code
stringlengths 122
4.99k
| label
int64 0
14
|
---|---|
diff --git a/client/src/components/views/Status/components/dilithiumStress.js b/client/src/components/views/Status/components/dilithiumStress.js @@ -20,27 +20,32 @@ const SUB = gql`
class DilithiumStress extends Component {
// This calculation came from client/src/components/views/DilithiumStress/dilithiumStress.js
- calcStressLevel = (reactor) => {
+ calcStressLevel = reactor => {
const { alphaTarget, betaTarget } = reactor;
const { alphaLevel, betaLevel } = reactor;
const alphaDif = Math.abs(alphaTarget - alphaLevel);
const betaDif = Math.abs(betaTarget - betaLevel);
const stressLevel = alphaDif + betaDif > 100 ? 100 : alphaDif + betaDif;
return stressLevel;
- }
+ };
- getDilithiumStressCard = (station) => {
- if (!station) return null;
- return station.cards.find(card => card.component === 'DilithiumStress');
- }
+ getDilithiumStressCard = stations => {
+ if (!stations) return null;
+ return stations
+ .map(s => s.cards.find(card => card.component === "DilithiumStress"))
+ .filter(Boolean)[0];
+ };
render() {
if (this.props.data.loading || !this.props.data.reactors) return null;
- const dilithiumStressCard = this.getDilithiumStressCard(this.props.station)
+ console.log(this.props);
+ const dilithiumStressCard = this.getDilithiumStressCard(
+ this.props.simulator.stations
+ );
if (!dilithiumStressCard) return null;
const reactor = this.props.data.reactors && this.props.data.reactors[0];
if (!reactor) return null;
- const stressLevel = this.calcStressLevel(reactor) / 100
+ const stressLevel = this.calcStressLevel(reactor) / 100;
return (
<div>
<SubscriptionHelper
| 1 |
diff --git a/test/browser/http.test.js b/test/browser/http.test.js @@ -6,7 +6,10 @@ define(['ably', 'shared_helper', 'chai'], function (Ably, helper, chai) {
describe('rest/http/fetch', function () {
this.timeout(60 * 1000);
+ let initialXhrSupported, initialJsonpSupported;
before(function (done) {
+ initialXhrSupported = Ably.Rest.Platform.Config.xhrSupported;
+ initialJsonpSupported = Ably.Rest.Platform.Config.jsonpSupported;
Ably.Rest.Platform.Config.xhrSupported = false;
Ably.Rest.Platform.Config.jsonpSupported = false;
helper.setupApp(function () {
@@ -16,8 +19,8 @@ define(['ably', 'shared_helper', 'chai'], function (Ably, helper, chai) {
});
after((done) => {
- Ably.Rest.Platform.Config.xhrSupported = window.XMLHttpRequest && 'withCredentials' in new XMLHttpRequest();
- Ably.Rest.Platform.Config.jsonpSupported = typeof document !== 'undefined';
+ Ably.Rest.Platform.Config.xhrSupported = initialXhrSupported;
+ Ably.Rest.Platform.Config.jsonpSupported = initialJsonpSupported;
done();
});
| 12 |
diff --git a/publish/src/commands/remove-synths.js b/publish/src/commands/remove-synths.js 'use strict';
const fs = require('fs');
-const { gray, yellow, red, cyan } = require('chalk');
-const Web3 = require('web3');
-const w3utils = require('web3-utils');
+const { gray, yellow, red, cyan, green } = require('chalk');
+const ethers = require('ethers');
const {
toBytes32,
+ getUsers,
constants: { CONFIG_FILENAME, DEPLOYMENT_FILENAME },
} = require('../../..');
@@ -18,9 +18,10 @@ const {
loadConnections,
confirmAction,
stringify,
- performTransactionalStepWeb3,
} = require('../util');
+const { performTransactionalStep } = require('../command-utils/transact');
+
const DEFAULTS = {
network: 'kovan',
gasLimit: 3e5,
@@ -34,6 +35,8 @@ const removeSynths = async ({
gasLimit = DEFAULTS.gasLimit,
synthsToRemove = [],
yes,
+ useFork,
+ dryRun = false,
privateKey,
}) => {
ensureNetwork(network);
@@ -74,6 +77,7 @@ const removeSynths = async ({
const { providerUrl, privateKey: envPrivateKey, etherscanLinkPrefix } = loadConnections({
network,
+ useFork,
});
// allow local deployments to use the private key passed as a CLI option
@@ -81,12 +85,21 @@ const removeSynths = async ({
privateKey = envPrivateKey;
}
- const web3 = new Web3(new Web3.providers.HttpProvider(providerUrl));
- web3.eth.accounts.wallet.add(privateKey);
- const account = web3.eth.accounts.wallet[0].address;
- console.log(gray(`Using account with public key ${account}`));
+ const provider = new ethers.providers.JsonRpcProvider(providerUrl);
+ let wallet;
+ if (useFork) {
+ const account = getUsers({ network, user: 'owner' }).address; // protocolDAO
+ wallet = provider.getSigner(account);
+ } else {
+ wallet = new ethers.Wallet(privateKey, provider);
+ }
+ if (!wallet.address) wallet.address = wallet._address;
+
+ console.log(gray(`Using account with public key ${wallet.address}`));
console.log(gray(`Using gas of ${gasPrice} GWEI with a max of ${gasLimit}`));
+ console.log(gray('Dry-run:'), dryRun ? green('yes') : yellow('no'));
+
if (!yes) {
try {
await confirmAction(
@@ -104,14 +117,16 @@ const removeSynths = async ({
}
}
- const Synthetix = new web3.eth.Contract(
+ const Synthetix = new ethers.Contract(
+ deployment.targets['Synthetix'].address,
deployment.sources['Synthetix'].abi,
- deployment.targets['Synthetix'].address
+ wallet
);
- const Issuer = new web3.eth.Contract(
+ const Issuer = new ethers.Contract(
+ deployment.targets['Issuer'].address,
deployment.sources['Issuer'].abi,
- deployment.targets['Issuer'].address
+ wallet
);
// deep clone these configurations so we can mutate and persist them
@@ -124,9 +139,9 @@ const removeSynths = async ({
`Synth${currencyKey}`
];
const { abi: synthABI } = deployment.sources[synthSource];
- const Synth = new web3.eth.Contract(synthABI, synthAddress);
+ const Synth = new ethers.Contract(synthAddress, synthABI, wallet);
- const currentSynthInSNX = await Synthetix.methods.synths(toBytes32(currencyKey)).call();
+ const currentSynthInSNX = await Synthetix.synths(toBytes32(currencyKey));
if (synthAddress !== currentSynthInSNX) {
console.error(
@@ -141,7 +156,7 @@ const removeSynths = async ({
}
// now check total supply (is required in Synthetix.removeSynth)
- const totalSupply = w3utils.fromWei(await Synth.methods.totalSupply().call());
+ const totalSupply = ethers.utils.formatEther(await Synth.totalSupply());
if (Number(totalSupply) > 0) {
console.error(
red(
@@ -155,8 +170,11 @@ const removeSynths = async ({
}
// perform transaction if owner of Synthetix or append to owner actions list
- await performTransactionalStepWeb3({
- account,
+ if (dryRun) {
+ console.log(green('Would attempt to remove the synth:', currencyKey));
+ } else {
+ await performTransactionalStep({
+ account: wallet,
contract: 'Issuer',
target: Issuer,
write: 'removeSynth',
@@ -182,6 +200,7 @@ const removeSynths = async ({
updatedSynths = updatedSynths.filter(({ name }) => name !== currencyKey);
fs.writeFileSync(synthsFile, stringify(updatedSynths));
}
+ }
};
module.exports = {
@@ -197,6 +216,13 @@ module.exports = {
.option('-g, --gas-price <value>', 'Gas price in GWEI', 1)
.option('-l, --gas-limit <value>', 'Gas limit', 1e6)
.option('-n, --network <value>', 'The network to run off.', x => x.toLowerCase(), 'kovan')
+ .option('-r, --dry-run', 'Dry run - no changes transacted')
+ .option(
+ '-k, --use-fork',
+ 'Perform the deployment on a forked chain running on localhost (see fork command).',
+ false
+ )
+ .option('-y, --yes', 'Dont prompt, just reply yes.')
.option(
'-s, --synths-to-remove <value>',
'The list of synths to remove',
| 2 |
diff --git a/README.md b/README.md @@ -57,9 +57,7 @@ Built on top of [node-postgres], this library adds the following:
# Support & Sponsorship
-<a href='https://lisk.io' alt='LISK' style='outline : none;' target='_blank'>
- 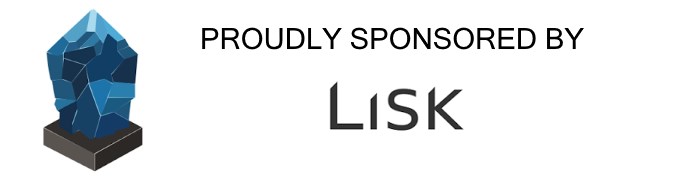
-</a>
+<a href='https://lisk.io' alt='LISK' style='outline : none;' target='_blank'>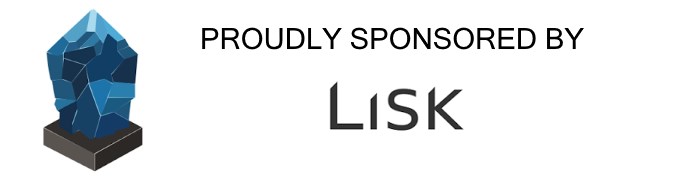</a>
If you like what we do, and would like to see it continue, then please consider donating:
| 1 |
diff --git a/docs/connections.jade b/docs/connections.jade @@ -211,7 +211,7 @@ block content
Mongoose creates a _default connection_ when you call `mongoose.connect()`.
You can access the default connection using `mongoose.connection`.
- <h3 id="connection_pools"><a href="connection_pools">Connection pools</a></h3>
+ <h3 id="connection_pools"><a href="#connection_pools">Connection pools</a></h3>
Each `connection`, whether created with `mongoose.connect` or
`mongoose.createConnection` are all backed by an internal configurable
| 1 |
diff --git a/src/OpenCloseMixin.js b/src/OpenCloseMixin.js @@ -37,7 +37,7 @@ export default function OpenCloseMixin(Base) {
* @type {boolean}
*/
get closed() {
- return !this.state.opened;
+ return this.state && !this.state.opened;
}
set closed(closed) {
const parsed = String(closed) === 'true';
@@ -121,7 +121,7 @@ export default function OpenCloseMixin(Base) {
* @type {boolean}
*/
get opened() {
- return this.state.opened;
+ return this.state && this.state.opened;
}
set opened(opened) {
const parsed = String(opened) === 'true';
| 11 |
diff --git a/test/sol5/networkHelper.js b/test/sol5/networkHelper.js @@ -309,7 +309,7 @@ module.exports.removeReservesFromNetwork = async function (networkInstance, rese
module.exports.genReserveID = genReserveID;
function genReserveID(reserveID, reserveAddress) {
- return reserveID + reserveAddress.substring(2,20) + "0".repeat(38);
+ return reserveID + reserveAddress.substring(2,20);
}
| 13 |
diff --git a/lib/assets/test/spec/cartodb/common/dialogs/change_privacy/change_privacy_view.spec.js b/lib/assets/test/spec/cartodb/common/dialogs/change_privacy/change_privacy_view.spec.js @@ -126,20 +126,6 @@ describe('dashboard/dialogs/change_privacy/change_privacy_view', function() {
});
});
- describe('_checkPrivacyChange', function () {
- it('should open PrivacyWarningView', function (done) {
- view._checkPrivacyChange();
-
- var dialogView = document.querySelector('.Dialog');
- expect(dialogView.innerText).toContain('components.modals.privacy-warning.title');
-
- view.clean();
-
- // Wait for the modal to close
- setTimeout(function () { done(); }, 250);
- });
- });
-
afterEach(function() {
if (this.view) {
this.view.clean();
| 2 |
diff --git a/scenes/classroom.scn b/scenes/classroom.scn 0
],
"start_url": "https://webaverse.github.io/classroom/"
+ },
+ {
+ "position": [1, 2, -4.5],
+ "quaternion": [0, 0, 0, 1],
+ "scale": [3, 3, 3],
+ "start_url": "https://https-vrchat-com.proxy.webaverse.com/"
}
]
}
\ No newline at end of file
| 0 |
diff --git a/assets/js/components/surveys/SurveyQuestionOpenText.js b/assets/js/components/surveys/SurveyQuestionOpenText.js @@ -34,6 +34,8 @@ import SurveyHeader from './SurveyHeader';
import { TextField, Input, HelperText } from '../../material-components';
import Button from '../Button';
+const MAXIMUM_CHARACTER_LIMIT = 100;
+
const SurveyQuestionOpenText = ( {
question,
answerQuestion,
@@ -48,7 +50,7 @@ const SurveyQuestionOpenText = ( {
};
const onChange = useCallback( ( event ) => {
- setValue( event.target.value );
+ setValue( event.target.value?.slice( 0, MAXIMUM_CHARACTER_LIMIT ) );
}, [ setValue ] );
return (
@@ -70,6 +72,8 @@ const SurveyQuestionOpenText = ( {
onChange={ onChange }
label={ placeholder }
noLabel
+ // docs are v11. we have v2. not sure what to do
+ // error
>
<Input
value={ value }
| 12 |
diff --git a/modules/xerte/parent_templates/Nottingham/models_html5/opinion.html b/modules/xerte/parent_templates/Nottingham/models_html5/opinion.html }
}
- this.weighting = x_currentPageXML.getAttribute("trackingWeight") != undefined ? x_currentPageXML.getAttribute("trackingWeight") != undefined : 1.0;
+ this.weighting = x_currentPageXML.getAttribute("trackingWeight") != undefined ? x_currentPageXML.getAttribute("trackingWeight") : 1.0;
XTSetPageType(x_currentPage, 'numeric', numberOfQuestions, this.weighting);
| 1 |
diff --git a/packages/node_modules/@node-red/editor-client/src/js/nodes.js b/packages/node_modules/@node-red/editor-client/src/js/nodes.js @@ -1235,6 +1235,9 @@ RED.nodes = (function() {
if (defaultWorkspace == null) {
defaultWorkspace = n;
}
+ if (activeWorkspace === 0) {
+ activeWorkspace = n.id;
+ }
if (createNewIds || options.importMap[n.id] === "copy") {
nid = getID();
workspace_map[n.id] = nid;
@@ -1490,6 +1493,9 @@ RED.nodes = (function() {
delete node.wires;
delete node.inputLabels;
delete node.outputLabels;
+ if (!n.z) {
+ delete node.z;
+ }
}
var orig = {};
for (var p in n) {
| 2 |
diff --git a/website/src/_posts/2016-09-0.10.md b/website/src/_posts/2016-09-0.10.md @@ -15,7 +15,7 @@ We have done a lot of research. We created [prototypes of Uppy React components]
<!-- more -->
-Finally, we are also discussing using [Redux](https://github.com/reactjs/redux) as the internal state management store within Uppy. You can see some drafts under the [`src/experimental` folder](https://github.com/transloadit/uppy/tree/master/src/experimental).
+Finally, we are also discussing using [Redux](https://github.com/reactjs/redux) as the internal state management store within Uppy. You can see some drafts under the `src/experimental` folder (EDIT: this folder has since been removed).
Have a look at the [Uppy Base repository here](https://github.com/hedgerh/uppy-base).
| 2 |
diff --git a/_labs/Genspace/Genspace.md b/_labs/Genspace/Genspace.md @@ -7,7 +7,7 @@ promotions:
- button: Take a Class
text: Genspace instructors come from top institutions around the New York
City area to teach advanced biology in an easy-to-understand, hands-on way.
- URL: https://www.genspace.org/classes-alt
+ URL: https://www.genspace.org/classes/
type-org: non-profit
address: 140 32nd Street
directions: Suite 108
| 1 |
diff --git a/src/services/verifiedProjectsGiversReport/verifiedPerojectsGiversReport.service.js b/src/services/verifiedProjectsGiversReport/verifiedPerojectsGiversReport.service.js @@ -8,7 +8,7 @@ const { findVerifiedTraces } = require('../../repositories/traceRepository');
const { getTokenByAddress } = require('../../utils/tokenHelper');
const { getTraceUrl, getCampaignUrl, getCommunityUrl } = require('../../utils/urlUtils');
-const fillProjectInfo = donation => {
+const extractProjectInfo = donation => {
const { campaign, trace, community } = donation;
if (campaign.length === 1) {
return {
@@ -82,7 +82,7 @@ module.exports = function aggregateDonations() {
// donations to communities may have both delegateId and ownerId but we should consider delegateId for them
donation.projectId = donation.delegateId || donation.ownerId;
- donation.projectInfo = fillProjectInfo(donation);
+ donation.projectInfo = extractProjectInfo(donation);
delete donation.delegateId;
delete donation.ownerId;
delete donation.campaign;
| 10 |
diff --git a/geometry-tool.js b/geometry-tool.js @@ -304,6 +304,7 @@ const shapeMaterial = (() => {
const map = new THREE.Texture();
map.wrapS = THREE.RepeatWrapping;
map.wrapT = THREE.RepeatWrapping;
+ map.anisotropy = 16;
{
const img = new Image();
img.onload = () => {
@@ -319,6 +320,7 @@ const shapeMaterial = (() => {
const normalMap = new THREE.Texture();
normalMap.wrapS = THREE.RepeatWrapping;
normalMap.wrapT = THREE.RepeatWrapping;
+ normalMap.anisotropy = 16;
{
const img = new Image();
img.onload = () => {
@@ -334,6 +336,7 @@ const shapeMaterial = (() => {
const heightMap = new THREE.Texture();
heightMap.wrapS = THREE.RepeatWrapping;
heightMap.wrapT = THREE.RepeatWrapping;
+ heightMap.anisotropy = 16;
{
const img = new Image();
img.onload = () => {
| 0 |
diff --git a/addon/components/base/bs-dropdown.js b/addon/components/base/bs-dropdown.js @@ -41,9 +41,10 @@ import layout from 'ember-bootstrap/templates/components/bs-dropdown';
contextual component rather than a standalone dropdown to ensure the correct styling
regardless of your Bootstrap version.
- > Note: the use of angle brackets `<ddm.linkTo>` as shown above is only supported for Ember 3.10, as it relies on its
- > own native implementation of the `LinkComponent`. For older Ember versions please use the legacy syntax with positional
- > arguments: `{{#ddm.link-to "bar" this.model}}Bar{{/ddm.link-to}}`
+ > Note: the use of angle brackets `<ddm.linkTo>` as shown above is only supported for Ember >= 3.10, as it relies on its
+ > Ember's native implementation of the [`LinkComponent`](https://api.emberjs.com/ember/3.12/classes/Ember.Templates.helpers/methods/link-to?anchor=link-to).
+ > For older Ember versions please use the legacy syntax with positional arguments:
+ > `{{#ddm.link-to "bar" this.model}}Bar{{/ddm.link-to}}`
### Button dropdowns
| 7 |
diff --git a/package.json b/package.json "main": "dist/mo.js",
"unpkg": "dist/mo.umd.js",
"scripts": {
+ "lint": "eslint \"**/*.js\"",
"dev": "webpack-dev-server --config webpack.dev.js",
"build": "webpack --config webpack.umd.js --progress",
"pretest": "webpack --config webpack.umd.js",
| 0 |
diff --git a/public/index.html b/public/index.html name="viewport"
content="width=device-width, height=device-height, initial-scale=1, shrink-to-fit=no, maximum-scale=1"
/>
- <meta name="theme-color" content="#000000" />
+ <meta name="theme-color" content="#00AFFF" />
<link rel="manifest" href="%PUBLIC_URL%/manifest.json" />
<link rel="apple-touch-icon" sizes="57x57" href="/apple-icon-57x57.png" />
<link rel="apple-touch-icon" sizes="60x60" href="/apple-icon-60x60.png" />
| 0 |
diff --git a/includes/Core/Util/Entity_Factory.php b/includes/Core/Util/Entity_Factory.php namespace Google\Site_Kit\Core\Util;
use Google\Site_Kit\Context;
+use Google\Site_Kit\Plugin;
use WP_Query;
use WP_Post;
use WP_Term;
@@ -62,11 +63,8 @@ final class Entity_Factory {
if ( $wp_the_query instanceof WP_Query ) {
$entity = self::from_wp_query( $wp_the_query );
- if ( is_null( $entity ) || ! defined( 'AMP__VERSION' ) ) {
- return $entity;
- }
-
- return self::maybe_convert_to_amp_entity($_SERVER['REQUEST_URI'], $entity); // phpcs:ignore
+ $request_uri = Plugin::instance()->context()->input()->filter( INPUT_SERVER, 'REQUEST_URI' );
+ return self::maybe_convert_to_amp_entity( $request_uri, $entity );
}
return null;
@@ -92,10 +90,6 @@ final class Entity_Factory {
$entity = self::from_wp_query( $query );
- if ( is_null( $entity ) || ! defined( 'AMP__VERSION' ) ) {
- return $entity;
- }
-
return self::maybe_convert_to_amp_entity( $url, $entity );
}
@@ -508,28 +502,52 @@ final class Entity_Factory {
* @return Entity The initial or new entity for the given URL.
*/
private static function maybe_convert_to_amp_entity( $url, $entity ) {
+ if ( is_null( $entity ) || ! defined( 'AMP__VERSION' ) ) {
+ return $entity;
+ }
+
$url_parts = wp_parse_url( $url );
- $new_url_tail = '';
+ $current_url = $entity->get_url();
+
+ if ( ! empty( $url_parts['query'] ) ) {
+ $url_query_params = array();
wp_parse_str( $url_parts['query'], $url_query_params );
// check if the $url has amp query param.
if ( array_key_exists( 'amp', $url_query_params ) ) {
- $new_url_tail = '?amp=1';
+ $new_url = add_query_arg( 'amp', '1', $current_url );
+ return self::create_updated_amp_entity( $new_url, $entity );
+ }
}
- // check if the $url has `/amp` in path.
- if ( '/amp' === substr( untrailingslashit( $url_parts['path'] ), -4 ) ) { // -strlen('/amp') is -4
- $new_url_tail = '/amp';
+ if ( ! empty( $url_parts['path'] ) ) {
+ // We need to correctly add trailing slash if the original url had trailing slash.
+ // That's the reason why we need to check for both version.
+ if ( '/amp' === substr( $url_parts['path'], -4 ) ) { // -strlen('/amp') is -4
+ $new_url = untrailingslashit( $url_parts['path'] ) . '/amp';
+ return self::create_updated_amp_entity( $new_url, $entity );
}
- if ( empty( $new_url_tail ) ) {
- return $entity;
+ if ( '/amp/' === substr( $url_parts['path'], -5 ) ) { // -strlen('/amp/') is -5
+ $new_url = untrailingslashit( $url_parts['path'] ) . '/amp/';
+ return self::create_updated_amp_entity( $new_url, $entity );
+ }
}
- $new_url = $entity->get_url();
- $new_url = $new_url . $new_url_tail;
+ return $entity;
+ }
+ /**
+ * Creates updated AMP Entity..
+ *
+ * @since n.e.x.t
+ *
+ * @param string $new_url URL of the new entity.
+ * @param Entity $entity The initial entity.
+ * @return Entity The new entity.
+ */
+ private static function create_updated_amp_entity( $new_url, $entity ) {
$new_entity = new Entity(
$new_url,
array(
| 7 |
diff --git a/Block/Menu.php b/Block/Menu.php @@ -133,18 +133,19 @@ class Menu extends Template implements IdentityInterface
* @param NodeRepositoryInterface|null $parent
* @return array
*/
- public function getNodesData($level = 0, $parent = null)
+ public function getNodesTree($level = 0, $parent = null)
{
- $nodesData = [];
+ $nodesTree = [];
$nodes = $this->getNodes($level, $parent);
foreach ($nodes as $node) {
- $nodeData = $node->getData();
- $nodeData['children'] = $this->getNodesData($level + 1, $node);
- $nodesData[] = $nodeData;
+ $nodesTree[] = [
+ 'node' => $node,
+ 'children' => $this->getNodesTree($level + 1, $node)
+ ];
}
- return $nodesData;
+ return $nodesTree;
}
/**
| 14 |
diff --git a/assets/js/modules/analytics/components/dashboard/DashboardSearchVisitorsWidget.js b/assets/js/modules/analytics/components/dashboard/DashboardSearchVisitorsWidget.js @@ -171,18 +171,6 @@ function DashboardSearchVisitorsWidget( {
{ type: 'number', label: 'Unique Visitors from Search' },
],
];
- sparkData[ 0 ].data = {
- dataLastRefreshed: null,
- isDataGolden: null,
- rowCount: null,
- samplesReadCounts: null,
- samplingSpaceSizes: null,
- totals: [
- {
- values: [ '0' ],
- },
- ],
- };
const dataRows = sparkData?.[ 0 ]?.data?.rows || [];
| 2 |
diff --git a/html/components/dropdown.stories.js b/html/components/dropdown.stories.js @@ -162,7 +162,7 @@ export const informational = () => {
<div
class="sprk-c-Dropdown__footer sprk-u-TextAlign--center"
>
- <a class="sprk-c-Button sprk-c-Button--tertiary" href="#nogo">
+ <a class="sprk-c-Button sprk-c-Button--secondary" href="#nogo">
Go Elsewhere
</a>
</div>
| 3 |
diff --git a/test/jasmine/tests/dragelement_test.js b/test/jasmine/tests/dragelement_test.js @@ -4,6 +4,7 @@ var d3 = require('d3');
var createGraphDiv = require('../assets/create_graph_div');
var destroyGraphDiv = require('../assets/destroy_graph_div');
var mouseEvent = require('../assets/mouse_event');
+var touchEvent = require('../assets/touch_event');
describe('dragElement', function() {
@@ -205,6 +206,30 @@ describe('dragElement', function() {
expect(mockObj.dummy).not.toHaveBeenCalled();
});
+
+ it('should not register move event handler when dragmode is false', function() {
+ var moveCalls = 0;
+ var options = {
+ element: this.element,
+ gd: this.gd,
+ dragmode: false,
+ moveFn: function() {
+ moveCalls++;
+ }
+ };
+ dragElement.init(options);
+ mouseEvent('mousedown', this.x, this.y);
+ mouseEvent('mousemove', this.x + 10, this.y + 10);
+ mouseEvent('mouseup', this.x, this.y);
+
+ expect(moveCalls).toBe(0);
+
+ touchEvent('touchstart', this.x, this.y);
+ touchEvent('touchmove', this.x + 10, this.y + 10);
+ touchEvent('touchend', this.x, this.y);
+
+ expect(moveCalls).toBe(0);
+ });
});
describe('dragElement.getCursor', function() {
| 0 |
diff --git a/js/bam/htsgetReader.js b/js/bam/htsgetReader.js import AlignmentContainer from "./alignmentContainer.js";
import BamUtils from "./bamUtils.js";
import {igvxhr, BGZip} from "../../node_modules/igv-utils/src/index.js";
+import {buildOptions} from "../util/igvUtils.js";
class HtsgetReader {
@@ -63,7 +64,7 @@ class HtsgetReader {
'&start=' + start +
'&end=' + end;
- const data = await igvxhr.loadJson(url, this.config);
+ const data = await igvxhr.loadJson(url, buildOptions(this.config));
const dataArr = await loadUrls(data.htsget.urls);
const compressedData = concatArrays(dataArr); // In essence a complete bam file
const unc = BGZip.unbgzf(compressedData.buffer);
@@ -94,14 +95,14 @@ async function loadUrls(urls) {
const promiseArray = [];
- urls.forEach(function (urlData) {
+ for(let urlData of urls) {
if (urlData.url.startsWith('data:')) {
// this is a data-uri
promiseArray.push(Promise.resolve(dataUriToBytes(urlData.url)));
} else {
- const options = {};
+ const options = buildOptions(this.config);
if (urlData.headers) {
options.headers = urlData.headers;
@@ -117,7 +118,7 @@ async function loadUrls(urls) {
});
}));
}
- });
+ }
return Promise.all(promiseArray)
}
| 4 |
diff --git a/main/windows/windowURL.js b/main/windows/windowURL.js @@ -11,7 +11,7 @@ const windowURL = page => {
}
const devPath = `http://localhost:8000/${page}`
let prodPath = format({
- pathname: resolve(`renderer/out/${page}/index.html`),
+ pathname: resolve(`renderer/out/${page}.html`),
protocol: 'file:',
slashes: true
})
| 4 |
diff --git a/__tests__/applyAtRule.test.js b/__tests__/applyAtRule.test.js @@ -206,7 +206,6 @@ test('you can apply utility classes without using the given prefix', () => {
...defaultConfig.options,
prefix: 'tw-',
},
- experiments: { shadowLookup: true },
}
return run(input, config, processPlugins(defaultPlugins(defaultConfig), config).utilities).then(
@@ -234,7 +233,6 @@ test('you can apply utility classes without using the given prefix when using a
return 'tw-'
},
},
- experiments: { shadowLookup: true },
}
return run(input, config, processPlugins(defaultPlugins(defaultConfig), config).utilities).then(
| 2 |
diff --git a/plugins/compute/app/views/compute/instances/index.html.haml b/plugins/compute/app/views/compute/instances/index.html.haml = render "intro"
-
-.toolbar
- / / seach form
%form
+ .toolbar.toolbar-controlcenter
+ / / seach form
.inputwrapper
- = select_tag 'searchfor', options_for_select(['Name', 'ID', 'IP', 'Status'], @searchfor)
- .toolbar-input-divider
- .input-group
- .has-feedback.has-feedback-searchable
+ = select_tag 'searchfor', options_for_select(['Name', 'ID', 'IP', 'Status'], @searchfor), class: "form-control"
+
+ .inputwrapper
+ .input-group.has-feedback.has-feedback-searchable
%input.form-control{:id => "search", :name => 'search', :placeholder => "Name, ID, IP or Status", :type => "text", :size => 40, :value => @search }
- .span.form-control-feedback.not-empty
- %button.btn.btn-sm{:type => 'button', :onclick => "$('#search').val('');"}
- %i.fa.fa-times-circle
.span.input-group-btn
%button.btn.btn-default{type: "submit"}
%i.fa.fa-search
- %a.help-link.has-feedback-help{href: "#", data: {toggle: "popover", "popover-type": "help-hint", content: 'ID and Status (Active, Shutoff, ...) are searched exactly with the given term. A Name and Fixed IP search gives as result all Servers where the term is contained'}}
+ %a.help-link.has-feedback-help{href: "#", data: {toggle: "popover", "popover-type": "help-hint", content: 'Search by name and fixed IP will find exact or partial matches. ID and status have to be exact matches to be found.'}}
%i.fa.fa-question-circle
-
- if current_user.is_allowed?("compute:instance_create", {target: { project: @active_project, scoped_domain_name: @scoped_domain_name}})
.main-buttons
= link_to 'Create new', plugin('compute').new_instance_path, data: {modal: true}, class: 'btn btn-primary'
| 1 |
diff --git a/src/platform/web/ui/general/utils.ts b/src/platform/web/ui/general/utils.ts @@ -14,10 +14,10 @@ See the License for the specific language governing permissions and
limitations under the License.
*/
-import {UIView, IMountArgs} from "./types";
+import {UIView, IMountArgs, ViewNode} from "./types";
import {tag} from "./html";
-export function mountView(view: UIView, mountArgs?: IMountArgs): HTMLElement {
+export function mountView(view: UIView, mountArgs?: IMountArgs): ViewNode {
let node;
try {
node = view.mount(mountArgs);
@@ -27,7 +27,7 @@ export function mountView(view: UIView, mountArgs?: IMountArgs): HTMLElement {
return node;
}
-export function errorToDOM(error: Error): HTMLElement {
+export function errorToDOM(error: Error): Element {
const stack = new Error().stack;
let callee: string | null = null;
if (stack) {
@@ -38,10 +38,10 @@ export function errorToDOM(error: Error): HTMLElement {
tag.h3(error.message),
tag.p(`This occurred while running ${callee}.`),
tag.pre(error.stack),
- ]) as HTMLElement;
+ ]);
}
-export function insertAt(parentNode: Element, idx: number, childNode: Element): void {
+export function insertAt(parentNode: Element, idx: number, childNode: Node): void {
const isLast = idx === parentNode.childElementCount;
if (isLast) {
parentNode.appendChild(childNode);
| 7 |
diff --git a/src/scripts/interaction.js b/src/scripts/interaction.js @@ -54,6 +54,22 @@ const isStaticLibrary = function (library, parameters) {
return staticLibraryTitles.indexOf(library) !== -1 || isGotoType(parameters, gotoType.TIME_CODE);
};
+/**
+ * @const {string[]}
+ */
+const scrollableLibraries = ['H5P.Text', 'H5P.Table'];
+
+/**
+ * Returns true if the given library is on the static libraries list or is goto type "timecode"
+ *
+ * @param {string} library
+ * @param {Parameters} parameters
+ * @return {boolean}
+ */
+const isScrollableLibrary = function (library) {
+ return staticLibraryTitles.indexOf(library) !== -1;
+};
+
/**
* @typedef {Object} Parameters Interaction settings
* @property {Object} action Library
@@ -265,7 +281,7 @@ function Interaction(parameters, player, previousState) {
const createLabel = (label, classes = '') => {
return $('<div/>', {
'class': `h5p-interaction-label ${classes}`,
- 'html': `<div class="h5p-interaction-label-text" tabindex="0">${label}</div>`
+ 'html': `<div class="h5p-interaction-label-text">${label}</div>`
});
};
@@ -455,7 +471,8 @@ function Interaction(parameters, player, previousState) {
// Create wrapper for dialog content
var $dialogContent = $(isGotoClickable ? '<a>' : '<div>', {
- 'class': 'h5p-dialog-interaction h5p-frame'
+ 'class': 'h5p-dialog-interaction h5p-frame',
+ 'tabindex': isScrollableLibrary(library) ? '0' : '' // Make content scrollable
});
$dialogContent.on('keyup', disableGlobalVideoControls);
@@ -463,7 +480,7 @@ function Interaction(parameters, player, previousState) {
if (player.editor !== undefined) {
$dialogContent.attr('tabindex', -1);
}
- // If tabbales no tabbable elements exist, make the dialog content tabbable
+ // If no tabbable elements exist, make the dialog content tabbable
else if (tabbableElements.length === 0) {
$dialogContent.attr('tabindex', 0);
}
@@ -780,7 +797,8 @@ function Interaction(parameters, player, previousState) {
}).appendTo($interaction);
$inner = $(isGotoClickable ? '<a>' : '<div>', {
- 'class': 'h5p-interaction-inner h5p-frame'
+ 'class': 'h5p-interaction-inner h5p-frame',
+ 'tabindex': isScrollableLibrary(library) ? '0' : '' // Make content scrollable
}).appendTo($outer);
if (player.editor !== undefined && instance.disableAutoPlay) {
| 12 |
diff --git a/src/components/EditableTextarea/EditableTextarea.tsx b/src/components/EditableTextarea/EditableTextarea.tsx @@ -229,6 +229,37 @@ export class EditableTextarea extends React.PureComponent<
}
}
+ handleOnKeyUp = e => {
+ const code = e.key
+
+ /* istanbul ignore next */
+ const stop = () => e.preventDefault() && e.stopPropagation()
+
+ if (code === key.ESCAPE) {
+ stop()
+
+ this.setState(
+ {
+ value: this.state.prevValue,
+ },
+ () => {
+ const { id } = this.props
+ const { value } = this.state
+ const item = {
+ value,
+ id,
+ }
+ this.props.onEscape({
+ name: id,
+ value: [item],
+ event: e,
+ })
+ this.textArea.current.blur()
+ }
+ )
+ }
+ }
+
handleOnBlur = e => {
const { id, onCommit, onInputBlur, validate } = this.props
const { prevValue, value, validated } = this.state
@@ -411,6 +442,7 @@ export class EditableTextarea extends React.PureComponent<
onClick={this.handleOnClick}
onHeightChange={this.handleTextareaHeightChange}
onKeyDown={this.handleOnKeyDown}
+ onKeyUp={this.handleOnKeyUp}
/>
<MaskUI
className={maskClasses}
| 9 |
diff --git a/bin/browsertimeWebPageReplay.js b/bin/browsertimeWebPageReplay.js @@ -7,12 +7,31 @@ const browsertime = require('browsertime');
const merge = require('lodash.merge');
const getURLs = require('../lib/cli/util').getURLs;
const get = require('lodash.get');
+const findUp = require('find-up');
+const fs = require('fs');
const browsertimeConfig = require('../lib/plugins/browsertime/index').config;
const iphone6UserAgent =
'Mozilla/5.0 (iPhone; CPU iPhone OS 6_1_3 like Mac OS X) AppleWebKit/536.26 ' +
'(KHTML, like Gecko) Version/6.0 Mobile/10B329 Safari/8536.25';
+const configPath = findUp.sync(['.sitespeed.io.json']);
+let config;
+
+try {
+ config = configPath ? JSON.parse(fs.readFileSync(configPath)) : {};
+} catch (e) {
+ if (e instanceof SyntaxError) {
+ /* eslint no-console: off */
+ console.error(
+ 'Could not parse the config JSON file ' +
+ configPath +
+ '. Is the file really valid JSON?'
+ );
+ }
+ throw e;
+}
+
async function testURLs(engine, urls) {
try {
await engine.start();
@@ -81,12 +100,18 @@ async function runBrowsertime() {
'Enables CPU throttling to emulate slow CPUs. Throttling rate as a slowdown factor (1 is no throttle, 2 is 2x slowdown, etc)',
group: 'chrome'
})
+ .option('config', {
+ describe:
+ 'Path to JSON config file. You can also use a .browsertime.json file that will automatically be found by Browsertime using find-up.',
+ config: 'config'
+ })
.option('browsertime.viewPort', {
alias: 'viewPort',
default: browsertimeConfig.viewPort,
describe: 'The browser view port size WidthxHeight like 400x300',
group: 'Browser'
- });
+ })
+ .config(config);
const defaultConfig = {
iterations: 1,
| 4 |
diff --git a/test/template.js b/test/template.js @@ -15,37 +15,37 @@ describe('TemplateGenerator', function () {
it('return correct zip filename for https link', function () {
let result = templateGenerator.getExternalProject("https://github.com/embark-framework/embark");
assert.strictEqual(result.url, "https://github.com/embark-framework/embark/archive/master.zip");
- assert.strictEqual(result.filePath, ".embark/templates/embark-framework/embark/archive.zip");
+ assert.strictEqual(result.filePath.replace(/\\/g,'/'), ".embark/templates/embark-framework/embark/archive.zip");
});
it('return correct zip filename for http link', function () {
let result = templateGenerator.getExternalProject("http://github.com/embark-framework/embark");
assert.strictEqual(result.url, "http://github.com/embark-framework/embark/archive/master.zip");
- assert.strictEqual(result.filePath, ".embark/templates/embark-framework/embark/archive.zip");
+ assert.strictEqual(result.filePath.replace(/\\/g,'/'), ".embark/templates/embark-framework/embark/archive.zip");
});
it('return correct zip filename without protocol specified ', function () {
let result = templateGenerator.getExternalProject("github.com/embark-framework/embark");
assert.strictEqual(result.url, "https://github.com/embark-framework/embark/archive/master.zip");
- assert.strictEqual(result.filePath, ".embark/templates/embark-framework/embark/archive.zip");
+ assert.strictEqual(result.filePath.replace(/\\/g,'/'), ".embark/templates/embark-framework/embark/archive.zip");
});
it('return correct zip filename without protocol specified ', function () {
let result = templateGenerator.getExternalProject("github.com/embark-framework/embark");
assert.strictEqual(result.url, "https://github.com/embark-framework/embark/archive/master.zip");
- assert.strictEqual(result.filePath, ".embark/templates/embark-framework/embark/archive.zip");
+ assert.strictEqual(result.filePath.replace(/\\/g,'/'), ".embark/templates/embark-framework/embark/archive.zip");
});
it('return correct zip filename with just username/repo specified', function () {
let result = templateGenerator.getExternalProject("embark-framework/embark");
assert.strictEqual(result.url, "https://github.com/embark-framework/embark/archive/master.zip");
- assert.strictEqual(result.filePath, ".embark/templates/embark-framework/embark/archive.zip");
+ assert.strictEqual(result.filePath.replace(/\\/g,'/'), ".embark/templates/embark-framework/embark/archive.zip");
});
it('return correct zip filename with just embark template specified', function () {
let result = templateGenerator.getExternalProject("react");
assert.strictEqual(result.url, "https://github.com/embark-framework/embark-react-template/archive/master.zip");
- assert.strictEqual(result.filePath, ".embark/templates/embark-framework/embark-react-template/archive.zip");
+ assert.strictEqual(result.filePath.replace(/\\/g,'/'), ".embark/templates/embark-framework/embark-react-template/archive.zip");
});
});
| 3 |
diff --git a/.codeclimate.yml b/.codeclimate.yml @@ -141,11 +141,13 @@ exclude_patterns:
- "test/"
- "tmp/"
- "workshops/"
+ - "**/_tools/"
- "**/benchmark/"
- "**/build/"
- "**/docs/"
- "**/etc/"
- "**/examples/"
+ - "**/namespace/lib/"
- "**/scripts/"
- "**/test/"
- "**/tmp/"
| 8 |
diff --git a/helpers/PrerequisiteChecker.php b/helpers/PrerequisiteChecker.php @@ -4,7 +4,7 @@ class ERequirementNotMet extends Exception
{
}
-const REQUIRED_PHP_EXTENSIONS = ['fileinfo', 'pdo_sqlite', 'gd', 'ctype', 'json', 'intl', 'zlib',
+const REQUIRED_PHP_EXTENSIONS = ['fileinfo', 'pdo_sqlite', 'gd', 'ctype', 'json', 'intl', 'zlib', 'mbstring',
// These are core extensions, so normally can't be missing, but seems to be the case, however, on FreeBSD
'filter', 'iconv', 'tokenizer'
];
| 0 |
diff --git a/assets/src/libraries/Library.js b/assets/src/libraries/Library.js @@ -65,9 +65,7 @@ class Library extends Component {
</div>
)}
>
- <div className="book-list">
<BookList books={this.state.books} />
- </div>
</InfiniteScroll>
</React.Fragment>
);
| 2 |
diff --git a/docs/articles/documentation/using-testcafe/using-testcafe-docker-image.md b/docs/articles/documentation/using-testcafe/using-testcafe-docker-image.md @@ -8,6 +8,15 @@ permalink: /documentation/using-testcafe/using-testcafe-docker-image.html
TestCafe provides a preconfigured Docker image with Chromium and Firefox installed.
Therefore, you can avoid manual installation of browsers and the testing framework on the server.
+* [Install Docker and Download TestCafe Image](#install-docker-and-download-testcafe-image)
+* [Test in Docker Containers](#test-in-docker-containers)
+* [Test on the Host Machine](#test-on-the-host-machine)
+* [Test on Remote Devices](#test-on-remote-devices)
+* [Test Heavy Websites](#test-heavy-websites)
+* [Proxy Settings](#proxy-settings)
+
+## Install Docker and Download TestCafe Image
+
To learn how to install Docker on your system, see [Install Docker](https://docs.docker.com/engine/installation/).
After Docker is installed, download the TestCafe Docker image from the repository.
@@ -22,7 +31,9 @@ The command above installs a stable version of the image. If you need an alpha v
docker pull testcafe/testcafe:alpha
```
-Now you can run TestCafe from the docker image.
+## Test in Docker Containers
+
+Use the `docker run` command to run TestCafe in the Docker container:
```sh
docker run -v ${TEST_FOLDER}:/tests -it testcafe/testcafe ${TESTCAFE_ARGS}
@@ -32,9 +43,13 @@ This command takes the following parameters:
* `-v ${TEST_FOLDER}:/tests` - maps the `TEST_FOLDER` directory on the host machine to the `/tests` directory in the container. You can map any host directory to any container directory:
- `-v //c/Users/Username/tests:/tests`
+ ```sh
+ -v //c/Users/Username/tests:/tests
+ ```
- `-v //d/tests:/myTests`
+ ```sh
+ -v //d/tests:/myTests
+ ```
Files referenced in tests (page models, utilities, Node.js modules) should be located in the mapped host directory or its subdirectories. Otherwise, they could not be accessed from the container.
@@ -45,13 +60,17 @@ This command takes the following parameters:
* `-it testcafe/testcafe` - runs TestCafe in the interactive mode with the console enabled;
* `${TESTCAFE_ARGS}` - arguments passed to the `testcafe` command. You can use any arguments from the TestCafe [command line interface](command-line-interface.md);
- `-it testcafe/testcafe 'chromium --no-sandbox,firefox' /tests/test.js`
+ ```sh
+ -it testcafe/testcafe 'chromium --no-sandbox,firefox' /tests/test.js
+ ```
You can run tests in the Chromium and Firefox browsers preinstalled to the Docker image. Add the `--no-sandbox` flag to Chromium if the container is run in the unprivileged mode.
You can pass a glob instead of the directory path in TestCafe parameters.
- `docker run -v //d/tests:/tests -it testcafe/testcafe firefox /tests/**/*.js`
+ ```sh
+ docker run -v //d/tests:/tests -it testcafe/testcafe firefox /tests/**/*.js
+ ```
> If tests use other Node.js modules, these modules should be located in the tests directory or its child directories. TestCafe will not be able to find modules in the parent directories.
@@ -101,7 +120,7 @@ docker run --add-host=${EXTERNAL_HOSTNAME}:127.0.0.1 -p ${PORT1}:${PORT1} -p ${P
where `${PORT1}` and `${PORT2}` are vacant container's ports, `${EXTERNAL_HOSTNAME}` is the host machine name.
-## Testing Heavy Websites
+## Test Heavy Websites
If you are testing a heavy website, you may need to allocate extra resources for the Docker image.
@@ -112,3 +131,13 @@ docker run --shm-size=1g -v /d/tests:/tests -it testcafe/testcafe firefox /tests
```
You can find a complete list of options that manage runtime constraints on resources in the [Docker documentation](https://docs.docker.com/engine/reference/run/#runtime-constraints-on-resources).
+
+## Proxy Settings
+
+Use the TestCafe [--proxy](command-line-interface.md#--proxy-host) and [--proxy-bypass](command-line-interface.md#--proxy-bypass-rules) options to configure proxy settings.
+
+```sh
+docker run -v /d/tests:/tests -it testcafe/testcafe remote /tests/test.js --proxy proxy.mycorp.com
+```
+
+> TestCafe ignores the [container's proxy settings](https://docs.docker.com/network/proxy/) specified in the Docker configuration file (`httpProxy`, `httpsProxy` and `noProxy`) or the environment variables (`HTTP_PROXY`, `HTTPS_PROXY` and `NO_PROXY`).
\ No newline at end of file
| 0 |
diff --git a/css/main.css b/css/main.css }
.bd-pfbtn {
- position: absolute;
- top: 60px;
- left: 120px;
+ position: relative;
+ top: -40px;
+ left: 80px;
+ margin-bottom: -19px;
background: #7289da;
color: #FFF;
border-radius: 5px;
| 1 |
diff --git a/src/utilities/text.less b/src/utilities/text.less .text-no-wrap { white-space: nowrap; }
.text-force-wrap { word-wrap: break-word; }
.text-smooth { -webkit-font-smoothing: antialiased; }
-.text-spaced { letter-spacing: 0.05em; }
.text-strike { text-decoration: line-through; }
.text-shadow-solid { text-shadow: 0 2px 0 rgba(0,0,0,0.15); }
.text-mono { font-family: @font-family-mono; }
&text-no-wrap { .text-no-wrap; }
&text-force-wrap { .text-force-wrap; }
&text-smooth { .text-smooth; }
- &text-spaced { .text-spaced; }
&text-strike { .text-strike; }
&text-shadow-solid { .text-shadow-solid; }
&text-mono { .text-mono; }
| 2 |
diff --git a/lib/Backend/EmitBuffer.cpp b/lib/Backend/EmitBuffer.cpp @@ -234,7 +234,11 @@ EmitBufferManager<TAlloc, TPreReservedAlloc, SyncObject>::FreeAllocation(void* a
{
if (!JITManager::GetJITManager()->IsJITServer() || CONFIG_FLAG(OOPCFGRegistration))
{
- threadContext->SetValidCallTargetForCFG(address, false);
+ void* callTarget = address;
+#if _M_ARM
+ callTarget = (void*)((uintptr_t)callTarget | 0x1); // add the thumb bit back, so we CFG-unregister the actual call target
+#endif
+ threadContext->SetValidCallTargetForCFG(callTarget, false);
}
}
VerboseHeapTrace(_u("Freeing 0x%p, allocation: 0x%p\n"), address, allocation->allocation->address);
| 12 |
diff --git a/admin-base/materialize/custom/_vue-form-generator.scss b/admin-base/materialize/custom/_vue-form-generator.scss .preview-list {
> li.preview-item {
clear: both;
- padding-bottom: 0;
.singlefield {
float: left;
padding-bottom: 0;
| 7 |
diff --git a/src/server/util/search.js b/src/server/util/search.js @@ -107,15 +107,16 @@ SearchClient.prototype.shouldIndexed = function(page) {
return page.creator != null && page.revision != null && page.redirectTo == null;
};
-// SEARCHBOX_SSL_URL is following format:
-// => https://{ID}:{PASSWORD}@{HOST}
SearchClient.prototype.parseUri = function(uri) {
let indexName = 'crowi';
let host = uri;
- const match = uri.match(/^(https?:\/\/[^/]+)\/(.+)$/);
+
+ // https://regex101.com/r/eKT4Db/4
+ const match = uri.match(/^(https?:\/\/[^/]+)\/?(.+)?$/);
+
if (match) {
host = match[1];
- indexName = match[2];
+ indexName = match[2] || 'growi';
}
return {
| 7 |
diff --git a/src/extras/config.js b/src/extras/config.js @@ -27,15 +27,24 @@ if(process.argv[2].endsWith('.json')){
//Try to load configuration
//TODO: create a lock file to prevent starting twice the same config file?
+let rawFile = null;
+try {
+ rawFile = fs.readFileSync(`data/${configName}.json`, 'utf8');
+} catch (error) {
+ logError(`Unnable to load configuration file 'data/${configName}.json'. (cannot read file, please read the documentation)`, context);
+ process.exit(0)
+}
+
let configFile = null;
try {
- let raw = fs.readFileSync(`data/${configName}.json`, 'utf8');
- configFile = JSON.parse(raw);
+ configFile = JSON.parse(rawFile);
} catch (error) {
- logError(`Unnable to load configuration file 'data/${configName}.json'`, context);
+ logError(`Unnable to load configuration file 'data/${configName}.json'. (json parse error, please read the documentation)`, context);
+ if(rawFile.includes('\\')) logError(`Note: your '${configName}.json' file contains '\\', make sure all your paths use only '/'.`, context)
process.exit(0)
}
+
let cfg = {
global: null,
logger: null,
| 7 |
diff --git a/accessibility-checker-engine/help/WCAG20_Label_RefValid.mdx b/accessibility-checker-engine/help/WCAG20_Label_RefValid.mdx @@ -8,7 +8,7 @@ import { CodeSnippet, Tag } from "carbon-components-react";
<Row>
<Column colLg={16} colMd={8} colSm={4} className="toolHead">
-### The value of the `<label>` `for` attribute is not the `id` of a valid `<input>` element
+### The value of the `<label>` `for` attribute does not match the value of the `id` of a valid `<input>` element
<div id="locLevel"></div>
| 2 |
diff --git a/lib/Client.js b/lib/Client.js @@ -346,6 +346,7 @@ class Client extends EventEmitter {
* @arg {String} [options.icon] The icon of the channel as a base64 data URI (group channels only). Note: base64 strings alone are not base64 data URI strings
* @arg {String} [options.ownerID] The ID of the channel owner (group channels only)
* @arg {String} [options.topic] The topic of the channel (guild text channels only)
+ * @arg {Boolean} [options.nsfw] The nsfw status of the channel (guild channels only)
* @arg {Number} [options.bitrate] The bitrate of the channel (guild voice channels only)
* @arg {Number} [options.userLimit] The channel user limit (guild voice channels only)
* @arg {String} [reason] The reason to be displayed in audit logs
@@ -357,6 +358,7 @@ class Client extends EventEmitter {
icon: options.icon,
owner_id: options.ownerID,
topic: options.topic,
+ nsfw: options.nsfw,
bitrate: options.bitrate,
user_limit: options.userLimit,
reason: reason
| 11 |
diff --git a/articles/connections/calling-an-external-idp-api.md b/articles/connections/calling-an-external-idp-api.md @@ -203,38 +203,3 @@ Your client will invoke the webtask with a simple HTTP request and manipulate th
:::note
You can find a sample [in this GitHub repository](https://github.com/vikasjayaram/ext-idp-api-webtask/tree/master/RS256). Review carefully before you use it since this is not officially maintained by Auth0 and could be outdated.
:::
-
-### Option 3: Use Auth0.js
-
-[Auth0.js](/libraries/auth0js) is a client-side library for Auth0. It enables you to authenticate a user and access the [Auth0 APIs](/api/info).
-
-For this scenario, we will use this library in order to access the Auth0 Management API and retrieve the full user's profile.
-
-The first step is to install the library. Pick your preferable option at [Auth0.js > Installation options](/libraries/auth0js#installation-options).
-
-Then we will instantiate the Management API client:
-
-```javascript
-var auth0Manage = new auth0.Management({
- domain: "${account.namespace}",
- token: "{USER_ID_TOKEN}"
-});
-```
-
-Parameters:
-- **domain**: your Auth0 domain (you can find it at your [Dashboard > Client > Settings](${manage_url}/#/clients/${account.clientId}/settings))
-- **token**: the [ID token](/tokens/id-token) you got from Auth0 when you authenticated your user
-
-Once the client is instantiated, you are ready to call the API and retrieve your user's profile:
-
-```javascript
-auth0Manage.getUser(userId, cb);
-```
-
-Parameters:
-- **userId**: your user's ID (see the **Where do I find the User ID?** panel if you don't have it)
-- **cb**: callback parameter
-
-Extract the IdP's access token from the response.
-
-You are now ready to call their API. Please refer to the IdP's documentation for specifics on how to do so.
| 2 |
diff --git a/_data/conferences.yml b/_data/conferences.yml place: Online
sub: NLP
-- title: INLG
- year: 2020
- id: inlg20
- link: https://www.inlg2020.org/
- deadline: '2020-08-15 23:59:00'
- timezone: UTC-12
- date: December 15-18, 2020
- place: Dublin, Ireland
- sub: NLP
-
- title: EACL
hindex: 36
year: 2021
| 2 |
diff --git a/packages/core/__tests__/interpreter/put.js b/packages/core/__tests__/interpreter/put.js import { createStore, applyMiddleware } from 'redux'
import * as io from '../../src/effects'
import deferred from '@redux-saga/deferred'
-import createSagaMiddleware, { channel, END } from '../../src'
+import createSagaMiddleware, { channel, END, stdChannel } from '../../src'
const thunk = () => next => action => {
if (typeof action.then === 'function') {
@@ -38,6 +38,7 @@ test('saga put handling', () => {
expect(actual).toEqual(expected)
})
})
+
test('saga put in a channel', () => {
const buffer = []
const spyBuffer = {
@@ -61,6 +62,7 @@ test('saga put in a channel', () => {
expect(buffer).toEqual(expected)
})
})
+
test("saga async put's response handling", () => {
let actual = []
const sagaMiddleware = createSagaMiddleware()
@@ -78,6 +80,7 @@ test("saga async put's response handling", () => {
expect(actual).toEqual(expected)
})
})
+
test("saga error put's response handling", () => {
let actual = []
const error = new Error('error')
@@ -111,6 +114,7 @@ test("saga error put's response handling", () => {
expect(actual).toEqual(expected)
})
})
+
test("saga error putResolve's response handling", () => {
let actual = []
@@ -134,6 +138,7 @@ test("saga error putResolve's response handling", () => {
expect(actual).toEqual(expected)
})
})
+
test('saga nested puts handling', () => {
let actual = []
const sagaMiddleware = createSagaMiddleware()
@@ -169,6 +174,7 @@ test('saga nested puts handling', () => {
expect(actual).toEqual(expected)
})
})
+
test('puts emitted while dispatching saga need not to cause stack overflow', () => {
function* root() {
yield io.put({
@@ -179,23 +185,24 @@ test('puts emitted while dispatching saga need not to cause stack overflow', ()
const reducer = (state, action) => action.type
- const sagaMiddleware = createSagaMiddleware({
- emitter: emit => () => {
- for (var i = 0; i < 32768; i++) {
- emit({
- type: 'test',
- })
+ const chan = stdChannel()
+ const rawPut = chan.put
+ chan.put = () => {
+ for (let i = 0; i < 32768; i++) {
+ rawPut({ type: 'test' })
}
- },
- })
- const store = createStore(reducer, applyMiddleware(sagaMiddleware))
- store.subscribe(() => {})
+ }
+
+ const sagaMiddleware = createSagaMiddleware({ channel: chan })
+ createStore(reducer, applyMiddleware(sagaMiddleware))
+
const task = sagaMiddleware.run(root)
return task.toPromise().then(() => {
// this saga needs to run without stack overflow
expect(true).toBe(true)
})
})
+
test('puts emitted directly after creating a task (caused by another put) should not be missed by that task', () => {
const actual = []
@@ -237,6 +244,7 @@ test('puts emitted directly after creating a task (caused by another put) should
expect(actual).toEqual(expected)
})
})
+
test('END should reach tasks created after it gets dispatched', () => {
const actual = []
| 1 |
diff --git a/src/windshaft/response.js b/src/windshaft/response.js @@ -12,7 +12,7 @@ function Response (windshaftSettings, serverResponse) {
this._layerGroupId = serverResponse.layergroupid;
this._layers = serverResponse.metadata.layers;
this._dataviews = serverResponse.metadata.dataviews;
- this._analyses = serverResponse.metadata.dataviews;
+ this._analyses = serverResponse.metadata.analyses;
this._cdnUrl = serverResponse.metadata.cdn_url;
}
| 1 |
diff --git a/generators/heroku/index.js b/generators/heroku/index.js @@ -778,7 +778,7 @@ module.exports = class extends BaseBlueprintGenerator {
this.log(chalk.yellow('After you have installed jq execute ./provision-okta-addon.sh manually.'));
}
if (curlAvailable && jqAvailable) {
- this.log(chalk.green('Running ./provision-okta-addon.sh to create all required roles and users to use with jhipster.'));
+ this.log(chalk.green('Running ./provision-okta-addon.sh to create all required roles and users for JHipster.'));
try {
await execCmd('./provision-okta-addon.sh');
this.log(chalk.bold('\nOkta configured successfully!'));
@@ -849,7 +849,7 @@ module.exports = class extends BaseBlueprintGenerator {
this.log(chalk.yellow('After you have installed jq execute ./provision-okta-addon.sh manually.'));
}
if (curlAvailable && jqAvailable) {
- this.log(chalk.green('Running ./provision-okta-addon.sh to create all required roles and users to use with JHipster.'));
+ this.log(chalk.green('Running ./provision-okta-addon.sh to create all required roles and users for JHipster.'));
try {
await execCmd('./provision-okta-addon.sh');
this.log(chalk.bold('\nOkta configured successfully!'));
| 3 |
diff --git a/store/buildMatch.js b/store/buildMatch.js @@ -102,7 +102,7 @@ async function getMatch(matchId) {
}
} catch (e) {
console.error(e);
- if (e.message.startsWith('Server failure during read query') || e.message.startsWith('no players found') || e.message.startsWith('Unexpected end of JSON input')) {
+ if (e.message.startsWith('Server failure during read query') || e.message.startsWith('no players found') || e.message.startsWith('Unexpected end of JSON input') || e.message.startsWith('Unexpected token')) {
// Delete and request new
await cassandra.execute('DELETE FROM player_matches where match_id = ?', [Number(matchId)], { prepare: true });
const match = {
| 9 |
diff --git a/src/components/Region.js b/src/components/Region.js @@ -20,7 +20,7 @@ const Region = ({ region, countries, search, onSearchChange, flagList }) => {
<ul className="list">{countryNames}</ul>
<a
className="f6 link dim br-pill ph4 pv2 mb2 dib white bg-purple"
- href="/"
+ href="/travel-guide"
>
BACK
</a>
| 1 |
diff --git a/articles/api-auth/tutorials/implicit-grant.md b/articles/api-auth/tutorials/implicit-grant.md @@ -26,10 +26,10 @@ Where:
In order to improve compatibility for client applications, Auth0 will now return profile information in a [structured claim format as defined by the OIDC specification](https://openid.net/specs/openid-connect-core-1_0.html#StandardClaims). This means that in order to add custom claims to ID tokens or access tokens, they must conform to a namespaced format to avoid possible collisions with standard OIDC claims. For example, if you choose the namespace `https://foo.com/` and you want to add a custom claim named `myclaim`, you would name the claim `https://foo.com/myclaim`, instead of `myclaim`.
:::
-* `response_type`: The response type. For this flow you can either use `token` or `id_token token`. This will specify the type of token you will receive at the end of the flow. Use `token` to get only an `access_token`, or `id_token token` to get both an `id_token` and an `access_token`.
+* `response_type`: Indicates the type of credentials returned in the response. For this flow you can either use `token`, to get only an `access_token`, or `id_token token`, to get both an `id_token` and an `access_token`.
* `client_id`: Your application's Client ID.
-* `redirect_uri`: The URL to which the Authorization Server (Auth0) will redirect the User Agent (Browser) after authorization has been granted by the User. The `access_token` (and optionally an `id_token`) will be available in the hash fragment of this URL. This URL must be specified as a valid callback URL under the Client Settings of your application.
-* `state`: An opaque value the clients adds to the initial request that the authorization server includes when redirecting the back to the client. This value must be used by the client to prevent CSRF attacks.
+* `redirect_uri`: The URL to which the Auth0 will redirect the user's browser after authorization has been granted by the user. The `access_token` (and optionally an `id_token`) will be available in the hash fragment of this URL. This URL must be specified as a valid callback URL under the Client Settings of your application.
+* `state`: An opaque value the clients adds to the initial request that Auth0 includes when redirecting the back to the client. This value must be used by the client to prevent CSRF attacks.
* `nonce`: A string value which will be included in the ID token response from Auth0, [used to prevent token replay attacks](/api-auth/tutorials/nonce). It is required for `response_type=id_token token`.
For example:
@@ -42,7 +42,7 @@ For example:
## Extract the Access Token
-After the Authorization Server has redirected back to the Client, you can extract the `access_token` from the hash fragment of the URL:
+After Auth0 has redirected back to the Client, you can extract the `access_token` from the hash fragment of the URL:
```js
function getParameterByName(name) {
| 14 |
diff --git a/aura-integration-test/src/test/java/org/auraframework/integration/test/util/WebDriverTestCase.java b/aura-integration-test/src/test/java/org/auraframework/integration/test/util/WebDriverTestCase.java @@ -163,6 +163,14 @@ public abstract class WebDriverTestCase extends IntegrationTestCase {
needsFreshBrowser = true;
}
+ @Override
+ public void setUp() throws Exception {
+ super.setUp();
+ if (currentBrowserType == null) {
+ beforeWebDriverTestCase();
+ }
+ }
+
@After
public void afterWebDriverTestCase() {
if (currentDriver != null && needsFreshBrowser) {
| 9 |
diff --git a/packages/@lwc/template-compiler/src/shared/types.ts b/packages/@lwc/template-compiler/src/shared/types.ts @@ -41,7 +41,7 @@ export interface SourceLocation {
export interface ElementSourceLocation extends SourceLocation {
startTag: SourceLocation;
- endTag?: SourceLocation;
+ endTag: SourceLocation;
}
export interface Literal<Value = string | boolean> {
| 1 |
diff --git a/assets/js/modules/analytics/components/setup/SetupFormUA.stories.js b/assets/js/modules/analytics/components/setup/SetupFormUA.stories.js import { STORE_NAME } from '../../datastore/constants';
import { MODULES_ANALYTICS_4 } from '../../../analytics-4/datastore/constants';
import { provideModules, provideModuleRegistrations } from '../../../../../../tests/js/utils';
-import { enabledFeatures } from '../../../../features';
import ModuleSetup from '../../../../components/setup/ModuleSetup';
import WithRegistrySetup from '../../../../../../tests/js/WithRegistrySetup';
import * as fixtures from '../../datastore/__fixtures__';
@@ -56,15 +55,17 @@ Ready.decorators = [
);
},
];
+Ready.parameters = {
+ features: [
+ 'ga4setup',
+ ],
+};
export default {
title: 'Modules/Analytics/Setup/SetupFormUA',
decorators: [
( Story ) => {
const setupRegistry = ( registry ) => {
- enabledFeatures.clear();
- enabledFeatures.add( 'ga4setup' );
-
provideModules( registry, [
{
slug: 'analytics',
| 2 |
diff --git a/src/sdk/conference/streamutils.js b/src/sdk/conference/streamutils.js @@ -117,7 +117,8 @@ export function convertToSubscriptionCapabilities(mediaInfo) {
frameRates.sort(sortNumbers);
const keyFrameIntervals = JSON.parse(
JSON.stringify(mediaInfo.video.optional.parameters.keyFrameInterval));
- if (mediaInfo.video && mediaInfo.video.parameters) {
+ if (mediaInfo.video && mediaInfo.video.parameters && mediaInfo.video.parameters
+ .keyFrameInterval) {
keyFrameIntervals.push(mediaInfo.video.parameters.keyFrameInterval);
}
keyFrameIntervals.sort(sortNumbers);
| 8 |
diff --git a/src/js/components/Search.js b/src/js/components/Search.js @@ -26,6 +26,7 @@ export default class Search extends Component {
this._onRemoveDrop = this._onRemoveDrop.bind(this);
this._onFocusInput = this._onFocusInput.bind(this);
this._onChangeInput = this._onChangeInput.bind(this);
+ this._onClickBody = this._onClickBody.bind(this);
this._onNextSuggestion = this._onNextSuggestion.bind(this);
this._onPreviousSuggestion = this._onPreviousSuggestion.bind(this);
this._announceSuggestion = this._announceSuggestion.bind(this);
@@ -87,7 +88,7 @@ export default class Search extends Component {
};
if (! dropActive && prevState.dropActive) {
- document.removeEventListener('click', this._onRemoveDrop);
+ document.removeEventListener('click', this._onClickBody);
KeyboardAccelerators.stopListeningToKeyboard(this,
activeKeyboardHandlers);
if (this._drop) {
@@ -97,12 +98,7 @@ export default class Search extends Component {
}
if (dropActive && ! prevState.dropActive) {
- // Slow down adding the click handler,
- // otherwise the drop will close when the mouse is released.
- // Not observable in Safari, 1ms is sufficient for Chrome,
- // Firefox needs 100ms though. :(
- // TODO: re-evaluate how to solve this without a timeout.
- document.addEventListener('click', this._onRemoveDrop);
+ document.addEventListener('click', this._onClickBody);
KeyboardAccelerators.startListeningToKeyboard(this,
activeKeyboardHandlers);
@@ -118,7 +114,7 @@ export default class Search extends Component {
};
this._drop = new Drop(baseElement, this._renderDropContent(), {
align: align,
- focusControl: true,
+ focusControl: ! inline,
responsive: false // so suggestion changes don't re-align
});
@@ -143,7 +139,7 @@ export default class Search extends Component {
}
componentWillUnmount () {
- document.removeEventListener('click', this._onRemoveDrop);
+ document.removeEventListener('click', this._onClickBody);
KeyboardAccelerators.stopListeningToKeyboard(this);
if (this._responsive) {
this._responsive.stop();
@@ -177,7 +173,7 @@ export default class Search extends Component {
event.preventDefault();
if (event.keyCode === down && !dropActive && inline) {
- this._onAddDrop(event);
+ this._onAddDrop();
}
}
}
@@ -186,8 +182,14 @@ export default class Search extends Component {
}
}
- _onAddDrop (event) {
- event.preventDefault();
+ _onClickBody (event) {
+ // don't close drop when clicking on input
+ if (event.target !== this._inputRef) {
+ this._onRemoveDrop();
+ }
+ }
+
+ _onAddDrop () {
this.setState({ dropActive: true, activeSuggestionIndex: -1 });
}
@@ -197,12 +199,10 @@ export default class Search extends Component {
_onFocusInput (event) {
const { onFocus } = this.props;
- this.setState({
- activeSuggestionIndex: -1
- });
if (onFocus) {
onFocus(event);
}
+ this._onAddDrop();
}
_fireDOMChange () {
| 1 |
diff --git a/fluent/src/bundle.js b/fluent/src/bundle.js @@ -199,19 +199,16 @@ export default class FluentBundle {
* const hello = bundle.getMessage('hello');
* if (hello.value) {
* bundle.formatPattern(hello.value, { name: 'Jane' }, errors);
- * }
- *
* // Returns 'Hello, Jane!' and `errors` is empty.
*
* bundle.formatPattern(hello.value, undefined, errors);
- *
- * // Returns 'Hello, $name!' and `errors` is now:
- *
- * [<ReferenceError: Unknown variable: name>]
+ * // Returns 'Hello, {$name}!' and `errors` is now:
+ * // [<ReferenceError: Unknown variable: name>]
+ * }
*
* @param {string|Array} pattern
* @param {?Object} args
- * @param {Array} errors
+ * @param {?Array} errors
* @returns {string}
*/
formatPattern(pattern, args, errors) {
| 7 |
diff --git a/data/networks.js b/data/networks.js @@ -218,7 +218,7 @@ module.exports = [
action_proposal: false,
default: false,
stakingDenom: 'KSM',
- enabled: true,
+ enabled: false,
icon: 'https://app.lunie.io/img/networks/polkadot-testnet.png',
slug: 'kusama'
}
| 12 |
diff --git a/policykit/policyengine/views.py b/policykit/policyengine/views.py @@ -8,8 +8,6 @@ from actstream.models import Action
from policyengine.filter import *
from policykit.settings import SERVER_URL, METAGOV_ENABLED
import integrations.metagov.api as MetagovAPI
-from pylint.lint import Run
-from pylint.reporters.text import TextReporter
from django.conf import settings
from urllib.parse import quote
import integrations.metagov.api as MetagovAPI
| 2 |
diff --git a/articles/getting-started/the-basics.md b/articles/getting-started/the-basics.md @@ -23,7 +23,7 @@ The term is borrowed from "software multitenancy". This refers to an architectur
Some characteristics:
-- It has to be unique (we will see in the next paragraph that it is used to create you own personal domain)
+- It has to be unique (we will see in the next paragraph that it is used to create your own personal domain)
- It cannot be changed afterwards
- You can create more than one tenants (you are actually encouraged to do so for each separate environment you have: Development/Staging/Production)
- If you chose to host your data in Europe or Australia, then your tenant will have a suffix (`eu` or `au`). In our example, if `Example-Co` picked the name `example-co`, then depending on where the data are stored, the tenant name could eventually be `example-co-eu` or `example-co-au`.
| 1 |
diff --git a/locales/pt.json b/locales/pt.json "webserver available at": "servidor web disponivel em",
"Webserver": "Servidor Web",
" already deployed at ": " already deployed at ",
- "Deployed": "Deployed",
+ "Deployed": "Publicado",
"Name must be only letters, spaces, or dashes": "Name must be only letters, spaces, or dashes",
"Name your app (default is %s)": "Name your app (default is %s)",
"Invalid name": "Invalid name",
"Error while downloading the file": "Error while downloading the file",
"Plugin {{name}} can only be loaded in the context of \"{{contextes}}\"": "Plugin {{name}} can only be loaded in the context of \"{{contextes}}\"",
"error running service check": "error running service check",
- "help": "help",
- "quit": "quit",
+ "help": "ajuda",
+ "quit": "sair",
"Type": "Type",
"to see the list of available commands": "to see the list of available commands",
"Asset Pipeline": "Asset Pipeline",
"Ethereum node detected": "Ethereum node detected",
"Deployment Done": "Deployment Done",
- "Looking for documentation? You can find it at": "Looking for documentation? You can find it at",
+ "Looking for documentation? You can find it at": "A procura de Documentacao? pode encontra-la em",
"Ready": "Ready",
"tip: you can resize the terminal or disable the dashboard with": "tip: you can resize the terminal or disable the dashboard with",
"finished building": "finished building",
"no webserver is currently running": "no webserver is currently running",
"couldn't find file": "couldn't find file",
"errors found while generating": "errors found while generating",
- "writing file": "writing file",
+ "writing file": "escrevendo ficheiro",
"Simulator not found; Please install it with \"%s\"": "Simulator not found; Please install it with \"%s\"",
"Tried to load testrpc but an error occurred. This is a problem with testrpc": "Tried to load testrpc but an error occurred. This is a problem with testrpc",
"IMPORTANT: if you using a NodeJS version older than 6.9.1 then you need to install an older version of testrpc \"%s\". Alternatively install node 6.9.1 and the testrpc 3.0": "IMPORTANT: if you using a NodeJS version older than 6.9.1 then you need to install an older version of testrpc \"%s\". Alternatively install node 6.9.1 and the testrpc 3.0",
| 3 |
diff --git a/packages/vue/src/components/atoms/SfButton/SfButton.stories.js b/packages/vue/src/components/atoms/SfButton/SfButton.stories.js @@ -223,7 +223,7 @@ export const WithDefaultSlot = (args, { argTypes }) => ({
<SfButton
:class="classes"
:disabled="disabled"
- @click="onClick"
+ @click="click"
:link="link">
<template>
<div v-html="content"/>
| 1 |
diff --git a/public/src/game/Chat.jsx b/public/src/game/Chat.jsx @@ -22,7 +22,7 @@ export default class Chat extends Component{
// must be mounted to receive messages
return (
<div className={`chat-container ${App.state.chat ? "" : "chat-container-hidden"}`}>
- <div className='chat'>
+ <div className='chat' onClick={this.onClickChat}>
<div className='messages' ref={(ref) => this.messageElement = ref}>
{this.state.messages.map(this.Message)}
</div>
@@ -31,6 +31,11 @@ export default class Chat extends Component{
</div>
);
}
+
+ onClickChat() {
+ document.getElementById("chat-input").focus();
+ }
+
hear(msg) {
this.state.messages.push(msg);
this.forceUpdate(this.scrollChat);
@@ -60,7 +65,7 @@ export default class Chat extends Component{
);
}
Entry() {
- return <input autoFocus className='chat-input' type='text' onKeyDown={this.key.bind(this)} placeholder='/nick name' />;
+ return <input id='chat-input' autoFocus className='chat-input' type='text' onKeyDown={this.key.bind(this)} placeholder='/nick name' />;
}
key(e) {
if (e.key !== "Enter")
| 11 |
diff --git a/src/components/signin/MnemonicInput.js b/src/components/signin/MnemonicInput.js @@ -6,6 +6,7 @@ import { View, StyleSheet } from 'react-native'
import logger from '../../lib/logger/pino-logger'
const log = logger.child({ from: 'MnemonicInput' })
+const MAX_WORDS = 12
type Props = {
onChange: Function
@@ -18,25 +19,29 @@ const isValidWord = word => {
const MnemonicInput = (props: Props) => {
const [state, setState] = useState({})
const refs = {}
- for (let i = 0; i < 12; i++) {
+ for (let i = 0; i < MAX_WORDS; i++) {
refs[i] = useRef(null)
}
const handleChange = () => {
- log.info({ state })
+ // Each time the state is updated we check if there is a valid mnemonic and execute onChange callback
const wordsArray = Object.values(state)
- if (wordsArray.length === 12 && wordsArray.every(isValidWord)) {
+ if (wordsArray.length === MAX_WORDS && wordsArray.every(isValidWord)) {
props.onChange(wordsArray)
}
}
const setWord = index => text => {
+ // If there is more than one word we want to put each word in his own input
+ // We also want to move focus to next word
const words = text.split(' ')
if (words.length > 1) {
const newState = { ...state }
- for (let i = index; i < words.length && i < 12; i++) {
+ for (let i = index; i < words.length && i < MAX_WORDS; i++) {
newState[i] = words[i]
}
setState(newState)
+
+ // If last word is empty means we need to consider length-1 as pos to focus on next
const pos = words[words.length - 1] === '' ? words.length - 1 : words.length
const next = Math.min(pos + index, 11)
refs[next].current.focus()
@@ -50,7 +55,7 @@ const MnemonicInput = (props: Props) => {
return (
<View style={styles.inputsContainer}>
- {[...Array(12).keys()].map(key => (
+ {[...Array(MAX_WORDS).keys()].map(key => (
<TextInput
value={state[key] || ''}
label={key + 1}
| 0 |
diff --git a/test/jasmine/tests/select_test.js b/test/jasmine/tests/select_test.js @@ -2121,7 +2121,7 @@ describe('Test select box and lasso per trace:', function() {
.then(done);
}, LONG_TIMEOUT_INTERVAL);
- it('@flaky should work for waterfall traces', function(done) {
+ it('@noCI @flaky should work for waterfall traces', function(done) {
var assertPoints = makeAssertPoints(['curveNumber', 'x', 'y']);
var assertSelectedPoints = makeAssertSelectedPoints();
var assertRanges = makeAssertRanges();
| 12 |
diff --git a/core/connection.js b/core/connection.js @@ -628,8 +628,7 @@ Blockly.Connection.prototype.targetBlock = function() {
* Returns whether type expression on the two connections can be unified with
* each other and also variables on both of connection's block can be resolved
* when they are connected.
- * @param {Blockly.Connection} otherConnection Connection to compare against.
- * If null, check if variables only on the block contains can be resolved.
+ * @param {!Blockly.Connection} otherConnection Connection to compare against.
* @param {Object=} opt_settings Object to specify settings for connection
* check with the following properties.
* - finalCheck: True if two connections will be connected immediately if
@@ -643,14 +642,10 @@ Blockly.Connection.prototype.checkTypeExprAndVariables_ = function(
if (dx) {
return Blockly.Connection.REASON_TYPE_UNIFICATION;
}
- if (otherConnection) {
var superior = this.isSuperior() ? this : otherConnection;
var inferior = superior == this ? otherConnection : this;
var childBlock = inferior.getSourceBlock();
- } else {
- var superior = null;
- var childBlock = this.getSourceBlock();
- }
+
var bindNewly = settings.finalCheck === true;
var resolved = childBlock.resolveReference(superior, bindNewly);
if (!resolved) {
| 2 |
diff --git a/scripts/avalanchego-installer.sh b/scripts/avalanchego-installer.sh @@ -94,7 +94,7 @@ then
echo "Available versions:"
wget -q -O - https://api.github.com/repos/ava-labs/avalanchego/releases \
| grep tag_name \
- | sed 's/.*: "(.*)".*/\1/' \
+ | sed 's/.*: "\(.*\)".*/\1/' \
| head
exit 0
;;
@@ -155,7 +155,7 @@ cd /tmp/avalanchego-install
version=${version:-latest}
echo "Looking for $getArch version $version..."
if [ "$version" = "latest" ]; then
- fileName="$(curl -s https://api.github.com/repos/ava-labs/avalanchego/releases/latest | grep "avalanchego-linux-$getArch.*tar(.gz)*\"" | cut -d : -f 2,3 | tr -d \" | cut -d , -f 2)"
+ fileName="$(curl -s https://api.github.com/repos/ava-labs/avalanchego/releases/latest | grep "avalanchego-linux-$getArch.*tar\(.gz\)*\"" | cut -d : -f 2,3 | tr -d \" | cut -d , -f 2)"
else
fileName="https://github.com/ava-labs/avalanchego/releases/download/$version/avalanchego-linux-$getArch-$version.tar.gz"
fi
| 13 |
diff --git a/src/ui/treeview/TreeViewDnD.hx b/src/ui/treeview/TreeViewDnD.hx @@ -42,7 +42,7 @@ class TreeViewDnD {
static var rxCanDrag = new RegExp(rsCanDrop + ".+", "i");
static function hasType(e:DragEvent, t:String):Bool {
var dtTypes = e.dataTransfer.types;
- return untyped (dtTypes.indexOf)
+ return untyped (dtTypes:Dynamic).indexOf
? dtTypes.indexOf(t) >= 0
: dtTypes.contains(t);
}
| 1 |
diff --git a/package.json b/package.json "create-secrets": "create-secrets",
"start-opa": "opa run deploy/helm/internal-charts/opa/policies",
"test-opa": "opa test deploy/helm/internal-charts/opa/policies -v",
- "cleanup": "rm -rf pancake magda-web-client/src/pancake; for dir in magda-*; do echo \"Removing node_modules from $dir\"; rm -rf $dir/node_modules; done"
+ "clean": "rm -rf pancake magda-web-client/src/pancake; for dir in magda-*; do echo \"Removing node_modules from $dir\"; rm -rf $dir/node_modules; done"
},
"pancake": {
"auto-save": true,
| 10 |
diff --git a/magda-csw-connector/src/Csw.ts b/magda-csw-connector/src/Csw.ts @@ -8,6 +8,7 @@ import formatServiceError from "@magda/typescript-common/dist/formatServiceError
import * as xmldom from "xmldom";
import * as xml2js from "xml2js";
import * as jsonpath from "jsonpath";
+import { groupBy } from "lodash";
export default class Csw implements ConnectorSource {
public readonly baseUrl: uri.URI;
@@ -263,6 +264,24 @@ export default class Csw implements ConnectorSource {
if (altOrgs.length != 0) {
orgData = altOrgs[0]["value"];
+ } else {
+ /**
+ * This means there ISN'T a proper pointOfContact section.
+ * This probably should be considered as a mistake
+ * and only very small amount of data like this can be found during my tests.
+ * We should now try pick one fron a list relevant entities.
+ */
+ const byRole = groupBy(datasetOrgs, node => node.role);
+ if (byRole["pointOfContact"]) {
+ const firstPointOfContactNode: any = byRole.pointOfContact[0];
+ orgData = firstPointOfContactNode.value;
+ }
+ /**
+ * Otherwise, don't touch orgData --- use the first one from the initial shorter list would be the best
+ * Main contributor usually will be listed as the first.
+ * We can be more accurate if we drill down into all possible roles.
+ * But it probably won't be necessary as dataset reaches here should be lower than 1%~2% based on my tests.
+ */
}
return Promise.resolve(orgData);
| 7 |
diff --git a/package.json b/package.json "name": "find-and-replace",
"main": "./lib/find",
"description": "Find and replace within buffers and across the project.",
- "version": "0.208.3",
+ "version": "0.209.0",
"license": "MIT",
"activationCommands": {
"atom-workspace": [
| 6 |
diff --git a/test/jasmine/tests/select_test.js b/test/jasmine/tests/select_test.js @@ -1279,6 +1279,147 @@ describe('Test select box and lasso per trace:', function() {
});
});
+describe('Test that selections persist:', function() {
+ var gd;
+
+ beforeEach(function() {
+ gd = createGraphDiv();
+ });
+
+ afterEach(destroyGraphDiv);
+
+ function assertPtOpacity(selector, expected) {
+ d3.selectAll(selector).each(function(_, i) {
+ var style = Number(this.style.opacity);
+ expect(style).toBe(expected.style[i], 'style for pt ' + i);
+ });
+ }
+
+ it('should persist for scatter', function(done) {
+ function _assert(expected) {
+ var selected = gd.calcdata[0].map(function(d) { return d.selected; });
+ expect(selected).toBeCloseToArray(expected.selected, 'selected vals');
+ assertPtOpacity('.point', expected);
+ }
+
+ Plotly.plot(gd, [{
+ x: [1, 2, 3],
+ y: [1, 2, 1]
+ }], {
+ dragmode: 'select',
+ width: 400,
+ height: 400,
+ margin: {l: 0, t: 0, r: 0, b: 0}
+ })
+ .then(function() {
+ resetEvents(gd);
+ drag([[5, 5], [250, 350]]);
+ return selectedPromise;
+ })
+ .then(function() {
+ _assert({
+ selected: [0, 1, 0],
+ style: [0.2, 1, 0.2]
+ });
+
+ // trigger a recalc
+ Plotly.restyle(gd, 'x', [[10, 20, 30]]);
+ })
+ .then(function() {
+ _assert({
+ selected: [undefined, 1, undefined],
+ style: [0.2, 1, 0.2]
+ });
+ })
+ .catch(fail)
+ .then(done);
+ });
+
+ it('should persist for box', function(done) {
+ function _assert(expected) {
+ var selected = gd.calcdata[0][0].pts.map(function(d) { return d.selected; });
+ expect(selected).toBeCloseToArray(expected.selected, 'selected vals');
+ assertPtOpacity('.point', expected);
+ }
+
+ Plotly.plot(gd, [{
+ type: 'box',
+ x0: 0,
+ y: [1, 2, 2, 2, 2, 2, 3, 3, 3, 4, 4, 5],
+ boxpoints: 'all'
+ }], {
+ dragmode: 'select',
+ width: 400,
+ height: 400,
+ margin: {l: 0, t: 0, r: 0, b: 0}
+ })
+ .then(function() {
+ resetEvents(gd);
+ drag([[5, 5], [400, 150]]);
+ return selectedPromise;
+ })
+ .then(function() {
+ _assert({
+ selected: [0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1],
+ style: [0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 1, 1, 1],
+ });
+
+ // trigger a recalc
+ Plotly.restyle(gd, 'x0', 1);
+ })
+ .then(function() {
+ _assert({
+ selected: [undefined, undefined, undefined, undefined, undefined, undefined, undefined, undefined, undefined, 1, 1, 1],
+ style: [0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 1, 1, 1],
+ });
+ })
+ .catch(fail)
+ .then(done);
+ });
+
+ it('should persist for histogram', function(done) {
+ function _assert(expected) {
+ var selected = gd.calcdata[0].map(function(d) { return d.selected; });
+ expect(selected).toBeCloseToArray(expected.selected, 'selected vals');
+ assertPtOpacity('.point > path', expected);
+ }
+
+ Plotly.plot(gd, [{
+ type: 'histogram',
+ x: [1, 2, 2, 2, 2, 2, 3, 3, 3, 4, 4, 5],
+ boxpoints: 'all'
+ }], {
+ dragmode: 'select',
+ width: 400,
+ height: 400,
+ margin: {l: 0, t: 0, r: 0, b: 0}
+ })
+ .then(function() {
+ resetEvents(gd);
+ drag([[5, 5], [400, 150]]);
+ return selectedPromise;
+ })
+ .then(function() {
+ _assert({
+ selected: [0, 1, 0, 0, 0],
+ style: [0.2, 1, 0.2, 0.2, 0.2],
+ });
+
+ // trigger a recalc
+ Plotly.restyle(gd, 'histfunc', 'sum');
+ })
+ .then(done)
+ .then(function() {
+ _assert({
+ selected: [undefined, 1, undefined, undefined, undefined],
+ style: [0.2, 1, 0.2, 0.2, 0.2],
+ });
+ })
+ .catch(fail)
+ .then(done);
+ });
+});
+
// to make sure none of the above tests fail with extraneous invisible traces,
// add a bunch of them here
function addInvisible(fig, canHaveLegend) {
| 0 |
diff --git a/README.md b/README.md @@ -358,7 +358,7 @@ Also check out [No Whiteboards](https://nowhiteboards.io) to search for jobs at
- [InfoSum](https://www.infosum.com) | Basingstoke, UK | On-site unsupervised exercise & discussion.
- [inKind Capital](https://inkindcapital.com) | Boulder, CO | Discussing real-world problems, pair programming, dinner & drinks with the team
- [Inmar](https://www.inmar.com/careers) | Winston-Salem, NC; Austin, TX & Remote | Take-home project and conversation-style interviews
-- [Innoplexus](https://www.innoplexus.com/careers/) | Pune, India; Frankfurt, Germany | Take-home projects and On-site pair programming assignment.
+- [Innoplexus](https://jobs.innoplexus.com/) | Pune, India; Frankfurt, Germany | Take-home projects and On-site pair programming assignment.
- [Instacart](https://careers.instacart.com) | San Francisco, CA | Take-home real world project, pair programming on-site
- [InstantPost](https://internshala.com/internships/internship-at-InstantPost) | Bangaluru, India | Remote assignment followed by Technical and Team round interview
- [Integral.](https://www.integral.io) | Detroit, MI | Initial remote technical screen featuring test-driven development & pair programming, then on-site full day interview that involves pair programming on production code using test-driven development.
| 1 |
diff --git a/src/index.js b/src/index.js @@ -214,6 +214,9 @@ function drawTrendChart(sheetTrend) {
}
},
y: {
+ padding: {
+ bottom: 0
+ },
tick: {
values: [0, 100, 500, 1000, 1500, 2000, 2500, 3000, 3500, 4000, 4500, 5000]
}
| 12 |
diff --git a/aleph/logic/graph.py b/aleph/logic/graph.py @@ -5,8 +5,10 @@ from followthemoney import model
from followthemoney.graph import Graph
from followthemoney.types import registry
-from aleph.index import entities as index
+# from aleph.index import entities as index
from aleph.logic.entities import entity_expand_nodes
+from aleph.model import Entity
+from aleph.logic import resolver
log = logging.getLogger(__name__)
@@ -16,15 +18,14 @@ class AlephGraph(Graph):
def queue(self, id_, proxy=None):
if id_ not in self.proxies:
self.proxies[id_] = proxy
+ resolver.queue(self, Entity, id_)
def resolve(self):
- entities_to_fetch = []
+ resolver.resolve(self)
for id_, proxy in self.proxies.items():
if proxy is None:
- entities_to_fetch.append(id_)
- for entity in index.entities_by_ids(entities_to_fetch, cached=True):
- self.proxies[entity.get('id')] = model.get_proxy(entity)
- for id_, proxy in self.proxies.items():
+ entity = resolver.get(self, Entity, id_)
+ proxy = model.get_proxy(entity)
node_id = registry.entity.node_id_safe(id_)
node = self.nodes.get(node_id)
if node is not None:
| 4 |
diff --git a/server/views/topics/stories.py b/server/views/topics/stories.py @@ -385,6 +385,8 @@ def _topic_story_page_with_media(user_key, topics_id, link_id, **kwargs):
story_page = apicache.topic_story_list_by_page(user_key, topics_id, link_id=link_id, **kwargs)
+ if len(story_page['stories']) > 0: # be careful to not construct malformed query if no story ids
+
story_ids = [str(s['stories_id']) for s in story_page['stories']]
stories_with_tags = apicache.story_list(user_key, 'stories_id:(' + " ".join(story_ids) + ")", kwargs['limit'])
| 9 |
diff --git a/README.md b/README.md @@ -140,3 +140,6 @@ On non Okta based deployments, you should run either `dokku run kamu /bin/bash`
```shell
python manage.py createsuperuser
```
+See #74
+
+
| 0 |
diff --git a/components/Article/PayNote.js b/components/Article/PayNote.js @@ -314,6 +314,7 @@ const withCounts = (text, replacements) => {
const BuyButton = ({ payNote, payload, darkMode }) => (
<Button
primary
+ href={payNote.button.link}
white={darkMode}
onClick={trackEventOnClick(
['PayNote', `pledge ${payload.position}`, payload.variation],
@@ -326,7 +327,10 @@ const BuyButton = ({ payNote, payload, darkMode }) => (
const SecondaryCta = ({ payNote, payload, darkMode }) =>
payNote.secondary && payNote.secondary.link ? (
- <div {...merge(styles.aside, darkMode && styles.asideDark)}>
+ <div
+ {...merge(styles.aside, darkMode && styles.asideDark)}
+ {...(darkMode ? styles.linksDark : styles.links)}
+ >
<span>{payNote.secondary.prefix} </span>
<a
key='secondary'
@@ -382,7 +386,10 @@ const PayNoteCta = ({ payNote, payload, darkMode }) =>
<BuyNoteCta darkMode={darkMode} payNote={payNote} payload={payload} />
)}
{payNote.note && (
- <div style={{ marginTop: 10, marginBottom: 5 }}>
+ <div
+ style={{ marginTop: 10, marginBottom: 5 }}
+ {...(darkMode ? styles.linksDark : styles.links)}
+ >
<Label
dangerouslySetInnerHTML={{
__html: payNote.note
@@ -396,6 +403,7 @@ const PayNoteCta = ({ payNote, payload, darkMode }) =>
const PayNoteP = ({ content, darkMode }) => (
<Interaction.P
{...styles.content}
+ {...(darkMode ? styles.linksDark : styles.links)}
style={{ color: darkMode ? colors.negative.text : '#000000' }}
dangerouslySetInnerHTML={{
__html: content
@@ -494,7 +502,6 @@ export const PayNote = compose(
style={{
backgroundColor: isBefore ? colors.error : colors.primaryBg
}}
- {...(darkMode ? styles.linksDark : styles.links)}
>
<Center>
<PayNoteContent
| 12 |
diff --git a/server/preprocessing/other-scripts/postprocess.R b/server/preprocessing/other-scripts/postprocess.R @@ -22,27 +22,6 @@ create_overview_output <- function(named_clusters, layout, metadata) {
output_json = toJSON(output)
- if(exists("DEBUG") && DEBUG == TRUE) {
- library(ggplot2)
- # Plot results from multidimensional scaling, highlight clusters with symbols
- temp <- fromJSON(output_json)
- temp$x <- as.numeric(temp$x)
- temp$y <- as.numeric(temp$y)
- temp$title <- unlist(lapply(temp$title, substr, start=0, stop=15))
- g <- ggplot(temp, aes(x, y, label=title)) +
- geom_point(aes(colour=area_uri)) +
- geom_text(size=2)
- ggsave(file = "debug_nmds.svg", plot = g, width = 15, height = 15)
- }
-
- # NEEDS FIX
- # if(exists("DEBUG") && DEBUG == TRUE) {
- # # Write output to file
- # file_handle = file("output_file.csv", open="w")
- # write.csv(output, file=file_handle, row.names=FALSE)
- # close(file_handle)
- # }
-
return(output_json)
}
| 2 |
diff --git a/ui/page/show/index.js b/ui/page/show/index.js +import { DOMAIN } from 'config';
import { connect } from 'react-redux';
import { PAGE_SIZE } from 'constants/claim';
import {
@@ -15,10 +16,25 @@ import { selectBlackListedOutpoints } from 'lbryinc';
import ShowPage from './view';
const select = (state, props) => {
- const { pathname, hash } = props.location;
+ const { pathname, hash, search } = props.location;
const urlPath = pathname + hash;
// Remove the leading "/" added by the browser
- const path = urlPath.slice(1).replace(/:/g, '#');
+ let path = urlPath.slice(1).replace(/:/g, '#');
+
+ // Google cache url
+ // ex: webcache.googleusercontent.com/search?q=cache:MLwN3a8fCbYJ:https://lbry.tv/%40Bombards_Body_Language:f+&cd=12&hl=en&ct=clnk&gl=us
+ // Extract the lbry url and use that instead
+ // Without this it will try to render lbry://search
+ if (search && search.startsWith('?q=cache:')) {
+ const googleCacheRegex = new RegExp(`(https://${DOMAIN}/)([^+]*)`);
+ const [x, y, googleCachedUrl] = search.match(googleCacheRegex); // eslint-disable-line
+ if (googleCachedUrl) {
+ const actualUrl = decodeURIComponent(googleCachedUrl);
+ if (actualUrl) {
+ path = actualUrl;
+ }
+ }
+ }
let uri;
try {
| 9 |
diff --git a/packages/@uppy/dashboard/src/index.js b/packages/@uppy/dashboard/src/index.js @@ -8,7 +8,7 @@ const ThumbnailGenerator = require('@uppy/thumbnail-generator')
const findAllDOMElements = require('@uppy/utils/lib/findAllDOMElements')
const toArray = require('@uppy/utils/lib/toArray')
const prettyBytes = require('prettier-bytes')
-const throttle = require('lodash.throttle')
+const ResizeObserver = require('resize-observer-polyfill')
const { defaultTabIcon } = require('./components/icons')
// Some code for managing focus was adopted from https://github.com/ghosh/micromodal
@@ -159,8 +159,6 @@ module.exports = class Dashboard extends Plugin {
this.handleDrop = this.handleDrop.bind(this)
this.handlePaste = this.handlePaste.bind(this)
this.handleInputChange = this.handleInputChange.bind(this)
- this.updateDashboardElWidth = this.updateDashboardElWidth.bind(this)
- this.throttledUpdateDashboardElWidth = throttle(this.updateDashboardElWidth, 500, { leading: true, trailing: true })
this.render = this.render.bind(this)
this.install = this.install.bind(this)
}
@@ -319,8 +317,7 @@ module.exports = class Dashboard extends Plugin {
// handle ESC and TAB keys in modal dialog
document.addEventListener('keydown', this.onKeydown)
- this.rerender(this.uppy.getState())
- this.updateDashboardElWidth()
+ // this.rerender(this.uppy.getState())
this.setFocusToBrowse()
}
@@ -441,8 +438,21 @@ module.exports = class Dashboard extends Plugin {
this.handleDrop(files)
})
- this.updateDashboardElWidth()
- window.addEventListener('resize', this.throttledUpdateDashboardElWidth)
+ // Watch for Dashboard container (`.uppy-Dashboard-inner`) resize
+ // and update containerWidth/containerHeight in plugin state accordingly
+ this.ro = new ResizeObserver((entries, observer) => {
+ for (const entry of entries) {
+ const { width, height } = entry.contentRect
+
+ this.uppy.log(`[Dashboard] resized: ${width} / ${height}`)
+
+ this.setPluginState({
+ containerWidth: width,
+ containerHeight: height
+ })
+ }
+ })
+ this.ro.observe(this.el.querySelector('.uppy-Dashboard-inner'))
this.uppy.on('plugin-remove', this.removeTarget)
this.uppy.on('file-added', (ev) => this.toggleAddFilesPanel(false))
@@ -454,24 +464,15 @@ module.exports = class Dashboard extends Plugin {
showModalTrigger.forEach(trigger => trigger.removeEventListener('click', this.openModal))
}
+ this.ro.unobserve(this.el.querySelector('.uppy-Dashboard-inner'))
+
this.removeDragDropListener()
- window.removeEventListener('resize', this.throttledUpdateDashboardElWidth)
+ // window.removeEventListener('resize', this.throttledUpdateDashboardElWidth)
window.removeEventListener('popstate', this.handlePopState, false)
this.uppy.off('plugin-remove', this.removeTarget)
this.uppy.off('file-added', (ev) => this.toggleAddFilesPanel(false))
}
- updateDashboardElWidth () {
- const dashboardEl = this.el.querySelector('.uppy-Dashboard-inner')
- if (!dashboardEl) return
-
- this.uppy.log(`Dashboard width: ${dashboardEl.offsetWidth}`)
-
- this.setPluginState({
- containerWidth: dashboardEl.offsetWidth
- })
- }
-
toggleFileCard (fileId) {
this.setPluginState({
fileCardFor: fileId || false
@@ -615,7 +616,6 @@ module.exports = class Dashboard extends Plugin {
toggleAddFilesPanel: this.toggleAddFilesPanel,
showAddFilesPanel: pluginState.showAddFilesPanel,
saveFileCard: saveFileCard,
- updateDashboardElWidth: this.updateDashboardElWidth,
width: this.opts.width,
height: this.opts.height,
showLinkToFileUploadResult: this.opts.showLinkToFileUploadResult,
| 14 |
diff --git a/src/registry/domain/nested-renderer.ts b/src/registry/domain/nested-renderer.ts @@ -15,7 +15,7 @@ interface Options {
}
export default function nestedRenderer(
- rendererCb: (
+ rendererWithCallback: (
options: RendererOptions,
cb: (result: GetComponentResult) => void
) => void,
@@ -23,7 +23,7 @@ export default function nestedRenderer(
) {
const renderer = (options: RendererOptions) =>
new Promise<string>((res, rej) => {
- rendererCb(options, result => {
+ rendererWithCallback(options, result => {
if (result.response.error) {
rej(result.response.error);
} else {
| 10 |
diff --git a/src/client/js/components/Admin/Security/OidcSecuritySetting.jsx b/src/client/js/components/Admin/Security/OidcSecuritySetting.jsx @@ -61,6 +61,7 @@ class OidcSecurityManagement extends React.Component {
)}
</div>
</div>
+
{adminGeneralSecurityContainer.state.isOidcEnabled && (
<React.Fragment>
@@ -193,6 +194,28 @@ class OidcSecurityManagement extends React.Component {
</div>
</div>
+ <div className="row mb-5">
+ <label className="col-xs-3 text-right">{ t('security_setting.callback_URL') }</label>
+ <div className="col-xs-6">
+ <input
+ className="form-control"
+ type="text"
+ value={adminOidcSecurityContainer.state.callbackUrl}
+ readOnly
+ />
+ <p className="help-block small">{ t('security_setting.desc_of_callback_URL', { AuthName: 'OAuth' }) }</p>
+ {!adminGeneralSecurityContainer.state.appSiteUrl && (
+ <div className="alert alert-danger">
+ <i
+ className="icon-exclamation"
+ // eslint-disable-next-line max-len
+ dangerouslySetInnerHTML={{ __html: t('security_setting.alert_siteUrl_is_not_set', { link: `<a href="/admin/app">${t('App settings')}<i class="icon-login"></i></a>` }) }}
+ />
+ </div>
+ )}
+ </div>
+ </div>
+
</React.Fragment>
)}
</React.Fragment>
| 12 |
diff --git a/aleph/search/parser.py b/aleph/search/parser.py @@ -3,7 +3,7 @@ from normality import stringify
from werkzeug.datastructures import MultiDict, OrderedMultiDict
# cf. https://www.elastic.co/guide/en/elasticsearch/reference/current/search-request-from-size.html # noqa
-MAX_RESULT_WINDOW = 1000
+MAX_RESULT_WINDOW = 9999
class QueryParser(object):
| 11 |
diff --git a/app/controllers/api/avatars.php b/app/controllers/api/avatars.php @@ -364,10 +364,10 @@ App::get('/v1/avatars/qr')
$download = ($download === '1' || $download === 'true' || $download === 1 || $download === true);
$qropts = new QROptions([
- 'quietzone' => $size
+ 'quietzone' => $size,
+ 'outputType' => QRCode::OUTPUT_IMAGICK
]);
$qrcode = new QRCode($qropts);
- $qrcode->render($text);
if ($download) {
$response->addHeader('Content-Disposition', 'attachment; filename="qr.png"');
| 12 |
diff --git a/avatars/avatars.js b/avatars/avatars.js @@ -15,6 +15,8 @@ import CBOR from '../cbor.js';
import Simplex from '../simplex-noise.js';
import {crouchMaxTime, useMaxTime, aimMaxTime, avatarInterpolationFrameRate, avatarInterpolationTimeDelay, avatarInterpolationNumFrames} from '../constants.js';
import {FixedTimeStep} from '../interpolants.js';
+import * as avatarCruncher from '../avatar-cruncher.js';
+import * as avatarSpriter from '../avatar-spriter.js';
import metaversefile from 'metaversefile';
import {
getSkinnedMeshes,
@@ -27,9 +29,9 @@ import {
// cloneModelBones,
decorateAnimation,
// retargetAnimation,
- animationBoneToModelBone,
+ // animationBoneToModelBone,
} from './util.mjs';
-import {scene, getRenderer} from '../renderer.js';
+// import {scene, getRenderer} from '../renderer.js';
const localVector = new THREE.Vector3();
const localVector2 = new THREE.Vector3();
@@ -1683,6 +1685,9 @@ class Avatar {
);
return localEuler.y;
}
+ setQuality(quality) {
+ console.log('set avatar quality', quality);
+ }
update(timestamp, timeDiff) {
const now = timestamp;
const timeDiffS = timeDiff / 1000;
| 0 |
diff --git a/test/test.js b/test/test.js @@ -224,31 +224,6 @@ module.exports = function (redom) {
onunmount: 2
});
});
- t.test('lifecycle with shadow root', function (t) {
- t.plan(2);
- var div = document.createElement('div');
- var root = div.createShadowRoot();
- var eventsFired = {};
-
- function Test () {
- this.el = el('div');
- this.onmount = function () {
- eventsFired.onmount = true;
- };
- this.onunmount = function () {
- eventsFired.onunmount = true;
- };
- }
-
- var test = new Test();
-
- mount(root, test);
-
- t.equals(eventsFired.onmount, true);
- unmount(root, test);
-
- t.equals(eventsFired.onunmount, true);
- });
t.test('component lifecycle events inside node element', function (t) {
t.plan(1);
var eventsFired = {};
| 2 |
diff --git a/components/bases-locales/validator/file-handler.js b/components/bases-locales/validator/file-handler.js @@ -15,14 +15,15 @@ class FileHandler extends React.Component {
]),
isLoading: PropTypes.bool,
onFileDrop: PropTypes.func.isRequired,
- onSubmit: PropTypes.func.isRequired
+ onSubmit: PropTypes.func
}
static defaultProps = {
defaultValue: '',
file: null,
error: null,
- isLoading: false
+ isLoading: false,
+ onSubmit: null
}
state = {inputValue: this.props.defaultValue}
@@ -53,6 +54,8 @@ class FileHandler extends React.Component {
file={file}
onDrop={onFileDrop}
/>
+ {this.props.onSubmit && (
+ <>
<div className='else'>ou</div>
<InputForm
placeholder='Entrer une url vers un fichier CSV'
@@ -62,6 +65,8 @@ class FileHandler extends React.Component {
buttonText='Utiliser'
loading={isLoading}
/>
+ </>
+ )}
</div>
{error && (
| 2 |
diff --git a/lib/app/reload-actions.js b/lib/app/reload-actions.js @@ -40,11 +40,11 @@ module.exports = function reloadActions(options, cb) {
sails._actions = {};
// Reload the actions.
- async.each(hooksToReload, function(hookIdentity, cb) {
+ async.each(hooksToReload, function(hookIdentity, next) {
if (_.isFunction(sails.hooks[hookIdentity].registerActions)) {
- sails.hooks[hookIdentity].registerActions(cb);
+ sails.hooks[hookIdentity].registerActions(next);
} else {
- return cb();
+ return next();
}
}, function doneReloadingActions(err) {
if (err) {return cb(err);}
| 10 |
diff --git a/articles/quickstart/backend/golang/01-authorization.md b/articles/quickstart/backend/golang/01-authorization.md @@ -38,8 +38,8 @@ Configure the **checkJwt** middleware to use the remote JWKS for your Auth0 acco
```go
// main.go
-const JWKS_URI = "https://{DOMAIN}/.well-known/jwks.json"
-const AUTH0_API_ISSUER = "https://{DOMAIN}.auth0.com/"
+const JWKS_URI = "https://${account.namespace}/.well-known/jwks.json"
+const AUTH0_API_ISSUER = "https://${account.namespace}"
var AUTH0_API_AUDIENCE = []string{"${apiIdentifier}"}
| 4 |
diff --git a/articles/connections/database/password-options.md b/articles/connections/database/password-options.md @@ -31,6 +31,8 @@ The Password Dictionary option, when enabled, allows the use of a password dicti
Additionally, you can use the text area here and add your own prohibited passwords, one per line. These can be items that are specific to your company, or passwords that your own research has shown you are commonly used in general or at your company in specific.
+Note that Auth0 uses case-insensitive comparison with the Password Dictionary feature.
+
## Personal Data
Enabling this option will force a user that is setting their password to not set passwords that contain any part of the user's personal data. This includes:
| 0 |
diff --git a/app/hotels/src/navigation/NavigationStack.js b/app/hotels/src/navigation/NavigationStack.js @@ -85,6 +85,7 @@ export default StackNavigator(
screen: withMappedProps(Payment),
navigationOptions: {
mode: 'modal',
+ headerTitle: 'Hotel',
},
},
},
| 0 |
diff --git a/modules/@apostrophecms/ui/ui/apos/components/AposTreeRows.vue b/modules/@apostrophecms/ui/ui/apos/components/AposTreeRows.vue <div class="apos-tree__row-data">
<button
v-if="row.children && row.children.length > 0"
- class="apos-tree__row__toggle"
+ class="apos-tree__row__toggle" data-apos-tree-toggle
aria-label="Toggle section"
@click="toggleSection($event)"
>
@@ -166,9 +166,6 @@ export default {
set(val) {
this.$emit('change', val);
}
- },
- isOpen() {
- return true;
}
},
mounted() {
@@ -200,24 +197,27 @@ export default {
toggleSection(event) {
const row = event.target.closest('[data-apos-tree-row]');
const rowList = row.querySelector('[data-apos-branch-height]');
+ const toggle = event.target.closest('[data-apos-tree-toggle]');
if (rowList && rowList.style.maxHeight === '0px') {
rowList.style.maxHeight = rowList.getAttribute('data-apos-branch-height');
- row.classList.remove('is-collapsed');
+ toggle.setAttribute('aria-expanded', true);
+ rowList.classList.remove('is-collapsed');
} else if (rowList) {
rowList.style.maxHeight = 0;
- row.classList.add('is-collapsed');
+ toggle.setAttribute('aria-expanded', false);
+ rowList.classList.add('is-collapsed');
}
},
selectRow(event) {
- let rowId;
- if (event.target.dataset.rowId) {
- rowId = event.target.dataset.rowId;
- } else {
- const row = event.target.closest('[data-row-id]');
- rowId = row.dataset.rowId;
+ const buttonParent = event.target.closest('[data-apos-tree-toggle]');
+
+ if (buttonParent) {
+ return;
}
- this.$emit('change', [ rowId ]);
+
+ const row = event.target.closest('[data-row-id]');
+ this.$emit('change', [ row.dataset.rowId ]);
},
getRowClasses(row) {
return [
@@ -262,7 +262,7 @@ export default {
.apos-tree__list {
transition: max-height 0.3s ease;
- .apos-tree__row.is-collapsed & {
+ &.is-collapsed {
overflow-y: auto;
}
}
@@ -279,7 +279,7 @@ export default {
.apos-tree__row__toggle-icon {
transition: transform 0.3s ease;
- .apos-tree__row.is-collapsed & {
+ [aria-expanded=false] > & {
transform: rotate(-90deg) translateY(0.25em);
}
}
| 9 |
diff --git a/lib/assertions.js b/lib/assertions.js @@ -1800,18 +1800,6 @@ module.exports = expect => {
const keys = valueType.getKeys(value);
const subjectKeys = subjectType.getKeys(subject);
- if (!subjectIsArrayLike) {
- // Find all non-enumerable subject keys present in value, but not returned by subjectType.getKeys:
- keys.forEach(key => {
- if (
- Object.prototype.hasOwnProperty.call(subject, key) &&
- subjectKeys.indexOf(key) === -1
- ) {
- subjectKeys.push(key);
- }
- });
- }
-
keys.forEach((key, index) => {
promiseByKey[key] = expect.promise(() => {
const subjectKey = subjectType.valueForKey(subject, key);
| 2 |
diff --git a/src/UserConfig.js b/src/UserConfig.js @@ -610,7 +610,7 @@ class UserConfig {
addExtension(fileExtension, options = {}) {
console.log(
chalk.yellow(
- "Warning: Configuration API `addExtension` is an undocumented experimental feature of Eleventy with an unstable API. Be careful!"
+ "Warning: Configuration API `addExtension` is an experimental Eleventy feature with an unstable API. Be careful!"
)
);
this.extensionMap.add(
| 3 |
diff --git a/test/unit/modules/tableEmbed.js b/test/unit/modules/tableEmbed.js @@ -454,7 +454,7 @@ describe('Delta', () => {
{
retain: {
table: {
- rows: [{ remove: { id: '22222222' } }],
+ rows: [{ delete: 1 }],
columns: [{ retain: 1 }, { delete: 1 }],
},
},
@@ -466,6 +466,7 @@ describe('Delta', () => {
{
retain: {
table: {
+ rows: [{ insert: { id: '11111111' } }],
columns: [
{ retain: 1 },
{ insert: { id: '44444444' }, attributes: { width: 100 } },
| 1 |
diff --git a/src/map/handler/Map.Drag.js b/src/map/handler/Map.Drag.js -import { now, sign } from '../../core/util';
+import { now } from '../../core/util';
import { preventDefault, getEventContainerPoint } from '../../core/util/dom';
import Handler from '../../handler/Handler';
import DragHandler from '../../handler/Drag';
@@ -92,7 +92,6 @@ class MapDragHandler extends Handler {
}
delete this.startDragTime;
delete this.startBearing;
- delete this.db;//bearing distance
}
_start(param) {
@@ -146,6 +145,7 @@ class MapDragHandler extends Handler {
delete this._rotateMode;
this.startBearing = this.target.getBearing();
this.target.onDragRotateStart(param);
+ this._db = 0;
}
_rotating(param) {
@@ -174,19 +174,19 @@ class MapDragHandler extends Handler {
}
if (this._rotateMode.indexOf('rotate') >= 0 && map.options['dragRotate']) {
- let bearing;
+
+ let db = 0;
if (map.options['dragPitch'] || dx > dy) {
- bearing = map.getBearing() - 0.6 * (this.preX - mx);
+ db = -0.6 * (this.preX - mx);
} else if (mx > map.width / 2) {
- bearing = map.getBearing() + 0.6 * (this.preY - my);
+ db = 0.6 * (this.preY - my);
} else {
- bearing = map.getBearing() - 0.6 * (this.preY - my);
+ db = -0.6 * (this.preY - my);
}
+ const bearing = map.getBearing() + db;
+ this._db = this._db || 0;
+ this._db += db;
- if (!this.db) {
- const d = bearing - this.startBearing;
- this.db = Math.abs(d) > 5 ? sign(d) : 0;
- }
map.setBearing(bearing);
}
if (this._rotateMode.indexOf('pitch') >= 0 && map.options['dragPitch']) {
@@ -208,10 +208,10 @@ class MapDragHandler extends Handler {
if (Math.abs(bearing - this.startBearing) > 20 && (this._rotateMode === 'rotate' || this._rotateMode === 'rotate_pitch') && !param.interupted && t < 400) {
const bearing = map.getBearing();
map.animateTo({
- 'bearing' : bearing + this.db * 10
+ 'bearing' : bearing + this._db / 2
}, {
'easing' : 'out',
- 'duration' : 160
+ 'duration' : 800
});
}
}
| 7 |
diff --git a/docs/SETUP.md b/docs/SETUP.md If you are using module bundlers such as Webpack, Rollup, Laravel elixir/mix, etc you may prefer directly include package
into your project. To get started use yarn or npm to get latest version.
+1- Download dependencies:
-1. Download dependencies:
```bash
# Using YARN
yarn add bootstrap-vue
@@ -16,7 +16,8 @@ yarn add -D style-loader
npm install --save bootstrap-vue
```
-2. Register BootstrapVue in your app entrypoint:
+2- Register BootstrapVue in your app entry point:
+
```js
import Vue from 'vue'
import BootstrapVue from 'bootstrap-vue';
@@ -24,21 +25,23 @@ import BootstrapVue from 'bootstrap-vue';
Vue.use(BootstrapVue);
```
-3. Import styles using style-loader:
+3- Import styles using style-loader:
```js
import 'bootstrap/dist/css/bootstrap.css'
import 'bootstrap-vue/dist/bootstrap-vue.css'
```
##### For users of Webpack or Webpack-Simple from `vue-cli` follow these instructions:
-1. Download the dependencies:
+1 - Download the dependencies:
+
```bash
yarn add bootstrap-vue
yarn add [email protected]
yarn add -D style-loader
```
-2. In `src/main.js`, add the following lines, in priority order:
+2 - In `src/main.js`, add the following lines, in priority order:
+
```js
import Vue from 'vue';
/* ( there may be other imports here ) */
| 1 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.