code
stringlengths 122
4.99k
| label
int64 0
14
|
---|---|
diff --git a/controllers/BaseController.php b/controllers/BaseController.php @@ -203,6 +203,7 @@ class BaseController
if (self::$htmlPurifierInstance == null)
{
$htmlPurifierConfig = \HTMLPurifier_Config::createDefault();
+ $htmlPurifierConfig->set('Cache.SerializerPath', GROCY_DATAPATH . '/viewcache');
$htmlPurifierConfig->set('HTML.Allowed', 'div,b,strong,i,em,u,a[href|title],ul,ol,li,p[style],br,span[style],img[width|height|alt|src],table[border|width|style],tbody,tr,td,th,blockquote');
$htmlPurifierConfig->set('CSS.AllowedProperties', 'font,font-size,font-weight,font-style,font-family,text-decoration,padding-left,color,background-color,text-align');
$htmlPurifierConfig->set('URI.AllowedSchemes', ['data' => true]);
| 12 |
diff --git a/server/tests/interpreter_test.js b/server/tests/interpreter_test.js @@ -75,11 +75,11 @@ function runTest(t, name, src, expected) {
* native functions into the interpreter. initFunc is called
* with the interpreter instance to be configured as its
* parameter.
- * @param {Function(Interpreter)=} asyncFunc Optional function to be
+ * @param {Function(Interpreter)=} waitFunc Optional function to be
* called if .run() returns true. Can be used to fake completion
* of asynchronous events for testing purposes.
*/
-function runComplexTest(t, name, src, expected, initFunc, asyncFunc) {
+function runComplexTest(t, name, src, expected, initFunc, waitFunc) {
var intrp = getInterpreter();
if (initFunc) {
initFunc(intrp);
@@ -88,8 +88,8 @@ function runComplexTest(t, name, src, expected, initFunc, asyncFunc) {
try {
var thread = intrp.createThreadForSrc(src).thread;
while (intrp.run()) {
- if (asyncFunc) {
- asyncFunc(intrp);
+ if (waitFunc) {
+ waitFunc(intrp);
}
}
} catch (e) {
@@ -784,7 +784,7 @@ exports.testNumberToString = function(t) {
* @param {!T} t The test runner object.
*/
exports.testAsync = function(t) {
- var resolve, reject, arg, name, asyncFunc;
+ var resolve, reject, arg, name, waitFunc;
var initFunc = function(intrp) {
intrp.addVariableToScope(intrp.global, 'async', new intrp.NativeFunction({
name: 'async', length: 0,
@@ -800,7 +800,7 @@ exports.testAsync = function(t) {
// Test ordinary return.
name = 'testAsyncResolve';
- asyncFunc = function(intrp) {
+ waitFunc = function(intrp) {
resolve(arg);
};
var src = `
@@ -809,11 +809,11 @@ exports.testAsync = function(t) {
'between';
async('af') + 'ter';
`;
- runComplexTest(t, name, src, 'after', initFunc, asyncFunc);
+ runComplexTest(t, name, src, 'after', initFunc, waitFunc);
// Test throwing an exception.
name ='testAsyncReject';
- asyncFunc = function(intrp) {
+ waitFunc = function(intrp) {
reject('except');
};
src = `
@@ -825,13 +825,13 @@ exports.testAsync = function(t) {
e;
}
`;
- runComplexTest(t, name, src, 'except', initFunc, asyncFunc);
+ runComplexTest(t, name, src, 'except', initFunc, waitFunc);
// Extra check to verify async function can't resolve/reject more
// than once without an asertion failure.
name = 'testAsyncSafetyCheck';
var ok;
- asyncFunc = function(intrp) {
+ waitFunc = function(intrp) {
resolve(ok);
// Call reject; this is expected to blow up.
try {
@@ -844,7 +844,7 @@ exports.testAsync = function(t) {
async();
async();
`;
- runComplexTest(t, name, src, 'ok', initFunc, asyncFunc);
+ runComplexTest(t, name, src, 'ok', initFunc, waitFunc);
// A test of unwind_, to make sure it unwinds and kills the correct
// thread when an async function throws.
| 10 |
diff --git a/src/update-views.js b/src/update-views.js @@ -14,7 +14,6 @@ async function main(){
async function updateViews(){
for(const uuid of await db.collection('views').distinct('uuid')){
- const profiles = await db.collection('views').distinct('profile_id', { uuid });
const { username } = await db.collection('usernames').findOne({ uuid });
const profileViews = await db.collection('views').find({ uuid }).toArray();
| 8 |
diff --git a/modules/command/common/keep-alive-command.js b/modules/command/common/keep-alive-command.js @@ -54,7 +54,7 @@ class KeepAliveCommand extends Command {
const config = {
method: 'post',
- url: 'http://localhost:3000/signal',
+ url: 'https://signum.origintrail.io:3000/signal',
headers: {
'Content-Type': 'application/json'
},
| 3 |
diff --git a/src/sdk/p2p/peerconnection-channel.js b/src/sdk/p2p/peerconnection-channel.js @@ -342,6 +342,13 @@ class P2PPeerConnectionChannel extends EventDispatcher {
Logger.debug('About to set remote description. Signaling state: ' +
this._pc.signalingState);
sdp.sdp = this._setRtpSenderOptions(sdp.sdp, this._config);
+ // Firefox only has one codec in answer, which does not truly reflect its
+ // decoding capability. So we set codec preference to remote offer, and let
+ // Firefox choose its preferred codec.
+ // Reference: https://bugzilla.mozilla.org/show_bug.cgi?id=814227.
+ if (Utils.isFirefox()) {
+ sdp.sdp = this._setCodecOrder(sdp.sdp);
+ }
const sessionDescription = new RTCSessionDescription(sdp);
this._pc.setRemoteDescription(sessionDescription).then(() => {
this._createAndSendAnswer();
| 12 |
diff --git a/app/controllers/data_library_controller.rb b/app/controllers/data_library_controller.rb @@ -10,7 +10,7 @@ class DataLibraryController < ApplicationController
render_404 and return if @viewed_user.nil? || (Cartodb.get_config(:data_library, 'username') && (Cartodb.config[:data_library]['username'] != @viewed_user.username))
@dataset_base_url = (Rails.env.production? || Rails.env.staging?) ? "#{request.protocol}#{CartoDB.account_host}/dataset/" : "#{@viewed_user.public_url(nil, request.protocol == "https://" ? "https" : "http")}/tables/"
- @has_new_dashboard = @viewed_user.builder_enabled? && @viewed_user.has_feature_flag?('dashboard_migration')
+ @has_new_dashboard = @viewed_user.has_feature_flag?('dashboard_migration')
respond_to do |format|
format.html { render 'index' }
| 4 |
diff --git a/src/utils/innerSliderUtils.js b/src/utils/innerSliderUtils.js @@ -121,8 +121,9 @@ export const initializedState = spec => {
ReactDOM.findDOMNode(spec.listRef).querySelector('[data-index="0"]')
)
let listHeight = slideHeight * spec.slidesToShow
- let currentSlide = spec.currentSlide || spec.initialSlide
- if (spec.rtl && !spec.currentSlide) {
+ let currentSlide = spec.currentSlide === undefined
+ ? spec.initialSlide : spec.currentSlide
+ if (spec.rtl && spec.currentSlide === undefined) {
currentSlide = slideCount - 1 - spec.initialSlide
}
let lazyLoadedList = spec.lazyLoadedList || []
@@ -150,7 +151,6 @@ export const changeSlideHelper = spec => {
}
if (lazyLoad && lazyLoadedList.indexOf(animationSlide) < 0) {
lazyLoadedList.push(animationSlide)
- //onLazyLoad && onLazyLoad(animationSlide)
}
state = {
animating: true,
@@ -162,7 +162,7 @@ export const changeSlideHelper = spec => {
finalSlide = animationSlide
if (animationSlide < 0) {
finalSlide = animationSlide + slideCount
- if (!inifinite) finalSlide = 0
+ if (!infinite) finalSlide = 0
else if(slideCount % slidesToScroll !== 0)
finalSlide = slideCount - slideCount % slidesToScroll
} else if (centerMode && animationSlide >= slideCount) {
@@ -171,10 +171,11 @@ export const changeSlideHelper = spec => {
} else if (animationSlide >= slideCount) {
finalSlide = animationSlide - slideCount
if (!infinite) finalSlide = slideCount - slidesToShow
- else if(slideCount % slidesToScroll !== 0) finalSlide = 0
+ else if((slideCount % slidesToScroll) !== 0) finalSlide = 0
} else if (currentSlide + slidesToShow < slideCount &&
animationSlide + slidesToShow >= slideCount) {
- if (!infinite || (slideCount - animationSlide) % slidesToScroll !== 0)
+ if (!infinite) finalSlide = slideCount - slidesToShow
+ else if ((slideCount - animationSlide) % slidesToScroll !== 0)
finalSlide = slideCount - slidesToShow
}
animationLeft = getTrackLeft({...spec, slideIndex: animationSlide})
| 1 |
diff --git a/requirements.txt b/requirements.txt @@ -26,7 +26,7 @@ networkx==1.9.1
XlsxWriter==0.6.6
krauler>=0.2.2
polyglot==16.7.4
-langid==1.1.5
+langid==1.1.6
blinker==1.4
Babel==2.3.4
xlrd==0.9.3
@@ -49,6 +49,10 @@ countrynames>=1.4
dalet>=1.1
ingestors>=0.3.7
+# Workarounds
+cryptography>=1.9
+cffi>=1.10.0
+
# Python 3 backports
functools32>=3.2
| 12 |
diff --git a/packages/idyll-cli/bin/cmds/build.js b/packages/idyll-cli/bin/cmds/build.js @@ -105,6 +105,10 @@ function buildOptions (yargs) {
.describe('theme', 'Name of (or path to) the theme to use')
.default('theme', 'github')
.describe('alias', 'A list of component aliases')
+ .describe('outputCSS', 'Name of CSS file to generate')
+ .default('outputCSS', 'idyll_styles.css')
+ .describe('outputJS', 'Name of JS file to generate')
+ .default('outputJS', 'idyll_index.js')
.alias('h', 'help')
}
| 1 |
diff --git a/articles/troubleshoot/concepts/auth-issues.md b/articles/troubleshoot/concepts/auth-issues.md @@ -14,7 +14,6 @@ useCase:
# Troubleshoot Authentication Issues
<%= include('../../_includes/_topic-links', { links: [
- 'monitoring/guides/test-testall-endpoints',
'troubleshoot/guides/check-api-calls',
'troubleshoot/guides/check-login-logout-issues',
'troubleshoot/guides/check-user-profiles',
| 2 |
diff --git a/assets/js/modules/analytics/components/dashboard-details/AnalyticsDashboardDetailsWidgetTopAcquisitionSources.js b/assets/js/modules/analytics/components/dashboard-details/AnalyticsDashboardDetailsWidgetTopAcquisitionSources.js * WordPress dependencies
*/
import { Fragment } from '@wordpress/element';
-import { __, _x, sprintf } from '@wordpress/i18n';
+import { __, _x } from '@wordpress/i18n';
/**
* Internal dependencies
@@ -68,12 +68,6 @@ export default function AnalyticsDashboardDetailsWidgetTopAcquisitionSources() {
<Layout
className="googlesitekit-analytics-acquisition-sources"
footer
- headerCtaLink="https://analytics.google.com"
- headerCtaLabel={ sprintf(
- /* translators: %s: module name. */
- __( 'See full stats in %s', 'google-site-kit' ),
- _x( 'Analytics', 'Service name', 'google-site-kit' )
- ) }
footerCtaLabel={ _x( 'Analytics', 'Service name', 'google-site-kit' ) }
footerCtaLink={ serviceURL }
>
| 2 |
diff --git a/articles/appliance/dashboard/index.md b/articles/appliance/dashboard/index.md @@ -39,8 +39,6 @@ For additional information about the pages contained in the PSaaS Appliance conf
[Activity](/appliance/dashboard/activity)
-[Instrumentation](/appliance/dashboard/instrumentation)
-
[Rate Limiting](/appliance/dashboard/rate-limiting)
[CLI](/appliance/dashboard/cli)
| 2 |
diff --git a/.travis.yml b/.travis.yml @@ -72,6 +72,7 @@ before_install:
- sudo add-apt-repository ppa:openjdk-r/ppa -y
- sudo apt-get update
- sudo apt-get install -y openjdk-11-jdk
+ - sudo update-java-alternatives -s java-1.11.0-openjdk-amd64
- java -version
- export TZ=Australia/Canberra
- date
| 4 |
diff --git a/packages/titus-components/src/navigation/navigation.js b/packages/titus-components/src/navigation/navigation.js @@ -91,7 +91,8 @@ class Navigation extends Component {
classes: PropTypes.object.isRequired,
theme: PropTypes.object.isRequired,
items: PropTypes.func,
- main: PropTypes.func.isRequired
+ main: PropTypes.func.isRequired,
+ customComponent: PropTypes.func
}
state = {
@@ -103,7 +104,7 @@ class Navigation extends Component {
render () {
const { handleMenuOpen, handleMenuClose } = this
- const { classes, title, main, items, theme } = this.props
+ const { classes, title, main, items, theme, customComponent: CustomComponent } = this.props
const { menuOpen } = this.state
return (
@@ -128,6 +129,9 @@ class Navigation extends Component {
<Typography variant='title' color='inherit' noWrap>
{title}
</Typography>
+
+ {CustomComponent && <CustomComponent />}
+
</Toolbar>
</AppBar>
<Drawer
| 11 |
diff --git a/src/views/enterprise/components/enterpriseCta.js b/src/views/enterprise/components/enterpriseCta.js @@ -22,26 +22,24 @@ const EnterpriseCTA = ({toggleBookMeetingModal}) => (
<style jsx>{`
/* = SECTION
* ==================================================================== */
- .Section {
- margin-top: 60px;
- margin-bottom: 60px;
- position: relative;
- }
-
- @media screen and (min-width: 560px) {
.Section {
margin-top: 90px;
margin-bottom: 90px;
- }
+ position: relative;
}
@media screen and (min-width: 960px) {
.Section {
- margin-top: 190px;
- margin-bottom: 190px;
+ margin-top: 120px;
+ margin-bottom: 160px;
}
}
+ .u-subtitle {
+ max-width: 545px;
+ margin: 10px auto;
+ }
+
/* = SPLIT
* ==================================================================== */
.Split {
| 7 |
diff --git a/lib/parselink.js b/lib/parselink.js @@ -113,7 +113,7 @@ export default function parselink(str, htmldesc, clrdmods = false) {
// Straight roll 4d, 2d-1, etc. Is "damage" if it includes a damage type. Allows "!" suffix to indicate minimum of 1.
// Supports: 2d+1x3(5), 4dX2(0.5), etc
- parse = str.replace(/^(\d+)d([-+]\d+)?([xX\*]\d+)?(\([.\d]+\))?(!)? ?(.*)$/g, "$1~$2~$3~$4~$5~$6")
+ parse = str.replace(/^(\d+)d([-+]\d+)?([xX\*]\d+)? ?(\([.\d]+\))?(!)? ?(.*)$/g, "$1~$2~$3~$4~$5~$6")
if (parse != str) {
let a = parse.split("~");
let d = a[5].trim();
| 11 |
diff --git a/src/react/projects/spark-core-react/src/SprkTable/SprkTable.js b/src/react/projects/spark-core-react/src/SprkTable/SprkTable.js @@ -23,10 +23,8 @@ const SprkTable = (props) => {
}
SprkTable.propTypes = {
- // The children that will be rendered inside the link
+ // The children that will be rendered inside the table
children: PropTypes.node,
- // The table variant that determines the class names
- variant: PropTypes.oneOf([]),
// The string to use for the data-id attribute
idString: PropTypes.string,
// Any additional classes to add to the table wrapper
| 3 |
diff --git a/edit.js b/edit.js @@ -316,9 +316,11 @@ scene.add(floorMesh); */
scene.add(mesh);
})(); */
+let rayMesh = null;
{
- const rayMesh = makeRayMesh();
+ rayMesh = makeRayMesh();
rayMesh.visible = false;
+ rayMesh.target = new THREE.Vector3();
scene.add(rayMesh);
window.addEventListener('mousedown', e => {
@@ -327,21 +329,31 @@ scene.add(floorMesh); */
const result = physicsManager.raycast(position, quaternion);
if (result) { // world geometry raycast
- result.point = new THREE.Vector3().fromArray(result.point);
+ rayMesh.target.fromArray(result.point);
rayMesh.position.copy(position);
- rayMesh.quaternion.setFromUnitVectors(new THREE.Vector3(0, 0, -1), result.point.clone().sub(position).normalize());
- rayMesh.scale.z = result.point.distanceTo(position);
+ rayMesh.quaternion.setFromUnitVectors(new THREE.Vector3(0, 0, -1), rayMesh.target.clone().sub(position).normalize());
+ rayMesh.scale.z = rayMesh.target.distanceTo(position);
rayMesh.visible = true;
- /* raycastChunkSpec.normal = new THREE.Vector3().fromArray(raycastChunkSpec.normal);
- raycastChunkSpec.objectPosition = new THREE.Vector3().fromArray(raycastChunkSpec.objectPosition);
- raycastChunkSpec.objectQuaternion = new THREE.Quaternion().fromArray(raycastChunkSpec.objectQuaternion);
- cubeMesh.position.copy(raycastChunkSpec.point); */
+ physicsManager.setGravity(false);
+ physicsManager.velocity.setScalar(0);
} else {
rayMesh.visible = false;
+
+ physicsManager.setGravity(true);
}
});
+ window.addEventListener('mouseup', e => {
+ rayMesh.visible = false;
+
+ physicsManager.setGravity(true);
+
+ const transforms = rigManager.getRigTransforms();
+ const {position} = transforms[0];
+ const direction = rayMesh.target.clone().sub(position).normalize();
+ physicsManager.velocity.copy(direction).multiplyScalar(10);
+ });
}
(async () => {
@@ -857,6 +869,20 @@ function animate(timestamp, frame) {
skybox.position.copy(rigManager.localRig.inputs.hmd.position);
skybox.update();
+ if (rayMesh.visible) {
+ const transforms = rigManager.getRigTransforms();
+ const {position} = transforms[0];
+ const direction = rayMesh.target.clone().sub(position).normalize();
+ direction.multiplyScalar(10 * timeDiff);
+ // physicsManager.velocity.add(direction);
+
+ dolly.matrix
+ // .premultiply(localMatrix2.makeTranslation(-xrCamera.position.x, -xrCamera.position.y, -xrCamera.position.z))
+ .premultiply(localMatrix3.makeTranslation(direction.x, direction.y, direction.z))
+ // .premultiply(localMatrix2.getInverse(localMatrix2))
+ .decompose(dolly.position, dolly.quaternion, dolly.scale);
+ }
+
ioManager.update(timeDiff, frame);
physicsManager.update(timeDiff, frame);
uiManager.update(timeDiff, frame);
| 0 |
diff --git a/src/components/container.js b/src/components/container.js @@ -44,11 +44,15 @@ module.exports = function(app) {
$templateCache.put('formio/formbuilder/container.html',
'<fieldset>' +
- '<label ng-if="component.label" class="control-label">' +
+ '<label ng-if="component.label && component.labelPosition !== \'bottom\'" class="control-label" ng-style="getLabelStyles(component)">' +
+ '{{ component.label }} ' +
+ '<formio-component-tooltip></formio-component-tooltip>' +
+ '</label>' +
+ '<form-builder-list component="component" form="form" options="options" formio="::formio" ng-style="getInputGroupStyles(component)"></form-builder-list>' +
+ '<label ng-if="component.label && component.labelPosition === \'bottom\'" class="control-label control-label--bottom" ng-style="getLabelStyles(component)">' +
'{{ component.label }} ' +
'<formio-component-tooltip></formio-component-tooltip>' +
'</label>' +
- '<form-builder-list component="component" form="form" options="options" formio="::formio"></form-builder-list>' +
'</fieldset>'
);
}
| 7 |
diff --git a/core/src/modules/configLoaders/destination.ts b/core/src/modules/configLoaders/destination.ts @@ -68,7 +68,7 @@ export async function loadDestination(
}
await destination.setDestinationGroupMemberships(destinationGroupMemberships);
- if (destination.groupGuid !== group.guid) {
+ if (group && destination.groupGuid !== group.guid) {
await destination.trackGroup(group);
}
| 11 |
diff --git a/app/scripts/HiGlassComponent.js b/app/scripts/HiGlassComponent.js @@ -2681,6 +2681,9 @@ class HiGlassComponent extends React.Component {
}
}
+ if ('created' in track) { delete track.created; }
+ if ('project' in track) { delete track.project; }
+ if ('project_name' in track) { delete.track.project_name; }
if ('serverUidKey' in track) { delete track.serverUidKey; }
if ('uuid' in track) { delete track.uuid; }
if ('private' in track) { delete track.private; }
| 2 |
diff --git a/website/lexonomy.py b/website/lexonomy.py @@ -173,7 +173,8 @@ def entrydelete(dictID: str, user: User, dictDB: Connection, configs: Configs):
@authDict([])
def entryread(dictID: str, user: User, dictDB: Connection, configs: Configs):
entryID = int(request.forms.id)
- entry = ops.readEntries(dictDB, configs, entryID, html = True, titlePlain = True)[0]
+ entries = ops.readEntries(dictDB, configs, entryID, html = True, titlePlain = True)
+ entry = entries[0] if len(entries) else None
if entry:
# interop between database and old frontend code
entry["success"] = True
| 1 |
diff --git a/shared/data/constants.js b/shared/data/constants.js @@ -5,7 +5,7 @@ module.exports = {
],
"entityList": "https://duckduckgo.com/contentblocking.js?l=entitylist2",
"entityMap": "data/tracker_lists/entityMap.json",
- "easylists": ["privacy", "general"],
+ "easylists": [],
"blocking": ["Advertising", "Analytics"],
"requestListenerTypes": ["main_frame","sub_frame","stylesheet","script","image","object","xmlhttprequest","other"],
"trackersWhitelistTemporary": "https://duckduckgo.com/contentblocking/trackers-whitelist-temporary.txt",
| 2 |
diff --git a/vaadin-date-picker.html b/vaadin-date-picker.html @@ -165,6 +165,10 @@ If you want to replace the default input field with a custom implementation, you
display: none;
}
+ :host [slot=suffix] {
+ display: flex;
+ }
+
/* The same code than paper-input and combo-box */
input {
position: relative; /* to make a stacking context */
@@ -198,7 +202,7 @@ If you want to replace the default input field with a custom implementation, you
disabled$="[[disabled]]"
role="application"
always-float-label="[[_computeAlwaysFloatLabel(placeholder)]]">
- <label id="label" aria-hidden="true">[[label]]</label>
+ <label id="label" slot="label" aria-hidden="true" for="input">[[label]]</label>
<iron-input slot="input" id="ironinput"
bind-value="{{_userInputValue}}"
| 12 |
diff --git a/token-metadata/0x7031AB87DCC46818806EC07aF46fa8c2aD2A2BFC/metadata.json b/token-metadata/0x7031AB87DCC46818806EC07aF46fa8c2aD2A2BFC/metadata.json "symbol": "TRBT",
"address": "0x7031AB87DCC46818806EC07aF46fa8c2aD2A2BFC",
"decimals": 18,
- "dharmaVerificationStatus": {
"dharmaVerificationStatus": "VERIFIED"
}
\ No newline at end of file
-}
\ No newline at end of file
| 3 |
diff --git a/build.html b/build.html <input type=file id=bake-file>
<input type=submit>
</form>
+ <form>
+ <h1>Bake mesh</h1>
+ <pre><code>TOKTX_PATH=./bin/toktx ./bin/gltfpack -v -kn -cc -tb -i assets/model.glb -o assets/model2.glb</code></pre>
+ </form>
<script src="./bin/geometry.js"></script>
<script type=module>
import * as THREE from './three.module.js';
| 0 |
diff --git a/docs/content/examples/charts/bar/Timeline.js b/docs/content/examples/charts/bar/Timeline.js -import { HtmlElement, DetachedScope } from 'cx/widgets';
+import { HtmlElement, DetachedScope, Content, Tab } from 'cx/widgets';
import { Controller } from 'cx/ui';
import { Svg, Rectangle, ClipRect } from 'cx/svg';
import { Chart, TimeAxis, NumericAxis, Range, Marker, Gridlines, ColumnGraph } from 'cx/charts';
@@ -110,7 +110,13 @@ export const Timeline = <cx>
</Svg>
</DetachedScope>
- <CodeSnippet putInto="code" fiddle="XzIyI8pv">{`
+ <Content name="code">
+ <div>
+ <Tab value-bind="$page.code.tab" tab="controller" mod="code"><code>Controller</code></Tab>
+ <Tab value-bind="$page.code.tab" tab="chart" mod="code" default><code>Chart</code></Tab>
+ </div>
+
+ <CodeSnippet fiddle="XzIyI8pv" visible-expr="{$page.code.tab}=='controller'">{`
class PageController extends Controller {
init() {
super.init();
@@ -128,7 +134,8 @@ export const Timeline = <cx>
})));
}
}
- ...
+ `}</CodeSnippet>
+ <CodeSnippet fiddle="XzIyI8pv" visible-expr="{$page.code.tab}=='chart'">{`
<div controller={PageController}>
<Svg style="width:100%;height:600px;" margin="60 10 60 60">
<Chart
@@ -207,6 +214,7 @@ export const Timeline = <cx>
</Svg>
</div>
`}</CodeSnippet>
+ </Content>
</CodeSplit>
</Md>
</cx>;
| 0 |
diff --git a/src/backends/backend.js b/src/backends/backend.js @@ -51,9 +51,9 @@ const slugFormatter = (template = "{{slug}}", entryData) => {
case "day":
return (`0${ date.getDate() }`).slice(-2);
case "slug":
- return sanitize(getIdentifier(entryData).trim().toLowerCase(), {replacement: "-"});
+ return sanitize(getIdentifier(entryData).trim().toLowerCase(), {replacement: "-"}).replace('.', '-');
default:
- return sanitize(entryData.get(field, "").trim().toLowerCase(), {replacement: "-"});
+ return sanitize(entryData.get(field, "").trim().toLowerCase(), {replacement: "-"}).replace('.', '-');
}
});
};
| 2 |
diff --git a/src/reducers/ledger/index.js b/src/reducers/ledger/index.js @@ -132,7 +132,16 @@ const ledger = handleActions({
signInWithLedgerStatus: undefined,
signInWithLedger: undefined
}
+ },
+ [showLedgerModal]: (state, { payload }) => {
+ return {
+ ...state,
+ modal: {
+ ...state.modal,
+ show: !state.signInWithLedgerStatus && payload.show,
+ }
}
+ },
}, initialState)
export default reduceReducers(
| 9 |
diff --git a/public/javascripts/Admin/src/Admin.js b/public/javascripts/Admin/src/Admin.js @@ -5,7 +5,7 @@ function Admin(_, $, difficultRegionIds) {
var mapData = InitializeMapLayerContainer();
var map;
var auditedStreetLayer;
- var params = {
+ var analyticsTabMapParams = {
regionColors: [
'#08306b', '#08519c', '#08719c', '#2171b5', '#4292c6',
'#6baed6', '#82badb', '#9ecae1', '#b3d3e8', '#c6dbef'
@@ -32,7 +32,7 @@ function Admin(_, $, difficultRegionIds) {
mapStyle: "mapbox.light",
accessToken: 'pk.eyJ1IjoibWlzYXVnc3RhZCIsImEiOiJjajN2dTV2Mm0wMDFsMndvMXJiZWcydDRvIn0.IXE8rQNF--HikYDjccA7Ug'
};
- var mapParams = {
+ var mapTabMapParams = {
choroplethType: 'labelMap',
neighborhoodPolygonStyle: {
color: '#888',
@@ -211,7 +211,7 @@ function Admin(_, $, difficultRegionIds) {
var loadSubmittedLabels = $.getJSON('/labels/all');
// When the polygons, polygon rates, and map params are all loaded the polygon regions can be rendered.
var renderPolygons = $.when(loadPolygons, loadPolygonRates, loadMapParams).done(function(data1, data2, data3) {
- map = Choropleth(_, $, difficultRegionIds, mapParams, [], data1[0], data2[0], data3[0]);
+ map = Choropleth(_, $, difficultRegionIds, mapTabMapParams, [], data1[0], data2[0], data3[0]);
});
// When the polygons have been rendered and the audited streets have loaded,
// the audited streets can be rendered.
@@ -398,7 +398,7 @@ function Admin(_, $, difficultRegionIds) {
var loadPolygonRates = $.getJSON('/adminapi/neighborhoodCompletionRate');
var loadMapParams = $.getJSON('/cityMapParams');
$.when(loadPolygons, loadPolygonRates, loadMapParams).done(function(data1, data2, data3) {
- Choropleth(_, $, difficultRegionIds, params, [], data1[0], data2[0], data3[0]);
+ Choropleth(_, $, difficultRegionIds, analyticsTabMapParams, [], data1[0], data2[0], data3[0]);
});
// Make charts showing neighborhood completion rate.
| 3 |
diff --git a/packages/composer-cli/lib/cmds/network/lib/loglevel.js b/packages/composer-cli/lib/cmds/network/lib/loglevel.js @@ -32,7 +32,6 @@ class LogLevel {
*/
static handler(argv) {
let adminConnection;
- let businessNetworkName;
let newlevel = argv.newlevel;
let cardName = argv.card;
@@ -47,7 +46,7 @@ class LogLevel {
})
.then((result) => {
if (newlevel) {
- cmdUtil.log(chalk.blue.bold('The logging level was successfully changed for: ')+businessNetworkName);
+ cmdUtil.log(chalk.blue.bold('The logging level was successfully changed to: ') + newlevel);
} else {
cmdUtil.log(chalk.blue.bold('The current logging level is: ') + result);
}
| 1 |
diff --git a/src/components/NavigationDesktop/NavigationItemDesktop.js b/src/components/NavigationDesktop/NavigationItemDesktop.js @@ -120,7 +120,7 @@ class NavigationItemDesktop extends Component {
<Grid item key={index}>
<MenuList disablePadding>
<MenuItem>
- <Link onClick={this.onClose} href={`${this.linkPath(item)}`} linkItem={item}>
+ <Link onClick={this.onClose} href={this.linkPath(item)} linkItem={item}>
<ListItemText primary={item.navigationItem.data.content[0].value} />
</Link>
</MenuItem>
| 2 |
diff --git a/OurUmbraco.Client/src/scss/elements/_markdown-syntax.scss b/OurUmbraco.Client/src/scss/elements/_markdown-syntax.scss }
-.highlight {
+.markdown-syntax .highlight {
&.csharp {
color: #333;
color: #333;
font-size: 12px;
line-height: 16px;
- .cdata { color: #df5000; }
- .cdatavalue { color: #df5000; }
- .element { color: #63a35c; }
- .attribute { color: #795da3; }
- .string { color: #df5000; }
- .quot { color: #df5000; }
- .comment { color: #969896; }
+ pre { color: #858585; }
+ .cdata { color: #ce9178; }
+ .cdatavalue { color: #ce9178; }
+ .element { color: #569cd6; }
+ .attribute { color: #8cdcfe; }
+ .string { color: #ce9178; }
+ .quot { color: #ce9178; }
+ .comment { color: #6a9955; }
}
&.javascript {
| 7 |
diff --git a/public/pocketsphinx.js/webapp/js/recognizer.js b/public/pocketsphinx.js/webapp/js/recognizer.js @@ -366,7 +366,7 @@ const _getLastWord = function(hyp, pred = () => true) {
};
const result = new Float32Array(VOWELS.length);
function _updateResult(hyp) {
- const lastWord = _getLastWord(hyp);
+ const lastWord = _getLastWord(hyp, word => word !== 'SIL');
const vowelIndex = VOWELS.findIndex(v => lastWord.includes(v)) ?? '';
if (vowelIndex !== -1) {
result[vowelIndex] += 1;
| 8 |
diff --git a/src/module.d.ts b/src/module.d.ts @@ -41,11 +41,9 @@ export interface ColumnDefinitionProps {
//Determines whether or not the user can disable this column from the settings.
locked?: boolean,
- // TODO: Unused? Rename to headingCssClassName?
//The css class name to apply to the header for the column
headerCssClassName?: string,
- // TODO: Unused?
//The css class name to apply to this column.
cssClassName?: string,
| 2 |
diff --git a/packages/spark-core/components/_alerts.scss b/packages/spark-core/components/_alerts.scss border: $sprk-alert-border-info;
color: $sprk-alert-text-color-info;
- .sprk-c-Alert__icon {
+ .sprk-c-Alert__icon:first-child {
color: $sprk-alert-icon-color-info;
}
border: $sprk-alert-border-success;
color: $sprk-alert-text-color-success;
- .sprk-c-Alert__icon {
+ .sprk-c-Alert__icon:first-child {
color: $sprk-alert-icon-color-success;
}
border: $sprk-alert-border-fail;
color: $sprk-alert-text-color-fail;
- .sprk-c-Alert__icon {
+ .sprk-c-Alert__icon:first-child {
color: $sprk-alert-icon-color-fail;
}
| 3 |
diff --git a/_data/conferences.yml b/_data/conferences.yml - title: AAMAS
hindex: 25
- year: 2020
- id: aamas20
- link: https://aamas2020.conference.auckland.ac.nz/
- deadline: '2019-11-15 23:59:59'
- abstract_deadline: '2019-11-12 23:59:59'
+ year: 2021
+ id: aamas21
+ link: https://aamas2021.soton.ac.uk/
+ deadline: '2020-10-09 23:59:59'
+ abstract_deadline: '2020-10-02 23:59:59'
timezone: UTC-12
- date: May 9-13, 2020
- place: Auckland, New Zealand
+ date: May 3-7, 2021
+ place: London, UK
sub: ML
- note: '<b>NOTE</b>: Mandatory abstract deadline on Nov 12, 2019. More info <a href=''https://aamas2020.conference.auckland.ac.nz/call-for-papers/''>here</a>.'
+ note: '<b>NOTE</b>: Mandatory abstract deadline on Oct 02, 2020. More info <a href=''https://aamas2021.soton.ac.uk/calls/papers/''>here</a>.'
- title: ECAI
hindex: 26
| 3 |
diff --git a/runtime.js b/runtime.js @@ -70,6 +70,9 @@ const startMonetization = (instanceId, monetizationPointer, ownerAddress) => {
const _importMapUrl = u => new URL(u, location.protocol + '//' + location.host).href;
const importMap = {
three: _importMapUrl('./three.module.js'),
+ BufferGeometryUtils: _importMapUrl('./BufferGeometryUtils.js'),
+ GLTFLoader: _importMapUrl('./GLTFLoader.js'),
+ GLTF1Loader: _importMapUrl('./GLTF1Loader.js'),
app: _importMapUrl('./app-object.js'),
world: _importMapUrl('./world.js'),
universe: _importMapUrl('./universe.js'),
@@ -80,9 +83,7 @@ const importMap = {
notifications: _importMapUrl('./notifications.js'),
popovers: _importMapUrl('./popovers.js'),
crypto: _importMapUrl('./crypto.js'),
- BufferGeometryUtils: _importMapUrl('./BufferGeometryUtils.js'),
- GLTFLoader: _importMapUrl('./GLTFLoader.js'),
- GLTF1Loader: _importMapUrl('./GLTF1Loader.js'),
+ constants: _importMapUrl('./constants.js'),
};
const _clone = o => JSON.parse(JSON.stringify(o));
@@ -451,6 +452,7 @@ const _makeAppUrl = appId => {
import * as notifications from ${JSON.stringify(importMap.notifications)};
import * as _popovers from ${JSON.stringify(importMap.popovers)};
import * as crypto from ${JSON.stringify(importMap.crypto)};
+ import * as constants from ${JSON.stringify(importMap.constants)};
const renderer = Object.create(_renderer);
renderer.setAnimationLoop = function(fn) {
@@ -558,7 +560,7 @@ const _makeAppUrl = appId => {
rig.localRig.model.visible = false;
}
};
- export {renderer, scene, camera, runtime, world, universe, physics, ui, notifications, popovers, crypto, rig, app, appManager};
+ export {renderer, scene, camera, runtime, world, universe, physics, ui, notifications, popovers, crypto, constants, rig, app, appManager};
`;
const b = new Blob([s], {
type: 'application/javascript',
| 0 |
diff --git a/editor/js/vendor/ckeditor/plugins/xotrecorder/dialogs/xotrecorder.js b/editor/js/vendor/ckeditor/plugins/xotrecorder/dialogs/xotrecorder.js //input.connect(audioContext.destination) //stop the input from playing back through the speakers
//disable controls
- getElementById('recordingDevicesSelect').disable();
- getElementById('encodingTypeSelect').disable();
+ enableDisable('recordingDevicesSelect', 'disable');
+ enableDisable('encodingTypeSelect', 'disable');
getButtonById('uploadButton').disable();
getButtonById('insertButton').disable();
}).catch(function(err) { console.log('The recorder stopped prematurely, with this error', err);
//enable the record button if getUSerMedia() fails
setRecordButton(permissionState.granted, false); //enabled??, but not red
- getElementById('stopButton').disable();
+ enableDisable('stopButton', 'disable');
recording = false;
if (err.toString().indexOf('Permission denied')) {
startUpdatingDevicesList();
//disable the record button
setRecordButton(false, true); //disable, red
- getElementById('stopButton').enable();
+ enableDisable('stopButton', 'enable');
}
- function stopRecording() { console.log("stop recording");
+ function stopRecording() {
//stop microphone access
- gumStream.getAudioTracks()[0].stop();
+ if (gumStream && gumStream.getAudioTracks) gumStream.getAudioTracks()[0].stop();
recording = false;
//disable the stop button, enable record
- getElementById('stopButton').disable();
+ enableDisable('stopButton', 'disable');
setRecordButton(permissionState.granted, false); //enabled??, not red
- getElementById('recordingDevicesSelect').enable();
- getElementById('encodingTypeSelect').enable();
+ enableDisable('recordingDevicesSelect', 'enable');
+ enableDisable('encodingTypeSelect', 'enable');
//tell the recorder to finish the recording (stop recording + encode the recorded audio)
recorder.finishRecording();
}
+ function enableDisable(identifier, en_di) {
+ let element = getElementById(identifier);
+ let state = (en_di === true || en_di === 'enable') ? true : false;
+ if (element) element[state ? 'enable' : 'disable']();
+ }
+
function setupAudioPlayer() {
//webkitURL is deprecated but nevertheless
var url = (window.URL || window.webkitURL).createObjectURL(blob);
}
//helper function
- function __log(e, data) {
+ function __log(e, data) { return;
console.log(e + " " + (data || '') );
}
// *** Plugin Helper Functions ***
- function initialiseRecorder() { console.log("initialise recorder")
- getButtonById('insertButton').disable();
- getButtonById('uploadButton').disable();
+ function initialiseRecorder() {
+ enableDisable('insertButton', 'disable');
+ enableDisable('uploadButton', 'disable');
getElementById('audioPlayer').getElement().setAttribute('src', '');
setRecordButton(permissionState.granted, false); //enable, not red
- getElementById('stopButton').disable();
- getElementById('recordingDevicesSelect').enable();
- getElementById('encodingTypeSelect').enable();
+ enableDisable('stopButton', 'disable');
+ enableDisable('recordingDevicesSelect', 'enable');
+ enableDisable('encodingTypeSelect', 'enable');
blob = undefined;
}
}
function setRecordButton(enable, recording) {
- let rec = getElementById('recordButton');
- rec[enable ? 'enable' : 'disable']();
- document.getElementById(rec.domId).classList[recording ? 'add' : 'remove']("recording");
+ enableDisable('recordButton', enable);
+ document.getElementById(getElementById('recordButton').domId).classList[recording ? 'add' : 'remove']("recording");
}
function swapTabTitlesAndLabels() {
| 1 |
diff --git a/templates/docs/getting-started.md b/templates/docs/getting-started.md <%= title("Installation", {}) %>
+Before installing make sure you have the required dependecies installed:
+ - gcc for [mattn/go-sqlite3](https://github.com/mattn/go-sqlite3).
+ - [node](https://github.com/nodejs/node) and [npm](https://github.com/npm/npm) for the [asset pipeline](/docs/assets) built upon [webpack](https://github.com/webpack/webpack).
+
+Buffalo uses *go-sqlite3* by default wich requires gcc to be built.
+Either install gcc from source/package manager or run before installing Buffalo:
+
+<%= code("text") { %>
+ $ go install github.com/mattn/go-sqlite3
+<% } %>
+
+You can now install buffalo with:
+
<%= code("text") { %>
$ go get -u github.com/gobuffalo/buffalo/buffalo
<% } %>
+For a verbose output use the -v flag instead:
+
+<%= code("text") { %>
+$ go get -u -v github.com/gobuffalo/buffalo/buffalo
+<% } %>
+
+
<%= title("Generating a New Project", {name: "new-project"}) %>
Buffalo aims to make building new web applications in Go as quick and simple as possible, and what could be more simple than a *new application* generator? Start by going to your `$GOPATH` and create your new application!
| 7 |
diff --git a/lib/v2/zhihu/utils.js b/lib/v2/zhihu/utils.js @@ -14,7 +14,7 @@ module.exports = {
$('a').each((_, elem) => {
const href = $(elem).attr('href');
- if (href.startsWith('https://link.zhihu.com/?target=')) {
+ if (href?.startsWith('https://link.zhihu.com/?target=')) {
const url = new URL(href);
const target = url.searchParams.get('target');
$(elem).attr('href', decodeURIComponent(target));
| 1 |
diff --git a/package.json b/package.json "author": "Khronos Group Inc.",
"license": "Apache-2.0",
"dependencies": {
- "argparse": "^1.0.10",
- "gl-matrix": "^3.2.1",
- "open": "7.0.2"
+ "gl-matrix": "^3.2.1"
},
"devDependencies": {
- "acorn": "^7.1.0",
"concurrently": "^5.1.0",
"eslint": "^6.8.0",
"rollup": "^1.31.1",
| 2 |
diff --git a/src/QuickMenu.jsx b/src/QuickMenu.jsx @@ -177,6 +177,7 @@ export default function QuickMenu() {
/* const emote = _getSelectedEmote();
emote && _triggerEmote(emote); */
setOpen(false);
+ setDown(false);
}
}
registerIoEventHandler('keyup', keyup);
| 0 |
diff --git a/apps/landing/landing.html b/apps/landing/landing.html </div>
</section>
<section class="post">
- <a target="_blank" href="https://github.com/camicroscope/" class="image"><img src="./code.jpg" alt=""/></a>
+ <a target="_blank" href="https://camicroscope.github.io/docs/" class="image"><img src="./code.jpg" alt=""/></a>
<div class="content">
- <h3>Source Code</h3>
- <p>Get and use the code, or file issues on our github repositories.</p>
- <a target="_blank" href="https://github.com/camicroscope/" class="button">More</a>
+ <h3>Documentation</h3>
+ <p>Read documentation for using and developing caMicroscope.</p>
+ <a target="_blank" href="https://camicroscope.github.io/docs/" class="button">More</a>
</div>
</section>
</div>
| 14 |
diff --git a/src/layer/VectorLayer.js b/src/layer/VectorLayer.js @@ -152,7 +152,7 @@ class VectorLayer extends OverlayLayer {
identify(coordinate, options = {}) {
const renderer = this._getRenderer();
// only iterate drawn geometries when onlyVisible is true.
- if (options['onlyVisible'] && renderer) {
+ if (options['onlyVisible'] && renderer && renderer.identify) {
return renderer.identify(coordinate, options);
}
return super.identify(coordinate, options);
| 1 |
diff --git a/lib/build/tasks/jasmine.js b/lib/build/tasks/jasmine.js @@ -13,11 +13,6 @@ module.exports = {
summary: true,
display: 'short',
specs: js_files.specs,
- tempDir: '.grunt/grunt-contrib-jasmine',
- vendor: [
- 'node_modules/jasmine-ajax/lib/mock-ajax.js',
- 'node_modules/underscore/underscore-min.js'
- ],
helpers: ['http://maps.googleapis.com/maps/api/js?sensor=false&v=3.12'].concat(js_files._spec_helpers)
// '--remote-debugger-port': 9000
}
| 2 |
diff --git a/src/pages/Component/resource.js b/src/pages/Component/resource.js @@ -77,11 +77,13 @@ export default class Index extends PureComponent {
rainbondUtil.OauthEnterpriseEnable(enterprise)
) {
enterprise.oauth_services.value.map(item => {
- const { oauth_type, service_id } = item;
+ const { oauth_type, service_id, is_git } = item;
+ if (is_git) {
tabList.push({
type: oauth_type,
- id: `${service_id}`,
+ id: `${service_id}`
});
+ }
});
this.setState({
tabList,
| 1 |
diff --git a/src/services/location.js b/src/services/location.js @@ -83,6 +83,20 @@ ngeo.Location = function(location, history) {
};
+/**
+ * @param {History} history History.
+ * @param {string} state State.
+ */
+ngeo.Location.replaceState = function(history, state) {
+ try {
+ history.replaceState(null, '', state);
+ } catch (error) {
+ // replaceState fails on some browser if the domain in the state
+ // is not the same as location.origin
+ }
+};
+
+
/**
* Get the location's current path.
* @return {string|undefined} The path.
@@ -328,7 +342,7 @@ ngeo.Location.prototype.deleteFragmentParam = function(key) {
* @export
*/
ngeo.Location.prototype.refresh = function() {
- this.history_.replaceState(null, '', this.getUriString());
+ ngeo.Location.replaceState(this.history_, this.getUriString());
};
@@ -361,7 +375,7 @@ ngeo.LocationFactory = function($rootScope, $window) {
$rootScope.$evalAsync(() => {
lastUri = newUri;
if (history !== undefined && history.replaceState !== undefined) {
- history.replaceState(null, '', newUri);
+ ngeo.Location.replaceState(history, newUri);
}
$rootScope.$broadcast('ngeoLocationChange');
});
| 8 |
diff --git a/assets/js/googlesitekit/modules/datastore/settings.test.js b/assets/js/googlesitekit/modules/datastore/settings.test.js @@ -84,7 +84,7 @@ describe( 'core/modules settings', () => {
describe( 'selectors', () => {
describe( 'isDoingSubmitChanges', () => {
- it( 'it proxies the selector call to the module with the given slug', async () => {
+ it( 'proxies the selector call to the module with the given slug', async () => {
expect( registry.select( STORE_NAME ).isDoingSubmitChanges( nonExistentModuleSlug ) ).toBe( false );
expect( registry.select( STORE_NAME ).isDoingSubmitChanges( slug ) ).toBe( false );
@@ -94,7 +94,7 @@ describe( 'core/modules settings', () => {
} );
describe( 'canSubmitChanges', () => {
- it( 'it proxies the selector call to the module with the given slug', () => {
+ it( 'proxies the selector call to the module with the given slug', () => {
expect( registry.select( STORE_NAME ).canSubmitChanges( slug ) ).toBe( false );
moduleCanSubmitChanges = true;
expect( registry.select( STORE_NAME ).canSubmitChanges( slug ) ).toBe( true );
| 2 |
diff --git a/accessibility-checker-extension/src/ts/usingAC/UsingACApp.tsx b/accessibility-checker-extension/src/ts/usingAC/UsingACApp.tsx @@ -550,7 +550,6 @@ class UsingACApp extends React.Component<{}, UsingACAppState> {
violations, items that need review or recommendations,
deselect the checkbox by the issue type in the issue count region below the
'Scan' button to exclude the issue type from the results. Select the checkbox by the issue type to include the issue type in the results.
- issue types.
</p>
</li>
<li>
| 2 |
diff --git a/features/step_definitions/tx-history-steps.js b/features/step_definitions/tx-history-steps.js @@ -4,6 +4,7 @@ import chai from 'chai';
import moment from 'moment';
import { getLovefieldTxs, getMockData } from '../support/mockDataBuilder';
import i18n from '../support/helpers/i18n-helpers';
+import { awaitExpression } from 'babel-types';
function verifyAllTxsFields(txType, txAmount, txTime, txStatus, txFromList, txToList,
txId, expectedTx, txConfirmations) {
@@ -107,6 +108,7 @@ async function (txsNumber) {
break;
}
await this.click(`//button[contains(@class, 'primary WalletTransactionsList_showMoreTransactionsButton')]`, By.xpath);
+ await this.driver.sleep(500);
}
const displayedTransactions = await this.driver.findElements(By.xpath(`//div[contains(@class, 'Transaction_component')]`));
await this.driver.findElement(By.xpath(`//div[contains(@class, 'WalletSummary_numberOfTransactions')]//span`)).getText().then(function(numberOfTransactions){
| 7 |
diff --git a/icons.js b/icons.js @@ -3,3 +3,6 @@ const _svg2Url = s => `data:image/svg+xml;base64,${btoa(s.replace(/currentColor/
export const code = _svg2Url(`<svg aria-hidden="true" focusable="false" data-prefix="far" data-icon="code" class="svg-inline--fa fa-code fa-w-18" role="img" xmlns="http://www.w3.org/2000/svg" viewBox="0 0 576 512"><path fill="currentColor" d="M234.8 511.7L196 500.4c-4.2-1.2-6.7-5.7-5.5-9.9L331.3 5.8c1.2-4.2 5.7-6.7 9.9-5.5L380 11.6c4.2 1.2 6.7 5.7 5.5 9.9L244.7 506.2c-1.2 4.3-5.6 6.7-9.9 5.5zm-83.2-121.1l27.2-29c3.1-3.3 2.8-8.5-.5-11.5L72.2 256l106.1-94.1c3.4-3 3.6-8.2.5-11.5l-27.2-29c-3-3.2-8.1-3.4-11.3-.4L2.5 250.2c-3.4 3.2-3.4 8.5 0 11.7L140.3 391c3.2 3 8.2 2.8 11.3-.4zm284.1.4l137.7-129.1c3.4-3.2 3.4-8.5 0-11.7L435.7 121c-3.2-3-8.3-2.9-11.3.4l-27.2 29c-3.1 3.3-2.8 8.5.5 11.5L503.8 256l-106.1 94.1c-3.4 3-3.6 8.2-.5 11.5l27.2 29c3.1 3.2 8.1 3.4 11.3.4z"></path></svg>`);
export const boxOpen = _svg2Url(`<svg aria-hidden="true" focusable="false" data-prefix="fas" data-icon="box-open" class="svg-inline--fa fa-box-open fa-w-20" role="img" xmlns="http://www.w3.org/2000/svg" viewBox="0 0 640 512"><path fill="currentColor" d="M425.7 256c-16.9 0-32.8-9-41.4-23.4L320 126l-64.2 106.6c-8.7 14.5-24.6 23.5-41.5 23.5-4.5 0-9-.6-13.3-1.9L64 215v178c0 14.7 10 27.5 24.2 31l216.2 54.1c10.2 2.5 20.9 2.5 31 0L551.8 424c14.2-3.6 24.2-16.4 24.2-31V215l-137 39.1c-4.3 1.3-8.8 1.9-13.3 1.9zm212.6-112.2L586.8 41c-3.1-6.2-9.8-9.8-16.7-8.9L320 64l91.7 152.1c3.8 6.3 11.4 9.3 18.5 7.3l197.9-56.5c9.9-2.9 14.7-13.9 10.2-23.1zM53.2 41L1.7 143.8c-4.6 9.2.3 20.2 10.1 23l197.9 56.5c7.1 2 14.7-1 18.5-7.3L320 64 69.8 32.1c-6.9-.8-13.5 2.7-16.6 8.9z"></path></svg>`);
export const waveform = _svg2Url(`<svg aria-hidden="true" focusable="false" data-prefix="far" data-icon="waveform" class="svg-inline--fa fa-waveform fa-w-20" role="img" xmlns="http://www.w3.org/2000/svg" viewBox="0 0 640 512"><path fill="currentColor" d="M328 0h-16a16 16 0 0 0-16 16v480a16 16 0 0 0 16 16h16a16 16 0 0 0 16-16V16a16 16 0 0 0-16-16zm-96 96h-16a16 16 0 0 0-16 16v288a16 16 0 0 0 16 16h16a16 16 0 0 0 16-16V112a16 16 0 0 0-16-16zm192 32h-16a16 16 0 0 0-16 16v224a16 16 0 0 0 16 16h16a16 16 0 0 0 16-16V144a16 16 0 0 0-16-16zm96-64h-16a16 16 0 0 0-16 16v352a16 16 0 0 0 16 16h16a16 16 0 0 0 16-16V80a16 16 0 0 0-16-16zM136 192h-16a16 16 0 0 0-16 16v96a16 16 0 0 0 16 16h16a16 16 0 0 0 16-16v-96a16 16 0 0 0-16-16zm-96 32H24a16 16 0 0 0-16 16v32a16 16 0 0 0 16 16h16a16 16 0 0 0 16-16v-32a16 16 0 0 0-16-16zm576 0h-16a16 16 0 0 0-16 16v32a16 16 0 0 0 16 16h16a16 16 0 0 0 16-16v-32a16 16 0 0 0-16-16z"></path></svg>`);
+export const vrCardboard = _svg2Url(`<svg aria-hidden="true" focusable="false" data-prefix="fas" data-icon="vr-cardboard" class="svg-inline--fa fa-vr-cardboard fa-w-20" role="img" xmlns="http://www.w3.org/2000/svg" viewBox="0 0 640 512"><path fill="currentColor" d="M608 64H32C14.33 64 0 78.33 0 96v320c0 17.67 14.33 32 32 32h160.22c25.19 0 48.03-14.77 58.36-37.74l27.74-61.64C286.21 331.08 302.35 320 320 320s33.79 11.08 41.68 28.62l27.74 61.64C399.75 433.23 422.6 448 447.78 448H608c17.67 0 32-14.33 32-32V96c0-17.67-14.33-32-32-32zM160 304c-35.35 0-64-28.65-64-64s28.65-64 64-64 64 28.65 64 64-28.65 64-64 64zm320 0c-35.35 0-64-28.65-64-64s28.65-64 64-64 64 28.65 64 64-28.65 64-64 64z"></path></svg>`);
+export const tShirt = _svg2Url(`<svg aria-hidden="true" focusable="false" data-prefix="far" data-icon="tshirt" class="svg-inline--fa fa-tshirt fa-w-20" role="img" xmlns="http://www.w3.org/2000/svg" viewBox="0 0 640 512"><path fill="currentColor" d="M638 121c-3.3-9.8-10.2-17.8-19.5-22.4L420.2 0c-9.5 13.2-28.4 50.3-100.2 50.3-72.4 0-91.1-37.7-100.2-50.3L21.6 98.6C12.3 103.2 5.3 111.2 2 121c-3.3 9.9-2.6 20.4 2.1 29.7l53 106.2c9.6 19.2 33 27 51.6 17.7l24-11.3c5.3-2.5 11.4 1.4 11.4 7.2v185.3c0 31 25.1 56.2 56 56.2h240c30.9 0 56-25.2 56-56.2V270.6c0-5.9 6.1-9.7 11.4-7.2l23.5 11.1c19.1 9.7 42.5 1.8 52.1-17.4l53-106.2c4.4-9.5 5.2-20 1.9-29.9zm-94 106.4l-73.2-34.6c-10.6-5-22.8 2.7-22.8 14.5v248.6c0 4.4-3.6 8-8 8H200c-4.4 0-8-3.6-8-8V207.3c0-11.7-12.2-19.5-22.8-14.5L96 227.4l-44.8-89.9 155.5-77.3c26.4 24 67.8 38.3 113.3 38.3s86.9-14.3 113.2-38.2l155.5 77.3-44.7 89.8z"></path></svg>`);
+export const cube = _svg2Url(`<svg aria-hidden="true" focusable="false" data-prefix="far" data-icon="cube" class="svg-inline--fa fa-cube fa-w-16" role="img" xmlns="http://www.w3.org/2000/svg" viewBox="0 0 512 512"><path fill="currentColor" d="M239.1 7.5l-208 78c-18.7 7-31.1 25-31.1 45v225.1c0 18.2 10.3 34.8 26.5 42.9l208 104c13.5 6.8 29.4 6.8 42.9 0l208-104c16.3-8.1 26.5-24.8 26.5-42.9V130.5c0-20-12.4-37.9-31.1-44.9l-208-78C262 3.4 250 3.4 239.1 7.5zm16.9 45l208 78v.3l-208 84.5-208-84.5v-.3l208-78zM48 182.6l184 74.8v190.2l-184-92v-173zm232 264.9V257.4l184-74.8v172.9l-184 92z"></path></svg>`);
\ No newline at end of file
| 0 |
diff --git a/src/items/vehicles/ultimate_hover_carrier.json b/src/items/vehicles/ultimate_hover_carrier.json "special": []
}
},
-
- "flags": {
-
- },
+ "flags": {},
"token": {
"flags": {},
"name": "Motorcycle",
},
"items": [
{
-
"name": "Collision (Ram)",
"type": "vehicleAttack",
"data": {
"parts": []
}
},
-
"flags": {},
"img": "icons/svg/mystery-man.svg",
"effects": []
},
{
-
"name": "Collision (Run Over)",
"type": "vehicleAttack",
"data": {
"parts": []
}
},
-
"flags": {},
"img": "icons/svg/mystery-man.svg",
"effects": []
},
{
-
"name": "Unlimited comm unit",
"type": "vehicleSystem",
"data": {
"parts": []
}
},
-
"flags": {},
"img": "systems/sfrpg/icons/equipment/technological%20items/comm-unit-personal.jpg",
"effects": []
},
{
-
"name": "Autopilot",
"type": "vehicleSystem",
"data": {
"parts": []
}
},
-
"flags": {},
"img": "icons/svg/mystery-man.svg",
"effects": []
},
{
-
"name": "Enhanced sensors (darkvision 20 miles)",
"type": "vehicleSystem",
"data": {
"parts": []
}
},
-
"flags": {},
"img": "systems/sfrpg/icons/equipment/technological%20items/emergency_defense_sphere.png",
"effects": []
},
{
-
"name": "Reaction cannon, paragon",
"type": "weapon",
"data": {
"parts": []
}
},
-
"flags": {},
"img": "systems/sfrpg/icons/equipment/weapons/advanced_reaction_cannon.jpg",
"effects": [],
]
},
{
-
"name": "Shock caster, tempest",
"type": "weapon",
"data": {
"parts": []
}
},
-
"flags": {},
"img": "systems/sfrpg/icons/equipment/weapons/aurora_shock_caster.jpg",
"effects": [],
]
},
{
-
"name": "Artillery laser, zenith",
"type": "weapon",
"data": {
"parts": []
}
},
-
"flags": {},
"img": "systems/sfrpg/icons/equipment/weapons/artillery-laser-azimuth.jpg",
"effects": [],
]
},
{
-
"name": "Hangar Bay (Ex)",
"type": "vehicleSystem",
"data": {
"img": "icons/svg/mystery-man.svg"
}
],
- "effects": [],
+ "effects": []
}
| 2 |
diff --git a/experimental/adaptive-tool/README.md b/experimental/adaptive-tool/README.md @@ -82,7 +82,7 @@ This is an example of a typical `launch.json`:
### Start bot runtime and complete initialization
-Start a bot runtime. For example, start a bot project: `todobot` in [SampleBots](https://github.com/microsoft/botbuilder-dotnet/tree/hond/debugger/tests/Microsoft.Bot.Builder.TestBot.Json). Then make sure the debugger port has been registered in `BotFrameworkHttpAdapter` with `UseDebugger` method.
+Start a bot runtime. For example, start a bot project: `todobot` in [SampleBots](https://github.com/microsoft/botbuilder-dotnet/tree/main/tests/Microsoft.Bot.Builder.TestBot.Json). Then make sure the debugger port has been registered in `BotFrameworkHttpAdapter` with `UseDebugger` method.
There are several ways to initialize a bot, and [BotFremawork Emulator](https://github.com/microsoft/BotFramework-Emulator) is a typical approach. Open the emulator and attach it to the bot to finish the initialization.
| 1 |
diff --git a/buildroot-external/package/neoserver/overlay/opt/mediola/www/bin/hmFunc.cgi b/buildroot-external/package/neoserver/overlay/opt/mediola/www/bin/hmFunc.cgi @@ -6,11 +6,6 @@ source session.tcl
if {[session_requestisvalid 0] < 0 } {
exit
}
+puts "Content-Type: application/json\r"
+puts "\r"
puts "{\"session\":\"valid\"}"
-
-
-
-
-
-
-
| 12 |
diff --git a/.travis.yml b/.travis.yml @@ -6,3 +6,11 @@ notifications:
sudo: false
script:
- npm test
+deploy:
+ # Deploy to a review app, with a nodejs buildpack - to preview PRs before they are merged.
+ - script: echo "Deploying to govuk-frontend-review.herokuapp.com ..."
+ - provider: heroku
+ app: govuk-frontend-review
+ buildpack: heroku/nodejs
+ api_key:
+ secure: OL58snlcd9Z4ge6m4N9VpvD9ZAYon8RZze16nMHifiHyIP98xwKY14WjXIRe68yaKplHINzJBTZTjkUqKugr4o+I1T28rhSqm/QQJa+5E2sQTgORV/4FccGCtR6hiC0kS2cq26KlV1zVFxO/ayXVtRat0vXB6faPdevaJM5p5lO4htW9sOFH6Nwj+Ig7+Noj7QugSiDJJqdAWxBZe5kZ4aw1spusNEFEI09UAVLJq+OI82taQl03AFR07Wxind8uJmslKMtYQUaAw/9C57w9i7jmtpv3tHuXIC1jAaWqNMnOJRB15MMias6oaT/bX0rBTE1MVe43YckLu5F1lhWkG6/FP7X6iMS3BgEShOGZFuKtczPCKUnPu6TANEXKPXnhIiAMbp/UUWe/7hRO8c/e8bVEOJ1URUWBvC9zWbVUyyeyOICVxmeRCZbTLizJQ6flRI+QuDgl6MCr3SKQ04J7YsXr2fb6j/CQh/axsVLb31At3fHcPGCdelPzpQCFG1oW/JUjrHV6E8k8VKuJiHPk/wF5Fs7HKY3E6mFG5iSqrMNl3VPE00TAmR9nXj464Z2zBNeaXngMDXBYwfukCeH825HfmnM2sFzZVR7YD+9ubiss8Qt5jDGtpIvJWH7FLIITuiXOM7cpXyb5i9HNYdZOYUkF4G+E0BEMJ1UvX0TnSN4=
| 12 |
diff --git a/server/game/cards/07-WotW/ShinjoTrailblazer.js b/server/game/cards/07-WotW/ShinjoTrailblazer.js @@ -8,8 +8,7 @@ class ShinjoTrailblazer extends DrawCard {
onCardRevealed: (event, context) => event.card.isProvince && event.card.controller === context.source.controller.opponent && this.game.isDuringConflict()
},
gameAction: ability.actions.cardLastingEffect({ effect: ability.effects.modifyBothSkills(2) }),
- effect: 'to give {0} +2{1}, +2{2}',
- effectArgs: () => ['military', 'political']
+ effect: 'to give {0} +2military, +2political'
});
}
}
| 2 |
diff --git a/packages/@uppy/aws-s3/src/index.js b/packages/@uppy/aws-s3/src/index.js @@ -97,8 +97,8 @@ module.exports = class AwsS3 extends Plugin {
throw new Error('Expected a `companionUrl` option containing a Companion address.')
}
- const filename = encodeURIComponent(file.meta.name)
- const type = encodeURIComponent(file.meta.type)
+ const filename = file.meta.name
+ const type = file.meta.type
const metadata = {}
this.opts.metaFields.forEach((key) => {
if (file.meta[key] != null) {
| 2 |
diff --git a/PostHeadersQuestionToc.user.js b/PostHeadersQuestionToc.user.js // @description Sticky post headers while you view each post (helps for long posts). Question ToC of Answers in sidebar.
// @homepage https://github.com/samliew/SO-mod-userscripts
// @author @samliew
-// @version 2.8
+// @version 2.8.1
//
// @include https://*stackoverflow.com/questions/*
// @include https://*serverfault.com/questions/*
@@ -549,13 +549,9 @@ body:not(.no-grid-post-layout) .post-layout--full .question-status {
padding-top: 2px;
width: calc(100% - 48px);
margin-bottom: 0;
- color: #0C0D0E;
line-height: 1.3;
font-size: 13px;
}
-#qtoc .post-hyperlink:hover {
- color: #9c1724;
-}
#qtoc .deleted-user {
margin: -3px 0;
}
| 2 |
diff --git a/src/utils/gh-auth.js b/src/utils/gh-auth.js @@ -60,7 +60,7 @@ async function getGitHubToken(opts) {
const parameters = querystring.parse(req.url.slice(req.url.indexOf('?') + 1))
if (parameters.token) {
deferredResolve(parameters)
- res.end("<html><head><style>html{font-family:sans-serif;background:#0e1e25}body{overflow:hidden;position:relative;display:flex;flex-direction:column;align-items:center;justify-content:center;height:100vh;width:100vw;}h3{margin:0}.card{position:relative;display:flex;flex-direction:column;width:75%;max-width:364px;padding:24px;background:white;color:rgb(14,30,37);border-radius:8px;box-shadow:0 2px 4px 0 rgba(14,30,37,.16);}</style></head>" +
+ res.end("<html><head><script>if(history.replaceState){history.replaceState({},'','/')}</script><style>html{font-family:sans-serif;background:#0e1e25}body{overflow:hidden;position:relative;display:flex;flex-direction:column;align-items:center;justify-content:center;height:100vh;width:100vw;}h3{margin:0}.card{position:relative;display:flex;flex-direction:column;width:75%;max-width:364px;padding:24px;background:white;color:rgb(14,30,37);border-radius:8px;box-shadow:0 2px 4px 0 rgba(14,30,37,.16);}</style></head>" +
"<body><div class=card><h3>Logged In</h3><p>You're now logged into Netlify CLI with your " +
parameters.provider + " credentials. Please close this window.</p></div>")
server.close()
| 2 |
diff --git a/lib/routes/prototype-admin-routes.js b/lib/routes/prototype-admin-routes.js @@ -311,8 +311,7 @@ router.post('/templates/install', async (req, res) => {
renderError('exists')
return
}
-
- if (!chosenUrl.match(/^(\/[a-zA-Z0-9-_]+)?$/)) {
+ if (`!#$&'()*+,:;=?@[].% `.split('').some((char) => chosenUrl.includes(char))) {
renderError('invalid')
return
}
| 14 |
diff --git a/examples/babel-webpack-4/.vscode/launch.json b/examples/babel-webpack-4/.vscode/launch.json {
"type": "node",
"request": "launch",
- "name": "Launch Dev Offline",
+ "name": "Launch Serverless Offline",
"program": "${workspaceRoot}/node_modules/serverless/bin/serverless",
"args": [ "offline", "--noTimeout", "--dontPrintOutput", "--stage=dev" ],
"sourceMaps": true,
| 7 |
diff --git a/src/parser/templateStrings.js b/src/parser/templateStrings.js @@ -35,14 +35,24 @@ const getScalingValue = (feature) => {
* @param {*} match
* @param {*} feature
*/
+// eslint-disable-next-line complexity
let parseMatch = (ddb, character, match, feature) => {
const splitMatchAt = match.split("@");
let result = splitMatchAt[0];
const characterAbilities = character.flags.ddbimporter.dndbeyond.effectAbilities;
+ const classOption = [ddb.character.options.race, ddb.character.options.class, ddb.character.options.feat]
+ .flat()
+ .find((option) => option.definition.id === feature.componentId);
// scalevalue
if (result.includes("scalevalue")) {
- const feat = feature.levelScale ? feature : utils.findComponentByComponentId(ddb, feature.componentId);
+ let feat = feature.levelScale ? feature : utils.findComponentByComponentId(ddb, feature.componentId);
+ if (!feat && hasProperty(feature, "flags.ddbimporter.dndbeyond.choice")) {
+ feat = utils.findComponentByComponentId(ddb, feature.flags.ddbimporter.dndbeyond.choice.componentId);
+ }
+ if (!feat && classOption) {
+ feat = utils.findComponentByComponentId(ddb, classOption.componentId);
+ }
const scaleValue = getScalingValue(feat);
result = result.replace("scalevalue", scaleValue);
}
@@ -91,24 +101,21 @@ let parseMatch = (ddb, character, match, feature) => {
: utils.findClassByFeatureId(ddb, feature.componentId);
if (cls) {
result = result.replace("classlevel", cls.level);
- } else {
+ } else if (classOption) {
// still not found a cls? could be an option
- const classOption = [ddb.character.options.race, ddb.character.options.class, ddb.character.options.feat]
- .flat()
- .find((option) => option.definition.id === feature.componentId);
- if (!classOption) {
- if (!feature.componentId) {
- logger.debug("Feature failed componentID parse", feature);
- }
- logger.error(`Unable to parse option class info. ComponentId is ${feature.componentId}`);
- } else {
const optionCls = utils.findClassByFeatureId(ddb, classOption.componentId);
if (optionCls) {
result = result.replace("classlevel", optionCls.level);
} else {
- logger.error(`Unable to parse option class info. classOption ComponentId is: ${classOption.componentId}. ComponentId is ${feature.componentId}`);
+ logger.error(
+ `Unable to parse option class info. classOption ComponentId is: ${classOption.componentId}. ComponentId is ${feature.componentId}`
+ );
}
+ } else {
+ if (!feature.componentId) {
+ logger.debug("Feature failed componentID parse", feature);
}
+ logger.error(`Unable to parse option class info. ComponentId is ${feature.componentId}`);
}
}
@@ -232,7 +239,9 @@ function parseSRDLinks(text) {
CONFIG.DDBI.SRD_LOOKUP.lookups.skills,
CONFIG.DDBI.SRD_LOOKUP.lookups.senses,
// CONFIG.DDBI.SRD_LOOKUP.lookups.weaponproperties,
- ].flat().forEach((entry) => {
+ ]
+ .flat()
+ .forEach((entry) => {
const linkRegEx = new RegExp(`${entry.name}`, "ig");
function replaceRule(match) {
return `@Compendium[${entry.compendium}.${entry.documentName}]{${match}}`;
@@ -265,12 +274,15 @@ export function parseTags(text) {
*/
export default function parseTemplateString(ddb, character, text, feature) {
if (!text) return text;
+ if (typeof text.replaceAll === "function") {
+ text = text.replaceAll(/\r?\n/g, "<br />");
+ }
let result = {
id: feature.id,
entityTypeId: feature.entityTypeId,
- componentId: (feature.componentId) ? feature.componentId : null,
- componentTypeId: (feature.componentTypeId) ? feature.componentTypeId : null,
- damageTypeId: (feature.damageTypeId) ? feature.damageTypeId : null,
+ componentId: feature.componentId ? feature.componentId : null,
+ componentTypeId: feature.componentTypeId ? feature.componentTypeId : null,
+ damageTypeId: feature.damageTypeId ? feature.damageTypeId : null,
text: text,
resultString: "",
definitions: [],
@@ -293,7 +305,7 @@ export default function parseTemplateString(ddb, character, text, feature) {
const splitMatchAt = splitRemoveUnsigned.split("@");
const parsedMatch = parseMatch(ddb, character, splitRemoveUnsigned, feature);
const dicePattern = /\d*d\d\d*/;
- const typeSplit = splitMatchAt[0].split(':');
+ const typeSplit = splitMatchAt[0].split(":");
entry.type = typeSplit[0];
if (typeSplit.length > 1) entry.subType = typeSplit[1];
// do we have a dice string, e.g. sneak attack?
| 7 |
diff --git a/js/inputmask.js b/js/inputmask.js }
resetMaskSet();
- getMaskSet().p = seekNext(-1);
- // if (writeOut) input.inputmask._valueSet(""); //initial clear
+ while (inputValue[0] === getPlaceholder(initialNdx)) {
+ inputValue.shift();
+ initialNdx++;
+ }
+ getMaskSet().p = initialNdx;
if (!strict) {
if (opts.autoUnmask !== true) {
writeBuffer(input, getBuffer(), selection);
if (frontPart.charCodeAt(frontPart.length - 1) !== frontBufferPart.charCodeAt(frontBufferPart.length - 1)) {
e.which = frontPart.charCodeAt(frontPart.length - 1);
+ ignorable = false; //make sure ignorable is ignored ;-)
EventHandlers.keypressEvent.call(input, e);
} else {
if (selection.begin == selection.end - 1)
| 9 |
diff --git a/rollup.config.js b/rollup.config.js @@ -10,7 +10,11 @@ import nodeResolve from "@rollup/plugin-node-resolve";
*/
const umd_config = {
onwarn(message) {
- if (message.code === "EVAL") { return; }
+ // Avoid this warning: "Use of eval is strongly discouraged, as it poses
+ // security risks and may cause issues with minification"
+ if (message.code === "EVAL") {
+ return;
+ }
console.error(message);
},
@@ -40,7 +44,14 @@ const browser_test_config = {
onwarn(message) {
// Avoid this warning: "Use of eval is strongly discouraged, as it poses
// security risks and may cause issues with minification"
- if (message.code === "EVAL") { return; }
+ if (message.code === "EVAL") {
+ return;
+ }
+
+ // Sinon has a circular dependency. Ignore it - everything seems to work
+ // in spite of the issue.
+ if ((message.code === "CIRCULAR_DEPENDENCY")
+ && message.importer.includes("node_modules/@sinonjs")) { return; }
console.error(message);
},
@@ -69,7 +80,11 @@ const browser_test_config = {
*/
const browser_benchmark_config = {
onwarn(message) {
- if (message.code === "EVAL") { return; }
+ // Avoid this warning: "Use of eval is strongly discouraged, as it poses
+ // security risks and may cause issues with minification"
+ if (message.code === "EVAL") {
+ return;
+ }
console.error(message);
},
| 8 |
diff --git a/assets/src/edit-story/components/library/panes/text/karma/textPane.karma.js b/assets/src/edit-story/components/library/panes/text/karma/textPane.karma.js @@ -54,6 +54,8 @@ describe('CUJ: Creator can Add and Write Text: Consecutive text presets', () =>
'Lorem ipsum dolor sit amet, consectetur adipiscing elit.';
let lastY;
let lastHeight;
+ let nextY;
+ let nextHeight;
let storyContext;
const verifyDefaultPosition = async (name, content) => {
@@ -64,29 +66,37 @@ describe('CUJ: Creator can Add and Write Text: Consecutive text presets', () =>
const preset = PRESETS.find(({ title }) => name === title);
expect(element.y).toEqual(preset.element.y);
});
- lastY = element.y;
- lastHeight = element.height;
+ nextY = element.y;
+ nextHeight = element.height;
};
const verifyStaggeredPosition = async (content) => {
+ // Store both last and next value to ensure incorrect value isn't used within waitFor.
+ lastY = nextY;
+ lastHeight = nextHeight;
storyContext = await fixture.renderHook(() => useStory());
const element = storyContext.state.selectedElements[0];
await waitFor(() => {
expect(stripHTML(element.content)).toEqual(content);
expect(element.y).toEqual(lastY + lastHeight + POSITION_MARGIN);
});
- lastY = element.y;
- lastHeight = element.height;
+ nextY = element.y;
+ nextHeight = element.height;
};
await fixture.editor.library.textTab.click();
+
+ // @todo Remove this once #4094 gets fixed!
+ // Wait until the history has changed to its initial (incorrect due to a bug) position.
+ await fixture.events.sleep(300);
+
// Stagger all different text presets.
await fixture.events.click(fixture.editor.library.text.preset('Heading 1'));
await verifyDefaultPosition('Heading 1', 'Heading 1');
await fixture.events.click(fixture.editor.library.text.preset('Paragraph'));
- await verifyStaggeredPosition('Paragraph');
+ await verifyStaggeredPosition(PARAGRAPH_TEXT);
await fixture.events.click(fixture.editor.library.text.preset('Heading 2'));
await verifyStaggeredPosition('Heading 2');
| 7 |
diff --git a/_layouts/home.html b/_layouts/home.html @@ -23,15 +23,16 @@ layout: default
<div class="u-textCenter u-padding-r-all u-textCenter">
<h3 class="u-text-r-m u-textWeight-300 u-color-white u-margin-top-l u-lineHeight-l">
{{ t.home_newsletter_title }}</h3>
- <form class="Form u-padding-r-top u-md-size7of12 u-lg-size5of12 u-layoutCenter" action="https://a0x0b4.mailupclient.com/frontend/subscribe.aspx" method="get" target="_blank">
+ <form class="Form u-padding-r-top u-md-size7of12 u-lg-size5of12 u-layoutCenter" action="https://italia.us19.list-manage.com/subscribe/post?u=5d9c606fb9659198899d9310b&id=3806dd80a5" method="post" target="_blank">
<div class="Form-field Form-field--withPlaceholder Grid u-background-white u-color-grey-30 u-borderRadius-s u-borderShadow-xxl">
<button class="Grid-cell u-sizeFit Icon-mail u-color-grey-40 u-text-r-m u-padding-all-s u-textWeight-400">
</button>
<input class="Form-input Form-input--ultraLean Grid-cell u-sizeFill u-text-r-s u-color-black u-text-r-xs u-borderHideFocus "
- required id="newsletter" name="email" />
+ required id="newsletter" name="EMAIL" />
<label class="Form-label u-color-grey-50 u-padding-left-xxl" for="newsletter"><span class="u-hidden u-md-inline u-lg-inline">
{{t.home_newsletter_placeholder }}</span></label>
- <input type="hidden" name="list" value="1">
+ <!-- real people should not fill this in and expect good things - do not remove this or risk form bot signups-->
+ <div style="position: absolute; left: -5000px;" aria-hidden="true"><input type="text" name="b_5d9c606fb9659198899d9310b_3806dd80a5" tabindex="-1" value=""></div>
<button type="submit" class="Grid-cell u-sizeFit u-background-teal-30 u-color-white u-textWeight-600 u-padding-r-left u-padding-r-right u-textUppercase u-borderRadius-s">
{{ t.home_newsletter_button }}
</button>
| 14 |
diff --git a/History.md b/History.md [4.0.3 / 2017-01-30](https://github.com/jakubpawlowicz/clean-css/compare/v4.0.2...v4.0.3)
==================
-* Fixed issue [#875](https://github.com/jakubpawlowicz/clean-css/issues/875) - invalid traversing in semanting merging.
+* Fixed issue [#875](https://github.com/jakubpawlowicz/clean-css/issues/875) - invalid traversing in semantic merging.
[4.0.2 / 2017-01-26](https://github.com/jakubpawlowicz/clean-css/compare/v4.0.1...v4.0.2)
==================
| 1 |
diff --git a/src/index.js b/src/index.js @@ -160,7 +160,7 @@ class Dayjs {
default:
break
}
- this.init()
+ this.init(this.$L)
return this
}
@@ -287,6 +287,11 @@ class Dayjs {
return this.endOf(C.M).$D
}
+ setLocale (l) {
+ Object.assign(this.$L, l)
+ return this
+ }
+
clone() {
return new Dayjs(this)
}
| 0 |
diff --git a/packages/@vue/cli-service/generator/template/src/components/HelloWorld.vue b/packages/@vue/cli-service/generator/template/src/components/HelloWorld.vue <li><a href="https://router.vuejs.org/en/essentials/getting-started.html" target="_blank">vue-router</a></li>
<li><a href="https://vuex.vuejs.org/en/intro.html" target="_blank">vuex</a></li>
<li><a href="https://github.com/vuejs/vue-devtools#vue-devtools" target="_blank">vue-devtools</a></li>
- <li><a href="https://vue-loader.vuejs.org/en" target="_blank">vue-loader</a></li>
+ <li><a href="https://vue-loader.vuejs.org" target="_blank">vue-loader</a></li>
<li><a href="https://github.com/vuejs/awesome-vue" target="_blank">awesome-vue</a></li>
</ul>
</div>
| 1 |
diff --git a/src/actor.js b/src/actor.js @@ -90,6 +90,7 @@ const getDefaultSequentialStoreIdOrThrow = () => {
* environment variable, or `application/json` if not set.
* This feature is useful for local development and debugging of your acts.
* </p>
+ * @param {String} key Key of the record.
* @param {Function} callback Optional callback.
* @returns {Promise} Returns a promise if no callback was provided.
*/
| 1 |
diff --git a/source/Table/Table.js b/source/Table/Table.js @@ -406,11 +406,11 @@ export default class Table extends React.PureComponent {
className: cn('ReactVirtualized__Table__headerRow', rowClass),
columns: this._getHeaderColumns(),
style: {
- ...rowStyleObject,
height: headerHeight,
overflow: 'hidden',
paddingRight: scrollbarWidth,
width: width,
+ ...rowStyleObject,
},
})}
@@ -635,10 +635,10 @@ export default class Table extends React.PureComponent {
const className = cn('ReactVirtualized__Table__row', rowClass);
const flattenedStyle = {
...style,
- ...rowStyleObject,
height: this._getRowHeight(index),
overflow: 'hidden',
paddingRight: scrollbarWidth,
+ ...rowStyleObject,
};
return rowRenderer({
| 11 |
diff --git a/token-metadata/0x12f649A9E821F90BB143089a6e56846945892ffB/metadata.json b/token-metadata/0x12f649A9E821F90BB143089a6e56846945892ffB/metadata.json "symbol": "UDOO",
"address": "0x12f649A9E821F90BB143089a6e56846945892ffB",
"decimals": 18,
- "dharmaVerificationStatus": {
"dharmaVerificationStatus": "VERIFIED"
}
\ No newline at end of file
-}
\ No newline at end of file
| 3 |
diff --git a/README.md b/README.md <br />
-<img src="https://swap.tech/images/airswap-high-res.png" width="500"/>
+<img src="https://www.airswap.io/airswap-blue-transparent.png" width="500"/>
<br />
[AirSwap](https://www.airswap.io/) is a peer-to-peer trading network for Ethereum tokens, initially built on the [Swap Protocol](https://swap.tech/whitepaper/). The AirSwap product family includes [Explorer](https://explorer.airswap.io/), [DexIndex](https://dexindex.io/), [AirSwap Instant](https://instant.airswap.io/), and [AirSwap Trader](https://trader.airswap.io/). This repository contains smart contracts and JavaScript packages for use by developers and traders on the AirSwap network.
| 3 |
diff --git a/package.json b/package.json {
"name": "kuzzle",
"author": "The Kuzzle Team <[email protected]>",
- "version": "1.0.0-RC9.6",
+ "version": "1.0.0-RC10",
"description": "Kuzzle is an open-source solution that handles all the data management through a secured API, with a large choice of protocols.",
"main": "./lib/index.js",
"bin": {
| 13 |
diff --git a/src/encoded/types/experiment.py b/src/encoded/types/experiment.py @@ -331,11 +331,11 @@ class Experiment(Dataset,
break
elif 'depleted_in_term_name' in library_object and \
'polyadenylated mRNA' in library_object['depleted_in_term_name']:
- preferred_name = 'polyA depleted RNA-seq'
+ preferred_name = 'polyA minus RNA-seq'
break
elif 'nucleic_acid_term_name' in library_object and \
library_object['nucleic_acid_term_name'] == 'polyadenylated mRNA':
- preferred_name = 'polyA RNA-seq'
+ preferred_name = 'polyA plus RNA-seq'
break
else:
continue
| 3 |
diff --git a/userscript.user.js b/userscript.user.js @@ -15048,7 +15048,7 @@ var $$IMU_EXPORT$$;
return options.cb({
url: decode_entities(match[1]),
headers: {
- Referer: null
+ Referer: result.finalUrl
},
extra: {
page: result.finalUrl
| 7 |
diff --git a/token-metadata/0xC9cE70A381910D0a90B30d408CC9C7705ee882de/metadata.json b/token-metadata/0xC9cE70A381910D0a90B30d408CC9C7705ee882de/metadata.json "symbol": "NYAN",
"address": "0xC9cE70A381910D0a90B30d408CC9C7705ee882de",
"decimals": 18,
- "dharmaVerificationStatus": {
"dharmaVerificationStatus": "VERIFIED"
}
\ No newline at end of file
-}
\ No newline at end of file
| 3 |
diff --git a/assets/js/googlesitekit/data/create-settings-store.js b/assets/js/googlesitekit/data/create-settings-store.js @@ -396,18 +396,15 @@ export const createSettingsStore = ( type, identifier, datapoint, {
};
};
- resolvers[ `get${ pascalCaseSlug }` ] = resolvers.getSettings;
-
/**
* Gets the current value for the setting indicated by the selector name.
*
* @since n.e.x.t
*
- * @param {Object} state Data store's state.
* @return {*} Setting value, or undefined.
*/
- selectors[ `get${ pascalCaseSlug }` ] = ( state ) => {
- const { settings } = state;
+ selectors[ `get${ pascalCaseSlug }` ] = () => {
+ const settings = registry.select( STORE_NAME ).getSettings();
if ( 'undefined' === typeof settings ) {
return settings;
| 14 |
diff --git a/packages/openneuro-app/src/scripts/refactor_2021/dataset/files/file-view.jsx b/packages/openneuro-app/src/scripts/refactor_2021/dataset/files/file-view.jsx import React, { useState, useEffect } from 'react'
-import Spinner from '../../../common/partials/spinner.jsx'
+import { Loading } from '@openneuro/components/loading'
import { apiPath } from './file.jsx'
import FileViewerType from './file-viewer-type.jsx'
@@ -22,7 +22,7 @@ const FileView = ({ datasetId, snapshotTag, path }) => {
})
if (loading) {
- return <Spinner active text="Loading File" />
+ return <Loading />
} else {
return <FileViewerType path={path} url={url} data={data} />
}
| 14 |
diff --git a/src/functional.js b/src/functional.js @@ -341,6 +341,22 @@ function insertAt(state, listId, index, value) {
return setField(newState, listId, newElem, value)
}
+function setListIndex(state, listId, index, value) {
+ const obj = state.getIn(['objects', listId])
+ let i = -1, elem = obj.getIn(['_head', 'next'])
+ while (elem) {
+ if (!obj.get(elem).get('actions', List()).isEmpty()) i += 1
+ if (i === index) break
+ elem = obj.getIn([elem, 'next'])
+ }
+
+ if (elem) {
+ return setField(state, listId, elem, value)
+ } else {
+ return insertAt(state, listId, index, value)
+ }
+}
+
function deleteField(state, targetId, key) {
const obj = state.getIn(['objects', targetId])
if (obj.get('_type') === 'list' && typeof key === 'number') {
@@ -378,6 +394,10 @@ function init(storeId) {
function set(target, key, value) {
if (!target || !target._id) throw 'Cannot modify object that does not exist'
+ if (target._type === 'list') {
+ if (typeof key !== 'number' || key < 0) throw 'Invalid index'
+ return makeStore(setListIndex(target._state, target._id, key, value))
+ }
if (key.startsWith('_')) throw 'Invalid key'
return makeStore(setField(target._state, target._id, key, value))
}
| 11 |
diff --git a/src/middleware/packages/triplestore/index.js b/src/middleware/packages/triplestore/index.js @@ -39,7 +39,8 @@ const TripleStoreService = {
});
}
- const response = await fetch(this.settings.sparqlEndpoint + this.settings.mainDataset + '/update', {
+ const url = this.settings.sparqlEndpoint + this.settings.mainDataset + '/update';
+ const response = await fetch(url, {
method: 'POST',
body: `INSERT DATA { ${rdf} }`,
headers: {
@@ -49,7 +50,7 @@ const TripleStoreService = {
}
});
- if (!response.ok) throw new Error(response.statusText);
+ if (!response.ok) throw new Error(`Unable to reach SPARQL endpoint ${url}. Error message: ${response.statusText}`);
}
},
countTriplesOfSubject: {
@@ -92,7 +93,9 @@ const TripleStoreService = {
async handler(ctx) {
const webId = ctx.params.webId || ctx.meta.webId;
const query = ctx.params.query;
- const response = await fetch(this.settings.sparqlEndpoint + this.settings.mainDataset + '/update', {
+
+ const url = this.settings.sparqlEndpoint + this.settings.mainDataset + '/update';
+ const response = await fetch(url, {
method: 'POST',
body: query,
headers: {
@@ -101,7 +104,8 @@ const TripleStoreService = {
Authorization: this.Authorization
}
});
- if (!response.ok) throw new Error(response.statusText);
+
+ if (!response.ok) throw new Error(`Unable to reach SPARQL endpoint ${url}. Error message: ${response.statusText}`);
}
},
query: {
@@ -130,12 +134,13 @@ const TripleStoreService = {
Accept: fusekiAccept
};
- const response = await fetch(this.settings.sparqlEndpoint + this.settings.mainDataset + '/query', {
+ const url = this.settings.sparqlEndpoint + this.settings.mainDataset + '/query';
+ const response = await fetch(url, {
method: 'POST',
body: query,
headers
});
- if (!response.ok) throw new Error(response.statusText);
+ if (!response.ok) throw new Error(`Unable to reach SPARQL endpoint ${url}. Error message: ${response.statusText}`);
// console.log(response.headers.get('content-type'));
ctx.meta.$responseType = response.headers.get('content-type');
@@ -181,7 +186,9 @@ const TripleStoreService = {
},
async handler(ctx) {
const webId = ctx.params.webId || ctx.meta.webId;
- const response = await fetch(this.settings.sparqlEndpoint + this.settings.mainDataset + '/update', {
+
+ const url = this.settings.sparqlEndpoint + this.settings.mainDataset + '/update';
+ const response = await fetch(url, {
method: 'POST',
body: 'update=DROP+ALL',
headers: {
@@ -191,7 +198,7 @@ const TripleStoreService = {
}
});
- if (!response.ok) throw new Error(response.statusText);
+ if (!response.ok) throw new Error(`Unable to reach SPARQL endpoint ${url}. Error message: ${response.statusText}`);
return response;
}
| 7 |
diff --git a/src/traces/parcoords/lines.js b/src/traces/parcoords/lines.js @@ -168,6 +168,59 @@ function emptyAttributes(regl) {
return attributes;
}
+function makeItem(model, leftmost, rightmost, itemNumber, i0, i1, x, y, panelSizeX, panelSizeY, crossfilterDimensionIndex, constraints, drwLayer) {
+ var dims = [[], []];
+ for(var k = 0; k < 64; k++) {
+ dims[0][k] = (k === i0) ? 1 : 0;
+ dims[1][k] = (k === i1) ? 1 : 0;
+ }
+
+ var overdrag = model.lines.canvasOverdrag;
+ var domain = model.domain;
+ var canvasWidth = model.canvasWidth;
+ var canvasHeight = model.canvasHeight;
+
+ var itemModel = Lib.extendFlat({
+ key: crossfilterDimensionIndex,
+ resolution: [canvasWidth, canvasHeight],
+ viewBoxPos: [x + overdrag, y],
+ viewBoxSize: [panelSizeX, panelSizeY],
+ i0: i0,
+ i1: i1,
+
+ dim0A: dims[0].slice(0, 16),
+ dim0B: dims[0].slice(16, 32),
+ dim0C: dims[0].slice(32, 48),
+ dim0D: dims[0].slice(48, 64),
+ dim1A: dims[1].slice(0, 16),
+ dim1B: dims[1].slice(16, 32),
+ dim1C: dims[1].slice(32, 48),
+ dim1D: dims[1].slice(48, 64),
+
+ drwLayer: drwLayer,
+
+ scissorX: (itemNumber === leftmost ? 0 : x + overdrag) + (model.pad.l - overdrag) + model.layoutWidth * domain.x[0],
+ scissorWidth: (itemNumber === rightmost ? canvasWidth - x + overdrag : panelSizeX + 0.5) + (itemNumber === leftmost ? x + overdrag : 0),
+ scissorY: y + model.pad.b + model.layoutHeight * domain.y[0],
+ scissorHeight: panelSizeY,
+
+ viewportX: model.pad.l - overdrag + model.layoutWidth * domain.x[0],
+ viewportY: model.pad.b + model.layoutHeight * domain.y[0],
+ viewportWidth: canvasWidth,
+ viewportHeight: canvasHeight
+ }, constraints);
+
+ return itemModel;
+}
+
+function expandedPixelRange(bounds) {
+ var dh = maskHeight - 1;
+ return [
+ Math.max(0, Math.floor(bounds[0] * dh), 0),
+ Math.min(dh, Math.ceil(bounds[1] * dh), dh)
+ ];
+}
+
module.exports = function(canvasGL, d) {
// context & pick describe which canvas we're talking about - won't change with new data
var context = d.context;
@@ -190,6 +243,8 @@ module.exports = function(canvasGL, d) {
var maskTexture;
var paletteTexture = regl.texture(paletteTextureConfig);
+ var prevAxisOrder = [];
+
update(d);
var glAes = regl({
@@ -299,53 +354,6 @@ module.exports = function(canvasGL, d) {
}
}
- var prevAxisOrder = [];
-
- function makeItem(leftmost, rightmost, itemNumber, i0, i1, x, y, panelSizeX, panelSizeY, crossfilterDimensionIndex, constraints, drwLayer) {
- var dims = [[], []];
- for(var k = 0; k < 64; k++) {
- dims[0][k] = (k === i0) ? 1 : 0;
- dims[1][k] = (k === i1) ? 1 : 0;
- }
-
- var overdrag = model.lines.canvasOverdrag;
- var domain = model.domain;
- var canvasWidth = model.canvasWidth;
- var canvasHeight = model.canvasHeight;
-
- var itemModel = Lib.extendFlat({
- key: crossfilterDimensionIndex,
- resolution: [canvasWidth, canvasHeight],
- viewBoxPos: [x + overdrag, y],
- viewBoxSize: [panelSizeX, panelSizeY],
- i0: i0,
- i1: i1,
-
- dim0A: dims[0].slice(0, 16),
- dim0B: dims[0].slice(16, 32),
- dim0C: dims[0].slice(32, 48),
- dim0D: dims[0].slice(48, 64),
- dim1A: dims[1].slice(0, 16),
- dim1B: dims[1].slice(16, 32),
- dim1C: dims[1].slice(32, 48),
- dim1D: dims[1].slice(48, 64),
-
- drwLayer: drwLayer,
-
- scissorX: (itemNumber === leftmost ? 0 : x + overdrag) + (model.pad.l - overdrag) + model.layoutWidth * domain.x[0],
- scissorWidth: (itemNumber === rightmost ? canvasWidth - x + overdrag : panelSizeX + 0.5) + (itemNumber === leftmost ? x + overdrag : 0),
- scissorY: y + model.pad.b + model.layoutHeight * domain.y[0],
- scissorHeight: panelSizeY,
-
- viewportX: model.pad.l - overdrag + model.layoutWidth * domain.x[0],
- viewportY: model.pad.b + model.layoutHeight * domain.y[0],
- viewportWidth: canvasWidth,
- viewportHeight: canvasHeight
- }, constraints);
-
- return itemModel;
- }
-
function makeConstraints(isContext) {
var i, j, k;
@@ -358,14 +366,6 @@ module.exports = function(canvasGL, d) {
limits[1][k] = p[1];
}
- function expandedPixelRange(bounds) {
- var dh = maskHeight - 1;
- return [
- Math.max(0, Math.floor(bounds[0] * dh), 0),
- Math.min(dh, Math.ceil(bounds[1] * dh), dh)
- ];
- }
-
var mask = [];
for(i = 0; i < maskHeight * 8; i++) {
mask[i] = 255;
@@ -458,6 +458,7 @@ module.exports = function(canvasGL, d) {
prevAxisOrder[i0] = [x, nextX];
var item = makeItem(
+ model,
leftmost, rightmost, i, i0, i1, x, y,
p.panelSizeX, p.panelSizeY,
p.dim0.crossfilterDimensionIndex,
| 5 |
diff --git a/features/cooldown.js b/features/cooldown.js @@ -2,7 +2,7 @@ const util = require('../lib/util');
//cooldown automod
exports.message = async (message, channels, database) => {
- if (!message.guild || message.author.bot)
+ if (!message.guild || message.author.bot || message.member.hasPermission('MANAGE_MESSAGES'))
return;
if (channels.get(message.channel.id) && channels.get(message.channel.id).cooldown) {
| 8 |
diff --git a/src/legacy.js b/src/legacy.js @@ -31,7 +31,10 @@ const runningComponents = () => {
// load components if user runs a keyword command, or "sls --all" or "sls --target" (that last one for china)
if (
componentKeywords.has(process.argv[2]) ||
- (process.argv[2] === 'deploy' && utils.runningTemplate(process.cwd())) ||
+ // only allow deploy & remove commands for nested templates
+ // to save up on extensive FS operations for all the other possible framework v1 commands
+ ((process.argv[2] === 'deploy' || process.argv[2] === 'remove') &&
+ utils.runningTemplate(process.cwd())) ||
args.target
) {
return true;
| 11 |
diff --git a/components/base-adresse-nationale/languages-preview.js b/components/base-adresse-nationale/languages-preview.js @@ -19,7 +19,15 @@ function LanguagesPreview({nomAlt}) {
message={
<ul>
{altNames.map(voieName => (
- <li color='white' key={voieName}>{nomAlt[voieName]}</li>
+ <li color='white' key={voieName}>
+ <Image
+ src={isFlagExist ? `/images/icons/flags/${voieName}.svg` : '/images/icons/flags/ntr.svg'}
+ height={20}
+ width={20}
+ onLoadingComplete={result => result.naturalHeight <= 1 ? setIsFlagExist(false) : setIsFlagExist(true)}
+ />
+ {nomAlt[voieName]}
+ </li>
))}
</ul>
}
@@ -66,6 +74,14 @@ function LanguagesPreview({nomAlt}) {
text-align: left;
}
+ li {
+ list-style-type: none;
+ display: flex;
+ align-items: center;
+ gap: 10px;
+ margin: 10px 0;
+ }
+
.language-with-icon {
font-style: italic;
font-weight: lighter;
| 0 |
diff --git a/contracts/contracts/RaidenMicroTransferChannels.sol b/contracts/contracts/RaidenMicroTransferChannels.sol @@ -34,12 +34,34 @@ contract RaidenMicroTransferChannels {
* Events
*/
- event ChannelCreated(address indexed _sender, address indexed _receiver, uint192 _deposit);
- event ChannelToppedUp (address indexed _sender, address indexed _receiver, uint32 indexed _open_block_number, uint192 _added_deposit, uint192 _deposit);
- event ChannelCloseRequested(address indexed _sender, address indexed _receiver, uint32 indexed _open_block_number, uint192 _balance);
- event ChannelSettled(address indexed _sender, address indexed _receiver, uint32 indexed _open_block_number);
- event TokenFallback(address indexed _sender, address indexed _receiver, uint192 _deposit, bytes indexed _data);
- event GasCost(string _function_name, uint _gaslimit, uint _gas);
+ event ChannelCreated(
+ address indexed _sender,
+ address indexed _receiver,
+ uint192 _deposit);
+ event ChannelToppedUp (
+ address indexed _sender,
+ address indexed _receiver,
+ uint32 indexed _open_block_number,
+ uint192 _added_deposit,
+ uint192 _deposit);
+ event ChannelCloseRequested(
+ address indexed _sender,
+ address indexed _receiver,
+ uint32 indexed _open_block_number,
+ uint192 _balance);
+ event ChannelSettled(
+ address indexed _sender,
+ address indexed _receiver,
+ uint32 indexed _open_block_number);
+ event TokenFallback(
+ address indexed _sender,
+ address indexed _receiver,
+ uint192 _deposit,
+ bytes indexed _data);
+ event GasCost(
+ string indexed _function_name,
+ uint _gaslimit,
+ uint _gas);
/*
* Constructor
| 7 |
diff --git a/src/plots/cartesian/set_convert.js b/src/plots/cartesian/set_convert.js @@ -99,19 +99,18 @@ module.exports = function setConvert(ax, fullLayout) {
if(ms === BADNUM) {
if(isNumeric(v)) {
v = +v;
- // keep track of tenths of ms, that `new Date` will drop
- // same logic as in Lib.ms2DateTime
- var msecTenths = Math.floor(Lib.mod(v + 0.05, 1) * 10);
- var msRounded = Math.round(v - msecTenths / 10);
-
- var d = new Date(msRounded);
- ms = dateTime2ms(d) + msecTenths / 10;
if(msUTC) {
// For now it is only used
// to fix bar length in milliseconds.
// It could be applied in other places in v2
- ms += d.getTimezoneOffset() * ONEMIN;
+ return v;
}
+
+ // keep track of tenths of ms, that `new Date` will drop
+ // same logic as in Lib.ms2DateTime
+ var msecTenths = Math.floor(Lib.mod(v + 0.05, 1) * 10);
+ var msRounded = Math.round(v - msecTenths / 10);
+ ms = dateTime2ms(new Date(msRounded)) + msecTenths / 10;
} else return BADNUM;
}
return ms;
| 0 |
diff --git a/stubs/defaultConfig.stub.js b/stubs/defaultConfig.stub.js -const _ = require('lodash')
-
module.exports = {
prefix: '',
important: false,
@@ -310,9 +308,10 @@ module.exports = {
margin: theme => {
const negativeSpacing = Object.keys(theme('spacing'))
.filter(key => theme('spacing')[key] !== '0')
- .reduce((negativeSpacing, key) => {
- return { ...negativeSpacing, [`-${key}`]: `-${theme('spacing')[key]}` }
- }, {})
+ .reduce((negativeSpacing, key) => ({
+ ...negativeSpacing,
+ [`-${key}`]: `-${theme('spacing')[key]}`
+ }), {})
return {
auto: 'auto',
| 2 |
diff --git a/src/pipeline/bundle-js.js b/src/pipeline/bundle-js.js @@ -14,7 +14,7 @@ const getTransform = (opts, paths) => {
return (opts[name] || []).map(d => require(d));
};
- return [[ babelify, { presets: [ reactPreset, es2015Preset ], ignore: ['node_modules/!(idyll)/**/*'] } ]]
+ return [[ babelify, { presets: [ reactPreset, es2015Preset ] } ]]
.concat(_getTransform('transform'))
.concat([[ brfs ]]);
};
| 13 |
diff --git a/javascript/components/MapView.js b/javascript/components/MapView.js @@ -225,7 +225,6 @@ class MapView extends NativeBridgeComponent {
regionDidChangeDebounceTime: 500,
};
- _mounted = false;
constructor(props) {
super(props);
@@ -258,12 +257,12 @@ class MapView extends NativeBridgeComponent {
}
componentDidMount() {
- this._mounted = true;
this._setHandledMapChangedEvents(this.props);
}
componentWillUnmount() {
- this._mounted = false;
+ this._onDebouncedRegionWillChange.cancel();
+ this._onDebouncedRegionDidChange.cancel();
}
componentWillReceiveProps(nextProps) {
@@ -672,8 +671,6 @@ class MapView extends NativeBridgeComponent {
if (isFunction(this.props.onRegionWillChange)) {
this.props.onRegionWillChange(payload);
}
- if (!this._mounted) return;
-
this.setState({isUserInteraction: payload.properties.isUserInteraction});
}
@@ -681,8 +678,6 @@ class MapView extends NativeBridgeComponent {
if (isFunction(this.props.onRegionDidChange)) {
this.props.onRegionDidChange(payload);
}
- if (!this._mounted) return;
-
this.setState({region: payload});
}
@@ -755,8 +750,6 @@ class MapView extends NativeBridgeComponent {
}
_onLayout(e) {
- if (!this._mounted) return;
-
this.setState({
isReady: true,
width: e.nativeEvent.layout.width,
| 2 |
diff --git a/README.md b/README.md @@ -75,7 +75,7 @@ Head over to the **interactive playground** at [codesandbox](https://codesandbox
### Why i18next?
- **Simplicity:** no need to change your webpack configuration or add additional babel transpilers, just use create-react-app and go.
-- **Production ready** we know there are more needs for production than just doing i18n on the clientside, so we offer wider support on [serverside](https://www.i18next.com/supported-frameworks.html) too (nodejs, php, ruby, .net, ...). **Learn once - translate everywhere**.
+- **Production ready** we know there are more needs for production than just doing i18n on the clientside, so we offer wider support on [serverside](https://www.i18next.com/overview/supported-frameworks) too (nodejs, php, ruby, .net, ...). **Learn once - translate everywhere**.
- **Beyond i18n** comes with [locize](https://locize.com) bridging the gap between developement and translations - covering the whole translation process.
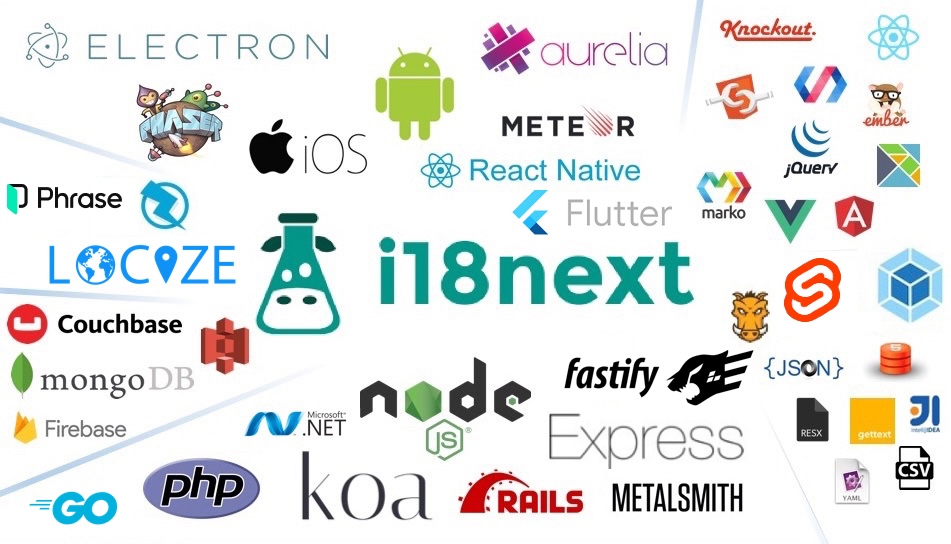
| 1 |
diff --git a/game.js b/game.js @@ -1024,6 +1024,18 @@ _setFirstPersonAction(lastFirstPerson);
_setFirstPersonAction(firstPerson);
}); */
+const _listenLocalPlayerPickupAction = () => {
+ const localPlayer = getLocalPlayer();
+ localPlayer.addEventListener('actionadd', e => {
+ const {action} = e;
+ const {type} = action;
+ if (type === 'pickUp') {
+ ioManager.setMovementEnabled(false);
+ }
+ });
+};
+_listenLocalPlayerPickupAction();
+
let lastMouseEvent = null;
class GameManager extends EventTarget {
constructor() {
| 0 |
diff --git a/config/app_config.yml.sample b/config/app_config.yml.sample @@ -764,7 +764,7 @@ defaults: &defaults
isolines: false
routing: false
data_observatory: false
- disable_email_mx_check: # true / false (default). Disables MX DNS record check and just performs syntax validation.
+ disable_email_mx_check: false # Disables MX DNS record check and just performs syntax validation.
development:
<<: *defaults
| 7 |
diff --git a/modules/Cockpit/Controller/Assets.php b/modules/Cockpit/Controller/Assets.php @@ -81,12 +81,17 @@ class Assets extends \Cockpit\AuthController {
$info = getimagesize($target);
$asset['width'] = $info[0];
$asset['height'] = $info[1];
- $asset['colors'] = \ColorThief\ColorThief::getPalette($target, 5);
+ $asset['colors'] = [];
+
+ if ($asset['width'] && $asset['height']) {
+
+ $asset['colors'] = \ColorThief\ColorThief::getPalette($target, 5, ceil(($asset['width'] * $asset['height']) / 10000));
foreach($asset['colors'] as &$color) {
$color = sprintf("%02x%02x%02x", $color[0], $color[1], $color[2]);
}
}
+ }
$assets[] = $asset;
| 7 |
diff --git a/angular/projects/spark-angular/src/lib/components/sprk-card/sprk-card.stories.ts b/angular/projects/spark-angular/src/lib/components/sprk-card/sprk-card.stories.ts @@ -161,6 +161,11 @@ export const teaser = () => ({
invenirevix te. Facilisi perpetua.
</p>
+ <sprk-stack
+ splitAt="tiny"
+ additionalClasses="sprk-o-Stack--end-row"
+ sprkStackItem
+ >
<div sprkStackItem>
<a
sprkLink
@@ -173,6 +178,7 @@ export const teaser = () => ({
</a>
</div>
</sprk-stack>
+ </sprk-stack>
</sprk-card>
`,
});
@@ -291,6 +297,11 @@ export const teaserWithDifferentElementOrder = () => ({
invenirevix te. Facilisi perpetua.
</p>
+ <sprk-stack
+ splitAt="tiny"
+ additionalClasses="sprk-o-Stack--end-row"
+ sprkStackItem
+ >
<div sprkStackItem>
<a
sprkLink
@@ -303,6 +314,7 @@ export const teaserWithDifferentElementOrder = () => ({
</a>
</div>
</sprk-stack>
+ </sprk-stack>
</sprk-card>
`,
});
@@ -364,6 +376,11 @@ export const twoUpCards = () => ({
tantas signiferumque at. Numquam.
</p>
+ <sprk-stack
+ splitAt="tiny"
+ additionalClasses="sprk-o-Stack--end-row"
+ sprkStackItem
+ >
<div sprkStackItem>
<a
sprkLink
@@ -376,6 +393,7 @@ export const twoUpCards = () => ({
</a>
</div>
</sprk-stack>
+ </sprk-stack>
</sprk-card>
</div>
@@ -409,6 +427,11 @@ export const twoUpCards = () => ({
tantas signiferumque at. Numquam.
</p>
+ <sprk-stack
+ splitAt="tiny"
+ additionalClasses="sprk-o-Stack--end-row"
+ sprkStackItem
+ >
<div sprkStackItem>
<a
sprkLink
@@ -421,6 +444,7 @@ export const twoUpCards = () => ({
</a>
</div>
</sprk-stack>
+ </sprk-stack>
</sprk-card>
</div>
</sprk-stack>
@@ -482,6 +506,11 @@ export const threeUpCards = () => ({
tantas signiferumque at. Numquam.
</p>
+ <sprk-stack
+ splitAt="tiny"
+ additionalClasses="sprk-o-Stack--end-row"
+ sprkStackItem
+ >
<div sprkStackItem>
<a
sprkLink
@@ -494,6 +523,7 @@ export const threeUpCards = () => ({
</a>
</div>
</sprk-stack>
+ </sprk-stack>
</sprk-card>
</div>
@@ -527,6 +557,11 @@ export const threeUpCards = () => ({
tantas signiferumque at. Numquam.
</p>
+ <sprk-stack
+ splitAt="tiny"
+ additionalClasses="sprk-o-Stack--end-row"
+ sprkStackItem
+ >
<div sprkStackItem>
<a
sprkLink
@@ -539,6 +574,7 @@ export const threeUpCards = () => ({
</a>
</div>
</sprk-stack>
+ </sprk-stack>
</sprk-card>
</div>
@@ -572,6 +608,11 @@ export const threeUpCards = () => ({
tantas signiferumque at. Numquam.
</p>
+ <sprk-stack
+ splitAt="tiny"
+ additionalClasses="sprk-o-Stack--end-row"
+ sprkStackItem
+ >
<div sprkStackItem>
<a
sprkLink
@@ -584,6 +625,7 @@ export const threeUpCards = () => ({
</a>
</div>
</sprk-stack>
+ </sprk-stack>
</sprk-card>
</div>
</sprk-stack>
@@ -645,6 +687,11 @@ export const fourUpCards = () => ({
tantas signiferumque at. Numquam.
</p>
+ <sprk-stack
+ splitAt="tiny"
+ additionalClasses="sprk-o-Stack--end-row"
+ sprkStackItem
+ >
<div sprkStackItem>
<a
sprkLink
@@ -657,6 +704,7 @@ export const fourUpCards = () => ({
</a>
</div>
</sprk-stack>
+ </sprk-stack>
</sprk-card>
</div>
@@ -690,6 +738,11 @@ export const fourUpCards = () => ({
tantas signiferumque at. Numquam.
</p>
+ <sprk-stack
+ splitAt="tiny"
+ additionalClasses="sprk-o-Stack--end-row"
+ sprkStackItem
+ >
<div sprkStackItem>
<a
sprkLink
@@ -702,6 +755,7 @@ export const fourUpCards = () => ({
</a>
</div>
</sprk-stack>
+ </sprk-stack>
</sprk-card>
</div>
@@ -735,6 +789,11 @@ export const fourUpCards = () => ({
tantas signiferumque at. Numquam.
</p>
+ <sprk-stack
+ splitAt="tiny"
+ additionalClasses="sprk-o-Stack--end-row"
+ sprkStackItem
+ >
<div sprkStackItem>
<a
sprkLink
@@ -747,6 +806,7 @@ export const fourUpCards = () => ({
</a>
</div>
</sprk-stack>
+ </sprk-stack>
</sprk-card>
</div>
@@ -780,6 +840,11 @@ export const fourUpCards = () => ({
tantas signiferumque at. Numquam.
</p>
+ <sprk-stack
+ splitAt="tiny"
+ additionalClasses="sprk-o-Stack--end-row"
+ sprkStackItem
+ >
<div sprkStackItem>
<a
sprkLink
@@ -792,6 +857,7 @@ export const fourUpCards = () => ({
</a>
</div>
</sprk-stack>
+ </sprk-stack>
</sprk-card>
</div>
</sprk-stack>
| 3 |
diff --git a/README.md b/README.md <p align="center">
+ <img src="https://raw.githubusercontent.com/hopsoft/stimulus_reflex/master/assets/stimulus-reflex-logo.svg?sanitize=true" height="100" />
<a href="https://codefund.io/properties/525/visit-sponsor">
<img src="https://codefund.io/properties/525/sponsor" />
</a>


-<img src="https://raw.githubusercontent.com/hopsoft/stimulus_reflex/master/assets/stimulus-reflex-logo.svg?sanitize=true" height="100" />
-
# StimulusReflex
_reflex_ - an action that is performed as a response to a stimulus
| 5 |
diff --git a/src/components/appSwitch/AppSwitch.js b/src/components/appSwitch/AppSwitch.js @@ -10,6 +10,7 @@ import GDStore from '../../lib/undux/GDStore'
import { checkAuthStatus } from '../../lib/login/checkAuthStatus'
import { CustomDialog } from '../common'
import LoadingIndicator from '../common/LoadingIndicator'
+import zoomSdkLoader from '../dashboard/FaceRecognition/ZoomSdkLoader'
type LoadingProps = {
navigation: any,
@@ -83,6 +84,10 @@ class AppSwitch extends React.Component<LoadingProps, {}> {
delay(TIMEOUT)
]).then(([authResult]) => authResult)
let destDetails = await this.getParams()
+ if (!isLoggedInCitizen) {
+ // load Zoom SDK at start so it will be loaded when user gets to FR screen
+ await zoomSdkLoader.load()
+ }
if (isLoggedIn) {
if (isLoggedInCitizen) {
API.verifyTopWallet()
| 0 |
diff --git a/activity/settings/production.py b/activity/settings/production.py @@ -74,7 +74,10 @@ GOOGLE_MAP_API_KEY = environ.get('ACTIVITY_GOOGLE_MAP_API_KEY', '')
# CACHE CONFIGURATION
# See: https://docs.djangoproject.com/en/dev/ref/settings/#caches
-CACHES = {}
+CACHES = {
+ "default":
+ {"BACKEND": "django.core.cache.backends.locmem.LocMemCache"}
+}
# END CACHE CONFIGURATION
| 12 |
diff --git a/routes/serve-routes.js b/routes/serve-routes.js const errorHandlers = require('../helpers/libraries/errorHandlers.js')
const serveController = require('../controllers/serveController.js')
-function serveFile ({ fileType, filePath }, res) {
+function serveFile ({ file_type, file_path }, res) {
// set default options
const options = {
headers: {
'X-Content-Type-Options': 'nosniff',
- 'Content-Type' : fileType,
+ 'Content-Type' : file_type,
},
}
// adjust default options as needed
- switch (fileType) {
+ // eslint-disable-next-line camelcase
+ switch (file_type) {
case 'image/jpeg':
break
case 'image/gif':
@@ -25,7 +26,7 @@ function serveFile ({ fileType, filePath }, res) {
break
}
// send file
- res.status(200).sendFile(filePath, options)
+ res.status(200).sendFile(file_path, options)
}
module.exports = (app, ua, googleAnalyticsId) => {
| 1 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.