intercept = d_tangent*x - d_tangent * x[0] + s[0]
tangent = intercept + 0.12
v_line_tan = np.linspace(0, sigmoid(1.3), 100)
v_line_start = np.linspace(0, s[0], 100)
v_line_end = np.linspace(0, s[-1], 100)
plt.xticks([])
plt.yticks([])
plt.plot(x_long, sigmoid(x_long), "k", x, intercept, "b--", x, tangent, "b", np.zeros_like(v_line_start)+start, v_line_start, "r--",
np.zeros_like(v_line_tan)+1.3, v_line_tan, "g--", np.zeros_like(v_line_end)+end, v_line_end, "r--")
plt.axhline(y=0, color='k', alpha=0.25)
plt.axvline(x=0, color='k', alpha=0.25)
plt.axis((-5, 5, -0.2, 1.2))
plt.grid(True, which='both')
plt.xlabel("z")
plt.ylabel("y")
###Output
_____no_output_____ |
notebooks/S16D_CNN_Notes.ipynb | ###Markdown
CNN for MNIST digits
###Code
%matplotlib inline
import matplotlib.pyplot as plt
import numpy as np
import warnings
warnings.simplefilter(action='ignore', category=FutureWarning)
import h5py
warnings.resetwarnings()
warnings.simplefilter(action='ignore', category=ImportWarning)
warnings.simplefilter(action='ignore', category=RuntimeWarning)
warnings.simplefilter(action='ignore', category=DeprecationWarning)
warnings.simplefilter(action='ignore', category=ResourceWarning)
from keras import models
from keras import layers
from keras.utils.np_utils import to_categorical, normalize
from keras.datasets import mnist
(train_data, train_labels), (test_data, test_labels) = mnist.load_data()
for i in range(10):
plt.subplot(2,5,i+1)
plt.imshow(train_data[i], cmap='gray')
plt.xticks([])
plt.yticks([])
plt.tight_layout()
model = models.Sequential()
model.add(layers.Conv2D(32, (3,3), activation='relu', input_shape=(28,28,1)))
model.add(layers.MaxPooling2D((2,2)))
model.add(layers.Conv2D(64, (3,3), activation='relu'))
model.add(layers.MaxPooling2D((2,2)))
model.add(layers.Conv2D(64, (3,3), activation='relu'))
model.add(layers.Flatten())
model.add(layers.Dense(64, activation='relu'))
model.add(layers.Dense(10, activation='softmax'))
model.summary()
model.compile('rmsprop', 'categorical_crossentropy', ['accuracy'])
train_data = train_data.reshape((-1,28,28,1)).astype('float32')/255
test_data = test_data.reshape((-1,28,28,1)).astype('float32')/255
train_labels = to_categorical(train_labels)
test_labels = to_categorical(test_labels)
%%time
model.fit(train_data, train_labels, batch_size=64, epochs=5)
model.evaluate(test_data, test_labels)
pred = np.argmax(model.predict(test_data[:10]), 1)
pred
for i in range(10):
plt.subplot(2,5,i+1)
plt.imshow(test_data[i].squeeze(), cmap='gray')
plt.xticks([])
plt.yticks([])
plt.title("pred=%d" % pred[i], fontsize=14)
plt.tight_layout()
###Output
_____no_output_____ |
Fuzzy.ipynb | ###Markdown
Implementation
###Code
import skfuzzy as fuzz
import numpy as np
from skfuzzy import control as ctrl
weight = ctrl.Antecedent(np.arange(0, 1001, 150), 'weight')
density = ctrl.Antecedent(np.arange(0, 12, 1), 'density')
speed = ctrl.Consequent(np.arange(0, 120, 6), 'speed')
weight.automf(3)
density.automf(3)
speed['low'] = fuzz.trimf(speed.universe, [0, 0, 40])
speed['medium'] = fuzz.trimf(speed.universe, [0, 40, 100])
speed['high'] = fuzz.trimf(speed.universe, [40, 100, 120])
rule1 = ctrl.Rule(weight['poor'] | density['poor'], speed['high'])
rule2 = ctrl.Rule(density['average'], speed['medium'])
rule3 = ctrl.Rule(density['good'] | weight['good'], speed['low'])
# rule1.view()
velocity_ctrl = ctrl.ControlSystem([rule1, rule2, rule3])
velocity = ctrl.ControlSystemSimulation(velocity_ctrl)
velocity.input['weight'] = 530
velocity.input['density'] = 8.6
velocity.compute()
print(velocity.output['speed'])
speed.view(sim=velocity)
import skfuzzy as fuzz
import numpy as np
from skfuzzy import control as ctrl
weight = ctrl.Antecedent(np.arange(0, 10, 1), 'weight')
height = ctrl.Antecedent(np.arange(0, 1, 0.1), 'height')
speed = ctrl.Consequent(np.arange(0, 1000, 200), 'speed')
weight.automf(3)
height.automf(3)
speed['low'] = fuzz.trimf(speed.universe, [0, 0, 200])
speed['medium'] = fuzz.trimf(speed.universe, [0, 200, 400])
speed['high'] = fuzz.trimf(speed.universe, [200, 400, 800])
rule1 = ctrl.Rule(weight['poor'] | height['poor'], speed['low'])
rule2 = ctrl.Rule(height['average'], speed['medium'])
rule3 = ctrl.Rule(height['good'] | weight['good'], speed['high'])
velocity_ctrl = ctrl.ControlSystem([rule1, rule2, rule3])
velocity = ctrl.ControlSystemSimulation(velocity_ctrl)
velocity.input['weight'] = 3
velocity.input['height'] = 0.8
velocity.compute()
print(velocity.output['speed'])
speed.view(sim=velocity)
###Output
394.6446446446446
###Markdown
fuzzy code for implementation
###Code
import skfuzzy as fuzz
from skfuzzy import control as ctrl
import numpy as np
import matplotlib
matplotlib.use('Qt5Agg')
weight = ctrl.Antecedent(np.arange(0, 1001, 150), 'weight')
density = ctrl.Antecedent(np.arange(0, 12, 1), 'density')
speed = ctrl.Consequent(np.arange(0, 1400, 200), 'speed')
temp = ctrl.Consequent(np.arange(0, 2000, 200), 'temp')
weight.automf(3)
density.automf(3)
speed['low'] = fuzz.trimf(speed.universe, [0, 300, 600])
speed['medium'] = fuzz.trimf(speed.universe, [400, 700, 1000])
speed['high'] = fuzz.trimf(speed.universe, [800, 1100, 1400])
temp['low'] = fuzz.trimf(temp.universe, [0, 400, 800])
temp['medium'] = fuzz.trimf(temp.universe, [600, 1000, 1400])
temp['high'] = fuzz.trimf(temp.universe, [1200, 1600, 2000])
rule1 = ctrl.Rule(weight['poor'] | density['poor'], speed['low'])
rule2 = ctrl.Rule(density['average']| weight['average'], speed['medium'])
rule3 = ctrl.Rule(density['good'] | weight['good'], speed['high'])
rule4 = ctrl.Rule(density['poor'] | weight['good'], speed['low'])
rule5 = ctrl.Rule(density['good'] | weight['poor'], speed['high'])
rule6 = ctrl.Rule(density['good'] | weight['poor'], temp['low'])
rule7 = ctrl.Rule(density['poor'] | weight['good'], temp['high'])
rule8 = ctrl.Rule(density['poor'] | weight['poor'], temp['low'])
rule9 = ctrl.Rule(density['good'] | weight['poor'], temp['low'])
rule10 = ctrl.Rule(density['average'] | weight['average'], temp['medium'])
rule11 = ctrl.Rule(density['good'] | weight['good'], temp['high'])
velocity_ctrl = ctrl.ControlSystem([rule1, rule2, rule3])
velocity = ctrl.ControlSystemSimulation(velocity_ctrl)
velocity.input['weight'] = 530
velocity.input['density'] = 8.6
velocity.compute()
print(velocity.output['speed'])
temperature_ctrl = ctrl.ControlSystem([rule11, rule8, rule10])
temperature = ctrl.ControlSystemSimulation(temperature_ctrl)
temperature.input['weight'] = 530
temperature.input['density'] = 8.6
temperature.compute()
print(temperature.output['temp'])
# speed.view(sim=velocity)
speed.view()
temp.view()
###Output
_____no_output_____
###Markdown
PLOT FOR FOLLOWER
###Code
# THE FOLLOWERS'S VALUE AND NAME
plt.plot(markFollower[:3], [1, 1, 0])
plt.suptitle("FOLLOWER - NANO")
plt.show()
plt.plot(markFollower[1:5], [0, 1, 1,0])
plt.suptitle("FOLLOWER - MICRO")
plt.show()
plt.plot(markFollower[3:], [0, 1, 1])
plt.suptitle("FOLLOWER - MEDIUM")
plt.show()
plt.plot(markFollower[:3], [1, 1, 0], label="NANO")
plt.plot(markFollower[1:5], [0, 1, 1,0], label="MICRO")
plt.plot(markFollower[3:], [0, 1, 1], label="MEDIUM")
plt.suptitle("FOLLOWER")
plt.show()
###Output
_____no_output_____
###Markdown
PLOT FOR LINGUSITIC
###Code
# THE LINGUISTIC'S VALUE AND NAME
plt.plot(markEngagement[:3], [1, 1, 0])
plt.suptitle("ENGAGEMENT - NANO")
plt.show()
plt.plot(markEngagement[1:5], [0, 1, 1,0])
plt.suptitle("ENGAGEMENT - MICRO")
plt.show()
plt.plot(markEngagement[3:], [0, 1, 1])
plt.suptitle("ENGAGEMENT - MEDIUM")
plt.show()
plt.plot(markEngagement[:3], [1, 1, 0], label="NANO")
plt.plot(markEngagement[1:5], [0, 1, 1,0], label="MICRO")
plt.plot(markEngagement[3:], [0, 1, 1], label="MEIUM")
plt.suptitle("ENGAGEMENT")
plt.show()
###Output
_____no_output_____
###Markdown
Fuzzification
###Code
# FOLLOWER=========================================
# membership function
def fuzzyFollower(countFol):
follower = []
# STABLE GRAPH
if (markFollower[0] <= countFol and countFol < markFollower[1]):
scoreFuzzy = 1
follower.append(Datafuzzy(scoreFuzzy, "NANO"))
# GRAPH DOWN
elif (markFollower[1] <= countFol and countFol <= markFollower[2]):
scoreFuzzy = np.absolute((markFollower[2] - countFol) / (markFollower[2] - markFollower[1]))
follower.append(Datafuzzy(scoreFuzzy, "NANO"))
# MICRO
# GRAPH UP
if (markFollower[1] <= countFol and countFol <= markFollower[2]):
scoreFuzzy = 1 - np.absolute((markFollower[2] - countFol) / (markFollower[2] - markFollower[1]))
follower.append(Datafuzzy(scoreFuzzy, "MICRO"))
# STABLE GRAPH
elif (markFollower[2] < countFol and countFol < markFollower[3]):
scoreFuzzy = 1
follower.append(Datafuzzy(scoreFuzzy, "MICRO"))
# GRAPH DOWN
elif (markFollower[3] <= countFol and countFol <= markFollower[4]):
scoreFuzzy = np.absolute((markFollower[4] - countFol) / (markFollower[4] - markFollower[3]))
follower.append(Datafuzzy(scoreFuzzy, "MICRO"))
# MEDIUM
# GRAPH UP
if (markFollower[3] <= countFol and countFol <= markFollower[4]):
scoreFuzzy = 1 - scoreFuzzy
follower.append(Datafuzzy(scoreFuzzy, "MEDIUM"))
# STABLE GRAPH
elif (countFol > markFollower[4]):
scoreFuzzy = 1
follower.append(Datafuzzy(scoreFuzzy, "MEDIUM"))
return follower
# ENGAGEMENT RATE =========================================
# membership function
def fuzzyEngagement(countEng):
engagement = []
# STABLE GRAPH
if (markEngagement[0] < countEng and countEng < markEngagement[1]):
scoreFuzzy = 1
engagement.append(Datafuzzy(scoreFuzzy, "NANO"))
# GRAPH DOWN
elif (markEngagement[1] <= countEng and countEng <= markEngagement[2]):
scoreFuzzy = np.absolute((markEngagement[2] - countEng) / (markEngagement[2] - markEngagement[1]))
engagement.append(Datafuzzy(scoreFuzzy, "NANO"))
# MICRO
# THE GRAPH GOES UP
if (markEngagement[1] <= countEng and countEng <= markEngagement[2]):
scoreFuzzy = 1 - scoreFuzzy
engagement.append(Datafuzzy(scoreFuzzy, "MICRO"))
# STABLE GRAPH
elif (markEngagement[2] < countEng and countEng < markEngagement[3]):
scoreFuzzy = 1
engagement.append(Datafuzzy(scoreFuzzy, "MICRO"))
# GRAPH DOWN
elif (markEngagement[3] <= countEng and countEng <= markEngagement[4]):
scoreFuzzy = np.absolute((markEngagement[4] - countEng) / (markEngagement[4] - markEngagement[3]))
engagement.append(Datafuzzy(scoreFuzzy, "MICRO"))
# MEDIUM
# THE GRAPH GOES UP
if (markEngagement[3] <= countEng and countEng <= markEngagement[4]):
scoreFuzzy = 1 - scoreFuzzy
engagement.append(Datafuzzy(scoreFuzzy, "MEDIUM"))
# STABLE GRAPH
elif (countEng > markEngagement[4]):
scoreFuzzy = 1
engagement.append(Datafuzzy(scoreFuzzy, "MEDIUM"))
return engagement
###Output
_____no_output_____
###Markdown
Inference
###Code
# Fuzzy Rules
def fuzzyRules(follower, engagement):
temp_yes = []
temp_no = []
# First decision Follower test is Nano
if (follower[0].decission == "NANO"):
# Get minimal score fuzzy every decision NO or YES
temp_yes.append(min(follower[0].score,engagement[0].score))
# if get 2 data fuzzy Engagement
if (len(engagement) > 1):
temp_yes.append(min(follower[0].score,engagement[1].score))
# First decision of Follower is Micro or Medium
else:
if (engagement[0].decission == "NANO"):
temp_no.append(min(follower[0].score, engagement[0].score))
elif (engagement[0].decission == "MICRO"):
if (follower[0].decission == "MICRO"):
temp_yes.append(min(follower[0].score, engagement[0].score))
else:
temp_no.append(min(follower[0].score,engagement[0].score))
else:
temp_yes.append(min(follower[0].score, engagement[0].score))
# if get 2 data fuzzy engagement
if (len(engagement) > 1):
if (engagement[1].decission == "NANO"):
temp_no.append(min(follower[0].score, engagement[1].score))
elif (engagement[0].decission == "MICRO"):
if (follower[0].decission == "MICRO"):
temp_yes.append(min(follower[0].score, engagement[1].score))
else:
temp_no.append(min(follower[0].score,engagement[1].score))
else:
temp_yes.append(min(follower[0].score, engagement[1].score))
# if get 2 data fuzzy Follower
if (len(follower) > 1):
# Second decision Follower is Nano
if (follower[1].decission == "NANO"):
temp_yes.append(min(engagement[0].score,follower[1].score))
if (len(engagement) > 1):
temp_yes.append(min(engagement[1].score,follower[1].score))
# Second decision follower is Micro or Medium
else:
if (engagement[0].decission == "NANO"):
temp_no.append(min(follower[1].score, engagement[0].score))
elif (engagement[0].decission == "MICRO"):
if (follower[1].decission == "MICRO"):
temp_yes.append(min(follower[1].score, engagement[0].score))
else:
temp_no.append(min(follower[1].score,engagement[0].score))
#if get 2 data fuzzy Engagement
if (len(engagement) > 1):
if (engagement[1].decission == "NANO"):
temp_no.append(min(follower[1].score, engagement[1].score))
elif (engagement[1].decission == "MICRO"):
if (follower[1].decission == "MICRO"):
temp_yes.append(min(follower[1].score, engagement[1].score))
else:
temp_no.append(min(follower[1].score,engagement[1].score))
return temp_yes, temp_no
###Output
_____no_output_____
###Markdown
Result
###Code
# Result
def getResult(resultYes, resultNo):
yes = 0
no = 0
if(resultNo):
no = max(resultNo)
if(resultYes):
yes = max(resultYes)
return yes, no
###Output
_____no_output_____
###Markdown
Defuzzification
###Code
def finalDecission(yes, no):
mamdani = (((10 + 20 + 30 + 40 + 50 + 60 + 70) * no) + ((80 + 90 + 100) * yes)) / ((7 * no) + (yes * 3))
return mamdani
###Output
_____no_output_____
###Markdown
Main Function
###Code
def mainFunction(followerCount, engagementRate):
follower = fuzzyFollower(followerCount)
engagement = fuzzyEngagement(engagementRate)
resultYes, resultNo = fuzzyRules(follower, engagement)
yes, no = getResult(resultYes, resultNo)
return finalDecission(yes, no)
data = pd.read_csv('influencers.csv')
data
hasil = []
result = []
idd = []
for i in range (len(data)):
# Insert ID and the score into the list
hasil.append([data.loc[i, 'id'], mainFunction(data.loc[i, 'followerCount'], data.loc[i, 'engagementRate'])])
result.append([data.loc[i, 'id'], (data.loc[i, 'followerCount'] * data.loc[i, 'engagementRate'] / 100)])
# Sorted list of hasil by fuzzy score DECREMENT
hasil.sort(key=lambda x:x[1], reverse=True)
result.sort(key=lambda x:x[1], reverse=True)
result = result[:20]
hasil = hasil[:20]
idd = [row[0] for row in result]
hasil
idd
def cekAkurasi(hasil, result):
count = 0
for i in range(len(hasil)):
if (hasil[i][0] in idd):
count += 1
return count
print("AKURASI : ", cekAkurasi(hasil, result)/20*100, " %")
chosen = pd.DataFrame(hasil[:20], columns=['ID', 'Score'])
chosen
chosen.to_csv('choosen.csv')
###Output
_____no_output_____
###Markdown
This notebook is used for the Fuzzy TOPSIS implementation. The main idea is to firstly rate the attributes for each alternative by the following scale: 1. Very Low (1, 1, 3)2. Low (1, 3, 5)3. Average (3, 5, 7)4. High (5, 7, 9)5. Very High (7, 9, 9)And the numbers in the brackets represent the Fuzzy Numbers. There could be several deceision makers, in our use case, several surgeons could rate the attributes by the scale and the average of their ratings will be used. As for how it will be calculated, the word representation of the rating is substituted with the appropriate fuzzy numbers from the brackets. Then, as there will be several decicison matrices (if there are several decision makers - doctors), therefore, to build a combined decision matrix the average will be calculated according to the following logic: x = (a, b, c), where a, b, and c are fuzzy numbers in the brackets; a = is the minimum of all a's from all decision matrices; b = the mean of all b componenets; c = maximum of all c components. Now, a combined decision matrix is built and the weights are going to be generated by the same logic - the rating will be provided for each attribute in general and then be substituted by the fuzzy numbers.Next, the benefit/cost criterias are calculated. The benefit criteria: r = (a/c*, b/c*, c/c*), where c* is the maximum of all the c components for the given attribute. And for the cost criteria: r = (a*/c, a*/b, a*/a), where a* is the minimum of all the a components for the given attribute. Next, the weighted normalized fuzzy decision matrix is calculated, where a * a_w, b * b_w, c * c_w and a_w, b_w, c_w are the components of the weight for the current attribute. Compute the Fuzzy Positive Ideal Solution (FPIS) and Fuzzy Negative Ideal Solution (FNIS). For FPIS, labeled as A*, the value is the maximum from all the c components within the attribute, if c components are equal, then the values are compared by the b component. As for the FNIS, labeled as A-, the value is the minimum from all a components within the attribute. Next, we calculate the distance from all the matrix cell values to the A* and A- values by the formula *sqrt(1/3 * [(a1-a2)^2 + (b1-b2)^2 + (c1-c2)^2])*. And this distance value is then used to calculate the closeness coefficient (CCi) = d-/(d- + d*).The rank is then generated according to the closeness coefficient, the highest will get higher rank.The calculation of the ranks is started by uploading the .xslx document with the table of attributes-alternatives' rating, according to the scale above (Very Low - Very High), including the rating of weights. The example of the table is provided below:
###Code
import numpy as np
import pandas as pd
import warnings
import copy
import csv
import matplotlib.pyplot as plt
import heapq
class FuzzyTopsis():
evaluation_matrix = np.array([]) # Matrix
weighted_normalized = np.array([]) # Weight matrix
normalized_decision = np.array([]) # Normalisation matrix
fuzzy_matrix = np.array([]) # Fuzzy matrix
fuzzy_weights = np.array([])
M = 0 # Number of rows
N = 0 # Number of columns
definitions_matrix = {'very low': [1, 1, 3],
'low': [1, 3, 5],
'average': [3, 5, 7],
'high': [5, 7, 9],
'very high': [7, 9, 9]}
definitions_weights = {'very low': [1, 1, 3],
'low': [1, 3, 5],
'average': [3, 5, 7],
'high': [5, 7, 9],
'very high': [7, 9, 9]}
'''
Create an evaluation matrix consisting of m alternatives and n attributes.
'''
def __init__(self, evaluation_matrix, weight_matrix, criteria):
# M×N matrix
self.evaluation_matrix = np.array(evaluation_matrix, dtype="object")
# M alternatives (options)
self.row_size = len(self.evaluation_matrix)
# N attributes/criteria
self.column_size = len(self.evaluation_matrix[0])
# N size weight matrix
self.weight_matrix = np.array(weight_matrix, dtype="object")
#self.weight_matrix = self.weight_matrix/sum(self.weight_matrix)
self.criteria = np.array(criteria, dtype="object")
'''
# Step 2
The word representation of ratings converted to fuzzy numbers
'''
def step_2(self):
self.fuzzy_matrix = np.empty(shape=(self.row_size,self.column_size),dtype="object")
self.fuzzy_weights = np.empty(shape=(1, self.column_size),dtype="object")
#self.fuzzy_matrix = np.copy(self.evaluation_matrix)
# Substituting words with fuzzy numbers for fuzzy matrix
for i in range(self.row_size):
for j in range(self.column_size):
if self.evaluation_matrix[i,j].lower() in self.definitions_matrix:
self.fuzzy_matrix[i,j] = self.definitions_matrix[self.evaluation_matrix[i,j].lower()]
else:
print("Wrong rating for cell [", i, j, "]")
break
# Substituting words with fuzzy numbers for fuzzy weights
for i in range(len(self.weight_matrix)):
if self.weight_matrix[i].lower() in self.definitions_weights:
self.fuzzy_weights[0, i] = self.definitions_weights[self.weight_matrix[i].lower()]
else:
print("Wrong rating for cell [", i, j, "]")
break
'''
df_orig_matrix = pd.DataFrame(self.evaluation_matrix)
df_orig_matrix.loc[df_orig_matrix.shape[0]] = [None]*self.column_size
df_orig_matrix.to_csv("./step1_matrix.csv", index = False, header = False)
text = [None] * self.column_size
text[0] = "Step 2. Fuzzy matrix"
with open(r'./step1_matrix.csv', 'a') as f:
writer = csv.writer(f)
writer.writerow(text)
df_matrix = pd.DataFrame(self.fuzzy_matrix)
df_matrix.loc[df_matrix.shape[0]] = [None]*self.column_size
df_matrix.to_csv("./step1_matrix.csv", mode = 'a', index = False, header = False)
text[0] = "Fuzzy weights"
with open(r'./step1_matrix.csv', 'a') as f:
writer = csv.writer(f)
writer.writerow(text)
text[0] = ["Step 3. Normalization matrix"]
df_weights = pd.DataFrame(self.fuzzy_weights)
df_weights.loc[df_weights.shape[0]] = [None]*self.column_size
df_weights.to_csv("./step1_matrix.csv", mode = 'a', index = False, header = False)
with open(r'./step1_matrix.csv', 'a') as f:
writer = csv.writer(f)
writer.writerow(text)
print("Fuzzy matrix: ", self.fuzzy_matrix)
print("\nFuzzy weights: ", self.fuzzy_weights)
'''
'''
# Step 3
Compute normalized fuzzy decision matrix
'''
def step_3(self):
# normalized scores
self.normalized_decision = copy.deepcopy(self.fuzzy_matrix)
for j in range(self.column_size):
for i in range(self.row_size):
# finding benefit and cost criterias for each attribute
benefit_criteria = max(x[2] for x in self.fuzzy_matrix[:, j]) # max better
cost_criteria = min(x[0] for x in self.fuzzy_matrix[:, j]) # min better
# calculating normalized matrix according to criteria
val = []
if self.criteria[j]:
for k in range(3):
val.append(self.fuzzy_matrix[i,j][k] / benefit_criteria)
else:
for k in range(3):
val.append(cost_criteria / self.fuzzy_matrix[i,j][-(k+1)])
self.normalized_decision[i,j] = val
#print("\nNormalized decision matrix: ", self.normalized_decision)
'''
df_norm = pd.DataFrame(self.normalized_decision)
df_norm.loc[df_norm.shape[0]] = [None]*self.column_size
df_norm.to_csv("./step3_normalization.csv", index = False, header = False)
text = [None] * self.column_size
text[0] = "Step 4. Weighted Normalization matrix"
with open(r'./step3_normalization.csv', 'a') as f:
writer = csv.writer(f)
writer.writerow(text)
'''
'''
# Step 4
Calculate the weighted normalised decision matrix
'''
def step_4(self):
self.weighted_normalized = copy.deepcopy(self.normalized_decision)
for i in range(self.row_size):
for j in range(self.column_size):
value = []
for val, weight in zip(self.normalized_decision[i,j], self.fuzzy_weights[0,j]):
value.append(val * weight)
self.weighted_normalized[i, j] = value
'''
print("\nStep 4")
print("\nWeighted Normalization matrix:", self.weighted_normalized)
df_weighted = pd.DataFrame(self.weighted_normalized)
df_weighted.loc[df_weighted.shape[0]] = [None]*self.column_size
df_weighted.to_csv("./step3_normalization.csv", mode = 'a', index = False, header = False)
text = [None] * self.column_size
text[0] = "Step 5. Fuzzy Positive Ideal Solution"
with open(r'./step3_normalization.csv', 'a') as f:
writer = csv.writer(f)
writer.writerow(text)
'''
'''
# Step 5
Determine the ideal worst alternative and the ideal best alternative:
'''
def step_5(self):
self.worst_alternatives = np.zeros(self.column_size, dtype="object")
self.best_alternatives = np.zeros(self.column_size, dtype="object")
self.weighted_normalized_copy = copy.deepcopy(self.weighted_normalized)
for j in range(self.column_size):
max_best = []
min_worst = []
for i in range(self.row_size):
#print("max best", max_best)
self.weighted_normalized_copy[i,j].reverse()
#print("reversed",self.weighted_normalized_copy[i,j])
heapq.heappush(max_best, tuple(np.negative(self.weighted_normalized_copy[i, j])))
heapq.heappush(min_worst, self.weighted_normalized[i,j])
best = list(heapq.heappop(max_best))
best.reverse()
self.best_alternatives[j] = np.negative(best)
self.worst_alternatives[j] = heapq.heappop(min_worst)
'''
print("\nStep 5")
print("\nA*:", self.best_alternatives)
print("\nA-:", self.worst_alternatives)
df_aa = pd.DataFrame(self.best_alternatives)
df_aa.loc[df_aa.shape[0]] = [None]
df_aa.to_csv("./step5_AA.csv", index = False, header = False)
text = [None]
text[0] = "Fuzzy Negative Ideal Solution"
with open(r'./step5_AA.csv', 'a') as f:
writer = csv.writer(f)
writer.writerow(text)
df_aa = pd.DataFrame(self.worst_alternatives)
df_aa.loc[df_aa.shape[0]] = [None]
df_aa.to_csv("./step5_AA.csv", mode = 'a', index = False, header = False)
text[0] = "Step 6. Distance values. d*:"
with open(r'./step5_AA.csv', 'a') as f:
writer = csv.writer(f)
writer.writerow(text)
'''
'''
# Step 6
Calculate the L2-distance between the target alternative with the ideal worst
value and the ideal best value.
'''
def step_6(self):
self.worst_distance = np.zeros(self.row_size)
self.best_distance = np.zeros(self.row_size)
self.worst_distance_mat = copy.deepcopy(self.weighted_normalized)
self.best_distance_mat = copy.deepcopy(self.weighted_normalized)
for i in range(self.row_size):
for j in range(self.column_size):
current_worst_distance = 0
current_best_distance = 0
for k in range(3):
current_worst_distance += (self.weighted_normalized[i,j][k]-self.worst_alternatives[j][k])**2
current_best_distance += (self.weighted_normalized[i,j][k]-self.best_alternatives[j][k])**2
self.worst_distance_mat[i, j] = (current_worst_distance / 3)**0.5
self.best_distance_mat[i, j] = (current_best_distance / 3)**0.5
self.worst_distance[i] += self.worst_distance_mat[i,j]
self.best_distance[i] += self.best_distance_mat[i,j]
'''
print("\nStep 6")
print("\nbest matrix", self.best_distance_mat)
print("\nworst matrix", self.worst_distance_mat)
print("\nd*:", self.best_distance)
print("\nd-:", self.worst_distance)
df_weighted = pd.DataFrame(self.best_distance)
df_weighted.loc[df_weighted.shape[0]] = [None]
df_weighted.to_csv("./step6_dd.csv", index = False, header = False)
text = [None]
text[0] = "d-:"
with open(r'./step6_dd.csv', 'a') as f:
writer = csv.writer(f)
writer.writerow(text)
df_weighted = pd.DataFrame(self.worst_distance)
df_weighted.loc[df_weighted.shape[0]] = [None]
df_weighted.to_csv("./step6_dd.csv", mode = 'a', index = False, header = False)
text[0] = "Closeness coefficients:"
with open(r'./step6_dd.csv', 'a') as f:
writer = csv.writer(f)
writer.writerow(text)
'''
'''
# Step 7
Calculate the closeness coefficient
'''
def step_7(self):
np.seterr(all='ignore')
self.closeness_coefficient = np.zeros(self.row_size)
for i in range(self.row_size):
self.closeness_coefficient[i] = self.worst_distance[i] / \
(self.worst_distance[i]+self.best_distance[i])
'''
df_cc = pd.DataFrame(self.worst_distance)
df_cc.loc[df_cc.shape[0]] = [None]
df_cc.to_csv("./step6_dd.csv", mode = 'a', index = False, header = False)
'''
def ranking(self, data):
return [i+1 for i in np.argsort(-1*data)]
'''
# Step 8
Calculate and visualize the ranking
'''
def rank_to_closeness_coefficient(self):
return self.ranking(self.closeness_coefficient)
def get_closeness_coefficient(self):
return self.closeness_coefficient
def visualization(self, ranking):
axes = []
for i, rank in enumerate(ranking):
axes.append(((rank), (i + 1)))
axes.sort(key=lambda x: x[0])
x_axis,y_axis = ['A' + str(val[0]) for val in axes],[val[1] for val in axes]
plt.figure(figsize=(12, 6))
ax = pd.Series(y_axis).plot(kind='bar')
ax.set_xticklabels(x_axis)
rects = ax.patches
for rect, label in zip(rects, y_axis):
height = rect.get_height()
ax.text(rect.get_x() + rect.get_width()/2, height, label, ha='center', va='bottom')
ax.bar(x_axis,y_axis)
def calc(self):
#print("Step 1\n", self.evaluation_matrix, end="\n\n")
self.step_2()
#print("Step 2\n", self.normalized_decision, end="\n\n")
self.step_3()
#print("Step 3\n", self.weighted_normalized, end="\n\n")
self.step_4()
self.step_5()
self.step_6()
self.step_7()
#print("Step 7\ncloseness coefficients\n", self.closeness_coefficient, end="\n\n")
###Output
_____no_output_____
###Markdown
The driver code for testing is presented below. The execution plan is as follows: 1. Read the excel file, where the file name and sheet_name should be provided to specify which case user wants to test. 2. Read the excel file to store the weights and criteria values. 3. Create Fuzzy Topsis instance with the read values 4. Calculate the rankingsFor the input file, the format should be:1. First row are the criterias: put either B - for beneficial or NB - for non beneficial2. Second row are weights3. Input matrix with attribute names and alternatives' names
###Code
import numpy as np
import pandas as pd
filename = "Fuzzy TOPSIS with custom weights.xlsx"
sheetname = "Beginning"
xl = pd.read_excel(filename, sheet_name=sheetname)
xl = pd.read_excel(filename, sheet_name=sheetname, usecols=range(1, len(xl.columns)+1))
# initializing input matrix, weights, criterias
input_matrix = xl.tail(n=len(xl.index) - 2).values.tolist()
weights = xl.loc[0].values
criterias = xl.head(n=0).columns.values
new_criterias = []
for criteria in criterias:
# if the attribute is non beneficiary, meaning min value is better -> False,
# otherwise, the criteria is True
new_criterias.append(False if criteria[0] == 'N' else True)
print('input matrix: ', input_matrix)
print('weights: ', weights)
print('criterias: ', new_criterias)
f = FuzzyTopsis(input_matrix, weights, new_criterias)
# calculating the ranking
f.calc()
ranks = f.rank_to_closeness_coefficient()
print("\nRanks\n", ranks)
print("\nVisualization graph:")
f.visualization(ranks)
'''import os
import glob
import pandas as pd
os.chdir("/content/")
extension = 'csv'
all_filenames = [i for i in glob.glob('*.{}'.format(extension))]
#combine all files in the list
li = []
for filename in all_filenames:
df = pd.read_csv(filename, index_col=None, header=None)
li.append(df)
combined_csv = pd.concat(li, axis=0, ignore_index=True)
#combined_csv = pd.concat([pd.read_csv(f) for f in all_filenames],axis=1, join='inner')
#export to csv
combined_csv.to_csv( "combined_csv.csv", index=False, encoding='utf-8-sig')
'''
###Output
_____no_output_____
###Markdown
A fuzzy approach to support decision-making in the triage processes for suspected COVID-19 patients in Brazil Authors* Nadya Regina Galo ([email protected]) * Marcos Paulino Roriz Junior ([email protected])* Rodrigo Pinheiro Tóffano Pereira ([email protected])----* Faculty of Science and Technology* Federal University of Goiás Dependencies* matplotlib, seaborn, pandas, numpy, **scikit-fuzzy** Data Source* https://coronavirus.es.gov.br/painel-covid-19-es Preamble
###Code
# Jupyter Imports
import warnings
from IPython.display import SVG
from IPython.display import display
warnings.simplefilter('ignore')
%matplotlib inline
# Basic Imports
import pandas as pd
import numpy as np
# Graphical Imports
import matplotlib as mpl
import matplotlib.pyplot as plt
import seaborn as sns
from matplotlib import rc
from matplotlib.ticker import PercentFormatter
sns.reset_defaults()
sns.reset_orig()
sns.set_palette("Set2", 8)
sns.color_palette("Set2")
rc('font', **{"size": 12})
# Fuzzy
import skfuzzy as fuzz
from skfuzzy import control as ctrl
###Output
_____no_output_____
###Markdown
Reading Input Data
###Code
rawdf = pd.read_csv("MICRODADOS.csv", error_bad_lines=False, sep=";", encoding="iso-8859-1")
rawdf.head()
# Number of records
rawdf.shape
# Removing none responses
df = rawdf
df = df[df["Febre"] != "-"]
df = df[df["DificuldadeRespiratoria"] != "-"]
df = df[df["Tosse"] != "-"]
df = df[df["Coriza"] != "-"]
df = df[df["DorGarganta"] != "-"]
df = df[df["Diarreia"] != "-"]
df = df[df["Cefaleia"] != "-"]
df = df[df["ComorbidadePulmao"] != "-"]
df = df[df["ComorbidadeCardio"] != "-"]
df = df[df["ComorbidadeRenal"] != "-"]
df = df[df["ComorbidadeDiabetes"] != "-"]
df = df[df["ComorbidadeObesidade"] != "-"]
df = df[df["FicouInternado"] != "-"]
###Output
_____no_output_____
###Markdown
Preprocessing input variables Vital Signs
###Code
# Febre = Fever
df["Febre"].value_counts()
# VS - Vital Signs
# Sim = Yes
def sv(row):
if row["Febre"] == "Sim":
return 2
else:
return 0
df["VS"] = df.apply(sv, axis=1)
df["VS"].value_counts()
###Output
_____no_output_____
###Markdown
Breathing Difficulty
###Code
# Dificuldade Respiratória = Breathing Difficulty
df["DificuldadeRespiratoria"].value_counts()
# BD - Breathing Difficulty
def bd(row):
if row["DificuldadeRespiratoria"] == "Sim":
return 0.5
else:
return 0
df["BD"] = df.apply(bd, axis=1)
df["BD"].value_counts()
###Output
_____no_output_____
###Markdown
Risk Factors
###Code
# Age Group
df["FaixaEtaria"].value_counts()
# Pregnant?
df["Gestante"].value_counts()
def rf(row):
if (row["FaixaEtaria"] == "60 a 69 anos" or
row["FaixaEtaria"] == "70 a 79 anos" or
row["FaixaEtaria"] == "80 a 89 anos" or
row["FaixaEtaria"] == "90 anos ou mais" or
row["ComorbidadePulmao"] == "Sim" or
row["ComorbidadeCardio"] == "Sim" or
row["ComorbidadeRenal"] == "Sim" or
row["ComorbidadeDiabetes"] == "Sim" or
row["ComorbidadeTabagismo"] == "Sim" or
row["ComorbidadeObesidade"] == "Sim" or
row["Gestante"] == "1º trimeste" or
row["Gestante"] == "2º trimeste" or
row["Gestante"] == "3º trimeste"):
return 1
else:
return 0
df["RF"] = df.apply(rf, axis=1)
df["RF"].value_counts()
###Output
_____no_output_____
###Markdown
Other Relevant Syntoms
###Code
# RS - Relevant Syntoms
# Tosse = Cough
# Coriza = Runny nose
# Dor Garganta = Sore throat
# Diarreia = Diarrhea
# Cefaleia = Headache
def rs(row):
if (row["Tosse"] == "Sim" or
row["Coriza"] == "Sim" or
row["DorGarganta"] == "Sim" or
row["Diarreia"] == "Sim" or
row["Cefaleia"] == "Sim"):
return 1
else:
return 0
df["RS"] = df.apply(rs, axis=1)
df["RS"].value_counts()
###Output
_____no_output_____
###Markdown
Fuzzy Model Variables and Membership
###Code
fuzzy_vs = ctrl.Antecedent(np.arange(0, 19, 1), 'VS')
fuzzy_vs["low"] = fuzz.trapmf(fuzzy_vs.universe, [0, 0, 4, 5])
fuzzy_vs['medium'] = fuzz.trapmf(fuzzy_vs.universe, [4, 5, 6, 7])
fuzzy_vs['high'] = fuzz.trapmf(fuzzy_vs.universe, [6, 7, 18, 18])
fuzzy_vs.view()
fuzzy_bd = ctrl.Antecedent(np.arange(0, 1.01, 0.05), 'BD')
fuzzy_bd["minimal"] = fuzz.trapmf(fuzzy_bd.universe, [0, 0, 0.2, 0.25])
fuzzy_bd['high'] = fuzz.trimf(fuzzy_bd.universe, [0.25, 0.5, 0.75])
fuzzy_bd['veryhigh'] = fuzz.trapmf(fuzzy_bd.universe, [0.75, 0.8, 1, 1])
fuzzy_bd.view()
fuzzy_rf = ctrl.Antecedent(np.arange(0, 1.01, 0.05), 'RF')
fuzzy_rf["minimal"] = fuzz.trapmf(fuzzy_rf.universe, [0, 0, 0.4, 0.6])
fuzzy_rf['meaningful'] = fuzz.trapmf(fuzzy_rf.universe, [0.4, 0.6, 1, 1])
fuzzy_rf.view()
fuzzy_rs = ctrl.Antecedent(np.arange(0, 1.01, 0.05), 'RS')
fuzzy_rs["minimal"] = fuzz.trapmf(fuzzy_rs.universe, [0, 0, 0.4, 0.6])
fuzzy_rs['meaningful'] = fuzz.trapmf(fuzzy_rs.universe, [0.4, 0.6, 1, 1])
fuzzy_rs.view()
fuzzy_sv = ctrl.Consequent(np.arange(0, 1.01, 0.05), 'SV', defuzzify_method="centroid")
fuzzy_sv["mild"] = fuzz.trimf(fuzzy_sv.universe, [0, 0, 0.3])
fuzzy_sv['moderate'] = fuzz.trimf(fuzzy_sv.universe, [0.2, 0.5, 0.8])
fuzzy_sv['severe'] = fuzz.trimf(fuzzy_sv.universe, [0.7, 1, 1])
fuzzy_sv.view()
###Output
_____no_output_____
###Markdown
Control
###Code
r1 = ctrl.Rule(fuzzy_vs['low'] & fuzzy_bd["minimal"] & fuzzy_rf["minimal"] & fuzzy_rs["minimal"], fuzzy_sv["mild"])
r2 = ctrl.Rule(fuzzy_vs['low'] & fuzzy_bd["minimal"] & fuzzy_rf["minimal"] & fuzzy_rs["meaningful"], fuzzy_sv["mild"])
r3 = ctrl.Rule(fuzzy_vs['low'] & fuzzy_bd["minimal"] & fuzzy_rf["meaningful"] & fuzzy_rs["minimal"], fuzzy_sv["mild"])
r4 = ctrl.Rule(fuzzy_vs['low'] & fuzzy_bd["minimal"] & fuzzy_rf["meaningful"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
r5 = ctrl.Rule(fuzzy_vs['low'] & fuzzy_bd["high"] & fuzzy_rf["minimal"] & fuzzy_rs["minimal"], fuzzy_sv["moderate"])
r6 = ctrl.Rule(fuzzy_vs['low'] & fuzzy_bd["high"] & fuzzy_rf["minimal"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
r7 = ctrl.Rule(fuzzy_vs['low'] & fuzzy_bd["high"] & fuzzy_rf["meaningful"] & fuzzy_rs["minimal"], fuzzy_sv["severe"])
r8 = ctrl.Rule(fuzzy_vs['low'] & fuzzy_bd["high"] & fuzzy_rf["meaningful"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
r9 = ctrl.Rule(fuzzy_vs['low'] & fuzzy_bd["veryhigh"] & fuzzy_rf["minimal"] & fuzzy_rs["minimal"], fuzzy_sv["severe"])
r10 = ctrl.Rule(fuzzy_vs['low'] & fuzzy_bd["veryhigh"] & fuzzy_rf["minimal"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
r11 = ctrl.Rule(fuzzy_vs['low'] & fuzzy_bd["veryhigh"] & fuzzy_rf["meaningful"] & fuzzy_rs["minimal"], fuzzy_sv["severe"])
r12 = ctrl.Rule(fuzzy_vs['low'] & fuzzy_bd["veryhigh"] & fuzzy_rf["meaningful"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
r13 = ctrl.Rule(fuzzy_vs['medium'] & fuzzy_bd["minimal"] & fuzzy_rf["minimal"] & fuzzy_rs["minimal"], fuzzy_sv["mild"])
r14 = ctrl.Rule(fuzzy_vs['medium'] & fuzzy_bd["minimal"] & fuzzy_rf["minimal"] & fuzzy_rs["meaningful"], fuzzy_sv["moderate"])
r15 = ctrl.Rule(fuzzy_vs['medium'] & fuzzy_bd["minimal"] & fuzzy_rf["meaningful"] & fuzzy_rs["minimal"], fuzzy_sv["moderate"])
r16 = ctrl.Rule(fuzzy_vs['medium'] & fuzzy_bd["minimal"] & fuzzy_rf["meaningful"] & fuzzy_rs["meaningful"], fuzzy_sv["moderate"])
r17 = ctrl.Rule(fuzzy_vs['medium'] & fuzzy_bd["high"] & fuzzy_rf["minimal"] & fuzzy_rs["minimal"], fuzzy_sv["moderate"])
r18 = ctrl.Rule(fuzzy_vs['medium'] & fuzzy_bd["high"] & fuzzy_rf["minimal"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
r19 = ctrl.Rule(fuzzy_vs['medium'] & fuzzy_bd["high"] & fuzzy_rf["meaningful"] & fuzzy_rs["minimal"], fuzzy_sv["severe"])
r20 = ctrl.Rule(fuzzy_vs['medium'] & fuzzy_bd["high"] & fuzzy_rf["meaningful"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
r21 = ctrl.Rule(fuzzy_vs['medium'] & fuzzy_bd["veryhigh"] & fuzzy_rf["minimal"] & fuzzy_rs["minimal"], fuzzy_sv["severe"])
r22 = ctrl.Rule(fuzzy_vs['medium'] & fuzzy_bd["veryhigh"] & fuzzy_rf["minimal"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
r23 = ctrl.Rule(fuzzy_vs['medium'] & fuzzy_bd["veryhigh"] & fuzzy_rf["meaningful"] & fuzzy_rs["minimal"], fuzzy_sv["severe"])
r24 = ctrl.Rule(fuzzy_vs['medium'] & fuzzy_bd["veryhigh"] & fuzzy_rf["meaningful"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
r25 = ctrl.Rule(fuzzy_vs['high'] & fuzzy_bd["minimal"] & fuzzy_rf["minimal"] & fuzzy_rs["minimal"], fuzzy_sv["moderate"])
r26 = ctrl.Rule(fuzzy_vs['high'] & fuzzy_bd["minimal"] & fuzzy_rf["minimal"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
r27 = ctrl.Rule(fuzzy_vs['high'] & fuzzy_bd["minimal"] & fuzzy_rf["meaningful"] & fuzzy_rs["minimal"], fuzzy_sv["severe"])
r28 = ctrl.Rule(fuzzy_vs['high'] & fuzzy_bd["minimal"] & fuzzy_rf["meaningful"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
r29 = ctrl.Rule(fuzzy_vs['high'] & fuzzy_bd["high"] & fuzzy_rf["minimal"] & fuzzy_rs["minimal"], fuzzy_sv["severe"])
r30 = ctrl.Rule(fuzzy_vs['high'] & fuzzy_bd["high"] & fuzzy_rf["minimal"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
r31 = ctrl.Rule(fuzzy_vs['high'] & fuzzy_bd["high"] & fuzzy_rf["meaningful"] & fuzzy_rs["minimal"], fuzzy_sv["severe"])
r32 = ctrl.Rule(fuzzy_vs['high'] & fuzzy_bd["high"] & fuzzy_rf["meaningful"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
r33 = ctrl.Rule(fuzzy_vs['high'] & fuzzy_bd["veryhigh"] & fuzzy_rf["minimal"] & fuzzy_rs["minimal"], fuzzy_sv["severe"])
r34 = ctrl.Rule(fuzzy_vs['high'] & fuzzy_bd["veryhigh"] & fuzzy_rf["minimal"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
r35 = ctrl.Rule(fuzzy_vs['high'] & fuzzy_bd["veryhigh"] & fuzzy_rf["meaningful"] & fuzzy_rs["minimal"], fuzzy_sv["severe"])
r36 = ctrl.Rule(fuzzy_vs['high'] & fuzzy_bd["veryhigh"] & fuzzy_rf["meaningful"] & fuzzy_rs["meaningful"], fuzzy_sv["severe"])
fuzzy_ctrl = ctrl.ControlSystem([r1, r2, r3, r4, r5, r6, r7, r8, r9, r10, r11, r12,
r13, r14, r15, r16, r17, r18, r19, r20, r21, r22, r23, r24,
r25, r26, r27, r28, r29, r30, r31, r32, r33, r34, r35, r36 ])
fuzzy_system = ctrl.ControlSystemSimulation(fuzzy_ctrl)
###Output
_____no_output_____
###Markdown
Sample Execution
###Code
fuzzy_system.input['VS'] = 2
fuzzy_system.input['BD'] = 0.5
fuzzy_system.input['RF'] = 1
fuzzy_system.input['RS'] = 1
fuzzy_system.compute()
fuzzy_system.output
fuzzy_sv.view(sim=fuzzy_system)
###Output
_____no_output_____
###Markdown
Apply to the dataset
###Code
def fuzzify(row):
fuzzy_system.input['VS'] = row["VS"]
fuzzy_system.input['BD'] = row["BD"]
fuzzy_system.input['RF'] = row["RF"]
fuzzy_system.input['RS'] = row["RS"]
fuzzy_system.compute()
return fuzzy_system.output["SV"]
df["FUZZY"] = df.apply(fuzzify, axis=1)
df["FUZZY"].value_counts()
df["FUZZYSTR"] = np.where(df["FUZZY"] >= 0.75, "Sim", "Não")
df["FUZZYSTR"].value_counts(normalize=True)
###Output
_____no_output_____
###Markdown
Plot
###Code
def plotComparison(df_fuzzy, df_manual, ax):
labels = ["Yes", "No"]
df_fuzzy_yes = 0
df_fuzzy_no = 0
df_manual_yes = 0
df_manual_no = 0
if "Sim" in df_fuzzy.index:
df_fuzzy_yes = df_fuzzy.loc["Sim"]["percentage"]
if "Não" in df_fuzzy.index:
df_fuzzy_no = df_fuzzy.loc["Não"]["percentage"]
if "Sim" in df_manual.index:
df_manual_yes = df_manual.loc["Sim"]["percentage"]
if "Não" in df_manual.index:
df_manual_no = df_manual.loc["Não"]["percentage"]
data_yes = [df_fuzzy_yes, df_fuzzy_no]
data_no = [df_manual_yes, df_manual_no]
x = np.arange(len(labels))
width = 0.3 # the width of the bars
rects1 = ax.bar(x - width/2, data_yes, width, label='Yes')
rects2 = ax.bar(x + width/2, data_no, width, label='No')
return ax
def processData(indf, column):
outdf = indf[indf["Evolucao"] == column]
outdf = outdf[(df["FicouInternado"] == "Sim") | (df["FicouInternado"] == "Não")]
a = outdf["FUZZYSTR"].value_counts(normalize=True)
b = outdf["FUZZYSTR"].value_counts()
df_fuzzy = pd.concat([a, b], axis=1)
df_fuzzy.columns = ["percentage", "raw"]
a = outdf["FicouInternado"].value_counts(normalize=True)
b = outdf["FicouInternado"].value_counts()
df_manual = pd.concat([a, b], axis=1)
df_manual.columns = ["percentage", "raw"]
return df_fuzzy, df_manual
def putLabels(ax, xticks, label, addYLabel=True):
for rect in ax.patches:
# Find where everything is located
height = rect.get_height()
width = rect.get_width()
x = rect.get_x()
y = rect.get_y()
# print(x, y, width, height)
label_x = x + width - 0.04 # adjust 0.2 to center the label
label_y = y + height + 0.04
if (label_y > 1):
label_y = label_y - 0.1
# The width of the bar is the data value and can used as the label
label_text = str(round(height * 100)) + "%"
ax.text(label_x, label_y, label_text, ha='right', va='center', fontsize=12)
if addYLabel:
ax.set_ylabel('Percentage')
ax.set_xlabel(label)
ax.set_xticks(np.arange(len(xticks)))
ax.set_xticklabels(xticks)
ax.yaxis.set_major_formatter(PercentFormatter(1, decimals=0))
ax.set_ylim(0, 1)
# Graph related to all cases in the state
fig, ax = plt.subplots(1, 2, figsize=(10, 4))
ax0, ax1 = ax.flatten()
ylabels=["Our approach", "Current procedure"]
xlabels=["Yes", "No"]
df_fuzzy, df_manual = processData(df, "Óbito pelo COVID-19")
plotComparison(df_fuzzy, df_manual, ax0)
putLabels(ax0, xlabels, "Hospitalization (patients that died by COVID-19)")
df_fuzzy, df_manual = processData(df, "Óbito por outras causas")
plotComparison(df_fuzzy, df_manual, ax1)
putLabels(ax1, xlabels, "Hospitalization (patients that died by other causes)", False)
lgd = fig.legend(ax0, labels=ylabels, ncol=4, loc='lower center', bbox_transform = plt.gcf().transFigure)
fig.tight_layout(w_pad=2.5, h_pad=1, pad=3.5)
for lh in lgd.legendHandles:
lh.set_alpha(1)
fig.savefig('fig6.png', bbox_inches='tight', dpi=300)
# Graph related to cases in Vila Valério
casedf = df[df["Municipio"] == "VILA VALERIO"]
fig, ax = plt.subplots(1, 2, figsize=(10, 4))
ax0, ax1 = ax.flatten()
ylabels=["Our approach", "Current procedure"]
xlabels=["Yes", "No"]
df_fuzzy, df_manual = processData(casedf, "Óbito pelo COVID-19")
plotComparison(df_fuzzy, df_manual, ax0)
putLabels(ax0, xlabels, "Hospitalization (patients that died by COVID-19)")
df_fuzzy, df_manual = processData(casedf, "Óbito por outras causas")
plotComparison(df_fuzzy, df_manual, ax1)
putLabels(ax1, xlabels, "Hospitalization (patients that died by other causes)", False)
lgd = fig.legend(ax0, labels=ylabels, ncol=4, loc='lower center', bbox_transform = plt.gcf().transFigure)
fig.tight_layout(w_pad=2.5, h_pad=1, pad=3.5)
for lh in lgd.legendHandles:
lh.set_alpha(1)
fig.savefig('fig7.png', bbox_inches='tight', dpi=300)
###Output
_____no_output_____ |
invent-prediction/main_feature_engine.ipynb | ###Markdown
Model to forecast inventory demand based on historical sales data.
###Code
%matplotlib inline
import numpy as np
import pandas as pd
from scipy import stats
import matplotlib.pyplot as plt
import time
import random
import pickle
import math
import warnings
warnings.filterwarnings("ignore")
###Output
_____no_output_____
###Markdown
Model accuracy is RMSLE
###Code
def rmsle(y, y_pred):
assert len(y) == len(y_pred)
terms_to_sum = [(math.log(y_pred[i] + 1) - math.log(y[i] + 1)) ** 2.0 for i,pred in enumerate(y_pred)]
return (sum(terms_to_sum) * (1.0/len(y))) ** 0.5
###Output
_____no_output_____
###Markdown
Load Training Data The size of the training data is quite large (~4 GB). Large datasets require significant amount of memory to process. Instead, we will sample the data randomly for our initial data analysis and visualization.
###Code
def load_samp_data(filename='train.csv', columns=[], load_pkl=1):
"""
Function returns a dataframe containing the training data sampled randomly.
The data is also stored in a pickle file for later processing.
"""
if load_pkl:
inputfile = open('train_samp_data.pkl', 'rb')
data = pickle.load(inputfile)
inputfile.close()
return data
chunksize= 10 ** 6
datasize = 74180464 #datasize = sum(1 for line in open(filename)) - 1 #number of records in file (excludes header)
samplesize = 10 ** 3 # samples per chunk of data read from the file.
data = pd.DataFrame([],columns=columns)
chunks = pd.read_csv(filename, iterator=True, chunksize=chunksize)
for chunk in chunks:
chunk.columns = columns
data = data.append(chunk.sample(samplesize))
# write data to a pickle file.
outputfile = open('train_samp_data.pkl','wb')
pickle.dump(data,outputfile)
outputfile.close()
return data
load_pkl = 0
columns = ['week_num', 'sales_depot_id', 'sales_chan_id', 'route_id', 'client_id', 'prod_id', 'saleunit_curr_wk', 'saleamt_curr_wk', 'retunit_next_week', 'retamt_next_wk', 'y_pred_demand']
tic = time.time()
train_data_samp = load_samp_data('train.csv', columns, load_pkl)
toc = time.time()
print '*********'
print 'Time to load: ', toc-tic, 'sec'
print
print train_data_samp.describe()
print '*********'
print train_data_samp[['week_num', 'sales_depot_id', 'sales_chan_id', 'route_id', 'client_id', 'prod_id']]
features_train = train_data_samp[['week_num', 'sales_depot_id', 'sales_chan_id', 'route_id', 'client_id', 'prod_id']].values
labels_train_sale = train_data_samp[['saleunit_curr_wk']].values
labels_train_return = train_data_samp[['retunit_next_week']].values
labels_train = train_data_samp[['y_pred_demand']].values
###Output
*********
Time to load: 75.1288080215 sec
week_num sales_depot_id sales_chan_id route_id \
count 75000.000000 75000.000000 75000.000000 75000.000000
mean 5.982800 2761.836040 1.378893 2114.386307
std 2.027004 4603.625646 1.455427 1492.045185
min 3.000000 1110.000000 1.000000 1.000000
25% 4.000000 1312.000000 1.000000 1161.000000
50% 6.000000 1614.000000 1.000000 1283.000000
75% 8.000000 2040.000000 1.000000 2802.000000
max 9.000000 25759.000000 11.000000 9935.000000
client_id prod_id saleunit_curr_wk saleamt_curr_wk \
count 7.500000e+04 75000.000000 75000.000000 75000.000000
mean 1.798237e+06 20879.108587 7.275120 67.941156
std 1.832623e+06 18659.089843 19.898198 258.362483
min 1.050000e+02 72.000000 0.000000 0.000000
25% 3.525328e+05 1242.000000 2.000000 16.760000
50% 1.192627e+06 30549.000000 3.000000 30.000000
75% 2.370167e+06 37519.000000 7.000000 56.580000
max 1.169326e+07 49994.000000 1920.000000 15724.800000
retunit_next_week retamt_next_wk y_pred_demand
count 75000.000000 75000.000000 75000.00000
mean 0.130547 1.179629 7.19192
std 3.653853 16.583161 19.76362
min 0.000000 0.000000 0.00000
25% 0.000000 0.000000 2.00000
50% 0.000000 0.000000 3.00000
75% 0.000000 0.000000 6.00000
max 880.000000 1882.580000 1920.00000
*********
week_num sales_depot_id sales_chan_id route_id client_id prod_id
603469 3.0 1122.0 1.0 1072.0 80777.0 1109.0
616160 3.0 1122.0 1.0 1225.0 2404123.0 1284.0
900348 3.0 1126.0 1.0 1439.0 2344751.0 1240.0
265531 3.0 1117.0 1.0 1274.0 58433.0 1109.0
980436 3.0 1129.0 2.0 91.0 20626.0 34469.0
374339 3.0 1118.0 1.0 1436.0 174077.0 1238.0
809719 3.0 1124.0 1.0 1487.0 1269824.0 1230.0
576696 3.0 1121.0 1.0 2110.0 4102682.0 36745.0
401710 3.0 1119.0 1.0 1252.0 61120.0 1212.0
547145 3.0 1121.0 1.0 1436.0 833293.0 1242.0
911606 3.0 1127.0 1.0 1001.0 427990.0 1125.0
876703 3.0 1126.0 1.0 1412.0 694607.0 1212.0
927400 3.0 1127.0 1.0 1203.0 433950.0 1232.0
678649 3.0 1123.0 1.0 1208.0 4381733.0 31423.0
849482 3.0 1126.0 1.0 1202.0 1048736.0 1240.0
853790 3.0 1126.0 1.0 1207.0 694019.0 41938.0
389931 3.0 1119.0 1.0 1073.0 413854.0 1146.0
62658 3.0 1111.0 1.0 2110.0 61646.0 36748.0
135061 3.0 1113.0 1.0 1402.0 18505.0 32819.0
312260 3.0 1117.0 1.0 1604.0 311478.0 40217.0
305331 3.0 1117.0 1.0 1480.0 4182885.0 1212.0
194110 3.0 1116.0 1.0 1458.0 4181549.0 1284.0
617067 3.0 1122.0 1.0 1228.0 4389655.0 1278.0
546132 3.0 1121.0 1.0 1433.0 885310.0 1242.0
834210 3.0 1126.0 1.0 1022.0 595177.0 1125.0
47702 3.0 1111.0 1.0 1620.0 4353154.0 4259.0
677824 3.0 1123.0 1.0 1208.0 409837.0 35651.0
724090 3.0 1123.0 1.0 1456.0 1123212.0 1242.0
972101 3.0 1127.0 1.0 4450.0 4427233.0 43285.0
545002 3.0 1121.0 1.0 1432.0 32683.0 1278.0
... ... ... ... ... ... ...
101366 9.0 23899.0 1.0 4501.0 7897208.0 1309.0
27435 9.0 23669.0 1.0 1110.0 250172.0 1309.0
174774 9.0 25759.0 1.0 2122.0 1090879.0 31471.0
5244 9.0 22560.0 1.0 1260.0 2126508.0 3270.0
42172 9.0 23669.0 1.0 2832.0 892995.0 37361.0
33660 9.0 23669.0 1.0 1222.0 436729.0 1250.0
68348 9.0 23719.0 1.0 2813.0 8012178.0 37058.0
31810 9.0 23669.0 1.0 1220.0 246772.0 1250.0
110113 9.0 24049.0 1.0 1205.0 1895047.0 41938.0
87675 9.0 23899.0 1.0 1235.0 2067518.0 1240.0
180384 9.0 25759.0 1.0 5517.0 4358772.0 43159.0
51471 9.0 23719.0 1.0 1166.0 153159.0 1220.0
93783 9.0 23899.0 1.0 2810.0 2210613.0 30531.0
52075 9.0 23719.0 1.0 1166.0 4643704.0 1687.0
156596 9.0 25699.0 1.0 2007.0 2023508.0 36610.0
47824 9.0 23719.0 1.0 1063.0 506341.0 1109.0
155877 9.0 25699.0 1.0 2006.0 655030.0 37058.0
178431 9.0 25759.0 1.0 5507.0 54143.0 40886.0
33386 9.0 23669.0 1.0 1221.0 4223242.0 1309.0
47828 9.0 23719.0 1.0 1063.0 506363.0 1109.0
24807 9.0 23669.0 1.0 1011.0 250102.0 1125.0
108525 9.0 24049.0 1.0 1203.0 2491991.0 1242.0
134417 9.0 24669.0 1.0 1201.0 4247222.0 43118.0
149301 9.0 24669.0 1.0 5505.0 4592929.0 36598.0
131958 9.0 24669.0 1.0 1054.0 186848.0 31393.0
103212 9.0 23899.0 1.0 5006.0 2501968.0 31031.0
94955 9.0 23899.0 1.0 2812.0 1752067.0 5000.0
140145 9.0 24669.0 1.0 1213.0 442488.0 36711.0
3153 9.0 22560.0 1.0 1230.0 158892.0 1242.0
175686 9.0 25759.0 1.0 2128.0 4554772.0 31586.0
[75000 rows x 6 columns]
###Markdown
Feature Engineering
###Code
train_data_samp.groupby(['client_id', 'prod_id']).sum()
###Output
_____no_output_____
###Markdown
Predict sale units $y_{sale}$ and returns $y_{return}$ using two different classifiers. We will use xgboost to fit $y_{sale}$ and $y_{return}$ with the input data.
###Code
# Utility function to report best scores
def report(grid_scores, n_top=3):
top_scores = sorted(grid_scores, key=itemgetter(1), reverse=True)[:n_top]
for i, score in enumerate(top_scores):
print("Model with rank: {0}".format(i + 1))
print("Mean validation score: {0:.3f} (std: {1:.3f})".format(
score.mean_validation_score,
np.std(score.cv_validation_scores)))
print("Parameters: {0}".format(score.parameters))
print("")
import warnings
warnings.filterwarnings("ignore")
from sklearn.ensemble import RandomForestClassifier
from sklearn.grid_search import RandomizedSearchCV
from scipy.stats import randint as sp_randint
from operator import itemgetter
clf = RandomForestClassifier(n_estimators=10)
# specify parameters and distributions to sample from
param_dist = {"max_depth": [10],
"max_features": sp_randint(4, 7),
}
# run randomized search
n_iter_search = 10
random_search_sale = RandomizedSearchCV(clf, param_distributions=param_dist,
n_iter=n_iter_search, n_jobs=4, cv=5)
start = time.time()
random_search_sale.fit(features_train, np.ravel(labels_train_sale))
predict = random_search_sale.predict(features_train)
print 'Model Report ********'
print 'Accuracy : ', rmsle(np.ravel(labels_train_sale), predict)
print 'Model Report ********'
print("RandomizedSearchCV took %.2f seconds for %d candidates"
" parameter settings." % ((time.time() - start), n_iter_search))
report(random_search_sale.grid_scores_)
print random_search_sale.best_score_
print random_search_sale.best_estimator_
feat_imp = pd.Series(random_search_sale.best_estimator_.feature_importances_).sort_values(ascending=False)
feat_imp.plot(kind='bar', title='Feature Importances')
import warnings
warnings.filterwarnings("ignore")
from sklearn.ensemble import RandomForestClassifier
from sklearn.grid_search import RandomizedSearchCV
from scipy.stats import randint as sp_randint
from operator import itemgetter
clf = RandomForestClassifier(n_estimators=15)
# specify parameters and distributions to sample from
param_dist = {"max_depth": [10],
"max_features": sp_randint(3, 5),
}
# run randomized search
n_iter_search = 10
random_search_return = RandomizedSearchCV(clf, param_distributions=param_dist,
n_iter=n_iter_search, n_jobs=4, cv=5)
start = time.time()
random_search_return.fit(features_train, np.ravel(labels_train_return))
predict = random_search_return.predict(features_train)
print 'Model Report ********'
print 'Accuracy : ', rmsle(np.ravel(labels_train_return), predict)
print 'Model Report ********'
print("RandomizedSearchCV took %.2f seconds for %d candidates"
" parameter settings." % ((time.time() - start), n_iter_search))
report(random_search_return.grid_scores_)
print random_search_return.best_score_
print random_search_return.best_estimator_
feat_imp = pd.Series(random_search_return.best_estimator_.feature_importances_).sort_values(ascending=False)
feat_imp.plot(kind='bar', title='Feature Importances')
predict_sale = random_search_sale.predict(features_train)
predict_return = random_search_return.predict(features_train)
y_pred = [max(0,(predict_sale[i]-predict_return[i])) for i in xrange(len(predict_return))]
plt.scatter(y_pred,np.ravel(labels_train))
print 'Model Report ********'
print 'Accuracy : ', rmsle(y_pred, np.ravel(labels_train))
print 'Model Report ********'
###Output
_____no_output_____
###Markdown
3. Gradient Boosting
###Code
import xgboost as xgb
from xgboost.sklearn import XGBClassifier
from matplotlib.pylab import rcParams
rcParams['figure.figsize'] = 12, 4
from sklearn import metrics
def modelfit(alg, Xtrain, ytrain, useTrainCV=True, cv_folds=5, early_stopping_rounds=50):
if useTrainCV:
xgb_param = alg.get_xgb_params()
xgtrain = xgb.DMatrix(Xtrain, label=ytrain)
print alg.get_params()['n_estimators']
cvresult = xgb.cv(xgb_param, xgtrain, num_boost_round = alg.get_params()['n_estimators'], early_stopping_rounds=early_stopping_rounds)
alg.set_params(n_estimators=cvresult.shape[0])
alg.fit(Xtrain, ytrain, eval_metric='auc')
predict = alg.predict(Xtrain)
return predict
###Output
_____no_output_____
###Markdown
Step 1 Fix learning rate and number of estimators for tuning tree-based parameters
###Code
xgb1 = XGBClassifier(
learning_rate =0.05,
n_estimators=100,
max_depth=15,
min_child_weight=4,
gamma=0,
subsample=0.8,
colsample_bytree=0.8,
objective= 'reg:linear',
scale_pos_weight=1,
seed=27)
predict = modelfit(xgb1, features_train, np.ravel(labels_train))
#print model report:
print '\nModel Report ********'
print "Accuracy : %.4g" % rmsle(np.ravel(labels_train), predict)
print '\nModel Report ********'
feat_imp = pd.Series(xgb1.booster().get_fscore()).sort_values(ascending=False)
feat_imp.plot(kind='bar', title='Feature Importances')
plt.ylabel('Feature Importance Score')
###Output
_____no_output_____
###Markdown
Step 2: Tune max_depth and min_child_weight
###Code
from sklearn.grid_search import GridSearchCV
param_test1 = {
'max_depth':range(3,10,2),
'min_child_weight':range(1,6,2)
}
gsearch1 = GridSearchCV(estimator = XGBClassifier( learning_rate =0.1, n_estimators=100, max_depth=5, min_child_weight=1, gamma=0, subsample=0.8, colsample_bytree=0.8, scale_pos_weight=1, seed=27), param_grid = param_test1, scoring='roc_auc', n_jobs=4,iid=False)
gsearch1.fit(features_train,np.ravel(labels_train))
gsearch1.grid_scores_, gsearch1.best_params_, gsearch1.best_score_
###Output
_____no_output_____
###Markdown
Data CleaningThere are duplicate client ids in cliente_table, which means one client id may have multiple client name that are very similar. We will cluster them based on a hash function and use a clustering algorithm to evaluate similarity.
###Code
import re
def hash_eval(s):
hash_base = 4
s = re.sub('[., ]', '', s)
seqlen = len(s)
n = seqlen - 1
h = 0
for c in s:
h += ord(c) * (hash_base ** n)
n -= 1
curhash = h
return curhash
# In the client table, same clients are assigned different client ID. We create a new client table where clients are assigned unique ID.
clientid_hash = dict()
new_client_id = [-1]
for idx, s in enumerate(clientnameid_data.NombreCliente):
t = hash_eval(s)
clientid_hash.setdefault(t, []).append(clientnameid_data.Cliente_ID[idx])
if t in clientid_hash:
a = clientid_hash[t]
new_client_id.append(a[0])
# In the agency table, same agencies (town, state) are assigned different agency ID. We create a new agency table where agencies (town, state) are assigned unique ID.
agencyid_hash = dict()
new_agency_id = [-1]
for idx, s in enumerate(townstate_data.Town+townstate_data.State):
t = hash_eval(s)
agencyid_hash.setdefault(t, []).append(townstate_data.Agencia_ID[idx])
if t in agencyid_hash:
a = agencyid_hash[t]
new_agency_id.append(a[0])
clientnameid_data['New_Cliente_ID'] = new_client_id[1:]
townstate_data['New_Agencia_ID'] = new_agency_id[1:]
print clientnameid_data.head(10)
print '---'
print townstate_data.head()
print '---'
print train_data_samp.head(10)
print train_data_samp.head(10)
print '------'
for idx, cid in enumerate(train_data_samp.client_id):
train_data_samp.client_id.values[idx] = clientnameid_data.New_Cliente_ID[train_data_samp.client_id.values[idx] == clientnameid_data.Cliente_ID.values].values[0]
train_data_samp.sales_depot_id.values[idx] = townstate_data.New_Agencia_ID[train_data_samp.sales_depot_id.values[idx] == townstate_data.Agencia_ID.values].values[0]
print '-----'
print train_data_samp.head()
###Output
_____no_output_____
###Markdown
Load Test Data
###Code
test_data = pd.read_csv('test.csv')
test_data.columns = ['id', 'week_num', 'sales_depot_id', 'sales_chan_id', 'route_id', 'client id', 'prod_id']
test_labels = pd.read_csv('sample_submission.csv')
test_data = test_data.drop('id', 1)
print test_data.head()
g = sns.PairGrid(data_t)
g.map(plt.scatter)
a = [[1, 2, 3, 4]]
print a
np.array(a)
print np.array(a)
a = np.array(a)
a = sp_randint(10,2)
range(3,10,2)
sp_randint(1, 6)
import subprocess
subprocess.call(['ec2kill'])
from subprocess import call
call(["ec2-terminate-instances", "i-308b33ed "])
# Utility function to report best scores
def report(grid_scores, n_top=3):
top_scores = sorted(grid_scores, key=itemgetter(1), reverse=True)[:n_top]
for i, score in enumerate(top_scores):
print("Model with rank: {0}".format(i + 1))
print("Mean validation score: {0:.3f} (std: {1:.3f})".format(
score.mean_validation_score,
np.std(score.cv_validation_scores)))
print("Parameters: {0}".format(score.parameters))
print("")
from sklearn.ensemble import RandomForestClassifier
from sklearn.grid_search import RandomizedSearchCV
from scipy.stats import randint as sp_randint
from operator import itemgetter
clf = RandomForestClassifier(n_estimators=30)
# specify parameters and distributions to sample from
param_dist = {"max_depth": [10, None],
"max_features": sp_randint(1, 6),
"min_samples_split": sp_randint(1, 6),
"min_samples_leaf": sp_randint(1, 6),
"bootstrap": [True, False],
"criterion": ["gini", "entropy"]}
# run randomized search
n_iter_search = 20
random_search = RandomizedSearchCV(clf, param_distributions=param_dist,
n_iter=n_iter_search, n_jobs=4, cv=3)
start = time.time()
random_search.fit(features_train, np.ravel(labels_train))
print("RandomizedSearchCV took %.2f seconds for %d candidates"
" parameter settings." % ((time.time() - start), n_iter_search))
report(random_search.grid_scores_)
print random_search.best_score_
print random_search.best_estimator_
###Output
_____no_output_____ |
Week12/StreamClustering.ipynb | ###Markdown
###Code
!pip install river
!pip install -U numpy
###Output
Requirement already satisfied: numpy in /usr/local/lib/python3.7/dist-packages (1.19.5)
Collecting numpy
Downloading numpy-1.21.4-cp37-cp37m-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (15.7 MB)
[K |████████████████████████████████| 15.7 MB 59 kB/s
[?25hInstalling collected packages: numpy
Attempting uninstall: numpy
Found existing installation: numpy 1.19.5
Uninstalling numpy-1.19.5:
Successfully uninstalled numpy-1.19.5
[31mERROR: pip's dependency resolver does not currently take into account all the packages that are installed. This behaviour is the source of the following dependency conflicts.
tensorflow 2.6.0 requires numpy~=1.19.2, but you have numpy 1.21.4 which is incompatible.
datascience 0.10.6 requires folium==0.2.1, but you have folium 0.8.3 which is incompatible.
albumentations 0.1.12 requires imgaug<0.2.7,>=0.2.5, but you have imgaug 0.2.9 which is incompatible.[0m
Successfully installed numpy-1.21.4
###Markdown
1. Clustream
###Code
from river import cluster
from river import stream
X = [
[1, 2],
[1, 4],
[1, 0],
[4, 2],
[4, 4],
[4, 0]
]
clustream = cluster.CluStream( time_window=1,
max_micro_clusters=3,
n_macro_clusters=2,
seed=0,
halflife=0.4)
for i, (x, _) in enumerate(stream.iter_array(X)):
clustream = clustream.learn_one(x)
ans1 = clustream.predict_one({0: 1, 1: 1})
print(ans1)
ans2 = clustream.predict_one({0: 4, 1: 3})
print(ans2)
ans3 = clustream.predict_one({0: 1, 1: 3})
print(ans3)
###Output
1
0
1
###Markdown
2. Denstream
###Code
from river import cluster
from river import stream
X = [
[-1, -0.5], [-1, -0.625], [-1, -0.75], [-1, -1], [-1, -1.125], [-1, -1.25],
[-1.5, -0.5], [-1.5, -0.625], [-1.5, -0.75], [-1.5, -1], [-1.5, -1.125], [-1.5, -1.25],
[1, 1.5], [1, 1.75], [1, 2], [4, 1.25], [4, 1.5], [4, 2.25],
[4, 2.5], [4, 3], [4, 3.25], [4, 3.5], [4, 3.75], [4, 4],
]
denstream = cluster.DenStream(decaying_factor = 0.01,
core_weight_threshold = 1.01,
tolerance_factor = 1.0005,
radius = 0.5)
for x, _ in stream.iter_array(X):
denstream = denstream.learn_one(x)
print(denstream.predict_one({0: -1, 1: -2}))
print(denstream.predict_one({0: 5, 1: 4}))
print("Number of clusters = ",denstream.n_clusters)
###Output
0
1
Number of clusters = 2
###Markdown
Stream K-Means
###Code
from river import cluster
from river import stream
X = [
[1, 0.5], [1, 0.625], [1, 0.75], [1, 1.125], [1, 1.5], [1, 1.75],
[4, 1.5], [4, 2.25], [4, 2.5], [4, 3], [4, 3.25], [4, 3.5]
]
streamkmeans = cluster.STREAMKMeans(chunk_size=3, n_clusters=2, halflife=0.5, sigma=1.5, seed=0)
for x, _ in stream.iter_array(X):
streamkmeans = streamkmeans.learn_one(x)
print(streamkmeans.predict_one({0:1, 1:0}))
print(streamkmeans.predict_one({0:5, 1:2}))
###Output
1
0
|
data_cleaning_function.ipynb | ###Markdown
Data Cleaning Function def data_cleaning (dataframe,replace_missing_value) return dataframe Written by : Loh Khai Shyang Scripted Date : 22 Nov 2021
###Code
import numpy as np
import pandas as pd
import seaborn as sns
import matplotlib.pyplot as plt
def data_cleaning(df,replace_missing_value = value to be filled into missing rows):
### 1. search_remove_individual_value_features
### 2. replace_missing_data
### 3. blank_space_repalcement
### return dataframe thru data cleaning process
### Hyper- Parameter
# 1. df = Dataframe to be clean
# 2. replace_missing_value = value to be filled into missing rows
def basic_data_info(df):
# check dataset overall information
print('\nData Cleaning Report - Basic Data Informations\n')
print(df.info())
print('\nData Cleaning Report - Summary of total "NAN" rows\n')
# identify amount of "NAN" row
print(df.isna().sum())
def search_remove_individual_value_features(df):
# remove features if :
# X[i].unique() == 1
# X[i].unique() == 2 & np.nan isin( X[i].unique() )
# X[i].unique() == 2 & ' ' isin( X[i].unique()) ### ' ' == empty space
column_name = df.columns
remove_single_value_features_list=[]
for name in column_name:
if len(df[name].unique())==1: # X[i].unique() == 1
remove_single_value_features_list=np.append(name,remove_single_value_features_list)
elif len(df[name].unique())==2 and np.nan in(df[name].unique()): # X[i].unique() == 2 & np.nan isin( X[i].unique() )
remove_single_value_features_list=np.append(name,remove_single_value_features_list)
elif len(df[name].unique())==2 and ' ' in(df[name].unique()): # X[i].unique() == 2 & ' ' isin( X[i].unique()) ### ' ' == empty space
remove_single_value_features_list=np.append(name,remove_single_value_features_list)
if len(remove_single_value_features_list)>=1:
print('\nData Cleaning Report - Individual Value feature removed : ',remove_single_value_features_list)
df.drop(remove_single_value_features_list,axis=1,inplace=True)
else:
print("\nData Cleaning Report - No Individual Value feature Found !!\n")
return df
def replace_missing_data(df,replace_missing_value):
# user can define what value to fill in to missing data
# "nan" and "blank space" consider missing data
column_list= df.columns
missing_EmptySpace_data_dict_list={} # Store "Empty Space" row in dictionary by column name
missing_NAN_data_dict_list={} # # Store "nan" row in dictionary by column name
for column in column_list:
if len(df.loc[df[column]==' '])!= 0 :
missing_EmptySpace_data_dict_list[column]=df.loc[df[column]==' '].index # index
elif len(df.loc[df[column]==np.nan])!=0:
missing_NAN_data_dict_list[column]=df.loc[df[column]==np.nan].index
## replace missing data function
if len(missing_EmptySpace_data_dict_list)!=0 : # check empty_space_column dictionary is empty or not
print('\nData Cleaning Report - Have Missing "Empty Space" Data :\n',missing_EmptySpace_data_dict_list)
for key in missing_EmptySpace_data_dict_list.keys():
df.loc[missing_EmptySpace_data_dict_list[key],key]=replace_missing_value # df.loc[idx list, column name] = 0
elif len(missing_NAN_data_dict_list)!=0 : # check empty_space_column dictionary is empty or not:
print('\nData Cleaning Report - Have Missing "NaN" Data :\n',missing_NAN_data_dict_list)
for key in missing_NAN_data_dict_list.keys():
df.loc[missing_NAN_data_dict_list[key],key]=replace_missing_value # df.loc[idx list, column name] = 0
else:
print('\nData Cleaning Report - No Missing Data or "Nan" row Found !!\n')
return df
def blank_space_repalcement(df):
# Dataframe Column Name Blank Space Replacement
df.columns=df.columns.str.replace(' ','_')
df.replace(' ','_',regex=True,inplace=True)
return df
basic_data_info(df)
df=search_remove_individual_value_features(df)
df=replace_missing_data(df,replace_missing_value)
df=blank_space_repalcement(df)
return df
def imbalance_data_check(df,label_name):
# SuitabLe for categorical class label [both ordinal or nominal data]
## Frequency Table of Label data ##
# print('\n Imbalance Data Check - Label vs Features Table \n',df.groupby([label_name]).count(),'\n')
freq_table=df.groupby([label_name]).size().reset_index(name='Count')
print('\n Imbalance Data Check - Frequency Table of Label Data :\n',freq_table,'\n')
## % of label's class distribution data Summary ##
summary={}
unique_class=freq_table[label_name].unique()
total_count=freq_table['Count'].sum()
for i in range(len(unique_class)):
summary[unique_class[i]]= [(freq_table['Count'][i]/total_count)*100]
summary_df=pd.DataFrame(data=summary)
print(f'Imbalance Data Check - Label Data Distribution % :\n{summary_df}')
## Plot Figure of Label class data distribution ##
print(f'\nImbalance Data Check - Data Distribution % Summary Plot :\n') # Imbalance data check summary
sns.set_theme(style="whitegrid")
ax=sns.barplot(x=label_name,y="Count", data=freq_table)
for i in unique_class:
num=round(summary[i][0],2)
# value=str(num)+'%'
ax.text(i,num,round(num,2), color='black', ha="center")
return
def numeric_histogram_plot_for_classification_model(df,label_feature,save_photo):
# label_feature = input label feature name
# df = input dataframe to be process
# save_photo = True ( auto saved), Default=="False"
# sns.displot(daframe,x="column name to plot on x-axis",hue=label_feature, element="step")
# subplot link : https://datavizpyr.com/seaborn-join-two-plots-with-shared-y-axis/
import matplotlib.pyplot as plt
import seaborn as sns
plot_columns=list(df.select_dtypes(include=['int32','int64','float64','float32']).columns)
if label_feature in(plot_columns):
plot_columns.remove(label_feature)
for i,column in zip( range(len(plot_columns)), plot_columns ):
plt.figure(i)
sns_plot=sns.displot(data=df,x=column,hue=label_feature, element="step",kde=True)
plt.title(column+" - Displot Plot")
# sns.histplot(df, x=column,hue=label_feature, element="step", kde=True)
# plt.title(column+" - Histogram Plot")
# plt.show()
if save_photo==True:
sns_plot.savefig(column+".png") # auto Saved figure file
return
def numeric_histplot(df,label):
# label must vbe a list of feature to plot
for i, feature in label:
plt.figure(i)
sns_plot=sns.displot(data=df,x=feature, element="step",kde=True)
plt.title(feature+" - Displot Plot")
return
def numeric_compare_histplot(df_1,df_2,compare_label,compare_name):
# Plot two dataframe histplot for in one graph df_1 and df_2 for comparison plot
# compare_label must be a list of feature to plot
# compare_name = show name of dataframe compare plot legend
from scipy.stats import norm
for i, feature in zip(range(len(compare_label)), compare_label) :
fig,ax=plt.subplots(figsize=(11.7,8.27)) # figsize = in inch
sns.distplot(df_1[feature], ax=ax, color='r',kde=True)
sns.distplot(df_2[feature], ax=ax, color='b', kde=True)
plt.title(feature+" - Distribution Count Plot")
plt.legend(title='Distribution', loc='upper left', labels=[compare_name[0],compare_name[1]])
fig,ax1=plt.subplots(figsize=(11.7,8.27))
sns.distplot(df_1[feature], ax=ax1,color='r', kde=True)
sns.distplot(df_2[feature], ax=ax1,color='b', kde=True)
ax1.set_ylim([0,0.5])
plt.title(feature+" - Distribution Density Plot")
plt.legend(title='Distribution', loc='upper left', labels=[compare_name[0],compare_name[1]])
plt.show()
return
def z_score_outlier_detection_numeric(df,label,gt_value,numeric_feature_column,outlier_threshold_rate,,feature_remove_decision=False,save_plot=False):
### 1. Calcualte z-score of whole df
### 2. Detect consider outlier if [- 3sigma >= Z-score >= +3 sigma == consider outlier ]
### 3. Remove outlier column from df if:
# [ outlier rate > outlier_threshold_rate ]
# feature_remove_decision = True
### 4. Plot Z-score Box plot for each feature column
### 5. Plot output features distribution graph
### Hyper Parameter
# 1. outlier_density_threshold, % = acceptable z-score density threshold before remove
# 2. label is used to plot
# 3. gt_value= Label Ground Truth value
# 3. Z_score_outlier_threshold = z_score removal threhold
# 4. numeric_feature_column = feature to calculate z-score analysis (user need to exclude categorical number column out of the list)
# 5. outlier_removal_rate = column removal % if outlier rate exceed threshold
# 6. save_photo = True = Auto saved photo
# return ploted z-score box plot
# return outlier cleaned dataframe ( clean column outlier of exceed outlier rate threshold)
import matplotlib.pyplot as plt
import seaborn as sns
from scipy import stats
## Dataframe Describe Info ##
print(label+' - Data Attribute\n',df[label].describe())
### Plot Distrubution Label Data Analysis Graph ###
stdev=round(df[label].std(),2) # label stdev
mean=round(df[label].mean(),2) # label mean value
min_value=round(min(df[label]),2) # min label value
max_value=round(max(df[label]),2) # max label value
UCL= mean+3*stdev # label negative 3-sigma
LCL = mean-3*stdev # label positive 3-sigma
no_outof_gt_value_spec=len(df[df[label]<gt_value]) # numbers of label value smaller than groundtruth value
no_within_gt_value_spec=len(df)-len(df[df[label]<gt_value]) # numbers of label value within ground truth value
no_within_3sigma_spec=len(df)-len(df[(df[label]<LCL) | ( df[label]>UCL)]) # numbers of label value within UCL & LCL
## Plot Label data Histogram ##
sns.set(rc={'figure.figsize':(20,15)})
plt.figure(100)
sns.distplot(df[label],kde=True)
plt.axvline(gt_value,linestyle='--',color='red') # Numeric label Ground Truth line
plt.axvline(UCL,linestyle='--',color='blue') # positive 3-sigma
plt.axvline(LCL,linestyle='--',color='green') # negative 3-sigma
plt.title(label+ 'Distribution Plot [Within '+label+' Spec:'+str(no_within_gt_value_spec)+', OutofSpec:'+str(no_outof_gt_value_spec)+'] [ Within UCL/LCL:'+str(no_within_3sigma_spec)+'/'+str(len(df))+' ]',fontsize=12)
plt.legend(['Dataset N:'+str(len(df))+' Mean:'+str(mean)+' Max:'+str(max_value)+' Min:'+str(min_value),
label+' Ground Truth',
'UCL',
'LCL'],
fancybox=True,
framealpha=1,
shadow=True,
borderpad=1,fontsize=12)
plt.xlabel(str(label)+' value',fontsize=12)
plt.ylabel('Counts',fontsize=12)
if save_plot==True:
plt.savefig("Label_Distribution_plot.png") # auto Saved figure file
############################################################################################################################################################
## Calcualte Z-score dataframe ##
df_feature_column=df.columns
new_numeric_feature_column=list() # create a new list to store exist numeric feature column after data cleaning
for i in df_feature_column:
if i in(numeric_feature_column):
new_numeric_feature_column.append(i)
df_z_score=df[new_numeric_feature_column] # form new dataframe of new_numeric_feature_column
for feature in df_z_score:
stdev=df_z_score[feature].std()
mean=df_z_score[feature].mean()
df_z_score[feature]=(df_z_score[feature]-mean)/stdev
def outlier_removal(df,df_z_score,outlier_threshold_rate,feature_remove_decision,save_plot):
# - 3sigma >= Z-score >= +3 sigma == consider outlier
outlier_rate_dict={}
total_outlier =0
for column in df_z_score:
outlier_number =len(df_z_score[df_z_score[column]>3.0]) + len(df_z_score[df_z_score[column]<-3.0])
outlier_rate=outlier_number/len(df_z_score[column])*100 # outlier rate in %
outlier_rate_dict[column]=outlier_rate
total_outlier=total_outlier + outlier_number
overall_outlier_rate=total_outlier/ (len(df_z_score)*len(df_z_score.columns))*100 # overall outlier rate of whole dataframe in %
outlier_remove_column=[]
for key in outlier_rate_dict:
if outlier_rate_dict[key] > outlier_threshold_rate:
outlier_remove_column.append(key)
outlier_df=pd.DataFrame(outlier_rate_dict.items(),columns=["Features","Outlier_rate"])
outlier_df.sort_values(by=["Outlier_rate"], ascending=True, inplace=True)
if feature_remove_decision = True : # if feature_remove_decision is True it will remove the outlier column from df
df.drop(outlier_remove_column,axis=1,inplace=True) # Remove train data outlier column
print('Outlier Remove Decision "Enable" ')
else:
print('Outlier Remove Decision "Disable" ')
print("outlier_remove_column[>"+str(outlier_threshold_rate)+"] : ",outlier_remove_column) # show outlier removed column
plt.figure()
ax1=sns.lineplot(data=outlier_df, x="Features", y="Outlier_rate", marker='o', sort=True)
ax1.tick_params(axis='x',rotation=90) # rotate label x by 90 degree
plt.xlabel('Features')
plt.ylabel("Outlier Percentage %")
plt.title("Outlier Rate Plot -- Data Shape:"+str(df_z_score.shape)+" Overall Outlier Rate[%]:"+str(overall_outlier_rate)+" Feature Reduction:"+str(len(outlier_remove_column))+"(<"+str(outlier_threshold_rate)+"%)")
if save_plot==True:
plt.savefig("Outlier_Rate_plot.png")
return df
def z_score_boxplot(df,save_plot):
# input
### Each graph boxplot contains only 20 features
start_index=0 # plot_column_index start index value
final_index=len(df.columns)
plot_column_list=[]
plot_column_index=[]
max_limit_value=max(df.max())
min_limit_value=min(df.min())
### Form index list of column to seperate different graph per box plot ###
for i in range(0,len(list(df.columns)),20): # seperate 20 features per graph of box plot
plot_column_index.append(i)
plot_column_index.remove(0)
### Form Column list of each graph of box plot ###
for i in plot_column_index :
plot_column_list.append(list(df.columns[start_index:i]))
start_index=i
if start_index<final_index:
plot_column_list.append(list(df.columns[start_index:final_index]))
pic_no=1
### Plot box plot ###
for column in plot_column_list:
sns.set(rc={'figure.figsize':(20,15)})
plt.figure()
sns.boxplot(data=df[column],orient="h",fliersize=6)
plt.xlim(min_limit_value-2,max_limit_value+2)
plt.xlabel('Z-Score')
plt.ylabel("Features")
plt.title("Z-score vs Features BoxPlot")
### Save Plot ###
if save_plot==True:
plt.savefig("Boxplot_"+str(pic_no)+".png") # auto Saved figure file
pic_no+=1
return
z_score_boxplot(df_z_score,save_plot) # Call z_score_boxplot function
df = outlier_removal(df,df_z_score,outlier_threshold_rate,feature_remove_decision,save_plot)
return
def correlation_filter_function(dataset,corr_dataset,label,corr_method,corr_threshold,remove_feature_decision=False,save_figure=False):
''' Function will Calculate Correlation Coefficient
Plot Correlation Coeffiecent as Heat map
Show Total Rejected Features ( > corr_threshold )
Show High correlated features pair and corr value ( > corr_threshold )
Plot Top 20 Features to Features Correlation Coeficent Heatmap --> label column
Plot Top 20 Features vs Label Features
return : Dataframe ( remove or not highly correlated feature )
'''
""" Function Variables """
# dataset = original dataframe
# corr_dataset: Consist of numeric feature from dataframe only
# label = Label Feature column
# corr_method : "pearson", "kendall", "spearman"
# corr_threshold : threshold to reject "corr value > corr_threshold"
# remove_feature_decision : True/ False decide to remove or not highly correlated feature from dataframe
import seaborn as sns
import matplotlib.pyplot as plt
def _get_diagonal_pairs(corr_dataset):
''' Get diagonal and lower triangular pairs of correlation matrix '''
pairs_to_drop=set()
cols=corr_dataset.columns
for i in range(len(corr_dataset.columns)):
for j in range(0,i+1):
pairs_to_drop.add((cols[i],cols[j])) # diagonal label col name [ it store index type of pandas series ]
return pairs_to_drop
def _get_reject_corr_features(corr_dataset,label,corr_method,corr_threshold,top_features_no):
''' Calculate/Show Features to Features Correlation Coefficient Value '''
corr_df = corr_dataset.corr(method=corr_method).unstack() # corr value non-absolute
corr_abs_df = corr_dataset.corr(method=corr_method).abs().unstack() # absolute corr Series, from "Dataframe" unstack to a list of "Pandas Series"
labels_to_drop = _get_diagonal_pairs(corr_dataset)
corr_abs_df = corr_abs_df.drop(labels=labels_to_drop).sort_values(ascending=False) # drop repeated pairs corr value and sort by descending order
corr_idx = corr_abs_df.index # get pandas series index of descending order
corr_df=corr_df.reindex(index=corr_idx) # re-arrange without absolute corr value to descending order
print(f'All Correlation Features Pairs (Corr Features Pair Values>{str(corr_threshold)}) :\n\n {corr_df[ corr_df > corr_threshold]}')
# corr_df = corr_df.drop(labels=labels_to_drop) # Drop "pandas series" by (column_name_1, column_name_2)
# # argsort returns only index of ascending order [::-1] re-arrange index inversly = sort in descending order
# sort_corr_abs_idx = corr_abs_df.argsort().[::-1] # return descending sort order index = Highest Correlation cooeficient index
""" Plot Top Features vs Label Features """
top_highest_corr_features_index=list(corr_dataset.corr()[label].abs().sort_values(ascending=False)[1:top_features_no+1].index) # Sort Corr abs value as descending order and set as list of index
plt.figure()
# Heatmap require input 2D
# plot single column dataframe into heatmap need to df[['columns']] will result in 2D suitable for heatmap input
sns.heatmap(corr_dataset.corr()[[label]].loc[top_highest_corr_features_index],annot=True, fmt=".2f", cmap='Blues')
plt.title(f' Top {top_features_no} Features vs {label} {corr_method} Corrleation ')
# Save Figure
if save_figure == True:
plt.savefig(f'Top_{top_features_no}_Features_vs_{label}_{corr_method}_Corrleation.png')
""" Plot Top Features to Features Correlation Coeficent Heatmap --> label column """
corr_matrix=corr_dataset[top_highest_corr_features_index].corr()
plt.figure(figsize=(15,15))
# "annot" = True = show readings
# "fmt" = use to set decimal .2f = 2 float decimal
sns.heatmap(corr_matrix,annot=True, fmt=".2f", cmap='Blues')
plt.title(f' Top {top_features_no} {label} - Features to Features {corr_method} Corrleation ')
# Save Figure
if save_figure == True:
plt.savefig(f'Top_{top_features_no}_{label}_Features_to_Features_{corr_method}_Corrleation.png')
""" Correlation Plot and return high correlated features as "col_corr" """
reject_col_corr=set() # set is use because it does not add duplicate same value in a set() variables
corr_matrix= corr_dataset.corr(method=corr_method)
# Plot figure
plt.figure(figsize=(12,10))
sns.heatmap(corr_matrix,annot=True)
plt.title(f'{corr_method} Correlation Coeficient All Features [Feature Size={corr_matrix.shape}]')
# Save Figure
if save_figure == True:
plt.savefig('Correlation_Coefficient_All_Features.png')
# Extract Out of corr_threshold features
col=corr_matrix.columns
for i in range(len(corr_matrix.columns)):
for j in range(i):
if (corr_matrix.iloc[i,j])>corr_threshold:
# add remove features to set for correlation value of features vs label is the smallest
if corr_matrix.loc[col[i],label]< corr_matrix.loc[col[j],label]:
reject_col_corr.add(col[i])
else:
reject_col_corr.add(col[j])
print(f'\nSize of Rejected Corr features :{len(reject_col_corr)} (>{str(corr_threshold)}) ')
print(f'\n Rejected Corr Features : \n\n {reject_col_corr}')
return reject_col_corr
""" Show Correlation Values of Pair Features " """
# Run Correlation Coefficient analaysis
reject_col_corr = _get_reject_corr_features(corr_dataset,label,corr_method,corr_threshold,20)
# Remove out of corr_threshold features
if remove_feature_decision == True:
dataset = dataset.drop(reject_col_corr, axis=1)
return dataset
###Output
_____no_output_____ |
mytest/MachineLearn/ensemble_prep.ipynb | ###Markdown
앙상블 학습과 랜덤 포레스트 대중의 지혜 : 무작위로 선택된 수천 명의 사람에게 복잡한 질문하고 대답을 모은다고 가정합시다.많은 경우 이렇게 모은 답이 전문가의 답보다 낫다는 것을 알 수 있음.이처럼 일련의 예측기로부터 예측을 수집하면 가장 좋은 모델하나보다 더 좋은 예측을 얻을 수 있음.|일련의 예측기를 앙상블이라고 부르기 때문에 앙상블 학습이라고 함.앙상블의 방법의 예를 들면 훈련세트로부터 무작위로 각기 다른 서브셋을 만들어 일련의 결정트리 분류기를훈련시킬 수 있음. 예측을 하려면 모든 개별트리의 예측을 구하면 됨.|결정트리의 앙상블을 랜덤 포레스트라고 함. (6장 마지막 연습문제 참고) 간단한 방법임에도 랜덤포레스트는 오늘날 가장 강력한 머신러닝 알고리즘 중 하나임. 사실 머신러닝 대회에서 우승하는 솔루션은 여러가지앙상블 방법을 사용한 경우가 많음. 특히 넷플릭스 대회에서 가장 인기있음. 이 장에서는 배깅, 부스팅, 스태킹 등 가장 인기있는 앙상블을 설명합니다. (+ 랜덤포레스트) 투표 기반 분류기정확도가 80%인 분류기 여러개를 훈련시켰다고 가정하고,아마도 로지스틱 회귀 분류기, SVM 분류기, 랜덤 포레스트 분류기, K-최근접 이웃 분류기 등을 가지고 있음.더 좋은 분류기를 만드는 매우 간단한 방법은 각 분류기의 예측을 모아서 가장 많이 선택된 클래스를 예측.이렇게 다수결 투표로 정해지는 분류기를 직접투표 분류기라고 합니다. 조금 놀랍게도 이 다수결 투표 분류기가 앙상블에 포함된 개별 분류기 중 가장 뛰어난 것보다도 정확도가 높을 것. 사실 각 분류기가 약한 학습기일지라도 충분하게 많고 다양하다면앙상블은 강한 학습기가 될 수 있음.|이게 어떻게 가능할까요? 다음 설명이 이 미스터리를 조금 밝혀줄 겁니다.동전을 던졌을 때 앞면이 51%, 뒷면이 49%가 나오는 균형이 맞지 않는 동전이 있다고 가정하겠습니다.이 동전을 1,000번 던진다면 대략 510번은 앞면, 490번은 뒷면이 나올 것이므로 다수는 앞면이 됨.수학적으로 계산하면, 1,000번 던진 후 앞면에 있을 확률은 75%에 가깝다는 것을 확인할 수 있음.더 많이 던질수록 확률은 증가합니다. (예를 들어 10,000번 던지면 확률이 97%이상으로 올라감.)
###Code
# 그림을 저장할 위치
PROJECT_ROOT_DIR = "."
CHAPTER_ID = "ensembles"
IMAGES_PATH = os.path.join(PROJECT_ROOT_DIR, "images", CHAPTER_ID)
os.makedirs(IMAGES_PATH, exist_ok=True)
def save_fig(fig_id, tight_layout=True, fig_extension="png", resolution=300):
path = os.path.join(IMAGES_PATH, fig_id + "." + fig_extension)
print("그림 저장:", fig_id)
if tight_layout:
plt.tight_layout()
plt.savefig(path, format=fig_extension, dpi=resolution)
%matplotlib inline
import matplotlib as mpl
import matplotlib.pyplot as plt
import numpy as np
import sys
import os
###Output
_____no_output_____
###Markdown
이는 큰 수의 법칙 때문인데, 이로 인해 동전을 자꾸 던질수록 앞면이 나올 비율은 점점 더 앞면이 나올 확률 (51%)에 가까워짐. [그림 7-3]은 균형이 틀어진 동전을 10번 실험한 그래프.던진 횟수가 증가할수록 앞면이 나올 확률 51%에 가까워지고, 결국 10번의 실험 모두 50%보다 높게유지되며 51%에 수렴하면서 끝나고 있음.
###Code
heads_proba = 0.51
coin_tosses = (np.random.rand(10000, 10) < heads_proba).astype(np.int32)
cumulative_heads_ratio = np.cumsum(coin_tosses, axis=0) / np.arange(1, 10001).reshape(-1, 1)
###Output
_____no_output_____
###Markdown
** 생성 코드**
###Code
plt.figure(figsize=(8,3.5))
plt.plot(cumulative_heads_ratio)
plt.plot([0, 10000], [0.51, 0.51], "k--", linewidth=2, label="51%")
plt.plot([0, 10000], [0.5, 0.5], "k-", label="50%")
plt.xlabel("Number of coin tosses")
plt.ylabel("Heads ratio")
plt.legend(loc="lower right")
plt.axis([0, 10000, 0.42, 0.58])
save_fig("[7-3] law_of_large_numbers_plot")
plt.show()
###Output
그림 저장: [7-3] law_of_large_numbers_plot
###Markdown
이와 비슷하게 (무작위 추측보다 좀 더 나은) 51% 정확도를 가진 1,000개의 분류기로앙상블 모델을 구축한다고 가정. 가장 많은 클래스를 예측으로 삼는다면 75%의 정확도를 기대가능!하지만 이런 가정은 모든 분류기가 완벽하게 독립적이고 오차에 상관관계가 없어야 가능.하지만 여기서는 같은 데이터로 훈련시키기 때문에 이런 가정이 맞지 않음.분류기들은 같은 종류의 오차를 만들기 쉽기 때문에 잘못된 클래스가 다수인 경우가 많고 앙상블의 정확도가 낮아짐. TIP_앙상블 방법은 각기 다른 알고리즘으로 학습시키면 앙상블 모델의 정확도 향상에 도움됨. 다음은 여러분류기를 조합해 사이킷런의 투표기반 분류기(VotingClassifier)를 만들어 훈련시키는 코드.(데이터셋은 5장에서 설명한 moons.)
###Code
from sklearn.model_selection import train_test_split
from sklearn.datasets import make_moons
X, y = make_moons(n_samples=500, noise=0.30, random_state=42)
X_train, X_test, y_train, y_test = train_test_split(X, y, random_state=42)
from sklearn.ensemble import RandomForestClassifier
from sklearn.ensemble import VotingClassifier
from sklearn.linear_model import LogisticRegression
from sklearn.svm import SVC
log_clf = LogisticRegression(solver="lbfgs", random_state=42)
rnd_clf = RandomForestClassifier(n_estimators=100, random_state=42)
svm_clf = SVC(gamma="scale", random_state=42)
voting_clf = VotingClassifier(
estimators=[('lr', log_clf), ('rf', rnd_clf), ('svc', svm_clf)],
voting='hard')
voting_clf.fit(X_train, y_train)
###Output
_____no_output_____
###Markdown
각 분류기의 테스트셋 정확도를 확인해봅시다.
###Code
from sklearn.metrics import accuracy_score
for clf in (log_clf, rnd_clf, svm_clf, voting_clf):
clf.fit(X_train, y_train)
y_pred = clf.predict(X_test)
print(clf.__class__.__name__, accuracy_score(y_test, y_pred))
###Output
LogisticRegression 0.864
RandomForestClassifier 0.896
SVC 0.896
VotingClassifier 0.912
###Markdown
예상대로 투표기반 분류기가 다른 개별 분류기보다 성능이 조금 높음.모든 분류기가 클래스의 확률을 예측할 수 있으면 (즉, predict_proba() 메서드가 있으면).개별 분류기의 예측을 평균 내어 확률이 가장 높은 클래스를 예측할 수 있음. => 간접투표|이 방식은 확률이 높은 투표에 비중을 더 두기 때문에 직접투표 방식보다 성능이 높음.이 방식을 사용하려면 voting="hard" -> voting="soft"로 바꾸고 모든 분류기가 클래스의 확률을 추정할 수 있으면 됨. SVC는 기본값에서는 클래스 확률을 제공하지 않으므로 probability 매개변수를True로 지정해야 함.(이렇게 하면 클래스 확률을 추정하기 위해 교차검증을 사용하므로 훈련속도가 느려지지만 SVC에서 predict_proba() 메서드를 사용할 수 있음.)앞의 코드를 간접 투표 방식으로 사용하도록 변경하면 좀 더 높은 정확도를 얻을 것. 간접 투표 방식
###Code
log_clf = LogisticRegression(solver="lbfgs", random_state=42)
rnd_clf = RandomForestClassifier(n_estimators=100, random_state=42)
svm_clf = SVC(gamma="scale", probability=True, random_state=42)
voting_clf = VotingClassifier(
estimators=[('lr', log_clf), ('rf', rnd_clf), ('svc', svm_clf)],
voting='soft')
voting_clf.fit(X_train, y_train)
from sklearn.metrics import accuracy_score
for clf in (log_clf, rnd_clf, svm_clf, voting_clf):
clf.fit(X_train, y_train)
y_pred = clf.predict(X_test)
print(clf.__class__.__name__, accuracy_score(y_test, y_pred))
###Output
LogisticRegression 0.864
RandomForestClassifier 0.896
SVC 0.896
VotingClassifier 0.92
###Markdown
배깅과 페이스팅* 배깅 : 훈련세트에서 중복을 허용하여 샘플링하는 방식* 페이스팅 : 중복을 허용하지 않고 샘플링하는 방식배깅과 페이스팅에서는 같은 훈련 샘플을 여러개의 예측기에 걸쳐 사용할 수 있음.하지만 배깅만이 한 예측기를 위해 같은 훈련샘플을 여러 번 샘플링할 수 있음. 모든 예측기가 훈련을 마치면 앙상블은 모든 예측기의 예측을 모아 새로운 샘플에 대한 예측을 만듦.수집함수는 전형적으로 분류일 때는 통계적 최빈값 (즉, 직접투표 분류기처럼 가장 많은 예측결과)이고회귀에 대해서는 평균을 계산. |개별 예측기는 원본훈련세트로 훈련시킨 것보다 훨씬 크게 편향되어 있지만수집함수를 통과하면 편향과 분산이 모두 감소함. 일반적으로 앙상블의 결과는 원본 데이터셋으로하나의 예측기를 훈련시킬때와 비교해 편향은 비슷하지만 분산은 줄어듦. 예측기는 모두 동시에 다른 CPU코어나 서버에서 병렬로 학습시킬 수 있음.이와 유사하게 예측도 병렬로 수행가능. 이런 확장성 덕분에 배깅과 페이스팅이 인기가 높음. 사이킷런의 배깅과 페이스팅사이킷런은 배깅과 페이스팅을 위해 간편한 API로 구성된 BaggingClassifier(회귀의 경우에는 BaggingRegressor)를 제공. 다음은 결정트리 분류기 500개의 앙상블을 훈련시키는 코드.각 분류기는 훈련세트에서 중복을 허용하여 무작위로 선택된 100개의 샘플로 훈련됨.(이는 배깅의 경우이고, 대신 페이스팅을 사용하려면 bootstrap=False로 지정하면 됨.)n_jobs 매개변수는 사이킷런이 훈련과 예측에 사용할 CPU 코어 수를 지정함.(-1로 지정하면 가용한 모든 코어 사용.)
###Code
from sklearn.ensemble import BaggingClassifier
from sklearn.tree import DecisionTreeClassifier
bag_clf = BaggingClassifier(
DecisionTreeClassifier(), n_estimators=500,
max_samples=100, bootstrap=True, random_state=42, n_jobs=-1)
bag_clf.fit(X_train, y_train)
y_pred = bag_clf.predict(X_test)
print(accuracy_score(y_test, y_pred))
tree_clf = DecisionTreeClassifier(random_state=42)
tree_clf.fit(X_train, y_train)
y_pred_tree = tree_clf.predict(X_test)
print(accuracy_score(y_test, y_pred_tree))
from matplotlib.colors import ListedColormap
def plot_decision_boundary(clf, X, y, axes=[-1.5, 2.45, -1, 1.5], alpha=0.5, contour=True):
x1s = np.linspace(axes[0], axes[1], 100)
x2s = np.linspace(axes[2], axes[3], 100)
x1, x2 = np.meshgrid(x1s, x2s)
X_new = np.c_[x1.ravel(), x2.ravel()]
y_pred = clf.predict(X_new).reshape(x1.shape)
custom_cmap = ListedColormap(['#fafab0','#9898ff','#a0faa0'])
plt.contourf(x1, x2, y_pred, alpha=0.3, cmap=custom_cmap)
if contour:
custom_cmap2 = ListedColormap(['#7d7d58','#4c4c7f','#507d50'])
plt.contour(x1, x2, y_pred, cmap=custom_cmap2, alpha=0.8)
plt.plot(X[:, 0][y==0], X[:, 1][y==0], "yo", alpha=alpha)
plt.plot(X[:, 0][y==1], X[:, 1][y==1], "bs", alpha=alpha)
plt.axis(axes)
plt.xlabel(r"$x_1$", fontsize=18)
plt.ylabel(r"$x_2$", fontsize=18, rotation=0)
fig, axes = plt.subplots(ncols=2, figsize=(10,4), sharey=True)
plt.sca(axes[0])
plot_decision_boundary(tree_clf, X, y)
plt.title("Decision Tree", fontsize=14)
plt.sca(axes[1])
plot_decision_boundary(bag_clf, X, y)
plt.title("Decision Trees with Bagging", fontsize=14)
plt.ylabel("")
save_fig("[7-5] decision_tree_without_and_with_bagging_plot")
plt.show()
###Output
그림 저장: [7-5] decision_tree_without_and_with_bagging_plot
###Markdown
NOTE_BaggingClassifier는 기반이 되는 분류기가 결정트리 분류기처럼 클래스 확률을 추정할 수 있으면(즉, predict_proba()가 있으면) 직접 투표 대신 자동으로 간접 투표 방식을 사용. [7-5]는 단일 결정트리의 결정경계와 500개의 트리를 사용한 배깅 앙상블(이전코드로 만든 모델)의결정경계를 비교할 것임. 둘 다 moons 데이터셋에 훈련시킴. 여기서 볼 수 있듯이 앙상블의 예측이결정트리 하나의 예측보다 일반화가 훨씬 잘된 것 같음. 앙상블은 비슷한 편향에서더 작은 분산을 만듦.(훈련세트의 오차 수가 거의 비슷하나 결정경계는 덜 불규칙함.)|부트스트래핑은 각 예측기가 학습하는 서브셋에 다양성을 증가시키므로 배깅이 페이스팅보다 편향이 조금 더 높음. 하지만 다양성을 추가한다는 것은 예측기들의 상관관계를줄이므로 앙상블의 분산을 감소시킴. |전반적으로 배깅이 더 나은 모델을 만들기 때문에 일반적으로더 선호. 그러나 시간과 CPU파워에 여유가 있다면 교차검증으로 배깅과 페이스팅을모두 평가해서 더 나은 쪽을 선택하는 것이 좋음. oob 평가배깅을 사용하면 특정 샘플만 샘플링되고 다른 것은 전혀 선택되지 않을 수 있음.BaggingClassifier는 기본값으로 중복을 허용해(bootstrap=True) 훈현세트의 크기만큼 m개의 샘플을 선택이는 평균적으로 63%정도만 샘플링 된다는 의미. |이렇게 선택되지 않은 샘플의 나머지 37%를 oob 샘플이라고 함.예측기마다 남겨진 37%는 모두 다름. 예측기가 훈련되는 동안에는 oob샘플을 사용하지 않으므로 oob 샘플을 사용해 평가할 수 있음.앙상블의 평가는 각 예측기의 oob평가를 평균하여 얻음.|사이킷런에서 BaggingClassifier를 만들 때 oob_score=True로 지정하면 훈련이 끝난 후자동으로 oob평가를 수행. 다음코드는 그 과정을 보여줌. 평과점수결과는 accuracy_score 변수에 저장.
###Code
bag_clf = BaggingClassifier(
DecisionTreeClassifier(), n_estimators=500,
bootstrap=True, oob_score=True, random_state=40, n_jobs=-1)
bag_clf.fit(X_train, y_train)
bag_clf.oob_score_
###Output
_____no_output_____
###Markdown
oob평가 결과를 보면 이 BaggingClassifier는 테스트 세트에서 약 90.1%의 정확도를 얻을 것.
###Code
from sklearn.metrics import accuracy_score
y_pred = bag_clf.predict(X_test)
accuracy_score(y_test, y_pred)
###Output
_____no_output_____
###Markdown
테스트세트에서 91.2%의 정확도를 얻었음. 매우 비슷!oob 샘플에 대한 결정함수의 값도 oob_decision_function_ 변수에서 확인가능.이 경우 결정함수는 각 훈련샘플의 클래스 확률을 반환함.(기반이 되는 예측기가 predict_proba()를 가지고 있기 때문에)다음 예를 보면 oob평가는 첫번째 훈련샘플이 양성클래스에 속할 확률을 67.72%로 추정하고 있음.(음성클래스에 속할 확률은 32.27%)
###Code
bag_clf.oob_decision_function_
###Output
_____no_output_____
###Markdown
랜덤 패치와 랜덤 서브스페이스BaggingClassifier는 특성 샘플링도 지원. 샘플링은 max_features, bootstrap_features 두 매개변수로 조절됨. 작동방식은 max_samples, bootstrap과 동일하지만 샘플이 아니고 특성에 대한 샘플링.따라서 각 예측기는 무작위로 선택한 입력 특서으이 일부분으로 훈련됨.|이 기법은 특히 (이미지와 같은) 매우 고차원의 데이터셋을 다룰 때 유용함.훈련특성과 샘플을 모두 샘플링하는 것을 랜덤패치방식이라고 함.훈련샘플을 모두 사용하고(bootstrap=False이고 max_samples=1.0로 설정) 특성은 샘플링하는(bootstrap_features=True 그리고/또는 max_features < 1.0) 것을 랜덤 서브스페이스 방식.특성 샘플링은 더 다양한 예측기를 만들면 편향을 늘리는 대신 분산을 낮춤. 랜덤 포레스트앞서 언급했듯이 랜덤 포레스트는 일반적으로 배깅 방법(또는 페이스팅)을 적용한 결정트리의 앙상블.전형적으로 max_samples를 훈련세트의 크기로 지정. BaggingClassifier에 DecisionTreeClassifier를넣어 만드는 대신 결정트리에 최적화되어 사용하기 편리한 RandomForestClassifier를 사용할 수 있음.(비슷하게 회귀 문제를 위한 RandomForestRegressor가 있음)|다음은 (최대 16개의 리프노드를 갖는) 500개 트리로 이뤄진 랜덤 포레스트 분류기를 여러 CPU 코어에서 훈련시키는 코드.
###Code
from sklearn.ensemble import RandomForestClassifier
rnd_clf = RandomForestClassifier(n_estimators=500, max_leaf_nodes=16, random_state=42)
rnd_clf.fit(X_train, y_train)
y_pred_rf = rnd_clf.predict(X_test)
###Output
_____no_output_____
###Markdown
랜덤 포레스트는 결정 트리의 배깅과 비슷합니다 :
###Code
'''
bag_clf = BaggingClassifier(
DecisionTreeClassifier(max_features="sqrt", max_leaf_nodes=16),
n_estimators=500, random_state=42)'''
bag_clf.fit(X_train, y_train)
y_pred = bag_clf.predict(X_test)
np.sum(y_pred == y_pred_rf) / len(y_pred) # 거의 에측이 동일합니다.
###Output
_____no_output_____
###Markdown
RandomForestClassifier는 몇 가지 예외가 있지만 (트리 성장의 조절을 위한) Decision Tree Classifier의 매개변수와 앙상블 자체를 제어하는데 필요한 BaggingClassifier의 매개변수를 모두 가지고 있음.|랜덤 포레스트 알고리즘은 트리의 노드를 분할할 때 전체특성 중에서 최선의 특성을 찾는 대신무작위로 선택한 특성 후보 중에서 최적의 특성을 찾는 식으로 무작위성을 더 주입함.(6장 참조)이는 결국 트리를 더욱 다양하게 만들고 (다시 한번) 편향을 손해보는 대신 분산을 낮추어 전체적으로 더 훌륭한 모델을 만들어냄.다음은 BaggingClassifier를 사용해 앞의 RandomForestClassifier와 거의 유사하게 만든 것.
###Code
bag_clf = BaggingClassifier(
DecisionTreeClassifier(max_features="sqrt", max_leaf_nodes=16),
n_estimators=500, random_state=42)
###Output
_____no_output_____
###Markdown
엑스트라 트리랜덤 포레스트에서 트리를 만들 때 각 노드는 무작위로 특성의 서브셋을 만들어 분할에 사용.트리를 더욱 무작위하게 만들기 위해 최적의 임곗값을 찾는 대신 후보 특성을 사용해 무작위로분할한 다음 그중에서 최상의 분할을 선택.|극단적으로 무작위한 트리의 랜덤포레스트를 익스트림 랜덤 트리 앙상블(또는 엑스트라 트리)라고 함.여기서도 역시 편향이 늘어나지만 대신 분산을 낮추게 됨. 모든 노드에서 특성마다 가장 최적의 임곗값을 찾는 것이 트리 알고리즘에서 가장 시간이 많이 소요되는 작업 중 하나이므로일반적인 랜덤 포레스트보다 엑스트라트리가 훨씬 빠름.|엑스트라 트리를 만들려면 사이킷런의 ExtraTreesClassifier를 사용함.사용법은 RandomForestClassifier와 같음. 마찬가지로 ExtraTreesClassifier도 RandomForestRegressor와 같은 API를 제공함. 특성 중요도랜덤포레스트의 또 다른 장점은 특성의 상대적 중요도를 측정하기 쉬움.사이킷런은 어떤 특성을 사용한 노드가 평균적으로 불순도를 얼마나 감소시키는지 확인해 특성의 중요도 측정.더 정확히 말하면 가중치 평균이며 각 노드의 가중치는 연관된 훈련 샘플 수와 같음.|사이킷런은 훈련이 끝난 뒤 특성마다 자동으로 이 점수를 계산하고 중요도의 전체 합이 1이 되도록결괏값을 정규화함. 이 값은 feature_importances_ 변수에 저장되어 있음.예를 들어 다음 코드는 (4장에서 소개한) iris 데이터셋에 RandomForestClassifier를 훈련시키고 각 특성을 출력함.|가장 중요한 특성은 꽃잎의 길이(44%)와 너비(42%)이고 꽃받침의 길이와 너비는 비교적 덜 중요해 보임.
###Code
from sklearn.datasets import load_iris
iris = load_iris()
rnd_clf = RandomForestClassifier(n_estimators=500, random_state=42)
rnd_clf.fit(iris["data"], iris["target"])
for name, score in zip(iris["feature_names"], rnd_clf.feature_importances_):
print(name, score)
rnd_clf.feature_importances_
###Output
_____no_output_____
###Markdown
이와 유사하게 (3장에) MNIST 데이터셋에 랜덤포레스트 분류기를 훈련시키고 각 픽셀의 중요도를 그래프로 나타내면 [7-6]과 같은 이미지가 나옴.랜덤포레스트는 특히 특성을 선택해야 할 때 어떤 특성이 중요한지 빠르게 확인할 수 있어 매우 편리.
###Code
'''from sklearn.datasets import fetch_openml
mnist = fetch_openml('mnist_784', version=1)
mnist.target = mnist.target.astype(np.uint8)
rnd_clf = RandomForestClassifier(n_estimators=100, random_state=42)
rnd_clf.fit(mnist["data"], mnist["target"])'''
'''def plot_digit(data):
image = data.reshape(28, 28)
plt.imshow(image, cmap = mpl.cm.hot,
interpolation="nearest")
plt.axis("off")
plot_digit(rnd_clf.feature_importances_)
cbar = plt.colorbar(ticks=[rnd_clf.feature_importances_.min(), rnd_clf.feature_importances_.max()])
cbar.ax.set_yticklabels(['Not important', 'Very important'])
save_fig("[7-6] mnist_feature_importance_plot")
plt.show()'''
###Output
_____no_output_____
###Markdown
부스팅부스팅은 약한 학습기를 여러 개 연결하여 강한 학습기를 만드는 앙상블 방법을 말함.부스팅의 아이디어는 앞의 모델을 보완해나가면서 일련의 예측기를 학습시키는 것.부스팅에는 여러가지가 있지만 가장 인기있는 것은 에이다부스트와 그레디언트 부스팅.에이다부스트부터 시작해보자. 에이다부스트에이다부스트에서 사용하는 방식은 이전 예측기를 보완하는 새로운 예측기를 만드는 방법은 이전 모델이 과소적합했던훈련샘플의 가중치를 더 높이는 것. 이렇게 하면 새로운 예측기는 학습하기 어려운 샘플에 점점 더 맞춰지게 됨. 예를 들어 에이다부스트 분류기를 만들 때 먼저 알고리즘이 기반이 되는 첫 번째 분류기(ex.결정트리)를 훈련세트에서 훈련시키고 예측을 만듦. 그 다음에 알고리즘이 잘못 분류된 훈련샘플의 가중치를 상대적으로 높임.두 번째 분류기는 업데이트된 가중치를 사용해 훈련세트에서 훈련하고 다시 예측을 만듦.그 다음에 다시 가중치를 업데이트하는 식으로 계속됨. [그림 7-8]은 moons 데이터셋에 훈련시킨 다섯 개의 연속된 예측기의 결정경계(이 모델은 규제를 강하게 한 RBF 커널 SVM 분류기임).첫 번째 분류기가 많은 샘플을 잘못 분류해서 이 샘플드르이 가중치가 높아짐. 따라서두 번째 분류기는 이 샘플들을 더 정확히 예측하게 됨.|오른쪽 그래프는 학습률을 반으로 낮춘 것만 빼고 똑같은 일련의 예측기를 나타냄.(즉, 잘못 분류된 샘플의 가중치는 반복마다 절반 정도만 높아짐.) 아래 그림에서 볼 수 있듯이 이런 연속된 학습 기법은 경사하강법과 비슷한 면이 있음.경사하강법은 비용함수를 최소화하기 위해 한 예측기의 모델 파라미터를 조정해가는 반면에이다부스트는 점차 더 좋아지도록 앙상블에 예측기를 추가함.
###Code
from sklearn.ensemble import AdaBoostClassifier
ada_clf = AdaBoostClassifier(
DecisionTreeClassifier(max_depth=1), n_estimators=200,
algorithm="SAMME.R", learning_rate=0.5, random_state=42)
ada_clf.fit(X_train, y_train)
m = len(X_train)
fig, axes = plt.subplots(ncols=2, figsize=(10,4), sharey=True)
for subplot, learning_rate in ((0, 1), (1, 0.5)):
sample_weights = np.ones(m) / m
plt.sca(axes[subplot])
for i in range(5):
svm_clf = SVC(kernel="rbf", C=0.2, gamma=0.6, random_state=42)
svm_clf.fit(X_train, y_train, sample_weight=sample_weights * m)
y_pred = svm_clf.predict(X_train)
r = sample_weights[y_pred != y_train].sum() / sample_weights.sum() # equation 7-1
alpha = learning_rate * np.log((1 - r) / r) # equation 7-2
sample_weights[y_pred != y_train] *= np.exp(alpha) # equation 7-3
sample_weights /= sample_weights.sum() # normalization step
plot_decision_boundary(svm_clf, X, y, alpha=0.2)
plt.title("learning_rate = {}".format(learning_rate), fontsize=16)
if subplot == 0:
plt.text(-0.7, -0.65, "1", fontsize=14)
plt.text(-0.6, -0.10, "2", fontsize=14)
plt.text(-0.5, 0.10, "3", fontsize=14)
plt.text(-0.4, 0.55, "4", fontsize=14)
plt.text(-0.3, 0.90, "5", fontsize=14)
else:
plt.ylabel("")
save_fig("[7-8] boosting_plot")
plt.show()
###Output
그림 저장: [7-8] boosting_plot
###Markdown
모든 예측기가 훈련을 마치면 이 앙상블은 배깅이나 페이스팅과 비슷한 방식으로 예측을 만듦.하지만 가중치가 적용된 훈련세트의 전반적인 정확도에 따라 예측기마다 다른 가중치가 적용됨. 더 자세히각 샘플의 가중치 $w^{(i)}$ 는 초기에 $\dfrac{1}{m}$ 로 초기화 됨.첫번째 예측기가 학습되고, 가중치가 적용된 에러율 $r_1$ 이 훈련세트에 대해 계산됨. (아래식 참고) **Equation 7-1: Weighted error rate of the $j^\text{th}$ predictor**$r_j = \dfrac{\displaystyle \sum\limits_{\textstyle {i=1 \atop \hat{y}_j^{(i)} \ne y^{(i)}}}^{m}{w^{(i)}}}{\displaystyle \sum\limits_{i=1}^{m}{w^{(i)}}} \quad\text{where }\hat{y}_j^{(i)}\text{ is the }j^{\text{th}}\text{ predictor's prediction for the }i^{\text{th}}\text{ instance.}$ 예측기의 가중치 $\alpha_j$ 는 [식 7-2]를 사용해 계산됨.여기서는 $\eta$ 는 학습률 하이퍼파라미터(기본값 1). 예측기가 정확할수록 가중치가 더 높아지게 됨.만약 무작위로 예측하는 정도라면 가중치가 0에 가까울 것. 그러나 나쁘면 (무작위 추측보다 정확도가 낮으면) 가중치는 음수가 됨. **Equation 7-2: Predictor weight**$\begin{split}\alpha_j = \eta \log{\dfrac{1 - r_j}{r_j}}\end{split}$ 그 다음 에이다부스트 알고리즘이 [식 7-3]을 사용해 샘플의 가중치를 업데이트.즉, 잘못 분류된 샘플의 가중치가 증가됨. **Equation 7-3: Weight update rule**$\begin{split}& \text{ for } i = 1, 2, \dots, m \\& w^{(i)} \leftarrow\begin{cases}w^{(i)} & \text{if }\hat{y_j}^{(i)} = y^{(i)}\\w^{(i)} \exp(\alpha_j) & \text{if }\hat{y_j}^{(i)} \ne y^{(i)}\end{cases}\end{split}$Then all the instance weights are normalized (i.e., divided by $ \sum_{i=1}^{m}{w^{(i)}} $). 마지막으로 새 예측기가 업데이트된 가중치를 사용해 훈련되고 전체과정이 반복됨.(새 예측기의 가중치가 계산되고 샘플의 가중치를 업데이트해서 또다른 예측기를 훈련시키는 식)이 알고리즘은 지정된 예측기 수에 도달하거나 완벽한 예측기가 만들어지면 중지됨.예측할 때 에이다부스트는 단순히 모든 예측기의 예측을 계산하고 예측기 가중치 $\alpha_j$ 를더해 예측 결과를 만듦. 가중치 합이 가장 큰 클래스가 예측 결과가 됨. ([식 7-4] 참조) **Equation 7-4: AdaBoost predictions**$\hat{y}(\mathbf{x}) = \underset{k}{\operatorname{argmax}}{\sum\limits_{\scriptstyle j=1 \atop \scriptstyle \hat{y}_j(\mathbf{x}) = k}^{N}{\alpha_j}} \quad \text{where }N\text{ is the number of predictors.}$ 사이킷런은 SAMME라는 에이다부스트의 다중클래스 버전을 사용. 클래스가 2개 뿐일때는 SAMME가 에이다부스트와 동일. 예측기가 클래스의 확률을 추정할 수 있다면 (predict_proba()가 있다면)사이킷런은 SAMME.R(R은 Real)이라는 SAMME변종을 사용.이 알고리즘은 예측값 대신 클래스 확률에 기반해 일반적으로 성능이 더 좋음. 다음 코드는 사이킷런의 AdaBoostClassifier를 사용하여 200개의 아주 얕은 결정트리를 기반으로 하는에이다부스트 분류기를 훈련시킴. 여기에서 사용한 결정트리는 max_depth=1.다시말해 결정노드 하나와 리프노드 2개로 이뤄진 트리.
###Code
'''
from sklearn.ensemble import AdaBoostClassifier
ada_clf = AdaBoostClassifier(
DecisionTreeClassifier(max_depth=1), n_estimators=200,
algorithm="SAMME.R", learning_rate=0.5, random_state=42)
ada_clf.fit(X_train, y_train)
'''
###Output
_____no_output_____
###Markdown
TIP에이다부스트 앙상블이 훈련세트에 과대적합되면 추정기 수를 줄이거나 추정기의 규제를 더 강하게 해봅시다. 그레디언트 부스팅 에이다부스트처럼 앙상블에 이전까지의 오차를 보정하도록 예측기를 순차적으로 추가.하지만 에이다부스트처럼 반복마다 샘플의 가중치를 수정하는 대신 이전 예측기가 만든 잔여오차에 새로운 예측기를 학습시킴. 간단하게 회귀 문제를 풀어봅시다. 그레디언트 부스티드 회귀 트리 (GBRT)라고 함.먼저 DecisionTreeRegressor를 훈련세트(잡음 섞인 2차 곡선 형태의) 에 학습시킵니다.
###Code
np.random.seed(42)
X = np.random.rand(100, 1) - 0.5
y = 3*X[:, 0]**2 + 0.05 * np.random.randn(100)
###Output
_____no_output_____
###Markdown
이제 이 데이터셋에 결정 트리 회귀 모델을 훈련시킵니다:
###Code
from sklearn.tree import DecisionTreeRegressor
tree_reg1 = DecisionTreeRegressor(max_depth=2, random_state=42)
tree_reg1.fit(X, y)
###Output
_____no_output_____
###Markdown
이제 첫번째 예측기에서 생긴 잔여오차에 2번째 DecisionTreeRegressor를 훈련시킵니다.
###Code
y2 = y - tree_reg1.predict(X)
tree_reg2 = DecisionTreeRegressor(max_depth=2, random_state=42)
tree_reg2.fit(X, y2)
###Output
_____no_output_____
###Markdown
그런 다음 두번째 예측기가 만든 잔여오차에 세번째 회귀 모델을 훈련시킴.
###Code
y3 = y2 - tree_reg2.predict(X)
tree_reg3 = DecisionTreeRegressor(max_depth=2, random_state=42)
tree_reg3.fit(X, y3)
###Output
_____no_output_____
###Markdown
이제 3개의 트리를 포함하는 앙상블 모델이 생김. 새로운 샘플에 대한 예측을 만들려면 모든 트리의 예측을 더하면 됨.
###Code
X_new = np.array([[0.8]])
y_pred = sum(tree.predict(X_new) for tree in (tree_reg1, tree_reg2, tree_reg3))
y_pred
###Output
_____no_output_____
###Markdown
[7-9]의 왼쪽 열은 이 3개 트리의 예측이고 오른쪽 열은 앙상블의 예측.첫번째 행에서는 앙상블에 트리가 하나만 있어서 첫번째 트리의 예측과 완전히 같음.두번쨰 행에서는 새로운 트리가 첫번째 트리의 잔여오차에 대해 학습됨.오른쪽의 앙상블 예측이 2개의 트리 예측의 합과 같은 것을 볼 수 있음.비슷하게 세번째 행에서는 또 다른 트리가 두번째 잔여오차에 훈련됨.트리가 앙상블에 추가될수록 앙상블의 예측이 점차 좋아지는 것을 알 수 있음. 사이킷런의 GradientBoostingRegressor을 사용하면 GBRT 앙상블을 간단하게 훈련시킬 수 있음.트리 수(n_estimators)와 같이 앙상블의 훈련을 제어하는 매개변수는 물론RendomForestRegressor와 아주 비슷하게 결정트리의 성장 제어 매개변수(max_depth, min_split_leaf)도 있음. ** 생성 코드**
###Code
def plot_predictions(regressors, X, y, axes, label=None, style="r-", data_style="b.", data_label=None):
x1 = np.linspace(axes[0], axes[1], 500)
y_pred = sum(regressor.predict(x1.reshape(-1, 1)) for regressor in regressors)
plt.plot(X[:, 0], y, data_style, label=data_label)
plt.plot(x1, y_pred, style, linewidth=2, label=label)
if label or data_label:
plt.legend(loc="upper center", fontsize=16)
plt.axis(axes)
plt.figure(figsize=(11,11))
plt.subplot(321)
plot_predictions([tree_reg1], X, y, axes=[-0.5, 0.5, -0.1, 0.8], label="$h_1(x_1)$", style="g-", data_label="Training set")
plt.ylabel("$y$", fontsize=16, rotation=0)
plt.title("Residuals and tree predictions", fontsize=16)
plt.subplot(322)
plot_predictions([tree_reg1], X, y, axes=[-0.5, 0.5, -0.1, 0.8], label="$h(x_1) = h_1(x_1)$", data_label="Training set")
plt.ylabel("$y$", fontsize=16, rotation=0)
plt.title("Ensemble predictions", fontsize=16)
plt.subplot(323)
plot_predictions([tree_reg2], X, y2, axes=[-0.5, 0.5, -0.5, 0.5], label="$h_2(x_1)$", style="g-", data_style="k+", data_label="Residuals")
plt.ylabel("$y - h_1(x_1)$", fontsize=16)
plt.subplot(324)
plot_predictions([tree_reg1, tree_reg2], X, y, axes=[-0.5, 0.5, -0.1, 0.8], label="$h(x_1) = h_1(x_1) + h_2(x_1)$")
plt.ylabel("$y$", fontsize=16, rotation=0)
plt.subplot(325)
plot_predictions([tree_reg3], X, y3, axes=[-0.5, 0.5, -0.5, 0.5], label="$h_3(x_1)$", style="g-", data_style="k+")
plt.ylabel("$y - h_1(x_1) - h_2(x_1)$", fontsize=16)
plt.xlabel("$x_1$", fontsize=16)
plt.subplot(326)
plot_predictions([tree_reg1, tree_reg2, tree_reg3], X, y, axes=[-0.5, 0.5, -0.1, 0.8], label="$h(x_1) = h_1(x_1) + h_2(x_1) + h_3(x_1)$")
plt.xlabel("$x_1$", fontsize=16)
plt.ylabel("$y$", fontsize=16, rotation=0)
save_fig("gradient_boosting_plot")
plt.show()
###Output
그림 저장: gradient_boosting_plot
###Markdown
이제 그레이디언트 부스팅 회귀 모델을 사용해 보죠:
###Code
from sklearn.ensemble import GradientBoostingRegressor
gbrt = GradientBoostingRegressor(max_depth=2, n_estimators=3, learning_rate=1.0, random_state=42)
gbrt.fit(X, y)
###Output
_____no_output_____
###Markdown
learning_rate 매개변수가 각 트리의 기여 정도를 조절함. 이를 0.1처럼 낮게 설정하면앙상블을 훈련세트에 학습시키기 위해 많은 트리가 필요하지만 일반적으로 예측의 성능은 좋아짐.=> 축소 (규제 방법)[7-10]은 작은 학습률로 훈련시킨 2개의 GBRT 앙상블을 보여줌.왼쪽은 훈련세트를 학습하기에 충분하지 않은 반면에, 오른쪽은 트리가 너무 많아 과대적합 됨. ** 생성 코드**
###Code
gbrt_slow = GradientBoostingRegressor(max_depth=2, n_estimators=200, learning_rate=0.1, random_state=42)
gbrt_slow.fit(X, y)
fig, axes = plt.subplots(ncols=2, figsize=(10,4), sharey=True)
plt.sca(axes[0])
plot_predictions([gbrt], X, y, axes=[-0.5, 0.5, -0.1, 0.8], label="Ensemble predictions")
plt.title("learning_rate={}, n_estimators={}".format(gbrt.learning_rate, gbrt.n_estimators), fontsize=14)
plt.xlabel("$x_1$", fontsize=16)
plt.ylabel("$y$", fontsize=16, rotation=0)
plt.sca(axes[1])
plot_predictions([gbrt_slow], X, y, axes=[-0.5, 0.5, -0.1, 0.8])
plt.title("learning_rate={}, n_estimators={}".format(gbrt_slow.learning_rate, gbrt_slow.n_estimators), fontsize=14)
plt.xlabel("$x_1$", fontsize=16)
save_fig("[7-10] gbrt_learning_rate_plot")
plt.show()
###Output
그림 저장: [7-10] gbrt_learning_rate_plot
###Markdown
최적의 트리 수를 찾기 위해선 조기종료(4장참조)를 사용할 수 있음.간단하게 구현하려면 staged_predict()를 사용함. 해당 메서드는 훈련의 각 단계(트리 하나, 두 개 등)에서앙상블에 의해 만들어진 예측기를 순회하는 반복자를 반환함. 다음 코드는 120개의 트리로 GBRT 앙상블을 훈련시키고 최적의 트리 수를 찾기 위해 각 훈련단계에서검증오차를 측정. 마지막에 트리 수를 사용해 GBRT 앙상블을 훈련시킴. **조기 종료를 사용한 그래디언트 부스팅**
###Code
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
X_train, X_val, y_train, y_val = train_test_split(X, y, random_state=49)
gbrt = GradientBoostingRegressor(max_depth=2, n_estimators=120, random_state=42)
gbrt.fit(X_train, y_train)
errors = [mean_squared_error(y_val, y_pred)
for y_pred in gbrt.staged_predict(X_val)]
bst_n_estimators = np.argmin(errors) + 1
gbrt_best = GradientBoostingRegressor(max_depth=2, n_estimators=bst_n_estimators, random_state=42)
gbrt_best.fit(X_train, y_train)
###Output
_____no_output_____
###Markdown
** 생성 코드**
###Code
min_error = np.min(errors)
plt.figure(figsize=(10, 4))
plt.subplot(121)
plt.plot(np.arange(1, len(errors) + 1), errors, "b.-")
plt.plot([bst_n_estimators, bst_n_estimators], [0, min_error], "k--")
plt.plot([0, 120], [min_error, min_error], "k--")
plt.plot(bst_n_estimators, min_error, "ko")
plt.text(bst_n_estimators, min_error*1.2, "Minimum", ha="center", fontsize=14)
plt.axis([0, 120, 0, 0.01])
plt.xlabel("Number of trees")
plt.ylabel("Error", fontsize=16)
plt.title("Validation error", fontsize=14)
plt.subplot(122)
plot_predictions([gbrt_best], X, y, axes=[-0.5, 0.5, -0.1, 0.8])
plt.title("Best model (%d trees)" % bst_n_estimators, fontsize=14)
plt.ylabel("$y$", fontsize=16, rotation=0)
plt.xlabel("$x_1$", fontsize=16)
save_fig("[7-11] early_stopping_gbrt_plot")
plt.show()
###Output
그림 저장: [7-11] early_stopping_gbrt_plot
###Markdown
(많은 수의 트리를 먼저 훈련시키고 최적의 수를 찾기 위해 살펴보는 대신)실제로 훈련을 중지하는 방법으로 조기 종료를 구현할 수도 있음.warm_start=True로 설정해 사이킷런이 fit()이 호출될 때 기존 트리를 유지하고 훈련을 추가할 수 있도록 함.다음코드는 연속해서 다섯번의 반복동안 검증 오차가 향상되지 않으면 훈련을 멈춤.
###Code
gbrt = GradientBoostingRegressor(max_depth=2, warm_start=True, random_state=42)
min_val_error = float("inf")
error_going_up = 0
for n_estimators in range(1, 120):
gbrt.n_estimators = n_estimators
gbrt.fit(X_train, y_train)
y_pred = gbrt.predict(X_val)
val_error = mean_squared_error(y_val, y_pred)
if val_error < min_val_error:
min_val_error = val_error
error_going_up = 0
else:
error_going_up += 1
if error_going_up == 5:
break # early stopping
print(gbrt.n_estimators)
print("Minimum validation MSE:", min_val_error)
###Output
Minimum validation MSE: 0.002712853325235463
###Markdown
GradientBoostingRegressor는 각 트리가 훈련할 때 사용할 훈련샘플의 비율을 지정할 수 있는subsample 매개변수도 지원함. 예를 들어 subsamples=0.25라고 하면 각 트리는 무작위로 선택된 25%의 훈련샘플로 학습됨. 아마 추측할 수 있겠지만 편향이 높아지는 대신 분산이 낮아지게 됨. => 확률적 그레디언트 부스팅 NOTE_그레디언트 부스팅에 다른 비용함수를 사용할 수 있음. loss 매개변수를 이용해 지정 최적화된 그레디언트 부스팅 구현으로 XGBoost 파이썬 라이브러리가 유명.XGBoost는 익스트림 그레디언트 부스팅의 약자. 이 패키지는 원래 DMLC 커뮤니티의 일원인 톈치 천이 개발.이 패키지의 목표는 매우 빠른속도, 확장성, 이식성임.사실 XGBoost는 머신러닝 경연 대회에서 우승후보들이 사용하는 중요도구 중 하나임.XGBoost API는 사이킷런과 매우 비슷함. **XGBoost 사용하기**
###Code
try:
import xgboost
except ImportError as ex:
print("에러: xgboost 라이브러리 설치되지 않았습니다.")
xgboost = None
if xgboost is not None: # 책에 없음
xgb_reg = xgboost.XGBRegressor(random_state=42)
xgb_reg.fit(X_train, y_train)
y_pred = xgb_reg.predict(X_val)
val_error = mean_squared_error(y_val, y_pred) # 책에 없음
print("Validation MSE:", val_error) # 책에 없음
###Output
Validation MSE: 0.004000408205406276
###Markdown
XGBoost는 자동 조기 종료와 같은 여러 좋은 기능도 제공
###Code
if xgboost is not None: # 책에 없음
xgb_reg.fit(X_train, y_train,
eval_set=[(X_val, y_val)], early_stopping_rounds=2)
y_pred = xgb_reg.predict(X_val)
val_error = mean_squared_error(y_val, y_pred) # 책에 없음
print("Validation MSE:", val_error) # 책에 없음
%timeit xgboost.XGBRegressor().fit(X_train, y_train) if xgboost is not None else None
%timeit GradientBoostingRegressor().fit(X_train, y_train)
###Output
22.1 ms ± 620 µs per loop (mean ± std. dev. of 7 runs, 10 loops each)
|
notebook/Python-2.ipynb | ###Markdown
Introduction to Python programming [Gerard Gorman](http://www.imperial.ac.uk/people/g.gorman), [Christian Jacobs](http://christianjacobs.uk/) Updated for MPECDT by [David Ham](http://www.imperial.ac.uk/people/david.ham) Lecture 2: Computing with basic language constructionsLearning objectives:* Introduction to two different forms of loops which are used to repeat operations on data: either until a condition is satisfied (*while-loop*) or for a fixed number of iterations (*for-loop*).* Form a *condition* using a *boolean expression*. This is used to either terminate loops or control if a specific block of code is executed via the *if-statement*.* Learn two different methods for storing many data elements within a single Python type.* Introduction to developing your own Python *functions* which allows you to execute the same block of code, with different inputs and outputs, from anywhere in the code. Boolean expressionsAn expression with value *true* or *false* is called a boolean expression. Example expressions for what you would write mathematically as$C=40$, $C\ne40$, $C\ge40$, $C\gt40$ and $C\lt40$ are:
###Code
C == 40 # Note: the double == checks for equality, but C=40 is an assignment!
C != 40 # This could also be written as 'not C == 4' (i.e. the opposite of what C == 4 gives)
C >= 40
C > 40
C < 40
###Output
_____no_output_____
###Markdown
We can test boolean expressions in a Python shell:
###Code
C = 41
print("C != 40: ", C != 40)
print("C < 40: ", C < 40)
print("C == 41: ", C == 41)
###Output
_____no_output_____
###Markdown
Several conditions can be combined with the special 'and' and 'or' operators into a single boolean expression:
###Code
condition1 and condition2
condition1 or condition2
###Output
_____no_output_____
###Markdown
* Rule 1: (**C1** *and* **C2**) is *True* only if both **C1** and **C2** are *True** Rule 2: (**C1** *or* **C2**) is *True* if either **C1** or **C2** are *True*Examples:
###Code
x=0; y=1.2
print (x >= 0 and y < 1)
###Output
_____no_output_____
###Markdown
Exercise: Values of boolean expressionsAdd a comment to the code below to explain the outcome of each of the boolean expressions:
###Code
C = 41
print("Case 1: ", C == 40)
print("Case 2: ", C != 40 and C < 41)
print("Case 3: ", C != 40 or C < 41)
print("Case 4: ", not C == 40)
print("Case 5: ", not C > 40)
print("Case 6: ", C <= 41)
print("Case 7: ", not False)
print("Case 8: ", True and False)
print("Case 9: ", False or True)
print("Case 10: ", False or False or False)
print("Case 11: ", True and True and False)
print("Case 12: ", False == 0)
print("Case 13: ", True == 0)
print("Case 14: ", True == 1)
###Output
Case 1: False
Case 2: False
Case 3: True
Case 4: True
Case 5: False
Case 6: True
Case 7: True
Case 8: False
Case 9: True
Case 10: False
Case 11: False
Case 12: True
Case 13: False
Case 14: True
###Markdown
LoopsSuppose we want to make a table of Celsius and Fahrenheitdegrees:
###Code
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Output
_____no_output_____
###Markdown
How do we write a program that prints out such a table?We know from the last lecture how to make one line in this table:
###Code
C = -20
F = 9/5*C + 32
print(C, F)
###Output
_____no_output_____
###Markdown
We can just repeat these statements:
###Code
C=-20; F=9/5*C+32; print(C,F)
C=-15; F=9/5*C+32; print(C,F)
C=-10; F=9/5*C+32; print(C,F)
C=-5; F=9/5*C+32; print(C,F)
C=0; F=9/5*C+32; print(C,F)
C=5; F=9/5*C+32; print(C,F)
C=10; F=9/5*C+32; print(C,F)
C=15; F=9/5*C+32; print(C,F)
C=20; F=9/5*C+32; print(C,F)
C=25; F=9/5*C+32; print(C,F)
C=30; F=9/5*C+32; print(C,F)
C=35; F=9/5*C+32; print(C,F)
C=40; F=9/5*C+32; print(C,F)
###Output
_____no_output_____
###Markdown
So we can see that works but its **very boring** to write and very easy to introduce a misprint.**You really should not be doing boring repetitive tasks like this.** Spend one time instead looking for a smarter solution. When programming becomes boring, there is usually a construct that automates the writing. Computers are very good at performing repetitive tasks. For this purpose we use **loops**. The while loop (and the significance of indentation)A while loop executes repeatedly a set of statements as long as a **boolean** (i.e. *True* / *False*) condition is *True*
###Code
while condition:
<statement 1>
<statement 2>
...
<first statement after loop>
###Output
_____no_output_____
###Markdown
Note that all statements to be executed within the loop must be indented by the same amount! The loop ends when an unindented statement is encountered.At this point it is worth noticing that **blank spaces may or may not be important** in Python programs. These statements are equivalent (blanks do not matter):
###Code
v0=3
v0 = 3
v0= 3
# The computer does not care but this formatting style is
# considered clearest for the human reader.
v0 = 3
###Output
_____no_output_____
###Markdown
Here is a while loop example where blank spaces really do matter:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
11
###Markdown
Let's take a look at what happens when we forget to indent correctly:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
_____no_output_____
###Markdown
Let's use the while loop to create the table above:
###Code
C = -20 # Initialise C
dC = 5 # Increment for C within the loop
while C <= 40: # Loop heading with condition
F = (9/5)*C + 32 # 1st statement inside loop
print ("%3d %6.1f" % (C, F)) # 2nd statement inside loop
C = C + dC # Increment C for the next iteration of the loop.
###Output
_____no_output_____
###Markdown
Exercise: Make a Fahrenheit-Celsius conversion tableWrite a program that prints out a table with Fahrenheit degrees 0, 10, 20, ..., 100 in the first column and the corresponding Celsius degrees in the second column. Exercise: Write an approximate Fahrenheit-Celsius conversion tableMany people use an approximate formula for quickly converting Fahrenheit ($F$) to Celsius ($C$) degrees:$C \approx \hat{C} = \frac{F − 30}{2}$Modify the program from the previous exercise so that it prints three columns: $F$, $C$, and the approximate value $\hat{C}$. ListsSo far, one variable has referred to one number (or string). Sometimes however we naturally have a collection of numbers, saydegrees −20, −15, −10, −5, 0, ..., 40. One way to store these values in a computer program would be to have one variable per value, i.e.
###Code
C1 = -20
C2 = -15
C3 = -10
# ...
C13 = 40
###Output
_____no_output_____
###Markdown
This is clearly a terrible solution, particularly if we have lots of values. A better way of doing this is to collect values together in a list:
###Code
C = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
###Output
_____no_output_____
###Markdown
Now there is just one variable, **C**, holding all the values. Elements in a list are accessed via an index. List indices are always numbered as 0, 1, 2, and so forth up to the number of elements minus one:
###Code
mylist = [4, 6, -3.5]
print(mylist[0])
print(mylist[1])
print(mylist[2])
print(len(mylist)) # length of list
###Output
4
6
-3.5
3
###Markdown
Here are a few example of operations that you can perform on lists:
###Code
C = [-10, -5, 0, 5, 10, 15, 20, 25, 30]
C.append(35) # add new element 35 at the end
print(C)
C = C + [40,45] # And another list to the end of C
print(C)
C.insert(0, -15) # Insert -15 as index 0
print(C)
del C[2] # delete 3rd element
print(C)
del C[2] # delete what is now 3rd element
print(C)
print(len(C)) # length of list
print(C.index(10)) # Find the index of the list with the value 10
print (10 in C) # True only if the value 10 is stored in the list
print(C[-1]) # The last value in the list.
print(C[-2]) # The second last value in the list.
###Output
40
###Markdown
You can also extract sublists using ":"
###Code
print(C[5:]) # From index 5 to the end of the list.
print(C[5:7]) # From index 5 up to, but not including index 7.
###Output
[15, 20]
###Markdown
Many languages (especially Matlab and Fortran) have would include the endpoint of a slice (7 in this case). One way to think of what Python does is that the slice is a half-open interval: $[5,7)$. This makes cutting a list in half particularly easy. For example:
###Code
print(C[:5] + ["Hello"] + C[5:])
print(C[7:-1]) # From index 7 up to the second last element.
print(C[:]) # [:] specifies the whole list.
somelist = ['Curly', 'Larry', 'Moe']
stooge1, stooge2, stooge3 = somelist # Unpack the entries in a list.
print(stooge3, stooge2, stooge1)
###Output
Moe Larry Curly
###Markdown
Of course this only works if `somelist` contains exactly the number of items on the left hand side of the equals. Another useful operator is `*` (pronounced "splat"). It turns a list (or any other sequence) into the set of arguments of a function. For example:
###Code
print(somelist) # Prints the list.
print(*somelist) # Prints the items in the list.
###Output
['Curly', 'Larry', 'Moe']
Curly Larry Moe
###Markdown
Exercise: Store odd numbers in a listStep 1: Write a program that generates all odd numbers from 1 to *n*. Set *n* in the beginning of the program and use a while loop to compute the numbers. (Make sure that if *n* is an even number, the largest generated odd number is *n*-1.).Step 2: Store the generated odd numbers in a list. Start with an empty list and use the same while loop where you generate each odd number, ao append the new number to the list.Finally, print the list elements to the screen.
###Code
a=[]
n=20
i=1
while i<=20:
a=a+[i]
i=i+2
print(a)
###Output
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
###Markdown
For loopsWe can visit each element in a list and process the element with some statements using a *for* loop, for example:
###Code
degrees = [0, 10, 20, 40, 100]
for C in degrees:
print('list element:', C)
print('The degrees list has', len(degrees), 'elements')
###Output
_____no_output_____
###Markdown
Notice again how the statements to be executed within the loop must be indented! Let's now revisit the conversion table example using the *for* loop:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
for C in Cdegrees:
F = (9/5)*C + 32
print("%3d %6.2f" % (C, F))
###Output
_____no_output_____
###Markdown
It is also possible to rewrite the *for* loop as a *while* loop, i.e.,
###Code
for element in somelist:
# process element
###Output
_____no_output_____
###Markdown
can always be transformed to a *while* loop
###Code
index = 0
while index < len(somelist):
element = somelist[index]
# process element
index += 1
###Output
_____no_output_____
###Markdown
Taking the previous table example:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
index = 0
while index < len(Cdegrees):
C = Cdegrees[index]
F = (9/5)*C + 32
print('%5d %5.1f' % (C, F))
index += 1
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Rather than just printing out the Fahrenheit values, let's also store these computed values in a list of their own:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
Fdegrees = [] # start with empty list
for C in Cdegrees:
F = (9/5)*C + 32
Fdegrees.append(F) # add new element to Fdegrees
print(Fdegrees)
###Output
_____no_output_____
###Markdown
Iterators: things you can iterate over.We've seen that a `for` loop can iterate over list values. In fact you can iterate over any object that is capable of providing values one at a time. In python, these objects are called `iterators`.A particularly useful python function which creates an iterator is `range`. For example, we can use range to iterate over the *indices* of a list:
###Code
for i in range(0, len(somelist), 1):
element = somelist[i]
... process element or somelist[i] directly
###Output
_____no_output_____
###Markdown
The statement *range(start, stop, inc)* generates an iterator over the integers *start*, *start+inc*, *start+2\*inc*, and so on up to, but not including, *stop*. We can also write *range(stop)* as an abbreviation for *range(0, stop, 1)*:
###Code
print(range(3)) # same as range(0, 3, 1)
###Output
range(0, 3)
###Markdown
Oh, that wasn't very informative. If we want to see all the numbers which the `range` will iterate over, we can cast the range to a list:
###Code
print(list(range(3)))
print(list(range(2, 8, 3)))
###Output
_____no_output_____
###Markdown
List comprehensionsConsider this example where we compute two lists in a *for* loop:
###Code
n = 16
Cdegrees = []; Fdegrees = [] # empty lists
for i in range(n):
Cdegrees.append(-5 + i*0.5)
Fdegrees.append((9/5)*Cdegrees[i] + 32)
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
As constructing lists is a very common requirement, the above way of doing it can become very tedious to both write and read. Therefore, Python has a compact construct, called list comprehension for generating lists from a *for* loop:
###Code
n = 16
Cdegrees = [-5 + i*0.5 for i in range(n)]
Fdegrees = [(9/5)*C + 32 for C in Cdegrees]
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
The general form of a list comprehension is:
###Code
somelist = [expression for element in somelist]
###Output
_____no_output_____
###Markdown
Exercise: Repeat the previous exercise of creating a list of odd numbers using using: a for loop, list comprehension and the range function
###Code
# Using for loop
# Using list comprehension
# Using the function range
###Output
_____no_output_____
###Markdown
Changing elements in a listSay we want to add $2$ to all the numbers in a list:
###Code
v = [-1, 1, 10]
for e in v:
e = e + 2
print(v)
###Output
_____no_output_____
###Markdown
We can see that the list *v* is unaltered! The reason for this is that inside the loop, *e* is an ordinary (int) variable. At the start of the iteration *e* is assigned a *copy* of the next element in the list. Inside the loop we can change *e* but *v* itself is unaltered. If we want to change *v* we have to use an index to access and modify its elements:
###Code
v[1] = 4 # assign 4 to 2nd element (index 1) in v
print(v)
###Output
_____no_output_____
###Markdown
To add 2 to all values we need a *for* loop over indices:
###Code
for i in range(len(v)):
v[i] = v[i] + 2
print(v)
###Output
_____no_output_____
###Markdown
Traversing multiple lists simultaneously - *zip(list1, list2, ...)*Consider how we can loop over elements in both Cdegrees and Fdegrees at the same time. One approach would be to use list indices:
###Code
for i in range(len(Cdegrees)):
print(Cdegrees[i], Fdegrees[i])
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
An alternative construct (regarded as more ”Pythonic”) uses the *zip* function:
###Code
for C, F in zip(Cdegrees, Fdegrees):
print(C, F)
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
`zip` creates an *iterator* over the corresponding items of its arguments in order.Another example with three lists:
###Code
l1 = [3, 6, 1]; l2 = [1.5, 1, 0]; l3 = [9.1, 3, 2]
for e in zip(l1, l2, l3):
print(*e)
###Output
3 1.5 9.1
6 1 3
1 0 2
###Markdown
If the lists are of unequal length then the loop stops when the end of the shortest list is reached. Experiment with this:
###Code
l1 = [3, 6, 1, 4, 6]; l2 = [1.5, 1, 0, 7]; l3 = [9.1, 3, 2, 0, 9]
for e in zip(l1, l2, l3):
print(*e)
###Output
_____no_output_____
###Markdown
Nested lists: list of listsA *list* can contain **any** object, including another *list*. To illustrate this, consider how to store the conversion table as a single Python list rather than two separate lists.
###Code
Cdegrees = range(-20, 41, 5)
Fdegrees = [(9.0/5)*C + 32 for C in Cdegrees]
table1 = [Cdegrees, Fdegrees] # List of two lists
print("table1 = ", table1)
print("table1[0] = ", table1[0])
print("table1[1] = ", table1[1])
print("table1[1][3] = ", table1[1][3])
###Output
table1 = [range(-20, 41, 5), [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]]
table1[0] = range(-20, 41, 5)
table1[1] = [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]
table1[1][3] = 23.0
###Markdown
This gives us a table of two columns. How do we create a table of rows instead:
###Code
table2 = []
for C, F in zip(Cdegrees, Fdegrees):
row = [C, F]
table2.append(row)
print(table2)
###Output
_____no_output_____
###Markdown
We can use `zip` to do this more elegantly:
###Code
table2 = list(zip(Cdegrees, Fdegrees))
print(table2)
###Output
_____no_output_____
###Markdown
And you can loop through this list as before:
###Code
for C, F in table2:
print(C, F)
###Output
_____no_output_____
###Markdown
Tuples: sequences that cannot be changedWe've already learned that lists are not the only thing which can be iterated over, but that there is a general category of `iterator` types. Similarly, lists are not the only objects which can be indexed. The general category of types which can be indexed are the `sequence` types.Tuples are **constant** sequences. You can use them in much the same way as lists except you cannot modify them. They are an example of an [**immutable**](http://en.wikipedia.org/wiki/Immutable_object) type.
###Code
t = (2, 4, 6, 'temp.pdf') # Define a tuple.
t = 2, 4, 6, 'temp.pdf' # Can skip parenthesis as it is assumed in this context.
###Output
_____no_output_____
###Markdown
Let's see what happens when we try to modify the tuple like we did with a list:
###Code
t[1] = -1
t.append(0)
del t[1]
###Output
_____no_output_____
###Markdown
However, we can use the tuple to compose a new tuple:
###Code
t = t + (-1.0, -2.0)
print(t)
###Output
_____no_output_____
###Markdown
So, why would we use tuples when lists have more functionality?* Tuples are constant and thus protected against accidental changes.* Tuples are faster than lists.* Tuples are widely used in Python software (so you need to know about tuples to understand other people's code!)* Tuples (but not lists) can be used as keys in dictionaries (more about dictionaries later). Exercise: Make a table of function valuesStep 1: Write a program that prints a table with $t$ values in the first column and the corresponding $y(t) = v_0 t − 0.5gt^2$ values in the second column. Use $n$ uniformly spaced $t$ values throughout the interval [0, $2v_0/g$]. Set $v0 = 1$, $g = 9.81$, and $n = 11$.Step 2: Once step 1 is working, modify the program so that the $t$ and $y$ values are stored in two lists *t* and *y*. Thereafter, transverse the lists with a *for* loop and write out a nicely formatted table of *t* and *y* values using a *zip* construction. FunctionsWe have already used many Python functions, including mathematical functions:
###Code
from math import *
x = pi
print cos(x)
###Output
_____no_output_____
###Markdown
Other functions you used include *len* and *range*:
###Code
n = len(somelist)
ints = range(5, n, 2)
###Output
_____no_output_____
###Markdown
You have also used functions that are executed with the dot syntax (called *methods*):
###Code
C = [5, 10, 40, 45]
i = C.index(10)
C.append(50)
C.insert(2, 20)
###Output
_____no_output_____
###Markdown
A function is a collection of statements we can execute wherever and whenever we want. Functions can take any number of inputs (called *arguments*) to produce outputs. Functions help to organize programs, make them more understandable, shorter, and easier to extend. For our first example we will turn our temperature conversion code into a function:
###Code
def C2F(C):
return (9/5)*C + 32
###Output
_____no_output_____
###Markdown
Functions start with *def*, then the name you want to give your function, then a list of arguments (here C). This is referred to as the *function header*. Inside the function there is a block of statements called the *function body*. Notice that the function body is indented - as was the case for the *for* / *while* loop the indentation indicates where the function ends. At any point within the function, we can "stop thefunction" and return as many values/variables as required. Local and global variablesVariables defined within a function are said to have *local scope*. That is to say that they can only be referenced within that function. Let's consider an example (and look carefully at the indentation!!):
###Code
def sumint(start, stop):
s = 0 # variable for accumulating the sum
i = start # counter
while i <= stop:
s += i
i += 1
return s
print(sumint(0, 5))
###Output
_____no_output_____
###Markdown
Variables *i* and *s* are local variables in *sumint*. They are destroyed at the end (return) of the function and never visible outside the function (in the calling program); in fact, *start* and *stop* are also local variables. So let's see what happens if we now try to print one of these variables:
###Code
print(stop)
###Output
_____no_output_____
###Markdown
Functions can also return multiple values. Let's recycle another of our previous examples - compute $y(t)$ and $y'(t)=v_0-gt$:
###Code
def yfunc(t, v0):
g = 9.81
y = v0*t - 0.5*g*t**2
dydt = v0 - g*t
return y, dydt
# call:
position, velocity = yfunc(0.6, 3)
print(position, velocity)
###Output
_____no_output_____
###Markdown
Remember that a series of comma separated variables implies a tuple - therefore "return y, dydt" is the same as writing "return (y, dydt)". Therefore, in general what is returned is a tuple. Let's take a look at another example illustrating this:
###Code
def f(x):
return x, x**2, x**4
s = f(2)
print(s)
print(type(s)) # The function type() tells us what type a variable it is.
###Output
_____no_output_____
###Markdown
No return value implies that `None` is returned. `None` is a special Python object that represents an ”empty” or undefined value. It is surprisingly useful and we will use it a lot later.
###Code
def message(course):
print("%s rocks!"% course)
message("Python")
r = message("Geo")
print("r = ", r)
###Output
_____no_output_____
###Markdown
Keyword arguments and default argument valuesIt's possible to specify default values that function arguments should take if no values are provided when the function is called:
###Code
def somefunc(arg1, arg2, kwarg1=True, kwarg2=0):
print(arg1, arg2, kwarg1, kwarg2)
somefunc("Hello", [1,2]) # Note that we have not specified values for kwarg1 and kwarg2
###Output
_____no_output_____
###Markdown
You can override the default values by passing additional arguments in the correct position:
###Code
somefunc("Hello", [1,2], "Hi")
###Output
_____no_output_____
###Markdown
... or by naming the argument to which the value should be passed. These are called keyword arguments:
###Code
somefunc("Hello", [1,2], kwarg2="Hi")
somefunc("Hello", [1,2], kwarg2="Hi", kwarg1=6)
###Output
_____no_output_____
###Markdown
If we use variable_name=value for all arguments, their sequence in the function header can be in any order.
###Code
somefunc(kwarg2="Hello", arg1="Hi", kwarg1=6, arg2=[2])
###Output
_____no_output_____
###Markdown
Let's look at another example - consider a function of $t$, with parameters $A$, $a$, and $\omega$:$$f(t; A,a, \omega) = Ae^{-at}\sin (\omega t)$$. (The choice of equation is actually pretty random - but it serves to show you that it is easy to translate formulae you encounter into Python code). We can implement $f$ in a Python function with $t$ as positional argument and $A$, $a$, and $\omega$ as keyword arguments.
###Code
from math import pi, exp, sin
def f(t, A=1, a=1, ω=2*pi):
return A*exp(-a*t)*sin(ω*t)
v1 = f(0.2)
v2 = f(0.2, ω=1)
v2 = f(0.2, 1, 3) # same as f(0.2, A=1, a=3)
v3 = f(0.2, ω=1, A=2.5)
v4 = f(A=5, a=0.1, ω=1, t=1.3)
v5 = f(t=0.2, A=9)
print(v1, v2, v3, v4, v5)
###Output
_____no_output_____
###Markdown
Exercise: Implement a Gaussian functionMake a Python function *gauss*( *x*, *m*=0, *s*=1) for computing the Gaussian function $$f(x)=\frac{1}{\sqrt{2\pi}s}\exp\left(-\frac{1}{2} \left(\frac{x-m}{s}\right)^2\right)$$Call the function and print out the result for x equal to −5, −4.9, −4.8, ..., 4.8, 4.9, 5, using default values for *m* and *s*. The *if* statementConsider this simple example:
###Code
def f(x):
if 0 <= x <= pi:
return sin(x)
else:
return 0
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
In general (the *else* block can be skipped if there are no statements to be executed when False) we can put together multiple conditions. Only the first condition that is True is executed.
###Code
if condition1:
<block of statements, executed if condition1 is True>
elif condition2:
<block of statements>
elif condition3:
<block of statements>
else:
<block of statements>
<next statement of the program>
###Output
_____no_output_____
###Markdown
The ternary operatorThere is another form of *if* which works as an operator, rather than a statement. If you've used the *if* function in a spreadsheet then you'll be familiar with this approach.
###Code
def f(x):
return (sin(x) if 0 <= x <= pi else 0)
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
The ternary operator has the following form:
###Code
<expression if condition is True> if <condition> else <expression if condition is false>
###Output
_____no_output_____
###Markdown
Introduction to Python programming [Gerard Gorman](http://www.imperial.ac.uk/people/g.gorman), [Christian Jacobs](http://christianjacobs.uk/) Updated for MPECDT by [David Ham](http://www.imperial.ac.uk/people/david.ham) Lecture 2: Computing with basic language constructionsLearning objectives:* Introduction to two different forms of loops which are used to repeat operations on data: either until a condition is satisfied (*while-loop*) or for a fixed number of iterations (*for-loop*).* Form a *condition* using a *boolean expression*. This is used to either terminate loops or control if a specific block of code is executed via the *if-statement*.* Learn two different methods for storing many data elements within a single Python type.* Introduction to developing your own Python *functions* which allows you to execute the same block of code, with different inputs and outputs, from anywhere in the code. Boolean expressionsAn expression with value *true* or *false* is called a boolean expression. Example expressions for what you would write mathematically as$C=40$, $C\ne40$, $C\ge40$, $C\gt40$ and $C\lt40$ are:
###Code
C == 40 # Note: the double == checks for equality, but C=40 is an assignment!
C != 40 # This could also be written as 'not C == 4' (i.e. the opposite of what C == 4 gives)
C >= 40
C > 40
C < 40
###Output
_____no_output_____
###Markdown
We can test boolean expressions in a Python shell:
###Code
C = 41
print("C != 40: ", C != 40)
print("C < 40: ", C < 40)
print("C == 41: ", C == 41)
###Output
_____no_output_____
###Markdown
Several conditions can be combined with the special 'and' and 'or' operators into a single boolean expression:
###Code
condition1 and condition2
condition1 or condition2
###Output
_____no_output_____
###Markdown
* Rule 1: (**C1** *and* **C2**) is *True* only if both **C1** and **C2** are *True** Rule 2: (**C1** *or* **C2**) is *True* if either **C1** or **C2** are *True*Examples:
###Code
x=0; y=1.2
print (x >= 0 and y < 1)
###Output
_____no_output_____
###Markdown
Exercise: Values of boolean expressionsAdd a comment to the code below to explain the outcome of each of the boolean expressions:
###Code
C = 41
print("Case 1: ", C == 40)
print("Case 2: ", C != 40 and C < 41)
print("Case 3: ", C != 40 or C < 41)
print("Case 4: ", not C == 40)
print("Case 5: ", not C > 40)
print("Case 6: ", C <= 41)
print("Case 7: ", not False)
print("Case 8: ", True and False)
print("Case 9: ", False or True)
print("Case 10: ", False or False or False)
print("Case 11: ", True and True and False)
print("Case 12: ", False == 0)
print("Case 13: ", True == 0)
print("Case 14: ", True == 1)
###Output
_____no_output_____
###Markdown
LoopsSuppose we want to make a table of Celsius and Fahrenheitdegrees:
###Code
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Output
_____no_output_____
###Markdown
How do we write a program that prints out such a table?We know from the last lecture how to make one line in this table:
###Code
C = -20
F = 9/5*C + 32
print(C, F)
###Output
_____no_output_____
###Markdown
We can just repeat these statements:
###Code
C=-20; F=9/5*C+32; print(C,F)
C=-15; F=9/5*C+32; print(C,F)
C=-10; F=9/5*C+32; print(C,F)
C=-5; F=9/5*C+32; print(C,F)
C=0; F=9/5*C+32; print(C,F)
C=5; F=9/5*C+32; print(C,F)
C=10; F=9/5*C+32; print(C,F)
C=15; F=9/5*C+32; print(C,F)
C=20; F=9/5*C+32; print(C,F)
C=25; F=9/5*C+32; print(C,F)
C=30; F=9/5*C+32; print(C,F)
C=35; F=9/5*C+32; print(C,F)
C=40; F=9/5*C+32; print(C,F)
###Output
_____no_output_____
###Markdown
So we can see that works but its **very boring** to write and very easy to introduce a misprint.**You really should not be doing boring repetitive tasks like this.** Spend one time instead looking for a smarter solution. When programming becomes boring, there is usually a construct that automates the writing. Computers are very good at performing repetitive tasks. For this purpose we use **loops**. The while loop (and the significance of indentation)A while loop executes repeatedly a set of statements as long as a **boolean** (i.e. *True* / *False*) condition is *True*
###Code
while condition:
<statement 1>
<statement 2>
...
<first statement after loop>
###Output
_____no_output_____
###Markdown
Note that all statements to be executed within the loop must be indented by the same amount! The loop ends when an unindented statement is encountered.At this point it is worth noticing that **blank spaces may or may not be important** in Python programs. These statements are equivalent (blanks do not matter):
###Code
v0=3
v0 = 3
v0= 3
# The computer does not care but this formatting style is
# considered clearest for the human reader.
v0 = 3
###Output
_____no_output_____
###Markdown
Here is a while loop example where blank spaces really do matter:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
11
###Markdown
Let's take a look at what happens when we forget to indent correctly:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
_____no_output_____
###Markdown
Let's use the while loop to create the table above:
###Code
C = -20 # Initialise C
dC = 5 # Increment for C within the loop
while C <= 40: # Loop heading with condition
F = (9/5)*C + 32 # 1st statement inside loop
print ("%3d %6.1f" % (C, F)) # 2nd statement inside loop
C = C + dC # Increment C for the next iteration of the loop.
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Exercise: Make a Fahrenheit-Celsius conversion tableWrite a program that prints out a table with Fahrenheit degrees 0, 10, 20, ..., 100 in the first column and the corresponding Celsius degrees in the second column. Exercise: Write an approximate Fahrenheit-Celsius conversion tableMany people use an approximate formula for quickly converting Fahrenheit ($F$) to Celsius ($C$) degrees:$C \approx \hat{C} = \frac{F − 30}{2}$Modify the program from the previous exercise so that it prints three columns: $F$, $C$, and the approximate value $\hat{C}$. ListsSo far, one variable has referred to one number (or string). Sometimes however we naturally have a collection of numbers, saydegrees −20, −15, −10, −5, 0, ..., 40. One way to store these values in a computer program would be to have one variable per value, i.e.
###Code
C1 = -20
C2 = -15
C3 = -10
# ...
C13 = 40
###Output
_____no_output_____
###Markdown
This is clearly a terrible solution, particularly if we have lots of values. A better way of doing this is to collect values together in a list:
###Code
C = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
###Output
_____no_output_____
###Markdown
Now there is just one variable, **C**, holding all the values. Elements in a list are accessed via an index. List indices are always numbered as 0, 1, 2, and so forth up to the number of elements minus one:
###Code
mylist = [4, 6, -3.5]
print(mylist[0])
print(mylist[1])
print(mylist[2])
print(len(mylist)) # length of list
###Output
4
6
-3.5
3
###Markdown
Here are a few example of operations that you can perform on lists:
###Code
C = [-10, -5, 0, 5, 10, 15, 20, 25, 30]
C.append(35) # add new element 35 at the end
print(C)
C = C + [40,45] # And another list to the end of C
print(C)
C.insert(0, -15) # Insert -15 as index 0
print(C)
del C[2] # delete 3rd element
print(C)
del C[2] # delete what is now 3rd element
print(C)
print(len(C)) # length of list
print(C.index(10)) # Find the index of the list with the value 10
print (10 in C) # True only if the value 10 is stored in the list
print(C[-1]) # The last value in the list.
print(C[-2]) # The second last value in the list.
###Output
40
###Markdown
You can also extract sublists using ":"
###Code
print(C[5:]) # From index 5 to the end of the list.
print(C[5:7]) # From index 5 up to, but not including index 7.
print(C[1:3])
###Output
[-10, 0]
###Markdown
Many languages (especially Matlab and Fortran) have would include the endpoint of a slice (7 in this case). One way to think of what Python does is that the slice is a half-open interval: $[5,7)$. This makes cutting a list in half particularly easy. For example:
###Code
print(C[:5] + ["Hello"] + C[5:])
print(C[7:-1]) # From index 7 up to the second last element.
print(C[:]) # [:] specifies the whole list.
somelist = ['Curly', 'Larry', 'Moe']
stooge1, stooge2, stooge3 = somelist # Unpack the entries in a list.
print(stooge3, stooge2, stooge1)
###Output
Moe Larry Curly
###Markdown
Of course this only works if `somelist` contains exactly the number of items on the left hand side of the equals. Another useful operator is `*` (pronounced "splat"). It turns a list (or any other sequence) into the set of arguments of a function. For example:
###Code
print(somelist) # Prints the list.
print(*somelist) # Prints the items in the list.
###Output
['Curly', 'Larry', 'Moe']
Curly Larry Moe
###Markdown
Exercise: Store odd numbers in a listStep 1: Write a program that generates all odd numbers from 1 to *n*. Set *n* in the beginning of the program and use a while loop to compute the numbers. (Make sure that if *n* is an even number, the largest generated odd number is *n*-1.).Step 2: Store the generated odd numbers in a list. Start with an empty list and use the same while loop where you generate each odd number, ao append the new number to the list.Finally, print the list elements to the screen.
###Code
1%2
2%2
3%2
lista=[]
n=10
counts=0
while counts<= n:
if counts%2 ==1:
lista.append(counts)
print(counts)
counts=counts+1
print(lista)
###Output
[1, 3, 5, 7, 9]
###Markdown
For loopsWe can visit each element in a list and process the element with some statements using a *for* loop, for example:
###Code
degrees = [0, 10, 20, 40, 100]
for C in degrees:
print('list element:', C)
print('The degrees list has', len(degrees), 'elements')
###Output
_____no_output_____
###Markdown
Notice again how the statements to be executed within the loop must be indented! Let's now revisit the conversion table example using the *for* loop:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
for C in Cdegrees:
F = (9/5)*C + 32
print("%3d %6.2f" % (C, F))
###Output
_____no_output_____
###Markdown
It is also possible to rewrite the *for* loop as a *while* loop, i.e.,
###Code
for element in somelist:
# process element
###Output
_____no_output_____
###Markdown
can always be transformed to a *while* loop
###Code
index = 0
while index < len(somelist):
element = somelist[index]
# process element
index += 1
###Output
_____no_output_____
###Markdown
Taking the previous table example:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
index = 0
while index < len(Cdegrees):
C = Cdegrees[index]
F = (9/5)*C + 32
print('%5d %5.1f' % (C, F))
index += 1
###Output
_____no_output_____
###Markdown
Rather than just printing out the Fahrenheit values, let's also store these computed values in a list of their own:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
Fdegrees = [] # start with empty list
for C in Cdegrees:
F = (9/5)*C + 32
Fdegrees.append(F) # add new element to Fdegrees
print(Fdegrees)
###Output
_____no_output_____
###Markdown
Iterators: things you can iterate over.We've seen that a `for` loop can iterate over list values. In fact you can iterate over any object that is capable of providing values one at a time. In python, these objects are called `iterators`.A particularly useful python function which creates an iterator is `range`. For example, we can use range to iterate over the *indices* of a list:
###Code
for i in range(0, len(somelist), 1):
element = somelist[i]
... process element or somelist[i] directly
###Output
_____no_output_____
###Markdown
The statement *range(start, stop, inc)* generates an iterator over the integers *start*, *start+inc*, *start+2\*inc*, and so on up to, but not including, *stop*. We can also write *range(stop)* as an abbreviation for *range(0, stop, 1)*:
###Code
print(range(3)) # same as range(0, 3, 1)
###Output
_____no_output_____
###Markdown
Oh, that wasn't very informative. If we want to see all the numbers which the `range` will iterate over, we can cast the range to a list:
###Code
print(list(range(3)))
print(list(range(2, 8, 3)))
###Output
_____no_output_____
###Markdown
List comprehensionsConsider this example where we compute two lists in a *for* loop:
###Code
n = 16
Cdegrees = []; Fdegrees = [] # empty lists
for i in range(n):
Cdegrees.append(-5 + i*0.5)
Fdegrees.append((9/5)*Cdegrees[i] + 32)
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
_____no_output_____
###Markdown
As constructing lists is a very common requirement, the above way of doing it can become very tedious to both write and read. Therefore, Python has a compact construct, called list comprehension for generating lists from a *for* loop:
###Code
n = 16
Cdegrees = [-5 + i*0.5 for i in range(n)]
Fdegrees = [(9/5)*C + 32 for C in Cdegrees]
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
_____no_output_____
###Markdown
The general form of a list comprehension is:
###Code
somelist = [expression for element in somelist]
###Output
_____no_output_____
###Markdown
Exercise: Repeat the previous exercise of creating a list of odd numbers using using: a for loop, list comprehension and the range function
###Code
# Using for loop
# Using list comprehension
# Using the function range
###Output
_____no_output_____
###Markdown
Changing elements in a listSay we want to add $2$ to all the numbers in a list:
###Code
v = [-1, 1, 10]
for e in v:
e = e + 2
print(v)
###Output
_____no_output_____
###Markdown
We can see that the list *v* is unaltered! The reason for this is that inside the loop, *e* is an ordinary (int) variable. At the start of the iteration *e* is assigned a *copy* of the next element in the list. Inside the loop we can change *e* but *v* itself is unaltered. If we want to change *v* we have to use an index to access and modify its elements:
###Code
v[1] = 4 # assign 4 to 2nd element (index 1) in v
print(v)
###Output
_____no_output_____
###Markdown
To add 2 to all values we need a *for* loop over indices:
###Code
for i in range(len(v)):
v[i] = v[i] + 2
print(v)
###Output
_____no_output_____
###Markdown
Traversing multiple lists simultaneously - *zip(list1, list2, ...)*Consider how we can loop over elements in both Cdegrees and Fdegrees at the same time. One approach would be to use list indices:
###Code
for i in range(len(Cdegrees)):
print(Cdegrees[i], Fdegrees[i])
###Output
_____no_output_____
###Markdown
An alternative construct (regarded as more ”Pythonic”) uses the *zip* function:
###Code
for C, F in zip(Cdegrees, Fdegrees):
print(C, F)
###Output
_____no_output_____
###Markdown
`zip` creates an *iterator* over the corresponding items of its arguments in order.Another example with three lists:
###Code
l1 = [3, 6, 1]; l2 = [1.5, 1, 0]; l3 = [9.1, 3, 2]
for e in zip(l1, l2, l3):
print(*e)
###Output
_____no_output_____
###Markdown
If the lists are of unequal length then the loop stops when the end of the shortest list is reached. Experiment with this:
###Code
l1 = [3, 6, 1, 4, 6]; l2 = [1.5, 1, 0, 7]; l3 = [9.1, 3, 2, 0, 9]
for e in zip(l1, l2, l3):
print(*e)
###Output
_____no_output_____
###Markdown
Nested lists: list of listsA *list* can contain **any** object, including another *list*. To illustrate this, consider how to store the conversion table as a single Python list rather than two separate lists.
###Code
Cdegrees = range(-20, 41, 5)
Fdegrees = [(9.0/5)*C + 32 for C in Cdegrees]
table1 = [Cdegrees, Fdegrees] # List of two lists
print("table1 = ", table1)
print("table1[0] = ", table1[0])
print("table1[1] = ", table1[1])
print("table1[2][3] = ", table1[1][3])
###Output
_____no_output_____
###Markdown
This gives us a table of two columns. How do we create a table of rows instead:
###Code
table2 = []
for C, F in zip(Cdegrees, Fdegrees):
row = [C, F]
table2.append(row)
print(table2)
###Output
_____no_output_____
###Markdown
We can use `zip` to do this more elegantly:
###Code
table2 = list(zip(Cdegrees, Fdegrees))
print(table2)
###Output
_____no_output_____
###Markdown
And you can loop through this list as before:
###Code
for C, F in table2:
print(C, F)
###Output
_____no_output_____
###Markdown
Tuples: sequences that cannot be changedWe've already learned that lists are not the only thing which can be iterated over, but that there is a general category of `iterator` types. Similarly, lists are not the only objects which can be indexed. The general category of types which can be indexed are the `sequence` types.Tuples are **constant** sequences. You can use them in much the same way as lists except you cannot modify them. They are an example of an [**immutable**](http://en.wikipedia.org/wiki/Immutable_object) type.
###Code
t = (2, 4, 6, 'temp.pdf') # Define a tuple.
t = 2, 4, 6, 'temp.pdf' # Can skip parenthesis as it is assumed in this context.
###Output
_____no_output_____
###Markdown
Let's see what happens when we try to modify the tuple like we did with a list:
###Code
t[1] = -1
t.append(0)
del t[1]
list(t)
###Output
_____no_output_____
###Markdown
However, we can use the tuple to compose a new tuple:
###Code
t = t + (-1.0, -2.0)
print(t)
###Output
(2, 4, 6, 'temp.pdf', -1.0, -2.0)
###Markdown
So, why would we use tuples when lists have more functionality?* Tuples are constant and thus protected against accidental changes.* Tuples are faster than lists.* Tuples are widely used in Python software (so you need to know about tuples to understand other people's code!)* Tuples (but not lists) can be used as keys in dictionaries (more about dictionaries later). Exercise: Make a table of function valuesStep 1: Write a program that prints a table with $t$ values in the first column and the corresponding $y(t) = v_0 t − 0.5gt^2$ values in the second column. Use $n$ uniformly spaced $t$ values throughout the interval [0, $2v_0/g$]. Set $v0 = 1$, $g = 9.81$, and $n = 11$.Step 2: Once step 1 is working, modify the program so that the $t$ and $y$ values are stored in two lists *t* and *y*. Thereafter, transverse the lists with a *for* loop and write out a nicely formatted table of *t* and *y* values using a *zip* construction. FunctionsWe have already used many Python functions, including mathematical functions:
###Code
from math import *
x = pi
print cos(x)
###Output
_____no_output_____
###Markdown
Other functions you used include *len* and *range*:
###Code
n = len(somelist)
ints = range(5, n, 2)
###Output
_____no_output_____
###Markdown
You have also used functions that are executed with the dot syntax (called *methods*):
###Code
C = [5, 10, 40, 45]
i = C.index(10)
C.append(50)
C.insert(2, 20)
###Output
_____no_output_____
###Markdown
A function is a collection of statements we can execute wherever and whenever we want. Functions can take any number of inputs (called *arguments*) to produce outputs. Functions help to organize programs, make them more understandable, shorter, and easier to extend. For our first example we will turn our temperature conversion code into a function:
###Code
def C2F(C):
return (9/5)*C + 32
###Output
_____no_output_____
###Markdown
Functions start with *def*, then the name you want to give your function, then a list of arguments (here C). This is referred to as the *function header*. Inside the function there is a block of statements called the *function body*. Notice that the function body is indented - as was the case for the *for* / *while* loop the indentation indicates where the function ends. At any point within the function, we can "stop thefunction" and return as many values/variables as required. Local and global variablesVariables defined within a function are said to have *local scope*. That is to say that they can only be referenced within that function. Let's consider an example (and look carefully at the indentation!!):
###Code
def sumint(start, stop):
s = 0 # variable for accumulating the sum
i = start # counter
while i <= stop:
s += i
i += 1
return s
print(sumint(0, 5))
###Output
_____no_output_____
###Markdown
Variables *i* and *s* are local variables in *sumint*. They are destroyed at the end (return) of the function and never visible outside the function (in the calling program); in fact, *start* and *stop* are also local variables. So let's see what happens if we now try to print one of these variables:
###Code
print(stop)
###Output
_____no_output_____
###Markdown
Functions can also return multiple values. Let's recycle another of our previous examples - compute $y(t)$ and $y'(t)=v_0-gt$:
###Code
def yfunc(t, v0):
g = 9.81
y = v0*t - 0.5*g*t**2
dydt = v0 - g*t
return y, dydt
# call:
position, velocity = yfunc(0.6, 3)
print(position, velocity)
###Output
_____no_output_____
###Markdown
Remember that a series of comma separated variables implies a tuple - therefore "return y, dydt" is the same as writing "return (y, dydt)". Therefore, in general what is returned is a tuple. Let's take a look at another example illustrating this:
###Code
def f(x):
return x, x**2, x**4
s = f(2)
print(s)
print(type(s)) # The function type() tells us what type a variable it is.
###Output
(2, 4, 16)
<class 'tuple'>
###Markdown
No return value implies that `None` is returned. `None` is a special Python object that represents an ”empty” or undefined value. It is surprisingly useful and we will use it a lot later.
###Code
def message(course):
print("%s rocks!"% course)
message("Python")
r = message("Geo")
print("r = ", r)
def foo(bar):
return(bar+1)
b=3
print(foo(b))
print(b)
###Output
4
3
###Markdown
Keyword arguments and default argument valuesIt's possible to specify default values that function arguments should take if no values are provided when the function is called:
###Code
def somefunc(arg1, arg2, kwarg1=True, kwarg2=0):
print(arg1, arg2, kwarg1, kwarg2)
somefunc("Hello", [1,2]) # Note that we have not specified values for kwarg1 and kwarg2
###Output
_____no_output_____
###Markdown
You can override the default values by passing additional arguments in the correct position:
###Code
somefunc("Hello", [1,2], "Hi")
###Output
_____no_output_____
###Markdown
... or by naming the argument to which the value should be passed. These are called keyword arguments:
###Code
somefunc("Hello", [1,2], kwarg2="Hi")
somefunc("Hello", [1,2], kwarg2="Hi", kwarg1=6)
###Output
_____no_output_____
###Markdown
If we use variable_name=value for all arguments, their sequence in the function header can be in any order.
###Code
somefunc(kwarg2="Hello", arg1="Hi", kwarg1=6, arg2=[2])
###Output
_____no_output_____
###Markdown
Let's look at another example - consider a function of $t$, with parameters $A$, $a$, and $\omega$:$$f(t; A,a, \omega) = Ae^{-at}\sin (\omega t)$$. (The choice of equation is actually pretty random - but it serves to show you that it is easy to translate formulae you encounter into Python code). We can implement $f$ in a Python function with $t$ as positional argument and $A$, $a$, and $\omega$ as keyword arguments.
###Code
from math import pi, exp, sin
def f(t, A=1, a=1, ω=2*pi):
return A*exp(-a*t)*sin(ω*t)
v1 = f(0.2)
v2 = f(0.2, ω=1)
v2 = f(0.2, 1, 3) # same as f(0.2, A=1, a=3)
v3 = f(0.2, ω=1, A=2.5)
v4 = f(A=5, a=0.1, ω=1, t=1.3)
v5 = f(t=0.2, A=9)
print(v1, v2, v3, v4, v5)
###Output
0.778659217806053 0.5219508827258282 0.40664172703834794 4.230480200204721 7.007932960254476
###Markdown
Exercise: Implement a Gaussian functionMake a Python function *gauss*( *x*, *m*=0, *s*=1) for computing the Gaussian function $$f(x)=\frac{1}{\sqrt{2\pi}s}\exp\left(-\frac{1}{2} \left(\frac{x-m}{s}\right)^2\right)$$Call the function and print out the result for x equal to −5, −4.9, −4.8, ..., 4.8, 4.9, 5, using default values for *m* and *s*.
###Code
from math import pi
from math import sqrt
from math import exp
def gauss(x,m=0,s=1):
return( 1/sqrt(2*pi*s)*exp(-0.5*((x-m)/s)**2 ))
lista=[(-50+i)/10 for i in range(101)]
lista
for i in lista:
print(gauss(i))
###Output
1.4867195147342977e-06
2.438960745893352e-06
3.961299091032075e-06
6.36982517886709e-06
1.0140852065486758e-05
1.5983741106905475e-05
2.4942471290053535e-05
3.853519674208713e-05
5.8943067756539855e-05
8.926165717713293e-05
0.00013383022576488537
0.00019865547139277272
0.00029194692579146027
0.00042478027055075143
0.0006119019301137719
0.0008726826950457602
0.00123221916847302
0.0017225689390536812
0.0023840882014648404
0.003266819056199918
0.0044318484119380075
0.005952532419775854
0.007915451582979969
0.010420934814422592
0.013582969233685613
0.01752830049356854
0.0223945302948429
0.028327037741601186
0.035474592846231424
0.04398359598042719
0.05399096651318806
0.0656158147746766
0.07895015830089415
0.09404907737688695
0.11092083467945554
0.12951759566589174
0.14972746563574488
0.17136859204780736
0.19418605498321295
0.21785217703255053
0.24197072451914337
0.2660852498987548
0.28969155276148273
0.31225393336676127
0.33322460289179967
0.3520653267642995
0.36827014030332333
0.38138781546052414
0.3910426939754559
0.3969525474770118
0.3989422804014327
0.3969525474770118
0.3910426939754559
0.38138781546052414
0.36827014030332333
0.3520653267642995
0.33322460289179967
0.31225393336676127
0.28969155276148273
0.2660852498987548
0.24197072451914337
0.21785217703255053
0.19418605498321295
0.17136859204780736
0.14972746563574488
0.12951759566589174
0.11092083467945554
0.09404907737688695
0.07895015830089415
0.0656158147746766
0.05399096651318806
0.04398359598042719
0.035474592846231424
0.028327037741601186
0.0223945302948429
0.01752830049356854
0.013582969233685613
0.010420934814422592
0.007915451582979969
0.005952532419775854
0.0044318484119380075
0.003266819056199918
0.0023840882014648404
0.0017225689390536812
0.00123221916847302
0.0008726826950457602
0.0006119019301137719
0.00042478027055075143
0.00029194692579146027
0.00019865547139277272
0.00013383022576488537
8.926165717713293e-05
5.8943067756539855e-05
3.853519674208713e-05
2.4942471290053535e-05
1.5983741106905475e-05
1.0140852065486758e-05
6.36982517886709e-06
3.961299091032075e-06
2.438960745893352e-06
1.4867195147342977e-06
###Markdown
The *if* statementConsider this simple example:
###Code
def f(x):
if 0 <= x <= pi:
return sin(x)
else:
return 0
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
In general (the *else* block can be skipped if there are no statements to be executed when False) we can put together multiple conditions. Only the first condition that is True is executed.
###Code
if condition1:
<block of statements, executed if condition1 is True>
elif condition2:
<block of statements>
elif condition3:
<block of statements>
else:
<block of statements>
<next statement of the program>
###Output
_____no_output_____
###Markdown
The ternary operatorThere is another form of *if* which works as an operator, rather than a statement. If you've used the *if* function in a spreadsheet then you'll be familiar with this approach.
###Code
def f(x):
return (sin(x) if 0 <= x <= pi else 0)
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
The ternary operator has the following form:
###Code
<expression if condition is True> if <condition> else <expression if condition is false>
###Output
_____no_output_____
###Markdown
Exercise: Express a step function as a Python functionThe following "step" function is known as the Heaviside function andis widely used in mathematics:$$H(x)=\begin{cases}0, & \text{if $x<0$}.\\\\1, & \text{if $x\ge 0$}.\end{cases}$$Write a Python function H(x) that computes H(x).
###Code
def Heavside(x):
return(0 if x<0 else 1)
print(Heavside(2))
print(Heavside(1))
print(Heavside(0))
print(Heavside(-0.2))
import numpy
x_vals=list(numpy.arange(-5,5,0.1))
print(x_vals)
import matplotlib
###Output
_____no_output_____
###Markdown
Introduction to Python programming [Gerard Gorman](http://www.imperial.ac.uk/people/g.gorman), [Christian Jacobs](http://christianjacobs.uk/) Updated for MPECDT by [David Ham](http://www.imperial.ac.uk/people/david.ham) Lecture 2: Computing with basic language constructionsLearning objectives:* Introduction to two different forms of loops which are used to repeat operations on data: either until a condition is satisfied (*while-loop*) or for a fixed number of iterations (*for-loop*).* Form a *condition* using a *boolean expression*. This is used to either terminate loops or control if a specific block of code is executed via the *if-statement*.* Learn two different methods for storing many data elements within a single Python type.* Introduction to developing your own Python *functions* which allows you to execute the same block of code, with different inputs and outputs, from anywhere in the code. Boolean expressionsAn expression with value *true* or *false* is called a boolean expression. Example expressions for what you would write mathematically as$C=40$, $C\ne40$, $C\ge40$, $C\gt40$ and $C\lt40$ are:
###Code
C == 40 # Note: the double == checks for equality, but C=40 is an assignment!
C != 40 # This could also be written as 'not C == 4' (i.e. the opposite of what C == 4 gives)
C >= 40
C > 40
C < 40
###Output
_____no_output_____
###Markdown
We can test boolean expressions in a Python shell:
###Code
C = 41
print("C != 40: ", C != 40)
print("C < 40: ", C < 40)
print("C == 41: ", C == 41)
###Output
_____no_output_____
###Markdown
Several conditions can be combined with the special 'and' and 'or' operators into a single boolean expression:
###Code
condition1 and condition2
condition1 or condition2
###Output
_____no_output_____
###Markdown
* Rule 1: (**C1** *and* **C2**) is *True* only if both **C1** and **C2** are *True** Rule 2: (**C1** *or* **C2**) is *True* if either **C1** or **C2** are *True*Examples:
###Code
x=0; y=1.2
print (x >= 0 and y < 1)
###Output
_____no_output_____
###Markdown
Exercise: Values of boolean expressionsAdd a comment to the code below to explain the outcome of each of the boolean expressions:
###Code
C = 41
print("Case 1: ", C == 40)
print("Case 2: ", C != 40 and C < 41)
print("Case 3: ", C != 40 or C < 41)
print("Case 4: ", not C == 40)
print("Case 5: ", not C > 40)
print("Case 6: ", C <= 41)
print("Case 7: ", not False)
print("Case 8: ", True and False)
print("Case 9: ", False or True)
print("Case 10: ", False or False or False)
print("Case 11: ", True and True and False)
print("Case 12: ", False == 0)
print("Case 13: ", True == 0)
print("Case 14: ", True == 1)
###Output
_____no_output_____
###Markdown
LoopsSuppose we want to make a table of Celsius and Fahrenheitdegrees:
###Code
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Output
_____no_output_____
###Markdown
How do we write a program that prints out such a table?We know from the last lecture how to make one line in this table:
###Code
C = -20
F = 9/5*C + 32
print(C, F)
###Output
_____no_output_____
###Markdown
We can just repeat these statements:
###Code
C=-20; F=9/5*C+32; print(C,F)
C=-15; F=9/5*C+32; print(C,F)
C=-10; F=9/5*C+32; print(C,F)
C=-5; F=9/5*C+32; print(C,F)
C=0; F=9/5*C+32; print(C,F)
C=5; F=9/5*C+32; print(C,F)
C=10; F=9/5*C+32; print(C,F)
C=15; F=9/5*C+32; print(C,F)
C=20; F=9/5*C+32; print(C,F)
C=25; F=9/5*C+32; print(C,F)
C=30; F=9/5*C+32; print(C,F)
C=35; F=9/5*C+32; print(C,F)
C=40; F=9/5*C+32; print(C,F)
###Output
_____no_output_____
###Markdown
So we can see that works but its **very boring** to write and very easy to introduce a misprint.**You really should not be doing boring repetitive tasks like this.** Spend one time instead looking for a smarter solution. When programming becomes boring, there is usually a construct that automates the writing. Computers are very good at performing repetitive tasks. For this purpose we use **loops**. The while loop (and the significance of indentation)A while loop executes repeatedly a set of statements as long as a **boolean** (i.e. *True* / *False*) condition is *True*
###Code
while condition:
<statement 1>
<statement 2>
...
<first statement after loop>
###Output
_____no_output_____
###Markdown
Note that all statements to be executed within the loop must be indented by the same amount! The loop ends when an unindented statement is encountered.At this point it is worth noticing that **blank spaces may or may not be important** in Python programs. These statements are equivalent (blanks do not matter):
###Code
v0=3
v0 = 3
v0= 3
# The computer does not care but this formatting style is
# considered clearest for the human reader.
v0 = 3
###Output
_____no_output_____
###Markdown
Here is a while loop example where blank spaces really do matter:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
11
###Markdown
Let's take a look at what happens when we forget to indent correctly:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
11
###Markdown
Let's use the while loop to create the table above:
###Code
C = -20 # Initialise C
dC = 5 # Increment for C within the loop
while C <= 40: # Loop heading with condition
F = (9/5)*C + 32 # 1st statement inside loop
print ("%3d %6.1f" % (C, F)) # 2nd statement inside loop
C = C + dC # Increment C for the next iteration of the loop.
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Exercise: Make a Fahrenheit-Celsius conversion tableWrite a program that prints out a table with Fahrenheit degrees 0, 10, 20, ..., 100 in the first column and the corresponding Celsius degrees in the second column. Exercise: Write an approximate Fahrenheit-Celsius conversion tableMany people use an approximate formula for quickly converting Fahrenheit ($F$) to Celsius ($C$) degrees:$C \approx \hat{C} = \frac{F − 30}{2}$Modify the program from the previous exercise so that it prints three columns: $F$, $C$, and the approximate value $\hat{C}$. ListsSo far, one variable has referred to one number (or string). Sometimes however we naturally have a collection of numbers, saydegrees −20, −15, −10, −5, 0, ..., 40. One way to store these values in a computer program would be to have one variable per value, i.e.
###Code
C1 = -20
C2 = -15
C3 = -10
# ...
C13 = 40
###Output
_____no_output_____
###Markdown
This is clearly a terrible solution, particularly if we have lots of values. A better way of doing this is to collect values together in a list:
###Code
C = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
###Output
_____no_output_____
###Markdown
Now there is just one variable, **C**, holding all the values. Elements in a list are accessed via an index. List indices are always numbered as 0, 1, 2, and so forth up to the number of elements minus one:
###Code
mylist = [4, 6, -3.5]
print(mylist[0])
print(mylist[1])
print(mylist[2])
print(len(mylist)) # length of list
###Output
4
6
-3.5
3
###Markdown
Here are a few example of operations that you can perform on lists:
###Code
C = [-10, -5, 0, 5, 10, 15, 20, 25, 30]
C.append(35) # add new element 35 at the end
print(C)
C = C + [40,45] # And another list to the end of C
print(C)
C.insert(0, -15) # Insert -15 as index 0
print(C)
del C[2] # delete 3rd element
print(C)
del C[2] # delete what is now 3rd element
print(C)
print(len(C)) # length of list
print(C.index(10)) # Find the index of the list with the value 10
print (10 in C) # True only if the value 10 is stored in the list
print(C[-1]) # The last value in the list.
print(C[-2]) # The second last value in the list.
###Output
40
###Markdown
You can also extract sublists using ":"
###Code
print(C[5:]) # From index 5 to the end of the list.
print(C[5:7]) # From index 5 up to, but not including index 7.
###Output
[20, 25]
###Markdown
Many languages (especially Matlab and Fortran) have would include the endpoint of a slice (7 in this case). One way to think of what Python does is that the slice is a half-open interval: $[5,7)$. This makes cutting a list in half particularly easy. For example:
###Code
print(C[:5] + ["Hello"] + C[5:])
print(C[7:-1]) # From index 7 up to the second last element.
print(C[:]) # [:] specifies the whole list.
somelist = ['Curly', 'Larry', 'Moe']
stooge1, stooge2, stooge3 = somelist # Unpack the entries in a list.
print(stooge3, stooge2, stooge1)
###Output
Moe Larry Curly
###Markdown
Of course this only works if `somelist` contains exactly the number of items on the left hand side of the equals. Another useful operator is `*` (pronounced "splat"). It turns a list (or any other sequence) into the set of arguments of a function. For example:
###Code
print(somelist) # Prints the list.
print(*somelist) # Prints the items in the list.
###Output
['Curly', 'Larry', 'Moe']
Curly Larry Moe
###Markdown
Exercise: Store odd numbers in a listStep 1: Write a program that generates all odd numbers from 1 to *n*. Set *n* in the beginning of the program and use a while loop to compute the numbers. (Make sure that if *n* is an even number, the largest generated odd number is *n*-1.).Step 2: Store the generated odd numbers in a list. Start with an empty list and use the same while loop where you generate each odd number, ao append the new number to the list.Finally, print the list elements to the screen.
###Code
a = []
n = 8
i = 1
while i <= n:
a.append(i)
i += 2
print(a)
###Output
[1, 3, 5, 7]
###Markdown
For loopsWe can visit each element in a list and process the element with some statements using a *for* loop, for example:
###Code
degrees = [0, 10, 20, 40, 100]
for C in degrees:
print('list element:', C)
print('The degrees list has', len(degrees), 'elements')
###Output
list element: 0
list element: 10
list element: 20
list element: 40
list element: 100
The degrees list has 5 elements
###Markdown
Notice again how the statements to be executed within the loop must be indented! Let's now revisit the conversion table example using the *for* loop:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
for C in Cdegrees:
F = (9/5)*C + 32
print("%3d %6.2f" % (C, F))
###Output
-20 -4.00
-15 5.00
-10 14.00
-5 23.00
0 32.00
5 41.00
10 50.00
15 59.00
20 68.00
25 77.00
30 86.00
35 95.00
40 104.00
###Markdown
It is also possible to rewrite the *for* loop as a *while* loop, i.e.,
###Code
for element in somelist:
# process element
###Output
_____no_output_____
###Markdown
can always be transformed to a *while* loop
###Code
index = 0
while index < len(somelist):
element = somelist[index]
# process element
index += 1
###Output
_____no_output_____
###Markdown
Taking the previous table example:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
index = 0
while index < len(Cdegrees):
C = Cdegrees[index]
F = (9/5)*C + 32
print('%5d %5.1f' % (C, F))
index += 1
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Rather than just printing out the Fahrenheit values, let's also store these computed values in a list of their own:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
Fdegrees = [] # start with empty list
for C in Cdegrees:
F = (9/5)*C + 32
Fdegrees.append(F) # add new element to Fdegrees
print(Fdegrees)
###Output
_____no_output_____
###Markdown
Iterators: things you can iterate over.We've seen that a `for` loop can iterate over list values. In fact you can iterate over any object that is capable of providing values one at a time. In python, these objects are called `iterators`.A particularly useful python function which creates an iterator is `range`. For example, we can use range to iterate over the *indices* of a list:
###Code
for i in range(0, len(somelist), 1):
element = somelist[i]
... process element or somelist[i] directly
###Output
_____no_output_____
###Markdown
The statement *range(start, stop, inc)* generates an iterator over the integers *start*, *start+inc*, *start+2\*inc*, and so on up to, but not including, *stop*. We can also write *range(stop)* as an abbreviation for *range(0, stop, 1)*:
###Code
print(range(3)) # same as range(0, 3, 1)
###Output
range(0, 3)
###Markdown
Oh, that wasn't very informative. If we want to see all the numbers which the `range` will iterate over, we can cast the range to a list:
###Code
print(list(range(3)))
print(list(range(2, 8, 3)))
###Output
[2, 5]
###Markdown
List comprehensionsConsider this example where we compute two lists in a *for* loop:
###Code
n = 16
Cdegrees = []; Fdegrees = [] # empty lists
for i in range(n):
Cdegrees.append(-5 + i*0.5)
Fdegrees.append((9/5)*Cdegrees[i] + 32)
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
As constructing lists is a very common requirement, the above way of doing it can become very tedious to both write and read. Therefore, Python has a compact construct, called list comprehension for generating lists from a *for* loop:
###Code
n = 16
Cdegrees = [-5 + i*0.5 for i in range(n)]
Fdegrees = [(9/5)*C + 32 for C in Cdegrees]
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
_____no_output_____
###Markdown
The general form of a list comprehension is:
###Code
somelist = [expression for element in somelist]
###Output
_____no_output_____
###Markdown
Exercise: Repeat the previous exercise of creating a list of odd numbers using using: a for loop, list comprehension and the range function
###Code
# Using for loop
# Using list comprehension
# Using the function range
###Output
_____no_output_____
###Markdown
Changing elements in a listSay we want to add $2$ to all the numbers in a list:
###Code
v = [-1, 1, 10]
for e in v:
e = e + 2
print(v)
###Output
_____no_output_____
###Markdown
We can see that the list *v* is unaltered! The reason for this is that inside the loop, *e* is an ordinary (int) variable. At the start of the iteration *e* is assigned a *copy* of the next element in the list. Inside the loop we can change *e* but *v* itself is unaltered. If we want to change *v* we have to use an index to access and modify its elements:
###Code
v[1] = 4 # assign 4 to 2nd element (index 1) in v
print(v)
###Output
_____no_output_____
###Markdown
To add 2 to all values we need a *for* loop over indices:
###Code
for i in range(len(v)):
v[i] = v[i] + 2
print(v)
###Output
_____no_output_____
###Markdown
Traversing multiple lists simultaneously - *zip(list1, list2, ...)*Consider how we can loop over elements in both Cdegrees and Fdegrees at the same time. One approach would be to use list indices:
###Code
for i in range(len(Cdegrees)):
print(Cdegrees[i], Fdegrees[i])
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
An alternative construct (regarded as more ”Pythonic”) uses the *zip* function:
###Code
for C, F in zip(Cdegrees, Fdegrees):
print(C, F)
print(list(zip(Cdegrees, Fdegrees[:-1])))
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
[(-5.0, 23.0), (-4.5, 23.9), (-4.0, 24.8), (-3.5, 25.7), (-3.0, 26.6), (-2.5, 27.5), (-2.0, 28.4), (-1.5, 29.3), (-1.0, 30.2), (-0.5, 31.1), (0.0, 32.0), (0.5, 32.9), (1.0, 33.8), (1.5, 34.7), (2.0, 35.6)]
###Markdown
`zip` creates an *iterator* over the corresponding items of its arguments in order.Another example with three lists:
###Code
l1 = [3, 6, 1]; l2 = [1.5, 1, 0]; l3 = [9.1, 3, 2]
for e in zip(l1, l2, l3):
print(*e)
###Output
3 1.5 9.1
6 1 3
1 0 2
###Markdown
If the lists are of unequal length then the loop stops when the end of the shortest list is reached. Experiment with this:
###Code
l1 = [3, 6, 1, 4, 6]; l2 = [1.5, 1, 0, 7]; l3 = [9.1, 3, 2, 0, 9]
for e in zip(l1, l2, l3):
print(*e)
###Output
_____no_output_____
###Markdown
Nested lists: list of listsA *list* can contain **any** object, including another *list*. To illustrate this, consider how to store the conversion table as a single Python list rather than two separate lists.
###Code
Cdegrees = range(-20, 41, 5)
Fdegrees = [(9.0/5)*C + 32 for C in Cdegrees]
table1 = [Cdegrees, Fdegrees] # List of two lists
print("table1 = ", table1)
print("table1[0] = ", table1[0])
print("table1[1] = ", table1[1])
print("table1[1][3] = ", table1[1][3])
###Output
table1 = [range(-20, 41, 5), [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]]
table1[0] = range(-20, 41, 5)
table1[1] = [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]
table1[1][3] = 23.0
###Markdown
This gives us a table of two columns. How do we create a table of rows instead:
###Code
table2 = []
for C, F in zip(Cdegrees, Fdegrees):
row = [C, F]
table2.append(row)
print(table2)
###Output
_____no_output_____
###Markdown
We can use `zip` to do this more elegantly:
###Code
table2 = list(zip(Cdegrees, Fdegrees))
print(table2)
###Output
_____no_output_____
###Markdown
And you can loop through this list as before:
###Code
for C, F in table2:
print(C, F)
###Output
_____no_output_____
###Markdown
Tuples: sequences that cannot be changedWe've already learned that lists are not the only thing which can be iterated over, but that there is a general category of `iterator` types. Similarly, lists are not the only objects which can be indexed. The general category of types which can be indexed are the `sequence` types.Tuples are **constant** sequences. You can use them in much the same way as lists except you cannot modify them. They are an example of an [**immutable**](http://en.wikipedia.org/wiki/Immutable_object) type.
###Code
t = (2, 4, 6, 'temp.pdf') # Define a tuple.
t = 2, 4, 6, 'temp.pdf' # Can skip parenthesis as it is assumed in this context.
###Output
_____no_output_____
###Markdown
Let's see what happens when we try to modify the tuple like we did with a list:
###Code
t[1] = -1
t.append(0)
del t[1]
###Output
_____no_output_____
###Markdown
However, we can use the tuple to compose a new tuple:
###Code
t = t + (-1.0, -2.0)
print(t)
###Output
(2, 4, 6, 'temp.pdf', -1.0, -2.0)
###Markdown
So, why would we use tuples when lists have more functionality?* Tuples are constant and thus protected against accidental changes.* Tuples are faster than lists.* Tuples are widely used in Python software (so you need to know about tuples to understand other people's code!)* Tuples (but not lists) can be used as keys in dictionaries (more about dictionaries later). Exercise: Make a table of function valuesStep 1: Write a program that prints a table with $t$ values in the first column and the corresponding $y(t) = v_0 t − 0.5gt^2$ values in the second column. Use $n$ uniformly spaced $t$ values throughout the interval [0, $2v_0/g$]. Set $v0 = 1$, $g = 9.81$, and $n = 11$.Step 2: Once step 1 is working, modify the program so that the $t$ and $y$ values are stored in two lists *t* and *y*. Thereafter, transverse the lists with a *for* loop and write out a nicely formatted table of *t* and *y* values using a *zip* construction. FunctionsWe have already used many Python functions, including mathematical functions:
###Code
from math import *
x = pi
print cos(x)
###Output
_____no_output_____
###Markdown
Other functions you used include *len* and *range*:
###Code
n = len(somelist)
ints = range(5, n, 2)
###Output
_____no_output_____
###Markdown
You have also used functions that are executed with the dot syntax (called *methods*):
###Code
C = [5, 10, 40, 45]
i = C.index(10)
C.append(50)
C.insert(2, 20)
###Output
_____no_output_____
###Markdown
A function is a collection of statements we can execute wherever and whenever we want. Functions can take any number of inputs (called *arguments*) to produce outputs. Functions help to organize programs, make them more understandable, shorter, and easier to extend. For our first example we will turn our temperature conversion code into a function:
###Code
def C2F(C):
return (9/5)*C + 32
###Output
_____no_output_____
###Markdown
Functions start with *def*, then the name you want to give your function, then a list of arguments (here C). This is referred to as the *function header*. Inside the function there is a block of statements called the *function body*. Notice that the function body is indented - as was the case for the *for* / *while* loop the indentation indicates where the function ends. At any point within the function, we can "stop thefunction" and return as many values/variables as required. Local and global variablesVariables defined within a function are said to have *local scope*. That is to say that they can only be referenced within that function. Let's consider an example (and look carefully at the indentation!!):
###Code
def sumint(start, stop):
s = 0 # variable for accumulating the sum
i = start # counter
while i <= stop:
s += i
i += 1
return s
print(sumint(0, 5))
###Output
_____no_output_____
###Markdown
Variables *i* and *s* are local variables in *sumint*. They are destroyed at the end (return) of the function and never visible outside the function (in the calling program); in fact, *start* and *stop* are also local variables. So let's see what happens if we now try to print one of these variables:
###Code
print(stop)
###Output
_____no_output_____
###Markdown
Functions can also return multiple values. Let's recycle another of our previous examples - compute $y(t)$ and $y'(t)=v_0-gt$:
###Code
def yfunc(t, v0):
g = 9.81
y = v0*t - 0.5*g*t**2
dydt = v0 - g*t
return y, dydt
# call:
position, velocity = yfunc(0.6, 3)
print(position, velocity)
###Output
_____no_output_____
###Markdown
Remember that a series of comma separated variables implies a tuple - therefore "return y, dydt" is the same as writing "return (y, dydt)". Therefore, in general what is returned is a tuple. Let's take a look at another example illustrating this:
###Code
def f(x):
return x, x**2, x**4
s = f(2)
print(s)
print(type(s)) # The function type() tells us what type a variable it is.
###Output
_____no_output_____
###Markdown
No return value implies that `None` is returned. `None` is a special Python object that represents an ”empty” or undefined value. It is surprisingly useful and we will use it a lot later.
###Code
def message(course):
print("%s rocks!"% course)
message("Python")
r = message("Geo")
print("r = ", r)
###Output
_____no_output_____
###Markdown
Keyword arguments and default argument valuesIt's possible to specify default values that function arguments should take if no values are provided when the function is called:
###Code
def somefunc(arg1, arg2, kwarg1=True, kwarg2=0):
print(arg1, arg2, kwarg1, kwarg2)
somefunc("Hello", [1,2]) # Note that we have not specified values for kwarg1 and kwarg2
###Output
Hello [1, 2] True 0
###Markdown
You can override the default values by passing additional arguments in the correct position:
###Code
somefunc("Hello", [1,2], "Hi")
###Output
Hello [1, 2] Hi 0
###Markdown
... or by naming the argument to which the value should be passed. These are called keyword arguments:
###Code
somefunc("Hello", [1,2], kwarg2="Hi")
def foo(*args):
print(args)
foo(1,2)
foo("hello", "world", "today")
somefunc("Hello", [1,2], kwarg2="Hi", kwarg1=6)
###Output
_____no_output_____
###Markdown
If we use variable_name=value for all arguments, their sequence in the function header can be in any order.
###Code
somefunc(kwarg2="Hello", arg1="Hi", kwarg1=6, arg2=[2])
###Output
_____no_output_____
###Markdown
Let's look at another example - consider a function of $t$, with parameters $A$, $a$, and $\omega$:$$f(t; A,a, \omega) = Ae^{-at}\sin (\omega t)$$. (The choice of equation is actually pretty random - but it serves to show you that it is easy to translate formulae you encounter into Python code). We can implement $f$ in a Python function with $t$ as positional argument and $A$, $a$, and $\omega$ as keyword arguments.
###Code
from math import pi, exp, sin
def f(t, A=1, a=1, ω=2*pi):
return A*exp(-a*t)*sin(ω*t)
v1 = f(0.2)
v2 = f(0.2, ω=1)
v2 = f(0.2, 1, 3) # same as f(0.2, A=1, a=3)
v3 = f(0.2, ω=1, A=2.5)
v4 = f(A=5, a=0.1, ω=1, t=1.3)
v5 = f(t=0.2, A=9)
print(v1, v2, v3, v4, v5)
###Output
0.778659217806053 0.5219508827258282 0.40664172703834794 4.230480200204721 7.007932960254476
###Markdown
Exercise: Implement a Gaussian functionMake a Python function *gauss*( *x*, *m*=0, *s*=1) for computing the Gaussian function $$f(x)=\frac{1}{\sqrt{2\pi}s}\exp\left(-\frac{1}{2} \left(\frac{x-m}{s}\right)^2\right)$$Call the function and print out the result for x equal to −5, −4.9, −4.8, ..., 4.8, 4.9, 5, using default values for *m* and *s*.
###Code
from math import pi, exp
def gauss(x, m = 0, s = 1):
return (2*pi)**(-.5)*s**(-1)*exp(-.5*((x-m)/s)**2)
i = -5
while i <= 5:
print("f({:.1f}) = {}".format(i, gauss(i)))
i += .1
numpy linespace
###Output
_____no_output_____
###Markdown
The *if* statementConsider this simple example:
###Code
def f(x):
if 0 <= x <= pi:
return sin(x)
else:
return 0
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
In general (the *else* block can be skipped if there are no statements to be executed when False) we can put together multiple conditions. Only the first condition that is True is executed.
###Code
if condition1:
<block of statements, executed if condition1 is True>
elif condition2:
<block of statements>
elif condition3:
<block of statements>
else:
<block of statements>
<next statement of the program>
###Output
_____no_output_____
###Markdown
The ternary operatorThere is another form of *if* which works as an operator, rather than a statement. If you've used the *if* function in a spreadsheet then you'll be familiar with this approach.
###Code
def f(x):
return (sin(x) if 0 <= x <= pi else 0)
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
0 1.0 0
###Markdown
The ternary operator has the following form:
###Code
<expression if condition is True> if <condition> else <expression if condition is false>
###Output
_____no_output_____
###Markdown
Exercise: Express a step function as a Python functionThe following "step" function is known as the Heaviside function andis widely used in mathematics:$$H(x)=\begin{cases}0, & \text{if $x<0$}.\\\\1, & \text{if $x\ge 0$}.\end{cases}$$Write a Python function H(x) that computes H(x).
###Code
def H(x):
return (0 if x < 0 else 1)
print(H(-3), H(0), H(6))
###Output
0 1 1
###Markdown
Introduction to Python programming [Gerard Gorman](http://www.imperial.ac.uk/people/g.gorman), [Christian Jacobs](http://christianjacobs.uk/) Updated for MPECDT by [David Ham](http://www.imperial.ac.uk/people/david.ham) Lecture 2: Computing with basic language constructionsLearning objectives:* Introduction to two different forms of loops which are used to repeat operations on data: either until a condition is satisfied (*while-loop*) or for a fixed number of iterations (*for-loop*).* Form a *condition* using a *boolean expression*. This is used to either terminate loops or control if a specific block of code is executed via the *if-statement*.* Learn two different methods for storing many data elements within a single Python type.* Introduction to developing your own Python *functions* which allows you to execute the same block of code, with different inputs and outputs, from anywhere in the code. Boolean expressionsAn expression with value *true* or *false* is called a boolean expression. Example expressions for what you would write mathematically as$C=40$, $C\ne40$, $C\ge40$, $C\gt40$ and $C\lt40$ are:
###Code
C == 40 # Note: the double == checks for equality, but C=40 is an assignment!
C != 40 # This could also be written as 'not C == 4' (i.e. the opposite of what C == 4 gives)
C >= 40
C > 40
C < 40
###Output
_____no_output_____
###Markdown
We can test boolean expressions in a Python shell:
###Code
C = 41
print("C != 40: ", C != 40)
print("C < 40: ", C < 40)
print("C == 41: ", C == 41)
###Output
C != 40: True
C < 40: False
C == 41: True
###Markdown
Several conditions can be combined with the special 'and' and 'or' operators into a single boolean expression:
###Code
condition1 and condition2
condition1 or condition2
###Output
_____no_output_____
###Markdown
* Rule 1: (**C1** *and* **C2**) is *True* only if both **C1** and **C2** are *True** Rule 2: (**C1** *or* **C2**) is *True* if either **C1** or **C2** are *True*Examples:
###Code
x=0; y=1.2
print (x >= 0 or y < 1)
z = y*(x != 0)
print(z)
###Output
True
0.0
###Markdown
Exercise: Values of boolean expressionsAdd a comment to the code below to explain the outcome of each of the boolean expressions:
###Code
C = 41
print("Case 1: ", C == 40)
print("Case 2: ", C != 40 and C < 41)
print("Case 3: ", C != 40 or C < 41)
print("Case 4: ", not C == 40)
print("Case 5: ", not C > 40)
print("Case 6: ", C <= 41)
print("Case 7: ", not False)
print("Case 8: ", True and False)
print("Case 9: ", False or True)
print("Case 10: ", False or False or False)
print("Case 11: ", True and True and False)
print("Case 12: ", False == 0)
print("Case 13: ", True == 0)
print("Case 14: ", True == 1)
###Output
Case 1: False
Case 2: False
Case 3: True
Case 4: True
Case 5: False
Case 6: True
Case 7: True
Case 8: False
Case 9: True
Case 10: False
Case 11: False
Case 12: True
Case 13: False
Case 14: True
###Markdown
LoopsSuppose we want to make a table of Celsius and Fahrenheitdegrees:
###Code
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Output
_____no_output_____
###Markdown
How do we write a program that prints out such a table?We know from the last lecture how to make one line in this table:
###Code
C = -20
F = 9/5*C + 32
print(C, F)
print('T(Celsius) =', C , ';', 'T(Fahrenheit) =', F)
###Output
-20 -4.0
T(Celsius) = -20 ; T(Fahrenheit) = -4.0
###Markdown
We can just repeat these statements:
###Code
C=-20; F=9/5*C+32; print(C,F)
C=-15; F=9/5*C+32; print(C,F)
C=-10; F=9/5*C+32; print(C,F)
C=-5; F=9/5*C+32; print(C,F)
C=0; F=9/5*C+32; print(C,F)
C=5; F=9/5*C+32; print(C,F)
C=10; F=9/5*C+32; print(C,F)
C=15; F=9/5*C+32; print(C,F)
C=20; F=9/5*C+32; print(C,F)
C=25; F=9/5*C+32; print(C,F)
C=30; F=9/5*C+32; print(C,F)
C=35; F=9/5*C+32; print(C,F)
C=40; F=9/5*C+32; print(C,F)
###Output
_____no_output_____
###Markdown
So we can see that works but its **very boring** to write and very easy to introduce a misprint.**You really should not be doing boring repetitive tasks like this.** Spend one time instead looking for a smarter solution. When programming becomes boring, there is usually a construct that automates the writing. Computers are very good at performing repetitive tasks. For this purpose we use **loops**. The while loop (and the significance of indentation)A while loop executes repeatedly a set of statements as long as a **boolean** (i.e. *True* / *False*) condition is *True*
###Code
while condition:
<statement 1>
<statement 2>
...
<first statement after loop>
###Output
_____no_output_____
###Markdown
Note that all statements to be executed within the loop must be indented by the same amount! The loop ends when an unindented statement is encountered.At this point it is worth noticing that **blank spaces may or may not be important** in Python programs. These statements are equivalent (blanks do not matter):
###Code
v0=3
v0 = 3
v0= 3
# The computer does not care but this formatting style is
# considered clearest for the human reader.
v0 = 3
###Output
_____no_output_____
###Markdown
Here is a while loop example where blank spaces really do matter:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
11
###Markdown
Let's take a look at what happens when we forget to indent correctly:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
_____no_output_____
###Markdown
Let's use the while loop to create the table above:
###Code
C = -20 # Initialise C
dC = 5 # Increment for C within the loop
while C <= 40: # Loop heading with condition
F = (9/5)*C + 32 # 1st statement inside loop
print ("%3d %6.1f" % (C, F)) # 2nd statement inside loop
C = C + dC # Increment C for the next iteration of the loop.
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Exercise: Make a Fahrenheit-Celsius conversion tableWrite a program that prints out a table with Fahrenheit degrees 0, 10, 20, ..., 100 in the first column and the corresponding Celsius degrees in the second column.
###Code
F = 0
dF = 10
while F <= 90:
F = F+dF
C = (F-32)*5/9
print("%3d %6.1f" % (F,C))
###Output
10 -12.2
20 -6.7
30 -1.1
40 4.4
50 10.0
60 15.6
70 21.1
80 26.7
90 32.2
100 37.8
###Markdown
Exercise: Write an approximate Fahrenheit-Celsius conversion tableMany people use an approximate formula for quickly converting Fahrenheit ($F$) to Celsius ($C$) degrees:$C \approx \hat{C} = \frac{F − 30}{2}$Modify the program from the previous exercise so that it prints three columns: $F$, $C$, and the approximate value $\hat{C}$. ListsSo far, one variable has referred to one number (or string). Sometimes however we naturally have a collection of numbers, saydegrees −20, −15, −10, −5, 0, ..., 40. One way to store these values in a computer program would be to have one variable per value, i.e.
###Code
C1 = -20
C2 = -15
C3 = -10
# ...
C13 = 40
###Output
_____no_output_____
###Markdown
This is clearly a terrible solution, particularly if we have lots of values. A better way of doing this is to collect values together in a list:
###Code
C = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
###Output
_____no_output_____
###Markdown
Now there is just one variable, **C**, holding all the values. Elements in a list are accessed via an index. List indices are always numbered as 0, 1, 2, and so forth up to the number of elements minus one:
###Code
mylist = [4, 6, -3.5]
print(mylist[0])
print(mylist[1])
print(mylist[2])
print(len(mylist)) # length of list
mylist.append('ciccio')
print(mylist)
###Output
4
6
-3.5
3
[4, 6, -3.5, 'ciccio']
###Markdown
Here are a few example of operations that you can perform on lists:
###Code
C = [-10, -5, 0, 5, 10, 15, 20, 25, 30]
C.append(35) # add new element 35 at the end
print(C)
C.append('verbatim')
C=C+C
print(C)
del C[len(C)-1]
print(C)
C.insert(3,'caccabubu')
print(C)
print(C.index('caccabubu'))
print(C.index(-5))
print(C[1])
C = C + [40,45] # And another list to the end of C
print(C)
C.insert(0, -15) # Insert -15 as index 0
print(C)
del C[2] # delete 3rd element
print(C)
del C[2] # delete what is now 3rd element
print(C)
print(len(C)) # length of list
print(C.index(10)) # Find the index of the list with the value 10
print (10 in C) # True only if the value 10 is stored in the list
print(C[-1]) # The last value in the list.
print(C[-2]) # The second last value in the list.
###Output
_____no_output_____
###Markdown
You can also extract sublists using ":"
###Code
print(C[5:]) # From index 5 to the end of the list.
print(C[0:7]) # From index 5 up to, but not including index 7.
###Output
[-15, -10, 5, 10, 15, 20, 25]
###Markdown
Many languages (especially Matlab and Fortran) have would include the endpoint of a slice (7 in this case). One way to think of what Python does is that the slice is a half-open interval: $[5,7)$. This makes cutting a list in half particularly easy. For example:
###Code
print(C[:5] + ["Hello"] + C[5:])
print(C[7:-1]) # From index 7 up to the second last element.
print(C[:]) # [:] specifies the whole list.
somelist = ['Curly', 'Larry', 'Moe']
stooge1, stooge2, stooge3 = somelist # Unpack the entries in a list.
print(stooge3, stooge2, stooge1)
###Output
Moe Larry Curly
###Markdown
Of course this only works if `somelist` contains exactly the number of items on the left hand side of the equals. Another useful operator is `*` (pronounced "splat"). It turns a list (or any other sequence) into the set of arguments of a function. For example:
###Code
print(somelist) # Prints the list.
print(*somelist) # Prints the items in the list.
###Output
['Curly', 'Larry', 'Moe']
Curly Larry Moe
###Markdown
Exercise: Store odd numbers in a listStep 1: Write a program that generates all odd numbers from 1 to *n*. Set *n* in the beginning of the program and use a while loop to compute the numbers. (Make sure that if *n* is an even number, the largest generated odd number is *n*-1.).Step 2: Store the generated odd numbers in a list. Start with an empty list and use the same while loop where you generate each odd number, ao append the new number to the list.Finally, print the list elements to the screen.
###Code
n = 12
counter = 0
lista=[]
while counter < 10:
counter = counter + 1
lista= lista + [2*counter-1]
print(lista)
###Output
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
###Markdown
For loopsWe can visit each element in a list and process the element with some statements using a *for* loop, for example:
###Code
degrees = [0, 10, 20, 40, 100]
for C in degrees:
print('list element:', C)
print('The degrees list has', len(degrees), 'elements')
###Output
list element: 0
list element: 10
list element: 20
list element: 40
list element: 100
The degrees list has 5 elements
###Markdown
Notice again how the statements to be executed within the loop must be indented! Let's now revisit the conversion table example using the *for* loop:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
for C in Cdegrees:
F = (9/5)*C + 32
print("%3d %6.2f" % (C, F))
###Output
-20 -4.00
-15 5.00
-10 14.00
-5 23.00
0 32.00
5 41.00
10 50.00
15 59.00
20 68.00
25 77.00
30 86.00
35 95.00
40 104.00
###Markdown
It is also possible to rewrite the *for* loop as a *while* loop, i.e.,
###Code
for element in somelist:
# process element
###Output
_____no_output_____
###Markdown
can always be transformed to a *while* loop
###Code
index = 0
while index < len(somelist):
element = somelist[index]
# process element
index += 1
###Output
_____no_output_____
###Markdown
Taking the previous table example:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
index = 0
while index < len(Cdegrees):
C = Cdegrees[index]
F = (9/5)*C + 32
print('%5d %5.1f' % (C, F))
index += 1
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Rather than just printing out the Fahrenheit values, let's also store these computed values in a list of their own:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
Fdegrees = [] # start with empty list
for C in Cdegrees:
F = (9/5)*C + 32
Fdegrees.append(F) # add new element to Fdegrees
print(Fdegrees)
###Output
[-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]
###Markdown
Iterators: things you can iterate over.We've seen that a `for` loop can iterate over list values. In fact you can iterate over any object that is capable of providing values one at a time. In python, these objects are called `iterators`.A particularly useful python function which creates an iterator is `range`. For example, we can use range to iterate over the *indices* of a list:
###Code
for i in range(0, len(somelist), 1):
element = somelist[i]
... process element or somelist[i] directly
###Output
_____no_output_____
###Markdown
The statement *range(start, stop, inc)* generates an iterator over the integers *start*, *start+inc*, *start+2\*inc*, and so on up to, but not including, *stop*. We can also write *range(stop)* as an abbreviation for *range(0, stop, 1)*:
###Code
print(range(3)) # same as range(0, 3, 1)
###Output
range(0, 3)
###Markdown
Oh, that wasn't very informative. If we want to see all the numbers which the `range` will iterate over, we can cast the range to a list:
###Code
print(list(range(3)))
print(list(range(2, 8, 3)))
###Output
[2, 5]
###Markdown
List comprehensionsConsider this example where we compute two lists in a *for* loop:
###Code
n = 16
Cdegrees = []; Fdegrees = [] # empty lists
for i in range(n):
Cdegrees.append(-5 + i*0.5)
Fdegrees.append((9/5)*Cdegrees[i] + 32)
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
As constructing lists is a very common requirement, the above way of doing it can become very tedious to both write and read. Therefore, Python has a compact construct, called list comprehension for generating lists from a *for* loop:
###Code
n = 16
Cdegrees = [-5 + i*0.5 for i in range(n)]
Fdegrees = [(9/5)*C + 32 for C in Cdegrees]
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
The general form of a list comprehension is:
###Code
somelist = [expression for element in somelist]
###Output
_____no_output_____
###Markdown
Exercise: Repeat the previous exercise of creating a list of odd numbers using using: a for loop, list comprehension and the range function
###Code
# Using for loop
# Using list comprehension
# Using the function range
###Output
_____no_output_____
###Markdown
Changing elements in a listSay we want to add $2$ to all the numbers in a list:
###Code
v = [-1, 1, 10]
for e in v:
e = e + 2
print(v)
###Output
_____no_output_____
###Markdown
We can see that the list *v* is unaltered! The reason for this is that inside the loop, *e* is an ordinary (int) variable. At the start of the iteration *e* is assigned a *copy* of the next element in the list. Inside the loop we can change *e* but *v* itself is unaltered. If we want to change *v* we have to use an index to access and modify its elements:
###Code
v[1] = 4 # assign 4 to 2nd element (index 1) in v
print(v)
###Output
_____no_output_____
###Markdown
To add 2 to all values we need a *for* loop over indices:
###Code
for i in range(len(v)):
v[i] = v[i] + 2
print(v)
###Output
_____no_output_____
###Markdown
Traversing multiple lists simultaneously - *zip(list1, list2, ...)*Consider how we can loop over elements in both Cdegrees and Fdegrees at the same time. One approach would be to use list indices:
###Code
for i in range(len(Cdegrees)):
print(Cdegrees[i], Fdegrees[i])
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
An alternative construct (regarded as more ”Pythonic”) uses the *zip* function:
###Code
for C, F in zip(Cdegrees, Fdegrees):
print(C, F)
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
`zip` creates an *iterator* over the corresponding items of its arguments in order.Another example with three lists:
###Code
l1 = [3, 6, 1]; l2 = [1.5, 1, 0]; l3 = [9.1, 3, 2]
for e in zip(l1, l2, l3):
print(*e)
###Output
3 1.5 9.1
6 1 3
1 0 2
###Markdown
If the lists are of unequal length then the loop stops when the end of the shortest list is reached. Experiment with this:
###Code
l1 = [3, 6, 1, 4, 6]; l2 = [1.5, 1, 0, 7]; l3 = [9.1, 3, 2, 0, 9]
for e in zip(l1, l2, l3):
print(*e)
###Output
3 1.5 9.1
6 1 3
1 0 2
4 7 0
###Markdown
Nested lists: list of listsA *list* can contain **any** object, including another *list*. To illustrate this, consider how to store the conversion table as a single Python list rather than two separate lists.
###Code
Cdegrees = range(-20, 41, 5)
Fdegrees = [(9.0/5)*C + 32 for C in Cdegrees]
table1 = [Cdegrees, Fdegrees] # List of two lists
print("table1 = ", table1)
print("table1[0] = ", table1[0])
print("table1[1] = ", table1[1])
print("table1[1][3] = ", table1[1][3])
###Output
table1 = [range(-20, 41, 5), [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]]
table1[0] = range(-20, 41, 5)
table1[1] = [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]
table1[1][3] = 23.0
###Markdown
This gives us a table of two columns. How do we create a table of rows instead:
###Code
table2 = []
for C, F in zip(Cdegrees, Fdegrees):
row = [C, F]
table2.append(row)
print(table2)
###Output
_____no_output_____
###Markdown
We can use `zip` to do this more elegantly:
###Code
table2 = list(zip(Cdegrees, Fdegrees))
print(table2)
###Output
_____no_output_____
###Markdown
And you can loop through this list as before:
###Code
for C, F in table2:
print(C, F)
###Output
_____no_output_____
###Markdown
Tuples: sequences that cannot be changedWe've already learned that lists are not the only thing which can be iterated over, but that there is a general category of `iterator` types. Similarly, lists are not the only objects which can be indexed. The general category of types which can be indexed are the `sequence` types.Tuples are **constant** sequences. You can use them in much the same way as lists except you cannot modify them. They are an example of an [**immutable**](http://en.wikipedia.org/wiki/Immutable_object) type.
###Code
t = (2, 4, 6, 'temp.pdf') # Define a tuple.
t = 2, 4, 6, 'temp.pdf' # Can skip parenthesis as it is assumed in this context.
###Output
_____no_output_____
###Markdown
Let's see what happens when we try to modify the tuple like we did with a list:
###Code
t[1] = -1
t.append(0)
del t[1]
###Output
_____no_output_____
###Markdown
However, we can use the tuple to compose a new tuple:
###Code
t = t + (-1.0, -2.0)
print(t)
###Output
_____no_output_____
###Markdown
So, why would we use tuples when lists have more functionality?* Tuples are constant and thus protected against accidental changes.* Tuples are faster than lists.* Tuples are widely used in Python software (so you need to know about tuples to understand other people's code!)* Tuples (but not lists) can be used as keys in dictionaries (more about dictionaries later). Exercise: Make a table of function valuesStep 1: Write a program that prints a table with $t$ values in the first column and the corresponding $y(t) = v_0 t − 0.5gt^2$ values in the second column. Use $n$ uniformly spaced $t$ values throughout the interval [0, $2v_0/g$]. Set $v0 = 1$, $g = 9.81$, and $n = 11$.Step 2: Once step 1 is working, modify the program so that the $t$ and $y$ values are stored in two lists *t* and *y*. Thereafter, transverse the lists with a *for* loop and write out a nicely formatted table of *t* and *y* values using a *zip* construction. FunctionsWe have already used many Python functions, including mathematical functions:
###Code
from math import *
x = pi
print cos(x)
###Output
_____no_output_____
###Markdown
Other functions you used include *len* and *range*:
###Code
n = len(somelist)
ints = range(5, n, 2)
###Output
_____no_output_____
###Markdown
You have also used functions that are executed with the dot syntax (called *methods*):
###Code
C = [5, 10, 40, 45]
i = C.index(10)
C.append(50)
C.insert(2, 20)
###Output
_____no_output_____
###Markdown
A function is a collection of statements we can execute wherever and whenever we want. Functions can take any number of inputs (called *arguments*) to produce outputs. Functions help to organize programs, make them more understandable, shorter, and easier to extend. For our first example we will turn our temperature conversion code into a function:
###Code
def C2F(C):
return (9/5)*C + 32
C2F(3)
###Output
_____no_output_____
###Markdown
Functions start with *def*, then the name you want to give your function, then a list of arguments (here C). This is referred to as the *function header*. Inside the function there is a block of statements called the *function body*. Notice that the function body is indented - as was the case for the *for* / *while* loop the indentation indicates where the function ends. At any point within the function, we can "stop thefunction" and return as many values/variables as required. Local and global variablesVariables defined within a function are said to have *local scope*. That is to say that they can only be referenced within that function. Let's consider an example (and look carefully at the indentation!!):
###Code
def sumint(start, stop):
s = 0 # variable for accumulating the sum
i = start # counter
while i <= stop:
s += i
i += 1
return s
print(sumint(0, 5))
###Output
_____no_output_____
###Markdown
Variables *i* and *s* are local variables in *sumint*. They are destroyed at the end (return) of the function and never visible outside the function (in the calling program); in fact, *start* and *stop* are also local variables. So let's see what happens if we now try to print one of these variables:
###Code
print(stop)
###Output
_____no_output_____
###Markdown
Functions can also return multiple values. Let's recycle another of our previous examples - compute $y(t)$ and $y'(t)=v_0-gt$:
###Code
def yfunc(t, v0):
g = 9.81
y = v0*t - 0.5*g*t**2
dydt = v0 - g*t
return y, dydt
# call:
position, velocity = yfunc(0.6, 3)
print(position, velocity)
###Output
_____no_output_____
###Markdown
Remember that a series of comma separated variables implies a tuple - therefore "return y, dydt" is the same as writing "return (y, dydt)". Therefore, in general what is returned is a tuple. Let's take a look at another example illustrating this:
###Code
def f(x):
return x, x**2, x**4
s = f(2)
print(s)
print(type(s)) # The function type() tells us what type a variable it is.
###Output
_____no_output_____
###Markdown
No return value implies that `None` is returned. `None` is a special Python object that represents an ”empty” or undefined value. It is surprisingly useful and we will use it a lot later.
###Code
def message(course):
print("%s rocks!"% course)
message("Python")
r = message("Geo")
print("r = ", r)
###Output
_____no_output_____
###Markdown
Keyword arguments and default argument valuesIt's possible to specify default values that function arguments should take if no values are provided when the function is called:
###Code
def somefunc(arg1, arg2, kwarg1=True, kwarg2=0):
print(arg1, arg2, kwarg1, kwarg2)
somefunc("Hello", [1,2]) # Note that we have not specified values for kwarg1 and kwarg2
###Output
Hello [1, 2] True 0
###Markdown
You can override the default values by passing additional arguments in the correct position:
###Code
somefunc("Hello", [1,2], "Hi")
###Output
_____no_output_____
###Markdown
... or by naming the argument to which the value should be passed. These are called keyword arguments:
###Code
somefunc("Hello", [1,2], kwarg2="Hi")
somefunc("Hello", [1,2], kwarg2="Hi", kwarg1=6)
###Output
_____no_output_____
###Markdown
If we use variable_name=value for all arguments, their sequence in the function header can be in any order.
###Code
somefunc(kwarg2="Hello", arg1="Hi", kwarg1=6, arg2=[2])
###Output
_____no_output_____
###Markdown
Let's look at another example - consider a function of $t$, with parameters $A$, $a$, and $\omega$:$$f(t; A,a, \omega) = Ae^{-at}\sin (\omega t)$$. (The choice of equation is actually pretty random - but it serves to show you that it is easy to translate formulae you encounter into Python code). We can implement $f$ in a Python function with $t$ as positional argument and $A$, $a$, and $\omega$ as keyword arguments.
###Code
from math import pi, exp, sin
def f(t, A=1, a=1, ω=2*pi):
return A*exp(-a*t)*sin(ω*t)
v1 = f(0.2)
v2 = f(0.2, ω=1)
v2 = f(0.2, 1, 3) # same as f(0.2, A=1, a=3)
v3 = f(0.2, ω=1, A=2.5)
v4 = f(A=5, a=0.1, ω=1, t=1.3)
v5 = f(t=0.2, A=9)
print(v1, v2, v3, v4, v5)
###Output
_____no_output_____
###Markdown
Exercise: Implement a Gaussian functionMake a Python function *gauss*( *x*, *m*=0, *s*=1) for computing the Gaussian function $$f(x)=\frac{1}{\sqrt{2\pi}s}\exp\left(-\frac{1}{2} \left(\frac{x-m}{s}\right)^2\right)$$Call the function and print out the result for x equal to −5, −4.9, −4.8, ..., 4.8, 4.9, 5, using default values for *m* and *s*.
###Code
from math import pi, exp
m=0
s=1
x = [1,2,3]
def gauss(x,m,s):
return exp(-0.5*((x-m)/s)**2)/(2*pi*s)**0.5
for i in range(0, len(x), 1):
print(gauss(x[i],m,s))
###Output
0.24197072451914337
0.05399096651318806
0.0044318484119380075
###Markdown
The *if* statementConsider this simple example:
###Code
def f(x):
if 0 <= x <= pi:
return sin(x)
else:
return 0
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
In general (the *else* block can be skipped if there are no statements to be executed when False) we can put together multiple conditions. Only the first condition that is True is executed.
###Code
if condition1:
<block of statements, executed if condition1 is True>
elif condition2:
<block of statements>
elif condition3:
<block of statements>
else:
<block of statements>
<next statement of the program>
###Output
_____no_output_____
###Markdown
The ternary operatorThere is another form of *if* which works as an operator, rather than a statement. If you've used the *if* function in a spreadsheet then you'll be familiar with this approach.
###Code
from math import *
def f(x):
return (sin(x) if 0 <= x <= pi else 0)
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
0 1.0 0
###Markdown
The ternary operator has the following form:
###Code
<expression if condition is True> if <condition> else <expression if condition is false>
###Output
_____no_output_____
###Markdown
Exercise: Express a step function as a Python functionThe following "step" function is known as the Heaviside function andis widely used in mathematics:$$H(x)=\begin{cases}0, & \text{if $x<0$}.\\\\1, & \text{if $x\ge 0$}.\end{cases}$$Write a Python function H(x) that computes H(x).
###Code
def step(x):
return 1 if x >= 0 else 0
print(step(-115))
###Output
0
###Markdown
Introduction to Python programming [Gerard Gorman](http://www.imperial.ac.uk/people/g.gorman), [Christian Jacobs](http://christianjacobs.uk/) Updated for MPECDT by [David Ham](http://www.imperial.ac.uk/people/david.ham) Lecture 2: Computing with basic language constructionsLearning objectives:* Introduction to two different forms of loops which are used to repeat operations on data: either until a condition is satisfied (*while-loop*) or for a fixed number of iterations (*for-loop*).* Form a *condition* using a *boolean expression*. This is used to either terminate loops or control if a specific block of code is executed via the *if-statement*.* Learn two different methods for storing many data elements within a single Python type.* Introduction to developing your own Python *functions* which allows you to execute the same block of code, with different inputs and outputs, from anywhere in the code. Boolean expressionsAn expression with value *true* or *false* is called a boolean expression. Example expressions for what you would write mathematically as$C=40$, $C\ne40$, $C\ge40$, $C\gt40$ and $C\lt40$ are:
###Code
C == 40 # Note: the double == checks for equality, but C=40 is an assignment!
C != 40 # This could also be written as 'not C == 4' (i.e. the opposite of what C == 4 gives)
C >= 40
C > 40
C < 40
###Output
_____no_output_____
###Markdown
We can test boolean expressions in a Python shell:
###Code
C = 41
print("C != 40: ", C != 40)
print("C < 40: ", C < 40)
print("C == 41: ", C == 41)
###Output
_____no_output_____
###Markdown
Several conditions can be combined with the special 'and' and 'or' operators into a single boolean expression:
###Code
condition1 and condition2
condition1 or condition2
###Output
_____no_output_____
###Markdown
* Rule 1: (**C1** *and* **C2**) is *True* only if both **C1** and **C2** are *True** Rule 2: (**C1** *or* **C2**) is *True* if either **C1** or **C2** are *True*Examples:
###Code
x=0; y=1.2
print (x >= 0 and y < 1)
###Output
_____no_output_____
###Markdown
Exercise: Values of boolean expressionsAdd a comment to the code below to explain the outcome of each of the boolean expressions:
###Code
C = 41
print("Case 1: ", C == 40)
print("Case 2: ", C != 40 and C < 41)
print("Case 3: ", C != 40 or C < 41)
print("Case 4: ", not C == 40)
print("Case 5: ", not C > 40)
print("Case 6: ", C <= 41)
print("Case 7: ", not False)
print("Case 8: ", True and False)
print("Case 9: ", False or True)
print("Case 10: ", False or False or False)
print("Case 11: ", True and True and False)
print("Case 12: ", False == 0)
print("Case 13: ", True == 0)
print("Case 14: ", True == 1)
###Output
_____no_output_____
###Markdown
LoopsSuppose we want to make a table of Celsius and Fahrenheitdegrees:
###Code
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Output
_____no_output_____
###Markdown
How do we write a program that prints out such a table?We know from the last lecture how to make one line in this table:
###Code
C = -20
F = 9/5*C + 32
print(C, F)
###Output
_____no_output_____
###Markdown
We can just repeat these statements:
###Code
C=-20; F=9/5*C+32; print(C,F)
C=-15; F=9/5*C+32; print(C,F)
C=-10; F=9/5*C+32; print(C,F)
C=-5; F=9/5*C+32; print(C,F)
C=0; F=9/5*C+32; print(C,F)
C=5; F=9/5*C+32; print(C,F)
C=10; F=9/5*C+32; print(C,F)
C=15; F=9/5*C+32; print(C,F)
C=20; F=9/5*C+32; print(C,F)
C=25; F=9/5*C+32; print(C,F)
C=30; F=9/5*C+32; print(C,F)
C=35; F=9/5*C+32; print(C,F)
C=40; F=9/5*C+32; print(C,F)
###Output
_____no_output_____
###Markdown
So we can see that works but its **very boring** to write and very easy to introduce a misprint.**You really should not be doing boring repetitive tasks like this.** Spend one time instead looking for a smarter solution. When programming becomes boring, there is usually a construct that automates the writing. Computers are very good at performing repetitive tasks. For this purpose we use **loops**. The while loop (and the significance of indentation)A while loop executes repeatedly a set of statements as long as a **boolean** (i.e. *True* / *False*) condition is *True*
###Code
while condition:
<statement 1>
<statement 2>
...
<first statement after loop>
###Output
_____no_output_____
###Markdown
Note that all statements to be executed within the loop must be indented by the same amount! The loop ends when an unindented statement is encountered.At this point it is worth noticing that **blank spaces may or may not be important** in Python programs. These statements are equivalent (blanks do not matter):
###Code
v0=3
v0 = 3
v0= 3
# The computer does not care but this formatting style is
# considered clearest for the human reader.
v0 = 3
###Output
_____no_output_____
###Markdown
Here is a while loop example where blank spaces really do matter:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
_____no_output_____
###Markdown
Let's take a look at what happens when we forget to indent correctly:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
_____no_output_____
###Markdown
Let's use the while loop to create the table above:
###Code
C = -20 # Initialise C
dC = 5 # Increment for C within the loop
while C <= 40: # Loop heading with condition
F = (9/5)*C + 32 # 1st statement inside loop
print ("%3d %6.1f" % (C, F)) # 2nd statement inside loop
C = C + dC # Increment C for the next iteration of the loop.
###Output
_____no_output_____
###Markdown
Exercise: Make a Fahrenheit-Celsius conversion tableWrite a program that prints out a table with Fahrenheit degrees 0, 10, 20, ..., 100 in the first column and the corresponding Celsius degrees in the second column. Exercise: Write an approximate Fahrenheit-Celsius conversion tableMany people use an approximate formula for quickly converting Fahrenheit ($F$) to Celsius ($C$) degrees:$C \approx \hat{C} = \frac{F − 30}{2}$Modify the program from the previous exercise so that it prints three columns: $F$, $C$, and the approximate value $\hat{C}$. ListsSo far, one variable has referred to one number (or string). Sometimes however we naturally have a collection of numbers, saydegrees −20, −15, −10, −5, 0, ..., 40. One way to store these values in a computer program would be to have one variable per value, i.e.
###Code
C1 = -20
C2 = -15
C3 = -10
# ...
C13 = 40
###Output
_____no_output_____
###Markdown
This is clearly a terrible solution, particularly if we have lots of values. A better way of doing this is to collect values together in a list:
###Code
C = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
###Output
_____no_output_____
###Markdown
Now there is just one variable, **C**, holding all the values. Elements in a list are accessed via an index. List indices are always numbered as 0, 1, 2, and so forth up to the number of elements minus one:
###Code
mylist = [4, 6, -3.5]
print(mylist[0])
print(mylist[1])
print(mylist[2])
print(len(mylist)) # length of list
###Output
_____no_output_____
###Markdown
Here are a few example of operations that you can perform on lists:
###Code
C = [-10, -5, 0, 5, 10, 15, 20, 25, 30]
C.append(35) # add new element 35 at the end
print(C)
C = C + [40,45] # And another list to the end of C
print(C)
C.insert(0, -15) # Insert -15 as index 0
print(C)
del C[2] # delete 3rd element
print(C)
del C[2] # delete what is now 3rd element
print(C)
print(len(C)) # length of list
print(C.index(10)) # Find the index of the list with the value 10
print (10 in C) # True only if the value 10 is stored in the list
print(C[-1]) # The last value in the list.
print(C[-2]) # The second last value in the list.
###Output
_____no_output_____
###Markdown
You can also extract sublists using ":"
###Code
print(C[5:]) # From index 5 to the end of the list.
print(C[5:7]) # From index 5 up to, but not including index 7.
###Output
_____no_output_____
###Markdown
Many languages (especially Matlab and Fortran) have would include the endpoint of a slice (7 in this case). One way to think of what Python does is that the slice is a half-open interval: $[5,7)$. This makes cutting a list in half particularly easy. For example:
###Code
print(C[:5] + ["Hello"] + C[5:])
print(C[7:-1]) # From index 7 up to the second last element.
print(C[:]) # [:] specifies the whole list.
somelist = ['Curly', 'Larry', 'Moe']
stooge1, stooge2, stooge3 = somelist # Unpack the entries in a list.
print(stooge3, stooge2, stooge1)
###Output
_____no_output_____
###Markdown
Of course this only works if `somelist` contains exactly the number of items on the left hand side of the equals. Another useful operator is `*` (pronounced "splat"). It turns a list (or any other sequence) into the set of arguments of a function. For example:
###Code
print(somelist) # Prints the list.
print(*somelist) # Prints the items in the list.
###Output
_____no_output_____
###Markdown
Exercise: Store odd numbers in a listStep 1: Write a program that generates all odd numbers from 1 to *n*. Set *n* in the beginning of the program and use a while loop to compute the numbers. (Make sure that if *n* is an even number, the largest generated odd number is *n*-1.).Step 2: Store the generated odd numbers in a list. Start with an empty list and use the same while loop where you generate each odd number, ao append the new number to the list.Finally, print the list elements to the screen. For loopsWe can visit each element in a list and process the element with some statements using a *for* loop, for example:
###Code
degrees = [0, 10, 20, 40, 100]
for C in degrees:
print('list element:', C)
print('The degrees list has', len(degrees), 'elements')
###Output
_____no_output_____
###Markdown
Notice again how the statements to be executed within the loop must be indented! Let's now revisit the conversion table example using the *for* loop:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
for C in Cdegrees:
F = (9/5)*C + 32
print("%3d %6.2f" % (C, F))
###Output
_____no_output_____
###Markdown
It is also possible to rewrite the *for* loop as a *while* loop, i.e.,
###Code
for element in somelist:
# process element
###Output
_____no_output_____
###Markdown
can always be transformed to a *while* loop
###Code
index = 0
while index < len(somelist):
element = somelist[index]
# process element
index += 1
###Output
_____no_output_____
###Markdown
Taking the previous table example:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
index = 0
while index < len(Cdegrees):
C = Cdegrees[index]
F = (9/5)*C + 32
print('%5d %5.1f' % (C, F))
index += 1
###Output
_____no_output_____
###Markdown
Rather than just printing out the Fahrenheit values, let's also store these computed values in a list of their own:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
Fdegrees = [] # start with empty list
for C in Cdegrees:
F = (9/5)*C + 32
Fdegrees.append(F) # add new element to Fdegrees
print(Fdegrees)
###Output
_____no_output_____
###Markdown
Iterators: things you can iterate over.We've seen that a `for` loop can iterate over list values. In fact you can iterate over any object that is capable of providing values one at a time. In python, these objects are called `iterators`.A particularly useful python function which creates an iterator is `range`. For example, we can use range to iterate over the *indices* of a list:
###Code
for i in range(0, len(somelist), 1):
element = somelist[i]
... process element or somelist[i] directly
###Output
_____no_output_____
###Markdown
The statement *range(start, stop, inc)* generates an iterator over the integers *start*, *start+inc*, *start+2\*inc*, and so on up to, but not including, *stop*. We can also write *range(stop)* as an abbreviation for *range(0, stop, 1)*:
###Code
print(range(3)) # same as range(0, 3, 1)
###Output
_____no_output_____
###Markdown
Oh, that wasn't very informative. If we want to see all the numbers which the `range` will iterate over, we can cast the range to a list:
###Code
print(list(range(3)))
print(list(range(2, 8, 3)))
###Output
_____no_output_____
###Markdown
List comprehensionsConsider this example where we compute two lists in a *for* loop:
###Code
n = 16
Cdegrees = []; Fdegrees = [] # empty lists
for i in range(n):
Cdegrees.append(-5 + i*0.5)
Fdegrees.append((9/5)*Cdegrees[i] + 32)
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
_____no_output_____
###Markdown
As constructing lists is a very common requirement, the above way of doing it can become very tedious to both write and read. Therefore, Python has a compact construct, called list comprehension for generating lists from a *for* loop:
###Code
n = 16
Cdegrees = [-5 + i*0.5 for i in range(n)]
Fdegrees = [(9/5)*C + 32 for C in Cdegrees]
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
_____no_output_____
###Markdown
The general form of a list comprehension is:
###Code
somelist = [expression for element in somelist]
###Output
_____no_output_____
###Markdown
Exercise: Repeat the previous exercise of creating a list of odd numbers using using: a for loop, list comprehension and the range function
###Code
# Using for loop
# Using list comprehension
# Using the function range
###Output
_____no_output_____
###Markdown
Changing elements in a listSay we want to add $2$ to all the numbers in a list:
###Code
v = [-1, 1, 10]
for e in v:
e = e + 2
print(v)
###Output
_____no_output_____
###Markdown
We can see that the list *v* is unaltered! The reason for this is that inside the loop, *e* is an ordinary (int) variable. At the start of the iteration *e* is assigned a *copy* of the next element in the list. Inside the loop we can change *e* but *v* itself is unaltered. If we want to change *v* we have to use an index to access and modify its elements:
###Code
v[1] = 4 # assign 4 to 2nd element (index 1) in v
print(v)
###Output
_____no_output_____
###Markdown
To add 2 to all values we need a *for* loop over indices:
###Code
for i in range(len(v)):
v[i] = v[i] + 2
print(v)
###Output
_____no_output_____
###Markdown
Traversing multiple lists simultaneously - *zip(list1, list2, ...)*Consider how we can loop over elements in both Cdegrees and Fdegrees at the same time. One approach would be to use list indices:
###Code
for i in range(len(Cdegrees)):
print(Cdegrees[i], Fdegrees[i])
###Output
_____no_output_____
###Markdown
An alternative construct (regarded as more ”Pythonic”) uses the *zip* function:
###Code
for C, F in zip(Cdegrees, Fdegrees):
print(C, F)
###Output
_____no_output_____
###Markdown
`zip` creates an *iterator* over the corresponding items of its arguments in order.Another example with three lists:
###Code
l1 = [3, 6, 1]; l2 = [1.5, 1, 0]; l3 = [9.1, 3, 2]
for e in zip(l1, l2, l3):
print(*e)
###Output
_____no_output_____
###Markdown
If the lists are of unequal length then the loop stops when the end of the shortest list is reached. Experiment with this:
###Code
l1 = [3, 6, 1, 4, 6]; l2 = [1.5, 1, 0, 7]; l3 = [9.1, 3, 2, 0, 9]
for e in zip(l1, l2, l3):
print(*e)
###Output
_____no_output_____
###Markdown
Nested lists: list of listsA *list* can contain **any** object, including another *list*. To illustrate this, consider how to store the conversion table as a single Python list rather than two separate lists.
###Code
Cdegrees = range(-20, 41, 5)
Fdegrees = [(9.0/5)*C + 32 for C in Cdegrees]
table1 = [Cdegrees, Fdegrees] # List of two lists
print("table1 = ", table1)
print("table1[0] = ", table1[0])
print("table1[1] = ", table1[1])
print("table1[2][3] = ", table1[1][3])
###Output
_____no_output_____
###Markdown
This gives us a table of two columns. How do we create a table of rows instead:
###Code
table2 = []
for C, F in zip(Cdegrees, Fdegrees):
row = [C, F]
table2.append(row)
print(table2)
###Output
_____no_output_____
###Markdown
We can use `zip` to do this more elegantly:
###Code
table2 = list(zip(Cdegrees, Fdegrees))
print(table2)
###Output
_____no_output_____
###Markdown
And you can loop through this list as before:
###Code
for C, F in table2:
print(C, F)
###Output
_____no_output_____
###Markdown
Tuples: sequences that cannot be changedWe've already learned that lists are not the only thing which can be iterated over, but that there is a general category of `iterator` types. Similarly, lists are not the only objects which can be indexed. The general category of types which can be indexed are the `sequence` types.Tuples are **constant** sequences. You can use them in much the same way as lists except you cannot modify them. They are an example of an [**immutable**](http://en.wikipedia.org/wiki/Immutable_object) type.
###Code
t = (2, 4, 6, 'temp.pdf') # Define a tuple.
t = 2, 4, 6, 'temp.pdf' # Can skip parenthesis as it is assumed in this context.
###Output
_____no_output_____
###Markdown
Let's see what happens when we try to modify the tuple like we did with a list:
###Code
t[1] = -1
t.append(0)
del t[1]
###Output
_____no_output_____
###Markdown
However, we can use the tuple to compose a new tuple:
###Code
t = t + (-1.0, -2.0)
print(t)
###Output
_____no_output_____
###Markdown
So, why would we use tuples when lists have more functionality?* Tuples are constant and thus protected against accidental changes.* Tuples are faster than lists.* Tuples are widely used in Python software (so you need to know about tuples to understand other people's code!)* Tuples (but not lists) can be used as keys in dictionaries (more about dictionaries later). Exercise: Make a table of function valuesStep 1: Write a program that prints a table with $t$ values in the first column and the corresponding $y(t) = v_0 t − 0.5gt^2$ values in the second column. Use $n$ uniformly spaced $t$ values throughout the interval [0, $2v_0/g$]. Set $v0 = 1$, $g = 9.81$, and $n = 11$.Step 2: Once step 1 is working, modify the program so that the $t$ and $y$ values are stored in two lists *t* and *y*. Thereafter, transverse the lists with a *for* loop and write out a nicely formatted table of *t* and *y* values using a *zip* construction. FunctionsWe have already used many Python functions, including mathematical functions:
###Code
from math import *
x = pi
print (cos(x))
###Output
-1.0
###Markdown
Other functions you used include *len* and *range*:
###Code
n = len(somelist)
ints = range(5, n, 2)
###Output
_____no_output_____
###Markdown
You have also used functions that are executed with the dot syntax (called *methods*):
###Code
C = [5, 10, 40, 45]
i = C.index(10)
C.append(50)
C.insert(2, 20)
###Output
_____no_output_____
###Markdown
A function is a collection of statements we can execute wherever and whenever we want. Functions can take any number of inputs (called *arguments*) to produce outputs. Functions help to organize programs, make them more understandable, shorter, and easier to extend. For our first example we will turn our temperature conversion code into a function:
###Code
def C2F(C):
return (9/5)*C + 32
###Output
_____no_output_____
###Markdown
Functions start with *def*, then the name you want to give your function, then a list of arguments (here C). This is referred to as the *function header*. Inside the function there is a block of statements called the *function body*. Notice that the function body is indented - as was the case for the *for* / *while* loop the indentation indicates where the function ends. At any point within the function, we can "stop thefunction" and return as many values/variables as required. Local and global variablesVariables defined within a function are said to have *local scope*. That is to say that they can only be referenced within that function. Let's consider an example (and look carefully at the indentation!!):
###Code
def sumint(start, stop):
s = 0 # variable for accumulating the sum
i = start # counter
while i <= stop:
s += i
i += 1
return s
print(sumint(0, 5))
###Output
_____no_output_____
###Markdown
Variables *i* and *s* are local variables in *sumint*. They are destroyed at the end (return) of the function and never visible outside the function (in the calling program); in fact, *start* and *stop* are also local variables. So let's see what happens if we now try to print one of these variables:
###Code
print(stop)
###Output
_____no_output_____
###Markdown
Functions can also return multiple values. Let's recycle another of our previous examples - compute $y(t)$ and $y'(t)=v_0-gt$:
###Code
def yfunc(t, v0):
g = 9.81
y = v0*t - 0.5*g*t**2
dydt = v0 - g*t
return y, dydt
# call:
position, velocity = yfunc(0.6, 3)
print(position, velocity)
###Output
_____no_output_____
###Markdown
Remember that a series of comma separated variables implies a tuple - therefore "return y, dydt" is the same as writing "return (y, dydt)". Therefore, in general what is returned is a tuple. Let's take a look at another example illustrating this:
###Code
def f(x):
return x, x**2, x**4
s = f(2)
print(s)
print(type(s)) # The function type() tells us what type a variable it is.
###Output
_____no_output_____
###Markdown
No return value implies that `None` is returned. `None` is a special Python object that represents an ”empty” or undefined value. It is surprisingly useful and we will use it a lot later.
###Code
def message(course):
print("%s rocks!"% course)
message("Python")
r = message("Geo")
print("r = ", r)
###Output
_____no_output_____
###Markdown
Keyword arguments and default argument valuesIt's possible to specify default values that function arguments should take if no values are provided when the function is called:
###Code
def somefunc(arg1, arg2, kwarg1=True, kwarg2=0):
print(arg1, arg2, kwarg1, kwarg2)
somefunc("Hello", [1,2]) # Note that we have not specified values for kwarg1 and kwarg2
###Output
Hello [1, 2] True 0
###Markdown
You can override the default values by passing additional arguments in the correct position:
###Code
somefunc("Hello", [1,2], "Hi")
###Output
_____no_output_____
###Markdown
... or by naming the argument to which the value should be passed. These are called keyword arguments:
###Code
somefunc("Hello", [1,2], kwarg2="Hi")
somefunc("Hello", [1,2], kwarg2="Hi", kwarg1=6)
###Output
Hello [1, 2] 6 Hi
###Markdown
If we use variable_name=value for all arguments, their sequence in the function header can be in any order.
###Code
somefunc(kwarg2="Hello", arg1="Hi", kwarg1=6, arg2=[2])
###Output
_____no_output_____
###Markdown
Let's look at another example - consider a function of $t$, with parameters $A$, $a$, and $\omega$:$$f(t; A,a, \omega) = Ae^{-at}\sin (\omega t)$$. (The choice of equation is actually pretty random - but it serves to show you that it is easy to translate formulae you encounter into Python code). We can implement $f$ in a Python function with $t$ as positional argument and $A$, $a$, and $\omega$ as keyword arguments.
###Code
from math import pi, exp, sin
def f(t, A=1, a=1, ω=2*pi):
return A*exp(-a*t)*sin(ω*t)
v1 = f(0.2)
v2 = f(0.2, ω=1)
v2 = f(0.2, 1, 3) # same as f(0.2, A=1, a=3)
v3 = f(0.2, ω=1, A=2.5)
v4 = f(A=5, a=0.1, ω=1, t=1.3)
v5 = f(t=0.2, A=9)
print(v1, v2, v3, v4, v5)
###Output
_____no_output_____
###Markdown
Exercise: Implement a Gaussian functionMake a Python function *gauss*( *x*, *m*=0, *s*=1) for computing the Gaussian function $$f(x)=\frac{1}{\sqrt{2\pi}s}\exp\left(-\frac{1}{2} \left(\frac{x-m}{s}\right)^2\right)$$Call the function and print out the result for x equal to −5, −4.9, −4.8, ..., 4.8, 4.9, 5, using default values for *m* and *s*.
###Code
from math import exp, pi
def gauss(x, m=0, s=1) :
return ((pi*2*s)**(-0.5))*exp(-0.5*((((x-m)/s))**2))
import numpy
num = numpy.linspace(-5, 5, num=101, endpoint=True, retstep=False, dtype=None)
print(num)
for n in num:
print(gauss(n))
###Output
1.4867195147342977e-06
2.438960745893352e-06
3.961299091032075e-06
6.36982517886709e-06
1.0140852065486758e-05
1.5983741106905475e-05
2.4942471290053535e-05
3.853519674208713e-05
5.8943067756539855e-05
8.926165717713293e-05
0.00013383022576488537
0.00019865547139277272
0.00029194692579146027
0.00042478027055075143
0.000611901930113773
0.0008726826950457602
0.00123221916847302
0.0017225689390536812
0.0023840882014648404
0.0032668190561999247
0.0044318484119380075
0.005952532419775854
0.007915451582979969
0.010420934814422605
0.013582969233685634
0.01752830049356854
0.0223945302948429
0.028327037741601186
0.03547459284623146
0.04398359598042723
0.05399096651318806
0.0656158147746766
0.07895015830089418
0.09404907737688697
0.11092083467945563
0.12951759566589174
0.14972746563574488
0.1713685920478074
0.19418605498321304
0.21785217703255064
0.24197072451914337
0.266085249898755
0.2896915527614828
0.3122539333667612
0.3332246028917997
0.3520653267642995
0.3682701403033234
0.38138781546052414
0.391042693975456
0.3969525474770118
0.3989422804014327
0.39695254747701175
0.3910426939754559
0.381387815460524
0.3682701403033233
0.3520653267642995
0.33322460289179956
0.3122539333667612
0.28969155276148256
0.26608524989875476
0.24197072451914337
0.21785217703255041
0.19418605498321292
0.1713685920478072
0.1497274656357448
0.12951759566589174
0.11092083467945546
0.0940490773768869
0.07895015830089407
0.06561581477467655
0.05399096651318806
0.043983595980427156
0.035474592846231424
0.02832703774160112
0.022394530294842882
0.01752830049356854
0.013582969233685602
0.010420934814422592
0.007915451582979946
0.005952532419775849
0.0044318484119380075
0.0032668190561999247
0.0023840882014648343
0.0017225689390536767
0.0012322191684730175
0.0008726826950457602
0.000611901930113773
0.00042478027055074997
0.0002919469257914595
0.00019865547139277237
0.00013383022576488537
8.926165717713293e-05
5.8943067756539645e-05
3.8535196742086994e-05
2.4942471290053535e-05
1.5983741106905475e-05
1.014085206548667e-05
6.369825178867068e-06
3.961299091032061e-06
2.438960745893352e-06
1.4867195147342977e-06
###Markdown
The *if* statementConsider this simple example:
###Code
def f(x):
if 0 <= x <= pi:
return sin(x)
else:
return 0
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
In general (the *else* block can be skipped if there are no statements to be executed when False) we can put together multiple conditions. Only the first condition that is True is executed.
###Code
if condition1:
<block of statements, executed if condition1 is True>
elif condition2:
<block of statements>
elif condition3:
<block of statements>
else:
<block of statements>
<next statement of the program>
###Output
_____no_output_____
###Markdown
The ternary operatorThere is another form of *if* which works as an operator, rather than a statement. If you've used the *if* function in a spreadsheet then you'll be familiar with this approach.
###Code
def f(x):
return (sin(x) if 0 <= x <= pi else 0)
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
0 1.0 0
###Markdown
The ternary operator has the following form:
###Code
<expression if condition is True> if <condition> else <expression if condition is false>
###Output
_____no_output_____
###Markdown
Exercise: Express a step function as a Python functionThe following "step" function is known as the Heaviside function andis widely used in mathematics:$$H(x)=\begin{cases}0, & \text{if $x<0$}.\\\\1, & \text{if $x\ge 0$}.\end{cases}$$Write a Python function H(x) that computes H(x).
###Code
def f(x):
return (0 if x<0 else 1)
print(f(-0.5), f(2), f(0))
numpy.linspace?
###Output
_____no_output_____
###Markdown
Introduction to Python programming [Gerard Gorman](http://www.imperial.ac.uk/people/g.gorman), [Christian Jacobs](http://christianjacobs.uk/) Updated for MPECDT by [David Ham](http://www.imperial.ac.uk/people/david.ham) Lecture 2: Computing with basic language constructionsLearning objectives:* Introduction to two different forms of loops which are used to repeat operations on data: either until a condition is satisfied (*while-loop*) or for a fixed number of iterations (*for-loop*).* Form a *condition* using a *boolean expression*. This is used to either terminate loops or control if a specific block of code is executed via the *if-statement*.* Learn two different methods for storing many data elements within a single Python type.* Introduction to developing your own Python *functions* which allows you to execute the same block of code, with different inputs and outputs, from anywhere in the code. Boolean expressionsAn expression with value *true* or *false* is called a boolean expression. Example expressions for what you would write mathematically as$C=40$, $C\ne40$, $C\ge40$, $C\gt40$ and $C\lt40$ are:
###Code
C == 40 # Note: the double == checks for equality, but C=40 is an assignment!
C != 40 # This could also be written as 'not C == 4' (i.e. the opposite of what C == 4 gives)
C >= 40
C > 40
C < 40
###Output
_____no_output_____
###Markdown
We can test boolean expressions in a Python shell:
###Code
C = 41
print("C != 40: ", C != 40)
print("C < 40: ", C < 40)
print("C == 41: ", C == 41)
###Output
_____no_output_____
###Markdown
Several conditions can be combined with the special 'and' and 'or' operators into a single boolean expression:
###Code
condition1 and condition2
condition1 or condition2
###Output
_____no_output_____
###Markdown
* Rule 1: (**C1** *and* **C2**) is *True* only if both **C1** and **C2** are *True** Rule 2: (**C1** *or* **C2**) is *True* if either **C1** or **C2** are *True*Examples:
###Code
x=0; y=1.2
print (x >= 0 and y < 1)
###Output
_____no_output_____
###Markdown
Exercise: Values of boolean expressionsAdd a comment to the code below to explain the outcome of each of the boolean expressions:
###Code
C = 41
print("Case 1: ", C == 40)
print("Case 2: ", C != 40 and C < 41)
print("Case 3: ", C != 40 or C < 41)
print("Case 4: ", not C == 40)
print("Case 5: ", not C > 40)
print("Case 6: ", C <= 41)
print("Case 7: ", not False)
print("Case 8: ", True and False)
print("Case 9: ", False or True)
print("Case 10: ", False or False or False)
print("Case 11: ", True and True and False)
print("Case 12: ", False == 0)
print("Case 13: ", True == 0)
print("Case 14: ", True == 1)
###Output
Case 1: False
Case 2: False
Case 3: True
Case 4: True
Case 5: False
Case 6: True
Case 7: True
Case 8: False
Case 9: True
Case 10: False
Case 11: False
Case 12: True
Case 13: False
Case 14: True
###Markdown
LoopsSuppose we want to make a table of Celsius and Fahrenheitdegrees:
###Code
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Output
_____no_output_____
###Markdown
How do we write a program that prints out such a table?We know from the last lecture how to make one line in this table:
###Code
C = -20
F = 9/5*C + 32
print(C, F)
###Output
_____no_output_____
###Markdown
We can just repeat these statements:
###Code
C=-20; F=9/5*C+32; print(C,F)
C=-15; F=9/5*C+32; print(C,F)
C=-10; F=9/5*C+32; print(C,F)
C=-5; F=9/5*C+32; print(C,F)
C=0; F=9/5*C+32; print(C,F)
C=5; F=9/5*C+32; print(C,F)
C=10; F=9/5*C+32; print(C,F)
C=15; F=9/5*C+32; print(C,F)
C=20; F=9/5*C+32; print(C,F)
C=25; F=9/5*C+32; print(C,F)
C=30; F=9/5*C+32; print(C,F)
C=35; F=9/5*C+32; print(C,F)
C=40; F=9/5*C+32; print(C,F)
###Output
_____no_output_____
###Markdown
So we can see that works but its **very boring** to write and very easy to introduce a misprint.**You really should not be doing boring repetitive tasks like this.** Spend one time instead looking for a smarter solution. When programming becomes boring, there is usually a construct that automates the writing. Computers are very good at performing repetitive tasks. For this purpose we use **loops**. The while loop (and the significance of indentation)A while loop executes repeatedly a set of statements as long as a **boolean** (i.e. *True* / *False*) condition is *True*
###Code
while condition:
<statement 1>
<statement 2>
...
<first statement after loop>
###Output
_____no_output_____
###Markdown
Note that all statements to be executed within the loop must be indented by the same amount! The loop ends when an unindented statement is encountered.At this point it is worth noticing that **blank spaces may or may not be important** in Python programs. These statements are equivalent (blanks do not matter):
###Code
v0=3
v0 = 3
v0= 3
# The computer does not care but this formatting style is
# considered clearest for the human reader.
v0 = 3
###Output
_____no_output_____
###Markdown
Here is a while loop example where blank spaces really do matter:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
11
###Markdown
Let's take a look at what happens when we forget to indent correctly:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
1
2
3
4
5
6
7
8
9
10
11
###Markdown
Let's use the while loop to create the table above:
###Code
C = -20 # Initialise C
dC = 5 # Increment for C within the loop
while C <= 40: # Loop heading with condition
F = (9/5)*C + 32 # 1st statement inside loop
print ("%3d %6.1f" % (C, F)) # 2nd statement inside loop
C = C + dC # Increment C for the next iteration of the loop.
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Exercise: Make a Fahrenheit-Celsius conversion tableWrite a program that prints out a table with Fahrenheit degrees 0, 10, 20, ..., 100 in the first column and the corresponding Celsius degrees in the second column. Exercise: Write an approximate Fahrenheit-Celsius conversion tableMany people use an approximate formula for quickly converting Fahrenheit ($F$) to Celsius ($C$) degrees:$C \approx \hat{C} = \frac{F − 30}{2}$Modify the program from the previous exercise so that it prints three columns: $F$, $C$, and the approximate value $\hat{C}$. ListsSo far, one variable has referred to one number (or string). Sometimes however we naturally have a collection of numbers, saydegrees −20, −15, −10, −5, 0, ..., 40. One way to store these values in a computer program would be to have one variable per value, i.e.
###Code
C1 = -20
C2 = -15
C3 = -10
# ...
C13 = 40
###Output
_____no_output_____
###Markdown
This is clearly a terrible solution, particularly if we have lots of values. A better way of doing this is to collect values together in a list:
###Code
C = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
###Output
_____no_output_____
###Markdown
Now there is just one variable, **C**, holding all the values. Elements in a list are accessed via an index. List indices are always numbered as 0, 1, 2, and so forth up to the number of elements minus one:
###Code
mylist = [4, 6, -3.5]
print(mylist[0])
print(mylist[1])
print(mylist[2])
print(len(mylist))# length of list
print(mylist[0:2])
print(mylist[-1])
###Output
4
6
-3.5
3
[4, 6]
-3.5
###Markdown
Here are a few example of operations that you can perform on lists:
###Code
C = [-10, -5, 0, 5, 10, 15, 20, 25, 30]
C.append(35) # add new element 35 at the end
print(C)
print(C[-1])
C = C + [40,45] # And another list to the end of C
print(C)
C.insert(0, -15) # Insert -15 as index 0
print(C)
del C[2] # delete 3rd element
print(C)
del C[2] # delete what is now 3rd element
print(C)
print(len(C)) # length of list
print(C.index(10)) # Find the index of the list with the value 10
print (10 in C) # True only if the value 10 is stored in the list
print(C[-1]) # The last value in the list.
print(C[-2]) # The second last value in the list.
###Output
_____no_output_____
###Markdown
You can also extract sublists using ":"
###Code
print(C[5:]) # From index 5 to the end of the list.
print(C[5:7]) # From index 5 up to, but not including index 7.
###Output
_____no_output_____
###Markdown
Many languages (especially Matlab and Fortran) have would include the endpoint of a slice (7 in this case). One way to think of what Python does is that the slice is a half-open interval: $[5,7)$. This makes cutting a list in half particularly easy. For example:
###Code
print(C[:5] + ["Hello"] + C[5:])
print(C[7:-1]) # From index 7 up to the second last element.
print(C[:]) # [:] specifies the whole list.
somelist = ['Curly', 'Larry', 'Moe', 'dsbh']
stooge1, stooge2, stooge3 = somelist # Unpack the entries in a list.
print(stooge3, stooge2, stooge1)
###Output
_____no_output_____
###Markdown
Of course this only works if `somelist` contains exactly the number of items on the left hand side of the equals. Another useful operator is `*` (pronounced "splat"). It turns a list (or any other sequence) into the set of arguments of a function. For example:
###Code
print(somelist) # Prints the list.
print(*somelist) # Prints the items in the list.
###Output
['Curly', 'Larry', 'Moe']
Curly Larry Moe
###Markdown
Exercise: Store odd numbers in a listStep 1: Write a program that generates all odd numbers from 1 to *n*. Set *n* in the beginning of the program and use a while loop to compute the numbers. (Make sure that if *n* is an even number, the largest generated odd number is *n*-1.).Step 2: Store the generated odd numbers in a list. Start with an empty list and use the same while loop where you generate each odd number, ao append the new number to the list.Finally, print the list elements to the screen.
###Code
odds = []
n = 99
i = 1
while i<=n:
if i%2==1:
odds = odds + [i]
i=i+1
print(odds)
###Output
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21, 23, 25, 27, 29, 31, 33, 35, 37, 39, 41, 43, 45, 47, 49, 51, 53, 55, 57, 59, 61, 63, 65, 67, 69, 71, 73, 75, 77, 79, 81, 83, 85, 87, 89, 91, 93, 95, 97, 99]
###Markdown
For loopsWe can visit each element in a list and process the element with some statements using a *for* loop, for example:
###Code
degrees = [0, 10, 20, 40, 100]
for C in degrees:
print('list element:', C)
print('The degrees list has', len(degrees), 'elements')
###Output
list element: 0
list element: 10
list element: 20
list element: 40
list element: 100
The degrees list has 5 elements
###Markdown
Notice again how the statements to be executed within the loop must be indented! Let's now revisit the conversion table example using the *for* loop:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
for C in Cdegrees:
F = (9/5)*C + 32
print("%3d %6.2f" % (C, F))
###Output
_____no_output_____
###Markdown
It is also possible to rewrite the *for* loop as a *while* loop, i.e.,
###Code
for element in somelist:
# process element
###Output
_____no_output_____
###Markdown
can always be transformed to a *while* loop
###Code
index = 0
while index < len(somelist):
element = somelist[index]
# process element
index += 1
###Output
_____no_output_____
###Markdown
Taking the previous table example:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
index = 0
while index < len(Cdegrees):
C = Cdegrees[index]
F = (9/5)*C + 32
print('%5d %5.1f' % (C, F))
index += 1
###Output
_____no_output_____
###Markdown
Rather than just printing out the Fahrenheit values, let's also store these computed values in a list of their own:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
Fdegrees = [] # start with empty list
for C in Cdegrees:
F = (9/5)*C + 32
Fdegrees.append(F) # add new element to Fdegrees
print(Fdegrees)
###Output
_____no_output_____
###Markdown
Iterators: things you can iterate over.We've seen that a `for` loop can iterate over list values. In fact you can iterate over any object that is capable of providing values one at a time. In python, these objects are called `iterators`.A particularly useful python function which creates an iterator is `range`. For example, we can use range to iterate over the *indices* of a list:
###Code
for i in range(0, len(somelist), 1):
element = somelist[i]
... process element or somelist[i] directly
###Output
_____no_output_____
###Markdown
The statement *range(start, stop, inc)* generates an iterator over the integers *start*, *start+inc*, *start+2\*inc*, and so on up to, but not including, *stop*. We can also write *range(stop)* as an abbreviation for *range(0, stop, 1)*:
###Code
print(range(3)) # same as range(0, 3, 1)
###Output
_____no_output_____
###Markdown
Oh, that wasn't very informative. If we want to see all the numbers which the `range` will iterate over, we can cast the range to a list:
###Code
print(list(range(3)))
print(list(range(2, 9, 3)))
###Output
[2, 5, 8]
###Markdown
List comprehensionsConsider this example where we compute two lists in a *for* loop:
###Code
n = 16
Cdegrees = []; Fdegrees = [] # empty lists
for i in range(n):
Cdegrees.append(-5 + i*0.5)
Fdegrees.append((9/5)*Cdegrees[i] + 32)
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
_____no_output_____
###Markdown
As constructing lists is a very common requirement, the above way of doing it can become very tedious to both write and read. Therefore, Python has a compact construct, called list comprehension for generating lists from a *for* loop:
###Code
n = 16
Cdegrees = [-5 + i*0.5 for i in range(n)]
Fdegrees = [(9/5)*C + 32 for C in Cdegrees]
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
The general form of a list comprehension is:
###Code
somelist = [expression for element in somelist]
###Output
_____no_output_____
###Markdown
Exercise: Repeat the previous exercise of creating a list of odd numbers using using: a for loop, list comprehension and the range function
###Code
# Using for loop
# Using list comprehension
# Using the function range
###Output
_____no_output_____
###Markdown
Changing elements in a listSay we want to add $2$ to all the numbers in a list:
###Code
v = [-1, 1, 10]
for e in v:
e = e + 2
print(v)
###Output
_____no_output_____
###Markdown
We can see that the list *v* is unaltered! The reason for this is that inside the loop, *e* is an ordinary (int) variable. At the start of the iteration *e* is assigned a *copy* of the next element in the list. Inside the loop we can change *e* but *v* itself is unaltered. If we want to change *v* we have to use an index to access and modify its elements:
###Code
v[1] = 4 # assign 4 to 2nd element (index 1) in v
print(v)
###Output
_____no_output_____
###Markdown
To add 2 to all values we need a *for* loop over indices:
###Code
for i in range(len(v)):
v[i] = v[i] + 2
print(v)
###Output
_____no_output_____
###Markdown
Traversing multiple lists simultaneously - *zip(list1, list2, ...)*Consider how we can loop over elements in both Cdegrees and Fdegrees at the same time. One approach would be to use list indices:
###Code
for i in range(len(Cdegrees)):
print(Cdegrees[i], Fdegrees[i])
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
An alternative construct (regarded as more ”Pythonic”) uses the *zip* function:
###Code
for C, F in zip(Cdegrees, Fdegrees):
print(C, F)
print(list(zip(Cdegrees,Fdegrees[12:-1])))
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
[(-5.0, 33.8), (-4.5, 34.7), (-4.0, 35.6)]
###Markdown
`zip` creates an *iterator* over the corresponding items of its arguments in order.Another example with three lists:
###Code
l1 = [3, 6, 1]; l2 = [1.5, 1, 0]; l3 = [9.1, 3, 2]
for e in zip(l1, l2, l3):
print(*e)
###Output
3 1.5 9.1
6 1 3
1 0 2
###Markdown
If the lists are of unequal length then the loop stops when the end of the shortest list is reached. Experiment with this:
###Code
l1 = [3, 6, 1, 4, 6]; l2 = [1.5, 1, 0, 7]; l3 = [9.1, 3, 2, 0, 9]
for e in zip(l1, l2, l3):
print(*e)
###Output
3 1.5 9.1
6 1 3
1 0 2
4 7 0
###Markdown
Nested lists: list of listsA *list* can contain **any** object, including another *list*. To illustrate this, consider how to store the conversion table as a single Python list rather than two separate lists.
###Code
Cdegrees = range(-20, 41, 5)
Fdegrees = [(9.0/5)*C + 32 for C in Cdegrees]
table1 = [Cdegrees, Fdegrees] # List of two lists
print("table1 = ", table1)
print("table1[0] = ", table1[0])
print("table1[1] = ", table1[1])
print("table1[1][3] = ", table1[1][3])
###Output
table1 = [range(-20, 41, 5), [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]]
table1[0] = range(-20, 41, 5)
table1[1] = [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]
table1[1][3] = 23.0
###Markdown
This gives us a table of two columns. How do we create a table of rows instead:
###Code
table2 = []
for C, F in zip(Cdegrees, Fdegrees):
row = [C, F]
table2.append(row)
print(table2)
###Output
_____no_output_____
###Markdown
We can use `zip` to do this more elegantly:
###Code
table2 = list(zip(Cdegrees, Fdegrees))
print(table2)
###Output
_____no_output_____
###Markdown
And you can loop through this list as before:
###Code
for C, F in table2:
print(C, F)
###Output
_____no_output_____
###Markdown
Tuples: sequences that cannot be changedWe've already learned that lists are not the only thing which can be iterated over, but that there is a general category of `iterator` types. Similarly, lists are not the only objects which can be indexed. The general category of types which can be indexed are the `sequence` types.Tuples are **constant** sequences. You can use them in much the same way as lists except you cannot modify them. They are an example of an [**immutable**](http://en.wikipedia.org/wiki/Immutable_object) type.
###Code
t = (2, 4, 6, 'temp.pdf') # Define a tuple.
t = 2, 4, 6, 'temp.pdf' # Can skip parenthesis as it is assumed in this context.
print(t[1])
###Output
4
###Markdown
Let's see what happens when we try to modify the tuple like we did with a list:
###Code
s = (1,)
print(s)
t[1] = -1
t.append(0)
del t[1]
###Output
_____no_output_____
###Markdown
However, we can use the tuple to compose a new tuple:
###Code
t = t + (-1.0, -2.0)
print(t)
###Output
_____no_output_____
###Markdown
So, why would we use tuples when lists have more functionality?* Tuples are constant and thus protected against accidental changes.* Tuples are faster than lists.* Tuples are widely used in Python software (so you need to know about tuples to understand other people's code!)* Tuples (but not lists) can be used as keys in dictionaries (more about dictionaries later). Exercise: Make a table of function valuesStep 1: Write a program that prints a table with $t$ values in the first column and the corresponding $y(t) = v_0 t − 0.5gt^2$ values in the second column. Use $n$ uniformly spaced $t$ values throughout the interval [0, $2v_0/g$]. Set $v0 = 1$, $g = 9.81$, and $n = 11$.Step 2: Once step 1 is working, modify the program so that the $t$ and $y$ values are stored in two lists *t* and *y*. Thereafter, transverse the lists with a *for* loop and write out a nicely formatted table of *t* and *y* values using a *zip* construction. FunctionsWe have already used many Python functions, including mathematical functions:
###Code
from math import *
x = pi
print cos(x)
###Output
_____no_output_____
###Markdown
Other functions you used include *len* and *range*:
###Code
n = len(somelist)
ints = range(5, n, 2)
###Output
_____no_output_____
###Markdown
You have also used functions that are executed with the dot syntax (called *methods*):
###Code
C = [5, 10, 40, 45]
i = C.index(10)
C.append(50)
C.insert(2, 20)
###Output
_____no_output_____
###Markdown
A function is a collection of statements we can execute wherever and whenever we want. Functions can take any number of inputs (called *arguments*) to produce outputs. Functions help to organize programs, make them more understandable, shorter, and easier to extend. For our first example we will turn our temperature conversion code into a function:
###Code
def C2F(C):
return (9/5)*C + 32
print(C2F(0))
###Output
32.0
###Markdown
Functions start with *def*, then the name you want to give your function, then a list of arguments (here C). This is referred to as the *function header*. Inside the function there is a block of statements called the *function body*. Notice that the function body is indented - as was the case for the *for* / *while* loop the indentation indicates where the function ends. At any point within the function, we can "stop thefunction" and return as many values/variables as required. Local and global variablesVariables defined within a function are said to have *local scope*. That is to say that they can only be referenced within that function. Let's consider an example (and look carefully at the indentation!!):
###Code
def sumint(start, stop):
s = 0 # variable for accumulating the sum
i = start # counter
while i <= stop:
s += i
i += 1
return s
print(sumint(0, 5))
###Output
_____no_output_____
###Markdown
Variables *i* and *s* are local variables in *sumint*. They are destroyed at the end (return) of the function and never visible outside the function (in the calling program); in fact, *start* and *stop* are also local variables. So let's see what happens if we now try to print one of these variables:
###Code
print(stop)
###Output
_____no_output_____
###Markdown
Functions can also return multiple values. Let's recycle another of our previous examples - compute $y(t)$ and $y'(t)=v_0-gt$:
###Code
def yfunc(t, v0):
g = 9.81
y = v0*t - 0.5*g*t**2
dydt = v0 - g*t
return y, dydt
# call:
position, velocity = yfunc(0.6, 3)
print(position, velocity)
###Output
_____no_output_____
###Markdown
Remember that a series of comma separated variables implies a tuple - therefore "return y, dydt" is the same as writing "return (y, dydt)". Therefore, in general what is returned is a tuple. Let's take a look at another example illustrating this:
###Code
def f(x):
return x, x**2, x**4
s = f(2)
print(s)
print(type(s)) # The function type() tells us what type a variable it is.
###Output
_____no_output_____
###Markdown
No return value implies that `None` is returned. `None` is a special Python object that represents an ”empty” or undefined value. It is surprisingly useful and we will use it a lot later.
###Code
def message(course):
print("%s rocks!"% course)
message("Python")
r = message("Geo")
print("r = ", r)
###Output
_____no_output_____
###Markdown
Keyword arguments and default argument valuesIt's possible to specify default values that function arguments should take if no values are provided when the function is called:
###Code
def somefunc(arg1, arg2, kwarg1=True, kwarg2=0):
print(arg1, arg2, kwarg1, kwarg2)
somefunc("Hello", [1,2]) # Note that we have not specified values for kwarg1 and kwarg2
###Output
Hello [1, 2] True 0
###Markdown
You can override the default values by passing additional arguments in the correct position:
###Code
somefunc("Hello", [1,2], "Hi")
###Output
Hello [1, 2] Hi 0
###Markdown
... or by naming the argument to which the value should be passed. These are called keyword arguments:
###Code
somefunc("Hello", [1,2], kwarg2="Hi")
def foo(*args):
print(args)
foo(1,2)
foo("hello", "world")
somefunc("Hello", [1,2], kwarg2="Hi", kwarg1=6)
###Output
Hello [1, 2] 6 Hi
###Markdown
If we use variable_name=value for all arguments, their sequence in the function header can be in any order.
###Code
somefunc(kwarg2="Hello", arg1="Hi", kwarg1=6, arg2=[2])
###Output
Hi [2] 6 Hello
###Markdown
Let's look at another example - consider a function of $t$, with parameters $A$, $a$, and $\omega$:$$f(t; A,a, \omega) = Ae^{-at}\sin (\omega t)$$. (The choice of equation is actually pretty random - but it serves to show you that it is easy to translate formulae you encounter into Python code). We can implement $f$ in a Python function with $t$ as positional argument and $A$, $a$, and $\omega$ as keyword arguments.
###Code
from math import pi, exp, sin
def f(t, A=1, a=1, ω=2*pi):
return A*exp(-a*t)*sin(ω*t)
v1 = f(0.2)
v2 = f(0.2, ω=1)
v2 = f(0.2, 1, 3) # same as f(0.2, A=1, a=3)
v3 = f(0.2, ω=1, A=2.5)
v4 = f(A=5, a=0.1, ω=1, t=1.3)
v5 = f(t=0.2, A=9)
print(v1, v2, v3, v4, v5)
###Output
0.778659217806053 0.5219508827258282 0.40664172703834794 4.230480200204721 7.007932960254476
###Markdown
Exercise: Implement a Gaussian functionMake a Python function *gauss*( *x*, *m*=0, *s*=1) for computing the Gaussian function $$f(x)=\frac{1}{\sqrt{2\pi}s}\exp\left(-\frac{1}{2} \left(\frac{x-m}{s}\right)^2\right)$$Call the function and print out the result for x equal to −5, −4.9, −4.8, ..., 4.8, 4.9, 5, using default values for *m* and *s*.
###Code
import matplotlib.pyplot as plt
from math import pi,sqrt,exp
def gauss(x,m,s=1):
return (1/(sqrt(2*pi)*s))*exp(-0.5*(x-m)*(x-m)/(s*s))
x = -5
X =[]
G = []
while x<=5:
print("%4.1f %10f" % (x,gauss(x,2)))
X = X + [x]
G = G + [gauss(x,2)]
x = x + 0.1
plt.plot(X,G)
plt.show()
###Output
-5.0 0.000000
-4.9 0.000000
-4.8 0.000000
-4.7 0.000000
-4.6 0.000000
-4.5 0.000000
-4.4 0.000000
-4.3 0.000000
-4.2 0.000000
-4.1 0.000000
-4.0 0.000000
-3.9 0.000000
-3.8 0.000000
-3.7 0.000000
-3.6 0.000000
-3.5 0.000000
-3.4 0.000000
-3.3 0.000000
-3.2 0.000001
-3.1 0.000001
-3.0 0.000001
-2.9 0.000002
-2.8 0.000004
-2.7 0.000006
-2.6 0.000010
-2.5 0.000016
-2.4 0.000025
-2.3 0.000039
-2.2 0.000059
-2.1 0.000089
-2.0 0.000134
-1.9 0.000199
-1.8 0.000292
-1.7 0.000425
-1.6 0.000612
-1.5 0.000873
-1.4 0.001232
-1.3 0.001723
-1.2 0.002384
-1.1 0.003267
-1.0 0.004432
-0.9 0.005953
-0.8 0.007915
-0.7 0.010421
-0.6 0.013583
-0.5 0.017528
-0.4 0.022395
-0.3 0.028327
-0.2 0.035475
-0.1 0.043984
-0.0 0.053991
0.1 0.065616
0.2 0.078950
0.3 0.094049
0.4 0.110921
0.5 0.129518
0.6 0.149727
0.7 0.171369
0.8 0.194186
0.9 0.217852
1.0 0.241971
1.1 0.266085
1.2 0.289692
1.3 0.312254
1.4 0.333225
1.5 0.352065
1.6 0.368270
1.7 0.381388
1.8 0.391043
1.9 0.396953
2.0 0.398942
2.1 0.396953
2.2 0.391043
2.3 0.381388
2.4 0.368270
2.5 0.352065
2.6 0.333225
2.7 0.312254
2.8 0.289692
2.9 0.266085
3.0 0.241971
3.1 0.217852
3.2 0.194186
3.3 0.171369
3.4 0.149727
3.5 0.129518
3.6 0.110921
3.7 0.094049
3.8 0.078950
3.9 0.065616
4.0 0.053991
4.1 0.043984
4.2 0.035475
4.3 0.028327
4.4 0.022395
4.5 0.017528
4.6 0.013583
4.7 0.010421
4.8 0.007915
4.9 0.005953
5.0 0.004432
###Markdown
The *if* statementConsider this simple example:
###Code
def f(x):
if 0 <= x <= pi:
return sin(x)
else:
return 0
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
0 1.0 0
###Markdown
In general (the *else* block can be skipped if there are no statements to be executed when False) we can put together multiple conditions. Only the first condition that is True is executed.
###Code
if condition1:
<block of statements, executed if condition1 is True>
elif condition2:
<block of statements>
elif condition3:
<block of statements>
else:
<block of statements>
<next statement of the program>
###Output
_____no_output_____
###Markdown
The ternary operatorThere is another form of *if* which works as an operator, rather than a statement. If you've used the *if* function in a spreadsheet then you'll be familiar with this approach.
###Code
def f(x):
return (sin(x) if 0 <= x <= pi else 0)
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
0 1.0 0
###Markdown
The ternary operator has the following form:
###Code
<expression if condition is True> if <condition> else <expression if condition is false>
###Output
_____no_output_____
###Markdown
Exercise: Express a step function as a Python functionThe following "step" function is known as the Heaviside function andis widely used in mathematics:$$H(x)=\begin{cases}0, & \text{if $x<0$}.\\\\1, & \text{if $x\ge 0$}.\end{cases}$$Write a Python function H(x) that computes H(x).
###Code
import matplotlib.pyplot as plt
def H(x):
return (1 if x>=0 else 0)
x = -10
X = []
Y = []
while x<=10:
X = X + [x]
Y = Y + [H(x)]
x += 0.1
plt.plot(X,Y)
plt.show()
###Output
_____no_output_____
###Markdown
Introduction to Python programming [Gerard Gorman](http://www.imperial.ac.uk/people/g.gorman), [Christian Jacobs](http://christianjacobs.uk/) Updated for MPECDT by [David Ham](http://www.imperial.ac.uk/people/david.ham) Lecture 2: Computing with basic language constructionsLearning objectives:* Introduction to two different forms of loops which are used to repeat operations on data: either until a condition is satisfied (*while-loop*) or for a fixed number of iterations (*for-loop*).* Form a *condition* using a *boolean expression*. This is used to either terminate loops or control if a specific block of code is executed via the *if-statement*.* Learn two different methods for storing many data elements within a single Python type.* Introduction to developing your own Python *functions* which allows you to execute the same block of code, with different inputs and outputs, from anywhere in the code. Boolean expressionsAn expression with value *true* or *false* is called a boolean expression. Example expressions for what you would write mathematically as$C=40$, $C\ne40$, $C\ge40$, $C\gt40$ and $C\lt40$ are:
###Code
C == 40 # Note: the double == checks for equality, but C=40 is an assignment!
C != 40 # This could also be written as 'not C == 4' (i.e. the opposite of what C == 4 gives)
C >= 40
C > 40
C < 40
###Output
_____no_output_____
###Markdown
We can test boolean expressions in a Python shell:
###Code
C = 41
print("C != 40: ", C != 40)
print("C < 40: ", C < 40)
print("C == 41: ", C == 41)
###Output
C != 40: True
C < 40: False
C == 41: True
###Markdown
Several conditions can be combined with the special 'and' and 'or' operators into a single boolean expression:
###Code
condition1 and condition2
condition1 or condition2
###Output
_____no_output_____
###Markdown
* Rule 1: (**C1** *and* **C2**) is *True* only if both **C1** and **C2** are *True** Rule 2: (**C1** *or* **C2**) is *True* if either **C1** or **C2** are *True*Examples:
###Code
x=0; y=1.2
print (x >= 0 and y < 1)
###Output
False
###Markdown
Exercise: Values of boolean expressionsAdd a comment to the code below to explain the outcome of each of the boolean expressions:
###Code
C = 41
print("Case 1: ", C == 40)
print("Case 2: ", C != 40 and C < 41)
print("Case 3: ", C != 40 or C < 41)
print("Case 4: ", not C == 40)
print("Case 5: ", not C > 40)
print("Case 6: ", C <= 41)
print("Case 7: ", not False)
print("Case 8: ", True and False)
print("Case 9: ", False or True)
print("Case 10: ", False or False or False)
print("Case 11: ", True and True and False)
print("Case 12: ", False == 0)
print("Case 13: ", True == 0)
print("Case 14: ", True == 1)
###Output
Case 1: False
Case 2: False
Case 3: True
Case 4: True
Case 5: False
Case 6: True
Case 7: True
Case 8: False
Case 9: True
Case 10: False
Case 11: False
Case 12: True
Case 13: False
Case 14: True
###Markdown
LoopsSuppose we want to make a table of Celsius and Fahrenheitdegrees:
###Code
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Output
_____no_output_____
###Markdown
How do we write a program that prints out such a table?We know from the last lecture how to make one line in this table:
###Code
C = -20
F = 9/5*C + 32
print(C, F)
###Output
-20 -4.0
###Markdown
We can just repeat these statements:
###Code
C=-20; F=9/5*C+32; print(C,F)
C=-15; F=9/5*C+32; print(C,F)
C=-10; F=9/5*C+32; print(C,F)
C=-5; F=9/5*C+32; print(C,F)
C=0; F=9/5*C+32; print(C,F)
C=5; F=9/5*C+32; print(C,F)
C=10; F=9/5*C+32; print(C,F)
C=15; F=9/5*C+32; print(C,F)
C=20; F=9/5*C+32; print(C,F)
C=25; F=9/5*C+32; print(C,F)
C=30; F=9/5*C+32; print(C,F)
C=35; F=9/5*C+32; print(C,F)
C=40; F=9/5*C+32; print(C,F)
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
So we can see that works but its **very boring** to write and very easy to introduce a misprint.**You really should not be doing boring repetitive tasks like this.** Spend one time instead looking for a smarter solution. When programming becomes boring, there is usually a construct that automates the writing. Computers are very good at performing repetitive tasks. For this purpose we use **loops**. The while loop (and the significance of indentation)A while loop executes repeatedly a set of statements as long as a **boolean** (i.e. *True* / *False*) condition is *True*
###Code
while condition:
<statement 1>
<statement 2>
...
<first statement after loop>
###Output
_____no_output_____
###Markdown
Note that all statements to be executed within the loop must be indented by the same amount! The loop ends when an unindented statement is encountered.At this point it is worth noticing that **blank spaces may or may not be important** in Python programs. These statements are equivalent (blanks do not matter):
###Code
v0=3
v0 = 3
v0= 3
# The computer does not care but this formatting style is
# considered clearest for the human reader.
v0 = 3
###Output
_____no_output_____
###Markdown
Here is a while loop example where blank spaces really do matter:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
11
###Markdown
Let's take a look at what happens when we forget to indent correctly:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
_____no_output_____
###Markdown
Let's use the while loop to create the table above:
###Code
C = -20 # Initialise C
dC = 5 # Increment for C within the loop
while C <= 40: # Loop heading with condition
F = (9/5)*C + 32 # 1st statement inside loop
print ("%3d %6.1f" % (C, F)) # 2nd statement inside loop
C = C + dC # Increment C for the next iteration of the loop.
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Exercise: Make a Fahrenheit-Celsius conversion tableWrite a program that prints out a table with Fahrenheit degrees 0, 10, 20, ..., 100 in the first column and the corresponding Celsius degrees in the second column.
###Code
F = 0 # Initialise F
dF = 10 # Increment for F within the loop
while F <= 100:
C = (F - 32)*5/9 # Loop heading with condition
print ("%5.1f %4d" % (F, C)) # 2nd statement inside loop
F = F + dF # Increment F for the next iteration of the loop
###Output
0.0 -17
10.0 -12
20.0 -6
30.0 -1
40.0 4
50.0 10
60.0 15
70.0 21
80.0 26
90.0 32
100.0 37
###Markdown
Exercise: Write an approximate Fahrenheit-Celsius conversion tableMany people use an approximate formula for quickly converting Fahrenheit ($F$) to Celsius ($C$) degrees:$C \approx \hat{C} = \frac{F − 30}{2}$Modify the program from the previous exercise so that it prints three columns: $F$, $C$, and the approximate value $\hat{C}$.
###Code
F = 0
dF = 10
while F <= 100:
C = (F - 32)*5/9
C_m = (F - 30)/2
print("""%5.1f %5d %5d""" % (F, C, C_m))
F = F + dF
###Output
0.0 -17 -15
10.0 -12 -10
20.0 -6 -5
30.0 -1 0
40.0 4 5
50.0 10 10
60.0 15 15
70.0 21 20
80.0 26 25
90.0 32 30
100.0 37 35
###Markdown
ListsSo far, one variable has referred to one number (or string). Sometimes however we naturally have a collection of numbers, saydegrees −20, −15, −10, −5, 0, ..., 40. One way to store these values in a computer program would be to have one variable per value, i.e.
###Code
C1 = -20
C2 = -15
C3 = -10
# ...
C13 = 40
###Output
_____no_output_____
###Markdown
This is clearly a terrible solution, particularly if we have lots of values. A better way of doing this is to collect values together in a list:
###Code
C = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
###Output
_____no_output_____
###Markdown
Now there is just one variable, **C**, holding all the values. Elements in a list are accessed via an index. List indices are always numbered as 0, 1, 2, and so forth up to the number of elements minus one:
###Code
mylist = [4, 6, -3.5]
print(mylist[0])
print(mylist[1])
print(mylist[2])
print(len(mylist)) # length of list
###Output
4
6
-3.5
3
###Markdown
Here are a few example of operations that you can perform on lists:
###Code
C = [-10, -5, 0, 5, 10, 15, 20, 25, 30]
C.append(35) # add new element 35 at the end
print(C)
C = C + [40,45] # And another list to the end of C
print(C)
C.insert(0, -15) # Insert -15 as index 0
print(C)
del C[2] # delete 3rd element
print(C)
del C[2] # delete what is now 3rd element
print(C)
print(len(C)) # length of list
print(C.index(10)) # Find the index of the list with the value 10
print (10 in C) # True only if the value 10 is stored in the list
print(C[-1]) # The last value in the list.
print(C[-2]) # The second last value in the list.
###Output
40
###Markdown
You can also extract sublists using ":"
###Code
print(C[5:]) # From index 5 to the end of the list.
print(C[5:7]) # From index 5 up to, but not including index 7.
###Output
[20, 25]
###Markdown
Many languages (especially Matlab and Fortran) have would include the endpoint of a slice (7 in this case). One way to think of what Python does is that the slice is a half-open interval: $[5,7)$. This makes cutting a list in half particularly easy. For example:
###Code
print(C[:5] + ["Hello"] + C[5:])
print(C[7:-1]) # From index 7 up to the second last element.
print(C[:]) # [:] specifies the whole list.
somelist = ['Curly', 'Larry', 'Moe']
stooge1, stooge2, stooge3 = somelist # Unpack the entries in a list.
print(stooge3, stooge2, stooge1)
###Output
Moe Larry Curly
###Markdown
Of course this only works if `somelist` contains exactly the number of items on the left hand side of the equals. Another useful operator is `*` (pronounced "splat"). It turns a list (or any other sequence) into the set of arguments of a function. For example:
###Code
print(somelist) # Prints the list.
print(*somelist) # Prints the items in the list.
###Output
['Curly', 'Larry', 'Moe']
Curly Larry Moe
###Markdown
Exercise: Store odd numbers in a listStep 1: Write a program that generates all odd numbers from 1 to *n*. Set *n* in the beginning of the program and use a while loop to compute the numbers. (Make sure that if *n* is an even number, the largest generated odd number is *n*-1.).Step 2: Store the generated odd numbers in a list. Start with an empty list and use the same while loop where you generate each odd number, ao append the new number to the list.Finally, print the list elements to the screen.
###Code
N = 20
cnt = -1
List = []
while(cnt <= N-3):
cnt = cnt + 2
List = List + [cnt]
print(List)
###Output
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
###Markdown
For loopsWe can visit each element in a list and process the element with some statements using a *for* loop, for example:
###Code
degrees = [0, 10, 20, 40, 100]
for C in degrees:
print('list element:', C)
print('The degrees list has', len(degrees), 'elements')
###Output
list element: 0
list element: 10
list element: 20
list element: 40
list element: 100
The degrees list has 5 elements
###Markdown
Notice again how the statements to be executed within the loop must be indented! Let's now revisit the conversion table example using the *for* loop:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
for C in Cdegrees:
F = (9/5)*C + 32
print("%3d %6.2f" % (C, F))
###Output
-20 -4.00
-15 5.00
-10 14.00
-5 23.00
0 32.00
5 41.00
10 50.00
15 59.00
20 68.00
25 77.00
30 86.00
35 95.00
40 104.00
###Markdown
It is also possible to rewrite the *for* loop as a *while* loop, i.e.,
###Code
for element in somelist:
# process element
###Output
_____no_output_____
###Markdown
can always be transformed to a *while* loop
###Code
index = 0
while index < len(somelist):
element = somelist[index]
# process element
index += 1
###Output
_____no_output_____
###Markdown
Taking the previous table example:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
index = 0
while index < len(Cdegrees):
C = Cdegrees[index]
F = (9/5)*C + 32
print('%5d %5.1f' % (C, F))
index += 1
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Rather than just printing out the Fahrenheit values, let's also store these computed values in a list of their own:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
Fdegrees = [] # start with empty list
for C in Cdegrees:
F = (9/5)*C + 32
Fdegrees.append(F) # add new element to Fdegrees
print(Fdegrees)
###Output
[-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]
###Markdown
Iterators: things you can iterate over.We've seen that a `for` loop can iterate over list values. In fact you can iterate over any object that is capable of providing values one at a time. In python, these objects are called `iterators`.A particularly useful python function which creates an iterator is `range`. For example, we can use range to iterate over the *indices* of a list:
###Code
for i in range(0, len(somelist), 1):
element = somelist[i]
... process element or somelist[i] directly
###Output
_____no_output_____
###Markdown
The statement *range(start, stop, inc)* generates an iterator over the integers *start*, *start+inc*, *start+2\*inc*, and so on up to, but not including, *stop*. We can also write *range(stop)* as an abbreviation for *range(0, stop, 1)*:
###Code
print(range(3)) # same as range(0, 3, 1)
###Output
range(0, 3)
###Markdown
Oh, that wasn't very informative. If we want to see all the numbers which the `range` will iterate over, we can cast the range to a list:
###Code
print(list(range(3)))
print(list(range(2, 8, 3)))
###Output
[2, 5]
###Markdown
List comprehensionsConsider this example where we compute two lists in a *for* loop:
###Code
n = 16
Cdegrees = []; Fdegrees = [] # empty lists
for i in range(n):
Cdegrees.append(-5 + i*0.5)
Fdegrees.append((9/5)*Cdegrees[i] + 32)
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
As constructing lists is a very common requirement, the above way of doing it can become very tedious to both write and read. Therefore, Python has a compact construct, called list comprehension for generating lists from a *for* loop:
###Code
n = 16
Cdegrees = [-5 + i*0.5 for i in range(n)]
Fdegrees = [(9/5)*C + 32 for C in Cdegrees]
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
The general form of a list comprehension is:
###Code
somelist = [expression for element in somelist]
###Output
_____no_output_____
###Markdown
Exercise: Repeat the previous exercise of creating a list of odd numbers using using: a for loop, list comprehension and the range function
###Code
# Using for loop
N = 10
List = []
for i in range(N):
List = List + [2*i + 1]
print(List)
# Using list comprehension
N = 10
List = []
List = [2*i + 1 for i in range(N)]
print(List)
# Using the function range
N = 10
List = []
List = [2*i + 1 for i in range(N)]
print(List)
###Output
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
###Markdown
Changing elements in a listSay we want to add $2$ to all the numbers in a list:
###Code
v = [-1, 1, 10]
for e in v:
e = e + 2
print(v)
###Output
[-1, 1, 10]
###Markdown
We can see that the list *v* is unaltered! The reason for this is that inside the loop, *e* is an ordinary (int) variable. At the start of the iteration *e* is assigned a *copy* of the next element in the list. Inside the loop we can change *e* but *v* itself is unaltered. If we want to change *v* we have to use an index to access and modify its elements:
###Code
v[1] = 4 # assign 4 to 2nd element (index 1) in v
print(v)
###Output
[-1, 4, 10]
###Markdown
To add 2 to all values we need a *for* loop over indices:
###Code
for i in range(len(v)):
v[i] = v[i] + 2
print(v)
###Output
[1, 3, 12]
###Markdown
Traversing multiple lists simultaneously - *zip(list1, list2, ...)*Consider how we can loop over elements in both Cdegrees and Fdegrees at the same time. One approach would be to use list indices:
###Code
for i in range(len(Cdegrees)):
print(Cdegrees[i], Fdegrees[i])
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
An alternative construct (regarded as more ”Pythonic”) uses the *zip* function:
###Code
for C, F in zip(Cdegrees, Fdegrees):
print(C, F)
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
`zip` creates an *iterator* over the corresponding items of its arguments in order.Another example with three lists:
###Code
l1 = [3, 6, 1]; l2 = [1.5, 1, 0]; l3 = [9.1, 3, 2]
for e in zip(l1, l2, l3):
print(*e)
###Output
3 1.5 9.1
6 1 3
1 0 2
###Markdown
If the lists are of unequal length then the loop stops when the end of the shortest list is reached. Experiment with this:
###Code
l1 = [3, 6, 1, 4, 6]; l2 = [1.5, 1, 0, 7]; l3 = [9.1, 3, 2, 0, 9]
for e in zip(l1, l2, l3):
print(*e)
###Output
3 1.5 9.1
6 1 3
1 0 2
4 7 0
###Markdown
Nested lists: list of listsA *list* can contain **any** object, including another *list*. To illustrate this, consider how to store the conversion table as a single Python list rather than two separate lists.
###Code
Cdegrees = range(-20, 41, 5)
Fdegrees = [(9.0/5)*C + 32 for C in Cdegrees]
table1 = [Cdegrees, Fdegrees] # List of two lists
print("table1 = ", table1)
print("table1[0] = ", table1[0])
print("table1[1] = ", table1[1])
print("table1[2][3] = ", table1[1][3])
###Output
table1 = [range(-20, 41, 5), [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]]
table1[0] = range(-20, 41, 5)
table1[1] = [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]
table1[2][3] = 23.0
###Markdown
This gives us a table of two columns. How do we create a table of rows instead:
###Code
table2 = []
for C, F in zip(Cdegrees, Fdegrees):
row = [C, F]
table2.append(row)
print(table2)
###Output
[[-20, -4.0], [-15, 5.0], [-10, 14.0], [-5, 23.0], [0, 32.0], [5, 41.0], [10, 50.0], [15, 59.0], [20, 68.0], [25, 77.0], [30, 86.0], [35, 95.0], [40, 104.0]]
###Markdown
We can use `zip` to do this more elegantly:
###Code
table2 = list(zip(Cdegrees, Fdegrees))
print(table2)
###Output
[(-20, -4.0), (-15, 5.0), (-10, 14.0), (-5, 23.0), (0, 32.0), (5, 41.0), (10, 50.0), (15, 59.0), (20, 68.0), (25, 77.0), (30, 86.0), (35, 95.0), (40, 104.0)]
###Markdown
And you can loop through this list as before:
###Code
for C, F in table2:
print(C, F)
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Tuples: sequences that cannot be changedWe've already learned that lists are not the only thing which can be iterated over, but that there is a general category of `iterator` types. Similarly, lists are not the only objects which can be indexed. The general category of types which can be indexed are the `sequence` types.Tuples are **constant** sequences. You can use them in much the same way as lists except you cannot modify them. They are an example of an [**immutable**](http://en.wikipedia.org/wiki/Immutable_object) type.
###Code
t = (2, 4, 6, 'temp.pdf') # Define a tuple.
t = 2, 4, 6, 'temp.pdf' # Can skip parenthesis as it is assumed in this context.
###Output
_____no_output_____
###Markdown
Let's see what happens when we try to modify the tuple like we did with a list:
###Code
t[1] = -1
t.append(0)
del t[1]
###Output
_____no_output_____
###Markdown
However, we can use the tuple to compose a new tuple:
###Code
t = t + (-1.0, -2.0)
print(t)
###Output
(2, 4, 6, 'temp.pdf', -1.0, -2.0)
###Markdown
So, why would we use tuples when lists have more functionality?* Tuples are constant and thus protected against accidental changes.* Tuples are faster than lists.* Tuples are widely used in Python software (so you need to know about tuples to understand other people's code!)* Tuples (but not lists) can be used as keys in dictionaries (more about dictionaries later). Exercise: Make a table of function valuesStep 1: Write a program that prints a table with $t$ values in the first column and the corresponding $y(t) = v_0 t − 0.5gt^2$ values in the second column. Use $n$ uniformly spaced $t$ values throughout the interval [0, $2v_0/g$]. Set $v0 = 1$, $g = 9.81$, and $n = 11$.Step 2: Once step 1 is working, modify the program so that the $t$ and $y$ values are stored in two lists *t* and *y*. Thereafter, transverse the lists with a *for* loop and write out a nicely formatted table of *t* and *y* values using a *zip* construction.
###Code
# Step 1
def v(t, v0):
g = 9.81
y = v0*t - 0.5*g*t**2
return t, y
v0 = 1
tmax =
t, y = v(t, v0)
print(t, y)
# Step 2
###Output
0.001 0.000995095
###Markdown
We have already used many Python functions, including mathematical functions:
###Code
import numpy as np
x = np.pi
print cos(x)
###Output
_____no_output_____
###Markdown
Other functions you used include *len* and *range*:
###Code
n = len(somelist)
ints = range(5, n, 2)
###Output
_____no_output_____
###Markdown
You have also used functions that are executed with the dot syntax (called *methods*):
###Code
C = [5, 10, 40, 45]
i = C.index(10)
C.append(50)
C.insert(2, 20)
###Output
_____no_output_____
###Markdown
A function is a collection of statements we can execute wherever and whenever we want. Functions can take any number of inputs (called *arguments*) to produce outputs. Functions help to organize programs, make them more understandable, shorter, and easier to extend. For our first example we will turn our temperature conversion code into a function:
###Code
def C2F(C):
return (9/5)*C + 32
###Output
_____no_output_____
###Markdown
Functions start with *def*, then the name you want to give your function, then a list of arguments (here C). This is referred to as the *function header*. Inside the function there is a block of statements called the *function body*. Notice that the function body is indented - as was the case for the *for* / *while* loop the indentation indicates where the function ends. At any point within the function, we can "stop thefunction" and return as many values/variables as required. Local and global variablesVariables defined within a function are said to have *local scope*. That is to say that they can only be referenced within that function. Let's consider an example (and look carefully at the indentation!!):
###Code
def sumint(start, stop):
s = 0 # variable for accumulating the sum
i = start # counter
while i <= stop:
s += i
i += 1
return s
print(sumint(0, 5))
###Output
15
###Markdown
Variables *i* and *s* are local variables in *sumint*. They are destroyed at the end (return) of the function and never visible outside the function (in the calling program); in fact, *start* and *stop* are also local variables. So let's see what happens if we now try to print one of these variables:
###Code
print(stop)
###Output
_____no_output_____
###Markdown
Functions can also return multiple values. Let's recycle another of our previous examples - compute $y(t)$ and $y'(t)=v_0-gt$:
###Code
def yfunc(t, v0):
g = 9.81
y = v0*t - 0.5*g*t**2
dydt = v0 - g*t
return y, dydt
# call:
position, velocity = yfunc(0.6, 3)
print(position, velocity)
###Output
0.034199999999999786 -2.886
###Markdown
Remember that a series of comma separated variables implies a tuple - therefore "return y, dydt" is the same as writing "return (y, dydt)". Therefore, in general what is returned is a tuple. Let's take a look at another example illustrating this:
###Code
def f(x):
return x, x**2, x**4
s = f(2)
print(s)
print(type(s)) # The function type() tells us what type a variable it is.
###Output
(2, 4, 16)
<class 'tuple'>
###Markdown
No return value implies that `None` is returned. `None` is a special Python object that represents an ”empty” or undefined value. It is surprisingly useful and we will use it a lot later.
###Code
def message(course):
print("%s rocks!"% course)
message("Python")
r = message("Geo")
print("r = ", r)
###Output
Python rocks!
Geo rocks!
r = None
###Markdown
Keyword arguments and default argument valuesIt's possible to specify default values that function arguments should take if no values are provided when the function is called:
###Code
def somefunc(arg1, arg2, kwarg1=True, kwarg2=0):
print(arg1, arg2, kwarg1, kwarg2)
somefunc("Hello", [1,2]) # Note that we have not specified values for kwarg1 and kwarg2
###Output
Hello [1, 2] True 0
###Markdown
You can override the default values by passing additional arguments in the correct position:
###Code
somefunc("Hello", [1,2], "Hi")
###Output
Hello [1, 2] Hi 0
###Markdown
... or by naming the argument to which the value should be passed. These are called keyword arguments:
###Code
somefunc("Hello", [1,2], kwarg2="Hi")
somefunc("Hello", [1,2], kwarg2="Hi", kwarg1=6)
###Output
Hello [1, 2] 6 Hi
###Markdown
If we use variable_name=value for all arguments, their sequence in the function header can be in any order.
###Code
somefunc(kwarg2="Hello", arg1="Hi", kwarg1=6, arg2=[2])
###Output
Hi [2] 6 Hello
###Markdown
Let's look at another example - consider a function of $t$, with parameters $A$, $a$, and $\omega$:$$f(t; A,a, \omega) = Ae^{-at}\sin (\omega t)$$. (The choice of equation is actually pretty random - but it serves to show you that it is easy to translate formulae you encounter into Python code). We can implement $f$ in a Python function with $t$ as positional argument and $A$, $a$, and $\omega$ as keyword arguments.
###Code
from math import pi, exp, sin
def f(t, A=1, a=1, ω=2*pi):
return A*exp(-a*t)*sin(ω*t)
v1 = f(0.2)
v2 = f(0.2, ω=1)
v2 = f(0.2, 1, 3) # same as f(0.2, A=1, a=3)
v3 = f(0.2, ω=1, A=2.5)
v4 = f(A=5, a=0.1, ω=1, t=1.3)
v5 = f(t=0.2, A=9)
print(v1, v2, v3, v4, v5)
###Output
0.778659217806053 0.5219508827258282 0.40664172703834794 4.230480200204721 7.007932960254476
###Markdown
Exercise: Implement a Gaussian functionMake a Python function *gauss*( *x*, *m*=0, *s*=1) for computing the Gaussian function $$f(x)=\frac{1}{\sqrt{2\pi}s}\exp\left(-\frac{1}{2} \left(\frac{x-m}{s}\right)^2\right)$$Call the function and print out the result for x equal to −5, −4.9, −4.8, ..., 4.8, 4.9, 5, using default values for *m* and *s*.
###Code
import numpy as np
def gauss(x, m = 0, s = 1):
return np.exp(-0.5*((x - m)/s)**2)/np.sqrt(2*np.pi)/s
answers = [gauss(i) for i in np.arange(-5, 5.1, 0.1)]
print("\n".join(str(a) for a in answers))
###Output
1.4867195147342979e-06
2.438960745893352e-06
3.961299091032062e-06
6.369825178867069e-06
1.014085206548667e-05
1.5983741106905332e-05
2.4942471290053356e-05
3.853519674208672e-05
5.8943067756539116e-05
8.926165717713167e-05
0.00013383022576488347
0.00019865547139276957
0.00029194692579145534
0.0004247802705507443
0.0006119019301137612
0.0008726826950457439
0.0012322191684729959
0.0017225689390536463
0.0023840882014647936
0.0032668190561998523
0.004431848411937913
0.005952532419775727
0.007915451582979793
0.010420934814422364
0.013582969233685318
0.017528300493568152
0.022394530294842407
0.028327037741600544
0.03547459284623067
0.043983595980426234
0.05399096651318691
0.06561581477467522
0.07895015830089255
0.09404907737688507
0.11092083467945342
0.12951759566588933
0.1497274656357422
0.17136859204780444
0.19418605498320982
0.21785217703254722
0.24197072451913992
0.2660852498987513
0.28969155276147934
0.31225393336675794
0.33322460289179656
0.3520653267642967
0.36827014030332095
0.3813878154605222
0.39104269397545455
0.3969525474770111
0.3989422804014327
0.39695254747701253
0.3910426939754574
0.38138781546052625
0.36827014030332617
0.35206532676430297
0.33322460289180367
0.3122539333667657
0.28969155276148756
0.26608524989875987
0.2419707245191485
0.21785217703255577
0.1941860549832181
0.17136859204781235
0.14972746563574965
0.1295175956658962
0.11092083467945973
0.09404907737689075
0.07895015830089759
0.06561581477467965
0.05399096651319074
0.04398359598042952
0.035474592846233444
0.02832703774160286
0.02239453029484431
0.017528300493569706
0.013582969233686572
0.010420934814423364
0.007915451582980581
0.00595253241977634
0.004431848411938386
0.003266819056200212
0.002384088201465065
0.001722568939053848
0.0012322191684731446
0.0008726826950458523
0.0006119019301138393
0.00042478027055080017
0.00029194692579149475
0.00019865547139279709
0.00013383022576490247
8.926165717714466e-05
5.894306775654792e-05
3.853519674209261e-05
2.4942471290057255e-05
1.5983741106907917e-05
1.0140852065488327e-05
6.369825178868133e-06
3.961299091032737e-06
2.438960745893777e-06
1.486719514734562e-06
###Markdown
The *if* statementConsider this simple example:
###Code
def f(x):
if 0 <= x <= pi:
return sin(x)
else:
return 0
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
0 1.0 0
###Markdown
In general (the *else* block can be skipped if there are no statements to be executed when False) we can put together multiple conditions. Only the first condition that is True is executed.
###Code
if condition1:
<block of statements, executed if condition1 is True>
elif condition2:
<block of statements>
elif condition3:
<block of statements>
else:
<block of statements>
<next statement of the program>
###Output
_____no_output_____
###Markdown
The ternary operatorThere is another form of *if* which works as an operator, rather than a statement. If you've used the *if* function in a spreadsheet then you'll be familiar with this approach.
###Code
def f(x):
return (sin(x) if 0 <= x <= pi else 0)
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
0 1.0 0
###Markdown
The ternary operator has the following form:
###Code
<expression if condition is True> if <condition> else <expression if condition is false>
###Output
_____no_output_____
###Markdown
Introduction to Python programming [Gerard Gorman](http://www.imperial.ac.uk/people/g.gorman), [Christian Jacobs](http://christianjacobs.uk/) Updated for MPECDT by [David Ham](http://www.imperial.ac.uk/people/david.ham) Lecture 2: Computing with basic language constructionsLearning objectives:* Introduction to two different forms of loops which are used to repeat operations on data: either until a condition is satisfied (*while-loop*) or for a fixed number of iterations (*for-loop*).* Form a *condition* using a *boolean expression*. This is used to either terminate loops or control if a specific block of code is executed via the *if-statement*.* Learn two different methods for storing many data elements within a single Python type.* Introduction to developing your own Python *functions* which allows you to execute the same block of code, with different inputs and outputs, from anywhere in the code. Boolean expressionsAn expression with value *true* or *false* is called a boolean expression. Example expressions for what you would write mathematically as$C=40$, $C\ne40$, $C\ge40$, $C\gt40$ and $C\lt40$ are:
###Code
C == 40 # Note: the double == checks for equality, but C=40 is an assignment!
C != 40 # This could also be written as 'not C == 4' (i.e. the opposite of what C == 4 gives)
C >= 40
C > 40
C < 40
###Output
_____no_output_____
###Markdown
We can test boolean expressions in a Python shell:
###Code
C = 41
print("C != 40: ", C != 40)
print("C < 40: ", C < 40)
print("C == 41: ", C == 41)
###Output
C != 40: True
C < 40: False
C == 41: True
###Markdown
Several conditions can be combined with the special 'and' and 'or' operators into a single boolean expression:
###Code
condition1 and condition2
condition1 or condition2
###Output
_____no_output_____
###Markdown
* Rule 1: (**C1** *and* **C2**) is *True* only if both **C1** and **C2** are *True** Rule 2: (**C1** *or* **C2**) is *True* if either **C1** or **C2** are *True*Examples:
###Code
x=0; y=1.2
print (x >= 0 and y < 1)
###Output
False
###Markdown
Exercise: Values of boolean expressionsAdd a comment to the code below to explain the outcome of each of the boolean expressions:
###Code
C = 41
print("Case 1: ", C == 40)
print("Case 2: ", C != 40 and C < 41)
print("Case 3: ", C != 40 or C < 41)
print("Case 4: ", not C == 40)
print("Case 5: ", not C > 40)
print("Case 6: ", C <= 41)
print("Case 7: ", not False)
print("Case 8: ", True and False)
print("Case 9: ", False or True)
print("Case 10: ", False or False or False)
print("Case 11: ", True and True and False)
print("Case 12: ", False == 0)
print("Case 13: ", True == 0)
print("Case 14: ", True == 1)
###Output
Case 1: False
Case 2: False
Case 3: True
Case 4: True
Case 5: False
Case 6: True
Case 7: True
Case 8: False
Case 9: True
Case 10: False
Case 11: False
Case 12: True
Case 13: False
Case 14: True
###Markdown
LoopsSuppose we want to make a table of Celsius and Fahrenheitdegrees:
###Code
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Output
_____no_output_____
###Markdown
How do we write a program that prints out such a table?We know from the last lecture how to make one line in this table:
###Code
C = -20
F = 9/5*C + 32
print(C, F)
###Output
_____no_output_____
###Markdown
We can just repeat these statements:
###Code
C=-20; F=9/5*C+32; print(C,F)
C=-15; F=9/5*C+32; print(C,F)
C=-10; F=9/5*C+32; print(C,F)
C=-5; F=9/5*C+32; print(C,F)
C=0; F=9/5*C+32; print(C,F)
C=5; F=9/5*C+32; print(C,F)
C=10; F=9/5*C+32; print(C,F)
C=15; F=9/5*C+32; print(C,F)
C=20; F=9/5*C+32; print(C,F)
C=25; F=9/5*C+32; print(C,F)
C=30; F=9/5*C+32; print(C,F)
C=35; F=9/5*C+32; print(C,F)
C=40; F=9/5*C+32; print(C,F)
###Output
_____no_output_____
###Markdown
So we can see that works but its **very boring** to write and very easy to introduce a misprint.**You really should not be doing boring repetitive tasks like this.** Spend one time instead looking for a smarter solution. When programming becomes boring, there is usually a construct that automates the writing. Computers are very good at performing repetitive tasks. For this purpose we use **loops**. The while loop (and the significance of indentation)A while loop executes repeatedly a set of statements as long as a **boolean** (i.e. *True* / *False*) condition is *True*
###Code
while condition:
<statement 1>
<statement 2>
...
<first statement after loop>
###Output
_____no_output_____
###Markdown
Note that all statements to be executed within the loop must be indented by the same amount! The loop ends when an unindented statement is encountered.At this point it is worth noticing that **blank spaces may or may not be important** in Python programs. These statements are equivalent (blanks do not matter):
###Code
v0=3
v0 = 3
v0= 3
# The computer does not care but this formatting style is
# considered clearest for the human reader.
v0 = 3
###Output
_____no_output_____
###Markdown
Here is a while loop example where blank spaces really do matter:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
11
###Markdown
Let's take a look at what happens when we forget to indent correctly:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
_____no_output_____
###Markdown
Let's use the while loop to create the table above:
###Code
C = -20 # Initialise C
dC = 5 # Increment for C within the loop
while C <= 40: # Loop heading with condition
F = (9/5)*C + 32 # 1st statement inside loop
print ("%3d %6.1f" % (C, F)) # 2nd statement inside loop
C = C + dC # Increment C for the next iteration of the loop.
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Exercise: Make a Fahrenheit-Celsius conversion tableWrite a program that prints out a table with Fahrenheit degrees 0, 10, 20, ..., 100 in the first column and the corresponding Celsius degrees in the second column.
###Code
F = 0
dF = 10
###Output
_____no_output_____
###Markdown
Exercise: Write an approximate Fahrenheit-Celsius conversion tableMany people use an approximate formula for quickly converting Fahrenheit ($F$) to Celsius ($C$) degrees:$C \approx \hat{C} = \frac{F − 30}{2}$Modify the program from the previous exercise so that it prints three columns: $F$, $C$, and the approximate value $\hat{C}$. ListsSo far, one variable has referred to one number (or string). Sometimes however we naturally have a collection of numbers, saydegrees −20, −15, −10, −5, 0, ..., 40. One way to store these values in a computer program would be to have one variable per value, i.e.
###Code
C1 = -20
C2 = -15
C3 = -10
# ...
C13 = 40
###Output
_____no_output_____
###Markdown
This is clearly a terrible solution, particularly if we have lots of values. A better way of doing this is to collect values together in a list:
###Code
C = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
###Output
_____no_output_____
###Markdown
Now there is just one variable, **C**, holding all the values. Elements in a list are accessed via an index. List indices are always numbered as 0, 1, 2, and so forth up to the number of elements minus one:
###Code
mylist = [4, 6, -3.5]
print(mylist[0])
print(mylist[1])
print(mylist[2])
print(mylist[-1]) # get end of list
print(len(mylist)) # length of list
###Output
4
6
-3.5
-3.5
3
###Markdown
Here are a few example of operations that you can perform on lists:
###Code
C = [-10, -5, 0, 5, 10, 15, 20, 25, 30]
C.append(35) # add new element 35 at the end
print(C)
C = C + [40,45] # And another list to the end of C
print(C)
# have to be careful with C.append([40, 45])
C.insert(0, -15) # Insert -15 as index 0
print(C)
del C[2] # delete 3rd element
print(C)
del C[2] # delete what is now 3rd element
print(C)
print(len(C)) # length of list
print(C.index(10)) # Find the index of the list with the value 10
print (10 in C) # True only if the value 10 is stored in the list
print(C[-1]) # The last value in the list.
print(C[-2]) # The second last value in the list.
###Output
40
###Markdown
You can also extract sublists using ":"
###Code
print(C[5:]) # From index 5 to the end of the list.
print(C[5:7]) # From index 5 up to, but not including index 7.
###Output
[20, 25]
###Markdown
Many languages (especially Matlab and Fortran) have would include the endpoint of a slice (7 in this case). One way to think of what Python does is that the slice is a half-open interval: $[5,7)$. This makes cutting a list in half particularly easy. For example:
###Code
print(C[:5] + ["Hello"] + C[5:])
print(C[7:-1]) # From index 7 up to the second last element.
print(C[:]) # [:] specifies the whole list.
somelist = ['Curly', 'Larry', 'Moe']
stooge1, stooge2, stooge3 = somelist # Unpack the entries in a list.
print(stooge3, stooge2, stooge1)
###Output
Moe Larry Curly
###Markdown
Of course this only works if `somelist` contains exactly the number of items on the left hand side of the equals. Another useful operator is `*` (pronounced "splat"). It turns a list (or any other sequence) into the set of arguments of a function. For example:
###Code
print(somelist) # Prints the list.
print(*somelist) # Prints the items in the list.
###Output
['Curly', 'Larry', 'Moe']
Curly Larry Moe
###Markdown
Exercise: Store odd numbers in a listStep 1: Write a program that generates all odd numbers from 1 to *n*. Set *n* in the beginning of the program and use a while loop to compute the numbers. (Make sure that if *n* is an even number, the largest generated odd number is *n*-1.).Step 2: Store the generated odd numbers in a list. Start with an empty list and use the same while loop where you generate each odd number, ao append the new number to the list.Finally, print the list elements to the screen.
###Code
odd = []
n = 23
i = 1
while i <= n:
odd.append(i)
i = i + 2
print(*odd)
###Output
1 3 5 7 9 11 13 15 17 19 21 23
###Markdown
For loopsWe can visit each element in a list and process the element with some statements using a *for* loop, for example:
###Code
degrees = [0, 10, 20, 40, 100]
for C in degrees:
print('list element:', C)
print('The degrees list has', len(degrees), 'elements')
###Output
list element: 0
list element: 10
list element: 20
list element: 40
list element: 100
The degrees list has 5 elements
###Markdown
Notice again how the statements to be executed within the loop must be indented! Let's now revisit the conversion table example using the *for* loop:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
for C in Cdegrees:
F = (9/5)*C + 32
print("%3d %6.2f" % (C, F))
###Output
-20 -4.00
-15 5.00
-10 14.00
-5 23.00
0 32.00
5 41.00
10 50.00
15 59.00
20 68.00
25 77.00
30 86.00
35 95.00
40 104.00
###Markdown
It is also possible to rewrite the *for* loop as a *while* loop, i.e.,
###Code
for element in somelist:
# process element
###Output
_____no_output_____
###Markdown
can always be transformed to a *while* loop
###Code
index = 0
while index < len(somelist):
element = somelist[index]
# process element
index += 1
###Output
_____no_output_____
###Markdown
Taking the previous table example:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
index = 0
while index < len(Cdegrees):
C = Cdegrees[index]
F = (9/5)*C + 32
print('%5d %5.1f' % (C, F))
index += 1
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Rather than just printing out the Fahrenheit values, let's also store these computed values in a list of their own:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
Fdegrees = [] # start with empty list
for C in Cdegrees:
F = (9/5)*C + 32
Fdegrees.append(F) # add new element to Fdegrees
print(Fdegrees)
###Output
[-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]
###Markdown
Iterators: things you can iterate over.We've seen that a `for` loop can iterate over list values. In fact you can iterate over any object that is capable of providing values one at a time. In python, these objects are called `iterators`.A particularly useful python function which creates an iterator is `range`. For example, we can use range to iterate over the *indices* of a list:
###Code
for i in range(0, len(somelist), 1):
element = somelist[i]
... process element or somelist[i] directly
###Output
_____no_output_____
###Markdown
The statement *range(start, stop, inc)* generates an iterator over the integers *start*, *start+inc*, *start+2\*inc*, and so on up to, but not including, *stop*. We can also write *range(stop)* as an abbreviation for *range(0, stop, 1)*:
###Code
print(range(3)) # same as range(0, 3, 1)
###Output
range(0, 3)
###Markdown
Oh, that wasn't very informative. If we want to see all the numbers which the `range` will iterate over, we can cast the range to a list:
###Code
print(list(range(3)))
print(list(range(2, 8, 3)))
###Output
[2, 5]
###Markdown
List comprehensionsConsider this example where we compute two lists in a *for* loop:
###Code
n = 16
Cdegrees = []; Fdegrees = [] # empty lists
for i in range(n):
Cdegrees.append(-5 + i*0.5)
Fdegrees.append((9/5)*Cdegrees[i] + 32)
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
As constructing lists is a very common requirement, the above way of doing it can become very tedious to both write and read. Therefore, Python has a compact construct, called list comprehension for generating lists from a *for* loop:
###Code
n = 16
Cdegrees = [-5 + i*0.5 for i in range(n)]
Fdegrees = [(9/5)*C + 32 for C in Cdegrees]
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
The general form of a list comprehension is:
###Code
somelist = [expression for element in somelist]
###Output
_____no_output_____
###Markdown
Exercise: Repeat the previous exercise of creating a list of odd numbers using using: a for loop, list comprehension and the range function
###Code
# Using for loop
# Using list comprehension
# Using the function range
###Output
_____no_output_____
###Markdown
Changing elements in a listSay we want to add $2$ to all the numbers in a list:
###Code
v = [-1, 1, 10]
for e in v:
e = e + 2
print(v)
###Output
_____no_output_____
###Markdown
We can see that the list *v* is unaltered! The reason for this is that inside the loop, *e* is an ordinary (int) variable. At the start of the iteration *e* is assigned a *copy* of the next element in the list. Inside the loop we can change *e* but *v* itself is unaltered. If we want to change *v* we have to use an index to access and modify its elements:
###Code
v[1] = 4 # assign 4 to 2nd element (index 1) in v
print(v)
###Output
_____no_output_____
###Markdown
To add 2 to all values we need a *for* loop over indices:
###Code
for i in range(len(v)):
v[i] = v[i] + 2
print(v)
###Output
_____no_output_____
###Markdown
Traversing multiple lists simultaneously - *zip(list1, list2, ...)*Consider how we can loop over elements in both Cdegrees and Fdegrees at the same time. One approach would be to use list indices:
###Code
for i in range(len(Cdegrees)):
print(Cdegrees[i], Fdegrees[i])
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
An alternative construct (regarded as more ”Pythonic”) uses the *zip* function:
###Code
for C, F in zip(Cdegrees, Fdegrees):
print(C, F)
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
`zip` creates an *iterator* over the corresponding items of its arguments in order.Another example with three lists:
###Code
l1 = [3, 6, 1]; l2 = [1.5, 1, 0]; l3 = [9.1, 3, 2]
for e in zip(l1, l2, l3):
print(*e)
###Output
_____no_output_____
###Markdown
If the lists are of unequal length then the loop stops when the end of the shortest list is reached. Experiment with this:
###Code
l1 = [3, 6, 1, 4, 6]; l2 = [1.5, 1, 0, 7]; l3 = [9.1, 3, 2, 0, 9]
for e in zip(l1, l2, l3):
print(*e)
###Output
_____no_output_____
###Markdown
Nested lists: list of listsA *list* can contain **any** object, including another *list*. To illustrate this, consider how to store the conversion table as a single Python list rather than two separate lists.
###Code
Cdegrees = range(-20, 41, 5)
Fdegrees = [(9.0/5)*C + 32 for C in Cdegrees]
table1 = [Cdegrees, Fdegrees] # List of two lists
print("table1 = ", table1)
print("table1[0] = ", table1[0])
print("table1[1] = ", table1[1])
print("table1[1][3] = ", table1[1][3])
###Output
table1 = [range(-20, 41, 5), [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]]
table1[0] = range(-20, 41, 5)
table1[1] = [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]
table1[1][3] = 23.0
###Markdown
This gives us a table of two columns. How do we create a table of rows instead:
###Code
table2 = []
for C, F in zip(Cdegrees, Fdegrees):
row = [C, F]
table2.append(row)
print(table2)
###Output
_____no_output_____
###Markdown
We can use `zip` to do this more elegantly:
###Code
table2 = list(zip(Cdegrees, Fdegrees))
print(table2)
###Output
_____no_output_____
###Markdown
And you can loop through this list as before:
###Code
for C, F in table2:
print(C, F)
###Output
_____no_output_____
###Markdown
Tuples: sequences that cannot be changedWe've already learned that lists are not the only thing which can be iterated over, but that there is a general category of `iterator` types. Similarly, lists are not the only objects which can be indexed. The general category of types which can be indexed are the `sequence` types.Tuples are **constant** sequences. You can use them in much the same way as lists except you cannot modify them. They are an example of an [**immutable**](http://en.wikipedia.org/wiki/Immutable_object) type.
###Code
t = (2, 4, 6, 'temp.pdf') # Define a tuple.
t = 2, 4, 6, 'temp.pdf' # Can skip parenthesis as it is assumed in this context.
print((1,)) # this is a tuple
###Output
(1,)
###Markdown
Let's see what happens when we try to modify the tuple like we did with a list:
###Code
t[1] = -1
t.append(0)
del t[1]
###Output
_____no_output_____
###Markdown
However, we can use the tuple to compose a new tuple:
###Code
t = t + (-1.0, -2.0)
print(t)
###Output
(2, 4, 6, 'temp.pdf', -1.0, -2.0)
###Markdown
So, why would we use tuples when lists have more functionality?* Tuples are constant and thus protected against accidental changes.* Tuples are faster than lists.* Tuples are widely used in Python software (so you need to know about tuples to understand other people's code!)* Tuples (but not lists) can be used as keys in dictionaries (more about dictionaries later). Exercise: Make a table of function valuesStep 1: Write a program that prints a table with $t$ values in the first column and the corresponding $y(t) = v_0 t − 0.5gt^2$ values in the second column. Use $n$ uniformly spaced $t$ values throughout the interval [0, $2v_0/g$]. Set $v0 = 1$, $g = 9.81$, and $n = 11$.Step 2: Once step 1 is working, modify the program so that the $t$ and $y$ values are stored in two lists *t* and *y*. Thereafter, transverse the lists with a *for* loop and write out a nicely formatted table of *t* and *y* values using a *zip* construction. FunctionsWe have already used many Python functions, including mathematical functions:
###Code
from math import *
x = pi
print (cos(x))
###Output
-1.0
###Markdown
Other functions you used include *len* and *range*:
###Code
n = len(somelist)
ints = range(5, n, 2)
###Output
_____no_output_____
###Markdown
You have also used functions that are executed with the dot syntax (called *methods*):
###Code
C = [5, 10, 40, 45]
i = C.index(10)
C.append(50)
C.insert(2, 20)
###Output
_____no_output_____
###Markdown
A function is a collection of statements we can execute wherever and whenever we want. Functions can take any number of inputs (called *arguments*) to produce outputs. Functions help to organize programs, make them more understandable, shorter, and easier to extend. For our first example we will turn our temperature conversion code into a function:
###Code
def C2F(C):
return (9/5)*C + 32
###Output
_____no_output_____
###Markdown
Functions start with *def*, then the name you want to give your function, then a list of arguments (here C). This is referred to as the *function header*. Inside the function there is a block of statements called the *function body*. Notice that the function body is indented - as was the case for the *for* / *while* loop the indentation indicates where the function ends. At any point within the function, we can "stop thefunction" and return as many values/variables as required. Local and global variablesVariables defined within a function are said to have *local scope*. That is to say that they can only be referenced within that function. Let's consider an example (and look carefully at the indentation!!):
###Code
def sumint(start, stop):
s = 0 # variable for accumulating the sum
i = start # counter
while i <= stop:
s += i
i += 1
return s
print(sumint(0, 5))
###Output
15
###Markdown
Variables *i* and *s* are local variables in *sumint*. They are destroyed at the end (return) of the function and never visible outside the function (in the calling program); in fact, *start* and *stop* are also local variables. So let's see what happens if we now try to print one of these variables:
###Code
print(stop)
###Output
_____no_output_____
###Markdown
Functions can also return multiple values. Let's recycle another of our previous examples - compute $y(t)$ and $y'(t)=v_0-gt$:
###Code
def yfunc(t, v0):
g = 9.81
y = v0*t - 0.5*g*t**2
dydt = v0 - g*t
return y, dydt
# call:
position, velocity = yfunc(0.6, 3)
print(position, velocity)
###Output
0.034199999999999786 -2.886
###Markdown
Remember that a series of comma separated variables implies a tuple - therefore "return y, dydt" is the same as writing "return (y, dydt)". Therefore, in general what is returned is a tuple. Let's take a look at another example illustrating this:
###Code
def f(x):
return x, x**2, x**4
s = f(2)
print(s)
print(type(s)) # The function type() tells us what type a variable it is.
###Output
(2, 4, 16)
<class 'tuple'>
###Markdown
No return value implies that `None` is returned. `None` is a special Python object that represents an ”empty” or undefined value. It is surprisingly useful and we will use it a lot later.
###Code
def message(course):
print("%s rocks!"% course)
message("Python")
r = message("Geo")
print("r = ", r)
###Output
Python rocks!
Geo rocks!
r = None
###Markdown
Keyword arguments and default argument valuesIt's possible to specify default values that function arguments should take if no values are provided when the function is called:
###Code
def somefunc(arg1, arg2, kwarg1=True, kwarg2=0):
print(arg1, arg2, kwarg1, kwarg2)
somefunc("Hello", [1,2]) # Note that we have not specified values for kwarg1 and kwarg2
###Output
Hello [1, 2] True 0
###Markdown
You can override the default values by passing additional arguments in the correct position:
###Code
somefunc("Hello", [1,2], "Hi")
###Output
_____no_output_____
###Markdown
... or by naming the argument to which the value should be passed. These are called keyword arguments:
###Code
somefunc("Hello", [1,2], kwarg2="Hi")
somefunc("Hello", [1,2], kwarg2="Hi", kwarg1=6)
def foo (*args):
print(args)
foo(1,2)
foo (3,4,5)
###Output
(1, 2)
(3, 4, 5)
###Markdown
If we use variable_name=value for all arguments, their sequence in the function header can be in any order.
###Code
somefunc(kwarg2="Hello", arg1="Hi", kwarg1=6, arg2=[2])
###Output
_____no_output_____
###Markdown
Let's look at another example - consider a function of $t$, with parameters $A$, $a$, and $\omega$:$$f(t; A,a, \omega) = Ae^{-at}\sin (\omega t)$$. (The choice of equation is actually pretty random - but it serves to show you that it is easy to translate formulae you encounter into Python code). We can implement $f$ in a Python function with $t$ as positional argument and $A$, $a$, and $\omega$ as keyword arguments.
###Code
from math import pi, exp, sin
def f(t, A=1, a=1, ω=2*pi):
return A*exp(-a*t)*sin(ω*t)
v1 = f(0.2)
v2 = f(0.2, ω=1)
v2 = f(0.2, 1, 3) # same as f(0.2, A=1, a=3)
v3 = f(0.2, ω=1, A=2.5)
v4 = f(A=5, a=0.1, ω=1, t=1.3)
v5 = f(t=0.2, A=9)
print(v1, v2, v3, v4, v5)
###Output
_____no_output_____
###Markdown
Exercise: Implement a Gaussian functionMake a Python function *gauss*( *x*, *m*=0, *s*=1) for computing the Gaussian function $$f(x)=\frac{1}{\sqrt{2\pi}s}\exp\left(-\frac{1}{2} \left(\frac{x-m}{s}\right)^2\right)$$Call the function and print out the result for x equal to −5, −4.9, −4.8, ..., 4.8, 4.9, 5, using default values for *m* and *s*.
###Code
from math import sqrt, pi, exp
def gauss(x, m=0, s=1):
return 1/(sqrt(2*pi)*s)*exp(-0.5*((x - m)/s)**2)
i = -5
while i <= 5:
print(gauss(i))
i = i + 0.1
###Output
1.4867195147342977e-06
2.438960745893352e-06
3.961299091032061e-06
6.369825178867068e-06
1.014085206548667e-05
1.5983741106905363e-05
2.4942471290053356e-05
3.8535196742086716e-05
5.8943067756539116e-05
8.926165717713165e-05
0.00013383022576488347
0.0001986554713927699
0.00029194692579145637
0.0004247802705507465
0.0006119019301137654
0.0008726826950457507
0.0012322191684730067
0.0017225689390536628
0.0023840882014648213
0.0032668190561998926
0.004431848411937972
0.005952532419775811
0.007915451582979908
0.010420934814422526
0.013582969233685536
0.017528300493568447
0.02239453029484278
0.028327037741601037
0.03547459284623128
0.04398359598042702
0.05399096651318786
0.06561581477467639
0.07895015830089394
0.0940490773768867
0.11092083467945532
0.12951759566589147
0.1497274656357446
0.1713685920478071
0.1941860549832127
0.2178521770325503
0.24197072451914314
0.2660852498987546
0.28969155276148256
0.3122539333667611
0.33322460289179945
0.35206532676429936
0.36827014030332317
0.38138781546052397
0.3910426939754558
0.39695254747701175
0.3989422804014327
0.39695254747701186
0.391042693975456
0.3813878154605242
0.36827014030332345
0.3520653267642997
0.3332246028917999
0.3122539333667615
0.289691552761483
0.2660852498987551
0.24197072451914364
0.2178521770325508
0.19418605498321317
0.17136859204780758
0.14972746563574502
0.12951759566589185
0.11092083467945565
0.094049077376887
0.07895015830089423
0.06561581477467664
0.053990966513188084
0.04398359598042723
0.03547459284623146
0.028327037741601186
0.0223945302948429
0.01752830049356854
0.013582969233685613
0.010420934814422592
0.007915451582979956
0.005952532419775849
0.004431848411938004
0.0032668190561999156
0.0023840882014648382
0.0017225689390536767
0.0012322191684730154
0.0008726826950457579
0.0006119019301137703
0.00042478027055074997
0.00029194692579145897
0.0001986554713927717
0.00013383022576488488
8.926165717713262e-05
5.8943067756539855e-05
3.853519674208713e-05
2.494247129005362e-05
1.5983741106905532e-05
1.0140852065486796e-05
6.369825178867147e-06
3.961299091032111e-06
2.438960745893387e-06
1.4867195147343189e-06
###Markdown
The *if* statementConsider this simple example:
###Code
def f(x):
if 0 <= x <= pi:
return sin(x)
else:
return 0
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
In general (the *else* block can be skipped if there are no statements to be executed when False) we can put together multiple conditions. Only the first condition that is True is executed.
###Code
if condition1:
<block of statements, executed if condition1 is True>
elif condition2:
<block of statements>
elif condition3:
<block of statements>
else:
<block of statements>
<next statement of the program>
###Output
_____no_output_____
###Markdown
The ternary operatorThere is another form of *if* which works as an operator, rather than a statement. If you've used the *if* function in a spreadsheet then you'll be familiar with this approach.
###Code
def f(x):
return (sin(x) if 0 <= x <= pi else 0)
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
The ternary operator has the following form:
###Code
<expression if condition is True> if <condition> else <expression if condition is false>
###Output
_____no_output_____
###Markdown
Exercise: Express a step function as a Python functionThe following "step" function is known as the Heaviside function andis widely used in mathematics:$$H(x)=\begin{cases}0, & \text{if $x<0$}.\\\\1, & \text{if $x\ge 0$}.\end{cases}$$Write a Python function H(x) that computes H(x).
###Code
def H(x):
return (1 if x>=1 else 0)
for i in np.arange(-4, 4, 1):
print((i, H(i)))
###Output
(-4, 0)
(-3, 0)
(-2, 0)
(-1, 0)
(0, 0)
(1, 1)
(2, 1)
(3, 1)
###Markdown
Introduction to Python programming [Gerard Gorman](http://www.imperial.ac.uk/people/g.gorman), [Christian Jacobs](http://christianjacobs.uk/) Updated for MPECDT by [David Ham](http://www.imperial.ac.uk/people/david.ham) Lecture 2: Computing with basic language constructionsLearning objectives:* Introduction to two different forms of loops which are used to repeat operations on data: either until a condition is satisfied (*while-loop*) or for a fixed number of iterations (*for-loop*).* Form a *condition* using a *boolean expression*. This is used to either terminate loops or control if a specific block of code is executed via the *if-statement*.* Learn two different methods for storing many data elements within a single Python type.* Introduction to developing your own Python *functions* which allows you to execute the same block of code, with different inputs and outputs, from anywhere in the code. Boolean expressionsAn expression with value *true* or *false* is called a boolean expression. Example expressions for what you would write mathematically as$C=40$, $C\ne40$, $C\ge40$, $C\gt40$ and $C\lt40$ are:
###Code
C == 40 # Note: the double == checks for equality, but C=40 is an assignment!
C != 40 # This could also be written as 'not C == 4' (i.e. the opposite of what C == 4 gives)
C >= 40
C > 40
C < 40
###Output
_____no_output_____
###Markdown
We can test boolean expressions in a Python shell:
###Code
C = 41
print("C != 40: ", C != 40)
print("C < 40: ", C < 40)
print("C == 41: ", C == 41)
###Output
C != 40: True
C < 40: False
C == 41: True
###Markdown
Several conditions can be combined with the special 'and' and 'or' operators into a single boolean expression:
###Code
condition1 and condition2
condition1 or condition2
###Output
_____no_output_____
###Markdown
* Rule 1: (**C1** *and* **C2**) is *True* only if both **C1** and **C2** are *True** Rule 2: (**C1** *or* **C2**) is *True* if either **C1** or **C2** are *True*Examples:
###Code
x=0; y=1.2
print (x >= 0 and y < 1)
###Output
_____no_output_____
###Markdown
Exercise: Values of boolean expressionsAdd a comment to the code below to explain the outcome of each of the boolean expressions:
###Code
C = 41
print("Case 1: ", C == 40)
print("Case 2: ", C != 40 and C < 41)
print("Case 3: ", C != 40 or C < 41)
print("Case 4: ", not C == 40)
print("Case 5: ", not C > 40)
print("Case 6: ", C <= 41)
print("Case 7: ", not False)
print("Case 8: ", True and False)
print("Case 9: ", False or True)
print("Case 10: ", False or False or False)
print("Case 11: ", True and True and False)
print("Case 12: ", False == 0)
print("Case 13: ", True == 0)
print("Case 14: ", True == 1)
###Output
_____no_output_____
###Markdown
LoopsSuppose we want to make a table of Celsius and Fahrenheitdegrees:
###Code
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Output
_____no_output_____
###Markdown
How do we write a program that prints out such a table?We know from the last lecture how to make one line in this table:
###Code
C = -20
F = 9/5*C + 32
print(C, F)
###Output
_____no_output_____
###Markdown
We can just repeat these statements:
###Code
C=-20; F=9/5*C+32; print(C,F)
C=-15; F=9/5*C+32; print(C,F)
C=-10; F=9/5*C+32; print(C,F)
C=-5; F=9/5*C+32; print(C,F)
C=0; F=9/5*C+32; print(C,F)
C=5; F=9/5*C+32; print(C,F)
C=10; F=9/5*C+32; print(C,F)
C=15; F=9/5*C+32; print(C,F)
C=20; F=9/5*C+32; print(C,F)
C=25; F=9/5*C+32; print(C,F)
C=30; F=9/5*C+32; print(C,F)
C=35; F=9/5*C+32; print(C,F)
C=40; F=9/5*C+32; print(C,F)
###Output
_____no_output_____
###Markdown
So we can see that works but its **very boring** to write and very easy to introduce a misprint.**You really should not be doing boring repetitive tasks like this.** Spend one time instead looking for a smarter solution. When programming becomes boring, there is usually a construct that automates the writing. Computers are very good at performing repetitive tasks. For this purpose we use **loops**. The while loop (and the significance of indentation)A while loop executes repeatedly a set of statements as long as a **boolean** (i.e. *True* / *False*) condition is *True*
###Code
while condition:
<statement 1>
<statement 2>
...
<first statement after loop>
###Output
_____no_output_____
###Markdown
Note that all statements to be executed within the loop must be indented by the same amount! The loop ends when an unindented statement is encountered.At this point it is worth noticing that **blank spaces may or may not be important** in Python programs. These statements are equivalent (blanks do not matter):
###Code
v0=3
v0 = 3
v0= 3
# The computer does not care but this formatting style is
# considered clearest for the human reader.
v0 = 3
###Output
_____no_output_____
###Markdown
Here is a while loop example where blank spaces really do matter:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
_____no_output_____
###Markdown
Let's take a look at what happens when we forget to indent correctly:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
_____no_output_____
###Markdown
Let's use the while loop to create the table above:
###Code
C = -20 # Initialise C
dC = 5 # Increment for C within the loop
while C <= 40: # Loop heading with condition
F = (9/5)*C + 32 # 1st statement inside loop
print ("%3d %6.1f" % (C, F)) # 2nd statement inside loop
C = C + dC # Increment C for the next iteration of the loop.
###Output
_____no_output_____
###Markdown
Exercise: Make a Fahrenheit-Celsius conversion tableWrite a program that prints out a table with Fahrenheit degrees 0, 10, 20, ..., 100 in the first column and the corresponding Celsius degrees in the second column. Exercise: Write an approximate Fahrenheit-Celsius conversion tableMany people use an approximate formula for quickly converting Fahrenheit ($F$) to Celsius ($C$) degrees:$C \approx \hat{C} = \frac{F − 30}{2}$Modify the program from the previous exercise so that it prints three columns: $F$, $C$, and the approximate value $\hat{C}$. ListsSo far, one variable has referred to one number (or string). Sometimes however we naturally have a collection of numbers, saydegrees −20, −15, −10, −5, 0, ..., 40. One way to store these values in a computer program would be to have one variable per value, i.e.
###Code
C1 = -20
C2 = -15
C3 = -10
# ...
C13 = 40
###Output
_____no_output_____
###Markdown
This is clearly a terrible solution, particularly if we have lots of values. A better way of doing this is to collect values together in a list:
###Code
C = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
###Output
_____no_output_____
###Markdown
Now there is just one variable, **C**, holding all the values. Elements in a list are accessed via an index. List indices are always numbered as 0, 1, 2, and so forth up to the number of elements minus one:
###Code
mylist = [4, 6, -3.5]
print(mylist[0])
print(mylist[1])
print(mylist[2])
print(len(mylist)) # length of list
###Output
_____no_output_____
###Markdown
Here are a few example of operations that you can perform on lists:
###Code
C = [-10, -5, 0, 5, 10, 15, 20, 25, 30]
C.append(35) # add new element 35 at the end
print(C)
C = C + [40,45] # And another list to the end of C
print(C)
C.insert(0, -15) # Insert -15 as index 0
print(C)
del C[2] # delete 3rd element
print(C)
del C[2] # delete what is now 3rd element
print(C)
print(len(C)) # length of list
print(C.index(10)) # Find the index of the list with the value 10
print (10 in C) # True only if the value 10 is stored in the list
print(C[-1]) # The last value in the list.
print(C[-2]) # The second last value in the list.
###Output
_____no_output_____
###Markdown
You can also extract sublists using ":"
###Code
print(C[5:]) # From index 5 to the end of the list.
print(C[5:7]) # From index 5 up to, but not including index 7.
###Output
_____no_output_____
###Markdown
Many languages (especially Matlab and Fortran) have would include the endpoint of a slice (7 in this case). One way to think of what Python does is that the slice is a half-open interval: $[5,7)$. This makes cutting a list in half particularly easy. For example:
###Code
print(C[:5] + ["Hello"] + C[5:])
print(C[7:-1]) # From index 7 up to the second last element.
print(C[:]) # [:] specifies the whole list.
somelist = ['Curly', 'Larry', 'Moe']
stooge1, stooge2, stooge3 = somelist # Unpack the entries in a list.
print(stooge3, stooge2, stooge1)
###Output
Moe Larry Curly
###Markdown
Of course this only works if `somelist` contains exactly the number of items on the left hand side of the equals. Another useful operator is `*` (pronounced "splat"). It turns a list (or any other sequence) into the set of arguments of a function. For example:
###Code
print(somelist) # Prints the list.
print(*somelist) # Prints the items in the list.
###Output
['Curly', 'Larry', 'Moe']
Curly Larry Moe
###Markdown
Exercise: Store odd numbers in a listStep 1: Write a program that generates all odd numbers from 1 to *n*. Set *n* in the beginning of the program and use a while loop to compute the numbers. (Make sure that if *n* is an even number, the largest generated odd number is *n*-1.).Step 2: Store the generated odd numbers in a list. Start with an empty list and use the same while loop where you generate each odd number, ao append the new number to the list.Finally, print the list elements to the screen.
###Code
n = 8
a = []
i=1
while (2*i-1 <= n):
a.append(2*i -1)
i = i+1
print(*a)
###Output
1 3 5 7
###Markdown
For loopsWe can visit each element in a list and process the element with some statements using a *for* loop, for example:
###Code
degrees = [0, 10, 20, 40, 100]
for C in degrees:
print('list element:', C)
print('The degrees list has', len(degrees), 'elements')
###Output
list element: 0
list element: 10
list element: 20
list element: 40
list element: 100
The degrees list has 5 elements
###Markdown
Notice again how the statements to be executed within the loop must be indented! Let's now revisit the conversion table example using the *for* loop:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
for C in Cdegrees:
F = (9/5)*C + 32
print("%3d %6.2f" % (C, F))
###Output
_____no_output_____
###Markdown
It is also possible to rewrite the *for* loop as a *while* loop, i.e.,
###Code
for element in somelist:
# process element
###Output
_____no_output_____
###Markdown
can always be transformed to a *while* loop
###Code
index = 0
while index < len(somelist):
element = somelist[index]
# process element
index += 1
###Output
_____no_output_____
###Markdown
Taking the previous table example:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
index = 0
while index < len(Cdegrees):
C = Cdegrees[index]
F = (9/5)*C + 32
print('%5d %5.1f' % (C, F))
index += 1
###Output
_____no_output_____
###Markdown
Rather than just printing out the Fahrenheit values, let's also store these computed values in a list of their own:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
Fdegrees = [] # start with empty list
for C in Cdegrees:
F = (9/5)*C + 32
Fdegrees.append(F) # add new element to Fdegrees
print(Fdegrees)
###Output
_____no_output_____
###Markdown
Iterators: things you can iterate over.We've seen that a `for` loop can iterate over list values. In fact you can iterate over any object that is capable of providing values one at a time. In python, these objects are called `iterators`.A particularly useful python function which creates an iterator is `range`. For example, we can use range to iterate over the *indices* of a list:
###Code
for i in range(0, len(somelist), 1):
element = somelist[i]
... process element or somelist[i] directly
###Output
_____no_output_____
###Markdown
The statement *range(start, stop, inc)* generates an iterator over the integers *start*, *start+inc*, *start+2\*inc*, and so on up to, but not including, *stop*. We can also write *range(stop)* as an abbreviation for *range(0, stop, 1)*:
###Code
print(range(3)) # same as range(0, 3, 1)
###Output
_____no_output_____
###Markdown
Oh, that wasn't very informative. If we want to see all the numbers which the `range` will iterate over, we can cast the range to a list:
###Code
print(list(range(3)))
print(list(range(2, 8, 3)))
###Output
_____no_output_____
###Markdown
List comprehensionsConsider this example where we compute two lists in a *for* loop:
###Code
n = 16
Cdegrees = []; Fdegrees = [] # empty lists
for i in range(n):
Cdegrees.append(-5 + i*0.5)
Fdegrees.append((9/5)*Cdegrees[i] + 32)
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
_____no_output_____
###Markdown
As constructing lists is a very common requirement, the above way of doing it can become very tedious to both write and read. Therefore, Python has a compact construct, called list comprehension for generating lists from a *for* loop:
###Code
n = 16
Cdegrees = [-5 + i*0.5 for i in range(n)]
Fdegrees = [(9/5)*C + 32 for C in Cdegrees]
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
The general form of a list comprehension is:
###Code
somelist = [expression for element in somelist]
###Output
_____no_output_____
###Markdown
Exercise: Repeat the previous exercise of creating a list of odd numbers using using: a for loop, list comprehension and the range function
###Code
# Using for loop
# Using list comprehension
# Using the function range
###Output
_____no_output_____
###Markdown
Changing elements in a listSay we want to add $2$ to all the numbers in a list:
###Code
v = [-1, 1, 10]
for e in v:
e = e + 2
print(v)
###Output
_____no_output_____
###Markdown
We can see that the list *v* is unaltered! The reason for this is that inside the loop, *e* is an ordinary (int) variable. At the start of the iteration *e* is assigned a *copy* of the next element in the list. Inside the loop we can change *e* but *v* itself is unaltered. If we want to change *v* we have to use an index to access and modify its elements:
###Code
v[1] = 4 # assign 4 to 2nd element (index 1) in v
print(v)
###Output
_____no_output_____
###Markdown
To add 2 to all values we need a *for* loop over indices:
###Code
for i in range(len(v)):
v[i] = v[i] + 2
print(v)
###Output
_____no_output_____
###Markdown
Traversing multiple lists simultaneously - *zip(list1, list2, ...)*Consider how we can loop over elements in both Cdegrees and Fdegrees at the same time. One approach would be to use list indices:
###Code
for i in range(len(Cdegrees)):
print(Cdegrees[i], Fdegrees[i])
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
An alternative construct (regarded as more ”Pythonic”) uses the *zip* function:
###Code
for C, F in zip(Cdegrees, Fdegrees):
print(C, F)
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
`zip` creates an *iterator* over the corresponding items of its arguments in order.Another example with three lists:
###Code
l1 = [3, 6, 1]; l2 = [1.5, 1, 0]; l3 = [9.1, 3, 2]
for e in zip(l1, l2, l3):
print(*e)
###Output
3 1.5 9.1
6 1 3
1 0 2
###Markdown
If the lists are of unequal length then the loop stops when the end of the shortest list is reached. Experiment with this:
###Code
l1 = [3, 6, 1, 4, 11]; l2 = [1.5, 1, 0, 7]; l3 = [9.1, 3, 2, 0, 9]
for e in zip(l1, l2, l3):
print(*e)
for e in zip(*zip(l1, l2, l3)):
print(e)
###Output
3 1.5 9.1
6 1 3
1 0 2
4 7 0
(3, 6, 1, 4)
(1.5, 1, 0, 7)
(9.1, 3, 2, 0)
###Markdown
Nested lists: list of listsA *list* can contain **any** object, including another *list*. To illustrate this, consider how to store the conversion table as a single Python list rather than two separate lists.
###Code
Cdegrees = range(-20, 41, 5)
Fdegrees = [(9.0/5)*C + 32 for C in Cdegrees]
table1 = [Cdegrees, Fdegrees] # List of two lists
print("table1 = ", table1)
print("table1[0] = ", table1[0])
print("table1[1] = ", table1[1])
print("table1[2][3] = ", table1[1][3])
###Output
_____no_output_____
###Markdown
This gives us a table of two columns. How do we create a table of rows instead:
###Code
table2 = []
for C, F in zip(Cdegrees, Fdegrees):
row = [C, F]
table2.append(row)
print(table2)
###Output
_____no_output_____
###Markdown
We can use `zip` to do this more elegantly:
###Code
table2 = list(zip(Cdegrees, Fdegrees))
print(table2)
###Output
_____no_output_____
###Markdown
And you can loop through this list as before:
###Code
for C, F in table2:
print(C, F)
###Output
_____no_output_____
###Markdown
Tuples: sequences that cannot be changedWe've already learned that lists are not the only thing which can be iterated over, but that there is a general category of `iterator` types. Similarly, lists are not the only objects which can be indexed. The general category of types which can be indexed are the `sequence` types.Tuples are **constant** sequences. You can use them in much the same way as lists except you cannot modify them. They are an example of an [**immutable**](http://en.wikipedia.org/wiki/Immutable_object) type.
###Code
t = (2, 4, 6, 'temp.pdf') # Define a tuple.
t = 2, 4, 6, 'temp.pdf' # Can skip parenthesis as it is assumed in this context.
###Output
_____no_output_____
###Markdown
Let's see what happens when we try to modify the tuple like we did with a list:
###Code
t[1] = -1
t.append(0)
del t[1]
###Output
_____no_output_____
###Markdown
However, we can use the tuple to compose a new tuple:
###Code
t = t + (-1.0, -2.0)
print(t)
###Output
_____no_output_____
###Markdown
So, why would we use tuples when lists have more functionality?* Tuples are constant and thus protected against accidental changes.* Tuples are faster than lists.* Tuples are widely used in Python software (so you need to know about tuples to understand other people's code!)* Tuples (but not lists) can be used as keys in dictionaries (more about dictionaries later). Exercise: Make a table of function valuesStep 1: Write a program that prints a table with $t$ values in the first column and the corresponding $y(t) = v_0 t − 0.5gt^2$ values in the second column. Use $n$ uniformly spaced $t$ values throughout the interval [0, $2v_0/g$]. Set $v0 = 1$, $g = 9.81$, and $n = 11$.Step 2: Once step 1 is working, modify the program so that the $t$ and $y$ values are stored in two lists *t* and *y*. Thereafter, transverse the lists with a *for* loop and write out a nicely formatted table of *t* and *y* values using a *zip* construction. FunctionsWe have already used many Python functions, including mathematical functions:
###Code
from math import *
x = pi
print cos(x)
###Output
_____no_output_____
###Markdown
Other functions you used include *len* and *range*:
###Code
n = len(somelist)
ints = range(5, n, 2)
###Output
_____no_output_____
###Markdown
You have also used functions that are executed with the dot syntax (called *methods*):
###Code
C = [5, 10, 40, 45]
i = C.index(10)
C.append(50)
C.insert(2, 20)
###Output
_____no_output_____
###Markdown
A function is a collection of statements we can execute wherever and whenever we want. Functions can take any number of inputs (called *arguments*) to produce outputs. Functions help to organize programs, make them more understandable, shorter, and easier to extend. For our first example we will turn our temperature conversion code into a function:
###Code
def C2F(C):
return (9/5)*C + 32
###Output
_____no_output_____
###Markdown
Functions start with *def*, then the name you want to give your function, then a list of arguments (here C). This is referred to as the *function header*. Inside the function there is a block of statements called the *function body*. Notice that the function body is indented - as was the case for the *for* / *while* loop the indentation indicates where the function ends. At any point within the function, we can "stop thefunction" and return as many values/variables as required. Local and global variablesVariables defined within a function are said to have *local scope*. That is to say that they can only be referenced within that function. Let's consider an example (and look carefully at the indentation!!):
###Code
def sumint(start, stop):
s = 0 # variable for accumulating the sum
i = start # counter
while i <= stop:
s += i
i += 1
return s
print(sumint(0, 5))
###Output
_____no_output_____
###Markdown
Variables *i* and *s* are local variables in *sumint*. They are destroyed at the end (return) of the function and never visible outside the function (in the calling program); in fact, *start* and *stop* are also local variables. So let's see what happens if we now try to print one of these variables:
###Code
print(stop)
###Output
_____no_output_____
###Markdown
Functions can also return multiple values. Let's recycle another of our previous examples - compute $y(t)$ and $y'(t)=v_0-gt$:
###Code
def yfunc(t, v0):
g = 9.81
y = v0*t - 0.5*g*t**2
dydt = v0 - g*t
return y, dydt
# call:
position, velocity = yfunc(0.6, 3)
print(position, velocity)
###Output
_____no_output_____
###Markdown
Remember that a series of comma separated variables implies a tuple - therefore "return y, dydt" is the same as writing "return (y, dydt)". Therefore, in general what is returned is a tuple. Let's take a look at another example illustrating this:
###Code
def f(x):
return x, x**2, x**4
s = f(2)
print(s)
print(type(s)) # The function type() tells us what type a variable it is.
###Output
_____no_output_____
###Markdown
No return value implies that `None` is returned. `None` is a special Python object that represents an ”empty” or undefined value. It is surprisingly useful and we will use it a lot later.
###Code
def message(course):
print("%s rocks!"% course)
message("Python")
r = message("Geo")
print("r = ", r)
###Output
_____no_output_____
###Markdown
Keyword arguments and default argument valuesIt's possible to specify default values that function arguments should take if no values are provided when the function is called:
###Code
def somefunc(arg1, arg2, kwarg1=True, kwarg2=0):
print(arg1, arg2, kwarg1, kwarg2)
somefunc("Hello", [1,2]) # Note that we have not specified values for kwarg1 and kwarg2
###Output
_____no_output_____
###Markdown
You can override the default values by passing additional arguments in the correct position:
###Code
somefunc("Hello", [1,2], "Hi")
###Output
_____no_output_____
###Markdown
... or by naming the argument to which the value should be passed. These are called keyword arguments:
###Code
somefunc("Hello", [1,2], kwarg2="Hi")
somefunc("Hello", [1,2], kwarg2="Hi", kwarg1=6)
###Output
_____no_output_____
###Markdown
If we use variable_name=value for all arguments, their sequence in the function header can be in any order.
###Code
somefunc(kwarg2="Hello", arg1="Hi", kwarg1=6, arg2=[2])
###Output
_____no_output_____
###Markdown
Let's look at another example - consider a function of $t$, with parameters $A$, $a$, and $\omega$:$$f(t; A,a, \omega) = Ae^{-at}\sin (\omega t)$$. (The choice of equation is actually pretty random - but it serves to show you that it is easy to translate formulae you encounter into Python code). We can implement $f$ in a Python function with $t$ as positional argument and $A$, $a$, and $\omega$ as keyword arguments.
###Code
from math import pi, exp, sin
def f(t, A=1, a=1, ω=2*pi):
return A*exp(-a*t)*sin(ω*t)
v1 = f(0.2)
v2 = f(0.2, ω=1)
v2 = f(0.2, 1, 3) # same as f(0.2, A=1, a=3)
v3 = f(0.2, ω=1, A=2.5)
v4 = f(A=5, a=0.1, ω=1, t=1.3)
v5 = f(t=0.2, A=9)
print(v1, v2, v3, v4, v5)
###Output
_____no_output_____
###Markdown
Exercise: Implement a Gaussian functionMake a Python function *gauss*( *x*, *m*=0, *s*=1) for computing the Gaussian function $$f(x)=\frac{1}{\sqrt{2\pi}s}\exp\left(-\frac{1}{2} \left(\frac{x-m}{s}\right)^2\right)$$Call the function and print out the result for x equal to −5, −4.9, −4.8, ..., 4.8, 4.9, 5, using default values for *m* and *s*.
###Code
def gauss(x, m=0, s=1):
from math import pi, exp
return exp(-0.5*((x-m)/s)**2)*((2*pi*s)**-0.5)
import numpy
numpy.linspace?
values = numpy.linspace(-5, 5, 101)
for e in values:
print(gauss(e))
###Output
1.4867195147342977e-06
2.438960745893352e-06
3.961299091032075e-06
6.36982517886709e-06
1.0140852065486758e-05
1.5983741106905475e-05
2.4942471290053535e-05
3.853519674208713e-05
5.8943067756539855e-05
8.926165717713293e-05
0.00013383022576488537
0.00019865547139277272
0.00029194692579146027
0.00042478027055075143
0.000611901930113773
0.0008726826950457602
0.00123221916847302
0.0017225689390536812
0.0023840882014648404
0.0032668190561999247
0.0044318484119380075
0.005952532419775854
0.007915451582979969
0.010420934814422605
0.013582969233685634
0.01752830049356854
0.0223945302948429
0.028327037741601186
0.03547459284623146
0.04398359598042723
0.05399096651318806
0.0656158147746766
0.07895015830089418
0.09404907737688697
0.11092083467945563
0.12951759566589174
0.14972746563574488
0.1713685920478074
0.19418605498321304
0.21785217703255064
0.24197072451914337
0.266085249898755
0.2896915527614828
0.3122539333667612
0.3332246028917997
0.3520653267642995
0.3682701403033234
0.38138781546052414
0.391042693975456
0.3969525474770118
0.3989422804014327
0.39695254747701175
0.3910426939754559
0.381387815460524
0.3682701403033233
0.3520653267642995
0.33322460289179956
0.3122539333667612
0.28969155276148256
0.26608524989875476
0.24197072451914337
0.21785217703255041
0.19418605498321292
0.1713685920478072
0.1497274656357448
0.12951759566589174
0.11092083467945546
0.0940490773768869
0.07895015830089407
0.06561581477467655
0.05399096651318806
0.043983595980427156
0.035474592846231424
0.02832703774160112
0.022394530294842882
0.01752830049356854
0.013582969233685602
0.010420934814422592
0.007915451582979946
0.005952532419775849
0.0044318484119380075
0.0032668190561999247
0.0023840882014648343
0.0017225689390536767
0.0012322191684730175
0.0008726826950457602
0.000611901930113773
0.00042478027055074997
0.0002919469257914595
0.00019865547139277237
0.00013383022576488537
8.926165717713293e-05
5.8943067756539645e-05
3.8535196742086994e-05
2.4942471290053535e-05
1.5983741106905475e-05
1.014085206548667e-05
6.369825178867068e-06
3.961299091032061e-06
2.438960745893352e-06
1.4867195147342977e-06
###Markdown
The *if* statementConsider this simple example:
###Code
def f(x):
if 0 <= x <= pi:
return sin(x)
else:
return 0
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
In general (the *else* block can be skipped if there are no statements to be executed when False) we can put together multiple conditions. Only the first condition that is True is executed.
###Code
if condition1:
<block of statements, executed if condition1 is True>
elif condition2:
<block of statements>
elif condition3:
<block of statements>
else:
<block of statements>
<next statement of the program>
###Output
_____no_output_____
###Markdown
The ternary operatorThere is another form of *if* which works as an operator, rather than a statement. If you've used the *if* function in a spreadsheet then you'll be familiar with this approach.
###Code
from math import pi, sin
def f(x):
return sin(x) if 0 <= x <= pi else 0
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
0 1.0 0
###Markdown
The ternary operator has the following form:
###Code
<expression if condition is True> if <condition> else <expression if condition is false>
###Output
_____no_output_____
###Markdown
Exercise: Express a step function as a Python functionThe following "step" function is known as the Heaviside function andis widely used in mathematics:$$H(x)=\begin{cases}0, & \text{if $x<0$}.\\\\1, & \text{if $x\ge 0$}.\end{cases}$$Write a Python function H(x) that computes H(x).
###Code
def H(x):
return 0 if x < 0 else 1
print(H(-0.0005))
###Output
0
###Markdown
Introduction to Python programming [Gerard Gorman](http://www.imperial.ac.uk/people/g.gorman), [Christian Jacobs](http://christianjacobs.uk/) Updated for MPECDT by [David Ham](http://www.imperial.ac.uk/people/david.ham) Lecture 2: Computing with basic language constructionsLearning objectives:* Introduction to two different forms of loops which are used to repeat operations on data: either until a condition is satisfied (*while-loop*) or for a fixed number of iterations (*for-loop*).* Form a *condition* using a *boolean expression*. This is used to either terminate loops or control if a specific block of code is executed via the *if-statement*.* Learn two different methods for storing many data elements within a single Python type.* Introduction to developing your own Python *functions* which allows you to execute the same block of code, with different inputs and outputs, from anywhere in the code. Boolean expressionsAn expression with value *true* or *false* is called a boolean expression. Example expressions for what you would write mathematically as$C=40$, $C\ne40$, $C\ge40$, $C\gt40$ and $C\lt40$ are:
###Code
C == 40 # Note: the double == checks for equality, but C=40 is an assignment!
C != 40 # This could also be written as 'not C == 4' (i.e. the opposite of what C == 4 gives)
C >= 40
C > 40
C < 40
###Output
_____no_output_____
###Markdown
We can test boolean expressions in a Python shell:
###Code
C = 41
print("C != 40: ", C != 40)
print("C < 40: ", C < 40)
print("C == 41: ", C == 41)
###Output
_____no_output_____
###Markdown
Several conditions can be combined with the special 'and' and 'or' operators into a single boolean expression:
###Code
condition1 and condition2
condition1 or condition2
###Output
_____no_output_____
###Markdown
* Rule 1: (**C1** *and* **C2**) is *True* only if both **C1** and **C2** are *True** Rule 2: (**C1** *or* **C2**) is *True* if either **C1** or **C2** are *True*Examples:
###Code
x=0; y=1.2
print (x >= 0 and y < 1)
###Output
_____no_output_____
###Markdown
Exercise: Values of boolean expressionsAdd a comment to the code below to explain the outcome of each of the boolean expressions:
###Code
C = 41
print("Case 1: ", C == 40)
print("Case 2: ", C != 40 and C < 41)
print("Case 3: ", C != 40 or C < 41)
print("Case 4: ", not C == 40)
print("Case 5: ", not C > 40)
print("Case 6: ", C <= 41)
print("Case 7: ", not False)
print("Case 8: ", True and False)
print("Case 9: ", False or True)
print("Case 10: ", False or False or False)
print("Case 11: ", True and True and False)
print("Case 12: ", False == 0)
print("Case 13: ", True == 0)
print("Case 14: ", True == 1)
###Output
_____no_output_____
###Markdown
LoopsSuppose we want to make a table of Celsius and Fahrenheitdegrees:
###Code
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Output
_____no_output_____
###Markdown
How do we write a program that prints out such a table?We know from the last lecture how to make one line in this table:
###Code
C = -20
F = 9/5*C + 32
print(C, F)
###Output
_____no_output_____
###Markdown
We can just repeat these statements:
###Code
C=-20; F=9/5*C+32; print(C,F)
C=-15; F=9/5*C+32; print(C,F)
C=-10; F=9/5*C+32; print(C,F)
C=-5; F=9/5*C+32; print(C,F)
C=0; F=9/5*C+32; print(C,F)
C=5; F=9/5*C+32; print(C,F)
C=10; F=9/5*C+32; print(C,F)
C=15; F=9/5*C+32; print(C,F)
C=20; F=9/5*C+32; print(C,F)
C=25; F=9/5*C+32; print(C,F)
C=30; F=9/5*C+32; print(C,F)
C=35; F=9/5*C+32; print(C,F)
C=40; F=9/5*C+32; print(C,F)
###Output
_____no_output_____
###Markdown
So we can see that works but its **very boring** to write and very easy to introduce a misprint.**You really should not be doing boring repetitive tasks like this.** Spend one time instead looking for a smarter solution. When programming becomes boring, there is usually a construct that automates the writing. Computers are very good at performing repetitive tasks. For this purpose we use **loops**. The while loop (and the significance of indentation)A while loop executes repeatedly a set of statements as long as a **boolean** (i.e. *True* / *False*) condition is *True*
###Code
while condition:
<statement 1>
<statement 2>
...
<first statement after loop>
###Output
_____no_output_____
###Markdown
Note that all statements to be executed within the loop must be indented by the same amount! The loop ends when an unindented statement is encountered.At this point it is worth noticing that **blank spaces may or may not be important** in Python programs. These statements are equivalent (blanks do not matter):
###Code
v0=3
v0 = 3
v0= 3
# The computer does not care but this formatting style is
# considered clearest for the human reader.
v0 = 3
###Output
_____no_output_____
###Markdown
Here is a while loop example where blank spaces really do matter:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
_____no_output_____
###Markdown
Let's take a look at what happens when we forget to indent correctly:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
_____no_output_____
###Markdown
Let's use the while loop to create the table above:
###Code
C = -20 # Initialise C
dC = 5 # Increment for C within the loop
while C <= 40: # Loop heading with condition
F = (9/5)*C + 32 # 1st statement inside loop
print ("%3d %6.1f" % (C, F)) # 2nd statement inside loop
C = C + dC # Increment C for the next iteration of the loop.
###Output
_____no_output_____
###Markdown
Exercise: Make a Fahrenheit-Celsius conversion tableWrite a program that prints out a table with Fahrenheit degrees 0, 10, 20, ..., 100 in the first column and the corresponding Celsius degrees in the second column. Exercise: Write an approximate Fahrenheit-Celsius conversion tableMany people use an approximate formula for quickly converting Fahrenheit ($F$) to Celsius ($C$) degrees:$C \approx \hat{C} = \frac{F − 30}{2}$Modify the program from the previous exercise so that it prints three columns: $F$, $C$, and the approximate value $\hat{C}$. ListsSo far, one variable has referred to one number (or string). Sometimes however we naturally have a collection of numbers, saydegrees −20, −15, −10, −5, 0, ..., 40. One way to store these values in a computer program would be to have one variable per value, i.e.
###Code
C1 = -20
C2 = -15
C3 = -10
# ...
C13 = 40
###Output
_____no_output_____
###Markdown
This is clearly a terrible solution, particularly if we have lots of values. A better way of doing this is to collect values together in a list:
###Code
C = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
###Output
_____no_output_____
###Markdown
Now there is just one variable, **C**, holding all the values. Elements in a list are accessed via an index. List indices are always numbered as 0, 1, 2, and so forth up to the number of elements minus one:
###Code
mylist = [4, 6, -3.5]
print(mylist[0])
print(mylist[1])
print(mylist[2])
print(len(mylist)) # length of list
###Output
4
6
-3.5
3
###Markdown
Here are a few example of operations that you can perform on lists:
###Code
C = [-10, -5, 0, 5, 10, 15, 20, 25, 30]
C.append(35) # add new element 35 at the end
print(C)
C = C + [40,45] # And another list to the end of C
print(C)
C.insert(0, -15) # Insert -15 as index 0
print(C)
print(C[:3])
del C[2] # delete 3rd element
print(C)
del C[2] # delete what is now 3rd element
print(C)
print(len(C)) # length of list
print(C.index(10)) # Find the index of the list with the value 10
print (10 in C) # True only if the value 10 is stored in the list
print(C[-1]) # The last value in the list.
print(C[-2]) # The second last value in the list.
###Output
_____no_output_____
###Markdown
You can also extract sublists using ":"
###Code
print(C[5:]) # From index 5 to the end of the list.
print(C[5:7]) # From index 5 up to, but not including index 7.
###Output
_____no_output_____
###Markdown
Many languages (especially Matlab and Fortran) have would include the endpoint of a slice (7 in this case). One way to think of what Python does is that the slice is a half-open interval: $[5,7)$. This makes cutting a list in half particularly easy. For example:
###Code
print(C[:5] + ["Hello"] + C[5:])
print(C[7:-1]) # From index 7 up to the second last element.
print(C[:]) # [:] specifies the whole list.
somelist = ['Curly', 'Larry', 'Moe']
stooge1, stooge2, stooge3 = somelist # Unpack the entries in a list.
print(stooge3, stooge2, stooge1)
###Output
Moe Larry Curly
###Markdown
Of course this only works if `somelist` contains exactly the number of items on the left hand side of the equals. Another useful operator is `*` (pronounced "splat"). It turns a list (or any other sequence) into the set of arguments of a function. For example:
###Code
print(somelist) # Prints the list.
print(*somelist) # Prints the items in the list.
###Output
['Curly', 'Larry', 'Moe']
Curly Larry Moe
###Markdown
Exercise: Store odd numbers in a listStep 1: Write a program that generates all odd numbers from 1 to *n*. Set *n* in the beginning of the program and use a while loop to compute the numbers. (Make sure that if *n* is an even number, the largest generated odd number is *n*-1.).Step 2: Store the generated odd numbers in a list. Start with an empty list and use the same while loop where you generate each odd number, ao append the new number to the list.Finally, print the list elements to the screen.
###Code
list_odd = []
n = 100
for i in range(n):
if i%2 != 0:
list_odd = list_odd + [i]
ind = 0
list_odd2= []
while ind < n:
if ind %2 != 0:
list_odd2 = list_odd2 + [ind]
ind += 1
print(list_odd2)
#print(list_odd)
###Output
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21, 23, 25, 27, 29, 31, 33, 35, 37, 39, 41, 43, 45, 47, 49, 51, 53, 55, 57, 59, 61, 63, 65, 67, 69, 71, 73, 75, 77, 79, 81, 83, 85, 87, 89, 91, 93, 95, 97, 99]
###Markdown
For loopsWe can visit each element in a list and process the element with some statements using a *for* loop, for example:
###Code
degrees = [0, 10, 20, 40, 100]
for C in degrees:
print('list element:', C)
print('The degrees list has', len(degrees), 'elements')
###Output
list element: 0
list element: 10
list element: 20
list element: 40
list element: 100
The degrees list has 5 elements
###Markdown
Notice again how the statements to be executed within the loop must be indented! Let's now revisit the conversion table example using the *for* loop:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
for C in Cdegrees:
F = (9/5)*C + 32
print("%3d %6.2f" % (C, F))
###Output
_____no_output_____
###Markdown
It is also possible to rewrite the *for* loop as a *while* loop, i.e.,
###Code
for element in somelist:
# process element
###Output
_____no_output_____
###Markdown
can always be transformed to a *while* loop
###Code
index = 0
while index < len(somelist):
element = somelist[index]
# process element
index += 1
###Output
_____no_output_____
###Markdown
Taking the previous table example:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
index = 0
while index < len(Cdegrees):
C = Cdegrees[index]
F = (9/5)*C + 32
print('%5d %5.1f' % (C, F))
index += 1
###Output
_____no_output_____
###Markdown
Rather than just printing out the Fahrenheit values, let's also store these computed values in a list of their own:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
Fdegrees = [] # start with empty list
for C in Cdegrees:
F = (9/5)*C + 32
Fdegrees.append(F) # add new element to Fdegrees
print(Fdegrees)
###Output
_____no_output_____
###Markdown
Iterators: things you can iterate over.We've seen that a `for` loop can iterate over list values. In fact you can iterate over any object that is capable of providing values one at a time. In python, these objects are called `iterators`.A particularly useful python function which creates an iterator is `range`. For example, we can use range to iterate over the *indices* of a list:
###Code
for i in range(0, len(somelist), 1):
element = somelist[i]
... process element or somelist[i] directly
###Output
_____no_output_____
###Markdown
The statement *range(start, stop, inc)* generates an iterator over the integers *start*, *start+inc*, *start+2\*inc*, and so on up to, but not including, *stop*. We can also write *range(stop)* as an abbreviation for *range(0, stop, 1)*:
###Code
print(range(3)) # same as range(0, 3, 1)
###Output
_____no_output_____
###Markdown
Oh, that wasn't very informative. If we want to see all the numbers which the `range` will iterate over, we can cast the range to a list:
###Code
print(list(range(3)))
print(list(range(2, 8, 3)))
###Output
_____no_output_____
###Markdown
List comprehensionsConsider this example where we compute two lists in a *for* loop:
###Code
n = 16
Cdegrees = []; Fdegrees = [] # empty lists
for i in range(n):
Cdegrees.append(-5 + i*0.5)
Fdegrees.append((9/5)*Cdegrees[i] + 32)
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
As constructing lists is a very common requirement, the above way of doing it can become very tedious to both write and read. Therefore, Python has a compact construct, called list comprehension for generating lists from a *for* loop:
###Code
n = 16
Cdegrees = [-5 + i*0.5 for i in range(n)]
Fdegrees = [(9/5)*C + 32 for C in Cdegrees]
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
_____no_output_____
###Markdown
The general form of a list comprehension is:
###Code
somelist = [expression for element in somelist]
###Output
_____no_output_____
###Markdown
Exercise: Repeat the previous exercise of creating a list of odd numbers using using: a for loop, list comprehension and the range function
###Code
# Using list comprehension
# Using the function range
###Output
_____no_output_____
###Markdown
Changing elements in a listSay we want to add $2$ to all the numbers in a list:
###Code
v = [-1, 1, 10]
for e in v:
e = e + 2
print(v)
###Output
[-1, 1, 10]
###Markdown
We can see that the list *v* is unaltered! The reason for this is that inside the loop, *e* is an ordinary (int) variable. At the start of the iteration *e* is assigned a *copy* of the next element in the list. Inside the loop we can change *e* but *v* itself is unaltered. If we want to change *v* we have to use an index to access and modify its elements:
###Code
v[1] = 4 # assign 4 to 2nd element (index 1) in v
print(v)
###Output
[-1, 4, 10]
###Markdown
To add 2 to all values we need a *for* loop over indices:
###Code
for i in range(len(v)):
v[i] = v[i] + 2
print(v)
###Output
[1, 6, 12]
###Markdown
Traversing multiple lists simultaneously - *zip(list1, list2, ...)*Consider how we can loop over elements in both Cdegrees and Fdegrees at the same time. One approach would be to use list indices:
###Code
for i in range(len(Cdegrees)):
print(Cdegrees[i], Fdegrees[i])
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
An alternative construct (regarded as more ”Pythonic”) uses the *zip* function:
###Code
for C, F in zip(Cdegrees, Fdegrees):
print(C, F)
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
`zip` creates an *iterator* over the corresponding items of its arguments in order.Another example with three lists:
###Code
l1 = [3, 6, 1]; l2 = [1.5, 1, 0]; l3 = [9.1, 3, 2]
for e in zip(l1, l2, l3):
print(*e)
###Output
3 1.5 9.1
6 1 3
1 0 2
###Markdown
If the lists are of unequal length then the loop stops when the end of the shortest list is reached. Experiment with this:
###Code
l1 = [3, 6, 1, 4, 6]; l2 = [1.5, 1, 0, 7]; l3 = [9.1, 3, 2, 0, 9]
for e in zip(l1, l2, l3):
print(*e)
###Output
3 1.5 9.1
6 1 3
1 0 2
4 7 0
###Markdown
Nested lists: list of listsA *list* can contain **any** object, including another *list*. To illustrate this, consider how to store the conversion table as a single Python list rather than two separate lists.
###Code
Cdegrees = range(-20, 41, 5)
Fdegrees = [(9.0/5)*C + 32 for C in Cdegrees]
table1 = [Cdegrees, Fdegrees] # List of two lists
print("table1 = ", table1)
print("table1[0] = ", table1[0])
print("table1[1] = ", table1[1])
print("table1[2][3] = ", table1[1][3])
###Output
table1 = [range(-20, 41, 5), [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]]
table1[0] = range(-20, 41, 5)
table1[1] = [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]
table1[2][3] = 23.0
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
###Markdown
This gives us a table of two columns. How do we create a table of rows instead:
###Code
table2 = []
for C, F in zip(Cdegrees, Fdegrees):
row = [C, F]
table2.append(row)
print(table2)
###Output
[[-20, -4.0], [-15, 5.0], [-10, 14.0], [-5, 23.0], [0, 32.0], [5, 41.0], [10, 50.0], [15, 59.0], [20, 68.0], [25, 77.0], [30, 86.0], [35, 95.0], [40, 104.0]]
###Markdown
We can use `zip` to do this more elegantly:
###Code
table2 = list(zip(Cdegrees, Fdegrees))
print(table2)
###Output
_____no_output_____
###Markdown
And you can loop through this list as before:
###Code
for C, F in table2:
print(C, F)
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Tuples: sequences that cannot be changedWe've already learned that lists are not the only thing which can be iterated over, but that there is a general category of `iterator` types. Similarly, lists are not the only objects which can be indexed. The general category of types which can be indexed are the `sequence` types.Tuples are **constant** sequences. You can use them in much the same way as lists except you cannot modify them. They are an example of an [**immutable**](http://en.wikipedia.org/wiki/Immutable_object) type.
###Code
t = (2, 4, 6, 'temp.pdf') # Define a tuple.
t = 2, 4, 6, 'temp.pdf' # Can skip parenthesis as it is assumed in this context.
###Output
_____no_output_____
###Markdown
Let's see what happens when we try to modify the tuple like we did with a list:
###Code
t[1] = -1
t.append(0)
del t[1]
###Output
_____no_output_____
###Markdown
However, we can use the tuple to compose a new tuple:
###Code
t = t + (-1.0, -2.0)
print(t)
###Output
_____no_output_____
###Markdown
So, why would we use tuples when lists have more functionality?* Tuples are constant and thus protected against accidental changes.* Tuples are faster than lists.* Tuples are widely used in Python software (so you need to know about tuples to understand other people's code!)* Tuples (but not lists) can be used as keys in dictionaries (more about dictionaries later). Exercise: Make a table of function valuesStep 1: Write a program that prints a table with $t$ values in the first column and the corresponding $y(t) = v_0 t − 0.5gt^2$ values in the second column. Use $n$ uniformly spaced $t$ values throughout the interval [0, $2v_0/g$]. Set $v0 = 1$, $g = 9.81$, and $n = 11$.Step 2: Once step 1 is working, modify the program so that the $t$ and $y$ values are stored in two lists *t* and *y*. Thereafter, transverse the lists with a *for* loop and write out a nicely formatted table of *t* and *y* values using a *zip* construction. FunctionsWe have already used many Python functions, including mathematical functions:
###Code
from math import *
x = pi
print cos(x)
###Output
_____no_output_____
###Markdown
Other functions you used include *len* and *range*:
###Code
n = len(somelist)
ints = range(5, n, 2)
###Output
_____no_output_____
###Markdown
You have also used functions that are executed with the dot syntax (called *methods*):
###Code
C = [5, 10, 40, 45]
i = C.index(10)
C.append(50)
C.insert(2, 20)
###Output
_____no_output_____
###Markdown
A function is a collection of statements we can execute wherever and whenever we want. Functions can take any number of inputs (called *arguments*) to produce outputs. Functions help to organize programs, make them more understandable, shorter, and easier to extend. For our first example we will turn our temperature conversion code into a function:
###Code
def C2F(C):
return (9/5)*C + 32
###Output
_____no_output_____
###Markdown
Functions start with *def*, then the name you want to give your function, then a list of arguments (here C). This is referred to as the *function header*. Inside the function there is a block of statements called the *function body*. Notice that the function body is indented - as was the case for the *for* / *while* loop the indentation indicates where the function ends. At any point within the function, we can "stop thefunction" and return as many values/variables as required. Local and global variablesVariables defined within a function are said to have *local scope*. That is to say that they can only be referenced within that function. Let's consider an example (and look carefully at the indentation!!):
###Code
def sumint(start, stop):
s = 0 # variable for accumulating the sum
i = start # counter
while i <= stop:
s += i
i += 1
return s
print(sumint(0, 5))
###Output
_____no_output_____
###Markdown
Variables *i* and *s* are local variables in *sumint*. They are destroyed at the end (return) of the function and never visible outside the function (in the calling program); in fact, *start* and *stop* are also local variables. So let's see what happens if we now try to print one of these variables:
###Code
print(stop)
###Output
_____no_output_____
###Markdown
Functions can also return multiple values. Let's recycle another of our previous examples - compute $y(t)$ and $y'(t)=v_0-gt$:
###Code
def yfunc(t, v0):
g = 9.81
y = v0*t - 0.5*g*t**2
dydt = v0 - g*t
return y, dydt
# call:
position, velocity = yfunc(0.6, 3)
print(position, velocity)
###Output
_____no_output_____
###Markdown
Remember that a series of comma separated variables implies a tuple - therefore "return y, dydt" is the same as writing "return (y, dydt)". Therefore, in general what is returned is a tuple. Let's take a look at another example illustrating this:
###Code
def f(x):
return x, x**2, x**4
s = f(2)
print(s)
print(type(s)) # The function type() tells us what type a variable it is.
###Output
_____no_output_____
###Markdown
No return value implies that `None` is returned. `None` is a special Python object that represents an ”empty” or undefined value. It is surprisingly useful and we will use it a lot later.
###Code
def message(course):
print("%s rocks!"% course)
message("Python")
r = message("Geo")
print("r = ", r)
###Output
_____no_output_____
###Markdown
Keyword arguments and default argument valuesIt's possible to specify default values that function arguments should take if no values are provided when the function is called:
###Code
def somefunc(arg1, arg2, kwarg1=True, kwarg2=0):
print(arg1, arg2, kwarg1, kwarg2)
somefunc("Hello", [1,2]) # Note that we have not specified values for kwarg1 and kwarg2
###Output
_____no_output_____
###Markdown
You can override the default values by passing additional arguments in the correct position:
###Code
somefunc("Hello", [1,2], "Hi")
###Output
_____no_output_____
###Markdown
... or by naming the argument to which the value should be passed. These are called keyword arguments:
###Code
somefunc("Hello", [1,2], kwarg2="Hi")
somefunc("Hello", [1,2], kwarg2="Hi", kwarg1=6)
###Output
_____no_output_____
###Markdown
If we use variable_name=value for all arguments, their sequence in the function header can be in any order.
###Code
somefunc(kwarg2="Hello", arg1="Hi", kwarg1=6, arg2=[2])
###Output
_____no_output_____
###Markdown
Let's look at another example - consider a function of $t$, with parameters $A$, $a$, and $\omega$:$$f(t; A,a, \omega) = Ae^{-at}\sin (\omega t)$$. (The choice of equation is actually pretty random - but it serves to show you that it is easy to translate formulae you encounter into Python code). We can implement $f$ in a Python function with $t$ as positional argument and $A$, $a$, and $\omega$ as keyword arguments.
###Code
from math import pi, exp, sin
def f(t, A=1, a=1, ω=2*pi):
return A*exp(-a*t)*sin(ω*t)
v1 = f(0.2)
v2 = f(0.2, ω=1)
v2 = f(0.2, 1, 3) # same as f(0.2, A=1, a=3)
v3 = f(0.2, ω=1, A=2.5)
v4 = f(A=5, a=0.1, ω=1, t=1.3)
v5 = f(t=0.2, A=9)
print(v1, v2, v3, v4, v5)
###Output
_____no_output_____
###Markdown
Exercise: Implement a Gaussian functionMake a Python function *gauss*( *x*, *m*=0, *s*=1) for computing the Gaussian function $$f(x)=\frac{1}{\sqrt{2\pi}s}\exp\left(-\frac{1}{2} \left(\frac{x-m}{s}\right)^2\right)$$Call the function and print out the result for x equal to −5, −4.9, −4.8, ..., 4.8, 4.9, 5, using default values for *m* and *s*. The *if* statementConsider this simple example:
###Code
def f(x):
if 0 <= x <= pi:
return sin(x)
else:
return 0
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
In general (the *else* block can be skipped if there are no statements to be executed when False) we can put together multiple conditions. Only the first condition that is True is executed.
###Code
if condition1:
<block of statements, executed if condition1 is True>
elif condition2:
<block of statements>
elif condition3:
<block of statements>
else:
<block of statements>
<next statement of the program>
###Output
_____no_output_____
###Markdown
The ternary operatorThere is another form of *if* which works as an operator, rather than a statement. If you've used the *if* function in a spreadsheet then you'll be familiar with this approach.
###Code
def f(x):
return (sin(x) if 0 <= x <= pi else 0)
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
The ternary operator has the following form:
###Code
<expression if condition is True> if <condition> else <expression if condition is false>
###Output
_____no_output_____
###Markdown
Introduction to Python programming [Gerard Gorman](http://www.imperial.ac.uk/people/g.gorman), [Christian Jacobs](http://christianjacobs.uk/) Updated for MPECDT by [David Ham](http://www.imperial.ac.uk/people/david.ham) Lecture 2: Computing with basic language constructionsLearning objectives:* Introduction to two different forms of loops which are used to repeat operations on data: either until a condition is satisfied (*while-loop*) or for a fixed number of iterations (*for-loop*).* Form a *condition* using a *boolean expression*. This is used to either terminate loops or control if a specific block of code is executed via the *if-statement*.* Learn two different methods for storing many data elements within a single Python type.* Introduction to developing your own Python *functions* which allows you to execute the same block of code, with different inputs and outputs, from anywhere in the code. Boolean expressionsAn expression with value *true* or *false* is called a boolean expression. Example expressions for what you would write mathematically as$C=40$, $C\ne40$, $C\ge40$, $C\gt40$ and $C\lt40$ are:
###Code
C == 40 # Note: the double == checks for equality, but C=40 is an assignment!
C != 40 # This could also be written as 'not C == 4' (i.e. the opposite of what C == 4 gives)
C >= 40
C > 40
C < 40
###Output
_____no_output_____
###Markdown
We can test boolean expressions in a Python shell:
###Code
C = 41
print("C != 40: ", C != 40)
print("C < 40: ", C < 40)
print("C == 41: ", C == 41)
###Output
_____no_output_____
###Markdown
Several conditions can be combined with the special 'and' and 'or' operators into a single boolean expression:
###Code
condition1 and condition2
condition1 or condition2
###Output
_____no_output_____
###Markdown
* Rule 1: (**C1** *and* **C2**) is *True* only if both **C1** and **C2** are *True** Rule 2: (**C1** *or* **C2**) is *True* if either **C1** or **C2** are *True*Examples:
###Code
x=0; y=1.2
print (x >= 0 and y < 1)
###Output
_____no_output_____
###Markdown
Exercise: Values of boolean expressionsAdd a comment to the code below to explain the outcome of each of the boolean expressions:
###Code
C = 41
print("Case 1: ", C == 40)
print("Case 2: ", C != 40 and C < 41)
print("Case 3: ", C != 40 or C < 41)
print("Case 4: ", not C == 40)
print("Case 5: ", not C > 40)
print("Case 6: ", C <= 41)
print("Case 7: ", not False)
print("Case 8: ", True and False)
print("Case 9: ", False or True)
print("Case 10: ", False or False or False)
print("Case 11: ", True and True and False)
print("Case 12: ", False == 0)
print("Case 13: ", True == 0)
print("Case 14: ", True == 1)
###Output
_____no_output_____
###Markdown
LoopsSuppose we want to make a table of Celsius and Fahrenheitdegrees:
###Code
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Output
_____no_output_____
###Markdown
How do we write a program that prints out such a table?We know from the last lecture how to make one line in this table:
###Code
C = -20
F = 9/5*C + 32
print(C, F)
###Output
_____no_output_____
###Markdown
We can just repeat these statements:
###Code
C=-20; F=9/5*C+32; print(C,F)
C=-15; F=9/5*C+32; print(C,F)
C=-10; F=9/5*C+32; print(C,F)
C=-5; F=9/5*C+32; print(C,F)
C=0; F=9/5*C+32; print(C,F)
C=5; F=9/5*C+32; print(C,F)
C=10; F=9/5*C+32; print(C,F)
C=15; F=9/5*C+32; print(C,F)
C=20; F=9/5*C+32; print(C,F)
C=25; F=9/5*C+32; print(C,F)
C=30; F=9/5*C+32; print(C,F)
C=35; F=9/5*C+32; print(C,F)
C=40; F=9/5*C+32; print(C,F)
###Output
_____no_output_____
###Markdown
So we can see that works but its **very boring** to write and very easy to introduce a misprint.**You really should not be doing boring repetitive tasks like this.** Spend one time instead looking for a smarter solution. When programming becomes boring, there is usually a construct that automates the writing. Computers are very good at performing repetitive tasks. For this purpose we use **loops**. The while loop (and the significance of indentation)A while loop executes repeatedly a set of statements as long as a **boolean** (i.e. *True* / *False*) condition is *True*
###Code
while condition:
<statement 1>
<statement 2>
...
<first statement after loop>
###Output
_____no_output_____
###Markdown
Note that all statements to be executed within the loop must be indented by the same amount! The loop ends when an unindented statement is encountered.At this point it is worth noticing that **blank spaces may or may not be important** in Python programs. These statements are equivalent (blanks do not matter):
###Code
v0=3
v0 = 3
v0= 3
# The computer does not care but this formatting style is
# considered clearest for the human reader.
v0 = 3
###Output
_____no_output_____
###Markdown
Here is a while loop example where blank spaces really do matter:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
_____no_output_____
###Markdown
Let's take a look at what happens when we forget to indent correctly:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
_____no_output_____
###Markdown
Let's use the while loop to create the table above:
###Code
C = -20 # Initialise C
dC = 5 # Increment for C within the loop
while C <= 40: # Loop heading with condition
F = (9/5)*C + 32 # 1st statement inside loop
print ("%3d %6.1f" % (C, F)) # 2nd statement inside loop
C = C + dC # Increment C for the next iteration of the loop.
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Exercise: Make a Fahrenheit-Celsius conversion tableWrite a program that prints out a table with Fahrenheit degrees 0, 10, 20, ..., 100 in the first column and the corresponding Celsius degrees in the second column. Exercise: Write an approximate Fahrenheit-Celsius conversion tableMany people use an approximate formula for quickly converting Fahrenheit ($F$) to Celsius ($C$) degrees:$C \approx \hat{C} = \frac{F − 30}{2}$Modify the program from the previous exercise so that it prints three columns: $F$, $C$, and the approximate value $\hat{C}$. ListsSo far, one variable has referred to one number (or string). Sometimes however we naturally have a collection of numbers, saydegrees −20, −15, −10, −5, 0, ..., 40. One way to store these values in a computer program would be to have one variable per value, i.e.
###Code
C1 = -20
C2 = -15
C3 = -10
# ...
C13 = 40
###Output
_____no_output_____
###Markdown
This is clearly a terrible solution, particularly if we have lots of values. A better way of doing this is to collect values together in a list:
###Code
C = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
###Output
_____no_output_____
###Markdown
Now there is just one variable, **C**, holding all the values. Elements in a list are accessed via an index. List indices are always numbered as 0, 1, 2, and so forth up to the number of elements minus one:
###Code
mylist = [4, 6, -3.5]
print(mylist[0])
print(mylist[1])
print(mylist[2])
print(len(mylist)) # length of list
###Output
4
6
-3.5
3
###Markdown
Here are a few example of operations that you can perform on lists:
###Code
C = [-10, -5, 0, 5, 10, 15, 20, 25, 30]
C.append(35) # add new element 35 at the end
print(C)
C = C + [40,45] # And another list to the end of C
print(C)
C.insert(0, -15) # Insert -15 as index 0
print(C)
del C[2] # delete 3rd element
print(C)
del C[2] # delete what is now 3rd element
print(C)
print(len(C)) # length of list
print(C.index(10)) # Find the index of the list with the value 10
print (10 in C) # True only if the value 10 is stored in the list
print(C[-1]) # The last value in the list.
print(C[-2]) # The second last value in the list.
###Output
_____no_output_____
###Markdown
You can also extract sublists using ":"
###Code
print(C[5:]) # From index 5 to the end of the list.
print(C[5:7]) # From index 5 up to, but not including index 7.
###Output
_____no_output_____
###Markdown
Many languages (especially Matlab and Fortran) have would include the endpoint of a slice (7 in this case). One way to think of what Python does is that the slice is a half-open interval: $[5,7)$. This makes cutting a list in half particularly easy. For example:
###Code
print(C[:5] + ["Hello"] + C[5:])
print(C[7:-1]) # From index 7 up to the second last element.
print(C[:]) # [:] specifies the whole list.
somelist = ['Curly', 'Larry', 'Moe']
stooge1, stooge2 = somelist # Unpack the entries in a list.
print(stooge2, stooge1)
###Output
_____no_output_____
###Markdown
Of course this only works if `somelist` contains exactly the number of items on the left hand side of the equals. Another useful operator is `*` (pronounced "splat"). It turns a list (or any other sequence) into the set of arguments of a function. For example:
###Code
print(somelist) # Prints the list.
print(*somelist) # Prints the items in the list.
###Output
_____no_output_____
###Markdown
Exercise: Store odd numbers in a listStep 1: Write a program that generates all odd numbers from 1 to *n*. Set *n* in the beginning of the program and use a while loop to compute the numbers. (Make sure that if *n* is an even number, the largest generated odd number is *n*-1.).Step 2: Store the generated odd numbers in a list. Start with an empty list and use the same while loop where you generate each odd number, ao append the new number to the list.Finally, print the list elements to the screen.
###Code
N=11
cnt=1
list_of_nr=[]
while cnt<=N:
if(cnt%2)!=0: list_of_nr.append(cnt)
cnt=cnt+1
print(*list_of_nr)
###Output
1 3 5 7 9 11
###Markdown
For loopsWe can visit each element in a list and process the element with some statements using a *for* loop, for example:
###Code
degrees = [0, 10, 20, 40, 100]
for C in degrees:
print('list element:', C)
print('The degrees list has', len(degrees), 'elements')
###Output
_____no_output_____
###Markdown
Notice again how the statements to be executed within the loop must be indented! Let's now revisit the conversion table example using the *for* loop:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
for C in Cdegrees:
F = (9/5)*C + 32
print("%3d %6.2f" % (C, F))
###Output
_____no_output_____
###Markdown
It is also possible to rewrite the *for* loop as a *while* loop, i.e.,
###Code
for element in somelist:
# process element
###Output
_____no_output_____
###Markdown
can always be transformed to a *while* loop
###Code
index = 0
while index < len(somelist):
element = somelist[index]
# process element
index += 1
###Output
_____no_output_____
###Markdown
Taking the previous table example:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
index = 0
while index < len(Cdegrees):
C = Cdegrees[index]
F = (9/5)*C + 32
print('%5d %5.1f' % (C, F))
index += 1
###Output
_____no_output_____
###Markdown
Rather than just printing out the Fahrenheit values, let's also store these computed values in a list of their own:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
Fdegrees = [] # start with empty list
for C in Cdegrees:
F = (9/5)*C + 32
Fdegrees.append(F) # add new element to Fdegrees
print(Fdegrees)
###Output
_____no_output_____
###Markdown
Iterators: things you can iterate over.We've seen that a `for` loop can iterate over list values. In fact you can iterate over any object that is capable of providing values one at a time. In python, these objects are called `iterators`.A particularly useful python function which creates an iterator is `range`. For example, we can use range to iterate over the *indices* of a list:
###Code
for i in range(0, len(somelist), 1):
element = somelist[i]
... process element or somelist[i] directly
###Output
_____no_output_____
###Markdown
The statement *range(start, stop, inc)* generates an iterator over the integers *start*, *start+inc*, *start+2\*inc*, and so on up to, but not including, *stop*. We can also write *range(stop)* as an abbreviation for *range(0, stop, 1)*:
###Code
print(range(3)) # same as range(0, 3, 1)
###Output
range(0, 3)
###Markdown
Oh, that wasn't very informative. If we want to see all the numbers which the `range` will iterate over, we can cast the range to a list:
###Code
print(list(range(3)))
print(list(range(2, 8, 3)))
###Output
[2, 5]
###Markdown
List comprehensionsConsider this example where we compute two lists in a *for* loop:
###Code
n = 16
Cdegrees = []; Fdegrees = [] # empty lists
for i in range(n):
Cdegrees.append(-5 + i*0.5)
Fdegrees.append((9/5)*Cdegrees[i] + 32)
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
As constructing lists is a very common requirement, the above way of doing it can become very tedious to both write and read. Therefore, Python has a compact construct, called list comprehension for generating lists from a *for* loop:
###Code
n = 16
Cdegrees = [-5 + i*0.5 for i in range(n)]
Fdegrees = [(9/5)*C + 32 for C in Cdegrees]
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
The general form of a list comprehension is:
###Code
somelist = [expression for element in somelist]
###Output
_____no_output_____
###Markdown
Exercise: Repeat the previous exercise of creating a list of odd numbers using using: a for loop, list comprehension and the range function
###Code
# Using for loop
n=16
odds=[]
for i in range(n):
if (i%2) != 0:
odds.append(i)
print(*odds)
# Using list comprehension
# Using the function range
###Output
_____no_output_____
###Markdown
Changing elements in a listSay we want to add $2$ to all the numbers in a list:
###Code
v = [-1, 1, 10]
for e in v:
e = e + 2
print(v)
###Output
_____no_output_____
###Markdown
We can see that the list *v* is unaltered! The reason for this is that inside the loop, *e* is an ordinary (int) variable. At the start of the iteration *e* is assigned a *copy* of the next element in the list. Inside the loop we can change *e* but *v* itself is unaltered. If we want to change *v* we have to use an index to access and modify its elements:
###Code
v[1] = 4 # assign 4 to 2nd element (index 1) in v
print(v)
###Output
_____no_output_____
###Markdown
To add 2 to all values we need a *for* loop over indices:
###Code
for i in range(len(v)):
v[i] = v[i] + 2
print(v)
###Output
_____no_output_____
###Markdown
Traversing multiple lists simultaneously - *zip(list1, list2, ...)*Consider how we can loop over elements in both Cdegrees and Fdegrees at the same time. One approach would be to use list indices:
###Code
for i in range(len(Cdegrees)):
print(Cdegrees[i], Fdegrees[i])
###Output
_____no_output_____
###Markdown
An alternative construct (regarded as more ”Pythonic”) uses the *zip* function:
###Code
for C, F in zip(Cdegrees, Fdegrees):
print(C, F)
###Output
_____no_output_____
###Markdown
`zip` creates an *iterator* over the corresponding items of its arguments in order.Another example with three lists:
###Code
l1 = [3, 6, 1]; l2 = [1.5, 1, 0]; l3 = [9.1, 3, 2]
for e in zip(l1, l2, l3):
print(*e)
###Output
_____no_output_____
###Markdown
If the lists are of unequal length then the loop stops when the end of the shortest list is reached. Experiment with this:
###Code
l1 = [3, 6, 1, 4, 6]; l2 = [1.5, 1, 0, 7]; l3 = [9.1, 3, 2, 0, 9]
for e in zip(l1, l2, l3):
print(*e)
###Output
_____no_output_____
###Markdown
Nested lists: list of listsA *list* can contain **any** object, including another *list*. To illustrate this, consider how to store the conversion table as a single Python list rather than two separate lists.
###Code
Cdegrees = range(-20, 41, 5)
Fdegrees = [(9.0/5)*C + 32 for C in Cdegrees]
table1 = [Cdegrees, Fdegrees] # List of two lists
print("table1 = ", table1)
print("table1[0] = ", table1[0])
print("table1[1] = ", table1[1])
print("table1[2][3] = ", table1[1][3])
###Output
_____no_output_____
###Markdown
This gives us a table of two columns. How do we create a table of rows instead:
###Code
table2 = []
for C, F in zip(Cdegrees, Fdegrees):
row = [C, F]
table2.append(row)
print(table2)
###Output
_____no_output_____
###Markdown
We can use `zip` to do this more elegantly:
###Code
table2 = list(zip(Cdegrees, Fdegrees))
print(table2)
###Output
_____no_output_____
###Markdown
And you can loop through this list as before:
###Code
for C, F in table2:
print(C, F)
###Output
_____no_output_____
###Markdown
Tuples: sequences that cannot be changedWe've already learned that lists are not the only thing which can be iterated over, but that there is a general category of `iterator` types. Similarly, lists are not the only objects which can be indexed. The general category of types which can be indexed are the `sequence` types.Tuples are **constant** sequences. You can use them in much the same way as lists except you cannot modify them. They are an example of an [**immutable**](http://en.wikipedia.org/wiki/Immutable_object) type.
###Code
t = (2, 4, 6, 'temp.pdf') # Define a tuple.
t = 2, 4, 6, 'temp.pdf' # Can skip parenthesis as it is assumed in this context.
###Output
_____no_output_____
###Markdown
Let's see what happens when we try to modify the tuple like we did with a list:
###Code
t[1] = -1
t.append(0)
del t[1]
###Output
_____no_output_____
###Markdown
However, we can use the tuple to compose a new tuple:
###Code
t = t + (-1.0, -2.0)
print(tuple(t))
print(*list(t))
###Output
(2, 4, 6, 'temp.pdf', -1.0, -2.0, -1.0, -2.0, -1.0, -2.0, -1.0, -2.0)
2 4 6 temp.pdf -1.0 -2.0 -1.0 -2.0 -1.0 -2.0 -1.0 -2.0
###Markdown
So, why would we use tuples when lists have more functionality?* Tuples are constant and thus protected against accidental changes.* Tuples are faster than lists.* Tuples are widely used in Python software (so you need to know about tuples to understand other people's code!)* Tuples (but not lists) can be used as keys in dictionaries (more about dictionaries later). Exercise: Make a table of function valuesStep 1: Write a program that prints a table with $t$ values in the first column and the corresponding $y(t) = v_0 t − 0.5gt^2$ values in the second column. Use $n$ uniformly spaced $t$ values throughout the interval [0, $2v_0/g$]. Set $v0 = 1$, $g = 9.81$, and $n = 11$.Step 2: Once step 1 is working, modify the program so that the $t$ and $y$ values are stored in two lists *t* and *y*. Thereafter, transverse the lists with a *for* loop and write out a nicely formatted table of *t* and *y* values using a *zip* construction. FunctionsWe have already used many Python functions, including mathematical functions:
###Code
from math import *
x = pi
print cos(x)
###Output
_____no_output_____
###Markdown
Other functions you used include *len* and *range*:
###Code
n = len(somelist)
ints = range(5, n, 2)
###Output
_____no_output_____
###Markdown
You have also used functions that are executed with the dot syntax (called *methods*):
###Code
C = [5, 10, 40, 45]
i = C.index(10)
C.append(50)
C.insert(2, 20)
###Output
_____no_output_____
###Markdown
A function is a collection of statements we can execute wherever and whenever we want. Functions can take any number of inputs (called *arguments*) to produce outputs. Functions help to organize programs, make them more understandable, shorter, and easier to extend. For our first example we will turn our temperature conversion code into a function:
###Code
def C2F(C):
return (9/5)*C + 32
###Output
_____no_output_____
###Markdown
Functions start with *def*, then the name you want to give your function, then a list of arguments (here C). This is referred to as the *function header*. Inside the function there is a block of statements called the *function body*. Notice that the function body is indented - as was the case for the *for* / *while* loop the indentation indicates where the function ends. At any point within the function, we can "stop thefunction" and return as many values/variables as required. Local and global variablesVariables defined within a function are said to have *local scope*. That is to say that they can only be referenced within that function. Let's consider an example (and look carefully at the indentation!!):
###Code
def sumint(start, stop):
s = 0 # variable for accumulating the sum
i = start # counter
while i <= stop:
s += i
i += 1
return s
print(sumint(0, 5))
###Output
_____no_output_____
###Markdown
Variables *i* and *s* are local variables in *sumint*. They are destroyed at the end (return) of the function and never visible outside the function (in the calling program); in fact, *start* and *stop* are also local variables. So let's see what happens if we now try to print one of these variables:
###Code
print(stop)
###Output
_____no_output_____
###Markdown
Functions can also return multiple values. Let's recycle another of our previous examples - compute $y(t)$ and $y'(t)=v_0-gt$:
###Code
def yfunc(t, v0):
g = 9.81
y = v0*t - 0.5*g*t**2
dydt = v0 - g*t
return y, dydt
# call:
position, velocity = yfunc(0.6, 3)
print(position, velocity)
###Output
_____no_output_____
###Markdown
Remember that a series of comma separated variables implies a tuple - therefore "return y, dydt" is the same as writing "return (y, dydt)". Therefore, in general what is returned is a tuple. Let's take a look at another example illustrating this:
###Code
def f(x):
return x, x**2, x**4
s = f(2)
print(s)
print(type(s)) # The function type() tells us what type a variable it is.
###Output
_____no_output_____
###Markdown
No return value implies that `None` is returned. `None` is a special Python object that represents an ”empty” or undefined value. It is surprisingly useful and we will use it a lot later.
###Code
def message(course):
print("%s rocks!"% course)
message("Python")
r = message("Geo")
print("r = ", r)
###Output
_____no_output_____
###Markdown
Keyword arguments and default argument valuesIt's possible to specify default values that function arguments should take if no values are provided when the function is called:
###Code
def somefunc(arg1, arg2, kwarg1=True, kwarg2=0):
print(arg1, arg2, kwarg1, kwarg2)
somefunc("Hello", [1,2]) # Note that we have not specified values for kwarg1 and kwarg2
###Output
_____no_output_____
###Markdown
You can override the default values by passing additional arguments in the correct position:
###Code
somefunc("Hello", [1,2], "Hi")
###Output
_____no_output_____
###Markdown
... or by naming the argument to which the value should be passed. These are called keyword arguments:
###Code
somefunc("Hello", [1,2], kwarg2="Hi")
somefunc("Hello", [1,2], kwarg2="Hi", kwarg1=6)
###Output
_____no_output_____
###Markdown
If we use variable_name=value for all arguments, their sequence in the function header can be in any order.
###Code
#somefunc(kwarg2="Hello", arg1="Hi", kwarg1=6, arg2=[2])
def foo(*Args):
print(Args)
foo(list([1,2]))
###Output
([1, 2],)
###Markdown
Let's look at another example - consider a function of $t$, with parameters $A$, $a$, and $\omega$:$$f(t; A,a, \omega) = Ae^{-at}\sin (\omega t)$$. (The choice of equation is actually pretty random - but it serves to show you that it is easy to translate formulae you encounter into Python code). We can implement $f$ in a Python function with $t$ as positional argument and $A$, $a$, and $\omega$ as keyword arguments.
###Code
from math import pi, exp, sin
def f(t, A=1, a=1, ω=2*pi):
return A*exp(-a*t)*sin(ω*t)
v1 = f(0.2)
v2 = f(0.2, ω=1)
v2 = f(0.2, 1, 3) # same as f(0.2, A=1, a=3)
v3 = f(0.2, ω=1, A=2.5)
v4 = f(A=5, a=0.1, ω=1, t=1.3)
v5 = f(t=0.2, A=9)
print(v1, v2, v3, v4, v5)
###Output
_____no_output_____
###Markdown
Exercise: Implement a Gaussian functionMake a Python function *gauss*( *x*, *m*=0, *s*=1) for computing the Gaussian function $$f(x)=\frac{1}{\sqrt{2\pi}s}\exp\left(-\frac{1}{2} \left(\frac{x-m}{s}\right)^2\right)$$Call the function and print out the result for x equal to −5, −4.9, −4.8, ..., 4.8, 4.9, 5, using default values for *m* and *s*.
###Code
from math import exp,pi, sqrt
def Gaussian(x, m=1, s=2):
_2pis_sqrt=sqrt(2*pi*s)
exponent=(.5)*(((x-m)/s)**2)
return (1/_2pis_sqrt)*exp(exponent)
values_big=range(-50, 51, 1)
values=[i/10 for i in values_big]
GaussianValues=[Gaussian(i) for i in values]
#Cdegrees = [-5 + i*0.5 for i in range(n)]
print(GaussianValues)
###Output
[25.393363910302547, 21.883608286772443, 18.90616154323709, 16.3747068081522, 14.21770297497922, 12.375737176705808, 10.799370836086927, 9.447383987999473, 8.285341561441621, 7.284420405642213, 6.420447867203734, 5.673112316135481, 5.025313683205685, 4.462628206831973, 3.972866508182827, 3.5457080658072, 3.1723983417499233, 2.8454973748974584, 2.558670727371146, 2.306515344041403, 2.084414241633346, 1.8884150446129384, 1.715128279761469, 1.5616420698187319, 1.4254504606656841, 1.3043931018062283, 1.1966043969645093, 1.1004705670049395, 1.014593334470125, 0.9377591586133843, 0.8689131306139231, 0.8071367877841907, 0.7516292287576888, 0.7016910135535245, 0.6567104168553983, 0.6161516729193676, 0.5795449087699274, 0.5464775108296526, 0.5165867105510866, 0.48955320837308103, 0.4650956835513164, 0.4429660610555187, 0.4229454265595686, 0.40484049721539744, 0.3884805699263782, 0.3737148806588267, 0.36041031831440123, 0.348449445133179, 0.3377287827579185, 0.3281573291735386, 0.3196552769167785, 0.3121529073754365, 0.3055896397871025, 0.2999132168070127, 0.29507901133062486, 0.2910494417025309, 0.2877934845822791, 0.28528627662349254, 0.2835087978019731, 0.282447630742008, 0.28209479177387814, 0.282447630742008, 0.2835087978019731, 0.28528627662349254, 0.2877934845822791, 0.2910494417025309, 0.29507901133062486, 0.2999132168070127, 0.3055896397871025, 0.3121529073754365, 0.3196552769167785, 0.3281573291735386, 0.3377287827579185, 0.348449445133179, 0.36041031831440123, 0.3737148806588267, 0.3884805699263782, 0.4048404972153975, 0.42294542655956857, 0.4429660610555187, 0.4650956835513164, 0.48955320837308103, 0.5165867105510866, 0.5464775108296526, 0.5795449087699274, 0.6161516729193676, 0.6567104168553983, 0.7016910135535245, 0.7516292287576888, 0.8071367877841907, 0.8689131306139231, 0.937759158613384, 1.014593334470125, 1.1004705670049395, 1.1966043969645097, 1.3043931018062283, 1.4254504606656837, 1.5616420698187319, 1.715128279761469, 1.8884150446129393, 2.084414241633346]
###Markdown
The *if* statementConsider this simple example:
###Code
def f(x):
if 0 <= x <= pi:
return sin(x)
else:
return 0
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
In general (the *else* block can be skipped if there are no statements to be executed when False) we can put together multiple conditions. Only the first condition that is True is executed.
###Code
if condition1:
<block of statements, executed if condition1 is True>
elif condition2:
<block of statements>
elif condition3:
<block of statements>
else:
<block of statements>
<next statement of the program>
###Output
_____no_output_____
###Markdown
The ternary operatorThere is another form of *if* which works as an operator, rather than a statement. If you've used the *if* function in a spreadsheet then you'll be familiar with this approach.
###Code
def f(x):
return (sin(x) if 0 <= x <= pi else 0)
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
The ternary operator has the following form:
###Code
<expression if condition is True> if <condition> else <expression if condition is false>
###Output
_____no_output_____
###Markdown
So, why would we use tuples when lists have more functionality?* Tuples are constant and thus protected against accidental changes.* Tuples are faster than lists.* Tuples are widely used in Python software (so you need to know about tuples to understand other people's code!)* Tuples (but not lists) can be used as keys in dictionaries (more about dictionaries later). Exercise: Make a table of function valuesStep 1: Write a program that prints a table with $t$ values in the first column and the corresponding $y(t) = v_0 t − 0.5gt^2$ values in the second column. Use $n$ uniformly spaced $t$ values throughout the interval [0, $2v_0/g$]. Set $v0 = 1$, $g = 9.81$, and $n = 11$.Step 2: Once step 1 is working, modify the program so that the $t$ and $y$ values are stored in two lists *t* and *y*. Thereafter, transverse the lists with a *for* loop and write out a nicely formatted table of *t* and *y* values using a *zip* construction. FunctionsWe have already used many Python functions, including mathematical functions:
###Code
from math import *
x = pi
print cos(x)
###Output
_____no_output_____
###Markdown
Other functions you used include *len* and *range*:
###Code
n = len(somelist)
ints = range(5, n, 2)
###Output
_____no_output_____
###Markdown
You have also used functions that are executed with the dot syntax (called *methods*):
###Code
C = [5, 10, 40, 45]
i = C.index(10)
C.append(50)
C.insert(2, 20)
###Output
_____no_output_____
###Markdown
A function is a collection of statements we can execute wherever and whenever we want. Functions can take any number of inputs (called *arguments*) to produce outputs. Functions help to organize programs, make them more understandable, shorter, and easier to extend. For our first example we will turn our temperature conversion code into a function:
###Code
def C2F(C):
return (9/5)*C + 32
###Output
_____no_output_____
###Markdown
Functions start with *def*, then the name you want to give your function, then a list of arguments (here C). This is referred to as the *function header*. Inside the function there is a block of statements called the *function body*. Notice that the function body is indented - as was the case for the *for* / *while* loop the indentation indicates where the function ends. At any point within the function, we can "stop thefunction" and return as many values/variables as required.
###Code
print(C2F(100))
###Output
212.0
###Markdown
Local and global variablesVariables defined within a function are said to have *local scope*. That is to say that they can only be referenced within that function. Let's consider an example (and look carefully at the indentation!!):
###Code
def sumint(start, stop):
s = 0 # variable for accumulating the sum
i = start # counter
while i <= stop:
s += i
i += 1
return s
print(sumint(0, 5))
###Output
_____no_output_____
###Markdown
Variables *i* and *s* are local variables in *sumint*. They are destroyed at the end (return) of the function and never visible outside the function (in the calling program); in fact, *start* and *stop* are also local variables. So let's see what happens if we now try to print one of these variables:
###Code
print(stop)
###Output
_____no_output_____
###Markdown
Functions can also return multiple values. Let's recycle another of our previous examples - compute $y(t)$ and $y'(t)=v_0-gt$:
###Code
def yfunc(t, v0):
g = 9.81
y = v0*t - 0.5*g*t**2
dydt = v0 - g*t
return y, dydt
# call:
position, velocity = yfunc(0.6, 3)
print(position, velocity)
###Output
_____no_output_____
###Markdown
Remember that a series of comma separated variables implies a tuple - therefore "return y, dydt" is the same as writing "return (y, dydt)". Therefore, in general what is returned is a tuple. Let's take a look at another example illustrating this:
###Code
def f(x):
return x, x**2, x**4
s = f(2)
print(s)
print(type(s)) # The function type() tells us what type a variable it is.
###Output
_____no_output_____
###Markdown
No return value implies that `None` is returned. `None` is a special Python object that represents an ”empty” or undefined value. It is surprisingly useful and we will use it a lot later.
###Code
def message(course):
print("%s rocks!"% course)
message("Python")
r = message("Geo")
print("r = ", r)
###Output
_____no_output_____
###Markdown
Keyword arguments and default argument valuesIt's possible to specify default values that function arguments should take if no values are provided when the function is called:
###Code
def somefunc(arg1, arg2, kwarg1=True, kwarg2=0):
print(arg1, arg2, kwarg1, kwarg2)
somefunc("Hello", [1,2]) # Note that we have not specified values for kwarg1 and kwarg2
###Output
Hello [1, 2] True 0
###Markdown
You can override the default values by passing additional arguments in the correct position:
###Code
somefunc("Hello", [1,2], "Hi")
###Output
Hello [1, 2] Hi 0
###Markdown
... or by naming the argument to which the value should be passed. These are called keyword arguments:
###Code
def foo(*args):
print(args)
foo(1,2)
foo("Hello","World","Today")
somefunc("Hello", [1,2], kwarg2="Hi")
somefunc("Hello", [1,2], kwarg2="Hi", kwarg1=6)
###Output
Hello [1, 2] 6 Hi
###Markdown
If we use variable_name=value for all arguments, their sequence in the function header can be in any order.
###Code
somefunc(kwarg2="Hello", arg1="Hi", kwarg1=6, arg2=[2])
###Output
_____no_output_____
###Markdown
Let's look at another example - consider a function of $t$, with parameters $A$, $a$, and $\omega$:$$f(t; A,a, \omega) = Ae^{-at}\sin (\omega t)$$. (The choice of equation is actually pretty random - but it serves to show you that it is easy to translate formulae you encounter into Python code). We can implement $f$ in a Python function with $t$ as positional argument and $A$, $a$, and $\omega$ as keyword arguments.
###Code
from math import pi, exp, sin
def f(t, A=1, a=1, ω=2*pi):
return A*exp(-a*t)*sin(ω*t)
v1 = f(0.2)
v2 = f(0.2, ω=1)
v2 = f(0.2, 1, 3) # same as f(0.2, A=1, a=3)
v3 = f(0.2, ω=1, A=2.5)
v4 = f(A=5, a=0.1, ω=1, t=1.3)
v5 = f(t=0.2, A=9)
print(v1, v2, v3, v4, v5)
###Output
0.778659217806053 0.5219508827258282 0.40664172703834794 4.230480200204721 7.007932960254476
###Markdown
Exercise: Implement a Gaussian functionMake a Python function *gauss*( *x*, *m*=0, *s*=1) for computing the Gaussian function $$f(x)=\frac{1}{\sqrt{2\pi}s}\exp\left(-\frac{1}{2} \left(\frac{x-m}{s}\right)^2\right)$$Call the function and print out the result for x equal to −5, −4.9, −4.8, ..., 4.8, 4.9, 5, using default values for *m* and *s*.
###Code
from math import pi, exp, sqrt
def gauss(x, m=0, s=1):
return 1/(sqrt(2*pi)*s)*exp(-0.5*((x-m)/s)**2)
a = -5
while(a <= 5):
print(gauss(a))
a=a+0.1
###Output
1.4867195147342977e-06
2.438960745893352e-06
3.961299091032061e-06
6.369825178867068e-06
1.014085206548667e-05
1.5983741106905332e-05
2.4942471290053356e-05
3.8535196742086716e-05
5.8943067756539116e-05
8.926165717713165e-05
0.00013383022576488347
0.0001986554713927699
0.00029194692579145637
0.0004247802705507465
0.0006119019301137654
0.0008726826950457507
0.0012322191684730067
0.0017225689390536628
0.0023840882014648213
0.0032668190561998926
0.004431848411937972
0.005952532419775811
0.007915451582979908
0.010420934814422526
0.013582969233685536
0.017528300493568436
0.02239453029484278
0.028327037741601037
0.03547459284623128
0.04398359598042702
0.05399096651318786
0.06561581477467639
0.07895015830089394
0.0940490773768867
0.11092083467945532
0.12951759566589147
0.1497274656357446
0.1713685920478071
0.1941860549832127
0.2178521770325503
0.24197072451914314
0.2660852498987546
0.28969155276148256
0.3122539333667611
0.33322460289179945
0.35206532676429936
0.36827014030332317
0.38138781546052397
0.3910426939754558
0.39695254747701175
0.3989422804014327
0.39695254747701186
0.391042693975456
0.3813878154605242
0.36827014030332345
0.3520653267642997
0.3332246028917999
0.3122539333667615
0.289691552761483
0.2660852498987551
0.24197072451914364
0.2178521770325508
0.19418605498321317
0.17136859204780758
0.14972746563574502
0.12951759566589185
0.11092083467945565
0.094049077376887
0.07895015830089422
0.06561581477467664
0.053990966513188084
0.04398359598042723
0.03547459284623146
0.028327037741601186
0.0223945302948429
0.01752830049356854
0.013582969233685613
0.010420934814422592
0.007915451582979956
0.005952532419775849
0.004431848411938
0.0032668190561999156
0.0023840882014648382
0.0017225689390536767
0.0012322191684730154
0.000872682695045757
0.0006119019301137703
0.00042478027055074997
0.00029194692579145897
0.0001986554713927717
0.00013383022576488488
8.926165717713262e-05
5.8943067756539855e-05
3.853519674208713e-05
2.494247129005362e-05
1.5983741106905532e-05
1.0140852065486796e-05
6.369825178867147e-06
3.961299091032111e-06
2.438960745893387e-06
1.4867195147343189e-06
###Markdown
The *if* statementConsider this simple example:
###Code
def f(x):
if 0 <= x <= pi:
return sin(x)
else:
return 0
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
In general (the *else* block can be skipped if there are no statements to be executed when False) we can put together multiple conditions. Only the first condition that is True is executed.
###Code
if condition1:
<block of statements, executed if condition1 is True>
elif condition2:
<block of statements>
elif condition3:
<block of statements>
else:
<block of statements>
<next statement of the program>
###Output
_____no_output_____
###Markdown
The ternary operatorThere is another form of *if* which works as an operator, rather than a statement. If you've used the *if* function in a spreadsheet then you'll be familiar with this approach.
###Code
def f(x):
return (sin(x) if 0 <= x <= pi else 0)
print(f(-pi/2), f(pi/2), f(3*pi/2))
###Output
_____no_output_____
###Markdown
The ternary operator has the following form:
###Code
<expression if condition is True> if <condition> else <expression if condition is false>
###Output
_____no_output_____
###Markdown
Exercise: Express a step function as a Python functionThe following "step" function is known as the Heaviside function andis widely used in mathematics:$$H(x)=\begin{cases}0, & \text{if $x<0$}.\\\\1, & \text{if $x\ge 0$}.\end{cases}$$Write a Python function H(x) that computes H(x).
###Code
def H(x):
return 0 if x<0 else 1
print(H(2), H(0), H(-2))
###Output
1 1 0
###Markdown
Introduction to Python programming [Gerard Gorman](http://www.imperial.ac.uk/people/g.gorman), [Christian Jacobs](http://www.imperial.ac.uk/people/c.jacobs10) Lecture 2: Computing with basic language constructionsLearning objectives:* Introduction to two different forms of loops which are used to repeat operations on data: either until a condition is satisfied (*while-loop*) or for a fixed number of iterations (*for-loop*).* Form a *condition* using a *boolean expression*. This is used to either terminate loops or control if a specific block of code is executed via the *if-statement*.* Learn two different methods for storing many data elements within a single Python type.* Introduction to developing your own Python *functions* which allows you to execute the same block of code, with different inputs and outputs, from anywhere in the code. Boolean expressionsAn expression with value *true* or *false* is called a boolean expression. Example expressions for what you would write mathematically as$C=40$, $C\ne40$, $C\ge40$, $C\gt40$ and $C\lt40$ are:
###Code
C == 40 # Note: the double == checks for equality, but C=40 is an assignment!
C != 40 # This could also be written as 'not C == 4' (i.e. the opposite of what C == 4 gives)
C >= 40
C > 40
C < 40
###Output
_____no_output_____
###Markdown
We can test boolean expressions in a Python shell:
###Code
from math import sin
print True or sin(0)
C = 41
print "C != 40: ", C != 40
print "C < 40: ", C < 40
print "C == 41: ", C == 41
###Output
True
C != 40: True
C < 40: False
C == 41: True
###Markdown
Several conditions can be combined with the special 'and' and 'or' operators into a single boolean expression:
###Code
condition1 and condition2
condition1 or condition2
###Output
_____no_output_____
###Markdown
* Rule 1: (**C1** *and* **C2**) is *True* only if both **C1** and **C2** are *True** Rule 2: (**C1** *or* **C2**) is *True* if either **C1** or **C2** are *True*Examples:
###Code
x=0; y=1.2
print (x >= 0 and y < 1)
###Output
False
###Markdown
Exercise: Values of boolean expressionsAdd a comment to the code below to explain the outcome of each of the boolean expressions:
###Code
C = 41
print "Case 1: ", C == 40
print "Case 2: ", C != 40 and C < 41
print "Case 3: ", C != 40 or C < 41
print "Case 4: ", not C == 40
print "Case 5: ", not C > 40
print "Case 6: ", C <= 41
print "Case 7: ", not False
print "Case 8: ", True and False
print "Case 9: ", False or True
print "Case 10: ", False or False or False
print "Case 11: ", True and True and False
print "Case 12: ", False == 0
print "Case 13: ", True == 0
print "Case 14: ", True == 1
print False or True or True
print False or False==0.
print True and True or False
###Output
Case 1: False
Case 2: False
Case 3: True
Case 4: True
Case 5: False
Case 6: True
Case 7: True
Case 8: False
Case 9: True
Case 10: False
Case 11: False
Case 12: True
Case 13: False
Case 14: True
True
True
True
###Markdown
LoopsSuppose we want to make a table of Celsius and Fahrenheitdegrees:
###Code
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Output
_____no_output_____
###Markdown
How do we write a program that prints out such a table?We know from the last lecture how to make one line in this table:
###Code
C = -20
F = 9.0/5*C + 32
print C, F
###Output
-20 -4.0
###Markdown
We can just repeat these statements:
###Code
C=-20; F=9.0/5*C+32; print C,F
C=-15; F=9.0/5*C+32; print C,F
C=-10; F=9.0/5*C+32; print C,F
C=-5; F=9.0/5*C+32; print C,F
C=0; F=9.0/5*C+32; print C,F
C=5; F=9.0/5*C+32; print C,F
C=10; F=9.0/5*C+32; print C,F
C=15; F=9.0/5*C+32; print C,F
C=20; F=9.0/5*C+32; print C,F
C=25; F=9.0/5*C+32; print C,F
C=30; F=9.0/5*C+32; print C,F
C=35; F=9.0/5*C+32; print C,F
C=40; F=9.0/5*C+32; print C,F
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
So we can see that works but its **very boring** to write and very easy to introduce a misprint.**You really should not be doing boring repetitive tasks like this.** Spend one time instead looking for a smarter solution. When programming becomes boring, there is usually a construct that automates the writing. Computers are very good at performing repetitive tasks. For this purpose we use **loops**. The while loop (and the significance of indentation)A while loop executes repeatedly a set of statements as long as a **boolean** (i.e. *True* / *False*) condition is *True*
###Code
while condition:
<statement 1>
<statement 2>
...
<first statement after loop>
###Output
_____no_output_____
###Markdown
Note that all statements to be executed within the loop must be indented by the same amount! The loop ends when an unindented statement is encountered.At this point it is worth noticing that **blank spaces may or may not be important** in Python programs. These statements are equivalent (blanks do not matter):
###Code
v0=3
v0 = 3
v0= 3
# The computer does not care but this formatting style is
# considered clearest for the human reader.
v0 = 3
###Output
_____no_output_____
###Markdown
Here is a while loop example where blank spaces really do matter:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print counter
###Output
11
###Markdown
Let's take a look at what happens when we forget to indent correctly:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print counter
###Output
_____no_output_____
###Markdown
Let's use the while loop to create the table above:
###Code
C = -20 # Initialise C
dC = 5 # Increment for C within the loop
while C <= 40: # Loop heading with condition
F = (9.0/5)*C + 32 # 1st statement inside loop
print C, F # 2nd statement inside loop
C = C + dC # Increment C for the next iteration of the loop.
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Exercise: Make a Fahrenheit-Celsius conversion tableWrite a program that prints out a table with Fahrenheit degrees 0, 10, 20, ..., 100 in the first column and the corresponding Celsius degrees in the second column. Exercise: Write an approximate Fahrenheit-Celsius conversion tableMany people use an approximate formula for quickly converting Fahrenheit ($F$) to Celsius ($C$) degrees:$C \approx \hat{C} = \frac{F − 30}{2}$Modify the program from the previous exercise so that it prints three columns: $F$, $C$, and the approximate value $\hat{C}$. ListsSo far, one variable has referred to one number (or string). Sometimes however we naturally have a collection of numbers, saydegrees −20, −15, −10, −5, 0, ..., 40. One way to store these values in a computer program would be to have one variable per value, i.e.
###Code
C1 = -20
C2 = -15
C3 = -10
# ...
C13 = 40
###Output
_____no_output_____
###Markdown
This is clearly a terrible solution, particularly if we have lots of values. A better way of doing this is to collect values together in a list:
###Code
C = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
###Output
_____no_output_____
###Markdown
Now there is just one variable, **C**, holding all the values. Elements in a list are accessed via an index. List indices are always numbered as 0, 1, 2, and so forth up to the number of elements minus one:
###Code
mylist = [4, 6, -3.5]
print mylist[0]
print mylist[1]
print mylist[2]
print len(mylist) # length of list
###Output
4
6
-3.5
3
###Markdown
Here are a few example of operations that you can perform on lists:
###Code
C = [-10, -5, 0, 5, 10, 15, 20, 25, 30]
C.append(35) # add new element 35 at the end
print C
C=C+[40,45] # And another list to the end of C
print C
C.insert(0, -15) # Insert -15 as index 0
print C
del C[2] # delete 3rd element
print C
del C[2] # delete what is now 3rd element
print C
print len(C) # length of list
print C.index(10) # Find the index of the list with the value 10
print (10 in C) # True only if the value 10 is stored in the list
print C[-1] # The last value in the list.
print C[-2] # The second last value in the list.
###Output
40
###Markdown
You can also extract sublists using ":"
###Code
print C[5:] # From index 5 to the end of the list.
print C[5:7] # From index 5 up to, but not including index 7.
###Output
[20, 25]
###Markdown
Many languages (especially Matlab and Fortran) have would include the endpoint of a slice (7 in this case). One way to think of what Python does is that the slice is a half-open interval: $[5,7)$. This makes cutting a list in half particularly easy. For example:
###Code
print C[:5] + ["Hello"] + C[5:]
print C[7:-1] # From index 7 up to the second last element.
print C[:] # [:] specifies the whole list.
somelist = ['Curly', 'Larry', 'Moe']
stooge1, stooge2, stooge3 = somelist
print stooge3, stooge2, stooge1
###Output
Moe Larry Curly
###Markdown
Exercise: Store odd numbers in a listStep 1: Write a program that generates all odd numbers from 1 to *n*. Set *n* in the beginning of the program and use a while loop to compute the numbers. (Make sure that if *n* is an even number, the largest generated odd number is *n*-1.).Step 2: Store the generated odd numbers in a list. Start with an empty list and use the same while loop where you generate each odd number, ao append the new number to the list.Finally, print the list elements to the screen.
###Code
n = 30
odd_numbers = []
i = 1
while i <= n:
odd_numbers.append(i)
i+=2
print odd_numbers
odd_numbers = []
for k in range(1,n+1,2):
odd_numbers.append(k)
print odd_numbers
n = 30
odd_numbers = range(1,n,2)
print odd_numbers
###Output
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21, 23, 25, 27, 29]
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21, 23, 25, 27, 29]
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21, 23, 25, 27, 29]
###Markdown
For loopsWe can visit each element in a list and process the element with some statements using a *for* loop, for example:
###Code
degrees = [0, 10, 20, 40, 100]
for C in degrees:
print 'list element:', C
print 'The degrees list has', len(degrees), 'elements'
###Output
list element: 0
list element: 10
list element: 20
list element: 40
list element: 100
The degrees list has 5 elements
###Markdown
Notice again how the statements to be executed within the loop must be indented! Let's now revisit the conversion table example using the *for* loop:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
for C in Cdegrees:
F = (9.0/5)*C + 32
print C, F
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
We can easily beautify the table using the printf syntax that we encountered in the last lecture:
###Code
for C in Cdegrees:
F = (9.0/5)*C + 32
print '%5d %5.1f' % (C, F)
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
It is also possible to rewrite the *for* loop as a *while* loop, i.e.,
###Code
for element in somelist:
# process element
###Output
_____no_output_____
###Markdown
can always be transformed to a *while* loop
###Code
index = 0
while index < len(somelist):
element = somelist[index]
# process element
index += 1
###Output
_____no_output_____
###Markdown
Taking the previous table example:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
index = 0
while index < len(Cdegrees):
C = Cdegrees[index]
F = (9.0/5)*C + 32
print '%5d %5.1f' % (C, F)
index += 1
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Rather than just printing out the Fahrenheit values, let's also store these computed values in a list of their own:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
Fdegrees = [] # start with empty list
for C in Cdegrees:
F = (9.0/5)*C + 32
Fdegrees.append(F) # add new element to Fdegrees
print Fdegrees
###Output
[-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]
###Markdown
In Python *for* loops usually loop over list values (elements), i.e.,
###Code
for element in somelist:
...process variable element
###Output
_____no_output_____
###Markdown
However, we can also loop over list indices:
###Code
for i in range(0, len(somelist), 1):
element = somelist[i]
... process element or somelist[i] directly
###Output
_____no_output_____
###Markdown
The statement *range(start, stop, inc)* generates a list of integers *start*, *start+inc*, *start+2\*inc*, and so on up to, but not including, *stop*. We can also write *range(stop)* as an abbreviation for *range(0, stop, 1)*:
###Code
print range(3) # same as range(0, 3, 1)
print range(2, 8, 3)
###Output
[2, 5]
###Markdown
List comprehensionsConsider this example where we compute two lists in a *for* loop:
###Code
n = 16
Cdegrees = []; Fdegrees = [] # empty lists
for i in range(n):
Cdegrees.append(-5 + i*0.5)
Fdegrees.append((9.0/5)*Cdegrees[i] + 32)
print "Cdegrees = ", Cdegrees
print "Fdegrees = ", Fdegrees
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
As constructing lists is a very common requirement, the above way of doing it can become very tedious to both write and read. Therefore, Python has a compact construct, called list comprehension for generating lists from a *for* loop:
###Code
n = 16
Cdegrees = [-5 + i*0.5 for i in range(n)]
Fdegrees = [(9.0/5)*C + 32 for C in Cdegrees]
print "Cdegrees = ", Cdegrees
print "Fdegrees = ", Fdegrees
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
The general form of a list comprehension is:
###Code
somelist = [expression for element in somelist]
###Output
_____no_output_____
###Markdown
Exercise: Repeat the previous exercise using: a for loop, list comprehension and the range function
###Code
# Using for loop
# Using list comprehension
# Using the function range
###Output
_____no_output_____
###Markdown
Changing elements in a listSay we want to add $2$ to all the numbers in a list:
###Code
v = [-1, 1, 10]
for e in v:
e=e+2
print v
###Output
[-1, 1, 10]
###Markdown
We can see that the list *v* is unaltered! The reason for this is that inside the loop, *e* is an ordinary (int) variable. At the start of the iteration *e* is assigned a *copy* of the next element in the list. Inside the loop we can change *e* but *v* itself is unaltered. If we want to change *v* we have to use an index to access and modify its elements:
###Code
v[1] = 4 # assign 4 to 2nd element (index 1) in v
print v
###Output
[-1, 4, 10]
###Markdown
To add 2 to all values we need a *for* loop over indices:
###Code
for i in range(len(v)):
v[i] = v[i] + 2
print v
###Output
[1, 6, 12]
###Markdown
Traversing multiple lists simultaneously - *zip(list1, list2, ...)*Consider how we can loop over elements in both Cdegrees and Fdegrees at the same time. One approach would be to use list indices:
###Code
for i in range(len(Cdegrees)):
print Cdegrees[i], Fdegrees[i]
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
An alternative construct (regarded as more ”Pythonic”) uses the *zip* function:
###Code
for C, F in zip(Cdegrees, Fdegrees):
print C, F
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
Another example with three lists:
###Code
l1 = [3, 6, 1]; l2 = [1.5, 1, 0]; l3 = [9.1, 3, 2]
for e1, e2, e3 in zip(l1, l2, l3):
print e1, e2, e3
###Output
3 1.5 9.1
6 1 3
1 0 2
###Markdown
If the lists are of unequal length then the loop stops when the end of the shortest list is reached. Experiment with this:
###Code
l1 = [3, 6, 1, 4, 6]; l2 = [1.5, 1, 0, 7]; l3 = [9.1, 3, 2, 0, 9]
for e1, e2, e3 in zip(l1, l2, l3):
print e1, e2, e3
###Output
3 1.5 9.1
6 1 3
1 0 2
4 7 0
###Markdown
Nested lists: list of listsA *list* can contain **any** object, including another *list*. To illustrate this, consider how to store the conversion table as a single Python list rather than two separate lists.
###Code
Cdegrees = range(-20, 41, 5)
Fdegrees = [(9.0/5)*C + 32 for C in Cdegrees]
table1 = [Cdegrees, Fdegrees] # List of two lists
print "table1 = ", table1
print "table1[0] = ", table1[0]
print "table1[1] = ", table1[1]
print "table1[2][3] = ", table1[1][3]
###Output
table1 = [[-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40], [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]]
table1[0] = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
table1[1] = [-4.0, 5.0, 14.0, 23.0, 32.0, 41.0, 50.0, 59.0, 68.0, 77.0, 86.0, 95.0, 104.0]
table1[2][3] = 23.0
###Markdown
This gives us a table of two columns. How do we create a table of rows instead:
###Code
table2 = []
for C, F in zip(Cdegrees, Fdegrees):
row = [C, F]
table2.append(row)
print table2
###Output
[[-20, -4.0], [-15, 5.0], [-10, 14.0], [-5, 23.0], [0, 32.0], [5, 41.0], [10, 50.0], [15, 59.0], [20, 68.0], [25, 77.0], [30, 86.0], [35, 95.0], [40, 104.0]]
###Markdown
We can use list comprehension to do this more elegantly:
###Code
table2 = [[C, F] for C, F in zip(Cdegrees, Fdegrees)]
print table2
###Output
[[-20, -4.0], [-15, 5.0], [-10, 14.0], [-5, 23.0], [0, 32.0], [5, 41.0], [10, 50.0], [15, 59.0], [20, 68.0], [25, 77.0], [30, 86.0], [35, 95.0], [40, 104.0]]
###Markdown
And you can loop through this list as before:
###Code
for C, F in table2:
print C, F
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Tuples: lists that cannot be changedTuples are **constant** lists, i.e. you can use them in much the same way as lists except you cannot modify them. They are an example of an [**immutable**](http://en.wikipedia.org/wiki/Immutable_object) type.
###Code
t = (2, 4, 6, 'temp.pdf') # Define a tuple.
t = 2, 4, 6, 'temp.pdf' # Can skip parenthesis as it is assumed in this context.
###Output
_____no_output_____
###Markdown
Let's see what happens when we try to modify the tuple like we did with a list:
###Code
t[1] = -1
t.append(0)
del t[1]
###Output
_____no_output_____
###Markdown
However, we can use the tuple to compose a new tuple:
###Code
t = t + (-1.0, -2.0)
print t
###Output
(2, 4, 6, 'temp.pdf', -1.0, -2.0)
###Markdown
So, why would we use tuples when lists have more functionality?* Tuples are constant and thus protected against accidental changes.* Tuples are faster than lists.* Tuples are widely used in Python software (so you need to know about tuples to understand other people's code!)* Tuples (but not lists) can be used as keys in dictionaries (more about dictionaries later). Exercise: Make a table of function valuesStep 1: Write a program that prints a table with $t$ values in the first column and the corresponding $y(t) = v_0 t − 0.5gt^2$ values in the second column. Use $n$ uniformly spaced $t$ values throughout the interval [0, $2v_0/g$]. Set $v0 = 1$, $g = 9.81$, and $n = 11$.Step 2: Once step 1 is working, modify the program so that the $t$ and $y$ values are stored in two lists *t* and *y*. Thereafter, transverse the lists with a *for* loop and write out a nicely formatted table of *t* and *y* values using a *zip* construction. FunctionsWe have already used many Python functions, e.g. mathematical functions:
###Code
from math import *
x = pi
print cos(x)
###Output
-1.0
###Markdown
Other functions you used include *len* and *range*:
###Code
n = len(somelist)
ints = range(5, n, 2)
###Output
_____no_output_____
###Markdown
You have also used functions that are executed with the dot syntax (called *methods*):
###Code
C = [5, 10, 40, 45]
i = C.index(10)
C.append(50)
C.insert(2, 20)
###Output
_____no_output_____
###Markdown
A function is a collection of statements we can execute wherever and whenever we want. Functions can take any number of inputs (called *arguments*) to produce outputs. Functions help to organize programs, make them more understandable, shorter, and easier to extend. For our first example we will turn our temperature conversion code into a function:
###Code
def C2F(C):
return (9.0/5)*C + 32
###Output
_____no_output_____
###Markdown
Functions start with *def*, then the name you want to give your function, then a list of arguments (here C). This is referred to as the *function header*. Inside the function there is a block of statements called the *function body*. Notice that the function body is indented - as was the case for the *for* / *while* loop the indentation indicates where the function ends. At any point within the function, we can "stop thefunction" and return as many values/variables as required. Local and global variablesVariables defined within a function are said to have *local scope*. That is to say that they can only be referenced within that function. Let's consider an example (and look carefully at the indentation!!):
###Code
def sumint(start, stop):
s = 0 # variable for accumulating the sum
i = start # counter
while i <= stop:
s += i
i += 1
return s
print sumint(0, 5)
###Output
15
###Markdown
Variables *i* and *s* are local variables in *sumint*. They are destroyed at the end (return) of the function and never visible outside the function (in the calling program); in fact, *start* and *stop* are also local variables. So let's see what happens if we now try to print one of these variables:
###Code
print stop
###Output
_____no_output_____
###Markdown
Functions can also return multiple values. Let's recycle another of our previous examples - compute $y(t)$ and $y'(t)=v_0-gt$:
###Code
def yfunc(t, v0):
g = 9.81
y = v0*t - 0.5*g*t**2
dydt = v0 - g*t
return y, dydt
# call:
position, velocity = yfunc(0.6, 3)
print position, velocity
###Output
0.0342 -2.886
###Markdown
Remember that a series of comma separated variables implies a tuple - therefore "return y, dydt" is the same as writing "return (y, dydt)". Therefore, in general what is returned is a tuple. Let's take a look at another example illustrating this:
###Code
def f(x):
return x, x**2, x**4
s = f(2)
print s
print type(s) # The function type() tells us what type a variable it is.
###Output
(2, 4, 16)
<type 'tuple'>
###Markdown
No return value implies that *None* is returned. *None* is a special Python object that represents an ”empty” or undefined value. It is surprisingly useful and we will use it a lot later.
###Code
def message(course):
print "%s rocks!"% course
message("Python")
r = message("Geo")
print "r = ", r
###Output
Python rocks!
Geo rocks!
r = None
###Markdown
Keyword arguments and default input valuesFunctions can have arguments of the form variable_name=value and are called keyword arguments:
###Code
def somefunc(arg1, arg2, kwarg1=True, kwarg2=0):
print arg1, arg2, kwarg1, kwarg2
somefunc("Hello", [1,2]) # Note that we have not specified inputs for kwarg1 and kwarg2
somefunc("Hello", [1,2], kwarg1="Hi")
somefunc("Hello", [1,2], kwarg2="Hi")
somefunc("Hello", [1,2], kwarg2="Hi", kwarg1=6)
###Output
Hello [1, 2] 6 Hi
###Markdown
If we use variable_name=value for all arguments, their sequence in the function header can be in any order.
###Code
def golo(*args):
print args
golo("frog")
def golo2(**kwargs):
print kwargs
golo2(species="frog")
###Output
('frog',)
{'species': 'frog'}
###Markdown
Let's look at another example - consider a function of $t$, with parameters $A$, $a$, and $\omega$:$$f(t; A,a, \omega) = Ae^{-at}\sin (\omega t)$$. (The choice of equation is actually pretty random - but it serves to show you that it is easy to translate formulae you encounter into Python code). We can implement $f$ in a Python function with $t$ as positional argument and $A$, $a$, and $\omega$ as keyword arguments.
###Code
from math import pi, exp, sin
def f(t, A=1, a=1, omega=2*pi):
return A*exp(-a*t)*sin(omega*t)
v1 = f(0.2)
v2 = f(0.2, omega=1)
v2 = f(0.2, 1, 3) # same as f(0.2, A=1, a=3)
v3 = f(0.2, omega=1, A=2.5)
v4 = f(A=5, a=0.1, omega=1, t=1.3)
v5 = f(t=0.2, A=9)
print v1, v2, v3, v4, v5
###Output
0.778659217806 0.521950882726 0.406641727038 4.2304802002 7.00793296025
###Markdown
Convention for input and output data in functionsA function can have three types of input and output data:* Input data specified through positional/keyword arguments.* Input/output data given as positional/keyword arguments that will be modified and returned.* Output data created inside the function.* All output data are returned, all input data are arguments.* Sketch of a general Python function:
###Code
def somefunc(i1, i2, i3, io4, io5, i6=value1, io7=value2):
# modify io4, io5, io7; compute o1, o2, o3
return o1, o2, o3, io4, io5, io7
###Output
_____no_output_____
###Markdown
* i1, i2, i3, i6: pure input data* io4, io5, io7: input and output data* o1, o2, o3: pure output data Exercise: Implement a Gaussian functionMake a Python function *gauss*( *x*, *m*=0, *s*=1) for computing the Gaussian function $$f(x)=\frac{1}{\sqrt{2\pi}s}\exp\left(-\frac{1}{2} \left(\frac{x-m}{s}\right)^2\right)$$Call the function and print out the result for x equal to −5, −4.9, −4.8, ..., 4.8, 4.9, 5, using default values for *m* and *s*.
###Code
from math import pi, sqrt, exp
import numpy as np
def gauss(x,m=0,s=1):
f=(1/(sqrt((2*pi))*s))*(exp(-0.5*((x-m)/s)**2))
return f
print map(gauss, np.linspace(-5,5,101))
for i in np.arange(-5,5,0.1):
print gauss(i)
for i in np.linspace(-5,5,101):
print gauss(i)
###Output
[1.4867195147342977e-06, 2.438960745893352e-06, 3.961299091032075e-06, 6.36982517886709e-06, 1.0140852065486758e-05, 1.5983741106905475e-05, 2.4942471290053535e-05, 3.853519674208713e-05, 5.8943067756539855e-05, 8.926165717713293e-05, 0.00013383022576488537, 0.00019865547139277272, 0.00029194692579146027, 0.00042478027055075143, 0.000611901930113773, 0.0008726826950457602, 0.00123221916847302, 0.0017225689390536812, 0.0023840882014648404, 0.0032668190561999247, 0.0044318484119380075, 0.005952532419775854, 0.007915451582979969, 0.010420934814422605, 0.013582969233685634, 0.01752830049356854, 0.0223945302948429, 0.028327037741601186, 0.03547459284623146, 0.04398359598042723, 0.05399096651318806, 0.0656158147746766, 0.07895015830089418, 0.09404907737688697, 0.11092083467945563, 0.12951759566589174, 0.14972746563574488, 0.1713685920478074, 0.19418605498321304, 0.21785217703255064, 0.24197072451914337, 0.266085249898755, 0.2896915527614828, 0.3122539333667612, 0.3332246028917997, 0.3520653267642995, 0.3682701403033234, 0.38138781546052414, 0.391042693975456, 0.3969525474770118, 0.3989422804014327, 0.39695254747701175, 0.3910426939754559, 0.381387815460524, 0.3682701403033233, 0.3520653267642995, 0.33322460289179956, 0.3122539333667612, 0.28969155276148256, 0.26608524989875476, 0.24197072451914337, 0.21785217703255041, 0.19418605498321292, 0.1713685920478072, 0.1497274656357448, 0.12951759566589174, 0.11092083467945546, 0.0940490773768869, 0.07895015830089407, 0.06561581477467655, 0.05399096651318806, 0.043983595980427156, 0.035474592846231424, 0.02832703774160112, 0.022394530294842882, 0.01752830049356854, 0.013582969233685602, 0.010420934814422592, 0.007915451582979946, 0.005952532419775849, 0.0044318484119380075, 0.0032668190561999247, 0.0023840882014648343, 0.0017225689390536767, 0.0012322191684730175, 0.0008726826950457602, 0.000611901930113773, 0.00042478027055074997, 0.0002919469257914595, 0.00019865547139277237, 0.00013383022576488537, 8.926165717713293e-05, 5.8943067756539645e-05, 3.8535196742086994e-05, 2.4942471290053535e-05, 1.5983741106905475e-05, 1.014085206548667e-05, 6.369825178867068e-06, 3.961299091032061e-06, 2.438960745893352e-06, 1.4867195147342977e-06]
1.48671951473e-06
2.43896074589e-06
3.96129909103e-06
6.36982517887e-06
1.01408520655e-05
1.59837411069e-05
2.49424712901e-05
3.85351967421e-05
5.89430677565e-05
8.92616571771e-05
0.000133830225765
0.000198655471393
0.000291946925791
0.000424780270551
0.000611901930114
0.000872682695046
0.00123221916847
0.00172256893905
0.00238408820146
0.0032668190562
0.00443184841194
0.00595253241978
0.00791545158298
0.0104209348144
0.0135829692337
0.0175283004936
0.0223945302948
0.0283270377416
0.0354745928462
0.0439835959804
0.0539909665132
0.0656158147747
0.0789501583009
0.0940490773769
0.110920834679
0.129517595666
0.149727465636
0.171368592048
0.194186054983
0.217852177033
0.241970724519
0.266085249899
0.289691552761
0.312253933367
0.333224602892
0.352065326764
0.368270140303
0.381387815461
0.391042693975
0.396952547477
0.398942280401
0.396952547477
0.391042693975
0.381387815461
0.368270140303
0.352065326764
0.333224602892
0.312253933367
0.289691552761
0.266085249899
0.241970724519
0.217852177033
0.194186054983
0.171368592048
0.149727465636
0.129517595666
0.110920834679
0.0940490773769
0.0789501583009
0.0656158147747
0.0539909665132
0.0439835959804
0.0354745928462
0.0283270377416
0.0223945302948
0.0175283004936
0.0135829692337
0.0104209348144
0.00791545158298
0.00595253241978
0.00443184841194
0.0032668190562
0.00238408820147
0.00172256893905
0.00123221916847
0.000872682695046
0.000611901930114
0.000424780270551
0.000291946925791
0.000198655471393
0.000133830225765
8.92616571771e-05
5.89430677565e-05
3.85351967421e-05
2.49424712901e-05
1.59837411069e-05
1.01408520655e-05
6.36982517887e-06
3.96129909103e-06
2.43896074589e-06
1.48671951473e-06
2.43896074589e-06
3.96129909103e-06
6.36982517887e-06
1.01408520655e-05
1.59837411069e-05
2.49424712901e-05
3.85351967421e-05
5.89430677565e-05
8.92616571771e-05
0.000133830225765
0.000198655471393
0.000291946925791
0.000424780270551
0.000611901930114
0.000872682695046
0.00123221916847
0.00172256893905
0.00238408820146
0.0032668190562
0.00443184841194
0.00595253241978
0.00791545158298
0.0104209348144
0.0135829692337
0.0175283004936
0.0223945302948
0.0283270377416
0.0354745928462
0.0439835959804
0.0539909665132
0.0656158147747
0.0789501583009
0.0940490773769
0.110920834679
0.129517595666
0.149727465636
0.171368592048
0.194186054983
0.217852177033
0.241970724519
0.266085249899
0.289691552761
0.312253933367
0.333224602892
0.352065326764
0.368270140303
0.381387815461
0.391042693975
0.396952547477
0.398942280401
0.396952547477
0.391042693975
0.381387815461
0.368270140303
0.352065326764
0.333224602892
0.312253933367
0.289691552761
0.266085249899
0.241970724519
0.217852177033
0.194186054983
0.171368592048
0.149727465636
0.129517595666
0.110920834679
0.0940490773769
0.0789501583009
0.0656158147747
0.0539909665132
0.0439835959804
0.0354745928462
0.0283270377416
0.0223945302948
0.0175283004936
0.0135829692337
0.0104209348144
0.00791545158298
0.00595253241978
0.00443184841194
0.0032668190562
0.00238408820146
0.00172256893905
0.00123221916847
0.000872682695046
0.000611901930114
0.000424780270551
0.000291946925791
0.000198655471393
0.000133830225765
8.92616571771e-05
5.89430677565e-05
3.85351967421e-05
2.49424712901e-05
1.59837411069e-05
1.01408520655e-05
6.36982517887e-06
3.96129909103e-06
2.43896074589e-06
1.48671951473e-06
###Markdown
The *if* statementConsider this simple example:
###Code
def f(x):
if 0 <= x <= pi:
return sin(x)
else:
return 0
print f(-pi/2), f(pi/2), f(3*pi/2)
###Output
0 1.0 0
###Markdown
In general (the *else* block can be skipped if there are no statements to be executed when False) we can put together multiple conditions. Only the first condition that is True is executed.
###Code
if condition1:
<block of statements, executed if condition1 is True>
elif condition2:
<block of statements>
elif condition3:
<block of statements>
else:
<block of statements>
<next statement of the program>
###Output
_____no_output_____
###Markdown
The ternary operatorThere is another form of *if* which works as an operator, rather than a statement. If you've used the *if* function in a spreadsheet then you'll be familiar with this approach.
###Code
def f(x):
return (sin(x) if 0 <= x <= pi else 0)
print f(-pi/2), f(pi/2), f(3*pi/2)
a=-0.1
print sqrt(a) if a>=0 else None
###Output
0 1.0 0
None
###Markdown
The ternary operator has the following form:
###Code
<expression if condition is True> if <condition> else <expression if condition is false>
###Output
_____no_output_____
###Markdown
Exercise: Express a step function as a Python functionThe following "step" function is known as the Heaviside function andis widely used in mathematics:$$H(x)=\begin{cases}0, & \text{if $x<0$}.\\\\1, & \text{if $x\ge 0$}.\end{cases}$$Write a Python function H(x) that computes H(x).
###Code
def H(x):
if x<0:
return 0
else:
return 1
print map(H,range(-5,5))
def H(x):
return (0 if x<0 else 1)
print map(H,range(-5,5))
###Output
[0, 0, 0, 0, 0, 1, 1, 1, 1, 1]
[0, 0, 0, 0, 0, 1, 1, 1, 1, 1]
###Markdown
Introduction to Python programming [Gerard Gorman](http://www.imperial.ac.uk/people/g.gorman), [Christian Jacobs](http://christianjacobs.uk/) Updated for MPECDT by [David Ham](http://www.imperial.ac.uk/people/david.ham) Lecture 2: Computing with basic language constructionsLearning objectives:* Introduction to two different forms of loops which are used to repeat operations on data: either until a condition is satisfied (*while-loop*) or for a fixed number of iterations (*for-loop*).* Form a *condition* using a *boolean expression*. This is used to either terminate loops or control if a specific block of code is executed via the *if-statement*.* Learn two different methods for storing many data elements within a single Python type.* Introduction to developing your own Python *functions* which allows you to execute the same block of code, with different inputs and outputs, from anywhere in the code. Boolean expressionsAn expression with value *true* or *false* is called a boolean expression. Example expressions for what you would write mathematically as$C=40$, $C\ne40$, $C\ge40$, $C\gt40$ and $C\lt40$ are:
###Code
C == 40 # Note: the double == checks for equality, but C=40 is an assignment!
C != 40 # This could also be written as 'not C == 4' (i.e. the opposite of what C == 4 gives)
C >= 40
C > 40
C < 40
###Output
_____no_output_____
###Markdown
We can test boolean expressions in a Python shell:
###Code
C = 41
print("C != 40: ", C != 40)
print("C < 40: ", C < 40)
print("C == 41: ", C == 41)
###Output
_____no_output_____
###Markdown
Several conditions can be combined with the special 'and' and 'or' operators into a single boolean expression:
###Code
condition1 and condition2
condition1 or condition2
###Output
_____no_output_____
###Markdown
* Rule 1: (**C1** *and* **C2**) is *True* only if both **C1** and **C2** are *True** Rule 2: (**C1** *or* **C2**) is *True* if either **C1** or **C2** are *True*Examples:
###Code
x=0; y=1.2
print (x >= 0 and y < 1)
###Output
_____no_output_____
###Markdown
Exercise: Values of boolean expressionsAdd a comment to the code below to explain the outcome of each of the boolean expressions:
###Code
C = 41
print("Case 1: ", C == 40)
print("Case 2: ", C != 40 and C < 41)
print("Case 3: ", C != 40 or C < 41)
print("Case 4: ", not C == 40)
print("Case 5: ", not C > 40)
print("Case 6: ", C <= 41)
print("Case 7: ", not False)
print("Case 8: ", True and False)
print("Case 9: ", False or True)
print("Case 10: ", False or False or False)
print("Case 11: ", True and True and False)
print("Case 12: ", False == 0)
print("Case 13: ", True == 0)
print("Case 14: ", True == 1)
###Output
_____no_output_____
###Markdown
LoopsSuppose we want to make a table of Celsius and Fahrenheitdegrees:
###Code
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Output
_____no_output_____
###Markdown
How do we write a program that prints out such a table?We know from the last lecture how to make one line in this table:
###Code
C = -20
F = 9/5*C + 32
print(C, F)
###Output
_____no_output_____
###Markdown
We can just repeat these statements:
###Code
C=-20; F=9/5*C+32; print(C,F)
C=-15; F=9/5*C+32; print(C,F)
C=-10; F=9/5*C+32; print(C,F)
C=-5; F=9/5*C+32; print(C,F)
C=0; F=9/5*C+32; print(C,F)
C=5; F=9/5*C+32; print(C,F)
C=10; F=9/5*C+32; print(C,F)
C=15; F=9/5*C+32; print(C,F)
C=20; F=9/5*C+32; print(C,F)
C=25; F=9/5*C+32; print(C,F)
C=30; F=9/5*C+32; print(C,F)
C=35; F=9/5*C+32; print(C,F)
C=40; F=9/5*C+32; print(C,F)
###Output
_____no_output_____
###Markdown
So we can see that works but its **very boring** to write and very easy to introduce a misprint.**You really should not be doing boring repetitive tasks like this.** Spend one time instead looking for a smarter solution. When programming becomes boring, there is usually a construct that automates the writing. Computers are very good at performing repetitive tasks. For this purpose we use **loops**. The while loop (and the significance of indentation)A while loop executes repeatedly a set of statements as long as a **boolean** (i.e. *True* / *False*) condition is *True*
###Code
while condition:
<statement 1>
<statement 2>
...
<first statement after loop>
###Output
_____no_output_____
###Markdown
Note that all statements to be executed within the loop must be indented by the same amount! The loop ends when an unindented statement is encountered.At this point it is worth noticing that **blank spaces may or may not be important** in Python programs. These statements are equivalent (blanks do not matter):
###Code
v0=3
v0 = 3
v0= 3
# The computer does not care but this formatting style is
# considered clearest for the human reader.
v0 = 3
###Output
_____no_output_____
###Markdown
Here is a while loop example where blank spaces really do matter:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
1
2
3
4
5
6
7
8
9
10
11
###Markdown
Let's take a look at what happens when we forget to indent correctly:
###Code
counter = 0
while counter <= 10:
counter = counter + 1
print(counter)
###Output
_____no_output_____
###Markdown
Let's use the while loop to create the table above:
###Code
C = -20 # Initialise C
dC = 5 # Increment for C within the loop
while C <= 40: # Loop heading with condition
F = (9/5)*C + 32 # 1st statement inside loop
print ("%3d %6.1f" % (C, F)) # 2nd statement inside loop
C = C + dC # Increment C for the next iteration of the loop.
###Output
-20 -4.0
-15 5.0
-10 14.0
-5 23.0
0 32.0
5 41.0
10 50.0
15 59.0
20 68.0
25 77.0
30 86.0
35 95.0
40 104.0
###Markdown
Exercise: Make a Fahrenheit-Celsius conversion tableWrite a program that prints out a table with Fahrenheit degrees 0, 10, 20, ..., 100 in the first column and the corresponding Celsius degrees in the second column. Exercise: Write an approximate Fahrenheit-Celsius conversion tableMany people use an approximate formula for quickly converting Fahrenheit ($F$) to Celsius ($C$) degrees:$C \approx \hat{C} = \frac{F − 30}{2}$Modify the program from the previous exercise so that it prints three columns: $F$, $C$, and the approximate value $\hat{C}$. ListsSo far, one variable has referred to one number (or string). Sometimes however we naturally have a collection of numbers, saydegrees −20, −15, −10, −5, 0, ..., 40. One way to store these values in a computer program would be to have one variable per value, i.e.
###Code
C1 = -20
C2 = -15
C3 = -10
# ...
C13 = 40
###Output
_____no_output_____
###Markdown
This is clearly a terrible solution, particularly if we have lots of values. A better way of doing this is to collect values together in a list:
###Code
C = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
###Output
_____no_output_____
###Markdown
Now there is just one variable, **C**, holding all the values. Elements in a list are accessed via an index. List indices are always numbered as 0, 1, 2, and so forth up to the number of elements minus one:
###Code
mylist = [4, 6, -3.5]
print(mylist[0])
print(mylist[1])
print(mylist[2])
print(len(mylist)) # length of list
###Output
4
6
-3.5
3
###Markdown
Here are a few example of operations that you can perform on lists:
###Code
C = [-10, -5, 0, 5, 10, 15, 20, 25, 30]
C.append(35) # add new element 35 at the end
print(C)
C = C + [40,45] # And another list to the end of C
print(C)
C.insert(0, -15) # Insert -15 as index 0
print(C)
del C[2] # delete 3rd element
print(C)
del C[2] # delete what is now 3rd element
print(C)
print(len(C)) # length of list
print(C.index(10)) # Find the index of the list with the value 10
print (10 in C) # True only if the value 10 is stored in the list
print(C[-1]) # The last value in the list.
print(C[-2]) # The second last value in the list.
###Output
40
###Markdown
You can also extract sublists using ":"
###Code
print(C[5:]) # From index 5 to the end of the list.
print(C[5:7]) # From index 5 up to, but not including index 7.
###Output
_____no_output_____
###Markdown
Many languages (especially Matlab and Fortran) have would include the endpoint of a slice (7 in this case). One way to think of what Python does is that the slice is a half-open interval: $[5,7)$. This makes cutting a list in half particularly easy. For example:
###Code
print(C[:5] + ["Hello"] + C[5:])
print(C[7:-1]) # From index 7 up to the second last element.
print(C[:]) # [:] specifies the whole list.
somelist = ['Curly', 'Larry', 'Moe']
stooge1, stooge2, stooge3 = somelist # Unpack the entries in a list.
print(stooge3, stooge2, stooge1)
###Output
Moe Larry Curly
###Markdown
Of course this only works if `somelist` contains exactly the number of items on the left hand side of the equals. Another useful operator is `*` (pronounced "splat"). It turns a list (or any other sequence) into the set of arguments of a function. For example:
###Code
print(somelist) # Prints the list.
print(*somelist) # Prints the items in the list.
###Output
['Curly', 'Larry', 'Moe']
Curly Larry Moe
###Markdown
Exercise: Store odd numbers in a listStep 1: Write a program that generates all odd numbers from 1 to *n*. Set *n* in the beginning of the program and use a while loop to compute the numbers. (Make sure that if *n* is an even number, the largest generated odd number is *n*-1.).Step 2: Store the generated odd numbers in a list. Start with an empty list and use the same while loop where you generate each odd number, ao append the new number to the list.Finally, print the list elements to the screen.
###Code
a = []
n = 20
counter = 0
while counter <= n:
if (counter%2)==1:
a.append(counter)
counter = counter+1
print(a)
###Output
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
###Markdown
For loopsWe can visit each element in a list and process the element with some statements using a *for* loop, for example:
###Code
degrees = [0, 10, 20, 40, 100]
for C in degrees:
print('list element:', C)
print('The degrees list has', len(degrees), 'elements')
###Output
_____no_output_____
###Markdown
Notice again how the statements to be executed within the loop must be indented! Let's now revisit the conversion table example using the *for* loop:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
for C in Cdegrees:
F = (9/5)*C + 32
print("%3d %6.2f" % (C, F))
###Output
_____no_output_____
###Markdown
It is also possible to rewrite the *for* loop as a *while* loop, i.e.,
###Code
for element in somelist:
# process element
###Output
_____no_output_____
###Markdown
can always be transformed to a *while* loop
###Code
index = 0
while index < len(somelist):
element = somelist[index]
# process element
index += 1
###Output
_____no_output_____
###Markdown
Taking the previous table example:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
index = 0
while index < len(Cdegrees):
C = Cdegrees[index]
F = (9/5)*C + 32
print('%5d %5.1f' % (C, F))
index += 1
###Output
_____no_output_____
###Markdown
Rather than just printing out the Fahrenheit values, let's also store these computed values in a list of their own:
###Code
Cdegrees = [-20, -15, -10, -5, 0, 5, 10, 15, 20, 25, 30, 35, 40]
Fdegrees = [] # start with empty list
for C in Cdegrees:
F = (9/5)*C + 32
Fdegrees.append(F) # add new element to Fdegrees
print(Fdegrees)
###Output
_____no_output_____
###Markdown
Iterators: things you can iterate over.We've seen that a `for` loop can iterate over list values. In fact you can iterate over any object that is capable of providing values one at a time. In python, these objects are called `iterators`.A particularly useful python function which creates an iterator is `range`. For example, we can use range to iterate over the *indices* of a list:
###Code
for i in range(0, len(somelist), 1):
element = somelist[i]
... process element or somelist[i] directly
###Output
_____no_output_____
###Markdown
The statement *range(start, stop, inc)* generates an iterator over the integers *start*, *start+inc*, *start+2\*inc*, and so on up to, but not including, *stop*. We can also write *range(stop)* as an abbreviation for *range(0, stop, 1)*:
###Code
print(range(3)) # same as range(0, 3, 1)
###Output
range(0, 3)
###Markdown
Oh, that wasn't very informative. If we want to see all the numbers which the `range` will iterate over, we can cast the range to a list:
###Code
print(list(range(3)))
print(list(range(2, 8, 3)))
###Output
[2, 5]
###Markdown
List comprehensionsConsider this example where we compute two lists in a *for* loop:
###Code
n = 16
Cdegrees = []; Fdegrees = [] # empty lists
for i in range(n):
Cdegrees.append(-5 + i*0.5)
Fdegrees.append((9/5)*Cdegrees[i] + 32)
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
As constructing lists is a very common requirement, the above way of doing it can become very tedious to both write and read. Therefore, Python has a compact construct, called list comprehension for generating lists from a *for* loop:
###Code
n = 16
Cdegrees = [-5 + i*0.5 for i in range(n)]
Fdegrees = [(9/5)*C + 32 for C in Cdegrees]
print("Cdegrees = ", Cdegrees)
print("Fdegrees = ", Fdegrees)
###Output
Cdegrees = [-5.0, -4.5, -4.0, -3.5, -3.0, -2.5, -2.0, -1.5, -1.0, -0.5, 0.0, 0.5, 1.0, 1.5, 2.0, 2.5]
Fdegrees = [23.0, 23.9, 24.8, 25.7, 26.6, 27.5, 28.4, 29.3, 30.2, 31.1, 32.0, 32.9, 33.8, 34.7, 35.6, 36.5]
###Markdown
The general form of a list comprehension is:
###Code
somelist = [expression for element in somelist]
###Output
_____no_output_____
###Markdown
Exercise: Repeat the previous exercise of creating a list of odd numbers using using: a for loop, list comprehension and the range function
###Code
# Using for loop
# Using list comprehension
# Using the function range
###Output
_____no_output_____
###Markdown
Changing elements in a listSay we want to add $2$ to all the numbers in a list:
###Code
v = [-1, 1, 10]
for e in v:
e = e + 2
print(v)
###Output
_____no_output_____
###Markdown
We can see that the list *v* is unaltered! The reason for this is that inside the loop, *e* is an ordinary (int) variable. At the start of the iteration *e* is assigned a *copy* of the next element in the list. Inside the loop we can change *e* but *v* itself is unaltered. If we want to change *v* we have to use an index to access and modify its elements:
###Code
v[1] = 4 # assign 4 to 2nd element (index 1) in v
print(v)
###Output
_____no_output_____
###Markdown
To add 2 to all values we need a *for* loop over indices:
###Code
for i in range(len(v)):
v[i] = v[i] + 2
print(v)
###Output
_____no_output_____
###Markdown
Traversing multiple lists simultaneously - *zip(list1, list2, ...)*Consider how we can loop over elements in both Cdegrees and Fdegrees at the same time. One approach would be to use list indices:
###Code
for i in range(len(Cdegrees)):
print(Cdegrees[i], Fdegrees[i])
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
An alternative construct (regarded as more ”Pythonic”) uses the *zip* function:
###Code
for C, F in zip(Cdegrees, Fdegrees):
print(C, F)
###Output
-5.0 23.0
-4.5 23.9
-4.0 24.8
-3.5 25.7
-3.0 26.6
-2.5 27.5
-2.0 28.4
-1.5 29.3
-1.0 30.2
-0.5 31.1
0.0 32.0
0.5 32.9
1.0 33.8
1.5 34.7
2.0 35.6
2.5 36.5
###Markdown
`zip` creates an *iterator* over the corresponding items of its arguments in order.Another example with three lists:
###Code
l1 = [3, 6, 1]; l2 = [1.5, 1, 0]; l3 = [9.1, 3, 2]
for e in zip(l1, l2, l3):
print(*e)
###Output
_____no_output_____
###Markdown
If the lists are of unequal length then the loop stops when the end of the shortest list is reached. Experiment with this:
###Code
l1 = [3, 6, 1, 4, 6]; l2 = [1.5, 1, 0, 7]; l3 = [9.1, 3, 2, 0, 9]
for e in zip(l1, l2, l3):
print(*e)
###Output
_____no_output_____
###Markdown
Nested lists: list of listsA *list* can contain **any** object, including another *list*. To illustrate this, consider how to store the conversion table as a single Python list rather than two separate lists.
###Code
Cdegrees = range(-20, 41, 5)
Fdegrees = [(9.0/5)*C + 32 for C in Cdegrees]
table1 = [Cdegrees, Fdegrees] # List of two lists
print("table1 = ", table1)
print("table1[0] = ", table1[0])
print("table1[1] = ", table1[1])
print("table1[2][3] = ", table1[1][3])
###Output
_____no_output_____
###Markdown
This gives us a table of two columns. How do we create a table of rows instead:
###Code
table2 = []
for C, F in zip(Cdegrees, Fdegrees):
row = [C, F]
table2.append(row)
print(table2)
###Output
_____no_output_____
###Markdown
We can use `zip` to do this more elegantly:
###Code
table2 = list(zip(Cdegrees, Fdegrees))
print(table2)
###Output
_____no_output_____
###Markdown
And you can loop through this list as before:
###Code
for C, F in table2:
print(C, F)
###Output
_____no_output_____
###Markdown
Tuples: sequences that cannot be changedWe've already learned that lists are not the only thing which can be iterated over, but that there is a general category of `iterator` types. Similarly, lists are not the only objects which can be indexed. The general category of types which can be indexed are the `sequence` types.Tuples are **constant** sequences. You can use them in much the same way as lists except you cannot modify them. They are an example of an [**immutable**](http://en.wikipedia.org/wiki/Immutable_object) type.
###Code
t = (2, 4, 6, 'temp.pdf') # Define a tuple.
t = 2, 4, 6, 'temp.pdf' # Can skip parenthesis as it is assumed in this context.
###Output
_____no_output_____
###Markdown
Let's see what happens when we try to modify the tuple like we did with a list:
###Code
t[1] = -1
t.append(0)
del t[1]
###Output
_____no_output_____
###Markdown
However, we can use the tuple to compose a new tuple:
###Code
t = t + (-1.0, -2.0)
print(t)
###Output
_____no_output_____ |
scikit-learn/machine-learning-course-notebooks/hierarchical-clustering/ML0101EN-Clus-Hierarchical-Cars.ipynb | ###Markdown
Hierarchical ClusteringEstimated time needed: **25** minutes ObjectivesAfter completing this lab you will be able to:* Use scikit-learn to do Hierarchical clustering* Create dendograms to visualize the clustering Table of contents Hierarchical Clustering - Agglomerative Generating Random Data Agglomerative Clustering Dendrogram Associated for the Agglomerative Hierarchical Clustering Clustering on the Vehicle Dataset Data Cleaning Clustering Using Scipy Clustering using scikit-learn Hierarchical Clustering - AgglomerativeWe will be looking at a clustering technique, which is Agglomerative Hierarchical Clustering. Remember that agglomerative is the bottom up approach. In this lab, we will be looking at Agglomerative clustering, which is more popular than Divisive clustering. We will also be using Complete Linkage as the Linkage Criteria. NOTE: You can also try using Average Linkage wherever Complete Linkage would be used to see the difference!
###Code
import numpy as np
import pandas as pd
from scipy import ndimage
from scipy.cluster import hierarchy
from scipy.spatial import distance_matrix
from matplotlib import pyplot as plt
from sklearn import manifold, datasets
from sklearn.cluster import AgglomerativeClustering
from sklearn.datasets._samples_generator import make_blobs
%matplotlib inline
###Output
_____no_output_____
###Markdown
Generating Random DataWe will be generating a set of data using the make_blobs class. Input these parameters into make_blobs: n_samples: The total number of points equally divided among clusters. Choose a number from 10-1500 centers: The number of centers to generate, or the fixed center locations. Choose arrays of x,y coordinates for generating the centers. Have 1-10 centers (ex. centers=[[1,1], [2,5]]) cluster_std: The standard deviation of the clusters. The larger the number, the further apart the clusters Choose a number between 0.5-1.5 Save the result to X1 and y1.
###Code
X1, y1 = make_blobs(n_samples=50, centers=[[4,4], [-2, -1], [1, 1], [10,4]], cluster_std=0.9)
###Output
_____no_output_____
###Markdown
Plot the scatter plot of the randomly generated data.
###Code
plt.scatter(X1[:, 0], X1[:, 1], marker='o');
###Output
_____no_output_____
###Markdown
Agglomerative ClusteringWe will start by clustering the random data points we just created. The Agglomerative Clustering class will require two inputs: n_clusters: The number of clusters to form as well as the number of centroids to generate. Value will be: 4 linkage: Which linkage criterion to use. The linkage criterion determines which distance to use between sets of observation. The algorithm will merge the pairs of cluster that minimize this criterion. Value will be: 'complete' Note: It is recommended you try everything with 'average' as well Save the result to a variable called agglom .
###Code
agglom = AgglomerativeClustering(n_clusters = 4, linkage = 'average')
###Output
_____no_output_____
###Markdown
Fit the model with X2 and y2 from the generated data above.
###Code
agglom.fit(X1,y1)
###Output
_____no_output_____
###Markdown
Run the following code to show the clustering! Remember to read the code and comments to gain more understanding on how the plotting works.
###Code
# Create a figure of size 6 inches by 4 inches.
plt.figure(figsize=(6,4))
# These two lines of code are used to scale the data points down,
# Or else the data points will be scattered very far apart.
# Create a minimum and maximum range of X1.
x_min, x_max = np.min(X1, axis=0), np.max(X1, axis=0)
# Get the average distance for X1.
X1 = (X1 - x_min) / (x_max - x_min)
# This loop displays all of the datapoints.
for i in range(X1.shape[0]):
# Replace the data points with their respective cluster value
# (ex. 0) and is color coded with a colormap (plt.cm.spectral)
plt.text(X1[i, 0], X1[i, 1], str(y1[i]),
color=plt.cm.nipy_spectral(agglom.labels_[i] / 10.),
fontdict={'weight': 'bold', 'size': 9})
# Remove the x ticks, y ticks, x and y axis
plt.xticks([])
plt.yticks([])
#plt.axis('off')
# Display the plot of the original data before clustering
plt.scatter(X1[:, 0], X1[:, 1], marker='.')
# Display the plot
plt.show()
###Output
_____no_output_____
###Markdown
Dendrogram Associated for the Agglomerative Hierarchical ClusteringRemember that a distance matrix contains the distance from each point to every other point of a dataset .Use the function distance_matrix, which requires two inputs. Use the Feature Matrix, X1 as both inputs and save the distance matrix to a variable called dist_matrix Remember that the distance values are symmetric, with a diagonal of 0's. This is one way of making sure your matrix is correct. (print out dist_matrix to make sure it's correct)
###Code
dist_matrix = distance_matrix(X1,X1)
print(dist_matrix)
###Output
[[0. 0.10561226 0.40896614 ... 0.60334667 0.43765978 0.06119911]
[0.10561226 0. 0.49168134 ... 0.51772935 0.52749445 0.08342776]
[0.40896614 0.49168134 0. ... 0.80857268 0.06331084 0.40826692]
...
[0.60334667 0.51772935 0.80857268 ... 0. 0.86810301 0.54596991]
[0.43765978 0.52749445 0.06331084 ... 0.86810301 0. 0.44476534]
[0.06119911 0.08342776 0.40826692 ... 0.54596991 0.44476534 0. ]]
###Markdown
Using the linkage class from hierarchy, pass in the parameters: The distance matrix 'complete' for complete linkage Save the result to a variable called Z .
###Code
Z = hierarchy.linkage(dist_matrix, 'complete')
###Output
/var/folders/qt/f27x0vsd4bvdhbv_smscjygw0000gp/T/ipykernel_7884/3518085107.py:1: ClusterWarning: scipy.cluster: The symmetric non-negative hollow observation matrix looks suspiciously like an uncondensed distance matrix
Z = hierarchy.linkage(dist_matrix, 'complete')
###Markdown
A Hierarchical clustering is typically visualized as a dendrogram as shown in the following cell. Each merge is represented by a horizontal line. The y-coordinate of the horizontal line is the similarity of the two clusters that were merged, where cities are viewed as singleton clusters.By moving up from the bottom layer to the top node, a dendrogram allows us to reconstruct the history of merges that resulted in the depicted clustering.Next, we will save the dendrogram to a variable called dendro. In doing this, the dendrogram will also be displayed.Using the dendrogram class from hierarchy, pass in the parameter: Z
###Code
dendro = hierarchy.dendrogram(Z)
###Output
_____no_output_____
###Markdown
PracticeWe used **complete** linkage for our case, change it to **average** linkage to see how the dendogram changes.
###Code
Z = hierarchy.linkage(dist_matrix, 'average')
dendro = hierarchy.dendrogram(Z)
###Output
/var/folders/qt/f27x0vsd4bvdhbv_smscjygw0000gp/T/ipykernel_7884/3726426701.py:1: ClusterWarning: scipy.cluster: The symmetric non-negative hollow observation matrix looks suspiciously like an uncondensed distance matrix
Z = hierarchy.linkage(dist_matrix, 'average')
###Markdown
Click here for the solution```pythonZ = hierarchy.linkage(dist_matrix, 'average')dendro = hierarchy.dendrogram(Z)``` Clustering on Vehicle datasetImagine that an automobile manufacturer has developed prototypes for a new vehicle. Before introducing the new model into its range, the manufacturer wants to determine which existing vehicles on the market are most like the prototypes--that is, how vehicles can be grouped, which group is the most similar with the model, and therefore which models they will be competing against.Our objective here, is to use clustering methods, to find the most distinctive clusters of vehicles. It will summarize the existing vehicles and help manufacturers to make decision about the supply of new models. Download dataTo download the data, we will use **`!wget`** to download it from IBM Object Storage.\**Did you know?** When it comes to Machine Learning, you will likely be working with large datasets. As a business, where can you host your data? IBM is offering a unique opportunity for businesses, with 10 Tb of IBM Cloud Object Storage: [Sign up now for free](http://cocl.us/ML0101EN-IBM-Offer-CC)
###Code
!wget -O cars_clus.csv https://cf-courses-data.s3.us.cloud-object-storage.appdomain.cloud/IBMDeveloperSkillsNetwork-ML0101EN-SkillsNetwork/labs/Module%204/data/cars_clus.csv
###Output
_____no_output_____
###Markdown
Read dataLet's read dataset to see what features the manufacturer has collected about the existing models.
###Code
filename = 'cars_clus.csv'
#Read csv
pdf = pd.read_csv(filename)
print ("Shape of dataset: ", pdf.shape)
pdf.head(5)
###Output
Shape of dataset: (159, 16)
###Markdown
The feature sets include price in thousands (price), engine size (engine_s), horsepower (horsepow), wheelbase (wheelbas), width (width), length (length), curb weight (curb_wgt), fuel capacity (fuel_cap) and fuel efficiency (mpg). Data CleaningLet's clean the dataset by dropping the rows that have null value:
###Code
print ("Shape of dataset before cleaning: ", pdf.size)
pdf[[ 'sales', 'resale', 'type', 'price', 'engine_s',
'horsepow', 'wheelbas', 'width', 'length', 'curb_wgt', 'fuel_cap',
'mpg', 'lnsales']] = pdf[['sales', 'resale', 'type', 'price', 'engine_s',
'horsepow', 'wheelbas', 'width', 'length', 'curb_wgt', 'fuel_cap',
'mpg', 'lnsales']].apply(pd.to_numeric, errors='coerce')
pdf = pdf.dropna()
pdf = pdf.reset_index(drop=True)
print ("Shape of dataset after cleaning: ", pdf.size)
pdf.head(5)
###Output
Shape of dataset before cleaning: 2544
Shape of dataset after cleaning: 1872
###Markdown
Feature selectionLet's select our feature set:
###Code
featureset = pdf[['engine_s', 'horsepow', 'wheelbas', 'width', 'length', 'curb_wgt', 'fuel_cap', 'mpg']]
###Output
_____no_output_____
###Markdown
NormalizationNow we can normalize the feature set. **MinMaxScaler** transforms features by scaling each feature to a given range. It is by default (0, 1). That is, this estimator scales and translates each feature individually such that it is between zero and one.
###Code
from sklearn.preprocessing import MinMaxScaler
x = featureset.values #returns a numpy array
min_max_scaler = MinMaxScaler()
feature_mtx = min_max_scaler.fit_transform(x)
feature_mtx [0:5]
###Output
_____no_output_____
###Markdown
Clustering using ScipyIn this part we use Scipy package to cluster the dataset.First, we calculate the distance matrix.
###Code
import scipy
leng = feature_mtx.shape[0]
D = scipy.zeros([leng,leng])
for i in range(leng):
for j in range(leng):
D[i,j] = scipy.spatial.distance.euclidean(feature_mtx[i], feature_mtx[j])
D
###Output
/var/folders/qt/f27x0vsd4bvdhbv_smscjygw0000gp/T/ipykernel_7884/458117257.py:3: DeprecationWarning: scipy.zeros is deprecated and will be removed in SciPy 2.0.0, use numpy.zeros instead
D = scipy.zeros([leng,leng])
###Markdown
In agglomerative clustering, at each iteration, the algorithm must update the distance matrix to reflect the distance of the newly formed cluster with the remaining clusters in the forest.The following methods are supported in Scipy for calculating the distance between the newly formed cluster and each:\- single\- complete\- average\- weighted\- centroidWe use **complete** for our case, but feel free to change it to see how the results change.
###Code
import pylab
import scipy.cluster.hierarchy
Z = hierarchy.linkage(D, 'complete')
###Output
/var/folders/qt/f27x0vsd4bvdhbv_smscjygw0000gp/T/ipykernel_7884/227076933.py:3: ClusterWarning: scipy.cluster: The symmetric non-negative hollow observation matrix looks suspiciously like an uncondensed distance matrix
Z = hierarchy.linkage(D, 'complete')
###Markdown
Essentially, Hierarchical clustering does not require a pre-specified number of clusters. However, in some applications we want a partition of disjoint clusters just as in flat clustering.So you can use a cutting line:
###Code
from scipy.cluster.hierarchy import fcluster
max_d = 3
clusters = fcluster(Z, max_d, criterion='distance')
clusters
###Output
_____no_output_____
###Markdown
Also, you can determine the number of clusters directly:
###Code
from scipy.cluster.hierarchy import fcluster
k = 5
clusters = fcluster(Z, k, criterion='maxclust')
clusters
###Output
_____no_output_____
###Markdown
Now, plot the dendrogram:
###Code
fig = pylab.figure(figsize=(18,50))
def llf(id):
return '[%s %s %s]' % (pdf['manufact'][id], pdf['model'][id], int(float(pdf['type'][id])) )
dendro = hierarchy.dendrogram(Z, leaf_label_func=llf, leaf_rotation=0, leaf_font_size =12, orientation = 'right')
###Output
_____no_output_____
###Markdown
Clustering using scikit-learnLet's redo it again, but this time using the scikit-learn package:
###Code
from sklearn.metrics.pairwise import euclidean_distances
dist_matrix = euclidean_distances(feature_mtx,feature_mtx)
print(dist_matrix)
Z_using_dist_matrix = hierarchy.linkage(dist_matrix, 'complete')
fig = pylab.figure(figsize=(18,50))
def llf(id):
return '[%s %s %s]' % (pdf['manufact'][id], pdf['model'][id], int(float(pdf['type'][id])) )
dendro = hierarchy.dendrogram(Z_using_dist_matrix, leaf_label_func=llf, leaf_rotation=0, leaf_font_size =12, orientation = 'right')
###Output
_____no_output_____
###Markdown
Now, we can use the 'AgglomerativeClustering' function from scikit-learn library to cluster the dataset. The AgglomerativeClustering performs a hierarchical clustering using a bottom up approach. The linkage criteria determines the metric used for the merge strategy:* Ward minimizes the sum of squared differences within all clusters. It is a variance-minimizing approach and in this sense is similar to the k-means objective function but tackled with an agglomerative hierarchical approach.* Maximum or complete linkage minimizes the maximum distance between observations of pairs of clusters.* Average linkage minimizes the average of the distances between all observations of pairs of clusters.
###Code
agglom = AgglomerativeClustering(n_clusters = 6, linkage = 'complete')
agglom.fit(dist_matrix)
agglom.labels_
###Output
/Users/elkin.guerra/Documents/Documents Elkin Guerra/PRAGMA LEARNING/data-science-dojo/venv/lib/python3.8/site-packages/sklearn/cluster/_agglomerative.py:542: ClusterWarning: scipy.cluster: The symmetric non-negative hollow observation matrix looks suspiciously like an uncondensed distance matrix
out = hierarchy.linkage(X, method=linkage, metric=affinity)
###Markdown
We can add a new field to our dataframe to show the cluster of each row:
###Code
pdf['cluster_'] = agglom.labels_
pdf.head()
import matplotlib.cm as cm
n_clusters = max(agglom.labels_)+1
colors = cm.rainbow(np.linspace(0, 1, n_clusters))
cluster_labels = list(range(0, n_clusters))
# Create a figure of size 6 inches by 4 inches.
plt.figure(figsize=(16,14))
for color, label in zip(colors, cluster_labels):
subset = pdf[pdf.cluster_ == label]
for i in subset.index:
plt.text(subset.horsepow[i], subset.mpg[i],str(subset['model'][i]), rotation=25)
plt.scatter(subset.horsepow, subset.mpg, s= subset.price*10, c=color, label='cluster'+str(label),alpha=0.5)
# plt.scatter(subset.horsepow, subset.mpg)
plt.legend()
plt.title('Clusters')
plt.xlabel('horsepow')
plt.ylabel('mpg')
###Output
*c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points.
*c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points.
*c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points.
*c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points.
*c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points.
*c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points.
###Markdown
As you can see, we are seeing the distribution of each cluster using the scatter plot, but it is not very clear where is the centroid of each cluster. Moreover, there are 2 types of vehicles in our dataset, "truck" (value of 1 in the type column) and "car" (value of 0 in the type column). So, we use them to distinguish the classes, and summarize the cluster. First we count the number of cases in each group:
###Code
pdf.groupby(['cluster_','type'])['cluster_'].count()
###Output
_____no_output_____
###Markdown
Now we can look at the characteristics of each cluster:
###Code
agg_cars = pdf.groupby(['cluster_','type'])['horsepow','engine_s','mpg','price'].mean()
agg_cars
###Output
/var/folders/qt/f27x0vsd4bvdhbv_smscjygw0000gp/T/ipykernel_7884/3307995906.py:1: FutureWarning: Indexing with multiple keys (implicitly converted to a tuple of keys) will be deprecated, use a list instead.
agg_cars = pdf.groupby(['cluster_','type'])['horsepow','engine_s','mpg','price'].mean()
###Markdown
It is obvious that we have 3 main clusters with the majority of vehicles in those.**Cars**:* Cluster 1: with almost high mpg, and low in horsepower.* Cluster 2: with good mpg and horsepower, but higher price than average.* Cluster 3: with low mpg, high horsepower, highest price.**Trucks**:* Cluster 1: with almost highest mpg among trucks, and lowest in horsepower and price.* Cluster 2: with almost low mpg and medium horsepower, but higher price than average.* Cluster 3: with good mpg and horsepower, low price.Please notice that we did not use **type** and **price** of cars in the clustering process, but Hierarchical clustering could forge the clusters and discriminate them with quite a high accuracy.
###Code
plt.figure(figsize=(16,10))
for color, label in zip(colors, cluster_labels):
subset = agg_cars.loc[(label,),]
for i in subset.index:
plt.text(subset.loc[i][0]+5, subset.loc[i][2], 'type='+str(int(i)) + ', price='+str(int(subset.loc[i][3]))+'k')
plt.scatter(subset.horsepow, subset.mpg, s=subset.price*20, c=color, label='cluster'+str(label))
plt.legend()
plt.title('Clusters')
plt.xlabel('horsepow')
plt.ylabel('mpg')
###Output
*c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points.
*c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points.
*c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points.
*c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points.
*c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points.
*c* argument looks like a single numeric RGB or RGBA sequence, which should be avoided as value-mapping will have precedence in case its length matches with *x* & *y*. Please use the *color* keyword-argument or provide a 2D array with a single row if you intend to specify the same RGB or RGBA value for all points.
|
examples/15_trajectory_module.ipynb | ###Markdown
pyscal `Trajectory``Trajectory` is a `pyscal` module intended for working with molecular dynamics trajectories which contain more than one time slice. Currently, the module only supports [LAMMPS dump](https://lammps.sandia.gov/doc/dump.html) text file formats. It can be used to get a single or slices from a trajectory, trim the trajectory or even combine multiple trajectories. The example below illustrates various uses of the module.**`Trajectory` is an experimental feature at the moment and may undergo significant changes in future releases** Start with importing the module
###Code
from pyscal import Trajectory
###Output
_____no_output_____
###Markdown
Read in a trajectory.
###Code
traj = Trajectory("traj.light")
###Output
_____no_output_____
###Markdown
When using the above statement, the trajectory is not yet read in to memory. Just the basic information is available now.
###Code
traj
###Output
_____no_output_____
###Markdown
We can know the number of slices in the trajectory and the number of atoms. `Trajectory` only works with fixed number of atoms. Now, one can get a single slice or multiple slices just as is done with a python list. Getting the 2nd slice (counting starts from 0!).
###Code
sl = traj[2]
sl
###Output
_____no_output_____
###Markdown
This slice can now be converted to a more usable format, either to a pyscal `System` or just written to another text file. Convert to a pyscal `System` object,
###Code
sys = sl.to_system()
sys
###Output
_____no_output_____
###Markdown
`System` objects contain all the information. The atomic positions, simulation box and so on are easily accessible.
###Code
sys[0].box
sys[0].atoms[0].pos
###Output
_____no_output_____
###Markdown
If information other than positions are required, the `customkeys` keyword can be used. For example, for velocity in the x direction,
###Code
sys = sl.to_system(customkeys=["vx"])
sys
sys[0].atoms[0].custom["vx"]
###Output
_____no_output_____
###Markdown
Instead of creating a System object, the slice can also be written to a file directly.
###Code
sl.to_file("test.dump")
###Output
_____no_output_____
###Markdown
Like normal python lists, multiple slices can also be accessed directly
###Code
sl1 = traj[0:4]
sl1
###Output
_____no_output_____
###Markdown
`to_system` and `to_file` methods can be used on this object too. Multiple slices can be added together
###Code
sl2 = traj[5:7]
sl2
slnew = sl1+sl2
slnew
###Output
_____no_output_____
###Markdown
pyscal `Trajectory` `Trajectory` is a `pyscal` module intended for working with molecular dynamics trajectories which contain more than one time slice. Currently, the module only supports [LAMMPS dump](https://lammps.sandia.gov/doc/dump.html) text file formats. It can be used to get a single or slices from a trajectory, trim the trajectory or even combine multiple trajectories. The example below illustrates various uses of the module.**`Trajectory` is an experimental feature at the moment and may undergo significant changes in future releases** Start with importing the module
###Code
from pyscal import Trajectory
###Output
_____no_output_____
###Markdown
Read in a trajectory.
###Code
traj = Trajectory("traj.light")
###Output
_____no_output_____
###Markdown
When using the above statement, the trajectory is not yet read in to memory. Just the basic information is available now.
###Code
traj
###Output
_____no_output_____
###Markdown
We can know the number of slices in the trajectory and the number of atoms. `Trajectory` only works with fixed number of atoms. Now, one can get a single slice or multiple slices just as is done with a python list. Getting the 2nd slice (counting starts from 0!).
###Code
sl = traj[2]
sl
###Output
_____no_output_____
###Markdown
This slice can now be converted to a more usable format, either to a pyscal `System` or just written to another text file. Convert to a pyscal `System` object,
###Code
sys = sl.to_system()
sys
###Output
_____no_output_____
###Markdown
`System` objects contain all the information. The atomic positions, simulation box and so on are easily accessible.
###Code
sys[0].box
sys[0].atoms[0].pos
###Output
_____no_output_____
###Markdown
If information other than positions are required, the `customkeys` keyword can be used. For example, for velocity in the x direction,
###Code
sys = sl.to_system(customkeys=["vx"])
sys
sys[0].atoms[0].custom["vx"]
###Output
_____no_output_____
###Markdown
Instead of creating a System object, the slice can also be written to a file directly.
###Code
sl.to_file("test.dump")
###Output
_____no_output_____
###Markdown
Like normal python lists, multiple slices can also be accessed directly
###Code
sl1 = traj[0:4]
sl1
###Output
_____no_output_____
###Markdown
`to_system` and `to_file` methods can be used on this object too. Multiple slices can be added together
###Code
sl2 = traj[5:7]
sl2
slnew = sl1+sl2
slnew
###Output
_____no_output_____ |
Task 1/Task 1 Linear Regression .ipynb | ###Markdown
**The Sparks Foundation Internship Program****Data Science & Business Analytics Internship****Technical TASK 1 :- Prediction using Supervised ML**In this task, we will predict the score of student based upon the number of hours they studied. This is a simple linear regression task as it involves just two variables.Author: NOMAN SAEED SOOMRO**Step 1: Importing Required Libraries**
###Code
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
###Output
_____no_output_____
###Markdown
**Step 2 : Reading Data from online source**
###Code
url="https://raw.githubusercontent.com/AdiPersonalWorks/Random/master/student_scores%20-%20student_scores.csv"
data=pd.read_csv(url)
data.head()
data.isnull().sum()
###Output
_____no_output_____
###Markdown
**Step 3: Plotting the distribution of scores**
###Code
data.plot(x='Hours', y='Scores', style='+')
plt.title('Hours vs Percentage')
plt.xlabel('Hours Studied')
plt.ylabel('Percentage Score')
plt.show()
###Output
_____no_output_____
###Markdown
**Step 4 : Preparing The Data and splitting the data** The next step is to divide the data into "attributes"/"X" (inputs) and "labels"/"Y" (outputs).Splitting the data into training data-set and test data-set.
###Code
X= data.iloc[:, :-1].values
Y= data.iloc[:, 1].values
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X,Y,test_size=0.2, random_state=0)
###Output
_____no_output_____
###Markdown
**Step 5 : Model Training**
###Code
from sklearn.linear_model import LinearRegression
regressor = LinearRegression()
regressor.fit(X_train, y_train)
print("Model Trained")
# Plotting the regression line
line = regressor.coef_*X+regressor.intercept_
# Plotting for the test data
plt.scatter(X, y)
plt.plot(X, line);
plt.show()
print(X_test) # Testing data - In Hours
y_pred = regressor.predict(X_test) # Predicting the scores
###Output
[[1.5]
[3.2]
[7.4]
[2.5]
[5.9]]
###Markdown
**Step 6 : Comparing Actual vs Predicted**
###Code
df = pd.DataFrame({'Actual': y_test, 'Predicted': y_pred})
df
###Output
_____no_output_____
###Markdown
**Step 7:Predicting the output on the New Data.**
###Code
### Testing your own data.
hours = 9.25
test = np.array([hours])
test = test.reshape(-1,1)
own_pred = regressor.predict(test)
print ("No. of Hours = {}".format(hours))
print ("Predicted Score = {}".format(own_pred[0]))
###Output
No. of Hours = 9.25
Predicted Score = 93.69173248737535
###Markdown
**Step 8 : Evaluating the model**The final step is to evaluate the performance of algorithm. This step is particularly important to compare how well different algorithms perform on a particular dataset. For simplicity here, we have chosen the mean square error. There are many such metrics.
###Code
from sklearn import metrics
print('Mean Absolute Error:', metrics.mean_absolute_error(y_test, y_pred))
print('Mean Squared Error:', metrics.mean_squared_error(y_test, y_pred))
print('Root mean squared Error:', np.sqrt(metrics.mean_squared_error(y_test, y_pred)))
###Output
Mean Absolute Error: 4.183859899002975
Mean Squared Error: 21.598769307217406
Root mean squared Error: 4.647447612100367
|
online.ipynb | ###Markdown
Demo NotebookRun the pretrained visual navigation models.Currently available: SAVN, GCN, Non-Adaptive-A3C
###Code
import numpy as np
import h5py
import main
from models.savn import SAVN
from models.basemodel import BaseModel
from models.gcn import GCN
import torch
import torch.nn as nn
import torchvision.models as models
from torchvision import transforms
from runners.train_util import get_params
from models.model_io import ModelOptions, ModelInput
from utils.net_util import gpuify
from utils.net_util import resnet_input_transform
import torch.nn.functional as F
import time
from runners.train_util import compute_learned_loss, SGD_step
#import matplotlib.pyplot as plt
MODEL_PATH_DICT = {'SAVN' : 'pretrained_models/savn_pretrained.dat',
'NON_ADAPTIVE_A3C': 'pretrained_models/nonadaptivea3c_pretrained.dat',
'GCN':'pretrained_models/gcn_pretrained.dat' }
GLOVE_FILE = './data/thor_glove/glove_map300d.hdf5'
ACTION_LIST = ['MoveAhead', 'RotateLeft', 'RotateRight', 'LookUp', 'LookDown', 'Done']
class FakeArgs():
def __init__(self, model='SAVN',glove_file=GLOVE_FILE,inner_lr=0.0001):
self.action_space = 6
self.glove_dim = 300
self.hidden_state_sz = 512
self.dropout_rate = 0.25
self.num_steps = 6 # initialized in main_eval.py
self.gpu_id = -1
self.learned_loss = True if model=='SAVN' else False
self.inner_lr = inner_lr
self.model = model
self.glove_file = GLOVE_FILE
class Agent():
def __init__(self,args, model):
self.gpu_id = args.gpu_id
self.model = model
self.hidden = None #initialized in function call
self.last_action_probs = None #initialized in function call
self.resnet18 = None #initialized in function call
self.hidden_state_sz = args.hidden_state_sz
self.action_space = args.action_space
self.learned_loss = args.learned_loss
self.learned_input = None #initialized in function call
def set_target(self,target_glove_embedding):
self.target_glove_embedding = target_glove_embedding
def eval_at_state(self, model_options,frame):
model_input = ModelInput()
# if self.episode.current_frame is None:
# model_input.state = self.state()
# else:
# model_input.state = self.episode.current_frame
#process_frame to shape [1,3,224,224], for input to resnet18
processed_frame = self.preprocess_frame(resnet_input_transform(frame, 224).unsqueeze(0))
resnet18_features = self.resnet18(processed_frame)
model_input.state = resnet18_features
model_input.hidden = self.hidden
model_input.target_class_embedding = gpuify(torch.Tensor(self.target_glove_embedding),gpu_id=self.gpu_id)
model_input.action_probs = self.last_action_probs
return model_input, self.model.forward(model_input, model_options)
def reset_hidden(self):
if self.gpu_id >= 0:
with torch.cuda.device(self.gpu_id):
self.hidden = (
torch.zeros(1, self.hidden_state_sz).cuda(),
torch.zeros(1, self.hidden_state_sz).cuda(),
)
else:
self.hidden = (
torch.zeros(1, self.hidden_state_sz),
torch.zeros(1, self.hidden_state_sz),
)
self.last_action_probs = gpuify(
torch.zeros((1, self.action_space)), self.gpu_id
)
def action(self, model_options, frame,training=False):
if training:
self.model.train() #torch.nn
else:
self.model.eval()
model_input, out = self.eval_at_state(model_options,frame)
self.hidden = out.hidden
prob = F.softmax(out.logit, dim=1)
#print(prob)
action = prob.multinomial(1).data
#log_prob = F.log_softmax(out.logit, dim=1)
self.last_action_probs = prob
if self.learned_loss:
res = torch.cat((self.hidden[0], self.last_action_probs), dim=1)
#if DEBUG: print("agent/action learned loss", res.size())
if self.learned_input is None:
self.learned_input = res
else:
self.learned_input = torch.cat((self.learned_input, res), dim=0)
return out.value, prob, action
def preprocess_frame(self, frame):
""" Preprocess the current frame for input into the model. """
state = torch.Tensor(frame)
return gpuify(state, self.gpu_id)
def init_resnet18(self):
resnet18 = models.resnet18(pretrained=True)
modules = list(resnet18.children())[:-2]
self.resnet18 = nn.Sequential(*modules)
for p in self.resnet18.parameters():
p.requires_grad = False
def load_glove_embedding(glove_file):
glove_embedding_dict = {}
with h5py.File(glove_file, "r") as f:
for key in f.keys():
glove_embedding_dict[key] = f[key].value
return glove_embedding_dict
def load_model(model_path,args):
if args.model=='NON_ADAPTIVE_A3C':
model = BaseModel(args)
elif args.model == 'GCN':
model = GCN(args)
else:
model = SAVN(args)
saved_state = torch.load(
model_path, map_location=lambda storage, loc: storage
)
model.load_state_dict(saved_state)
model_options = ModelOptions()
params_list = [get_params(model, args.gpu_id)]
model_options.params = params_list[-1]
return model, model_options
def init_agent(args, model):
agent = Agent(args, model)
agent.reset_hidden()
agent.init_resnet18()
return agent
def find_target(args,agent, controller, model_options, target, glove_embedding_dict, action_list, max_step=10):
agent.set_target(glove_embedding_dict[target])
event = controller.step(action='Initialize')
action = None
for i in range(max_step):
frame = event.frame
_,_, action = agent.action(model_options, frame)
print(i, action_list[action[0,0]])
if action[0,0] == 5:
print("Agent stopped after move ", i)
break
event = controller.step(action=action_list[action[0,0]])
#print(event.metadata['lastActionSuccess'])
#use gradient from interaction loss
if args.learned_loss:
if i % args.num_steps == 5 and i/args.num_steps < 4:
learned_loss = compute_learned_loss(args, agent, args.gpu_id, model_options)
inner_gradient = torch.autograd.grad(
learned_loss["learned_loss"],
[v for _, v in model_options.params.items()],
create_graph=True,
retain_graph=True,
allow_unused=True,
)
print("gradient update")
model_options.params = SGD_step(model_options.params, inner_gradient, args.inner_lr)
time.sleep(1)
return event.frame
###Output
_____no_output_____
###Markdown
Initialize the ai2thor controller. If you are on a local machine, a display may pop up. (If running in Docker container, the display may not show up)
###Code
from ai2thor.controller import Controller
# Kitchens: FloorPlan1 - FloorPlan30
# Living rooms: FloorPlan201 - FloorPlan230
# Bedrooms: FloorPlan301 - FloorPlan330
# Bathrooms: FloorPLan401 - FloorPlan430
# KITCHEN_OBJECT_CLASS_LIST = [
# "Toaster",
# "Microwave",
# "Fridge",
# "CoffeeMaker",
# "GarbageCan",
# "Box",
# "Bowl",
# ]
# LIVING_ROOM_OBJECT_CLASS_LIST = [
# "Pillow",
# "Laptop",
# "Television",
# "GarbageCan",
# "Box",
# "Bowl",
# ]
# BEDROOM_OBJECT_CLASS_LIST = ["HousePlant", "Lamp", "Book", "AlarmClock"]
# BATHROOM_OBJECT_CLASS_LIST = ["Sink", "ToiletPaper", "SoapBottle", "LightSwitch"]
controller = Controller(scene='FloorPlan1', gridSize=0.25)
#
###Output
_____no_output_____
###Markdown
Specify the target object, model name, and maximum number of steps the agent can attempt.
###Code
TARGET = 'Toaster'
MODEL = 'GCN' #available 'SAVN', GCN', 'NON_ADAPTIVE_A3C'
MAX_STEP = 50
args = FakeArgs(model=MODEL,inner_lr=0.0001)
glove_embedding_dict = load_glove_embedding(args.glove_file)
model, model_options = load_model(MODEL_PATH_DICT[args.model],args)
agent = init_agent(args, model)
final_frame = find_target(args, agent, controller, model_options,
target=TARGET, glove_embedding_dict = glove_embedding_dict,
action_list = ACTION_LIST,max_step=MAX_STEP)
controller.stop()
glove_embedding_dict.keys()
###Output
_____no_output_____ |
doc/handbook/BoundaryAndInitialData/BoundaryAndInitialData.ipynb | ###Markdown
Boundary and Initial data
###Code
#r "BoSSSpad.dll"
using System;
using System.Collections.Generic;
using System.Linq;
using ilPSP;
using ilPSP.Utils;
using BoSSS.Platform;
using BoSSS.Foundation;
using BoSSS.Foundation.Grid;
using BoSSS.Foundation.Grid.Classic;
using BoSSS.Foundation.IO;
using BoSSS.Solution;
using BoSSS.Solution.Control;
using BoSSS.Solution.GridImport;
using BoSSS.Solution.Statistic;
using BoSSS.Solution.Utils;
using BoSSS.Solution.Gnuplot;
using BoSSS.Application.BoSSSpad;
using BoSSS.Application.XNSE_Solver;
using static BoSSS.Application.BoSSSpad.BoSSSshell;
Init();
###Output
_____no_output_____
###Markdown
This tutorial demostrates the **definition**, resp. the **import** of data for **boundary and initial values**. In order to demonstrate the usage, we employ the exemplaric **Poisson solver**.
###Code
using BoSSS.Application.SipPoisson;
###Output
_____no_output_____
###Markdown
We use a **temporary database** for this tutorial:
###Code
var tempDb = CreateTempDatabase();
###Output
Creating database 'C:\Users\jenkinsci\AppData\Local\Temp\4\1584059917'.
###Markdown
We use the following helper function to create a **template for the multiple solver runs**.
###Code
Func<SipControl> PreDefinedControl = delegate() {
SipControl c = new SipControl();
c.SetDGdegree(2);
c.GridFunc = delegate() {
// define a grid of 10x10 cells
double[] nodes = GenericBlas.Linspace(-1, 1, 11);
var grd = Grid2D.Cartesian2DGrid(nodes, nodes);
// set the entire boundary to Dirichlet b.c.
grd.DefineEdgeTags(delegate (double[] X) {
return BoundaryType.Dirichlet.ToString();
});
return grd;
};
c.SetDatabase(tempDb);
c.savetodb = true;
return c;
};
###Output
_____no_output_____
###Markdown
Again, we are using the **workflow management**
###Code
BoSSSshell.WorkflowMgm.Init("Demo_BoundaryAndInitialData");
###Output
Project name is set to 'Demo_BoundaryAndInitialData'.
###Markdown
Textual and Embedded formulas
###Code
SipControl c1 = PreDefinedControl();
###Output
_____no_output_____
###Markdown
Provide **initial data** as a text:
###Code
c1.AddInitialValue("RHS","X => Math.Sin(X[0])*Math.Cos(X[1])",
TimeDependent:false);
###Output
_____no_output_____
###Markdown
Finally, all initial data is stored in the ***AppControl.InitialVa*** dictionary and all boundary data is stored in the ***AppControl.BoundaryValues*** dictionary. The common interface for all varinats to specify boundaryand initial data is ***IBoundaryAndInitialData***.The snippet above is only a shortcut to add a ***Formula*** object,which implements the ***IBoundaryAndInitialData*** interface.
###Code
c1.InitialValues
c1.InitialValues["RHS"]
###Output
_____no_output_____
###Markdown
In **BoSSSpad**, such objects can also be extracted from static methods of classes; note that these should not depend on any otherobject in the worksheet.
###Code
Formula BndyFormula = new Formula(
"BndyValue.BndyFunction",
false,
"static class BndyValue {"+
" public static double BndyFunction(double[] X) {"+
" return 1.0;"+
" }"+
"}");
c1.AddBoundaryValue(BoundaryType.Dirichlet.ToString(),
"T",
BndyFormula);
###Output
_____no_output_____
###Markdown
Creates a Job named **J1** and runs it
###Code
var J1 = c1.RunBatch();
###Output
Control object contains grid function. Trying to Serialize the grid...
Grid Edge Tags changed.
Control object modified.
Deploying job UnnamedJob_1 ...
Deploying executables and additional files ...
Deployment directory: C:\BoSSStests\Demo_BoundaryAndInitialData-ipPoisson2021Oct20_133955
copied 42 files.
written file: control.obj
deployment finished.
###Markdown
The next line prints the Status of the Job **J1**.
###Code
BoSSSshell.WorkflowMgm.BlockUntilAllJobsTerminate(3600*4);
###Output
All jobs finished.
###Markdown
We can print the Status of the Job **J1**.
###Code
J1.Status
###Output
_____no_output_____
###Markdown
We can also check via a method if the Job **J1** is truly finished
###Code
NUnit.Framework.Assert.IsTrue(J1.Status == JobStatus.FinishedSuccessful);
###Output
_____no_output_____
###Markdown
1D Splines**Splines** can be used to interpolate nodal data onto a DG field;currently, only 1D is supported.
###Code
SipControl c2 = PreDefinedControl();
// create test data for the spline
double[] xNodes = GenericBlas.Linspace(-2,2,13);
double[] yNodes = xNodes.Select(x => x*0.4).ToArray();
var rhsSpline = new Spline1D(xNodes, yNodes,
0,
Spline1D.OutOfBoundsBehave.Extrapolate);
/// BoSSScmdSilent
double err = 0;
// test the spline: a line must be interpolated exactly.
foreach(double xtst in GenericBlas.Linspace(-3,3,77)) {
double sVal = rhsSpline.Evaluate(new double[] {xtst , 0, 0 }, 0.0);
double rVal = xtst*0.4;
err += Math.Abs(sVal - rVal);
}
NUnit.Framework.Assert.Less(err, 1.0e-10, "Slpine implementation fail.");
c2.AddInitialValue("RHS", rhsSpline);
var J2 = c2.RunBatch();
BoSSSshell.WorkflowMgm.BlockUntilAllJobsTerminate(3600*4);
J2.Status
/// BoSSScmdSilent
NUnit.Framework.Assert.IsTrue(J2.Status == JobStatus.FinishedSuccessful);
###Output
_____no_output_____
###Markdown
Interpolating values from other CalculationsFor demonstrational purposes, we use the result (i.e. the last time-step) of a previous calculation as a right-hand-side for the next calculation.
###Code
var j2Sess = J2.LatestSession;
j2Sess
j2Sess.Timesteps
var lastTimeStep = j2Sess.Timesteps.Last();
###Output
_____no_output_____
###Markdown
We encapsulate the value **T** in the **ForeignGridValue** object,which allows interpolation between different meshes:
###Code
var newForeignMesh = new ForeignGridValue(lastTimeStep,"T");
/// Use different mesh in the control file:
SipControl c3 = PreDefinedControl();
c3.GridFunc = delegate() {
// define a grid of *triangle* cells
double[] nodes = GenericBlas.Linspace(-1, 1, 11);
var grd = Grid2D.UnstructuredTriangleGrid(nodes, nodes);
// set the entire boundary to Dirichlet b.c.
grd.DefineEdgeTags(delegate (double[] X) {
return BoundaryType.Dirichlet.ToString();
});
return grd;
};
// we also save the RHS in the database
c3.AddFieldOption("RHS", SaveOpt: FieldOpts.SaveToDBOpt.TRUE);
/// finally, we define the RHS:
c3.AddInitialValue("RHS", newForeignMesh);
/// BoSSScmdSilent
double orgProbe = newForeignMesh.Evaluate(new double[] {0.5,0.5}, 0.0);
double newProbe = lastTimeStep.GetField("T").ProbeAt(new double[] {0.5,0.5});
NUnit.Framework.Assert.Less(Math.Abs(orgProbe - newProbe), 1.0e-10, "Check (1) on ForeignGridValue failed");
var J3 = c3.RunBatch();
BoSSSshell.WorkflowMgm.BlockUntilAllJobsTerminate(3600*4);
J3.Status
/// BoSSScmdSilent
NUnit.Framework.Assert.IsTrue(J3.Status == JobStatus.FinishedSuccessful);
###Output
_____no_output_____
###Markdown
Since the quadrilateral mesh used for the originalright-hand-side is geometrically embedded in the triangular mesh the **interpolation error should be zero** (up to machine precision).
###Code
var firstTimeStep = J3.LatestSession.Timesteps.First();
DGField RhsOnTriangles = firstTimeStep.GetField("rhs"); // case-insensitive!
DGField RhsOriginal = lastTimeStep.GetField("T");
// note: we have to cast DGField to ConventionalDGField in order to use
// the 'L2Distance' function:
((ConventionalDGField)RhsOnTriangles).L2Distance((ConventionalDGField)RhsOriginal)
/// BoSSScmdSilent
var H1err = ((ConventionalDGField)RhsOnTriangles).H1Distance((ConventionalDGField)RhsOriginal);
NUnit.Framework.Assert.Less(H1err, 1.0e-10, "Check (2) on ForeignGridValue failed.");
###Output
_____no_output_____
###Markdown
Restart from Dummy-SessionsDummy sessions are kind of fake siolver runs, with the only purpose of using them for a restart.
###Code
DGField RHSforRestart = firstTimeStep.GetField("RHS");
###Output
_____no_output_____
###Markdown
We save the DG field ***RHSforRestart*** in the database;This automatically creates a timestep and a session which host the DG field:
###Code
var RestartTimestep = tempDb.SaveTimestep(RHSforRestart);
RestartTimestep
RestartTimestep.Session
###Output
_____no_output_____
###Markdown
This time step can be used as a restart value.:
###Code
var c4 = PreDefinedControl();
c4.InitialValues.Clear();
c4.SetRestart(RestartTimestep);
var J4 = c4.RunBatch();
BoSSSshell.WorkflowMgm.BlockUntilAllJobsTerminate(3600*4);
J4.Status
/// BoSSScmdSilent
NUnit.Framework.Assert.IsTrue(J4.Status == JobStatus.FinishedSuccessful);
###Output
_____no_output_____ |
examples/5_QAOAUtilities.ipynb | ###Markdown
Utility functions for QAOAThis section walks through some of the key features provided in EntropicaQAOA, all of which are contained in the `utilities.py` file. In particular, it illustrates the integration of functionalities from common graph and data analysis packages such as NetworkX and Pandas. We also provide two examples that bring together the functionalities to solve real problems. Contents1. [Methods for creating and converting Hamiltonians and graphs](hamiltonians_and_graphs) - [Hyperparameters to Hamiltonians](hyperparams_to_ham) - [Random Hamiltonians](random_hamiltonian) - [Hamiltonians to Graphs](graph_from_hamiltonian) - [Graphs to Hamiltonians](hamiltonian_from_graph) - [Hyperparameters to Graphs](graph_from_hyperparams) - [Random, regular Graphs](random_k_regular_graph)2. [Methods to create Hamiltonians from input datasets](hamiltonians_and_data) - [Random cluster generation and distance datasets](gaussian_2Dclusters) - [Distance datasets to Hamiltonians](hamiltonian_from_distance_matrix)3. [Some more miscellanous utilities](miscellaneous) - [Use different initial states for QAOA](prepare_classical_state) - [Get the bitstring corresponding to the maximum probability state](max_probability_bitstring) - [Accuracy scores for QAOA](cluster_accuracy) - [Nice plots of probabilites and energies](plot_probabilities)4. [Example 1: Using QAOA to solve the Maxut problem for clustering](qaoa_clustering)5. [Example 2: The Ring of Disagrees](ring_of_disagrees)6. [References](references)
###Code
# The usual combination of imports from numpy, scipy and matplotlib
from scipy.optimize import minimize
import numpy as np
import matplotlib.pyplot as plt
# import QAOA Parameter classes
from entropica_qaoa.qaoa.parameters import ExtendedParams, StandardParams
# Cost functions and all the utilities
from entropica_qaoa.qaoa.cost_function import QAOACostFunctionOnWFSim, QAOACostFunctionOnQVM
from entropica_qaoa.utilities import *
# Pyquil import
from pyquil.api import get_qc
# Matplotlib raises errors about NetworkX using outdated methods. Nothing we can change, so we suppress the messages.
import warnings
warnings.filterwarnings('ignore')
###Output
_____no_output_____
###Markdown
Hamiltonians and graphsIn QAOA, a problem instance is defined by its corresponding *hyperparameters*, which refers to a specification of the total number of qubits `nqubits`, and one or both of the following:1. The single qubits that have a bias term (denoted `singles`) and the corresponding bias coefficients (denoted `biases`).2. The pairs of qubits that are coupled (denoted `pairs`), and the corresponding coupling coefficients (denoted `couplings`).Equivalently, when viewed as a network graph problem, a QAOA instance is defined by specifying the total number of vertices or nodes in the graph, and one or both of the following: 1. The vertices that have a bias term, and the corresponding bias coefficients.2. The pairs of vertices that are connected by an edge, and the corresponding edge weight.The following sections explain how EntropicaQAOA's utility functions allow for the simple creation of, and conversion between, Hamiltonians and graphs. Hyperparameters to HamiltonianIf we have a known set of problem hyperparameters, the `hamiltonian_from_hyperparams()` method allows us to easily create the corresponding Hamiltonian.
###Code
# Specify some hyperparameters
nqubits = 3
singles = [1]
biases = [0.3]
pairs = [[0,1], [1,2]]
couplings = [0.4, 0.6]
# Create the Hamiltonian
h0 = hamiltonian_from_hyperparams(nqubits,singles,biases,pairs,couplings)
print(h0)
###Output
(0.4+0j)*Z0*Z1 + (0.6+0j)*Z1*Z2 + (0.3+0j)*Z1
###Markdown
Random HamiltonianThe `.random_hamiltonian()` method allows us to generate a random Hamiltonian (problem instance) for a specified number of qubits. It randomly selects a number of biases and number of couplings, then assigns each of them a random value between zero and one. For instance, let's create two 4-qubit Hamiltonians.
###Code
h1 = random_hamiltonian(range(4))
h2 = random_hamiltonian(range(4))
print("h1 =",h1)
print()
print("h2 =",h2)
###Output
h1 = (0.22426675667765372+0j)*Z3 + (0.1501986539217275+0j)*Z0*Z1 + (0.9707501010061365+0j)*Z2*Z3
h2 = (0.33295405269057887+0j)*Z0 + (0.9418311774355997+0j)*Z3 + (0.24919377260659348+0j)*Z0*Z2 + (0.298367175101687+0j)*Z0*Z3 + (0.2604542179761158+0j)*Z1*Z3
###Markdown
Hamiltonians to GraphsWe can create a `NetworkX` graph corresponding to the qubit couplings in `h1` using the `graph_from_hamiltonian` method and then plot it using `plot_graph()`:
###Code
g1 = graph_from_hamiltonian(h1)
plot_graph(g1)
###Output
_____no_output_____
###Markdown
Graphs to HamiltoniansAlternatively, we can work backwards, creating a graph first, then the corresponding Hamiltonian using the `hamiltonian_from_graph()` method. Let's take the graph we have just produced (`g1`) and convert it back to its corresponding Hamiltonian, which we called `h1` above.
###Code
H1 = hamiltonian_from_graph(g1)
print('From graph:', H1)
print('')
print('Original:', h1)
###Output
From graph: (0.22426675667765372+0j)*Z3 + (0.9707501010061365+0j)*Z3*Z2 + (0.1501986539217275+0j)*Z0*Z1
Original: (0.22426675667765372+0j)*Z3 + (0.1501986539217275+0j)*Z0*Z1 + (0.9707501010061365+0j)*Z2*Z3
###Markdown
Hyperparameters to GraphsWe can also create a graph directly from hyperparameters, using the `graph_from_hyperparams()` method. Here we use the Hamiltonian created [above](hyperparams_to_ham).
###Code
g0 = graph_from_hyperparams(nqubits, singles, biases, pairs, couplings)
plot_graph(g0)
###Output
_____no_output_____
###Markdown
Random, regular GraphsIn recent research on QAOA, there has been interest in the performance of the algorithm on $k$-regular graphs, i.e. graphs where every node is connected to exactly $k$ other nodes. We can generate such graphs easily using the `random_k_regular_graph()` function. For instance, let's create a 3-regular graph with 8 nodes:
###Code
G_3_reg = random_k_regular_graph(3, range(8), weighted=True)
plot_graph(G_3_reg)
###Output
_____no_output_____
###Markdown
Hamiltonians and dataOne prominent application of QAOA is to solve the weighted MaxCut problem, which may be used as a clustering technique - see, for example, [Ref 1](references). Here, the pairwise distance between a given pair of data points in a dataset is used as the weight on the corresponding graph, and enters the Hamiltonian as the corresponding coupling coefficient between the corresponding qubits.In the following, we demo some steps of a workflow to use QAOA to solve such a MaxCut problem for clustering. We use simple toy data generated by the `gaussian_2Dclusters()` function. Cluster generation and distance calculations Let's create a data set of two clusters, where the points in each cluster follow Gaussian statistics.
###Code
n_clusters = 2 # Number of clusters we want
n_points = [3,3] # Number of data points in each cluster
means = [[0,0], [2,2]] # Cluster means (the [x,y] coordinates of each cluster centre)
# Covariance matrix: we will use the same one for each of the two clusters here,
# but more generally they could be different
cov_matrix = [[0.1, 0], [0, 0.1]]
cov_matrices = [cov_matrix,cov_matrix]
cluster_data = gaussian_2Dclusters(n_clusters,n_points,means,cov_matrices)
plot_cluster_data(cluster_data)
###Output
_____no_output_____
###Markdown
The next step in setting up the MaxCut problem is to compute the pairwise distances of the points in the dataset, which we can do using the `distances_dataset()` function. Here we will use the Euclidean distance, but more generally we can ask for any distance metric included in Scipy's [cdist](https://docs.scipy.org/doc/scipy/reference/generated/scipy.spatial.distance.cdist.html) function.
###Code
dists = distances_dataset(cluster_data, metric='euclidean')
dists
###Output
_____no_output_____
###Markdown
Note that `distances_dataset()` can also take and return data in the Pandas dataframe format. Distance datasets to HamiltoniansNow that we have the distances between all points in the dataset, we want to generate the corresponding MaxCut Hamiltonian. We can do this easily with the `hamiltonian_from_distances()` method.
###Code
hData = hamiltonian_from_distances(dists)
print(hData)
###Output
(0.8920869530576089+0j)*Z0*Z1 + (0.6213793918993751+0j)*Z0*Z2 + (2.6458987711536555+0j)*Z0*Z3 + (3.5713519713338515+0j)*Z0*Z4 + (3.244710235054523+0j)*Z0*Z5 + (1.4728630670136837+0j)*Z1*Z2 + (1.938791326007791+0j)*Z1*Z3 + (2.784531176657113+0j)*Z1*Z4 + (2.4655850590944866+0j)*Z1*Z5 + (3.019437590479767+0j)*Z2*Z3 + (3.987331765143783+0j)*Z2*Z4 + (3.6594755517875734+0j)*Z2*Z5 + (0.9922590043101441+0j)*Z3*Z4 + (0.6766019064351919+0j)*Z3*Z5 + (0.3280598229772459+0j)*Z4*Z5
###Markdown
For simplicity here we have omitted terms proportional to the identity matrix, which are commonly included in the definition of the MaxCut cost function. Since such terms only introduce a global energy shift, they do not affect the optimal configuration that we find as a solution. Example 1: Using QAOA to solve MaxCut for the clustering problemNow that we have the Hamiltonian, we can go ahead and run the QAOA to check that the points are clustered correctly. We will use the `ExtendedParams` class, and three timesteps (p=3). We don't include any single-qubit bias terms.
###Code
n_qubits = 6
p = 3
# Specify some angles
betas = np.random.rand(n_qubits,p)
gammas_singles = []
gammas_pairs = np.random.rand(len(hData),p)
parameters = (betas,gammas_singles,gammas_pairs)
extended_params = ExtendedParams([hData,p],parameters)
# NOTE - the optimiser will reach its maximum number of iterations, but for the parameters being used here,
# the choice maxiter=200 seems to be more than sufficient to get to the optimum with high probability.
cost_function = QAOACostFunctionOnWFSim(hData,
params=extended_params,
scalar_cost_function=False)
res = minimize(cost_function, extended_params.raw(),
tol=1e-3, method="Cobyla", options={"maxiter": 200})
res
###Output
_____no_output_____
###Markdown
Let us plot the probabilities of the different bitstrings. Since the energies are invariant under a bit flip on all qubits, each bitstring and its complement have identical outcome probabilities.
###Code
opt_wfn = cost_function.get_wavefunction(res.x)
probs = opt_wfn.probabilities()
plt.bar(range(len(probs)), probs)
plt.show()
###Output
_____no_output_____
###Markdown
Now we want to find the string corresponding to the optimal solution. Numpy's `argmax` function will return the first of the two degenerate solutions. As expected, we find that the first three qubits are in one class, and the second three qubits in another (this is the way the data was constructed above, in two distinct clusters).
###Code
optimal_string = np.argmax(probs)
"{0:06b}".format(optimal_string)
###Output
_____no_output_____
###Markdown
We can check that the other optimal solution found is the complement bitstring, i.e. 111000:
###Code
probs[optimal_string] = 0 # Sets the solution 000111 to have zero probability
optimal_string_complement = np.argmax(probs)
"{0:06b}".format(optimal_string_complement)
###Output
_____no_output_____
###Markdown
Example 2: The Ring of Disagrees The _Ring of Diasgrees_ is a 2-regular graph on a given number of nodes $n$. Its simple structure has allowed a number of extremely useful benchmarking results for QAOA to be derived. The ground state has energy $-n$ for even $n$, and $-n+1$ for odd $n$, and neighbouring nodes have opposite values (i.e. if a given node has value 1, its neighbour has value 0). In the paper that originally introduced the QAOA ([Ref 2](references)), it was shown numerically that this graph provides a simple example of how the approximation ratio returned by QAOA can be made arbitrarily close to 1 by increasing the parameter $p$. For the MaxCut problem, the optimal cost function value returned for a given $n$ and $p$ was found to be $$C(n,p) = \left(\frac{2p + 1}{2p + 2}\right)n$$This result assumes the `StandardParams` parameterisation, and that the graph is unweighted (all edge weights equal to 1). Here we verify this result using the `ring_of_disagrees()` function. Note that subsequent to [Ref 2](references), this result has been derived using analytic methods in [Ref 3](references).
###Code
n_nodes = 8
h_disagrees = ring_of_disagrees(n_nodes)
g_disagrees = graph_from_hamiltonian(h_disagrees)
plot_graph(g_disagrees)
def optimise_ring_of_disagrees(pval):
# Initialise angles
betas = np.random.rand(pval)
gammas = np.random.rand(pval)
parameters = (betas, gammas)
# Set up (hyper)parameters
disagrees_params = StandardParams([h_disagrees,pval],parameters)
# Cost function and optimisation
cost_function = QAOACostFunctionOnWFSim(h_disagrees, params=disagrees_params)
res = minimize(cost_function, disagrees_params.raw(),
tol=1e-3, method="BFGS", options={"maxiter": 500})
return res.fun, res.x
p_vals = np.arange(1,5) # p range to consider
output_val = np.zeros((len(p_vals),))
for i in p_vals:
output_val[i-1] = optimise_ring_of_disagrees(i)[0]
###Output
_____no_output_____
###Markdown
Since we have 8 qubits, according to Farhi's formula we should find the maximum energy to be $-8 \cdot (3/4,5/6,7/8,9/10) = -(6, 6.67, 7, 7.2)$ for $p = (1,2,3,4)$:
###Code
output_val
###Output
_____no_output_____
###Markdown
For the case $p=1$, the optimal angles can be computed analytically, and are given by $(\beta_{opt}, \gamma_{opt}) = (\pi/8, \pi/4$) - see [Ref 4](references). We can see that the optimiser does indeed return these angles:
###Code
opt_angles = optimise_ring_of_disagrees(1)[1]
opt_angles
###Output
_____no_output_____
###Markdown
Let's finish off by running an example of the Ring of Disagrees on the QVM; we would follow a similar method to run the computation on the QPU. We'll use the optimal angles we have just found to check that the probability distribution of samples we obtain does indeed return the bitstring [0,1,0,1], or its complement [1,0,1,0], with high probability. Here, we make use of the `sample_qaoa_bitstrings` function, which executes the circuit defined by the QAOA instance and samples from output multiple times. We can plot the output conveniently using `bitstring_histogram`.
###Code
qvm = get_qc("4q-qvm")
ham_disagrees_4 = ring_of_disagrees(4)
params_disagrees_4 = StandardParams([ham_disagrees_4,1], opt_angles)
bitstrings = sample_qaoa_bitstrings(params_disagrees_4, qvm)
bitstring_histogram(bitstrings)
###Output
_____no_output_____
###Markdown
More miscellaneous utilitiesHere we demonstrate the functionality of some additional methods that may be useful in certain contexts. Different initial states for QAOAWe can easily use an initial state different from $\left|+ \cdots +\right>$ for QAOA, by passing a state preparation program for the `initial_state` argument of the QAOA cost functions. For purely classical states (i.e. not a quantum superposition state) such as $\left|10 \cdots 10\right>$, these programs cane be created via `prepare_classical_state`.
###Code
register = [0, 1, 2, 3, 4, 5] # the register to create the state on
state = [1, 0, 1, 0, 1, 0] # the |42> state (encodes the decimal number 42)
prepare42_circuit = prepare_classical_state(register, state)
print(prepare42_circuit)
###Output
X 0
X 2
X 4
###Markdown
Get the bitstring corresponding to the maximum probability stateThe `max_probability_bitstring()` method returns the bitstring corresponding to the maximum probability state of a wavefunction.
###Code
probs = np.exp(-np.linspace(-5, 10, 16)**2) # just an array of length 16 (corresponds to a 4-qubit system)
probs = probs/probs.sum() # normalise to represent a proper probability distribution
max_prob_state = max_probability_bitstring(probs)
print(max_prob_state)
###Output
[0, 1, 0, 1]
###Markdown
Accuracy scores for QAOA `cluster_accuracy()` gives accuary scores for a QAOA result, if the true solution is known. The accuracy here is defined as the percentage of bits that are correct compared to the known solution.
###Code
cluster_accuracy(max_prob_state, true_labels=[1, 1, 0, 0])
###Output
True Labels of samples: [1, 1, 0, 0]
Lowest QAOA State: [0, 1, 0, 1]
Accuracy of Original State: 50.0 %
Accuracy of Complement State: 50.0 %
###Markdown
Get nice plots of probabiltiesIf the true energies of all states are known, we can also obtain a nice side-by-side plot of the energies and probabilites using `plot_probabilities()`.
###Code
energies = np.sin(np.linspace(0, 10, 16))
fig, ax = plt.subplots(figsize=(10,5))
plot_probabilities(probs, energies, ax=ax)
###Output
_____no_output_____
###Markdown
Utility functions for QAOAThis section walks through some of the key features provided in EntropicaQAOA, all of which are contained in the `utilities.py` file. In particular, it illustrates the integration of functionalities from common graph and data analysis packages such as NetworkX and Pandas. We also provide two examples that bring together the functionalities to solve real problems. Contents1. [Methods for creating and converting Hamiltonians and graphs](hamiltonians_and_graphs) - [Hyperparameters to Hamiltonians](hyperparams_to_ham) - [Random Hamiltonians](random_hamiltonian) - [Hamiltonians to Graphs](graph_from_hamiltonian) - [Graphs to Hamiltonians](hamiltonian_from_graph) - [Hyperparameters to Graphs](graph_from_hyperparams) - [Random, regular Graphs](random_k_regular_graph)2. [Methods to create Hamiltonians from input datasets](hamiltonians_and_data) - [Random cluster generation and distance datasets](gaussian_2Dclusters) - [Distance datasets to Hamiltonians](hamiltonian_from_distance_matrix)3. [Some more miscellanous utilities](miscellaneous) - [Use different initial states for QAOA](prepare_classical_state) - [Get the bitstring corresponding to the maximum probability state](max_probability_bitstring) - [Accuracy scores for QAOA](cluster_accuracy) - [Nice plots of probabilites and energies](plot_probabilities)4. [Example 1: Using QAOA to solve the Maxut problem for clustering](qaoa_clustering)5. [Example 2: The Ring of Disagrees](ring_of_disagrees)6. [References](references)
###Code
# The usual combination of imports from numpy, scipy and matplotlib
from scipy.optimize import minimize
import numpy as np
import matplotlib.pyplot as plt
# import QAOA Parameter classes
from entropica_qaoa.qaoa.parameters import ExtendedParams, StandardParams
# Cost functions and all the utilities
from entropica_qaoa.qaoa.cost_function import QAOACostFunctionOnWFSim, QAOACostFunctionOnQVM
from entropica_qaoa.utilities import *
# Pyquil import
from pyquil.api import get_qc
# Matplotlib raises errors about NetworkX using outdated methods. Nothing we can change, so we suppress the messages.
import warnings
warnings.filterwarnings('ignore')
###Output
_____no_output_____
###Markdown
Hamiltonians and graphsIn QAOA, a problem instance is defined by its corresponding *hyperparameters*, which refers to a specification of the total number of qubits `nqubits`, and one or both of the following:1. The single qubits that have a bias term (denoted `singles`) and the corresponding bias coefficients (denoted `biases`).2. The pairs of qubits that are coupled (denoted `pairs`), and the corresponding coupling coefficients (denoted `couplings`).Equivalently, when viewed as a network graph problem, a QAOA instance is defined by specifying the total number of vertices or nodes in the graph, and one or both of the following: 1. The vertices that have a bias term, and the corresponding bias coefficients.2. The pairs of vertices that are connected by an edge, and the corresponding edge weight.The following sections explain how EntropicaQAOA's utility functions allow for the simple creation of, and conversion between, Hamiltonians and graphs. Hyperparameters to HamiltonianIf we have a known set of problem hyperparameters, the `hamiltonian_from_hyperparams()` method allows us to easily create the corresponding Hamiltonian.
###Code
# Specify some hyperparameters
nqubits = 3
singles = [1]
biases = [0.3]
pairs = [[0,1], [1,2]]
couplings = [0.4, 0.6]
# Create the Hamiltonian
h0 = hamiltonian_from_hyperparams(nqubits,singles,biases,pairs,couplings)
print(h0)
###Output
(0.4+0j)*Z0*Z1 + (0.6+0j)*Z1*Z2 + (0.3+0j)*Z1
###Markdown
Random HamiltonianThe `.random_hamiltonian()` method allows us to generate a random Hamiltonian (problem instance) for a specified number of qubits. It randomly selects a number of biases and number of couplings, then assigns each of them a random value between zero and one. For instance, let's create two 4-qubit Hamiltonians.
###Code
h1 = random_hamiltonian(range(4))
h2 = random_hamiltonian(range(4))
print("h1 =",h1)
print()
print("h2 =",h2)
###Output
h1 = (0.3444223975387848+0j)*Z0*Z1 + (0.5665300480074544+0j)*Z1*Z2
h2 = (0.22600458675338964+0j)*Z0*Z3 + (0.6716304577317461+0j)*Z1*Z2 + (0.044073757302479644+0j)*Z1*Z3 + (0.5442489227939361+0j)*Z2*Z3
###Markdown
Hamiltonians to GraphsWe can create a `NetworkX` graph corresponding to the qubit couplings in `h1` using the `graph_from_hamiltonian` method and then plot it using `plot_graph()`:
###Code
g1 = graph_from_hamiltonian(h1)
plot_graph(g1)
###Output
_____no_output_____
###Markdown
Graphs to HamiltoniansAlternatively, we can work backwards, creating a graph first, then the corresponding Hamiltonian using the `hamiltonian_from_graph()` method. Let's take the graph we have just produced (`g1`) and convert it back to its corresponding Hamiltonian, which we called `h1` above.
###Code
H1 = hamiltonian_from_graph(g1)
print('From graph:', H1)
print('')
print('Original:', h1)
###Output
From graph: (0.3444223975387848+0j)*Z0*Z1 + (0.5665300480074544+0j)*Z1*Z2
Original: (0.3444223975387848+0j)*Z0*Z1 + (0.5665300480074544+0j)*Z1*Z2
###Markdown
Hyperparameters to GraphsWe can also create a graph directly from hyperparameters, using the `graph_from_hyperparams()` method. Here we use the Hamiltonian created [above](hyperparams_to_ham).
###Code
g0 = graph_from_hyperparams(nqubits, singles, biases, pairs, couplings)
plot_graph(g0)
###Output
_____no_output_____
###Markdown
Random, regular GraphsIn recent research on QAOA, there has been interest in the performance of the algorithm on $k$-regular graphs, i.e. graphs where every node is connected to exactly $k$ other nodes. We can generate such graphs easily using the `random_k_regular_graph()` function. For instance, let's create a 3-regular graph with 8 nodes:
###Code
G_3_reg = random_k_regular_graph(3, range(8), weighted=True)
plot_graph(G_3_reg)
###Output
_____no_output_____
###Markdown
Hamiltonians and dataOne prominent application of QAOA is to solve the weighted MaxCut problem, which may be used as a clustering technique - see, for example, [Ref 1](references). Here, the pairwise distance between a given pair of data points in a dataset is used as the weight on the corresponding graph, and enters the Hamiltonian as the corresponding coupling coefficient between the corresponding qubits.In the following, we demo some steps of a workflow to use QAOA to solve such a MaxCut problem for clustering. We use simple toy data generated by the `gaussian_2Dclusters()` function. Cluster generation and distance calculations Let's create a data set of two clusters, where the points in each cluster follow Gaussian statistics.
###Code
n_clusters = 2 # Number of clusters we want
n_points = [3,3] # Number of data points in each cluster
means = [[0,0], [2,2]] # Cluster means (the [x,y] coordinates of each cluster centre)
# Covariance matrix: we will use the same one for each of the two clusters here,
# but more generally they could be different
cov_matrix = [[0.1, 0], [0, 0.1]]
cov_matrices = [cov_matrix,cov_matrix]
cluster_data = gaussian_2Dclusters(n_clusters,n_points,means,cov_matrices)
plot_cluster_data(cluster_data)
###Output
_____no_output_____
###Markdown
The next step in setting up the MaxCut problem is to compute the pairwise distances of the points in the dataset, which we can do using the `distances_dataset()` function. Here we will use the Euclidean distance, but more generally we can ask for any distance metric included in Scipy's [cdist](https://docs.scipy.org/doc/scipy/reference/generated/scipy.spatial.distance.cdist.html) function.
###Code
dists = distances_dataset(cluster_data, metric='euclidean')
dists
###Output
_____no_output_____
###Markdown
Note that `distances_dataset()` can also take and return data in the Pandas dataframe format. Distance datasets to HamiltoniansNow that we have the distances between all points in the dataset, we want to generate the corresponding MaxCut Hamiltonian. We can do this easily with the `hamiltonian_from_distances()` method.
###Code
hData = hamiltonian_from_distances(dists)
print(hData)
###Output
(0.6215198423525486+0j)*Z0*Z1 + (0.2957232451342951+0j)*Z0*Z2 + (2.4763685409019853+0j)*Z0*Z3 + (2.9314470110607784+0j)*Z0*Z4 + (2.9659054882278806+0j)*Z0*Z5 + (0.7327122400924281+0j)*Z1*Z2 + (2.4230943434886276+0j)*Z1*Z3 + (2.9000186289816763+0j)*Z1*Z4 + (2.8453353972391864+0j)*Z1*Z5 + (2.7718880270988357+0j)*Z2*Z3 + (3.227137405390538+0j)*Z2*Z4 + (3.25947297597396+0j)*Z2*Z5 + (0.4785230180612345+0j)*Z3*Z4 + (0.5438067902631225+0j)*Z3*Z5 + (0.42072433194663644+0j)*Z4*Z5
###Markdown
For simplicity here we have omitted terms proportional to the identity matrix, which are commonly included in the definition of the MaxCut cost function. Since such terms only introduce a global energy shift, they do not affect the optimal configuration that we find as a solution. Example 1: Using QAOA to solve MaxCut for the clustering problemNow that we have the Hamiltonian, we can go ahead and run the QAOA to check that the points are clustered correctly. We will use the `ExtendedParams` class, and three timesteps (p=3). We don't include any single-qubit bias terms.
###Code
n_qubits = 6
p = 3
# Specify some angles
betas = np.random.rand(n_qubits,p)
gammas_singles = []
gammas_pairs = np.random.rand(len(hData),p)
parameters = (betas,gammas_singles,gammas_pairs)
extended_params = ExtendedParams([hData,p],parameters)
# NOTE - the optimiser will reach its maximum number of iterations, but for the parameters being used here,
# the choice maxiter=200 seems to be more than sufficient to get to the optimum with high probability.
cost_function = QAOACostFunctionOnWFSim(hData,
params=extended_params,
scalar_cost_function=False)
res = minimize(cost_function, extended_params.raw(),
tol=1e-3, method="Cobyla", options={"maxiter": 200})
res
###Output
_____no_output_____
###Markdown
Let us plot the probabilities of the different bitstrings. Since the energies are invariant under a bit flip on all qubits, each bitstring and its complement have identical outcome probabilities.
###Code
opt_wfn = cost_function.get_wavefunction(res.x)
probs = opt_wfn.probabilities()
plt.bar(range(len(probs)), probs)
plt.show()
###Output
_____no_output_____
###Markdown
Now we want to find the string corresponding to the optimal solution. Numpy's `argmax` function will return the first of the two degenerate solutions. As expected, we find that the first three qubits are in one class, and the second three qubits in another (this is the way the data was constructed above, in two distinct clusters).
###Code
optimal_string = np.argmax(probs)
"{0:06b}".format(optimal_string)
###Output
_____no_output_____
###Markdown
We can check that the other optimal solution found is the complement bitstring, i.e. 111000:
###Code
probs[optimal_string] = 0 # Sets the solution 000111 to have zero probability
optimal_string_complement = np.argmax(probs)
"{0:06b}".format(optimal_string_complement)
###Output
_____no_output_____
###Markdown
Example 2: The Ring of Disagrees The _Ring of Diasgrees_ is a 2-regular graph on a given number of nodes $n$. Its simple structure has allowed a number of extremely useful benchmarking results for QAOA to be derived. The ground state has energy $-n$ for even $n$, and $-n+1$ for odd $n$, and neighbouring nodes have opposite values (i.e. if a given node has value 1, its neighbour has value 0). In the paper that originally introduced the QAOA ([Ref 2](references)), it was shown numerically that this graph provides a simple example of how the approximation ratio returned by QAOA can be made arbitrarily close to 1 by increasing the parameter $p$. For the MaxCut problem, the optimal cost function value returned for a given $n$ and $p$ was found to be $$C(n,p) = \left(\frac{2p + 1}{2p + 2}\right)n$$This result assumes the `StandardParams` parameterisation, and that the graph is unweighted (all edge weights equal to 1). Here we verify this result using the `ring_of_disagrees()` function. Note that subsequent to [Ref 2](references), this result has been derived using analytic methods in [Ref 3](references).
###Code
n_nodes = 8
h_disagrees = ring_of_disagrees(n_nodes)
g_disagrees = graph_from_hamiltonian(h_disagrees)
plot_graph(g_disagrees)
def optimise_ring_of_disagrees(pval):
# Initialise angles
betas = np.random.rand(pval)
gammas = np.random.rand(pval)
parameters = (betas, gammas)
# Set up (hyper)parameters
disagrees_params = StandardParams([h_disagrees,pval],parameters)
# Cost function and optimisation
cost_function = QAOACostFunctionOnWFSim(h_disagrees, params=disagrees_params)
res = minimize(cost_function, disagrees_params.raw(),
tol=1e-3, method="BFGS", options={"maxiter": 500})
return res.fun, res.x
p_vals = np.arange(1,5) # p range to consider
output_val = np.zeros((len(p_vals),))
for i in p_vals:
output_val[i-1] = optimise_ring_of_disagrees(i)[0]
###Output
_____no_output_____
###Markdown
Since we have 8 qubits, according to Farhi's formula we should find the maximum energy to be $-8 \cdot (3/4,5/6,7/8,9/10) = -(6, 6.67, 7, 7.2)$ for $p = (1,2,3,4)$:
###Code
output_val
###Output
_____no_output_____
###Markdown
For the case $p=1$, the optimal angles can be computed analytically, and are given by $(\beta_{opt}, \gamma_{opt}) = (\pi/8, \pi/4$) - see [Ref 4](references). We can see that the optimiser does indeed return these angles:
###Code
opt_angles = optimise_ring_of_disagrees(1)[1]
opt_angles
###Output
_____no_output_____
###Markdown
Let's finish off by running an example of the Ring of Disagrees on the QVM; we would follow a similar method to run the computation on the QPU. We'll use the optimal angles we have just found to check that the probability distribution of samples we obtain does indeed return the bitstring [0,1,0,1], or its complement [1,0,1,0], with high probability. Here, we make use of the `sample_qaoa_bitstrings` function, which executes the circuit defined by the QAOA instance and samples from output multiple times. We can plot the output conveniently using `bitstring_histogram`.
###Code
qvm = get_qc("4q-qvm")
ham_disagrees_4 = ring_of_disagrees(4)
params_disagrees_4 = StandardParams([ham_disagrees_4,1], opt_angles)
bitstrings = sample_qaoa_bitstrings(params_disagrees_4, qvm)
bitstring_histogram(bitstrings)
###Output
_____no_output_____
###Markdown
More miscellaneous utilitiesHere we demonstrate the functionality of some additional methods that may be useful in certain contexts. Different initial states for QAOAWe can easily use an initial state different from $\left|+ \cdots +\right>$ for QAOA, by passing a state preparation program for the `initial_state` argument of the QAOA cost functions. For purely classical states (i.e. not a quantum superposition state) such as $\left|10 \cdots 10\right>$, these programs cane be created via `prepare_classical_state`.
###Code
register = [0, 1, 2, 3, 4, 5] # the register to create the state on
state = [1, 0, 1, 0, 1, 0] # the |42> state (encodes the decimal number 42)
prepare42_circuit = prepare_classical_state(register, state)
print(prepare42_circuit)
###Output
X 0
X 2
X 4
###Markdown
Get the bitstring corresponding to the maximum probability stateThe `max_probability_bitstring()` method returns the bitstring corresponding to the maximum probability state of a wavefunction.
###Code
probs = np.exp(-np.linspace(-5, 10, 16)**2) # just an array of length 16 (corresponds to a 4-qubit system)
probs = probs/probs.sum() # normalise to represent a proper probability distribution
max_prob_state = max_probability_bitstring(probs)
print(max_prob_state)
###Output
[0, 1, 0, 1]
###Markdown
Accuracy scores for QAOA `cluster_accuracy()` gives accuary scores for a QAOA result, if the true solution is known. The accuracy here is defined as the percentage of bits that are correct compared to the known solution.
###Code
cluster_accuracy(max_prob_state, true_labels=[1, 1, 0, 0])
###Output
True Labels of samples: [1, 1, 0, 0]
Lowest QAOA State: [0, 1, 0, 1]
Accuracy of Original State: 50.0 %
Accuracy of Complement State: 50.0 %
###Markdown
Get nice plots of probabiltiesIf the true energies of all states are known, we can also obtain a nice side-by-side plot of the energies and probabilites using `plot_probabilities()`.
###Code
energies = np.sin(np.linspace(0, 10, 16))
fig, ax = plt.subplots(figsize=(10,5))
plot_probabilities(probs, energies, ax=ax)
###Output
_____no_output_____
###Markdown
Utility functions for QAOAThis section walks through some of the key features provided in EntropicaQAOA, all of which are contained in the `utilities.py` file. In particular, it illustrates the integration of functionalities from common graph and data analysis packages such as NetworkX and Pandas. We also provide two examples that bring together the functionalities to solve real problems. Contents1. [Methods for creating and converting Hamiltonians and graphs](hamiltonians_and_graphs) - [Hyperparameters to Hamiltonians](hyperparams_to_ham) - [Random Hamiltonians](random_hamiltonian) - [Hamiltonians to Graphs](graph_from_hamiltonian) - [Graphs to Hamiltonians](hamiltonian_from_graph) - [Hyperparameters to Graphs](graph_from_hyperparams) - [Random, regular Graphs](random_k_regular_graph)2. [Methods to create Hamiltonians from input datasets](hamiltonians_and_data) - [Random cluster generation and distance datasets](gaussian_2Dclusters) - [Distance datasets to Hamiltonians](hamiltonian_from_distance_matrix)3. [Some more miscellanous utilities](miscellaneous) - [Use different initial states for QAOA](prepare_classical_state) - [Get the bitstring corresponding to the maximum probability state](max_probability_bitstring) - [Accuracy scores for QAOA](cluster_accuracy) - [Nice plots of probabilites and energies](plot_probabilities)4. [All in action: Using QAOA to solve the Maxut problem for clustering](qaoa_clustering)5. [Another example: The ring of disagrees](ring_of_disagrees)6. [References](references)
###Code
# The usual combination of imports from numpy, scipy and matplotlib
from scipy.optimize import minimize
import numpy as np
import matplotlib.pyplot as plt
# import QAOA Parameter classes
from entropica_qaoa.qaoa.parameters import ExtendedParams, StandardParams
# Cost functions and all the utilities
from entropica_qaoa.qaoa.cost_function import QAOACostFunctionOnWFSim
from entropica_qaoa.utilities import *
# Matplotlib raises errors about NetworkX using outdated methods. Nothing we can change, so we suppress the messages.
import warnings
warnings.filterwarnings('ignore')
###Output
_____no_output_____
###Markdown
Hamiltonians and graphsIn QAOA, a problem instance is defined by its corresponding *hyperparameters*, which refers to a specification of the total number of qubits `nqubits`, and one or both of the following:1. The single qubits that have a bias term (denoted `singles`) and the corresponding bias coefficients (denoted `biases`).2. The pairs of qubits that are coupled (denoted `pairs`), and the corresponding coupling coefficients (denoted `couplings`).Equivalently, when viewed as a network graph problem, a QAOA instance is defined by specifying the total number of vertices or nodes in the graph, and one or both of the following: 1. The vertices that have a bias term, and the corresponding bias coefficients.2. The pairs of vertices that are connected by an edge, and the corresponding edge weight.The following sections explain how EntropicaQAOA's utility functions allow for the simple creation of, and conversion between, Hamiltonians and graphs. Hyperparameters to HamiltonianIf we have a known set of problem hyperparameters, the `hamiltonian_from_hyperparams()` method allows us to easily create the corresponding Hamiltonian.
###Code
# Specify some hyperparameters
nqubits = 3
singles = [1]
biases = [0.3]
pairs = [[0,1], [1,2]]
couplings = [0.4, 0.6]
# Create the Hamiltonian
h0 = hamiltonian_from_hyperparams(nqubits,singles,biases,pairs,couplings)
print(h0)
###Output
(0.4+0j)*Z0*Z1 + (0.6+0j)*Z1*Z2 + (0.3+0j)*Z1
###Markdown
Random HamiltonianThe `.random_hamiltonian()` method allows us to generate a random Hamiltonian (problem instance) for a specified number of qubits. It randomly selects a number of biases and number of couplings, then assigns each of them a random value between zero and one. For instance, let's create two 4-qubit Hamiltonians.
###Code
h1 = random_hamiltonian(range(4))
h2 = random_hamiltonian(range(4))
print("h1 =",h1)
print()
print("h2 =",h2)
###Output
h1 = (0.13843254520170012+0j)*Z0*Z2 + (0.11442349279762465+0j)*Z0*Z3 + (0.6094957253483044+0j)*Z1*Z2
h2 = (0.6617028567587064+0j)*Z3 + (0.2768760456730499+0j)*Z2 + (0.06712400939511054+0j)*Z0*Z1 + (0.45817871396579035+0j)*Z0*Z2 + (0.8381365781397312+0j)*Z1*Z2 + (0.2757354248566338+0j)*Z2*Z3
###Markdown
Hamiltonians to GraphsWe can create a `NetworkX` graph corresponding to the qubit couplings in `h1` using the `graph_from_hamiltonian` method and then plot it using `plot_graph()`:
###Code
g1 = graph_from_hamiltonian(h1)
plot_graph(g1)
###Output
_____no_output_____
###Markdown
Graphs to HamiltoniansAlternatively, we can work backwards, creating a graph first, then the corresponding Hamiltonian using the `hamiltonian_from_graph()` method. Let's take the graph we have just produced (`g1`) and convert it back to its corresponding Hamiltonian, which we called `h1` above.
###Code
H1 = hamiltonian_from_graph(g1)
print('From graph:', H1)
print('')
print('Original:', h1)
###Output
From graph: (0.13843254520170012+0j)*Z0*Z2 + (0.11442349279762465+0j)*Z0*Z3 + (0.6094957253483044+0j)*Z2*Z1
Original: (0.13843254520170012+0j)*Z0*Z2 + (0.11442349279762465+0j)*Z0*Z3 + (0.6094957253483044+0j)*Z1*Z2
###Markdown
Hyperparameters to GraphsWe can also create a graph directly from hyperparameters, using the `graph_from_hyperparams()` method. Here we use the Hamiltonian created [above](hyperparams_to_ham).
###Code
g0 = graph_from_hyperparams(nqubits, singles, biases, pairs, couplings)
plot_graph(g0)
###Output
_____no_output_____
###Markdown
Random, regular GraphsIn recent research on QAOA, there has been interest in the performance of the algorithm on $k$-regular graphs, i.e. graphs where every node is connected to exactly $k$ other nodes. We can generate such graphs easily using the `random_k_regular_graph()` function. For instance, let's create a 3-regular graph with 8 nodes:
###Code
G_3_reg = random_k_regular_graph(3, range(8), weighted=True)
plot_graph(G_3_reg)
###Output
_____no_output_____
###Markdown
Hamiltonians and dataOne prominent application of QAOA is to solve the weighted MaxCut problem, which may be used as a clustering technique - see, for example, [Ref 1](references). Here, the pairwise distance between a given pair of data points in a dataset is used as the weight on the corresponding graph, and enters the Hamiltonian as the corresponding coupling coefficient between the corresponding qubits.In the following, we demo some steps of a workflow to use QAOA to solve such a MaxCut problem for clustering. We use simple toy data generated by the `gaussian_2Dclusters()` function. Cluster generation and distance calculations Let's create a data set of two clusters, where the points in each cluster follow Gaussian statistics.
###Code
n_clusters = 2 # Number of clusters we want
n_points = [3,3] # Number of data points in each cluster
means = [[0,0], [2,2]] # Cluster means (the [x,y] coordinates of each cluster centre)
# Covariance matrix: we will use the same one for each of the two clusters here,
# but more generally they could be different
cov_matrix = [[0.1, 0], [0, 0.1]]
cov_matrices = [cov_matrix,cov_matrix]
cluster_data = gaussian_2Dclusters(n_clusters,n_points,means,cov_matrices)
plot_cluster_data(cluster_data)
###Output
_____no_output_____
###Markdown
The next step in setting up the MaxCut problem is to compute the pairwise distances of the points in the dataset, which we can do using the `distances_dataset()` function. Here we will use the Euclidean distance, but more generally we can ask for any distance metric included in Scipy's [cdist](https://docs.scipy.org/doc/scipy/reference/generated/scipy.spatial.distance.cdist.html) function.
###Code
dists = distances_dataset(cluster_data, metric='euclidean')
dists
###Output
_____no_output_____
###Markdown
Note that `distances_dataset()` can also take and return data in the Pandas dataframe format. Distance datasets to HamiltoniansNow that we have the distances between all points in the dataset, we want to generate the corresponding MaxCut Hamiltonian. We can do this easily with the `hamiltonian_from_distances()` method.
###Code
hData = hamiltonian_from_distances(dists)
print(hData)
###Output
(0.858084893781957+0j)*Z0*Z1 + (0.5333823908775777+0j)*Z0*Z2 + (2.6247855313110726+0j)*Z0*Z3 + (3.0263893988392088+0j)*Z0*Z4 + (2.7701090503087755+0j)*Z0*Z5 + (0.5512942721134212+0j)*Z1*Z2 + (3.4790579365937346+0j)*Z1*Z3 + (3.8834051215129337+0j)*Z1*Z4 + (3.6262316383445024+0j)*Z1*Z5 + (3.0891206803693088+0j)*Z2*Z3 + (3.47582319401406+0j)*Z2*Z4 + (3.181704587650603+0j)*Z2*Z5 + (0.42755383601779606+0j)*Z3*Z4 + (0.5215227654746857+0j)*Z3*Z5 + (0.4646633272264256+0j)*Z4*Z5
###Markdown
For simplicity here we have omitted terms proportional to the identity matrix, which are commonly included in the definition of the MaxCut cost function. Since such terms only introduce a global energy shift, they do not affect the optimal configuration that we find as a solution. Example 1: Using QAOA to solve MaxCut for the clustering problemNow that we have the Hamiltonian, we can go ahead and run the QAOA to check that the points are clustered correctly. We will use the `ExtendedParams` class, and three timesteps (p=3). We don't include any single-qubit bias terms.
###Code
n_qubits = 6
p = 3
# Specify some angles
betas = np.random.rand(n_qubits,p)
gammas_singles = []
gammas_pairs = np.random.rand(len(hData),p)
parameters = (betas,gammas_singles,gammas_pairs)
extended_params = ExtendedParams([hData,p],parameters)
# NOTE - the optimiser will reach its maximum number of iterations, but for the parameters being used here,
# the choice maxiter=200 seems to be more than sufficient to get to the optimum with high probability.
cost_function = QAOACostFunctionOnWFSim(hData,
params=extended_params,
scalar_cost_function=False)
res = minimize(cost_function, extended_params.raw(),
tol=1e-3, method="Cobyla", options={"maxiter": 200})
res
###Output
_____no_output_____
###Markdown
Let us plot the probabilities of the different bitstrings. Since the energies are invariant under a bit flip on all qubits, each bitstring and its complement have identical outcome probabilities.
###Code
opt_wfn = cost_function.get_wavefunction(res.x)
probs = opt_wfn.probabilities()
plt.bar(range(len(probs)), probs)
plt.show()
###Output
_____no_output_____
###Markdown
Now we want to find the string corresponding to the optimal solution. Numpy's `argmax` function will return the first of the two degenerate solutions. As expected, we find that the first three qubits are in one class, and the second three qubits in another (this is the way the data was constructed above, in two distinct clusters).
###Code
optimal_string = np.argmax(probs)
"{0:06b}".format(optimal_string)
###Output
_____no_output_____
###Markdown
We can check that the other optimal solution found is the complement bitstring, i.e. 111000:
###Code
probs[optimal_string] = 0 # Sets the solution 000111 to have zero probability
optimal_string_complement = np.argmax(probs)
"{0:06b}".format(optimal_string_complement)
###Output
_____no_output_____
###Markdown
Example 2: Ring of disagreesThe _Ring of Diasgrees_ is a 2-regular graph on a given number of nodes $n$. The ground state has energy $-n$ for even $n$, and $-n+1$ for odd $n$.In the paper that originally introduced the QAOA ([Ref 2](references)), it was shown numerically that this graph provides a simple example of how the approximation ratio returned by QAOA can be made arbitrarily close to 1 by increasing the parameter $p$. For the MaxCut problem, the optimal cost function value returned for a given $n$ and $p$ was found to be $$C(n,p) = \left(\frac{2p + 1}{2p + 2}\right)n$$This result assumes the `StandardParams` parameterisation, and that the graph is unweighted (all edge weights equal to 1). Here we verify this result using the `ring_of_disagrees()` function. Note that subsequent to [Ref 2](references), this result has been derived using analytic methods in [Ref 3](references).
###Code
n_nodes = 8
h_disagrees = ring_of_disagrees(n_nodes)
g_disagrees = graph_from_hamiltonian(h_disagrees)
plot_graph(g_disagrees)
p_vals = np.arange(1,5) # p range to consider
output_val = np.zeros((len(p_vals),))
for i in p_vals:
# Initialise angles
betas = np.random.rand(i)
gammas_singles = np.zeros((i,)) # Remove this when issue is fixed
gammas_pairs = np.random.rand(i)
parameters = (betas, gammas_singles, gammas_pairs)
# Set up (hyper)parameters
diasagrees_params = StandardParams([h_disagrees,i],parameters)
# Cost function and optimisation
cost_function = QAOACostFunctionOnWFSim(h_disagrees,
params=diasagrees_params,
scalar_cost_function=True,
nshots=0)
res = minimize(cost_function, diasagrees_params.raw(),
tol=1e-3, method="BFGS", options={"maxiter": 500})
output_val[i-1] = res.fun
###Output
_____no_output_____
###Markdown
Since we have 8 qubits, according to Farhi's formula we should find the maximum energy to be $-8 \cdot (3/4,5/6,7/8,9/10) = -(6, 6.67, 7, 7.2)$ for $p = (1,2,3,4)$:
###Code
output_val
###Output
_____no_output_____
###Markdown
The lowest energy state corresponds to the situation where neighbouring qubits have opposite orientations:
###Code
wf = cost_function.get_wavefunction(res.x)
probs = wf.probabilities()
print(max_probability_bitstring(probs))
###Output
[0, 1, 0, 1, 0, 1, 0, 1]
###Markdown
More miscellaneous utilitiesHere we demonstrate the functionality of some additional methods that may be useful in certain contexts. Different initial states for QAOAWe can easily use an initial state different from $\left|+ \cdots +\right>$ for QAOA, by passing a state preparation program for the `initial_state` argument of the QAOA cost functions. For purely classical states (i.e. not a quantum superposition state) such as $\left|10 \cdots 10\right>$, these programs cane be created via `prepare_classical_state`.
###Code
register = [0, 1, 2, 3, 4, 5] # the register to create the state on
state = [1, 0, 1, 0, 1, 0] # the |42> state (encodes the decimal number 42)
prepare42_circuit = prepare_classical_state(register, state)
print(prepare42_circuit)
###Output
X 0
X 2
X 4
###Markdown
Get the bitstring corresponding to the maximum probability stateThe `max_probability_bitstring()` method returns the bitstring corresponding to the maximum probability state of a wavefunction.
###Code
probs = np.exp(-np.linspace(-5, 10, 16)**2) # just an array of length 16 (corresponds to a 4-qubit system)
probs = probs/probs.sum() # normalise to represent a proper probability distribution
max_prob_state = max_probability_bitstring(probs)
print(max_prob_state)
###Output
[0, 1, 0, 1]
###Markdown
Accuracy scores for QAOA `cluster_accuracy()` gives accuary scores for a QAOA result, if the true solution is known. The accuracy here is defined as the percentage of bits that are correct compared to the known solution.
###Code
cluster_accuracy(max_prob_state, true_labels=[1, 1, 0, 0])
###Output
True Labels of samples: [1, 1, 0, 0]
Lowest QAOA State: [0, 1, 0, 1]
Accuracy of Original State: 50.0 %
Accuracy of Complement State: 50.0 %
###Markdown
Get nice plots of probabiltiesIf the true energies of all states are known, we can also obtain a nice side-by-side plot of the energies and probabilites using `plot_probabilities()`.
###Code
energies = np.sin(np.linspace(0, 10, 16))
fig, ax = plt.subplots(figsize=(10,5))
plot_probabilities(probs, energies, ax=ax)
###Output
_____no_output_____ |
99_pca.ipynb | ###Markdown
PCA
###Code
# example of pca for dimensionality reduction
from sklearn.datasets import make_classification
from sklearn.decomposition import PCA
# define dataset
X, y = make_classification(n_samples=1000, n_features=10, n_informative=3, n_redundant=7,
random_state=1)
# summarize data before the transform
print(X[:3, :])
# define the transform
trans = PCA(n_components=10)
# transform the data
X_dim = trans.fit_transform(X)
# summarize data after the transform
print(X_dim[:3, :])
print(X_dim)
import pandas as pd
df_X = pd.DataFrame(X_dim)
df_X.describe().round()
df_X_org = pd.DataFrame(X)
df_X_org.describe().round()
X_dim.shape
print("\nFirstprincipal axis :", trans.components_[0])
print("Second principal axis", trans.components_[1])
print("Third principal axis", trans.components_[2])
print(trans.explained_variance_ratio_)
###Output
_____no_output_____ |
1. Custom Models, Layers, and Loss Functions with TensorFlow/Week 1/C1_W1_Lab_1_functional-practice.ipynb | ###Markdown
Ungraded Lab: Practice with the Keras Functional APIThis lab will demonstrate how to build models with the Functional syntax. You'll build one using the Sequential API and see how you can do the same with the Functional API. Both will arrive at the same architecture and you can train and evaluate it as usual. Imports
###Code
try:
# %tensorflow_version only exists in Colab.
%tensorflow_version 2.x
except Exception:
pass
import tensorflow as tf
from tensorflow.python.keras.utils.vis_utils import plot_model
import pydot
from tensorflow.keras.models import Model
###Output
_____no_output_____
###Markdown
Sequential APIHere is how we use the `Sequential()` class to build a model.
###Code
def build_model_with_sequential():
# instantiate a Sequential class and linearly stack the layers of your model
seq_model = tf.keras.models.Sequential([tf.keras.layers.Flatten(input_shape=(28, 28)),
tf.keras.layers.Dense(128, activation=tf.nn.relu),
tf.keras.layers.Dense(10, activation=tf.nn.softmax)])
return seq_model
###Output
_____no_output_____
###Markdown
Functional APIAnd here is how you build the same model above with the functional syntax.
###Code
def build_model_with_functional():
# instantiate the input Tensor
input_layer = tf.keras.Input(shape=(28, 28))
# stack the layers using the syntax: new_layer()(previous_layer)
flatten_layer = tf.keras.layers.Flatten()(input_layer)
first_dense = tf.keras.layers.Dense(128, activation=tf.nn.relu)(flatten_layer)
output_layer = tf.keras.layers.Dense(10, activation=tf.nn.softmax)(first_dense)
# declare inputs and outputs
func_model = Model(inputs=input_layer, outputs=output_layer)
return func_model
###Output
_____no_output_____
###Markdown
Build the model and visualize the model graph You can choose how to build your model below. Just uncomment which function you'd like to use. You'll notice that the plot will look the same.
###Code
model = build_model_with_functional()
#model = build_model_with_sequential()
# Plot model graph
plot_model(model, show_shapes=True, show_layer_names=True, to_file='model.png')
#model = build_model_with_functional()
model2 = build_model_with_sequential()
# Plot model graph
plot_model(model2, show_shapes=True, show_layer_names=True, to_file='model2.png')
###Output
_____no_output_____
###Markdown
Training the modelRegardless if you built it with the Sequential or Functional API, you'll follow the same steps when training and evaluating your model.
###Code
# prepare fashion mnist dataset
mnist = tf.keras.datasets.fashion_mnist
(training_images, training_labels), (test_images, test_labels) = mnist.load_data()
training_images = training_images / 255.0
test_images = test_images / 255.0
# configure, train, and evaluate the model
model.compile(optimizer=tf.optimizers.Adam(),
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
model.fit(training_images, training_labels, epochs=5)
model.evaluate(test_images, test_labels)
# prepare fashion mnist dataset
mnist = tf.keras.datasets.fashion_mnist
(training_images, training_labels), (test_images, test_labels) = mnist.load_data()
training_images = training_images / 255.0
test_images = test_images / 255.0
# configure, train, and evaluate the model
model2.compile(optimizer=tf.optimizers.Adam(),
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
model2.fit(training_images, training_labels, epochs=5)
model2.evaluate(test_images, test_labels)
###Output
Train on 60000 samples
Epoch 1/5
60000/60000 [==============================] - 4s 74us/sample - loss: 0.5017 - accuracy: 0.8238
Epoch 2/5
60000/60000 [==============================] - 4s 73us/sample - loss: 0.3795 - accuracy: 0.8631
Epoch 3/5
60000/60000 [==============================] - 4s 72us/sample - loss: 0.3356 - accuracy: 0.8771
Epoch 4/5
60000/60000 [==============================] - 4s 72us/sample - loss: 0.3125 - accuracy: 0.8853
Epoch 5/5
60000/60000 [==============================] - 4s 73us/sample - loss: 0.2925 - accuracy: 0.8914
10000/10000 [==============================] - 0s 30us/sample - loss: 0.3664 - accuracy: 0.8677
|
docs/tutorials/08_property_framework.ipynb | ###Markdown
The Property Framework
###Code
import numpy as np
###Output
_____no_output_____
###Markdown
Qiskit Nature 0.2.0 introduces the _Property_ framework. This framework replaces the legacy driver return types like `QMolecule` and `WatsonHamiltonian` with a more modular and extensible approach. In this tutorial, we will walk through the framework, explain its most important components and show you, how you can extend it with a custom _property_ yourself. What is a `Property`? At its core, a `Property` is an object complementing some raw data with functions that allow you to transform/manipulate/interpret this raw data. This "definition" is kept rather abstract on purpose, but what it means is essentially the following.A `Property`:* represents a physical observable (that's the raw data)* can be expressed as an operator* can be evaluated with a wavefunction* provides an `interpret` method which gives meaning to the eigenvalue of the evaluated qubit operator
###Code
from qiskit_nature.properties import Property, GroupedProperty
###Output
_____no_output_____
###Markdown
The `qiskit_nature.properties` module provides two classes:1. `Property`: this is the basic interface. It requires only a `name` and an `interpret` method to be implemented.2. `GroupedProperty`: this class is an implementation of the [Composite pattern](https://en.wikipedia.org/wiki/Composite_pattern) which allows you to _group_ multiple properties into one.**Note:** Grouped properties must have unique `name` attributes! Second Quantization Properties At the time of writing, Qiskit Nature ships with a single variant of properties: the `SecondQuantizedProperty` objects.This sub-type adds one additional requirement because any `SecondQuantizedProperty`* **must** implement a `second_q_ops` method which constructs a (list of) `SecondQuantizedOp`s. The `qiskit_nature.properties.second_quantization` module is further divided into `electronic` and `vibrational` modules (similar to other modules of Qiskit Nature).Let us dive into the `electronic` sub-module first. Electronic Second Quantization Properties Out-of-the-box Qiskit Nature ships the following electronic properties:
###Code
from qiskit_nature.properties.second_quantization.electronic import (
ElectronicEnergy,
ElectronicDipoleMoment,
ParticleNumber,
AngularMomentum,
Magnetization,
)
###Output
_____no_output_____
###Markdown
`ElectronicEnergy` is arguably the most important property because it contains the _Hamiltonian_ representing the electronic structure problem whose eigenvalues we are interested in when solving an `ElectronicStructureProblem`.The way in which it stores this Hamiltonian is, just like the rest of the framework, highly modular. The initializer of the `ElectronicEnergy` has a single required argument, `electronic_integrals`, which is a `List[ElectronicIntegrals]`. ElectronicIntegrals
###Code
from qiskit_nature.properties.second_quantization.electronic.integrals import (
ElectronicIntegrals,
OneBodyElectronicIntegrals,
TwoBodyElectronicIntegrals,
IntegralProperty,
)
###Output
_____no_output_____
###Markdown
The `ElectronicIntegrals` are a container class for _n-body_ interactions in a given basis, which can be any of the following:
###Code
from qiskit_nature.properties.second_quantization.electronic.bases import ElectronicBasis
list(ElectronicBasis)
###Output
_____no_output_____
###Markdown
Let us take the `OneBodyElectronicIntegrals` as an example. As the name suggests, this container is for 1-body interaction integrals. You can construct an instance of it like so:
###Code
one_body_ints = OneBodyElectronicIntegrals(
ElectronicBasis.MO,
(
np.eye(2),
2 * np.eye(2),
),
)
print(one_body_ints)
###Output
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 1.0
[1, 1] = 1.0
Beta
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 2.0
[1, 1] = 2.0
###Markdown
As you can see, the first argument simply specifies the basis of the integrals.The second argument requires a little further explanation:1. In the case of the `AO` or `MO` basis, this argument **must** be a pair of numpy arrays, where the first and second one are the alpha- and beta-spin integrals, respectively.**Note:** The second argument may be `None`, for the case where the beta-spin integrals are the same as the alpha-spin integrals (so there is no need to provide the same values twice).2. In the case of the `SO` basis, this argument **must** be a single numpy array, storing the alpha- and beta-spin integrals in blocked order, i.e. like so:```pythonspin_basis = np.block([[alpha_spin, zeros], [zeros, beta_spin]])``` The `TwoBodyElectronicIntegrals` work pretty much the same except that they contain all possible spin-combinations in the tuple of numpy arrays. For example:
###Code
two_body_ints = TwoBodyElectronicIntegrals(
ElectronicBasis.MO,
(
np.arange(1, 17).reshape((2, 2, 2, 2)),
np.arange(16, 32).reshape((2, 2, 2, 2)),
np.arange(-16, 0).reshape((2, 2, 2, 2)),
None,
),
)
print(two_body_ints)
###Output
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 16.0
[0, 0, 0, 1] = 17.0
[0, 0, 1, 0] = 18.0
[0, 0, 1, 1] = 19.0
[0, 1, 0, 0] = 20.0
... skipping 11 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = -16.0
[0, 0, 0, 1] = -15.0
[0, 0, 1, 0] = -14.0
[0, 0, 1, 1] = -13.0
[0, 1, 0, 0] = -12.0
... skipping 11 entries
Alpha-Beta
Same values as the corresponding primary-spin data.
###Markdown
We should take note of a few observations:* the numpy arrays shall be ordered as `("alpha-alpha", "beta-alpha", "beta-beta", "alpha-beta")`* the `alpha-alpha` matrix may **not** be `None`* if the `alpha-beta` matrix is `None`, but `beta-alpha` is not, its transpose will be used (like above)* in any other case, matrices which are `None` will be filled with the `alpha-alpha` matrix* in the `SO` basis case, a single numpy array must be specified. Refer to `TwoBodyElectronicIntegrals.to_spin()` for its exact formatting. ElectronicEnergy Now we are ready to construct an `ElectronicEnergy` instance:
###Code
electronic_energy = ElectronicEnergy(
[one_body_ints, two_body_ints],
)
print(electronic_energy)
###Output
ElectronicEnergy
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 1.0
[1, 1] = 1.0
Beta
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 2.0
[1, 1] = 2.0
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 16.0
[0, 0, 0, 1] = 17.0
[0, 0, 1, 0] = 18.0
[0, 0, 1, 1] = 19.0
[0, 1, 0, 0] = 20.0
... skipping 11 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = -16.0
[0, 0, 0, 1] = -15.0
[0, 0, 1, 0] = -14.0
[0, 0, 1, 1] = -13.0
[0, 1, 0, 0] = -12.0
... skipping 11 entries
Alpha-Beta
Same values as the corresponding primary-spin data.
###Markdown
This property can now be used to construct a `SecondQuantizedOp` (which can then be mapped to a `QubitOperator`):
###Code
hamiltonian = electronic_energy.second_q_ops()[0] # here, output length is always 1
print(hamiltonian)
###Output
Fermionic Operator
register length=4, number terms=20
(22+0j) * ( +_0 -_1 +_2 -_3 )
+ (-26+0j) * ( +_0 -_1 -_2 +_3 )
+ (30+0j) * ( +_0 -_1 +_3 -_3 )
+ (18+0j) * ( +_0 -_1 +_2 -_2 )
+ (-21+0j) * ( -_0 +_1 +_2 -_3 )
+ (25+0j) * ( -_0 +_1 -_2 +_3 )
+ ...
###Markdown
Result interpretation An additional benefit which we gain from the `Property` framework, is that the result interpretation of a computed eigenvalue can be handled by each property itself. This results in nice and logically consistent classes because the result gets interpreted in the same context where the raw data is available.
###Code
from qiskit_nature.results import ElectronicStructureResult
# some dummy result
result = ElectronicStructureResult()
result.eigenenergies = np.asarray([-1])
result.computed_energies = np.asarray([-1])
# now, let's interpret it
electronic_energy.interpret(result)
print(result)
###Output
=== GROUND STATE ENERGY ===
* Electronic ground state energy (Hartree): -1.
- computed part: -1.
###Markdown
While this particular example is not yet very impressive, wait until we use more properties at once. ParticleNumber The `ParticleNumber` property also takes a special place among the builtin properties because it serves a dual purpose of:* storing the number of particles in the electronic system* providing the operators to evaluate the number of particles for a given eigensolution of the problemTherefore, this property is required if you want to use additional functionality like the `ActiveSpaceTransformer` or the `ElectronicStructureProblem.default_filter_criterion()`.
###Code
particle_number = ParticleNumber(
num_spin_orbitals=4,
num_particles=(1, 1),
)
print(particle_number)
###Output
ParticleNumber:
4 SOs
1 alpha electrons
orbital occupation: [1. 0.]
1 beta electrons
orbital occupation: [1. 0.]
###Markdown
GroupedProperty Rather than iterating all of the other properties one by one, let us simply consider a group of properties as provided by any `ElectronicStructureDriver` from the `qiskit_nature.drivers.second_quantization` module.
###Code
from qiskit_nature.drivers.second_quantization.pyscfd import PySCFDriver
electronic_driver = PySCFDriver(atom="H 0 0 0; H 0 0 0.735", basis="sto3g")
electronic_driver_result = electronic_driver.run()
print(electronic_driver_result)
###Output
ElectronicStructureDriverResult:
DriverMetadata:
Program: PYSCF
Version: 2.0.1
Config:
atom=H 0 0 0; H 0 0 0.735
unit=Angstrom
charge=0
spin=0
basis=sto3g
method=rhf
conv_tol=1e-09
max_cycle=50
init_guess=minao
max_memory=4000
ElectronicBasisTransform:
Initial basis: atomic
Final basis: molecular
Alpha coefficients:
[0, 0] = 0.5483020229014732
[0, 1] = 1.2183273138546826
[1, 0] = 0.548302022901473
[1, 1] = -1.2183273138546826
ParticleNumber:
4 SOs
1 alpha electrons
orbital occupation: [1. 0.]
1 beta electrons
orbital occupation: [1. 0.]
ElectronicEnergy
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = -1.1242175791954514
[0, 1] = -0.9652573993472754
[1, 0] = -0.9652573993472754
[1, 1] = -1.1242175791954514
Beta
Same values as the corresponding primary-spin data.
(AO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 0.7746059439198978
[0, 0, 0, 1] = 0.4474457245330949
[0, 0, 1, 0] = 0.447445724533095
[0, 0, 1, 1] = 0.5718769760004512
[0, 1, 0, 0] = 0.4474457245330951
... skipping 11 entries
Beta-Alpha
Same values as the corresponding primary-spin data.
Beta-Beta
Same values as the corresponding primary-spin data.
Alpha-Beta
Same values as the corresponding primary-spin data.
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = -1.2563390730032498
[1, 1] = -0.47189600728114245
Beta
Same values as the corresponding primary-spin data.
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 8 non-zero entries>
[0, 0, 0, 0] = 0.6757101548035161
[0, 0, 1, 1] = 0.6645817302552968
[0, 1, 0, 1] = 0.18093119978423136
[0, 1, 1, 0] = 0.18093119978423106
[1, 0, 0, 1] = 0.18093119978423144
... skipping 3 entries
Beta-Alpha
Same values as the corresponding primary-spin data.
Beta-Beta
Same values as the corresponding primary-spin data.
Alpha-Beta
Same values as the corresponding primary-spin data.
ElectronicDipoleMoment:
DipoleMomentX
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
Same values as the corresponding primary-spin data.
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
Same values as the corresponding primary-spin data.
DipoleMomentY
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
Same values as the corresponding primary-spin data.
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
Same values as the corresponding primary-spin data.
DipoleMomentZ
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 3 non-zero entries>
[0, 1] = 0.46053770796603194
[1, 0] = 0.46053770796603194
[1, 1] = 1.3889487015553206
Beta
Same values as the corresponding primary-spin data.
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = 0.6944743507776598
[0, 1] = -0.927833470459232
[1, 0] = -0.9278334704592321
[1, 1] = 0.6944743507776604
Beta
Same values as the corresponding primary-spin data.
AngularMomentum:
4 SOs
Magnetization:
4 SOs
Molecule:
Multiplicity: 1
Charge: 0
Unit: Angstrom
Geometry:
H [0.0, 0.0, 0.0]
H [0.0, 0.0, 0.735]
Masses:
H 1
H 1
###Markdown
There is a lot going on but with the explanations above you should not have any problems with understanding this output. Constructing a Hamiltonian from raw integrals If you have obtained some raw one- and two-body integrals by means other than through a driver provided by Qiskit Nature, you can still easily construct an `ElectronicEnergy` property to serve as your access point into the stack:
###Code
one_body_ints = np.arange(1, 5).reshape((2, 2))
two_body_ints = np.arange(1, 17).reshape((2, 2, 2, 2))
electronic_energy_from_ints = ElectronicEnergy.from_raw_integrals(
ElectronicBasis.MO, one_body_ints, two_body_ints
)
print(electronic_energy_from_ints)
###Output
ElectronicEnergy
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = 1.0
[0, 1] = 2.0
[1, 0] = 3.0
[1, 1] = 4.0
Beta
Same values as the corresponding primary-spin data.
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Alpha
Same values as the corresponding primary-spin data.
Beta-Beta
Same values as the corresponding primary-spin data.
Alpha-Beta
Same values as the corresponding primary-spin data.
###Markdown
Vibrational Second Quantization Properties The `vibrational` stack for vibrational structure calculations also integrates with the Property framework. After the above introduction you should be able to understand the following directly:
###Code
from qiskit_nature.drivers.second_quantization.gaussiand import GaussianForcesDriver
# if you ran Gaussian elsewhere and already have the output file
vibrational_driver = GaussianForcesDriver(logfile="aux_files/CO2_freq_B3LYP_631g.log")
vibrational_driver_result = vibrational_driver.run()
###Output
_____no_output_____
###Markdown
For vibrational structure calculations we always need to define the basis which we want to work in, separately:
###Code
from qiskit_nature.properties.second_quantization.vibrational.bases import HarmonicBasis
harmonic_basis = HarmonicBasis([2] * 4)
vibrational_driver_result.basis = harmonic_basis
print(vibrational_driver_result)
###Output
VibrationalStructureDriverResult:
HarmonicBasis:
Modals: [2, 2, 2, 2]:
VibrationalEnergy:
HarmonicBasis:
Modals: [2, 2, 2, 2]
1-Body Terms:
<sparse integral list with 13 entries>
(2, 2) = 352.3005875
(-2, -2) = -352.3005875
(1, 1) = 631.6153975
(-1, -1) = -631.6153975
(4, 4) = 115.653915
... skipping 8 entries
2-Body Terms:
<sparse integral list with 11 entries>
(1, 1, 2) = -88.2017421687633
(4, 4, 2) = 42.675273102831454
(3, 3, 2) = 42.675273102831454
(1, 1, 2, 2) = 4.9425425
(4, 4, 2, 2) = -4.194299375
... skipping 6 entries
3-Body Terms:
<sparse integral list with 0 entries>
OccupiedModals:
HarmonicBasis:
Modals: [2, 2, 2, 2]
###Markdown
Writing custom Properties You can extend the Property framework with your own implementations. Here, we will walk through the simple example of constructing a Property for the _electronic density_. Since this observable is essentially based on matrices, we will be leveraging the `OneBodyElectronicIntegrals` container to store the actual density matrix.
###Code
from itertools import product
from typing import List
import h5py
from qiskit_nature.drivers import QMolecule
from qiskit_nature.operators.second_quantization import FermionicOp
from qiskit_nature.properties.second_quantization.electronic.bases import ElectronicBasis
from qiskit_nature.properties.second_quantization.electronic.types import ElectronicProperty
from qiskit_nature.properties.second_quantization.electronic.integrals import (
OneBodyElectronicIntegrals,
)
class ElectronicDensity(ElectronicProperty):
"""A simple electronic density property.
This basic example works only in the MO basis!
"""
def __init__(self, num_molecular_orbitals: int) -> None:
super().__init__(self.__class__.__name__)
self._num_molecular_orbitals = num_molecular_orbitals
def __str__(self) -> str:
string = [super().__str__() + ":"]
string += [f"\t{self._num_molecular_orbitals} MOs"]
return "\n".join(string)
def to_hdf5(self, parent: h5py.Group) -> None:
super().to_hdf5(parent)
group = parent.require_group(self.name)
group.attrs["num_molecular_orbitals"] = self._num_molecular_orbitals
@classmethod
def from_hdf5(cls, h5py_group: h5py.Group) -> "ElectronicDensity":
return ElectronicDensity(h5py_group.attrs["num_molecular_orbitals"])
@classmethod
def from_legacy_driver_result(cls, result) -> "ElectronicDensity":
cls._validate_input_type(result, QMolecule)
qmol = cast(QMolecule, result)
return cls(qmol.num_molecular_orbitals)
def second_q_ops(self) -> List[FermionicOp]:
ops = []
# iterate all pairs of molecular orbitals
for mo_i, mo_j in product(range(self._num_molecular_orbitals), repeat=2):
# construct an auxiliary matrix where the only non-zero entry is at the current pair of MOs
number_op_matrix = np.zeros(
(self._num_molecular_orbitals, self._num_molecular_orbitals)
)
number_op_matrix[mo_i, mo_j] = 1
# leverage the OneBodyElectronicIntegrals to construct the corresponding FermionicOp
one_body_ints = OneBodyElectronicIntegrals(
ElectronicBasis.MO, (number_op_matrix, number_op_matrix)
)
ops.append(one_body_ints.to_second_q_op())
return ops
def interpret(self, result) -> None:
# here goes the code which interprets the eigenvalues returned for the auxiliary operators
pass
density = ElectronicDensity(2)
print(density)
for idx, op in enumerate(density.second_q_ops()):
print(idx, ":", op)
###Output
0 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_0 -_0 )
+ (1+0j) * ( +_2 -_2 )
1 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_0 -_1 )
+ (1+0j) * ( +_2 -_3 )
2 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_1 -_0 )
+ (1+0j) * ( +_3 -_2 )
3 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_1 -_1 )
+ (1+0j) * ( +_3 -_3 )
###Markdown
Of course, the above example is very minimal and can be extended at will. **Note:** as of Qiskit Nature version 0.2.0, the direct integration of custom Property objects into the stack is not implemented yet, due to limitations of the auxiliary operator parsing. See https://github.com/Qiskit/qiskit-nature/issues/312 for more details.For the time being, you can still evaluate a custom Property, by passing it's generated operators directly to the `Eigensolver.solve` method by means of constructing the `aux_operators`. Note, however, that you will have to deal with transformations applied to your properties manually, until the above issue is resolved.
###Code
# set up some problem
problem = ...
# set up a solver
solver = ...
# when solving the problem, pass additional operators in like so:
aux_ops = density.second_q_ops()
# solver.solve(problem, aux_ops)
import qiskit.tools.jupyter
%qiskit_version_table
%qiskit_copyright
###Output
_____no_output_____
###Markdown
The Property Framework
###Code
import numpy as np
###Output
_____no_output_____
###Markdown
Qiskit Nature 0.2.0 introduces the _Property_ framework. This framework replaces the legacy driver return types like `QMolecule` and `WatsonHamiltonian` with a more modular and extensible approach. In this tutorial, we will walk through the framework, explain its most important components and show you, how you can extend it with a custom _property_ yourself. What is a `Property`? At its core, a `Property` is an object complementing some raw data with functions that allow you to transform/manipulate/interpret this raw data. This "definition" is kept rather abstract on purpose, but what it means is essentially the following.A `Property`:* represents a physical observable (that's the raw data)* can be expressed as an operator* can be evaluated with a wavefunction* provides an `interpret` method which gives meaning to the eigenvalue of the evaluated qubit operator
###Code
from qiskit_nature.properties import Property, GroupedProperty
###Output
_____no_output_____
###Markdown
The `qiskit_nature.properties` module provides two classes:1. `Property`: this is the basic interface. It requires only a `name` and an `interpret` method to be implemented.2. `GroupedProperty`: this class is an implementation of the [Composite pattern](https://en.wikipedia.org/wiki/Composite_pattern) which allows you to _group_ multiple properties into one.**Note:** Grouped properties must have unique `name` attributes! Second Quantization Properties At the time of writing, Qiskit Nature ships with a single variant of properties: the `SecondQuantizedProperty` objects.This sub-type adds one additional requirement because any `SecondQuantizedProperty`* **must** implement a `second_q_ops` method which constructs a (list of) `SecondQuantizedOp`s. The `qiskit_nature.properties.second_quantization` module is further divided into `electronic` and `vibrational` modules (similar to other modules of Qiskit Nature).Let us dive into the `electronic` sub-module first. Electronic Second Quantization Properties Out-of-the-box Qiskit Nature ships the following electronic properties:
###Code
from qiskit_nature.properties.second_quantization.electronic import (
ElectronicEnergy,
ElectronicDipoleMoment,
ParticleNumber,
AngularMomentum,
Magnetization,
)
###Output
_____no_output_____
###Markdown
`ElectronicEnergy` is arguably the most important property because it contains the _Hamiltonian_ representing the electronic structure problem whose eigenvalues we are interested in when solving an `ElectronicStructureProblem`.The way in which it stores this Hamiltonian is, just like the rest of the framework, highly modular. The initializer of the `ElectronicEnergy` has a single required argument, `electronic_integrals`, which is a `List[ElectronicIntegrals]`. ElectronicIntegrals
###Code
from qiskit_nature.properties.second_quantization.electronic.integrals import (
ElectronicIntegrals,
OneBodyElectronicIntegrals,
TwoBodyElectronicIntegrals,
IntegralProperty,
)
###Output
_____no_output_____
###Markdown
The `ElectronicIntegrals` are a container class for _n-body_ interactions in a given basis, which can be any of the following:
###Code
from qiskit_nature.properties.second_quantization.electronic.bases import ElectronicBasis
list(ElectronicBasis)
###Output
_____no_output_____
###Markdown
Let us take the `OneBodyElectronicIntegrals` as an example. As the name suggests, this container is for 1-body interaction integrals. You can construct an instance of it like so:
###Code
one_body_ints = OneBodyElectronicIntegrals(
ElectronicBasis.MO,
(
np.eye(2),
2 * np.eye(2),
),
)
print(one_body_ints)
###Output
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 1.0
[1, 1] = 1.0
Beta
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 2.0
[1, 1] = 2.0
###Markdown
As you can see, the first argument simply specifies the basis of the integrals.The second argument requires a little further explanation:1. In the case of the `AO` or `MO` basis, this argument **must** be a pair of numpy arrays, where the first and second one are the alpha- and beta-spin integrals, respectively.**Note:** The second argument may be `None`, for the case where the beta-spin integrals are the same as the alpha-spin integrals (so there is no need to provide the same values twice).2. In the case of the `SO` basis, this argument **must** be a single numpy array, storing the alpha- and beta-spin integrals in blocked order, i.e. like so:```pythonspin_basis = np.block([[alpha_spin, zeros], [zeros, beta_spin]])``` The `TwoBodyElectronicIntegrals` work pretty much the same except that they contain all possible spin-combinations in the tuple of numpy arrays. For example:
###Code
two_body_ints = TwoBodyElectronicIntegrals(
ElectronicBasis.MO,
(
np.arange(1, 17).reshape((2, 2, 2, 2)),
np.arange(16, 32).reshape((2, 2, 2, 2)),
np.arange(-16, 0).reshape((2, 2, 2, 2)),
None,
),
)
print(two_body_ints)
###Output
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 16.0
[0, 0, 0, 1] = 17.0
[0, 0, 1, 0] = 18.0
[0, 0, 1, 1] = 19.0
[0, 1, 0, 0] = 20.0
... skipping 11 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = -16.0
[0, 0, 0, 1] = -15.0
[0, 0, 1, 0] = -14.0
[0, 0, 1, 1] = -13.0
[0, 1, 0, 0] = -12.0
... skipping 11 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 16.0
[0, 0, 0, 1] = 24.0
[0, 0, 1, 0] = 20.0
[0, 0, 1, 1] = 28.0
[0, 1, 0, 0] = 18.0
... skipping 11 entries
###Markdown
We should take note of a few observations:* the numpy arrays shall be ordered as `("alpha-alpha", "beta-alpha", "beta-beta", "alpha-beta")`* the `alpha-alpha` matrix may **not** be `None`* if the `alpha-beta` matrix is `None`, but `beta-alpha` is not, its transpose will be used (like above)* in any other case, matrices which are `None` will be filled with the `alpha-alpha` matrix* in the `SO` basis case, a single numpy array must be specified. Refer to `TwoBodyElectronicIntegrals.to_spin()` for its exact formatting. ElectronicEnergy Now we are ready to construct an `ElectronicEnergy` instance:
###Code
electronic_energy = ElectronicEnergy(
[one_body_ints, two_body_ints],
)
print(electronic_energy)
###Output
ElectronicEnergy
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 1.0
[1, 1] = 1.0
Beta
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 2.0
[1, 1] = 2.0
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 16.0
[0, 0, 0, 1] = 17.0
[0, 0, 1, 0] = 18.0
[0, 0, 1, 1] = 19.0
[0, 1, 0, 0] = 20.0
... skipping 11 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = -16.0
[0, 0, 0, 1] = -15.0
[0, 0, 1, 0] = -14.0
[0, 0, 1, 1] = -13.0
[0, 1, 0, 0] = -12.0
... skipping 11 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 16.0
[0, 0, 0, 1] = 24.0
[0, 0, 1, 0] = 20.0
[0, 0, 1, 1] = 28.0
[0, 1, 0, 0] = 18.0
... skipping 11 entries
###Markdown
This property can now be used to construct a `SecondQuantizedOp` (which can then be mapped to a `QubitOperator`):
###Code
hamiltonian = electronic_energy.second_q_ops()[0] # here, output length is always 1
print(hamiltonian)
###Output
Fermionic Operator
register length=4, number terms=20
(22+0j) * ( +_0 -_1 +_2 -_3 )
+ (-26+0j) * ( +_0 -_1 -_2 +_3 )
+ (30+0j) * ( +_0 -_1 +_3 -_3 )
+ (18+0j) * ( +_0 -_1 +_2 -_2 )
+ (-21+0j) * ( -_0 +_1 +_2 -_3 )
+ (25+0j) * ( -_0 +_1 -_2 +_3 )
+ ...
###Markdown
Result interpretation An additional benefit which we gain from the `Property` framework, is that the result interpretation of a computed eigenvalue can be handled by each property itself. This results in nice and logically consistent classes because the result gets interpreted in the same context where the raw data is available.
###Code
from qiskit_nature.results import ElectronicStructureResult
# some dummy result
result = ElectronicStructureResult()
result.eigenenergies = np.asarray([-1])
result.computed_energies = np.asarray([-1])
# now, let's interpret it
electronic_energy.interpret(result)
print(result)
###Output
=== GROUND STATE ENERGY ===
* Electronic ground state energy (Hartree): -1.
- computed part: -1.
###Markdown
While this particular example is not yet very impressive, wait until we use more properties at once. ParticleNumber The `ParticleNumber` property also takes a special place among the builtin properties because it serves a dual purpose of:* storing the number of particles in the electronic system* providing the operators to evaluate the number of particles for a given eigensolution of the problemTherefore, this property is required if you want to use additional functionality like the `ActiveSpaceTransformer` or the `ElectronicStructureProblem.default_filter_criterion()`.
###Code
particle_number = ParticleNumber(
num_spin_orbitals=4,
num_particles=(1, 1),
)
print(particle_number)
###Output
ParticleNumber:
4 SOs
1 alpha electrons
orbital occupation: [1. 0.]
1 beta electrons
orbital occupation: [1. 0.]
###Markdown
GroupedProperty Rather than iterating all of the other properties one by one, let us simply consider a group of properties as provided by any `ElectronicStructureDriver` from the `qiskit_nature.drivers.second_quantization` module.
###Code
from qiskit_nature.drivers.second_quantization.pyscfd import PySCFDriver
electronic_driver = PySCFDriver(atom="H 0 0 0; H 0 0 0.735", basis="sto3g")
electronic_driver_result = electronic_driver.run()
print(electronic_driver_result)
###Output
ElectronicStructureDriverResult:
DriverMetadata:
Program: PYSCF
Version: 2.0.1
Config:
atom=H 0 0 0; H 0 0 0.735
unit=Angstrom
charge=0
spin=0
basis=sto3g
method=rhf
conv_tol=1e-09
max_cycle=50
init_guess=minao
max_memory=4000
ElectronicBasisTransform:
Initial basis: atomic
Final basis: molecular
Alpha coefficients:
[0, 0] = 0.5483020229014732
[0, 1] = 1.2183273138546826
[1, 0] = 0.548302022901473
[1, 1] = -1.2183273138546826
Beta coefficients:
[0, 0] = 0.5483020229014732
[0, 1] = 1.2183273138546826
[1, 0] = 0.548302022901473
[1, 1] = -1.2183273138546826
ParticleNumber:
4 SOs
1 alpha electrons
orbital occupation: [1. 0.]
1 beta electrons
orbital occupation: [1. 0.]
ElectronicEnergy
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = -1.1242175791954514
[0, 1] = -0.9652573993472754
[1, 0] = -0.9652573993472754
[1, 1] = -1.1242175791954514
Beta
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = -1.1242175791954514
[0, 1] = -0.9652573993472754
[1, 0] = -0.9652573993472754
[1, 1] = -1.1242175791954514
(AO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 0.7746059439198978
[0, 0, 0, 1] = 0.4474457245330949
[0, 0, 1, 0] = 0.447445724533095
[0, 0, 1, 1] = 0.5718769760004512
[0, 1, 0, 0] = 0.4474457245330951
... skipping 11 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 0.7746059439198978
[0, 0, 0, 1] = 0.4474457245330949
[0, 0, 1, 0] = 0.447445724533095
[0, 0, 1, 1] = 0.5718769760004512
[0, 1, 0, 0] = 0.4474457245330951
... skipping 11 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 0.7746059439198978
[0, 0, 0, 1] = 0.4474457245330949
[0, 0, 1, 0] = 0.447445724533095
[0, 0, 1, 1] = 0.5718769760004512
[0, 1, 0, 0] = 0.4474457245330951
... skipping 11 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 0.7746059439198978
[0, 0, 0, 1] = 0.4474457245330949
[0, 0, 1, 0] = 0.447445724533095
[0, 0, 1, 1] = 0.5718769760004512
[0, 1, 0, 0] = 0.4474457245330951
... skipping 11 entries
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = -1.2563390730032498
[1, 1] = -0.47189600728114245
Beta
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = -1.2563390730032498
[1, 1] = -0.47189600728114245
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 8 non-zero entries>
[0, 0, 0, 0] = 0.6757101548035161
[0, 0, 1, 1] = 0.6645817302552968
[0, 1, 0, 1] = 0.18093119978423136
[0, 1, 1, 0] = 0.18093119978423106
[1, 0, 0, 1] = 0.18093119978423144
... skipping 3 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 8 non-zero entries>
[0, 0, 0, 0] = 0.6757101548035161
[0, 0, 1, 1] = 0.6645817302552968
[0, 1, 0, 1] = 0.18093119978423136
[0, 1, 1, 0] = 0.18093119978423106
[1, 0, 0, 1] = 0.18093119978423144
... skipping 3 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 8 non-zero entries>
[0, 0, 0, 0] = 0.6757101548035161
[0, 0, 1, 1] = 0.6645817302552968
[0, 1, 0, 1] = 0.18093119978423136
[0, 1, 1, 0] = 0.18093119978423106
[1, 0, 0, 1] = 0.18093119978423144
... skipping 3 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 8 non-zero entries>
[0, 0, 0, 0] = 0.6757101548035161
[0, 0, 1, 1] = 0.6645817302552968
[0, 1, 0, 1] = 0.18093119978423136
[0, 1, 1, 0] = 0.18093119978423106
[1, 0, 0, 1] = 0.18093119978423144
... skipping 3 entries
ElectronicDipoleMoment:
DipoleMomentX
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
<(2, 2) matrix with 0 non-zero entries>
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
<(2, 2) matrix with 0 non-zero entries>
DipoleMomentY
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
<(2, 2) matrix with 0 non-zero entries>
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
<(2, 2) matrix with 0 non-zero entries>
DipoleMomentZ
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 3 non-zero entries>
[0, 1] = 0.46053770796603194
[1, 0] = 0.46053770796603194
[1, 1] = 1.3889487015553206
Beta
<(2, 2) matrix with 3 non-zero entries>
[0, 1] = 0.46053770796603194
[1, 0] = 0.46053770796603194
[1, 1] = 1.3889487015553206
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = 0.6944743507776598
[0, 1] = -0.927833470459232
[1, 0] = -0.9278334704592321
[1, 1] = 0.6944743507776604
Beta
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = 0.6944743507776598
[0, 1] = -0.927833470459232
[1, 0] = -0.9278334704592321
[1, 1] = 0.6944743507776604
AngularMomentum:
4 SOs
Magnetization:
4 SOs
Molecule:
Multiplicity: 1
Charge: 0
Unit: Angstrom
Geometry:
H [0.0, 0.0, 0.0]
H [0.0, 0.0, 0.735]
Masses:
H 1
H 1
###Markdown
There is a lot going on but with the explanations above you should not have any problems with understanding this output. Constructing a Hamiltonian from raw integrals If you have obtained some raw one- and two-body integrals by means other than through a driver provided by Qiskit Nature, you can still easily construct an `ElectronicEnergy` property to serve as your access point into the stack:
###Code
one_body_ints = np.arange(1, 5).reshape((2, 2))
two_body_ints = np.arange(1, 17).reshape((2, 2, 2, 2))
electronic_energy_from_ints = ElectronicEnergy.from_raw_integrals(
ElectronicBasis.MO, one_body_ints, two_body_ints
)
print(electronic_energy_from_ints)
###Output
ElectronicEnergy
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = 1.0
[0, 1] = 2.0
[1, 0] = 3.0
[1, 1] = 4.0
Beta
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = 1.0
[0, 1] = 2.0
[1, 0] = 3.0
[1, 1] = 4.0
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
###Markdown
Vibrational Second Quantization Properties The `vibrational` stack for vibrational structure calculations also integrates with the Property framework. After the above introduction you should be able to understand the following directly:
###Code
from qiskit_nature.drivers.second_quantization.gaussiand import GaussianForcesDriver
# if you ran Gaussian elsewhere and already have the output file
vibrational_driver = GaussianForcesDriver(logfile="aux_files/CO2_freq_B3LYP_631g.log")
vibrational_driver_result = vibrational_driver.run()
###Output
_____no_output_____
###Markdown
For vibrational structure calculations we always need to define the basis which we want to work in, separately:
###Code
from qiskit_nature.properties.second_quantization.vibrational.bases import HarmonicBasis
harmonic_basis = HarmonicBasis([2] * 4)
vibrational_driver_result.basis = harmonic_basis
print(vibrational_driver_result)
###Output
VibrationalStructureDriverResult:
HarmonicBasis:
Modals: [2, 2, 2, 2]:
VibrationalEnergy:
HarmonicBasis:
Modals: [2, 2, 2, 2]
1-Body Terms:
<sparse integral list with 13 entries>
(2, 2) = 352.3005875
(-2, -2) = -352.3005875
(1, 1) = 631.6153975
(-1, -1) = -631.6153975
(4, 4) = 115.653915
... skipping 8 entries
2-Body Terms:
<sparse integral list with 11 entries>
(1, 1, 2) = -88.2017421687633
(4, 4, 2) = 42.675273102831454
(3, 3, 2) = 42.675273102831454
(1, 1, 2, 2) = 4.9425425
(4, 4, 2, 2) = -4.194299375
... skipping 6 entries
3-Body Terms:
<sparse integral list with 0 entries>
OccupiedModals:
HarmonicBasis:
Modals: [2, 2, 2, 2]
###Markdown
Writing custom Properties You can extend the Property framework with your own implementations. Here, we will walk through the simple example of constructing a Property for the _electronic density_. Since this observable is essentially based on matrices, we will be leveraging the `OneBodyElectronicIntegrals` container to store the actual density matrix.
###Code
from itertools import product
from typing import List
import h5py
from qiskit_nature.drivers import QMolecule
from qiskit_nature.operators.second_quantization import FermionicOp
from qiskit_nature.properties.second_quantization.electronic.bases import ElectronicBasis
from qiskit_nature.properties.second_quantization.electronic.types import ElectronicProperty
from qiskit_nature.properties.second_quantization.electronic.integrals import (
OneBodyElectronicIntegrals,
)
class ElectronicDensity(ElectronicProperty):
"""A simple electronic density property.
This basic example works only in the MO basis!
"""
def __init__(self, num_molecular_orbitals: int) -> None:
super().__init__(self.__class__.__name__)
self._num_molecular_orbitals = num_molecular_orbitals
def __str__(self) -> str:
string = [super().__str__() + ":"]
string += [f"\t{self._num_molecular_orbitals} MOs"]
return "\n".join(string)
def to_hdf5(self, parent: h5py.Group) -> None:
super().to_hdf5(parent)
group = parent.require_group(self.name)
group.attrs["num_molecular_orbitals"] = self._num_molecular_orbitals
@classmethod
def from_hdf5(cls, h5py_group: h5py.Group) -> "ElectronicDensity":
return ElectronicDensity(h5py_group.attrs["num_molecular_orbitals"])
@classmethod
def from_legacy_driver_result(cls, result) -> "ElectronicDensity":
cls._validate_input_type(result, QMolecule)
qmol = cast(QMolecule, result)
return cls(qmol.num_molecular_orbitals)
def second_q_ops(self) -> List[FermionicOp]:
ops = []
# iterate all pairs of molecular orbitals
for mo_i, mo_j in product(range(self._num_molecular_orbitals), repeat=2):
# construct an auxiliary matrix where the only non-zero entry is at the current pair of MOs
number_op_matrix = np.zeros(
(self._num_molecular_orbitals, self._num_molecular_orbitals)
)
number_op_matrix[mo_i, mo_j] = 1
# leverage the OneBodyElectronicIntegrals to construct the corresponding FermionicOp
one_body_ints = OneBodyElectronicIntegrals(
ElectronicBasis.MO, (number_op_matrix, number_op_matrix)
)
ops.append(one_body_ints.to_second_q_op())
return ops
def interpret(self, result) -> None:
# here goes the code which interprets the eigenvalues returned for the auxiliary operators
pass
density = ElectronicDensity(2)
print(density)
for idx, op in enumerate(density.second_q_ops()):
print(idx, ":", op)
###Output
0 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_0 -_0 )
+ (1+0j) * ( +_2 -_2 )
1 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_0 -_1 )
+ (1+0j) * ( +_2 -_3 )
2 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_1 -_0 )
+ (1+0j) * ( +_3 -_2 )
3 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_1 -_1 )
+ (1+0j) * ( +_3 -_3 )
###Markdown
Of course, the above example is very minimal and can be extended at will. **Note:** as of Qiskit Nature version 0.2.0, the direct integration of custom Property objects into the stack is not implemented yet, due to limitations of the auxiliary operator parsing. See https://github.com/Qiskit/qiskit-nature/issues/312 for more details.For the time being, you can still evaluate a custom Property, by passing it's generated operators directly to the `Eigensolver.solve` method by means of constructing the `aux_operators`. Note, however, that you will have to deal with transformations applied to your properties manually, until the above issue is resolved.
###Code
# set up some problem
problem = ...
# set up a solver
solver = ...
# when solving the problem, pass additional operators in like so:
aux_ops = density.second_q_ops()
# solver.solve(problem, aux_ops)
import qiskit.tools.jupyter
%qiskit_version_table
%qiskit_copyright
###Output
_____no_output_____
###Markdown
The Property Framework
###Code
import numpy as np
###Output
_____no_output_____
###Markdown
Qiskit Nature 0.2.0 introduces the _Property_ framework. This framework replaces the legacy driver return types like `QMolecule` and `WatsonHamiltonian` with a more modular and extensible approach. In this tutorial, we will walk through the framework, explain its most important components and show you, how you can extend it with a custom _property_ yourself. What is a `Property`? At its core, a `Property` is an object complementing some raw data with functions that allow you to transform/manipulate/interpret this raw data. This "definition" is kept rather abstract on purpose, but what it means is essentially the following.A `Property`:* represents a physical observable (that's the raw data)* can be expressed as an operator* can be evaluated with a wavefunction* provides an `interpret` method which gives meaning to the eigenvalue of the evaluated qubit operator
###Code
from qiskit_nature.properties import Property, GroupedProperty
###Output
_____no_output_____
###Markdown
The `qiskit_nature.properties` module provides two classes:1. `Property`: this is the basic interface. It requires only a `name` and an `interpret` method to be implemented.2. `GroupedProperty`: this class is an implementation of the [Composite pattern](https://en.wikipedia.org/wiki/Composite_pattern) which allows you to _group_ multiple properties into one.**Note:** Grouped properties must have unique `name` attributes! Second Quantization Properties At the time of writing, Qiskit Nature ships with a single variant of properties: the `SecondQuantizedProperty` objects.This sub-type adds one additional requirement because any `SecondQuantizedProperty`* **must** implement a `second_q_ops` method which constructs a (list of) `SecondQuantizedOp`s. The `qiskit_nature.properties.second_quantization` module is further divided into `electronic` and `vibrational` modules (similar to other modules of Qiskit Nature).Let us dive into the `electronic` sub-module first. Electronic Second Quantization Properties Out-of-the-box Qiskit Nature ships the following electronic properties:
###Code
from qiskit_nature.properties.second_quantization.electronic import (
ElectronicEnergy,
ElectronicDipoleMoment,
ParticleNumber,
AngularMomentum,
Magnetization,
)
###Output
/home/oss/Files/Qiskit/.direnv/python-3.9.6/lib/python3.9/site-packages/pyscf/lib/misc.py:46: H5pyDeprecationWarning: Using default_file_mode other than 'r' is deprecated. Pass the mode to h5py.File() instead.
h5py.get_config().default_file_mode = 'a'
###Markdown
`ElectronicEnergy` is arguably the most important property because it contains the _Hamiltonian_ representing the electronic structure problem whose eigenvalues we are interested in when solving an `ElectronicStructureProblem`.The way in which it stores this Hamiltonian is, just like the rest of the framework, highly modular. The initializer of the `ElectronicEnergy` has a single required argument, `electronic_integrals`, which is a `List[ElectronicIntegrals]`. ElectronicIntegrals
###Code
from qiskit_nature.properties.second_quantization.electronic.integrals import (
ElectronicIntegrals,
OneBodyElectronicIntegrals,
TwoBodyElectronicIntegrals,
IntegralProperty,
)
###Output
_____no_output_____
###Markdown
The `ElectronicIntegrals` are a container class for _n-body_ interactions in a given basis, which can be any of the following:
###Code
from qiskit_nature.properties.second_quantization.electronic.bases import ElectronicBasis
list(ElectronicBasis)
###Output
_____no_output_____
###Markdown
Let us take the `OneBodyElectronicIntegrals` as an example. As the name suggests, this container is for 1-body interaction integrals. You can construct an instance of it like so:
###Code
one_body_ints = OneBodyElectronicIntegrals(
ElectronicBasis.MO,
(
np.eye(2),
2 * np.eye(2),
),
)
print(one_body_ints)
###Output
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 1.0
[1, 1] = 1.0
Beta
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 2.0
[1, 1] = 2.0
###Markdown
As you can see, the first argument simply specifies the basis of the integrals.The second argument requires a little further explanation:1. In the case of the `AO` or `MO` basis, this argument **must** be a pair of numpy arrays, where the first and second one are the alpha- and beta-spin integrals, respectively.**Note:** The second argument may be `None`, for the case where the beta-spin integrals are the same as the alpha-spin integrals (so there is no need to provide the same values twice).2. In the case of the `SO` basis, this argument **must** be a single numpy array, storing the alpha- and beta-spin integrals in blocked order, i.e. like so:```pythonspin_basis = np.block([[alpha_spin, zeros], [zeros, beta_spin]])``` The `TwoBodyElectronicIntegrals` work pretty much the same except that they contain all possible spin-combinations in the tuple of numpy arrays. For example:
###Code
two_body_ints = TwoBodyElectronicIntegrals(
ElectronicBasis.MO,
(
np.arange(1, 17).reshape((2, 2, 2, 2)),
np.arange(16, 32).reshape((2, 2, 2, 2)),
np.arange(-16, 0).reshape((2, 2, 2, 2)),
None,
),
)
print(two_body_ints)
###Output
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 16.0
[0, 0, 0, 1] = 17.0
[0, 0, 1, 0] = 18.0
[0, 0, 1, 1] = 19.0
[0, 1, 0, 0] = 20.0
... skipping 11 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = -16.0
[0, 0, 0, 1] = -15.0
[0, 0, 1, 0] = -14.0
[0, 0, 1, 1] = -13.0
[0, 1, 0, 0] = -12.0
... skipping 11 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 16.0
[0, 0, 0, 1] = 24.0
[0, 0, 1, 0] = 20.0
[0, 0, 1, 1] = 28.0
[0, 1, 0, 0] = 18.0
... skipping 11 entries
###Markdown
We should take note of a few observations:* the numpy arrays shall be ordered as `("alpha-alpha", "beta-alpha", "beta-beta", "alpha-beta")`* the `alpha-alpha` matrix may **not** be `None`* if the `alpha-beta` matrix is `None`, but `beta-alpha` is not, its transpose will be used (like above)* in any other case, matrices which are `None` will be filled with the `alpha-alpha` matrix* in the `SO` basis case, a single numpy array must be specified. Refer to `TwoBodyElectronicIntegrals.to_spin()` for its exact formatting. ElectronicEnergy Now we are ready to construct an `ElectronicEnergy` instance:
###Code
electronic_energy = ElectronicEnergy(
[one_body_ints, two_body_ints],
)
print(electronic_energy)
###Output
ElectronicEnergy
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 1.0
[1, 1] = 1.0
Beta
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 2.0
[1, 1] = 2.0
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 16.0
[0, 0, 0, 1] = 17.0
[0, 0, 1, 0] = 18.0
[0, 0, 1, 1] = 19.0
[0, 1, 0, 0] = 20.0
... skipping 11 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = -16.0
[0, 0, 0, 1] = -15.0
[0, 0, 1, 0] = -14.0
[0, 0, 1, 1] = -13.0
[0, 1, 0, 0] = -12.0
... skipping 11 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 16.0
[0, 0, 0, 1] = 24.0
[0, 0, 1, 0] = 20.0
[0, 0, 1, 1] = 28.0
[0, 1, 0, 0] = 18.0
... skipping 11 entries
###Markdown
This property can now be used to construct a `SecondQuantizedOp` (which can then be mapped to a `QubitOperator`):
###Code
hamiltonian = electronic_energy.second_q_ops()[0] # here, output length is always 1
print(hamiltonian)
###Output
Fermionic Operator
register length=4, number terms=20
(22+0j) * ( +_0 -_1 +_2 -_3 )
+ (-26+0j) * ( +_0 -_1 -_2 +_3 )
+ (30+0j) * ( +_0 -_1 +_3 -_3 )
+ (18+0j) * ( +_0 -_1 +_2 -_2 )
+ (-21+0j) * ( -_0 +_1 +_2 -_3 )
+ (25+0j) * ( -_0 +_1 -_2 +_3 )
+ ...
###Markdown
Result interpretation An additional benefit which we gain from the `Property` framework, is that the result interpretation of a computed eigenvalue can be handled by each property itself. This results in nice and logically consistent classes because the result gets interpreted in the same context where the raw data is available.
###Code
from qiskit_nature.results import ElectronicStructureResult
# some dummy result
result = ElectronicStructureResult()
result.eigenenergies = np.asarray([-1])
result.computed_energies = np.asarray([-1])
# now, let's interpret it
electronic_energy.interpret(result)
print(result)
###Output
=== GROUND STATE ENERGY ===
* Electronic ground state energy (Hartree): -1
- computed part: -1
###Markdown
While this particular example is not yet very impressive, wait until we use more properties at once. ParticleNumber The `ParticleNumber` property also takes a special place among the builtin properties because it serves a dual purpose of:* storing the number of particles in the electronic system* providing the operators to evaluate the number of particles for a given eigensolution of the problemTherefore, this property is required if you want to use additional functionality like the `ActiveSpaceTransformer` or the `ElectronicStructureProblem.default_filter_criterion()`.
###Code
particle_number = ParticleNumber(
num_spin_orbitals = 4,
num_particles = (1, 1),
)
print(particle_number)
###Output
ParticleNumber:
4 SOs
1 alpha electrons
orbital occupation: [1. 0.]
1 beta electrons
orbital occupation: [1. 0.]
###Markdown
GroupedProperty Rather than iterating all of the other properties one by one, let us simply consider a group of properties as provided by any `ElectronicStructureDriver` from the `qiskit_nature.drivers.second_quantization` module.
###Code
from qiskit_nature.drivers.second_quantization.pyscfd import PySCFDriver
electronic_driver = PySCFDriver(atom="H 0 0 0; H 0 0 0.735", basis="sto3g")
electronic_driver_result = electronic_driver.run()
print(electronic_driver_result)
###Output
ElectronicStructureDriverResult:
DriverMetadata:
Program: PYSCF
Version: 1.7.6
Config:
atom=H 0 0 0; H 0 0 0.735
unit=Angstrom
charge=0
spin=0
basis=sto3g
method=rhf
conv_tol=1e-09
max_cycle=50
init_guess=minao
max_memory=4000
ElectronicBasisTransform:
Initial basis: atomic
Final basis: molecular
Alpha coefficients:
[0, 0] = 0.5483020229014736
[0, 1] = -1.2183273138546826
[1, 0] = 0.548302022901473
[1, 1] = 1.2183273138546828
Beta coefficients:
[0, 0] = 0.5483020229014736
[0, 1] = -1.2183273138546826
[1, 0] = 0.548302022901473
[1, 1] = 1.2183273138546828
ParticleNumber:
4 SOs
1 alpha electrons
orbital occupation: [1. 0.]
1 beta electrons
orbital occupation: [1. 0.]
ElectronicEnergy
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = -1.1242175791954514
[0, 1] = -0.9652573993472758
[1, 0] = -0.9652573993472758
[1, 1] = -1.1242175791954512
Beta
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = -1.1242175791954514
[0, 1] = -0.9652573993472758
[1, 0] = -0.9652573993472758
[1, 1] = -1.1242175791954512
(AO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 0.7746059439198978
[0, 0, 0, 1] = 0.4474457245330949
[0, 0, 1, 0] = 0.447445724533095
[0, 0, 1, 1] = 0.5718769760004512
[0, 1, 0, 0] = 0.4474457245330951
... skipping 11 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 0.7746059439198978
[0, 0, 0, 1] = 0.4474457245330949
[0, 0, 1, 0] = 0.447445724533095
[0, 0, 1, 1] = 0.5718769760004512
[0, 1, 0, 0] = 0.4474457245330951
... skipping 11 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 0.7746059439198978
[0, 0, 0, 1] = 0.4474457245330949
[0, 0, 1, 0] = 0.447445724533095
[0, 0, 1, 1] = 0.5718769760004512
[0, 1, 0, 0] = 0.4474457245330951
... skipping 11 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 0.7746059439198978
[0, 0, 0, 1] = 0.4474457245330949
[0, 0, 1, 0] = 0.447445724533095
[0, 0, 1, 1] = 0.5718769760004512
[0, 1, 0, 0] = 0.4474457245330951
... skipping 11 entries
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = -1.2563390730032507
[1, 1] = -0.47189600728114073
Beta
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = -1.2563390730032507
[1, 1] = -0.47189600728114073
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 8 non-zero entries>
[0, 0, 0, 0] = 0.675710154803517
[0, 0, 1, 1] = 0.6645817302552974
[0, 1, 0, 1] = 0.18093119978423153
[0, 1, 1, 0] = 0.18093119978423136
[1, 0, 0, 1] = 0.18093119978423164
... skipping 3 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 8 non-zero entries>
[0, 0, 0, 0] = 0.675710154803517
[0, 0, 1, 1] = 0.6645817302552974
[0, 1, 0, 1] = 0.18093119978423153
[0, 1, 1, 0] = 0.18093119978423136
[1, 0, 0, 1] = 0.18093119978423164
... skipping 3 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 8 non-zero entries>
[0, 0, 0, 0] = 0.675710154803517
[0, 0, 1, 1] = 0.6645817302552974
[0, 1, 0, 1] = 0.18093119978423153
[0, 1, 1, 0] = 0.18093119978423136
[1, 0, 0, 1] = 0.18093119978423164
... skipping 3 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 8 non-zero entries>
[0, 0, 0, 0] = 0.675710154803517
[0, 0, 1, 1] = 0.6645817302552974
[0, 1, 0, 1] = 0.18093119978423153
[0, 1, 1, 0] = 0.18093119978423136
[1, 0, 0, 1] = 0.18093119978423164
... skipping 3 entries
ElectronicDipoleMoment:
DipoleMomentX
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
<(2, 2) matrix with 0 non-zero entries>
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
<(2, 2) matrix with 0 non-zero entries>
DipoleMomentY
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
<(2, 2) matrix with 0 non-zero entries>
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
<(2, 2) matrix with 0 non-zero entries>
DipoleMomentZ
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 3 non-zero entries>
[0, 1] = 0.4605377079660319
[1, 0] = 0.4605377079660319
[1, 1] = 1.3889487015553204
Beta
<(2, 2) matrix with 3 non-zero entries>
[0, 1] = 0.4605377079660319
[1, 0] = 0.4605377079660319
[1, 1] = 1.3889487015553204
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = 0.69447435077766
[0, 1] = 0.9278334704592323
[1, 0] = 0.9278334704592324
[1, 1] = 0.6944743507776605
Beta
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = 0.69447435077766
[0, 1] = 0.9278334704592323
[1, 0] = 0.9278334704592324
[1, 1] = 0.6944743507776605
AngularMomentum:
4 SOs
Magnetization:
4 SOs
Molecule:
Multiplicity: 1
Charge: 0
Geometry:
H [0.0, 0.0, 0.0]
H [0.0, 0.0, 1.3889487015553204]
Masses:
H 1
H 1
###Markdown
There is a lot going on but with the explanations above you should not have any problems with understanding this output. Constructing a Hamiltonian from raw integrals If you have obtained some raw one- and two-body integrals by means other than through a driver provided by Qiskit Nature, you can still easily construct an `ElectronicEnergy` property to serve as your access point into the stack:
###Code
one_body_ints = np.arange(1, 5).reshape((2, 2))
two_body_ints = np.arange(1, 17).reshape((2, 2, 2, 2))
electronic_energy_from_ints = ElectronicEnergy.from_raw_integrals(ElectronicBasis.MO, one_body_ints, two_body_ints)
print(electronic_energy_from_ints)
###Output
ElectronicEnergy
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = 1.0
[0, 1] = 2.0
[1, 0] = 3.0
[1, 1] = 4.0
Beta
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = 1.0
[0, 1] = 2.0
[1, 0] = 3.0
[1, 1] = 4.0
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
###Markdown
Vibrational Second Quantization Properties The `vibrational` stack for vibrational structure calculations also integrates with the Property framework. After the above introduction you should be able to understand the following directly:
###Code
from qiskit_nature.drivers.second_quantization.gaussiand import GaussianForcesDriver
# if you ran Gaussian elsewhere and already have the output file
vibrational_driver = GaussianForcesDriver(logfile='aux_files/CO2_freq_B3LYP_ccpVDZ.log')
vibrational_driver_result = vibrational_driver.run()
###Output
_____no_output_____
###Markdown
For vibrational structure calculations we always need to define the basis which we want to work in, separately:
###Code
from qiskit_nature.properties.second_quantization.vibrational.bases import HarmonicBasis
harmonic_basis = HarmonicBasis([2] * 4)
vibrational_driver_result.basis = harmonic_basis
print(vibrational_driver_result)
###Output
VibrationalStructureDriverResult:
HarmonicBasis:
Modals: [2, 2, 2, 2]:
VibrationalEnergy:
HarmonicBasis:
Modals: [2, 2, 2, 2]
1-Body Terms:
<sparse integral list with 13 entries>
(1, 1) = 605.3643675
(-1, -1) = -605.3643675
(2, 2) = 340.5950575
(-2, -2) = -340.5950575
(3, 3) = 163.7595125
... skipping 8 entries
2-Body Terms:
<sparse integral list with 15 entries>
(2, 1, 1) = -89.09086530649508
(3, 3, 2) = 21.644966371722838
(4, 4, 2) = 6.412754934114705
(2, 2, 1, 1) = 5.03965375
(3, 1, 1, 1) = -2.4473854166666666
... skipping 10 entries
3-Body Terms:
<sparse integral list with 9 entries>
(3, 2, 1) = 44.01468537435673
(4, 2, 1) = -78.71701132125833
(4, 3, 2) = 17.15529085952822
(3, 2, 2, 1) = -3.73513125
(4, 2, 2, 1) = 6.68000625
... skipping 4 entries
OccupiedModals:
HarmonicBasis:
Modals: [2, 2, 2, 2]
###Markdown
Writing custom Properties You can extend the Property framework with your own implementations. Here, we will walk through the simple example of constructing a Property for the _electronic density_. Since this observable is essentially based on matrices, we will be leveraging the `OneBodyElectronicIntegrals` container to store the actual density matrix.
###Code
from itertools import product
from typing import List
from qiskit_nature.drivers import QMolecule
from qiskit_nature.operators.second_quantization import FermionicOp
from qiskit_nature.properties.second_quantization.electronic.bases import ElectronicBasis
from qiskit_nature.properties.second_quantization.electronic.types import ElectronicProperty
from qiskit_nature.properties.second_quantization.electronic.integrals import OneBodyElectronicIntegrals
class ElectronicDensity(ElectronicProperty):
"""A simple electronic density property.
This basic example works only in the MO basis!
"""
def __init__(self, num_molecular_orbitals: int) -> None:
super().__init__(self.__class__.__name__)
self._num_molecular_orbitals = num_molecular_orbitals
def __str__(self) -> str:
string = [super().__str__() + ":"]
string += [f"\t{self._num_molecular_orbitals} MOs"]
return "\n".join(string)
@classmethod
def from_legacy_driver_result(cls, result) -> "ElectronicDensity":
cls._validate_input_type(result, QMolecule)
qmol = cast(QMolecule, result)
return cls(qmol.num_molecular_orbitals)
def second_q_ops(self) -> List[FermionicOp]:
ops = []
# iterate all pairs of molecular orbitals
for mo_i, mo_j in product(range(self._num_molecular_orbitals), repeat=2):
# construct an auxiliary matrix where the only non-zero entry is at the current pair of MOs
number_op_matrix = np.zeros((self._num_molecular_orbitals, self._num_molecular_orbitals))
number_op_matrix[mo_i, mo_j] = 1
# leverage the OneBodyElectronicIntegrals to construct the corresponding FermionicOp
one_body_ints = OneBodyElectronicIntegrals(ElectronicBasis.MO, (number_op_matrix, number_op_matrix))
ops.append(one_body_ints.to_second_q_op())
return ops
def interpret(self, result) -> None:
# here goes the code which interprets the eigenvalues returned for the auxiliary operators
pass
density = ElectronicDensity(2)
print(density)
for idx, op in enumerate(density.second_q_ops()):
print(idx, ":", op)
###Output
0 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_0 -_0 )
+ (1+0j) * ( +_2 -_2 )
1 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_0 -_1 )
+ (1+0j) * ( +_2 -_3 )
2 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_1 -_0 )
+ (1+0j) * ( +_3 -_2 )
3 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_1 -_1 )
+ (1+0j) * ( +_3 -_3 )
###Markdown
Of course, the above example is very minimal and can be extended at will. **Note:** as of Qiskit Nature version 0.2.0, the direct integration of custom Property objects into the stack is not implemented yet, due to limitations of the auxiliary operator parsing. See https://github.com/Qiskit/qiskit-nature/issues/312 for more details.For the time being, you can still evaluate a custom Property, by passing it's generated operators directly to the `Eigensolver.solve` method by means of constructing the `aux_operators`. Note, however, that you will have to deal with transformations applied to your properties manually, until the above issue is resolved.
###Code
# set up some problem
problem = ...
# set up a solver
solver = ...
# when solving the problem, pass additional operators in like so:
aux_ops = density.second_q_ops()
# solver.solve(problem, aux_ops)
import qiskit.tools.jupyter
%qiskit_version_table
%qiskit_copyright
###Output
_____no_output_____
###Markdown
The Property Framework
###Code
import numpy as np
###Output
_____no_output_____
###Markdown
Qiskit Nature 0.2.0 introduces the _Property_ framework. This framework replaces the legacy driver return types like `QMolecule` and `WatsonHamiltonian` with a more modular and extensible approach. In this tutorial, we will walk through the framework, explain its most important components and show you, how you can extend it with a custom _property_ yourself. What is a `Property`? At its core, a `Property` is an object complementing some raw data with functions that allow you to transform/manipulate/interpret this raw data. This "definition" is kept rather abstract on purpose, but what it means is essentially the following.A `Property`:* represents a physical observable (that's the raw data)* can be expressed as an operator* can be evaluated with a wavefunction* provides an `interpret` method which gives meaning to the eigenvalue of the evaluated qubit operator
###Code
from qiskit_nature.properties import Property, GroupedProperty
###Output
_____no_output_____
###Markdown
The `qiskit_nature.properties` module provides two classes:1. `Property`: this is the basic interface. It requires only a `name` and an `interpret` method to be implemented.2. `GroupedProperty`: this class is an implementation of the [Composite pattern](https://en.wikipedia.org/wiki/Composite_pattern) which allows you to _group_ multiple properties into one.**Note:** Grouped properties must have unique `name` attributes! Second Quantization Properties At the time of writing, Qiskit Nature ships with a single variant of properties: the `SecondQuantizedProperty` objects.This sub-type adds one additional requirement because any `SecondQuantizedProperty`* **must** implement a `second_q_ops` method which constructs a (list of) `SecondQuantizedOp`s. The `qiskit_nature.properties.second_quantization` module is further divided into `electronic` and `vibrational` modules (similar to other modules of Qiskit Nature).Let us dive into the `electronic` sub-module first. Electronic Second Quantization Properties Out-of-the-box Qiskit Nature ships the following electronic properties:
###Code
from qiskit_nature.properties.second_quantization.electronic import (
ElectronicEnergy,
ElectronicDipoleMoment,
ParticleNumber,
AngularMomentum,
Magnetization,
)
###Output
/home/oss/Files/Qiskit/.direnv/python-3.9.6/lib/python3.9/site-packages/pyscf/lib/misc.py:46: H5pyDeprecationWarning: Using default_file_mode other than 'r' is deprecated. Pass the mode to h5py.File() instead.
h5py.get_config().default_file_mode = 'a'
###Markdown
`ElectronicEnergy` is arguably the most important property because it contains the _Hamiltonian_ representing the electronic structure problem whose eigenvalues we are interested in when solving an `ElectronicStructureProblem`.The way in which it stores this Hamiltonian is, just like the rest of the framework, highly modular. The initializer of the `ElectronicEnergy` has a single required argument, `electronic_integrals`, which is a `List[ElectronicIntegrals]`. ElectronicIntegrals
###Code
from qiskit_nature.properties.second_quantization.electronic.integrals import (
ElectronicIntegrals,
OneBodyElectronicIntegrals,
TwoBodyElectronicIntegrals,
IntegralProperty,
)
###Output
_____no_output_____
###Markdown
The `ElectronicIntegrals` are a container class for _n-body_ interactions in a given basis, which can be any of the following:
###Code
from qiskit_nature.properties.second_quantization.electronic.bases import ElectronicBasis
list(ElectronicBasis)
###Output
_____no_output_____
###Markdown
Let us take the `OneBodyElectronicIntegrals` as an example. As the name suggests, this container is for 1-body interaction integrals. You can construct an instance of it like so:
###Code
one_body_ints = OneBodyElectronicIntegrals(
ElectronicBasis.MO,
(
np.eye(2),
2 * np.eye(2),
),
)
print(one_body_ints)
###Output
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 1.0
[1, 1] = 1.0
Beta
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 2.0
[1, 1] = 2.0
###Markdown
As you can see, the first argument simply specifies the basis of the integrals.The second argument requires a little further explanation:1. In the case of the `AO` or `MO` basis, this argument **must** be a pair of numpy arrays, where the first and second one are the alpha- and beta-spin integrals, respectively.**Note:** The second argument may be `None`, for the case where the beta-spin integrals are the same as the alpha-spin integrals (so there is no need to provide the same values twice).2. In the case of the `SO` basis, this argument **must** be a single numpy array, storing the alpha- and beta-spin integrals in blocked order, i.e. like so:```pythonspin_basis = np.block([[alpha_spin, zeros], [zeros, beta_spin]])``` The `TwoBodyElectronicIntegrals` work pretty much the same except that they contain all possible spin-combinations in the tuple of numpy arrays. For example:
###Code
two_body_ints = TwoBodyElectronicIntegrals(
ElectronicBasis.MO,
(
np.arange(1, 17).reshape((2, 2, 2, 2)),
np.arange(16, 32).reshape((2, 2, 2, 2)),
np.arange(-16, 0).reshape((2, 2, 2, 2)),
None,
),
)
print(two_body_ints)
###Output
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 16.0
[0, 0, 0, 1] = 17.0
[0, 0, 1, 0] = 18.0
[0, 0, 1, 1] = 19.0
[0, 1, 0, 0] = 20.0
... skipping 11 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = -16.0
[0, 0, 0, 1] = -15.0
[0, 0, 1, 0] = -14.0
[0, 0, 1, 1] = -13.0
[0, 1, 0, 0] = -12.0
... skipping 11 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 16.0
[0, 0, 0, 1] = 24.0
[0, 0, 1, 0] = 20.0
[0, 0, 1, 1] = 28.0
[0, 1, 0, 0] = 18.0
... skipping 11 entries
###Markdown
We should take note of a few observations:* the numpy arrays shall be ordered as `("alpha-alpha", "beta-alpha", "beta-beta", "alpha-beta")`* the `alpha-alpha` matrix may **not** be `None`* if the `alpha-beta` matrix is `None`, but `beta-alpha` is not, its transpose will be used (like above)* in any other case, matrices which are `None` will be filled with the `alpha-alpha` matrix* in the `SO` basis case, a single numpy array must be specified. Refer to `TwoBodyElectronicIntegrals.to_spin()` for its exact formatting. ElectronicEnergy Now we are ready to construct an `ElectronicEnergy` instance:
###Code
electronic_energy = ElectronicEnergy(
[one_body_ints, two_body_ints],
)
print(electronic_energy)
###Output
ElectronicEnergy
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 1.0
[1, 1] = 1.0
Beta
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = 2.0
[1, 1] = 2.0
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 16.0
[0, 0, 0, 1] = 17.0
[0, 0, 1, 0] = 18.0
[0, 0, 1, 1] = 19.0
[0, 1, 0, 0] = 20.0
... skipping 11 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = -16.0
[0, 0, 0, 1] = -15.0
[0, 0, 1, 0] = -14.0
[0, 0, 1, 1] = -13.0
[0, 1, 0, 0] = -12.0
... skipping 11 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 16.0
[0, 0, 0, 1] = 24.0
[0, 0, 1, 0] = 20.0
[0, 0, 1, 1] = 28.0
[0, 1, 0, 0] = 18.0
... skipping 11 entries
###Markdown
This property can now be used to construct a `SecondQuantizedOp` (which can then be mapped to a `QubitOperator`):
###Code
hamiltonian = electronic_energy.second_q_ops()[0] # here, output length is always 1
print(hamiltonian)
###Output
Fermionic Operator
register length=4, number terms=20
(22+0j) * ( +_0 -_1 +_2 -_3 )
+ (-26+0j) * ( +_0 -_1 -_2 +_3 )
+ (30+0j) * ( +_0 -_1 +_3 -_3 )
+ (18+0j) * ( +_0 -_1 +_2 -_2 )
+ (-21+0j) * ( -_0 +_1 +_2 -_3 )
+ (25+0j) * ( -_0 +_1 -_2 +_3 )
+ ...
###Markdown
Result interpretation An additional benefit which we gain from the `Property` framework, is that the result interpretation of a computed eigenvalue can be handled by each property itself. This results in nice and logically consistent classes because the result gets interpreted in the same context where the raw data is available.
###Code
from qiskit_nature.results import ElectronicStructureResult
# some dummy result
result = ElectronicStructureResult()
result.eigenenergies = np.asarray([-1])
result.computed_energies = np.asarray([-1])
# now, let's interpret it
electronic_energy.interpret(result)
print(result)
###Output
=== GROUND STATE ENERGY ===
* Electronic ground state energy (Hartree): -1
- computed part: -1
###Markdown
While this particular example is not yet very impressive, wait until we use more properties at once. ParticleNumber The `ParticleNumber` property also takes a special place among the builtin properties because it serves a dual purpose of:* storing the number of particles in the electronic system* providing the operators to evaluate the number of particles for a given eigensolution of the problemTherefore, this property is required if you want to use additional functionality like the `ActiveSpaceTransformer` or the `ElectronicStructureProblem.default_filter_criterion()`.
###Code
particle_number = ParticleNumber(
num_spin_orbitals=4,
num_particles=(1, 1),
)
print(particle_number)
###Output
ParticleNumber:
4 SOs
1 alpha electrons
orbital occupation: [1. 0.]
1 beta electrons
orbital occupation: [1. 0.]
###Markdown
GroupedProperty Rather than iterating all of the other properties one by one, let us simply consider a group of properties as provided by any `ElectronicStructureDriver` from the `qiskit_nature.drivers.second_quantization` module.
###Code
from qiskit_nature.drivers.second_quantization.pyscfd import PySCFDriver
electronic_driver = PySCFDriver(atom="H 0 0 0; H 0 0 0.735", basis="sto3g")
electronic_driver_result = electronic_driver.run()
print(electronic_driver_result)
###Output
ElectronicStructureDriverResult:
DriverMetadata:
Program: PYSCF
Version: 1.7.6
Config:
atom=H 0 0 0; H 0 0 0.735
unit=Angstrom
charge=0
spin=0
basis=sto3g
method=rhf
conv_tol=1e-09
max_cycle=50
init_guess=minao
max_memory=4000
ElectronicBasisTransform:
Initial basis: atomic
Final basis: molecular
Alpha coefficients:
[0, 0] = 0.5483020229014736
[0, 1] = -1.2183273138546826
[1, 0] = 0.548302022901473
[1, 1] = 1.2183273138546828
Beta coefficients:
[0, 0] = 0.5483020229014736
[0, 1] = -1.2183273138546826
[1, 0] = 0.548302022901473
[1, 1] = 1.2183273138546828
ParticleNumber:
4 SOs
1 alpha electrons
orbital occupation: [1. 0.]
1 beta electrons
orbital occupation: [1. 0.]
ElectronicEnergy
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = -1.1242175791954514
[0, 1] = -0.9652573993472758
[1, 0] = -0.9652573993472758
[1, 1] = -1.1242175791954512
Beta
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = -1.1242175791954514
[0, 1] = -0.9652573993472758
[1, 0] = -0.9652573993472758
[1, 1] = -1.1242175791954512
(AO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 0.7746059439198978
[0, 0, 0, 1] = 0.4474457245330949
[0, 0, 1, 0] = 0.447445724533095
[0, 0, 1, 1] = 0.5718769760004512
[0, 1, 0, 0] = 0.4474457245330951
... skipping 11 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 0.7746059439198978
[0, 0, 0, 1] = 0.4474457245330949
[0, 0, 1, 0] = 0.447445724533095
[0, 0, 1, 1] = 0.5718769760004512
[0, 1, 0, 0] = 0.4474457245330951
... skipping 11 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 0.7746059439198978
[0, 0, 0, 1] = 0.4474457245330949
[0, 0, 1, 0] = 0.447445724533095
[0, 0, 1, 1] = 0.5718769760004512
[0, 1, 0, 0] = 0.4474457245330951
... skipping 11 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 0.7746059439198978
[0, 0, 0, 1] = 0.4474457245330949
[0, 0, 1, 0] = 0.447445724533095
[0, 0, 1, 1] = 0.5718769760004512
[0, 1, 0, 0] = 0.4474457245330951
... skipping 11 entries
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = -1.2563390730032507
[1, 1] = -0.47189600728114073
Beta
<(2, 2) matrix with 2 non-zero entries>
[0, 0] = -1.2563390730032507
[1, 1] = -0.47189600728114073
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 8 non-zero entries>
[0, 0, 0, 0] = 0.675710154803517
[0, 0, 1, 1] = 0.6645817302552974
[0, 1, 0, 1] = 0.18093119978423153
[0, 1, 1, 0] = 0.18093119978423136
[1, 0, 0, 1] = 0.18093119978423164
... skipping 3 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 8 non-zero entries>
[0, 0, 0, 0] = 0.675710154803517
[0, 0, 1, 1] = 0.6645817302552974
[0, 1, 0, 1] = 0.18093119978423153
[0, 1, 1, 0] = 0.18093119978423136
[1, 0, 0, 1] = 0.18093119978423164
... skipping 3 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 8 non-zero entries>
[0, 0, 0, 0] = 0.675710154803517
[0, 0, 1, 1] = 0.6645817302552974
[0, 1, 0, 1] = 0.18093119978423153
[0, 1, 1, 0] = 0.18093119978423136
[1, 0, 0, 1] = 0.18093119978423164
... skipping 3 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 8 non-zero entries>
[0, 0, 0, 0] = 0.675710154803517
[0, 0, 1, 1] = 0.6645817302552974
[0, 1, 0, 1] = 0.18093119978423153
[0, 1, 1, 0] = 0.18093119978423136
[1, 0, 0, 1] = 0.18093119978423164
... skipping 3 entries
ElectronicDipoleMoment:
DipoleMomentX
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
<(2, 2) matrix with 0 non-zero entries>
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
<(2, 2) matrix with 0 non-zero entries>
DipoleMomentY
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
<(2, 2) matrix with 0 non-zero entries>
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 0 non-zero entries>
Beta
<(2, 2) matrix with 0 non-zero entries>
DipoleMomentZ
(AO) 1-Body Terms:
Alpha
<(2, 2) matrix with 3 non-zero entries>
[0, 1] = 0.4605377079660319
[1, 0] = 0.4605377079660319
[1, 1] = 1.3889487015553204
Beta
<(2, 2) matrix with 3 non-zero entries>
[0, 1] = 0.4605377079660319
[1, 0] = 0.4605377079660319
[1, 1] = 1.3889487015553204
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = 0.69447435077766
[0, 1] = 0.9278334704592323
[1, 0] = 0.9278334704592324
[1, 1] = 0.6944743507776605
Beta
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = 0.69447435077766
[0, 1] = 0.9278334704592323
[1, 0] = 0.9278334704592324
[1, 1] = 0.6944743507776605
AngularMomentum:
4 SOs
Magnetization:
4 SOs
Molecule:
Multiplicity: 1
Charge: 0
Geometry:
H [0.0, 0.0, 0.0]
H [0.0, 0.0, 1.3889487015553204]
Masses:
H 1
H 1
###Markdown
There is a lot going on but with the explanations above you should not have any problems with understanding this output. Constructing a Hamiltonian from raw integrals If you have obtained some raw one- and two-body integrals by means other than through a driver provided by Qiskit Nature, you can still easily construct an `ElectronicEnergy` property to serve as your access point into the stack:
###Code
one_body_ints = np.arange(1, 5).reshape((2, 2))
two_body_ints = np.arange(1, 17).reshape((2, 2, 2, 2))
electronic_energy_from_ints = ElectronicEnergy.from_raw_integrals(
ElectronicBasis.MO, one_body_ints, two_body_ints
)
print(electronic_energy_from_ints)
###Output
ElectronicEnergy
(MO) 1-Body Terms:
Alpha
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = 1.0
[0, 1] = 2.0
[1, 0] = 3.0
[1, 1] = 4.0
Beta
<(2, 2) matrix with 4 non-zero entries>
[0, 0] = 1.0
[0, 1] = 2.0
[1, 0] = 3.0
[1, 1] = 4.0
(MO) 2-Body Terms:
Alpha-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Alpha
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Beta-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
Alpha-Beta
<(2, 2, 2, 2) matrix with 16 non-zero entries>
[0, 0, 0, 0] = 1.0
[0, 0, 0, 1] = 2.0
[0, 0, 1, 0] = 3.0
[0, 0, 1, 1] = 4.0
[0, 1, 0, 0] = 5.0
... skipping 11 entries
###Markdown
Vibrational Second Quantization Properties The `vibrational` stack for vibrational structure calculations also integrates with the Property framework. After the above introduction you should be able to understand the following directly:
###Code
from qiskit_nature.drivers.second_quantization.gaussiand import GaussianForcesDriver
# if you ran Gaussian elsewhere and already have the output file
vibrational_driver = GaussianForcesDriver(logfile="aux_files/CO2_freq_B3LYP_ccpVDZ.log")
vibrational_driver_result = vibrational_driver.run()
###Output
_____no_output_____
###Markdown
For vibrational structure calculations we always need to define the basis which we want to work in, separately:
###Code
from qiskit_nature.properties.second_quantization.vibrational.bases import HarmonicBasis
harmonic_basis = HarmonicBasis([2] * 4)
vibrational_driver_result.basis = harmonic_basis
print(vibrational_driver_result)
###Output
VibrationalStructureDriverResult:
HarmonicBasis:
Modals: [2, 2, 2, 2]:
VibrationalEnergy:
HarmonicBasis:
Modals: [2, 2, 2, 2]
1-Body Terms:
<sparse integral list with 13 entries>
(1, 1) = 605.3643675
(-1, -1) = -605.3643675
(2, 2) = 340.5950575
(-2, -2) = -340.5950575
(3, 3) = 163.7595125
... skipping 8 entries
2-Body Terms:
<sparse integral list with 15 entries>
(2, 1, 1) = -89.09086530649508
(3, 3, 2) = 21.644966371722838
(4, 4, 2) = 6.412754934114705
(2, 2, 1, 1) = 5.03965375
(3, 1, 1, 1) = -2.4473854166666666
... skipping 10 entries
3-Body Terms:
<sparse integral list with 9 entries>
(3, 2, 1) = 44.01468537435673
(4, 2, 1) = -78.71701132125833
(4, 3, 2) = 17.15529085952822
(3, 2, 2, 1) = -3.73513125
(4, 2, 2, 1) = 6.68000625
... skipping 4 entries
OccupiedModals:
HarmonicBasis:
Modals: [2, 2, 2, 2]
###Markdown
Writing custom Properties You can extend the Property framework with your own implementations. Here, we will walk through the simple example of constructing a Property for the _electronic density_. Since this observable is essentially based on matrices, we will be leveraging the `OneBodyElectronicIntegrals` container to store the actual density matrix.
###Code
from itertools import product
from typing import List
from qiskit_nature.drivers import QMolecule
from qiskit_nature.operators.second_quantization import FermionicOp
from qiskit_nature.properties.second_quantization.electronic.bases import ElectronicBasis
from qiskit_nature.properties.second_quantization.electronic.types import ElectronicProperty
from qiskit_nature.properties.second_quantization.electronic.integrals import (
OneBodyElectronicIntegrals,
)
class ElectronicDensity(ElectronicProperty):
"""A simple electronic density property.
This basic example works only in the MO basis!
"""
def __init__(self, num_molecular_orbitals: int) -> None:
super().__init__(self.__class__.__name__)
self._num_molecular_orbitals = num_molecular_orbitals
def __str__(self) -> str:
string = [super().__str__() + ":"]
string += [f"\t{self._num_molecular_orbitals} MOs"]
return "\n".join(string)
@classmethod
def from_legacy_driver_result(cls, result) -> "ElectronicDensity":
cls._validate_input_type(result, QMolecule)
qmol = cast(QMolecule, result)
return cls(qmol.num_molecular_orbitals)
def second_q_ops(self) -> List[FermionicOp]:
ops = []
# iterate all pairs of molecular orbitals
for mo_i, mo_j in product(range(self._num_molecular_orbitals), repeat=2):
# construct an auxiliary matrix where the only non-zero entry is at the current pair of MOs
number_op_matrix = np.zeros(
(self._num_molecular_orbitals, self._num_molecular_orbitals)
)
number_op_matrix[mo_i, mo_j] = 1
# leverage the OneBodyElectronicIntegrals to construct the corresponding FermionicOp
one_body_ints = OneBodyElectronicIntegrals(
ElectronicBasis.MO, (number_op_matrix, number_op_matrix)
)
ops.append(one_body_ints.to_second_q_op())
return ops
def interpret(self, result) -> None:
# here goes the code which interprets the eigenvalues returned for the auxiliary operators
pass
density = ElectronicDensity(2)
print(density)
for idx, op in enumerate(density.second_q_ops()):
print(idx, ":", op)
###Output
0 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_0 -_0 )
+ (1+0j) * ( +_2 -_2 )
1 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_0 -_1 )
+ (1+0j) * ( +_2 -_3 )
2 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_1 -_0 )
+ (1+0j) * ( +_3 -_2 )
3 : Fermionic Operator
register length=4, number terms=2
(1+0j) * ( +_1 -_1 )
+ (1+0j) * ( +_3 -_3 )
###Markdown
Of course, the above example is very minimal and can be extended at will. **Note:** as of Qiskit Nature version 0.2.0, the direct integration of custom Property objects into the stack is not implemented yet, due to limitations of the auxiliary operator parsing. See https://github.com/Qiskit/qiskit-nature/issues/312 for more details.For the time being, you can still evaluate a custom Property, by passing it's generated operators directly to the `Eigensolver.solve` method by means of constructing the `aux_operators`. Note, however, that you will have to deal with transformations applied to your properties manually, until the above issue is resolved.
###Code
# set up some problem
problem = ...
# set up a solver
solver = ...
# when solving the problem, pass additional operators in like so:
aux_ops = density.second_q_ops()
# solver.solve(problem, aux_ops)
import qiskit.tools.jupyter
%qiskit_version_table
%qiskit_copyright
###Output
_____no_output_____ |
Phase_3/ds-k-nearest_neighbors-main/knn.ipynb | ###Markdown
Table of Contents1 Objectives2 Concept of the $k$-Nearest Neighbors Algorithm2.1 Who's Nearby?2.2 Summary of $k$NN2.3 Implementing in Scikit-Learn2.3.1 Training the KNN2.3.2 Make Some Predictions3 The Pros and Cons3.1 Advantages3.2 Disadvantages4 Classification with sklearn.neighbors4.1 Train-Test Split4.2 Validation Split4.3 Different $k$ Values4.3.1 $k=1$4.3.2 $k=3$4.3.3 $k=5$4.3.4 Observing Different $k$ Values4.4 Scaling4.4.1 More Resources on Scaling5 $k$ and the Bias-Variance Tradeoff5.1 The Relation Between $k$ and Bias/Variance6 Level Up: Distance Metrics 
###Code
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.metrics import f1_score, confusion_matrix,\
recall_score, precision_score, accuracy_score
from src.confusion import plot_confusion_matrix
from src.k_classify import predict_one
from src.plot_train import *
from src.euclid import *
from sklearn import datasets
from sklearn.preprocessing import StandardScaler, MinMaxScaler, LabelEncoder
from sklearn.neighbors import KNeighborsClassifier, NearestNeighbors
from sklearn.model_selection import train_test_split, KFold
###Output
_____no_output_____
###Markdown
Objectives - Describe the $k$-nearest neighbors algorithm- Identify multiple common distance metrics- Tune $k$ appropriately in response to models with high bias or variance Concept of the $k$-Nearest Neighbors Algorithm
###Code
#KNN can be used with more than one target.
###Output
_____no_output_____
###Markdown
First let's recall what is **supervised learning**.> In **supervised learning** we use example data (_training data_) to inform our predictions of future data Note that this definition includes _classification_ and _regression_ problems. And there are a variety of ways we can make predictions from past data.$k$-nearest neighbors is one such method of making predictions. Who's Nearby? One strategy to make predictions on a new data is to just look at what _similar_ data points are like.  We can say _nearby_ points are _similar_ to one another. There are a few different wasy to determine how "close" data points are to one another. Check out the [Level Up section on distance metrics](Level-Up:-Distance-Metrics) for some more detail. Summary of $k$NN  Implementing in Scikit-Learn > [`KNeighborsClassifier`](https://scikit-learn.org/stable/modules/generated/sklearn.neighbors.KNeighborsClassifier.html) & [`KNeighborsRegressor`](https://scikit-learn.org/stable/modules/generated/sklearn.neighbors.KNeighborsRegressor.html) Let's try doing some basic classification on some data using the KNN algorithms.
###Code
iris = sns.load_dataset('iris')
display(iris)
# Let's convert this over to NumPy array
X = iris.iloc[:,:2].to_numpy()
# Let's convert classes to numerical values
y = LabelEncoder().fit_transform(iris['species'])
y
f, ax = plt.subplots()
sns.scatterplot(x=X[:,0], y=X[:,1], ax=ax, hue=y, palette='colorblind')
ax.get_legend().remove()
###Output
_____no_output_____
###Markdown
Training the KNN
###Code
neigh = KNeighborsClassifier(n_neighbors=3, metric='euclidean')
neigh.fit(X, y)
###Output
_____no_output_____
###Markdown
Make Some Predictions
###Code
# Made up data points
pred_pts = np.array([
[7.0, 3.0],
[8.0, 3.5],
[7.0, 4.0],
[4.0, 3.0],
[5.0, 3.0],
[5.5, 4.0],
[5.0, 2.0],
[6.0, 2.5],
[5.8, 3.5],
])
###Output
_____no_output_____
###Markdown
Let's see these new points against the training data. Think about how they'll be made classified.
###Code
f, ax = plt.subplots()
sns.scatterplot(x=X[:,0], y=X[:,1], ax=ax, hue=y, palette='colorblind')
sns.scatterplot(x=pred_pts[:,0], ax=ax, y=pred_pts[:,1], marker="*", s=200, edgecolor='black', color='magenta')
ax.get_legend().remove()
# Make predictions
pred_y = neigh.predict(pred_pts)
print(pred_y)
# Probabilities for KNN (how they voted)
for p,prob in zip(pred_y,neigh.predict_proba(pred_pts)):
print(f'{p}: {prob}')
f, ax = plt.subplots()
sns.scatterplot(x=X[:,0],y=X[:,1], ax=ax, hue=y, palette='colorblind')
sns.scatterplot(x=pred_pts[:,0], ax=ax, y=pred_pts[:,1], hue=pred_y, palette='colorblind', marker="*", s=200, edgecolor='black')
ax.get_legend().remove()
###Output
_____no_output_____
###Markdown
Let's see those predictions plotted with the other points after the classification. The Pros and Cons Models have different use cases and it helps to understand the strengths and weaknesses Advantages - Lazy learning (no training phase)- Simple algorithm to understand and implement Disadvantages - Has to be kept in memory (small data with few features)- Not robust; doesn't generalize well- Soft boundaries are troublesome- "Curse of Dimensionality" Classification with `sklearn.neighbors` $k$-Nearest Neighbors is a modeling technique that works for both regression and classification problems. Here we'll apply it to a version of the Titanic dataset.
###Code
titanic = pd.read_csv('data/cleaned_titanic.csv')
titanic = titanic.iloc[:, :-2]
titanic.head()
###Output
_____no_output_____
###Markdown
**For visualization purposes, we will use only two features for our first model.**
###Code
X = titanic[['Age', 'Fare']]
y = titanic['Survived']
y.value_counts()
###Output
_____no_output_____
###Markdown
Train-Test Split This dataset of course presents a binary classification problem, with our target being the `Survived` feature.
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
###Output
_____no_output_____
###Markdown
Validation Split
###Code
X_t, X_val, y_t, y_val = train_test_split(X_train, y_train,
random_state=42,
test_size=0.25)
knn = KNeighborsClassifier()
knn.fit(X_t, y_t)
print(f"training accuracy: {knn.score(X_t, y_t)}")
print(f"validation accuracy: {knn.score(X_val, y_val)}")
y_hat = knn.predict(X_val)
plot_confusion_matrix(confusion_matrix(y_val, y_hat), classes=['Perished', 'Survived'])
X_for_viz = X_t.sample(15, random_state=40)
y_for_viz = y_t[X_for_viz.index]
fig, ax = plt.subplots(figsize=(10, 10))
sns.scatterplot(X_for_viz['Age'], X_for_viz['Fare'],
hue=y_for_viz, palette={0: 'red', 1: 'green'},
s=200, ax=ax)
ax.set_xlim(0, 80)
ax.set_ylim(0, 80)
plt.legend()
plt.title('Subsample of Training Data');
###Output
C:\Users\IM\anaconda3\lib\site-packages\seaborn\_decorators.py:36: FutureWarning: Pass the following variables as keyword args: x, y. From version 0.12, the only valid positional argument will be `data`, and passing other arguments without an explicit keyword will result in an error or misinterpretation.
warnings.warn(
###Markdown
The $k$-NN algorithm works by simply storing the training set in memory, then measuring the distance from the training points to a new point.Let's drop a point from our validation set into the plot above.
###Code
X_for_viz = X_t.sample(15, random_state=40)
y_for_viz = y_t[X_for_viz.index]
fig, ax = plt.subplots(figsize=(10, 10))
sns.scatterplot(X_for_viz['Age'], X_for_viz['Fare'],
hue=y_for_viz, palette={0: 'red', 1: 'green'},
s=200, ax=ax)
plt.legend()
#################^^^Old code^^^##############
####################New code#################
# Let's take one sample from our validation set and plot it
new_x = pd.DataFrame(X_val.loc[484]).T
new_y = y_val[new_x.index]
sns.scatterplot(new_x['Age'], new_x['Fare'], color='blue',
s=200, ax=ax, label='New', marker='P')
ax.set_xlim(0, 100)
ax.set_ylim(0, 100);
new_x
###Output
_____no_output_____
###Markdown
Then, $k$-NN finds the $k$ nearest points. $k$ corresponds to the `n_neighbors` parameter defined when we instantiate the classifier object. **If $k$ = 1, then the prediction for a point will simply be the value of the target for the nearest point.** Different $k$ Values A big factor in this algorithm is choosing $k$  $k=1$
###Code
knn = KNeighborsClassifier(n_neighbors=1)
###Output
_____no_output_____
###Markdown
Let's fit our training data, then predict what our validation point will be based on the (one) closest neighbor.
###Code
knn.fit(X_for_viz, y_for_viz)
knn.predict(new_x)
###Output
_____no_output_____
###Markdown
**When we raise the value of $k$, $k$-NN will act democratically: It will find the $k$ closest points, and take a vote based on the labels.** $k=3$ Let's raise $k$ to 3.
###Code
knn3 = KNeighborsClassifier(n_neighbors=3)
knn3.fit(X_for_viz, y_for_viz)
knn3.predict(new_x)
###Output
_____no_output_____
###Markdown
It's not easy to tell what which points are closest by eye.Let's update our plot to add indices.
###Code
X_for_viz = X_t.sample(15, random_state=40)
y_for_viz = y_t[X_for_viz.index]
fig, ax = plt.subplots(figsize=(10,10))
sns.scatterplot(X_for_viz['Age'], X_for_viz['Fare'], hue=y_for_viz,
palette={0: 'red', 1: 'green'}, s=200, ax=ax)
# Now let's take another sample
# new_x = X_val.sample(1, random_state=33)
new_x = pd.DataFrame(X_val.loc[484]).T
new_x.columns = ['Age', 'Fare']
new_y = y_val[new_x.index]
print(new_x)
sns.scatterplot(new_x['Age'], new_x['Fare'], color='blue',
s=200, ax=ax, label='New', marker='P')
ax.set_xlim(0, 100)
ax.set_ylim(0, 100)
plt.legend()
#################^^^Old code^^^##############
####################New code#################
# add annotations one by one with a loop
for index in X_for_viz.index:
ax.text(X_for_viz.Age[index]+0.7, X_for_viz.Fare[index],
s=index, horizontalalignment='left', size='medium',
color='black', weight='semibold')
###Output
C:\Users\IM\anaconda3\lib\site-packages\seaborn\_decorators.py:36: FutureWarning: Pass the following variables as keyword args: x, y. From version 0.12, the only valid positional argument will be `data`, and passing other arguments without an explicit keyword will result in an error or misinterpretation.
warnings.warn(
C:\Users\IM\anaconda3\lib\site-packages\seaborn\_decorators.py:36: FutureWarning: Pass the following variables as keyword args: x, y. From version 0.12, the only valid positional argument will be `data`, and passing other arguments without an explicit keyword will result in an error or misinterpretation.
warnings.warn(
###Markdown
We can use `sklearn`'s NearestNeighors object to see the exact calculations.
###Code
df_for_viz = pd.merge(X_for_viz, y_for_viz, left_index=True, right_index=True)
neighbor = NearestNeighbors(3)
neighbor.fit(X_for_viz)
nearest = neighbor.kneighbors(new_x)
nearest
df_for_viz.iloc[nearest[1][0]]
new_x
# Use Euclidean distance to see how close they are to this point
print(((29-24)**2 + (33-25.4667)**2)**0.5)
print(((26-24)**2 + (16.1-25.4667)**2)**0.5)
print(((20-24)**2 + (15.7417-25.4667)**2)**0.5)
###Output
9.041604331643805
9.57784260102451
10.515494519992865
###Markdown
$k=5$ And with five neighbors?
###Code
knn = KNeighborsClassifier(n_neighbors=5)
knn.fit(X_for_viz, y_for_viz)
knn.predict(new_x)
###Output
_____no_output_____
###Markdown
Observing Different $k$ Values Let's iterate through $k$, odd numbers 1 through 10, and see the predictions.
###Code
for k in range(1, 10, 2):
knn = KNeighborsClassifier(n_neighbors=k)
knn.fit(X_for_viz, y_for_viz)
print(f'k={k}', knn.predict(new_x))
###Output
k=1 [1]
k=3 [1]
k=5 [0]
k=7 [0]
k=9 [0]
###Markdown
Which models were correct?
###Code
new_y
###Output
_____no_output_____
###Markdown
Scaling You may have suspected that we were leaving something out. For any distance-based algorithms, scaling is very important. Look at how the shape of the array changes before and after scaling.   Let's look at our data_for_viz dataset:
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
X_t, X_val, y_t, y_val = train_test_split(X_train, y_train,
random_state=42,
test_size=0.25)
knn = KNeighborsClassifier(n_neighbors=5)
ss = StandardScaler()
X_ind = X_t.index
X_col = X_t.columns
X_t_s = pd.DataFrame(ss.fit_transform(X_t))
X_t_s.index = X_ind
X_t_s.columns = X_col
X_v_ind = X_val.index
X_val_s = pd.DataFrame(ss.transform(X_val))
X_val_s.index = X_v_ind
X_val_s.columns = X_col
knn.fit(X_t_s, y_t)
print(f"training accuracy: {knn.score(X_t_s, y_t)}")
print(f"Val accuracy: {knn.score(X_val_s, y_val)}")
y_hat = knn.predict(X_val_s)
# The plot_train() function just does what we did above.
plot_train(X_t, y_t, X_val, y_val)
plot_train(X_t_s, y_t, X_val_s, y_val, -2, 2, text_pos=0.1 )
###Output
Age Fare
484 24.0 25.4667
###Markdown
Look at how much that changes things.Look at points 166 and 150. Look at the group 621, 143, and 191. Now let's run our classifier on scaled data and compare to unscaled.
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
X_t, X_val, y_t, y_val = train_test_split(X_train, y_train,
random_state=42,
test_size=0.25)
# The predict_one() function prints predictions on a given point
# (#484) for k-nn models with k ranging from 1 to 10.
predict_one(X_t, X_val, y_t, y_val)
mm = MinMaxScaler()
X_t_s = pd.DataFrame(mm.fit_transform(X_t))
X_t_s.index = X_t.index
X_t_s.columns = X_t.columns
X_val_s = pd.DataFrame(mm.transform(X_val))
X_val_s.index = X_val.index
X_val_s.columns = X_val.columns
predict_one(X_t_s, X_val_s, y_t, y_val)
###Output
[0]
[0]
[0]
[0]
[1]
[1]
[1]
[1]
[1]
[1]
###Markdown
More Resources on Scaling https://sebastianraschka.com/Articles/2014_about_feature_scaling.html http://datareality.blogspot.com/2016/11/scaling-normalizing-standardizing-which.html $k$ and the Bias-Variance Tradeoff
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
# Let's slowly increase k and see what happens to our accuracy scores.
kf = KFold(n_splits=5)
k_scores_train = {}
k_scores_val = {}
for k in range(1, 20):
knn = KNeighborsClassifier(n_neighbors=k)
accuracy_score_t = []
accuracy_score_v = []
for train_ind, val_ind in kf.split(X_train, y_train):
X_t, y_t = X_train.iloc[train_ind], y_train.iloc[train_ind]
X_v, y_v = X_train.iloc[val_ind], y_train.iloc[val_ind]
mm = MinMaxScaler()
X_t_ind = X_t.index
X_v_ind = X_v.index
X_t = pd.DataFrame(mm.fit_transform(X_t))
X_t.index = X_t_ind
X_v = pd.DataFrame(mm.transform(X_v))
X_v.index = X_v_ind
knn.fit(X_t, y_t)
y_pred_t = knn.predict(X_t)
y_pred_v = knn.predict(X_v)
accuracy_score_t.append(accuracy_score(y_t, y_pred_t))
accuracy_score_v.append(accuracy_score(y_v, y_pred_v))
k_scores_train[k] = np.mean(accuracy_score_t)
k_scores_val[k] = np.mean(accuracy_score_v)
k_scores_train
k_scores_val
fig, ax = plt.subplots(figsize=(15, 15))
ax.plot(list(k_scores_train.keys()), list(k_scores_train.values()),
color='red', linestyle='dashed', marker='o',
markerfacecolor='blue', markersize=10, label='Train')
ax.plot(list(k_scores_val.keys()), list(k_scores_val.values()),
color='green', linestyle='dashed', marker='o',
markerfacecolor='blue', markersize=10, label='Val')
ax.set_xlabel('k')
ax.set_ylabel('Accuracy')
plt.legend();
###Output
_____no_output_____
###Markdown
The Relation Between $k$ and Bias/Variance > Small $k$ values leads to overfitting, but larger $k$ values tend towards underfitting  > From [Machine Learning Flashcards](https://machinelearningflashcards.com/) by Chris Albon
###Code
mm = MinMaxScaler()
X_train_ind = X_train.index
X_train = pd.DataFrame(mm.fit_transform(X_train))
X_train.index = X_train_ind
X_test_ind = X_test.index
X_test = pd.DataFrame(mm.transform(X_test))
X_test.index = X_test_ind
knn = KNeighborsClassifier(n_neighbors=9)
knn.fit(X_train, y_train)
print(f"training accuracy: {knn.score(X_train, y_train)}")
print(f"Test accuracy: {knn.score(X_test, y_test)}")
y_hat = knn.predict(X_test)
plot_confusion_matrix(confusion_matrix(y_test, y_hat), classes=['Perished', 'Survived'])
recall_score(y_test, y_hat)
precision_score(y_test, y_hat)
###Output
_____no_output_____
###Markdown
Level Up: Distance Metrics > The "closeness" of data points → proxy for similarity  **Minkowski Distance**:$$dist(A,B) = (\sum_{k=1}^{N} |a_k - b_k|^c)^\frac{1}{c} $$
###Code
#Minkowski is a generalization. With a value of 2 it is the same as Euclidean
###Output
_____no_output_____
###Markdown
Table of Contents1 Objectives2 Concept of the $k$-Nearest Neighbors Algorithm2.1 Who's Nearby?2.2 Summary of $k$NN2.3 Implementing in Scikit-Learn2.3.1 Training the KNN2.3.2 Make Some Predictions3 The Pros and Cons3.1 Advantages3.2 Disadvantages4 Classification with sklearn.neighbors4.1 Train-Test Split4.2 Validation Split4.3 Different $k$ Values4.3.1 $k=1$4.3.2 $k=3$4.3.3 $k=5$4.3.4 Observing Different $k$ Values4.4 Scaling4.4.1 More Resources on Scaling5 $k$ and the Bias-Variance Tradeoff5.1 The Relation Between $k$ and Bias/Variance6 Level Up: Distance Metrics 
###Code
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.metrics import f1_score, confusion_matrix,\
recall_score, precision_score, accuracy_score
from src.confusion import plot_confusion_matrix
from src.k_classify import predict_one
from src.plot_train import *
from src.euclid import *
from sklearn import datasets
from sklearn.preprocessing import StandardScaler, MinMaxScaler, LabelEncoder
from sklearn.neighbors import KNeighborsClassifier, NearestNeighbors
from sklearn.model_selection import train_test_split, KFold
###Output
_____no_output_____
###Markdown
Objectives - Describe the $k$-nearest neighbors algorithm- Identify multiple common distance metrics- Tune $k$ appropriately in response to models with high bias or variance Concept of the $k$-Nearest Neighbors Algorithm First let's recall what is **supervised learning**.> In **supervised learning** we use example data (_training data_) to inform our predictions of future data Note that this definition includes _classification_ and _regression_ problems. And there are a variety of ways we can make predictions from past data.$k$-nearest neighbors is one such method of making predictions. Who's Nearby? One strategy to make predictions on a new data is to just look at what _similar_ data points are like.  We can say _nearby_ points are _similar_ to one another. There are a few different wasy to determine how "close" data points are to one another. Check out the [Level Up section on distance metrics](Level-Up:-Distance-Metrics) for some more detail. Summary of $k$NN  Implementing in Scikit-Learn > [`KNeighborsClassifier`](https://scikit-learn.org/stable/modules/generated/sklearn.neighbors.KNeighborsClassifier.html) & [`KNeighborsRegressor`](https://scikit-learn.org/stable/modules/generated/sklearn.neighbors.KNeighborsRegressor.html) Let's try doing some basic classification on some data using the KNN algorithms.
###Code
iris = sns.load_dataset('iris')
display(iris)
# Let's convert this over to NumPy array
X = iris.iloc[:,:2].to_numpy()
# Let's convert classes to numerical values
y = LabelEncoder().fit_transform(iris['species'])
y
f, ax = plt.subplots()
sns.scatterplot(x=X[:,0], y=X[:,1], ax=ax, hue=y, palette='colorblind')
ax.get_legend().remove()
###Output
_____no_output_____
###Markdown
Training the KNN
###Code
neigh = KNeighborsClassifier(n_neighbors=3, metric='euclidean')
neigh.fit(X, y)
###Output
_____no_output_____
###Markdown
Make Some Predictions
###Code
# Made up data points
pred_pts = np.array([
[7.0, 3.0],
[8.0, 3.5],
[7.0, 4.0],
[4.0, 3.0],
[5.0, 3.0],
[5.5, 4.0],
[5.0, 2.0],
[6.0, 2.5],
[5.8, 3.5],
])
###Output
_____no_output_____
###Markdown
Let's see these new points against the training data. Think about how they'll be made classified.
###Code
f, ax = plt.subplots()
sns.scatterplot(x=X[:,0], y=X[:,1], ax=ax, hue=y, palette='colorblind')
sns.scatterplot(x=pred_pts[:,0], ax=ax, y=pred_pts[:,1], marker="*", s=200, edgecolor='black', color='magenta')
ax.get_legend().remove()
# Make predictions
pred_y = neigh.predict(pred_pts)
print(pred_y)
# Probabilities for KNN (how they voted)
for p,prob in zip(pred_y,neigh.predict_proba(pred_pts)):
print(f'{p}: {prob}')
f, ax = plt.subplots()
sns.scatterplot(x=X[:,0],y=X[:,1], ax=ax, hue=y, palette='colorblind')
sns.scatterplot(x=pred_pts[:,0], ax=ax, y=pred_pts[:,1], hue=pred_y, palette='colorblind', marker="*", s=200, edgecolor='black')
ax.get_legend().remove()
###Output
_____no_output_____
###Markdown
Let's see those predictions plotted with the other points after the classification. The Pros and Cons Models have different use cases and it helps to understand the strengths and weaknesses Advantages - Lazy learning (no training phase)- Simple algorithm to understand and implement Disadvantages - Has to be kept in memory (small data with few features)- Not robust; doesn't generalize well- Soft boundaries are troublesome- "Curse of Dimensionality" Classification with `sklearn.neighbors` $k$-Nearest Neighbors is a modeling technique that works for both regression and classification problems. Here we'll apply it to a version of the Titanic dataset.
###Code
titanic = pd.read_csv('data/cleaned_titanic.csv')
titanic = titanic.iloc[:, :-2]
titanic.head()
###Output
_____no_output_____
###Markdown
**For visualization purposes, we will use only two features for our first model.**
###Code
X = titanic[['Age', 'Fare']]
y = titanic['Survived']
y.value_counts()
###Output
_____no_output_____
###Markdown
Train-Test Split This dataset of course presents a binary classification problem, with our target being the `Survived` feature.
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
###Output
_____no_output_____
###Markdown
Validation Split
###Code
X_t, X_val, y_t, y_val = train_test_split(X_train, y_train,
random_state=42,
test_size=0.25)
knn = KNeighborsClassifier()
knn.fit(X_t, y_t)
print(f"training accuracy: {knn.score(X_t, y_t)}")
print(f"validation accuracy: {knn.score(X_val, y_val)}")
y_hat = knn.predict(X_val)
plot_confusion_matrix(confusion_matrix(y_val, y_hat), classes=['Perished', 'Survived'])
X_for_viz = X_t.sample(15, random_state=40)
y_for_viz = y_t[X_for_viz.index]
fig, ax = plt.subplots(figsize=(10, 10))
sns.scatterplot(X_for_viz['Age'], X_for_viz['Fare'],
hue=y_for_viz, palette={0: 'red', 1: 'green'},
s=200, ax=ax)
ax.set_xlim(0, 80)
ax.set_ylim(0, 80)
plt.legend()
plt.title('Subsample of Training Data');
###Output
_____no_output_____
###Markdown
The $k$-NN algorithm works by simply storing the training set in memory, then measuring the distance from the training points to a new point.Let's drop a point from our validation set into the plot above.
###Code
X_for_viz = X_t.sample(15, random_state=40)
y_for_viz = y_t[X_for_viz.index]
fig, ax = plt.subplots(figsize=(10, 10))
sns.scatterplot(X_for_viz['Age'], X_for_viz['Fare'],
hue=y_for_viz, palette={0: 'red', 1: 'green'},
s=200, ax=ax)
plt.legend()
#################^^^Old code^^^##############
####################New code#################
# Let's take one sample from our validation set and plot it
new_x = pd.DataFrame(X_val.loc[484]).T
new_y = y_val[new_x.index]
sns.scatterplot(new_x['Age'], new_x['Fare'], color='blue',
s=200, ax=ax, label='New', marker='P')
ax.set_xlim(0, 100)
ax.set_ylim(0, 100);
new_x
###Output
_____no_output_____
###Markdown
Then, $k$-NN finds the $k$ nearest points. $k$ corresponds to the `n_neighbors` parameter defined when we instantiate the classifier object. **If $k$ = 1, then the prediction for a point will simply be the value of the target for the nearest point.** Different $k$ Values A big factor in this algorithm is choosing $k$  $k=1$
###Code
knn = KNeighborsClassifier(n_neighbors=1)
###Output
_____no_output_____
###Markdown
Let's fit our training data, then predict what our validation point will be based on the (one) closest neighbor.
###Code
knn.fit(X_for_viz, y_for_viz)
knn.predict(new_x)
###Output
_____no_output_____
###Markdown
**When we raise the value of $k$, $k$-NN will act democratically: It will find the $k$ closest points, and take a vote based on the labels.** $k=3$ Let's raise $k$ to 3.
###Code
knn3 = KNeighborsClassifier(n_neighbors=3)
knn3.fit(X_for_viz, y_for_viz)
knn3.predict(new_x)
###Output
_____no_output_____
###Markdown
It's not easy to tell what which points are closest by eye.Let's update our plot to add indices.
###Code
X_for_viz = X_t.sample(15, random_state=40)
y_for_viz = y_t[X_for_viz.index]
fig, ax = plt.subplots(figsize=(10,10))
sns.scatterplot(X_for_viz['Age'], X_for_viz['Fare'], hue=y_for_viz,
palette={0: 'red', 1: 'green'}, s=200, ax=ax)
# Now let's take another sample
# new_x = X_val.sample(1, random_state=33)
new_x = pd.DataFrame(X_val.loc[484]).T
new_x.columns = ['Age', 'Fare']
new_y = y_val[new_x.index]
print(new_x)
sns.scatterplot(new_x['Age'], new_x['Fare'], color='blue',
s=200, ax=ax, label='New', marker='P')
ax.set_xlim(0, 100)
ax.set_ylim(0, 100)
plt.legend()
#################^^^Old code^^^##############
####################New code#################
# add annotations one by one with a loop
for index in X_for_viz.index:
ax.text(X_for_viz.Age[index]+0.7, X_for_viz.Fare[index],
s=index, horizontalalignment='left', size='medium',
color='black', weight='semibold')
###Output
_____no_output_____
###Markdown
We can use `sklearn`'s NearestNeighors object to see the exact calculations.
###Code
df_for_viz = pd.merge(X_for_viz, y_for_viz, left_index=True, right_index=True)
neighbor = NearestNeighbors(3)
neighbor.fit(X_for_viz)
nearest = neighbor.kneighbors(new_x)
nearest
df_for_viz.iloc[nearest[1][0]]
new_x
# Use Euclidean distance to see how close they are to this point
print(((29-24)**2 + (33-25.4667)**2)**0.5)
print(((26-24)**2 + (16.1-25.4667)**2)**0.5)
print(((20-24)**2 + (15.7417-25.4667)**2)**0.5)
###Output
_____no_output_____
###Markdown
$k=5$ And with five neighbors?
###Code
knn = KNeighborsClassifier(n_neighbors=5)
knn.fit(X_for_viz, y_for_viz)
knn.predict(new_x)
###Output
_____no_output_____
###Markdown
Observing Different $k$ Values Let's iterate through $k$, odd numbers 1 through 10, and see the predictions.
###Code
for k in range(1, 10, 2):
knn = KNeighborsClassifier(n_neighbors=k)
knn.fit(X_for_viz, y_for_viz)
print(f'k={k}', knn.predict(new_x))
###Output
_____no_output_____
###Markdown
Which models were correct?
###Code
new_y
###Output
_____no_output_____
###Markdown
Scaling You may have suspected that we were leaving something out. For any distance-based algorithms, scaling is very important. Look at how the shape of the array changes before and after scaling.   Let's look at our data_for_viz dataset:
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
X_t, X_val, y_t, y_val = train_test_split(X_train, y_train,
random_state=42,
test_size=0.25)
knn = KNeighborsClassifier(n_neighbors=5)
ss = StandardScaler()
X_ind = X_t.index
X_col = X_t.columns
X_t_s = pd.DataFrame(ss.fit_transform(X_t))
X_t_s.index = X_ind
X_t_s.columns = X_col
X_v_ind = X_val.index
X_val_s = pd.DataFrame(ss.transform(X_val))
X_val_s.index = X_v_ind
X_val_s.columns = X_col
knn.fit(X_t_s, y_t)
print(f"training accuracy: {knn.score(X_t_s, y_t)}")
print(f"Val accuracy: {knn.score(X_val_s, y_val)}")
y_hat = knn.predict(X_val_s)
# The plot_train() function just does what we did above.
plot_train(X_t, y_t, X_val, y_val)
plot_train(X_t_s, y_t, X_val_s, y_val, -2, 2, text_pos=0.1 )
###Output
_____no_output_____
###Markdown
Look at how much that changes things.Look at points 166 and 150. Look at the group 621, 143, and 191. Now let's run our classifier on scaled data and compare to unscaled.
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
X_t, X_val, y_t, y_val = train_test_split(X_train, y_train,
random_state=42,
test_size=0.25)
# The predict_one() function prints predictions on a given point
# (#484) for k-nn models with k ranging from 1 to 10.
predict_one(X_t, X_val, y_t, y_val)
mm = MinMaxScaler()
X_t_s = pd.DataFrame(mm.fit_transform(X_t))
X_t_s.index = X_t.index
X_t_s.columns = X_t.columns
X_val_s = pd.DataFrame(mm.transform(X_val))
X_val_s.index = X_val.index
X_val_s.columns = X_val.columns
predict_one(X_t_s, X_val_s, y_t, y_val)
###Output
_____no_output_____
###Markdown
More Resources on Scaling https://sebastianraschka.com/Articles/2014_about_feature_scaling.html http://datareality.blogspot.com/2016/11/scaling-normalizing-standardizing-which.html $k$ and the Bias-Variance Tradeoff
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
# Let's slowly increase k and see what happens to our accuracy scores.
kf = KFold(n_splits=5)
k_scores_train = {}
k_scores_val = {}
for k in range(1, 20):
knn = KNeighborsClassifier(n_neighbors=k)
accuracy_score_t = []
accuracy_score_v = []
for train_ind, val_ind in kf.split(X_train, y_train):
X_t, y_t = X_train.iloc[train_ind], y_train.iloc[train_ind]
X_v, y_v = X_train.iloc[val_ind], y_train.iloc[val_ind]
mm = MinMaxScaler()
X_t_ind = X_t.index
X_v_ind = X_v.index
X_t = pd.DataFrame(mm.fit_transform(X_t))
X_t.index = X_t_ind
X_v = pd.DataFrame(mm.transform(X_v))
X_v.index = X_v_ind
knn.fit(X_t, y_t)
y_pred_t = knn.predict(X_t)
y_pred_v = knn.predict(X_v)
accuracy_score_t.append(accuracy_score(y_t, y_pred_t))
accuracy_score_v.append(accuracy_score(y_v, y_pred_v))
k_scores_train[k] = np.mean(accuracy_score_t)
k_scores_val[k] = np.mean(accuracy_score_v)
k_scores_train
k_scores_val
fig, ax = plt.subplots(figsize=(15, 15))
ax.plot(list(k_scores_train.keys()), list(k_scores_train.values()),
color='red', linestyle='dashed', marker='o',
markerfacecolor='blue', markersize=10, label='Train')
ax.plot(list(k_scores_val.keys()), list(k_scores_val.values()),
color='green', linestyle='dashed', marker='o',
markerfacecolor='blue', markersize=10, label='Val')
ax.set_xlabel('k')
ax.set_ylabel('Accuracy')
plt.legend();
###Output
_____no_output_____
###Markdown
The Relation Between $k$ and Bias/Variance > Small $k$ values leads to overfitting, but larger $k$ values tend towards underfitting  > From [Machine Learning Flashcards](https://machinelearningflashcards.com/) by Chris Albon
###Code
mm = MinMaxScaler()
X_train_ind = X_train.index
X_train = pd.DataFrame(mm.fit_transform(X_train))
X_train.index = X_train_ind
X_test_ind = X_test.index
X_test = pd.DataFrame(mm.transform(X_test))
X_test.index = X_test_ind
knn = KNeighborsClassifier(n_neighbors=9)
knn.fit(X_train, y_train)
print(f"training accuracy: {knn.score(X_train, y_train)}")
print(f"Test accuracy: {knn.score(X_test, y_test)}")
y_hat = knn.predict(X_test)
plot_confusion_matrix(confusion_matrix(y_test, y_hat), classes=['Perished', 'Survived'])
recall_score(y_test, y_hat)
precision_score(y_test, y_hat)
###Output
_____no_output_____
###Markdown
Table of Contents1 Objectives2 Concept of the $k$-Nearest Neighbors Algorithm2.1 Who's Nearby?2.2 Summary of $k$NN2.3 Implementing in Scikit-Learn2.3.1 Training the KNN2.3.2 Make Some Predictions3 The Pros and Cons3.1 Advantages3.2 Disadvantages4 Classification with sklearn.neighbors4.1 Train-Test Split4.2 Validation Split4.3 Different $k$ Values4.3.1 $k=1$4.3.2 $k=3$4.3.3 $k=5$4.3.4 Observing Different $k$ Values4.4 Scaling4.4.1 More Resources on Scaling5 $k$ and the Bias-Variance Tradeoff5.1 The Relation Between $k$ and Bias/Variance6 Level Up: Distance Metrics 
###Code
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.metrics import f1_score, confusion_matrix,\
recall_score, precision_score, accuracy_score
from src.confusion import plot_confusion_matrix
from src.k_classify import predict_one
from src.plot_train import *
from src.euclid import *
from sklearn import datasets
from sklearn.preprocessing import StandardScaler, MinMaxScaler, LabelEncoder
from sklearn.neighbors import KNeighborsClassifier, NearestNeighbors
from sklearn.model_selection import train_test_split, KFold
###Output
_____no_output_____
###Markdown
Objectives - Describe the $k$-nearest neighbors algorithm- Identify multiple common distance metrics- Tune $k$ appropriately in response to models with high bias or variance Concept of the $k$-Nearest Neighbors Algorithm First let's recall what is **supervised learning**.> In **supervised learning** we use example data (_training data_) to inform our predictions of future data Note that this definition includes _classification_ and _regression_ problems. And there are a variety of ways we can make predictions from past data.$k$-nearest neighbors is one such method of making predictions. Who's Nearby? One strategy to make predictions on a new data is to just look at what _similar_ data points are like.  We can say _nearby_ points are _similar_ to one another. There are a few different wasy to determine how "close" data points are to one another. Check out the [Level Up section on distance metrics](Level-Up:-Distance-Metrics) for some more detail. Summary of $k$NN  Implementing in Scikit-Learn > [`KNeighborsClassifier`](https://scikit-learn.org/stable/modules/generated/sklearn.neighbors.KNeighborsClassifier.html) & [`KNeighborsRegressor`](https://scikit-learn.org/stable/modules/generated/sklearn.neighbors.KNeighborsRegressor.html) Let's try doing some basic classification on some data using the KNN algorithms.
###Code
iris = sns.load_dataset('iris')
display(iris)
# Let's convert this over to NumPy array
X = iris.iloc[:,:2].to_numpy()
# Let's convert classes to numerical values
y = LabelEncoder().fit_transform(iris['species'])
y
f, ax = plt.subplots()
sns.scatterplot(x=X[:,0], y=X[:,1], ax=ax, hue=y, palette='colorblind')
ax.get_legend().remove()
###Output
_____no_output_____
###Markdown
Training the KNN
###Code
neigh = KNeighborsClassifier(n_neighbors=3, metric='euclidean')
neigh.fit(X, y)
###Output
_____no_output_____
###Markdown
Make Some Predictions
###Code
# Made up data points
pred_pts = np.array([
[7.0, 3.0],
[8.0, 3.5],
[7.0, 4.0],
[4.0, 3.0],
[5.0, 3.0],
[5.5, 4.0],
[5.0, 2.0],
[6.0, 2.5],
[5.8, 3.5],
])
###Output
_____no_output_____
###Markdown
Let's see these new points against the training data. Think about how they'll be made classified.
###Code
f, ax = plt.subplots()
sns.scatterplot(x=X[:,0], y=X[:,1], ax=ax, hue=y, palette='colorblind')
sns.scatterplot(x=pred_pts[:,0], ax=ax, y=pred_pts[:,1], marker="*", s=200, edgecolor='black', color='magenta')
ax.get_legend().remove()
# Make predictions
pred_y = neigh.predict(pred_pts)
print(pred_y)
# Probabilities for KNN (how they voted)
for p,prob in zip(pred_y,neigh.predict_proba(pred_pts)):
print(f'{p}: {prob}')
f, ax = plt.subplots()
sns.scatterplot(x=X[:,0],y=X[:,1], ax=ax, hue=y, palette='colorblind')
sns.scatterplot(x=pred_pts[:,0], ax=ax, y=pred_pts[:,1], hue=pred_y, palette='colorblind', marker="*", s=200, edgecolor='black')
ax.get_legend().remove()
###Output
_____no_output_____
###Markdown
Let's see those predictions plotted with the other points after the classification. The Pros and Cons Models have different use cases and it helps to understand the strengths and weaknesses Advantages - Lazy learning (no training phase)- Simple algorithm to understand and implement Disadvantages - Has to be kept in memory (small data with few features)- Not robust; doesn't generalize well- Soft boundaries are troublesome- "Curse of Dimensionality" Classification with `sklearn.neighbors` $k$-Nearest Neighbors is a modeling technique that works for both regression and classification problems. Here we'll apply it to a version of the Titanic dataset.
###Code
titanic = pd.read_csv('data/cleaned_titanic.csv')
titanic = titanic.iloc[:, :-2]
titanic.head()
###Output
_____no_output_____
###Markdown
**For visualization purposes, we will use only two features for our first model.**
###Code
X = titanic[['Age', 'Fare']]
y = titanic['Survived']
y.value_counts()
###Output
_____no_output_____
###Markdown
Train-Test Split This dataset of course presents a binary classification problem, with our target being the `Survived` feature.
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
###Output
_____no_output_____
###Markdown
Validation Split
###Code
X_t, X_val, y_t, y_val = train_test_split(X_train, y_train,
random_state=42,
test_size=0.25)
knn = KNeighborsClassifier()
knn.fit(X_t, y_t)
print(f"training accuracy: {knn.score(X_t, y_t)}")
print(f"validation accuracy: {knn.score(X_val, y_val)}")
y_hat = knn.predict(X_val)
plot_confusion_matrix(confusion_matrix(y_val, y_hat), classes=['Perished', 'Survived'])
X_for_viz = X_t.sample(15, random_state=40)
y_for_viz = y_t[X_for_viz.index]
fig, ax = plt.subplots(figsize=(10, 10))
sns.scatterplot(X_for_viz['Age'], X_for_viz['Fare'],
hue=y_for_viz, palette={0: 'red', 1: 'green'},
s=200, ax=ax)
ax.set_xlim(0, 80)
ax.set_ylim(0, 80)
plt.legend()
plt.title('Subsample of Training Data');
###Output
C:\Users\bmcca\anaconda3\envs\learn-env-bmc\lib\site-packages\seaborn\_decorators.py:36: FutureWarning: Pass the following variables as keyword args: x, y. From version 0.12, the only valid positional argument will be `data`, and passing other arguments without an explicit keyword will result in an error or misinterpretation.
warnings.warn(
###Markdown
The $k$-NN algorithm works by simply storing the training set in memory, then measuring the distance from the training points to a new point.Let's drop a point from our validation set into the plot above.
###Code
X_for_viz = X_t.sample(15, random_state=40)
y_for_viz = y_t[X_for_viz.index]
fig, ax = plt.subplots(figsize=(10, 10))
sns.scatterplot(X_for_viz['Age'], X_for_viz['Fare'],
hue=y_for_viz, palette={0: 'red', 1: 'green'},
s=200, ax=ax)
plt.legend()
#################^^^Old code^^^##############
####################New code#################
# Let's take one sample from our validation set and plot it
new_x = pd.DataFrame(X_val.loc[484]).T
new_y = y_val[new_x.index]
sns.scatterplot(new_x['Age'], new_x['Fare'], color='blue',
s=200, ax=ax, label='New', marker='P')
ax.set_xlim(0, 100)
ax.set_ylim(0, 100);
new_x
###Output
_____no_output_____
###Markdown
Then, $k$-NN finds the $k$ nearest points. $k$ corresponds to the `n_neighbors` parameter defined when we instantiate the classifier object. **If $k$ = 1, then the prediction for a point will simply be the value of the target for the nearest point.** Different $k$ Values A big factor in this algorithm is choosing $k$  $k=1$
###Code
knn = KNeighborsClassifier(n_neighbors=1)
###Output
_____no_output_____
###Markdown
Let's fit our training data, then predict what our validation point will be based on the (one) closest neighbor.
###Code
knn.fit(X_for_viz, y_for_viz)
knn.predict(new_x)
###Output
_____no_output_____
###Markdown
**When we raise the value of $k$, $k$-NN will act democratically: It will find the $k$ closest points, and take a vote based on the labels.** $k=3$ Let's raise $k$ to 3.
###Code
knn3 = KNeighborsClassifier(n_neighbors=3)
knn3.fit(X_for_viz, y_for_viz)
knn3.predict(new_x)
###Output
_____no_output_____
###Markdown
It's not easy to tell what which points are closest by eye.Let's update our plot to add indices.
###Code
X_for_viz = X_t.sample(15, random_state=40)
y_for_viz = y_t[X_for_viz.index]
fig, ax = plt.subplots(figsize=(10,10))
sns.scatterplot(X_for_viz['Age'], X_for_viz['Fare'], hue=y_for_viz,
palette={0: 'red', 1: 'green'}, s=200, ax=ax)
# Now let's take another sample
# new_x = X_val.sample(1, random_state=33)
new_x = pd.DataFrame(X_val.loc[484]).T
new_x.columns = ['Age', 'Fare']
new_y = y_val[new_x.index]
print(new_x)
sns.scatterplot(new_x['Age'], new_x['Fare'], color='blue',
s=200, ax=ax, label='New', marker='P')
ax.set_xlim(0, 100)
ax.set_ylim(0, 100)
plt.legend()
#################^^^Old code^^^##############
####################New code#################
# add annotations one by one with a loop
for index in X_for_viz.index:
ax.text(X_for_viz.Age[index]+0.7, X_for_viz.Fare[index],
s=index, horizontalalignment='left', size='medium',
color='black', weight='semibold')
###Output
C:\Users\bmcca\anaconda3\envs\learn-env-bmc\lib\site-packages\seaborn\_decorators.py:36: FutureWarning: Pass the following variables as keyword args: x, y. From version 0.12, the only valid positional argument will be `data`, and passing other arguments without an explicit keyword will result in an error or misinterpretation.
warnings.warn(
C:\Users\bmcca\anaconda3\envs\learn-env-bmc\lib\site-packages\seaborn\_decorators.py:36: FutureWarning: Pass the following variables as keyword args: x, y. From version 0.12, the only valid positional argument will be `data`, and passing other arguments without an explicit keyword will result in an error or misinterpretation.
warnings.warn(
###Markdown
We can use `sklearn`'s NearestNeighors object to see the exact calculations.
###Code
df_for_viz = pd.merge(X_for_viz, y_for_viz, left_index=True, right_index=True)
neighbor = NearestNeighbors(3)
neighbor.fit(X_for_viz)
nearest = neighbor.kneighbors(new_x)
nearest
df_for_viz.iloc[nearest[1][0]]
new_x
# Use Euclidean distance to see how close they are to this point
print(((29-24)**2 + (33-25.4667)**2)**0.5)
print(((26-24)**2 + (16.1-25.4667)**2)**0.5)
print(((20-24)**2 + (15.7417-25.4667)**2)**0.5)
###Output
9.041604331643805
9.57784260102451
10.515494519992865
###Markdown
$k=5$ And with five neighbors?
###Code
knn = KNeighborsClassifier(n_neighbors=5)
knn.fit(X_for_viz, y_for_viz)
knn.predict(new_x)
###Output
_____no_output_____
###Markdown
Observing Different $k$ Values Let's iterate through $k$, odd numbers 1 through 10, and see the predictions.
###Code
for k in range(1, 10, 2):
knn = KNeighborsClassifier(n_neighbors=k)
knn.fit(X_for_viz, y_for_viz)
print(f'k={k}', knn.predict(new_x))
###Output
k=1 [1]
k=3 [1]
k=5 [0]
k=7 [0]
k=9 [0]
###Markdown
Which models were correct?
###Code
new_y
###Output
_____no_output_____
###Markdown
Scaling You may have suspected that we were leaving something out. For any distance-based algorithms, scaling is very important. Look at how the shape of the array changes before and after scaling.   Let's look at our data_for_viz dataset:
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
X_t, X_val, y_t, y_val = train_test_split(X_train, y_train,
random_state=42,
test_size=0.25)
knn = KNeighborsClassifier(n_neighbors=5)
ss = StandardScaler()
X_ind = X_t.index
X_col = X_t.columns
X_t_s = pd.DataFrame(ss.fit_transform(X_t))
X_t_s.index = X_ind
X_t_s.columns = X_col
X_v_ind = X_val.index
X_val_s = pd.DataFrame(ss.transform(X_val))
X_val_s.index = X_v_ind
X_val_s.columns = X_col
knn.fit(X_t_s, y_t)
print(f"training accuracy: {knn.score(X_t_s, y_t)}")
print(f"Val accuracy: {knn.score(X_val_s, y_val)}")
y_hat = knn.predict(X_val_s)
# The plot_train() function just does what we did above.
plot_train(X_t, y_t, X_val, y_val)
plot_train(X_t_s, y_t, X_val_s, y_val, -2, 2, text_pos=0.1 )
###Output
Age Fare
484 24.0 25.4667
Age Fare
484 -0.4055 -0.154222
###Markdown
Look at how much that changes things.Look at points 166 and 150. Look at the group 621, 143, and 191. Now let's run our classifier on scaled data and compare to unscaled.
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
X_t, X_val, y_t, y_val = train_test_split(X_train, y_train,
random_state=42,
test_size=0.25)
# The predict_one() function prints predictions on a given point
# (#484) for k-nn models with k ranging from 1 to 10.
predict_one(X_t, X_val, y_t, y_val)
mm = MinMaxScaler()
X_t_s = pd.DataFrame(mm.fit_transform(X_t))
X_t_s.index = X_t.index
X_t_s.columns = X_t.columns
X_val_s = pd.DataFrame(mm.transform(X_val))
X_val_s.index = X_val.index
X_val_s.columns = X_val.columns
predict_one(X_t_s, X_val_s, y_t, y_val)
###Output
[0]
[0]
[0]
[0]
[1]
[1]
[1]
[1]
[1]
[1]
###Markdown
More Resources on Scaling https://sebastianraschka.com/Articles/2014_about_feature_scaling.html http://datareality.blogspot.com/2016/11/scaling-normalizing-standardizing-which.html $k$ and the Bias-Variance Tradeoff
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
# Let's slowly increase k and see what happens to our accuracy scores.
kf = KFold(n_splits=5)
k_scores_train = {}
k_scores_val = {}
for k in range(1, 20):
knn = KNeighborsClassifier(n_neighbors=k)
accuracy_score_t = []
accuracy_score_v = []
for train_ind, val_ind in kf.split(X_train, y_train):
X_t, y_t = X_train.iloc[train_ind], y_train.iloc[train_ind]
X_v, y_v = X_train.iloc[val_ind], y_train.iloc[val_ind]
mm = MinMaxScaler()
X_t_ind = X_t.index
X_v_ind = X_v.index
X_t = pd.DataFrame(mm.fit_transform(X_t))
X_t.index = X_t_ind
X_v = pd.DataFrame(mm.transform(X_v))
X_v.index = X_v_ind
knn.fit(X_t, y_t)
y_pred_t = knn.predict(X_t)
y_pred_v = knn.predict(X_v)
accuracy_score_t.append(accuracy_score(y_t, y_pred_t))
accuracy_score_v.append(accuracy_score(y_v, y_pred_v))
k_scores_train[k] = np.mean(accuracy_score_t)
k_scores_val[k] = np.mean(accuracy_score_v)
k_scores_train
k_scores_val
fig, ax = plt.subplots(figsize=(15, 15))
ax.plot(list(k_scores_train.keys()), list(k_scores_train.values()),
color='red', linestyle='dashed', marker='o',
markerfacecolor='blue', markersize=10, label='Train')
ax.plot(list(k_scores_val.keys()), list(k_scores_val.values()),
color='green', linestyle='dashed', marker='o',
markerfacecolor='blue', markersize=10, label='Val')
ax.set_xlabel('k')
ax.set_ylabel('Accuracy')
plt.legend();
###Output
_____no_output_____
###Markdown
The Relation Between $k$ and Bias/Variance > Small $k$ values leads to overfitting, but larger $k$ values tend towards underfitting  > From [Machine Learning Flashcards](https://machinelearningflashcards.com/) by Chris Albon
###Code
mm = MinMaxScaler()
X_train_ind = X_train.index
X_train = pd.DataFrame(mm.fit_transform(X_train))
X_train.index = X_train_ind
X_test_ind = X_test.index
X_test = pd.DataFrame(mm.transform(X_test))
X_test.index = X_test_ind
knn = KNeighborsClassifier(n_neighbors=9)
knn.fit(X_train, y_train)
print(f"training accuracy: {knn.score(X_train, y_train)}")
print(f"Test accuracy: {knn.score(X_test, y_test)}")
y_hat = knn.predict(X_test)
plot_confusion_matrix(confusion_matrix(y_test, y_hat), classes=['Perished', 'Survived'])
recall_score(y_test, y_hat)
precision_score(y_test, y_hat)
###Output
_____no_output_____
###Markdown
Table of Contents1 Objectives2 Concept of the $k$-Nearest Neighbors Algorithm2.1 Who's Nearby?2.2 Summary of $k$NN2.3 Implementing in Scikit-Learn2.3.1 Training the KNN2.3.2 Make Some Predictions3 The Pros and Cons3.1 Advantages3.2 Disadvantages4 Classification with sklearn.neighbors4.1 Train-Test Split4.2 Validation Split4.3 Different $k$ Values4.3.1 $k=1$4.3.2 $k=3$4.3.3 $k=5$4.3.4 Observing Different $k$ Values4.4 Scaling4.4.1 More Resources on Scaling5 $k$ and the Bias-Variance Tradeoff5.1 The Relation Between $k$ and Bias/Variance6 Level Up: Distance Metrics 
###Code
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.metrics import f1_score, confusion_matrix,\
recall_score, precision_score, accuracy_score
from src.confusion import plot_confusion_matrix
from src.k_classify import predict_one
from src.plot_train import *
from src.euclid import *
from sklearn import datasets
from sklearn.preprocessing import StandardScaler, MinMaxScaler, LabelEncoder
from sklearn.neighbors import KNeighborsClassifier, NearestNeighbors
from sklearn.model_selection import train_test_split, KFold
###Output
_____no_output_____
###Markdown
Objectives - Describe the $k$-nearest neighbors algorithm- Identify multiple common distance metrics- Tune $k$ appropriately in response to models with high bias or variance Concept of the $k$-Nearest Neighbors Algorithm First let's recall what is **supervised learning**.> In **supervised learning** we use example data (_training data_) to inform our predictions of future data Note that this definition includes _classification_ and _regression_ problems. And there are a variety of ways we can make predictions from past data.$k$-nearest neighbors is one such method of making predictions. Who's Nearby? One strategy to make predictions on a new data is to just look at what _similar_ data points are like.  We can say _nearby_ points are _similar_ to one another. There are a few different wasy to determine how "close" data points are to one another. Check out the [Level Up section on distance metrics](Level-Up:-Distance-Metrics) for some more detail. Summary of $k$NN  Implementing in Scikit-Learn > [`KNeighborsClassifier`](https://scikit-learn.org/stable/modules/generated/sklearn.neighbors.KNeighborsClassifier.html) & [`KNeighborsRegressor`](https://scikit-learn.org/stable/modules/generated/sklearn.neighbors.KNeighborsRegressor.html) Let's try doing some basic classification on some data using the KNN algorithms.
###Code
iris = sns.load_dataset('iris')
display(iris)
# Let's convert this over to NumPy array
X = iris.iloc[:,:2].to_numpy()
# Let's convert classes to numerical values
y = LabelEncoder().fit_transform(iris['species'])
y
f, ax = plt.subplots()
sns.scatterplot(x=X[:,0], y=X[:,1], ax=ax, hue=y, palette='colorblind')
ax.get_legend().remove()
###Output
_____no_output_____
###Markdown
Training the KNN
###Code
neigh = KNeighborsClassifier(n_neighbors=3, metric='euclidean')
neigh.fit(X, y)
###Output
_____no_output_____
###Markdown
Make Some Predictions
###Code
# Made up data points
pred_pts = np.array([
[7.0, 3.0],
[8.0, 3.5],
[7.0, 4.0],
[4.0, 3.0],
[5.0, 3.0],
[5.5, 4.0],
[5.0, 2.0],
[6.0, 2.5],
[5.8, 3.5],
])
###Output
_____no_output_____
###Markdown
Let's see these new points against the training data. Think about how they'll be made classified.
###Code
f, ax = plt.subplots()
sns.scatterplot(x=X[:,0], y=X[:,1], ax=ax, hue=y, palette='colorblind')
sns.scatterplot(x=pred_pts[:,0], ax=ax, y=pred_pts[:,1], marker="*", s=200, edgecolor='black', color='magenta')
ax.get_legend().remove()
# Make predictions
pred_y = neigh.predict(pred_pts)
print(pred_y)
# Probabilities for KNN (how they voted)
for p,prob in zip(pred_y,neigh.predict_proba(pred_pts)):
print(f'{p}: {prob}')
f, ax = plt.subplots()
sns.scatterplot(x=X[:,0],y=X[:,1], ax=ax, hue=y, palette='colorblind')
sns.scatterplot(x=pred_pts[:,0], ax=ax, y=pred_pts[:,1], hue=pred_y, palette='colorblind', marker="*", s=200, edgecolor='black')
ax.get_legend().remove()
###Output
_____no_output_____
###Markdown
Let's see those predictions plotted with the other points after the classification. The Pros and Cons Models have different use cases and it helps to understand the strengths and weaknesses Advantages - Lazy learning (no training phase)- Simple algorithm to understand and implement Disadvantages - Has to be kept in memory (small data with few features)- Not robust; doesn't generalize well- Soft boundaries are troublesome- "Curse of Dimensionality" Classification with `sklearn.neighbors` $k$-Nearest Neighbors is a modeling technique that works for both regression and classification problems. Here we'll apply it to a version of the Titanic dataset.
###Code
titanic = pd.read_csv('data/cleaned_titanic.csv')
titanic = titanic.iloc[:, :-2]
titanic.head()
###Output
_____no_output_____
###Markdown
**For visualization purposes, we will use only two features for our first model.**
###Code
X = titanic[['Age', 'Fare']]
y = titanic['Survived']
y.value_counts()
###Output
_____no_output_____
###Markdown
Train-Test Split This dataset of course presents a binary classification problem, with our target being the `Survived` feature.
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
###Output
_____no_output_____
###Markdown
Validation Split
###Code
X_t, X_val, y_t, y_val = train_test_split(X_train, y_train,
random_state=42,
test_size=0.25)
knn = KNeighborsClassifier()
knn.fit(X_t, y_t)
print(f"training accuracy: {knn.score(X_t, y_t)}")
print(f"validation accuracy: {knn.score(X_val, y_val)}")
y_hat = knn.predict(X_val)
plot_confusion_matrix(confusion_matrix(y_val, y_hat), classes=['Perished', 'Survived'])
X_for_viz = X_t.sample(15, random_state=40)
y_for_viz = y_t[X_for_viz.index]
fig, ax = plt.subplots(figsize=(10, 10))
sns.scatterplot(X_for_viz['Age'], X_for_viz['Fare'],
hue=y_for_viz, palette={0: 'red', 1: 'green'},
s=200, ax=ax)
ax.set_xlim(0, 80)
ax.set_ylim(0, 80)
plt.legend()
plt.title('Subsample of Training Data');
###Output
_____no_output_____
###Markdown
The $k$-NN algorithm works by simply storing the training set in memory, then measuring the distance from the training points to a new point.Let's drop a point from our validation set into the plot above.
###Code
X_for_viz = X_t.sample(15, random_state=40)
y_for_viz = y_t[X_for_viz.index]
fig, ax = plt.subplots(figsize=(10, 10))
sns.scatterplot(X_for_viz['Age'], X_for_viz['Fare'],
hue=y_for_viz, palette={0: 'red', 1: 'green'},
s=200, ax=ax)
plt.legend()
#################^^^Old code^^^##############
####################New code#################
# Let's take one sample from our validation set and plot it
new_x = pd.DataFrame(X_val.loc[484]).T
new_y = y_val[new_x.index]
sns.scatterplot(new_x['Age'], new_x['Fare'], color='blue',
s=200, ax=ax, label='New', marker='P')
ax.set_xlim(0, 100)
ax.set_ylim(0, 100);
new_x
###Output
_____no_output_____
###Markdown
Then, $k$-NN finds the $k$ nearest points. $k$ corresponds to the `n_neighbors` parameter defined when we instantiate the classifier object. **If $k$ = 1, then the prediction for a point will simply be the value of the target for the nearest point.** Different $k$ Values A big factor in this algorithm is choosing $k$  $k=1$
###Code
knn = KNeighborsClassifier(n_neighbors=1)
###Output
_____no_output_____
###Markdown
Let's fit our training data, then predict what our validation point will be based on the (one) closest neighbor.
###Code
knn.fit(X_for_viz, y_for_viz)
knn.predict(new_x)
###Output
_____no_output_____
###Markdown
**When we raise the value of $k$, $k$-NN will act democratically: It will find the $k$ closest points, and take a vote based on the labels.** $k=3$ Let's raise $k$ to 3.
###Code
knn3 = KNeighborsClassifier(n_neighbors=3)
knn3.fit(X_for_viz, y_for_viz)
knn3.predict(new_x)
###Output
_____no_output_____
###Markdown
It's not easy to tell what which points are closest by eye.Let's update our plot to add indices.
###Code
X_for_viz = X_t.sample(15, random_state=40)
y_for_viz = y_t[X_for_viz.index]
fig, ax = plt.subplots(figsize=(10,10))
sns.scatterplot(X_for_viz['Age'], X_for_viz['Fare'], hue=y_for_viz,
palette={0: 'red', 1: 'green'}, s=200, ax=ax)
# Now let's take another sample
# new_x = X_val.sample(1, random_state=33)
new_x = pd.DataFrame(X_val.loc[484]).T
new_x.columns = ['Age', 'Fare']
new_y = y_val[new_x.index]
print(new_x)
sns.scatterplot(new_x['Age'], new_x['Fare'], color='blue',
s=200, ax=ax, label='New', marker='P')
ax.set_xlim(0, 100)
ax.set_ylim(0, 100)
plt.legend()
#################^^^Old code^^^##############
####################New code#################
# add annotations one by one with a loop
for index in X_for_viz.index:
ax.text(X_for_viz.Age[index]+0.7, X_for_viz.Fare[index],
s=index, horizontalalignment='left', size='medium',
color='black', weight='semibold')
###Output
_____no_output_____
###Markdown
We can use `sklearn`'s NearestNeighors object to see the exact calculations.
###Code
df_for_viz = pd.merge(X_for_viz, y_for_viz, left_index=True, right_index=True)
neighbor = NearestNeighbors(3)
neighbor.fit(X_for_viz)
nearest = neighbor.kneighbors(new_x)
nearest
df_for_viz.iloc[nearest[1][0]]
new_x
# Use Euclidean distance to see how close they are to this point
print(((29-24)**2 + (33-25.4667)**2)**0.5)
print(((26-24)**2 + (16.1-25.4667)**2)**0.5)
print(((20-24)**2 + (15.7417-25.4667)**2)**0.5)
###Output
_____no_output_____
###Markdown
$k=5$ And with five neighbors?
###Code
knn = KNeighborsClassifier(n_neighbors=5)
knn.fit(X_for_viz, y_for_viz)
knn.predict(new_x)
###Output
_____no_output_____
###Markdown
Observing Different $k$ Values Let's iterate through $k$, odd numbers 1 through 10, and see the predictions.
###Code
for k in range(1, 10, 2):
knn = KNeighborsClassifier(n_neighbors=k)
knn.fit(X_for_viz, y_for_viz)
print(f'k={k}', knn.predict(new_x))
###Output
_____no_output_____
###Markdown
Which models were correct?
###Code
new_y
###Output
_____no_output_____
###Markdown
Scaling You may have suspected that we were leaving something out. For any distance-based algorithms, scaling is very important. Look at how the shape of the array changes before and after scaling.   Let's look at our data_for_viz dataset:
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
X_t, X_val, y_t, y_val = train_test_split(X_train, y_train,
random_state=42,
test_size=0.25)
knn = KNeighborsClassifier(n_neighbors=5)
ss = StandardScaler()
X_ind = X_t.index
X_col = X_t.columns
X_t_s = pd.DataFrame(ss.fit_transform(X_t))
X_t_s.index = X_ind
X_t_s.columns = X_col
X_v_ind = X_val.index
X_val_s = pd.DataFrame(ss.transform(X_val))
X_val_s.index = X_v_ind
X_val_s.columns = X_col
knn.fit(X_t_s, y_t)
print(f"training accuracy: {knn.score(X_t_s, y_t)}")
print(f"Val accuracy: {knn.score(X_val_s, y_val)}")
y_hat = knn.predict(X_val_s)
# The plot_train() function just does what we did above.
plot_train(X_t, y_t, X_val, y_val)
plot_train(X_t_s, y_t, X_val_s, y_val, -2, 2, text_pos=0.1 )
###Output
_____no_output_____
###Markdown
Look at how much that changes things.Look at points 166 and 150. Look at the group 621, 143, and 191. Now let's run our classifier on scaled data and compare to unscaled.
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
X_t, X_val, y_t, y_val = train_test_split(X_train, y_train,
random_state=42,
test_size=0.25)
# The predict_one() function prints predictions on a given point
# (#484) for k-nn models with k ranging from 1 to 10.
predict_one(X_t, X_val, y_t, y_val)
mm = MinMaxScaler()
X_t_s = pd.DataFrame(mm.fit_transform(X_t))
X_t_s.index = X_t.index
X_t_s.columns = X_t.columns
X_val_s = pd.DataFrame(mm.transform(X_val))
X_val_s.index = X_val.index
X_val_s.columns = X_val.columns
predict_one(X_t_s, X_val_s, y_t, y_val)
###Output
_____no_output_____
###Markdown
More Resources on Scaling https://sebastianraschka.com/Articles/2014_about_feature_scaling.html http://datareality.blogspot.com/2016/11/scaling-normalizing-standardizing-which.html $k$ and the Bias-Variance Tradeoff
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y,
random_state=42,
test_size=0.25)
# Let's slowly increase k and see what happens to our accuracy scores.
kf = KFold(n_splits=5)
k_scores_train = {}
k_scores_val = {}
for k in range(1, 20):
knn = KNeighborsClassifier(n_neighbors=k)
accuracy_score_t = []
accuracy_score_v = []
for train_ind, val_ind in kf.split(X_train, y_train):
X_t, y_t = X_train.iloc[train_ind], y_train.iloc[train_ind]
X_v, y_v = X_train.iloc[val_ind], y_train.iloc[val_ind]
mm = MinMaxScaler()
X_t_ind = X_t.index
X_v_ind = X_v.index
X_t = pd.DataFrame(mm.fit_transform(X_t))
X_t.index = X_t_ind
X_v = pd.DataFrame(mm.transform(X_v))
X_v.index = X_v_ind
knn.fit(X_t, y_t)
y_pred_t = knn.predict(X_t)
y_pred_v = knn.predict(X_v)
accuracy_score_t.append(accuracy_score(y_t, y_pred_t))
accuracy_score_v.append(accuracy_score(y_v, y_pred_v))
k_scores_train[k] = np.mean(accuracy_score_t)
k_scores_val[k] = np.mean(accuracy_score_v)
k_scores_train
k_scores_val
fig, ax = plt.subplots(figsize=(15, 15))
ax.plot(list(k_scores_train.keys()), list(k_scores_train.values()),
color='red', linestyle='dashed', marker='o',
markerfacecolor='blue', markersize=10, label='Train')
ax.plot(list(k_scores_val.keys()), list(k_scores_val.values()),
color='green', linestyle='dashed', marker='o',
markerfacecolor='blue', markersize=10, label='Val')
ax.set_xlabel('k')
ax.set_ylabel('Accuracy')
plt.legend();
###Output
_____no_output_____
###Markdown
The Relation Between $k$ and Bias/Variance > Small $k$ values leads to overfitting, but larger $k$ values tend towards underfitting  > From [Machine Learning Flashcards](https://machinelearningflashcards.com/) by Chris Albon
###Code
mm = MinMaxScaler()
X_train_ind = X_train.index
X_train = pd.DataFrame(mm.fit_transform(X_train))
X_train.index = X_train_ind
X_test_ind = X_test.index
X_test = pd.DataFrame(mm.transform(X_test))
X_test.index = X_test_ind
knn = KNeighborsClassifier(n_neighbors=9)
knn.fit(X_train, y_train)
print(f"training accuracy: {knn.score(X_train, y_train)}")
print(f"Test accuracy: {knn.score(X_test, y_test)}")
y_hat = knn.predict(X_test)
plot_confusion_matrix(confusion_matrix(y_test, y_hat), classes=['Perished', 'Survived'])
recall_score(y_test, y_hat)
precision_score(y_test, y_hat)
###Output
_____no_output_____ |
tutorials/05_rrt_motion_planning.ipynb | ###Markdown
RRT-star Motion Planning Tutorial We'll show rrt-star motion planning on a panda robot.If you want to see a simulation demo, check out the examples directory.
###Code
import sys, os
import yaml
import trimesh
%matplotlib inline
parent_dir = os.path.dirname(os.getcwd())
pykin_path = parent_dir
sys.path.append(pykin_path)
from pykin.robots.single_arm import SingleArm
from pykin.planners.rrt_star_planner import RRTStarPlanner
from pykin.collision.collision_manager import CollisionManager
from pykin.kinematics.transform import Transform
from pykin.utils.object_utils import ObjectManager
from pykin.utils import plot_utils as plt
file_path = '../asset/urdf/panda/panda.urdf'
mesh_path = pykin_path+"/asset/urdf/panda/"
yaml_path = '../asset/config/panda_init_params.yaml'
with open(yaml_path) as f:
controller_config = yaml.safe_load(f)
robot = SingleArm(file_path, Transform(rot=[0.0, 0.0, 0], pos=[0, 0, 0]))
robot.setup_link_name("panda_link_0", "panda_right_hand")
init_qpos = controller_config["init_qpos"]
init_fk = robot.forward_kin(init_qpos)
goal_eef_pose = controller_config["goal_pose"]
###Output
_____no_output_____
###Markdown
Apply to robot using CollisionManager
###Code
c_manager = CollisionManager(mesh_path)
c_manager.setup_robot_collision(robot, init_fk)
milk_path = pykin_path+"/asset/objects/meshes/milk.stl"
milk_mesh = trimesh.load_mesh(milk_path)
###Output
_____no_output_____
###Markdown
Apply to Object using CollisionManager
###Code
obs = ObjectManager()
o_manager = CollisionManager(milk_path)
for i in range(6):
name = "miik_" + str(i)
obs_pos = [0.6, -0.2+i*0.1, 0.3]
o_manager.add_object(name, gtype="mesh", gparam=milk_mesh, transform=Transform(pos=obs_pos).h_mat)
obs(name=name, gtype="mesh", gparam=milk_mesh, transform=Transform(pos=obs_pos).h_mat)
###Output
_____no_output_____
###Markdown
Use RRTStarPlanner- delta_distance(float): distance between nearest vertex and new vertex- epsilon(float): 1-epsilon is probability of random sampling- gamma_RRT_star(int): factor used for search radius- max_iter(int): maximum number of iterations- dimension(int): robot arm's dof- n_step(int): for n equal divisions between waypoints
###Code
planner = RRTStarPlanner(
robot=robot,
self_collision_manager=c_manager,
object_collision_manager=o_manager,
delta_distance=0.5,
epsilon=0.4,
max_iter=600,
gamma_RRT_star=1,
dimension=7,
n_step=10
)
###Output
_____no_output_____
###Markdown
interpolated_path : joint path divided equally by n_steps between waypointsjoint_path : actual joint path
###Code
interpolated_path, joint_path = planner.get_path_in_joinst_space(cur_q=init_qpos, goal_pose=goal_eef_pose, resolution=0.3)
if joint_path is None :
print("Cannot Visulization Path")
exit()
joint_trajectory = []
eef_poses = []
for step, joint in enumerate(interpolated_path):
transformations = robot.forward_kin(joint)
joint_trajectory.append(transformations)
eef_poses.append(transformations[robot.eef_name].pos)
###Output
_____no_output_____
###Markdown
Visualization
###Code
fig, ax = plt.init_3d_figure(figsize=(10,6), dpi= 100)
plt.plot_trajectories(ax, eef_poses, size=1)
plt.plot_robot(
robot,
transformations=joint_trajectory[0],
ax=ax,
visible_text=False)
plt.plot_robot(
robot,
transformations=joint_trajectory[-1],
ax=ax,
visible_text=False,
visible_basis=False)
plt.plot_objects(
ax, objects=obs
)
plt.show_figure()
###Output
_____no_output_____ |
site/en/r2/tutorials/distribute/keras.ipynb | ###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training with Keras View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see the [tf.distribute.Strategy with training loops](training_loops.ipynb) tutorial. Import dependencies
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
# Import TensorFlow and TensorFlow Datasets
try:
# %tensorflow_version only exists in Colab.
%tensorflow_version 2.x
except Exception:
pass
import tensorflow_datasets as tfds
import tensorflow as tf
tfds.disable_progress_bar()
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define distribution strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup input pipeline When training a model with multiple GPUs, you can use the extra computing power effectively by increasing the batch size. In general, use the largest batch size that fits the GPU memory, and tune the learning rate accordingly.
###Code
# You can also do info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = info.splits['train'].num_examples
num_test_examples = info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks The callbacks used here are:* *TensorBoard*: This callback writes a log for TensorBoard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=12, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel Export the graph and the variables to the platform-agnostic SavedModel format. After your model is saved, you can load it with or without the scope.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed Training in TensorFlow View on TensorFlow.org Run in Google Colab View source on GitHub OverviewThe `tf.distribute.Strategy` API is an easy way to distribute your trainingacross multiple devices/machines. Our goal is to allow users to use existingmodels and training code with minimal changes to enable distributed training.Currently, core TensorFlow includes `tf.distribute.MirroredStrategy`. Thisdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it create copies of all variables in the model's layers on eachdevice. It then use all-reduce to combine gradients across the devices beforeapplying them to the variables to keep them in sync.Many other strategies will soon beavailable in core TensorFlow. You can find more information about them in the[README](https://github.com/tensorflow/tensorflow/tree/master/tensorflow/contrib/distribute). Example with Keras APIThe easiest way to get started with multiple GPUs on one machine using `MirroredStrategy` is with `tf.keras`.
###Code
from __future__ import absolute_import, division, print_function
# Import TensorFlow
!pip install tf-nightly-gpu-2.0-preview
import tensorflow_datasets as tfds
import tensorflow as tf
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset to train our model on. Use [TensorFlow Datasets](https://www.tensorflow.org/datasets) to load the dataset. This returns a dataset in `tf.data` format.
###Code
datasets, ds_info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
`with_info=True` returns the metadata for the entire dataset.In this example, `ds_info.splits.total_num_examples = 70000`.
###Code
num_train_examples = ds_info.splits['train'].num_examples
num_test_examples = ds_info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE = 64
###Output
_____no_output_____
###Markdown
Input data pipeline
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label[..., tf.newaxis]
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Define Distribution Strategy To distribute a Keras model on multiple GPUs using `MirroredStrategy`, we first instantiate a `MirroredStrategy` object.
###Code
strategy = tf.distribute.MirroredStrategy()
print ('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks. The callbacks used here are:* *Tensorboard*: This callback writes a log for Tensorboard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can use a complicated decay equation too.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print ('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate To train the model call Keras `fit` API using the input dataset that wascreated earlier, same as how it would be called in a non-distributed case.
###Code
model.fit(train_dataset, epochs=10, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Let's load the latest checkpoint and see how the model performs on the test dataset.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss = model.evaluate(eval_dataset)
print ('Eval loss: {}'.format(eval_loss))
###Output
_____no_output_____
###Markdown
You can download the tensorboard logs and then use the following command to see the output.```tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training with Keras View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see the [tf.distribute.Strategy with training loops](training_loops.ipynb) tutorial. Import dependencies
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
# Import TensorFlow and TensorFlow Datasets
try:
%tensorflow_version 2.x # Colab only.
except Exception:
pass
import tensorflow_datasets as tfds
import tensorflow as tf
tfds.disable_progress_bar()
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define distribution strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup input pipeline When training a model with multiple GPUs, you can use the extra computing power effectively by increasing the batch size. In general, use the largest batch size that fits the GPU memory, and tune the learning rate accordingly.
###Code
# You can also do info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = info.splits['train'].num_examples
num_test_examples = info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks The callbacks used here are:* *TensorBoard*: This callback writes a log for TensorBoard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=12, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel Export the graph and the variables to the platform-agnostic SavedModel format. After your model is saved, you can load it with or without the scope.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training in TensorFlow View on TensorFlow.org Run in Google Colab View source on GitHub OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see [this tutorial](training_loops.ipynb). Import Dependencies
###Code
from __future__ import absolute_import, division, print_function
# Import TensorFlow
!pip install tensorflow-gpu==2.0.0-alpha0
import tensorflow_datasets as tfds
import tensorflow as tf
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `ds_info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, ds_info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define Distribution Strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print ('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup Input pipeline If a model is trained on multiple GPUs, the batch size should be increased accordingly so as to make effective use of the extra computing power. Moreover, the learning rate should be tuned accordingly.
###Code
# You can also do ds_info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = ds_info.splits['train'].num_examples
num_test_examples = ds_info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks. The callbacks used here are:* *Tensorboard*: This callback writes a log for Tensorboard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print ('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=10, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel If you want to export the graph and the variables, SavedModel is the best way of doing this. The model can be loaded back with or without the scope. Moreover, SavedModel is platform agnostic.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training with Keras View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see the [tf.distribute.Strategy with training loops](training_loops.ipynb) tutorial. Import dependencies
###Code
# Import TensorFlow and TensorFlow Datasets
!pip install tensorflow-gpu==2.0.0-beta0
!pip install tensorflow_datasets
from __future__ import absolute_import, division, print_function, unicode_literals
import tensorflow as tf
import tensorflow_datasets as tfds
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define distribution strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup input pipeline When training a model with multiple GPUs, you can use the extra computing power effectively by increasing the batch size. In general, use the largest batch size that fits the GPU memory, and tune the learning rate accordingly.
###Code
# You can also do info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = info.splits['train'].num_examples
num_test_examples = info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks The callbacks used here are:* *TensorBoard*: This callback writes a log for TensorBoard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=12, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel Export the graph and the variables to the platform-agnostic SavedModel format. After your model is saved, you can load it with or without the scope.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training in TensorFlow View on TensorFlow.org Run in Google Colab View source on GitHub OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see [this tutorial](training_loops.ipynb). Import Dependencies
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
# Import TensorFlow
!pip install tensorflow-gpu==2.0.0-alpha0
import tensorflow_datasets as tfds
import tensorflow as tf
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `ds_info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, ds_info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define Distribution Strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print ('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup Input pipeline If a model is trained on multiple GPUs, the batch size should be increased accordingly so as to make effective use of the extra computing power. Moreover, the learning rate should be tuned accordingly.
###Code
# You can also do ds_info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = ds_info.splits['train'].num_examples
num_test_examples = ds_info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks. The callbacks used here are:* *Tensorboard*: This callback writes a log for Tensorboard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print ('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=10, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel If you want to export the graph and the variables, SavedModel is the best way of doing this. The model can be loaded back with or without the scope. Moreover, SavedModel is platform agnostic.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training with Keras View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see the [tf.distribute.Strategy with training loops](training_loops.ipynb) tutorial. Import dependencies
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
# Import TensorFlow and TensorFlow Datasets
!pip install tensorflow-gpu==2.0.0-beta1
import tensorflow_datasets as tfds
import tensorflow as tf
tfds.disable_progress_bar()
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define distribution strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup input pipeline When training a model with multiple GPUs, you can use the extra computing power effectively by increasing the batch size. In general, use the largest batch size that fits the GPU memory, and tune the learning rate accordingly.
###Code
# You can also do info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = info.splits['train'].num_examples
num_test_examples = info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks The callbacks used here are:* *TensorBoard*: This callback writes a log for TensorBoard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=12, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel Export the graph and the variables to the platform-agnostic SavedModel format. After your model is saved, you can load it with or without the scope.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training in TensorFlow View on TensorFlow.org Run in Google Colab View source on GitHub OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see [this tutorial](training_loops.ipynb). Import Dependencies
###Code
from __future__ import absolute_import, division, print_function
# Import TensorFlow
!pip install tf-nightly-gpu-2.0-preview
import tensorflow_datasets as tfds
import tensorflow as tf
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `ds_info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, ds_info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define Distribution Strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print ('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup Input pipeline If a model is trained on multiple GPUs, the batch size should be increased accordingly so as to make effective use of the extra computing power. Moreover, the learning rate should be tuned accordingly.
###Code
# You can also do ds_info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = ds_info.splits['train'].num_examples
num_test_examples = ds_info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks. The callbacks used here are:* *Tensorboard*: This callback writes a log for Tensorboard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print ('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=10, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel If you want to export the graph and the variables, SavedModel is the best way of doing this. The model can be loaded back with or without the scope. Moreover, SavedModel is platform agnostic.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss = model.evaluate(eval_dataset)
print ('Eval loss: {}'.format(eval_loss))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training with Keras View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see the [tf.distribute.Strategy with training loops](training_loops.ipynb) tutorial. Import dependencies
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
# Import TensorFlow and TensorFlow Datasets
!pip install tensorflow-gpu==2.0.0-beta1
import tensorflow_datasets as tfds
import tensorflow as tf
tfds.disable_progress_bar()
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define distribution strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup input pipeline When training a model with multiple GPUs, you can use the extra computing power effectively by increasing the batch size. In general, use the largest batch size that fits the GPU memory, and tune the learning rate accordingly.
###Code
# You can also do info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = info.splits['train'].num_examples
num_test_examples = info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks The callbacks used here are:* *TensorBoard*: This callback writes a log for TensorBoard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=12, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel Export the graph and the variables to the platform-agnostic SavedModel format. After your model is saved, you can load it with or without the scope.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training in TensorFlow View on TensorFlow.org Run in Google Colab View source on GitHub OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see [this tutorial](training_loops.ipynb). Import Dependencies
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
# Import TensorFlow
!pip install tensorflow-gpu==2.0.0-alpha0
import tensorflow_datasets as tfds
import tensorflow as tf
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define Distribution Strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print ('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup Input pipeline If a model is trained on multiple GPUs, the batch size should be increased accordingly so as to make effective use of the extra computing power. Moreover, the learning rate should be tuned accordingly.
###Code
# You can also do info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = info.splits['train'].num_examples
num_test_examples = info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks. The callbacks used here are:* *Tensorboard*: This callback writes a log for Tensorboard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print ('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=10, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel If you want to export the graph and the variables, SavedModel is the best way of doing this. The model can be loaded back with or without the scope. Moreover, SavedModel is platform agnostic.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2018 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed Training in TensorFlow View on TensorFlow.org Run in Google Colab View source on GitHub OverviewThe `tf.distribute.Strategy` API is an easy way to distribute your trainingacross multiple devices/machines. Our goal is to allow users to use existingmodels and training code with minimal changes to enable distributed training.Currently, core TensorFlow includes `tf.distribute.MirroredStrategy`. Thisdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it create copies of all variables in the model's layers on eachdevice. It then use all-reduce to combine gradients across the devices beforeapplying them to the variables to keep them in sync.Many other strategies will soon beavailable in core TensorFlow. You can find more information about them in the[README](https://github.com/tensorflow/tensorflow/tree/master/tensorflow/contrib/distribute). Example with Keras APIThe easiest way to get started with multiple GPUs on one machine using `MirroredStrategy` is with `tf.keras`.
###Code
from __future__ import absolute_import, division, print_function
# Import TensorFlow
!pip install tf-nightly-gpu-2.0-preview
import tensorflow_datasets as tfds
import tensorflow as tf
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset to train our model on. Use [TensorFlow Datasets](https://www.tensorflow.org/datasets) to load the dataset. This returns a dataset in `tf.data` format.
###Code
datasets, ds_info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
`with_info=True` returns the metadata for the entire dataset.In this example, `ds_info.splits.total_num_examples = 70000`.
###Code
num_train_examples = ds_info.splits['train'].num_examples
num_test_examples = ds_info.splits['test'].num_examples
BUFFER_SIZE = num_train_examples
BATCH_SIZE = 64
###Output
_____no_output_____
###Markdown
Input data pipeline
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Define Distribution Strategy To distribute a Keras model on multiple GPUs using `MirroredStrategy`, we first instantiate a `MirroredStrategy` object.
###Code
strategy = tf.distribute.MirroredStrategy()
print ('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# TODO(yashkatariya): Add accuracy when b/122371345 is fixed.
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam())
#metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks. The callbacks used here are:* *Tensorboard*: This callback writes a log for Tensorboard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can use a complicated decay equation too.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print ('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate To train the model call Keras `fit` API using the input dataset that wascreated earlier, same as how it would be called in a non-distributed case.
###Code
model.fit(train_dataset, epochs=10, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
Let's load the latest checkpoint and see how the model performs on the test dataset.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss = model.evaluate(eval_dataset)
print ('Eval loss: {}'.format(eval_loss))
###Output
_____no_output_____
###Markdown
You can download the tensorboard logs and then use the following command to see the output.```tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training with Keras View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see the [tf.distribute.Strategy with training loops](training_loops.ipynb) tutorial. Import dependencies
###Code
# Import TensorFlow and TensorFlow Datasets
!pip install tensorflow-gpu==2.0.0-beta1
!pip install tensorflow_datasets
from __future__ import absolute_import, division, print_function, unicode_literals
import tensorflow as tf
import tensorflow_datasets as tfds
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define distribution strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup input pipeline When training a model with multiple GPUs, you can use the extra computing power effectively by increasing the batch size. In general, use the largest batch size that fits the GPU memory, and tune the learning rate accordingly.
###Code
# You can also do info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = info.splits['train'].num_examples
num_test_examples = info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks The callbacks used here are:* *TensorBoard*: This callback writes a log for TensorBoard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=12, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel Export the graph and the variables to the platform-agnostic SavedModel format. After your model is saved, you can load it with or without the scope.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training with Keras View on TensorFlow.org Run in Google Colab View source on GitHub OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see the [tf.distribute.Strategy with training loops](training_loops.ipynb) tutorial. Import dependencies
###Code
# Import TensorFlow and TensorFlow Datasets
!pip install tensorflow-gpu==2.0.0-beta0
!pip install tensorflow_datasets
from __future__ import absolute_import, division, print_function, unicode_literals
import tensorflow as tf
import tensorflow_datasets as tfds
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define distribution strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup input pipeline When training a model with multiple GPUs, you can use the extra computing power effectively by increasing the batch size. In general, use the largest batch size that fits the GPU memory, and tune the learning rate accordingly.
###Code
# You can also do info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = info.splits['train'].num_examples
num_test_examples = info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks The callbacks used here are:* *TensorBoard*: This callback writes a log for TensorBoard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=12, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel Export the graph and the variables to the platform-agnostic SavedModel format. After your model is saved, you can load it with or without the scope.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training with Keras View on TensorFlow.org Run in Google Colab View source on GitHub OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see the [tf.distribute.Strategy with training loops](training_loops.ipynb) tutorial. Import dependencies
###Code
# Import TensorFlow and TensorFlow Datasets
!pip install tensorflow-gpu==2.0.0-alpha0
!pip install tensorflow_datasets
from __future__ import absolute_import, division, print_function, unicode_literals
import tensorflow as tf
import tensorflow_datasets as tfds
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define distribution strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup input pipeline When training a model with multiple GPUs, you can use the extra computing power effectively by increasing the batch size. In general, use the largest batch size that fits the GPU memory, and tune the learning rate accordingly.
###Code
# You can also do info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = info.splits['train'].num_examples
num_test_examples = info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks The callbacks used here are:* *TensorBoard*: This callback writes a log for TensorBoard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=12, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel Export the graph and the variables to the platform-agnostic SavedModel format. After your model is saved, you can load it with or without the scope.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training in TensorFlow View on TensorFlow.org Run in Google Colab View source on GitHub OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. Advanced users can implement the `tf.distribute.Strategy` API to create custom strategies.For more details, see the [Distribution Strategy README](https://github.com/tensorflow/tensorflow/tree/master/tensorflow/contrib/distribute). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see [this tutorial](training_loops.ipynb). Import Dependencies
###Code
from __future__ import absolute_import, division, print_function
# Import TensorFlow
!pip install tf-nightly-gpu-2.0-preview
import tensorflow_datasets as tfds
import tensorflow as tf
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format.
###Code
datasets, ds_info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `ds_info`.Among other things, this metadata object includes the number of train and test examples.
###Code
num_train_examples = ds_info.splits['train'].num_examples
num_test_examples = ds_info.splits['test'].num_examples
# You can also get ds_info.splits.total_num_examples
BUFFER_SIZE = 10000
BATCH_SIZE = 64
###Output
_____no_output_____
###Markdown
Input data pipelinePixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Define Distribution Strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print ('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks. The callbacks used here are:* *Tensorboard*: This callback writes a log for Tensorboard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print ('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=10, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel If you want to export the graph and the variables, SavedModel is the best way of doing this. The model can be loaded back with or without the scope. Moreover, SavedModel is platform agnostic.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training in TensorFlow View on TensorFlow.org Run in Google Colab View source on GitHub OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see [this tutorial](training_loops.ipynb). Import Dependencies
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
# Import TensorFlow
!pip install tensorflow-gpu==2.0.0-alpha0
import tensorflow_datasets as tfds
import tensorflow as tf
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define Distribution Strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print ('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup Input pipeline If a model is trained on multiple GPUs, the batch size should be increased accordingly so as to make effective use of the extra computing power. Moreover, the learning rate should be tuned accordingly.
###Code
# You can also do info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = info.splits['train'].num_examples
num_test_examples = info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks. The callbacks used here are:* *Tensorboard*: This callback writes a log for Tensorboard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print ('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=10, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel If you want to export the graph and the variables, SavedModel is the best way of doing this. The model can be loaded back with or without the scope. Moreover, SavedModel is platform agnostic.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training in TensorFlow View on TensorFlow.org Run in Google Colab View source on GitHub OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. Advanced users can implement the `tf.distribute.Strategy` API to create custom strategies.For more details, see the [Distribution Strategy README](https://github.com/tensorflow/tensorflow/tree/master/tensorflow/contrib/distribute). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see [this tutorial](training_loops.ipynb). Import Dependencies
###Code
from __future__ import absolute_import, division, print_function
# Import TensorFlow
!pip install tf-nightly-gpu-2.0-preview
import tensorflow_datasets as tfds
import tensorflow as tf
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `ds_info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, ds_info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define Distribution Strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print ('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup Input pipeline If a model is trained on multiple GPUs, the batch size should be increased accordingly so as to make effective use of the extra computing power. Moreover, the learning rate should be tuned accordingly.
###Code
# You can also do ds_info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = ds_info.splits['train'].num_examples
num_test_examples = ds_info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks. The callbacks used here are:* *Tensorboard*: This callback writes a log for Tensorboard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print ('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=10, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel If you want to export the graph and the variables, SavedModel is the best way of doing this. The model can be loaded back with or without the scope. Moreover, SavedModel is platform agnostic.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training with Keras View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see the [tf.distribute.Strategy with training loops](training_loops.ipynb) tutorial. Import dependencies
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
# Import TensorFlow and TensorFlow Datasets
!pip install tensorflow-gpu==2.0.0-beta1
import tensorflow_datasets as tfds
import tensorflow as tf
tfds.disable_progress_bar()
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define distribution strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup input pipeline When training a model with multiple GPUs, you can use the extra computing power effectively by increasing the batch size. In general, use the largest batch size that fits the GPU memory, and tune the learning rate accordingly.
###Code
# You can also do info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = info.splits['train'].num_examples
num_test_examples = info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks The callbacks used here are:* *TensorBoard*: This callback writes a log for TensorBoard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=12, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel Export the graph and the variables to the platform-agnostic SavedModel format. After your model is saved, you can load it with or without the scope.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training in TensorFlow View on TensorFlow.org Run in Google Colab View source on GitHub OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see the [tf.distribute.Strategy with training loops](training_loops.ipynb) tutorial. Import Dependencies
###Code
# Import TensorFlow and TensorFlow Datasets
!pip install tensorflow-gpu==2.0.0-alpha0
!pip install tensorflow_datasets
from __future__ import absolute_import, division, print_function, unicode_literals
import tensorflow as tf
import tensorflow_datasets as tfds
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define Distribution Strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup Input pipeline If a model is trained on multiple GPUs, the batch size should be increased accordingly so as to make effective use of the extra computing power. Moreover, the learning rate should be tuned accordingly.
###Code
# You can also do info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = info.splits['train'].num_examples
num_test_examples = info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks. The callbacks used here are:* *Tensorboard*: This callback writes a log for Tensorboard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=12, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel If you want to export the graph and the variables, SavedModel is the best way of doing this. The model can be loaded back with or without the scope. Moreover, SavedModel is platform agnostic.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training in TensorFlow View on TensorFlow.org Run in Google Colab View source on GitHub OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. Advanced users can implement the `tf.distribute.Strategy` API to create custom strategies.For more details, see the [Distribution Strategy README](https://github.com/tensorflow/tensorflow/tree/master/tensorflow/contrib/distribute). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see [this tutorial](training_loops.ipynb). Import Dependencies
###Code
from __future__ import absolute_import, division, print_function
# Import TensorFlow
!pip install tf-nightly-gpu-2.0-preview
import tensorflow_datasets as tfds
import tensorflow as tf
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format.
###Code
datasets, ds_info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `ds_info`.Among other things, this metadata object includes the number of train and test examples.
###Code
num_train_examples = ds_info.splits['train'].num_examples
num_test_examples = ds_info.splits['test'].num_examples
# You can also get ds_info.splits.total_num_examples
BUFFER_SIZE = 10000
BATCH_SIZE = 64
###Output
_____no_output_____
###Markdown
Input data pipelinePixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Define Distribution Strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print ('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks. The callbacks used here are:* *Tensorboard*: This callback writes a log for Tensorboard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print ('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=10, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Distributed training in TensorFlow View on TensorFlow.org Run in Google Colab View source on GitHub OverviewThe `tf.distribute.Strategy` API provides an abstraction for distributing your trainingacross multiple processing units. The goal is to allow users to enable distributed training using existing models and training code, with minimal changes.This tutorial uses the `tf.distribute.MirroredStrategy`, whichdoes in-graph replication with synchronous training on many GPUs on one machine.Essentially, it copies all of the model's variables to each processor.Then, it uses [all-reduce](http://mpitutorial.com/tutorials/mpi-reduce-and-allreduce/) to combine the gradients from all processors and applies the combined value to all copies of the model.`MirroredStategy` is one of several distribution strategy available in TensorFlow core. You can read about more strategies at [distribution strategy guide](../../guide/distribute_strategy.ipynb). Keras APIThis example uses the `tf.keras` API to build the model and training loop. For custom training loops, see the [tf.distribute.Strategy with training loops](training_loops.ipynb) tutorial. Import Dependencies
###Code
# Import TensorFlow and TensorFlow Datasets
!pip install tensorflow-gpu==2.0.0-alpha0
!pip install tensorflow_datasets
from __future__ import absolute_import, division, print_function, unicode_literals
import tensorflow as tf
import tensorflow_datasets as tfds
import os
###Output
_____no_output_____
###Markdown
Download the dataset Download the MNIST dataset and load it from [TensorFlow Datasets](https://www.tensorflow.org/datasets). This returns a dataset in `tf.data` format. Setting `with_info` to `True` includes the metadata for the entire dataset, which is being saved here to `info`.Among other things, this metadata object includes the number of train and test examples.
###Code
datasets, info = tfds.load(name='mnist', with_info=True, as_supervised=True)
mnist_train, mnist_test = datasets['train'], datasets['test']
###Output
_____no_output_____
###Markdown
Define Distribution Strategy Create a `MirroredStrategy` object. This will handle distribution, and provides a context manager (`tf.distribute.MirroredStrategy.scope`) to build your model inside.
###Code
strategy = tf.distribute.MirroredStrategy()
print('Number of devices: {}'.format(strategy.num_replicas_in_sync))
###Output
_____no_output_____
###Markdown
Setup Input pipeline If a model is trained on multiple GPUs, the batch size should be increased accordingly so as to make effective use of the extra computing power. Moreover, the learning rate should be tuned accordingly.
###Code
# You can also do info.splits.total_num_examples to get the total
# number of examples in the dataset.
num_train_examples = info.splits['train'].num_examples
num_test_examples = info.splits['test'].num_examples
BUFFER_SIZE = 10000
BATCH_SIZE_PER_REPLICA = 64
BATCH_SIZE = BATCH_SIZE_PER_REPLICA * strategy.num_replicas_in_sync
###Output
_____no_output_____
###Markdown
Pixel values, which are 0-255, [have to be normalized to the 0-1 range](https://en.wikipedia.org/wiki/Feature_scaling). Define this scale in a function.
###Code
def scale(image, label):
image = tf.cast(image, tf.float32)
image /= 255
return image, label
###Output
_____no_output_____
###Markdown
Apply this function to the training and test data, shuffle the training data, and [batch it for training](https://www.tensorflow.org/api_docs/python/tf/data/Datasetbatch).
###Code
train_dataset = mnist_train.map(scale).shuffle(BUFFER_SIZE).batch(BATCH_SIZE)
eval_dataset = mnist_test.map(scale).batch(BATCH_SIZE)
###Output
_____no_output_____
###Markdown
Create the model Create and compile the Keras model in the context of `strategy.scope`.
###Code
with strategy.scope():
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Define the callbacks. The callbacks used here are:* *Tensorboard*: This callback writes a log for Tensorboard which allows you to visualize the graphs.* *Model Checkpoint*: This callback saves the model after every epoch.* *Learning Rate Scheduler*: Using this callback, you can schedule the learning rate to change after every epoch/batch.For illustrative purposes, add a print callback to display the *learning rate* in the notebook.
###Code
# Define the checkpoint directory to store the checkpoints
checkpoint_dir = './training_checkpoints'
# Name of the checkpoint files
checkpoint_prefix = os.path.join(checkpoint_dir, "ckpt_{epoch}")
# Function for decaying the learning rate.
# You can define any decay function you need.
def decay(epoch):
if epoch < 3:
return 1e-3
elif epoch >= 3 and epoch < 7:
return 1e-4
else:
return 1e-5
# Callback for printing the LR at the end of each epoch.
class PrintLR(tf.keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs=None):
print('\nLearning rate for epoch {} is {}'.format(epoch + 1,
model.optimizer.lr.numpy()))
callbacks = [
tf.keras.callbacks.TensorBoard(log_dir='./logs'),
tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_prefix,
save_weights_only=True),
tf.keras.callbacks.LearningRateScheduler(decay),
PrintLR()
]
###Output
_____no_output_____
###Markdown
Train and evaluate Now, train the model in the usual way, calling `fit` on the model and passing in the dataset created at the beginning of the tutorial. This step is the same whether you are distributing the training or not.
###Code
model.fit(train_dataset, epochs=10, callbacks=callbacks)
###Output
_____no_output_____
###Markdown
As you can see below, the checkpoints are getting saved.
###Code
# check the checkpoint directory
!ls {checkpoint_dir}
###Output
_____no_output_____
###Markdown
To see how the model perform, load the latest checkpoint and call `evaluate` on the test data.Call `evaluate` as before using appropriate datasets.
###Code
model.load_weights(tf.train.latest_checkpoint(checkpoint_dir))
eval_loss, eval_acc = model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
To see the output, you can download and view the TensorBoard logs at the terminal.```$ tensorboard --logdir=path/to/log-directory```
###Code
!ls -sh ./logs
###Output
_____no_output_____
###Markdown
Export to SavedModel If you want to export the graph and the variables, SavedModel is the best way of doing this. The model can be loaded back with or without the scope. Moreover, SavedModel is platform agnostic.
###Code
path = 'saved_model/'
tf.keras.experimental.export_saved_model(model, path)
###Output
_____no_output_____
###Markdown
Load the model without `strategy.scope`.
###Code
unreplicated_model = tf.keras.experimental.load_from_saved_model(path)
unreplicated_model.compile(
loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = unreplicated_model.evaluate(eval_dataset)
print('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____
###Markdown
Load the model with `strategy.scope`.
###Code
with strategy.scope():
replicated_model = tf.keras.experimental.load_from_saved_model(path)
replicated_model.compile(loss='sparse_categorical_crossentropy',
optimizer=tf.keras.optimizers.Adam(),
metrics=['accuracy'])
eval_loss, eval_acc = replicated_model.evaluate(eval_dataset)
print ('Eval loss: {}, Eval Accuracy: {}'.format(eval_loss, eval_acc))
###Output
_____no_output_____ |
notebooks/1-Low-D-viz.ipynb | ###Markdown
This is an unsuccessful attempt to do some nonlinear dim reduction
###Code
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
import os
from copy import copy
MOVIE_LENS_PATH = os.path.abspath(os.path.join(os.getcwd(), '..', 'data', 'raw', 'movie_lense'))
MOVIE_LENS_PATH
genome_scores = pd.read_csv(os.path.join(MOVIE_LENS_PATH, 'genome-scores.csv'))
wide_genome_scores = genome_scores.pivot(index='movieId', columns='tagId')
wide_genome_scores.columns = ['TagId_' + str(i) for i in wide_genome_scores.columns.droplevel().rename(None)]
wide_genome_scores.head()
from sklearn.decomposition import PCA
reduced_genome = PCA(n_components=10).fit_transform(wide_genome_scores)
def visualize_embedding(method, X, hyperparams, clusters, **kwargs):
sns.set_style("whitegrid", {'axes.grid' : False})
default_args = copy(kwargs)
embeddings_results = {}
for range_key in hyperparams.keys():
#Set to default kwargs:
kwargs = copy(default_args)
X_embs = []
for hyperparam in hyperparams[range_key]:
print(range_key, '=', hyperparam)
kwargs[range_key] = hyperparam
model = method(**kwargs)
# model.fit(X)
X_embs.append(model.fit_transform(X))
embeddings_results[range_key] = X_embs
for key in embeddings_results.keys():
X_embs = embeddings_results[key]
fig, axs = plt.subplots(2, len(X_embs)//2, figsize=(30,15))#
fig.suptitle(method.__name__)
plt.subplots_adjust(top=0.93)
for num, ax_ in enumerate(axs.flatten()):
ax_.scatter(X_embs[num][:, 0], X_embs[num][:, 1], c = clusters)
ax_.title.set_text('{} {}'.format(key, hyperparams[key][num]))
return
from sklearn.manifold import TSNE
method = TSNE
hyperparams = {'perplexity': [5, 20, 30, 50],
'early_exaggeration': [10.0, 12.0, 15.0, 20.0]}
visualize_embedding(method, wide_genome_scores, hyperparams, None, **{'perplexity': 30, 'early_exaggeration': 20.0,
'n_components': 2,
'n_iter': 300,
'n_jobs': -1})
from sklearn.manifold import Isomap
method = Isomap
hyperparams = {'n_neighbors': [5, 10, 20, 30]}#, 30, 40, 50, 100, 200]}
visualize_embedding(method, reduced_genome, hyperparams, None, **{'n_neighbors': 50,
'n_components': 2,
'max_iter': 1000,
'n_jobs': -1})
from sklearn.manifold import SpectralEmbedding
method = SpectralEmbedding
hyperparams = {'n_neighbors': [5, 10, 20]}#, 30, 40, 50, 100, 200]}
visualize_embedding(method, wide_genome_scores, hyperparams, None, **{'n_neighbors': 50,
'n_components': 2,
'n_jobs': -1})
%matplotlib inline
from sklearn.decomposition import PCA
method = PCA
hyperparams = {'n_components': [2, 2]}
visualize_embedding(method, wide_genome_scores, hyperparams, None, **{'n_components': 2})
from time import time
import numpy as np
import matplotlib.pyplot as plt
from sklearn import metrics
from sklearn.cluster import KMeans
from sklearn.datasets import load_digits
from sklearn.decomposition import PCA
from sklearn.preprocessing import scale
np.random.seed(42)
# X_digits, y_digits = load_digits(return_X_y=True)
X_digits = wide_genome_scores
data = scale(X_digits)
n_samples, n_features = data.shape
n_digits = 10#len(np.unique(y_digits))
# labels = y_digits
sample_size = 300
# print("n_digits: %d, \t n_samples %d, \t n_features %d"
# % (n_digits, n_samples, n_features))
# print(82 * '_')
# print('init\t\ttime\tinertia\thomo\tcompl\tv-meas\tARI\tAMI\tsilhouette')
# def bench_k_means(estimator, name, data):
# t0 = time()
# estimator.fit(data)
# # print('%-9s\t%.2fs\t%i\t%.3f\t%.3f\t%.3f\t%.3f\t%.3f\t%.3f'
# # % (name, (time() - t0), estimator.inertia_,
# # # metrics.homogeneity_score(labels, estimator.labels_),
# # # metrics.completeness_score(labels, estimator.labels_),
# # # metrics.v_measure_score(labels, estimator.labels_),
# # # metrics.adjusted_rand_score(labels, estimator.labels_),
# # # metrics.adjusted_mutual_info_score(labels, estimator.labels_),
# # metrics.silhouette_score(data, estimator.labels_,
# # metric='euclidean',
# # sample_size=sample_size)))
# bench_k_means(KMeans(init='k-means++', n_clusters=n_digits, n_init=10),
# name="k-means++", data=data)
# bench_k_means(KMeans(init='random', n_clusters=n_digits, n_init=10),
# name="random", data=data)
# in this case the seeding of the centers is deterministic, hence we run the
# kmeans algorithm only once with n_init=1
pca = PCA(n_components=n_digits).fit(data)
# bench_k_means(KMeans(init=pca.components_, n_clusters=n_digits, n_init=1),
# name="PCA-based",
# data=data)
# print(82 * '_')
# #############################################################################
# Visualize the results on PCA-reduced data
reduced_data = PCA(n_components=2).fit_transform(data)
kmeans = KMeans(init='k-means++', n_clusters=n_digits, n_init=10)
kmeans.fit(reduced_data)
# Step size of the mesh. Decrease to increase the quality of the VQ.
h = .02 # point in the mesh [x_min, x_max]x[y_min, y_max].
# Plot the decision boundary. For that, we will assign a color to each
x_min, x_max = reduced_data[:, 0].min() - 1, reduced_data[:, 0].max() + 1
y_min, y_max = reduced_data[:, 1].min() - 1, reduced_data[:, 1].max() + 1
xx, yy = np.meshgrid(np.arange(x_min, x_max, h), np.arange(y_min, y_max, h))
# Obtain labels for each point in mesh. Use last trained model.
Z = kmeans.predict(np.c_[xx.ravel(), yy.ravel()])
# Put the result into a color plot
Z = Z.reshape(xx.shape)
plt.figure(1)
plt.clf()
plt.imshow(Z, interpolation='nearest',
extent=(xx.min(), xx.max(), yy.min(), yy.max()),
cmap=plt.cm.Paired,
aspect='auto', origin='lower')
plt.plot(reduced_data[:, 0], reduced_data[:, 1], 'k.', markersize=2, alpha = 0.1)
# Plot the centroids as a white X
centroids = kmeans.cluster_centers_
plt.scatter(centroids[:, 0], centroids[:, 1],
marker='x', s=169, linewidths=3,
color='w', zorder=10)
plt.title('K-means clustering on the Genome Scores (PCA-reduced data)\n'
'Centroids are marked with white cross')
plt.xlim(x_min, x_max)
plt.ylim(y_min, y_max)
plt.xticks(())
plt.yticks(())
plt.show()
X_digits.shape
from time import time
import numpy as np
import matplotlib.pyplot as plt
from sklearn import metrics
from sklearn.cluster import KMeans
from sklearn.datasets import load_digits
from sklearn.decomposition import PCA
from sklearn.preprocessing import scale
np.random.seed(42)
# X_digits, y_digits = load_digits(return_X_y=True)
X_digits = wide_genome_scores
data = scale(X_digits)
n_samples, n_features = data.shape
n_digits = 10#len(np.unique(y_digits))
# labels = y_digits
sample_size = 300
# print("n_digits: %d, \t n_samples %d, \t n_features %d"
# % (n_digits, n_samples, n_features))
# print(82 * '_')
# print('init\t\ttime\tinertia\thomo\tcompl\tv-meas\tARI\tAMI\tsilhouette')
# def bench_k_means(estimator, name, data):
# t0 = time()
# estimator.fit(data)
# # print('%-9s\t%.2fs\t%i\t%.3f\t%.3f\t%.3f\t%.3f\t%.3f\t%.3f'
# # % (name, (time() - t0), estimator.inertia_,
# # # metrics.homogeneity_score(labels, estimator.labels_),
# # # metrics.completeness_score(labels, estimator.labels_),
# # # metrics.v_measure_score(labels, estimator.labels_),
# # # metrics.adjusted_rand_score(labels, estimator.labels_),
# # # metrics.adjusted_mutual_info_score(labels, estimator.labels_),
# # metrics.silhouette_score(data, estimator.labels_,
# # metric='euclidean',
# # sample_size=sample_size)))
# bench_k_means(KMeans(init='k-means++', n_clusters=n_digits, n_init=10),
# name="k-means++", data=data)
# bench_k_means(KMeans(init='random', n_clusters=n_digits, n_init=10),
# name="random", data=data)
# in this case the seeding of the centers is deterministic, hence we run the
# kmeans algorithm only once with n_init=1
pca = PCA(n_components=n_digits).fit(data)
# bench_k_means(KMeans(init=pca.components_, n_clusters=n_digits, n_init=1),
# name="PCA-based",
# data=data)
# print(82 * '_')
# #############################################################################
# Visualize the results on PCA-reduced data
reduced_PCA = PCA(n_components=2).fit(data)
reduced_data = reduced_PCA.transform(data)
kmeans = KMeans(init='k-means++', n_clusters=n_digits, n_init=10)
kmeans.fit(reduced_data)
kmeans1 = KMeans(init='k-means++', n_clusters=n_digits, n_init=10)
kmeans1.fit(data)
# Step size of the mesh. Decrease to increase the quality of the VQ.
h = .02 # point in the mesh [x_min, x_max]x[y_min, y_max].
# Plot the decision boundary. For that, we will assign a color to each
x_min, x_max = reduced_data[:, 0].min() - 1, reduced_data[:, 0].max() + 1
y_min, y_max = reduced_data[:, 1].min() - 1, reduced_data[:, 1].max() + 1
xx, yy = np.meshgrid(np.arange(x_min, x_max, h), np.arange(y_min, y_max, h))
# Obtain labels for each point in mesh. Use last trained model.
Z = kmeans.predict(np.c_[xx.ravel(), yy.ravel()])
# Put the result into a color plot
Z = Z.reshape(xx.shape)
plt.figure(1)
plt.clf()
plt.imshow(Z, interpolation='nearest',
extent=(xx.min(), xx.max(), yy.min(), yy.max()),
cmap=plt.cm.Paired,
aspect='auto', origin='lower')
plt.plot(reduced_data[:, 0], reduced_data[:, 1], 'k.', markersize=2, alpha = 0.1)
# Plot the centroids as a white X
centroids = reduced_PCA.transform(kmeans1.cluster_centers_)
plt.scatter(centroids[:, 0], centroids[:, 1],
marker='x', s=169, linewidths=3,
color='w', zorder=10)
plt.title('K-means clustering on the Genome Scores (PCA-reduced data)\n'
'Centroids are marked with white cross')
plt.xlim(x_min, x_max)
plt.ylim(y_min, y_max)
plt.xticks(())
plt.yticks(())
reduced_PCA.explained_variance_
###Output
_____no_output_____ |
Sorting Algorithms/Radix_Sort.ipynb | ###Markdown
Radix Sort(c) 2020, Joe James
###Code
# get number of digits in largest item
def __get_num_digits(A):
m = 0
for item in A:
m = max(m, item)
return len(str(m))
# flatten into a 1D List
from functools import reduce
def __flatten(A):
return reduce(lambda x, y: x + y, A)
###Output
_____no_output_____
###Markdown
Changed from YouTube video:It's much cleaner to put the _get_num_digits call inside the radix function rather than in main as shown in the video. That way you only need to pass a List to the radix function. Thanks to Brother Lui for this suggestion.
###Code
def radix(A):
num_digits = __get_num_digits(A)
for digit in range(0, num_digits):
B = [[] for i in range(10)]
for item in A:
# num is the bucket number that the item will be put into
num = item // 10 ** (digit) % 10
B[num].append(item)
A = __flatten(B)
return A
def main():
A = [55, 45, 3, 289, 213, 1, 288, 53, 2]
A = radix(A)
print(A)
B = [i for i in range(1000000)]
from random import shuffle
shuffle(B)
B = radix(B)
print(B[:6], B[-6:])
main()
###Output
[1, 2, 3, 45, 53, 55, 213, 288, 289]
[0, 1, 2, 3, 4, 5] [999994, 999995, 999996, 999997, 999998, 999999]
###Markdown
Radix Sort(c) 2020, Joe James
###Code
# get number of digits in largest item
def __get_num_digits(A):
m = 0
for item in A:
m = max(m, item)
return len(str(m))
# flatten into a 1D List
from functools import reduce
def __flatten(A):
return reduce(lambda x, y: x + y, A)
###Output
_____no_output_____
###Markdown
Changed from YouTube video:It's much cleaner to put the _get_num_digits call inside the radix function rather than in main as shown in the video. That way you only need to pass a List to the radix function. Thanks to Brother Lui for this suggestion.
###Code
def radix(A):
num_digits = __get_num_digits(A)
for digit in range(0, num_digits):
B = [[] for i in range(10)]
for item in A:
# num is the bucket number that the item will be put into
num = item // 10 ** (digit) % 10
B[num].append(item)
A = __flatten(B)
return A
def main():
A = [55, 45, 3, 289, 213, 1, 288, 53, 2]
A = radix(A)
print(A)
B = [i for i in range(1000000)]
from random import shuffle
shuffle(B)
B = radix(B)
print(B[:6], B[-6:])
main()
###Output
[1, 2, 3, 45, 53, 55, 213, 288, 289]
[0, 1, 2, 3, 4, 5] [999994, 999995, 999996, 999997, 999998, 999999]
|
exercises/word2vec_fasttext_demo.ipynb | ###Markdown
Word Embeddings with word2vec, fastText and GloVe
###Code
!pip install gensim plotly
%matplotlib inline
import os
import urllib.request
import gensim
from sklearn.manifold import TSNE
import matplotlib.pyplot as plt
import numpy as np
###Output
_____no_output_____
###Markdown
Q: Name two advantages of using dense parametrised word representations compared to one-hot vectors A: Give your answer here1) We can define a similarity measure between different words, for example using the cosine of the scalar product (which would be zero for the one-hot encoding since all vectors are orthogonal)2) Can try to embedd in lower-dimensional space (do not need a separate dimension for each word) Play around with pre-trained Word2Vec
###Code
#convenience function for plotting embeddings into 2-dimensional space
def plot_word_embeddings(model, search_list):
words = []
for term in search_list:
words += [w[0] for w in model.wv.most_similar([term], topn=6)]
words += search_list
words = list(dict.fromkeys(words)) # remove duplicates
vectors = model.wv[words]
tsne = TSNE(n_components=2, random_state=0, n_iter=10000, perplexity=7)
T = tsne.fit_transform(vectors)
plt.figure(figsize=(16, 10))
plt.scatter(T[:, 0], T[:, 1])
for label, x, y in zip(words, T[:, 0], T[:, 1]):
plt.annotate(label, xy=(x+2, y+2), xytext=(0, 0), textcoords='offset points')
import gensim.downloader as api
corpus = api.load('text8')
api.info("text8")
# inspect the training corpus
corpus_list = [doc for doc in corpus]
print(corpus_list[102])
from gensim.models.word2vec import Word2Vec
print("Training model on corpus ...")
# Documentation:
# https://radimrehurek.com/gensim/models/word2vec.html
# sg ({0, 1}, optional) – Training algorithm: 1 for skip-gram; otherwise CBOW.
w2v_trained = Word2Vec(corpus, vector_size=100, window=5, min_count=1, sg=0, workers=4)
print("Finished training.")
# Check the number of embedded words and their embedding size
w2v_trained.wv.vectors.shape
# Get the vector to a word
queen_vec = w2v_trained.wv['queen']
print(queen_vec)
print('queen_vec.shape is', queen_vec.shape)
w2v_trained.wv['king']
###Output
_____no_output_____
###Markdown
We can use the cosine-smililarity metric to compare different embeddings.
###Code
w2v_trained.wv.similarity('queen', 'king')
###Output
_____no_output_____
###Markdown
or even find the most similar words to a given word
###Code
w2v_trained.wv.most_similar('queen')
w2v_trained.wv.most_similar('good')
#plot to see clustering
search_list = ['queen', 'king', 'man', 'cheap', 'west', 'good']
plot_word_embeddings(w2v_trained, search_list)
###Output
/opt/homebrew/Caskroom/miniforge/base/envs/2022-02-10_rosetta_py3.8_new/lib/python3.7/site-packages/sklearn/manifold/_t_sne.py:783: FutureWarning: The default initialization in TSNE will change from 'random' to 'pca' in 1.2.
FutureWarning,
/opt/homebrew/Caskroom/miniforge/base/envs/2022-02-10_rosetta_py3.8_new/lib/python3.7/site-packages/sklearn/manifold/_t_sne.py:793: FutureWarning: The default learning rate in TSNE will change from 200.0 to 'auto' in 1.2.
FutureWarning,
###Markdown
Find interesting relationships between words: A is to B as C is to ? (Take a look into the lecture slides if you don't know)B - A + C
###Code
B = 'king'
A = 'man'
C = 'woman'
w2v_trained.wv.most_similar(positive=[B, C], negative=[A])
B = 'brother'
A = 'man'
C = 'woman'
w2v_trained.wv.most_similar(positive=[B, C], negative=[A])
B = 'france'
A = 'paris'
C = 'germany'
w2v_trained.wv.most_similar(positive=[B, C], negative=[A])
###Output
_____no_output_____
###Markdown
We can naively compare sentences by adding up all word embeddings of the respective sentences and diving by the length
###Code
from sklearn.metrics.pairwise import cosine_similarity
from copy import deepcopy
#TODO: Generalize the similarity function to be able to compare sentences using cosine-similarity
def sentence_similarity(embeddings, sentence1, sentence2):
# TODO: Your code here
return
print(sentence_similarity(w2v_trained.wv, 'apple is more tasty than orange', 'fruit'))
print(sentence_similarity(w2v_trained.wv, 'apple is more tasty than orange', 'computer'))
###Output
_____no_output_____
###Markdown
fastText A big issue of Word2Vec is the fixed vocabulary size, an alternative solution is provided by fastText
###Code
# not in the vocabulary
try:
w2v_trained.wv['kingg']
except:
print("Error: This word is not in the vocabulary!")
###Output
Error: This word is not in the vocabulary!
###Markdown
fasttext using gensim
###Code
from gensim.models.fasttext import FastText
# Train your model using the FastText method
trained_ft = FastText(corpus, vector_size=100, window=5, min_count=1, epochs=1)
# Sanity check if trained word-embeddings capture similarities as before
print(trained_ft.wv.most_similar('west'))
print(trained_ft.wv.most_similar('king'))
for index, word in enumerate(trained_ft.wv.index_to_key):
if index == 10:
break
print(f"word #{index}/{len(trained_ft.wv.index_to_key)} is {word}")
search_list = ['cheap', 'west', 'italian']
plot_word_embeddings(trained_ft, search_list)
# FastText lets us do comparisons for words outside of the vocabulary in contrast to Word2Vec
trained_ft.wv.most_similar('kingg')
trained_ft.wv.most_similar('expensif')
trained_ft.wv.vectors.shape
from sklearn.decomposition import IncrementalPCA # inital reduction
from sklearn.manifold import TSNE # final reduction
import plotly.offline as plt
from plotly.offline import init_notebook_mode, iplot, plot
import plotly.graph_objs as go
import plotly.express as px
plt.init_notebook_mode(connected=True)
import plotly.io as pio
pio.renderers.default = "iframe"
def reduce_dimensions(model, num_dimensions = 2, number_of_vectors = 2000):
# extract the words & their vectors, as numpy arrays
vectors = np.asarray(model.wv.vectors)[:number_of_vectors]
labels = np.asarray(model.wv.index_to_key)[:number_of_vectors] # fixed-width numpy strings
# reduce using t-SNE
print("Dimension reduction using tSNE ...")
tsne = TSNE(n_components=num_dimensions, random_state=0)
vectors = tsne.fit_transform(vectors)
print("Dimension reduction using tSNE Done")
x_vals = [v[0] for v in vectors]
y_vals = [v[1] for v in vectors]
z_vals = None
if num_dimensions == 3:
z_vals = [v[2] for v in vectors]
return x_vals, y_vals, z_vals, labels
def plot_2d_with_plotly(x_vals, y_vals, labels):
trace = go.Scatter(x=x_vals, y=y_vals, mode='text', text=labels)
data = [trace]
iplot(data, filename='word-embedding-plot-2d')
return
def plot_3d_with_plotly(x_vals, y_vals, z_vals, labels):
trace = go.Scatter3d(x=x_vals, y=y_vals, z=z_vals, mode='text', text=labels)
data = [trace]
iplot(data, filename='word-embedding-plot-3d')
return
def plot_with_matplotlib(x_vals, y_vals, labels):
import matplotlib.pyplot as plt
import random
random.seed(0)
plt.figure(figsize=(12, 12))
plt.scatter(x_vals, y_vals)
#
# Label randomly subsampled 25 data points
#
indices = list(range(len(labels)))
selected_indices = random.sample(indices, 25)
for i in selected_indices:
plt.annotate(labels[i], (x_vals[i], y_vals[i]))
return
model = trained_ft
x_vals, y_vals, z_vals, labels = reduce_dimensions(model, 3, 1500)
# plot_with_matplotlib(x_vals, y_vals, labels)
# plot_2d_with_plotly(x_vals, y_vals, labels)
plot_3d_with_plotly(x_vals, y_vals, z_vals, labels)
###Output
_____no_output_____
###Markdown
GloVe
###Code
# TODO
###Output
_____no_output_____ |
REPP_custom_loop_01.ipynb | ###Markdown
RandomForestRegressor
###Code
test_data = pd.read_csv('test.csv')
train_data = pd.read_csv('train.csv')
prepare_data(train_data)
prepare_data(test_data)
X = pd.get_dummies(train_data)
X.drop("Price", axis=1, inplace=True)
X.drop("Id", axis=1, inplace=True)
y = train_data.Price
X_train, X_valid, y_train, y_valid = train_test_split(X, y, test_size=0.13, random_state=42)
model = RandomForestRegressor(n_estimators=2500, max_depth=17, random_state=42, max_features=8,
n_jobs=3)
model.fit(X_train, y_train)
y_pred = model.predict(X_valid)
y_pred_train = model.predict(X_train)
print(r2_score(y_train, y_pred_train))
r2_score(y_valid, y_pred)
# Предсказываем цены для тестовых данных и выгружаем в файл
X_test = pd.get_dummies(test_data)
X_test.drop("Id", axis=1, inplace=True)
test_data["Price"] = model.predict(X_test)
# экспорт в файл
test_data.loc[:, ['Id', 'Price']].to_csv('kag_best_loop_cust_01.csv', index=False)
# md=0
# for number in range(50):
# mf=0
# md=md+1
# for j in range(21):
# mf = mf+1
# X_train, X_valid, y_train, y_valid = train_test_split(X, y, test_size=0.13, random_state=42)
# model = RandomForestRegressor(n_estimators=50, max_depth=md, random_state=42, max_features=mf,
# n_jobs=-1)
# model.fit(X_train, y_train)
# y_pred = model.predict(X_valid)
# y_pred_train = model.predict(X_train)
# print('r2: ', r2_score(y_valid, y_pred),', max_depth: ',md,', max_features: ',mf)
# ts=0.16
# for number in range(20):
# ts=round(ts+0.001,3)
# test_size_x=ts
# X_train, X_valid, y_train, y_valid = train_test_split(X, y, test_size=ts, random_state=42)
# model = RandomForestRegressor(n_estimators=50, max_depth=18, random_state=42, max_features=7,
# n_jobs=-1)
# model.fit(X_train, y_train)
# y_pred = model.predict(X_valid)
# y_pred_train = model.predict(X_train)
# print('r2: ', r2_score(y_valid, y_pred),', test_size: ',ts)
###Output
_____no_output_____
###Markdown
model = RandomForestRegressor(n_estimators=50, max_depth=18, random_state=42, max_features=7, n_jobs=-1)r2: 0.7314039075184304 , test_size: 0.11r2: 0.7348465702732343 , test_size: 0.12r2: 0.7531442873674634 , test_size: 0.13r2: 0.7397530855670512 , test_size: 0.14r2: 0.7402901437028434 , test_size: 0.15r2: 0.7461407454357274 , test_size: 0.16r2: 0.7417489594981834 , test_size: 0.17r2: 0.733112333462109 , test_size: 0.18r2: 0.7379151448519967 , test_size: 0.19r2: 0.7271108078624069 , test_size: 0.2r2: 0.7358392774554288 , test_size: 0.21r2: 0.7234253045558205 , test_size: 0.22r2: 0.7310595191785009 , test_size: 0.23r2: 0.7302756236955046 , test_size: 0.24r2: 0.732396858741041 , test_size: 0.25r2: 0.7281463330409553 , test_size: 0.26r2: 0.7413437772544844 , test_size: 0.27r2: 0.7378859359412591 , test_size: 0.28r2: 0.7270656783365747 , test_size: 0.29r2: 0.7347855953292995 , test_size: 0.3r2: 0.7277362296138763 , test_size: 0.31r2: 0.7350732907642259 , test_size: 0.32r2: 0.7319064529608187 , test_size: 0.33r2: 0.7245733301519215 , test_size: 0.34r2: 0.722668945883973 , test_size: 0.35r2: 0.7242921775304727 , test_size: 0.36r2: 0.7226587707008562 , test_size: 0.37r2: 0.7242812897957027 , test_size: 0.38r2: 0.7204556250684372 , test_size: 0.39r2: 0.7174929275491773 , test_size: 0.4r2: 0.7167735052675073 , test_size: 0.41r2: 0.7250472082776203 , test_size: 0.42r2: 0.7208011475443763 , test_size: 0.43r2: 0.7201513439285026 , test_size: 0.44r2: 0.724039687786426 , test_size: 0.45r2: 0.7239343522492809 , test_size: 0.46r2: 0.7197914138383095 , test_size: 0.47r2: 0.7157873372544823 , test_size: 0.48r2: 0.7157699102768378 , test_size: 0.49r2: 0.7109548234637448 , test_size: 0.5r2: 0.7154723688673392 , test_size: 0.101r2: 0.7150860959936948 , test_size: 0.102r2: 0.7194436389645893 , test_size: 0.103r2: 0.7086587409342243 , test_size: 0.104r2: 0.7216106036389413 , test_size: 0.105r2: 0.7267645760361496 , test_size: 0.106r2: 0.7315304388773025 , test_size: 0.107r2: 0.725592743198056 , test_size: 0.108r2: 0.719997562335243 , test_size: 0.109r2: 0.7314039075184304 , test_size: 0.11r2: 0.7334681695906031 , test_size: 0.111r2: 0.7299438116600453 , test_size: 0.112r2: 0.7313718018153201 , test_size: 0.113r2: 0.719559585242212 , test_size: 0.114r2: 0.7256418719593536 , test_size: 0.115r2: 0.7331049804512098 , test_size: 0.116r2: 0.7381470883685923 , test_size: 0.117r2: 0.73699810216503 , test_size: 0.118r2: 0.7372264882855786 , test_size: 0.119r2: 0.7348465702732343 , test_size: 0.12r2: 0.7352555999683252 , test_size: 0.121r2: 0.7336662528990658 , test_size: 0.122r2: 0.7363564586004046 , test_size: 0.123r2: 0.7446621996596268 , test_size: 0.124 +++r2: 0.739625944018613 , test_size: 0.125r2: 0.7425696328139295 , test_size: 0.126r2: 0.7444803068169805 , test_size: 0.127 ++r2: 0.7497990211063045 , test_size: 0.128 +++r2: 0.7419879244263697 , test_size: 0.129r2: 0.7531442873674634 , test_size: 0.13 ++++++++++++++r2: 0.7443487016817805 , test_size: 0.131 ++r2: 0.7468140732304189 , test_size: 0.132 +++r2: 0.7391109476743771 , test_size: 0.133r2: 0.7401260883101677 , test_size: 0.134r2: 0.7461989869665404 , test_size: 0.135 +++r2: 0.7438869499057317 , test_size: 0.136 +r2: 0.7466217661476909 , test_size: 0.137 +++r2: 0.7401647431176883 , test_size: 0.138r2: 0.7398109353047051 , test_size: 0.139r2: 0.7397530855670512 , test_size: 0.14r2: 0.7379659825685931 , test_size: 0.141r2: 0.743543193166815 , test_size: 0.142 +r2: 0.7383156972179791 , test_size: 0.143r2: 0.7376905217448939 , test_size: 0.144r2: 0.7402547929820429 , test_size: 0.145r2: 0.744002942220638 , test_size: 0.146 ++r2: 0.7375024111540858 , test_size: 0.147r2: 0.7398681607223119 , test_size: 0.148r2: 0.7362831202564167 , test_size: 0.149r2: 0.7402901437028434 , test_size: 0.15r2: 0.7366235999497139 , test_size: 0.151r2: 0.7350029874622135 , test_size: 0.152r2: 0.7391484997284079 , test_size: 0.153r2: 0.7394627104085587 , test_size: 0.154r2: 0.7430961846394291 , test_size: 0.155 +r2: 0.7407583434490533 , test_size: 0.156r2: 0.740140543546047 , test_size: 0.157r2: 0.7449067350859468 , test_size: 0.158 +++r2: 0.7466785317388027 , test_size: 0.159 +++r2: 0.7461407454357274 , test_size: 0.16 +++r2: 0.7443250354808149 , test_size: 0.161 +r2: 0.7447491432465998 , test_size: 0.162 +r2: 0.7404569502581667 , test_size: 0.163r2: 0.7359500072674392 , test_size: 0.164r2: 0.7406613409752312 , test_size: 0.165r2: 0.7371751055558255 , test_size: 0.166r2: 0.740769588221069 , test_size: 0.167r2: 0.7384351537775957 , test_size: 0.168r2: 0.7449509359690494 , test_size: 0.169 +++r2: 0.7417489594981834 , test_size: 0.17 +r2: 0.7424241798336026 , test_size: 0.171 +r2: 0.7386520309148124 , test_size: 0.172r2: 0.7385811145018726 , test_size: 0.173r2: 0.7334773548989142 , test_size: 0.174r2: 0.7284566857469685 , test_size: 0.175r2: 0.7288804941700773 , test_size: 0.176r2: 0.7320631476873077 , test_size: 0.177r2: 0.7277549013101753 , test_size: 0.178r2: 0.731905283280081 , test_size: 0.179r2: 0.733112333462109 , test_size: 0.18
###Code
X_train, X_valid, y_train, y_valid = train_test_split(X, y, test_size=0.13, random_state=42)
model = RandomForestRegressor(n_estimators=500, max_depth=17, random_state=42, max_features=8,
n_jobs=-1)
model.fit(X_train, y_train)
y_pred = model.predict(X_valid)
y_pred_train = model.predict(X_train)
r2_score(y_valid, y_pred)
# ne=1000
# for number in range(200):
# ne=ne+100
# XX_train, X_valid, y_train, y_valid = train_test_split(X, y, test_size=0.13, random_state=42)
# model = RandomForestRegressor(n_estimators=ne, max_depth=17, random_state=42, max_features=8,
# n_jobs=-1)
# model.fit(X_train, y_train)
# y_pred = model.predict(X_valid)
# y_pred_train = model.predict(X_train)
# print('r2: ', r2_score(y_valid, y_pred),', n_estimators: ',ne)
###Output
_____no_output_____
###Markdown
r2: 0.7558277451333204 , n_estimators: 40
###Code
# Предсказываем на valid и train данных и проверяем метрики
# Для train
r2_score(y_train, y_pred_train)
###Output
_____no_output_____
###Markdown
0.7395022017238742 - test_size=0.27, max_features=60.7403280251584794 10 - минимальная жилая площадь0.7410478387471598 - n_estimators=26000.7481240761275991 - 0.160.7493928705312163 - 0.16 и n_estimators=5000.7461407454357274 - 0.16 и n_estimators=500.7396502626673827 - после моей обработки!!! Хуже!!!0.7388456750277941 - минимальная площадь 15 ХУЖЕ!
###Code
# importances = model.feature_importances_
# # Sort feature importances in descending order
# indices = np.argsort(importances)[::-1]
# # Rearrange feature names so they match the sorted feature importances
# names = [X_train.columns[i] for i in indices]
# # Create plot
# plt.figure()
# # Create plot title
# plt.title("Feature Importance")
# # Add bars
# plt.bar(range(X.shape[1]), importances[indices])
# # Add feature names as x-axis labels
# plt.xticks(range(X.shape[1]), names, rotation=90)
# # plt.barh(x_train.columns, model.feature_importances_)
# plt.show
# X_train.describe()
# Предсказываем цены для тестовых данных и выгружаем в файл
X_test = pd.get_dummies(test_data)
X_test.drop("Id", axis=1, inplace=True)
test_data["Price"] = model.predict(X_test)
# экспорт в файл
test_data.loc[:, ['Id', 'Price']].to_csv('kaggle_loop_01.csv', index=False)
###Output
_____no_output_____
###Markdown
GradientBoostingRegressor
###Code
test_data = pd.read_csv('test.csv')
train_data = pd.read_csv('train.csv')
prepare_data(train_data)
prepare_data(test_data)
X = pd.get_dummies(train_data)
X.drop("Price", axis=1, inplace=True)
X.drop("Id", axis=1, inplace=True)
y = train_data.Price
X_train, X_valid, y_train, y_valid = train_test_split(X, y, test_size=0.16, random_state=42)
# переобучение и оценка модели
from sklearn.ensemble import GradientBoostingRegressor
final_model = GradientBoostingRegressor(n_estimators=200, max_depth=5, random_state=42,
min_samples_leaf=4
)
# min_samples_split=5, subsample=0.5
final_model.fit(X_train, y_train)
y_pred_gbr = final_model.predict(X_valid)
y_pred_train_gbr = final_model.predict(X_train)
r2_score(y_valid, y_pred_gbr)
# Предсказываем цены для тестовых данных и выгружаем в файл
X_test = pd.get_dummies(test_data)
X_test.drop("Id", axis=1, inplace=True)
test_data["Price"] = final_model.predict(X_test)
# экспорт в файл
test_data.loc[:, ['Id', 'Price']].to_csv('kaggle_example_gbr_04.csv', index=False)
y_pred_gbr = final_model.predict(X_valid)
y_pred_train_gbr = final_model.predict(X_train)
r2_score(y_train, y_pred_train_gbr)
# Для valid
r2_score(y_valid, y_pred_gbr)
###Output
_____no_output_____
###Markdown
0.72124439625655480.72374688185723370.72856776176773710.7333433435335188 - test_size=0.250.7342160493130999 - test_size=0.220.7473503024824598 - test_size=0.190.7490824550201877 - test_size=0.160.7583256877639833 - n_estimators=2000.7606112262441324 - subsample=1 Кросс0.7562788223657668 - n_estimators=3500.7586189801660074 - n_estimators=3800.7581203326572028 - n_estimators=385
###Code
from sklearn.metrics import r2_score as r2, mean_absolute_error as mae, mean_squared_error as mse
import seaborn as sns
def evaluate_preds(true_values, pred_values):
print("R2:\t" + str(round(r2(true_values, pred_values), 9)) + "\n" +
"MAE:\t" + str(round(mae(true_values, pred_values), 9)) + "\n" +
"MSE:\t" + str(round(mse(true_values, pred_values), 9)))
plt.figure(figsize=(10,10))
sns.scatterplot(x=pred_values, y=true_values)
plt.xlabel('Predicted values')
plt.ylabel('True values')
plt.title('True vs Predicted values')
plt.show()
# y_train_preds = final_model.predict(X_train)
# evaluate_preds(y_train, y_train_preds)
###Output
_____no_output_____
###Markdown
нейросеть
###Code
from keras.utils import to_categorical
from keras import models
from keras import layers
from keras.datasets import imdb
(training_data, training_targets), (testing_data, testing_targets) = imdb.load_data(num_words=10000)
data = np.concatenate((training_data, testing_data), axis=0)
targets = np.concatenate((training_targets, testing_targets), axis=0)
def vectorize(sequences, dimension = 10000):
results = np.zeros((len(sequences), dimension))
for i, sequence in enumerate(sequences):
results[i, sequence] = 1
return results
data = vectorize(data)
targets = np.array(targets).astype("float32")
test_x = data[:10000]
test_y = targets[:10000]
train_x = data[10000:]
train_y = targets[10000:]
model = models.Sequential()
# Input - Layer
model.add(layers.Dense(50, activation = "relu", input_shape=(10000, )))
# Hidden - Layers
model.add(layers.Dropout(0.3, noise_shape=None, seed=None))
model.add(layers.Dense(50, activation = "relu"))
model.add(layers.Dropout(0.2, noise_shape=None, seed=None))
model.add(layers.Dense(50, activation = "relu"))
# Output- Layer
model.add(layers.Dense(1, activation = "sigmoid"))
model.summary()
# compiling the model
model.compile(
optimizer = "adam",
loss = "binary_crossentropy",
metrics = ["accuracy"]
)
results = model.fit(
train_x, train_y,
epochs= 2,
batch_size = 500,
validation_data = (test_x, test_y)
)
print("Test-Accuracy:", np.mean(results.history["val_acc"]))
###Output
_____no_output_____ |
notebooks/3.2 Skills, Wrangling.ipynb | ###Markdown
3.2 Skills/WranglingIn this notebook, we will focus on two essential skills in data analysis:1. The ability to add select, aggregate and transform data in a dataframe (**part 1**)2. The ability to get insights about a dataset by means of plotting and summary statistics (**part 2**) Part 1 Imports
###Code
import pandas as pd
###Output
_____no_output_____
###Markdown
Load dataset Let's read in a CSV file containing an export of [Elon Musk's tweets](https://twitter.com/elonmusk), exported from Twitter's API.
###Code
dataset_path = '../data/musk_tweets/elonmusk_tweets.csv'
df = pd.read_csv(dataset_path)
df.info()
df.set_index('id', drop=True, inplace=True)
###Output
_____no_output_____
###Markdown
Let's give this dataset a bit more structure:- the `id` column can be transformed into the dataframe's index, thus enabling us e.g. to select a tweet by id;- `created_at` contains a timestamp, thus it can easily be converted into a `datetime` value- but what's going on with the text column ??
###Code
df.created_at = pd.to_datetime(df.created_at)
df.info()
###Output
<class 'pandas.core.frame.DataFrame'>
Int64Index: 2819 entries, 849636868052275200 to 15434727182
Data columns (total 2 columns):
created_at 2819 non-null datetime64[ns]
text 2819 non-null object
dtypes: datetime64[ns](1), object(1)
memory usage: 66.1+ KB
###Markdown
Selection Renaming columns An operation on dataframes that you'll find yourself doing very often is to rename the columns. The first way of renaming columns is by manipulating directly the dataframe's index via the `columns` property.
###Code
df.columns
###Output
_____no_output_____
###Markdown
We can change the column names by assigning to `columns` a list having as values the new column names.**NB**: the size of the list and new number of colums must match!
###Code
# here we renamed the column `text` => `tweet`
df.columns = ['created_at', 'tweet']
# let's check that the change did take place
df.head()
###Output
_____no_output_____
###Markdown
The second way of renaming colums is to use the method `rename()` of a dataframe. The `columns` parameter takes a dictionary of mappings between old and new column names.```pythonmapping_dict = { "old_column_name": "new_column_name"}```
###Code
# let's change column `tweet` => `text`
df = df.rename(columns={"tweet": "text"})
df.head()
###Output
_____no_output_____
###Markdown
**Question**: in which cases is it more convenient to use the second method over the first? Selecting columns
###Code
# this selects one single column and returns as a Series
df["created_at"].head()
type(df["created_at"])
# whereas this syntax selects one single column
# but returns a Dataframe
df[["created_at"]].head()
type(df[["created_at"]])
###Output
_____no_output_____
###Markdown
Selecting rowsFiltering rows in `pandas` is done by means of `[ ]`, which can contain the row number as well as a condition for the selection.
###Code
df[0:2]
###Output
_____no_output_____
###Markdown
Numerical values
###Code
# equivalent of `df.query('n_mentions > 0')`
df[df.n_mentions > 0].shape
df[df.n_mentions <= 0].shape
###Output
_____no_output_____
###Markdown
Strings
###Code
df[df.week_day_name == 'Saturday'].shape
df[df.week_day_name.str.startswith('S')].shape
###Output
_____no_output_____
###Markdown
Multiple conditions
###Code
# AND condition with `&`
df[
(df.week_day_name == 'Saturday') & (df.n_mentions == 0)
].shape
# equivalent expression with `query()`
df.query("week_day_name == 'Saturday' and n_mentions == 0").shape
# OR condition with `|`
df[
(df.week_day_name == 'Saturday') | (df.n_mentions == 0)
].shape
###Output
_____no_output_____
###Markdown
TransformationThe two main functions used to manipulate and transform values in a dataframe are:- `map()`- `apply()`In this section we'll be using both to enrich our datasets with useful information (useful for exploration, for later visualizations, etc.). Add link to original tweet The `map()` method can be called on a column, as well as on the dataframe's index.When passed as a parameter to `map`, the functional programming-stlye function `lambda` can be used to transform any value from that column into another one.
###Code
df['tweet_link'] = df.index.map(lambda x: f'https://twitter.com/i/web/status/{x}')
pd.set_option("display.max_colwidth", 10000)
def make_clickable(val):
# target _blank to open new window
return '<a target="_blank" href="{}">{}</a>'.format(val, val)
df.head().style.format({'tweet_link': make_clickable})
# to apply the style to the entire dataframe just remove
# `.head` from the line above
###Output
_____no_output_____
###Markdown
Add colums with mentions
###Code
import re
def find_mentions(tweet_text):
handle_regexp = r'@[a-zA-Z0-9_]{1,15}'
return re.findall(handle_regexp, tweet_text)
df['tweet_mentions'] = df.text.apply(find_mentions)
df['n_mentions'] = df.tweet_mentions.apply(len)
df.head()
###Output
_____no_output_____
###Markdown
Add column with week day
###Code
df.created_at.dt.weekday_name.head()
df["week_day_name"] = df.created_at.dt.weekday_name
df["week_day"] = df.created_at.dt.weekday
df.head(3)
###Output
_____no_output_____
###Markdown
Add column with day hour
###Code
df.created_at.dt?
df.created_at.dt.hour.head()
df["day_hour"] = df.created_at.dt.hour
display_cols = ['created_at', 'week_day', 'day_hour']
df[display_cols].head(4)
###Output
_____no_output_____
###Markdown
Aggregation(Figure taken from W. Mckinney's *Python for Data Analysis* 2013, p. 252)
###Code
df.agg({'n_mentions': ['min', 'max', 'sum']})
###Output
_____no_output_____
###Markdown
Grouping
###Code
df.groupby?
grp_by_day = df.groupby('week_day')
###Output
_____no_output_____
###Markdown
The object returned by `gropuby` is a `DataFrameGroupBy` **not** a normal `DataFrame`.However, some methods of the latter work also on the former, e.g. `head` and `tail`
###Code
# the head of a DataFrameGroupBy consists of the first
# n records for each group (see `help(grp_by_day.head)`)
grp_by_day.head(1)
###Output
_____no_output_____
###Markdown
`agg` is used to pass an aggregation function to be applied to each group resulting from `groupby`.
###Code
# here we are interested in how many tweets
# there are for each group, so we pass `len()`
grp_by_day.agg(len)
# however, we are not interested in having the count for all
# columns. rather we want to create a new dataframe with renamed
# column names
grp_by_day.agg({'text': len}).rename({'text': 'tweet_count'}, axis='columns')
###Output
_____no_output_____
###Markdown
By label (column) Previously we've added a column indicating on which day of the week a given tweet appeared.
###Code
grpby_result_as_series = df.groupby('day_hour')['text'].count()
grpby_result_as_series.head()
grpby_result_as_df = df.groupby('day_hour')[['text']]\
.count()\
.rename({'text': 'count'}, axis='columns')
grpby_result_as_df.head()
###Output
_____no_output_____
###Markdown
By series or dict
###Code
df.groupby?
for group, rows in df.groupby(df.created_at.dt.day):
print(group, type(rows))
# here we pass the groups as a series
df.groupby(df.created_at.dt.day).agg({'text':len}).head()
# here we pass the groups as a series
df.groupby(df.created_at.dt.day)[['text']].count().head()
df.groupby(df.created_at.dt.hour)[['text']].count().head()
###Output
_____no_output_____
###Markdown
By multiple labels (columns)
###Code
# here we group based on the values of two columns
# instead of one
x = df.groupby(['week_day', 'day_hour'])[['text']].count()
x.head()
###Output
_____no_output_____
###Markdown
Aggregation methods**Summary**:- `count`: Number of non-NA values- `sum`: Sum of non-NA values- `mean`: Mean of non-NA values- `median`: Arithmetic median of non-NA values- `std`, `var`: standard deviation and variance- `min`, `max`: Minimum and maximum of non-NA values They can be used on a single series:
###Code
df.n_mentions.max()
###Output
_____no_output_____
###Markdown
On the entire dataframe:
###Code
df.mean()
###Output
_____no_output_____
###Markdown
Or also as aggregation functions within a groupby:
###Code
df.groupby('week_day').agg(
{
# each key in this dict specifies
# a given column
'n_mentions':[
# the list contains aggregation functions
# to be applied to this column
'count',
'mean',
'min',
'max',
'std',
'var'
]
}
)
###Output
_____no_output_____
###Markdown
Sorting To sort the values of a dataframe we use its `sort_values` method:- `by`: specifies the name of the column to be used for sorting- `ascending` (default = `True`): specifies whether the sorting should be *ascending* (A-Z, 0-9) or `descending` (Z-A, 9-0)
###Code
df.sort_values(by='created_at', ascending=True).head()
df.sort_values(by='n_mentions', ascending=False).head()
###Output
_____no_output_____
###Markdown
SaveBefore continuing with the plotting, let's save our enhanced dataframe, so that we can come back to it without having to redo the same manipulations on it.`pandas` provides a number of handy functions to export dataframes in a variety of formats. Here we use `to_pickle` to serialize the dataframe into a binary format, by using behind the scenes Python's `pickle` library.
###Code
df.to_pickle("./musk_tweets_enhanced.pkl")
ls -al | grep pkl
###Output
-rw-r--r-- 1 matteo staff 602215 Jul 21 17:42 musk_tweets_enhanced.pkl
###Markdown
Part 2 `describe()`
###Code
# the default behavior is to include only
# column with numerical values
df.describe()
# in this case fails as pandas does not
# now how to handle a column with values of type list (fair enough)
df.describe(include='all')
# that's a workaround to include all other columns
df.describe(exclude=[list])
df.created_at.describe()
df['week_day_name'] = df['week_day_name'].astype('category')
df.describe(exclude=['object'])
###Output
_____no_output_____
###Markdown
Plotting
###Code
%matplotlib inline
import matplotlib.pyplot as plt
###Output
_____no_output_____
###Markdown
HistogramsThey are useful to see the distribution of a certain variable in your dataset.
###Code
df.groupby(['n_mentions'])[['text']].count()
%matplotlib inline
plt.figure(figsize=(10, 6))
plt.hist(df.n_mentions, bins='auto', rwidth=1.0)
plt.title('Distribution of the number of mentions per tweet')
plt.ylabel("Tweets")
plt.xlabel("Mentions (per tweet)")
plt.show()
%matplotlib inline
plt.figure(figsize=(10, 6))
plt.hist(df.day_hour, bins='auto', rwidth=0.6)
plt.title('Distribution of the number of mentions per tweet')
plt.ylabel("Tweets")
plt.xlabel("Hour of the day")
plt.show()
df_2017 = df[df.created_at.dt.year == 2017]
%matplotlib inline
plt.figure(figsize=(10, 6))
plt.hist(df_2017.day_hour, bins='auto', rwidth=0.6)
plt.title('Year 2017')
plt.ylabel("Tweets")
plt.xlabel("Hour of the day")
plt.show()
###Output
_____no_output_____
###Markdown
So far we have used directly `matplotlib` to generate our plots.`pandas`'s dataframes provide some methods that directly call `matplotlib`'s API behind the scenes:- `hist()` for histograms- `boxplot()` for boxplots- `plot()` for other types of plots (specified with e.g. `any='scatter'`) By passing the `by` parameter to e.g. `hist()` it is possible to produce one histogram plot of a given variable for each value in another column. Let's see how we can plot the number of mentions by year:
###Code
df['year'] = df.created_at.dt.year
axes = df.hist(column='day_hour', by='year', figsize=(10,10))
###Output
_____no_output_____
###Markdown
Scatter plotsThey are useful to plot the relation between two variables in your dataset.
###Code
df.head(3)
%matplotlib inline
plt.figure(figsize=(10, 6))
# specify the type of plot and the two
# variables to be plotted against one another
plt.scatter(df.n_mentions, df.day_hour)
# give a title to the plot
plt.title('Number of mentions vs hour of the day')
# give a label to the axes
plt.ylabel("Day hour")
plt.xlabel("Number of mentions")
plt.show()
###Output
_____no_output_____
###Markdown
Bar chartsThey are useful to plot categorical data.
###Code
plt.bar?
tweets_by_weekday = df.groupby(df.created_at.dt.weekday)[['text']].count()
week_days = [
"Mon",
"Tue",
"Wed",
"Thur",
"Fri",
"Sat",
"Sun"
]
%matplotlib inline
plt.figure(figsize=(8, 6))
# specify the type of plot and the labels
# for the y axis (the bars)
plt.bar(
tweets_by_weekday.index,
tweets_by_weekday.text,
tick_label=week_days,
width=0.5
)
# give a title to the plot
plt.title('Elon Musk\'s week on Twitter')
# give a label to the axes
plt.ylabel("Number of tweets")
plt.xlabel("Week day")
plt.show()
###Output
_____no_output_____
###Markdown
Box plots
###Code
tweets_by_weekday
tweets_by_weekday.describe()
tweets_by_weekday.boxplot()
plt.bar?
df.head(3)
df[['day_hour']].describe()
df[['day_hour']].quantile(.25)
df.boxplot?
%matplotlib inline
df[['day_hour', 'week_day_name']].boxplot(
by='week_day_name',
grid=False,
figsize=(8,6),
fontsize=10
)
# give a title to the plot
plt.title('')
# give a label to the axes
plt.xlabel("Day of the week")
plt.show()
%matplotlib inline
df[['day_hour', 'week_day']].boxplot(
by='week_day',
grid=True, # just to show the difference with/without
figsize=(8,6),
fontsize=10
)
# give a title to the plot
plt.title('')
# give a label to the axes
plt.xlabel("Day of the week")
plt.show()
###Output
_____no_output_____ |
code_listings/03.11-Working-with-Time-Series.ipynb | ###Markdown
Working with Time Series
###Code
from datetime import datetime
datetime(year=2015, month=7, day=4)
from dateutil import parser
date = parser.parse("4th of July, 2015")
date
date.strftime('%A')
import numpy as np
date = np.array('2015-07-04', dtype=np.datetime64)
date
date + np.arange(12)
np.datetime64('2015-07-04')
np.datetime64('2015-07-04 12:00')
np.datetime64('2015-07-04 12:59:59.50', 'ns')
import pandas as pd
date = pd.to_datetime("4th of July, 2015")
date
date.strftime('%A')
date + pd.to_timedelta(np.arange(12), 'D')
index = pd.DatetimeIndex(['2014-07-04', '2014-08-04',
'2015-07-04', '2015-08-04'])
data = pd.Series([0, 1, 2, 3], index=index)
data
data['2014-07-04':'2015-07-04']
data['2015']
dates = pd.to_datetime([datetime(2015, 7, 3), '4th of July, 2015',
'2015-Jul-6', '07-07-2015', '20150708'])
dates
dates.to_period('D')
dates - dates[0]
pd.date_range('2015-07-03', '2015-07-10')
pd.date_range('2015-07-03', periods=8)
pd.date_range('2015-07-03', periods=8, freq='H')
pd.period_range('2015-07', periods=8, freq='M')
pd.timedelta_range(0, periods=10, freq='H')
pd.timedelta_range(0, periods=9, freq="2H30T")
from pandas.tseries.offsets import BDay
pd.date_range('2015-07-01', periods=5, freq=BDay())
from pandas_datareader import data
goog = data.DataReader('GOOG', start='2004', end='2016',
data_source='google')
goog.head()
goog = goog['Close']
%matplotlib inline
import matplotlib.pyplot as plt
import seaborn; seaborn.set()
goog.plot();
goog.plot(alpha=0.5, style='-')
goog.resample('BA').mean().plot(style=':')
goog.asfreq('BA').plot(style='--');
plt.legend(['input', 'resample', 'asfreq'],
loc='upper left');
fig, ax = plt.subplots(2, sharex=True)
data = goog.iloc[:10]
data.asfreq('D').plot(ax=ax[0], marker='o')
data.asfreq('D', method='bfill').plot(ax=ax[1], style='-o')
data.asfreq('D', method='ffill').plot(ax=ax[1], style='--o')
ax[1].legend(["back-fill", "forward-fill"]);
fig, ax = plt.subplots(3, sharey=True)
# apply a frequency to the data
goog = goog.asfreq('D', method='pad')
goog.plot(ax=ax[0])
goog.shift(900).plot(ax=ax[1])
goog.tshift(900).plot(ax=ax[2])
# legends and annotations
local_max = pd.to_datetime('2007-11-05')
offset = pd.Timedelta(900, 'D')
ax[0].legend(['input'], loc=2)
ax[0].get_xticklabels()[4].set(weight='heavy', color='red')
ax[0].axvline(local_max, alpha=0.3, color='red')
ax[1].legend(['shift(900)'], loc=2)
ax[1].get_xticklabels()[4].set(weight='heavy', color='red')
ax[1].axvline(local_max + offset, alpha=0.3, color='red')
ax[2].legend(['tshift(900)'], loc=2)
ax[2].get_xticklabels()[1].set(weight='heavy', color='red')
ax[2].axvline(local_max + offset, alpha=0.3, color='red');
ROI = 100 * (goog.tshift(-365) / goog - 1)
ROI.plot()
plt.ylabel('% Return on Investment');
rolling = goog.rolling(365, center=True)
data = pd.DataFrame({'input': goog,
'one-year rolling_mean': rolling.mean(),
'one-year rolling_std': rolling.std()})
ax = data.plot(style=['-', '--', ':'])
ax.lines[0].set_alpha(0.3)
# !curl -o FremontBridge.csv https://data.seattle.gov/api/views/65db-xm6k/rows.csv?accessType=DOWNLOAD
data = pd.read_csv('FremontBridge.csv', index_col='Date', parse_dates=True)
data.head()
data.columns = ['West', 'East']
data['Total'] = data.eval('West + East')
data.dropna().describe()
%matplotlib inline
import seaborn; seaborn.set()
data.plot()
plt.ylabel('Hourly Bicycle Count');
weekly = data.resample('W').sum()
weekly.plot(style=[':', '--', '-'])
plt.ylabel('Weekly bicycle count');
daily = data.resample('D').sum()
daily.rolling(30, center=True).sum().plot(style=[':', '--', '-'])
plt.ylabel('mean hourly count');
daily.rolling(50, center=True,
win_type='gaussian').sum(std=10).plot(style=[':', '--', '-']);
by_time = data.groupby(data.index.time).mean()
hourly_ticks = 4 * 60 * 60 * np.arange(6)
by_time.plot(xticks=hourly_ticks, style=[':', '--', '-']);
by_weekday = data.groupby(data.index.dayofweek).mean()
by_weekday.index = ['Mon', 'Tues', 'Wed', 'Thurs', 'Fri', 'Sat', 'Sun']
by_weekday.plot(style=[':', '--', '-']);
weekend = np.where(data.index.weekday < 5, 'Weekday', 'Weekend')
by_time = data.groupby([weekend, data.index.time]).mean()
import matplotlib.pyplot as plt
fig, ax = plt.subplots(1, 2, figsize=(14, 5))
by_time.ix['Weekday'].plot(ax=ax[0], title='Weekdays',
xticks=hourly_ticks, style=[':', '--', '-'])
by_time.ix['Weekend'].plot(ax=ax[1], title='Weekends',
xticks=hourly_ticks, style=[':', '--', '-']);
###Output
_____no_output_____ |
dev/17_callback_tracker.ipynb | ###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves ShortEpochCallback -
###Code
#export
class ShortEpochCallback(Callback):
"Fit just `pct` of an epoch, then stop"
def __init__(self,pct=0.01,short_valid=True): self.pct,self.short_valid = pct,short_valid
def after_batch(self):
if self.iter/self.n_iter < self.pct: return
if self.training: raise CancelTrainException
if self.short_valid: raise CancelValidException
learn = synth_learner()
learn.fit(1, cbs=ShortEpochCallback())
learn = synth_learner()
learn.fit(1, cbs=ShortEpochCallback(short_valid=False))
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.dbunch.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = list(self.recorder.metric_names[1:]).index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
if hasattr(self, "lr_finder"): return
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = list(self.recorder.metric_names[1:]).index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if hasattr(self, "lr_finder"): return
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that saves the model's best during training and loads it at the end."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., fname='model', every_epoch=False, add_save=None, with_opt=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
store_attr(self, 'fname,every_epoch,add_save,with_opt')
def _save(self, name):
self.learn.save(name, with_opt=self.with_opt)
if self.add_save is not None:
with self.add_save.open('wb') as f: self.learn.save(f, with_opt=self.with_opt)
def after_epoch(self):
"Compare the value monitored to its best score and save if best."
if hasattr(self, "lr_finder"): return
if self.every_epoch: self._save(f'{self.fname}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self._save(f'{self.fname}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch: self.learn.load(f'{self.fname}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity.
###Code
learn = synth_learner(n_trn=2, path=Path.cwd()/'tmp')
learn.fit(n_epoch=2, cbs=SaveModelCallback())
assert (Path.cwd()/'tmp/models/model.pth').exists()
learn.fit(n_epoch=2, cbs=SaveModelCallback(every_epoch=True))
for i in range(2): assert (Path.cwd()/f'tmp/models/model_{i}.pth').exists()
shutil.rmtree(Path.cwd()/'tmp')
###Output
_____no_output_____
###Markdown
ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and reduce LR by `factor` if no improvement."
super().after_epoch()
if hasattr(self, "lr_finder"): return
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core_foundation.ipynb.
Converted 01a_core_utils.ipynb.
Converted 01b_core_dispatch.ipynb.
Converted 01c_core_transform.ipynb.
Converted 02_core_script.ipynb.
Converted 03_torchcore.ipynb.
Converted 03a_layers.ipynb.
Converted 04_data_load.ipynb.
Converted 05_data_core.ipynb.
Converted 06_data_transforms.ipynb.
Converted 07_data_block.ipynb.
Converted 08_vision_core.ipynb.
Converted 09_vision_augment.ipynb.
Converted 09a_vision_data.ipynb.
Converted 09b_vision_utils.ipynb.
Converted 10_pets_tutorial.ipynb.
Converted 11_vision_models_xresnet.ipynb.
Converted 12_optimizer.ipynb.
Converted 13_learner.ipynb.
Converted 13a_metrics.ipynb.
Converted 14_callback_schedule.ipynb.
Converted 14a_callback_data.ipynb.
Converted 15_callback_hook.ipynb.
Converted 15a_vision_models_unet.ipynb.
Converted 16_callback_progress.ipynb.
Converted 17_callback_tracker.ipynb.
Converted 18_callback_fp16.ipynb.
Converted 19_callback_mixup.ipynb.
Converted 20_interpret.ipynb.
Converted 20a_distributed.ipynb.
Converted 21_vision_learner.ipynb.
Converted 22_tutorial_imagenette.ipynb.
Converted 23_tutorial_transfer_learning.ipynb.
Converted 30_text_core.ipynb.
Converted 31_text_data.ipynb.
Converted 32_text_models_awdlstm.ipynb.
Converted 33_text_models_core.ipynb.
Converted 34_callback_rnn.ipynb.
Converted 35_tutorial_wikitext.ipynb.
Converted 36_text_models_qrnn.ipynb.
Converted 37_text_learner.ipynb.
Converted 38_tutorial_ulmfit.ipynb.
Converted 40_tabular_core.ipynb.
Converted 41_tabular_model.ipynb.
Converted 42_tabular_rapids.ipynb.
Converted 50_data_block_examples.ipynb.
Converted 60_medical_imaging.ipynb.
Converted 65_medical_text.ipynb.
Converted 70_callback_wandb.ipynb.
Converted 71_callback_tensorboard.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_notebook_test.ipynb.
Converted 95_index.ipynb.
Converted 96_data_external.ipynb.
Converted 97_utils_test.ipynb.
Converted notebook2jekyll.ipynb.
Converted xse_resnext.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves
###Code
from local.utils.test import *
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.data.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., name='model', every_epoch=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.name,self.every_epoch = name,every_epoch
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
if self.every_epoch: self.learn.save(f'{self.name}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self.learn.save(f'{self.name}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch and (self.learn.path/f'{self.learn.model_dir}/{self.name}.pth').is_file():
self.learn.load(f'{self.name}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity. TODO: test when `Learner.save` is implemented. ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core.ipynb.
Converted 02_data_pipeline.ipynb.
Converted 03_data_external.ipynb.
Converted 04_data_core.ipynb.
Converted 05_data_source.ipynb.
Converted 06_vision_core.ipynb.
Converted 07_pets_tutorial.ipynb.
Converted 08_augmentation.ipynb.
Converted 10_layers.ipynb.
Converted 11_optimizer.ipynb.
Converted 12_learner.ipynb.
Converted 13_callback_schedule.ipynb.
Converted 14_callback_hook.ipynb.
Converted 15_callback_progress.ipynb.
Converted 16_callback_tracker.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_index.ipynb.
Converted 95_synth_learner.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves
###Code
from local.utils.test import *
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.data.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., name='model', every_epoch=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.name,self.every_epoch = name,every_epoch
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
if self.every_epoch: self.learn.save(f'{self.name}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self.learn.save(f'{self.name}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch and (self.learn.path/f'{self.learn.model_dir}/{self.name}.pth').is_file():
self.learn.load(f'{self.name}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity. TODO: test when `Learner.save` is implemented. ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core.ipynb.
Converted 02_data_pipeline.ipynb.
Converted 03_data_external.ipynb.
Converted 04_data_core.ipynb.
Converted 05_data_source.ipynb.
Converted 06_vision_core.ipynb.
Converted 07_pets_tutorial.ipynb.
Converted 08_augmentation.ipynb.
Converted 10_layers.ipynb.
Converted 11_optimizer.ipynb.
Converted 12_learner.ipynb.
Converted 13_callback_schedule.ipynb.
Converted 14_callback_hook.ipynb.
Converted 15_callback_progress.ipynb.
Converted 16_callback_tracker.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_index.ipynb.
Converted 95_synth_learner.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves
###Code
from local.utils.test import *
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.data.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., name='model', every_epoch=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.name,self.every_epoch = name,every_epoch
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
if self.every_epoch: self.learn.save(f'{self.name}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self.learn.save(f'{self.name}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch and (self.learn.path/f'{self.learn.model_dir}/{self.name}.pth').is_file():
self.learn.load(f'{self.name}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity. TODO: test when `Learner.save` is implemented. ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core.ipynb.
Converted 02_data_pipeline.ipynb.
Converted 03_data_external.ipynb.
Converted 04_data_core.ipynb.
Converted 05_data_source.ipynb.
Converted 06_vision_core.ipynb.
Converted 07_pets_tutorial.ipynb.
Converted 08_augmentation.ipynb.
Converted 10_layers.ipynb.
Converted 11_optimizer.ipynb.
Converted 12_learner.ipynb.
Converted 13_callback_schedule.ipynb.
Converted 14_callback_hook.ipynb.
Converted 15_callback_progress.ipynb.
Converted 16_callback_tracker.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_index.ipynb.
Converted 95_synth_learner.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves
###Code
from local.utils.test import *
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.data.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., name='model', every_epoch=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.name,self.every_epoch = name,every_epoch
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
if self.every_epoch: self.learn.save(f'{self.name}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self.learn.save(f'{self.name}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch and (self.learn.path/f'{self.learn.model_dir}/{self.name}.pth').is_file():
self.learn.load(f'{self.name}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity. TODO: test when `Learner.save` is implemented. ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core.ipynb.
Converted 02_data_pipeline.ipynb.
Converted 03_data_external.ipynb.
Converted 04_data_core.ipynb.
Converted 05_data_source.ipynb.
Converted 06_vision_core.ipynb.
Converted 07_pets_tutorial.ipynb.
Converted 08_augmentation.ipynb.
Converted 10_layers.ipynb.
Converted 11_optimizer.ipynb.
Converted 12_learner.ipynb.
Converted 13_callback_schedule.ipynb.
Converted 14_callback_hook.ipynb.
Converted 15_callback_progress.ipynb.
Converted 16_callback_tracker.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_index.ipynb.
Converted 95_synth_learner.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves
###Code
from local.utils.test import *
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.dbunch.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., fname='model', every_epoch=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
store_attr(self, 'fname,every_epoch')
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
if self.every_epoch: self.learn.save(f'{self.fname}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self.learn.save(f'{self.fname}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch and (self.learn.path/f'{self.learn.model_dir}/{self.fname}.pth').is_file():
self.learn.load(f'{self.fname}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity.
###Code
learn = synth_learner(n_trn=2, path=Path.cwd()/'tmp')
learn.fit(n_epoch=2, cbs=SaveModelCallback())
assert (Path.cwd()/'tmp/models/model.pth').exists()
learn.fit(n_epoch=2, cbs=SaveModelCallback(every_epoch=True))
for i in range(2): assert (Path.cwd()/f'tmp/models/model_{i}.pth').exists()
shutil.rmtree(Path.cwd()/'tmp')
###Output
_____no_output_____
###Markdown
ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core.ipynb.
Converted 01a_torch_core.ipynb.
Converted 02_script.ipynb.
Converted 03_dataloader.ipynb.
Converted 04_transform.ipynb.
Converted 05_data_core.ipynb.
Converted 06_data_transforms.ipynb.
Converted 07_vision_core.ipynb.
Converted 08_pets_tutorial.ipynb.
Converted 09_vision_augment.ipynb.
Converted 11_layers.ipynb.
Converted 11a_vision_models_xresnet.ipynb.
Converted 12_optimizer.ipynb.
Converted 13_learner.ipynb.
Converted 14_callback_schedule.ipynb.
Converted 15_callback_hook.ipynb.
Converted 16_callback_progress.ipynb.
Converted 17_callback_tracker.ipynb.
Converted 18_callback_fp16.ipynb.
Converted 19_callback_mixup.ipynb.
Converted 20_metrics.ipynb.
Converted 21_tutorial_imagenette.ipynb.
Converted 22_vision_learner.ipynb.
Converted 23_tutorial_transfer_learning.ipynb.
Converted 30_text_core.ipynb.
Converted 31_text_data.ipynb.
Converted 32_text_models_awdlstm.ipynb.
Converted 33_text_models_core.ipynb.
Converted 34_callback_rnn.ipynb.
Converted 35_tutorial_wikitext.ipynb.
Converted 36_text_models_qrnn.ipynb.
Converted 37_text_learner.ipynb.
Converted 38_tutorial_ulmfit.ipynb.
Converted 40_tabular_core.ipynb.
Converted 41_tabular_model.ipynb.
Converted 42_tabular_rapids.ipynb.
Converted 50_data_block.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_index.ipynb.
Converted 95_utils_test.ipynb.
Converted 96_data_external.ipynb.
Converted notebook2jekyll.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves ShortEpochCallback -
###Code
#export
class ShortEpochCallback(Callback):
"Fit just `pct` of an epoch, then stop"
def __init__(self,pct=0.01,short_valid=True): self.pct,self.short_valid = pct,short_valid
def after_batch(self):
if self.iter/self.n_iter < self.pct: return
if self.training: raise CancelTrainException
if self.short_valid: raise CancelValidException
learn = synth_learner()
learn.fit(1, cbs=ShortEpochCallback())
learn = synth_learner()
learn.fit(1, cbs=ShortEpochCallback(short_valid=False))
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.dbunch.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that saves the model's best during training and loads it at the end."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., fname='model', every_epoch=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
store_attr(self, 'fname,every_epoch')
def after_epoch(self):
"Compare the value monitored to its best score and save if best."
if self.every_epoch: self.learn.save(f'{self.fname}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self.learn.save(f'{self.fname}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch and (self.learn.path/f'{self.learn.model_dir}/{self.fname}.pth').is_file():
self.learn.load(f'{self.fname}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity.
###Code
learn = synth_learner(n_trn=2, path=Path.cwd()/'tmp')
learn.fit(n_epoch=2, cbs=SaveModelCallback())
assert (Path.cwd()/'tmp/models/model.pth').exists()
learn.fit(n_epoch=2, cbs=SaveModelCallback(every_epoch=True))
for i in range(2): assert (Path.cwd()/f'tmp/models/model_{i}.pth').exists()
shutil.rmtree(Path.cwd()/'tmp')
###Output
_____no_output_____
###Markdown
ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and reduce LR by `factor` if no improvement."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core.ipynb.
Converted 01a_utils.ipynb.
Converted 01b_dispatch.ipynb.
Converted 01c_transform.ipynb.
Converted 02_script.ipynb.
Converted 03_torch_core.ipynb.
Converted 03a_layers.ipynb.
Converted 04_dataloader.ipynb.
Converted 05_data_core.ipynb.
Converted 06_data_transforms.ipynb.
Converted 07_data_block.ipynb.
Converted 08_vision_core.ipynb.
Converted 09_vision_augment.ipynb.
Converted 10_pets_tutorial.ipynb.
Converted 11_vision_models_xresnet.ipynb.
Converted 12_optimizer.ipynb.
Converted 13_learner.ipynb.
Converted 13a_metrics.ipynb.
Converted 14_callback_schedule.ipynb.
Converted 14a_callback_data.ipynb.
Converted 15_callback_hook.ipynb.
Converted 15a_vision_models_unet.ipynb.
Converted 16_callback_progress.ipynb.
Converted 17_callback_tracker.ipynb.
Converted 18_callback_fp16.ipynb.
Converted 19_callback_mixup.ipynb.
Converted 21_vision_learner.ipynb.
Converted 22_tutorial_imagenette.ipynb.
Converted 23_tutorial_transfer_learning.ipynb.
Converted 30_text_core.ipynb.
Converted 31_text_data.ipynb.
Converted 32_text_models_awdlstm.ipynb.
Converted 33_text_models_core.ipynb.
Converted 34_callback_rnn.ipynb.
Converted 35_tutorial_wikitext.ipynb.
Converted 36_text_models_qrnn.ipynb.
Converted 37_text_learner.ipynb.
Converted 38_tutorial_ulmfit.ipynb.
Converted 40_tabular_core.ipynb.
Converted 41_tabular_model.ipynb.
Converted 42_tabular_rapids.ipynb.
Converted 50_data_block_examples.ipynb.
Converted 60_medical_imaging.ipynb.
Converted 65_medical_text.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_notebook_test.ipynb.
Converted 95_index.ipynb.
Converted 96_data_external.ipynb.
Converted 97_utils_test.ipynb.
Converted notebook2jekyll.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves ShortEpochCallback -
###Code
#export
class ShortEpochCallback(Callback):
"Fit just `pct` of an epoch, then stop"
def __init__(self,pct=0.01,short_valid=True): self.pct,self.short_valid = pct,short_valid
def after_batch(self):
if self.iter/self.n_iter < self.pct: return
if self.training: raise CancelTrainException
if self.short_valid: raise CancelValidException
learn = synth_learner()
learn.fit(1, cbs=ShortEpochCallback())
learn = synth_learner()
learn.fit(1, cbs=ShortEpochCallback(short_valid=False))
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.dbunch.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = list(self.recorder.metric_names[1:]).index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = list(self.recorder.metric_names[1:]).index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that saves the model's best during training and loads it at the end."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., fname='model', every_epoch=False, add_save=None, with_opt=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
store_attr(self, 'fname,every_epoch,add_save,with_opt')
def _save(self, name):
self.learn.save(name, with_opt=self.with_opt)
if self.add_save is not None:
with self.add_save.open('wb') as f: self.learn.save(f, with_opt=self.with_opt)
def after_epoch(self):
"Compare the value monitored to its best score and save if best."
if self.every_epoch: self._save(f'{self.fname}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self._save(f'{self.fname}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch and (self.learn.path/f'{self.learn.model_dir}/{self.fname}.pth').is_file():
self.learn.load(f'{self.fname}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity.
###Code
learn = synth_learner(n_trn=2, path=Path.cwd()/'tmp')
learn.fit(n_epoch=2, cbs=SaveModelCallback())
assert (Path.cwd()/'tmp/models/model.pth').exists()
learn.fit(n_epoch=2, cbs=SaveModelCallback(every_epoch=True))
for i in range(2): assert (Path.cwd()/f'tmp/models/model_{i}.pth').exists()
shutil.rmtree(Path.cwd()/'tmp')
###Output
_____no_output_____
###Markdown
ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and reduce LR by `factor` if no improvement."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core.ipynb.
Converted 01a_utils.ipynb.
Converted 01b_dispatch.ipynb.
Converted 01c_transform.ipynb.
Converted 02_script.ipynb.
Converted 03_torch_core.ipynb.
Converted 03a_layers.ipynb.
Converted 04_dataloader.ipynb.
Converted 05_data_core.ipynb.
Converted 06_data_transforms.ipynb.
Converted 07_data_block.ipynb.
Converted 08_vision_core.ipynb.
Converted 09_vision_augment.ipynb.
Converted 09a_vision_data.ipynb.
Converted 10_pets_tutorial.ipynb.
Converted 11_vision_models_xresnet.ipynb.
Converted 12_optimizer.ipynb.
Converted 13_learner.ipynb.
Converted 13a_metrics.ipynb.
Converted 14_callback_schedule.ipynb.
Converted 14a_callback_data.ipynb.
Converted 15_callback_hook.ipynb.
Converted 15a_vision_models_unet.ipynb.
Converted 16_callback_progress.ipynb.
Converted 17_callback_tracker.ipynb.
Converted 18_callback_fp16.ipynb.
Converted 19_callback_mixup.ipynb.
Converted 20_interpret.ipynb.
Converted 20a_distributed.ipynb.
Converted 21_vision_learner.ipynb.
Converted 22_tutorial_imagenette.ipynb.
Converted 23_tutorial_transfer_learning.ipynb.
Converted 30_text_core.ipynb.
Converted 31_text_data.ipynb.
Converted 32_text_models_awdlstm.ipynb.
Converted 33_text_models_core.ipynb.
Converted 34_callback_rnn.ipynb.
Converted 35_tutorial_wikitext.ipynb.
Converted 36_text_models_qrnn.ipynb.
Converted 37_text_learner.ipynb.
Converted 38_tutorial_ulmfit.ipynb.
Converted 40_tabular_core.ipynb.
Converted 41_tabular_model.ipynb.
Converted 42_tabular_rapids.ipynb.
Converted 50_data_block_examples.ipynb.
Converted 60_medical_imaging.ipynb.
Converted 65_medical_text.ipynb.
Converted 70_callback_wandb.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_notebook_test.ipynb.
Converted 95_index.ipynb.
Converted 96_data_external.ipynb.
Converted 97_utils_test.ipynb.
Converted notebook2jekyll.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves ShortEpochCallback -
###Code
#export
class ShortEpochCallback(Callback):
"Fit just `pct` of an epoch, then stop"
def __init__(self,pct=0.01,short_valid=True): self.pct,self.short_valid = pct,short_valid
def after_batch(self):
if self.iter/self.n_iter < self.pct: return
if self.training: raise CancelTrainException
if self.short_valid: raise CancelValidException
learn = synth_learner()
learn.fit(1, cbs=ShortEpochCallback())
learn = synth_learner()
learn.fit(1, cbs=ShortEpochCallback(short_valid=False))
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.dbunch.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.run = not hasattr(self, "lr_finder") and not hasattr(self, "gather_preds")
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = list(self.recorder.metric_names[1:]).index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
def after_fit(self): self.run=True
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = list(self.recorder.metric_names[1:]).index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#hide
#A tracker callback is not run during an lr_find
from local.callback.schedule import *
learn = synth_learner(n_trn=2, cbs=TrackerCallback(monitor='tst_metric'), metrics=tst_metric)
learn.lr_find(num_it=5, show_plot=False)
assert not hasattr(learn, 'new_best')
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that saves the model's best during training and loads it at the end."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., fname='model', every_epoch=False, add_save=None, with_opt=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
store_attr(self, 'fname,every_epoch,add_save,with_opt')
def _save(self, name):
self.learn.save(name, with_opt=self.with_opt)
if self.add_save is not None:
with self.add_save.open('wb') as f: self.learn.save(f, with_opt=self.with_opt)
def after_epoch(self):
"Compare the value monitored to its best score and save if best."
if self.every_epoch: self._save(f'{self.fname}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self._save(f'{self.fname}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch: self.learn.load(f'{self.fname}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity.
###Code
learn = synth_learner(n_trn=2, path=Path.cwd()/'tmp')
learn.fit(n_epoch=2, cbs=SaveModelCallback())
assert (Path.cwd()/'tmp/models/model.pth').exists()
learn.fit(n_epoch=2, cbs=SaveModelCallback(every_epoch=True))
for i in range(2): assert (Path.cwd()/f'tmp/models/model_{i}.pth').exists()
shutil.rmtree(Path.cwd()/'tmp')
###Output
_____no_output_____
###Markdown
ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and reduce LR by `factor` if no improvement."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core_foundation.ipynb.
Converted 01a_core_utils.ipynb.
Converted 01b_core_dispatch.ipynb.
Converted 01c_core_transform.ipynb.
Converted 02_core_script.ipynb.
Converted 03_torchcore.ipynb.
Converted 03a_layers.ipynb.
Converted 04_data_load.ipynb.
Converted 05_data_core.ipynb.
Converted 06_data_transforms.ipynb.
Converted 07_data_block.ipynb.
Converted 08_vision_core.ipynb.
Converted 09_vision_augment.ipynb.
Converted 09a_vision_data.ipynb.
Converted 09b_vision_utils.ipynb.
Converted 10_pets_tutorial.ipynb.
Converted 11_vision_models_xresnet.ipynb.
Converted 12_optimizer.ipynb.
Converted 13_learner.ipynb.
Converted 13a_metrics.ipynb.
Converted 14_callback_schedule.ipynb.
Converted 14a_callback_data.ipynb.
Converted 15_callback_hook.ipynb.
Converted 15a_vision_models_unet.ipynb.
Converted 16_callback_progress.ipynb.
Converted 17_callback_tracker.ipynb.
Converted 18_callback_fp16.ipynb.
Converted 19_callback_mixup.ipynb.
Converted 20_interpret.ipynb.
Converted 20a_distributed.ipynb.
Converted 21_vision_learner.ipynb.
Converted 22_tutorial_imagenette.ipynb.
Converted 23_tutorial_transfer_learning.ipynb.
Converted 30_text_core.ipynb.
Converted 31_text_data.ipynb.
Converted 32_text_models_awdlstm.ipynb.
Converted 33_text_models_core.ipynb.
Converted 34_callback_rnn.ipynb.
Converted 35_tutorial_wikitext.ipynb.
Converted 36_text_models_qrnn.ipynb.
Converted 37_text_learner.ipynb.
Converted 38_tutorial_ulmfit.ipynb.
Converted 40_tabular_core.ipynb.
Converted 41_tabular_model.ipynb.
Converted 42_tabular_rapids.ipynb.
Converted 50_data_block_examples.ipynb.
Converted 60_medical_imaging.ipynb.
Converted 65_medical_text.ipynb.
Converted 70_callback_wandb.ipynb.
Converted 71_callback_tensorboard.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_notebook_test.ipynb.
Converted 95_index.ipynb.
Converted 96_data_external.ipynb.
Converted 97_utils_test.ipynb.
Converted notebook2jekyll.ipynb.
Converted xse_resnext.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves ShortEpochCallback -
###Code
#export
class ShortEpochCallback(Callback):
"Fit just `pct` of an epoch, then stop"
def __init__(self,pct=0.01,short_valid=True): self.pct,self.short_valid = pct,short_valid
def after_batch(self):
if self.iter/self.n_iter < self.pct: return
if self.training: raise CancelTrainException
if self.short_valid: raise CancelValidException
learn = synth_learner()
learn.fit(1, cbs=ShortEpochCallback())
learn = synth_learner()
learn.fit(1, cbs=ShortEpochCallback(short_valid=False))
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.dbunch.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.run = not hasattr(self, "lr_finder") and not hasattr(self, "gather_preds")
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = list(self.recorder.metric_names[1:]).index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
def after_fit(self): self.run=True
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = list(self.recorder.metric_names[1:]).index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#hide
#A tracker callback is not run during an lr_find
from fastai2.callback.schedule import *
learn = synth_learner(n_trn=2, cbs=TrackerCallback(monitor='tst_metric'), metrics=tst_metric)
learn.lr_find(num_it=5, show_plot=False)
assert not hasattr(learn, 'new_best')
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that saves the model's best during training and loads it at the end."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., fname='model', every_epoch=False, add_save=None, with_opt=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
store_attr(self, 'fname,every_epoch,add_save,with_opt')
def _save(self, name):
self.learn.save(name, with_opt=self.with_opt)
if self.add_save is not None:
with self.add_save.open('wb') as f: self.learn.save(f, with_opt=self.with_opt)
def after_epoch(self):
"Compare the value monitored to its best score and save if best."
if self.every_epoch: self._save(f'{self.fname}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self._save(f'{self.fname}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch: self.learn.load(f'{self.fname}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity.
###Code
learn = synth_learner(n_trn=2, path=Path.cwd()/'tmp')
learn.fit(n_epoch=2, cbs=SaveModelCallback())
assert (Path.cwd()/'tmp/models/model.pth').exists()
learn.fit(n_epoch=2, cbs=SaveModelCallback(every_epoch=True))
for i in range(2): assert (Path.cwd()/f'tmp/models/model_{i}.pth').exists()
shutil.rmtree(Path.cwd()/'tmp')
###Output
_____no_output_____
###Markdown
ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and reduce LR by `factor` if no improvement."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from nbdev.export import notebook2script
notebook2script()
###Output
Converted 00_test.ipynb.
Converted 01_core_foundation.ipynb.
Converted 01a_core_utils.ipynb.
Converted 01b_core_dispatch.ipynb.
Converted 01c_core_transform.ipynb.
Converted 02_core_script.ipynb.
Converted 03_torchcore.ipynb.
Converted 03a_layers.ipynb.
Converted 04_data_load.ipynb.
Converted 05_data_core.ipynb.
Converted 06_data_transforms.ipynb.
Converted 07_data_block.ipynb.
Converted 08_vision_core.ipynb.
Converted 09_vision_augment.ipynb.
Converted 09a_vision_data.ipynb.
Converted 09b_vision_utils.ipynb.
Converted 10_pets_tutorial.ipynb.
Converted 11_vision_models_xresnet.ipynb.
Converted 12_optimizer.ipynb.
Converted 13_learner.ipynb.
Converted 13a_metrics.ipynb.
Converted 14_callback_schedule.ipynb.
Converted 14a_callback_data.ipynb.
Converted 15_callback_hook.ipynb.
Converted 15a_vision_models_unet.ipynb.
Converted 16_callback_progress.ipynb.
Converted 17_callback_tracker.ipynb.
Converted 18_callback_fp16.ipynb.
Converted 19_callback_mixup.ipynb.
Converted 20_interpret.ipynb.
Converted 20a_distributed.ipynb.
Converted 21_vision_learner.ipynb.
Converted 22_tutorial_imagenette.ipynb.
Converted 23_tutorial_transfer_learning.ipynb.
Converted 30_text_core.ipynb.
Converted 31_text_data.ipynb.
Converted 32_text_models_awdlstm.ipynb.
Converted 33_text_models_core.ipynb.
Converted 34_callback_rnn.ipynb.
Converted 35_tutorial_wikitext.ipynb.
Converted 36_text_models_qrnn.ipynb.
Converted 37_text_learner.ipynb.
Converted 38_tutorial_ulmfit.ipynb.
Converted 40_tabular_core.ipynb.
Converted 41_tabular_model.ipynb.
Converted 42_tabular_rapids.ipynb.
Converted 50_data_block_examples.ipynb.
Converted 60_medical_imaging.ipynb.
Converted 65_medical_text.ipynb.
Converted 70_callback_wandb.ipynb.
Converted 71_callback_tensorboard.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_notebook_test.ipynb.
Converted 95_index.ipynb.
Converted 96_data_external.ipynb.
Converted 97_utils_test.ipynb.
Converted notebook2jekyll.ipynb.
Converted xse_resnext.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves
###Code
from local.test_utils import *
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.dbunch.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., fname='model', every_epoch=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
store_attr(self, 'fname,every_epoch')
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
if self.every_epoch: self.learn.save(f'{self.fname}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self.learn.save(f'{self.fname}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch and (self.learn.path/f'{self.learn.model_dir}/{self.fname}.pth').is_file():
self.learn.load(f'{self.fname}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity.
###Code
learn = synth_learner(n_trn=2, path=Path.cwd()/'tmp')
learn.fit(n_epoch=2, cbs=SaveModelCallback())
assert (Path.cwd()/'tmp/models/model.pth').exists()
learn.fit(n_epoch=2, cbs=SaveModelCallback(every_epoch=True))
for i in range(2): assert (Path.cwd()/f'tmp/models/model_{i}.pth').exists()
shutil.rmtree(Path.cwd()/'tmp')
###Output
_____no_output_____
###Markdown
ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core.ipynb.
Converted 01a_torch_core.ipynb.
Converted 02_script.ipynb.
Converted 03_dataloader.ipynb.
Converted 04_transform.ipynb.
Converted 05_data_core.ipynb.
Converted 06_data_transforms.ipynb.
Converted 07_vision_core.ipynb.
Converted 08_pets_tutorial.ipynb.
Converted 09_vision_augment.ipynb.
Converted 11_layers.ipynb.
Converted 11a_vision_models_xresnet.ipynb.
Converted 12_optimizer.ipynb.
Converted 13_learner.ipynb.
Converted 14_callback_schedule.ipynb.
Converted 15_callback_hook.ipynb.
Converted 16_callback_progress.ipynb.
Converted 17_callback_tracker.ipynb.
Converted 18_callback_fp16.ipynb.
Converted 19_callback_mixup.ipynb.
Converted 20_metrics.ipynb.
Converted 21_tutorial_imagenette.ipynb.
Converted 22_vision_learner.ipynb.
Converted 23_tutorial_transfer_learning.ipynb.
Converted 30_text_core.ipynb.
Converted 31_text_data.ipynb.
Converted 32_text_models_awdlstm.ipynb.
Converted 33_text_models_core.ipynb.
Converted 34_callback_rnn.ipynb.
Converted 35_tutorial_wikitext.ipynb.
Converted 36_text_models_qrnn.ipynb.
Converted 37_text_learner.ipynb.
Converted 38_tutorial_ulmfit.ipynb.
Converted 40_tabular_core.ipynb.
Converted 41_tabular_model.ipynb.
Converted 42_tabular_rapids.ipynb.
Converted 50_data_block.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_index.ipynb.
Converted 95_utils_test.ipynb.
Converted 96_data_external.ipynb.
Converted notebook2jekyll.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves
###Code
from local.test_utils import *
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.dbunch.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that saves the model's best during training and loads it at the end."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., fname='model', every_epoch=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
store_attr(self, 'fname,every_epoch')
def after_epoch(self):
"Compare the value monitored to its best score and save if best."
if self.every_epoch: self.learn.save(f'{self.fname}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self.learn.save(f'{self.fname}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch and (self.learn.path/f'{self.learn.model_dir}/{self.fname}.pth').is_file():
self.learn.load(f'{self.fname}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity.
###Code
learn = synth_learner(n_trn=2, path=Path.cwd()/'tmp')
learn.fit(n_epoch=2, cbs=SaveModelCallback())
assert (Path.cwd()/'tmp/models/model.pth').exists()
learn.fit(n_epoch=2, cbs=SaveModelCallback(every_epoch=True))
for i in range(2): assert (Path.cwd()/f'tmp/models/model_{i}.pth').exists()
shutil.rmtree(Path.cwd()/'tmp')
###Output
_____no_output_____
###Markdown
ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and reduce LR by `factor` if no improvement."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core.ipynb.
Converted 01a_utils.ipynb.
Converted 01b_dispatch.ipynb.
Converted 01c_transform.ipynb.
Converted 02_script.ipynb.
Converted 03_torch_core.ipynb.
Converted 03a_layers.ipynb.
Converted 04_dataloader.ipynb.
Converted 05_data_core.ipynb.
Converted 06_data_transforms.ipynb.
Converted 07_data_block.ipynb.
Converted 08_vision_core.ipynb.
Converted 09_vision_augment.ipynb.
Converted 10_pets_tutorial.ipynb.
Converted 11_vision_models_xresnet.ipynb.
Converted 12_optimizer.ipynb.
Converted 13_learner.ipynb.
Converted 13a_metrics.ipynb.
Converted 14_callback_schedule.ipynb.
Converted 14a_callback_data.ipynb.
Converted 15_callback_hook.ipynb.
Converted 15a_vision_models_unet.ipynb.
Converted 16_callback_progress.ipynb.
Converted 17_callback_tracker.ipynb.
Converted 18_callback_fp16.ipynb.
Converted 19_callback_mixup.ipynb.
Converted 21_vision_learner.ipynb.
Converted 22_tutorial_imagenette.ipynb.
Converted 23_tutorial_transfer_learning.ipynb.
Converted 30_text_core.ipynb.
Converted 31_text_data.ipynb.
Converted 32_text_models_awdlstm.ipynb.
Converted 33_text_models_core.ipynb.
Converted 34_callback_rnn.ipynb.
Converted 35_tutorial_wikitext.ipynb.
Converted 36_text_models_qrnn.ipynb.
Converted 37_text_learner.ipynb.
Converted 38_tutorial_ulmfit.ipynb.
Converted 40_tabular_core.ipynb.
Converted 41_tabular_model.ipynb.
Converted 42_tabular_rapids.ipynb.
Converted 50_data_block_examples.ipynb.
Converted 60_medical_imaging.ipynb.
Converted 65_medical_text.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_notebook_test.ipynb.
Converted 95_index.ipynb.
Converted 96_data_external.ipynb.
Converted 97_utils_test.ipynb.
Converted Untitled.ipynb.
Converted notebook2jekyll.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves
###Code
from local.utils.test import *
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.dbunch.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., fname='model', every_epoch=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
store_attr(self, 'fname,every_epoch')
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
if self.every_epoch: self.learn.save(f'{self.fname}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self.learn.save(f'{self.fname}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch and (self.learn.path/f'{self.learn.model_dir}/{self.fname}.pth').is_file():
self.learn.load(f'{self.fname}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity.
###Code
learn = synth_learner(n_trn=2, path=Path.cwd()/'tmp')
learn.fit(n_epoch=2, cbs=SaveModelCallback())
assert (Path.cwd()/'tmp/models/model.pth').exists()
learn.fit(n_epoch=2, cbs=SaveModelCallback(every_epoch=True))
for i in range(2): assert (Path.cwd()/f'tmp/models/model_{i}.pth').exists()
shutil.rmtree(Path.cwd()/'tmp')
###Output
_____no_output_____
###Markdown
ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core.ipynb.
Converted 01a_torch_core.ipynb.
Converted 02_script.ipynb.
Converted 03_dataloader.ipynb.
Converted 04_transform.ipynb.
Converted 05_data_core.ipynb.
Converted 06_data_transforms.ipynb.
Converted 07_vision_core.ipynb.
Converted 08_pets_tutorial.ipynb.
Converted 09_vision_augment.ipynb.
Converted 11_layers.ipynb.
Converted 11a_vision_models_xresnet.ipynb.
Converted 12_optimizer.ipynb.
Converted 13_learner.ipynb.
Converted 14_callback_schedule.ipynb.
Converted 15_callback_hook.ipynb.
Converted 16_callback_progress.ipynb.
Converted 17_callback_tracker.ipynb.
Converted 18_callback_fp16.ipynb.
Converted 19_callback_mixup.ipynb.
Converted 20_metrics.ipynb.
Converted 21_tutorial_imagenette.ipynb.
Converted 22_vision_learner.ipynb.
Converted 23_tutorial_transfer_learning.ipynb.
Converted 30_text_core.ipynb.
Converted 31_text_data.ipynb.
Converted 32_text_models_awdlstm.ipynb.
Converted 33_text_models_core.ipynb.
Converted 34_callback_rnn.ipynb.
Converted 35_tutorial_wikitext.ipynb.
Converted 36_text_models_qrnn.ipynb.
Converted 37_text_learner.ipynb.
Converted 38_tutorial_ulmfit.ipynb.
Converted 40_tabular_core.ipynb.
Converted 41_tabular_model.ipynb.
Converted 42_tabular_rapids.ipynb.
Converted 50_data_block.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_index.ipynb.
Converted 95_utils_test.ipynb.
Converted 96_data_external.ipynb.
Converted notebook2jekyll.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves ShortEpochCallback -
###Code
#export
class ShortEpochCallback(Callback):
"Fit just `pct` of an epoch, then stop"
def __init__(self,pct=0.01,short_valid=True): self.pct,self.short_valid = pct,short_valid
def after_batch(self):
if self.iter/self.n_iter < self.pct: return
if self.training: raise CancelTrainException
if self.short_valid: raise CancelValidException
learn = synth_learner()
learn.fit(1, cbs=ShortEpochCallback())
learn = synth_learner()
learn.fit(1, cbs=ShortEpochCallback(short_valid=False))
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.dbunch.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = list(self.recorder.metric_names[1:]).index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = list(self.recorder.metric_names[1:]).index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that saves the model's best during training and loads it at the end."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., fname='model', every_epoch=False, add_save=None, with_opt=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
store_attr(self, 'fname,every_epoch,add_save,with_opt')
def _save(self, name):
self.learn.save(name, with_opt=self.with_opt)
if self.add_save is not None:
with self.add_save.open('wb') as f: self.learn.save(f, with_opt=self.with_opt)
def after_epoch(self):
"Compare the value monitored to its best score and save if best."
if self.every_epoch: self._save(f'{self.fname}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self._save(f'{self.fname}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch: self.learn.load(f'{self.fname}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity.
###Code
learn = synth_learner(n_trn=2, path=Path.cwd()/'tmp')
learn.fit(n_epoch=2, cbs=SaveModelCallback())
assert (Path.cwd()/'tmp/models/model.pth').exists()
learn.fit(n_epoch=2, cbs=SaveModelCallback(every_epoch=True))
for i in range(2): assert (Path.cwd()/f'tmp/models/model_{i}.pth').exists()
shutil.rmtree(Path.cwd()/'tmp')
###Output
_____no_output_____
###Markdown
ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and reduce LR by `factor` if no improvement."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core.ipynb.
Converted 01a_utils.ipynb.
Converted 01b_dispatch.ipynb.
Converted 01c_transform.ipynb.
Converted 02_script.ipynb.
Converted 03_torch_core.ipynb.
Converted 03a_layers.ipynb.
Converted 04_dataloader.ipynb.
Converted 05_data_core.ipynb.
Converted 06_data_transforms.ipynb.
Converted 07_data_block.ipynb.
Converted 08_vision_core.ipynb.
Converted 09_vision_augment.ipynb.
Converted 09a_vision_data.ipynb.
Converted 10_pets_tutorial.ipynb.
Converted 11_vision_models_xresnet.ipynb.
Converted 12_optimizer.ipynb.
Converted 13_learner.ipynb.
Converted 13a_metrics.ipynb.
Converted 14_callback_schedule.ipynb.
Converted 14a_callback_data.ipynb.
Converted 15_callback_hook.ipynb.
Converted 15a_vision_models_unet.ipynb.
Converted 16_callback_progress.ipynb.
Converted 17_callback_tracker.ipynb.
Converted 18_callback_fp16.ipynb.
Converted 19_callback_mixup.ipynb.
Converted 20_interpret.ipynb.
Converted 20a_distributed.ipynb.
Converted 21_vision_learner.ipynb.
Converted 22_tutorial_imagenette.ipynb.
Converted 23_tutorial_transfer_learning.ipynb.
Converted 30_text_core.ipynb.
Converted 31_text_data.ipynb.
Converted 32_text_models_awdlstm.ipynb.
Converted 33_text_models_core.ipynb.
Converted 34_callback_rnn.ipynb.
Converted 35_tutorial_wikitext.ipynb.
Converted 36_text_models_qrnn.ipynb.
Converted 37_text_learner.ipynb.
Converted 38_tutorial_ulmfit.ipynb.
Converted 40_tabular_core.ipynb.
Converted 41_tabular_model.ipynb.
Converted 42_tabular_rapids.ipynb.
Converted 50_data_block_examples.ipynb.
Converted 60_medical_imaging.ipynb.
Converted 65_medical_text.ipynb.
Converted 70_callback_wandb.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_notebook_test.ipynb.
Converted 95_index.ipynb.
Converted 96_data_external.ipynb.
Converted 97_utils_test.ipynb.
Converted notebook2jekyll.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves
###Code
from local.utils.test import *
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.dbunch.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., name='model', every_epoch=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.name,self.every_epoch = name,every_epoch
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
if self.every_epoch: self.learn.save(f'{self.name}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self.learn.save(f'{self.name}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch and (self.learn.path/f'{self.learn.model_dir}/{self.name}.pth').is_file():
self.learn.load(f'{self.name}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity. TODO: test when `Learner.save` is implemented. ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core.ipynb.
Converted 01a_torch_core.ipynb.
Converted 01b_script.ipynb.
Converted 01c_dataloader.ipynb.
Converted 02_data_transforms.ipynb.
Converted 03_data_pipeline.ipynb.
Converted 05_data_core.ipynb.
Converted 06_data_source.ipynb.
Converted 07_vision_core.ipynb.
Converted 08_pets_tutorial.ipynb.
Converted 09_vision_augment.ipynb.
Converted 11_layers.ipynb.
Converted 11a_vision_models_xresnet.ipynb.
Converted 12_optimizer.ipynb.
Converted 13_learner.ipynb.
Converted 14_callback_schedule.ipynb.
Converted 15_callback_hook.ipynb.
Converted 16_callback_progress.ipynb.
Converted 17_callback_tracker.ipynb.
Converted 18_callback_fp16.ipynb.
Converted 19_callback_mixup.ipynb.
Converted 20_metrics.ipynb.
Converted 21_tutorial_imagenette.ipynb.
Converted 22_vision_learner.ipynb.
Converted 23_tutorial_transfer_learning.ipynb.
Converted 30_text_core.ipynb.
Converted 31_text_data.ipynb.
Converted 32_text_models_awdlstm.ipynb.
Converted 33_text_models_core.ipynb.
Converted 34_callback_rnn.ipynb.
Converted 35_tutorial_wikitext.ipynb.
Converted 36_text_models_qrnn.ipynb.
Converted 40_tabular_core.ipynb.
Converted 41_tabular_model.ipynb.
Converted 42_tabular_rapids.ipynb.
Converted 50_data_block.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_index.ipynb.
Converted 95_utils_test.ipynb.
Converted 96_data_external.ipynb.
Converted notebook2jekyll.ipynb.
###Markdown
Tracking callbacks> Callbacks that make decisions depending how a monitored metric/loss behaves
###Code
from local.test_utils import *
###Output
_____no_output_____
###Markdown
TerminateOnNaNCallback -
###Code
# export
class TerminateOnNaNCallback(Callback):
"A `Callback` that terminates training if loss is NaN."
run_before=Recorder
def after_batch(self):
"Test if `last_loss` is NaN and interrupts training."
if torch.isinf(self.loss) or torch.isnan(self.loss): raise CancelFitException
learn = synth_learner()
learn.fit(10, lr=100, cbs=TerminateOnNaNCallback())
assert len(learn.recorder.losses) < 10 * len(learn.dbunch.train_dl)
for l in learn.recorder.losses:
assert not torch.isinf(l) and not torch.isnan(l)
###Output
_____no_output_____
###Markdown
TrackerCallback -
###Code
# export
class TrackerCallback(Callback):
"A `Callback` that keeps track of the best value in `monitor`."
run_after=Recorder
def __init__(self, monitor='valid_loss', comp=None, min_delta=0.):
if comp is None: comp = np.less if 'loss' in monitor else np.greater
if comp == np.less: min_delta *= -1
self.monitor,self.comp,self.min_delta = monitor,comp,min_delta
def begin_fit(self):
"Prepare the monitored value"
self.best = float('inf') if self.comp == np.less else -float('inf')
assert self.monitor in self.recorder.metric_names[1:]
self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self):
"Compare the last value to the best up to know"
val = self.recorder.values[-1][self.idx]
if self.comp(val - self.min_delta, self.best): self.best,self.new_best = val,True
else: self.new_best = False
###Output
_____no_output_____
###Markdown
When implementing a `Callback` that has behavior that depends on the best value of a metric or loss, subclass this `Callback` and use its `best` (for best value so far) and `new_best` (there was a new best value this epoch) attributes. `comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount.
###Code
#hide
class FakeRecords(Callback):
run_after=Recorder
run_before=TrackerCallback
def __init__(self, monitor, values): self.monitor,self.values = monitor,values
def begin_fit(self): self.idx = self.recorder.metric_names[1:].index(self.monitor)
def after_epoch(self): self.recorder.values[-1][self.idx] = self.values[self.epoch]
class TestTracker(Callback):
run_after=TrackerCallback
def begin_fit(self): self.bests,self.news = [],[]
def after_epoch(self):
self.bests.append(self.tracker.best)
self.news.append(self.tracker.new_best)
#hide
learn = synth_learner(n_trn=2, cbs=TestTracker())
cbs=[TrackerCallback(monitor='valid_loss'), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
#With a min_delta
cbs=[TrackerCallback(monitor='valid_loss', min_delta=0.15), FakeRecords('valid_loss', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#hide
#By default metrics have to be bigger at each epoch.
def tst_metric(out,targ): return F.mse_loss(out,targ)
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric'), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.2])
test_eq(learn.test_tracker.news, [True,False])
#This can be overwritten by passing `comp=np.less`.
learn = synth_learner(n_trn=2, cbs=TestTracker(), metrics=tst_metric)
cbs=[TrackerCallback(monitor='tst_metric', comp=np.less), FakeRecords('tst_metric', [0.2,0.1])]
with learn.no_logging(): learn.fit(2, cbs=cbs)
test_eq(learn.test_tracker.bests, [0.2, 0.1])
test_eq(learn.test_tracker.news, [True,True])
###Output
_____no_output_____
###Markdown
EarlyStoppingCallback -
###Code
# export
class EarlyStoppingCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience = patience
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
print(f'No improvement since epoch {self.epoch-self.wait}: early stopping')
raise CancelFitException()
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. `patience` is the number of epochs you're willing to wait without improvement.
###Code
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=200, lr=1e-7, cbs=EarlyStoppingCallback(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(len(learn.recorder.values), 3)
###Output
_____no_output_____
###Markdown
SaveModelCallback -
###Code
# export
class SaveModelCallback(TrackerCallback):
"A `TrackerCallback` that terminates training when monitored quantity stops improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., fname='model', every_epoch=False):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
store_attr(self, 'fname,every_epoch')
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
if self.every_epoch: self.learn.save(f'{self.fname}_{self.epoch}')
else: #every improvement
super().after_epoch()
if self.new_best: self.learn.save(f'{self.fname}')
def on_train_end(self, **kwargs):
"Load the best model."
if not self.every_epoch and (self.learn.path/f'{self.learn.model_dir}/{self.fname}.pth').is_file():
self.learn.load(f'{self.fname}')
###Output
_____no_output_____
###Markdown
`comp` is the comparison operator used to determine if a value is best than another (defaults to `np.less` if 'loss' is in the name passed in `monitor`, `np.greater` otherwise) and `min_delta` is an optional float that requires a new value to go over the current best (depending on `comp`) by at least that amount. Model will be saved in `learn.path/learn.model_dir/name.pth`, maybe `every_epoch` or at each improvement of the monitored quantity.
###Code
learn = synth_learner(n_trn=2, path=Path.cwd()/'tmp')
learn.fit(n_epoch=2, cbs=SaveModelCallback())
assert (Path.cwd()/'tmp/models/model.pth').exists()
learn.fit(n_epoch=2, cbs=SaveModelCallback(every_epoch=True))
for i in range(2): assert (Path.cwd()/f'tmp/models/model_{i}.pth').exists()
shutil.rmtree(Path.cwd()/'tmp')
###Output
_____no_output_____
###Markdown
ReduceLROnPlateau
###Code
# export
class ReduceLROnPlateau(TrackerCallback):
"A `TrackerCallback` that reduces learning rate when a metric has stopped improving."
def __init__(self, monitor='valid_loss', comp=None, min_delta=0., patience=1, factor=10.):
super().__init__(monitor=monitor, comp=comp, min_delta=min_delta)
self.patience,self.factor = patience,factor
def begin_fit(self): self.wait = 0; super().begin_fit()
def after_epoch(self):
"Compare the value monitored to its best score and maybe stop training."
super().after_epoch()
if self.new_best: self.wait = 0
else:
self.wait += 1
if self.wait >= self.patience:
for h in self.opt.hypers: h['lr'] /= self.factor
self.wait = 0
print(f'Epoch {self.epoch}: reducing lr to {self.opt.hypers[-1]["lr"]}')
learn = synth_learner(n_trn=2)
learn.fit(n_epoch=4, lr=1e-7, cbs=ReduceLROnPlateau(monitor='valid_loss', min_delta=0.1, patience=2))
#hide
test_eq(learn.opt.hypers[-1]['lr'], 1e-8)
###Output
_____no_output_____
###Markdown
Export -
###Code
#hide
from local.notebook.export import notebook2script
notebook2script(all_fs=True)
###Output
Converted 00_test.ipynb.
Converted 01_core.ipynb.
Converted 01a_torch_core.ipynb.
Converted 02_script.ipynb.
Converted 03_dataloader.ipynb.
Converted 04_transform.ipynb.
Converted 05_data_core.ipynb.
Converted 06_data_transforms.ipynb.
Converted 07_vision_core.ipynb.
Converted 08_pets_tutorial.ipynb.
Converted 09_vision_augment.ipynb.
Converted 11_layers.ipynb.
Converted 11a_vision_models_xresnet.ipynb.
Converted 12_optimizer.ipynb.
Converted 13_learner.ipynb.
Converted 14_callback_schedule.ipynb.
Converted 15_callback_hook.ipynb.
Converted 16_callback_progress.ipynb.
Converted 17_callback_tracker.ipynb.
Converted 18_callback_fp16.ipynb.
Converted 19_callback_mixup.ipynb.
Converted 20_metrics.ipynb.
Converted 21_tutorial_imagenette.ipynb.
Converted 22_vision_learner.ipynb.
Converted 23_tutorial_transfer_learning.ipynb.
Converted 30_text_core.ipynb.
Converted 31_text_data.ipynb.
Converted 32_text_models_awdlstm.ipynb.
Converted 33_text_models_core.ipynb.
Converted 34_callback_rnn.ipynb.
Converted 35_tutorial_wikitext.ipynb.
Converted 36_text_models_qrnn.ipynb.
Converted 37_text_learner.ipynb.
Converted 38_tutorial_ulmfit.ipynb.
Converted 40_tabular_core.ipynb.
Converted 41_tabular_model.ipynb.
Converted 42_tabular_rapids.ipynb.
Converted 50_data_block.ipynb.
Converted 90_notebook_core.ipynb.
Converted 91_notebook_export.ipynb.
Converted 92_notebook_showdoc.ipynb.
Converted 93_notebook_export2html.ipynb.
Converted 94_index.ipynb.
Converted 95_utils_test.ipynb.
Converted 96_data_external.ipynb.
Converted notebook2jekyll.ipynb.
|
Project01/Data_ETL/profile_data_extraction.ipynb | ###Markdown
Basic
###Code
basic = []
for i in range(1):
# print("Serial :,"i)
all_projects = []
url = pl[1]
print(url)
driver = webdriver.Chrome(r'C:\Users\USER\chromedriver_win32\chromedriver.exe')
driver.get(url)
time.sleep(3)
source_code = driver.page_source
soup = BeautifulSoup(source_code, 'html.parser')
name = soup.find_all('strong', class_ = 'userName--1ZA07')
for n in name:
basic.append(n.text)
category = soup.find_all('strong', class_ = 'introCategory--F81Ky')
for e in category:
basic.append(e.text)
sp = soup.find_all('div', class_ = 'categoryName--1zWtA')
for m in sp:
basic.append(m.text)
rating = soup.find_all('div', class_ = 'itemRating--360UA itemRating--2-rFv typeLarge--1cEMN')
for k in rating:
basic.append(k.text)
reviews = soup.find_all('span', class_ = 'statsNum--32OX2')
for kk in reviews:
basic.append(kk.text)
driver.quit()
print(basic)
basic
###Output
_____no_output_____
###Markdown
Projects
###Code
projects = soup.find_all('div', class_ = 'listArea--peDdh')
projects
### Project data for one user
projects = soup.find_all('div', class_ = 'listArea--peDdh')
all_projects = []
for y in projects:
yy = y.find_all('div', class_ = 'item--1ZJSx')
for t in yy:
project_item = []
tdiv = t.find_all('div', class_ =['itemTitle--2vWBq','elip2--nFWXY'])
for td in tdiv:
project_title = td.text
project_item.append(project_title)
ratdiv = t.find_all('div', class_ =['itemGroup--2RnIL','ItemGroup_itemGroup--1f-on'])
for rd in ratdiv:
rating = rd.text
project_item.append(rating)
feediv = t.find_all('span', class_ ='priceInner--1HE2v')
for fd in feediv:
fee = fd.text
project_item.append(fee)
all_projects.append(project_item)
all_projects
temp = []
for item in all_projects:
for i in item:
hh = ["평균평점","후기","판매가","원할인률","원할인률-", "할인 전 가격"]
for yt in hh:
if yt in i:
temp.append(i.replace(yt ," "))
temp
for item in project_item:
print("명쾌하고" in item)
###Output
명쾌하고 꼼꼼한 20분 심층 전화상담
True
평균 평점4.8후기92
False
판매가20,000원할인률60%할인 전 가격50,000
False
###Markdown
product and review number
###Code
products = []
tt = soup.find_all('div', class_ = "list--e6w5E")
for t in tt:
cc = t.find_all('div', class_='count--2w5o6')
for cd in cc:
cd.find_all('div', class_ = "count--2w5o6")
ce = cd.text
print(ce)
products.append(ce)
products
###Output
3
715
###Markdown
Seller main information
###Code
### Project data for one user
maininfo = []
infos = soup.find_all('ul', class_ = 'productInfoList--1-H-D')
for f in infos:
li = f.find_all('li')
for ll in li:
uh = ["대표자","상호명","사업자등록번호","통신판매업번호-", "사업장 주소", "고객센터",'메일']
for u in range(len(uh)):
if uh[u] in ll.text:
b = uh[u]
la = ll.text
maininfo.append(la.replace(b , ""))
maininfo
###Output
_____no_output_____
###Markdown
Reviews
###Code
### review data for one user
rdiv = soup.find_all('div', class_ = ['titleSection--1lnfz','SubTitle_titleSection--2YQaq'])
for i in rdiv:
ii = i.find_all('div', class_ = "titleArea--s3-rN")
for each in ii:
ai = each.find_all('a', class_ = "titleLink--2BV8L")
for a in ai:
print(a)
rdiv = soup.find_all('div', class_ = "listSection--kViCl")
reviews_rating = []
reviews_heading = []
reviews_text = []
for eachr in rdiv:
ee = eachr.find_all('div', class_ = "reviewItem--1OwNO")
for each in ee:
rating = each.find_all('div', class_ = ["expertPoint--2Zrvr","expertPoint--13H3V"])
for r in rating:
reviews_rating.append(r.text)
head = each.find_all('div', class_ = "reviewTitle--qv3Pk")
for r in head:
reviews_heading.append(r.text)
commentdiv = each.find_all('p', class_ = "reviewText--28mzN")
for ecom in commentdiv:
reviews_text.append(ecom.text)
review_obj = []
for i in range(len(reviews_heading)):
review_obj.append(reviews_heading[i])
review_obj.append(reviews_rating[i])
review_obj.append(reviews_text[i])
reviews_rating
reviews_heading
reviews_text
review_obj = []
for i in range(len(reviews_heading)):
review_obj.append(reviews_heading[i])
review_obj.append(reviews_rating[i])
review_obj.append(reviews_text[i])
review_obj
# all project dataframe section
# allprojectdf = pd.DataFrame(all_project)
# allprojectdf.columns =["Representative", "Company_name", "Business_registration_number", "Mail_order_number", "Business_address", "Customer_Center",'Mail']
# allprojectdf
###Output
_____no_output_____ |
ICP/ICP-12/ICP12.ipynb | ###Markdown
Let's start out by importing all the libraries we're going to use.We need numpy to transform our input data into arrays our network can use, and we'll obviously be using several functions from Keras.We'll also need to use some functions from the Natural Language Toolkit (NLTK) to preprocess our text and get it ready to train on. Finally, we'll need the sys library to handle the printing of our text.
###Code
import numpy
import requests
import sys
import nltk
nltk.download('stopwords')
from nltk.tokenize import RegexpTokenizer
from nltk.corpus import stopwords
from keras.models import Sequential
from keras.layers import Dense, Dropout, LSTM
from keras.utils import np_utils
from keras.callbacks import ModelCheckpoint
###Output
[nltk_data] Downloading package stopwords to /root/nltk_data...
[nltk_data] Unzipping corpora/stopwords.zip.
###Markdown
We need to have data to train our model on. You can use any text file you'd like , but for this ICP we'll be using part of Mary Shelley's Frankenstein, which is available at the following Github link.
###Code
txt = "/content/aadr.us.txt"
text_string = open(txt, "r")
file = text_string.read()
###Output
_____no_output_____
###Markdown
Lets verify the data is proprely read.
###Code
file
###Output
_____no_output_____
###Markdown
Let's start by loading in our text data and doing some preprocessing of the data. We're going to need to apply some transformations to the text so everything is standardized and our model can work with it.We're going to lowercase everything so and not worry about capitalization in this example. We're also going to use NLTK to make tokens out of the words in the input file. Let's create an instance of the tokenizer and use it on our input file.Finally, we're going to filter our list of tokens and only keep the tokens that aren't in a list of Stop Words, or common words that provide little information about the sentence in question. We'll do this by using lambda to make a quick throwaway function and only assign the words to our variable if they aren't in a list of Stop Words provided by NLTK.Let's create a function to handle all that:
###Code
def tokenize_words(input):
# lowercase everything to standardize it
input = input.lower()
# instantiate the tokenizer
tokenizer = RegexpTokenizer(r'\w+')
tokens = tokenizer.tokenize(input)
# if the created token isn't in the stop words, make it part of "filtered"
filtered = filter(lambda token: token not in stopwords.words('english'), tokens)
return " ".join(filtered)
###Output
_____no_output_____
###Markdown
Now we call the function on our file:
###Code
# preprocess the input data, make tokens
processed_inputs = tokenize_words(file)
print(len(file))
print(len(processed_inputs))
###Output
70908
70907
###Markdown
A neural network works with numbers, not text characters. So well need to convert the characters in our input to numbers. We'll sort the list of the set of all characters that appear in our input text, then use the enumerate function to get numbers which represent the characters. We then create a dictionary that stores the keys and values, or the characters and the numbers that represent them:
###Code
chars = sorted(list(set(processed_inputs)))
char_to_num = dict((c, i) for i, c in enumerate(chars))
char_to_num
###Output
_____no_output_____
###Markdown
lets store the total length of our inputs and total length of our set of characters in a variable. Just so we get an idea of if our process of converting words to characters has worked thus far, let's print the length of our variables:
###Code
input_len = len(processed_inputs)
vocab_len = len(chars)
print ("Total number of characters:", input_len)
print ("Total vocab:", vocab_len)
###Output
Total number of characters: 70907
Total vocab: 28
###Markdown
Now that we've transformed the data into the form it needs to be in, we can begin making a dataset out of it, which we'll feed into our network. We need to define how long we want an individual sequence (one complete mapping of inputs characters as integers) to be. We'll set a length of 100 for now, and declare empty lists to store our input and output data:
###Code
seq_length = 100
x_data = []
y_data = []
###Output
_____no_output_____
###Markdown
Now we need to go through the entire list of inputs and convert the characters to numbers. We'll do this with a for loop. This will create a bunch of sequences where each sequence starts with the next character in the input data, beginning with the first character:
###Code
# loop through inputs, start at the beginning and go until we hit
# the final character we can create a sequence out of
for i in range(0, input_len - seq_length, 1):
# Define input and output sequences
# Input is the current character plus desired sequence length
in_seq = processed_inputs[i:i + seq_length]
# Out sequence is the initial character plus total sequence length
out_seq = processed_inputs[i + seq_length]
# We now convert list of characters to integers based on
# previously and add the values to our lists
x_data.append([char_to_num[char] for char in in_seq])
y_data.append(char_to_num[out_seq])
###Output
_____no_output_____
###Markdown
Now we have our input sequences of characters and our output, which is the character that should come after the sequence ends. We now have our training data features and labels, stored as x_data and y_data. Let's save our total number of sequences and check to see how many total input sequences we have:
###Code
n_patterns = len(x_data)
print ("Total Patterns:", n_patterns)
###Output
Total Patterns: 70807
###Markdown
Now we'll go ahead and convert our input sequences into a processed numpy array that our network can use. We'll also need to convert the numpy array values into floats so that the sigmoid activation function our network uses can interpret them and output probabilities from 0 to 1:
###Code
X = numpy.reshape(x_data, (n_patterns, seq_length, 1))
X = X/float(vocab_len)
###Output
_____no_output_____
###Markdown
We'll now one-hot encode our label data:
###Code
y = np_utils.to_categorical(y_data)
###Output
_____no_output_____
###Markdown
Since our features and labels are now ready for the network to use, let's go ahead and create our LSTM model. We specify the kind of model we want to make (a sequential one), and then add our first layer.We'll do dropout to prevent overfitting, followed by another layer or two. Then we'll add the final layer, a densely connected layer that will output a probability about what the next character in the sequence will be:
###Code
model = Sequential()
model.add(LSTM(256, input_shape=(X.shape[1], X.shape[2]), return_sequences=True))
model.add(Dropout(0.2))
model.add(LSTM(256, return_sequences=True))
model.add(Dropout(0.2))
model.add(LSTM(128))
model.add(Dropout(0.2))
model.add(Dense(y.shape[1], activation='softmax'))
###Output
_____no_output_____
###Markdown
We compile the model now, and it is ready for training:
###Code
model.compile(loss='categorical_crossentropy', optimizer='adam')
desired_callbacks= ModelCheckpoint('model_saved_weights.hd5', monitor='loss', verbose=1, save_best_only=True, mode='min')
###Output
_____no_output_____
###Markdown
Now we'll fit the model and let it train
###Code
model.fit(X, y, epochs=4, batch_size=256, callbacks=desired_callbacks)
model.load_weights()
###Output
_____no_output_____
###Markdown
Since we converted the characters to numbers earlier, we need to define a dictionary variable that will convert the output of the model back into numbers:
###Code
num_to_char = dict((i, c) for i, c in enumerate(chars))
###Output
_____no_output_____
###Markdown
To generate characters, we need to provide our trained model with a random seed character that it can generate a sequence of characters from:
###Code
start = numpy.random.randint(0, len(x_data) - 1)
pattern = x_data[start]
print("Random Seed:")
print("\"", ''.join([num_to_char[value] for value in pattern]), "\"")
###Output
Random Seed:
" 126 36 126 270 0 2014 10 07 36 059 36 059 35 805 35 805 571 0 2014 10 08 36 078 36 078 36 078 36 07 "
###Markdown
Now to finally generate text, we're going to iterate through our chosen number of characters and convert our input (the random seed) into float values.We'll ask the model to predict what comes next based off of the random seed, convert the output numbers to characters and then append it to the pattern, which is our list of generated characters plus the initial seed:
###Code
for i in range(1000):
x = numpy.reshape(pattern, (1, len(pattern), 1))
x = x / float(vocab_len)
prediction = model.predict(x, verbose=0)
print(prediction)
index = numpy.argmax(prediction)
result = num_to_char[index]
print(result)
print("---------")
# sys.stdout.write(result)
pattern.append(index)
pattern = pattern[1:len(pattern)]
#print(len(result))
#print(type(result))
model.predict(pattern)
x = [1,2,3,4]
for i in range(len(x)- 1 ):
print(i)
print(x[i])
print("---------")
###Output
_____no_output_____ |
capstone/DataExploration.ipynb | ###Markdown
Starbucks Capstone Challenge Data ExplorationThis notebook aims to load files and present some data visualization in order to understand the distribution and characteristics of the data, and possibly identify inconsistencies. Data exploration is one of the most important parts of the machine learning workflow because it allows you to notice any initial patterns in data distribution and features that may inform how to proceed with modeling and clustering the data. Data exploration uses visual exploration to understand what is in a dataset and the characteristics of the data. These characteristics can include size or amount of data, completeness and correctness of the data, and possible relationships amongst data elements.
###Code
## Import all the necessary libraries
import base64
import os
from io import BytesIO
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
from IPython.display import display_html
from matplotlib.figure import Figure
from sklearn.preprocessing import robust_scale, quantile_transform, scale
## Global definitions
data_dir = 'data'
pd.set_option('display.precision', 2)
pd.set_option('display.max_colwidth', None)
pd.set_option('display.max_columns', None)
portfolio_data_path = os.path.join(data_dir, 'portfolio.json')
profile_data_path = os.path.join(data_dir, 'profile.json')
transcript_data_path = os.path.join(data_dir, 'transcript.json')
## global functions
def load_dataframe(data_path):
"""Create a dataframe from a json file"""
return pd.read_json(data_path, orient='records', lines=True)
def convert_fig_to_html(figure):
"""
Convert a given matplotlib figure to HTML code
Attributes:
figure (matplotlib.figure.Figure): Generated without using pyplot.
Return:
return: img-html tag containing the figure data
"""
# Save figure to a temporary buffer
buf = BytesIO()
figure.savefig(buf, format="png")
# Embed the result in the html output
data = base64.b64encode(buf.getbuffer()).decode("ascii")
return f'<img src="data:image/png;base64,{data}" ' \
'style="display:inline;top:0%"/>'
###Output
_____no_output_____
###Markdown
Portfolio data setData set containing information about the offers which can be sent to customers. Overview
###Code
portfolio_df = load_dataframe(portfolio_data_path)
display(portfolio_df)
###Output
_____no_output_____
###Markdown
There are three types of offers that can be sent: buy-one-get-one(BOGO), discount, and informational:* In a BOGO offer, a user needs to spend a certain amount to get a reward equal to that threshold amount. * In a discount, a user gains a reward equal to a fraction of the amount spent. * In an informational offer, there is no reward, but neither is there a requisite amount that the user is expected to spend.Offers can be delivered via multiple channels:* email* social media* on the web* via the Starbucks’s app.Every offer has a validity period (*duration*) before the offer expires. We see that informational offers have a validity period even though these ads are merely providing information about a product. Here, the duration is the assumed period in which the customer is feeling the influence of the offer after receiving the advertisement. Analysis
###Code
print('Missing data: {}\n'.format(portfolio_df.isna().any().any()))
print('Dataset description:')
display(pd.DataFrame(portfolio_df.describe()))
###Output
Missing data: False
Dataset description:
###Markdown
In this portfolio, any offer is sent by email, so this is an informative feature and might be filtered out when feeding the neural networks. Apart from that, no issue is noticeable in this dataset. Scale valuesFeatures *reward*, *difficulty*, and *duration* present values in different ranges. It is a good practice to scale then to the same range than other features.
###Code
fig, axs = plt.subplots(figsize=(15,4), nrows=1, ncols=2)
rdd_df = portfolio_df[['reward','difficulty','duration']]
rdd_df.plot.density(ax=axs[0])
axs[0].set_title('Original data')
axs[0].set_xlabel('Original value')
data_transformed = robust_scale(rdd_df)
rdd_df = pd.DataFrame(data_transformed, columns=rdd_df.columns, index=rdd_df.index)
rdd_df.plot.density(ax=axs[1])
axs[1].set_title('Transformed data')
axs[1].set_xlabel('Scaled value')
plt.show()
###Output
_____no_output_____
###Markdown
Profile data setDataset containing demographic data for each one of the reward program users. Overview
###Code
profile_df = load_dataframe(profile_data_path)
display(profile_df.head())
###Output
_____no_output_____
###Markdown
Exploration Analyzing the missing valuesAt a glance, it is possible to notice empty values in columns **income** and **gender**, as well as missing value encoded as 118 in the column **age**. Also, aparently, those values are present in the same rows. It would be productive to verify if they always accur together.
###Code
display(pd.DataFrame(profile_df.count()).T \
.style.set_caption('Counting not null features'))
display(profile_df.describe().T \
.style.set_caption('Dataframe description'))
display(pd.DataFrame(profile_df.gender.value_counts()).T \
.style.set_caption('Gender by user'))
print('\nInvestigating whether all missing values are in the same rows.')
gender_isna = profile_df.gender.isna()
income_isna = profile_df.income.isna()
age_missing = profile_df.age == 118
print('Gender:\tnumber of NA: \t', gender_isna.sum())
print('Income:\tnumber of NA: \t', income_isna.sum())
print('Age:\tmissing values:\t', age_missing.sum())
print('\nAre gender and income missing in the same rows?\t{}' \
.format(gender_isna.isin(income_isna).all()))
print('Are gender and age missing in the same rows?\t{}' \
.format(gender_isna.isin(age_missing).all()))
print('Are income and age missing in the same rows?\t{}' \
.format(income_isna.isin(age_missing).all()))
display(pd.DataFrame(profile_df.became_member_on[gender_isna])\
.describe().T.style.set_caption('When did they register?'))
display(pd.DataFrame(profile_df.became_member_on[~gender_isna])\
.describe().T.style.set_caption('When did other users register?'))
###Output
_____no_output_____
###Markdown
By analyzing data above, it is possible to conclude that all the missing values occur in the same rows. This may have several possible causes, for instance: * the interface where those profiles were created might not have requested those information to be input, or* those users may not have agreeded with the privacy policy. In addition to that, those users have been signing up all over the analyzed period in the same fashion than the other users. Altough those users could be filtered out of the data set, they might have some peculiar behaviour which would be interesting to be analyzed. Thus, my approach with those record is:* maintain those records in the data set* mark those users as belonging to a particular class, by creating a new feature for that* since gender is a discrete feature, create another gender category* as age and income are continuous features, fill them with the respective mean values Explore ages
###Code
## Select registers with valid age
age_df = pd.DataFrame(profile_df.age[~age_missing])
## Generate the registers' description
description_pdstyle = \
pd.DataFrame(age_df.describe()).style \
.set_caption('Ages description') \
.set_table_attributes('style="display:inline;' \
'vertical-align:top"')
## Generate box and violin plots
fig1 = Figure(figsize=(10,4))
axs = fig1.subplots(nrows=1, ncols=2)
axs = axs.flatten()
axs[0].set_title('Box Plot')
age_df.plot.box(ax=axs[0])
axs[1].violinplot(age_df.values)
axs[1].set_title('Violin Plot')
## Generate histograms
bins = 84
fig2, axs = plt.subplots(nrows=2, ncols=2, figsize=(15, 10))
axs = axs.flatten()
# Cumulative distribution
age_df.hist(bins=bins, ax=axs[0], cumulative=True, density=True)
axs[0].set_title('Cumulative distribution')
axs[0].set_ylabel('users (%)')
axs[0].set_yticklabels((axs[0].get_yticks()*100).round(0))
axs[0].set_xscale('linear')
axs[0].set_xlabel('years')
axs[0].grid(False)
# Histogram
age_df.hist(bins=bins, ax=axs[1])
axs[1].set_title('Density')
axs[1].set_ylabel('users')
axs[1].set_xscale('linear')
axs[1].set_xlabel('years')
axs[1].grid(False)
# density
age_df.plot.density(ax=axs[2])
axs[2].legend()
axs[2].set_title('Density plot')
axs[2].set_ylabel('density')
axs[2].grid(False)
age_gender_df = profile_df[~age_missing].groupby('gender').age
age_gender_df.plot.density(ax=axs[3])
axs[3].set_title('Density by gender')
axs[3].set_ylabel('density')
axs[3].legend()
axs[3].grid(False)
## Display the information generated above
display_html('<center>' \
+ description_pdstyle._repr_html_() \
+ convert_fig_to_html(fig1) \
+ '</center>',
raw=True)
plt.show()
###Output
_____no_output_____
###Markdown
Analyzing the graphs above, we can see that although the age is normally distributed among the population, there is a local peak around the interval 20 ~ 25 for both male and female genders. Apparently, this deviation is not a problem to be handled beforehand. However, this is a point to be taken into consideration if networks have difficulty to converge. Hence, simply standardization for this feature seems to be good enough, as shown in the graphs below.
###Code
fig, axs = plt.subplots(figsize=(15,4), nrows=1, ncols=2)
age_df.plot.density(ax=axs[0])
axs[0].set_title('Original data')
axs[0].set_xlabel('Age')
data_transformed = robust_scale(age_df)
age_df = pd.DataFrame(data_transformed, columns=age_df.columns, index=age_df.index)
age_df.plot.density(ax=axs[1])
axs[1].set_title('Transformed data')
axs[1].set_xlabel('Age (scaled)')
plt.show()
###Output
_____no_output_____
###Markdown
Explore income
###Code
## Select registers with valid income
income_df = pd.DataFrame(profile_df.income[~income_isna])
## Generate the registers' description
description_pdstyle = \
pd.DataFrame(income_df.describe()).style \
.set_caption('Income description') \
.set_table_attributes('style="display:inline;' \
'vertical-align:top"')
## Generate box and violin plots
fig1 = Figure(figsize=(10,4))
axs = fig1.subplots(nrows=1, ncols=2)
axs = axs.flatten()
axs[0].set_title('Box Plot')
income_df.plot.box(ax=axs[0])
axs[1].violinplot(income_df.values)
axs[1].set_title('Violin Plot')
## Generate histograms
bins = 19
logbins = np.logspace(np.log10(income_df.min().min()),
np.log10(income_df.max().max()),
num=bins)
fig2, axs = plt.subplots(nrows=3, ncols=2, figsize=(18, 18))
axs = axs.flatten()
# linear scale
income_df.hist(bins=bins, ax=axs[0])
axs[0].set_title('Histogram (linear base)')
axs[0].set_ylabel('users')
axs[0].set_xscale('linear')
axs[0].set_xlabel('$\n(linear scale)')
axs[0].grid(False)
# log scale
income_df.hist(bins=logbins, ax=axs[1])
axs[1].set_title('Histogram (log base)')
axs[1].set_ylabel('users')
axs[1].set_xscale('log')
axs[1].set_xlabel('$\n(log scale)')
axs[1].grid(False)
# density
income_df.plot.density(ax=axs[2])
axs[2].set_title('Density plot')
axs[2].set_ylabel('density')
axs[2].set_xlabel('$')
axs[2].grid(False)
# Group income by gender
income_gender_df = profile_df[~income_isna].groupby('gender')
income_gender_df.income.plot.density(ax=axs[3])
axs[3].set_title('Density by gender')
axs[3].set_ylabel('density')
axs[3].legend()
axs[3].grid(False)
income_gender_df.get_group('F') \
.income.hist(bins=bins, ax=axs[4], alpha=0.5, label='F')
income_gender_df.get_group('F') \
.plot.scatter(x='age', y='income', ax=axs[5], alpha=0.5, label='F')
income_gender_df.get_group('M') \
.income.hist(bins=bins, ax=axs[4], alpha=0.4, label='M')
income_gender_df.get_group('M') \
.plot.scatter(x='age', y='income', c='orange',
ax=axs[5], alpha=0.2, label='M')
income_gender_df.get_group('O') \
.income.hist(bins=bins, ax=axs[4], color='green', alpha=0.3, label='O')
income_gender_df.get_group('O') \
.plot.scatter(x='age', y='income', c='lightgreen',
ax=axs[5], alpha=0.5, label='O')
axs[4].set_title('Histogram by gender')
axs[4].set_ylabel('users')
axs[4].set_xlabel('income')
axs[4].legend()
axs[4].grid(False)
axs[5].set_title('Scatter plot: income by gender')
axs[5].legend()
axs[5].grid(False)
# Display the information generated above
display_html('<center>' \
+ description_pdstyle._repr_html_() \
+ convert_fig_to_html(fig1) \
+ '</center>',
raw=True)
plt.show()
###Output
_____no_output_____
###Markdown
These graphs show us that income is not a well distributed feature. The density plot is bimodal, including some other local peaks. Splitting the income by gender, we note that the number of men presenting income below $80,000 is considerably higher than women. The scatter plot shows us a strong correlation between some ranges of age and income, with well defined steps.
###Code
fig, axs = plt.subplots(figsize=(15,4), nrows=1, ncols=2)
income_df.plot.density(ax=axs[0])
axs[0].set_title('Original data')
axs[0].set_xlabel('Income')
data_transformed = robust_scale(income_df)
income_df = pd.DataFrame(data_transformed, columns=income_df.columns, index=income_df.index)
income_df.plot.density(ax=axs[1])
axs[1].set_title('Transformed data')
axs[1].set_xlabel('Income (scaled)')
plt.show()
###Output
_____no_output_____
###Markdown
Explore membership
###Code
## Select registers
membership_df = profile_df.became_member_on
# Generate box plot
fig1 = Figure(figsize=(7,4))
ax = fig1.subplots()
ax.set_title('Box Plot')
membership_df.plot.box(ax=ax, vert=False)
ax.set_yticklabels('')
# Generate registers' description
member_desc_integers_pdstyle = \
pd.DataFrame(membership_df.describe()).style \
.set_caption('Membership description') \
.set_table_attributes('style="display:inline;' \
'vertical-align:top"')
## Convert data to datetime format
membership_df = pd.to_datetime(membership_df, format='%Y%m%d')
# Generate registers' description
member_desc_datetime_pdstyle = \
pd.DataFrame(membership_df.describe()).style \
.set_caption('Membership description') \
.set_table_attributes('style="display:inline;' \
'vertical-align:top"')
## Generate histograms
bins = 12
fig2, axs = plt.subplots(nrows=1, ncols=2, figsize=(15, 4))
membership_df.groupby(membership_df.dt.year) \
.hist(bins=bins, ax=axs[0])
axs[0].set_ylabel('users')
axs[0].set_xlabel('date\n(year/month)')
axs[0].grid(False)
membership_df.astype(np.long).plot.density(ax=axs[1])
## Display the information generated above
display_html('<center>' \
+ member_desc_integers_pdstyle._repr_html_() \
+ ' '
+ member_desc_datetime_pdstyle._repr_html_() \
+ convert_fig_to_html(fig1) \
+ '</center>',
raw=True)
plt.show()
fig, axs = plt.subplots(figsize=(15,4), nrows=1, ncols=2)
membership_values = membership_df.astype(np.long)
membership_values.plot.density(ax=axs[0])
axs[0].set_title('Original data')
axs[0].set_xlabel('Became Member On')
membership_values = quantile_transform(membership_values.values.reshape(-1,1),
output_distribution='normal',
copy=True)
membership_df = pd.DataFrame(membership_values,
index=membership_df.index)
membership_df.plot.density(ax=axs[1])
axs[1].set_title('Transformed data')
axs[1].set_xlabel('Became Member On (scaled)')
plt.show()
###Output
_____no_output_____
###Markdown
Transcript data setEvent log containing records for transactions, offers received, offers viewed, and offers completed. Overview
###Code
transcript_df = load_dataframe(transcript_data_path)
display(transcript_df.head())
display(pd.DataFrame(transcript_df.event.value_counts()).T \
.assign(missing=transcript_df.event.isna().sum()) \
.assign(total=transcript_df.count()) \
.style.set_caption('Count events'))
###Output
_____no_output_____
###Markdown
Exploration Initial transformationThe first step to understand this data set is splitting the value column, so that it is possible to analyze the relationship between events and results.
###Code
transcript_df = transcript_df.join(
pd.DataFrame.from_records(transcript_df.pop('value')))
transcript_df.offer_id.update(transcript_df.pop('offer id'))
display(pd.DataFrame().append([
transcript_df.query('event=="offer received"').head(),
transcript_df.query('event=="offer viewed"').head(),
transcript_df.query('event=="transaction"').head(),
transcript_df.query('event=="offer completed"').head(),
transcript_df.query('person=="78afa995795e4d85b5d9ceeca43f5fef"').head()]))
###Output
_____no_output_____
###Markdown
In the representative dataset above, we notice that:* *offer received* and *offer viewed* have an associated *offer id** *transaction* has an amount value that indicates how much the customer spent* *offer completed* is associated to a reward value* every event is informed with person and time* distinct events can occur to the same person at the same time (see registers 47582 and 47583)So that, we need to check if these statements are always true. Search for missing values
###Code
display(pd.DataFrame(
{'offer received':
transcript_df.query('event=="offer received"').notna().all(),
'offer viewed':
transcript_df.query('event=="offer viewed"').notna().all(),
'transaction':
transcript_df.query('event=="transaction"').notna().all(),
'offer completed':
transcript_df.query('event=="offer completed"').notna().all()
}).T.style.set_caption('Is there missing information?'))
display(pd.DataFrame(
{'offer received':
transcript_df.query('event=="offer received"').isna().all(),
'offer viewed':
transcript_df.query('event=="offer viewed"').isna().all(),
'transaction':
transcript_df.query('event=="transaction"').isna().all(),
'offer completed':
transcript_df.query('event=="offer completed"').isna().all()
}).T.style.set_caption('Is there event with extraneous information provided?'))
###Output
_____no_output_____
###Markdown
According to these verifications, there is no missing nor extraneous information in this dataset. Now, we neeed to verify if there is some inconsistent values in it. Explore events
###Code
cmap = plt.get_cmap("Set3")
colors = cmap(range(10))
## Plot the number of events that occurred.
display(pd.DataFrame(transcript_df.event.value_counts()).T)
ax = transcript_df.event.value_counts().plot.pie(colors=colors)
ax.set_ylabel('')
plt.show()
## Count offers received, viewed, and completed
offer_event = load_dataframe(portfolio_data_path).set_index('id')
offer_event = offer_event.assign(
received=pd.DataFrame(
transcript_df.query('event == "offer received"')
.offer_id.value_counts()))
offer_event = offer_event.assign(
viewed=pd.DataFrame(
transcript_df.query('event == "offer viewed"')
.offer_id.value_counts()))
offer_event = offer_event.assign(
completed=pd.DataFrame(
transcript_df.query('event == "offer completed"')
.offer_id.value_counts()))
display(offer_event)
# Plot
fig, axs = plt.subplots(figsize=(16,4), nrows=1, ncols=3)
offer_event.received.plot.pie(ax=axs[0], labels=None, colors=colors)
offer_event.viewed.plot.pie(ax=axs[1], labels=None, colors=colors)
offer_event.completed.plot.pie(ax=axs[2], labels=None, colors=colors)
axs[0].set_xlabel('offers')
axs[1].set_xlabel('offers')
axs[2].set_xlabel('offers')
plt.show()
## Group events by reward, difficulty, and duration
fig, axs = plt.subplots(figsize=(16,10), nrows=3, ncols=3)
offer_event = offer_event.query('offer_type != "informational"')
event_by_reward = offer_event.groupby('reward').sum()
event_by_reward.received.plot.pie(ax=axs[0][0], colors=colors)
event_by_reward.viewed.plot.pie(ax=axs[1][0], colors=colors)
event_by_reward.completed.plot.pie(ax=axs[2][0], colors=colors)
axs[0][0].set_xlabel('reward')
axs[1][0].set_xlabel('reward')
axs[2][0].set_xlabel('reward')
event_by_difficulty = offer_event.groupby('difficulty').sum()
event_by_difficulty.received.plot.pie(ax=axs[0][1], colors=colors)
event_by_difficulty.viewed.plot.pie(ax=axs[1][1], colors=colors)
event_by_difficulty.completed.plot.pie(ax=axs[2][1], colors=colors)
axs[0][1].set_xlabel('difficulty')
axs[1][1].set_xlabel('difficulty')
axs[2][1].set_xlabel('difficulty')
event_by_duration = offer_event.groupby('duration').sum()
event_by_duration.received.plot.pie(ax=axs[0][2], colors=colors)
event_by_duration.viewed.plot.pie(ax=axs[1][2], colors=colors)
event_by_duration.completed.plot.pie(ax=axs[2][2], colors=colors)
axs[0][2].set_xlabel('duration')
axs[1][2].set_xlabel('duration')
axs[2][2].set_xlabel('duration')
plt.show()
###Output
_____no_output_____
###Markdown
There is no anomaly detected in the data related to events. Besides that, we note a correlation between views and channels. Not surprisingly, the more channels used to deliver the offer, the better. Additionally, using social media seems to be the most effective channel. Furthermore, there is some correlation between offer completion and features reward, difficulty, and duration. However, it seems more related to the number of offers received. Explore Transaction
###Code
amount_desc = pd.DataFrame(transcript_df.amount.describe()).style \
.set_caption('Total') \
.set_table_attributes('style="display:inline;' \
'vertical-align:top"')
transcript_by_person = transcript_df.groupby('person')
amount_count_desc = pd.DataFrame(transcript_by_person.amount.count().describe()).style \
.set_caption('Count by person') \
.set_table_attributes('style="display:inline;' \
'vertical-align:top"')
amount_count_sum = pd.DataFrame(transcript_by_person.amount.sum().describe()).style \
.set_caption('Sum by person') \
.set_table_attributes('style="display:inline;' \
'vertical-align:top"')
display_html(
'<center>' \
+ amount_desc._repr_html_() \
+ ' ' \
+ amount_count_desc._repr_html_() \
+ ' ' \
+ amount_count_sum._repr_html_() \
+ '</center>',
raw=True)
###Output
_____no_output_____
###Markdown
These description tables show us some interesting points. * There are customers who did not make one single purchase in the period* There are anomalous values in the amount feature No purchase
###Code
customers_no_transaction = transcript_by_person.count() \
.query('amount == 0').index
print('Customers who did not make a transaction in this period:',
customers_no_transaction.nunique())
## Offers sent to them
offer_received = transcript_df.query(
'person in @customers_no_transaction ' \
'and event == "offer received"') \
.groupby('person') \
.count()
boxplot_rec_fig = Figure(figsize=(4,4))
ax_rec = boxplot_rec_fig.subplots()
offer_received_desc = offer_received.event.describe()
offer_received_desc = pd.DataFrame(offer_received_desc) \
.style.set_caption('Offers received') \
.set_table_attributes('style="display:inline;' \
'vertical-align:top"')
offer_received.event.plot.box(ax=ax_rec)
ax_rec.set_xticklabels(labels=['Offer received'])
## Offers viewed by them
offer_viewed = transcript_df.query(
'person in @customers_no_transaction ' \
'and event == "offer viewed"') \
.groupby('person') \
.count()
boxplot_view_fig = Figure(figsize=(4,4))
ax_view = boxplot_view_fig.subplots()
offer_viewed_desc = offer_viewed.event.describe()
offer_viewed_desc = pd.DataFrame(offer_viewed_desc) \
.style.set_caption('Offers viewed') \
.set_table_attributes('style="display:inline;' \
'vertical-align:top"')
offer_viewed.event.plot.box(ax=ax_view)
ax_view.set_xticklabels(labels=['Offer viewed'])
display_html('<center>' \
+ offer_received_desc._repr_html_() \
+ convert_fig_to_html(boxplot_rec_fig) \
+ offer_viewed_desc._repr_html_() \
+ convert_fig_to_html(boxplot_view_fig) \
+ '</center>',
raw=True)
###Output
Customers who did not make a transaction in this period: 422
###Markdown
There are 422 customers who did not make transactions in this period, even though they received and viewed offers. Admittedly, these customers may be analyzed in order to verify why they did not buy anything in the studied period. It is possible that they are opposed to marketing campaigns. Perhaps, they should be filtered out of the dataset. Anyways, this is a point to be aware and analyze while training and evaluating the networks. Anomalous values
###Code
display(pd.DataFrame(transcript_df.amount.describe()).T)
fig, axs = plt.subplots(figsize=(16,6), nrows=1, ncols=2)
transcript_df.amount.plot.box(ax=axs[0], vert=False)
axs[0].set_yticklabels('')
transcript_df.amount.plot.density(ax=axs[1])
plt.show()
###Output
_____no_output_____
###Markdown
Anomalous values in transactions are so high that they deform completely the plots above.
###Code
fig, axs = plt.subplots(figsize=(16,4), nrows=1, ncols=3)
n_users = []
values = range(40,1100)
for v in values:
n_users.append(transcript_df[transcript_df.amount > v] \
.person.duplicated().sum())
axs[0].plot(values, n_users)
axs[0].set_title('range: \$40 - \$1100')
axs[0].set_xlabel('amount ($)')
axs[0].set_ylabel('customers')
n_users = []
values = range(45,100)
for v in values:
n_users.append(transcript_df[transcript_df.amount > v] \
.person.duplicated().sum())
axs[1].plot(values, n_users)
axs[1].set_title('range: \$45 - \$100')
axs[1].set_xlabel('amount ($)')
axs[1].set_ylabel('customers')
n_users = []
values = range(45,55)
for v in values:
n_users.append(transcript_df[transcript_df.amount > v] \
.person.duplicated().sum())
axs[2].plot(values, n_users)
axs[2].set_title('range: \$45 - \$55')
axs[2].set_xlabel('amount ($)')
axs[2].set_ylabel('customers')
plt.show()
print('Number of customers with more than 1 transaction with a value >= $50,00:',
transcript_df[transcript_df.amount >= 50].person.duplicated().sum())
print('Number of customers with transactions with a value >= $50,00:',
transcript_df[transcript_df.amount >= 50].person.nunique())
fig, axs = plt.subplots(figsize=(16,4), nrows=1, ncols=3)
transcript_df.amount.clip(lower=0.05, upper=50).plot.box(ax=axs[0], vert=False)
axs[0].set_yticklabels('')
transcript_df.amount.clip(lower=0.05, upper=50).plot.density(ax=axs[1])
bins = 100
logbins = np.logspace(np.log10(transcript_df.amount.min().min()),
np.log10(transcript_df.amount.max().max()),
num=bins)
transcript_df.amount.clip(0.05, 50).hist(bins=logbins, ax=axs[2])
axs[2].set_ylabel('transactions')
axs[2].set_xscale('log')
axs[2].set_xlabel('log scale')
axs[2].grid(False)
plt.show()
###Output
_____no_output_____ |
TEMA-1/Clase8_SimulacionMontecarlo.ipynb | ###Markdown
Simulación Montecarlo> El método de Montecarlo es un método no determinista o estadístico numérico, usado para aproximar expresiones matemáticas complejas y costosas de evaluar con exactitud. El método se llamó así en referencia al Casino de Montecarlo (Mónaco) por ser “la capital del juego de azar”, al ser la ruleta un generador simple de números aleatorios. El nombre y el desarrollo sistemático de los métodos de Montecarlo datan aproximadamente de 1944 y se mejoraron enormemente con el desarrollo de la computadora.Referencia:- https://es.wikipedia.org/wiki/M%C3%A9todo_de_Montecarlo ___ 1. Introducción- Inventado por Stanislaw Ulam y a John von Neumann. Ulam ha explicado cómo se le ocurrió la idea mientras jugaba un solitario durante una enfermedad en 1946. - Advirtió que resulta mucho más simple tener una idea del resultado general del solitario haciendo pruebas múltiples con las cartas y contando las proporciones de los resultados que computar todas las posibilidades de combinación formalmente.- Se le ocurrió que esta misma observación debía aplicarse a su trabajo de Los Álamos sobre difusión de neutrones, para la cual resulta prácticamente imposible solucionar las ecuaciones íntegro-diferenciales que gobiernan la dispersión, la absorción y la fisión.- Dado que ya empezaban a estar disponibles máquinas de computación para efectuar las pruebas numéricas, el método cobró mucha fuerza.- El método de Montecarlo proporciona soluciones aproximadas a una gran variedad de problemas matemáticos posibilitando la realización de experimentos con muestreos de números pseudoaleatorios en una computadora. El método es aplicable a cualquier tipo de problema, ya sea estocástico o determinista. - El método de Montecarlo tiene un error absoluto de la estimación que decrece como $\frac{1}{\sqrt{N}}$ en virtud del teorema del límite central. EjemploTodos alguna vez hemos aplicado el método Montecarlo (inconscientemente). Como ejemplo, consideremos el juego de Astucia Naval.Normalmente, primero se realizan una serie de tiros a puntos aleatorios. Una vez se impacta en un barco, se puede utilizar un algoritmo determinista para identificar la posición del barco y así terminar de derrumbarlo. ___ 2. Caminata aleatoriaUna caminata aleatoria (*random walk* en inglés) es una formalización matemática de la trayectoria que resulta al hacer pasos sucesivos aleatorios. Un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y en cada paso se mueve $+1$ o $-1$ con igual probabilidad.Otros ejemplos:- Trayectoria de una molécula al viajar en un fluido (líquido o gas).- El camino que sigue un animal en su búsqueda de comida.- El precio fluctuante de una acción.- La situación de un apostador en un juego de azar.Todos pueden ser aproximados por caminatas aleatorias, aunque no sean en verdad procesos aleatorios. Caminata aleatoria en una dimensiónComo dijimos, un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y a cada paso se mueve $+1$ o $-1$ con igual probabilidad.Esta caminata se puede ilustrar como sigue:- Se posiciona en $0$ en la línea de números enteros y una moneda justa se tira.- Si cae en **sol** nos moveremos una unidad a la derecha.- Si cae en **águila** nos moveremos una unidad a la izquierda.Notemos que después de $5$ pasos podremos estar en 1, −1, 3, −3, 5, or −5. Las posibilidades son las siguientes:Referencia:- https://en.wikipedia.org/wiki/Random_walk**Importante:** librería random.Referencia:- https://docs.python.org/3/library/random.html Caminata aleatoria
###Code
from IPython.display import YouTubeVideo
YouTubeVideo('Y77WnkLbT2Q')
###Output
_____no_output_____
###Markdown
¿Qué es una simulación montecarlo?Revisitamos el concepto de simulación montecarlo.> La idea de una simulación montecarlo es probar muchos resultados posibles. En la realidad, solo uno de esos resultados posibles se dará, pero, en términos de evaluación de riesgos, cualquiera de las posibilidades podría ocurrir.Los simuladores montecarlo se usan usualmente para evaluar el riesgo de una estrategia de negocios dada con opciones y acciones.Los simuladores montecarlo pueden ayudar a tomar decisiones exitosas, y que el resultado de una decisión no sea la única medida de si dicha decisión fue buena. Las decisiones no deben ser evaluadas después del resultado. Por el contrario, los riesgos y beneficios solo deben ser considerados en el momento en que se debe tomar la decisión, sin prejuicios retrospectivos. Un **simulador montecarlo puede ayudar a visualizar muchos (o en algunos casos, todos) de los resultados potenciales** para tener una mejor idea de los riesgos de una decisión. Usamos montecarlo para evaluar el resultado de la caminata aleatoria- Ver el valor esperado de la caminata después de N pasos.- Luego, evaluar el proceso utilizando montecarlo y comparar resultados.
###Code
# Hacer código acá
import random
import numpy as np
import matplotlib.pyplot as plt
from functools import reduce
# Caminata aleatoria método convencional
# Crear una función que te regrese todo el recorrido de una caminata aleatoria empezando desde 0
def randon_walk(N):
return x
# Caminata aleatoria vectorizada
def randon_walk_vect(N):
return x
# Usando el método ineficiente
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
print('En promedio el caminante esta en :',np.mean(final))
# Usando el método vectorizado
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
x0 = 0 # Condición inicial
# np.asmatrix
###Output
_____no_output_____
###Markdown
EjemploAhora analicemos el ejemplo básico del apostador.Referencia:- https://pythonprogramming.net/monte-carlo-simulator-python/Supongamos que estamos en un casino especial, donde el usuario puede tirar un *dado metafórico* que puede dar como resultado un número del uno (1) al número cien (100).Si el usuario tira cualquier número entre 1 y 50, el casino gana. Si el usuario tira cualquier número entre 51 y 99, el usuario gana. Si el usuario tira 100, pierde.Con esto, el casino mantiene un margen del 1%, el cual es mucho más pequeño que el margen típico en casinos, al igual que el margen de mercado cuando se incorporan costos por transacción.Por ejemplo, [Scottrade](https://www.scottrade.com/) cobra $\$7$ USD por transacción. Si se invierten $\$1000$ USD por acción, esto significa que tienes que pagar $\$7$ USD para entrar, y $\$7$ USD para salir, para un total de $\$14$ USD.Esto pone el margen en $1.4\%$ . Esto significa, que a largo plazo, las ganancias tienen que ser mayores a $1.4\%$ en promedio, de otra manera se estará perdiendo dinero. De nuevo, con nuestro ejemplo en mente, 1-50, la casa gana. 51-99 el usuario gana. Un 100 significa que la casa gana.Ahora, comencemos. Primero tenemos que crear nuestro dado.
###Code
# Crear una función para que devuelva simplemente ganar(true) o perder(false)
def dado():
x = np.random.randint(1,101)
if 0<x<=50 or x == 100:
return False
else:
return True
dado()
# Probar la función creada para ver que funcione
N = 1000000
print("Ganamos", contador_ganar, " veces y perdimos", contador_perder, " veces.")
###Output
Ganamos 490461 veces y perdimos 509539 veces.
###Markdown
Ahora, necesitamos crear un **apostador**. Empezaremos con uno extremadamente básico por ahora. Veremos, que aún con un apostador muy básico, veremos cosas muy reveladoras usando un simulador montecarlo.
###Code
# Comando para interactuar con las gráficas
# %matplotlib notebook
# Comando para no interactuar mas con las gráficas
%matplotlib inline
###Output
_____no_output_____
###Markdown
Forma ineficiente
###Code
# Crearemos un apostador simple MÉTODO CONVENCIONAL
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador2(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
capital = cap_inicial
c_capital = [capital]
for i in range(n_apuestas-1):
if dado():
capital += apuesta
c_capital.append(capital)
else:
capital -= apuesta
ccapital.append(capital)
return ccapital
###Output
_____no_output_____
###Markdown
Forma eficiente
###Code
# Crearemos un apostador simple VECTORIZADOA ----- FUNCIÓN ANIDADA
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador_nested_f(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
return c_capital
apostador(10000, 100, 10)
# Crearemos un apostador simple VECTORIZADO
# Proponer otra forma de obtener el mismo resultado (CUMSUM)
def apostador_vect(cap_inicial, apuesta, n_apuestas):
return
###Output
_____no_output_____
###Markdown
1. Probar tu función `apostador2`
###Code
# Ver como evolucionan los fondos de nuestro apostador al jugar 100 veces
# %matplotlib inline
ccapital = apostador2(10000, 100, 1000)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
El capital luego del juego fue 8900
###Markdown
2. Probar tu función `apostador_nested_f`
###Code
ccapital = apostador_nested_f(10000, 100, 1000)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
El capital luego del juego fue 8700
###Markdown
3. Probar tu función `apostador_vect`
###Code
ccapital = apostador2(10000, 100, 1000)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
_____no_output_____
###Markdown
Ahora vamos a simular 100 escenarios en donde cada apuesta es 100, 50, 100, 1000 y 10000 veces. ¿Qué pasa?
###Code
# (Montecarlo)
n = [50,100,1000,10000]
capital = 10000
apuesta = 100
# Deseo correr la función creada para cada elemento de la lista n,
# donde el capital es siempre 10000 y la apuesta es 100.
# Luego quiero graficar el comportamiento de mi capital cuando juego
# Graficar histograma para ver el comportamiento del de los fondos para 1000 juegos distintos
plt.hist(resul[3],10)
plt.show()
print(np.mean(resultado[3]))
###Output
_____no_output_____
###Markdown
Simulación Montecarlo> El método de Montecarlo es un método no determinista o estadístico numérico, usado para aproximar expresiones matemáticas complejas y costosas de evaluar con exactitud. El método se llamó así en referencia al Casino de Montecarlo (Mónaco) por ser “la capital del juego de azar”, al ser la ruleta un generador simple de números aleatorios. El nombre y el desarrollo sistemático de los métodos de Montecarlo datan aproximadamente de 1944 y se mejoraron enormemente con el desarrollo de la computadora.Referencia:- https://es.wikipedia.org/wiki/M%C3%A9todo_de_Montecarlo ___ 1. Introducción- Inventado por Stanislaw Ulam y a John von Neumann. Ulam ha explicado cómo se le ocurrió la idea mientras jugaba un solitario durante una enfermedad en 1946. - Advirtió que resulta mucho más simple tener una idea del resultado general del solitario haciendo pruebas múltiples con las cartas y contando las proporciones de los resultados que computar todas las posibilidades de combinación formalmente.- Se le ocurrió que esta misma observación debía aplicarse a su trabajo de Los Álamos sobre difusión de neutrones, para la cual resulta prácticamente imposible solucionar las ecuaciones íntegro-diferenciales que gobiernan la dispersión, la absorción y la fisión.- Dado que ya empezaban a estar disponibles máquinas de computación para efectuar las pruebas numéricas, el método cobró mucha fuerza.- El método de Montecarlo proporciona soluciones aproximadas a una gran variedad de problemas matemáticos posibilitando la realización de experimentos con muestreos de números pseudoaleatorios en una computadora. El método es aplicable a cualquier tipo de problema, ya sea estocástico o determinista. - El método de Montecarlo tiene un error absoluto de la estimación que decrece como $\frac{1}{\sqrt{N}}$ en virtud del teorema del límite central. EjemploTodos alguna vez hemos aplicado el método Montecarlo (inconscientemente). Como ejemplo, consideremos el juego de Astucia Naval.Normalmente, primero se realizan una serie de tiros a puntos aleatorios. Una vez se impacta en un barco, se puede utilizar un algoritmo determinista para identificar la posición del barco y así terminar de derrumbarlo. ___ 2. Caminata aleatoriaUna caminata aleatoria (*random walk* en inglés) es una formalización matemática de la trayectoria que resulta al hacer pasos sucesivos aleatorios. Un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y en cada paso se mueve $+1$ o $-1$ con igual probabilidad.Otros ejemplos:- Trayectoria de una molécula al viajar en un fluido (líquido o gas).- El camino que sigue un animal en su búsqueda de comida.- El precio fluctuante de una acción.- La situación de un apostador en un juego de azar.Todos pueden ser aproximados por caminatas aleatorias, aunque no sean en verdad procesos aleatorios. Caminata aleatoria en una dimensiónComo dijimos, un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y a cada paso se mueve $+1$ o $-1$ con igual probabilidad.Esta caminata se puede ilustrar como sigue:- Se posiciona en $0$ en la línea de números enteros y una moneda justa se tira.- Si cae en **sol** nos moveremos una unidad a la derecha.- Si cae en **águila** nos moveremos una unidad a la izquierda.Notemos que después de $5$ pasos podremos estar en 1, −1, 3, −3, 5, or −5. Las posibilidades son las siguientes:Referencia:- https://en.wikipedia.org/wiki/Random_walk**Importante:** librería random.Referencia:- https://docs.python.org/3/library/random.html Caminata aleatoria
###Code
from IPython.display import YouTubeVideo
YouTubeVideo('Y77WnkLbT2Q')
###Output
_____no_output_____
###Markdown
¿Qué es una simulación montecarlo?Revisitamos el concepto de simulación montecarlo.> La idea de una simulación montecarlo es probar muchos resultados posibles. En la realidad, solo uno de esos resultados posibles se dará, pero, en términos de evaluación de riesgos, cualquiera de las posibilidades podría ocurrir.Los simuladores montecarlo se usan usualmente para evaluar el riesgo de una estrategia de negocios dada con opciones y acciones.Los simuladores montecarlo pueden ayudar a tomar decisiones exitosas, y que el resultado de una decisión no sea la única medida de si dicha decisión fue buena. Las decisiones no deben ser evaluadas después del resultado. Por el contrario, los riesgos y beneficios solo deben ser considerados en el momento en que se debe tomar la decisión, sin prejuicios retrospectivos. Un **simulador montecarlo puede ayudar a visualizar muchos (o en algunos casos, todos) de los resultados potenciales** para tener una mejor idea de los riesgos de una decisión. Usamos montecarlo para evaluar el resultado de la caminata aleatoria- Ver el valor esperado de la caminata después de N pasos.- Luego, evaluar el proceso utilizando montecarlo y comparar resultados.
###Code
# Hacer código acá
import random
import numpy as np
import matplotlib.pyplot as plt
from functools import reduce
# Caminata aleatoria método convencional
# Crear una función que te regrese todo el recorrido de una caminata aleatoria empezando desde 0
def randon_walk(N):
# np.random.seed(123)
x0 = 0
x = [x0]
for i in range(N):
x0 += np.random.choice([1, -1])
x.append(x0)
return x
plt.plot(randon_walk(1000))
# Caminata aleatoria vectorizada
def randon_walk_vect(N):
# np.random.seed(123)
x = np.random.choice([1, -1], N)
x[0] = 0
return x.cumsum()
plt.plot(randon_walk_vect(1000))
# Usando el método ineficiente
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
tray1 = np.asmatrix([randon_walk(N) for i in range(n)])
plt.plot(tray1.T);
# print('En promedio el caminante esta en :',np.mean(final))
# Usando el método vectorizado
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
x0 = 0 # Condición inicial
tray2 = np.asmatrix([randon_walk_vect(N) for i in range(n)])
plt.plot(tray2.T);
###Output
_____no_output_____
###Markdown
EjemploAhora analicemos el ejemplo básico del apostador.Referencia:- https://pythonprogramming.net/monte-carlo-simulator-python/Supongamos que estamos en un casino especial, donde el usuario puede tirar un *dado metafórico* que puede dar como resultado un número del uno (1) al número cien (100).Si el usuario tira cualquier número entre 1 y 50, el casino gana. Si el usuario tira cualquier número entre 51 y 99, el usuario gana. Si el usuario tira 100, pierde.Con esto, el casino mantiene un margen del 1%, el cual es mucho más pequeño que el margen típico en casinos, al igual que el margen de mercado cuando se incorporan costos por transacción.Por ejemplo, [Scottrade](https://www.scottrade.com/) cobra $\$7$ USD por transacción. Si se invierten $\$1000$ USD por acción, esto significa que tienes que pagar $\$7$ USD para entrar, y $\$7$ USD para salir, para un total de $\$14$ USD.Esto pone el margen en $1.4\%$ . Esto significa, que a largo plazo, las ganancias tienen que ser mayores a $1.4\%$ en promedio, de otra manera se estará perdiendo dinero. De nuevo, con nuestro ejemplo en mente, 1-50, la casa gana. 51-99 el usuario gana. Un 100 significa que la casa gana.Ahora, comencemos. Primero tenemos que crear nuestro dado.
###Code
# Crear una función para que devuelva simplemente ganar(true) o perder(false)
def dado():
x = np.random.randint(1,101)
if 0<x<=50 or x == 100:
return False
else:
return True
dado()
# Probar la función creada para ver que funcione
N = 10000
contador_ganar = sum(list(map(lambda x: dado(), range(N))))
contador_perder = N - contador_ganar
print("Ganamos", contador_ganar, " veces y perdimos", contador_perder, " veces.")
contador_ganar / N
###Output
Ganamos 4895 veces y perdimos 5105 veces.
###Markdown
Ahora, necesitamos crear un **apostador**. Empezaremos con uno extremadamente básico por ahora. Veremos, que aún con un apostador muy básico, veremos cosas muy reveladoras usando un simulador montecarlo.
###Code
# Comando para interactuar con las gráficas
# %matplotlib notebook
# Comando para no interactuar mas con las gráficas
%matplotlib inline
###Output
_____no_output_____
###Markdown
Forma ineficiente
###Code
# Crearemos un apostador simple MÉTODO CONVENCIONAL
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador2(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
c_capital = [cap_inicial]
capital = cap_inicial
for i in range(n_apuestas):
if dado():
capital += apuesta
else:
capital -= apuesta
c_capital.append(capital)
return c_capital
apostador2(10000, 100, 10)
###Output
_____no_output_____
###Markdown
Forma eficiente
###Code
# Crearemos un apostador simple VECTORIZADOA ----- FUNCIÓN ANIDADA
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador_nested_f(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
c_capital = np.zeros(n_apuestas + 1, dtype=int)
c_capital[0] = cap_inicial
def fill_vect(i, apuesta_i):
nonlocal c_capital
c_capital[i] = c_capital[i - 1] + apuesta_i
[fill_vect(i, apuesta) if dado() else fill_vect(i, -apuesta)
for i in range(1, n_apuestas + 1)]
return c_capital
apostador_nested_f(10000, 100, 10)
# Crearemos un apostador simple VECTORIZADO
# Proponer otra forma de obtener el mismo resultado (CUMSUM)
def apostador_vect(cap_inicial, apuesta, n_apuestas):
# np.random.seed(516)
c_capital = apuesta * np.array([1 if dado() else -1 for i in range(n_apuestas + 1)])
c_capital[0] = cap_inicial
return c_capital.cumsum()
apostador_nested_f(10000, 100, 10)
import time
###Output
_____no_output_____
###Markdown
1. Probar tu función `apostador2`
###Code
%%time
# Ver como evolucionan los fondos de nuestro apostador al jugar 100 veces
# %matplotlib inline
N = int(1e6)
ccapital = apostador2(10000, 100, N)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
El capital al final del juego fue -1883400
###Markdown
2. Probar tu función `apostador_nested_f`
###Code
%%time
ccapital = apostador_nested_f(10000, 100, N)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
El capital al final del juego fue -1883400
###Markdown
3. Probar tu función `apostador_vect`
###Code
%%time
ccapital = apostador_vect(10000, 100, N)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
El capital al final del juego fue -1803600
###Markdown
Ahora vamos a simular 100 escenarios en donde cada apuesta es 100, 50, 100, 1000 y 10000 veces. ¿Qué pasa?
###Code
# (Montecarlo)
n = np.array([50,100,1000,10000]) * 10
capital = 10000
apuesta = 100
# Deseo correr la función creada para cada elemento de la lista n,
# donde el capital es siempre 10000 y la apuesta es 100.
# Luego quiero graficar el comportamiento de mi capital cuando juego
def graph(ni):
cap = apostador_vect(capital, apuesta, ni)
plt.plot(cap, label=f'se apostó {ni} veces')
plt.legend()
plt.show()
[graph(ni) for ni in n]
# Graficar histograma para ver el comportamiento del de los fondos para 1000 juegos distintos
plt.hist(cap,10)
plt.show()
print(np.mean(cap))
###Output
_____no_output_____
###Markdown
Simulación Montecarlo> El método de Montecarlo es un método no determinista o estadístico numérico, usado para aproximar expresiones matemáticas complejas y costosas de evaluar con exactitud. El método se llamó así en referencia al Casino de Montecarlo (Mónaco) por ser “la capital del juego de azar”, al ser la ruleta un generador simple de números aleatorios. El nombre y el desarrollo sistemático de los métodos de Montecarlo datan aproximadamente de 1944 y se mejoraron enormemente con el desarrollo de la computadora.Referencia:- https://es.wikipedia.org/wiki/M%C3%A9todo_de_Montecarlo ___ 1. Introducción- Inventado por Stanislaw Ulam y a John von Neumann. Ulam ha explicado cómo se le ocurrió la idea mientras jugaba un solitario durante una enfermedad en 1946. - Advirtió que resulta mucho más simple tener una idea del resultado general del solitario haciendo pruebas múltiples con las cartas y contando las proporciones de los resultados que computar todas las posibilidades de combinación formalmente.- Se le ocurrió que esta misma observación debía aplicarse a su trabajo de Los Álamos sobre difusión de neutrones, para la cual resulta prácticamente imposible solucionar las ecuaciones íntegro-diferenciales que gobiernan la dispersión, la absorción y la fisión.- Dado que ya empezaban a estar disponibles máquinas de computación para efectuar las pruebas numéricas, el método cobró mucha fuerza.- El método de Montecarlo proporciona soluciones aproximadas a una gran variedad de problemas matemáticos posibilitando la realización de experimentos con muestreos de números pseudoaleatorios en una computadora. El método es aplicable a cualquier tipo de problema, ya sea estocástico o determinista. - El método de Montecarlo tiene un error absoluto de la estimación que decrece como $\frac{1}{\sqrt{N}}$ en virtud del teorema del límite central. EjemploTodos alguna vez hemos aplicado el método Montecarlo (inconscientemente). Como ejemplo, consideremos el juego de Astucia Naval.Normalmente, primero se realizan una serie de tiros a puntos aleatorios. Una vez se impacta en un barco, se puede utilizar un algoritmo determinista para identificar la posición del barco y así terminar de derrumbarlo. ___ 2. Caminata aleatoriaUna caminata aleatoria (*random walk* en inglés) es una formalización matemática de la trayectoria que resulta al hacer pasos sucesivos aleatorios. Un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y en cada paso se mueve $+1$ o $-1$ con igual probabilidad.Otros ejemplos:- Trayectoria de una molécula al viajar en un fluido (líquido o gas).- El camino que sigue un animal en su búsqueda de comida.- El precio fluctuante de una acción.- La situación de un apostador en un juego de azar.Todos pueden ser aproximados por caminatas aleatorias, aunque no sean en verdad procesos aleatorios. Caminata aleatoria en una dimensiónComo dijimos, un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y a cada paso se mueve $+1$ o $-1$ con igual probabilidad.Esta caminata se puede ilustrar como sigue:- Se posiciona en $0$ en la línea de números enteros y una moneda justa se tira.- Si cae en **sol** nos moveremos una unidad a la derecha.- Si cae en **águila** nos moveremos una unidad a la izquierda.Notemos que después de $5$ pasos podremos estar en 1, −1, 3, −3, 5, or −5. Las posibilidades son las siguientes:Referencia:- https://en.wikipedia.org/wiki/Random_walk**Importante:** librería random.Referencia:- https://docs.python.org/3/library/random.html Caminata aleatoria
###Code
from IPython.display import YouTubeVideo
YouTubeVideo('Y77WnkLbT2Q')
###Output
_____no_output_____
###Markdown
¿Qué es una simulación montecarlo?Revisitamos el concepto de simulación montecarlo.> La idea de una simulación montecarlo es probar muchos resultados posibles. En la realidad, solo uno de esos resultados posibles se dará, pero, en términos de evaluación de riesgos, cualquiera de las posibilidades podría ocurrir.Los simuladores montecarlo se usan usualmente para evaluar el riesgo de una estrategia de negocios dada con opciones y acciones.Los simuladores montecarlo pueden ayudar a tomar decisiones exitosas, y que el resultado de una decisión no sea la única medida de si dicha decisión fue buena. Las decisiones no deben ser evaluadas después del resultado. Por el contrario, los riesgos y beneficios solo deben ser considerados en el momento en que se debe tomar la decisión, sin prejuicios retrospectivos. Un **simulador montecarlo puede ayudar a visualizar muchos (o en algunos casos, todos) de los resultados potenciales** para tener una mejor idea de los riesgos de una decisión. Usamos montecarlo para evaluar el resultado de la caminata aleatoria- Ver el valor esperado de la caminata después de N pasos.- Luego, evaluar el proceso utilizando montecarlo y comparar resultados.
###Code
# Hacer código acá
import random
import numpy as np
import matplotlib.pyplot as plt
from functools import reduce
# Caminata aleatoria método convencional
# Crear una función que te regrese todo el recorrido de una caminata aleatoria empezando desde 0
def randon_walk(N):
x0 = 0
x = [x0]
for i in range(N - 1):
rnd = np.random.choice([1, -1])
x.append(x[-1] + rnd)
return x
randon_walk(10)
rnd = np.random.choice([1, -1],5)
rnd
# Caminata aleatoria vectorizada
def randon_walk_vect(N):
x0 = 0
x = np.random.choice([1, -1], N)
x[0] = x0
return x.cumsum()
randon_walk_vect(10)
# Usando el método ineficiente
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
np.random.seed(2)
tray1 = np.array([randon_walk(N) for i in range(n)])
plt.plot(tray1.T);
#print('En promedio el caminante esta en :',np.mean(final))
# Usando el método vectorizado
np.random.seed(2)
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
x0 = 0 # Condición inicial
# np.asmatrix
tray2 = np.array([randon_walk_vect(N) for i in range(n)])
plt.plot(tray2.T);
###Output
_____no_output_____
###Markdown
EjemploAhora analicemos el ejemplo básico del apostador.Referencia:- https://pythonprogramming.net/monte-carlo-simulator-python/Supongamos que estamos en un casino especial, donde el usuario puede tirar un *dado metafórico* que puede dar como resultado un número del uno (1) al número cien (100).Si el usuario tira cualquier número entre 1 y 50, el casino gana. Si el usuario tira cualquier número entre 51 y 99, el usuario gana. Si el usuario tira 100, pierde.Con esto, el casino mantiene un margen del 1%, el cual es mucho más pequeño que el margen típico en casinos, al igual que el margen de mercado cuando se incorporan costos por transacción.Por ejemplo, [Scottrade](https://www.scottrade.com/) cobra $\$7$ USD por transacción. Si se invierten $\$1000$ USD por acción, esto significa que tienes que pagar $\$7$ USD para entrar, y $\$7$ USD para salir, para un total de $\$14$ USD.Esto pone el margen en $1.4\%$ . Esto significa, que a largo plazo, las ganancias tienen que ser mayores a $1.4\%$ en promedio, de otra manera se estará perdiendo dinero. De nuevo, con nuestro ejemplo en mente, 1-50, la casa gana. 51-99 el usuario gana. Un 100 significa que la casa gana.Ahora, comencemos. Primero tenemos que crear nuestro dado.
###Code
# Crear una función para que devuelva simplemente ganar(true) o perder(false)
def dado():
x = np.random.randint(1,101)
if 0<x<=50 or x == 100:
return False
else:
return True
sum([True, False, True])
# Probar la función creada para ver que funcione
N = 1000000
juegos = [dado() for i in range(N)]
contador_ganar = sum(juegos)
contador_perder = N - contador_ganar
print("Ganamos", contador_ganar, " veces y perdimos", contador_perder, " veces.")
print('Probabilidad de ganar', contador_ganar / N)
###Output
Ganamos 490152 veces y perdimos 509848 veces.
Probabilidad de ganar 0.490152
###Markdown
Ahora, necesitamos crear un **apostador**. Empezaremos con uno extremadamente básico por ahora. Veremos, que aún con un apostador muy básico, veremos cosas muy reveladoras usando un simulador montecarlo.
###Code
# Comando para interactuar con las gráficas
# %matplotlib notebook
# Comando para no interactuar mas con las gráficas
%matplotlib inline
###Output
_____no_output_____
###Markdown
Forma ineficiente
###Code
# Crearemos un apostador simple MÉTODO CONVENCIONAL
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador2(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
capital = cap_inicial
c_capital = [capital]
for i in range(n_apuestas-1):
if dado():
capital += apuesta
c_capital.append(capital)
else:
capital -= apuesta
c_capital.append(capital)
return c_capital
###Output
_____no_output_____
###Markdown
Forma eficiente
###Code
# Crearemos un apostador simple VECTORIZADOA ----- FUNCIÓN ANIDADA
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador_nested_f(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
c_capital = np.zeros(n_apuestas)
c_capital[0] = cap_inicial
def llenar_vector(i):
nonlocal c_capital
c_capital[i + 1] = c_capital[i] + apuesta if dado() else c_capital[i] - apuesta
[llenar_vector(i) for i in range(n_apuestas - 1)]
return c_capital
apostador_nested_f(10000, 100, 10)
# Crearemos un apostador simple VECTORIZADO
# Proponer otra forma de obtener el mismo resultado (CUMSUM)
def apostador_vect(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
c_capital = np.array([apuesta if dado() else -apuesta for i in range(n_apuestas)])
c_capital[0] = cap_inicial
return c_capital.cumsum()
apostador_vect(10000, 100, 10)
###Output
_____no_output_____
###Markdown
1. Probar tu función `apostador2`
###Code
# Ver como evolucionan los fondos de nuestro apostador al jugar 100 veces
# %matplotlib inline
ccapital = apostador2(10000, 100, 1000)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
El capital al final del juego fue 8900
###Markdown
2. Probar tu función `apostador_nested_f`
###Code
ccapital = apostador_nested_f(10000, 100, 1000)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
El capital al final del juego fue 8900.0
###Markdown
3. Probar tu función `apostador_vect`
###Code
ccapital = apostador_vect(10000, 100, 1000)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
El capital al final del juego fue 8700
###Markdown
Ahora vamos a simular 100 escenarios en donde cada apuesta es 100, 50, 100, 1000 y 10000 veces. ¿Qué pasa?
###Code
# (Montecarlo)
n = [50,100,1000,100000]
capital = 10000
apuesta = 100
# Deseo correr la función creada para cada elemento de la lista n,
# donde el capital es siempre 10000 y la apuesta es 100.
# Luego quiero graficar el comportamiento de mi capital cuando juego
def plot_dinero(n):
plt.plot(apostador_vect(10000, 100, n), label=f'aposté {n} veces')
plt.legend()
plt.show()
[plot_dinero(ni) for ni in n]
# Graficar histograma para ver el comportamiento del de los fondos para 1000 juegos distintos
plt.hist(resul[3],10)
plt.show()
print(np.mean(resultado[3]))
###Output
_____no_output_____
###Markdown
Simulación Montecarlo> El método de Montecarlo es un método no determinista o estadístico numérico, usado para aproximar expresiones matemáticas complejas y costosas de evaluar con exactitud. El método se llamó así en referencia al Casino de Montecarlo (Mónaco) por ser “la capital del juego de azar”, al ser la ruleta un generador simple de números aleatorios. El nombre y el desarrollo sistemático de los métodos de Montecarlo datan aproximadamente de 1944 y se mejoraron enormemente con el desarrollo de la computadora.Referencia:- https://es.wikipedia.org/wiki/M%C3%A9todo_de_Montecarlo ___ 1. Introducción- Inventado por Stanislaw Ulam y a John von Neumann. Ulam ha explicado cómo se le ocurrió la idea mientras jugaba un solitario durante una enfermedad en 1946. - Advirtió que resulta mucho más simple tener una idea del resultado general del solitario haciendo pruebas múltiples con las cartas y contando las proporciones de los resultados que computar todas las posibilidades de combinación formalmente.- Se le ocurrió que esta misma observación debía aplicarse a su trabajo de Los Álamos sobre difusión de neutrones, para la cual resulta prácticamente imposible solucionar las ecuaciones íntegro-diferenciales que gobiernan la dispersión, la absorción y la fisión.- Dado que ya empezaban a estar disponibles máquinas de computación para efectuar las pruebas numéricas, el método cobró mucha fuerza.- El método de Montecarlo proporciona soluciones aproximadas a una gran variedad de problemas matemáticos posibilitando la realización de experimentos con muestreos de números pseudoaleatorios en una computadora. El método es aplicable a cualquier tipo de problema, ya sea estocástico o determinista. - El método de Montecarlo tiene un error absoluto de la estimación que decrece como $\frac{1}{\sqrt{N}}$ en virtud del teorema del límite central. EjemploTodos alguna vez hemos aplicado el método Montecarlo (inconscientemente). Como ejemplo, consideremos el juego de Astucia Naval.Normalmente, primero se realizan una serie de tiros a puntos aleatorios. Una vez se impacta en un barco, se puede utilizar un algoritmo determinista para identificar la posición del barco y así terminar de derrumbarlo. ___ 2. Caminata aleatoriaUna caminata aleatoria (*random walk* en inglés) es una formalización matemática de la trayectoria que resulta al hacer pasos sucesivos aleatorios. Un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y en cada paso se mueve $+1$ o $-1$ con igual probabilidad.Otros ejemplos:- Trayectoria de una molécula al viajar en un fluido (líquido o gas).- El camino que sigue un animal en su búsqueda de comida.- El precio fluctuante de una acción.- La situación de un apostador en un juego de azar.Todos pueden ser aproximados por caminatas aleatorias, aunque no sean en verdad procesos aleatorios. Caminata aleatoria en una dimensiónComo dijimos, un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y a cada paso se mueve $+1$ o $-1$ con igual probabilidad.Esta caminata se puede ilustrar como sigue:- Se posiciona en $0$ en la línea de números enteros y una moneda justa se tira.- Si cae en **sol** nos moveremos una unidad a la derecha.- Si cae en **águila** nos moveremos una unidad a la izquierda.Notemos que después de $5$ pasos podremos estar en 1, −1, 3, −3, 5, or −5. Las posibilidades son las siguientes:Referencia:- https://en.wikipedia.org/wiki/Random_walk**Importante:** librería random.Referencia:- https://docs.python.org/3/library/random.html Caminata aleatoria
###Code
from IPython.display import YouTubeVideo
YouTubeVideo('Y77WnkLbT2Q')
###Output
_____no_output_____
###Markdown
¿Qué es una simulación montecarlo?Revisitamos el concepto de simulación montecarlo.> La idea de una simulación montecarlo es probar muchos resultados posibles. En la realidad, solo uno de esos resultados posibles se dará, pero, en términos de evaluación de riesgos, cualquiera de las posibilidades podría ocurrir.Los simuladores montecarlo se usan usualmente para evaluar el riesgo de una estrategia de negocios dada con opciones y acciones.Los simuladores montecarlo pueden ayudar a tomar decisiones exitosas, y que el resultado de una decisión no sea la única medida de si dicha decisión fue buena. Las decisiones no deben ser evaluadas después del resultado. Por el contrario, los riesgos y beneficios solo deben ser considerados en el momento en que se debe tomar la decisión, sin prejuicios retrospectivos. Un **simulador montecarlo puede ayudar a visualizar muchos (o en algunos casos, todos) de los resultados potenciales** para tener una mejor idea de los riesgos de una decisión. Usamos montecarlo para evaluar el resultado de la caminata aleatoria- Ver el valor esperado de la caminata después de N pasos.- Luego, evaluar el proceso utilizando montecarlo y comparar resultados.
###Code
# Hacer código acá
import random
import numpy as np
import matplotlib.pyplot as plt
from functools import reduce
# Caminata aleatoria método convencional
# Crear una función que te regrese todo el recorrido de una caminata aleatoria empezando desde 0
def randon_walk(N):
# np.random.seed(123)
x0 = 0
x = [x0]
for i in range(N):
x0 += np.random.choice([1, -1])
x.append(x0)
return x
plt.plot(randon_walk(1000))
# Caminata aleatoria vectorizada
def randon_walk_vect(N):
# np.random.seed(123)
x = np.random.choice([1, -1], N)
x[0] = 0
return x.cumsum()
plt.plot(randon_walk_vect(1000))
# Usando el método ineficiente
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
tray1 = np.asmatrix([randon_walk(N) for i in range(n)])
plt.plot(tray1.T);
# print('En promedio el caminante esta en :',np.mean(final))
# Usando el método vectorizado
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
x0 = 0 # Condición inicial
tray2 = np.asmatrix([randon_walk_vect(N) for i in range(n)])
plt.plot(tray2.T);
###Output
_____no_output_____
###Markdown
EjemploAhora analicemos el ejemplo básico del apostador.Referencia:- https://pythonprogramming.net/monte-carlo-simulator-python/Supongamos que estamos en un casino especial, donde el usuario puede tirar un *dado metafórico* que puede dar como resultado un número del uno (1) al número cien (100).Si el usuario tira cualquier número entre 1 y 50, el casino gana. Si el usuario tira cualquier número entre 51 y 99, el usuario gana. Si el usuario tira 100, pierde.Con esto, el casino mantiene un margen del 1%, el cual es mucho más pequeño que el margen típico en casinos, al igual que el margen de mercado cuando se incorporan costos por transacción.Por ejemplo, [Scottrade](https://www.scottrade.com/) cobra $\$7$ USD por transacción. Si se invierten $\$1000$ USD por acción, esto significa que tienes que pagar $\$7$ USD para entrar, y $\$7$ USD para salir, para un total de $\$14$ USD.Esto pone el margen en $1.4\%$ . Esto significa, que a largo plazo, las ganancias tienen que ser mayores a $1.4\%$ en promedio, de otra manera se estará perdiendo dinero. De nuevo, con nuestro ejemplo en mente, 1-50, la casa gana. 51-99 el usuario gana. Un 100 significa que la casa gana.Ahora, comencemos. Primero tenemos que crear nuestro dado.
###Code
# Crear una función para que devuelva simplemente ganar(true) o perder(false)
def dado():
x = np.random.randint(1,101)
if 0<x<=50 or x == 100:
return False
else:
return True
dado()
# Probar la función creada para ver que funcione
N = 10000
contador_ganar = sum(list(map(lambda x: dado(), range(N))))
contador_perder = N - contador_ganar
print("Ganamos", contador_ganar, " veces y perdimos", contador_perder, " veces.")
contador_ganar / N
###Output
Ganamos 4895 veces y perdimos 5105 veces.
###Markdown
Ahora, necesitamos crear un **apostador**. Empezaremos con uno extremadamente básico por ahora. Veremos, que aún con un apostador muy básico, veremos cosas muy reveladoras usando un simulador montecarlo.
###Code
# Comando para interactuar con las gráficas
# %matplotlib notebook
# Comando para no interactuar mas con las gráficas
%matplotlib inline
###Output
_____no_output_____
###Markdown
Forma ineficiente
###Code
# Crearemos un apostador simple MÉTODO CONVENCIONAL
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador2(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
c_capital = [cap_inicial]
capital = cap_inicial
for i in range(n_apuestas):
if dado():
capital += apuesta
else:
capital -= apuesta
c_capital.append(capital)
return c_capital
apostador2(10000, 100, 10)
###Output
_____no_output_____
###Markdown
Forma eficiente
###Code
# Crearemos un apostador simple VECTORIZADOA ----- FUNCIÓN ANIDADA
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador_nested_f(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
c_capital = np.zeros(n_apuestas + 1, dtype=int)
c_capital[0] = cap_inicial
def fill_vect(i, apuesta_i):
nonlocal c_capital
c_capital[i] = c_capital[i - 1] + apuesta_i
[fill_vect(i, apuesta) if dado() else fill_vect(i, -apuesta)
for i in range(1, n_apuestas + 1)]
return c_capital
apostador_nested_f(10000, 100, 10)
# Crearemos un apostador simple VECTORIZADO
# Proponer otra forma de obtener el mismo resultado (CUMSUM)
def apostador_vect(cap_inicial, apuesta, n_apuestas):
# np.random.seed(516)
c_capital = apuesta * np.array([1 if dado() else -1 for i in range(n_apuestas + 1)])
c_capital[0] = cap_inicial
return c_capital.cumsum()
apostador_nested_f(10000, 100, 10)
import time
###Output
_____no_output_____
###Markdown
1. Probar tu función `apostador2`
###Code
%%time
# Ver como evolucionan los fondos de nuestro apostador al jugar 100 veces
# %matplotlib inline
N = int(1e6)
ccapital = apostador2(10000, 100, N)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
El capital al final del juego fue -1883400
###Markdown
2. Probar tu función `apostador_nested_f`
###Code
%%time
ccapital = apostador_nested_f(10000, 100, N)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
El capital al final del juego fue -1883400
###Markdown
3. Probar tu función `apostador_vect`
###Code
%%time
ccapital = apostador_vect(10000, 100, N)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
El capital al final del juego fue -1803600
###Markdown
Ahora vamos a simular 100 escenarios en donde cada apuesta es 100, 50, 100, 1000 y 10000 veces. ¿Qué pasa?
###Code
# (Montecarlo)
n = np.array([50,100,1000,10000]) * 10
capital = 10000
apuesta = 100
# Deseo correr la función creada para cada elemento de la lista n,
# donde el capital es siempre 10000 y la apuesta es 100.
# Luego quiero graficar el comportamiento de mi capital cuando juego
def graph(ni):
cap = apostador_vect(capital, apuesta, ni)
plt.plot(cap, label=f'se apostó {ni} veces')
plt.legend()
plt.show()
[graph(ni) for ni in n]
# Graficar histograma para ver el comportamiento del de los fondos para 1000 juegos distintos
plt.hist(cap,10)
plt.show()
print(np.mean(cap))
###Output
_____no_output_____
###Markdown
Simulación Montecarlo> El método de Montecarlo es un método no determinista o estadístico numérico, usado para aproximar expresiones matemáticas complejas y costosas de evaluar con exactitud. El método se llamó así en referencia al Casino de Montecarlo (Mónaco) por ser “la capital del juego de azar”, al ser la ruleta un generador simple de números aleatorios. El nombre y el desarrollo sistemático de los métodos de Montecarlo datan aproximadamente de 1944 y se mejoraron enormemente con el desarrollo de la computadora.Referencia:- https://es.wikipedia.org/wiki/M%C3%A9todo_de_Montecarlo ___ 1. Introducción- Inventado por Stanislaw Ulam y a John von Neumann. Ulam ha explicado cómo se le ocurrió la idea mientras jugaba un solitario durante una enfermedad en 1946. - Advirtió que resulta mucho más simple tener una idea del resultado general del solitario haciendo pruebas múltiples con las cartas y contando las proporciones de los resultados que computar todas las posibilidades de combinación formalmente.- Se le ocurrió que esta misma observación debía aplicarse a su trabajo de Los Álamos sobre difusión de neutrones, para la cual resulta prácticamente imposible solucionar las ecuaciones íntegro-diferenciales que gobiernan la dispersión, la absorción y la fisión.- Dado que ya empezaban a estar disponibles máquinas de computación para efectuar las pruebas numéricas, el método cobró mucha fuerza.- El método de Montecarlo proporciona soluciones aproximadas a una gran variedad de problemas matemáticos posibilitando la realización de experimentos con muestreos de números pseudoaleatorios en una computadora. El método es aplicable a cualquier tipo de problema, ya sea estocástico o determinista. - El método de Montecarlo tiene un error absoluto de la estimación que decrece como $\frac{1}{\sqrt{N}}$ en virtud del teorema del límite central. EjemploTodos alguna vez hemos aplicado el método Montecarlo (inconscientemente). Como ejemplo, consideremos el juego de Astucia Naval.Normalmente, primero se realizan una serie de tiros a puntos aleatorios. Una vez se impacta en un barco, se puede utilizar un algoritmo determinista para identificar la posición del barco y así terminar de derrumbarlo. ___ 2. Caminata aleatoriaUna caminata aleatoria (*random walk* en inglés) es una formalización matemática de la trayectoria que resulta al hacer pasos sucesivos aleatorios. Un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y en cada paso se mueve $+1$ o $-1$ con igual probabilidad.Otros ejemplos:- Trayectoria de una molécula al viajar en un fluido (líquido o gas).- El camino que sigue un animal en su búsqueda de comida.- El precio fluctuante de una acción.- La situación de un apostador en un juego de azar.Todos pueden ser aproximados por caminatas aleatorias, aunque no sean en verdad procesos aleatorios. Caminata aleatoria en una dimensiónComo dijimos, un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y a cada paso se mueve $+1$ o $-1$ con igual probabilidad.Esta caminata se puede ilustrar como sigue:- Se posiciona en $0$ en la línea de números enteros y una moneda justa se tira.- Si cae en **sol** nos moveremos una unidad a la derecha.- Si cae en **águila** nos moveremos una unidad a la izquierda.Notemos que después de $5$ pasos podremos estar en 1, −1, 3, −3, 5, or −5. Las posibilidades son las siguientes:Referencia:- https://en.wikipedia.org/wiki/Random_walk**Importante:** librería random.Referencia:- https://docs.python.org/3/library/random.html Caminata aleatoria
###Code
from IPython.display import YouTubeVideo
YouTubeVideo('Y77WnkLbT2Q')
###Output
_____no_output_____
###Markdown
¿Qué es una simulación montecarlo?Revisitamos el concepto de simulación montecarlo.> La idea de una simulación montecarlo es probar muchos resultados posibles. En la realidad, solo uno de esos resultados posibles se dará, pero, en términos de evaluación de riesgos, cualquiera de las posibilidades podría ocurrir.Los simuladores montecarlo se usan usualmente para evaluar el riesgo de una estrategia de negocios dada con opciones y acciones.Los simuladores montecarlo pueden ayudar a tomar decisiones exitosas, y que el resultado de una decisión no sea la única medida de si dicha decisión fue buena. Las decisiones no deben ser evaluadas después del resultado. Por el contrario, los riesgos y beneficios solo deben ser considerados en el momento en que se debe tomar la decisión, sin prejuicios retrospectivos. Un **simulador montecarlo puede ayudar a visualizar muchos (o en algunos casos, todos) de los resultados potenciales** para tener una mejor idea de los riesgos de una decisión. Usamos montecarlo para evaluar el resultado de la caminata aleatoria- Ver el valor esperado de la caminata después de N pasos.- Luego, evaluar el proceso utilizando montecarlo y comparar resultados.
###Code
# Hacer código acá
import random
import numpy as np
import matplotlib.pyplot as plt
from functools import reduce
# Caminata aleatorio método convencional
def randon_walk(N):
x = 0
xx = [x]
add_el = xx.append
for i in range(N):
z = random.choice([-1,1])
x += z
add_el(x)
return xx,x
# Caminata aleatoria vectorizada
def randon_walk2(N):
x = 0
z = np.random.choice([-1,1],N)
z[0] = x
x = np.cumsum(z)
return x
N = 1000
x = np.array(list(map(lambda i: randon_walk2(N), range(8)))) # Lambda por que queremos iterar la funcion randon walk 8 veces
plt.plot(x.T);
# Usando el método ineficiente
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
final = []
# %matplotlib inline
for j in range(n):
xx,x = randon_walk(N)
final.append(x)
plt.plot(xx)
plt.show()
print('En promedio el caminante esta en :',np.mean(final))
# Usando el método vectorizado
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
x0 = 0 # Condición inicial
x2 = np.asmatrix([randon_walk2(N) for i in range(n)])
x2.shape
plt.plot(x2.T)
plt.show()
# np.mean(x2[:,-1])
###Output
_____no_output_____
###Markdown
EjemploAhora analicemos el ejemplo básico del apostador.Referencia:- https://pythonprogramming.net/monte-carlo-simulator-python/Supongamos que estamos en un casino especial, donde el usuario puede tirar un *dado metafórico* que puede dar como resultado un número del uno (1) al número cien (100).Si el usuario tira cualquier número entre 1 y 50, el casino gana. Si el usuario tira cualquier número entre 51 y 99, el usuario gana. Si el usuario tira 100, pierde.Con esto, el casino mantiene un margen del 1%, el cual es mucho más pequeño que el margen típico en casinos, al igual que el margen de mercado cuando se incorporan costos por transacción.Por ejemplo, [Scottrade](https://www.scottrade.com/) cobra $\$7$ USD por transacción. Si se invierten $\$1000$ USD por acción, esto significa que tienes que pagar $\$7$ USD para entrar, y $\$7$ USD para salir, para un total de $\$14$ USD.Esto pone el margen en $1.4\%$ . Esto significa, que a largo plazo, las ganancias tienen que ser mayores a $1.4\%$ en promedio, de otra manera se estará perdiendo dinero. De nuevo, con nuestro ejemplo en mente, 1-50, la casa gana. 51-99 el usuario gana. Un 100 significa que la casa gana.Ahora, comencemos. Primero tenemos que crear nuestro dado.
###Code
N = 10000000
sum([dado() for i in range(N)])/N
# Crear una función para que devuelva simplemente ganar(true) o perder(false)
def dado():
x = np.random.randint(1,101)
if 0<x<=50 or x == 100:
return False
else:
return True
dado()
# Probar la función creada para ver que funcione
N = 1000000
resultado = [1 if dado() else 0 for i in range(N)]
contador_ganar = sum(resultado)
contador_perder = N-contador_ganar
# resultado
print("Ganamos", contador_ganar, " veces y perdimos", contador_perder, " veces.")
###Output
Ganamos 490461 veces y perdimos 509539 veces.
###Markdown
Ahora, necesitamos crear un **apostador**. Empezaremos con uno extremadamente básico por ahora. Veremos, que aún con un apostador muy básico, veremos cosas muy reveladoras usando un simulador montecarlo.
###Code
# Comando para interactuar con las gráficas
# %matplotlib notebook
# Comando para no interactuar mas con las gráficas
%matplotlib inline
###Output
_____no_output_____
###Markdown
Forma ineficiente
###Code
# Crearemos un apostador simple MÉTODO CONVENCIONAL
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador2(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
capital = cap_inicial
ccapital = [capital]
for i in range(n_apuestas-1):
if dado():
capital += apuesta
ccapital.append(capital)
else:
capital -= apuesta
ccapital.append(capital)
return ccapital#,capital
ccapital = apostador2(10000, 100, 1000)
plt.plot(ccapital);
###Output
_____no_output_____
###Markdown
Forma eficiente
###Code
# Crearemos un apostador simple VECTORIZADOA ----- FUNCIÓN ANIDADA
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador_vect1(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
ccapital = np.zeros(n_apuestas)
ccapital[0] = cap_inicial
def llenar_vect(i):
nonlocal ccapital
if dado():
ccapital[i + 1] = apuesta + ccapital[i]
else:
ccapital[i + 1] = -apuesta + ccapital[i]
[llenar_vect(i) for i in range(n_apuestas-1)]
return ccapital
ccapital = apostador_vect1(10000, 100, 1000)
plt.plot(ccapital);
# Crearemos un apostador simple VECTORIZADO
# Proponer otra forma de obtener el mismo resultado (CUMSUM)
def apostador_vect2(cap_inicial, apuesta, n_apuestas):
# np.random.seed(516)
resultado = np.array([1 if dado() else -1 for i in range(n_apuestas)]) * apuesta
resultado[0] = cap_inicial
return resultado.cumsum()
n = [50, 100, 1000, 100000]
ccapital = list(map(lambda ni: apostador_vect2(10000, 100, ni), n))
# plt.plot(ccapital);
for i in range(len(n)):
plt.plot(ccapital[i],label = f'con n={n[i]}')
plt.legend()
plt.show()
# Ver como evolucionan los fondos de nuestro apostador al jugar 100 veces
# %matplotlib inline
ccapital = apostador2(10000, 100, 1000)
print('El capital luego del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
ccapital = apostador(10000, 100, 1000)
print('El capital luego del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
# (Montecarlo) Simular 100 escenarios en donde cada apuesta es (100)
# 50, 100, 1000 y 10000 veces. ¿Qué pasa?
n = [50,100,1000,100000]
capital = 10000
apuesta = 100
# Deseo correr la función creada para cada elemento de la lista n,
# donde el capital es siempre 10000 y la apuesta es 100.
# Luego quiero graficar el comportamiento de mi capital cuando juego
# n = [50,100,1000,10000]
resul = np.array([apostador_vect2(capital,apuesta,N_apuesta) for N_apuesta in n])
for i in range(4):
plt.plot(resul[i],label = 'se corrio %i veces'% n[i])
plt.legend()
plt.show()
# Graficar histograma para ver el comportamiento del de los fondos para 1000 juegos distintos
plt.hist(resul[3],10)
plt.show()
print(np.mean(resul[3]))
###Output
_____no_output_____
###Markdown
Simulación Montecarlo> El método de Montecarlo es un método no determinista o estadístico numérico, usado para aproximar expresiones matemáticas complejas y costosas de evaluar con exactitud. El método se llamó así en referencia al Casino de Montecarlo (Mónaco) por ser “la capital del juego de azar”, al ser la ruleta un generador simple de números aleatorios. El nombre y el desarrollo sistemático de los métodos de Montecarlo datan aproximadamente de 1944 y se mejoraron enormemente con el desarrollo de la computadora.Referencia:- https://es.wikipedia.org/wiki/M%C3%A9todo_de_Montecarlo ___ 1. Introducción- Inventado por Stanislaw Ulam y a John von Neumann. Ulam ha explicado cómo se le ocurrió la idea mientras jugaba un solitario durante una enfermedad en 1946. - Advirtió que resulta mucho más simple tener una idea del resultado general del solitario haciendo pruebas múltiples con las cartas y contando las proporciones de los resultados que computar todas las posibilidades de combinación formalmente.- Se le ocurrió que esta misma observación debía aplicarse a su trabajo de Los Álamos sobre difusión de neutrones, para la cual resulta prácticamente imposible solucionar las ecuaciones íntegro-diferenciales que gobiernan la dispersión, la absorción y la fisión.- Dado que ya empezaban a estar disponibles máquinas de computación para efectuar las pruebas numéricas, el método cobró mucha fuerza.- El método de Montecarlo proporciona soluciones aproximadas a una gran variedad de problemas matemáticos posibilitando la realización de experimentos con muestreos de números pseudoaleatorios en una computadora. El método es aplicable a cualquier tipo de problema, ya sea estocástico o determinista. - El método de Montecarlo tiene un error absoluto de la estimación que decrece como $\frac{1}{\sqrt{N}}$ en virtud del teorema del límite central. EjemploTodos alguna vez hemos aplicado el método Montecarlo (inconscientemente). Como ejemplo, consideremos el juego de Astucia Naval.Normalmente, primero se realizan una serie de tiros a puntos aleatorios. Una vez se impacta en un barco, se puede utilizar un algoritmo determinista para identificar la posición del barco y así terminar de derrumbarlo. ___ 2. Caminata aleatoriaUna caminata aleatoria (*random walk* en inglés) es una formalización matemática de la trayectoria que resulta al hacer pasos sucesivos aleatorios. Un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y en cada paso se mueve $+1$ o $-1$ con igual probabilidad.Otros ejemplos:- Trayectoria de una molécula al viajar en un fluido (líquido o gas).- El camino que sigue un animal en su búsqueda de comida.- El precio fluctuante de una acción.- La situación de un apostador en un juego de azar.Todos pueden ser aproximados por caminatas aleatorias, aunque no sean en verdad procesos aleatorios. Caminata aleatoria en una dimensiónComo dijimos, un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y a cada paso se mueve $+1$ o $-1$ con igual probabilidad.Esta caminata se puede ilustrar como sigue:- Se posiciona en $0$ en la línea de números enteros y una moneda justa se tira.- Si cae en **sol** nos moveremos una unidad a la derecha.- Si cae en **águila** nos moveremos una unidad a la izquierda.Notemos que después de $5$ pasos podremos estar en 1, −1, 3, −3, 5, or −5. Las posibilidades son las siguientes:Referencia:- https://en.wikipedia.org/wiki/Random_walk**Importante:** librería random.Referencia:- https://docs.python.org/3/library/random.html Caminata aleatoria
###Code
from IPython.display import YouTubeVideo
YouTubeVideo('Y77WnkLbT2Q')
###Output
_____no_output_____
###Markdown
¿Qué es una simulación montecarlo?Revisitamos el concepto de simulación montecarlo.> La idea de una simulación montecarlo es probar muchos resultados posibles. En la realidad, solo uno de esos resultados posibles se dará, pero, en términos de evaluación de riesgos, cualquiera de las posibilidades podría ocurrir.Los simuladores montecarlo se usan usualmente para evaluar el riesgo de una estrategia de negocios dada con opciones y acciones.Los simuladores montecarlo pueden ayudar a tomar decisiones exitosas, y que el resultado de una decisión no sea la única medida de si dicha decisión fue buena. Las decisiones no deben ser evaluadas después del resultado. Por el contrario, los riesgos y beneficios solo deben ser considerados en el momento en que se debe tomar la decisión, sin prejuicios retrospectivos. Un **simulador montecarlo puede ayudar a visualizar muchos (o en algunos casos, todos) de los resultados potenciales** para tener una mejor idea de los riesgos de una decisión. Usamos montecarlo para evaluar el resultado de la caminata aleatoria- Ver el valor esperado de la caminata después de N pasos.- Luego, evaluar el proceso utilizando montecarlo y comparar resultados.
###Code
# Hacer código acá
import random
import numpy as np
import matplotlib.pyplot as plt
from functools import reduce
# Caminata aleatorio método convencional
def randon_walk(N):
x = 0
xx = [x]
add_el = xx.append
for i in range(N):
z = random.choice([-1,1])
x += z
add_el(x)
return xx,x
# Caminata aleatoria vectorizada
def randon_walk2(N):
x = 0
z = np.random.choice([-1,1],N)
z[0] = x
x = np.cumsum(z)
return x
N = 1000
x = np.array(list(map(lambda i: randon_walk2(N), range(10))))
# plt.plot(x.T);
# Usando el método ineficiente
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
final = []
# %matplotlib inline
for j in range(n):
xx,x = randon_walk(N)
final.append(x)
plt.plot(xx)
plt.show()
print('En promedio el caminante esta en :',np.mean(final))
# Usando el método vectorizado
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
x0 = 0 # Condición inicial
x2 = np.asmatrix([randon_walk2(N) for i in range(n)])
x2.shape
plt.plot(x2.T)
plt.show()
np.mean(x2[:,-1])
###Output
_____no_output_____
###Markdown
EjemploAhora analicemos el ejemplo básico del apostador.Referencia:- https://pythonprogramming.net/monte-carlo-simulator-python/Supongamos que estamos en un casino especial, donde el usuario puede tirar un *dado metafórico* que puede dar como resultado un número del uno (1) al número cien (100).Si el usuario tira cualquier número entre 1 y 50, el casino gana. Si el usuario tira cualquier número entre 51 y 99, el usuario gana. Si el usuario tira 100, pierde.Con esto, el casino mantiene un margen del 1%, el cual es mucho más pequeño que el margen típico en casinos, al igual que el margen de mercado cuando se incorporan costos por transacción.Por ejemplo, [Scottrade](https://www.scottrade.com/) cobra $\$7$ USD por transacción. Si se invierten $\$1000$ USD por acción, esto significa que tienes que pagar $\$7$ USD para entrar, y $\$7$ USD para salir, para un total de $\$14$ USD.Esto pone el margen en $1.4\%$ . Esto significa, que a largo plazo, las ganancias tienen que ser mayores a $1.4\%$ en promedio, de otra manera se estará perdiendo dinero. De nuevo, con nuestro ejemplo en mente, 1-50, la casa gana. 51-99 el usuario gana. Un 100 significa que la casa gana.Ahora, comencemos. Primero tenemos que crear nuestro dado.
###Code
# Crear una función para que devuelva simplemente ganar(true) o perder(false)
def dado():
x = np.random.randint(1,101)
if 0<x<=50 or x == 100:
return False
else:
return True
dado()
# Probar la función creada para ver que funcione
N = 1000000
resultado = [1 if dado() else 0 for i in range(N)]
contador_ganar = sum(resultado)
contador_perder = N-contador_ganar
# resultado
print("Ganamos", contador_ganar, " veces y perdimos", contador_perder, " veces.")
###Output
Ganamos 490227 veces y perdimos 509773 veces.
###Markdown
Ahora, necesitamos crear un **apostador**. Empezaremos con uno extremadamente básico por ahora. Veremos, que aún con un apostador muy básico, veremos cosas muy reveladoras usando un simulador montecarlo.
###Code
# Comando para interactuar con las gráficas
# %matplotlib notebook
# Comando para no interactuar mas con las gráficas
%matplotlib inline
###Output
_____no_output_____
###Markdown
Forma ineficiente
###Code
# Crearemos un apostador simple MÉTODO CONVENCIONAL
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador2(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
capital = cap_inicial
ccapital = [capital]
for i in range(n_apuestas-1):
if dado():
capital += apuesta
ccapital.append(capital)
else:
capital -= apuesta
ccapital.append(capital)
return ccapital#,capital
###Output
_____no_output_____
###Markdown
Forma eficiente
###Code
# Crearemos un apostador simple VECTORIZADOA ----- FUNCIÓN ANIDADA
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
ccapital = np.zeros(n_apuestas)
ccapital[0] = cap_inicial
def llenado(i):
nonlocal ccapital
if dado():
ccapital[i] = ccapital[i-1] + apuesta
else:
ccapital[i] = ccapital[i-1] - apuesta
[llenado(i) for i in range(1, n_apuestas)]
return ccapital
ccapital = apostador(10000, 100, 1000)
plt.plot(ccapital);
# Crearemos un apostador simple VECTORIZADO
# Proponer otra forma de obtener el mismo resultado (CUMSUM)
def apostador_vec2(cap_inicial, apuesta, n_apuestas):
# np.random.seed(516)
ccapital = np.array([1 if dado() else -1 for i in range(n_apuestas)]) * apuesta
ccapital[0] = cap_inicial
return ccapital.cumsum()
ccapital = apostador_vec2(10000, 100, 1000)
plt.plot(ccapital);
# Ver como evolucionan los fondos de nuestro apostador al jugar 100 veces
# %matplotlib inline
ccapital = apostador2(10000, 100, 1000)
print('El capital luego del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
ccapital = apostador(10000, 100, 1000)
print('El capital luego del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
# (Montecarlo) Simular 100 escenarios en donde cada apuesta es (100)
# 50, 100, 1000 y 10000 veces. ¿Qué pasa?
n = [50,100,1000,100000]
capital = 10000
apuesta = 100
# Deseo correr la función creada para cada elemento de la lista n,
# donde el capital es siempre 10000 y la apuesta es 100.
# Luego quiero graficar el comportamiento de mi capital cuando juego
# n = [50,100,1000,10000]
resul = [apostador_vec2(capital,apuesta,N_apuesta) for N_apuesta in n]
for i in range(4):
plt.plot(resul[i],label = 'se corrio %i veces'% n[i])
plt.legend()
plt.show()
# Graficar histograma para ver el comportamiento del de los fondos para 1000 juegos distintos
plt.hist(resul[3],10)
plt.show()
print(np.mean(resultado[3]))
###Output
_____no_output_____
###Markdown
Simulación Montecarlo> El método de Montecarlo es un método no determinista o estadístico numérico, usado para aproximar expresiones matemáticas complejas y costosas de evaluar con exactitud. El método se llamó así en referencia al Casino de Montecarlo (Mónaco) por ser “la capital del juego de azar”, al ser la ruleta un generador simple de números aleatorios. El nombre y el desarrollo sistemático de los métodos de Montecarlo datan aproximadamente de 1944 y se mejoraron enormemente con el desarrollo de la computadora.Referencia:- https://es.wikipedia.org/wiki/M%C3%A9todo_de_Montecarlo ___ 1. Introducción- Inventado por Stanislaw Ulam y a John von Neumann. Ulam ha explicado cómo se le ocurrió la idea mientras jugaba un solitario durante una enfermedad en 1946. - Advirtió que resulta mucho más simple tener una idea del resultado general del solitario haciendo pruebas múltiples con las cartas y contando las proporciones de los resultados que computar todas las posibilidades de combinación formalmente.- Se le ocurrió que esta misma observación debía aplicarse a su trabajo de Los Álamos sobre difusión de neutrones, para la cual resulta prácticamente imposible solucionar las ecuaciones íntegro-diferenciales que gobiernan la dispersión, la absorción y la fisión.- Dado que ya empezaban a estar disponibles máquinas de computación para efectuar las pruebas numéricas, el método cobró mucha fuerza.- El método de Montecarlo proporciona soluciones aproximadas a una gran variedad de problemas matemáticos posibilitando la realización de experimentos con muestreos de números pseudoaleatorios en una computadora. El método es aplicable a cualquier tipo de problema, ya sea estocástico o determinista. - El método de Montecarlo tiene un error absoluto de la estimación que decrece como $\frac{1}{\sqrt{N}}$ en virtud del teorema del límite central. EjemploTodos alguna vez hemos aplicado el método Montecarlo (inconscientemente). Como ejemplo, consideremos el juego de Astucia Naval.Normalmente, primero se realizan una serie de tiros a puntos aleatorios. Una vez se impacta en un barco, se puede utilizar un algoritmo determinista para identificar la posición del barco y así terminar de derrumbarlo. ___ 2. Caminata aleatoriaUna caminata aleatoria (*random walk* en inglés) es una formalización matemática de la trayectoria que resulta al hacer pasos sucesivos aleatorios. Un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y en cada paso se mueve $+1$ o $-1$ con igual probabilidad.Otros ejemplos:- Trayectoria de una molécula al viajar en un fluido (líquido o gas).- El camino que sigue un animal en su búsqueda de comida.- El precio fluctuante de una acción.- La situación de un apostador en un juego de azar.Todos pueden ser aproximados por caminatas aleatorias, aunque no sean en verdad procesos aleatorios. Caminata aleatoria en una dimensiónComo dijimos, un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y a cada paso se mueve $+1$ o $-1$ con igual probabilidad.Esta caminata se puede ilustrar como sigue:- Se posiciona en $0$ en la línea de números enteros y una moneda justa se tira.- Si cae en **sol** nos moveremos una unidad a la derecha.- Si cae en **águila** nos moveremos una unidad a la izquierda.Notemos que después de $5$ pasos podremos estar en 1, −1, 3, −3, 5, or −5. Las posibilidades son las siguientes:Referencia:- https://en.wikipedia.org/wiki/Random_walk**Importante:** librería random.Referencia:- https://docs.python.org/3/library/random.html Caminata aleatoria
###Code
from IPython.display import YouTubeVideo
YouTubeVideo('Y77WnkLbT2Q')
###Output
_____no_output_____
###Markdown
¿Qué es una simulación montecarlo?Revisitamos el concepto de simulación montecarlo.> La idea de una simulación montecarlo es probar muchos resultados posibles. En la realidad, solo uno de esos resultados posibles se dará, pero, en términos de evaluación de riesgos, cualquiera de las posibilidades podría ocurrir.Los simuladores montecarlo se usan usualmente para evaluar el riesgo de una estrategia de negocios dada con opciones y acciones.Los simuladores montecarlo pueden ayudar a tomar decisiones exitosas, y que el resultado de una decisión no sea la única medida de si dicha decisión fue buena. Las decisiones no deben ser evaluadas después del resultado. Por el contrario, los riesgos y beneficios solo deben ser considerados en el momento en que se debe tomar la decisión, sin prejuicios retrospectivos. Un **simulador montecarlo puede ayudar a visualizar muchos (o en algunos casos, todos) de los resultados potenciales** para tener una mejor idea de los riesgos de una decisión. Usamos montecarlo para evaluar el resultado de la caminata aleatoria- Ver el valor esperado de la caminata después de N pasos.- Luego, evaluar el proceso utilizando montecarlo y comparar resultados.
###Code
# Hacer código acá
import random
import numpy as np
import matplotlib.pyplot as plt
from functools import reduce
# Caminata aleatoria método convencional
# Crear una función que te regrese todo el recorrido de una caminata aleatoria empezando desde 0
def randon_walk(N):
x0 = 0
x = [x0]
for i in range(N):
rnd = np.random.choice([1, -1])
x.append(x[-1] + rnd)
return x
randon_walk(10)
rnd = np.random.choice([1, -1], 5)
rnd
# Caminata aleatoria vectorizada
def randon_walk_vect(N):
x0 = 0
x = np.random.choice([1, -1], N)
x[0] = x0
return x.cumsum()
randon_walk_vect(10)
# Usando el método ineficiente
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
np.random.seed(2)
tray1 = np.array([randon_walk(N) for i in range(n)])
plt.plot(tray1.T);
#print('En promedio el caminante esta en :',np.mean(final))
# Usando el método vectorizado
np.random.seed(2)
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
x0 = 0 # Condición inicial
# np.asmatrix
tray2 = np.array([randon_walk_vect(N) for i in range(n)])
plt.plot(tray2.T);
###Output
_____no_output_____
###Markdown
EjemploAhora analicemos el ejemplo básico del apostador.Referencia:- https://pythonprogramming.net/monte-carlo-simulator-python/Supongamos que estamos en un casino especial, donde el usuario puede tirar un *dado metafórico* que puede dar como resultado un número del uno (1) al número cien (100).Si el usuario tira cualquier número entre 1 y 50, el casino gana. Si el usuario tira cualquier número entre 51 y 99, el usuario gana. Si el usuario tira 100, pierde.Con esto, el casino mantiene un margen del 1%, el cual es mucho más pequeño que el margen típico en casinos, al igual que el margen de mercado cuando se incorporan costos por transacción.Por ejemplo, [Scottrade](https://www.scottrade.com/) cobra $\$7$ USD por transacción. Si se invierten $\$1000$ USD por acción, esto significa que tienes que pagar $\$7$ USD para entrar, y $\$7$ USD para salir, para un total de $\$14$ USD.Esto pone el margen en $1.4\%$ . Esto significa, que a largo plazo, las ganancias tienen que ser mayores a $1.4\%$ en promedio, de otra manera se estará perdiendo dinero. De nuevo, con nuestro ejemplo en mente, 1-50, la casa gana. 51-99 el usuario gana. Un 100 significa que la casa gana.Ahora, comencemos. Primero tenemos que crear nuestro dado.
###Code
# Crear una función para que devuelva simplemente ganar(true) o perder(false)
def dado():
x = np.random.randint(1,101)
if 0<x<=50 or x == 100:
return False
else:
return True
dado()
# Probar la función creada para ver que funcione
N = 1000000
juegos = [dado() for i in range(N)]
contador_ganar = sum(juegos)
contador_perder = N - contador_ganar
print("Ganamos", contador_ganar, " veces y perdimos", contador_perder, " veces.")
print("Probabilidad de ganar:", 100 * contador_ganar / N, "%")
###Output
Ganamos 489645 veces y perdimos 510355 veces.
Probabilidad de ganar: 48.9645 %
###Markdown
Ahora, necesitamos crear un **apostador**. Empezaremos con uno extremadamente básico por ahora. Veremos, que aún con un apostador muy básico, veremos cosas muy reveladoras usando un simulador montecarlo.
###Code
# Comando para interactuar con las gráficas
# %matplotlib notebook
# Comando para no interactuar mas con las gráficas
%matplotlib inline
###Output
_____no_output_____
###Markdown
Forma ineficiente
###Code
# Crearemos un apostador simple MÉTODO CONVENCIONAL
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador2(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
capital = cap_inicial
c_capital = [capital]
for i in range(n_apuestas-1):
if dado():
capital += apuesta
c_capital.append(capital)
else:
capital -= apuesta
c_capital.append(capital)
return c_capital
###Output
_____no_output_____
###Markdown
Forma eficiente
###Code
# Crearemos un apostador simple VECTORIZADOA ----- FUNCIÓN ANIDADA
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador_nested_f(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
c_capital = np.zeros(n_apuestas)
c_capital[0] = cap_inicial
def llenar_vector(i):
nonlocal c_capital
c_capital[i + 1] = c_capital[i] + apuesta if dado() else c_capital[i] - apuesta
[llenar_vector(i) for i in range(n_apuestas - 1)]
return c_capital
apostador_nested_f(10000, 100, 10)
# Crearemos un apostador simple VECTORIZADO
# Proponer otra forma de obtener el mismo resultado
#(CUMSUM)
def apostador_vect(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
c_capital = np.array([apuesta if dado() else -apuesta for i in range(n_apuestas)])
c_capital[0] = cap_inicial
return c_capital.cumsum()
apostador_vect(10000, 100, 10)
###Output
_____no_output_____
###Markdown
1. Probar tu función `apostador2`
###Code
# Ver como evolucionan los fondos de nuestro apostador al jugar 100 veces
# %matplotlib inline
c_capital = apostador2(10000, 100, 1000)
print('El capital al final del juego fue',c_capital[-1]);
plt.plot(c_capital)
plt.show()
###Output
El capital al final del juego fue 8900
###Markdown
2. Probar tu función `apostador_nested_f`
###Code
c_capital = apostador_nested_f(10000, 100, 1000)
print('El capital al final del juego fue',c_capital[-1]);
plt.plot(c_capital)
plt.show()
###Output
El capital al final del juego fue 8900.0
###Markdown
3. Probar tu función `apostador_vect`
###Code
c_capital = apostador_vect(10000, 100, 1000)
print('El capital al final del juego fue',c_capital[-1]);
plt.plot(c_capital)
plt.show()
###Output
El capital al final del juego fue 8700
###Markdown
Ahora vamos a simular 100 escenarios en donde cada apuesta es 100, 50, 100, 1000 y 10000 veces. ¿Qué pasa?
###Code
# (Montecarlo)
n = [50,100,1000,100000]
capital = 10000
apuesta = 100
# Deseo correr la función creada para cada elemento de la lista n,
# donde el capital es siempre 10000 y la apuesta es 100.
# Luego quiero graficar el comportamiento de mi capital cuando juego
def plot_dinero(n):
plt.plot(apostador_vect(1000,100,n), label=f'aposté {n} veces')
plt.legend()
plt.show()
[plot_dinero(ni) for ni in n]
# Graficar histograma para ver el comportamiento del de los fondos para 1000 juegos distintos
###Output
_____no_output_____
###Markdown
Simulación Montecarlo> El método de Montecarlo es un método no determinista o estadístico numérico, usado para aproximar expresiones matemáticas complejas y costosas de evaluar con exactitud. El método se llamó así en referencia al Casino de Montecarlo (Mónaco) por ser “la capital del juego de azar”, al ser la ruleta un generador simple de números aleatorios. El nombre y el desarrollo sistemático de los métodos de Montecarlo datan aproximadamente de 1944 y se mejoraron enormemente con el desarrollo de la computadora.Referencia:- https://es.wikipedia.org/wiki/M%C3%A9todo_de_Montecarlo ___ 1. Introducción- Inventado por Stanislaw Ulam y a John von Neumann. Ulam ha explicado cómo se le ocurrió la idea mientras jugaba un solitario durante una enfermedad en 1946. - Advirtió que resulta mucho más simple tener una idea del resultado general del solitario haciendo pruebas múltiples con las cartas y contando las proporciones de los resultados que computar todas las posibilidades de combinación formalmente.- Se le ocurrió que esta misma observación debía aplicarse a su trabajo de Los Álamos sobre difusión de neutrones, para la cual resulta prácticamente imposible solucionar las ecuaciones íntegro-diferenciales que gobiernan la dispersión, la absorción y la fisión.- Dado que ya empezaban a estar disponibles máquinas de computación para efectuar las pruebas numéricas, el método cobró mucha fuerza.- El método de Montecarlo proporciona soluciones aproximadas a una gran variedad de problemas matemáticos posibilitando la realización de experimentos con muestreos de números pseudoaleatorios en una computadora. El método es aplicable a cualquier tipo de problema, ya sea estocástico o determinista. - El método de Montecarlo tiene un error absoluto de la estimación que decrece como $\frac{1}{\sqrt{N}}$ en virtud del teorema del límite central. EjemploTodos alguna vez hemos aplicado el método Montecarlo (inconscientemente). Como ejemplo, consideremos el juego de Astucia Naval.Normalmente, primero se realizan una serie de tiros a puntos aleatorios. Una vez se impacta en un barco, se puede utilizar un algoritmo determinista para identificar la posición del barco y así terminar de derrumbarlo. ___ 2. Caminata aleatoriaUna caminata aleatoria (*random walk* en inglés) es una formalización matemática de la trayectoria que resulta al hacer pasos sucesivos aleatorios. Un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y en cada paso se mueve $+1$ o $-1$ con igual probabilidad.Otros ejemplos:- Trayectoria de una molécula al viajar en un fluido (líquido o gas).- El camino que sigue un animal en su búsqueda de comida.- El precio fluctuante de una acción.- La situación de un apostador en un juego de azar.Todos pueden ser aproximados por caminatas aleatorias, aunque no sean en verdad procesos aleatorios. Caminata aleatoria en una dimensiónComo dijimos, un ejemplo elemental de caminata aleatoria es la caminata aleatoria en la línea de números enteros $\mathbb{Z}$, la cual comienza en $0$ y a cada paso se mueve $+1$ o $-1$ con igual probabilidad.Esta caminata se puede ilustrar como sigue:- Se posiciona en $0$ en la línea de números enteros y una moneda justa se tira.- Si cae en **sol** nos moveremos una unidad a la derecha.- Si cae en **águila** nos moveremos una unidad a la izquierda.Notemos que después de $5$ pasos podremos estar en 1, −1, 3, −3, 5, or −5. Las posibilidades son las siguientes:Referencia:- https://en.wikipedia.org/wiki/Random_walk**Importante:** librería random.Referencia:- https://docs.python.org/3/library/random.html Caminata aleatoria
###Code
from IPython.display import YouTubeVideo
YouTubeVideo('Y77WnkLbT2Q')
###Output
_____no_output_____
###Markdown
¿Qué es una simulación montecarlo?Revisitamos el concepto de simulación montecarlo.> La idea de una simulación montecarlo es probar muchos resultados posibles. En la realidad, solo uno de esos resultados posibles se dará, pero, en términos de evaluación de riesgos, cualquiera de las posibilidades podría ocurrir.Los simuladores montecarlo se usan usualmente para evaluar el riesgo de una estrategia de negocios dada con opciones y acciones.Los simuladores montecarlo pueden ayudar a tomar decisiones exitosas, y que el resultado de una decisión no sea la única medida de si dicha decisión fue buena. Las decisiones no deben ser evaluadas después del resultado. Por el contrario, los riesgos y beneficios solo deben ser considerados en el momento en que se debe tomar la decisión, sin prejuicios retrospectivos. Un **simulador montecarlo puede ayudar a visualizar muchos (o en algunos casos, todos) de los resultados potenciales** para tener una mejor idea de los riesgos de una decisión. Usamos montecarlo para evaluar el resultado de la caminata aleatoria- Ver el valor esperado de la caminata después de N pasos.- Luego, evaluar el proceso utilizando montecarlo y comparar resultados.
###Code
# Hacer código acá
import random
import numpy as np
import matplotlib.pyplot as plt
from functools import reduce
# Caminata aleatoria método convencional
# Crear una función que te regrese todo el recorrido de una caminata aleatoria empezando desde 0
def randon_walk(N):
x = [0]
for i in range(N):
x.append(x[-1] + np.random.choice([1, -1]))
return x
plt.plot(randon_walk(100))
# Caminata aleatoria vectorizada
def randon_walk_vect(N):
x = np.zeros(N)
def fill_vector(i):
nonlocal x
x[i + 1] = x[i] + np.random.choice([1, -1])
[fill_vector(i) for i in range(N - 1)]
return x
plt.plot(randon_walk_vect(100))
def random_walk_optimal(N):
x = np.random.choice([1, -1], N)
x[0] = 0
return x.cumsum()
random_walk_optimal(100)
# Usando el método ineficiente
N = 1000 # número de pasos
n = 30000 # cantidad de trayectorias
x = np.array([random_walk_optimal(N) for i in range(n)])
# plt.plot(x.T);
x.mean(axis=0)[-1], x
# print('En promedio el caminante esta en :',np.mean(final))
# Usando el método vectorizado
N = 1000 # número de pasos
n = 10 # cantidad de trayectorias
x0 = 0 # Condición inicial
# np.asmatrix
###Output
_____no_output_____
###Markdown
EjemploAhora analicemos el ejemplo básico del apostador.Referencia:- https://pythonprogramming.net/monte-carlo-simulator-python/Supongamos que estamos en un casino especial, donde el usuario puede tirar un *dado metafórico* que puede dar como resultado un número del uno (1) al número cien (100).Si el usuario tira cualquier número entre 1 y 50, el casino gana. Si el usuario tira cualquier número entre 51 y 99, el usuario gana. Si el usuario tira 100, pierde.Con esto, el casino mantiene un margen del 1%, el cual es mucho más pequeño que el margen típico en casinos, al igual que el margen de mercado cuando se incorporan costos por transacción.Por ejemplo, [Scottrade](https://www.scottrade.com/) cobra $\$7$ USD por transacción. Si se invierten $\$1000$ USD por acción, esto significa que tienes que pagar $\$7$ USD para entrar, y $\$7$ USD para salir, para un total de $\$14$ USD.Esto pone el margen en $1.4\%$ . Esto significa, que a largo plazo, las ganancias tienen que ser mayores a $1.4\%$ en promedio, de otra manera se estará perdiendo dinero. De nuevo, con nuestro ejemplo en mente, 1-50, la casa gana. 51-99 el usuario gana. Un 100 significa que la casa gana.Ahora, comencemos. Primero tenemos que crear nuestro dado.
###Code
# Crear una función para que devuelva simplemente ganar(true) o perder(false)
def dado():
x = np.random.randint(1,101)
if 0<x<=50 or x == 100:
return False
else:
return True
dado()
# Probar la función creada para ver que funcione
N = 1000000
len([1 for i in range(N) if dado()]) / N
# print("Ganamos", contador_ganar, " veces y perdimos", contador_perder, " veces.")
###Output
_____no_output_____
###Markdown
Ahora, necesitamos crear un **apostador**. Empezaremos con uno extremadamente básico por ahora. Veremos, que aún con un apostador muy básico, veremos cosas muy reveladoras usando un simulador montecarlo.
###Code
# Comando para interactuar con las gráficas
# %matplotlib notebook
# Comando para no interactuar mas con las gráficas
%matplotlib inline
###Output
_____no_output_____
###Markdown
Forma ineficiente
###Code
# Crearemos un apostador simple MÉTODO CONVENCIONAL
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador2(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
capital = cap_inicial
c_capital = [capital]
for i in range(n_apuestas-1):
if dado():
capital += apuesta
c_capital.append(capital)
else:
capital -= apuesta
c_capital.append(capital)
return c_capital
apostador2(10000, 100, 10)
###Output
_____no_output_____
###Markdown
Forma eficiente
###Code
# Crearemos un apostador simple VECTORIZADOA ----- FUNCIÓN ANIDADA
# Las caracterísitcas son: se empieza con un capital inicial,
# siempre se apuesta lo mismo, y se va a apostar un número determinado de veces.
def apostador_nested_f(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
c_capital = np.zeros(n_apuestas)
c_capital[0] = cap_inicial
def fill_vector(i):
nonlocal c_capital
if dado():
c_capital[i + 1] = c_capital[i] + apuesta
else:
c_capital[i + 1] = c_capital[i] - apuesta
[fill_vector(i) for i in range(n_apuestas - 1)]
return c_capital
apostador_nested_f(10000, 100, 10)
# Crearemos un apostador simple VECTORIZADO
# Proponer otra forma de obtener el mismo resultado (CUMSUM)
def apostador_vect(cap_inicial, apuesta, n_apuestas):
np.random.seed(516)
x = [cap_inicial] + [apuesta if dado() else -apuesta for i in range(n_apuestas - 1)]
return np.cumsum(x)
apostador_vect(10000, 100, 10)
###Output
_____no_output_____
###Markdown
1. Probar tu función `apostador2`
###Code
# Ver como evolucionan los fondos de nuestro apostador al jugar 100 veces
# %matplotlib inline
ccapital = apostador2(10000, 100, 1000)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
El capital luego del juego fue 8900
###Markdown
2. Probar tu función `apostador_nested_f`
###Code
ccapital = apostador_nested_f(10000, 100, 1000)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
El capital luego del juego fue 8700
###Markdown
3. Probar tu función `apostador_vect`
###Code
ccapital = apostador2(10000, 100, 1000)
print('El capital al final del juego fue',ccapital[-1]);
plt.plot(ccapital)
plt.show()
###Output
_____no_output_____
###Markdown
Ahora vamos a simular 100 escenarios en donde cada apuesta es 100, 50, 100, 1000 y 10000 veces. ¿Qué pasa?
###Code
# (Montecarlo)
n = [50,100,1000,100000]
capital = 10000
apuesta = 100
# Deseo correr la función creada para cada elemento de la lista n,
# donde el capital es siempre 10000 y la apuesta es 100.
# Luego quiero graficar el comportamiento de mi capital cuando juego
plot = [apostador_vect(capital, apuesta, apuesta_i) for apuesta_i in n]
for graph, apuesta_i in zip(plot, n):
plt.plot(graph, label=f'la apuesta fue:{apuesta_i}')
plt.legend()
plt.show()
# Graficar histograma para ver el comportamiento del de los fondos para 1000 juegos distintos
plt.hist(plot[3],10)
plt.show()
print(np.mean(plot[3]))
###Output
_____no_output_____ |
lesson-5-Building-Evaluating-and-Interpreting-Models-for-Bias-and-Uncertainty/exercises/solution/Lesson_Modeling_Exercise_1_Starter_Code.ipynb | ###Markdown
L4 - 1: Regression Model with TF DenseFeatures Instructions- Build regression model to predict resting blood pressure (trestbps field in the dataset) using Tensorflow DenseFeatures- Please include the age and sex features and create a TF cross feature(https://www.tensorflow.org/api_docs/python/tf/feature_column/crossed_column) with them by binning the age field - Evaluate with common regression(MSE, MAE) and classification metrics(accuracy, F1, precision and recall across classes, AUC). No ROC or PR curve needed since this is a regression model that was converted to have a binary output and does not have the confidence in a given prediction.
###Code
import pandas as pd
import numpy as np
import tensorflow as tf
from sklearn.metrics import accuracy_score, f1_score, classification_report, roc_auc_score
#from https://archive.ics.uci.edu/ml/datasets/Heart+Disease
swiss_dataset_path = "./data/heart_disease_data/processed_swiss.csv"
swiss_df = pd.read_csv(swiss_dataset_path).replace('?', np.nan)
column_list = ['age', 'sex', 'cp', 'trestbps', 'chol', 'fbs', 'restecg', 'thalach',
'exang', 'oldpeak', 'slope', 'ca', 'thal', 'num_label']
cleveland_df = pd.read_csv("./data/heart_disease_data/processed.cleveland.txt", names=column_list).replace('?', np.nan)
combined_heart_df = pd.concat([swiss_df, cleveland_df])
len(combined_heart_df)
# Review the data
combined_heart_df.head()
# It would appear that we have some na values to deal with
combined_heart_df.isna().sum()
# Based on the solution, it would appear that we're only trainin on specific features.
# Subset?
combined_heart_df = combined_heart_df[['sex', 'age', 'trestbps', 'thalach']]
# The simple method is just to drop em'. Based on what I see here, this might be
# severe...
print(combined_heart_df.shape)
clean_df = combined_heart_df.dropna()
print(clean_df.shape)
clean_df.dtypes
# Skipping ahead, it looks as though we're being encouraged to recast sex as a string
clean_df["sex"] = np.where(clean_df["sex"] == 1, "male", "female")
clean_df.head()
# It's unclear to my why some of these features are reading as object. So, recast
clean_df['trestbps'] = clean_df['trestbps'].astype("float")
clean_df['thalach'] = clean_df['thalach'].astype("float")
clean_df.dtypes
# Define the train and test groups
training_data = clean_df.sample(frac=0.8)
test_data = clean_df.drop(training_data.index)
#adapted from https://www.tensorflow.org/tutorials/structured_data/feature_columns
def df_to_dataset(df, predictor, batch_size=32):
df = df.copy()
labels = df.pop(predictor)
ds = tf.data.Dataset.from_tensor_slices((dict(df), labels))
ds = ds.shuffle(buffer_size=len(df))
ds = ds.batch(batch_size)
return ds
# Convert to tensors
PREDICTOR_FIELD = 'trestbps'
batch_size = 128
train_ds = df_to_dataset(training_data, PREDICTOR_FIELD, batch_size=batch_size)
test_ds = df_to_dataset(test_data, PREDICTOR_FIELD, batch_size=batch_size)
# Preprocess numerical features -- there is only one
tf_numeric_age_feature = tf.feature_column.numeric_column(key="age",
default_value=0,
dtype=tf.float64)
# Based on what I'm seeing, they want us to bucket age, too?
b_list = [ 0, 18, 25, 40, 55, 65, 80, 100]
#create TF bucket feature from numeric feature
tf_numeric_age_feature
tf_bucket_age_feature = tf.feature_column.bucketized_column(source_column=tf_numeric_age_feature, boundaries= b_list)
# And then one-hot encode the gender column... Which, given cardinality, doesn't really
# require a vocab.
# Here, save in memory as oppose to writtin to file
# I think it's also relevant to mention here that we're running this on the WHOLE file,
# not just the splits
gender_vocab = tf.feature_column.categorical_column_with_vocabulary_list(
'sex', clean_df['sex'].unique())
gender_one_hot = tf.feature_column.indicator_column(gender_vocab)
# Now, this is new: cross features...
# More here: https://www.tensorflow.org/api_docs/python/tf/feature_column/crossed_column
# A way to combine categorical features? Here, we combine the age and the gender features.
crossed_age_gender_feature = tf.feature_column.crossed_column([tf_bucket_age_feature, gender_vocab], hash_bucket_size=1000)
tf_crossed_age_gender_feature = tf.feature_column.indicator_column(crossed_age_gender_feature)
feature_columns = [ tf_crossed_age_gender_feature, tf_bucket_age_feature, gender_one_hot ]
# Create the densefeatures layer
dense_feature_layer = tf.keras.layers.DenseFeatures(feature_columns)
# Use same architecture as example
def build_model(dense_feature_layer):
model = tf.keras.Sequential([
dense_feature_layer,
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(1)
])
optimizer = tf.keras.optimizers.RMSprop(0.001)
model.compile(loss='mse',
optimizer=optimizer,
metrics=['mae', 'mse'])
return model
# Build the model
model = build_model(dense_feature_layer)
# Train
# No validation set b/c need to build separate TF dataset
EPOCHS = 2000
# Set to patience to 100 so it trains to end of epochs
early_stop = tf.keras.callbacks.EarlyStopping(monitor='mse', patience=10)
history = model.fit(train_ds, callbacks=[early_stop], epochs=EPOCHS, verbose=1)
loss, mae, mse = model.evaluate(test_ds, verbose=2)
print("MAE:{}\nMSE:{}".format(mae, mse))
test_labels = test_dataset[PREDICTOR_FIELD].values
test_predictions = model.predict(test_ds).flatten()
model_pred_outputs = {
"pred": test_predictions,
"actual_value": test_labels,
}
model_output_df = pd.DataFrame(model_pred_output
# Convert Regression Output to binary classification output
model_output_df.tail()
#convert to binary prediction for Brier score - resting bps above 130
def convert_to_binary(df, pred_field, actual_field):
# score is the field name we will use for predictions and is what Aequitas uses
df['score'] = df[pred_field].apply(lambda x: 1 if x>=130 else 0 )
# label_value is the field name we will use for the truth value and is what Aequitas uses
df['label_value'] = df[actual_field].apply(lambda x: 1 if x>=130 else 0)
return df
binary_df = convert_to_binary(model_output_df, 'pred', 'actual_value')
binary_df.head()
from sklearn.metrics import accuracy_score, f1_score, classification_report, roc_auc_score
y_true = binary_df['label_value'].values
y_pred = binary_df['score'].values
accuracy_score(y_true, y_pred)
print(classification_report(y_true, y_pred))
roc_auc_score(y_true, y_pred)
###Output
_____no_output_____ |
20200823/Experiment2/discreteOptimization.ipynb | ###Markdown
Agent state and action definition* State Variable: x = [w, n, g_lag, e, s, (H, Ms, rh, m), O_lag], actions variable a = [c, b, k, i, q] both of them are numpy array.
###Code
%pylab inline
from scipy.interpolate import interpn
from helpFunctions import surfacePlot
import numpy as np
from multiprocessing import Pool
from functools import partial
import warnings
import math
warnings.filterwarnings("ignore")
np.printoptions(precision=2)
# time line
T_min = 0
T_max = 70
T_R = 45
# discounting factor
beta = 1/(1+0.02)
# utility function parameter
gamma = 2
# relative importance of housing consumption and non durable consumption
alpha = 0.8
# parameter used to calculate the housing consumption
kappa = 0.3
# depreciation parameter
delta = 0.025
# housing parameter
chi = 0.3
# uB associated parameter
B = 2
# # minimum consumption
# c_bar = 3
# constant cost
c_h = 0.5
# All the money amount are denoted in thousand dollars
earningShock = [0.8,1.2]
# Define transition matrix of economical states
# GOOD -> GOOD 0.8, BAD -> BAD 0.6
Ps = np.array([[0.6, 0.4],[0.2, 0.8]])
# current risk free interest rate
r_b = np.array([0.01 ,0.03])
# r_b = np.array([0.05 ,0.07])
# stock return depends on current and future econ states
# r_k = np.array([[-0.2, 0.15],[-0.15, 0.2]])
r_k = np.array([[-0.15, 0.20],[-0.15, 0.20]])
# expected return on stock market
# r_bar = 0.0667
r_bar = 0.02
# probability of survival
Pa = np.load("prob.npy")
# deterministic income
detEarning = np.load("detEarning.npy")
# probability of employment transition Pe[s, s_next, e, e_next]
Pe = np.array([[[[0.3, 0.7], [0.1, 0.9]], [[0.25, 0.75], [0.05, 0.95]]],
[[[0.25, 0.75], [0.05, 0.95]], [[0.2, 0.8], [0.01, 0.99]]]])
# tax rate before and after retirement
tau_L = 0.2
tau_R = 0.1
# constant state variables: Purchase value 250k, down payment 50k, mortgage 200k, interest rate 3.6%,
# 55 payment period, 8.4k per period. One housing unit is roughly 1 square feet. Housing price 0.25k/sf
# some variables associate with 401k amount
Nt = [np.sum(Pa[t:]) for t in range(T_max-T_min)]
Dt = [np.ceil(((1+r_bar)**N - 1)/(r_bar*(1+r_bar)**N)) for N in Nt]
# mortgate rate
rh = 0.036
D = [((1+rh)**N - 1)/(rh*(1+rh)**N) for N in range(T_max-T_min)]
# 401k contribution
yi = 0.01
# owning a house
O_lag = 1
# housing unit
H = 1000
# housing price constant
pt = 250/1000
# MS starting mortgate amount is set to 80 percent mortgage
MS = H * 0.8 * pt
# mortgate payment
m = MS / D[-1]
# Mt is the Mortgage balance at time t
Mt = [MS]
for t in range(T_max - T_min -1):
Mt.append(Mt[-1]*(1+rh) - m)
# 30k rent 1000 sf
pr = 30/1000
#Define the utility function
def u(c):
# shift utility function to the left, so it only takes positive value
return (np.float_power(c, 1-gamma) - 1)/(1 - gamma)
#Define the bequeath function, which is a function of wealth
def uB(tb):
return B*u(tb)
#Calcualte HE
def calHE(x, t):
# change input x as numpy array
# w, n, g_lag, e, s = x
HE = (H+(1-chi)*(1-delta)*x[:,2])*pt - Mt[t]
return HE
#Calculate TB
def calTB(x, t):
# change input x as numpy array
# w, n, g_lag, e, s = x
TB = x[:,0] + x[:,1] + calHE(x, t)
return TB
def R(x, a):
'''
Input:
state x: w, n, g_lag, e, s
action a: c, b, k, i, q = a which is a np array
Output:
reward value: the length of return should be equal to the length of a
'''
w, n, g_lag, e, s = x
# The number of reward should be the number of actions taken
reward = np.zeros(a.shape[0])
i_index = (a[:,4]==1)
ni_index = (a[:,4]!=1)
i_h = H + (1-delta)*g_lag + a[i_index][:,3]
i_Vh = (1+kappa)*i_h
ni_h = H + (1-delta)*g_lag
ni_Vh = (1-kappa)*(ni_h-(1-a[ni_index][:,4])*H)
i_C = np.float_power(a[i_index][:,0], alpha) * np.float_power(i_Vh, 1-alpha)
ni_C = np.float_power(a[ni_index][:,0], alpha) * np.float_power(ni_Vh, 1-alpha)
reward[i_index] = u(i_C)
reward[ni_index] = u(ni_C)
return reward
#Define the earning function, which applies for both employment and unemployment, good econ state and bad econ state
def y(t, x):
w, n, g_lag, e, s = x
if t <= T_R:
welfare = 5
return detEarning[t] * earningShock[int(s)] * e + (1-e) * welfare
else:
return detEarning[t]
#Earning after tax and fixed by transaction in and out from 401k account
def yAT(t,x):
yt = y(t, x)
w, n, g_lag, e, s = x
if t <= T_R and e == 1:
# 5% of the income will be put into the 401k
return (1-tau_L)*(yt * (1-yi))
if t <= T_R and e == 0:
return yt
else:
# t > T_R, n/discounting amount will be withdraw from the 401k
return (1-tau_R)*yt + n/Dt[t]
#Define the evolution of the amount in 401k account
def gn(t, n, x, s_next):
w, n, g_lag, e, s = x
if t <= T_R and e == 1:
# if the person is employed, then 5 percent of his income goes into 401k
n_cur = n + y(t, x) * yi
elif t <= T_R and e == 0:
# if the perons is unemployed, then n does not change
n_cur = n
else:
# t > T_R, n/discounting amount will be withdraw from the 401k
n_cur = n - n/Dt[t]
return (1+r_k[int(s), s_next])*n_cur
def transition(x, a, t):
'''
Input: state and action and time
Output: possible future states and corresponding probability
'''
w, n, g_lag, e, s = x
# variables used to collect possible states and probabilities
x_next = []
prob_next = []
for aa in a:
c,b,k,i,q = aa
if q == 1:
g = (1-delta)*g_lag + i
else:
g = (1-delta)*g_lag
for s_next in [0,1]:
w_next = b*(1+r_b[int(s)]) + k*(1+r_k[int(s), s_next])
n_next = gn(t, n, x, s_next)
if t >= T_R:
e_next = 0
x_next.append([w_next, n_next, g, e_next, s_next])
prob_next.append(Ps[int(s),s_next])
else:
for e_next in [0,1]:
x_next.append([w_next, n_next, g, e_next, s_next])
prob_next.append(Ps[int(s),s_next] * Pe[int(s),s_next,int(e),e_next])
return np.array(x_next), np.array(prob_next)
# used to calculate dot product
def dotProduct(p_next, uBTB, t):
if t >= 45:
return (p_next*uBTB).reshape((len(p_next)//2,2)).sum(axis = 1)
else:
return (p_next*uBTB).reshape((len(p_next)//4,4)).sum(axis = 1)
# Value function is a function of state and time t < T
def V(x, t, NN):
w, n, g_lag, e, s = x
yat = yAT(t,x)
if t == T_max-1:
# The objective functions of terminal state
def obj(actions):
# Not renting out case
# a = [c, b, k, i, q]
x_next, p_next = transition(x, actions, t)
uBTB = uB(calTB(x_next, t)) # conditional on being dead in the future
return R(x, actions) + beta * dotProduct(uBTB, p_next, t)
else:
def obj(actions):
# Renting out case
# a = [c, b, k, i, q]
x_next, p_next = transition(x, actions, t)
V_tilda = NN.predict(x_next) # V_{t+1} conditional on being alive, approximation here
uBTB = uB(calTB(x_next, t)) # conditional on being dead in the future
return R(x, actions) + beta * (Pa[t] * dotProduct(V_tilda, p_next, t) + (1 - Pa[t]) * dotProduct(uBTB, p_next, t))
def obj_solver(obj):
# Constrain: yat + w - m = c + b + k + (1+chi)*i*pt + I{i>0}*c_h
# i_portion takes [0:0.05:0.95]
# c_portion takes remaining [0:0.05:0.95]
# b_portion takes reamining [0:0.05:0.95]
# k is the remainder
actions = []
for ip in np.linspace(0,0.99,20):
budget1 = yat + w - m
if ip*budget1 > c_h:
i = (budget1*ip - c_h)/((1+chi)*pt)
budget2 = budget1 * (1-ip)
else:
i = 0
budget2 = budget1
for cp in np.linspace(0,1,11):
c = budget2*cp
budget3 = budget2 * (1-cp)
for bp in np.linspace(0,1,11):
b = budget3* bp
k = budget3 * (1-bp)
# q = 1 not renting in this case
actions.append([c,b,k,i,1])
# Constrain: yat + w - m + (1-q)*H*pr = c + b + k
# q takes value [0:0.05:0.95]
# c_portion takes remaining [0:0.05:0.95]
# b_portion takes reamining [0:0.05:0.95]
# k is the remainder
for q in np.linspace(0,0.99,20):
budget1 = yat + w - m + (1-q)*H*pr
for cp in np.linspace(0,1,11):
c = budget1*cp
budget2 = budget1 * (1-cp)
for bp in np.linspace(0,1,11):
b = budget2* bp
k = budget2 * (1-bp)
# i = 0, no housing improvement when renting out
actions.append([c,b,k,0,q])
actions = np.array(actions)
values = obj(actions)
fun = np.max(values)
ma = actions[np.argmax(values)]
return fun, ma
fun, action = obj_solver(obj)
return np.array([fun, action])
# wealth discretization
ws = np.array([10,25,50,75,100,125,150,175,200,250,500,750,1000,1500,3000])
w_grid_size = len(ws)
# 401k amount discretization
ns = np.array([1, 5, 10, 15, 25, 40, 65, 100, 150, 300, 400, 1000, 2000])
n_grid_size = len(ns)
# Improvement amount
gs = np.array([0,25,50,75,100,200,500,800])
g_grid_size = len(gs)
xgrid = np.array([[w, n, g_lag, e, s]
for w in ws
for n in ns
for g_lag in gs
for e in [0,1]
for s in [0,1]
]).reshape((w_grid_size, n_grid_size,g_grid_size,2,2,5))
Vgrid = np.zeros((w_grid_size, n_grid_size, g_grid_size,2,2, T_max))
cgrid = np.zeros((w_grid_size, n_grid_size, g_grid_size,2,2, T_max))
bgrid = np.zeros((w_grid_size, n_grid_size, g_grid_size,2,2, T_max))
kgrid = np.zeros((w_grid_size, n_grid_size, g_grid_size,2,2, T_max))
igrid = np.zeros((w_grid_size, n_grid_size, g_grid_size,2,2, T_max))
qgrid = np.zeros((w_grid_size, n_grid_size, g_grid_size,2,2, T_max))
###Output
_____no_output_____
###Markdown
multidimensional interpolation
###Code
class iApproxy(object):
def __init__(self, points, Vgrid):
self.V = Vgrid
self.p = points
def predict(self, xx):
pvalues = np.zeros(xx.shape[0])
index00 = (xx[:,3] == 0) & (xx[:,4] == 0)
index01 = (xx[:,3] == 0) & (xx[:,4] == 1)
index10 = (xx[:,3] == 1) & (xx[:,4] == 0)
index11 = (xx[:,3] == 1) & (xx[:,4] == 1)
pvalues[index00]=interpn(self.p, self.V[:,:,:,0,0], xx[index00][:,:3], bounds_error = False, fill_value = None)
pvalues[index01]=interpn(self.p, self.V[:,:,:,0,1], xx[index01][:,:3], bounds_error = False, fill_value = None)
pvalues[index10]=interpn(self.p, self.V[:,:,:,1,0], xx[index10][:,:3], bounds_error = False, fill_value = None)
pvalues[index11]=interpn(self.p, self.V[:,:,:,1,1], xx[index11][:,:3], bounds_error = False, fill_value = None)
return pvalues
###Output
_____no_output_____
###Markdown
Value iteration with interpolation approximation
###Code
%%time
# value iteration part
xs = xgrid.reshape((w_grid_size*n_grid_size*g_grid_size*2*2,5))
pool = Pool()
points = (ws,ns,gs)
for t in range(T_max-1,T_min-1, -1):
print(t)
if t == T_max - 1:
f = partial(V, t = t, NN = None)
results = np.array(pool.map(f, xs))
else:
approx = iApproxy(points,Vgrid[:,:,:,:,:,t+1])
f = partial(V, t = t, NN = approx)
results = np.array(pool.map(f, xs))
Vgrid[:,:,:,:,:,t] = results[:,0].reshape((w_grid_size,n_grid_size,g_grid_size,2,2))
cgrid[:,:,:,:,:,t] = np.array([r[0] for r in results[:,1]]).reshape((w_grid_size,n_grid_size,g_grid_size,2,2))
bgrid[:,:,:,:,:,t] = np.array([r[1] for r in results[:,1]]).reshape((w_grid_size,n_grid_size,g_grid_size,2,2))
kgrid[:,:,:,:,:,t] = np.array([r[2] for r in results[:,1]]).reshape((w_grid_size,n_grid_size,g_grid_size,2,2))
igrid[:,:,:,:,:,t] = np.array([r[3] for r in results[:,1]]).reshape((w_grid_size,n_grid_size,g_grid_size,2,2))
qgrid[:,:,:,:,:,t] = np.array([r[4] for r in results[:,1]]).reshape((w_grid_size,n_grid_size,g_grid_size,2,2))
pool.close()
np.save("Vgrid_i", Vgrid)
np.save("cgrid_i", cgrid)
np.save("bgrid_i", bgrid)
np.save("kgrid_i", kgrid)
np.save("igrid_i", igrid)
np.save("qgrid_i", qgrid)
###Output
69
68
67
66
65
64
63
62
61
60
59
58
57
56
55
54
53
52
51
50
49
48
47
46
45
44
43
42
41
40
39
38
37
36
35
34
33
32
31
30
29
28
27
26
25
24
23
22
21
20
19
18
17
16
15
14
13
12
11
10
9
8
7
6
5
4
3
2
1
0
CPU times: user 23.5 s, sys: 6.03 s, total: 29.5 s
Wall time: 58min 25s
|
bar-on-twinned-ax-with-lines.ipynb | ###Markdown
-->-->
###Code
# Based on: https://stackoverflow.com/a/48621177/10749432
import pandas as pd
import matplotlib.pyplot as plt
data = {
2011: [3.8, 3, 99],
2012: [3.6, 2.2, 116.6],
2013: [5.5, 3.8, 191],
2014: [4.2, 2.6, 165],
2015: [3.2, 1.2, 152.8],
2016: [2.6, 1.7, 142.3],
2017: [2.4, 1.3, 160.7],
2018: [3.4, 2.2, 263.1],
2019: [4.2, 2.5, 275.5],
}
df = pd.DataFrame(
data,
index=['Bank nonperforming loans to total gross loans (%)',
'Bank nonperforming loans to total net loans (%)',
'Bank Total gross loans (LK Bn)'],
columns=data.keys()
)
years = list(data.keys())
fig, ax = plt.subplots()
# axis for bar
ax2 = ax.twinx()
ax2.set_ylim([90, 280])
# creating a cloned axis from ax for lines
# wee need this to put line plots on bars
ax1 = fig.add_axes(ax.get_position(), sharex=ax, sharey=ax)
ax1.set_facecolor('none')
ax1.set_axis_off()
ax1.set_xticks(years)
bar = ax2.bar(
years,
df.loc['Bank Total gross loans (LK Bn)'], color='brown',
label='chk',
)
line1, = ax1.plot(
df.loc['Bank nonperforming loans to total gross loans (%)', years],
color='mediumvioletred',
marker='o',
markerfacecolor='blue',
markersize=9,
label='NPL %',
)
line2, = ax1.plot(
df.loc['Bank nonperforming loans to total net loans (%)', years],
color='blue',
label='SL',
)
ax.set_title('Immigration from Afghanistan')
ax.set_ylabel('NPL %')
ax.set_xlabel('years')
ax2.legend([bar, line1, line2], ['chk', 'NPL %', 'SL'])
###Output
_____no_output_____ |
teaching_material/session_4/module_4_solution.ipynb | ###Markdown
Session 4: Data Structuring - data typesIn this combined teaching module and exercise set you will be working with structuring data. We will start out with some material about how write [readable code](Readable-code). Then we will focus on data cleaning, in particular working with pandas data types and new data types:1. [string data](String-data)1. [categorical data](Categorical-data)1. [temporal data](Temporal-data)1. [missing data](Missing-data) and [duplicates](Duplicated-data) PackagesFirst load in the required modules and set up the plotting library:
###Code
%matplotlib inline
import pandas as pd
import matplotlib.pyplot as plt
###Output
_____no_output_____
###Markdown
Welcome back to pandasAs mentioned earlier, data structuring skills are necessary to become a great data scientist. There is no way around it. In this video we will motivate why learning to structure data is important (irrespective of the program you use!).
###Code
from IPython.display import YouTubeVideo
YouTubeVideo('XMlCLdzz43w', width=640, height=360)
###Output
_____no_output_____
###Markdown
Readable codeIf we have lots of code it may be very difficult for others or ourselves to read. Therefore, providing some structure and meta text can help reading the code. CommentingWhen making code it's good practice to document different parts of the code. In particular describing functions and complex code. The example below shows how to make multi-line comments (as a string, which is not assigned) and in-line comments using the `` character.```pythondef my_fct(x,y): ''' Computes the sum of input values (multi-line comment as string) ''' z = x+y Here we perform the summation (in-line comment) return z``` Method chainingWe can write multiple operations together by putting them one after the other, which is known as `method chaining`. Using this, we only need to assign them once to a new object and therefore we save a lot of code. We change the example below into one using a method chain:Example without method chain```pythondf_temp1 = df.loc[selection]df_temp2 = df_temp1.sort_values(by=X)df_out = df_temp2.reset_index()```Example with method chain - one line```pythondf_out = df.loc[selection].sort_values(by=X).reset_index()```As seen in the example, although using less code, our method chain will get more and more difficult to read if we include two or more operations. We can overcome this problem of long chains by splitting into multiple lines with line breaks:Example with method chain - line break```pythondf_out = df\ .loc[selection]\ .sort_values(by=X)\ .reset_index()```Note that the backslash allows us to make a visual line break, but the code is read as one line. Weather data structuring - part 2We continue with the exercise that analyzes NOAA data. The first part was Assignment Part 0.4. The last part will come in Exercise Section 5.2. We start out reviewing what we did in Assignment 0. > **Ex. 4.1.1:** The code below runs through all the steps we completed in Assignment 0.4 step by step. Your task is to document this code in your own words. You should also make your own annotation of parts. You should also make the code more readable by applying method chaining.```pythonimport pandas as pddef load_weather(year): url = f"ftp://ftp.ncdc.noaa.gov/pub/data/ghcn/daily/by_year/{year}.csv.gz" df_weather = pd.read_csv(url, header=None) df_weather = df_weather.iloc[:,:4] column_names = ['station', 'datetime', 'obs_type', 'obs_value'] df_weather.columns = column_names df_weather['obs_value'] = df_weather['obs_value'] / 10 selection_tmax = df_weather.obs_type == 'TMAX' df_select = df_weather.loc[selection_tmax] df_sorted = df_select.sort_values(by=['station', 'datetime']) df_reset = df_sorted.reset_index(drop=True) df_out = df_reset.copy() return df_out```
###Code
# [Answer to Ex. 4.1.1]
# This is an annotated answer using method chaining
import pandas as pd
def load_weather(year):
'''
The input value is an integer.
This functions loads the data for a selected year and then structures and cleans it.
- Structuring includes removing unused columns, renaming and selecting only observations
of maximum temperature.
- Cleaning includes inserting missing decimal, sorting and resetting index.
'''
url = f"ftp://ftp.ncdc.noaa.gov/pub/data/ghcn/daily/by_year/{year}.csv.gz"
# loads the data
df_weather = pd.read_csv(url, header=None)\
.iloc[:,:4]
# structure and clean data using methods chaining
df_out = \
df_weather\
.rename(columns={0: 'station', 1: 'datetime', 2: 'obs_type', 3: 'obs_value'})\
.query("obs_type == 'TMAX'")\
.assign(obs_value=lambda df: df['obs_value']/10)\
.sort_values(by=['station', 'datetime'])\
.reset_index(drop=True)\
.copy()
return df_out
load_weather(1884)
###Output
_____no_output_____
###Markdown
Data types String dataWe will proceed with learning about processing string data. We have already seen this in Python (session). See the video with a short recap and how we do things in Python.
###Code
YouTubeVideo('bMGRna-iLFM', width=640, height=360)
###Output
_____no_output_____
###Markdown
> **Ex. 4.1.2:** Load the data for year 1864. Extract the area code (country and state) from the station name into a separate column.> _Hint:_ The station column contains a GHCND ID, given to each weather station by NOAA. The format of these ID's is a 2 letter country/state code, followed by possible additional information on the third character and then an integer identifying the specific station. A simple approach is to assume a fixed length of the country ID. A more complex way would be to use the [`re`](https://docs.python.org/3.8/library/re.html) module.
###Code
# [Answer to Ex. 4.1.2]
# HANDIN
###Output
_____no_output_____
###Markdown
Categorical dataWatch the video below introducing categorical data and how to work with it.
###Code
YouTubeVideo('qGAYwb8NHPE', width=640, height=360)
###Output
_____no_output_____
###Markdown
> **Ex. 4.1.3:** Convert the `area` column to a categorical variable. Transform the `obs_value` column from a continuous to a categorical variable by partitioning it into `3` intervals. Call this new column for `obs_value_cat`. This can be done using the `pd.cut()` method of pandas. Make another column with `obs_value` as a categorical variable but this time label the 3 intervals as `["cold", "medium", "hot"]`. This can be done by specifying the `labels` parameter in the `pd.cut()` method of pandas. Call this new column for `obs_value_cat_labeled`.
###Code
# [Answer to Ex. 4.1.3]
# HANDIN
###Output
_____no_output_____
###Markdown
Temporal dataOur coverage of basic Python did not include time. This is another elementary datatypes, that has its own native structure or maybe converted to an integer using a smart method. See more below.
###Code
YouTubeVideo('BGnxa6mT94g', width=640, height=360)
###Output
_____no_output_____
###Markdown
> **Ex. 4.1.4:** Convert the date formatted as string to datetime. Call this column `datetime_dt`. Make a new column named `month` with the month for each observation. Set the datetime variable as temporal index. > Hint: Look up `.set_index()` setting the index.
###Code
# [Answer to Ex. 4.1.4]
# datetime column
df['datetime_dt'] = pd.to_datetime(df['datetime'], format = '%Y%m%d')
# month column
df['month'] = df.datetime_dt.dt.month
# set datetime index
df = df.set_index('datetime_dt')
df.head()
###Output
_____no_output_____
###Markdown
> **Ex. 4.1.5:** Update your annotated function above with processing of area and temporal data.
###Code
# [Answer to Ex. 4.1.5]
def load_weather(year):
'''
This functions loads the data for selected year and then structures and cleans it.
- Structuring includes removing unused columns, renaming and selecting only observations
of maximum temperature.
- Cleaning includes inserting missing decimal, sorting and resetting index as well as
processing temporal and spatial information.
'''
url = f"ftp://ftp.ncdc.noaa.gov/pub/data/ghcn/daily/by_year/{year}.csv.gz"
# loads the data
df_weather = pd.read_csv(url, header=None)\
.iloc[:,:4]
# structure and clean data using methods chaining
df_out = \
df_weather\
.rename(columns={0: 'station', 1: 'datetime', 2: 'obs_type', 3: 'obs_value'})\
.query("obs_type == 'TMAX'")\
.assign(obs_value=lambda df: df['obs_value']/10)\
.sort_values(by=['station', 'datetime'])\
.reset_index(drop=True)\
.copy()
# area process
df_out['area'] = df_out['station'].str[0:2]
#categorical
df.area = df.area.astype('category')
df['obs_value_cat'] = pd.cut(df.obs_value, 3)
df['obs_value_cat_labeled'] = pd.cut(df.obs_value, 3,
labels=["cold", "medium", "hot"])
# datetime process
df_out['datetime_dt'] = pd.to_datetime(df_out['datetime'], format = '%Y%m%d')
df_out = df_out.set_index('datetime_dt')
return df_out
###Output
_____no_output_____
###Markdown
> **Ex. 4.1.6:** Make a timeseries plot for the stations called `AU000005901`.> _Hint:_ for this you need to know a few methods of the pandas Series objects. `.plot()`.
###Code
# [Answer to Ex. 4.1.6]
df\
.query('station=="AU000005901"')\
.obs_value\
.plot(figsize=[11,6])
plt.show()
###Output
_____no_output_____
###Markdown
Working with US census dataIn this section we will use [this dataset](https://archive.ics.uci.edu/ml/datasets/Adult) from the [UCI Machine Learning Repository](https://archive.ics.uci.edu/ml/datasets.html) to practice some basic operations on pandas dataframes. > **Ex. 4.2.1:** This link `'https://archive.ics.uci.edu/ml/machine-learning-databases/adult/adult.data'` leads to a comma-separated file with income data from a US census. Load the data into a pandas dataframe and show the 25th to 35th row.> _Hint 1:_ There are no column names in the dataset. Use the list `['age','workclass', 'fnlwgt', 'educ', 'educ_num', 'marital_status', 'occupation','relationship', 'race', 'sex','capital_gain', 'capital_loss', 'hours_per_week', 'native_country', 'wage']` as names. > _Hint 2:_ When you read in the csv, you might find that pandas includes whitespace in all of the cells. To get around this include the argument `skipinitialspace = True` to `read_csv()`.
###Code
# [Answer to Ex. 4.2.1]
url = 'https://archive.ics.uci.edu/ml/machine-learning-databases/adult/adult.data'
head = ['age','workclass', 'fnlwgt', 'educ', 'educ_num', 'marital_status',
'occupation','relationship', 'race', 'sex','capital_gain', 'capital_loss',
'hours_per_week', 'native_country', 'wage']
df2 = pd.read_csv(url,
sep = ',',
skipinitialspace = True, # Removes whitespace
names = head,
index_col = False # dont use 'age' as the index (!)
)
df2.iloc[24:35]
###Output
_____no_output_____
###Markdown
Missing dataOften our data having information missing, e.g. one row lacks data on education for a specific person. Watch the video below about missing data type and get some simple tools to deal with the problem.
###Code
YouTubeVideo('GDaxQig-qCU', width=640, height=360)
###Output
_____no_output_____
###Markdown
> **Ex. 4.2.2:** What is the missing value sign in this dataset? Replace all missing values with NA's understood by pandas. Then proceed to drop all rows containing any missing values with the `dropna` method. How many rows are removed in this operation?> _Hint 1:_ if this doesn't work as expected you might want to take a look at the hint for 4.2.1 again. > _Hint 2:_ The NaN method from NumPy might be useful
###Code
# [Answer to Ex. 4.2.2]
# The data uses '?' for NA's. To replace them we can simply run
from numpy import NaN
df2_clean = \
df2.replace('?', NaN)\
.dropna()
print(f"We have dropped {len(df2) - len(df2_clean)} rows")
###Output
We have dropped 2399 rows
###Markdown
Duplicated dataWatch the video below about duplicated data - in particular duplicated rows and how to handle them.
###Code
YouTubeVideo('BLLQofon9Ug', width=640, height=360)
###Output
_____no_output_____
###Markdown
> **Ex. 4.2.3:** Determine whether or not duplicated rows is a problem in the NOAA weather data and the US census data in the module. You should come up with arguments from the structure of the rows.
###Code
# [Answer to Ex. 4.2.3]
# Duplicates is not a problem in the first dataset.
# In the second there are duplicates, but it's most likely that they are identical
# in terms of these observed characteristics but they refer to different individuals.
n_duplicates_1 = df.duplicated(['station','datetime']).sum()
n_duplicates_2 = df2.duplicated().sum()
print(f'Number of duplicates in the datasets are respectively: {n_duplicates_1, n_duplicates_2}')
###Output
Number of duplicates in the datasets are respectively: (0, 24)
###Markdown
Summary of data typesWatch the video below where we conclude the lecturing material for this teaching module.
###Code
YouTubeVideo('mohBV7crmsU', width=640, height=360)
###Output
_____no_output_____
###Markdown
Additional exercises> **_Note_**: to solve the bonus exercises below, you will need to apply the `.groupby()` method a few times. This has not yet been covered in the lectures (you will see it in on of the next lectures). >> `.groupby()` is a method of pandas dataframes, meaning we can call it like so: `data.groupby('colname')`. The method groups your dataset by a specified column, and applies any following changes within each of these groups. For a more detailed explanation see [this link](https://www.tutorialspoint.com/python_pandas/python_pandas_groupby.htm). The [documentation](https://pandas.pydata.org/pandas-docs/stable/generated/pandas.DataFrame.groupby.html) might also be useful. > **Ex. 4.2.4:** (_Bonus_) Is there any evidence of a gender-wage-gap in the data? Create a table showing the percentage of men and women earning more than 50K a year.
###Code
# [Answer to Ex. 4.2.4]
# first we create a dummy for earning more than 50K
df2_clean['HighWage'] = (df2_clean['wage'] == '>50K').astype(int)
# then group by sex and calculate the mean
df2_clean[['sex', 'HighWage']]\
.groupby('sex')\
.mean()
###Output
_____no_output_____
###Markdown
> **Ex. 4.2.5:** (_Bonus_) Group the data by years of education (`educ_num`) and marital status. Now plot the share of individuals who earn more than 50K for the two groups 'Divorced' and 'Married-civ-spouse' (normal marriage). Your final result should look like this: > _Hint:_ remember the `.query()` method is extremely useful for filtering data.
###Code
# [Answer to Ex. 4.2.5]
df2_clean[['marital_status', 'HighWage', 'educ_num']]\
.groupby(['marital_status', 'educ_num'])\
.mean()\
.reset_index()\
.query("marital_status == 'Divorced' | marital_status == 'Married-civ-spouse'")\
.set_index('educ_num')\
.groupby('marital_status')\
.HighWage\
.plot()
plt.xlabel('Years of education')
plt.ylabel('Share earning more than 50K')
plt.legend()
###Output
_____no_output_____ |
assets/code/2020-06-18-vscode-intro-for-python.ipynb | ###Markdown
Example using Jupyter Notebook with PythonTo demonstrate the compatibility of vscode with Python I will use some basic data manipulation codes with the `pandas` package. This brief example comes from Lesson 1 of GitHub user guipsamora repository [pandas_exercises](https://github.com/guipsamora/pandas_exercises). To work on similar exercises yourself I recommend forking this repository.test ChipotleDatasets and materials made available thanks to (https://github.com/justmarkham). Step 1Importing the needed libraries
###Code
import numpy as np
import pandas as pd
print("Pandas version: {0}".format(pd.__version__))
print("Numpy version: {0}".format(np.__version__))
###Output
Pandas version: 1.0.4
Numpy version: 1.18.5
###Markdown
Step 2. Import the dataset from [here](https://raw.githubusercontent.com/justmarkham/DAT8/master/data/chipotle.tsv) and assign it as a an object named chipo
###Code
url = "https://raw.githubusercontent.com/justmarkham/DAT8/master/data/chipotle.tsv"
chipo = pd.read_csv(url, sep="\t")
###Output
_____no_output_____
###Markdown
Step 3. Peek at the first 10 entries
###Code
chipo.head(10)
###Output
_____no_output_____
###Markdown
This dataset is an item level description of orders placed at the restaurant Chipotle. It is in long format where each `order_id` contains the `quantity` and `item_name` for each unique order item. Note that if the item name has different choices then it is listed as a separate order item. Step 4. Convert the item_price to numeric and calculate the total revenue
###Code
chipo.item_price.dtype
###Output
_____no_output_____
###Markdown
The original variable is considered as a character since it has the dollar sign appended so we need to remove this and convert to float.
###Code
dollar_scrub = lambda x: float(x[1:-1])
chipo.item_price = chipo.item_price.apply(dollar_scrub)
###Output
_____no_output_____
###Markdown
Now we check to make sure that the type has been changed.
###Code
chipo.item_price.dtype
###Output
_____no_output_____
###Markdown
We have successfuly converted this variable, so now if we look at the first 10 entries again the `item_price` does not contain the $ sign.
###Code
chipo.head(10)
###Output
_____no_output_____
###Markdown
Now with our float `item_price` we can calculate the total revenue as $\text{revenue}=\text{quantity}\times\text{price}$.
###Code
rev = (chipo.quantity * chipo.item_price).sum()
print("Total Revenue: $" + str(np.round(rev, decimals=2)))
###Output
Total Revenue: $39237.02
|
data/synthesis/mwem_sample/MNIST MWEM (Image Data).ipynb | ###Markdown
MNIST classification (drawn from sklearn example)=====================================================MWEM is not particularly well suited for image data (where there are tons of features with relatively large ranges) but it is still able to capture some important information about the underlying distributions if tuned correctly.We use a feature included with MWEM that allows a column to be specified for a custom bin count, if we are capping every other bin count at a small value. In this case, we specify that the numerical column (784) has 10 possible values. We do this with the dict {'784': 10}.Here we borrow from a scikit-learn example, and insert MWEM synthetic data into their training example/visualization, to understand the tradeoffs.https://scikit-learn.org/stable/auto_examples/linear_model/plot_sparse_logistic_regression_mnist.htmlsphx-glr-download-auto-examples-linear-model-plot-sparse-logistic-regression-mnist-py
###Code
import time
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
from sklearn.datasets import fetch_openml
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.utils import check_random_state
# pip install scikit-image
from skimage import data, color
from skimage.transform import rescale
# Author: Arthur Mensch <[email protected]>
# License: BSD 3 clause
# Turn down for faster convergence
t0 = time.time()
train_samples = 5000
# Load data from https://www.openml.org/d/554
data = fetch_openml('mnist_784', version=1, return_X_y=False)
data_np = np.hstack((data.data,np.reshape(data.target.astype(int), (-1, 1))))
from opendp.smartnoise.synthesizers.mwem import MWEMSynthesizer
# Here we set max bin count to be 10, so that we retain the numeric labels
synth = MWEMSynthesizer(40, 10.0, 15, 10, split_factor=1, max_bin_count = 128, custom_bin_count={'784':10})
synth.fit(data_np)
sample_size = 2000
synthetic = synth.sample(sample_size)
from sklearn.linear_model import RidgeClassifier
import utils
real = pd.DataFrame(data_np[:sample_size])
model_real, model_fake = utils.test_real_vs_synthetic_data(real, synthetic, RidgeClassifier, tsne=True)
# Classification
coef = model_real.coef_.copy()
plt.figure(figsize=(10, 5))
scale = np.abs(coef).max()
for i in range(10):
l1_plot = plt.subplot(2, 5, i + 1)
l1_plot.imshow(coef[i].reshape(28, 28), interpolation='nearest',
cmap=plt.cm.RdBu, vmin=-scale, vmax=scale)
l1_plot.set_xticks(())
l1_plot.set_yticks(())
l1_plot.set_xlabel('Class %i' % i)
plt.suptitle('Classification vector for...')
run_time = time.time() - t0
print('Example run in %.3f s' % run_time)
plt.show()
coef = model_fake.coef_.copy()
plt.figure(figsize=(10, 5))
scale = np.abs(coef).max()
for i in range(10):
l1_plot = plt.subplot(2, 5, i + 1)
l1_plot.imshow(coef[i].reshape(28, 28), interpolation='nearest',
cmap=plt.cm.RdBu, vmin=-scale, vmax=scale)
l1_plot.set_xticks(())
l1_plot.set_yticks(())
l1_plot.set_xlabel('Class %i' % i)
plt.suptitle('Classification vector for...')
run_time = time.time() - t0
print('Example run in %.3f s' % run_time)
plt.show()
###Output
Example run in 288.237 s
###Markdown
MNIST classification (drawn from sklearn example)=====================================================MWEM is not particularly well suited for image data (where there are tons of features with relatively large ranges) but it is still able to capture some important information about the underlying distributions if tuned correctly.We use a feature included with MWEM that allows a column to be specified for a custom bin count, if we are capping every other bin count at a small value. In this case, we specify that the numerical column (784) has 10 possible values. We do this with the dict {'784': 10}.Here we borrow from a scikit-learn example, and insert MWEM synthetic data into their training example/visualization, to understand the tradeoffs.https://scikit-learn.org/stable/auto_examples/linear_model/plot_sparse_logistic_regression_mnist.htmlsphx-glr-download-auto-examples-linear-model-plot-sparse-logistic-regression-mnist-py
###Code
import time
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
from sklearn.datasets import fetch_openml
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.utils import check_random_state
# pip install scikit-image
from skimage import data, color
from skimage.transform import rescale
# Author: Arthur Mensch <[email protected]>
# License: BSD 3 clause
# Turn down for faster convergence
t0 = time.time()
train_samples = 5000
# Load data from https://www.openml.org/d/554
data = fetch_openml('mnist_784', version=1, return_X_y=False)
data_np = np.hstack((data.data,np.reshape(data.target.astype(int), (-1, 1))))
from opendp.smartnoise.synthesizers.mwem import MWEMSynthesizer
# Here we set max bin count to be 10, so that we retain the numeric labels
synth = MWEMSynthesizer(10.0, 40, 15, 10, split_factor=1, max_bin_count = 128, custom_bin_count={'784':10})
synth.fit(data_np)
sample_size = 2000
synthetic = synth.sample(sample_size)
from sklearn.linear_model import RidgeClassifier
import utils
real = pd.DataFrame(data_np[:sample_size])
model_real, model_fake = utils.test_real_vs_synthetic_data(real, synthetic, RidgeClassifier, tsne=True)
# Classification
coef = model_real.coef_.copy()
plt.figure(figsize=(10, 5))
scale = np.abs(coef).max()
for i in range(10):
l1_plot = plt.subplot(2, 5, i + 1)
l1_plot.imshow(coef[i].reshape(28, 28), interpolation='nearest',
cmap=plt.cm.RdBu, vmin=-scale, vmax=scale)
l1_plot.set_xticks(())
l1_plot.set_yticks(())
l1_plot.set_xlabel('Class %i' % i)
plt.suptitle('Classification vector for...')
run_time = time.time() - t0
print('Example run in %.3f s' % run_time)
plt.show()
coef = model_fake.coef_.copy()
plt.figure(figsize=(10, 5))
scale = np.abs(coef).max()
for i in range(10):
l1_plot = plt.subplot(2, 5, i + 1)
l1_plot.imshow(coef[i].reshape(28, 28), interpolation='nearest',
cmap=plt.cm.RdBu, vmin=-scale, vmax=scale)
l1_plot.set_xticks(())
l1_plot.set_yticks(())
l1_plot.set_xlabel('Class %i' % i)
plt.suptitle('Classification vector for...')
run_time = time.time() - t0
print('Example run in %.3f s' % run_time)
plt.show()
###Output
Example run in 95.536 s
|
Homework/2019/Task2/11/code/Malicious_url_detection.ipynb | ###Markdown
使用一些URL的特征来作为属性进行判断:
###Code
from __future__ import division
import os
import sys
import re
import matplotlib
import pandas as pd
import numpy as np
from os.path import splitext
import ipaddress as ip
import tldextract
import whois
import datetime
from urllib.parse import urlparse
import matplotlib.pyplot as plt
import pandas as pd
import seaborn as sns
import pickle as pkl
#2016's top most suspicious TLD and words
Suspicious_TLD=['zip','cricket','link','work','party','gq','kim','country','science','tk']
Suspicious_Domain=['luckytime.co.kr','mattfoll.eu.interia.pl','trafficholder.com','dl.baixaki.com.br','bembed.redtube.comr','tags.expo9.exponential.com','deepspacer.com','funad.co.kr','trafficconverter.biz']
#trend micro's top malicious domains
# Method to count number of dots
def countdots(url):
return url.count('.')
# Method to count number of delimeters
def countdelim(url):
count = 0
delim=[';','_','?','=','&']
for each in url:
if each in delim:
count = count + 1
return count
# Is IP addr present as th hostname, let's validate
import ipaddress as ip #works only in python 3
def isip(uri):
try:
if ip.ip_address(uri):
return 1
except:
return 0
#method to check the presence of @
def isPresentAt(url):
return url.count('@')
def isPresentDSlash(url):
return url.count('//')
def countSubDir(url):
return url.count('/')
def get_ext(url):
"""Return the filename extension from url, or ''."""
root, ext = splitext(url)
return ext
def countSubDomain(subdomain):
if not subdomain:
return 0
else:
return len(subdomain.split('.'))
def countQueries(query):
if not query:
return 0
else:
return len(query.split('&'))
###Output
_____no_output_____
###Markdown
导入数据
###Code
df = pd.read_csv("dataset.csv", encoding='utf-8')
#df=df.sample(frac=1)
df = df.sample(frac=1).reset_index(drop=True)
df.head()
len(df)
###Output
_____no_output_____
###Markdown
利用以上函数统计出每个url的属性
###Code
featureSet = pd.DataFrame(columns=('url','no of dots','len of url','presence of at',\
'presence of double slash','no of subdir','no of subdomain','len of domain','no of queries','is IP','presence of Suspicious_TLD',\
'presence of suspicious domain','label'))
from urllib.parse import urlparse
import tldextract
def getFeatures(url, label):
result = []
url = str(url)
#add the url to feature set
result.append(url)
#parse the URL and extract the domain information
path = urlparse(url)
ext = tldextract.extract(url)
# print("1:"+path.scheme)
# print("2:"+path.netloc)
# print("3:"+path.path)
# print("4:"+ext.subdomain)
# print("5:"+ext.suffix)
#counting number of dots in subdomain
result.append(countdots(ext.subdomain))
#length of URL
result.append(len(url))
#checking @ in the url
result.append(isPresentAt(path.netloc))
#checking presence of double slash
result.append(isPresentDSlash(path.path))
#Count number of subdir
result.append(countSubDir(path.path))
#number of sub domain
result.append(countSubDomain(ext.subdomain))
#length of domain name
result.append(len(path.netloc))
#count number of queries
result.append(len(path.query))
#Adding domain information
#if IP address is being used as a URL
result.append(isip(ext.domain))
#presence of Suspicious_TLD
result.append(1 if ext.suffix in Suspicious_TLD else 0)
#presence of suspicious domain
result.append(1 if '.'.join(ext[1:]) in Suspicious_Domain else 0 )
#result.append(get_ext(path.path))
result.append(str(label))
return result
for i in range(len(df)):
features = getFeatures(df["url"].loc[i], df["label"].loc[i])
featureSet.loc[i] = features
featureSet.head(10)
###Output
_____no_output_____
###Markdown
做一个数据样本的恶意网址和正常网址的统计
###Code
featureSet.groupby(featureSet['label']).size()
###Output
_____no_output_____
###Markdown
开始训练
###Code
import sklearn.ensemble as ek
from sklearn.model_selection import train_test_split
from sklearn import tree, linear_model
from sklearn.feature_selection import SelectFromModel
from sklearn.externals import joblib
from sklearn.metrics import confusion_matrix
from sklearn.pipeline import make_pipeline
from sklearn import preprocessing
from sklearn import svm
###Output
/Users/dqy/anaconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.
warnings.warn(msg, category=DeprecationWarning)
###Markdown
把属性存在 X,类别标签存在 y,drop函数作用为删除列
###Code
X = featureSet.drop(['url','label'],axis=1).values
y = featureSet['label'].values
###Output
_____no_output_____
###Markdown
把数据集分为训练集和测试集,其中 test_size=0.2,即测试集占总数据的 20%:
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y ,test_size=0.2)
###Output
_____no_output_____
###Markdown
用决策树训练模型
###Code
clf = tree.DecisionTreeClassifier(max_depth=10)
clf.fit(X_train,y_train)
res = clf.predict(X)
mt = confusion_matrix(y, res)
print("%f,%f" % (mt[0][0],mt[0][1]))
print("%f,%f" % (mt[1][0],mt[1][1]))
print("Accuracy rate : %f %%" % (((mt[0][0]+mt[1][1]) / float(mt.sum()))*100))
print('Recall rate : %f %%' % ( (mt[0][0] / float(mt.sum(axis=1)[0])*100)))
###Output
3654.000000,345.000000
250.000000,3749.000000
Accuracy rate : 92.560640 %
Recall rate : 91.372843 %
###Markdown
测试
###Code
result = pd.DataFrame(columns=('url','no of dots','len of url','presence of at',\
'presence of double slash','no of subdir','no of subdomain','len of domain','no of queries','is IP','presence of Suspicious_TLD',\
'presence of suspicious domain','label'))
results = getFeatures('www.baidu.com', '0')
result.loc[0] = results
result = result.drop(['url','label'],axis=1).values
if(clf.predict(result)=="0"):
print("Benign URL")
else:
print("Malicious URL")
result = pd.DataFrame(columns=('url','no of dots','len of url','presence of at',\
'presence of double slash','no of subdir','no of subdomain','len of domain','no of queries','is IP','presence of Suspicious_TLD',\
'presence of suspicious domain','label'))
results = getFeatures('dinas.tomsk.ru/err/?paypal.ch/ch/cgi-bin/webscr1.htm?cmd=_login-run&dispatch=5885d80a13c0db1f1ff80d546411d7f8a8350c132bc41e0934cfc023d4r4ere32132', '1')
result.loc[0] = results
result = result.drop(['url','label'],axis=1).values
if(clf.predict(result)=="0"):
print("Benign URL")
else:
print("Malicious URL")
###Output
Malicious URL
###Markdown
使用一些URL的特征来作为属性进行判断:
###Code
from __future__ import division
import os
import sys
import re
import matplotlib
import pandas as pd
import numpy as np
from os.path import splitext
import ipaddress as ip
import tldextract
import whois
import datetime
from urllib.parse import urlparse
import matplotlib.pyplot as plt
import pandas as pd
import seaborn as sns
import pickle as pkl
#2016's top most suspicious TLD and words
Suspicious_TLD=['zip','cricket','link','work','party','gq','kim','country','science','tk']
Suspicious_Domain=['luckytime.co.kr','mattfoll.eu.interia.pl','trafficholder.com','dl.baixaki.com.br','bembed.redtube.comr','tags.expo9.exponential.com','deepspacer.com','funad.co.kr','trafficconverter.biz']
#trend micro's top malicious domains
# Method to count number of dots
def countdots(url):
return url.count('.')
# Method to count number of delimeters
def countdelim(url):
count = 0
delim=[';','_','?','=','&']
for each in url:
if each in delim:
count = count + 1
return count
# Is IP addr present as th hostname, let's validate
import ipaddress as ip #works only in python 3
def isip(uri):
try:
if ip.ip_address(uri):
return 1
except:
return 0
#method to check the presence of @
def isPresentAt(url):
return url.count('@')
def isPresentDSlash(url):
return url.count('//')
def countSubDir(url):
return url.count('/')
def get_ext(url):
"""Return the filename extension from url, or ''."""
root, ext = splitext(url)
return ext
def countSubDomain(subdomain):
if not subdomain:
return 0
else:
return len(subdomain.split('.'))
def countQueries(query):
if not query:
return 0
else:
return len(query.split('&'))
###Output
_____no_output_____
###Markdown
导入数据
###Code
df = pd.read_csv("dataset.csv", encoding='utf-8')
#df=df.sample(frac=1)
df = df.sample(frac=1).reset_index(drop=True)
df.head()
len(df)
###Output
_____no_output_____
###Markdown
利用以上函数统计出每个url的属性
###Code
featureSet = pd.DataFrame(columns=('url','no of dots','len of url','presence of at',\
'presence of double slash','no of subdir','no of subdomain','len of domain','no of queries','is IP','presence of Suspicious_TLD',\
'presence of suspicious domain','label'))
from urllib.parse import urlparse
import tldextract
def getFeatures(url, label):
result = []
url = str(url)
#add the url to feature set
result.append(url)
#parse the URL and extract the domain information
path = urlparse(url)
ext = tldextract.extract(url)
# print("1:"+path.scheme)
# print("2:"+path.netloc)
# print("3:"+path.path)
# print("4:"+ext.subdomain)
# print("5:"+ext.suffix)
#counting number of dots in subdomain
result.append(countdots(ext.subdomain))
#length of URL
result.append(len(url))
#checking @ in the url
result.append(isPresentAt(path.netloc))
#checking presence of double slash
result.append(isPresentDSlash(path.path))
#Count number of subdir
result.append(countSubDir(path.path))
#number of sub domain
result.append(countSubDomain(ext.subdomain))
#length of domain name
result.append(len(path.netloc))
#count number of queries
result.append(len(path.query))
#Adding domain information
#if IP address is being used as a URL
result.append(isip(ext.domain))
#presence of Suspicious_TLD
result.append(1 if ext.suffix in Suspicious_TLD else 0)
#presence of suspicious domain
result.append(1 if '.'.join(ext[1:]) in Suspicious_Domain else 0 )
#result.append(get_ext(path.path))
result.append(str(label))
return result
for i in range(len(df)):
features = getFeatures(df["url"].loc[i], df["label"].loc[i])
featureSet.loc[i] = features
featureSet.head(10)
###Output
_____no_output_____
###Markdown
做一个数据样本的恶意网址和正常网址的统计
###Code
featureSet.groupby(featureSet['label']).size()
###Output
_____no_output_____
###Markdown
开始训练
###Code
import sklearn.ensemble as ek
from sklearn.model_selection import train_test_split
from sklearn import tree, linear_model
from sklearn.feature_selection import SelectFromModel
from sklearn.externals import joblib
from sklearn.metrics import confusion_matrix
from sklearn.pipeline import make_pipeline
from sklearn import preprocessing
from sklearn import svm
###Output
/Users/dqy/anaconda3/lib/python3.7/site-packages/sklearn/externals/joblib/__init__.py:15: DeprecationWarning: sklearn.externals.joblib is deprecated in 0.21 and will be removed in 0.23. Please import this functionality directly from joblib, which can be installed with: pip install joblib. If this warning is raised when loading pickled models, you may need to re-serialize those models with scikit-learn 0.21+.
warnings.warn(msg, category=DeprecationWarning)
###Markdown
把属性存在 X,类别标签存在 y,drop函数作用为删除列
###Code
X = featureSet.drop(['url','label'],axis=1).values
y = featureSet['label'].values
###Output
_____no_output_____
###Markdown
把数据集分为训练集和测试集,其中 test_size=0.2,即测试集占总数据的 20%:
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y ,test_size=0.2)
###Output
_____no_output_____
###Markdown
用决策树训练模型
###Code
clf = tree.DecisionTreeClassifier(max_depth=10)
clf.fit(X_train,y_train)
res = clf.predict(X)
mt = confusion_matrix(y, res)
print("%f,%f" % (mt[0][0],mt[0][1]))
print("%f,%f" % (mt[1][0],mt[1][1]))
print("Accuracy rate : %f %%" % (((mt[0][0]+mt[1][1]) / float(mt.sum()))*100))
print('Recall rate : %f %%' % ( (mt[0][0] / float(mt.sum(axis=1)[0])*100)))
###Output
3654.000000,345.000000
250.000000,3749.000000
Accuracy rate : 92.560640 %
Recall rate : 91.372843 %
###Markdown
测试
###Code
result = pd.DataFrame(columns=('url','no of dots','len of url','presence of at',\
'presence of double slash','no of subdir','no of subdomain','len of domain','no of queries','is IP','presence of Suspicious_TLD',\
'presence of suspicious domain','label'))
results = getFeatures('www.baidu.com', '0')
result.loc[0] = results
result = result.drop(['url','label'],axis=1).values
if(clf.predict(result)=="0"):
print("Benign URL")
else:
print("Malicious URL")
result = pd.DataFrame(columns=('url','no of dots','len of url','presence of at',\
'presence of double slash','no of subdir','no of subdomain','len of domain','no of queries','is IP','presence of Suspicious_TLD',\
'presence of suspicious domain','label'))
results = getFeatures('dinas.tomsk.ru/err/?paypal.ch/ch/cgi-bin/webscr1.htm?cmd=_login-run&dispatch=5885d80a13c0db1f1ff80d546411d7f8a8350c132bc41e0934cfc023d4r4ere32132', '1')
result.loc[0] = results
result = result.drop(['url','label'],axis=1).values
if(clf.predict(result)=="0"):
print("Benign URL")
else:
print("Malicious URL")
###Output
Malicious URL
|
Image_Resize.ipynb | ###Markdown
###Code
from PIL import Image
img = Image.open('./sample_data/11_2.jpg')
img.size
img.info
img.save('./sample_data/11_2_1.jpg',dpi=[72,72])
img = Image.open('./sample_data/11_2_1.jpg')
img.info
###Output
_____no_output_____
###Markdown
Final Solution for resizing an Image while retaining maximum quality
###Code
img = Image.open('./sample_data/11_2.jpg')
img = img.resize((612,792),Image.ANTIALIAS)
img.save("./sample_data/11_2_2.jpg",optimize=True,quality=95)
img = Image.open('./sample_data/11_2_2.jpg')
img.size
img.info
###Output
_____no_output_____ |
savenadrestore.ipynb | ###Markdown
###Code
# MLP for Pima Indians Dataset Serialize to JSON and HDF5
from keras.models import Sequential
from keras.layers import Dense
from keras.models import model_from_json
import numpy
import os
# fix random seed for reproducibility
numpy.random.seed(7)
# load pima indians dataset
dataset = numpy.loadtxt("/content/pima-indians-diabetes.csv", delimiter=",")
# split into input (X) and output (Y) variables
X = dataset[:,0:8]
Y = dataset[:,8]
# create model
model = Sequential()
model.add(Dense(12, input_dim=8, activation='relu'))
model.add(Dense(8, activation='relu'))
model.add(Dense(1, activation='sigmoid'))
# Compile model
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
# Fit the model
model.fit(X, Y, epochs=150, batch_size=10, verbose=0)
# evaluate the model
scores = model.evaluate(X, Y, verbose=0)
print("%s: %.2f%%" % (model.metrics_names[1], scores[1]*100))
# serialize model to JSON
model_json = model.to_json()
with open("model.json", "w") as json_file:
json_file.write(model_json)
# serialize weights to HDF5
model.save_weights("model.h5")
print("Saved model to disk")
# later...
# load json and create model
json_file = open('model.json', 'r')
loaded_model_json = json_file.read()
json_file.close()
loaded_model = model_from_json(loaded_model_json)
# load weights into new model
loaded_model.load_weights("model.h5")
print("Loaded model from disk")
# evaluate loaded model on test data
loaded_model.compile(loss='binary_crossentropy', optimizer='rmsprop', metrics=['accuracy'])
score = loaded_model.evaluate(X, Y, verbose=0)
print("%s: %.2f%%" % (loaded_model.metrics_names[1], score[1]*100))
from numpy import loadtxt
from keras.models import load_model
# load model
model.load_weights('/content/model.h5')
# summarize model.
model.summary()
# load dataset
dataset = loadtxt("/content/pima-indians-diabetes.csv", delimiter=",")
# split into input (X) and output (Y) variables
X = dataset[:,0:8]
Y = dataset[:,8]
# evaluate the model
score = model.evaluate(X, Y, verbose=0)
print("%s: %.2f%%" % (model.metrics_names[1], score[1]*100))
###Output
Model: "sequential_1"
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
dense_1 (Dense) (None, 12) 108
_________________________________________________________________
dense_2 (Dense) (None, 8) 104
_________________________________________________________________
dense_3 (Dense) (None, 1) 9
=================================================================
Total params: 221
Trainable params: 221
Non-trainable params: 0
_________________________________________________________________
acc: 77.99%
|
2020_12_05_Assignment/Assignment_1_Solution/Assignment 1.ipynb | ###Markdown
Assignment 1 Initial Setup---This sets up the code which reads the file and stores all the data each in its appropriate variable
###Code
# === Variables =========================================
code = ''
title = ''
fieldCount = 0
f = []
studentCount = 0
students = []
# === Functions =========================================
def sortDATA(filename):
'''Gets & reads the .txt file provided in the input and sorts its data into its relevant variables'''
global code, title, fieldCount, f, studentCount, students
with open(filename, 'rt') as sub:
for line in sub.readlines():
line = line.replace('\n','').replace(' ','').split('|')
for entry in line:
if entry == '':
line.pop(line.index(entry))
try:
line[line.index(entry)] = int(entry)
except:
try:
line[line.index(entry)] = float(entry)
except:
pass
if line[0] == 'Code':
code = line[1]
elif line[0] == 'Title':
title = line[1]
elif line[0] == 'FieldCount':
fieldCount = line[1]
elif line[0] == 'F':
for i in range(1,4):
f.append(line[i])
elif line[0] == 'StudentCount':
studentCount = line[1]
elif line[0] == 'S':
line.pop(0)
stuDetails = []
marks = []
for entry in line:
if type(entry) == float:
marks.append(entry)
else:
stuDetails.append(entry)
stuDetails.append(marks)
students.append(tuple(stuDetails))
students = tuple(students)
def printAllDATA():
'''Prints all the sorted Data for the subject loaded through the sortDATA() funtion.'''
print(code)
print(title)
print(fieldCount)
print(f)
print(studentCount)
print(students)
# Run the function
sortDATA('subject.txt')
###Output
_____no_output_____
###Markdown
Assignment 1 (a)
###Code
for student in students:
print(student)### Assignment 1 (a)
###Output
(18447565, 'VANDERGRAFF', 'T', [74.0, 42.5, 57.0])
(18907347, 'MARS', 'V', [80.5, 60.0, 72.0])
(18981993, 'FENNEL', 'WG', [77.5, 55.0, 69.0])
(18983070, 'ECHOLLS', 'L', [69.0, 40.0, 71.0])
(18930300, 'KANE', 'DS', [65.0, 47.5, 80.0])
(18915430, 'KANE', 'L', [0.0, 0.0, 34.0])
(18917104, 'NAVARRO', 'EW', [58.0, 35.0, 66.5])
(18928929, 'CASABLANCAS', 'D', [50.5, 57.5, 64.0])
(18982917, 'CASABLANCAS', 'C', [88.5, 62.5, 87.5])
(18971724, 'COOK', 'J', [61.0, 37.5, 63.5])
(18982863, 'MANNING', 'M', [51.5, 52.5, 79.0])
(18928875, 'GANT', 'C', [66.5, 32.5, 43.0])
(18927829, 'HAMILTON', 'Y', [69.0, 35.0, 48.0])
(18963759, 'TOOMBS', 'F', [83.0, 60.0, 74.0])
(18917386, 'DAMATO', 'L', [56.5, 70.0, 73.0])
(18961542, 'LAMB', 'D', [87.0, 42.5, 74.0])
(18130217, 'WEIDMAN', 'C', [25.0, 57.5, 86.0])
(18966580, 'CLEMMONS', 'V', [46.5, 32.5, 77.5])
(18934516, 'VANLOWE', 'V', [71.0, 17.5, 74.5])
(18933190, 'MCCORMACK', 'C', [71.5, 77.5, 70.5])
(18930122, 'POMEROY', 'S', [57.0, 57.5, 78.0])
(18940511, 'SINCLAIR', 'M', [75.5, 65.0, 60.5])
(18927590, 'GOODMAN', 'W', [89.5, 70.0, 84.5])
(18529509, 'MORAN', 'C', [51.0, 30.0, 77.5])
(18965595, 'GRIFFITH', 'H', [76.0, 50.0, 49.0])
(18933014, 'FITZPATRICK', 'M', [51.0, 32.5, 55.5])
###Markdown
Assignment 1 (b)
###Code
with open('finalmarks.txt', 'wt') as fMark:
fMark.write('') # Makes sure the 'finalmarks.txt' file is empty on each run of the script (won't replicate data)
for student in students:
name = student[1]+" "+student[2]
finMark = round(student[3][0]*(f[0]/100)) + round(student[3][1]*(f[1]/100)) + round(student[3][2]*(f[2]/100))
with open('finalmarks.txt', 'at') as fMark:
fMark.write(name+" "+str(finMark)+'\n')
###Output
_____no_output_____
###Markdown
Assignment 1 (c)
###Code
assignment1 = []
assignment2 = []
final = []
def avg(num):
sumOfNumbers = 0
for t in num:
sumOfNumbers = sumOfNumbers + t
avg = sumOfNumbers / len(num)
return round(avg, 2)
for student in students:
assignment1.append(student[3][0])
assignment2.append(student[3][1])
final.append(student[3][2])
print('| Assignment 1 |', str(min(assignment1)), " |", str(max(assignment1)), "|", str(avg(assignment1)), "|")
print('| Assignment 2 |', str(min(assignment2)), " |", str(max(assignment2)), "|", str(avg(assignment2)), "|")
print('| Final |', str(min(final)), "|", str(max(final)), "|", str(avg(final)), "|")
###Output
| Assignment 1 | 0.0 | 89.5 | 63.52 |
| Assignment 2 | 0.0 | 77.5 | 46.92 |
| Final | 34.0 | 87.5 | 68.04 |
|
mini_book/_build/jupyter_execute/docs/course_schedule_notebook.ipynb | ###Markdown
Course scheduleHere is the current week-by-week schedule. We may adjust as we go along. To get started, we're going to create the calendar of weeks for the course programmatically rather than manually!
###Code
## import modules
import pandas as pd
import re
## display output
from IPython.display import display, HTML
## create range b/t start and end date
## of course
start_date = pd.to_datetime("2021-03-29")
end_date = pd.to_datetime("2021-06-02")
st_alldates = pd.date_range(start_date, end_date)
## subset to days in that range equal to Tuesday or Thursday
st_tuth = st_alldates[st_alldates.day_name().isin(['Tuesday', 'Thursday'])]
## create data frame with that information
st_dates = [re.sub("2021\\-", "", str(day.date())) for day in st_tuth]
course_sched = pd.DataFrame({'dow': st_tuth.day_name(), 'st_tuth': st_dates})
course_sched['date_toprint'] = course_sched.dow.astype(str) + " " + \
course_sched.st_tuth.astype(str)
course_sched = course_sched['date_toprint']
## display the resulting date sequence
display(course_sched)
###Output
_____no_output_____ |
week3/day3/theory/statistics.ipynb | ###Markdown
Estadística Es la rama de las matemáticas que estudia la variabilidad, así como el proceso aleatorio que la genera siguiendo leyes de probabilidad.La estadística es útil para una amplia variedad de ciencias empíricas (la que entiende los hechos creando representaciones de la realidad), desde la física hasta las ciencias sociales, desde las ciencias de la salud hasta el control de calidad. Además, se usa en áreas de negocios o instituciones gubernamentales con el objetivo de describir el conjunto de datos obtenidos para la toma de decisiones, o bien para realizar generalizaciones sobre las características observadas. La estadística se divide en dos grandes áreas:- **Estadística descriptiva**: Se dedica a la descripción, visualización y resumen de datos originados a partir de los fenómenos de estudio. Los datos pueden ser resumidos numérica o gráficamente. Su objetivo es organizar y describir las características sobre un conjunto de datos con el propósito de facilitar su aplicación, generalmente con el apoyo de gráficas, tablas o medidas numéricas. - Ejemplos básicos de parámetros estadísticos son: la media y la desviación estándar. - Ejemplos gráficos son: histograma, pirámide poblacional, gráfico circular, entre otros.- **Estadística inferencial**: Se dedica a la generación de los modelos, inferencias y predicciones asociadas a los fenómenos en cuestión teniendo en cuenta la aleatoriedad de las observaciones. Se usa para modelar patrones en los datos y extraer inferencias acerca de la población bajo estudio. Estas inferencias pueden tomar la forma de respuestas a preguntas sí/no (prueba de hipótesis), estimaciones de unas características numéricas (estimación), pronósticos de futuras observaciones, descripciones de asociación (correlación) o modelamiento de relaciones entre variables (análisis de regresión). Otras técnicas de modelamiento incluyen análisis de varianza, series de tiempo y minería de datos. Su objetivo es obtener conclusiones útiles para lograr hacer deducciones acerca de la totalidad de todas las observaciones hechas, basándose en la información numérica. SamplingOne reason we need statistics is because we'd like to make statements about a general population based only on a subset - a *sample* - of the data. This can be for practical reasons, to reduce costs, or can be inherently necessary because of the nature of the problem. For example, it's not possible to collect data on "everyone who ever had a headache", so people wanting to study headaches will have to somehow get a group of people and try to generalize based on that.What's the right way to build that group? If a drug company decides to only ask its employees if their headache drug works, does that have any problems?*Yes* - let's discuss!
###Code
import random
population = range(100)
sample = random.sample(population, 10)
print(sample)
###Output
[92, 25, 83, 8, 30, 31, 48, 13, 88, 77]
###Markdown
--> Tarea Crear población a partir de lista de alturas de alumnos y con clases Conceptos básicos de la estadística descriptivaEn *[estadística descriptiva](https://es.wikipedia.org/wiki/Estad%C3%ADstica_descriptiva)* se utilizan distintas medidas para intentar describir las propiedades de nuestros datos, algunos de los conceptos básicos, son:* **Media aritmética**: La [media aritmética](https://es.wikipedia.org/wiki/Media_aritm%C3%A9tica) es el valor obtenido al sumar todos los *[datos](https://es.wikipedia.org/wiki/Dato)* y dividir el resultado entre el número total elementos. Se suele representar con la letra griega $\mu$. Si tenemos una [muestra](https://es.wikipedia.org/wiki/Muestra_estad%C3%ADstica) de $n$ valores, $x_i$, la *media aritmética*, $\mu$, es la suma de los valores divididos por el numero de elementos; en otras palabras:$$\mu = \frac{1}{n} \sum_{i}x_i$$* **Desviación respecto a la media**: La desviación respecto a la media es la diferencia en valor absoluto entre cada valor de la variable estadística y la media aritmética.$$D_i = |x_i - \mu|$$* **Varianza**: La [varianza](https://es.wikipedia.org/wiki/Varianza) es la media aritmética del cuadrado de las desviaciones respecto a la media de una distribución estadística. La varianza intenta describir la dispersión de los *[datos](https://es.wikipedia.org/wiki/Dato). Básicamente representa lo que varían los datos.* Se representa como $\sigma^2$. $$\sigma^2 = \frac{\sum\limits_{i=1}^n(x_i - \mu)^2}{n} $$* **Desviación típica**: La [desviación típica](https://es.wikipedia.org/wiki/Desviaci%C3%B3n_t%C3%ADpica) es la raíz cuadrada de la varianza. Se representa con la letra griega $\sigma$.$$\sigma = \sqrt{\frac{\sum\limits_{i=1}^n(x_i - \mu)^2}{n}} $$* **Moda**: La moda es el valor que tiene mayor frecuencia absoluta. Se representa con $M_0$* **Mediana**: La mediana es el valor que ocupa el lugar central de todos los datos cuando éstos están ordenados de menor a mayor. Se representa con $\widetilde{x}$.* **Correlación**: La [correlación](https://es.wikipedia.org/wiki/Correlaci%C3%B3n) trata de establecer la relación o dependencia que existe entre las dos variables que intervienen en una distribución bidimensional. Es decir, determinar si los cambios en una de las variables influyen en los cambios de la otra. En caso de que suceda, diremos que las variables están correlacionadas o que hay correlación entre ellas. La correlación es positiva cuando los valores de las variables aumenta juntos; y es negativa cuando un valor de una variable se reduce cuando el valor de la otra variable aumenta.* **Covarianza**: La [covarianza](https://es.wikipedia.org/wiki/Covarianza) es el equivalente de la varianza aplicado a una variable bidimensional. Es la media aritmética de los productos de las desviaciones de cada una de las variables respecto a sus medias respectivas.La covarianza indica el sentido de la correlación entre las variables; Si $\sigma_{xy} > 0$ la correlación es directa; Si $\sigma_{xy} < 0$ la correlación es inversa.$$\sigma_{xy} = \frac{\sum\limits_{i=1}^n(x_i - \mu_x)(y_i -\mu_y)}{n}$$* **Valor atípico (Outlier)**: Un [valor atípico](https://es.wikipedia.org/wiki/Valor_at%C3%ADpico) es una observación que se aleja demasiado de la moda; esta muy lejos de la tendencia principal del resto de los *[datos](https://es.wikipedia.org/wiki/Dato)*. Pueden ser causados por errores en la recolección de *[datos](https://es.wikipedia.org/wiki/Dato)* o medidas inusuales. Generalmente se recomienda eliminarlos del [conjunto de datos](https://es.wikipedia.org/wiki/Conjunto_de_datos). 1. https://towardsdatascience.com/a-quick-guide-on-descriptive-statistics-using-pandas-and-seaborn-2aadc7395f322. https://www.tutorialspoint.com/python_pandas/python_pandas_descriptive_statistics.htm Ejemplos en PythonCalcular los principales indicadores de la *[estadística descriptiva](https://es.wikipedia.org/wiki/Estad%C3%ADstica_descriptiva)* con [Python](http://python.org/) es muy fácil!.
###Code
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
print(np.abs(-2))
import numpy as np
abs()
lista = [2, 4, 6, 8]
print(type(lista))
lista_array = np.asarray(lista)
print(type(lista_array))
max(lista)
lista_array.argmax()
lista_array.max()
max(lista_array)
x = list(lista_array)
from scipy import unique, stats
# Ejemplos de estadistica descriptiva con python
import numpy as np # importando numpy
from scipy import stats # importando scipy.stats
import pandas as pd # importando pandas
np.random.seed(4) # para poder replicar el random
lista = [2., 1.76, 1.8, 1.6]
a = np.array(lista)
a
datos = np.random.randn(5, 4) # datos normalmente distribuidos
datos
# media arítmetica
datos.mean() # Calcula la media aritmetica de los datos
x = np.mean(datos) # Mismo resultado desde la funcion de numpy
x
datos
datos.mean(axis=1) # media aritmetica de cada fila
datos.mean(axis=0) # media aritmetica de cada columna
# mediana
np.median(datos)
np.median(datos, axis=0) # media aritmetica de cada columna
import numpy as np
# Desviación típica
np.std(datos)
type(datos)
datos.std()
np.std(datos, 0) # Desviación típica de cada columna
lista = [1, 3, 5, 7]
np.mean(a=lista)
x = np.array(lista)
x
x.mean()
# varianza
np.var(datos)
datos.var()
np.var(datos, axis=0) # varianza de cada columna
# moda
stats.mode(datos) # Calcula la moda de cada columna
# el 2do array devuelve la frecuencia.
datos2 = np.array([1, 2, 3, 6, 6, 1, 2, 4, 2, 2, 6, 6, 8, 10, 6])
from scipy import stats # importando scipy.stats
stats.mode(datos2) # aqui la moda es el 6 porque aparece 5 veces en el vector.
# correlacion
np.corrcoef(datos) # Crea matriz de correlación.
# calculando la correlación entre dos vectores.
np.corrcoef(datos[0], datos[1])
# covarianza
np.cov(datos) # calcula matriz de covarianza
# covarianza de dos vectores
np.cov(datos[0], datos[1])
datos
datos
def f1(index):
return index[0]
l = ['a', 'b', 'c', 'd', 'e']
f1(index=['a', 'b', 'c', 'd', 'e'])
import pandas as pd
# usando pandas
dataframe = pd.DataFrame(data=datos, index=['a', 'b', 'c', 'd', 'e'],
columns=['col1', 'col2', 'col3', 'col4'])
dataframe
df = pd.DataFrame(data=datos)
df
dataframe["col1"].values
# resumen estadistadistico con pandas
dataframe.describe()
lista = [2., 1.76, 1.8, 1.6]
lista_array = np.array(lista)
print(lista_array)
dataframe2 = pd.DataFrame(lista_array, index=['a', 'b', 'c', 'd'],
columns=['col1'])
dataframe2
dataframe
# sumando las columnas
dataframe.sum()
# sumando filas
dataframe.sum(axis=1)
dataframe.cumsum() # acumulados
# media aritmética de cada columna con pandas
dataframe.mean()
# media aritmética de cada fila con pandas
dataframe.mean(axis=1)
altura1 = [1.78, 1.63, 1.75, 1.68]
altura2 = [2.00, 1.82, 1.76, 1.66]
altura3 = [1.65, 1.73, 1.75, 1.76]
altura4 = [1.72, 1.71, 1.71, 1.62]
lista_alturas = [altura1, altura2, altura3, altura4]
class Humano():
def __init__(self, altura):
self.altura = altura
def crece(self):
self.altura = self.altura + 2.0
lista_humanos = []
for col_alt in lista_alturas:
for alt in col_alt:
humano = Humano(altura=alt)
lista_humanos.append(humano)
lista_humanos
for humano in lista_humanos:
print(humano.altura)
humano.crece()
print(humano.altura)
print("----------")
lista_alturas
x = np.arange(0, 15)
x
lista = [2, 5, 7]
print(sum(lista))
import numpy as np
def axis_x(limit):
return np.arange(0, limit)
total_elements_x = axis_x(limit=sum([len(x) for x in lista_alturas]))
total_elements_x
lista_alturas_total = []
for humano in lista_humanos:
lista_alturas_total.append(humano.altura)
array_alturas_completo = np.array(lista_alturas_total).T
array_alturas_completo
array_alturas_completo.shape
import pandas as pd
df = pd.DataFrame({'Alturas':array_alturas_completo})
df
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
plt.plot(lista_alturas_alumnos, linewidth=2, marker='o', color="g", linestyle='dashed', markersize=12)
plt.ylabel("Alturas")
plt.show()
import mi_libreria as ml
ml.grafica_verde(lista=lista_alturas_alumnos, ylabel="Alturas 2")
def create_array_with_same_value(value, limit):
return np.full(limit, value)
a = create_array_with_same_value(2, 80)
a
a = np.arange(4)
a
media = np.mean(lista_alturas_alumnos)
media_graph = create_array_with_same_value(value=media, limit=len(lista_alturas_alumnos))
print(lista_alturas_alumnos)
media_graph
print(len(lista_alturas_alumnos))
print(len(media_graph))
# Añadimos la media a la gráfica
plt.plot(lista_alturas_alumnos, "ro")
media = np.mean(lista_alturas_alumnos)
print("Media:", media)
media_graph = create_array_with_same_value(value=media, limit=len(lista_alturas_alumnos))
plt.ylabel("Alturas")
plt.plot(media_graph, "b--")
plt.show()
lista_alturas_total = np.array(lista_alturas_total)
std = np.std(lista_alturas_total)
std
std_superior = media + std
std_inferior = media - std
plt.plot(lista_alturas_total, "ro")
media = np.mean(lista_alturas_total)
print("Media:", media)
std_superior_total = create_array_with_same_value(value=std_superior, limit=numero_de_alturas)
std_inferior_total = create_array_with_same_value(value=std_inferior, limit=numero_de_alturas)
plt.ylabel("Alturas")
plt.plot(std_superior_total, "b--")
plt.plot(std_inferior_total, "g--")
plt.show()
lista = [[[[2,4,6,8], [5,6,7,8]]]]
lista_array = np.array(lista)
lista_array
lista_array.shape
arrays_alturas = np.array([np.array(x) for x in lista_alturas])
arrays_alturas
np.mean(arrays_alturas)
np.mean(arrays_alturas, 1) # Fila
np.mean(arrays_alturas, 0) # Columna
lista = [2, 2, 2, 4, 6, 10, 10]
2 --> 3
4 --> 1
6 --> 1
10 -> 2
import pandas as pd
import numpy as np
df = pd.DataFrame({'Alturas':np.array(lista_alturas_alumnos)})
df
df.hist(bins=10)
###Output
_____no_output_____
###Markdown
Histogramas y DistribucionesMuchas veces los indicadores de la *[estadística descriptiva](https://es.wikipedia.org/wiki/Estad%C3%ADstica_descriptiva)* no nos proporcionan una imagen clara de nuestros *[datos](https://es.wikipedia.org/wiki/Dato)*. Por esta razón, siempre es útil complementarlos con gráficos de las distribuciones de los *[datos](https://es.wikipedia.org/wiki/Dato)*, que describan con qué frecuencia aparece cada valor. La representación más común de una distribución es un [histograma](https://es.wikipedia.org/wiki/Histograma), que es un gráfico que muestra la frecuencia o probabilidad de cada valor. El [histograma](https://es.wikipedia.org/wiki/Histograma) muestra las frecuencias como un gráfico de barras que indica cuan frecuente un determinado valor ocurre en el [conjunto de datos](https://es.wikipedia.org/wiki/Conjunto_de_datos). El eje horizontal representa los valores del [conjunto de datos](https://es.wikipedia.org/wiki/Conjunto_de_datos) y el eje vertical representa la frecuencia con que esos valores ocurren.Las distribuciones se pueden clasificar en dos grandes grupos:1. Las **[distribuciones continuas](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_de_probabilidad_continua)**, que son aquellas que presentan un número infinito de posibles soluciones. Dentro de este grupo vamos a encontrar a las distribuciones: * [normal](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_normal), * [gamma](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_gamma), * [chi cuadrado](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_%CF%87%C2%B2), * [t de Student](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_t_de_Student), * [pareto](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_de_Pareto), * entre otras2. Las **distribuciones discretas**, que son aquellas en las que la variable puede pude tomar un número determinado de valores. Los principales exponenetes de este grupo son las distribuciones: * [poisson](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_de_Poisson), * [binomial](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_binomial), * [hipergeométrica](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_hipergeom%C3%A9trica), * [bernoulli](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_de_Bernoulli) * entre otrasVeamos algunos ejemplos graficados con la ayuda de [Python](http://python.org/).
###Code
# Histogram
df.hist()
###Output
_____no_output_____
###Markdown
Distribución normalLa [distribución normal](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_normal) es una de las principales distribuciones, ya que es la que con más frecuencia aparece aproximada en los fenómenos reales. Tiene una forma acampanada y es simétrica respecto de un determinado parámetro estadístico. Con la ayuda de [Python](http://python.org/) la podemos graficar de la siguiente manera:
###Code
# Graficos embebidos.
%matplotlib inline
import matplotlib.pyplot as plt # importando matplotlib
import seaborn as sns # importando seaborn
# parametros esteticos de seaborn
sns.set_palette("deep", desat=.6)
sns.set_context(rc={"figure.figsize": (8, 4)})
mu, sigma = 0, 0.1 # media y desvio estandar
s = np.random.normal(mu, sigma, 1000) #creando muestra de datos
# histograma de distribución normal.
cuenta, cajas, ignorar = plt.hist(s, 30, normed=True)
normal = plt.plot(cajas, 1/(sigma * np.sqrt(2 * np.pi)) *
np.exp( - (cajas - mu)**2 / (2 * sigma**2) ),
linewidth=2, color='r')
###Output
_____no_output_____
###Markdown
Distribuciones simétricas y asimétricasUna distribución es simétrica cuando moda, mediana y media coinciden aproximadamente en sus valores. Si una distribución es simétrica, existe el mismo número de valores a la derecha que a la izquierda de la media, por tanto, el mismo número de desviaciones con signo positivo que con signo negativo.Una distribución tiene [asimetria](https://es.wikipedia.org/wiki/Asimetr%C3%ADa_estad%C3%ADstica) positiva (o a la derecha) si la "cola" a la derecha de la media es más larga que la de la izquierda, es decir, si hay valores más separados de la media a la derecha. De la misma forma una distribución tiene [asimetria](https://es.wikipedia.org/wiki/Asimetr%C3%ADa_estad%C3%ADstica) negativa (o a la izquierda) si la "cola" a la izquierda de la media es más larga que la de la derecha, es decir, si hay valores más separados de la media a la izquierda.Las distribuciones asimétricas suelen ser problemáticas, ya que la mayoría de los métodos estadísticos suelen estar desarrollados para distribuciones del tipo [normal](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_normal). Para salvar estos problemas se suelen realizar transformaciones a los datos para hacer a estas distribuciones más simétricas y acercarse a la [distribución normal](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_normal).
###Code
# Dibujando la distribucion Gamma
x = stats.gamma(3).rvs(5000)
gamma = plt.hist(x, 70, histtype="stepfilled", alpha=.7)
###Output
_____no_output_____
###Markdown
En este ejemplo podemos ver que la [distribución gamma](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_gamma) que dibujamos tiene una [asimetria](https://es.wikipedia.org/wiki/Asimetr%C3%ADa_estad%C3%ADstica) positiva.
###Code
# Calculando la simetria con scipy
stats.skew(x)
###Output
_____no_output_____
###Markdown
Cuartiles y diagramas de cajasLos **[cuartiles](https://es.wikipedia.org/wiki/Cuartil)** son los tres valores de la variable estadística que dividen a un [conjunto de datos](https://es.wikipedia.org/wiki/Conjunto_de_datos) ordenados en cuatro partes iguales. Q1, Q2 y Q3 determinan los valores correspondientes al 25%, al 50% y al 75% de los datos. Q2 coincide con la mediana.Los [diagramas de cajas](https://es.wikipedia.org/wiki/Diagrama_de_caja) son una presentación visual que describe varias características importantes al mismo tiempo, tales como la dispersión y simetría. Para su realización se representan los tres cuartiles y los valores mínimo y máximo de los datos, sobre un rectángulo, alineado horizontal o verticalmente. Estos gráficos nos proporcionan abundante información y son sumamente útiles para encontrar [valores atípicos](https://es.wikipedia.org/wiki/Valor_at%C3%ADpico) y comparar dos [conjunto de datos](https://es.wikipedia.org/wiki/Conjunto_de_datos).
###Code
lista = [2, 3, 4, 5,6,7,9,10,11, 1000]
import matplotlib.pyplot as plt
# Ejemplo de grafico de cajas en python
datos_1 = np.random.normal(100, 10, 200)
#datos_2 = np.random.normal(80, 30, 200)
datos_3 = np.random.normal(90, 20, 200)
datos_4 = np.random.normal(70, 25, 200)
datos_graf = [datos_1, datos_2, datos_3, datos_4]
# Creando el objeto figura
fig = plt.figure(1, figsize=(9, 6))
# Creando el subgrafico
ax = fig.add_subplot(111)
# creando el grafico de cajas
bp = ax.boxplot(datos_graf)
# visualizar mas facile los atípicos
for flier in bp['fliers']:
flier.set(marker='o', color='red', alpha=0.5)
# los puntos aislados son valores atípicos
df = pd.DataFrame(datos_2)
df
es_menor_a_80 = 0
for value in datos_2:
if value <= 80:
es_menor_a_80 += 1
print(es_menor_a_80)
df.hist(bins=2)
x = list(datos_2)
x.append(500)
datos_2 = np.array(x)
df = pd.DataFrame(datos_2)
df.hist()
# usando seaborn
sns.boxplot(datos_graf, names=["grupo1", "grupo2", "grupo3", "grupo 4"],
color="PaleGreen");
###Output
_____no_output_____
###Markdown
Estadística Es la rama de las matemáticas que estudia la variabilidad, así como el proceso aleatorio que la genera siguiendo leyes de probabilidad.La estadística es útil para una amplia variedad de ciencias empíricas (la que entiende los hechos creando representaciones de la realidad), desde la física hasta las ciencias sociales, desde las ciencias de la salud hasta el control de calidad. Además, se usa en áreas de negocios o instituciones gubernamentales con el objetivo de describir el conjunto de datos obtenidos para la toma de decisiones, o bien para realizar generalizaciones sobre las características observadas. La estadística se divide en dos grandes áreas:- **Estadística descriptiva**: Se dedica a la descripción, visualización y resumen de datos originados a partir de los fenómenos de estudio. Los datos pueden ser resumidos numérica o gráficamente. Su objetivo es organizar y describir las características sobre un conjunto de datos con el propósito de facilitar su aplicación, generalmente con el apoyo de gráficas, tablas o medidas numéricas. - Ejemplos básicos de parámetros estadísticos son: la media y la desviación estándar. - Ejemplos gráficos son: histograma, pirámide poblacional, gráfico circular, entre otros.- **Estadística inferencial**: Se dedica a la generación de los modelos, inferencias y predicciones asociadas a los fenómenos en cuestión teniendo en cuenta la aleatoriedad de las observaciones. Se usa para modelar patrones en los datos y extraer inferencias acerca de la población bajo estudio. Estas inferencias pueden tomar la forma de respuestas a preguntas sí/no (prueba de hipótesis), estimaciones de unas características numéricas (estimación), pronósticos de futuras observaciones, descripciones de asociación (correlación) o modelamiento de relaciones entre variables (análisis de regresión). Otras técnicas de modelamiento incluyen análisis de varianza, series de tiempo y minería de datos. Su objetivo es obtener conclusiones útiles para lograr hacer deducciones acerca de la totalidad de todas las observaciones hechas, basándose en la información numérica. SamplingOne reason we need statistics is because we'd like to make statements about a general population based only on a subset - a *sample* - of the data. This can be for practical reasons, to reduce costs, or can be inherently necessary because of the nature of the problem. For example, it's not possible to collect data on "everyone who ever had a headache", so people wanting to study headaches will have to somehow get a group of people and try to generalize based on that.What's the right way to build that group? If a drug company decides to only ask its employees if their headache drug works, does that have any problems?*Yes* - let's discuss!
###Code
import random
population = range(100)
sample = random.sample(population, 10) #sample crea una muestra aleatorio dentro del rango
print(sample)
###Output
[2, 78, 40, 59, 13, 31, 24, 43, 29, 68]
###Markdown
--> Tarea Crear población a partir de lista de alturas de alumnos y con clases Conceptos básicos de la estadística descriptivaEn *[estadística descriptiva](https://es.wikipedia.org/wiki/Estad%C3%ADstica_descriptiva)* se utilizan distintas medidas para intentar describir las propiedades de nuestros datos, algunos de los conceptos básicos, son:* **Media aritmética**: La [media aritmética](https://es.wikipedia.org/wiki/Media_aritm%C3%A9tica) es el valor obtenido al sumar todos los *[datos](https://es.wikipedia.org/wiki/Dato)* y dividir el resultado entre el número total elementos. Se suele representar con la letra griega $\mu$. Si tenemos una [muestra](https://es.wikipedia.org/wiki/Muestra_estad%C3%ADstica) de $n$ valores, $x_i$, la *media aritmética*, $\mu$, es la suma de los valores divididos por el numero de elementos; en otras palabras:$$\mu = \frac{1}{n} \sum_{i}x_i$$* **Desviación respecto a la media**: La desviación respecto a la media es la diferencia en valor absoluto entre cada valor de la variable estadística y la media aritmética.$$D_i = |x_i - \mu|$$* **Varianza**: La [varianza](https://es.wikipedia.org/wiki/Varianza) es la media aritmética del cuadrado de las desviaciones respecto a la media de una distribución estadística. La varianza intenta describir la dispersión de los *[datos](https://es.wikipedia.org/wiki/Dato). Básicamente representa lo que varían los datos.* Se representa como $\sigma^2$. $$\sigma^2 = \frac{\sum\limits_{i=1}^n(x_i - \mu)^2}{n} $$* **Desviación típica**: La [desviación típica](https://es.wikipedia.org/wiki/Desviaci%C3%B3n_t%C3%ADpica) es la raíz cuadrada de la varianza. Se representa con la letra griega $\sigma$.$$\sigma = \sqrt{\frac{\sum\limits_{i=1}^n(x_i - \mu)^2}{n}} $$* **Moda**: La moda es el valor que tiene mayor frecuencia absoluta. Se representa con $M_0$* **Mediana**: La mediana es el valor que ocupa el lugar central de todos los datos cuando éstos están ordenados de menor a mayor. Se representa con $\widetilde{x}$.* **Correlación**: La [correlación](https://es.wikipedia.org/wiki/Correlaci%C3%B3n) trata de establecer la relación o dependencia que existe entre las dos variables que intervienen en una distribución bidimensional. Es decir, determinar si los cambios en una de las variables influyen en los cambios de la otra. En caso de que suceda, diremos que las variables están correlacionadas o que hay correlación entre ellas. La correlación es positiva cuando los valores de las variables aumenta juntos; y es negativa cuando un valor de una variable se reduce cuando el valor de la otra variable aumenta.* **Covarianza**: La [covarianza](https://es.wikipedia.org/wiki/Covarianza) es el equivalente de la varianza aplicado a una variable bidimensional. Es la media aritmética de los productos de las desviaciones de cada una de las variables respecto a sus medias respectivas.La covarianza indica el sentido de la correlación entre las variables; Si $\sigma_{xy} > 0$ la correlación es directa; Si $\sigma_{xy} < 0$ la correlación es inversa.$$\sigma_{xy} = \frac{\sum\limits_{i=1}^n(x_i - \mu_x)(y_i -\mu_y)}{n}$$* **Valor atípico (Outlier)**: Un [valor atípico](https://es.wikipedia.org/wiki/Valor_at%C3%ADpico) es una observación que se aleja demasiado de la moda; esta muy lejos de la tendencia principal del resto de los *[datos](https://es.wikipedia.org/wiki/Dato)*. Pueden ser causados por errores en la recolección de *[datos](https://es.wikipedia.org/wiki/Dato)* o medidas inusuales. Generalmente se recomienda eliminarlos del [conjunto de datos](https://es.wikipedia.org/wiki/Conjunto_de_datos). 1. https://towardsdatascience.com/a-quick-guide-on-descriptive-statistics-using-pandas-and-seaborn-2aadc7395f322. https://www.tutorialspoint.com/python_pandas/python_pandas_descriptive_statistics.htm Ejemplos en PythonCalcular los principales indicadores de la *[estadística descriptiva](https://es.wikipedia.org/wiki/Estad%C3%ADstica_descriptiva)* con [Python](http://python.org/) es muy fácil!.
###Code
import numpy as np #np y pd es un alias. son librerias de estadistica y tratamientos de datos
import pandas as pd
import matplotlib.pyplot as pyplot
#se instalan en terminal
#pip3 install numpy
from numpy import *
#importo las funciones de numpy a jupiter y se puede usar sin np.
#no se recomienda
lista = [2, 4, 6, 8]
print(type(lista))
lista_array = np.asarray(lista) #numpy trabaja con arrays. cambio de lista a array con asarray(lista) ARRAY tiene sus propios metodos incluidos en np
#el equivalente a max(lista) q nos da el numero mas alto es lista_array.argmax()
#list(lista_array) paso de array a lista
print(type(lista_array))
np.arange()
from scipy import unique, stats
# Ejemplos de estadistica descriptiva con python
import numpy as np # importando numpy
from scipy import stats # importando scipy.stats
import pandas as pd # importando pandas
np.random.seed(4) # para poder replicar el random. fichero semilla seed
lista = [2., 1.76, 1.8, 1.6]
a = np.array(lista)
a
datos = np.random.randn(5, 4) # datos normalmente distribuidos. 5 filas y 4 columnas
datos #ya es un array de numpy
# media arítmetica
datos.mean() # Calcula la media aritmetica de los datos. no le alado np pq es un array q ya es de numpy
x = np.mean(datos) # Mismo resultado desde la funcion de numpy
x
datos
datos.mean(axis=1) # media aritmetica de cada fila
datos.mean(axis=0) # media aritmetica de cada columna
# mediana
np.median(datos)
np.median(datos, axis=0) # media aritmetica de cada columna
import numpy as np
# Desviación típica
np.std(datos)
type(datos)
datos.std()
np.std(datos, 0) # Desviación típica de cada columna
lista = [1, 3, 5, 7]
np.mean(a=lista)
x = np.array(lista)
x
x.mean()
# varianza
np.var(datos)
datos.var()
np.var(datos, 0) # varianza de cada columna
# moda
stats.mode(datos) # Calcula la moda de cada columna
# el 2do array devuelve la frecuencia.
datos2 = np.array([1, 2, 3, 6, 6, 1, 2, 4, 2, 2, 6, 6, 8, 10, 6])
from scipy import stats # importando scipy.stats
stats.mode(datos2) # aqui la moda es el 6 porque aparece 5 veces en el vector.
# correlacion
np.corrcoef(datos) # Crea matriz de correlación.
# calculando la correlación entre dos vectores.
np.corrcoef(datos[0], datos[1])
# covarianza
np.cov(datos) # calcula matriz de covarianza
# covarianza de dos vectores
np.cov(datos[0], datos[1])
datos
import pandas as pd
# usando pandas
dataframe = pd.DataFrame(datos, index=['a', 'b', 'c', 'd', 'e'],
columns=['col1', 'col2', 'col3', 'col4'])
dataframe
dataframe["col1"].values
# resumen estadistadistico con pandas
dataframe.describe()
lista = [2., 1.76, 1.8, 1.6]
lista_array = np.array(lista)
print(lista_array)
dataframe2 = pd.DataFrame(lista_array, index=['a', 'b', 'c', 'd'],
columns=['col1'])
dataframe2
dataframe
# sumando las columnas
dataframe.sum()
# sumando filas
dataframe.sum(axis=1)
dataframe.cumsum() # acumulados
# media aritmética de cada columna con pandas
dataframe.mean()
# media aritmética de cada fila con pandas
dataframe.mean(axis=1)
altura1 = [1.78, 1.63, 1.75, 1.68]
altura2 = [2.00, 1.82, 1.76, 1.66]
altura3 = [1.65, 1.73, 1.75, 1.76]
altura4 = [1.72, 1.71, 1.71, 1.62]
lista_alturas = [altura1, altura2, altura3, altura4]
class Humano():
def __init__(self, altura):
self.altura = altura
def crece(self):
self.altura = self.altura + 2.0
lista_humanos = []
for col_alt in lista_alturas:
for alt in col_alt:
humano = Humano(altura=alt)
lista_humanos.append(humano)
lista_humanos
for humano in lista_humanos:
print(humano.altura)
humano.crece()
print(humano.altura)
print("----------")
lista_alturas
x = np.arange(0, 15)
x
lista = [2, 5, 7]
print(sum(lista))
import numpy as np
def axis_x(limit):
return np.arange(0, limit)
total_elements_x = axis_x(limit=sum([len(x) for x in lista_alturas]))
total_elements_x
lista_alturas_total = []
for humano in lista_humanos:
lista_alturas_total.append(humano.altura)
array_alturas_completo = np.array(lista_alturas_total).T
array_alturas_completo
array_alturas_completo.shape
import pandas as pd
df = pd.DataFrame({'Alturas':array_alturas_completo})
df
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
plt.plot(lista_alturas_alumnos, linewidth=2, marker='o', color="g", linestyle='dashed', markersize=12)
plt.ylabel("Alturas")
plt.show()
import mi_libreria as ml
ml.grafica_verde(lista=lista_alturas_alumnos, ylabel="Alturas 2")
def create_array_with_same_value(value, limit):
return np.full(limit, value)
a = create_array_with_same_value(2, 80)
a
a = np.arange(4)
a
media = np.mean(lista_alturas_alumnos)
media_graph = create_array_with_same_value(value=media, limit=len(lista_alturas_alumnos))
print(lista_alturas_alumnos)
media_graph
print(len(lista_alturas_alumnos))
print(len(media_graph))
# Añadimos la media a la gráfica
plt.plot(lista_alturas_alumnos, "ro")
media = np.mean(lista_alturas_alumnos)
print("Media:", media)
media_graph = create_array_with_same_value(value=media, limit=len(lista_alturas_alumnos))
plt.ylabel("Alturas")
plt.plot(media_graph, "b--")
plt.show()
lista_alturas_total = np.array(lista_alturas_total)
std = np.std(lista_alturas_total)
std
std_superior = media + std
std_inferior = media - std
plt.plot(lista_alturas_total, "ro")
media = np.mean(lista_alturas_total)
print("Media:", media)
std_superior_total = create_array_with_same_value(value=std_superior, limit=numero_de_alturas)
std_inferior_total = create_array_with_same_value(value=std_inferior, limit=numero_de_alturas)
plt.ylabel("Alturas")
plt.plot(std_superior_total, "b--")
plt.plot(std_inferior_total, "g--")
plt.show()
lista = [[[[2,4,6,8], [5,6,7,8]]]]
lista_array = np.array(lista)
lista_array
lista_array.shape
arrays_alturas = np.array([np.array(x) for x in lista_alturas])
arrays_alturas
np.mean(arrays_alturas)
np.mean(arrays_alturas, 1) # Fila
np.mean(arrays_alturas, 0) # Columna
lista = [2, 2, 2, 4, 6, 10, 10]
2 --> 3
4 --> 1
6 --> 1
10 -> 2
import pandas as pd
import numpy as np
df = pd.DataFrame({'Alturas':np.array(lista_alturas_alumnos)})
df
df.hist(bins=10)
###Output
_____no_output_____
###Markdown
Histogramas y DistribucionesMuchas veces los indicadores de la *[estadística descriptiva](https://es.wikipedia.org/wiki/Estad%C3%ADstica_descriptiva)* no nos proporcionan una imagen clara de nuestros *[datos](https://es.wikipedia.org/wiki/Dato)*. Por esta razón, siempre es útil complementarlos con gráficos de las distribuciones de los *[datos](https://es.wikipedia.org/wiki/Dato)*, que describan con qué frecuencia aparece cada valor. La representación más común de una distribución es un [histograma](https://es.wikipedia.org/wiki/Histograma), que es un gráfico que muestra la frecuencia o probabilidad de cada valor. El [histograma](https://es.wikipedia.org/wiki/Histograma) muestra las frecuencias como un gráfico de barras que indica cuan frecuente un determinado valor ocurre en el [conjunto de datos](https://es.wikipedia.org/wiki/Conjunto_de_datos). El eje horizontal representa los valores del [conjunto de datos](https://es.wikipedia.org/wiki/Conjunto_de_datos) y el eje vertical representa la frecuencia con que esos valores ocurren.Las distribuciones se pueden clasificar en dos grandes grupos:1. Las **[distribuciones continuas](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_de_probabilidad_continua)**, que son aquellas que presentan un número infinito de posibles soluciones. Dentro de este grupo vamos a encontrar a las distribuciones: * [normal](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_normal), * [gamma](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_gamma), * [chi cuadrado](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_%CF%87%C2%B2), * [t de Student](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_t_de_Student), * [pareto](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_de_Pareto), * entre otras2. Las **distribuciones discretas**, que son aquellas en las que la variable puede pude tomar un número determinado de valores. Los principales exponenetes de este grupo son las distribuciones: * [poisson](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_de_Poisson), * [binomial](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_binomial), * [hipergeométrica](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_hipergeom%C3%A9trica), * [bernoulli](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_de_Bernoulli) * entre otrasVeamos algunos ejemplos graficados con la ayuda de [Python](http://python.org/).
###Code
# Histogram
df.hist()
###Output
_____no_output_____
###Markdown
Distribución normalLa [distribución normal](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_normal) es una de las principales distribuciones, ya que es la que con más frecuencia aparece aproximada en los fenómenos reales. Tiene una forma acampanada y es simétrica respecto de un determinado parámetro estadístico. Con la ayuda de [Python](http://python.org/) la podemos graficar de la siguiente manera:
###Code
# Graficos embebidos.
%matplotlib inline
import matplotlib.pyplot as plt # importando matplotlib
import seaborn as sns # importando seaborn
# parametros esteticos de seaborn
sns.set_palette("deep", desat=.6)
sns.set_context(rc={"figure.figsize": (8, 4)})
mu, sigma = 0, 0.1 # media y desvio estandar
s = np.random.normal(mu, sigma, 1000) #creando muestra de datos
# histograma de distribución normal.
cuenta, cajas, ignorar = plt.hist(s, 30, normed=True)
normal = plt.plot(cajas, 1/(sigma * np.sqrt(2 * np.pi)) *
np.exp( - (cajas - mu)**2 / (2 * sigma**2) ),
linewidth=2, color='r')
###Output
_____no_output_____
###Markdown
Distribuciones simétricas y asimétricasUna distribución es simétrica cuando moda, mediana y media coinciden aproximadamente en sus valores. Si una distribución es simétrica, existe el mismo número de valores a la derecha que a la izquierda de la media, por tanto, el mismo número de desviaciones con signo positivo que con signo negativo.Una distribución tiene [asimetria](https://es.wikipedia.org/wiki/Asimetr%C3%ADa_estad%C3%ADstica) positiva (o a la derecha) si la "cola" a la derecha de la media es más larga que la de la izquierda, es decir, si hay valores más separados de la media a la derecha. De la misma forma una distribución tiene [asimetria](https://es.wikipedia.org/wiki/Asimetr%C3%ADa_estad%C3%ADstica) negativa (o a la izquierda) si la "cola" a la izquierda de la media es más larga que la de la derecha, es decir, si hay valores más separados de la media a la izquierda.Las distribuciones asimétricas suelen ser problemáticas, ya que la mayoría de los métodos estadísticos suelen estar desarrollados para distribuciones del tipo [normal](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_normal). Para salvar estos problemas se suelen realizar transformaciones a los datos para hacer a estas distribuciones más simétricas y acercarse a la [distribución normal](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_normal).
###Code
# Dibujando la distribucion Gamma
x = stats.gamma(3).rvs(5000)
gamma = plt.hist(x, 70, histtype="stepfilled", alpha=.7)
###Output
_____no_output_____
###Markdown
En este ejemplo podemos ver que la [distribución gamma](https://es.wikipedia.org/wiki/Distribuci%C3%B3n_gamma) que dibujamos tiene una [asimetria](https://es.wikipedia.org/wiki/Asimetr%C3%ADa_estad%C3%ADstica) positiva.
###Code
# Calculando la simetria con scipy
stats.skew(x)
###Output
_____no_output_____
###Markdown
Cuartiles y diagramas de cajasLos **[cuartiles](https://es.wikipedia.org/wiki/Cuartil)** son los tres valores de la variable estadística que dividen a un [conjunto de datos](https://es.wikipedia.org/wiki/Conjunto_de_datos) ordenados en cuatro partes iguales. Q1, Q2 y Q3 determinan los valores correspondientes al 25%, al 50% y al 75% de los datos. Q2 coincide con la mediana.Los [diagramas de cajas](https://es.wikipedia.org/wiki/Diagrama_de_caja) son una presentación visual que describe varias características importantes al mismo tiempo, tales como la dispersión y simetría. Para su realización se representan los tres cuartiles y los valores mínimo y máximo de los datos, sobre un rectángulo, alineado horizontal o verticalmente. Estos gráficos nos proporcionan abundante información y son sumamente útiles para encontrar [valores atípicos](https://es.wikipedia.org/wiki/Valor_at%C3%ADpico) y comparar dos [conjunto de datos](https://es.wikipedia.org/wiki/Conjunto_de_datos).
###Code
lista = [2, 3, 4, 5,6,7,9,10,11, 1000]
import matplotlib.pyplot as plt
# Ejemplo de grafico de cajas en python
datos_1 = np.random.normal(100, 10, 200)
#datos_2 = np.random.normal(80, 30, 200)
datos_3 = np.random.normal(90, 20, 200)
datos_4 = np.random.normal(70, 25, 200)
datos_graf = [datos_1, datos_2, datos_3, datos_4]
# Creando el objeto figura
fig = plt.figure(1, figsize=(9, 6))
# Creando el subgrafico
ax = fig.add_subplot(111)
# creando el grafico de cajas
bp = ax.boxplot(datos_graf)
# visualizar mas facile los atípicos
for flier in bp['fliers']:
flier.set(marker='o', color='red', alpha=0.5)
# los puntos aislados son valores atípicos
df = pd.DataFrame(datos_2)
df
es_menor_a_80 = 0
for value in datos_2:
if value <= 80:
es_menor_a_80 += 1
print(es_menor_a_80)
df.hist(bins=2)
x = list(datos_2)
x.append(500)
datos_2 = np.array(x)
df = pd.DataFrame(datos_2)
df.hist()
# usando seaborn
sns.boxplot(datos_graf, names=["grupo1", "grupo2", "grupo3", "grupo 4"],
color="PaleGreen");
###Output
_____no_output_____ |
ipynbs/introduction.ipynb | ###Markdown
`print('Hello!')`
###Code
import this
###Output
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
|
Python-API/pal/notebooks/bankDirectMarketing.ipynb | ###Markdown
Logistic Regression Example Bank dataset to determine if a customer would buy a CDThe data is related with direct marketing campaigns of a Portuguese banking institution. The marketing campaigns were based on phone calls. A number of features such as age, kind of job, marital status, education level, credit default, existence of housing loan, etc. were considered. The classification goal is to predict if the client will subscribe (yes/no) a term deposit.More information regarding the data set is at https://archive.ics.uci.edu/ml/datasets/bank+marketing. For tutorials use only.__ The objective is to demonstrate the use of logistic regression and to tune hyperparameters enet_lamba and enet_alpha. __ Attribute Information: Input variables: Bank client data:1. age (numeric)2. job : type of job (categorical: 'admin.','blue-collar','entrepreneur','housemaid','management','retired','self-employed','services','student','technician','unemployed','unknown')3. marital : marital status (categorical: 'divorced','married','single','unknown'; note: 'divorced' means divorced or widowed)4. education (categorical: 'basic.4y','basic.6y','basic.9y','high.school','illiterate','professional.course','university.degree','unknown')5. default: has credit in default? (categorical: 'no','yes','unknown')6. housing: has housing loan? (categorical: 'no','yes','unknown')7. loan: has personal loan? (categorical: 'no','yes','unknown') Related with the last contact of the current campaign:8. contact: contact communication type (categorical: 'cellular','telephone') 9. month: last contact month of year (categorical: 'jan', 'feb', 'mar', ..., 'nov', 'dec')10. day_of_week: last contact day of the week (categorical: 'mon','tue','wed','thu','fri')11. duration: last contact duration, in seconds (numeric). Important note: this attribute highly affects the output target (e.g., if duration=0 then y='no'). Yet, the duration is not known before a call is performed. Also, after the end of the call y is obviously known. Thus, this input should only be included for benchmark purposes and should be discarded if the intention is to have a realistic predictive model. Other attributes:12. campaign: number of contacts performed during this campaign and for this client (numeric, includes last contact)13. pdays: number of days that passed by after the client was last contacted from a previous campaign (numeric; 999 means client was not previously contacted)14. previous: number of contacts performed before this campaign and for this client (numeric)15. poutcome: outcome of the previous marketing campaign (categorical: 'failure','nonexistent','success') Social and economic context attributes:16. emp.var.rate: employment variation rate - quarterly indicator (numeric)17. cons.price.idx: consumer price index - monthly indicator (numeric) 18. cons.conf.idx: consumer confidence index - monthly indicator (numeric) 19. euribor3m: euribor 3 month rate - daily indicator (numeric)20. nr.employed: number of employees - quarterly indicator (numeric) Output variable (desired target):21. y - has the client subscribed a term deposit? (binary: 'yes','no') InitializationSet up the imports, logging, and loading of the data sets.
###Code
from hana_ml import dataframe
from hana_ml.algorithms.pal import linear_model
from hana_ml.algorithms.pal import clustering
from hana_ml.algorithms.pal import trees
import numpy as np
import matplotlib.pyplot as plt
import logging
from IPython.core.display import Image, display
###Output
_____no_output_____
###Markdown
Load data
The data is loaded into 3 tables, one for the test set, another for the training set, and finally the validation set:
DBM2_RTEST_TBL
DBM2_RTRAINING_TBL
DBM2_RVALIDATION_TBL
To do that, a connection is created and passed to the loader.
There is a config file, config/e2edata.ini that controls the connection parameters and whether or not to reload the data from scratch. In case the data is already loaded, there would be no need to load the data. A sample section is below. If the config parameter, reload_data is true then the tables for test, training, and validation are (re-)created and data inserted into them.
Although this ini file has other sections, please do not modify them. Only the [hana] section should be modified.
[hana]
url=host.sjc.sap.corp
user=username
passwd=userpassword
port=3xx15
Define Datasets - Training, validation, and test sets
Data frames are used keep references to data so computation on large data sets in HANA can happen in HANA. Trying to bring the entire data set into the client will likely result in out of memory exceptions.
The original/full dataset is split into training, test and validation sets. In the example below, they reside in different tables.
###Code
from hana_ml.algorithms.pal.utility import DataSets, Settings
url, port, user, pwd = Settings.load_config("../../config/e2edata.ini")
connection_context = dataframe.ConnectionContext(url, port, user, pwd)
full_set, training_set, validation_set, test_set = DataSets.load_bank_data(connection_context)
###Output
_____no_output_____
###Markdown
Simple ExplorationLet us look at the number of rows in the data set
###Code
print('Number of rows in full set: {}'.format(full_set.count()))
print('Number of rows in training set: {}'.format(training_set.count()))
print('Number of rows in validation set: {}'.format(validation_set.count()))
print('Number of rows in test set: {}'.format(test_set.count()))
###Output
_____no_output_____
###Markdown
Let's look at the columns
###Code
print(full_set.columns)
###Output
_____no_output_____
###Markdown
Let's look at the data types
###Code
full_set.dtypes()
features = ['AGE','JOB','MARITAL','EDUCATION','DBM_DEFAULT', 'HOUSING','LOAN','CONTACT','DBM_MONTH','DAY_OF_WEEK','DURATION','CAMPAIGN','PDAYS','PREVIOUS','POUTCOME','EMP_VAR_RATE','CONS_PRICE_IDX','CONS_CONF_IDX','EURIBOR3M','NREMPLOYED']
label = "LABEL"
###Output
_____no_output_____
###Markdown
Let us look at some rows
###Code
training_set.head(5).collect()
training_set.filter("\"LABEL\"='yes'").head(5).collect()
###Output
_____no_output_____
###Markdown
Create Model and Tune HyperparametersTry different hyperparameters and see what parameter is best.The results are stored in a list called res which can then be used to visualize the results._The variable "quick" is to run the tests for only a few values to avoid running the code below for a long time._
###Code
quick = True
enet_lambdas = np.linspace(0.01,0.02, endpoint=False, num=1) if quick else np.append(np.linspace(0.01,0.02, endpoint=False, num=4), np.linspace(0.02,0.02, num=5))
enet_alphas = np.linspace(0, 1, num=4) if quick else np.linspace(0, 1, num=40)
res = []
for enet_alpha in enet_alphas:
for enet_lambda in enet_lambdas:
lr = linear_model.LogisticRegression(solver='Cyclical', tol=0.000001, max_iter=10000,
stat_inf=True,pmml_export='multi-row', enet_lambda=enet_lambda, enet_alpha=enet_alpha,
class_map0='no', class_map1='yes')
lr.fit(training_set, features=features, label=label)
accuracy_val = lr.score(validation_set, 'ID', features, label)
res.append((enet_alpha, enet_lambda, accuracy_val, lr.coef_))
###Output
_____no_output_____
###Markdown
Graph the resultsPlot the accuracy on the validation set against the hyperparameters.This is only done if all the combinations are tried.
###Code
%matplotlib inline
if not quick:
arry = np.asarray(res)
fig = plt.figure(figsize=(10,10))
plt.title("Validation accuracy for training set with different lambdas")
ax = fig.add_subplot(111)
most_accurate_lambda = arry[np.argmax(arry[:,2]),1]
best_accuracy_arg = np.argmax(arry[:,2])
for lamda in enet_lambdas:
if lamda == most_accurate_lambda:
ax.plot(arry[arry[:,1]==lamda][:,0], arry[arry[:,1]==lamda][:,2], label="%.3f" % round(lamda,3), linewidth=5, c='r')
else:
ax.plot(arry[arry[:,1]==lamda][:,0], arry[arry[:,1]==lamda][:,2], label="%.3f" % round(lamda,3))
plt.legend(loc=1, title="Legend (Lambda)", fancybox=True, fontsize=12)
ax.set_xlabel('Alpha', fontsize=12)
ax.set_ylabel('Accuracy', fontsize=12)
plt.xticks(fontsize=12)
plt.yticks(fontsize=12)
plt.grid()
plt.show()
print("Best accuracy: %.4f" % (arry[best_accuracy_arg][2]))
print("Value of alpha for maximum accuracy: %.3f\nValue of lambda for maximum accuracy: %.3f\n" % (arry[best_accuracy_arg][0], arry[best_accuracy_arg][1]))
else:
display(Image('images/bank-data-hyperparameter-tuning.png', width=800, unconfined=True))
print("Best accuracy: 0.9148")
print("Value of alpha for maximum accuracy: 0.769")
print("Value of lambda for maximum accuracy: 0.010")
###Output
_____no_output_____
###Markdown
Predictions on test setLet us do the predictions on the test set using these values of alpha and lambda
###Code
alpha = 0.769
lamda = 0.01
lr = linear_model.LogisticRegression(solver='Cyclical', tol=0.000001, max_iter=10000,
stat_inf=True,pmml_export='multi-row', enet_lambda=lamda, enet_alpha=alpha,
class_map0='no', class_map1='yes')
lr.fit(training_set, features=features, label=label)
###Output
_____no_output_____
###Markdown
Look at the coefficientsThe coefficients are again a data frame. So, we sort and get the top 5.
###Code
lr.coef_.sort("COEFFICIENT", desc=True).head(5).collect()
###Output
_____no_output_____
###Markdown
Look at the predictions
###Code
result_df = lr.predict(test_set, 'ID')
result_df.filter('"CLASS"=\'no\'').head(5).collect()
result_df.filter('"CLASS"=\'yes\'').head(5).collect()
###Output
_____no_output_____
###Markdown
What about the final score?
###Code
lr.score(test_set, 'ID')
###Output
_____no_output_____
###Markdown
Logistic Regression Example Bank dataset to determine if a customer would buy a CDThe data is related with direct marketing campaigns of a Portuguese banking institution. The marketing campaigns were based on phone calls. A number of features such as age, kind of job, marital status, education level, credit default, existence of housing loan, etc. were considered. The classification goal is to predict if the client will subscribe (yes/no) a term deposit.More information regarding the data set is at https://archive.ics.uci.edu/ml/datasets/bank+marketing. For tutorials use only.__ The objective is to demonstrate the use of logistic regression and to tune hyperparameters enet_lamba and enet_alpha. __ Attribute Information: Input variables: Bank client data:1. age (numeric)2. job : type of job (categorical: 'admin.','blue-collar','entrepreneur','housemaid','management','retired','self-employed','services','student','technician','unemployed','unknown')3. marital : marital status (categorical: 'divorced','married','single','unknown'; note: 'divorced' means divorced or widowed)4. education (categorical: 'basic.4y','basic.6y','basic.9y','high.school','illiterate','professional.course','university.degree','unknown')5. default: has credit in default? (categorical: 'no','yes','unknown')6. housing: has housing loan? (categorical: 'no','yes','unknown')7. loan: has personal loan? (categorical: 'no','yes','unknown') Related with the last contact of the current campaign:8. contact: contact communication type (categorical: 'cellular','telephone') 9. month: last contact month of year (categorical: 'jan', 'feb', 'mar', ..., 'nov', 'dec')10. day_of_week: last contact day of the week (categorical: 'mon','tue','wed','thu','fri')11. duration: last contact duration, in seconds (numeric). Important note: this attribute highly affects the output target (e.g., if duration=0 then y='no'). Yet, the duration is not known before a call is performed. Also, after the end of the call y is obviously known. Thus, this input should only be included for benchmark purposes and should be discarded if the intention is to have a realistic predictive model. Other attributes:12. campaign: number of contacts performed during this campaign and for this client (numeric, includes last contact)13. pdays: number of days that passed by after the client was last contacted from a previous campaign (numeric; 999 means client was not previously contacted)14. previous: number of contacts performed before this campaign and for this client (numeric)15. poutcome: outcome of the previous marketing campaign (categorical: 'failure','nonexistent','success') Social and economic context attributes:16. emp.var.rate: employment variation rate - quarterly indicator (numeric)17. cons.price.idx: consumer price index - monthly indicator (numeric) 18. cons.conf.idx: consumer confidence index - monthly indicator (numeric) 19. euribor3m: euribor 3 month rate - daily indicator (numeric)20. nr.employed: number of employees - quarterly indicator (numeric) Output variable (desired target):21. y - has the client subscribed a term deposit? (binary: 'yes','no') InitializationSet up the imports, logging, and loading of the data sets.
###Code
from hana_ml import dataframe
from hana_ml.algorithms.pal import linear_model
from hana_ml.algorithms.pal import clustering
from hana_ml.algorithms.pal import trees
import numpy as np
import matplotlib.pyplot as plt
import logging
from IPython.core.display import Image, display
###Output
_____no_output_____
###Markdown
Load dataThe data is loaded into 3 tables, one for the test set, another for the training set, and finally the validation set:DBM2_RTEST_TBLDBM2_RTRAINING_TBLDBM2_RVALIDATION_TBLTo do that, a connection is created and passed to the loader.There is a config file, config/e2edata.ini that controls the connection parameters and whether or not to reload the data from scratch. In case the data is already loaded, there would be no need to load the data. A sample section is below. If the config parameter, reload_data is true then the tables for test, training, and validation are (re-)created and data inserted into them.Although this ini file has other sections, please do not modify them. Only the [hana] section should be modified.[hana]url=host.sjc.sap.corpuser=usernamepasswd=userpasswordport=3xx15
###Code
from data_load_utils import DataSets, Settings
url, port, user, pwd = Settings.load_config("../../config/e2edata.ini")
connection_context = dataframe.ConnectionContext(url, port, user, pwd)
full_tbl, training_tbl, validation_tbl, test_tbl = DataSets.load_bank_data(connection_context)
###Output
Table DBM2_RFULL_TBL exists and data exists
###Markdown
Create Data FramesCreate the data frames for the full, test, training, and validation sets.Let us also do some data exploration. Define Datasets - Training, validation, and test setsData frames are used keep references to data so computation on large data sets in HANA can happen in HANA. Trying to bring the entire data set into the client will likely result in out of memory exceptions.The original/full dataset is split into training, test and validation sets. In the example below, they reside in different tables.
###Code
full_set = connection_context.table(full_tbl)
training_set = connection_context.table(training_tbl)
validation_set = connection_context.table(validation_tbl)
test_set = connection_context.table(test_tbl)
###Output
_____no_output_____
###Markdown
Simple ExplorationLet us look at the number of rows in the data set
###Code
print('Number of rows in full set: {}'.format(full_set.count()))
print('Number of rows in training set: {}'.format(training_set.count()))
print('Number of rows in validation set: {}'.format(validation_set.count()))
print('Number of rows in test set: {}'.format(test_set.count()))
###Output
Number of rows in full set: 41188
Number of rows in training set: 20594
Number of rows in validation set: 16475
Number of rows in test set: 4119
###Markdown
Let's look at the columns
###Code
print(full_set.columns)
###Output
['ID', 'AGE', 'JOB', 'MARITAL', 'EDUCATION', 'DBM_DEFAULT', 'HOUSING', 'LOAN', 'CONTACT', 'DBM_MONTH', 'DAY_OF_WEEK', 'DURATION', 'CAMPAIGN', 'PDAYS', 'PREVIOUS', 'POUTCOME', 'EMP_VAR_RATE', 'CONS_PRICE_IDX', 'CONS_CONF_IDX', 'EURIBOR3M', 'NREMPLOYED', 'LABEL']
###Markdown
Let's look at the data types
###Code
full_set.dtypes()
features = ['AGE','JOB','MARITAL','EDUCATION','DBM_DEFAULT', 'HOUSING','LOAN','CONTACT','DBM_MONTH','DAY_OF_WEEK','DURATION','CAMPAIGN','PDAYS','PREVIOUS','POUTCOME','EMP_VAR_RATE','CONS_PRICE_IDX','CONS_CONF_IDX','EURIBOR3M','NREMPLOYED']
label = "LABEL"
###Output
_____no_output_____
###Markdown
Let us look at some rows
###Code
training_set.head(5).collect()
training_set.filter("\"LABEL\"='yes'").head(5).collect()
###Output
_____no_output_____
###Markdown
Create Model and Tune HyperparametersTry different hyperparameters and see what parameter is best.The results are stored in a list called res which can then be used to visualize the results._The variable "quick" is to run the tests for only a few values to avoid running the code below for a long time._
###Code
quick = True
enet_lambdas = np.linspace(0.01,0.02, endpoint=False, num=1) if quick else np.append(np.linspace(0.01,0.02, endpoint=False, num=4), np.linspace(0.02,0.02, num=5))
enet_alphas = np.linspace(0, 1, num=4) if quick else np.linspace(0, 1, num=40)
res = []
for enet_alpha in enet_alphas:
for enet_lambda in enet_lambdas:
lr = linear_model.LogisticRegression(solver='Cyclical', tol=0.000001, max_iter=10000,
stat_inf=True,pmml_export='multi-row', enet_lambda=enet_lambda, enet_alpha=enet_alpha,
class_map0='no', class_map1='yes')
lr.fit(training_set, features=features, label=label)
accuracy_val = lr.score(validation_set, 'ID', features, label)
res.append((enet_alpha, enet_lambda, accuracy_val, lr.coef_))
###Output
_____no_output_____
###Markdown
Graph the resultsPlot the accuracy on the validation set against the hyperparameters.This is only done if all the combinations are tried.
###Code
%matplotlib inline
if not quick:
arry = np.asarray(res)
fig = plt.figure(figsize=(10,10))
plt.title("Validation accuracy for training set with different lambdas")
ax = fig.add_subplot(111)
most_accurate_lambda = arry[np.argmax(arry[:,2]),1]
best_accuracy_arg = np.argmax(arry[:,2])
for lamda in enet_lambdas:
if lamda == most_accurate_lambda:
ax.plot(arry[arry[:,1]==lamda][:,0], arry[arry[:,1]==lamda][:,2], label="%.3f" % round(lamda,3), linewidth=5, c='r')
else:
ax.plot(arry[arry[:,1]==lamda][:,0], arry[arry[:,1]==lamda][:,2], label="%.3f" % round(lamda,3))
plt.legend(loc=1, title="Legend (Lambda)", fancybox=True, fontsize=12)
ax.set_xlabel('Alpha', fontsize=12)
ax.set_ylabel('Accuracy', fontsize=12)
plt.xticks(fontsize=12)
plt.yticks(fontsize=12)
plt.grid()
plt.show()
print("Best accuracy: %.4f" % (arry[best_accuracy_arg][2]))
print("Value of alpha for maximum accuracy: %.3f\nValue of lambda for maximum accuracy: %.3f\n" % (arry[best_accuracy_arg][0], arry[best_accuracy_arg][1]))
else:
display(Image('images/bank-data-hyperparameter-tuning.png', width=800, unconfined=True))
print("Best accuracy: 0.9148")
print("Value of alpha for maximum accuracy: 0.769")
print("Value of lambda for maximum accuracy: 0.010")
###Output
_____no_output_____
###Markdown
Predictions on test setLet us do the predictions on the test set using these values of alpha and lambda
###Code
alpha = 0.769
lamda = 0.01
lr = linear_model.LogisticRegression(solver='Cyclical', tol=0.000001, max_iter=10000,
stat_inf=True,pmml_export='multi-row', enet_lambda=lamda, enet_alpha=alpha,
class_map0='no', class_map1='yes')
lr.fit(training_set, features=features, label=label)
###Output
_____no_output_____
###Markdown
Look at the coefficientsThe coefficients are again a data frame. So, we sort and get the top 5.
###Code
lr.coef_.sort("COEFFICIENT", desc=True).head(5).collect()
###Output
_____no_output_____
###Markdown
Look at the predictions
###Code
result_df = lr.predict(test_set, 'ID')
result_df.filter('"CLASS"=\'no\'').head(5).collect()
result_df.filter('"CLASS"=\'yes\'').head(5).collect()
###Output
_____no_output_____
###Markdown
What about the final score?
###Code
lr.score(test_set, 'ID')
###Output
_____no_output_____
###Markdown
Logistic Regression Example Bank dataset to determine if a customer would buy a CDThe data is related with direct marketing campaigns of a Portuguese banking institution. The marketing campaigns were based on phone calls. A number of features such as age, kind of job, marital status, education level, credit default, existence of housing loan, etc. were considered. The classification goal is to predict if the client will subscribe (yes/no) a term deposit.More information regarding the data set is at https://archive.ics.uci.edu/ml/datasets/bank+marketing.__ The objective is to demonstrate the use of logistic regression and to tune hyperparameters enet_lamba and enet_alpha. __ Attribute Information: Input variables: Bank client data:1. age (numeric)2. job : type of job (categorical: 'admin.','blue-collar','entrepreneur','housemaid','management','retired','self-employed','services','student','technician','unemployed','unknown')3. marital : marital status (categorical: 'divorced','married','single','unknown'; note: 'divorced' means divorced or widowed)4. education (categorical: 'basic.4y','basic.6y','basic.9y','high.school','illiterate','professional.course','university.degree','unknown')5. default: has credit in default? (categorical: 'no','yes','unknown')6. housing: has housing loan? (categorical: 'no','yes','unknown')7. loan: has personal loan? (categorical: 'no','yes','unknown') Related with the last contact of the current campaign:8. contact: contact communication type (categorical: 'cellular','telephone') 9. month: last contact month of year (categorical: 'jan', 'feb', 'mar', ..., 'nov', 'dec')10. day_of_week: last contact day of the week (categorical: 'mon','tue','wed','thu','fri')11. duration: last contact duration, in seconds (numeric). Important note: this attribute highly affects the output target (e.g., if duration=0 then y='no'). Yet, the duration is not known before a call is performed. Also, after the end of the call y is obviously known. Thus, this input should only be included for benchmark purposes and should be discarded if the intention is to have a realistic predictive model. Other attributes:12. campaign: number of contacts performed during this campaign and for this client (numeric, includes last contact)13. pdays: number of days that passed by after the client was last contacted from a previous campaign (numeric; 999 means client was not previously contacted)14. previous: number of contacts performed before this campaign and for this client (numeric)15. poutcome: outcome of the previous marketing campaign (categorical: 'failure','nonexistent','success') Social and economic context attributes:16. emp.var.rate: employment variation rate - quarterly indicator (numeric)17. cons.price.idx: consumer price index - monthly indicator (numeric) 18. cons.conf.idx: consumer confidence index - monthly indicator (numeric) 19. euribor3m: euribor 3 month rate - daily indicator (numeric)20. nr.employed: number of employees - quarterly indicator (numeric) Output variable (desired target):21. y - has the client subscribed a term deposit? (binary: 'yes','no') InitializationSet up the imports, logging, and loading of the data sets.
###Code
from hana_ml import dataframe
from hana_ml.algorithms.pal import linear_model
from hana_ml.algorithms.pal import clustering
from hana_ml.algorithms.pal import trees
import numpy as np
import matplotlib.pyplot as plt
import logging
from IPython.core.display import Image, display
###Output
_____no_output_____
###Markdown
Load dataThe data is loaded into 3 tables, one for the test set, another for the training set, and finally the validation set:DBM2_RTEST_TBLDBM2_RTRAINING_TBLDBM2_RVALIDATION_TBLTo do that, a connection is created and passed to the loader.There is a config file, config/e2edata.ini that controls the connection parameters and whether or not to reload the data from scratch. In case the data is already loaded, there would be no need to load the data. A sample section is below. If the config parameter, reload_data is true then the tables for test, training, and validation are (re-)created and data inserted into them.Although this ini file has other sections, please do not modify them. Only the [hana] section should be modified.[hana]url=host.sjc.sap.corpuser=usernamepasswd=userpasswordport=3xx15
###Code
from data_load_utils import DataSets, Settings
url, port, user, pwd = Settings.load_config("../../config/e2edata.ini")
connection_context = dataframe.ConnectionContext(url, port, user, pwd)
full_tbl, training_tbl, validation_tbl, test_tbl = DataSets.load_bank_data(connection_context)
###Output
_____no_output_____
###Markdown
Create Data FramesCreate the data frames for the full, test, training, and validation sets.Let us also do some data exploration. Define Datasets - Training, validation, and test setsData frames are used keep references to data so computation on large data sets in HANA can happen in HANA. Trying to bring the entire data set into the client will likely result in out of memory exceptions.The original/full dataset is split into training, test and validation sets. In the example below, they reside in different tables.
###Code
full_set = connection_context.table(full_tbl)
training_set = connection_context.table(training_tbl)
validation_set = connection_context.table(validation_tbl)
test_set = connection_context.table(test_tbl)
###Output
_____no_output_____
###Markdown
Simple ExplorationLet us look at the number of rows in the data set
###Code
print('Number of rows in full set: {}'.format(full_set.count()))
print('Number of rows in training set: {}'.format(training_set.count()))
print('Number of rows in validation set: {}'.format(validation_set.count()))
print('Number of rows in test set: {}'.format(test_set.count()))
###Output
Number of rows in full set: 41188
Number of rows in training set: 16895
Number of rows in validation set: 12096
Number of rows in test set: 12197
###Markdown
Let's look at the columns
###Code
print(full_set.columns)
###Output
['ID', 'AGE', 'JOB', 'MARITAL', 'EDUCATION', 'DBM_DEFAULT', 'HOUSING', 'LOAN', 'CONTACT', 'DBM_MONTH', 'DAY_OF_WEEK', 'DURATION', 'CAMPAIGN', 'PDAYS', 'PREVIOUS', 'POUTCOME', 'EMP_VAR_RATE', 'CONS_PRICE_IDX', 'CONS_CONF_IDX', 'EURIBOR3M', 'NREMPLOYED', 'LABEL']
###Markdown
Let's look at the data types
###Code
full_set.dtypes()
features = ['AGE','JOB','MARITAL','EDUCATION','DBM_DEFAULT', 'HOUSING','LOAN','CONTACT','DBM_MONTH','DAY_OF_WEEK','DURATION','CAMPAIGN','PDAYS','PREVIOUS','POUTCOME','EMP_VAR_RATE','CONS_PRICE_IDX','CONS_CONF_IDX','EURIBOR3M','NREMPLOYED']
label = "LABEL"
###Output
_____no_output_____
###Markdown
Let us look at some rows
###Code
training_set.head(5).collect()
training_set.filter("\"LABEL\"='yes'").head(5).collect()
###Output
_____no_output_____
###Markdown
Create Model and Tune HyperparametersTry different hyperparameters and see what parameter is best.The results are stored in a list called res which can then be used to visualize the results._The variable "quick" is to run the tests for only a few values to avoid running the code below for a long time._
###Code
quick = True
enet_lambdas = np.linspace(0.01,0.02, endpoint=False, num=1) if quick else np.append(np.linspace(0.01,0.02, endpoint=False, num=4), np.linspace(0.02,0.02, num=5))
enet_alphas = np.linspace(0, 1, num=4) if quick else np.linspace(0, 1, num=40)
res = []
for enet_alpha in enet_alphas:
for enet_lambda in enet_lambdas:
lr = linear_model.LogisticRegression(connection_context, solver='Cyclical', tol=0.000001, max_iter=10000,
stat_inf=True,pmml_export='multi-row', lamb=enet_lambda, alpha=enet_alpha,
class_map0='no', class_map1='yes')
lr.fit(training_set, features=features, label=label)
accuracy_val = lr.score(validation_set, 'ID', features, label)
res.append((enet_alpha, enet_lambda, accuracy_val, lr.coef_))
###Output
_____no_output_____
###Markdown
Graph the resultsPlot the accuracy on the validation set against the hyperparameters.This is only done if all the combinations are tried.
###Code
%matplotlib inline
if not quick:
arry = np.asarray(res)
fig = plt.figure(figsize=(10,10))
plt.title("Validation accuracy for training set with different lambdas")
ax = fig.add_subplot(111)
most_accurate_lambda = arry[np.argmax(arry[:,2]),1]
best_accuracy_arg = np.argmax(arry[:,2])
for lamda in enet_lambdas:
if lamda == most_accurate_lambda:
ax.plot(arry[arry[:,1]==lamda][:,0], arry[arry[:,1]==lamda][:,2], label="%.3f" % round(lamda,3), linewidth=5, c='r')
else:
ax.plot(arry[arry[:,1]==lamda][:,0], arry[arry[:,1]==lamda][:,2], label="%.3f" % round(lamda,3))
plt.legend(loc=1, title="Legend (Lambda)", fancybox=True, fontsize=12)
ax.set_xlabel('Alpha', fontsize=12)
ax.set_ylabel('Accuracy', fontsize=12)
plt.xticks(fontsize=12)
plt.yticks(fontsize=12)
plt.grid()
plt.show()
print("Best accuracy: %.4f" % (arry[best_accuracy_arg][2]))
print("Value of alpha for maximum accuracy: %.3f\nValue of lambda for maximum accuracy: %.3f\n" % (arry[best_accuracy_arg][0], arry[best_accuracy_arg][1]))
else:
display(Image('images/bank-data-hyperparameter-tuning.png', width=800, unconfined=True))
print("Best accuracy: 0.9148")
print("Value of alpha for maximum accuracy: 0.769")
print("Value of lambda for maximum accuracy: 0.010")
###Output
_____no_output_____
###Markdown
Predictions on test setLet us do the predictions on the test set using these values of alpha and lambda
###Code
alpha = 0.769
lamda = 0.01
lr = linear_model.LogisticRegression(connection_context, solver='Cyclical', tol=0.000001, max_iter=10000,
stat_inf=True,pmml_export='multi-row', lamb=lamda, alpha=alpha,
class_map0='no', class_map1='yes')
lr.fit(training_set, features=features, label=label)
###Output
_____no_output_____
###Markdown
Look at the coefficientsThe coefficients are again a data frame. So, we sort and get the top 5.
###Code
lr.coef_.sort("COEFFICIENT", desc=True).head(5).collect()
###Output
_____no_output_____
###Markdown
Look at the predictions
###Code
result_df = lr.predict(test_set, 'ID')
result_df.filter('"CLASS"=\'no\'').head(5).collect()
result_df.filter('"CLASS"=\'yes\'').head(5).collect()
###Output
_____no_output_____
###Markdown
What about the final score?
###Code
lr.score(test_set, 'ID')
###Output
_____no_output_____
###Markdown
Logistic Regression Example Bank dataset to determine if a customer would buy a CDThe data is related with direct marketing campaigns of a Portuguese banking institution. The marketing campaigns were based on phone calls. A number of features such as age, kind of job, marital status, education level, credit default, existence of housing loan, etc. were considered. The classification goal is to predict if the client will subscribe (yes/no) a term deposit.More information regarding the data set is at https://archive.ics.uci.edu/ml/datasets/bank+marketing. For tutorials use only.__ The objective is to demonstrate the use of logistic regression and to tune hyperparameters enet_lamba and enet_alpha. __ Attribute Information: Input variables: Bank client data:1. age (numeric)2. job : type of job (categorical: 'admin.','blue-collar','entrepreneur','housemaid','management','retired','self-employed','services','student','technician','unemployed','unknown')3. marital : marital status (categorical: 'divorced','married','single','unknown'; note: 'divorced' means divorced or widowed)4. education (categorical: 'basic.4y','basic.6y','basic.9y','high.school','illiterate','professional.course','university.degree','unknown')5. default: has credit in default? (categorical: 'no','yes','unknown')6. housing: has housing loan? (categorical: 'no','yes','unknown')7. loan: has personal loan? (categorical: 'no','yes','unknown') Related with the last contact of the current campaign:8. contact: contact communication type (categorical: 'cellular','telephone') 9. month: last contact month of year (categorical: 'jan', 'feb', 'mar', ..., 'nov', 'dec')10. day_of_week: last contact day of the week (categorical: 'mon','tue','wed','thu','fri')11. duration: last contact duration, in seconds (numeric). Important note: this attribute highly affects the output target (e.g., if duration=0 then y='no'). Yet, the duration is not known before a call is performed. Also, after the end of the call y is obviously known. Thus, this input should only be included for benchmark purposes and should be discarded if the intention is to have a realistic predictive model. Other attributes:12. campaign: number of contacts performed during this campaign and for this client (numeric, includes last contact)13. pdays: number of days that passed by after the client was last contacted from a previous campaign (numeric; 999 means client was not previously contacted)14. previous: number of contacts performed before this campaign and for this client (numeric)15. poutcome: outcome of the previous marketing campaign (categorical: 'failure','nonexistent','success') Social and economic context attributes:16. emp.var.rate: employment variation rate - quarterly indicator (numeric)17. cons.price.idx: consumer price index - monthly indicator (numeric) 18. cons.conf.idx: consumer confidence index - monthly indicator (numeric) 19. euribor3m: euribor 3 month rate - daily indicator (numeric)20. nr.employed: number of employees - quarterly indicator (numeric) Output variable (desired target):21. y - has the client subscribed a term deposit? (binary: 'yes','no') InitializationSet up the imports, logging, and loading of the data sets.
###Code
from hana_ml import dataframe
from hana_ml.algorithms.pal import linear_model
from hana_ml.algorithms.pal import clustering
from hana_ml.algorithms.pal import trees
import numpy as np
import matplotlib.pyplot as plt
import logging
from IPython.core.display import Image, display
###Output
_____no_output_____
###Markdown
Load dataThe data is loaded into 3 tables, one for the test set, another for the training set, and finally the validation set:DBM2_RTEST_TBLDBM2_RTRAINING_TBLDBM2_RVALIDATION_TBLTo do that, a connection is created and passed to the loader.There is a config file, config/e2edata.ini that controls the connection parameters and whether or not to reload the data from scratch. In case the data is already loaded, there would be no need to load the data. A sample section is below. If the config parameter, reload_data is true then the tables for test, training, and validation are (re-)created and data inserted into them.Although this ini file has other sections, please do not modify them. Only the [hana] section should be modified.[hana]url=host.sjc.sap.corpuser=usernamepasswd=userpasswordport=3xx15
###Code
from data_load_utils import DataSets, Settings
url, port, user, pwd = Settings.load_config("../../config/e2edata.ini")
connection_context = dataframe.ConnectionContext(url, port, user, pwd)
full_tbl, training_tbl, validation_tbl, test_tbl = DataSets.load_bank_data(connection_context)
###Output
_____no_output_____
###Markdown
Create Data FramesCreate the data frames for the full, test, training, and validation sets.Let us also do some data exploration. Define Datasets - Training, validation, and test setsData frames are used keep references to data so computation on large data sets in HANA can happen in HANA. Trying to bring the entire data set into the client will likely result in out of memory exceptions.The original/full dataset is split into training, test and validation sets. In the example below, they reside in different tables.
###Code
full_set = connection_context.table(full_tbl)
training_set = connection_context.table(training_tbl)
validation_set = connection_context.table(validation_tbl)
test_set = connection_context.table(test_tbl)
###Output
_____no_output_____
###Markdown
Simple ExplorationLet us look at the number of rows in the data set
###Code
print('Number of rows in full set: {}'.format(full_set.count()))
print('Number of rows in training set: {}'.format(training_set.count()))
print('Number of rows in validation set: {}'.format(validation_set.count()))
print('Number of rows in test set: {}'.format(test_set.count()))
###Output
Number of rows in full set: 41188
Number of rows in training set: 16895
Number of rows in validation set: 12096
Number of rows in test set: 12197
###Markdown
Let's look at the columns
###Code
print(full_set.columns)
###Output
['ID', 'AGE', 'JOB', 'MARITAL', 'EDUCATION', 'DBM_DEFAULT', 'HOUSING', 'LOAN', 'CONTACT', 'DBM_MONTH', 'DAY_OF_WEEK', 'DURATION', 'CAMPAIGN', 'PDAYS', 'PREVIOUS', 'POUTCOME', 'EMP_VAR_RATE', 'CONS_PRICE_IDX', 'CONS_CONF_IDX', 'EURIBOR3M', 'NREMPLOYED', 'LABEL']
###Markdown
Let's look at the data types
###Code
full_set.dtypes()
features = ['AGE','JOB','MARITAL','EDUCATION','DBM_DEFAULT', 'HOUSING','LOAN','CONTACT','DBM_MONTH','DAY_OF_WEEK','DURATION','CAMPAIGN','PDAYS','PREVIOUS','POUTCOME','EMP_VAR_RATE','CONS_PRICE_IDX','CONS_CONF_IDX','EURIBOR3M','NREMPLOYED']
label = "LABEL"
###Output
_____no_output_____
###Markdown
Let us look at some rows
###Code
training_set.head(5).collect()
training_set.filter("\"LABEL\"='yes'").head(5).collect()
###Output
_____no_output_____
###Markdown
Create Model and Tune HyperparametersTry different hyperparameters and see what parameter is best.The results are stored in a list called res which can then be used to visualize the results._The variable "quick" is to run the tests for only a few values to avoid running the code below for a long time._
###Code
quick = True
enet_lambdas = np.linspace(0.01,0.02, endpoint=False, num=1) if quick else np.append(np.linspace(0.01,0.02, endpoint=False, num=4), np.linspace(0.02,0.02, num=5))
enet_alphas = np.linspace(0, 1, num=4) if quick else np.linspace(0, 1, num=40)
res = []
for enet_alpha in enet_alphas:
for enet_lambda in enet_lambdas:
lr = linear_model.LogisticRegression(connection_context, solver='Cyclical', tol=0.000001, max_iter=10000,
stat_inf=True,pmml_export='multi-row', lamb=enet_lambda, alpha=enet_alpha,
class_map0='no', class_map1='yes')
lr.fit(training_set, features=features, label=label)
accuracy_val = lr.score(validation_set, 'ID', features, label)
res.append((enet_alpha, enet_lambda, accuracy_val, lr.coef_))
###Output
_____no_output_____
###Markdown
Graph the resultsPlot the accuracy on the validation set against the hyperparameters.This is only done if all the combinations are tried.
###Code
%matplotlib inline
if not quick:
arry = np.asarray(res)
fig = plt.figure(figsize=(10,10))
plt.title("Validation accuracy for training set with different lambdas")
ax = fig.add_subplot(111)
most_accurate_lambda = arry[np.argmax(arry[:,2]),1]
best_accuracy_arg = np.argmax(arry[:,2])
for lamda in enet_lambdas:
if lamda == most_accurate_lambda:
ax.plot(arry[arry[:,1]==lamda][:,0], arry[arry[:,1]==lamda][:,2], label="%.3f" % round(lamda,3), linewidth=5, c='r')
else:
ax.plot(arry[arry[:,1]==lamda][:,0], arry[arry[:,1]==lamda][:,2], label="%.3f" % round(lamda,3))
plt.legend(loc=1, title="Legend (Lambda)", fancybox=True, fontsize=12)
ax.set_xlabel('Alpha', fontsize=12)
ax.set_ylabel('Accuracy', fontsize=12)
plt.xticks(fontsize=12)
plt.yticks(fontsize=12)
plt.grid()
plt.show()
print("Best accuracy: %.4f" % (arry[best_accuracy_arg][2]))
print("Value of alpha for maximum accuracy: %.3f\nValue of lambda for maximum accuracy: %.3f\n" % (arry[best_accuracy_arg][0], arry[best_accuracy_arg][1]))
else:
display(Image('images/bank-data-hyperparameter-tuning.png', width=800, unconfined=True))
print("Best accuracy: 0.9148")
print("Value of alpha for maximum accuracy: 0.769")
print("Value of lambda for maximum accuracy: 0.010")
###Output
_____no_output_____
###Markdown
Predictions on test setLet us do the predictions on the test set using these values of alpha and lambda
###Code
alpha = 0.769
lamda = 0.01
lr = linear_model.LogisticRegression(connection_context, solver='Cyclical', tol=0.000001, max_iter=10000,
stat_inf=True,pmml_export='multi-row', lamb=lamda, alpha=alpha,
class_map0='no', class_map1='yes')
lr.fit(training_set, features=features, label=label)
###Output
_____no_output_____
###Markdown
Look at the coefficientsThe coefficients are again a data frame. So, we sort and get the top 5.
###Code
lr.coef_.sort("COEFFICIENT", desc=True).head(5).collect()
###Output
_____no_output_____
###Markdown
Look at the predictions
###Code
result_df = lr.predict(test_set, 'ID')
result_df.filter('"CLASS"=\'no\'').head(5).collect()
result_df.filter('"CLASS"=\'yes\'').head(5).collect()
###Output
_____no_output_____
###Markdown
What about the final score?
###Code
lr.score(test_set, 'ID')
###Output
_____no_output_____ |
chapter_09_recurrent-modern/1_lstm.ipynb | ###Markdown
长短期记忆网络(LSTM)
###Code
import sys
sys.path.append('..')
import mindspore
import numpy as np
import mindspore.nn as nn
import mindspore.ops as ops
import mindspore.numpy as mnp
from d2l import mindspore as d2l
batch_size, num_steps = 32, 35
train_iter, vocab = d2l.load_data_time_machine(batch_size, num_steps)
###Output
_____no_output_____
###Markdown
初始化模型参数
###Code
from mindspore import Tensor, Parameter, ParameterTuple
def get_lstm_params(vocab_size, num_hiddens):
num_inputs = num_outputs = vocab_size
def normal(shape):
return Parameter(Tensor(np.random.randn(*shape) * 0.01, mindspore.float32))
def three():
return (normal((num_inputs, num_hiddens)),
normal((num_hiddens, num_hiddens)),
Parameter(Tensor(np.zeros(num_hiddens), mindspore.float32)))
W_xi, W_hi, b_i = three()
W_xf, W_hf, b_f = three()
W_xo, W_ho, b_o = three()
W_xc, W_hc, b_c = three()
W_hq = normal((num_hiddens, num_outputs))
b_q = Parameter(Tensor(np.zeros(num_outputs), mindspore.float32))
params = [W_xi, W_hi, b_i, W_xf, W_hf, b_f, W_xo, W_ho, b_o, W_xc, W_hc,
b_c, W_hq, b_q]
return ParameterTuple(params)
###Output
_____no_output_____
###Markdown
初始化函数
###Code
def init_lstm_state(batch_size, num_hiddens):
return (mnp.zeros((batch_size, num_hiddens)),
mnp.zeros((batch_size, num_hiddens)))
###Output
_____no_output_____
###Markdown
实际模型
###Code
def lstm(inputs, state, params):
W_xi, W_hi, b_i, W_xf, W_hf, b_f, W_xo, W_ho, b_o, W_xc, W_hc, b_c, W_hq, b_q = params
(H, C) = state
outputs = []
for i in range(inputs.shape[0]):
X = inputs[i]
I = ops.Sigmoid()(ops.matmul(X, W_xi) + ops.matmul(H, W_hi) + b_i)
F = ops.Sigmoid()(ops.matmul(X, W_xf) + ops.matmul(H, W_hf) + b_f)
O = ops.Sigmoid()(ops.matmul(X, W_xo) + ops.matmul(H, W_ho) + b_o)
C_tilda = ops.Tanh()(ops.matmul(X, W_xc) + ops.matmul(H, W_hc) + b_c)
C = F * C + I * C_tilda
H = O * ops.Tanh()(C)
Y = ops.matmul(H, W_hq) + b_q
outputs.append(Y)
return ops.Concat(axis=0)(outputs), (H, C)
###Output
_____no_output_____
###Markdown
训练
###Code
vocab_size, num_hiddens = len(vocab), 256
num_epochs, lr = 500, 1
model = d2l.RNNModelScratch(len(vocab), num_hiddens, get_lstm_params,
init_lstm_state, lstm)
d2l.train_ch8(model, train_iter, vocab, lr, num_epochs)
###Output
困惑度 1.7, 33790.5 词元/秒
time traveller thand thinithenethepone medow the i you a sut the
traveller thand thinithenethepone medow the i you a sut the
###Markdown
简洁实现
###Code
num_inputs = vocab_size
lstm_layer = nn.LSTM(num_inputs, num_hiddens)
model = d2l.RNNModel(lstm_layer, len(vocab), num_hiddens)
d2l.train_ch8(model, train_iter, vocab, lr, num_epochs)
###Output
困惑度 1.5, 149846.7 词元/秒
time travellerit would be remarkably conveniett for the historia
traveller after the pauserequired for the cracll thes caris
|
Infection_vs_Inflammation/Code/01-Process_Data_Josh.ipynb | ###Markdown
Goal: Differentiate Infections, sterile inflammation, and healthy tissue using MRIThe following methods were used in this study:1. T2 relaxation of the tissue without a contrast agent2. Dynamic contrast-enhanced (DCE) MRI using Maltose as a T2-ex contrast agent3. Chemical Exchange Saturation Transfer (CEST) MRI without a contrast agent**Author**: Julio Cárdenas-Rodríguez, Ph.D. **email**: [email protected] Description of the dataA total of **XX** mice were used in this study. Each mouse was infected as follows:- Right thigh: with approximatley 100 uL of a solution of XX CFU/mL of *E. Coli*.- Left thigh: same dose but using a solution that contain heat-inactivated *E. Coli*.Both thighs can be seen in each image, and a total of of five imaging slices were collected around the center of infection. The average signal for the following region of interest (ROIS) were collected for all slices:1. Infected Site2. Apparently Healthy Tissue on the right thigh3. Sterile inflammation on the left thigh4. Apparently Healthy Tissue on the left thigh
###Code
# Import Python Modules
import numpy as np
#import seaborn as sn
import matplotlib.pyplot as plt
%matplotlib inline
from pylab import *
import pandas as pd
# Import LOCAL functions written by me
from mylocal_functions import *
###Output
_____no_output_____
###Markdown
T2 relaxation
###Code
# Make list of all T2.txt files
T2_list = get_ipython().getoutput('ls ../Study_03_CBA/*T2.txt')
# Allocate variables needed for analysis
T2DF=pd.DataFrame()
TR=np.linspace(.012,.012*12,12)
# Fit T2 for all ROIs, slices and mice. construct dataframe
for names in T2_list:
#Convert txt file to array
YDataMatrix=txt_2_array(names)
#Estimate T2
T2time=fitT2(TR,YDataMatrix)
#convert to data frame
df_T2=pd.DataFrame(T2time.T,columns=["Infected","Healthy_Right","Sterile_Inflammation","Healthy_Left"])
#df_T2=pd.DataFrame(T2time.T,columns=["ROI-1","ROI-2","ROI-3","ROI-4"])
df_info=name_2_df(names)
df_final=pd.concat([df_T2,df_info], axis=1)
T2DF=T2DF.append(df_final,ignore_index=True)
# Plot distribution of estimated T2 for each slice
T2DF[T2DF.Slice==1].iloc[:,:4].plot.density(); title("Slice 01"); xlim((0.025,.15))
T2DF[T2DF.Slice==2].iloc[:,:4].plot.density(); title("Slice 02"); xlim((0.025,.15))
T2DF[T2DF.Slice==3].iloc[:,:4].plot.density(); title("Slice 03"); xlim((0.025,.15))
T2DF[T2DF.Slice==4].iloc[:,:4].plot.density(); title("Slice 04"); xlim((0.025,.15))
T2DF[T2DF.Slice==5].iloc[:,:4].plot.density(); title("Slice 05"); xlim((0.025,.15))
# Make list of all T2.txt files
CEST_list = get_ipython().getoutput('ls ../Study_03_CBA/*CEST.txt')
CEST_list[0]
# Allocate variables needed for analysis
YDataMatrix=txt_2_array(CEST_list)
# Fit T2 for all ROIs, slices and mice. construct dataframe
for names in T2_list:
#Convert txt file to array
YDataMatrix=txt_2_array(names)
#Estimate T2
T2time=fitT2(TR,YDataMatrix)
#convert to data frame
df_T2=pd.DataFrame(T2time.T,columns=["Infected","Healthy_Right","Sterile_Inflammation","Healthy_Left"])
#df_T2=pd.DataFrame(T2time.T,columns=["ROI-1","ROI-2","ROI-3","ROI-4"])
df_info=name_2_df(names)
df_final=pd.concat([df_T2,df_info], axis=1)
T2DF=T2DF.append(df_final,ignore_index=True)
###Output
_____no_output_____ |
2_data_manipulation_with_pandas/2_data_manipulation_with_pandas.ipynb | ###Markdown
*This notebook was created by [Jean de Dieu Nyandwi](https://twitter.com/jeande_d) for the love of machine learning community. For any feedback, errors or suggestion, he can be reached on email (johnjw7084 at gmail dot com), [Twitter](https://twitter.com/jeande_d), or [LinkedIn](https://linkedin.com/in/nyandwi).* Data Manipulation with Pandas In this lab, you will learn how to manipulate data with Pandas. Here is an overview:* [1. Basics of Pandas for data manipulation:](1) * [A. Series and DataFrames](1-1) * [B. Data Indexing and Selection, and Iteration (Add Iteration)](1-2) * [C. Dealing with Missing data](1-3) * [D. Basic operations and Functions](1-4) * [E. Aggregation Methods](1-5) * [F. Groupby](1-6) * [G. Merging, Joining and Concatenate](1-7) * [H. Beyond Dataframes: Working with CSV, and Excel](1-8) * [2. Real World Exploratory Data Analysis (EDA)](2) 1. Basics of Pandas for data manipulation A. Series and DataFramesBoth series and DataFrames are Pandas Data structures. Series is like one dimensional NumPy array with axis labels.DataFrame is multidimensional NumPy array with labels on rows and columns. Working with NumPy, we saw that it supports numeric type data. Pandas on other hand supports whole range of data types, from numeric to strings, etc.. Since we are using python notebook, we do not need to install Pandas. We only just have to import it. ```import pandas as pd```
###Code
# importing numpy and pandas
import numpy as np
import pandas as pd
###Output
_____no_output_____
###Markdown
Creating SeriesSeries can be created from a Python list, dictionary, and NumPy array.
###Code
# Creating the series from a Python list
num_list = [1,2,3,4,5]
pd.Series(num_list)
week_days = ['Mon','Tues','Wed','Thur','Fri']
pd.Series(week_days, index=["a", "b", "c", "d", "e"])
###Output
_____no_output_____
###Markdown
Note the data types `int64` and `object`.
###Code
# Creating the Series from dictionary
countries_code = { 1:"United States",
91:"India",
49:"Germany",
86:"China",
250:"Rwanda"}
pd.Series(countries_code)
d = {1:'a', 2:'b', 3:'c', 4:'d'}
pd.Series(d)
# Creating the Series from NumPy array
# We peovide the list of indexes
# if we don't provide the indexes, the default indexes are numbers...starts from 0,1,2..
arr = np.array ([1, 2, 3, 4, 5])
pd.Series(arr)
pd.Series(arr, index=['a', 'b', 'c', 'd', 'e'])
###Output
_____no_output_____
###Markdown
Creating DataFramesDataFrames are the most used Pandas data structure. It can be created from a dictionary, 2D array, and Series.
###Code
# Creating DataFrame from a dictionary
countries = {'Name': ['USA', 'India', 'German', 'Rwanda'],
'Codes':[1, 91, 49, 250] }
pd.DataFrame(countries)
# Creating a dataframe from a 2D array
# You pass the list of columns
array_2d = np.array ([[1,2,3], [4,5,6], [7,8,9]])
pd.DataFrame(array_2d, columns = ['column 1', 'column 2', 'column 3'])
# Creating a dataframe from Pandas series
# Pass the columns in a list
countries_code = { "United States": 1,
"India": 91,
"Germany": 49,
"China": 86,
"Rwanda":250}
pd_series = pd.Series(countries_code)
pd.Series(countries_code)
df = pd.DataFrame(pd_series, columns = ['Codes'])
df
# Adding a column
# Number in population are pretty random
df ['Population'] = [100, 450, 575, 5885, 533]
df
# Removing a column
df.drop('Population', axis =1)
df.columns
df.keys
df.index
###Output
_____no_output_____
###Markdown
B. Data Indexing and SelectionIndexing and selection works in both Series and Dataframe.Because DataFrame is made of Series, let's focus on how to select data in DataFrame.
###Code
# Creating DataFrame from a dictionary
countries = {'Name': ['USA', 'India', 'German', 'Rwanda'],
'Codes':[1, 91, 49, 250] }
df = pd.DataFrame(countries, index=['a', 'b', 'c', 'd'])
df
df['Name']
df.Name
df ['Codes']
## When you have many columns, columns in list will be selected
df [['Name', 'Codes']]
# This will return the first two rows
df [0:2]
###Output
_____no_output_____
###Markdown
You can also use `loc` to select data by the label indexes and `iloc` to select by default integer index (or by the position of the row)
###Code
df.loc['a']
df.loc['b':'d']
df [:'b']
df.iloc[2]
df.iloc[1:3]
df.iloc[2:]
###Output
_____no_output_____
###Markdown
Conditional Selection
###Code
df
#Let's select a country with code 49
df [df['Codes'] == 49 ]
df [df['Codes'] < 250 ]
df [df['Name'] == 'USA' ]
# You can use and (&) or (|) for more than conditions
#df [(condition 1) & (condition 2)]
df [(df['Codes'] == 91 ) & (df['Name'] == 'India') ]
###Output
_____no_output_____
###Markdown
You can also use `isin()` and `where()` to select data in a series or dataframe.
###Code
# isin() return false or true when provided value is included in dataframe
sample_codes_names=[1,3,250, 'USA', 'India', 'England']
df.isin(sample_codes_names)
###Output
_____no_output_____
###Markdown
As you can see, it returned `True` wherever a country code or name was found. Otherwise, `False`. You can use a dictinary to match search by columns. A key must be a column and values are passed in list.
###Code
sample_codes_names = {'Codes':[1,3,250], 'Name':['USA', 'India', 'England']}
df.isin(sample_codes_names)
df2 = pd.DataFrame(np.array ([[1,2,3], [4,5,6], [7,8,9]]),
columns = ['column 1', 'column 2', 'column 3'])
df2
df2.isin([0,3,4,5,7])
df2 [df2 > 4]
df2.where(df2 > 4)
###Output
_____no_output_____
###Markdown
Where the condition is false, `where` allows you to replace values. In this case, all values less than 4 will be 0.
###Code
df2.where(df2 > 4, 0)
# The above does the same as
df2 [df2 > 4 ] = 0
df2
###Output
_____no_output_____
###Markdown
Iteration```df.items() Iterate over (column name, Series) pairs.df.iteritems() Iterate over (column name, Series) pairs.DataFrame.iterrows() Iterate over DataFrame rows as (index, Series) pairs.DataFrame.itertuples([index, name]) Iterate over DataFrame rows as namedtuples.```
###Code
# Iterate over (column name, Series) pairs.
for col_name, content in df2.items():
print(col_name)
print(content)
# Iterate over (column name, Series) pairs.
# Same as df.items()
for col_name, content in df2.iteritems():
print(col_name)
print(content)
# Iterate over DataFrame rows as (index, Series) pairs
for row in df2.iterrows():
print(row)
# Iterate over DataFrame rows as namedtuples
for row in df2.itertuples():
print(row)
###Output
Pandas(Index=0, _1=1, _2=2, _3=3)
Pandas(Index=1, _1=4, _2=0, _3=0)
Pandas(Index=2, _1=0, _2=0, _3=0)
###Markdown
C. Dealing with Missing dataReal world datasets are messy, often with missing values. Pandas replace NaN with missing values by default. NaN stands for not a number. Missing values can either be ignored, droped or filled.
###Code
# Creating a dataframe
df3 = pd.DataFrame(np.array ([[1,2,3], [4,np.nan,6], [7,np.nan,np.nan]]),
columns = ['column 1', 'column 2', 'column 3'])
###Output
_____no_output_____
###Markdown
Checking Missing values
###Code
# Recognizing the missing values
df3.isnull()
# Calculating number of the missing values in each feature
df3.isnull().sum()
# Recognizng non missig values
df3.notna()
df3.notna().sum()
###Output
_____no_output_____
###Markdown
Removing the missing values
###Code
## Dropping missing values
df3.dropna()
###Output
_____no_output_____
###Markdown
All rows are deleted because dropna() will remove each row which have missing value.
###Code
# you can drop NaNs in specific column(s)
df3['column 3'].dropna()
# You can drop data by axis
# Axis = 1...drop all columns with Nans
# df3.dropna(axis='columns')
df3.dropna(axis=1)
# axis = 0...drop all rows with Nans
# df3.dropna(axis='rows') is same
df3.dropna(axis=0)
###Output
_____no_output_____
###Markdown
Filling the missing values
###Code
# Filling Missing values
df3.fillna(10)
df3.fillna('fillme')
# You can forward fill (ffill) or backward fill(bfill)
# Or fill a current value with previous or next value
df3.fillna(method='ffill')
# Won't change it because the last values are NaNs, so it backward it
df3.fillna(method='bfill')
# If we change the axis to columns, you can see that Nans at row 2 and col 2 is backfilled with 6
df3.fillna(method='bfill', axis='columns')
###Output
_____no_output_____
###Markdown
D. More Operations and FunctionsThis section will show the more and most useful functions of Pandas.
###Code
df4 = pd.DataFrame({'Product Name':['Shirt','Boot','Bag'],
'Order Number':[45,56,64],
'Total Quantity':[10,5,9]},
columns = ['Product Name', 'Order Number', 'Total Quantity'])
###Output
_____no_output_____
###Markdown
Retrieving basic info about the Dataframe
###Code
# Return a summary about the dataframe
df4.info()
# Return dataframe columns
df4.columns
# Return dataframe data
df4.keys
# Return the head of the dataframe ....could make sense if you have long frame
# Choose how many rows you want in head()
df4.head(1)
# Return the tail of the dataframe
df4.tail(1)
# Return NumPy array of the dataframe
df4.values
# Return the size or number of elements in a dataframe
df4.size
# Return the shape
df4.shape
# Return the length of the dataframe/the number of rows in a dataframe
df4.shape[0]
# Return the length of the dataframe/the number of columns in a dataframe
df4.shape[1]
###Output
_____no_output_____
###Markdown
Unique Values
###Code
# Return unique values in a given column
df4['Product Name'].unique()
# Return a number of unique values
df4['Product Name'].nunique()
# Counting the occurence of each value in a column
df4['Product Name'].value_counts()
###Output
_____no_output_____
###Markdown
Applying a Function to Dataframe
###Code
# Double the quantity product
def double_quantity(x):
return x * x
df4['Total Quantity'].apply(double_quantity)
# You can also apply an anonymous function to a dataframe
# Squaring each value in dataframe
df5 = pd.DataFrame([[1,2], [4,5]], columns=['col1', 'col2'])
df5.applymap(lambda x: x**2)
###Output
_____no_output_____
###Markdown
Sorting values in dataframe
###Code
# Sort the df4 by the order number
df4.sort_values(['Order Number'])
df4.sort_values(['Order Number'], ascending=False)
###Output
_____no_output_____
###Markdown
E. Aggregation Methods
###Code
df4
# summary statistics
df4.describe()
df4.describe().transpose()
# Mode of the dataframe
# Mode is the most recurring values
df4['Total Quantity'].mode()
# The maximum value
df4['Total Quantity'].max()
# The minimum value
df4['Total Quantity'].min()
# The mean
df4['Total Quantity'].mean()
# The median value in a dataframe
df4['Total Quantity'].median()
# Standard deviation
df4['Total Quantity'].std()
# Variance
df4['Total Quantity'].var()
# Sum of all values in a column
df4['Total Quantity'].sum()
# Product of all values in dataframe
df4['Total Quantity'].prod()
###Output
_____no_output_____
###Markdown
F. Groupby`Group by` involves splitting data into groups, applying function to each group, and combining the results.
###Code
df4 = pd.DataFrame({'Product Name':['Shirt','Boot','Bag', 'Ankle', 'Pullover', 'Boot', 'Ankle', 'Tshirt', 'Shirt'],
'Order Number':[45,56,64, 34, 67, 56, 34, 89, 45],
'Total Quantity':[10,5,9, 11, 11, 8, 14, 23, 10]},
columns = ['Product Name', 'Order Number', 'Total Quantity'])
df4
# Let's group the df by product name
df4.groupby('Product Name').mean()
df4.groupby('Product Name').sum()
df4.groupby('Product Name').min()
df4.groupby('Product Name').max()
df4.groupby(['Product Name', 'Order Number']).max()
df4.groupby(['Product Name', 'Order Number']).sum()
###Output
_____no_output_____
###Markdown
You can also use `aggregation()` after groupby.
###Code
df4.groupby('Product Name').aggregate(['min', 'max', 'sum'])
###Output
_____no_output_____
###Markdown
G. Combining Datasets: Concatenating, Joining and Merging Concatenation
###Code
# Creating dataframes
df1 = pd.DataFrame({'Col1':['A','B','C'],
'Col2':[1,2,3]},
index=['a','b','c'])
df2 = pd.DataFrame({'Col1':['D','E','F'],
'Col2':[4,5,6]},
index=['d','e','f'])
df3 = pd.DataFrame({'Col1':['G','I','J'],
'Col2':[7,8,9]},
index=['g', 'i','j'])
df1
df2
df3
# Concatenating: Adding one dataset to another
pd.concat([df1, df2, df3])
###Output
_____no_output_____
###Markdown
The default axis is `0`. This is how the combined dataframes will look like if we change the `axis to 1`.
###Code
pd.concat([df1, df2, df3], axis=1)
# We can also use append()
df1.append([df2, df3])
###Output
_____no_output_____
###Markdown
MergingIf you have worked with SQL, what `pd.merge()` does may be familiar. It links data from different sources (different features) and you have a control on the structure of the combined dataset. *Pandas Merge method(`how`): SQL Join Name : Description*```* left : LEFT OUTER JOIN : Use keys or columns from left frame only* right : RIGHT OUTER JOIN : Use keys or columns from right frame only* outer : FULL OUTER JOIN : Use union of keys or columns from both frames* inner : INNER JOIN : Use intersection of keys or columns from both frames```
###Code
df1 = pd.DataFrame({'Name': ['Joe', 'Joshua', 'Jeanne', 'David'],
'Role': ['Manager', 'Developer', 'Engineer', 'Scientist']})
df2 = pd.DataFrame({'Name': ['David', 'Joshua', 'Joe', 'Jeanne'],
'Year Hired': [2018, 2017, 2020, 2018]})
df3 = pd.DataFrame({'Name': ['David', 'Joshua', 'Joe', 'Jeanne'],
'No of Leaves': [15, 3, 10, 12]})
df1
df2
pd.merge(df1, df2)
## Let's merge on Role being a key
pd.merge(df1, df2, how='inner', on="Name")
df1 = pd.DataFrame({'col1': ['K0', 'K0', 'K1', 'K2'],
'col2': ['K0', 'K1', 'K0', 'K1'],
'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3']})
df2 = pd.DataFrame({'col1': ['K0', 'K1', 'K1', 'K2'],
'col2': ['K0', 'K0', 'K0', 'K0'],
'C': ['C0', 'C1', 'C2', 'C3'],
'D': ['D0', 'D1', 'D2', 'D3']})
df1
df2
pd.merge(df1, df2, how='inner', on=['col1', 'col2'])
pd.merge(df1, df2, how='outer', on=['col1', 'col2'])
pd.merge(df1, df2, how='left')
pd.merge(df1, df2, how='right')
###Output
_____no_output_____
###Markdown
JoiningJoining is a simple way to combine columns of two dataframes with different indexes.
###Code
df1 = pd.DataFrame({'Col1': ['A', 'B', 'C'],
'Col2': [11, 12, 13]},
index=['a', 'b', 'c'])
df2 = pd.DataFrame({'Col3': ['D', 'E', 'F'],
'Col4': [14, 14, 16]},
index=['a', 'c', 'd'])
df1
df2
df1.join(df2)
df2.join(df1)
###Output
_____no_output_____
###Markdown
You can see that with `df.join()`, the alignment of data is on indexes.
###Code
df1.join(df2, how='inner')
df1.join(df2, how='outer')
###Output
_____no_output_____
###Markdown
Learn more about Merging, Joining, and Concatenating the Pandas Dataframes [here](https://pandas.pydata.org/docs/user_guide/merging.html). H. Beyond Dataframes: Working with CSV and ExcelIn this last section of Pandas' fundamentals, we will see how to read real world data with different formats: CSV and Excel CSV and ExcelLet's use california housing dataset.
###Code
# Let's download the data
!curl -O https://raw.githubusercontent.com/nyandwi/public_datasets/master/housing.csv
data = pd.read_csv('housing.csv')
data.head()
type(data)
## Exporting dataframe back to csv
data.to_csv('housing_dataset', index=False)
###Output
_____no_output_____
###Markdown
If you look into the folder sidebar, you can see `Housing Dataset`.
###Code
## Exporting CSV to Excel
data.to_excel('housing_excel.xlsx', index=False)
## Reading the Excel file back
excel_data = pd.read_excel('housing_excel.xlsx')
excel_data.head()
###Output
_____no_output_____
###Markdown
Real World: Exploratory Data Analysis (EDA)All above was the basics. Let us apply some of these techniques to the real world dataset, `Red wine quality`.
###Code
!curl -O https://raw.githubusercontent.com/nyandwi/public_datasets/master/winequality-red.csv
wine_data = pd.read_csv('winequality-red.csv')
# Displaying the head of the dataset
wine_data.head()
# Displaying the tail of the dataset
wine_data.tail()
# Displaying summary statistics
wine_data.describe().transpose()
# Displaying quick information about the dataset
wine_data.info()
# Checking missing values
wine_data.isnull().sum()
# wine quality range from 0 to 10. The higher the quality value, the good wine is
wine_data['quality'].value_counts()
wine_data.groupby(['fixed acidity', 'volatile acidity', 'citric acid']).sum()
wine_data.groupby(['free sulfur dioxide', 'total sulfur dioxide']).sum()
###Output
_____no_output_____
###Markdown
*This notebook was created by [Jean de Dieu Nyandwi](https://twitter.com/jeande_d) for the love of machine learning community. For any feedback, errors or suggestion, he can be reached on email (johnjw7084 at gmail dot com), [Twitter](https://twitter.com/jeande_d), or [LinkedIn](https://linkedin.com/in/nyandwi).* Data Manipulation with Pandas In this lab, you will learn how to manipulate data with Pandas. Here is an overview:* [1. Basics of Pandas for data manipulation:](1) * [A. Series and DataFrames](1-1) * [B. Data Indexing and Selection, and Iteration (Add Iteration)](1-2) * [C. Dealing with Missing data](1-3) * [D. Basic operations and Functions](1-4) * [E. Aggregation Methods](1-5) * [F. Groupby](1-6) * [G. Merging, Joining and Concatenate](1-7) * [H. Beyond Dataframes: Working with CSV, and Excel](1-8) * [2. Real World Exploratory Data Analysis (EDA)](2) 1. Basics of Pandas for data manipulation A. Series and DataFramesBoth series and DataFrames are Pandas Data structures. Series is like one dimensional NumPy array with axis labels.DataFrame is multidimensional NumPy array with labels on rows and columns. Working with NumPy, we saw that it supports numeric type data. Pandas on other hand supports whole range of data types, from numeric to strings, etc.. Since we are using python notebook, we do not need to install Pandas. We only just have to import it. ```import pandas as pd```
###Code
# importing numpy and pandas
import numpy as np
import pandas as pd
###Output
_____no_output_____
###Markdown
Creating SeriesSeries can be created from a Python list, dictionary, and NumPy array.
###Code
# Creating the series from a Python list
num_list = [1,2,3,4,5]
pd.Series(num_list)
week_days = ['Mon','Tues','Wed','Thur','Fri']
pd.Series(week_days, index=["a", "b", "c", "d", "e"])
###Output
_____no_output_____
###Markdown
Note the data types `int64` and `object`.
###Code
# Creating the Series from dictionary
countries_code = { 1:"United States",
91:"India",
49:"Germany",
86:"China",
250:"Rwanda"}
pd.Series(countries_code)
d = {1:'a', 2:'b', 3:'c', 4:'d'}
pd.Series(d)
# Creating the Series from NumPy array
# We peovide the list of indexes
# if we don't provide the indexes, the default indexes are numbers...starts from 0,1,2..
arr = np.array ([1, 2, 3, 4, 5])
pd.Series(arr)
pd.Series(arr, index=['a', 'b', 'c', 'd', 'e'])
###Output
_____no_output_____
###Markdown
Creating DataFramesDataFrames are the most used Pandas data structure. It can be created from a dictionary, 2D array, and Series.
###Code
# Creating DataFrame from a dictionary
countries = {'Name': ['USA', 'India', 'German', 'Rwanda'],
'Codes':[1, 91, 49, 250] }
pd.DataFrame(countries)
# Creating a dataframe from a 2D array
# You pass the list of columns
array_2d = np.array ([[1,2,3], [4,5,6], [7,8,9]])
pd.DataFrame(array_2d, columns = ['column 1', 'column 2', 'column 3'])
# Creating a dataframe from Pandas series
# Pass the columns in a list
countries_code = { "United States": 1,
"India": 91,
"Germany": 49,
"China": 86,
"Rwanda":250}
pd_series = pd.Series (countries_code)
pd.Series(countries_code)
df = pd.DataFrame(pd_series, columns = ['Codes'])
df
# Adding a column
# Number in population are pretty random
df ['Population'] = [100, 450, 575, 5885, 533]
df
# Removing a column
df.drop('Population', axis =1)
df.columns
df.keys
df.index
###Output
_____no_output_____
###Markdown
B. Data Indexing and SelectionIndexing and selection works in both Series and Dataframe.Because DataFrame is made of Series, let's focus on how to select data in DataFrame.
###Code
# Creating DataFrame from a dictionary
countries = {'Name': ['USA', 'India', 'German', 'Rwanda'],
'Codes':[1, 91, 49, 250] }
df = pd.DataFrame(countries, index=['a', 'b', 'c', 'd'])
df
df['Name']
df.Name
df ['Codes']
## When you have many columns, columns in list will be selected
df [['Name', 'Codes']]
# This will return the first two rows
df [0:2]
###Output
_____no_output_____
###Markdown
You can also use `loc` to select data by the label indexes and `iloc` to select by default integer index (or by the position of the row)
###Code
df.loc['a']
df.loc['b':'d']
df [:'b']
df.iloc[2]
df.iloc[1:3]
df.iloc[2:]
###Output
_____no_output_____
###Markdown
Conditional Selection
###Code
df
#Let's select a country with code 49
df [df['Codes'] ==49 ]
df [df['Codes'] < 250 ]
df [df['Name'] =='USA' ]
# You can use and (&) or (|) for more than conditions
#df [(condition 1) & (condition 2)]
df [(df['Codes'] == 91 ) & (df['Name'] == 'India') ]
###Output
_____no_output_____
###Markdown
You can also use `isin()` and `where()` to select data in a series or dataframe.
###Code
# isin() return false or true when provided value is included in dataframe
sample_codes_names=[1,3,250, 'USA', 'India', 'England']
df.isin(sample_codes_names)
###Output
_____no_output_____
###Markdown
As you can see, it returned `True` wherever a country code or name was found. Otherwise, `False`. You can use a dictinary to match search by columns. A key must be a column and values are passed in list.
###Code
sample_codes_names = {'Codes':[1,3,250], 'Name':['USA', 'India', 'England']}
df.isin(sample_codes_names)
df2 = pd.DataFrame(np.array ([[1,2,3], [4,5,6], [7,8,9]]),
columns = ['column 1', 'column 2', 'column 3'])
df2
df2.isin([0,3,4,5,7])
df2 [df2 > 4]
df2.where(df2 > 4)
###Output
_____no_output_____
###Markdown
Where the condition is false, `where` allows you to replace values. In this case, all values less than 4 will be 0.
###Code
df2.where(df2 > 4, 0)
# The above do the same as as
df2 [df2 >4 ] = 0
df2
###Output
_____no_output_____
###Markdown
Iteration```df.items() Iterate over (column name, Series) pairs.df.iteritems() Iterate over (column name, Series) pairs.DataFrame.iterrows() Iterate over DataFrame rows as (index, Series) pairs.DataFrame.itertuples([index, name]) Iterate over DataFrame rows as namedtuples.```
###Code
# Iterate over (column name, Series) pairs.
for col_name, content in df2.items():
print(col_name)
print(content)
# Iterate over (column name, Series) pairs.
# Same as df.items()
for col_name, content in df2.iteritems():
print(col_name)
print(content)
# Iterate over DataFrame rows as (index, Series) pairs
for row in df2.iterrows():
print(row)
# Iterate over DataFrame rows as namedtuples
for row in df2.itertuples():
print(row)
###Output
Pandas(Index=0, _1=1, _2=2, _3=3)
Pandas(Index=1, _1=4, _2=0, _3=0)
Pandas(Index=2, _1=0, _2=0, _3=0)
###Markdown
C. Dealing with Missing dataReal world datasets are messy, often with missing values. Pandas replace NaN with missing values by default. NaN stands for not a number. Missing values can either be ignored, droped or filled.
###Code
# Creating a dataframe
df3 = pd.DataFrame(np.array ([[1,2,3], [4,np.nan,6], [7,np.nan,np.nan]]),
columns = ['column 1', 'column 2', 'column 3'])
###Output
_____no_output_____
###Markdown
Checking Missing values
###Code
# Recognizing the missing values
df3.isnull()
# Calculating number of the missing values in each feature
df3.isnull().sum()
# Recognizng non missig values
df3.notna()
df3.notna().sum()
###Output
_____no_output_____
###Markdown
Removing the missing values
###Code
## Dropping missing values
df3.dropna()
###Output
_____no_output_____
###Markdown
All rows are deleted because dropna() will remove each row which have missing value.
###Code
# you can drop NaNs in specific column(s)
df3['column 3'].dropna()
# You can drop data by axis
# Axis = 1...drop all columns with Nans
# df3.dropna(axis='columns')
df3.dropna(axis=1)
# axis = 0...drop all rows with Nans
# df3.dropna(axis='rows') is same
df3.dropna(axis=0)
###Output
_____no_output_____
###Markdown
Filling the missing values
###Code
# Filling Missing values
df3.fillna(10)
df3.fillna('fillme')
# You can forward fill (ffill) or backward fill(bfill)
# Or fill a current value with previous or next value
df3.fillna(method='ffill')
# Won't change it because the last values are NaNs, so it backward it
df3.fillna(method='bfill')
# If we change the axis to columns, you can see that Nans at row 2 and col 2 is backfilled with 6
df3.fillna(method='bfill', axis='columns')
###Output
_____no_output_____
###Markdown
D. More Operations and FunctionsThis section will show the more and most useful functions of Pandas.
###Code
df4 = pd.DataFrame({'Product Name':['Shirt','Boot','Bag'],
'Order Number':[45,56,64],
'Total Quantity':[10,5,9]},
columns = ['Product Name', 'Order Number', 'Total Quantity'])
###Output
_____no_output_____
###Markdown
Retrieving basic info about the Dataframe
###Code
# Return a summary about the dataframe
df4.info()
# Return dataframe columns
df4.columns
# Return dataframe data
df4.keys
# Return the head of the dataframe ....could make sense if you have long frame
# Choose how many rows you want in head()
df4.head(1)
# Return the tail of the dataframe
df4.tail(1)
# Return NumPy array of the dataframe
df4.values
# Return the size or number of elements in a dataframe
df4.size
# Return the shape
df4.shape
# Return the length of the dataframe/the number of rows in a dataframe
df4.shape[0]
# Return the length of the dataframe/the number of columns in a dataframe
df4.shape[1]
###Output
_____no_output_____
###Markdown
Unique Values
###Code
# Return unique values in a given column
df4['Product Name'].unique()
# Return a number of unique values
df4['Product Name'].nunique()
# Counting the occurence of each value in a column
df4['Product Name'].value_counts()
###Output
_____no_output_____
###Markdown
Applying a Function to Dataframe
###Code
# Double the quantity product
def double_quantity(x):
return x * x
df4['Total Quantity'].apply(double_quantity)
# You can also apply an anonymous function to a dataframe
# Squaring each value in dataframe
df5 = pd.DataFrame([[1,2], [4,5]], columns=['col1', 'col2'])
df5.applymap(lambda x: x**2)
###Output
_____no_output_____
###Markdown
Sorting values in dataframe
###Code
# Sort the df4 by the order number
df4.sort_values(['Order Number'])
df4.sort_values(['Order Number'], ascending=False)
###Output
_____no_output_____
###Markdown
E. Aggregation Methods
###Code
df4
# summary statistics
df4.describe()
df4.describe().transpose()
# Mode of the dataframe
# Mode is the most recurring values
df4['Total Quantity'].mode()
# The maximum value
df4['Total Quantity'].max()
# The minimum value
df4['Total Quantity'].min()
# The mean
df4['Total Quantity'].mean()
# The median value in a dataframe
df4['Total Quantity'].median()
# Standard deviation
df4['Total Quantity'].std()
# Variance
df4['Total Quantity'].var()
# Sum of all values in a column
df4['Total Quantity'].sum()
# Product of all values in dataframe
df4['Total Quantity'].prod()
###Output
_____no_output_____
###Markdown
F. Groupby`Group by` involves splitting data into groups, applying function to each group, and combining the results.
###Code
df4 = pd.DataFrame({'Product Name':['Shirt','Boot','Bag', 'Ankle', 'Pullover', 'Boot', 'Ankle', 'Tshirt', 'Shirt'],
'Order Number':[45,56,64, 34, 67, 56, 34, 89, 45],
'Total Quantity':[10,5,9, 11, 11, 8, 14, 23, 10]},
columns = ['Product Name', 'Order Number', 'Total Quantity'])
df4
# Let's group the df by product name
df4.groupby('Product Name').mean()
df4.groupby('Product Name').sum()
df4.groupby('Product Name').min()
df4.groupby('Product Name').max()
df4.groupby(['Product Name', 'Order Number']).max()
df4.groupby(['Product Name', 'Order Number']).sum()
###Output
_____no_output_____
###Markdown
You can also use `aggregation()` after groupby.
###Code
df4.groupby('Product Name').aggregate(['min', 'max', 'sum'])
###Output
_____no_output_____
###Markdown
G. Combining Datasets: Concatenating, Joining and Merging Concatenation
###Code
# Creating dataframes
df1 = pd.DataFrame({'Col1':['A','B','C'],
'Col2':[1,2,3]},
index=['a','b','c'])
df2 = pd.DataFrame({'Col1':['D','E','F'],
'Col2':[4,5,6]},
index=['d','e','f'])
df3 = pd.DataFrame({'Col1':['G','I','J'],
'Col2':[7,8,9]},
index=['g', 'i','j'])
df1
df2
df3
# Concatenating: Adding one dataset to another
pd.concat([df1, df2, df3])
###Output
_____no_output_____
###Markdown
The default axis is `0`. This is how the combined dataframes will look like if we change the `axis to 1`.
###Code
pd.concat([df1, df2, df3], axis=1)
# We can also use append()
df1.append([df2, df3])
###Output
_____no_output_____
###Markdown
MergingIf you have worked with SQL, what `pd.merge()` does may be familiar. It links data from different sources (different features) and you have a control on the structure of the combined dataset. *Pandas Merge method(`how`): SQL Join Name : Description*```* left : LEFT OUTER JOIN : Use keys or columns from left frame only* right : RIGHT OUTER JOIN : Use keys or columns from right frame only* outer : FULL OUTER JOIN : Use union of keys or columns from both frames* inner : INNER JOIN : Use intersection of keys or columns from both frames```
###Code
df1 = pd.DataFrame({'Name': ['Joe', 'Joshua', 'Jeanne', 'David'],
'Role': ['Manager', 'Developer', 'Engineer', 'Scientist']})
df2 = pd.DataFrame({'Name': ['David', 'Joshua', 'Joe', 'Jeanne'],
'Year Hired': [2018, 2017, 2020, 2018]})
df3 = pd.DataFrame({'Name': ['David', 'Joshua', 'Joe', 'Jeanne'],
'No of Leaves': [15, 3, 10, 12]})
df1
df2
pd.merge(df1, df2)
## Let's merge on Role being a key
pd.merge(df1, df2, how='inner', on="Name")
df1 = pd.DataFrame({'col1': ['K0', 'K0', 'K1', 'K2'],
'col2': ['K0', 'K1', 'K0', 'K1'],
'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3']})
df2 = pd.DataFrame({'col1': ['K0', 'K1', 'K1', 'K2'],
'col2': ['K0', 'K0', 'K0', 'K0'],
'C': ['C0', 'C1', 'C2', 'C3'],
'D': ['D0', 'D1', 'D2', 'D3']})
df1
df2
pd.merge(df1, df2, how='inner', on=['col1', 'col2'])
pd.merge(df1, df2, how='outer', on=['col1', 'col2'])
pd.merge(df1, df2, how='left')
pd.merge(df1, df2, how='right')
###Output
_____no_output_____
###Markdown
JoiningJoining is a simple way to combine columns of two dataframes with different indexes.
###Code
df1 = pd.DataFrame({'Col1': ['A', 'B', 'C'],
'Col2': [11, 12, 13]},
index=['a', 'b', 'c'])
df2 = pd.DataFrame({'Col3': ['D', 'E', 'F'],
'Col4': [14, 14, 16]},
index=['a', 'c', 'd'])
df1
df2
df1.join(df2)
df2.join(df1)
###Output
_____no_output_____
###Markdown
You can see that with `df.join()`, the alignment of data is on indexes.
###Code
df1.join(df2, how='inner')
df1.join(df2, how='outer')
###Output
_____no_output_____
###Markdown
Learn more about Merging, Joining, and Concatenating the Pandas Dataframes [here](https://pandas.pydata.org/docs/user_guide/merging.html). H. Beyond Dataframes: Working with CSV and ExcelIn this last section of Pandas' fundamentals, we will see how to read real world data with different formats: CSV and Excel CSV and ExcelLet's use california housing dataset.
###Code
# Let's download the data
!curl -O https://raw.githubusercontent.com/nyandwi/public_datasets/master/housing.csv
data = pd.read_csv('housing.csv')
data.head()
type(data)
## Exporting dataframe back to csv
data.to_csv('housing_dataset', index=False)
###Output
_____no_output_____
###Markdown
If you look into the folder sidebar, you can see `Housing Dataset`.
###Code
## Exporting CSV to Excel
data.to_excel('housing_excel.xlsx', index=False)
## Reading the Excel file back
excel_data = pd.read_excel('housing_excel.xlsx')
excel_data.head()
###Output
_____no_output_____
###Markdown
Real World: Exploratory Data Analysis (EDA)All above was the basics. Let us apply some of these techniques to the real world dataset, `Red wine quality`.
###Code
!curl -O https://raw.githubusercontent.com/nyandwi/public_datasets/master/winequality-red.csv
wine_data = pd.read_csv('winequality-red.csv')
# Displaying the head of the dataset
wine_data.head()
# Displaying the tail of the dataset
wine_data.tail()
# Displaying summary statistics
wine_data.describe().transpose()
# Displaying quick information about the dataset
wine_data.info()
# Checking missing values
wine_data.isnull().sum()
# wine quality range from 0 to 10. The higher the quality value, the good wine is
wine_data['quality'].value_counts()
wine_data.groupby(['fixed acidity', 'volatile acidity', 'citric acid']).sum()
wine_data.groupby(['free sulfur dioxide', 'total sulfur dioxide']).sum()
###Output
_____no_output_____
###Markdown
*This notebook was created by [Jean de Dieu Nyandwi](https://twitter.com/jeande_d) for the love of machine learning community. For any feedback, errors or suggestion, he can be reached on email (johnjw7084 at gmail dot com), [Twitter](https://twitter.com/jeande_d), or [LinkedIn](https://linkedin.com/in/nyandwi).* Data Manipulation with Pandas In this lab, you will learn how to manipulate data with Pandas. Here is an overview:* [1. Basics of Pandas for data manipulation:](1) * [A. Series and DataFrames](1-1) * [B. Data Indexing and Selection, and Iteration](1-2) * [C. Dealing with Missing data](1-3) * [D. Basic operations and Functions](1-4) * [E. Aggregation Methods](1-5) * [F. Groupby](1-6) * [G. Merging, Joining and Concatenate](1-7) * [H. Beyond Dataframes: Working with CSV, and Excel](1-8) * [2. Real World Exploratory Data Analysis (EDA)](2) 1. Basics of Pandas for data manipulation A. Series and DataFramesBoth series and DataFrames are Pandas Data structures. Series is like one dimensional NumPy array with axis labels.DataFrame is multidimensional NumPy array with labels on rows and columns. Working with NumPy, we saw that it supports numeric type data. Pandas on other hand supports whole range of data types, from numeric to strings, etc.. Since we are using python notebook, we do not need to install Pandas. We only just have to import it. ```import pandas as pd```
###Code
# importing numpy and pandas
import numpy as np
import pandas as pd
###Output
_____no_output_____
###Markdown
Creating SeriesSeries can be created from a Python list, dictionary, and NumPy array.
###Code
# Creating the series from a Python list
num_list = [1,2,3,4,5]
pd.Series(num_list)
week_days = ['Mon','Tues','Wed','Thur','Fri']
pd.Series(week_days, index=["a", "b", "c", "d", "e"])
###Output
_____no_output_____
###Markdown
Note the data types `int64` and `object`.
###Code
# Creating the Series from dictionary
countries_code = { 1:"United States",
91:"India",
49:"Germany",
86:"China",
250:"Rwanda"}
pd.Series(countries_code)
d = {1:'a', 2:'b', 3:'c', 4:'d'}
pd.Series(d)
# Creating the Series from NumPy array
# We peovide the list of indexes
# if we don't provide the indexes, the default indexes are numbers...starts from 0,1,2..
arr = np.array ([1, 2, 3, 4, 5])
pd.Series(arr)
pd.Series(arr, index=['a', 'b', 'c', 'd', 'e'])
###Output
_____no_output_____
###Markdown
Creating DataFramesDataFrames are the most used Pandas data structure. It can be created from a dictionary, 2D array, and Series.
###Code
# Creating DataFrame from a dictionary
countries = {'Name': ['USA', 'India', 'German', 'Rwanda'],
'Codes':[1, 91, 49, 250] }
pd.DataFrame(countries)
# Creating a dataframe from a 2D array
# You pass the list of columns
array_2d = np.array ([[1,2,3], [4,5,6], [7,8,9]])
pd.DataFrame(array_2d, columns = ['column 1', 'column 2', 'column 3'])
# Creating a dataframe from Pandas series
# Pass the columns in a list
countries_code = { "United States": 1,
"India": 91,
"Germany": 49,
"China": 86,
"Rwanda":250}
pd_series = pd.Series (countries_code)
pd.Series(countries_code)
df = pd.DataFrame(pd_series, columns = ['Codes'])
df
# Adding a column
# Number in population are pretty random
df ['Population'] = [100, 450, 575, 5885, 533]
df
# Removing a column
df.drop('Population', axis =1)
df.columns
df.keys
df.index
###Output
_____no_output_____
###Markdown
B. Data Indexing, Selection and IterationIndexing and selection works in both Series and Dataframe.Because DataFrame is made of Series, let's focus on how to select data in DataFrame.
###Code
# Creating DataFrame from a dictionary
countries = {'Name': ['USA', 'India', 'German', 'Rwanda'],
'Codes':[1, 91, 49, 250] }
df = pd.DataFrame(countries, index=['a', 'b', 'c', 'd'])
df
df['Name']
df.Name
df ['Codes']
## When you have many columns, columns in list will be selected
df [['Name', 'Codes']]
# This will return the first two rows
df [0:2]
###Output
_____no_output_____
###Markdown
You can also use `loc` to select data by the label indexes and `iloc` to select by default integer index (or by the position of the row)
###Code
df.loc['a']
df.loc['b':'d']
df [:'b']
df.iloc[2]
df.iloc[1:3]
df.iloc[2:]
###Output
_____no_output_____
###Markdown
Conditional Selection
###Code
df
#Let's select a country with code 49
df [df['Codes'] ==49 ]
df [df['Codes'] < 250 ]
df [df['Name'] =='USA' ]
# You can use and (&) or (|) for more than conditions
#df [(condition 1) & (condition 2)]
df [(df['Codes'] == 91 ) & (df['Name'] == 'India') ]
###Output
_____no_output_____
###Markdown
You can also use `isin()` and `where()` to select data in a series or dataframe.
###Code
# isin() return false or true when provided value is included in dataframe
sample_codes_names=[1,3,250, 'USA', 'India', 'England']
df.isin(sample_codes_names)
###Output
_____no_output_____
###Markdown
As you can see, it returned `True` wherever a country code or name was found. Otherwise, `False`. You can use a dictinary to match search by columns. A key must be a column and values are passed in list.
###Code
sample_codes_names = {'Codes':[1,3,250], 'Name':['USA', 'India', 'England']}
df.isin(sample_codes_names)
df2 = pd.DataFrame(np.array ([[1,2,3], [4,5,6], [7,8,9]]),
columns = ['column 1', 'column 2', 'column 3'])
df2
df2.isin([0,3,4,5,7])
df2 [df2 > 4]
df2.where(df2 > 4)
###Output
_____no_output_____
###Markdown
`where` allows you to replace the values that doesn't meet the provided condition with any other value. So, if we do `df2.where(df2 > 4, 0)` as follows, all values less than `4` will be replaced by `0`.
###Code
df2.where(df2 > 4, 0)
###Output
_____no_output_____
###Markdown
Similarly, we can achieve the above by...
###Code
df2 [df2 <= 4] = 0
df2
###Output
_____no_output_____
###Markdown
Iteration```df.items() Iterate over (column name, Series) pairs.df.iteritems() Iterate over (column name, Series) pairs.DataFrame.iterrows() Iterate over DataFrame rows as (index, Series) pairs.DataFrame.itertuples([index, name]) Iterate over DataFrame rows as namedtuples.```
###Code
# Iterate over (column name, Series) pairs.
for col_name, content in df2.items():
print(col_name)
print(content)
# Iterate over (column name, Series) pairs.
# Same as df.items()
for col_name, content in df2.iteritems():
print(col_name)
print(content)
# Iterate over DataFrame rows as (index, Series) pairs
for row in df2.iterrows():
print(row)
# Iterate over DataFrame rows as namedtuples
for row in df2.itertuples():
print(row)
###Output
Pandas(Index=0, _1=0, _2=0, _3=0)
Pandas(Index=1, _1=0, _2=5, _3=6)
Pandas(Index=2, _1=7, _2=8, _3=9)
###Markdown
Notes: Thanks to [Prit Kalariya](https://twitter.com/pritkalariya) for Contributing the Iteration part! C. Dealing with Missing dataReal world datasets are messy, often with missing values. Pandas replace NaN with missing values by default. NaN stands for not a number. Missing values can either be ignored, droped or filled.
###Code
# Creating a dataframe
df3 = pd.DataFrame(np.array ([[1,2,3], [4,np.nan,6], [7,np.nan,np.nan]]),
columns = ['column 1', 'column 2', 'column 3'])
###Output
_____no_output_____
###Markdown
Checking Missing values
###Code
# Recognizing the missing values
df3.isnull()
# Calculating number of the missing values in each feature
df3.isnull().sum()
# Recognizng non missig values
df3.notna()
df3.notna().sum()
###Output
_____no_output_____
###Markdown
Removing the missing values
###Code
## Dropping missing values
df3.dropna()
###Output
_____no_output_____
###Markdown
All rows are deleted because dropna() will remove each row which have missing value.
###Code
# you can drop NaNs in specific column(s)
df3['column 3'].dropna()
# You can drop data by axis
# Axis = 1...drop all columns with Nans
# df3.dropna(axis='columns')
df3.dropna(axis=1)
# axis = 0...drop all rows with Nans
# df3.dropna(axis='rows') is same
df3.dropna(axis=0)
###Output
_____no_output_____
###Markdown
Filling the missing values
###Code
# Filling Missing values
df3.fillna(10)
df3.fillna('fillme')
# You can forward fill (ffill) or backward fill(bfill)
# Or fill a current value with previous or next value
df3.fillna(method='ffill')
# Won't change it because the last values are NaNs, so it backward it
df3.fillna(method='bfill')
# If we change the axis to columns, you can see that Nans at row 2 and col 2 is backfilled with 6
df3.fillna(method='bfill', axis='columns')
###Output
_____no_output_____
###Markdown
D. More Operations and FunctionsThis section will show the more and most useful functions of Pandas.
###Code
df4 = pd.DataFrame({'Product Name':['Shirt','Boot','Bag'],
'Order Number':[45,56,64],
'Total Quantity':[10,5,9]},
columns = ['Product Name', 'Order Number', 'Total Quantity'])
###Output
_____no_output_____
###Markdown
Retrieving basic info about the Dataframe
###Code
# Return a summary about the dataframe
df4.info()
# Return dataframe columns
df4.columns
# Return dataframe data
df4.keys
# Return the head of the dataframe ....could make sense if you have long frame
# Choose how many rows you want in head()
df4.head(1)
# Return the tail of the dataframe
df4.tail(1)
# Return NumPy array of the dataframe
df4.values
# Return the size or number of elements in a dataframe
df4.size
# Return the shape
df4.shape
# Return the length of the dataframe/the number of rows in a dataframe
df4.shape[0]
# Return the length of the dataframe/the number of columns in a dataframe
df4.shape[1]
###Output
_____no_output_____
###Markdown
Unique Values
###Code
# Return unique values in a given column
df4['Product Name'].unique()
# Return a number of unique values
df4['Product Name'].nunique()
# Counting the occurence of each value in a column
df4['Product Name'].value_counts()
###Output
_____no_output_____
###Markdown
Applying a Function to Dataframe
###Code
# Double the quantity product
def double_quantity(x):
return x * x
df4['Total Quantity'].apply(double_quantity)
# You can also apply an anonymous function to a dataframe
# Squaring each value in dataframe
df5 = pd.DataFrame([[1,2], [4,5]], columns=['col1', 'col2'])
df5.applymap(lambda x: x**2)
###Output
_____no_output_____
###Markdown
Sorting values in dataframe
###Code
# Sort the df4 by the order number
df4.sort_values(['Order Number'])
df4.sort_values(['Order Number'], ascending=False)
###Output
_____no_output_____
###Markdown
E. Aggregation Methods
###Code
df4
# summary statistics
df4.describe()
df4.describe().transpose()
# Mode of the dataframe
# Mode is the most recurring values
df4['Total Quantity'].mode()
# The maximum value
df4['Total Quantity'].max()
# The minimum value
df4['Total Quantity'].min()
# The mean
df4['Total Quantity'].mean()
# The median value in a dataframe
df4['Total Quantity'].median()
# Standard deviation
df4['Total Quantity'].std()
# Variance
df4['Total Quantity'].var()
# Sum of all values in a column
df4['Total Quantity'].sum()
# Product of all values in dataframe
df4['Total Quantity'].prod()
###Output
_____no_output_____
###Markdown
F. Groupby`Group by` involves splitting data into groups, applying function to each group, and combining the results.
###Code
df4 = pd.DataFrame({'Product Name':['Shirt','Boot','Bag', 'Ankle', 'Pullover', 'Boot', 'Ankle', 'Tshirt', 'Shirt'],
'Order Number':[45,56,64, 34, 67, 56, 34, 89, 45],
'Total Quantity':[10,5,9, 11, 11, 8, 14, 23, 10]},
columns = ['Product Name', 'Order Number', 'Total Quantity'])
df4
# Let's group the df by product name
df4.groupby('Product Name').mean()
df4.groupby('Product Name').sum()
df4.groupby('Product Name').min()
df4.groupby('Product Name').max()
df4.groupby(['Product Name', 'Order Number']).max()
df4.groupby(['Product Name', 'Order Number']).sum()
###Output
_____no_output_____
###Markdown
You can also use `aggregation()` after groupby.
###Code
df4.groupby('Product Name').aggregate(['min', 'max', 'sum'])
###Output
_____no_output_____
###Markdown
G. Combining Datasets: Concatenating, Joining and Merging Concatenation
###Code
# Creating dataframes
df1 = pd.DataFrame({'Col1':['A','B','C'],
'Col2':[1,2,3]},
index=['a','b','c'])
df2 = pd.DataFrame({'Col1':['D','E','F'],
'Col2':[4,5,6]},
index=['d','e','f'])
df3 = pd.DataFrame({'Col1':['G','I','J'],
'Col2':[7,8,9]},
index=['g', 'i','j'])
df1
df2
df3
# Concatenating: Adding one dataset to another
pd.concat([df1, df2, df3])
###Output
_____no_output_____
###Markdown
The default axis is `0`. This is how the combined dataframes will look like if we change the `axis to 1`.
###Code
pd.concat([df1, df2, df3], axis=1)
# We can also use append()
df1.append([df2, df3])
###Output
_____no_output_____
###Markdown
MergingIf you have worked with SQL, what `pd.merge()` does may be familiar. It links data from different sources (different features) and you have a control on the structure of the combined dataset. *Pandas Merge method(`how`): SQL Join Name : Description*```* left : LEFT OUTER JOIN : Use keys or columns from left frame only* right : RIGHT OUTER JOIN : Use keys or columns from right frame only* outer : FULL OUTER JOIN : Use union of keys or columns from both frames* inner : INNER JOIN : Use intersection of keys or columns from both frames```
###Code
df1 = pd.DataFrame({'Name': ['Joe', 'Joshua', 'Jeanne', 'David'],
'Role': ['Manager', 'Developer', 'Engineer', 'Scientist']})
df2 = pd.DataFrame({'Name': ['David', 'Joshua', 'Joe', 'Jeanne'],
'Year Hired': [2018, 2017, 2020, 2018]})
df3 = pd.DataFrame({'Name': ['David', 'Joshua', 'Joe', 'Jeanne'],
'No of Leaves': [15, 3, 10, 12]})
df1
df2
pd.merge(df1, df2)
## Let's merge on Role being a key
pd.merge(df1, df2, how='inner', on="Name")
df1 = pd.DataFrame({'col1': ['K0', 'K0', 'K1', 'K2'],
'col2': ['K0', 'K1', 'K0', 'K1'],
'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3']})
df2 = pd.DataFrame({'col1': ['K0', 'K1', 'K1', 'K2'],
'col2': ['K0', 'K0', 'K0', 'K0'],
'C': ['C0', 'C1', 'C2', 'C3'],
'D': ['D0', 'D1', 'D2', 'D3']})
df1
df2
pd.merge(df1, df2, how='inner', on=['col1', 'col2'])
pd.merge(df1, df2, how='outer', on=['col1', 'col2'])
pd.merge(df1, df2, how='left')
pd.merge(df1, df2, how='right')
###Output
_____no_output_____
###Markdown
JoiningJoining is a simple way to combine columns of two dataframes with different indexes.
###Code
df1 = pd.DataFrame({'Col1': ['A', 'B', 'C'],
'Col2': [11, 12, 13]},
index=['a', 'b', 'c'])
df2 = pd.DataFrame({'Col3': ['D', 'E', 'F'],
'Col4': [14, 14, 16]},
index=['a', 'c', 'd'])
df1
df2
df1.join(df2)
df2.join(df1)
###Output
_____no_output_____
###Markdown
You can see that with `df.join()`, the alignment of data is on indexes.
###Code
df1.join(df2, how='inner')
df1.join(df2, how='outer')
###Output
_____no_output_____
###Markdown
Learn more about Merging, Joining, and Concatenating the Pandas Dataframes [here](https://pandas.pydata.org/docs/user_guide/merging.html). H. Beyond Dataframes: Working with CSV and ExcelIn this last section of Pandas' fundamentals, we will see how to read real world data with different formats: CSV and Excel CSV and ExcelLet's use california housing dataset.
###Code
# Let's download the data
!curl -O https://raw.githubusercontent.com/nyandwi/public_datasets/master/housing.csv
data = pd.read_csv('housing.csv')
data.head()
type(data)
## Exporting dataframe back to csv
data.to_csv('housing_dataset', index=False)
###Output
_____no_output_____
###Markdown
If you look into the folder sidebar, you can see `Housing Dataset`.
###Code
## Exporting CSV to Excel
data.to_excel('housing_excel.xlsx', index=False)
## Reading the Excel file back
excel_data = pd.read_excel('housing_excel.xlsx')
excel_data.head()
###Output
_____no_output_____
###Markdown
Real World: Exploratory Data Analysis (EDA)All above was the basics. Let us apply some of these techniques to the real world dataset, `Red wine quality`.
###Code
!curl -O https://raw.githubusercontent.com/nyandwi/public_datasets/master/winequality-red.csv
wine_data = pd.read_csv('winequality-red.csv')
# Displaying the head of the dataset
wine_data.head()
# Displaying the tail of the dataset
wine_data.tail()
# Displaying summary statistics
wine_data.describe().transpose()
# Displaying quick information about the dataset
wine_data.info()
# Checking missing values
wine_data.isnull().sum()
# wine quality range from 0 to 10. The higher the quality value, the good wine is
wine_data['quality'].value_counts()
wine_data.groupby(['fixed acidity', 'volatile acidity', 'citric acid']).sum()
wine_data.groupby(['free sulfur dioxide', 'total sulfur dioxide']).sum()
###Output
_____no_output_____ |
Custom_Mask_RCNN/mask_rcnn_custom_images.ipynb | ###Markdown
Object Detection DemoWelcome to the object detection inference walkthrough! This notebook will walk you step by step through the process of using a pre-trained model to detect objects in an image. Make sure to follow the [installation instructions](https://github.com/tensorflow/models/blob/master/research/object_detection/g3doc/installation.md) before you start. Imports
###Code
import numpy as np
import os
import six.moves.urllib as urllib
import sys
import tarfile
import tensorflow as tf
import zipfile
from collections import defaultdict
from io import StringIO
from matplotlib import pyplot as plt
from PIL import Image
# This is needed since the notebook is stored in the object_detection folder.
sys.path.append("..")
from object_detection.utils import ops as utils_ops
if tf.__version__ < '1.4.0':
raise ImportError('Please upgrade your tensorflow installation to v1.4.* or later!')
###Output
_____no_output_____
###Markdown
Env setup
###Code
# This is needed to display the images.
%matplotlib inline
###Output
_____no_output_____
###Markdown
Object detection importsHere are the imports from the object detection module.
###Code
from utils import label_map_util
from utils import visualization_utils as vis_util
###Output
_____no_output_____
###Markdown
Model preparation VariablesAny model exported using the `export_inference_graph.py` tool can be loaded here simply by changing `PATH_TO_CKPT` to point to a new .pb file. By default we use an "SSD with Mobilenet" model here. See the [detection model zoo](https://github.com/tensorflow/models/blob/master/research/object_detection/g3doc/detection_model_zoo.md) for a list of other models that can be run out-of-the-box with varying speeds and accuracies.
###Code
# What model to download.
# Path to frozen detection graph. This is the actual model that is used for the object detection.
PATH_TO_CKPT = '/home/priya/Documents/mask_rcnn/exported_graphs_rcnn_inception' + '/frozen_inference_graph.pb'
# List of the strings that is used to add correct label for each box.
PATH_TO_LABELS = os.path.join('data', 'toy_label_map.pbtxt')
NUM_CLASSES = 1
###Output
_____no_output_____
###Markdown
Load a (frozen) Tensorflow model into memory.
###Code
detection_graph = tf.Graph()
with detection_graph.as_default():
od_graph_def = tf.GraphDef()
with tf.gfile.GFile(PATH_TO_CKPT, 'rb') as fid:
serialized_graph = fid.read()
od_graph_def.ParseFromString(serialized_graph)
tf.import_graph_def(od_graph_def, name='')
###Output
_____no_output_____
###Markdown
Loading label mapLabel maps map indices to category names, so that when our convolution network predicts `5`, we know that this corresponds to `airplane`. Here we use internal utility functions, but anything that returns a dictionary mapping integers to appropriate string labels would be fine
###Code
label_map = label_map_util.load_labelmap(PATH_TO_LABELS)
categories = label_map_util.convert_label_map_to_categories(label_map, max_num_classes=NUM_CLASSES, use_display_name=True)
category_index = label_map_util.create_category_index(categories)
print(category_index)
###Output
{1: {'id': 1, 'name': u'toy'}}
###Markdown
Helper code
###Code
def load_image_into_numpy_array(image):
(im_width, im_height) = image.size
return np.array(image.getdata()).reshape(
(im_height, im_width, 3)).astype(np.uint8)
###Output
_____no_output_____
###Markdown
Detection
###Code
# For the sake of simplicity we will use only 2 images:
# image1.jpg
# image2.jpg
# If you want to test the code with your images, just add path to the images to the TEST_IMAGE_PATHS.
PATH_TO_TEST_IMAGES_DIR = 'test_images'
TEST_IMAGE_PATHS = [ os.path.join(PATH_TO_TEST_IMAGES_DIR, 'image{}.jpg'.format(i)) for i in range(1, 4) ]
# Size, in inches, of the output images.
IMAGE_SIZE = (12, 8)
def run_inference_for_single_image(image, graph):
with graph.as_default():
with tf.Session() as sess:
# Get handles to input and output tensors
ops = tf.get_default_graph().get_operations()
all_tensor_names = {output.name for op in ops for output in op.outputs}
tensor_dict = {}
for key in [
'num_detections', 'detection_boxes', 'detection_scores',
'detection_classes', 'detection_masks'
]:
tensor_name = key + ':0'
if tensor_name in all_tensor_names:
tensor_dict[key] = tf.get_default_graph().get_tensor_by_name(
tensor_name)
if 'detection_masks' in tensor_dict:
# The following processing is only for single image
detection_boxes = tf.squeeze(tensor_dict['detection_boxes'], [0])
detection_masks = tf.squeeze(tensor_dict['detection_masks'], [0])
# Reframe is required to translate mask from box coordinates to image coordinates and fit the image size.
real_num_detection = tf.cast(tensor_dict['num_detections'][0], tf.int32)
detection_boxes = tf.slice(detection_boxes, [0, 0], [real_num_detection, -1])
detection_masks = tf.slice(detection_masks, [0, 0, 0], [real_num_detection, -1, -1])
detection_masks_reframed = utils_ops.reframe_box_masks_to_image_masks(
detection_masks, detection_boxes, image.shape[0], image.shape[1])
detection_masks_reframed = tf.cast(
tf.greater(detection_masks_reframed, 0.5), tf.uint8)
# Follow the convention by adding back the batch dimension
tensor_dict['detection_masks'] = tf.expand_dims(
detection_masks_reframed, 0)
image_tensor = tf.get_default_graph().get_tensor_by_name('image_tensor:0')
# Run inference
output_dict = sess.run(tensor_dict,
feed_dict={image_tensor: np.expand_dims(image, 0)})
# all outputs are float32 numpy arrays, so convert types as appropriate
output_dict['num_detections'] = int(output_dict['num_detections'][0])
output_dict['detection_classes'] = output_dict[
'detection_classes'][0].astype(np.uint8)
output_dict['detection_boxes'] = output_dict['detection_boxes'][0]
output_dict['detection_scores'] = output_dict['detection_scores'][0]
if 'detection_masks' in output_dict:
output_dict['detection_masks'] = output_dict['detection_masks'][0]
return output_dict
for image_path in TEST_IMAGE_PATHS:
image = Image.open(image_path)
# the array based representation of the image will be used later in order to prepare the
# result image with boxes and labels on it.
image_np = load_image_into_numpy_array(image)
# Expand dimensions since the model expects images to have shape: [1, None, None, 3]
image_np_expanded = np.expand_dims(image_np, axis=0)
# Actual detection.
output_dict = run_inference_for_single_image(image_np, detection_graph)
# Visualization of the results of a detection.
vis_util.visualize_boxes_and_labels_on_image_array(
image_np,
output_dict['detection_boxes'],
output_dict['detection_classes'],
output_dict['detection_scores'],
category_index,
instance_masks=output_dict.get('detection_masks'),
use_normalized_coordinates=True,
line_thickness=8)
plt.figure(figsize=IMAGE_SIZE)
plt.imshow(image_np)
###Output
_____no_output_____ |
docs_src/callbacks.hooks.ipynb | ###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.docs import *
from fastai import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
learn = ConvLearner(get_mnist(), tvm.resnet18, callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_batches,num_modules)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 5th last layer (`axis1==-5`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-5].numpy());
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a `DynamicUnet`), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
show_doc(ActivationStats.hook)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_train_end)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
###Output
_____no_output_____
###Markdown
This method only works on a [`Learner`](/basic_train.htmlLearner) object with `train_ds` in it. If it was created as a result of [`load_learner`](/basic_train.htmlload_learner), there is no [`data`](/vision.data.htmlvision.data) to run through the model and therefore it's not possible to create such summary.A sample `summary` looks like:```======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________ReLU [64, 176, 176] 0 False ______________________________________________________________________MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False ...```Column definition:1. **Layer (type)** is the name of the corresponding [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module).2. **Output Shape** is the shape of the output of the corresponding layer (minus the batch dimension, which is always the same and has no impact on the model params).3. **Param ** is the number of weights (and optionally bias), and it will vary for each layer. The number of params is calculated differently for each layer type. Here is how it's calculated for some of the most common layer types: * Conv: `kernel_size*kernel_size*ch_in*ch_out` * Linear: `(n_in+bias) * n_out` * Batchnorm: `2 * n_out` * Embeddings: `n_embed * emb_sz`4. **Trainable** indicates whether a layer is trainable or not. * Layers with `0` parameters are always Untrainable (e.g., `ReLU` and `MaxPool2d`). * Other layers are either Trainable or not, usually depending on whether they are frozen or not. See [Discriminative layer training](https://docs.fast.ai/basic_train.htmlDiscriminative-layer-training).To better understand this summary it helps to also execute `learn.model` and correlate the two outputs.Example:Let's feed to a [`Learner`](/basic_train.htmlLearner) a dataset of 3-channel images size 352x352 and look at the model and its summary:```data.train_ds[0][0].data.shapelearn = cnn_learner(data, models.resnet34, ...)print(learn.model)print(learn.summary())```Here are the outputs with everything but the relevant to the example lines removed:```torch.Size([3, 352, 352]) [...] (0): Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False) (1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True) [...] (3): MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False) [...] (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False) (8): Linear(in_features=512, out_features=37, bias=True)======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________[...]MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False [...]______________________________________________________________________Linear [37] 18,981 True```**So let's calculate some params:**For the `Conv2d` layers, multiply the first 4 numbers from the corresponding layer definition:```Conv2d(3, 64, kernel_size=(7, 7), ...)3*64*7*7 = 9,408Conv2d(64, 64, kernel_size=(3, 3), ...)64*64*3*3 = 36,864```For the `BatchNorm2d` layer, multiply the first number by 2:```BatchNorm2d(64, ...)64*2 = 128```For `Linear` we multiply the first 2 and include the bias if it's `True`:```Linear(in_features=512, out_features=37, bias=True)(512+1)*37 = 18,981```**Now let's calculate some output shapes:**We started with 3x352x352 image and run it through this layer:`Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False)`How did we get: `[64, 176, 176]`The number of output channels is `64`, that's the first dimension in the number above. And then our image of `352x352` got convolved into `176x176` because of stride `2x2` (`352/2`).Then we had:`MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False)`which reduced `[64, 176, 176]` to `[64, 88, 88]` again because of stride 2.And so on, finishing with:`Linear(in_features=512, out_features=37, bias=True)`which reduced everything to just `[37]`.
###Code
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
###Output
_____no_output_____
###Markdown
This method only works on a [`Learner`](/basic_train.htmlLearner) object with `train_ds` in it. If it was created as a result of [`load_learner`](/basic_train.htmlload_learner), there is no [`data`](/vision.data.htmlvision.data) to run through the model and therefore it's not possible to create such summary.A sample `summary` looks like:```======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________ReLU [64, 176, 176] 0 False ______________________________________________________________________MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False ...```Column definition:1. **Layer (type)** is the name of the corresponding [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module).2. **Output Shape** is the shape of the output of the corresponding layer (minus the batch dimension, which is always the same and has no impact on the model params).3. **Param ** is the number of weights (and optionally bias), and it will vary for each layer. The number of params is calculated differently for each layer type. Here is how it's calculated for some of the most common layer types: * Conv: `kernel_size*kernel_size*ch_in*ch_out` * Linear: `(n_in+bias) * n_out` * Batchnorm: `2 * n_out` * Embeddings: `n_embed * emb_sz`4. **Trainable** indicates whether a layer is trainable or not. * Layers with `0` parameters are always Untrainable (e.g., `ReLU` and `MaxPool2d`). * Other layers are either Trainable or not, usually depending on whether they are frozen or not. See [Discriminative layer training](https://docs.fast.ai/basic_train.htmlDiscriminative-layer-training).To better understand this summary it helps to also execute `learn.model` and correlate the two outputs.Example:Let's feed to a [`Learner`](/basic_train.htmlLearner) a dataset of 3-channel images size 352x352 and look at the model and its summary:```data.train_ds[0][0].data.shapelearn = cnn_learner(data, models.resnet34, ...)print(learn.model)print(learn.summary())```Here are the outputs with everything but the relevant to the example lines removed:```torch.Size([3, 352, 352]) [...] (0): Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False) (1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True) [...] (3): MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False) [...] (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False) (8): Linear(in_features=512, out_features=37, bias=True)======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________[...]MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False [...]______________________________________________________________________Linear [37] 18,981 True```**So let's calculate some params:**For the `Conv2d` layers, multiply the first 4 numbers from the corresponding layer definition:```Conv2d(3, 64, kernel_size=(7, 7), ...)3*64*7*7 = 9,408Conv2d(64, 64, kernel_size=(3, 3), ...)64*64*3*3 = 36,864```For the `BatchNorm2d` layer, multiply the first number by 2:```BatchNorm2d(64, ...)64*2 = 128```For `Linear` we multiply the first 2 and include the bias if it's `True`:```Linear(in_features=512, out_features=37, bias=True)(512+1)*37 = 18,981```**Now let's calculate some output shapes:**We started with 3x352x352 image and run it through this layer:`Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False)`How did we get: `[64, 176, 176]`The number of output channels is `64`, that's the first dimension in the number above. And then our image of `352x352` got convolved into `176x176` because of stride `2x2` (`352/2`).Then we had:`MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False)`which reduced `[64, 176, 176]` to `[64, 88, 88]` again because of stride 2.And so on, finishing with:`Linear(in_features=512, out_features=37, bias=True)`which reduced everything to just `[37]`.
###Code
jekyll_warn("Known issue: `model_summary` and `Learner.summary` don't work with the AWD LSTM in text models.")
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
learn = ConvLearner(data, models.resnet18, callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_batches,num_modules)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 5th last layer (`axis1==-5`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-5].numpy());
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a `DynamicUnet`), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
show_doc(ActivationStats.hook)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_train_end)
###Output
_____no_output_____
###Markdown
New Methods - Please document or move to the undocumented section
###Code
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 5th last layer (`axis1==-5`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-5].numpy());
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
show_doc(ActivationStats.hook)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_train_end)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 5th last layer (`axis1==-5`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-5].numpy());
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
show_doc(ActivationStats.hook)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_train_end)
###Output
_____no_output_____
###Markdown
New Methods - Please document or move to the undocumented section
###Code
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
###Output
_____no_output_____
###Markdown
This method only works on a [`Learner`](/basic_train.htmlLearner) object with `train_ds` in it. If it was created as a result of [`load_learner`](/basic_train.htmlload_learner), there is no [`data`](/vision.data.htmlvision.data) to run through the model and therefore it's not possible to create such summary.A sample `summary` looks like:```======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________ReLU [64, 176, 176] 0 False ______________________________________________________________________MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False ...```Column definition:1. **Layer (type)** is the name of the corresponding [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module).2. **Output Shape** is the shape of the output of the corresponding layer (minus the batch dimension, which is always the same and has no impact on the model params).3. **Param ** is the number of weights (and optionally bias), and it will vary for each layer. The number of params is calculated differently for each layer type. Here is how it's calculated for some of the most common layer types: * Conv: `kernel_size*kernel_size*ch_in*ch_out` * Linear: `(n_in+bias) * n_out` * Batchnorm: `2 * n_out` * Embeddings: `n_embed * emb_sz`4. **Trainable** indicates whether a layer is trainable or not. * Layers with `0` parameters are always Untrainable (e.g., `ReLU` and `MaxPool2d`). * Other layers are either Trainable or not, usually depending on whether they are frozen or not. See [Discriminative layer training](https://docs.fast.ai/basic_train.htmlDiscriminative-layer-training).To better understand this summary it helps to also execute `learn.model` and correlate the two outputs.Example:Let's feed to a [`Learner`](/basic_train.htmlLearner) a dataset of 3-channel images size 352x352 and look at the model and its summary:```data.train_ds[0][0].data.shapelearn = cnn_learner(data, models.resnet34, ...)print(learn.model)print(learn.summary())```Here are the outputs with everything but the relevant to the example lines removed:```torch.Size([3, 352, 352]) [...] (0): Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False) (1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True) [...] (3): MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False) [...] (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False) (8): Linear(in_features=512, out_features=37, bias=True)======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________[...]MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False [...]______________________________________________________________________Linear [37] 18,981 True```**So let's calculate some params:**For the `Conv2d` layers, multiply the first 4 numbers from the corresponding layer definition:```Conv2d(3, 64, kernel_size=(7, 7), ...)3*64*7*7 = 9,408Conv2d(64, 64, kernel_size=(3, 3), ...)64*64*3*3 = 36,864```For the `BatchNorm2d` layer, multiply the first number by 2:```BatchNorm2d(64, ...)64*2 = 128```For `Linear` we multiply the first 2 and include the bias if it's `True`:```Linear(in_features=512, out_features=37, bias=True)(512+1)*37 = 18,981```**Now let's calculate some output shapes:**We started with 3x352x352 image and run it through this layer:`Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False)`How did we get: `[64, 176, 176]`The number of output channels is `64`, that's the first dimension in the number above. And then our image of `352x352` got convolved into `176x176` because of stride `2x2` (`352/2`).Then we had:`MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False)`which reduced `[64, 176, 176]` to `[64, 88, 88]` again because of stride 2.And so on, finishing with:`Linear(in_features=512, out_features=37, bias=True)`which reduced everything to just `[37]`.
###Code
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 5th last layer (`axis1==-5`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-5].numpy());
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
show_doc(ActivationStats.hook)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_train_end)
###Output
_____no_output_____
###Markdown
New Methods - Please document or move to the undocumented section
###Code
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
###Output
_____no_output_____
###Markdown
This method only works on a [`Learner`](/basic_train.htmlLearner) object with `train_ds` in it. If it was created as a result of [`load_learner`](/basic_train.htmlload_learner), there is no [`data`](/vision.data.htmlvision.data) to run through the model and therefore it's not possible to create such summary.A sample `summary` looks like:```======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________ReLU [64, 176, 176] 0 False ______________________________________________________________________MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False ...```Column definition:1. **Layer (type)** is the name of the corresponding [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module).2. **Output Shape** is the shape of the output of the corresponding layer (minus the batch dimension, which is always the same and has no impact on the model params).3. **Param ** is the number of weights (and optionally bias), and it will vary for each layer. The number of params is calculated differently for each layer type. Here is how it's calculated for some of the most common layer types: * Conv: `kernel_size*kernel_size*ch_in*ch_out` * Linear: `(n_in+bias) * n_out` * Batchnorm: `2 * n_out` * Embeddings: `n_embed * emb_sz`4. **Trainable** indicates whether a layer is trainable or not. * Layers with `0` parameters are always Untrainable (e.g., `ReLU` and `MaxPool2d`). * Other layers are either Trainable or not, usually depending on whether they are frozen or not. See [Discriminative layer training](https://docs.fast.ai/basic_train.htmlDiscriminative-layer-training).To better understand this summary it helps to also execute `learn.model` and correlate the two outputs.Example:Let's feed to a [`Learner`](/basic_train.htmlLearner) a dataset of 3-channel images size 352x352 and look at the model and its summary:```data.train_ds[0][0].data.shapelearn = cnn_learner(data, models.resnet34, ...)print(learn.model)print(learn.summary())```Here are the outputs with everything but the relevant to the example lines removed:```torch.Size([3, 352, 352]) [...] (0): Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False) (1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True) [...] (3): MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False) [...] (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False) (8): Linear(in_features=512, out_features=37, bias=True)======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________[...]MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False [...]______________________________________________________________________Linear [37] 18,981 True```**So let's calculate some params:**For the `Conv2d` layers, multiply the first 4 numbers from the corresponding layer definition:```Conv2d(3, 64, kernel_size=(7, 7), ...)3*64*7*7 = 9,408Conv2d(64, 64, kernel_size=(3, 3), ...)64*64*3*3 = 36,864```For the `BatchNorm2d` layer, multiply the first number by 2:```BatchNorm2d(64, ...)64*2 = 128```For `Linear` we multiply the first 2 and include the bias if it's `True`:```Linear(in_features=512, out_features=37, bias=True)(512+1)*37 = 18,981```**Now let's calculate some output shapes:**We started with 3x352x352 image and run it through this layer:`Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False)`How did we get: `[64, 176, 176]`The number of output channels is `64`, that's the first dimension in the number above. And then our image of `352x352` got convolved into `176x176` because of stride `2x2` (`352/2`).Then we had:`MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False)`which reduced `[64, 176, 176]` to `[64, 88, 88]` again because of stride 2.And so on, finishing with:`Linear(in_features=512, out_features=37, bias=True)`which reduced everything to just `[37]`.
###Code
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
###Output
_____no_output_____
###Markdown
This method only works on a [`Learner`](/basic_train.htmlLearner) object with `train_ds` in it. If it was created as a result of [`load_learner`](/basic_train.htmlload_learner), there is no [`data`](/vision.data.htmlvision.data) to run through the model and therefore it's not possible to create such summary.A sample `summary` looks like:```======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________ReLU [64, 176, 176] 0 False ______________________________________________________________________MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False ...```Column definition:1. **Layer (type)** is the name of the corresponding [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module).2. **Output Shape** is the shape of the output of the corresponding layer (minus the batch dimension, which is always the same and has no impact on the model params).3. **Param ** is the number of weights (and optionally bias), and it will vary for each layer. The number of params is calculated differently for each layer type. Here is how it's calculated for some of the most common layer types: * Conv: `kernel_size*kernel_size*ch_in*ch_out` * Linear: `(n_in+bias) * n_out` * Batchnorm: `2 * n_out` * Embeddings: `n_embed * emb_sz`4. **Trainable** indicates whether a layer is trainable or not. * Layers with `0` parameters are always Untrainable (e.g., `ReLU` and `MaxPool2d`). * Other layers are either Trainable or not, usually depending on whether they are frozen or not. See [Discriminative layer training](https://docs.fast.ai/basic_train.htmlDiscriminative-layer-training).To better understand this summary it helps to also execute `learn.model` and correlate the two outputs.Example:Let's feed to a [`Learner`](/basic_train.htmlLearner) a dataset of 3-channel images size 352x352 and look at the model and its summary:```data.train_ds[0][0].data.shapelearn = cnn_learner(data, models.resnet34, ...)print(learn.model)print(learn.summary())```Here are the outputs with everything but the relevant to the example lines removed:```torch.Size([3, 352, 352]) [...] (0): Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False) (1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True) [...] (3): MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False) [...] (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False) (8): Linear(in_features=512, out_features=37, bias=True)======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________[...]MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False [...]______________________________________________________________________Linear [37] 18,981 True```**So let's calculate some params:**For the `Conv2d` layers, multiply the first 4 numbers from the corresponding layer definition:```Conv2d(3, 64, kernel_size=(7, 7), ...)3*64*7*7 = 9,408Conv2d(64, 64, kernel_size=(3, 3), ...)64*64*3*3 = 36,864```For the `BatchNorm2d` layer, multiply the first number by 2:```BatchNorm2d(64, ...)64*2 = 128```For `Linear` we multiply the first 2 and include the bias if it's `True`:```Linear(in_features=512, out_features=37, bias=True)(512+1)*37 = 18,981```**Now let's calculate some output shapes:**We started with 3x352x352 image and run it through this layer:`Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False)`How did we get: `[64, 176, 176]`The number of output channels is `64`, that's the first dimension in the number above. And then our image of `352x352` got convolved into `176x176` because of stride `2x2` (`352/2`).Then we had:`MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False)`which reduced `[64, 176, 176]` to `[64, 88, 88]` again because of stride 2.And so on, finishing with:`Linear(in_features=512, out_features=37, bias=True)`which reduced everything to just `[37]`.
###Code
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
callbacks.hooks Type an introduction of the package here.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
###Output
_____no_output_____
###Markdown
Global Variable Definitions:
###Code
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[ActivationStats](http://docs.fast.ai/callbacks.hooks.htmlActivationStats)
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
`ActivationStats.hook`
###Code
show_doc(ActivationStats.on_batch_end)
###Output
_____no_output_____
###Markdown
`ActivationStats.on_batch_end`
###Code
show_doc(ActivationStats.on_train_begin)
###Output
_____no_output_____
###Markdown
`ActivationStats.on_train_begin`
###Code
show_doc(ActivationStats.on_train_end)
###Output
_____no_output_____
###Markdown
`ActivationStats.on_train_end`
###Code
show_doc(ClassificationInterpretation)
###Output
_____no_output_____
###Markdown
[ClassificationInterpretation](http://docs.fast.ai/callbacks.hooks.htmlClassificationInterpretation)
###Code
show_doc(ClassificationInterpretation.confusion_matrix)
###Output
_____no_output_____
###Markdown
`ClassificationInterpretation.confusion_matrix`
###Code
show_doc(ClassificationInterpretation.plot_confusion_matrix)
###Output
_____no_output_____
###Markdown
`ClassificationInterpretation.plot_confusion_matrix`
###Code
show_doc(ClassificationInterpretation.plot_top_losses)
###Output
_____no_output_____
###Markdown
`ClassificationInterpretation.plot_top_losses`
###Code
show_doc(ClassificationInterpretation.top_losses)
###Output
_____no_output_____
###Markdown
`ClassificationInterpretation.top_losses`
###Code
show_doc(Hook)
###Output
_____no_output_____
###Markdown
[Hook](http://docs.fast.ai/callbacks.hooks.htmlHook)
###Code
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
`Hook.hook_fn`
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
`Hook.remove`
###Code
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
[HookCallback](http://docs.fast.ai/callbacks.hooks.htmlHookCallback)
###Code
show_doc(HookCallback.on_train_begin)
###Output
_____no_output_____
###Markdown
`HookCallback.on_train_begin`
###Code
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
`HookCallback.on_train_end`
###Code
show_doc(HookCallback.remove)
###Output
_____no_output_____
###Markdown
`HookCallback.remove`
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
[Hooks](http://docs.fast.ai/callbacks.hooks.htmlHooks)
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
`Hooks.remove`
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
[hook_output](http://docs.fast.ai/callbacks.hooks.htmlhook_output)
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
[hook_outputs](http://docs.fast.ai/callbacks.hooks.htmlhook_outputs)
###Code
show_doc(model_sizes)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 5th last layer (`axis1==-5`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-5].numpy());
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
show_doc(ActivationStats.hook)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_train_end)
###Output
_____no_output_____
###Markdown
New Methods - Please document or move to the undocumented section
###Code
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
###Output
_____no_output_____
###Markdown
This method only works on a [`Learner`](/basic_train.htmlLearner) object with `train_ds` in it. If it was created as a result of [`load_learner`](/basic_train.htmlload_learner), there is no [`data`](/vision.data.htmlvision.data) to run through the model and therefore it's not possible to create such summary.A sample `summary` looks like:```======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________ReLU [64, 176, 176] 0 False ______________________________________________________________________MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False ...```Column definition:1. **Layer (type)** is the name of the corresponding [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module).2. **Output Shape** is the shape of the output of the corresponding layer (minus the batch dimension, which is always the same and has no impact on the model params).3. **Param ** is the number of weights (and optionally bias), and it will vary for each layer. The number of params is calculated differently for each layer type. Here is how it's calculated for some of the most common layer types: * Conv: `kernel_size*kernel_size*ch_in*ch_out` * Linear: `(n_in+bias) * n_out` * Batchnorm: `2 * n_out` * Embeddings: `n_embed * emb_sz`4. **Trainable** indicates whether a layer is trainable or not. * Layers with `0` parameters are always Untrainable (e.g., `ReLU` and `MaxPool2d`). * Other layers are either Trainable or not, usually depending on whether they are frozen or not. See [Discriminative layer training](https://docs.fast.ai/basic_train.htmlDiscriminative-layer-training).To better understand this summary it helps to also execute `learn.model` and correlate the two outputs.Example:Let's feed to a [`Learner`](/basic_train.htmlLearner) a dataset of 3-channel images size 352x352 and look at the model and its summary:```data.train_ds[0][0].data.shapelearn = cnn_learner(data, models.resnet34, ...)print(learn.model)print(learn.summary())```Here are the outputs with everything but the relevant to the example lines removed:```torch.Size([3, 352, 352]) [...] (0): Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False) (1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True) [...] (3): MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False) [...] (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False) (8): Linear(in_features=512, out_features=37, bias=True)======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________[...]MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False [...]______________________________________________________________________Linear [37] 18,981 True```**So let's calculate some params:**For the `Conv2d` layers, multiply the first 4 numbers from the corresponding layer definition:```Conv2d(3, 64, kernel_size=(7, 7), ...)3*64*7*7 = 9,408Conv2d(64, 64, kernel_size=(3, 3), ...)64*64*3*3 = 36,864```For the `BatchNorm2d` layer, multiply the first number by 2:```BatchNorm2d(64, ...)64*2 = 128```For `Linear` we multiply the first 2 and include the bias if it's `True`:```Linear(in_features=512, out_features=37, bias=True)(512+1)*37 = 18,981```**Now let's calculate some output shapes:**We started with 3x352x352 image and run it through this layer:`Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False)`How did we get: `[64, 176, 176]`The number of output channels is `64`, that's the first dimension in the number above. And then our image of `352x352` got convolved into `176x176` because of stride `2x2` (`352/2`).Then we had:`MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False)`which reduced `[64, 176, 176]` to `[64, 88, 88]` again because of stride 2.And so on, finishing with:`Linear(in_features=512, out_features=37, bias=True)`which reduced everything to just `[37]`.
###Code
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
###Output
_____no_output_____
###Markdown
This method only works on a [`Learner`](/basic_train.htmlLearner) object with `train_ds` in it. If it was created as a result of [`load_learner`](/basic_train.htmlload_learner), there is no [`data`](/vision.data.htmlvision.data) to run through the model and therefore it's not possible to create such summary.A sample `summary` looks like:```======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________ReLU [64, 176, 176] 0 False ______________________________________________________________________MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False ...```Column definition:1. **Layer (type)** is the name of the corresponding [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module).2. **Output Shape** is the shape of the output of the corresponding layer (minus the batch dimension, which is always the same and has no impact on the model params).3. **Param ** is the number of weights (and optionally bias), and it will vary for each layer. The number of params is calculated differently for each layer type. Here is how it's calculated for some of the most common layer types: * Conv: `kernel_size*kernel_size*ch_in*ch_out` * Linear: `(n_in+bias) * n_out` * Batchnorm: `2 * n_out` * Embeddings: `n_embed * emb_sz`4. **Trainable** indicates whether a layer is trainable or not. * Layers with `0` parameters are always Untrainable (e.g., `ReLU` and `MaxPool2d`). * Other layers are either Trainable or not, usually depending on whether they are frozen or not. See [Discriminative layer training](https://docs.fast.ai/basic_train.htmlDiscriminative-layer-training).To better understand this summary it helps to also execute `learn.model` and correlate the two outputs.Example:Let's feed to a [`Learner`](/basic_train.htmlLearner) a dataset of 3-channel images size 352x352 and look at the model and its summary:```data.train_ds[0][0].data.shapelearn = cnn_learner(data, models.resnet34, ...)print(learn.model)print(learn.summary())```Here are the outputs with everything but the relevant to the example lines removed:```torch.Size([3, 352, 352]) [...] (0): Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False) (1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True) [...] (3): MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False) [...] (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False) (8): Linear(in_features=512, out_features=37, bias=True)======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________[...]MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False [...]______________________________________________________________________Linear [37] 18,981 True```**So let's calculate some params:**For the `Conv2d` layers, multiply the first 4 numbers from the corresponding layer definition:```Conv2d(3, 64, kernel_size=(7, 7), ...)3*64*7*7 = 9,408Conv2d(64, 64, kernel_size=(3, 3), ...)64*64*3*3 = 36,864```For the `BatchNorm2d` layer, multiply the first number by 2:```BatchNorm2d(64, ...)64*2 = 128```For `Linear` we multiply the first 2 and include the bias if it's `True`:```Linear(in_features=512, out_features=37, bias=True)(512+1)*37 = 18,981```**Now let's calculate some output shapes:**We started with 3x352x352 image and run it through this layer:`Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False)`How did we get: `[64, 176, 176]`The number of output channels is `64`, that's the first dimension in the number above. And then our image of `352x352` got convolved into `176x176` because of stride `2x2` (`352/2`).Then we had:`MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False)`which reduced `[64, 176, 176]` to `[64, 88, 88]` again because of stride 2.And so on, finishing with:`Linear(in_features=512, out_features=37, bias=True)`which reduced everything to just `[37]`.
###Code
jekyll_warn("Known issue: `model_summary` and `Learner.summary` don't work with the AWD LSTM in text models.")
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
###Output
_____no_output_____
###Markdown
This method only works on a [`Learner`](/basic_train.htmlLearner) object with `train_ds` in it. If it was created as a result of [`load_learner`](/basic_train.htmlload_learner), there is no [`data`](/vision.data.htmlvision.data) to run through the model and therefore it's not possible to create such summary.A sample `summary` looks like:```======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________ReLU [64, 176, 176] 0 False ______________________________________________________________________MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False ...```Column definition:1. **Layer (type)** is the name of the corresponding [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module).2. **Output Shape** is the shape of the output of the corresponding layer (minus the batch dimension, which is always the same and has no impact on the model params).3. **Param ** is the number of weights (and optionally bias), and it will vary for each layer. The number of params is calculated differently for each layer type. Here is how it's calculated for some of the most common layer types: * Conv: `kernel_size*kernel_size*ch_in*ch_out` * Linear: `(n_in+bias) * n_out` * Batchnorm: `2 * n_out` * Embeddings: `n_embed * emb_sz`4. **Trainable** indicates whether a layer is trainable or not. * Layers with `0` parameters are always Untrainable (e.g., `ReLU` and `MaxPool2d`). * Other layers are either Trainable or not, usually depending on whether they are frozen or not. See [Discriminative layer training](https://docs.fast.ai/basic_train.htmlDiscriminative-layer-training).To better understand this summary it helps to also execute `learn.model` and correlate the two outputs.Example:Let's feed to a [`Learner`](/basic_train.htmlLearner) a dataset of 3-channel images size 352x352 and look at the model and its summary:```data.train_ds[0][0].data.shapelearn = cnn_learner(data, models.resnet34, ...)print(learn.model)print(learn.summary())```Here are the outputs with everything but the relevant to the example lines removed:```torch.Size([3, 352, 352]) [...] (0): Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False) (1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True) [...] (3): MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False) [...] (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False) (8): Linear(in_features=512, out_features=37, bias=True)======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________[...]MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False [...]______________________________________________________________________Linear [37] 18,981 True```**So let's calculate some params:**For the `Conv2d` layers, multiply the first 4 numbers from the corresponding layer definition:```Conv2d(3, 64, kernel_size=(7, 7), ...)3*64*7*7 = 9,408Conv2d(64, 64, kernel_size=(3, 3), ...)64*64*3*3 = 36,864```For the `BatchNorm2d` layer, multiply the first number by 2:```BatchNorm2d(64, ...)64*2 = 128```For `Linear` we multiply the first 2 and include the bias if it's `True`:```Linear(in_features=512, out_features=37, bias=True)(512+1)*37 = 18,981```**Now let's calculate some output shapes:**We started with 3x352x352 image and run it through this layer:`Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False)`How did we get: `[64, 176, 176]`The number of output channels is `64`, that's the first dimension in the number above. And then our image of `352x352` got convolved into `176x176` because of stride `2x2` (`352/2`).Then we had:`MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False)`which reduced `[64, 176, 176]` to `[64, 88, 88]` again because of stride 2.And so on, finishing with:`Linear(in_features=512, out_features=37, bias=True)`which reduced everything to just `[37]`.
###Code
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 5th last layer (`axis1==-5`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-5].numpy());
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
show_doc(ActivationStats.hook)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_train_end)
###Output
_____no_output_____
###Markdown
New Methods - Please document or move to the undocumented section
###Code
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any `nn.Module`, for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.docs import *
from fastai import *
from fastai.train import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
learn = ConvLearner(get_mnist(), tvm.resnet18, callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_batches,num_modules)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 5th last layer (`axis1==-5`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-5].numpy());
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a `DynamicUnet`), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(HookCallback.on_train_begin)
###Output
_____no_output_____
###Markdown
`HookCallback.on_train_begin`
###Code
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
`HookCallback.on_train_end`
###Code
show_doc(ActivationStats.hook)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_train_end)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 5th last layer (`axis1==-5`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-5].numpy());
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
show_doc(ActivationStats.hook)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_train_end)
###Output
_____no_output_____
###Markdown
New Methods - Please document or move to the undocumented section
###Code
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
###Output
_____no_output_____
###Markdown
This method only works on a [`Learner`](/basic_train.htmlLearner) object with `train_ds` in it. If it was created as a result of [`load_learner`](/basic_train.htmlload_learner), there is no [`data`](/vision.data.htmlvision.data) to run through the model and therefore it's not possible to create such summary.A sample `summary` looks like:```======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________ReLU [64, 176, 176] 0 False ______________________________________________________________________MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False ...```Column definition:1. **Layer (type)** is the name of the corresponding [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module).2. **Output Shape** is the shape of the output of the corresponding layer (minus the batch dimension, which is always the same and has no impact on the model params).3. **Param ** is the number of weights (and optionally bias), and it will vary for each layer. The number of params is calculated differently for each layer type. Here is how it's calculated for some of the most common layer types: * Conv: `kernel_size*kernel_size*ch_in*ch_out` * Linear: `(n_in+bias) * n_out` * Batchnorm: `2 * n_out` * Embeddings: `n_embed * emb_sz`4. **Trainable** indicates whether a layer is trainable or not. * Layers with `0` parameters are always Untrainable (e.g., `ReLU` and `MaxPool2d`). * Other layers are either Trainable or not, usually depending on whether they are frozen or not. See [Discriminative layer training](https://docs.fast.ai/basic_train.htmlDiscriminative-layer-training).To better understand this summary it helps to also execute `learn.model` and correlate the two outputs.Example:Let's feed to a [`Learner`](/basic_train.htmlLearner) a dataset of 3-channel images size 352x352 and look at the model and its summary:```data.train_ds[0][0].data.shapelearn = cnn_learner(data, models.resnet34, ...)print(learn.model)print(learn.summary())```Here are the outputs with everything but the relevant to the example lines removed:```torch.Size([3, 352, 352]) [...] (0): Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False) (1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True) [...] (3): MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False) [...] (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False) (8): Linear(in_features=512, out_features=37, bias=True)======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________[...]MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False [...]______________________________________________________________________Linear [37] 18,981 True```**So let's calculate some params:**For the `Conv2d` layers, multiply the first 4 numbers from the corresponding layer definition:```Conv2d(3, 64, kernel_size=(7, 7), ...)3*64*7*7 = 9,408Conv2d(64, 64, kernel_size=(3, 3), ...)64*64*3*3 = 36,864```For the `BatchNorm2d` layer, multiply the first number by 2:```BatchNorm2d(64, ...)64*2 = 128```For `Linear` we multiply the first 2 and include the bias if it's `True`:```Linear(in_features=512, out_features=37, bias=True)(512+1)*37 = 18,981```**Now let's calculate some output shapes:**We started with 3x352x352 image and run it through this layer:`Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False)`How did we get: `[64, 176, 176]`The number of output channels is `64`, that's the first dimension in the number above. And then our image of `352x352` got convolved into `176x176` because of stride `2x2` (`352/2`).Then we had:`MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False)`which reduced `[64, 176, 176]` to `[64, 88, 88]` again because of stride 2.And so on, finishing with:`Linear(in_features=512, out_features=37, bias=True)`which reduced everything to just `[37]`.
###Code
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 5th last layer (`axis1==-5`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-5].numpy());
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
show_doc(ActivationStats.hook)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_train_end)
###Output
_____no_output_____
###Markdown
New Methods - Please document or move to the undocumented section
###Code
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 5th last layer (`axis1==-5`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-5].numpy());
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
show_doc(ActivationStats.hook)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_train_end)
###Output
_____no_output_____
###Markdown
New Methods - Please document or move to the undocumented section
###Code
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
###Output
_____no_output_____
###Markdown
This method only works on a [`Learner`](/basic_train.htmlLearner) object with `train_ds` in it. If it was created as a result of [`load_learner`](/basic_train.htmlload_learner), there is no [`data`](/vision.data.htmlvision.data) to run through the model and therefore it's not possible to create such summary.A sample `summary` looks like:```======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________ReLU [64, 176, 176] 0 False ______________________________________________________________________MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False ...```Column definition:1. **Layer (type)** is the name of the corresponding [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module).2. **Output Shape** is the shape of the output of the corresponding layer (minus the batch dimension, which is always the same and has no impact on the model params).3. **Param ** is the number of weights (and optionally bias), and it will vary for each layer. The number of params is calculated differently for each layer type. Here is how it's calculated for some of the most common layer types: * Conv: `kernel_size*kernel_size*ch_in*ch_out` * Linear: `(n_in+bias) * n_out` * Batchnorm: `2 * n_out` * Embeddings: `n_embed * emb_sz`4. **Trainable** indicates whether a layer is trainable or not. * Layers with `0` parameters are always Untrainable (e.g., `ReLU` and `MaxPool2d`). * Other layers are either Trainable or not, usually depending on whether they are frozen or not. See [Discriminative layer training](https://docs.fast.ai/basic_train.htmlDiscriminative-layer-training).To better understand this summary it helps to also execute `learn.model` and correlate the two outputs.Example:Let's feed to a [`Learner`](/basic_train.htmlLearner) a dataset of 3-channel images size 352x352 and look at the model and its summary:```data.train_ds[0][0].data.shapelearn = cnn_learner(data, models.resnet34, ...)print(learn.model)print(learn.summary())```Here are the outputs with everything but the relevant to the example lines removed:```torch.Size([3, 352, 352]) [...] (0): Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False) (1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True) [...] (3): MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False) [...] (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False) (8): Linear(in_features=512, out_features=37, bias=True)======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________[...]MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False [...]______________________________________________________________________Linear [37] 18,981 True```**So let's calculate some params:**For the `Conv2d` layers, multiply the first 4 numbers from the corresponding layer definition:```Conv2d(3, 64, kernel_size=(7, 7), ...)3*64*7*7 = 9,408Conv2d(64, 64, kernel_size=(3, 3), ...)64*64*3*3 = 36,864```For the `BatchNorm2d` layer, multiply the first number by 2:```BatchNorm2d(64, ...)64*2 = 128```For `Linear` we multiply the first 2 and include the bias if it's `True`:```Linear(in_features=512, out_features=37, bias=True)(512+1)*37 = 18,981```**Now let's calculate some output shapes:**We started with 3x352x352 image and run it through this layer:`Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False)`How did we get: `[64, 176, 176]`The number of output channels is `64`, that's the first dimension in the number above. And then our image of `352x352` got convolved into `176x176` because of stride `2x2` (`352/2`).Then we had:`MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False)`which reduced `[64, 176, 176]` to `[64, 88, 88]` again because of stride 2.And so on, finishing with:`Linear(in_features=512, out_features=37, bias=True)`which reduced everything to just `[37]`.
###Code
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
###Output
_____no_output_____
###Markdown
This method only works on a [`Learner`](/basic_train.htmlLearner) object with `train_ds` in it. If it was created as a result of [`load_learner`](/basic_train.htmlload_learner), there is no [`data`](/vision.data.htmlvision.data) to run through the model and therefore it's not possible to create such summary.A sample `summary` looks like:```======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________ReLU [64, 176, 176] 0 False ______________________________________________________________________MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False ...```Column definition:1. **Layer (type)** is the name of the corresponding [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module).2. **Output Shape** is the shape of the output of the corresponding layer (minus the batch dimension, which is always the same and has no impact on the model params).3. **Param ** is the number of weights (and optionally bias), and it will vary for each layer. The number of params is calculated differently for each layer type. Here is how it's calculated for some of the most common layer types: * Conv: `kernel_size*kernel_size*ch_in*ch_out` * Linear: `(n_in+bias) * n_out` * Batchnorm: `2 * n_out` * Embeddings: `n_embed * emb_sz`4. **Trainable** indicates whether a layer is trainable or not. * Layers with `0` parameters are always Untrainable (e.g., `ReLU` and `MaxPool2d`). * Other layers are either Trainable or not, usually depending on whether they are frozen or not. See [Discriminative layer training](https://docs.fast.ai/basic_train.htmlDiscriminative-layer-training).To better understand this summary it helps to also execute `learn.model` and correlate the two outputs.Example:Let's feed to a [`Learner`](/basic_train.htmlLearner) a dataset of 3-channel images size 352x352 and look at the model and its summary:```data.train_ds[0][0].data.shapelearn = cnn_learner(data, models.resnet34, ...)print(learn.model)print(learn.summary())```Here are the outputs with everything but the relevant to the example lines removed:```torch.Size([3, 352, 352]) [...] (0): Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False) (1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True) [...] (3): MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False) [...] (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False) (8): Linear(in_features=512, out_features=37, bias=True)======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________[...]MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False [...]______________________________________________________________________Linear [37] 18,981 True```**So let's calculate some params:**For the `Conv2d` layers, multiply the first 4 numbers from the corresponding layer definition:```Conv2d(3, 64, kernel_size=(7, 7), ...)3*64*7*7 = 9,408Conv2d(64, 64, kernel_size=(3, 3), ...)64*64*3*3 = 36,864```For the `BatchNorm2d` layer, multiply the first number by 2:```BatchNorm2d(64, ...)64*2 = 128```For `Linear` we multiply the first 2 and include the bias if it's `True`:```Linear(in_features=512, out_features=37, bias=True)(512+1)*37 = 18,981```**Now let's calculate some output shapes:**We started with 3x352x352 image and run it through this layer:`Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False)`How did we get: `[64, 176, 176]`The number of output channels is `64`, that's the first dimension in the number above. And then our image of `352x352` got convolved into `176x176` because of stride `2x2` (`352/2`).Then we had:`MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False)`which reduced `[64, 176, 176]` to `[64, 88, 88]` again because of stride 2.And so on, finishing with:`Linear(in_features=512, out_features=37, bias=True)`which reduced everything to just `[37]`.
###Code
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = cnn_learner(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
###Output
_____no_output_____
###Markdown
This method only works on a [`Learner`](/basic_train.htmlLearner) object with `train_ds` in it. If it was created as a result of [`load_learner`](/basic_train.htmlload_learner), there is no [`data`](/vision.data.htmlvision.data) to run through the model and therefore it's not possible to create such summary.A sample `summary` looks like:```======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________ReLU [64, 176, 176] 0 False ______________________________________________________________________MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False ...```Column definition:1. **Layer (type)** is the name of the corresponding [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module).2. **Output Shape** is the shape of the output of the corresponding layer (minus the batch dimension, which is always the same and has no impact on the model params).3. **Param ** is the number of weights (and optionally bias), and it will vary for each layer. The number of params is calculated differently for each layer type. Here is how it's calculated for some of the most common layer types: * Conv: `kernel_size*kernel_size*ch_in*ch_out` * Linear: `(n_in+bias) * n_out` * Batchnorm: `2 * n_out` * Embeddings: `n_embed * emb_sz`4. **Trainable** indicates whether a layer is trainable or not. * Layers with `0` parameters are always Untrainable (e.g., `ReLU` and `MaxPool2d`). * Other layers are either Trainable or not, usually depending on whether they are frozen or not. See [Discriminative layer training](https://docs.fast.ai/basic_train.htmlDiscriminative-layer-training).To better understand this summary it helps to also execute `learn.model` and correlate the two outputs.Example:Let's feed to a [`Learner`](/basic_train.htmlLearner) a dataset of 3-channel images size 352x352 and look at the model and its summary:```data.train_ds[0][0].data.shapelearn = cnn_learner(data, models.resnet34, ...)print(learn.model)print(learn.summary())```Here are the outputs with everything but the relevant to the example lines removed:```torch.Size([3, 352, 352]) [...] (0): Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False) (1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True) [...] (3): MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False) [...] (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False) (8): Linear(in_features=512, out_features=37, bias=True)======================================================================Layer (type) Output Shape Param Trainable ======================================================================Conv2d [64, 176, 176] 9,408 False ______________________________________________________________________BatchNorm2d [64, 176, 176] 128 True ______________________________________________________________________[...]MaxPool2d [64, 88, 88] 0 False ______________________________________________________________________Conv2d [64, 88, 88] 36,864 False [...]______________________________________________________________________Linear [37] 18,981 True```**So let's calculate some params:**For the `Conv2d` layers, multiply the first 4 numbers from the corresponding layer definition:```Conv2d(3, 64, kernel_size=(7, 7), ...)3*64*7*7 = 9,408Conv2d(64, 64, kernel_size=(3, 3), ...)64*64*3*3 = 36,864```For the `BatchNorm2d` layer, multiply the first number by 2:```BatchNorm2d(64, ...)64*2 = 128```For `Linear` we multiply the first 2 and include the bias if it's `True`:```Linear(in_features=512, out_features=37, bias=True)(512+1)*37 = 18,981```**Now let's calculate some output shapes:**We started with 3x352x352 image and run it through this layer:`Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False)`How did we get: `[64, 176, 176]`The number of output channels is `64`, that's the first dimension in the number above. And then our image of `352x352` got convolved into `176x176` because of stride `2x2` (`352/2`).Then we had:`MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False)`which reduced `[64, 176, 176]` to `[64, 88, 88]` again because of stride 2.And so on, finishing with:`Linear(in_features=512, out_features=37, bias=True)`which reduced everything to just `[37]`.
###Code
jekyll_warn("Known issue: `model_summary` and `Learner.summary` don't work with the AWD LSTM in text models.")
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 5th last layer (`axis1==-5`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-5].numpy());
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
show_doc(ActivationStats.hook)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_train_end)
show_doc(Hook.hook_fn)
###Output
_____no_output_____
###Markdown
Hook callbacks This provides both a standalone class and a callback for registering and automatically deregistering [PyTorch hooks](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks), along with some pre-defined hooks. Hooks can be attached to any [`nn.Module`](https://pytorch.org/docs/stable/nn.htmltorch.nn.Module), for either the forward or the backward pass.We'll start by looking at the pre-defined hook [`ActivationStats`](/callbacks.hooks.htmlActivationStats), then we'll see how to create our own.
###Code
from fastai.gen_doc.nbdoc import *
from fastai.callbacks.hooks import *
from fastai.train import *
from fastai.vision import *
show_doc(ActivationStats)
###Output
_____no_output_____
###Markdown
[`ActivationStats`](/callbacks.hooks.htmlActivationStats) saves the layer activations in `self.stats` for all `modules` passed to it. By default it will save activations for *all* modules. For instance:
###Code
path = untar_data(URLs.MNIST_SAMPLE)
data = ImageDataBunch.from_folder(path)
#learn = create_cnn(data, models.resnet18, callback_fns=ActivationStats)
learn = Learner(data, simple_cnn((3,16,16,2)), callback_fns=ActivationStats)
learn.fit(1)
###Output
_____no_output_____
###Markdown
The saved `stats` is a `FloatTensor` of shape `(2,num_modules,num_batches)`. The first axis is `(mean,stdev)`.
###Code
len(learn.data.train_dl),len(learn.activation_stats.modules)
learn.activation_stats.stats.shape
###Output
_____no_output_____
###Markdown
So this shows the standard deviation (`axis0==1`) of 2th last layer (`axis1==-2`) for each batch (`axis2`):
###Code
plt.plot(learn.activation_stats.stats[1][-2].numpy());
###Output
_____no_output_____
###Markdown
Internal implementation
###Code
show_doc(ActivationStats.hook)
###Output
_____no_output_____
###Markdown
Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(ActivationStats.on_train_begin)
show_doc(ActivationStats.on_batch_end)
show_doc(ActivationStats.on_train_end)
show_doc(Hook)
###Output
_____no_output_____
###Markdown
Registers and manually deregisters a [PyTorch hook](https://pytorch.org/tutorials/beginner/former_torchies/nn_tutorial.htmlforward-and-backward-function-hooks). Your `hook_func` will be called automatically when forward/backward (depending on `is_forward`) for your module `m` is run, and the result of that function is placed in `self.stored`.
###Code
show_doc(Hook.remove)
###Output
_____no_output_____
###Markdown
Deregister the hook, if not called already.
###Code
show_doc(Hooks)
###Output
_____no_output_____
###Markdown
Acts as a `Collection` (i.e. `len(hooks)` and `hooks[i]`) and an `Iterator` (i.e. `for hook in hooks`) of a group of hooks, one for each module in `ms`, with the ability to remove all as a group. Use `stored` to get all hook results. `hook_func` and `is_forward` behavior is the same as [`Hook`](/callbacks.hooks.htmlHook). See the source code for [`HookCallback`](/callbacks.hooks.htmlHookCallback) for a simple example.
###Code
show_doc(Hooks.remove)
###Output
_____no_output_____
###Markdown
Deregister all hooks created by this class, if not previously called. Convenience functions for hooks
###Code
show_doc(hook_output)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for `module` that simply stores the output of the layer.
###Code
show_doc(hook_outputs)
###Output
_____no_output_____
###Markdown
Function that creates a [`Hook`](/callbacks.hooks.htmlHook) for all passed `modules` that simply stores the output of the layers. For example, the (slightly simplified) source code of [`model_sizes`](/callbacks.hooks.htmlmodel_sizes) is:```pythondef model_sizes(m, size): x = m(torch.zeros(1, in_channels(m), *size)) return [o.stored.shape for o in hook_outputs(m)]```
###Code
show_doc(model_sizes)
show_doc(model_summary)
show_doc(num_features_model)
###Output
_____no_output_____
###Markdown
It can be useful to get the size of each layer of a model (e.g. for printing a summary, or for generating cross-connections for a [`DynamicUnet`](/vision.models.unet.htmlDynamicUnet)), however they depend on the size of the input. This function calculates the layer sizes by passing in a minimal tensor of `size`.
###Code
show_doc(dummy_batch)
show_doc(dummy_eval)
show_doc(HookCallback)
###Output
_____no_output_____
###Markdown
For all `modules`, uses a callback to automatically register a method `self.hook` (that you must define in an inherited class) as a hook. This method must have the signature:```pythondef hook(self, m:Model, input:Tensors, output:Tensors)```If `do_remove` then the hook is automatically deregistered at the end of training. See [`ActivationStats`](/callbacks.hooks.htmlActivationStats) for a simple example of inheriting from this class. Callback methods You don't call these yourself - they're called by fastai's [`Callback`](/callback.htmlCallback) system automatically to enable the class's functionality.
###Code
show_doc(HookCallback.on_train_begin)
show_doc(HookCallback.on_train_end)
###Output
_____no_output_____
###Markdown
Undocumented Methods - Methods moved below this line will intentionally be hidden
###Code
show_doc(HookCallback.remove)
show_doc(Hook.hook_fn)
###Output
_____no_output_____ |
cycleGAN.ipynb | ###Markdown
CycleGAN> Implementing cycleGAN for unsupervised image to image domain translation. See https://junyanz.github.io/CycleGAN/.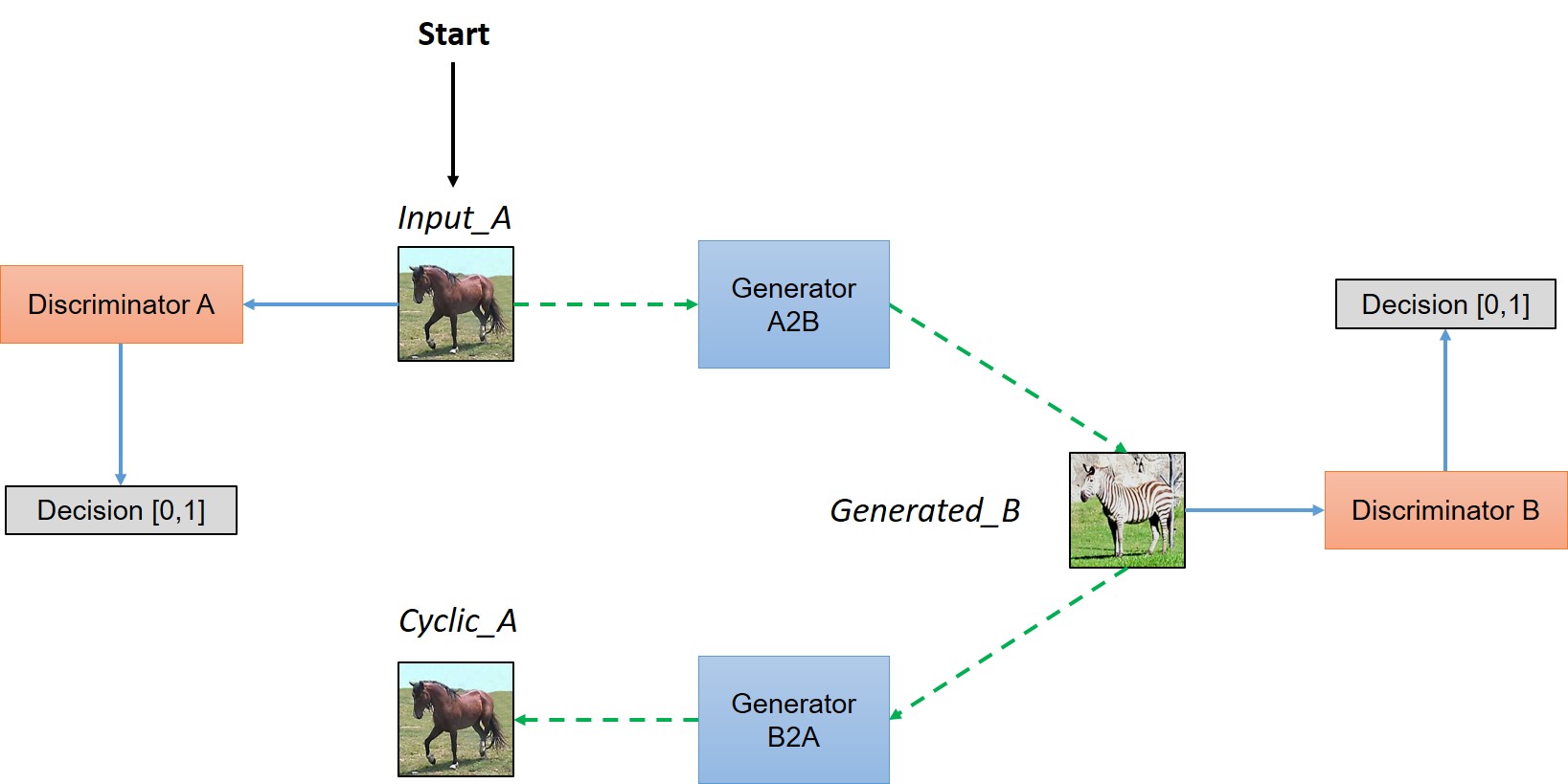 Building Blocks
###Code
#export
class AutoTransConv(nn.Module):
"Automatic padding of transpose convolution for input-output feature size"
def __init__(self, n_in, n_out, ks, stride, bias=True):
super().__init__()
padding = ks // 2
self.conv = nn.ConvTranspose2d(n_in, n_out, ks, stride, padding=padding, output_padding=padding, bias=bias)
#export
def trans_conv_norm_relu(n_in, n_out, norm_layer, bias, ks=3, stride=2):
"Transpose convolutional layer"
return [AutoTransConv(n_in, n_out, ks=ks, stride=stride, bias=bias),
norm_layer(n_out),
nn.ReLU()
]
#export
def pad_conv_norm_relu(n_in, n_out, norm_layer, padding_mode="zeros", pad=1, ks=3, stride=1, activ=True, bias=True):
"Adding ability to specify different paddings to convolutional layer"
layers = []
if padding_mode != "zeros":
pad = 0
if padding_mode == "reflection": layers.append(nn.ReflectionPad2d(pad))
elif padding_mode == "border": layers.append(nn.ReplicationPad2d(pad))
layers.append(AutoConv(n_in, n_out, ks, stride=stride, padding_mode=padding_mode, bias=bias))
layers.append(norm_layer(n_out))
if activ: layers.append(nn.ReLU(inplace=True))
return layers
#export
def conv_norm_relu(n_in, n_out, norm_layer=None, ks=3, bias:bool=True, pad=1, stride=1, activ=True, a=0.2):
"Convolutional layer"
layers = []
layers.append(nn.Conv2d(n_in, n_out, ks, stride=stride, padding=pad))
if norm_layer != None: layers.append(norm_layer(n_out))
if activ: layers.append(nn.LeakyReLU(a, True))
return nn.Sequential(*layers)
#export
class ResBlock(nn.Module):
def __init__(self, dim, padding_mode, bias, dropout, norm_layer=nn.InstanceNorm2d):
"Residual connections for middle section of generator"
super().__init__()
layers = []
layers += pad_conv_norm_relu(dim, dim, norm_layer, padding_mode, bias=bias)
if dropout > 0: layers.append(nn.Dropout(dropout))
layers += (pad_conv_norm_relu(dim, dim, norm_layer, padding_mode, bias=bias, activ=False))
self.xb = nn.Sequential(*layers)
def forward(self, xb): return xb + self.conv(xb)
###Output
_____no_output_____
###Markdown
Generator
###Code
#export
def generator(n_in, n_out, n_f=64, norm_layer=None, dropout=0., n_blocks=6, pad_mode="reflection"):
"Generator that maps an input of one domain to the other"
norm_layer = norm_layer if norm_layer is not None else nn.InstanceNorm2d
bias = (norm_layer == nn.InstanceNorm2d)
layers = []
layers += pad_conv_norm_relu(n_in, n_f, norm_layer, pad_mode, pad=3, ks=7, bias=bias)
for i in range(2):
layers += pad_conv_norm_relu(n_f, n_f*2, norm_layer, 'zeros', stride=2, bias=bias)
n_f*=2
layers += [ResBlock(n_f, pad_mode, bias, dropout, norm_layer) for _ in range(n_blocks)]
for i in range(2):
layers += trans_conv_norm_relu(n_f, n_f//2, norm_layer, bias=bias)
n_f //= 2
layers.append(nn.ReflectionPad2d(3))
layers.append(nn.Conv2d(n_f, n_out, kernel_size=7, padding=0))
layers.append(nn.Tanh())
return nn.Sequential(*layers)
generator(3,10)
###Output
_____no_output_____
###Markdown
Discriminator
###Code
#export
def discriminator(c_in, n_f, n_layers, norm_layer=None, sigmoid=False):
"Discrminator to classify input as belonging to one class or the other"
norm_layer = nn.InstanceNorm2d if norm_layer is None else norm_layer
bias = (norm_layer == nn.InstanceNorm2d)
layers = []
layers += (conv_norm_relu(c_in, n_f, ks=4, stride=2, pad=1))
for i in range(n_layers-1):
new_f = 2*n_f if i <= 3 else n_f
layers += (conv_norm_relu(n_f, new_f, norm_layer, ks=4, stride=2, pad=1, bias=bias))
n_f = new_f
new_f = 2*n_f if n_layers <= 3 else n_f
layers += (conv_norm_relu(n_f, new_f, norm_layer, ks=4, stride=1, pad=1, bias=bias))
layers.append(nn.Conv2d(new_f, 1, kernel_size=4, stride=1, padding=1))
if sigmoid: layers.append(nn.Sigmoid())
return nn.Sequential(*layers)
discriminator(3, 6, 3)
###Output
_____no_output_____
###Markdown
Loss and Trainer
###Code
#export
class cycleGAN(nn.Module):
def __init__(self, c_in, c_out, n_f=64, disc_layers=3, gen_blocks=6, drop=0., norm_layer=None, sigmoid=False):
super().__init__()
self.a_discriminator = discriminator(c_in, n_f, disc_layers, norm_layer, sigmoid)
self.b_discriminator = discriminator(c_in, n_f, disc_layers, norm_layer, sigmoid)
self.generate_a = generator(c_in, c_out, n_f, norm_layer, drop, gen_blocks)
self.generate_b = generator(c_in, c_out, n_f, norm_layer, drop, gen_blocks)
def forward(self, real_A, real_B):
generated_a, generated_B = self.generate_a(real_B), self.generate_b(real_A)
if not self.training: return generated_a, generated_b
id_a, id_b = self.generate_a(real_A), self.generate_b(real_B)
return [generated_a, generated_b, id_a, id_b]
#export
class DynamicLoss(nn.Module):
def __init__(self, loss_fn):
"Loss allowing for dynamic resizing of prediction based on shape of output"
super().__init__()
self.loss_fn = loss_fn
def forward(self, pred, targ, **kwargs):
targ = output.new_ones(*pred.shape) if targ == 1 else output.new_zeros(*pred.shape)
return self.loss_fn(pred, targ, **kwargs)
#export
class CycleGANLoss(nn.Module):
def __init__(self, model, loss_fn=F.mse_loss, la=10., lb=10, lid=0.5):
"CycleGAN loss"
super().__init__()
self.model,self.la,self.lb,self.lid = model,la,lb,lid
self.loss_fn = DynamicLoss(loss_fn)
def store_inputs(self, inputs):
self.reala,self.realb = inputs
def forward(self, pred, target):
gena, genb, ida, idb = pred
self.id_loss = self.lid * (self.la * F.l1_loss(ida, self.reala) + self.lb * F.l1_loss(idb,self.realb))
self.gen_loss = self.crit(self.model.a_discriminator(gena), True) + self.crit(self.model.b_discriminator(genb), True)
self.cyc_loss = self.la* F.l1_loss(self.model.generate_a(genb), self.reala) + self.lb*F.l1_loss(self.model.generate_b(gena), self.realb)
return self.id_loss+ self.gen_loss + self.cyc_loss
###Output
_____no_output_____
###Markdown
CycleGAN loss is composed of 3 parts:1. Identity, an image that has gone through its own domain should remain the same2. Generator, the output images should fool the discriminator into thinking they belong to that class3. Cyclical loss, and image that has been mapped to the other domain then mapped back to itself should resemble the original input
###Code
#export
class cycleGANTrainer(Callback):
"Trainer to sequence timing of training both the discriminator as well as the generator's"
_order = -20
def set_grad(self, da=False, db=False):
in_gen = (not da) and (not db)
requires_grad(self.learn.model.generate_a, in_gen)
requires_grad(self.learn.generate_b, in_gen)
requires_grad(self.learn.a_discriminator, da)
requires_grad(self.learn.b_discriminator, db)
if not gen:
self.opt_D_A.lr, self.opt_D_A.mom = self.learn.opt.lr, self.learn.opt.mom
self.opt_D_A.wd, self.opt_D_A.beta = self.learn.opt.wd, self.learn.opt.beta
self.opt_D_B.lr, self.opt_D_B.mom = self.learn.opt.lr, self.learn.opt.mom
self.opt_D_B.wd, self.opt_D_B.beta = self.learn.opt.wd, self.learn.opt.beta
def before_fit(self, **kwargs):
self.ga = self.learn.model.generate_a
self.gb = self.learn.generate_b
self.da = self.learn.a_discriminator
self.db = self.learn.b_discriminator
self.loss_fn = self.learn.loss_func.loss_func
if not getattr(self,'opt_gen',None):
self.opt_gen = self.learn.opt.new([nn.Sequential(*flatten_model(self.ga), *flatten_model(self.gb))])
else:
self.opt_gen.lr,self.opt_gen.wd = self.opt.lr,self.opt.wd
self.opt_gen.mom,self.opt_gen.beta = self.opt.mom,self.opt.beta
if not getattr(self,'opt_da',None):
self.opt_da = self.learn.opt.new([nn.Sequential(*flatten_model(self.da))])
if not getattr(self,'opt_db',None):
self.opt_db = self.learn.opt.new([nn.Sequential(*flatten_model(self.db))])
self.learn.opt.opt = self.opt_gen
self.set_grad()
def before_batch(self, last_input, **kwargs):
self.learn.loss_func.store_inputs(last_input)
def after_batch(self, last_input, last_output, **kwags):
#Discriminator loss
self.ga.zero_grad(), self.gb.zero_grad()
fakea, fakeb = last_output[0].detach(), last_output[1].detach()
reala,realb = last_input
self.set_grad(da=True)
self.da.zero_grad()
lossda = 0.5 * (self.loss_fn(self.da(reala), True) + self.loss_fn(self.da(fakea), False))
lossda.backward()
self.opt_da.step()
self.set_grad(db=True)
self.opt_db.zero_grad()
lossdb = 0.5 * (self.loss_fn(self.db(realb), True) + self.loss_fn(self.da(fakeb), False))
lossdb.backward()
self.opt_db.step()
self.set_grad()
###Output
_____no_output_____
###Markdown
Training
###Code
cgan = cycleGAN(3, 3, gen_blocks=9)
learn = get_learner(cgan, loss=CycleGANLoss(cgan))
run = get_runner(learn, [cycleGANTrainer()])
cgan
###Output
_____no_output_____ |
20202/pcd_20202_0902_conway.ipynb | ###Markdown
Conway's problem
###Code
!sudo apt install golang-go
%%writefile 1.go
package main
import (
"fmt"
)
const (
MAX = 9
K = 4
)
func compress(inC, pipe chan rune) {
n := 0
previous := <-inC
for c := range inC {
if c == previous && n < MAX-1 {
n++
} else {
if n > 0 {
pipe<- rune(n+1 + 48)
n = 0
}
pipe<- previous
previous = c
}
}
close(pipe)
}
func output(pipe, outC chan rune) {
m := 0
for c := range pipe {
outC<- c
m++
if m == K {
outC <- '\n'
m = 0
}
}
close(outC)
}
func main() {
inC := make(chan rune)
pipe := make(chan rune)
outC := make(chan rune)
go compress(inC, pipe)
go output(pipe, outC)
go func() {
str := "aasbdsssdbsbdjjjfffskskkjsjhsshss."
for _, c := range str {
inC<- c
}
close(inC)
}()
for c := range outC {
fmt.Printf("%c", c)
}
fmt.Println()
}
!go run 1.go
package main
import (
"fmt"
)
var end chan bool
func zero(n int, west chan float64) {
for i := 0; i < n; i++ {
west <- 0.0
}
close(west)
}
func source(row []float64, south chan float64) {
for _, element := range row {
south <- element
}
close(south)
}
func sink(north chan float64) {
for range north {
}
}
func result(c [][]float64, i int, east chan float64) {
j := 0
for element := range east {
c[i][j] = element
j++
}
end <- true
}
func multiplier(firstElement float64, north, east, south, west chan float64) {
for secondElement := range north {
sum := <-east
sum = sum + firstElement*secondElement
south <- secondElement
west <- sum
}
close(south)
close(west)
}
func main() {
a := [][]float64{{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}}
b := [][]float64{{1, 0, 2}, {0, 1, 2}, {1, 0, 0}}
c := [][]float64{{0, 0, 0}, {0, 0, 0}, {0, 0, 0}}
end = make(chan bool)
nra := len(a)
nca := len(a[0])
ns := make([][]chan float64, nra+1) // canales norte sur, matrix de 4x3
for i := range ns {
ns[i] = make([]chan float64, nca)
for j := range ns[i] {
ns[i][j] = make(chan float64)
}
}
ew := make([][]chan float64, nra) // canales easte oeste, matrix de 3x4
for i := range ew {
ew[i] = make([]chan float64, nca+1)
for j := range ew[i] {
ew[i][j] = make(chan float64)
}
}
for i := 0; i < nra; i++ {
go zero(nra, ew[i][nca])
go result(c, i, ew[i][0])
}
for i := 0; i < nca; i++ {
go source(b[i], ns[0][i])
go sink(ns[nra][i])
}
for i := 0; i < nra; i++ {
for j := 0; j < nca; j++ {
go multiplier(a[i][j],
ns[i][j], ew[i][j+1],
ns[i+1][j], ew[i][j])
}
}
for i := 0; i < nra; i++ {
<-end
}
fmt.Println(c)
}
%%writefile 3.go
package main
import (
"fmt"
)
var end chan bool
func zero(n int, west chan float64) {
for i := 0; i < n; i++ {
west <- 0.0
}
close(west)
}
func source(row []float64, south chan float64) {
for _, element := range row {
south <- element
}
close(south)
}
func sink(north chan float64) {
for range north {
}
}
func result(c [][]float64, i int, east chan float64) {
j := 0
for element := range east {
c[i][j] = element
j++
}
end <- true
}
func multiplier(firstElement float64, north, east, south, west chan float64) {
for secondElement := range north {
sum := <-east
sum = sum + firstElement*secondElement
south <- secondElement
west <- sum
}
close(south)
close(west)
}
func main() {
a := [][]float64{{ 1, 2, 3, 4},
{ 5, 6, 7, 8}}
b := [][]float64{{ 1, 2},
{ 5, 6},
{ 9, 10},
{13, 14}}
c := [][]float64{{0, 0},
{0, 0}}
end = make(chan bool)
nra := len(a)
nca := len(a[0])
ns := make([][]chan float64, nra+1) // canales norte sur, matrix de 4x3
for i := range ns {
ns[i] = make([]chan float64, nca)
for j := range ns[i] {
ns[i][j] = make(chan float64)
}
}
ew := make([][]chan float64, nra) // canales easte oeste, matrix de 3x4
for i := range ew {
ew[i] = make([]chan float64, nca+1)
for j := range ew[i] {
ew[i][j] = make(chan float64)
}
}
for i := 0; i < nra; i++ {
go zero(nra, ew[i][nca])
go result(c, i, ew[i][0])
}
for i := 0; i < nca; i++ {
go source(b[i], ns[0][i])
go sink(ns[nra][i])
}
for i := 0; i < nra; i++ {
for j := 0; j < nca; j++ {
go multiplier(a[i][j],
ns[i][j], ew[i][j+1],
ns[i+1][j], ew[i][j])
}
}
for i := 0; i < nra; i++ {
<-end
}
fmt.Println(c)
}
!go run 3.go
import numpy as np
a = np.array([[ 1, 2, 3, 4],
[ 5, 6, 7, 8]])
b = np.array([[ 1, 2],
[ 5, 6],
[ 9, 10],
[13, 14]])
a.dot(b)
###Output
_____no_output_____ |
ensemble-methods-notebooks-master/Ch5.1-gradient-descent-for-minimization.ipynb | ###Markdown
_This notebook contains code and comments from Section 5.1 of the book [Ensemble Methods for Machine Learning](https://www.manning.com/books/ensemble-methods-for-machine-learning). Please see the book for additional details on this topic. This notebook and code are released under the [MIT license](https://github.com/gkunapuli/ensemble-methods-notebooks/blob/master/LICENSE)._ 5.1 Gradient Descent for MinimizationWhen learning a machine learning model, we typically aim to find the model that fits the training data. The "goodness of this fit" is measured using the loss function. Model training is essentially finding the model parameters that minimize the loss function. Training of most machine learning algorithms can ultimately be cast into this framework, and attemtp to minimize the loss function in many different ways.[Gradient descent](https://en.wikipedia.org/wiki/Gradient_descent) is one such way. It is an iterative technique that can be used to find the (local) minimimum of an objective function. It is an example of a first-order optimization technique as it uses first-derivative information, that is, the gradient.--- 5.1.1 Gradient Descent with an Illustrative ExampleWe will use the [Branin function](https://uqworld.org/t/branin-function/53) as a test function to visualize how gradient descent works. The Branin function is a function of two variables $w_1$ and $w_2$:\\[f(w_1, w_2) = a (w_2 - b w_1^2 + c w_1 - r)^2 + s (1-t) \cos{w_1} + s\\]Since we will be performing gradient descent, we will need the gradient of $f(w_1, w_2)$ with respect to both $w_1$ and $w_2$, which we collect into a two dimensional vector:\\[g(w_1, w_2) = \left[ \begin{array}{c} \frac{\partial f(w_1, w_2)}{\partial w_1} \\ \frac{\partial f(w_1, w_2)}{\partial w_2} \end{array} \right] = \left[ \begin{array}{c} 2a(w_2 - b w_1^2 + c w_1 - r) \cdot (-2 b w_1 + c) - s (1 - t) \sin{w_1} \\ 2a(w_2 - b w_1^2 + c w_1 - r) \end{array} \right]\\]
###Code
import numpy as np
def branin(w, a, b, c, r, s, t):
return a * (w[1] - b * w[0] ** 2 + c * w[0] - r) ** 2 + s * (1 - t) * np.cos(w[0]) + s
def branin_gradient(w, a, b, c, r, s, t):
return np.array([2 * a * (w[1] - b * w[0] ** 2 + c * w[0] - r) * (-2 * b * w[0] + c) - s * (1 - t) * np.sin(w[0]),
2 * a * (w[1] - b * w[0] ** 2 + c * w[0] - r)])
###Output
_____no_output_____
###Markdown
We can visualize the function in three dimensions, as well as its contours in two dimensions.
###Code
import matplotlib.pyplot as plt
%matplotlib inline
# Set the constants of the Branin function
a, b, c, r, s, t = 1, 5.1 / (4 * np.pi ** 2), 5 / np.pi, 6, 10, 1 / (8 * np.pi)
# Set plotting boundaries and generate the mesh
w1Min, w1Max, w2Min, w2Max = -5, 18, -10, 18
w1, w2 = np.meshgrid(np.arange(w1Min, w1Max, 0.1),
np.arange(w2Min, w2Max, 0.1))
# Compute the Branin function over this mesh
z = np.apply_along_axis(branin, 1, np.c_[w1.ravel(), w2.ravel()], a, b, c, r, s, t)
z = z.reshape(w1.shape)
# Visualize the Branin function in 3d
fig = plt.figure(figsize=(9, 5))
ax = fig.add_subplot(1, 2, 1, projection='3d')
ax.set_position([0.025, 0.15, 0.5, 0.9])
ax.plot_surface(w1, w2, z, rstride=20, cstride=20, alpha=0.6, linewidth=0.25, edgecolors='k', cmap='viridis')
ax.view_init(elev=25.0, azim=-100.0)
ax.contour(w1, w2, z, zdir='z', levels=np.array([1, 2, 4, 7, 12, 20, 50, 75, 125, 175]),
offset=-50, cmap='viridis', alpha=0.5)
ax.set_xlabel('$w_1$')
ax.set_xlim(w1Min, w1Max)
ax.set_ylabel('$w_2$')
ax.set_ylim(w2Min, w2Max)
ax.set_zlabel('$f(w_1, w_2)$')
ax.set_zlim(-50, 400)
ax.dist = 8
ax.set_title('Branin function: surface')
# Visualize the Branin function in 2d
ax = fig.add_subplot(1, 2, 2)
# ax.set_position([0.55, 0.11, 0.425, 0.85])
ctr = ax.contour(w1, w2, z, levels=np.array([1, 2, 4, 7, 12, 20, 50, 75, 125, 175]), cmap='viridis', alpha=0.75)
ax.clabel(ctr, inline=1, fontsize=6)
ax.set_xlabel('$w_1$')
ax.set_ylabel('$w_2$')
ax.set_title('Branin function: contours')
fig.tight_layout()
pngFile = './figures/CH05_F02_Kunapuli.png'
plt.savefig(pngFile, dpi=300, bbox_inches='tight', pad_inches=0)
###Output
_____no_output_____
###Markdown
This function has **four global minima**, which are the centers of the elliptical regions in contours. Gradient descent will aim to find one of these four global minima. Gradient descent performs the following steps:Initialize: ``x_old`` = initial guesswhile not converged: 1. compute the negative gradient and normalize to unit length (direction) 2. compute the step length using line search (distance) 3. update the solution: x_new = x_old + distance * direction 4. check for convergence: if amount of change between x_new and x_old is below our tolerance threshold We can implement a basic version of gradient descent that can take a function $$f$$ and corresponding gradient $$g$$ as input and returns a locally optimal solution.**Listing 5.1**: Gradient Descent
###Code
from scipy.optimize import line_search
# Gradient descent with function f, and gradient g
def gradient_descent(f, g, x_init, max_iter=100, args=()):
converged = False
n_iter = 0
x_old, x_new = np.array(x_init), None
descent_path = np.full((max_iter + 1, 2), fill_value=np.nan) # Save the descent path
descent_path[n_iter] = x_old
while not converged:
n_iter += 1
gradient = -g(x_old, *args) # Compute the negative gradient
direction = gradient / np.linalg.norm(gradient) # Normalize the gradient
step = line_search(f, g, x_old, direction, args=args) # Compute the step length using line search
if step[0] is None: # If step length doesn't return a useful value, make it 1.0
distance = 1.0
else:
distance = step[0]
x_new = x_old + distance * direction # Compute the update
descent_path[n_iter] = x_new
# Update status
# print('Iter {0:02d}: obj value = {1} (step={2}, dir={3}'.format(n_iter, step[3], step[0], direction))
err = np.linalg.norm(x_new - x_old) # Compute amount of change between x_new and x_old
if err <= 1e-3 or n_iter >= max_iter: # Check for convergence
converged = True
x_old = x_new # Get ready for the next iteration
return x_new, descent_path
###Output
_____no_output_____
###Markdown
We perform gradient descent on the Branin function, intializing our solution at $w = [-4, -5]$, and visualize our solution path.
###Code
%matplotlib inline
# Set the constants of the Branin function
a, b, c, r, s, t = 1, 5.1 / (4 * np.pi ** 2), 5 / np.pi, 6, 10, 1 / (8 * np.pi)
# Initialize and perform gradient descent
w_init = np.array([-4, -5])
w_optimal, w_path = gradient_descent(branin, branin_gradient, w_init, args=(a, b, c, r, s, t))
# Plot optimization path over all iterations
fig, ax = plt.subplots(nrows=1, ncols=2, figsize=(9, 4))
ax[0].contour(w1, w2, z, levels=np.array([1, 2, 4, 7, 12, 20, 50, 75, 125, 175]), cmap='viridis', alpha=0.75)
ax[0].plot(w_path[:, 0], w_path[:, 1], 'k', linewidth=2)
ax[0].scatter(w_init[0], w_init[1], s=50, marker='s')
ax[0].scatter(w_optimal[0], w_optimal[1], s=50, c='r')
ax[0].set_xlabel('$w_1$')
ax[0].set_ylabel('$w_2$')
ax[0].set_title('Gradient descent')
# Plot optimization path zoomed in
ax[1].contour(w1, w2, z, levels=np.array([1, 2, 4, 7, 12, 20, 50, 75, 125, 175]), cmap='viridis', alpha=0.75)
ax[1].plot(w_path[:, 0], w_path[:, 1], 'k', linewidth=2)
ax[1].scatter(w_optimal[0], w_optimal[1], s=50, c='r')
ax[1].set_xlim(1.5, 5.5)
ax[1].set_ylim(-1, 3)
ax[1].set_xlabel('$w_1$')
ax[1].set_ylabel('$w_2$')
ax[1].set_title('Gradient descent (zoomed in)')
fig.tight_layout()
pngFile = './figures/CH05_F03_Kunapuli.png'
plt.savefig(pngFile, dpi=300, bbox_inches='tight', pad_inches=0)
###Output
_____no_output_____
###Markdown
Two important things to note here:1. **Gradient descent typically demonstrates zig-zagging behavior**, especially in narrow valleys. This is a consequence of rapidly changing gradient direction. The magnitude of the gradient also becomes smaller as we approach the minima.2. Of the four minima, which one will gradient descent converge to? That depends on the initial guess. As we see below, **different initializations will cause gradient descent to reach different minima**.
###Code
%matplotlib inline
n = 20
inits = np.r_[np.c_[np.random.uniform(0, 15, n), np.random.uniform(10, 16, n)],
np.c_[np.random.uniform(-5, 0, n), np.random.uniform(-10, 0, n)],
np.c_[np.random.uniform(12, 17, n), np.random.uniform(-10, 0, n)]]
plt.figure()
plt.contour(w1, w2, z, levels=np.array([1, 2, 4, 7, 12, 20, 50, 75, 125, 175]), cmap='viridis', alpha=0.5)
# For each initialization, optimize and plot the path
for i, w_init in enumerate(inits):
w, path = gradient_descent(branin, branin_gradient, w_init, args=(a, b, c, r, s, t))
plt.plot(path[:, 0], path[:, 1], 'r', linewidth=1)
plt.scatter(inits[:, 0], inits[:, 1], s=20)
plt.xlabel('$w_1$')
plt.ylabel('$w_2$')
plt.title('Gradient descent with different initializations');
fig.tight_layout()
pngFile = './figures/CH05_F04_Kunapuli.png'
plt.savefig(pngFile, dpi=300, bbox_inches='tight', pad_inches=0)
###Output
c:\users\gauta\appdata\local\programs\python\python36\lib\site-packages\scipy\optimize\linesearch.py:314: LineSearchWarning: The line search algorithm did not converge
warn('The line search algorithm did not converge', LineSearchWarning)
c:\users\gauta\appdata\local\programs\python\python36\lib\site-packages\scipy\optimize\linesearch.py:314: LineSearchWarning: The line search algorithm did not converge
warn('The line search algorithm did not converge', LineSearchWarning)
c:\users\gauta\appdata\local\programs\python\python36\lib\site-packages\scipy\optimize\linesearch.py:314: LineSearchWarning: The line search algorithm did not converge
warn('The line search algorithm did not converge', LineSearchWarning)
###Markdown
--- 5.1.2 Gradient Descent over Loss Functions for TrainingLet's consider a simple classification problem in a two-dimensional feature space
###Code
%matplotlib inline
from sklearn.datasets import make_blobs
X, y = make_blobs(n_samples=200, n_features=2,
centers=[[-1.5, -1.5], [1.5, 1.5]], random_state=42)
from visualization import plot_2d_data
fig, ax = plt.subplots(nrows=1, ncols=1, figsize=(5, 4))
plot_2d_data(ax, X, y, xlabel='x', ylabel='y',
title='Simple classification problem',
legend=['pos', 'neg'], colormap='RdBu')
fig.tight_layout()
pngFile = './figures/CH05_F05_Kunapuli.png'
plt.savefig(pngFile, dpi=300, bbox_inches='tight')
###Output
_____no_output_____
###Markdown
Now, let's say that we want to learn a linear classifier $h(\mathbf{x})$ of the form shown below:\\[h_\mathbf{w}(\mathbf{x}) = w_1 x_1 + w_2 x_2,\\]which takes a training example $\mathbf{x} = [x_1, x_2]^T$. The classifier is parameterized by $\mathbf{w} = [w_1, w_2]^T$, which we have to learn using the training examples. In order to train a classifier, we'll need a loss function. For this problem, we will chose the **squared loss** of the classifier $h_\mathbf{w}(\mathbf{x})$ over the training set of $n$ training examples $\mathbf{x}_i = [x_1^i, x_2^i]^T$, $i=1, ..., n$, with corresponding labels $y_i$.\\[f_{loss}(w_1, w_2) = \frac{1}{2} \sum_{i=1}^n \left( y_i - h_\mathbf{w}(\mathbf{x}_i) \right)^2 = \frac{1}{2} \sum_{i=1}^n \left( y_i - w_1 x_1^i - w_2 x_2^i \right)^2 = \frac{1}{2} (\mathbf{y} - X\mathbf{w})^T (\mathbf{y} - X\mathbf{w}).\\] Similar to the Branin function in Section 5.2.1, we can compute the gradient of this loss function with respect to $w_1$ and $w_2$.\\[g(w_1, w_2) = \left[ \begin{array}{c} \frac{\partial f_{loss}(w_1, w_2)}{\partial w_1} \\ \frac{\partial f_{loss}(w_1, w_2)}{\partial w_2} \end{array} \right] = \left[ \begin{array}{c} - \sum_{i=1}^n \left( y_i - w_1 x_1 - w_2 x_2 \right) x_1\\ - \sum_{i=1}^n \left( y_i - w_1 x_1 - w_2 x_2 \right) x_2 \end{array} \right] = -X^T (\mathbf{y} - X\mathbf{w})\\]In both the equations above, the expressions on the far right are the vectorized versions of the loss function, where $X$ is the data matrix and $\mathbf{y}$ is the label vector. The vectorized version is more compact and easier and more efficient to implement as it avoids explicit loops for summation.
###Code
def squared_loss(w, X, y):
return 0.5 * np.sum((y - np.dot(X, w))**2)
def squared_loss_gradient(w, X, y):
return -np.dot(X.T, (y - np.dot(X, w)))
###Output
_____no_output_____
###Markdown
As before, we visualize the function we want to optimize.
###Code
%matplotlib inline
fig = plt.figure(figsize=(9, 5))
# Plot the loss function
w1Min, w1Max, w2Min, w2Max = -1, 1, -1, 1
w1, w2 = np.meshgrid(np.arange(w1Min, w1Max, 0.05),
np.arange(w2Min, w2Max, 0.05))
z = np.apply_along_axis(squared_loss, 1, np.c_[w1.ravel(), w2.ravel()], X, y)
z = z.reshape(w1.shape)
ax = fig.add_subplot(1, 2, 1, projection='3d')
ax.plot_surface(w1, w2, z, rstride=5, cstride=5, alpha=0.5, linewidth=0.25, edgecolors='k', cmap='viridis')
ax.view_init(elev=34, azim=-40.0)
ax.contour(w1, w2, z, zdir='z', levels=np.array([50, 100, 150, 200, 300, 400, 600, 800, 1000]),
offset=-50, cmap='viridis', alpha=0.5)
ax.set_xlabel('$w_1$, weight for $x_1$')
ax.set_ylabel('$w_2$, weight for $x_2$')
ax.set_zlabel('$L(w)$ ')
ax.set_title('Loss function, $f_{loss}(w_1, w_2)$: surface')
# pos1 = ax.get_position() # get the original position
# pos2 = [pos1.x0 - 0.1, pos1.y0, pos1.width, pos1.height]
# ax.set_position(pos2) # set a new position
# Plot the contour
ax = fig.add_subplot(1, 2, 2)
ctr = ax.contour(w1, w2, z, levels=np.array([50, 100, 150, 200, 300, 400, 600, 800, 1000]),
cmap='viridis', alpha=0.75)
ax.clabel(ctr, inline=1, fontsize=6)
ax.set_xlabel('$w_1$, weight for $x_1$')
ax.set_ylabel('$w_2$, weight for $x_2$')
ax.set_title('Loss function, $f_{loss}(w_1, w_2)$: contours')
pos1 = ax.get_position() # get the original position
pos2 = [pos1.x0 + 0.1, pos1.y0, pos1.width, pos1.height]
ax.set_position(pos2) # set a new position
# fig.tight_layout()
pngFile = './figures/CH05_F06_Kunapuli.png'
plt.savefig(pngFile, dpi=300, bbox_inches='tight')
###Output
_____no_output_____
###Markdown
As before, we perform gradient descent, this time initializing at $\mathbf{w} = [0.0, -0.99]$.
###Code
%matplotlib inline
w_init = np.array([0.0, -0.99])
w, path = gradient_descent(squared_loss, squared_loss_gradient, w_init, args=(X, y))
# Compute the Loss function in this domain
w1Min, w1Max, w2Min, w2Max = -1, 1, -1, 1
w1, w2 = np.meshgrid(np.arange(w1Min, w1Max, 0.05),
np.arange(w2Min, w2Max, 0.05))
z = np.apply_along_axis(squared_loss, 1, np.c_[w1.ravel(), w2.ravel()], X, y)
z = z.reshape(w1.shape)
# Plot optimization path over all iterations
fig, ax = plt.subplots(nrows=1, ncols=2, figsize=(9, 4))
ax[0].contour(w1, w2, z, levels=np.array([25, 50, 100, 150, 200, 300, 400, 600, 800, 1000]),
cmap='viridis', alpha=0.75)
ax[0].scatter(w_init[0], w_init[1], s=50, marker='s')
ax[0].scatter(w[0], w[1], c='r', s=50)
ax[0].plot(path[:, 0], path[:, 1], 'k', linewidth=2)
ax[0].set_xlabel('$w_1$')
ax[0].set_ylabel('$w_2$')
ax[0].set_title('Gradient descent over the loss function')
# Plot the solution
xMin, xMax = X[:, 0].min() - 0.25, X[:, 0].max() + 0.25
yMin, yMax = X[:, 1].min() - 0.25, X[:, 1].max() + 0.25
xMesh, yMesh = np.meshgrid(np.arange(xMin, xMax, 0.05),
np.arange(yMin, yMax, 0.05))
zMesh = np.dot(np.c_[xMesh.ravel(), yMesh.ravel()], w)
zMesh = (zMesh.reshape(xMesh.shape) + 1) / 2
ax[1].contourf(xMesh, yMesh, zMesh, cmap='RdBu', alpha=0.75)
ax[1].contour(xMesh, yMesh, zMesh, levels=[0.5])
plot_2d_data(ax[1], X, y, colormap='RdBu')
ax[1].set_xlabel('$x_1$, first feature')
ax[1].set_ylabel('$x_2$, second feature')
ax[1].set_title('Simple classification problem');
# fig.tight_layout()
pngFile = './figures/CH05_F07_Kunapuli.png'
plt.savefig(pngFile, dpi=300, bbox_inches='tight')
print(w)
###Output
[0.13643511 0.13862275]
###Markdown
The final $\mathbf{w}$ is trained by performing gradient descent on the loss function defined over the training examples. From the figure on the right above, we can see that gradient descent does, in fact, produce a nice fit.
###Code
ypred = (np.dot(X, w) >= 0).astype(int)
from sklearn.metrics import accuracy_score
accuracy_score(y, ypred)
###Output
_____no_output_____ |
0-pre-tutorial-exercises.ipynb | ###Markdown
Pre-Tutorial ExercisesIf you've arrived early for the tutorial, please feel free to attempt the following exercises to warm-up.
###Code
# 1. Basic Python data structures
# I have a list of dictionaries as such:
names = [{'name': 'Eric',
'surname': 'Ma'},
{'name': 'Jeffrey',
'surname': 'Elmer'},
{'name': 'Mike',
'surname': 'Lee'},
{'name': 'Jennifer',
'surname': 'Elmer'}]
# Write a function that takes in a list of dictionaries and a query surname,
# and searches it for all individuals with a given surname.
def find_persons_with_surname(persons, query_surname):
# Assert that the persons parameter is a list.
# This is a good defensive programming practice.
assert isinstance(persons, list)
results = []
for ______ in ______:
if ___________ == __________:
results.append(________)
return results
# Test your result below.
results = find_persons_with_surname(names, 'Lee')
assert len(results) == 1
results = find_persons_with_surname(names, 'Elmer')
assert len(results) == 2
###Output
_____no_output_____
###Markdown
Pre-Tutorial ExercisesIf you've arrived early for the tutorial, please feel free to attempt the following exercises to warm-up.
###Code
# 1. Basic Python data structures
# I have a list of dictionaries as such:
names = [{'name': 'Eric',
'surname': 'Ma'},
{'name': 'Jeffrey',
'surname': 'Elmer'},
{'name': 'Mike',
'surname': 'Lee'},
{'name': 'Jennifer',
'surname': 'Elmer'}]
# Write a function that takes in a list of dictionaries and a query surname,
# and searches it for all individuals with a given surname.
def find_persons_with_surname(persons, query_surname):
# Assert that the persons parameter is a list.
# This is a good defensive programming practice.
assert isinstance(persons, list)
results = []
for ______ in ______:
if ___________ == __________:
results.append(________)
return results
# my solution
# 1. Basic Python data structures
# I have a list of dictionaries as such:
names = [{'name': 'Eric',
'surname': 'Ma'},
{'name': 'Jeffrey',
'surname': 'Elmer'},
{'name': 'Mike',
'surname': 'Lee'},
{'name': 'Jennifer',
'surname': 'Elmer'}]
# Write a function that takes in a list of dictionaries and a query surname,
# and searches it for all individuals with a given surname.
def find_persons_with_surname(persons, query_surname):
# Assert that the persons parameter is a list.
# This is a good defensive programming practice.
assert isinstance(persons, list)
results = []
for person in persons:
if person['surname'] == query_surname:
results.append(person)
return results
# Test your result below.
results = find_persons_with_surname(names, 'Lee')
assert len(results) == 1
results = find_persons_with_surname(names, 'Elmer')
assert len(results) == 2
###Output
_____no_output_____
###Markdown
Pre-Tutorial ExercisesIf you've arrived early for the tutorial, please feel free to attempt the following exercises to warm-up.
###Code
# 1. Basic Python data structures
# I have a list of dictionaries as such:
names = [{'name': 'Eric',
'surname': 'Ma'},
{'name': 'Jeffrey',
'surname': 'Elmer'},
{'name': 'Mike',
'surname': 'Lee'},
{'name': 'Jennifer',
'surname': 'Elmer'}]
# Write a function that takes in a list of dictionaries and a query surname,
# and searches it for all individuals with a given surname.
def find_persons_with_surname(persons, query_surname):
# Assert that the persons parameter is a list.
# This is a good defensive programming practice.
assert isinstance(persons, list)
results = []
for ______ in ______:
if ___________ == __________:
results.append(________)
return results
# Test your result below.
results = find_persons_with_surname(names, 'Lee')
assert len(results) == 1
results = find_persons_with_surname(names, 'Elmer')
assert len(results) == 2
###Output
_____no_output_____
###Markdown
Pre-Tutorial ExercisesIf you've arrived early for the tutorial, please feel free to attempt the following exercises to warm-up.
###Code
# 1. Basic Python data structures
# I have a list of dictionaries as such:
names = [{'name': 'Eric',
'surname': 'Ma'},
{'name': 'Jeffrey',
'surname': 'Elmer'},
{'name': 'Mike',
'surname': 'Lee'},
{'name': 'Jennifer',
'surname': 'Elmer'}]
# Write a function that takes in a list of dictionaries and a query surname,
# and searches it for all individuals with a given surname.
def find_persons_with_surname(persons, query_surname):
# Assert that the persons parameter is a list.
# This is a good defensive programming practice.
assert isinstance(persons, list)
results = []
for name in names:
if name['surname'] == query_surname:
results.append(name['name'])
return results
# Test your result below.
results = find_persons_with_surname(names, 'Lee')
assert len(results) == 1
results = find_persons_with_surname(names, 'Elmer')
assert len(results) == 2
###Output
_____no_output_____
###Markdown
Pre-Tutorial ExercisesIf you've arrived early for the tutorial, please feel free to attempt the following exercises to warm-up.
###Code
# 1. Basic Python data structures
# I have a list of dictionaries as such:
names = [{'name': 'Eric',
'surname': 'Ma'},
{'name': 'Jeffrey',
'surname': 'Elmer'},
{'name': 'Mike',
'surname': 'Lee'},
{'name': 'Jennifer',
'surname': 'Elmer'}]
# Write a function that takes in a list of dictionaries and a query surname,
# and searches it for all individuals with a given surname.
def find_persons_with_surname(persons, query_surname):
# Assert that the persons parameter is a list.
# This is a good defensive programming practice.
assert isinstance(persons, list)
results = []
for data in persons:
if data["surname"] == query_surname:
results.append(data)
return results
# Test your result below.
results = find_persons_with_surname(names, 'Lee')
assert len(results) == 1
results = find_persons_with_surname(names, 'Elmer')
assert len(results) == 2
###Output
_____no_output_____
###Markdown
Pre-Tutorial ExercisesIf you've arrived early for the tutorial, please feel free to attempt the following exercises to warm-up.
###Code
# 1. Basic Python data structures
# I have a list of dictionaries as such:
names = [{'name': 'Eric',
'surname': 'Ma'},
{'name': 'Jeffrey',
'surname': 'Elmer'},
{'name': 'Mike',
'surname': 'Lee'},
{'name': 'Jennifer',
'surname': 'Elmer'}]
# Write a function that takes in a list of dictionaries and a query surname,
# and searches it for all individuals with a given surname.
def find_persons_with_surname(persons, query_surname):
# Assert that the persons parameter is a list.
# This is a good defensive programming practice.
assert isinstance(persons, list)
results = []
for dude in persons:
if dude['surname'] == query_surname:
results.append(dude['name'])
return results
# Test your result below.
results = find_persons_with_surname(names, 'Lee')
assert len(results) == 1
results = find_persons_with_surname(names, 'Elmer')
assert len(results) == 2
###Output
_____no_output_____
###Markdown
Pre-Tutorial ExercisesIf you've arrived early for the tutorial, please feel free to attempt the following exercises to warm-up.
###Code
# 1. Basic Python data structures
# I have a list of dictionaries as such:
names = [{'name': 'Eric',
'surname': 'Ma'},
{'name': 'Jeffrey',
'surname': 'Elmer'},
{'name': 'Mike',
'surname': 'Lee'},
{'name': 'Jennifer',
'surname': 'Elmer'}]
# Write a function that takes in a list of dictionaries and a query surname,
# and searches it for all individuals with a given surname.
def find_persons_with_surname(persons, query_surname):
# Assert that the persons parameter is a list.
# This is a good defensive programming practice.
assert isinstance(persons, list)
results = []
for ______ in ______:
if ___________ == __________:
results.append(________)
return results
# Test your result below.
results = find_persons_with_surname(names, 'Lee')
assert len(results) == 1
results = find_persons_with_surname(names, 'Elmer')
assert len(results) == 2
###Output
_____no_output_____ |
Lab2/prepare_lab/prepare_lab2.ipynb | ###Markdown
Prepare Lab of 2nd Exercise Speech Recognition using HMMs and RNNs Description Our goal is the implementation of a speech recognition system, that recognizes isolated words. The first part involves the extraction of the appropriate acoustic features from our recordings and their further analysis. These features are the cepstral coefficients, that are computed using a filterbank (inspired by psychoacoustic methods). More specifically, the system will recognize isolated digits in English. Our dataset contains dictations of 9 digits from 15 different speakers in separate .wav files. In total, there are 133 files, since 2 dictations are missing. The name of each file (e.g. eight8.wav) declares both the dictated digit (e.g. eight) and the speaker (speakers are numbered from 1 to 15). The sampling rate is Fs=16k and the duration of each dictation differs. Implementation Import necessary libraries
###Code
import librosa as l
import os
import re
import IPython.display as ipd
import numpy as np
import random
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# sklearn
from sklearn.decomposition import PCA
from sklearn.model_selection import train_test_split
from sklearn.base import BaseEstimator, ClassifierMixin
from sklearn.naive_bayes import GaussianNB
from sklearn import preprocessing
from sklearn.svm import SVC
from sklearn.neighbors import KNeighborsClassifier
from sklearn.linear_model import LogisticRegression
# pytorch
from torch.utils.data import Dataset, DataLoader
import torch
from torch import nn
from torch import optim
# pandas
import pandas as pd
import warnings
warnings.simplefilter(action='ignore', category=FutureWarning)
###Output
_____no_output_____
###Markdown
__Step 1:__ Sound analysis using [Praat](http://www.fon.hum.uva.nl/praat/). - Open __onetwothree1.wav__ and __onetwothree8.wav__ using Praat. These files contain the phrase "one two three" from speakers 1 and 8 (male and female respectively). - Waveforms of male and female speaker - Spectogram of male speaker Spectogram of female speaker - Extract the mean value of the pitch for the vowels "α" "ου" and "ι" (in praat select the part of the waveform that the vowel exists and press "F5"). | File | Gender | Vowel | Pitch (Hz) || -----| ----- | ----- | ----- || onetwothree1.wav | male | α | 133.66 || onetwothree1.wav | male | ου | 128.51 || onetwothree1.wav | male | ι | 130.3 || onetwothree8.wav | female | α | 176.83 || onetwothree8.wav | female | ου | 179.81 || onetwothree8.wav | female | ι | 174.59 | We observe that the pitch of the female speaker is higher than the pitch of the male speaker in all vowels. As a result, we can use pitch in order to distinguish the gender of the speaker. However, we can't use pitch in a digit recognition task, since for a single speaker the pitch of different vowels is close to each other. - Extract the first 3 formants for each vowel (in praat select the central point in the part of the waveform that the vowel exists and press F1, F2 and F3 respectively). | File | Gender | Vowel | F1 | F2 | F3 || -----| ----- | ----- | -- | -- | -- || onetwothree1.wav | male | α | 782 | 1069 | 2243 || onetwothree8.wav | female | α | 951 | 1584 | 2999 || onetwothree1.wav | male | ου | 359 | 1818 | 2443 || onetwothree8.wav | female | ου | 370 | 2150 | 2663 || onetwothree1.wav | male | ι | 386 | 1981 | 2447 || onetwothree8.wav | female | ι | 356 | 2456 | 2552 | We observe that the formants differ from each other in different vowels and are not influenced a lot when the speaker is changed. So, these peaks (F1, F2, F3) can be used in speech recognition to distinguish vowels. __Step 2:__ Create a function (data parser) that reads the sound files in __digits/__ folder and returns 3 Python lists: the .wav file read in librosa, the respective speaker and the digit.
###Code
# Define useful variables
n_samples = 133
digits_dir = "../pr_lab2_2019-20_data/digits"
sr = 16000
# Dictionary to convert string digits in their numerical format.
str2num = {'one':1, 'two':2, 'three':3, 'four':4, 'five':5, 'six':6,
'seven':7, 'eight':8, 'nine':9}
# Dictionary to convert digits from numerical format in their
# string representation.
num2str = {v: k for k, v in str2num.items()}
# Simple parser that split a string in the first digit
def digit_split(s):
return re.split(r'(\d+)', s)[:2]
print(digit_split("six13.wav"))
# Main data parser method
def data_parser(digits_dir):
wav = [None] * n_samples
speaker = np.zeros(n_samples, dtype=int)
digit = np.zeros(n_samples, dtype=int)
for i, file in enumerate(os.listdir(digits_dir)):
filename = os.fsdecode(file)
# Read wav file with librosa
wav[i],_ = l.load(os.path.join(digits_dir, filename), sr=16000)
# Keep speaker and digit
decoded_name = digit_split(filename.split('.')[0])
if decoded_name[0] in str2num:
digit[i] = str2num[decoded_name[0]]
else:
print("Error in decoding " + str(decoded_name[0]))
speaker[i] = int(decoded_name[1])
return wav, speaker, digit
wav, speaker, digit = data_parser(digits_dir)
###Output
_____no_output_____
###Markdown
Check data parser before continue on step 3.
###Code
# Print first three files
for i in range(3):
print("Sample " + str(i))
print("Waveform: " + str(wav[i][:3]))
print("Speaker: " + str(speaker[i]))
print("Digit: " + str(digit[i]))
print()
# Sample a random file
sample = random.randint(0,n_samples-1)
# Construct its correct filename
name = num2str[digit[sample]] + str(speaker[sample]) + '.wav'
print(name)
# Play it and check that parser is correct.
ipd.Audio("../pr_lab2_2019-20_data/digits/" + name)
###Output
four11.wav
###Markdown
__Step 3:__ Extract Mel-Frequency Cepstral Coefficients (MFCCs) from each sound file using librosa (13 features per file). Use 25 ms window size and 10 ms step size. Also, compute delta and delta-deltas of the features.
###Code
# Convert window and step size from ms to number os points.
n_fft = int(sr * 0.025)
hop_length = int(sr * 0.01)
mfccs = []
delta = []
delta_deltas = []
# For each sample compute the mfccs, the deltas and the delta-deltas.
for i in range(n_samples):
mfcc = l.feature.mfcc(wav[i], sr=sr, n_mfcc=13, hop_length=hop_length, n_fft=n_fft)
mfccs.append(mfcc)
delta.append(l.feature.delta(mfcc))
delta_deltas.append(l.feature.delta(mfcc, order=2))
###Output
_____no_output_____
###Markdown
__Step 4:__ Display a histogram for the 1st and the 2nd MFCC of digits n1 and n2 for all recordings. In my case, n1 = 0 and n2 = 9. Since n1 = 0, we define n1 = 9-1 = 8.
###Code
# Extract 1st and 2nd mfcc of digit 8
mfcc1_d8 = [mfccs[i][0] for i in range(n_samples) if digit[i] == 8]
mfcc2_d8 = [mfccs[i][1] for i in range(n_samples) if digit[i] == 8]
# Extract 1st and 2nd mfcc of digit 9
mfcc1_d9 = [mfccs[i][0] for i in range(n_samples) if digit[i] == 9]
mfcc2_d9 = [mfccs[i][1] for i in range(n_samples) if digit[i] == 9]
# Define a function that convert a list of lists in a global list
def flat_list(l):
return [item for sublist in l for item in sublist]
# Flattening
mfcc1_d8_flat = flat_list(mfcc1_d8)
mfcc2_d8_flat = flat_list(mfcc2_d8)
mfcc1_d9_flat = flat_list(mfcc1_d9)
mfcc2_d9_flat = flat_list(mfcc2_d9)
# Plot the histograms
fig = plt.figure(figsize=(15,12))
fig.add_subplot(2, 2, 1)
plt.hist(mfcc1_d8_flat, bins=20)
fig.add_subplot(2, 2, 2)
plt.hist(mfcc2_d8_flat, bins=20)
fig.add_subplot(2, 2, 3)
plt.hist(mfcc1_d9_flat, bins=20)
fig.add_subplot(2, 2, 4)
plt.hist(mfcc2_d9_flat, bins=20)
plt.show()
###Output
_____no_output_____
###Markdown
The divergence between 8 and 9 is small in both featured. In order to classify them, we need more MFCC features. Choose two recordings for each digit from two different speaker and compute Mel Filterbank Spectral Coefficients (MFSCs). Then, plot the correlation of the MFSCs and MFCCs for each recording.
###Code
spk1 = 1
spk2 = 2
n1 = 8
n2 = 9
for i in range(n_samples):
if speaker[i] == spk1 and digit[i] == n1:
s1_n1 = i
if speaker[i] == spk1 and digit[i] == n2:
s1_n2 = i
if speaker[i] == spk2 and digit[i] == n1:
s2_n1 = i
if speaker[i] == spk2 and digit[i] == n2:
s2_n2 = i
print("Speaker 1 - Digit 8: " + str(s1_n1))
print("Speaker 1 - Digit 9: " + str(s1_n2))
print("Speaker 2 - Digit 8: " + str(s2_n1))
print("Speaker 2 - Digit 9: " + str(s2_n2))
mfscs_1_1 = l.feature.melspectrogram(wav[s1_n1], sr=sr, hop_length=hop_length, n_fft=n_fft, n_mels=13)
mfscs_1_2 = l.feature.melspectrogram(wav[s1_n2], sr=sr, hop_length=hop_length, n_fft=n_fft, n_mels=13)
mfscs_2_1 = l.feature.melspectrogram(wav[s2_n1], sr=sr, hop_length=hop_length, n_fft=n_fft, n_mels=13)
mfscs_2_2 = l.feature.melspectrogram(wav[s2_n2], sr=sr, hop_length=hop_length, n_fft=n_fft, n_mels=13)
###Output
_____no_output_____
###Markdown
In order to construct the correlation plot easily, we convert out data in a dataframe and use the function df.corr by pandas.
###Code
fig = plt.figure(figsize=(15,12))
fig.add_subplot(2, 2, 1)
mfsc_df_1_1 = pd.DataFrame.from_records(mfscs_1_1.T)
plt.imshow(mfsc_df_1_1.corr())
fig.add_subplot(2, 2, 2)
mfsc_df_1_2 = pd.DataFrame.from_records(mfscs_1_2.T)
plt.imshow(mfsc_df_1_2.corr())
fig.add_subplot(2, 2, 3)
mfsc_df_2_1 = pd.DataFrame.from_records(mfscs_2_1.T)
plt.imshow(mfsc_df_2_1.corr())
fig.add_subplot(2, 2, 4)
mfsc_df_2_2 = pd.DataFrame.from_records(mfscs_2_2.T)
plt.imshow(mfsc_df_2_2.corr())
plt.show()
fig = plt.figure(figsize=(15,12))
fig.add_subplot(2, 2, 1)
mfcc_df_1_1 = pd.DataFrame.from_records(mfccs[s1_n1].T)
plt.imshow(mfcc_df_1_1.corr())
fig.add_subplot(2, 2, 2)
mfcc_df_1_2 = pd.DataFrame.from_records(mfccs[s1_n2].T)
plt.imshow(mfcc_df_1_2.corr())
fig.add_subplot(2, 2, 3)
mfcc_df_2_1 = pd.DataFrame.from_records(mfccs[s2_n1].T)
plt.imshow(mfcc_df_2_1.corr())
fig.add_subplot(2, 2, 4)
mfcc_df_2_2 = pd.DataFrame.from_records(mfccs[s2_n2].T)
plt.imshow(mfcc_df_2_2.corr())
plt.show()
###Output
_____no_output_____
###Markdown
We observe that MFSCs coefficients are higly correlated, while MSCCs are not. This explains the fact that the Discrete Cosine Transform (DCT) is used to decorrelate the mel filter bank coefficients, a process also referred to as whitening. __Step 5:__ Extraction of a global feature vector for each recording, by combining the mean value and the variance of the mfccs – deltas – delta-deltas for all the windows.
###Code
# X = mean mffc - mean delta - mean delta-deltas- var mfcc - var delta - var delta-deltas
X = np.zeros((n_samples, 78))
for i in range(n_samples):
X[i, :13] = np.mean(mfccs[i], axis=1)
X[i, 13:26] = np.mean(delta[i], axis=1)
X[i, 26:39] = np.mean(delta_deltas[i], axis=1)
X[i, 39:52] = np.std(mfccs[i], axis=1)
X[i, 52:65] = np.std(delta[i], axis=1)
X[i, 65:] = np.std(delta_deltas[i], axis=1)
###Output
_____no_output_____
###Markdown
Plot the first two dimensions in a 2D scatter plot.
###Code
# Define a function that plots the decision surface of 2D-dimensional data
def scatter_2d(X, y, labels):
fig, ax = plt.subplots()
# title for the plots
# Set-up grid for plotting.
X0, X1 = X[:, 0], X[:, 1]
one = ax.scatter(
X0[y == 1], X1[y == 1],
c='red', label=labels[0],
s=50, alpha=0.9, edgecolors='k')
two = ax.scatter(
X0[y == 2], X1[y == 2],
c='purple', label=labels[1],
s=50, alpha=0.9, edgecolors='k')
three = ax.scatter(
X0[y == 3], X1[y == 3],
c='green', label=labels[2],
s=50, alpha=0.9, edgecolors='k')
four = ax.scatter(
X0[y == 4], X1[y == 4],
c='gray', label=labels[3],
s=50, alpha=0.9, edgecolors='k')
five = ax.scatter(
X0[y == 5], X1[y == 5],
c='orange', label=labels[4],
s=50, alpha=0.9, edgecolors='k')
six = ax.scatter(
X0[y == 6], X1[y == 6],
c='black', label=labels[5],
s=50, alpha=0.9, edgecolors='k')
seven = ax.scatter(
X0[y == 7], X1[y == 7],
c='pink', label=labels[6],
s=50, alpha=0.9, edgecolors='k')
eight = ax.scatter(
X0[y == 8], X1[y == 8],
c='white', label=labels[7],
s=50, alpha=0.9, edgecolors='k')
nine = ax.scatter(
X0[y == 9], X1[y == 9],
c='yellow', label=labels[8],
s=50, alpha=0.9, edgecolors='k')
ax.set_xticks(())
ax.set_yticks(())
ax.legend()
plt.show()
plt.rcParams['figure.figsize'] = [20, 15]
scatter_2d(X, digit, [i for i in range(1,10)])
###Output
_____no_output_____
###Markdown
We observe that if we take into account only the first two dimensions of the global feature vector, the points of the same class are not always close to each other and their class is easily separable among the other classes. As a result, a classifier would perform poorly in these 2D data. __Step 6:__ Apply PCA to reduce the dimensions of the feature vector from 78 to 2 or 3 and plot the data in 2D and 3D respectively.
###Code
# Define PCA
pca_2d = PCA(n_components=2)
# Apply PCA on data
X_2d = pca_2d.fit_transform(X)
scatter_2d(X_2d, digit, [i for i in range(1, 10)])
###Output
_____no_output_____
###Markdown
After applying PCA, the scatter plot is much better since we kept more information from all the 78 dimensions in our 2D data.
###Code
# Define a function that plots the decision surface of 3-dimensional data
def scatter_3d(X, y, labels):
fig, ax = plt.subplots()
ax = fig.add_subplot(111, projection='3d')
# title for the plots
# Set-up grid for plotting.
X0, X1, X2 = X[:, 0], X[:, 1], X[:,2]
one = ax.scatter(
X0[y == 1], X1[y == 1], X2[y == 1],
c='red', label=labels[0],
s=50, alpha=0.9, edgecolors='k')
two = ax.scatter(
X0[y == 2], X1[y == 2], X2[y == 2],
c='purple', label=labels[1],
s=50, alpha=0.9, edgecolors='k')
three = ax.scatter(
X0[y == 3], X1[y == 3], X2[y == 3],
c='green', label=labels[2],
s=50, alpha=0.9, edgecolors='k')
four = ax.scatter(
X0[y == 4], X1[y == 4], X2[y == 4],
c='gray', label=labels[3],
s=50, alpha=0.9, edgecolors='k')
five = ax.scatter(
X0[y == 5], X1[y == 5], X2[y == 5],
c='orange', label=labels[4],
s=50, alpha=0.9, edgecolors='k')
six = ax.scatter(
X0[y == 6], X1[y == 6], X2[y == 6],
c='black', label=labels[5],
s=50, alpha=0.9, edgecolors='k')
seven = ax.scatter(
X0[y == 7], X1[y == 7], X2[y == 7],
c='pink', label=labels[6],
s=50, alpha=0.9, edgecolors='k')
eight = ax.scatter(
X0[y == 8], X1[y == 8], X2[y == 8],
c='white', label=labels[7],
s=50, alpha=0.9, edgecolors='k')
nine = ax.scatter(
X0[y == 9], X1[y == 9], X2[y == 9],
c='yellow', label=labels[8],
s=50, alpha=0.9, edgecolors='k')
ax.set_xticks(())
ax.set_yticks(())
ax.legend()
plt.show()
# Define PCA
pca_3d = PCA(n_components=3)
# Apply PCA on data
X_3d = pca_3d.fit_transform(X)
scatter_3d(X_3d, digit, [i for i in range(1,10)])
print(pca_2d.explained_variance_ratio_)
print(pca_3d.explained_variance_ratio_)
###Output
[0.58803289 0.11856749]
[0.58803289 0.11856749 0.10831836]
###Markdown
We observe that after dimensionality reduction we lost a lot of variance, that means that the whole procedure did not go well and we have lost useful information. __Step 7:__ Classification - Split data in train and test set in proportion 70%-30%
###Code
y = digit
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42, stratify=y)
###Output
_____no_output_____
###Markdown
- Normalize our data
###Code
X_train_norm = preprocessing.normalize(X_train)
X_test_norm = preprocessing.normalize(X_test)
###Output
_____no_output_____
###Markdown
- Define the numpy based Gaussian classifier used in the 1st Lab.
###Code
class GaussianNB_np(BaseEstimator, ClassifierMixin):
"""Classify samples based on the Gaussian Naive Bayes"""
def __init__(self):
self.X_mean_ = None
self.X_var_ = None
self.prior = None
self.classes = None
self.n_classes = None
def fit(self, X, y):
"""
This should fit classifier. All the "work" should be done here.
Calculates self.X_mean_ and self.X_var_ based on the mean
feature values in X for each class. Also, calculates self.prior
that contains the prior probability of each class.
self.X_mean_ becomes a numpy.ndarray of shape
(n_classes, n_features)
self.X_var_ becomes a numpy.ndarray of shape
(n_classes, n_features)
self.prior becomes a numpy.array of shape
(n_classes)
fit always returns self.
"""
# Initialize useful variables
self.classes = np.unique(y)
train_size, n_features = X.shape
self.n_classes = len(self.classes)
self.X_mean_ = np.zeros((self.n_classes, n_features))
self.X_var_ = np.zeros((self.n_classes, n_features))
# Compute mean and variance values for each class
for k, c in enumerate(self.classes):
idx_i = [i for i in range(train_size) if y[i] == c]
X_k = np.take(X, idx_i, axis=0)
self.X_mean_[k, :] = np.mean(X_k, axis=0, keepdims=True)
self.X_var_[k, :] = np.var(X_k, axis=0, keepdims=True)
# Compute prior probabilities for each class
self.prior = np.zeros(self.n_classes)
for k, c in enumerate(self.classes):
self.prior[k] = np.count_nonzero(y == c) / train_size
return self
def predict(self, X, smooth=None):
"""
Make predictions for X based on
the highest posterior probability
"""
# Compute likelihood
like = np.zeros((self.n_classes, len(X)))
# Define e for calculation stability (division by zero).
if smooth:
e = smooth
else:
e = 10**(-9)
for i in range(self.n_classes):
like[i] = np.prod(1/(np.sqrt(2*np.pi*self.X_var_[i]+ e)) * \
np.exp(-0.5*((X - self.X_mean_[i])**2 / (self.X_var_[i] + e))), axis=1)
return np.argmax(like.T * self.prior, axis=1) + 1
def score(self, X, y, smooth=None):
"""
Return accuracy score on the predictions
for X based on ground truth y
"""
corr = 0
y_pred = self.predict(X, smooth)
corr = sum(int(y[i] == y_pred[i]) for i in range(len(y)))
acc = corr / len(y)
return acc
###Output
_____no_output_____
###Markdown
- Classify data using our custom Bayes from Lab 1.
###Code
# Define a custom scorer
def my_scorer(clf, X, y_true):
return clf.score(X, y_true)
# Define the classifier
gaussNB_np = GaussianNB_np()
gaussNB_np.fit(X_train, y_train)
print("Accuracy of custom NumPy GaussianNB classifier")
print()
# Predict using default smoothing.
print("Smoothing 1e-9: " + str(gaussNB_np.score(X_test, y_test)))
# Predict using 1e-6 smoothing.
print("Smoothing 1e-6: " + str(gaussNB_np.score(X_test, y_test, smooth=10**(-6))))
# Predict using 1e-3 smoothing.
print("Smoothing 1e-3: " + str(gaussNB_np.score(X_test, y_test, smooth=10**(-3))))
# Predict using 1 smoothing.
print("Smoothing 1: " + str(gaussNB_np.score(X_test, y_test, smooth=1)))
# Define a custom scorer
def my_scorer(clf, X, y_true):
return clf.score(X, y_true)
# Define the classifier
gaussNB_np = GaussianNB_np()
gaussNB_np.fit(X_train_norm, y_train)
print("Accuracy of custom NumPy GaussianNB classifier in normalized data")
print()
# Predict using default smoothing.
print("Smoothing 1e-9: " + str(gaussNB_np.score(X_test_norm, y_test)))
# Predict using 1e-6 smoothing.
print("Smoothing 1e-6: " + str(gaussNB_np.score(X_test_norm, y_test, smooth=10**(-6))))
# Predict using 1e-3 smoothing.
print("Smoothing 1e-3: " + str(gaussNB_np.score(X_test_norm, y_test, smooth=10**(-3))))
# Predict using 1 smoothing.
print("Smoothing 1: " + str(gaussNB_np.score(X_test_norm, y_test, smooth=1)))
###Output
Accuracy of custom NumPy GaussianNB classifier in normalized data
Smoothing 1e-9: 0.6
Smoothing 1e-6: 0.675
Smoothing 1e-3: 0.45
Smoothing 1: 0.15
###Markdown
- Classify data using Naive Bayes of sklearn.
###Code
gaussNB = GaussianNB()
gaussNB.fit(X_train, y_train)
print("Accuracy of sklearn GaussianNB classifier")
print()
print(gaussNB.score(X_test, y_test))
gaussNB = GaussianNB()
gaussNB.fit(X_train_norm, y_train)
print("Accuracy of sklearn GaussianNB classifier in normalized data")
print()
print(gaussNB.score(X_test_norm, y_test))
###Output
Accuracy of sklearn GaussianNB classifier in normalized data
0.6
###Markdown
- Classify data using Nearest Neighbors classifier
###Code
neigh = KNeighborsClassifier(n_neighbors=3)
neigh.fit(X_train, y_train)
print("Accuracy of Nearest Neihbors classifier")
print()
print(neigh.score(X_test, y_test))
neigh = KNeighborsClassifier(n_neighbors=3)
neigh.fit(X_train_norm, y_train)
print("Accuracy of Nearest Neihbors classifier in normalized data")
print()
print(neigh.score(X_test_norm, y_test))
###Output
Accuracy of Nearest Neihbors classifier in normalized data
0.55
###Markdown
- Classify data using SVM classifier with linear kernel.
###Code
svm_linear = SVC(kernel="linear", probability=True)
svm_linear.fit(X_train, y_train)
print("Accuracy of SVM classifier, using linear kernel")
print()
print(svm_linear.score(X_test, y_test))
svm_linear = SVC(kernel="linear", probability=True)
svm_linear.fit(X_train_norm, y_train)
print("Accuracy of SVM classifier, using linear kernel in normalized data")
print()
print(svm_linear.score(X_test_norm, y_test))
###Output
Accuracy of SVM classifier, using linear kernel in normalized data
0.25
###Markdown
- Classify data using SVM classifier with poly kernel.
###Code
svm_poly = SVC(kernel="poly", probability=True)
svm_poly.fit(X_train, y_train)
print("Accuracy of SVM classifier, using poly kernel")
print()
print(svm_poly.score(X_test, y_test))
svm_poly = SVC(kernel="poly", probability=True)
svm_poly.fit(X_train_norm, y_train)
print("Accuracy of SVM classifier, using poly kernel in normalized data")
print()
print(svm_poly.score(X_test_norm, y_test))
###Output
Accuracy of SVM classifier, using poly kernel in normalized data
0.25
###Markdown
- Classify data using Logistic Regression.
###Code
log_reg = LogisticRegression()
log_reg.fit(X_train, y_train)
print("Accuracy of Logistic Regression classifier")
print()
print(log_reg.score(X_test, y_test))
log_reg = LogisticRegression()
log_reg.fit(X_train_norm, y_train)
print("Accuracy of Logistic Regression classifier in normalized data")
print()
print(log_reg.score(X_test_norm, y_test))
###Output
Accuracy of Logistic Regression classifier in normalized data
0.25
###Markdown
- Summarize our results in the following table: | Classifier | Parameter | Normalized | Accuracy || ---------------- | --------------- | ---------- | -------- || Bayes_np | smooth=1e-6 | No | 65 || Bayes_np | smooth=1e-6 | Yes | 67.5 || Bayes_sklearn | smooth=1e-9 | No | 65 || Bayes_sklearn | smooth=1e-9 | Yes | 60 || Nearest Neighbor | n = 3 | No | 62.5 || Nearest Neighbor | n = 3 | Yes | 55 || SVM | kernel = linear | No | 87.5 || SVM | kernel = linear | Yes | 25 || SVM | kernel = poly | No | 85 || SVM | kernel = poly | Yes | 25 || Logistic | - | No | 82.5 || Logistic | - | Yes | 25 | __Step 8:__ Pytorch introduction - Generate 10-point sin and cosine waves with f = 40 Hz and random amplitude.
###Code
f = 40
step = 0.001
X = np.zeros((1000, 10))
y = np.zeros((1000, 10))
for i in range(1000):
# Random amplitude in range [0, 10]
A = np.random.rand()*10
# Random starting point in range [0, 40T]
start = np.random.rand() * (40/f)
time = np.linspace(start, start+step*10, num=10)
X[i] = A*np.sin(2*np.pi*f*time)
y[i] = A*np.cos(2*np.pi*f*time)
###Output
_____no_output_____
###Markdown
- Plot some samples from our generated dataset
###Code
# Define a figure with 10 plots.
fig = plt.figure(figsize=(25,6))
columns = 9
samples = [100, 200, 300, 400, 500, 600, 700, 800, 900]
for i in range(9):
# Display the randomly selected image in a subplot
fig.add_subplot(2, columns, i+1)
plt.plot(np.arange(10), X[samples[i]])
for i in range(9):
# Display the randomly selected image in a subplot
fig.add_subplot(2, columns, i+10)
plt.plot(np.arange(10), y[samples[i]])
plt.show()
###Output
_____no_output_____
###Markdown
- Split data in train and test set and convert them in tensors.
###Code
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
X_train = torch.from_numpy(X_train)
y_train = torch.from_numpy(y_train)
X_test = torch.from_numpy(X_test)
y_test = torch.from_numpy(y_test)
###Output
_____no_output_____
###Markdown
- Define an LSTM
###Code
class LSTMNet(nn.Module):
def __init__(self, input_size=1, hidden_layer_size=100, output_size=1):
super().__init__()
self.hidden_layer_size = hidden_layer_size
self.lstm = nn.LSTMCell(1, hidden_layer_size)
self.linear = nn.Linear(hidden_layer_size, 1)
def forward(self, input, future = 0):
outputs = []
h_t = torch.zeros(input.size(0), self.hidden_layer_size, dtype=torch.double)
c_t = torch.zeros(input.size(0), self.hidden_layer_size, dtype=torch.double)
for i, input_t in enumerate(input.chunk(input.size(1), dim=1)):
h_t, c_t = self.lstm(input_t, (h_t, c_t))
output = self.linear(h_t)
outputs += [output]
outputs = torch.stack(outputs, 1).squeeze(2)
return outputs
###Output
_____no_output_____
###Markdown
- Define model parameters
###Code
model = LSTMNet().double()
criterion = nn.MSELoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
###Output
_____no_output_____
###Markdown
- Train the model
###Code
train_losses = []
test_losses = []
epochs = 1500
for i in range(epochs):
optimizer.zero_grad()
out = model(X_train)
loss = criterion(out, y_train)
if i%100 == 0:
print('Train loss', loss.item())
loss.backward()
optimizer.step()
train_losses.append(loss.item())
with torch.no_grad():
pred = model(X_test)
loss = criterion(pred, y_test)
if i%100 == 0:
print('Test loss:', loss.item())
print()
test_losses.append(loss.item())
train_losses
plt.rcParams['figure.figsize'] = [10, 5]
plt.plot(np.arange(len(train_losses)), train_losses)
plt.plot(np.arange(len(test_losses)), test_losses)
###Output
_____no_output_____
###Markdown
- Plot some test predictions
###Code
# Define a figure with 10 plots.
fig = plt.figure(figsize=(25,10))
columns = 5
rows = 3
samples = np.random.randint(0, 200, 15)
for i in range(15):
# Display the randomly selected image in a subplot
fig.add_subplot(rows, columns, i+1)
plt.axis('off')
with torch.no_grad():
pred = model(X_test[samples[i]].view(1,-1))
plt.plot(np.arange(10), pred[0])
plt.plot(np.arange(10), y_test[samples[i]])
###Output
_____no_output_____ |
13-Sharpening.ipynb | ###Markdown
[](https://notebooks.azure.com/import/gh/Alireza-Akhavan/class.vision) Sharpening By altering our kernels we can implement sharpening, which has the effects of in strengthening or emphasizing edges in an image.
###Code
import cv2
import numpy as np
image = cv2.imread('images/input.jpg')
cv2.imshow('Original', image)
# Create our shapening kernel, we don't normalize since the
# the values in the matrix sum to 1
kernel_sharpening = np.array([[-1,-1,-1],
[-1,9,-1],
[-1,-1,-1]])
# applying different kernels to the input image
sharpened = cv2.filter2D(image, -1, kernel_sharpening)
cv2.imshow('Image Sharpening', sharpened)
cv2.waitKey(0)
cv2.destroyAllWindows()
###Output
_____no_output_____ |
Homework Numpy 1.ipynb | ###Markdown
Семинар по numpyВ этом семинаре мы познакомимся с первой библиотекой, которая понадобится нам в курсе - библиотекой numpy. Это библиотека для работы с матрицами, и она может быть также полезна для матричных вычислений в других дисциплинах. Но сначала познакомимся с интерфейсом jupyter notebook. Это инструмент, позволяющий писать код в интерактивном режиме, рисовать графики и оформлять материалы. Очень краткий обзор интерфейса jupyter notebookJupyter notebook состоит из ячеек.В ячейку можно записывать код, выполнять, а потом использовать созданные переменные / определенные функции и т. д. в других ячейках:
###Code
x = [1, 2, 3]
print(x)
x = 9
y = 3
###Output
_____no_output_____
###Markdown
Выполнить ячейку: shift + enter, показать аргументы функции: shift + tab. Есть много других горячих клавиш, см. Help -> Keyboard Shortcuts (вверху интерфейса). Там же есть Help -> User Interface Tool!Обратите внимание на кнопки + (добавить ячейку), ножницы (удалить ячейку), стрелки, меняющие ячейки местами, кнопку остановить выполнение.Ноутбук сохраняется автоматически. Чтобы скачать: File -> Download as -> ipynb.Этот текст написан в ячейке типа Markdown, она позволяет красиво оформлять код. Переключение типа ячейки справа от кнопки стоп (черный квадрат). Ячейки с кодом имеют тип Code. Создание массивов в numpyNumpy - библиотека для работы с матрицами. Импортируем библиотеку:
###Code
import numpy as np
###Output
_____no_output_____
###Markdown
Предположим, что мы провели опрос, в котором было четыре вопроса, на каждый можно ответить Да, Нет, Воздержался. В итоге получим таблицу размера 4 на 3. Создадим такую таблицу в numpy:
###Code
l = [[30, 2, 0], [3, 27, 1], [28, 1, 1], [6, 17, 5]]
ar = np.array(l)
ar # выводится созданное в последней строке, если в ней нет присваивания (знак =)
###Output
_____no_output_____
###Markdown
Можно создавать массивы из нулей (np.zeros) или из единиц (np.ones):
###Code
import numpy as np
A = np.ones((7, 9))
###Output
_____no_output_____
###Markdown
Также часто используется создание векторов из натуральных чисел:
###Code
vec = np.arange(15)
###Output
_____no_output_____
###Markdown
И случайно сгенерированные массивы:
###Code
r = np.random.rand(3, 4)
r
###Output
_____no_output_____
###Markdown
Размерности массивов Размерности:
###Code
ar.shape
A.shape
vec.shape
###Output
_____no_output_____
###Markdown
В numpy нулевая размерность отвечает за строки, первая - за столбцы. В нашей матрице ar 4 строки и 3 столбца. Вообще в numpy можно создавать массивы любых размерностей точно таким же образом, как мы сделали выше - numpy не различает векторы, матрицы и тензоры, все они называются массивами. Например, vec имеет длину 15 только по одной размерности, поэтому его shape равен (15,). Shape - это обычный кортеж языка python. Можно вытянуть все ответы в одну строку:
###Code
ar.ravel()
###Output
_____no_output_____
###Markdown
Можно наоборот: преобразовать вектор в матрицу:
###Code
vec.reshape(3, 5)
###Output
_____no_output_____
###Markdown
Обратите внимание, что числа записываются по строкам.
###Code
vec.reshape(3, 5).shape
###Output
_____no_output_____
###Markdown
По одной из осей можно указывать -1, тогда библиотека сама посчитает число элементов по этой размерности:
###Code
vec.reshape(3, -1)
###Output
_____no_output_____
###Markdown
Аналогичным образом можно дублироать функционал функции ravel:
###Code
vec.reshape(-1)
###Output
_____no_output_____
###Markdown
Операции с массивами Можно выделить три группы операций с массивами в numpy:* поэлементные* матричные* агрегирующие Поэлементные выполняются между массивами одной формы (shape), хотя ниже мы обсудим некое обощение правила одной формы.
###Code
vec + vec
A * 10
x = np.array([1, 2, 3])
y = np.array([-1, 1, -1])
x * y
###Output
_____no_output_____
###Markdown
Обратите внимание, что * - это поэлементное умножение, а не матричное!
###Code
np.exp(x)
###Output
_____no_output_____
###Markdown
Матричные операции - операции из линейной алгебры. Например, матричное произведение:
###Code
A = np.random.rand(7, 8)
B = np.random.rand(8, 3)
A.dot(B)
###Output
_____no_output_____
###Markdown
Можно писать и так:
###Code
np.dot(A, B)
###Output
_____no_output_____
###Markdown
И так:
###Code
A @ B
###Output
_____no_output_____
###Markdown
Проверим форму:
###Code
A.dot(B).shape
###Output
_____no_output_____
###Markdown
Обращение матрицы:
###Code
np.linalg.inv(np.random.rand(3, 3))
###Output
_____no_output_____
###Markdown
Модуль np.linalg содержит много полезных матричных функций, их можно посмотреть в документации модуля. Агрегирующие операции агрерируют информацию в троках, столбцах, во всем массиве и т. д. Самые популярные такие операции - суммирование np.sum, усреднение np.mean, медиана np.median, максимум np.max и минимум np.min. Число полученных ответов на вопросы (всего):
###Code
np.sum(ar)
###Output
_____no_output_____
###Markdown
Пробуем выяснить число респондентов. Для этого просуммируем матрицу по строкам (это делается с помощью указания axis=1):
###Code
np.sum(ar, axis = 1)
np.sum(ar, axis = 1).shape
###Output
_____no_output_____
###Markdown
По столбцам: axis=0, по строкам: axis=1.В результате суммирования получился вектор (размерность на 1 меньше, чем у исходной матрицы). Можно указать keepdims=True, чтобы сохранть размерности:
###Code
np.sum(ar, axis = 1, keepdims=True).shape
###Output
_____no_output_____
###Markdown
Задание для студентов: посчитать сумму по строкам, используя матричное произведение.
###Code
# student's code here
###Output
_____no_output_____
###Markdown
Считаем число ответов "да", "нет", "воздержался" двумя способами:
###Code
np.sum(ar, axis=0)
ones = np.ones(4)
np.dot(ones, ar)
###Output
_____no_output_____
###Markdown
ИндексацияДля индексации ставим [ ] и через запятую перечисляем действия с осями. В матрице 0 - по вертикали, 1 - по горизонтали
###Code
ar[2, 1] # выбрать 1 элемент
ar # вывели для проверки
ar[:, 2] # выделить столбец
ar[:, -1] # выделить последний столбец
ar[1] # выделить строку
ar[:, ::2] # выделить все столбы с четными номерами
###Output
_____no_output_____
###Markdown
Можно делать логическую индексацию, чтобы выбирались только те элементы, для которых стоит True. Выберем ответы на вопросы с номерами, кратными 2:
###Code
ar[np.arange(ar.shape[0])%2==0]
###Output
_____no_output_____
###Markdown
Добавление осиДля удобного перехода между размерностями используют добавление оси. Вектор можно сделать матрицей с размером 1 по одной из размерностей.
###Code
ones[:, np.newaxis]
ones[:, np.newaxis].shape
# вместо вектора с формой (4,) стала матрциа с формой (4, 1)
ones[np.newaxis, :]
# вместо вектора с формой (4,) стала матрциа с формой (1, 4)
ones[np.newaxis, :].shape
###Output
_____no_output_____
###Markdown
Добавление оси в поэлементных операциях В поэлементных операциях можно использовать не только массивы в одинаковым в точности размером. В общем виде условие такое: по каждой размерности либо размер совпадает, либо в одном из массивов размер 1. Например, матрица размера (4, 3) и вектор размера (4, 1). В этом случае при выполнении операции столбец будет как бы "дублироваться" для каждого столбца в первой матрице. Воспользуемся этим, чтобы найти долю каждого ответа на все вопросы:
###Code
sums = ar.sum(axis=1) # всего ответов на каждый вопрос
sums.shape
sums[:, np.newaxis].shape # добавили ось
ar / sums[:, np.newaxis] # поделили число каждого варианта на общее число ответов на вопрос
###Output
_____no_output_____
###Markdown
Объединение массивов Добавляем новый вопрос в таблицу:
###Code
row = np.array([5, 12, 15])
row = row[np.newaxis, :]
# конкретно тут можно без увеличения размерности
# но в других случаях может быть ошибка, лучше добавлять
ar = np.vstack((ar, row))
###Output
_____no_output_____
###Markdown
Добавляем новый столбец в таблицу - максимальное число ответов:
###Code
mx = np.max(ar, 1)
mx
mx.shape
mx = mx[:, np.newaxis]
ar = np.hstack ((ar, mx))
ar
###Output
_____no_output_____
###Markdown
Удаление строки (аналогично можно удалять столбец):
###Code
np.delete(ar, np.arange(3, 5), axis=0)
###Output
_____no_output_____
###Markdown
Задания для студентов Выделите строки, у которых ответов "нет" больше, чем ответов "да":
###Code
# student's code here
import numpy as np
arr = np.array([[0, 1, 1, 0], [0, 0, 0, 1], [1, 1, 0, 1], [1, 1, 1, 1]])
ar = np.array(arr, dtype=bool)
a = np.sum(ar[0, :])
if a > 2:
print(ar[0, :])
b = np.sum(ar[1, :])
if b > 2:
print(ar[1, :])
c = np.sum(ar[2, :])
if c > 2:
print(ar[2, :])
d = np.sum(ar[3, :])
if d > 2:
print(ar[3, :])
###Output
[ True True False True]
[ True True True True]
###Markdown
Вывести квадраты первых десяти натуральных чисел:
###Code
# student's code here
c = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
c2 = c*c
print(c2)
###Output
[ 1 4 9 16 25 36 49 64 81 100]
###Markdown
Перемешать числа натуральные числа от 1 до 10 (воспользуйтесь np.random.permutation):
###Code
# помощь по функции
?np.random.permutation
# student's code here
d = np.random.permutation(10)
print(d)
###Output
_____no_output_____
###Markdown
Составить таблицу умножения от 1 до 10:
###Code
# student's code here
r = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
col = np.copy(r)
col = col.reshape(10, 1)
table = r * col
print(table)
###Output
[[ 1 2 3 4 5 6 7 8 9 10]
[ 2 4 6 8 10 12 14 16 18 20]
[ 3 6 9 12 15 18 21 24 27 30]
[ 4 8 12 16 20 24 28 32 36 40]
[ 5 10 15 20 25 30 35 40 45 50]
[ 6 12 18 24 30 36 42 48 54 60]
[ 7 14 21 28 35 42 49 56 63 70]
[ 8 16 24 32 40 48 56 64 72 80]
[ 9 18 27 36 45 54 63 72 81 90]
[ 10 20 30 40 50 60 70 80 90 100]]
|
examples/notebooks/cartpole_swing_up.ipynb | ###Markdown
III. Integrate the modelAfter creating DAM for the cartpole system. We need to create an Integrated Action Model (IAM). Remenber that an IAM converts the continuos-time action model into a discrete-time action model. For this exercise we'll use a simpletic Euler integrator.
###Code
# %load solutions/cartpole_integration.py
###########################################################################
################## TODO: Create an IAM with from the DAM ##################
###########################################################################
# Hint:
# Use IntegratedActionModelEuler
###Output
_____no_output_____
###Markdown
IV. Write the problem, create the solverFirst, you need to describe your shooting problem. For that, you have to indicate the number of knots and their time step. For this exercise we want to use 50 knots with $dt=$5e-2.Here is how we create the problem.
###Code
# Fill the number of knots (T) and the time step (dt)
x0 = np.matrix([ 0., 3.14, 0., 0. ]).T
T = 50
problem = crocoddyl.ShootingProblem(x0, [ cartpoleIAM ]*T, cartpoleIAM)
###Output
_____no_output_____
###Markdown
Problem can not solve, just integrate:
###Code
us = [ pinocchio.utils.zero(cartpoleIAM.differential.nu) ]*T
xs = problem.rollout(us)
###Output
_____no_output_____
###Markdown
In cartpole_utils, we provite a plotCartpole and a animateCartpole methods.
###Code
%%capture
%matplotlib inline
from cartpole_utils import animateCartpole
anim = animateCartpole(xs)
# If you encounter problems probably you need to install ffmpeg/libav-tools:
# sudo apt-get install ffmpeg
# or
# sudo apt-get install libav-tools
###Output
_____no_output_____
###Markdown
And let's display this rollout!Note that to_jshtml spawns the video control commands.
###Code
from IPython.display import HTML
# HTML(anim.to_jshtml())
HTML(anim.to_html5_video())
###Output
_____no_output_____
###Markdown
Now we want to create the solver (SolverDDP class) and run it. Display the result. **Do you like it?**
###Code
# %load solutions/cartpole_ddp.py
##########################################################################
################## TODO: Create the DDP solver and run it ###############
###########################################################################
%%capture
%matplotlib inline
# Create animation
anim = animateCartpole(xs)
# HTML(anim.to_jshtml())
HTML(anim.to_html5_video())
###Output
_____no_output_____
###Markdown
Tune the problem, solve itGive some indication about what should be tried for solving the problem. - Without a terminal model, we can see some swings but we cannot stabilize. What should we do? - The most important is to reach the standing position. Can we also nullify the velocity? - Increasing all the weights is not working. How to slowly increase the penalty?
###Code
# %load solutions/cartpole_tuning.py
###########################################################################
################## TODO: Tune the weights for each cost ###################
###########################################################################
terminalCartpole = DifferentialActionModelCartpole()
terminalCartpoleDAM = crocoddyl.DifferentialActionModelNumDiff(terminalCartpole, True)
terminalCartpoleIAM = crocoddyl.IntegratedActionModelEuler(terminalCartpoleDAM)
terminalCartpole.costWeights[0] = 0 # fix me :)
terminalCartpole.costWeights[1] = 0 # fix me :)
terminalCartpole.costWeights[2] = 0 # fix me :)
terminalCartpole.costWeights[3] = 0 # fix me :)
terminalCartpole.costWeights[4] = 0 # fix me :)
terminalCartpole.costWeights[5] = 0 # fix me :)
problem = crocoddyl.ShootingProblem(x0, [ cartpoleIAM ]*T, terminalCartpoleIAM)
# Creating the DDP solver
ddp = crocoddyl.SolverDDP(problem)
ddp.setCallbacks([ crocoddyl.CallbackVerbose() ])
# Solving this problem
done = ddp.solve([], [], 300)
print done
%%capture
%matplotlib inline
# Create animation
anim = animateCartpole(xs)
# HTML(anim.to_jshtml())
HTML(anim.to_html5_video())
###Output
_____no_output_____
###Markdown
Cartpole optimal control problemA cartpole is another classical example of control. In this system, an underactuated pole is attached on top of a 1D actuacted cart. The game is to raise the pole to a standing position.The model is here:https://en.wikipedia.org/wiki/Inverted_pendulumWe denote by $m_1$ the cart mass, $m_2$ the pole mass ($m=m_1+m_2$), $l$ the pole length, $\theta$ the pole angle w.r.t. the vertical axis, $p$ the cart position, and $g=$9.81 the gravity.The system acceleration can be rewritten as:$$\ddot{\theta} = \frac{1}{\mu(\theta)} \big( \frac{\cos \theta}{l} f + \frac{mg}{l} \sin(\theta) - m_2 \cos(\theta) \sin(\theta) \dot{\theta}^2\big),$$$$\ddot{p} = \frac{1}{\mu(\theta)} \big( f + m_2 \cos(\theta) \sin(\theta) g -m_2 l \sin(\theta) \dot{\theta} \big),$$$\hspace{12em}$with $$\mu(\theta) = m_1+m_2 \sin(\theta)^2,$$where $f$ represents the input command (i.e $f=u$) and $m=m_1+m_2$ is the total mass. I. Differential Action ModelA Differential Action Model (DAM) describes the action (control/dynamics) in continous-time. In this exercise, we ask you to write the equation of motions for the cartpole.For more details, see the instructions inside the DifferentialActionModelCartpole class.
###Code
import crocoddyl
import pinocchio
import numpy as np
from IPython.display import HTML
from cartpole_utils import animateCartpole
class DifferentialActionModelCartpole(crocoddyl.DifferentialActionModelAbstract):
def __init__(self):
crocoddyl.DifferentialActionModelAbstract.__init__(self, crocoddyl.StateVector(4), 1, 6) # nu = 1; nr = 6
self.unone = np.zeros(self.nu)
self.m1 = 1.
self.m2 = .1
self.l = .5
self.g = 9.81
self.costWeights = [1., 1., 0.1, 0.001, 0.001, 1.] # sin, 1-cos, x, xdot, thdot, f
def calc(self, data, x, u=None):
if u is None:
u = model.unone
# Getting the state and control variables
y, th, ydot, thdot = x[0].item(), x[1].item(), x[2].item(), x[3].item()
f = u[0].item()
# Shortname for system parameters
m1, m2, l, g = self.m1, self.m2, self.l, self.g
s, c = np.sin(th), np.cos(th)
m = m1 + m2
mu = m1 + m2 * s**2
xddot = (f + m2 * c * s * g - m2 * l * s * thdot**2 ) / mu
thddot = (c * f / l + m * g * s / l - m2 * c * s * thdot**2 ) / mu
data.xout = np.matrix([ xddot, thddot ]).T
# Computing the cost residual and value
data.r = np.matrix(self.costWeights * np.array([ s, 1 - c, y, ydot, thdot, f ])).T
data.cost = .5 * sum(np.asarray(data.r) ** 2).item()
def calcDiff(model,data,x,u=None):
# Advance user might implement the derivatives in cartpole_analytical_derivatives
cartpole_analytical_derivatives(model, data, x, u)
###Output
_____no_output_____
###Markdown
You can get the solution of the cartpole dynamics by uncommenting the following line:
###Code
cartpoleDAM = DifferentialActionModelCartpole()
cartpoleData = cartpoleDAM.createData()
x = cartpoleDAM.state.rand()
u = np.zeros(1)
cartpoleDAM.calc(cartpoleData, x, u)
###Output
_____no_output_____
###Markdown
You may want to check your computation. Here is how to create the model and run the calc method.
###Code
cartpoleDAM = DifferentialActionModelCartpole()
cartpoleData = cartpoleDAM.createData()
x = cartpoleDAM.state.rand()
u = np.zeros(1)
cartpoleDAM.calc(cartpoleData, x, u)
###Output
_____no_output_____
###Markdown
II. Write the derivatives with DAMNumDiffIn the previous exercise, we didn't define the derivatives of the cartpole system. In crocoddyl, we can compute them without any additional code thanks to the DifferentialActionModelNumDiff class. This class computes the derivatives through numerical differentiation.In the following cell, you need to create a cartpole DAM that computes the derivates using NumDiff.
###Code
# Creating the carpole DAM using NumDiff for computing the derivatives.
# We specify the withGaussApprox=True to have approximation of the
# Hessian based on the Jacobian of the cost residuals.
cartpoleND = crocoddyl.DifferentialActionModelNumDiff(cartpoleDAM, True)
###Output
_____no_output_____
###Markdown
After creating your cartpole DAM with NumDiff. We would like that you answer the follows: - 2 columns of Fx are null. Wich ones? Why? - can you double check the values of Fu?
###Code
timeStep = 5e-2
cartpoleIAM = crocoddyl.IntegratedActionModelEuler(cartpoleND, timeStep)
###Output
_____no_output_____
###Markdown
III. Integrate the modelAfter creating DAM for the cartpole system. We need to create an Integrated Action Model (IAM). Remenber that an IAM converts the continuos-time action model into a discrete-time action model. For this exercise we'll use a simpletic Euler integrator.
###Code
# Fill the number of knots (T) and the time step (dt)
x0 = np.matrix([ 0., 3.14, 0., 0. ]).T
T = 50
problem = crocoddyl.ShootingProblem(x0, [ cartpoleIAM ]*T, cartpoleIAM)
###Output
_____no_output_____
###Markdown
IV. Write the problem, create the solverFirst, you need to describe your shooting problem. For that, you have to indicate the number of knots and their time step. For this exercise we want to use 50 knots with $dt=$5e-2.Here is how we create the problem.
###Code
# Fill the number of knots (T) and the time step (dt)
x0 = np.array([0., 3.14, 0., 0.])
T = 50
problem = crocoddyl.ShootingProblem(x0, [cartpoleIAM] * T, cartpoleIAM)
###Output
_____no_output_____
###Markdown
Problem can not solve, just integrate:
###Code
us = [np.zeros(cartpoleIAM.differential.nu)] * T
xs = problem.rollout(us)
###Output
_____no_output_____
###Markdown
In cartpole_utils, we provite a plotCartpole and a animateCartpole methods.
###Code
%%capture
%matplotlib inline
from cartpole_utils import animateCartpole
anim = animateCartpole(xs)
Note that to_jshtml spawns the video control commands.
HTML(animateCartpole(xs).to_jshtml())
# %load solutions/cartpole_ddp.py
# #########################################################################
# ################# TODO: Create the DDP solver and run it ###############
# ##########################################################################
HTML(animateCartpole(ddp.xs.tolist()).to_jshtml())
# HTML(anim.to_jshtml())
HTML(anim.to_html5_video())
###Output
_____no_output_____
###Markdown
Tune the problem, solve itGive some indication about what should be tried for solving the problem. - Without a terminal model, we can see some swings but we cannot stabilize. What should we do? - The most important is to reach the standing position. Can we also nullify the velocity? - Increasing all the weights is not working. How to slowly increase the penalty?
###Code
terminalCartpole = DifferentialActionModelCartpole()
terminalCartpoleDAM = crocoddyl.DifferentialActionModelNumDiff(terminalCartpole, True)
terminalCartpoleIAM = crocoddyl.IntegratedActionModelEuler(terminalCartpoleDAM)
terminalCartpole.costWeights[0] = 100
terminalCartpole.costWeights[1] = 100
terminalCartpole.costWeights[2] = 1
terminalCartpole.costWeights[3] = 0.1
terminalCartpole.costWeights[4] = 0.01
terminalCartpole.costWeights[5] = 0.001
problem = crocoddyl.ShootingProblem(x0, [ cartpoleIAM ]*T, terminalCartpoleIAM)
#Solution:
#Q1: Set weights
#Q2: Standing position with high w[0], w[1]; 0 velocity by slighthly increasing w[3]=xdot
# Creating the DDP solver
ddp = crocoddyl.SolverDDP(problem)
ddp.setCallbacks([crocoddyl.CallbackVerbose()])
# Solving this problem
done = ddp.solve([], [], 300)
print(done)
HTML(animateCartpole(ddp.xs.tolist()).to_jshtml())
###Output
_____no_output_____
###Markdown
Using analytical derivatives Create animationanim = animateCartpole(ddp.xs)
###Code
# %load solutions/cartpole_analytical_derivatives.py
def cartpole_analytical_derivatives(model, data, x, u=None):
pass
###Output
_____no_output_____
###Markdown
Cartpole optimal control problemA cartpole is another classical example of control. In this system, an underactuated pole is attached on top of a 1D actuacted cart. The game is to raise the pole to a standing position.The model is here:https://en.wikipedia.org/wiki/Inverted_pendulumWe denote by $m_1$ the cart mass, $m_2$ the pole mass ($m=m_1+m_2$), $l$ the pole length, $\theta$ the pole angle w.r.t. the vertical axis, $p$ the cart position, and $g=$9.81 the gravity.The system acceleration can be rewritten as:$$\ddot{\theta} = \frac{1}{\mu(\theta)} \big( \frac{\cos \theta}{l} f + \frac{mg}{l} \sin(\theta) - m_2 \cos(\theta) \sin(\theta) \dot{\theta}^2\big),$$$$\ddot{p} = \frac{1}{\mu(\theta)} \big( f + m_2 \cos(\theta) \sin(\theta) g -m_2 l \sin(\theta) \dot{\theta} \big),$$$\hspace{12em}$with $$\mu(\theta) = m_1+m_2 \sin(\theta)^2,$$where $f$ represents the input command (i.e $f=u$) and $m=m_1+m_2$ is the total mass. I. Differential Action ModelA Differential Action Model (DAM) describes the action (control/dynamics) in continous-time. In this exercise, we ask you to write the equation of motions for the cartpole.For more details, see the instructions inside the DifferentialActionModelCartpole class.
###Code
import crocoddyl
import pinocchio
import numpy as np
from IPython.display import HTML
from cartpole_utils import animateCartpole
class DifferentialActionModelCartpole(crocoddyl.DifferentialActionModelAbstract):
def __init__(self):
crocoddyl.DifferentialActionModelAbstract.__init__(self, crocoddyl.StateVector(4), 1, 6) # nu = 1; nr = 6
self.unone = np.zeros(self.nu)
self.m1 = 1.
self.m2 = .1
self.l = .5
self.g = 9.81
self.costWeights = [1., 1., 0.1, 0.001, 0.001, 1.] # sin, 1-cos, x, xdot, thdot, f
def calc(self, data, x, u=None):
if u is None:
u = model.unone
# Getting the state and control variables
y, th, ydot, thdot = x[0].item(), x[1].item(), x[2].item(), x[3].item()
f = u[0].item()
# Shortname for system parameters
m1, m2, l, g = self.m1, self.m2, self.l, self.g
s, c = np.sin(th), np.cos(th)
###########################################################################
############ TODO: Write the dynamics equation of your system #############
###########################################################################
# Hint:
# You don't need to implement integration rules for your dynamic system.
# Remember that DAM implemented action models in continuous-time.
m = m1 + m2
mu = m1 + m2 * s ** 2
xddot, thddot = cartpole_dynamics(self, data, x, u) # Write the cartpole dynamics here
data.xout = np.matrix([ xddot, thddot ]).T
# Computing the cost residual and value
data.r = np.matrix(self.costWeights * np.array([ s, 1 - c, y, ydot, thdot, f ])).T
data.cost = .5 * sum(np.asarray(data.r) ** 2).item()
def calcDiff(model,data,x,u=None):
# Advance user might implement the derivatives in cartpole_analytical_derivatives
cartpole_analytical_derivatives(model, data, x, u)
###Output
_____no_output_____
###Markdown
You can get the solution of the cartpole dynamics by uncommenting the following line:
###Code
# %load solutions/cartpole_dyn.py
###Output
_____no_output_____
###Markdown
You may want to check your computation. Here is how to create the model and run the calc method.
###Code
cartpoleDAM = DifferentialActionModelCartpole()
cartpoleData = cartpoleDAM.createData()
x = cartpoleDAM.state.rand()
u = np.zeros(1)
cartpoleDAM.calc(cartpoleData, x, u)
###Output
_____no_output_____
###Markdown
II. Write the derivatives with DAMNumDiffIn the previous exercise, we didn't define the derivatives of the cartpole system. In crocoddyl, we can compute them without any additional code thanks to the DifferentialActionModelNumDiff class. This class computes the derivatives through numerical differentiation.In the following cell, you need to create a cartpole DAM that computes the derivates using NumDiff.
###Code
# Creating the carpole DAM using NumDiff for computing the derivatives.
# We specify the withGaussApprox=True to have approximation of the
# Hessian based on the Jacobian of the cost residuals.
cartpoleND = crocoddyl.DifferentialActionModelNumDiff(cartpoleDAM, True)
###Output
_____no_output_____
###Markdown
After creating your cartpole DAM with NumDiff. We would like that you answer the follows: - 2 columns of Fx are null. Wich ones? Why? - can you double check the values of Fu?
###Code
# %load solutions/cartpole_fxfu.py
###Output
_____no_output_____
###Markdown
III. Integrate the modelAfter creating DAM for the cartpole system. We need to create an Integrated Action Model (IAM). Remenber that an IAM converts the continuos-time action model into a discrete-time action model. For this exercise we'll use a simpletic Euler integrator.
###Code
# %load solutions/cartpole_integration.py
###########################################################################
################## TODO: Create an IAM with from the DAM ##################
###########################################################################
# Hint:
# Use IntegratedActionModelEuler
###Output
_____no_output_____
###Markdown
IV. Write the problem, create the solverFirst, you need to describe your shooting problem. For that, you have to indicate the number of knots and their time step. For this exercise we want to use 50 knots with $dt=$5e-2.Here is how we create the problem.
###Code
# Fill the number of knots (T) and the time step (dt)
x0 = np.array([0., 3.14, 0., 0.])
T = 50
problem = crocoddyl.ShootingProblem(x0, [cartpoleIAM] * T, cartpoleIAM)
###Output
_____no_output_____
###Markdown
Problem can not solve, just integrate:
###Code
us = [np.zeros(cartpoleIAM.differential.nu)] * T
xs = problem.rollout(us)
###Output
_____no_output_____
###Markdown
In cartpole_utils, we provite a plotCartpole and a animateCartpole methods. Let's display this rollout!Note that to_jshtml spawns the video control commands.
###Code
HTML(animateCartpole(xs).to_jshtml())
# %load solutions/cartpole_ddp.py
# #########################################################################
# ################# TODO: Create the DDP solver and run it ###############
# ##########################################################################
HTML(animateCartpole(ddp.xs.tolist()).to_jshtml())
###Output
_____no_output_____
###Markdown
Tune the problem, solve itGive some indication about what should be tried for solving the problem. - Without a terminal model, we can see some swings but we cannot stabilize. What should we do? - The most important is to reach the standing position. Can we also nullify the velocity? - Increasing all the weights is not working. How to slowly increase the penalty?
###Code
# %load solutions/cartpole_tuning.py
# ##########################################################################
# ################# TODO: Tune the weights for each cost ###################
# ##########################################################################
terminalCartpole = DifferentialActionModelCartpole()
terminalCartpoleDAM = crocoddyl.DifferentialActionModelNumDiff(terminalCartpole, True)
terminalCartpoleIAM = crocoddyl.IntegratedActionModelEuler(terminalCartpoleDAM)
terminalCartpole.costWeights[0] = 0 # fix me :)
terminalCartpole.costWeights[1] = 0 # fix me :)
terminalCartpole.costWeights[2] = 0 # fix me :)
terminalCartpole.costWeights[3] = 0 # fix me :)
terminalCartpole.costWeights[4] = 0 # fix me :)
terminalCartpole.costWeights[5] = 0 # fix me :)
problem = crocoddyl.ShootingProblem(x0, [cartpoleIAM] * T, terminalCartpoleIAM)
# Creating the DDP solver
ddp = crocoddyl.SolverDDP(problem)
ddp.setCallbacks([crocoddyl.CallbackVerbose()])
# Solving this problem
done = ddp.solve([], [], 300)
print(done)
HTML(animateCartpole(ddp.xs.tolist()).to_jshtml())
###Output
_____no_output_____
###Markdown
Using analytical derivativesYou can get the solution of the analytical derivatives by uncommenting the following line:
###Code
# %load solutions/cartpole_analytical_derivatives.py
def cartpole_analytical_derivatives(model, data, x, u=None):
pass
###Output
_____no_output_____
###Markdown
The analytical derivatives being defined, we do not need to use DAMNumDiff to numerically approximate the derivatives.
###Code
timeStep = 5e-2
cartpoleIAM = crocoddyl.IntegratedActionModelEuler(cartpoleDAM, timeStep)
###Output
_____no_output_____
###Markdown
Cartpole optimal control problemA cartpole is another classical example of control. In this system, an underactuated pole is attached on top of a 1D actuacted cart. The game is to raise the pole to a standing position.The model is here:https://en.wikipedia.org/wiki/Inverted_pendulumWe denote by $m_1$ the cart mass, $m_2$ the pole mass ($m=m_1+m_2$), $l$ the pole length, $\theta$ the pole angle w.r.t. the vertical axis, $p$ the cart position, and $g=$9.81 the gravity.The system acceleration can be rewritten as:$$\ddot{\theta} = \frac{1}{\mu(\theta)} \big( \frac{\cos \theta}{l} f + \frac{mg}{l} \sin(\theta) - m_2 \cos(\theta) \sin(\theta) \dot{\theta}^2\big),$$$$\ddot{p} = \frac{1}{\mu(\theta)} \big( f + m_2 \cos(\theta) \sin(\theta) g -m_2 l \sin(\theta) \dot{\theta} \big),$$$\hspace{12em}$with $$\mu(\theta) = m_1+m_2 \sin(\theta)^2,$$where $f$ represents the input command (i.e $f=u$) and $m=m_1+m_2$ is the total mass. I. Differential Action ModelA Differential Action Model (DAM) describes the action (control/dynamics) in continous-time. In this exercise, we ask you to write the equation of motions for the cartpole.For more details, see the instructions inside the DifferentialActionModelCartpole class.
###Code
import crocoddyl
import pinocchio
import numpy as np
class DifferentialActionModelCartpole(crocoddyl.DifferentialActionModelAbstract):
def __init__(self):
crocoddyl.DifferentialActionModelAbstract.__init__(self, crocoddyl.StateVector(4), 1, 6) # nu = 1; nr = 6
self.unone = np.zeros(self.nu)
self.m1 = 1.
self.m2 = .1
self.l = .5
self.g = 9.81
self.costWeights = [ 1., 1., 0.1, 0.001, 0.001, 1. ] # sin, 1-cos, x, xdot, thdot, f
def calc(self, data, x, u=None):
if u is None: u=model.unone
# Getting the state and control variables
y, th, ydot, thdot = np.asscalar(x[0]), np.asscalar(x[1]), np.asscalar(x[2]), np.asscalar(x[3])
f = np.asscalar(u[0])
# Shortname for system parameters
m1, m2, l, g = self.m1, self.m2, self.l, self.g
s, c = np.sin(th), np.cos(th)
###########################################################################
############ TODO: Write the dynamics equation of your system #############
###########################################################################
# Hint:
# You don't need to implement integration rules for your dynamic system.
# Remember that DAM implemented action models in continuous-time.
m = m1 + m2
mu = m1 + m2 * s**2
xddot, thddot = cartpole_dynamics(self, data, x, u) ### Write the cartpole dynamics here
data.xout = np.matrix([ xddot, thddot ]).T
# Computing the cost residual and value
data.r = np.matrix(self.costWeights * np.array([ s, 1-c, y, ydot, thdot, f ])).T
data.cost = .5* np.asscalar(sum(np.asarray(data.r)**2))
def calcDiff(model,data,x,u=None,recalc=True):
# Advance user might implement the derivatives
pass
###Output
_____no_output_____
###Markdown
You can get the solution by uncommenting the following line:
###Code
# %load solutions/cartpole_dyn.py
###Output
_____no_output_____
###Markdown
You may want to check your computation. Here is how to create the model and run the calc method.
###Code
cartpoleDAM = DifferentialActionModelCartpole()
cartpoleData = cartpoleDAM.createData()
x = cartpoleDAM.State.rand()
u = np.zeros(1)
cartpoleDAM.calc(cartpoleData, x, u)
###Output
_____no_output_____
###Markdown
II. Write the derivatives with DAMNumDiffIn the previous exercise, we didn't define the derivatives of the cartpole system. In crocoddyl, we can compute them without any additional code thanks to the DifferentialActionModelNumDiff class. This class computes the derivatives through numerical differentiation.In the following cell, you need to create a cartpole DAM that computes the derivates using NumDiff.
###Code
# Creating the carpole DAM using NumDiff for computing the derivatives.
# We specify the withGaussApprox=True to have approximation of the
# Hessian based on the Jacobian of the cost residuals.
cartpoleND = crocoddyl.DifferentialActionModelNumDiff(cartpoleDAM, True)
###Output
_____no_output_____
###Markdown
After creating your cartpole DAM with NumDiff. We would like that you answer the follows: - 2 columns of Fx are null. Wich ones? Why? - can you double check the values of Fu?
###Code
# %load solutions/cartpole_fxfu.py
###Output
_____no_output_____
###Markdown
III. Integrate the modelAfter creating DAM for the cartpole system. We need to create an Integrated Action Model (IAM). Remenber that an IAM converts the continuos-time action model into a discrete-time action model. For this exercise we'll use a simpletic Euler integrator.
###Code
# %load solutions/cartpole_integration.py
###########################################################################
################## TODO: Create an IAM with from the DAM ##################
###########################################################################
# Hint:
# Use IntegratedActionModelEuler
###Output
_____no_output_____
###Markdown
IV. Write the problem, create the solverFirst, you need to describe your shooting problem. For that, you have to indicate the number of knots and their time step. For this exercise we want to use 50 knots with $dt=$5e-2.Here is how we create the problem.
###Code
# Fill the number of knots (T) and the time step (dt)
x0 = np.matrix([ 0., 3.14, 0., 0. ]).T
T = 50
problem = crocoddyl.ShootingProblem(x0, [ cartpoleIAM ]*T, cartpoleIAM)
###Output
_____no_output_____
###Markdown
Problem can not solve, just integrate:
###Code
us = [ pinocchio.utils.zero(cartpoleIAM.differential.nu) ]*T
xs = problem.rollout(us)
###Output
_____no_output_____
###Markdown
In cartpole_utils, we provite a plotCartpole and a animateCartpole methods.
###Code
%%capture
%matplotlib inline
from cartpole_utils import animateCartpole
anim = animateCartpole(xs)
# If you encounter problems probably you need to install ffmpeg/libav-tools:
# sudo apt-get install ffmpeg
# or
# sudo apt-get install libav-tools
###Output
_____no_output_____
###Markdown
And let's display this rollout!Note that to_jshtml spawns the video control commands.
###Code
from IPython.display import HTML
# HTML(anim.to_jshtml())
HTML(anim.to_html5_video())
###Output
_____no_output_____
###Markdown
Now we want to create the solver (SolverDDP class) and run it. Display the result. **Do you like it?**
###Code
# %load solutions/cartpole_ddp.py
##########################################################################
################## TODO: Create the DDP solver and run it ###############
###########################################################################
%%capture
%matplotlib inline
# Create animation
anim = animateCartpole(xs)
# HTML(anim.to_jshtml())
HTML(anim.to_html5_video())
###Output
_____no_output_____
###Markdown
Tune the problem, solve itGive some indication about what should be tried for solving the problem. - Without a terminal model, we can see some swings but we cannot stabilize. What should we do? - The most important is to reach the standing position. Can we also nullify the velocity? - Increasing all the weights is not working. How to slowly increase the penalty?
###Code
# %load solutions/cartpole_tuning.py
###########################################################################
################## TODO: Tune the weights for each cost ###################
###########################################################################
terminalCartpole = DifferentialActionModelCartpole()
terminalCartpoleDAM = crocoddyl.DifferentialActionModelNumDiff(terminalCartpole, True)
terminalCartpoleIAM = crocoddyl.IntegratedActionModelEuler(terminalCartpoleDAM)
terminalCartpole.costWeights[0] = 0 # fix me :)
terminalCartpole.costWeights[1] = 0 # fix me :)
terminalCartpole.costWeights[2] = 0 # fix me :)
terminalCartpole.costWeights[3] = 0 # fix me :)
terminalCartpole.costWeights[4] = 0 # fix me :)
terminalCartpole.costWeights[5] = 0 # fix me :)
problem = crocoddyl.ShootingProblem(x0, [ cartpoleIAM ]*T, terminalCartpoleIAM)
# Creating the DDP solver
ddp = crocoddyl.SolverDDP(problem)
ddp.setCallbacks([ crocoddyl.CallbackVerbose() ])
# Solving this problem
done = ddp.solve([], [], 300)
print done
%%capture
%matplotlib inline
# Create animation
anim = animateCartpole(xs)
# HTML(anim.to_jshtml())
HTML(anim.to_html5_video())
###Output
_____no_output_____
###Markdown
Cartpole optimal control problemA cartpole is another classical example of control. In this system, an underactuated pole is attached on top of a 1D actuacted cart. The game is to raise the pole to a standing position.The model is here:https://en.wikipedia.org/wiki/Inverted_pendulumWe denote by $m_1$ the cart mass, $m_2$ the pole mass ($m=m_1+m_2$), $l$ the pole length, $\theta$ the pole angle w.r.t. the vertical axis, $p$ the cart position, and $g=$9.81 the gravity.The system acceleration can be rewritten as:$$\ddot{\theta} = \frac{1}{\mu(\theta)} \big( \frac{\cos \theta}{l} f + \frac{mg}{l} \sin(\theta) - m_2 \cos(\theta) \sin(\theta) \dot{\theta}^2\big),$$$$\ddot{p} = \frac{1}{\mu(\theta)} \big( f + m_2 \cos(\theta) \sin(\theta) g -m_2 l \sin(\theta) \dot{\theta} \big),$$$\hspace{12em}$with $$\mu(\theta) = m_1+m_2 \sin(\theta)^2,$$where $f$ represents the input command (i.e $f=u$) and $m=m_1+m_2$ is the total mass. I. Differential Action ModelA Differential Action Model (DAM) describes the action (control/dynamics) in continous-time. In this exercise, we ask you to write the equation of motions for the cartpole.For more details, see the instructions inside the DifferentialActionModelCartpole class.
###Code
import crocoddyl
import pinocchio
import numpy as np
class DifferentialActionModelCartpole(crocoddyl.DifferentialActionModelAbstract):
def __init__(self):
crocoddyl.DifferentialActionModelAbstract.__init__(self, crocoddyl.StateVector(4), 1, 6) # nu = 1; nr = 6
self.unone = np.zeros(self.nu)
self.m1 = 1.
self.m2 = .1
self.l = .5
self.g = 9.81
self.costWeights = [ 1., 1., 0.1, 0.001, 0.001, 1. ] # sin, 1-cos, x, xdot, thdot, f
def calc(self, data, x, u=None):
if u is None: u=model.unone
# Getting the state and control variables
y, th, ydot, thdot = np.asscalar(x[0]), np.asscalar(x[1]), np.asscalar(x[2]), np.asscalar(x[3])
f = np.asscalar(u[0])
# Shortname for system parameters
m1, m2, l, g = self.m1, self.m2, self.l, self.g
s, c = np.sin(th), np.cos(th)
###########################################################################
############ TODO: Write the dynamics equation of your system #############
###########################################################################
# Hint:
# You don't need to implement integration rules for your dynamic system.
# Remember that DAM implemented action models in continuous-time.
m = m1 + m2
mu = m1 + m2 * s**2
xddot, thddot = cartpole_dynamics(self, data, x, u) ### Write the cartpole dynamics here
data.xout = np.matrix([ xddot, thddot ]).T
# Computing the cost residual and value
data.r = np.matrix(self.costWeights * np.array([ s, 1-c, y, ydot, thdot, f ])).T
data.cost = .5* np.asscalar(sum(np.asarray(data.r)**2))
def calcDiff(model,data,x,u=None):
# Advance user might implement the derivatives
pass
###Output
_____no_output_____
###Markdown
You can get the solution by uncommenting the following line:
###Code
# %load solutions/cartpole_dyn.py
###Output
_____no_output_____
###Markdown
You may want to check your computation. Here is how to create the model and run the calc method.
###Code
cartpoleDAM = DifferentialActionModelCartpole()
cartpoleData = cartpoleDAM.createData()
x = cartpoleDAM.State.rand()
u = np.zeros(1)
cartpoleDAM.calc(cartpoleData, x, u)
###Output
_____no_output_____
###Markdown
II. Write the derivatives with DAMNumDiffIn the previous exercise, we didn't define the derivatives of the cartpole system. In crocoddyl, we can compute them without any additional code thanks to the DifferentialActionModelNumDiff class. This class computes the derivatives through numerical differentiation.In the following cell, you need to create a cartpole DAM that computes the derivates using NumDiff.
###Code
# Creating the carpole DAM using NumDiff for computing the derivatives.
# We specify the withGaussApprox=True to have approximation of the
# Hessian based on the Jacobian of the cost residuals.
cartpoleND = crocoddyl.DifferentialActionModelNumDiff(cartpoleDAM, True)
###Output
_____no_output_____
###Markdown
After creating your cartpole DAM with NumDiff. We would like that you answer the follows: - 2 columns of Fx are null. Wich ones? Why? - can you double check the values of Fu?
###Code
# %load solutions/cartpole_fxfu.py
###Output
_____no_output_____
###Markdown
III. Integrate the modelAfter creating DAM for the cartpole system. We need to create an Integrated Action Model (IAM). Remenber that an IAM converts the continuos-time action model into a discrete-time action model. For this exercise we'll use a simpletic Euler integrator.
###Code
# %load solutions/cartpole_integration.py
###########################################################################
################## TODO: Create an IAM with from the DAM ##################
###########################################################################
# Hint:
# Use IntegratedActionModelEuler
###Output
_____no_output_____
###Markdown
IV. Write the problem, create the solverFirst, you need to describe your shooting problem. For that, you have to indicate the number of knots and their time step. For this exercise we want to use 50 knots with $dt=$5e-2.Here is how we create the problem.
###Code
# Fill the number of knots (T) and the time step (dt)
x0 = np.matrix([ 0., 3.14, 0., 0. ]).T
T = 50
problem = crocoddyl.ShootingProblem(x0, [ cartpoleIAM ]*T, cartpoleIAM)
###Output
_____no_output_____
###Markdown
Problem can not solve, just integrate:
###Code
us = [ pinocchio.utils.zero(cartpoleIAM.differential.nu) ]*T
xs = problem.rollout(us)
###Output
_____no_output_____
###Markdown
In cartpole_utils, we provite a plotCartpole and a animateCartpole methods.
###Code
%%capture
%matplotlib inline
from cartpole_utils import animateCartpole
anim = animateCartpole(xs)
# If you encounter problems probably you need to install ffmpeg/libav-tools:
# sudo apt-get install ffmpeg
# or
# sudo apt-get install libav-tools
###Output
_____no_output_____
###Markdown
And let's display this rollout!Note that to_jshtml spawns the video control commands.
###Code
from IPython.display import HTML
# HTML(anim.to_jshtml())
HTML(anim.to_html5_video())
###Output
_____no_output_____
###Markdown
Now we want to create the solver (SolverDDP class) and run it. Display the result. **Do you like it?**
###Code
# %load solutions/cartpole_ddp.py
##########################################################################
################## TODO: Create the DDP solver and run it ###############
###########################################################################
%%capture
%matplotlib inline
# Create animation
anim = animateCartpole(xs)
# HTML(anim.to_jshtml())
HTML(anim.to_html5_video())
###Output
_____no_output_____
###Markdown
Tune the problem, solve itGive some indication about what should be tried for solving the problem. - Without a terminal model, we can see some swings but we cannot stabilize. What should we do? - The most important is to reach the standing position. Can we also nullify the velocity? - Increasing all the weights is not working. How to slowly increase the penalty?
###Code
# %load solutions/cartpole_tuning.py
###########################################################################
################## TODO: Tune the weights for each cost ###################
###########################################################################
terminalCartpole = DifferentialActionModelCartpole()
terminalCartpoleDAM = crocoddyl.DifferentialActionModelNumDiff(terminalCartpole, True)
terminalCartpoleIAM = crocoddyl.IntegratedActionModelEuler(terminalCartpoleDAM)
terminalCartpole.costWeights[0] = 0 # fix me :)
terminalCartpole.costWeights[1] = 0 # fix me :)
terminalCartpole.costWeights[2] = 0 # fix me :)
terminalCartpole.costWeights[3] = 0 # fix me :)
terminalCartpole.costWeights[4] = 0 # fix me :)
terminalCartpole.costWeights[5] = 0 # fix me :)
problem = crocoddyl.ShootingProblem(x0, [ cartpoleIAM ]*T, terminalCartpoleIAM)
# Creating the DDP solver
ddp = crocoddyl.SolverDDP(problem)
ddp.setCallbacks([ crocoddyl.CallbackVerbose() ])
# Solving this problem
done = ddp.solve([], [], 300)
print done
%%capture
%matplotlib inline
# Create animation
anim = animateCartpole(xs)
# HTML(anim.to_jshtml())
HTML(anim.to_html5_video())
###Output
_____no_output_____
###Markdown
Cartpole optimal control problemA cartpole is another classical example of control. In this system, an underactuated pole is attached on top of a 1D actuacted cart. The game is to raise the pole to a standing position.The model is here:https://en.wikipedia.org/wiki/Inverted_pendulumWe denote by $m_1$ the cart mass, $m_2$ the pole mass ($m=m_1+m_2$), $l$ the pole length, $\theta$ the pole angle w.r.t. the vertical axis, $p$ the cart position, and $g=$9.81 the gravity.The system acceleration can be rewritten as:$$\ddot{\theta} = \frac{1}{\mu(\theta)} \big( \frac{\cos \theta}{l} f + \frac{mg}{l} \sin(\theta) - m_2 \cos(\theta) \sin(\theta) \dot{\theta}^2\big),$$$$\ddot{p} = \frac{1}{\mu(\theta)} \big( f + m_2 \cos(\theta) \sin(\theta) g -m_2 l \sin(\theta) \dot{\theta} \big),$$$\hspace{12em}$with $$\mu(\theta) = m_1+m_2 \sin(\theta)^2,$$where $f$ represents the input command (i.e $f=u$) and $m=m_1+m_2$ is the total mass. I. Differential Action ModelA Differential Action Model (DAM) describes the action (control/dynamics) in continous-time. In this exercise, we ask you to write the equation of motions for the cartpole.For more details, see the instructions inside the DifferentialActionModelCartpole class.
###Code
import crocoddyl
import pinocchio
import numpy as np
class DifferentialActionModelCartpole(crocoddyl.DifferentialActionModelAbstract):
def __init__(self):
crocoddyl.DifferentialActionModelAbstract.__init__(self, crocoddyl.StateVector(4), 1, 6) # nu = 1; nr = 6
self.unone = np.zeros(self.nu)
self.m1 = 1.
self.m2 = .1
self.l = .5
self.g = 9.81
self.costWeights = [ 1., 1., 0.1, 0.001, 0.001, 1. ] # sin, 1-cos, x, xdot, thdot, f
def calc(self, data, x, u=None):
if u is None: u=model.unone
# Getting the state and control variables
x, th, xdot, thdot = np.asscalar(x[0]), np.asscalar(x[1]), np.asscalar(x[2]), np.asscalar(x[3])
f = np.asscalar(u[0])
# Shortname for system parameters
m1, m2, l, g = self.m1, self.m2, self.l, self.g
s, c = np.sin(th), np.cos(th)
###########################################################################
############ TODO: Write the dynamics equation of your system #############
###########################################################################
# Hint:
# You don't need to implement integration rules for your dynamic system.
# Remember that DAM implemented action models in continuous-time.
m = m1 + m2
mu = m1 + m2 * s**2
xddot, thddot = cartpole_dynamics(self, data, x, u) ### Write the cartpole dynamics here
data.xout = np.matrix([ xddot, thddot ]).T
# Computing the cost residual and value
data.r = np.matrix(self.costWeights * np.array([ s, 1-c, x, xdot, thdot, f ])).T
data.cost = .5* np.asscalar(sum(np.asarray(data.r)**2))
def calcDiff(model,data,x,u=None,recalc=True):
# Advance user might implement the derivatives
pass
###Output
_____no_output_____
###Markdown
You can get the solution by uncommenting the following line:
###Code
# %load solutions/cartpole_dyn.py
###Output
_____no_output_____
###Markdown
You may want to check your computation. Here is how to create the model and run the calc method.
###Code
cartpoleDAM = DifferentialActionModelCartpole()
cartpoleData = cartpoleDAM.createData()
x = cartpoleDAM.State.rand()
u = np.zeros(1)
cartpoleDAM.calc(cartpoleData,x,u)
###Output
_____no_output_____
###Markdown
II. Write the derivatives with DAMNumDiffIn the previous exercise, we didn't define the derivatives of the cartpole system. In crocoddyl, we can compute them without any additional code thanks to the DifferentialActionModelNumDiff class. This class computes the derivatives through numerical differentiation.In the following cell, you need to create a cartpole DAM that computes the derivates using NumDiff.
###Code
# Creating the carpole DAM using NumDiff for computing the derivatives.
# We specify the withGaussApprox=True to have approximation of the
# Hessian based on the Jacobian of the cost residuals.
cartpoleND = crocoddyl.DifferentialActionModelNumDiff(cartpoleDAM, True)
###Output
_____no_output_____
###Markdown
After creating your cartpole DAM with NumDiff. We would like that you answer the follows: - 2 columns of Fx are null. Wich ones? Why? - can you double check the values of Fu?
###Code
# %load solutions/cartpole_fxfu.py
###Output
_____no_output_____ |
notebooks/noise/Phase Correlations.ipynb | ###Markdown
Phase correlationsIn this notebook we play around with linear phase correlations of white noise.
###Code
from plotly import tools
from plotly import offline as py
from plotly import graph_objs as go
from plotly import figure_factory as ff
py.init_notebook_mode(connected=True)
###Output
_____no_output_____
###Markdown
In order to create linear phase correlated white noise we start with normal white noise $(x_1,\dots,x_n)$. The real fourier transform is given through $(X_0,\dots,X_{n/2})$. $X_0$ equals the signal mean and should not be modified. We can write $X_i=|X_i|e^{i\phi_i}$. Now we apply the transform,$$\phi_i=\phi_{i+j}+\phi+\eta_i$$,wherein $phi\in[-\pi,+\pi[$ is a constant, $\eta_i$ a noise term. With regard to the index shift $i+j$ we apply the circular condition $i+j = i+j-n/2$ if $i+j>n/2-1$.
###Code
def correlate_phases(x, offset, phase, noise):
X = np.fft.rfft(x)
phi = np.arctan2(X.imag, X.real)
phi[1:] = np.roll(phi[1:], -offset) + phase + noise
X = np.abs(X) * np.exp(1j * phi)
return np.fft.irfft(X)
x = np.random.normal(0, 1, 100)
n = np.random.normal(0, 0.5, 50)
y1 = correlate_phases(x, 1, 0, n)
y2 = correlate_phases(x, 1, np.pi, n)
layout = go.Layout(
legend=dict(orientation='h')
)
figure = go.Figure([
go.Scatter(y=x, name='white noise'),
go.Scatter(y=y1, name='phase correlated (δ=0)'),
# go.Scatter(y=y2, name='phase correlated (δ=π)'),
], layout)
py.iplot(figure)
###Output
_____no_output_____
###Markdown
If we apply the linear phase correlation without constant phase offset. We find that there are areas where the signal has large overlap with the original uncorrelated white noise.
###Code
layout = go.Layout(
legend=dict(orientation='h')
)
figure = go.Figure([
go.Scatter(y=x, name='white noise'),
go.Scatter(y=y2, name='phase correlated (δ=π)'),
], layout)
py.iplot(figure)
###Output
_____no_output_____
###Markdown
When using a constant phase offset to obtain the linear phase correlated white noise the local similarity is reduced compared to the previous time series. However, global structural features are still similar.
###Code
z1 = correlate_phases(x, 1, 0, n)
z2 = correlate_phases(x, 2, 0, n)
layout = go.Layout(
legend=dict(orientation='h')
)
figure = go.Figure([
go.Scatter(y=x, name='white noise'),
go.Scatter(y=z1, name='phase correlated (i=1)'),
go.Scatter(y=z2, name='phase correlated (i=2)'),
], layout)
py.iplot(figure)
###Output
_____no_output_____ |
KDDCUP99.ipynb | ###Markdown
KDD Cup 1999 Data http://kdd.ics.uci.edu/databases/kddcup99/kddcup99.html
###Code
import pandas as pd
import matplotlib.pyplot as pyplot
from sklearn import datasets
import sklearn.preprocessing as sp
from sklearn.externals import joblib
% matplotlib inline
###Output
_____no_output_____
###Markdown
|ファイル名|ファイル内容||---|---||kddcup.data|フルデータ||kddcup.data_10_percent|フルデータの10%を抽出した学習用データ||corrected|正常・攻撃のラベル付けがなされた評価用データ||kddcup.testdata.unlabeled|正常・攻撃のラベル付けがなされていないデータ||kddcup.testdata.unlabeled_10_percent|正常・攻撃のラベル付けがなされていないデータの10%サブセット||kddcup.newtestdata_10_percent_unlabeled|正常・攻撃のラベル付けがなされていないデータの10%サブセット|
###Code
col_names = ["duration","protocol_type","service","flag","src_bytes",
"dst_bytes","land","wrong_fragment","urgent","hot","num_failed_logins",
"logged_in","num_compromised","root_shell","su_attempted","num_root","num_file_creations",
"num_shells","num_access_files","num_outbound_cmds","is_host_login","is_guest_login","count",
"srv_count","serror_rate","srv_serror_rate","rerror_rate","srv_rerror_rate","same_srv_rate",
"diff_srv_rate","srv_diff_host_rate","dst_host_count","dst_host_srv_count",
"dst_host_same_srv_rate","dst_host_diff_srv_rate","dst_host_same_src_port_rate",
"dst_host_srv_diff_host_rate","dst_host_serror_rate","dst_host_srv_serror_rate",
"dst_host_rerror_rate","dst_host_srv_rerror_rate","label"]
#kdd_data = pd.read_csv("kddcup.data", header=None, names = col_names)
kdd_data_10percent = pd.read_csv("kddcup.data_10_percent", header=None, names = col_names)
kdd_data_10percent.head()
###Output
_____no_output_____
###Markdown
Transform Objects Into Categories
###Code
kdd_data_10percent.dtypes
kdd_data_10percent['label'].value_counts()
kdd_data_10percent.protocol_type = kdd_data_10percent.protocol_type.astype("category")
kdd_data_10percent.service = kdd_data_10percent.service.astype("category")
kdd_data_10percent.flag = kdd_data_10percent.flag.astype("category")
kdd_data_10percent.label = kdd_data_10percent.label.astype("category")
kdd_data_10percent.dtypes
kdd_data_10percent['protocol_type'].value_counts()
kdd_data_10percent['service'].value_counts()
kdd_data_10percent['flag'].value_counts()
###Output
_____no_output_____
###Markdown
Transform Categories Into Integers
###Code
le_protocol_type=sp.LabelEncoder()
le_protocol_type.fit(kdd_data_10percent['protocol_type'])
joblib.dump(le_protocol_type, 'dump/le_protocol_type.pkl')
kdd_data_10percent.protocol_type=le_protocol_type.transform(kdd_data_10percent['protocol_type'])
kdd_data_10percent.protocol_type.value_counts()
le_service=sp.LabelEncoder()
le_service.fit(kdd_data_10percent['service'])
joblib.dump(le_service, 'dump/le_service.pkl')
kdd_data_10percent.service=le_service.transform(kdd_data_10percent['service'])
kdd_data_10percent.service.value_counts()
le_flag=sp.LabelEncoder()
le_flag.fit(kdd_data_10percent['flag'])
joblib.dump(le_flag, 'dump/le_flag.pkl')
kdd_data_10percent.flag=le_flag.transform(kdd_data_10percent['flag'])
kdd_data_10percent['flag'].value_counts()
train_labels = kdd_data_10percent['label'].copy()
train_features = kdd_data_10percent.drop('label',axis=1)
train_labels.head()
train_features.head()
###Output
_____no_output_____
###Markdown
SVM training
###Code
from sklearn import svm
clf = svm.SVC()
clf.fit(train_features, train_labels)
#joblib.dump(clf, 'dump/clf.pkl')
#clf = joblib.load('dump/clf.pkl')
test_pred = clf.predict(train_features)
test_pred
from sklearn.metrics import classification_report, accuracy_score
print(classification_report(train_labels, test_pred))
print(accuracy_score(train_labels, test_pred))
train_labels = kdd_data_10percent['label'].copy()
###Output
_____no_output_____ |
Prediticing EC2 Spot Prices.ipynb | ###Markdown
Prediticing spot prices for AWS EC2 Instances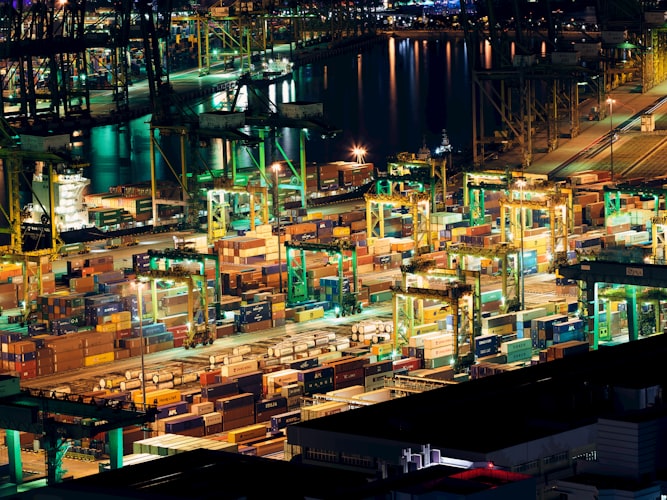 Table of Contents* Introduction* Background* Import libraries* EDA (Exploratory Data Analysis)* Cleaning* Implement Model* Conculsion on results IntroductionThe purpose of this experiment is to train a deep learning model to predict an outcome on time series data. I will be using the Fast.ai library for the model. More specifically, we will be predicting the Spot prices for specifc regions. BackgroundAmazon Web Services [(AWS)](https://aws.amazon.com) provides virtual computing environments via their EC2 service. You can launch instances with your favourite operating system, select pre-configured instance images or create your own. Why this is revelant to data sciensits is because generally to run deep learning models you need a machine with a good GPU. EC2 can be configured with a P2/P3 instance and can be configured with up to 8 or 16 GPUs respectively! However, you can request Spot Instance Pricing. Which basically charges you for the spot price that is in effect for the duration of your instance running time. They are adjusted based on long-term trends in supply and demand for Spot instance capacity. Spot instances can be discounted at up to 90% off compared to On-Demand pricing. Our goal will be to predict Spot pricing for the different global regions on offer:* US East* US West* South America (East)* EU (European Union) West * EU Central* Canda * Asia Pacific North East 1* Asia Pacific North East 2* Asia Pacific South* Asia Pacific Southeast 1 * Asia Pacific Southeast 2 Import Libraries
###Code
import seaborn as sns
%reload_ext autoreload
%autoreload 2
%matplotlib inline
from IPython.display import HTML, display
from fastai.structured import *
from fastai.column_data import *
np.set_printoptions(threshold=50, edgeitems=20)
import numpy as np # linear algebra
import pandas as pd # data processing, CSV file I/O (e.g. pd.read_csv)
import os
print(os.listdir("../input"))
###Output
_____no_output_____
###Markdown
Lets import all the tables
###Code
PATH = "../input/"
PATH_WRITE = "/kaggle/working/"
ls {PATH}
table_names = ['ap-southeast-2', 'ap-northeast-2', 'ca-central-1', 'us-east-1',
'ap-northeast-1', 'ap-south-1', 'sa-east-1', 'eu-west-1',
'ap-southeast-1', 'us-west-1', 'eu-central-1']
tables = [pd.read_csv(f'{PATH}{fname}.csv', low_memory=False) for fname in table_names]
###Output
_____no_output_____
###Markdown
EDA Lets call head and take a look at what our data looks like.
###Code
for t in tables: display(t.head())
###Output
_____no_output_____
###Markdown
Lets call summary
###Code
for t in tables: display(DataFrameSummary(t).summary())
###Output
_____no_output_____
###Markdown
I think we need to change some of the columns names
###Code
new_labels = ['Date', 'Instance Type', 'OS', 'Region', 'Price ($)']
for t in tables:
t.columns = new_labels
for t in tables: display(t.head())
for t in tables:
plt.figure(figsize=(12,8))
sns.countplot(t['Instance Type'], order=t['Instance Type'].value_counts().iloc[:20].index)
plt.xticks(rotation=90);
###Output
_____no_output_____
###Markdown
List of questions to ask:* Average price for certain instances in each region* Frequent instance type* Seasonlity of instances* Determine if there are any stationary variables* Which instance type is frequently link with what OS?* Need to plot instances in time-intervalse eg: between 21:00 - 22:00Also need to figure out how to give each region a table name for the graphs. Lets look at the tables seperately: US East
###Code
us_east = pd.read_csv("../input/us-east-1.csv")
PATH_USEAST = "../input/us-east-1.csv"
us_east.columns = new_labels
us_east.head()
us_east['Date'].head()
###Output
_____no_output_____
###Markdown
We need to parse the dates otherwise the dates will not apear on axis. The format string needs to match the format in the column EXACTLY! For more info look [here](http://strftime.org/) and [here](https://codeburst.io/dealing-with-datetimes-like-a-pro-in-pandas-b80d3d808a7f)
###Code
us_east['Date'] = pd.to_datetime(us_east['Date'], format='%Y-%m-%d %H:%M:%S+00:00', utc=False)
us_east.info()
us_east['Date'].head(500)
###Output
_____no_output_____
###Markdown
Instance: d2.xlarge
###Code
d2_= us_east[us_east['Instance Type'] == "d2.xlarge"].set_index('Date')
d2_Unix = us_east[us_east['OS'] == "Linux/UNIX"].set_index('Date')
d2_Suse = us_east[us_east['OS'] == "SUSE Linux"].set_index('Date')
d2_Win = us_east[us_east['OS'] == "Windows"].set_index('Date')
d2.head()
d2.head()
d2.head(100).plot(title="d2.xlarge Instances", figsize=(15,10))
d2_Suse.head(100).plot(title="d2.xlarge Instances OS:SUSE Linux", figsize=(15,10))
d2_Unix.head(100).plot(title="d2.xlarge Instances OS:Linux/UNIX", figsize=(15,10))
d2_Win.head(100).plot(title="d2.xlarge Instances OS:Windows", figsize=(15,10))
###Output
_____no_output_____
###Markdown
Looks like windows Instances can get quite pricey with highs of around roughly `$` 7 dollars - `$` 29!🤷♀️ Cleaning Lets go over the steps for checking for signs that we need to clean our dataset.
###Code
us_east['Instance Type'].value_counts(dropna=False)
us_east['OS'].value_counts(dropna=False)
len(us_east.isnull())
###Output
_____no_output_____
###Markdown
Out of 3 721 999 entries none have null values Lets train on another dataset
###Code
eu_west = pd.read_csv("../input/eu-west-1.csv")
PATH_euwest = "../input/"
eu_west.columns = new_labels
eu_west.info()
eu_west['Instance Type'].value_counts(dropna=False)
len(eu_west.isnull())
###Output
_____no_output_____
###Markdown
Implement Model Things to do:* Len the Instance type [done]* Add date part [done] * Create cat & continous vars [done] - do not have any other kind continous var!!* Process datasets [done]* Split Dataset - via datetime [done]* Create RMSE metric* Create model data object* calculate embeddings* Train model
###Code
add_datepart(eu_west, 'Date', drop=False)
eu_west.reset_index(inplace=True)
eu_west.to_feather(f'{PATH_WRITE}eu_west')
eu_west.shape
eu_west=pd.read_feather(f'{PATH_WRITE}eu_west')
eu_west.columns
joined = eu_west
joined_test = eu_west
joined.to_feather(f'{PATH_WRITE}joined')
joined_test.to_feather(f'{PATH_WRITE}joined_test')
joined = pd.read_feather(f'{PATH_WRITE}joined')
joined_test = pd.read_feather(f'{PATH_WRITE}joined_test')
joined.head()
cat_vars = [
'Instance Type',
'OS',
'Region',
'Year' ,
'Month' ,
'Week' ,
'Day',
'Dayofweek',
'Dayofyear'
]
contin_vars = ['Elapsed']
n = len(joined); n
dep = 'Price ($)'
joined = joined[cat_vars+contin_vars+[dep,'Date']].copy()
joined_test[dep] = 0
joined_test = joined_test[cat_vars+contin_vars+[dep,'Date',]].copy()
for cat in cat_vars: joined[cat] = joined[cat].astype('category').cat.as_ordered()
eu_west['Price ($)'] = eu_west['Price ($)'].astype('float32')
for contin in contin_vars:
joined[contin] = joined[contin].astype('float32')
joined_test[contin] = joined_test[contin].astype('float32')
idxs = get_cv_idxs(n, val_pct=150000/n)
joined_sample = joined.iloc[idxs].set_index("Date")
samp_size = len(joined_sample); samp_size
samp_size = n
joined_sample.head()
df_train, y, nas, mapper = proc_df(joined_sample,'Price ($)', do_scale=True)
yl = np.log(y)
joined_test = joined_test.set_index("Date")
df_test, _, nas, mapper = proc_df(joined_test,'Price ($)', do_scale=True,mapper=mapper, na_dict=nas )
%debug
df_train.info()
train_val_split = 0.80
train_size = int(2383999 * train_val_split);train_size
val_idx = list(range(train_size, len(df_train)))
val_idx = np.flatnonzero(
(df_train.index<=datetime.datetime(2017,4,12)) & (df_train.index>=datetime.datetime(2017,4,12)))
val_idx=[0]
len(val_idx)
###Output
_____no_output_____
###Markdown
We can put our Model.
###Code
def inv_y(a): return np.exp(a)
def exp_rmspe(y_pred, targ):
targ = inv_y(targ)
pct_var = (targ - inv_y(y_pred))/targ
return math.sqrt((pct_var**2).mean())
max_log_y = np.max(yl)
y_range = (0, max_log_y*1.2)
md = ColumnarModelData.from_data_frame(PATH_euwest, val_idx, df_train, yl.astype(np.float32),
cat_flds=cat_vars, bs=128, test_df=df_test)
cat_sz = [(c, len(df_train[c].cat.categories)+1) for c in cat_vars]
###Output
_____no_output_____ |
ibd_tp_01_mike.ipynb | ###Markdown
Universidade Federal de Minas GeraisDepartamento de Ciência da ComputaçãoIntrodução a Banco de Dados - Trabalho Prático 1 1. Introdução 1.1. Contexto Nesse trabalho prático utilizaremos o banco de dados representado pelo schema abaixo: 1.2. Entrega 1. Você deve completar esse notebook, executar todas as células e submetê-lo no **Minha UFMG**.2. Você pode entregar até o dia 29/07/2021 às 23:59.3. O trabalho é individual e vale 15 pontos. 2. Preparação do Ambiente
###Code
# importando os packages necessários
import io
import sqlite3
import pandas as pd
import matplotlib.pyplot as plt
# download do dump do banco de dados:
!wget https://raw.githubusercontent.com/claudiovaliense/ibd/master/data/despesas_publicas_tp1.sql
###Output
--2021-07-29 19:17:23-- https://raw.githubusercontent.com/claudiovaliense/ibd/master/data/despesas_publicas_tp1.sql
Resolving raw.githubusercontent.com (raw.githubusercontent.com)... 185.199.108.133, 185.199.109.133, 185.199.110.133, ...
Connecting to raw.githubusercontent.com (raw.githubusercontent.com)|185.199.108.133|:443... connected.
HTTP request sent, awaiting response... 200 OK
Length: 277046 (271K) [text/plain]
Saving to: ‘despesas_publicas_tp1.sql’
despesas_ 0%[ ] 0 --.-KB/s
despesas_publicas_t 100%[===================>] 270.55K --.-KB/s in 0.01s
2021-07-29 19:17:23 (18.8 MB/s) - ‘despesas_publicas_tp1.sql’ saved [277046/277046]
###Markdown
2.1. Criação da conexão com o banco* No momento da conexão, é informado o nome do arquivo onde será montado o banco de dados. No nosso caso, este arquivo não precisa ser pré-existente, porque iremos carregar as informações de um dump;* Se este comando for executado com um arquivo que já existe e possui as mesmas tabelas que serão criadas, ocorrerá um erro no momento da execução do script mais à frente.
###Code
conn = sqlite3.connect('despesas.db')
cursor = conn.cursor()
###Output
_____no_output_____
###Markdown
2.2. Execução do script para inicializar e popular o banco de dados* Criada a conexão, iremos utilizar o arquivo .sql disponibilizado para recuperar o schema e os dados do banco
###Code
f = io.open('despesas_publicas_tp1.sql', 'r', encoding='ISO-8859-1')
sql = f.read()
cursor.executescript(sql)
###Output
_____no_output_____
###Markdown
2.3. Realizando consultas no banco de dados* Criada a conexão e realizada a carga dos dados no banco, pode ser executada qualquer consulta SQL;* O SQLite3 permite que o retorno dos dados seja feito em vários formatos, entretanto, utilizaremos o retorno em um dataframe do Pandas.**CONSULTA EXEMPLO:** todos os órgãos ordenados pela quantidade de orgãos subordinados de forma decrescente.
###Code
df = pd.read_sql_query("SELECT * FROM ORGAO ORDER BY QTD_ORGAOS_SUBORDINADOS DESC", conn)
df
###Output
_____no_output_____
###Markdown
3. Consultas Expresse cada consulta abaixo utilizando SQL: **1.** Retorne a quantidade de palavras em cada registro da coluna nome_orgao da tabela orgão.
###Code
df = pd.read_sql_query("SELECT NOME_ORGAO, (length(rtrim(NOME_ORGAO)) - length(replace(rtrim(NOME_ORGAO), ' ', '')) + 1) AS QTD_PALAVRAS FROM ORGAO", conn)
df
###Output
_____no_output_____
###Markdown
**2.** Dado a coluna nome_orgao, retorne a primeira posição que a string 'da' aparece da tabela orgão
###Code
df = pd.read_sql_query("SELECT * FROM ORGAO WHERE NOME_ORGAO LIKE '%da%' LIMIT 1", conn)
df
###Output
_____no_output_____
###Markdown
**3.** Liste todos os nomes dos órgãos e o valor das despesas totais no ano de 2018 para os mesmos, ordenando de forma decrescente pelo valor.
###Code
df = pd.read_sql_query("SELECT NOME_ORGAO, SUM(VALOR_DESPESA) AS VALOR_DESP_2018 FROM DESPESA_PUBLICA NATURAL JOIN ORGAO WHERE ANO_DESPESA='2018' GROUP BY NOME_ORGAO ORDER BY VALOR_DESP_2018 DESC", conn)
df
###Output
_____no_output_____
###Markdown
**4.** Liste o mês e ano, modalidade, programa de governo e valor de todas as despesas do órgão Ministério da Fazenda no primeiro semestre de 2018.
###Code
df = pd.read_sql_query("SELECT MES_DESPESA, ANO_DESPESA, MODALIDADE_DESPESA, NOME_PROGRAMA_GOVERNO, VALOR_DESPESA FROM (DESPESA_PUBLICA NATURAL JOIN ORGAO NATURAL JOIN PROGRAMA_GOVERNO) WHERE NOME_ORGAO='Ministério da Fazenda' AND ANO_DESPESA=2018 AND MES_DESPESA<7", conn)
df
###Output
_____no_output_____
###Markdown
**5.** Liste o código e nome dos programas de governo que possuíram os 3 maiores valores de despesas totais na função de educação durante o ano de 2018.
###Code
df = pd.read_sql_query("SELECT COD_PROGRAMA_GOVERNO, NOME_PROGRAMA_GOVERNO, SUM(VALOR_DESPESA) AS DESP_TOTAIS FROM (DESPESA_PUBLICA NATURAL JOIN FUNCAO NATURAL JOIN PROGRAMA_GOVERNO) WHERE NOME_FUNCAO='Educação' AND ANO_DESPESA='2018' GROUP BY COD_PROGRAMA_GOVERNO ORDER BY DESP_TOTAIS DESC LIMIT 3", conn)
df
###Output
_____no_output_____
###Markdown
**6.** Transforme os caracteres em minúsculo e remova todos os termos 'da' na coluna nome_orgao da tabela orgão.
###Code
df = pd.read_sql_query("SELECT COD_ORGAO, lower(replace(NOME_ORGAO, ' da ', ' ')) AS NOME_ORGAO, QTD_ORGAOS_SUBORDINADOS FROM ORGAO", conn)
df
###Output
_____no_output_____
###Markdown
**7.** Liste os órgãos governamentais e a quantidade de programas de governo distintos envolvidos nas despesas públicas desses órgãos. Nesta consulta, desconsidere valores de despesa negativos ou iguais a zero no período.
###Code
df = pd.read_sql_query("SELECT NOME_ORGAO, COUNT(DISTINCT NOME_PROGRAMA_GOVERNO) AS QTD_PROG FROM (DESPESA_PUBLICA NATURAL JOIN ORGAO NATURAL JOIN PROGRAMA_GOVERNO) WHERE VALOR_DESPESA>0 GROUP BY NOME_ORGAO", conn)
df
###Output
_____no_output_____
###Markdown
**8.** Liste os nomes das subfunções das despesas públicas envolvidas para cada programa de governo. Sua consulta deve retornar o nome do programa de governo, a subfunção da despesa, a quantidade de entradas de despesas públicas e o valor total dessas despesas.
###Code
df = pd.read_sql_query("SELECT NOME_PROGRAMA_GOVERNO, NOME_SUBFUNCAO, COUNT(*) AS QTD_DESP, SUM(VALOR_DESPESA) AS VALOR_TOTAL_DESP FROM (DESPESA_PUBLICA NATURAL JOIN PROGRAMA_GOVERNO NATURAL JOIN DETALHAMENTO_FUNCAO NATURAL JOIN SUBFUNCAO) GROUP BY NOME_PROGRAMA_GOVERNO, NOME_SUBFUNCAO", conn)
df
###Output
_____no_output_____
###Markdown
**9.** Liste todas as despesas públicas (código da despesa, mês e ano da despesa, modalidade, valor) em que o órgão responsável é aquele que possui o maior número de órgãos subordinados.
###Code
df = pd.read_sql_query("SELECT COD_DESPESA, MES_DESPESA, ANO_DESPESA, MODALIDADE_DESPESA, VALOR_DESPESA FROM DESPESA_PUBLICA WHERE COD_ORGAO IN (SELECT COD_ORGAO FROM ORGAO ORDER BY QTD_ORGAOS_SUBORDINADOS DESC LIMIT 1)", conn)
df
###Output
_____no_output_____
###Markdown
**10.** Liste todas as despesas públicas (código da despesa, mês e ano da despesa, modalidade, valor) em que o órgão responsável é aquele que possui o maior valor total em despesas durante o ano de 2018.
###Code
df = pd.read_sql_query("SELECT COD_DESPESA, MES_DESPESA, ANO_DESPESA, MODALIDADE_DESPESA, VALOR_DESPESA FROM DESPESA_PUBLICA WHERE COD_ORGAO IN (SELECT COD_ORGAO FROM DESPESA_PUBLICA NATURAL JOIN ORGAO WHERE ANO_DESPESA='2018' GROUP BY NOME_ORGAO ORDER BY SUM(VALOR_DESPESA) DESC LIMIT 1)", conn)
df
###Output
_____no_output_____
###Markdown
**11.** Liste os códigos e nomes dos programas de governo relacionados ao órgão (ou órgãos, caso haja empate) que teve menos registros de despesas públicas durante o ano.
###Code
df = pd.read_sql_query("SELECT COD_PROGRAMA_GOVERNO, NOME_PROGRAMA_GOVERNO FROM DESPESA_PUBLICA NATURAL JOIN PROGRAMA_GOVERNO WHERE COD_ORGAO IN (SELECT COD_ORGAO FROM (SELECT COD_ORGAO, NOME_ORGAO, COUNT(*) AS NUM_DESP FROM DESPESA_PUBLICA NATURAL JOIN ORGAO WHERE ANO_DESPESA='2018' GROUP BY COD_ORGAO ORDER BY COUNT(*) ASC) WHERE NUM_DESP IN (SELECT min(NUM_DESP) FROM (SELECT COD_ORGAO, NOME_ORGAO, COUNT(*) AS NUM_DESP FROM DESPESA_PUBLICA NATURAL JOIN ORGAO WHERE ANO_DESPESA='2018' GROUP BY COD_ORGAO ORDER BY COUNT(*) ASC)))", conn)
df
###Output
_____no_output_____
###Markdown
**12.** Álgebra relacional: projeção do NOME_FUNCAO e NOME_SUBFUNCAO da junção natural das tabelas FUNCAO, DETALHAMENTO_FUNCAO e SUBFUNCAO RESULT = 𝜋 NOME_FUNCAO, NOME_SUBFUNCAO (FUNCAO ⋈ DETALHAMENTO_FUNCAO ⋈ SUBFUNCAO)
###Code
df = pd.read_sql_query("SELECT NOME_FUNCAO, NOME_SUBFUNCAO FROM (FUNCAO NATURAL JOIN DETALHAMENTO_FUNCAO NATURAL JOIN SUBFUNCAO)", conn)
df
###Output
_____no_output_____
###Markdown
**13.** Álgebra relacional: projeção de MODALIDADE_DESPESA, VALOR_DESPESA e NOME_PROGRAMA_GOVERNO da junção natural das tabelas PROGRAMA_GOVERNO e DESPESA_PUBLICA onde a MODALIDADE_DESPESA = 'Reserva de Contingência' RESULT = 𝜋 (MODALIDADE_DESPESA, VALOR_DESPESA, NOME_PROGRAMA_GOVERNO) 𝜎 MODALIDADE_DESPESA='Reserva de Contingência' (PROGRAMA_GOVERNO ⋈ DESPESA_PUBLICA)
###Code
df = pd.read_sql_query("SELECT MODALIDADE_DESPESA, VALOR_DESPESA, NOME_PROGRAMA_GOVERNO FROM (DESPESA_PUBLICA NATURAL JOIN PROGRAMA_GOVERNO) WHERE MODALIDADE_DESPESA='Reserva de Contingência'", conn)
df
###Output
_____no_output_____
###Markdown
**14.** Álgebra relacional: projeção de VALOR_DESPESA e NOME_ORGAO da junção natural das tabelas DESPESA_PUBLICA e ORGAO onde o NOME_ORGAO = 'Ministério da Fazenda' RESULT = 𝜋 (VALOR_DESPESA, NOME_ORGAO) 𝜎 NOME_ORGAO='Ministério da Fazenda' (DESPESA_PUBLICA ⋈ ORGAO)
###Code
df = pd.read_sql_query("SELECT VALOR_DESPESA, NOME_ORGAO FROM (DESPESA_PUBLICA NATURAL JOIN ORGAO) WHERE NOME_ORGAO='Ministério da Fazenda'", conn)
df
###Output
_____no_output_____
###Markdown
**15.** Álgebra relacional: projeção de NOME_PROGRAMA_GOVERNO e VALOR_DESPESA da junção natural das tabelas DESPESA_PUBLICA, PROGRAMA_GOVERNO e ORGAO onde o NOME_ORGAO = 'Ministério da Saúde' RESULT = 𝜋 (NOME_PROGRAMA_GOVERNO, VALOR_DESPESA) 𝜎 NOME_ORGAO='Ministério da Saúde' (DESPESA_PUBLICA ⋈ PROGRAMA_GOVERNO ⋈ ORGAO)
###Code
df = pd.read_sql_query("SELECT NOME_PROGRAMA_GOVERNO, VALOR_DESPESA FROM (DESPESA_PUBLICA NATURAL JOIN PROGRAMA_GOVERNO NATURAL JOIN ORGAO) WHERE NOME_ORGAO='Ministério da Saúde'", conn)
df
###Output
_____no_output_____
###Markdown
4. Fechamento da conexão com o banco* Após serem realizadas todas as consultas necessárias, é aconselhavel encerrar formalmente a conexão com o banco de dados
###Code
conn.close()
###Output
_____no_output_____ |
08_Learning_SEIR.ipynb | ###Markdown
Define SEIR
###Code
def diff_eqns(y, t, beta, sigma, gamma, N):
St, Et, It, Rt = y
dSdt = -beta * St * It / N
dEdt = beta * St * It / N - sigma * Et
dIdt = sigma * Et - gamma * It
dRdt = gamma * It
return ([dSdt, dEdt, dIdt, dRdt])
def seir_model(t, beta, sigma, gamma, E0, N):
S0 = N - E0
I0 = R0 = 0
out = integrate.odeint(diff_eqns, (S0, E0, I0, R0), t, args=(beta, sigma, gamma, N))
return out.T # S, E, I, R
###Output
_____no_output_____
###Markdown
Sample SEIR plot
###Code
N = 1000
days = np.arange(100)
beta = 1.5
sigma = 1 / 5.2 # 5.2 days incubation, i.e. from exposed to infectious
gamma = 1 / 2.9 # 2.9 days from infectious to removal
S, E, I, R = seir_model(days, beta, sigma, gamma, 1, N)
df = pd.DataFrame({
"Days": days,
"S": S,
"E": E,
"I": I,
"R": R
})
df.plot(
x='Days',
y=['S', 'E', 'I', 'R'],
grid=True,
title="SEIR sample"
)
###Output
_____no_output_____
###Markdown
Fit Italy's data
###Code
it_df = pd.read_csv("csv/italy.csv", parse_dates=['date'])
it_df.sample()
def seir_model_italy_i(t, beta):
sigma = 1 / 5.2
gamma = 1 / 2.9
E0 = 19
N = 60461826
return seir_model(t, beta, sigma, gamma, E0, N)[2]
params, covar = optimize.curve_fit(seir_model_italy_i, it_df.index, it_df.active)
it_df['fitted'] = seir_model_italy_i(it_df.index, *params)
print("Params:", params)
ax = it_df.plot(
x='date',
y=['active', 'fitted'],
grid=True,
title="Fitting active cases to I"
)
###Output
Params: [0.73755627]
|
Old/graph_builder.ipynb | ###Markdown
Convert CSV to a graph
###Code
import networkx as nx
import pandas as pd
def import_csv(filename):
""" import csv file into a Pandas dataframe """
return pd.read_csv(filename)
def preprocessing(filename):
""" make Pandas dataframe easier to work with by:
- deleting timestamp column
- making the names column into the row labels
"""
data = import_csv(filename)
del data['Timestamp'] #delete timestamp column
data = data.set_index('Name') # set names column to row labels
data.index.names = [None]
return data
data = preprocessing("Survey.csv")
data.head(3)
def initialize_graph(data):
""" build a graph with the name/identifiers as nodes """
num_rows = data.shape[0]
G = nx.Graph()
row_names = []
for (name, b) in data.iterrows():
row_names.append(name)
G.add_node(name)
return G
def build_graph(data):
""" iterates through all question answers and adds an edge when people agree
"""
for question, answers in data.iteritems():
print(answers)
for curr_name in row_names:
for compare_name in row_names:
if answers[curr_name] == answers[compare_name] and curr_name != compare_name:
G.add_edge(curr_name, compare_name)
return G
print(G.edges)
###Output
[('Ghost', 'Turkey'), ('Ghost', 'Cactus'), ('Ghost', 'Zombie'), ('Ghost', 'Santa Claus'), ('Ghost', 'Cat'), ('Zombie', 'Santa Claus'), ('Zombie', 'Cat'), ('Zombie', 'Turkey'), ('Zombie', 'Cactus'), ('Turkey', 'Cactus'), ('Turkey', 'Santa Claus'), ('Turkey', 'Cat'), ('Santa Claus', 'Cat'), ('Santa Claus', 'Cactus'), ('Cat', 'Cactus')]
|
ml-lec/simple_linear.ipynb | ###Markdown
###Code
import tensorflow.compat.v1 as tf
tf.disable_v2_behavior()
x_train = [1,2,3]
y_train = [1,2,3]
W = tf.Variable(tf.random_normal([1]), name='weight') #shape를 설정하면 random값을 추출
b = tf.Variable(tf.random_normal([1]), name='bias') #shape를 설정하면 random값을 추출
#XW+b
hypothesis = x_train * W + b
cost = tf.reduce_mean(tf.square(hypothesis - y_train))
optimizer = tf.train.GradientDescentOptimizer(learning_rate=0.01)
train = optimizer.minimize(cost) # 가중치들을 조정함 (W, b)
sess = tf.Session() #실행하기위한 세션
sess.run(tf.global_variables_initializer())
#fit the line
for step in range(2001):
sess.run(train)
if step % 20 == 0:
print(step, sess.run(cost), sess.run(W), sess.run(b)) # 확인
X = tf.placeholder(tf.float32)
Y = tf.placeholder(tf.float32)
# X, Y 값의 타입을 설정
W = tf.Variable(tf.random_normal([1]), name='weight') #shape를 설정하면 random값을 추출
b = tf.Variable(tf.random_normal([1]), name='bias') #shape를 설정하면 random값을 추출
#XW+b
hypothesis = X * W + b
#cost function
cost = tf.reduce_mean(tf.square(hypothesis - Y))
#minimize
optimizer = tf.train.GradientDescentOptimizer(learning_rate=0.01)
train = optimizer.minimize(cost) # 가중치들을 조정함 (W, b)
""" 1. 텐서플로우 연산자로 그래프를 만듦
2. feed_dict로 데이터를 입력
3. W, b를 업데이트 시킴
4. 검증해보기
"""
#tf 세션을 열고 global variable을 초기화
sess = tf.Session()
sess.run(tf.global_variables_initializer())
for step in range(2001):
cost_val, W_val, b_val, _ = sess.run([cost, W, b, train],
feed_dict={X:[1,2,3,4,5], Y:[2.1,3.1,4.1,5.1,6.1]}) # 예상 값 W = 1, b = 1.1
if step % 20 == 0:
print(step, cost_val, W_val, b_val)
print(sess.run(hypothesis, feed_dict={X:5}))
print(sess.run(hypothesis, feed_dict={X:2.5}))
print(sess.run(hypothesis, feed_dict={X:[1.5, 3.5]}))
###Output
_____no_output_____ |
docs/notebooks/flax_basics.ipynb | ###Markdown
Flax BasicsThis notebook will walk you through the following workflow:* Instantiating a model from Flax built-in layers or third-party models.* Initializing parameters of the model and manually written training.* Using optimizers provided by Flax to ease training.* Serialization of parameters and other objects.* Creating your own models and managing state. Setting up our environmentHere we provide the code needed to set up the environment for our notebook.
###Code
# Install the latest JAXlib version.
!pip install --upgrade -q pip jax jaxlib
# Install Flax at head:
!pip install --upgrade -q git+https://github.com/google/flax.git
import jax
from typing import Any, Callable, Sequence, Optional
from jax import lax, random, numpy as jnp
import flax
from flax.core import freeze, unfreeze
from flax import linen as nn
from jax.config import config
config.enable_omnistaging() # Linen requires enabling omnistaging
###Output
_____no_output_____
###Markdown
Linear regression with FlaxIn the previous *JAX for the impatient* notebook, we finished up with a linear regression example. As we know, linear regression can also be written as a single dense neural network layer, which we will show in the following so that we can compare how it's done.A dense layer is a layer that has a kernel parameter $W\in\mathcal{M}_{m,n}(\mathbb{R})$ where $m$ is the number of features as an output of the model, and $n$ the dimensionality of the input, and a bias parameter $b\in\mathbb{R}^m$. The dense layers returns $Wx+b$ from an input $x\in\mathbb{R}^n$.This dense layer is already provided by Flax in the `flax.linen` module (here imported as `nn`).
###Code
# We create one dense layer instance (taking 'features' parameter as input)
model = nn.Dense(features=5)
###Output
_____no_output_____
###Markdown
Layers (and models in general, we'll use that word from now on) are subclasses of the `linen.Module` class. Model parameters & initializationParameters are not stored with the models themselves. You need to initialize parameters by calling the `init` function, using a PRNGKey and a dummy input parameter.
###Code
key1, key2 = random.split(random.PRNGKey(0))
x = random.normal(key1, (10,)) # Dummy input
params = model.init(key2, x) # Initialization call
jax.tree_map(lambda x: x.shape, params) # Checking output shapes
###Output
WARNING:absl:No GPU/TPU found, falling back to CPU. (Set TF_CPP_MIN_LOG_LEVEL=0 and rerun for more info.)
###Markdown
*Note: JAX and Flax, like NumPy, are row-based systems, meaning that vectors are represented as row vectors and not column vectors. This can be seen in the shape of the kernel here.*The result is what we expect: bias and kernel parameters of the correct size. Under the hood:* The dummy input variable `x` is used to trigger shape inference: we only declared the number of features we wanted in the output of the model, not the size of the input. Flax finds out by itself the correct size of the kernel.* The random PRNG key is used to trigger the initialization functions (those have default values provided by the module here).* Initialization functions are called to generate the initial set of parameters that the model will use. Those are functions that take as arguments `(PRNG Key, shape, dtype)` and return an Array of shape `shape`.* The init function returns the initialized set of parameters (you can also get the output of the evaluation on the dummy input with the same syntax but using the `init_with_output` method instead of `init`. We see in the output that parameters are stored in a `FrozenDict` instance which helps deal with the functional nature of JAX by preventing any mutation of the underlying dict and making the user aware of it. Read more about it in the Flax docs. As a consequence, the following doesn't work:
###Code
try:
params['new_key'] = jnp.ones((2,2))
except ValueError as e:
print("Error: ", e)
###Output
Error: FrozenDict is immutable.
###Markdown
To evaluate the model with a given set of parameters (never stored with the model), we just use the `apply` method by providing it the parameters to use as well as the input:
###Code
model.apply(params, x)
###Output
_____no_output_____
###Markdown
Gradient descentIf you jumped here directly without going through the JAX part, here is the linear regression formulation we're going to use: from a set of data points $\{(x_i,y_i), i\in \{1,\ldots, k\}, x_i\in\mathbb{R}^n,y_i\in\mathbb{R}^m\}$, we try to find a set of parameters $W\in \mathcal{M}_{m,n}(\mathbb{R}), b\in\mathbb{R}^m$ such that the function $f_{W,b}(x)=Wx+b$ minimizes the mean squared error:$$\mathcal{L}(W,b)\rightarrow\frac{1}{k}\sum_{i=1}^{k} \frac{1}{2}\|y_i-f_{W,b}(x_i)\|^2_2$$Here, we see that the tuple $(W,b)$ matches the parameters of the Dense layer. We'll perform gradient descent using those. Let's first generate the fake data we'll use.
###Code
# Set problem dimensions
nsamples = 20
xdim = 10
ydim = 5
# Generate random ground truth W and b
key = random.PRNGKey(0)
k1, k2 = random.split(key)
W = random.normal(k1, (xdim, ydim))
b = random.normal(k2, (ydim,))
true_params = freeze({'params': {'bias': b, 'kernel': W}})
# Generate samples with additional noise
ksample, knoise = random.split(k1)
x_samples = random.normal(ksample, (nsamples, xdim))
y_samples = jnp.dot(x,W) + b
y_samples += 0.1*random.normal(knoise,(nsamples, ydim)) # Adding noise
print('x shape:', x_samples.shape, '; y shape:', y_samples.shape)
###Output
x shape: (20, 10) ; y shape: (20, 5)
###Markdown
Now let's generate the loss function (mean squared error) with that data.
###Code
def make_mse_func(x_batched, y_batched):
def mse(params):
# Define the squared loss for a single pair (x,y)
def squared_error(x, y):
pred = model.apply(params, x)
return jnp.inner(y-pred, y-pred)/2.0
# We vectorize the previous to compute the average of the loss on all samples.
return jnp.mean(jax.vmap(squared_error)(x_batched,y_batched), axis=0)
return jax.jit(mse) # And finally we jit the result.
# Get the sampled loss
loss = make_mse_func(x_samples, y_samples)
###Output
_____no_output_____
###Markdown
And finally perform the gradient descent.
###Code
alpha = 0.3 # Gradient step size
print('Loss for "true" W,b: ', loss(true_params))
grad_fn = jax.value_and_grad(loss)
for i in range(101):
# We perform one gradient update
loss_val, grad = grad_fn(params)
params = jax.tree_multimap(lambda old, grad: old - alpha * grad,
params, grad)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss for "true" W,b: 29.070158
Loss step 0: 23.618902
Loss step 10: 0.30728564
Loss step 20: 0.06495677
Loss step 30: 0.025215296
Loss step 40: 0.015619493
Loss step 50: 0.012849321
Loss step 60: 0.011984843
Loss step 70: 0.011705536
Loss step 80: 0.011613827
Loss step 90: 0.011583473
Loss step 100: 0.011573391
###Markdown
Build-in optimization APIFlax provides an optimization package in `flax.optim` to make your life easier when training models. The process is:1. You choose an optimization method (e.g. `optim.GradientDescent`, `optim.Adam`)2. From the previous optimization method, you create a wrapper around the parameters you're going to optimize for with the `create` method. Your parameters are accessible through the `target` field.3. You compute the gradients of your loss with `jax.value_and_grad()`.4. At every iteration, you compute the gradients at the current point, then use the `apply_gradient()` method on the optimizer to return a new optimizer with updated parameters.
###Code
from flax import optim
optimizer_def = optim.GradientDescent(learning_rate=alpha) # Choose the method
optimizer = optimizer_def.create(params) # Create the wrapping optimizer with initial parameters
loss_grad_fn = jax.value_and_grad(loss)
for i in range(101):
loss_val, grad = loss_grad_fn(optimizer.target)
optimizer = optimizer.apply_gradient(grad) # Return the updated optimizer with parameters.
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss step 0: 0.011572863
Loss step 10: 0.011569859
Loss step 20: 0.011568859
Loss step 30: 0.011568523
Loss step 40: 0.011568412
Loss step 50: 0.011568374
Loss step 60: 0.011568364
Loss step 70: 0.011568359
Loss step 80: 0.01156836
Loss step 90: 0.011568356
Loss step 100: 0.011568357
###Markdown
Serializing the resultNow that we're happy with the result of our training, we might want to save the model parameters to load them back later. Flax provides a serialization package to enable you to do that.
###Code
from flax import serialization
bytes_output = serialization.to_bytes(optimizer.target)
dict_output = serialization.to_state_dict(optimizer.target)
print('Dict output')
print(dict_output)
print('Bytes output')
print(bytes_output)
###Output
Dict output
{'params': {'bias': DeviceArray([-3.023082 , 0.5307182, 3.7256303, 1.4638226, -3.2100437], dtype=float32), 'kernel': DeviceArray([[-1.4092493e-02, 4.8609809e-03, 1.1460093e-02,
-6.0927689e-02, 2.0413438e-05],
[-3.3569761e-02, -1.5614161e-03, 4.3190460e-04,
-7.9035060e-03, -1.9851506e-02],
[-1.8882388e-02, -2.1366426e-03, -1.8663550e-02,
-3.0001188e-02, 5.1880259e-02],
[-4.8119370e-02, -2.9280247e-02, -1.1992223e-02,
-1.0111435e-02, -8.3459895e-03],
[-1.7368369e-02, -1.7084973e-02, 6.0279824e-02,
9.2046618e-02, -1.5414236e-02],
[-3.0089449e-02, -5.5370983e-03, -9.1237156e-03,
2.1827107e-02, -2.0405082e-02],
[-5.6748122e-02, -4.2654604e-02, -1.1436724e-02,
7.5801805e-02, -2.0075133e-02],
[-1.4368590e-03, -1.6048675e-02, 1.5781123e-02,
2.8437756e-03, -8.5009886e-03],
[ 1.7892396e-02, 5.7572998e-02, 4.1483097e-02,
-9.9685444e-03, -2.1875760e-02],
[-2.1158390e-02, -1.3853005e-02, 2.5077526e-02,
3.2925244e-02, 3.8115401e-02]], dtype=float32)}}
Bytes output
b'\x81\xa6params\x82\xa4bias\xc7!\x01\x93\x91\x05\xa7float32\xc4\x14-zA\xc0&\xdd\x07?\xbapn@\x8a^\xbb?[qM\xc0\xa6kernel\xc7\xd6\x01\x93\x92\n\x05\xa7float32\xc4\xc83\xe4f\xbc\xddH\x9f;\x1d\xc3;<P\x8fy\xbd\x86=\xab7r\x80\t\xbdn\xa8\xcc\xbaAq\xe29\xb5}\x01\xbc\xa0\x9f\xa2\xbc=\xaf\x9a\xbc\xea\x06\x0c\xbbM\xe4\x98\xbc\r\xc5\xf5\xbce\x80T=\xd1\x18E\xbd!\xdd\xef\xbc\x07{D\xbco\xaa%\xbc\x9e\xbd\x08\xbc\x1cH\x8e\xbc\xc9\xf5\x8b\xbc\xfa\xe7v=\xf0\x82\xbc=\xfe\x8b|\xbc&~\xf6\xbc\x8cp\xb5\xbb\xa3{\x15\xbc\xc3\xce\xb2<\x8f(\xa7\xbc\xb8ph\xbd\x98\xb6.\xbd\x19a;\xbc\xfa=\x9b=\x9bt\xa4\xbc\xfdT\xbc\xba\x83x\x83\xbcjG\x81<\xa3^:;\xbbG\x0b\xbc\x13\x93\x92<\xaa\xd1k=.\xea)=\x1bS#\xbc\xcb4\xb3\xbc\\T\xad\xbc\xb7\xf7b\xbcbo\xcd<\x9f\xdc\x06=\xe5\x1e\x1c='
###Markdown
To load the model back, you'll need to use as a template the model parameter structure, like the one you would get from the model initialization. Here, we use the previously generated `params` as a template. Note that this will produce a new variable structure, and not mutate in-place.*The point of enforcing structure through template is to avoid users issues downstream, so you need to first have the right model that generates the parameters structure.*
###Code
serialization.from_bytes(params, bytes_output)
###Output
_____no_output_____
###Markdown
The serialization utils provided by Flax work on objects beyond parameters, for example you might want to serialize the optimizer and it's states, which we show in the following cell:
###Code
serialization.to_state_dict(optimizer)
###Output
_____no_output_____
###Markdown
Defining your own modelsFlax allows you to define your own models, which should be a bit more complicated than a linear regression. In this section, we'll show you how to build simple models. To do so, you'll need to create subclasses of the base `nn.Module` class.*Keep in mind that we imported* `linen as nn` *and this only works with the new linen API* Module basicsThe base abstraction for models is the `nn.Module` class, and every type of predefined layers in Flax (like the previous `Dense`) is a subclass of `nn.Module`. Let's take a look and start by defining a simple but custom multi-layer perceptron i.e. a sequence of Dense layers interleaved with calls to a non-linear activation function.
###Code
class ExplicitMLP(nn.Module):
features: Sequence[int]
def setup(self):
# we automatically know what to do with lists, dicts of submodules
self.layers = [nn.Dense(feat) for feat in self.features]
# for single submodules, we would just write:
# self.layer1 = nn.Dense(self, feat1)
def __call__(self, inputs):
x = inputs
for i, lyr in enumerate(self.layers):
x = lyr(x)
if i != len(self.layers) - 1:
x = nn.relu(x)
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = ExplicitMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292822e-02 -4.3807123e-02 2.9323796e-02 6.5492545e-03
-1.7147183e-02]
[ 1.2967804e-01 -1.4551792e-01 9.4432175e-02 1.2521386e-02
-4.5417294e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
As we can see, a `nn.Module` subclass is made of:* A collection of data fields (`nn.Module` are Python dataclasses) - here we only have the `features` field of type `Sequence[int]`.* A `setup()` method that is being called at the end of the `__postinit__` where you can register submodules, variables, parameters you will need in your model.* A `__call__` function that returns the output of the model from a given input.* The model structure defines a pytree of parameters following the same tree structure as the model: the params tree contains one `layers_n` sub dict per layer, and each of those contain the parameters of the associated Dense layer. The layout is very explicit.*Note: lists are mostly managed as you would expect (WIP), there are corner cases you should be aware of as pointed out [here](https://github.com/google/flax/issues/524)*Since the module structure and its parameters are not tied to each other, you can't call directly `model(x)` on a given input as it will return an error. The `__call__` function is being wrapped up in the `apply` one, which is the one to call on an input:
###Code
try:
y = model(x) # Returns an error
except ValueError as e:
print(e)
###Output
Can't call methods on orphaned modules
###Markdown
Since here we have a very simple model, we could have used an alternative (but equivalent) way of declaring the submodules inline in the `__call__` using the `@nn.compact` annotation like so:
###Code
class SimpleMLP(nn.Module):
features: Sequence[int]
@nn.compact
def __call__(self, inputs):
x = inputs
for i, feat in enumerate(self.features):
x = nn.Dense(feat, name=f'layers_{i}')(x)
if i != len(self.features) - 1:
x = nn.relu(x)
# providing a name is optional though!
# the default autonames would be "Dense_0", "Dense_1", ...
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292822e-02 -4.3807123e-02 2.9323796e-02 6.5492545e-03
-1.7147183e-02]
[ 1.2967804e-01 -1.4551792e-01 9.4432175e-02 1.2521386e-02
-4.5417294e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
There are, however, a few differences you should be aware of between the two declaration modes:* In `setup`, you are able to name some sublayers and keep them around for further use (e.g. encoder/decoder methods in autoencoders).* If you want to have multiple methods, then you **need** to declare the module using `setup`, as the `@nn.compact` annotation only allows one method to be annotated.* The last initialization will be handled differently see these notes for more details (TODO: add notes link) Module parametersIn the previous MLP example, we relied only on predefined layers and operators (`Dense`, `relu`). Let's imagine that you didn't have a Dense layer provided by Flax and you wanted to write it on your own. Here is what it would look like using the `@nn.compact` way to declare a new modules:
###Code
class SimpleDense(nn.Module):
features: int
kernel_init: Callable = nn.initializers.lecun_normal()
bias_init: Callable = nn.initializers.zeros
@nn.compact
def __call__(self, inputs):
kernel = self.param('kernel',
self.kernel_init, # Initialization function
(inputs.shape[-1], self.features)) # shape info.
y = lax.dot_general(inputs, kernel,
(((inputs.ndim - 1,), (0,)), ((), ())),) # TODO Why not jnp.dot?
bias = self.param('bias', self.bias_init, (self.features,))
y = y + bias
return y
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleDense(features=3)
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameters:\n', params)
print('output:\n', y)
###Output
initialized parameters:
FrozenDict({'params': {'kernel': DeviceArray([[ 0.6503669 , 0.8678979 , 0.46042678],
[ 0.05673932, 0.9909285 , -0.63536596],
[ 0.76134115, -0.3250529 , -0.6522163 ],
[-0.8243032 , 0.4150194 , 0.19405058]], dtype=float32), 'bias': DeviceArray([0., 0., 0.], dtype=float32)}})
output:
[[ 0.50355184 1.8548559 -0.4270196 ]
[ 0.02790972 0.5589246 -0.43061778]
[ 0.35471287 1.5740999 -0.32865524]
[ 0.52648634 1.2928859 0.10089307]]
###Markdown
Here, we see how both declare and assign a parameter to the model using the `self.param` method. It takes as input `(name, init_fn, *init_args)` : * `name` is simply the name of the parameter that will end up in the parameter structure.* `init_fun` is a function with input `(PRNGKey, *init_args)` returning an Array with `init_args` the arguments needed to call the initialisation function* `init_args` the arguments to provide to the initialization function.Such params can also be declared in the `setup` method, it won't be able to use shape inference because Flax is using lazy initialization at the first call site. Variables and collections of variablesAs we've seen so far, working with models means working with:* A subclass of `nn.Module`;* A pytree of parameters for the model (typically from `model.init()`);However this is not enough to cover everything that we would need for machine learning, especially neural networks. In some cases, you might want your neural network to keep track of some internal state while it runs (e.g. batch normalization layers). There is a way to declare variables beyond the parameters of the model with the `variable` method.For demonstration purposes, we'll implement a simplified but similar mechanism to batch normalization: we'll store running averages and subtract those to the input at training time. For proper batchnorm, you should use (and look at) the implementation [here](https://github.com/google/flax/blob/master/flax/linen/normalization.py).
###Code
class BiasAdderWithRunningMean(nn.Module):
decay: float = 0.99
@nn.compact
def __call__(self, x):
# easy pattern to detect if we're initializing via empty variable tree
is_initialized = self.has_variable('batch_stats', 'mean')
ra_mean = self.variable('batch_stats', 'mean',
lambda s: jnp.zeros(s),
x.shape[1:])
mean = ra_mean.value # This will get either the value, or trigger init
bias = self.param('bias', lambda rng, shape: jnp.zeros(shape), x.shape[1:])
if is_initialized:
ra_mean.value = self.decay * ra_mean.value + (1.0 - self.decay) * jnp.mean(x, axis=0, keepdims=True)
return x - ra_mean.value + bias
key1, key2 = random.split(random.PRNGKey(0), 2)
x = jnp.ones((10,5))
model = BiasAdderWithRunningMean()
variables = model.init(key1, x)
print('initialized variables:\n', variables)
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
print('updated state:\n', updated_state)
###Output
initialized variables:
FrozenDict({'batch_stats': {'mean': DeviceArray([0., 0., 0., 0., 0.], dtype=float32)}, 'params': {'bias': DeviceArray([0., 0., 0., 0., 0.], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
###Markdown
Here, `updated_state` returns only the state variables that are being mutated by the model while applying it on data. To update the variables and get the new parameters of the model, we can use the following pattern:
###Code
for val in [1.0, 2.0, 3.0]:
x = val * jnp.ones((10,5))
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
old_state, params = variables.pop('params')
variables = freeze({'params': params, **updated_state})
print('updated state:\n', updated_state) # Shows only the mutable part
###Output
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.0299, 0.0299, 0.0299, 0.0299, 0.0299]], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.059601, 0.059601, 0.059601, 0.059601, 0.059601]], dtype=float32)}})
###Markdown
From this simplified example, you should be able to derive a full BatchNorm implementation, or any layer involving a state. To finish, let's add an optimizer to see how to play with both parameters updated by an optimizer and state variables.*This example isn't doing anything and is only for demonstration purposes.*
###Code
def update_step(apply_fun, x, optimizer, state):
def loss(params):
y, updated_state = apply_fun({'params': params, **state},
x, mutable=list(state.keys()))
l = ((x - y) ** 2).sum()
return l, updated_state
(l, updated_state), grads = jax.value_and_grad(
loss, has_aux=True)(optimizer.target)
optimizer = optimizer.apply_gradient(grads)
return optimizer, updated_state
variables = model.init(random.PRNGKey(0), x)
state, params = variables.pop('params')
del variables
optimizer = optim.sgd.GradientDescent(learning_rate=0.02).create(params)
x = jnp.ones((10,5))
for _ in range(3):
optimizer, state = update_step(model.apply, x, optimizer, state)
print('Updated state: ', state)
###Output
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.0199, 0.0199, 0.0199, 0.0199, 0.0199]], dtype=float32)}})
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.029701, 0.029701, 0.029701, 0.029701, 0.029701]], dtype=float32)}})
###Markdown
Flax BasicsThis notebook will walk you through the following workflow:* Instantiating a model from Flax built-in layers or third-party models.* Initializing parameters of the model and manually written training.* Using optimizers provided by Flax to ease training.* Serialization of parameters and other objects.* Creating your own models and managing state. Setting up our environmentHere we provide the code needed to set up the environment for our notebook.
###Code
# Install the latest JAXlib version.
!pip install --upgrade -q pip jax jaxlib
# Install Flax at head:
!pip install --upgrade -q git+https://github.com/google/flax.git
import jax
from typing import Any, Callable, Sequence, Optional
from jax import lax, random, numpy as jnp
import flax
from flax.core import freeze, unfreeze
from flax import linen as nn
###Output
_____no_output_____
###Markdown
Linear regression with FlaxIn the previous *JAX for the impatient* notebook, we finished up with a linear regression example. As we know, linear regression can also be written as a single dense neural network layer, which we will show in the following so that we can compare how it's done.A dense layer is a layer that has a kernel parameter $W\in\mathcal{M}_{m,n}(\mathbb{R})$ where $m$ is the number of features as an output of the model, and $n$ the dimensionality of the input, and a bias parameter $b\in\mathbb{R}^m$. The dense layers returns $Wx+b$ from an input $x\in\mathbb{R}^n$.This dense layer is already provided by Flax in the `flax.linen` module (here imported as `nn`).
###Code
# We create one dense layer instance (taking 'features' parameter as input)
model = nn.Dense(features=5)
###Output
_____no_output_____
###Markdown
Layers (and models in general, we'll use that word from now on) are subclasses of the `linen.Module` class. Model parameters & initializationParameters are not stored with the models themselves. You need to initialize parameters by calling the `init` function, using a PRNGKey and a dummy input parameter.
###Code
key1, key2 = random.split(random.PRNGKey(0))
x = random.normal(key1, (10,)) # Dummy input
params = model.init(key2, x) # Initialization call
jax.tree_map(lambda x: x.shape, params) # Checking output shapes
###Output
WARNING:absl:No GPU/TPU found, falling back to CPU. (Set TF_CPP_MIN_LOG_LEVEL=0 and rerun for more info.)
###Markdown
*Note: JAX and Flax, like NumPy, are row-based systems, meaning that vectors are represented as row vectors and not column vectors. This can be seen in the shape of the kernel here.*The result is what we expect: bias and kernel parameters of the correct size. Under the hood:* The dummy input variable `x` is used to trigger shape inference: we only declared the number of features we wanted in the output of the model, not the size of the input. Flax finds out by itself the correct size of the kernel.* The random PRNG key is used to trigger the initialization functions (those have default values provided by the module here).* Initialization functions are called to generate the initial set of parameters that the model will use. Those are functions that take as arguments `(PRNG Key, shape, dtype)` and return an Array of shape `shape`.* The init function returns the initialized set of parameters (you can also get the output of the evaluation on the dummy input with the same syntax but using the `init_with_output` method instead of `init`. We see in the output that parameters are stored in a `FrozenDict` instance which helps deal with the functional nature of JAX by preventing any mutation of the underlying dict and making the user aware of it. Read more about it in the Flax docs. As a consequence, the following doesn't work:
###Code
try:
params['new_key'] = jnp.ones((2,2))
except ValueError as e:
print("Error: ", e)
###Output
Error: FrozenDict is immutable.
###Markdown
To evaluate the model with a given set of parameters (never stored with the model), we just use the `apply` method by providing it the parameters to use as well as the input:
###Code
model.apply(params, x)
###Output
_____no_output_____
###Markdown
Gradient descentIf you jumped here directly without going through the JAX part, here is the linear regression formulation we're going to use: from a set of data points $\{(x_i,y_i), i\in \{1,\ldots, k\}, x_i\in\mathbb{R}^n,y_i\in\mathbb{R}^m\}$, we try to find a set of parameters $W\in \mathcal{M}_{m,n}(\mathbb{R}), b\in\mathbb{R}^m$ such that the function $f_{W,b}(x)=Wx+b$ minimizes the mean squared error:$$\mathcal{L}(W,b)\rightarrow\frac{1}{k}\sum_{i=1}^{k} \frac{1}{2}\|y_i-f_{W,b}(x_i)\|^2_2$$Here, we see that the tuple $(W,b)$ matches the parameters of the Dense layer. We'll perform gradient descent using those. Let's first generate the fake data we'll use.
###Code
# Set problem dimensions
nsamples = 20
xdim = 10
ydim = 5
# Generate random ground truth W and b
key = random.PRNGKey(0)
k1, k2 = random.split(key)
W = random.normal(k1, (xdim, ydim))
b = random.normal(k2, (ydim,))
true_params = freeze({'params': {'bias': b, 'kernel': W}})
# Generate samples with additional noise
ksample, knoise = random.split(k1)
x_samples = random.normal(ksample, (nsamples, xdim))
y_samples = jnp.dot(x_samples, W) + b
y_samples += 0.1*random.normal(knoise,(nsamples, ydim)) # Adding noise
print('x shape:', x_samples.shape, '; y shape:', y_samples.shape)
###Output
x shape: (20, 10) ; y shape: (20, 5)
###Markdown
Now let's generate the loss function (mean squared error) with that data.
###Code
def make_mse_func(x_batched, y_batched):
def mse(params):
# Define the squared loss for a single pair (x,y)
def squared_error(x, y):
pred = model.apply(params, x)
return jnp.inner(y-pred, y-pred)/2.0
# We vectorize the previous to compute the average of the loss on all samples.
return jnp.mean(jax.vmap(squared_error)(x_batched,y_batched), axis=0)
return jax.jit(mse) # And finally we jit the result.
# Get the sampled loss
loss = make_mse_func(x_samples, y_samples)
###Output
_____no_output_____
###Markdown
And finally perform the gradient descent.
###Code
alpha = 0.3 # Gradient step size
print('Loss for "true" W,b: ', loss(true_params))
grad_fn = jax.value_and_grad(loss)
for i in range(101):
# We perform one gradient update
loss_val, grads = grad_fn(params)
params = jax.tree_multimap(lambda p, g: p - alpha * g,
params, grads)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss for "true" W,b: 0.023639778
Loss step 0: 38.094772
Loss step 10: 0.44692168
Loss step 20: 0.10053458
Loss step 30: 0.035822745
Loss step 40: 0.018846875
Loss step 50: 0.013864839
Loss step 60: 0.012312559
Loss step 70: 0.011812928
Loss step 80: 0.011649306
Loss step 90: 0.011595251
Loss step 100: 0.0115773035
###Markdown
Optimizing with OptaxFlax used to use its own `flax.optim` package for optimization, but with[FLIP 1009](https://github.com/google/flax/blob/main/docs/flip/1009-optimizer-api.md)this was deprecated in favor of[Optax](https://github.com/deepmind/optax).Basic usage of Optax is straightforward:1. You choose an optimization method (e.g. `optax.sgd`).2. Create optimizer state from parameters.3. You compute the gradients of your loss with `jax.value_and_grad()`.4. At every iteration, call the Optax update function to update the internal optimizer state and create an update to the parameters. Then add the update to the parameters.Note that Optax can do a lot more: It's designed for composing simple gradienttransformations into more complex transformations that allows to implement awide range of optimizers. There is also support for changing optimizerhyperparameters over time ("schedules"), applying different udpates to differentparts of the parameter tree ("masking") and much more. For details please referto the[official documentation](https://optax.readthedocs.io/en/latest/).
###Code
import optax
tx = optax.sgd(learning_rate=alpha)
opt_state = tx.init(params)
loss_grad_fn = jax.value_and_grad(loss)
for i in range(101):
loss_val, grads = loss_grad_fn(params)
updates, opt_state = tx.update(grads, opt_state)
params = optax.apply_updates(params, updates)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss step 0: 0.011576377
Loss step 10: 0.0115710115
Loss step 20: 0.011569244
Loss step 30: 0.011568661
Loss step 40: 0.011568454
Loss step 50: 0.011568379
Loss step 60: 0.011568358
Loss step 70: 0.01156836
Loss step 80: 0.01156835
Loss step 90: 0.011568353
Loss step 100: 0.011568348
###Markdown
Serializing the resultNow that we're happy with the result of our training, we might want to save the model parameters to load them back later. Flax provides a serialization package to enable you to do that.
###Code
from flax import serialization
bytes_output = serialization.to_bytes(params)
dict_output = serialization.to_state_dict(params)
print('Dict output')
print(dict_output)
print('Bytes output')
print(bytes_output)
###Output
Dict output
{'params': {'bias': DeviceArray([-1.4540135, -2.0262308, 2.0806582, 1.2201802, -0.9964547], dtype=float32), 'kernel': DeviceArray([[ 1.0106664 , 0.19014716, 0.04533899, -0.92722285,
0.34720102],
[ 1.7320251 , 0.9901233 , 1.1662225 , 1.1027892 ,
-0.10574618],
[-1.2009128 , 0.28837162, 1.4176372 , 0.12073109,
-1.3132601 ],
[-1.1944956 , -0.18993308, 0.03379077, 1.3165942 ,
0.07996067],
[ 0.14103189, 1.3737966 , -1.3162128 , 0.53401774,
-2.239638 ],
[ 0.5643044 , 0.813604 , 0.31888172, 0.5359193 ,
0.90352124],
[-0.37948322, 1.7408353 , 1.0788013 , -0.5041964 ,
0.9286919 ],
[ 0.9701384 , -1.3158673 , 0.33630812, 0.80941117,
-1.202457 ],
[ 1.0198247 , -0.6198277 , 1.0822718 , -1.8385581 ,
-0.45790705],
[-0.64384323, 0.4564892 , -1.1331053 , -0.68556863,
0.17010891]], dtype=float32)}}
Bytes output
b'\x81\xa6params\x82\xa4bias\xc7!\x01\x93\x91\x05\xa7float32\xc4\x14\x1d\x1d\xba\xbf\xc4\xad\x01\xc0\x81)\x05@\xdd.\x9c?\xa8\x17\x7f\xbf\xa6kernel\xc7\xd6\x01\x93\x92\n\x05\xa7float32\xc4\xc8\x84]\x81?\xf0\xb5B>`\xb59=z^m\xbfU\xc4\xb1>\x00\xb3\xdd?\xb8x}?\xc7F\x95?2(\x8d?t\x91\xd8\xbd\x83\xb7\x99\xbfr\xa5\x93>#u\xb5?\xdcA\xf7=\xe8\x18\xa8\xbf;\xe5\x98\xbf\xd1}B\xbe0h\n=)\x86\xa8?k\xc2\xa3=\xaaj\x10>\x91\xd8\xaf?\xa9y\xa8\xbfc\xb5\x08?;V\x0f\xc0Av\x10?ZHP?wD\xa3>\x022\t?+Mg?\xa0K\xc2\xbe\xb1\xd3\xde?)\x16\x8a?\x04\x13\x01\xbf\xc1\xbem?\xfdZx?Wn\xa8\xbf\x940\xac>\x925O?\x1c\xea\x99\xbf\x9e\x89\x82?\x07\xad\x1e\xbf\xe2\x87\x8a?\xdfU\xeb\xbf\xcbr\xea\xbe\xe9\xd2$\xbf\xf4\xb8\xe9>\x98\t\x91\xbfm\x81/\xbf\x081.>'
###Markdown
To load the model back, you'll need to use as a template the model parameter structure, like the one you would get from the model initialization. Here, we use the previously generated `params` as a template. Note that this will produce a new variable structure, and not mutate in-place.*The point of enforcing structure through template is to avoid users issues downstream, so you need to first have the right model that generates the parameters structure.*
###Code
serialization.from_bytes(params, bytes_output)
###Output
_____no_output_____
###Markdown
Defining your own modelsFlax allows you to define your own models, which should be a bit more complicated than a linear regression. In this section, we'll show you how to build simple models. To do so, you'll need to create subclasses of the base `nn.Module` class.*Keep in mind that we imported* `linen as nn` *and this only works with the new linen API* Module basicsThe base abstraction for models is the `nn.Module` class, and every type of predefined layers in Flax (like the previous `Dense`) is a subclass of `nn.Module`. Let's take a look and start by defining a simple but custom multi-layer perceptron i.e. a sequence of Dense layers interleaved with calls to a non-linear activation function.
###Code
class ExplicitMLP(nn.Module):
features: Sequence[int]
def setup(self):
# we automatically know what to do with lists, dicts of submodules
self.layers = [nn.Dense(feat) for feat in self.features]
# for single submodules, we would just write:
# self.layer1 = nn.Dense(feat1)
def __call__(self, inputs):
x = inputs
for i, lyr in enumerate(self.layers):
x = lyr(x)
if i != len(self.layers) - 1:
x = nn.relu(x)
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = ExplicitMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292815e-02 -4.3807115e-02 2.9323792e-02 6.5492536e-03
-1.7147182e-02]
[ 1.2967806e-01 -1.4551792e-01 9.4432183e-02 1.2521387e-02
-4.5417298e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
As we can see, a `nn.Module` subclass is made of:* A collection of data fields (`nn.Module` are Python dataclasses) - here we only have the `features` field of type `Sequence[int]`.* A `setup()` method that is being called at the end of the `__postinit__` where you can register submodules, variables, parameters you will need in your model.* A `__call__` function that returns the output of the model from a given input.* The model structure defines a pytree of parameters following the same tree structure as the model: the params tree contains one `layers_n` sub dict per layer, and each of those contain the parameters of the associated Dense layer. The layout is very explicit.*Note: lists are mostly managed as you would expect (WIP), there are corner cases you should be aware of as pointed out* [here](https://github.com/google/flax/issues/524)Since the module structure and its parameters are not tied to each other, you can't call directly `model(x)` on a given input as it will return an error. The `__call__` function is being wrapped up in the `apply` one, which is the one to call on an input:
###Code
try:
y = model(x) # Returns an error
except AttributeError as e:
print(e)
###Output
"ExplicitMLP" object has no attribute "layers"
###Markdown
Since here we have a very simple model, we could have used an alternative (but equivalent) way of declaring the submodules inline in the `__call__` using the `@nn.compact` annotation like so:
###Code
class SimpleMLP(nn.Module):
features: Sequence[int]
@nn.compact
def __call__(self, inputs):
x = inputs
for i, feat in enumerate(self.features):
x = nn.Dense(feat, name=f'layers_{i}')(x)
if i != len(self.features) - 1:
x = nn.relu(x)
# providing a name is optional though!
# the default autonames would be "Dense_0", "Dense_1", ...
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292815e-02 -4.3807115e-02 2.9323792e-02 6.5492536e-03
-1.7147182e-02]
[ 1.2967806e-01 -1.4551792e-01 9.4432183e-02 1.2521387e-02
-4.5417298e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
There are, however, a few differences you should be aware of between the two declaration modes:* In `setup`, you are able to name some sublayers and keep them around for further use (e.g. encoder/decoder methods in autoencoders).* If you want to have multiple methods, then you **need** to declare the module using `setup`, as the `@nn.compact` annotation only allows one method to be annotated.* The last initialization will be handled differently. See these notes for more details (TODO: add notes link). Module parametersIn the previous MLP example, we relied only on predefined layers and operators (`Dense`, `relu`). Let's imagine that you didn't have a Dense layer provided by Flax and you wanted to write it on your own. Here is what it would look like using the `@nn.compact` way to declare a new modules:
###Code
class SimpleDense(nn.Module):
features: int
kernel_init: Callable = nn.initializers.lecun_normal()
bias_init: Callable = nn.initializers.zeros
@nn.compact
def __call__(self, inputs):
kernel = self.param('kernel',
self.kernel_init, # Initialization function
(inputs.shape[-1], self.features)) # shape info.
y = lax.dot_general(inputs, kernel,
(((inputs.ndim - 1,), (0,)), ((), ())),) # TODO Why not jnp.dot?
bias = self.param('bias', self.bias_init, (self.features,))
y = y + bias
return y
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleDense(features=3)
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameters:\n', params)
print('output:\n', y)
###Output
initialized parameters:
FrozenDict({
params: {
kernel: DeviceArray([[ 0.6503669 , 0.86789787, 0.4604268 ],
[ 0.05673932, 0.9909285 , -0.63536596],
[ 0.76134115, -0.3250529 , -0.65221626],
[-0.82430327, 0.4150194 , 0.19405058]], dtype=float32),
bias: DeviceArray([0., 0., 0.], dtype=float32),
},
})
output:
[[ 0.5035518 1.8548558 -0.4270195 ]
[ 0.0279097 0.5589246 -0.43061772]
[ 0.3547128 1.5740999 -0.32865518]
[ 0.5264864 1.2928858 0.10089308]]
###Markdown
Here, we see how to both declare and assign a parameter to the model using the `self.param` method. It takes as input `(name, init_fn, *init_args)` : * `name` is simply the name of the parameter that will end up in the parameter structure.* `init_fn` is a function with input `(PRNGKey, *init_args)` returning an Array, with `init_args` being the arguments needed to call the initialisation function.* `init_args` are the arguments to provide to the initialization function.Such params can also be declared in the `setup` method, it won't be able to use shape inference because Flax is using lazy initialization at the first call site. Variables and collections of variablesAs we've seen so far, working with models means working with:* A subclass of `nn.Module`;* A pytree of parameters for the model (typically from `model.init()`);However this is not enough to cover everything that we would need for machine learning, especially neural networks. In some cases, you might want your neural network to keep track of some internal state while it runs (e.g. batch normalization layers). There is a way to declare variables beyond the parameters of the model with the `variable` method.For demonstration purposes, we'll implement a simplified but similar mechanism to batch normalization: we'll store running averages and subtract those to the input at training time. For proper batchnorm, you should use (and look at) the implementation [here](https://github.com/google/flax/blob/main/flax/linen/normalization.py).
###Code
class BiasAdderWithRunningMean(nn.Module):
decay: float = 0.99
@nn.compact
def __call__(self, x):
# easy pattern to detect if we're initializing via empty variable tree
is_initialized = self.has_variable('batch_stats', 'mean')
ra_mean = self.variable('batch_stats', 'mean',
lambda s: jnp.zeros(s),
x.shape[1:])
mean = ra_mean.value # This will either get the value or trigger init
bias = self.param('bias', lambda rng, shape: jnp.zeros(shape), x.shape[1:])
if is_initialized:
ra_mean.value = self.decay * ra_mean.value + (1.0 - self.decay) * jnp.mean(x, axis=0, keepdims=True)
return x - ra_mean.value + bias
key1, key2 = random.split(random.PRNGKey(0), 2)
x = jnp.ones((10,5))
model = BiasAdderWithRunningMean()
variables = model.init(key1, x)
print('initialized variables:\n', variables)
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
print('updated state:\n', updated_state)
###Output
initialized variables:
FrozenDict({
batch_stats: {
mean: DeviceArray([0., 0., 0., 0., 0.], dtype=float32),
},
params: {
bias: DeviceArray([0., 0., 0., 0., 0.], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
###Markdown
Here, `updated_state` returns only the state variables that are being mutated by the model while applying it on data. To update the variables and get the new parameters of the model, we can use the following pattern:
###Code
for val in [1.0, 2.0, 3.0]:
x = val * jnp.ones((10,5))
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
old_state, params = variables.pop('params')
variables = freeze({'params': params, **updated_state})
print('updated state:\n', updated_state) # Shows only the mutable part
###Output
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.0299, 0.0299, 0.0299, 0.0299, 0.0299]], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.059601, 0.059601, 0.059601, 0.059601, 0.059601]], dtype=float32),
},
})
###Markdown
From this simplified example, you should be able to derive a full BatchNorm implementation, or any layer involving a state. To finish, let's add an optimizer to see how to play with both parameters updated by an optimizer and state variables.*This example isn't doing anything and is only for demonstration purposes.*
###Code
def update_step(tx, apply_fn, x, opt_state, params, state):
def loss(params):
y, updated_state = apply_fn({'params': params, **state},
x, mutable=list(state.keys()))
l = ((x - y) ** 2).sum()
return l, updated_state
(l, state), grads = jax.value_and_grad(loss, has_aux=True)(params)
updates, opt_state = tx.update(grads, opt_state)
params = optax.apply_updates(params, updates)
return opt_state, params, state
x = jnp.ones((10,5))
variables = model.init(random.PRNGKey(0), x)
state, params = variables.pop('params')
del variables
tx = optax.sgd(learning_rate=0.02)
opt_state = tx.init(params)
for _ in range(3):
opt_state, params, state = update_step(tx, model.apply, x, opt_state, params, state)
print('Updated state: ', state)
###Output
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.0199, 0.0199, 0.0199, 0.0199, 0.0199]], dtype=float32),
},
})
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.029701, 0.029701, 0.029701, 0.029701, 0.029701]], dtype=float32),
},
})
###Markdown
Flax BasicsThis notebook will walk you through the following workflow:* Instantiating a model from Flax built-in layers or third-party models.* Initializing parameters of the model and manually written training.* Using optimizers provided by Flax to ease training.* Serialization of parameters and other objects.* Creating your own models and managing state. Setting up our environmentHere we provide the code needed to set up the environment for our notebook.
###Code
# Install the latest JAXlib version.
!pip install --upgrade -q pip jax jaxlib
# Install Flax at head:
!pip install --upgrade -q git+https://github.com/google/flax.git
import jax
from typing import Any, Callable, Sequence, Optional
from jax import lax, random, numpy as jnp
import flax
from flax.core import freeze, unfreeze
from flax import linen as nn
###Output
_____no_output_____
###Markdown
Linear regression with FlaxIn the previous *JAX for the impatient* notebook, we finished up with a linear regression example. As we know, linear regression can also be written as a single dense neural network layer, which we will show in the following so that we can compare how it's done.A dense layer is a layer that has a kernel parameter $W\in\mathcal{M}_{m,n}(\mathbb{R})$ where $m$ is the number of features as an output of the model, and $n$ the dimensionality of the input, and a bias parameter $b\in\mathbb{R}^m$. The dense layers returns $Wx+b$ from an input $x\in\mathbb{R}^n$.This dense layer is already provided by Flax in the `flax.linen` module (here imported as `nn`).
###Code
# We create one dense layer instance (taking 'features' parameter as input)
model = nn.Dense(features=5)
###Output
_____no_output_____
###Markdown
Layers (and models in general, we'll use that word from now on) are subclasses of the `linen.Module` class. Model parameters & initializationParameters are not stored with the models themselves. You need to initialize parameters by calling the `init` function, using a PRNGKey and a dummy input parameter.
###Code
key1, key2 = random.split(random.PRNGKey(0))
x = random.normal(key1, (10,)) # Dummy input
params = model.init(key2, x) # Initialization call
jax.tree_map(lambda x: x.shape, params) # Checking output shapes
###Output
WARNING:absl:No GPU/TPU found, falling back to CPU. (Set TF_CPP_MIN_LOG_LEVEL=0 and rerun for more info.)
###Markdown
*Note: JAX and Flax, like NumPy, are row-based systems, meaning that vectors are represented as row vectors and not column vectors. This can be seen in the shape of the kernel here.*The result is what we expect: bias and kernel parameters of the correct size. Under the hood:* The dummy input variable `x` is used to trigger shape inference: we only declared the number of features we wanted in the output of the model, not the size of the input. Flax finds out by itself the correct size of the kernel.* The random PRNG key is used to trigger the initialization functions (those have default values provided by the module here).* Initialization functions are called to generate the initial set of parameters that the model will use. Those are functions that take as arguments `(PRNG Key, shape, dtype)` and return an Array of shape `shape`.* The init function returns the initialized set of parameters (you can also get the output of the evaluation on the dummy input with the same syntax but using the `init_with_output` method instead of `init`. We see in the output that parameters are stored in a `FrozenDict` instance which helps deal with the functional nature of JAX by preventing any mutation of the underlying dict and making the user aware of it. Read more about it in the Flax docs. As a consequence, the following doesn't work:
###Code
try:
params['new_key'] = jnp.ones((2,2))
except ValueError as e:
print("Error: ", e)
###Output
Error: FrozenDict is immutable.
###Markdown
To evaluate the model with a given set of parameters (never stored with the model), we just use the `apply` method by providing it the parameters to use as well as the input:
###Code
model.apply(params, x)
###Output
_____no_output_____
###Markdown
Gradient descentIf you jumped here directly without going through the JAX part, here is the linear regression formulation we're going to use: from a set of data points $\{(x_i,y_i), i\in \{1,\ldots, k\}, x_i\in\mathbb{R}^n,y_i\in\mathbb{R}^m\}$, we try to find a set of parameters $W\in \mathcal{M}_{m,n}(\mathbb{R}), b\in\mathbb{R}^m$ such that the function $f_{W,b}(x)=Wx+b$ minimizes the mean squared error:$$\mathcal{L}(W,b)\rightarrow\frac{1}{k}\sum_{i=1}^{k} \frac{1}{2}\|y_i-f_{W,b}(x_i)\|^2_2$$Here, we see that the tuple $(W,b)$ matches the parameters of the Dense layer. We'll perform gradient descent using those. Let's first generate the fake data we'll use. The data is exactly the same as in the JAX part's linear regression pytree example.
###Code
# Set problem dimensions.
n_samples = 20
x_dim = 10
y_dim = 5
# Generate random ground truth W and b.
key = random.PRNGKey(0)
k1, k2 = random.split(key)
W = random.normal(k1, (x_dim, y_dim))
b = random.normal(k2, (y_dim,))
# Store the parameters in a pytree.
true_params = freeze({'params': {'bias': b, 'kernel': W}})
# Generate samples with additional noise.
key_sample, key_noise = random.split(k1)
x_samples = random.normal(key_sample, (n_samples, x_dim))
y_samples = jnp.dot(x_samples, W) + b + 0.1 * random.normal(key_noise,(n_samples, y_dim))
print('x shape:', x_samples.shape, '; y shape:', y_samples.shape)
###Output
x shape: (20, 10) ; y shape: (20, 5)
###Markdown
We copy the same training loop that we used in the JAX pytree linear regression example with `jax.value_and_grad()`, but here we can use `model.apply()` instead of having to define our own feed-forward function (`predict_pytree()` in the JAX example).
###Code
# Same as JAX version but using model.apply().
def mse(params, x_batched, y_batched):
# Define the squared loss for a single pair (x,y)
def squared_error(x, y):
pred = model.apply(params, x)
return jnp.inner(y-pred, y-pred) / 2.0
# Vectorize the previous to compute the average of the loss on all samples.
return jnp.mean(jax.vmap(squared_error)(x_batched,y_batched), axis=0)
###Output
_____no_output_____
###Markdown
And finally perform the gradient descent.
###Code
learning_rate = 0.3 # Gradient step size.
print('Loss for "true" W,b: ', mse(true_params, x_samples, y_samples))
loss_grad_fn = jax.value_and_grad(mse)
@jax.jit
def update_params(params, learning_rate, grads):
params = jax.tree_map(
lambda p, g: p - learning_rate * g, params, grads)
return params
for i in range(101):
# Perform one gradient update.
loss_val, grads = loss_grad_fn(params, x_samples, y_samples)
params = update_params(params, learning_rate, grads)
if i % 10 == 0:
print(f'Loss step {i}: ', loss_val)
###Output
Loss for "true" W,b: 0.023639778
Loss step 0: 38.094772
Loss step 10: 0.44692168
Loss step 20: 0.10053458
Loss step 30: 0.035822745
Loss step 40: 0.018846875
Loss step 50: 0.013864839
Loss step 60: 0.012312559
Loss step 70: 0.011812928
Loss step 80: 0.011649306
Loss step 90: 0.011595251
Loss step 100: 0.0115773035
###Markdown
Optimizing with OptaxFlax used to use its own `flax.optim` package for optimization, but with[FLIP 1009](https://github.com/google/flax/blob/main/docs/flip/1009-optimizer-api.md)this was deprecated in favor of[Optax](https://github.com/deepmind/optax).Basic usage of Optax is straightforward:1. Choose an optimization method (e.g. `optax.sgd`).2. Create optimizer state from parameters.3. Compute the gradients of your loss with `jax.value_and_grad()`.4. At every iteration, call the Optax `update` function to update the internal optimizer state and create an update to the parameters. Then add the update to the parameters with Optax's `apply_updates` method.Note that Optax can do a lot more: it's designed for composing simple gradienttransformations into more complex transformations that allows to implement awide range of optimizers. There is also support for changing optimizerhyperparameters over time ("schedules"), applying different updates to differentparts of the parameter tree ("masking") and much more. For details please referto the[official documentation](https://optax.readthedocs.io/en/latest/).
###Code
import optax
tx = optax.sgd(learning_rate=alpha)
opt_state = tx.init(params)
loss_grad_fn = jax.value_and_grad(loss)
for i in range(101):
loss_val, grads = loss_grad_fn(params)
updates, opt_state = tx.update(grads, opt_state)
params = optax.apply_updates(params, updates)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss step 0: 0.011576377
Loss step 10: 0.0115710115
Loss step 20: 0.011569244
Loss step 30: 0.011568661
Loss step 40: 0.011568454
Loss step 50: 0.011568379
Loss step 60: 0.011568358
Loss step 70: 0.01156836
Loss step 80: 0.01156835
Loss step 90: 0.011568353
Loss step 100: 0.011568348
###Markdown
Serializing the resultNow that we're happy with the result of our training, we might want to save the model parameters to load them back later. Flax provides a serialization package to enable you to do that.
###Code
from flax import serialization
bytes_output = serialization.to_bytes(params)
dict_output = serialization.to_state_dict(params)
print('Dict output')
print(dict_output)
print('Bytes output')
print(bytes_output)
###Output
Dict output
{'params': {'bias': DeviceArray([-1.4540135, -2.0262308, 2.0806582, 1.2201802, -0.9964547], dtype=float32), 'kernel': DeviceArray([[ 1.0106664 , 0.19014716, 0.04533899, -0.92722285,
0.34720102],
[ 1.7320251 , 0.9901233 , 1.1662225 , 1.1027892 ,
-0.10574618],
[-1.2009128 , 0.28837162, 1.4176372 , 0.12073109,
-1.3132601 ],
[-1.1944956 , -0.18993308, 0.03379077, 1.3165942 ,
0.07996067],
[ 0.14103189, 1.3737966 , -1.3162128 , 0.53401774,
-2.239638 ],
[ 0.5643044 , 0.813604 , 0.31888172, 0.5359193 ,
0.90352124],
[-0.37948322, 1.7408353 , 1.0788013 , -0.5041964 ,
0.9286919 ],
[ 0.9701384 , -1.3158673 , 0.33630812, 0.80941117,
-1.202457 ],
[ 1.0198247 , -0.6198277 , 1.0822718 , -1.8385581 ,
-0.45790705],
[-0.64384323, 0.4564892 , -1.1331053 , -0.68556863,
0.17010891]], dtype=float32)}}
Bytes output
b'\x81\xa6params\x82\xa4bias\xc7!\x01\x93\x91\x05\xa7float32\xc4\x14\x1d\x1d\xba\xbf\xc4\xad\x01\xc0\x81)\x05@\xdd.\x9c?\xa8\x17\x7f\xbf\xa6kernel\xc7\xd6\x01\x93\x92\n\x05\xa7float32\xc4\xc8\x84]\x81?\xf0\xb5B>`\xb59=z^m\xbfU\xc4\xb1>\x00\xb3\xdd?\xb8x}?\xc7F\x95?2(\x8d?t\x91\xd8\xbd\x83\xb7\x99\xbfr\xa5\x93>#u\xb5?\xdcA\xf7=\xe8\x18\xa8\xbf;\xe5\x98\xbf\xd1}B\xbe0h\n=)\x86\xa8?k\xc2\xa3=\xaaj\x10>\x91\xd8\xaf?\xa9y\xa8\xbfc\xb5\x08?;V\x0f\xc0Av\x10?ZHP?wD\xa3>\x022\t?+Mg?\xa0K\xc2\xbe\xb1\xd3\xde?)\x16\x8a?\x04\x13\x01\xbf\xc1\xbem?\xfdZx?Wn\xa8\xbf\x940\xac>\x925O?\x1c\xea\x99\xbf\x9e\x89\x82?\x07\xad\x1e\xbf\xe2\x87\x8a?\xdfU\xeb\xbf\xcbr\xea\xbe\xe9\xd2$\xbf\xf4\xb8\xe9>\x98\t\x91\xbfm\x81/\xbf\x081.>'
###Markdown
To load the model back, you'll need to use as a template the model parameter structure, like the one you would get from the model initialization. Here, we use the previously generated `params` as a template. Note that this will produce a new variable structure, and not mutate in-place.*The point of enforcing structure through template is to avoid users issues downstream, so you need to first have the right model that generates the parameters structure.*
###Code
serialization.from_bytes(params, bytes_output)
###Output
_____no_output_____
###Markdown
Defining your own modelsFlax allows you to define your own models, which should be a bit more complicated than a linear regression. In this section, we'll show you how to build simple models. To do so, you'll need to create subclasses of the base `nn.Module` class.*Keep in mind that we imported* `linen as nn` *and this only works with the new linen API* Module basicsThe base abstraction for models is the `nn.Module` class, and every type of predefined layers in Flax (like the previous `Dense`) is a subclass of `nn.Module`. Let's take a look and start by defining a simple but custom multi-layer perceptron i.e. a sequence of Dense layers interleaved with calls to a non-linear activation function.
###Code
class ExplicitMLP(nn.Module):
features: Sequence[int]
def setup(self):
# we automatically know what to do with lists, dicts of submodules
self.layers = [nn.Dense(feat) for feat in self.features]
# for single submodules, we would just write:
# self.layer1 = nn.Dense(feat1)
def __call__(self, inputs):
x = inputs
for i, lyr in enumerate(self.layers):
x = lyr(x)
if i != len(self.layers) - 1:
x = nn.relu(x)
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = ExplicitMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292815e-02 -4.3807115e-02 2.9323792e-02 6.5492536e-03
-1.7147182e-02]
[ 1.2967806e-01 -1.4551792e-01 9.4432183e-02 1.2521387e-02
-4.5417298e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
As we can see, a `nn.Module` subclass is made of:* A collection of data fields (`nn.Module` are Python dataclasses) - here we only have the `features` field of type `Sequence[int]`.* A `setup()` method that is being called at the end of the `__postinit__` where you can register submodules, variables, parameters you will need in your model.* A `__call__` function that returns the output of the model from a given input.* The model structure defines a pytree of parameters following the same tree structure as the model: the params tree contains one `layers_n` sub dict per layer, and each of those contain the parameters of the associated Dense layer. The layout is very explicit.*Note: lists are mostly managed as you would expect (WIP), there are corner cases you should be aware of as pointed out* [here](https://github.com/google/flax/issues/524)Since the module structure and its parameters are not tied to each other, you can't directly call `model(x)` on a given input as it will return an error. The `__call__` function is being wrapped up in the `apply` one, which is the one to call on an input:
###Code
try:
y = model(x) # Returns an error
except AttributeError as e:
print(e)
###Output
"ExplicitMLP" object has no attribute "layers"
###Markdown
Since here we have a very simple model, we could have used an alternative (but equivalent) way of declaring the submodules inline in the `__call__` using the `@nn.compact` annotation like so:
###Code
class SimpleMLP(nn.Module):
features: Sequence[int]
@nn.compact
def __call__(self, inputs):
x = inputs
for i, feat in enumerate(self.features):
x = nn.Dense(feat, name=f'layers_{i}')(x)
if i != len(self.features) - 1:
x = nn.relu(x)
# providing a name is optional though!
# the default autonames would be "Dense_0", "Dense_1", ...
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292815e-02 -4.3807115e-02 2.9323792e-02 6.5492536e-03
-1.7147182e-02]
[ 1.2967806e-01 -1.4551792e-01 9.4432183e-02 1.2521387e-02
-4.5417298e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
There are, however, a few differences you should be aware of between the two declaration modes:* In `setup`, you are able to name some sublayers and keep them around for further use (e.g. encoder/decoder methods in autoencoders).* If you want to have multiple methods, then you **need** to declare the module using `setup`, as the `@nn.compact` annotation only allows one method to be annotated.* The last initialization will be handled differently. See these notes for more details (TODO: add notes link). Module parametersIn the previous MLP example, we relied only on predefined layers and operators (`Dense`, `relu`). Let's imagine that you didn't have a Dense layer provided by Flax and you wanted to write it on your own. Here is what it would look like using the `@nn.compact` way to declare a new modules:
###Code
class SimpleDense(nn.Module):
features: int
kernel_init: Callable = nn.initializers.lecun_normal()
bias_init: Callable = nn.initializers.zeros
@nn.compact
def __call__(self, inputs):
kernel = self.param('kernel',
self.kernel_init, # Initialization function
(inputs.shape[-1], self.features)) # shape info.
y = lax.dot_general(inputs, kernel,
(((inputs.ndim - 1,), (0,)), ((), ())),) # TODO Why not jnp.dot?
bias = self.param('bias', self.bias_init, (self.features,))
y = y + bias
return y
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleDense(features=3)
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameters:\n', params)
print('output:\n', y)
###Output
initialized parameters:
FrozenDict({
params: {
kernel: DeviceArray([[ 0.6503669 , 0.86789787, 0.4604268 ],
[ 0.05673932, 0.9909285 , -0.63536596],
[ 0.76134115, -0.3250529 , -0.65221626],
[-0.82430327, 0.4150194 , 0.19405058]], dtype=float32),
bias: DeviceArray([0., 0., 0.], dtype=float32),
},
})
output:
[[ 0.5035518 1.8548558 -0.4270195 ]
[ 0.0279097 0.5589246 -0.43061772]
[ 0.3547128 1.5740999 -0.32865518]
[ 0.5264864 1.2928858 0.10089308]]
###Markdown
Here, we see how to both declare and assign a parameter to the model using the `self.param` method. It takes as input `(name, init_fn, *init_args)` : * `name` is simply the name of the parameter that will end up in the parameter structure.* `init_fn` is a function with input `(PRNGKey, *init_args)` returning an Array, with `init_args` being the arguments needed to call the initialisation function.* `init_args` are the arguments to provide to the initialization function.Such params can also be declared in the `setup` method; it won't be able to use shape inference because Flax is using lazy initialization at the first call site. Variables and collections of variablesAs we've seen so far, working with models means working with:* A subclass of `nn.Module`;* A pytree of parameters for the model (typically from `model.init()`);However this is not enough to cover everything that we would need for machine learning, especially neural networks. In some cases, you might want your neural network to keep track of some internal state while it runs (e.g. batch normalization layers). There is a way to declare variables beyond the parameters of the model with the `variable` method.For demonstration purposes, we'll implement a simplified but similar mechanism to batch normalization: we'll store running averages and subtract those to the input at training time. For proper batchnorm, you should use (and look at) the implementation [here](https://github.com/google/flax/blob/main/flax/linen/normalization.py).
###Code
class BiasAdderWithRunningMean(nn.Module):
decay: float = 0.99
@nn.compact
def __call__(self, x):
# easy pattern to detect if we're initializing via empty variable tree
is_initialized = self.has_variable('batch_stats', 'mean')
ra_mean = self.variable('batch_stats', 'mean',
lambda s: jnp.zeros(s),
x.shape[1:])
mean = ra_mean.value # This will either get the value or trigger init
bias = self.param('bias', lambda rng, shape: jnp.zeros(shape), x.shape[1:])
if is_initialized:
ra_mean.value = self.decay * ra_mean.value + (1.0 - self.decay) * jnp.mean(x, axis=0, keepdims=True)
return x - ra_mean.value + bias
key1, key2 = random.split(random.PRNGKey(0), 2)
x = jnp.ones((10,5))
model = BiasAdderWithRunningMean()
variables = model.init(key1, x)
print('initialized variables:\n', variables)
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
print('updated state:\n', updated_state)
###Output
initialized variables:
FrozenDict({
batch_stats: {
mean: DeviceArray([0., 0., 0., 0., 0.], dtype=float32),
},
params: {
bias: DeviceArray([0., 0., 0., 0., 0.], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
###Markdown
Here, `updated_state` returns only the state variables that are being mutated by the model while applying it on data. To update the variables and get the new parameters of the model, we can use the following pattern:
###Code
for val in [1.0, 2.0, 3.0]:
x = val * jnp.ones((10,5))
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
old_state, params = variables.pop('params')
variables = freeze({'params': params, **updated_state})
print('updated state:\n', updated_state) # Shows only the mutable part
###Output
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.0299, 0.0299, 0.0299, 0.0299, 0.0299]], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.059601, 0.059601, 0.059601, 0.059601, 0.059601]], dtype=float32),
},
})
###Markdown
From this simplified example, you should be able to derive a full BatchNorm implementation, or any layer involving a state. To finish, let's add an optimizer to see how to play with both parameters updated by an optimizer and state variables.*This example isn't doing anything and is only for demonstration purposes.*
###Code
def update_step(tx, apply_fn, x, opt_state, params, state):
def loss(params):
y, updated_state = apply_fn({'params': params, **state},
x, mutable=list(state.keys()))
l = ((x - y) ** 2).sum()
return l, updated_state
(l, state), grads = jax.value_and_grad(loss, has_aux=True)(params)
updates, opt_state = tx.update(grads, opt_state)
params = optax.apply_updates(params, updates)
return opt_state, params, state
x = jnp.ones((10,5))
variables = model.init(random.PRNGKey(0), x)
state, params = variables.pop('params')
del variables
tx = optax.sgd(learning_rate=0.02)
opt_state = tx.init(params)
for _ in range(3):
opt_state, params, state = update_step(tx, model.apply, x, opt_state, params, state)
print('Updated state: ', state)
###Output
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.0199, 0.0199, 0.0199, 0.0199, 0.0199]], dtype=float32),
},
})
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.029701, 0.029701, 0.029701, 0.029701, 0.029701]], dtype=float32),
},
})
###Markdown
Flax basicsThis notebook will walk you through the following workflow:* Instantiating a model from Flax built-in layers or third-party models.* Initializing parameters of the model and manually written training.* Using optimizers provided by Flax to ease training.* Serialization of parameters and other objects.* Creating your own models and managing state. Setting up our environmentHere we provide the code needed to set up the environment for our notebook.
###Code
# Install the latest JAXlib version.
!pip install --upgrade -q pip jax jaxlib
# Install Flax at head:
!pip install --upgrade -q git+https://github.com/google/flax.git
import jax
from typing import Any, Callable, Sequence, Optional
from jax import lax, random, numpy as jnp
import flax
from flax.core import freeze, unfreeze
from flax import linen as nn
from jax.config import config
config.enable_omnistaging() # Linen requires enabling omnistaging
###Output
_____no_output_____
###Markdown
Linear regression with FlaxIn the previous *JAX for the impatient* notebook, we finished up with a linear regression example. As we know, linear regression can also be written as a single dense neural network layer, which we will show in the following so that we can compare how it's done.A dense layer is a layer that has a kernel parameter $W\in\mathcal{M}_{m,n}(\mathbb{R})$ where $m$ is the number of features as an output of the model, and $n$ the dimensionality of the input, and a bias parameter $b\in\mathbb{R}^m$. The dense layers returns $Wx+b$ from an input $x\in\mathbb{R}^n$.This dense layer is already provided by Flax in the `flax.linen` module (here imported as `nn`).
###Code
# We create one dense layer instance (taking 'features' parameter as input)
model = nn.Dense(features=5)
###Output
_____no_output_____
###Markdown
Layers (and models in general, we'll use that word from now on) are subclasses of the `linen.Module` class. Model parameters & initializationParameters are not stored with the models themselves. You need to initialize parameters by calling the `init` function, using a PRNGKey and a dummy input parameter.
###Code
key1, key2 = random.split(random.PRNGKey(0))
x = random.normal(key1, (10,)) # Dummy input
params = model.init(key2, x) # Initialization call
jax.tree_map(lambda x: x.shape, params) # Checking output shapes
###Output
WARNING:absl:No GPU/TPU found, falling back to CPU. (Set TF_CPP_MIN_LOG_LEVEL=0 and rerun for more info.)
###Markdown
*Note: JAX and Flax, like NumPy, are row-based systems, meaning that vectors are represented as row vectors and not column vectors. This can be seen in the shape of the kernel here.*The result is what we expect: bias and kernel parameters of the correct size. Under the hood:* The dummy input variable `x` is used to trigger shape inference: we only declared the number of features we wanted in the output of the model, not the size of the input. Flax finds out by itself the correct size of the kernel.* The random PRNG key is used to trigger the initialization functions (those have default values provided by the module here).* Initialization functions are called to generate the initial set of parameters that the model will use. Those are functions that take as arguments `(PRNG Key, shape, dtype)` and return an Array of shape `shape`.* The init function returns the initialized set of parameters (you can also get the output of the evaluation on the dummy input with the same syntax but using the `init_with_output` method instead of `init`. We see in the output that parameters are stored in a `FrozenDict` instance which helps deal with the functional nature of JAX by preventing any mutation of the underlying dict and making the user aware of it. Read more about it in the Flax docs. As a consequence, the following doesn't work:
###Code
try:
params['new_key'] = jnp.ones((2,2))
except ValueError as e:
print("Error: ", e)
###Output
Error: FrozenDict is immutable.
###Markdown
To evaluate the model with a given set of parameters (never stored with the model), we just use the `apply` method by providing it the parameters to use as well as the input:
###Code
model.apply(params, x)
###Output
_____no_output_____
###Markdown
Gradient descentIf you jumped here directly without going through the JAX part, here is the linear regression formulation we're going to use: from a set of data points $\{(x_i,y_i), i\in \{1,\ldots, k\}, x_i\in\mathbb{R}^n,y_i\in\mathbb{R}^m\}$, we try to find a set of parameters $W\in \mathcal{M}_{m,n}(\mathbb{R}), b\in\mathbb{R}^m$ such that the function $f_{W,b}(x)=Wx+b$ minimizes the mean squared error:$$\mathcal{L}(W,b)\rightarrow\frac{1}{k}\sum_{i=1}^{k} \frac{1}{2}\|y_i-f_{W,b}(x_i)\|^2_2$$Here, we see that the tuple $(W,b)$ matches the parameters of the Dense layer. We'll perform gradient descent using those. Let's first generate the fake data we'll use.
###Code
# Set problem dimensions
nsamples = 20
xdim = 10
ydim = 5
# Generate random ground truth W and b
key = random.PRNGKey(0)
k1, k2 = random.split(key)
W = random.normal(k1, (xdim, ydim))
b = random.normal(k2, (ydim,))
true_params = freeze({'params': {'bias': b, 'kernel': W}})
# Generate samples with additional noise
ksample, knoise = random.split(k1)
x_samples = random.normal(ksample, (nsamples, xdim))
y_samples = jnp.dot(x,W) + b
y_samples += 0.1*random.normal(knoise,(nsamples, ydim)) # Adding noise
print('x shape:', x_samples.shape, '; y shape:', y_samples.shape)
###Output
x shape: (20, 10) ; y shape: (20, 5)
###Markdown
Now let's generate the loss function (mean squared error) with that data.
###Code
def make_mse_func(x_batched, y_batched):
def mse(params):
# Define the squared loss for a single pair (x,y)
def squared_error(x, y):
pred = model.apply(params, x)
return jnp.inner(y-pred, y-pred)/2.0
# We vectorize the previous to compute the average of the loss on all samples.
return jnp.mean(jax.vmap(squared_error)(x_batched,y_batched), axis=0)
return jax.jit(mse) # And finally we jit the result.
# Get the sampled loss
loss = make_mse_func(x_samples, y_samples)
###Output
_____no_output_____
###Markdown
And finally perform the gradient descent.
###Code
alpha = 0.3 # Gradient step size
print('Loss for "true" W,b: ', loss(true_params))
grad_fn = jax.value_and_grad(loss)
for i in range(101):
# We perform one gradient update
loss_val, grad = grad_fn(params)
params = jax.tree_multimap(lambda old, grad: old - alpha * grad,
params, grad)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss for "true" W,b: 29.070158
Loss step 0: 23.618902
Loss step 10: 0.30728564
Loss step 20: 0.06495677
Loss step 30: 0.025215296
Loss step 40: 0.015619493
Loss step 50: 0.012849321
Loss step 60: 0.011984843
Loss step 70: 0.011705536
Loss step 80: 0.011613827
Loss step 90: 0.011583473
Loss step 100: 0.011573391
###Markdown
Build-in optimization APIFlax provides an optimization package in `flax.optim` to make your life easier when training models. The process is:1. You choose an optimization method (e.g. `optim.GradientDescent`, `optim.Adam`)2. From the previous optimization method, you create a wrapper around the parameters you're going to optimize for with the `create` method. Your parameters are accessible through the `target` field.3. You compute the gradients of your loss with `jax.value_and_grad()`.4. At every iteration, you compute the gradients at the current point, then use the `apply_gradient()` method on the optimizer to return a new optimizer with updated parameters.
###Code
from flax import optim
optimizer_def = optim.GradientDescent(learning_rate=alpha) # Choose the method
optimizer = optimizer_def.create(params) # Create the wrapping optimizer with initial parameters
loss_grad_fn = jax.value_and_grad(loss)
for i in range(101):
loss_val, grad = loss_grad_fn(optimizer.target)
optimizer = optimizer.apply_gradient(grad) # Return the updated optimizer with parameters.
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss step 0: 0.011572863
Loss step 10: 0.011569859
Loss step 20: 0.011568859
Loss step 30: 0.011568523
Loss step 40: 0.011568412
Loss step 50: 0.011568374
Loss step 60: 0.011568364
Loss step 70: 0.011568359
Loss step 80: 0.01156836
Loss step 90: 0.011568356
Loss step 100: 0.011568357
###Markdown
Serializing the resultNow that we're happy with the result of our training, we might want to save the model parameters to load them back later. Flax provides a serialization package to enable you to do that.
###Code
from flax import serialization
bytes_output = serialization.to_bytes(optimizer.target)
dict_output = serialization.to_state_dict(optimizer.target)
print('Dict output')
print(dict_output)
print('Bytes output')
print(bytes_output)
###Output
Dict output
{'params': {'bias': DeviceArray([-3.023082 , 0.5307182, 3.7256303, 1.4638226, -3.2100437], dtype=float32), 'kernel': DeviceArray([[-1.4092493e-02, 4.8609809e-03, 1.1460093e-02,
-6.0927689e-02, 2.0413438e-05],
[-3.3569761e-02, -1.5614161e-03, 4.3190460e-04,
-7.9035060e-03, -1.9851506e-02],
[-1.8882388e-02, -2.1366426e-03, -1.8663550e-02,
-3.0001188e-02, 5.1880259e-02],
[-4.8119370e-02, -2.9280247e-02, -1.1992223e-02,
-1.0111435e-02, -8.3459895e-03],
[-1.7368369e-02, -1.7084973e-02, 6.0279824e-02,
9.2046618e-02, -1.5414236e-02],
[-3.0089449e-02, -5.5370983e-03, -9.1237156e-03,
2.1827107e-02, -2.0405082e-02],
[-5.6748122e-02, -4.2654604e-02, -1.1436724e-02,
7.5801805e-02, -2.0075133e-02],
[-1.4368590e-03, -1.6048675e-02, 1.5781123e-02,
2.8437756e-03, -8.5009886e-03],
[ 1.7892396e-02, 5.7572998e-02, 4.1483097e-02,
-9.9685444e-03, -2.1875760e-02],
[-2.1158390e-02, -1.3853005e-02, 2.5077526e-02,
3.2925244e-02, 3.8115401e-02]], dtype=float32)}}
Bytes output
b'\x81\xa6params\x82\xa4bias\xc7!\x01\x93\x91\x05\xa7float32\xc4\x14-zA\xc0&\xdd\x07?\xbapn@\x8a^\xbb?[qM\xc0\xa6kernel\xc7\xd6\x01\x93\x92\n\x05\xa7float32\xc4\xc83\xe4f\xbc\xddH\x9f;\x1d\xc3;<P\x8fy\xbd\x86=\xab7r\x80\t\xbdn\xa8\xcc\xbaAq\xe29\xb5}\x01\xbc\xa0\x9f\xa2\xbc=\xaf\x9a\xbc\xea\x06\x0c\xbbM\xe4\x98\xbc\r\xc5\xf5\xbce\x80T=\xd1\x18E\xbd!\xdd\xef\xbc\x07{D\xbco\xaa%\xbc\x9e\xbd\x08\xbc\x1cH\x8e\xbc\xc9\xf5\x8b\xbc\xfa\xe7v=\xf0\x82\xbc=\xfe\x8b|\xbc&~\xf6\xbc\x8cp\xb5\xbb\xa3{\x15\xbc\xc3\xce\xb2<\x8f(\xa7\xbc\xb8ph\xbd\x98\xb6.\xbd\x19a;\xbc\xfa=\x9b=\x9bt\xa4\xbc\xfdT\xbc\xba\x83x\x83\xbcjG\x81<\xa3^:;\xbbG\x0b\xbc\x13\x93\x92<\xaa\xd1k=.\xea)=\x1bS#\xbc\xcb4\xb3\xbc\\T\xad\xbc\xb7\xf7b\xbcbo\xcd<\x9f\xdc\x06=\xe5\x1e\x1c='
###Markdown
To load the model back, you'll need to use as a template the model parameter structure, like the one you would get from the model initialization. Here, we use the previously generated `params` as a template. Note that this will produce a new variable structure, and not mutate in-place.*The point of enforcing structure through template is to avoid users issues downstream, so you need to first have the right model that generates the parameters structure.*
###Code
serialization.from_bytes(params, bytes_output)
###Output
_____no_output_____
###Markdown
The serialization utils provided by Flax work on objects beyond parameters, for example you might want to serialize the optimizer and it's states, which we show in the following cell:
###Code
serialization.to_state_dict(optimizer)
###Output
_____no_output_____
###Markdown
Defining your own modelsFlax allows you to define your own models, which should be a bit more complicated than a linear regression. In this section, we'll show you how to build simple models. To do so, you'll need to create subclasses of the base `nn.Module` class.*Keep in mind that we imported* `linen as nn` *and this only works with the new linen API* Module basicsThe base abstraction for models is the `nn.Module` class, and every type of predefined layers in Flax (like the previous `Dense`) is a subclass of `nn.Module`. Let's take a look and start by defining a simple but custom multi-layer perceptron i.e. a sequence of Dense layers interleaved with calls to a non-linear activation function.
###Code
class ExplicitMLP(nn.Module):
features: Sequence[int]
def setup(self):
# we automatically know what to do with lists, dicts of submodules
self.layers = [nn.Dense(feat) for feat in self.features]
# for single submodules, we would just write:
# self.layer1 = nn.Dense(self, feat1)
def __call__(self, inputs):
x = inputs
for i, lyr in enumerate(self.layers):
x = lyr(x)
if i != len(self.layers) - 1:
x = nn.relu(x)
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = ExplicitMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292822e-02 -4.3807123e-02 2.9323796e-02 6.5492545e-03
-1.7147183e-02]
[ 1.2967804e-01 -1.4551792e-01 9.4432175e-02 1.2521386e-02
-4.5417294e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
As we can see, a `nn.Module` subclass is made of:* A collection of data fields (`nn.Module` are Python dataclasses) - here we only have the `features` field of type `Sequence[int]`.* A `setup()` method that is being called at the end of the `__postinit__` where you can register submodules, variables, parameters you will need in your model.* A `__call__` function that returns the output of the model from a given input.* The model structure defines a pytree of parameters following the same tree structure as the model: the params tree contains one `layers_n` sub dict per layer, and each of those contain the parameters of the associated Dense layer. The layout is very explicit.*Note: lists are mostly managed as you would expect (WIP), there are corner cases you should be aware of as pointed out [here](https://github.com/google/flax/issues/524)*Since the module structure and its parameters are not tied to each other, you can't call directly `model(x)` on a given input as it will return an error. The `__call__` function is being wrapped up in the `apply` one, which is the one to call on an input:
###Code
try:
y = model(x) # Returns an error
except ValueError as e:
print(e)
###Output
Can't call methods on orphaned modules
###Markdown
Since here we have a very simple model, we could have used an alternative (but equivalent) way of declaring the submodules inline in the `__call__` using the `@nn.compact` annotation like so:
###Code
class SimpleMLP(nn.Module):
features: Sequence[int]
@nn.compact
def __call__(self, inputs):
x = inputs
for i, feat in enumerate(self.features):
x = nn.Dense(feat, name=f'layers_{i}')(x)
if i != len(self.features) - 1:
x = nn.relu(x)
# providing a name is optional though!
# the default autonames would be "Dense_0", "Dense_1", ...
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292822e-02 -4.3807123e-02 2.9323796e-02 6.5492545e-03
-1.7147183e-02]
[ 1.2967804e-01 -1.4551792e-01 9.4432175e-02 1.2521386e-02
-4.5417294e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
There are, however, a few differences you should be aware of between the two declaration modes:* In `setup`, you are able to name some sublayers and keep them around for further use (e.g. encoder/decoder methods in autoencoders).* If you want to have multiple methods, then you **need** to declare the module using `setup`, as the `@nn.compact` annotation only allows one method to be annotated.* The last initialization will be handled differently see these notes for more details (TODO: add notes link) Module parametersIn the previous MLP example, we relied only on predefined layers and operators (`Dense`, `relu`). Let's imagine that you didn't have a Dense layer provided by Flax and you wanted to write it on your own. Here is what it would look like using the `@nn.compact` way to declare a new modules:
###Code
class SimpleDense(nn.Module):
features: int
kernel_init: Callable = nn.initializers.lecun_normal()
bias_init: Callable = nn.initializers.zeros
@nn.compact
def __call__(self, inputs):
kernel = self.param('kernel',
self.kernel_init, # Initialization function
(inputs.shape[-1], self.features)) # shape info.
y = lax.dot_general(inputs, kernel,
(((inputs.ndim - 1,), (0,)), ((), ())),) # TODO Why not jnp.dot?
bias = self.param('bias', self.bias_init, (self.features,))
y = y + bias
return y
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleDense(features=3)
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameters:\n', params)
print('output:\n', y)
###Output
initialized parameters:
FrozenDict({'params': {'kernel': DeviceArray([[ 0.6503669 , 0.8678979 , 0.46042678],
[ 0.05673932, 0.9909285 , -0.63536596],
[ 0.76134115, -0.3250529 , -0.6522163 ],
[-0.8243032 , 0.4150194 , 0.19405058]], dtype=float32), 'bias': DeviceArray([0., 0., 0.], dtype=float32)}})
output:
[[ 0.50355184 1.8548559 -0.4270196 ]
[ 0.02790972 0.5589246 -0.43061778]
[ 0.35471287 1.5740999 -0.32865524]
[ 0.52648634 1.2928859 0.10089307]]
###Markdown
Here, we see how both declare and assign a parameter to the model using the `self.param` method. It takes as input `(name, init_fn, *init_args)` : * `name` is simply the name of the parameter that will end up in the parameter structure.* `init_fun` is a function with input `(PRNGKey, *init_args)` returning an Array with `init_args` the arguments needed to call the initialisation function* `init_args` the arguments to provide to the initialization function.Such params can also be declared in the `setup` method, it won't be able to use shape inference because Flax is using lazy initialization at the first call site. Variables and collections of variablesAs we've seen so far, working with models means working with:* A subclass of `nn.Module`;* A pytree of parameters for the model (typically from `model.init()`);However this is not enough to cover everything that we would need for machine learning, especially neural networks. In some cases, you might want your neural network to keep track of some internal state while it runs (e.g. batch normalization layers). There is a way to declare variables beyond the parameters of the model with the `variable` method.For demonstration purposes, we'll implement a simplified but similar mechanism to batch normalization: we'll store running averages and subtract those to the input at training time. For proper batchnorm, you should use (and look at) the implementation [here](https://github.com/google/flax/blob/master/flax/linen/normalization.py).
###Code
class BiasAdderWithRunningMean(nn.Module):
decay: float = 0.99
@nn.compact
def __call__(self, x):
# easy pattern to detect if we're initializing via empty variable tree
is_initialized = self.has_variable('batch_stats', 'mean')
ra_mean = self.variable('batch_stats', 'mean',
lambda s: jnp.zeros(s),
x.shape[1:])
mean = ra_mean.value # This will get either the value, or trigger init
bias = self.param('bias', lambda rng, shape: jnp.zeros(shape), x.shape[1:])
if is_initialized:
ra_mean.value = self.decay * ra_mean.value + (1.0 - self.decay) * jnp.mean(x, axis=0, keepdims=True)
return x - ra_mean.value + bias
key1, key2 = random.split(random.PRNGKey(0), 2)
x = jnp.ones((10,5))
model = BiasAdderWithRunningMean()
variables = model.init(key1, x)
print('initialized variables:\n', variables)
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
print('updated state:\n', updated_state)
###Output
initialized variables:
FrozenDict({'batch_stats': {'mean': DeviceArray([0., 0., 0., 0., 0.], dtype=float32)}, 'params': {'bias': DeviceArray([0., 0., 0., 0., 0.], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
###Markdown
Here, `updated_state` returns only the state variables that are being mutated by the model while applying it on data. To update the variables and get the new parameters of the model, we can use the following pattern:
###Code
for val in [1.0, 2.0, 3.0]:
x = val * jnp.ones((10,5))
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
old_state, params = variables.pop('params')
variables = freeze({'params': params, **updated_state})
print('updated state:\n', updated_state) # Shows only the mutable part
###Output
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.0299, 0.0299, 0.0299, 0.0299, 0.0299]], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.059601, 0.059601, 0.059601, 0.059601, 0.059601]], dtype=float32)}})
###Markdown
From this simplified example, you should be able to derive a full BatchNorm implementation, or any layer involving a state. To finish, let's add an optimizer to see how to play with both parameters updated by an optimizer and state variables.*This example isn't doing anything and is only for demonstration purposes.*
###Code
def update_step(apply_fun, x, optimizer, state):
def loss(params):
y, updated_state = apply_fun({'params': params, **state},
x, mutable=list(state.keys()))
l = ((x - y) ** 2).sum()
return l, updated_state
(l, updated_state), grads = jax.value_and_grad(
loss, has_aux=True)(optimizer.target)
optimizer = optimizer.apply_gradient(grads)
return optimizer, updated_state
variables = model.init(random.PRNGKey(0), x)
state, params = variables.pop('params')
del variables
optimizer = optim.sgd.GradientDescent(learning_rate=0.02).create(params)
x = jnp.ones((10,5))
for _ in range(3):
optimizer, state = update_step(model.apply, x, optimizer, state)
print('Updated state: ', state)
###Output
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.0199, 0.0199, 0.0199, 0.0199, 0.0199]], dtype=float32)}})
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.029701, 0.029701, 0.029701, 0.029701, 0.029701]], dtype=float32)}})
###Markdown
Flax BasicsThis notebook will walk you through the following workflow:* Instantiating a model from Flax built-in layers or third-party models.* Initializing parameters of the model and manually written training.* Using optimizers provided by Flax to ease training.* Serialization of parameters and other objects.* Creating your own models and managing state. Setting up our environmentHere we provide the code needed to set up the environment for our notebook.
###Code
# Install the latest JAXlib version.
!pip install --upgrade -q pip jax jaxlib
# Install Flax at head:
!pip install --upgrade -q git+https://github.com/google/flax.git
import jax
from typing import Any, Callable, Sequence, Optional
from jax import lax, random, numpy as jnp
import flax
from flax.core import freeze, unfreeze
from flax import linen as nn
from jax.config import config
config.enable_omnistaging() # Linen requires enabling omnistaging
###Output
_____no_output_____
###Markdown
Linear regression with FlaxIn the previous *JAX for the impatient* notebook, we finished up with a linear regression example. As we know, linear regression can also be written as a single dense neural network layer, which we will show in the following so that we can compare how it's done.A dense layer is a layer that has a kernel parameter $W\in\mathcal{M}_{m,n}(\mathbb{R})$ where $m$ is the number of features as an output of the model, and $n$ the dimensionality of the input, and a bias parameter $b\in\mathbb{R}^m$. The dense layers returns $Wx+b$ from an input $x\in\mathbb{R}^n$.This dense layer is already provided by Flax in the `flax.linen` module (here imported as `nn`).
###Code
# We create one dense layer instance (taking 'features' parameter as input)
model = nn.Dense(features=5)
###Output
_____no_output_____
###Markdown
Layers (and models in general, we'll use that word from now on) are subclasses of the `linen.Module` class. Model parameters & initializationParameters are not stored with the models themselves. You need to initialize parameters by calling the `init` function, using a PRNGKey and a dummy input parameter.
###Code
key1, key2 = random.split(random.PRNGKey(0))
x = random.normal(key1, (10,)) # Dummy input
params = model.init(key2, x) # Initialization call
jax.tree_map(lambda x: x.shape, params) # Checking output shapes
###Output
WARNING:absl:No GPU/TPU found, falling back to CPU. (Set TF_CPP_MIN_LOG_LEVEL=0 and rerun for more info.)
###Markdown
*Note: JAX and Flax, like NumPy, are row-based systems, meaning that vectors are represented as row vectors and not column vectors. This can be seen in the shape of the kernel here.*The result is what we expect: bias and kernel parameters of the correct size. Under the hood:* The dummy input variable `x` is used to trigger shape inference: we only declared the number of features we wanted in the output of the model, not the size of the input. Flax finds out by itself the correct size of the kernel.* The random PRNG key is used to trigger the initialization functions (those have default values provided by the module here).* Initialization functions are called to generate the initial set of parameters that the model will use. Those are functions that take as arguments `(PRNG Key, shape, dtype)` and return an Array of shape `shape`.* The init function returns the initialized set of parameters (you can also get the output of the evaluation on the dummy input with the same syntax but using the `init_with_output` method instead of `init`. We see in the output that parameters are stored in a `FrozenDict` instance which helps deal with the functional nature of JAX by preventing any mutation of the underlying dict and making the user aware of it. Read more about it in the Flax docs. As a consequence, the following doesn't work:
###Code
try:
params['new_key'] = jnp.ones((2,2))
except ValueError as e:
print("Error: ", e)
###Output
Error: FrozenDict is immutable.
###Markdown
To evaluate the model with a given set of parameters (never stored with the model), we just use the `apply` method by providing it the parameters to use as well as the input:
###Code
model.apply(params, x)
###Output
_____no_output_____
###Markdown
Gradient descentIf you jumped here directly without going through the JAX part, here is the linear regression formulation we're going to use: from a set of data points $\{(x_i,y_i), i\in \{1,\ldots, k\}, x_i\in\mathbb{R}^n,y_i\in\mathbb{R}^m\}$, we try to find a set of parameters $W\in \mathcal{M}_{m,n}(\mathbb{R}), b\in\mathbb{R}^m$ such that the function $f_{W,b}(x)=Wx+b$ minimizes the mean squared error:$$\mathcal{L}(W,b)\rightarrow\frac{1}{k}\sum_{i=1}^{k} \frac{1}{2}\|y_i-f_{W,b}(x_i)\|^2_2$$Here, we see that the tuple $(W,b)$ matches the parameters of the Dense layer. We'll perform gradient descent using those. Let's first generate the fake data we'll use.
###Code
# Set problem dimensions
nsamples = 20
xdim = 10
ydim = 5
# Generate random ground truth W and b
key = random.PRNGKey(0)
k1, k2 = random.split(key)
W = random.normal(k1, (xdim, ydim))
b = random.normal(k2, (ydim,))
true_params = freeze({'params': {'bias': b, 'kernel': W}})
# Generate samples with additional noise
ksample, knoise = random.split(k1)
x_samples = random.normal(ksample, (nsamples, xdim))
y_samples = jnp.dot(x,W) + b
y_samples += 0.1*random.normal(knoise,(nsamples, ydim)) # Adding noise
print('x shape:', x_samples.shape, '; y shape:', y_samples.shape)
###Output
x shape: (20, 10) ; y shape: (20, 5)
###Markdown
Now let's generate the loss function (mean squared error) with that data.
###Code
def make_mse_func(x_batched, y_batched):
def mse(params):
# Define the squared loss for a single pair (x,y)
def squared_error(x, y):
pred = model.apply(params, x)
return jnp.inner(y-pred, y-pred)/2.0
# We vectorize the previous to compute the average of the loss on all samples.
return jnp.mean(jax.vmap(squared_error)(x_batched,y_batched), axis=0)
return jax.jit(mse) # And finally we jit the result.
# Get the sampled loss
loss = make_mse_func(x_samples, y_samples)
###Output
_____no_output_____
###Markdown
And finally perform the gradient descent.
###Code
alpha = 0.3 # Gradient step size
print('Loss for "true" W,b: ', loss(true_params))
grad_fn = jax.value_and_grad(loss)
for i in range(101):
# We perform one gradient update
loss_val, grad = grad_fn(params)
params = jax.tree_multimap(lambda old, grad: old - alpha * grad,
params, grad)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss for "true" W,b: 29.070158
Loss step 0: 23.618902
Loss step 10: 0.30728564
Loss step 20: 0.06495677
Loss step 30: 0.025215296
Loss step 40: 0.015619493
Loss step 50: 0.012849321
Loss step 60: 0.011984843
Loss step 70: 0.011705536
Loss step 80: 0.011613827
Loss step 90: 0.011583473
Loss step 100: 0.011573391
###Markdown
Build-in optimization APIFlax provides an optimization package in `flax.optim` to make your life easier when training models. The process is:1. You choose an optimization method (e.g. `optim.GradientDescent`, `optim.Adam`)2. From the previous optimization method, you create a wrapper around the parameters you're going to optimize for with the `create` method. Your parameters are accessible through the `target` field.3. You compute the gradients of your loss with `jax.value_and_grad()`.4. At every iteration, you compute the gradients at the current point, then use the `apply_gradient()` method on the optimizer to return a new optimizer with updated parameters.
###Code
from flax import optim
optimizer_def = optim.GradientDescent(learning_rate=alpha) # Choose the method
optimizer = optimizer_def.create(params) # Create the wrapping optimizer with initial parameters
loss_grad_fn = jax.value_and_grad(loss)
for i in range(101):
loss_val, grad = loss_grad_fn(optimizer.target)
optimizer = optimizer.apply_gradient(grad) # Return the updated optimizer with parameters.
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss step 0: 0.011572863
Loss step 10: 0.011569859
Loss step 20: 0.011568859
Loss step 30: 0.011568523
Loss step 40: 0.011568412
Loss step 50: 0.011568374
Loss step 60: 0.011568364
Loss step 70: 0.011568359
Loss step 80: 0.01156836
Loss step 90: 0.011568356
Loss step 100: 0.011568357
###Markdown
Serializing the resultNow that we're happy with the result of our training, we might want to save the model parameters to load them back later. Flax provides a serialization package to enable you to do that.
###Code
from flax import serialization
bytes_output = serialization.to_bytes(optimizer.target)
dict_output = serialization.to_state_dict(optimizer.target)
print('Dict output')
print(dict_output)
print('Bytes output')
print(bytes_output)
###Output
Dict output
{'params': {'bias': DeviceArray([-3.023082 , 0.5307182, 3.7256303, 1.4638226, -3.2100437], dtype=float32), 'kernel': DeviceArray([[-1.4092493e-02, 4.8609809e-03, 1.1460093e-02,
-6.0927689e-02, 2.0413438e-05],
[-3.3569761e-02, -1.5614161e-03, 4.3190460e-04,
-7.9035060e-03, -1.9851506e-02],
[-1.8882388e-02, -2.1366426e-03, -1.8663550e-02,
-3.0001188e-02, 5.1880259e-02],
[-4.8119370e-02, -2.9280247e-02, -1.1992223e-02,
-1.0111435e-02, -8.3459895e-03],
[-1.7368369e-02, -1.7084973e-02, 6.0279824e-02,
9.2046618e-02, -1.5414236e-02],
[-3.0089449e-02, -5.5370983e-03, -9.1237156e-03,
2.1827107e-02, -2.0405082e-02],
[-5.6748122e-02, -4.2654604e-02, -1.1436724e-02,
7.5801805e-02, -2.0075133e-02],
[-1.4368590e-03, -1.6048675e-02, 1.5781123e-02,
2.8437756e-03, -8.5009886e-03],
[ 1.7892396e-02, 5.7572998e-02, 4.1483097e-02,
-9.9685444e-03, -2.1875760e-02],
[-2.1158390e-02, -1.3853005e-02, 2.5077526e-02,
3.2925244e-02, 3.8115401e-02]], dtype=float32)}}
Bytes output
b'\x81\xa6params\x82\xa4bias\xc7!\x01\x93\x91\x05\xa7float32\xc4\x14-zA\xc0&\xdd\x07?\xbapn@\x8a^\xbb?[qM\xc0\xa6kernel\xc7\xd6\x01\x93\x92\n\x05\xa7float32\xc4\xc83\xe4f\xbc\xddH\x9f;\x1d\xc3;<P\x8fy\xbd\x86=\xab7r\x80\t\xbdn\xa8\xcc\xbaAq\xe29\xb5}\x01\xbc\xa0\x9f\xa2\xbc=\xaf\x9a\xbc\xea\x06\x0c\xbbM\xe4\x98\xbc\r\xc5\xf5\xbce\x80T=\xd1\x18E\xbd!\xdd\xef\xbc\x07{D\xbco\xaa%\xbc\x9e\xbd\x08\xbc\x1cH\x8e\xbc\xc9\xf5\x8b\xbc\xfa\xe7v=\xf0\x82\xbc=\xfe\x8b|\xbc&~\xf6\xbc\x8cp\xb5\xbb\xa3{\x15\xbc\xc3\xce\xb2<\x8f(\xa7\xbc\xb8ph\xbd\x98\xb6.\xbd\x19a;\xbc\xfa=\x9b=\x9bt\xa4\xbc\xfdT\xbc\xba\x83x\x83\xbcjG\x81<\xa3^:;\xbbG\x0b\xbc\x13\x93\x92<\xaa\xd1k=.\xea)=\x1bS#\xbc\xcb4\xb3\xbc\\T\xad\xbc\xb7\xf7b\xbcbo\xcd<\x9f\xdc\x06=\xe5\x1e\x1c='
###Markdown
To load the model back, you'll need to use as a template the model parameter structure, like the one you would get from the model initialization. Here, we use the previously generated `params` as a template. Note that this will produce a new variable structure, and not mutate in-place.*The point of enforcing structure through template is to avoid users issues downstream, so you need to first have the right model that generates the parameters structure.*
###Code
serialization.from_bytes(params, bytes_output)
###Output
_____no_output_____
###Markdown
The serialization utils provided by Flax work on objects beyond parameters, for example you might want to serialize the optimizer and it's states, which we show in the following cell:
###Code
serialization.to_state_dict(optimizer)
###Output
_____no_output_____
###Markdown
Defining your own modelsFlax allows you to define your own models, which should be a bit more complicated than a linear regression. In this section, we'll show you how to build simple models. To do so, you'll need to create subclasses of the base `nn.Module` class.*Keep in mind that we imported* `linen as nn` *and this only works with the new linen API* Module basicsThe base abstraction for models is the `nn.Module` class, and every type of predefined layers in Flax (like the previous `Dense`) is a subclass of `nn.Module`. Let's take a look and start by defining a simple but custom multi-layer perceptron i.e. a sequence of Dense layers interleaved with calls to a non-linear activation function.
###Code
class ExplicitMLP(nn.Module):
features: Sequence[int]
def setup(self):
# we automatically know what to do with lists, dicts of submodules
self.layers = [nn.Dense(feat) for feat in self.features]
# for single submodules, we would just write:
# self.layer1 = nn.Dense(self, feat1)
def __call__(self, inputs):
x = inputs
for i, lyr in enumerate(self.layers):
x = lyr(x)
if i != len(self.layers) - 1:
x = nn.relu(x)
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = ExplicitMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292822e-02 -4.3807123e-02 2.9323796e-02 6.5492545e-03
-1.7147183e-02]
[ 1.2967804e-01 -1.4551792e-01 9.4432175e-02 1.2521386e-02
-4.5417294e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
As we can see, a `nn.Module` subclass is made of:* A collection of data fields (`nn.Module` are Python dataclasses) - here we only have the `features` field of type `Sequence[int]`.* A `setup()` method that is being called at the end of the `__postinit__` where you can register submodules, variables, parameters you will need in your model.* A `__call__` function that returns the output of the model from a given input.* The model structure defines a pytree of parameters following the same tree structure as the model: the params tree contains one `layers_n` sub dict per layer, and each of those contain the parameters of the associated Dense layer. The layout is very explicit.*Note: lists are mostly managed as you would expect (WIP), there are corner cases you should be aware of as pointed out* [here](https://github.com/google/flax/issues/524)Since the module structure and its parameters are not tied to each other, you can't call directly `model(x)` on a given input as it will return an error. The `__call__` function is being wrapped up in the `apply` one, which is the one to call on an input:
###Code
try:
y = model(x) # Returns an error
except ValueError as e:
print(e)
###Output
Can't call methods on orphaned modules
###Markdown
Since here we have a very simple model, we could have used an alternative (but equivalent) way of declaring the submodules inline in the `__call__` using the `@nn.compact` annotation like so:
###Code
class SimpleMLP(nn.Module):
features: Sequence[int]
@nn.compact
def __call__(self, inputs):
x = inputs
for i, feat in enumerate(self.features):
x = nn.Dense(feat, name=f'layers_{i}')(x)
if i != len(self.features) - 1:
x = nn.relu(x)
# providing a name is optional though!
# the default autonames would be "Dense_0", "Dense_1", ...
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292822e-02 -4.3807123e-02 2.9323796e-02 6.5492545e-03
-1.7147183e-02]
[ 1.2967804e-01 -1.4551792e-01 9.4432175e-02 1.2521386e-02
-4.5417294e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
There are, however, a few differences you should be aware of between the two declaration modes:* In `setup`, you are able to name some sublayers and keep them around for further use (e.g. encoder/decoder methods in autoencoders).* If you want to have multiple methods, then you **need** to declare the module using `setup`, as the `@nn.compact` annotation only allows one method to be annotated.* The last initialization will be handled differently see these notes for more details (TODO: add notes link) Module parametersIn the previous MLP example, we relied only on predefined layers and operators (`Dense`, `relu`). Let's imagine that you didn't have a Dense layer provided by Flax and you wanted to write it on your own. Here is what it would look like using the `@nn.compact` way to declare a new modules:
###Code
class SimpleDense(nn.Module):
features: int
kernel_init: Callable = nn.initializers.lecun_normal()
bias_init: Callable = nn.initializers.zeros
@nn.compact
def __call__(self, inputs):
kernel = self.param('kernel',
self.kernel_init, # Initialization function
(inputs.shape[-1], self.features)) # shape info.
y = lax.dot_general(inputs, kernel,
(((inputs.ndim - 1,), (0,)), ((), ())),) # TODO Why not jnp.dot?
bias = self.param('bias', self.bias_init, (self.features,))
y = y + bias
return y
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleDense(features=3)
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameters:\n', params)
print('output:\n', y)
###Output
initialized parameters:
FrozenDict({'params': {'kernel': DeviceArray([[ 0.6503669 , 0.8678979 , 0.46042678],
[ 0.05673932, 0.9909285 , -0.63536596],
[ 0.76134115, -0.3250529 , -0.6522163 ],
[-0.8243032 , 0.4150194 , 0.19405058]], dtype=float32), 'bias': DeviceArray([0., 0., 0.], dtype=float32)}})
output:
[[ 0.50355184 1.8548559 -0.4270196 ]
[ 0.02790972 0.5589246 -0.43061778]
[ 0.35471287 1.5740999 -0.32865524]
[ 0.52648634 1.2928859 0.10089307]]
###Markdown
Here, we see how both declare and assign a parameter to the model using the `self.param` method. It takes as input `(name, init_fn, *init_args)` : * `name` is simply the name of the parameter that will end up in the parameter structure.* `init_fun` is a function with input `(PRNGKey, *init_args)` returning an Array with `init_args` the arguments needed to call the initialisation function* `init_args` the arguments to provide to the initialization function.Such params can also be declared in the `setup` method, it won't be able to use shape inference because Flax is using lazy initialization at the first call site. Variables and collections of variablesAs we've seen so far, working with models means working with:* A subclass of `nn.Module`;* A pytree of parameters for the model (typically from `model.init()`);However this is not enough to cover everything that we would need for machine learning, especially neural networks. In some cases, you might want your neural network to keep track of some internal state while it runs (e.g. batch normalization layers). There is a way to declare variables beyond the parameters of the model with the `variable` method.For demonstration purposes, we'll implement a simplified but similar mechanism to batch normalization: we'll store running averages and subtract those to the input at training time. For proper batchnorm, you should use (and look at) the implementation [here](https://github.com/google/flax/blob/master/flax/linen/normalization.py).
###Code
class BiasAdderWithRunningMean(nn.Module):
decay: float = 0.99
@nn.compact
def __call__(self, x):
# easy pattern to detect if we're initializing via empty variable tree
is_initialized = self.has_variable('batch_stats', 'mean')
ra_mean = self.variable('batch_stats', 'mean',
lambda s: jnp.zeros(s),
x.shape[1:])
mean = ra_mean.value # This will get either the value, or trigger init
bias = self.param('bias', lambda rng, shape: jnp.zeros(shape), x.shape[1:])
if is_initialized:
ra_mean.value = self.decay * ra_mean.value + (1.0 - self.decay) * jnp.mean(x, axis=0, keepdims=True)
return x - ra_mean.value + bias
key1, key2 = random.split(random.PRNGKey(0), 2)
x = jnp.ones((10,5))
model = BiasAdderWithRunningMean()
variables = model.init(key1, x)
print('initialized variables:\n', variables)
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
print('updated state:\n', updated_state)
###Output
initialized variables:
FrozenDict({'batch_stats': {'mean': DeviceArray([0., 0., 0., 0., 0.], dtype=float32)}, 'params': {'bias': DeviceArray([0., 0., 0., 0., 0.], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
###Markdown
Here, `updated_state` returns only the state variables that are being mutated by the model while applying it on data. To update the variables and get the new parameters of the model, we can use the following pattern:
###Code
for val in [1.0, 2.0, 3.0]:
x = val * jnp.ones((10,5))
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
old_state, params = variables.pop('params')
variables = freeze({'params': params, **updated_state})
print('updated state:\n', updated_state) # Shows only the mutable part
###Output
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.0299, 0.0299, 0.0299, 0.0299, 0.0299]], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.059601, 0.059601, 0.059601, 0.059601, 0.059601]], dtype=float32)}})
###Markdown
From this simplified example, you should be able to derive a full BatchNorm implementation, or any layer involving a state. To finish, let's add an optimizer to see how to play with both parameters updated by an optimizer and state variables.*This example isn't doing anything and is only for demonstration purposes.*
###Code
def update_step(apply_fun, x, optimizer, state):
def loss(params):
y, updated_state = apply_fun({'params': params, **state},
x, mutable=list(state.keys()))
l = ((x - y) ** 2).sum()
return l, updated_state
(l, updated_state), grads = jax.value_and_grad(
loss, has_aux=True)(optimizer.target)
optimizer = optimizer.apply_gradient(grads)
return optimizer, updated_state
variables = model.init(random.PRNGKey(0), x)
state, params = variables.pop('params')
del variables
optimizer = optim.sgd.GradientDescent(learning_rate=0.02).create(params)
x = jnp.ones((10,5))
for _ in range(3):
optimizer, state = update_step(model.apply, x, optimizer, state)
print('Updated state: ', state)
###Output
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.0199, 0.0199, 0.0199, 0.0199, 0.0199]], dtype=float32)}})
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.029701, 0.029701, 0.029701, 0.029701, 0.029701]], dtype=float32)}})
###Markdown
Flax BasicsThis notebook will walk you through the following workflow:* Instantiating a model from Flax built-in layers or third-party models.* Initializing parameters of the model and manually written training.* Using optimizers provided by Flax to ease training.* Serialization of parameters and other objects.* Creating your own models and managing state. Setting up our environmentHere we provide the code needed to set up the environment for our notebook.
###Code
# Install the latest JAXlib version.
!pip install --upgrade -q pip jax jaxlib
# Install Flax at head:
!pip install --upgrade -q git+https://github.com/google/flax.git
import jax
from typing import Any, Callable, Sequence, Optional
from jax import lax, random, numpy as jnp
import flax
from flax.core import freeze, unfreeze
from flax import linen as nn
###Output
_____no_output_____
###Markdown
Linear regression with FlaxIn the previous *JAX for the impatient* notebook, we finished up with a linear regression example. As we know, linear regression can also be written as a single dense neural network layer, which we will show in the following so that we can compare how it's done.A dense layer is a layer that has a kernel parameter $W\in\mathcal{M}_{m,n}(\mathbb{R})$ where $m$ is the number of features as an output of the model, and $n$ the dimensionality of the input, and a bias parameter $b\in\mathbb{R}^m$. The dense layers returns $Wx+b$ from an input $x\in\mathbb{R}^n$.This dense layer is already provided by Flax in the `flax.linen` module (here imported as `nn`).
###Code
# We create one dense layer instance (taking 'features' parameter as input)
model = nn.Dense(features=5)
###Output
_____no_output_____
###Markdown
Layers (and models in general, we'll use that word from now on) are subclasses of the `linen.Module` class. Model parameters & initializationParameters are not stored with the models themselves. You need to initialize parameters by calling the `init` function, using a PRNGKey and a dummy input parameter.
###Code
key1, key2 = random.split(random.PRNGKey(0))
x = random.normal(key1, (10,)) # Dummy input
params = model.init(key2, x) # Initialization call
jax.tree_map(lambda x: x.shape, params) # Checking output shapes
###Output
WARNING:absl:No GPU/TPU found, falling back to CPU. (Set TF_CPP_MIN_LOG_LEVEL=0 and rerun for more info.)
###Markdown
*Note: JAX and Flax, like NumPy, are row-based systems, meaning that vectors are represented as row vectors and not column vectors. This can be seen in the shape of the kernel here.*The result is what we expect: bias and kernel parameters of the correct size. Under the hood:* The dummy input variable `x` is used to trigger shape inference: we only declared the number of features we wanted in the output of the model, not the size of the input. Flax finds out by itself the correct size of the kernel.* The random PRNG key is used to trigger the initialization functions (those have default values provided by the module here).* Initialization functions are called to generate the initial set of parameters that the model will use. Those are functions that take as arguments `(PRNG Key, shape, dtype)` and return an Array of shape `shape`.* The init function returns the initialized set of parameters (you can also get the output of the evaluation on the dummy input with the same syntax but using the `init_with_output` method instead of `init`. We see in the output that parameters are stored in a `FrozenDict` instance which helps deal with the functional nature of JAX by preventing any mutation of the underlying dict and making the user aware of it. Read more about it in the Flax docs. As a consequence, the following doesn't work:
###Code
try:
params['new_key'] = jnp.ones((2,2))
except ValueError as e:
print("Error: ", e)
###Output
Error: FrozenDict is immutable.
###Markdown
To evaluate the model with a given set of parameters (never stored with the model), we just use the `apply` method by providing it the parameters to use as well as the input:
###Code
model.apply(params, x)
###Output
_____no_output_____
###Markdown
Gradient descentIf you jumped here directly without going through the JAX part, here is the linear regression formulation we're going to use: from a set of data points $\{(x_i,y_i), i\in \{1,\ldots, k\}, x_i\in\mathbb{R}^n,y_i\in\mathbb{R}^m\}$, we try to find a set of parameters $W\in \mathcal{M}_{m,n}(\mathbb{R}), b\in\mathbb{R}^m$ such that the function $f_{W,b}(x)=Wx+b$ minimizes the mean squared error:$$\mathcal{L}(W,b)\rightarrow\frac{1}{k}\sum_{i=1}^{k} \frac{1}{2}\|y_i-f_{W,b}(x_i)\|^2_2$$Here, we see that the tuple $(W,b)$ matches the parameters of the Dense layer. We'll perform gradient descent using those. Let's first generate the fake data we'll use.
###Code
# Set problem dimensions
nsamples = 20
xdim = 10
ydim = 5
# Generate random ground truth W and b
key = random.PRNGKey(0)
k1, k2 = random.split(key)
W = random.normal(k1, (xdim, ydim))
b = random.normal(k2, (ydim,))
true_params = freeze({'params': {'bias': b, 'kernel': W}})
# Generate samples with additional noise
ksample, knoise = random.split(k1)
x_samples = random.normal(ksample, (nsamples, xdim))
y_samples = jnp.dot(x_samples, W) + b
y_samples += 0.1*random.normal(knoise,(nsamples, ydim)) # Adding noise
print('x shape:', x_samples.shape, '; y shape:', y_samples.shape)
###Output
x shape: (20, 10) ; y shape: (20, 5)
###Markdown
Now let's generate the loss function (mean squared error) with that data.
###Code
def make_mse_func(x_batched, y_batched):
def mse(params):
# Define the squared loss for a single pair (x,y)
def squared_error(x, y):
pred = model.apply(params, x)
return jnp.inner(y-pred, y-pred)/2.0
# We vectorize the previous to compute the average of the loss on all samples.
return jnp.mean(jax.vmap(squared_error)(x_batched,y_batched), axis=0)
return jax.jit(mse) # And finally we jit the result.
# Get the sampled loss
loss = make_mse_func(x_samples, y_samples)
###Output
_____no_output_____
###Markdown
And finally perform the gradient descent.
###Code
alpha = 0.3 # Gradient step size
print('Loss for "true" W,b: ', loss(true_params))
grad_fn = jax.value_and_grad(loss)
for i in range(101):
# We perform one gradient update
loss_val, grads = grad_fn(params)
params = jax.tree_multimap(lambda p, g: p - alpha * g,
params, grads)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss for "true" W,b: 0.023639778
Loss step 0: 38.094772
Loss step 10: 0.44692168
Loss step 20: 0.10053458
Loss step 30: 0.035822745
Loss step 40: 0.018846875
Loss step 50: 0.013864839
Loss step 60: 0.012312559
Loss step 70: 0.011812928
Loss step 80: 0.011649306
Loss step 90: 0.011595251
Loss step 100: 0.0115773035
###Markdown
Optimizing with OptaxFlax used to use its own `flax.optim` package for optimization, but with[FLIP 1009](https://github.com/google/flax/blob/main/docs/flip/1009-optimizer-api.md)this was deprecated in favor of[Optax](https://github.com/deepmind/optax).Basic usage of Optax is straightforward:1. Choose an optimization method (e.g. `optax.sgd`).2. Create optimizer state from parameters.3. Compute the gradients of your loss with `jax.value_and_grad()`.4. At every iteration, call the Optax `update` function to update the internal optimizer state and create an update to the parameters. Then add the update to the parameters with Optax's `apply_updates` method.Note that Optax can do a lot more: it's designed for composing simple gradienttransformations into more complex transformations that allows to implement awide range of optimizers. There is also support for changing optimizerhyperparameters over time ("schedules"), applying different updates to differentparts of the parameter tree ("masking") and much more. For details please referto the[official documentation](https://optax.readthedocs.io/en/latest/).
###Code
import optax
tx = optax.sgd(learning_rate=alpha)
opt_state = tx.init(params)
loss_grad_fn = jax.value_and_grad(loss)
for i in range(101):
loss_val, grads = loss_grad_fn(params)
updates, opt_state = tx.update(grads, opt_state)
params = optax.apply_updates(params, updates)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss step 0: 0.011576377
Loss step 10: 0.0115710115
Loss step 20: 0.011569244
Loss step 30: 0.011568661
Loss step 40: 0.011568454
Loss step 50: 0.011568379
Loss step 60: 0.011568358
Loss step 70: 0.01156836
Loss step 80: 0.01156835
Loss step 90: 0.011568353
Loss step 100: 0.011568348
###Markdown
Serializing the resultNow that we're happy with the result of our training, we might want to save the model parameters to load them back later. Flax provides a serialization package to enable you to do that.
###Code
from flax import serialization
bytes_output = serialization.to_bytes(params)
dict_output = serialization.to_state_dict(params)
print('Dict output')
print(dict_output)
print('Bytes output')
print(bytes_output)
###Output
Dict output
{'params': {'bias': DeviceArray([-1.4540135, -2.0262308, 2.0806582, 1.2201802, -0.9964547], dtype=float32), 'kernel': DeviceArray([[ 1.0106664 , 0.19014716, 0.04533899, -0.92722285,
0.34720102],
[ 1.7320251 , 0.9901233 , 1.1662225 , 1.1027892 ,
-0.10574618],
[-1.2009128 , 0.28837162, 1.4176372 , 0.12073109,
-1.3132601 ],
[-1.1944956 , -0.18993308, 0.03379077, 1.3165942 ,
0.07996067],
[ 0.14103189, 1.3737966 , -1.3162128 , 0.53401774,
-2.239638 ],
[ 0.5643044 , 0.813604 , 0.31888172, 0.5359193 ,
0.90352124],
[-0.37948322, 1.7408353 , 1.0788013 , -0.5041964 ,
0.9286919 ],
[ 0.9701384 , -1.3158673 , 0.33630812, 0.80941117,
-1.202457 ],
[ 1.0198247 , -0.6198277 , 1.0822718 , -1.8385581 ,
-0.45790705],
[-0.64384323, 0.4564892 , -1.1331053 , -0.68556863,
0.17010891]], dtype=float32)}}
Bytes output
b'\x81\xa6params\x82\xa4bias\xc7!\x01\x93\x91\x05\xa7float32\xc4\x14\x1d\x1d\xba\xbf\xc4\xad\x01\xc0\x81)\x05@\xdd.\x9c?\xa8\x17\x7f\xbf\xa6kernel\xc7\xd6\x01\x93\x92\n\x05\xa7float32\xc4\xc8\x84]\x81?\xf0\xb5B>`\xb59=z^m\xbfU\xc4\xb1>\x00\xb3\xdd?\xb8x}?\xc7F\x95?2(\x8d?t\x91\xd8\xbd\x83\xb7\x99\xbfr\xa5\x93>#u\xb5?\xdcA\xf7=\xe8\x18\xa8\xbf;\xe5\x98\xbf\xd1}B\xbe0h\n=)\x86\xa8?k\xc2\xa3=\xaaj\x10>\x91\xd8\xaf?\xa9y\xa8\xbfc\xb5\x08?;V\x0f\xc0Av\x10?ZHP?wD\xa3>\x022\t?+Mg?\xa0K\xc2\xbe\xb1\xd3\xde?)\x16\x8a?\x04\x13\x01\xbf\xc1\xbem?\xfdZx?Wn\xa8\xbf\x940\xac>\x925O?\x1c\xea\x99\xbf\x9e\x89\x82?\x07\xad\x1e\xbf\xe2\x87\x8a?\xdfU\xeb\xbf\xcbr\xea\xbe\xe9\xd2$\xbf\xf4\xb8\xe9>\x98\t\x91\xbfm\x81/\xbf\x081.>'
###Markdown
To load the model back, you'll need to use as a template the model parameter structure, like the one you would get from the model initialization. Here, we use the previously generated `params` as a template. Note that this will produce a new variable structure, and not mutate in-place.*The point of enforcing structure through template is to avoid users issues downstream, so you need to first have the right model that generates the parameters structure.*
###Code
serialization.from_bytes(params, bytes_output)
###Output
_____no_output_____
###Markdown
Defining your own modelsFlax allows you to define your own models, which should be a bit more complicated than a linear regression. In this section, we'll show you how to build simple models. To do so, you'll need to create subclasses of the base `nn.Module` class.*Keep in mind that we imported* `linen as nn` *and this only works with the new linen API* Module basicsThe base abstraction for models is the `nn.Module` class, and every type of predefined layers in Flax (like the previous `Dense`) is a subclass of `nn.Module`. Let's take a look and start by defining a simple but custom multi-layer perceptron i.e. a sequence of Dense layers interleaved with calls to a non-linear activation function.
###Code
class ExplicitMLP(nn.Module):
features: Sequence[int]
def setup(self):
# we automatically know what to do with lists, dicts of submodules
self.layers = [nn.Dense(feat) for feat in self.features]
# for single submodules, we would just write:
# self.layer1 = nn.Dense(feat1)
def __call__(self, inputs):
x = inputs
for i, lyr in enumerate(self.layers):
x = lyr(x)
if i != len(self.layers) - 1:
x = nn.relu(x)
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = ExplicitMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292815e-02 -4.3807115e-02 2.9323792e-02 6.5492536e-03
-1.7147182e-02]
[ 1.2967806e-01 -1.4551792e-01 9.4432183e-02 1.2521387e-02
-4.5417298e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
As we can see, a `nn.Module` subclass is made of:* A collection of data fields (`nn.Module` are Python dataclasses) - here we only have the `features` field of type `Sequence[int]`.* A `setup()` method that is being called at the end of the `__postinit__` where you can register submodules, variables, parameters you will need in your model.* A `__call__` function that returns the output of the model from a given input.* The model structure defines a pytree of parameters following the same tree structure as the model: the params tree contains one `layers_n` sub dict per layer, and each of those contain the parameters of the associated Dense layer. The layout is very explicit.*Note: lists are mostly managed as you would expect (WIP), there are corner cases you should be aware of as pointed out* [here](https://github.com/google/flax/issues/524)Since the module structure and its parameters are not tied to each other, you can't directly call `model(x)` on a given input as it will return an error. The `__call__` function is being wrapped up in the `apply` one, which is the one to call on an input:
###Code
try:
y = model(x) # Returns an error
except AttributeError as e:
print(e)
###Output
"ExplicitMLP" object has no attribute "layers"
###Markdown
Since here we have a very simple model, we could have used an alternative (but equivalent) way of declaring the submodules inline in the `__call__` using the `@nn.compact` annotation like so:
###Code
class SimpleMLP(nn.Module):
features: Sequence[int]
@nn.compact
def __call__(self, inputs):
x = inputs
for i, feat in enumerate(self.features):
x = nn.Dense(feat, name=f'layers_{i}')(x)
if i != len(self.features) - 1:
x = nn.relu(x)
# providing a name is optional though!
# the default autonames would be "Dense_0", "Dense_1", ...
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292815e-02 -4.3807115e-02 2.9323792e-02 6.5492536e-03
-1.7147182e-02]
[ 1.2967806e-01 -1.4551792e-01 9.4432183e-02 1.2521387e-02
-4.5417298e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
There are, however, a few differences you should be aware of between the two declaration modes:* In `setup`, you are able to name some sublayers and keep them around for further use (e.g. encoder/decoder methods in autoencoders).* If you want to have multiple methods, then you **need** to declare the module using `setup`, as the `@nn.compact` annotation only allows one method to be annotated.* The last initialization will be handled differently. See these notes for more details (TODO: add notes link). Module parametersIn the previous MLP example, we relied only on predefined layers and operators (`Dense`, `relu`). Let's imagine that you didn't have a Dense layer provided by Flax and you wanted to write it on your own. Here is what it would look like using the `@nn.compact` way to declare a new modules:
###Code
class SimpleDense(nn.Module):
features: int
kernel_init: Callable = nn.initializers.lecun_normal()
bias_init: Callable = nn.initializers.zeros
@nn.compact
def __call__(self, inputs):
kernel = self.param('kernel',
self.kernel_init, # Initialization function
(inputs.shape[-1], self.features)) # shape info.
y = lax.dot_general(inputs, kernel,
(((inputs.ndim - 1,), (0,)), ((), ())),) # TODO Why not jnp.dot?
bias = self.param('bias', self.bias_init, (self.features,))
y = y + bias
return y
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleDense(features=3)
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameters:\n', params)
print('output:\n', y)
###Output
initialized parameters:
FrozenDict({
params: {
kernel: DeviceArray([[ 0.6503669 , 0.86789787, 0.4604268 ],
[ 0.05673932, 0.9909285 , -0.63536596],
[ 0.76134115, -0.3250529 , -0.65221626],
[-0.82430327, 0.4150194 , 0.19405058]], dtype=float32),
bias: DeviceArray([0., 0., 0.], dtype=float32),
},
})
output:
[[ 0.5035518 1.8548558 -0.4270195 ]
[ 0.0279097 0.5589246 -0.43061772]
[ 0.3547128 1.5740999 -0.32865518]
[ 0.5264864 1.2928858 0.10089308]]
###Markdown
Here, we see how to both declare and assign a parameter to the model using the `self.param` method. It takes as input `(name, init_fn, *init_args)` : * `name` is simply the name of the parameter that will end up in the parameter structure.* `init_fn` is a function with input `(PRNGKey, *init_args)` returning an Array, with `init_args` being the arguments needed to call the initialisation function.* `init_args` are the arguments to provide to the initialization function.Such params can also be declared in the `setup` method; it won't be able to use shape inference because Flax is using lazy initialization at the first call site. Variables and collections of variablesAs we've seen so far, working with models means working with:* A subclass of `nn.Module`;* A pytree of parameters for the model (typically from `model.init()`);However this is not enough to cover everything that we would need for machine learning, especially neural networks. In some cases, you might want your neural network to keep track of some internal state while it runs (e.g. batch normalization layers). There is a way to declare variables beyond the parameters of the model with the `variable` method.For demonstration purposes, we'll implement a simplified but similar mechanism to batch normalization: we'll store running averages and subtract those to the input at training time. For proper batchnorm, you should use (and look at) the implementation [here](https://github.com/google/flax/blob/main/flax/linen/normalization.py).
###Code
class BiasAdderWithRunningMean(nn.Module):
decay: float = 0.99
@nn.compact
def __call__(self, x):
# easy pattern to detect if we're initializing via empty variable tree
is_initialized = self.has_variable('batch_stats', 'mean')
ra_mean = self.variable('batch_stats', 'mean',
lambda s: jnp.zeros(s),
x.shape[1:])
mean = ra_mean.value # This will either get the value or trigger init
bias = self.param('bias', lambda rng, shape: jnp.zeros(shape), x.shape[1:])
if is_initialized:
ra_mean.value = self.decay * ra_mean.value + (1.0 - self.decay) * jnp.mean(x, axis=0, keepdims=True)
return x - ra_mean.value + bias
key1, key2 = random.split(random.PRNGKey(0), 2)
x = jnp.ones((10,5))
model = BiasAdderWithRunningMean()
variables = model.init(key1, x)
print('initialized variables:\n', variables)
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
print('updated state:\n', updated_state)
###Output
initialized variables:
FrozenDict({
batch_stats: {
mean: DeviceArray([0., 0., 0., 0., 0.], dtype=float32),
},
params: {
bias: DeviceArray([0., 0., 0., 0., 0.], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
###Markdown
Here, `updated_state` returns only the state variables that are being mutated by the model while applying it on data. To update the variables and get the new parameters of the model, we can use the following pattern:
###Code
for val in [1.0, 2.0, 3.0]:
x = val * jnp.ones((10,5))
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
old_state, params = variables.pop('params')
variables = freeze({'params': params, **updated_state})
print('updated state:\n', updated_state) # Shows only the mutable part
###Output
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.0299, 0.0299, 0.0299, 0.0299, 0.0299]], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.059601, 0.059601, 0.059601, 0.059601, 0.059601]], dtype=float32),
},
})
###Markdown
From this simplified example, you should be able to derive a full BatchNorm implementation, or any layer involving a state. To finish, let's add an optimizer to see how to play with both parameters updated by an optimizer and state variables.*This example isn't doing anything and is only for demonstration purposes.*
###Code
def update_step(tx, apply_fn, x, opt_state, params, state):
def loss(params):
y, updated_state = apply_fn({'params': params, **state},
x, mutable=list(state.keys()))
l = ((x - y) ** 2).sum()
return l, updated_state
(l, state), grads = jax.value_and_grad(loss, has_aux=True)(params)
updates, opt_state = tx.update(grads, opt_state)
params = optax.apply_updates(params, updates)
return opt_state, params, state
x = jnp.ones((10,5))
variables = model.init(random.PRNGKey(0), x)
state, params = variables.pop('params')
del variables
tx = optax.sgd(learning_rate=0.02)
opt_state = tx.init(params)
for _ in range(3):
opt_state, params, state = update_step(tx, model.apply, x, opt_state, params, state)
print('Updated state: ', state)
###Output
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.0199, 0.0199, 0.0199, 0.0199, 0.0199]], dtype=float32),
},
})
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.029701, 0.029701, 0.029701, 0.029701, 0.029701]], dtype=float32),
},
})
###Markdown
Flax BasicsThis notebook will walk you through the following workflow:* Instantiating a model from Flax built-in layers or third-party models.* Initializing parameters of the model and manually written training.* Using optimizers provided by Flax to ease training.* Serialization of parameters and other objects.* Creating your own models and managing state. Setting up our environmentHere we provide the code needed to set up the environment for our notebook.
###Code
# Install the latest JAXlib version.
!pip install --upgrade -q pip jax jaxlib
# Install Flax at head:
!pip install --upgrade -q git+https://github.com/google/flax.git
import jax
from typing import Any, Callable, Sequence, Optional
from jax import lax, random, numpy as jnp
import flax
from flax.core import freeze, unfreeze
from flax import linen as nn
###Output
_____no_output_____
###Markdown
Linear regression with FlaxIn the previous *JAX for the impatient* notebook, we finished up with a linear regression example. As we know, linear regression can also be written as a single dense neural network layer, which we will show in the following so that we can compare how it's done.A dense layer is a layer that has a kernel parameter $W\in\mathcal{M}_{m,n}(\mathbb{R})$ where $m$ is the number of features as an output of the model, and $n$ the dimensionality of the input, and a bias parameter $b\in\mathbb{R}^m$. The dense layers returns $Wx+b$ from an input $x\in\mathbb{R}^n$.This dense layer is already provided by Flax in the `flax.linen` module (here imported as `nn`).
###Code
# We create one dense layer instance (taking 'features' parameter as input)
model = nn.Dense(features=5)
###Output
_____no_output_____
###Markdown
Layers (and models in general, we'll use that word from now on) are subclasses of the `linen.Module` class. Model parameters & initializationParameters are not stored with the models themselves. You need to initialize parameters by calling the `init` function, using a PRNGKey and a dummy input parameter.
###Code
key1, key2 = random.split(random.PRNGKey(0))
x = random.normal(key1, (10,)) # Dummy input
params = model.init(key2, x) # Initialization call
jax.tree_map(lambda x: x.shape, params) # Checking output shapes
###Output
WARNING:absl:No GPU/TPU found, falling back to CPU. (Set TF_CPP_MIN_LOG_LEVEL=0 and rerun for more info.)
###Markdown
*Note: JAX and Flax, like NumPy, are row-based systems, meaning that vectors are represented as row vectors and not column vectors. This can be seen in the shape of the kernel here.*The result is what we expect: bias and kernel parameters of the correct size. Under the hood:* The dummy input variable `x` is used to trigger shape inference: we only declared the number of features we wanted in the output of the model, not the size of the input. Flax finds out by itself the correct size of the kernel.* The random PRNG key is used to trigger the initialization functions (those have default values provided by the module here).* Initialization functions are called to generate the initial set of parameters that the model will use. Those are functions that take as arguments `(PRNG Key, shape, dtype)` and return an Array of shape `shape`.* The init function returns the initialized set of parameters (you can also get the output of the evaluation on the dummy input with the same syntax but using the `init_with_output` method instead of `init`. We see in the output that parameters are stored in a `FrozenDict` instance which helps deal with the functional nature of JAX by preventing any mutation of the underlying dict and making the user aware of it. Read more about it in the Flax docs. As a consequence, the following doesn't work:
###Code
try:
params['new_key'] = jnp.ones((2,2))
except ValueError as e:
print("Error: ", e)
###Output
Error: FrozenDict is immutable.
###Markdown
To evaluate the model with a given set of parameters (never stored with the model), we just use the `apply` method by providing it the parameters to use as well as the input:
###Code
model.apply(params, x)
###Output
_____no_output_____
###Markdown
Gradient descentIf you jumped here directly without going through the JAX part, here is the linear regression formulation we're going to use: from a set of data points $\{(x_i,y_i), i\in \{1,\ldots, k\}, x_i\in\mathbb{R}^n,y_i\in\mathbb{R}^m\}$, we try to find a set of parameters $W\in \mathcal{M}_{m,n}(\mathbb{R}), b\in\mathbb{R}^m$ such that the function $f_{W,b}(x)=Wx+b$ minimizes the mean squared error:$$\mathcal{L}(W,b)\rightarrow\frac{1}{k}\sum_{i=1}^{k} \frac{1}{2}\|y_i-f_{W,b}(x_i)\|^2_2$$Here, we see that the tuple $(W,b)$ matches the parameters of the Dense layer. We'll perform gradient descent using those. Let's first generate the fake data we'll use. The data is exactly the same as in the JAX part's linear regression pytree example.
###Code
# Set problem dimensions.
n_samples = 20
x_dim = 10
y_dim = 5
# Generate random ground truth W and b.
key = random.PRNGKey(0)
k1, k2 = random.split(key)
W = random.normal(k1, (x_dim, y_dim))
b = random.normal(k2, (y_dim,))
# Store the parameters in a pytree.
true_params = freeze({'params': {'bias': b, 'kernel': W}})
# Generate samples with additional noise.
key_sample, key_noise = random.split(k1)
x_samples = random.normal(key_sample, (n_samples, x_dim))
y_samples = jnp.dot(x_samples, W) + b + 0.1 * random.normal(key_noise,(n_samples, y_dim))
print('x shape:', x_samples.shape, '; y shape:', y_samples.shape)
###Output
x shape: (20, 10) ; y shape: (20, 5)
###Markdown
We copy the same training loop that we used in the JAX pytree linear regression example with `jax.value_and_grad()`, but here we can use `model.apply()` instead of having to define our own feed-forward function (`predict_pytree()` in the JAX example).
###Code
# Same as JAX version but using model.apply().
def mse(params, x_batched, y_batched):
# Define the squared loss for a single pair (x,y)
def squared_error(x, y):
pred = model.apply(params, x)
return jnp.inner(y-pred, y-pred) / 2.0
# Vectorize the previous to compute the average of the loss on all samples.
return jnp.mean(jax.vmap(squared_error)(x_batched,y_batched), axis=0)
###Output
_____no_output_____
###Markdown
And finally perform the gradient descent.
###Code
learning_rate = 0.3 # Gradient step size.
print('Loss for "true" W,b: ', mse(true_params, x_samples, y_samples))
loss_grad_fn = jax.value_and_grad(mse)
@jax.jit
def update_params(params, learning_rate, grads):
params = jax.tree_map(
lambda p, g: p - learning_rate * g, params, grads)
return params
for i in range(101):
# Perform one gradient update.
loss_val, grads = loss_grad_fn(params, x_samples, y_samples)
params = update_params(params, learning_rate, grads)
if i % 10 == 0:
print(f'Loss step {i}: ', loss_val)
###Output
Loss for "true" W,b: 0.023639778
Loss step 0: 38.094772
Loss step 10: 0.44692168
Loss step 20: 0.10053458
Loss step 30: 0.035822745
Loss step 40: 0.018846875
Loss step 50: 0.013864839
Loss step 60: 0.012312559
Loss step 70: 0.011812928
Loss step 80: 0.011649306
Loss step 90: 0.011595251
Loss step 100: 0.0115773035
###Markdown
Optimizing with OptaxFlax used to use its own `flax.optim` package for optimization, but with[FLIP 1009](https://github.com/google/flax/blob/main/docs/flip/1009-optimizer-api.md)this was deprecated in favor of[Optax](https://github.com/deepmind/optax).Basic usage of Optax is straightforward:1. Choose an optimization method (e.g. `optax.sgd`).2. Create optimizer state from parameters.3. Compute the gradients of your loss with `jax.value_and_grad()`.4. At every iteration, call the Optax `update` function to update the internal optimizer state and create an update to the parameters. Then add the update to the parameters with Optax's `apply_updates` method.Note that Optax can do a lot more: it's designed for composing simple gradienttransformations into more complex transformations that allows to implement awide range of optimizers. There is also support for changing optimizerhyperparameters over time ("schedules"), applying different updates to differentparts of the parameter tree ("masking") and much more. For details please referto the[official documentation](https://optax.readthedocs.io/en/latest/).
###Code
import optax
tx = optax.sgd(learning_rate=learning_rate)
opt_state = tx.init(params)
loss_grad_fn = jax.value_and_grad(mse)
for i in range(101):
loss_val, grads = loss_grad_fn(params, x_samples, y_samples)
updates, opt_state = tx.update(grads, opt_state)
params = optax.apply_updates(params, updates)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss step 0: 0.011576377
Loss step 10: 0.0115710115
Loss step 20: 0.011569244
Loss step 30: 0.011568661
Loss step 40: 0.011568454
Loss step 50: 0.011568379
Loss step 60: 0.011568358
Loss step 70: 0.01156836
Loss step 80: 0.01156835
Loss step 90: 0.011568353
Loss step 100: 0.011568348
###Markdown
Serializing the resultNow that we're happy with the result of our training, we might want to save the model parameters to load them back later. Flax provides a serialization package to enable you to do that.
###Code
from flax import serialization
bytes_output = serialization.to_bytes(params)
dict_output = serialization.to_state_dict(params)
print('Dict output')
print(dict_output)
print('Bytes output')
print(bytes_output)
###Output
Dict output
{'params': {'bias': DeviceArray([-1.4540135, -2.0262308, 2.0806582, 1.2201802, -0.9964547], dtype=float32), 'kernel': DeviceArray([[ 1.0106664 , 0.19014716, 0.04533899, -0.92722285,
0.34720102],
[ 1.7320251 , 0.9901233 , 1.1662225 , 1.1027892 ,
-0.10574618],
[-1.2009128 , 0.28837162, 1.4176372 , 0.12073109,
-1.3132601 ],
[-1.1944956 , -0.18993308, 0.03379077, 1.3165942 ,
0.07996067],
[ 0.14103189, 1.3737966 , -1.3162128 , 0.53401774,
-2.239638 ],
[ 0.5643044 , 0.813604 , 0.31888172, 0.5359193 ,
0.90352124],
[-0.37948322, 1.7408353 , 1.0788013 , -0.5041964 ,
0.9286919 ],
[ 0.9701384 , -1.3158673 , 0.33630812, 0.80941117,
-1.202457 ],
[ 1.0198247 , -0.6198277 , 1.0822718 , -1.8385581 ,
-0.45790705],
[-0.64384323, 0.4564892 , -1.1331053 , -0.68556863,
0.17010891]], dtype=float32)}}
Bytes output
b'\x81\xa6params\x82\xa4bias\xc7!\x01\x93\x91\x05\xa7float32\xc4\x14\x1d\x1d\xba\xbf\xc4\xad\x01\xc0\x81)\x05@\xdd.\x9c?\xa8\x17\x7f\xbf\xa6kernel\xc7\xd6\x01\x93\x92\n\x05\xa7float32\xc4\xc8\x84]\x81?\xf0\xb5B>`\xb59=z^m\xbfU\xc4\xb1>\x00\xb3\xdd?\xb8x}?\xc7F\x95?2(\x8d?t\x91\xd8\xbd\x83\xb7\x99\xbfr\xa5\x93>#u\xb5?\xdcA\xf7=\xe8\x18\xa8\xbf;\xe5\x98\xbf\xd1}B\xbe0h\n=)\x86\xa8?k\xc2\xa3=\xaaj\x10>\x91\xd8\xaf?\xa9y\xa8\xbfc\xb5\x08?;V\x0f\xc0Av\x10?ZHP?wD\xa3>\x022\t?+Mg?\xa0K\xc2\xbe\xb1\xd3\xde?)\x16\x8a?\x04\x13\x01\xbf\xc1\xbem?\xfdZx?Wn\xa8\xbf\x940\xac>\x925O?\x1c\xea\x99\xbf\x9e\x89\x82?\x07\xad\x1e\xbf\xe2\x87\x8a?\xdfU\xeb\xbf\xcbr\xea\xbe\xe9\xd2$\xbf\xf4\xb8\xe9>\x98\t\x91\xbfm\x81/\xbf\x081.>'
###Markdown
To load the model back, you'll need to use as a template the model parameter structure, like the one you would get from the model initialization. Here, we use the previously generated `params` as a template. Note that this will produce a new variable structure, and not mutate in-place.*The point of enforcing structure through template is to avoid users issues downstream, so you need to first have the right model that generates the parameters structure.*
###Code
serialization.from_bytes(params, bytes_output)
###Output
_____no_output_____
###Markdown
Defining your own modelsFlax allows you to define your own models, which should be a bit more complicated than a linear regression. In this section, we'll show you how to build simple models. To do so, you'll need to create subclasses of the base `nn.Module` class.*Keep in mind that we imported* `linen as nn` *and this only works with the new linen API* Module basicsThe base abstraction for models is the `nn.Module` class, and every type of predefined layers in Flax (like the previous `Dense`) is a subclass of `nn.Module`. Let's take a look and start by defining a simple but custom multi-layer perceptron i.e. a sequence of Dense layers interleaved with calls to a non-linear activation function.
###Code
class ExplicitMLP(nn.Module):
features: Sequence[int]
def setup(self):
# we automatically know what to do with lists, dicts of submodules
self.layers = [nn.Dense(feat) for feat in self.features]
# for single submodules, we would just write:
# self.layer1 = nn.Dense(feat1)
def __call__(self, inputs):
x = inputs
for i, lyr in enumerate(self.layers):
x = lyr(x)
if i != len(self.layers) - 1:
x = nn.relu(x)
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = ExplicitMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292815e-02 -4.3807115e-02 2.9323792e-02 6.5492536e-03
-1.7147182e-02]
[ 1.2967806e-01 -1.4551792e-01 9.4432183e-02 1.2521387e-02
-4.5417298e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
As we can see, a `nn.Module` subclass is made of:* A collection of data fields (`nn.Module` are Python dataclasses) - here we only have the `features` field of type `Sequence[int]`.* A `setup()` method that is being called at the end of the `__postinit__` where you can register submodules, variables, parameters you will need in your model.* A `__call__` function that returns the output of the model from a given input.* The model structure defines a pytree of parameters following the same tree structure as the model: the params tree contains one `layers_n` sub dict per layer, and each of those contain the parameters of the associated Dense layer. The layout is very explicit.*Note: lists are mostly managed as you would expect (WIP), there are corner cases you should be aware of as pointed out* [here](https://github.com/google/flax/issues/524)Since the module structure and its parameters are not tied to each other, you can't directly call `model(x)` on a given input as it will return an error. The `__call__` function is being wrapped up in the `apply` one, which is the one to call on an input:
###Code
try:
y = model(x) # Returns an error
except AttributeError as e:
print(e)
###Output
"ExplicitMLP" object has no attribute "layers"
###Markdown
Since here we have a very simple model, we could have used an alternative (but equivalent) way of declaring the submodules inline in the `__call__` using the `@nn.compact` annotation like so:
###Code
class SimpleMLP(nn.Module):
features: Sequence[int]
@nn.compact
def __call__(self, inputs):
x = inputs
for i, feat in enumerate(self.features):
x = nn.Dense(feat, name=f'layers_{i}')(x)
if i != len(self.features) - 1:
x = nn.relu(x)
# providing a name is optional though!
# the default autonames would be "Dense_0", "Dense_1", ...
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292815e-02 -4.3807115e-02 2.9323792e-02 6.5492536e-03
-1.7147182e-02]
[ 1.2967806e-01 -1.4551792e-01 9.4432183e-02 1.2521387e-02
-4.5417298e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
There are, however, a few differences you should be aware of between the two declaration modes:* In `setup`, you are able to name some sublayers and keep them around for further use (e.g. encoder/decoder methods in autoencoders).* If you want to have multiple methods, then you **need** to declare the module using `setup`, as the `@nn.compact` annotation only allows one method to be annotated.* The last initialization will be handled differently. See these notes for more details (TODO: add notes link). Module parametersIn the previous MLP example, we relied only on predefined layers and operators (`Dense`, `relu`). Let's imagine that you didn't have a Dense layer provided by Flax and you wanted to write it on your own. Here is what it would look like using the `@nn.compact` way to declare a new modules:
###Code
class SimpleDense(nn.Module):
features: int
kernel_init: Callable = nn.initializers.lecun_normal()
bias_init: Callable = nn.initializers.zeros
@nn.compact
def __call__(self, inputs):
kernel = self.param('kernel',
self.kernel_init, # Initialization function
(inputs.shape[-1], self.features)) # shape info.
y = lax.dot_general(inputs, kernel,
(((inputs.ndim - 1,), (0,)), ((), ())),) # TODO Why not jnp.dot?
bias = self.param('bias', self.bias_init, (self.features,))
y = y + bias
return y
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleDense(features=3)
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameters:\n', params)
print('output:\n', y)
###Output
initialized parameters:
FrozenDict({
params: {
kernel: DeviceArray([[ 0.6503669 , 0.86789787, 0.4604268 ],
[ 0.05673932, 0.9909285 , -0.63536596],
[ 0.76134115, -0.3250529 , -0.65221626],
[-0.82430327, 0.4150194 , 0.19405058]], dtype=float32),
bias: DeviceArray([0., 0., 0.], dtype=float32),
},
})
output:
[[ 0.5035518 1.8548558 -0.4270195 ]
[ 0.0279097 0.5589246 -0.43061772]
[ 0.3547128 1.5740999 -0.32865518]
[ 0.5264864 1.2928858 0.10089308]]
###Markdown
Here, we see how to both declare and assign a parameter to the model using the `self.param` method. It takes as input `(name, init_fn, *init_args)` : * `name` is simply the name of the parameter that will end up in the parameter structure.* `init_fn` is a function with input `(PRNGKey, *init_args)` returning an Array, with `init_args` being the arguments needed to call the initialisation function.* `init_args` are the arguments to provide to the initialization function.Such params can also be declared in the `setup` method; it won't be able to use shape inference because Flax is using lazy initialization at the first call site. Variables and collections of variablesAs we've seen so far, working with models means working with:* A subclass of `nn.Module`;* A pytree of parameters for the model (typically from `model.init()`);However this is not enough to cover everything that we would need for machine learning, especially neural networks. In some cases, you might want your neural network to keep track of some internal state while it runs (e.g. batch normalization layers). There is a way to declare variables beyond the parameters of the model with the `variable` method.For demonstration purposes, we'll implement a simplified but similar mechanism to batch normalization: we'll store running averages and subtract those to the input at training time. For proper batchnorm, you should use (and look at) the implementation [here](https://github.com/google/flax/blob/main/flax/linen/normalization.py).
###Code
class BiasAdderWithRunningMean(nn.Module):
decay: float = 0.99
@nn.compact
def __call__(self, x):
# easy pattern to detect if we're initializing via empty variable tree
is_initialized = self.has_variable('batch_stats', 'mean')
ra_mean = self.variable('batch_stats', 'mean',
lambda s: jnp.zeros(s),
x.shape[1:])
mean = ra_mean.value # This will either get the value or trigger init
bias = self.param('bias', lambda rng, shape: jnp.zeros(shape), x.shape[1:])
if is_initialized:
ra_mean.value = self.decay * ra_mean.value + (1.0 - self.decay) * jnp.mean(x, axis=0, keepdims=True)
return x - ra_mean.value + bias
key1, key2 = random.split(random.PRNGKey(0), 2)
x = jnp.ones((10,5))
model = BiasAdderWithRunningMean()
variables = model.init(key1, x)
print('initialized variables:\n', variables)
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
print('updated state:\n', updated_state)
###Output
initialized variables:
FrozenDict({
batch_stats: {
mean: DeviceArray([0., 0., 0., 0., 0.], dtype=float32),
},
params: {
bias: DeviceArray([0., 0., 0., 0., 0.], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
###Markdown
Here, `updated_state` returns only the state variables that are being mutated by the model while applying it on data. To update the variables and get the new parameters of the model, we can use the following pattern:
###Code
for val in [1.0, 2.0, 3.0]:
x = val * jnp.ones((10,5))
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
old_state, params = variables.pop('params')
variables = freeze({'params': params, **updated_state})
print('updated state:\n', updated_state) # Shows only the mutable part
###Output
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.0299, 0.0299, 0.0299, 0.0299, 0.0299]], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.059601, 0.059601, 0.059601, 0.059601, 0.059601]], dtype=float32),
},
})
###Markdown
From this simplified example, you should be able to derive a full BatchNorm implementation, or any layer involving a state. To finish, let's add an optimizer to see how to play with both parameters updated by an optimizer and state variables.*This example isn't doing anything and is only for demonstration purposes.*
###Code
def update_step(tx, apply_fn, x, opt_state, params, state):
def loss(params):
y, updated_state = apply_fn({'params': params, **state},
x, mutable=list(state.keys()))
l = ((x - y) ** 2).sum()
return l, updated_state
(l, state), grads = jax.value_and_grad(loss, has_aux=True)(params)
updates, opt_state = tx.update(grads, opt_state)
params = optax.apply_updates(params, updates)
return opt_state, params, state
x = jnp.ones((10,5))
variables = model.init(random.PRNGKey(0), x)
state, params = variables.pop('params')
del variables
tx = optax.sgd(learning_rate=0.02)
opt_state = tx.init(params)
for _ in range(3):
opt_state, params, state = update_step(tx, model.apply, x, opt_state, params, state)
print('Updated state: ', state)
###Output
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.0199, 0.0199, 0.0199, 0.0199, 0.0199]], dtype=float32),
},
})
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.029701, 0.029701, 0.029701, 0.029701, 0.029701]], dtype=float32),
},
})
###Markdown
Flax BasicsThis notebook will walk you through the following workflow:* Instantiating a model from Flax built-in layers or third-party models.* Initializing parameters of the model and manually written training.* Using optimizers provided by Flax to ease training.* Serialization of parameters and other objects.* Creating your own models and managing state. Setting up our environmentHere we provide the code needed to set up the environment for our notebook.
###Code
# Install the latest JAXlib version.
!pip install --upgrade -q pip jax jaxlib
# Install Flax at head:
!pip install --upgrade -q git+https://github.com/google/flax.git
import jax
from typing import Any, Callable, Sequence, Optional
from jax import lax, random, numpy as jnp
import flax
from flax.core import freeze, unfreeze
from flax import linen as nn
###Output
_____no_output_____
###Markdown
Linear regression with FlaxIn the previous *JAX for the impatient* notebook, we finished up with a linear regression example. As we know, linear regression can also be written as a single dense neural network layer, which we will show in the following so that we can compare how it's done.A dense layer is a layer that has a kernel parameter $W\in\mathcal{M}_{m,n}(\mathbb{R})$ where $m$ is the number of features as an output of the model, and $n$ the dimensionality of the input, and a bias parameter $b\in\mathbb{R}^m$. The dense layers returns $Wx+b$ from an input $x\in\mathbb{R}^n$.This dense layer is already provided by Flax in the `flax.linen` module (here imported as `nn`).
###Code
# We create one dense layer instance (taking 'features' parameter as input)
model = nn.Dense(features=5)
###Output
_____no_output_____
###Markdown
Layers (and models in general, we'll use that word from now on) are subclasses of the `linen.Module` class. Model parameters & initializationParameters are not stored with the models themselves. You need to initialize parameters by calling the `init` function, using a PRNGKey and a dummy input parameter.
###Code
key1, key2 = random.split(random.PRNGKey(0))
x = random.normal(key1, (10,)) # Dummy input
params = model.init(key2, x) # Initialization call
jax.tree_map(lambda x: x.shape, params) # Checking output shapes
###Output
WARNING:absl:No GPU/TPU found, falling back to CPU. (Set TF_CPP_MIN_LOG_LEVEL=0 and rerun for more info.)
###Markdown
*Note: JAX and Flax, like NumPy, are row-based systems, meaning that vectors are represented as row vectors and not column vectors. This can be seen in the shape of the kernel here.*The result is what we expect: bias and kernel parameters of the correct size. Under the hood:* The dummy input variable `x` is used to trigger shape inference: we only declared the number of features we wanted in the output of the model, not the size of the input. Flax finds out by itself the correct size of the kernel.* The random PRNG key is used to trigger the initialization functions (those have default values provided by the module here).* Initialization functions are called to generate the initial set of parameters that the model will use. Those are functions that take as arguments `(PRNG Key, shape, dtype)` and return an Array of shape `shape`.* The init function returns the initialized set of parameters (you can also get the output of the evaluation on the dummy input with the same syntax but using the `init_with_output` method instead of `init`. We see in the output that parameters are stored in a `FrozenDict` instance which helps deal with the functional nature of JAX by preventing any mutation of the underlying dict and making the user aware of it. Read more about it in the Flax docs. As a consequence, the following doesn't work:
###Code
try:
params['new_key'] = jnp.ones((2,2))
except ValueError as e:
print("Error: ", e)
###Output
Error: FrozenDict is immutable.
###Markdown
To evaluate the model with a given set of parameters (never stored with the model), we just use the `apply` method by providing it the parameters to use as well as the input:
###Code
model.apply(params, x)
###Output
_____no_output_____
###Markdown
Gradient descentIf you jumped here directly without going through the JAX part, here is the linear regression formulation we're going to use: from a set of data points $\{(x_i,y_i), i\in \{1,\ldots, k\}, x_i\in\mathbb{R}^n,y_i\in\mathbb{R}^m\}$, we try to find a set of parameters $W\in \mathcal{M}_{m,n}(\mathbb{R}), b\in\mathbb{R}^m$ such that the function $f_{W,b}(x)=Wx+b$ minimizes the mean squared error:$$\mathcal{L}(W,b)\rightarrow\frac{1}{k}\sum_{i=1}^{k} \frac{1}{2}\|y_i-f_{W,b}(x_i)\|^2_2$$Here, we see that the tuple $(W,b)$ matches the parameters of the Dense layer. We'll perform gradient descent using those. Let's first generate the fake data we'll use.
###Code
# Set problem dimensions
nsamples = 20
xdim = 10
ydim = 5
# Generate random ground truth W and b
key = random.PRNGKey(0)
k1, k2 = random.split(key)
W = random.normal(k1, (xdim, ydim))
b = random.normal(k2, (ydim,))
true_params = freeze({'params': {'bias': b, 'kernel': W}})
# Generate samples with additional noise
ksample, knoise = random.split(k1)
x_samples = random.normal(ksample, (nsamples, xdim))
y_samples = jnp.dot(x_samples, W) + b
y_samples += 0.1*random.normal(knoise,(nsamples, ydim)) # Adding noise
print('x shape:', x_samples.shape, '; y shape:', y_samples.shape)
###Output
x shape: (20, 10) ; y shape: (20, 5)
###Markdown
Now let's generate the loss function (mean squared error) with that data.
###Code
def make_mse_func(x_batched, y_batched):
def mse(params):
# Define the squared loss for a single pair (x,y)
def squared_error(x, y):
pred = model.apply(params, x)
return jnp.inner(y-pred, y-pred)/2.0
# We vectorize the previous to compute the average of the loss on all samples.
return jnp.mean(jax.vmap(squared_error)(x_batched,y_batched), axis=0)
return jax.jit(mse) # And finally we jit the result.
# Get the sampled loss
loss = make_mse_func(x_samples, y_samples)
###Output
_____no_output_____
###Markdown
And finally perform the gradient descent.
###Code
alpha = 0.3 # Gradient step size
print('Loss for "true" W,b: ', loss(true_params))
grad_fn = jax.value_and_grad(loss)
for i in range(101):
# We perform one gradient update
loss_val, grads = grad_fn(params)
params = jax.tree_multimap(lambda p, g: p - alpha * g,
params, grads)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss for "true" W,b: 0.023639778
Loss step 0: 38.094772
Loss step 10: 0.44692168
Loss step 20: 0.10053458
Loss step 30: 0.035822745
Loss step 40: 0.018846875
Loss step 50: 0.013864839
Loss step 60: 0.012312559
Loss step 70: 0.011812928
Loss step 80: 0.011649306
Loss step 90: 0.011595251
Loss step 100: 0.0115773035
###Markdown
Optimizing with OptaxFlax used to use its own `flax.optim` package for optimization, but with[FLIP 1009](https://github.com/google/flax/blob/main/docs/flip/1009-optimizer-api.md)this was deprecated in favor of[Optax](https://github.com/deepmind/optax).Basic usage of Optax is straightforward:1. You choose an optimization method (e.g. `optax.sgd`).2. Create optimizer state from parameters.3. You compute the gradients of your loss with `jax.value_and_grad()`.4. At every iteration, call the Optax update function to update the internal optimizer state and create an update to the parameters. Then add the update to the parameters.Note that Optax can do a lot more: It's designed for composing simple gradienttransformations into more complex transformations that allows to implement awide range of optimizers. There is also support for changing optimizerhyperparameters over time ("schedules"), applying different udpates to differentparts of the parameter tree ("masking") and much more. For details please referto the[official documentation](https://optax.readthedocs.io/en/latest/).
###Code
import optax
tx = optax.sgd(learning_rate=alpha)
opt_state = tx.init(params)
loss_grad_fn = jax.value_and_grad(loss)
for i in range(101):
loss_val, grads = loss_grad_fn(params)
updates, opt_state = tx.update(grads, opt_state)
params = optax.apply_updates(params, updates)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss step 0: 0.011576377
Loss step 10: 0.0115710115
Loss step 20: 0.011569244
Loss step 30: 0.011568661
Loss step 40: 0.011568454
Loss step 50: 0.011568379
Loss step 60: 0.011568358
Loss step 70: 0.01156836
Loss step 80: 0.01156835
Loss step 90: 0.011568353
Loss step 100: 0.011568348
###Markdown
Serializing the resultNow that we're happy with the result of our training, we might want to save the model parameters to load them back later. Flax provides a serialization package to enable you to do that.
###Code
from flax import serialization
bytes_output = serialization.to_bytes(params)
dict_output = serialization.to_state_dict(params)
print('Dict output')
print(dict_output)
print('Bytes output')
print(bytes_output)
###Output
Dict output
{'params': {'bias': DeviceArray([-1.4540135, -2.0262308, 2.0806582, 1.2201802, -0.9964547], dtype=float32), 'kernel': DeviceArray([[ 1.0106664 , 0.19014716, 0.04533899, -0.92722285,
0.34720102],
[ 1.7320251 , 0.9901233 , 1.1662225 , 1.1027892 ,
-0.10574618],
[-1.2009128 , 0.28837162, 1.4176372 , 0.12073109,
-1.3132601 ],
[-1.1944956 , -0.18993308, 0.03379077, 1.3165942 ,
0.07996067],
[ 0.14103189, 1.3737966 , -1.3162128 , 0.53401774,
-2.239638 ],
[ 0.5643044 , 0.813604 , 0.31888172, 0.5359193 ,
0.90352124],
[-0.37948322, 1.7408353 , 1.0788013 , -0.5041964 ,
0.9286919 ],
[ 0.9701384 , -1.3158673 , 0.33630812, 0.80941117,
-1.202457 ],
[ 1.0198247 , -0.6198277 , 1.0822718 , -1.8385581 ,
-0.45790705],
[-0.64384323, 0.4564892 , -1.1331053 , -0.68556863,
0.17010891]], dtype=float32)}}
Bytes output
b'\x81\xa6params\x82\xa4bias\xc7!\x01\x93\x91\x05\xa7float32\xc4\x14\x1d\x1d\xba\xbf\xc4\xad\x01\xc0\x81)\x05@\xdd.\x9c?\xa8\x17\x7f\xbf\xa6kernel\xc7\xd6\x01\x93\x92\n\x05\xa7float32\xc4\xc8\x84]\x81?\xf0\xb5B>`\xb59=z^m\xbfU\xc4\xb1>\x00\xb3\xdd?\xb8x}?\xc7F\x95?2(\x8d?t\x91\xd8\xbd\x83\xb7\x99\xbfr\xa5\x93>#u\xb5?\xdcA\xf7=\xe8\x18\xa8\xbf;\xe5\x98\xbf\xd1}B\xbe0h\n=)\x86\xa8?k\xc2\xa3=\xaaj\x10>\x91\xd8\xaf?\xa9y\xa8\xbfc\xb5\x08?;V\x0f\xc0Av\x10?ZHP?wD\xa3>\x022\t?+Mg?\xa0K\xc2\xbe\xb1\xd3\xde?)\x16\x8a?\x04\x13\x01\xbf\xc1\xbem?\xfdZx?Wn\xa8\xbf\x940\xac>\x925O?\x1c\xea\x99\xbf\x9e\x89\x82?\x07\xad\x1e\xbf\xe2\x87\x8a?\xdfU\xeb\xbf\xcbr\xea\xbe\xe9\xd2$\xbf\xf4\xb8\xe9>\x98\t\x91\xbfm\x81/\xbf\x081.>'
###Markdown
To load the model back, you'll need to use as a template the model parameter structure, like the one you would get from the model initialization. Here, we use the previously generated `params` as a template. Note that this will produce a new variable structure, and not mutate in-place.*The point of enforcing structure through template is to avoid users issues downstream, so you need to first have the right model that generates the parameters structure.*
###Code
serialization.from_bytes(params, bytes_output)
###Output
_____no_output_____
###Markdown
Defining your own modelsFlax allows you to define your own models, which should be a bit more complicated than a linear regression. In this section, we'll show you how to build simple models. To do so, you'll need to create subclasses of the base `nn.Module` class.*Keep in mind that we imported* `linen as nn` *and this only works with the new linen API* Module basicsThe base abstraction for models is the `nn.Module` class, and every type of predefined layers in Flax (like the previous `Dense`) is a subclass of `nn.Module`. Let's take a look and start by defining a simple but custom multi-layer perceptron i.e. a sequence of Dense layers interleaved with calls to a non-linear activation function.
###Code
class ExplicitMLP(nn.Module):
features: Sequence[int]
def setup(self):
# we automatically know what to do with lists, dicts of submodules
self.layers = [nn.Dense(feat) for feat in self.features]
# for single submodules, we would just write:
# self.layer1 = nn.Dense(self, feat1)
def __call__(self, inputs):
x = inputs
for i, lyr in enumerate(self.layers):
x = lyr(x)
if i != len(self.layers) - 1:
x = nn.relu(x)
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = ExplicitMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292815e-02 -4.3807115e-02 2.9323792e-02 6.5492536e-03
-1.7147182e-02]
[ 1.2967806e-01 -1.4551792e-01 9.4432183e-02 1.2521387e-02
-4.5417298e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
As we can see, a `nn.Module` subclass is made of:* A collection of data fields (`nn.Module` are Python dataclasses) - here we only have the `features` field of type `Sequence[int]`.* A `setup()` method that is being called at the end of the `__postinit__` where you can register submodules, variables, parameters you will need in your model.* A `__call__` function that returns the output of the model from a given input.* The model structure defines a pytree of parameters following the same tree structure as the model: the params tree contains one `layers_n` sub dict per layer, and each of those contain the parameters of the associated Dense layer. The layout is very explicit.*Note: lists are mostly managed as you would expect (WIP), there are corner cases you should be aware of as pointed out* [here](https://github.com/google/flax/issues/524)Since the module structure and its parameters are not tied to each other, you can't call directly `model(x)` on a given input as it will return an error. The `__call__` function is being wrapped up in the `apply` one, which is the one to call on an input:
###Code
try:
y = model(x) # Returns an error
except AttributeError as e:
print(e)
###Output
"ExplicitMLP" object has no attribute "layers"
###Markdown
Since here we have a very simple model, we could have used an alternative (but equivalent) way of declaring the submodules inline in the `__call__` using the `@nn.compact` annotation like so:
###Code
class SimpleMLP(nn.Module):
features: Sequence[int]
@nn.compact
def __call__(self, inputs):
x = inputs
for i, feat in enumerate(self.features):
x = nn.Dense(feat, name=f'layers_{i}')(x)
if i != len(self.features) - 1:
x = nn.relu(x)
# providing a name is optional though!
# the default autonames would be "Dense_0", "Dense_1", ...
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292815e-02 -4.3807115e-02 2.9323792e-02 6.5492536e-03
-1.7147182e-02]
[ 1.2967806e-01 -1.4551792e-01 9.4432183e-02 1.2521387e-02
-4.5417298e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
There are, however, a few differences you should be aware of between the two declaration modes:* In `setup`, you are able to name some sublayers and keep them around for further use (e.g. encoder/decoder methods in autoencoders).* If you want to have multiple methods, then you **need** to declare the module using `setup`, as the `@nn.compact` annotation only allows one method to be annotated.* The last initialization will be handled differently see these notes for more details (TODO: add notes link) Module parametersIn the previous MLP example, we relied only on predefined layers and operators (`Dense`, `relu`). Let's imagine that you didn't have a Dense layer provided by Flax and you wanted to write it on your own. Here is what it would look like using the `@nn.compact` way to declare a new modules:
###Code
class SimpleDense(nn.Module):
features: int
kernel_init: Callable = nn.initializers.lecun_normal()
bias_init: Callable = nn.initializers.zeros
@nn.compact
def __call__(self, inputs):
kernel = self.param('kernel',
self.kernel_init, # Initialization function
(inputs.shape[-1], self.features)) # shape info.
y = lax.dot_general(inputs, kernel,
(((inputs.ndim - 1,), (0,)), ((), ())),) # TODO Why not jnp.dot?
bias = self.param('bias', self.bias_init, (self.features,))
y = y + bias
return y
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleDense(features=3)
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameters:\n', params)
print('output:\n', y)
###Output
initialized parameters:
FrozenDict({
params: {
kernel: DeviceArray([[ 0.6503669 , 0.86789787, 0.4604268 ],
[ 0.05673932, 0.9909285 , -0.63536596],
[ 0.76134115, -0.3250529 , -0.65221626],
[-0.82430327, 0.4150194 , 0.19405058]], dtype=float32),
bias: DeviceArray([0., 0., 0.], dtype=float32),
},
})
output:
[[ 0.5035518 1.8548558 -0.4270195 ]
[ 0.0279097 0.5589246 -0.43061772]
[ 0.3547128 1.5740999 -0.32865518]
[ 0.5264864 1.2928858 0.10089308]]
###Markdown
Here, we see how both declare and assign a parameter to the model using the `self.param` method. It takes as input `(name, init_fn, *init_args)` : * `name` is simply the name of the parameter that will end up in the parameter structure.* `init_fun` is a function with input `(PRNGKey, *init_args)` returning an Array with `init_args` the arguments needed to call the initialisation function* `init_args` the arguments to provide to the initialization function.Such params can also be declared in the `setup` method, it won't be able to use shape inference because Flax is using lazy initialization at the first call site. Variables and collections of variablesAs we've seen so far, working with models means working with:* A subclass of `nn.Module`;* A pytree of parameters for the model (typically from `model.init()`);However this is not enough to cover everything that we would need for machine learning, especially neural networks. In some cases, you might want your neural network to keep track of some internal state while it runs (e.g. batch normalization layers). There is a way to declare variables beyond the parameters of the model with the `variable` method.For demonstration purposes, we'll implement a simplified but similar mechanism to batch normalization: we'll store running averages and subtract those to the input at training time. For proper batchnorm, you should use (and look at) the implementation [here](https://github.com/google/flax/blob/main/flax/linen/normalization.py).
###Code
class BiasAdderWithRunningMean(nn.Module):
decay: float = 0.99
@nn.compact
def __call__(self, x):
# easy pattern to detect if we're initializing via empty variable tree
is_initialized = self.has_variable('batch_stats', 'mean')
ra_mean = self.variable('batch_stats', 'mean',
lambda s: jnp.zeros(s),
x.shape[1:])
mean = ra_mean.value # This will get either the value, or trigger init
bias = self.param('bias', lambda rng, shape: jnp.zeros(shape), x.shape[1:])
if is_initialized:
ra_mean.value = self.decay * ra_mean.value + (1.0 - self.decay) * jnp.mean(x, axis=0, keepdims=True)
return x - ra_mean.value + bias
key1, key2 = random.split(random.PRNGKey(0), 2)
x = jnp.ones((10,5))
model = BiasAdderWithRunningMean()
variables = model.init(key1, x)
print('initialized variables:\n', variables)
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
print('updated state:\n', updated_state)
###Output
initialized variables:
FrozenDict({
batch_stats: {
mean: DeviceArray([0., 0., 0., 0., 0.], dtype=float32),
},
params: {
bias: DeviceArray([0., 0., 0., 0., 0.], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
###Markdown
Here, `updated_state` returns only the state variables that are being mutated by the model while applying it on data. To update the variables and get the new parameters of the model, we can use the following pattern:
###Code
for val in [1.0, 2.0, 3.0]:
x = val * jnp.ones((10,5))
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
old_state, params = variables.pop('params')
variables = freeze({'params': params, **updated_state})
print('updated state:\n', updated_state) # Shows only the mutable part
###Output
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.0299, 0.0299, 0.0299, 0.0299, 0.0299]], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.059601, 0.059601, 0.059601, 0.059601, 0.059601]], dtype=float32),
},
})
###Markdown
From this simplified example, you should be able to derive a full BatchNorm implementation, or any layer involving a state. To finish, let's add an optimizer to see how to play with both parameters updated by an optimizer and state variables.*This example isn't doing anything and is only for demonstration purposes.*
###Code
def update_step(tx, apply_fun, x, opt_state, params, state):
def loss(params):
y, updated_state = apply_fun({'params': params, **state},
x, mutable=list(state.keys()))
l = ((x - y) ** 2).sum()
return l, updated_state
(l, state), grads = jax.value_and_grad(loss, has_aux=True)(params)
updates, opt_state = tx.update(grads, opt_state)
params = optax.apply_updates(params, updates)
return opt_state, params, state
x = jnp.ones((10,5))
variables = model.init(random.PRNGKey(0), x)
state, params = variables.pop('params')
del variables
tx = optax.sgd(learning_rate=0.02)
opt_state = tx.init(params)
for _ in range(3):
opt_state, params, state = update_step(tx, model.apply, x, opt_state, params, state)
print('Updated state: ', state)
###Output
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.0199, 0.0199, 0.0199, 0.0199, 0.0199]], dtype=float32),
},
})
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.029701, 0.029701, 0.029701, 0.029701, 0.029701]], dtype=float32),
},
})
###Markdown
Flax BasicsThis notebook will walk you through the following workflow:* Instantiating a model from Flax built-in layers or third-party models.* Initializing parameters of the model and manually written training.* Using optimizers provided by Flax to ease training.* Serialization of parameters and other objects.* Creating your own models and managing state. Setting up our environmentHere we provide the code needed to set up the environment for our notebook.
###Code
# Install the latest JAXlib version.
!pip install --upgrade -q pip jax jaxlib
# Install Flax at head:
!pip install --upgrade -q git+https://github.com/google/flax.git
import jax
from typing import Any, Callable, Sequence, Optional
from jax import lax, random, numpy as jnp
import flax
from flax.core import freeze, unfreeze
from flax import linen as nn
from jax.config import config
config.enable_omnistaging() # Linen requires enabling omnistaging
###Output
_____no_output_____
###Markdown
Linear regression with FlaxIn the previous *JAX for the impatient* notebook, we finished up with a linear regression example. As we know, linear regression can also be written as a single dense neural network layer, which we will show in the following so that we can compare how it's done.A dense layer is a layer that has a kernel parameter $W\in\mathcal{M}_{m,n}(\mathbb{R})$ where $m$ is the number of features as an output of the model, and $n$ the dimensionality of the input, and a bias parameter $b\in\mathbb{R}^m$. The dense layers returns $Wx+b$ from an input $x\in\mathbb{R}^n$.This dense layer is already provided by Flax in the `flax.linen` module (here imported as `nn`).
###Code
# We create one dense layer instance (taking 'features' parameter as input)
model = nn.Dense(features=5)
###Output
_____no_output_____
###Markdown
Layers (and models in general, we'll use that word from now on) are subclasses of the `linen.Module` class. Model parameters & initializationParameters are not stored with the models themselves. You need to initialize parameters by calling the `init` function, using a PRNGKey and a dummy input parameter.
###Code
key1, key2 = random.split(random.PRNGKey(0))
x = random.normal(key1, (10,)) # Dummy input
params = model.init(key2, x) # Initialization call
jax.tree_map(lambda x: x.shape, params) # Checking output shapes
###Output
WARNING:absl:No GPU/TPU found, falling back to CPU. (Set TF_CPP_MIN_LOG_LEVEL=0 and rerun for more info.)
###Markdown
*Note: JAX and Flax, like NumPy, are row-based systems, meaning that vectors are represented as row vectors and not column vectors. This can be seen in the shape of the kernel here.*The result is what we expect: bias and kernel parameters of the correct size. Under the hood:* The dummy input variable `x` is used to trigger shape inference: we only declared the number of features we wanted in the output of the model, not the size of the input. Flax finds out by itself the correct size of the kernel.* The random PRNG key is used to trigger the initialization functions (those have default values provided by the module here).* Initialization functions are called to generate the initial set of parameters that the model will use. Those are functions that take as arguments `(PRNG Key, shape, dtype)` and return an Array of shape `shape`.* The init function returns the initialized set of parameters (you can also get the output of the evaluation on the dummy input with the same syntax but using the `init_with_output` method instead of `init`. We see in the output that parameters are stored in a `FrozenDict` instance which helps deal with the functional nature of JAX by preventing any mutation of the underlying dict and making the user aware of it. Read more about it in the Flax docs. As a consequence, the following doesn't work:
###Code
try:
params['new_key'] = jnp.ones((2,2))
except ValueError as e:
print("Error: ", e)
###Output
Error: FrozenDict is immutable.
###Markdown
To evaluate the model with a given set of parameters (never stored with the model), we just use the `apply` method by providing it the parameters to use as well as the input:
###Code
model.apply(params, x)
###Output
_____no_output_____
###Markdown
Gradient descentIf you jumped here directly without going through the JAX part, here is the linear regression formulation we're going to use: from a set of data points $\{(x_i,y_i), i\in \{1,\ldots, k\}, x_i\in\mathbb{R}^n,y_i\in\mathbb{R}^m\}$, we try to find a set of parameters $W\in \mathcal{M}_{m,n}(\mathbb{R}), b\in\mathbb{R}^m$ such that the function $f_{W,b}(x)=Wx+b$ minimizes the mean squared error:$$\mathcal{L}(W,b)\rightarrow\frac{1}{k}\sum_{i=1}^{k} \frac{1}{2}\|y_i-f_{W,b}(x_i)\|^2_2$$Here, we see that the tuple $(W,b)$ matches the parameters of the Dense layer. We'll perform gradient descent using those. Let's first generate the fake data we'll use.
###Code
# Set problem dimensions
nsamples = 20
xdim = 10
ydim = 5
# Generate random ground truth W and b
key = random.PRNGKey(0)
k1, k2 = random.split(key)
W = random.normal(k1, (xdim, ydim))
b = random.normal(k2, (ydim,))
true_params = freeze({'params': {'bias': b, 'kernel': W}})
# Generate samples with additional noise
ksample, knoise = random.split(k1)
x_samples = random.normal(ksample, (nsamples, xdim))
y_samples = jnp.dot(x_samples, W) + b
y_samples += 0.1*random.normal(knoise,(nsamples, ydim)) # Adding noise
print('x shape:', x_samples.shape, '; y shape:', y_samples.shape)
###Output
x shape: (20, 10) ; y shape: (20, 5)
###Markdown
Now let's generate the loss function (mean squared error) with that data.
###Code
def make_mse_func(x_batched, y_batched):
def mse(params):
# Define the squared loss for a single pair (x,y)
def squared_error(x, y):
pred = model.apply(params, x)
return jnp.inner(y-pred, y-pred)/2.0
# We vectorize the previous to compute the average of the loss on all samples.
return jnp.mean(jax.vmap(squared_error)(x_batched,y_batched), axis=0)
return jax.jit(mse) # And finally we jit the result.
# Get the sampled loss
loss = make_mse_func(x_samples, y_samples)
###Output
_____no_output_____
###Markdown
And finally perform the gradient descent.
###Code
alpha = 0.3 # Gradient step size
print('Loss for "true" W,b: ', loss(true_params))
grad_fn = jax.value_and_grad(loss)
for i in range(101):
# We perform one gradient update
loss_val, grad = grad_fn(params)
params = jax.tree_multimap(lambda old, grad: old - alpha * grad,
params, grad)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss for "true" W,b: 29.070158
Loss step 0: 23.618902
Loss step 10: 0.30728564
Loss step 20: 0.06495677
Loss step 30: 0.025215296
Loss step 40: 0.015619493
Loss step 50: 0.012849321
Loss step 60: 0.011984843
Loss step 70: 0.011705536
Loss step 80: 0.011613827
Loss step 90: 0.011583473
Loss step 100: 0.011573391
###Markdown
Build-in optimization APIFlax provides an optimization package in `flax.optim` to make your life easier when training models. The process is:1. You choose an optimization method (e.g. `optim.GradientDescent`, `optim.Adam`)2. From the previous optimization method, you create a wrapper around the parameters you're going to optimize for with the `create` method. Your parameters are accessible through the `target` field.3. You compute the gradients of your loss with `jax.value_and_grad()`.4. At every iteration, you compute the gradients at the current point, then use the `apply_gradient()` method on the optimizer to return a new optimizer with updated parameters.
###Code
from flax import optim
optimizer_def = optim.GradientDescent(learning_rate=alpha) # Choose the method
optimizer = optimizer_def.create(params) # Create the wrapping optimizer with initial parameters
loss_grad_fn = jax.value_and_grad(loss)
for i in range(101):
loss_val, grad = loss_grad_fn(optimizer.target)
optimizer = optimizer.apply_gradient(grad) # Return the updated optimizer with parameters.
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss step 0: 0.011572863
Loss step 10: 0.011569859
Loss step 20: 0.011568859
Loss step 30: 0.011568523
Loss step 40: 0.011568412
Loss step 50: 0.011568374
Loss step 60: 0.011568364
Loss step 70: 0.011568359
Loss step 80: 0.01156836
Loss step 90: 0.011568356
Loss step 100: 0.011568357
###Markdown
Serializing the resultNow that we're happy with the result of our training, we might want to save the model parameters to load them back later. Flax provides a serialization package to enable you to do that.
###Code
from flax import serialization
bytes_output = serialization.to_bytes(optimizer.target)
dict_output = serialization.to_state_dict(optimizer.target)
print('Dict output')
print(dict_output)
print('Bytes output')
print(bytes_output)
###Output
Dict output
{'params': {'bias': DeviceArray([-3.023082 , 0.5307182, 3.7256303, 1.4638226, -3.2100437], dtype=float32), 'kernel': DeviceArray([[-1.4092493e-02, 4.8609809e-03, 1.1460093e-02,
-6.0927689e-02, 2.0413438e-05],
[-3.3569761e-02, -1.5614161e-03, 4.3190460e-04,
-7.9035060e-03, -1.9851506e-02],
[-1.8882388e-02, -2.1366426e-03, -1.8663550e-02,
-3.0001188e-02, 5.1880259e-02],
[-4.8119370e-02, -2.9280247e-02, -1.1992223e-02,
-1.0111435e-02, -8.3459895e-03],
[-1.7368369e-02, -1.7084973e-02, 6.0279824e-02,
9.2046618e-02, -1.5414236e-02],
[-3.0089449e-02, -5.5370983e-03, -9.1237156e-03,
2.1827107e-02, -2.0405082e-02],
[-5.6748122e-02, -4.2654604e-02, -1.1436724e-02,
7.5801805e-02, -2.0075133e-02],
[-1.4368590e-03, -1.6048675e-02, 1.5781123e-02,
2.8437756e-03, -8.5009886e-03],
[ 1.7892396e-02, 5.7572998e-02, 4.1483097e-02,
-9.9685444e-03, -2.1875760e-02],
[-2.1158390e-02, -1.3853005e-02, 2.5077526e-02,
3.2925244e-02, 3.8115401e-02]], dtype=float32)}}
Bytes output
b'\x81\xa6params\x82\xa4bias\xc7!\x01\x93\x91\x05\xa7float32\xc4\x14-zA\xc0&\xdd\x07?\xbapn@\x8a^\xbb?[qM\xc0\xa6kernel\xc7\xd6\x01\x93\x92\n\x05\xa7float32\xc4\xc83\xe4f\xbc\xddH\x9f;\x1d\xc3;<P\x8fy\xbd\x86=\xab7r\x80\t\xbdn\xa8\xcc\xbaAq\xe29\xb5}\x01\xbc\xa0\x9f\xa2\xbc=\xaf\x9a\xbc\xea\x06\x0c\xbbM\xe4\x98\xbc\r\xc5\xf5\xbce\x80T=\xd1\x18E\xbd!\xdd\xef\xbc\x07{D\xbco\xaa%\xbc\x9e\xbd\x08\xbc\x1cH\x8e\xbc\xc9\xf5\x8b\xbc\xfa\xe7v=\xf0\x82\xbc=\xfe\x8b|\xbc&~\xf6\xbc\x8cp\xb5\xbb\xa3{\x15\xbc\xc3\xce\xb2<\x8f(\xa7\xbc\xb8ph\xbd\x98\xb6.\xbd\x19a;\xbc\xfa=\x9b=\x9bt\xa4\xbc\xfdT\xbc\xba\x83x\x83\xbcjG\x81<\xa3^:;\xbbG\x0b\xbc\x13\x93\x92<\xaa\xd1k=.\xea)=\x1bS#\xbc\xcb4\xb3\xbc\\T\xad\xbc\xb7\xf7b\xbcbo\xcd<\x9f\xdc\x06=\xe5\x1e\x1c='
###Markdown
To load the model back, you'll need to use as a template the model parameter structure, like the one you would get from the model initialization. Here, we use the previously generated `params` as a template. Note that this will produce a new variable structure, and not mutate in-place.*The point of enforcing structure through template is to avoid users issues downstream, so you need to first have the right model that generates the parameters structure.*
###Code
serialization.from_bytes(params, bytes_output)
###Output
_____no_output_____
###Markdown
The serialization utils provided by Flax work on objects beyond parameters, for example you might want to serialize the optimizer and it's states, which we show in the following cell:
###Code
serialization.to_state_dict(optimizer)
###Output
_____no_output_____
###Markdown
Defining your own modelsFlax allows you to define your own models, which should be a bit more complicated than a linear regression. In this section, we'll show you how to build simple models. To do so, you'll need to create subclasses of the base `nn.Module` class.*Keep in mind that we imported* `linen as nn` *and this only works with the new linen API* Module basicsThe base abstraction for models is the `nn.Module` class, and every type of predefined layers in Flax (like the previous `Dense`) is a subclass of `nn.Module`. Let's take a look and start by defining a simple but custom multi-layer perceptron i.e. a sequence of Dense layers interleaved with calls to a non-linear activation function.
###Code
class ExplicitMLP(nn.Module):
features: Sequence[int]
def setup(self):
# we automatically know what to do with lists, dicts of submodules
self.layers = [nn.Dense(feat) for feat in self.features]
# for single submodules, we would just write:
# self.layer1 = nn.Dense(self, feat1)
def __call__(self, inputs):
x = inputs
for i, lyr in enumerate(self.layers):
x = lyr(x)
if i != len(self.layers) - 1:
x = nn.relu(x)
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = ExplicitMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292822e-02 -4.3807123e-02 2.9323796e-02 6.5492545e-03
-1.7147183e-02]
[ 1.2967804e-01 -1.4551792e-01 9.4432175e-02 1.2521386e-02
-4.5417294e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
As we can see, a `nn.Module` subclass is made of:* A collection of data fields (`nn.Module` are Python dataclasses) - here we only have the `features` field of type `Sequence[int]`.* A `setup()` method that is being called at the end of the `__postinit__` where you can register submodules, variables, parameters you will need in your model.* A `__call__` function that returns the output of the model from a given input.* The model structure defines a pytree of parameters following the same tree structure as the model: the params tree contains one `layers_n` sub dict per layer, and each of those contain the parameters of the associated Dense layer. The layout is very explicit.*Note: lists are mostly managed as you would expect (WIP), there are corner cases you should be aware of as pointed out* [here](https://github.com/google/flax/issues/524)Since the module structure and its parameters are not tied to each other, you can't call directly `model(x)` on a given input as it will return an error. The `__call__` function is being wrapped up in the `apply` one, which is the one to call on an input:
###Code
try:
y = model(x) # Returns an error
except ValueError as e:
print(e)
###Output
Can't call methods on orphaned modules
###Markdown
Since here we have a very simple model, we could have used an alternative (but equivalent) way of declaring the submodules inline in the `__call__` using the `@nn.compact` annotation like so:
###Code
class SimpleMLP(nn.Module):
features: Sequence[int]
@nn.compact
def __call__(self, inputs):
x = inputs
for i, feat in enumerate(self.features):
x = nn.Dense(feat, name=f'layers_{i}')(x)
if i != len(self.features) - 1:
x = nn.relu(x)
# providing a name is optional though!
# the default autonames would be "Dense_0", "Dense_1", ...
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292822e-02 -4.3807123e-02 2.9323796e-02 6.5492545e-03
-1.7147183e-02]
[ 1.2967804e-01 -1.4551792e-01 9.4432175e-02 1.2521386e-02
-4.5417294e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
There are, however, a few differences you should be aware of between the two declaration modes:* In `setup`, you are able to name some sublayers and keep them around for further use (e.g. encoder/decoder methods in autoencoders).* If you want to have multiple methods, then you **need** to declare the module using `setup`, as the `@nn.compact` annotation only allows one method to be annotated.* The last initialization will be handled differently see these notes for more details (TODO: add notes link) Module parametersIn the previous MLP example, we relied only on predefined layers and operators (`Dense`, `relu`). Let's imagine that you didn't have a Dense layer provided by Flax and you wanted to write it on your own. Here is what it would look like using the `@nn.compact` way to declare a new modules:
###Code
class SimpleDense(nn.Module):
features: int
kernel_init: Callable = nn.initializers.lecun_normal()
bias_init: Callable = nn.initializers.zeros
@nn.compact
def __call__(self, inputs):
kernel = self.param('kernel',
self.kernel_init, # Initialization function
(inputs.shape[-1], self.features)) # shape info.
y = lax.dot_general(inputs, kernel,
(((inputs.ndim - 1,), (0,)), ((), ())),) # TODO Why not jnp.dot?
bias = self.param('bias', self.bias_init, (self.features,))
y = y + bias
return y
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleDense(features=3)
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameters:\n', params)
print('output:\n', y)
###Output
initialized parameters:
FrozenDict({'params': {'kernel': DeviceArray([[ 0.6503669 , 0.8678979 , 0.46042678],
[ 0.05673932, 0.9909285 , -0.63536596],
[ 0.76134115, -0.3250529 , -0.6522163 ],
[-0.8243032 , 0.4150194 , 0.19405058]], dtype=float32), 'bias': DeviceArray([0., 0., 0.], dtype=float32)}})
output:
[[ 0.50355184 1.8548559 -0.4270196 ]
[ 0.02790972 0.5589246 -0.43061778]
[ 0.35471287 1.5740999 -0.32865524]
[ 0.52648634 1.2928859 0.10089307]]
###Markdown
Here, we see how both declare and assign a parameter to the model using the `self.param` method. It takes as input `(name, init_fn, *init_args)` : * `name` is simply the name of the parameter that will end up in the parameter structure.* `init_fun` is a function with input `(PRNGKey, *init_args)` returning an Array with `init_args` the arguments needed to call the initialisation function* `init_args` the arguments to provide to the initialization function.Such params can also be declared in the `setup` method, it won't be able to use shape inference because Flax is using lazy initialization at the first call site. Variables and collections of variablesAs we've seen so far, working with models means working with:* A subclass of `nn.Module`;* A pytree of parameters for the model (typically from `model.init()`);However this is not enough to cover everything that we would need for machine learning, especially neural networks. In some cases, you might want your neural network to keep track of some internal state while it runs (e.g. batch normalization layers). There is a way to declare variables beyond the parameters of the model with the `variable` method.For demonstration purposes, we'll implement a simplified but similar mechanism to batch normalization: we'll store running averages and subtract those to the input at training time. For proper batchnorm, you should use (and look at) the implementation [here](https://github.com/google/flax/blob/master/flax/linen/normalization.py).
###Code
class BiasAdderWithRunningMean(nn.Module):
decay: float = 0.99
@nn.compact
def __call__(self, x):
# easy pattern to detect if we're initializing via empty variable tree
is_initialized = self.has_variable('batch_stats', 'mean')
ra_mean = self.variable('batch_stats', 'mean',
lambda s: jnp.zeros(s),
x.shape[1:])
mean = ra_mean.value # This will get either the value, or trigger init
bias = self.param('bias', lambda rng, shape: jnp.zeros(shape), x.shape[1:])
if is_initialized:
ra_mean.value = self.decay * ra_mean.value + (1.0 - self.decay) * jnp.mean(x, axis=0, keepdims=True)
return x - ra_mean.value + bias
key1, key2 = random.split(random.PRNGKey(0), 2)
x = jnp.ones((10,5))
model = BiasAdderWithRunningMean()
variables = model.init(key1, x)
print('initialized variables:\n', variables)
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
print('updated state:\n', updated_state)
###Output
initialized variables:
FrozenDict({'batch_stats': {'mean': DeviceArray([0., 0., 0., 0., 0.], dtype=float32)}, 'params': {'bias': DeviceArray([0., 0., 0., 0., 0.], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
###Markdown
Here, `updated_state` returns only the state variables that are being mutated by the model while applying it on data. To update the variables and get the new parameters of the model, we can use the following pattern:
###Code
for val in [1.0, 2.0, 3.0]:
x = val * jnp.ones((10,5))
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
old_state, params = variables.pop('params')
variables = freeze({'params': params, **updated_state})
print('updated state:\n', updated_state) # Shows only the mutable part
###Output
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.0299, 0.0299, 0.0299, 0.0299, 0.0299]], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.059601, 0.059601, 0.059601, 0.059601, 0.059601]], dtype=float32)}})
###Markdown
From this simplified example, you should be able to derive a full BatchNorm implementation, or any layer involving a state. To finish, let's add an optimizer to see how to play with both parameters updated by an optimizer and state variables.*This example isn't doing anything and is only for demonstration purposes.*
###Code
def update_step(apply_fun, x, optimizer, state):
def loss(params):
y, updated_state = apply_fun({'params': params, **state},
x, mutable=list(state.keys()))
l = ((x - y) ** 2).sum()
return l, updated_state
(l, updated_state), grads = jax.value_and_grad(
loss, has_aux=True)(optimizer.target)
optimizer = optimizer.apply_gradient(grads)
return optimizer, updated_state
variables = model.init(random.PRNGKey(0), x)
state, params = variables.pop('params')
del variables
optimizer = optim.sgd.GradientDescent(learning_rate=0.02).create(params)
x = jnp.ones((10,5))
for _ in range(3):
optimizer, state = update_step(model.apply, x, optimizer, state)
print('Updated state: ', state)
###Output
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.0199, 0.0199, 0.0199, 0.0199, 0.0199]], dtype=float32)}})
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.029701, 0.029701, 0.029701, 0.029701, 0.029701]], dtype=float32)}})
###Markdown
Flax basicsThis notebook will walk you through the following workflow:* Instanciating a model from Flax built-in layers or third-party models.* Initializing parameters of the model and manually written training.* Using optimizers provided by Flax to ease training.* Serialization of parameters and other objects.* Creating your own models and managing state. Setting up our environmentHere we provide the code needed to set up the environment for our notebook.
###Code
# Install the newest JAXlib version.
!pip install --upgrade -q pip jax jaxlib
# Install Flax at head:
!pip install --upgrade -q git+https://github.com/google/flax.git
import jax
from typing import Any, Callable, Sequence, Optional
from jax import lax, random, numpy as jnp
import flax
from flax.core import freeze, unfreeze
from flax import linen as nn
from jax.config import config
config.enable_omnistaging() # Linen requires enabling omnistaging
###Output
_____no_output_____
###Markdown
Linear regression with FlaxIn the previous *JAX for the impatient* notebook, we finished up with a linear regression example. As we know, linear regression can also be written as a single dense neural network layer, which we will show in the following so that we can compare how it's done.A dense layer is a layer that has a kernel parameter $W\in\mathcal{M}_{m,n}(\mathbb{R})$ where $m$ is the number of features as an output of the model, and $n$ the dimensionality of the input, and a bias parameter $b\in\mathbb{R}^m$. The dense layers returns $Wx+b$ from an input $x\in\mathbb{R}^n$.This dense layer is already provided by Flax in the `flax.linen` module (here imported as `nn`).
###Code
# We create one dense layer instance (taking 'features' parameter as input)
model = nn.Dense(features=5)
###Output
_____no_output_____
###Markdown
Layers (and models in general, we'll use that word from now on) are subclasses of the `linen.Module` class. Model parameters & initializationParameters are not stored with the models themselves. You need to initialize parameters by calling the `init` function, using a PRNGKey and a dummy input parameter.
###Code
key1, key2 = random.split(random.PRNGKey(0))
x = random.normal(key1, (10,)) # Dummy input
params = model.init(key2, x) # Initialization call
jax.tree_map(lambda x: x.shape, params) # Checking output shapes
###Output
WARNING:absl:No GPU/TPU found, falling back to CPU. (Set TF_CPP_MIN_LOG_LEVEL=0 and rerun for more info.)
###Markdown
*Note: JAX and Flax, like numpy are row-based systems, meaning that vectors a represented as row vectors and not a column vector. This can be seen in the shape of the kernel here.*The result is what we expect: bias and kernel parameters of the correct size. Under the hood:* The dummy input variable `x` is used to trigger shape inference: we only declared the number of features we wanted in the output of the model, not the size of the input. Flax finds out by itself the correct size of the kernel.* The random PRNG key is used to trigger the initialization functions (those have default values provided by the module here).* Initialization functions are called to generate the intial set of parameters that the model will use. Those are functions that take as arguments `(PRNG Key, shape, dtype)` and return an Array of shape `shape`.* The init function returns the initalized set of parameter (you can also get the output of the evaluation on the dummy input with the same syntax but using the `init_with_output` method instead of `init`. We see in the output that parameters are stored in a `FrozenDict` instance which helps deal with the functional nature of JAX by preventing any mutation of the underlying dict and making the user aware of it. Read more about it in the Flax docs. As a consequence, the following doesn't work:
###Code
try:
params['new_key'] = jnp.ones((2,2))
except ValueError as e:
print("Error: ", e)
###Output
Error: FrozenDict is immutable.
###Markdown
To evaluate the model with a given set of parameters (never stored with the model), we just use the `apply` method by providing it the parameters to use as well as the input:
###Code
model.apply(params, x)
###Output
_____no_output_____
###Markdown
Gradient descentIf you jumped here directly without going through the JAX part, here is the linear regression formulation we're going to use: from a set of data points $\{(x_i,y_i), i\in \{1,\ldots, k\}, x_i\in\mathbb{R}^n,y_i\in\mathbb{R}^m\}$, we try to find a set of parameters $W\in \mathcal{M}_{m,n}(\mathbb{R}), b\in\mathbb{R}^m$ such that the function $f_{W,b}(x)=Wx+b$ minimizes the mean squared error:$$\mathcal{L}(W,b)\rightarrow\frac{1}{k}\sum_{i=1}^{k} \frac{1}{2}\|y_i-f_{W,b}(x_i)\|^2_2$$Here, we see that the tuple $(W,b)$ matches the parameters of the Dense layer. We'll perform gradient descent using those. Let's first generate the fake data we'll use.
###Code
# Set problem dimensions
nsamples = 20
xdim = 10
ydim = 5
# Generate random ground truth W and b
key = random.PRNGKey(0)
k1, k2 = random.split(key)
W = random.normal(k1, (xdim, ydim))
b = random.normal(k2, (ydim,))
true_params = freeze({'params': {'bias': b, 'kernel': W}})
# Generate samples with additional noise
ksample, knoise = random.split(k1)
x_samples = random.normal(ksample, (nsamples, xdim))
y_samples = jnp.dot(x,W) + b
y_samples += 0.1*random.normal(knoise,(nsamples, ydim)) # Adding noise
print('x shape:', x_samples.shape, '; y shape:', y_samples.shape)
###Output
x shape: (20, 10) ; y shape: (20, 5)
###Markdown
Now let's generate the loss function (mean squarred error) with that data.
###Code
def make_mse_func(x_batched, y_batched):
def mse(params):
# Define the squared loss for a single pair (x,y)
def squared_error(x, y):
pred = model.apply(params, x)
return jnp.inner(y-pred, y-pred)/2.0
# We vectorize the previous to compute the average of the loss on all samples.
return jnp.mean(jax.vmap(squared_error)(x_batched,y_batched), axis=0)
return jax.jit(mse) # And finally we jit the result.
# Get the sampled loss
loss = make_mse_func(x_samples, y_samples)
###Output
_____no_output_____
###Markdown
And finally perform the gradient descent.
###Code
alpha = 0.3 # Gradient step size
print('Loss for "true" W,b: ', loss(true_params))
grad_fn = jax.value_and_grad(loss)
for i in range(101):
# We perform one gradient update
loss_val, grad = grad_fn(params)
params = jax.tree_multimap(lambda old, grad: old - alpha * grad,
params, grad)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss for "true" W,b: 29.070158
Loss step 0: 23.618902
Loss step 10: 0.30728564
Loss step 20: 0.06495677
Loss step 30: 0.025215296
Loss step 40: 0.015619493
Loss step 50: 0.012849321
Loss step 60: 0.011984843
Loss step 70: 0.011705536
Loss step 80: 0.011613827
Loss step 90: 0.011583473
Loss step 100: 0.011573391
###Markdown
Build-in optimization APIFlax provides an optimization package in `flax.optim` to make your life easier when training models. The process is:1. You choose an optimization method (e.g. `optim.GradientDescent`, `optim.Adam`)2. From the previous optimization method, you create a wrapper around the parameters you're going to optimize for with the `create` method. Your parameters are accessible through the `target` field.3. You compute the gradients of your loss with `jax.value_and_grad()`.4. At every iteration, you compute the gradients at the current point, then use the `apply_gradient()` method on the optimizer to return a new optimizer with updated parameters.
###Code
from flax import optim
optimizer_def = optim.GradientDescent(learning_rate=alpha) # Choose the method
optimizer = optimizer_def.create(params) # Create the wrapping optimizer with initial parameters
loss_grad_fn = jax.value_and_grad(loss)
for i in range(101):
loss_val, grad = loss_grad_fn(optimizer.target)
optimizer = optimizer.apply_gradient(grad) # Return the updated optimizer with parameters.
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss step 0: 0.011572863
Loss step 10: 0.011569859
Loss step 20: 0.011568859
Loss step 30: 0.011568523
Loss step 40: 0.011568412
Loss step 50: 0.011568374
Loss step 60: 0.011568364
Loss step 70: 0.011568359
Loss step 80: 0.01156836
Loss step 90: 0.011568356
Loss step 100: 0.011568357
###Markdown
Serializing the resultNow that we're happy with the result of our training, we might want to save the model parameters to load them back later. Flax provides a serialization package to enable you to do that.
###Code
from flax import serialization
bytes_output = serialization.to_bytes(optimizer.target)
dict_output = serialization.to_state_dict(optimizer.target)
print('Dict output')
print(dict_output)
print('Bytes output')
print(bytes_output)
###Output
Dict output
{'params': {'bias': DeviceArray([-3.023082 , 0.5307182, 3.7256303, 1.4638226, -3.2100437], dtype=float32), 'kernel': DeviceArray([[-1.4092493e-02, 4.8609809e-03, 1.1460093e-02,
-6.0927689e-02, 2.0413438e-05],
[-3.3569761e-02, -1.5614161e-03, 4.3190460e-04,
-7.9035060e-03, -1.9851506e-02],
[-1.8882388e-02, -2.1366426e-03, -1.8663550e-02,
-3.0001188e-02, 5.1880259e-02],
[-4.8119370e-02, -2.9280247e-02, -1.1992223e-02,
-1.0111435e-02, -8.3459895e-03],
[-1.7368369e-02, -1.7084973e-02, 6.0279824e-02,
9.2046618e-02, -1.5414236e-02],
[-3.0089449e-02, -5.5370983e-03, -9.1237156e-03,
2.1827107e-02, -2.0405082e-02],
[-5.6748122e-02, -4.2654604e-02, -1.1436724e-02,
7.5801805e-02, -2.0075133e-02],
[-1.4368590e-03, -1.6048675e-02, 1.5781123e-02,
2.8437756e-03, -8.5009886e-03],
[ 1.7892396e-02, 5.7572998e-02, 4.1483097e-02,
-9.9685444e-03, -2.1875760e-02],
[-2.1158390e-02, -1.3853005e-02, 2.5077526e-02,
3.2925244e-02, 3.8115401e-02]], dtype=float32)}}
Bytes output
b'\x81\xa6params\x82\xa4bias\xc7!\x01\x93\x91\x05\xa7float32\xc4\x14-zA\xc0&\xdd\x07?\xbapn@\x8a^\xbb?[qM\xc0\xa6kernel\xc7\xd6\x01\x93\x92\n\x05\xa7float32\xc4\xc83\xe4f\xbc\xddH\x9f;\x1d\xc3;<P\x8fy\xbd\x86=\xab7r\x80\t\xbdn\xa8\xcc\xbaAq\xe29\xb5}\x01\xbc\xa0\x9f\xa2\xbc=\xaf\x9a\xbc\xea\x06\x0c\xbbM\xe4\x98\xbc\r\xc5\xf5\xbce\x80T=\xd1\x18E\xbd!\xdd\xef\xbc\x07{D\xbco\xaa%\xbc\x9e\xbd\x08\xbc\x1cH\x8e\xbc\xc9\xf5\x8b\xbc\xfa\xe7v=\xf0\x82\xbc=\xfe\x8b|\xbc&~\xf6\xbc\x8cp\xb5\xbb\xa3{\x15\xbc\xc3\xce\xb2<\x8f(\xa7\xbc\xb8ph\xbd\x98\xb6.\xbd\x19a;\xbc\xfa=\x9b=\x9bt\xa4\xbc\xfdT\xbc\xba\x83x\x83\xbcjG\x81<\xa3^:;\xbbG\x0b\xbc\x13\x93\x92<\xaa\xd1k=.\xea)=\x1bS#\xbc\xcb4\xb3\xbc\\T\xad\xbc\xb7\xf7b\xbcbo\xcd<\x9f\xdc\x06=\xe5\x1e\x1c='
###Markdown
To load the model back, you'll need to use as a template the model parameter structure, like the one you would get from the model initialization. Here we use the previously generated `params` as template. Note that this will produce a new variable structure, and not mutate in-place.*The point of enforcing structure through template is to avoid users issues downstream, so you need to first have the right model that generated the parameters structure.*
###Code
serialization.from_bytes(params, bytes_output)
###Output
_____no_output_____
###Markdown
The serialization utils provided by Flax work on objects beyond parameters, for example you might want to serialize the optimizer and it's states, which we show in the following cell:
###Code
serialization.to_state_dict(optimizer)
###Output
_____no_output_____
###Markdown
Defining your own modelsFlax allows you to define your own models, which should be a bit more complicated than a linear regression. In this section, we'll show you how to build simple models. To do so, you'll need to create subclasses of the base `nn.Module` class.*Keep in mind that we imported* `linen as nn` *and this only works with the new linen API* Module basicsThe base abstraction for models is the `nn.Module` class, and every type of predefined layers in Flax (like the previous `Dense`) is a subclass of `nn.Module`. Let's take a look and start by defining a simple, but custom multi-layer perceptron i.e. a sequence of Dense layer interleaved with calls to a non-linear activation function.
###Code
class ExplicitMLP(nn.Module):
features: Sequence[int]
def setup(self):
# we automatically know what to do with lists, dicts of submodules
self.layers = [nn.Dense(feat) for feat in self.features]
# for single submodules, we would just write:
# self.layer1 = nn.Dense(self, feat1)
def __call__(self, inputs):
x = inputs
for i, lyr in enumerate(self.layers):
x = lyr(x)
if i != len(self.layers) - 1:
x = nn.relu(x)
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = ExplicitMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292822e-02 -4.3807123e-02 2.9323796e-02 6.5492545e-03
-1.7147183e-02]
[ 1.2967804e-01 -1.4551792e-01 9.4432175e-02 1.2521386e-02
-4.5417294e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
As we can see, a `nn.Module` subclass is made of:* A collection of data fields (`nn.Module` are Python dataclasses) - here we only have the `features` field of type `Sequence[int]`.* A `setup()` method that is being called at the end of the `__postinit__` where you can register submodules, variables, parameters you will need in your model.* A `__call__` function that returns the output of the model from a given input.* The model structure defines a pytree of parameters following the same tree structure as the model: the params tree contains one `layers_n` sub dict per layer, and each of those contain the parameters of the associated Dense layer. The layout is very explicit.*Note: lists are mostly managed as you would expect (WIP), there are corner cases you should be aware of as pointed out [here](https://github.com/google/flax/issues/524)*Since the module structure and its parameters are not tied to each other, you can't call directly `model(x)` on a given input as it will return an error. The `__call__` function is being wrapped up in the `apply` one, which is the one to call on an input:
###Code
try:
y = model(x) # Returns an error
except ValueError as e:
print(e)
###Output
Can't call methods on orphaned modules
###Markdown
Since here we have a very simple model, we could have used an alternative (but equivalent) way of declaring the submodules inline in the `__call__` using the `@nn.compact` annotation like so:
###Code
class SimpleMLP(nn.Module):
features: Sequence[int]
@nn.compact
def __call__(self, inputs):
x = inputs
for i, feat in enumerate(self.features):
x = nn.Dense(feat, name=f'layers_{i}')(x)
if i != len(self.features) - 1:
x = nn.relu(x)
# providing a name is optional though!
# the default autonames would be "Dense_0", "Dense_1", ...
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292822e-02 -4.3807123e-02 2.9323796e-02 6.5492545e-03
-1.7147183e-02]
[ 1.2967804e-01 -1.4551792e-01 9.4432175e-02 1.2521386e-02
-4.5417294e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
There are however a few differences you should be aware of between the two declaration modes:* In `setup`, you are able to name some sublayers and keep them around for further use (e.g. encoder/decoder methods in autoencoders).* If you want to have multiple methods, then you **need** to declare the module using `setup`, as the `@nn.compact` annotation only allow one method to be annotated.* Lazt initialization will be handled differently see these notes for more details (TODO: add notes link) Module parametersIn the previous MLP example, we relied only on predefined layers and operators (`Dense`, `relu`). Let's imagine that you didn't have a Dense layer provided by Flax and you wanted to write it on your own. Here is what it would look like using the `@nn.compact` way to declare a new modules:
###Code
class SimpleDense(nn.Module):
features: int
kernel_init: Callable = nn.initializers.lecun_normal()
bias_init: Callable = nn.initializers.zeros
@nn.compact
def __call__(self, inputs):
kernel = self.param('kernel',
self.kernel_init, # Initialization function
(inputs.shape[-1], self.features)) # shape info.
y = lax.dot_general(inputs, kernel,
(((inputs.ndim - 1,), (0,)), ((), ())),) # TODO Why not jnp.dot?
bias = self.param('bias', self.bias_init, (self.features,))
y = y + bias
return y
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleDense(features=3)
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameters:\n', params)
print('output:\n', y)
###Output
initialized parameters:
FrozenDict({'params': {'kernel': DeviceArray([[ 0.6503669 , 0.8678979 , 0.46042678],
[ 0.05673932, 0.9909285 , -0.63536596],
[ 0.76134115, -0.3250529 , -0.6522163 ],
[-0.8243032 , 0.4150194 , 0.19405058]], dtype=float32), 'bias': DeviceArray([0., 0., 0.], dtype=float32)}})
output:
[[ 0.50355184 1.8548559 -0.4270196 ]
[ 0.02790972 0.5589246 -0.43061778]
[ 0.35471287 1.5740999 -0.32865524]
[ 0.52648634 1.2928859 0.10089307]]
###Markdown
Here, we see how both declare and assign a parameter to the model using the `self.param` method. It takes as input `(name, init_fn, *init_args)` : * `name` is simply the name of the parameter that will end up in the parameter structure.* `init_fun` is a function with input `(PRNGKey, *init_args)` returning an Array with `init_args` the arguments needed to call the initialisation function* `init_args` the arguments to provide to the initialization function.Such params can also be declared in the `setup` method, it won't be able to use shape inference as Flax is using lazy initialization at first call site. Variables and collections of variablesAs we've seen so far, working with models means working with:* A subclass of `nn.Module`;* A pytree of parameters for the model (typically from `model.init()`);However this is not enough to cover everything that we would need for machine learning, especially neural networks. In some cases, you might want your neural network to keep track of some internal state while it runs (e.g. batch normlization layers). There is a way to declare variables beyond the parameters of the model with the `variable` method.For demonstration purpose, we'll implement a simplified but similar mechanism to batch normalization: we'll store running averages and substract those to the input at training time. For proper batchnorm, you should use (and look at) the implementation [here](https://github.com/google/flax/blob/master/flax/linen/normalization.py).
###Code
class BiasAdderWithRunningMean(nn.Module):
decay: float = 0.99
@nn.compact
def __call__(self, x):
# easy pattern to detect if we're initializing via empty variable tree
is_initialized = self.has_variable('batch_stats', 'mean')
ra_mean = self.variable('batch_stats', 'mean',
lambda s: jnp.zeros(s),
x.shape[1:])
mean = ra_mean.value # This will get either the value, or trigger init
bias = self.param('bias', lambda rng, shape: jnp.zeros(shape), x.shape[1:])
if is_initialized:
ra_mean.value = self.decay * ra_mean.value + (1.0 - self.decay) * jnp.mean(x, axis=0, keepdims=True)
return x - ra_mean.value + bias
key1, key2 = random.split(random.PRNGKey(0), 2)
x = jnp.ones((10,5))
model = BiasAdderWithRunningMean()
variables = model.init(key1, x)
print('initialized variables:\n', variables)
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
print('updated state:\n', updated_state)
###Output
initialized variables:
FrozenDict({'batch_stats': {'mean': DeviceArray([0., 0., 0., 0., 0.], dtype=float32)}, 'params': {'bias': DeviceArray([0., 0., 0., 0., 0.], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
###Markdown
Here, `updated_state` returns only the state variables that are being mutated by the model while applying it on data. To update the variables and get the new parameters of the model, we can use the following pattern:
###Code
for val in [1.0, 2.0, 3.0]:
x = val * jnp.ones((10,5))
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
old_state, params = variables.pop('params')
variables = freeze({'params': params, **updated_state})
print('updated state:\n', updated_state) # Shows only the mutable part
###Output
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.0299, 0.0299, 0.0299, 0.0299, 0.0299]], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.059601, 0.059601, 0.059601, 0.059601, 0.059601]], dtype=float32)}})
###Markdown
From this simplified example, you should be able to derive a full BatchNorm implementation, or any layer involving a state. To finish, let's add an optimizer to see how to play with both parameters updated by an optimizer and state variables.*This example isn't doing anything and is only for demonstration purpose.*
###Code
def update_step(apply_fun, x, optimizer, state):
def loss(params):
y, updated_state = apply_fun({'params': params, **state},
x, mutable=list(state.keys()))
l = ((x - y) ** 2).sum()
return l, updated_state
(l, updated_state), grads = jax.value_and_grad(
loss, has_aux=True)(optimizer.target)
optimizer = optimizer.apply_gradient(grads)
return optimizer, updated_state
variables = model.init(random.PRNGKey(0), x)
state, params = variables.pop('params')
del variables
optimizer = optim.sgd.GradientDescent(learning_rate=0.02).create(params)
x = jnp.ones((10,5))
for _ in range(3):
optimizer, state = update_step(model.apply, x, optimizer, state)
print('Updated state: ', state)
###Output
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.0199, 0.0199, 0.0199, 0.0199, 0.0199]], dtype=float32)}})
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.029701, 0.029701, 0.029701, 0.029701, 0.029701]], dtype=float32)}})
###Markdown
Flax BasicsThis notebook will walk you through the following workflow:* Instantiating a model from Flax built-in layers or third-party models.* Initializing parameters of the model and manually written training.* Using optimizers provided by Flax to ease training.* Serialization of parameters and other objects.* Creating your own models and managing state. Setting up our environmentHere we provide the code needed to set up the environment for our notebook.
###Code
# Install the latest JAXlib version.
!pip install --upgrade -q pip jax jaxlib
# Install Flax at head:
!pip install --upgrade -q git+https://github.com/google/flax.git
import jax
from typing import Any, Callable, Sequence, Optional
from jax import lax, random, numpy as jnp
import flax
from flax.core import freeze, unfreeze
from flax import linen as nn
###Output
_____no_output_____
###Markdown
Linear regression with FlaxIn the previous *JAX for the impatient* notebook, we finished up with a linear regression example. As we know, linear regression can also be written as a single dense neural network layer, which we will show in the following so that we can compare how it's done.A dense layer is a layer that has a kernel parameter $W\in\mathcal{M}_{m,n}(\mathbb{R})$ where $m$ is the number of features as an output of the model, and $n$ the dimensionality of the input, and a bias parameter $b\in\mathbb{R}^m$. The dense layers returns $Wx+b$ from an input $x\in\mathbb{R}^n$.This dense layer is already provided by Flax in the `flax.linen` module (here imported as `nn`).
###Code
# We create one dense layer instance (taking 'features' parameter as input)
model = nn.Dense(features=5)
###Output
_____no_output_____
###Markdown
Layers (and models in general, we'll use that word from now on) are subclasses of the `linen.Module` class. Model parameters & initializationParameters are not stored with the models themselves. You need to initialize parameters by calling the `init` function, using a PRNGKey and a dummy input parameter.
###Code
key1, key2 = random.split(random.PRNGKey(0))
x = random.normal(key1, (10,)) # Dummy input
params = model.init(key2, x) # Initialization call
jax.tree_map(lambda x: x.shape, params) # Checking output shapes
###Output
WARNING:absl:No GPU/TPU found, falling back to CPU. (Set TF_CPP_MIN_LOG_LEVEL=0 and rerun for more info.)
###Markdown
*Note: JAX and Flax, like NumPy, are row-based systems, meaning that vectors are represented as row vectors and not column vectors. This can be seen in the shape of the kernel here.*The result is what we expect: bias and kernel parameters of the correct size. Under the hood:* The dummy input variable `x` is used to trigger shape inference: we only declared the number of features we wanted in the output of the model, not the size of the input. Flax finds out by itself the correct size of the kernel.* The random PRNG key is used to trigger the initialization functions (those have default values provided by the module here).* Initialization functions are called to generate the initial set of parameters that the model will use. Those are functions that take as arguments `(PRNG Key, shape, dtype)` and return an Array of shape `shape`.* The init function returns the initialized set of parameters (you can also get the output of the evaluation on the dummy input with the same syntax but using the `init_with_output` method instead of `init`. We see in the output that parameters are stored in a `FrozenDict` instance which helps deal with the functional nature of JAX by preventing any mutation of the underlying dict and making the user aware of it. Read more about it in the Flax docs. As a consequence, the following doesn't work:
###Code
try:
params['new_key'] = jnp.ones((2,2))
except ValueError as e:
print("Error: ", e)
###Output
Error: FrozenDict is immutable.
###Markdown
To evaluate the model with a given set of parameters (never stored with the model), we just use the `apply` method by providing it the parameters to use as well as the input:
###Code
model.apply(params, x)
###Output
_____no_output_____
###Markdown
Gradient descentIf you jumped here directly without going through the JAX part, here is the linear regression formulation we're going to use: from a set of data points $\{(x_i,y_i), i\in \{1,\ldots, k\}, x_i\in\mathbb{R}^n,y_i\in\mathbb{R}^m\}$, we try to find a set of parameters $W\in \mathcal{M}_{m,n}(\mathbb{R}), b\in\mathbb{R}^m$ such that the function $f_{W,b}(x)=Wx+b$ minimizes the mean squared error:$$\mathcal{L}(W,b)\rightarrow\frac{1}{k}\sum_{i=1}^{k} \frac{1}{2}\|y_i-f_{W,b}(x_i)\|^2_2$$Here, we see that the tuple $(W,b)$ matches the parameters of the Dense layer. We'll perform gradient descent using those. Let's first generate the fake data we'll use.
###Code
# Set problem dimensions
nsamples = 20
xdim = 10
ydim = 5
# Generate random ground truth W and b
key = random.PRNGKey(0)
k1, k2 = random.split(key)
W = random.normal(k1, (xdim, ydim))
b = random.normal(k2, (ydim,))
true_params = freeze({'params': {'bias': b, 'kernel': W}})
# Generate samples with additional noise
ksample, knoise = random.split(k1)
x_samples = random.normal(ksample, (nsamples, xdim))
y_samples = jnp.dot(x_samples, W) + b
y_samples += 0.1*random.normal(knoise,(nsamples, ydim)) # Adding noise
print('x shape:', x_samples.shape, '; y shape:', y_samples.shape)
###Output
x shape: (20, 10) ; y shape: (20, 5)
###Markdown
Now let's generate the loss function (mean squared error) with that data.
###Code
def make_mse_func(x_batched, y_batched):
def mse(params):
# Define the squared loss for a single pair (x,y)
def squared_error(x, y):
pred = model.apply(params, x)
return jnp.inner(y-pred, y-pred)/2.0
# We vectorize the previous to compute the average of the loss on all samples.
return jnp.mean(jax.vmap(squared_error)(x_batched,y_batched), axis=0)
return jax.jit(mse) # And finally we jit the result.
# Get the sampled loss
loss = make_mse_func(x_samples, y_samples)
###Output
_____no_output_____
###Markdown
And finally perform the gradient descent.
###Code
alpha = 0.3 # Gradient step size
print('Loss for "true" W,b: ', loss(true_params))
grad_fn = jax.value_and_grad(loss)
for i in range(101):
# We perform one gradient update
loss_val, grads = grad_fn(params)
params = jax.tree_multimap(lambda p, g: p - alpha * g,
params, grads)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss for "true" W,b: 0.023639778
Loss step 0: 38.094772
Loss step 10: 0.44692168
Loss step 20: 0.10053458
Loss step 30: 0.035822745
Loss step 40: 0.018846875
Loss step 50: 0.013864839
Loss step 60: 0.012312559
Loss step 70: 0.011812928
Loss step 80: 0.011649306
Loss step 90: 0.011595251
Loss step 100: 0.0115773035
###Markdown
Optimizing with OptaxFlax used to use its own `flax.optim` package for optimization, but with[FLIP 1009](https://github.com/google/flax/blob/master/docs/flip/1009-optimizer-api.md)this was deprecated in favor of[Optax](https://github.com/deepmind/optax).Basic usage of Optax is straightforward:1. You choose an optimization method (e.g. `optax.sgd`).2. Create optimizer state from parameters.3. You compute the gradients of your loss with `jax.value_and_grad()`.4. At every iteration, call the Optax update function to update the internal optimizer state and create an update to the parameters. Then add the update to the parameters.Note that Optax can do a lot more: It's designed for composing simple gradienttransformations into more complex transformations that allows to implement awide range of optimizers. There is also support for changing optimizerhyperparameters over time ("schedules"), applying different udpates to differentparts of the parameter tree ("masking") and much more. For details please referto the[official documentation](https://optax.readthedocs.io/en/latest/).
###Code
import optax
tx = optax.sgd(learning_rate=alpha)
opt_state = tx.init(params)
loss_grad_fn = jax.value_and_grad(loss)
for i in range(101):
loss_val, grads = loss_grad_fn(params)
updates, opt_state = tx.update(grads, opt_state)
params = optax.apply_updates(params, updates)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss step 0: 0.011576377
Loss step 10: 0.0115710115
Loss step 20: 0.011569244
Loss step 30: 0.011568661
Loss step 40: 0.011568454
Loss step 50: 0.011568379
Loss step 60: 0.011568358
Loss step 70: 0.01156836
Loss step 80: 0.01156835
Loss step 90: 0.011568353
Loss step 100: 0.011568348
###Markdown
Serializing the resultNow that we're happy with the result of our training, we might want to save the model parameters to load them back later. Flax provides a serialization package to enable you to do that.
###Code
from flax import serialization
bytes_output = serialization.to_bytes(params)
dict_output = serialization.to_state_dict(params)
print('Dict output')
print(dict_output)
print('Bytes output')
print(bytes_output)
###Output
Dict output
{'params': {'bias': DeviceArray([-1.4540135, -2.0262308, 2.0806582, 1.2201802, -0.9964547], dtype=float32), 'kernel': DeviceArray([[ 1.0106664 , 0.19014716, 0.04533899, -0.92722285,
0.34720102],
[ 1.7320251 , 0.9901233 , 1.1662225 , 1.1027892 ,
-0.10574618],
[-1.2009128 , 0.28837162, 1.4176372 , 0.12073109,
-1.3132601 ],
[-1.1944956 , -0.18993308, 0.03379077, 1.3165942 ,
0.07996067],
[ 0.14103189, 1.3737966 , -1.3162128 , 0.53401774,
-2.239638 ],
[ 0.5643044 , 0.813604 , 0.31888172, 0.5359193 ,
0.90352124],
[-0.37948322, 1.7408353 , 1.0788013 , -0.5041964 ,
0.9286919 ],
[ 0.9701384 , -1.3158673 , 0.33630812, 0.80941117,
-1.202457 ],
[ 1.0198247 , -0.6198277 , 1.0822718 , -1.8385581 ,
-0.45790705],
[-0.64384323, 0.4564892 , -1.1331053 , -0.68556863,
0.17010891]], dtype=float32)}}
Bytes output
b'\x81\xa6params\x82\xa4bias\xc7!\x01\x93\x91\x05\xa7float32\xc4\x14\x1d\x1d\xba\xbf\xc4\xad\x01\xc0\x81)\x05@\xdd.\x9c?\xa8\x17\x7f\xbf\xa6kernel\xc7\xd6\x01\x93\x92\n\x05\xa7float32\xc4\xc8\x84]\x81?\xf0\xb5B>`\xb59=z^m\xbfU\xc4\xb1>\x00\xb3\xdd?\xb8x}?\xc7F\x95?2(\x8d?t\x91\xd8\xbd\x83\xb7\x99\xbfr\xa5\x93>#u\xb5?\xdcA\xf7=\xe8\x18\xa8\xbf;\xe5\x98\xbf\xd1}B\xbe0h\n=)\x86\xa8?k\xc2\xa3=\xaaj\x10>\x91\xd8\xaf?\xa9y\xa8\xbfc\xb5\x08?;V\x0f\xc0Av\x10?ZHP?wD\xa3>\x022\t?+Mg?\xa0K\xc2\xbe\xb1\xd3\xde?)\x16\x8a?\x04\x13\x01\xbf\xc1\xbem?\xfdZx?Wn\xa8\xbf\x940\xac>\x925O?\x1c\xea\x99\xbf\x9e\x89\x82?\x07\xad\x1e\xbf\xe2\x87\x8a?\xdfU\xeb\xbf\xcbr\xea\xbe\xe9\xd2$\xbf\xf4\xb8\xe9>\x98\t\x91\xbfm\x81/\xbf\x081.>'
###Markdown
To load the model back, you'll need to use as a template the model parameter structure, like the one you would get from the model initialization. Here, we use the previously generated `params` as a template. Note that this will produce a new variable structure, and not mutate in-place.*The point of enforcing structure through template is to avoid users issues downstream, so you need to first have the right model that generates the parameters structure.*
###Code
serialization.from_bytes(params, bytes_output)
###Output
_____no_output_____
###Markdown
Defining your own modelsFlax allows you to define your own models, which should be a bit more complicated than a linear regression. In this section, we'll show you how to build simple models. To do so, you'll need to create subclasses of the base `nn.Module` class.*Keep in mind that we imported* `linen as nn` *and this only works with the new linen API* Module basicsThe base abstraction for models is the `nn.Module` class, and every type of predefined layers in Flax (like the previous `Dense`) is a subclass of `nn.Module`. Let's take a look and start by defining a simple but custom multi-layer perceptron i.e. a sequence of Dense layers interleaved with calls to a non-linear activation function.
###Code
class ExplicitMLP(nn.Module):
features: Sequence[int]
def setup(self):
# we automatically know what to do with lists, dicts of submodules
self.layers = [nn.Dense(feat) for feat in self.features]
# for single submodules, we would just write:
# self.layer1 = nn.Dense(self, feat1)
def __call__(self, inputs):
x = inputs
for i, lyr in enumerate(self.layers):
x = lyr(x)
if i != len(self.layers) - 1:
x = nn.relu(x)
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = ExplicitMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292815e-02 -4.3807115e-02 2.9323792e-02 6.5492536e-03
-1.7147182e-02]
[ 1.2967806e-01 -1.4551792e-01 9.4432183e-02 1.2521387e-02
-4.5417298e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
As we can see, a `nn.Module` subclass is made of:* A collection of data fields (`nn.Module` are Python dataclasses) - here we only have the `features` field of type `Sequence[int]`.* A `setup()` method that is being called at the end of the `__postinit__` where you can register submodules, variables, parameters you will need in your model.* A `__call__` function that returns the output of the model from a given input.* The model structure defines a pytree of parameters following the same tree structure as the model: the params tree contains one `layers_n` sub dict per layer, and each of those contain the parameters of the associated Dense layer. The layout is very explicit.*Note: lists are mostly managed as you would expect (WIP), there are corner cases you should be aware of as pointed out* [here](https://github.com/google/flax/issues/524)Since the module structure and its parameters are not tied to each other, you can't call directly `model(x)` on a given input as it will return an error. The `__call__` function is being wrapped up in the `apply` one, which is the one to call on an input:
###Code
try:
y = model(x) # Returns an error
except AttributeError as e:
print(e)
###Output
"ExplicitMLP" object has no attribute "layers"
###Markdown
Since here we have a very simple model, we could have used an alternative (but equivalent) way of declaring the submodules inline in the `__call__` using the `@nn.compact` annotation like so:
###Code
class SimpleMLP(nn.Module):
features: Sequence[int]
@nn.compact
def __call__(self, inputs):
x = inputs
for i, feat in enumerate(self.features):
x = nn.Dense(feat, name=f'layers_{i}')(x)
if i != len(self.features) - 1:
x = nn.relu(x)
# providing a name is optional though!
# the default autonames would be "Dense_0", "Dense_1", ...
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292815e-02 -4.3807115e-02 2.9323792e-02 6.5492536e-03
-1.7147182e-02]
[ 1.2967806e-01 -1.4551792e-01 9.4432183e-02 1.2521387e-02
-4.5417298e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
There are, however, a few differences you should be aware of between the two declaration modes:* In `setup`, you are able to name some sublayers and keep them around for further use (e.g. encoder/decoder methods in autoencoders).* If you want to have multiple methods, then you **need** to declare the module using `setup`, as the `@nn.compact` annotation only allows one method to be annotated.* The last initialization will be handled differently see these notes for more details (TODO: add notes link) Module parametersIn the previous MLP example, we relied only on predefined layers and operators (`Dense`, `relu`). Let's imagine that you didn't have a Dense layer provided by Flax and you wanted to write it on your own. Here is what it would look like using the `@nn.compact` way to declare a new modules:
###Code
class SimpleDense(nn.Module):
features: int
kernel_init: Callable = nn.initializers.lecun_normal()
bias_init: Callable = nn.initializers.zeros
@nn.compact
def __call__(self, inputs):
kernel = self.param('kernel',
self.kernel_init, # Initialization function
(inputs.shape[-1], self.features)) # shape info.
y = lax.dot_general(inputs, kernel,
(((inputs.ndim - 1,), (0,)), ((), ())),) # TODO Why not jnp.dot?
bias = self.param('bias', self.bias_init, (self.features,))
y = y + bias
return y
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleDense(features=3)
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameters:\n', params)
print('output:\n', y)
###Output
initialized parameters:
FrozenDict({
params: {
kernel: DeviceArray([[ 0.6503669 , 0.86789787, 0.4604268 ],
[ 0.05673932, 0.9909285 , -0.63536596],
[ 0.76134115, -0.3250529 , -0.65221626],
[-0.82430327, 0.4150194 , 0.19405058]], dtype=float32),
bias: DeviceArray([0., 0., 0.], dtype=float32),
},
})
output:
[[ 0.5035518 1.8548558 -0.4270195 ]
[ 0.0279097 0.5589246 -0.43061772]
[ 0.3547128 1.5740999 -0.32865518]
[ 0.5264864 1.2928858 0.10089308]]
###Markdown
Here, we see how both declare and assign a parameter to the model using the `self.param` method. It takes as input `(name, init_fn, *init_args)` : * `name` is simply the name of the parameter that will end up in the parameter structure.* `init_fun` is a function with input `(PRNGKey, *init_args)` returning an Array with `init_args` the arguments needed to call the initialisation function* `init_args` the arguments to provide to the initialization function.Such params can also be declared in the `setup` method, it won't be able to use shape inference because Flax is using lazy initialization at the first call site. Variables and collections of variablesAs we've seen so far, working with models means working with:* A subclass of `nn.Module`;* A pytree of parameters for the model (typically from `model.init()`);However this is not enough to cover everything that we would need for machine learning, especially neural networks. In some cases, you might want your neural network to keep track of some internal state while it runs (e.g. batch normalization layers). There is a way to declare variables beyond the parameters of the model with the `variable` method.For demonstration purposes, we'll implement a simplified but similar mechanism to batch normalization: we'll store running averages and subtract those to the input at training time. For proper batchnorm, you should use (and look at) the implementation [here](https://github.com/google/flax/blob/master/flax/linen/normalization.py).
###Code
class BiasAdderWithRunningMean(nn.Module):
decay: float = 0.99
@nn.compact
def __call__(self, x):
# easy pattern to detect if we're initializing via empty variable tree
is_initialized = self.has_variable('batch_stats', 'mean')
ra_mean = self.variable('batch_stats', 'mean',
lambda s: jnp.zeros(s),
x.shape[1:])
mean = ra_mean.value # This will get either the value, or trigger init
bias = self.param('bias', lambda rng, shape: jnp.zeros(shape), x.shape[1:])
if is_initialized:
ra_mean.value = self.decay * ra_mean.value + (1.0 - self.decay) * jnp.mean(x, axis=0, keepdims=True)
return x - ra_mean.value + bias
key1, key2 = random.split(random.PRNGKey(0), 2)
x = jnp.ones((10,5))
model = BiasAdderWithRunningMean()
variables = model.init(key1, x)
print('initialized variables:\n', variables)
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
print('updated state:\n', updated_state)
###Output
initialized variables:
FrozenDict({
batch_stats: {
mean: DeviceArray([0., 0., 0., 0., 0.], dtype=float32),
},
params: {
bias: DeviceArray([0., 0., 0., 0., 0.], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
###Markdown
Here, `updated_state` returns only the state variables that are being mutated by the model while applying it on data. To update the variables and get the new parameters of the model, we can use the following pattern:
###Code
for val in [1.0, 2.0, 3.0]:
x = val * jnp.ones((10,5))
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
old_state, params = variables.pop('params')
variables = freeze({'params': params, **updated_state})
print('updated state:\n', updated_state) # Shows only the mutable part
###Output
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.0299, 0.0299, 0.0299, 0.0299, 0.0299]], dtype=float32),
},
})
updated state:
FrozenDict({
batch_stats: {
mean: DeviceArray([[0.059601, 0.059601, 0.059601, 0.059601, 0.059601]], dtype=float32),
},
})
###Markdown
From this simplified example, you should be able to derive a full BatchNorm implementation, or any layer involving a state. To finish, let's add an optimizer to see how to play with both parameters updated by an optimizer and state variables.*This example isn't doing anything and is only for demonstration purposes.*
###Code
def update_step(tx, apply_fun, x, opt_state, params, state):
def loss(params):
y, updated_state = apply_fun({'params': params, **state},
x, mutable=list(state.keys()))
l = ((x - y) ** 2).sum()
return l, updated_state
(l, state), grads = jax.value_and_grad(loss, has_aux=True)(params)
updates, opt_state = tx.update(grads, opt_state)
params = optax.apply_updates(params, updates)
return opt_state, params, state
x = jnp.ones((10,5))
variables = model.init(random.PRNGKey(0), x)
state, params = variables.pop('params')
del variables
tx = optax.sgd(learning_rate=0.02)
opt_state = tx.init(params)
for _ in range(3):
opt_state, params, state = update_step(tx, model.apply, x, opt_state, params, state)
print('Updated state: ', state)
###Output
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32),
},
})
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.0199, 0.0199, 0.0199, 0.0199, 0.0199]], dtype=float32),
},
})
Updated state: FrozenDict({
batch_stats: {
mean: DeviceArray([[0.029701, 0.029701, 0.029701, 0.029701, 0.029701]], dtype=float32),
},
})
###Markdown
Flax BasicsThis notebook will walk you through the following workflow:* Instantiating a model from Flax built-in layers or third-party models.* Initializing parameters of the model and manually written training.* Using optimizers provided by Flax to ease training.* Serialization of parameters and other objects.* Creating your own models and managing state. Setting up our environmentHere we provide the code needed to set up the environment for our notebook.
###Code
# Install the latest JAXlib version.
!pip install --upgrade -q pip jax jaxlib
# Install Flax at head:
!pip install --upgrade -q git+https://github.com/google/flax.git
import jax
from typing import Any, Callable, Sequence, Optional
from jax import lax, random, numpy as jnp
import flax
from flax.core import freeze, unfreeze
from flax import linen as nn
###Output
_____no_output_____
###Markdown
Linear regression with FlaxIn the previous *JAX for the impatient* notebook, we finished up with a linear regression example. As we know, linear regression can also be written as a single dense neural network layer, which we will show in the following so that we can compare how it's done.A dense layer is a layer that has a kernel parameter $W\in\mathcal{M}_{m,n}(\mathbb{R})$ where $m$ is the number of features as an output of the model, and $n$ the dimensionality of the input, and a bias parameter $b\in\mathbb{R}^m$. The dense layers returns $Wx+b$ from an input $x\in\mathbb{R}^n$.This dense layer is already provided by Flax in the `flax.linen` module (here imported as `nn`).
###Code
# We create one dense layer instance (taking 'features' parameter as input)
model = nn.Dense(features=5)
###Output
_____no_output_____
###Markdown
Layers (and models in general, we'll use that word from now on) are subclasses of the `linen.Module` class. Model parameters & initializationParameters are not stored with the models themselves. You need to initialize parameters by calling the `init` function, using a PRNGKey and a dummy input parameter.
###Code
key1, key2 = random.split(random.PRNGKey(0))
x = random.normal(key1, (10,)) # Dummy input
params = model.init(key2, x) # Initialization call
jax.tree_map(lambda x: x.shape, params) # Checking output shapes
###Output
WARNING:absl:No GPU/TPU found, falling back to CPU. (Set TF_CPP_MIN_LOG_LEVEL=0 and rerun for more info.)
###Markdown
*Note: JAX and Flax, like NumPy, are row-based systems, meaning that vectors are represented as row vectors and not column vectors. This can be seen in the shape of the kernel here.*The result is what we expect: bias and kernel parameters of the correct size. Under the hood:* The dummy input variable `x` is used to trigger shape inference: we only declared the number of features we wanted in the output of the model, not the size of the input. Flax finds out by itself the correct size of the kernel.* The random PRNG key is used to trigger the initialization functions (those have default values provided by the module here).* Initialization functions are called to generate the initial set of parameters that the model will use. Those are functions that take as arguments `(PRNG Key, shape, dtype)` and return an Array of shape `shape`.* The init function returns the initialized set of parameters (you can also get the output of the evaluation on the dummy input with the same syntax but using the `init_with_output` method instead of `init`. We see in the output that parameters are stored in a `FrozenDict` instance which helps deal with the functional nature of JAX by preventing any mutation of the underlying dict and making the user aware of it. Read more about it in the Flax docs. As a consequence, the following doesn't work:
###Code
try:
params['new_key'] = jnp.ones((2,2))
except ValueError as e:
print("Error: ", e)
###Output
Error: FrozenDict is immutable.
###Markdown
To evaluate the model with a given set of parameters (never stored with the model), we just use the `apply` method by providing it the parameters to use as well as the input:
###Code
model.apply(params, x)
###Output
_____no_output_____
###Markdown
Gradient descentIf you jumped here directly without going through the JAX part, here is the linear regression formulation we're going to use: from a set of data points $\{(x_i,y_i), i\in \{1,\ldots, k\}, x_i\in\mathbb{R}^n,y_i\in\mathbb{R}^m\}$, we try to find a set of parameters $W\in \mathcal{M}_{m,n}(\mathbb{R}), b\in\mathbb{R}^m$ such that the function $f_{W,b}(x)=Wx+b$ minimizes the mean squared error:$$\mathcal{L}(W,b)\rightarrow\frac{1}{k}\sum_{i=1}^{k} \frac{1}{2}\|y_i-f_{W,b}(x_i)\|^2_2$$Here, we see that the tuple $(W,b)$ matches the parameters of the Dense layer. We'll perform gradient descent using those. Let's first generate the fake data we'll use.
###Code
# Set problem dimensions
nsamples = 20
xdim = 10
ydim = 5
# Generate random ground truth W and b
key = random.PRNGKey(0)
k1, k2 = random.split(key)
W = random.normal(k1, (xdim, ydim))
b = random.normal(k2, (ydim,))
true_params = freeze({'params': {'bias': b, 'kernel': W}})
# Generate samples with additional noise
ksample, knoise = random.split(k1)
x_samples = random.normal(ksample, (nsamples, xdim))
y_samples = jnp.dot(x_samples, W) + b
y_samples += 0.1*random.normal(knoise,(nsamples, ydim)) # Adding noise
print('x shape:', x_samples.shape, '; y shape:', y_samples.shape)
###Output
x shape: (20, 10) ; y shape: (20, 5)
###Markdown
Now let's generate the loss function (mean squared error) with that data.
###Code
def make_mse_func(x_batched, y_batched):
def mse(params):
# Define the squared loss for a single pair (x,y)
def squared_error(x, y):
pred = model.apply(params, x)
return jnp.inner(y-pred, y-pred)/2.0
# We vectorize the previous to compute the average of the loss on all samples.
return jnp.mean(jax.vmap(squared_error)(x_batched,y_batched), axis=0)
return jax.jit(mse) # And finally we jit the result.
# Get the sampled loss
loss = make_mse_func(x_samples, y_samples)
###Output
_____no_output_____
###Markdown
And finally perform the gradient descent.
###Code
alpha = 0.3 # Gradient step size
print('Loss for "true" W,b: ', loss(true_params))
grad_fn = jax.value_and_grad(loss)
for i in range(101):
# We perform one gradient update
loss_val, grad = grad_fn(params)
params = jax.tree_multimap(lambda old, grad: old - alpha * grad,
params, grad)
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss for "true" W,b: 29.070158
Loss step 0: 23.618902
Loss step 10: 0.30728564
Loss step 20: 0.06495677
Loss step 30: 0.025215296
Loss step 40: 0.015619493
Loss step 50: 0.012849321
Loss step 60: 0.011984843
Loss step 70: 0.011705536
Loss step 80: 0.011613827
Loss step 90: 0.011583473
Loss step 100: 0.011573391
###Markdown
Build-in optimization APIFlax provides an optimization package in `flax.optim` to make your life easier when training models. The process is:1. You choose an optimization method (e.g. `optim.GradientDescent`, `optim.Adam`)2. From the previous optimization method, you create a wrapper around the parameters you're going to optimize for with the `create` method. Your parameters are accessible through the `target` field.3. You compute the gradients of your loss with `jax.value_and_grad()`.4. At every iteration, you compute the gradients at the current point, then use the `apply_gradient()` method on the optimizer to return a new optimizer with updated parameters.
###Code
from flax import optim
optimizer_def = optim.GradientDescent(learning_rate=alpha) # Choose the method
optimizer = optimizer_def.create(params) # Create the wrapping optimizer with initial parameters
loss_grad_fn = jax.value_and_grad(loss)
for i in range(101):
loss_val, grad = loss_grad_fn(optimizer.target)
optimizer = optimizer.apply_gradient(grad) # Return the updated optimizer with parameters.
if i % 10 == 0:
print('Loss step {}: '.format(i), loss_val)
###Output
Loss step 0: 0.011572863
Loss step 10: 0.011569859
Loss step 20: 0.011568859
Loss step 30: 0.011568523
Loss step 40: 0.011568412
Loss step 50: 0.011568374
Loss step 60: 0.011568364
Loss step 70: 0.011568359
Loss step 80: 0.01156836
Loss step 90: 0.011568356
Loss step 100: 0.011568357
###Markdown
Serializing the resultNow that we're happy with the result of our training, we might want to save the model parameters to load them back later. Flax provides a serialization package to enable you to do that.
###Code
from flax import serialization
bytes_output = serialization.to_bytes(optimizer.target)
dict_output = serialization.to_state_dict(optimizer.target)
print('Dict output')
print(dict_output)
print('Bytes output')
print(bytes_output)
###Output
Dict output
{'params': {'bias': DeviceArray([-3.023082 , 0.5307182, 3.7256303, 1.4638226, -3.2100437], dtype=float32), 'kernel': DeviceArray([[-1.4092493e-02, 4.8609809e-03, 1.1460093e-02,
-6.0927689e-02, 2.0413438e-05],
[-3.3569761e-02, -1.5614161e-03, 4.3190460e-04,
-7.9035060e-03, -1.9851506e-02],
[-1.8882388e-02, -2.1366426e-03, -1.8663550e-02,
-3.0001188e-02, 5.1880259e-02],
[-4.8119370e-02, -2.9280247e-02, -1.1992223e-02,
-1.0111435e-02, -8.3459895e-03],
[-1.7368369e-02, -1.7084973e-02, 6.0279824e-02,
9.2046618e-02, -1.5414236e-02],
[-3.0089449e-02, -5.5370983e-03, -9.1237156e-03,
2.1827107e-02, -2.0405082e-02],
[-5.6748122e-02, -4.2654604e-02, -1.1436724e-02,
7.5801805e-02, -2.0075133e-02],
[-1.4368590e-03, -1.6048675e-02, 1.5781123e-02,
2.8437756e-03, -8.5009886e-03],
[ 1.7892396e-02, 5.7572998e-02, 4.1483097e-02,
-9.9685444e-03, -2.1875760e-02],
[-2.1158390e-02, -1.3853005e-02, 2.5077526e-02,
3.2925244e-02, 3.8115401e-02]], dtype=float32)}}
Bytes output
b'\x81\xa6params\x82\xa4bias\xc7!\x01\x93\x91\x05\xa7float32\xc4\x14-zA\xc0&\xdd\x07?\xbapn@\x8a^\xbb?[qM\xc0\xa6kernel\xc7\xd6\x01\x93\x92\n\x05\xa7float32\xc4\xc83\xe4f\xbc\xddH\x9f;\x1d\xc3;<P\x8fy\xbd\x86=\xab7r\x80\t\xbdn\xa8\xcc\xbaAq\xe29\xb5}\x01\xbc\xa0\x9f\xa2\xbc=\xaf\x9a\xbc\xea\x06\x0c\xbbM\xe4\x98\xbc\r\xc5\xf5\xbce\x80T=\xd1\x18E\xbd!\xdd\xef\xbc\x07{D\xbco\xaa%\xbc\x9e\xbd\x08\xbc\x1cH\x8e\xbc\xc9\xf5\x8b\xbc\xfa\xe7v=\xf0\x82\xbc=\xfe\x8b|\xbc&~\xf6\xbc\x8cp\xb5\xbb\xa3{\x15\xbc\xc3\xce\xb2<\x8f(\xa7\xbc\xb8ph\xbd\x98\xb6.\xbd\x19a;\xbc\xfa=\x9b=\x9bt\xa4\xbc\xfdT\xbc\xba\x83x\x83\xbcjG\x81<\xa3^:;\xbbG\x0b\xbc\x13\x93\x92<\xaa\xd1k=.\xea)=\x1bS#\xbc\xcb4\xb3\xbc\\T\xad\xbc\xb7\xf7b\xbcbo\xcd<\x9f\xdc\x06=\xe5\x1e\x1c='
###Markdown
To load the model back, you'll need to use as a template the model parameter structure, like the one you would get from the model initialization. Here, we use the previously generated `params` as a template. Note that this will produce a new variable structure, and not mutate in-place.*The point of enforcing structure through template is to avoid users issues downstream, so you need to first have the right model that generates the parameters structure.*
###Code
serialization.from_bytes(params, bytes_output)
###Output
_____no_output_____
###Markdown
The serialization utils provided by Flax work on objects beyond parameters, for example you might want to serialize the optimizer and it's states, which we show in the following cell:
###Code
serialization.to_state_dict(optimizer)
###Output
_____no_output_____
###Markdown
Defining your own modelsFlax allows you to define your own models, which should be a bit more complicated than a linear regression. In this section, we'll show you how to build simple models. To do so, you'll need to create subclasses of the base `nn.Module` class.*Keep in mind that we imported* `linen as nn` *and this only works with the new linen API* Module basicsThe base abstraction for models is the `nn.Module` class, and every type of predefined layers in Flax (like the previous `Dense`) is a subclass of `nn.Module`. Let's take a look and start by defining a simple but custom multi-layer perceptron i.e. a sequence of Dense layers interleaved with calls to a non-linear activation function.
###Code
class ExplicitMLP(nn.Module):
features: Sequence[int]
def setup(self):
# we automatically know what to do with lists, dicts of submodules
self.layers = [nn.Dense(feat) for feat in self.features]
# for single submodules, we would just write:
# self.layer1 = nn.Dense(self, feat1)
def __call__(self, inputs):
x = inputs
for i, lyr in enumerate(self.layers):
x = lyr(x)
if i != len(self.layers) - 1:
x = nn.relu(x)
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = ExplicitMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292822e-02 -4.3807123e-02 2.9323796e-02 6.5492545e-03
-1.7147183e-02]
[ 1.2967804e-01 -1.4551792e-01 9.4432175e-02 1.2521386e-02
-4.5417294e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
As we can see, a `nn.Module` subclass is made of:* A collection of data fields (`nn.Module` are Python dataclasses) - here we only have the `features` field of type `Sequence[int]`.* A `setup()` method that is being called at the end of the `__postinit__` where you can register submodules, variables, parameters you will need in your model.* A `__call__` function that returns the output of the model from a given input.* The model structure defines a pytree of parameters following the same tree structure as the model: the params tree contains one `layers_n` sub dict per layer, and each of those contain the parameters of the associated Dense layer. The layout is very explicit.*Note: lists are mostly managed as you would expect (WIP), there are corner cases you should be aware of as pointed out* [here](https://github.com/google/flax/issues/524)Since the module structure and its parameters are not tied to each other, you can't call directly `model(x)` on a given input as it will return an error. The `__call__` function is being wrapped up in the `apply` one, which is the one to call on an input:
###Code
try:
y = model(x) # Returns an error
except ValueError as e:
print(e)
###Output
Can't call methods on orphaned modules
###Markdown
Since here we have a very simple model, we could have used an alternative (but equivalent) way of declaring the submodules inline in the `__call__` using the `@nn.compact` annotation like so:
###Code
class SimpleMLP(nn.Module):
features: Sequence[int]
@nn.compact
def __call__(self, inputs):
x = inputs
for i, feat in enumerate(self.features):
x = nn.Dense(feat, name=f'layers_{i}')(x)
if i != len(self.features) - 1:
x = nn.relu(x)
# providing a name is optional though!
# the default autonames would be "Dense_0", "Dense_1", ...
return x
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleMLP(features=[3,4,5])
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameter shapes:\n', jax.tree_map(jnp.shape, unfreeze(params)))
print('output:\n', y)
###Output
initialized parameter shapes:
{'params': {'layers_0': {'bias': (3,), 'kernel': (4, 3)}, 'layers_1': {'bias': (4,), 'kernel': (3, 4)}, 'layers_2': {'bias': (5,), 'kernel': (4, 5)}}}
output:
[[ 4.2292822e-02 -4.3807123e-02 2.9323796e-02 6.5492545e-03
-1.7147183e-02]
[ 1.2967804e-01 -1.4551792e-01 9.4432175e-02 1.2521386e-02
-4.5417294e-02]
[ 0.0000000e+00 0.0000000e+00 0.0000000e+00 0.0000000e+00
0.0000000e+00]
[ 9.3024032e-04 2.7864395e-05 2.4478821e-04 8.1344310e-04
-1.0110770e-03]]
###Markdown
There are, however, a few differences you should be aware of between the two declaration modes:* In `setup`, you are able to name some sublayers and keep them around for further use (e.g. encoder/decoder methods in autoencoders).* If you want to have multiple methods, then you **need** to declare the module using `setup`, as the `@nn.compact` annotation only allows one method to be annotated.* The last initialization will be handled differently see these notes for more details (TODO: add notes link) Module parametersIn the previous MLP example, we relied only on predefined layers and operators (`Dense`, `relu`). Let's imagine that you didn't have a Dense layer provided by Flax and you wanted to write it on your own. Here is what it would look like using the `@nn.compact` way to declare a new modules:
###Code
class SimpleDense(nn.Module):
features: int
kernel_init: Callable = nn.initializers.lecun_normal()
bias_init: Callable = nn.initializers.zeros
@nn.compact
def __call__(self, inputs):
kernel = self.param('kernel',
self.kernel_init, # Initialization function
(inputs.shape[-1], self.features)) # shape info.
y = lax.dot_general(inputs, kernel,
(((inputs.ndim - 1,), (0,)), ((), ())),) # TODO Why not jnp.dot?
bias = self.param('bias', self.bias_init, (self.features,))
y = y + bias
return y
key1, key2 = random.split(random.PRNGKey(0), 2)
x = random.uniform(key1, (4,4))
model = SimpleDense(features=3)
params = model.init(key2, x)
y = model.apply(params, x)
print('initialized parameters:\n', params)
print('output:\n', y)
###Output
initialized parameters:
FrozenDict({'params': {'kernel': DeviceArray([[ 0.6503669 , 0.8678979 , 0.46042678],
[ 0.05673932, 0.9909285 , -0.63536596],
[ 0.76134115, -0.3250529 , -0.6522163 ],
[-0.8243032 , 0.4150194 , 0.19405058]], dtype=float32), 'bias': DeviceArray([0., 0., 0.], dtype=float32)}})
output:
[[ 0.50355184 1.8548559 -0.4270196 ]
[ 0.02790972 0.5589246 -0.43061778]
[ 0.35471287 1.5740999 -0.32865524]
[ 0.52648634 1.2928859 0.10089307]]
###Markdown
Here, we see how both declare and assign a parameter to the model using the `self.param` method. It takes as input `(name, init_fn, *init_args)` : * `name` is simply the name of the parameter that will end up in the parameter structure.* `init_fun` is a function with input `(PRNGKey, *init_args)` returning an Array with `init_args` the arguments needed to call the initialisation function* `init_args` the arguments to provide to the initialization function.Such params can also be declared in the `setup` method, it won't be able to use shape inference because Flax is using lazy initialization at the first call site. Variables and collections of variablesAs we've seen so far, working with models means working with:* A subclass of `nn.Module`;* A pytree of parameters for the model (typically from `model.init()`);However this is not enough to cover everything that we would need for machine learning, especially neural networks. In some cases, you might want your neural network to keep track of some internal state while it runs (e.g. batch normalization layers). There is a way to declare variables beyond the parameters of the model with the `variable` method.For demonstration purposes, we'll implement a simplified but similar mechanism to batch normalization: we'll store running averages and subtract those to the input at training time. For proper batchnorm, you should use (and look at) the implementation [here](https://github.com/google/flax/blob/master/flax/linen/normalization.py).
###Code
class BiasAdderWithRunningMean(nn.Module):
decay: float = 0.99
@nn.compact
def __call__(self, x):
# easy pattern to detect if we're initializing via empty variable tree
is_initialized = self.has_variable('batch_stats', 'mean')
ra_mean = self.variable('batch_stats', 'mean',
lambda s: jnp.zeros(s),
x.shape[1:])
mean = ra_mean.value # This will get either the value, or trigger init
bias = self.param('bias', lambda rng, shape: jnp.zeros(shape), x.shape[1:])
if is_initialized:
ra_mean.value = self.decay * ra_mean.value + (1.0 - self.decay) * jnp.mean(x, axis=0, keepdims=True)
return x - ra_mean.value + bias
key1, key2 = random.split(random.PRNGKey(0), 2)
x = jnp.ones((10,5))
model = BiasAdderWithRunningMean()
variables = model.init(key1, x)
print('initialized variables:\n', variables)
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
print('updated state:\n', updated_state)
###Output
initialized variables:
FrozenDict({'batch_stats': {'mean': DeviceArray([0., 0., 0., 0., 0.], dtype=float32)}, 'params': {'bias': DeviceArray([0., 0., 0., 0., 0.], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
###Markdown
Here, `updated_state` returns only the state variables that are being mutated by the model while applying it on data. To update the variables and get the new parameters of the model, we can use the following pattern:
###Code
for val in [1.0, 2.0, 3.0]:
x = val * jnp.ones((10,5))
y, updated_state = model.apply(variables, x, mutable=['batch_stats'])
old_state, params = variables.pop('params')
variables = freeze({'params': params, **updated_state})
print('updated state:\n', updated_state) # Shows only the mutable part
###Output
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.0299, 0.0299, 0.0299, 0.0299, 0.0299]], dtype=float32)}})
updated state:
FrozenDict({'batch_stats': {'mean': DeviceArray([[0.059601, 0.059601, 0.059601, 0.059601, 0.059601]], dtype=float32)}})
###Markdown
From this simplified example, you should be able to derive a full BatchNorm implementation, or any layer involving a state. To finish, let's add an optimizer to see how to play with both parameters updated by an optimizer and state variables.*This example isn't doing anything and is only for demonstration purposes.*
###Code
def update_step(apply_fun, x, optimizer, state):
def loss(params):
y, updated_state = apply_fun({'params': params, **state},
x, mutable=list(state.keys()))
l = ((x - y) ** 2).sum()
return l, updated_state
(l, updated_state), grads = jax.value_and_grad(
loss, has_aux=True)(optimizer.target)
optimizer = optimizer.apply_gradient(grads)
return optimizer, updated_state
variables = model.init(random.PRNGKey(0), x)
state, params = variables.pop('params')
del variables
optimizer = optim.sgd.GradientDescent(learning_rate=0.02).create(params)
x = jnp.ones((10,5))
for _ in range(3):
optimizer, state = update_step(model.apply, x, optimizer, state)
print('Updated state: ', state)
###Output
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.01, 0.01, 0.01, 0.01, 0.01]], dtype=float32)}})
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.0199, 0.0199, 0.0199, 0.0199, 0.0199]], dtype=float32)}})
Updated state: FrozenDict({'batch_stats': {'mean': DeviceArray([[0.029701, 0.029701, 0.029701, 0.029701, 0.029701]], dtype=float32)}})
|
Demo Codes/ch08.ipynb | ###Markdown
Chapter 8 Analyzing Sentence Structure 8.1 Some Grammatical Dilemmas Ubiquitous Ambiguity
###Code
import nltk
groucho_grammar = nltk.CFG.fromstring("""
S -> NP VP
PP -> P NP
NP -> Det N | Det N PP | 'I'
VP -> V NP | VP PP
Det -> 'an' | 'my'
N -> 'elephant' | 'pajamas'
V -> 'shot'
P -> 'in'
""")
sent = ['I', 'shot', 'an', 'elephant', 'in', 'my', 'pajamas']
parser = nltk.ChartParser(groucho_grammar)
for tree in parser.parse(sent):
print(tree)
###Output
(S
(NP I)
(VP
(VP (V shot) (NP (Det an) (N elephant)))
(PP (P in) (NP (Det my) (N pajamas)))))
(S
(NP I)
(VP
(V shot)
(NP (Det an) (N elephant) (PP (P in) (NP (Det my) (N pajamas))))))
###Markdown
8.3 Context-Free Grammar A Simple Grammar
###Code
grammar1 = nltk.CFG.fromstring("""
S -> NP VP
VP -> V NP | V NP PP
PP -> P NP
V -> "saw" | "ate" | "walked"
NP -> "John" | "Mary" | "Bob" | Det N | Det N PP
Det -> "a" | "an" | "the" | "my"
N -> "man" | "dog" | "cat" | "telescope" | "park"
P -> "in" | "on" | "by" | "with"
""")
sent = "Mary saw Bob".split()
rd_parser = nltk.RecursiveDescentParser(grammar1)
for tree in rd_parser.parse(sent):
print(tree)
###Output
(S (NP Mary) (VP (V saw) (NP Bob)))
###Markdown
Recursion in Syntactic Structure
###Code
grammar2 = nltk.CFG.fromstring("""
S -> NP VP
NP -> Det Nom | PropN
Nom -> Adj Nom | N
VP -> V Adj | V NP | V S | V NP PP
PP -> P NP
PropN -> 'Buster' | 'Chatterer' | 'Joe'
Det -> 'the' | 'a'
N -> 'bear' | 'squirrel' | 'tree' | 'fish' | 'log'
Adj -> 'angry' | 'frightened' | 'little' | 'tall'
V -> 'chased' | 'saw' | 'said' | 'thought' | 'was' | 'put'
P -> 'on'
""")
###Output
_____no_output_____
###Markdown
8.4 Parsing with Context-Free Grammar Recursive Descent Parsing
###Code
rd_parser = nltk.RecursiveDescentParser(grammar1)
sent = 'Mary saw a dog'.split()
for t in rd_parser.parse(sent):
print(t)
###Output
(S (NP Mary) (VP (V saw) (NP (Det a) (N dog))))
###Markdown
Shift-Reduce Parsing
###Code
sr_parser = nltk.ShiftReduceParser(grammar1)
sent = 'Mary saw a dog'.split()
for tree in sr_parser.parse(sent):
print(tree)
###Output
(S (NP Mary) (VP (V saw) (NP (Det a) (N dog))))
###Markdown
Well-Formed Substring Tables
###Code
def init_wfst(tokens, grammar):
numtokens = len(tokens)
wfst = [[None for i in range(numtokens+1)] for j in range(numtokens+1)]
for i in range(numtokens):
productions = grammar.productions(rhs=tokens[i])
wfst[i][i+1] = productions[0].lhs()
return wfst
def complete_wfst(wfst, tokens, grammar, trace=False):
index = dict((p.rhs(), p.lhs()) for p in grammar.productions())
numtokens = len(tokens)
for span in range(2, numtokens+1):
for start in range(numtokens+1-span):
end = start + span
for mid in range(start+1, end):
nt1, nt2 = wfst[start][mid], wfst[mid][end]
if nt1 and nt2 and (nt1,nt2) in index:
wfst[start][end] = index[(nt1,nt2)]
if trace:
print("[%s] %3s [%s] %3s [%s] ==> [%s] %3s [%s]" % \
(start, nt1, mid, nt2, end, start, index[(nt1,nt2)], end))
return wfst
def display(wfst, tokens):
print('\nWFST ' + ' '.join(("%-4d" % i) for i in range(1, len(wfst))))
for i in range(len(wfst)-1):
print("%d " % i, end=" ")
for j in range(1, len(wfst)):
print("%-4s" % (wfst[i][j] or '.'), end=" ")
print()
tokens = "I shot an elephant in my pajamas".split()
wfst0 = init_wfst(tokens, groucho_grammar)
display(wfst0, tokens)
wfst1 = complete_wfst(wfst0, tokens, groucho_grammar)
display(wfst1, tokens)
wfst1 = complete_wfst(wfst0, tokens, groucho_grammar, trace=True)
###Output
[2] Det [3] N [4] ==> [2] NP [4]
[5] Det [6] N [7] ==> [5] NP [7]
[1] V [2] NP [4] ==> [1] VP [4]
[4] P [5] NP [7] ==> [4] PP [7]
[0] NP [1] VP [4] ==> [0] S [4]
[1] VP [4] PP [7] ==> [1] VP [7]
[0] NP [1] VP [7] ==> [0] S [7]
###Markdown
8.5 Dependencies and Dependency Grammar
###Code
groucho_dep_grammar = nltk.DependencyGrammar.fromstring("""
'shot' -> 'I' | 'elephant' | 'in'
'elephant' -> 'an' | 'in'
'in' -> 'pajamas'
'pajamas' -> 'my'
""")
print(groucho_dep_grammar)
pdp = nltk.ProjectiveDependencyParser(groucho_dep_grammar)
sent = 'I shot an elephant in my pajamas'.split()
trees = pdp.parse(sent)
for tree in trees:
print(tree)
###Output
(shot I (elephant an (in (pajamas my))))
(shot I (elephant an) (in (pajamas my)))
###Markdown
8.6 Grammar Development Treebanks and Grammars
###Code
from nltk.corpus import treebank
t = treebank.parsed_sents('wsj_0001.mrg')[0]
print(t)
def filter(tree):
child_nodes = [child.label() for child in tree
if isinstance(child, nltk.Tree)]
return (tree.label() == 'VP') and ('S' in child_nodes)
[subtree for tree in treebank.parsed_sents()
for subtree in tree.subtrees(filter)]
from collections import defaultdict
entries = nltk.corpus.ppattach.attachments('training')
table = defaultdict(lambda: defaultdict(set))
for entry in entries:
key = entry.noun1 + '-' + entry.prep + '-' + entry.noun2
table[key][entry.attachment].add(entry.verb)
for key in sorted(table):
if len(table[key]) > 1:
print(key, 'N:', sorted(table[key]['N']), 'V:', sorted(table[key]['V']))
nltk.corpus.sinica_treebank.parsed_sents()[3450].draw()
###Output
_____no_output_____
###Markdown
Pernicious Ambiguity
###Code
grammar = nltk.CFG.fromstring("""
S -> NP V NP
NP -> NP Sbar
Sbar -> NP V
NP -> 'fish'
V -> 'fish'
""")
tokens = ["fish"] * 5
cp = nltk.ChartParser(grammar)
for tree in cp.parse(tokens):
print(tree)
###Output
(S (NP fish) (V fish) (NP (NP fish) (Sbar (NP fish) (V fish))))
(S (NP (NP fish) (Sbar (NP fish) (V fish))) (V fish) (NP fish))
###Markdown
Weighted Grammar
###Code
def give(t):
return t.label() == 'VP' and len(t) > 2 and t[1].label() == 'NP'\
and (t[2].label() == 'PP-DTV' or t[2].label() == 'NP')\
and ('give' in t[0].leaves() or 'gave' in t[0].leaves())
def sent(t):
return ' '.join(token for token in t.leaves() if token[0] not in '*-0')
def print_node(t, width):
output = "%s %s: %s / %s: %s" %\
(sent(t[0]), t[1].label(), sent(t[1]), t[2].label(), sent(t[2]))
if len(output) > width:
output = output[:width] + "..."
print(output)
for tree in nltk.corpus.treebank.parsed_sents():
for t in tree.subtrees(give):
print_node(t, 72)
grammar = nltk.PCFG.fromstring("""
S -> NP VP [1.0]
VP -> TV NP [0.4]
VP -> IV [0.3]
VP -> DatV NP NP [0.3]
TV -> 'saw' [1.0]
IV -> 'ate' [1.0]
DatV -> 'gave' [1.0]
NP -> 'telescopes' [0.8]
NP -> 'Jack' [0.2]
""")
print(grammar)
viterbi_parser = nltk.ViterbiParser(grammar)
for tree in viterbi_parser.parse(['Jack', 'saw', 'telescopes']):
print(tree)
###Output
(S (NP Jack) (VP (TV saw) (NP telescopes))) (p=0.064)
|
notebooks/day09-ProbabilisticLogisticRegressionAndMAPEstimation.ipynb | ###Markdown
COMP 135 day09: MAP estimation for Logistic Regression Outline* **Part 1: Understanding sigmoids and Logistic Regression as a model*** **Part 2: Computing the MAP objective*** **Part 3: Gradient descent for the MAP: Comparing 1st and 2nd order GD** Takeaways* First-order methods are cheap but require many iterations* Second-order methods are awesome, but still require careful step-size selection* For all gradient descent methods, selecting step sizes is super important. Line search is needed!
###Code
import numpy as np
import pandas as pd
import scipy.stats
np.set_printoptions(precision=3, suppress=False)
pd.options.display.float_format = '{:,.3g}'.format # show 3 digits of precision
import matplotlib.pyplot as plt
import seaborn as sns
sns.set_style("whitegrid")
sns.set_context("notebook", font_scale=1.25)
###Output
_____no_output_____
###Markdown
Part 1: The Probabilistic view of logistic regression Task: Binary classificationGiven $N$ observations of *paired* feature-outcome observations: $\{ x_n, t_n \}$.* Each input feature $x_n$ is a scalar real: $x_n \in \mathbb{R}$* Each output or "label" or "outcome" $t_n$ is a scalar binary value: $t_n \in \{0, 1\}$We're also given a feature transform function $\phi$ which maps each $x_n$ to a vector in $M$-dimensional space. This function is known in advance.We want to make good predictions of new outcomes $t_*$ given new features $x_*$. Feature transformationFor now, we'll assume that the "feature transform" $\phi(x_n)$ just simply passes along the features $x_n$, while adding an additional offset or "intercept" feature that is always 1. This is a *simplifying* assumption for today.
###Code
def calc_features(x_N1, M=2):
''' Transform raw features into complete features useful for prediction
Could do any non-linear transformations thought relevant for the problem.
Here we'll just do an identity transform with an extra intercept feature.
Args
----
x_N1 : 2D array, shape (N, 1) = (n_examples,)
Returns
-------
phi_NM : 2D array, shape (N, M) = (n_examples, n_transformed_features)
First column will contain all ones (a bias or intercept feature)
Second column will just include the raw features
'''
assert x_N1.ndim == 2
assert x_N1.shape[1] == 1
N = x_N1.shape[0]
phi_NM = np.zeros((N, M))
phi_NM[:,0] = 1
phi_NM[:,1] = x_N1[:,0]
return phi_NM
x_N1 = np.linspace(-1, 1, 5)[:,np.newaxis]
# Get transformed features using our "calc_features" function
# * first column will be all 1s, an "intercept"
# * second column will be the x values
calc_features(x_N1)
###Output
_____no_output_____
###Markdown
Understanding the logistic sigmoid functionAs discussed in your pre-recorded lectures, the *logistic sigmoid function* is:\begin{align}\sigma(r) = \frac{1}{1 + e^{-r}}\end{align}It maps real inputs $r \in (-\infty, +\infty)$ to the probability interval $(0, 1)$.We call it a "sigmoid" function because it has an S-shaped curve, which you'll plot below.This function is also sometimes called the "expit" function. We can use an existing implementation of this function available in SciPy:* expit: https://docs.scipy.org/doc/scipy/reference/generated/scipy.special.expit.html
###Code
from scipy.special import expit as sigmoid
sigmoid(0)
sigmoid(-4)
sigmoid(4)
sigmoid(np.asarray([-6, -4, -2, 0, 2, 4, 6]))
###Output
_____no_output_____
###Markdown
Exercise 1a: Plot the logistic sigmoid function We give you an array of G candidate $r$ values below.
###Code
G = 101
r_G = np.linspace(-8, 8, G)
sigmoid_of_r_G = sigmoid(np.asarray(r_G)) # TODO evaluate sigmoid at each r value
plt.plot(r_G, sigmoid_of_r_G, 'k.-');
plt.xlabel('r'); plt.ylabel('$\sigma(r)$');
plt.ylim([-0.001, 1.001])
###Output
_____no_output_____
###Markdown
Define the LikelihoodEach observation (indexed by $n$) is drawn iid from a Bernoulli as follows:$$t_n | w \sim \text{BernPMF}\left( t_n | \sigma(w^T \phi(x_n)) \right)$$where $w \in \mathbb{R}^M$ is a weight vector, the same size as our feature vector $\phi(x_n) \in \mathbb{R}^M$The key properties here are:* The *mean* of $t_n$ is a *non-linear activation* of a linear function of the transformed features. Define the PriorFor now, we'll assume that weights come from a zero mean prior with some covariance determined by a scalar parameter $\alpha$:$$w \sim \mathcal{N}( 0, \alpha^{-1} I_M )$$A zero mean prior makes sense if we don't know if the slope should be negative or positive. Parameter we'll treat as a random variable: $w$* Weights vector: $w = [w_1, w_2, \ldots w_M]^T$, so $w \in \mathbb{R}^M$ Parameters we'll treat as fixed: $\alpha$ * Prior precision $\alpha > 0$The larger $\alpha$ is, the more confident we are in the weight values before seeing any data. Create a simple toy data for analysisJust execute the cells below to get the sense of how to generate toy data from this modelWe'll manually intervene to set the weight vector to a known value. This makes it easy to tell if our learning is working later on.
###Code
N = 10 # Number of examples we observe
M = 2 # Number of transformed features
###Output
_____no_output_____
###Markdown
Create the weight vector we'll use to generate our dataset. Set an intercept of 1.2 and a slope of -0.75
###Code
true_w_M = np.asarray([0.1, -0.25])
###Output
_____no_output_____
###Markdown
Create a "true" alpha value which controls the prior precision
###Code
true_alpha = 0.01
###Output
_____no_output_____
###Markdown
Create observed features $x$ and observed outputs $t$ manually
###Code
x_N1 = np.asarray([-5, -0.8, -0.7, -0.6, -0.4, 0.5, 0.8, 0.9, 4.3, 4.1]).reshape((N, 1))
phi_NM = calc_features(x_N1)
prng = np.random.RandomState(101) # reproducible random seed
t_N = (prng.rand(N) < sigmoid(np.dot(phi_NM, true_w_M))).astype(np.float64)
###Output
_____no_output_____
###Markdown
Visualize the toy dataset
###Code
plt.plot(x_N1, t_N, 'k.');
ax_h = plt.gca()
ax_h.set_xlim([-8, 8]); ax_h.set_xticks([-6, -4, -2, 0, 2, 4, 6]);
ax_h.set_ylim([-.1, 1.1]);
xgrid_G1 = np.linspace(-8, 8, 100)[:,np.newaxis]
plt.plot(xgrid_G1, sigmoid(np.dot(calc_features(xgrid_G1), true_w_M)), 'c-', linewidth=3);
plt.xlabel('input: x');
plt.ylabel('output: t');
plt.title("Toy Data\n true_slope %.2f \n true intercept %.2f" % (
true_w_M[1], true_w_M[0]));
###Output
_____no_output_____
###Markdown
Discussion 1b: What about this observed dataset of 10 points would prefer a *negative* slope vs. a positive slope?
###Code
# TODO discuss
###Output
_____no_output_____
###Markdown
Part 2: MAP estimation : View as optimization problem There is NO closed form for the posterior over weights $p( w | t)$. However, we can evaluate (and thus optimize) the MAP objective, since this doesn't require knowing the full posterior.Let's see how. Begin with the MAP optimization problem:\begin{align} w^* = \arg \max_{w \in \mathbb{R}^M} ~~ p( w | t_{1:N} )\end{align}Rewriting using the log of the objective for tractability and simplifying via Bayes rule, we get the objective function to maximize is:\begin{align}\mathcal{M}(w) &= \log p( w | t_{1:N}) \\ &= \log p( w ) + \log p( t_{1:N} | w ) - \underbrace{\log p(t_{1:N})}_{\text{const wrt}~ w}\end{align}Thus, we can simply ignore the constant term, and maximize the following alternative objective:\begin{align} \mathcal{M}'(w) &= \log \text{MVNormPDF}( w | 0, \alpha^{-1} I_M ) + \sum_{n=1}^N \log \text{BernPMF}( t_n | \sigma(w^T \phi(x_n) ) \end{align}Finally, we can *standardize* our problem by transforming so we *minimize* rather than *maximize*, just by multiplying by -1. Now the *loss* function we wish to minimize is:\begin{align} \mathcal{L}(w) &= - \log \text{MVNormPDF}( w | 0, \alpha^{-1} I_M ) - \sum_{n=1}^N \log \text{BernPMF}( t_n | \sigma(w^T \phi(x_n) ) \end{align}Thus, we can find our optimal weights $w^*$ via:\begin{align} w^* = \arg \min_{w \in \mathbb{R}^M} ~~ \mathcal{L}(w)\end{align} How can we compute each of these terms?* Use `scipy.stats.multivariate_normal.logpdf` to evaluate the log prior PDF $\log \text{MVNormPDF}(\cdot)$* For the likelihood pdf, use this formula:$$\sum_{n=1}^N \log \text{BernPMF}(t_n | p_n ) = \sum_{n=1}^N t_n \log p_n + (1-t_n) \log (1 - p_n)$$This is translated into the code below.
###Code
def calc_sum_of_log_bern_pmf(t_N, p_N):
''' Calculate the log of the bernoulli pmf for N observations
Args
----
t_N : 1D array, shape (N,)
Binary value (0 or 1) for each example n
p_N : 1D array, shape (N,)
Probability parameter of the Bernoulli for each example n
Returns
-------
summed_logpmf : scalar float
Summed log PMF over all N examples given
'''
# Make sure provided probabilities are not hard 0 or hard 1
# so that the log values will not be numerically bad
safe_p_N = np.minimum(np.maximum(p_N, 1e-100), 1 - 1e-13)
return np.sum(np.log(safe_p_N)[t_N==1]) + np.sum(np.log(1-safe_p_N)[t_N==0])
###Output
_____no_output_____
###Markdown
Exercise 2a: Compute the objective of our minimization problemTranslate the formula for $\mathcal{L}(w)$ above into concrete NumPy expressions
###Code
def calc_loss(wguess_M, phi_NM, t_N, alpha=0.1):
''' Compute the MAP loss objective function.
The loss is equal to the negative log prior plus negative log likelihood
Args
----
w_M : 1D array, shape (M,)
Weight parameter at which we want to evaluate the loss
phi_NM : 2D array, shape (N,M)
Observed input features
Each row is a feature vector for one example
t_N : 1D array, shape (N,)
Observed outputs
Each row is a output scalar value for one example
alpha : positive scalar
Prior precision
Returns
-------
loss : scalar float
The value of the loss function at provided w value
'''
log_prior_pdf = scipy.stats.multivariate_normal.logpdf(wguess_M, mean=np.zeros_like(wguess_M * 1/alpha),
cov=np.eye(len(wguess_M))/alpha) # TODO compute log prior pdf value
log_lik_pdf = calc_sum_of_log_bern_pmf(t_N,sigmoid(np.dot(phi_NM,wguess_M))) # TODO compute log likelihood pdf value
return -1 * log_prior_pdf + -1 * log_lik_pdf
###Output
_____no_output_____
###Markdown
Exercise 2b: Evaluate the MAP objective (aka MAP loss function) at possible w values
###Code
phi_NM
t_N
np.log(0.5)
# Try with all zero weights
w1_M = np.zeros(M)
calc_loss(w1_M, phi_NM, t_N, true_alpha)
# Try with all weights set to 10
w2_M = 10 * np.ones(M)
calc_loss(w2_M, phi_NM, t_N, true_alpha)
# Try with all weights set to TRUE values
# TODO write code using calc_loss(...)
###Output
_____no_output_____
###Markdown
Discussion 2c: Which value of the weight vector out of the 3 tried had the "best" loss value? Does that agree with what you expect?Use what you know about how this toy dataset was generated (hint: we know which weights were used to make the true observations).
###Code
# TODO discuss
###Output
_____no_output_____
###Markdown
Demo: Visualizing the MAP objective as a contour plotStep through the code below to see how we create a 2d contour plot visualization of our MAP optimization problem.
###Code
# Create a 2-dim grid of possible w values
G = 51 # G possible values for intercept
w0_grid_G = np.linspace(-2, 2, G)
H = 51 # H possible values for slope
w1_grid_H = np.linspace(-2, 2, H)
w0_GH, w1_GH = np.meshgrid(w0_grid_G, w1_grid_H,)
# Compute loss at each possible value in our grid
loss_GH = np.zeros((G, H))
for gg in range(G):
for hh in range(H):
cur_w_M = np.hstack([w0_GH[gg,hh], w1_GH[gg, hh]])
loss_GH[gg, hh] = calc_loss(cur_w_M, phi_NM, t_N, true_alpha)
# Create a pretty contour plot over the grid of w[0], w[1], loss values
levels = np.linspace(0, 40, 51) # 50 evenly spaced levels
fig_handle, ax_handle = plt.subplots(nrows=1, ncols=1, figsize=(8,8));
ax_handle.contour(w0_GH, w1_GH, loss_GH, levels=levels, linewidths=0, colors='k')
cntrf_handle = ax_handle.contourf(w0_GH, w1_GH, loss_GH, levels=levels, cmap='RdBu_r', vmin=levels[0], vmax=levels[-1]);
cbar = plt.colorbar(cntrf_handle, ax=ax_handle)
cbar.set_label('MAP loss objective (lower is better)', fontsize=16);
cbar.set_ticks(levels[::10]);
plt.xlabel('intercept $w_1$');
plt.ylabel('slope $w_2$');
plt.gca().set_aspect('equal', 'box');
###Output
_____no_output_____
###Markdown
Exercise 2d: Visually interpret the plot above. By inspection, which intercept and slope values are optimal? What is the loss at this optimal point?
###Code
# TODO interpret the plot and discuss with your group
###Output
_____no_output_____
###Markdown
Exercise 2e: Numerically, search the grid of computed loss values `loss_GH` and determine the MAP value of weight vector
###Code
# TODO solve this cell
# Hint: you might find it easier to flatten each array of shape (G,H) into shape (L,) where L=G*H
loss_L = loss_GH.flatten()# new shape (G*H,)
w0_L = w0_GH.flatten() # new shape (G*H,)
w1_L = w1_GH.flatten() # new shape (G*H,)
# TODO find values of w0 (intercept) and w1 (slope) that minimize the loss
###Output
_____no_output_____
###Markdown
Part 3: Gradients, Hessians, and Gradient Descent Gradient and Hessian formulasWe saw in lecture that we can compute the gradient and Hessian as:\begin{align}\nabla_w \mathcal{L} &= \Phi^T ( \sigma(\Phi w) - t ) + \alpha w\\\nabla_w \nabla_w \mathcal{L} &= \Phi^T R(w) \Phi + \alpha I_M\end{align}where $R$ is a diagonal matrix given by $$R = \text{diag}( \sigma(\Phi w) \sigma(- \Phi w ) )$$The functions below compute the gradient and Hessian. You don't need to do anything, just inspect them to gain understanding.
###Code
def calc_R(w_M, phi_NM):
s_N = np.dot(phi_NM, w_M)
R_NN = np.diag( sigmoid(s_N) * sigmoid(-s_N) )
return R_NN
def calc_gradient_of_map_loss(w_M, phi_NM, t_N, alpha):
''' Calculate the gradient.
Returns
-------
g_M : 1D array, shape (M,)
Gradient vector evaluated at current weights w
'''
# Compute predicted probability of positive class
yproba_N = sigmoid( np.dot(phi_NM, w_M) )
return np.dot(phi_NM.T, (yproba_N - t_N)) + alpha * w_M
def calc_hessian_of_map_loss(w_M, phi_NM, t_N, alpha):
''' Calculate the Hessian.
Returns
-------
H_MM : 2D array, shape (M,M)
Hessian matrix evaluated at current weights w
'''
R_NN = calc_R(w_M, phi_NM)
return np.dot(phi_NM.T, np.dot(R_NN, phi_NM)) + alpha * np.eye(M)
###Output
_____no_output_____
###Markdown
First-order gradient descentThe code below performs 1st-order GD. While not converged, we perform the updates:$$w_{t+1} \gets w_t - \epsilon g( w_t )$$
###Code
max_n_steps = 100
w_M = 1.5 * np.ones(M)
step_size = 0.2 # Selected by starting at 1.0, and trying smaller values until first 5 steps made loss better
GD1_history_of_w = [w_M]
GD1_history_of_loss = [calc_loss(w_M, phi_NM, t_N, true_alpha)]
for step in range(max_n_steps):
# Compute gradient
g_M = calc_gradient_of_map_loss(w_M, phi_NM, t_N, true_alpha)
# Update the weights by taking a step downhill
w_M = w_M - step_size * g_M
# Print out progress
cur_loss = calc_loss(w_M, phi_NM, t_N, true_alpha)
print("step %3d/%d loss %11.4f | gradient_norm %9.4f | intercept %9.3f | slope %9.3f" % (
step, max_n_steps, cur_loss, np.sum(np.abs(g_M)), w_M[0], w_M[1]))
GD1_history_of_loss.append(cur_loss)
GD1_history_of_w.append(w_M)
if step % 10:
step_size = 0.95 * step_size # slowly decay the step size
bestw_fromGD_M = w_M
###Output
step 0/100 loss 13.3582 | gradient_norm 12.4385 | intercept 1.231 | slope -0.719
step 1/100 loss 12.9273 | gradient_norm 2.8867 | intercept 1.098 | slope -0.275
step 2/100 loss 12.8019 | gradient_norm 2.4277 | intercept 0.878 | slope -0.516
step 3/100 loss 12.6890 | gradient_norm 1.7594 | intercept 0.815 | slope -0.262
step 4/100 loss 12.6510 | gradient_norm 1.5637 | intercept 0.708 | slope -0.423
step 5/100 loss 12.6150 | gradient_norm 1.0530 | intercept 0.683 | slope -0.278
step 6/100 loss 12.6012 | gradient_norm 0.8692 | intercept 0.634 | slope -0.363
step 7/100 loss 12.5921 | gradient_norm 0.5274 | intercept 0.620 | slope -0.299
step 8/100 loss 12.5887 | gradient_norm 0.3724 | intercept 0.599 | slope -0.330
step 9/100 loss 12.5872 | gradient_norm 0.2076 | intercept 0.590 | slope -0.311
step 10/100 loss 12.5866 | gradient_norm 0.1210 | intercept 0.581 | slope -0.317
step 11/100 loss 12.5863 | gradient_norm 0.0752 | intercept 0.575 | slope -0.313
step 12/100 loss 12.5862 | gradient_norm 0.0413 | intercept 0.571 | slope -0.313
step 13/100 loss 12.5861 | gradient_norm 0.0350 | intercept 0.568 | slope -0.313
step 14/100 loss 12.5860 | gradient_norm 0.0247 | intercept 0.565 | slope -0.312
step 15/100 loss 12.5860 | gradient_norm 0.0202 | intercept 0.563 | slope -0.312
step 16/100 loss 12.5860 | gradient_norm 0.0161 | intercept 0.562 | slope -0.312
step 17/100 loss 12.5860 | gradient_norm 0.0130 | intercept 0.561 | slope -0.312
step 18/100 loss 12.5860 | gradient_norm 0.0107 | intercept 0.560 | slope -0.312
step 19/100 loss 12.5860 | gradient_norm 0.0089 | intercept 0.560 | slope -0.311
step 20/100 loss 12.5860 | gradient_norm 0.0074 | intercept 0.559 | slope -0.311
step 21/100 loss 12.5860 | gradient_norm 0.0063 | intercept 0.559 | slope -0.311
step 22/100 loss 12.5860 | gradient_norm 0.0053 | intercept 0.558 | slope -0.311
step 23/100 loss 12.5860 | gradient_norm 0.0045 | intercept 0.558 | slope -0.311
step 24/100 loss 12.5860 | gradient_norm 0.0039 | intercept 0.558 | slope -0.311
step 25/100 loss 12.5860 | gradient_norm 0.0034 | intercept 0.558 | slope -0.311
step 26/100 loss 12.5860 | gradient_norm 0.0030 | intercept 0.557 | slope -0.311
step 27/100 loss 12.5860 | gradient_norm 0.0026 | intercept 0.557 | slope -0.311
step 28/100 loss 12.5860 | gradient_norm 0.0023 | intercept 0.557 | slope -0.311
step 29/100 loss 12.5860 | gradient_norm 0.0021 | intercept 0.557 | slope -0.311
step 30/100 loss 12.5860 | gradient_norm 0.0019 | intercept 0.557 | slope -0.311
step 31/100 loss 12.5860 | gradient_norm 0.0017 | intercept 0.557 | slope -0.311
step 32/100 loss 12.5860 | gradient_norm 0.0015 | intercept 0.557 | slope -0.311
step 33/100 loss 12.5860 | gradient_norm 0.0014 | intercept 0.557 | slope -0.311
step 34/100 loss 12.5860 | gradient_norm 0.0013 | intercept 0.557 | slope -0.311
step 35/100 loss 12.5860 | gradient_norm 0.0011 | intercept 0.557 | slope -0.311
step 36/100 loss 12.5860 | gradient_norm 0.0011 | intercept 0.557 | slope -0.311
step 37/100 loss 12.5860 | gradient_norm 0.0010 | intercept 0.557 | slope -0.311
step 38/100 loss 12.5860 | gradient_norm 0.0009 | intercept 0.557 | slope -0.311
step 39/100 loss 12.5860 | gradient_norm 0.0008 | intercept 0.557 | slope -0.311
step 40/100 loss 12.5860 | gradient_norm 0.0008 | intercept 0.557 | slope -0.311
step 41/100 loss 12.5860 | gradient_norm 0.0007 | intercept 0.557 | slope -0.311
step 42/100 loss 12.5860 | gradient_norm 0.0007 | intercept 0.556 | slope -0.311
step 43/100 loss 12.5860 | gradient_norm 0.0007 | intercept 0.556 | slope -0.311
step 44/100 loss 12.5860 | gradient_norm 0.0006 | intercept 0.556 | slope -0.311
step 45/100 loss 12.5860 | gradient_norm 0.0006 | intercept 0.556 | slope -0.311
step 46/100 loss 12.5860 | gradient_norm 0.0006 | intercept 0.556 | slope -0.311
step 47/100 loss 12.5860 | gradient_norm 0.0005 | intercept 0.556 | slope -0.311
step 48/100 loss 12.5860 | gradient_norm 0.0005 | intercept 0.556 | slope -0.311
step 49/100 loss 12.5860 | gradient_norm 0.0005 | intercept 0.556 | slope -0.311
step 50/100 loss 12.5860 | gradient_norm 0.0005 | intercept 0.556 | slope -0.311
step 51/100 loss 12.5860 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 52/100 loss 12.5860 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 53/100 loss 12.5860 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 54/100 loss 12.5860 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 55/100 loss 12.5860 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 56/100 loss 12.5860 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 57/100 loss 12.5860 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 58/100 loss 12.5860 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 59/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 60/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 61/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 62/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 63/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 64/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 65/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 66/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 67/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 68/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 69/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 70/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 71/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 72/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 73/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 74/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 75/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 76/100 loss 12.5860 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 77/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 78/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 79/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 80/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 81/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 82/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 83/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 84/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 85/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 86/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 87/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 88/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 89/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 90/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 91/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 92/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 93/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 94/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 95/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 96/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 97/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 98/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 99/100 loss 12.5860 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
###Markdown
Discussion 3a: Compare the GD estimate of the best weights $w$ to those found via grid search
###Code
print("Optimal weights via grid search")
bestid = np.argmin(loss_GH.flatten())
bestw_fromgridsearch_M = np.asarray([w0_GH.flatten()[bestid], w1_GH.flatten()[bestid]])
print(bestw_fromgridsearch_M)
print("Optimal weights via 1st order gradient descent")
print(w_M)
calc_loss(bestw_fromgridsearch_M, phi_NM, t_N, true_alpha)
calc_loss(bestw_fromGD_M, phi_NM, t_N, true_alpha)
# TODO discuss: which is better? are they similar?
###Output
_____no_output_____
###Markdown
Second order gradient descent
###Code
max_n_steps = 100
w_M = 1.5 * np.ones(M)
step_size = 0.15 # Selected by starting at 1.0, and trying smaller values until first 5 steps made loss better
GD2_history_of_w = [w_M]
GD2_history_of_loss = [calc_loss(w_M, phi_NM, t_N, true_alpha)]
for step in range(max_n_steps):
g_M = calc_gradient_of_map_loss(w_M, phi_NM, t_N, true_alpha)
H_MM = calc_hessian_of_map_loss(w_M, phi_NM, t_N, true_alpha)
w_M = w_M - step_size * np.linalg.solve(H_MM, g_M) # compute H^1 times g
cur_loss = calc_loss(w_M, phi_NM, t_N, true_alpha)
print("step %3d/%d loss %11.4f | gradient_norm %9.4f | intercept %9.3f | slope %9.3f" % (
step, max_n_steps, cur_loss, np.sum(np.abs(g_M)), w_M[0], w_M[1]))
GD2_history_of_loss.append(cur_loss)
GD2_history_of_w.append(w_M)
if step % 10:
step_size = 0.95 * step_size # slowly decay step size
whistory_GD1_T2 = np.vstack(GD1_history_of_w)
whistory_GD2_T2 = np.vstack(GD2_history_of_w)
# Create a pretty contour plot over the grid of w[0], w[1], loss values
levels = np.linspace(0, 40, 51) # 50 evenly spaced levels
fig_handle, ax_handle = plt.subplots(nrows=1, ncols=1, figsize=(8,8));
ax_handle.contour(w0_GH, w1_GH, loss_GH, levels=levels, linewidths=0, colors='k')
cntrf_handle = ax_handle.contourf(w0_GH, w1_GH, loss_GH, levels=levels, cmap='RdBu_r', vmin=levels[0], vmax=levels[-1]);
cbar = plt.colorbar(cntrf_handle, ax=ax_handle)
cbar.set_label('MAP loss objective (lower is better)', fontsize=16);
cbar.set_ticks(levels[::10]);
# Show the first 10 iterates of GD
ax_handle.plot(whistory_GD1_T2[:1,0], whistory_GD1_T2[:1,1], 'kx', markersize=15, label='Initial w value')
ax_handle.plot(whistory_GD1_T2[:10,0], whistory_GD1_T2[:10,1], 'ks-', label='First 10 steps of 1st-order GD')
ax_handle.plot(whistory_GD2_T2[:10,0], whistory_GD2_T2[:10,1], 'ms-', label='First 10 steps of 2st-order GD')
plt.xlabel('intercept $w_1$');
plt.ylabel('slope $w_2$');
plt.gca().set_aspect('equal', 'box');
plt.ylim([-3, 2]);
plt.legend(loc='upper left');
###Output
_____no_output_____
###Markdown
COMP 135 day09: MAP estimation for Logistic Regression Outline* **Part 1: Understanding sigmoids and Logistic Regression as a model*** **Part 2: Computing the MAP objective*** **Part 3: Gradient descent for the MAP: Comparing 1st and 2nd order GD** Takeaways* First-order methods are cheap but require many iterations* Second-order methods are awesome, but still require careful step-size selection* For all gradient descent methods, selecting step sizes is super important. Line search is needed!
###Code
import numpy as np
import pandas as pd
import scipy.stats
np.set_printoptions(precision=3, suppress=False)
pd.options.display.float_format = '{:,.3g}'.format # show 3 digits of precision
import matplotlib.pyplot as plt
import seaborn as sns
sns.set_style("whitegrid")
sns.set_context("notebook", font_scale=1.25)
###Output
_____no_output_____
###Markdown
Part 1: The Probabilistic view of logistic regression Task: Binary classificationGiven $N$ observations of *paired* feature-outcome observations: $\{ x_n, t_n \}$.* Each input feature $x_n$ is a scalar real: $x_n \in \mathbb{R}$* Each output or "label" or "outcome" $t_n$ is a scalar binary value: $t_n \in \{0, 1\}$We're also given a feature transform function $\phi$ which maps each $x_n$ to a vector in $M$-dimensional space. This function is known in advance.We want to make good predictions of new outcomes $t_*$ given new features $x_*$. Feature transformationFor now, we'll assume that the "feature transform" $\phi(x_n)$ just simply passes along the features $x_n$, while adding an additional offset or "intercept" feature that is always 1. This is a *simplifying* assumption for today.
###Code
def calc_features(x_N1, M=2):
''' Transform raw features into complete features useful for prediction
Could do any non-linear transformations thought relevant for the problem.
Here we'll just do an identity transform with an extra intercept feature.
Args
----
x_N1 : 2D array, shape (N, 1) = (n_examples,)
Returns
-------
phi_NM : 2D array, shape (N, M) = (n_examples, n_transformed_features)
First column will contain all ones (a bias or intercept feature)
Second column will just include the raw features
'''
assert x_N1.ndim == 2
assert x_N1.shape[1] == 1
N = x_N1.shape[0]
phi_NM = np.zeros((N, M))
phi_NM[:,0] = 1
phi_NM[:,1] = x_N1[:,0]
return phi_NM
x_N1 = np.linspace(-1, 1, 5)[:,np.newaxis]
# Get transformed features using our "calc_features" function
# * first column will be all 1s, an "intercept"
# * second column will be the x values
calc_features(x_N1)
###Output
_____no_output_____
###Markdown
Understanding the logistic sigmoid functionAs discussed in your pre-recorded lectures, the *logistic sigmoid function* is:\begin{align}\sigma(r) = \frac{1}{1 + e^{-r}}\end{align}It maps real inputs $r \in (-\infty, +\infty)$ to the probability interval $(0, 1)$.We call it a "sigmoid" function because it has an S-shaped curve, which you'll plot below.This function is also sometimes called the "expit" function. We can use an existing implementation of this function available in SciPy:* expit: https://docs.scipy.org/doc/scipy/reference/generated/scipy.special.expit.html
###Code
from scipy.special import expit as sigmoid
sigmoid(0)
sigmoid(-4)
sigmoid(4)
sigmoid(np.asarray([-6, -4, -2, 0, 2, 4, 6]))
###Output
_____no_output_____
###Markdown
Exercise 1a: Plot the logistic sigmoid function We give you an array of G candidate $r$ values below.
###Code
G = 101
r_G = np.linspace(-8, 8, G)
sigmoid_of_r_G = np.zeros(G) # TODO evaluate sigmoid at each r value
plt.plot(r_G, sigmoid_of_r_G, 'k.-');
plt.xlabel('r'); plt.ylabel('$\sigma(r)$');
plt.ylim([-0.001, 1.001])
###Output
_____no_output_____
###Markdown
Define the LikelihoodEach observation (indexed by $n$) is drawn iid from a Bernoulli as follows:$$t_n | w \sim \text{BernPMF}\left( t_n | \sigma(w^T \phi(x_n)) \right)$$where $w \in \mathbb{R}^M$ is a weight vector, the same size as our feature vector $\phi(x_n) \in \mathbb{R}^M$The key properties here are:* The *mean* of $t_n$ is a *non-linear activation* of a linear function of the transformed features. Define the PriorFor now, we'll assume that weights come from a zero mean prior with some covariance determined by a scalar parameter $\alpha$:$$w \sim \mathcal{N}( 0, \alpha^{-1} I_M )$$A zero mean prior makes sense if we don't know if the slope should be negative or positive. Parameter we'll treat as a random variable: $w$* Weights vector: $w = [w_1, w_2, \ldots w_M]^T$, so $w \in \mathbb{R}^M$ Parameters we'll treat as fixed: $\alpha$ * Prior precision $\alpha > 0$The larger $\alpha$ is, the more confident we are in the weight values before seeing any data. Create a simple toy data for analysisJust execute the cells below to get the sense of how to generate toy data from this modelWe'll manually intervene to set the weight vector to a known value. This makes it easy to tell if our learning is working later on.
###Code
N = 10 # Number of examples we observe
M = 2 # Number of transformed features
###Output
_____no_output_____
###Markdown
Create the weight vector we'll use to generate our dataset. Set an intercept of 1.2 and a slope of -0.75
###Code
true_w_M = np.asarray([0.1, -0.25])
###Output
_____no_output_____
###Markdown
Create a "true" alpha value which controls the prior precision
###Code
true_alpha = 0.01
###Output
_____no_output_____
###Markdown
Create observed features $x$ and observed outputs $t$ manually
###Code
x_N1 = np.asarray([-5, -0.8, -0.7, -0.6, -0.4, 0.5, 0.8, 0.9, 4.3, 4.1]).reshape((N, 1))
phi_NM = calc_features(x_N1)
prng = np.random.RandomState(101) # reproducible random seed
t_N = (prng.rand(N) < sigmoid(np.dot(phi_NM, true_w_M))).astype(np.float64)
###Output
_____no_output_____
###Markdown
Visualize the toy dataset
###Code
plt.plot(x_N1, t_N, 'k.');
ax_h = plt.gca()
ax_h.set_xlim([-8, 8]); ax_h.set_xticks([-6, -4, -2, 0, 2, 4, 6]);
ax_h.set_ylim([-.1, 1.1]);
xgrid_G1 = np.linspace(-8, 8, 100)[:,np.newaxis]
plt.plot(xgrid_G1, sigmoid(np.dot(calc_features(xgrid_G1), true_w_M)), 'c-', linewidth=3);
plt.xlabel('input: x');
plt.ylabel('output: t');
plt.title("Toy Data\n true_slope %.2f \n true intercept %.2f" % (
true_w_M[1], true_w_M[0]));
###Output
_____no_output_____
###Markdown
Discussion 1b: What about this observed dataset of 10 points would prefer a *negative* slope vs. a positive slope?
###Code
# TODO discuss
###Output
_____no_output_____
###Markdown
Part 2: MAP estimation : View as optimization problem There is NO closed form for the posterior over weights $p( w | t)$. However, we can evaluate (and thus optimize) the MAP objective, since this doesn't require knowing the full posterior.Let's see how. Begin with the MAP optimization problem:\begin{align} w^* = \arg \max_{w \in \mathbb{R}^M} ~~ p( w | t_{1:N} )\end{align}Rewriting using the log of the objective for tractability and simplifying via Bayes rule, we get the objective function to maximize is:\begin{align}\mathcal{M}(w) &= \log p( w | t_{1:N}) \\ &= \log p( w ) + \log p( t_{1:N} | w ) - \underbrace{\log p(t_{1:N})}_{\text{const wrt}~ w}\end{align}Thus, we can simply ignore the constant term, and maximize the following alternative objective:\begin{align} \mathcal{M}'(w) &= \log \text{MVNormPDF}( w | 0, \alpha^{-1} I_M ) + \sum_{n=1}^N \log \text{BernPMF}( t_n | \sigma(w^T \phi(x_n) ) \end{align}Finally, we can *standardize* our problem by transforming so we *minimize* rather than *maximize*, just by multiplying by -1. Now the *loss* function we wish to minimize is:\begin{align} \mathcal{L}(w) &= - \log \text{MVNormPDF}( w | 0, \alpha^{-1} I_M ) - \sum_{n=1}^N \log \text{BernPMF}( t_n | \sigma(w^T \phi(x_n) ) \end{align}Thus, we can find our optimal weights $w^*$ via:\begin{align} w^* = \arg \min_{w \in \mathbb{R}^M} ~~ \mathcal{L}(w)\end{align} How can we compute each of these terms?* Use `scipy.stats.multivariate_normal.logpdf` to evaluate the log prior PDF $\log \text{MVNormPDF}(\cdot)$* For the likelihood pdf, use this formula:$$\sum_{n=1}^N \log \text{BernPMF}(t_n | p_n ) = \sum_{n=1}^N t_n \log p_n + (1-t_n) \log (1 - p_n)$$This is translated into the code below.
###Code
def calc_sum_of_log_bern_pmf(t_N, p_N):
''' Calculate the log of the bernoulli pmf for N observations
Args
----
t_N : 1D array, shape (N,)
Binary value (0 or 1) for each example n
p_N : 1D array, shape (N,)
Probability parameter of the Bernoulli for each example n
Returns
-------
summed_logpmf : scalar float
Summed log PMF over all N examples given
'''
# Make sure provided probabilities are not hard 0 or hard 1
# so that the log values will not be numerically bad
safe_p_N = np.minimum(np.maximum(p_N, 1e-100), 1 - 1e-13)
return np.sum(np.log(safe_p_N)[t_N==1]) + np.sum(np.log(1-safe_p_N)[t_N==0])
###Output
_____no_output_____
###Markdown
Exercise 2a: Compute the objective of our minimization problemTranslate the formula for $\mathcal{L}(w)$ above into concrete NumPy expressions
###Code
def calc_loss(wguess_M, phi_NM, t_N, alpha=0.1):
''' Compute the MAP loss objective function.
The loss is equal to the negative log prior plus negative log likelihood
Args
----
w_M : 1D array, shape (M,)
Weight parameter at which we want to evaluate the loss
phi_NM : 2D array, shape (N,M)
Observed input features
Each row is a feature vector for one example
t_N : 1D array, shape (N,)
Observed outputs
Each row is a output scalar value for one example
alpha : positive scalar
Prior precision
Returns
-------
loss : scalar float
The value of the loss function at provided w value
'''
log_prior_pdf = 0.0 # TODO compute log prior pdf value
log_lik_pdf = 0.0 # TODO compute log likelihood pdf value
return -1 * log_prior_pdf + -1 * log_lik_pdf
###Output
_____no_output_____
###Markdown
Exercise 2b: Evaluate the MAP objective (aka MAP loss function) at possible w values
###Code
phi_NM
t_N
np.log(0.5)
# Try with all zero weights
w1_M = np.zeros(M)
calc_loss(w1_M, phi_NM, t_N, true_alpha)
# Try with all weights set to 10
w2_M = 10 * np.ones(M)
calc_loss(w2_M, phi_NM, t_N, true_alpha)
# Try with all weights set to TRUE values
# TODO write code using calc_loss(...)
###Output
_____no_output_____
###Markdown
Discussion 2c: Which value of the weight vector out of the 3 tried had the "best" loss value? Does that agree with what you expect?Use what you know about how this toy dataset was generated (hint: we know which weights were used to make the true observations).
###Code
# TODO discuss
###Output
_____no_output_____
###Markdown
Demo: Visualizing the MAP objective as a contour plotStep through the code below to see how we create a 2d contour plot visualization of our MAP optimization problem.
###Code
# Create a 2-dim grid of possible w values
G = 51 # G possible values for intercept
w0_grid_G = np.linspace(-2, 2, G)
H = 51 # H possible values for slope
w1_grid_H = np.linspace(-2, 2, H)
w0_GH, w1_GH = np.meshgrid(w0_grid_G, w1_grid_H,)
# Compute loss at each possible value in our grid
loss_GH = np.zeros((G, H))
for gg in range(G):
for hh in range(H):
cur_w_M = np.hstack([w0_GH[gg,hh], w1_GH[gg, hh]])
loss_GH[gg, hh] = calc_loss(cur_w_M, phi_NM, t_N, true_alpha)
# Create a pretty contour plot over the grid of w[0], w[1], loss values
levels = np.linspace(0, 40, 51) # 50 evenly spaced levels
fig_handle, ax_handle = plt.subplots(nrows=1, ncols=1, figsize=(8,8));
ax_handle.contour(w0_GH, w1_GH, loss_GH, levels=levels, linewidths=0, colors='k')
cntrf_handle = ax_handle.contourf(w0_GH, w1_GH, loss_GH, levels=levels, cmap='RdBu_r', vmin=levels[0], vmax=levels[-1]);
cbar = plt.colorbar(cntrf_handle, ax=ax_handle)
cbar.set_label('MAP loss objective (lower is better)', fontsize=16);
cbar.set_ticks(levels[::10]);
plt.xlabel('intercept $w_1$');
plt.ylabel('slope $w_2$');
plt.gca().set_aspect('equal', 'box');
###Output
/Users/mhughes/miniconda3/envs/semimarkov_forecaster/lib/python3.7/site-packages/ipykernel_launcher.py:7: UserWarning: No contour levels were found within the data range.
import sys
###Markdown
Exercise 2d: Visually interpret the plot above. By inspection, which intercept and slope values are optimal? What is the loss at this optimal point?
###Code
# TODO interpret the plot and discuss with your group
###Output
_____no_output_____
###Markdown
Exercise 2e: Numerically, search the grid of computed loss values `loss_GH` and determine the MAP value of weight vector
###Code
# TODO solve this cell
# Hint: you might find it easier to flatten each array of shape (G,H) into shape (L,) where L=G*H
loss_L = loss_GH.flatten()# new shape (G*H,)
w0_L = w0_GH.flatten() # new shape (G*H,)
w1_L = w1_GH.flatten() # new shape (G*H,)
# TODO find values of w0 (intercept) and w1 (slope) that minimize the loss
###Output
_____no_output_____
###Markdown
Part 3: Gradients, Hessians, and Gradient Descent Gradient and Hessian formulasWe saw in lecture that we can compute the gradient and Hessian as:\begin{align}\nabla_w \mathcal{L} &= \Phi^T ( \sigma(\Phi w) - t ) + \alpha w\\\nabla_w \nabla_w \mathcal{L} &= \Phi^T R(w) \Phi + \alpha I_M\end{align}where $R$ is a diagonal matrix given by $$R = \text{diag}( \sigma(\Phi w) \sigma(- \Phi w ) )$$The functions below compute the gradient and Hessian. You don't need to do anything, just inspect them to gain understanding.
###Code
def calc_R(w_M, phi_NM):
s_N = np.dot(phi_NM, w_M)
R_NN = np.diag( sigmoid(s_N) * sigmoid(-s_N) )
return R_NN
def calc_gradient_of_map_loss(w_M, phi_NM, t_N, alpha):
''' Calculate the gradient.
Returns
-------
g_M : 1D array, shape (M,)
Gradient vector evaluated at current weights w
'''
# Compute predicted probability of positive class
yproba_N = sigmoid( np.dot(phi_NM, w_M) )
return np.dot(phi_NM.T, (yproba_N - t_N)) + alpha * w_M
def calc_hessian_of_map_loss(w_M, phi_NM, t_N, alpha):
''' Calculate the Hessian.
Returns
-------
H_MM : 2D array, shape (M,M)
Hessian matrix evaluated at current weights w
'''
R_NN = calc_R(w_M, phi_NM)
return np.dot(phi_NM.T, np.dot(R_NN, phi_NM)) + alpha * np.eye(M)
###Output
_____no_output_____
###Markdown
First-order gradient descentThe code below performs 1st-order GD. While not converged, we perform the updates:$$w_{t+1} \gets w_t - \epsilon g( w_t )$$
###Code
max_n_steps = 100
w_M = 1.5 * np.ones(M)
step_size = 0.2 # Selected by starting at 1.0, and trying smaller values until first 5 steps made loss better
GD1_history_of_w = [w_M]
GD1_history_of_loss = [calc_loss(w_M, phi_NM, t_N, true_alpha)]
for step in range(max_n_steps):
# Compute gradient
g_M = calc_gradient_of_map_loss(w_M, phi_NM, t_N, true_alpha)
# Update the weights by taking a step downhill
w_M = w_M - step_size * g_M
# Print out progress
cur_loss = calc_loss(w_M, phi_NM, t_N, true_alpha)
print("step %3d/%d loss %11.4f | gradient_norm %9.4f | intercept %9.3f | slope %9.3f" % (
step, max_n_steps, cur_loss, np.sum(np.abs(g_M)), w_M[0], w_M[1]))
GD1_history_of_loss.append(cur_loss)
GD1_history_of_w.append(w_M)
if step % 10:
step_size = 0.95 * step_size # slowly decay the step size
bestw_fromGD_M = w_M
###Output
step 0/100 loss -0.0000 | gradient_norm 12.4385 | intercept 1.231 | slope -0.719
step 1/100 loss -0.0000 | gradient_norm 2.8867 | intercept 1.098 | slope -0.275
step 2/100 loss -0.0000 | gradient_norm 2.4277 | intercept 0.878 | slope -0.516
step 3/100 loss -0.0000 | gradient_norm 1.7594 | intercept 0.815 | slope -0.262
step 4/100 loss -0.0000 | gradient_norm 1.5637 | intercept 0.708 | slope -0.423
step 5/100 loss -0.0000 | gradient_norm 1.0530 | intercept 0.683 | slope -0.278
step 6/100 loss -0.0000 | gradient_norm 0.8692 | intercept 0.634 | slope -0.363
step 7/100 loss -0.0000 | gradient_norm 0.5274 | intercept 0.620 | slope -0.299
step 8/100 loss -0.0000 | gradient_norm 0.3724 | intercept 0.599 | slope -0.330
step 9/100 loss -0.0000 | gradient_norm 0.2076 | intercept 0.590 | slope -0.311
step 10/100 loss -0.0000 | gradient_norm 0.1210 | intercept 0.581 | slope -0.317
step 11/100 loss -0.0000 | gradient_norm 0.0752 | intercept 0.575 | slope -0.313
step 12/100 loss -0.0000 | gradient_norm 0.0413 | intercept 0.571 | slope -0.313
step 13/100 loss -0.0000 | gradient_norm 0.0350 | intercept 0.568 | slope -0.313
step 14/100 loss -0.0000 | gradient_norm 0.0247 | intercept 0.565 | slope -0.312
step 15/100 loss -0.0000 | gradient_norm 0.0202 | intercept 0.563 | slope -0.312
step 16/100 loss -0.0000 | gradient_norm 0.0161 | intercept 0.562 | slope -0.312
step 17/100 loss -0.0000 | gradient_norm 0.0130 | intercept 0.561 | slope -0.312
step 18/100 loss -0.0000 | gradient_norm 0.0107 | intercept 0.560 | slope -0.312
step 19/100 loss -0.0000 | gradient_norm 0.0089 | intercept 0.560 | slope -0.311
step 20/100 loss -0.0000 | gradient_norm 0.0074 | intercept 0.559 | slope -0.311
step 21/100 loss -0.0000 | gradient_norm 0.0063 | intercept 0.559 | slope -0.311
step 22/100 loss -0.0000 | gradient_norm 0.0053 | intercept 0.558 | slope -0.311
step 23/100 loss -0.0000 | gradient_norm 0.0045 | intercept 0.558 | slope -0.311
step 24/100 loss -0.0000 | gradient_norm 0.0039 | intercept 0.558 | slope -0.311
step 25/100 loss -0.0000 | gradient_norm 0.0034 | intercept 0.558 | slope -0.311
step 26/100 loss -0.0000 | gradient_norm 0.0030 | intercept 0.557 | slope -0.311
step 27/100 loss -0.0000 | gradient_norm 0.0026 | intercept 0.557 | slope -0.311
step 28/100 loss -0.0000 | gradient_norm 0.0023 | intercept 0.557 | slope -0.311
step 29/100 loss -0.0000 | gradient_norm 0.0021 | intercept 0.557 | slope -0.311
step 30/100 loss -0.0000 | gradient_norm 0.0019 | intercept 0.557 | slope -0.311
step 31/100 loss -0.0000 | gradient_norm 0.0017 | intercept 0.557 | slope -0.311
step 32/100 loss -0.0000 | gradient_norm 0.0015 | intercept 0.557 | slope -0.311
step 33/100 loss -0.0000 | gradient_norm 0.0014 | intercept 0.557 | slope -0.311
step 34/100 loss -0.0000 | gradient_norm 0.0013 | intercept 0.557 | slope -0.311
step 35/100 loss -0.0000 | gradient_norm 0.0011 | intercept 0.557 | slope -0.311
step 36/100 loss -0.0000 | gradient_norm 0.0011 | intercept 0.557 | slope -0.311
step 37/100 loss -0.0000 | gradient_norm 0.0010 | intercept 0.557 | slope -0.311
step 38/100 loss -0.0000 | gradient_norm 0.0009 | intercept 0.557 | slope -0.311
step 39/100 loss -0.0000 | gradient_norm 0.0008 | intercept 0.557 | slope -0.311
step 40/100 loss -0.0000 | gradient_norm 0.0008 | intercept 0.557 | slope -0.311
step 41/100 loss -0.0000 | gradient_norm 0.0007 | intercept 0.557 | slope -0.311
step 42/100 loss -0.0000 | gradient_norm 0.0007 | intercept 0.556 | slope -0.311
step 43/100 loss -0.0000 | gradient_norm 0.0007 | intercept 0.556 | slope -0.311
step 44/100 loss -0.0000 | gradient_norm 0.0006 | intercept 0.556 | slope -0.311
step 45/100 loss -0.0000 | gradient_norm 0.0006 | intercept 0.556 | slope -0.311
step 46/100 loss -0.0000 | gradient_norm 0.0006 | intercept 0.556 | slope -0.311
step 47/100 loss -0.0000 | gradient_norm 0.0005 | intercept 0.556 | slope -0.311
step 48/100 loss -0.0000 | gradient_norm 0.0005 | intercept 0.556 | slope -0.311
step 49/100 loss -0.0000 | gradient_norm 0.0005 | intercept 0.556 | slope -0.311
step 50/100 loss -0.0000 | gradient_norm 0.0005 | intercept 0.556 | slope -0.311
step 51/100 loss -0.0000 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 52/100 loss -0.0000 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 53/100 loss -0.0000 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 54/100 loss -0.0000 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 55/100 loss -0.0000 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 56/100 loss -0.0000 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 57/100 loss -0.0000 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 58/100 loss -0.0000 | gradient_norm 0.0004 | intercept 0.556 | slope -0.311
step 59/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 60/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 61/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 62/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 63/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 64/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 65/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 66/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 67/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 68/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 69/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 70/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 71/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 72/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 73/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 74/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 75/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 76/100 loss -0.0000 | gradient_norm 0.0003 | intercept 0.556 | slope -0.311
step 77/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 78/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 79/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 80/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 81/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 82/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 83/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 84/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 85/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 86/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 87/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 88/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 89/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 90/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 91/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 92/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 93/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 94/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 95/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 96/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 97/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 98/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
step 99/100 loss -0.0000 | gradient_norm 0.0002 | intercept 0.556 | slope -0.311
###Markdown
Discussion 3a: Compare the GD estimate of the best weights $w$ to those found via grid search
###Code
print("Optimal weights via grid search")
bestid = np.argmin(loss_GH.flatten())
bestw_fromgridsearch_M = np.asarray([w0_GH.flatten()[bestid], w1_GH.flatten()[bestid]])
print(bestw_fromgridsearch_M)
print("Optimal weights via 1st order gradient descent")
print(w_M)
calc_loss(bestw_fromgridsearch_M, phi_NM, t_N, true_alpha)
calc_loss(bestw_fromGD_M, phi_NM, t_N, true_alpha)
# TODO discuss: which is better? are they similar?
###Output
_____no_output_____
###Markdown
Second order gradient descent
###Code
max_n_steps = 100
w_M = 1.5 * np.ones(M)
step_size = 0.15 # Selected by starting at 1.0, and trying smaller values until first 5 steps made loss better
GD2_history_of_w = [w_M]
GD2_history_of_loss = [calc_loss(w_M, phi_NM, t_N, true_alpha)]
for step in range(max_n_steps):
g_M = calc_gradient_of_map_loss(w_M, phi_NM, t_N, true_alpha)
H_MM = calc_hessian_of_map_loss(w_M, phi_NM, t_N, true_alpha)
w_M = w_M - step_size * np.linalg.solve(H_MM, g_M) # compute H^1 times g
cur_loss = calc_loss(w_M, phi_NM, t_N, true_alpha)
print("step %3d/%d loss %11.4f | gradient_norm %9.4f | intercept %9.3f | slope %9.3f" % (
step, max_n_steps, cur_loss, np.sum(np.abs(g_M)), w_M[0], w_M[1]))
GD2_history_of_loss.append(cur_loss)
GD2_history_of_w.append(w_M)
if step % 10:
step_size = 0.95 * step_size # slowly decay step size
whistory_GD1_T2 = np.vstack(GD1_history_of_w)
whistory_GD2_T2 = np.vstack(GD2_history_of_w)
# Create a pretty contour plot over the grid of w[0], w[1], loss values
levels = np.linspace(0, 40, 51) # 50 evenly spaced levels
fig_handle, ax_handle = plt.subplots(nrows=1, ncols=1, figsize=(8,8));
ax_handle.contour(w0_GH, w1_GH, loss_GH, levels=levels, linewidths=0, colors='k')
cntrf_handle = ax_handle.contourf(w0_GH, w1_GH, loss_GH, levels=levels, cmap='RdBu_r', vmin=levels[0], vmax=levels[-1]);
cbar = plt.colorbar(cntrf_handle, ax=ax_handle)
cbar.set_label('MAP loss objective (lower is better)', fontsize=16);
cbar.set_ticks(levels[::10]);
# Show the first 10 iterates of GD
ax_handle.plot(whistory_GD1_T2[:1,0], whistory_GD1_T2[:1,1], 'kx', markersize=15, label='Initial w value')
ax_handle.plot(whistory_GD1_T2[:10,0], whistory_GD1_T2[:10,1], 'ks-', label='First 10 steps of 1st-order GD')
ax_handle.plot(whistory_GD2_T2[:10,0], whistory_GD2_T2[:10,1], 'ms-', label='First 10 steps of 2st-order GD')
plt.xlabel('intercept $w_1$');
plt.ylabel('slope $w_2$');
plt.gca().set_aspect('equal', 'box');
plt.ylim([-3, 2]);
plt.legend(loc='upper left');
###Output
/Users/mhughes/miniconda3/envs/semimarkov_forecaster/lib/python3.7/site-packages/ipykernel_launcher.py:7: UserWarning: No contour levels were found within the data range.
import sys
|
notebooks/1_ML.ipynb | ###Markdown
Predictive maintenance for turbofan engine example Part 2: Linear RegressionBased on open dataset provided by NASA at:https://data.nasa.gov/widgets/vrks-gjiedataset can be downloaded at: http://ti.arc.nasa.gov/c/6/
###Code
import os, time
import datetime
import numpy as np
import matplotlib
import matplotlib.pyplot as plt
import pandas as pd
import tensorflow as tf
from tensorflow import keras
# Load the TensorBoard notebook extension (optional, can be started from the command line)
#%load_ext tensorboard
# Select a plotting style
#plt.style.use('dark_background')
plt.style.use('seaborn')
#plt.style.available
SCALE = 1
SEED = 1
EPOCHS = 20
###Output
_____no_output_____
###Markdown
Data Preparation
###Code
# Load the data
data_root = 'data/'
original_dir = data_root + 'original/'
dataset_dir = data_root + 'dataset/'
train_data = pd.read_csv(dataset_dir + 'train_data.csv')
test_data = pd.read_csv(dataset_dir + 'test_data.csv')
train_data
###Output
_____no_output_____
###Markdown
Quick EDA
###Code
# Plot the lifecycles
one_engine = []
for i, r in train_data.iterrows():
rul = r['RUL']
one_engine.append(rul)
if rul == 0:
plt.plot(one_engine)
one_engine = []
#plt.grid()
plt.xlabel('Cycles')
plt.ylabel('RUL')
###Output
_____no_output_____
###Markdown
Machine Learning ApplicationWe will split the data in 4 parts: x_train, y_train, x_test, y_test.(actually the dataset is already split)- x is for the sensor data- y is for the known Remaining Useful Life- train is for data we will use to train the model (we will use the known RUL in the training)- test is for data validation... we will apply predictions and compute models performance metrics using the known RUL
###Code
# Shuffle train data frame and apply scaling factor
train_data = train_data.sample(frac=SCALE, random_state=SEED).reset_index(drop=True)
# prepare a x frame with useful data and a y frame with RUL value
x_train = train_data.drop(columns=['Unit', 'Cycle', 'RUL'])
y_train = train_data['RUL']
x_test = test_data.drop(columns=['Cycle', 'RUL'])
y_test = test_data['RUL']
# data normalization
mean = x_train.mean()
std = x_train.std()
x_train = (x_train - mean) / std
x_test = (x_test - mean) / std
x_train = x_train.dropna(axis=1, how='any')
x_test = x_test.dropna(axis=1, how='any')
#x_test = np.asarray(x_test).astype('float32')
# what's the shape now we dropped some columns? create a variable to use in
# get_model_v1 function call
(lines,shape) = x_train.shape
# Build a ML model
def get_model_v1(shape):
model = keras.models.Sequential()
model.add(keras.layers.Input(shape, name='input_layer'))
model.add(keras.layers.Dense(128, activation='relu', name='dense_n1'))
model.add(keras.layers.Dense(128, activation='relu', name='dense_n2'))
model.add(keras.layers.Dense(128, activation='relu', name='dense_n3'))
model.add(keras.layers.Dense(128, activation='relu', name='dense_n4'))
model.add(keras.layers.Dense(128, activation='relu', name='dense_n5'))
model.add(keras.layers.Dense(128, activation='relu', name='dense_n6'))
model.add(keras.layers.Dense(128, activation='relu', name='dense_n7'))
model.add(keras.layers.Dense(128, activation='relu', name='dense_n8'))
model.add(keras.layers.Dense(128, activation='relu', name='dense_n9'))
model.add(keras.layers.Dense(1, name='output'))
model.compile(optimizer = 'adam',
loss = 'mse',
metrics = ['mae', 'mse'],
)
return model
# Instanciate the model
model = get_model_v1((shape,))
model.summary()
# Train the model
# Configure callback for vizualization of the training data in tensorboard
if not os.path.exists('logs/'):
os.mkdir('logs')
log_dir = 'logs/fit/' + f'S{SCALE}_E{EPOCHS}_' + datetime.datetime.now().strftime('%Y%m%d-%H%M%S')
tensorboard_callback = tf.keras.callbacks.TensorBoard(log_dir=log_dir, histogram_freq=1)
#%tensorboard --logdir ./logs
start_time = time.perf_counter()
history = model.fit(x_train,
y_train,
epochs = EPOCHS,
batch_size = 20,
verbose = 1,
validation_data = (x_test, y_test),
callbacks = [tensorboard_callback],)
end_time = time.perf_counter()
print(f"\n\nTraining time: {end_time-start_time}")
# Evaluate the model
score = model.evaluate(x_test, y_test, verbose=1)
###Output
_____no_output_____
###Markdown
Training History
###Code
df = pd.DataFrame(data=history.history)
display(df)
print("min(val_mae) : {:.4f}".format(min(history.history['val_mae'])))
def plot_history(history, figsize=(8,6),
plot={"Accuracy":['accuracy','val_accuracy'], 'Loss':['loss', 'val_loss']},
save_as='auto'):
"""
Show history
args:
history: history
figsize: fig size
plot: list of data to plot : {<title>:[<metrics>,...], ...}
"""
fig_id=0
for title,curves in plot.items():
plt.figure(figsize=figsize)
plt.title(title)
plt.ylabel(title)
plt.xlabel('Epoch')
for c in curves:
plt.plot(history.history[c])
plt.legend(curves, loc='upper left')
plt.show()
plot_history(history, plot={'MSE' :['mse', 'val_mse'],
'MAE' :['mae', 'val_mae'],
'LOSS':['loss','val_loss']}, save_as='01-history')
###Output
_____no_output_____
###Markdown
Make a prediction
###Code
# Make a prediction
selection = 56
engine = x_test.iloc[selection]
engine_rul = y_test.iat[selection]
print('Data (denormalized):\n\n', engine.dropna(axis=0, how='any') * std + mean, '\n\n')
print('RUL = ', engine_rul)
engine = np.array(engine).reshape(1, shape)
print('\n\n---\n\n')
predictions = model.predict(engine)
print('Prediction : {:.0f} Cycles'.format(predictions[0][0]))
print('Real RUL : {:.0f} Cycles'.format(engine_rul))
# TODO confusion matrix
predictions = []
for i in range(len(x_test)):
engine = x_test.iloc[i]
engine = np.array(engine).reshape(1, shape)
prediction = model.predict(engine)
predictions.append(prediction[0][0])
plt.figure(figsize=(12,12))
plt.scatter(predictions, y_test);
# Add a line
x = [0, 150]
y = x
plt.plot(x,y, color='lightgreen');
# Layout
plt.xlabel('Predictions');
plt.ylabel('Reality');
# Obviously the ML algo doesn't do much... but this was for benchmarking the DOKS infrastructures anyway :)
###Output
_____no_output_____ |
START_HERE.ipynb | ###Markdown
Find the Repos Available in your Database, and What Repository Groups They Are In Connect to your database
###Code
import psycopg2
import pandas as pd
import sqlalchemy as salc
import numpy as np
import seaborn as sns
import matplotlib.pyplot as plt
import warnings
import datetime
import json
warnings.filterwarnings('ignore')
with open("config.json") as config_file:
config = json.load(config_file)
database_connection_string = 'postgres+psycopg2://{}:{}@{}:{}/{}'.format(config['user'], config['password'], config['host'], config['port'], config['database'])
dbschema='augur_data'
engine = salc.create_engine(
database_connection_string,
connect_args={'options': '-csearch_path={}'.format(dbschema)})
###Output
_____no_output_____
###Markdown
Retrieve Available Respositories
###Code
repolist = pd.DataFrame()
repo_query = salc.sql.text(f"""
SELECT a.rg_name,
a.repo_group_id,
b.repo_name,
b.repo_id,
b.forked_from,
b.repo_archived
FROM
repo_groups a,
repo b
WHERE
a.repo_group_id = b.repo_group_id
ORDER BY
rg_name,
repo_name;
""")
repolist = pd.read_sql(repo_query, con=engine)
display(repolist)
repolist.dtypes
###Output
_____no_output_____
###Markdown
Create a Simpler List for quickly Identifying repo_group_id's and repo_id's for other queries
###Code
repolist = pd.DataFrame()
repo_query = salc.sql.text(f"""
SELECT b.repo_id,
a.repo_group_id,
b.repo_name,
a.rg_name
FROM
repo_groups a,
repo b
WHERE
a.repo_group_id = b.repo_group_id
ORDER BY
rg_name,
repo_name;
""")
repolist = pd.read_sql(repo_query, con=engine)
display(repolist)
repolist.dtypes
###Output
_____no_output_____
###Markdown
Part 1 intuition behind elastic weight colsolidationIn the figure below, $\theta^{*}$ are the weights (ie parameters, synaptic strengths) learned by the neural network (NN) to solve old task A, shown as a vector in vector space. ie if this neural network as 100 total weights then this is a 2D representation of $\mathbb{R}^{100}$ space. The blue horizontal arrow shows an example of catastrophic forgetting, whereby $\theta^{*}$ moves out of the region that allows the NN to perform well at task A (grey), and into the center of a region that allows the NN to perform well at task B (cream). The downward green arrow is the update to $\theta^{*}$ regularized by L2 penalty $\alpha (\theta_{i} - \theta_{A , i}^{*})^{2}$ that causes it to move toward the cream region irrespective of the shape of the grey region. The desired update vector is the red arrow that moves the NN weights into a region capable of performing well at both tasks A and B. How Elastic Weight Cosolidation Changes Learning New Weights $\theta^{*}$EWC encourages movement of weights along the red path by modifying the loss function when re-training a NN that has already been trained to convergence using the loss function for task A, $L_{A}$, which has settled on weights $\theta_{A}$. When re-training the NN on task B using $L_{B}$, we add a term which penalizes changes to weights that are both far from $\theta_{A}$, ie $(\theta_{i} - \theta_{A , i}^{*})^{2}$, and also high in $F_{i}$ which encodes the shape of the grey region.$$L \left(\right. \theta \left.\right) = L_{B} \left(\right. \theta \left.\right) + \underset{i}{\sum} \frac{\lambda}{2} F_{i} \left(\theta_{i} - \theta_{A , i}^{*}\right)^{2} $$ But what is F_i? F_i is the diagonal of the Fisher information matrix. We want to use the diagonal components in Fisher Information Matrix to identify which parameters are more important to task A and apply higher weights to them (the direction of the short axis of grey oval). To learn B we should instead change those weights where F_i is small (long axis of grey oval). In the EWC paper:"we approximate the posterior as a Gaussian distribution with mean given by the parameters θ∗A and a diagonal precision given by the diagonal of the Fisher information matrix F. F has three key properties (20): (i) It is equivalent to the second derivative of the loss near a minimum, (ii) it can be computed from first-order derivatives alone and is thus easy to calculate even for large models, and (iii) it is guaranteed to be positive semidefinite. Note that this approach is similar to expectation propagation where each subtask is seen as a factor of the posterior (21). where LB(θ) is the loss for task B only, λ sets how important the old task is compared with the new one, and i labels each parameter.When moving to a third task, task C, EWC will try to keep the network parameters close to the learned parameters of both tasks A and B. This can be enforced either with two separate penalties or as one by noting that the sum of two quadratic penalties is itself a quadratic penalty." Lets learn what F is in the exampleThis article gives a very good explaination of F in the context of EWC: [Fisher Information Matrix by Yuan-Hong Liao](https://andrewliao11.github.io/blog/fisher-info-matrix/)To compute F_i, we sample the data from task A once and calculate the empirical Fisher Information Matrix. $$I_{\theta_\mathcal{A}^*} = \frac{1}{N} \sum_{i=1}^{N} \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*) \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)^T$$This is just to say that the above equation is how you calculate the below equation from the data. For each pair of parameters in $\theta$ ($\theta_i$ and $\theta_j$), the Fisher Information matrix at position ij is$$I(\theta)_{ij} = E\left[ \left( \frac{\partial}{\partial\theta_i}\log f(X;\theta) \right)\left( \frac{\partial}{\partial\theta_j}\log f(X;\theta) \right) \mid \theta\right]$$If this equation is hard to understand, then the code should make it clearer, dont worry, we will match parts of the code to the equation above so it becomes more tangible. Part 2 A look at the data and the task MNISTThe MNIST data set contains 70,000 images of handwritten digits and their corresponding labels. The images are 28x28 with pixel values from 0 to 255. The labels are the digits from 0 to 9. By default 60,000 of these images belong to a training set and 10,000 of these images belong to a test set. Fashion-MNISTFashion-MNIST is a dataset of Zalando's article images—consisting of a training set of 60,000 examples and a test set of 10,000 examples. Each example is a 28x28 grayscale image, associated with a label from 10 classes. Zalando intends Fashion-MNIST to serve as a direct drop-in replacement for the original MNIST dataset for benchmarking machine learning algorithms. It shares the same image size and structure of training and testing splits.Each training and test example is assigned to one of the following labels:- 0 T-shirt/top- 1 Trouser- 2 Pullover- 3 Dress- 4 Coat- 5 Sandal- 6 Shirt- 7 Sneaker- 8 Bag- 9 Ankle boot Taskas you might guess, our goal is to train an NN that retains it's ability to perform well on MNIST after being retrained on only Fashion-MNIST
###Code
import numpy as np
from PIL import Image
from matplotlib import pyplot as plt
from matplotlib.pyplot import imshow
from contlearn.getdata import getMNIST, getFashionMNIST
%load_ext autoreload
%autoreload 2
%matplotlib inline
# task A training and test set
train_loader_mnist, test_loader_mnist = getMNIST(batch_size=32)
# task B training and test set
train_loader_fashion, test_loader_fashion = getFashionMNIST(batch_size=32)
input_image, target_label = next(iter(train_loader_mnist))
print(target_label[0])
print(input_image[0][0].shape)
img = Image.fromarray(input_image[0][0].detach().cpu().numpy()*255)
plt.imshow(img)
input_image, target_label = next(iter(train_loader_fashion))
fashion_key = {
0: "T-shirt/top",
1: "Trouser",
2: "Pullover",
3: "Dress",
4: "Coat",
5: "Sandal",
6: "Shirt",
7: "Sneaker",
8: "Bag",
9: "Ankle boot",
}
print(fashion_key[int(target_label[0].detach().cpu().numpy())])
print(input_image[0][0].shape)
img = Image.fromarray(input_image[0][0].detach().cpu().numpy()*255)
plt.imshow(img)
###Output
Coat
torch.Size([28, 28])
###Markdown
Part 3 Baseline resultsfirst we train on MNIST and the we will observe the drop in performance once we retrain on Fashion-MNIST, WITHOUT Elastic Weight Consolidation
###Code
import math
import random
import numpy as np
import torch
from matplotlib import pyplot as plt
from matplotlib.pyplot import imshow
from tqdm.notebook import tqdm
from contlearn.getmodels import MLP
from contlearn.gettrainer import one_epoch_baseline, test, var2device
%load_ext autoreload
%autoreload 2
%matplotlib inline
print('you are using PyTorch version ',torch.__version__)
if torch.cuda.is_available():
use_cuda = True
print("you have", torch.cuda.device_count(), "GPUs")
device = torch.device("cuda:0")
print(device)
else:
use_cuda = False
print('no GPUs detected')
device = torch.device("cpu")
# initialize a new model
model = MLP(hidden_size=256)
if torch.cuda.is_available() and use_cuda:
model.cuda()
# push an image through it
input_image, target_label = next(iter(train_loader_fashion))
input_image = var2device(input_image).squeeze(1)
print(input_image.shape)
output = model(input_image)
print(output.shape)
def baseline_training(
model,
epochs,
train_loader,
test_loader,
test2_loader = None,
use_cuda=True,
):
"""
This function saves the training curve data consisting
training set loss and validation set accuracy over the
course of the epochs of training.
I set this up such that if you provide 2 test sets,you
can watch the test accuracy change together during training
on train_loder
"""
if torch.cuda.is_available() and use_cuda:
model.cuda()
train_loss, val_acc, val2_acc = [], [], []
for epoch in tqdm(range(epochs)):
epoch_loss = one_epoch_baseline(model,train_loader)
train_loss.append(epoch_loss)
acc = test(model,test_loader)
val_acc.append(acc.detach().cpu().numpy())
if test2_loader is not None:
acc2 = test(model,test2_loader)
val2_acc.append(acc2.detach().cpu().numpy())
return train_loss, val_acc, val2_acc, model
# set seeds for reproducibility and train the model using the training loop called
# baseline_training
torch.manual_seed(0)
np.random.seed(0)
random.seed(0)
train_loss, val_acc, val2_acc, model = baseline_training(
model,
epochs = 25,
train_loader = train_loader_mnist,
test_loader = test_loader_mnist,
)
# save the trained model
model = model.cpu()
torch.save(model, "files/basemodel.pth")
plt.figure()
plt.xlabel('epochs', fontsize=25)
plt.ylabel('validation accuracy', fontsize=25)
plt.plot(val_acc, label='mnist')
plt.legend()
plt.show()
###Output
_____no_output_____
###Markdown
learning curveyou should get something like this where the accuracy starts to plateau at around 75% for this simple feed forward model
###Code
# you can use this to load the model instead of training it from scratch like above
model = torch.load("files/basemodel.pth")
if torch.cuda.is_available() and use_cuda:
model.cuda()
# tensor(0.8238, device='cuda:0') means that the test set accuracy was 82.4%
# compared to a baseline accuracy of 10% if the model is choosing randomly
print('mnist accuracy', test(model,test_loader_mnist))
print('fashion accuracy', test(model,test_loader_fashion))
###Output
mnist accuracy tensor(0.8238)
fashion accuracy tensor(0.0704)
###Markdown
Catastrophic ForgettingThis is one of the main problems we are trying to solve in the continual learning, aka lifelong learning, aka sequential learning, research field. As you can see, learning Fashion MNIST also degrades performance on original MNIST. In part this is because some of the useful parameters, aka weights, used for original MNIST, are overwritten or updated past what is useful for original MNIST in order to become useful for Fashion MNISTWe expect something like this in which the fashion accuracy increases from it's random performance at around 10% to almost 40% and the mnist test accuracy drops to below 30% from a previous performance of around 80%
###Code
torch.manual_seed(0)
np.random.seed(0)
random.seed(0)
train_loss, val_acc, val2_acc, model = baseline_training(
model,
epochs = 25,
train_loader = train_loader_fashion,
test_loader = test_loader_fashion,
test2_loader = test_loader_mnist,
)
plt.figure()
plt.xlabel('epochs', fontsize=25)
plt.ylabel('validation accuracy', fontsize=25)
plt.plot(val_acc, label='fashion')
plt.plot(val2_acc, label='mnist')
plt.legend()
plt.show()
###Output
_____no_output_____
###Markdown
Part 4 Elastic Weight Consolidationnow that we have implemented the control group, lets implement the experimental groupinstead of revisiting every old MNIST example to build our Fisher Information Matrix, we will visit num_samples of them to form a approximate of the matrixplease read the comments in the EWC class, which explains each step of the math neededto calculate the EWC loss function, dont worry if it doesnt make sense, we will then go through it step by stepAfter gathering your examples of task A in the cell below, mnist, run the below cells from the second cell all the way to sum the squares of the gradientscomparing the norm of the precision _matrices when you use a pretrained model and using a randomly initialized model
###Code
# instead of revisiting every old MNIST example to build our Fisher Information Matrix
# use num_samples of them to calculate a approximate of the matrix
torch.manual_seed(0)
np.random.seed(0)
random.seed(0)
num_batches = 16
old_tasks = []
for sample in range(num_batches):
input_batch, target_batch = next(iter(train_loader_mnist))
for image in input_batch:
old_tasks.append(image)
print("num_samples", len(old_tasks))
from copy import deepcopy
import torch
from torch import nn
from torch.nn import functional as F
from torch.autograd import Variable
from torch import optim
import torch.utils.data
from contlearn.getmodels import MLP
from contlearn.gettrainer import var2device
%load_ext autoreload
%autoreload 2
%matplotlib inline
# Uncomment one of the two below lines of code that instantiates a model
### birth a new randomly initiated model ###
# model = MLP(hidden_size=256)
### load a model previously trained on one task, task A ###
model = torch.load("files/basemodel.pth")
#####
if torch.cuda.is_available() and use_cuda:
model.cuda()
# tensor(0.8238, device='cuda:0') means that the test set accuracy was 82.4%
# compared to a baseline accuracy of 10% if the model is choosing randomly
acc = test(model,test_loader_mnist)
print("accuracy on mnist test set", acc.item())
class EWC(object):
"""
Class to calculate the Fisher Information Matrix
used in the Elastic Weight Consolidation portion
of the loss function
"""
def __init__(self, model: nn.Module, dataset: list):
self.model = model #pretrained model
self.dataset = dataset #samples from the old task or tasks
# n is the string name of the parameter matrix p, aka theta, aka weights
# in self.params we reference all of those weights that are open to
# being updated by the gradient
self.params = {n: p for n, p in self.model.named_parameters() if p.requires_grad}
# make a copy of the old weights, ie theta_A,star, ie 𝜃∗A, in the loss equation
# we need this to calculate (𝜃 - 𝜃∗A)^2 because self.params will be changing
# upon every backward pass and parameter update by the optimizer
self._means = {}
for n, p in deepcopy(self.params).items():
self._means[n] = var2device(p.data)
# calculate the fisher information matrix
self._precision_matrices = self._diag_fisher()
def _diag_fisher(self):
# save a copy of the zero'd out version of
# each layer's parameters of the same shape
# to precision_matrices[n]
precision_matrices = {}
for n, p in deepcopy(self.params).items():
p.data.zero_()
precision_matrices[n] = var2device(p.data)
# we need the model to calculate the gradient but
# we have no intention in this step to actually update the model
# that will have to wait for the combining of this EWC loss term
# with the new task's loss term
self.model.eval()
for input in self.dataset:
self.model.zero_grad()
# remove channel dim, these are greyscale, not color rgb images
# bs,1,h,w -> bs,h,w
input = input.squeeze(1)
input = var2device(input)
output = self.model(input).view(1, -1)
label = output.max(1)[1].view(-1)
# calculate loss and backprop
loss = F.nll_loss(F.log_softmax(output, dim=1), label)
loss.backward()
for n, p in self.model.named_parameters():
precision_matrices[n].data += p.grad.data ** 2 / len(self.dataset)
precision_matrices = {n: p for n, p in precision_matrices.items()}
return precision_matrices
def penalty(self, model: nn.Module):
loss = 0
for n, p in model.named_parameters():
_loss = self._precision_matrices[n] * (p - self._means[n]) ** 2
loss += _loss.sum()
return loss
# place the model pretrained on mnist, but not fashion-mnist, along with some mnist examples
# into the Elastic Weight Consolidation object to perform EWC related tasks like calculating the
# Fisher Matrix
ewc = EWC(model, old_tasks)
###Output
_____no_output_____
###Markdown
Empirical Estimate of the Fisher Information MatrixLets work through the _diag_fisher() method that calculates the fisher matrix, together, step by stepas a reminder here is the equation for the fisher matrix one more time$$I_{\theta_\mathcal{A}^*} = \frac{1}{N} \sum_{i=1}^{N} \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*) \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)^T$$To my understanding, the equation is saying, sum N of these matrices on top of each other such that the resultant matrix is the same shape of the individual matrices. The gradient term $\nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*$ is a vector and the expression $ \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*) \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)^T$ represents the [outer product](https://en.wikipedia.org/wiki/Outer_product) of these two vectors to produce a matrix where the elements of that matrix we are interested in is the diagonal F_ii. ie we are interested in $I_{ii}$ not $I_{ij}$. note: does this mean that in addition to penalizing moving $\theta_i$ when $F_{ii}$ is high, we could also penalize moving both $\theta_i$ and $\theta_j$ together when $F_{ij}$ is high? The Fisher Information Matrix is w.r.t the log probability of the NN prediction p(y|x,theta), rather than w.r.t the log likelihood of the data p(x|theta)notice that the term grad log p(D|theta)$$\nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)$$looks alot like the formula for the gradient of the loss function with respect to the parameters.Thats because for the purposes of EWC, it is!from the [Overcoming catastrophic forgetting in neural networks paper](https://www.pnas.org/content/114/13/3521):"""Note that the log probability of the data given the parameters logp(D|θ) is simply the negative of the loss function for the problem at hand −L(θ). """The key mental leap we need to make is that in our machine learning model, p(D|θ) is the probability of the target label given the parameters rather than the p(X|θ) we are used to seeing that represents the likelihood of observing X given θ. Had the neural netword ended in a softmax layer the term $p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)$ would be the softmax output, but in the code this is combined with the log using the F.log_softmax function. $ log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)$ is the log likelihood. In the code below this is:```F.log_softmax(output, dim=1)```from [the pytorch docs](https://pytorch.org/docs/stable/generated/torch.nn.NLLLoss.htmltorch.nn.NLLLoss)"""Obtaining log-probabilities in a neural network is easily achieved by adding a LogSoftmax layer in the last layer of your network. You may use CrossEntropyLoss instead, if you prefer not to add an extra layer."""Meaning that the full line of code:```F.nll_loss(F.log_softmax(output, dim=1), label)```is essentially the cross entropy loss
###Code
precision_matrices = {}
for n, p in deepcopy(ewc.params).items():
print("name of this layer's weights:", n)
p.data.zero_()
precision_matrices[n] = var2device(p.data)
print("shape of this matrix W", precision_matrices[n].shape)
#print(precision_matrices[n])
print("norm of the matrix",torch.norm(precision_matrices[n]).item())
break
###Output
name of this layer's weights: fc1.weight
shape of this matrix W torch.Size([256, 784])
norm of the matrix 0.0
###Markdown
the code aboveAs a reminder, your input shape x is (batch_size, 784) becasue 28*28 = 784. The matrix W of this first linear, aka affine, aka fully connect layer, is(256, 784) because xW^T = a of shape (batch_size, 256) which is the shape of the first layer's activations the code belowwe pass just one image through our model, and examine the tensors being createdas they are applied to just the first layer of parameters ofcourse thereal thing accumulates this update through all the samples and all the layers
###Code
ewc.model.eval()
for input in ewc.dataset:
ewc.model.zero_grad()
# no need for the channel dim, these are greyscale, not color rgb images
# bs,1,h,w -> bs,h,w
input = input.squeeze(1)
input = var2device(input)
output = ewc.model(input).view(1, -1)
label = output.max(1)[1].view(-1)
loss = F.nll_loss(F.log_softmax(output, dim=1), label)
loss.backward()
break
print("input.shape", input.shape)
img = Image.fromarray(input[0].detach().cpu().numpy()*255)
plt.imshow(img)
print(list(output.detach().cpu().numpy()[0]))
print("predicted number",label)
print("loss",loss.item())
###Output
input.shape torch.Size([1, 28, 28])
[0.0, 0.0, 4.682543, 0.0, 4.328751, 0.0, 7.281813, 0.0, 0.0, 0.0]
predicted number tensor([6], device='cuda:0')
loss 0.12338782846927643
###Markdown
sum the squares of the gradientsthe cell below is the last one you should run when comparing the [norm](https://pytorch.org/docs/stable/generated/torch.norm.html) of the matrix between when the model is pretrained or not.noticed that when you ran the above steps with a pretrained model, the norm is larger than when run with a randomly initialized model, why is this?
###Code
for n, p in ewc.params.items():
print("name of this layer's weights:", n)
print("shape of p.grad.data", p.grad.data.shape)
print("shape of p.grad.data ** 2", (p.grad.data ** 2).shape)
precision_matrices[n].data += p.grad.data ** 2 / len(ewc.dataset)
#print(precision_matrices[n])
print("norm of the matrix",torch.norm(precision_matrices[n]).item())
break
###Output
name of this layer's weights: fc1.weight
shape of p.grad.data torch.Size([256, 784])
shape of p.grad.data ** 2 torch.Size([256, 784])
norm of the matrix 0.0003205974353477359
###Markdown
The Math in the CodeOne clarification that I think needs to be made that was brought to my attention on [Reddit](https://www.reddit.com/r/MachineLearning/comments/t2riby/p_i_made_the_kind_of_tutorial_i_wish_someone_had/?utm_source=share&utm_medium=web2x&context=3). The equation for the empirical fisher below suggests we are calculating the full Fisher Matrix:$$I_{\theta_\mathcal{A}^*} = \frac{1}{N} \sum_{i=1}^{N} \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*) \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)^T$$But in the code we are not, this line is where the diagonal terms are calculated```p.grad.data ** 2```the above line of code corresponds only to the diagonal component of the matrix $I_{\theta_\mathcal{A}^*}$ above.Notice `p.grad.data ** 2` is the same shape as the W for each layer, thats becasue `** 2` is an element-wise operation where we square every element. which is essentially the gradient of the loss function w.r.t. just one parameter, aka weight, multiplied by itself. If we took all the layer's `p.grad.data ** 2`'s and flattened them out into a very long vector, then that would be the just diagonal of the fisher matrix we see in the above equation. So we have only calculated the F_ii terms of the matrix and none of the F_ij terms. note: the diagonal therefore must all be positive valuedThe fisher diagonals are summed over all the N examples and divided by N, aka we average them to get the mean, aka the expectation, which is why in the actual EWS class, the code is written```+= p.grad.data ** 2 / len(self.dataset)```and placed in the inner loop of:```for input in self.dataset:```After the code accumulates over all N examples, it completes the rest of the equation:$$\frac{1}{N} \sum_{i=1}^{N} \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*) \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)^T$$ using the F_i in the overall loss functionthis line of code in the `penalty()` method of the EWS class```_loss = self._precision_matrices[n] * (p - self._means[n]) ** 2```corresponds to this formula$$ F_{i} \left(\theta_{i} - \theta_{A , i}^{*}\right)^{2} $$where `self._precision_matrices[n]` = $ F_{i}$ and `(p - self._means[n]) ** 2` = $\left(\theta_{i} - \theta_{A , i}^{*}\right)^{2} $this is then summed over all parameters `loss += _loss.sum()`and done for each layer, which is why the `penaly()` method places this line of code in the inner loop of `for n, p in model.named_parameters():`later in the code for EWC training, this has to be added to the loss for task B `F.cross_entropy(output, target)` and scaled by $\lambda$ = importance, for the full line of code:`loss = F.cross_entropy(output, target) + importance * ewc.penalty(model)`which is the equivalent of our EWC loss function$$L \left(\right. \theta \left.\right) = L_{B} \left(\right. \theta \left.\right) + \underset{i}{\sum} \frac{\lambda}{2} F_{i} \left(\theta_{i} - \theta_{A , i}^{*}\right)^{2} $$ ExplainationFor the full proof read [Fisher Information Matrix by Agustinus Kristiadi](https://agustinus.kristia.de/techblog/2018/03/11/fisher-information/) or [Fisher Information Matrix by Yuan-Hong Liao](https://andrewliao11.github.io/blog/fisher-info-matrix/). The end result of the proof is that "The Fisher is the negative expectation of the Hessian of the log-likelihood"The Hessian is a square matrix of second-order partial derivatives of a scalar-valued function, or scalar field. It describes the local curvature of a function of many variables. So the diagonal of the Hessian is the 2nd derivative of the loss (negative log likelihood) with respect to the parameters. Imagine if you only had 2 parameters, the Fisher Information over a patch of values for $\theta_i$ adn $\theta_j$ might look like this:Hypothetical F_ij Surface, ignore the vertical axis label for nowThe warmer areas (more red) are the areas of higher curvature, the areas of higher curvature are the areas of local minima and maxima. If you turn the above figure upside down, this would look just like the topological manifold, aka surface, of the loss function, And what is gradient descent trying to do? it is trying to find a local minima on this loss surface, aka a local maxima on the fisher information surface. In intuitive terms, both the Hessian and Fisher Matrix describe how sharp, ie curved, each point in $\theta$ space is, with respect to the loss.But wait a minute, cant we have a valley with a very flat and wide floor? yes we can, and it very well might be that some of our parameters have settled into such a region while training on task A. However, if that is the case, then while $\theta$ is in the center of such a region, it can move in various directions and still contribute to good performance on task A, which means we should not penalize changes in this region until $\theta$ reaches the edge of such a region, in which case, the Fisher information should start to increase. Basically the Fisher Matrix is the negative of this matrix below, where you replace f with log[p(x|theta)] and replace x with theta*The $F_i$ is higher for those $\theta_i$ that are already optimized into a narrow region and thus if changed, would cause an increase in the loss for task A, thereby reducing the performance on task A described by that loss. More of these "important and just right" parameters will exist for trained models and thus the norm on the fisher diagonals will be higher. For models not yet trained on task A, there are fewer parameters that have been optimized to help solve task A, therefore fewer parameters that when changed will have a significant effect on the loss for task A*
###Code
# since the parameters have not changed yet, we expect (𝜃 - 𝜃∗)2 to be zero throughout
ewc.penalty(model)
###Output
_____no_output_____
###Markdown
EWC TrainingLoad a model pretrained on MNIST, then train it on Fashion-MNIST while observing the new training's effect on MNIST performance
###Code
# verify that the model you are about to retrain is indeed pretrained
acc = test(model,test_loader_mnist)
print("accuracy on mnist test set", acc.item())
def one_epoch_ewc(
ewc: EWC,
importance: float,
model: nn.Module,
data_loader: torch.utils.data.DataLoader,
lr = 1e-3,
):
model.train()
epoch_loss = 0
optimizer = optim.SGD(params=model.parameters(), lr=lr)
for input, target in data_loader:
# no need for the channel dim
# bs,1,h,w -> bs,h,w
input = input.squeeze(1)
input, target = var2device(input), var2device(target)
optimizer.zero_grad()
output = model(input)
loss = F.cross_entropy(output, target) + importance * ewc.penalty(model)
epoch_loss += loss.item()
loss.backward()
optimizer.step()
return epoch_loss / len(data_loader)
def ewc_training(
ewc,
importance,
model,
epochs,
train_loader,
test_loader,
test2_loader = None,
use_cuda=True,
):
"""
This function saves the training curve data consisting
training set loss and validation set accuracy over the
course of the epochs of training.
I set this up such that if you provide 2 test sets,you
can watch the test accuracy change together during training
on train_loder
"""
if torch.cuda.is_available() and use_cuda:
model.cuda()
train_loss, val_acc, val2_acc = [], [], []
for epoch in tqdm(range(epochs)):
epoch_loss = one_epoch_ewc(ewc,importance, model,train_loader)
train_loss.append(epoch_loss)
acc = test(model,test_loader)
val_acc.append(acc.detach().cpu().numpy())
if test2_loader is not None:
acc2 = test(model,test2_loader)
val2_acc.append(acc2.detach().cpu().numpy())
return train_loss, val_acc, val2_acc, model
torch.manual_seed(0)
np.random.seed(0)
random.seed(0)
train_loss, val_acc, val2_acc, model = ewc_training(
ewc = ewc,
importance = 1000,
model = model,
epochs = 25,
train_loader = train_loader_fashion,
test_loader = test_loader_fashion,
test2_loader = test_loader_mnist,
)
plt.figure()
plt.xlabel('epochs', fontsize=25)
plt.ylabel('validation accuracy', fontsize=25)
plt.plot(val_acc, label='fashion')
plt.plot(val2_acc, label='mnist')
plt.legend()
plt.show()
# save the trained model
model = model.cpu()
torch.save(model, "files/ewcmodel.pth")
###Output
_____no_output_____
###Markdown
Find the Repos Available in your Database, and What Repository Groups They Are In Connect to your database
###Code
import psycopg2
import pandas as pd
import sqlalchemy as salc
import numpy as np
import seaborn as sns
import matplotlib.pyplot as plt
import warnings
import datetime
import json
warnings.filterwarnings('ignore')
with open("config.json") as config_file:
config = json.load(config_file)
database_connection_string = 'postgres+psycopg2://{}:{}@{}:{}/{}'.format(config['user'], config['password'], config['host'], config['port'], config['database'])
dbschema='augur_data'
engine = salc.create_engine(
database_connection_string,
connect_args={'options': '-csearch_path={}'.format(dbschema)})
###Output
_____no_output_____
###Markdown
Retrieve Available Respositories
###Code
repolist = pd.DataFrame()
repo_query = salc.sql.text(f"""
SELECT a.rg_name,
a.repo_group_id,
b.repo_name,
b.repo_id,
b.forked_from,
b.repo_archived
FROM
repo_groups a,
repo b
WHERE
a.repo_group_id = b.repo_group_id
ORDER BY
rg_name,
repo_name;
""")
repolist = pd.read_sql(repo_query, con=engine)
display(repolist)
repolist.dtypes
###Output
_____no_output_____
###Markdown
Create a Simpler List for quickly Identifying repo_group_id's and repo_id's for other queries
###Code
repolist = pd.DataFrame()
repo_query = salc.sql.text(f"""
SELECT b.repo_id,
a.repo_group_id,
b.repo_name,
a.rg_name
FROM
repo_groups a,
repo b
WHERE
a.repo_group_id = b.repo_group_id
ORDER BY
rg_name,
repo_name;
""")
repolist = pd.read_sql(repo_query, con=engine)
display(repolist)
repolist.dtypes
###Output
_____no_output_____
###Markdown
Part 1 intuition behind elastic weight colsolidationIn the figure below, $\theta^{*}$ are the weights (ie parameters, synaptic strengths) learned by the neural network (NN) to solve old task A, shown as a vector in vector space. ie if this neural network as 100 total weights then this is a 2D representation of $\mathbb{R}^{100}$ space. The blue horizontal arrow shows an example of catastrophic forgetting, whereby $\theta^{*}$ moves out of the region that allows the NN to perform well at task A (grey), and into the center of a region that allows the NN to perform well at task B (cream). The downward green arrow is the update to $\theta^{*}$ regularized by L2 penalty $\alpha (\theta_{i} - \theta_{A , i}^{*})^{2}$ that causes it to move toward the cream region irrespective of the shape of the grey region. The desired update vector is the red arrow that moves the NN weights into a region capable of performing well at both tasks A and B. How Elastic Weight Cosolidation Changes Learning New Weights $\theta^{*}$EWC encourages movement of weights along the red path by modifying the loss function when re-training a NN that has already been trained to convergence using the loss function for task A, $L_{A}$, which has settled on weights $\theta_{A}$. When re-training the NN on task B using $L_{B}$, we add a term which penalizes changes to weights that are both far from $\theta_{A}$, ie $(\theta_{i} - \theta_{A , i}^{*})^{2}$, and also high in $F_{i}$ which encodes the shape of the grey region.$$L \left(\right. \theta \left.\right) = L_{B} \left(\right. \theta \left.\right) + \underset{i}{\sum} \frac{\lambda}{2} F_{i} \left(\theta_{i} - \theta_{A , i}^{*}\right)^{2} $$ But what is F_i? F_i is the diagonal of the Fisher information matrix. We want to use the diagonal components in Fisher Information Matrix to identify which parameters are more important to task A and apply higher weights to them (the direction of the short axis of grey oval). To learn B we should instead change those weights where F_i is small (long axis of grey oval). In the EWC paper:"we approximate the posterior as a Gaussian distribution with mean given by the parameters θ∗A and a diagonal precision given by the diagonal of the Fisher information matrix F. F has three key properties (20): (i) It is equivalent to the second derivative of the loss near a minimum, (ii) it can be computed from first-order derivatives alone and is thus easy to calculate even for large models, and (iii) it is guaranteed to be positive semidefinite. Note that this approach is similar to expectation propagation where each subtask is seen as a factor of the posterior (21). where LB(θ) is the loss for task B only, λ sets how important the old task is compared with the new one, and i labels each parameter.When moving to a third task, task C, EWC will try to keep the network parameters close to the learned parameters of both tasks A and B. This can be enforced either with two separate penalties or as one by noting that the sum of two quadratic penalties is itself a quadratic penalty." Lets learn what F is in the exampleThis article gives a very good explaination of F in the context of EWC: [Fisher Information Matrix by Yuan-Hong Liao](https://andrewliao11.github.io/blog/fisher-info-matrix/)To compute F_i, we sample the data from task A once and calculate the empirical Fisher Information Matrix. $$I_{\theta_\mathcal{A}^*} = \frac{1}{N} \sum_{i=1}^{N} \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*) \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)^T$$This is just to say that the above equation is how you calculate the below equation from the data. For each pair of parameters in $\theta$ ($\theta_i$ and $\theta_j$), the Fisher Information matrix at position ij is$$I(\theta)_{ij} = E\left[ \left( \frac{\partial}{\partial\theta_i}\log f(X;\theta) \right)\left( \frac{\partial}{\partial\theta_j}\log f(X;\theta) \right) \mid \theta\right]$$If this equation is hard to understand, then the code should make it clearer, dont worry, we will match parts of the code to the equation above so it becomes more tangible. Part 2 A look at the data and the task MNISTThe MNIST data set contains 70,000 images of handwritten digits and their corresponding labels. The images are 28x28 with pixel values from 0 to 255. The labels are the digits from 0 to 9. By default 60,000 of these images belong to a training set and 10,000 of these images belong to a test set. Fashion-MNISTFashion-MNIST is a dataset of Zalando's article images—consisting of a training set of 60,000 examples and a test set of 10,000 examples. Each example is a 28x28 grayscale image, associated with a label from 10 classes. Zalando intends Fashion-MNIST to serve as a direct drop-in replacement for the original MNIST dataset for benchmarking machine learning algorithms. It shares the same image size and structure of training and testing splits.Each training and test example is assigned to one of the following labels:- 0 T-shirt/top- 1 Trouser- 2 Pullover- 3 Dress- 4 Coat- 5 Sandal- 6 Shirt- 7 Sneaker- 8 Bag- 9 Ankle boot Taskas you might guess, our goal is to train an NN that retains it's ability to perform well on MNIST after being retrained on only Fashion-MNIST
###Code
import numpy as np
from PIL import Image
from matplotlib import pyplot as plt
from matplotlib.pyplot import imshow
from contlearn.getdata import getMNIST, getFashionMNIST
%load_ext autoreload
%autoreload 2
%matplotlib inline
# task A training and test set
train_loader_mnist, test_loader_mnist = getMNIST(batch_size=32)
# task B training and test set
train_loader_fashion, test_loader_fashion = getFashionMNIST(batch_size=32)
input_image, target_label = next(iter(train_loader_mnist))
print(target_label[0])
print(input_image[0][0].shape)
img = Image.fromarray(input_image[0][0].detach().cpu().numpy()*255)
plt.imshow(img)
input_image, target_label = next(iter(train_loader_fashion))
fashion_key = {
0: "T-shirt/top",
1: "Trouser",
2: "Pullover",
3: "Dress",
4: "Coat",
5: "Sandal",
6: "Shirt",
7: "Sneaker",
8: "Bag",
9: "Ankle boot",
}
print(fashion_key[int(target_label[0].detach().cpu().numpy())])
print(input_image[0][0].shape)
img = Image.fromarray(input_image[0][0].detach().cpu().numpy()*255)
plt.imshow(img)
###Output
Coat
torch.Size([28, 28])
###Markdown
Part 3 Baseline resultsfirst we train on MNIST and the we will observe the drop in performance once we retrain on Fashion-MNIST, WITHOUT Elastic Weight Consolidation
###Code
import math
import random
import numpy as np
import torch
from matplotlib import pyplot as plt
from matplotlib.pyplot import imshow
from tqdm.notebook import tqdm
from contlearn.getmodels import MLP
from contlearn.gettrainer import one_epoch_baseline, test, var2device
%load_ext autoreload
%autoreload 2
%matplotlib inline
print('you are using PyTorch version ',torch.__version__)
if torch.cuda.is_available():
use_cuda = True
print("you have", torch.cuda.device_count(), "GPUs")
device = torch.device("cuda:0")
print(device)
else:
use_cuda = False
print('no GPUs detected')
device = torch.device("cpu")
# initialize a new model
model = MLP(hidden_size=256)
if torch.cuda.is_available() and use_cuda:
model.cuda()
# push an image through it
input_image, target_label = next(iter(train_loader_fashion))
input_image = var2device(input_image).squeeze(1)
print(input_image.shape)
output = model(input_image)
print(output.shape)
def baseline_training(
model,
epochs,
train_loader,
test_loader,
test2_loader = None,
use_cuda=True,
):
"""
This function saves the training curve data consisting
training set loss and validation set accuracy over the
course of the epochs of training.
I set this up such that if you provide 2 test sets,you
can watch the test accuracy change together during training
on train_loder
"""
if torch.cuda.is_available() and use_cuda:
model.cuda()
train_loss, val_acc, val2_acc = [], [], []
for epoch in tqdm(range(epochs)):
epoch_loss = one_epoch_baseline(model,train_loader)
train_loss.append(epoch_loss)
acc = test(model,test_loader)
val_acc.append(acc.detach().cpu().numpy())
if test2_loader is not None:
acc2 = test(model,test2_loader)
val2_acc.append(acc2.detach().cpu().numpy())
return train_loss, val_acc, val2_acc, model
# set seeds for reproducibility and train the model using the training loop called
# baseline_training
torch.manual_seed(0)
np.random.seed(0)
random.seed(0)
train_loss, val_acc, val2_acc, model = baseline_training(
model,
epochs = 25,
train_loader = train_loader_mnist,
test_loader = test_loader_mnist,
)
# save the trained model
model = model.cpu()
torch.save(model, "files/basemodel.pth")
plt.figure()
plt.xlabel('epochs', fontsize=25)
plt.ylabel('validation accuracy', fontsize=25)
plt.plot(val_acc, label='mnist')
plt.legend()
plt.show()
###Output
_____no_output_____
###Markdown
learning curveyou should get something like this where the accuracy starts to plateau at around 75% for this simple feed forward model
###Code
# you can use this to load the model instead of training it from scratch like above
model = torch.load("files/basemodel.pth")
if torch.cuda.is_available() and use_cuda:
model.cuda()
# tensor(0.8238, device='cuda:0') means that the test set accuracy was 82.4%
# compared to a baseline accuracy of 10% if the model is choosing randomly
print('mnist accuracy', test(model,test_loader_mnist))
print('fashion accuracy', test(model,test_loader_fashion))
###Output
mnist accuracy tensor(0.8238)
fashion accuracy tensor(0.0704)
###Markdown
Catastrophic ForgettingThis is one of the main problems we are trying to solve in the continual learning, aka lifelong learning, aka sequential learning, research field. As you can see, learning Fashion MNIST also degrades performance on original MNIST. In part this is because some of the useful parameters, aka weights, used for original MNIST, are overwritten or updated past what is useful for original MNIST in order to become useful for Fashion MNISTWe expect something like this in which the fashion accuracy increases from it's random performance at around 10% to almost 40% and the mnist test accuracy drops to below 30% from a previous performance of around 80%
###Code
torch.manual_seed(0)
np.random.seed(0)
random.seed(0)
train_loss, val_acc, val2_acc, model = baseline_training(
model,
epochs = 25,
train_loader = train_loader_fashion,
test_loader = test_loader_fashion,
test2_loader = test_loader_mnist,
)
plt.figure()
plt.xlabel('epochs', fontsize=25)
plt.ylabel('validation accuracy', fontsize=25)
plt.plot(val_acc, label='fashion')
plt.plot(val2_acc, label='mnist')
plt.legend()
plt.show()
###Output
_____no_output_____
###Markdown
Part 4 Elastic Weight Consolidationnow that we have implemented the control group, lets implement the experimental groupinstead of revisiting every old MNIST example to build our Fisher Information Matrix, we will visit num_samples of them to form a approximate of the matrixplease read the comments in the EWC class, which explains each step of the math neededto calculate the EWC loss function, dont worry if it doesnt make sense, we will then go through it step by stepAfter gathering your examples of task A in the cell below, mnist, run the below cells from the second cell all the way to sum the squares of the gradientscomparing the norm of the precision _matrices when you use a pretrained model and using a randomly initialized model
###Code
# instead of revisiting every old MNIST example to build our Fisher Information Matrix
# use num_samples of them to calculate a approximate of the matrix
torch.manual_seed(0)
np.random.seed(0)
random.seed(0)
num_batches = 16
old_tasks = []
for sample in range(num_batches):
input_batch, target_batch = next(iter(train_loader_mnist))
for image in input_batch:
old_tasks.append(image)
print("num_samples", len(old_tasks))
from copy import deepcopy
import torch
from torch import nn
from torch.nn import functional as F
from torch.autograd import Variable
from torch import optim
import torch.utils.data
from contlearn.getmodels import MLP
from contlearn.gettrainer import var2device
%load_ext autoreload
%autoreload 2
%matplotlib inline
# Uncomment one of the two below lines of code that instantiates a model
### birth a new randomly initiated model ###
# model = MLP(hidden_size=256)
### load a model previously trained on one task, task A ###
model = torch.load("files/basemodel.pth")
#####
if torch.cuda.is_available() and use_cuda:
model.cuda()
# tensor(0.8238, device='cuda:0') means that the test set accuracy was 82.4%
# compared to a baseline accuracy of 10% if the model is choosing randomly
acc = test(model,test_loader_mnist)
print("accuracy on mnist test set", acc.item())
class EWC(object):
"""
Class to calculate the Fisher Information Matrix
used in the Elastic Weight Consolidation portion
of the loss function
"""
def __init__(self, model: nn.Module, dataset: list):
self.model = model #pretrained model
self.dataset = dataset #samples from the old task or tasks
# n is the string name of the parameter matrix p, aka theta, aka weights
# in self.params we reference all of those weights that are open to
# being updated by the gradient
self.params = {n: p for n, p in self.model.named_parameters() if p.requires_grad}
# make a copy of the old weights, ie theta_A,star, ie 𝜃∗A, in the loss equation
# we need this to calculate (𝜃 - 𝜃∗A)^2 because self.params will be changing
# upon every backward pass and parameter update by the optimizer
self._means = {}
for n, p in deepcopy(self.params).items():
self._means[n] = var2device(p.data)
# calculate the fisher information matrix
self._precision_matrices = self._diag_fisher()
def _diag_fisher(self):
# save a copy of the zero'd out version of
# each layer's parameters of the same shape
# to precision_matrices[n]
precision_matrices = {}
for n, p in deepcopy(self.params).items():
p.data.zero_()
precision_matrices[n] = var2device(p.data)
# we need the model to calculate the gradient but
# we have no intention in this step to actually update the model
# that will have to wait for the combining of this EWC loss term
# with the new task's loss term
self.model.eval()
for input in self.dataset:
self.model.zero_grad()
# remove channel dim, these are greyscale, not color rgb images
# bs,1,h,w -> bs,h,w
input = input.squeeze(1)
input = var2device(input)
output = self.model(input).view(1, -1)
label = output.max(1)[1].view(-1)
# calculate loss and backprop
loss = F.nll_loss(F.log_softmax(output, dim=1), label)
loss.backward()
for n, p in self.model.named_parameters():
precision_matrices[n].data += p.grad.data ** 2 / len(self.dataset)
precision_matrices = {n: p for n, p in precision_matrices.items()}
return precision_matrices
def penalty(self, model: nn.Module):
loss = 0
for n, p in model.named_parameters():
_loss = self._precision_matrices[n] * (p - self._means[n]) ** 2
loss += _loss.sum()
return loss
# place the model pretrained on mnist, but not fashion-mnist, along with some mnist examples
# into the Elastic Weight Consolidation object to perform EWC related tasks like calculating the
# Fisher Matrix
ewc = EWC(model, old_tasks)
###Output
_____no_output_____
###Markdown
Empirical Estimate of the Fisher Information MatrixLets work through the _diag_fisher() method that calculates the fisher matrix, together, step by stepas a reminder here is the equation for the fisher matrix one more time$$I_{\theta_\mathcal{A}^*} = \frac{1}{N} \sum_{i=1}^{N} \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*) \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)^T$$To my understanding, the equation is saying, sum N of these matrices on top of each other such that the resultant matrix is the same shape of the individual matrices. The gradient term $\nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)$ is a vector and the expression $ \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*) \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)^T$ represents the [outer product](https://en.wikipedia.org/wiki/Outer_product) of these two vectors to produce a matrix where the elements of that matrix we are interested in is the diagonal $F_{ii}$. ie we are interested in $I_{ii}$, not $I_{ij}$. note: does this mean that in addition to penalizing moving $\theta_i$ when $F_{ii}$ is high, we could also penalize moving both $\theta_i$ and $\theta_j$ together when $F_{ij}$ is high? The Fisher Information Matrix is w.r.t the log probability of the NN prediction p(y|x,theta), rather than w.r.t the log likelihood of the data p(x|theta)notice that the term grad log p(D|theta)$$\nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)$$looks alot like the formula for the gradient of the loss function with respect to the parameters.Thats because for the purposes of EWC, it is!from the [Overcoming catastrophic forgetting in neural networks paper](https://www.pnas.org/content/114/13/3521):"""Note that the log probability of the data given the parameters logp(D|θ) is simply the negative of the loss function for the problem at hand −L(θ). """The key mental leap we need to make is that in our machine learning model, p(D|θ) is the probability of the target label given the parameters rather than the p(X|θ) we are used to seeing that represents the likelihood of observing X given θ. Had the neural netword ended in a softmax layer the term $p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)$ would be the softmax output, but in the code this is combined with the log using the F.log_softmax function. $ log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)$ is the log likelihood. In the code below this is:```F.log_softmax(output, dim=1)```from [the pytorch docs](https://pytorch.org/docs/stable/generated/torch.nn.NLLLoss.htmltorch.nn.NLLLoss)"""Obtaining log-probabilities in a neural network is easily achieved by adding a LogSoftmax layer in the last layer of your network. You may use CrossEntropyLoss instead, if you prefer not to add an extra layer."""Meaning that the full line of code:```F.nll_loss(F.log_softmax(output, dim=1), label)```is essentially the cross entropy loss
###Code
precision_matrices = {}
for n, p in deepcopy(ewc.params).items():
print("name of this layer's weights:", n)
p.data.zero_()
precision_matrices[n] = var2device(p.data)
print("shape of this matrix W", precision_matrices[n].shape)
#print(precision_matrices[n])
print("norm of the matrix",torch.norm(precision_matrices[n]).item())
break
###Output
name of this layer's weights: fc1.weight
shape of this matrix W torch.Size([256, 784])
norm of the matrix 0.0
###Markdown
the code aboveAs a reminder, your input shape x is (batch_size, 784) becasue 28*28 = 784. The matrix W of this first linear, aka affine, aka fully connect layer, is(256, 784) because xW^T = a of shape (batch_size, 256) which is the shape of the first layer's activations the code belowwe pass just one image through our model, and examine the tensors being createdas they are applied to just the first layer of parameters ofcourse thereal thing accumulates this update through all the samples and all the layers
###Code
ewc.model.eval()
for input in ewc.dataset:
ewc.model.zero_grad()
# no need for the channel dim, these are greyscale, not color rgb images
# bs,1,h,w -> bs,h,w
input = input.squeeze(1)
input = var2device(input)
output = ewc.model(input).view(1, -1)
label = output.max(1)[1].view(-1)
loss = F.nll_loss(F.log_softmax(output, dim=1), label)
loss.backward()
break
print("input.shape", input.shape)
img = Image.fromarray(input[0].detach().cpu().numpy()*255)
plt.imshow(img)
print(list(output.detach().cpu().numpy()[0]))
print("predicted number",label)
print("loss",loss.item())
###Output
input.shape torch.Size([1, 28, 28])
[0.0, 0.0, 4.682543, 0.0, 4.328751, 0.0, 7.281813, 0.0, 0.0, 0.0]
predicted number tensor([6], device='cuda:0')
loss 0.12338782846927643
###Markdown
sum the squares of the gradientsthe cell below is the last one you should run when comparing the [norm](https://pytorch.org/docs/stable/generated/torch.norm.html) of the matrix between when the model is pretrained or not.noticed that when you ran the above steps with a pretrained model, the norm is larger than when run with a randomly initialized model, why is this?
###Code
for n, p in ewc.params.items():
print("name of this layer's weights:", n)
print("shape of p.grad.data", p.grad.data.shape)
print("shape of p.grad.data ** 2", (p.grad.data ** 2).shape)
precision_matrices[n].data += p.grad.data ** 2 / len(ewc.dataset)
#print(precision_matrices[n])
print("norm of the matrix",torch.norm(precision_matrices[n]).item())
break
###Output
name of this layer's weights: fc1.weight
shape of p.grad.data torch.Size([256, 784])
shape of p.grad.data ** 2 torch.Size([256, 784])
norm of the matrix 0.0003205974353477359
###Markdown
The Math in the CodeOne clarification that I think needs to be made that was brought to my attention on [Reddit](https://www.reddit.com/r/MachineLearning/comments/t2riby/p_i_made_the_kind_of_tutorial_i_wish_someone_had/?utm_source=share&utm_medium=web2x&context=3). The equation for the empirical fisher below suggests we are calculating the full Fisher Matrix:$$I_{\theta_\mathcal{A}^*} = \frac{1}{N} \sum_{i=1}^{N} \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*) \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)^T$$But in the code we are not, this line is where the diagonal terms are calculated```p.grad.data ** 2```the above line of code corresponds only to the diagonal component of the matrix $I_{\theta_\mathcal{A}^*}$ above.Notice `p.grad.data ** 2` is the same shape as the W for each layer, thats becasue `** 2` is an element-wise operation where we square every element. which is essentially the gradient of the loss function w.r.t. just one parameter, aka weight, multiplied by itself. If we took all the layer's `p.grad.data ** 2`'s and flattened them out into a very long vector, then that would be the just diagonal of the fisher matrix we see in the above equation. So we have only calculated the F_ii terms of the matrix and none of the F_ij terms. note: the diagonal therefore must all be positive valuedThe fisher diagonals are summed over all the N examples and divided by N, aka we average them to get the mean, aka the expectation, which is why in the actual EWS class, the code is written```+= p.grad.data ** 2 / len(self.dataset)```and placed in the inner loop of:```for input in self.dataset:```After the code accumulates over all N examples, it completes the rest of the equation:$$\frac{1}{N} \sum_{i=1}^{N} \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*) \nabla_\theta log \ p(x_{\mathcal{A}, i}|\theta_\mathcal{A}^*)^T$$ using the F_i in the overall loss functionthis line of code in the `penalty()` method of the EWS class```_loss = self._precision_matrices[n] * (p - self._means[n]) ** 2```corresponds to this formula$$ F_{i} \left(\theta_{i} - \theta_{A , i}^{*}\right)^{2} $$where `self._precision_matrices[n]` = $ F_{i}$ and `(p - self._means[n]) ** 2` = $\left(\theta_{i} - \theta_{A , i}^{*}\right)^{2} $this is then summed over all parameters `loss += _loss.sum()`and done for each layer, which is why the `penaly()` method places this line of code in the inner loop of `for n, p in model.named_parameters():`later in the code for EWC training, this has to be added to the loss for task B `F.cross_entropy(output, target)` and scaled by $\lambda$ = importance, for the full line of code:`loss = F.cross_entropy(output, target) + importance * ewc.penalty(model)`which is the equivalent of our EWC loss function$$L \left(\right. \theta \left.\right) = L_{B} \left(\right. \theta \left.\right) + \underset{i}{\sum} \frac{\lambda}{2} F_{i} \left(\theta_{i} - \theta_{A , i}^{*}\right)^{2} $$ ExplainationFor the full proof read [Fisher Information Matrix by Agustinus Kristiadi](https://agustinus.kristia.de/techblog/2018/03/11/fisher-information/) or [Fisher Information Matrix by Yuan-Hong Liao](https://andrewliao11.github.io/blog/fisher-info-matrix/). The end result of the proof is that "The Fisher is the negative expectation of the Hessian of the log-likelihood"The Hessian is a square matrix of second-order partial derivatives of a scalar-valued function, or scalar field. It describes the local curvature of a function of many variables. So the diagonal of the Hessian is the 2nd derivative of the loss (negative log likelihood) with respect to the parameters. Imagine if you only had 2 parameters, the Fisher Information over a patch of values for $\theta_i$ adn $\theta_j$ might look like this:Hypothetical F_ij Surface, ignore the vertical axis label for nowThe warmer areas (more red) are the areas of higher curvature, the areas of higher curvature are the areas of local minima and maxima. If you turn the above figure upside down, this would look just like the topological manifold, aka surface, of the loss function, And what is gradient descent trying to do? it is trying to find a local minima on this loss surface, aka a local maxima on the fisher information surface. In intuitive terms, both the Hessian and Fisher Matrix describe how sharp, ie curved, each point in $\theta$ space is, with respect to the loss.But wait a minute, cant we have a valley with a very flat and wide floor? yes we can, and it very well might be that some of our parameters have settled into such a region while training on task A. However, if that is the case, then while $\theta$ is in the center of such a region, it can move in various directions and still contribute to good performance on task A, which means we should not penalize changes in this region until $\theta$ reaches the edge of such a region, in which case, the Fisher information should start to increase. Basically the Fisher Matrix is the negative of this matrix below, where you replace f with log[p(x|theta)] and replace x with theta*The $F_i$ is higher for those $\theta_i$ that are already optimized into a narrow region and thus if changed, would cause an increase in the loss for task A, thereby reducing the performance on task A described by that loss. More of these "important and just right" parameters will exist for trained models and thus the norm on the fisher diagonals will be higher. For models not yet trained on task A, there are fewer parameters that have been optimized to help solve task A, therefore fewer parameters that when changed will have a significant effect on the loss for task A*
###Code
# since the parameters have not changed yet, we expect (𝜃 - 𝜃∗)2 to be zero throughout
ewc.penalty(model)
###Output
_____no_output_____
###Markdown
EWC TrainingLoad a model pretrained on MNIST, then train it on Fashion-MNIST while observing the new training's effect on MNIST performance
###Code
# verify that the model you are about to retrain is indeed pretrained
acc = test(model,test_loader_mnist)
print("accuracy on mnist test set", acc.item())
def one_epoch_ewc(
ewc: EWC,
importance: float,
model: nn.Module,
data_loader: torch.utils.data.DataLoader,
lr = 1e-3,
):
model.train()
epoch_loss = 0
optimizer = optim.SGD(params=model.parameters(), lr=lr)
for input, target in data_loader:
# no need for the channel dim
# bs,1,h,w -> bs,h,w
input = input.squeeze(1)
input, target = var2device(input), var2device(target)
optimizer.zero_grad()
output = model(input)
loss = F.cross_entropy(output, target) + importance * ewc.penalty(model)
epoch_loss += loss.item()
loss.backward()
optimizer.step()
return epoch_loss / len(data_loader)
def ewc_training(
ewc,
importance,
model,
epochs,
train_loader,
test_loader,
test2_loader = None,
use_cuda=True,
):
"""
This function saves the training curve data consisting
training set loss and validation set accuracy over the
course of the epochs of training.
I set this up such that if you provide 2 test sets,you
can watch the test accuracy change together during training
on train_loder
"""
if torch.cuda.is_available() and use_cuda:
model.cuda()
train_loss, val_acc, val2_acc = [], [], []
for epoch in tqdm(range(epochs)):
epoch_loss = one_epoch_ewc(ewc,importance, model,train_loader)
train_loss.append(epoch_loss)
acc = test(model,test_loader)
val_acc.append(acc.detach().cpu().numpy())
if test2_loader is not None:
acc2 = test(model,test2_loader)
val2_acc.append(acc2.detach().cpu().numpy())
return train_loss, val_acc, val2_acc, model
torch.manual_seed(0)
np.random.seed(0)
random.seed(0)
train_loss, val_acc, val2_acc, model = ewc_training(
ewc = ewc,
importance = 1000,
model = model,
epochs = 25,
train_loader = train_loader_fashion,
test_loader = test_loader_fashion,
test2_loader = test_loader_mnist,
)
plt.figure()
plt.xlabel('epochs', fontsize=25)
plt.ylabel('validation accuracy', fontsize=25)
plt.plot(val_acc, label='fashion')
plt.plot(val2_acc, label='mnist')
plt.legend()
plt.show()
# save the trained model
model = model.cpu()
torch.save(model, "files/ewcmodel.pth")
###Output
_____no_output_____
###Markdown
Find the Repos Available in your Database, and What Repository Groups They Are In Connect to your database
###Code
import psycopg2
import pandas as pd
import sqlalchemy as salc
import numpy as np
import seaborn as sns
import matplotlib.pyplot as plt
import warnings
import datetime
import json
warnings.filterwarnings('ignore')
with open("config.json") as config_file:
config = json.load(config_file)
database_connection_string = 'postgres+psycopg2://{}:{}@{}:{}/{}'.format(config['user'], config['password'], config['host'], config['port'], config['database'])
dbschema='augur_data'
engine = salc.create_engine(
database_connection_string,
connect_args={'options': '-csearch_path={}'.format(dbschema)})
###Output
_____no_output_____
###Markdown
Retrieve Available Respositories
###Code
repolist = pd.DataFrame()
repo_query = salc.sql.text(f"""
SELECT a.rg_name,
a.repo_group_id,
b.repo_name,
b.repo_id,
b.forked_from,
b.repo_archived
FROM
repo_groups a,
repo b
WHERE
a.repo_group_id = b.repo_group_id
ORDER BY
rg_name,
repo_name;
""")
repolist = pd.read_sql(repo_query, con=engine)
display(repolist)
repolist.dtypes
###Output
_____no_output_____
###Markdown
Create a Simpler List for quickly Identifying repo_group_id's and repo_id's for other queries
###Code
repolist = pd.DataFrame()
repo_query = salc.sql.text(f"""
SELECT b.repo_id,
a.repo_group_id,
b.repo_name,
a.rg_name
FROM
repo_groups a,
repo b
WHERE
a.repo_group_id = b.repo_group_id
ORDER BY
rg_name,
repo_name;
""")
repolist = pd.read_sql(repo_query, con=engine)
display(repolist)
repolist.dtypes
###Output
_____no_output_____ |
Functions and Methods/Methods.ipynb | ###Markdown
Methods The distinction between methods and functions is somewhat sublte. They are functions that are built into objects, and are a subclass of functions. Functions are "general purpose" and do not belong to objects. We've talked about list, tuple, string, and dictionary methods. Even numbers themselves have methods. Any call to a function that belongs to an object is called a method. The format is the following: object.method(arg1, arg2..) We can observe that there is an object here. Let's create a list object and try out some built-in methods (we can even create our own methods, coming in the next chapter). Number Methods
###Code
# Numbers have methods, too
my_num = 2
print(my_num.__add__(3))
print(my_num.__sub__(2))
print(my_num.__mul__(2))
print(my_num.__truediv__(2))
###Output
5
0
4
1.0
###Markdown
List Methods (Recap)
###Code
# These are examples of list methods.
my_list = ["Bonet", "Triggly", "Ross"]
my_list.append("Triggly")
print("After appending {}".format(my_list))
another_list = my_list.copy()
print("After copying {}".format(another_list))
my_list.clear()
print("After clearing {}".format(my_list))
another_list.insert(2, "Triggly")
print("After insertion: {}".format(another_list))
the_count = another_list.count("Triggly")
print("Count of specific word: {}".format(the_count))
###Output
After appending ['Bonet', 'Triggly', 'Ross', 'Triggly']
After copying ['Bonet', 'Triggly', 'Ross', 'Triggly']
After clearing []
After insertion: ['Bonet', 'Triggly', 'Triggly', 'Ross', 'Triggly']
Count of specific word: 3
###Markdown
Tuple Methods (Recap)
###Code
# These are some tuple methods
my_tuple = ("hate", 19.0, "redemption", "hate")
print(my_tuple.count("hate"))
print(my_tuple.index("redemption"))
###Output
2
2
###Markdown
Dictionary Methods (Recap)
###Code
# These are Dictionary Methods
my_dict = {"Sally":19, "Robert":[1, 48, 29], "Wallace":0}
keys = list(my_dict.keys())
values = list(my_dict.values())
popped_value = my_dict.pop("Robert")
print(popped_key)
###Output
[1, 48, 29]
|
slim/slim_walkthough.ipynb | ###Markdown
TF-Slim WalkthroughThis notebook will walk you through the basics of using TF-Slim to define, train and evaluate neural networks on various tasks. It assumes a basic knowledge of neural networks. Table of contentsInstallation and setupCreating your first neural network with TF-SlimReading Data with TF-SlimTraining a convolutional neural network (CNN)Using pre-trained models Installation and setupAs of 8/28/16, the latest stable release of TF is r0.10, which does not contain the latest version of slim.To obtain the latest version of TF-Slim, please install the most recent nightly build of TFas explained [here](https://github.com/tensorflow/models/tree/master/sliminstalling-latest-version-of-tf-slim).To use TF-Slim for image classification (as we do in this notebook), you also have to install the TF-Slim image models library from [here](https://github.com/tensorflow/models/tree/master/slim). Let's suppose you install this into a directory called TF_MODELS. Then you should change directory to TF_MODELS/slim **before** running this notebook, so that these files are in your python path.To check you've got these two steps to work, just execute the cell below. If it complains about unknown modules, restart the notebook after moving to the TF-Slim models directory.
###Code
import matplotlib
%matplotlib inline
import matplotlib.pyplot as plt
import math
import numpy as np
import tensorflow as tf
import time
from datasets import dataset_utils
# Main slim library
slim = tf.contrib.slim
###Output
_____no_output_____
###Markdown
Creating your first neural network with TF-SlimBelow we give some code to create a simple multilayer perceptron (MLP) which can be usedfor regression problems. The model has 2 hidden layers.The output is a single node. When this function is called, it will create various nodes, and silently add them to whichever global TF graph is currently in scope. When a node which corresponds to a layer with adjustable parameters (eg., a fully connected layer) is created, additional parameter variable nodes are silently created, and added to the graph. (We will discuss how to train the parameters later.)We use variable scope to put all the nodes under a common name,so that the graph has some hierarchical structure.This is useful when we want to visualize the TF graph in tensorboard, or if we want to query relatedvariables. The fully connected layers all use the same L2 weight decay and ReLu activations, as specified by **arg_scope**. (However, the final layer overrides these defaults, and uses an identity activation function.)We also illustrate how to add a dropout layer after the first fully connected layer (FC1). Note that at test time, we do not drop out nodes, but instead use the average activations; hence we need to know whether the model is beingconstructed for training or testing, since the computational graph will be different in the two cases(although the variables, storing the model parameters, will be shared, since they have the same name/scope).
###Code
def regression_model(inputs, is_training=True, scope="deep_regression"):
"""Creates the regression model.
Args:
inputs: A node that yields a `Tensor` of size [batch_size, dimensions].
is_training: Whether or not we're currently training the model.
scope: An optional variable_op scope for the model.
Returns:
predictions: 1-D `Tensor` of shape [batch_size] of responses.
end_points: A dict of end points representing the hidden layers.
"""
with tf.variable_scope(scope, 'deep_regression', [inputs]):
end_points = {}
# Set the default weight _regularizer and acvitation for each fully_connected layer.
with slim.arg_scope([slim.fully_connected],
activation_fn=tf.nn.relu,
weights_regularizer=slim.l2_regularizer(0.01)):
# Creates a fully connected layer from the inputs with 32 hidden units.
net = slim.fully_connected(inputs, 32, scope='fc1')
end_points['fc1'] = net
# Adds a dropout layer to prevent over-fitting.
net = slim.dropout(net, 0.8, is_training=is_training)
# Adds another fully connected layer with 16 hidden units.
net = slim.fully_connected(net, 16, scope='fc2')
end_points['fc2'] = net
# Creates a fully-connected layer with a single hidden unit. Note that the
# layer is made linear by setting activation_fn=None.
predictions = slim.fully_connected(net, 1, activation_fn=None, scope='prediction')
end_points['out'] = predictions
return predictions, end_points
###Output
_____no_output_____
###Markdown
Let's create the model and examine its structure.We create a TF graph and call regression_model(), which adds nodes (tensors) to the graph. We then examine their shape, and print the names of all the model variables which have been implicitly created inside of each layer. We see that the names of the variables follow the scopes that we specified.
###Code
with tf.Graph().as_default():
# Dummy placeholders for arbitrary number of 1d inputs and outputs
inputs = tf.placeholder(tf.float32, shape=(None, 1))
outputs = tf.placeholder(tf.float32, shape=(None, 1))
# Build model
predictions, end_points = regression_model(inputs)
# Print name and shape of each tensor.
print "Layers"
for k, v in end_points.iteritems():
print 'name = {}, shape = {}'.format(v.name, v.get_shape())
# Print name and shape of parameter nodes (values not yet initialized)
print "\n"
print "Parameters"
for v in slim.get_model_variables():
print 'name = {}, shape = {}'.format(v.name, v.get_shape())
###Output
_____no_output_____
###Markdown
Let's create some 1d regression data .We will train and test the model on some noisy observations of a nonlinear function.
###Code
def produce_batch(batch_size, noise=0.3):
xs = np.random.random(size=[batch_size, 1]) * 10
ys = np.sin(xs) + 5 + np.random.normal(size=[batch_size, 1], scale=noise)
return [xs.astype(np.float32), ys.astype(np.float32)]
x_train, y_train = produce_batch(200)
x_test, y_test = produce_batch(200)
plt.scatter(x_train, y_train)
###Output
_____no_output_____
###Markdown
Let's fit the model to the dataThe user has to specify the loss function and the optimizer, and slim does the rest.In particular, the slim.learning.train function does the following:- For each iteration, evaluate the train_op, which updates the parameters using the optimizer applied to the current minibatch. Also, update the global_step.- Occasionally store the model checkpoint in the specified directory. This is useful in case your machine crashes - then you can simply restart from the specified checkpoint.
###Code
def convert_data_to_tensors(x, y):
inputs = tf.constant(x)
inputs.set_shape([None, 1])
outputs = tf.constant(y)
outputs.set_shape([None, 1])
return inputs, outputs
# The following snippet trains the regression model using a sum_of_squares loss.
ckpt_dir = '/tmp/regression_model/'
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
inputs, targets = convert_data_to_tensors(x_train, y_train)
# Make the model.
predictions, nodes = regression_model(inputs, is_training=True)
# Add the loss function to the graph.
loss = slim.losses.sum_of_squares(predictions, targets)
# The total loss is the uers's loss plus any regularization losses.
total_loss = slim.losses.get_total_loss()
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.005)
train_op = slim.learning.create_train_op(total_loss, optimizer)
# Run the training inside a session.
final_loss = slim.learning.train(
train_op,
logdir=ckpt_dir,
number_of_steps=5000,
save_summaries_secs=5,
log_every_n_steps=500)
print("Finished training. Last batch loss:", final_loss)
print("Checkpoint saved in %s" % ckpt_dir)
###Output
_____no_output_____
###Markdown
Training with multiple loss functions.Sometimes we have multiple objectives we want to simultaneously optimize.In slim, it is easy to add more losses, as we show below. (We do not optimize the total loss in this example,but we show how to compute it.)
###Code
with tf.Graph().as_default():
inputs, targets = convert_data_to_tensors(x_train, y_train)
predictions, end_points = regression_model(inputs, is_training=True)
# Add multiple loss nodes.
sum_of_squares_loss = slim.losses.sum_of_squares(predictions, targets)
absolute_difference_loss = slim.losses.absolute_difference(predictions, targets)
# The following two ways to compute the total loss are equivalent
regularization_loss = tf.add_n(slim.losses.get_regularization_losses())
total_loss1 = sum_of_squares_loss + absolute_difference_loss + regularization_loss
# Regularization Loss is included in the total loss by default.
# This is good for training, but not for testing.
total_loss2 = slim.losses.get_total_loss(add_regularization_losses=True)
init_op = tf.global_variables_initializer()
with tf.Session() as sess:
sess.run(init_op) # Will initialize the parameters with random weights.
total_loss1, total_loss2 = sess.run([total_loss1, total_loss2])
print('Total Loss1: %f' % total_loss1)
print('Total Loss2: %f' % total_loss2)
print('Regularization Losses:')
for loss in slim.losses.get_regularization_losses():
print(loss)
print('Loss Functions:')
for loss in slim.losses.get_losses():
print(loss)
###Output
_____no_output_____
###Markdown
Let's load the saved model and use it for prediction.
###Code
with tf.Graph().as_default():
inputs, targets = convert_data_to_tensors(x_test, y_test)
# Create the model structure. (Parameters will be loaded below.)
predictions, end_points = regression_model(inputs, is_training=False)
# Make a session which restores the old parameters from a checkpoint.
sv = tf.train.Supervisor(logdir=ckpt_dir)
with sv.managed_session() as sess:
inputs, predictions, targets = sess.run([inputs, predictions, targets])
plt.scatter(inputs, targets, c='r');
plt.scatter(inputs, predictions, c='b');
plt.title('red=true, blue=predicted')
###Output
_____no_output_____
###Markdown
Let's compute various evaluation metrics on the test set.In TF-Slim termiology, losses are optimized, but metrics (which may not be differentiable, e.g., precision and recall) are just measured. As an illustration, the code below computes mean squared error and mean absolute error metrics on the test set.Each metric declaration creates several local variables (which must be initialized via tf.initialize_local_variables()) and returns both a value_op and an update_op. When evaluated, the value_op returns the current value of the metric. The update_op loads a new batch of data, runs the model, obtains the predictions and accumulates the metric statistics appropriately before returning the current value of the metric. We store these value nodes and update nodes in 2 dictionaries.After creating the metric nodes, we can pass them to slim.evaluation.evaluation, which repeatedly evaluates these nodes the specified number of times. (This allows us to compute the evaluation in a streaming fashion across minibatches, which is usefulf for large datasets.) Finally, we print the final value of each metric.
###Code
with tf.Graph().as_default():
inputs, targets = convert_data_to_tensors(x_test, y_test)
predictions, end_points = regression_model(inputs, is_training=False)
# Specify metrics to evaluate:
names_to_value_nodes, names_to_update_nodes = slim.metrics.aggregate_metric_map({
'Mean Squared Error': slim.metrics.streaming_mean_squared_error(predictions, targets),
'Mean Absolute Error': slim.metrics.streaming_mean_absolute_error(predictions, targets)
})
# Make a session which restores the old graph parameters, and then run eval.
sv = tf.train.Supervisor(logdir=ckpt_dir)
with sv.managed_session() as sess:
metric_values = slim.evaluation.evaluation(
sess,
num_evals=1, # Single pass over data
eval_op=names_to_update_nodes.values(),
final_op=names_to_value_nodes.values())
names_to_values = dict(zip(names_to_value_nodes.keys(), metric_values))
for key, value in names_to_values.iteritems():
print('%s: %f' % (key, value))
###Output
_____no_output_____
###Markdown
Reading Data with TF-SlimReading data with TF-Slim has two main components: A[Dataset](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset.py) and a [DatasetDataProvider](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset_data_provider.py). The former is a descriptor of a dataset, while the latter performs the actions necessary for actually reading the data. Lets look at each one in detail: DatasetA TF-Slim[Dataset](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset.py)contains descriptive information about a dataset necessary for reading it, such as the list of data files and how to decode them. It also contains metadata including class labels, the size of the train/test splits and descriptions of the tensors that the dataset provides. For example, some datasets contain images with labels. Others augment this data with bounding box annotations, etc. The Dataset object allows us to write generic code using the same API, regardless of the data content and encoding type.TF-Slim's Dataset works especially well when the data is stored as a (possibly sharded)[TFRecords file](https://www.tensorflow.org/versions/r0.10/how_tos/reading_data/index.htmlfile-formats), where each record contains a [tf.train.Example protocol buffer](https://github.com/tensorflow/tensorflow/blob/r0.10/tensorflow/core/example/example.proto).TF-Slim uses a consistent convention for naming the keys and values inside each Example record. DatasetDataProviderA[DatasetDataProvider](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset_data_provider.py) is a class which actually reads the data from a dataset. It is highly configurable to read the data in various ways that may make a big impact on the efficiency of your training process. For example, it can be single or multi-threaded. If your data is sharded across many files, it can read each files serially, or from every file simultaneously. Demo: The Flowers DatasetFor convenience, we've include scripts to convert several common image datasets into TFRecord format and have providedthe Dataset descriptor files necessary for reading them. We demonstrate how easy it is to use these dataset via the Flowers dataset below. Download the Flowers DatasetWe've made available a tarball of the Flowers dataset which has already been converted to TFRecord format.
###Code
import tensorflow as tf
from datasets import dataset_utils
url = "http://download.tensorflow.org/data/flowers.tar.gz"
flowers_data_dir = '/tmp/flowers'
if not tf.gfile.Exists(flowers_data_dir):
tf.gfile.MakeDirs(flowers_data_dir)
dataset_utils.download_and_uncompress_tarball(url, flowers_data_dir)
###Output
_____no_output_____
###Markdown
Display some of the data.
###Code
from datasets import flowers
import tensorflow as tf
slim = tf.contrib.slim
with tf.Graph().as_default():
dataset = flowers.get_split('train', flowers_data_dir)
data_provider = slim.dataset_data_provider.DatasetDataProvider(
dataset, common_queue_capacity=32, common_queue_min=1)
image, label = data_provider.get(['image', 'label'])
with tf.Session() as sess:
with slim.queues.QueueRunners(sess):
for i in xrange(4):
np_image, np_label = sess.run([image, label])
height, width, _ = np_image.shape
class_name = name = dataset.labels_to_names[np_label]
plt.figure()
plt.imshow(np_image)
plt.title('%s, %d x %d' % (name, height, width))
plt.axis('off')
plt.show()
###Output
_____no_output_____
###Markdown
Convolutional neural nets (CNNs).In this section, we show how to train an image classifier using a simple CNN. Define the model.Below we define a simple CNN. Note that the output layer is linear function - we will apply softmax transformation externally to the model, either in the loss function (for training), or in the prediction function (during testing).
###Code
def my_cnn(images, num_classes, is_training): # is_training is not used...
with slim.arg_scope([slim.max_pool2d], kernel_size=[3, 3], stride=2):
net = slim.conv2d(images, 64, [5, 5])
net = slim.max_pool2d(net)
net = slim.conv2d(net, 64, [5, 5])
net = slim.max_pool2d(net)
net = slim.flatten(net)
net = slim.fully_connected(net, 192)
net = slim.fully_connected(net, num_classes, activation_fn=None)
return net
###Output
_____no_output_____
###Markdown
Apply the model to some randomly generated images.
###Code
import tensorflow as tf
with tf.Graph().as_default():
# The model can handle any input size because the first layer is convolutional.
# The size of the model is determined when image_node is first passed into the my_cnn function.
# Once the variables are initialized, the size of all the weight matrices is fixed.
# Because of the fully connected layers, this means that all subsequent images must have the same
# input size as the first image.
batch_size, height, width, channels = 3, 28, 28, 3
images = tf.random_uniform([batch_size, height, width, channels], maxval=1)
# Create the model.
num_classes = 10
logits = my_cnn(images, num_classes, is_training=True)
probabilities = tf.nn.softmax(logits)
# Initialize all the variables (including parameters) randomly.
init_op = tf.global_variables_initializer()
with tf.Session() as sess:
# Run the init_op, evaluate the model outputs and print the results:
sess.run(init_op)
probabilities = sess.run(probabilities)
print('Probabilities Shape:')
print(probabilities.shape) # batch_size x num_classes
print('\nProbabilities:')
print(probabilities)
print('\nSumming across all classes (Should equal 1):')
print(np.sum(probabilities, 1)) # Each row sums to 1
###Output
_____no_output_____
###Markdown
Train the model on the Flowers dataset.Before starting, make sure you've run the code to Download the Flowers dataset. Now, we'll get a sense of what it looks like to use TF-Slim's training functions found in[learning.py](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/learning.py). First, we'll create a function, `load_batch`, that loads batches of dataset from a dataset. Next, we'll train a model for a single step (just to demonstrate the API), and evaluate the results.
###Code
from preprocessing import inception_preprocessing
import tensorflow as tf
slim = tf.contrib.slim
def load_batch(dataset, batch_size=32, height=299, width=299, is_training=False):
"""Loads a single batch of data.
Args:
dataset: The dataset to load.
batch_size: The number of images in the batch.
height: The size of each image after preprocessing.
width: The size of each image after preprocessing.
is_training: Whether or not we're currently training or evaluating.
Returns:
images: A Tensor of size [batch_size, height, width, 3], image samples that have been preprocessed.
images_raw: A Tensor of size [batch_size, height, width, 3], image samples that can be used for visualization.
labels: A Tensor of size [batch_size], whose values range between 0 and dataset.num_classes.
"""
data_provider = slim.dataset_data_provider.DatasetDataProvider(
dataset, common_queue_capacity=32,
common_queue_min=8)
image_raw, label = data_provider.get(['image', 'label'])
# Preprocess image for usage by Inception.
image = inception_preprocessing.preprocess_image(image_raw, height, width, is_training=is_training)
# Preprocess the image for display purposes.
image_raw = tf.expand_dims(image_raw, 0)
image_raw = tf.image.resize_images(image_raw, [height, width])
image_raw = tf.squeeze(image_raw)
# Batch it up.
images, images_raw, labels = tf.train.batch(
[image, image_raw, label],
batch_size=batch_size,
num_threads=1,
capacity=2 * batch_size)
return images, images_raw, labels
from datasets import flowers
# This might take a few minutes.
train_dir = '/tmp/tfslim_model/'
print('Will save model to %s' % train_dir)
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset)
# Create the model:
logits = my_cnn(images, num_classes=dataset.num_classes, is_training=True)
# Specify the loss function:
one_hot_labels = slim.one_hot_encoding(labels, dataset.num_classes)
slim.losses.softmax_cross_entropy(logits, one_hot_labels)
total_loss = slim.losses.get_total_loss()
# Create some summaries to visualize the training process:
tf.scalar_summary('losses/Total Loss', total_loss)
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.01)
train_op = slim.learning.create_train_op(total_loss, optimizer)
# Run the training:
final_loss = slim.learning.train(
train_op,
logdir=train_dir,
number_of_steps=1, # For speed, we just do 1 epoch
save_summaries_secs=1)
print('Finished training. Final batch loss %d' % final_loss)
###Output
_____no_output_____
###Markdown
Evaluate some metrics.As we discussed above, we can compute various metrics besides the loss.Below we show how to compute prediction accuracy of the trained model, as well as top-5 classification accuracy. (The difference between evaluation and evaluation_loop is that the latter writes the results to a log directory, so they can be viewed in tensorboard.)
###Code
from datasets import flowers
# This might take a few minutes.
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.DEBUG)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset)
logits = my_cnn(images, num_classes=dataset.num_classes, is_training=False)
predictions = tf.argmax(logits, 1)
# Define the metrics:
names_to_values, names_to_updates = slim.metrics.aggregate_metric_map({
'eval/Accuracy': slim.metrics.streaming_accuracy(predictions, labels),
'eval/Recall@5': slim.metrics.streaming_recall_at_k(logits, labels, 5),
})
print('Running evaluation Loop...')
checkpoint_path = tf.train.latest_checkpoint(train_dir)
metric_values = slim.evaluation.evaluate_once(
master='',
checkpoint_path=checkpoint_path,
logdir=train_dir,
eval_op=names_to_updates.values(),
final_op=names_to_values.values())
names_to_values = dict(zip(names_to_values.keys(), metric_values))
for name in names_to_values:
print('%s: %f' % (name, names_to_values[name]))
###Output
_____no_output_____
###Markdown
Using pre-trained modelsNeural nets work best when they have many parameters, making them very flexible function approximators.However, this means they must be trained on big datasets. Since this process is slow, we provide various pre-trained models - see the list [here](https://github.com/tensorflow/models/tree/master/slimpre-trained-models).You can either use these models as-is, or you can perform "surgery" on them, to modify them for some other task. For example, it is common to "chop off" the final pre-softmax layer, and replace it with a new set of weights corresponding to some new set of labels. You can then quickly fine tune the new model on a small new dataset. We illustrate this below, using inception-v1 as the base model. While models like Inception V3 are more powerful, Inception V1 is used for speed purposes.Take into account that VGG and ResNet final layers have only 1000 outputs rather than 1001. The ImageNet dataset provied has an empty background class which can be used to fine-tune the model to other tasks. VGG and ResNet models provided here don't use that class. We provide two examples of using pretrained models: Inception V1 and VGG-19 models to highlight this difference. Download the Inception V1 checkpoint
###Code
from datasets import dataset_utils
url = "http://download.tensorflow.org/models/inception_v1_2016_08_28.tar.gz"
checkpoints_dir = '/tmp/checkpoints'
if not tf.gfile.Exists(checkpoints_dir):
tf.gfile.MakeDirs(checkpoints_dir)
dataset_utils.download_and_uncompress_tarball(url, checkpoints_dir)
###Output
_____no_output_____
###Markdown
Apply Pre-trained Inception V1 model to Images.We have to convert each image to the size expected by the model checkpoint.There is no easy way to determine this size from the checkpoint itself.So we use a preprocessor to enforce this.
###Code
import numpy as np
import os
import tensorflow as tf
import urllib2
from datasets import imagenet
from nets import inception
from preprocessing import inception_preprocessing
slim = tf.contrib.slim
image_size = inception.inception_v1.default_image_size
with tf.Graph().as_default():
url = 'https://upload.wikimedia.org/wikipedia/commons/7/70/EnglishCockerSpaniel_simon.jpg'
image_string = urllib2.urlopen(url).read()
image = tf.image.decode_jpeg(image_string, channels=3)
processed_image = inception_preprocessing.preprocess_image(image, image_size, image_size, is_training=False)
processed_images = tf.expand_dims(processed_image, 0)
# Create the model, use the default arg scope to configure the batch norm parameters.
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(processed_images, num_classes=1001, is_training=False)
probabilities = tf.nn.softmax(logits)
init_fn = slim.assign_from_checkpoint_fn(
os.path.join(checkpoints_dir, 'inception_v1.ckpt'),
slim.get_model_variables('InceptionV1'))
with tf.Session() as sess:
init_fn(sess)
np_image, probabilities = sess.run([image, probabilities])
probabilities = probabilities[0, 0:]
sorted_inds = [i[0] for i in sorted(enumerate(-probabilities), key=lambda x:x[1])]
plt.figure()
plt.imshow(np_image.astype(np.uint8))
plt.axis('off')
plt.show()
names = imagenet.create_readable_names_for_imagenet_labels()
for i in range(5):
index = sorted_inds[i]
print('Probability %0.2f%% => [%s]' % (probabilities[index], names[index]))
###Output
_____no_output_____
###Markdown
Download the VGG-16 checkpoint
###Code
from datasets import dataset_utils
import tensorflow as tf
url = "http://download.tensorflow.org/models/vgg_16_2016_08_28.tar.gz"
checkpoints_dir = '/tmp/checkpoints'
if not tf.gfile.Exists(checkpoints_dir):
tf.gfile.MakeDirs(checkpoints_dir)
dataset_utils.download_and_uncompress_tarball(url, checkpoints_dir)
###Output
_____no_output_____
###Markdown
Apply Pre-trained VGG-16 model to Images.We have to convert each image to the size expected by the model checkpoint.There is no easy way to determine this size from the checkpoint itself.So we use a preprocessor to enforce this. Pay attention to the difference caused by 1000 classes instead of 1001.
###Code
import numpy as np
import os
import tensorflow as tf
import urllib2
from datasets import imagenet
from nets import vgg
from preprocessing import vgg_preprocessing
slim = tf.contrib.slim
image_size = vgg.vgg_16.default_image_size
with tf.Graph().as_default():
url = 'https://upload.wikimedia.org/wikipedia/commons/d/d9/First_Student_IC_school_bus_202076.jpg'
image_string = urllib2.urlopen(url).read()
image = tf.image.decode_jpeg(image_string, channels=3)
processed_image = vgg_preprocessing.preprocess_image(image, image_size, image_size, is_training=False)
processed_images = tf.expand_dims(processed_image, 0)
# Create the model, use the default arg scope to configure the batch norm parameters.
with slim.arg_scope(vgg.vgg_arg_scope()):
# 1000 classes instead of 1001.
logits, _ = vgg.vgg_16(processed_images, num_classes=1000, is_training=False)
probabilities = tf.nn.softmax(logits)
init_fn = slim.assign_from_checkpoint_fn(
os.path.join(checkpoints_dir, 'vgg_16.ckpt'),
slim.get_model_variables('vgg_16'))
with tf.Session() as sess:
init_fn(sess)
np_image, probabilities = sess.run([image, probabilities])
probabilities = probabilities[0, 0:]
sorted_inds = [i[0] for i in sorted(enumerate(-probabilities), key=lambda x:x[1])]
plt.figure()
plt.imshow(np_image.astype(np.uint8))
plt.axis('off')
plt.show()
names = imagenet.create_readable_names_for_imagenet_labels()
for i in range(5):
index = sorted_inds[i]
# Shift the index of a class name by one.
print('Probability %0.2f%% => [%s]' % (probabilities[index], names[index+1]))
###Output
_____no_output_____
###Markdown
Fine-tune the model on a different set of labels.We will fine tune the inception model on the Flowers dataset.
###Code
# Note that this may take several minutes.
import os
from datasets import flowers
from nets import inception
from preprocessing import inception_preprocessing
slim = tf.contrib.slim
image_size = inception.inception_v1.default_image_size
def get_init_fn():
"""Returns a function run by the chief worker to warm-start the training."""
checkpoint_exclude_scopes=["InceptionV1/Logits", "InceptionV1/AuxLogits"]
exclusions = [scope.strip() for scope in checkpoint_exclude_scopes]
variables_to_restore = []
for var in slim.get_model_variables():
excluded = False
for exclusion in exclusions:
if var.op.name.startswith(exclusion):
excluded = True
break
if not excluded:
variables_to_restore.append(var)
return slim.assign_from_checkpoint_fn(
os.path.join(checkpoints_dir, 'inception_v1.ckpt'),
variables_to_restore)
train_dir = '/tmp/inception_finetuned/'
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset, height=image_size, width=image_size)
# Create the model, use the default arg scope to configure the batch norm parameters.
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(images, num_classes=dataset.num_classes, is_training=True)
# Specify the loss function:
one_hot_labels = slim.one_hot_encoding(labels, dataset.num_classes)
slim.losses.softmax_cross_entropy(logits, one_hot_labels)
total_loss = slim.losses.get_total_loss()
# Create some summaries to visualize the training process:
tf.scalar_summary('losses/Total Loss', total_loss)
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.01)
train_op = slim.learning.create_train_op(total_loss, optimizer)
# Run the training:
final_loss = slim.learning.train(
train_op,
logdir=train_dir,
init_fn=get_init_fn(),
number_of_steps=2)
print('Finished training. Last batch loss %f' % final_loss)
###Output
_____no_output_____
###Markdown
Apply fine tuned model to some images.
###Code
import numpy as np
import tensorflow as tf
from datasets import flowers
from nets import inception
slim = tf.contrib.slim
image_size = inception.inception_v1.default_image_size
batch_size = 3
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, images_raw, labels = load_batch(dataset, height=image_size, width=image_size)
# Create the model, use the default arg scope to configure the batch norm parameters.
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(images, num_classes=dataset.num_classes, is_training=True)
probabilities = tf.nn.softmax(logits)
checkpoint_path = tf.train.latest_checkpoint(train_dir)
init_fn = slim.assign_from_checkpoint_fn(
checkpoint_path,
slim.get_variables_to_restore())
with tf.Session() as sess:
with slim.queues.QueueRunners(sess):
sess.run(tf.initialize_local_variables())
init_fn(sess)
np_probabilities, np_images_raw, np_labels = sess.run([probabilities, images_raw, labels])
for i in xrange(batch_size):
image = np_images_raw[i, :, :, :]
true_label = np_labels[i]
predicted_label = np.argmax(np_probabilities[i, :])
predicted_name = dataset.labels_to_names[predicted_label]
true_name = dataset.labels_to_names[true_label]
plt.figure()
plt.imshow(image.astype(np.uint8))
plt.title('Ground Truth: [%s], Prediction [%s]' % (true_name, predicted_name))
plt.axis('off')
plt.show()
###Output
_____no_output_____
###Markdown
TF-Slim WalkthroughThis notebook will walk you through the basics of using TF-Slim to define, train and evaluate neural networks on various tasks. It assumes a basic knowledge of neural networks. Table of contentsInstallation and setupCreating your first neural network with TF-SlimReading Data with TF-SlimTraining a convolutional neural network (CNN)Using pre-trained models Installation and setupAs of 8/28/16, the latest stable release of TF is r0.10, which does not contain the latest version of slim.To obtain the latest version of TF-Slim, please install the most recent nightly build of TFas explained [here](https://github.com/tensorflow/models/tree/master/sliminstalling-latest-version-of-tf-slim).To use TF-Slim for image classification (as we do in this notebook), you also have to install the TF-Slim image models library from [here](https://github.com/tensorflow/models/tree/master/slim). Let's suppose you install this into a directory called TF_MODELS. Then you should change directory to TF_MODELS/slim **before** running this notebook, so that these files are in your python path.To check you've got these two steps to work, just execute the cell below. If it complains about unknown modules, restart the notebook after moving to the TF-Slim models directory.
###Code
from __future__ import print_function
import matplotlib
%matplotlib inline
import matplotlib.pyplot as plt
import math
import numpy as np
import os
import tensorflow as tf
import time
from datasets import dataset_utils
# Main slim library
slim = tf.contrib.slim
from six.moves import xrange
from six import iteritems
###Output
_____no_output_____
###Markdown
Creating your first neural network with TF-SlimBelow we give some code to create a simple multilayer perceptron (MLP) which can be usedfor regression problems. The model has 2 hidden layers.The output is a single node. When this function is called, it will create various nodes, and silently add them to whichever global TF graph is currently in scope. When a node which corresponds to a layer with adjustable parameters (eg., a fully connected layer) is created, additional parameter variable nodes are silently created, and added to the graph. (We will discuss how to train the parameters later.)We use variable scope to put all the nodes under a common name,so that the graph has some hierarchical structure.This is useful when we want to visualize the TF graph in tensorboard, or if we want to query relatedvariables. The fully connected layers all use the same L2 weight decay and ReLu activations, as specified by **arg_scope**. (However, the final layer overrides these defaults, and uses an identity activation function.)We also illustrate how to add a dropout layer after the first fully connected layer (FC1). Note that at test time, we do not drop out nodes, but instead use the average activations; hence we need to know whether the model is beingconstructed for training or testing, since the computational graph will be different in the two cases(although the variables, storing the model parameters, will be shared, since they have the same name/scope).
###Code
def regression_model(inputs, is_training=True, scope="deep_regression"):
"""Creates the regression model.
Args:
inputs: A node that yields a `Tensor` of size [batch_size, dimensions].
is_training: Whether or not we're currently training the model.
scope: An optional variable_op scope for the model.
Returns:
predictions: 1-D `Tensor` of shape [batch_size] of responses.
end_points: A dict of end points representing the hidden layers.
"""
with tf.variable_scope(scope, 'deep_regression', [inputs]):
end_points = {}
# Set the default weight _regularizer and acvitation for each fully_connected layer.
with slim.arg_scope([slim.fully_connected],
activation_fn=tf.nn.relu,
weights_regularizer=slim.l2_regularizer(0.01)):
# Creates a fully connected layer from the inputs with 32 hidden units.
net = slim.fully_connected(inputs, 32, scope='fc1')
end_points['fc1'] = net
# Adds a dropout layer to prevent over-fitting.
net = slim.dropout(net, 0.8, is_training=is_training)
# Adds another fully connected layer with 16 hidden units.
net = slim.fully_connected(net, 16, scope='fc2')
end_points['fc2'] = net
# Creates a fully-connected layer with a single hidden unit. Note that the
# layer is made linear by setting activation_fn=None.
predictions = slim.fully_connected(net, 1, activation_fn=None, scope='prediction')
end_points['out'] = predictions
return predictions, end_points
###Output
_____no_output_____
###Markdown
Let's create the model and examine its structure.We create a TF graph and call regression_model(), which adds nodes (tensors) to the graph. We then examine their shape, and print the names of all the model variables which have been implicitly created inside of each layer. We see that the names of the variables follow the scopes that we specified.
###Code
with tf.Graph().as_default():
# Dummy placeholders for arbitrary number of 1d inputs and outputs
inputs = tf.placeholder(tf.float32, shape=(None, 1))
outputs = tf.placeholder(tf.float32, shape=(None, 1))
# Build model
predictions, end_points = regression_model(inputs)
# Print name and shape of each tensor.
print("Layers")
for k, v in iteritems(end_points):
print('name = {}, shape = {}'.format(v.name, v.get_shape()))
# Print name and shape of parameter nodes (values not yet initialized)
print("\n")
print("Parameters")
for v in slim.get_model_variables():
print('name = {}, shape = {}'.format(v.name, v.get_shape()))
###Output
_____no_output_____
###Markdown
Let's create some 1d regression data .We will train and test the model on some noisy observations of a nonlinear function.
###Code
def produce_batch(batch_size, noise=0.3):
xs = np.random.random(size=[batch_size, 1]) * 10
ys = np.sin(xs) + 5 + np.random.normal(size=[batch_size, 1], scale=noise)
return [xs.astype(np.float32), ys.astype(np.float32)]
x_train, y_train = produce_batch(200)
x_test, y_test = produce_batch(200)
plt.scatter(x_train, y_train)
###Output
_____no_output_____
###Markdown
Let's fit the model to the dataThe user has to specify the loss function and the optimizer, and slim does the rest.In particular, the slim.learning.train function does the following:- For each iteration, evaluate the train_op, which updates the parameters using the optimizer applied to the current minibatch. Also, update the global_step.- Occasionally store the model checkpoint in the specified directory. This is useful in case your machine crashes - then you can simply restart from the specified checkpoint.
###Code
def convert_data_to_tensors(x, y):
inputs = tf.constant(x)
inputs.set_shape([None, 1])
outputs = tf.constant(y)
outputs.set_shape([None, 1])
return inputs, outputs
# The following snippet trains the regression model using a sum_of_squares loss.
ckpt_dir = '/tmp/regression_model/'
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
inputs, targets = convert_data_to_tensors(x_train, y_train)
# Make the model.
predictions, nodes = regression_model(inputs, is_training=True)
# Add the loss function to the graph.
loss = tf.losses.mean_squared_error(targets, predictions)
# The total loss is the uers's loss plus any regularization losses.
total_loss = slim.losses.get_total_loss()
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.005)
train_op = slim.learning.create_train_op(total_loss, optimizer)
# Run the training inside a session.
final_loss = slim.learning.train(
train_op,
logdir=ckpt_dir,
number_of_steps=5000,
save_summaries_secs=5,
log_every_n_steps=500)
print("Finished training. Last batch loss:", final_loss)
print("Checkpoint saved in %s" % ckpt_dir)
###Output
_____no_output_____
###Markdown
Training with multiple loss functions.Sometimes we have multiple objectives we want to simultaneously optimize.In slim, it is easy to add more losses, as we show below. (We do not optimize the total loss in this example,but we show how to compute it.)
###Code
with tf.Graph().as_default():
inputs, targets = convert_data_to_tensors(x_train, y_train)
predictions, end_points = regression_model(inputs, is_training=True)
# Add multiple loss nodes.
sum_of_squares_loss = tf.losses.mean_squared_error(targets, predictions)
absolute_difference_loss = slim.losses.absolute_difference(predictions, targets)
# The following two ways to compute the total loss are equivalent
regularization_loss = tf.add_n(slim.losses.get_regularization_losses())
total_loss1 = sum_of_squares_loss + absolute_difference_loss + regularization_loss
# Regularization Loss is included in the total loss by default.
# This is good for training, but not for testing.
total_loss2 = slim.losses.get_total_loss(add_regularization_losses=True)
init_op = tf.global_variables_initializer()
with tf.Session() as sess:
sess.run(init_op) # Will initialize the parameters with random weights.
total_loss1, total_loss2 = sess.run([total_loss1, total_loss2])
print('Total Loss1: %f' % total_loss1)
print('Total Loss2: %f' % total_loss2)
print('Regularization Losses:')
for loss in slim.losses.get_regularization_losses():
print(loss)
print('Loss Functions:')
for loss in slim.losses.get_losses():
print(loss)
###Output
_____no_output_____
###Markdown
Let's load the saved model and use it for prediction.
###Code
with tf.Graph().as_default():
inputs, targets = convert_data_to_tensors(x_test, y_test)
# Create the model structure. (Parameters will be loaded below.)
predictions, end_points = regression_model(inputs, is_training=False)
# Make a session which restores the old parameters from a checkpoint.
sv = tf.train.Supervisor(logdir=ckpt_dir)
with sv.managed_session() as sess:
inputs, predictions, targets = sess.run([inputs, predictions, targets])
plt.scatter(inputs, targets, c='r');
plt.scatter(inputs, predictions, c='b');
plt.title('red=true, blue=predicted')
###Output
_____no_output_____
###Markdown
Let's compute various evaluation metrics on the test set.In TF-Slim termiology, losses are optimized, but metrics (which may not be differentiable, e.g., precision and recall) are just measured. As an illustration, the code below computes mean squared error and mean absolute error metrics on the test set.Each metric declaration creates several local variables (which must be initialized via tf.initialize_local_variables()) and returns both a value_op and an update_op. When evaluated, the value_op returns the current value of the metric. The update_op loads a new batch of data, runs the model, obtains the predictions and accumulates the metric statistics appropriately before returning the current value of the metric. We store these value nodes and update nodes in 2 dictionaries.After creating the metric nodes, we can pass them to slim.evaluation.evaluation, which repeatedly evaluates these nodes the specified number of times. (This allows us to compute the evaluation in a streaming fashion across minibatches, which is usefulf for large datasets.) Finally, we print the final value of each metric.
###Code
# Updated with code from section "Evaluating a Checkpointed Model with Metrics"
# of https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/training/python/training/evaluation.py
with tf.Graph().as_default():
inputs, targets = convert_data_to_tensors(x_test, y_test)
predictions, end_points = regression_model(inputs, is_training=False)
# Specify metrics to evaluate:
names_to_value_nodes, names_to_update_nodes = slim.metrics.aggregate_metric_map({
'Mean Squared Error': slim.metrics.streaming_mean_squared_error(predictions, targets),
'Mean Absolute Error': slim.metrics.streaming_mean_absolute_error(predictions, targets)
})
metric_values = tf.contrib.training.evaluate_once(
os.path.join(ckpt_dir, 'model.ckpt'),
eval_ops=list(names_to_update_nodes.values()),
final_ops=list(names_to_value_nodes.values()),
hooks=[
tf.contrib.training.StopAfterNEvalsHook(1)
],)
names_to_values = dict(zip(names_to_value_nodes.keys(), metric_values))
for key, value in iteritems(names_to_values):
print('%s: %f' % (key, value))
###Output
_____no_output_____
###Markdown
Reading Data with TF-SlimReading data with TF-Slim has two main components: A[Dataset](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset.py) and a [DatasetDataProvider](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset_data_provider.py). The former is a descriptor of a dataset, while the latter performs the actions necessary for actually reading the data. Lets look at each one in detail: DatasetA TF-Slim[Dataset](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset.py)contains descriptive information about a dataset necessary for reading it, such as the list of data files and how to decode them. It also contains metadata including class labels, the size of the train/test splits and descriptions of the tensors that the dataset provides. For example, some datasets contain images with labels. Others augment this data with bounding box annotations, etc. The Dataset object allows us to write generic code using the same API, regardless of the data content and encoding type.TF-Slim's Dataset works especially well when the data is stored as a (possibly sharded)[TFRecords file](https://www.tensorflow.org/versions/r0.10/how_tos/reading_data/index.htmlfile-formats), where each record contains a [tf.train.Example protocol buffer](https://github.com/tensorflow/tensorflow/blob/r0.10/tensorflow/core/example/example.proto).TF-Slim uses a consistent convention for naming the keys and values inside each Example record. DatasetDataProviderA[DatasetDataProvider](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset_data_provider.py) is a class which actually reads the data from a dataset. It is highly configurable to read the data in various ways that may make a big impact on the efficiency of your training process. For example, it can be single or multi-threaded. If your data is sharded across many files, it can read each files serially, or from every file simultaneously. Demo: The Flowers DatasetFor convenience, we've include scripts to convert several common image datasets into TFRecord format and have providedthe Dataset descriptor files necessary for reading them. We demonstrate how easy it is to use these dataset via the Flowers dataset below. Download the Flowers DatasetWe've made available a tarball of the Flowers dataset which has already been converted to TFRecord format.
###Code
import tensorflow as tf
from datasets import dataset_utils
url = "http://download.tensorflow.org/data/flowers.tar.gz"
flowers_data_dir = '/tmp/flowers'
if not tf.gfile.Exists(flowers_data_dir):
tf.gfile.MakeDirs(flowers_data_dir)
dataset_utils.download_and_uncompress_tarball(url, flowers_data_dir)
###Output
_____no_output_____
###Markdown
Display some of the data.
###Code
from datasets import flowers
import tensorflow as tf
slim = tf.contrib.slim
with tf.Graph().as_default():
dataset = flowers.get_split('train', flowers_data_dir)
data_provider = slim.dataset_data_provider.DatasetDataProvider(
dataset, common_queue_capacity=32, common_queue_min=1)
image, label = data_provider.get(['image', 'label'])
with tf.Session() as sess:
with slim.queues.QueueRunners(sess):
for i in xrange(4):
np_image, np_label = sess.run([image, label])
height, width, _ = np_image.shape
class_name = name = dataset.labels_to_names[np_label]
plt.figure()
plt.imshow(np_image)
plt.title('%s, %d x %d' % (name, height, width))
plt.axis('off')
plt.show()
###Output
_____no_output_____
###Markdown
Convolutional neural nets (CNNs).In this section, we show how to train an image classifier using a simple CNN. Define the model.Below we define a simple CNN. Note that the output layer is linear function - we will apply softmax transformation externally to the model, either in the loss function (for training), or in the prediction function (during testing).
###Code
def my_cnn(images, num_classes, is_training): # is_training is not used...
with slim.arg_scope([slim.max_pool2d], kernel_size=[3, 3], stride=2):
net = slim.conv2d(images, 64, [5, 5])
net = slim.max_pool2d(net)
net = slim.conv2d(net, 64, [5, 5])
net = slim.max_pool2d(net)
net = slim.flatten(net)
net = slim.fully_connected(net, 192)
net = slim.fully_connected(net, num_classes, activation_fn=None)
return net
###Output
_____no_output_____
###Markdown
Apply the model to some randomly generated images.
###Code
import tensorflow as tf
with tf.Graph().as_default():
# The model can handle any input size because the first layer is convolutional.
# The size of the model is determined when image_node is first passed into the my_cnn function.
# Once the variables are initialized, the size of all the weight matrices is fixed.
# Because of the fully connected layers, this means that all subsequent images must have the same
# input size as the first image.
batch_size, height, width, channels = 3, 28, 28, 3
images = tf.random_uniform([batch_size, height, width, channels], maxval=1)
# Create the model.
num_classes = 10
logits = my_cnn(images, num_classes, is_training=True)
probabilities = tf.nn.softmax(logits)
# Initialize all the variables (including parameters) randomly.
init_op = tf.global_variables_initializer()
with tf.Session() as sess:
# Run the init_op, evaluate the model outputs and print the results:
sess.run(init_op)
probabilities = sess.run(probabilities)
print('Probabilities Shape:')
print(probabilities.shape) # batch_size x num_classes
print('\nProbabilities:')
print(probabilities)
print('\nSumming across all classes (Should equal 1):')
print(np.sum(probabilities, 1)) # Each row sums to 1
###Output
_____no_output_____
###Markdown
Train the model on the Flowers dataset.Before starting, make sure you've run the code to Download the Flowers dataset. Now, we'll get a sense of what it looks like to use TF-Slim's training functions found in[learning.py](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/learning.py). First, we'll create a function, `load_batch`, that loads batches of dataset from a dataset. Next, we'll train a model for a single step (just to demonstrate the API), and evaluate the results.
###Code
from preprocessing import inception_preprocessing
import tensorflow as tf
slim = tf.contrib.slim
def load_batch(dataset, batch_size=32, height=299, width=299, is_training=False):
"""Loads a single batch of data.
Args:
dataset: The dataset to load.
batch_size: The number of images in the batch.
height: The size of each image after preprocessing.
width: The size of each image after preprocessing.
is_training: Whether or not we're currently training or evaluating.
Returns:
images: A Tensor of size [batch_size, height, width, 3], image samples that have been preprocessed.
images_raw: A Tensor of size [batch_size, height, width, 3], image samples that can be used for visualization.
labels: A Tensor of size [batch_size], whose values range between 0 and dataset.num_classes.
"""
data_provider = slim.dataset_data_provider.DatasetDataProvider(
dataset, common_queue_capacity=32,
common_queue_min=8)
image_raw, label = data_provider.get(['image', 'label'])
# Preprocess image for usage by Inception.
image = inception_preprocessing.preprocess_image(image_raw, height, width, is_training=is_training)
# Preprocess the image for display purposes.
image_raw = tf.expand_dims(image_raw, 0)
image_raw = tf.image.resize_images(image_raw, [height, width])
image_raw = tf.squeeze(image_raw)
# Batch it up.
images, images_raw, labels = tf.train.batch(
[image, image_raw, label],
batch_size=batch_size,
num_threads=1,
capacity=2 * batch_size)
return images, images_raw, labels
from datasets import flowers
# This might take a few minutes.
train_dir = '/tmp/tfslim_model/'
print('Will save model to %s' % train_dir)
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset)
# Create the model:
logits = my_cnn(images, num_classes=dataset.num_classes, is_training=True)
# Specify the loss function:
one_hot_labels = slim.one_hot_encoding(labels, dataset.num_classes)
slim.losses.softmax_cross_entropy(logits, one_hot_labels)
total_loss = slim.losses.get_total_loss()
# Create some summaries to visualize the training process:
tf.summary.scalar('losses/Total Loss', total_loss)
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.01)
train_op = slim.learning.create_train_op(total_loss, optimizer)
# Run the training:
final_loss = slim.learning.train(
train_op,
logdir=train_dir,
number_of_steps=10, # For speed, we just do 1 epoch
save_summaries_secs=1)
print('Finished training. Final batch loss %d' % final_loss)
###Output
_____no_output_____
###Markdown
Evaluate some metrics.As we discussed above, we can compute various metrics besides the loss.Below we show how to compute prediction accuracy of the trained model, as well as top-5 classification accuracy. (The difference between evaluation and evaluation_loop is that the latter writes the results to a log directory, so they can be viewed in tensorboard.)
###Code
from datasets import flowers
# This might take a few minutes.
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.DEBUG)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset)
logits = my_cnn(images, num_classes=dataset.num_classes, is_training=False)
predictions = tf.argmax(logits, 1)
# Define the metrics:
names_to_values, names_to_updates = slim.metrics.aggregate_metric_map({
'eval/Accuracy': slim.metrics.streaming_accuracy(predictions, labels),
'eval/Recall@5': slim.metrics.streaming_recall_at_k(logits, labels, 5),
})
print('Running evaluation Loop...')
checkpoint_path = tf.train.latest_checkpoint(train_dir)
metric_values = slim.evaluation.evaluate_once(
master='',
checkpoint_path=checkpoint_path,
logdir=train_dir,
eval_op=list(names_to_updates.values()),
final_op=list(names_to_values.values()))
names_to_values = dict(zip(names_to_values.keys(), metric_values))
for name in names_to_values:
print('%s: %f' % (name, names_to_values[name]))
###Output
_____no_output_____
###Markdown
Using pre-trained modelsNeural nets work best when they have many parameters, making them very flexible function approximators.However, this means they must be trained on big datasets. Since this process is slow, we provide various pre-trained models - see the list [here](https://github.com/tensorflow/models/tree/master/slimpre-trained-models).You can either use these models as-is, or you can perform "surgery" on them, to modify them for some other task. For example, it is common to "chop off" the final pre-softmax layer, and replace it with a new set of weights corresponding to some new set of labels. You can then quickly fine tune the new model on a small new dataset. We illustrate this below, using inception-v1 as the base model. While models like Inception V3 are more powerful, Inception V1 is used for speed purposes. Download the Inception V1 checkpoint
###Code
from datasets import dataset_utils
url = "http://download.tensorflow.org/models/inception_v1_2016_08_28.tar.gz"
checkpoints_dir = '/tmp/checkpoints'
if not tf.gfile.Exists(checkpoints_dir):
tf.gfile.MakeDirs(checkpoints_dir)
dataset_utils.download_and_uncompress_tarball(url, checkpoints_dir)
###Output
_____no_output_____
###Markdown
Apply Pre-trained model to Images.We have to convert each image to the size expected by the model checkpoint.There is no easy way to determine this size from the checkpoint itself.So we use a preprocessor to enforce this.
###Code
import numpy as np
import tensorflow as tf
#import urllib2
from datasets import imagenet
from nets import inception
from preprocessing import inception_preprocessing
slim = tf.contrib.slim
batch_size = 3
image_size = inception.inception_v1.default_image_size
with tf.Graph().as_default():
#url = 'https://upload.wikimedia.org/wikipedia/commons/7/70/EnglishCockerSpaniel_simon.jpg'
#image_string = urllib2.urlopen(url).read()
image_string = open("/tmp/EnglishCockerSpaniel_simon.jpg", mode='rb').read()
image = tf.image.decode_jpeg(image_string, channels=3)
processed_image = inception_preprocessing.preprocess_image(image, image_size, image_size, is_training=False)
processed_images = tf.expand_dims(processed_image, 0)
# Create the model, use the default arg scope to configure the batch norm parameters.
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(processed_images, num_classes=1001, is_training=False)
probabilities = tf.nn.softmax(logits)
init_fn = slim.assign_from_checkpoint_fn(
os.path.join(checkpoints_dir, 'inception_v1.ckpt'),
slim.get_model_variables('InceptionV1'))
with tf.Session() as sess:
init_fn(sess)
np_image, probabilities = sess.run([image, probabilities])
probabilities = probabilities[0, 0:]
sorted_inds = [i[0] for i in sorted(enumerate(-probabilities), key=lambda x:x[1])]
plt.figure()
plt.imshow(np_image.astype(np.uint8))
plt.axis('off')
plt.show()
names = imagenet.create_readable_names_for_imagenet_labels()
for i in xrange(5):
index = sorted_inds[i]
print('Probability %0.2f%% => [%s]' % (probabilities[index]*100, names[index]))
###Output
_____no_output_____
###Markdown
Fine-tune the model on a different set of labels.We will fine tune the inception model on the Flowers dataset.
###Code
# Note that this may take several minutes.
import os
from datasets import flowers
from nets import inception
from preprocessing import inception_preprocessing
slim = tf.contrib.slim
image_size = inception.inception_v1.default_image_size
def get_init_fn():
"""Returns a function run by the chief worker to warm-start the training."""
checkpoint_exclude_scopes=["InceptionV1/Logits", "InceptionV1/AuxLogits"]
exclusions = [scope.strip() for scope in checkpoint_exclude_scopes]
variables_to_restore = []
for var in slim.get_model_variables():
excluded = False
for exclusion in exclusions:
if var.op.name.startswith(exclusion):
excluded = True
break
if not excluded:
variables_to_restore.append(var)
return slim.assign_from_checkpoint_fn(
os.path.join(checkpoints_dir, 'inception_v1.ckpt'),
variables_to_restore)
train_dir = '/tmp/inception_finetuned/'
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset, height=image_size, width=image_size)
# Create the model, use the default arg scope to configure the batch norm parameters.
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(images, num_classes=dataset.num_classes, is_training=True)
# Specify the loss function:
one_hot_labels = slim.one_hot_encoding(labels, dataset.num_classes)
slim.losses.softmax_cross_entropy(logits, one_hot_labels)
total_loss = slim.losses.get_total_loss()
# Create some summaries to visualize the training process:
tf.summary.scalar('losses/Total Loss', total_loss)
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.01)
train_op = slim.learning.create_train_op(total_loss, optimizer)
# Run the training:
final_loss = slim.learning.train(
train_op,
logdir=train_dir,
init_fn=get_init_fn(),
number_of_steps=100)
print('Finished training. Last batch loss %f' % final_loss)
###Output
_____no_output_____
###Markdown
Apply fine tuned model to some images.
###Code
import numpy as np
import tensorflow as tf
from datasets import flowers
from nets import inception
slim = tf.contrib.slim
image_size = inception.inception_v1.default_image_size
batch_size = 3
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, images_raw, labels = load_batch(dataset, height=image_size, width=image_size)
# Create the model, use the default arg scope to configure the batch norm parameters.
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(images, num_classes=dataset.num_classes, is_training=True)
probabilities = tf.nn.softmax(logits)
checkpoint_path = tf.train.latest_checkpoint(train_dir)
init_fn = slim.assign_from_checkpoint_fn(
checkpoint_path,
slim.get_variables_to_restore())
with tf.Session() as sess:
with slim.queues.QueueRunners(sess):
sess.run(tf.initialize_local_variables())
init_fn(sess)
np_probabilities, np_images_raw, np_labels = sess.run([probabilities, images_raw, labels])
for i in xrange(batch_size):
image = np_images_raw[i, :, :, :]
true_label = np_labels[i]
predicted_label = np.argmax(np_probabilities[i, :])
predicted_name = dataset.labels_to_names[predicted_label]
true_name = dataset.labels_to_names[true_label]
plt.figure()
plt.imshow(image.astype(np.uint8))
plt.title('Ground Truth: [%s], Prediction [%s]' % (true_name, predicted_name))
plt.axis('off')
plt.show()
###Output
_____no_output_____
###Markdown
TF-Slim WalkthroughThis notebook will walk you through the basics of using TF-Slim to define, train and evaluate neural networks on various tasks. It assumes a basic knowledge of neural networks. Table of contentsInstallation and setupCreating your first neural network with TF-SlimReading Data with TF-SlimTraining a convolutional neural network (CNN)Using pre-trained models Installation and setupAs of 8/28/16, the latest stable release of TF is r0.10, which does not contain the latest version of slim.To obtain the latest version of TF-Slim, please install the most recent nightly build of TFas explained [here](https://github.com/tensorflow/models/tree/master/sliminstalling-latest-version-of-tf-slim).To use TF-Slim for image classification (as we do in this notebook), you also have to install the TF-Slim image models library from [here](https://github.com/tensorflow/models/tree/master/slim). Let's suppose you install this into a directory called TF_MODELS. Then you should change directory to TF_MODELS/slim **before** running this notebook, so that these files are in your python path.To check you've got these two steps to work, just execute the cell below. If it complains about unknown modules, restart the notebook after moving to the TF-Slim models directory.
###Code
import matplotlib
%matplotlib inline
import matplotlib.pyplot as plt
import math
import numpy as np
import tensorflow as tf
import time
from datasets import dataset_utils
# Main slim library
slim = tf.contrib.slim
###Output
_____no_output_____
###Markdown
Creating your first neural network with TF-SlimBelow we give some code to create a simple multilayer perceptron (MLP) which can be usedfor regression problems. The model has 2 hidden layers.The output is a single node. When this function is called, it will create various nodes, and silently add them to whichever global TF graph is currently in scope. When a node which corresponds to a layer with adjustable parameters (eg., a fully connected layer) is created, additional parameter variable nodes are silently created, and added to the graph. (We will discuss how to train the parameters later.)We use variable scope to put all the nodes under a common name,so that the graph has some hierarchical structure.This is useful when we want to visualize the TF graph in tensorboard, or if we want to query relatedvariables. The fully connected layers all use the same L2 weight decay and ReLu activations, as specified by **arg_scope**. (However, the final layer overrides these defaults, and uses an identity activation function.)We also illustrate how to add a dropout layer after the first fully connected layer (FC1). Note that at test time, we do not drop out nodes, but instead use the average activations; hence we need to know whether the model is beingconstructed for training or testing, since the computational graph will be different in the two cases(although the variables, storing the model parameters, will be shared, since they have the same name/scope).
###Code
def regression_model(inputs, is_training=True, scope="deep_regression"):
"""Creates the regression model.
Args:
inputs: A node that yields a `Tensor` of size [batch_size, dimensions].
is_training: Whether or not we're currently training the model.
scope: An optional variable_op scope for the model.
Returns:
predictions: 1-D `Tensor` of shape [batch_size] of responses.
end_points: A dict of end points representing the hidden layers.
"""
with tf.variable_scope(scope, 'deep_regression', [inputs]):
end_points = {}
# Set the default weight _regularizer and acvitation for each fully_connected layer.
with slim.arg_scope([slim.fully_connected],
activation_fn=tf.nn.relu,
weights_regularizer=slim.l2_regularizer(0.01)):
# Creates a fully connected layer from the inputs with 32 hidden units.
net = slim.fully_connected(inputs, 32, scope='fc1')
end_points['fc1'] = net
# Adds a dropout layer to prevent over-fitting.
net = slim.dropout(net, 0.8, is_training=is_training)
# Adds another fully connected layer with 16 hidden units.
net = slim.fully_connected(net, 16, scope='fc2')
end_points['fc2'] = net
# Creates a fully-connected layer with a single hidden unit. Note that the
# layer is made linear by setting activation_fn=None.
predictions = slim.fully_connected(net, 1, activation_fn=None, scope='prediction')
end_points['out'] = predictions
return predictions, end_points
###Output
_____no_output_____
###Markdown
Let's create the model and examine its structure.We create a TF graph and call regression_model(), which adds nodes (tensors) to the graph. We then examine their shape, and print the names of all the model variables which have been implicitly created inside of each layer. We see that the names of the variables follow the scopes that we specified.
###Code
with tf.Graph().as_default():
# Dummy placeholders for arbitrary number of 1d inputs and outputs
inputs = tf.placeholder(tf.float32, shape=(None, 1))
outputs = tf.placeholder(tf.float32, shape=(None, 1))
# Build model
predictions, end_points = regression_model(inputs)
# Print name and shape of each tensor.
print "Layers"
for k, v in end_points.iteritems():
print 'name = {}, shape = {}'.format(v.name, v.get_shape())
# Print name and shape of parameter nodes (values not yet initialized)
print "\n"
print "Parameters"
for v in slim.get_model_variables():
print 'name = {}, shape = {}'.format(v.name, v.get_shape())
###Output
_____no_output_____
###Markdown
Let's create some 1d regression data .We will train and test the model on some noisy observations of a nonlinear function.
###Code
def produce_batch(batch_size, noise=0.3):
xs = np.random.random(size=[batch_size, 1]) * 10
ys = np.sin(xs) + 5 + np.random.normal(size=[batch_size, 1], scale=noise)
return [xs.astype(np.float32), ys.astype(np.float32)]
x_train, y_train = produce_batch(200)
x_test, y_test = produce_batch(200)
plt.scatter(x_train, y_train)
###Output
_____no_output_____
###Markdown
Let's fit the model to the dataThe user has to specify the loss function and the optimizer, and slim does the rest.In particular, the slim.learning.train function does the following:- For each iteration, evaluate the train_op, which updates the parameters using the optimizer applied to the current minibatch. Also, update the global_step.- Occasionally store the model checkpoint in the specified directory. This is useful in case your machine crashes - then you can simply restart from the specified checkpoint.
###Code
def convert_data_to_tensors(x, y):
inputs = tf.constant(x)
inputs.set_shape([None, 1])
outputs = tf.constant(y)
outputs.set_shape([None, 1])
return inputs, outputs
# The following snippet trains the regression model using a sum_of_squares loss.
ckpt_dir = '/tmp/regression_model/'
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
inputs, targets = convert_data_to_tensors(x_train, y_train)
# Make the model.
predictions, nodes = regression_model(inputs, is_training=True)
# Add the loss function to the graph.
loss = slim.losses.sum_of_squares(predictions, targets)
# The total loss is the uers's loss plus any regularization losses.
total_loss = slim.losses.get_total_loss()
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.005)
train_op = slim.learning.create_train_op(total_loss, optimizer)
# Run the training inside a session.
final_loss = slim.learning.train(
train_op,
logdir=ckpt_dir,
number_of_steps=5000,
save_summaries_secs=5,
log_every_n_steps=500)
print("Finished training. Last batch loss:", final_loss)
print("Checkpoint saved in %s" % ckpt_dir)
###Output
_____no_output_____
###Markdown
Training with multiple loss functions.Sometimes we have multiple objectives we want to simultaneously optimize.In slim, it is easy to add more losses, as we show below. (We do not optimize the total loss in this example,but we show how to compute it.)
###Code
with tf.Graph().as_default():
inputs, targets = convert_data_to_tensors(x_train, y_train)
predictions, end_points = regression_model(inputs, is_training=True)
# Add multiple loss nodes.
sum_of_squares_loss = slim.losses.sum_of_squares(predictions, targets)
absolute_difference_loss = slim.losses.absolute_difference(predictions, targets)
# The following two ways to compute the total loss are equivalent
regularization_loss = tf.add_n(slim.losses.get_regularization_losses())
total_loss1 = sum_of_squares_loss + absolute_difference_loss + regularization_loss
# Regularization Loss is included in the total loss by default.
# This is good for training, but not for testing.
total_loss2 = slim.losses.get_total_loss(add_regularization_losses=True)
init_op = tf.global_variables_initializer()
with tf.Session() as sess:
sess.run(init_op) # Will initialize the parameters with random weights.
total_loss1, total_loss2 = sess.run([total_loss1, total_loss2])
print('Total Loss1: %f' % total_loss1)
print('Total Loss2: %f' % total_loss2)
print('Regularization Losses:')
for loss in slim.losses.get_regularization_losses():
print(loss)
print('Loss Functions:')
for loss in slim.losses.get_losses():
print(loss)
###Output
_____no_output_____
###Markdown
Let's load the saved model and use it for prediction.
###Code
with tf.Graph().as_default():
inputs, targets = convert_data_to_tensors(x_test, y_test)
# Create the model structure. (Parameters will be loaded below.)
predictions, end_points = regression_model(inputs, is_training=False)
# Make a session which restores the old parameters from a checkpoint.
sv = tf.train.Supervisor(logdir=ckpt_dir)
with sv.managed_session() as sess:
inputs, predictions, targets = sess.run([inputs, predictions, targets])
plt.scatter(inputs, targets, c='r');
plt.scatter(inputs, predictions, c='b');
plt.title('red=true, blue=predicted')
###Output
_____no_output_____
###Markdown
Let's compute various evaluation metrics on the test set.In TF-Slim termiology, losses are optimized, but metrics (which may not be differentiable, e.g., precision and recall) are just measured. As an illustration, the code below computes mean squared error and mean absolute error metrics on the test set.Each metric declaration creates several local variables (which must be initialized via tf.initialize_local_variables()) and returns both a value_op and an update_op. When evaluated, the value_op returns the current value of the metric. The update_op loads a new batch of data, runs the model, obtains the predictions and accumulates the metric statistics appropriately before returning the current value of the metric. We store these value nodes and update nodes in 2 dictionaries.After creating the metric nodes, we can pass them to slim.evaluation.evaluation, which repeatedly evaluates these nodes the specified number of times. (This allows us to compute the evaluation in a streaming fashion across minibatches, which is usefulf for large datasets.) Finally, we print the final value of each metric.
###Code
with tf.Graph().as_default():
inputs, targets = convert_data_to_tensors(x_test, y_test)
predictions, end_points = regression_model(inputs, is_training=False)
# Specify metrics to evaluate:
names_to_value_nodes, names_to_update_nodes = slim.metrics.aggregate_metric_map({
'Mean Squared Error': slim.metrics.streaming_mean_squared_error(predictions, targets),
'Mean Absolute Error': slim.metrics.streaming_mean_absolute_error(predictions, targets)
})
# Make a session which restores the old graph parameters, and then run eval.
sv = tf.train.Supervisor(logdir=ckpt_dir)
with sv.managed_session() as sess:
metric_values = slim.evaluation.evaluation(
sess,
num_evals=1, # Single pass over data
eval_op=names_to_update_nodes.values(),
final_op=names_to_value_nodes.values())
names_to_values = dict(zip(names_to_value_nodes.keys(), metric_values))
for key, value in names_to_values.iteritems():
print('%s: %f' % (key, value))
###Output
_____no_output_____
###Markdown
Reading Data with TF-SlimReading data with TF-Slim has two main components: A[Dataset](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset.py) and a [DatasetDataProvider](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset_data_provider.py). The former is a descriptor of a dataset, while the latter performs the actions necessary for actually reading the data. Lets look at each one in detail: DatasetA TF-Slim[Dataset](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset.py)contains descriptive information about a dataset necessary for reading it, such as the list of data files and how to decode them. It also contains metadata including class labels, the size of the train/test splits and descriptions of the tensors that the dataset provides. For example, some datasets contain images with labels. Others augment this data with bounding box annotations, etc. The Dataset object allows us to write generic code using the same API, regardless of the data content and encoding type.TF-Slim's Dataset works especially well when the data is stored as a (possibly sharded)[TFRecords file](https://www.tensorflow.org/versions/r0.10/how_tos/reading_data/index.htmlfile-formats), where each record contains a [tf.train.Example protocol buffer](https://github.com/tensorflow/tensorflow/blob/r0.10/tensorflow/core/example/example.proto).TF-Slim uses a consistent convention for naming the keys and values inside each Example record. DatasetDataProviderA[DatasetDataProvider](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset_data_provider.py) is a class which actually reads the data from a dataset. It is highly configurable to read the data in various ways that may make a big impact on the efficiency of your training process. For example, it can be single or multi-threaded. If your data is sharded across many files, it can read each files serially, or from every file simultaneously. Demo: The Flowers DatasetFor convenience, we've include scripts to convert several common image datasets into TFRecord format and have providedthe Dataset descriptor files necessary for reading them. We demonstrate how easy it is to use these dataset via the Flowers dataset below. Download the Flowers DatasetWe've made available a tarball of the Flowers dataset which has already been converted to TFRecord format.
###Code
import tensorflow as tf
from datasets import dataset_utils
url = "http://download.tensorflow.org/data/flowers.tar.gz"
flowers_data_dir = '/tmp/flowers'
if not tf.gfile.Exists(flowers_data_dir):
tf.gfile.MakeDirs(flowers_data_dir)
dataset_utils.download_and_uncompress_tarball(url, flowers_data_dir)
###Output
_____no_output_____
###Markdown
Display some of the data.
###Code
from datasets import flowers
import tensorflow as tf
slim = tf.contrib.slim
with tf.Graph().as_default():
dataset = flowers.get_split('train', flowers_data_dir)
data_provider = slim.dataset_data_provider.DatasetDataProvider(
dataset, common_queue_capacity=32, common_queue_min=1)
image, label = data_provider.get(['image', 'label'])
with tf.Session() as sess:
with slim.queues.QueueRunners(sess):
for i in xrange(4):
np_image, np_label = sess.run([image, label])
height, width, _ = np_image.shape
class_name = name = dataset.labels_to_names[np_label]
plt.figure()
plt.imshow(np_image)
plt.title('%s, %d x %d' % (name, height, width))
plt.axis('off')
plt.show()
###Output
_____no_output_____
###Markdown
Convolutional neural nets (CNNs).In this section, we show how to train an image classifier using a simple CNN. Define the model.Below we define a simple CNN. Note that the output layer is linear function - we will apply softmax transformation externally to the model, either in the loss function (for training), or in the prediction function (during testing).
###Code
def my_cnn(images, num_classes, is_training): # is_training is not used...
with slim.arg_scope([slim.max_pool2d], kernel_size=[3, 3], stride=2):
net = slim.conv2d(images, 64, [5, 5])
net = slim.max_pool2d(net)
net = slim.conv2d(net, 64, [5, 5])
net = slim.max_pool2d(net)
net = slim.flatten(net)
net = slim.fully_connected(net, 192)
net = slim.fully_connected(net, num_classes, activation_fn=None)
return net
###Output
_____no_output_____
###Markdown
Apply the model to some randomly generated images.
###Code
import tensorflow as tf
with tf.Graph().as_default():
# The model can handle any input size because the first layer is convolutional.
# The size of the model is determined when image_node is first passed into the my_cnn function.
# Once the variables are initialized, the size of all the weight matrices is fixed.
# Because of the fully connected layers, this means that all subsequent images must have the same
# input size as the first image.
batch_size, height, width, channels = 3, 28, 28, 3
images = tf.random_uniform([batch_size, height, width, channels], maxval=1)
# Create the model.
num_classes = 10
logits = my_cnn(images, num_classes, is_training=True)
probabilities = tf.nn.softmax(logits)
# Initialize all the variables (including parameters) randomly.
init_op = tf.global_variables_initializer()
with tf.Session() as sess:
# Run the init_op, evaluate the model outputs and print the results:
sess.run(init_op)
probabilities = sess.run(probabilities)
print('Probabilities Shape:')
print(probabilities.shape) # batch_size x num_classes
print('\nProbabilities:')
print(probabilities)
print('\nSumming across all classes (Should equal 1):')
print(np.sum(probabilities, 1)) # Each row sums to 1
###Output
_____no_output_____
###Markdown
Train the model on the Flowers dataset.Before starting, make sure you've run the code to Download the Flowers dataset. Now, we'll get a sense of what it looks like to use TF-Slim's training functions found in[learning.py](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/learning.py). First, we'll create a function, `load_batch`, that loads batches of dataset from a dataset. Next, we'll train a model for a single step (just to demonstrate the API), and evaluate the results.
###Code
from preprocessing import inception_preprocessing
import tensorflow as tf
slim = tf.contrib.slim
def load_batch(dataset, batch_size=32, height=299, width=299, is_training=False):
"""Loads a single batch of data.
Args:
dataset: The dataset to load.
batch_size: The number of images in the batch.
height: The size of each image after preprocessing.
width: The size of each image after preprocessing.
is_training: Whether or not we're currently training or evaluating.
Returns:
images: A Tensor of size [batch_size, height, width, 3], image samples that have been preprocessed.
images_raw: A Tensor of size [batch_size, height, width, 3], image samples that can be used for visualization.
labels: A Tensor of size [batch_size], whose values range between 0 and dataset.num_classes.
"""
data_provider = slim.dataset_data_provider.DatasetDataProvider(
dataset, common_queue_capacity=32,
common_queue_min=8)
image_raw, label = data_provider.get(['image', 'label'])
# Preprocess image for usage by Inception.
image = inception_preprocessing.preprocess_image(image_raw, height, width, is_training=is_training)
# Preprocess the image for display purposes.
image_raw = tf.expand_dims(image_raw, 0)
image_raw = tf.image.resize_images(image_raw, [height, width])
image_raw = tf.squeeze(image_raw)
# Batch it up.
images, images_raw, labels = tf.train.batch(
[image, image_raw, label],
batch_size=batch_size,
num_threads=1,
capacity=2 * batch_size)
return images, images_raw, labels
from datasets import flowers
# This might take a few minutes.
train_dir = '/tmp/tfslim_model/'
print('Will save model to %s' % train_dir)
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset)
# Create the model:
logits = my_cnn(images, num_classes=dataset.num_classes, is_training=True)
# Specify the loss function:
one_hot_labels = slim.one_hot_encoding(labels, dataset.num_classes)
slim.losses.softmax_cross_entropy(logits, one_hot_labels)
total_loss = slim.losses.get_total_loss()
# Create some summaries to visualize the training process:
tf.scalar_summary('losses/Total Loss', total_loss)
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.01)
train_op = slim.learning.create_train_op(total_loss, optimizer)
# Run the training:
final_loss = slim.learning.train(
train_op,
logdir=train_dir,
number_of_steps=1, # For speed, we just do 1 epoch
save_summaries_secs=1)
print('Finished training. Final batch loss %d' % final_loss)
###Output
_____no_output_____
###Markdown
Evaluate some metrics.As we discussed above, we can compute various metrics besides the loss.Below we show how to compute prediction accuracy of the trained model, as well as top-5 classification accuracy. (The difference between evaluation and evaluation_loop is that the latter writes the results to a log directory, so they can be viewed in tensorboard.)
###Code
from datasets import flowers
# This might take a few minutes.
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.DEBUG)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset)
logits = my_cnn(images, num_classes=dataset.num_classes, is_training=False)
predictions = tf.argmax(logits, 1)
# Define the metrics:
names_to_values, names_to_updates = slim.metrics.aggregate_metric_map({
'eval/Accuracy': slim.metrics.streaming_accuracy(predictions, labels),
'eval/Recall@5': slim.metrics.streaming_recall_at_k(logits, labels, 5),
})
print('Running evaluation Loop...')
checkpoint_path = tf.train.latest_checkpoint(train_dir)
metric_values = slim.evaluation.evaluate_once(
master='',
checkpoint_path=checkpoint_path,
logdir=train_dir,
eval_op=names_to_updates.values(),
final_op=names_to_values.values())
names_to_values = dict(zip(names_to_values.keys(), metric_values))
for name in names_to_values:
print('%s: %f' % (name, names_to_values[name]))
###Output
_____no_output_____
###Markdown
Using pre-trained modelsNeural nets work best when they have many parameters, making them very flexible function approximators.However, this means they must be trained on big datasets. Since this process is slow, we provide various pre-trained models - see the list [here](https://github.com/tensorflow/models/tree/master/slimpre-trained-models).You can either use these models as-is, or you can perform "surgery" on them, to modify them for some other task. For example, it is common to "chop off" the final pre-softmax layer, and replace it with a new set of weights corresponding to some new set of labels. You can then quickly fine tune the new model on a small new dataset. We illustrate this below, using inception-v1 as the base model. While models like Inception V3 are more powerful, Inception V1 is used for speed purposes. Download the Inception V1 checkpoint
###Code
from datasets import dataset_utils
url = "http://download.tensorflow.org/models/inception_v1_2016_08_28.tar.gz"
checkpoints_dir = '/tmp/checkpoints'
if not tf.gfile.Exists(checkpoints_dir):
tf.gfile.MakeDirs(checkpoints_dir)
dataset_utils.download_and_uncompress_tarball(url, checkpoints_dir)
###Output
_____no_output_____
###Markdown
Apply Pre-trained model to Images.We have to convert each image to the size expected by the model checkpoint.There is no easy way to determine this size from the checkpoint itself.So we use a preprocessor to enforce this.
###Code
import numpy as np
import os
import tensorflow as tf
import urllib2
from datasets import imagenet
from nets import inception
from preprocessing import inception_preprocessing
slim = tf.contrib.slim
batch_size = 3
image_size = inception.inception_v1.default_image_size
with tf.Graph().as_default():
url = 'https://upload.wikimedia.org/wikipedia/commons/7/70/EnglishCockerSpaniel_simon.jpg'
image_string = urllib2.urlopen(url).read()
image = tf.image.decode_jpeg(image_string, channels=3)
processed_image = inception_preprocessing.preprocess_image(image, image_size, image_size, is_training=False)
processed_images = tf.expand_dims(processed_image, 0)
# Create the model, use the default arg scope to configure the batch norm parameters.
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(processed_images, num_classes=1001, is_training=False)
probabilities = tf.nn.softmax(logits)
init_fn = slim.assign_from_checkpoint_fn(
os.path.join(checkpoints_dir, 'inception_v1.ckpt'),
slim.get_model_variables('InceptionV1'))
with tf.Session() as sess:
init_fn(sess)
np_image, probabilities = sess.run([image, probabilities])
probabilities = probabilities[0, 0:]
sorted_inds = [i[0] for i in sorted(enumerate(-probabilities), key=lambda x:x[1])]
plt.figure()
plt.imshow(np_image.astype(np.uint8))
plt.axis('off')
plt.show()
names = imagenet.create_readable_names_for_imagenet_labels()
for i in range(5):
index = sorted_inds[i]
print('Probability %0.2f%% => [%s]' % (probabilities[index], names[index]))
###Output
_____no_output_____
###Markdown
Fine-tune the model on a different set of labels.We will fine tune the inception model on the Flowers dataset.
###Code
# Note that this may take several minutes.
import os
from datasets import flowers
from nets import inception
from preprocessing import inception_preprocessing
slim = tf.contrib.slim
image_size = inception.inception_v1.default_image_size
def get_init_fn():
"""Returns a function run by the chief worker to warm-start the training."""
checkpoint_exclude_scopes=["InceptionV1/Logits", "InceptionV1/AuxLogits"]
exclusions = [scope.strip() for scope in checkpoint_exclude_scopes]
variables_to_restore = []
for var in slim.get_model_variables():
excluded = False
for exclusion in exclusions:
if var.op.name.startswith(exclusion):
excluded = True
break
if not excluded:
variables_to_restore.append(var)
return slim.assign_from_checkpoint_fn(
os.path.join(checkpoints_dir, 'inception_v1.ckpt'),
variables_to_restore)
train_dir = '/tmp/inception_finetuned/'
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset, height=image_size, width=image_size)
# Create the model, use the default arg scope to configure the batch norm parameters.
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(images, num_classes=dataset.num_classes, is_training=True)
# Specify the loss function:
one_hot_labels = slim.one_hot_encoding(labels, dataset.num_classes)
slim.losses.softmax_cross_entropy(logits, one_hot_labels)
total_loss = slim.losses.get_total_loss()
# Create some summaries to visualize the training process:
tf.scalar_summary('losses/Total Loss', total_loss)
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.01)
train_op = slim.learning.create_train_op(total_loss, optimizer)
# Run the training:
final_loss = slim.learning.train(
train_op,
logdir=train_dir,
init_fn=get_init_fn(),
number_of_steps=2)
print('Finished training. Last batch loss %f' % final_loss)
###Output
_____no_output_____
###Markdown
Apply fine tuned model to some images.
###Code
import numpy as np
import tensorflow as tf
from datasets import flowers
from nets import inception
slim = tf.contrib.slim
image_size = inception.inception_v1.default_image_size
batch_size = 3
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, images_raw, labels = load_batch(dataset, height=image_size, width=image_size)
# Create the model, use the default arg scope to configure the batch norm parameters.
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(images, num_classes=dataset.num_classes, is_training=True)
probabilities = tf.nn.softmax(logits)
checkpoint_path = tf.train.latest_checkpoint(train_dir)
init_fn = slim.assign_from_checkpoint_fn(
checkpoint_path,
slim.get_variables_to_restore())
with tf.Session() as sess:
with slim.queues.QueueRunners(sess):
sess.run(tf.initialize_local_variables())
init_fn(sess)
np_probabilities, np_images_raw, np_labels = sess.run([probabilities, images_raw, labels])
for i in xrange(batch_size):
image = np_images_raw[i, :, :, :]
true_label = np_labels[i]
predicted_label = np.argmax(np_probabilities[i, :])
predicted_name = dataset.labels_to_names[predicted_label]
true_name = dataset.labels_to_names[true_label]
plt.figure()
plt.imshow(image.astype(np.uint8))
plt.title('Ground Truth: [%s], Prediction [%s]' % (true_name, predicted_name))
plt.axis('off')
plt.show()
###Output
_____no_output_____
###Markdown
TF-Slim WalkthroughThis notebook will walk you through the basics of using TF-Slim to define, train and evaluate neural networks on various tasks. It assumes a basic knowledge of neural networks. Table of contentsInstallation and setupCreating your first neural network with TF-SlimReading Data with TF-SlimTraining a convolutional neural network (CNN)Using pre-trained models Installation and setupAs of 8/28/16, the latest stable release of TF is r0.10, which does not contain the latest version of slim.To obtain the latest version of TF-Slim, please install the most recent nightly build of TFas explained [here](https://github.com/tensorflow/models/tree/master/sliminstalling-latest-version-of-tf-slim).To use TF-Slim for image classification (as we do in this notebook), you also have to install the TF-Slim image models library from [here](https://github.com/tensorflow/models/tree/master/slim). Let's suppose you install this into a directory called TF_MODELS. Then you should change directory to TF_MODELS/slim **before** running this notebook, so that these files are in your python path.To check you've got these two steps to work, just execute the cell below. If it complains about unknown modules, restart the notebook after moving to the TF-Slim models directory.
###Code
import matplotlib
%matplotlib inline
import matplotlib.pyplot as plt
import math
import numpy as np
import tensorflow as tf
import time
from datasets import dataset_utils
# Main slim library
slim = tf.contrib.slim
###Output
_____no_output_____
###Markdown
Creating your first neural network with TF-SlimBelow we give some code to create a simple multilayer perceptron (MLP) which can be usedfor regression problems. The model has 2 hidden layers.The output is a single node. When this function is called, it will create various nodes, and silently add them to whichever global TF graph is currently in scope. When a node which corresponds to a layer with adjustable parameters (eg., a fully connected layer) is created, additional parameter variable nodes are silently created, and added to the graph. (We will discuss how to train the parameters later.)We use variable scope to put all the nodes under a common name,so that the graph has some hierarchical structure.This is useful when we want to visualize the TF graph in tensorboard, or if we want to query relatedvariables. The fully connected layers all use the same L2 weight decay and ReLu activations, as specified by **arg_scope**. (However, the final layer overrides these defaults, and uses an identity activation function.)We also illustrate how to add a dropout layer after the first fully connected layer (FC1). Note that at test time, we do not drop out nodes, but instead use the average activations; hence we need to know whether the model is beingconstructed for training or testing, since the computational graph will be different in the two cases(although the variables, storing the model parameters, will be shared, since they have the same name/scope).
###Code
def regression_model(inputs, is_training=True, scope="deep_regression"):
"""Creates the regression model.
Args:
inputs: A node that yields a `Tensor` of size [batch_size, dimensions].
is_training: Whether or not we're currently training the model.
scope: An optional variable_op scope for the model.
Returns:
predictions: 1-D `Tensor` of shape [batch_size] of responses.
end_points: A dict of end points representing the hidden layers.
"""
with tf.variable_scope(scope, 'deep_regression', [inputs]):
end_points = {}
# Set the default weight _regularizer and acvitation for each fully_connected layer.
with slim.arg_scope([slim.fully_connected],
activation_fn=tf.nn.relu,
weights_regularizer=slim.l2_regularizer(0.01)):
# Creates a fully connected layer from the inputs with 32 hidden units.
net = slim.fully_connected(inputs, 32, scope='fc1')
end_points['fc1'] = net
# Adds a dropout layer to prevent over-fitting.
net = slim.dropout(net, 0.8, is_training=is_training)
# Adds another fully connected layer with 16 hidden units.
net = slim.fully_connected(net, 16, scope='fc2')
end_points['fc2'] = net
# Creates a fully-connected layer with a single hidden unit. Note that the
# layer is made linear by setting activation_fn=None.
predictions = slim.fully_connected(net, 1, activation_fn=None, scope='prediction')
end_points['out'] = predictions
return predictions, end_points
###Output
_____no_output_____
###Markdown
Let's create the model and examine its structure.We create a TF graph and call regression_model(), which adds nodes (tensors) to the graph. We then examine their shape, and print the names of all the model variables which have been implicitly created inside of each layer. We see that the names of the variables follow the scopes that we specified.
###Code
with tf.Graph().as_default():
# Dummy placeholders for arbitrary number of 1d inputs and outputs
inputs = tf.placeholder(tf.float32, shape=(None, 1))
outputs = tf.placeholder(tf.float32, shape=(None, 1))
# Build model
predictions, end_points = regression_model(inputs)
# Print name and shape of each tensor.
print "Layers"
for k, v in end_points.iteritems():
print 'name = {}, shape = {}'.format(v.name, v.get_shape())
# Print name and shape of parameter nodes (values not yet initialized)
print "\n"
print "Parameters"
for v in slim.get_model_variables():
print 'name = {}, shape = {}'.format(v.name, v.get_shape())
###Output
_____no_output_____
###Markdown
Let's create some 1d regression data .We will train and test the model on some noisy observations of a nonlinear function.
###Code
def produce_batch(batch_size, noise=0.3):
xs = np.random.random(size=[batch_size, 1]) * 10
ys = np.sin(xs) + 5 + np.random.normal(size=[batch_size, 1], scale=noise)
return [xs.astype(np.float32), ys.astype(np.float32)]
x_train, y_train = produce_batch(200)
x_test, y_test = produce_batch(200)
plt.scatter(x_train, y_train)
###Output
_____no_output_____
###Markdown
Let's fit the model to the dataThe user has to specify the loss function and the optimizer, and slim does the rest.In particular, the slim.learning.train function does the following:- For each iteration, evaluate the train_op, which updates the parameters using the optimizer applied to the current minibatch. Also, update the global_step.- Occasionally store the model checkpoint in the specified directory. This is useful in case your machine crashes - then you can simply restart from the specified checkpoint.
###Code
def convert_data_to_tensors(x, y):
inputs = tf.constant(x)
inputs.set_shape([None, 1])
outputs = tf.constant(y)
outputs.set_shape([None, 1])
return inputs, outputs
# The following snippet trains the regression model using a sum_of_squares loss.
ckpt_dir = '/tmp/regression_model/'
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
inputs, targets = convert_data_to_tensors(x_train, y_train)
# Make the model.
predictions, nodes = regression_model(inputs, is_training=True)
# Add the loss function to the graph.
loss = slim.losses.sum_of_squares(predictions, targets)
# The total loss is the uers's loss plus any regularization losses.
total_loss = slim.losses.get_total_loss()
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.005)
train_op = slim.learning.create_train_op(total_loss, optimizer)
# Run the training inside a session.
final_loss = slim.learning.train(
train_op,
logdir=ckpt_dir,
number_of_steps=5000,
save_summaries_secs=5,
log_every_n_steps=500)
print("Finished training. Last batch loss:", final_loss)
print("Checkpoint saved in %s" % ckpt_dir)
###Output
_____no_output_____
###Markdown
Training with multiple loss functions.Sometimes we have multiple objectives we want to simultaneously optimize.In slim, it is easy to add more losses, as we show below. (We do not optimize the total loss in this example,but we show how to compute it.)
###Code
with tf.Graph().as_default():
inputs, targets = convert_data_to_tensors(x_train, y_train)
predictions, end_points = regression_model(inputs, is_training=True)
# Add multiple loss nodes.
sum_of_squares_loss = slim.losses.sum_of_squares(predictions, targets)
absolute_difference_loss = slim.losses.absolute_difference(predictions, targets)
# The following two ways to compute the total loss are equivalent
regularization_loss = tf.add_n(slim.losses.get_regularization_losses())
total_loss1 = sum_of_squares_loss + absolute_difference_loss + regularization_loss
# Regularization Loss is included in the total loss by default.
# This is good for training, but not for testing.
total_loss2 = slim.losses.get_total_loss(add_regularization_losses=True)
init_op = tf.initialize_all_variables()
with tf.Session() as sess:
sess.run(init_op) # Will initialize the parameters with random weights.
total_loss1, total_loss2 = sess.run([total_loss1, total_loss2])
print('Total Loss1: %f' % total_loss1)
print('Total Loss2: %f' % total_loss2)
print('Regularization Losses:')
for loss in slim.losses.get_regularization_losses():
print(loss)
print('Loss Functions:')
for loss in slim.losses.get_losses():
print(loss)
###Output
_____no_output_____
###Markdown
Let's load the saved model and use it for prediction.
###Code
with tf.Graph().as_default():
inputs, targets = convert_data_to_tensors(x_test, y_test)
# Create the model structure. (Parameters will be loaded below.)
predictions, end_points = regression_model(inputs, is_training=False)
# Make a session which restores the old parameters from a checkpoint.
sv = tf.train.Supervisor(logdir=ckpt_dir)
with sv.managed_session() as sess:
inputs, predictions, targets = sess.run([inputs, predictions, targets])
plt.scatter(inputs, targets, c='r');
plt.scatter(inputs, predictions, c='b');
plt.title('red=true, blue=predicted')
###Output
_____no_output_____
###Markdown
Let's compute various evaluation metrics on the test set.In TF-Slim termiology, losses are optimized, but metrics (which may not be differentiable, e.g., precision and recall) are just measured. As an illustration, the code below computes mean squared error and mean absolute error metrics on the test set.Each metric declaration creates several local variables (which must be initialized via tf.initialize_local_variables()) and returns both a value_op and an update_op. When evaluated, the value_op returns the current value of the metric. The update_op loads a new batch of data, runs the model, obtains the predictions and accumulates the metric statistics appropriately before returning the current value of the metric. We store these value nodes and update nodes in 2 dictionaries.After creating the metric nodes, we can pass them to slim.evaluation.evaluation, which repeatedly evaluates these nodes the specified number of times. (This allows us to compute the evaluation in a streaming fashion across minibatches, which is usefulf for large datasets.) Finally, we print the final value of each metric.
###Code
with tf.Graph().as_default():
inputs, targets = convert_data_to_tensors(x_test, y_test)
predictions, end_points = regression_model(inputs, is_training=False)
# Specify metrics to evaluate:
names_to_value_nodes, names_to_update_nodes = slim.metrics.aggregate_metric_map({
'Mean Squared Error': slim.metrics.streaming_mean_squared_error(predictions, targets),
'Mean Absolute Error': slim.metrics.streaming_mean_absolute_error(predictions, targets)
})
# Make a session which restores the old graph parameters, and then run eval.
sv = tf.train.Supervisor(logdir=ckpt_dir)
with sv.managed_session() as sess:
metric_values = slim.evaluation.evaluation(
sess,
num_evals=1, # Single pass over data
eval_op=names_to_update_nodes.values(),
final_op=names_to_value_nodes.values())
names_to_values = dict(zip(names_to_value_nodes.keys(), metric_values))
for key, value in names_to_values.iteritems():
print('%s: %f' % (key, value))
###Output
_____no_output_____
###Markdown
Reading Data with TF-SlimReading data with TF-Slim has two main components: A[Dataset](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset.py) and a [DatasetDataProvider](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset_data_provider.py). The former is a descriptor of a dataset, while the latter performs the actions necessary for actually reading the data. Lets look at each one in detail: DatasetA TF-Slim[Dataset](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset.py)contains descriptive information about a dataset necessary for reading it, such as the list of data files and how to decode them. It also contains metadata including class labels, the size of the train/test splits and descriptions of the tensors that the dataset provides. For example, some datasets contain images with labels. Others augment this data with bounding box annotations, etc. The Dataset object allows us to write generic code using the same API, regardless of the data content and encoding type.TF-Slim's Dataset works especially well when the data is stored as a (possibly sharded)[TFRecords file](https://www.tensorflow.org/versions/r0.10/how_tos/reading_data/index.htmlfile-formats), where each record contains a [tf.train.Example protocol buffer](https://github.com/tensorflow/tensorflow/blob/r0.10/tensorflow/core/example/example.proto).TF-Slim uses a consistent convention for naming the keys and values inside each Example record. DatasetDataProviderA[DatasetDataProvider](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset_data_provider.py) is a class which actually reads the data from a dataset. It is highly configurable to read the data in various ways that may make a big impact on the efficiency of your training process. For example, it can be single or multi-threaded. If your data is sharded across many files, it can read each files serially, or from every file simultaneously. Demo: The Flowers DatasetFor convenience, we've include scripts to convert several common image datasets into TFRecord format and have providedthe Dataset descriptor files necessary for reading them. We demonstrate how easy it is to use these dataset via the Flowers dataset below. Download the Flowers DatasetWe've made available a tarball of the Flowers dataset which has already been converted to TFRecord format.
###Code
import tensorflow as tf
from datasets import dataset_utils
url = "http://download.tensorflow.org/data/flowers.tar.gz"
flowers_data_dir = '/tmp/flowers'
if not tf.gfile.Exists(flowers_data_dir):
tf.gfile.MakeDirs(flowers_data_dir)
dataset_utils.download_and_uncompress_tarball(url, flowers_data_dir)
###Output
_____no_output_____
###Markdown
Display some of the data.
###Code
from datasets import flowers
import tensorflow as tf
slim = tf.contrib.slim
with tf.Graph().as_default():
dataset = flowers.get_split('train', flowers_data_dir)
data_provider = slim.dataset_data_provider.DatasetDataProvider(
dataset, common_queue_capacity=32, common_queue_min=1)
image, label = data_provider.get(['image', 'label'])
with tf.Session() as sess:
with slim.queues.QueueRunners(sess):
for i in xrange(4):
np_image, np_label = sess.run([image, label])
height, width, _ = np_image.shape
class_name = name = dataset.labels_to_names[np_label]
plt.figure()
plt.imshow(np_image)
plt.title('%s, %d x %d' % (name, height, width))
plt.axis('off')
plt.show()
###Output
_____no_output_____
###Markdown
Convolutional neural nets (CNNs).In this section, we show how to train an image classifier using a simple CNN. Define the model.Below we define a simple CNN. Note that the output layer is linear function - we will apply softmax transformation externally to the model, either in the loss function (for training), or in the prediction function (during testing).
###Code
def my_cnn(images, num_classes, is_training): # is_training is not used...
with slim.arg_scope([slim.max_pool2d], kernel_size=[3, 3], stride=2):
net = slim.conv2d(images, 64, [5, 5])
net = slim.max_pool2d(net)
net = slim.conv2d(net, 64, [5, 5])
net = slim.max_pool2d(net)
net = slim.flatten(net)
net = slim.fully_connected(net, 192)
net = slim.fully_connected(net, num_classes, activation_fn=None)
return net
###Output
_____no_output_____
###Markdown
Apply the model to some randomly generated images.
###Code
import tensorflow as tf
with tf.Graph().as_default():
# The model can handle any input size because the first layer is convolutional.
# The size of the model is determined when image_node is first passed into the my_cnn function.
# Once the variables are initialized, the size of all the weight matrices is fixed.
# Because of the fully connected layers, this means that all subsequent images must have the same
# input size as the first image.
batch_size, height, width, channels = 3, 28, 28, 3
images = tf.random_uniform([batch_size, height, width, channels], maxval=1)
# Create the model.
num_classes = 10
logits = my_cnn(images, num_classes, is_training=True)
probabilities = tf.nn.softmax(logits)
# Initialize all the variables (including parameters) randomly.
init_op = tf.initialize_all_variables()
with tf.Session() as sess:
# Run the init_op, evaluate the model outputs and print the results:
sess.run(init_op)
probabilities = sess.run(probabilities)
print('Probabilities Shape:')
print(probabilities.shape) # batch_size x num_classes
print('\nProbabilities:')
print(probabilities)
print('\nSumming across all classes (Should equal 1):')
print(np.sum(probabilities, 1)) # Each row sums to 1
###Output
_____no_output_____
###Markdown
Train the model on the Flowers dataset.Before starting, make sure you've run the code to Download the Flowers dataset. Now, we'll get a sense of what it looks like to use TF-Slim's training functions found in[learning.py](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/learning.py). First, we'll create a function, `load_batch`, that loads batches of dataset from a dataset. Next, we'll train a model for a single step (just to demonstrate the API), and evaluate the results.
###Code
from preprocessing import inception_preprocessing
import tensorflow as tf
slim = tf.contrib.slim
def load_batch(dataset, batch_size=32, height=299, width=299, is_training=False):
"""Loads a single batch of data.
Args:
dataset: The dataset to load.
batch_size: The number of images in the batch.
height: The size of each image after preprocessing.
width: The size of each image after preprocessing.
is_training: Whether or not we're currently training or evaluating.
Returns:
images: A Tensor of size [batch_size, height, width, 3], image samples that have been preprocessed.
images_raw: A Tensor of size [batch_size, height, width, 3], image samples that can be used for visualization.
labels: A Tensor of size [batch_size], whose values range between 0 and dataset.num_classes.
"""
data_provider = slim.dataset_data_provider.DatasetDataProvider(
dataset, common_queue_capacity=32,
common_queue_min=8)
image_raw, label = data_provider.get(['image', 'label'])
# Preprocess image for usage by Inception.
image = inception_preprocessing.preprocess_image(image_raw, height, width, is_training=is_training)
# Preprocess the image for display purposes.
image_raw = tf.expand_dims(image_raw, 0)
image_raw = tf.image.resize_images(image_raw, [height, width])
image_raw = tf.squeeze(image_raw)
# Batch it up.
images, images_raw, labels = tf.train.batch(
[image, image_raw, label],
batch_size=batch_size,
num_threads=1,
capacity=2 * batch_size)
return images, images_raw, labels
from datasets import flowers
# This might take a few minutes.
train_dir = '/tmp/tfslim_model/'
print('Will save model to %s' % train_dir)
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset)
# Create the model:
logits = my_cnn(images, num_classes=dataset.num_classes, is_training=True)
# Specify the loss function:
one_hot_labels = slim.one_hot_encoding(labels, dataset.num_classes)
slim.losses.softmax_cross_entropy(logits, one_hot_labels)
total_loss = slim.losses.get_total_loss()
# Create some summaries to visualize the training process:
tf.scalar_summary('losses/Total Loss', total_loss)
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.01)
train_op = slim.learning.create_train_op(total_loss, optimizer)
# Run the training:
final_loss = slim.learning.train(
train_op,
logdir=train_dir,
number_of_steps=1, # For speed, we just do 1 epoch
save_summaries_secs=1)
print('Finished training. Final batch loss %d' % final_loss)
###Output
_____no_output_____
###Markdown
Evaluate some metrics.As we discussed above, we can compute various metrics besides the loss.Below we show how to compute prediction accuracy of the trained model, as well as top-5 classification accuracy. (The difference between evaluation and evaluation_loop is that the latter writes the results to a log directory, so they can be viewed in tensorboard.)
###Code
from datasets import flowers
# This might take a few minutes.
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.DEBUG)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset)
logits = my_cnn(images, num_classes=dataset.num_classes, is_training=False)
predictions = tf.argmax(logits, 1)
# Define the metrics:
names_to_values, names_to_updates = slim.metrics.aggregate_metric_map({
'eval/Accuracy': slim.metrics.streaming_accuracy(predictions, labels),
'eval/Recall@5': slim.metrics.streaming_recall_at_k(logits, labels, 5),
})
print('Running evaluation Loop...')
checkpoint_path = tf.train.latest_checkpoint(train_dir)
metric_values = slim.evaluation.evaluate_once(
master='',
checkpoint_path=checkpoint_path,
logdir=train_dir,
eval_op=names_to_updates.values(),
final_op=names_to_values.values())
names_to_values = dict(zip(names_to_values.keys(), metric_values))
for name in names_to_values:
print('%s: %f' % (name, names_to_values[name]))
###Output
_____no_output_____
###Markdown
Using pre-trained modelsNeural nets work best when they have many parameters, making them very flexible function approximators.However, this means they must be trained on big datasets. Since this process is slow, we provide various pre-trained models - see the list [here](https://github.com/tensorflow/models/tree/master/slimpre-trained-models).You can either use these models as-is, or you can perform "surgery" on them, to modify them for some other task. For example, it is common to "chop off" the final pre-softmax layer, and replace it with a new set of weights corresponding to some new set of labels. You can then quickly fine tune the new model on a small new dataset. We illustrate this below, using inception-v1 as the base model. While models like Inception V3 are more powerful, Inception V1 is used for speed purposes. Download the Inception V1 checkpoint
###Code
from datasets import dataset_utils
url = "http://download.tensorflow.org/models/inception_v1_2016_08_28.tar.gz"
checkpoints_dir = '/tmp/checkpoints'
if not tf.gfile.Exists(checkpoints_dir):
tf.gfile.MakeDirs(checkpoints_dir)
dataset_utils.download_and_uncompress_tarball(url, checkpoints_dir)
###Output
_____no_output_____
###Markdown
Apply Pre-trained model to Images.We have to convert each image to the size expected by the model checkpoint.There is no easy way to determine this size from the checkpoint itself.So we use a preprocessor to enforce this.
###Code
import numpy as np
import os
import tensorflow as tf
import urllib2
from datasets import imagenet
from nets import inception
from preprocessing import inception_preprocessing
slim = tf.contrib.slim
batch_size = 3
image_size = inception.inception_v1.default_image_size
with tf.Graph().as_default():
url = 'https://upload.wikimedia.org/wikipedia/commons/7/70/EnglishCockerSpaniel_simon.jpg'
image_string = urllib2.urlopen(url).read()
image = tf.image.decode_jpeg(image_string, channels=3)
processed_image = inception_preprocessing.preprocess_image(image, image_size, image_size, is_training=False)
processed_images = tf.expand_dims(processed_image, 0)
# Create the model, use the default arg scope to configure the batch norm parameters.
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(processed_images, num_classes=1001, is_training=False)
probabilities = tf.nn.softmax(logits)
init_fn = slim.assign_from_checkpoint_fn(
os.path.join(checkpoints_dir, 'inception_v1.ckpt'),
slim.get_model_variables('InceptionV1'))
with tf.Session() as sess:
init_fn(sess)
np_image, probabilities = sess.run([image, probabilities])
probabilities = probabilities[0, 0:]
sorted_inds = [i[0] for i in sorted(enumerate(-probabilities), key=lambda x:x[1])]
plt.figure()
plt.imshow(np_image.astype(np.uint8))
plt.axis('off')
plt.show()
names = imagenet.create_readable_names_for_imagenet_labels()
for i in range(5):
index = sorted_inds[i]
print('Probability %0.2f%% => [%s]' % (probabilities[index], names[index]))
###Output
_____no_output_____
###Markdown
Fine-tune the model on a different set of labels.We will fine tune the inception model on the Flowers dataset.
###Code
# Note that this may take several minutes.
import os
from datasets import flowers
from nets import inception
from preprocessing import inception_preprocessing
slim = tf.contrib.slim
image_size = inception.inception_v1.default_image_size
def get_init_fn():
"""Returns a function run by the chief worker to warm-start the training."""
checkpoint_exclude_scopes=["InceptionV1/Logits", "InceptionV1/AuxLogits"]
exclusions = [scope.strip() for scope in checkpoint_exclude_scopes]
variables_to_restore = []
for var in slim.get_model_variables():
excluded = False
for exclusion in exclusions:
if var.op.name.startswith(exclusion):
excluded = True
break
if not excluded:
variables_to_restore.append(var)
return slim.assign_from_checkpoint_fn(
os.path.join(checkpoints_dir, 'inception_v1.ckpt'),
variables_to_restore)
train_dir = '/tmp/inception_finetuned/'
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset, height=image_size, width=image_size)
# Create the model, use the default arg scope to configure the batch norm parameters.
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(images, num_classes=dataset.num_classes, is_training=True)
# Specify the loss function:
one_hot_labels = slim.one_hot_encoding(labels, dataset.num_classes)
slim.losses.softmax_cross_entropy(logits, one_hot_labels)
total_loss = slim.losses.get_total_loss()
# Create some summaries to visualize the training process:
tf.scalar_summary('losses/Total Loss', total_loss)
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.01)
train_op = slim.learning.create_train_op(total_loss, optimizer)
# Run the training:
final_loss = slim.learning.train(
train_op,
logdir=train_dir,
init_fn=get_init_fn(),
number_of_steps=2)
print('Finished training. Last batch loss %f' % final_loss)
###Output
_____no_output_____
###Markdown
Apply fine tuned model to some images.
###Code
import numpy as np
import tensorflow as tf
from datasets import flowers
from nets import inception
slim = tf.contrib.slim
image_size = inception.inception_v1.default_image_size
batch_size = 3
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, images_raw, labels = load_batch(dataset, height=image_size, width=image_size)
# Create the model, use the default arg scope to configure the batch norm parameters.
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(images, num_classes=dataset.num_classes, is_training=True)
probabilities = tf.nn.softmax(logits)
checkpoint_path = tf.train.latest_checkpoint(train_dir)
init_fn = slim.assign_from_checkpoint_fn(
checkpoint_path,
slim.get_variables_to_restore())
with tf.Session() as sess:
with slim.queues.QueueRunners(sess):
sess.run(tf.initialize_local_variables())
init_fn(sess)
np_probabilities, np_images_raw, np_labels = sess.run([probabilities, images_raw, labels])
for i in xrange(batch_size):
image = np_images_raw[i, :, :, :]
true_label = np_labels[i]
predicted_label = np.argmax(np_probabilities[i, :])
predicted_name = dataset.labels_to_names[predicted_label]
true_name = dataset.labels_to_names[true_label]
plt.figure()
plt.imshow(image.astype(np.uint8))
plt.title('Ground Truth: [%s], Prediction [%s]' % (true_name, predicted_name))
plt.axis('off')
plt.show()
###Output
_____no_output_____
###Markdown
TF-Slim WalkthroughThis notebook will walk you through the basics of using TF-Slim to define, train and evaluate neural networks on various tasks. It assumes a basic knowledge of neural networks. Table of contentsInstallation and setupCreating your first neural network with TF-SlimReading Data with TF-SlimTraining a convolutional neural network (CNN)Using pre-trained models Installation and setupAs of 8/28/16, the latest stable release of TF is r0.10, which does not contain the latest version of slim.To obtain the latest version of TF-Slim, please install the most recent nightly build of TFas explained [here](https://github.com/nathansilberman/models/tree/master/slimgetting-started).To use TF-Slim for image classification (as we do in this notebook), you also have to install the TF-Slim image models library from [here](https://github.com/tensorflow/models/tree/master/slim). Let's suppose you install this into a directory called TF_MODELS. Then you should change directory to TF_MODELS/slim **before** running this notebook, so that all the files are on the path.To check you've got these two steps to work, just execute the cell below. It it complains about unknown modules, restart the notebook after moving to the TF-Slim models directory.
###Code
import matplotlib
%matplotlib inline
import matplotlib.pyplot as plt
import math
import numpy as np
import tensorflow as tf
import time
from datasets import dataset_utils
# Main slim library
slim = tf.contrib.slim
###Output
_____no_output_____
###Markdown
Creating your first neural network with TF-SlimBelow we give some code to create a simple multilayer perceptron (MLP) which can be usedfor regression problems. The model has 2 hidden layers.The output is a single node. When this function is called, it will create various nodes, and silently add them to whichever global TF graph is currently in scope. When a node which corresponds to a layer with adjustable parameters (eg., a fully connected layer) is created, additional parameter variable nodes are silently created, and added to the graph. (We will discuss how to train the parameters later.)We use variable scope to put all the nodes under a common name,so that the graph has some hierarchical structure.This is useful when we want to visualize the TF graph in tensorboard, or if we want to query relatedvariables. The fully connected layers all use the same L2 weight decay and ReLu activations, as specified by **arg_scope**. (However, the final layer overrides these defaults, and uses an identity activation function.)We also illustrate how to add a dropout layer after the first fully connected layer (FC1). Note that at test time, we do not drop out nodes, but instead use the average activations; hence we need to know whether the model is beingconstructed for training or testing, since the computational graph will be different in the two cases(although the variables, storing the model parameters, will be shared, since they have the same name/scope).
###Code
def regression_model(inputs, is_training=True, scope="deep_regression"):
"""Creates the regression model.
Args:
input_node: A node that yields a `Tensor` of size [batch_size, dimensions].
is_training: Whether or not we're currently training the model.
scope: An optional variable_op scope for the model.
Returns:
output_node: 1-D `Tensor` of shape [batch_size] of responses.
nodes: A dict of nodes representing the hidden layers.
"""
with tf.variable_scope(scope, 'deep_regression', [input_node]):
nodes = {}
# Set the default weight _regularizer and acvitation for each fully_connected layer.
with slim.arg_scope([slim.fully_connected],
activation_fn=tf.nn.relu,
weights_regularizer=slim.l2_regularizer(0.01)):
# Creates a fully connected layer from the inputs with 10 hidden units.
fc1_node = slim.fully_connected(inputs, 10, scope='fc1')
nodes['fc1'] = fc1_node
# Adds a dropout layer to prevent over-fitting.
dropout_node = slim.dropout(fc1_node, 0.8, is_training=is_training)
# Adds another fully connected layer with 5 hidden units.
fc2_node = slim.fully_connected(dropout_node, 5, scope='fc2')
nodes['fc2'] = fc2_node
# Creates a fully-connected layer with a single hidden unit. Note that the
# layer is made linear by setting activation_fn=None.
prediction_node = slim.fully_connected(fc2_node, 1, activation_fn=None, scope='prediction')
nodes['out'] = prediction_node
return prediction_node, nodes
###Output
_____no_output_____
###Markdown
Let's create the model and examine its structure.We create a TF graph and call regression_model(), which adds nodes (tensors) to the graph. We then examine their shape, and print the names of all the model variables which have been implicitly created inside of each layer. We see that the names of the variables follow the scopes that we specified.
###Code
with tf.Graph().as_default():
# Dummy placeholders for arbitrary number of 1d inputs and outputs
input_node = tf.placeholder(tf.float32, shape=(None, 1))
output_node = tf.placeholder(tf.float32, shape=(None, 1))
# Build model
prediction_node, all_nodes = regression_model(input_node)
# Print name and shape of each tensor.
print "Layers"
for k, v in all_nodes.iteritems():
print 'name = {}, shape = {}'.format(v.name, v.get_shape())
# Print name and shape of parameter nodes (values not yet initialized)
print "\n"
print "Parameters"
for v in slim.get_model_variables():
print 'name = {}, shape = {}'.format(v.name, v.get_shape())
###Output
_____no_output_____
###Markdown
Let's create some 1d regression data .We will train and test the model on some noisy observations of a nonlinear function.
###Code
def produce_batch(batch_size, noise=0.3):
xs = np.random.random(size=[batch_size, 1]) * 10
ys = np.sin(xs) + 5 + np.random.normal(size=[batch_size, 1], scale=noise)
return [xs.astype(np.float32), ys.astype(np.float32)]
x_train, y_train = produce_batch(100)
x_test, y_test = produce_batch(100)
plt.scatter(x_train, y_train)
###Output
_____no_output_____
###Markdown
Let's fit the model to the dataThe user has to specify the loss function and the optimizer, and slim does the rest.In particular, the slim.learning.train function does the following:- For each iteration, evaluate the train_op, which updates the parameters using the optimizer applied to the current minibatch. Also, update the global_step.- Occasionally store the model checkpoint in the specified directory. This is useful in case your machine crashes - then you can simply restart from the specified checkpoint.
###Code
# Everytime we run training, we need to store the model checkpoint in a new directory,
# in case anything has changed.
import time
ts = time.time()
ckpt_dir = '/tmp/tf/regression_model/model{}'.format(ts) # Place to store the checkpoint.
print('Saving to {}'.format(ckpt_dir))
def convert_data_to_tensors(x, y):
input_tensor = tf.constant(x)
input_tensor.set_shape([None, 1])
output_tensor = tf.constant(y)
output_tensor.set_shape([None, 1])
return input_tensor, output_tensor
graph = tf.Graph() # new graph
with graph.as_default():
input_node, output_node = convert_data_to_tensors(x_train, y_train)
# Make the model.
prediction_node, nodes = regression_model(input_node, is_training=True)
# Add the loss function to the graph.
loss_node = slim.losses.sum_of_squares(prediction_node, output_node)
# The total loss is the uers's loss plus any regularization losses.
total_loss_node = slim.losses.get_total_loss()
# Create some summaries to visualize the training process:
## TODO: add summaries.py to 3p
#slim.summaries.add_scalar_summary(total_loss, 'Total Loss', print_summary=True)
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.01)
train_op_node = slim.learning.create_train_op(total_loss_node, optimizer)
# Run the training inside a session.
final_loss = slim.learning.train(
train_op_node,
logdir=ckpt_dir,
number_of_steps=500,
save_summaries_secs=1)
print("Finished training. Last batch loss:", final_loss)
print("Checkpoint saved in %s" % ckpt_dir)
###Output
_____no_output_____
###Markdown
Training with multiple loss functions.Sometimes we have multiple objectives we want to simultaneously optimize.In slim, it is easy to add more losses, as we show below. (We do not optimize the total loss in this example,but we show how to compute it.)
###Code
graph = tf.Graph() # Make a new graph
with graph.as_default():
input_node, output_node = convert_data_to_tensors(x_train, y_train)
prediction_node, nodes = regression_model(input_node, is_training=True)
# Add multiple loss nodes.
sum_of_squares_loss_node = slim.losses.sum_of_squares(prediction_node, output_node)
absolute_difference_loss_node = slim.losses.absolute_difference(prediction_node, output_node)
# The following two ways to compute the total loss are equivalent
regularization_loss_node = tf.add_n(slim.losses.get_regularization_losses())
total_loss1_node = sum_of_squares_loss_node + absolute_difference_loss_node + regularization_loss_node
# Regularization Loss is included in the total loss by default.
# This is good for training, but not for testing.
total_loss2_node = slim.losses.get_total_loss(add_regularization_losses=True)
init_node = tf.initialize_all_variables()
with tf.Session() as sess:
sess.run(init_node) # Will randomize the parameters.
total_loss1, total_loss2 = sess.run([total_loss1_node, total_loss2_node])
print('Total Loss1: %f' % total_loss1)
print('Total Loss2: %f' % total_loss2)
print('Regularization Losses:')
for loss_node in slim.losses.get_regularization_losses():
print(loss_node)
print('Loss Functions:')
for loss_node in slim.losses.get_losses():
print(loss_node)
###Output
_____no_output_____
###Markdown
Let's load the saved model and use it for prediction.The predictive accuracy is not very good, because we used a small model,and only trained for 500 steps, to keep the demo fast. Running for 5000 steps improves performance a lot.
###Code
with tf.Graph().as_default():
input_node, output_node = convert_data_to_tensors(x_test, y_test)
# Create the model structure. (Parameters will be loaded below.)
prediction_node, nodes = regression_model(input_node, is_training=False)
# Make a session which restores the old parameters from a checkpoint.
sv = tf.train.Supervisor(logdir=ckpt_dir)
with sv.managed_session() as sess:
inputs, predictions, true_outputs = sess.run([input_node, prediction_node, output_node])
plt.scatter(inputs, true_outputs, c='r');
plt.scatter(inputs, predictions, c='b');
plt.title('red=true, blue=predicted')
###Output
_____no_output_____
###Markdown
Let's examine the learned parameters.
###Code
with tf.Graph().as_default():
input_node = tf.placeholder(tf.float32, shape=(None, 1))
output_node = tf.placeholder(tf.float32, shape=(None, 1))
prediction_node, nodes = regression_model(input_node, is_training=False)
sv = tf.train.Supervisor(logdir=ckpt_dir)
with sv.managed_session() as sess:
model_variables = slim.get_model_variables()
for v in model_variables:
val = sess.run(v)
print v.name, val.shape, val
###Output
_____no_output_____
###Markdown
Let's compute various evaluation metrics on the test set.In slim termiology, losses are optimized, but metrics (which may not be differentiable, e.g., precision and recall) are just measured.As an illustration, the code below computes mean squared error and mean absolute error metrics on the test set.Each metric declaration creates several local variables (which must be initialized via tf.initialize_local_variables()) and returns both a value_op and an update_op. When evaluated, the value_op returns the current value of the metric. The update_op loads a new batch of data, runs the model, obtains the predictions and accumulates the metric statistics appropriately before returning the current value of the metric. We store these value nodes and update nodes in 2 dictionaries.After creating the metric nodes, we can pass them to slim.evaluation.evaluation, which repeatedly evaluates these nodes the specified number of times. (This allows us to compute the evaluation in a streaming fashion across minibatches, which is usefulf for large datasets.) Finally, we print the final value of each metric.
###Code
with tf.Graph().as_default():
input_node, output_node = convert_data_to_tensors(x_test, y_test)
prediction_node, nodes = regression_model(input_node, is_training=False)
# Specify metrics to evaluate:
names_to_value_nodes, names_to_update_nodes = slim.metrics.aggregate_metric_map({
'Mean Squared Error': slim.metrics.streaming_mean_squared_error(prediction_node, output_node),
'Mean Absolute Error': slim.metrics.streaming_mean_absolute_error(prediction_node, output_node)
})
init_node = tf.group(
tf.initialize_all_variables(),
tf.initialize_local_variables())
# Make a session which restores the old graph parameters, and then run eval.
sv = tf.train.Supervisor(logdir=ckpt_dir)
with sv.managed_session() as sess:
metric_values = slim.evaluation.evaluation(
sess,
num_evals=1, # Single pass over data
init_op=init_node,
eval_op=names_to_update_nodes.values(),
final_op=names_to_value_nodes.values())
names_to_values = dict(zip(names_to_value_nodes.keys(), metric_values))
for key, value in names_to_values.iteritems():
print('%s: %f' % (key, value))
###Output
_____no_output_____
###Markdown
Reading Data with TF-SlimReading data with TF-Slim has two main components: A[Dataset](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset.py) and a [DatasetDataProvider](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset_data_provider.py). The former is a descriptor of a dataset, while the latter performs the actions necessary for actually reading the data. Lets look at each one in detail: DatasetA TF-Slim[Dataset](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset.py)contains descriptive information about a dataset necessary for reading it, such as the list of data files and how to decode them. It also contains metadata including class labels, the size of the train/test splits and descriptions of the tensors that the dataset provides. For example, some datasets contain images with labels. Others augment this data with bounding box annotations, etc. The Dataset object allows us to write generic code using the same API, regardless of the data content and encoding type.TF-Slim's Dataset works especially well when the data is stored as a (possibly sharded)[TFRecords file](https://www.tensorflow.org/versions/r0.10/how_tos/reading_data/index.htmlfile-formats), where each record contains a [tf.train.Example protocol buffer](https://github.com/tensorflow/tensorflow/blob/r0.10/tensorflow/core/example/example.proto).TF-Slim uses a consistent convention for naming the keys and values inside each Example record. DatasetDataProviderA[DatasetDataProvider](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/data/dataset_data_provider.py) is a class which actually reads the data from a dataset. It is highly configurable to read the data in various ways that may make a big impact on the efficiency of your training process. For example, it can be single or multi-threaded. If your data is sharded across many files, it can read each files serially, or from every file simultaneously. Demo: The Flowers DatasetFor convenience, we've include scripts to convert several common image datasets into TFRecord format and have providedthe Dataset descriptor files necessary for reading them. We demonstrate how easy it is to use these dataset via the Flowers dataset below. Download the Flowers DatasetWe've made available a tarball of the Flowers dataset which has already been converted to TFRecord format.
###Code
from datasets import dataset_utils
url = "http://download.tensorflow.org/data/flowers.tar.gz"
flowers_data_dir = '/tmp/flowers'
dataset_utils.download_and_uncompress_tarball(url, flowers_data_dir)
###Output
_____no_output_____
###Markdown
Display some of the data.
###Code
from datasets import flowers
import tensorflow as tf
slim = tf.contrib.slim
with tf.Graph().as_default():
dataset = flowers.get_split('train', flowers_data_dir)
data_provider = slim.dataset_data_provider.DatasetDataProvider(
dataset, common_queue_capacity=32, common_queue_min=1)
image, label = data_provider.get(['image', 'label'])
with tf.Session() as sess:
with slim.queues.QueueRunners(sess):
for i in xrange(4):
np_image, np_label = sess.run([image, label])
height, width, _ = np_image.shape
class_name = name = dataset.labels_to_names[np_label]
plt.figure()
plt.imshow(np_image)
plt.title('%s, %d x %d' % (name, height, width))
plt.axis('off')
plt.show()
###Output
_____no_output_____
###Markdown
Convolutional neural nets (CNNs).In this section, we show how to train an image classifier using a simple CNN. Define the model.Below we define a simple CNN. Note that the output layer is linear function - we will apply softmax transformation externally to the model, either in the loss function (for training), or in the prediction function (during testing).
###Code
def my_cnn(images, num_classes, is_training): # is_training is not used...
with slim.arg_scope([slim.max_pool2d], kernel_size=[3, 3], stride=2):
net = slim.conv2d(images, 64, [5, 5])
net = slim.max_pool2d(net)
net = slim.conv2d(net, 64, [5, 5])
net = slim.max_pool2d(net)
net = slim.flatten(net)
net = slim.fully_connected(net, 192)
net = slim.fully_connected(net, num_classes, activation_fn=None)
return net
###Output
_____no_output_____
###Markdown
Apply the model to some randomly generated images.
###Code
import tensorflow as tf
with tf.Graph().as_default():
# The model can handle any input size because the first layer is convolutional.
# The size of the model is determined when image_node is first passed into the my_cnn function.
# Once the variables are initialized, the size of all the weight matrices is fixed.
# Because of the fully connected layers, this means that all subsequent images must have the same
# input size as the first image.
batch_size, height, width, channels = 3, 28, 28, 3
images = tf.random_uniform([batch_size, height, width, channels], maxval=1)
# Create the model.
num_classes = 10
logits = my_cnn(images, num_classes, is_training=True)
probabilities = tf.nn.softmax(logits)
# Initialize all the variables (including parameters) randomly.
init_op = tf.initialize_all_variables()
with tf.Session() as sess:
# Run the init_op, evaluate the model outputs and print the results:
sess.run(init_op)
probabilities = sess.run(probabilities)
print('Probabilities Shape:')
print(probabilities.shape) # batch_size x num_classes
print('\nProbabilities:')
print(probabilities)
print('\nSumming across all classes (Should equal 1):')
print(np.sum(probabilities, 1)) # Each row sums to 1
###Output
_____no_output_____
###Markdown
Train the model on the Flowers dataset.Before starting, make sure you've run the code to Download the Flowers dataset. Now, we'll get a sense of what it looks like to use TF-Slim's training functions found in[learning.py](https://github.com/tensorflow/tensorflow/blob/master/tensorflow/contrib/slim/python/slim/learning.py). First, we'll create a function, `load_batch`, that loads batches of dataset from a dataset. Next, we'll train a model for a single step (just to demonstrate the API), and evaluate the results.
###Code
from preprocessing import inception_preprocessing
import tensorflow as tf
slim = tf.contrib.slim
def load_batch(dataset, batch_size=32, height=299, width=299, is_training=False):
"""Loads a single batch of data.
Args:
dataset: The dataset to load.
batch_size: The number of images in the batch.
height: The size of each image after preprocessing.
width: The size of each image after preprocessing.
is_training: Whether or not we're currently training or evaluating.
"""
data_provider = slim.dataset_data_provider.DatasetDataProvider(
dataset, common_queue_capacity=32,
common_queue_min=8)
image_raw, label = data_provider.get(['image', 'label'])
# Preprocess image for usage by Inception.
image = inception_preprocessing.preprocess_image(image_raw, height, width, is_training=is_training)
# Preprocess the image for display purposes.
image_raw = tf.expand_dims(image_raw, 0)
image_raw = tf.image.resize_images(image_raw, height, width)
image_raw = tf.squeeze(image_raw)
# Batch it up.
images, images_raw, labels = tf.train.batch(
[image, image_raw, label],
batch_size=batch_size,
num_threads=1,
capacity=2 * batch_size)
return images, images_raw, labels
from datasets import flowers
# This might take a few minutes.
train_dir = '/tmp/tfslim_model/'
print('Will save model to %s' % CHECKPOINT_DIR)
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset)
# Create the model:
logits = my_cnn(images, num_classes=dataset.num_classes, is_training=True)
# Specify the loss function:
one_hot_labels = slim.one_hot_encoding(labels, dataset.num_classes)
slim.losses.softmax_cross_entropy(logits, one_hot_labels)
total_loss = slim.losses.get_total_loss()
# Create some summaries to visualize the training process:
tf.scalar_summary('losses/Total Loss', total_loss)
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.01)
train_op = slim.learning.create_train_op(total_loss, optimizer)
# Run the training:
final_loss = slim.learning.train(
train_op,
logdir=train_dir,
number_of_steps=1, # For speed, we just do 1 epoch
save_summaries_secs=1)
print('Finished training. Final batch loss %d' % final_loss)
###Output
_____no_output_____
###Markdown
Evaluate some metrics.As we discussed above, we can compute various metrics besides the loss.Below we show how to compute prediction accuracy of the trained model, as well as top-5 classification accuracy. (The difference between evaluation and evaluation_loop is that the latter writes the results to a log directory, so they can be viewed in tensorboard.)
###Code
from datasets import flowers
# This might take a few minutes.
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.DEBUG)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset)
logits = my_cnn(images, num_classes=dataset.num_classes, is_training=False)
predictions = tf.argmax(logits, 1)
# Define the metrics:
names_to_values, names_to_updates = slim.metrics.aggregate_metric_map({
'eval/Accuracy': slim.metrics.streaming_accuracy(predictions, labels),
'eval/Recall@5': slim.metrics.streaming_recall_at_k(logits, labels, 5),
})
print('Running evaluation Loop...')
checkpoint_path = tf.train.latest_checkpoint(CHECKPOINT_DIR)
metric_values = slim.evaluation.evaluate_once(
master='',
checkpoint_path=checkpoint_path,
logdir=train_dir,
eval_op=names_to_updates.values(),
final_op=names_to_values.values())
names_to_values = dict(zip(names_to_values.keys(), metric_values))
for name in names_to_values:
print('%s: %f' % (name, names_to_values[name]))
###Output
_____no_output_____
###Markdown
Using pre-trained modelsNeural nets work best when they have many parameters, making them very flexible function approximators.However, this means they must be trained on big datasets. Since this process is slow, we provide various pre-trained models - see the list [here](https://github.com/tensorflow/models/tree/master/slimpre-trained-models).You can either use these models as-is, or you can perform "surgery" on them, to modify them for some other task. For example, it is common to "chop off" the final pre-softmax layer, and replace it with a new set of weights corresponding to some new set of labels. You can then quickly fine tune the new model on a small new dataset. We illustrate this below, using inception-v3 as the base model. Download the Inception V1 checkpoint
###Code
from datasets import dataset_utils
url = "http://download.tensorflow.org/models/inception_v1_2016_08_28.tar.gz"
checkpoints_dir = '/tmp/checkpoints'
dataset_utils.download_and_uncompress_tarball(url, checkpoints_dir)
###Output
_____no_output_____
###Markdown
Apply Pre-trained model to Images.We have to convert each image to the size expected by the model checkpoint.There is no easy way to determine this size from the checkpoint itself.So we use a preprocessor to enforce this.
###Code
import numpy as np
import os
import tensorflow as tf
import urllib2
from datasets import imagenet
from nets import inception
from preprocessing import inception_preprocessing
slim = tf.contrib.slim
batch_size = 3
with tf.Graph().as_default():
url = 'https://upload.wikimedia.org/wikipedia/commons/7/70/EnglishCockerSpaniel_simon.jpg'
image_string = urllib2.urlopen(url).read()
image = tf.image.decode_jpeg(image_string, channels=3)
processed_image = inception_preprocessing.preprocess_image(image, 224, 224, is_training=False)
processed_images = tf.expand_dims(processed_image, 0)
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(processed_images, num_classes=1001, is_training=False)
probabilities = tf.nn.softmax(logits)
init_fn = slim.assign_from_checkpoint_fn(
os.path.join(checkpoints_dir, 'inception_v1.ckpt'),
slim.get_model_variables('InceptionV1'))
with tf.Session() as sess:
init_fn(sess)
np_image, probabilities = sess.run([image, probabilities])
probabilities = probabilities[0, 0:]
sorted_inds = [i[0] for i in sorted(enumerate(probabilities), key=lambda x:x[1])]
plt.figure()
plt.imshow(np_image.astype(np.uint8))
plt.axis('off')
plt.show()
sorted_inds = [i[0] for i in sorted(enumerate(-probabilities), key=lambda x:x[1])]
names = imagenet.create_readable_names_for_imagenet_labels()
for i in range(5):
index = sorted_inds[i]
print('Probability %0.2f%% => [%s]' % (probabilities[index], names[index]))
###Output
_____no_output_____
###Markdown
Fine-tune the model on a different set of labels.We will fine tune the inception model on the Flowers dataset.
###Code
import os
from datasets import flowers
from nets import inception
from preprocessing import inception_preprocessing
slim = tf.contrib.slim
def get_init_fn():
"""Returns a function run by the chief worker to warm-start the training."""
checkpoint_exclude_scopes=["InceptionV1/Logits", "InceptionV1/AuxLogits"]
exclusions = [scope.strip() for scope in checkpoint_exclude_scopes]
variables_to_restore = []
for var in slim.get_model_variables():
excluded = False
for exclusion in exclusions:
if var.op.name.startswith(exclusion):
excluded = True
break
if not excluded:
variables_to_restore.append(var)
return slim.assign_from_checkpoint_fn(
os.path.join(checkpoints_dir, 'inception_v1.ckpt'),
variables_to_restore)
train_dir = '/tmp/inception_finetuned/'
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, _, labels = load_batch(dataset, height=224, width=224)
# Create the model:
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(images, num_classes=dataset.num_classes, is_training=True)
# Specify the loss function:
one_hot_labels = slim.one_hot_encoding(labels, dataset.num_classes)
slim.losses.softmax_cross_entropy(logits, one_hot_labels)
total_loss = slim.losses.get_total_loss()
# Create some summaries to visualize the training process:
tf.scalar_summary('losses/Total Loss', total_loss)
# Specify the optimizer and create the train op:
optimizer = tf.train.AdamOptimizer(learning_rate=0.01)
train_op = slim.learning.create_train_op(total_loss, optimizer)
# Run the training:
final_loss = slim.learning.train(
train_op,
logdir=train_dir,
init_fn=get_init_fn(),
number_of_steps=2)
print('Finished training. Last batch loss %f' % final_loss)
###Output
_____no_output_____
###Markdown
Apply fine tuned model to some images.
###Code
import numpy as np
import tensorflow as tf
from datasets import flowers
from nets import inception
slim = tf.contrib.slim
batch_size = 3
with tf.Graph().as_default():
tf.logging.set_verbosity(tf.logging.INFO)
dataset = flowers.get_split('train', flowers_data_dir)
images, images_raw, labels = load_batch(dataset, height=224, width=224)
# Create the model:
with slim.arg_scope(inception.inception_v1_arg_scope()):
logits, _ = inception.inception_v1(images, num_classes=dataset.num_classes, is_training=True)
probabilities = tf.nn.softmax(logits)
checkpoint_path = tf.train.latest_checkpoint(train_dir)
init_fn = slim.assign_from_checkpoint_fn(
checkpoint_path,
slim.get_variables_to_restore())
with tf.Session() as sess:
with slim.queues.QueueRunners(sess):
sess.run(tf.initialize_local_variables())
init_fn(sess)
np_probabilities, np_images_raw, np_labels = sess.run([probabilities, images_raw, labels])
for i in xrange(batch_size):
image = np_images_raw[i, :, :, :]
true_label = np_labels[i]
predicted_label = np.argmax(np_probabilities[i, :])
predicted_name = dataset.labels_to_names[predicted_label]
true_name = dataset.labels_to_names[true_label]
plt.figure()
plt.imshow(image.astype(np.uint8))
plt.title('Ground Truth: [%s], Prediction [%s]' % (true_name, predicted_name))
plt.axis('off')
plt.show()
###Output
_____no_output_____ |
notebooks/Math156_classification_MNIST_myCopy.ipynb | ###Markdown
Load and display MNIST handwritten digits dataset
###Code
# Load data from https://www.openml.org/d/554
X, y = fetch_openml('mnist_784', version=1, return_X_y=True)
# X = X.values ### Uncomment this line if you are having type errors in plotting. It is loading as a pandas dataframe, but our indexing is for numpy array.
X = X / 255.
print('X.shape', X.shape)
print('y.shape', y.shape)
'''
Each row of X is a vectroization of an image of 28 x 28 = 784 pixels.
The corresponding row of y holds the true class label from {0,1, .. , 9}.
'''
# see how many images are there for each digit
for j in np.arange(10):
idx = np.where(y==str(j))
idx = np.asarray(idx)[0,:]
print('digit %i length %i' % (j, len(idx)))
# Plot some sample images
ncols = 10
nrows = 4
fig, ax = plt.subplots(nrows=nrows, ncols=ncols, figsize=[15, 6.5])
for j in np.arange(ncols):
for i in np.arange(nrows):
idx = np.where(y==str(j)) # index of all images of digit 'j'
idx = np.asarray(idx)[0,:] # make idx from tuple to array
idx_subsampled = np.random.choice(idx, nrows)
# ax[i,j].imshow(X.loc[idx_subsampled[i],:].reshape(28,28))
ax[i,j].imshow(X.loc[idx_subsampled[i],:].values.reshape(28,28))
# ax[i,j].title.set_text("label=%s" % y[idx_subsampled[j]])
if i == 0:
# ax[j,i].set_ylabel("label=%s" % y[idx_subsampled[j]])
ax[i,j].set_title("label$=$%s" % y[idx_subsampled[i]], fontsize=14)
# ax[i].legend()
plt.subplots_adjust(wspace=0.3, hspace=-0.1)
plt.savefig('MNIST_ex1.pdf', bbox_inches='tight')
# Split the dataset into train and test sets
X_train = []
X_test = []
y_test = []
y_train = []
for i in np.arange(X.shape[0]):
# for each example i, make it into train set with probabiliy 0.8 and into test set otherwise
U = np.random.rand() # Uniform([0,1]) variable
if U<0.8:
X_train.append(X.loc[i,:])
y_train.append(y[i])
else:
X_test.append(X.loc[i,:])
y_test.append(y[i])
X_train = np.asarray(X_train)
X_test = np.asarray(X_test)
y_train = np.asarray(y_train)
y_test = np.asarray(y_test)
print('X_train.shape', X_train.shape)
print('X_test.shape', X_test.shape)
print('y_train.shape', y_train.shape)
print('y_test.shape', y_test.shape)
def sample_binary_MNIST(list_digits=['0','1'], full_MNIST=None, noise_rate=0):
# get train and test set from MNIST of given two digits
# e.g., list_digits = ['0', '1']
if full_MNIST is not None:
X, y = full_MNIST
else:
X, y = fetch_openml('mnist_784', version=1, return_X_y=True)
X = X / 255.
idx = [i for i in np.arange(len(y)) if y[i] in list_digits] # list of indices where the label y is in list_digits
X01 = X.iloc[idx,:]
# print(type(X01))
# y01 = y.iloc[idx]
y01 = y[idx]
X_train = []
X_test = []
y_test = [] # list of integers 0 and 1s
y_train = [] # list of integers 0 and 1s
for i in np.arange(X01.shape[0]):
# for each example i, make it into train set with probabiliy 0.8 and into test set otherwise
U = np.random.rand() # Uniform([0,1]) variable
label = 0
if y01.iloc[i] == str(list_digits[1]):
label = 1
if U<0.8:
# add noise to the sampled images
if noise_rate > 0:
for j in np.arange(X01.shape[1]):
U1 = np.random.rand()
if U1 < noise_rate:
X01.iloc[i,j] += np.random.rand()
X_train.append(X01.iloc[i,:])
y_train.append(label)
else:
X_test.append(X01.iloc[i,:])
y_test.append(label)
X_train = np.asarray(X_train)
X_test = np.asarray(X_test)
y_train = np.asarray(y_train).reshape(-1,1)
y_test = np.asarray(y_test).reshape(-1,1)
return X_train, X_test, y_train, y_test
# X_train, X_test, y_train, y_test = sample_binary_MNIST(list_digits=['0','1'], full_MNIST=[X, y], noise_rate=0.5)
X_train, X_test, y_train, y_test = sample_binary_MNIST(list_digits=['0','1'], full_MNIST=[X, y])
print('X_train.shape', X_train.shape)
print('X_test.shape', X_test.shape)
print('y_train.shape', y_train.shape)
print('y_test.shape', y_test.shape)
print('y_test', y_test)
# plot normal images
# corrupted images
ncols = 4
fig, ax = plt.subplots(nrows=1, ncols=ncols, figsize=[15, 6.5])
for j in np.arange(ncols):
id = np.random.choice(np.arange(X_train.shape[0]))
ax[j].imshow(X_train[id,:].reshape(28,28))
plt.savefig('MNIST_ex_corrupted1.pdf', bbox_inches='tight')
def onehot2list(y, list_classes=None):
"""
y = n x k array, i th row = one-hot encoding of y[i] (e.g., [0,0,1,0,0])
output = list of class lables of length n
"""
if list_classes is None:
list_classes = np.arange(y.shape[1])
y_list = []
for i in np.arange(y.shape[0]):
idx = np.where(y[i,:]==1)
idx = idx[0][0]
y_list.append(list_classes[idx])
return y_list
def list2onehot(y, list_classes):
"""
y = list of class lables of length n
output = n x k array, i th row = one-hot encoding of y[i] (e.g., [0,0,1,0,0])
"""
Y = np.zeros(shape = [len(y), len(list_classes)], dtype=int)
for i in np.arange(Y.shape[0]):
for j in np.arange(len(list_classes)):
if y[i] == list_classes[j]:
Y[i,j] = 1
return Y
def sample_multiclass_MNIST(list_digits=['0','1', '2'], full_MNIST=None):
# get train and test set from MNIST of given digits
# e.g., list_digits = ['0', '1', '2']
if full_MNIST is not None:
X, y = full_MNIST
else:
X, y = fetch_openml('mnist_784', version=1, return_X_y=True)
X = X / 255.
Y = list2onehot(y.tolist(), list_digits)
idx = [i for i in np.arange(len(y)) if y[i] in list_digits] # list of indices where the label y is in list_digits
X01 = X.iloc[idx,:]
y01 = Y[idx,:]
X_train = []
X_test = []
y_test = [] # list of one-hot encodings (indicator vectors) of each label
y_train = [] # list of one-hot encodings (indicator vectors) of each label
for i in np.arange(X01.shape[0]):
# for each example i, make it into train set with probabiliy 0.8 and into test set otherwise
U = np.random.rand() # Uniform([0,1]) variable
if U<0.8:
X_train.append(X01.iloc[i,:])
y_train.append(y01[i,:].copy())
else:
X_test.append(X01.iloc[i,:])
y_test.append(y01[i,:].copy())
X_train = np.asarray(X_train)
X_test = np.asarray(X_test)
y_train = np.asarray(y_train)
y_test = np.asarray(y_test)
return X_train, X_test, y_train, y_test
# test
X_train, X_test, y_train, y_test = sample_multiclass_MNIST(list_digits=['0','1', '2'], full_MNIST=[X, y])
print('X_train.shape', X_train.shape)
print('X_test.shape', X_test.shape)
print('y_train.shape', y_train.shape)
print('y_test.shape', y_test.shape)
print('y_test', y_test)
###Output
X_train.shape (17400, 784)
X_test.shape (4370, 784)
y_train.shape (17400, 3)
y_test.shape (4370, 3)
y_test [[0 0 1]
[0 1 0]
[0 0 1]
...
[0 0 1]
[0 1 0]
[1 0 0]]
###Markdown
Logistic Regression
###Code
# sigmoid and logit function
def sigmoid(x):
return np.exp(x)/(1+np.exp(x))
# plot sigmoid function
fig, ax = plt.subplots(nrows=1, ncols=1, figsize=[10,3])
x = np.linspace(-7, 7, 100)
ax.plot(x, sigmoid(x), color='blue', label="$y=\sigma(x)=\exp(x)/(1+\exp(x))$")
plt.axhline(y=1, color='g', linestyle='--')
plt.axvline(x=0, color='g', linestyle='--')
ax.legend()
plt.savefig('sigmoid_ex.pdf', bbox_inches='tight')
def fit_LR_GD(Y, H, W0=None, sub_iter=100, stopping_diff=0.01):
'''
Convex optimization algorithm for Logistic Regression using Gradient Descent
Y = (n x 1), H = (p x n) (\Phi in lecture note), W = (p x 1)
Logistic Regression: Y ~ Bernoulli(Q), Q = sigmoid(H.T @ W)
MLE -->
Find \hat{W} = argmin_W ( sum_j ( log(1+exp(H_j.T @ W) ) - Y.T @ H.T @ W ) )
'''
if W0 is None:
W0 = np.random.rand(H.shape[0],1) #If initial coefficients W0 is None, randomly initialize
W1 = W0.copy()
i = 0
grad = np.ones(W0.shape)
while (i < sub_iter) and (np.linalg.norm(grad) > stopping_diff):
Q = 1/(1+np.exp(-H.T @ W1)) # probability matrix, same shape as Y
# grad = H @ (Q - Y).T + alpha * np.ones(W0.shape[1])
grad = H @ (Q - Y)
W1 = W1 - (np.log(i+1) / (((i + 1) ** (0.5)))) * grad
i = i + 1
# print('iter %i, grad_norm %f' %(i, np.linalg.norm(grad)))
return W1
def fit_LR_NR(Y, H, W0=None, sub_iter=100, stopping_diff=0.01):
'''
Convex optimization algorithm for Logistic Regression using Newton-Ralphson algorithm.
Y = (n x 1), H = (p x n) (\Phi in lecture note), W = (p x 1)
Logistic Regression: Y ~ Bernoulli(Q), Q = sigmoid(H.T @ W)
MLE -->
Find \hat{W} = argmin_W ( sum_j ( log(1+exp(H_j.T @ W) ) - Y.T @ H.T @ W ) )
'''
### Implement by yourself.
# fit logistic regression using GD
X_train, X_test, y_train, y_test = sample_binary_MNIST(['0', '1'], full_MNIST = [X,y])
# Feature matrix of size (p x n) = (feature dim x samples)
print(X_train.shape)
H_train = np.vstack((np.ones(X_train.shape[0]), X_train.T)) # add first row of 1's for bias features
print(H_train.shape)
W = fit_LR_GD(Y=y_train, H=H_train)
plt.imshow(W[1:,:].reshape(28,28))
# plot fitted logistic regression curve
# digit_list_list = [['0','1'],['0','7'],['2','3'],['2', '8']] # list of list of two digits
digit_list_list = [['4','7']] # list of list of two digits
# fit LR for each cases
W_array = []
for i in np.arange(len(digit_list_list)):
L = digit_list_list[i]
X_train, X_test, y_train, y_test = sample_binary_MNIST(list_digits=L, full_MNIST = [X,y])
H_train = np.vstack((np.ones(X_train.shape[0]), X_train.T)) # add first row of 1's for bias features
W = fit_LR_GD(Y=y_train, H=H_train)
W = fit_LR_GD(Y=y_train, H=H_train)
W_array.append(W.copy())
W_array = np.asarray(W_array)
# make plot
fig, ax = plt.subplots(nrows=1, ncols=len(digit_list_list), figsize=[16, 4])
for i in np.arange(len(digit_list_list)):
L = digit_list_list[i]
W = W_array[i]
im = ax.imshow(W[1:,:].reshape(28,28), vmin=np.min(W_array), vmax=np.max(W_array))
ax.title.set_text("LR coeff. for %s vs. %s" % (L[0], L[1]))
# ax[i].legend()
fig.subplots_adjust(right=0.9)
cbar_ax = fig.add_axes([0.92, 0.15, 0.01, 0.7])
fig.colorbar(im, cax=cbar_ax)
plt.savefig('LR_MNIST_training_ex.pdf', bbox_inches='tight')
def compute_accuracy_metrics(Y_test, P_pred, use_opt_threshold=False, verbose=False):
# y_test = binary label
# P_pred = predicted probability for y_test
# compuate various binary classification accuracy metrics
fpr, tpr, thresholds = metrics.roc_curve(Y_test, P_pred, pos_label=None)
mythre = thresholds[np.argmax(tpr - fpr)]
myauc = metrics.auc(fpr, tpr)
# print('!!! auc', myauc)
# Compute classification statistics
threshold = 0.5
if use_opt_threshold:
threshold = mythre
Y_pred = P_pred.copy()
Y_pred[Y_pred < threshold] = 0
Y_pred[Y_pred >= threshold] = 1
mcm = confusion_matrix(Y_test, Y_pred)
tn = mcm[0, 0]
tp = mcm[1, 1]
fn = mcm[1, 0]
fp = mcm[0, 1]
accuracy = (tp + tn) / (tp + tn + fp + fn)
sensitivity = tn / (tn + fp)
specificity = tp / (tp + fn)
precision = tp / (tp + fp)
fall_out = fp / (fp + tn)
miss_rate = fn / (fn + tp)
# Save results
results_dict = {}
results_dict.update({'Y_test': Y_test})
results_dict.update({'Y_pred': Y_pred})
results_dict.update({'AUC': myauc})
results_dict.update({'Opt_threshold': mythre})
results_dict.update({'Accuracy': accuracy})
results_dict.update({'Sensitivity': sensitivity})
results_dict.update({'Specificity': specificity})
results_dict.update({'Precision': precision})
results_dict.update({'Fall_out': fall_out})
results_dict.update({'Miss_rate': miss_rate})
if verbose:
for key in [key for key in results_dict.keys()]:
print('% s ===> %.3f' % (key, results_dict.get(key)))
return results_dict
# fit logistic regression using GD and compute binary classification accuracies
# Get train and test data
digits_list = ['4', '7']
X_train, X_test, y_train, y_test = sample_binary_MNIST(digits_list, full_MNIST = [X,y])
# Feature matrix of size (p x n) = (feature dim x samples)
list_train_size = [1,10, 30, 100]
# train the regression coefficients for all cases
W_list = []
results_list = []
for i in np.arange(len(list_train_size)):
size = list_train_size[i]
idx = np.random.choice(np.arange(len(y_train)), size)
X_train0 = X_train[idx, :]
y_train0 = y_train[idx]
# Train the logistic regression model
H_train0 = np.vstack((np.ones(X_train0.shape[0]), X_train0.T)) # add first row of 1's for bias features
W = fit_LR_GD(Y=y_train0, H=H_train0)
W_list.append(W.copy()) # make sure use copied version of W since the same name is overrided in the loop
# Get predicted probabilities
H_test = np.vstack((np.ones(X_test.shape[0]), X_test.T))
Q = 1 / (1 + np.exp(-H_test.T @ W)) # predicted probabilities for y_test
# Compute binary classification accuracies
results_dict = compute_accuracy_metrics(Y_test=y_test, P_pred = Q)
results_dict.update({'train size':X_train0.shape[0]}) # add the train data size to the results dictionary
results_list.append(results_dict.copy())
# Print out the results
"""
keys_list = [i for i in results_dict.keys()]
for key in keys_list:
if key not in ['Y_test', 'Y_pred']:
print('%s = %f' % (key, results_dict.get(key)))
"""
# make plot
fig, ax = plt.subplots(nrows=1, ncols=len(list_train_size), figsize=[16, 4])
for i in np.arange(len(list_train_size)):
result_dict = results_list[i]
W = W_list[i][1:,:]
im = ax[i].imshow(W.copy().reshape(28,28), vmin=np.min(W_list), vmax=np.max(W_list))
subtitle = ""
keys_list = [i for i in results_list[i].keys()]
for key in keys_list:
if key not in ['Y_test', 'Y_pred', 'AUC', 'Opt_threshold']:
subtitle += "\n" + str(key) + " = " + str(np.round(results_list[i].get(key),3))
# print('%s = %f' % (key, results_list[i].get(key)))
ax[i].set_title('Opt. regression coeff.', fontsize=13)
ax[i].set_xlabel(subtitle, fontsize=20)
fig.subplots_adjust(right=0.9)
fig.suptitle("MNIST Binary Classification by LR for %s vs. %s" % (digits_list[0], digits_list[1]), fontsize=20, y=1.05)
cbar_ax = fig.add_axes([0.92, 0.15, 0.01, 0.7])
fig.colorbar(im, cax=cbar_ax)
plt.savefig('LR_MNIST_test_ex1.pdf', bbox_inches='tight')
###Output
<ipython-input-14-7ee600204434>:18: RuntimeWarning: overflow encountered in exp
Q = 1/(1+np.exp(-H.T @ W1)) # probability matrix, same shape as Y
<ipython-input-18-980e28468c77>:27: RuntimeWarning: overflow encountered in exp
Q = 1 / (1 + np.exp(-H_test.T @ W)) # predicted probabilities for y_test
###Markdown
Multiclass Logistic Regression
###Code
def sample_multiclass_MNIST(list_digits=['0','1', '2'], full_MNIST=None):
# get train and test set from MNIST of given digits
# e.g., list_digits = ['0', '1', '2']
if full_MNIST is not None:
X, y = full_MNIST
else:
X, y = fetch_openml('mnist_784', version=1, return_X_y=True)
X = X / 255.
Y = list2onehot(y.tolist(), list_digits)
idx = [i for i in np.arange(len(y)) if y[i] in list_digits] # list of indices where the label y is in list_digits
X01 = X.iloc[idx,:]
y01 = Y[idx,:]
X_train = []
X_test = []
y_test = [] # list of one-hot encodings (indicator vectors) of each label
y_train = [] # list of one-hot encodings (indicator vectors) of each label
for i in np.arange(X01.shape[0]):
# for each example i, make it into train set with probabiliy 0.8 and into test set otherwise
U = np.random.rand() # Uniform([0,1]) variable
if U<0.8:
X_train.append(X01.iloc[i,:])
y_train.append(y01[i,:].copy())
else:
X_test.append(X01.iloc[i,:])
y_test.append(y01[i,:].copy())
X_train = np.asarray(X_train)
X_test = np.asarray(X_test)
y_train = np.asarray(y_train)
y_test = np.asarray(y_test)
return X_train, X_test, y_train, y_test
# test
X_train, X_test, y_train, y_test = sample_multiclass_MNIST(list_digits=['0','1', '2'], full_MNIST=[X, y])
print('X_train.shape', X_train.shape)
print('X_test.shape', X_test.shape)
print('y_train.shape', y_train.shape)
print('y_test.shape', y_test.shape)
print('y_test', y_test)
def fit_MLR_GD(Y, H, W0=None, sub_iter=100, stopping_diff=0.01):
'''
Convex optimization algorithm for Multiclass Logistic Regression using Gradient Descent
Y = (n x k), H = (p x n) (\Phi in lecture note), W = (p x k)
Multiclass Logistic Regression: Y ~ vector of discrete RVs with PMF = sigmoid(H.T @ W)
MLE -->
Find \hat{W} = argmin_W ( sum_j ( log(1+exp(H_j.T @ W) ) - Y.T @ H.T @ W ) )
'''
k = Y.shape[1] # number of classes
if W0 is None:
W0 = np.random.rand(H.shape[0],k) #If initial coefficients W0 is None, randomly initialize
W1 = W0.copy()
i = 0
grad = np.ones(W0.shape)
while (i < sub_iter) and (np.linalg.norm(grad) > stopping_diff):
Q = 1/(1+np.exp(-H.T @ W1)) # probability matrix, same shape as Y
# grad = H @ (Q - Y).T + alpha * np.ones(W0.shape[1])
grad = H @ (Q - Y)
W1 = W1 - (np.log(i+1) / (((i + 1) ** (0.5)))) * grad
i = i + 1
# print('iter %i, grad_norm %f' %(i, np.linalg.norm(grad)))
return W1
def custom_softmax(a):
"""
given an array a = [a_1, .. a_k], compute the softmax distribution p = [p_1, .. , p_k] where p_i \propto exp(a_i)
"""
a1 = a - np.max(a)
p = np.exp(a1)
if type(a) is list:
p = p/np.sum(p)
else:
row_sum = np.sum(p, axis=1)
p = p/row_sum[:, np.newaxis]
return p
print(np.sum(custom_softmax([1,20,30,50])))
a= np.ones((2,3))
print(softmax(a))
def multiclass_accuracy_metrics(Y_test, P_pred, class_labels=None, use_opt_threshold=False):
# Y_test = multiclass one-hot encoding labels
# P_pred = predicted probability for y_test
# compuate various classification accuracy metrics
# print('len input test pred',len(Y_test),len(P_pred))
results_dict = {}
y_test = []
y_pred = []
for i in np.arange(Y_test.shape[0]):
for j in np.arange(Y_test.shape[1]):
if Y_test[i,j] == 1:
y_test.append(j) # actual value
if(P_pred[i,j] == np.max(P_pred[i,:])): # or np.isnan(P_pred[i,j])):
y_pred.append(j)
confusion_mx = metrics.confusion_matrix(y_test, y_pred)
print('!!! confusion_mx', confusion_mx)
results_dict.update({'confusion_mx':confusion_mx})
results_dict.update({'Accuracy':np.trace(confusion_mx)/np.sum(np.sum(confusion_mx))})
print('!!! Accuracy', results_dict.get('Accuracy'))
return results_dict
# fit multiclass logistic regression using GD
list_digits=['0', '1', '2']
X_train, X_test, y_train, y_test = sample_multiclass_MNIST(list_digits=list_digits, full_MNIST = [X,y])
# Feature matrix of size (p x n) = (feature dim x samples)
H_train = np.vstack((np.ones(X_train.shape[0]), X_train.T)) # add first row of 1's for bias features
W = fit_MLR_GD(Y=y_train, H=H_train)
print('!! W.shape', W.shape)
# Get predicted probabilities
H_test = np.vstack((np.ones(X_test.shape[0]), X_test.T))
Q = softmax(H_test.T @ W.copy()) # predicted probabilities for y_test # Uses sklearn's softmax for numerical stability
print('!!! y_test.shape', y_test.shape)
print('!!! Q', Q)
results_dict = multiclass_accuracy_metrics(Y_test=y_test, P_pred=Q)
confusion_mx = results_dict.get('results_dict')
# make plot
fig, ax = plt.subplots(nrows=1, ncols=len(list_digits), figsize=[12, 4])
for i in np.arange(len(list_digits)):
L = list_digits[i]
im = ax[i].imshow(W[1:,i].reshape(28,28), vmin=np.min(W), vmax=np.max(W))
ax[i].title.set_text("MLR coeff. for %s" % L )
# ax[i].legend()
# if i == len(list_digits) - 1:
cbar_ax = fig.add_axes([0.92, 0.15, 0.01, 0.7])
fig.colorbar(im, cax=cbar_ax)
plt.savefig('MLR_MNIST_ex1.pdf', bbox_inches='tight')
# fit multiclass logistic regression using GD and compute multiclass classification accuracies
# Get train and test data
digits_list = ['0', '1', '2', '3', '4']
X_train, X_test, y_train, y_test = sample_multiclass_MNIST(digits_list, full_MNIST = [X,y])
# Feature matrix of size (p x n) = (feature dim x samples)
list_train_size = [1,10, 30, 100]
# train the regression coefficients for all cases
W_list = []
results_list = []
for i in np.arange(len(list_train_size)):
size = list_train_size[i]
idx = np.random.choice(np.arange(len(y_train)), size)
X_train0 = X_train[idx, :]
y_train0 = y_train[idx, :]
# Train the multiclass logistic regression model
H_train0 = np.vstack((np.ones(X_train0.shape[0]), X_train0.T)) # add first row of 1's for bias features
W = fit_MLR_GD(Y=y_train0, H=H_train0)
W_list.append(W.copy()) # make sure use copied version of W since the same name is overrided in the loop
# Get predicted probabilities
H_test = np.vstack((np.ones(X_test.shape[0]), X_test.T))
Q = softmax(H_test.T @ W.copy()) # predicted probabilities for y_test # Uses sklearn's softmax for numerical stability
results_dict = multiclass_accuracy_metrics(Y_test=y_test, P_pred=Q)
results_dict.update({'train size':X_train0.shape[0]}) # add the train data size to the results dictionary
results_list.append(results_dict.copy())
# make plot
fig, ax = plt.subplots(nrows=len(list_train_size), ncols=len(digits_list)+1, figsize=[15, 10])
for i in np.arange(len(list_train_size)):
for j in np.arange(len(digits_list)+1):
if j < len(digits_list):
L = digits_list[j]
W = W_list[i]
im = ax[i,j].imshow(W[1:,j].reshape(28,28), vmin=np.min(W), vmax=np.max(W))
ax[i,j].title.set_text("MLR coeff. for %s" % L )
if j == 0:
ax[i,j].set_ylabel("train size = %i" % results_list[i].get("train size"), fontsize=13)
divider = make_axes_locatable(ax[i,j])
cax = divider.append_axes('right', size='5%', pad=0.05)
fig.colorbar(im, cax=cax)
else:
confusion_mx = results_list[i].get("confusion_mx")
im_confusion = ax[i,j].matshow(confusion_mx)
# ax[i,j].set_title("Confusion Matrix")
ax[i,j].set_xlabel("Confusion Matrix", fontsize=13)
# ax[i].legend()
# if i == len(list_digits) - 1:
divider = make_axes_locatable(ax[i,j])
cax = divider.append_axes('right', size='5%', pad=0.05)
fig.colorbar(im_confusion, cax=cax)
plt.subplots_adjust(wspace=0.3, hspace=0.3)
plt.savefig('MLR_MNIST_test_ex2.pdf', bbox_inches='tight')
print(X_train.shape)
###Output
(17437, 784)
###Markdown
HW 7 1
###Code
def random_padding(img, thickness=1):
# img = a x b image
[a,b] = img.shape
Y = np.zeros(shape=[a+thickness, b+thickness])
r_loc = np.random.choice(np.arange(thickness+1))
c_loc = np.random.choice(np.arange(thickness+1))
Y[r_loc:r_loc+a, c_loc:c_loc+b] = img
return Y
def sample_multiclass_MNIST_padding(list_digits=['0','1', '2'], full_MNIST=[X,y], padding_thickness=10):
# get train and test set from MNIST of given digits
# e.g., list_digits = ['0', '1', '2']
# pad each 28 x 28 image with zeros so that it has now "padding_thickness" more rows and columns
# The original image is superimposed at a uniformly chosen location
if full_MNIST is not None:
X, y = full_MNIST
else:
X, y = fetch_openml('mnist_784', version=1, return_X_y=True)
X = X / 255.
Y = list2onehot(y.tolist(), list_digits)
idx = [i for i in np.arange(len(y)) if y[i] in list_digits] # list of indices where the label y is in list_digits
X01 = X.iloc[idx,:]
y01 = Y[idx,:]
X_train = []
X_test = []
y_test = [] # list of one-hot encodings (indicator vectors) of each label
y_train = [] # list of one-hot encodings (indicator vectors) of each label
for i in trange(X01.shape[0]):
# for each example i, make it into train set with probabiliy 0.8 and into test set otherwise
U = np.random.rand() # Uniform([0,1]) variable
img_padded = random_padding(X01.iloc[i,:].values.reshape(28,28), thickness=padding_thickness)
img_padded_vec = img_padded.reshape(1,-1)
if U<0.8:
X_train.append(img_padded_vec[0,:].copy())
y_train.append(y01[i,:].copy())
else:
X_test.append(img_padded_vec[0,:].copy())
y_test.append(y01[i,:].copy())
X_train = np.asarray(X_train)
X_test = np.asarray(X_test)
y_train = np.asarray(y_train)
y_test = np.asarray(y_test)
return X_train, X_test, y_train, y_test
# compute comparative multiclass classification metrics on test data (LR)
padding_list = [0, 5, 10, 15, 20]
list_digits=['0','1','2','3','4']
## Train
train_size_list = [50, 100, 200]
# make plot
ncols = len(train_size_list)
fig, ax = plt.subplots(nrows=1, ncols=ncols, figsize=[13,5])
W_list = []
results_list = []
for t in np.arange(len(train_size_list)): # train size
print(t)
accuracy_list_test = []
accuracy_list_train = []
train_size = train_size_list[t]
for thickness in padding_list: # padding size
# Data preprocessing
X_train, X_test, y_train, y_test = sample_multiclass_MNIST_padding(list_digits=list_digits,
full_MNIST=[X,y],
padding_thickness=thickness)
idx = np.random.choice(np.arange(len(y_train)), train_size) # sample random indices of train size
X_train0 = X_train[idx, :]/np.max(X_train) #scale 0 to 1
y_train0 = y_train[idx, :]
idx = np.random.choice(np.arange(len(y_test)), 100) # sample 100 test
X_test0 = X_test[idx, :]/np.max(X_test)
y_test0 = y_test[idx, :]
# LR train
H_train0 = np.vstack((np.ones(X_train0.shape[0]), X_train0.T)) # add first row of 1's for bias features
# print(H_train0.shape)
# print(H_train0[0:6])
W = fit_MLR_GD(Y=y_train0, H=H_train0)
W_list.append(W.copy()) # make sure use copied version of W since the same name is overrided in the loop
# Get predicted probabilities for train and test
Q_train = softmax(H_train0.T @ W.copy())
H_test0= np.vstack((np.ones(X_test0.shape[0]), X_test0.T))
Q_test = softmax(H_test0.T @ W.copy())
# print(Q_train.shape, y_train0.shape, Q_test.shape,y_test0.shape)
# results_dict.update({'train size':train_size}) # add the train data size to the results dictionary
results_train = multiclass_accuracy_metrics(Y_test=y_train0, P_pred=Q_train)
results_test = multiclass_accuracy_metrics(Y_test=y_test0, P_pred=Q_test)
accuracy_list_train.append(results_train.get('Accuracy'))
accuracy_list_test.append(results_test.get('Accuracy'))
# print(accuracy_list_train)
# print(accuracy_list_test)
## Plot
ax[t].plot(padding_list, accuracy_list_train, color='blue', label="train accuracy")
ax[t].plot(padding_list, accuracy_list_test, color='red', label="test accuracy")
ax[t].set_xlabel('Padding thickness', fontsize=15)
ax[t].set_ylabel('Classification Accuracy', fontsize=15)
ax[t].title.set_text("num training ex = %i" % (train_size))
ax[t].legend(fontsize=15)
plt.tight_layout(rect=[0, 0.03, 1, 0.9])
plt.savefig('MNIST_LR_accuracy_padding_ex.pdf')
def fit_MNB(Y, H):
'''
Fit Multinomial Naive Bayes Calssifier
Use the Maximum Likelihood prior and class conditional probabilities (in closed forms)
Y = (n x k), H = (p x n) (\Phi in lecture note), W = (p x k)
prior on class labels = empirical PMF = [ # class i examples / total ]
class-conditional for class i = [ # word j in class i examples / # words in class i examples]
Output = prior (k, ), class_conditional_PMF = (k, p)
'''
k = Y.shape[1] # number of classes
# print(k)
prior = np.sum(Y, axis=0)/np.sum(np.sum(Y, axis=0)) # prior prob of digit
class_conditional_PMF = [] # prob of pixel per digit
for i in np.arange(Y.shape[1]): # for each digit
idx = np.where(Y[:,i]==1) # cols that equal digit
sub_H = H[:,idx[0]] + 0.01 # add psuedocount
pixel_ct_per_digit = np.sum(sub_H, axis=1) # sum across columns (images)
class_conditional_PMF.append(pixel_ct_per_digit/np.sum(pixel_ct_per_digit)) # turn into prob
return prior, np.asarray(class_conditional_PMF)
#test
prior, class_conditional_PMF = fit_MNB(Y=y_train0, H=X_train0.T)
print(prior)
print(class_conditional_PMF)
def predict_MNB(X_test, prior, class_conditional_PMF):
'''
Compute predicted PMF for the test data given prior and class_conditional_PMF
Simple use of Bayes' Theorem
X_test = (p x n) (words x docs)
'''
P = class_conditional_PMF / np.min(class_conditional_PMF) # normalize so that log(P) is not too small
# print(P)
Q = X_test @ P.T
# Q = np.exp((X_test @ np.log(P).T)/1000) # / 1000 to prevent inf
# print('Q1',Q)
Q = Q * np.repeat(prior[:, np.newaxis], repeats=Q.shape[0], axis=1).T
# print('Q2',Q)
sum_of_rows = Q.sum(axis=1)
return Q / sum_of_rows[:, np.newaxis]
# test
predictive_PMF = predict_MNB(X_test0, prior, class_conditional_PMF)
plt.plot(predictive_PMF[4])
print(predictive_PMF.shape)
# compute comparative multiclass classification metrics on test data (NB)
padding_list = [0, 5, 10, 15, 20]
list_digits=['0','1','2','3','4']
## Train
train_size_list = [50, 100, 200]
# train_size_list = [100, 200, 400]
# make plot
ncols = len(train_size_list)
fig, ax = plt.subplots(nrows=1, ncols=ncols, figsize=[13,5])
for t in np.arange(len(train_size_list)): # train size
# for t in np.arange(1): # train size
accuracy_list_test = []
accuracy_list_train = []
train_size = train_size_list[t]
for thickness in padding_list: # padding size
# Data preprocessing
X_train, X_test, y_train, y_test = sample_multiclass_MNIST_padding(list_digits=list_digits,
full_MNIST=[X,y],
padding_thickness=thickness)
idx = np.random.choice(np.arange(len(y_train)), train_size) # sample random indices of train size
X_train0 = X_train[idx, :]/np.max(X_train) #scale 0 to 1
y_train0 = y_train[idx, :]
idx = np.random.choice(np.arange(len(y_test)), 100) # sample 100 test
X_test0 = X_test[idx, :]/np.max(X_test)
y_test0 = y_test[idx, :]
# NBC train
prior, class_conditional_PMF = fit_MNB(Y=y_train0, H=X_train0.T)
# Get predicted probabilities for train and test
Q_train = predict_MNB(X_train0, prior, class_conditional_PMF)
Q_test = predict_MNB(X_test0, prior, class_conditional_PMF)
# print(Q_test[0:6])
# print(Q_train.shape,Q_test.shape)
results_train = multiclass_accuracy_metrics(Y_test=y_train0, P_pred=Q_train)
results_test = multiclass_accuracy_metrics(Y_test=y_test0, P_pred=Q_test)
accuracy_list_train.append(results_train.get('Accuracy'))
accuracy_list_test.append(results_test.get('Accuracy'))
## Plot
ax[t].plot(padding_list, accuracy_list_train, color='blue', label="train accuracy")
ax[t].plot(padding_list, accuracy_list_test, color='red', label="test accuracy")
ax[t].set_xlabel('Padding thickness', fontsize=15)
ax[t].set_ylabel('Classification Accuracy', fontsize=15)
ax[t].title.set_text("num training ex = %i" % (train_size))
ax[t].legend(fontsize=15)
plt.tight_layout(rect=[0, 0.03, 1, 0.9])
plt.savefig('MNIST_MNB_accuracy_padding_ex.pdf')
###Output
100%|██████████████████████████████████████████████████████████████████████████| 35735/35735 [00:05<00:00, 6448.37it/s]
###Markdown
Probit Regression
###Code
# probit function
from scipy.stats import norm
def probit(x):
return norm.cdf(x) # Yes, it is exactly the standard normal CDF.
# plot probit and sigmoid function
fig, ax = plt.subplots(nrows=1, ncols=1, figsize=[10,3])
x = np.linspace(-7, 7, 100)
ax.plot(x, sigmoid(x), color='blue', label="$y=\sigma(x)=\exp(x)/(1+\exp(x))$")
ax.plot(x, probit(x), color='red', label="$y=\psi(x)=Probit(x)$")
plt.axhline(y=1, color='g', linestyle='--')
plt.axvline(x=0, color='g', linestyle='--')
ax.legend()
plt.savefig('probit_ex.pdf', bbox_inches='tight')
def fit_PR_GD(Y, H, W0=None, sub_iter=100, stopping_diff=0.01):
'''
Convex optimization algorithm for Probit Regression using Gradient Descent
Y = (n x 1), H = (p x n) (\Phi in lecture note), W = (p x 1)
Logistic Regression: Y ~ Bernoulli(Q), Q = Probit(H.T @ W)
'''
if W0 is None:
W0 = 1-2*np.random.rand(H.shape[0],1) #If initial coefficients W0 is None, randomly initialize from [-1,1]
W1 = W0.copy()
i = 0
grad = np.ones(W0.shape)
while (i < sub_iter) and (np.linalg.norm(grad) > stopping_diff):
Q = norm.pdf(H.T @ W1) * ( (1-Y)/norm.cdf(-H.T @ W1) - Y/norm.cdf(H.T @ W1) )
grad = H @ Q
W1 = W1 - (np.log(i+1) / (((i + 1) ** (0.5)))) * grad
i = i + 1
# print('iter %i, grad_norm %f' %(i, np.linalg.norm(grad)))
return W1
# plot fitted probit regression curve
digit_list_list = [['0','1'],['0','7'],['2','3'],['2', '8']] # list of list of two digits
# fit LR for each cases
W_array = []
for i in np.arange(len(digit_list_list)):
L = digit_list_list[i]
X_train, X_test, y_train, y_test = sample_binary_MNIST(list_digits=L, full_MNIST = [X,y], noise_rate=0.5)
H_train = np.vstack((np.ones(X_train.shape[0]), X_train.T)) # add first row of 1's for bias features
W = fit_PR_GD(Y=y_train, H=H_train/1000)
W = fit_PR_GD(Y=y_train, H=H_train/1000)
W_array.append(W.copy())
W_array = np.asarray(W_array)
# make plot
fig, ax = plt.subplots(nrows=1, ncols=len(digit_list_list), figsize=[16, 4])
for i in np.arange(len(digit_list_list)):
L = digit_list_list[i]
W = W_array[i]
im = ax[i].imshow(W[1:,:].reshape(28,28), vmin=np.min(W_array), vmax=np.max(W_array))
ax[i].title.set_text("LR coeff. for %s vs. %s" % (L[0], L[1]))
# ax[i].legend()
fig.subplots_adjust(right=0.9)
cbar_ax = fig.add_axes([0.92, 0.15, 0.01, 0.7])
fig.colorbar(im, cax=cbar_ax)
plt.savefig('PR_MNIST_training_ex.pdf', bbox_inches='tight')
# fit probit regression using GD and compute binary classification accuracies
# Get train and test data
digits_list = ['4', '7']
X_train, X_test, y_train, y_test = sample_binary_MNIST(digits_list, full_MNIST = [X,y])
# Feature matrix of size (p x n) = (feature dim x samples)
list_train_size = [1,10, 30, 100]
# train the regression coefficients for all cases
W_list = []
results_list = []
for i in np.arange(len(list_train_size)):
size = list_train_size[i]
idx = np.random.choice(np.arange(len(y_train)), size)
X_train0 = X_train[idx, :]
y_train0 = y_train[idx]
# Train the logistic regression model
H_train0 = np.vstack((np.ones(X_train0.shape[0]), X_train0.T)) # add first row of 1's for bias features
W = fit_PR_GD(Y=y_train0, H=H_train0/100) # reduce the scale of H for numerical stability
W_list.append(W.copy()) # make sure use copied version of W since the same name is overrided in the loop
# Get predicted probabilities
H_test = np.vstack((np.ones(X_test.shape[0]), X_test.T))
Q = 1 / (1 + np.exp(-H_test.T @ W)) # predicted probabilities for y_test
# Compute binary classification accuracies
results_dict = compute_accuracy_metrics(Y_test=y_test, P_pred = Q)
results_dict.update({'train size':X_train0.shape[0]}) # add the train data size to the results dictionary
results_list.append(results_dict.copy())
# Print out the results
"""
keys_list = [i for i in results_dict.keys()]
for key in keys_list:
if key not in ['Y_test', 'Y_pred']:
print('%s = %f' % (key, results_dict.get(key)))
"""
# make plot
fig, ax = plt.subplots(nrows=1, ncols=len(list_train_size), figsize=[16, 4])
for i in np.arange(len(list_train_size)):
result_dict = results_list[i]
W = W_list[i][1:,:]
im = ax[i].imshow(W.copy().reshape(28,28), vmin=np.min(W_list), vmax=np.max(W_list))
subtitle = ""
keys_list = [i for i in results_list[i].keys()]
for key in keys_list:
if key not in ['Y_test', 'Y_pred', 'AUC', 'Opt_threshold']:
subtitle += "\n" + str(key) + " = " + str(np.round(results_list[i].get(key),3))
# print('%s = %f' % (key, results_list[i].get(key)))
ax[i].set_title('Opt. regression coeff.', fontsize=13)
ax[i].set_xlabel(subtitle, fontsize=20)
fig.subplots_adjust(right=0.9)
fig.suptitle("MNIST Binary Classification by Probit for %s vs. %s" % (digits_list[0], digits_list[1]), fontsize=20, y=1.05)
cbar_ax = fig.add_axes([0.92, 0.15, 0.01, 0.7])
fig.colorbar(im, cax=cbar_ax)
plt.savefig('PR_MNIST_test_ex1.pdf', bbox_inches='tight')
###Output
<class 'pandas.core.frame.DataFrame'>
0 th iter
1000 th iter
2000 th iter
3000 th iter
4000 th iter
5000 th iter
6000 th iter
7000 th iter
8000 th iter
9000 th iter
10000 th iter
11000 th iter
12000 th iter
13000 th iter
14000 th iter
###Markdown
HW5 1.1 MNB
###Code
def fit_MNB(Y, H, psuedocount=1):
'''
Fit Multinomial Naive Bayes Calssifier
Use the Maximum Likelihood prior and class conditional probabilities (in closed forms)
Y = (n x k), H = (p x n) (\Phi in lecture note), W = (p x k)
prior on class labels = empirical PMF = [ # class i examples / total ]
class-conditional for class i = [ # word j in class i examples / # words in class i examples]
Output = prior (k, ), class_conditional_PMF = (k, p)
'''
k = Y.shape[1] # number of classes (columns)
prior = np.sum(Y, axis=0)/np.sum(np.sum(Y, axis=0))
class_conditional_PMF = []
for i in np.arange(Y.shape[1]):
idx = np.where(Y[:,i]==1) # rows of class i
sub_H = H[:,idx[0]] + psuedocount # add psuedocount
pixel_value_per_class = np.sum(sub_H, axis=1) # sum pixel value across all imgs of class i
if np.sum(pixel_value_per_class) == 0:
print('div0')
class_conditional_PMF.append(pixel_value_per_class/np.sum(pixel_value_per_class)) # turn into probability
return prior, np.asarray(class_conditional_PMF)
#test
prior, class_conditional_PMF = fit_MNB(Y=y_train, H=X_train.T)
digits_list = ['4', '7']
X_train, X_test, y_train, y_test = sample_binary_MNIST(digits_list, full_MNIST = [X,y])
list_classes = [0,1] # 4 and 7
y_train = list2onehot(y_train.tolist(), list_classes)
y_test = list2onehot(y_test.tolist(), list_classes)
fig, ax = plt.subplots(nrows=1, ncols=1, figsize=[15, 5])
for i in [0,1]:
ax.plot(np.arange(X.shape[1]), class_conditional_PMF[i], label=digits_list[i])
ax.legend(fontsize='15')
plt.suptitle("Class conditional PMFs", fontsize='15')
def predict_MNB(X_test, prior, class_conditional_PMF):
'''
Compute predicted PMF for the test data given prior and class_conditional_PMF
Simple use of Bayes' Theorem
X_test = (p x n) (words x docs)
'''
# print(X_test.shape)
# print(class_conditional_PMF.shape)
P = class_conditional_PMF / np.min(class_conditional_PMF) # normalize so that log(P) is not too small
Q = np.exp(X_test @ np.log(P).T)
Q = Q * np.repeat(prior[:, np.newaxis], repeats=Q.shape[0], axis=1).T
sum_of_rows = Q.sum(axis=1)
return Q / sum_of_rows[:, np.newaxis]
# test
predictive_PMF = predict_MNB(X_test, prior, class_conditional_PMF)
plt.plot(predictive_PMF[4])
def list2onehot(y, list_classes):
"""
y = list of class lables of length n
output = n x k array, i th row = one-hot encoding of y[i] (e.g., [0,0,1,0,0])
"""
Y = np.zeros(shape = [len(y), len(list_classes)], dtype=int)
for i in np.arange(Y.shape[0]):
for j in np.arange(len(list_classes)):
if y[i][0] == list_classes[j]:
Y[i,j] = 1
break
return Y
def predict_MNB(X_test, prior, class_conditional_PMF):
'''
Compute predicted PMF for the test data given prior and class_conditional_PMF
Simple use of Bayes' Theorem
X_test = (p x n) (words x docs)
'''
print(X_test.shape)
print(class_conditional_PMF.shape)
P = class_conditional_PMF / np.min(class_conditional_PMF) # normalize so that log(P) is not too small
Q = np.exp(X_test @ np.log(P).T)
Q = Q * np.repeat(prior[:, np.newaxis], repeats=Q.shape[0], axis=1).T
sum_of_rows = Q.sum(axis=1)
return Q / sum_of_rows[:, np.newaxis]
# test
# predictive_PMF = predict_MNB(X_test, prior, class_conditional_PMF)
# plt.plot(predictive_PMF[4])
# plt.plot(predictive_PMF[2])
# Get train and test data
digits_list = ['4', '7']
X_train, X_test, y_train, y_test = sample_binary_MNIST(digits_list, full_MNIST = [X,y])
list_classes = [0,1] # 4 and 7
y_train = list2onehot(y_train.tolist(), list_classes)
y_test_onehot = list2onehot(y_test.tolist(), list_classes)
print(y_test[:3],y_test_onehot[:3])
y_test.shape
# fit MNB and compute binary classification accuracies
list_train_size = [3,10, 30, 100]
# train the coefficients
cc_PMF_list = []
results_list = []
for i in np.arange(len(list_train_size)):
size = list_train_size[i]
idx = np.random.choice(np.arange(len(y_train)), size) # sample size elts from X_train
X_train0 = X_train[idx, :]
y_train0 = y_train[idx]
# Train the MNB model
prior, class_conditional_PMF = fit_MNB(Y=y_train0, H=X_train0.T,psuedocount = 0.01)
cc_PMF_list.append(class_conditional_PMF.copy()) # make sure use copied version of W since the same name is overrided in the loop
# get predictive probabilities
Q = predict_MNB(X_test, prior, class_conditional_PMF)
# take max for prediction
Q_pred = np.asarray([np.flatnonzero(q == max(q))[0] for q in Q])
# print(Q_pred[:10])
# Compute binary classification accuracies
results_dict = compute_accuracy_metrics(Y_test=y_test, P_pred = Q_pred)
results_dict.update({'train size':X_train0.shape[0]}) # add the train data size to the results dictionary
results_list.append(results_dict.copy())
# print(results_list)
# make plot
fig, ax = plt.subplots(nrows=1, ncols=len(list_train_size), figsize=[16, 4])
for i in np.arange(len(list_train_size)):
# result_dict = results_list[i]
W = cc_PMF_list[i][1]
# print(np.min(cc_PMF_list), np.max(cc_PMF_list))
im = ax[i].imshow(W.copy().reshape(28,28), vmin=np.min(cc_PMF_list), vmax=np.max(cc_PMF_list))
subtitle = ""
keys_list = [i for i in results_list[i].keys()]
for key in keys_list:
if key not in ['Y_test', 'Y_pred', 'AUC', 'Opt_threshold']:
subtitle += "\n" + str(key) + " = " + str(np.round(results_list[i].get(key),3))
# print('%s = %f' % (key, results_list[i].get(key)))
ax[i].set_title('Opt. regression coeff.', fontsize=13)
ax[i].set_xlabel(subtitle, fontsize=20)
fig.subplots_adjust(right=0.9)
fig.suptitle("MNIST Binary Classification by MNB for %s vs. %s" % (digits_list[0], digits_list[1]), fontsize=20, y=1.05)
cbar_ax = fig.add_axes([0.92, 0.15, 0.01, 0.7])
fig.colorbar(im, cax=cbar_ax)
plt.savefig('PR_MNIST_test_ex2.pdf', bbox_inches='tight')
###Output
div0
div0
###Markdown
HW5 1.2
###Code
# Get train and test data
np.random.seed(2)
digits_list = ['0', '1', '2', '3', '4']
X_train, X_test, y_train, y_test = sample_multiclass_MNIST(digits_list, full_MNIST = [X,y])
# y_test = np.asarray(onehot2list(y_test))
list_train_size = [5, 30, 100, 500]
cc_PMF_list = [] # train for each size
results_list = []
for i in np.arange(len(list_train_size)):
size = list_train_size[i]
if size == 5:
idx = np.array([0,1,2,3,5])
else:
idx = np.random.choice(np.arange(len(y_train)), size)
X_train0 = X_train[idx, :]
y_train0 = y_train[idx, :]
# Train the MNB model
prior, class_conditional_PMF = fit_MNB(Y=y_train0, H=X_train0.T,psuedocount = 0.01)
cc_PMF_list.append(class_conditional_PMF.copy()) # make sure use copied version of W since the same name is overrided in the loop
# get predictive probabilities
Q = predict_MNB(X_test, prior, class_conditional_PMF)
print("pred-probs:",Q[0])
# take max for prediction
# Q_pred = np.asarray([np.flatnonzero(q == max(q))[0] for q in Q])
# print("predictions:",Q_pred[:10])
# Compute binary classification accuracies
results_dict = multiclass_accuracy_metrics(Y_test=y_test, P_pred = Q)
results_dict.update({'train size':X_train0.shape[0]}) # add the train data size to the results dictionary
results_list.append(results_dict.copy())
# print(len(cc_PMF_list[0])) # pixel pmfs for each of 5 digits of first train size
# print(len(cc_PMF_list[0][0])) # first pixel PMFs for first train size
# make plot
fig, ax = plt.subplots(nrows=len(list_train_size), ncols=len(digits_list)+1, figsize=[15, 10])
for i in np.arange(len(list_train_size)):
for j in np.arange(len(digits_list)+1):
if j < len(digits_list):
L = digits_list[j]
W = cc_PMF_list[i]
im = ax[i,j].imshow(W[j].reshape(28,28), vmin=np.min(W), vmax=np.max(W))
# im = ax[i,j].imshow(W[1:,j].reshape(28,28), vmin=np.min(W), vmax=np.max(W))
ax[i,j].title.set_text("MLR coeff. for %s" % L )
if j == 0:
ax[i,j].set_ylabel("train size = %i" % results_list[i].get("train size"), fontsize=13)
divider = make_axes_locatable(ax[i,j])
cax = divider.append_axes('right', size='5%', pad=0.05)
fig.colorbar(im, cax=cax)
else:
confusion_mx = results_list[i].get("confusion_mx")
im_confusion = ax[i,j].matshow(confusion_mx)
# ax[i,j].set_title("Confusion Matrix")
ax[i,j].set_xlabel("Confusion Matrix", fontsize=13)
# ax[i].legend()
# if i == len(list_digits) - 1:
divider = make_axes_locatable(ax[i,j])
cax = divider.append_axes('right', size='5%', pad=0.05)
fig.colorbar(im_confusion, cax=cax)
plt.subplots_adjust(wspace=0.3, hspace=0.3)
plt.savefig('MLR_MNIST_test_ex2.pdf', bbox_inches='tight')
###Output
pred-probs: [4.87077818e-067 1.94027057e-069 3.50108996e-046 1.00000000e+000
4.29159173e-108]
!!! confusion_mx [[1249 0 37 113 0]
[ 6 1047 98 461 0]
[ 37 124 835 415 1]
[ 60 4 59 1234 0]
[ 21 56 480 679 159]]
pred-probs: [3.33958073e-051 4.63817905e-105 1.00000000e+000 2.91119213e-026
1.03873086e-052]
|
apphub/image_generation/pggan_nihchestxray/pggan_nihchestxray.ipynb | ###Markdown
Synthetic Chest X-Ray Image Generation with PGGANIn this notebook, we will demonstrate the functionality of ``Scheduler`` which enables advanced training schemes such as a progressive training method as described in [Karras et al.](https://arxiv.org/pdf/1710.10196.pdf). We will train a PGGAN to produce high resolution synthetic frontal chest X-ray images where both the generator and the discriminator grows from $4\times4$ to $1024\times1024$.Stably training a GAN to produce realistic high resolution synthetic images is challenging.The discriminator can easily tell apart real images from generated images in the high resolution setting.This leads to instability in the training. Progressive Growing Strategy[Karras et al.](https://arxiv.org/pdf/1710.10196.pdf) propose a training scheme in which both the generator and the discriminator progressively grow from a low resolution to a high resolution.Both networks first start out training based on images of $4\times4$ as illustrated below.Then, both networks progress from $4\times4$ to $8\times8$ by an adding additional block that contains a couple of convolutional layers.Both the generator and the discriminator progressively grow until reaching the desired resolution of $1024\times 1024$.*Image Credit: [Presentation slide](https://drive.google.com/open?id=1jYlrX4DgTs2VAfRcyl3pcNI4ONkBg3-g)* Smooth Transition between ResolutionsHowever, when growing the networks, the new blocks are slowly faded into the networks in order to smoothly transition between different resolutions.For example, when growing the generator from $16\times16$ to $32\times32$, the newly added block of $32\times32$ is slowly faded into the already well trained $16\times16$ network by linearly increasing $\alpha$ from $0$ to $1$.Once the network is fully transitioned to $32\times32$, the network is trained on a bit further to stabilize before growing to $64\times64$.*Image Credit: [PGGAN Paper](https://arxiv.org/pdf/1710.10196.pdf)*With this progressive training strategy, PGGAN has achieved the state-of-the-art in producing synthetic images of high fidelity. Problem SettingIn this PGGAN example, we decide the following:* 560K images will be used when transitioning from a lower resolution to a higher resolution.* 560K images will be used when stabilizing the fully transitioned network.* Initial resolution will be $4\times4$.* Final resolution will be $1024\times1024$.The number of images for both transitioning and stabilizing is equivalent to 5 epochs; the networks would smoothly grow over 5 epochs and would stabilize for 5 epochs.This yields the following schedule of growing both networks:* Until $4^{th}$ epoch: train $4\times4$ resolution* From $5^{th}$ epoch to $9^{th}$ epoch: transition from $4\times4$ to $8\times8$* From $10^{th}$ epoch to $14^{th}$ epoch: stabilize $8\times8$* From $15^{th}$ epoch to $19^{th}$ epoch: transition from $8\times8$ to $16\times16$* From $20^{th}$ epoch to $24^{th}$ epoch: stabilize $16\times16$$\cdots$* From $80^{th}$ epoch to $84^{th}$ epoch: stabilize $1024\times1024$
###Code
import os
from pathlib import Path
import cv2
import matplotlib.pyplot as plt
import numpy as np
import tensorflow as tf
from tensorflow.keras import backend
import fastestimator as fe
###Output
_____no_output_____
###Markdown
Defining Input PipelineFirst, we need to download chest frontal X-ray dataset from the National Institute of Health (NIH); the dataset has over 112,000 images of $1024\times1024$. We use ``fastestimator.dataset.nih_chestxray.load_data`` to download images and create a csv file that contains relative paths to the training images. The parent path to the images returned in ``data_path`` .A detailed description of the dataset is available [here](https://www.nih.gov/news-events/news-releases/nih-clinical-center-provides-one-largest-publicly-available-chest-x-ray-datasets-scientific-community). Note: Please make sure to have a stable internet connection when downloading the dataset for the first time since the size of the dataset is over 40GB.
###Code
from fastestimator.dataset.nih_chestxray import load_data
train_csv, data_path = load_data()
###Output
_____no_output_____
###Markdown
We will create two instances of ``RecordWriter`` objects to create two sets of tfrecords to use throughout the training.``writer_128`` would contain training images of $128 \times 128$ to be used for the early phase of the training, and ``writer_1024`` would contain training images of $1024 \times 1024$ to be used for the later phase of the training.
###Code
from fastestimator.op.numpyop import ImageReader
from fastestimator.op.numpyop import Resize as ResizeRecord
from fastestimator.util.record_writer import RecordWriter
imreader = ImageReader(inputs="x", parent_path=data_path, grey_scale=True)
writer_128 = RecordWriter(save_dir=os.path.join(data_path, "tfrecord_128"),
train_data=train_csv,
ops=[imreader, ResizeRecord(target_size=(128, 128), outputs="x")])
writer_1024 = RecordWriter(save_dir=os.path.join(data_path, "tfrecord_1024"),
train_data=train_csv,
ops=[imreader, ResizeRecord(target_size=(1024, 1024), outputs="x")])
###Output
_____no_output_____
###Markdown
We need to define the following two custom ``TensorOp`` to process input images prior to feeding them to the network.* ``Rescale`` operation to rescale pixels values from $[0, 255]$ to $[-1, 1]$.* ``CreateLowRes`` operation to create images that are downsampled by a factor of 2 and upsampled by a factor of 2. The resulting images will be used for smooth transitioning between different resolutions.
###Code
from fastestimator.op import TensorOp
class Rescale(TensorOp):
"""Scale image values from uint8 to float32 between -1 and 1."""
def forward(self, data, state):
data = tf.cast(data, tf.float32)
data = (data - 127.5) / 127.5
return data
class CreateLowRes(TensorOp):
def forward(self, data, state):
data_shape = tf.shape(data)
height = data_shape[0]
width = data_shape[1]
data = tf.image.resize(data, (height / 2, width / 2))
data = tf.image.resize(data, (height, width))
return data
###Output
_____no_output_____
###Markdown
The resolution of images change at epoch $0$, $5$, $15$, $25$, $35$, $45$, $55$, and $65$ throughout the training.We can accomplish this using ``Scheduler`` which takes a dictionary as an input to express which operation (value) to perform at which epoch (key). Therefore, we specify to perform ``Resize`` operation to change the image resolution at those epoch. We resize images of $128\times128$ from the first set of tfrecords for the early training. Then, we resize $1024\times1024$ images for the later training.In addition, we wil define another ``Scheduler`` to denote how we change the batch size alongside the image resolution. The batch size will progressively decrease as the resolution of image grows; the batch size will decrease from $128$ for $4\times4$ images to $1$ for $1024\times1024$.Note that the batch size we specify here is the batch size per device. Therefore, the global batch size for $1024\times1024$ for 4 gpu machine will be $4$ whereas it will be $8$ for 8 gpu machine.
###Code
from fastestimator.schedule import Scheduler
from fastestimator.op.tensorop import Resize
# resize ops
resize_scheduler_128 = Scheduler({
0: Resize(inputs="x", size=(4, 4), outputs="x"),
5: Resize(inputs="x", size=(8, 8), outputs="x"),
15: Resize(inputs="x", size=(16, 16), outputs="x"),
25: Resize(inputs="x", size=(32, 32), outputs="x"),
35: Resize(inputs="x", size=(64, 64), outputs="x"),
45: None
})
resize_scheduler_1024 = Scheduler({
55: Resize(inputs="x", size=(256, 256), outputs="x"),
65: Resize(inputs="x", size=(512, 512), outputs="x"),
75: None
})
# We create a scheduler for batch_size with the epochs at which it will change and corresponding values.
batchsize_scheduler_128 = Scheduler({0: 128, 5: 64, 15: 32, 25: 16, 35: 8, 45: 4})
batchsize_scheduler_1024 = Scheduler({55: 4, 65: 2, 75: 1})
###Output
_____no_output_____
###Markdown
We will define two instances of ``Pipeline`` for each ``RecordWriter`` objects.Each ``Pipeline`` will perform the following operations:* Resize train images according to ``Scheduler`` defined above* Create lower resolution version of images* Rescale resized image* Rescale lower resolution imageThen, we create another ``Scheduler`` to control which tfrecords to use throughout the training.
###Code
lowres_op = CreateLowRes(inputs="x", outputs="x_lowres")
rescale_x = Rescale(inputs="x", outputs="x")
rescale_lowres = Rescale(inputs="x_lowres", outputs="x_lowres")
pipeline_128 = fe.Pipeline(batch_size=batchsize_scheduler_128,
data=writer_128,
ops=[resize_scheduler_128, lowres_op, rescale_x, rescale_lowres])
pipeline_1024 = fe.Pipeline(batch_size=batchsize_scheduler_1024,
data=writer_1024,
ops=[resize_scheduler_1024, lowres_op, rescale_x, rescale_lowres])
pipeline_scheduler = Scheduler({0: pipeline_128, 55: pipeline_1024})
###Output
_____no_output_____
###Markdown
Let's visualize how ``Pipeline``s change image resolutions at different epochs we specified in ``Scheduler``.We provide ``show_results`` methods to visaulize the resulting images of ``Pipeline``.In order to correctly visualize the output of ``Pipeline``, we need to provide epoch numbers to ``show_results``
###Code
def display_pipeline_result(pipeline, epoch, reuse, batch_idx=0, img_key="x"):
batch = pipeline.show_results(current_epoch=epoch, reuse=reuse)[0]
img = batch[img_key][batch_idx].numpy()
img = (img + 1) * 0.5
return img[..., 0]
# Visualizing pipeline_128
batch_idx = 0
epochs_128 = [0, 5, 15, 25, 35, 45]
plt.figure(figsize=(50,50))
for i, epoch in enumerate(epochs_128):
img_128 = display_pipeline_result(pipeline_128, epoch, epoch != epochs_128[-1])
plt.subplot(1, 9, i+1)
plt.imshow(img_128, cmap='gray')
# Visualizing pipeline_1024
epochs_1024 = [55, 65, 75]
for i, epoch in enumerate(epochs_1024):
img_1024 = display_pipeline_result(pipeline_1024, epoch, epoch != epochs_1024[-1])
plt.subplot(1, 9, i+7)
plt.imshow(img_1024, cmap='gray')
###Output
FastEstimator: Reading non-empty directory: /root/fastestimator_data/NIH_Chestxray/tfrecord_128
FastEstimator: Found 112120 examples for train in /root/fastestimator_data/NIH_Chestxray/tfrecord_128/train_summary0.json
FastEstimator: Reading non-empty directory: /root/fastestimator_data/NIH_Chestxray/tfrecord_1024
FastEstimator: Found 112120 examples for train in /root/fastestimator_data/NIH_Chestxray/tfrecord_1024/train_summary0.json
###Markdown
Defining Network Defining the generator and the discriminatorTo express the progressive growing of networks, we return a list of models that progressively grow from $4 \times 4$ to $1024 \times 1024$ such that $i^{th}$ model in the list is the superset previous models.We define a ``tf.Variable`` to allow models to grow smoothly.``fe.build`` bundles each model, optimizer, the name of the model, and the associated loss name.
###Code
from fastestimator.architecture.pggan import build_G, build_D
optimizer = tf.keras.optimizers.Adam(learning_rate=0.001, beta_1=0.0, beta_2=0.99, epsilon=1e-8)
fade_in_alpha = tf.Variable(initial_value=1.0, dtype='float32', trainable=False)
d2, d3, d4, d5, d6, d7, d8, d9, d10 = fe.build(
model_def=lambda: build_D(fade_in_alpha=fade_in_alpha, target_resolution=10, num_channels=1),
model_name=["d2", "d3", "d4", "d5", "d6", "d7", "d8", "d9", "d10"],
optimizer=[optimizer]*9,
loss_name=["dloss"]*9)
g2, g3, g4, g5, g6, g7, g8, g9, g10, G = fe.build(
model_def=lambda: build_G(fade_in_alpha=fade_in_alpha, target_resolution=10, num_channels=1),
model_name=["g2", "g3", "g4", "g5", "g6", "g7", "g8", "g9", "g10", "G"],
optimizer=[optimizer]*10,
loss_name=["gloss"]*10)
###Output
_____no_output_____
###Markdown
We need to define the following ``TensorOp``:* ``RandomInput`` to produce random variables drawn from $\mathcal{N}(0,\,1)$* ``ImageBlender`` to blend real images of $2^{i} \times 2^{i}$ and $2^{i-1} \times 2^{i-1}$ to allow smooth transition when growing from $2^{i-1} \times 2^{i-1}$ to $2^{i} \times 2^{i}$* ``Interpolate`` to interpolate between real images and generated images* ``GradientPenalty`` to compute the gradient penalty using the result of the previous TensorOp ``Interpolate``
###Code
class RandomInput(TensorOp):
def forward(self, data, state):
latent_dim = data
batch_size = state["local_batch_size"]
random_vector = tf.random.normal([batch_size, latent_dim])
return random_vector
class ImageBlender(TensorOp):
def __init__(self, alpha, inputs=None, outputs=None, mode=None):
super().__init__(inputs=inputs, outputs=outputs, mode=mode)
self.alpha = alpha
def forward(self, data, state):
image, image_lowres = data
new_img = self.alpha * image + (1 - self.alpha) * image_lowres
return new_img
class Interpolate(TensorOp):
def forward(self, data, state):
fake, real = data
batch_size = state["local_batch_size"]
coeff = tf.random.uniform(shape=[batch_size, 1, 1, 1], minval=0.0, maxval=1.0, dtype=tf.float32)
return real + (fake - real) * coeff
class GradientPenalty(TensorOp):
def __init__(self, inputs, outputs=None, mode=None):
super().__init__(inputs=inputs, outputs=outputs, mode=mode)
def forward(self, data, state):
x_interp, interp_score = data
interp_score = tf.reshape(interp_score, [-1])
tape = state['tape']
gradient_x_interp = tape.gradient(tf.reduce_sum(interp_score), x_interp)
grad_l2 = tf.math.sqrt(tf.reduce_sum(tf.math.square(gradient_x_interp), axis=[1, 2, 3]))
gp = tf.math.square(grad_l2 - 1.0)
return gp
###Output
_____no_output_____
###Markdown
We are now ready to define loss functions for the genrator and the discriminator.The loss functions are modified version of the [WGAN-GP](https://arxiv.org/pdf/1704.00028.pdf); the discriminator's loss function has an additional term to penalize the discriminator's output on real images deviating too much from 0.
###Code
from fastestimator.op.tensorop import Loss
class GLoss(Loss):
def forward(self, data, state):
return -data
class DLoss(Loss):
"""Compute discriminator loss."""
def __init__(self, inputs, outputs=None, mode=None, wgan_lambda=10, wgan_epsilon=0.001):
super().__init__(inputs=inputs, outputs=outputs, mode=mode)
self.wgan_lambda = wgan_lambda
self.wgan_epsilon = wgan_epsilon
def forward(self, data, state):
real_score, fake_score, gp = data
loss = fake_score - real_score + self.wgan_lambda * gp + self.wgan_epsilon * tf.math.square(real_score)
return loss
###Output
_____no_output_____
###Markdown
With the losses defined, we need to specify the forward pass of the networks.We utilize ``Scheduler`` to progressively train different generators and the discriminators to express progressive growing of the networks.
###Code
from fastestimator.op.tensorop import ModelOp
g_scheduler = Scheduler({
0: ModelOp(model=g2, outputs="x_fake"),
5: ModelOp(model=g3, outputs="x_fake"),
15: ModelOp(model=g4, outputs="x_fake"),
25: ModelOp(model=g5, outputs="x_fake"),
35: ModelOp(model=g6, outputs="x_fake"),
45: ModelOp(model=g7, outputs="x_fake"),
55: ModelOp(model=g8, outputs="x_fake"),
65: ModelOp(model=g9, outputs="x_fake"),
75: ModelOp(model=g10, outputs="x_fake")
})
fake_score_scheduler = Scheduler({
0: ModelOp(inputs="x_fake", model=d2, outputs="fake_score"),
5: ModelOp(inputs="x_fake", model=d3, outputs="fake_score"),
15: ModelOp(inputs="x_fake", model=d4, outputs="fake_score"),
25: ModelOp(inputs="x_fake", model=d5, outputs="fake_score"),
35: ModelOp(inputs="x_fake", model=d6, outputs="fake_score"),
45: ModelOp(inputs="x_fake", model=d7, outputs="fake_score"),
55: ModelOp(inputs="x_fake", model=d8, outputs="fake_score"),
65: ModelOp(inputs="x_fake", model=d9, outputs="fake_score"),
75: ModelOp(inputs="x_fake", model=d10, outputs="fake_score")
})
real_score_scheduler = Scheduler({
0: ModelOp(model=d2, outputs="real_score"),
5: ModelOp(model=d3, outputs="real_score"),
15: ModelOp(model=d4, outputs="real_score"),
25: ModelOp(model=d5, outputs="real_score"),
35: ModelOp(model=d6, outputs="real_score"),
45: ModelOp(model=d7, outputs="real_score"),
55: ModelOp(model=d8, outputs="real_score"),
65: ModelOp(model=d9, outputs="real_score"),
75: ModelOp(model=d10, outputs="real_score")
})
interp_score_scheduler = Scheduler({
0: ModelOp(inputs="x_interp", model=d2, outputs="interp_score", track_input=True),
5: ModelOp(inputs="x_interp", model=d3, outputs="interp_score", track_input=True),
15: ModelOp(inputs="x_interp", model=d4, outputs="interp_score", track_input=True),
25: ModelOp(inputs="x_interp", model=d5, outputs="interp_score", track_input=True),
35: ModelOp(inputs="x_interp", model=d6, outputs="interp_score", track_input=True),
45: ModelOp(inputs="x_interp", model=d7, outputs="interp_score", track_input=True),
55: ModelOp(inputs="x_interp", model=d8, outputs="interp_score", track_input=True),
65: ModelOp(inputs="x_interp", model=d9, outputs="interp_score", track_input=True),
75: ModelOp(inputs="x_interp", model=d10, outputs="interp_score", track_input=True)
})
network = fe.Network(ops=[
RandomInput(inputs=lambda: 512),
g_scheduler,
fake_score_scheduler,
ImageBlender(inputs=("x", "x_lowres"), alpha=fade_in_alpha),
real_score_scheduler,
Interpolate(inputs=("x_fake", "x"), outputs="x_interp"),
interp_score_scheduler,
GradientPenalty(inputs=("x_interp", "interp_score"), outputs="gp"),
GLoss(inputs="fake_score", outputs="gloss"),
DLoss(inputs=("real_score", "fake_score", "gp"), outputs="dloss")
])
###Output
_____no_output_____
###Markdown
Defining TraceGiven that ``Pipeline`` and ``Network`` are properly defined, we need to define the following ``Trace``s:* ``AlphaController`` to allow smooth transition from different resolutions.* ``ImageSaving`` to save intermediate outputs of the generator* ``ModelSaving`` to save the final generator model* ``ResetOptimizer`` to reset the internal state of the optimizer once the resolution is fully transitioned``AlphaController`` facilitates both the generator and the discriminator to smoothly grow by controlling the value of ``fade_in_alpha`` tensor created previously.
###Code
from fastestimator.trace import Trace
class AlphaController(Trace):
def __init__(self, alpha, fade_start, duration):
super().__init__(inputs=None, outputs=None, mode="train")
self.alpha = alpha
self.fade_start = fade_start
self.duration = duration
self.change_alpha = False
self._idx = 0
def on_epoch_begin(self, state):
# check whetehr the current epoch is in smooth transition of resolutions
fade_epoch = self.fade_start[self._idx]
if state["epoch"] == fade_epoch:
self.nimg_total = self.duration[self._idx] * state["num_examples"]
self.change_alpha = True
self.nimg_so_far = 0
print("FastEstimator-Alpha: Started fading in for size {}".format(2**(self._idx + 3)))
elif state["epoch"] == fade_epoch + self.duration[self._idx]:
print("FastEstimator-Alpha: Finished fading in for size {}".format(2**(self._idx + 3)))
self.change_alpha = False
self._idx += 1
backend.set_value(self.alpha, 1.0)
def on_batch_begin(self, state):
# if in resolution transition, smoothly change the alpha from 0 to 1
if self.change_alpha:
self.nimg_so_far += state["batch_size"]
current_alpha = np.float32(self.nimg_so_far / self.nimg_total)
backend.set_value(self.alpha, current_alpha)
class ImageSaving(Trace):
def __init__(self, epoch_model, save_dir, num_sample=16, latent_dim=512, num_channels=3):
super().__init__(inputs=None, outputs=None, mode="train")
self.epoch_model = epoch_model
self.save_dir = save_dir
self.latent_dim = latent_dim
self.num_sample = num_sample
self.num_channels = num_channels
self.eps = 1e-8
def on_epoch_end(self, state):
if state["epoch"] in self.epoch_model:
model = self.epoch_model[state["epoch"]]
for i in range(self.num_sample):
random_vectors = tf.random.normal([1, self.latent_dim])
pred = model(random_vectors)
disp_img = pred.numpy()
disp_img = np.squeeze(disp_img)
disp_img -= disp_img.min()
disp_img /= (disp_img.max() + self.eps)
disp_img = np.uint8(disp_img * 255)
cv2.imwrite(os.path.join(self.save_dir, 'image_at_{:08d}_{}.png').format(state["epoch"], i), disp_img)
print("on epoch {}, saving image to {}".format(state["epoch"], self.save_dir))
class ModelSaving(Trace):
def __init__(self, epoch_model, save_dir):
super().__init__(inputs=None, outputs=None, mode="train")
self.epoch_model = epoch_model
self.save_dir = save_dir
def on_epoch_end(self, state):
if state["epoch"] in self.epoch_model:
model = self.epoch_model[state["epoch"]]
save_path = os.path.join(self.save_dir, model.model_name + ".h5")
model.save(save_path, include_optimizer=False)
print("FastEstimator-ModelSaver: Saving model to {}".format(save_path))
class ResetOptimizer(Trace):
def __init__(self, reset_epochs, optimizer):
super().__init__(inputs=None, outputs=None, mode="train")
self.reset_epochs = reset_epochs
self.optimizer = optimizer
def on_epoch_begin(self, state):
if state["epoch"] in self.reset_epochs:
for weight in self.optimizer.weights:
backend.set_value(weight, weight - weight)
print("Resetting optimizer on epoch {}".format(state["epoch"]))
###Output
_____no_output_____
###Markdown
Defining EstimatorWe can now define ``Estimator`` putting ``Pipeline``, ``Network``, and ``Trace`` together.We will create an intermediate directory to save the intermediate outputs of the generator to keep track of the progress.
###Code
save_dir = os.path.join(str(Path.home()), 'fastestimator_results', 'NIH_CXR_PGGAN')
os.makedirs(save_dir, exist_ok=True)
traces = [AlphaController(alpha=fade_in_alpha,
fade_start=[5, 15, 25, 35, 45, 55, 65, 75, 85],
duration=[5, 5, 5, 5, 5, 5, 5, 5, 5]),
ResetOptimizer(reset_epochs=[5, 15, 25, 35, 45, 55, 65, 75], optimizer=optimizer),
ImageSaving(epoch_model={4: g2, 14: g3, 24: g4, 34: g5, 44: g6, 54: g7, 64: g8, 74: g9, 84: G},
save_dir=save_dir,
num_channels=1),
ModelSaving(epoch_model={84: G}, save_dir=save_dir)]
estimator = fe.Estimator(network=network,
pipeline=pipeline_scheduler,
epochs=85,
traces=traces)
###Output
_____no_output_____
###Markdown
Finally, we can start training by calling ``fit`` method.
###Code
estimator.fit()
###Output
______ __ ______ __ _ __
/ ____/___ ______/ /_/ ____/____/ /_(_)___ ___ ____ _/ /_____ _____
/ /_ / __ `/ ___/ __/ __/ / ___/ __/ / __ `__ \/ __ `/ __/ __ \/ ___/
/ __/ / /_/ (__ ) /_/ /___(__ ) /_/ / / / / / / /_/ / /_/ /_/ / /
/_/ \__,_/____/\__/_____/____/\__/_/_/ /_/ /_/\__,_/\__/\____/_/
FastEstimator: Reading non-empty directory: /root/fastestimator_data/NIH_Chestxray/tfrecord_128
FastEstimator: Found 112120 examples for train in /root/fastestimator_data/NIH_Chestxray/tfrecord_128/train_summary0.json
FastEstimator: Reading non-empty directory: /root/fastestimator_data/NIH_Chestxray/tfrecord_1024
FastEstimator: Found 112120 examples for train in /root/fastestimator_data/NIH_Chestxray/tfrecord_1024/train_summary0.json
WARNING:tensorflow:Using MirroredStrategy eagerly has significant overhead currently. We will be working on improving this in the future, but for now please wrap `call_for_each_replica` or `experimental_run` or `experimental_run_v2` inside a tf.function to get the best performance.
WARNING:tensorflow:Calling GradientTape.gradient on a persistent tape inside its context is significantly less efficient than calling it outside the context (it causes the gradient ops to be recorded on the tape, leading to increased CPU and memory usage). Only call GradientTape.gradient inside the context if you actually want to trace the gradient in order to compute higher order derivatives.
WARNING:tensorflow:Using MirroredStrategy eagerly has significant overhead currently. We will be working on improving this in the future, but for now please wrap `call_for_each_replica` or `experimental_run` or `experimental_run_v2` inside a tf.function to get the best performance.
|
notebooks/Classifier-viz.ipynb | ###Markdown
Training data visualization This notebook uses this Matplotlib plotting API: https://matplotlib.org/3.1.1/api/axes_api.html
###Code
import json
import matplotlib.pyplot as plt
import pandas as pd
with open('./stats.json', 'r') as fp:
data = json.load(fp)
hist = data.pop("history")
params = data.pop("params")
assert data == {}
df = pd.DataFrame(hist)
df.columns
###Output
_____no_output_____
###Markdown
Matplotlib plotting with `subplots`: https://matplotlib.org/3.1.1/api/axes_api.html
###Code
fig, ax = plt.subplots()
df.plot(x="epoch", y="score__loss", ax=ax)
ax.set_title("Progress")
ax.set_ylabel("Test loss")
ax.grid(alpha=0.6)
fig, ax = plt.subplots()
df.plot(x="epoch", y="score__acc", ax=ax)
ax.set_title("Progress")
ax.set_ylabel("Test loss")
ax.grid(alpha=0.6)
ax = df["partial_fit__time"].plot.hist()
ax.set_xlabel("Time for one epoch (s)")
ax = df["score__time"].plot.hist()
###Output
_____no_output_____ |
1_model_train_deploy.ipynb | ###Markdown
SageMaker Serverless Inference NLP ExampleAmazon SageMaker Serverless Inference is a purpose-built inference option that makes it easy for customers to deploy and scale ML models. Serverless Inference is ideal for workloads which have idle periods between traffic spurts and can tolerate cold starts. Serverless endpoints also automatically launch compute resources and scale them in and out depending on traffic, eliminating the need to choose instance types or manage scaling policies.We will use a binary text classification example, which borrows from this code sample: https://github.com/huggingface/notebooks/tree/master/sagemaker/01_getting_started_pytorchNotebook Setting- SageMaker Classic Notebook Instance: ml.m5.xlarge Notebook Instance & conda_python3 Kernel- SageMaker Studio: Python 3 (Data Science)- Regions Available: SageMaker Serverless Inference is currently available in the following regions: US East (Northern Virginia), US East (Ohio), US West (Oregon), EU (Ireland), Asia Pacific (Tokyo) and Asia Pacific (Sydney) Table of Contents- Setup- Model Training- Deployment - Model Creation - Endpoint Configuration (Adjust for Serverless) - Serverless Endpoint Creation - Endpoint Invocation- Cleanup Development Environment and Permissions SetupFor testing you need to properly configure your Notebook Role to have SageMaker Full Access. Let's start by installing preview wheels of the Python SDK, boto and aws cli Installation_*Note:* we only install the required libraries from Hugging Face and AWS. You also need PyTorch or Tensorflow, if you haven´t it installed_
###Code
%pip -q install torch boto3 sagemaker transformers datasets[s3] --user
###Output
_____no_output_____
###Markdown
Permissions _If you are going to use Sagemaker in a local environment. You need access to an IAM Role with the required permissions for Sagemaker. You can find [here](https://docs.aws.amazon.com/sagemaker/latest/dg/sagemaker-roles.html) more about it._ Make sure Sagemaker version is >= 2.70.0
###Code
import sagemaker
print(sagemaker.__version__)
sess = sagemaker.Session()
# sagemaker session bucket -> used for uploading data, models and logs
# sagemaker will automatically create this bucket if it not exists
sagemaker_session_bucket=None
if sagemaker_session_bucket is None and sess is not None:
# set to default bucket if a bucket name is not given
sagemaker_session_bucket = sess.default_bucket()
role = sagemaker.get_execution_role()
sess = sagemaker.Session(default_bucket=sagemaker_session_bucket)
print(f"sagemaker role arn: {role}")
print(f"sagemaker bucket: {sess.default_bucket()}")
print(f"sagemaker session region: {sess.boto_region_name}")
###Output
_____no_output_____
###Markdown
PreprocessingWe are using the `datasets` library to download and preprocess the `imdb` dataset. After preprocessing, the dataset will be uploaded to our `sagemaker_session_bucket` to be used within our training job. The [imdb](http://ai.stanford.edu/~amaas/data/sentiment/) dataset consists of 25000 training and 25000 testing highly polar movie reviews. Tokenization
###Code
from datasets import load_dataset
from transformers import AutoTokenizer
# tokenizer used in preprocessing
tokenizer_name = 'distilbert-base-uncased'
# dataset used
dataset_name = 'imdb'
# s3 key prefix for the data
s3_prefix = 'samples/datasets/imdb'
# load dataset
dataset = load_dataset(dataset_name)
# download tokenizer
tokenizer = AutoTokenizer.from_pretrained(tokenizer_name)
# tokenizer helper function
def tokenize(batch):
return tokenizer(batch['text'], padding='max_length', truncation=True)
# load dataset
train_dataset, test_dataset = load_dataset('imdb', split=['train', 'test'])
test_dataset = test_dataset.shuffle().select(range(10000)) # smaller the size for test dataset to 10k
# tokenize dataset
train_dataset = train_dataset.map(tokenize, batched=True)
test_dataset = test_dataset.map(tokenize, batched=True)
# set format for pytorch
train_dataset = train_dataset.rename_column("label", "labels")
train_dataset.set_format('torch', columns=['input_ids', 'attention_mask', 'labels'])
test_dataset = test_dataset.rename_column("label", "labels")
test_dataset.set_format('torch', columns=['input_ids', 'attention_mask', 'labels'])
###Output
_____no_output_____
###Markdown
Uploading data to `sagemaker_session_bucket`After we processed the `datasets` we are going to use the new `FileSystem` [integration](https://huggingface.co/docs/datasets/filesystems.html) to upload our dataset to S3.
###Code
import botocore
from datasets.filesystems import S3FileSystem
s3 = S3FileSystem()
# save train_dataset to s3
training_input_path = f's3://{sess.default_bucket()}/{s3_prefix}/train'
train_dataset.save_to_disk(training_input_path,fs=s3)
# save test_dataset to s3
test_input_path = f's3://{sess.default_bucket()}/{s3_prefix}/test'
test_dataset.save_to_disk(test_input_path,fs=s3)
###Output
_____no_output_____
###Markdown
Fine-tuning & starting Sagemaker Training JobIn order to create a sagemaker training job we need an `HuggingFace` Estimator. The Estimator handles end-to-end Amazon SageMaker training and deployment tasks. In a Estimator we define, which fine-tuning script should be used as `entry_point`, which `instance_type` should be used, which `hyperparameters` are passed in .....```pythonhuggingface_estimator = HuggingFace(entry_point='train.py', source_dir='./scripts', base_job_name='huggingface-sdk-extension', instance_type='ml.p3.2xlarge', instance_count=1, transformers_version='4.4', pytorch_version='1.6', py_version='py36', role=role, hyperparameters = {'epochs': 1, 'train_batch_size': 32, 'model_name':'distilbert-base-uncased' })```When we create a SageMaker training job, SageMaker takes care of starting and managing all the required ec2 instances for us with the `huggingface` container, uploads the provided fine-tuning script `train.py` and downloads the data from our `sagemaker_session_bucket` into the container at `/opt/ml/input/data`. Then, it starts the training job by running. ```python/opt/conda/bin/python train.py --epochs 1 --model_name distilbert-base-uncased --train_batch_size 32```The `hyperparameters` you define in the `HuggingFace` estimator are passed in as named arguments. Sagemaker is providing useful properties about the training environment through various environment variables, including the following:* `SM_MODEL_DIR`: A string that represents the path where the training job writes the model artifacts to. After training, artifacts in this directory are uploaded to S3 for model hosting.* `SM_NUM_GPUS`: An integer representing the number of GPUs available to the host.* `SM_CHANNEL_XXXX:` A string that represents the path to the directory that contains the input data for the specified channel. For example, if you specify two input channels in the HuggingFace estimator’s fit call, named `train` and `test`, the environment variables `SM_CHANNEL_TRAIN` and `SM_CHANNEL_TEST` are set.To run your training job locally you can define `instance_type='local'` or `instance_type='local_gpu'` for gpu usage. _Note: this does not working within SageMaker Studio_
###Code
!pygmentize ./scripts/train.py
###Output
_____no_output_____
###Markdown
Creating an Estimator and start a training job
###Code
from sagemaker.huggingface import HuggingFace
# hyperparameters, which are passed into the training job
hyperparameters={'epochs': 1,
'train_batch_size': 32,
'model_name':'distilbert-base-uncased'
}
huggingface_estimator = HuggingFace(entry_point='train.py',
source_dir='./scripts',
instance_type='ml.p3.2xlarge',
instance_count=1,
role=role,
transformers_version='4.6',
pytorch_version='1.7',
py_version='py36',
hyperparameters = hyperparameters)
# starting the train job with our uploaded datasets as input
huggingface_estimator.fit({'train': training_input_path, 'test': test_input_path})
###Output
_____no_output_____
###Markdown
Deployment After training the model, retrieve the model artifacts so that we can deploy the model to an endpoint.
###Code
# Retrieve model data from training job
model_artifacts = huggingface_estimator.model_data
model_artifacts
###Output
_____no_output_____
###Markdown
Model CreationCreate a model by providing your model artifacts, the container image URI, environment variables for the container (if applicable), a model name, and the SageMaker IAM role.
###Code
# Setup clients
import boto3
client = boto3.client(service_name="sagemaker")
runtime = boto3.client(service_name="sagemaker-runtime")
region = boto3.session.Session().region_name
image_uri = sagemaker.image_uris.retrieve(
framework='huggingface',
base_framework_version='pytorch1.7',
region=region,
version='4.6',
py_version='py36',
instance_type='ml.m5.large',
image_scope='inference'
)
image_uri
from time import gmtime, strftime
model_name = "hf-serverless" + strftime("%Y-%m-%d-%H-%M-%S", gmtime())
print("Model name: " + model_name)
create_model_response = client.create_model(
ModelName=model_name,
Containers=[
{
"Image": image_uri,
"Mode": "SingleModel",
"ModelDataUrl": model_artifacts,
}
],
ExecutionRoleArn=role,
)
print("Model Arn: " + create_model_response["ModelArn"])
###Output
_____no_output_____
###Markdown
Endpoint Configuration CreationThis is where you can adjust the Serverless Configuration for your endpoint. The current max concurrent invocations for a single endpoint, known as MaxConcurrency, can be any value from 1 to 50, and MemorySize can be any of the following: 1024 MB, 2048 MB, 3072 MB, 4096 MB, 5120 MB, or 6144 MB.
###Code
epc_name = "hf-serverless-epc" + strftime("%Y-%m-%d-%H-%M-%S", gmtime())
endpoint_config_response = client.create_endpoint_config(
EndpointConfigName=epc_name,
ProductionVariants=[
{
'VariantName': 'single-variant',
'ModelName': model_name,
'ServerlessConfig': {
'MemorySizeInMB': 6144,
'MaxConcurrency': 8,
},
},
],
)
print("Endpoint Configuration Arn: " + endpoint_config_response["EndpointConfigArn"])
###Output
_____no_output_____
###Markdown
Serverless Endpoint CreationNow that we have an endpoint configuration, we can create a serverless endpoint and deploy our model to it. When creating the endpoint, provide the name of your endpoint configuration and a name for the new endpoint.
###Code
endpoint_name = "hf-serverless-ep" + strftime("%Y-%m-%d-%H-%M-%S", gmtime())
create_endpoint_response = client.create_endpoint(
EndpointName=endpoint_name,
EndpointConfigName=epc_name,
)
print("Endpoint Arn: " + create_endpoint_response["EndpointArn"])
###Output
_____no_output_____
###Markdown
Wait until the endpoint status is InService before invoking the endpoint.
###Code
# wait for endpoint to reach a terminal state (InService) using describe endpoint
import time
describe_endpoint_response = client.describe_endpoint(EndpointName=endpoint_name)
while describe_endpoint_response["EndpointStatus"] == "Creating":
describe_endpoint_response = client.describe_endpoint(EndpointName=endpoint_name)
print(describe_endpoint_response["EndpointStatus"])
time.sleep(15)
describe_endpoint_response
###Output
_____no_output_____
###Markdown
Endpoint InvocationInvoke the endpoint by sending a request to it. The following is a sample data point grabbed from the CSV file downloaded from the public Abalone dataset.
###Code
import json
sentiment_input = {"inputs": "I love using the new Serverless Inference on SageMaker."}
response = runtime.invoke_endpoint(
EndpointName=endpoint_name,
Body=json.dumps(sentiment_input),
ContentType="application/json",
)
print(response["Body"].read())
###Output
_____no_output_____
###Markdown
Clean UpDelete any resources you created in this notebook that you no longer wish to use.
###Code
# client.delete_model(ModelName=model_name)
# client.delete_endpoint_config(EndpointConfigName=epc_name)
# client.delete_endpoint(EndpointName=endpoint_name)
###Output
_____no_output_____ |
Woche 4/2 Wir trainieren nur bergab.ipynb | ###Markdown
Wir trainieren nur bergab? Das Problem der RegressionBei der Regressionsanalyse muss eine Modellfunktion gefunden werden, die zu einem gegebenen Satz von Datenpunkten N möglichst **genau** passt. Ein häufig verwendetes Maß für die Genauigkeit der Approximation ist die **Methode der kleinsten Quadrate** (engl. least squares approach). Der vertikale Abstand zwischen jedem Datenpunkt $(x_n,y_n)$ und der Ausgabe der Modellfunktion $m(x_n)$ wird durch Subtraktion der y-Werte der Datenpunkte von den vorhergesagten y-Werten der Modellfunktion berechnet (3).\begin{align}d_n & = m(x_n)-y_n \;\;\;\;\;\;\;\;\;\;\; (3)\end{align}Diese Abstände werden dann quadriert und aufsummiert. Da wir die Qualität einer Approximation mit anderen Approximationen vergleichen wollen, die möglicherweise eine andere Anzahl von Datenpunkten haben, teilen wir die Summe noch durch die Gesamtzahl der Datenpunkte (**mittlerer quadratischer Fehler**). So erhalten wir unsere **Verlust**-Funktion (4). Unser Ziel ist es, diese Metrik so niedrig wie möglich zu halten, denn je niedriger der Verlust, desto besser die Approximation. Hier haben die Begriffe "Verlust" und "Fehler" die gleiche Bedeutung. Ein anderer häufig verwendeter Begriff ist "Kosten".\begin{align}Verlust & = \frac{1}{N} \sum_{n=0}^{N-1} {[m(x_n)-y_n]}^2 \;\;\;\;\;\;\;\;\;\;\;\; (4)\end{align}Hinweis: Beachten Sie, dass in Gl.(4) $x_0$ kein Merkmal wie in Gl.(1) aus W4V2 ist, sondern ein Datenpunkt des Datensatzes. Wenn wir eine genaue Regression erreicht haben, können wir damit **Vorhersagen** machen. Wir werden unsere Neuronen auf einen gegebenen Satz von Punkten trainieren und sie dann verwenden, um neue Punkte vorherzusagen. Dazu werden wir dem trainierten Neuron neue x-Werte geben, zu denen es die y-Werte vorhersagen soll. Abb. 3 - Visualisierung des Abstands zur Modellfunktion
###Code
# do not change
class SimpleNeuron:
def __init__(self, plot):
self.plot = plot #I am assigned the following plot
self.plot.register_neuron(self) #hey plot, remember me
def set_values(self, weight:float, bias:float):
self.weight = weight
self.bias = bias
self.plot.update() #hey plot, I have changed, redraw my output
def get_weight(self) -> float:
return self.weight
def get_bias(self) -> float:
return self.bias
def compute(self, x:float) -> float:
self.activation = self.weight * x + self.bias
return self.activation
###Output
_____no_output_____
###Markdown
Wir werden eine Funktion "loss" erstellen, die die Operation in Gleichung (4) durchführt. Als Argumente wird sie dazu ein Neuron-Objekt und einen Satz von Punkten erhalten.- Für jeden Punkt, den wir ihr geben, trennt sie zunächst x- und y-Werte. - Dann übergibt sie dem Neuron einen x-Wert und bittet das Neuron, eine Vorhersage für den y-Wert zu berechnen. (siehe $m(x_n)$) - Dann subtrahiert sie den realen y-Wert von dem vorhergesagten y-Wert, wie in Gleichung (3), und erhält einen Abstand- Anschließend quadriert sie den Abstand und summiert die quadrierten Abstände. - Im letzten Schritt wird die Summe der quadrierten Abstände durch die Anzahl der verglichenen Punkte dividiert. Führen Sie die nachfolgende Zelle aus, um eine Verlustfunktion zu definieren.
###Code
# do not change
def loss(neuron:SimpleNeuron, points:dict) -> float:
sum_squared_dist = 0
for point_x, point_y in zip(points["x"], points["y"]): # zip merges both points["x"] and points["y"]
predicted_point_y = neuron.compute(point_x)
dist = point_y - predicted_point_y
squared_dist = dist ** 2
sum_squared_dist += squared_dist
loss = sum_squared_dist / len(points["y"])
return loss
###Output
_____no_output_____
###Markdown
Vorbereiten eines interaktiven PlotsNachdem wir die notwendigen Bibliotheken importiert haben, werden wir nun eine interaktive Plot-Klasse einrichten. Diese soll die Ausgabe eines Neurons zeichnen, indem sie es auffordert, einen Satz von x-Werten zu berechnen. Das führt zu einem Satz von vorhergesagten y-Werten, die auf einer Ebene gezeichnet werden können. Wenn das Gewicht oder der Bias eines Neurons geändert wird, ruft das Neuron die "redraw"-Methode seines Plots auf, um ihn zu aktualisieren. Der Plot kann auch feste Punkte zeichnen. Interaktive Schieberegler werden verwendet, um die Gewichte und den Bias der Neuronenobjekten direkt zu ändern.Hinweis: Die Plot-Klassen sind nicht Teil des Lernstoffs für dieses Praktikum. Führen Sie die nachfolgenden Zellen aus, um die Bibliotheken zu importieren und einen interaktiven Plot zu definieren. Hinweis: Wechseln Sie in die tf1-Umgebung. Wenn Sie einen eigenen Rechner verwenden oder diese Environment kein Plotly enthält, installieren Sie es. Dazu öffnen Sie das Terminal in tf1 und verwenden conda install ...
###Code
# do not change
import numpy as np
import plotly.offline as plotly
import plotly.graph_objs as go
from ipywidgets import interact, Layout, HBox, FloatSlider
import time
import threading
# do not change
# an Interactive Plot monitors the activation of a neuron or a neural network
class Interactive2DPlot:
def __init__(self, points:dict, ranges:dict, width:int=800, height:int=400, margin:dict=dict(t=0, l=170), draw_time:float=0.05):
self.idle = True
self.points = points
self.x = np.arange(ranges["x"][0], ranges["x"][1], 0.1)
self.y = np.arange(ranges["y"][0], ranges["y"][1], 0.1)
self.draw_time = draw_time
self.layout = go.Layout(
xaxis=dict(title="Input: x", range=ranges["x"], fixedrange=True),
yaxis=dict(title="Output: y", range=ranges["y"], fixedrange=True),
width=width,
height=height,
showlegend=False,
autosize=False,
margin=margin,
)
self.trace = go.Scatter(x=self.x, y=self.y)
self.plot_points = go.Scatter(x=points["x"], y=points["y"], mode="markers")
self.data = [self.trace, self.plot_points]
self.plot = go.FigureWidget(self.data, self.layout)
# self.plot = plotly.iplot(self.data, self.layout,config={"displayModeBar": False})
def register_neuron(self, neuron:SimpleNeuron):
self.neuron = neuron
def redraw(self):
self.idle = False
time.sleep(self.draw_time)
self.plot.data[0].y = self.neuron.compute(self.x)
self.idle = True
def update(self):
print("Loss: {:0.2f}".format(loss(self.neuron, self.points)))
if self.idle:
thread = threading.Thread(target=self.redraw)
thread.start()
###Output
_____no_output_____
###Markdown
Aufgabe 4.2.1: Das Neuron trainierenSie erhalten einen Satz mit 3 Punkten und ein Neuron, um eine Kurvenanpassung durchzuführen. Führen Sie die folgende Zelle aus. Verändern Sie das Gewicht und den Bias des Neurons mit den Schiebereglern, um den Verlust zu minimieren. Tipp: Sie können die Schieberegler auch mit den Pfeiltasten auf Ihrer Tastatur verändern, nachdem Sie auf den Schieberegler geklickt haben.
###Code
# do not change
points_linreg = dict(x=[1, 2, 3], y=[1.5, 0.7, 1.2])
ranges_linreg = dict(x=[-4, 4], y=[-4, 4])
linreg_plot = Interactive2DPlot(points_linreg, ranges_linreg)
simple_neuron = SimpleNeuron(linreg_plot)
slider_layout = Layout(width="90%")
interact(
simple_neuron.set_values,
weight=FloatSlider(min=-3, max=3, step=0.1, value = 0, layout=slider_layout),
bias=FloatSlider(min=-3, max=3, step=0.1, value = 0, layout=slider_layout)
)
linreg_plot.plot
###Output
_____no_output_____
###Markdown
Frage 4.2.2: Was ist die optimale Kombination aus Gewicht und Bias? Ihre Antwort: Vorbereiten des 3D-PlotsWir sehen, dass die Suche nach dem geringsten Verlust ein **Parameteroptimierungsproblem** ist. Bisher können wir das Problem manuell lösen. Wollen wir aber neuronale Netze zur Lösung komplexerer Probleme verwenden, müssen wir einen Weg finden, diesen Prozess zu automatisieren.Die Verlustfunktion wird sowohl mit dem festgelegten Gewicht als auch mit dem festgelegten Bias geändert. Diese Beziehung kann dreidimensional visualisiert werden, was uns weitere Einblicke geben kann, um einen Algorithmus zu konstruieren, der das Optimierungsproblem löst. In dieser 3D-Ansicht werden logarithmische Skalen verwendet, um die Topographie hervorzuheben. Wir werden eine neue Funktion definieren, um den logarithmischen Verlust für einen Satz von Punkten zu berechnen.Der Plot wird wie folgt definiert:- Die **X-Achse** stellt die Gewichte dar. - Die **Y-Achse** stellt den Bias dar.- Die **Z-Achse** (Höhe) stellt den entsprechenden Verlustwert bei einer gegebenen Gewicht/Bias-Konfiguration dar. Zur besseren Verständlichkeit wird der Logarithmus des MSE-Verlusts angezeigt.- Die **schwarze Kugel** stellt die aktuelle Gewicht/Bias-Konfiguration. Seine Höhe zeigt den Verlust dieser Konfiguration an. Führen Sie die folgenden Zellen aus.
###Code
# do not change
def log_mse(neuron:SimpleNeuron, points:dict) -> float:
least_squares_loss = loss(neuron, points)
return np.log10(least_squares_loss)
# do not change
class Interactive3DPlot:
def __init__(self, points:dict, ranges:dict, width:int=600, height:int=600, draw_time:int=0.1):
self.idle = True
self.points = points
self.draw_time = draw_time
self.threading = threading
self.range_weights = np.arange( # Array with all possible weight values in the given range
ranges["x"][0], ranges["x"][1], 0.1
)
self.range_biases = np.arange( # Array with all possible bias values in the given range
ranges["y"][0], ranges["y"][1], 0.1
)
self.range_biases_t = self.range_biases[:, np.newaxis] # Bias array transposed
self.range_losses = [] # initialize z axis for 3D surface
self.ball = go.Scatter3d( # initialize ball
x=[], y=[], z=[], hoverinfo="none", mode="markers", marker=dict(size=12, color="black")
)
self.layout = go.Layout(
width=width,
height=height,
showlegend=False,
autosize=False,
margin=dict(t=0, l=0),
scene=dict(
xaxis=dict(title="Weight", range=ranges["x"], autorange=False, showticklabels=True),
yaxis=dict(title="Bias", range=ranges["y"], autorange=False, showticklabels=True),
zaxis=dict(title="Loss: log(MSE)", range=ranges["z"], autorange=True, showticklabels=False),
),
)
self.data = [
go.Surface(
z=self.range_losses,
x=self.range_weights,
y=self.range_biases,
colorscale="Viridis",
opacity=0.9,
showscale=False,
hoverinfo="none",
),
self.ball,
]
self.plot = go.FigureWidget(self.data, self.layout)
def register_neuron(self, neuron:SimpleNeuron):
self.neuron = neuron
self.calc_surface()
# height of 3d surface represents loss of weight/bias combination
# In the 2D plot, x is an array from e.g. -4 to +4. But the weights and biases only have a single value
# Here x will be the points to do regression and to calculate the loss on.
# The surface is spanned by the arrays of weight and bias.
def calc_surface(self):
self.neuron.weight = ( #instead of 1 weight and 1 bias, let Neuron have an array of all weights and biases
self.range_weights
)
self.neuron.bias = self.range_biases_t
self.range_losses = log_mse( # result: matrix of losses of all weight/bias combinations in the given range
self.neuron, self.points
)
self.plot.data[0].z = self.range_losses
def update(self):
if self.idle:
thread = threading.Thread(target=self.redraw)
thread.start()
def redraw(self): # when updating, only the ball is redrawn
self.idle = False
time.sleep(self.draw_time)
self.ball.x = [self.neuron.weight]
self.ball.y = [self.neuron.bias]
self.ball.z = [log_mse(self.neuron, self.points)]
self.plot.data[1].x = self.ball.x
self.plot.data[1].y = self.ball.y
self.plot.data[1].z = self.ball.z
self.idle = True
# do not change
class DualPlot:
def __init__(self, points:dict, ranges_3d:dict, ranges_2d:dict):
self.plot_3d = Interactive3DPlot(points, ranges_3d)
self.plot_2d = Interactive2DPlot(points, ranges_2d, width=400, height=500, margin=dict(t=200, l=30))
def register_neuron(self, neuron:SimpleNeuron):
self.plot_3d.register_neuron(neuron)
self.plot_2d.register_neuron(neuron)
def update(self):
self.plot_3d.update()
self.plot_2d.update()
###Output
_____no_output_____
###Markdown
Aufgabe 4.2.3: Das Neuron trainieren Sie erhalten den gleiche Satz von 3 Datenpunkten und erneut ein Neuron, um eine Kurvenanpassung durchzuführen. Führen Sie die folgende Zelle aus. Verändern Sie das Gewicht und den Bias des Neurons mit den Schiebereglern, um den Verlust zu minimieren. Beobachten Sie alle Änderungen. Hinweis: Sie können den 3D-Plot drehen, indem Sie auf ihn klicken und den Cursor bewegen. Dabei müssen Sie mit dem Cursor innerhalb des Widgets bleiben.
###Code
# do not change
ranges_3d = dict(x=[-2.5, 2.5], y=[-2.5, 2.5], z=[-1, 2.5]) # set up ranges for the 3d plot
plot_task2 = DualPlot(points_linreg, ranges_3d, ranges_linreg) # create a DualPlot object to mange plotting on two plots
neuron_task2 = SimpleNeuron(plot_task2) # create a new neuron for this task
interact(
neuron_task2.set_values,
weight=FloatSlider(min=-2, max=2, step=0.2, layout=slider_layout),
bias=FloatSlider(min=-2, max=2, step=0.2, layout=slider_layout),
)
HBox((plot_task2.plot_3d.plot, plot_task2.plot_2d.plot))
###Output
_____no_output_____
###Markdown
Frage 4.2.4: Wo liegt im Allgemeinen die optimale Kombination aus Gewicht und Bias im 3D-Plot?Ihre Antwort: Frage 4.2.5: Wie steil ist das Tal an der Stelle der optimalen Gewichts- und Bias Kombination?Ihre Antwort: *** AktivierungsfunktionenBis jetzt ist unser Neuronenmodell, das aus Gewichten und Bias besteht, nur in der Lage, lineare Funktionen zu imitieren. Folglich können wir damit nur lineare Regression durchführen. Aktivierungsfunktionen erweitern unsere Möglichkeiten, indem sie dem Neuron eine zusätzliche Nichtlinearität hinzufügen. Mit ihnen können wir komplexere Funktionen modellieren. Die heutzutage am häufigsten verwendete Aktivierungsfunktion ist die Rectified Linear Unit, auch **ReLU** genannt. Sie gibt nur den Eingabewert aus, solange er größer als 0 ist. Ist er kleiner als 0, gibt sie 0 aus. Wir können diese Funktion bequem beschreiben, indem wir das Maximum des Eingabewertes und 0 nehmen. Der größere Wert von beiden wird als Ausgabe gewählt (5).\begin{align}f_{relu}(x) & = max(0,x) \;\;\;\;\;\;\;\;\;\;\; (5)\end{align} Führen Sie die folgende Zelle aus, um die ReLU Funktion zu definieren.
###Code
# do not change
def relu(input_val:float) -> float:
return np.where(input_val > 0, input_val, 0.0)
###Output
_____no_output_____
###Markdown
Wir können ein Neuron mit einer ReLU-Aktivierungsfunktion folgendermaßen zeichnen: Abb. 5 - Neuron mit ReLU-Aktivierungsfunktion visualisiertLassen Sie uns eine neue Klasse erstellen, um dieses Neuron in Python zu implementieren. Wir werden alle Eigenschaften eines Neurons von SimpleNeuron erben.Wir ändern nur die Ausgabe, indem wir sie zunächst durch unsere ReLU-Funktion führen:Aufgabe 4.2.6: Implementieren Sie ein komplettes künstlichen Neurons mit ReLU-Aktivierungsfunktion Vervollständigen Sie den folgenden Code eines künstliches Neuron, indem Sie die ReLU-Funktion von oben verwenden, um seine Aktivierung zu berechnen (wie in Abbildung 5). Schauen Sie sich die einfache Neuronenklasse an und schreiben Sie eine ähnliche BerechnungsfunktionSie brauchen die relu-Funktion nicht neu zu implementieren und sollten nicht mehr als 1 Zeile hinzufügen müssen.
###Code
class ReluNeuron(SimpleNeuron): #inherit from SimpleNeuron class
def compute(self, inputs:list) -> float:
# STUDENT CODE HERE
# STUDENT CODE until HERE
return self.activation
###Output
_____no_output_____
###Markdown
*** Aufgabe: Nichtlineare KlimaregelungSie finden sich als Ingenieur bei der Firma "ClimaTronics" wieder. Ihre Firma möchte KI-Technologie implementieren, um ihr neues Klimasystem "Perfect Climate 9000" zu regeln. Obwohl das Problem leicht mit konventioneller Programmierung gelöst werden kann, möchte die Geschäftsleitung, dass Sie KI implementieren, um Investoren zu gewinnen. Sie müssen die folgenden Anforderungen erfüllen, die im Datenblattauszug visualisiert sind:`Bei Temperaturen unter 25°C soll die Klimasteuerung ausgeschaltet bleiben. Bei einer Temperatur von 30°C soll sie 10% ihrer Kühlleistung erreichen. Zwischen 30°C und 40°C soll die Kühlleistung quadratisch mit der Temperatur ansteigen. Die Kühlleistung soll bei 40°C ihr Maximum erreichen.` Führen Sie die nachfolgende Zelle aus, um ein interaktives Diagramm anzuzeigen.
###Code
# do not change
points_climate = dict(x=[25.0, 27.5, 30.0, 32.5, 35, 37.5, 40.0], y=[0.0, 2.0, 10.0, 23.7, 43, 68.7, 100.0])
ranges_climate = dict(x=[-4, 45], y=[-4, 105])
climate_plot = Interactive2DPlot(points_climate, ranges_climate)
our_relu_neuron = ReluNeuron(climate_plot)
interact(
our_relu_neuron.set_values,
weight=FloatSlider(min=-10, max=10, step=0.1, value=0, layout=slider_layout),
bias=FloatSlider(min=-200.0, max=200.0, step=1, value=0, layout=slider_layout),
)
climate_plot.plot
###Output
_____no_output_____
###Markdown
Frage 4.2.7: Wie wirkt sich eine Änderung des Gewichts auf die Ausgabefunktion aus, wenn der Bias auf 0,00 gesetzt wird? Ihre Antwort: Frage 4.2.8: Wie wirkt sich die Änderung des Bias auf die Ausgangsfunktion aus? Ihre Antwort: Frage 4.2.9: Bei welcher Temperatur beginnt die Klimatisierung, wenn sie das Gewicht auf 1,00 und den Bias auf -10, setzen? Ihre Antwort: Frage 4.2.10: Bei welcher Temperatur beginnt die Klimatisierung, wenn Sie das Gewicht auf 1,00 und den Bias auf -20 setzen?Ihre Antwort: Frage 4.2.11: Bei welcher Temperatur beginnt die Klimatisierung, wenn Sie das Gewicht auf 2,00 und des Bias auf -20 setzen?Ihre Antwort: Frage 4.2.12: Was ist die beste Gewicht/Bias-Konfiguration, die Sie finden konnten?Ihre Antwort: SchlussfolgerungMit nur einem Neuron können wir den Einfluss von Gewicht und Bias leicht verstehen und nachvollziehen.Aber unsere Ein-Neuron-Approximation reicht nicht aus, um die benötigte quadratische Beziehung genau zu approximieren. *** Neuronale NetzeDie Approximation kann durch die Verwendung mehrerer Neuronen verbessert werden. Anstatt nur ein Neuron für unsere Approximation zu verwenden, konstruieren wir ein neuronales Netzwerk. Wir werden zwei ReLU-Neuronen und ein Ausgangsneuron verwenden, das ebenfalls Gewichte hat. Nun können wir entscheiden, wie wir das Ergebnis der beiden ReLU-Neuronen in der Mitte gewichten wollen. Versteckte Schichten (engl. Hidden Layers)In dem nachfolgenden neuronalen Netzwerk stellen die beiden Neuronen in der Mitte eine **versteckte Schicht** dar.In der letzten Aufgabe hatten das Gewicht und der Bias einen leicht nachvollziehbaren Einfluss auf die Ausgabe.Durch das Hinzufügen weiterer Neuronen wird die Beziehung zwischen den einzelnen Gewichten und Bias und der Ausgabe jedoch weniger nachvollziehbar.Wir erhalten die Gewichte und Bias, indem wir sie einfach solange anpassen, bis das Ergebnis stimmt. Bei diesem Vorgang verlieren wir schnell den Überblick darüber, was wir eigentlich genau berechnen. Daher ist es schwierig, ein einzelnes Neuron aus diesem Netz zu isolieren und seine Verantwortung im System zu beschreiben.Der Eingabewert wird mit den ersten Gewichten multipliziert und nach dem Hinzufügen der Bias auf eine Aktivierungsfunktion gegeben. Anschließend wird dieser Ausgang mit den zweiten Gewichten multipliziert. Versteckte Schichten können mehrfach hintereinander gestapelt werden. Dies gibt Raum für mehrere Berechnungsschritte und ermöglicht die Approximation komplexerer Funktionen.Neuronale Netze, die mindestens eine versteckte Schicht verwenden, haben eine interessante Eigenschaft: Sie können zur Approximation einer beliebigen stetigen Funktion verwendet werden. _(Siehe "Weiterführende Literatur")_ Wir werden eine Klasse für neuronale Netze erstellen. Das Netzwerk wird vier Gewichte und zwei Bias haben. Hinweis: Der Einfachheit halber und zur Wiederverwendbarkeit des Codes werden wir neuronale Netze so behandeln, wie wir einzelne Neuronen in den bisherigen Beispielen behandelt haben. Denken Sie daran, dass ein künstliches Neuron nur eine mathematische Funktion ist. Ein ganzes neuronales Netzwerk kann auch vollständig durch eine einzelne Funktion beschrieben werden, wie es auch bei der Berechnung der Aktivierung geschieht. Die Neuronen müssen nicht die konkrete Form von einzelnen Datenobjekten annehmen. Führen Sie die folgende Zelle aus, um ein neuronales Netzwerk zu definieren.
###Code
# do not change
class NeuralNetwork:
def __init__(self, plot):
self.plot = plot #I am assigned the following plot
self.plot.register_neuron(self) #hey plot, remember me
def set_config(self, w_i1:float, w_o1:float, b1:float, w_i2:float, w_o2:float, b2:float):
self.w_i1 = w_i1
self.w_o1 = w_o1
self.b1 = b1
self.w_i2 = w_i2
self.w_o2 = w_o2
self.b2 = b2
self.show_config()
self.plot.update() # please redraw my output
def show_config(self):
print("w_i1:", self.w_i1, "\t| ", "w_o1:", self.w_o1,"\n")
print("b1:", self.b1, "\t| ", "w_i2:", self.w_i2,"\n")
print("w_o2:", self.w_o2, "\t| ", "b2:", self.b2,"\n")
def compute(self, x:float)->float:
self.prediction = (relu(self.w_i1 * x + self.b1) * self.w_o1
+ relu(self.w_i2 * x + self.b2) * self.w_o2)
return self.prediction
###Output
_____no_output_____
###Markdown
*** Aufgabe: Nichtlineare Klimaregelung mit einem neuronalen NetzwerkFühren Sie die folgende Zelle aus und passen Sie Gewichte und Bias an, um eine bessere Annäherung an die gewünschte Kurve als in der vorherigen Aufgabe zu erreichen.
###Code
# do not change
climate_plot_adv = Interactive2DPlot(points_climate, ranges_climate)
our_neural_net = NeuralNetwork(climate_plot_adv)
interact(
our_neural_net.set_config,
w_i1=FloatSlider(min=-10, max=10, step=0.1, layout=slider_layout),
w_o1=FloatSlider(min=-10, max=10, step=0.1, layout=slider_layout),
b1=FloatSlider(min=-200.0, max=200.0, step=1, layout=slider_layout),
w_i2=FloatSlider(min=-10, max=10, step=0.1, layout=slider_layout),
w_o2=FloatSlider(min=-10, max=10, step=0.1, layout=slider_layout),
b2=FloatSlider(min=-200.0, max=200.0, step=1,layout=slider_layout),
)
climate_plot_adv.plot
###Output
_____no_output_____
###Markdown
Frage 4.2.13: Was ist die beste Konfiguration, die Sie finden konnten? (Kopie von oberhalb des Plots)Ihre Antwort: SchlussfolgerungWir stellen fest, dass die quadratische Beziehung durch die Verwendung zusätzlicher Gewichte und Bias besser angenähert werden kann. Mit zwei ReLU Neuronen können wir eine Funktion mit zwei Knicken erstellen.Die Komplexität der Suche nach den optimalen Gewichten/Biases nimmt jedoch mit jeder Variablen drastisch zu. Je leistungsfähiger unsere neuronalen Netze sein sollen, desto schwieriger wird die Optimierung. *** BackpropagationDie Lösung für unser Optimierungsproblem lautet Backpropagation. Mit ihr können wir den Prozess der Anpassung von Gewichten und Bias automatisieren. In diesem Beispiel werden wir zu den Grundlagen zurückkehren und ein einfaches Neuron ohne Aktivierungsfunktion betrachten. Backpropagation funktioniert unter Hinzunahme der partiellen Ableitungen der Verlustfunktion in Bezug auf jedes Gewicht und jeden Bias im Netz. Diese können mit Hilfe der Kettenregel der Infinitesimalrechnung berechnet werden. Die Ausgabe des Netzwerks $\hat{y} = \hat{f}(x,\theta )$(wenn $\hat{y}$ den vom neuronalen Netz vorhergesagten y-Wert bezeichnet) wird in der Forwardpropagation unter Anwendung der gegebenen Rechenregeln (Multiplizieren mit Gewichten, Summieren mit Bias und Aktivierungsfunktion, bis Sie den Ausgang erreichen) berechnet. Der Verlust wird dann aus dem vorhergesagten und dem Ground-Truth-Wert (tatsächlichem Wert) mit der Verlustfunktion berechnet. Mit diesem Verlust können Sie einfach die partiellen Ableitungen in der so genannten Backpropagation berechnen. Siehe zum Beispiel: [BackpropagationExample](https://ml-cheatsheet.readthedocs.io/en/latest/backpropagation.html) An jedem Punkt zeigen der Bias- und der Gewichtsgradient in die Richtung des höheren Verlustes. Die Größe des Gradienten repräsentiert den Betrag der Verlustzunahme. Angenommen, wir würden den Verlust in Abb. 6 __maximieren__: Alles, was wir tun müssen, ist, den partiellen Ableitungen zu folgen, indem wir sie zu unserem aktuellen Gewicht/Bias-Punkt addieren. In diesem Beispiel bedeutet das, dass wir das Gewicht stark verringern (siehe die Achsen in Abb. 6) und den Bias um einen geringen Betrag verringern (da seine partielle Ableitung eine geringere Größe hat).Da wir aber mit dem Verlust nach unten gehen wollen, _subtrahieren_ wir den Gradienten von unserem aktuellen Punkt. Dadurch nähern wir uns dem Minimum entgegen. Im nächsten Schritt sind wir so weiter unten und nahe dem Tiefpunkt. Da wir dann aber immer noch nicht nah genug am Tiefpunkt sind, wiederholen wir diese Schritte einfach, bis wir das Minimum erreicht haben.Das Gute an neuronalen Netzwerken ist, dass wir den Gradienten **analytisch** für alle möglichen Datenpunkte bestimmen können. Wir müssen ihn nicht durch numerische Methoden schätzen, wie z. B. durch die Berechnung des Verlusts zweier Gewicht/Bias-Kombinationen dividiert durch den "Schrittabstand" ("Euler-Methode"). Dieses Vorwissen über den Gradienten macht die Backpropagation vergleichsweise schnell. Leider können wir aber nicht analytisch die Gewicht/Bias-Kombination bestimmen, die die Verlustfunktion auf ihr Minimum bringt. Wir müssen sie immer noch iterativ über viele Schritte finden.Jeder Schritt, den wir machen, wird eine **Epoche** genannt. (In diesem Fall sind _Trainingsschritte_ und _Epochen_ gleichwertig). Da es schwierig ist, festzustellen, ob das Minimum erreicht ist, geben wir die Anzahl der Epochen vor unserem Abstieg an und lassen das Programm dann einfach laufen.Ist die Größe der Gradienten zu groß, werden wir nie ein Minimum erreichen. Das liegt daran, dass unser Algorithmus die Kugel (also den aktuellen Punkt) bei jedem Schritt zu stark bewegen will. Er wird um das Minimum oszillieren, aber nie dort ankommen. Im Extremfall kann die Bewegung sogar bis in die Unendlichkeit oszillieren. Um die Kontrolle über die Größe der Bewegung zu haben, wird der Gradient mit einem Faktor multipliziert, der **Lernrate** (engl. learning rate) genannt wird (auch "Schrittgröße" genannt im Gradientenabstieg). Indem wir die Lernrate auf einen optimalen Wert einstellen, können wir Oszillationen verhindern. Ist die Lernrate allerdings zu klein, wird das Netzwerk sehr lange brauchen, um zu "lernen", da sich dann die Gewichte und Bias nur sehr langsam ändern.Die Anzahl der Epochen sowie die Lernrate sind so genannte **Hyperparameter**. Sie beeinflussen den Trainingsprozess, sind aber nicht Teil des Netzwerks selbst. Abb. 6 - Partielle Ableitungen der Verlustfunktion Backpropagation-Plot vorbereitenWir werden ein neues 3D-Diagramm erstellen, das die vergangenen Gewichts-/Bias-/Verlustwerte darstellt, während wir versuchen, den Verlust Schritt für Schritt zu optimieren. Die schwarze Kugel hinterlässt eine Spur ihrer vergangenen Werte. Führen Sie die nachfolgende Zelle aus, um das Plotten der Backpropagation-Schritte zu aktivieren.
###Code
# do not change
plot_backprop = DualPlot(points_linreg, ranges_3d, ranges_linreg)
trace_to_plot = go.Scatter3d(x=[], y=[], z=[], hoverinfo="none", mode="lines", line=dict(width=10, color="grey"))
plot_backprop.plot_3d.data.append(trace_to_plot) # Expand 3D Plot to also plot traces
plot_backprop.plot_3d.plot = go.FigureWidget(plot_backprop.plot_3d.data, plot_backprop.plot_3d.layout)
plot_backprop.plot_3d.draw_time = 0
def redraw_with_traces(plot_to_update:DualPlot, neuron:SimpleNeuron, trace_list:dict, points:dict): # executed every update step
plot_to_update.plot_3d.plot.data[2].x = trace_list["x"]
plot_to_update.plot_3d.plot.data[2].y = trace_list["y"]
plot_to_update.plot_3d.plot.data[2].z = trace_list["z"]
plot_to_update.plot_3d.plot.data[1].x = [neuron.weight]
plot_to_update.plot_3d.plot.data[1].y = [neuron.bias]
plot_to_update.plot_3d.plot.data[1].z = [log_mse(neuron, points)]
plot_to_update.update()
def add_traces(neuron:SimpleNeuron, points:dict, trace_list:dict): # executed every epoch
trace_list["x"].extend([neuron.weight])
trace_list["y"].extend([neuron.bias])
trace_list["z"].extend([log_mse(neuron, points)])
###Output
_____no_output_____
###Markdown
*** DIY BackpropagationUm Backpropagation durchzuführen, müssen Sie zunächst die partiellen Ableitungen der Verlustfunktion des "einfachen Neurons" in Abhängigkeit von Gewicht und Bias bestimmen. Danach müssen Sie herausfinden, wie Sie die Gewichte und Bias richtig an den auf die Lernrate skalierten Gradienten anpassen.Am Ende dieser Übung können Sie Ihre Ergebnisse durch Training verifizieren. Wenn Sie die erwartete Leistung (Benchmark) erreichen, ist Ihr Algorithmus korrekt.Der Algorithmus arbeitet mit einem Dictionary von Punkten mit der Form von: [points_linreg](points_linreg). Aufgabe 4.2.14: Bestimmen Sie die Steigung analytisch!! Vervollständigen Sie die folgende Funktion selbst.Es gibt mehrere Lösungen, Ihr Algorithmus kann das Gewicht und den Bias in die richtige Richtung anpassen, obwohl die Berechnung des Gradienten falsch ist. Benchmark: Wenn Sie nach 100 Epochen und mit einer Lernrate von 0,03 einen Verlust von 0,22 erreichen können, ist Ihre Lösung richtig
###Code
def simple_neuron_loss_gradient(neuron:SimpleNeuron, points:dict)->dict:
gradient_sum = dict(weight=0, bias=0) # contains the sum of the weight and bias gradient
for point_x, point_y in zip(points["x"], points["y"]): # for each point
# Hint: point_x and point_y are the current point values
gradient_sum["weight"] += ( # sum up the gradient for each point
# STUDENT CODE HERE
# STUDENT CODE until HERE
)
gradient_sum["bias"] += (
# STUDENT CODE HERE
# STUDENT CODE until HERE
)
gradient = dict(weight=gradient_sum["weight"] / len(points["x"]), bias=gradient_sum["bias"] / len(points["x"]))
return gradient
###Output
_____no_output_____
###Markdown
Aufgabe 4.2.15: Das Neuron anpassenNachdem Sie den Gradienten ermittelt haben, müssen Sie das Gewicht und den Bias des Neurons basierend auf den partiellen Ableitungen und der Lernrate anpassen. Sie sollten Ihre Ergebnisse überprüfen, indem Sie das Netz unten trainieren. Vervollständigen Sie die nachfolgende Funktion selbst.
###Code
def adjust_neuron(neuron:SimpleNeuron, gradient:dict, learning_rate:float):
# STUDENT CODE HERE
# STUDENT CODE until HERE
###Output
_____no_output_____
###Markdown
Trainingsprozess definieren
###Code
# do not change
def train(neuron:SimpleNeuron, points:dict, epochs:int, learning_rate:float, redraw_step:int, trace_list:dict):
redraw_with_traces(neuron.plot, neuron, trace_list, points)
for i in range(1, epochs + 1): # first Epoch is Epoch no.1
add_traces(neuron, points, trace_list)
gradient = simple_neuron_loss_gradient(neuron, points)
adjust_neuron(neuron, gradient, learning_rate)
if i % redraw_step == 0:
print("Epoch:{} \t".format(i), end="")
redraw_with_traces(neuron.plot, neuron_backprop, trace_list, points)
###Output
_____no_output_____
###Markdown
Aufgabe 4.2.16: Hyperparameter wählen und trainierenWählen Sie eine optimale Lernrate und Anzahl der Epochen, indem Sie verschiedene Werte einstellen und die beiden nachfolgenden Zellen ausführen.
###Code
learning_rate = 0.03 #keep this for benchmarking, change to play around
epochs = 100 # keep this for benchmarking, change to play around
redraw_step = 10 # update plot every n'th epoch. too slow? set this to a higher value (e.g. 100)
# these values are taken as parameters by the train function below
neuron_backprop = SimpleNeuron(plot_backprop)
HBox((plot_backprop.plot_3d.plot, plot_backprop.plot_2d.plot))
#run this cell to test algorithm
np.random.seed(4) # keep this for benchmarking, remove to play around # TODO: Use np.RandomState !!!!!
neuron_backprop.set_values( # set weight and bias randomly
(5 * np.random.random() - 2.5), (5 * np.random.random() - 2.5)
)
trace_list1 = dict(x=[], y=[], z=[])
train(neuron_backprop, points_linreg, epochs, learning_rate, redraw_step, trace_list1)
###Output
_____no_output_____ |
.ipynb_checkpoints/MT_environment_setup-checkpoint.ipynb | ###Markdown
Get Started---
###Code
%run nmt_translate.py
_ = predict(s=10000, num=1, plot=True)
_ = predict(s=10000, num=10)
_ = predict(s=0, num=10)
_ = predict(s=10000, num=10, r_filt=.5)
_ = predict(s=10000, num=10, p_filt=.5)
###Output
English predictions, s=10000, num=10:
--------------------------------------------------
Src | 彼 は ドイツ 生まれ の 人 だ 。
Ref | he is a german by origin .
Hyp | he is a of of . . _EOS
--------------------------------------------------
precision | 0.5000
recall | 0.5714
sentences matching filter = 1
|
notebooks/Deep Learning with TensorFlow/2.6_intermediate_net_in_tensorflow.ipynb | ###Markdown
Intermediate Neural Network in TensorFlow In this notebook, we convert our [intermediate-depth MNIST-classifying neural network](https://github.com/the-deep-learners/TensorFlow-LiveLessons/blob/master/notebooks/intermediate_net_in_keras.ipynb) from Keras to TensorFlow (compare them side by side) following Aymeric Damien's [Multi-Layer Perceptron Notebook](https://github.com/aymericdamien/TensorFlow-Examples/blob/master/notebooks/3_NeuralNetworks/multilayer_perceptron.ipynb) style. Load dependencies
###Code
import numpy as np
np.random.seed(42)
import tensorflow as tf
tf.set_random_seed(42)
###Output
_____no_output_____
###Markdown
Load dataSmall cheat here - it's easier to load the data from keras' libraries. TensorFlow previously had these common datasets in `tf.contrib` however that module is experimental and is likely to change heavily between TensorFlow versions. As such, it's easier to get the data from a stable source.You could, however, download the data yourself from (Yann LeCun's website)[http://yann.lecun.com/exdb/mnist/] and process it on your own. We just think this is the simplest approach here, since this notebook is not about how to process MNIST images.
###Code
from keras.datasets import mnist
(X_train, y_train), (X_test, y_test) = mnist.load_data()
# Reshape the data and cast as float
X_train = X_train.reshape(60000, 784).astype('float32')
X_test = X_test.reshape(10000, 784).astype('float32')
# Normalize the data so that each pixel value is in the range [0,1]
X_train /= 255
X_test /= 255
# One-hot encode the labels
from keras.utils import to_categorical
y_train = to_categorical(y_train,10)
y_test = to_categorical(y_test,10)
###Output
Using TensorFlow backend.
###Markdown
Set neural network hyperparameters (tidier at top of file!)
###Code
lr = 0.1
epochs = 20
train_batch_size = 128
weight_initializer = tf.contrib.layers.xavier_initializer()
###Output
_____no_output_____
###Markdown
Set number of neurons for each layer
###Code
n_input = 784
n_dense_1 = 64
n_dense_2 = 64
n_classes = 10
###Output
_____no_output_____
###Markdown
Define placeholders Tensors for inputs and labels
###Code
x_placeholder = tf.placeholder(tf.float32, [None, n_input])
y_placeholder = tf.placeholder(tf.int32, [None, n_classes])
batch_size = tf.placeholder(tf.int64)
###Output
_____no_output_____
###Markdown
Create TensorFlow data sets
###Code
mnist_data = tf.data.Dataset.from_tensor_slices((x_placeholder, y_placeholder)).repeat().batch(batch_size)
###Output
_____no_output_____
###Markdown
Initialize iterator for data
###Code
iterator = mnist_data.make_initializable_iterator()
x, y = iterator.get_next()
###Output
_____no_output_____
###Markdown
Define types of layers
###Code
# dense layer with ReLU activation:
def dense(x, W, b):
z = tf.add(tf.matmul(x, W), b)
a = tf.nn.relu(z)
return a
###Output
_____no_output_____
###Markdown
Design neural network architecture
###Code
def network(x, weights, biases):
# two dense hidden layers:
dense_1 = dense(x, weights['W1'], biases['b1'])
dense_2 = dense(dense_1, weights['W2'], biases['b2'])
# linear output layer (softmax)
out_layer_z = tf.add(tf.matmul(dense_2, weights['W_out']), biases['b_out'])
return out_layer_z
###Output
_____no_output_____
###Markdown
Define dictionaries for storing weights and biases for each layer -- and initialize
###Code
bias_dict = {
'b1': tf.Variable(tf.zeros([n_dense_1])),
'b2': tf.Variable(tf.zeros([n_dense_2])),
'b_out': tf.Variable(tf.zeros([n_classes]))
}
weight_dict = {
'W1': tf.get_variable('W1', [n_input, n_dense_1], initializer=weight_initializer),
'W2': tf.get_variable('W2', [n_dense_1, n_dense_2], initializer=weight_initializer),
'W_out': tf.get_variable('W_out', [n_dense_2, n_classes], initializer=weight_initializer)
}
###Output
_____no_output_____
###Markdown
Build model
###Code
predictions = network(x, weights=weight_dict, biases=bias_dict)
###Output
_____no_output_____
###Markdown
Define model's loss and its optimizer
###Code
cost = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits_v2(logits=predictions, labels=y))
optimizer = tf.train.GradientDescentOptimizer(learning_rate=lr).minimize(cost)
###Output
_____no_output_____
###Markdown
Define evaluation metrics
###Code
# calculate accuracy by identifying test cases where the model's highest-probability class matches the true y label:
correct_prediction = tf.equal(tf.argmax(predictions, 1), tf.argmax(y, 1))
accuracy_pct = tf.reduce_mean(tf.cast(correct_prediction, tf.float32)) * 100
###Output
_____no_output_____
###Markdown
Create op for variable initialization
###Code
initializer_op = tf.global_variables_initializer()
###Output
_____no_output_____
###Markdown
Train the network in a session
###Code
with tf.Session() as session:
# Initialize the session variables
session.run(initializer_op)
# Initialize the dataset iterator
session.run(iterator.initializer, feed_dict={x_placeholder: X_train,
y_placeholder: y_train,
batch_size: train_batch_size})
print("Training for", epochs, "epochs.")
# loop over epochs:
for epoch in range(epochs):
avg_cost = 0.0 # track cost to monitor performance during training
avg_accuracy_pct = 0.0
n_batches = int(round(X_train.shape[0] / train_batch_size))
# Loop over batches
for batch in range(n_batches):
# feed batch data to run optimization and fetch cost and accuracy:
_, batch_cost, batch_acc = session.run([optimizer, cost, accuracy_pct])
# accumulate mean loss and accuracy over epoch:
avg_cost += batch_cost / n_batches
avg_accuracy_pct += batch_acc / n_batches
# output logs at end of each epoch of training:
print("Epoch ", '%03d' % (epoch+1),
": cost = ", '{:.3f}'.format(avg_cost),
", accuracy = ", '{:.2f}'.format(avg_accuracy_pct), "%",
sep='')
print("Training Complete. Testing Model.\n")
# Re-initialize the dataset iterator with the test data
session.run(iterator.initializer, feed_dict={x_placeholder: X_test,
y_placeholder: y_test,
batch_size: X_test.shape[0]})
# Run the session using the test data and fetch the cost and accuracy
_, test_cost, test_accuracy_pct = session.run([optimizer, cost, accuracy_pct])
# Print the final results
print("Test Cost:", '{:.3f}'.format(test_cost))
print("Test Accuracy: ", '{:.2f}'.format(test_accuracy_pct), "%", sep='')
###Output
Training for 20 epochs.
Epoch 001: cost = 0.477, accuracy = 86.41%
Epoch 002: cost = 0.237, accuracy = 93.05%
Epoch 003: cost = 0.181, accuracy = 94.62%
Epoch 004: cost = 0.147, accuracy = 95.69%
Epoch 005: cost = 0.124, accuracy = 96.38%
Epoch 006: cost = 0.108, accuracy = 96.89%
Epoch 007: cost = 0.096, accuracy = 97.21%
Epoch 008: cost = 0.086, accuracy = 97.54%
Epoch 009: cost = 0.077, accuracy = 97.78%
Epoch 010: cost = 0.071, accuracy = 97.96%
Epoch 011: cost = 0.065, accuracy = 98.13%
Epoch 012: cost = 0.059, accuracy = 98.31%
Epoch 013: cost = 0.055, accuracy = 98.48%
Epoch 014: cost = 0.051, accuracy = 98.61%
Epoch 015: cost = 0.047, accuracy = 98.71%
Epoch 016: cost = 0.043, accuracy = 98.84%
Epoch 017: cost = 0.040, accuracy = 98.96%
Epoch 018: cost = 0.037, accuracy = 99.03%
Epoch 019: cost = 0.034, accuracy = 99.11%
Epoch 020: cost = 0.032, accuracy = 99.21%
Training Complete. Testing Model.
Test Cost: 0.088
Test Accuracy: 97.31%
|
Accessing_summative_code_appendix.ipynb | ###Markdown
Accessing Data paper codeThis code was used in my MSc to produce a paper about OSM. It then formed the basis for a more complete approach using only HTML web scraping (no RSS feed) and a different approach to storing data, as part of my MSc thesis - addressing many of the issues with this approach which are discussed in the paper. For more information see the [paper](https://docs.google.com/document/d/1ZZvyY366r5glFlgblz3Sf2mJO8DrmmfNOvVCcaETHnQ/edit?usp=sharing) and the [short write up](https://www.jamiefawcett.org.uk/project/masters-accessing-data).
###Code
import requests
from bs4 import BeautifulSoup as bs
import feedparser as fp
import pandas as pd
import re
import datetime
import numpy as np
# import pprint as pp
###Output
_____no_output_____
###Markdown
Data collection
###Code
#A way to extract relevant data from a single topic's RSS feed from URL
#get the rss feed from a url
def getrss(url):
feed = fp.parse(url)
entries = feed['entries']
return entries
#gets all the feeds from topic url
def getPosts(topic_url):
topic_id = topic_url.split("?")[1]
topic_rss = "https://forum.openstreetmap.org/extern.php?action=feed&t%s&type=atom"%topic_id
feed = fp.parse(topic_rss)
topic_feed = feed['entries']
if len(topic_feed) == 0:
error = "ERROR: Couldn't find anything for %s" %topic_id
print(error)
with open("error_log{}.txt".format(datetime.datetime.now().date()),"a") as errorfile:
errorfile.write("Topic Empty Error: " + topic_id + "-" + str(datetime.datetime.now().time()) + "\n")
return None
else:
try:
posts = []
for c,entry in enumerate(topic_feed):
post = {}
post["author"] = entry['author']
post["time"] = entry['updated_parsed']
post_df = pd.DataFrame([post.values()],index=["{}.{}".format(topic_id,c)], columns=post.keys()) #
posts.append(post_df)
except KeyError:
print("ERROR: Something went wrong with ID")
with open("error_log{}.txt".format(datetime.datetime.now().date()),"a") as errorfile:
errorfile.write("ID Error: " + topic_url + "-" + str(datetime.datetime.now().time()) + "\n")
if len(posts) == 0:
return None
else:
topic_df = pd.concat(posts,axis=0)
return topic_df
# A way to get all RSS feeds from any sub-forum page
#A function to extract topic titles and links from sub-forums
def getTopics(forum_page):
soup = bs(forum_page)
topics_stuff = []
try:
for row in soup.findAll('tr',{"class": ["roweven","rowodd"]}):
topic_link = row.find("div",{"class":"tclcon"}).a.get("href")
posts = getPosts(topic_link)
try:
posts["topic_link"] = topic_link
posts["topic_title"] = row.find("div",{"class":"tclcon"}).a.text
posts["topic_reply_count"] = row.find('td',{"class": "tc2"}).text.strip()
posts["topic_view_count"] = row.find('td',{"class": "tc3"}).text.strip()
except TypeError: #to catch where no posts in topic
print("Error, no posts in topic, skipping {}".format(topic_link))
with open("error_log{}.txt".format(datetime.datetime.now().date()),"a") as errorfile:
errorfile.write("Post Error: " + topic_link + "-" + str(datetime.datetime.now().time()) + "\n")
topics_stuff.append(posts)
except: #to catch where forum page has no topics
print("ERROR: forum has no topics")
with open("error_log{}.txt".format(datetime.datetime.now().date()),"w") as errorfile:
errorfile.write("Forum Error: " + str(datetime.datetime.now().time()) + "\n")
continue
return topics_stuff
#A function that gets the number of pages and then gets forum pages for each page
def getAllPages(sub_forum_id):
first_page = requests.get('https://forum.openstreetmap.org/%s'%(sub_forum_id)).text
soup = bs(first_page)
page_nums = soup.find('p',{"class":"pagelink conl"})
if len(page_nums.findAll("a")) == 0:
max_page = 1
else:
numbers = [int(item.text) for item in page_nums.find_all("a") if item.text.isdigit()]
max_page = max(numbers)
# print("Getting %s pages" % (max_page))
forum_topics =[]
for page in range(0,max_page):
page_num = page+1
if page_num % 5 == 0:
print('Processing %s pages...and counting'%(page_num))
full_page = requests.get('https://forum.openstreetmap.org/%s&p=%s'%(sub_forum_id,page_num)).text
topic_entries = getTopics(full_page)
forum_topics.extend(topic_entries)
# print("Processed all pages")
forum_df = pd.concat(forum_topics,axis=0)
return forum_df
# This function wraps up all the previous functions
# starting from the index page and moving through each of the sub-forums
def getForums(index_url,filename): #include filename to write to
index = requests.get(index_url).text
soup = bs(index)
#Catching file overwrite issues
try:
with open(filename,"rb") as filein:
print("Something in this writing file already")
filename = "{}_new.{}".format(filename.split(".")[0],filename.split(".")[1])
print("Changed filename to {}".format(filename))
except FileNotFoundError:
print("Good to go, writing to {}".format(filename))
try:
with open("error_log{}.txt".format(datetime.datetime.now().date()),"rb") as errorfile:
print("Something in this error file already")
filename = "{}_new.{}".format(filename.split(".")[0],filename.split(".")[1])
print("Changed filename to {}".format(filename))
except FileNotFoundError:
print("Good to go, writing errors")
for c,row in enumerate(soup.findAll('tr',{"class": ["roweven","rowodd"]})):
title = row.find('h3').text.strip()
# print("Getting pages for %s" % (title))
link = row.find('h3').a.get("href").strip()
forum_topics = getAllPages(link)
forum_topics["forum_topic_count"] = row.find('td',{"class": "tc2"}).text.strip()
forum_topics["Forum_post_count"] = row.find('td',{"class": "tc3"}).text.strip()
forum_topics["forum_link"] = link
forum_topics["forum_title"] = title
forum_topics.to_csv(filename, mode ='a')
completion = (c / len(soup.findAll('tr',{"class": ["roweven","rowodd"]})))*100
print("Completed {0:.0f}% of forums".format(completion))
#Running
getForums("https://forum.openstreetmap.org/index.php","real_{}.csv".format(datetime.datetime.now().date()))
###Output
_____no_output_____
###Markdown
additional HTML scraping
###Code
all_data = pd.read_csv('full_data_2019-02-12.csv',index_col=0)
all_data = all_data[all_data["topic_link"]!="topic_link"]
topic_items = ["topic_link","topic_title","topic_reply_count","forum_title","forum_link"]
topic_df = all_data[topic_items].drop_duplicates().reset_index()
del topic_df['index']
def removeDash(x):
if x == '-':
x = np.nan
else:
x = x.replace(",","")
return float(x)
topic_df["topic_reply_count"] = topic_df["topic_reply_count"].map(lambda x: removeDash(x))
#create a df of all topics
missing_num = len(topic_df[topic_df["topic_reply_count"]>15])
# display(missing_num)
missing_topics = topic_df[topic_df["topic_reply_count"]>15]
#gets all the HTML pages from topic url
def getaPosts(topic_url,page_num):
topic_page = requests.get('https://forum.openstreetmap.org/%s&p=%s'%(topic_url,page_num)).text
topic_id = topic_url.split("?")[1]
soup = bs(topic_page)
page_posts = []
for c,post in enumerate(soup.findAll("div",{"class":re.compile("(blockpost(?:.*))")})):
if page_num == 1: #for first page, skip first 15 entries
if c < 15:
continue
post_details = {}
post_details["author"] = post.find("div",{"class":"postleft"}).find("dt").find("strong").text
post_details["time"] = post.find("h2").span.a.text
post_df = pd.DataFrame([post_details.values()],index=["{}.{}.{}".format(topic_id,page_num,c)], columns=post_details.keys()) #
page_posts.append(post_df)
topic_df = pd.concat(page_posts,axis=0)
return topic_df
#A function that gets RSS feeds for the entire forum
def getMissingPosts(topic_url):
first_page = requests.get('https://forum.openstreetmap.org/%s'%(topic_url)).text
soup = bs(first_page)
page_nums = soup.find('p',{"class":"pagelink conl"})
if len(page_nums.findAll("a")) == 0:
max_page = 1
else:
numbers = [int(item.text) for item in page_nums.find_all("a") if item.text.isdigit()]
max_page = max(numbers)
topics_feeds = []
for page in range(0,max_page):
page_num = page+1
post_entries = getaPosts(topic_url,page_num)
topics_feeds.append(post_entries)
post_df = pd.concat(topics_feeds,axis=0)
post_df["topic_link"] = topic_url
# post_df.to_csv("missing_posts{}.csv".format(datetime.datetime.now().date()), mode ='a')
return post_df
missing_post_list = []
for c, topic_link in enumerate(missing_topics["topic_link"]):
try:
missing_post_list.append(getMissingPosts(topic_link))
except ValueError:
print("Error: no missing posts in {}".format(topic_link))
if c%100 == 0:
how = (c+1) / missing_num
print("{}%".format(how*100))
missing_df = pd.concat(missing_post_list,axis=0)
combined_df = missing_df.merge(topic_df, on = "topic_link")
display(len(combined_df))
combined_df.to_csv("missing_posts.csv")
###Output
_____no_output_____
###Markdown
Analysis
###Code
import matplotlib.pyplot as plt
import seaborn as sns
% matplotlib inline
###Output
UsageError: Line magic function `%` not found.
###Markdown
Checking and cleaning
###Code
#append only -- means it all goes in -- drop title rows
all_data = all_data[all_data["topic_link"]!="topic_link"]
#merge in missing data
all_data = pd.read_csv('full_data_2019-02-12.csv',index_col=0)
# print(len(all_data))
missing_data = pd.read_csv('missing_posts.csv',index_col=0)
# print(len(missing_data))
# print(len(missing_data)+len(all_data))
combined_df = pd.concat([all_data,missing_data])
# print(len(combined_df))
combined_df.to_csv("all_data_inc_missing2019-02-13.csv")
#Check number of topics identified
print(len(combined_df["topic_title"].unique()))
###Output
_____no_output_____
###Markdown
Contributor analysis
###Code
#getting number of contributions
all_contributors = combined_df["author"].value_counts()
print(len(all_contributors))
#Get stats on contributors
contrib_stats = pd.DataFrame(all_contributors.describe())
display(contrib_stats)
# contrib_stats.to_csv("contributor_stats.csv")
#Get the data required for plots and stats
contrib_count = pd.DataFrame(all_contributors.value_counts())
contrib_count.columns = ["num_contributors"]
contrib_count["num_contributions"] = contrib_count.index
contrib_count["contributions_by_group"] = contrib_count.apply(lambda x: x.loc["num_contributors"] * x.loc["num_contributions"],axis=1)
contrib_count["log_contributions"] = np.log(contrib_count["num_contributions"])
contrib_count["log_contributors"] = np.log(contrib_count["num_contributors"])
# contrib_count.to_csv("contribution_counts.csv")
##Contrib plot
contrib_plot = sns.distplot(all_contributors,color="blue",kde=False)
plt.yscale('log')
contrib_plot.set_title("Histogram of contributions by number of contributors") #(log)
contrib_plot.set(xlabel="Number of contributions",ylabel="Number of contributors")
fig = contrib_plot.get_figure()
fig.savefig("contributor_hist.png")
plt.show()
##Log-log plot
log_plot = sns.lmplot(x="log_contributors",y="log_contributions", data=contrib_count,aspect=1.1, fit_reg=False) #,color="blue"
log_plot.fig.suptitle("Log-Log plot of contributors by contributions")
plt.xlabel("Logarithm of contributors")
plt.ylabel("Logarithm of contributions")
plt.xlim(None, 10)
log_plot.savefig(fname ="contributor_scatter.png",dpi =500)
plt.show()
## contributor split -- what % of users account for what % of contributions
total_contributions = contrib_count["contributions_by_group"].sum()
total_contributors = contrib_count["num_contributors"].sum()
# print(total_contributions)
# print(total_contributors)
percents = [90,95,99]
contrib_percents = []
for percent in percents:
newdic = {}
#take all that value or less and sum contributions / total contributions = gives tail
percentile = np.percentile(all_contributors, percent)
newdic["percentile"] = percentile
newdic["tail"] = (contrib_count["contributions_by_group"][contrib_count["num_contributions"]<=percentile].sum()/total_contributions)*100
# take all that value or more and sum contributions / total contributions = gives head
newdic["head"] = (contrib_count["contributions_by_group"][contrib_count["num_contributions"]>=percentile].sum()/total_contributions)*100
temp_df = pd.DataFrame([newdic.values()],index=[percent] ,columns=newdic.keys())
contrib_percents.append(temp_df)
contrib_percents_df = pd.concat(contrib_percents,axis=0)
contrib_percents_df.to_csv("percentile_cuts.csv",index = True)
## head tail breaks
def htfunction(longlist, htlist=[]):
mean = longlist.mean()
head = longlist[longlist > longlist.mean()]
tail = longlist[longlist < longlist.mean()]
head_count = len(head)
tail_count = len(tail)
sumht = len(head) + len(tail)
newdic = {"sum":sumht,"head_count":head_count,"tail_count":tail_count,"mean":mean}
temp_df = pd.DataFrame([newdic.values()],columns=newdic.keys())
while (head_count/sumht) < 0.4:
htlist.append(temp_df)
htfunction(head)
return htlist
hts_list = htfunction(all_contributors)
htbreaks_df = pd.concat(hts_list,axis=0)
htbreaks_df["head_percent"] = htbreaks_df.apply(lambda x: ((x["head_count"]) / (x["sum"]))*100,axis=1)
htbreaks_df["tail_percent"] = htbreaks_df.apply(lambda x: ((x["tail_count"]) / (x["sum"]))*100,axis=1)
# display(htbreaks_df)
htbreaks_df.to_csv("head_tail_breaks.csv")
###Output
_____no_output_____ |
covid-19-data-analysis.ipynb | ###Markdown
Imports
###Code
import json
import folium
import requests
import mimetypes
import http.client
import pandas as pd
import streamlit as st
import plotly
import plotly.express as px
from streamlit_folium import folium_static
from folium.plugins import HeatMap
from pandas.io.json import json_normalize
import matplotlib.pyplot as plt
import seaborn as sns
import inflection
import datetime
from IPython.core.display import HTML
from IPython.display import Image
!pip install inflection
###Output
Collecting inflection
Using cached inflection-0.5.1-py2.py3-none-any.whl (9.5 kB)
Installing collected packages: inflection
Successfully installed inflection-0.5.1
[33mWARNING: You are using pip version 20.2.3; however, version 21.1.1 is available.
You should consider upgrading via the '/home/marxcerqueira/.pyenv/versions/3.9.1/envs/covid-dashboard/bin/python3.9 -m pip install --upgrade pip' command.[0m
###Markdown
Helper Functions
###Code
def jupyter_settings():
%matplotlib inline
%pylab inline
plt.style.use( 'bmh' )
plt.rcParams['figure.figsize'] = [25, 12]
plt.rcParams['font.size'] = 24
display( HTML( '<style>.container { width:100% !important; }</style>') )
pd.options.display.max_columns = None
pd.options.display.max_rows = None
pd.set_option( 'display.expand_frame_repr', False )
sns.set()
jupyter_settings()
###Output
Populating the interactive namespace from numpy and matplotlib
###Markdown
Loading Data
###Code
conn = http.client.HTTPSConnection("api.covid19api.com")
payload = ''
headers = {}
conn.request("GET","/summary",payload,headers)
res = conn.getresponse()
data = res.read().decode('UTF-8')
covid = json.loads(data)
# Gera o dataframe
data_raw = pd.DataFrame(covid['Countries'])
data_raw.head()
###Output
_____no_output_____
###Markdown
Data Description
###Code
df1 = data_raw.copy()
###Output
_____no_output_____
###Markdown
Rename Columns
###Code
list(df1)
cols_old = list(df1)
snakecase = lambda x: inflection.underscore(x)
col_news = list(map(snakecase, cols_old))
# Rename columns
df1.columns = col_news
df1.head()
###Output
_____no_output_____
###Markdown
Data Dimension
###Code
print('Number of rows: {}'.format(df1.shape[0]))
print('Number of columns: {}'.format(df1.shape[1]))
###Output
Number of rows: 190
Number of columns: 12
###Markdown
Check NA Values
###Code
#checking NA values, dtypes, dataset dimension, change dtypes
df1.isnull().sum()
###Output
_____no_output_____
###Markdown
Data Types
###Code
df.info()
###Output
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 190 entries, 0 to 189
Data columns (total 12 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 ID 190 non-null object
1 Country 190 non-null object
2 CountryCode 190 non-null object
3 Slug 190 non-null object
4 NewConfirmed 190 non-null int64
5 TotalConfirmed 190 non-null int64
6 NewDeaths 190 non-null int64
7 TotalDeaths 190 non-null int64
8 NewRecovered 190 non-null int64
9 TotalRecovered 190 non-null int64
10 Date 190 non-null object
11 Premium 190 non-null object
dtypes: int64(6), object(6)
memory usage: 17.9+ KB
###Markdown
Change Dtypes
###Code
# chage date dtype to datetime
df1['date'] = pd.to_datetime(df1['date'])
df1.dtypes
###Output
_____no_output_____
###Markdown
Feature Engineering
###Code
df2 = df1.copy()
# create features from date - day, week, month, year
#day
df2['day'] = df2['date'].dt.day
#week
df2['week_of_year'] = df2['date'].dt.isocalendar().week.astype('int64')
#month
df2['month'] = df2['date'].dt.month
#year
df2['year'] = df2['date'].dt.year
df2.dtypes
###Output
_____no_output_____
###Markdown
Data Cleaning
###Code
df3 = df2.copy()
df3.head()
# drop unnecessary features
df3 = df3.drop(['id', 'country_code', 'slug', 'premium'], axis = 1)
df3.head()
###Output
_____no_output_____
###Markdown
Data Visualization
###Code
# daily cases, daily deaths, daily recovery
#daily
daily_cases = df2[['total_confirmed', 'day']].groupby('day').sum()
daily_cases
###Output
_____no_output_____
###Markdown
teste
###Code
conn = http.client.HTTPSConnection("api.covid19api.com")
payload = ''
headers = {}
conn.request("GET","/summary",payload,headers)
res = conn.getresponse()
data = res.read().decode('UTF-8')
covid = json.loads(data)
# Gera o dataframe
df = pd.DataFrame(covid['Countries'])
# Data Cleaning
# Drop unnecessary features
covid1 = df.drop(columns = ['CountryCode', 'Slug', 'Premium'], axis = 1)
# Feature Engineering
covid1['ActiveCases'] = covid1['TotalConfirmed'] - covid1['TotalRecovered']
covid1['ActiveCases'] = covid1['ActiveCases'] - covid1['TotalDeaths']
# New Dataframes
dfn = covid1.drop(['NewConfirmed', 'NewDeaths', 'NewRecovered'], axis = 1)
dfn = dfn.groupby('Country')['TotalConfirmed','TotalDeaths','TotalRecovered','ActiveCases'].sum().sort_values(by = 'TotalConfirmed', ascending = False)
dfn.style.background_gradient(cmap = 'Oranges')
dfc = covid1.groupby('Country')['TotalConfirmed', 'TotalDeaths', 'TotalRecovered', 'ActiveCases'].max().sort_values(by = 'TotalConfirmed', ascending = False).reset_index()
# Obtém os totais consolidados
confirmed_tot = int(dfc['TotalConfirmed'].sum())
deaths_tot = int(dfc['TotalDeaths'].sum())
recovered_tot = int(dfc['TotalRecovered'].sum())
active_tot = int(dfc['ActiveCases'].sum())
d = {'total confirmed cases': confirmed_tot, 'total deaths': deaths_tot, 'total recovered': recovered_tot, 'total active cases': active_tot}
world_df = pd.DataFrame(d, index=['world'])
d
world_df
###Output
_____no_output_____ |
notebooks/FINAL.ipynb | ###Markdown
Bitcoin Model Processed DataExaming Bitcoin over the last 5 years starting from the first few bitcoins that emerged. More specifically, analysis will examine prospects in the development of bitcoin predictive models. Major trends and patterns can be determined in such a fluctuating currency by looking major events that resulted in rapid increase of decrease of the bitcoin value. This model will be compared and contrasted to a sentiment analysis algorithim.
###Code
import numpy as np
import pandas as pd
%matplotlib inline
import seaborn as sns; sns.set(style="ticks", color_codes=True)
import matplotlib.pyplot as plt
import datetime
###Output
_____no_output_____
###Markdown
Problem Statement:Bitcoin along with several other variations ushered in a new era of cyrptocurrency and how humans today understand the functions and socioeconomic implications of developing a new currency. Essentially, the main and intial coin, bitcoin, functions as a new and decentralized method currency exhange that is traded and protect by the block chain. Bitcoins can be either purchase by their equivalent conversion or "mined" through a series of complex computer based problems. The issue the financial capitals around the world are facing is determing whether or not Bitcoin is a fad and if it not, when is the best and most lucrative time to invest. Essentially, is it possible to predict such a dynamic and fluctuative currency that has only existed for less than half a decade? Hypothesis: The theory that these algorithim(s) is a two aspect approach of standard currency and stock analysis techniques. the first method is a simple numerical time series examination. Essentially can the past historical data of the currency help predict some sort of model for how the coin will behave in a few years. Such a model is often is more stable forms of stock(s) that can be purchased on the New York Stock Exchange. The second model that will be implemented is edited text block analysis which will compare the sentiments developed for a particular day from online twitter, facebook, reddit, etc. sources. The theory behind testing will see that both models will prove to be much more accurate together, but the the sentiment model will be much more accurate because of the dynamic nature of the coin and its sensitivity as a currency. Importing & Processing Data
###Code
import requests
from pandas.io.json import json_normalize
date1= '2013-09-05'
date2= '2014-09-06'
r = requests.get("https://api.coindesk.com/v1/bpi/historical/close.json?start=" + date1 + "&end=" + date2)
#df=pd.read_json("https://api.coindesk.com/v1/bpi/historical/close.json?start=" + date1 + "&end=" + date2)
b=requests.get('https://www.quandl.com/api/v3/datasets/BCHARTS/BITSTAMPUSD')
r=(r.json()) # if response type was set to JSON, then you'll automatically have a JSON response here...
#df.pivot(index='foo', columns='bar', values='baz')
A=json_normalize(b.json())#.transpose#.pivot(index='date', columns='bpi')
#df=pd.DataFrame(r.key(),index=r.values())
A
data= A['dataset.data'].all()
df = pd.DataFrame(data)
df.columns=A['dataset.column_names'].all()
df.index= df.Date
df=df.reindex(index=df.index[::-1])
del df['Open'],df['High'],df['Low'],df['Volume (Currency)'],df['Date']
sns.pairplot(df,diag_kind="kde")
df.head()
pd.read_csv('bit_data.csv').dropna()
#print(A)
#A['Date'] = pd.to_datetime(A['Date'], format = '%m-%d-%y')
date_range = pd.date_range(datetime.date(2010, 7, 18), datetime.date(2018, 2, 4)) #year, month, day
# df= pd.Series(A,index=date_range)
# df
#A.index=A['Date']
#del A['Date']
# date_rangex
#A['Date'] = pd.to_datetime(A['Date'], format = '%Y-%m-%d')
df.plot()
df.describe()
df['2017-09-13':'2018-02-08'].plot() #Add most reason date
b=requests.get('https://api.blockchain.info/charts/total-bitcoins?timespan=all&format=json')
bitcoin_num=json_normalize(b.json())
#print(A.columns)
bitcoin_num
df2 = pd.DataFrame(bitcoin_num['values'].all())
# df.index=df[0]
# del df[0]
#df=df.reindex(index=df.index[::-1])
df2.index=df2['x']
del df2['x']
df2.plot()
plt.xlabel('Time')
plt.ylabel('Bitcoin number')
#plt.plot(df.x,df.y)
date_range = pd.date_range(datetime.date(2010, 7, 18), datetime.date(2018, 2, 4)) #year, month, day
# bit =pd.read_csv('total-bitcoins.csv')
# bit.columns=['Date', 'Number of Coins']
# bit.index=bit.Date
# del bit['Date']
# fig, ax = plt.subplots(figsize=(15,7))
# bit.plot(kind='bar',ax=ax)
# ax.xaxis.set_major_formatter(bit.index.DateFormatter('%b %d'))
(df['Weighted Price']).plot()
plt.ylabel('Coin Value is USD($)')
df['Volume (BTC)'];
(df['Volume (BTC)']).plot()
###Output
_____no_output_____
###Markdown
Statistical Analysis:Examining the code through a series of various trends to see if linear/logistic/bayesian modelling is more intuitive and whether or not the data tells us anything statistically
###Code
import sys
from statsmodels.tsa.stattools import adfuller
def test_stationarity(timeseries):
#Determing rolling statistics
rolmean = timeseries.rolling(window=12,center=True).mean()
rolstd = timeseries.rolling(window=12,center=False).std()
#Plot rolling statistics:
orig = plt.plot(timeseries, color='blue',label='Bit Coin Data wrt Time')
mean = plt.plot(rolmean, color='red', label='Mean Over Time')
std = plt.plot(rolstd, color='black', label = 'Standard Deviation over Time')
plt.title('Bitcoin Rolling Mean & Standard Deviation')
plt.legend()
plt.show(block=False)
# #Perform Dickey-Fuller test:
# print('Results of Dickey-Fuller Test:')
# dftest = adfuller(timeseries, autolag='AIC')
# dfoutput = pd.Series(dftest[0:2], index=['Test Statistic','p-value'])
# print(dfoutput)
test_stationarity(df['Close'])
rolmean = timeseries.rolling(window=12,center=True).mean()
rolstd = timeseries.rolling(window=12,center=False).std()
#Plot rolling statistics:
orig = plt.plot(timeseries, color='blue',label='Bit Coin Data wrt Time')
mean = plt.plot(rolmean, color='red', label='Mean Over Time')
std = plt.plot(rolstd, color='black', label = 'Standard Deviation over Time')
plt.show
prices=df['Close']
prices= pd.DataFrame({'Close':prices.values,'Date':prices.index,})
prices.columns=['Close', 'Date']
prices['Date'] = pd.to_datetime(prices['Date'])
prices = prices.set_index('Date')
prices
prices_log = np.log(prices)
plt.plot(prices_log)
plt.title('Logistic Bit Coin Examination')
plt.ylabel('Logistic Coin Value')
plt.xlabel('Time (yr)')
#some data is lost here because of inf values
prices_log= prices_log[~prices_log.isin([np.nan, np.inf, -np.inf]).any(1)]
###Output
_____no_output_____
###Markdown
Seasonal Examination? Are there any seasonal trends?
###Code
from statsmodels.tsa.seasonal import seasonal_decompose
decomposition = seasonal_decompose(prices)
trend = decomposition.trend
seasonal = decomposition.seasonal
residual = decomposition.resid
plt.subplot(411)
plt.plot(prices, label='Original')
plt.legend(loc='best')
plt.subplot(412)
plt.plot(trend, label='Trend')
plt.legend(loc='best')
plt.subplot(413)
plt.plot(seasonal,label='Seasonality')
plt.legend(loc='best')
plt.subplot(414)
plt.plot(residual, label='Residuals')
plt.legend(loc='best')
plt.tight_layout()
prices=df['Close']
N=120
movmean=[]
movstd=[]
for p in range(N,len(prices)):
A= np.mean(prices[p-N:p])
movmean.append(A)
X=np.std(prices[p-N:p])
movstd.append(X)
plt.plot(movmean,label = 'Moving Mean')
plt.plot(movstd, label = 'Moving Standard Deviation')
#plt.plot(prices, label ='Price Data')
plt.legend()
plt.show()
fig = plt.figure(figsize=(9, 6))
ax = fig.add_subplot(111, xlabel='Coin Price in \$', ylabel='Price GLD in \$')
colors = np.linspace(0.1, 1, len(prices))
mymap = plt.get_cmap("winter")
sc = ax.scatter(prices.GFI, prices.GLD, c=colors, cmap=mymap, lw=0)
cb = plt.colorbar(sc)
cb.ax.set_yticklabels([str(p.date()) for p in prices[::len(prices)//10].index])
from pandas.plotting import lag_plot
lag_plot(prices)
from sklearn.model_selection import cross_val_predict
from sklearn import linear_model
import matplotlib.pyplot as plt
lr = linear_model.LinearRegression()
predicted = cross_val_predict(lr, , prices, cv=10)
fig, ax = plt.subplots()
ax.scatter(y, predicted, edgecolors=(0, 0, 0))
ax.plot([prices.min(), prices.max()], [prices.min(), prices.max()], 'k--', lw=4)
ax.set_xlabel('Measured')
ax.set_ylabel('Predicted')
plt.show()
date_list= [datetime(2017, 6, 30), datetime(2017, 7, 31), datetime(2017, 8, 31), datetime(2017, 9, 30),
datetime(2017, 10, 31), datetime(2017, 11, 30), datetime(2017, 12, 31), datetime(2018, 1, 31)]
###Output
_____no_output_____ |
Modulo_2/Problemas Diversos.ipynb | ###Markdown
PROBLEMAS DIVERSOS 1.Realizar una función que permita la carga de n alumnos. Por cada alumno se deberá preguntar el nombre completo y permitir el ingreso de 3 notas. Las notas deben estar comprendidas entre 0 y 10. Devolver el listado de alumnos.
###Code
cantidad = int(input("Ingrese la cantidad de alumnos a registrar : "))
cantidad
lista_alumnos = []
# solicito n veces un alumno por teclado
for i in range(cantidad):
# dicx vacio
dicx_alumnos = {}
# ingreso datos
dicx_alumnos['alumno'] = input(f'Ingrese el {i+1} alumno: ')
nota1 = int(input('ingrese la nota 1: '))
nota2 = int(input('ingrese la nota 2: '))
nota3 = int(input('ingrese la nota 3: '))
dicx_alumnos['notas'] = [nota1,nota2,nota3]
# añado alumno a listado
lista_alumnos.append(dicx_alumnos)
lista_alumnos
dicx_alumnos = {}
dicx_alumnos
dicx_alumnos['alumno'] = "Gonzalo"
dicx_alumnos['notas'] = [10,5,3]
###Output
_____no_output_____
###Markdown
2.Definir una función que dado un listado de alumnos evalúe cuántos aprobaron y cuántos desaprobaron, teniendo en cuenta que se aprueba con 4. La nota será el promedio de las 3 notas para cada alumno.
###Code
for alumno in lista_alumnos:
print(alumno['alumno'])
###Output
Gonzalo
Juan
Maria
|
notebooks/solutions/S05_Exercises_Solution.ipynb | ###Markdown
**1**.Using R magic in Jupyter, load the `iris` data set from R into a `pandas` DataFrame and use `seaborn` to generate the plot shown.
###Code
iris = %R iris
iris.head()
import seaborn as sns
sns.set_context('notebook', font_scale=1.5)
iris.columns = iris.columns.str.replace('\.Length', 'L').str.replace('\.Width', 'W')
g = sns.catplot(
data=iris.melt(id_vars='Species', var_name='Measurement', value_name='Value'),
x='Measurement',
y='Value',
col='Species',
kind='violin'
)
g.set_titles('{col_name}')
pass
g.savefig('iris.png')
###Output
_____no_output_____
###Markdown
**1**.Using R magic in Jupyter, load the `iris` data set from R into a `pandas` DataFrame and use `seaborn` to generate the plot shown.
###Code
iris = %R iris
iris.head()
import seaborn as sns
sns.set_context('notebook', font_scale=1.5)
iris.columns = iris.columns.str.replace('\.Length', 'L').str.replace('\.Width', 'W')
g = sns.catplot(
data=iris.melt(id_vars='Species', var_name='Measurement', value_name='Value'),
x='Measurement',
y='Value',
col='Species',
kind='violin'
)
g.set_titles('{col_name}')
pass
g.savefig('iris.png')
###Output
_____no_output_____
###Markdown
**1**.Using R magic in Jupyter, load the `iris` data set from R into a `pandas` DataFrame and use `seaborn` to generate the plot shown.
###Code
iris = %R iris
iris.head()
import seaborn as sns
sns.set_context('notebook', font_scale=1.5)
iris.columns = iris.columns.str.replace('\.Length', 'L').str.replace('\.Width', 'W')
g = sns.catplot(
data=iris.melt(id_vars='Species', var_name='Measurement', value_name='Value'),
x='Measurement',
y='Value',
col='Species',
kind='violin'
)
g.set_titles('{col_name}')
pass
g.savefig('iris.png')
###Output
_____no_output_____ |
Project Notes/Kaggle Learn/02 Intro to Machine Learning/exercise05 random forests.ipynb | ###Markdown
**[Machine Learning Micro-Course Home Page](https://www.kaggle.com/learn/intro-to-machine-learning)**--- RecapHere's the code you've written so far.
###Code
# Code you have previously used to load data
import pandas as pd
from sklearn.metrics import mean_absolute_error
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeRegressor
# Path of the file to read
iowa_file_path = '../input/home-data-for-ml-course/train.csv'
home_data = pd.read_csv(iowa_file_path)
# Create target object and call it y
y = home_data.SalePrice
# Create X
features = ['LotArea', 'YearBuilt', '1stFlrSF', '2ndFlrSF', 'FullBath', 'BedroomAbvGr', 'TotRmsAbvGrd']
X = home_data[features]
# Split into validation and training data
train_X, val_X, train_y, val_y = train_test_split(X, y, random_state=1)
# Specify Model
iowa_model = DecisionTreeRegressor(random_state=1)
# Fit Model
iowa_model.fit(train_X, train_y)
# Make validation predictions and calculate mean absolute error
val_predictions = iowa_model.predict(val_X)
val_mae = mean_absolute_error(val_predictions, val_y)
print("Validation MAE when not specifying max_leaf_nodes: {:,.0f}".format(val_mae))
# Using best value for max_leaf_nodes
iowa_model = DecisionTreeRegressor(max_leaf_nodes=100, random_state=1)
iowa_model.fit(train_X, train_y)
val_predictions = iowa_model.predict(val_X)
val_mae = mean_absolute_error(val_predictions, val_y)
print("Validation MAE for best value of max_leaf_nodes: {:,.0f}".format(val_mae))
# Set up code checking
from learntools.core import binder
binder.bind(globals())
from learntools.machine_learning.ex6 import *
print("\nSetup complete")
###Output
Validation MAE when not specifying max_leaf_nodes: 29,653
Validation MAE for best value of max_leaf_nodes: 27,283
Setup complete
###Markdown
ExercisesData science isn't always this easy. But replacing the decision tree with a Random Forest is going to be an easy win. Step 1: Use a Random Forest
###Code
from sklearn.ensemble import RandomForestRegressor
# Define the model. Set random_state to 1
rf_model = RandomForestRegressor(random_state=1)
# fit your model
rf_model.fit(train_X, train_y)
# Calculate the mean absolute error of your Random Forest model on the validation data
rf_val_mae = mean_absolute_error(rf_model.predict(val_X), val_y)
print("Validation MAE for Random Forest Model: {}".format(rf_val_mae))
step_1.check()
# The lines below will show you a hint or the solution.
# step_1.hint()
# step_1.solution()
###Output
_____no_output_____
###Markdown
So far, you have followed specific instructions at each step of your project. This helped learn key ideas and build your first model, but now you know enough to try things on your own. Machine Learning competitions are a great way to try your own ideas and learn more as you independently navigate a machine learning project.
###Code
# Keep Going
You are ready for **[Machine Learning Competitions](https://www.kaggle.com/kernels/fork/1259198).**
###Output
_____no_output_____ |
foundation/applied-statistics/notebooks/SM3 - Normal Distribution Example - Cereal Packs.ipynb | ###Markdown
Problem Statement - Normal DistributionThe mean weight of a morning breakfast cereal pack is 0.295 kg with a standard deviation of 0.025 kg. The random variable weight of the pack follows a normal distribution. a)What is the probability that the pack weighs less than 0.280 kg? b)What is the probability that the pack weighs more than 0.350 kg? c)What is the probability that the pack weighs between 0.260 kg to 0.340 kg?
###Code
import scipy.stats as stats
z=(0.28-0.295)/0.025
z
stats.norm.cdf(-0.6)
z=(0.35-0.295)/0.025
z
1 - stats.norm.cdf(2.2)
z1= (0.26-0.295)/ 0.025
z2 = (0.36-0.295)/0.025
stats.
###Output
_____no_output_____ |
1-Introduction/04-stats-and-probability/assignment.ipynb | ###Markdown
Introduction to Probability and Statistics AssignmentIn this assignment, we will use the dataset of diabetes patients taken [from here](https://www4.stat.ncsu.edu/~boos/var.select/diabetes.html).
###Code
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
df = pd.read_csv("../../data/diabetes.tsv",sep='\t')
df.head()
###Output
_____no_output_____
###Markdown
In this dataset, columns as the following:* Age and sex are self-explanatory* BMI is body mass index* BP is average blood pressure* S1 through S6 are different blood measurements* Y is the qualitative measure of disease progression over one yearLet's study this dataset using methods of probability and statistics. Task 1: Compute mean values and variance for all values
###Code
print("Mean: \n-------------\n", df.mean())
print("\n")
print("Variance: \n-------------\n", df.var())
###Output
Mean:
-------------
AGE 48.518100
SEX 1.468326
BMI 26.375792
BP 94.647014
S1 189.140271
S2 115.439140
S3 49.788462
S4 4.070249
S5 4.641411
S6 91.260181
Y 152.133484
dtype: float64
Variance:
-------------
AGE 171.846610
SEX 0.249561
BMI 19.519798
BP 191.304401
S1 1197.717241
S2 924.955494
S3 167.293585
S4 1.665261
S5 0.272892
S6 132.165712
Y 5943.331348
dtype: float64
###Markdown
Task 2: Plot boxplots for BMI, BP and Y depending on gender
###Code
plt.figure(figsize=(10,2))
df.boxplot(column='BMI', by='SEX')
plt.suptitle('')
plt.title('Boxplot for BMI grouped by SEX')
plt.ylabel('BMI')
plt.show()
plt.figure(figsize=(10,2))
df.boxplot(column='BP', by='SEX')
plt.suptitle('')
plt.title('Boxplot for BP grouped by SEX')
plt.ylabel('BP')
plt.show()
plt.figure(figsize=(10,2))
df.boxplot(column='Y', by='SEX')
plt.suptitle('')
plt.title('Boxplot for Y grouped by SEX')
plt.ylabel('Y')
plt.show()
###Output
_____no_output_____
###Markdown
Task 3: What is the the distribution of Age, Sex, BMI and Y variables?
###Code
plt.figure(figsize=(8,6))
df['AGE'].hist(bins=15)
plt.ylabel('AGE')
plt.show()
plt.figure(figsize=(8,6))
df['SEX'].hist(bins=15)
plt.ylabel('SEX')
plt.show()
plt.figure(figsize=(8,6))
df['BMI'].hist(bins=15)
plt.ylabel('BMI')
plt.show()
plt.figure(figsize=(8,6))
df['Y'].hist(bins=15)
plt.ylabel('Y')
plt.show()
###Output
_____no_output_____
###Markdown
Task 4: Test the correlation between different variables and disease progression (Y)> **Hint** Correlation matrix would give you the most useful information on which values are dependent.
###Code
pd.DataFrame(df.corr()['Y']).style.background_gradient(cmap='coolwarm', axis=0)
###Output
_____no_output_____
###Markdown
Task 5: Test the hypothesis that the degree of diabetes progression is different between men and women
###Code
from scipy.stats import ttest_ind
tval, pval = ttest_ind(df.loc[df['SEX']==1,['Y']],
df.loc[df['SEX']==2,['Y']],
equal_var=False)
print(f"T-value = {tval[0]:.2f}\nP-value: {pval[0]}")
###Output
T-value = -0.90
P-value: 0.3674449793083975
###Markdown
Introduction to Probability and Statistics
Assignment
In this assignment, we will use the dataset of diabetes patients taken [from here](https://www4.stat.ncsu.edu/~boos/var.select/diabetes.html).
###Code
import pandas as pd
import numpy as np
df = pd.read_csv("../../data/diabetes.tsv",sep='\t')
df.head()
###Output
_____no_output_____
###Markdown
Introduction to Probability and Statistics AssignmentIn this assignment, we will use the dataset of diabetes patients taken [from here](https://www4.stat.ncsu.edu/~boos/var.select/diabetes.html).
###Code
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
df = pd.read_csv("../../data/diabetes.tsv",sep='\t')
df.head()
###Output
_____no_output_____
###Markdown
In this dataset, columns as the following:* Age and sex are self-explanatory* BMI is body mass index* BP is average blood pressure* S1 through S6 are different blood measurements* Y is the qualitative measure of disease progression over one yearLet's study this dataset using methods of probability and statistics. Task 1: Compute mean values and variance for all values
###Code
df.describe()
###Output
_____no_output_____
###Markdown
Task 2: Plot boxplots for BMI, BP and Y depending on gender
###Code
df.boxplot(column=['BMI', 'BP', 'Y'],by='SEX')
plt.show()
for col in ['BMI', 'BP', 'Y']:
df.boxplot(column=col, by='SEX')
plt.show()
###Output
_____no_output_____
###Markdown
Task 3: What is the the distribution of Age, Sex, BMI and Y variables?
###Code
for col in ['AGE', 'SEX', 'BMI', 'Y']:
df[col].hist(bins=15)
plt.xlabel(col)
plt.ylabel('Count')
plt.show()
###Output
_____no_output_____
###Markdown
Task 4: Test the correlation between different variables and disease progression (Y)> **Hint** Correlation matrix would give you the most useful information on which values are dependent.
###Code
df.corr()
###Output
_____no_output_____
###Markdown
Task 5: Test the hypothesis that the degree of diabetes progression is different between men and women
###Code
from scipy.stats import ttest_ind
tval, pval = ttest_ind(df.loc[df['SEX']==1,['Y']], df.loc[df['SEX']==2,['Y']],equal_var=False)
print(f"T-value = {tval[0]:.2f}\nP-value: {pval[0]}")
###Output
T-value = -0.90
P-value: 0.3674449793083975
###Markdown
Introduction to Probability and Statistics AssignmentIn this assignment, we will use the dataset of diabetes patients taken [from here](https://www4.stat.ncsu.edu/~boos/var.select/diabetes.html).
###Code
import pandas as pd
import numpy as np
df = pd.read_csv("../../data/diabetes.tsv",sep='\t')
df.head()
###Output
_____no_output_____
###Markdown
In this dataset, columns as the following:* Age and sex are self-explanatory* BMI is body mass index* BP is average blood pressure* S1 through S6 are different blood measurements* Y is the qualitative measure of disease progression over one yearLet's study this dataset using methods of probability and statistics. Task 1: Compute mean values and variance for all values
###Code
df.mean()
df.var()
###Output
_____no_output_____
###Markdown
Task 2: Plot boxplots for BMI, BP and Y depending on gender
###Code
import matplotlib.pyplot as plt
df.boxplot(column='BMI',by='SEX')
plt.xticks(rotation='vertical')
plt.show()
df.boxplot(column='BP',by='SEX')
plt.xticks(rotation='vertical')
plt.show()
df.boxplot(column='Y',by='SEX')
plt.xticks(rotation='vertical')
plt.show()
###Output
_____no_output_____
###Markdown
Task 3: What is the the distribution of Age, Sex, BMI and Y variables?
###Code
df['AGE'].hist(bins=20)
plt.suptitle('Age distribution of diabetes patients')
plt.xlabel('Age')
plt.ylabel('Count')
plt.show()
df['SEX'].hist(bins=3)
plt.suptitle('Sex distribution of diabetes patients')
plt.xlabel('Sex')
plt.ylabel('Count')
plt.show()
df['BMI'].hist(bins=20)
plt.suptitle('BMI distribution of diabetes patients')
plt.xlabel('BMI')
plt.ylabel('Count')
plt.show()
df['Y'].hist(bins=30)
plt.suptitle('Y distribution of diabetes patients')
plt.xlabel('Y')
plt.ylabel('Count')
plt.show()
###Output
_____no_output_____
###Markdown
Task 4: Test the correlation between different variables and disease progression (Y)> **Hint** Correlation matrix would give you the most useful information on which values are dependent.
###Code
progression = df['Y']
bmi = df['BMI']
print(f"Correlation = {np.corrcoef(bmi,progression)[0,1]}")
progression = df['Y']
sex = df['SEX']
print(f"Correlation = {np.corrcoef(sex,progression)[0,1]}")
progression = df['Y']
bp = df['BP']
print(f"Correlation = {np.corrcoef(bp,progression)[0,1]}")
###Output
Correlation = 0.4414817585625712
###Markdown
Task 5: Test the hypothesis that the degree of diabetes progression is different between men and women
###Code
df.groupby('SEX').agg({ 'AGE' : 'mean', 'BP' : 'mean', 'Y' : 'mean', 'SEX' : 'count'}).rename(columns={ 'SEX' : 'Count'})
# Student t-test
# Testataan, eteneekö diabetes nopeammin sukupuolella 1 kuin sukupuolella 2
# P-value can be considered as the probability of two distributions having the same mean. Koska tässä tapauksessa
# se on hyvin pieni, sukupuolten välillä vaikuttaa olevan eroa siinä miten tauti etenee.
# Negatiivinen T-arvo ilmeisesti viittaa siihen, että diabetes etenee nopeammin sukupuolella 2 kuin sukupuolella 1?
from scipy.stats import ttest_ind
tval, pval = ttest_ind(df.loc[df['SEX']== 1,['Y']], df.loc[df['SEX']== 2,['Y']],equal_var=False)
print(f"T-value = {tval[0]:.2f}\nP-value: {pval[0]}")
###Output
T-value = -0.90
P-value: 0.3674449793083975
###Markdown
Introduction to Probability and Statistics AssignmentIn this assignment, we will use the dataset of diabetes patients taken [from here](https://www4.stat.ncsu.edu/~boos/var.select/diabetes.html).
###Code
import pandas as pd
import numpy as np
df = pd.read_csv("../../data/diabetes.tsv",sep='\t')
df.head()
###Output
_____no_output_____
###Markdown
In this dataset, columns as the following:* Age and sex are self-explanatory* BMI is body mass index* BP is average blood pressure* S1 through S6 are different blood measurements* Y is the qualitative measure of disease progression over one yearLet's study this dataset using methods of probability and statistics. Task 1: Compute mean values and variance for all values
###Code
means = df.mean()
vars = df.var()
print("means:")
print(means)
print("vars:")
print(vars)
###Output
means:
AGE 48.518100
SEX 1.468326
BMI 26.375792
BP 94.647014
S1 189.140271
S2 115.439140
S3 49.788462
S4 4.070249
S5 4.641411
S6 91.260181
Y 152.133484
dtype: float64
vars:
AGE 171.846610
SEX 0.249561
BMI 19.519798
BP 191.304401
S1 1197.717241
S2 924.955494
S3 167.293585
S4 1.665261
S5 0.272892
S6 132.165712
Y 5943.331348
dtype: float64
###Markdown
Task 2: Plot boxplots for BMI, BP and Y depending on gender
###Code
import matplotlib.pyplot as plt
# BMI
df.boxplot(column=['BMI'],by='SEX')
plt.show()
# BP
df.boxplot(column=['BP'],by='SEX')
plt.show()
# Y
df.boxplot(column=['Y'],by='SEX')
plt.show()
# not good to show
df.boxplot(column=['BMI','BP','Y'],by='SEX')
plt.show()
###Output
_____no_output_____
###Markdown
Task 3: What is the the distribution of Age, Sex, BMI and Y variables?
###Code
# AGE might be normal distribution
df['AGE'].hist()
plt.show()
# SEX might be uniform distribution
df['SEX'].hist()
plt.show()
# BMI hard to tell
df['BMI'].hist()
plt.show()
# Y hard to tell
df['Y'].hist()
plt.show()
###Output
_____no_output_____
###Markdown
Task 4: Test the correlation between different variables and disease progression (Y)> **Hint** Correlation matrix would give you the most useful information on which values are dependent.
###Code
df.corr()
###Output
_____no_output_____
###Markdown
Conclusion:* The strongest correlation of Y is BMI and S5 (blood sugar). This sounds reasonable.
###Code
fig, ax = plt.subplots(1,3,figsize=(10,5))
for i,n in enumerate(['BMI','S5','BP']):
ax[i].scatter(df['Y'],df[n])
ax[i].set_title(n)
plt.show()
###Output
_____no_output_____
###Markdown
Task 5: Test the hypothesis that the degree of diabetes progression is different between men and women
###Code
from scipy.stats import ttest_ind
tval, pval = ttest_ind(df.loc[df['SEX']==1,['Y']], df.loc[df['SEX']==2,['Y']],equal_var=False)
print(f"T-value = {tval[0]:.2f}\nP-value: {pval[0]}")
###Output
T-value = -0.90
P-value: 0.3674449793083975
###Markdown
Introduction to Probability and Statistics AssignmentIn this assignment, we will use the dataset of diabetes patients taken [from here](https://www4.stat.ncsu.edu/~boos/var.select/diabetes.html).
###Code
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
df = pd.read_csv("../../data/diabetes.tsv",sep='\t')
df.head()
###Output
_____no_output_____
###Markdown
In this dataset, columns as the following:* Age and sex are self-explanatory* BMI is body mass index* BP is average blood pressure* S1 through S6 are different blood measurements* Y is the qualitative measure of disease progression over one yearLet's study this dataset using methods of probability and statistics. Task 1: Compute mean values and variance for all values
###Code
pd.DataFrame([df.mean(), df.var()], index=['Mean', 'Variance'])
###Output
_____no_output_____
###Markdown
Task 2: Plot boxplots for BMI, BP and Y depending on gender
###Code
for col in ['BMI', 'BP', 'Y']:
df.boxplot(column=col, by='SEX')
plt.plot()
###Output
_____no_output_____
###Markdown
Task 3: What is the the distribution of Age, Sex, BMI and Y variables?
###Code
for col in ['AGE', 'SEX', 'BMI', 'Y']:
df.hist(column=col)
plt.plot()
###Output
_____no_output_____
###Markdown
Task 4: Test the correlation between different variables and disease progression (Y)> **Hint** Correlation matrix would give you the most useful information on which values are dependent.
###Code
correlations = {}
for col in ['AGE', 'SEX', 'BMI', 'BP', 'S1', 'S2', 'S3', 'S4', 'S5', 'S6']:
cc = {}
correlations[f'{col}'] = np.corrcoef(df[col], df['Y'])[0,1]
maxCorr = max(zip(correlations.values(), correlations.keys()))
print(f"Max correlation between variables and Y: {maxCorr[1]} with a correlation of {maxCorr[0]}")
###Output
Max correlation between variables and Y: BMI with a correlation of 0.5864501344746885
###Markdown
Task 5: Test the hypothesis that the degree of diabetes progression is different between men and women
###Code
from scipy.stats import ttest_ind
tval, pval = ttest_ind(df.loc[df['SEX'] == 1, ['Y']], df.loc[df['SEX'] == 2, ['Y']], equal_var=False)
print(f'T-value is: {tval[0]:.2f}\nP-value is: {pval[0]}')
###Output
T-value is: -0.90
P-value is: 0.3674449793083975
|
image_barycenters.ipynb | ###Markdown
Show data samples
###Code
toshow = []
for digit in range(0, 10):
ix = data.dataset.targets == digit
D = data.dataset.data[ix].view(-1, 28, 28).float()
toshow.append(D[np.random.choice(D.shape[0])])
plt.figure(figsize=(11, 2))
plt.imshow(torch.cat(toshow, dim=1).data.numpy(), cmap='gray_r')
plt.axis('off')
plt.show()
###Output
_____no_output_____
###Markdown
Compute barycenters
###Code
barys = []
for digit in range(0, 10):
start = time()
ix = data.dataset.targets == digit
D = data.dataset.data[ix].view(-1, 28, 28).float()
# for EMNIST
#D = data.dataset.train_data[ix].view(-1, 28, 28).transpose(-1, -2).float()
logit_q = torch.nn.Parameter(0*torch.rand(1, img_size*img_size))
temp = torch.nn.Parameter(torch.tensor(0.1))
optimizer = torch.optim.Adam([logit_q, temp], lr=0.03, amsgrad=True)
for i in range(500):
optimizer.zero_grad()
q = torch.softmax(logit_q / torch.exp(temp), dim=1).view(1, img_size, img_size)
p = utils.sample_and_resize(D[0:-1, ...], img_size, 32)
loss = gait.breg_sim_divergence(img_kernel, p, q).mean() #this is convex
if i % 100 == 0:
print("%d - %d : %.3e" % (digit, i, loss.item()))
if False and i % 100 == 0:
q = torch.softmax(logit_q / torch.exp(temp), dim=1).view(img_size, img_size).data
plt.figure(figsize=(1, 1))
plt.imshow(q, cmap='gray_r');
plt.show()
loss.backward()
optimizer.step()
q = torch.softmax(logit_q / torch.exp(temp), dim=1).view(img_size, img_size)
barys.append(q.data)
print('Class time: ', time()-start)
###Output
0 - 0 : 1.404e+00
0 - 100 : 5.290e-01
0 - 200 : 4.951e-01
0 - 300 : 5.135e-01
0 - 400 : 4.507e-01
Class time: 6.253126859664917
1 - 0 : 2.143e+00
1 - 100 : 5.374e-01
1 - 200 : 5.486e-01
1 - 300 : 5.100e-01
1 - 400 : 7.565e-01
Class time: 6.263250112533569
2 - 0 : 1.489e+00
2 - 100 : 5.569e-01
2 - 200 : 5.552e-01
2 - 300 : 6.365e-01
2 - 400 : 5.464e-01
Class time: 6.106867551803589
3 - 0 : 1.513e+00
3 - 100 : 5.855e-01
3 - 200 : 5.427e-01
3 - 300 : 4.787e-01
3 - 400 : 5.127e-01
Class time: 6.367943525314331
4 - 0 : 1.702e+00
4 - 100 : 5.804e-01
4 - 200 : 6.236e-01
4 - 300 : 6.343e-01
4 - 400 : 6.353e-01
Class time: 6.370590925216675
5 - 0 : 1.600e+00
5 - 100 : 5.988e-01
5 - 200 : 6.585e-01
5 - 300 : 7.309e-01
5 - 400 : 7.576e-01
Class time: 6.655850887298584
6 - 0 : 1.604e+00
6 - 100 : 5.395e-01
6 - 200 : 5.009e-01
6 - 300 : 6.186e-01
6 - 400 : 5.378e-01
Class time: 6.7001893520355225
7 - 0 : 1.788e+00
7 - 100 : 7.068e-01
7 - 200 : 6.484e-01
7 - 300 : 5.483e-01
7 - 400 : 6.800e-01
Class time: 6.570442199707031
8 - 0 : 1.473e+00
8 - 100 : 5.198e-01
8 - 200 : 4.701e-01
8 - 300 : 4.910e-01
8 - 400 : 4.852e-01
Class time: 6.43403697013855
9 - 0 : 1.641e+00
9 - 100 : 5.990e-01
9 - 200 : 6.613e-01
9 - 300 : 6.183e-01
9 - 400 : 6.405e-01
Class time: 6.658806085586548
###Markdown
Show computed barycenters
###Code
plt.figure(figsize=(1.1*len(barys), 2.5))
cat_barys = torch.cat( [ (_ - _.min()) / (_.max() - _.min()) for _ in barys], dim=1)
plt.imshow(cat_barys**1.2, cmap='gray_r')
plt.axis('off')
#plt.savefig('mnist_gray_barys_breg', dpi=300)
plt.show()
###Output
_____no_output_____ |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.