code
stringlengths 122
4.99k
| label
int64 0
14
|
---|---|
diff --git a/physics-manager.js b/physics-manager.js @@ -400,6 +400,7 @@ const _collideItems = matrix => {
geometryManager.currentChunkMesh.update(localVector3);
}; */
+physicsManager.getGravity = () => gravity;
physicsManager.setGravity = g => {
if (g === true) {
gravity.set(0, -9.8, 0);
| 0 |
diff --git a/src/runtime/plugin.ts b/src/runtime/plugin.ts @@ -81,8 +81,7 @@ export default defineNuxtPlugin(async nuxt => {
* If `initLocale` is not set, then the `vueI18n` option `locale` is respect!
* It means a mode that works only with simple vue-i18n, without nuxtjs/i18n routing, browser detection, SEO, and other features.
*/
- initialLocale ||= vueI18nOptions.locale || 'en-US'
-
+ initialLocale = initialLocale || vueI18nOptions.locale || 'en-US'
// create i18n instance
const i18n = createI18n({
...vueI18nOptions,
| 14 |
diff --git a/src/plots/polar/polar.js b/src/plots/polar/polar.js @@ -556,36 +556,25 @@ proto.updateAngularAxis = function(fullLayout, polarLayout) {
var out = Axes.makeLabelFns(ax, 0);
var labelStandoff = out.labelStandoff;
- var labelShift = out.labelShift;
- var offset4fontsize = (angularLayout.ticks !== 'outside' ? 0.7 : 0.5);
- var pad = (ax.linewidth || 1) / 2;
var labelXFn = function(d) {
var rad = t2g(d);
-
- var offset4tx = signSin(rad) === 0 ?
- 0 :
- Math.cos(rad) * (labelStandoff + pad + offset4fontsize * d.fontSize);
- var offset4tick = signCos(rad) * (d.dx + labelStandoff + pad);
-
- return offset4tx + offset4tick;
+ return Math.cos(rad) * labelStandoff;
};
var labelYFn = function(d) {
var rad = t2g(d);
-
- var offset4tx = d.dy + d.fontSize * MID_SHIFT - labelShift;
- var offset4tick = -Math.sin(rad) * (labelStandoff + pad + offset4fontsize * d.fontSize);
-
- return offset4tx + offset4tick;
+ var ff = Math.sin(rad) > 0 ? 0.2 : 1;
+ return -Math.sin(rad) * (labelStandoff + d.fontSize * ff) +
+ Math.abs(Math.cos(rad)) * (d.fontSize * MID_SHIFT);
};
- // TODO maybe switch angle, d ordering ??
var labelAnchorFn = function(angle, d) {
var rad = t2g(d);
- return signSin(rad) === 0 ?
- (signCos(rad) > 0 ? 'start' : 'end') :
- 'middle';
+ var cos = Math.cos(rad);
+ return Math.abs(cos) < 0.1 ?
+ 'middle' :
+ (cos > 0 ? 'start' : 'end');
};
var newTickLayout = strTickLayout(angularLayout);
@@ -623,6 +612,7 @@ proto.updateAngularAxis = function(fullLayout, polarLayout) {
if(ax.visible) {
var tickSign = ax.ticks === 'inside' ? -1 : 1;
+ var pad = (ax.linewidth || 1) / 2;
Axes.drawTicks(gd, ax, {
vals: vals,
@@ -1413,18 +1403,3 @@ function strTranslate(x, y) {
function strRotate(angle) {
return 'rotate(' + angle + ')';
}
-
-// because Math.sign(Math.cos(Math.PI / 2)) === 1
-// oh javascript ;)
-function sign(v) {
- return Math.abs(v) < 1e-10 ? 0 :
- v > 0 ? 1 : -1;
-}
-
-function signCos(v) {
- return sign(Math.cos(v));
-}
-
-function signSin(v) {
- return sign(Math.sin(v));
-}
| 7 |
diff --git a/accessibility-checker-engine/src/v4/rules/element_tabbable_role_valid.ts b/accessibility-checker-engine/src/v4/rules/element_tabbable_role_valid.ts @@ -37,13 +37,13 @@ export let element_tabbable_role_valid: Rule = {
},
rulesets: [{
"id": ["IBM_Accessibility"],
- "num": ["2.4.3"],
+ "num": ["4.1.2"],
"level": eRulePolicy.VIOLATION,
"toolkitLevel": eToolkitLevel.LEVEL_ONE
},
{
"id": ["WCAG_2_1", "WCAG_2_0"],
- "num": ["2.4.3"],
+ "num": ["4.1.2"],
"level": eRulePolicy.RECOMMENDATION,
"toolkitLevel": eToolkitLevel.LEVEL_ONE
}],
| 3 |
diff --git a/src/internal/proc.js b/src/internal/proc.js @@ -164,11 +164,6 @@ export default function proc(
) {
const { sagaMonitor, logger, onError, middleware } = options
const log = logger || _log
- const logError = err => {
- let message = err.sagaStack
-
- log('error', message || err)
- }
const taskContext = Object.create(parentContext)
@@ -286,7 +281,7 @@ export default function proc(
}
} catch (error) {
if (mainTask.isCancelled) {
- logError(error)
+ log('error', error)
}
mainTask.isMainRunning = false
mainTask.cont(error, true)
@@ -301,18 +296,12 @@ export default function proc(
iterator._result = result
iterator._deferredEnd && iterator._deferredEnd.resolve(result)
} else {
- if (result instanceof Error) {
- Object.defineProperty(result, 'sagaStack', {
- value: `at ${name} \n ${result.sagaStack || result.stack}`,
- configurable: true,
- })
- }
if (!task.cont) {
if (result instanceof Error && onError) {
onError(result)
} else {
// TODO: could we skip this when _deferredEnd is attached?
- logError(result)
+ log('error', result)
}
}
iterator._error = result
@@ -410,7 +399,7 @@ export default function proc(
try {
currCb.cancel()
} catch (err) {
- logError(err)
+ log('error', err)
}
currCb.cancel = noop // defensive measure
@@ -475,7 +464,7 @@ export default function proc(
try {
result = (channel ? channel.put : dispatch)(action)
} catch (error) {
- logError(error)
+ log('error', error)
// TODO: should such error here be passed to `onError`?
// or is it already if we dropped error swallowing
cb(error, true)
| 2 |
diff --git a/src/plots/gl2d/index.js b/src/plots/gl2d/index.js @@ -170,101 +170,3 @@ function makeSubplotData(gd) {
return subplotData;
}
-
-function makeSubplotLayer(plotinfo) {
- var plotgroup = plotinfo.plotgroup,
- id = plotinfo.id;
-
- // Layers to keep plot types in the right order.
- // from back to front:
- // 1. heatmaps, 2D histos and contour maps
- // 2. bars / 1D histos
- // 3. errorbars for bars and scatter
- // 4. scatter
- // 5. box plots
- function joinPlotLayers(parent) {
- joinLayer(parent, 'g', 'imagelayer');
- joinLayer(parent, 'g', 'maplayer');
- joinLayer(parent, 'g', 'barlayer');
- joinLayer(parent, 'g', 'carpetlayer');
- joinLayer(parent, 'g', 'boxlayer');
- joinLayer(parent, 'g', 'scatterlayer');
- }
-
- if(!plotinfo.mainplot) {
- var backLayer = joinLayer(plotgroup, 'g', 'layer-subplot');
- plotinfo.shapelayer = joinLayer(backLayer, 'g', 'shapelayer');
- plotinfo.imagelayer = joinLayer(backLayer, 'g', 'imagelayer');
-
- plotinfo.gridlayer = joinLayer(plotgroup, 'g', 'gridlayer');
- plotinfo.overgrid = joinLayer(plotgroup, 'g', 'overgrid');
-
- plotinfo.zerolinelayer = joinLayer(plotgroup, 'g', 'zerolinelayer');
- plotinfo.overzero = joinLayer(plotgroup, 'g', 'overzero');
-
- plotinfo.plot = joinLayer(plotgroup, 'g', 'plot');
- plotinfo.overplot = joinLayer(plotgroup, 'g', 'overplot');
-
- plotinfo.xlines = joinLayer(plotgroup, 'path', 'xlines');
- plotinfo.ylines = joinLayer(plotgroup, 'path', 'ylines');
- plotinfo.overlines = joinLayer(plotgroup, 'g', 'overlines');
-
- plotinfo.xaxislayer = joinLayer(plotgroup, 'g', 'xaxislayer');
- plotinfo.yaxislayer = joinLayer(plotgroup, 'g', 'yaxislayer');
- plotinfo.overaxes = joinLayer(plotgroup, 'g', 'overaxes');
- }
- else {
- var mainplotinfo = plotinfo.mainplotinfo;
-
- // now make the components of overlaid subplots
- // overlays don't have backgrounds, and append all
- // their other components to the corresponding
- // extra groups of their main plots.
-
- plotinfo.gridlayer = joinLayer(mainplotinfo.overgrid, 'g', id);
- plotinfo.zerolinelayer = joinLayer(mainplotinfo.overzero, 'g', id);
-
- plotinfo.plot = joinLayer(mainplotinfo.overplot, 'g', id);
- plotinfo.xlines = joinLayer(mainplotinfo.overlines, 'path', id);
- plotinfo.ylines = joinLayer(mainplotinfo.overlines, 'path', id);
- plotinfo.xaxislayer = joinLayer(mainplotinfo.overaxes, 'g', id);
- plotinfo.yaxislayer = joinLayer(mainplotinfo.overaxes, 'g', id);
- }
-
- // common attributes for all subplots, overlays or not
- plotinfo.plot.call(joinPlotLayers);
-
- plotinfo.xlines
- .style('fill', 'none')
- .classed('crisp', true);
-
- plotinfo.ylines
- .style('fill', 'none')
- .classed('crisp', true);
-}
-
-function purgeSubplotLayers(layers, fullLayout) {
- if(!layers) return;
-
- layers.each(function(subplot) {
- var plotgroup = d3.select(this),
- clipId = 'clip' + fullLayout._uid + subplot + 'plot';
-
- plotgroup.remove();
- fullLayout._draggers.selectAll('g.' + subplot).remove();
- fullLayout._defs.select('#' + clipId).remove();
-
- // do not remove individual axis <clipPath>s here
- // as other subplots may need them
- });
-}
-
-function joinLayer(parent, nodeType, className) {
- var layer = parent.selectAll('.' + className)
- .data([0]);
-
- layer.enter().append(nodeType)
- .classed(className, true);
-
- return layer;
-}
| 2 |
diff --git a/articles/support/matrix.md b/articles/support/matrix.md @@ -82,10 +82,6 @@ This section covers the browsers that Auth0 will support when using the Auth0 Da
<td>Apple Safari (Latest version)</td>
<td><div class="label label-warning">Sustained</div></td>
</tr>
- <tr>
- <td>Microsoft Internet Explorer 11</td>
- <td><div class="label label-warning">Sustained</div></td>
- </tr>
<tr>
<td>Microsoft Edge (Latest version)</td>
<td><div class="label label-warning">Sustained</div></td>
| 2 |
diff --git a/src/UnitTests/Core/Events/CommandHandlerTests/CommandReceivedHandlerShould.cs b/src/UnitTests/Core/Events/CommandHandlerTests/CommandReceivedHandlerShould.cs @@ -19,7 +19,7 @@ public void CallProcessOnCommandWhenEnabled()
var fakeCommand = new FakeCommand(GetTestRepository(), true);
CommandHandler commandHandler = GetTestCommandHandler(fakeCommand);
- commandHandler.CommandReceivedHandler(new FakeChatClient(),
+ commandHandler.CommandReceivedHandler(new Mock<IChatClient>().Object,
new CommandReceivedEventArgs { CommandWord = fakeCommand.CommandText});
Assert.True(fakeCommand.ProcessWasCalled);
@@ -31,7 +31,7 @@ public void NotCallProcessOnCommandWhenDisabled()
var fakeCommand = new FakeCommand(GetTestRepository(), false);
CommandHandler commandHandler = GetTestCommandHandler(fakeCommand);
- commandHandler.CommandReceivedHandler(new FakeChatClient(),
+ commandHandler.CommandReceivedHandler(new Mock<IChatClient>().Object,
new CommandReceivedEventArgs { CommandWord = fakeCommand.CommandText });
Assert.False(fakeCommand.ProcessWasCalled);
@@ -57,7 +57,7 @@ private static IRepository GetTestRepository()
private static CommandHandler GetTestCommandHandler(FakeCommand fakeCommand)
{
var commandUsageTracker = new CommandUsageTracker(new CommandHandlerSettings());
- var chatClients = new List<IChatClient> { new FakeChatClient() };
+ var chatClients = new List<IChatClient> { new Mock<IChatClient>().Object };
var commandMessages = new List<IBotCommand> { fakeCommand };
var commandHandler = new CommandHandler(commandUsageTracker, chatClients, new CommandList(commandMessages));
return commandHandler;
| 14 |
diff --git a/Libraries/Components/ScrollResponder.js b/Libraries/Components/ScrollResponder.js @@ -440,7 +440,7 @@ const ScrollResponderMixin = {
UIManager.dispatchViewManagerCommand(
nullthrows(this.scrollResponderGetScrollableNode()),
- UIManager.RCTScrollView.Commands.scrollTo,
+ commandID,
[x || 0, y || 0, animated !== false]
);
},
| 11 |
diff --git a/website/themes/uppy/layout/example.ejs b/website/themes/uppy/layout/example.ejs text = JSON.stringify(text);
}
- container.value += text;
+ container.value += text + '\n';
container.scrollTop = container.scrollHeight + '\n';
};
}(console.log.bind(console), document.getElementById("console-log")));
| 0 |
diff --git a/books/admin.py b/books/admin.py @@ -20,9 +20,9 @@ class LibraryAdmin(admin.ModelAdmin):
class BookAdmin(admin.ModelAdmin):
inlines = [BookCopyInline]
- list_display = ['title', 'author']
+ list_display = ['isbn', 'title', 'author']
list_per_page = 20
- search_fields = ['title', 'author']
+ search_fields = ['title', 'author', 'isbn']
class BookCopyAdmin(admin.ModelAdmin):
| 0 |
diff --git a/src/libraries/adapters/DatabaseMySql.php b/src/libraries/adapters/DatabaseMySql.php @@ -1857,7 +1857,7 @@ class DatabaseMySql implements DatabaseInterface
switch($name)
{
case 'album':
- $subquery = sprintf("`id` IN (SELECT element FROM `{$this->mySqlTablePrefix}elementAlbum` WHERE `{$this->mySqlTablePrefix}elementAlbum`.`owner`='%s' AND `type`='%s' AND `album`='%s')", $this->_($this->owner), 'photo', $this->_($value));
+ $subquery = sprintf("`id` IN (SELECT element FROM `{$this->mySqlTablePrefix}elementAlbum` WHERE `{$this->mySqlTablePrefix}elementAlbum`.`owner`='%s' AND `type`='%s' AND `album`='%s')", $this->_($this->owner), 'photo', $this->_($value),
$this->_($this->owner), 'photo', $value);
$where = $this->buildWhere($where, $subquery);
break;
@@ -2658,6 +2658,6 @@ class DatabaseMySql implements DatabaseInterface
*/
private function _($str)
{
- return mysql_real_escape_string($str);
+ return addslashes($str);
}
}
| 1 |
diff --git a/OurUmbraco.Site/config/uVersion.config b/OurUmbraco.Site/config/uVersion.config <configuration default="v750">
<versions>
<!-- version key="v5" name="Version 5.0.x" description="Compatible with version 5.0.x" vote="false"/ -->
+ <version key="v830" name="Version 8.3.x" description="Compatible with version 8.3.x" vote="true" voteDescription="Version 8.3.x" voteName="8.3.x"/>
<version key="v820" name="Version 8.2.x" description="Compatible with version 8.2.x" vote="true" voteDescription="Version 8.2.x" voteName="8.2.x"/>
<version key="v810" name="Version 8.1.x" description="Compatible with version 8.1.x" vote="true" voteDescription="Version 8.1.x" voteName="8.1.x"/>
<version key="v800" name="Version 8.0.x" description="Compatible with version 8.0.x" vote="true" voteDescription="Version 8.0.x" voteName="8.0.x"/>
| 11 |
diff --git a/assets/js/modules/analytics/datastore/accounts.test.js b/assets/js/modules/analytics/datastore/accounts.test.js @@ -89,7 +89,7 @@ describe( 'modules/analytics accounts', () => {
registry.dispatch( STORE_NAME ).createAccount( { accountName, propertyName, profileName, timezone } );
await subscribeUntil( registry,
() => (
- registry.select( STORE_NAME ).isDoingCreateAccount() !== true
+ registry.select( STORE_NAME ).isDoingCreateAccount() === false
),
);
| 7 |
diff --git a/local_modules/SendFundsTab/Views/SendFundsView.web.js b/local_modules/SendFundsTab/Views/SendFundsView.web.js @@ -491,7 +491,7 @@ class SendFundsView extends View
)
const estimatedTotalFee_JSBigInt = hostingServiceFee_JSBigInt.add(estimatedNetworkFee_JSBigInt)
const estimatedTotalFee_str = monero_utils.formatMoney(estimatedTotalFee_JSBigInt)
- var displayString = `+ ~${estimatedTotalFee_str} fee`
+ var displayString = `+ ${estimatedTotalFee_str} fee`
//
return displayString
}
| 2 |
diff --git a/src/modules/Graphics.js b/src/modules/Graphics.js @@ -774,9 +774,9 @@ class Graphics {
textObj.textContent = textString
if (textString.length > 0) {
// ellipsis is needed
- if (textObj.getComputedTextLength() >= width) {
+ if (textObj.getComputedTextLength() >= width / 1.1) {
for (let x = textString.length - 3; x > 0; x -= 3) {
- if (textObj.getSubStringLength(0, x) <= width) {
+ if (textObj.getSubStringLength(0, x) <= width / 1.1) {
textObj.textContent = textString.substring(0, x) + '...'
return
}
| 7 |
diff --git a/packages/app/src/server/service/config-loader.ts b/packages/app/src/server/service/config-loader.ts @@ -458,7 +458,7 @@ const ENV_VAR_NAME_TO_CONFIG_INFO = {
},
SLACKBOT_TYPE: {
ns: 'crowi',
- key: 'slackbot:currentType', // enum SlackbotType
+ key: 'slackbot:currentBotType', // enum SlackbotType
type: ValueType.STRING,
default: null,
},
| 13 |
diff --git a/components/explorer/explore-search.js b/components/explorer/explore-search.js @@ -2,6 +2,8 @@ import React, {useState, useCallback, useEffect} from 'react'
import Router from 'next/router'
import {debounce} from 'lodash'
+import theme from '@/styles/theme'
+
import {search} from '@/lib/explore/api'
import {useInput} from '../../hooks/input'
@@ -82,6 +84,8 @@ function ExploreSearch() {
return (
<>
+ <small className='example'>Rechercher une adresse, une voie, un lieu-dit ou une commune dans la Base Adresse Nationale</small>
+
<SearchInput
value={input}
results={orderResults}
@@ -99,6 +103,12 @@ function ExploreSearch() {
</div>}
<style jsx>{`
+ .example {
+ color: ${theme.colors.darkerGrey};
+ font-style: italic;
+ background-color: ${theme.colors.white};
+ }
+
.error {
margin: 1em 0;
}
| 0 |
diff --git a/src/track.js b/src/track.js @@ -33,7 +33,16 @@ const getSlideClasses = spec => {
spec.currentSlide <= index &&
index < spec.currentSlide + spec.slidesToShow;
}
- let slickCurrent = index === spec.targetSlide;
+
+ let focusedSlide;
+ if (spec.targetSlide < 0) {
+ focusedSlide = spec.targetSlide + spec.slideCount;
+ } else if (spec.targetSlide >= spec.slideCount) {
+ focusedSlide = spec.targetSlide - spec.slideCount;
+ } else {
+ focusedSlide = spec.targetSlide;
+ }
+ let slickCurrent = index === focusedSlide;
return {
"slick-slide": true,
"slick-active": slickActive,
| 1 |
diff --git a/extensions/README.md b/extensions/README.md -# Content Extensions
+# Extensions
This folder contains content extensions to the SpatioTemporal Asset Catalog specification. The core STAC specification
defines only a minimal core, but is designed for extension. It is expected that most real-world
| 2 |
diff --git a/generators/client/templates/vue/src/main/webapp/app/admin/configuration/configuration.service.ts.ejs b/generators/client/templates/vue/src/main/webapp/app/admin/configuration/configuration.service.ts.ejs @@ -12,11 +12,10 @@ export default class ConfigurationService {
}
}
- resolve(
properties.sort((propertyA, propertyB) => {
return propertyA.prefix === propertyB.prefix ? 0 : propertyA.prefix < propertyB.prefix ? -1 : 1;
- })
- );
+ });
+ resolve(properties);
});
});
}
| 7 |
diff --git a/src/main-blockchain/wallet/Main-Blockchain-Wallet.js b/src/main-blockchain/wallet/Main-Blockchain-Wallet.js @@ -190,12 +190,12 @@ class MainBlockchainWallet {
if (typeof address === "object" && address.hasOwnProperty("address"))
address = address.address;
- for (let i = 0; i < this.addresses.length; i++)
- if (address === this.addresses[i].address || address === this.addresses[i])
- return returnPos ? i : this.addresses[i];
- else
- if (typeof address ==="object" && (this.addresses[i].address === address.address || this.addresses[i].unencodedAddress.equals(address.unencodedAddress)))
- return returnPos ? i : this.addresses[i];
+ for (let i = 0; i < this.addresses.length; i++) {
+
+ if (typeof address === "string" && address === this.addresses[i].address) return returnPos ? i : this.addresses[i];
+ else if (Buffer.isBuffer(address) && address.equals( this.addresses[i].unencodedAddress) ) return returnPos ? i : this.addresses[i];
+ else if (typeof address === "object" && !Buffer.isBuffer(address) && (this.addresses[i].address === address.address || this.addresses[i].unencodedAddress.equals(address.unencodedAddress))) return returnPos ? i : this.addresses[i];
+ }
return returnPos ? -1 : null;
| 7 |
diff --git a/src/components/dashboard/Claim.js b/src/components/dashboard/Claim.js @@ -48,10 +48,9 @@ class Claim extends Component<ClaimProps, ClaimState> {
}
const { entitlement } = this.props.store.get('account')
- const goodWallet = this.goodWalletWrapped
const [claimedToday, nextClaimDate] = await Promise.all([
- goodWallet.getAmountAndQuantityClaimedToday(entitlement),
- goodWallet.getNextClaimTime()
+ this.goodWalletWrapped.getAmountAndQuantityClaimedToday(entitlement),
+ this.goodWalletWrapped.getNextClaimTime()
])
this.setState({ claimedToday })
this.interval = setInterval(async () => {
| 1 |
diff --git a/src/components/webView/iframe.embed.html b/src/components/webView/iframe.embed.html +<!-- IFrame DOMContentLoaded support -->
<script type="text/javascript">
(function () {
- var DOMReady = 'DOMContentLoaded';
- var messenger = (window.ReactNativeWebView || parent || {}).postMessage;
+ var messenger = window.ReactNativeWebView || parent;
- if ('function' === (typeof messenger)) {
+ if (!messenger || ('function' !== (typeof messenger.postMessage))) {
return;
}
- window.addEventListener(DOMReady, function() {
+ var documentUrl = location.href;
+ var DOMReady = 'DOMContentLoaded';
+
+ var onDOMContentLoaded = function() {
var messagePayload = {
event: DOMReady,
target: 'iframe',
- src: location.href
+ src: documentUrl
};
- parent.postMessage(messagePayload, '*')
- });
- })()
+ messenger.postMessage(messagePayload, '*');
+ }
+
+ if (document.readyState !== 'loading') {
+ onDOMContentLoaded();
+ return;
+ }
+
+ window.addEventListener(DOMReady, onDOMContentLoaded);
+ })();
</script>
+<!-- End IFrame DOMContentLoaded support -->
\ No newline at end of file
| 3 |
diff --git a/package.json b/package.json },
"devDependencies": {
"@11ty/eleventy-plugin-syntaxhighlight": "^2.0.3",
- "ava": "^2.2.0",
+ "ava": "^2.4.0",
"ink-docstrap": "1.3.2",
"jsdoc": "3.6.2",
"lint-staged": "^9.2.5",
| 3 |
diff --git a/packages/openneuro-app/src/scripts/utils/global-polyfill.ts b/packages/openneuro-app/src/scripts/utils/global-polyfill.ts @@ -4,16 +4,9 @@ interface Object {
global: object
}
-// eslint-disable-next-line @typescript-eslint/ban-types
-function polyfillGlobal(): object {
- if (typeof global !== 'undefined') return global
- Object.defineProperty(Object.prototype, 'global', {
- get: function () {
- delete Object.prototype.global
- this.global = this
- },
- configurable: true,
- })
- return global
+function polyfillGlobal(): void {
+ if (typeof global === 'object') return
+ Object.defineProperty(Object.prototype, 'global', globalThis)
}
+
polyfillGlobal()
| 7 |
diff --git a/docs/Air.md b/docs/Air.md @@ -16,6 +16,7 @@ The Air workflow allows you to do what most travel agents did in the past and wh
* [.importPNR(params)](#importPNR)
* [.flightInfo(params)](#flightInfo)
* [.getPNRByTicketNumber(params)](#getPNRByTicketNumber)
+* [.searchBookingsByPassengerName(params)](#searchBookingsByPassengerName)
* [.getTicket(params)](#getTicket)
* [.getTickets(params)](#getTickets)
* [.cancelTicket(params)](#cancelTicket)
| 0 |
diff --git a/spec/calculus.spec.js b/spec/calculus.spec.js @@ -181,5 +181,194 @@ describe('calculus', function () {
}
});
+ it('should integrate properly', function () {
+ // given
+ var testCases = [
+ {
+ given: 'integrate(sin(x), x)',
+ expected: '-cos(x)'
+ },
+ {
+ given: 'integrate(cos(x), x)',
+ expected: 'sin(x)'
+ },
+ {
+ given: 'integrate(2*x^2+x, x)',
+ expected: '(1/2)*x^2+(2/3)*x^3'
+ },
+ {
+ given: 'integrate(log(x), x)',
+ expected: '-x+log(x)*x'
+ },
+ {
+ given: 'integrate(sqrt(x), x)',
+ expected: '(2/3)*x^(3/2)'
+ },
+ {
+ given: 'integrate(asin(a*x), x)',
+ expected: 'a^(-1)*sqrt(-(a*x)^2+1)+asin(a*x)*x'
+ },
+ {
+ given: 'integrate(a/x, x)',
+ expected: 'a*log(x)'
+ },
+ {
+ given: 'integrate(x*e^x, x)',
+ expected: '-e^x+e^x*x'
+ },
+ {
+ given: 'integrate(x^3*log(x), x)',
+ expected: '(-1/16)*x^4+(1/4)*log(x)*x^4'
+ },
+ {
+ given: 'integrate(x^2*sin(x), x)',
+ expected: '-cos(x)*x^2+2*cos(x)+2*sin(x)*x'
+ },
+ {
+ given: 'integrate(sin(x)*log(cos(x)), x)',
+ expected: '-cos(x)*log(cos(x))+cos(x)'
+ },
+ {
+ given: 'integrate(x*asin(x), x)',
+ expected: '(-1/4)*asin(x)+(1/2)*asin(x)*x^2+(1/4)*sqrt(-x^2+1)*x'
+ },
+ {
+ given: 'integrate(q/((2-3*x^2)^(1/2)), x)',
+ expected: 'asin(sqrt(2)^(-1)*sqrt(3)*x)*q*sqrt(3)^(-1)'
+ },
+ {
+ given: 'integrate(1/(a^2+x^2), x)',
+ expected: 'a^(-1)*atan(a^(-1)*x)'
+ },
+ {
+ given: 'integrate(11/(a+5*r*x)^2,x)',
+ expected: '(-11/5)*(5*r*x+a)^(-1)*r^(-1)'
+ },
+ {
+ given: 'integrate(cos(x)*sin(x), x)',
+ expected: '(-1/2)*cos(x)^2'
+ },
+ {
+ given: 'integrate(x*cos(x)*sin(x), x)',
+ expected: '(-1/2)*cos(x)^2*x+(1/4)*cos(x)*sin(x)+(1/4)*x'
+ },
+ {
+ given: 'integrate(t/(a*x+b), x)',
+ expected: 'a^(-1)*log(a*x+b)*t'
+ },
+ {
+ given: 'integrate(x*(x+a)^3, x)',
+ expected: '((1/4)*x^4+(3/2)*a^2*x^2+a*x^3+a^3*x)*x+(-1/2)*a^2*x^3+(-1/2)*a^3*x^2+(-1/20)*x^5+(-1/4)*a*x^4'
+ },
+ {
+ given: 'integrate(4*x/(x^2+a^2), x)',
+ expected: '2*log(a^2+x^2)'
+ },
+ {
+ given: 'integrate(1/(x^2+3*a^2), x)',
+ expected: 'a^(-1)*atan(a^(-1)*sqrt(3)^(-1)*x)*sqrt(3)^(-1)'
+ },
+ {
+ given: 'integrate(8*x^3/(6*x^2+3*a^2), x)',
+ expected: '8*((-1/24)*a^2*log(3*a^2+x^2)+(1/12)*x^2)'
+ },
+ {
+ given: 'integrate(10*q/(4*x^2+24*x+20), x)',
+ expected: '(5/8)*log((1+x)*(5+x)^(-1))*q'
+ },
+ // TODO jiggzson: This test is produces result (a+x)^(-1)*a+log(a+x)
+ //{
+ // given: 'integrate(x/(x+a)^2, x)',
+ // expected: '-(a+x)^(-1)*x+log(a+x)'
+ //},
+ {
+ given: 'integrate(sqrt(x-a), x)',
+ expected: '(2/3)*(-a+x)^(3/2)'
+ },
+ {
+ given: 'integrate(x^n*log(x), x)',
+ expected: '(1+n)^(-1)*log(x)*x^(1+n)-(1+n)^(-2)*x^(1+n)'
+ },
+ {
+ given: 'integrate(3*a*sec(x)^2, x)',
+ expected: '3*a*tan(x)'
+ },
+ {
+ given: 'integrate(a/(x^2+b*x+a*x+a*b),x)',
+ expected: '(-b+a)^(-1)*a*log((a+x)^(-1)*(b+x))'
+ },
+ {
+ given: 'integrate(log(a*x+b),x)',
+ expected: '((a*x+b)*log(a*x+b)-a*x-b)*a^(-1)'
+ },
+ {
+ given: 'integrate(x*log(x),x)',
+ expected: '(-1/4)*x^2+(1/2)*log(x)*x^2'
+ },
+ {
+ given: 'integrate(log(a*x)/x,x)',
+ expected: '(1/2)*log(a*x)^2'
+ },
+ {
+ given: 'integrate(log(x)^2,x)',
+ expected: '-2*log(x)*x+2*x+log(x)^2*x'
+ },
+ {
+ given: 'integrate(t*log(x)^3,x)',
+ expected: '(-3*log(x)^2+6*log(x)+log(x)^3-6)*t*x'
+ },
+ {
+ given: 'integrate(x*log(x)^2,x)',
+ expected: '(-1/2)*log(x)*x^2+(1/2)*log(x)^2*x^2+(1/4)*x^2'
+ },
+ {
+ given: 'integrate(x^2*log(x)^2,x)',
+ expected: '(-2/9)*log(x)*x^3+(1/3)*log(x)^2*x^3+(2/27)*x^3'
+ },
+ {
+ given: 'integrate(x^2*e^(a*x),x)',
+ expected: '-2*(-a^(-2)*e^(a*x)+a^(-1)*e^(a*x)*x)*a^(-1)+a^(-1)*e^(a*x)*x^2'
+ },
+ {
+ given: 'integrate(8*e^(a*x^2),x)',
+ expected: '4*erf(sqrt(-a)*x)*sqrt(-a)^(-1)*sqrt(pi)'
+ },
+ {
+ given: 'integrate(5*x*e^(-8*a*x^2),x)',
+ expected: '(-5/16)*a^(-1)*e^(-8*a*x^2)*log(e)^(-1)'
+ },
+ {
+ given: 'integrate(x^2*sin(x),x)',
+ expected: '-cos(x)*x^2+2*cos(x)+2*sin(x)*x'
+ },
+ {
+ given: 'integrate(8*tan(b*x)^2,x)',
+ expected: '8*(-x+b^(-1)*tan(b*x))'
+ },
+ {
+ given: 'integrate(sec(a*x)^3,x)',
+ expected: '(1/2)*a^(-1)*log(sec(a*x)+tan(a*x))+(1/2)*a^(-1)*sec(a*x)*tan(a*x)'
+ },
+ {
+ given: 'integrate(sec(a*x)*tan(a*x),x)',
+ expected: 'a^(-1)*sec(a*x)'
+ },
+ {
+ given: 'integrate(3*a*cot(a*x)^4, x)',
+ expected: '3*((-1/3)*a^(-1)*cot(a*x)^3+a^(-1)*cot(a*x)+x)*a'
+ },
+ {
+ given: 'integrate(3*a*csc(a*x)^4, x)',
+ expected: '3*((-1/3)*a^(-1)*cot(a*x)*csc(a*x)^2+(-2/3)*a^(-1)*cot(a*x))*a'
+ }
+ ];
+
+ for (var i = 0; i < testCases.length; ++i) {
+ // when
+ var parsed = nerdamer(testCases[i].given);
+ // then
+ expect(parsed.toString()).toEqual(testCases[i].expected);
+ }
+ });
});
\ No newline at end of file
| 0 |
diff --git a/shared/data/constants.js b/shared/data/constants.js -let constants = {
+module.exports = {
"trackerListLoc": "data/tracker_lists",
"blockLists": [
"trackersWithParentCompany.json"
@@ -48,7 +48,3 @@ let constants = {
"automattic": 7
}
}
-
-if (module && module.exports) {
- module.exports = constants
-}
| 2 |
diff --git a/packages/composables/use-polyline-drawing/index.ts b/packages/composables/use-polyline-drawing/index.ts @@ -79,6 +79,13 @@ export default function (props, $services: VcViewerProvider, drawTipOpts) {
}
}
+ const { defined, Cartesian2 } = Cesium
+ lastClickPosition = lastClickPosition || new Cesium.Cartesian2(Number.POSITIVE_INFINITY, Number.POSITIVE_INFINITY)
+
+ if ((Cartesian2.magnitude(Cartesian2.subtract(lastClickPosition, movement, {} as any)) < mouseDelta)) {
+ return
+ }
+
if (options.button === 2 && drawStatus.value === DrawStatus.Drawing) {
if (tempPositions.length > 1) {
tempPositions.pop()
@@ -90,9 +97,6 @@ export default function (props, $services: VcViewerProvider, drawTipOpts) {
return
}
- const { defined, Cartesian2 } = Cesium
- lastClickPosition = lastClickPosition || new Cesium.Cartesian2(Number.POSITIVE_INFINITY, Number.POSITIVE_INFINITY)
- if (!(Cartesian2.magnitude(Cartesian2.subtract(lastClickPosition, movement, {} as any)) < mouseDelta)) {
const scene = viewer.scene
const position = getWorldPosition(scene, movement, {} as any)
let finished = false
@@ -127,7 +131,6 @@ export default function (props, $services: VcViewerProvider, drawTipOpts) {
}
}
}
- }
const handleMouseMove = (movement: Cesium.Cartesian2) => {
const { viewer, getWorldPosition } = $services
| 1 |
diff --git a/token-metadata/0xe61eECfDBa2aD1669cee138f1919D08cEd070B83/metadata.json b/token-metadata/0xe61eECfDBa2aD1669cee138f1919D08cEd070B83/metadata.json "symbol": "VGTG",
"address": "0xe61eECfDBa2aD1669cee138f1919D08cEd070B83",
"decimals": 18,
- "dharmaVerificationStatus": {
"dharmaVerificationStatus": "VERIFIED"
}
\ No newline at end of file
-}
\ No newline at end of file
| 3 |
diff --git a/token-metadata/0x4Ba012f6e411a1bE55b98E9E62C3A4ceb16eC88B/metadata.json b/token-metadata/0x4Ba012f6e411a1bE55b98E9E62C3A4ceb16eC88B/metadata.json "symbol": "CBR",
"address": "0x4Ba012f6e411a1bE55b98E9E62C3A4ceb16eC88B",
"decimals": 18,
- "dharmaVerificationStatus": {
"dharmaVerificationStatus": "VERIFIED"
}
\ No newline at end of file
-}
\ No newline at end of file
| 3 |
diff --git a/src/defaults.js b/src/defaults.js @@ -274,7 +274,7 @@ JSONEditor.defaults.resolvers.unshift(function(schema) {
// Use the table editor for arrays with the format set to `table`
JSONEditor.defaults.resolvers.unshift(function(schema) {
// Type `array` with format set to `table`
- if(schema.type == "array" && schema.format == "table") {
+ if(schema.type === "array" && schema.format === "table") {
return "table";
}
});
| 4 |
diff --git a/js/models/denseMatrix.js b/js/models/denseMatrix.js @@ -106,7 +106,7 @@ function dataToHeatmap(column, vizSettings, data, samples) {
function geneProbesToHeatmap(column, vizSettings, data, samples) {
var pos = parsePos(column.fields[0]); // disabled until we support the query
- if (!_.get(data, 'req') || !_.get(data, 'refGene')) {
+ if (!_.get(data, 'req') || _.isEmpty(_.get(data, 'refGene'))) {
return null;
}
var {req} = data,
| 9 |
diff --git a/Source/Core/Resource.js b/Source/Core/Resource.js @@ -1774,7 +1774,7 @@ define([
};
function loadWithHttpRequest(url, responseType, method, data, headers, deferred, overrideMimeType) {
- // Note: only the 'json' responseType transforms the loaded buffer
+ // Note: only the 'json' and 'text' responseTypes transforms the loaded buffer
var URL = require('url').parse(url);
var http_s = URL.protocol === 'https:' ? require('https') : require('http');
var zlib = require('zlib');
@@ -1806,12 +1806,16 @@ define([
deferred.reject(new RuntimeError('Error decompressing response.'));
} else if (responseType === 'json') {
deferred.resolve(JSON.parse(result.toString('utf8')));
+ } else if (responseType === 'text') {
+ deferred.resolve(result.toString('utf8'));
} else {
deferred.resolve(new Uint8Array(result).buffer); // Convert Buffer to ArrayBuffer
}
});
+ } else if (responseType === 'text') {
+ deferred.resolve(response.toString('utf8'));
} else {
- deferred.resolve(responseType === 'json' ? JSON.parse(response.toString('utf8')) : response);
+ deferred.resolve(responseType === 'json' ? JSON.parse(response.toString('utf8')) : new Uint8Array(response).buffer);
}
});
});
| 9 |
diff --git a/doc/README.md b/doc/README.md * **Tooltip queuing**
- The tooltip queue makes it a fundamental rule of the system that there will only ever be one tooltip visible on the screen. When the user moves their cursor to another element with a tooltip, the last tooltip will close gracefully before the next tooltip opens.
+ The tooltip queue makes it a fundamental rule of the system that there will only ever be one tooltip visible on the screen. When the user moves their cursor from one element with a tooltip to another element with a tooltip, the previous tooltip will close gracefully before the new tooltip opens.
### Features
| 7 |
diff --git a/ui/src/plugins/Notify.js b/ui/src/plugins/Notify.js @@ -129,8 +129,8 @@ const Notifications = {
if (notif.group === false) {
notif.group = void 0
}
- else if (notif.group !== void 0) {
- if (notif.group === true) {
+ else {
+ if (notif.group === void 0 || notif.group === true) {
// do not replace notifications with different buttons
notif.group = [
notif.message,
@@ -142,12 +142,14 @@ const Notifications = {
notif.group += '|' + notif.position
}
- const groupNotif = groups[notif.group]
+ console.log(notif.group)
if (notif.actions.length === 0) {
notif.actions = void 0
}
+ const groupNotif = groups[notif.group]
+
// wohoo, new notification
if (groupNotif === void 0) {
notif.__uid = uid++
@@ -164,6 +166,10 @@ const Notifications = {
const action = notif.position.indexOf('top') > -1 ? 'unshift' : 'push'
this.notifs[notif.position][action](notif)
}
+
+ if (notif.group !== void 0) {
+ groups[notif.group] = notif
+ }
}
// ok, so it's NOT a new one
else {
@@ -173,7 +179,7 @@ const Notifications = {
}
notif = Object.assign(groups[notif.group], notif)
- groups[notif.group].__badge++
+ notif.__badge++
}
notif.__close = () => {
@@ -194,7 +200,9 @@ const Notifications = {
const index = this.notifs[notif.position].indexOf(notif)
if (index !== -1) {
- groups[notif.group] = void 0
+ if (notif.group !== void 0) {
+ delete groups[notif.group]
+ }
const el = this.$refs[`notif_${notif.__uid}`]
| 1 |
diff --git a/src/regions/TextAreaRegion.js b/src/regions/TextAreaRegion.js @@ -13,6 +13,7 @@ import { TextAreaModel } from "../tags/control/TextArea";
import { guidGenerator } from "../core/Helpers";
import styles from "./TextAreaRegion/TextAreaRegion.module.scss";
+import { HtxTextBox } from "../components/HtxTextBox/HtxTextBox";
const { Paragraph } = Typography;
@@ -65,15 +66,15 @@ const HtxTextAreaRegionView = ({ item }) => {
}
if (parent.editable) {
- params["editable"] = {
- onChange: str => {
+ params.onChange = str => {
item.setValue(str);
- },
};
}
let divAttrs = {};
- if (!parent.perregion) {
+ if (parent.perregion) {
+ params.onDelete = () => item.parent.remove(item);
+ } else {
divAttrs = {
onMouseOver: () => {
if (relationMode) {
@@ -91,10 +92,13 @@ const HtxTextAreaRegionView = ({ item }) => {
return (
<div {...divAttrs} className={styles.row} data-testid="textarea-region">
- <Paragraph id={`TextAreaRegion-${item.id}`} className={classes.join(" ")} {...params}>
- {item._value}
- </Paragraph>
- {parent.perregion && <DeleteOutlined className={styles.delete} onClick={() => item.parent.remove(item)} />}
+ <HtxTextBox
+ id={`TextAreaRegion-${item.id}`}
+ className={classes.join(" ")}
+ rows={parent.rows}
+ text={item._value}
+ {...params}
+ />
</div>
);
};
| 4 |
diff --git a/components/FontSize/Sync.js b/components/FontSize/Sync.js @@ -2,25 +2,30 @@ import React, { useEffect } from 'react'
import { FONT_SIZE_KEY, useFontSize } from '../../lib/fontSize'
import { DEFAULT_FONT_SIZE } from '@project-r/styleguide'
import NextHead from 'next/head'
+import { css } from 'glamor'
const FontSizeSync = () => {
const [fontSize] = useFontSize(DEFAULT_FONT_SIZE)
useEffect(
() => {
document.documentElement.style.fontSize = fontSize + 'px'
+ document.documentElement.className = css({ fontSize }).toString()
},
[fontSize]
)
useEffect(
() => {
- return () => { document.documentElement.style.fontSize = DEFAULT_FONT_SIZE + 'px' }
+ return () => {
+ document.documentElement.style.fontSize = DEFAULT_FONT_SIZE + 'px'
+ document.documentElement.className = ''
+ }
},
[]
)
return <NextHead>
- <script dangerouslySetInnerHTML={{ __html: `try {
- document.documentElement.style.fontSize = (localStorage.getItem('${FONT_SIZE_KEY}') || ${DEFAULT_FONT_SIZE}) + 'px'
- } catch (e) {}` }} />
+ <script dangerouslySetInnerHTML={{
+ __html: `try {document.documentElement.style.fontSize = (localStorage.getItem('${FONT_SIZE_KEY}') || ${DEFAULT_FONT_SIZE}) + 'px'} catch (e) {}`
+ }} />
</NextHead>
}
| 12 |
diff --git a/src/utils/init/config-github.js b/src/utils/init/config-github.js @@ -101,6 +101,8 @@ async function configGithub(ctx, site, repo) {
}
}
+ // TODO: Generalize this so users can reset these automatically.
+ // Quick and dirty implementation
const ntlHooks = await ctx.netlify.listHooksBySiteId({ siteId: site.id })
const createdHook = ntlHooks.find(h => h.type === 'github_commit_status' && h.event === 'deploy_created')
@@ -108,12 +110,74 @@ async function configGithub(ctx, site, repo) {
const buildingHook = ntlHooks.find(h => h.type === 'github_commit_status' && h.event === 'deploy_building')
if (!createdHook) {
+ const h = await ctx.netlify.createHookBySiteId({
+ site_id: site.id,
+ body: {
+ type: 'github_commit_status',
+ event: 'deploy_created',
+ data: {
+ access_token: ctx.global.get('ghauth.token')
+ }
+ }
+ })
+ ctx.log(`Created Github Created Hook: ${h.id}`)
} else {
+ const h = await ctx.netlify.updateHook({
+ hook_id: createdHook.id,
+ body: {
+ data: {
+ access_token: ctx.global.get('ghauth.token')
+ }
+ }
+ })
+ ctx.log(`Updated Github Created Hook: ${h.id}`)
}
+
if (!failedHook) {
+ const h = await ctx.netlify.createHookBySiteId({
+ site_id: site.id,
+ body: {
+ type: 'github_commit_status',
+ event: 'deploy_failed',
+ data: {
+ access_token: ctx.global.get('ghauth.token')
+ }
+ }
+ })
+ ctx.log(`Created Github Failed Hook: ${h.id}`)
} else {
+ const h = await ctx.netlify.updateHook({
+ hook_id: failedHook.id,
+ body: {
+ data: {
+ access_token: ctx.global.get('ghauth.token')
}
+ }
+ })
+ ctx.log(`Updated Github Created Hook: ${h.id}`)
+ }
+
if (!buildingHook) {
+ const h = await ctx.netlify.createHookBySiteId({
+ site_id: site.id,
+ body: {
+ type: 'github_commit_status',
+ event: 'deploy_building',
+ data: {
+ access_token: ctx.global.get('ghauth.token')
+ }
+ }
+ })
+ ctx.log(`Created Github Building Hook: ${h.id}`)
} else {
+ const h = await ctx.netlify.updateHook({
+ hook_id: buildingHook.id,
+ body: {
+ data: {
+ access_token: ctx.global.get('ghauth.token')
+ }
+ }
+ })
+ ctx.log(`Updated Github Building Hook: ${h.id}`)
}
}
| 12 |
diff --git a/ui/component/markdownLink/view.jsx b/ui/component/markdownLink/view.jsx @@ -18,9 +18,13 @@ type Props = {
function MarkdownLink(props: Props) {
const { children, href, title, embed = false, parentCommentId, isMarkdownPost, simpleLinks = false } = props;
- const decodedUri = decodeURI(href);
- if (!href) {
+ let decodedUri;
+ try {
+ decodedUri = decodeURI(href);
+ } catch (e) {}
+
+ if (!href || !decodedUri) {
return children || null;
}
| 9 |
diff --git a/modules/Cockpit/webhooks.php b/modules/Cockpit/webhooks.php @@ -17,17 +17,23 @@ if (!function_exists('curl_init')) {
if ($cachepath = $this->path('#tmp:webhooks.cache.php')) {
$webhooks = include($cachepath);
} else {
- $webhooks = $app->storage->find('cockpit/webhooks');
- $this->helper('fs')->write('#tmp:webhooks.cache.php', '<?php return '.var_export($webhooks->toArray(), true ).';');
+ $webhooks = $app->storage->find('cockpit/webhooks')->toArray();
+ $this->helper('fs')->write('#tmp:webhooks.cache.php', '<?php return '.var_export($webhooks, true ).';');
}
-foreach ($webhooks as &$webhook) {
+if (!count($webhooks)) {
+ return;
+}
+
+$webHookCalls = new ArrayObject([]);
+
+foreach ($webhooks as $webhook) {
if ($webhook['active'] && $webhook['url'] && count($webhook['events'])) {
foreach ($webhook['events'] as $evt) {
- $app->on($evt, function() use($evt, $webhook) {
+ $app->on($evt, function() use($evt, $webhook, $webHookCalls) {
$url = trim($webhook['url']);
$data = json_encode([
@@ -54,7 +60,26 @@ foreach ($webhooks as &$webhook) {
}
}
- $ch = curl_init($url);
+ $auth = $webhook['auth'] ?? null;
+
+ $webHookCalls[] = compact('url', 'data', 'headers', 'auth');
+
+ }, -1000);
+ }
+ }
+}
+
+$app->on('shutdown', function() use($webHookCalls) {
+
+ if (!count($webHookCalls)) {
+ return;
+ }
+
+ \session_write_close();
+
+ foreach ($webHookCalls as $webhook) {
+
+ $ch = curl_init($webhook['url']);
// add basic http auth
if (isset($webhook['auth']) && $webhook['auth']['user'] && $webhook['auth']['pass']) {
@@ -65,14 +90,11 @@ foreach ($webhooks as &$webhook) {
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0);
curl_setopt($ch, CURLOPT_TIMEOUT, 1);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'POST');
- curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
+ curl_setopt($ch, CURLOPT_POSTFIELDS, $webhook['data']);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
- curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
+ curl_setopt($ch, CURLOPT_HTTPHEADER, $webhook['headers']);
curl_exec($ch);
curl_close($ch);
-
- }, -1000);
- }
- }
}
+});
\ No newline at end of file
| 7 |
diff --git a/src/main/webapp/render.html b/src/main/webapp/render.html <script src="lib/jquery.ba-resize.js"></script>
<script src="plugins/echart/china.js"></script>
<script src="plugins/ace/ace.js"></script>
- <script src="plugins/ui-ace/ui-ace.min.js"></script>
+ <script src="plugins/ui-ace/ui-ace.js"></script>
<script src="plugins/FineMap/d3.v3.js"></script>
<script src="plugins/FineMap/d3.tip.js"></script>
| 14 |
diff --git a/common/stores/organisation-store.js b/common/stores/organisation-store.js @@ -16,7 +16,9 @@ const controller = {
data.get(`${Project.api}organisations/${id}/invites/`),
] : [])).then((res) => {
if (id === store.id) {
- const [projects, users, invites, usage] = res;
+ // eslint-disable-next-line prefer-const
+ let [projects, users, invites, usage] = res;
+ projects = projects.results;
store.model = { users, invites: invites && invites.results };
if (AccountStore.getOrganisationRole(id) === 'ADMIN') {
| 9 |
diff --git a/deepfence_ui/app/scripts/components/integration-view/reports/reports.js b/deepfence_ui/app/scripts/components/integration-view/reports/reports.js @@ -579,8 +579,8 @@ const Reports = props => {
const hostName = host_name && host_name.map(v => v.value);
globalFilter = {
type: [compliance_provider.value],
- host_name: hostName,
- account_id: accountId,
+ node_name: hostName,
+ node_id: accountId,
};
} else if (node_type.value === 'host') {
const hostName = host_name && host_name.map(v => v.value);
| 3 |
diff --git a/source/setup/containers/AddArchivePage.js b/source/setup/containers/AddArchivePage.js @@ -117,9 +117,6 @@ export default connect(
onConnectWebDAVBasedSource: (type, url, username, password) => dispatch => {
let webdavURL;
switch (type) {
- case "nextcloud":
- webdavURL = joinURL(url, "remote.php/dav/files/admin");
- break;
case "owncloud":
/* falls-through */
case "nextcloud":
| 2 |
diff --git a/gulpfile.js b/gulpfile.js @@ -234,10 +234,8 @@ async function unpackPacks() {
* Cook db source json files into .db files with nedb
*/
var cookErrorCount = 0;
-var cookWarningCount = 0;
var cookAborted = false;
var packErrors = {};
-var packWarnings = {};
async function cookPacks() {
console.log(`Cooking db files`);
@@ -245,7 +243,6 @@ async function cookPacks() {
let allItems = [];
cookErrorCount = 0;
- cookWarningCount = 0;
cookAborted = false;
packErrors = {};
@@ -418,12 +415,12 @@ function isSourceValid(source) {
function addWarningForPack(warning, pack) {
- if (!(pack in packWarnings)) {
- packWarnings[pack] = [];
+ if (!(pack in packErrors)) {
+ packErrors[pack] = [];
}
- cookWarningCount += 1;
- packWarnings[pack].push(warning);
+ cookErrorCount += 1;
+ packErrors[pack].push(warning);
}
function consistencyCheck(allItems, compendiumMap) {
@@ -538,15 +535,6 @@ function consistencyCheck(allItems, compendiumMap) {
async function postCook() {
- if (Object.keys(packWarnings).length > 0) {
- for (let pack of Object.keys(packWarnings)) {
- console.log(`\n${packWarnings[pack].length} Warnings cooking ${pack}.db:`);
- for (let warning of packWarnings[pack]) {
- console.log(`> ${warning}`);
- }
- }
- }
-
if (Object.keys(packErrors).length > 0) {
for (let pack of Object.keys(packErrors)) {
console.log(`\n${packErrors[pack].length} Errors cooking ${pack}.db:`);
@@ -557,7 +545,7 @@ async function postCook() {
}
if (!cookAborted) {
- console.log(`\nCompendiums cooked with ${cookWarningCount} warnings, ${cookErrorCount} errors!\nDon't forget to restart Foundry to refresh compendium data!\n`);
+ console.log(`\nCompendiums cooked with ${cookErrorCount} errors!\nDon't forget to restart Foundry to refresh compendium data!\n`);
} else {
console.log(`\nCook aborted after ${cookErrorCount} critical errors!\n`);
}
| 2 |
diff --git a/src/workingtitle-vcockpits-instruments-cj4/html_ui/Pages/VCockpit/Instruments/Airliners/CJ4/Shared/Autopilot/WT_VnavAutopilot.js b/src/workingtitle-vcockpits-instruments-cj4/html_ui/Pages/VCockpit/Instruments/Airliners/CJ4/Shared/Autopilot/WT_VnavAutopilot.js @@ -228,12 +228,9 @@ class WT_VnavAutopilot {
if (Math.round(this._navModeSelector.selectedAlt2) != this._vnavTargetAltitude) {
this.setTargetAltitude();
}
- if (this._navModeSelector.currentAltSlotIndex !=2 && this._vnav._altDeviation > 0) {
- this._vnavAltSlot = 2;
- }
-
- // if (this._navModeSelector.currentVerticalActiveState != VerticalNavModeState.PATH) {
- // this.recalculate();
+ //ADDED for 0.9.0 and then reverted prior to release per CWB
+ // if (this._navModeSelector.currentAltSlotIndex !=2 && this._vnav._altDeviation > 0) {
+ // this._vnavAltSlot = 2;
// }
}
else if (this._pathArm) {
@@ -341,9 +338,10 @@ class WT_VnavAutopilot {
}
setVerticalSpeed = 100 * Math.ceil(setVerticalSpeed / 100);
Coherent.call("AP_VS_VAR_SET_ENGLISH", 2, setVerticalSpeed);
- if (SimVar.GetSimVarValue("AUTOPILOT VERTICAL HOLD", "number") != 1 && this._vnav._altDeviation > 0) {
- SimVar.SetSimVarValue("K:AP_PANEL_VS_HOLD", "number", 1);
- }
+ // ADDED for 0.9.0 but reverted per CWB
+ // if (SimVar.GetSimVarValue("AUTOPILOT VERTICAL HOLD", "number") != 1 && this._vnav._altDeviation > 0) {
+ // SimVar.SetSimVarValue("K:AP_PANEL_VS_HOLD", "number", 1);
+ // }
}
}
| 13 |
diff --git a/html/settings/_settings.scss b/html/settings/_settings.scss @@ -1638,7 +1638,7 @@ $sprk-toggle-trigger-transition: $sprk-link-transition !default;
/// The hover border bottom style for Toggle Trigger.
$sprk-toggle-trigger-hover-border-bottom: $sprk-link-has-icon-hover-border-bottom !default;
/// The hover text color for Toggle Trigger.
-$sprk-toggle-trigger-hover-color: $sprk-link-has-icon-hover-color-text !default;
+$sprk-toggle-trigger-hover-color: $sprk-purple !default;
/// The background color for Toggle Trigger.
$sprk-toggle-trigger-background-color: transparent !default;
/// The padding top for Toggle Trigger.
| 3 |
diff --git a/js/coreweb/bisweb_connectivityvis3d.js b/js/coreweb/bisweb_connectivityvis3d.js @@ -15,10 +15,6 @@ const globalParams={
const lobeoffset=20.0;
const axisoffset=[0,5.0,20.0];
-const brain_colors = [
- [ 1.0,1.0,1.0 ],
- [ 1.0,1.0,1.0 ]
-];
const color_modes = [ 'Uniform', 'PosDegree', 'NegDegree', 'Sum', 'Difference' ];
const display_modes = [ 'None', 'Left', 'Right', 'Both' ];
@@ -119,7 +115,7 @@ var createAndDisplayBrainSurface=function(index=0,color,opacity=0.8,attributeInd
if (attributeIndex<0) {
for (let i=0;i<parcels.length;i++) {
- attributes[i]=10+index*10;
+ attributes[i]=1;
}
} else {
for (let i=0;i<parcels.length;i++) {
@@ -226,7 +222,7 @@ var parsebrainsurface = function(textstring,filename) {
globalParams.braingeom[meshindex]=buf;
globalParams.brainindices[meshindex]=parcels;
- createAndDisplayBrainSurface(meshindex, brain_colors[meshindex],0.7,-1);
+ createAndDisplayBrainSurface(meshindex, [1.0,1.0,1.0],0.7,-1);
if (globalParams.internal.axisline[0]===null) {
// create axis line meshes
@@ -404,8 +400,8 @@ var update3DMeshes=function(opacity=0.5,modename='uniform',displaymode='Both') {
let mode=color_modes.indexOf(modename)-1;
let dmode=display_modes.indexOf(displaymode);
- createAndDisplayBrainSurface(0, brain_colors[0],opacity,mode);
- createAndDisplayBrainSurface(1, brain_colors[1],opacity,mode);
+ createAndDisplayBrainSurface(0, [1.0,1.0,1.0],opacity,mode);
+ createAndDisplayBrainSurface(1, [1.0,1.0,1.0],opacity,mode);
let show=[true,true];
if (dmode<=0)
@@ -430,7 +426,6 @@ module.exports = {
drawlines3d : drawlines3d,
lobeoffset : lobeoffset,
createAndDisplayBrainSurface : createAndDisplayBrainSurface,
- brain_colors : brain_colors,
color_modes : color_modes,
display_modes : display_modes,
update3DMeshes : update3DMeshes,
| 3 |
diff --git a/includes/Modules/Analytics.php b/includes/Modules/Analytics.php @@ -1421,7 +1421,6 @@ final class Analytics extends Module
$dimension_filter->setExpressions( array( rawurldecode( $args['page'] ) ) );
$dimension_filter_clause = new Google_Service_AnalyticsReporting_DimensionFilterClause();
$dimension_filter_clause->setFilters( array( $dimension_filter ) );
- $dimension_filter_clause->setOperator( 'AND' );
$dimension_filter_clauses[] = $dimension_filter_clause;
}
| 2 |
diff --git a/tests/unit/specs/store/session.spec.js b/tests/unit/specs/store/session.spec.js @@ -195,12 +195,31 @@ describe(`Module: Session`, () => {
const commit = jest.fn()
const dispatch = jest.fn()
state.signedIn = true
+ state.addresses = [
+ {
+ address: `123`,
+ type: `explore`
+ },
+ {
+ address: `456`,
+ type: `ledger`
+ }
+ ]
await actions.signIn(
{ state, commit, dispatch },
{ sessionType: `explore`, address }
)
expect(dispatch).toHaveBeenCalledWith(`persistAddresses`, {
- addresses: []
+ addresses: [
+ {
+ address: `123`,
+ type: `explore`
+ },
+ {
+ address: `456`,
+ type: `ledger`
+ }
+ ]
})
expect(dispatch).toHaveBeenCalledWith(`persistSession`, {
address: `cosmos1z8mzakma7vnaajysmtkwt4wgjqr2m84tzvyfkz`,
@@ -215,6 +234,22 @@ describe(`Module: Session`, () => {
})
})
+ it("should commit checkForPersistedAddresses when dispatch checkForPersistedAddresses ", async () => {
+ const address = `cosmos1z8mzakma7vnaajysmtkwt4wgjqr2m84tzvyfkz`
+ const commit = jest.fn()
+ state.signedIn = true
+ await actions.rememberAddress(
+ { state, commit },
+ { sessionType: `explore`, address }
+ )
+ expect(commit).toHaveBeenCalledWith(`setUserAddresses`, [
+ {
+ type: `explore`,
+ address
+ }
+ ])
+ })
+
it("should remember the address", async () => {
const address = `cosmos1z8mzakma7vnaajysmtkwt4wgjqr2m84tzvyfkz`
const commit = jest.fn()
| 7 |
diff --git a/docs/content/widgets/Grids.js b/docs/content/widgets/Grids.js @@ -65,7 +65,13 @@ export const Grids = <cx>
selection={{type: KeySelection, bind: '$page.selection', multiple: true}}
/>
</div>
- <CodeSnippet putInto="code" fiddle="kzHH3vkM">{`
+ <Content name="code">
+ <div>
+ <Tab value-bind="$page.code.tab" tab="controller" mod="code"><code>Controller</code></Tab>
+ <Tab value-bind="$page.code.tab" tab="grid" mod="code" default><code>Grid</code></Tab>
+ </div>
+ <CodeSnippet visible-expr="{$page.code.tab}=='controller'" fiddle="kzHH3vkM">
+ {`
class PageController extends Controller {
init() {
super.init();
@@ -81,7 +87,8 @@ export const Grids = <cx>
})));
}
}
- ...
+ `}</CodeSnippet>
+ <CodeSnippet visible-expr="{$page.code.tab}=='grid'" fiddle="kzHH3vkM">{`
<Grid records:bind='$page.records'
style={{width: "100%"}}
columns={[
@@ -93,7 +100,9 @@ export const Grids = <cx>
]}
selection={{type: KeySelection, bind:'$page.selection'}}
/>
- `}</CodeSnippet>
+ `}
+ </CodeSnippet>
+ </Content>
</CodeSplit>
## Examples:
| 0 |
diff --git a/tools/CommandLineTools.hx b/tools/CommandLineTools.hx @@ -928,7 +928,7 @@ class CommandLineTools {
LogHelper.println ("\x1b[37m 888 \x1b[0m");
LogHelper.println ("");
- LogHelper.println ("\x1b[1mOpenFL Command-Line Tools\x1b[0;1m (" + HaxelibHelper.getVersion (new Haxelib ("openfl")) + "-L" + StringHelper.generateUUID (5, null, StringHelper.generateHashCode (version)) + ")\x1b[0m");
+ LogHelper.println ("\x1b[1mOpenFL Command-Line Tools\x1b[0;1m (" + getToolsVersion () + ")\x1b[0m");
} else {
@@ -941,7 +941,7 @@ class CommandLineTools {
LogHelper.println ("\x1b[32m \\/____/ \\/_/\\/_/\\/_/\\/_/\\/____/\x1b[0m");
LogHelper.println ("");
- LogHelper.println ("\x1b[1mLime Command-Line Tools\x1b[0;1m (" + version + ")\x1b[0m");
+ LogHelper.println ("\x1b[1mLime Command-Line Tools\x1b[0;1m (" + getToolsVersion () + ")\x1b[0m");
}
@@ -1316,6 +1316,23 @@ class CommandLineTools {
}
+ private function getToolsVersion (version:String = null):String {
+
+ if (version == null) version = this.version;
+
+ if (targetFlags.exists ("openfl")) {
+
+ return HaxelibHelper.getVersion (new Haxelib ("openfl")) + "-L" + StringHelper.generateUUID (5, null, StringHelper.generateHashCode (version));
+
+ } else {
+
+ return version;
+
+ }
+
+ }
+
+
private function initializeProject (project:HXProject = null, targetName:String = ""):HXProject {
LogHelper.info ("", LogHelper.accentColor + "Initializing project..." + LogHelper.resetColor);
@@ -1607,17 +1624,40 @@ class CommandLineTools {
if (haxelib.name == "lime" && haxelib.version != null && haxelib.version != "" && haxelib.version != "dev" && haxelib.version != version) {
- LogHelper.info ("", LogHelper.accentColor + "Switching to Lime version " + Std.string (haxelib.version) + "...\x1b[0m");
+ if (!project.targetFlags.exists ("notoolscheck")) {
+
+ if (targetFlags.exists ("openfl")) {
+
+ for (haxelib in project.haxelibs) {
+
+ if (haxelib.name == "openfl") {
+
+ PathHelper.haxelibOverrides.set ("openfl", PathHelper.getHaxelib (haxelib));
+
+ }
+
+ }
+
+ }
+
+ LogHelper.info ("", LogHelper.accentColor + "Requesting tools version " + getToolsVersion (haxelib.version) + "...\x1b[0m");
var path = PathHelper.getHaxelib (haxelib);
var args = Sys.args ();
var workingDirectory = args.pop ();
args.push ("--haxelib-lime=" + path);
+ args.push ("-notoolscheck");
var args = [ "run", "lime:" + haxelib.version ].concat (args);
Sys.exit (Sys.command ("haxelib", args));
+ } else {
+
+ LogHelper.warn ("", LogHelper.accentColor + "Could not switch to requested tools version\x1b[0m");
+
+ }
+
}
}
| 7 |
diff --git a/packages/vue/docs/contributing/github-guidelines.md b/packages/vue/docs/contributing/github-guidelines.md @@ -219,7 +219,7 @@ For example: `#290-contribution-guide`, and description of the issue **should no
There are lots of way to create a local branch, below are two most common ways:
-### Using terminal (or command line for Windows)**
+### Using terminal (or command line for Windows)
* **Navigate** to your folder cloned from your forked repo.
@@ -252,19 +252,40 @@ git branch --set-upstream <your-branch-name> origin/<your-branch-name>
The good news is VS Code has Git support built-in.
-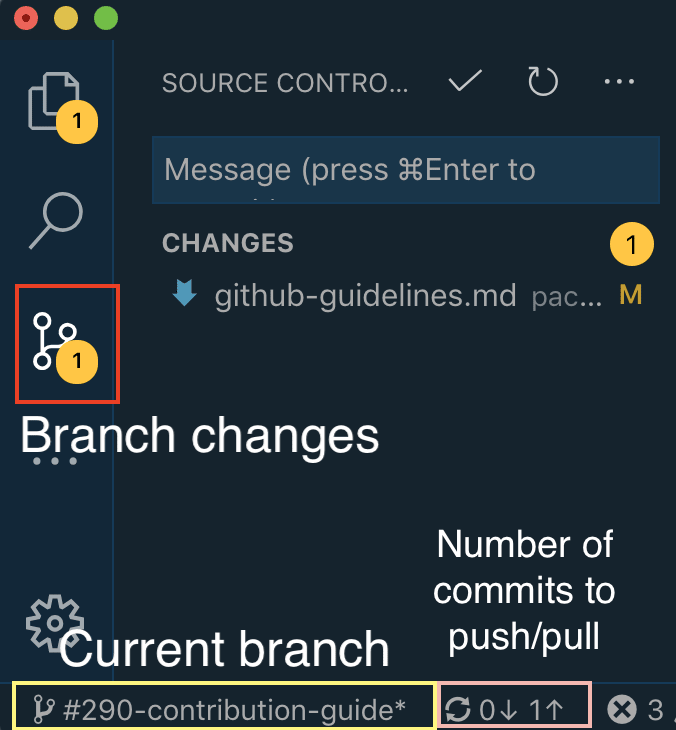
+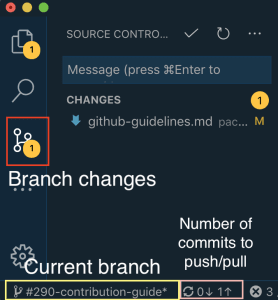
+
+#### Create branch
+
+* It can be done easily by clicking the **bottom left corner** of VS Code, where the current Github local branch is shown.
+
+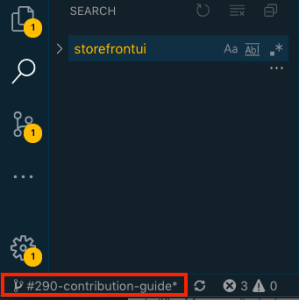
+
+* A new input dropdown will be opened at **the top** of VS Code with an input field for branch name and several options for selecting. After **typing the new branch name** and select whether to branch out from current branch (_first option_) or from a different branch (_second option_), a local branch will be created.
+
+
+
+:::tip SWITCH TO ANOTHER BRANCH
+There is **autocomplete enabled** for this branch name input field. You can also **check out** (switch to) another **existing** branch by do exact the same and **select the matched branch** shown in the dropdown instead of the above options.
+:::
+
+:::warning DO NOT FORGET
+Click on the **cloud icon** at the bottom, next to the branch name to set the remote branch and have it tied to the local one.
+:::
+
+![]()
+
It allows you to do basic features, such as:
* Initialize a repository.
* Clone a repository.
* Create branches and tags.
-
* Stage and commit changes.
* Push/pull/sync with a remote branch.
* Resolve merge conflicts.
* **View differences** made.
+
## 5. Create PR from branch
### Make a commit and push your branch
| 1 |
diff --git a/login.js b/login.js @@ -164,6 +164,10 @@ async function tryLogin() {
<i class="fal fa-sign-in"></i>
Switch account
</nav>
+ <nav class=subbutton id=logout-button>
+ <i class="fal fa-sign-out"></i>
+ Log out
+ </nav>
</div>
</nav>
</div>
@@ -236,6 +240,10 @@ async function tryLogin() {
loginForm.classList.add('phase-1');
loginEmail.focus();
});
+ document.getElementById('logout-button').addEventListener('click', async e => {
+ await storage.remove('loginToken');
+ window.location.reload();
+ });
const unregisteredWarning = document.getElementById('unregistered-warning');
// const userButton = document.getElementById('user-button');
| 0 |
diff --git a/test/unit/specs/components/staking/__snapshots__/PageValidator.spec.js.snap b/test/unit/specs/components/staking/__snapshots__/PageValidator.spec.js.snap @@ -989,9 +989,9 @@ exports[`PageValidator shows a validator profile information if user hasn't sign
</tmpage-stub>
`;
-exports[`PageValidator shows a validator profile information shows a validator as candidate if he has no voting_power 1`] = `"This validator does not have enough voting power yet and is inactive"`;
+exports[`PageValidator shows a validator profile information shows a validator as candidate if he has no voting_power 1`] = `"This validator is actively validating"`;
-exports[`PageValidator shows a validator profile information shows that a validator is revoked 1`] = `"This validator has been jailed and is not currently validating"`;
+exports[`PageValidator shows a validator profile information shows that a validator is jailed 1`] = `"This validator has been jailed and is not currently validating"`;
exports[`delegationTargetOptions always shows wallet in the first position 1`] = `
Array [
| 3 |
diff --git a/resources/prosody-plugins/token/util.lib.lua b/resources/prosody-plugins/token/util.lib.lua @@ -142,7 +142,7 @@ function Util:get_public_key(keyId)
end
--- Verifies issuer part of token
--- @param 'iss' claim from the token to verify
+-- @param 'issClaim' claim from the token to verify
-- @param 'acceptedIssuers' list of issuers to check
-- @return nil and error string or true for accepted claim
function Util:verify_issuer(issClaim, acceptedIssuers)
@@ -151,6 +151,10 @@ function Util:verify_issuer(issClaim, acceptedIssuers)
end
module:log("debug", "verify_issuer claim: %s against accepted: %s", issClaim, acceptedIssuers);
for i, iss in ipairs(acceptedIssuers) do
+ if iss == '*' then
+ -- "*" indicates to accept any issuer in the claims so return success
+ return true;
+ end
if issClaim == iss then
-- claim matches an accepted issuer so return success
return true;
@@ -161,13 +165,13 @@ function Util:verify_issuer(issClaim, acceptedIssuers)
end
--- Verifies audience part of token
--- @param 'aud' claim from the token to verify
+-- @param 'audClaim' claim from the token to verify
-- @return nil and error string or true for accepted claim
function Util:verify_audience(audClaim)
module:log("debug", "verify_audience claim: %s against accepted: %s", audClaim, self.acceptedAudiences);
for i, aud in ipairs(self.acceptedAudiences) do
if aud == '*' then
- --* indicates to accept any audience in the claims so return success
+ -- "*" indicates to accept any audience in the claims so return success
return true;
end
if audClaim == aud then
@@ -175,7 +179,7 @@ function Util:verify_audience(audClaim)
return true;
end
end
- --if issClaim not found in acceptedIssuers, fail claim
+ -- if audClaim not found in acceptedAudiences, fail claim
return nil, "Invalid audience ('aud' claim)";
end
| 11 |
diff --git a/README.md b/README.md @@ -24,7 +24,7 @@ If you want the ansible approach, you can find ansible role [here](https://githu
## Package Installation
If you want to install it on the Debian & Ubuntu based operating systems.
* Prerequisites:
-multiparty-meeting will run on nodejs v12.x and later versions.
+multiparty-meeting will run on nodejs v10.x and later versions. (v12.x has issues for now, please use another major version)
To install see here [here](https://github.com/nodesource/distributions/blob/master/README.md#debinstall).
* Download .deb package from [here](https://github.com/havfo/multiparty-meeting/actions?query=workflow%3ADeployer+branch%3Amaster+is%3Asuccess) (job artifact)
| 3 |
diff --git a/public/javascripts/Admin/src/Admin.js b/public/javascripts/Admin/src/Admin.js @@ -941,28 +941,60 @@ function Admin(_, $, c3, turf) {
});
});
$.getJSON('/adminapi/labels/all', function (data) {
+ for (var i = 0; i < data.features.length; i++) {
+ data.features[i].label_type = data.features[i].properties.label_type;
+ data.features[i].severity = data.features[i].properties.severity;
+ }
+ var curbRamps = data.features.filter(function(label) {return label.properties.label_type === "CurbRamp"});
+ var noCurbRamps = data.features.filter(function(label) {return label.properties.label_type === "NoCurbRamp"});
+ var surfaceProblems = data.features.filter(function(label) {return label.properties.label_type === "SurfaceProblem"});
+ var obstacles = data.features.filter(function(label) {return label.properties.label_type === "Obstacle"});
+
+ var subPlotHeight = 250;
+ var subPlotWidth = 150;
var chart = {
- "data": {
- "values": data.features, "format": {
- "type": "json"
+ "hconcat": [
+ {
+ "height": subPlotHeight,
+ "width": subPlotWidth,
+ "data": {"values": curbRamps},
+ "mark": "bar",
+ "encoding": {
+ "x": {"field": "severity", "type": "ordinal"},
+ "y": {"aggregate": "count", "type": "quantitative", "axis": {"title": "# of labels"}}
}
},
- "transform": [
- {"calculate": "datum.properties.severity", "as": "severity"},
- {"calculate": "datum.properties.label_type", "as": "label_type"}
- ],
+ {
+ "height": subPlotHeight,
+ "width": subPlotWidth,
+ "data": {"values": noCurbRamps},
"mark": "bar",
"encoding": {
- "x": {
- "field": "severity", "type": "ordinal"
+ "x": {"field": "severity", "type": "ordinal"},
+ "y": {"aggregate": "count", "type": "quantitative", "axis": {"title": "# of labels"}}
+ }
},
- "y": {
- "aggregate": "count", "type": "quantitative"
+ {
+ "height": subPlotHeight,
+ "width": subPlotWidth,
+ "data": {"values": surfaceProblems},
+ "mark": "bar",
+ "encoding": {
+ "x": {"field": "severity", "type": "ordinal"},
+ "y": {"aggregate": "count", "type": "quantitative", "axis": {"title": "# of labels"}}
+ }
},
- "row": {
- "field": "label_type", "type": "nominal"
+ {
+ "height": subPlotHeight,
+ "width": subPlotWidth,
+ "data": {"values": obstacles},
+ "mark": "bar",
+ "encoding": {
+ "x": {"field": "severity", "type": "ordinal"},
+ "y": {"aggregate": "count", "type": "quantitative", "axis": {"title": "# of labels"}}
}
}
+ ]
};
var opt = {
"mode": "vega-lite"
| 1 |
diff --git a/token-metadata/0xa0246c9032bC3A600820415aE600c6388619A14D/metadata.json b/token-metadata/0xa0246c9032bC3A600820415aE600c6388619A14D/metadata.json "symbol": "FARM",
"address": "0xa0246c9032bC3A600820415aE600c6388619A14D",
"decimals": 18,
- "dharmaVerificationStatus": {
"dharmaVerificationStatus": "VERIFIED"
}
\ No newline at end of file
-}
\ No newline at end of file
| 3 |
diff --git a/includes/Core/Modules/Module.php b/includes/Core/Modules/Module.php @@ -659,8 +659,6 @@ abstract class Module {
/**
* Sets whether or not to return raw requests and returns a callback to reset to the previous value.
*
- * This works similar to e.g. a higher-order component in JavaScript.
- *
* @since n.e.x.t
*
* @param bool $defer Whether or not to return raw requests.
| 2 |
diff --git a/src/core/background/object/song.js b/src/core/background/object/song.js @@ -103,17 +103,7 @@ define((require) => {
* Safe copy of initial parsed data.
* Must not be modified.
*/
- this.parsed = {
- artist: parsedData.artist,
- track: parsedData.track,
- album: parsedData.album,
- uniqueID: parsedData.uniqueID,
- duration: parsedData.duration,
- currentTime: parsedData.currentTime,
- isPlaying: parsedData.isPlaying,
- trackArt: parsedData.trackArt,
- sourceUrl: parsedData.sourceUrl
- };
+ this.parsed = Object.assign({}, parsedData);
/**
* Post-processed song data, for example auto-corrected.
@@ -121,10 +111,7 @@ define((require) => {
* as the object is processed in pipeline. Can be modified.
*/
this.processed = {
- artist: parsedData.artist,
- track: parsedData.track,
- album: parsedData.album,
- duration: parsedData.duration,
+ // Filled by invoking Song.setDefaults function
};
/**
@@ -158,6 +145,24 @@ define((require) => {
/**
* Various flags. Can be modified.
*/
+ this.flags = {
+ // Filled by invoking Song.setDefaults function
+ };
+
+ this.setDefaults();
+ }
+
+ /**
+ * Apply default song state (processed song info and song flags).
+ */
+ setDefaults() {
+ this.processed = {
+ track: this.parsed.track,
+ album: this.parsed.album,
+ artist: this.parsed.artist,
+ duration: this.parsed.duration,
+ };
+
this.flags = Object.assign({}, DEFAULT_FLAGS);
}
@@ -263,16 +268,7 @@ define((require) => {
* Set default song data.
*/
resetSongData() {
- this.processed = {
- artist: this.parsed.artist,
- track: this.parsed.track,
- album: this.parsed.album,
- duration: this.parsed.duration,
- };
-
- for (const flag in DEFAULT_FLAGS) {
- this.flags[flag] = DEFAULT_FLAGS[flag];
- }
+ this.setDefaults();
}
/**
| 12 |
diff --git a/src/types/components/a11y.d.ts b/src/types/components/a11y.d.ts @@ -51,4 +51,25 @@ export interface A11yOptions {
* @default 'swiper-notification'
*/
notificationClass?: string;
+
+ /**
+ * Message for screen readers for outer swiper container
+ *
+ * @default null
+ */
+ containerMessage?: string;
+
+ /**
+ * Message for screen readers describing the role of outer swiper container
+ *
+ * @default null
+ */
+ containerRoleDescriptionMessage?: string;
+
+ /**
+ * Message for screen readers describing the role of slide element
+ *
+ * @default null
+ */
+ itemRoleDescriptionMessage?: string;
}
| 0 |
diff --git a/packages/lib/src/core/presets/columnGallery.js b/packages/lib/src/core/presets/columnGallery.js @@ -13,7 +13,6 @@ const fixToColumn = (options) => {
presetOptions.isVertical = false;
presetOptions.groupSize = 1;
presetOptions.groupTypes = '1';
- presetOptions.slideshowLoop = false;
presetOptions.numberOfImagesPerCol = 1;
presetOptions.smartCrop = false;
presetOptions.galleryType = 'Strips';
| 11 |
diff --git a/docs-website/pages/cli-export.md b/docs-website/pages/cli-export.md @@ -31,20 +31,28 @@ Provide the `--output=/path/to/assets` argument to specify a custom output folde
## Layers
-Any layer can be exported with `sketchtool`. By default only slices and layers marked _Exportable_ are exported. Alternatively, provide one or more layer ids in `item` or `items` to only export specific layers.
+Any layer can be exported with `sketchtool`. By default only slices and layers marked _Exportable_ are exported.
```sh
sketchtool export layers /path/to/document.sketch
```
-For specific layers, get the layer id by [inspecting the document](/inspect-document).
+To export only specific layers provide one or more layer ids with the `item` or `items` command-line argument. Get the layer id by [inspecting the document](/cli/inspect-document).
+
+```sh
+sketchtool export layers /path/to/document.sketch --item=3E0A01F1-482E-4A32-AD5B-EDF0B98575EA
+```
+
+View all export options in the usage instructions.
+
+```sh
+sketchtool help export layers
+```
## Artboards
Artboards are a subset of layers and `sketchtool` provides a convenience command to export them. It automatically exports both artboards that have been made _Exportable_ and such that haven't.
-### All artboards
-
Export all artboards of a document running the following command:
```sh
@@ -57,26 +65,12 @@ Following is an example exporting all artboards overriding any presets, generati
sketchtool export artboards /path/to/document.sketch --formats=jpg,png,svg --scales=1,2
```
-For a list of all available options, see the command help:
+Provide specific artboard layer ids in `item` or `items` limit the export to certain artboards. For a list of all available options, see the command help:
```sh
sketchtool help export artboards
```
-### Specific artboards
-
-Specify an artboard's layer id in `item` or `items` for multiple to limit the export to certain artboards.
-
-```sh
-sketchtool export artboards /path/to/document.sketch --item=1B7FCC8E-5030-43A5-94BE-28A9C7ABFF72
-```
-
-Get the layer id of an artboard by [inspecting the document](/inspect-document) and list the JSON data.
-
-```sh
-sketchtool list artboards /path/to/document.sketch
-```
-
> **Note:** You can also use `sketchtool metadata` for artboards but `sketchtool list` works the same for artboards as well as any other document elements. See [Inspect a document](/cli/inspect-document) for details.
## Document preview
| 7 |
diff --git a/src/plugins/Dashboard/index.js b/src/plugins/Dashboard/index.js @@ -131,6 +131,7 @@ module.exports = class Dashboard extends Plugin {
this.isModalOpen = this.isModalOpen.bind(this)
this.addTarget = this.addTarget.bind(this)
+ this.removeTarget = this.removeTarget.bind(this)
this.hideAllPanels = this.hideAllPanels.bind(this)
this.showPanel = this.showPanel.bind(this)
this.getFocusableNodes = this.getFocusableNodes.bind(this)
@@ -149,6 +150,16 @@ module.exports = class Dashboard extends Plugin {
this.install = this.install.bind(this)
}
+ removeTarget (plugin) {
+ const pluginState = this.getPluginState()
+ // filter out the one we want to remove
+ const newTargets = pluginState.targets.filter(target => target.id !== plugin.id)
+
+ this.setPluginState({
+ targets: newTargets
+ })
+ }
+
addTarget (plugin) {
const callerPluginId = plugin.id || plugin.constructor.name
const callerPluginName = plugin.title || callerPluginId
@@ -362,6 +373,8 @@ module.exports = class Dashboard extends Plugin {
this.updateDashboardElWidth()
window.addEventListener('resize', this.updateDashboardElWidth)
+
+ this.uppy.on('plugin-removed', this.removeTarget)
}
removeEvents () {
@@ -372,6 +385,8 @@ module.exports = class Dashboard extends Plugin {
this.removeDragDropListener()
window.removeEventListener('resize', this.updateDashboardElWidth)
+
+ this.uppy.off('plugin-removed', this.removeTarget)
}
updateDashboardElWidth () {
| 0 |
diff --git a/api/test/utils/__snapshots__/create-graphql-error-formatter.test.js.snap b/api/test/utils/__snapshots__/create-graphql-error-formatter.test.js.snap exports[`createGraphQLErrorFormatter logs error path 1`] = `
Array [
"---GraphQL Error---",
- "GraphQLError: Cannot return null for non-nullable field Thread.channel.",
+ "Cannot return null for non-nullable field Thread.channel.
+
+GraphQL request (9:15)
+ 8: id
+ 9: channel {
+ ^
+10: id
+",
"path community.threadConnection.edges[0].node.channel",
"-------------------
",
@@ -13,7 +20,14 @@ Array [
exports[`createGraphQLErrorFormatter logs query and variables 1`] = `
Array [
"---GraphQL Error---",
- "GraphQLError: Cannot return null for non-nullable field Thread.channel.",
+ "Cannot return null for non-nullable field Thread.channel.
+
+GraphQL request (9:15)
+ 8: id
+ 9: channel {
+ ^
+10: id
+",
"query getCommunityThreadConnection( $id: ID )",
"variables {\\"id\\":1}",
"path community.threadConnection.edges[0].node.channel",
| 1 |
diff --git a/bin/language_scan.js b/bin/language_scan.js @@ -168,7 +168,7 @@ languages.forEach(language => {
log("==========");
var translations = JSON.parse(fs.readFileSync(BASEDIR+"/lang/"+language.url).toString());
translatedStrings.forEach(str => {
- if (!translations.GLOBAL[str])
+ if (!translations.GLOBAL[str.str])
console.log(`Missing translation for ${JSON.stringify(str)}`);
});
log("");
| 4 |
diff --git a/articles/email/liquid-syntax.md b/articles/email/liquid-syntax.md @@ -24,12 +24,46 @@ There are two types of markup in Liquid: **output** and **tag**.
`Hello {{ name }}!`
-You can further customize the appearance of the output by using [filters](https://github.com/Shopify/liquid/wiki/Liquid-for-Designers#standard-filters), which are simple methods.
+You can further customize the appearance of the output by using filters, which are simple methods. For example, the `upcase` filter will convert the text which is passed to the filter to upper case:
`Hello {{ name | upcase }}!`
Multiple filters are separated by `|` and are processed from left to right, applying the subsequent filter to the result of the previous one. The template will render the final result.
+The following filters are supported:
+
+* `append` - append a string *e.g.* `{{ 'foo' | append:'bar' }} #=> 'foobar'`
+* `capitalize` - capitalize words in the input sentence, *e.g.* `{{ "my great title" | capitalize }} #=> My great title`
+* `date` - reformat a date ([syntax reference](http://docs.shopify.com/themes/liquid-documentation/filters/additional-filters#date))
+* `default` - returns the given variable unless it is null or the empty string, when it will return the given value, *e.g.* `{{ undefined_variable | default: "Default value" }} #=> "Default value"`
+* `divided_by` - integer division *e.g.* `{{ 10 | divided_by:3 }} #=> 3`
+* `downcase` - convert an input string to lowercase, *e.g.* `{{ "Parker Moore" | downcase }} #=> parker moore`
+* `escape` - html escape a string, *e.g.* `{{ "Have you read 'James & the Giant Peach'?" | escape }} #=> Have you read 'James & the Giant Peach'?`
+* `escape_once` - returns an escaped version of html without affecting existing escaped entities, *e.g.* `{{ "1 < 2 & 3" | escape_once }} #=> 1 < 2 & 3`
+* `first` - get the first element of the passed in array
+* `join` - join elements of the array with certain character between them
+* `last` - get the last element of the passed in array
+* `map` - map/collect an array on a given property
+* `minus` - subtraction *e.g.* `{{ 4 | minus:2 }} #=> 2`
+* `modulo` - remainder, *e.g.* `{{ 3 | modulo:2 }} #=> 1`
+* `newline_to_br` - replace each newline (\n) with html break
+* `plus` - addition *e.g.* `{{ '1' | plus:'1' }} #=> 2`, `{{ 1 | plus:1 }} #=> 2`
+* `prepend` - prepend a string *e.g.* `{{ 'bar' | prepend:'foo' }} #=> 'foobar'`
+* `remove` - remove each occurrence *e.g.* `{{ 'foobarfoobar' | remove:'foo' }} #=> 'barbar'`
+* `remove_first` - remove the first occurrence *e.g.* `{{ 'barbar' | remove_first:'bar' }} #=> 'bar'`
+* `replace` - replace each occurrence *e.g.* `{{ 'foofoo' | replace:'foo','bar' }} #=> 'barbar'`
+* `replace_first` - replace the first occurrence *e.g.* `{{ 'barbar' | replace_first:'bar','foo' }} #=> 'foobar'`
+* `round` - rounds input to the nearest integer or specified number of decimals *e.g.* `{{ 4.5612 | round: 2 }} #=> 4.56`
+* `size` - return the size of an array or string, *e.g.* `{{ "Ground control to Major Tom." | size }} #=> 28`
+* `sort` - sort elements of the array
+* `split` - split a string on a matching pattern *e.g.* `{{ "a~b" | split:"~" }} #=> ['a','b']`
+* `strip_html` - strip html from string, *e.g.* `{{ "How <em>are</em> you?" | strip_html }} #=> How are you?`
+* `strip_newlines` - strip all newlines (\n) from string
+* `times` - multiplication *e.g* `{{ 5 | times:4 }} #=> 20`
+* `truncate` - truncate a string down to x characters. It also accepts a second parameter that will append to the string *e.g.* `{{ 'foobarfoobar' | truncate: 5, '.' }} #=> 'foob.'`
+* `truncatewords` - truncate a string down to x words
+* `upcase` - convert an input string to uppercase, *e.g* `{{ "Parker Moore" | upcase }} #=> PARKER MOORE`
+
### Liquid Markup: Tag
**Tag** markup does not resolve to text and is surrounded by a pair of matched curly braces and percent signs:
| 0 |
diff --git a/assets/data/landing_pages.json b/assets/data/landing_pages.json "name": "Congressional Plan"
},
{
- "id": "state_sen",
- "name": "State Senate Plan"
+ "id": "leg_dist",
+ "name": "Legislative Districts"
}
]
}
| 10 |
diff --git a/.eslintrc.js b/.eslintrc.js @@ -27,7 +27,7 @@ module.exports = {
],
"block-scoped-var": "error",
"block-spacing": "error",
- "brace-style": ["error", "1tbs"],
+ "brace-style": ["error", "1tbs", { "allowSingleLine": true }],
"callback-return": "error",
camelcase: "off",
"capitalized-comments": "off",
@@ -45,7 +45,7 @@ module.exports = {
"computed-property-spacing": ["error", "never"],
"consistent-return": "off",
"consistent-this": "error",
- curly: "error",
+ curly: ["error", "multi-line"],
"default-case": "error",
"dot-location": ["error", "property"],
"dot-notation": "error",
@@ -242,8 +242,6 @@ module.exports = {
"space-before-blocks": "error",
"space-before-function-paren": "off",
"space-in-parens": ["error", "never"],
- "space-infix-ops": "error",
- "space-unary-ops": "error",
"spaced-comment": ["error", "always"],
strict: "error",
"switch-colon-spacing": "error",
| 11 |
diff --git a/assets/images/expensify-wordmark.svg b/assets/images/expensify-wordmark.svg <?xml version="1.0" encoding="utf-8"?>
<!-- Generator: Adobe Illustrator 26.5.0, SVG Export Plug-In . SVG Version: 6.00 Build 0) -->
<svg version="1.1" id="Layer_1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" x="0px" y="0px"
- viewBox="0 0 78 18" style="enable-background:new 0 0 78 18;" xml:space="preserve">
+ viewBox="0 0 78 19" style="enable-background:new 0 0 78 19;" xml:space="preserve">
<style type="text/css">
.st0{fill:#FFFFFF;}
</style>
<g>
- <polygon class="st0" points="0,14.1 8.5,14.1 8.5,11.5 3.1,11.5 3.1,9.2 7.7,9.2 7.7,6.5 3.1,6.5 3.1,4.3 8.5,4.3 8.5,1.7 0,1.7
+ <polygon class="st0" points="0,14.7 8.7,14.7 8.7,12.1 3.2,12.1 3.2,9.8 7.6,9.8 7.6,7.2 3.2,7.2 3.2,4.9 8.7,4.9 8.7,2.4 0,2.4
"/>
- <path class="st0" d="M25.3,4.3c-1.3,0-2.2,0.4-2.9,1.4h-0.1l-0.4-1.2h-2.2v12.8h2.9v-4.1h0.1c0.6,0.6,1.4,0.9,2.5,0.9
- c2.6,0,4.5-2.2,4.5-4.9V9C29.6,6.1,27.4,4.3,25.3,4.3z M24.5,11.9c-1.4,0-2-1.1-2-2.6c0-1.4,0.6-2.6,2-2.6s2,1.2,2,2.6
- S25.8,11.9,24.5,11.9z"/>
- <path class="st0" d="M39.7,9c0-3.2-2.2-4.7-4.7-4.7c-2.7,0-4.9,1.9-4.9,4.9v0.3c0,3.1,2.3,4.9,5,4.9c2.2,0,4.1-1.1,4.6-3.2h-2.8
- c-0.3,0.7-0.9,1-1.7,1c-1.2,0-1.9-0.9-2.1-2.1h6.5C39.6,9.9,39.7,9.4,39.7,9z M33.2,8.2c0.1-1,0.7-1.7,1.9-1.7c1,0,1.7,0.7,1.7,1.7
- H33.2z"/>
- <path class="st0" d="M45.8,4.3c-1.2,0-2.1,0.4-2.8,1.5l0,0l-0.4-1.2h-2.3V14h3V8.5c0-1.2,0.6-1.8,1.6-1.8c0.8,0,1.4,0.5,1.4,1.8V14
- h3V7.7C49.3,5.9,48.2,4.3,45.8,4.3z"/>
- <path class="st0" d="M53.7,8c-0.5-0.1-0.9-0.3-0.9-0.9c0-0.4,0.3-0.7,1-0.7s1.1,0.4,1.1,1h2.7V7.2c0-2-1.8-2.8-3.9-2.8
- c-1.8,0-3.9,0.7-3.9,3v0.1c0,0.7,0.2,1.3,0.7,1.7c0.9,0.9,2.4,1.1,3.4,1.3c0.8,0.2,1,0.6,1,1c0,0.6-0.4,0.9-1.1,0.9
- c-0.8,0-1.3-0.4-1.4-1.4h-2.7v0.2c0.1,2.3,1.9,3.2,4.1,3.2c1.9,0,4.1-0.9,4.1-3.1V11c0-0.7-0.2-1.3-0.6-1.7
- C56.4,8.3,54.9,8.2,53.7,8z"/>
- <rect x="58.5" y="4.6" class="st0" width="3" height="9.5"/>
- <polygon class="st0" points="19,4.6 15.7,4.6 14.2,7 12.5,4.6 9.2,4.6 12.3,9 8.7,14.1 12,14.1 14,11.2 16,14.1 19.5,14.1 15.8,9
- "/>
- <path class="st0" d="M60,0c-1,0-1.8,0.8-1.8,1.8S59,3.6,60,3.6s1.8-0.8,1.8-1.8S61,0,60,0z"/>
- <path class="st0" d="M74.9,4.6l-2.1,5.7L71,4.6h-4.1V4.3c0-0.8,0.6-1.6,1.4-1.6c0.1,0,0.9,0,0.9,0V0.1h-1.1c-2.6,0.1-4.2,2-4.2,4.4
- v0.1h-1.7v2.2h1.7V14h3V6.9h1.9l2.5,6.5l-1.7,4.1h3.1L78,4.6H74.9z"/>
+ <polygon class="st0" points="19.4,5.1 15.6,5.1 14.4,7.4 13,5.1 9.1,5.1 12.3,9.7 8.9,14.7 12.7,14.7 14.2,12.1 15.7,14.7
+ 19.6,14.7 16.2,9.7 "/>
+ <path class="st0" d="M25.4,4.8L25.4,4.8c-1.1,0-2,0.4-2.6,1.2v-1h-2.9v13.7H23V14c0.6,0.6,1.4,1,2.4,1c2.5,0,4.3-2,4.3-5
+ C29.7,6.8,28.1,4.8,25.4,4.8z M24.8,12.4c-1.2,0-1.9-0.9-1.9-2.5c0-1.6,0.7-2.5,1.9-2.5c1.2,0,1.9,0.9,1.9,2.5
+ C26.6,11.5,25.9,12.4,24.8,12.4z"/>
+ <path class="st0" d="M35.2,12.5c-1,0-1.7-0.5-1.8-1.9H40v-0.4c0-3-1.7-5.3-4.9-5.3c-2.3,0-4.9,1.2-4.9,5c0,3.1,2,5,5.1,5
+ c1.8,0,4.1-0.7,4.8-3.5h-3.2C36.7,11.9,36.3,12.5,35.2,12.5z M35.1,7.1c0.9,0,1.5,0.5,1.6,1.6h-3.3C33.7,7.5,34.3,7.1,35.1,7.1z"/>
+ <path class="st0" d="M46.1,4.8c-1.1,0-2,0.5-2.6,1.4V5.1h-2.9v9.6h3.1V9.3c0-1.3,0.7-1.9,1.5-1.9c0.9,0,1.5,0.4,1.5,1.8v5.4h3.1
+ V9.1C49.7,6,48.5,4.8,46.1,4.8z"/>
+ <path class="st0" d="M61.1,0.7c-1,0-1.6,0.7-1.6,1.6c0,0.9,0.7,1.6,1.6,1.6c1,0,1.7-0.7,1.7-1.6C62.7,1.4,62.1,0.7,61.1,0.7z"/>
+ <rect x="59.6" y="5.1" class="st0" width="3.1" height="9.6"/>
+ <path class="st0" d="M55.5,8.7L55.5,8.7l-0.8-0.2c-0.8-0.2-1.1-0.5-1.1-0.9c0-0.5,0.4-0.9,1.2-0.9c0.9,0,1.4,0.3,1.4,1.2h2.7
+ c-0.1-2-1.2-3.2-4.2-3.2c-2.6,0-4.1,1-4.1,3.1c0,1.7,1.1,2.5,3.7,3L55,11c0.8,0.2,1.1,0.6,1.1,0.9c0,0.5-0.5,0.8-1.3,0.8
+ c-1.1,0-1.6-0.4-1.7-1.3h-3c0.2,2.3,1.8,3.5,4.6,3.5c2.8,0,4.5-1,4.5-3.2C59.2,10.1,58.1,9.3,55.5,8.7z"/>
+ <path class="st0" d="M74.8,5.1L74.8,5.1L73.1,11l-1.7-5.9h-4V4.8c0-0.8,0.6-1.6,1.5-1.6h0.4V0.7h-0.6C66,0.7,64.2,2.6,64.2,5v0.1
+ h-1.2v2.8h1.2v6.8h3.1V7.9h1.8l2.3,6.5l-0.4,1c-0.3,0.7-0.6,1-1.3,1c-0.3,0-0.7,0-1.1-0.1l-0.2,2.5c0.5,0.1,1,0.2,1.8,0.2
+ c1.9,0,2.8-0.8,3.8-3.3l4-10.6H74.8z"/>
</g>
</svg>
| 4 |
diff --git a/packages/spark/components/masthead.js b/packages/spark/components/masthead.js @@ -5,7 +5,7 @@ import { isEscPressed } from '../utilities/keypress';
import isElementVisible from '../utilities/isElementVisible';
import scrollYDirection from '../utilities/scrollYDirection';
import { hideDropDown, showDropDown } from './dropdown';
-import debounce from '../node_modules/lodash/debounce';
+import throttle from '../node_modules/lodash/throttle';
const addClassOnScroll = (element, scrollPos, scrollPoint, classToToggle) => {
// If user scrolls past the scrollPoint then add class
@@ -20,6 +20,7 @@ const addClassOnScroll = (element, scrollPos, scrollPoint, classToToggle) => {
* Add or remove the class depending
* on if the user is scrolling up or down
*/
+let direction = scrollYDirection();
const toggleMenu = scrollDirection => {
const masthead = document.querySelector('[data-sprk-masthead]');
if (scrollDirection === 'down') {
@@ -34,14 +35,13 @@ const toggleMenu = scrollDirection => {
* If the scroll direction changes
* toggle the masthead visibility
*/
-let direction = scrollYDirection();
-const checkScrollDirection = debounce(() => {
+const checkScrollDirection = throttle(() => {
const newDirection = scrollYDirection();
if (direction !== newDirection) {
toggleMenu(newDirection);
}
direction = newDirection;
-}, 30);
+}, 500);
/*
* If the mobile menu is visible
@@ -50,6 +50,10 @@ const checkScrollDirection = debounce(() => {
*/
const toggleScrollEvent = isMenuVisible => {
let attached = false;
+ if (!isMenuVisible) {
+ const masthead = document.querySelector('[data-sprk-masthead]');
+ masthead.classList.remove('sprk-c-Masthead--hidden');
+ }
if (isMenuVisible) {
window.addEventListener('scroll', checkScrollDirection);
attached = true;
@@ -137,14 +141,16 @@ const bindUIEvents = () => {
* If the mobile menu visibility changes
* toggle scroll event listener
*/
- window.addEventListener('resize', () => {
- console.log(isMenuVisible);
+ window.addEventListener(
+ 'resize',
+ throttle(() => {
const newMenuVisibility = isElementVisible('.sprk-c-Masthead__menu');
if (isMenuVisible !== newMenuVisibility) {
toggleScrollEvent(newMenuVisibility);
}
isMenuVisible = newMenuVisibility;
- });
+ }, 500),
+ );
element.addEventListener('click', e => {
e.preventDefault();
| 3 |
diff --git a/CONTRIBUTING.md b/CONTRIBUTING.md @@ -73,6 +73,41 @@ Markdown on this site conforms to the [CommonMark](http://commonmark.org/) spec.
### Headings
+```
+The headings are written in the following manner (the number of hashtags describes the level of the heading in the document.):
+# H1
+## H2
+### H3
+#### H4
+##### H5
+###### H6
+
+Alternative for H1 and H2, in a underline-ish manner:
+
+Alt H1
+======
+
+Alt H2
+------
+```
+The above text will give you an output like the one shown below:
+
+The headings are written in the following manner (the number of hashtags describes the level of the heading in the document.):
+# H1
+## H2
+### H3
+#### H4
+##### H5
+###### H6
+
+Alternative for H1 and H2, in a underline-ish manner:
+
+Alt H1
+======
+
+Alt H2
+------
+
One common mistake with formatting of headings is to not include a space between the hashes and the text. Some markdown processors allow this, but we do not. You must put a space as shown below.
INVALID: `#My Heading`
| 3 |
diff --git a/src/patterns/fundamentals/color/collection.yaml b/src/patterns/fundamentals/color/collection.yaml description:
These are the colors that have been chosen to represent the brand. The colors are set as
- variables in Spark and as document colors in the Design Toolkit.
+ variables in Spark.
sparkPackageCore: true
noCodeSwitch: true
| 3 |
diff --git a/docs/event-listener.rst b/docs/event-listener.rst @@ -79,14 +79,14 @@ To configure carrier-grade network address translation (CGNAT), use the followin
- The Large Scale NAT (LSN) Pool must use the Telemetry Streaming Log Publisher you created (**telemetry_publisher** if you used the AS3 example to configure TS logging). |br| If you have an existing pool, update the pool to use the TS Log Publisher:
- - TMSH: ``modify ltm lsn-pool cgnat_lsn_pool log-publisher telemetry_publisher``
- - GUI: **Carrier Grade NAT > LSN Pools > LSN Pools List**
+ - TMSH:|br| ``modify ltm lsn-pool cgnat_lsn_pool log-publisher telemetry_publisher``
+ - GUI:|br| **Carrier Grade NAT > LSN Pools > LSN Pools List** |br| |br|
- Create and attach a new CGNAT Logging Profile to the LSN pool. This determines what types of logs you wish to receive (optional).
- - TMSH-create: ``create ltm lsn-log-profile telemetry_lsn_log_profile { start-inbound-session { action enabled } }``
- - TMSH-attach: ``modify ltm lsn-pool cgnat_lsn_pool log-profile telemetry_lsn_log_profile``
- - GUI: **Carrier Grade NAT -> Logging Profiles -> LSN**
+ - TMSH-create:|br| ``create ltm lsn-log-profile telemetry_lsn_log_profile { start-inbound-session { action enabled } }``
+ - TMSH-attach:|br| ``modify ltm lsn-pool cgnat_lsn_pool log-profile telemetry_lsn_log_profile``
+ - GUI:|br| **Carrier Grade NAT -> Logging Profiles -> LSN**
Example output:
| 1 |
diff --git a/continuous_deployment/bs.js b/continuous_deployment/bs.js @@ -14,14 +14,14 @@ var iPhone = {
'browserstack.key': process.argv[3]
}
-// var android = {
-// browserName: 'android',
-// device: 'Samsung Galaxy S8',
-// realMobile: 'true',
-// os_version: '7.0',
-// 'browserstack.user': process.argv[2],
-// 'browserstack.key': process.argv[3]
-// }
+var android = {
+ browserName: 'android',
+ device: 'Samsung Galaxy S8',
+ realMobile: 'true',
+ os_version: '7.0',
+ 'browserstack.user': process.argv[2],
+ 'browserstack.key': process.argv[3]
+}
var desktopFF = {
browserName: 'Firefox',
@@ -53,15 +53,17 @@ var desktopIE = {
'browserstack.key': process.argv[3]
}
+/*
var iPhoneDriver = new webdriver.Builder()
.usingServer('http://hub-cloud.browserstack.com/wd/hub')
.withCapabilities(iPhone)
.build()
-// var androidDriver = new webdriver.Builder()
-// .usingServer('http://hub-cloud.browserstack.com/wd/hub')
-// .withCapabilities(android)
-// .build()
+ */
+var androidDriver = new webdriver.Builder()
+ .usingServer('http://hub-cloud.browserstack.com/wd/hub')
+ .withCapabilities(android)
+ .build()
var desktopFFDriver = new webdriver.Builder()
.usingServer('http://hub-cloud.browserstack.com/wd/hub')
@@ -78,8 +80,8 @@ var desktopIEDriver = new webdriver.Builder()
.withCapabilities(desktopIE)
.build()
-test.runTest(iPhoneDriver)
-//test.runTest(androidDriver)
+//test.runTest(iPhoneDriver)
+test.runTest(androidDriver)
test.runTest(desktopFFDriver)
test.runTest(desktopEdgeDriver)
test.runTest(desktopIEDriver)
| 2 |
diff --git a/token-metadata/0xbCa3C97837A39099eC3082DF97e28CE91BE14472/metadata.json b/token-metadata/0xbCa3C97837A39099eC3082DF97e28CE91BE14472/metadata.json "symbol": "DUST",
"address": "0xbCa3C97837A39099eC3082DF97e28CE91BE14472",
"decimals": 8,
- "dharmaVerificationStatus": {
"dharmaVerificationStatus": "VERIFIED"
}
\ No newline at end of file
-}
\ No newline at end of file
| 3 |
diff --git a/microraiden/microraiden/test/test_eth_ticker.py b/microraiden/microraiden/test/test_eth_ticker.py import pytest # noqa: F401
+from _pytest.monkeypatch import MonkeyPatch
+from flask import jsonify
from microraiden import DefaultHTTPClient
from microraiden.examples.eth_ticker import ETHTickerClient, ETHTickerProxy
from microraiden.proxy.paywalled_proxy import PaywalledProxy
+from microraiden.proxy.resources import PaywalledProxyUrl
@pytest.mark.needs_xorg
@@ -11,20 +14,30 @@ def test_eth_ticker(
default_http_client: DefaultHTTPClient,
sender_privkey: str,
receiver_privkey: str,
+ monkeypatch: MonkeyPatch
):
- proxy = ETHTickerProxy(receiver_privkey, proxy=empty_proxy) # noqa
- ticker = ETHTickerClient(sender_privkey, httpclient=default_http_client)
+ def get_patched(*args, **kwargs):
+ body = {
+ 'mid': '682.435', 'bid': '682.18', 'ask': '682.69', 'last_price': '683.16',
+ 'low': '532.97', 'high': '684.0', 'volume': '724266.25906224',
+ 'timestamp': '1513167820.721733'
+ }
+ return jsonify(body)
+
+ monkeypatch.setattr(PaywalledProxyUrl, 'get', get_patched)
+
+ ETHTickerProxy(receiver_privkey, proxy=empty_proxy)
+ ticker = ETHTickerClient(sender_privkey, httpclient=default_http_client, poll_interval=0.5)
def post():
ticker.close()
- # This test fails if ETH price is below 100 USD. But why even bother anymore if it does?
- assert float(ticker.pricevar.get().split()[0]) > 100
+ assert ticker.pricevar.get() == '683.16 USD'
client = default_http_client.client
assert len(client.get_open_channels()) == 0
ticker.success = True
ticker.success = False
- ticker.root.after(5000, post)
+ ticker.root.after(1500, post)
ticker.run()
assert ticker.success
| 7 |
diff --git a/javascripts/core/timestudies.js b/javascripts/core/timestudies.js @@ -281,7 +281,9 @@ var path = [0,0];
}
for (var i = 1; i < row; i++) {
var chosenPath = path[i > 11 ? 1 : 0];
+ if (row > 6 && row < 11) var secondPath = col;
if ((i > 6 && i < 11) || (i > 11 && i < 15)) buyTimeStudy(i * 10 + (chosenPath === 0 ? col : chosenPath), studyCosts[all.indexOf(i * 10 + (chosenPath === 0 ? col : chosenPath))], 0);
+ if ((i > 6 && i < 11) && player.timestudy.studies.includes(201)) buyTimeStudy(i * 10 + secondPath, studyCosts[all.indexOf(i * 10 + secondPath)], 0);
else for (var j = 1; all.includes(i * 10 + j) ; j++) buyTimeStudy(i * 10 + j, studyCosts[all.indexOf(i * 10 + j)], 0);
}
buyTimeStudy(id, studyCosts[all.indexOf(id)], 0);
| 11 |
diff --git a/assets/js/components/settings/SettingsApp.test.js b/assets/js/components/settings/SettingsApp.test.js @@ -30,7 +30,6 @@ import {
fireEvent,
createTestRegistry,
provideModules,
- act,
} from '../../../../tests/js/test-utils';
import { CORE_USER } from '../../googlesitekit/datastore/user/constants';
import { CORE_SITE } from '../../googlesitekit/datastore/site/constants';
@@ -101,10 +100,6 @@ describe( 'SettingsApp', () => {
registry,
} );
- act( () => {
- jest.advanceTimersByTime( 5 );
- } );
-
fireEvent.click(
getAllByRole( 'tab' )[ getTabID( 'connected-services' ) ]
);
| 2 |
diff --git a/api/services/KongService.js b/api/services/KongService.js @@ -166,11 +166,18 @@ var KongService = {
getData(data, (Utils.withoutTrailingSlash(req.kong_admin_url) || Utils.withoutTrailingSlash(req.connection.kong_admin_url)) + response.body.next);
}
else {
+ try {
response.body.data = data;
ProxyHooks.afterEntityList(endpoint.replace('/', '').split('?')[0], req, response.body, (err, finalData) => {
if (err) return cb(err);
return cb(null, finalData)
})
+ }catch(err) {
+ return cb(null, {
+ data: []
+ })
+ }
+
}
});
};
| 9 |
diff --git a/token-metadata/0x291FA2725d153bcc6C7E1C304bcaD47fdEf1EF84/metadata.json b/token-metadata/0x291FA2725d153bcc6C7E1C304bcaD47fdEf1EF84/metadata.json "symbol": "MX3",
"address": "0x291FA2725d153bcc6C7E1C304bcaD47fdEf1EF84",
"decimals": 18,
- "dharmaVerificationStatus": {
"dharmaVerificationStatus": "VERIFIED"
}
\ No newline at end of file
-}
\ No newline at end of file
| 3 |
diff --git a/packages/spark-core/settings/_settings.scss b/packages/spark-core/settings/_settings.scss @@ -486,7 +486,7 @@ $modal-shadow: 0 4px 5px rgba(0, 0, 0, 0.6) !default;
// Masthead / Main Nav
//
$masthead-bg-color: $white !default;
-$big-nav-breakpoint: 50rem !default;
+$big-nav-breakpoint: 54rem !default;
$masthead-column-width: 89rem !default;
$big-nav-dropdown-shadow: 0 2px 2px -2px rgba(0, 0, 0, 0.2) !default;
$big-nav-column-width: 64rem !default;
| 3 |
diff --git a/app-template/package-template.json b/app-template/package-template.json "bezier-easing": "^2.0.3",
"bhttp": "1.2.1",
"bitauth": "^0.2.1",
- "bitcore-wallet-client": "6.1.0",
+ "bitcore-wallet-client": "6.2.0",
"bower": "^1.7.9",
"cordova-android": "5.1.1",
"cordova-custom-config": "^3.0.5",
| 3 |
diff --git a/includes/Core/Permissions/Permissions.php b/includes/Core/Permissions/Permissions.php @@ -18,7 +18,6 @@ use Google\Site_Kit\Core\Modules\Module_With_Owner;
use Google\Site_Kit\Core\Modules\Modules;
use Google\Site_Kit\Core\Storage\User_Options;
use Google\Site_Kit\Core\Util\Feature_Flags;
-use WP_User;
/**
* Class managing plugin permissions.
@@ -393,7 +392,7 @@ final class Permissions {
private function check_view_shared_dashboard_capability( $user_id ) {
$module_sharing_settings = $this->modules->get_module_sharing_settings();
$shared_roles = $module_sharing_settings->get_all_shared_roles();
- $user = new WP_User( $user_id );
+ $user = get_userdata( $user_id );
$user_has_shared_role = ! empty( array_intersect( $shared_roles, $user->roles ) );
if ( ! $user_has_shared_role ) {
@@ -423,7 +422,7 @@ final class Permissions {
private function check_read_shared_module_data_capability( $user_id, $module_slug ) {
$module_sharing_settings = $this->modules->get_module_sharing_settings();
$sharing_settings = $module_sharing_settings->get();
- $user = new WP_User( $user_id );
+ $user = get_userdata( $user_id );
if ( ! isset( $sharing_settings[ $module_slug ]['sharedRoles'] ) ) {
return array( 'do_not_allow' );
| 2 |
diff --git a/shaders.js b/shaders.js @@ -975,6 +975,108 @@ const _ensureLoadMesh = p => {
transparent: true,
}); */
+/* const wallGeometry = (() => {
+ const panelGeometries = [];
+ for (let x = -1 / 2; x <= 1 / 2; x++) {
+ panelGeometries.push(
+ new THREE.BoxBufferGeometry(0.01, 1, 0.01)
+ .applyMatrix4(new THREE.Matrix4().makeTranslation(x, 0, -1/2)),
+ );
+ }
+ for (let h = 0; h <= 1; h++) {
+ panelGeometries.push(
+ new THREE.BoxBufferGeometry(1, 0.01, 0.01)
+ .applyMatrix4(new THREE.Matrix4().makeTranslation(0, h -1/2, -1/2)),
+ );
+ }
+ return BufferGeometryUtils.mergeBufferGeometries(panelGeometries);
+})();
+const topWallGeometry = wallGeometry.clone();
+// .applyMatrix(new THREE.Matrix4().makeTranslation(-0.5, 0, -0.5));
+const leftWallGeometry = wallGeometry.clone()
+ .applyMatrix4(new THREE.Matrix4().makeRotationAxis(new THREE.Vector3(0, 1, 0), Math.PI / 2));
+ // .applyMatrix(new THREE.Matrix4().makeTranslation(-0.5, 0, -0.5));
+const rightWallGeometry = wallGeometry.clone()
+ .applyMatrix4(new THREE.Matrix4().makeRotationAxis(new THREE.Vector3(0, 1, 0), -Math.PI / 2));
+ // .applyMatrix(new THREE.Matrix4().makeTranslation(-0.5, 0, -0.5));
+const bottomWallGeometry = wallGeometry.clone()
+ .applyMatrix4(new THREE.Matrix4().makeRotationAxis(new THREE.Vector3(0, 1, 0), Math.PI));
+ // .applyMatrix(new THREE.Matrix4().makeTranslation(-0.5, 0, -0.5));
+const distanceFactor = 64;
+export function GuardianMesh(extents, color) {
+ const geometry = (() => {
+ const geometries = [];
+ const [x1, y1, z1, x2, y2, z2] = extents;
+ const ax1 = (x1 + 0.5);
+ const ay1 = (y1 + 0.5);
+ const az1 = (z1 + 0.5);
+ const ax2 = (x2 + 0.5);
+ const ay2 = (y2 + 0.5);
+ const az2 = (z2 + 0.5);
+ for (let y = ay1; y < ay2; y++) {
+ for (let x = ax1; x < ax2; x++) {
+ geometries.push(
+ topWallGeometry.clone()
+ .applyMatrix4(new THREE.Matrix4().makeTranslation(x, y, az1)),
+ );
+ geometries.push(
+ bottomWallGeometry.clone()
+ .applyMatrix4(new THREE.Matrix4().makeTranslation(x, y, (az2 - 1))),
+ );
+ }
+ for (let z = az1; z < az2; z++) {
+ geometries.push(
+ leftWallGeometry.clone()
+ .applyMatrix4(new THREE.Matrix4().makeTranslation(ax1, y, z)),
+ );
+ geometries.push(
+ rightWallGeometry.clone()
+ .applyMatrix4(new THREE.Matrix4().makeTranslation((ax2 - 1), y, z)),
+ );
+ }
+ }
+ return BufferGeometryUtils.mergeBufferGeometries(geometries);
+ })();
+ const gridVsh = `
+ // varying vec3 vWorldPos;
+ // varying vec2 vUv;
+ varying float vDepth;
+ void main() {
+ gl_Position = projectionMatrix * modelViewMatrix * vec4(position, 1.);
+ // vUv = uv;
+ // vWorldPos = abs(position);
+ vDepth = gl_Position.z / ${distanceFactor.toFixed(8)};
+ }
+ `;
+ const gridFsh = `
+ // uniform sampler2D uTex;
+ uniform vec3 uColor;
+ // uniform float uAnimation;
+ // varying vec3 vWorldPos;
+ varying float vDepth;
+ void main() {
+ gl_FragColor = vec4(uColor, (1.0-vDepth));
+ }
+ `;
+ const material = new THREE.ShaderMaterial({
+ uniforms: {
+ uColor: {
+ type: 'c',
+ value: new THREE.Color(color),
+ },
+ },
+ vertexShader: gridVsh,
+ fragmentShader: gridFsh,
+ transparent: true,
+ });
+ const mesh = new THREE.Mesh(geometry, material);
+ mesh.frustumCulled = false;
+ mesh.setColor = c => {
+ mesh.material.uniforms.uColor.value.setHex(c);
+ };
+ return mesh;
+} */
+
export {
/* LAND_SHADER,
WATER_SHADER,
| 0 |
diff --git a/index.html b/index.html <input class="form__field" type="number"
placeholder="Enter Radius" id="inputradiushemisph"
oninput="solvehemisphere();">
- <p class="stopwrap" id="resultofvolhollowsp"
+ <p class="stopwrap" id="resultofvolhemisp"
style="font-family:mathfont;"></p>
- <p class="stopwrap" id="resultoftsahollowsp"
+ <p class="stopwrap" id="resultoftsahemisp"
style="font-family:mathfont;"></p>
</div>
<!---TILL-->
| 1 |
diff --git a/packages/diffhtml-website/public/styles/index.css b/packages/diffhtml-website/public/styles/index.css @import 'https://cdnjs.cloudflare.com/ajax/libs/highlight.js/9.12.0/styles/tomorrow-night.min.css';
-@import 'https://fonts.googleapis.com/css?family=Lato:300,400,bold';
+@import 'https://fonts.googleapis.com/css?family=Lato:300,400,bold&display=swap';
@import 'https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css';
html, body {
| 7 |
diff --git a/src/utils/wallet.js b/src/utils/wallet.js @@ -442,6 +442,7 @@ class Wallet {
async getLedgerAccountIds() {
const publicKey = await this.getLedgerPublicKey()
+ await store.dispatch(setLedgerTxSigned(true))
// TODO: getXXX methods shouldn't be modifying the state
await setKeyMeta(publicKey, { type: 'ledger' })
| 12 |
diff --git a/src/components/EditableField/EditableFieldComposite.tsx b/src/components/EditableField/EditableFieldComposite.tsx @@ -70,6 +70,9 @@ export class EditableFieldComposite extends React.PureComponent<
size: props.size,
onInputFocus: this.handleFieldFocus(child.props.onInputFocus),
onInputBlur: this.handleFieldBlur(child.props.onInputBlur),
+ onKeyDown: this.handleFieldKeyEvent(child.props.onInputKeyDown),
+ onKeyPress: this.handleFieldKeyEvent(child.props.onInputKeyPress),
+ onKeyUp: this.handleFieldKeyEvent(child.props.onInputKeyUp),
onChange: this.handleChange(child.props.onChange),
onEnter: this.handleEnter(child.props.onEnter),
onEscape: this.handleEscape(child.props.onEscape),
@@ -157,6 +160,12 @@ export class EditableFieldComposite extends React.PureComponent<
}
}
+ handleFieldKeyEvent = passedFn => {
+ return (...params) => {
+ passedFn && passedFn(...params)
+ }
+ }
+
handleFieldBlur = passedFn => {
return () => {
passedFn && passedFn()
| 9 |
diff --git a/scripts/get-quests.js b/scripts/get-quests.js @@ -3,7 +3,6 @@ const path = require('path');
const got = require('got');
-const allItems = require('../src/data/all-en.json');
const traders = require('../src/data/traders');
const formatName = (name) => {
@@ -45,8 +44,7 @@ const getTraderId = (name) => {
}
const findItem = {
- id: allItems.find(item => formatName(item.name) === formatName(objective.target))?.id,
- name: objective.target,
+ id: objective.target,
count: objective.number,
foundInRaid: objective.type === 'find' ? true : false,
};
| 4 |
diff --git a/articles/tokens/id-token.md b/articles/tokens/id-token.md @@ -96,8 +96,8 @@ Once issued, tokens can not be revoked in the same fashion as cookies with sessi
## More Information
* [Overview of JSON Web Tokens](/jwt)
-* [Silent Authentication for Single Page Apps](/api-auth/tutorials/silent-authentication).
-* [How to get, use and revoke a Refresh Token](/tokens/refresh-token).
+* [Silent Authentication for Single Page Apps](/api-auth/tutorials/silent-authentication)
+* [How to get, use and revoke a Refresh Token](/tokens/refresh-token)
* [A writeup on the contents of a JSON Web Token](https://scotch.io/tutorials/the-anatomy-of-a-json-web-token)
* [Wikipedia page on JSON Web Tokens](https://en.wikipedia.org/wiki/JSON_Web_Token)
* [IETF RFC for JWT](https://tools.ietf.org/html/rfc7519)
| 2 |
diff --git a/app/shared/components/ColdWallet/Wallet/Transaction.js b/app/shared/components/ColdWallet/Wallet/Transaction.js @@ -48,7 +48,7 @@ class ColdWalletTransaction extends Component<Props> {
const validContracts = ['eosio', 'eosio.token', 'eosio.msig'];
const validActions = actions.filter((action) => validContracts.indexOf(action.account) >= 0);
const invalidContract = validActions.length !== actions.length;
- const disabled = (expired || signed || invalidContract);
+ const disabled = (signed || invalidContract);
return (
<Segment basic>
<Segment attached="top">
| 11 |
diff --git a/token-metadata/0x49E833337ECe7aFE375e44F4E3e8481029218E5c/metadata.json b/token-metadata/0x49E833337ECe7aFE375e44F4E3e8481029218E5c/metadata.json "symbol": "VALUE",
"address": "0x49E833337ECe7aFE375e44F4E3e8481029218E5c",
"decimals": 18,
- "dharmaVerificationStatus": {
"dharmaVerificationStatus": "VERIFIED"
}
\ No newline at end of file
-}
\ No newline at end of file
| 3 |
diff --git a/token-metadata/0x035dF12E0F3ac6671126525f1015E47D79dFEDDF/metadata.json b/token-metadata/0x035dF12E0F3ac6671126525f1015E47D79dFEDDF/metadata.json "symbol": "0XMR",
"address": "0x035dF12E0F3ac6671126525f1015E47D79dFEDDF",
"decimals": 18,
- "dharmaVerificationStatus": {
"dharmaVerificationStatus": "VERIFIED"
}
\ No newline at end of file
-}
\ No newline at end of file
| 3 |
diff --git a/tests/mocha/block_test.js b/tests/mocha/block_test.js @@ -105,7 +105,7 @@ suite('Blocks', function() {
// Add extra input to middle block
blocks.B.appendValueInput("INPUT").setCheck(null);
blocks.B.unplug(true);
- assertUnpluggedNoheal(blocks);
+ assertUnpluggedHealed(blocks);
});
test('Child block has multiple inputs', function() {
| 1 |
diff --git a/README.md b/README.md @@ -74,24 +74,23 @@ $ npm install vue
## Build Setup
``` bash
-Once you have cloned this repo :
-# install dependencies
-npm install
+# Once you have cloned this repo, install dependencies
+$ npm install
# build for development and production with minification
-npm run build
+$ npm run build
```
## Run demo locally
``` bash
# Run demo at localhost:8080
-npm link
-cd examples
-npm link vue2-leaflet
-npm install
+$ npm link
+$ cd examples
+$ npm link vue2-leaflet
+$ npm install
# serve with hot reload at localhost:8080
-npm run dev
+$ npm run dev
```
Go to <http://localhost:8080/> to see a some examples
| 1 |
diff --git a/src/components/Main/Main.tsx b/src/components/Main/Main.tsx @@ -215,7 +215,9 @@ function Main() {
result={result}
caseCounts={empiricalCases}
scenarioUrl={scenarioUrl}
- openPrintPreview={() => setPrintable(true)}
+ openPrintPreview={() => {
+ setPrintable(true)
+ }}
/>
</Col>
</Row>
| 1 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.